text
stringlengths 184
4.48M
|
---|
<template>
<view class="smodel">
<el-form ref="form" :model="form" :rules="rules" label-width="200rpx" :status-icon=true>
<el-form-item label="模型标识" prop="name">
<el-input v-model="form.name"></el-input>
</el-form-item>
<el-form-item label="模型名称" prop="title">
<el-input v-model="form.title"></el-input>
</el-form-item>
<el-form-item label="模型类型" prop="type">
<el-radio-group v-model="form.type">
<el-tooltip content="真实数据表/集合,需要查库" placement="top">
<el-radio label="db">数据模型</el-radio>
</el-tooltip>
<el-tooltip content="JSON字段配置,只需要配置字段和表单即可" placement="top">
<el-radio label="json">JSON模型</el-radio>
</el-tooltip>
</el-radio-group>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="submitForm('form')">立即创建</el-button>
</el-form-item>
</el-form>
</view>
</template>
<script>
import {
addSmodel
} from './api/smodel_api.js'
export default {
data() {
return {
form: {
name: '',
title: '',
type: 'db',
},
rules: {
name: [{
required: true,
message: '请输入模型标识',
trigger: 'blur'
},
{
min: 3,
max: 20,
message: '长度在 3 到 20 个字符',
trigger: 'blur'
}
],
title: [{
required: true,
message: '模型名称',
trigger: 'blur'
}],
type: [{
required: true,
message: '模型类型',
trigger: 'blur'
}],
}
}
},
// 监听 - 页面每次【加载时】执行(如:前进)
onLoad(options = {}) {
this.init(options)
},
// 监听 - 页面【首次渲染完成时】执行。注意如果渲染速度快,会在页面进入动画完成前触发
onReady() {},
// 监听 - 页面每次【显示时】执行(如:前进和返回) (页面每次出现在屏幕上都触发,包括从下级页面点返回露出当前页面)
onShow() {},
// 监听 - 页面每次【隐藏时】执行(如:返回)
onHide() {},
// 函数
methods: {
// 页面数据初始化函数
async submitForm(formName) {
console.log('formName', formName)
this.$refs[formName].validate(async (valid) => {
if (valid) {
this.form.collection = this.form.name
addSmodel(this.form).then(res => {
this.$message.success('添加模型成功')
uni.$emit('smodel_add_ok', {})
uni.navigateBack({
animationDuration: 1500
})
}).catch(err => {
console.error('add model error:', err)
this.$message.error('添加模型错误');
})
console.log('submit!', this.form);
} else {
console.error('error submit!!', this.form);
return false;
}
});
},
resetForm(formName) {
this.$refs[formName].resetFields();
},
init(options) {},
},
// 过滤器
filters: {
},
// 计算属性
computed: {
}
}
</script>
<style lang="scss" scoped>
.smodel {
padding: 40rpx;
}
</style> |
export interface Recipe {
author:RecipeAuthor;
comments:RecipeComments[];
cookTime:PrepTime,
prepTime:PrepTime,
ingredients:Ingredients[],
cuisineType:string,
dietType:string,
mealType:string,
ratings:RecipeRatings,
slug:string,
steps:RecipeStep[],
thumbnailPhoto:string,
title:string,
description:string,
id?:string,
}
export interface NewRecipe {
author: RecipeAuthor
comments: RecipeComments[];
cookTime: PrepTime
cuisineType: string | undefined;
description: string;
dietType: string | undefined;
ingredients: Ingredients[];
mealType: string | undefined;
prepTime: PrepTime
ratings: RecipeRatings
slug: string;
steps: RecipeStep[];
thumbnailPhoto: string ;
title: string;
}
export interface RecipeFormValues {
title: string;
description: string;
servings: number;
cuisineType: string | undefined;
mealType: string | undefined;
dietType: string | undefined;
prepTimeHours: number;
prepTimeMinutes: number;
cookTimeHours: number;
cookTimeMinutes: number;
recipeThumbnail: File;
}
export interface RecipeRatings {
downvotes:number,
upvotes:number,
}
export interface RecipeAuthor {
name:string,
photo:string,
uid:string,
}
export interface RecipeComments {
author:RecipeAuthor;
content:string
}
export interface PrepTime {
hours: number;
minutes: number;
}
export interface Ingredients {
name:string,
quantity:string,
imageUrl: string
}
export interface RecipeStep {
step:number,
content:string,
} |
"use client";
import React, { useState } from "react";
import { Handle } from "reactflow";
import Image from "next/image";
import { useIfHorizontal } from "../constants/ifHorizontal";
import { useTheme } from "../../themeContext";
const CustomNode = ({ data, isHovered }) => {
const [showDetails, setShowDetails] = useState(false.style);
const { ifHorizontal } = useIfHorizontal();
const { theme, toggleTheme } = useTheme();
const handleMouseEnter = () => {
setShowDetails(true);
};
const handleMouseLeave = () => {
setShowDetails(false);
};
return (
<div
className={`w-[181px] h-[164 px] flex flex-col items-center p-2.5 rounded-[10px] shadow-md w-[160px] transition-border transition-shadow duration-300 max-md:w-[100px] max-md:h-[115px] ${
theme === "dark" ? "bg-[#373737]" : "bg-[#f8f8f8] "
}`}
onMouseEnter={handleMouseEnter}
onMouseLeave={handleMouseLeave}
>
{/* <div className="w-4 h-4 bg-red-700 rounded-full absolute left-3 top-3"></div> */}
<div className="w-[100px] h-[100px] mb-2 max-md:w-[70px] ">
<Image
src={data.imageUrl}
alt="Node"
width="100"
height="100"
className="w-full rounded-[50%] object-cover shadow-md "
/>
</div>
<div
className={`text-base font-bold items-center mb-[6px] max-md:text-[12px] text-[#333] ${
theme === "dark" && "dark:text-[#efefef]"
}`}
>
{data.label}
</div>
{isHovered && showDetails && (
<div className="details">
<p>{data.additionalInfo}</p>
</div>
)}
<div className="flex justify-center mt-[6px]">
<Handle type="target" position={ifHorizontal ? "left" : "top"} />
<Handle type="source" position={ifHorizontal ? "right" : "bottom"} />
</div>
</div>
);
};
export default CustomNode; |
class GetProfilePosts {
final int itemsReceived;
final int curPage;
final int? nextPage;
final int? prevPage;
final int offset;
final int itemsTotal;
final int pageTotal;
final List<ItemPostProfile> items;
GetProfilePosts({
required this.itemsReceived,
required this.curPage,
this.nextPage,
this.prevPage,
required this.offset,
required this.itemsTotal,
required this.pageTotal,
required this.items,
});
factory GetProfilePosts.fromJson(Map<String, dynamic> json) {
List<ItemPostProfile> itemsList = [];
if (json['items'] != null) {
var itemsArray = json['items'] as List;
itemsList = itemsArray
.map((item) => ItemPostProfile.fromJson(item as Map<String, dynamic>))
.toList();
}
return GetProfilePosts(
itemsReceived: json['itemsReceived'],
curPage: json['curPage'],
nextPage: json['nextPage'],
prevPage: json['prevPage'],
offset: json['offset'],
itemsTotal: json['itemsTotal'],
pageTotal: json['pageTotal'],
items: itemsList,
);
}
}
class ItemPostProfile {
final int id;
final int createdAt;
final String? content;
final int userId;
final ImagePostProfile? image;
final int commentsCount;
ItemPostProfile({
required this.id,
required this.createdAt,
required this.content,
required this.userId,
this.image,
required this.commentsCount,
});
factory ItemPostProfile.fromJson(Map<String, dynamic> json) {
return ItemPostProfile(
id: json['id'],
createdAt: json['created_at'],
content: json['content'],
userId: json['user_id'],
image: json['image'] != null ? ImagePostProfile.fromJson(json['image']) : null,
commentsCount: json['comments_count'],
);
}
}
class ImagePostProfile {
final String url;
ImagePostProfile({
required this.url,
});
factory ImagePostProfile.fromJson(Map<String, dynamic> json) {
return ImagePostProfile(
url: json['url'],
);
}
} |
<?php
namespace Civi\Osdi\ActionNetwork\Matcher;
use Civi\Osdi\ActionNetwork\DonationHelperTrait;
use Civi\Osdi\RemoteObjectInterface;
use Civi\OsdiClient;
use OsdiClient\ActionNetwork\PersonMatchingFixture as PersonMatchFixture;
use OsdiClient\ActionNetwork\TestUtils;
use PHPUnit;
/**
* @group headless
*/
class DonationBasicTest extends PHPUnit\Framework\TestCase implements
\Civi\Test\HeadlessInterface,
\Civi\Test\TransactionalInterface {
use DonationHelperTrait;
private static \Civi\Osdi\ActionNetwork\Matcher\DonationBasic $matcher;
public function setUpHeadless() {
return \Civi\Test::headless()->installMe(__DIR__)->apply();
}
public static function setUpBeforeClass(): void {
PersonMatchFixture::$personClass = \Civi\Osdi\ActionNetwork\Object\Person::class;
self::$system = TestUtils::createRemoteSystem();
$container = OsdiClient::container();
$container->register('Matcher', 'Donation', DonationBasic::class);
self::$matcher = $container->getSingle('Matcher', 'Donation');
static::$testFundraisingPage = static::getDefaultFundraisingPage();
static::$financialTypeId = static::getTestFinancialTypeId();
}
private static function makePair($input): \Civi\Osdi\LocalRemotePair {
$pair = new \Civi\Osdi\LocalRemotePair();
if (is_a($input, RemoteObjectInterface::class)) {
$pair->setRemoteObject($input)->setOrigin($pair::ORIGIN_REMOTE);
}
else {
$pair->setLocalObject($input)->setOrigin($pair::ORIGIN_LOCAL);
}
return $pair;
}
/**
* @return array{0: \Civi\Osdi\LocalObject\DonationBasic, 1: \Civi\Osdi\ActionNetwork\Object\Donation}
*/
private function makeSameDonationOnBothSides(): array {
$personPair = $this->createInSyncPerson();
$remotePerson = $personPair->getRemoteObject();
$localPerson = $personPair->getLocalObject();
$localDonationTime = '2020-03-04 05:06:07';
$remoteDonationTime = static::$system::formatDateTime(strtotime($localDonationTime));
$remoteDonation = new \Civi\Osdi\ActionNetwork\Object\Donation(self::$system);
$remoteDonation->setDonor($remotePerson);
$recipients = [['display_name' => 'Test recipient financial type', 'amount' => '5.55']];
$remoteDonation->recipients->set($recipients);
$remoteDonation->createdDate->set($remoteDonationTime);
$remoteDonation->setFundraisingPage(static::$testFundraisingPage);
$remoteDonation->save();
$localDonation = new \Civi\Osdi\LocalObject\DonationBasic();
$localDonation->setPerson($localPerson);
$localDonation->amount->set('5.55');
$localDonation->receiveDate->set($localDonationTime);
$localDonation->financialTypeId->set(static::$financialTypeId);
$localDonation->save();
return [$localDonation, $remoteDonation];
}
public function testRemoteMatch_Success_NoSavedMatches() {
[$localDonation, $remoteDonation] = $this->makeSameDonationOnBothSides();
self::assertNotNull($remoteDonation->getId());
$pair = $this->makePair($localDonation);
$matchFindResult = self::$matcher->tryToFindMatchFor($pair);
self::assertFalse($matchFindResult->isError());
self::assertTrue($matchFindResult->gotMatch());
self::assertEquals($remoteDonation->getId(), $matchFindResult->getMatch()->getId());
}
public function testLocalMatch_Success_NoSavedMatches() {
[$localDonation, $remoteDonation] = $this->makeSameDonationOnBothSides();
self::assertNotNull($localDonation->getId());
$pair = $this->makePair($remoteDonation);
$matchFindResult = self::$matcher->tryToFindMatchFor($pair);
self::assertFalse($matchFindResult->isError(),
print_r($matchFindResult->toArray(), TRUE));
self::assertTrue($matchFindResult->gotMatch());
self::assertEquals($localDonation->getId(), $matchFindResult->getMatch()->getId());
}
public function testLocalMatch_Success_WithSavedMatches() {
// could also be made part of preceding tests
self::markTestIncomplete('Todo');
}
} |
package com.HomeSahulat.config.otp;
import com.infobip.ApiClient;
import com.infobip.ApiException;
import com.infobip.ApiKey;
import com.infobip.BaseUrl;
import com.infobip.api.SmsApi;
import com.infobip.model.SmsAdvancedTextualRequest;
import com.infobip.model.SmsDestination;
import com.infobip.model.SmsTextualMessage;
import lombok.var;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
import java.util.Collections;
@Component
public class InfoBip {
@Value("${infobip.apiKey}")
private String apiKey;
@Value("${infobip.baseUrl}")
private String baseUrl;
public boolean sendSMS(String from, String to, String messageText) {
var client = ApiClient.forApiKey(ApiKey.from(apiKey))
.withBaseUrl(BaseUrl.from(baseUrl))
.build();
var api = new SmsApi(client);
var message = new SmsTextualMessage()
.from(from)
.destinations(Collections.singletonList(new SmsDestination().to(to)))
.text(messageText);
var request = new SmsAdvancedTextualRequest()
.messages(Collections.singletonList(message));
try {
var response = api.sendSmsMessage(request).execute();
System.out.println(response);
return true;
} catch (ApiException exception) {
exception.printStackTrace();
return false;
}
}
} |
from io import BytesIO
from datetime import datetime
from unittest.mock import MagicMock, patch
from mcap.records import Schema, Channel, Message
from foxglove.client import Client
from .generate import generate_json_data
def get_generated_data(url, **kwargs):
assert url == "the_link"
class Resp:
def __init__(self):
self.raw = BytesIO(generate_json_data())
def raise_for_status(self):
return None
return Resp()
@patch("requests.get", side_effect=get_generated_data)
def test_boot(arg):
client = Client("test")
client._make_stream_link = MagicMock(return_value="the_link")
count = 0
for schema, channel, message, decoded_message in client.iter_messages(
device_id="test_id", start=datetime.now(), end=datetime.now()
):
assert "level" in decoded_message
assert isinstance(schema, Schema)
assert isinstance(channel, Channel)
assert isinstance(message, Message)
count += 1
assert count == 10 |
const std = @import("std");
const Sha512 = std.crypto.hash.sha2.Sha512;
// TODO: add output param
// TODO: remove length from format
// TODO: add hash of the hashes at the end
// TODO: include filename in hashing
// Generates 'plugin_hash.bin' to be included in annodue.dll via @embedFile
// CLI Arguments:
// -Isrc_dir
// -Odest_dir
// -Ffile [repeatable]
// Output File Format
// u32 number of hashes in array
// [_][64]u8 sorted array of sha512 hashes
pub fn main() !void {
var gpa = std.heap.GeneralPurposeAllocator(.{}){};
const alloc = gpa.allocator();
// parse args
var args = try std.process.argsWithAllocator(alloc);
defer args.deinit();
var i_path: ?[:0]u8 = null;
var o_path: ?[:0]u8 = null;
var files = std.ArrayList([]u8).init(alloc);
defer files.deinit();
while (args.next()) |a| {
if (a.len > 2 and std.mem.eql(u8, "-I", a[0..2]))
i_path = @constCast(a[2..]);
if (a.len > 2 and std.mem.eql(u8, "-O", a[0..2]))
o_path = @constCast(a[2..]);
if (a.len > 2 and std.mem.eql(u8, "-F", a[0..2]))
try files.append(@constCast(a[2..]));
}
if (i_path == null) return error.NoInputPath;
if (o_path == null) return error.NoOutputPath;
std.fs.makeDirAbsolute(o_path.?) catch |err| switch(err) {
error.PathAlreadyExists => {},
else => return err,
};
// generate hash file
//std.debug.print("\n", .{});
//std.debug.print("Generating: plugin_hash.bin... ", .{});
const hash_filename = try std.fmt.allocPrint(alloc, "{s}/hashfile.bin", .{o_path.?});
const hash_file = try std.fs.cwd().createFile(hash_filename, .{});
defer hash_file.close();
_ = try hash_file.write(@as([*]u8, @ptrCast(&files.items.len))[0..4]);
var hashes = std.ArrayList([64]u8).init(alloc);
for (files.items) |f| {
const dll_filename = try std.fmt.allocPrint(alloc, "{s}/{s}", .{ i_path.?, f });
const digest = try getFileSha512(dll_filename);
try hashes.append(digest);
}
std.mem.sort([64]u8, hashes.items, {}, lessThanFnHash);
for (hashes.items) |h|
_ = try hash_file.write(&h);
std.debug.print("GENERATE HASHFILE SUCCESS\n", .{});
//std.debug.print("\n", .{});
}
// helpers
fn getFileSha512(filename: []u8) ![Sha512.digest_length]u8 {
const file = try std.fs.cwd().openFile(filename, .{});
defer file.close();
var sha512 = Sha512.init(.{});
const rdr = file.reader();
var buf: [std.mem.page_size]u8 = undefined;
var n = try rdr.read(&buf);
while (n != 0) {
sha512.update(buf[0..n]);
n = try rdr.read(&buf);
}
return sha512.finalResult();
}
fn lessThanFnHash(_: void, a: [64]u8, b: [64]u8) bool {
for (a, b) |a_v, b_v| {
if (a_v == b_v) continue;
return a_v < b_v;
}
return false;
} |
from django.conf import settings
from django.urls import include, path
from django.views.decorators.cache import cache_page
from rest_framework import routers
from .views import (
ContactsView,
ExperienceView,
FeedbackView,
GetAppProgramInterfaceView,
HobbyView,
HomePageView,
LoginUser,
RegisterUser,
SkillDetailView,
SkillsView,
logout_user
)
from .views_api import (
FeedbackViewSet,
SkillViewSet
)
router = routers.DefaultRouter()
router.register(r'skills_list', SkillViewSet)
router.register(r'feedbacks_list', FeedbackViewSet)
# override api-root name
router.urls[-1].name = router.urls[-2].name = 'ervand-api-root'
urlpatterns = [
# ======= URLS =====
path('', cache_page(5)(HomePageView.as_view()), name='home'),
path('contacts/', ContactsView.as_view(), name='contacts'),
path('experience/', ExperienceView.as_view(), name='experience'),
path('feedback/', FeedbackView.as_view(), name='feedback'),
path('get_api/', GetAppProgramInterfaceView.as_view(), name='get_api'),
path('hobby/', HobbyView.as_view(), name='hobby'),
path('register/', RegisterUser.as_view(), name='register'),
path('site_login/', LoginUser.as_view(), name='site_login'),
path('site_logout/', logout_user, name='site_logout'),
path('skills/', SkillsView.as_view(), name='skills'),
path('skills/<slug:skill_slug>/', SkillDetailView.as_view(), name='skill_detail'),
# ======= API URLS =====
path(f'{settings.API_V1_BASE_PREFIX}/', include(router.urls, )),
] |
package com.baeldung.jacksonannotation.general.reference;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.junit.Test;
import java.util.Collections;
import static io.restassured.path.json.JsonPath.from;
import static org.assertj.core.api.Assertions.assertThat;
/**
* Source code github.com/eugenp/tutorials
*
* @author Alex Theedom www.baeldung.com
* @version 1.0
*/
public class ReferenceUnitTest {
@Test
public void whenSerializingUsingReference_thenCorrect() throws JsonProcessingException {
// arrange
Author author = new Author("Alex", "Theedom");
Course course = new Course("Java EE Introduction", author);
author.setItems(Collections.singletonList(course));
course.setAuthors(Collections.singletonList(author));
// act
String result = new ObjectMapper().writeValueAsString(author);
// assert
assertThat(from(result).getString("items[0].authors")).isNull();
/*
Without references defined it throws StackOverflowError.
Authors excluded.
{
"id": "9c45d9b3-4888-4c24-8b74-65ef35627cd7",
"firstName": "Alex",
"lastName": "Theedom",
"items": [
{
"id": "f8309629-d178-4d67-93a4-b513ec4a7f47",
"title": "Java EE Introduction",
"price": 0,
"duration": 0,
"medium": null,
"level": null,
"prerequisite": null
}
]
}
*/
}
} |
import React, { useCallback, useEffect, useRef, useState } from 'react';
import { FontAwesomeIcon } from '@fortawesome/react-fontawesome';
import { faBars, faClose } from '@fortawesome/free-solid-svg-icons';
import { NavLink } from 'react-router-dom';
interface NavBarLinkProps {
text: string
url: string
}
const NavBar: React.FC = () => {
const [expanded, setExpanded] = useState(false);
const navRef = useRef<HTMLElement>(null);
const toggleExpanded = useCallback(() => {
setExpanded(!expanded);
}, [expanded, setExpanded]);
let navClasses = [
expanded ? 'block' : 'hidden',
'transition-all',
expanded ? '-translate-y-[20px]' : '-translate-y-[20px]',
'opacity-0',
'md:block',
'md:opacity-100',
'md:transition-none',
'md:transform-none',
'md:ml-3'
]
useEffect(() => {
const nav = navRef.current!;
requestAnimationFrame(() => {
if (expanded) {
nav.classList.remove('-translate-y-[20px]', 'opacity-0');
nav.classList.add('translate-y-0', 'opacity-100');
}
});
}, [expanded]);
const onNavClick = useCallback(() => {
setExpanded(false);
}, []);
return (
<header className='md:flex md:flex-row md:items-center'>
<div className='flex flex-row items-center'>
<h1 className='font-serif text-xl text-emerald-600'>
<NavLink to='/' className='block px-3 py-3'>Alex Duzik</NavLink>
</h1>
<div className='ml-auto md:hidden px-2'>
<button type='button' onClick={toggleExpanded} className='px-2'>
<span className='inline-block w-[20px] text-center'>
<FontAwesomeIcon icon={(expanded ? faClose : faBars)} />
</span>
<span className='sr-only'>Expand/Collapse</span>
</button>
</div>
</div>
<nav ref={navRef} className={navClasses.join(' ')} onClick={onNavClick}>
<ul className='md:flex md:flex-row'>
<li>
<NavBarLink
text="Profile"
url="/profile" />
</li>
<li>
<NavBarLink
text="Contact"
url="/contact" />
</li>
</ul>
</nav>
</header>
)
}
const NavBarLink: React.FC<NavBarLinkProps> = (props) => {
const {
text,
url
} = props;
type StyleProps = {
isActive: boolean
}
function styleLink(props: StyleProps) {
const { isActive } = props;
let styles = [
'inline-block', 'relative', 'px-2', 'py-2', 'hover:text-emerald-600', 'hover:after:content-[""]', 'after:bg-emerald-600', 'after:absolute', 'after:left-1/2', 'after:right-1/2', 'after:bottom-0', 'after:h-[2px]', 'hover:after:left-2', 'hover:after:right-2', 'after:transition-all', 'transition-colors'
];
if (isActive) {
styles.push('font-bold', 'text-emerald-600');
}
return styles.join(' ');
}
return (
<div className='block mx-2'>
<NavLink to={url} className={styleLink}>
{text}
</NavLink>
</div>)
}
export default NavBar; |
/* ************************************************************************** */
/* */
/* ::: :::::::: */
/* header.h :+: :+: :+: */
/* +:+ +:+ +:+ */
/* By: pdeshaye <marvin@42.fr> +#+ +:+ +#+ */
/* +#+#+#+#+#+ +#+ */
/* Created: 2022/01/22 23:43:10 by pdeshaye #+# #+# */
/* Updated: 2022/01/22 23:57:07 by pdeshaye ### ########.fr */
/* */
/* ************************************************************************** */
# ifndef HEADER_H
# define HEADER_H
# define EATING 1
# define SLEEPING 2
# define THINKING 3
# define DIE 4
# define GFORK 5
# include <stdlib.h>
# include <stdio.h>
# include <unistd.h>
# include <pthread.h>
# include <sys/time.h>
typedef struct s_philo
{
int id;
pthread_t self_thread;
int *sstop;
int lfork;
int rfork;
int is_eating;
uint64_t tbeforedie;
int countmeal;
struct s_data *data;
} t_philo;
typedef struct s_data
{
uint64_t time_to_die;
uint64_t time_to_eat;
uint64_t time_to_sleep;
int number_of_philosophers;
int philosopher_must_eat;
uint64_t time_start;
pthread_mutex_t talking;
t_philo *philo_l;
pthread_mutex_t *fork_l;
int stop;
} t_data;
void init_data(char **argv, t_data *data);
void init_philo(t_data *data);
void debug_philo(t_data *data);
uint64_t get_time(void);
char *message_type(int type);
uint64_t get_actual_time(uint64_t time);
void type_message(t_philo *philo, int type, uint64_t time);
void ft_usleep(uint64_t obj);
void philo_sleep(t_philo *philo);
uint64_t ft_atoi(const char *str);
void eat(t_philo *philo);
void *death_loop(void *data);
int stop_meal(t_data *data);
void free_all(t_data *data);
#endif |
package gradleproject;
import com.google.common.graph.*;
import java.awt.Color;
class Main {
public static void main(String[] args) {
// Mutable graphs can be changed after we build them
MutableGraph<String> graph =
GraphBuilder.directed().build();
//System.out.println(graph instanceof MutableGraph);
//System.out.println(graph instanceof Graph);
//System.out.println(graph instanceof ValueGraph);
//System.out.println(graph instanceof Network);
// Here we make changes:
graph.addNode("Joe");
graph.putEdge("Jordan", "Nick"); // if edge references nodes that don't exist, they get added
new GraphDisplay(graph);
//System.out.println(graph);
// Can add/remove anytime
graph.putEdge("Jordan", "Joe");
//System.out.println(graph);
// Immutable ones can't: you have to add everything before you build
ImmutableValueGraph<String,Integer> graph2 =
ValueGraphBuilder.undirected()
.<String,Integer>immutable()
.addNode("Ford")
.putEdgeValue("Bass", "McConnell", 1)
.putEdgeValue("Sabin-Reed", "McConnell", 0)
.putEdgeValue("Sabin-Reed", "Burton", 0) // edge values not necessarily unique
.build();
//System.out.println(graph2);
new GraphDisplay(graph2);
// Throws error
// graph2.removeEdge("Bass", "McConnell");
// Network allows objects on the edges
MutableNetwork<String,String> network = NetworkBuilder.undirected().build();
network.addEdge("Northampton","Boston","I-90");
network.addEdge("Northampton","New York","I-91");
network.addEdge("New York","Boston","I-95");
System.out.println(network);
//System.out.println(network.incidentEdges("Northampton"));
//System.out.println(network.successors("Northampton"));
//System.out.println(network.incidentNodes("I-95"));
GraphDisplay d3 = new GraphDisplay(network);
d3.setNodeColors(Color.GREEN);
d3.setEdgeColors(Color.BLUE);
d3.setColor("Northampton",Color.RED);
for (Object n : d3.getNodeSet()) {
System.out.println(n+" : "+d3.getLabel(n));
}
for (Object e : d3.getEdgeSet()) {
System.out.println(e+" : "+d3.getLabel(e));
}
//System.out.println(network instanceof MutableGraph);
//System.out.println(network instanceof Graph);
//System.out.println(network instanceof ValueGraph);
//System.out.println(network instanceof Network);
}
} |
import os
import re
from cs50 import SQL
from flask import Flask, flash, redirect, render_template, request, session, url_for
from flask_session import Session
from tempfile import mkdtemp
from werkzeug.security import check_password_hash, generate_password_hash
from helpers import apology, login_required
import json
# Configure application
app = Flask(__name__)
# Ensure templates are auto-reloaded
app.config["TEMPLATES_AUTO_RELOAD"] = True
# Configure session to use filesystem (instead of signed cookies)
app.config["SESSION_PERMANENT"] = False
app.config["SESSION_TYPE"] = "filesystem"
Session(app)
# Configure CS50 Library to use SQLite database
db = SQL("sqlite:///ideas.db")
valid_email = re.compile(r'([A-Za-z0-9]+[.-_])*[A-Za-z0-9]+@[A-Za-z0-9-]+(\.[A-Z|a-z]{2,})+')
DEPARTMENTS = [
"Finance",
"Sales",
"Marketing",
"Operations",
"Engineering",
"Product",
"Customer Experience",
"Legal",
"HR",
"Other"
]
@app.after_request
def after_request(response):
"""Ensure responses aren't cached"""
response.headers["Cache-Control"] = "no-cache, no-store, must-revalidate"
response.headers["Expires"] = 0
response.headers["Pragma"] = "no-cache"
return response
@app.route("/login", methods=["GET", "POST"])
def login():
# Forget any user_id
session.clear()
# User reached route via POST (as by submitting a form via POST)
if request.method == "POST":
# Ensure username was submitted
if not request.form.get("username"):
return apology("must provide username", 403)
# Ensure password was submitted
elif not request.form.get("password"):
return apology("must provide password", 403)
# Query database for username
rows = db.execute(
"SELECT id, hash FROM users WHERE username = ?", request.form.get("username"))
# Ensure username exists and password is correct
if len(rows) != 1 or not check_password_hash(rows[0]["hash"], request.form.get("password")):
return apology("invalid username and/or password", 403)
# Remember which user has logged in
session["user_id"] = rows[0]["ID"]
# Redirect user to home page
return redirect("/")
# User reached route via GET (as by clicking a link or via redirect)
else:
return render_template("login.html")
@app.route("/logout")
def logout():
"""Log user out"""
# Forget any user_id
session.clear()
# Redirect user to login form
return redirect("/")
@app.route("/register_company", methods=["GET", "POST"])
def register_company():
"""Register user"""
if request.method == "POST":
# collect info from form
username = request.form.get("username")
company = request.form.get("company")
size = request.form.get("size")
industry = request.form.get("industry")
password = request.form.get("password")
FirstName = request.form.get("firstname")
LastName = request.form.get("lastname")
email = request.form.get("email")
department = request.form.get("department")
confirmation = request.form.get("confirmation")
password_hash = generate_password_hash(password)
rows = db.execute("SELECT * FROM users WHERE username = ?", username)
emails = db.execute("SELECT email FROM users WHERE email = ?", email)
companies = db.execute("SELECT * FROM users WHERE company = ?", company)
# error check
if not username:
return apology("Username Required", 400)
if not company or not size or not industry:
return apology("Company Info missing", 400)
if len(companies) == 1:
return apology("Company already registered", 400)
if not FirstName:
return apology("First Name Required", 400)
if not LastName:
return apology("Last Name Required", 400)
if not email:
return apology("Email Required", 400)
if not department:
return apology("Department Required", 400)
if len(rows) == 1:
return apology("Username already exists", 400)
if len(emails) >= 1:
return apology("Email already exists", 400)
if not re.fullmatch(valid_email, email):
return apology("Invalid Email", 400)
if not password:
return apology("Password Required", 400)
if len(password) < 8:
return apology("Password must have at least 8 characters", 400)
if not any(c.isdigit() for c in password):
return apology("Password must contain numbers", 400)
if not confirmation:
return apology("Please re-enter password", 400)
if not password == confirmation:
return apology("Passwords do not match", 400)
# if valid then update the user db
db.execute("INSERT INTO users(username, hash, FirstName, LastName, email, department, permissions, company) VALUES(?, ?, ?, ?, ?, ?, ?, ?)",
username, password_hash, FirstName, LastName, email, department, 'admin', company)
db.execute("INSERT INTO companies(company, industry, size) VALUES(?, ?, ?)",
company, industry, size)
# automatically login the user after they have registered
rows = db.execute("SELECT * FROM users WHERE username = ?", username)
print(rows)
session["user_id"] = rows[0]["ID"]
print(session["user_id"])
return redirect("/")
else:
return render_template("register_company.html", departments=DEPARTMENTS)
@app.route("/register", methods=["GET", "POST"])
def register():
"""Register user"""
if request.method == "POST":
# ask the user for required info
username = request.form.get("username")
company = request.form.get("company")
password = request.form.get("password")
FirstName = request.form.get("firstname")
LastName = request.form.get("lastname")
email = request.form.get("email")
department = request.form.get("department")
confirmation = request.form.get("confirmation")
password_hash = generate_password_hash(password)
rows = db.execute("SELECT * FROM users WHERE username = ?", username)
emails = db.execute("SELECT email FROM users WHERE email = ?", email)
companies = db.execute("SELECT * FROM users WHERE company = ?", company)
# error check
if not username:
return apology("Username Required", 400)
if not company:
return apology("Company Required", 400)
if len(companies) == 0:
return render_template("registration_failed.html")
if not FirstName:
return apology("First Name Required", 400)
if not LastName:
return apology("Last Name Required", 400)
if not email:
return apology("Email Required", 400)
if not department:
return apology("Department Required", 400)
if len(rows) == 1:
return apology("Username already exists", 400)
if len(emails) >= 1:
return apology("Email already exists", 400)
if not re.fullmatch(valid_email, email):
return apology("Invalid Email", 400)
if not password:
return apology("Password Required", 400)
if len(password) < 8:
return apology("Password must have at least 8 characters", 400)
if not any(c.isdigit() for c in password):
return apology("Password must contain numbers", 400)
if not confirmation:
return apology("Please re-enter password", 400)
if not password == confirmation:
return apology("Passwords do not match", 400)
# if valid then update the user db
db.execute("INSERT INTO users(username, hash, FirstName, LastName, email, department, permissions, company) VALUES(?, ?, ?, ?, ?, ?, ?, ?)",
username, password_hash, FirstName, LastName, email, department, 'user', company)
# automatically login the user after they have registered
rows = db.execute("SELECT * FROM users WHERE username = ?", username)
print(rows)
session["user_id"] = rows[0]["ID"]
print(session["user_id"])
return redirect("/")
else:
return render_template("register.html", departments=DEPARTMENTS)
@app.route("/")
@login_required
def index():
# get user id
id = session.get("user_id")
# find users company
rows = db.execute("SELECT company FROM users WHERE id = ?", id)
company = rows[0]["company"]
# query db for all ideas submitted for current company
ideas = db.execute("SELECT * FROM ideas WHERE stage = ? AND company = ?", "idea", company)
inReview = db.execute("SELECT * FROM ideas WHERE stage = ? AND company = ?", "review", company)
accepted = db.execute("SELECT * FROM ideas WHERE stage = ? AND company = ?", "accept", company)
rejected = db.execute("SELECT * FROM ideas WHERE stage = ? AND company = ?", "reject", company)
return render_template("index.html", ideas=ideas, inReview=inReview, accepted=accepted, rejected=rejected)
@app.route("/vote")
@login_required
def ideas():
#get user id
id = session.get("user_id")
# query db for all ideas submitted for current company
rows = db.execute("SELECT company FROM users WHERE id = ?", id)
company = rows[0]["company"]
ideas = db.execute("SELECT * FROM ideas WHERE stage = ? AND company = ? ORDER BY title ASC", "idea", company)
return render_template("vote.html", ideas=ideas)
@app.route("/upvote/<int:idea_id>")
def upvote(idea_id):
# get user_id
id = session.get("user_id")
#Check if user has voted on idea before this
actions = db.execute("SELECT action FROM actions WHERE idea_id = ? AND user_id = ?", idea_id, id)
if len(actions) == 0:
#get current upvotes from current idea and increase count by 1
row = db.execute("SELECT upvotes FROM ideas WHERE idea_id = ?", idea_id)
votes = int(row[0]["upvotes"])
upvotes = votes + 1
#update db with new upvotes
db.execute("UPDATE ideas SET upvotes = ? WHERE idea_id = ?",upvotes, idea_id)
#add action to actions db
db.execute("INSERT INTO actions (idea_id, action, user_id) VALUES (?, ?, ?)",idea_id, "upvote", id)
#if there's more than 10 votes then update stage
if upvotes >= 10:
db.execute("UPDATE ideas SET stage = ? WHERE idea_id = ? AND stage = ?",'review', idea_id, 'idea')
return redirect("/vote")
else:
return render_template("voted.html")
@app.route("/downvote/<int:idea_id>")
def downvote(idea_id):
# get user_id
id = session.get("user_id")
#Check if user has voted on idea before this
actions = db.execute("SELECT action FROM actions WHERE idea_id = ? AND user_id = ?", idea_id, id)
if len(actions) == 0:
#get current upvotes from current idea and increase count by 1
row = db.execute("SELECT downvotes FROM ideas WHERE idea_id = ?", idea_id)
votes = int(row[0]["downvotes"])
downvotes = votes - 1
#update db with new upvotes
db.execute("UPDATE ideas SET downvotes = ? WHERE idea_id = ?",downvotes, idea_id)
#add action to actions db
db.execute("INSERT INTO actions (idea_id, action, user_id) VALUES (?, ?, ?)",idea_id, "downvote", id)
#if there's more than 10 votes then update stage
if downvotes <= -5:
db.execute("UPDATE ideas SET stage = ? WHERE idea_id = ? AND stage = ?",'rejected', idea_id, 'idea')
return redirect("/vote")
else:
return render_template("voted.html")
@app.route("/idea_page/<int:idea_id>", methods=["GET", "POST"])
def idea_page(idea_id):
if request.method == "POST":
# get submitted comment and add to comments db
print("POST")
id = session.get("user_id")
comment = request.form.get("comment")
db.execute("INSERT INTO comments(comment, user_id, idea_id) VALUES(?, ?, ?)",
comment, id, idea_id)
return redirect(url_for("idea_page", idea_id=idea_id))
else:
# get all idea info and comments for current idea
id = session.get("user_id")
ideas = db.execute("SELECT * FROM ideas WHERE idea_id = ?", idea_id)
comments = db.execute("SELECT * FROM comments JOIN users ON comments.user_id = users.id WHERE comments.idea_id = ?", idea_id)
# find users current permission and render relevant template based onn permissions
rows = db.execute("SELECT permissions FROM users WHERE id = ?", id)
permission = rows[0]["permissions"]
stages = db.execute("SELECT stage FROM ideas WHERE idea_id = ?", idea_id)
stage = stages[0]["stage"]
if permission == "admin" and stage == "review":
return render_template("decide.html", ideas=ideas, comments=comments, idea_id=idea_id)
elif stage == "idea":
return render_template("voting_page.html", ideas=ideas, idea_id=idea_id, comments=comments)
else:
return render_template("ideas_page.html", ideas=ideas, comments=comments, idea_id=idea_id)
@app.route("/accept/<int:idea_id>")
def accept(idea_id):
print('idea_id')
# find users permissions
id = session.get("user_id")
rows = db.execute("SELECT permissions FROM users WHERE id = ?", id)
permission = rows[0]["permissions"]
# if user is admin allow db to update idea stage
if permission == "admin":
db.execute("UPDATE ideas SET stage = ? WHERE idea_id = ?",
'accept', idea_id)
return redirect('/')
@app.route("/reject/<int:idea_id>")
def reject(idea_id):
print('idea_id')
# find user permissions
id = session.get("user_id")
rows = db.execute("SELECT permissions FROM users WHERE id = ?", id)
permission = rows[0]["permissions"]
# if user is an admin then allow db to update idea stage
if permission == "admin":
db.execute("UPDATE ideas SET stage = ? WHERE idea_id = ?",
'reject', idea_id)
return redirect('/')
@app.route("/history")
@login_required
def history():
# select all actions current user has taken
user_id = session.get("user_id")
ideas = db.execute(
"SELECT DISTINCT(ideas.title), ideas.time, actions.action FROM ideas JOIN actions ON ideas.idea_id = actions.idea_id WHERE ideas.user_id = ?",
user_id)
return render_template("history.html", ideas=ideas)
@app.route("/review", methods=["GET", "POST"])
@login_required
def review():
if request.method == "POST":
# get stage user wants to filter to
stage = request.form.get("stage")
print(stage)
# if they haven't choosen a stage view all ideas
if stage == 'Choose Stage':
return redirect('/review')
elif stage is None:
return redirect('/review')
# select ideas based on users selected stage
else:
stages = db.execute("SELECT DISTINCT(stage) FROM ideas")
ideas = db.execute("SELECT * FROM ideas WHERE stage = ? ORDER BY title ASC", stage)
return render_template("filter.html", ideas=ideas, stages=stages)
else:
# find user id and company
id = session.get("user_id")
rows = db.execute("SELECT company FROM users WHERE id = ?", id)
company = rows[0]["company"]
# select applicable idea stages for current company
stages = db.execute("SELECT DISTINCT(stage) FROM ideas ORDER BY title ASC")
# select all ideas submitted to current company
ideas = db.execute("SELECT * FROM ideas WHERE company = ? ORDER BY title ASC", company)
return render_template("ideas.html", ideas=ideas, stages=stages)
@app.route("/add", methods=["GET", "POST"])
@login_required
def add():
if request.method == "POST":
# check user id and current company
id = session.get("user_id")
rows = db.execute("SELECT company FROM users WHERE id = ?", id)
company = rows[0]["company"]
# get form data
title = request.form.get("title")
notes = request.form.get("notes")
rows = db.execute("SELECT * FROM ideas WHERE title = ?", title)
# error check
if not title or not notes:
return apology("Missing Input", 400)
if len(rows) >= 1:
return apology("Title already exists, please use another title", 400)
# update ideas and actions
db.execute("INSERT INTO ideas (user_id, title, notes, stage, company) VALUES (?, ?, ?, ?, ?)",
id, title, notes, "idea", company)
row = db.execute("SELECT idea_id FROM ideas WHERE user_id = ? AND title = ?",
id, title)
idea_id = row[0]["idea_id"]
db.execute("INSERT INTO actions (idea_id, action, user_id) VALUES (?, ?, ?)",
idea_id, "submit", id)
return render_template("submitted.html")
else:
return render_template("add.html", departments=DEPARTMENTS)
@app.route("/settings")
@login_required
def settings():
# check user id
id = session.get("user_id")
# check user permissions
rows = db.execute("SELECT permissions FROM users WHERE id = ?", id)
permission = rows[0]["permissions"]
# check user company
rows = db.execute("SELECT company FROM users WHERE id = ?", id)
company = rows[0]["company"]
# select all users from current company
users = db.execute("SELECT * FROM users WHERE company = ?", company)
permissions = db.execute("SELECT DISTINCT(permissions) FROM users")
if permission == "admin":
return render_template("settings.html", users=users, permissions=permissions)
else:
return render_template("permissions.html")
@app.route("/update_permissions/<int:user_id>")
@login_required
def update_permissions(user_id):
# check users persmissions
permissions = db.execute("SELECT permissions FROM users WHERE ID = ?", user_id)
permission = permissions[0]["permissions"]
# if admin change to user
if permission == 'admin':
db.execute("UPDATE users SET permissions = ? WHERE ID = ?",'user', user_id)
# if user change to admin
else:
db.execute("UPDATE users SET permissions = ? WHERE ID = ?",
'admin', user_id)
return redirect("/settings") |
// pages/add.tsx
"use client"
// pages/add.tsx
import React, { useState } from 'react';
import Image from 'next/image';
import { MdAddAPhoto, MdCancel } from 'react-icons/md';
import Swal from 'sweetalert2';
import { useRouter } from 'next/navigation';
import Sidebar from '@/components/Admin/Sidebar';
import Header from '@/components/Admin/Header';
import axios from 'axios';
import auth from '@/utils/withAuth';
const Add = () => {
const [articleHead, setArticleHead] = useState('');
const [articleDescription, setArticleDescription] = useState('');
const [selectedImage, setSelectedImage] = useState<File | null>(null);
const [imagePreview, setImagePreview] = useState<string | null>(null);
const [uploading, setUploading] = useState(false);
const router = useRouter();
const handleImageChange = (e: React.ChangeEvent<HTMLInputElement>) => {
const file = e.target.files?.[0];
if (file) {
setSelectedImage(file);
const reader = new FileReader();
reader.onload = () => {
setImagePreview(reader.result as string);
};
reader.readAsDataURL(file);
}
};
const handleClearImage = () => {
setSelectedImage(null);
setImagePreview(null);
};
const handleArticleHeadChange = (e: React.ChangeEvent<HTMLInputElement>) => {
setArticleHead(e.target.value);
};
const handleArticleDescriptionChange = (e: React.ChangeEvent<HTMLTextAreaElement>) => {
setArticleDescription(e.target.value);
};
const handleSubmit = async (e: React.FormEvent<HTMLFormElement>) => {
e.preventDefault();
// Validation
if (!articleHead || !articleDescription || !selectedImage) {
Swal.fire({
icon: 'error',
title: 'Validation Error',
text: 'All fields are required',
});
return;
}
setUploading(true);
const formData = new FormData();
formData.append('articleHead', articleHead);
formData.append('articleDescription', articleDescription);
formData.append('image', selectedImage);
try {
const response = await axios.post('http://localhost:5000/api/articles', formData, {
headers: {
'Content-Type': 'multipart/form-data',
},
});
setUploading(false);
Swal.fire({
icon: 'success',
title: 'Success!',
text: 'Article added successfully',
});
setSelectedImage(null);
setImagePreview(null);
router.push('/admin/dashboard');
} catch (error) {
console.error(error);
setUploading(false);
Swal.fire({
icon: 'error',
title: 'Error',
text: 'Failed to add article. Please try again later.',
});
}
};
return (
<div className="flex flex-col items-center justify-center min-h-screen bg-gray-100">
<Header />
<Sidebar />
<div className="w-full max-w-md mt-8 px-4">
<div className="bg-white rounded-lg shadow-lg p-6">
<form className="space-y-4" onSubmit={handleSubmit}>
<div className="flex flex-col">
<label htmlFor="title" className="text-lg font-medium text-gray-800">Title</label>
<input id="title" name="title" type="text" className="border border-gray-300 rounded-md px-4 py-2" value={articleHead} onChange={handleArticleHeadChange} />
</div>
<div className="flex flex-col">
<label htmlFor="image" className="text-lg font-medium flex items-center text-gray-800">
<MdAddAPhoto className="mr-2 text-accent" /> Image
</label>
<div className="relative border-2 border-gray-300 rounded-md px-4 py-3 focus-within:ring focus-within:border-accent-light overflow-hidden">
<input type="file" id="image" name="image" accept="image/*" className="absolute inset-0 opacity-0 w-full h-full cursor-pointer" onChange={handleImageChange} />
<div className="h-32 w-full">
{imagePreview && (
<div className="relative w-full h-full">
<Image
src={imagePreview}
alt="Uploaded"
layout="fill"
objectFit="contain"
className="max-w-full max-h-full"
/>
<button
type="button"
onClick={handleClearImage}
className="absolute top-0 right-0 bg-gray-800 text-white rounded-full p-1 m-2"
>
<MdCancel />
</button>
</div>
)}
{!imagePreview && (
<div className="flex items-center justify-center h-full">
<p className="text-sm text-gray-600">Upload an image for your article</p>
</div>
)}
</div>
</div>
</div>
<div className="flex flex-col">
<label htmlFor="content" className="text-lg font-medium text-gray-800">Content</label>
<textarea id="content" name="content" rows={3} className="border border-gray-300 rounded-md px-4 py-2" value={articleDescription} onChange={handleArticleDescriptionChange}></textarea>
</div>
<button type="submit" className="bg-[#FA2E56] text-white px-6 py-3 rounded-md hover:bg-accent-dark transition duration-300" disabled={uploading}>
{uploading ? 'Adding...' : 'Add Article'}
</button>
</form>
</div>
</div>
</div>
);
};
export default auth(Add); |
<!DOCTYPE html>
<html lang="es">
<head>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<!-- Bootstrap CSS -->
<link href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css" rel="stylesheet">
<link rel="stylesheet" href="ccs/clientes.css">
<title>CRM</title>
<style>
/* Estilos para el botón "Iniciar" */
input[type="submit"] {
background-color: #4CAF50;
/* Color de fondo */
color: white;
/* Color del texto */
padding: 10px 20px;
/* Espaciado interno */
border: none;
/* Sin borde */
border-radius: 5px;
/* Bordes redondeados */
cursor: pointer;
/* Cambia el cursor al pasar sobre el botón */
font-size: 16px;
/* Tamaño de fuente */
}
/* Estilos adicionales cuando el cursor pasa sobre el botón */
input[type="submit"]:hover {
background-color: #45a049;
/* Cambia el color de fondo al pasar sobre el botón */
}
.container_formulario_request {
height: 100vh;
width: 100vw;
display: flex;
padding-right: 20px;
justify-content: center;
align-items: center;
background-color: hsl(218, 41%, 15%);
overflow: hidden;
}
.container_formulario_text {
display: flex;
flex-direction: row;
justify-content: space-between;
align-items: center;
width: 80%;
/* Puedes ajustar según tus necesidades */
}
.text_info {
width: 50%;
text-align: right;
padding-right: 20px;
/* Espaciado a la derecha para separar del formulario */
}
.containerbtnIniciar {
width: 100%;
display: flex;
justify-content: center;
align-items: center;
}
#radius-shape-1 {
height: 220px;
width: 220px;
top: -60px;
left: -10px;
background: radial-gradient(#44006b, #ad1fff);
overflow: hidden;
}
#radius-shape-2 {
border-radius: 38% 62% 63% 37% / 70% 33% 67% 30%;
bottom: -60px;
right: -110px;
width: 300px;
height: 300px;
background: radial-gradient(#44006b, #ad1fff);
overflow: hidden;
}
.bg-glass {
background-color: hsla(0, 0%, 100%, 0.9) !important;
backdrop-filter: saturate(200%) blur(25px);
}
</style>
<script>
function validarFormulario() {
var email = document.getElementById('form3Example3').value;
var password = document.getElementById('form3Example4').value;
var emailPattern = /^[^ ]+@[^ ]+\.[a-z]{2,3}$/;
var passwordPattern = /^(?=.*\d)(?=.*[a-z])(?=.*[A-Z]).{8,}$/;
if (!email.match(emailPattern)) {
alert('Por favor, introduce un correo electrónico válido.');
return false;
}
if (!password.match(passwordPattern)) {
alert('La contraseña debe tener al menos 8 caracteres, incluyendo al menos una letra mayúscula, una letra minúscula y un número.');
return false;
}
// Si las validaciones son exitosas, puedes realizar alguna acción adicional aquí.
// Agrega la acción que deseas realizar después de la validación exitosa.
console.log('Formulario enviado con éxito!');
}
</script>
</head>
<body>
<main class="py-4">
@yield('content')
</main>
<!-- Optional JavaScript -->
<!-- jQuery first, then Popper.js, then Bootstrap JS -->
<script src="https://code.jquery.com/jquery-3.3.1.slim.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js"></script>
</body>
</html> |
import { homedir } from 'os';
import { existsSync, mkdirSync, readFileSync, writeFileSync } from 'fs';
import { normalize } from 'path';
import { BrowserWindow } from 'electron';
import { EditorSettings } from '../interfaces/Settings';
export class AppSettings {
private context: BrowserWindow;
private appPath: string;
private filePath: string;
private settings: EditorSettings = {
autoindent: false,
darkmode: false,
wordwrap: true,
whitespace: false,
minimap: true,
systemtheme: true,
};
public applied: EditorSettings | null = null;
constructor(context: BrowserWindow) {
this.context = context;
this.appPath = normalize(homedir() + '/.mkeditor/');
this.filePath = this.appPath + 'settings.json';
this.createFileIfNotExists(this.settings);
this.applied = this.loadFile() as EditorSettings;
}
loadFile() {
const file = readFileSync(this.filePath, {
encoding: 'utf-8',
});
return JSON.parse(file);
}
createFileIfNotExists(settings: EditorSettings) {
if (!existsSync(this.appPath)) {
mkdirSync(this.appPath);
}
if (!existsSync(this.filePath)) {
settings = { ...this.settings, ...settings };
this.saveSettingsToFile(settings, true);
}
}
saveSettingsToFile(settings: EditorSettings, init = false) {
try {
writeFileSync(this.filePath, JSON.stringify(settings, null, 4), {
encoding: 'utf-8',
});
if (!init) {
this.context.webContents.send('from:notification:display', {
status: 'success',
message: 'Settings successfully updated.',
});
}
} catch (err) {
const detail = err as { code: string };
const message =
detail.code === 'EPERM'
? 'Unable to save settings: permission denied.'
: 'Unable to save settings: unknown error.';
this.context.webContents.send('from:notification:display', {
status: 'error',
message,
});
}
}
} |
import React from "react";
import "antd/dist/antd.css";
// import { DeleteOutlined } from "@ant-design/icons";
import { useState } from "react";
import { Space, Table, Tag, Row, Col, Breadcrumb, Button, Input } from "antd";
import type { ColumnsType, TableProps } from "antd/lib/table";
import ModelCustom from "./ModelCustom";
const { Column } = Table;
// const products = [
// {
// key: "1",
// name: "Product 1",
// quantity: 32,
// supplier: "New York No. 1 Lake Park",
// tags: ["nice", "developer"],
// },
// {
// key: "2",
// name: "Product 2",
// quantity: 42,
// supplier: "London No. 1 Lake Park",
// tags: ["loser"],
// },
// {
// key: "3",
// name: "Product 3",
// quantity: 32,
// supplier: "Sidney No. 1 Lake Park",
// tags: ["cool", "teacher"],
// },
// ];
interface DataType {
key: React.Key;
name: string;
age: number;
address: string;
}
const columns: ColumnsType<DataType> = [
{
title: "Id",
dataIndex: "id",
},
{
title: "Title",
dataIndex: "title",
},
{
title: "Content",
dataIndex: "body",
},
];
const Product = () => {
const onSearch = (value: any) => console.log(value);
const [isModalVisible, setIsModalVisible] = useState(false);
const [products, setProducts] = useState([
{
key: "1",
name: "Product 1",
quantity: 32,
supplier: "New York No. 1 Lake Park",
tags: ["nice", "developer"],
},
{
key: "2",
name: "Product 2",
quantity: 42,
supplier: "London No. 1 Lake Park",
tags: ["loser"],
},
{
key: "3",
name: "Product 3",
quantity: 32,
supplier: "Sidney No. 1 Lake Park",
tags: ["cool", "teacher"],
},
]);
const [title, setTitle] = useState("");
const [dataRepair, setDataRepair] = useState([]);
const showModal = (title: any, data: any) => {
setIsModalVisible(true);
setTitle(title);
setDataRepair(data);
};
const handleAddNewProduct = (product: any) => {
setIsModalVisible(false);
if (product && product.tags) {
product.tags = product.tags.split(",");
product.key = products.length + 1;
setProducts([...products, product]);
}
};
const handleUpdateProductById = (id: number, product: any) => {
product.key = id;
const copyProducts = products;
copyProducts[id - 1] = product;
setProducts([...copyProducts]);
setIsModalVisible(false);
};
const handleDeleteProductById = (id: number) => {
const copyProducts = products;
copyProducts.splice(id, 1);
setProducts([...copyProducts]);
};
const handleCancel = () => {
setIsModalVisible(false);
};
return (
<Row className="w-[99%] mt-[1%] ml-[0.5%] bg-white">
<ModelCustom
isShowModal={isModalVisible}
isHandleAddNewProduct={handleAddNewProduct}
isHandleUpdateProductById={handleUpdateProductById}
isHandleCancel={handleCancel}
title={title}
data={dataRepair}
/>
<Col span={12} offset={2}>
<Button
type="primary"
className="float-right mt-3 mr-3"
onClick={() =>
showModal("add", {
key: "",
name: "",
quantity: 0,
supplier: "",
tags: [],
})
}
>
Add Product
</Button>
<Input
placeholder="Search..."
onChange={(e) => onSearch(e.target.value)}
style={{
width: 250,
height: 50,
marginRight: 12,
marginTop: 12,
float: "right",
}}
/>
</Col>
<Col span={23} className={`mt-3 ml-7`}>
<Table dataSource={products}>
<Column title="Key" dataIndex="key" key="key"></Column>
<Column title="Name" dataIndex="name" key="name" />
<Column
title="Quantity"
dataIndex="quantity"
key="quantity"
/>
<Column
title="Supplier"
dataIndex="supplier"
key="supplier"
/>
<Column
title="Tags"
dataIndex="tags"
key="tags"
render={(tags) => (
<>
{tags.map((tag: any) => (
<Tag color="blue" key={tag}>
{tag}
</Tag>
))}
</>
)}
/>
<Column
title="Action"
key="action"
render={(_, record: any) => (
<Space size="middle">
<a onClick={() => showModal("update", record)}>
Repair {record.name}
</a>
<a
onClick={() =>
handleDeleteProductById(record.key - 1)
}
>
Delete
</a>
</Space>
)}
/>
</Table>
</Col>
</Row>
);
};
export default Product; |
// CSS Reset, comment out if not required or using a different module
// Custom Theming for Angular Material
// For more information: https://material.angular.io/guide/theming
@import '~@angular/material/theming';
// Plus imports for other components in your app.
// Include the common styles for Angular Material. We include this here so that you only
// have to load a single css file for Angular Material in your app.
// Be sure that you only ever include this mixin once!
@include mat-core();
// Define the palettes for your theme using the Material Design palettes available in palette.scss
// (imported above). For each palette, you can optionally specify a default, lighter, and darker
// hue. Available color palettes: https://material.io/design/color/
$CustomerWebsite2-primary: mat-palette($mat-blue);
$CustomerWebsite2-accent: mat-palette($mat-light-blue, A200, A100, A400);
// The warn palette is optional (defaults to red).
$CustomerWebsite2-warn: mat-palette($mat-red);
// Create the theme object (a Sass map containing all of the palettes).
$CustomerWebsite2-theme: mat-light-theme($CustomerWebsite2-primary, $CustomerWebsite2-accent, $CustomerWebsite2-warn);
// Include theme styles for core and each component used in your app.
// Alternatively, you can import and @include the theme mixins for each component
// that you are using.
@include angular-material-theme($CustomerWebsite2-theme);
// Custom Theming for Angular Material
// For more information: https://material.angular.io/guide/theming
@import '~@angular/material/theming';
// Plus imports for other components in your app.
// Include the common styles for Angular Material. We include this here so that you only
// have to load a single css file for Angular Material in your app.
// Be sure that you only ever include this mixin once!
@include mat-core();
// Define the palettes for your theme using the Material Design palettes available in palette.scss
// (imported above). For each palette, you can optionally specify a default, lighter, and darker
// hue. Available color palettes: https://material.io/design/color/
$CustomerWebsite2-primary: mat-palette($mat-blue);
$CustomerWebsite2-accent: mat-palette($mat-light-blue, A200, A100, A400);
// The warn palette is optional (defaults to red).
$CustomerWebsite2-warn: mat-palette($mat-red);
// Create the theme object (a Sass map containing all of the palettes).
$CustomerWebsite2-theme: mat-light-theme($CustomerWebsite2-primary, $CustomerWebsite2-accent, $CustomerWebsite2-warn);
// Include theme styles for core and each component used in your app.
// Alternatively, you can import and @include the theme mixins for each component
// that you are using.
@include angular-material-theme($CustomerWebsite2-theme);
// Custom Theming for Angular Material
// For more information: https://material.angular.io/guide/theming
@import '~@angular/material/theming';
// Plus imports for other components in your app.
// Include the common styles for Angular Material. We include this here so that you only
// have to load a single css file for Angular Material in your app.
// Be sure that you only ever include this mixin once!
@include mat-core();
// Define the palettes for your theme using the Material Design palettes available in palette.scss
// (imported above). For each palette, you can optionally specify a default, lighter, and darker
// hue. Available color palettes: https://material.io/design/color/
$CustomerWebsite2-primary: mat-palette($mat-blue);
$CustomerWebsite2-accent: mat-palette($mat-light-blue, A200, A100, A400);
// The warn palette is optional (defaults to red).
$CustomerWebsite2-warn: mat-palette($mat-red);
// Create the theme object (a Sass map containing all of the palettes).
$CustomerWebsite2-theme: mat-light-theme($CustomerWebsite2-primary, $CustomerWebsite2-accent, $CustomerWebsite2-warn);
// Include theme styles for core and each component used in your app.
// Alternatively, you can import and @include the theme mixins for each component
// that you are using.
@include angular-material-theme($CustomerWebsite2-theme);
/* You can add global styles to this file, and also import other style files */
body { margin: 0; font-family: Roboto, "Helvetica Neue", sans-serif; }
@media (max-width: 868px) {
.content-wrapper {
padding-top: 0;
}
}
@keyframes fadeInUp {
from {
transform: translate(0, 50px);
}
to {
transform: translate(0,0);
opacity: 1
}
}
@-webkit-keyframes fadeInUp {
from {
transform: translate(0,50px)
}
to {
transform: translate(0,0);
opacity: 1
}
}
@-webkit-keyframes fadeIn {
from {
opacity: 0;
}
to {
opacity: 1;
}
}
@keyframes fadeIn {
from {
opacity: 0;
}
to {
opacity: 1;
}
}
.fadeIn {
animation-duration: 1s;
animation-fill-mode: both;
-webkit-animation-duration: 1s;
-webkit-animation-fill-mode: both
}
.fadeIn {
opacity: 0;
animation-name: fadeIn;
-webkit-animation-name: fadeIn;
} |
import { Directive, HostListener, ElementRef, Renderer2, HostBinding } from '@angular/core';
@Directive({
selector: '[appHighlightMouse]',
})
export class HighlightMouseDirective {
@HostListener('mouseenter') onMouseOver() {
// this.renderer.setStyle(
// this.elementRef.nativeElement,
// 'background-color',
// 'yellow'
// );
this.backgroundColor = 'yellow';
}
@HostListener('mouseleave') onMouseLeave() {
// this.renderer.setStyle(
// this.elementRef.nativeElement,
// 'background-color',
// 'initial'
// );
this.backgroundColor = 'initial';
}
@HostBinding('style.backgroundColor') backgroundColor: string = '';
constructor(private elementRef: ElementRef, private renderer: Renderer2) {}
} |
import re
import sys
from datetime import datetime
from enum import Enum
from typing import Final
DATE_TIME_FMT = '%b %d %H:%M:%S'
TIME_FMT = '%H:%M:%S'
DATE_FMT = '%b %d'
class Keys(Enum):
FLD_TIME = 'time'
FLD_COMPUTER = 'pc_name'
FLD_SERVICE = 'service_name'
FLD_MSG = 'message'
FLD_DATE = 'date'
LOGS: Final[list[str]] = [
'May 18 11:59:18 PC-00102 plasmashell[1312]: kf.plasma.core: findInCache with a lastModified timestamp of 0 is deprecated',
'May 18 13:06:54 ideapad kwin_x11[1273]: Qt Quick Layouts: Detected recursive rearrange. Aborting after two iterations.',
'May 20 09:16:28 PC0078 systemd[1]: Starting PackageKit Daemon...',
'May 20 11:01:12 PC-00102 PackageKit: daemon start',
'May 20 12:48:18 PC0078 systemd[1]: Starting Message of the Day...',
'May 21 14:33:55 PC0078 kernel: [221558.992188] usb 1-4: New USB device found, idVendor=1395, idProduct=0025, bcdDevice= 1.00',
'May 22 11:48:30 ideapad mtp-probe: checking bus 1, device 3: "/sys/devices/pci0000:00/0000:00:08.1/0000:03:00.3/usb1/1-4"',
'May 22 11:50:09 ideapad mtp-probe: bus: 1, device: 3 was not an MTP device',
'May 23 08:06:14 PC-00233 kernel: [221559.381614] usbcore: registered new interface driver snd-usb-audio',
'May 24 16:19:52 PC-00233 systemd[1116]: Reached target Sound Card.',
'May 24 19:26:40 PC-00102 rtkit-daemon[1131]: Supervising 5 threads of 2 processes of 1 users.',
]
def log_splitter(item: str) -> dict:
reg = re.compile(
r'^(?P<date_time>\w{3}\s+\d{2}\s+\d{2}:\d{2}:\d{2})\s+'
r'(?P<computer_name>[\w\-\[\]]+)(:+|\s+)*'
r'(?P<service_name>[\w\.\-[\]]+)(:+|\s+)*(?P<msg>[\W\w]+)$'
)
grp = reg.search(item).group
return {
Keys.FLD_TIME: grp('date_time'),
Keys.FLD_COMPUTER: grp('computer_name'),
Keys.FLD_SERVICE: grp('service_name'),
Keys.FLD_MSG: grp('msg')
}
def build_parsed_logs(logs):
if not isinstance(logs, list) or len(logs) < 1:
return []
return [log_splitter(item) for item in logs]
def split_time(logs_dics):
if not isinstance(logs_dics, list) or len(logs_dics) < 1:
return logs_dics
current_year = datetime.now().year
for item_dict in logs_dics:
date_time = datetime.strptime(
item_dict[Keys.FLD_TIME], DATE_TIME_FMT).replace(year=current_year)
item_dict[Keys.FLD_TIME] = date_time.time().strftime(TIME_FMT)
item_dict[Keys.FLD_DATE] = date_time.date().strftime(DATE_FMT)
def to_dic(lst, start_key):
return {k: value for k, value in enumerate(lst, start_key)}
def main():
# Создайте из него список словарей, используя ключи из того же задания. Напоминаю
list_dict_of_logs = build_parsed_logs(LOGS)
# Выведите на экран список значений <дата/время> всех словарей. Воспользуйтесь списковым включением.
print([log_dic[Keys.FLD_TIME] for log_dic in list_dict_of_logs])
# Измените словари в списке: создайте новый ключ 'date', перенеся в его значение дату из поля 'time'. В поле 'time' оставьте только время. Выведите значения для поля 'time' всех словарей в списке.
split_time(list_dict_of_logs)
print('-' * 20)
print([(log_dic[Keys.FLD_TIME], log_dic[Keys.FLD_DATE])
for log_dic in list_dict_of_logs])
print('-' * 20)
# Выведите список значений поля 'message' для всех логов, которые записал ПК с именем 'PC0078'. Воспользуйтесь списковым включением.
for line in [f'{i}. {log_item[Keys.FLD_MSG]}' for i, log_item in
enumerate([log_item for log_item in list_dict_of_logs if log_item[Keys.FLD_COMPUTER] == 'PC0078'], 1)]:
print(line)
print('-' * 20)
# Превратите список словарей логов (который вы сделали в пункте 2) в словарь. Ключами в нем будут целые числа от 100 до 110, а значениями - словари логов.
dic_of_dics = to_dic(list_dict_of_logs, 100)
# Выведите на экран словарь лога под ключом 104
print(dic_of_dics[104])
return 0
if __name__ == '__main__':
sys.exit(main()) |
package com.dji.sdk.cloudapi.map;
import com.dji.sdk.exception.CloudSDKException;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.v3.oas.annotations.media.Schema;
import java.util.Arrays;
/**
* @author sean
* @version 0.2
* @date 2021/11/30
*/
@Schema(enumAsRef = true, description = "<p>0: Point <p/><p>1: LineString <p/><p>2: Polygon</p>", allowableValues = {"0", "1", "2"})
public enum ElementResourceTypeEnum {
POINT(0, "Point"),
LINE_STRING(1, "LineString"),
POLYGON(2, "Polygon");
private final int type;
private final String typeName;
ElementResourceTypeEnum(int type, String typeName) {
this.type = type;
this.typeName = typeName;
}
public String getTypeName() {
return typeName;
}
@JsonValue
public int getType() {
return type;
}
@JsonCreator
public static ElementResourceTypeEnum find(int type) {
return Arrays.stream(values()).filter(typeEnum -> typeEnum.type == type).findAny()
.orElseThrow(() -> new CloudSDKException(ElementResourceTypeEnum.class, type));
}
public static ElementResourceTypeEnum find(String typeName) {
return Arrays.stream(values()).filter(typeEnum -> typeEnum.typeName.equals(typeName)).findAny()
.orElseThrow(() -> new CloudSDKException(ElementResourceTypeEnum.class, typeName));
}
} |
import { EntityManager, Repository } from "typeorm";
import { User } from "../entities/User";
import { ICreateUserDTO } from "../../../dto/ICreateUserDTO";
import { BaseRepository } from "@shared/infra/typeorm/repositories/BaseRepository";
import { injectable } from "inversify";
@injectable()
class UserRepository extends BaseRepository {
private _repository: Repository<User>;
constructor(manager?: EntityManager) {
super(manager);
this._repository = this.dataSource.getRepository(User);
}
async create({
name,
email,
password,
gender,
status,
type,
avatar,
cellPhone,
phone,
postalCode,
street,
number,
complement,
district,
city,
state,
}: ICreateUserDTO) {
const user = this._repository.create({
name,
email,
password,
gender,
status,
type,
avatar,
cellPhone,
phone,
postalCode,
street,
number,
district,
complement,
city,
state,
});
await this._repository.save(user);
return user;
}
async findByEmail(email: string) {
const user = await this._repository.findOne({
where: { email },
});
return user;
}
async findById(id: string) {
const user = await this._repository.findOneBy({
id,
});
return user;
}
async listUsers() {
const users = await this._repository.find();
return users;
}
}
export { UserRepository }; |
#include <stdio.h>
#include <stdlib.h>
#define TRACKS 100
//bubble sort
void sort(int tracks[], int n) {
int i, j;
for (i = 0; i < n - 1; i++) {
for (j = 0; j < n - i - 1; j++) {
if (tracks[j] > tracks[j + 1]) {
int temp = tracks[j];
tracks[j] = tracks[j + 1];
tracks[j + 1] = temp;
}
}
}
}
void c_scan(int tracks[], int n, int start) {
int i, j, seek_count = 0;
int current_track = start;
sort(tracks, n);
int start_index = -1;
for (i = 0; i < n; i++) {
if (tracks[i] >= start) {
start_index = i;
break;
}
}
printf("Sequence of tracks visited: ");
for (i = start_index; i < n; i++) {
printf("%d ", tracks[i]);
seek_count += abs(tracks[i] - current_track);
current_track = tracks[i];
}
printf("%d ", 0);
seek_count += abs(current_track);
current_track = 0;
for (i = 0; i < start_index; i++) {
printf("%d ", tracks[i]);
seek_count += abs(tracks[i] - current_track);
current_track = tracks[i];
}
printf("\nTotal number of track movements: %d\n", seek_count);
}
int main() {
int n, start_track;
printf("Enter the number of requests: ");
scanf("%d", &n);
int tracks[n];
printf("Enter the request numbers: ");
for (int i = 0; i < n; i++) {
scanf("%d", &tracks[i]);
}
printf("Enter the current position of Read/Write head: ");
scanf("%d", &start_track);
c_scan(tracks, n, start_track);
return 0;
} |
import io
import textwrap
def main() -> None:
with open("/tmp/_test_seek0.txt", "w+") as f:
f.write("hello\nworld\n")
f.seek(0)
assert f.readline().strip() == "hello"
assert f.readline().strip() == "world"
input_stream = textwrap.dedent(
"""\
hello
world\n""")
with io.StringIO(input_stream) as f:
# To move the pointer to the end of the file, you can use seek(0, 2).
# Here, 0 is the offset, and 2 means that the offset should be calculated from the end of the file.
f.seek(0, 2)
f.write("again\n")
f.seek(0)
assert f.readline().strip() == "hello"
assert f.readline().strip() == "world"
assert f.readline().strip() == "again"
main() |
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.19;
contract TestMultiCall {
function func1() external view returns (uint, uint) {
return (1, block.timestamp);
}
function func2() external view returns (uint, uint) {
return (2, block.timestamp);
}
function getData1() external pure returns(bytes memory) {
//abi.encodeWithSignature("func1()");
return abi.encodeWithSelector(this.func1.selector);
}
function getData2() external pure returns(bytes memory) {
return abi.encodeWithSelector(this.func2.selector);
}
}
contract MultiCall {
function multiCall(address[] calldata targets, bytes[] calldata data)
external
view
returns (bytes[] memory)
{
require(targets.length == data.length, "target length != data length");
bytes[] memory results = new bytes[](data.length);
for (uint256 i; i < targets.length; i++) {
(bool success,bytes memory result)=targets[i].staticcall(data[i]);
require(success,"call failed");
results[i] = result;
}
return results;
}
} |
// Join
1. Selezionare tutti gli studenti iscritti al Corso di Laurea in Economia
SELECT `students`.`id`,`students`.`name`, `students`.`surname`
FROM `students`
INNER JOIN `degrees`
ON `degrees`.`id` = `students`.`degree_id`
WHERE `degrees`.`name` = 'Corso di Laurea in Economia';
2. Selezionare tutti i Corsi di Laurea Magistrale del Dipartimento di
Neuroscienze
SELECT *
FROM `degrees`
INNER JOIN `departments`
ON `departments`.`id` = `degrees`.`department_id`
WHERE `degrees`.`level` = 'magistrale' AND `departments`.`name` = 'Dipartimento di neuroscienze';
3. Selezionare tutti i corsi in cui insegna Fulvio Amato (id=44)
SELECT `courses`.name
FROM `teachers`
INNER JOIN `course_teacher`
ON `course_teacher`.`teacher_id` = `teachers`.`id`
INNER JOIN `courses`
ON `courses`.`id` = `course_teacher`.`course_id`
WHERE `teachers`.`id` = 44;
4. Selezionare tutti gli studenti con i dati relativi al corso di laurea a cui
sono iscritti e il relativo dipartimento, in ordine alfabetico per cognome e
nome
SELECT `students`.`id`,`students`.`name`, `students`.`surname`, `degrees`. `name` , `departments`. `name`
FROM `students`
INNER JOIN `degrees`
ON `degrees`.`id` = `students`.`id`
INNER JOIN `departments`
ON `departments`.`id` = `degrees`.`department_id`
ORDER BY `students`.`name`, `students`.`surname`;
5. Selezionare tutti i corsi di laurea con i relativi corsi e insegnanti
SELECT `degrees`.`name` , `courses`. * , `teachers`.`id`, `teachers`.`name`, `teachers`.`surname`
FROM `degrees`
INNER JOIN `courses`
ON `courses`.`degree_id` = `degrees`.`id`
INNER JOIN `course_teacher`
ON `course_teacher`.`course_id` = `courses`.`id`
INNER JOIN `teachers`
ON `teachers`.`id` = `course_teacher`.`teacher_id`;
6. Selezionare tutti i docenti che insegnano nel Dipartimento di
Matematica (54)
SELECT DISTINCT `teachers`.`id`, `teachers`.`name`, `teachers`.`surname`
FROM `teachers`
INNER JOIN `course_teacher`
ON `course_teacher`.`teacher_id` = `teachers`.`id`
INNER JOIN `courses`
ON `courses`.`id` = `course_teacher`.`course_id`
INNER JOIN `degrees`
ON `degrees`.`id` = `courses`.`degree_id`
INNER JOIN `departments`
ON `departments`.`id` = `degrees`.`department_id`
WHERE `departments`.`name` = 'dipartimento di matematica';
7. BONUS: Selezionare per ogni studente il numero di tentativi sostenuti
per ogni esame, stampando anche il voto massimo. Successivamente,
filtrare i tentativi con voto minimo 18.
// Group by
1. Contare quanti iscritti ci sono stati ogni anno
SELECT YEAR(`enrolment_date`) AS anno_iscrizione, COUNT(*) AS numero_iscritti
FROM `students`
GROUP BY YEAR(`enrolment_date`);
2. Contare gli insegnanti che hanno l'ufficio nello stesso edificio
SELECT `office_address` AS edificio, COUNT(`id`) AS numero_insegnanti
FROM `teachers`
GROUP BY `office_address`;
3. Calcolare la media dei voti di ogni appello d'esame
SELECT `exam_id` as appello_esame_id, AVG(`vote`) as media_voto
FROM `exam_student`
GROUP BY `exam_id`;
4. Contare quanti corsi di laurea ci sono per ogni dipartimento
SELECT `department_id`, COUNT(`name`) AS corsi_laurea
FROM `degrees`
GROUP BY `department_id`; |
<?php
/*
add_action( 'wp_enqueue_scripts', 'my_theme_enqueue_styles' );
function my_theme_enqueue_styles() {
wp_enqueue_style( 'child-style',
get_stylesheet_uri(),
array( 'parenthandle' ),
wp_get_theme()->get( 'Version' ) // This only works if you have Version defined in the style header.
);
*/
function ot_register_theme_options_page() {
/* get the settings array */
$get_settings = get_option( 'option_tree_settings' );
/* sections array */
$sections = isset( $get_settings['sections'] ) ? $get_settings['sections'] : array();
/* settings array */
$settings = isset( $get_settings['settings'] ) ? $get_settings['settings'] : array();
/* contexual_help array */
$contextual_help = isset( $get_settings['contextual_help'] ) ? $get_settings['contextual_help'] : array();
/* build the Theme Options */
if ( function_exists( 'ot_register_settings' ) && OT_USE_THEME_OPTIONS ) {
ot_register_settings( array(
array(
'id' => 'option_tree',
'pages' => array(
array(
'id' => 'ot_theme_options',
'parent_slug' => apply_filters( 'ot_theme_options_parent_slug', 'themes.php' ),
'page_title' => apply_filters( 'ot_theme_options_page_title', __( 'Theme Options', 'option-tree' ) ),
'menu_title' => apply_filters( 'ot_theme_options_menu_title', __( 'Theme Options', 'option-tree' ) ),
'capability' => $caps = apply_filters( 'ot_theme_options_capability', 'edit_theme_options' ),
'menu_slug' => apply_filters( 'ot_theme_options_menu_slug', 'ot-theme-options' ),
'icon_url' => apply_filters( 'ot_theme_options_icon_url', null ),
'position' => apply_filters( 'ot_theme_options_position', null ),
'updated_message' => apply_filters( 'ot_theme_options_updated_message', __( 'Theme Options updated.', 'option-tree' ) ),
'reset_message' => apply_filters( 'ot_theme_options_reset_message', __( 'Theme Options reset.', 'option-tree' ) ),
'button_text' => apply_filters( 'ot_theme_options_button_text', __( 'Save Changes', 'option-tree' ) ),
'screen_icon' => 'themes',
'contextual_help' => $contextual_help,
'sections' => $sections,
'settings' => $settings
)
)
)
)
);
// Filters the options.php to add the minimum user capabilities.
// replace the below create_function with an anonymous function because create_function() is deprecated and causes
// fatal errors in PHP 8.0
//add_filter( 'option_page_capability_option_tree', create_function( '$caps', "return '$caps';" ), 999 );
add_filter( 'option_page_capability_option_tree',
function( $caps ) {
return $caps;
}
, 999 );
}
}
function flato_child_enqueue_styles() {
$theme = wp_get_theme();
$parenthandle = 'themememe-base';
wp_enqueue_style( 'themememe-child-base',
get_stylesheet_directory_uri() . '/css/base.css',
array( $parenthandle ),
$theme->get( 'Version' ) // This only works if you have Version defined in the style header.
);
$parenthandle = 'themememe-icons';
wp_enqueue_style( 'themememe-child-icons',
get_stylesheet_directory_uri() . '/css/font-awesome.min.css',
array( $parenthandle ),
$theme->get( 'Version' ) // This only works if you have Version defined in the style header.
);
$parenthandle = 'themememe-style'; // This is themememe-style for the Flato theme.
wp_enqueue_style( $parenthandle,
get_template_directory_uri() . '/style.css');
wp_enqueue_style( 'themememe-child-style',
get_stylesheet_uri(),
array( $parenthandle ),
$theme->get( 'Version' ) // This only works if you have Version defined in the style header.
);
}
add_action( 'wp_enqueue_scripts', 'flato_child_enqueue_styles');
function wpdocs_remove_website_field( $fields ) {
unset( $fields['url'] );
return $fields;
}
add_filter( 'comment_form_default_fields', 'wpdocs_remove_website_field' ); |
import fs from 'fs/promises'
import axios from 'axios'
const API_KEY = process.env.API_KEY
interface Locations {
order: number
province: string
name: string
}
async function load() {
const content = await fs.readFile('./_debug.csv', 'utf-8')
const locations: Locations[] = content
.split('\n')
.map((line) => line.replace(/\"/g, '').split(','))
.map(([name, province, order]) => ({
order: parseInt(order),
province,
name,
}))
for (let location of locations) {
try {
const locationQuery = `${location.name} ${location.province}`
console.log(`> ${location.name}, ${location.province}`)
const response = await axios.post(
`https://maps.googleapis.com/maps/api/place/textsearch/json?query=${locationQuery}&key=${API_KEY}`
)
const json = response.data.results?.[0]
const {lat, lng} = json.geometry.location
const {name, formatted_address, place_id, business_status} = json
const fileContent = `"${location.name}","${location.province}","${location.order}","${name}","${formatted_address}","${place_id}","${business_status}","${lat}","${lng}"\n`
await fs.appendFile('./_locations2.csv', fileContent)
console.log(`[ok] ${name} ${lat},${lng}`)
} catch (e) {
console.warn(`[fail] ${location?.name}`, e)
await fs.appendFile(
'./_fails.csv',
`"${location?.name}","${location?.province}","${location?.order}"\n`
)
continue
}
}
}
load() |
"""Demonstrating an example pick and place task"""
from datetime import datetime
import numpy as np
from reachbot_manipulation.core.env import Environment, EnvConfig
from reachbot_manipulation.utils.bullet_utils import initialize_pybullet
from reachbot_manipulation.optimization.stance_planner import StancePlanner
from reachbot_manipulation.optimization.tension_planner import TensionPlanner
from reachbot_manipulation.core.reachbot import Reachbot
from reachbot_manipulation.trajectories.traj_planner import plan_traj
from reachbot_manipulation.trajectories.trajectory import ReachbotTrajectory
from reachbot_manipulation.utils.bullet_utils import load_floor, load_rigid_object
from reachbot_manipulation.config.reachbot_config import (
LOCAL_CABLE_POSITIONS,
LOCAL_CONE_NORMALS,
)
RECOMPUTE = False
PRECOMPUTED_ASSIGNMENTS = [10, 1, 13, 16, 2, 17, 5, 8]
RECORD = False
SHOW_ENV = True
def main():
# Generate an environment
rng = np.random.default_rng(0)
env_config = EnvConfig(rng=rng)
env = Environment.from_config(env_config)
# Sizes
gripper_len = 1
robot_size = 1
object_size = 0.5
env_radius = 10
# Masses
robot_mass = 10
object_mass = 1
system_mass = robot_mass + object_mass
# Constants
g = 3.71
# Trajectory definition
x0 = -2
xf = 2
y = 0
z_grasp = -env_radius + object_size + gripper_len + robot_size / 2
z_lift = z_grasp + 1
orn = np.array([0, 0, 0, 1])
pick_pose = np.array([x0, y, z_grasp, *orn])
above_pick_pose = np.array([x0, y, z_lift, *orn])
above_place_pose = np.array([xf, y, z_lift, *orn])
place_pose = np.array([xf, y, z_grasp, *orn])
# Wrenches
robot_static_eq_wrench = np.array([0, 0, robot_mass * g, 0, 0, 0])
system_static_eq_wrench = np.array([0, 0, system_mass * g, 0, 0, 0])
pose_wrench_pairs = [
[above_pick_pose, robot_static_eq_wrench],
[pick_pose, robot_static_eq_wrench],
[pick_pose, system_static_eq_wrench],
[above_pick_pose, system_static_eq_wrench],
[above_place_pose, system_static_eq_wrench],
[place_pose, system_static_eq_wrench],
[place_pose, robot_static_eq_wrench],
[above_place_pose, robot_static_eq_wrench],
]
# Planner inputs
# HACK: in theory there would be more pose/wrench pairs but this subset will work
poses = np.array([above_pick_pose, pick_pose, above_place_pose, place_pose])
wrenches = np.array(
[
system_static_eq_wrench,
system_static_eq_wrench,
system_static_eq_wrench,
system_static_eq_wrench,
]
)
max_tension = 100 # Increase for ease of computation
end_pts = np.array([site.position for site in env.sites])
task_basis = np.vstack([np.eye(6), -np.eye(6)]) # +- standard basis
basis_weights = np.ones(12) # Unweighted (ball metric)
site_weights = np.ones(env.num_sites) # Assumes all sites are good
cone_angle = np.pi / 2
# Solve the problem
if RECOMPUTE:
print("Constructing and solving the problem")
prob = StancePlanner(
poses,
LOCAL_CABLE_POSITIONS,
end_pts,
LOCAL_CONE_NORMALS,
cone_angle,
wrenches,
max_tension,
task_basis,
basis_weights,
site_weights,
verbose=False,
)
prob.solve()
assignments = prob.optimal_assignments
print("Assignments: ", assignments)
else:
assignments = PRECOMPUTED_ASSIGNMENTS
attached_points = np.array([env.sites[i].position for i in assignments])
# Init tension prob
tension_prob = TensionPlanner(
poses[0, :3],
poses[0, 3:],
LOCAL_CABLE_POSITIONS,
attached_points,
wrenches[0],
max_tension,
verbose=False,
)
tensions = []
for pose, wrench in pose_wrench_pairs:
tension_prob.update_robot_pose(pose[:3], pose[3:])
tension_prob.update_applied_wrench(wrench)
tension_prob.solve()
tensions.append(tension_prob.optimal_tensions)
tensions = np.array(tensions)
traj_components = []
dt = 1 / 240
for i in range(len(pose_wrench_pairs) - 1):
pose_1 = pose_wrench_pairs[i][0]
pose_2 = pose_wrench_pairs[i + 1][0]
if np.allclose(pose_1, pose_2):
# Fixed position, just vary tensions
# Give it 3 seconds to adjust the tensions in the cables
duration = 3
n_timesteps = int(round(duration / dt))
times = np.arange(0, n_timesteps) * dt
traj_components.append(
ReachbotTrajectory(
positions=np.ones((n_timesteps, 1)) * pose_1[:3],
quats=np.ones((n_timesteps, 1)) * pose_1[3:],
lin_vels=np.zeros((n_timesteps, 3)),
ang_vels=np.zeros((n_timesteps, 3)),
lin_accels=np.zeros((n_timesteps, 3)),
ang_accels=np.zeros((n_timesteps, 3)),
times=times,
attached_points=attached_points,
init_tensions=tensions[i],
final_tensions=tensions[i + 1],
)
)
else:
traj_components.append(
plan_traj(
pose_1[:3],
pose_1[3:],
pose_2[:3],
pose_2[3:],
dt,
attached_points,
tensions[i],
tensions[i + 1],
)
)
# Simulate
client = initialize_pybullet(gravity=-g, bg_color=(0.5, 0.5, 0.5))
if SHOW_ENV:
env_id, sites_id = env.visualize()
client.resetDebugVisualizerCamera(6.40, 10.40, -4.60, (0.38, -1.18, -6.97))
floor_id = load_floor(z_pos=-env_radius, client=client)
client.changeVisualShape(
floor_id,
-1,
rgbaColor=[1, 1, 1, 0],
)
object_id = load_rigid_object(
"cube.urdf",
scale=object_size,
pos=(x0, 0, -env_radius + object_size / 2),
mass=object_mass,
)
robot = Reachbot(pose_wrench_pairs[0][0], client=client, kp=100)
robot.attach_to(attached_points, tensions[0], max_site_tensions=None)
if RECORD:
input("Press Enter to begin recording")
log_id = client.startStateLogging(
client.STATE_LOGGING_VIDEO_MP4,
f"artifacts/manip_task_{datetime.now().strftime('%Y%m%d_%H%M%S_%f')}.mp4",
)
cid = None
for j, traj in enumerate(traj_components):
for i in range(traj.num_timesteps):
robot.set_tensions(traj.tensions[i])
robot.set_nominal_lengths(traj.cable_lengths[i])
robot.set_nominal_speeds(traj.cable_speeds[i])
robot.step()
if j == 0:
cid = client.createConstraint(
robot.id,
0,
object_id,
-1,
client.JOINT_FIXED,
(0, 0, 0),
(0, 0, -0.5),
(0, 0, 0.25),
)
if j == len(traj_components) - 2:
client.removeConstraint(cid)
if RECORD:
client.stopStateLogging(log_id)
input("Press Enter to exit")
client.disconnect()
if __name__ == "__main__":
main() |
/**
* @license Apache-2.0
*
* Copyright (c) 2023 The Stdlib Authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
'use strict';
/**
* Slice constructor.
*
* @module @stdlib/slice/ctor
*
* @example
* var Slice = require( '@stdlib/slice/ctor' );
*
* var s = new Slice( 10 );
* // returns <Slice>
*
* var start = s.start;
* // returns null
*
* var stop = s.stop;
* // returns 10
*
* var step = s.step;
* // returns null
*
* @example
* var Slice = require( '@stdlib/slice/ctor' );
*
* var s = new Slice( 3, 10 );
* // returns <Slice>
*
* var start = s.start;
* // returns 3
*
* var stop = s.stop;
* // returns 10
*
* var step = s.step;
* // returns null
*
* @example
* var Slice = require( '@stdlib/slice/ctor' );
*
* var s = new Slice( 3, 10, 2 );
* // returns <Slice>
*
* var start = s.start;
* // returns 3
*
* var stop = s.stop;
* // returns 10
*
* var step = s.step;
* // returns 2
*/
// MODULES //
var main = require( './main.js' );
// EXPORTS //
module.exports = main; |
"""
Copyright (c) 2016 Keith Sterling
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated
documentation files (the "Software"), to deal in the Software without restriction, including without limitation
the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software,
and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO
THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
"""
import logging
import importlib
class ClassLoader(object):
@staticmethod
def instantiate_class(class_string):
processor_path = class_string.strip()
logging.debug("Processor path [%s]", processor_path)
last_dot = processor_path.rfind(".")
module_path = processor_path[:last_dot]
class_name = processor_path[last_dot+1:]
logging.debug("Importing module [%s]", module_path)
imported_module = importlib.import_module(module_path)
logging.debug("Instantiating class [%s]", class_name)
new_class = getattr(imported_module, class_name)
return new_class |
<template>
<v-container>
<v-row class="pr-5" justify="center" >
<v-col cols="12" md="11">
<v-alert type="info">
Please note that this is a log of the successful password resets made by staff's accounts. This log serves only for record purposes, to help the admin keep tracks of staff accounts whose passwords were reset,
so actions can be taken if resets were done illegaly.
</v-alert>
<v-progress-circular indeterminate color="primary" :width="4" :size="40" v-if="isLoading" justify="center" class="mx-auto"></v-progress-circular>
<v-card v-else light raised elevation="8" min-height="100" class="scroll">
<v-card-title class="primary white--text subtitle-1 justify-center">Password Resets Log </v-card-title>
<v-card-text>
<table class="table table-hover table-striped" v-if="resets.length > 0">
<thead>
<tr>
<th>Staff</th>
<th>Req Date</th>
<th>Reset Date</th>
<th>IP Address</th>
<th>Action</th>
</tr>
</thead>
<tbody class="resets_list">
<tr v-for="(res, i) in resets" :key="res.id">
<td class="text-truncate">{{ res.user && res.user.fullname }}</td>
<td>{{ res.request_date | moment('DD/MM/YYYY - HH:mma') }}</td>
<td>{{ res.reset_date | moment('DD/MM/YYYY - HH:mma') }}</td>
<td>{{ res.request_ip }} </td>
<td> <v-btn icon color="red darken-2" @click="confirmDel(res.id, i)"><i class="uil uil-trash-alt"></i></v-btn></td>
</tr>
</tbody>
</table>
<v-alert class="mt-5" type="info" border="left" v-else>
There are no reset requests to display at the moment.
</v-alert>
</v-card-text>
</v-card>
</v-col>
</v-row>
<v-dialog v-model="delConfDial" max-width="450">
<v-card min-height="120">
<v-card-title class="subtitle-1 white--text primary justify-center">Are you sure you want to delete this password request log?</v-card-title>
<v-card-text class="mt-4 grey_text--text">
Once deleted, this can't be recovered!
</v-card-text>
<v-card-actions class="pb-8 mt-3 justify-center">
<v-btn text width="40%" color="red darken--2" @click="delConfDial = false">Cancel</v-btn>
<v-btn dark width="40%" color="primary" :loading="isDeleting" @click="deleteReq">Yes, Delete</v-btn>
</v-card-actions>
</v-card>
</v-dialog>
<v-snackbar v-model="reqDeleted" :timeout="4000" top dark color="green darken-1">
A password reset request was deleted.
<v-btn text color="white--text" @click="reqDeleted = false">Close</v-btn>
</v-snackbar>
</v-container>
</template>
<script>
export default {
data(){
return{
resets: [],
isLoading: false,
resToDelInd: null,
resToDel: null,
delConfDial: false,
isDeleting: false,
reqDeleted: false
}
},
computed:{
authAdmin(){
return this.$store.getters.authAdmin
},
api(){
return this.$store.getters.api
},
adminHeaders(){
let headers = {
headers: {
"Authorization": `Bearer ${this.authAdmin.token}`
}
}
return headers
},
},
methods: {
getReqs(){
this.isLoading = true
axios.get(this.api + '/auth-admin/get_pswd_req_resets', this.adminHeaders)
.then((res) => {
this.isLoading = false
this.resets = res.data
})
},
confirmDel(res, i){
this.resToDel = res
this.resToDelInd = i
this.delConfDial = true
},
deleteReq(){
this.isDeleting = true
axios.post(this.api + `/auth-admin/del_pswd_req/${this.resToDel}`, {}, this.adminHeaders)
.then((res) => {
this.isDeleting = false
this.delConfDial = false
this.reqDeleted = true
this.resets.splice(this.resToDelInd, 1)
})
}
},
created() {
this.getReqs()
}
}
</script>
<style lang="css" scoped>
.table .resets_list tr td{
vertical-align: middle !important;
}
.v-btn i{
font-size: 1.1rem !important;
}
table .news_list tr{
cursor: pointer;
}
table tbody tr td{
white-space: nowrap !important;
}
.v-card.scroll .v-card__text{
overflow-x: scroll !important;
}
</style> |
/***************************************************************************
* Copyright (C) 2011 by H-Store Project *
* Brown University *
* Massachusetts Institute of Technology *
* Yale University *
* *
* Permission is hereby granted, free of charge, to any person obtaining *
* a copy of this software and associated documentation files (the *
* "Software"), to deal in the Software without restriction, including *
* without limitation the rights to use, copy, modify, merge, publish, *
* distribute, sublicense, and/or sell copies of the Software, and to *
* permit persons to whom the Software is furnished to do so, subject to *
* the following conditions: *
* *
* The above copyright notice and this permission notice shall be *
* included in all copies or substantial portions of the Software. *
* *
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, *
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF *
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.*
* IN NO EVENT SHALL THE AUTHORS BE LIABLE FOR ANY CLAIM, DAMAGES OR *
* OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, *
* ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR *
* OTHER DEALINGS IN THE SOFTWARE. *
***************************************************************************/
package edu.brown.hstore.txns;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.atomic.AtomicBoolean;
import org.apache.log4j.Logger;
import org.voltdb.ParameterSet;
import org.voltdb.VoltTable;
import org.voltdb.catalog.Procedure;
import org.voltdb.catalog.Table;
import org.voltdb.exceptions.SerializableException;
import org.voltdb.types.SpeculationType;
import org.voltdb.utils.NotImplementedException;
import com.google.protobuf.ByteString;
import com.google.protobuf.RpcCallback;
import edu.brown.hstore.HStoreConstants;
import edu.brown.hstore.HStoreSite;
import edu.brown.hstore.Hstoreservice.Status;
import edu.brown.hstore.Hstoreservice.UnevictDataResponse;
import edu.brown.hstore.Hstoreservice.WorkFragment;
import edu.brown.hstore.callbacks.PartitionCountingCallback;
import edu.brown.hstore.estimators.Estimate;
import edu.brown.hstore.estimators.EstimatorState;
import edu.brown.hstore.internal.FinishTxnMessage;
import edu.brown.hstore.internal.SetDistributedTxnMessage;
import edu.brown.hstore.internal.WorkFragmentMessage;
import edu.brown.interfaces.DebugContext;
import edu.brown.logging.LoggerUtil;
import edu.brown.logging.LoggerUtil.LoggerBoolean;
import edu.brown.pools.Poolable;
import edu.brown.utils.PartitionSet;
import edu.brown.utils.StringUtil;
/**
* Base Transaction State
* @author pavlo
*/
public abstract class AbstractTransaction implements Poolable, Comparable<AbstractTransaction> {
private static final Logger LOG = Logger.getLogger(AbstractTransaction.class);
private static final LoggerBoolean debug = new LoggerBoolean();
static {
LoggerUtil.attachObserver(LOG, debug);
}
/**
* Internal state for the transaction
*/
public enum RoundState {
INITIALIZED,
STARTED,
FINISHED;
}
protected final HStoreSite hstore_site;
// ----------------------------------------------------------------------------
// GLOBAL DATA MEMBERS
// ----------------------------------------------------------------------------
/**
* Catalog object of the Procedure that this transaction is currently executing
*/
private Procedure catalog_proc;
private boolean sysproc;
private boolean readonly;
private boolean allow_early_prepare = true;
protected Long txn_id = null;
protected Long last_txn_id = null; // FOR DEBUGGING
protected long client_handle;
protected int base_partition;
protected Status status;
protected SerializableException pending_error;
/**
* StoredProcedureInvocation Input Parameters
* These are the parameters that are sent from the client
*/
protected ParameterSet parameters;
/**
* Internal flag that is set to true once to tell whomever
* that this transaction handle can be deleted.
*/
private AtomicBoolean deletable = new AtomicBoolean(false);
// ----------------------------------------------------------------------------
// ATTACHED DATA STRUCTURES
// ----------------------------------------------------------------------------
/**
* Attached inputs for the current execution round.
* This cannot be in the ExecutionState because we may have attached inputs for
* transactions that aren't running yet.
*/
private Map<Integer, List<VoltTable>> attached_inputs;
/**
* Attached ParameterSets for the current execution round
* This is so that we don't have to marshal them over to different partitions on the same HStoreSite
*/
private ParameterSet attached_parameterSets[];
/**
* Internal state information for txns that request prefetch queries
* This is only needed for distributed transactions
*/
protected PrefetchState prefetch;
// ----------------------------------------------------------------------------
// INTERNAL MESSAGE WRAPPERS
// ----------------------------------------------------------------------------
private SetDistributedTxnMessage setdtxn_task;
private FinishTxnMessage finish_task;
private WorkFragmentMessage work_task[];
// ----------------------------------------------------------------------------
// GLOBAL PREDICTIONS FLAGS
// ----------------------------------------------------------------------------
/**
* Whether this transaction will only touch one partition
*/
protected boolean predict_singlePartition = false;
/**
* Whether this txn can abort
*/
protected boolean predict_abortable = true;
/**
* Whether we predict that this txn will be read-only
*/
protected boolean predict_readOnly = false;
/**
* EstimationState Handle
*/
private EstimatorState predict_tState;
/**
* The set of partitions that we expected this partition to touch.
*/
protected PartitionSet predict_touchedPartitions;
// ----------------------------------------------------------------------------
// PER PARTITION EXECUTION FLAGS
// ----------------------------------------------------------------------------
private final boolean released[];
private final boolean prepared[];
private final boolean finished[];
protected final RoundState round_state[];
protected final int round_ctr[];
/**
* The number of times that this transaction has been restarted
*/
protected int restart_ctr = 0;
/**
* The first undo token used at each local partition
* When we abort a txn we will need to give the EE this value
*/
private final long exec_firstUndoToken[];
/**
* The last undo token used at each local partition
* When we commit a txn we will need to give the EE this value
*/
private final long exec_lastUndoToken[];
/**
* This is set to true if the transaction did some work without an undo
* buffer at a local partition. This is used to just check that we aren't
* trying to rollback changes without having used the undo log.
*/
private final boolean exec_noUndoBuffer[];
/**
* Whether this transaction has been read-only so far on a local partition
*/
protected final boolean exec_readOnly[];
/**
* Whether this Transaction has queued work that may need to be removed
* from this partition
*/
protected final boolean exec_queueWork[];
/**
* Whether this Transaction has submitted work to the EE that may need to be
* rolled back on a local partition
*/
protected final boolean exec_eeWork[];
/**
* BitSets for whether this txn has read from a particular table on each
* local partition.
* PartitionId -> TableId
*/
protected final boolean readTables[][];
/**
* BitSets for whether this txn has executed a modifying query against a particular table
* on each partition. Note that it does not actually record whether any rows were changed,
* but just we executed a query that targeted that table.
* PartitionId -> TableId
*/
protected final boolean writeTables[][];
/**
* The table that this txn needs to merge the results for in the EE
* before it starts executing
*/
protected Table anticache_table = null;
/**
* Whether this txn was speculatively executed
*/
protected SpeculationType exec_specExecType = SpeculationType.NULL;
// ----------------------------------------------------------------------------
// INITIALIZATION
// ----------------------------------------------------------------------------
/**
* Constructor
* @param executor
*/
public AbstractTransaction(HStoreSite hstore_site) {
this.hstore_site = hstore_site;
int numPartitions = hstore_site.getCatalogContext().numberOfPartitions;
this.released = new boolean[numPartitions];
this.prepared = new boolean[numPartitions];
this.finished = new boolean[numPartitions];
this.round_state = new RoundState[numPartitions];
this.round_ctr = new int[numPartitions];
this.exec_readOnly = new boolean[numPartitions];
this.exec_queueWork = new boolean[numPartitions];
this.exec_eeWork = new boolean[numPartitions];
this.exec_firstUndoToken = new long[numPartitions];
this.exec_lastUndoToken = new long[numPartitions];
this.exec_noUndoBuffer = new boolean[numPartitions];
this.readTables = new boolean[numPartitions][];
this.writeTables = new boolean[numPartitions][];
Arrays.fill(this.exec_firstUndoToken, HStoreConstants.NULL_UNDO_LOGGING_TOKEN);
Arrays.fill(this.exec_lastUndoToken, HStoreConstants.NULL_UNDO_LOGGING_TOKEN);
Arrays.fill(this.exec_readOnly, true);
Arrays.fill(this.exec_queueWork, false);
Arrays.fill(this.exec_eeWork, false);
}
/**
* Initialize this TransactionState for a new Transaction invocation
* @param txn_id
* @param client_handle
* @param base_partition
* @param parameters TODO
* @param sysproc
* @param predict_singlePartition
* @param predict_readOnly
* @param predict_abortable
* @param exec_local
* @return
*/
protected final AbstractTransaction init(Long txn_id,
long client_handle,
int base_partition,
ParameterSet parameters,
Procedure catalog_proc,
PartitionSet predict_touchedPartitions,
boolean predict_readOnly,
boolean predict_abortable,
boolean exec_local) {
assert(predict_touchedPartitions != null);
assert(predict_touchedPartitions.isEmpty() == false);
assert(catalog_proc != null) : "Unexpected null Procedure catalog handle";
this.txn_id = txn_id;
this.last_txn_id = txn_id;
this.client_handle = client_handle;
this.base_partition = base_partition;
this.parameters = parameters;
this.catalog_proc = catalog_proc;
this.sysproc = this.catalog_proc.getSystemproc();
this.readonly = this.catalog_proc.getReadonly();
// Initialize the predicted execution properties for this transaction
this.predict_touchedPartitions = predict_touchedPartitions;
this.predict_singlePartition = (this.predict_touchedPartitions.size() == 1);
this.predict_readOnly = predict_readOnly;
this.predict_abortable = predict_abortable;
return (this);
}
@Override
public final boolean isInitialized() {
return (this.txn_id != null && this.catalog_proc != null);
}
/**
* <B>Note:</B> This should never be called by anything other than the TransactionInitializer
* @param txn_id
*/
public void setTransactionId(Long txn_id) {
this.txn_id = txn_id;
}
/**
* Returns the speculation type (i.e., stall point) that this txn was executed at.
* Will be null if this transaction was not speculatively executed
* @return
*/
public SpeculationType getSpeculationType() {
return (this.exec_specExecType);
}
/**
* Should be called once the TransactionState is finished and is
* being returned to the pool
*/
@Override
public void finish() {
this.predict_singlePartition = false;
this.predict_abortable = true;
this.predict_readOnly = false;
this.predict_tState = null;
this.allow_early_prepare = true;
this.pending_error = null;
this.status = null;
this.parameters = null;
if (this.attached_inputs != null) this.attached_inputs.clear();
this.attached_parameterSets = null;
for (int partition : this.hstore_site.getLocalPartitionIds().values()) {
this.released[partition] = false;
this.prepared[partition] = false;
this.finished[partition] = false;
this.round_state[partition] = null;
this.round_ctr[partition] = 0;
this.exec_readOnly[partition] = true;
this.exec_queueWork[partition] = false;
this.exec_eeWork[partition] = false;
this.exec_firstUndoToken[partition] = HStoreConstants.NULL_UNDO_LOGGING_TOKEN;
this.exec_lastUndoToken[partition] = HStoreConstants.NULL_UNDO_LOGGING_TOKEN;
this.exec_noUndoBuffer[partition] = false;
if (this.readTables[partition] != null) Arrays.fill(this.readTables[partition], false);
if (this.writeTables[partition] != null) Arrays.fill(this.writeTables[partition], false);
} // FOR
if (debug.val)
LOG.debug(String.format("Finished txn #%d and cleaned up internal state [hashCode=%d, finished=%s]",
this.txn_id, this.hashCode(), Arrays.toString(this.finished)));
this.deletable.lazySet(false);
this.catalog_proc = null;
this.sysproc = false;
this.readonly = false;
this.base_partition = HStoreConstants.NULL_PARTITION_ID;
this.txn_id = null;
}
/**
* Return the number of times that this transaction was restarted
* @return
*/
public int getRestartCounter() {
return (this.restart_ctr);
}
/**
* Set the number of times that this transaction has been restarted
* @param val
*/
public void setRestartCounter(int val) {
this.restart_ctr = val;
}
// ----------------------------------------------------------------------------
// DATA STORAGE
// ----------------------------------------------------------------------------
/**
* Store data from mapOutput tables to reduceInput table
* reduceInput table should merge all incoming data from the mapOutput tables.
*/
public Status storeData(int partition, VoltTable vt) {
throw new NotImplementedException("Not able to store data for non-MapReduce transactions");
}
// ----------------------------------------------------------------------------
// ROUND METHODS
// ----------------------------------------------------------------------------
/**
* Must be called once before one can add new WorkFragments for this txn
* This will always clear out any pending errors
* @param undoToken
*/
public void initRound(int partition, long undoToken) {
assert(this.round_state[partition] == null || this.round_state[partition] == RoundState.FINISHED) :
String.format("Invalid state %s for ROUND #%s on partition %d for %s [hashCode=%d]",
this.round_state[partition], this.round_ctr[partition],
partition, this, this.hashCode());
// If we get to this point, then we know that nobody cares about any
// errors from the previous round, therefore we can just clear it out
this.pending_error = null;
if (this.exec_lastUndoToken[partition] == HStoreConstants.NULL_UNDO_LOGGING_TOKEN ||
undoToken != HStoreConstants.DISABLE_UNDO_LOGGING_TOKEN) {
// LAST UNDO TOKEN
this.exec_lastUndoToken[partition] = undoToken;
// FIRST UNDO TOKEN
if (this.exec_firstUndoToken[partition] == HStoreConstants.NULL_UNDO_LOGGING_TOKEN ||
this.exec_firstUndoToken[partition] == HStoreConstants.DISABLE_UNDO_LOGGING_TOKEN) {
this.exec_firstUndoToken[partition] = undoToken;
}
}
// NO UNDO LOGGING
if (undoToken == HStoreConstants.DISABLE_UNDO_LOGGING_TOKEN) {
this.exec_noUndoBuffer[partition] = true;
}
this.round_state[partition] = RoundState.INITIALIZED;
if (debug.val)
LOG.debug(String.format("%s - Initializing ROUND %d at partition %d [undoToken=%d / first=%d / last=%d]",
this, this.round_ctr[partition], partition,
undoToken, this.exec_firstUndoToken[partition],
this.exec_lastUndoToken[partition]));
}
/**
* Called once all of the WorkFragments have been submitted for this txn
* @return
*/
public void startRound(int partition) {
assert(this.round_state[partition] == RoundState.INITIALIZED) :
String.format("Invalid state %s for ROUND #%s on partition %d for %s [hashCode=%d]",
this.round_state[partition], this.round_ctr[partition],
partition, this, this.hashCode());
this.round_state[partition] = RoundState.STARTED;
if (debug.val)
LOG.debug(String.format("%s - Starting batch ROUND #%d on partition %d",
this, this.round_ctr[partition], partition));
}
/**
* When a round is over, this must be called so that we can clean up the various
* dependency tracking information that we have
*/
public void finishRound(int partition) {
assert(this.round_state[partition] == RoundState.STARTED) :
String.format("Invalid batch round state %s for %s at partition %d",
this.round_state[partition], this, partition);
if (debug.val)
LOG.debug(String.format("%s - Finishing batch ROUND #%d on partition %d",
this, this.round_ctr[partition], partition));
this.round_state[partition] = RoundState.FINISHED;
this.round_ctr[partition]++;
}
// ----------------------------------------------------------------------------
// PREDICTIONS
// ----------------------------------------------------------------------------
/**
* Return the collection of the partitions that this transaction is expected
* to need during its execution. The transaction may choose to not use all of
* these but it is not allowed to use more.
*/
public final PartitionSet getPredictTouchedPartitions() {
return (this.predict_touchedPartitions);
}
/**
* Returns true if this Transaction was originally predicted as being able to abort
*/
public final boolean isPredictAbortable() {
return (this.predict_abortable);
}
/**
* Returns true if this transaction was originally predicted as read only
*/
public final boolean isPredictReadOnly() {
return (this.predict_readOnly);
}
/**
* Returns true if this Transaction was originally predicted to be single-partitioned
*/
public final boolean isPredictSinglePartition() {
return (this.predict_singlePartition);
}
/**
* Returns the EstimatorState for this txn
* @return
*/
public EstimatorState getEstimatorState() {
return (this.predict_tState);
}
/**
* Set the EstimatorState for this txn
* @param state
*/
public final void setEstimatorState(EstimatorState state) {
assert(this.predict_tState == null) :
String.format("Trying to set the %s for %s twice",
EstimatorState.class.getSimpleName(), this);
if (debug.val)
LOG.debug(String.format("%s - Setting %s for txn",
this, state.getClass().getSimpleName()));
this.predict_tState = state;
}
/**
* Get the last TransactionEstimate produced for this transaction.
* If there is no estimate, then the return result is null.
* @return
*/
public final Estimate getLastEstimate() {
if (this.predict_tState != null) {
return (this.predict_tState.getLastEstimate());
}
return (null);
}
/**
* Returns true if this transaction was executed speculatively
*/
public boolean isSpeculative() {
return (false);
}
// ----------------------------------------------------------------------------
// EXECUTION FLAG METHODS
// ----------------------------------------------------------------------------
/**
* Mark this transaction as have performed some modification on this partition
*/
public final void markExecNotReadOnly(int partition) {
assert(this.sysproc == true || this.readonly == false);
this.exec_readOnly[partition] = false;
}
/**
* Returns true if this transaction has not executed any modifying work at this partition
*/
public final boolean isExecReadOnly(int partition) {
if (this.readonly) return (true);
return (this.exec_readOnly[partition]);
}
/**
* Returns true if this transaction executed without undo buffers at some point
*/
public final boolean isExecNoUndoBuffer(int partition) {
return (this.exec_noUndoBuffer[partition]);
}
/**
* Mark that the transaction executed queries without using undo logging
* at the given partition.
* @param partition
*/
public final void markExecNoUndoBuffer(int partition) {
this.exec_noUndoBuffer[partition] = true;
}
/**
* Returns true if this transaction's control code running at this partition
*/
public final boolean isExecLocal(int partition) {
return (this.base_partition == partition);
}
/**
* Returns true if this transaction has done something at this partition and therefore
* the PartitionExecutor needs to be told that they are finished.
* This could be either executing a query or executing the transaction's control code
*/
public final boolean needsFinish(int partition) {
boolean ret = (this.exec_readOnly[partition] == false &&
(this.round_state[partition] != null ||
this.exec_eeWork[partition] ||
this.exec_queueWork[partition])
);
// if (this.isSpeculative()) {
// LOG.info(String.format(
// "%s - Speculative Execution Partition %d => %s\n" +
// " Round State: %s\n" +
// " Exec ReadOnly: %s\n" +
// " Exec Queue: %s\n" +
// " Exec EE: %s\n",
// this, partition, ret,
// this.round_state[offset],
// this.exec_readOnly[offset],
// this.exec_queueWork[offset],
// this.exec_eeWork[offset]));
// }
// This transaction needs to be "finished" down in the EE if:
// (1) It's not read-only
// (2) It has executed at least one batch round
// (3) It has invoked work directly down in the EE
// (4) It added work that may be waiting in this partition's queue
return (ret);
}
/**
* Returns true if we believe that this transaction can be deleted
* <B>Note:</B> This will only return true once and only once for each
* transaction invocation. So don't call this unless you are able to really
* delete the transaction now. If you just want to know whether the txn has
* been marked as deletable before, then use checkDeletableFlag()
* @return
*/
public boolean isDeletable() {
// if (this.isInitialized() == false) {
// return (false);
// }
return (this.deletable.compareAndSet(false, true));
}
/**
* Returns true if this txn has already been marked for deletion
* This will not change the internal state of the txn.
*/
public final boolean checkDeletableFlag() {
return (this.deletable.get());
}
// ----------------------------------------------------------------------------
// GENERAL METHODS
// ----------------------------------------------------------------------------
@Override
public final boolean equals(Object obj) {
if (obj instanceof AbstractTransaction) {
AbstractTransaction other = (AbstractTransaction)obj;
if (this.txn_id == null) {
return (this.hashCode() != other.hashCode());
}
return (this.txn_id.equals(other.txn_id));
}
return (false);
}
@Override
public final int compareTo(AbstractTransaction o) {
if (this.txn_id == null) return (1);
else if (o.txn_id == null) return (-1);
return this.txn_id.compareTo(o.txn_id);
}
/**
* Get this state's transaction id
*/
public final Long getTransactionId() {
return this.txn_id;
}
/**
* Get this state's client_handle
*/
public final long getClientHandle() {
return this.client_handle;
}
/**
* Get the base PartitionId where this txn's Java code is executing on
*/
public final int getBasePartition() {
return this.base_partition;
}
/**
* Return the underlying procedure catalog object
* @return
*/
public final Procedure getProcedure() {
return (this.catalog_proc);
}
/**
* Return the ParameterSet that contains the procedure input
* parameters for this transaction. These are the original parameters
* that were sent from the client for this txn.
* This can be null for distributed transactions on remote partitions
*/
public ParameterSet getProcedureParameters() {
return (this.parameters);
}
/**
* Returns true if this transaction is for a system procedure
*/
public final boolean isSysProc() {
return (this.sysproc);
}
public final boolean allowEarlyPrepare() {
return (this.allow_early_prepare);
}
public final void setAllowEarlyPrepare(boolean enable) {
this.allow_early_prepare = enable;
}
// ----------------------------------------------------------------------------
// CALLBACK METHODS
// ----------------------------------------------------------------------------
/**
* Return this handle's InitCallback
*/
public abstract <T extends PartitionCountingCallback<? extends AbstractTransaction>> T getInitCallback();
/**
* Return this handle's PrepareCallback
*/
public abstract <T extends PartitionCountingCallback<? extends AbstractTransaction>> T getPrepareCallback();
/**
* Return this handle's FinishCallback
*/
public abstract <T extends PartitionCountingCallback<? extends AbstractTransaction>> T getFinishCallback();
// ----------------------------------------------------------------------------
// ERROR METHODS
// ----------------------------------------------------------------------------
/**
* Returns true if this transaction has a pending error
*/
public final boolean hasPendingError() {
return (this.pending_error != null);
}
/**
* Return the pending error for this transaction.
* This does not clear it.
*/
public final SerializableException getPendingError() {
return (this.pending_error);
}
/**
* Return the message for the pending error of this transaction.
*/
public final String getPendingErrorMessage() {
return (this.pending_error != null ? this.pending_error.getMessage() : null);
}
/**
* Set the Exception that is causing this txn to abort. It will need
* to be processed later on.
* This is a thread-safe operation. Only the first error will be stored.
* @param error
*/
public synchronized void setPendingError(SerializableException error) {
assert(error != null) : "Trying to set a null error for txn #" + this.txn_id;
if (this.pending_error == null) {
if (debug.val)
LOG.warn(String.format("%s - Got %s error for txn: %s",
this, error.getClass().getSimpleName(), error.getMessage()));
this.pending_error = error;
}
}
// ----------------------------------------------------------------------------
// INTERNAL MESSAGE WRAPPERS
// ----------------------------------------------------------------------------
public final SetDistributedTxnMessage getSetDistributedTxnMessage() {
if (this.setdtxn_task == null) {
this.setdtxn_task = new SetDistributedTxnMessage(this);
}
return (this.setdtxn_task);
}
public final FinishTxnMessage getFinishTxnMessage(Status status) {
if (this.finish_task == null) {
synchronized (this) {
if (this.finish_task == null) {
this.finish_task = new FinishTxnMessage(this, status);
}
} // SYNCH
}
this.finish_task.setStatus(status);
return (this.finish_task);
}
public final WorkFragmentMessage getWorkFragmentMessage(WorkFragment fragment) {
if (this.work_task == null) {
this.work_task = new WorkFragmentMessage[hstore_site.getCatalogContext().numberOfPartitions];
}
int partition = fragment.getPartitionId();
if (this.work_task[partition] == null) {
this.work_task[partition] = new WorkFragmentMessage(this, fragment);
} else {
this.work_task[partition].setFragment(fragment);
}
return (this.work_task[partition]);
}
/**
* Set the current Status for this transaction
* This is not thread-safe.
* @param status
*/
public final void setStatus(Status status) {
this.status = status;
}
public final Status getStatus() {
return (this.status);
}
/**
* Returns true if this transaction has been aborted.
*/
public final boolean isAborted() {
return (this.status != null && this.status != Status.OK);
}
// ----------------------------------------------------------------------------
// ANTI-CACHING
// ----------------------------------------------------------------------------
public boolean hasAntiCacheMergeTable() {
return (this.anticache_table != null);
}
public Table getAntiCacheMergeTable() {
return (this.anticache_table);
}
public void setAntiCacheMergeTable(Table catalog_tbl) {
assert(this.anticache_table == null);
this.anticache_table = catalog_tbl;
}
// ----------------------------------------------------------------------------
// TRANSACTION STATE BOOKKEEPING
// ----------------------------------------------------------------------------
/**
* Mark this txn as being released from the lock queue at the given partition.
* This means that this txn was released to that partition and will
* need to be removed with a FinishTxnMessage
* @param partition - The partition to mark this txn as "released"
*/
public final void markReleased(int partition) {
if (debug.val)
LOG.debug(String.format("%s - Marking as released on partition %d %s [hashCode=%d]",
this, partition, Arrays.toString(this.released), this.hashCode()));
// assert(this.released[partition] == false) :
// String.format("Trying to mark %s as released to partition %d twice", this, partition);
this.released[partition] = true;
}
/**
* Is this TransactionState marked as released at the given partition
* @return
*/
public final boolean isMarkedReleased(int partition) {
return (this.released[partition]);
}
/**
* Mark this txn as prepared and return the original value
* This is a thread-safe operation
* @param partition - The partition to mark this txn as "prepared"
*/
public final boolean markPrepared(int partition) {
if (debug.val)
LOG.debug(String.format("%s - Marking as prepared on partition %d %s [hashCode=%d, offset=%d]",
this, partition, Arrays.toString(this.prepared),
this.hashCode(), partition));
boolean orig = false;
synchronized (this.prepared) {
orig = this.prepared[partition];
this.prepared[partition] = true;
} // SYNCH
return (orig == false);
}
/**
* Is this TransactionState marked as prepared at the given partition
* @return
*/
public final boolean isMarkedPrepared(int partition) {
return (this.prepared[partition]);
}
/**
* Mark this txn as finished (and thus ready for clean-up)
*/
public final void markFinished(int partition) {
if (debug.val)
LOG.debug(String.format("%s - Marking as finished on partition %d " +
"[finished=%s / hashCode=%d / offset=%d]",
this, partition, Arrays.toString(this.finished),
this.hashCode(), partition));
this.finished[partition] = true;
}
/**
* Is this TransactionState marked as finished
* @return
*/
public final boolean isMarkedFinished(int partition) {
return (this.finished[partition]);
}
/**
* Should be called whenever the txn submits work to the EE
*/
public final void markQueuedWork(int partition) {
if (debug.val) LOG.debug(String.format("%s - Marking as having queued work on partition %d [exec_queueWork=%s]",
this, partition, Arrays.toString(this.exec_queueWork)));
this.exec_queueWork[partition] = true;
}
/**
* Returns true if this txn has queued work at the given partition
* @return
*/
public final boolean hasQueuedWork(int partition) {
return (this.exec_queueWork[partition]);
}
/**
* Should be called whenever the txn submits work to the EE
*/
public final void markExecutedWork(int partition) {
if (debug.val) LOG.debug(String.format("%s - Marking as having submitted to the EE on partition %d [exec_eeWork=%s]",
this, partition, Arrays.toString(this.exec_eeWork)));
this.exec_eeWork[partition] = true;
}
/**
* Returns true if this txn has submitted work to the EE that needs to be rolled back
* @return
*/
public final boolean hasExecutedWork(int partition) {
return (this.exec_eeWork[partition]);
}
// ----------------------------------------------------------------------------
// READ/WRITE TABLE TRACKING
// ----------------------------------------------------------------------------
private final int[] getMarkedTableIds(boolean bitmap[]) {
int cnt = 0;
if (bitmap != null) {
for (int i = 0; i < bitmap.length; i++) {
if (bitmap[i]) cnt++;
} // FOR
}
int ret[] = new int[cnt];
if (bitmap != null) {
cnt = 0;
for (int i = 0; i < bitmap.length; i++) {
if (bitmap[i]) {
ret[cnt++] = i;
}
} // FOR
}
return (ret);
}
/**
* Return an array of the tableIds that are marked as read by the txn at
* the given partition id.
* @param partition
* @return
*/
public final int[] getTableIdsMarkedRead(int partition) {
return (this.getMarkedTableIds(this.readTables[partition]));
}
/**
* Mark that this txn read from the Table at the given partition
* @param partition
* @param catalog_tbl
*/
public final void markTableRead(int partition, Table catalog_tbl) {
if (this.readTables[partition] == null) {
this.readTables[partition] = new boolean[hstore_site.getCatalogContext().numberOfTables + 1];
}
this.readTables[partition][catalog_tbl.getRelativeIndex()] = true;
}
/**
* Mark that this txn read from the tableIds at the given partition
* @param partition
* @param tableIds
*/
public final void markTableIdsRead(int partition, int...tableIds) {
if (this.readTables[partition] == null) {
this.readTables[partition] = new boolean[hstore_site.getCatalogContext().numberOfTables + 1];
}
for (int id : tableIds) {
this.readTables[partition][id] = true;
} // FOR
}
/**
* Returns true if this txn has executed a query that read from the Table
* at the given partition.
* @param partition
* @param catalog_tbl
* @return
*/
public final boolean isTableRead(int partition, Table catalog_tbl) {
if (this.readTables[partition] != null) {
return (this.readTables[partition][catalog_tbl.getRelativeIndex()]);
}
return (false);
}
/**
* Return an array of the tableIds that are marked as written by the txn at
* the given partition id.
* @param partition
* @return
*/
public final int[] getTableIdsMarkedWritten(int partition) {
return (this.getMarkedTableIds(this.writeTables[partition]));
}
/**
* Mark that this txn has executed a modifying query for the Table at the given partition.
* <B>Note:</B> This is just tracking that we executed a query.
* It does not necessarily mean that the query actually changed anything.
* @param partition
* @param catalog_tbl
*/
public final void markTableWritten(int partition, Table catalog_tbl) {
if (this.writeTables[partition] == null) {
this.writeTables[partition] = new boolean[hstore_site.getCatalogContext().numberOfTables + 1];
}
this.writeTables[partition][catalog_tbl.getRelativeIndex()] = true;
}
/**
* Mark that this txn has executed a modifying query for the tableIds at the given partition.
* <B>Note:</B> This is just tracking that we executed a query.
* It does not necessarily mean that the query actually changed anything.
* @param partition
* @param tableIds
*/
public final void markTableIdsWritten(int partition, int...tableIds) {
if (this.writeTables[partition] == null) {
this.writeTables[partition] = new boolean[hstore_site.getCatalogContext().numberOfTables + 1];
}
for (int id : tableIds) {
this.writeTables[partition][id] = true;
} // FOR
}
/**
* Returns true if this txn has executed a non-readonly query that read from
* the Table at the given partition.
* @param partition
* @param catalog_tbl
* @return
*/
public final boolean isTableWritten(int partition, Table catalog_tbl) {
if (this.writeTables[partition] != null) {
return (this.writeTables[partition][catalog_tbl.getRelativeIndex()]);
}
return (false);
}
/**
* Returns true if this txn executed at query that either accessed or modified
* the Table at the given partition.
* @param partition
* @param catalog_tbl
* @return
*/
public final boolean isTableReadOrWritten(int partition, Table catalog_tbl) {
int tableId = catalog_tbl.getRelativeIndex();
if (this.readTables[partition] != null && this.readTables[partition][tableId]) {
return (true);
}
if (this.writeTables[partition] != null && this.writeTables[partition][tableId]) {
return (true);
}
return (false);
}
// ----------------------------------------------------------------------------
// GLOBAL STATE TRACKING
// ----------------------------------------------------------------------------
/**
* Get the current Round that this TransactionState is in
* Used only for testing
*/
protected RoundState getCurrentRoundState(int partition) {
return (this.round_state[partition]);
}
/**
* Get the first undo token used for this transaction
* When we ABORT a txn we will need to give the EE this value
*/
public long getFirstUndoToken(int partition) {
return this.exec_firstUndoToken[partition];
}
/**
* Get the last undo token used for this transaction
* When we COMMIT a txn we will need to give the EE this value
*/
public long getLastUndoToken(int partition) {
return this.exec_lastUndoToken[partition];
}
// ----------------------------------------------------------------------------
// ATTACHED DATA FOR NORMAL WORK FRAGMENTS
// ----------------------------------------------------------------------------
public final void attachParameterSets(ParameterSet parameterSets[]) {
this.attached_parameterSets = parameterSets;
}
public final ParameterSet[] getAttachedParameterSets() {
assert(this.attached_parameterSets != null) :
String.format("The attached ParameterSets for %s is null?", this);
return (this.attached_parameterSets);
}
public final void attachInputDependency(int input_dep_id, VoltTable vt) {
if (this.attached_inputs == null) {
this.attached_inputs = new HashMap<Integer, List<VoltTable>>();
}
List<VoltTable> l = this.attached_inputs.get(input_dep_id);
if (l == null) {
l = new ArrayList<VoltTable>();
this.attached_inputs.put(input_dep_id, l);
}
l.add(vt);
}
public final Map<Integer, List<VoltTable>> getAttachedInputDependencies() {
if (this.attached_inputs == null) {
this.attached_inputs = new HashMap<Integer, List<VoltTable>>();
}
return (this.attached_inputs);
}
// ----------------------------------------------------------------------------
// PREFETCH QUERIES
// ----------------------------------------------------------------------------
/**
* Grab a PrefetchState object for this LocalTransaction
* This must be called before you can send prefetch requests on behalf of this txn
*/
public final void initializePrefetch() {
if (this.prefetch == null) {
this.prefetch = new PrefetchState(this.hstore_site);
this.prefetch.init(this);
}
}
public final PrefetchState getPrefetchState() {
return (this.prefetch);
}
/**
* Returns true if this transaction has prefetched queries
*/
public final boolean hasPrefetchQueries() {
return (this.prefetch != null);
}
/**
* Attach prefetchable WorkFragments for this transaction
* This should be invoked on the remote side of the initialization request.
* That is, it is not the transaction's base partition that is storing this information,
* it's coming from over the network
* @param fragments
* @param rawParameters
*/
public void attachPrefetchQueries(List<WorkFragment> fragments, List<ByteString> rawParameters) {
assert(this.prefetch.fragments == null) :
"Trying to attach Prefetch WorkFragments more than once!";
// Simply copy the references so we don't allocate more objects
this.prefetch.fragments = fragments;
this.prefetch.paramsRaw = rawParameters;
}
public boolean hasPrefetchParameters() {
return (this.prefetch != null && this.prefetch.params != null);
}
public void attachPrefetchParameters(ParameterSet params[]) {
assert(this.prefetch.params == null) :
String.format("Trying to attach Prefetch %s more than once for %s",
ParameterSet.class.getSimpleName(), this);
this.prefetch.params = params;
}
public final boolean hasPrefetchFragments() {
return (this.prefetch != null && this.prefetch.fragments != null);
}
public final List<WorkFragment> getPrefetchFragments() {
return (this.prefetch.fragments);
}
/**
*
* @return
*/
public final List<ByteString> getPrefetchRawParameterSets() {
return (this.prefetch.paramsRaw);
}
/**
* Retrieve the ParameterSets for the prefetch queries.
* There should be one ParameterSet array per site, which means that this
* is shared across all partitions.
* @return
*/
public final ParameterSet[] getPrefetchParameterSets() {
assert(this.prefetch.params != null);
return (this.prefetch.params);
}
/**
* Mark this txn as having executed a prefetched query at the given partition
* @param partition
*/
public final void markExecPrefetchQuery(int partition) {
assert(this.prefetch != null);
this.prefetch.partitions.add(partition);
}
// ----------------------------------------------------------------------------
// DEBUG METHODS
// ----------------------------------------------------------------------------
/**
* Get the current round counter at the given partition.
* Note that a round is different than a batch. A "batch" contains multiple queries
* that the txn wants to execute, of which their PlanFragments are broken
* up into separate execution "rounds" in the PartitionExecutor.
*/
protected int getCurrentRound(int partition) {
return (this.round_ctr[partition]);
}
@Override
public final String toString() {
String str = null;
if (this.isInitialized()) {
str = this.toStringImpl();
} else {
str = String.format("<Uninitialized-%s>", this.getClass().getSimpleName());
if (this.last_txn_id != null) str += String.format(" {LAST:%d}", this.last_txn_id);
}
// Include hashCode for debugging
// str += "/" + this.hashCode();
return (str);
}
public abstract String toStringImpl();
public abstract String debug();
@SuppressWarnings("unchecked")
protected Map<String, Object>[] getDebugMaps() {
List<Map<String, Object>> maps = new ArrayList<Map<String,Object>>();
Map<String, Object> m;
m = new LinkedHashMap<String, Object>();
m.put("Transaction Id", this.txn_id);
m.put("Procedure", this.catalog_proc);
m.put("Base Partition", this.base_partition);
m.put("Hash Code", this.hashCode());
m.put("Pending Error", this.pending_error);
m.put("Allow Early Prepare", this.allow_early_prepare);
maps.add(m);
// Predictions
m = new LinkedHashMap<String, Object>();
m.put("Predict Single-Partitioned", this.predict_singlePartition);
m.put("Predict Touched Partitions", this.getPredictTouchedPartitions());
m.put("Predict Read Only", this.isPredictReadOnly());
m.put("Predict Abortable", this.isPredictAbortable());
maps.add(m);
// Global State
m = new LinkedHashMap<String, Object>();
m.put("Marked Released", PartitionSet.toString(this.released));
m.put("Marked Prepared", PartitionSet.toString(this.prepared));
m.put("Marked Finished", PartitionSet.toString(this.finished));
m.put("Marked Deletable", this.checkDeletableFlag());
maps.add(m);
// Prefetch State
if (this.prefetch != null) {
m = new LinkedHashMap<String, Object>();
m.put("Prefetch Partitions", this.prefetch.partitions);
m.put("Prefetch Fragments", StringUtil.join("\n", this.prefetch.fragments));
m.put("Prefetch Parameters", StringUtil.join("\n", this.prefetch.params));
m.put("Prefetch Raw Parameters", StringUtil.join("\n", this.prefetch.paramsRaw));
maps.add(m);
}
// Partition Execution State
m = new LinkedHashMap<String, Object>();
m.put("Current Round State", Arrays.toString(this.round_state));
m.put("Exec Read-Only", PartitionSet.toString(this.exec_readOnly));
m.put("First UndoToken", Arrays.toString(this.exec_firstUndoToken));
m.put("Last UndoToken", Arrays.toString(this.exec_lastUndoToken));
m.put("No Undo Buffer", PartitionSet.toString(this.exec_noUndoBuffer));
m.put("# of Rounds", Arrays.toString(this.round_ctr));
m.put("Executed Work", PartitionSet.toString(this.exec_eeWork));
maps.add(m);
return ((Map<String, Object>[])maps.toArray(new Map[0]));
}
public class Debug implements DebugContext {
/**
* Clear read/write table sets
* <B>NOTE:</B> This should only be used for testing
*/
public final void clearReadWriteSets() {
for (int i = 0; i < readTables.length; i++) {
if (readTables[i] != null) Arrays.fill(readTables[i], false);
if (writeTables[i] != null) Arrays.fill(writeTables[i], false);
} // FOR
}
}
private Debug cachedDebugContext;
private RpcCallback<UnevictDataResponse> unevict_callback;
private long new_transaction_id;
public Debug getDebugContext() {
if (this.cachedDebugContext == null) {
// We don't care if we're thread-safe here...
this.cachedDebugContext = new Debug();
}
return this.cachedDebugContext;
}
public void setUnevictCallback(RpcCallback<UnevictDataResponse> done) {
this.unevict_callback = done;
}
public RpcCallback<UnevictDataResponse> getUnevictCallback() {
return this.unevict_callback;
}
public void setNewTransactionId(long newTransactionId) {
this.new_transaction_id = newTransactionId;
}
public long getNewTransactionId(){
return this.new_transaction_id;
}
} |
<template>
<main aria-label="Sign-up form">
<section>
<article class="formulario">
<p>Sign up</p>
<form @submit.prevent="sendForm" aria-label="Sign-up form">
<label for="name">First name</label>
<input v-model="name" @blur="nameValidation" type="text" id="name" name="name" placeholder="First name"
aria-required="true">
<span class="error" aria-live="assertive">{{ nameError }}</span>
<label for="lastName">Last name</label>
<input v-model="lastName" @blur="lastNameValidation" type="text" id="lastName" name="lastName"
placeholder="Last name" aria-required="true">
<span class="error" aria-live="assertive">{{ lastNameError }}</span>
<label for="username">Username</label>
<input v-model="username" @blur="usernameValidation" type="text" id="username" name="username"
placeholder="Username" aria-required="true">
<span class="error" aria-live="assertive">{{ usernameError }}</span>
<label for="email">Email</label>
<input v-model="email" @blur="emailValidation" type="email" id="email" name="email" placeholder="Email"
aria-required="true">
<span class="error" aria-live="assertive">{{ emailError }}</span>
<label for="password">Password</label>
<input v-model="password" @blur="passwordValidation" type="password" id="password" name="password"
placeholder="Password" aria-required="true">
<span class="error" aria-live="assertive">{{ passwordError }}</span>
<span class="error" aria-live="assertive">{{ errorServidor }}</span>
<button type="submit" class="btn-signup" aria-label="Sign up for an account">SIGN UP</button>
</form>
</article>
</section>
<section>
<article class="info">
<h1>Welcome!</h1>
<p>Are you ready to join and explore the world of coffee with us?</p>
<p>Have you signed up already?</p>
<button class="btn-login" @click="navigationLogIn" aria-label="Log in to your account">LOG IN</button>
</article>
</section>
</main>
</template>
<script>
import router from '@/aromio/router/router';
import { userStore } from '@/aromio/stores/userStore'
export default {
props: ['loggedIn'],
data() {
return {
name: "",
lastName: "",
username: "",
email: "",
password: "",
cart: [],
nameError: "",
lastNameError: "",
usernameError: "",
emailError: "",
passwordError: "",
emailRegex: /^[\w-\.]+@([\w-]+\.)+[\w-]{2,4}$/,
errorServidor: ""
}
},
methods: {
//MÉTODOS CON VALIDACIONES PARA TODOS LOS CAMPOS: nombre, apellido, username, email y contraseña
nameValidation() {
if (this.name.trim() === "") {
this.nameError = "Please write your first name"
return false
}
this.nameError = ""
return true
},
lastNameValidation() {
if (this.lastName.trim() === "") {
this.lastNameError = "Please write your last name"
return false
}
this.lastNameError = ""
return true
},
usernameValidation() {
if (this.username.trim() === "") {
this.usernameError = "Please choose your username"
return false
}
this.usernameError = ""
return true
},
emailValidation() {
if (this.email.trim() === "" || !this.emailRegex.test(this.email)) {
this.emailError = "Please enter a valid email address"
return false
}
this.emailError = ""
return true
},
passwordValidation() {
if (this.password.trim() === "" || this.password.length < 5) {
this.passwordError = "Please provide a password that is 5 characters or longer"
return false
}
this.passwordError = ""
return true
},
//si las validaciones son correctas, primero comprueba que el usuario no está ya registrado en la bd
//si no lo está, crea un nuevo usuario y lo introduce en la base de datos en la tabla users
sendForm() {
if (this.nameValidation() && this.lastNameValidation() && this.usernameValidation() && this.emailValidation() && this.passwordValidation()) {
const user = userStore()
const newUser = {
first_name: this.name,
last_name: this.lastName,
username: this.username,
email: this.email,
password: this.password,
}
fetch('http://localhost:8000/api/signup', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(newUser),
})
.then(response => {
if (!response.ok) {
throw new Error('Your username or email already exist')
}
return response.json()
})
//y establece todos sus datos en la variable global
.then(data => {
user.setFirstName(this.name)
user.setLastName(this.lastName)
user.setUsername(this.username)
user.setEmail(this.email)
user.setPassword(this.password)
user.setLogin(true)
this.$router.push('/public/shop')
})
.catch((error) => {
this.errorServidor = "Your username or email already exist"
})
}
},
}
}
</script>
<style scoped>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
background-color: rgb(243, 239, 238);
}
main {
display: flex;
width: 100%;
flex-direction: row-reverse;
}
label {
color: transparent;
user-select: none;
}
section {
width: 50%;
}
.formulario,
.info {
display: flex;
height: 94.6vh;
justify-content: center;
}
.formulario {
align-items: center;
}
.btn-signup {
margin-top: 2vh;
font-family: 'Alata', sans-serif;
background-color: rgb(73, 30, 18);
color: white;
font-size: medium;
border-radius: 30vh;
height: 5vh;
width: 22vh;
border: none;
cursor: pointer;
letter-spacing: 0.13cm;
}
.formulario p {
position: absolute;
font-family: 'Alata', sans-serif;
font-size: 1.3vw;
color: rgb(73, 30, 18);
margin-bottom: 65vh;
}
.info {
background-color: rgb(73, 30, 18);
}
form {
display: flex;
flex-direction: column;
align-items: center;
width: 43vh;
}
input {
border: none;
border-bottom: 0.16vw solid rgb(186, 186, 186);
outline: none;
width: 20vw;
height: 2vw;
}
input::placeholder {
font-family: 'Alata', sans-serif;
color: rgb(186, 186, 186);
font-size: large;
}
.error {
padding: 1vw;
margin: 0 auto;
color: rgb(223, 67, 67);
font-family: 'Alata', sans-serif;
}
.info h1,
.info p,
.info button {
background-color: rgb(73, 30, 18);
}
.info {
display: flex;
flex-direction: column;
}
.btn-login {
border: 1px solid rgb(255, 255, 255);
color: white;
font-family: 'Alata', sans-serif;
height: 3vw;
width: 10vw;
justify-content: center;
font-size: 1vw;
border-radius: 50px;
letter-spacing: 5px;
cursor: pointer;
margin-left: 2vw;
margin-top: 2vw;
}
.info p,
.info h1 {
color: white;
}
.info p {
font-family: 'Alata', sans-serif;
margin-left: 2vw;
font-size: 1.5vw;
width: 60%;
}
.info h1 {
font-family: 'Archivo Black', sans-serif;
font-size: 4vw;
margin-left: 2vw;
}
.info p:nth-child(3) {
font-style: italic;
margin-top: 6vw;
font-size: 1.2vw;
}
@media screen and (max-width: 800px) {
section:nth-child(2) {
width: 100%;
}
main {
flex-direction: column;
}
.info h1 {
padding-top: 7vh;
}
.info p {
font-size: 3vw;
padding-bottom: 0px;
}
.info {
width: 100%;
align-self: flex-end;
justify-content: start;
height: 54.5vh;
}
.btn-login {
height: 50px;
padding-bottom: 15vh;
border: none;
font-size: 1.5vh;
width: 11vh;
}
.btn-signup {
background-color: rgb(243, 239, 238);
color: rgb(73, 30, 18);
}
.formulario p {
display: none;
}
.formulario input {
width: 30vh;
height: 6vh;
}
.formulario {
height: 45vh;
margin-bottom: 7vh;
margin-top: 5vh;
margin-left: 20vh;
}
.info p:nth-child(3) {
font-size: 2vh;
}
}
</style> |
import 'package:flutter/material.dart';
class GroceryItemTile extends StatelessWidget {
GroceryItemTile(
{Key? key,
required this.itemName,
required this.itemPrice,
required this.imagePath,
this.color,
required this.onPressed})
: super(key: key);
final String itemName;
final String itemPrice;
final String imagePath;
final color;
void Function()? onPressed;
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(12.0),
child: Container(
padding: const EdgeInsets.all(12),
decoration: BoxDecoration(
color: color[100],
borderRadius: BorderRadius.circular(12),
),
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
Image.asset(
imagePath,
height: 64,
),
//image
//name of item
Text(itemName),
TextButton(
onPressed: onPressed,
child: Text('\$ ' + itemPrice),
style: TextButton.styleFrom(
backgroundColor: color[400],
primary: Colors.white,
),
)
],
),
),
);
}
} |
import com.google.gson.JsonSyntaxException
import com.google.gson.reflect.TypeToken
import model.Film
import model.Session
import model.Ticket
import utils.exception.ExceptionHandler
import utils.extention.toRed
import presentation.CinemaController
import presentation.handler.InputHandler
import service.*
import java.io.File
fun main(args: Array<String>) {
val inputHandler = InputHandler()
val cinemaController = initializeServicesAndControllers(inputHandler, args)
while (true) {
println("1. View seats") // Посмотреть места на сеансе (3 пункт в задании)
println("2. Buy tickets") // Зафиксировать билеты (1 пункт в задании)
println("3. Return tickets") // Вернуть билет (2 пункт в задании)
println("4. Edit film") // Редактировать информацию о фильме (4 пункт в задании)
println("5. Edit sessions") // Редактировать информацию о сеансах (4 пункт в задании)
println("6. Mark a visit") // Отметка занятых мест в зале (5 пункт в задании)
println("7. View all films")// Опционально, для удобства тестирования
println("8. View all sessions")// Опционально, для удобства тестирования
println("9. Add Film")
println("10. Add Session")
println("11. Delete Film")
println("12. Delete Session")
println("13. Exit") // Выход
var answer: String = readLine()!!
when (answer) {
"1" -> {
try {
val filmName = inputHandler.getFilmName()
val sessionTime = inputHandler.getSessionTime()
cinemaController.displaySeats(filmName, sessionTime)
} catch (e: RuntimeException) {
ExceptionHandler.handleException(e)
} catch (e: java.text.ParseException) {
ExceptionHandler.handleException(e)
}
}
"2" -> {
try {
val filmName = inputHandler.getFilmName()
val sessionTime = inputHandler.getSessionTime()
val seats = inputHandler.getSeats()
cinemaController.buyTickets(filmName, sessionTime, seats)
} catch (e: RuntimeException) {
ExceptionHandler.handleException(e)
}
}
"3" -> {
try {
val filmName = inputHandler.getFilmName()
val sessionTime = inputHandler.getSessionTime()
val seats = inputHandler.getSeats()
cinemaController.returnTickets(filmName, sessionTime, seats)
} catch (e: RuntimeException) {
ExceptionHandler.handleException(e)
}
}
"4" -> {
try {
val filmId = inputHandler.getFilmId()
println("Input NEW data: ")
val newFilmName = inputHandler.getFilmName()
val newFilmDirector = inputHandler.getFilmDirector()
val newFilmDuration = inputHandler.getFilmDuration()
cinemaController.updateFilm(filmId, newFilmName, newFilmDuration, newFilmDirector)
} catch (e: RuntimeException) {
ExceptionHandler.handleException(e)
}
}
"5" -> {
try {
val filmName = inputHandler.getFilmId()
val sessionTime = inputHandler.getSessionTime()
cinemaController.updateSession(filmName, sessionTime)
} catch (e: RuntimeException) {
ExceptionHandler.handleException(e)
}
}
"6" -> {
try {
val filmName = inputHandler.getFilmName()
val sessionDate = inputHandler.getSessionTime()
val seats = inputHandler.getSeats()
cinemaController.markVisit(filmName, sessionDate, seats)
} catch (e: RuntimeException) {
ExceptionHandler.handleException(e)
}
}
"7" -> {
cinemaController.displayAllFilms()
}
"8" -> {
cinemaController.displayAllSessions()
}
"9" -> {
try {
val filmName = inputHandler.getFilmName()
val filmDirector = inputHandler.getFilmDirector()
val durationInMinutes = inputHandler.getFilmDuration()
val rentalDates = inputHandler.getRentalDates()
cinemaController.addFilm(filmName, filmDirector, durationInMinutes, rentalDates)
} catch (e: RuntimeException) {
ExceptionHandler.handleException(e)
}
}
"10" -> {
try {
val filmName = inputHandler.getFilmName()
val sessionTime = inputHandler.getSessionTime()
val seatsNumber = inputHandler.getSeatsNumber()
cinemaController.addSession(filmName, sessionTime, seatsNumber)
} catch (e: RuntimeException) {
ExceptionHandler.handleException(e)
}
}
"11" -> {
try {
val filmName = inputHandler.getFilmName()
val sessionTime = inputHandler.getSessionTime()
cinemaController.deleteFilm(filmName, sessionTime)
} catch (e: RuntimeException) {
ExceptionHandler.handleException(e)
}
}
"12" -> {
try {
val filmName = inputHandler.getFilmName()
val sessionTime = inputHandler.getSessionTime()
cinemaController.deleteSession(filmName, sessionTime)
} catch (e: RuntimeException) {
ExceptionHandler.handleException(e)
}
}
"13" -> {
break
}
else -> {
ExceptionHandler.handleException(RuntimeException("Wrong input"))
}
}
}
}
fun initializeServicesAndControllers(
inputHandler: InputHandler,
args: Array<String>,
isManualInput: Boolean = false
): CinemaController {
val filePathForFilm: String
val filePathForSession: String
val filePathForTicket: String
val filmService: FilmService
val sessionService: SessionService
val ticketService: TicketService
if (args.size == 3 && !isManualInput) {
filePathForFilm = args[0]
filePathForSession = args[1]
filePathForTicket = args[2]
} else {
filePathForFilm = inputHandler.getFilePath("Film")
filePathForSession = inputHandler.getFilePath("Sessions:")
filePathForTicket = inputHandler.getFilePath("Tickets:")
}
try {
filmService = FilmServiceImpl(
JsonRepository(
File(filePathForFilm),
object : TypeToken<List<Film>>() {}
)
)
sessionService = SessionServiceImpl(
JsonRepository(
File(filePathForSession),
object : TypeToken<List<Session>>() {})
)
ticketService = TicketServiceImpl(
sessionService,
JsonRepository(
File(filePathForTicket),
object : TypeToken<List<Ticket>>() {})
)
} catch (e: JsonSyntaxException) {
println("Error in deserializing the file. Please enter a different file name.".toRed())
return initializeServicesAndControllers(inputHandler, args, true)
} catch (e: RuntimeException) {
ExceptionHandler.handleException(e)
return initializeServicesAndControllers(inputHandler, args, true)
}
return CinemaController(
CinemaServiceImpl(
ticketService,
filmService,
sessionService
)
)
} |
constant DESCRIPTION = "Drag and Drop Between 2 Treeviews - by Vikram Ambrose";
/* Some sample data for treeview 1. A NULL row is added so we dont
need to pass around the size of the array */
array row_data = ({
({ "row0","item 12", 3, 4.3 }),
({ "row1","item 23", 44,34.4}),
({ "row2","item 33", 34,25.4}),
({ "row3","item 43", 37,64.4}),
({ "row4","item 53", 12,14.4}),
({ "row5","item 68", 42,34.4}),
({ "row6","item 75", 72,74.4}),
});
/* Sample data for treeview 2 */
array row2_data = ({
({"row7", "item 127", 105, 115.5}),
({"row8","item 124", 117, 118.6}),
({"row9", "item 123", 120, 121.73}),
});
constant drag_targets = ({
({"text/plain", GTK2.TARGET_SAME_APP, 0}),
});
/* Convenience function to print out the contents of a DATA struct onto stdout */
void print_DATA(array data){
write("DATA @ %x\n", hash_value(data));
write(" |->row = %s\n",data[0]);
write(" |->item = %s\n",data[1]);
write(" |->qty = %d\n",data[2]);
write(" +->price = %f\n",data[3]);
}
/* User callback for "get"ing the data out of the row that was DnD'd */
mixed on_drag_data_get(GTK2.Widget self, GDK2.DragContext drag_context,
GTK2.SelectionData sdata, int info, int time) {
write("on_drag_data_get: self %O, dc %O, sd %O\n", self, drag_context, sdata);
/* Get the selector widget from the treeview in question */
/* Get the tree model (list_store) and initialise the iterator */
[GTK2.TreeIter iter, GTK2.TreeModel list_store] = self->get_selection()->get_selected();
array temp = list_store->get_row(iter);
sdata->set_text(Standards.JSON.encode(temp));
print_DATA(temp);
}
/* User callback for putting the data into the other treeview */
void on_drag_data_received(GTK2.Widget self, GDK2.DragContext drag_context,
int x, int y, GTK2.SelectionData sdata, int info,
int time) {
write("on_drag_data_received:\n");
//Variation from the C version: ask what the source widget is, don't hard-code it!
GTK2.Widget source = drag_context->get_source_widget();
/* Remove row from the source treeview */
[GTK2.TreeIter iter, GTK2.TreeModel list_store] = source->get_selection()->get_selected();
list_store->remove(iter);
/* Now add to the other treeview */
GTK2.TreeModel list_store2 = self->get_model();
GTK2.TreeIter iter2 = list_store2->append();
/* Copy the pointer we received into a new struct */
array temp = Standards.JSON.decode(sdata->get_text());
/* Add the received data to the treeview model */
list_store2->set_row(iter2, temp);
}
/* User callback just to see which row was selected, doesnt affect DnD.
However it might be important to note that this signal and drag-data-received may occur at the same time. If you drag a row out of one view, your selection changes too */
void on_selection_changed(object self) {
[GTK2.TreeIter iter, GTK2.TreeModel list_store] = self->get_selected();
/* "changed" signal sometimes fires blanks, so make sure we actually
have a selection/
http://library.gnome.org/devel/gtk/stable/GtkTreeSelection.html#GtkTreeSelection-changed */
if (!iter) {
write("No row selected\n");
return;
}
write("on_selection_changed: ");
print_DATA(list_store->get_row(iter));
}
/* Create and populate a treeview */
GTK2.TreeView create_treeview(array data) {
/* Create the treeview and its list store */
GTK2.ListStore list_store = GTK2.ListStore(
({"string", "string", "int", "float"}));
GTK2.Widget tree_view = GTK2.TreeView(list_store);
/* Add the columns */
foreach (({"Row #", "Description", "Qty", "Price"}); int i; string hdr) {
tree_view->append_column(GTK2.TreeViewColumn(hdr,
GTK2.CellRendererText(), "text", i)
->set_sort_column_id(i)
);
}
/* Tell the theme engine we would like differentiated row colour */
tree_view->set_rules_hint(1);
/* Add the data */
foreach (data, array row) {
list_store->set_row(list_store->append(), row);
}
/* Attach the "changed" callback onto the tree's selector */
tree_view->get_selection()->signal_connect("changed", on_selection_changed);
return tree_view;
}
int main(int argc, array(string) argv){
GTK2.setup_gtk(argv);
/* Create the top level window and setup the quit callback */
GTK2.TreeView view1, view2;
GTK2.Window window = GTK2.Window(GTK.WINDOW_TOPLEVEL)
->add(GTK2.Vbox(0, 10)
->pack_start(GTK2.Label(DESCRIPTION), 0, 0, 1)
->pack_start(GTK2.Hbox(1, 1)
->pack_start(GTK2.ScrolledWindow()->set_policy(GTK2.POLICY_AUTOMATIC, GTK2.POLICY_AUTOMATIC)
->add(view1 = create_treeview(row_data))
, 1, 1, 2)
->pack_start(GTK2.ScrolledWindow()->set_policy(GTK2.POLICY_AUTOMATIC, GTK2.POLICY_AUTOMATIC)
->add(view2 = create_treeview(row2_data))
, 1, 1, 2)
, 1, 1, 1)
)
->set_default_size(666,266)->show_all();
window->signal_connect("destroy", lambda() {exit(0);});
view1->drag_source_set(GTK2.GDK_BUTTON1_MASK, drag_targets,
GTK2.GDK_ACTION_COPY|GTK2.GDK_ACTION_MOVE);
view1->signal_connect("drag-data-get", on_drag_data_get);
/* Set treeview 2 as the destination of the Drag-N-Drop operation */
view2->drag_dest_set(GTK2.DEST_DEFAULT_ALL,drag_targets,
GTK2.GDK_ACTION_COPY|GTK2.GDK_ACTION_MOVE);
/* Attach a "drag-data-received" signal to pull in the dragged data */
view2->signal_connect("drag-data-received", on_drag_data_received);
/* Rock'n Roll */
return -1;
} |
---
title: "[New] In 2024, Elevating Income with Mobile Video Monetization Techniques for YouTubers"
date: 2024-06-05T14:02:44.238Z
updated: 2024-06-06T14:02:44.238Z
tags:
- ai video
- ai youtube
categories:
- ai
- youtube
description: "This Article Describes [New] In 2024, Elevating Income with Mobile Video Monetization Techniques for YouTubers"
excerpt: "This Article Describes [New] In 2024, Elevating Income with Mobile Video Monetization Techniques for YouTubers"
keywords: "Earn Money Online (YouTube),YouTube Revenue Boost,Mobile Ad Revenue Growth,Profit From Video Content,Monetize Social Media Videos,Increase YouTube Income,Video Asset Marketability"
thumbnail: https://thmb.techidaily.com/cb670c879e89656e881160d22473efee38dda114df0401fe96c31b4cf2882857.jpg
---
## Elevating Income with Mobile Video Monetization Techniques for YouTubers
YouTube makes money easier and more accessible than ever in 2024\. Create videos from the convenience of your mobile device and earn money from your passion. Your dreams can become a reality when you explore them. The goal of every YouTube creator is to monetize their channel. They can start side businesses, turn YouTube into a full-time job, or sell physical products. With today's technology, the possibilities are endless!
This article is here to guide you on making money from your YouTube channel in 2024\. You'll discover steps and tips that can help boost your earnings. Whether you're a pro or just starting out, monetizing your content can work for you. Get ready to increase your YouTube income potential as your journey on the platform is set to get even better.
**YouTube Monetization Editor** A cross-platform helps create attention-grabbing thumbnails and enhance your videos on YouTube easy!
[Free Download](https://tools.techidaily.com/wondershare/filmora/download/) [Free Download](https://tools.techidaily.com/wondershare/filmora/download/) [Learn More](https://tools.techidaily.com/wondershare/filmora/download/)
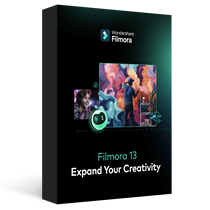
## Part 1\. What you need to know about YouTube Monetization
### Rules and requirements for YouTube Monetization
YouTube Monetization means making money from YouTube content. For monetization, you need to join the YouTube Partner program and for this, you need to follow some requirements. In order to benefit from YPP fan funding, such as Super Chats and Super Thanks, here are the minimum requirements:
* A subscriber base of 500
* 90-day history of public uploads
* Within the last 90 days, public YouTube Shorts have been viewed 3 million times.
Video advertisements can make you money if you meet the following requirements:
* Subscribers of 1,000
* Watches by the public of 4,000 hours or views of 10 million YouTube Shorts in 90 days.
Promote your channel and create quality content to increase these numbers.
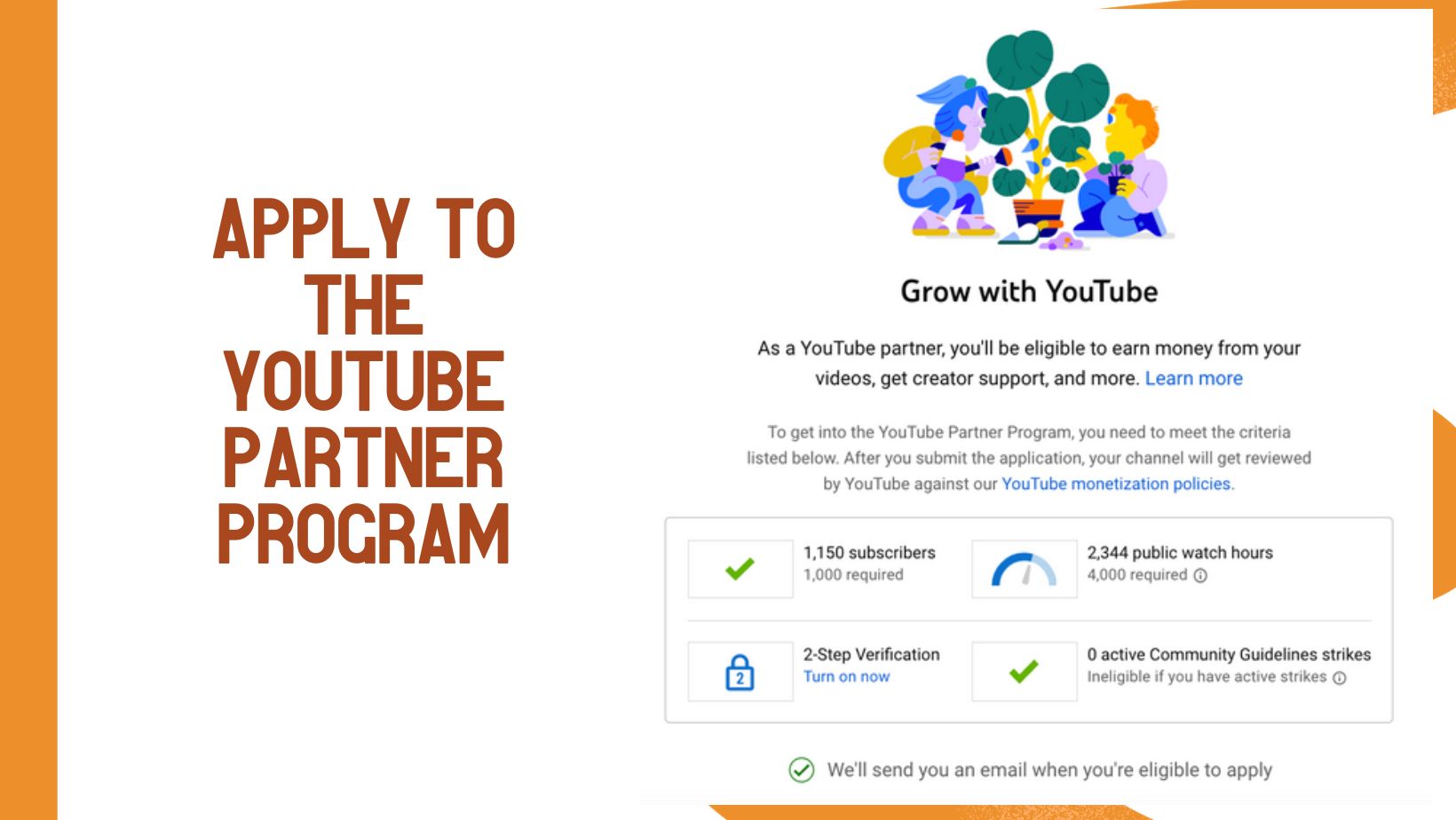
### Join the YouTube Partner Program
In order to participate in the YouTube Partner Program, you must apply (YPP). YouTube is the top platform for content creators, offering them the chance to monetize their work.
Applications are reviewed by YouTube for at least one month before a decision is made. Due to YouTube's actual specialists reviewing each application, it may take longer than a month.
To join the YPP, you must meet the following requirements:
* A minimum of 1,000 subscribers subscribe to your channel
* In the last 12 months, you've logged at least 4,000 public watch hours
* You live in an area that offers the YouTube Partner Program
* In terms of YouTube channel monetization policies, you follow them all
* Your YouTube channel must be secured with 2-Step Verification via your Google Account.
* Your YouTube channel is associated with an AdSense account
* The Community Guidelines strike on your channel is not active
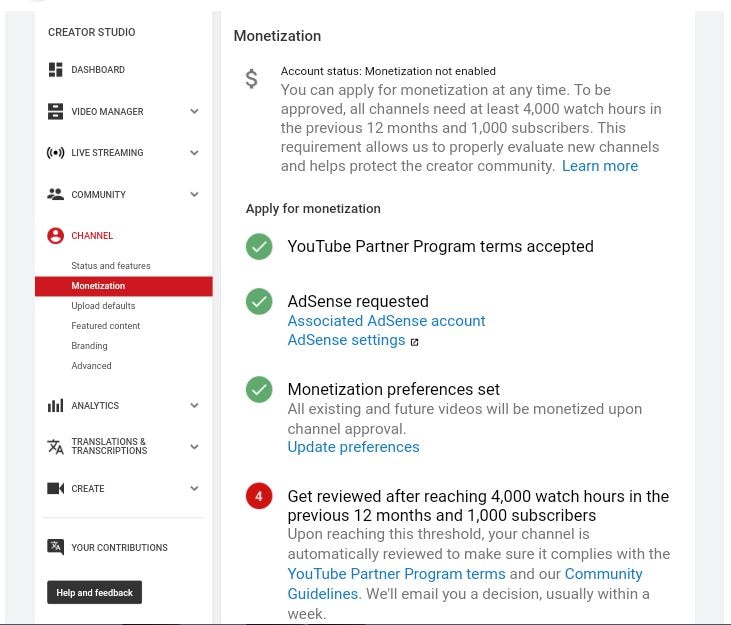
## Part 2\. The Step-by-Step Guide for Monetizing Your YouTube Channel on Your Phone
### YouTube Monetization Steps for Mobile
Following YPP's requirements, you should follow these steps. Several steps must be followed in order to enable YouTube monetization using a mobile device:
A Quick Navigation
* [Step 1 - Create an account on YouTube](#step1)
* [Step 2 - Get YouTube Studio access](#step2)
* [Step 3 -Monetize your site with Google Adsense](#step3)
* [Step 4 -Accept YouTube's terms and conditions](#step4)
* [Step 5 -Creating ad formats](#step5)
* [Step 6 -Monetizing videos](#step6)
* [Step 7 -Content Promotion](#step7)
* [Step 8 -Analyze the results](#step8)
* [Step 9 -Ensure compliance](#step9)
#### Step - 1 . Create an account on YouTube
Simply open YouTube on your mobile device, and sign in with your Google account right there on your device.
#### Step - 2 . Get YouTube Studio access
To access the YouTube Studio dashboard, click the icon of your channel or profile picture.
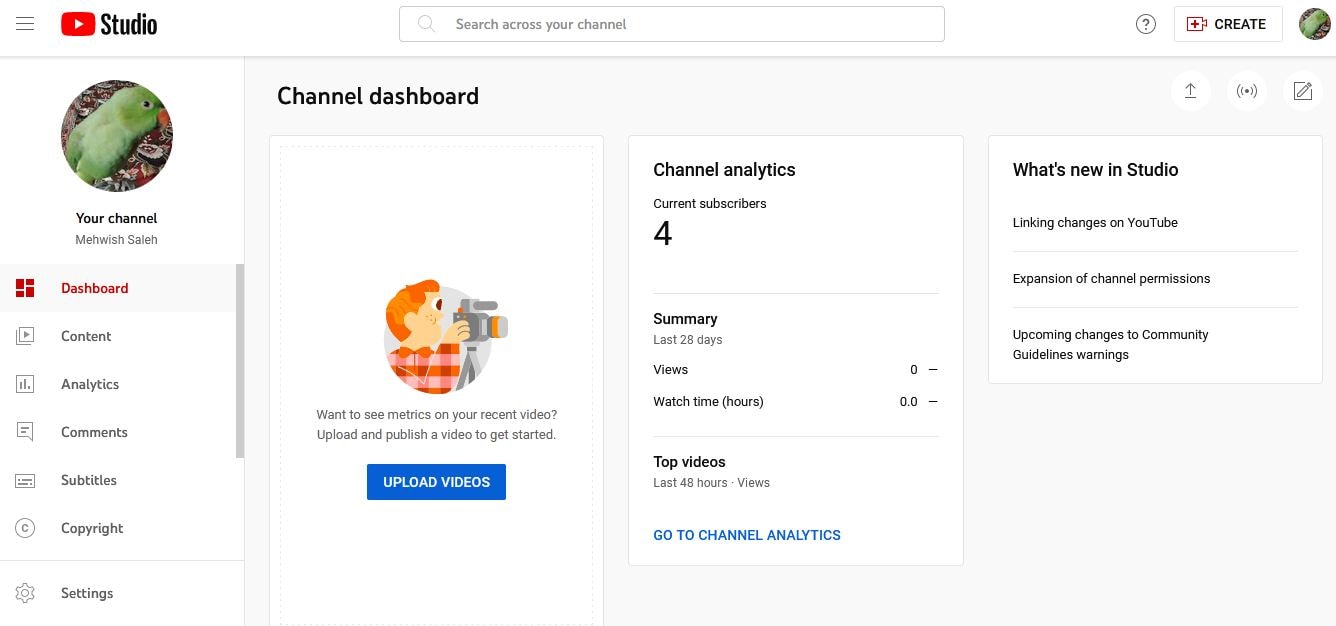
#### Step - 3 . Monetize your site with Google Adsense
To view YouTube Studio's monetization options, click on the "Monetization" tab. The "Enable" option will appear if you meet the eligibility criteria. To receive your earnings, follow the instructions onscreen to set up Google AdSense.
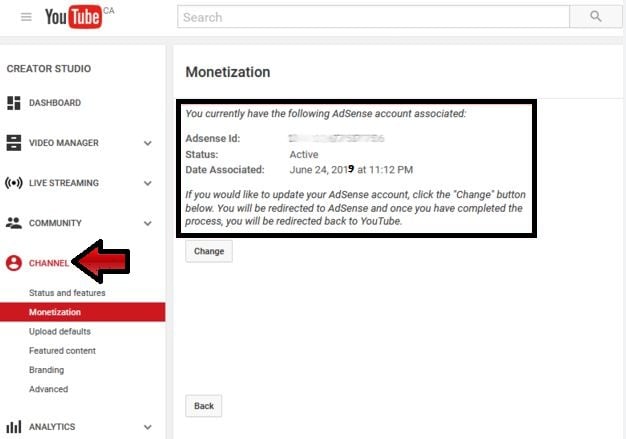
#### Step - 4 . Accept YouTube's terms and conditions
Read and accept YouTube's terms and conditions for the Partner Program.
#### Step - 5 . Creating ad formats
In your videos, you can choose whether you want skippable or non-skippable advertisements.
#### Step - 6 . Monetizing videos
To enable or disable monetization on specific videos, select "Videos" from the YouTube Studio menu.
#### Step - 7 . Content Promotion
Continue producing high-quality content that engages viewers. It is crucial to create engaging content in order to increase ad revenue.
#### Step - 8 . Analyze the results
YouTube Studio lets you monitor channel performance, identify improvements, and monitor ad revenue.
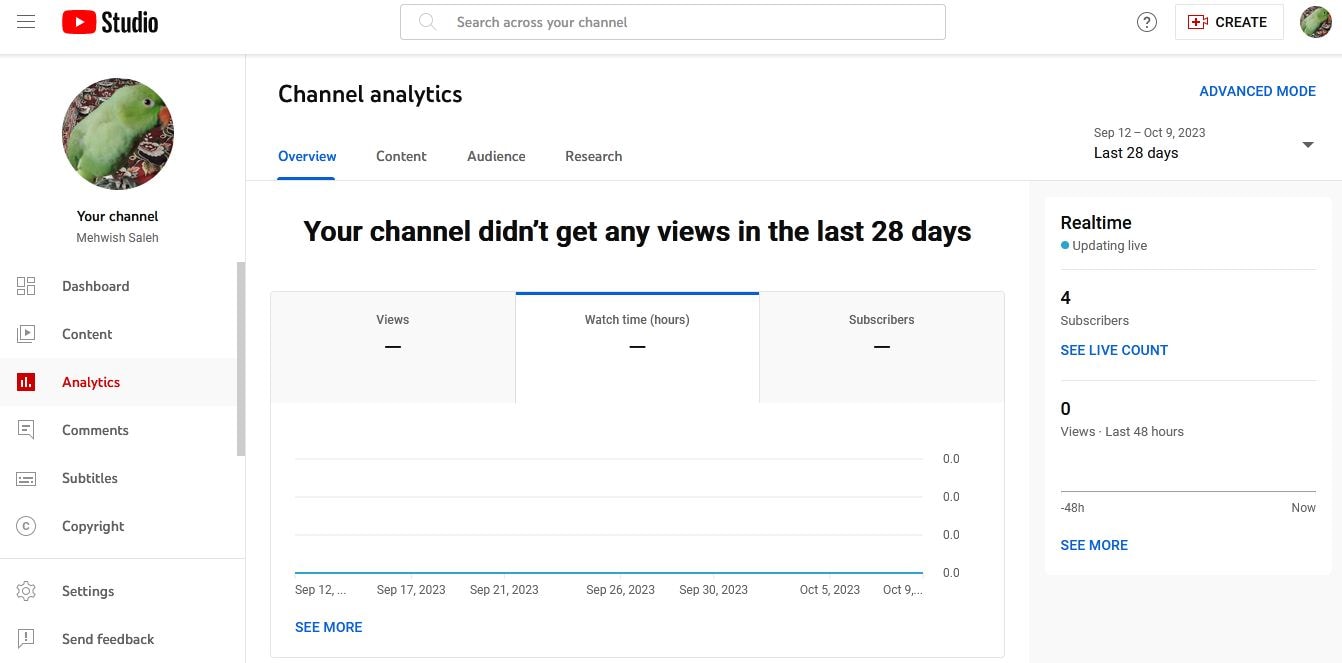
#### Step - 9 . Ensure compliance
Keep in mind YouTube's content policies if you want to maintain your monetization status.
Your YouTube channel may need some time to be approved for monetization. Engagement with your audience is the key to increasing viewership and revenue for your videos.
## Part 3\. Monetizing YouTube channels on mobile devices: Some Proven Ways
It is beneficial for creators to use YouTube AdSense, although it is not a reliable source of income. The advertising rates fluctuate, so making the same amount of money every year is hard. At last, here are some methods for monetizing YouTube:
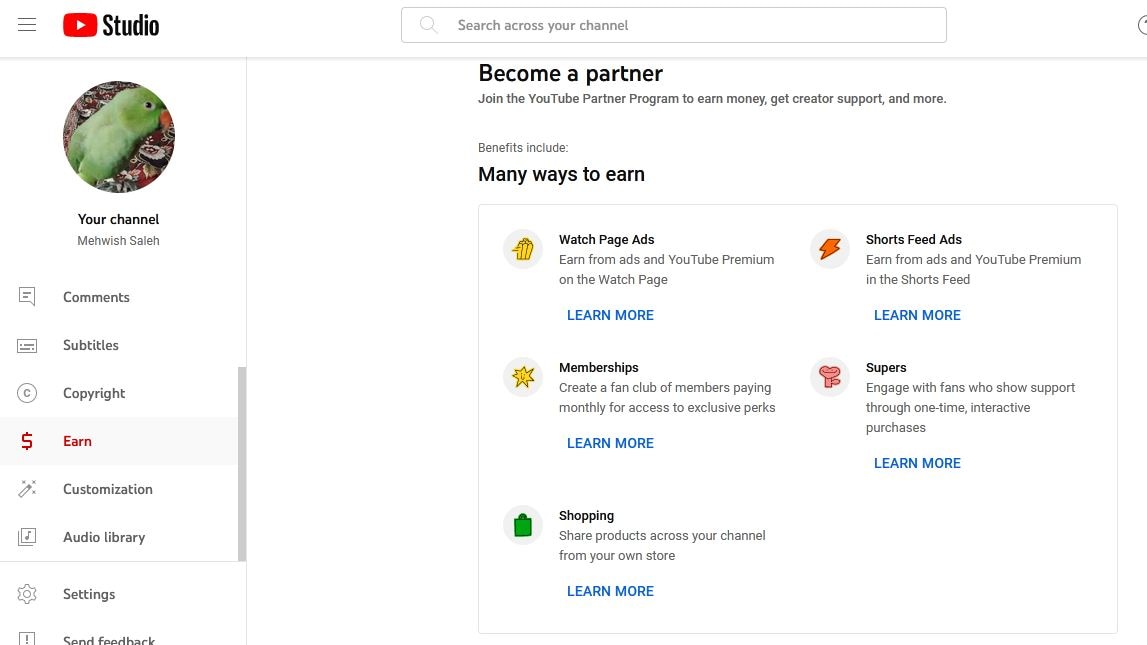
### Ads on YouTube
Monetizing a website has many methods, but this is the most basic and straightforward one. Various YouTube ad formats provide control over ad placement before or during a video.
### Sponsorships that are paid
Sponsorship involves paying a content creator to use, demonstrate, or test a brand's product in a video. It benefits both the sponsors and the viewers when brands sponsor creators.
### Affiliates
Using affiliate programs is another way of monetizing your YouTube videos. With affiliate marketing, your audience is directed to a brand's landing page or product by clicking an affiliate link. A commission is earned when the user purchases successfully. It is possible to earn extra income through affiliate programs. Offer discounts to your followers when you become an affiliate for a product you already use.
### The merchandise
A similar tactic has already been discussed. Your fans can buy branded goods from a wide range of merchant platforms available today.
### Premium YouTube subscriptions
Using YouTube Premium, users can watch videos ad-free and download videos. Fortunately, you can still earn money by uploading videos to this service. Video creators receive subscription revenue payments in the same manner as ad revenues. You earn money based on the number of times Premium members watch your videos.
### Crowdfunding on YouTube
Creators can unlock this feature once they reach 500 subscribers and watch 3000 hours. Through three main avenues, they can directly monetize their audience:
* Fans can earn exclusive loyalty badges and watch private streams with channel memberships. Offering YouTube monetization options that can be customized.
* Live streams on YouTube can also be monetized through Super Chats. Viewers send creators "donations" or "tips" between $1 and $500 during live streams.
* The Super Thanks feature on YouTube lets viewers show appreciation for your content by buying digital goods.
## Part 4\. Wondershare Filmora's Power: Elevating YouTube Content for Monetization
Keeping YouTube revenue high in 2024 means ensuring high-quality content. Introducing Wondershare Filmora, a tool that allows you to edit videos on your mobile device. You can use it to make your YouTube content stand out.
Creating attention-grabbing thumbnails and enhancing your videos is easy with Filmora. A package for trimming, adding music, overlaying text, and applying captivating effects to videos.
For your channel to succeed, you need to create eye-catching thumbnails. With Filmora, you can create thumbnails that pique interest and boost click-through rates.
You can elevate your content with Filmora whether you're experienced or starting out. You can maximise your YouTube earnings in 2024 if your videos connect with your audience.
[Free Download](https://tools.techidaily.com/wondershare/filmora/download/) For Win 7 or later(64-bit)
[Free Download](https://tools.techidaily.com/wondershare/filmora/download/) For macOS 10.14 or later
### Features for Editing Videos or Images for YouTube
#### 1\. [AI Copywriting](https://tools.techidaily.com/wondershare/filmora/download/)
Using the ChatGPT API, Filmora's AI Copywriting feature generates scripts based on AI. Assists in the generation of text during the editing of videos. Automates YouTube title and description creation and simplifies the process.
#### 2\. [Keyframing](https://tools.techidaily.com/wondershare/filmora/download/)
The keyframing capabilities of Filmora enhance the quality and energy of your videos. Adding smooth animated effects to capture your audience's attention.
#### 3\. [AI Image](https://tools.techidaily.com/wondershare/filmora/download/)
With Text to Image, you can use images directly in your video project in three resolutions. And explore limitless image styles, enhancing the visual appeal of your content.
#### 4\. [Video Templates for All Your Needs](https://tools.techidaily.com/wondershare/filmora/download/)
Start with Filmora's built-in video templates, creating creative videos with ease. You can also save templates on the cloud. Streamline your video production process by sharing them with fellow creators.
## Conclusion
You have now learned how to enable monetization on YouTube in mobile in 2024\. From your mobile device, you can turn your passion into income. The possibilities are endless once you achieve monetization. No matter if you're starting a side gig, launching a business, or going full-time on YouTube.
We have covered key steps for meeting YouTube's requirements and monetizing videos. Following these steps and using the available strategies will assist you in achieving your goals. Making monetization easy with quality content is easy with Filmora's above mentioned features. The road to success is open. Maximize your YouTube revenue potential today to make your YouTube journey truly rewarding.
[Free Download](https://tools.techidaily.com/wondershare/filmora/download/) [Free Download](https://tools.techidaily.com/wondershare/filmora/download/) [Learn More](https://tools.techidaily.com/wondershare/filmora/download/)
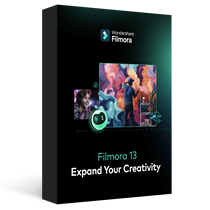
## Part 1\. What you need to know about YouTube Monetization
### Rules and requirements for YouTube Monetization
YouTube Monetization means making money from YouTube content. For monetization, you need to join the YouTube Partner program and for this, you need to follow some requirements. In order to benefit from YPP fan funding, such as Super Chats and Super Thanks, here are the minimum requirements:
* A subscriber base of 500
* 90-day history of public uploads
* Within the last 90 days, public YouTube Shorts have been viewed 3 million times.
Video advertisements can make you money if you meet the following requirements:
* Subscribers of 1,000
* Watches by the public of 4,000 hours or views of 10 million YouTube Shorts in 90 days.
Promote your channel and create quality content to increase these numbers.
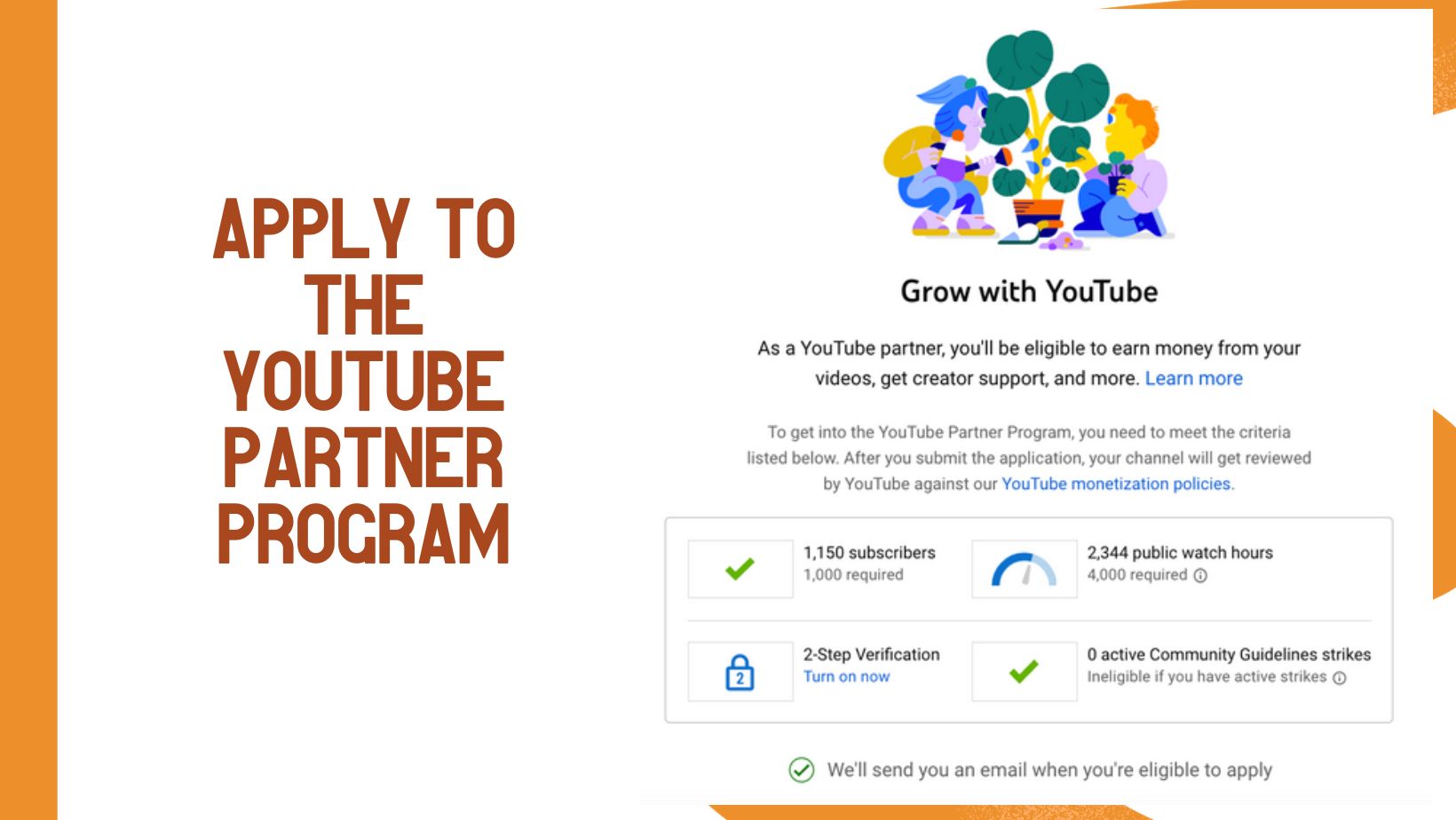
### Join the YouTube Partner Program
In order to participate in the YouTube Partner Program, you must apply (YPP). YouTube is the top platform for content creators, offering them the chance to monetize their work.
Applications are reviewed by YouTube for at least one month before a decision is made. Due to YouTube's actual specialists reviewing each application, it may take longer than a month.
To join the YPP, you must meet the following requirements:
* A minimum of 1,000 subscribers subscribe to your channel
* In the last 12 months, you've logged at least 4,000 public watch hours
* You live in an area that offers the YouTube Partner Program
* In terms of YouTube channel monetization policies, you follow them all
* Your YouTube channel must be secured with 2-Step Verification via your Google Account.
* Your YouTube channel is associated with an AdSense account
* The Community Guidelines strike on your channel is not active
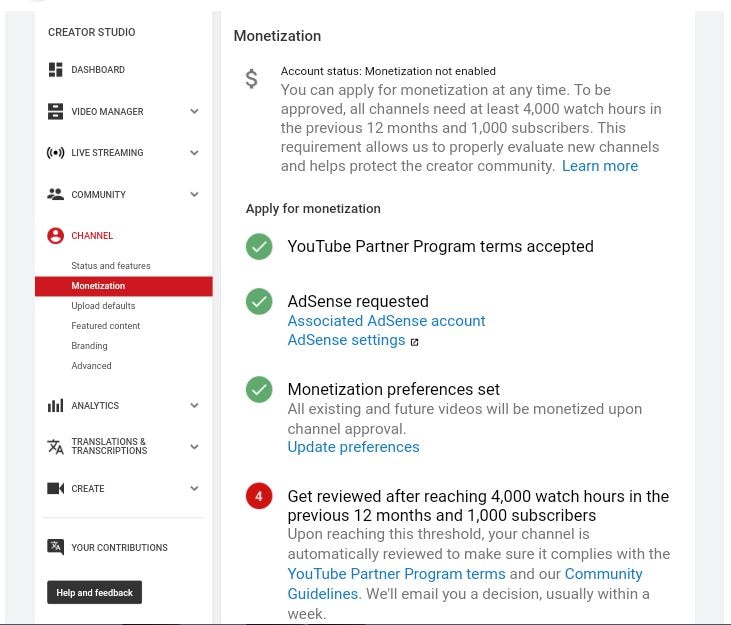
## Part 2\. The Step-by-Step Guide for Monetizing Your YouTube Channel on Your Phone
### YouTube Monetization Steps for Mobile
Following YPP's requirements, you should follow these steps. Several steps must be followed in order to enable YouTube monetization using a mobile device:
A Quick Navigation
* [Step 1 - Create an account on YouTube](#step1)
* [Step 2 - Get YouTube Studio access](#step2)
* [Step 3 -Monetize your site with Google Adsense](#step3)
* [Step 4 -Accept YouTube's terms and conditions](#step4)
* [Step 5 -Creating ad formats](#step5)
* [Step 6 -Monetizing videos](#step6)
* [Step 7 -Content Promotion](#step7)
* [Step 8 -Analyze the results](#step8)
* [Step 9 -Ensure compliance](#step9)
#### Step - 1 . Create an account on YouTube
Simply open YouTube on your mobile device, and sign in with your Google account right there on your device.
#### Step - 2 . Get YouTube Studio access
To access the YouTube Studio dashboard, click the icon of your channel or profile picture.
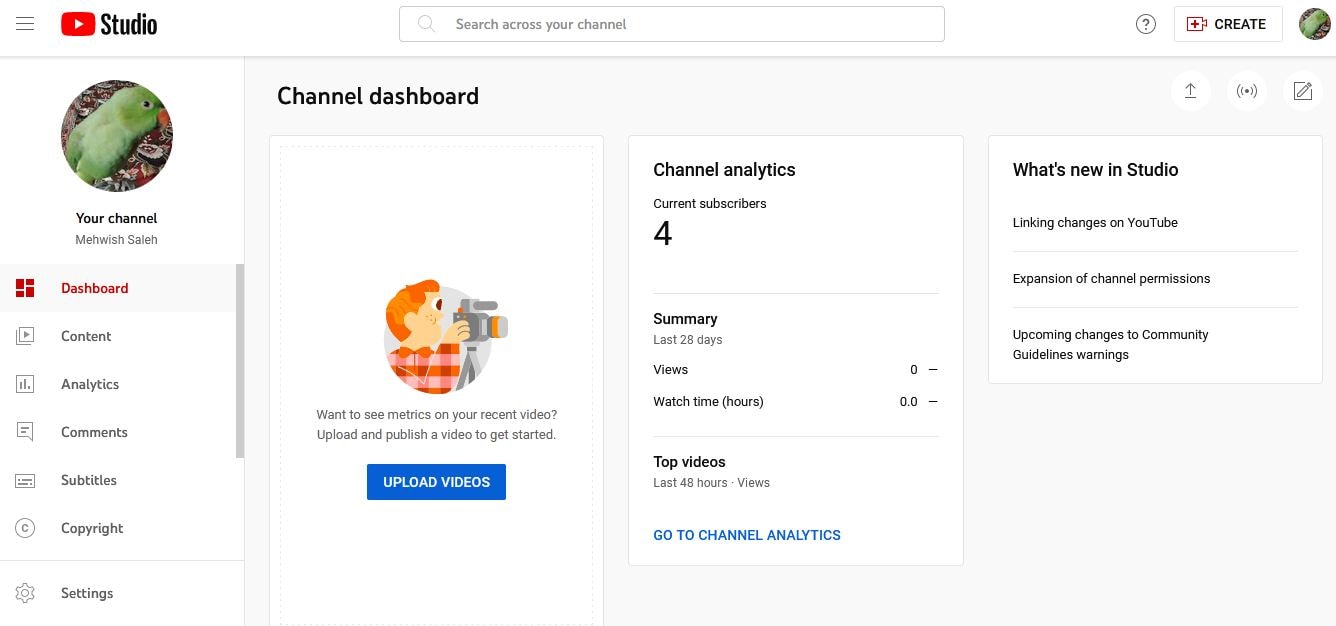
#### Step - 3 . Monetize your site with Google Adsense
To view YouTube Studio's monetization options, click on the "Monetization" tab. The "Enable" option will appear if you meet the eligibility criteria. To receive your earnings, follow the instructions onscreen to set up Google AdSense.
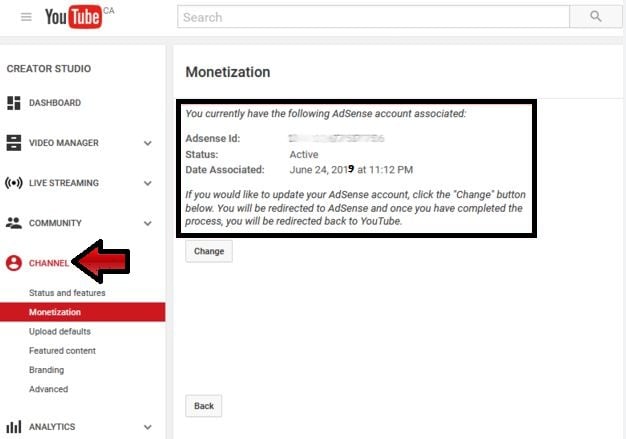
#### Step - 4 . Accept YouTube's terms and conditions
Read and accept YouTube's terms and conditions for the Partner Program.
#### Step - 5 . Creating ad formats
In your videos, you can choose whether you want skippable or non-skippable advertisements.
#### Step - 6 . Monetizing videos
To enable or disable monetization on specific videos, select "Videos" from the YouTube Studio menu.
#### Step - 7 . Content Promotion
Continue producing high-quality content that engages viewers. It is crucial to create engaging content in order to increase ad revenue.
#### Step - 8 . Analyze the results
YouTube Studio lets you monitor channel performance, identify improvements, and monitor ad revenue.
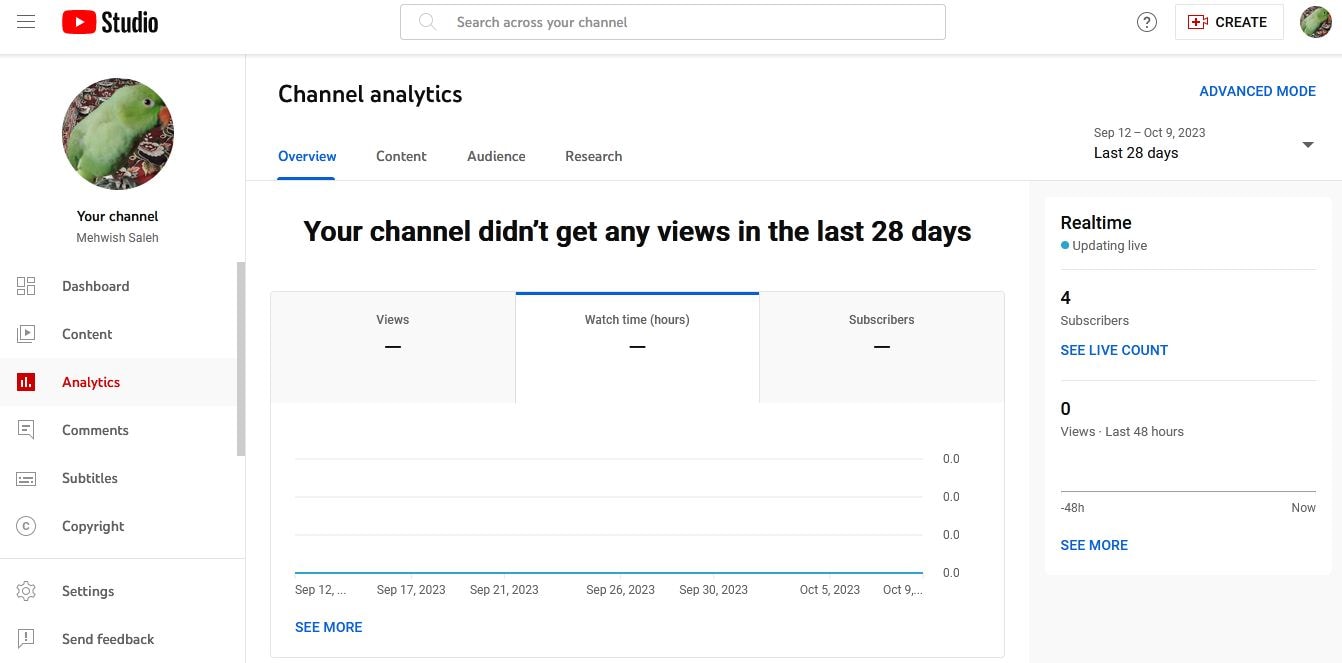
#### Step - 9 . Ensure compliance
Keep in mind YouTube's content policies if you want to maintain your monetization status.
Your YouTube channel may need some time to be approved for monetization. Engagement with your audience is the key to increasing viewership and revenue for your videos.
## Part 3\. Monetizing YouTube channels on mobile devices: Some Proven Ways
It is beneficial for creators to use YouTube AdSense, although it is not a reliable source of income. The advertising rates fluctuate, so making the same amount of money every year is hard. At last, here are some methods for monetizing YouTube:
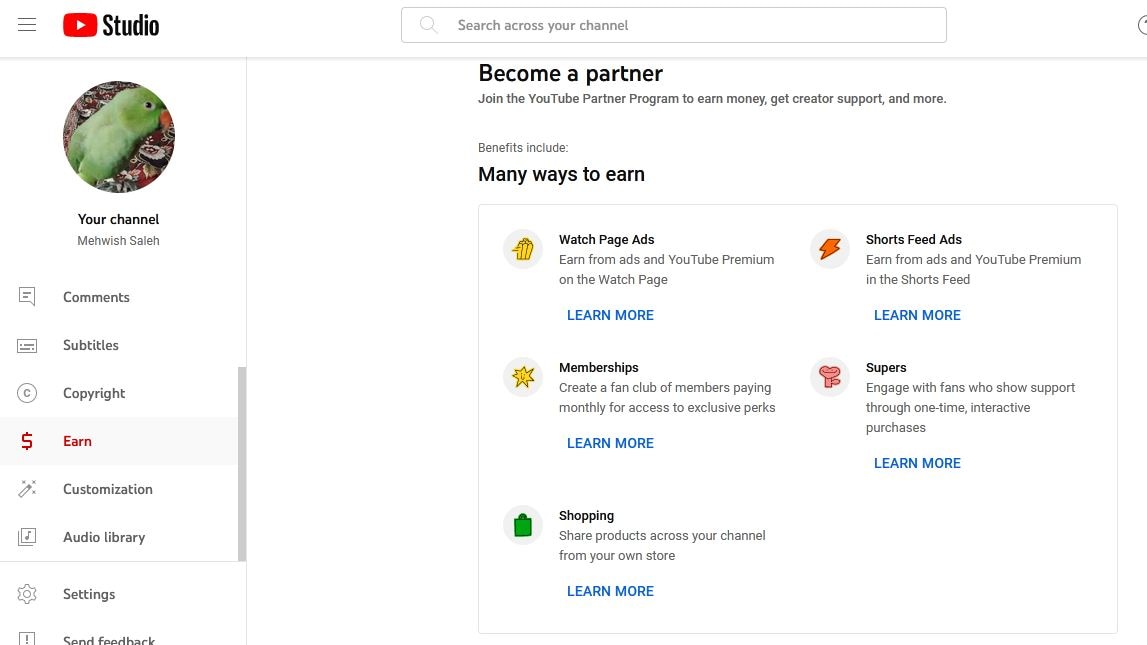
### Ads on YouTube
Monetizing a website has many methods, but this is the most basic and straightforward one. Various YouTube ad formats provide control over ad placement before or during a video.
### Sponsorships that are paid
Sponsorship involves paying a content creator to use, demonstrate, or test a brand's product in a video. It benefits both the sponsors and the viewers when brands sponsor creators.
### Affiliates
Using affiliate programs is another way of monetizing your YouTube videos. With affiliate marketing, your audience is directed to a brand's landing page or product by clicking an affiliate link. A commission is earned when the user purchases successfully. It is possible to earn extra income through affiliate programs. Offer discounts to your followers when you become an affiliate for a product you already use.
### The merchandise
A similar tactic has already been discussed. Your fans can buy branded goods from a wide range of merchant platforms available today.
### Premium YouTube subscriptions
Using YouTube Premium, users can watch videos ad-free and download videos. Fortunately, you can still earn money by uploading videos to this service. Video creators receive subscription revenue payments in the same manner as ad revenues. You earn money based on the number of times Premium members watch your videos.
### Crowdfunding on YouTube
Creators can unlock this feature once they reach 500 subscribers and watch 3000 hours. Through three main avenues, they can directly monetize their audience:
* Fans can earn exclusive loyalty badges and watch private streams with channel memberships. Offering YouTube monetization options that can be customized.
* Live streams on YouTube can also be monetized through Super Chats. Viewers send creators "donations" or "tips" between $1 and $500 during live streams.
* The Super Thanks feature on YouTube lets viewers show appreciation for your content by buying digital goods.
## Part 4\. Wondershare Filmora's Power: Elevating YouTube Content for Monetization
Keeping YouTube revenue high in 2024 means ensuring high-quality content. Introducing Wondershare Filmora, a tool that allows you to edit videos on your mobile device. You can use it to make your YouTube content stand out.
Creating attention-grabbing thumbnails and enhancing your videos is easy with Filmora. A package for trimming, adding music, overlaying text, and applying captivating effects to videos.
For your channel to succeed, you need to create eye-catching thumbnails. With Filmora, you can create thumbnails that pique interest and boost click-through rates.
You can elevate your content with Filmora whether you're experienced or starting out. You can maximise your YouTube earnings in 2024 if your videos connect with your audience.
[Free Download](https://tools.techidaily.com/wondershare/filmora/download/) For Win 7 or later(64-bit)
[Free Download](https://tools.techidaily.com/wondershare/filmora/download/) For macOS 10.14 or later
### Features for Editing Videos or Images for YouTube
#### 1\. [AI Copywriting](https://tools.techidaily.com/wondershare/filmora/download/)
Using the ChatGPT API, Filmora's AI Copywriting feature generates scripts based on AI. Assists in the generation of text during the editing of videos. Automates YouTube title and description creation and simplifies the process.
#### 2\. [Keyframing](https://tools.techidaily.com/wondershare/filmora/download/)
The keyframing capabilities of Filmora enhance the quality and energy of your videos. Adding smooth animated effects to capture your audience's attention.
#### 3\. [AI Image](https://tools.techidaily.com/wondershare/filmora/download/)
With Text to Image, you can use images directly in your video project in three resolutions. And explore limitless image styles, enhancing the visual appeal of your content.
#### 4\. [Video Templates for All Your Needs](https://tools.techidaily.com/wondershare/filmora/download/)
Start with Filmora's built-in video templates, creating creative videos with ease. You can also save templates on the cloud. Streamline your video production process by sharing them with fellow creators.
## Conclusion
You have now learned how to enable monetization on YouTube in mobile in 2024\. From your mobile device, you can turn your passion into income. The possibilities are endless once you achieve monetization. No matter if you're starting a side gig, launching a business, or going full-time on YouTube.
We have covered key steps for meeting YouTube's requirements and monetizing videos. Following these steps and using the available strategies will assist you in achieving your goals. Making monetization easy with quality content is easy with Filmora's above mentioned features. The road to success is open. Maximize your YouTube revenue potential today to make your YouTube journey truly rewarding.
<ins class="adsbygoogle"
style="display:block"
data-ad-format="autorelaxed"
data-ad-client="ca-pub-7571918770474297"
data-ad-slot="1223367746"></ins>
<ins class="adsbygoogle"
style="display:block"
data-ad-format="autorelaxed"
data-ad-client="ca-pub-7571918770474297"
data-ad-slot="1223367746"></ins>
## IGTV Vs. YouTube: Unveiling Key Distinctions & Best Platform for Sharing
# IGTV VS YouTube: Should Video Content Creators Use One Platform or The Other?
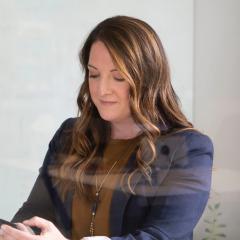
##### Shanoon Cox
Mar 27, 2024• Proven solutions
IGTV receives quite a lot of attention from marketers and content creators alike. Some even go so far to compare **IGTV to YouTube**, currently, the largest video sharing platform in the world that hosts 300 minutes of video each minute. IGTV may have fewer users than YouTube, but that doesn’t mean you can’t find a way to use it effectively for a wide array of different purposes. So, in this article, we are going to take a look at the advantages and disadvantages of each platform in order to help you discover how you can utilize each of these social networks to broaden the audience for the content you share online.
**You May Also Like:**
[Instagram Reels VS Instagram Stories--Everything You Need to Know >>](https://tools.techidaily.com/wondershare/filmora/download/)
[YouTube Shorts vs. TikTok: Which One Is Better? >>](https://tools.techidaily.com/wondershare/filmora/download/)
## IGTV VS YouTube: What's the difference
Comparison between IGTV and YouTube is somewhat unfair towards the former since IGTV is a much younger platform that still needs time to develop. YouTube, on the other hand, has a reputation as one of the most reliable video sharing platforms ever created that enables people from all walks of life to upload and share music, movies, gaming videos and virtually any other type of moving images. Even so, let’s take a look at major differences and similarities between IGTV and YouTube.
#### Have a Quick Glance of the Differences
01 [Interface Differences](#part1)
02 [The Upload Process](#part2)
03 [Discovering New Content](#part3)
03 [Monetization](#part4)
03 [Analytics and Insights](#part5)
#### 1. Interface Differences
Despite the fact that you can access IGTV from a web browser, this platform is predominantly dedicated to videos that are going to be watched on mobile devices. This also means that the thumbnails of the videos you uploaded to your IGTV channel are going to be displayed differently than those on your YouTube channel. The interfaces of these two social networks are entirely different, but if you are a newcomer to each of these platforms you won’t have much trouble getting used to them.
#### 2. The Upload Process
The fact that you can only upload vertically oriented videos to IGTV somewhat limits your options because you either have to record a video for IGTV specifically or you must edit a horizontally oriented video and change its aspect ratio to 9:16\. You can upload videos to YouTube and IGTV directly from your computer or you can use iPhone and Android-based apps to capture videos with your phone and upload them to either of these platforms. The maximum duration of an IGTV video can’t exceed sixty minutes, while the default duration of all videos on YouTube is set to 15 minutes. Nonetheless, if you own a verified YouTube account, you can upload videos that have 128GB and last up to 12 hours.
At the moment the videos you want to upload to your IGTV channel have to be saved in the MP4 file format because the platform still doesn’t offer support for any other video file format. YouTube grants much more freedom in this respect as it allows you to upload videos saved in AVI, FLV, MOV, MP4 and other commonly used file formats.
#### 3. Discovering New Content
IGTV is more than a decade younger than YouTube, so you shouldn’t be surprised if you’re having trouble discovering new content. Moreover, IGTV’s search engine is still relatively new and it is much less powerful than the one used by YouTube. This is the reason why the videos you upload to your YouTube channel are going to be much easier to find via a simple Google search than those you shared on IGTV. Besides, YouTube offers much more new content on a daily basis than IGTV, and it is clear that the Instagram’s new brainchild still has ways to go before it reaches the point when it can challenge the largest video sharing platform in the world in terms of diversity of the content that it delivers to its users.
#### 4. Monetization
At the moment, views and likes can’t be monetized on IGTV, so most influencers rely on sponsorships from brands. Things may change as the platform grows, but for the time being IGTV doesn’t provide the content creators with any compensation for the work they are putting in. YouTube allows content creators to monetize their videos through Google Adsense for years, and some of its most prominent users like PewDiePie or Logan Paul have earned hundreds of millions of dollars by posting new content on their YouTube channels. Keep in mind that you are going to need at least a thousand subscribers and 4,000 hours of viewing time before you can enable YouTube’s Monetize feature.
#### 5. Analytics and Insights
Knowing how many people watched the entire video or how many likes and comments certain types of videos get can help you produce content that attracts a larger audience on all social networks. The metrics such as engagement or view-through rates can be essential during online marketing campaigns as they can suggest how many people are watching your videos and for how long. The analytics tools YouTube provides are much more diverse than those offered by IGTV that only lets you see the audience retention graph and the number of views and comments a video currently has. YouTube is an obviously a much better option for anyone who wants to conduct an in-depth analysis of their content’s performance, although the tools on IGTV are still powerful enough to indicate which type of content your audience likes the most.
## Why Shouldn’t You Use YouTube and IGTV For the Same Purposes?
You shouldn’t assume that you can post the same type of videos on your YouTube and IGTV channels, just because they are both video sharing platforms. The content you create for your IGTV channel needs to be dynamic and entertaining in order to captivate the audience’s attention. Posting tutorials or hour-long gaming videos on IGTV won’t attract as many viewers as on YouTube, which is why businesses who want to use IGTV to promote their products and services need to choose the content carefully. Furthermore, the videos that last between two and five minutes are still by far the most popular on IGTV, so even though technically you can upload a video that lasts one hour to your IGTV channel, the chances are that such long videos won’t get a lot of views.
IGTV is probably best used as a tool that can help improve the engagement with your followers on Instagram, but if you want to become an established video content creator, then running a YouTube channel is simply a much better option. It still remains to be seen how will IGTV evolve in the years to come, but at the present moment this platform doesn’t really have any chance of challenging YouTube’s position as the world’s leading video sharing platform.
However, no matter which video platform you choose, you need to ensure the videos you create are of high quality. A good video editing software like Filmora can help you acheive this goal.
**Useful Features of Filmora**
* Cokor Matching: Apply color correction setting to multiple clips on one-click.
* Effects: Create blockbuster videos with preset effects.
* Motion Tracking: Track moving items and add elements to it at ease.
* And more waiting for you to discover.
[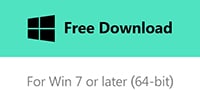](https://tools.techidaily.com/wondershare/filmora/download/)[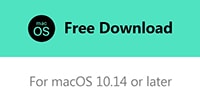](https://tools.techidaily.com/wondershare/filmora/download/)
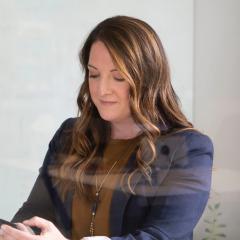
Shanoon Cox
Shanoon Cox is a writer and a lover of all things video.
Follow @Shanoon Cox
##### Shanoon Cox
Mar 27, 2024• Proven solutions
IGTV receives quite a lot of attention from marketers and content creators alike. Some even go so far to compare **IGTV to YouTube**, currently, the largest video sharing platform in the world that hosts 300 minutes of video each minute. IGTV may have fewer users than YouTube, but that doesn’t mean you can’t find a way to use it effectively for a wide array of different purposes. So, in this article, we are going to take a look at the advantages and disadvantages of each platform in order to help you discover how you can utilize each of these social networks to broaden the audience for the content you share online.
**You May Also Like:**
[Instagram Reels VS Instagram Stories--Everything You Need to Know >>](https://tools.techidaily.com/wondershare/filmora/download/)
[YouTube Shorts vs. TikTok: Which One Is Better? >>](https://tools.techidaily.com/wondershare/filmora/download/)
## IGTV VS YouTube: What's the difference
Comparison between IGTV and YouTube is somewhat unfair towards the former since IGTV is a much younger platform that still needs time to develop. YouTube, on the other hand, has a reputation as one of the most reliable video sharing platforms ever created that enables people from all walks of life to upload and share music, movies, gaming videos and virtually any other type of moving images. Even so, let’s take a look at major differences and similarities between IGTV and YouTube.
#### Have a Quick Glance of the Differences
01 [Interface Differences](#part1)
02 [The Upload Process](#part2)
03 [Discovering New Content](#part3)
03 [Monetization](#part4)
03 [Analytics and Insights](#part5)
#### 1. Interface Differences
Despite the fact that you can access IGTV from a web browser, this platform is predominantly dedicated to videos that are going to be watched on mobile devices. This also means that the thumbnails of the videos you uploaded to your IGTV channel are going to be displayed differently than those on your YouTube channel. The interfaces of these two social networks are entirely different, but if you are a newcomer to each of these platforms you won’t have much trouble getting used to them.
#### 2. The Upload Process
The fact that you can only upload vertically oriented videos to IGTV somewhat limits your options because you either have to record a video for IGTV specifically or you must edit a horizontally oriented video and change its aspect ratio to 9:16\. You can upload videos to YouTube and IGTV directly from your computer or you can use iPhone and Android-based apps to capture videos with your phone and upload them to either of these platforms. The maximum duration of an IGTV video can’t exceed sixty minutes, while the default duration of all videos on YouTube is set to 15 minutes. Nonetheless, if you own a verified YouTube account, you can upload videos that have 128GB and last up to 12 hours.
At the moment the videos you want to upload to your IGTV channel have to be saved in the MP4 file format because the platform still doesn’t offer support for any other video file format. YouTube grants much more freedom in this respect as it allows you to upload videos saved in AVI, FLV, MOV, MP4 and other commonly used file formats.
#### 3. Discovering New Content
IGTV is more than a decade younger than YouTube, so you shouldn’t be surprised if you’re having trouble discovering new content. Moreover, IGTV’s search engine is still relatively new and it is much less powerful than the one used by YouTube. This is the reason why the videos you upload to your YouTube channel are going to be much easier to find via a simple Google search than those you shared on IGTV. Besides, YouTube offers much more new content on a daily basis than IGTV, and it is clear that the Instagram’s new brainchild still has ways to go before it reaches the point when it can challenge the largest video sharing platform in the world in terms of diversity of the content that it delivers to its users.
#### 4. Monetization
At the moment, views and likes can’t be monetized on IGTV, so most influencers rely on sponsorships from brands. Things may change as the platform grows, but for the time being IGTV doesn’t provide the content creators with any compensation for the work they are putting in. YouTube allows content creators to monetize their videos through Google Adsense for years, and some of its most prominent users like PewDiePie or Logan Paul have earned hundreds of millions of dollars by posting new content on their YouTube channels. Keep in mind that you are going to need at least a thousand subscribers and 4,000 hours of viewing time before you can enable YouTube’s Monetize feature.
#### 5. Analytics and Insights
Knowing how many people watched the entire video or how many likes and comments certain types of videos get can help you produce content that attracts a larger audience on all social networks. The metrics such as engagement or view-through rates can be essential during online marketing campaigns as they can suggest how many people are watching your videos and for how long. The analytics tools YouTube provides are much more diverse than those offered by IGTV that only lets you see the audience retention graph and the number of views and comments a video currently has. YouTube is an obviously a much better option for anyone who wants to conduct an in-depth analysis of their content’s performance, although the tools on IGTV are still powerful enough to indicate which type of content your audience likes the most.
## Why Shouldn’t You Use YouTube and IGTV For the Same Purposes?
You shouldn’t assume that you can post the same type of videos on your YouTube and IGTV channels, just because they are both video sharing platforms. The content you create for your IGTV channel needs to be dynamic and entertaining in order to captivate the audience’s attention. Posting tutorials or hour-long gaming videos on IGTV won’t attract as many viewers as on YouTube, which is why businesses who want to use IGTV to promote their products and services need to choose the content carefully. Furthermore, the videos that last between two and five minutes are still by far the most popular on IGTV, so even though technically you can upload a video that lasts one hour to your IGTV channel, the chances are that such long videos won’t get a lot of views.
IGTV is probably best used as a tool that can help improve the engagement with your followers on Instagram, but if you want to become an established video content creator, then running a YouTube channel is simply a much better option. It still remains to be seen how will IGTV evolve in the years to come, but at the present moment this platform doesn’t really have any chance of challenging YouTube’s position as the world’s leading video sharing platform.
However, no matter which video platform you choose, you need to ensure the videos you create are of high quality. A good video editing software like Filmora can help you acheive this goal.
**Useful Features of Filmora**
* Cokor Matching: Apply color correction setting to multiple clips on one-click.
* Effects: Create blockbuster videos with preset effects.
* Motion Tracking: Track moving items and add elements to it at ease.
* And more waiting for you to discover.
[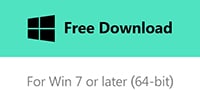](https://tools.techidaily.com/wondershare/filmora/download/)[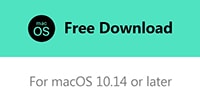](https://tools.techidaily.com/wondershare/filmora/download/)
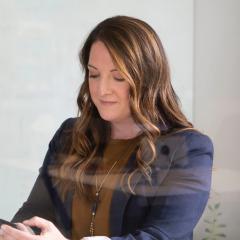
Shanoon Cox
Shanoon Cox is a writer and a lover of all things video.
Follow @Shanoon Cox
##### Shanoon Cox
Mar 27, 2024• Proven solutions
IGTV receives quite a lot of attention from marketers and content creators alike. Some even go so far to compare **IGTV to YouTube**, currently, the largest video sharing platform in the world that hosts 300 minutes of video each minute. IGTV may have fewer users than YouTube, but that doesn’t mean you can’t find a way to use it effectively for a wide array of different purposes. So, in this article, we are going to take a look at the advantages and disadvantages of each platform in order to help you discover how you can utilize each of these social networks to broaden the audience for the content you share online.
**You May Also Like:**
[Instagram Reels VS Instagram Stories--Everything You Need to Know >>](https://tools.techidaily.com/wondershare/filmora/download/)
[YouTube Shorts vs. TikTok: Which One Is Better? >>](https://tools.techidaily.com/wondershare/filmora/download/)
## IGTV VS YouTube: What's the difference
Comparison between IGTV and YouTube is somewhat unfair towards the former since IGTV is a much younger platform that still needs time to develop. YouTube, on the other hand, has a reputation as one of the most reliable video sharing platforms ever created that enables people from all walks of life to upload and share music, movies, gaming videos and virtually any other type of moving images. Even so, let’s take a look at major differences and similarities between IGTV and YouTube.
#### Have a Quick Glance of the Differences
01 [Interface Differences](#part1)
02 [The Upload Process](#part2)
03 [Discovering New Content](#part3)
03 [Monetization](#part4)
03 [Analytics and Insights](#part5)
#### 1. Interface Differences
Despite the fact that you can access IGTV from a web browser, this platform is predominantly dedicated to videos that are going to be watched on mobile devices. This also means that the thumbnails of the videos you uploaded to your IGTV channel are going to be displayed differently than those on your YouTube channel. The interfaces of these two social networks are entirely different, but if you are a newcomer to each of these platforms you won’t have much trouble getting used to them.
#### 2. The Upload Process
The fact that you can only upload vertically oriented videos to IGTV somewhat limits your options because you either have to record a video for IGTV specifically or you must edit a horizontally oriented video and change its aspect ratio to 9:16\. You can upload videos to YouTube and IGTV directly from your computer or you can use iPhone and Android-based apps to capture videos with your phone and upload them to either of these platforms. The maximum duration of an IGTV video can’t exceed sixty minutes, while the default duration of all videos on YouTube is set to 15 minutes. Nonetheless, if you own a verified YouTube account, you can upload videos that have 128GB and last up to 12 hours.
At the moment the videos you want to upload to your IGTV channel have to be saved in the MP4 file format because the platform still doesn’t offer support for any other video file format. YouTube grants much more freedom in this respect as it allows you to upload videos saved in AVI, FLV, MOV, MP4 and other commonly used file formats.
#### 3. Discovering New Content
IGTV is more than a decade younger than YouTube, so you shouldn’t be surprised if you’re having trouble discovering new content. Moreover, IGTV’s search engine is still relatively new and it is much less powerful than the one used by YouTube. This is the reason why the videos you upload to your YouTube channel are going to be much easier to find via a simple Google search than those you shared on IGTV. Besides, YouTube offers much more new content on a daily basis than IGTV, and it is clear that the Instagram’s new brainchild still has ways to go before it reaches the point when it can challenge the largest video sharing platform in the world in terms of diversity of the content that it delivers to its users.
#### 4. Monetization
At the moment, views and likes can’t be monetized on IGTV, so most influencers rely on sponsorships from brands. Things may change as the platform grows, but for the time being IGTV doesn’t provide the content creators with any compensation for the work they are putting in. YouTube allows content creators to monetize their videos through Google Adsense for years, and some of its most prominent users like PewDiePie or Logan Paul have earned hundreds of millions of dollars by posting new content on their YouTube channels. Keep in mind that you are going to need at least a thousand subscribers and 4,000 hours of viewing time before you can enable YouTube’s Monetize feature.
#### 5. Analytics and Insights
Knowing how many people watched the entire video or how many likes and comments certain types of videos get can help you produce content that attracts a larger audience on all social networks. The metrics such as engagement or view-through rates can be essential during online marketing campaigns as they can suggest how many people are watching your videos and for how long. The analytics tools YouTube provides are much more diverse than those offered by IGTV that only lets you see the audience retention graph and the number of views and comments a video currently has. YouTube is an obviously a much better option for anyone who wants to conduct an in-depth analysis of their content’s performance, although the tools on IGTV are still powerful enough to indicate which type of content your audience likes the most.
## Why Shouldn’t You Use YouTube and IGTV For the Same Purposes?
You shouldn’t assume that you can post the same type of videos on your YouTube and IGTV channels, just because they are both video sharing platforms. The content you create for your IGTV channel needs to be dynamic and entertaining in order to captivate the audience’s attention. Posting tutorials or hour-long gaming videos on IGTV won’t attract as many viewers as on YouTube, which is why businesses who want to use IGTV to promote their products and services need to choose the content carefully. Furthermore, the videos that last between two and five minutes are still by far the most popular on IGTV, so even though technically you can upload a video that lasts one hour to your IGTV channel, the chances are that such long videos won’t get a lot of views.
IGTV is probably best used as a tool that can help improve the engagement with your followers on Instagram, but if you want to become an established video content creator, then running a YouTube channel is simply a much better option. It still remains to be seen how will IGTV evolve in the years to come, but at the present moment this platform doesn’t really have any chance of challenging YouTube’s position as the world’s leading video sharing platform.
However, no matter which video platform you choose, you need to ensure the videos you create are of high quality. A good video editing software like Filmora can help you acheive this goal.
**Useful Features of Filmora**
* Cokor Matching: Apply color correction setting to multiple clips on one-click.
* Effects: Create blockbuster videos with preset effects.
* Motion Tracking: Track moving items and add elements to it at ease.
* And more waiting for you to discover.
[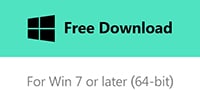](https://tools.techidaily.com/wondershare/filmora/download/)[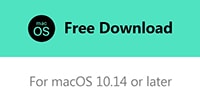](https://tools.techidaily.com/wondershare/filmora/download/)
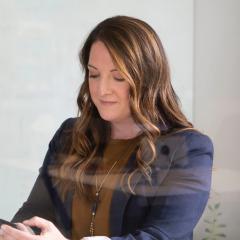
Shanoon Cox
Shanoon Cox is a writer and a lover of all things video.
Follow @Shanoon Cox
##### Shanoon Cox
Mar 27, 2024• Proven solutions
IGTV receives quite a lot of attention from marketers and content creators alike. Some even go so far to compare **IGTV to YouTube**, currently, the largest video sharing platform in the world that hosts 300 minutes of video each minute. IGTV may have fewer users than YouTube, but that doesn’t mean you can’t find a way to use it effectively for a wide array of different purposes. So, in this article, we are going to take a look at the advantages and disadvantages of each platform in order to help you discover how you can utilize each of these social networks to broaden the audience for the content you share online.
**You May Also Like:**
[Instagram Reels VS Instagram Stories--Everything You Need to Know >>](https://tools.techidaily.com/wondershare/filmora/download/)
[YouTube Shorts vs. TikTok: Which One Is Better? >>](https://tools.techidaily.com/wondershare/filmora/download/)
## IGTV VS YouTube: What's the difference
Comparison between IGTV and YouTube is somewhat unfair towards the former since IGTV is a much younger platform that still needs time to develop. YouTube, on the other hand, has a reputation as one of the most reliable video sharing platforms ever created that enables people from all walks of life to upload and share music, movies, gaming videos and virtually any other type of moving images. Even so, let’s take a look at major differences and similarities between IGTV and YouTube.
#### Have a Quick Glance of the Differences
01 [Interface Differences](#part1)
02 [The Upload Process](#part2)
03 [Discovering New Content](#part3)
03 [Monetization](#part4)
03 [Analytics and Insights](#part5)
#### 1. Interface Differences
Despite the fact that you can access IGTV from a web browser, this platform is predominantly dedicated to videos that are going to be watched on mobile devices. This also means that the thumbnails of the videos you uploaded to your IGTV channel are going to be displayed differently than those on your YouTube channel. The interfaces of these two social networks are entirely different, but if you are a newcomer to each of these platforms you won’t have much trouble getting used to them.
#### 2. The Upload Process
The fact that you can only upload vertically oriented videos to IGTV somewhat limits your options because you either have to record a video for IGTV specifically or you must edit a horizontally oriented video and change its aspect ratio to 9:16\. You can upload videos to YouTube and IGTV directly from your computer or you can use iPhone and Android-based apps to capture videos with your phone and upload them to either of these platforms. The maximum duration of an IGTV video can’t exceed sixty minutes, while the default duration of all videos on YouTube is set to 15 minutes. Nonetheless, if you own a verified YouTube account, you can upload videos that have 128GB and last up to 12 hours.
At the moment the videos you want to upload to your IGTV channel have to be saved in the MP4 file format because the platform still doesn’t offer support for any other video file format. YouTube grants much more freedom in this respect as it allows you to upload videos saved in AVI, FLV, MOV, MP4 and other commonly used file formats.
#### 3. Discovering New Content
IGTV is more than a decade younger than YouTube, so you shouldn’t be surprised if you’re having trouble discovering new content. Moreover, IGTV’s search engine is still relatively new and it is much less powerful than the one used by YouTube. This is the reason why the videos you upload to your YouTube channel are going to be much easier to find via a simple Google search than those you shared on IGTV. Besides, YouTube offers much more new content on a daily basis than IGTV, and it is clear that the Instagram’s new brainchild still has ways to go before it reaches the point when it can challenge the largest video sharing platform in the world in terms of diversity of the content that it delivers to its users.
#### 4. Monetization
At the moment, views and likes can’t be monetized on IGTV, so most influencers rely on sponsorships from brands. Things may change as the platform grows, but for the time being IGTV doesn’t provide the content creators with any compensation for the work they are putting in. YouTube allows content creators to monetize their videos through Google Adsense for years, and some of its most prominent users like PewDiePie or Logan Paul have earned hundreds of millions of dollars by posting new content on their YouTube channels. Keep in mind that you are going to need at least a thousand subscribers and 4,000 hours of viewing time before you can enable YouTube’s Monetize feature.
#### 5. Analytics and Insights
Knowing how many people watched the entire video or how many likes and comments certain types of videos get can help you produce content that attracts a larger audience on all social networks. The metrics such as engagement or view-through rates can be essential during online marketing campaigns as they can suggest how many people are watching your videos and for how long. The analytics tools YouTube provides are much more diverse than those offered by IGTV that only lets you see the audience retention graph and the number of views and comments a video currently has. YouTube is an obviously a much better option for anyone who wants to conduct an in-depth analysis of their content’s performance, although the tools on IGTV are still powerful enough to indicate which type of content your audience likes the most.
## Why Shouldn’t You Use YouTube and IGTV For the Same Purposes?
You shouldn’t assume that you can post the same type of videos on your YouTube and IGTV channels, just because they are both video sharing platforms. The content you create for your IGTV channel needs to be dynamic and entertaining in order to captivate the audience’s attention. Posting tutorials or hour-long gaming videos on IGTV won’t attract as many viewers as on YouTube, which is why businesses who want to use IGTV to promote their products and services need to choose the content carefully. Furthermore, the videos that last between two and five minutes are still by far the most popular on IGTV, so even though technically you can upload a video that lasts one hour to your IGTV channel, the chances are that such long videos won’t get a lot of views.
IGTV is probably best used as a tool that can help improve the engagement with your followers on Instagram, but if you want to become an established video content creator, then running a YouTube channel is simply a much better option. It still remains to be seen how will IGTV evolve in the years to come, but at the present moment this platform doesn’t really have any chance of challenging YouTube’s position as the world’s leading video sharing platform.
However, no matter which video platform you choose, you need to ensure the videos you create are of high quality. A good video editing software like Filmora can help you acheive this goal.
**Useful Features of Filmora**
* Cokor Matching: Apply color correction setting to multiple clips on one-click.
* Effects: Create blockbuster videos with preset effects.
* Motion Tracking: Track moving items and add elements to it at ease.
* And more waiting for you to discover.
[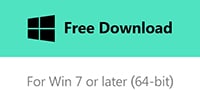](https://tools.techidaily.com/wondershare/filmora/download/)[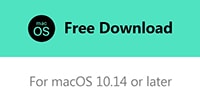](https://tools.techidaily.com/wondershare/filmora/download/)
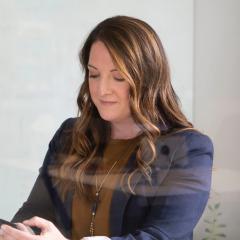
Shanoon Cox
Shanoon Cox is a writer and a lover of all things video.
Follow @Shanoon Cox
<ins class="adsbygoogle"
style="display:block"
data-ad-format="autorelaxed"
data-ad-client="ca-pub-7571918770474297"
data-ad-slot="1223367746"></ins>
<ins class="adsbygoogle"
style="display:block"
data-ad-client="ca-pub-7571918770474297"
data-ad-slot="8358498916"
data-ad-format="auto"
data-full-width-responsive="true"></ins>
<span class="atpl-alsoreadstyle">Also read:</span>
<div><ul>
<li><a href="https://facebook-video-share.techidaily.com/shegames-supreme-the-10-best-female-youtubers-for-2024/"><u>SheGames Supreme The 10 Best Female YouTubers for 2024</u></a></li>
<li><a href="https://facebook-video-share.techidaily.com/new-2024-approved-enhancing-youtube-visibility-with-tubebuddy/"><u>[New] 2024 Approved Enhancing YouTube Visibility with TubeBuddy</u></a></li>
<li><a href="https://facebook-video-share.techidaily.com/updated-iosplusandroid-tips-for-uninterrupted-youtube-viewing/"><u>[Updated] IOS+Android Tips for Uninterrupted YouTube Viewing</u></a></li>
<li><a href="https://facebook-video-share.techidaily.com/new-2024-approved-essential-insights-on-asmr-videos-unveiled/"><u>[New] 2024 Approved Essential Insights on ASMR Videos Unveiled</u></a></li>
<li><a href="https://facebook-video-share.techidaily.com/updated-keyword-mastery-the-10-best-online-resources-to-increase-views/"><u>[Updated] Keyword Mastery The 10 Best Online Resources to Increase Views</u></a></li>
<li><a href="https://facebook-video-share.techidaily.com/updated-break-through-the-crowd-key-youtube-seo-strategies-to-rank-high-for-2024/"><u>[Updated] Break Through the Crowd Key YouTube SEO Strategies to Rank High for 2024</u></a></li>
<li><a href="https://facebook-video-share.techidaily.com/new-culinary-craftsmanship-innovative-naming-for-food-shows-for-2024/"><u>[New] Culinary Craftsmanship Innovative Naming for Food Shows for 2024</u></a></li>
<li><a href="https://screen-mirror.techidaily.com/in-2024-best-3-xiaomi-redmi-12-emulator-for-mac-to-run-your-wanted-android-apps-drfone-by-drfone-android/"><u>In 2024, Best 3 Xiaomi Redmi 12 Emulator for Mac to Run Your Wanted Android Apps | Dr.fone</u></a></li>
<li><a href="https://facebook-video-content.techidaily.com/in-2024-12-facebook-marketing-tips-for-business-beginners-and-pros/"><u>In 2024, 12 Facebook Marketing Tips for Business (Beginners and Pros)</u></a></li>
<li><a href="https://extra-skills.techidaily.com/updated-photo-perfection-seamless-text-integration-on-pc-and-mac-systems/"><u>[Updated] Photo Perfection Seamless Text Integration on PC & Mac Systems</u></a></li>
<li><a href="https://android-unlock.techidaily.com/in-2024-still-using-pattern-locks-with-vivo-s17t-tips-tricks-and-helpful-advice-by-drfone-android/"><u>In 2024, Still Using Pattern Locks with Vivo S17t? Tips, Tricks and Helpful Advice</u></a></li>
<li><a href="https://extra-lessons.techidaily.com/perfect-your-videos-captions-with-10plus-top-free-converters/"><u>Perfect Your Videos' Captions with 10+ Top FREE Converters</u></a></li>
<li><a href="https://extra-support.techidaily.com/2024-approved-mastering-the-art-of-live-cricket-livestreams/"><u>2024 Approved Mastering the Art of Live Cricket Livestreams</u></a></li>
<li><a href="https://ios-unlock.techidaily.com/forgot-iphone-passcode-again-unlock-iphone-11-without-passcode-now-by-drfone-ios/"><u>Forgot iPhone Passcode Again? Unlock iPhone 11 Without Passcode Now</u></a></li>
<li><a href="https://phone-solutions.techidaily.com/best-android-data-recovery-undelete-lost-call-logs-from-realme-c67-5g-by-fonelab-android-recover-call-logs/"><u>Best Android Data Recovery - undelete lost call logs from Realme C67 5G</u></a></li>
<li><a href="https://remote-screen-capture.techidaily.com/updated-complete-guide-to-recording-live-tv-on-your-windows-pc-for-2024/"><u>[Updated] Complete Guide to Recording Live TV on Your Windows PC for 2024</u></a></li>
</ul></div> |
init();
function init() {
const $inputs = document.querySelectorAll(".validate-target");
// クラス名にvalidate-targetがついているもの全てをAllで見つけてinputsに格納する
for (const $input of $inputs) {
// inputsの中からinputを取り出しfor文で処理を行う
$input.addEventListener("input", function (event) {
const $target = event.currentTarget;
console.log($target.validity);
if ($target.checkValidity()) {
$target.classList.add("is-valid");
$target.classList.remove("is-invalid");
} else {
$target.classList.add("is-invalid");
$target.classList.remove("is-valid");
if ($target.validity.valueMissing) {
console.log("値の入力が必須です。");
} else if ($target.validity.tooShort) {
console.log(
$target.minLength +
"文字以上で入力してください。現在の文字数は" +
$target.value.length +
"文字です。"
);
} else if ($target.validity.tooLong) {
console.log(
$target.maxLength +
"文字以下で入力してください。現在の文字数は" +
$target.value.length +
"文字です。"
);
} else if ($target.validity.patternMismatch) {
console.log("半角英数字で入力してください。");
}
}
});
}
} |
/*
***** BEGIN LICENSE BLOCK *****
Copyright © 2020 Corporation for Digital Scholarship
Vienna, Virginia, USA
https://www.zotero.org
This file is part of Zotero.
Zotero is free software: you can redistribute it and/or modify
it under the terms of the GNU Affero General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
Zotero is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Affero General Public License for more details.
You should have received a copy of the GNU Affero General Public License
along with Zotero. If not, see <http://www.gnu.org/licenses/>.
***** END LICENSE BLOCK *****
*/
"use strict";
import { getCSSIcon } from 'components/icons';
{
class LibrariesCollectionsBox extends XULElementBase {
content = MozXULElement.parseXULToFragment(`
<collapsible-section data-l10n-id="section-libraries-collections" data-pane="libraries-collections" extra-buttons="add">
<html:div class="body"/>
</collapsible-section>
<popupset>
<menupopup class="add-popup" onpopupshowing="ZoteroPane_Local.buildAddToCollectionMenu(event)">
<menuitem label="&zotero.toolbar.newCollection.label;" oncommand="ZoteroPane_Local.addSelectedItemsToCollection(null, true)"/>
<menuseparator/>
</menupopup>
</popupset>
`, ['chrome://zotero/locale/zotero.dtd']);
_item = null;
_linkedItems = [];
_mode = null;
get item() {
return this._item;
}
set item(item) {
if (item?.isRegularItem()) {
this.hidden = false;
}
else {
this.hidden = true;
return;
}
this._item = item;
// Getting linked items is an async process, so start by rendering without them
this._linkedItems = [];
this.render();
this._updateLinkedItems();
}
get mode() {
return this._mode;
}
set mode(mode) {
this._mode = mode;
this.setAttribute('mode', mode);
this.render();
}
init() {
this._notifierID = Zotero.Notifier.registerObserver(this, ['item'], 'librariesCollectionsBox');
this._body = this.querySelector('.body');
this._section = this.querySelector('collapsible-section');
this._section.addEventListener('add', (event) => {
this.querySelector('.add-popup').openPopupAtScreen(
event.detail.button.screenX,
event.detail.button.screenY,
true
);
this._section.open = true;
});
this.render();
}
destroy() {
Zotero.Notifier.unregisterObserver(this._notifierID);
}
notify(action, type, ids) {
if (action == 'modify'
&& this._item
&& (ids.includes(this._item.id) || this._linkedItems.some(item => ids.includes(item.id)))) {
this.render();
}
}
_buildRow(obj, level, contextItem) {
let isContext = obj instanceof Zotero.Collection && !contextItem.inCollection(obj.id);
let row = document.createElement('div');
row.classList.add('row');
row.dataset.id = obj.treeViewID;
row.dataset.level = level;
row.style.setProperty('--level', level);
row.classList.toggle('context', isContext);
let box = document.createElement('div');
box.className = 'box keyboard_clickable';
box.setAttribute("tabindex", "0");
let iconName;
if (obj instanceof Zotero.Group) {
iconName = 'library-group';
}
else if (obj instanceof Zotero.Library) {
iconName = 'library';
}
else {
iconName = 'collection';
}
let icon = getCSSIcon(iconName);
box.append(icon);
let text = document.createElement('span');
text.classList.add('label');
text.textContent = obj.name;
box.append(text);
row.append(box);
if (this._mode == 'edit' && obj instanceof Zotero.Collection && !isContext) {
let remove = document.createXULElement('toolbarbutton');
remove.className = 'zotero-clicky zotero-clicky-minus';
remove.setAttribute("tabindex", "0");
remove.addEventListener('command', () => {
if (Services.prompt.confirm(
window,
Zotero.getString('pane.items.remove.title'),
Zotero.getString('pane.items.removeFromOther', [obj.name])
)) {
contextItem.removeFromCollection(obj.id);
contextItem.saveTx();
}
});
row.append(remove);
}
let isCurrent = ZoteroPane.collectionsView.selectedTreeRow?.id == obj.treeViewID;
box.classList.toggle('current', isCurrent);
// Disable clicky if this is a context row or we're already in the library/collection it points to
let disableClicky = isContext || isCurrent;
box.toggleAttribute('disabled', disableClicky);
if (!disableClicky) {
box.addEventListener('click', async () => {
await ZoteroPane.collectionsView.selectByID(obj.treeViewID);
await ZoteroPane.selectItem(contextItem.id);
});
}
return row;
}
_findRow(obj) {
return this._body.querySelector(`.row[data-id="${obj.treeViewID}"]`);
}
_getChildren(row = null, deep = false) {
let rows = Array.from(this._body.querySelectorAll('.row'));
let startIndex = row ? rows.indexOf(row) + 1 : 0;
let level = row ? parseInt(row.dataset.level) + 1 : 0;
let children = [];
for (let i = startIndex; i < rows.length; i++) {
let childLevel = parseInt(rows[i].dataset.level);
if (childLevel == level || deep && childLevel > level) {
children.push(rows[i]);
}
else if (childLevel < level) {
break;
}
}
return children;
}
_addObject(obj, contextItem) {
let existingRow = this._findRow(obj);
if (existingRow) {
return existingRow;
}
let parent = obj instanceof Zotero.Library
? null
: (obj.parentID ? Zotero.Collections.get(obj.parentID) : Zotero.Libraries.get(obj.libraryID));
let parentRow = parent && this._findRow(parent);
if (parent && !parentRow) {
parentRow = this._addObject(parent, contextItem);
}
let row = this._buildRow(obj, parentRow ? parseInt(parentRow.dataset.level) + 1 : 0, contextItem);
let siblings = this._getChildren(parentRow);
let added = false;
for (let sibling of siblings) {
if (Zotero.localeCompare(sibling.querySelector('.label').textContent, obj.name) > 0) {
sibling.before(row);
added = true;
break;
}
}
if (!added) {
if (siblings.length) {
let lastSibling = siblings[siblings.length - 1];
let childrenOfLastSibling = this._getChildren(lastSibling, true);
if (childrenOfLastSibling.length) {
childrenOfLastSibling[childrenOfLastSibling.length - 1].after(row);
}
else {
lastSibling.after(row);
}
}
else if (parentRow) {
parentRow.after(row);
}
else {
this._body.append(row);
}
}
return row;
}
async _updateLinkedItems() {
this._linkedItems = (await Promise.all(Zotero.Libraries.getAll()
.filter(lib => lib.libraryID !== this._item.libraryID)
.map(lib => this._item.getLinkedItem(lib.libraryID, true))))
.filter(Boolean);
this.render();
}
render() {
if (!this._item) {
return;
}
this._body.replaceChildren();
for (let item of [this._item, ...this._linkedItems]) {
this._addObject(Zotero.Libraries.get(item.libraryID), item);
for (let collection of Zotero.Collections.get(item.getCollections())) {
this._addObject(collection, item);
}
}
}
}
customElements.define("libraries-collections-box", LibrariesCollectionsBox);
} |
import { AbstractControl, ValidationErrors, ValidatorFn } from '@angular/forms';
export function soloTexto(): ValidatorFn {
return (control: AbstractControl): ValidationErrors | null => {
const textoIngresado: string = control.value || '';
const regex = /^[a-zA-Z\s]*$/;
if (!textoIngresado.match(regex)) {
return { 'soloTexto': { message: 'Este campo solo permite texto.' } };
}
return null;
};
}
export function validarCorreo(): ValidatorFn {
return (control: AbstractControl): { [key: string]: any } | null => {
const correoIngresado: string = control.value;
const regex = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/;
if (!correoIngresado.match(regex)) {
return { 'correoInvalido': true };
}
return null;
};
}
export function validarDecimalConDosDecimales(): ValidatorFn {
return (control: AbstractControl): { [key: string]: any } | null => {
const valorIngresado: string = control.value;
if (!valorIngresado) {
return null;
}
const regex = /^\d+/;
if (regex.test(valorIngresado)) {
return { 'decimalInvalido': true };
}
return null;
};
}
export function cantidadMayorQueCero(): ValidatorFn {
return (control: AbstractControl): ValidationErrors | null => {
const cantidad = control.value;
if (cantidad !== null && cantidad <= 0) {
return { 'cantidadInvalida': true };
}
return null;
};
} |
import { camelToSnakeCase } from '@helpers/string';
import knex from 'knex';
import {
DynamicMigrationConfig, DynamicMigrationBuilderConfig, DynamicMigration
} from './types';
// eslint-disable-next-line @typescript-eslint/explicit-module-boundary-types
const buildMakeDynamicMigration = ({ client, defaults, }: DynamicMigrationBuilderConfig) => {
const qb = knex({
client,
log: {
warn() {
//
},
},
});
const float = {
precision: 8,
scale: 2,
};
const decimal = {
precision: 12,
scale: 2,
};
if (defaults) {
if (defaults.float) {
float.precision = defaults.float.precision;
if (defaults.float.scale)
float.scale = defaults.float.scale;
}
if (defaults.decimal) {
decimal.precision = defaults.decimal.precision;
if (defaults.decimal.scale)
decimal.scale = defaults.decimal.scale;
}
}
return <S> ({
table: {
name,
schema,
columns,
constraints,
},
}: DynamicMigrationConfig<S>): DynamicMigration<S> => {
const up = qb.schema.withSchema(schema).createTableIfNotExists(name, (t) => {
for (const col in columns) {
let tmp: any;
const val = columns[col];
const name = camelToSnakeCase(col);
switch (val.type) {
case 'string':
if (val.max)
tmp = t.string(name, val.max);
else
tmp = t.text(name);
break;
case 'uuid':
tmp = t.uuid(name);
break;
case 'email':
tmp = t.string(name, 256);
break;
case 'uri':
tmp = t.string(name, val.max);
break;
case 'serial':
tmp = t.increments(name, { primaryKey: false, });
break;
case 'int':
tmp = t.integer(name);
break;
case 'float':
if (val.precision && val.scale) {
tmp = t.float(name, val.precision, val.scale);
} else if (val.precision) {
tmp = t.float(name, val.precision, float.scale);
} else {
tmp = t.float(name, float.precision, float.scale);
}
break;
case 'decimal':
if (val.precision && val.scale) {
tmp = t.decimal(name, val.precision, val.scale);
} else if (val.precision) {
tmp = t.decimal(name, val.precision, decimal.scale);
} else {
tmp = t.decimal(name, decimal.precision, decimal.scale);
}
break;
case 'boolean':
tmp = t.boolean(name);
break;
case 'date':
tmp = t.date(name);
break;
case 'time':
tmp = t.time(name);
break;
case 'timestamp':
tmp = t.timestamp(name, { useTz: true, });
break;
case 'array':
if (val.data !== 'string')
continue;
tmp = t.string(name);
break;
case 'binary':
tmp = t.binary(name);
break;
default:
throw new Error(`Invalid type: ${(val as any).type}`);
}
if (!val.nullable) {
tmp = tmp.notNullable();
}
if (val.default) {
if (val.default === true && val.type === 'uuid') {
tmp = tmp.default(qb.raw('uuid_generate_v4()'));
} else if (val.default === 'now()' && val.type === 'timestamp') {
tmp = tmp.default(qb.raw('now()'));
} else {
tmp = tmp.default(val.default);
}
}
// Always last
if (val.references) {
const {
col, table, name: n, del,
} = val.references;
tmp = t.foreign(name, n).references(col).inTable(table);
if (del) {
tmp = tmp.onDelete('cascade');
}
}
}
const { primary, unique, } = constraints;
if (primary) {
t.primary([ primary.col as string ], { constraintName: primary.name, });
}
if (unique) {
for (const { columns, name, } of unique) {
t.unique((columns as string[]).map((c) => camelToSnakeCase(c)), { indexName: name, });
}
}
}).toQuery() + ';';
const insQb = qb.withSchema(schema).from(name);
const del = qb.withSchema(schema).from(name).del().toQuery() + ';';
const down = qb.schema.withSchema(schema)
.dropTableIfExists(name)
.toQuery() + ';';
return Object.freeze({
makeUp: () => up,
makeIns: (data?: S[]) => {
if (!data) return '';
// TODO: Can be optimized but not really that important
const parsed: { [key: string]: any }[] = [];
for (const d of data) {
const p: { [key: string]: any } = {};
for (const key in d) {
p[camelToSnakeCase(key)] = d[key];
}
parsed.push(p);
}
return insQb.insert(parsed).toQuery() + ';';
},
makeDel: () => del,
makeDown: () => down,
});
};
};
export default buildMakeDynamicMigration; |
/**
* @license Copyright (c) 2003-2023, CKSource Holding sp. z o.o. All rights reserved.
* For licensing, see LICENSE.md or https://ckeditor.com/legal/ckeditor-oss-license
*/
/**
* @module typing/twostepcaretmovement
*/
import { Plugin, type Editor } from '@ckeditor/ckeditor5-core';
import { keyCodes } from '@ckeditor/ckeditor5-utils';
import {
MouseObserver,
type DocumentSelection,
type DocumentSelectionChangeRangeEvent,
type DomEventData,
type Model,
type Position,
type ViewDocumentArrowKeyEvent,
type ViewDocumentMouseDownEvent,
type ViewDocumentSelectionChangeEvent,
type ModelInsertContentEvent,
type ModelDeleteContentEvent
} from '@ckeditor/ckeditor5-engine';
import type { ViewDocumentDeleteEvent } from './deleteobserver';
/**
* This plugin enables the two-step caret (phantom) movement behavior for
* {@link module:typing/twostepcaretmovement~TwoStepCaretMovement#registerAttribute registered attributes}
* on arrow right (<kbd>→</kbd>) and left (<kbd>←</kbd>) key press.
*
* Thanks to this (phantom) caret movement the user is able to type before/after as well as at the
* beginning/end of an attribute.
*
* **Note:** This plugin support right–to–left (Arabic, Hebrew, etc.) content by mirroring its behavior
* but for the sake of simplicity examples showcase only left–to–right use–cases.
*
* # Forward movement
*
* ## "Entering" an attribute:
*
* When this plugin is enabled and registered for the `a` attribute and the selection is right before it
* (at the attribute boundary), pressing the right arrow key will not move the selection but update its
* attributes accordingly:
*
* * When enabled:
*
* ```xml
* foo{}<$text a="true">bar</$text>
* ```
*
* <kbd>→</kbd>
*
* ```xml
* foo<$text a="true">{}bar</$text>
* ```
*
* * When disabled:
*
* ```xml
* foo{}<$text a="true">bar</$text>
* ```
*
* <kbd>→</kbd>
*
* ```xml
* foo<$text a="true">b{}ar</$text>
* ```
*
*
* ## "Leaving" an attribute:
*
* * When enabled:
*
* ```xml
* <$text a="true">bar{}</$text>baz
* ```
*
* <kbd>→</kbd>
*
* ```xml
* <$text a="true">bar</$text>{}baz
* ```
*
* * When disabled:
*
* ```xml
* <$text a="true">bar{}</$text>baz
* ```
*
* <kbd>→</kbd>
*
* ```xml
* <$text a="true">bar</$text>b{}az
* ```
*
* # Backward movement
*
* * When enabled:
*
* ```xml
* <$text a="true">bar</$text>{}baz
* ```
*
* <kbd>←</kbd>
*
* ```xml
* <$text a="true">bar{}</$text>baz
* ```
*
* * When disabled:
*
* ```xml
* <$text a="true">bar</$text>{}baz
* ```
*
* <kbd>←</kbd>
*
* ```xml
* <$text a="true">ba{}r</$text>b{}az
* ```
*
* # Multiple attributes
*
* * When enabled and many attributes starts or ends at the same position:
*
* ```xml
* <$text a="true" b="true">bar</$text>{}baz
* ```
*
* <kbd>←</kbd>
*
* ```xml
* <$text a="true" b="true">bar{}</$text>baz
* ```
*
* * When enabled and one procedes another:
*
* ```xml
* <$text a="true">bar</$text><$text b="true">{}bar</$text>
* ```
*
* <kbd>←</kbd>
*
* ```xml
* <$text a="true">bar{}</$text><$text b="true">bar</$text>
* ```
*
*/
export default class TwoStepCaretMovement extends Plugin {
/**
* A set of attributes to handle.
*/
private attributes: Set<string>;
/**
* The current UID of the overridden gravity, as returned by
* {@link module:engine/model/writer~Writer#overrideSelectionGravity}.
*/
private _overrideUid: string | null;
/**
* A flag indicating that the automatic gravity restoration should not happen upon the next
* gravity restoration.
* {@link module:engine/model/selection~Selection#event:change:range} event.
*/
private _isNextGravityRestorationSkipped = false;
/**
* @inheritDoc
*/
public static get pluginName() {
return 'TwoStepCaretMovement' as const;
}
/**
* @inheritDoc
*/
constructor( editor: Editor ) {
super( editor );
this.attributes = new Set();
this._overrideUid = null;
}
/**
* @inheritDoc
*/
public init(): void {
const editor = this.editor;
const model = editor.model;
const view = editor.editing.view;
const locale = editor.locale;
const modelSelection = model.document.selection;
// Listen to keyboard events and handle the caret movement according to the 2-step caret logic.
this.listenTo<ViewDocumentArrowKeyEvent>( view.document, 'arrowKey', ( evt, data ) => {
// This implementation works only for collapsed selection.
if ( !modelSelection.isCollapsed ) {
return;
}
// When user tries to expand the selection or jump over the whole word or to the beginning/end then
// two-steps movement is not necessary.
if ( data.shiftKey || data.altKey || data.ctrlKey ) {
return;
}
const arrowRightPressed = data.keyCode == keyCodes.arrowright;
const arrowLeftPressed = data.keyCode == keyCodes.arrowleft;
// When neither left or right arrow has been pressed then do noting.
if ( !arrowRightPressed && !arrowLeftPressed ) {
return;
}
const contentDirection = locale.contentLanguageDirection;
let isMovementHandled = false;
if ( ( contentDirection === 'ltr' && arrowRightPressed ) || ( contentDirection === 'rtl' && arrowLeftPressed ) ) {
isMovementHandled = this._handleForwardMovement( data );
} else {
isMovementHandled = this._handleBackwardMovement( data );
}
// Stop the keydown event if the two-step caret movement handled it. Avoid collisions
// with other features which may also take over the caret movement (e.g. Widget).
if ( isMovementHandled === true ) {
evt.stop();
}
}, { context: '$text', priority: 'highest' } );
// The automatic gravity restoration logic.
this.listenTo<DocumentSelectionChangeRangeEvent>( modelSelection, 'change:range', ( evt, data ) => {
// Skipping the automatic restoration is needed if the selection should change
// but the gravity must remain overridden afterwards. See the #handleBackwardMovement
// to learn more.
if ( this._isNextGravityRestorationSkipped ) {
this._isNextGravityRestorationSkipped = false;
return;
}
// Skip automatic restore when the gravity is not overridden — simply, there's nothing to restore
// at this moment.
if ( !this._isGravityOverridden ) {
return;
}
// Skip automatic restore when the change is indirect AND the selection is at the attribute boundary.
// It means that e.g. if the change was external (collaboration) and the user had their
// selection around the link, its gravity should remain intact in this change:range event.
if ( !data.directChange && isBetweenDifferentAttributes( modelSelection.getFirstPosition()!, this.attributes ) ) {
return;
}
this._restoreGravity();
} );
// Handle a click at the beginning/end of a two-step element.
this._enableClickingAfterNode();
// Change the attributes of the selection in certain situations after the two-step node was inserted into the document.
this._enableInsertContentSelectionAttributesFixer();
// Handle removing the content after the two-step node.
this._handleDeleteContentAfterNode();
}
/**
* Registers a given attribute for the two-step caret movement.
*
* @param attribute Name of the attribute to handle.
*/
public registerAttribute( attribute: string ): void {
this.attributes.add( attribute );
}
/**
* Updates the document selection and the view according to the two–step caret movement state
* when moving **forwards**. Executed upon `keypress` in the {@link module:engine/view/view~View}.
*
* @param data Data of the key press.
* @returns `true` when the handler prevented caret movement.
*/
private _handleForwardMovement( data: DomEventData ): boolean {
const attributes = this.attributes;
const model = this.editor.model;
const selection = model.document.selection;
const position = selection.getFirstPosition()!;
// DON'T ENGAGE 2-SCM if gravity is already overridden. It means that we just entered
//
// <paragraph>foo<$text attribute>{}bar</$text>baz</paragraph>
//
// or left the attribute
//
// <paragraph>foo<$text attribute>bar</$text>{}baz</paragraph>
//
// and the gravity will be restored automatically.
if ( this._isGravityOverridden ) {
return false;
}
// DON'T ENGAGE 2-SCM when the selection is at the beginning of the block AND already has the
// attribute:
// * when the selection was initially set there using the mouse,
// * when the editor has just started
//
// <paragraph><$text attribute>{}bar</$text>baz</paragraph>
//
if ( position.isAtStart && hasAnyAttribute( selection, attributes ) ) {
return false;
}
// ENGAGE 2-SCM When at least one of the observed attributes changes its value (incl. starts, ends).
//
// <paragraph>foo<$text attribute>bar{}</$text>baz</paragraph>
// <paragraph>foo<$text attribute>bar{}</$text><$text otherAttribute>baz</$text></paragraph>
// <paragraph>foo<$text attribute=1>bar{}</$text><$text attribute=2>baz</$text></paragraph>
// <paragraph>foo{}<$text attribute>bar</$text>baz</paragraph>
//
if ( isBetweenDifferentAttributes( position, attributes ) ) {
preventCaretMovement( data );
// CLEAR 2-SCM attributes if we are at the end of one 2-SCM and before
// the next one with a different value of the same attribute.
//
// <paragraph>foo<$text attribute=1>bar{}</$text><$text attribute=2>bar</$text>baz</paragraph>
//
if (
hasAnyAttribute( selection, attributes ) &&
isBetweenDifferentAttributes( position, attributes, true )
) {
clearSelectionAttributes( model, attributes );
} else {
this._overrideGravity();
}
return true;
}
return false;
}
/**
* Updates the document selection and the view according to the two–step caret movement state
* when moving **backwards**. Executed upon `keypress` in the {@link module:engine/view/view~View}.
*
* @param data Data of the key press.
* @returns `true` when the handler prevented caret movement
*/
private _handleBackwardMovement( data: DomEventData ): boolean {
const attributes = this.attributes;
const model = this.editor.model;
const selection = model.document.selection;
const position = selection.getFirstPosition()!;
// When the gravity is already overridden (by this plugin), it means we are on the two-step position.
// Prevent the movement, restore the gravity and update selection attributes.
//
// <paragraph>foo<$text attribute=1>bar</$text><$text attribute=2>{}baz</$text></paragraph>
// <paragraph>foo<$text attribute>bar</$text><$text otherAttribute>{}baz</$text></paragraph>
// <paragraph>foo<$text attribute>{}bar</$text>baz</paragraph>
// <paragraph>foo<$text attribute>bar</$text>{}baz</paragraph>
//
if ( this._isGravityOverridden ) {
preventCaretMovement( data );
this._restoreGravity();
// CLEAR 2-SCM attributes if we are at the end of one 2-SCM and before
// the next one with a different value of the same attribute.
//
// <paragraph>foo<$text attribute=1>bar</$text><$text attribute=2>{}bar</$text>baz</paragraph>
//
if ( isBetweenDifferentAttributes( position, attributes, true ) ) {
clearSelectionAttributes( model, attributes );
} else {
setSelectionAttributesFromTheNodeBefore( model, attributes, position );
}
return true;
} else {
// REMOVE SELECTION ATTRIBUTE when restoring gravity towards a non-existent content at the
// beginning of the block.
//
// <paragraph>{}<$text attribute>bar</$text></paragraph>
//
if ( position.isAtStart ) {
if ( hasAnyAttribute( selection, attributes ) ) {
preventCaretMovement( data );
setSelectionAttributesFromTheNodeBefore( model, attributes, position );
return true;
}
return false;
}
// SET 2-SCM attributes if we are between nodes with the same attribute but with different values.
//
// <paragraph>foo<$text attribute=1>bar</$text>[]<$text attribute=2>bar</$text>baz</paragraph>
//
if (
!hasAnyAttribute( selection, attributes ) &&
isBetweenDifferentAttributes( position, attributes, true )
) {
preventCaretMovement( data );
setSelectionAttributesFromTheNodeBefore( model, attributes, position );
return true;
}
// When we are moving from natural gravity, to the position of the 2SCM, we need to override the gravity,
// and make sure it won't be restored. Unless it's at the end of the block and an observed attribute.
// We need to check if the caret is a one position before the attribute boundary:
//
// <paragraph>foo<$text attribute=1>bar</$text><$text attribute=2>b{}az</$text></paragraph>
// <paragraph>foo<$text attribute>bar</$text><$text otherAttribute>b{}az</$text></paragraph>
// <paragraph>foo<$text attribute>b{}ar</$text>baz</paragraph>
// <paragraph>foo<$text attribute>bar</$text>b{}az</paragraph>
//
if ( isStepAfterAnyAttributeBoundary( position, attributes ) ) {
// ENGAGE 2-SCM if the selection has no attribute. This may happen when the user
// left the attribute using a FORWARD 2-SCM.
//
// <paragraph><$text attribute>bar</$text>{}</paragraph>
//
if (
position.isAtEnd &&
!hasAnyAttribute( selection, attributes ) &&
isBetweenDifferentAttributes( position, attributes )
) {
preventCaretMovement( data );
setSelectionAttributesFromTheNodeBefore( model, attributes, position );
return true;
}
// Skip the automatic gravity restore upon the next selection#change:range event.
// If not skipped, it would automatically restore the gravity, which should remain
// overridden.
this._isNextGravityRestorationSkipped = true;
this._overrideGravity();
// Don't return "true" here because we didn't call _preventCaretMovement.
// Returning here will destabilize the filler logic, which also listens to
// keydown (and the event would be stopped).
return false;
}
}
return false;
}
/**
* Starts listening to {@link module:engine/view/document~Document#event:mousedown} and
* {@link module:engine/view/document~Document#event:selectionChange} and puts the selection before/after a 2-step node
* if clicked at the beginning/ending of the 2-step node.
*
* The purpose of this action is to allow typing around the 2-step node directly after a click.
*
* See https://github.com/ckeditor/ckeditor5/issues/1016.
*/
private _enableClickingAfterNode(): void {
const editor = this.editor;
const model = editor.model;
const selection = model.document.selection;
const document = editor.editing.view.document;
editor.editing.view.addObserver( MouseObserver );
let clicked = false;
// Detect the click.
this.listenTo<ViewDocumentMouseDownEvent>( document, 'mousedown', () => {
clicked = true;
} );
// When the selection has changed...
this.listenTo<ViewDocumentSelectionChangeEvent>( document, 'selectionChange', () => {
const attributes = this.attributes;
if ( !clicked ) {
return;
}
// ...and it was caused by the click...
clicked = false;
// ...and no text is selected...
if ( !selection.isCollapsed ) {
return;
}
// ...and clicked text is the 2-step node...
if ( !hasAnyAttribute( selection, attributes ) ) {
return;
}
const position = selection.getFirstPosition()!;
if ( !isBetweenDifferentAttributes( position, attributes ) ) {
return;
}
// The selection at the start of a block would use surrounding attributes
// from text after the selection so just clear 2-SCM attributes.
//
// Also, clear attributes for selection between same attribute with different values.
if (
position.isAtStart ||
isBetweenDifferentAttributes( position, attributes, true )
) {
clearSelectionAttributes( model, attributes );
}
else if ( !this._isGravityOverridden ) {
this._overrideGravity();
}
} );
}
/**
* Starts listening to {@link module:engine/model/model~Model#event:insertContent} and corrects the model
* selection attributes if the selection is at the end of a two-step node after inserting the content.
*
* The purpose of this action is to improve the overall UX because the user is no longer "trapped" by the
* two-step attribute of the selection, and they can type a "clean" (`linkHref`–less) text right away.
*
* See https://github.com/ckeditor/ckeditor5/issues/6053.
*/
private _enableInsertContentSelectionAttributesFixer(): void {
const editor = this.editor;
const model = editor.model;
const selection = model.document.selection;
const attributes = this.attributes;
this.listenTo<ModelInsertContentEvent>( model, 'insertContent', () => {
const position = selection.getFirstPosition()!;
if (
hasAnyAttribute( selection, attributes ) &&
isBetweenDifferentAttributes( position, attributes )
) {
clearSelectionAttributes( model, attributes );
}
}, { priority: 'low' } );
}
/**
* Starts listening to {@link module:engine/model/model~Model#deleteContent} and checks whether
* removing a content right after the tow-step attribute.
*
* If so, the selection should not preserve the two-step attribute. However, if
* the {@link module:typing/twostepcaretmovement~TwoStepCaretMovement} plugin is active and
* the selection has the two-step attribute due to overridden gravity (at the end), the two-step attribute should stay untouched.
*
* The purpose of this action is to allow removing the link text and keep the selection outside the link.
*
* See https://github.com/ckeditor/ckeditor5/issues/7521.
*/
private _handleDeleteContentAfterNode(): void {
const editor = this.editor;
const model = editor.model;
const selection = model.document.selection;
const view = editor.editing.view;
let isBackspace = false;
let shouldPreserveAttributes = false;
// Detect pressing `Backspace`.
this.listenTo<ViewDocumentDeleteEvent>( view.document, 'delete', ( evt, data ) => {
isBackspace = data.direction === 'backward';
}, { priority: 'high' } );
// Before removing the content, check whether the selection is inside a two-step attribute.
// If so, we want to preserve those attributes.
this.listenTo<ModelDeleteContentEvent>( model, 'deleteContent', () => {
if ( !isBackspace ) {
return;
}
const position = selection.getFirstPosition()!;
shouldPreserveAttributes = hasAnyAttribute( selection, this.attributes ) &&
!isStepAfterAnyAttributeBoundary( position, this.attributes );
}, { priority: 'high' } );
// After removing the content, check whether the current selection should preserve the `linkHref` attribute.
this.listenTo<ModelDeleteContentEvent>( model, 'deleteContent', () => {
if ( !isBackspace ) {
return;
}
isBackspace = false;
// Do not escape two-step attribute if it was inside it before content deletion.
if ( shouldPreserveAttributes ) {
return;
}
// Use `model.enqueueChange()` in order to execute the callback at the end of the changes process.
editor.model.enqueueChange( () => {
const position = selection.getFirstPosition()!;
if (
hasAnyAttribute( selection, this.attributes ) &&
isBetweenDifferentAttributes( position, this.attributes )
) {
if ( position.isAtStart || isBetweenDifferentAttributes( position, this.attributes, true ) ) {
clearSelectionAttributes( model, this.attributes );
}
else if ( !this._isGravityOverridden ) {
this._overrideGravity();
}
}
} );
}, { priority: 'low' } );
}
/**
* `true` when the gravity is overridden for the plugin.
*/
private get _isGravityOverridden(): boolean {
return !!this._overrideUid;
}
/**
* Overrides the gravity using the {@link module:engine/model/writer~Writer model writer}
* and stores the information about this fact in the {@link #_overrideUid}.
*
* A shorthand for {@link module:engine/model/writer~Writer#overrideSelectionGravity}.
*/
private _overrideGravity(): void {
this._overrideUid = this.editor.model.change( writer => {
return writer.overrideSelectionGravity();
} );
}
/**
* Restores the gravity using the {@link module:engine/model/writer~Writer model writer}.
*
* A shorthand for {@link module:engine/model/writer~Writer#restoreSelectionGravity}.
*/
private _restoreGravity(): void {
this.editor.model.change( writer => {
writer.restoreSelectionGravity( this._overrideUid! );
this._overrideUid = null;
} );
}
}
/**
* Checks whether the selection has any of given attributes.
*/
function hasAnyAttribute( selection: DocumentSelection, attributes: Set<string> ): boolean {
for ( const observedAttribute of attributes ) {
if ( selection.hasAttribute( observedAttribute ) ) {
return true;
}
}
return false;
}
/**
* Applies the given attributes to the current selection using using the
* values from the node before the current position. Uses
* the {@link module:engine/model/writer~Writer model writer}.
*/
function setSelectionAttributesFromTheNodeBefore( model: Model, attributes: Set<string>, position: Position ) {
const nodeBefore = position.nodeBefore;
model.change( writer => {
if ( nodeBefore ) {
const attributes: Array<[string, unknown]> = [];
const isInlineObject = model.schema.isObject( nodeBefore ) && model.schema.isInline( nodeBefore );
for ( const [ key, value ] of nodeBefore.getAttributes() ) {
if (
model.schema.checkAttribute( '$text', key ) &&
( !isInlineObject || model.schema.getAttributeProperties( key ).copyFromObject !== false )
) {
attributes.push( [ key, value ] );
}
}
writer.setSelectionAttribute( attributes );
} else {
writer.removeSelectionAttribute( attributes );
}
} );
}
/**
* Removes 2-SCM attributes from the selection.
*/
function clearSelectionAttributes( model: Model, attributes: Set<string> ) {
model.change( writer => {
writer.removeSelectionAttribute( attributes );
} );
}
/**
* Prevents the caret movement in the view by calling `preventDefault` on the event data.
*
* @alias data.preventDefault
*/
function preventCaretMovement( data: DomEventData ) {
data.preventDefault();
}
/**
* Checks whether the step before `isBetweenDifferentAttributes()`.
*/
function isStepAfterAnyAttributeBoundary( position: Position, attributes: Set<string> ): boolean {
const positionBefore = position.getShiftedBy( -1 );
return isBetweenDifferentAttributes( positionBefore, attributes );
}
/**
* Checks whether the given position is between different values of given attributes.
*/
function isBetweenDifferentAttributes( position: Position, attributes: Set<string>, isStrict: boolean = false ): boolean {
const { nodeBefore, nodeAfter } = position;
for ( const observedAttribute of attributes ) {
const attrBefore = nodeBefore ? nodeBefore.getAttribute( observedAttribute ) : undefined;
const attrAfter = nodeAfter ? nodeAfter.getAttribute( observedAttribute ) : undefined;
if ( isStrict && ( attrBefore === undefined || attrAfter === undefined ) ) {
continue;
}
if ( attrAfter !== attrBefore ) {
return true;
}
}
return false;
} |
# Hubitat API
import json
import logging
import requests
from datetime import datetime
logger = logging.getLogger(__name__)
class HubitatAPI:
DEVICE_INFO_URL = '{}/apps/api/{}/devices/{}?access_token={}'
SET_VARIABLE_URL = '{}/apps/api/{}/devices/{}/setVariable/{}?access_token={}'
def __init__(self, config_file):
with open(config_file) as f:
data = json.load(f)
self.host = data['host']
self.api_id = data['api-id']
self.token = data['token']
self.on_switch_id = data['automation-on-switch-id']
self.night_charge_switch_id = data['night-charge-switch-id']
self.info_device_id = data['info-device-id']
def get_switch_attribute(self, device_id, attribute):
url = self.DEVICE_INFO_URL.format(self.host, self.api_id, device_id, self.token)
logger.info('Reading switch ({}) state from Hubitat'.format(device_id))
response = requests.get(url)
if response.status_code != 200:
logger.error('Failed to make Hubitat request: {}'.format(response.text))
return
data = response.json()
value = None
for attr in data['attributes']:
if attr['name'] == attribute:
value = attr['currentValue']
return value
def get_switch_state(self, device_id):
return self.get_switch_attribute(device_id, attribute='switch')
def is_automation_on(self):
state = self.get_switch_state(self.on_switch_id)
return state == 'on'
def is_night_charging_on(self):
state = self.get_switch_state(self.night_charge_switch_id)
return state == 'on'
def get_night_charging_limit(self):
limit = self.get_switch_attribute(self.night_charge_switch_id, attribute='level')
return limit
def set_info_message(self, msg, amps, grid):
time = datetime.now()
message = '{} -- Amps: {} -- Grid: {}W -- Last update: {}'.format(
msg,
amps,
round(grid),
time.strftime("%Y-%m-%d %H:%M")
)
self.update_info_device_message(message)
def update_info_device_message(self, message):
url = self.SET_VARIABLE_URL.format(self.host, self.api_id, self.info_device_id, message, self.token)
logger.info('Sending info to Hubitat')
response = requests.get(url)
if response.status_code != 200:
logger.error('Failed to make Hubitat request: {}'.format(response.text)) |
package model;
import interfaces.Match;
import java.util.List;
/**
* ItemMatch class is responsible for finding an item within a given list based on the item's ID.
* It implements the Match interface and provides the matcher method to find items.
*/
public class ItemMatch implements Match {
/**
* Matches an item within the given list based on the provided item's ID.
* If the item is found in the list, it returns the item; otherwise, it returns null.
*
* @param searchItem The ItemDTO containing the ID of the item to match.
* @param itemList The list of ItemDTOs to search through.
* @return The matched ItemDTO if found, otherwise null.
*/
@Override
public ItemDTO matcher(ItemDTO searchItem, List<ItemDTO> itemList) {
for (ItemDTO item : itemList) {
if (item.getID() == searchItem.getID()) {
return item;
}
}
return null;
}
} |
<?php
/**
* Magento Enterprise Edition
*
* NOTICE OF LICENSE
*
* This source file is subject to the Magento Enterprise Edition License
* that is bundled with this package in the file LICENSE_EE.txt.
* It is also available through the world-wide-web at this URL:
* http://www.magentocommerce.com/license/enterprise-edition
* If you did not receive a copy of the license and are unable to
* obtain it through the world-wide-web, please send an email
* to license@magentocommerce.com so we can send you a copy immediately.
*
* DISCLAIMER
*
* Do not edit or add to this file if you wish to upgrade Magento to newer
* versions in the future. If you wish to customize Magento for your
* needs please refer to http://www.magentocommerce.com for more information.
*
* @category Mage
* @package Mage_Catalog
* @copyright Copyright (c) 2010 Magento Inc. (http://www.magentocommerce.com)
* @license http://www.magentocommerce.com/license/enterprise-edition
*/
/**
* Catalog navigation
*
* @category Mage
* @package Mage_Catalog
* @author Magento Core Team <core@magentocommerce.com>
*/
class SM_OverideCore_Block_Catalog_Navigation extends Mage_Catalog_Block_Navigation
{
/**
* Get Key pieces for caching block content
*
* @return array
*/
public function getCacheKeyInfo()
{
$shortCacheId = array(
'CATALOG_NAVIGATION',
Mage::app()->getStore()->getId(),
Mage::getDesign()->getPackageName(),
Mage::getDesign()->getTheme('template'),
Mage::getSingleton('customer/session')->getCustomerGroupId(),
'template' => $this->getTemplate(),
'name' => $this->getNameInLayout(),
$this->getCurrenCategoryKey(),
);
$cacheId = $shortCacheId;
$shortCacheId = array_values($shortCacheId);
$shortCacheId = implode('|', $shortCacheId);
$shortCacheId = md5($shortCacheId);
$cacheId['category_path'] = $this->getCurrenCategoryKey();
$cacheId['short_cache_id'] = $shortCacheId;
return $cacheId;
}
/**
* Render category to html
*
* @param Mage_Catalog_Model_Category $category
* @param int Nesting level number
* @param boolean Whether ot not this item is last, affects list item class
* @param boolean Whether ot not this item is first, affects list item class
* @param boolean Whether ot not this item is outermost, affects list item class
* @param string Extra class of outermost list items
* @param string If specified wraps children list in div with this class
* @param boolean Whether ot not to add on* attributes to list item
* @return string
*/
protected function _renderCategoryMenuItemHtml($category, $level = 2, $isLast = false, $isFirst = false,
$isOutermost = false, $outermostItemClass = '', $childrenWrapClass = '', $noEventAttributes = true)
{
if (!$category->getIsActive()) {
return '';
}
$html = array();
// get all children
if (Mage::helper('catalog/category_flat')->isEnabled()) {
$children = (array)$category->getChildrenNodes();
$childrenCount = count($children);
} else {
$children = $category->getChildren();
$childrenCount = $children->count();
}
$hasChildren = ($children && $childrenCount);
// select active children
$activeChildren = array();
foreach ($children as $child) {
if ($child->getIsActive()) {
$activeChildren[] = $child;
}
}
$activeChildrenCount = count($activeChildren);
$hasActiveChildren = ($activeChildrenCount > 0);
// prepare list item html classes
$classes = array();
$classes[] = 'level' . $level;
$classes[] = 'nav-' . $this->_getItemPosition($level);
if ($this->isCategoryActive($category)) {
$classes[] = 'active';
}
$linkClass = '';
if ($isOutermost && $outermostItemClass) {
$classes[] = $outermostItemClass;
$linkClass = ' class="' . $outermostItemClass . '"';
}
if ($isFirst) {
$classes[] = 'first';
}
if ($isLast) {
$classes[] = 'last';
}
if ($hasActiveChildren) {
$classes[] = 'parent';
}
$classes[] = $category->getUrlKey(); //add url key as class for styling
// prepare list item attributes
$attributes = array();
if (count($classes) > 0) {
$attributes['class'] = implode(' ', $classes);
}
if ($hasActiveChildren && !$noEventAttributes) {
$attributes['onmouseover'] = 'toggleMenu(this,1)';
$attributes['onmouseout'] = 'toggleMenu(this,0)';
}
// assemble list item with attributes
$htmlLi = '<li';
foreach ($attributes as $attrName => $attrValue) {
$htmlLi .= ' ' . $attrName . '="' . str_replace('"', '\"', $attrValue) . '"';
}
$htmlLi .= '>';
$html[] = $htmlLi;
$html[] = '<a href="' . $this->getCategoryUrl($category) . '"' . $linkClass . '>';
$html[] = '<span>' . $this->escapeHtml($category->getName()) . '</span>';
$html[] = '</a>';
// render children
$htmlChildren = '';
$j = 0;
foreach ($activeChildren as $child) {
$htmlChildren .= $this->_renderCategoryMenuItemHtml(
$child,
($level + 1),
($j == $activeChildrenCount - 1),
($j == 0),
false,
$outermostItemClass,
$childrenWrapClass,
$noEventAttributes
);
$j++;
}
if (!empty($htmlChildren)) {
if ($childrenWrapClass) {
$html[] = '<div class="' . $childrenWrapClass . '">';
}
$html[] = '<ul class="level' . $level . '">';
$html[] = $htmlChildren;
$html[] = '</ul>';
if ($childrenWrapClass) {
$html[] = '</div>';
}
}
$html[] = '</li>';
$html = implode("\n", $html);
return $html;
}
} |
import React, { useState, useEffect } from "react";
import { useMutation } from "@apollo/client";
import { Sheet, Typography, IconButton, Grid } from "@mui/joy";
import { DELETE_PRACTICE_PLAN } from "../../../utils/mutations";
import RegularModal from "../../common/Modal/RegularModal";
import DeleteModalContent from "../../common/Modal/DeleteModalContent";
import CreateAssignmentContainer from "./Assignments/CreateAssignmentContainer";
import DeleteIcon from "@mui/icons-material/Delete";
import PracticePlanTable from "./PracticePlanTable";
import CreateResourceContainer from "./Resources/CreateResourceContainer";
import ResourceContainer from "./Resources/ResourceContainer";
import KeyboardArrowDownIcon from "@mui/icons-material/KeyboardArrowDown";
import KeyboardControlKeyIcon from "@mui/icons-material/KeyboardControlKey";
import Auth from "../../../utils/auth";
import ProgressBar from "../../common/ProgressBar";
import EditIcon from "@mui/icons-material/Edit";
import EditPracticePlan from "./EditPracticePlan";
import SelectResourceContainer from "./Resources/SelectResourceContainer";
const styles = {
sheet: {
mt: 3,
p: 2,
backgroundColor: "lavender",
borderRadius: "4px",
boxShadow: "md",
},
};
// The card of an individual practice plan
const PracticePlanCard = ({ practicePlan, onDelete }) => {
const [open, setOpen] = useState(0); // for modal
const [deletePracticePlan] = useMutation(DELETE_PRACTICE_PLAN); // delete practice plan mutation
const [assignments, setAssignments] = useState(practicePlan.assignments); // assignments for the practice plan
const [planPoints, setPlanPoints] = useState(0); // total points available to earn for the plan
const [completedPoints, setCompletedPoints] = useState(0); // amount of completed points a student has earned by completing assignments
const [resources, setResources] = useState(practicePlan.resources); // resources for the practice plan
const [revealed, setRevealed] = useState(false); // for revealing the practice plan details
const [progressBarPercentage, setProgressBarPercentage] = useState(0); // progress bar percentage
const [activePlan, setActivePlan] = useState(practicePlan); // active practice plan
// Setting the total points available to earn for the plan
useEffect(() => {
if (assignments !== undefined) {
const pointsArr = assignments.map((assignment) => assignment.pointsWorth);
const total = pointsArr.reduce((acc, curr) => acc + curr, 0);
setPlanPoints(total);
}
}, [assignments]);
// Setting the amount of completed points a student has earned by completing assignments
useEffect(() => {
if (assignments !== undefined) {
const completedAssignments = assignments.filter(
(assignment) => assignment.completed === true
);
const pointsArr = completedAssignments.map(
(assignment) => assignment.pointsWorth
);
const total = pointsArr.reduce((acc, curr) => acc + curr, 0);
setCompletedPoints(total);
}
}, [assignments]);
// Setting the progress bar percentage
useEffect(() => {
const percentage = Math.floor((completedPoints / planPoints) * 100);
setProgressBarPercentage(percentage || 0);
}, [setProgressBarPercentage, completedPoints, planPoints]);
// Setting the assignments
useEffect(() => {
setAssignments(assignments || []);
}, [setAssignments, assignments]);
// Deleting the practice plan
const deletePracticePlanFunc = async () => {
try {
await deletePracticePlan({
variables: { planId: activePlan._id },
});
alert("Plan Deleted!");
setOpen(false);
onDelete();
} catch (err) {
console.error(err);
}
};
return (
<Sheet id="practicePlanCard" sx={styles.sheet}>
<Grid container>
<Grid xs={12} md={6}>
<Typography
level="h2"
endDecorator={
Auth.teacherLoggedIn() && (
<>
<IconButton onClick={() => setOpen(1)} color="danger">
<DeleteIcon />
</IconButton>
{/* Modal for deleting a practice plan */}
<RegularModal
name="deletePracticePlan"
open={open === 1}
onRequestClose={() => setOpen(0)}
>
<DeleteModalContent
onRequestClose={() => setOpen(0)}
confirmAction={() => deletePracticePlanFunc()}
resourceName="Practice Plan"
/>
</RegularModal>
<IconButton onClick={() => setOpen(2)}>
<EditIcon />
</IconButton>
{/* Modal for editing practice plan */}
<RegularModal
name="editPracticePlan"
open={open === 2}
onRequestClose={() => setOpen(0)}
>
<EditPracticePlan
onRequestClose={() => setOpen(0)}
setActivePlan={setActivePlan}
setOpen={setOpen}
practicePlan={activePlan}
/>
</RegularModal>
</>
)
}
>
{activePlan?.name}
</Typography>
<Typography>
{new Date(activePlan?.dateCreated).toLocaleString()}
</Typography>
<Typography level="h4">Plan Points: {planPoints}</Typography>
<Typography level="h4">Points Earned: {completedPoints}</Typography>
</Grid>
<Grid xs={12} md={6}>
<ProgressBar
percentage={progressBarPercentage}
width={"175px"}
height={"175px"}
/>
</Grid>
<Grid xs={12}>
<Typography level="body1">{activePlan?.planNotes}</Typography>
{!revealed ? (
<KeyboardArrowDownIcon
fontSize="large"
onClick={() => setRevealed(true)}
/>
) : (
<KeyboardControlKeyIcon
fontSize="large"
onClick={() => setRevealed(false)}
/>
)}
</Grid>
</Grid>
{revealed && (
<>
{/* Container for creating an assignment */}
<CreateAssignmentContainer
practicePlan={activePlan}
assignments={assignments}
setAssignments={setAssignments}
resources={resources}
setResources={setResources}
/>
{/* Container for creating a resource */}
<CreateResourceContainer
practicePlan={activePlan}
resources={resources}
setResources={setResources}
/>
{/* Container for selecting a resource from pool of teacher resources */}
<SelectResourceContainer
practicePlan={activePlan}
resources={resources}
setResources={setResources}
/>
{/* Table displaying list of all practice plans */}
<PracticePlanTable
assignments={assignments}
setAssignments={setAssignments}
/>
<ResourceContainer
practicePlan={activePlan}
resources={resources}
setResources={setResources}
/>
</>
)}
</Sheet>
);
};
export default PracticePlanCard; |
import { useAuthContext } from "./useAuthContext";
import { useState } from "react";
import ILogin from "../../models/db/Login";
import { Cookies } from 'react-cookie';
import { useNavigate } from "react-router-dom";
import { useMessageContext } from "./useMessageContext";
import User from "../../models/db/User";
export const useLogin = () => {
const navigate = useNavigate();
const [error, setError] = useState<string | null>(null)
const [isLoading, setIsLoading] = useState<boolean>(false)
const { dispatch } = useAuthContext();
const { dispatch : dispatch2 } = useMessageContext()
let cookie = new Cookies()
const login = async (loginCred: ILogin) => {
setIsLoading(true)
setError(null)
const response = await fetch('https://localhost:7112/api/User/Login', {
method: 'POST',
headers: { "Content-Type": "application/json" },
body: JSON.stringify(loginCred)
});
const json = await response.json()
if (!response.ok) {
setIsLoading(false)
setError(json.error)
}
if (response.status === 400) {
setIsLoading(false)
dispatch2({type: "ERROR", message: "Either the email or the password is incorrect."})
}
if (response.ok) {
let user: User = json.user
user.keepLoggedIn = loginCred.keepLoggedIn
localStorage.setItem('user', JSON.stringify(user))
cookie.set("token", json.jwt)
cookie.set("refresh", json.refreshToken.token)
dispatch({type: "LOGIN", payload: json.user})
setIsLoading(false)
navigate("/")
}
}
return { login, isLoading, error }
} |
import app from "../../salesflare.app.mjs";
export default {
props: {
app,
startDate: {
type: "string",
label: "Start Date",
description: "Start date. Must be in ISO format. e.g. `2019-08-24T14:15:22Z`",
optional: true,
},
probability: {
type: "string",
label: "Probability",
description: "Probability of the opportunity. Min `0`",
optional: true,
},
name: {
propDefinition: [
app,
"name",
],
description: "Name of the opportunity",
},
value: {
type: "integer",
label: "Value",
description: "Value of the opportunity. Min `0`",
optional: true,
},
closeDate: {
type: "string",
label: "Close Date",
description: "Close date of the opportunity. Must be in ISO format. e.g. `2019-08-24T14:15:22Z`",
optional: true,
},
closed: {
type: "boolean",
label: "Closed",
description: "Is closed",
optional: true,
},
tags: {
propDefinition: [
app,
"tags",
],
},
recurringPricePerUnit: {
type: "integer",
label: "Recurring Price Per Unit",
description: "Recurring price per unit",
optional: true,
},
frequency: {
type: "string",
label: "Frequency",
description: "Opportunity frequency",
optional: true,
options: [
"annually",
"weekly",
"monthly",
"daily",
],
},
contractStartDate: {
type: "string",
label: "Contract Start Date",
description: "Contract start date. Must be in ISO format. e.g. `2019-08-24T14:15:22Z`",
optional: true,
},
contractEndDate: {
type: "string",
label: "Contract End Date",
description: "Contract end date. Must be in ISO format. e.g. `2019-08-24T14:15:22Z`",
optional: true,
},
custom: {
propDefinition: [
app,
"custom",
],
},
},
}; |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<link rel="stylesheet" href="./styles/reset.css" />
<link rel="stylesheet" href="./styles/normalize.css" />
<link rel="stylesheet" href="./styles/header.css" />
<link rel="stylesheet" href="./styles/footer.css" />
<link rel="stylesheet" href="./styles/styles.css" />
</head>
<body>
<header class="header">
<div class="black_fon">
<div class="wrapperna">
<div class="info_block">
<img class="icon_email" src="./img/social_item/email.png" alt="" />
<div class="info_block_text_content">
<p class="info_block_text_1">Hello@colorlib.com</p>
<p>|</p>
<p class="info_block_text_2">Free Shipping for all order of $99</p>
</div>
</div>
<div class="social_block">
<div class="social_info">
<a href=""><img src="./img/social_item/face.png" alt="" /></a><a href=""><img src="./img/social_item/wats.png" alt="" /></a
><a href=""><img src="./img/social_item/inst.png" alt="" /></a><a href=""><img src="./img/social_item/twit.png" alt="" /></a>
</div>
<div class="lang_auto">
<div class="language">
<img src="./img/social_item/eng.png" alt="" />
<div class="language_block">
<select class="language_list">
<option value="1"><p>English</p></option>
<option value="2"><p>English</p></option>
<option value="3"><p>English</p></option>
<option value="4"><p>English</p></option>
<option value="5"><p>English</p></option>
<option value="6"><p>English</p></option>
</select>
</div>
</div>
<div>
<p>|</p>
</div>
<div class="authorization">
<img src="./img/social_item/log.png" alt="" />
<a href=""> <p>Login</p></a>
</div>
</div>
</div>
</div>
</div>
<div class="menu_block">
<div class="logo">
<a href="">LOGO </a>
<img src="./img/social_item/logo.png" alt="" />
</div>
<nav class="nav_menu">
<ul class="nav_menu_list">
<li class="nav_menu_obj" id="active"><a href="">HOME</a></li>
<li class="nav_menu_obj"><a href="">SHOP</a></li>
<li class="nav_menu_obj"><a href="">PAGES</a></li>
<li class="nav_menu_obj"><a href="">BLOG</a></li>
<li class="nav_menu_obj"><a href="">CONTACT</a></li>
</ul>
</nav>
<div class="nav_menu_list_item">
<div class="nav_menu_item">
<img src="./img/social_item/notification_heart.png" alt="" />
</div>
<div class="nav_menu_item">
<img src="./img/social_item/notification_shop.png" alt="" />
</div>
<div class="nav_menu_item_block">
<div class="nav_menu_item_block_gray_text">
<p>item:</p>
</div>
<div class="nav_menu_item_block_bold_text">
<p>$150.00</p>
</div>
</div>
</div>
</div>
</header>
<main class="main">
<div class="main_2">
<!-- Первая группа начало -->
<div class="group_one">
<div class="all_list">
<div class="all_list_block">
<div class="icon_department"><img src="./img/social_item/menu_black.png" alt="" /></div>
<div class="text_department">All Department</div>
<select class="department_list">
<div>
<option hidden></option>
<option value="1"><p>English</p></option>
<option value="2"><p>English</p></option>
<option value="3"><p>English</p></option>
<option value="4"><p>English</p></option>
<option value="5"><p>English</p></option>
</div>
</select>
</div>
</div>
<div class="all_category">
<div class="text_category">All Categories</div>
<div class="abc">
<select class="category_list">
<div>
<option hidden></option>
<option value="1"><p>English</p></option>
<option value="2"><p>English</p></option>
<option value="3"><p>English</p></option>
<option value="4"><p>English</p></option>
<option value="5"><p>English</p></option>
</div>
</select>
<p class="text_gray">|</p>
</div>
<form class="form_searh">
<p>
<input type="search" name="q" placeholder="What do you need?" />
</p>
</form>
<div class="button_searh">
<button>SEARCH</button>
</div>
</div>
<div class="social_frame">
<div class="icon_img">
<img src="./img/social_item/phone_in_talk_black.png" alt="" />
</div>
<div class="social_frame_info">
<div class="social_frame_info_text_1"><p>+65 11.188.888</p></div>
<div class="social_frame_info_text_2"><p>support 24/7 time</p></div>
</div>
</div>
</div>
<!-- Первая группа конец -->
<!-- Вторая группа начало -->
<div class="group_two">
<div class="easy_list">
<p>Fresh Meat</p>
<p>Vegetables</p>
<p>Fruit & Nut Gifts</p>
<p>Fresh Berries</p>
<p>Ocean Foods</p>
<p>Butter & Eggs</p>
<p>Fastfood</p>
<p>Fresh Onion</p>
<p>Papayaya & Crisps</p>
<p>Oatmeal</p>
<p>Fresh Bananas</p>
</div>
<div class="block_1">
<div class="block_1_info">
<p id="block_1_info_green">FRUIT FRESH</p>
<p id="block_1_info_bold">Vegetable 100% Organic</p>
<p id="block_1_info_gray_op">Free Pickup and Delivery Available</p>
<button class="block_1_info_button">SHOP NOW</button>
</div>
<div class="block_1_img"></div>
</div>
</div>
<!-- Второая группа конец -->
<!-- Третья группа начало -->
<div class="group_three">
<div class="block_2">
<div class="block_2_item">
<img src="./img/row_after_block_1/oblect_1_row_after_block_1.png" alt="" />
<div class="block_2_item_text">
<p>FRESH FRUIT</p>
</div>
</div>
<div class="block_2_item">
<img src="./img/row_after_block_1/oblect_2_row_after_block_1.png" alt="" />
<div class="block_2_item_text">
<p>DRIED FRUIT</p>
</div>
</div>
<div class="block_2_item">
<img src="./img/row_after_block_1/oblect_3_row_after_block_1.png" alt="" />
<div class="block_2_item_text">
<p>VEGETABLES</p>
</div>
</div>
<div class="block_2_item">
<img src="./img/row_after_block_1/oblect_4_row_after_block_1.png" alt="" />
<div class="block_2_item_text">
<p>DRINK FRUITS</p>
</div>
</div>
</div>
</div>
<!-- Третья группа конец -->
<!-- Четвертая группа начало -->
<div class="group_4">
<div class="group_4_img">
<div class="group_4_img_item">
<img src="./img/catalog/nav_catalog_summer_fruits.png" alt="" />
<div class="group_4_img_item_block_text">
<p class="group_4_img_item_block_text_bold">Summer Fruits</p>
<p class="group_4_img_item_block_text_normal">100% All Natural Fruit Juice</p>
<button class="group_4_img_item_block_button">SHOP NOW</button>
</div>
</div>
<div class="group_4_img_item">
<img src="./img/catalog/nav_catalog_dried_and_drink.png" alt="" />
<div class="group_4_img_item_block_text">
<p class="group_4_img_item_block_text_bold">Dried & Drink Fruits</p>
<p class="group_4_img_item_block_text_normal">100% All Natural Fruit Juice</p>
<button class="group_4_img_item_block_button">SHOP NOW</button>
</div>
</div>
</div>
<div class="group_4_catalog">
<div class="group_4_catalog_group" id="group_4_catalog_group_latest">
<div class="group_4_catalog_nav_item">
<div class="group_4_catalog_nav_item_text">Latest Product</div>
<div class="group_4_catalog_nav_item_buttons">
<button><img src="./img/social_item/Vector_left.png" alt="" /></button>
<button><img src="./img/social_item/Vector_right.png" alt="" /></button>
</div>
</div>
<div class="group_4_catalog_content">
<div class="group_4_catalog_content_obj">
<div class="group_4_catalog_content_obj_block_img"><img src="./img/catalog/catalog_object_1.png" alt="" /></div>
<div class="group_4_catalog_content_obj_text">
<p class="group_4_catalog_content_obj_text_normal">Cauliflower</p>
<p class="group_4_catalog_content_obj_text_bold">$30.00</p>
</div>
</div>
<div class="group_4_catalog_content_obj">
<div class="group_4_catalog_content_obj_block_img"><img src="./img/catalog/catalog_object_2.png" alt="" /></div>
<div class="group_4_catalog_content_obj_text">
<p class="group_4_catalog_content_obj_text_normal">Organic Quince</p>
<p class="group_4_catalog_content_obj_text_bold">$30.00</p>
</div>
</div>
<div class="group_4_catalog_content_obj">
<div class="group_4_catalog_content_obj_block_img"><img src="./img/catalog/catalog_object_3.png" alt="" /></div>
<div class="group_4_catalog_content_obj_text">
<p class="group_4_catalog_content_obj_text_normal">Tomato Hybrid</p>
<p class="group_4_catalog_content_obj_text_bold">$30.00</p>
</div>
</div>
</div>
</div>
<div class="group_4_catalog_group" id="group_4_catalog_group_popular">
<div class="group_4_catalog_nav_item">
<div class="group_4_catalog_nav_item">
<div class="group_4_catalog_nav_item_text">Top rated Products</div>
<div class="group_4_catalog_nav_item_buttons">
<button><img src="./img/social_item/Vector_left.png" alt="" /></button>
<button><img src="./img/social_item/Vector_right.png" alt="" /></button>
</div>
</div>
</div>
<div class="group_4_catalog_content">
<div class="group_4_catalog_content_obj">
<div class="group_4_catalog_content_obj_block_img"><img src="./img/catalog/catalog_object_4.png" alt="" /></div>
<div class="group_4_catalog_content_obj_text">
<p class="group_4_catalog_content_obj_text_normal">Bleuberry</p>
<p class="group_4_catalog_content_obj_text_bold">$30.00</p>
</div>
</div>
<div class="group_4_catalog_content_obj">
<div class="group_4_catalog_content_obj_block_img"><img src="./img/catalog/catalog_object_5.png" alt="" /></div>
<div class="group_4_catalog_content_obj_text">
<p class="group_4_catalog_content_obj_text_normal">Capsicum-Green</p>
<p class="group_4_catalog_content_obj_text_bold">$30.00</p>
</div>
</div>
<div class="group_4_catalog_content_obj">
<div class="group_4_catalog_content_obj_block_img"><img src="./img/catalog/catalog_object_6.png" alt="" /></div>
<div class="group_4_catalog_content_obj_text">
<p class="group_4_catalog_content_obj_text_normal">Tomato Hybrid</p>
<p class="group_4_catalog_content_obj_text_bold">$30.00</p>
</div>
</div>
</div>
</div>
<div class="group_4_catalog_group" id="group_4_catalog_group_lreview">
<div class="group_4_catalog_nav_item">
<div class="group_4_catalog_nav_item">
<div class="group_4_catalog_nav_item_text">Review Products</div>
<div class="group_4_catalog_nav_item_buttons">
<button><img src="./img/social_item/Vector_left.png" alt="" /></button>
<button><img src="./img/social_item/Vector_right.png" alt="" /></button>
</div>
</div>
</div>
<div class="group_4_catalog_content">
<div class="group_4_catalog_content_obj">
<div class="group_4_catalog_content_obj_block_img"><img src="./img/catalog/catalog_object_7.png" alt="" /></div>
<div class="group_4_catalog_content_obj_text">
<p class="group_4_catalog_content_obj_text_normal">Hazelnuts Filbert Nut</p>
<p class="group_4_catalog_content_obj_text_bold">$30.00</p>
</div>
</div>
<div class="group_4_catalog_content_obj">
<div class="group_4_catalog_content_obj_block_img"><img src="./img/catalog/catalog_object_8.png" alt="" /></div>
<div class="group_4_catalog_content_obj_text">
<p class="group_4_catalog_content_obj_text_normal">Green Apple</p>
<p class="group_4_catalog_content_obj_text_bold">$30.00</p>
</div>
</div>
<div class="group_4_catalog_content_obj">
<div class="group_4_catalog_content_obj_block_img"><img src="./img/catalog/catalog_object_9.png" alt="" /></div>
<div class="group_4_catalog_content_obj_text">
<p class="group_4_catalog_content_obj_text_normal">Fresh Raspberry</p>
<p class="group_4_catalog_content_obj_text_bold">$30.00</p>
</div>
</div>
</div>
</div>
</div>
</div>
<!-- Четвертая группа конец -->
<!-- Пятая группа начало -->
<div class="group_5">
<div class="group_5_nav">
<p class="group_5_nav_text">Featured Product</p>
<div class="group_5_nav_bottom_border_green"></div>
</div>
<div class="group_5_sort">
<div class="group_5_sort_nav">
<p class="group_5_sort_nav_bold">All</p>
<p class="group_5_sort_nav_gray_op">Oranges</p>
<p class="group_5_sort_nav_gray_op">Fresh Meat</p>
<p class="group_5_sort_nav_gray_op">Vegetables</p>
<p class="group_5_sort_nav_gray_op">Fastfood</p>
</div>
</div>
<div class="group_5_sort_content">
<div class="group_5_sort_content_block">
<div class="group_5_sort_content_block_img">
<img src="./img/block_sort/sort_fruit_1.png" alt="" />
</div>
<div class="group_5_sort_content_block_text_block">
<p class="group_5_sort_content_block_text_block_text">Bunc of bananas</p>
<p class="group_5_sort_content_block_text_block_price">$30.00</p>
</div>
</div>
<div class="group_5_sort_content_block">
<div class="group_5_sort_content_block_img">
<img src="./img/block_sort/sort_fruit_2.png" alt="" />
</div>
<div class="group_5_sort_content_block_text_block">
<p class="group_5_sort_content_block_text_block_text">Fresh fruit apricot</p>
<p class="group_5_sort_content_block_text_block_price">$30.00</p>
</div>
</div>
<div class="group_5_sort_content_block">
<div class="group_5_sort_content_block_img">
<img src="./img/block_sort/sort_fruit_3.png" alt="" />
</div>
<div class="group_5_sort_content_block_text_block">
<p class="group_5_sort_content_block_text_block_text">Avokado fruit</p>
<p class="group_5_sort_content_block_text_block_price">$30.00</p>
</div>
</div>
<div class="group_5_sort_content_block">
<div class="group_5_sort_content_block_img">
<img src="./img/block_sort/sort_fruit_4.png" alt="" />
</div>
<div class="group_5_sort_content_block_text_block">
<p class="group_5_sort_content_block_text_block_text">Red aplle</p>
<p class="group_5_sort_content_block_text_block_price">$30.00</p>
</div>
</div>
<div class="group_5_sort_content_block">
<div class="group_5_sort_content_block_img">
<img src="./img/block_sort/sort_fruit_5.png" alt="" />
</div>
<div class="group_5_sort_content_block_text_block">
<p class="group_5_sort_content_block_text_block_text">Two freesh figs</p>
<p class="group_5_sort_content_block_text_block_price">$30.00</p>
</div>
</div>
<div class="group_5_sort_content_block">
<div class="group_5_sort_content_block_img">
<img src="./img/block_sort/sort_fruit_6.png" alt="" />
</div>
<div class="group_5_sort_content_block_text_block">
<p class="group_5_sort_content_block_text_block_text">Cucumber</p>
<p class="group_5_sort_content_block_text_block_price">$30.00</p>
</div>
</div>
<div class="group_5_sort_content_block">
<div class="group_5_sort_content_block_img">
<img src="./img/block_sort/sort_fruit_7.png" alt="" />
</div>
<div class="group_5_sort_content_block_text_block">
<p class="group_5_sort_content_block_text_block_text">Beef steak</p>
<p class="group_5_sort_content_block_text_block_price">$30.00</p>
</div>
</div>
<div class="group_5_sort_content_block">
<div class="group_5_sort_content_block_img">
<img src="./img/block_sort/sort_fruit_8.png" alt="" />
</div>
<div class="group_5_sort_content_block_text_block">
<p class="group_5_sort_content_block_text_block_text">Raw Broccoli</p>
<p class="group_5_sort_content_block_text_block_price">$30.00</p>
</div>
</div>
</div>
</div>
<!-- Пятая группа конец -->
<!-- Шестой блок начало -->
<div class="group_6">
<div class="group_6_nav">
<p class="group_6_nav_text">From The Blog</p>
<div class="group_6_nav_bottom_border_green"></div>
</div>
<div class="group_6_content">
<div class="group_6_content_block">
<img src="./img/blog_img/blog_img_1.png" alt="" />\
<div class="group_6_nav_text_block">
<div class="group_6_nav_text_block_icons">
<div class="group_6_nav_text_block_icon_calendar">
<img src="./img/social_item/calendar.png" alt="" />
</div>
<p>May 4, 2021</p>
<div class="group_6_nav_text_block_icon_textsms">
<img src="./img/social_item/textsms.png" alt="" />
</div>
<p>54</p>
</div>
<div class="group_6_nav_text_block_bold">
<p>Busting Salad Myths: Eat A Well-Built Salad (If You Want To)</p>
</div>
<div class="group_6_nav_text_block_normal_and_button">
<p>
This is usually said when someone is trying to lose weight or be “super healthy.” first, to lose weight, it is widely understood that we must burn more calories
than we eat. thus <a href="">Read More</a>
</p>
</div>
</div>
</div>
<div class="group_6_content_block">
<img src="./img/blog_img/blog_img_2.png" alt="" />
<div class="group_6_nav_text_block">
<div class="group_6_nav_text_block_icons">
<div class="group_6_nav_text_block_icon_calendar">
<img src="./img/social_item/calendar.png" alt="" />
</div>
<p>May 4, 2021</p>
<div class="group_6_nav_text_block_icon_textsms">
<img src="./img/social_item/textsms.png" alt="" />
</div>
<p>25</p>
</div>
<div class="group_6_nav_text_block_bold">
<p>Vitamin c: how much and which sources are best for boosting immunity?</p>
</div>
<div class="group_6_nav_text_block_normal_and_button_2">
<p>
During cold and flu season, we try to do all we can to prevent illness or speed up how fast we recover from illness. One such strategy many employ is the use of
Vitamin C for a natural remedy. <a href="">Read More</a>
</p>
</div>
</div>
</div>
<div class="group_6_content_block">
<img src="./img/blog_img/blog_img_3.png" alt="" />
<div class="group_6_nav_text_block">
<div class="group_6_nav_text_block_icons">
<div class="group_6_nav_text_block_icon_calendar">
<img src="./img/social_item/calendar.png" alt="" />
</div>
<p>May 4, 2021</p>
<div class="group_6_nav_text_block_icon_textsms">
<img src="./img/social_item/textsms.png" alt="" />
</div>
<p>58</p>
</div>
<div class="group_6_nav_text_block_bold">
<p>Pump Up The Produce Power In Winter Meals</p>
</div>
<div class="group_6_nav_text_block_normal_and_button">
<p>
We’ve all been here before: When it seems the long, gray winter will never end and you make one more pot of hearty vegetable soup or another casserole;
<a href="">Read More</a>
</p>
</div>
</div>
</div>
</div>
</div>
<!-- Шестой блок конец -->
</div>
</main>
<footer class="footer">
<div class="footer_content">
<div class="footer_content_container">
<div class="footer_content_container_item" id="f_content_1">
<div class="f_content_1_text">
<div class="footer_logo">
<p>LOGO</p>
<img src="./img/social_item/logo_dark.png" alt="" />
</div>
<div class="footer_logo_text">
<p>Address: 60-49 Road 11378 New York</p>
<p>Phone: +65 11.188.888</p>
<p>Email: hello@colorlib.com</p>
</div>
</div>
</div>
<div class="footer_content_container_item" id="f_content_2">
<div class="f_content_2_text">
<div class="f_content_2_text_bold">
<p>Useful link</p>
</div>
<div class="f_content_2_text_normal">
<p>About Us</p>
<p>About Our Shop</p>
<p>Secure Shopping</p>
<p>Delivery infomation</p>
<p>Privacy Policy</p>
<p>Our Sitemap</p>
</div>
</div>
</div>
<div class="footer_content_container_item" id="f_content_3">
<div class="f_content_3_block">
<p>Who We Are</p>
<p>Our Services</p>
<p>Projects</p>
<p>Contact</p>
<p>Innovation</p>
<p>Testimonials</p>
</div>
</div>
<div class="footer_content_container_item" id="f_content_4">
<div class="f_content_4_block">
<div class="f_content_4_block_nav">
<div class="f_content_4_block_nav_text_bold"><p>Sign up for our newsletter</p></div>
<div class="f_content_4_block_nav_text_normal"><p>Get e-mail updates about our latest Shop and special offers.</p></div>
</div>
<div class="f_content_4_block_placebtn">
<input type="search" name="q" placeholder="Enter Email Addres" />
<button>SUBSCRIBE</button>
</div>
<div class="f_content_4_block_footer">
<div class="social_info">
<a href=""><img src="./img/social_item/face.png" alt="" /></a><a href=""><img src="./img/social_item/wats.png" alt="" /></a
><a href=""><img src="./img/social_item/inst.png" alt="" /></a><a href=""><img src="./img/social_item/twit.png" alt="" /></a>
</div>
</div>
</div>
</div>
</div>
<div class="footer_content_block">
<div class="footer_content_block_2_item_1"><p>Copyright ©2021 All rights reserved | This template is made with 💚 by LOGO</p></div>
<div class="footer_content_block_2_item_2">
<div class="footer_content_block_2_item_2_cards">
<img src="./img/social_item/Visa-light.png" alt="" />
<img src="./img/social_item/MasterCard-light.png" alt="" />
<img src="./img/social_item/Maestro-light.png" alt="" />
<img src="./img/social_item/Cirrus-light.png" alt="" />
<img src="./img/social_item/AmericanExpress-light.png" alt="" />
</div>
</div>
</div>
</div>
</footer>
</body>
</html> |
/* eslint-disable react/display-name */
import React from 'react';
import DatePicker from 'react-datepicker';
import Button, { ButtonProps } from '@material-ui/core/Button';
import 'react-datepicker/dist/react-datepicker.css';
interface Props {
selectedDate: Date | null;
startDate: Date | null;
endDate: Date | null;
openToDate: string;
disabled?: boolean;
onChange: (date: Date) => void;
}
const SelectCalendar: React.FC<Props> = ({
startDate,
endDate,
disabled,
openToDate,
onChange,
selectedDate,
}) => {
const ExampleCustomInput = React.forwardRef<HTMLButtonElement, ButtonProps>(
({ value, onClick, disabled }, ref) => (
<Button
variant="outlined"
onClick={onClick}
ref={ref}
disabled={disabled}>
<div>{value ? `${value} ` : 'date picker'}</div>
</Button>
),
);
const isWeekday = (date: Date) => {
const day = date.getDay();
return day !== 0 && day !== 6;
};
return (
<div className="relative w-full my-4">
<DatePicker
dateFormat="yyyy-MM-dd"
selected={!selectedDate ? null : selectedDate}
showDisabledMonthNavigation
minDate={startDate}
maxDate={endDate}
onChange={onChange}
filterDate={isWeekday}
// 공휴일 제외 로직 하나 추가해야함
openToDate={new Date(openToDate)}
disabled={disabled}
showYearDropdown
showMonthDropdown
useShortMonthInDropdown
customInput={<ExampleCustomInput disabled={disabled} />}
withPortal
/>
</div>
);
};
export default React.memo(SelectCalendar); |
import React, { RefObject, useEffect, useRef, useState } from 'react';
import styled, { keyframes } from 'styled-components';
import WorkCord, { WorkProps } from '../components/work/WorkCord';
import Me from '../assets/Images/profile.png';
import Netflix from '../assets/Images/netflix-logo.png';
import { useRecoilState } from 'recoil';
import { ClickValue, ToggleValue, WorkValue } from '../atom';
import { Colors } from '../Styled/Colors';
import { AiOutlineMenu, AiOutlineClose } from 'react-icons/ai';
import { motion } from 'framer-motion';
import BigTitle from '../components/BigTitle';
import { MdDoubleArrow } from 'react-icons/md';
const Work = () => {
const WorkData = [
{
id: 1,
title: 'Tem-Sellbar',
stack: ['Next.js,', 'Ts,', 'Sass'],
Info: '중고 의류 플랫폼 서비스',
visit:
'https://www.notion.so/Team-sellbar-5c4e9b57c3134aaca75d06464d612b18',
TitleColor: '#5352ed',
githubLink: 'https://github.com/beaever/team-project',
},
{
id: 2,
title: 'Wanted ON BOARDING',
stack: ['React,', 'vue,', 'JS,', 'Style-Component'],
Info: 'Wanted 주최 onboarding 프로그램 ',
visit: 'https://team4-nexon-kartrider.netlify.app/',
TitleColor: '#fff',
githubLink: 'https://github.com/wanted-Team4',
},
{
id: 3,
title: 'CATCHER-UIKIT',
stack: ['React,', 'TS,', 'Style-Component'],
Info: 'SIDE-PROJECT에서 사용되는 UIKit 제작',
visit: 'https://www.npmjs.com/package/react-uikit-catcher',
TitleColor: '#97511E',
githubLink: 'https://github.com/94-s/catcher-storybook',
},
{
id: 4,
title: 'TvMOVIE',
stack: ['React,', 'TS,', 'Style-Component'],
Info: '상영중인 영화 및 TV SHOW 를 알수있는 페이지',
visit: 'https://slobbie.github.io/TvMovie/',
TitleColor: 'red',
notionLink: '',
githubLink: 'https://github.com/slobbie/TvMovie',
},
{
id: 5,
title: 'PoKemon Card book',
stack: ['React,', 'TS,', 'Style-Component'],
Info: 'PoKemon API 를 이용한 Card Book',
visit: 'https://slobbie.github.io/PokemonCardBooks/',
TitleColor: 'yellow',
notionLink: '',
githubLink: 'https://github.com/slobbie/PokemonCardBooks',
},
{
id: 6,
title: 'REACT-CANVAS',
stack: ['React,', 'TS,', 'Style-Component'],
Info: 'REACT-CANVAS',
visit: 'https://slobbie.github.io/React-canvas/',
TitleColor: '#1E5DF7',
notionLink: '',
githubLink: 'https://github.com/slobbie/React-canvas',
},
];
const [click, setClick] = useRecoilState(ClickValue);
const [work, setWork] = useRecoilState(WorkValue);
const [active, setActive] = useRecoilState(ToggleValue);
useEffect(() => {
setClick(false);
}, []);
const onToggle = () => {
setActive(!active);
};
const Container = {
hidden: { scale: 0 },
show: {
opacity: 1,
scale: 1,
transition: {
staggerChildren: 0.7,
duration: 2,
type: 'spring',
},
},
};
return (
<Section
initial={{ x: 1000 }}
animate={{ x: 0 }}
transition={{ type: 'tween', duration: 1 }}
>
<Toggle onClick={() => onToggle()}>
{active ? (
<AiOutlineClose className='closeIcon' />
) : (
<AiOutlineMenu className='menuIcon' />
)}
</Toggle>
<BigTitle size='L' text='Work' top={8} left={10} position />
<Wrapper
className='page'
variants={Container}
initial='hidden'
animate='show'
style={{}}
>
{WorkData.map((item: WorkProps) => {
return (
<WorkCord
key={item.id}
title={item.title}
stack={item.stack}
Info={item.Info}
TitleColor={item.TitleColor}
visit={item.visit}
githubLink={item.githubLink}
/>
);
})}
</Wrapper>
<BottomTitle>
<BigTitle size='S' text='Scroll' bottom={0} right={10} />
{/* <MdDoubleArrow className='arrow' /> */}
</BottomTitle>
</Section>
);
};
export default Work;
const Section = styled(motion.section)`
background-color: white;
width: 100%;
height: 100vh;
display: flex;
flex-direction: row;
justify-content: center;
align-items: center;
overflow: auto;
scrollbar-width: none;
::-webkit-scrollbar {
display: none;
}
`;
const Wrapper = styled(motion.div)`
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
justify-content: space-between;
width: 100%;
position: relative;
left: 100px;
height: 100vh;
@media screen and (max-width: 768px) {
flex-direction: column;
align-items: flex-start;
top: 150px;
left: 0;
}
`;
const Toggle = styled.div`
width: 40px;
height: 40px;
display: flex;
align-items: center;
justify-content: center;
font-size: 2rem;
cursor: pointer;
position: absolute;
top: 0;
right: 0;
margin: 5px;
margin-right: 25px;
z-index: 1000;
.menuIcon,
.closeIcon {
transition: 0.3s ease-in-out;
width: 25px;
height: 25px;
fill: ${Colors.black};
}
`;
const animate = keyframes`
0% {
transform: translateX(0);
}
40% {
transform: translateX(-5px);
}
70% {
transform: translateX(5px);
}
100%{
transform: translateX(0);
}
`;
const BottomTitle = styled.div`
position: absolute;
bottom: 3px;
right: 90px;
display: flex;
align-items: center;
height: 100%;
.arrow {
position: absolute;
fill: ${Colors.gray200};
width: calc(3rem + 1vw);
height: calc(3rem + 1vw);
bottom: 6px;
left: 10px;
animation: ${animate} 3s linear infinite;
}
@media screen and (max-width: 768px) {
display: none;
}
`; |
syntax = "proto3";
package api;
option go_package = "/beverage";
service BeveragesManagement {
rpc CreateBeverage(CreateBeverageRequest) returns (Beverage);
rpc GetBeverages(GetBeveragesParams) returns (BeverageList);
}
enum BeverageType {
BEVERAGE_TYPE_UNSPECIFIED = 0;
BEVERAGE_TYPE_BEER = 1;
BEVERAGE_TYPE_VINE = 2;
BEVERAGE_TYPE_VODKA = 3;
BEVERAGE_TYPE_GIN = 4;
BEVERAGE_TYPE_RUM = 5;
}
message beverageMainAttributes {
BeverageType type = 1;
string name = 2;
int32 volume = 3;
}
message CreateBeverageRequest {
beverageMainAttributes attr = 1;
}
message Beverage {
uint64 id = 1;
beverageMainAttributes attr = 2;
int32 price = 3;
}
message GetBeveragesParams {
// Implement here getting users rules
}
message BeverageList {
repeated Beverage beverages = 1;
} |
'use client';
import { BarChart, Compass, Layout, List } from 'lucide-react';
import { SidebarItems } from './sidebar-item';
import { usePathname } from 'next/navigation';
const guestsRoutes = [
{
icon: Layout,
label: 'Dashboard',
href: '/',
},
{
icon: Compass,
label: 'Browse',
href: '/search',
},
];
const teacherRoutes = [
{
icon: List,
label: 'Courses',
href: '/teacher/courses',
},
{
icon: BarChart,
label: 'Analytics',
href: '/teacher/analytics',
},
];
export const SidebarRoutes = () => {
const pathName = usePathname();
const isTeacher = pathName?.includes('/teacher');
const routes = isTeacher ? teacherRoutes : guestsRoutes;
return (
<div className="flex flex-col w-full">
{routes.map(({ icon, label, href }) => (
<SidebarItems key={href} icon={icon} label={label} href={href} />
))}
</div>
);
}; |
import torch
import torch.nn as nn
import torch.nn.functional as F
# from torch.tensor import Tensor
from utils.test_env import EnvTest
from q4_schedule import LinearExploration, LinearSchedule
from core.deep_q_learning_torch import DQN
from configs.q6_nature import config
class NatureQN(DQN):
"""
Implementing DeepMind's Nature paper. Here are the relevant urls.
https://storage.googleapis.com/deepmind-data/assets/papers/DeepMindNature14236Paper.pdf
Model configuration can be found in the Methods section of the above paper.
"""
def initialize_models(self):
"""Creates the 2 separate networks (Q network and Target network). The input
to these models will be an img_height * img_width image
with channels = n_channels * self.config.state_history
1. Set self.q_network to be a model with num_actions as the output size
2. Set self.target_network to be the same configuration self.q_network but initialized from scratch
3. What is the input size of the model?
To simplify, we specify the paddings as:
(stride - 1) * img_height - stride + filter_size) // 2
Hints:
1. Simply setting self.target_network = self.q_network is incorrect.
2. The following functions might be useful
- nn.Sequential
- nn.Conv2d
- nn.ReLU
- nn.Flatten
- nn.Linear
"""
self.input_shape = list(self.env.observation_space.shape)
state_shape = self.input_shape
img_height, img_width, n_channels = state_shape
# print("img_height",img_height)
# print("img_width",img_width)
# print("n_channels",n_channels)
# print("n_channels * self.config.state_history",n_channels * self.config.state_history)
num_actions = self.env.action_space.n
##############################################################
################ YOUR CODE HERE - 25-30 lines lines ################
# 创建Q网络
self.q_network = nn.Sequential(
nn.Conv2d(in_channels=n_channels * self.config.state_history, out_channels=32, kernel_size=8, stride=4,
padding=((4 - 1) * img_height - 4 + 8) // 2),
nn.ReLU(),
nn.Conv2d(in_channels=32, out_channels=64, kernel_size=4, stride=2,
padding=((2 - 1) * img_height - 2 + 4) // 2),
nn.ReLU(),
nn.Conv2d(in_channels=64, out_channels=64, kernel_size=3, stride=1,
padding=((1 - 1) * img_height - 1 + 3) // 2),
nn.ReLU(),
nn.Flatten(),
nn.Linear(64 * img_height * img_width, 512),
nn.ReLU(),
nn.Linear(in_features=512, out_features=num_actions)
)
# 创建Target网络,与Q网络具有相同的配置但从头开始初始化
self.target_network = nn.Sequential(
nn.Conv2d(in_channels=n_channels * self.config.state_history, out_channels=32, kernel_size=8, stride=4,
padding=((4 - 1) * img_height - 4 + 8) // 2),
nn.ReLU(),
nn.Conv2d(in_channels=32, out_channels=64, kernel_size=4, stride=2,
padding=((2 - 1) * img_height - 2 + 4) // 2),
nn.ReLU(),
nn.Conv2d(in_channels=64, out_channels=64, kernel_size=3, stride=1,
padding=((1 - 1) * img_height - 1 + 3) // 2),
nn.ReLU(),
nn.Flatten(),
nn.Linear(64 * img_height * img_width, 512),
nn.ReLU(),
nn.Linear(in_features=512, out_features=num_actions)
)
# 模型的输入大小为img_height * img_width的图像,通道数为n_channels * self.config.state_history
input_size = (img_height, img_width, n_channels * self.config.state_history)
return input_size
##############################################################
######################## END YOUR CODE #######################
def feature_size(self):
# print(self.q_network.conv3(self.q_network.conv2(self.q_network.conv1(torch.zeros(1, *self.input_shape)))).view(1, -1).size(1))
return self.q_network.conv3(self.q_network.conv2(self.q_network.conv1(torch.zeros(1, *self.input_shape)))).view(1, -1).size(1)
def get_q_values(self, state, network):
"""
Returns Q values for all actions
Args:
state: (torch tensor)
shape = (batch_size, img height, img width, nchannels x config.state_history)
network: (str)
The name of the network, either "q_network" or "target_network"
Returns:
out: (torch tensor) of shape = (batch_size, num_actions)
Hint:
1. What are the input shapes to the network as compared to the "state" argument?
2. You can forward a tensor through a network by simply calling it (i.e. network(tensor))
"""
out = None
##############################################################
################ YOUR CODE HERE - 4-5 lines lines ################
state = state.permute(0,3,1,2)
# print(f'Input shape after flattening = {input.shape}')
if network == 'q_network':
out = self.q_network(state)
elif network == 'target_network':
out = self.target_network(state)
##############################################################
######################## END YOUR CODE #######################
return out
def update_target(self):
"""
update_target_op will be called periodically
to copy Q network weights to target Q network
Remember that in DQN, we maintain two identical Q networks with
2 different sets of weights.
Periodically, we need to update all the weights of the Q network
and assign them with the values from the regular network.
Hint:
1. look up saving and loading pytorch models
"""
##############################################################
################### YOUR CODE HERE - 1-2 lines ###############
self.target_network.load_state_dict(self.q_network.state_dict())
##############################################################
######################## END YOUR CODE #######################
# def calc_loss(self, q_values : Tensor, target_q_values : Tensor,
# actions : Tensor, rewards: Tensor, done_mask: Tensor) -> Tensor:
def calc_loss(self, q_values, target_q_values,
actions, rewards, done_mask):
"""
Calculate the MSE loss of this step.
The loss for an example is defined as:
Q_samp(s) = r if done
= r + gamma * max_a' Q_target(s', a')
loss = (Q_samp(s) - Q(s, a))^2
Args:
q_values: (torch tensor) shape = (batch_size, num_actions)
The Q-values that your current network estimates (i.e. Q(s, a') for all a')
target_q_values: (torch tensor) shape = (batch_size, num_actions)
The Target Q-values that your target network estimates (i.e. (i.e. Q_target(s', a') for all a')
actions: (torch tensor) shape = (batch_size,)
The actions that you actually took at each step (i.e. a)
rewards: (torch tensor) shape = (batch_size,)
The rewards that you actually got at each step (i.e. r)
done_mask: (torch tensor) shape = (batch_size,)
A boolean mask of examples where we reached the terminal state
Hint:
You may find the following functions useful
- torch.max
- torch.sum
- torch.nn.functional.one_hot
- torch.nn.functional.mse_loss
"""
# you may need this variable
num_actions = self.env.action_space.n
gamma = self.config.gamma
##############################################################
##################### YOUR CODE HERE - 3-5 lines #############
q_samp = rewards + gamma * torch.max(target_q_values, dim=1)[0] * (~done_mask)
actions = actions.to(torch.int64)
q_values = q_values.gather(1, actions.unsqueeze(1)).squeeze(1)
loss = torch.nn.functional.mse_loss(q_samp, q_values)
return loss
##############################################################
######################## END YOUR CODE #######################
def add_optimizer(self):
"""
Set self.optimizer to be an Adam optimizer optimizing only the self.q_network
parameters
Hint:
- Look up torch.optim.Adam
- What are the input to the optimizer's constructor?
"""
##############################################################
#################### YOUR CODE HERE - 1 line #############
self.optimizer = torch.optim.Adam(self.q_network.parameters(), lr=0.00025)
# self.optimizer = torch.optim.Adam(self.q_network.parameters(), lr=1e-6)
##############################################################
######################## END YOUR CODE #######################
"""
Use deep Q network for test environment.
"""
if __name__ == '__main__':
env = EnvTest((8, 8, 6))
# exploration strategy
exp_schedule = LinearExploration(env, config.eps_begin,
config.eps_end, config.eps_nsteps)
# learning rate schedule
lr_schedule = LinearSchedule(config.lr_begin, config.lr_end,
config.lr_nsteps)
# train model
model = NatureQN(env, config)
model.run(exp_schedule, lr_schedule) |
# Copyright (C) Internet Systems Consortium, Inc. ("ISC")
#
# SPDX-License-Identifier: MPL-2.0
#
# This Source Code Form is subject to the terms of the Mozilla Public
# License, v. 2.0. If a copy of the MPL was not distributed with this
# file, you can obtain one at https://mozilla.org/MPL/2.0/.
#
# See the COPYRIGHT file distributed with this work for additional
# information regarding copyright ownership.
import logging
from pathlib import Path
from typing import Dict, Optional
CONFTEST_LOGGER = logging.getLogger("conftest")
LOG_FORMAT = "%(asctime)s %(levelname)7s:%(name)s %(message)s"
LOGGERS = {
"conftest": None,
"module": None,
"test": None,
} # type: Dict[str, Optional[logging.Logger]]
def init_conftest_logger():
"""
This initializes the conftest logger which is used for pytest setup
and configuration before tests are executed -- aka any logging in this
file that is _not_ module-specific.
"""
LOGGERS["conftest"] = logging.getLogger("conftest")
LOGGERS["conftest"].setLevel(logging.DEBUG)
file_handler = logging.FileHandler("pytest.conftest.log.txt")
file_handler.setFormatter(logging.Formatter(LOG_FORMAT))
LOGGERS["conftest"].addHandler(file_handler)
def avoid_duplicated_logs():
"""
Remove direct root logger output to file descriptors.
This default is causing duplicates because all our messages go through
regular logging as well and are thus displayed twice.
"""
todel = []
for handler in logging.root.handlers:
if handler.__class__ == logging.StreamHandler:
# Beware: As for pytest 7.2.2, LiveLogging and LogCapture
# handlers inherit from logging.StreamHandler
todel.append(handler)
for handler in todel:
logging.root.handlers.remove(handler)
def init_module_logger(system_test_name: str, testdir: Path):
logger = logging.getLogger(system_test_name)
logger.handlers.clear()
logger.setLevel(logging.DEBUG)
handler = logging.FileHandler(testdir / "pytest.log.txt", mode="w")
handler.setFormatter(logging.Formatter(LOG_FORMAT))
logger.addHandler(handler)
LOGGERS["module"] = logger
def deinit_module_logger():
for handler in LOGGERS["module"].handlers:
handler.flush()
handler.close()
LOGGERS["module"] = None
def init_test_logger(system_test_name: str, test_name: str):
LOGGERS["test"] = logging.getLogger(f"{system_test_name}.{test_name}")
def deinit_test_logger():
LOGGERS["test"] = None
def log(lvl: int, msg: str, *args, **kwargs):
"""Log message with the most-specific logger currently available."""
logger = LOGGERS["test"]
if logger is None:
logger = LOGGERS["module"]
if logger is None:
logger = LOGGERS["conftest"]
assert logger is not None
logger.log(lvl, msg, *args, **kwargs)
def debug(msg: str, *args, **kwargs):
log(logging.DEBUG, msg, *args, **kwargs)
def info(msg: str, *args, **kwargs):
log(logging.INFO, msg, *args, **kwargs)
def warning(msg: str, *args, **kwargs):
log(logging.WARNING, msg, *args, **kwargs)
def error(msg: str, *args, **kwargs):
log(logging.ERROR, msg, *args, **kwargs)
def critical(msg: str, *args, **kwargs):
log(logging.CRITICAL, msg, *args, **kwargs) |
package com.sy.bhid.bk
import com.sy.bhid.utils.HidUtils
import android.annotation.SuppressLint
import android.bluetooth.BluetoothDevice
import android.bluetooth.BluetoothHidDevice
import android.bluetooth.BluetoothHidDeviceAppQosSettings
import android.bluetooth.BluetoothHidDeviceAppSdpSettings
import android.bluetooth.BluetoothManager
import android.bluetooth.BluetoothProfile
import android.content.Context
import android.util.Log
import com.sy.bhid.utils.Utils
import java.util.concurrent.Executors
class BluetoothClient private constructor(private val context: Context) :
BluetoothHidDevice.Callback() {
private var btHid: BluetoothHidDevice? = null
private val bluetoothDevice: BluetoothDevice? = null
private var hostDevice: BluetoothDevice? = null
private var mpluggedDevice: BluetoothDevice? = null
private val btAdapter = (context.getSystemService(Context.BLUETOOTH_SERVICE) as BluetoothManager).adapter
private val serviceListener: ServiceListener = ServiceListener()
private var listener: Listener? = null
private val sdpRecord = BluetoothHidDeviceAppSdpSettings(
HidUtils.NAME,
HidUtils.DESCRIPTION,
HidUtils.PROVIDER,
BluetoothHidDevice.SUBCLASS1_COMBO,
HidUtils.DESCRIPTOR
)
private val qosOut = BluetoothHidDeviceAppQosSettings(
BluetoothHidDeviceAppQosSettings.SERVICE_BEST_EFFORT,
800,
9,
0,
11250,
BluetoothHidDeviceAppQosSettings.MAX
)
interface Listener {
fun onConnected(name: String, mac: String)
fun onDisConnected()
}
init {
init()
}
@SuppressLint("MissingPermission")
private fun init() {
if (btHid != null) {
return
}
btAdapter.getProfileProxy(context, serviceListener, BluetoothProfile.HID_DEVICE)
}
companion object {
@SuppressLint("StaticFieldLeak")
private var instance: BluetoothClient? = null
fun bindContext(context: Context): BluetoothClient? {
if (instance != null) {
Utils.showLog("BluetoothClient already bind a Context")
return null
}
instance = BluetoothClient(context)
return instance
}
}
fun setListener(listener: Listener?) {
this.listener = listener
}
@SuppressLint("MissingPermission")
fun connect() {
btHid?.connect(mpluggedDevice)
}
@SuppressLint("MissingPermission")
fun sendData(id: Int, data: ByteArray?) {
btHid?.sendReport(hostDevice, id, data)
}
@SuppressLint("MissingPermission")
fun active() {
val status = btHid?.registerApp(
sdpRecord, null, qosOut,
Executors.newCachedThreadPool(), this@BluetoothClient
)
Utils.showLog(status.toString())
}
@SuppressLint("MissingPermission")
fun stop() {
btHid?.unregisterApp()
}
fun destory() {
btAdapter.closeProfileProxy(BluetoothProfile.HID_DEVICE, btHid)
}
@SuppressLint("MissingPermission")
private inner class ServiceListener : BluetoothProfile.ServiceListener {
override fun onServiceConnected(profile: Int, proxy: BluetoothProfile) {
Utils.showLog("Connected to service")
if (profile != BluetoothProfile.HID_DEVICE) {
Utils.showLog("WTF:$profile")
return
}
btHid = proxy as BluetoothHidDevice
active()
}
override fun onServiceDisconnected(profile: Int) {
Utils.showLog("Service disconnected!", level = Log.ERROR)
if (profile == BluetoothProfile.HID_DEVICE) {
btHid = null
}
}
}
@SuppressLint("MissingPermission")
override fun onConnectionStateChanged(device: BluetoothDevice, state: Int) {
super.onConnectionStateChanged(device, state)
Utils.showLog("onConnectionStateChanged:$device state:$state", level = Log.WARN)
when (state) {
BluetoothProfile.STATE_CONNECTED -> {
hostDevice = device
listener?.onConnected(device.name, device.address)
Utils.showLog("if connected")
}
else -> {
hostDevice = null
listener?.onDisConnected()
}
}
}
@SuppressLint("MissingPermission")
override fun onAppStatusChanged(pluggedDevice: BluetoothDevice?, registered: Boolean) {
super.onAppStatusChanged(pluggedDevice, registered)
if (pluggedDevice != null) {
Utils.showLog("onAppStatusChanged:" + pluggedDevice.getName() + " registered:" + registered, level = Log.DEBUG)
}
if (registered) {
val states = intArrayOf(
BluetoothProfile.STATE_DISCONNECTED,
BluetoothProfile.STATE_CONNECTING,
BluetoothProfile.STATE_CONNECTED,
BluetoothProfile.STATE_DISCONNECTING
)
val pairedDevices = btHid?.getDevicesMatchingConnectionStates(states)
Utils.showLog("paired devices: $pairedDevices")
mpluggedDevice = pluggedDevice
btHid?.apply {
val remoteDevice = btAdapter.getRemoteDevice("38:7A:0E:A4:05:78")
this.connect(remoteDevice)
// if (this.getConnectionState(pluggedDevice) == BluetoothProfile.STATE_DISCONNECTED)
// this.connect(pluggedDevice)
// else {
// val pairedDevice = pairedDevices?.get(0)
// val pairedDState = this.getConnectionState(pairedDevice)
// Utils.showLog("paired $pairedDState")
// if (pairedDState == BluetoothProfile.STATE_DISCONNECTED) {
// this.connect(pairedDevice)
// }
// }
}
}
}
} |
import { Modal, Popconfirm, Space, Table } from "antd";
import React, { useState } from "react";
import { toast } from "react-toastify";
import "react-toastify/dist/ReactToastify.css";
import { DeleteInsurance } from "../../../services/insurance";
import MyButton from "../../common/MyButton";
import EditInformation from "./edit-information/EditInformation";
import ViewInformation from "./view-information/ViewInformation";
const ResultTable = ({
rowSelection,
data,
allCompanyNames,
paramDiffNames,
loading,
getInsurance,
}) => {
const [isEditModalVisible, setIsEditModalVisible] = useState(false);
const [isViewModalVisible, setIsViewModalVisible] = useState(false);
const [curRecord, setCurRecord] = useState(null);
const handleEditCancel = () => {
setIsEditModalVisible(false);
};
const handleViewCancel = () => {
setIsViewModalVisible(false);
};
const handleConfirm = (id) => {
DeleteInsurance(id).then(() => {
toast.success("删除成功!");
getInsurance();
});
};
const columns = [
{
title: "序号",
dataIndex: "insurId",
key: "insurId",
width: 60,
},
{
title: "保险公司",
dataIndex: "companyId",
key: "companyId",
render: (record) =>
allCompanyNames.filter((_) => _.value === record)[0]?.label,
width: 100,
},
{
title: "险种代码",
dataIndex: "code",
key: "code",
width: 100,
},
{
title: "险种名称",
dataIndex: "insurFullName",
key: "insurFullName",
},
{
title: "险种简称",
dataIndex: "insurShortName",
key: "insurShortName",
},
{
title: "主附约",
dataIndex: "mainOrVice",
key: "mainOrVice",
width: 80,
},
{
title: "险种类别",
key: "insurType",
dataIndex: "insurType",
},
{
title: "启售日",
dataIndex: "startSaleTime",
key: "startSaleTime",
width: 120,
},
{
title: "停售日",
dataIndex: "stopSaleTime",
key: "stopSaleTime",
width: 120,
},
{
title: "操作",
key: "action",
width: 160,
render: (_, record) => (
<Space size="middle">
<a
className="text-blue-500"
onClick={() => {
setIsViewModalVisible(true);
setCurRecord(record);
}}
>
查看
</a>
<a
className="text-blue-500"
onClick={() => {
setIsEditModalVisible(true);
setCurRecord(record);
}}
>
编辑
</a>
<Popconfirm
title="删除"
description="确定要删除吗?"
onConfirm={() => {
handleConfirm(record.insurId);
}}
okType="default"
okText="是"
cancelText="否"
>
<a className="text-red-500">删除</a>
</Popconfirm>
</Space>
),
},
];
return (
<>
<Table
rowKey={"insurId"}
rowSelection={rowSelection}
loading={loading}
columns={columns}
dataSource={data}
scroll={{ y: 500 }}
pagination={{ pageSize: 5 }}
/>
{/* View Modal */}
<Modal
key={`view modal ${isViewModalVisible}`}
title={"险种信息详情"}
open={isViewModalVisible}
onCancel={handleViewCancel}
footer={[
<MyButton key="back" onClick={handleViewCancel}>
{"关闭"}
</MyButton>,
]}
>
<ViewInformation
record={curRecord}
allCompanyNames={allCompanyNames}
paramDiffNames={paramDiffNames}
/>
</Modal>
{/* Edit Modal */}
<Modal
key={`edit modal ${isEditModalVisible}`}
title={"编辑险种信息"}
open={isEditModalVisible}
onCancel={handleEditCancel}
footer={null}
>
<EditInformation
record={curRecord}
allCompanyNames={allCompanyNames}
paramDiffNames={paramDiffNames}
setIsModalVisible={setIsEditModalVisible}
getInsurance={getInsurance}
/>
</Modal>
</>
);
};
export default ResultTable; |
package bme.spoti.redflags.ui.ingame.screens.sabotage
import androidx.compose.animation.AnimatedVisibility
import androidx.compose.animation.core.animateFloatAsState
import androidx.compose.foundation.background
import androidx.compose.foundation.clickable
import androidx.compose.foundation.layout.*
import androidx.compose.material.MaterialTheme
import androidx.compose.material.Text
import androidx.compose.runtime.*
import androidx.compose.runtime.saveable.rememberSaveable
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.draw.alpha
import androidx.compose.ui.draw.clip
import androidx.compose.ui.res.colorResource
import androidx.compose.ui.text.style.TextAlign
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import bme.spoti.redflags.R
import bme.spoti.redflags.data.model.DoneIndicatorInfo
import bme.spoti.redflags.data.model.websocket.DateInfo
import bme.spoti.redflags.data.model.websocket.PlayerState
import bme.spoti.redflags.data.model.websocket.`in`.DateToSabotage
import bme.spoti.redflags.ui.common.views.PopUpFooter
import bme.spoti.redflags.ui.common.views.SmallProfilePicture
import bme.spoti.redflags.ui.ingame.component.TimeLeft
import bme.spoti.redflags.ui.theme.Colors
import bme.spoti.redflags.ui.theme.PaddingSizes
import bme.spoti.redflags.ui.theme.RedFlagsTheme
@Composable
fun DateToSabotageFooter(
personToSabotage: PlayerState?,
dateToSabotage: DateInfo?
) {
PopUpFooter(
extraVisibilityCondition = { dateToSabotage != null },
visible = { showAction ->
Row(
Modifier
.wrapContentHeight()
.padding(bottom = PaddingSizes.default)
.fillMaxWidth()
.background(Colors.Sand, MaterialTheme.shapes.large)
.clip(MaterialTheme.shapes.large)
.clickable {
showAction()
}
.padding(start = PaddingSizes.loose),
verticalAlignment = Alignment.CenterVertically
) {
SmallProfilePicture(url = personToSabotage?.imageURL)
personToSabotage?.username?.let { name ->
Text(
text = name,
style = MaterialTheme.typography.h2,
color = Colors.DarkRed,
modifier = Modifier.padding(start = 10.dp)
)
}
}
},
hidden = {
Column(
modifier = Modifier
.wrapContentHeight()
.fillMaxWidth()
.background(Colors.AlmostWhite, MaterialTheme.shapes.large)
.padding(
horizontal = PaddingSizes.loose,
vertical = PaddingSizes.looser
),
horizontalAlignment = Alignment.CenterHorizontally
) {
if (dateToSabotage?.positiveAttributes?.isNotEmpty() == true) {
Text(
text = dateToSabotage.positiveAttributes[0],
textAlign = TextAlign.Center,
color = Colors.DarkRed,
style = MaterialTheme.typography.body1
)
Text(
text = "&",
color = Colors.PrimaryRed,
style = MaterialTheme.typography.h2,
modifier = Modifier.padding(vertical = 2.dp)
)
Text(
text = dateToSabotage.positiveAttributes[1],
textAlign = TextAlign.Center,
color = colorResource(id = R.color.dark_red),
style = MaterialTheme.typography.body1
)
} else {
Text(
text = "This player did not hand in anything!\uD83E\uDD28",
textAlign = TextAlign.Center,
color = Colors.DarkRed,
style = MaterialTheme.typography.body1
)
}
}
})
} |
import React from 'react'
type Props = {
title: string
content: string
commentsQty: number
tags: string[]
}
const Destructuring = ({title, content, commentsQty, tags}: Props) => {
return (
<div>
<h2>{title}</h2>
<p>{content}</p>
<p>Quantidade de comentários: {commentsQty}</p>
<div>
{tags.map((tag)=>(
<span>#{tag} </span>
))}
</div>
</div>
)
}
export default Destructuring |
/*
* Copyright 2023 Roman Likhachev
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package ${packageName}
import androidx.compose.foundation.layout.Column
import androidx.compose.material3.SnackbarHostState
import androidx.compose.runtime.Composable
import androidx.compose.runtime.LaunchedEffect
import androidx.compose.runtime.getValue
import androidx.compose.ui.res.stringResource
import androidx.compose.ui.tooling.preview.PreviewParameter
import androidx.hilt.navigation.compose.hiltViewModel
import androidx.lifecycle.compose.collectAsStateWithLifecycle
import ${domainPackage}.model.${modelName}
import ${packageName}.${viewName}.Action
import ${packageName}.${viewName}.State.NavigationState
import ${packageName}.R
import ${uiKitPackage}.LoadingContent
import ${uiKitPackage}.WarningContent
import ${uiKitPackage}.common.ThemePreviews
import ${uiKitPackage}.component.QuizBackground
import ${uiKitPackage}.component.SimpleToolbar
import ${uiKitPackage}.theme.QuizApplicationTheme
import ${uiKitPackage}.R as UiKitR
@Composable
fun ${routeName}(
viewModel: ${viewModelName} = hiltViewModel(),
snackbarHostState: SnackbarHostState,
onBack: () -> Unit,
onNavigateToBrowser: (String) -> Unit
) {
val state by viewModel.state.collectAsStateWithLifecycle()
${screenName}(
uiState = state,
snackbarHostState = snackbarHostState,
onBackPressed = {
viewModel.onAction(Action.OnBackClicked)
},
onItemClicked = {
viewModel.onAction(Action.OnItemClicked(it))
},
onErrorDismissState = {
viewModel.onAction(Action.OnSnackbarDismissed)
},
onBack = onBack,
onNavigateToBrowser = onNavigateToBrowser,
onNavigationHandled = {
viewModel.onAction(Action.OnNavigationHandled)
},
)
}
@Composable
internal fun ${screenName}(
uiState: ${viewName}.State,
snackbarHostState: SnackbarHostState,
onBackPressed: () -> Unit,
onItemClicked: (${modelName}) -> Unit,
onErrorDismissState: () -> Unit,
onBack: () -> Unit,
onNavigateToBrowser: (String) -> Unit,
onNavigationHandled: () -> Unit,
) {
val errorMessage = stringResource(id = UiKitR.string.error_base)
LaunchedEffect(key1 = uiState.showErrorMessage) {
if (uiState.showErrorMessage) {
snackbarHostState.showSnackbar(message = errorMessage)
onErrorDismissState()
}
}
Column {
SimpleToolbar(
title = stringResource(id = R.string.${titleName}),
onBackPressed = onBackPressed,
)
when {
uiState.isLoading -> {
LoadingContent()
}
uiState.isWarning -> {
WarningContent()
}
else -> {
${contentName}(
item = uiState.item,
onItemClicked = onItemClicked,
)
}
}
}
NavigationHandler(
navigationState = uiState.navigationState,
onBack = onBack,
onNavigateToBrowser = onNavigateToBrowser,
onNavigationHandled = onNavigationHandled,
)
}
@Composable
internal fun ${contentName}(
item: ${modelName},
onItemClicked: (${modelName}) -> Unit,
) {}
@Composable
internal fun NavigationHandler(
navigationState: NavigationState?,
onBack: () -> Unit,
onNavigateToBrowser: (String) -> Unit,
onNavigationHandled: () -> Unit,
) {
LaunchedEffect(key1 = navigationState) {
when (navigationState) {
NavigationState.Back -> onBack()
is NavigationState.NavigateToExternalBrowser -> onNavigateToBrowser(navigationState.url)
null -> Unit
}
navigationState?.let { onNavigationHandled() }
}
}
@ThemePreviews
@Composable
private fun ContentPreview(
@PreviewParameter(${previewParameterProviderName}::class) item: ${modelName},
) {
QuizApplicationTheme {
QuizBackground {
${contentName}(
item = item,
onItemClicked = {},
)
}
}
} |
import React from 'react';
import { PAGES } from '../constants/PAGES';
import { useDispatch, useSelector } from 'react-redux';
import { selectPage } from '../utils/selectors';
import { definePage } from '../features/page.action';
export function SettingsSidebar() {
const dispatch = useDispatch();
const page = useSelector(selectPage());
const handlePageChange = (e) => {
if (e.currentTarget.dataset.page === page) return;
dispatch(definePage(e.currentTarget.dataset.page));
};
return <ul className='h-100 w-[140px] mr-3 flex flex-col flex-wrap border-r-2 border-solid border-gray-800'>
<li
className={'w-fit min-w-[71px] p-1 px-2 mb-1 text-sm text-gray-600 font-semibold cursor-pointer hover:text-white transition-all' + (page === PAGES.SETTINGS_ACCOUNT ? ' text-white' : '')}
onClick={handlePageChange} data-page={PAGES.SETTINGS_ACCOUNT}>Mon Compte</li>
<li
className={'w-fit min-w-[71px] p-1 px-2 mb-1 text-sm text-gray-600 font-semibold cursor-pointer hover:text-white transition-all' + (page === PAGES.SETTINGS_MINECRAFT ? ' text-white' : '')}
onClick={handlePageChange} data-page={PAGES.SETTINGS_MINECRAFT}>Lancement</li>
<li
className={'w-fit min-w-[71px] p-1 px-2 mb-1 text-sm text-gray-600 font-semibold cursor-pointer hover:text-white transition-all' + (page === PAGES.SETTINGS_MODS ? ' text-white' : '')}
onClick={handlePageChange} data-page={PAGES.SETTINGS_MODS}>Mods</li>
<li
className={'w-fit min-w-[71px] p-1 px-2 mb-1 text-sm text-gray-600 font-semibold cursor-pointer hover:text-white transition-all' + (page === PAGES.SETTINGS_JAVA ? ' text-white' : '')}
onClick={handlePageChange} data-page={PAGES.SETTINGS_JAVA}>Java</li>
<li
className={'w-fit min-w-[71px] p-1 px-2 mb-1 text-sm text-gray-600 font-semibold cursor-pointer hover:text-white transition-all' + (page === PAGES.SETTINGS_UPDATE ? ' text-white' : '')}
onClick={handlePageChange} data-page={PAGES.SETTINGS_UPDATE}>Mises à jour</li>
</ul>;
} |
package tests
import (
"bytes"
"context"
"encoding/json"
"fmt"
"net/http/httptest"
"testing"
"github.com/stretchr/testify/assert"
"github.com/volatiletech/sqlboiler/v4/boil"
"github.com/hyuabot-developers/hyuabot-backend-golang/database"
"github.com/hyuabot-developers/hyuabot-backend-golang/dto/responses"
"github.com/hyuabot-developers/hyuabot-backend-golang/models"
"github.com/hyuabot-developers/hyuabot-backend-golang/utils"
)
func TestSignUp(t *testing.T) {
setupDatabase()
test := assert.New(t)
testCases := []struct {
UserName string `json:"username"`
Password string `json:"password"`
Name string `json:"name"`
Email string `json:"email"`
Phone string `json:"phone"`
}{
// Provide invalid JSON
{
UserName: "test",
Password: "test",
Name: "test",
Email: "test@email.com",
},
// Provide successful request
{
UserName: "test",
Password: "test",
Name: "test",
Email: "test@email.com",
Phone: "010-1234-5678",
},
// Provide duplicated username
{
UserName: "test",
Password: "test",
Name: "test",
Email: "test@email.com",
Phone: "010-1234-5678",
},
}
expectedStatusCodes := []int{422, 201, 409}
for index, testCase := range testCases {
app := setup()
body, _ := json.Marshal(testCase)
req := httptest.NewRequest("POST", "/api/v1/auth/signup", bytes.NewBuffer(body))
req.Header.Set("Content-Type", "application/json")
response, _ := app.Test(req, 5000)
test.Equal(expectedStatusCodes[index], response.StatusCode)
}
tearDownDatabase()
}
func TestLogin(t *testing.T) {
setupDatabase()
// Insert test user
hashedPassword, _ := utils.HashPassword("test")
user := models.AdminUser{
UserID: "test",
Password: hashedPassword,
Name: "test",
Email: "test@email.com",
Phone: "010-1234-5678",
Active: false,
}
ctx := context.Background()
_ = user.Insert(ctx, database.DB, boil.Infer())
// Test login
test := assert.New(t)
testCases := []struct {
UserName string `json:"username"`
Password string `json:"password"`
}{
{
UserName: "test",
Password: "test",
},
{
UserName: "test",
Password: "test",
},
{
UserName: "test2",
Password: "test",
},
{
UserName: "test",
Password: "test2",
},
}
expectedStatusCodes := []int{401, 200, 401, 401}
for index, testCase := range testCases {
if index == 1 {
// Activate test user
user.Active = true
_, _ = user.Update(ctx, database.DB, boil.Infer())
}
app := setup()
body, _ := json.Marshal(testCase)
req := httptest.NewRequest("POST", "/api/v1/auth/login", bytes.NewBuffer(body))
req.Header.Set("Content-Type", "application/json")
response, _ := app.Test(req, 5000)
test.Equal(expectedStatusCodes[index], response.StatusCode)
test.Equal("application/json", response.Header.Get("Content-Type"))
if response.StatusCode == 200 {
var result responses.TokenResponse
_ = json.NewDecoder(response.Body).Decode(&result)
test.NotEmpty(result.AccessToken)
test.NotEmpty(result.RefreshToken)
} else {
var result responses.ErrorResponse
_ = json.NewDecoder(response.Body).Decode(&result)
test.NotEmpty(result.Message)
test.Equal("INVALID_LOGIN_CREDENTIALS", result.Message)
}
}
tearDownDatabase()
}
func TestLogout(t *testing.T) {
setupDatabase()
// Insert test user
hashedPassword, _ := utils.HashPassword("test")
user := models.AdminUser{
UserID: "test",
Password: hashedPassword,
Name: "test",
Email: "test@email.com",
Phone: "010-1234-5678",
Active: true,
}
ctx := context.Background()
_ = user.Insert(ctx, database.DB, boil.Infer())
// Test logout
test := assert.New(t)
testCase := struct {
UserName string `json:"username"`
Password string `json:"password"`
}{
UserName: "test",
Password: "test",
}
// Login
app := setup()
body, _ := json.Marshal(testCase)
req := httptest.NewRequest("POST", "/api/v1/auth/login", bytes.NewBuffer(body))
req.Header.Set("Content-Type", "application/json")
response, _ := app.Test(req, 5000)
test.Equal(200, response.StatusCode)
test.Equal("application/json", response.Header.Get("Content-Type"))
var result responses.TokenResponse
_ = json.NewDecoder(response.Body).Decode(&result)
// Logout
req = httptest.NewRequest("POST", "/api/v1/auth/logout", bytes.NewBuffer(body))
req.Header.Set("Content-Type", "application/json")
req.Header.Set("Authorization", fmt.Sprintf("Bearer %s", result.AccessToken))
response, _ = app.Test(req, 5000)
test.Equal(200, response.StatusCode)
test.Equal("application/json", response.Header.Get("Content-Type"))
var successRes responses.SuccessResponse
_ = json.NewDecoder(response.Body).Decode(&successRes)
test.NotEmpty(successRes.Message)
test.Equal("LOGGED_OUT", successRes.Message)
// Logout again
req = httptest.NewRequest("POST", "/api/v1/auth/logout", bytes.NewBuffer(body))
req.Header.Set("Content-Type", "application/json")
req.Header.Set("Authorization", fmt.Sprintf("Bearer %s", result.AccessToken))
response, _ = app.Test(req, 5000)
test.Equal(401, response.StatusCode)
test.Equal("application/json", response.Header.Get("Content-Type"))
var errorRes responses.ErrorResponse
_ = json.NewDecoder(response.Body).Decode(&errorRes)
test.NotEmpty(errorRes.Message)
test.Equal("UNAUTHORIZED", errorRes.Message)
tearDownDatabase()
}
func TestRefresh(t *testing.T) {
setupDatabase()
// Insert test user
hashedPassword, _ := utils.HashPassword("test")
user := models.AdminUser{
UserID: "test",
Password: hashedPassword,
Name: "test",
Email: "test@email.com",
Phone: "010-1234-5678",
Active: true,
}
ctx := context.Background()
_ = user.Insert(ctx, database.DB, boil.Infer())
// Test logout
test := assert.New(t)
testCase := struct {
UserName string `json:"username"`
Password string `json:"password"`
}{
UserName: "test",
Password: "test",
}
// Login
app := setup()
body, _ := json.Marshal(testCase)
req := httptest.NewRequest("POST", "/api/v1/auth/login", bytes.NewBuffer(body))
req.Header.Set("Content-Type", "application/json")
response, _ := app.Test(req, 5000)
test.Equal(200, response.StatusCode)
test.Equal("application/json", response.Header.Get("Content-Type"))
var result responses.TokenResponse
_ = json.NewDecoder(response.Body).Decode(&result)
// Refresh
tokenRequest := struct {
RefreshToken string `json:"refreshToken"`
}{
RefreshToken: result.RefreshToken,
}
body, _ = json.Marshal(tokenRequest)
req = httptest.NewRequest("POST", "/api/v1/auth/refresh", bytes.NewBuffer(body))
req.Header.Set("Content-Type", "application/json")
req.Header.Set("Authorization", fmt.Sprintf("Bearer %s", result.AccessToken))
response, _ = app.Test(req, 5000)
test.Equal(201, response.StatusCode)
test.Equal("application/json", response.Header.Get("Content-Type"))
var tokenRes responses.TokenResponse
_ = json.NewDecoder(response.Body).Decode(&tokenRes)
test.NotEmpty(tokenRes.AccessToken)
test.NotEmpty(tokenRes.RefreshToken)
tearDownDatabase()
} |
import clsx from "clsx";
import { ComponentPropsWithRef, ReactNode, forwardRef } from "react";
export interface ButtonProps extends ComponentPropsWithRef<"button"> {
variant?: "solid" | "outline" | "ghost" | "link";
/**
* Used for buttons which initiate an asynchronous action
* This can set a loading state and set the button to be busy
*/
isLoading?: boolean;
/**
* When `isLoading` is true, this will be the content within the button
*/
loadingText?: ReactNode;
/**
* @default "md"
*/
size?: "sm" | "md" | "lg";
}
const Button = forwardRef<HTMLButtonElement, ButtonProps>(
(
{
isLoading = false,
loadingText,
variant,
"aria-busy": ariaBusy,
disabled,
children,
className,
size = "md",
...props
},
ref,
) => {
return (
<button
{...props}
disabled={isLoading || disabled}
aria-busy={isLoading || ariaBusy}
className={clsx(
className,
"rounded",
"transition-all",
"text-white",
"bg-cyan-500",
"disabled:opacity-50",
"disabled:cursor-not-allowed",
{
"opacity-50": isLoading,
"px-4 py-2": size === "md",
"px-2 py-1": size === "sm",
},
)}
ref={ref}
>
{isLoading ? loadingText : children}
</button>
);
},
);
Button.displayName = "Button";
export default Button; |
package com.example.demo.service;
import com.example.demo.assembler.SalesAssembler;
import com.example.demo.domain.Client;
import com.example.demo.domain.Product;
import com.example.demo.domain.Sales;
import com.example.demo.dto.SaleDto;
import com.example.demo.dto.SaleReturnDto;
import com.example.demo.repository.ClientRepository;
import com.example.demo.repository.ProductRepository;
import com.example.demo.repository.SalesRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.PageRequest;
import org.springframework.http.HttpStatus;
import org.springframework.stereotype.Service;
import org.springframework.web.client.HttpClientErrorException;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
@Service
public class SaleService {
@Autowired
private ClientRepository clientRepository;
@Autowired
private ProductRepository productRepository;
@Autowired
private SalesRepository salesRepository;
@Autowired
private SalesAssembler assembler;
public SaleReturnDto sale(SaleDto dto) {
Optional<Client> client = clientRepository.findByCpf(dto.getClientCpf());
if (!client.isPresent()) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Client not found");
}
Optional<Product> product = productRepository.findByName(dto.getProduct());
if (!client.isPresent()) {
throw new HttpClientErrorException(HttpStatus.BAD_REQUEST, "Product not found");
}
salesRepository.save(assembler.tranformSale(dto, product.get().getId()));
return findProductsIndication(dto);
}
public List<SaleDto> findLastPurchases(String cpf) {
List<SaleDto> dtos = new ArrayList<>();
List<Sales> salesList = salesRepository.findByClientCpfOrderByCreatedAtDesc(cpf, PageRequest.of(0, 5));
for (Sales sale: salesList) {
Optional<Product> product = productRepository.findById(sale.getProductId());
Optional<Client> client = clientRepository.findByCpf(sale.getClientCpf());
SaleDto dto = assembler.buildSaleDto(sale, product.get(), client.get());
dtos.add(dto);
}
return dtos;
}
private SaleReturnDto findProductsIndication(SaleDto dto) {
Sales sale = salesRepository.findByMorePurchasedByCity(dto.getClientCity());
Optional<Product> product = productRepository.findById(sale.getProductId());
return new SaleReturnDto(product.get().getName());
}
} |
/*
* The MIT License (MIT)
*
* Copyright (c) 2017 Jakob Hendeß
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package org.xlrnet.metadict.core.services.aggregation.group;
import com.google.common.base.MoreObjects;
import org.xlrnet.metadict.core.api.aggregation.Group;
import java.util.Iterator;
import java.util.List;
/**
* Immutable implementation of {@link Group}.
*/
public class ImmutableGroup<T> implements Group<T> {
private static final long serialVersionUID = -3599681937843767303L;
private final String groupIdentifier;
private final List<T> resultEntries;
ImmutableGroup(String groupIdentifier, List<T> elements) {
this.groupIdentifier = groupIdentifier;
this.resultEntries = elements;
}
/**
* Returns a string representation of the group identifier. For example if the group is based on entry types the
* identifier might be "NOUNS" for a group that contains only nouns.
*
* @return a string representation of the group identifier.
*/
@Override
public String getGroupIdentifier() {
return this.groupIdentifier;
}
/**
* Returns the entries of this group.
*
* @return the entries of this group.
*/
@Override
public List<T> getResultEntries() {
return this.resultEntries;
}
/**
* Returns an iterator over elements of type {@code T}.
*
* @return an Iterator.
*/
@Override
public Iterator<T> iterator() {
return this.resultEntries.iterator();
}
@Override
public String toString() {
return MoreObjects.toStringHelper(this)
.add("groupIdentifier", this.groupIdentifier)
.add("elements", this.resultEntries)
.toString();
}
} |
<template>
<li class="cart__item product">
<div class="product__pic">
<img :src="item.product.image.file.url" width="120" height="120" :alt="item.product.title" />
</div>
<h3 class="product__title">{{ item.product.title }}</h3>
<p class="product__info product__info--color">
<span>
<!-- <i :style="`background-color: ???`" class="bordered"> </i> -->
</span>
</p>
<span class="product__code"> Артикул: {{ item.product.id }} </span>
<ProductInput v-model="amount" />
<b class="product__price"> <AnimatedNumber :number="item.product.price * item.amount" /> ₽ </b>
<button
class="product__del button-del"
type="button"
aria-label="Удалить товар из корзины"
@click.prevent="deleteProduct"
>
<svg width="20" height="20" fill="currentColor">
<use xlink:href="#icon-close"></use>
</svg>
</button>
</li>
</template>
<script>
import ProductInput from '@/components/ProductInput.vue';
import { getBgColorHEX, numberFormat } from '@/helpers/custom_function';
import { mapActions } from 'vuex';
import AnimatedNumber from '@/components/AnimatedNumber.vue';
export default {
components: {
ProductInput,
AnimatedNumber,
},
filters: {
numberFormat,
},
props: {
item: {
type: Object,
required: true,
},
},
computed: {
amount: {
get() {
return this.item.amount;
},
set(value) {
this.updateCartProductAmound({
productId: this.item.product.id,
amount: value,
});
},
},
},
methods: {
getBgColorHEX,
...mapActions(['updateCartProductAmound', 'deleteCartProduct']),
deleteProduct() {
this.deleteCartProduct({ productId: this.item.product.id });
},
},
};
</script>
<style scoped>
.button-del {
cursor: pointer;
}
.bordered {
border: 1px solid lightgray;
}
.product__price {
margin: 0 auto;
}
</style> |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<!-- REACT LIBRARY -->
<script src="https://unpkg.com/react@15.5.4/dist/react.js"></script>
<!-- REACT DOM LIBRARY -->
<script src="https://unpkg.com/react-dom@15.5.4/dist/react-dom.js"></script>
<!-- BABEL LIBRARY -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/babel-standalone/6.25.0/babel.min.js"></script>
<title>Meal Voter</title>
</head>
<body>
<div id="app">React has not rendered yet</div>
<script type="text/babel">
//CREATE DinnerVote class component
class DinnerVote extends React.Component {
constructor(props) {
super(props);
this.state = {
votes: 0
};
/*
this.add = this.add.bind(this);
this.minus = this.minus.bind(this);
*/
}
add() {
this.setState({
votes: this.state.votes + 1
});
}
minus() {
this.setState({
votes: this.state.votes - 1
});
}
render() {
var dinnerStyle = {
display: "inline-block",
margin: 20,
height: 500,
width: 300,
padding: 0,
textAlign: "center",
backgroundColor: "#fff",
WebkitFilter: "drop-shadow(0px 0px 5px #555)",
filter: "drop-shadow(0px 0px 5px #555)"
};
var imgStyle = {
width: 200,
height: 200,
marginTop: 20
}
return(
<div style={dinnerStyle}>
<img src={this.props.img} style={imgStyle} alt="food image" />
<h1>Dinner Time</h1>
<p>Vote Here If You Want This</p>
<button onClick={() => this.add()}>+1</button>
<p>Vote Here If You Do Not Want This</p>
<button onClick={() => this.minus()}>-1</button>
<p>Total: {this.state.votes}</p>
</div>
);
}
}
ReactDOM.render(
<div>
<DinnerVote img="https://static.pexels.com/photos/46239/salmon-dish-food-meal-46239.jpeg"/>
<DinnerVote img="https://static.pexels.com/photos/58722/pexels-photo-58722.jpeg"/>
<DinnerVote img="https://static.pexels.com/photos/70497/pexels-photo-70497.jpeg"/>
<br /><br /><br />
<h3><a href="https://github.com/Usheninte/meal-voter">View on Github</a></h3>
</div>,
document.getElementById("app")
);
</script>
<!-- Github Pages LINK === https://usheninte.github.io/meal-voter/index.html -->
</body>
</html> |
// @ts-nocheck
import axios, { AxiosError } from "axios";
import { BASE_URL } from "@constants/config";
import { getItem } from "@utils/storage";
import { logout } from "@actions/authAction";
import { store } from "@store";
import { navigate } from "@navigation";
import ROUTES from "@navigation/Routes";
export const getError = (error: AxiosError | any) => {
let message = "Failed to load";
if (error.response) {
// The request was made and the server responded with a status code
// that falls out of the range of 2xx
console.log("error res", error.response.data);
const serverMsg = error.response.data.length
? error.response.data[0]?.message
: error.response.data.message;
if (serverMsg) {
message = serverMsg;
}
} else if (error.request) {
// The request was made but no response was received
// `error.request` is an instance of XMLHttpRequest in the browser and an instance of
// http.ClientRequest in node.js
console.log(error.request);
} else {
// Something happened in setting up the request that triggered an Error
console.log("Error", error.message);
}
if (typeof message === "string") {
return message;
} else if (message.length !== 0) {
const msgObj = message[0];
if (msgObj && msgObj.messages) {
return msgObj.messages[0].message;
}
}
};
// Axios instance
const API = axios.create({
baseURL: BASE_URL,
headers: { "Content-Type": "application/json" },
});
// Request interceptor
API.interceptors.request.use(
async (config) => {
const token = await getItem("jwt");
if (token) {
config.headers.Authorization = `Bearer ${token}`;
}
return config;
},
(error) => {
return Promise.reject(error);
}
);
// Response interceptor
API.interceptors.response.use(
(res) => {
return res;
},
async (error) => {
// User Not Found
if (error.response.data.statusCode === 401) {
await store.dispatch(logout());
navigate(ROUTES.HOME_TAB);
}
return Promise.reject(error);
}
);
export default API; |
import React, { useEffect, useState } from 'react';
import { Link, useNavigate, useParams } from 'react-router-dom';
import { useQuery } from '@tanstack/react-query';
import useAxiosPrivate from '../../Hooks/useAxiosPrivate';
import { useDispatch, useSelector } from 'react-redux';
import { selectSelectedCategory } from '../../Store/reducers/SelectedCategory/selectedCategory.selector';
import { Breadcrumbs, Image, Select } from '@mantine/core';
import { formatNumber } from '../../Lib/Utils';
import { Check, MapPin } from 'lucide-react';
import { addToCart, removeFromCart } from '../../Store/reducers/cartReducer/cartReducer';
import { notifications } from '@mantine/notifications';
import { selectCartItems } from '../../Store/reducers/cartReducer/cart.selector';
import { endLoading, startLoading } from '../../Store/reducers/globalLoader/loaderSlice';
const ProductPage = () => {
const { id } = useParams();
const dispatch = useDispatch();
const navigate = useNavigate();
const axios = useAxiosPrivate();
const cartItems = useSelector(selectCartItems);
const selectedCategory = useSelector(selectSelectedCategory);
const [selectedQuantity, setSelectedQuantity] = useState({ label: `Quantity: 1`, value: `1` });
const { data: productData, isFetching: isLoading } = useQuery({
queryKey: [id],
queryFn: fetchProductData,
staleTime: Infinity,
retryDelay: Infinity,
refetchOnWindowFocus: false,
});
async function fetchProductData() {
const { data, status } = await axios.get(`products?id=${id}`);
if (status) {
return data?.data;
}
}
const breadCrumData = [
{
title: selectedCategory?.label,
to: -1,
},
{
title: productData?.name,
to: '#',
},
];
const quantityOptions = Array.from({ length: 50 }).map((_, i) => ({
label: `Quantity: ${i + 1}`,
value: `${i + 1}`,
}));
const isInCart = () => {
return cartItems.find(({ item }) => item._id === id) !== undefined;
};
const addToCartHandler = () => {
if (selectedQuantity && selectedQuantity?.value) {
const payload = {
item: productData,
quantity: selectedQuantity?.value,
};
dispatch(addToCart(payload));
notifications.show({
id: 'addedToCart',
withCloseButton: true,
autoClose: 2000,
title: <h4 className="font-bold text-lg capitalize">{productData.name}</h4>,
message: <p className="text-base">Added item to cart!</p>,
color: 'yellow',
icon: <Check size={40} className="p-1" key={'addedToCart'} />,
loading: false,
});
}
};
const removeFromCartHandler = () => {
if (selectedQuantity && selectedQuantity?.value) {
const payload = {
item: productData,
};
dispatch(removeFromCart(payload));
notifications.show({
id: 'removeFromCart',
withCloseButton: true,
autoClose: 2000,
title: <h4 className="font-bold text-lg capitalize">{productData.name}</h4>,
message: <p className="text-base">Removed from the cart!</p>,
color: 'yellow',
icon: <Check size={40} className="p-1" key={'removeFromCart'} />,
loading: false,
});
}
};
const buyNowHandler = () => {
addToCartHandler();
return navigate('/checkout');
};
useEffect(() => {
if (isLoading) {
dispatch(startLoading());
} else {
dispatch(endLoading());
}
}, [isLoading]);
return (
<section className="w-full">
<div className="mx-4 pt-2">
<Breadcrumbs separator=">" separatorMargin="xs">
{breadCrumData.map(({ title, to }, i) => (
<Link key={i} className="text-gray-500 text-sm capitalize" to={to}>
{title}
</Link>
))}
</Breadcrumbs>
</div>
<div className="grid lg:grid-cols-6 w-full p-4">
<div className="lg:col-span-2 rounded-md overflow-hidden w-full h-full">
<Image className="object-cover" src={productData?.image} />
</div>
<div className="lg:col-span-3 p-3 px-8 flex flex-col [&>*]:border-b [&>*]:border-gray-300 [&>*]:p-2">
<h3 className="text-3xl capitalize">{productData?.name}</h3>
<p>Ratings: 5 Stars | 1 Rating</p>
<p className="font-semibold text-lg">About this item:</p>
<p>Material: {productData?.productMaterial}</p>
<p className="border-none">Description:</p>
<p>{productData?.productDescription}</p>
</div>
<div className="flex flex-col gap-2 py-4 border-2 rounded-md shadow-md p-3">
<div className="flex gap-1">
<p className="text-xs mt-1">{productData?.currency?.code}</p>
<p className="text-3xl tracking-widest font-semibold">{`${productData?.currency?.symbol}${formatNumber(productData?.price)}`}</p>
</div>
<div className="flex min-w-fit rounded-sm pt-2 p-1 gap-1 cursor-pointer">
<MapPin size={20} className="self-center" />
<div className="flex flex-col items-center leading-3 text-blue-500">
<Link className="hover:text-amazon_yellow text-xs pr-3">
{'Deliver to Jishan - Ghaziabad 201007'}
</Link>
</div>
</div>
<div>
<p>
{productData?.stock > 0 ? (
<span className="text-2xl text-green-700">In stock</span>
) : (
<span className="text-2xl text-red-500">Out of stock</span>
)}
</p>
</div>
<div>
<Select
data={quantityOptions}
value={selectedQuantity?.value || null}
onChange={(_, opt) => setSelectedQuantity(opt)}
placeholder="Select a quantity"
/>
</div>
<div className="my-2 flex flex-col gap-3">
<button
onClick={isInCart() === true ? removeFromCartHandler : addToCartHandler}
disabled={!selectedQuantity}
className="w-full bg-amazon_yellow_dark rounded-full disabled:cursor-not-allowed disabled:opacity-80 hover:opacity-90 p-1"
>
{isInCart() ? 'Remove from cart' : 'Add to cart'}
</button>
<button
onClick={buyNowHandler}
className="w-full bg-orange_100 rounded-full hover:opacity-90 p-1"
>
Buy now
</button>
</div>
</div>
</div>
</section>
);
};
export default ProductPage; |
package com.atguigu.springcloud.controller;
import com.atguigu.springcloud.entities.CommonResult;
import com.atguigu.springcloud.entities.Payment;
import com.atguigu.springcloud.lb.LoadBalancer;
import lombok.extern.slf4j.Slf4j;
import org.springframework.cloud.client.ServiceInstance;
import org.springframework.cloud.client.discovery.DiscoveryClient;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.client.RestTemplate;
import javax.annotation.Resource;
import java.net.URI;
import java.util.List;
/**
* @ProjectName: cloud2020
* @Package: com.atguigu.springcloud.controller
* @Author: Lu Fang
* @Date: 2022/6/10 下午 11:04
*/
@RestController
@Slf4j
public class OrderController {
// public static final String PAYMENT_SRV = "http://localhost:8001";
// 通过在eureka上注册过的微服务名称调用
public static final String PAYMENT_SRV = "http://CLOUD-PAYMENT-SERVICE/";
@Resource
private RestTemplate restTemplate;
@Resource
private LoadBalancer loadBalancer;
@Resource
private DiscoveryClient discoveryClient;
@GetMapping(value = "/consumer/payment/create")
public CommonResult<Payment> create(@RequestBody Payment payment) {
return restTemplate.postForObject(PAYMENT_SRV + "payment/create", payment, CommonResult.class);
}
@GetMapping(value = "/consumer/payment/get/{id}")
public CommonResult<Payment> getPayment(@PathVariable("id") Long id) {
return restTemplate.getForObject(PAYMENT_SRV + "payment/get/" + id, CommonResult.class);
}
@GetMapping(value = "/consumer/payment/getForEntity/{id}")
public CommonResult<Payment> getPayment2(@PathVariable("id") Long id) {
ResponseEntity<CommonResult> entity = restTemplate.getForEntity(PAYMENT_SRV + "payment/get/" + id, CommonResult.class);
return entity.getStatusCode().is2xxSuccessful() ? entity.getBody() : new CommonResult<>(400, "Error");
}
@GetMapping(value = "/consumer/payment/lb")
public String getPaymentLB() {
List<ServiceInstance> instances = discoveryClient.getInstances("CLOUD-PAYMENT-SERVICE");
if (instances.isEmpty()) return null;
ServiceInstance serviceInstance = loadBalancer.instances(instances);
URI uri = serviceInstance.getUri();
return restTemplate.getForObject(uri + "/payment/lb", String.class);
}
@GetMapping("/consumer/payment/zipkin")
public String paymentZipkin() {
String result = restTemplate.getForObject("http://localhost:8001"+"/payment/zipkin/", String.class);
return result;
}
} |
---
solution: Journey Optimizer
product: journey optimizer
title: Adobe Campaign v7/v8 actions
description: Learn about Adobe Campaign v7/v8 actions
feature: Actions
topic: Administration
role: Admin
level: Intermediate
keywords: journey, integration, campaign, v7, v8, classic
exl-id: 3da712e7-0e08-4585-8ca4-b6ff79df0b68
---
# Adobe Campaign v7/v8 actions {#using_campaign_classic}
An integration is available if you have Adobe Campaign v7 or v8. It will allow you to send emails, push notifications and SMS using Adobe Campaign Transactional Messaging capabilities.
The connection between the Journey Optimizer and Campaign instances is setup by Adobe at provisioning time. Contact Adobe.
For this to work, you need to configure a dedicated action. Refer to this [section](../action/acc-action.md).
An end-to-end use case is presented in this [section](../building-journeys/ajo-ac.md).
1. Design your journey, starting with an event. See this [section](../building-journeys/journey.md).
1. In the **Action** section of the palette, select a Campaign action and add it to your journey.
1. In the **Action parameters**, all the fields expected in the message payload are displayed. You need to map each of these fields with the field you want to use, either from the event or from the data source. This is similar to custom actions. Refer to this [section](../building-journeys/using-custom-actions.md).
 |
/*
* Array with size
*
* @author: Gabriel-AB
* https://github.com/Gabriel-AB/
*
* Usage:
* call `ARRAY_TYPEDEF(type)` before all the code
* and use `typeArray` as your a array of type
*
* Ex:
* ARRAY_TYPEDEF(int);
* intArray array1 = ARRAY_CREATE(int, {1,2,3});
* intArray array2 = ARRAY_ALLOCATE(int, 10);
*
* Obs:
* > every array has fixed size
* > You must call `free(array)` later
*/
#pragma once
#include <stdlib.h>
#include <stdbool.h>
/**
* @brief Declares a array of type.
* The type `typeArray` is will be avaliable to you
*/
#define ARRAY_TYPEDEF(type)\
typedef struct type ## _array {\
const size_t size;\
struct { const size_t dsize; } internal;\
type at[];\
} *type##Array
// Declaring basic data arrays
ARRAY_TYPEDEF(char);
ARRAY_TYPEDEF(int);
ARRAY_TYPEDEF(float);
// ===== MACROS ===== //
/**
* @brief Create a new Array with zeros.
*
* Obs: you must call `free(array)` later
*/
#define ARRAY_ALLOCATE(type, size) (type##Array)array_create(sizeof(type), size, 0)
/**
* @brief Create a new Array based on a set of values.
*
* @param type: any defined type. ex: int, float, etc...
* @param __VA_ARGS__: values in curly braces. ex: {1,2,3}
*
* usage:
* ARRAY_CREATE(int, {0,2,4})
* ARRAY_CREATE(float, {2.3f, 0.1f})
*
* Obs: you must call `free(array)` later
*/
#define ARRAY_CREATE(type, ...) ({\
type _arr[] = __VA_ARGS__;\
(type##Array)array_create(sizeof(type), sizeof(_arr)/sizeof(type), _arr);\
})
// ===== FUNCTIONS ===== //
/**
* @brief Create a new Array with values
*
* @param dsize: size of each element in bytes
* @param size: initial size of the list. (non zero value)
* @param initial_values: array of data to be pushed first. (NULL set all elements to 0)
*/
void* array_create(size_t dsize, size_t size, void *initial_values);
/**
* @brief Reallocates an existing array
*/
void* array_resize(void* array, const size_t new_size);
/// @brief Create a new array combining `a` and `b`
void* array_join(void* a, void* b);
/**
* @brief Create a new array from another. Interval: [begin, end)
*/
void* array_slice(void* array, unsigned int begin, unsigned int end);
/**
* @brief Check equality between `a` and `b`
*/
bool array_equals(void* a, void* b); |
<template>
<div class="layout-sider h-full bg-gray-800">
<a-menu class="bg-gray-800" accordion :auto-open-selected="true" :level-indent="40" style="height: 100%">
<template v-for="route in menuList" :key="route.key">
<a-menu-item v-if="!route.children || !route.children.length" :key="route.key" @click="goto(route)">
<template #icon>
<!-- <component :is="icon" :style="{ color: 'rgba(0, 0, 0, 0.7)' }" /> -->
<icon-tag />
</template>
{{ route.title }}
</a-menu-item>
<a-sub-menu v-if="route.children && route.children.length" :key="route.key">
<template #icon>
<component :is="route.icon" :style="{ color: 'rgba(255, 255, 255, 0.7)' }" />
</template>
<template #title>
{{ route.title || '' }}
</template>
<template #expand-icon-down>
<icon-caret-down :size="10" :style="{ color: 'rgba(255, 255, 255, 0.7)' }" />
</template>
<a-menu-item v-for="_route in route.children || []" :key="_route.key" @click="goto(_route)">
{{ _route.title || '' }}
</a-menu-item>
</a-sub-menu>
</template>
</a-menu>
</div>
</template>
<script lang="ts" setup>
import { generatorMenu, MenuProps } from '@/utils/router'
const icon = resolveComponent('IconTag')
const menu = ['index', 'about', 'list', 'user']
const router = useRouter()
const menuList = computed(() => {
const routers = router.getRoutes().filter(item => menu.includes(item.name as string))
return generatorMenu([], routers)
})
const goto = (item: MenuProps) => {
console.log(item)
router.push({ path: item.path })
}
</script> |
import React, { useEffect, useRef, useState } from "react";
import MyButton from "../UI/button/MyButton.jsx";
import MyInput from "../UI/input/MyInput.jsx";
import TrackList from './TrackList.jsx'
const MusicPlayer = ({ tracks }) => {
const [trackID, setTrackID] = useState(null)
const [trackProgress, setTrackProgress] = useState({timePassed: '0:00', timeTotal: '0:00'})
const [isPlaying, setIsPlaying] = useState(false)
const [isActivePlayer, setIsActivePlayer] = useState(false)
const [trackInfo, setTrackInfo] = useState({ artist: 'Имя артиста', title: 'Название трека', audiosrc: null })
const audioRef = useRef(new Audio(null));
const intervalRef = useRef(null);
const isReady = useRef(false);
const { artist, title, mp3: audioSrc } = trackInfo
const changeFormatTime = (currentTime, duration) => {
const minutesPassed = Math.floor(currentTime / 60) || '0'
const secondsPassed = Math.floor(currentTime % 60) || '0'
const minutesDuration = Math.floor(duration / 60) || '0'
const secondsDuration = Math.floor(duration % 60) || '0'
return {
timePassed: `${minutesPassed}:${secondsPassed < 10 ? `0${secondsPassed}` : secondsPassed}`,
timeTotal: `${minutesDuration}:${secondsDuration < 10 ? `0${secondsDuration}` : secondsDuration}`
}
}
const changeVolume = e => {
audioRef.current.volume = e.currentTarget.value / 100
lastValVol = audioRef.current.volume
}
const mute = e => {
if (audioRef.current.volume) {
e.currentTarget.previousSibling.firstChild.value = 0
audioRef.current.volume = 0
} else {
audioRef.current.volume = 0.1
e.currentTarget.previousSibling.firstChild.value = 0.1
}
}
const startTimer = () => {
clearInterval(intervalRef.current);
intervalRef.current = setInterval(() => {
if (audioRef.current.ended) {
nextTrack();
} else {
setTrackProgress({trackProgress,...changeFormatTime(audioRef.current.currentTime, audioRef.current.duration)})
}
}, [1000]);
};
const onScrub = (value) => {
clearInterval(intervalRef.current);
audioRef.current.currentTime = value;
setTrackProgress(changeFormatTime(audioRef.current.currentTime, audioRef.current.duration));
};
const onScrubEnd = () => {
if (!isPlaying) {
setIsPlaying(true);
}
startTimer();
};
const prevTrack = () => {
if (trackID - 1 < 0) {
setTrackID(tracks.length - 1)
} else {
setTrackID(trackID - 1)
}
}
const nextTrack = () => {
if (trackID < tracks.length - 1) {
setTrackID(tracks.length + 1)
} else {
setTrackID(0)
}
}
const stopPlayer = () => {
setIsPlaying(!isPlaying)
setIsActivePlayer(!isActivePlayer)
}
useEffect(() => {
if (isPlaying) {
audioRef.current.play();
} else {
audioRef.current.pause();
}
}, [isPlaying]);
useEffect(() => {
if (!trackID) return
audioRef.current.pause();
audioRef.current = new Audio(audioSrc);
audioRef.current.volume = 0.1;
setTrackProgress(changeFormatTime(audioRef.current.currentTime, audioRef.current.duration));
if (isReady.current) {
audioRef.current.play();
setIsPlaying(true);
startTimer();
} else {
isReady.current = true;
}
}, [trackID]);
useEffect(() => {
return () => {
audioRef.current.pause();
clearInterval(intervalRef.current);
};
}, []);
return (
<>
<section className="catalog">
<h2 className="visually-hidden">Список треков</h2>
<div className="catalog__container">
<TrackList
data={tracks}
setTrackID={setTrackID}
setTrackInfo={setTrackInfo}
setIsActivePlayer={setIsActivePlayer}
/>
<MyButton className='catalog__btn-add'>
<span>Увидеть все</span>
<svg width="24" height="24" viewBox="0 0 24 24" fill="currentColor" xmlns="http://www.w3.org/2000/svg">
<path d="M8.59 16.59L13.17 12L8.59 7.41L10 6L16 12L10 18L8.59 16.59Z" />
</svg>
</MyButton>
</div>
</section>
<section className={`player ${isPlaying || isActivePlayer ? 'player_active' : ''}`}>
<h1 className="visually-hidden">Плеер</h1>
<div className="player__container">
<div className="player__track-info track-info track-info_mobile-center">
<p className="player-info__title">{artist}</p>
<p className="player-info__artist">{title}</p>
</div>
<div className="player__controller">
<MyButton
className="player__icon player__icon_stop"
onClick={stopPlayer}
></MyButton>
<MyButton
className="player__icon player__icon_prev"
onClick={prevTrack}
></MyButton>
<MyButton
onClick={() => { setIsPlaying(!isPlaying) }}
className={`player__icon
${isPlaying
? 'player__icon_pause'
: 'player__icon_play'
}`}></MyButton>
<MyButton className="player__icon player__icon_next" onClick={nextTrack}></MyButton>
<MyButton className="player__icon player__icon_like"></MyButton>
</div>
<div className="player__progress-wrap">
<div className="player__progress">
<MyInput
type="range"
className="player__progress-input"
min="0"
max="100"
onChange={(e) => onScrub(e.target.value)}
onMouseUp={onScrubEnd}
onKeyUp={onScrubEnd}
/>
</div>
<p className="player__time">
<span className="player__time-passed">{trackProgress.timePassed}</span>
<span>/</span>
<span className="player__time-total">{trackProgress.timeTotal}</span>
</p>
</div>
<div className="player__volume-wrap">
<div className="player__volume">
<MyInput
className="player__volume-input"
type="range"
min="0"
max="100"
onChange={changeVolume}
/>
</div>
<MyButton
className="player__icon player__icon_mute"
onClick={mute}
>
</MyButton>
</div>
</div>
</section>
</>
)
}
export default MusicPlayer |
import torch
from torch import nn
import torch.nn.functional as F
class ConvBlock(nn.Module):
def __init__(self, input_ch=3, output_ch=64, activf=nn.ReLU, bias=True):
super().__init__()
self.conv1 = nn.Conv2d(input_ch, output_ch, 3, 1, 1, bias=bias)
self.conv2 = nn.Conv2d(output_ch, output_ch, 3, 1, 1, bias=bias)
self.activf = activf
self.conv_block = nn.Sequential(
self.conv1,
self.activf(inplace=True),
self.conv2,
self.activf(inplace=True)
)
def forward(self, x):
return self.conv_block(x)
class UpConv(nn.Module):
def __init__(self, input_ch=64, output_ch=32, bias=True):
super().__init__()
self.conv = nn.ConvTranspose2d(input_ch, output_ch, 2, 2, bias=bias)
self.conv_block = nn.Sequential(self.conv)
def forward(self, x):
return self.conv_block(x)
class UNetModule(nn.Module):
def __init__(self, input_ch, output_ch, base_ch):
super().__init__()
self.conv1 = ConvBlock(input_ch, base_ch)
self.conv2 = ConvBlock(base_ch, 2* base_ch)
self.conv3 = ConvBlock(2 * base_ch, 4 * base_ch)
self.conv4 = ConvBlock(4 * base_ch, 8 * base_ch)
self.conv5 = ConvBlock(8 * base_ch, 16 * base_ch)
self.upconv1 = UpConv(16 * base_ch, 8 * base_ch)
self.conv6 = ConvBlock(16 * base_ch, 8 * base_ch)
self.upconv2 = UpConv(8 * base_ch, 4 * base_ch)
self.conv7 = ConvBlock(8 * base_ch, 4 * base_ch)
self.upconv3 = UpConv(4 * base_ch, 2 * base_ch)
self.conv8 = ConvBlock(4 * base_ch, 2 * base_ch)
self.upconv4 = UpConv(2 * base_ch, base_ch)
self.conv9 = ConvBlock(2 * base_ch, base_ch)
self.outconv = nn.Conv2d(base_ch, output_ch, 1, bias=True)
def forward(self, x):
x1 = self.conv1(x)
x = F.max_pool2d(x1, 2, 2)
x2 = self.conv2(x)
x = F.max_pool2d(x2, 2, 2)
x3 = self.conv3(x)
x = F.max_pool2d(x3, 2, 2)
x4 = self.conv4(x)
x = F.max_pool2d(x4, 2, 2)
x = self.conv5(x)
x = self.upconv1(x)
x = torch.cat((x4, x), dim=1)
x = self.conv6(x)
x = self.upconv2(x)
x = torch.cat((x3, x), dim=1)
x = self.conv7(x)
x = self.upconv3(x)
x = torch.cat((x2, x), dim=1)
x = self.conv8(x)
x = self.upconv4(x)
x = torch.cat((x1, x), dim=1)
x = self.conv9(x)
x = self.outconv(x)
return x
class RRWNet(nn.Module):
def __init__(self, input_ch=3, output_ch=3, base_ch=64, iterations=5):
super().__init__()
self.first_u = UNetModule(input_ch, output_ch, base_ch)
self.second_u = UNetModule(output_ch, 2, base_ch)
self.iterations = iterations
def forward(self, x):
predictions = []
pred_1 = self.first_u(x)
predictions.append(pred_1)
bv_logits = pred_1[:, 2:3, :, :]
pred_1 = torch.sigmoid(pred_1)
bv = pred_1[:, 2:3, :, :]
pred_2 = self.second_u(pred_1)
predictions.append(torch.cat((pred_2, bv_logits), dim=1))
for _ in range(self.iterations):
pred_2 = torch.sigmoid(pred_2)
pred_2 = torch.cat((pred_2, bv), dim=1)
pred_2 = self.second_u(pred_2)
predictions.append(torch.cat((pred_2, bv_logits), dim=1))
return predictions
def refine(self, x):
predictions = []
bv = x[:, 2:3, :, :]
pred_2 = self.second_u(x)
predictions.append(torch.cat((torch.sigmoid(pred_2), bv), dim=1))
for _ in range(self.iterations):
pred_2 = torch.sigmoid(pred_2)
pred_2 = torch.cat((pred_2, bv), dim=1)
pred_2 = self.second_u(pred_2)
predictions.append(torch.cat((torch.sigmoid(pred_2), bv), dim=1))
return predictions |
/**
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.apache.wss4j.policy.stax.test;
import org.apache.wss4j.common.ext.WSSecurityException;
import org.apache.wss4j.policy.stax.PolicyViolationException;
import org.apache.wss4j.policy.stax.enforcer.PolicyEnforcer;
import org.apache.wss4j.stax.ext.WSSConstants;
import org.apache.wss4j.stax.securityToken.WSSecurityTokenConstants;
import org.apache.wss4j.stax.impl.securityToken.HttpsSecurityTokenImpl;
import org.apache.wss4j.stax.securityEvent.*;
import org.apache.xml.security.stax.ext.XMLSecurityConstants;
import org.apache.xml.security.stax.securityEvent.EncryptedElementSecurityEvent;
import org.junit.jupiter.api.Test;
import javax.xml.namespace.QName;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertTrue;
import static org.junit.jupiter.api.Assertions.fail;
public class TransportBindingTest extends AbstractPolicyTestBase {
@Test
public void testPolicy() throws Exception {
String policyString =
"<sp:TransportBinding xmlns:sp=\"http://docs.oasis-open.org/ws-sx/ws-securitypolicy/200702\" xmlns:sp3=\"http://docs.oasis-open.org/ws-sx/ws-securitypolicy/200802\">\n" +
"<wsp:Policy xmlns:wsp=\"http://schemas.xmlsoap.org/ws/2004/09/policy\">\n" +
" <sp:AlgorithmSuite>\n" +
" <wsp:Policy>\n" +
" <sp:Basic256/>\n" +
" </wsp:Policy>\n" +
" </sp:AlgorithmSuite>\n" +
"<sp:IncludeTimestamp/>\n" +
"</wsp:Policy>\n" +
"</sp:TransportBinding>";
PolicyEnforcer policyEnforcer = buildAndStartPolicyEngine(policyString);
TimestampSecurityEvent timestampSecurityEvent = new TimestampSecurityEvent();
policyEnforcer.registerSecurityEvent(timestampSecurityEvent);
RequiredElementSecurityEvent requiredElementSecurityEvent = new RequiredElementSecurityEvent();
List<QName> headerPath = new ArrayList<>();
headerPath.addAll(WSSConstants.SOAP_11_WSSE_SECURITY_HEADER_PATH);
headerPath.add(WSSConstants.TAG_WSU_TIMESTAMP);
requiredElementSecurityEvent.setElementPath(headerPath);
policyEnforcer.registerSecurityEvent(requiredElementSecurityEvent);
HttpsTokenSecurityEvent httpsTokenSecurityEvent = new HttpsTokenSecurityEvent();
HttpsSecurityTokenImpl httpsSecurityToken = new HttpsSecurityTokenImpl(true, "username");
httpsSecurityToken.addTokenUsage(WSSecurityTokenConstants.TOKENUSAGE_MAIN_SIGNATURE);
httpsTokenSecurityEvent.setSecurityToken(httpsSecurityToken);
policyEnforcer.registerSecurityEvent(httpsTokenSecurityEvent);
OperationSecurityEvent operationSecurityEvent = new OperationSecurityEvent();
operationSecurityEvent.setOperation(new QName("definitions"));
policyEnforcer.registerSecurityEvent(operationSecurityEvent);
List<XMLSecurityConstants.ContentType> protectionOrder = new LinkedList<>();
protectionOrder.add(XMLSecurityConstants.ContentType.SIGNATURE);
protectionOrder.add(XMLSecurityConstants.ContentType.ENCRYPTION);
EncryptedElementSecurityEvent encryptedElementSecurityEvent = new EncryptedElementSecurityEvent(null, true, protectionOrder);
headerPath = new ArrayList<>();
headerPath.addAll(WSSConstants.SOAP_11_WSSE_SECURITY_HEADER_PATH);
headerPath.add(WSSConstants.TAG_dsig_Signature);
requiredElementSecurityEvent.setElementPath(headerPath);
policyEnforcer.registerSecurityEvent(encryptedElementSecurityEvent);
encryptedElementSecurityEvent = new EncryptedElementSecurityEvent(null, true, protectionOrder);
headerPath = new ArrayList<>();
headerPath.addAll(WSSConstants.SOAP_11_WSSE_SECURITY_HEADER_PATH);
headerPath.add(WSSConstants.TAG_WSSE11_SIG_CONF);
requiredElementSecurityEvent.setElementPath(headerPath);
policyEnforcer.registerSecurityEvent(encryptedElementSecurityEvent);
SignedPartSecurityEvent signedPartSecurityEvent = new SignedPartSecurityEvent(null, true, protectionOrder);
signedPartSecurityEvent.setElementPath(WSSConstants.SOAP_11_BODY_PATH);
policyEnforcer.registerSecurityEvent(signedPartSecurityEvent);
policyEnforcer.doFinal();
}
@Test
public void testPolicyNotIncludeTimestamp() throws Exception {
String policyString =
"<sp:TransportBinding xmlns:sp=\"http://docs.oasis-open.org/ws-sx/ws-securitypolicy/200702\" xmlns:sp3=\"http://docs.oasis-open.org/ws-sx/ws-securitypolicy/200802\">\n" +
"<wsp:Policy xmlns:wsp=\"http://schemas.xmlsoap.org/ws/2004/09/policy\">\n" +
" <sp:AlgorithmSuite>\n" +
" <wsp:Policy>\n" +
" <sp:Basic256/>\n" +
" </wsp:Policy>\n" +
" </sp:AlgorithmSuite>\n" +
"</wsp:Policy>\n" +
"</sp:TransportBinding>";
PolicyEnforcer policyEnforcer = buildAndStartPolicyEngine(policyString);
HttpsTokenSecurityEvent httpsTokenSecurityEvent = new HttpsTokenSecurityEvent();
HttpsSecurityTokenImpl httpsSecurityToken = new HttpsSecurityTokenImpl(true, "username");
httpsSecurityToken.addTokenUsage(WSSecurityTokenConstants.TOKENUSAGE_MAIN_SIGNATURE);
httpsTokenSecurityEvent.setSecurityToken(httpsSecurityToken);
policyEnforcer.registerSecurityEvent(httpsTokenSecurityEvent);
TimestampSecurityEvent timestampSecurityEvent = new TimestampSecurityEvent();
policyEnforcer.registerSecurityEvent(timestampSecurityEvent);
OperationSecurityEvent operationSecurityEvent = new OperationSecurityEvent();
operationSecurityEvent.setOperation(new QName("definitions"));
try {
policyEnforcer.registerSecurityEvent(operationSecurityEvent);
fail("Exception expected");
} catch (WSSecurityException e) {
assertTrue(e.getCause() instanceof PolicyViolationException);
assertEquals(e.getCause().getMessage(),
"Timestamp must not be present");
assertEquals(e.getFaultCode(), WSSecurityException.INVALID_SECURITY);
}
}
@Test
public void testPolicySignatureNotEncrypted() throws Exception {
String policyString =
"<sp:TransportBinding xmlns:sp=\"http://docs.oasis-open.org/ws-sx/ws-securitypolicy/200702\" xmlns:sp3=\"http://docs.oasis-open.org/ws-sx/ws-securitypolicy/200802\">\n" +
"<wsp:Policy xmlns:wsp=\"http://schemas.xmlsoap.org/ws/2004/09/policy\">\n" +
" <sp:AlgorithmSuite>\n" +
" <wsp:Policy>\n" +
" <sp:Basic256/>\n" +
" </wsp:Policy>\n" +
" </sp:AlgorithmSuite>\n" +
"<sp:IncludeTimestamp/>\n" +
"</wsp:Policy>\n" +
"</sp:TransportBinding>";
PolicyEnforcer policyEnforcer = buildAndStartPolicyEngine(policyString);
TimestampSecurityEvent timestampSecurityEvent = new TimestampSecurityEvent();
policyEnforcer.registerSecurityEvent(timestampSecurityEvent);
RequiredElementSecurityEvent requiredElementSecurityEvent = new RequiredElementSecurityEvent();
List<QName> headerPath = new ArrayList<>();
headerPath.addAll(WSSConstants.SOAP_11_WSSE_SECURITY_HEADER_PATH);
headerPath.add(WSSConstants.TAG_WSU_TIMESTAMP);
requiredElementSecurityEvent.setElementPath(headerPath);
policyEnforcer.registerSecurityEvent(requiredElementSecurityEvent);
HttpsTokenSecurityEvent httpsTokenSecurityEvent = new HttpsTokenSecurityEvent();
HttpsSecurityTokenImpl httpsSecurityToken = new HttpsSecurityTokenImpl(true, "username");
httpsSecurityToken.addTokenUsage(WSSecurityTokenConstants.TOKENUSAGE_MAIN_SIGNATURE);
httpsTokenSecurityEvent.setSecurityToken(httpsSecurityToken);
policyEnforcer.registerSecurityEvent(httpsTokenSecurityEvent);
List<XMLSecurityConstants.ContentType> protectionOrder = new LinkedList<>();
protectionOrder.add(XMLSecurityConstants.ContentType.SIGNATURE);
protectionOrder.add(XMLSecurityConstants.ContentType.ENCRYPTION);
EncryptedElementSecurityEvent encryptedElementSecurityEvent = new EncryptedElementSecurityEvent(null, false, protectionOrder);
headerPath = new ArrayList<>();
headerPath.addAll(WSSConstants.SOAP_11_WSSE_SECURITY_HEADER_PATH);
headerPath.add(WSSConstants.TAG_dsig_Signature);
encryptedElementSecurityEvent.setElementPath(headerPath);
policyEnforcer.registerSecurityEvent(encryptedElementSecurityEvent);
OperationSecurityEvent operationSecurityEvent = new OperationSecurityEvent();
operationSecurityEvent.setOperation(new QName("definitions"));
policyEnforcer.registerSecurityEvent(operationSecurityEvent);
policyEnforcer.doFinal();
}
@Test
public void testPolicyNotWholeBodySigned() throws Exception {
String policyString =
"<sp:TransportBinding xmlns:sp=\"http://docs.oasis-open.org/ws-sx/ws-securitypolicy/200702\" xmlns:sp3=\"http://docs.oasis-open.org/ws-sx/ws-securitypolicy/200802\">\n" +
"<wsp:Policy xmlns:wsp=\"http://schemas.xmlsoap.org/ws/2004/09/policy\">\n" +
" <sp:AlgorithmSuite>\n" +
" <wsp:Policy>\n" +
" <sp:Basic256/>\n" +
" </wsp:Policy>\n" +
" </sp:AlgorithmSuite>\n" +
"<sp:IncludeTimestamp/>\n" +
"</wsp:Policy>\n" +
"</sp:TransportBinding>";
PolicyEnforcer policyEnforcer = buildAndStartPolicyEngine(policyString);
TimestampSecurityEvent timestampSecurityEvent = new TimestampSecurityEvent();
policyEnforcer.registerSecurityEvent(timestampSecurityEvent);
RequiredElementSecurityEvent requiredElementSecurityEvent = new RequiredElementSecurityEvent();
List<QName> headerPath = new ArrayList<>();
headerPath.addAll(WSSConstants.SOAP_11_WSSE_SECURITY_HEADER_PATH);
headerPath.add(WSSConstants.TAG_WSU_TIMESTAMP);
requiredElementSecurityEvent.setElementPath(headerPath);
policyEnforcer.registerSecurityEvent(requiredElementSecurityEvent);
HttpsTokenSecurityEvent httpsTokenSecurityEvent = new HttpsTokenSecurityEvent();
HttpsSecurityTokenImpl httpsSecurityToken = new HttpsSecurityTokenImpl(true, "username");
httpsSecurityToken.addTokenUsage(WSSecurityTokenConstants.TOKENUSAGE_MAIN_SIGNATURE);
httpsTokenSecurityEvent.setSecurityToken(httpsSecurityToken);
policyEnforcer.registerSecurityEvent(httpsTokenSecurityEvent);
List<XMLSecurityConstants.ContentType> protectionOrder = new LinkedList<>();
protectionOrder.add(XMLSecurityConstants.ContentType.SIGNATURE);
protectionOrder.add(XMLSecurityConstants.ContentType.ENCRYPTION);
EncryptedElementSecurityEvent encryptedElementSecurityEvent = new EncryptedElementSecurityEvent(null, true, protectionOrder);
headerPath = new ArrayList<>();
headerPath.addAll(WSSConstants.SOAP_11_WSSE_SECURITY_HEADER_PATH);
headerPath.add(WSSConstants.TAG_dsig_Signature);
encryptedElementSecurityEvent.setElementPath(headerPath);
policyEnforcer.registerSecurityEvent(encryptedElementSecurityEvent);
encryptedElementSecurityEvent = new EncryptedElementSecurityEvent(null, true, protectionOrder);
headerPath = new ArrayList<>();
headerPath.addAll(WSSConstants.SOAP_11_WSSE_SECURITY_HEADER_PATH);
headerPath.add(WSSConstants.TAG_WSSE11_SIG_CONF);
encryptedElementSecurityEvent.setElementPath(headerPath);
policyEnforcer.registerSecurityEvent(encryptedElementSecurityEvent);
OperationSecurityEvent operationSecurityEvent = new OperationSecurityEvent();
operationSecurityEvent.setOperation(new QName("definitions"));
policyEnforcer.registerSecurityEvent(operationSecurityEvent);
SignedPartSecurityEvent signedPartSecurityEvent = new SignedPartSecurityEvent(null, false, protectionOrder);
signedPartSecurityEvent.setElementPath(WSSConstants.SOAP_11_BODY_PATH);
policyEnforcer.registerSecurityEvent(signedPartSecurityEvent);
policyEnforcer.doFinal();
}
} |
using Microsoft.EntityFrameworkCore;
using Microsoft.Extensions.Logging;
using PracticeCalendar.Domain.Entities;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace PracticeCalendar.Infrastructure.Persistence
{
public class ApplicationDbContextInitialiser
{
private readonly ILogger<ApplicationDbContextInitialiser> logger;
private readonly ApplicationDbContext context;
public ApplicationDbContextInitialiser(ILogger<ApplicationDbContextInitialiser> logger,
ApplicationDbContext context)
{
this.logger = logger;
this.context = context;
}
public async Task InitialiseAsync()
{
try
{
if (context.Database.IsSqlite())
{
await context.Database.MigrateAsync();
}
}
catch (Exception ex)
{
logger.LogError(ex, "An error occurred while initialising the database.");
throw;
}
}
public async Task SeedAsync()
{
try
{
await TrySeedAsync();
}
catch (Exception ex)
{
logger.LogError(ex, "An error occurred while seeding the database.");
throw;
}
}
public async Task TrySeedAsync()
{
// Default data
// Seed, if necessary
if (!context.PracticeEvents.Any())
{
context.PracticeEvents.Add(new PracticeEvent("Event 1", "Event 1 desc",
DateTime.Now.AddHours(-1),
DateTime.Now.AddHours(1)));
await context.SaveChangesAsync();
}
}
}
} |
<template>
<div id="nav" v-if="isAuthenticated">
<router-link to="/home">Home</router-link> |
<router-link to="/profile">Profile</router-link> |
<router-link to="/user/list">Daftar User</router-link> |
<router-link to="/" @click="logOut" >Logout</router-link>
</div>
<div id="nav" v-if="!isAuthenticated">
<router-link to="/">Login</router-link> |
<router-link to="/register">Register</router-link>
</div>
<router-view/>
</template>
<script>
export default {
computed: {
isAuthenticated() {
return this.$store.getters['auth/isAuthenticated'];
},
},
methods: {
logOut() {
this.$store.dispatch('auth/logout');
this.$router.push('/');
alert("Berhasil keluar!")
}
},
};
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
}
#nav {
padding: 30px;
}
#nav a {
font-weight: bold;
color: #2c3e50;
}
#nav a.router-link-exact-active {
color: #42b983;
}
.container {
max-width: 80%;
margin: auto;
}
.card {
padding: 16px;
box-shadow: 0 4px 8px 0 rgba(0,0,0,0.2);
transition: 0.3s;
border-radius: 5px; /* 5px rounded corners */
width: fit-content;
margin: auto;
}
/* On mouse-over, add a deeper shadow */
.card:hover {
box-shadow: 0 8px 16px 0 rgba(0,0,0,0.2);
}
.form-control {
margin-bottom: 16px;
display: flex;
}
.form-control label {
font-weight: bold;
width: 50%;
text-align: left;
}
.form-control input {
margin-left: 16px;
/* width: 50%; */
}
</style> |
import { FunctionComponent, useMemo, type CSSProperties } from "react";
import styles from "./Elements2on.module.css";
type Elements2onType = {
icon?: string;
vector?: string;
prop?: string;
/** Style props */
elements2onPosition?: CSSProperties["position"];
elements2onWidth?: CSSProperties["width"];
elements2onTop?: CSSProperties["top"];
elements2onRight?: CSSProperties["right"];
elements2onLeft?: CSSProperties["left"];
};
const Elements2on: FunctionComponent<Elements2onType> = ({
icon,
vector,
prop,
elements2onPosition,
elements2onWidth,
elements2onTop,
elements2onRight,
elements2onLeft,
}) => {
const elements2onStyle: CSSProperties = useMemo(() => {
return {
position: elements2onPosition,
width: elements2onWidth,
top: elements2onTop,
right: elements2onRight,
left: elements2onLeft,
};
}, [
elements2onPosition,
elements2onWidth,
elements2onTop,
elements2onRight,
elements2onLeft,
]);
return (
<div className={styles.elements2on} style={elements2onStyle}>
<div className={styles.rectangle} />
<img className={styles.icon} alt="" src={icon} />
<img className={styles.vectorIcon} alt="" src={vector} />
<b className={styles.b}>{prop}</b>
</div>
);
};
export default Elements2on; |
import {useDispatch, useSelector} from "react-redux";
import {AppDispatch} from "@store/index";
import {
selectIsPlaying,
selectNext,
selectPlayMode,
selectPrev,
selectSeek,
selectCurrent,
setSeek,
setCurrent,
addToQueue,
selectQueue,
setAudioList
} from "@store/slices/player-status.slice";
import React from "react";
import {
getAudioMeta,
getSettingItem,
rebuild,
setProgress,
setTitle,
getAudios
} from "../api";
import Player from "@plugins/player";
import debug from "@plugins/debug";
import {selectRefreshMusicLibraryTimeStamp} from "@store/slices/setting.slice";
const logger = debug("App:DataManager");
export const player = new Player();
export default function DataManager() {
const dispatch = useDispatch<AppDispatch>();
const seek = useSelector(selectSeek);
const prev = useSelector(selectPrev);
const next = useSelector(selectNext);
const current = useSelector(selectCurrent);
const queue = useSelector(selectQueue);
const isPlaying = useSelector(selectIsPlaying);
const playMode = useSelector(selectPlayMode);
const refreshMusicLibraryTimeStamp = useSelector(
selectRefreshMusicLibraryTimeStamp
);
// 每隔一秒刷新一次播放进度
React.useEffect(() => {
const timer = setInterval(() => {
dispatch(setSeek(player.seek));
}, 500);
// send progress to the status bar here
// because the progress should update per 1 second
setProgress(seek / current?.duration).then();
// set the title to the progress bar here
setTitle(`${current?.title} - ${current?.artist}`).then();
return () => clearInterval(timer);
}, [seek]);
// 通过生成新的时间戳来指示歌曲库的变动
// 这是一种取巧的方式,利用了 useEffect 这个 hook 的特性
React.useEffect(() => {
if (refreshMusicLibraryTimeStamp !== 0) {
logger("refresh library, timestamp: ", refreshMusicLibraryTimeStamp);
(async() => {
const paths = await _refreshLibrary();
const resp = await getAudios(paths);
if (resp.code === 1) dispatch(setAudioList(resp.data.lists));
})();
}
}, [refreshMusicLibraryTimeStamp]);
// 下一首
React.useEffect(() => {
logger("is playing: ", isPlaying);
// its behavior is similar to the prev button.
player.isAutoPlay = isPlaying;
logger("skip to the next, timestamp: ", next);
player.next();
}, [next]);
// 上一首
React.useEffect(() => {
logger("is playing: ", isPlaying);
// when you click the prev button
// the current track is playing determined
// the track to be playing is to play auto.
player.isAutoPlay = isPlaying;
logger("skip to the prev, timestamp: ", prev);
player.prev();
}, [prev]);
// 切换播放 & 暂停
// switch between playing or paused.
React.useEffect(() => {
logger("set the playing: ", isPlaying);
player.playOrPause();
}, [isPlaying]);
// 切换播放模式
// switch the play mode in [[PlayMode]]
// the type definition is in the plugins/player.ts
React.useEffect(() => {
logger("set the play mode: ", playMode);
player.playMode = playMode;
}, [playMode]);
React.useEffect(() => {
player.trackList = queue;
}, [queue?.length]);
React.useEffect(() => {
if (player?.track?.src) {
let exists = false;
for (const q of queue) {
if (q.src === player?.track?.src) exists = true;
}
getAudioMeta(player?.track?.src).then(res => {
if (res.code === 1) {
dispatch(setCurrent(res.data));
if (!exists) dispatch(addToQueue([res.data]));
}
})
}
}, [player?.track?.src]);
const _refreshLibrary = async () => {
const resp = await getSettingItem("libraries");
if (resp.code === 1) {
const paths = resp.data;
const res = await rebuild(paths);
logger("rebuild result: ", res);
return resp.data;
}
};
return <></>;
} |
import 'package:flutter/material.dart';
class Indicator extends StatelessWidget {
const Indicator({
super.key,
required this.color,
required this.title,
required this.description,
required this.isSquare,
this.size = 16,
this.textColor = const Color(0xff505050),
});
final Color color;
final String title;
final String description;
final bool isSquare;
final double size;
final Color textColor;
@override
Widget build(BuildContext context) {
return Row(
children: <Widget>[
Container(
width: size,
height: size,
decoration: BoxDecoration(
shape: isSquare ? BoxShape.rectangle : BoxShape.circle,
color: color,
),
),
const SizedBox(
width: 4,
),
Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
title,
style: TextStyle(
fontSize: 15,
fontWeight: FontWeight.bold,
color: textColor,
),
),
Text(
description,
style: TextStyle(
fontSize: 10,
fontWeight: FontWeight.normal,
color: textColor,
),
)
],
)
],
);
}
} |
---
description: "Bagaimana Menyiapkan Semur Telur Terong yang Enak Banget"
title: "Bagaimana Menyiapkan Semur Telur Terong yang Enak Banget"
slug: 954-bagaimana-menyiapkan-semur-telur-terong-yang-enak-banget
date: 2020-08-18T05:03:08.903Z
image: https://img-global.cpcdn.com/recipes/fd19c2c2024203fb/751x532cq70/semur-telur-terong-foto-resep-utama.jpg
thumbnail: https://img-global.cpcdn.com/recipes/fd19c2c2024203fb/751x532cq70/semur-telur-terong-foto-resep-utama.jpg
cover: https://img-global.cpcdn.com/recipes/fd19c2c2024203fb/751x532cq70/semur-telur-terong-foto-resep-utama.jpg
author: Jordan Cooper
ratingvalue: 3.3
reviewcount: 5
recipeingredient:
- "2 buah terong"
- "3 buah telur ayam"
- "2 siung bawang putih"
- "2 siung bawang merah"
- "1 buah tomat"
- "2,5 buah cabai"
- "2 sdm daun bawang iris"
- "3 sdm Bumbu Bakar Madu Sari Kurma lihat resep"
- " Air"
- " Garam"
- " Minyak goreng"
recipeinstructions:
- "Iris terong, tomat, daun bawang, dan bawang merah."
- "Panaskan minyak, goreng terong."
- "Goreng tomat"
- "Goreng bawang putih dan cabai. (Cabai perlu disayat-sayat biar aman pas digoreng)"
- "Goreng telur, lalu sisihkan."
- "Masukkan tomat yang sudah digoreng, lalu penyet tomat tersebut beserta cabai dan bawang putih."
- "Tambahkan air dan "bumbu bakar madu + sari kurma". (Lihat di referensi) (lihat resep)"
- "Aduk, tunggu sampai mendidih, lalu masukkan terong dan telur. Di akhir, tambahkan irisan daun bawang."
- "Selesai"
categories:
- Resep
tags:
- semur
- telur
- terong
katakunci: semur telur terong
nutrition: 160 calories
recipecuisine: Indonesian
preptime: "PT34M"
cooktime: "PT50M"
recipeyield: "3"
recipecategory: Dinner
---
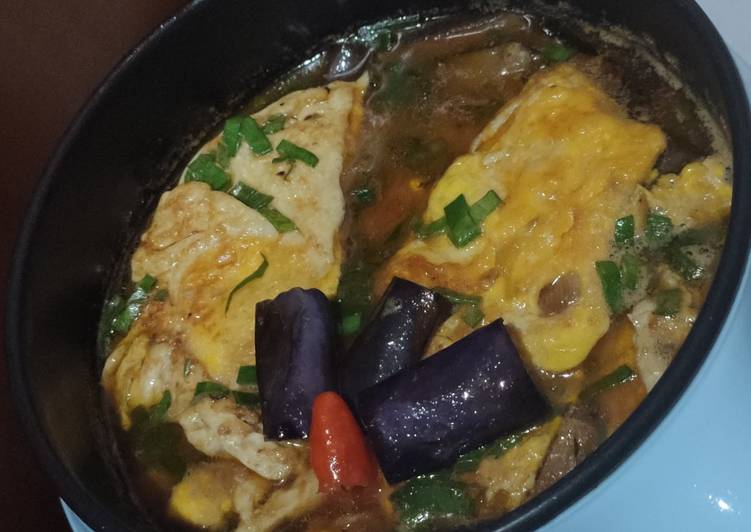
<b><i>semur telur terong</i></b>, Memasak adalah bentuk kegiatan yang seru dilakukan oleh berbagai komunitas. bukan hanya para bunda, sebagian laki laki juga cukup banyak yang suka dengan kegemaran ini. walau hanya untuk sekedar berpesta dengan kolega atau memang sudah menjadi kegemaran dalam dirinya. tak heran dalam dunia juru masak sekarang sedikit banyak ditemukan pria dengan ketrampilan memasak yang hebat, dan banyak juga kita jumpai di aneka kedai dan restoran yang menggunakan juru masak pria sebagai chef andalan nya.
Oke, kita mulai ke hal bumbu bumbu olahan <i>semur telur terong</i>. di sela sela pekerjaan kita, kemungkinan akan terasa membahagiakan jika sejenak anda menyisihkan sedikit waktu untuk meracik semur telur terong ini. dengan keberhasilan kita dalam membuat masakan tersebut, bisa menjadikan diri kita bangga oleh hasil olahan kalian sendiri. dan juga disini dengan situs ini kita akan dapat pedoman untuk memasak makanan <u>semur telur terong</u> tersebut menjadi menu yang endess dan sempurna, oleh sebab itu tandai alamat website ini di handphone anda sebagai sebagian referensi anda dalam meracik hidangan baru yang enak.
Sekarang langsung saja kita start untuk menyediakan bahan bahan yang diperlukan dalam memasak menu <u><i>semur telur terong</i></u> ini. seenggaknya dibutuhkan <b>11</b> bahan yang diperlukan di makanan ini. supaya nanti dapat tercapai rasa yang endess dan nikmat. dan juga sediakan waktu anda sebentar, karena anda akan memulainya paling tidak dengan <b>9</b> langkah. saya berharap semua yang dibutuhkan sudah kalian sediakan disini, oke mari kita olah dengan mencatat dulu bahan baku berikut ini.
<!--inarticleads1-->
##### Komposisi dan bumbu yang dibutuhkan untuk pembuatan Semur Telur Terong:
1. Ambil 2 buah terong
1. Gunakan 3 buah telur ayam
1. Gunakan 2 siung bawang putih
1. Ambil 2 siung bawang merah
1. Gunakan 1 buah tomat
1. Siapkan 2,5 buah cabai
1. Sediakan 2 sdm daun bawang iris
1. Ambil 3 sdm "Bumbu Bakar Madu + Sari Kurma" (lihat resep)
1. Ambil Air
1. Ambil Garam
1. Ambil Minyak goreng
<!--inarticleads2-->
##### Tahapan mengolah Semur Telur Terong:
1. Iris terong, tomat, daun bawang, dan bawang merah.
1. Panaskan minyak, goreng terong.
1. Goreng tomat
1. Goreng bawang putih dan cabai. (Cabai perlu disayat-sayat biar aman pas digoreng)
1. Goreng telur, lalu sisihkan.
1. Masukkan tomat yang sudah digoreng, lalu penyet tomat tersebut beserta cabai dan bawang putih.
1. Tambahkan air dan "bumbu bakar madu + sari kurma". (Lihat di referensi) - (lihat resep)
1. Aduk, tunggu sampai mendidih, lalu masukkan terong dan telur. Di akhir, tambahkan irisan daun bawang.
1. Selesai
Diatas adalah sedikit bahasan masakan tentang resep resep <u>semur telur terong</u> yang sempurna. saya ingin kalian dapat paham dengan pembahasan diatas, dan kamu dapat praktek ulang di masa datang untuk di hidangkan dalam saat saat kegiatan keluarga atau kolega kalian. kalian dapat menyesuaikan bumbu bumbu yang tersedia diatas sesuai dengan selera anda, sehingga masakan <b>semur telur terong</b> ini bs menjadi lebih enak dan sempurna lagi. berikut penjabaran singkat ini, sampai bertemu kembali di lain kesempatan. semoga hari kalian menyenangkan. |
import Element from "./DashElement";
import ebayLogo from '../images/ebay-logo.png';
import listed from './hooks/listed';
import time from './hooks/time'
const Table = ({currentRecords}) => {
return (
<>
{currentRecords.map((data, key) => {
return(
<tr key={key}>
<td>
<div className="accordion">
<div className="accordion-item">
<h2 className="accordion-header">
<button
className="accordion-button collapsed bg-dark"
type="button"
data-bs-toggle="collapse"
data-bs-target={`#flush-collapse${key}`}
aria-expanded="false"
aria-controls="flush-collapseOne"
>
{data.title}
</button>
</h2>
<div id={`flush-collapse${key}`} className="accordion-collapse collapse" data-bs-parent="#accordionFlushExample">
<div className="accordion-body">
<div className='container'>
<div className='row'>
<div className='col'>
<div className='row'>
<div className='col d-flex justify-content-center'>
<div className="card listing-box">
<div className="card-header listing-header">
View on
</div>
<div className="card-body listing-body text-body-secondary">
<a href={data.itemurl} target="_blank"><img src={ebayLogo} style={{height:"1.5rem", width:"auto"}} /> </a>
</div>
</div>
</div>
<div className='col d-flex justify-content-center'>
<div className="card listing-box">
<div className="card-header listing-header">
Listed on
</div>
<div className="card-body listing-body text-body-secondary">
{listed(data.starttime)}
</div>
</div>
</div>
<div className='col d-flex justify-content-center'>
<div className="card listing-box">
<div className="card-header listing-header">
Bids
</div>
<div className="card-body listing-body text-body-secondary">
{data.bidcount}
</div>
</div>
</div>
<div className='col d-flex justify-content-center'>
<div className="card listing-box">
<div className="card-header listing-header">
Watching:
</div>
<div className="card-body listing-body text-body-secondary">
{data.watchcount}
</div>
</div>
</div>
<div className='col d-flex justify-content-center'>
<div className="card listing-box">
<div className="card-header listing-header">
Time Left
</div>
<div className="card-body listing-body text-body-secondary">
{time(data.timeleft)}
</div>
</div>
</div>
<div className='col d-flex justify-content-center'>
<div className="card listing-box">
<div className="card-header listing-header">
Current Price:
</div>
<div className="card-body listing-body text-body-secondary">
$ {data.currentprice.toFixed(2)}
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</td>
<td>
<span className='time-left'>
{time(data.timeleft)}
</span>
</td>
<td>
<span className='price'>
$ {data.currentprice.toFixed(2)}
</span>
</td>
</tr>
)
})}
</>
)
}
export default Table; |
<html>
<head>
<title> Booth Sentiment</title>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=no">
<!--<script src="static/d3/d3.min.js" charset="utf-8"></script>
<script src="../static/jquery-2.1.3.min.js" charset="utf-8"></script> -->
<script src="http://d3js.org/d3.v3.min.js" charset="utf-8"></script>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.1/js/bootstrap.min.js"></script>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.1/css/bootstrap.min.css">
<!-- Optional theme -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.1/css/bootstrap-theme.min.css">
</head>
<body>
<div id="container" class="container">
<div class="col-md-2 visible-desktop"></div>
<div class="col-md-8" id="main_container">
<div class = "row"><h3>Booth Sentiments</h3></div>
<div id="sentiment_holder" class = 'row'></div>
</div>
<div class="col-md-2 visible-desktop"></div>
</div>
<script>
var margin = {top: 40, right: 30, bottom: 100, left: 40},
width = 760 - margin.left - margin.right,
height = 600 - margin.top - margin.bottom;
var graph_loaded = false;
colors = d3.scale.category20()
function create_chart(div_id,data){
var x_scale = d3.scale.ordinal()
.domain(data.map(function(d){return d.booth;}))
.rangeRoundBands([0,width],.1);
var y_scale = d3.scale.linear()
.domain([0, d3.max(data,function(d){return d.polarity;})])
.range([height,1]);
var xAxis = d3.svg.axis()
//.data(data)
.scale(x_scale)
.tickFormat(function(d){return d})
.orient("bottom");
var yAxis = d3.svg.axis()
.scale(y_scale)
.orient("left").ticks(10);
svg = d3.select(div_id).append("svg")
.attr("width",width + margin.left + margin.right)
.attr("height",height + margin.top + margin.bottom)
.append("g")
.attr("transform", "translate(" + margin.left + "," + margin.top + ")");
//vote_sum = d3.sum(data,function(d){return d.polarity;})
rects = svg.selectAll('rect')
.data(data)
.enter()
.append("rect")
.attr("class","rect")
.attr("x",function(d){return x_scale(d.booth);})
.attr("width",x_scale.rangeBand())
.attr("y",function(d){return y_scale(d.polarity);})
.attr("height",function(d){return height - y_scale(d.polarity);})
//.attr("fill","teal");
.attr("fill",function(d,i){return colors(i)})
texts = svg.selectAll("text")
.data(data)
.enter()
.append("text")
.text(function(d){return d.polarity;})
.attr("x",function(d){return x_scale(d.booth)+(width/data.length)*1/2;})
.attr("y",function(d){return y_scale(d.polarity)+15;})
.attr("height",function(d){return height - y_scale(d.polarity);})
.attr("font-family", "sans-serif")
.attr("font-size", "11px")
.attr("fill", "blck")
.attr("text-anchor","middle");
svg.append("g")
.attr("class","x axis")
.attr("transform","translate(0," + height + ")")
.call(xAxis)
.selectAll("text")
.style("text-anchor", "start")
.attr("x", "9")
.attr("y",0)
.attr("dy", ".15em")
.attr("transform", "rotate(90)");
svg.append("g")
.attr("class","y axis")
.call(yAxis);
}
function update_chart(div_id,data){
var y_scale = d3.scale.linear()
.domain([0, d3.max(data,function(d){return d.polarity;})])
.range([height,0]);
var x_scale = d3.scale.ordinal()
.domain(data.map(function(d){return d.booth;}))
.rangeRoundBands([0,width],.1);
svg = d3.select(div_id).select("svg")
//vote_sum = d3.sum(data,function(d){return d.polarity;})
rects = svg.selectAll('rect')
//.attr("transform", "translate(" + margin.left + "," + margin.top + ")")
.data(data)
.transition()
.duration(1000)
.attr("class","rect")
.attr("x",function(d){return x_scale(d.booth);})
.attr("width",x_scale.rangeBand())
.attr("y",function(d){return y_scale(d.polarity);})
.attr("height",function(d){return height - y_scale(d.polarity);})
//.attr("fill","teal");
.attr("fill",function(d,i){return colors(i)})
texts = svg.selectAll("text")
.data(data)
.transition()
.duration(1000)
.text(function(d){return d.polarity;})
.attr("x",function(d){return x_scale(d.booth)+(width/data.length)*1/2;})
.attr("y",function(d){return y_scale(d.polarity)+15;})
.attr("font-family", "sans-serif")
.attr("font-size", "11px")
.attr("fill", "black")
.attr("text-anchor","middle");
//Update axis
var xAxis = d3.svg.axis()
.scale(x_scale)
.tickFormat(function(d){return d})
.orient("bottom");
var yAxis = d3.svg.axis()
.scale(y_scale)
.orient("left");
svg.select(".x.axis")
.transition()
.duration(1000)
.call(xAxis)
.selectAll("text")
.style("text-anchor", "start")
.attr("x", "9")
.attr("y",0)
.attr("dy", ".15em")
.attr("transform", "rotate(90)");
svg.select(".y.axis")
.transition()
.duration(1000)
.call(yAxis);
}
</script>
<script>
var host = window.location.host
var ws = new WebSocket("ws://"+host+"/monitor");
ws.onerror = function(err){
alert(err);
}
ws.onmessage = function(msg){
console.log(msg.data);
sentiment_data = JSON.parse(msg.data)
if(graph_loaded){
update_chart("#sentiment_holder",sentiment_data["polarity_list"])
}else{
graph_loaded = true;
create_chart("#sentiment_holder",sentiment_data["polarity_list"])
}
}
</script>
</body>
</html> |
US010
Feature: Mapa GPS
Como <usuario> quiero visualizar el mapa
del centro recreacional para facilitar mi recorrido
Scenario:Usuario que compró entradas en la aplicación accede a "Mapa GPS"
Dado que el <usuario> se encuentra en el centro recreacional
Y presiona <Mapa GPS>
Y activa su <ubicación>
Entonces la aplicación despliega el <mapa> del centro recreacional
Examples:
|usuario|ubicación|mapa interactivo|
|Liliana|coordenadas de geolocalización|mapa del centro en tiempo real|
Scenario: Usuario que no compró entradas en la aplicación ni en el centro accede a "Mapa GPS"
Dado que el <usuario> se encuentra en el centro recreacional
Y presiona <Mapa GPS>
Y activa su <ubicación>
Entonces la aplicación le envía el <mensaje> "Error", con dos <opciones> "Escanear ticket", "No tengo Ticket"
Cuando el usuario presione <No tengo ticket>
Entonces el sistema despliega un formulario de compra
Cuando el usuario <rellene el formulario> de compra de forma correcta
Entonces la aplicación despliega el <mapa> del centro recreacional
Examples:
|opciones|
|Escanear Ticket|
|No tengo ticket|
|usuario|ubicación|mensaje|opción|Fomulario de compra|mapa interactivo|
|Liliana|coordenadas de geolocalización|"Error (No tiene entradas)"|No tengo ticket|formulario completo|mapa del centro en tiempo real|
Scenario:Usuario que no compró entradas en la aplicación, pero si en el centro accede a "Mapa GPS"
Dado que el <usuario> se encuentra en el centro recreacional
Y presiona <Mapa GPS>
Y activa su <ubicación>
Entonces la aplicación le envía el <mensaje> "Error", con dos opciones "Escanear ticket", "No tengo Ticket"
Cuando el usuario presione <Escanear ticket>
Entonces el sistema despliega Escanear Código QR
Cuando el usuario realice el <escaneo> del ticket
Entonces la aplicación despliega el <mapa> del centro recreacional
Examples:
|usuario|ubicación|mensaje|opción|escaneo|mapa interactivo|
|Liliana|coordenadas de geolocalización|"Error (No tiene entradas)"|Escanear ticket|ticket escaneado|mapa del centro en tiempo real| |
import 'package:flutter/material.dart';
import 'package:provider/provider.dart';
import '../providers/products.dart';
class ProductDetailScreen extends StatelessWidget {
static const routeName = '/product-detail';
@override
Widget build(BuildContext context) {
final productId =
ModalRoute.of(context)?.settings.arguments as String; // is the id!
final loadedProduct = Provider.of<Products>(
context,
listen: false,
).findById(productId);
return Scaffold(
appBar: AppBar(
title: Text(loadedProduct.title),
),
body: SingleChildScrollView(
child: Column(children: [
Container(
height: 300,
child: Image.network(
loadedProduct.imageUrl,
fit: BoxFit.cover,
),
),
const SizedBox(height: 10),
Text(
'${loadedProduct.price}',
style: TextStyle(color: Colors.grey, fontSize: 20),
),
const SizedBox(height: 20),
Container(
padding: EdgeInsets.all(5),
width: double.infinity,
child: Text(
loadedProduct.description,
textAlign: TextAlign.center,
softWrap: true,
),
)
]),
));
}
} |
import Head from "next/head";
import Image from "next/image";
import styles from "../styles/Home.module.css";
import Link from "next/link";
import React, { useEffect, useState, useRef } from "react";
import Web3Modal from "web3modal";
import {
GOVT_DAO_CONTRACT_ADDRESS,
GOVT_DAO_CONTRACT_ABI,
GD_TOKEN_ADDRESS,
GD_TOKEN_ABI,
} from "../Constants/pages";
import { formatEther } from "ethers/lib/utils";
import { providers, Contract } from "ethers";
function Home() {
// state variables declaration
const [loading, setLoading] = useState(false);
// set the DAO treasury
const [daoTreasury, setDaoTreasury] = useState("0");
// set no of proposals
const [proposals, setAllProposals] = useState([]);
// const set balance of tokens
const [tokenBalance, setTokenBalance] = useState(0);
// Check if the wallet connected
const [walletConnected, setWalletConnected] = useState(false);
// set the owner
const [owner, setIsOwner] = useState(false);
//
// const [proposalId, setProposalId] = useState("");
// hold the no of proposals:
const [noOfProposals, setNoOfProposals] = useState("0");
//
const [selectedTab, setSelectedTab] = useState("");
//
const [title, setTitle] = useState("");
//
const [description, setDescription] = useState("");
// An instance of web3Modal
const web3modalRef = useRef();
// begin components:
// helper fxn
async function getProviderOrSigner(Signer = false) {
const provider = await web3modalRef.current.connect();
const web3Provider = new providers.Web3Provider(provider);
const { chainId } = await web3Provider.getNetwork();
if (chainId !== 11155111) {
window.alert("Please change network to Sepolia!!!");
throw new Error("Change network to sepolia");
}
if (Signer) {
const signer = web3Provider.getSigner();
return signer;
}
return web3Provider;
}
// helper fxn
function getDaoContractInstance(providerOrSigner) {
return new Contract(
GOVT_DAO_CONTRACT_ADDRESS,
GOVT_DAO_CONTRACT_ABI,
providerOrSigner
);
}
// helper fxn
function getGDTokenContractInstance(providerOrSigner) {
return new Contract(GD_TOKEN_ADDRESS, GD_TOKEN_ABI, providerOrSigner);
}
// Get the owner of DAO contract
async function getDaoContractOwner() {
try {
const signer = await getProviderOrSigner(true);
const contract = getDaoContractInstance(signer);
const contractOwner = await contract.owner();
const callerAddress = await signer.getAddress();
if (contractOwner.toLowerCase() === callerAddress.toLowerCase()) {
setIsOwner(true);
}
} catch (err) {
console.error(err);
}
}
// helper fxn
async function connectWallet() {
try {
await getProviderOrSigner();
setWalletConnected(true);
} catch (err) {
console.error(err);
}
}
// withdraw ether from DAO
async function withdrawETHFromDao() {
try {
const signer = await getProviderOrSigner(true);
const daoContract = getDaoContractInstance(signer);
const tx = await daoContract.withdrawETHFromDAO();
setLoading(true);
await tx.wait();
setLoading(false);
await getDaoTreasuryBalance();
} catch (err) {
console.error(err);
}
}
async function getDaoTreasuryBalance() {
try {
const provider = await getProviderOrSigner();
const contractBalance = await provider.getBalance(
GOVT_DAO_CONTRACT_ADDRESS
);
setDaoTreasury(contractBalance.toString());
} catch (err) {
console.error(err);
}
}
// get no of proposals
async function getNoOfProposals() {
try {
const provider = await getProviderOrSigner();
const daoContract = getDaoContractInstance(provider);
const NoOfProposals = await daoContract.numProposals();
setNoOfProposals(NoOfProposals.toString());
} catch (err) {
console.error(err);
}
}
// get the user token balance
async function getTokenBalance() {
try {
const signer = await getProviderOrSigner(true);
const GDContract = getGDTokenContractInstance(signer);
const noOfTokens = await GDContract.balanceOf(signer.getAddress());
setTokenBalance(parseInt(noOfTokens.toString()));
} catch (err) {
console.error(err);
}
}
// create a proposal
async function createProposal() {
try {
const signer = await getProviderOrSigner(true);
const daoContract = getDaoContractInstance(signer);
const tx = await daoContract.createProposal(title, description);
setLoading(true);
await tx.wait();
await getNoOfProposals();
setLoading(false);
} catch (err) {
console.error(err);
window.alert(err.reason);
}
}
async function fetchProposalById(id) {
try {
const provider = await getProviderOrSigner();
const daoContract = getDaoContractInstance(provider);
const proposal = await daoContract.proposals(id);
const parsedProposal = {
id: id,
title: proposal.title.toString(),
description: proposal.description.toString(),
deadline: new Date(parseInt(proposal.deadline.toString()) * 1000),
yesVotes: proposal.yesVotes.toString(),
noVotes: proposal.noVotes.toString(),
executed: proposal.executed,
};
return parsedProposal;
} catch (err) {
console.error(err);
window.alert(err.reason);
}
}
// fetch all proposals
async function fetchAllProposals() {
try {
const allProposals = [];
for (let i = 0; i < noOfProposals; i++) {
const proposal = await fetchProposalById(i);
allProposals.push(proposal);
}
setAllProposals(allProposals);
return allProposals;
} catch (err) {
console.error(err);
window.alert(err.reason);
}
}
// vote on a proposal
async function voteOnProposal(proposalId, _vote) {
try {
const signer = await getProviderOrSigner(true);
const daoContract = getDaoContractInstance(signer);
let vote = _vote === "YES" ? 0 : 1;
const tx = await daoContract.voteOnProposal(proposalId, vote);
setLoading(true);
await tx.wait();
setLoading(false);
await fetchAllProposals();
} catch (err) {
console.error(err);
window.alert(err.reason);
}
}
// execute the proposal
async function executeProposal(proposalId) {
try {
const signer = await getProviderOrSigner(true);
const daoContract = getDaoContractInstance(signer);
const tx = await daoContract.executeProposal(proposalId);
setLoading(true);
await tx.wait();
setLoading(false);
await fetchAllProposals();
await getDaoTreasuryBalance();
} catch (err) {
console.error(err);
window.alert(err.reason);
}
}
useEffect(() => {
if (!walletConnected) {
web3modalRef.current = new Web3Modal({
network: "sepolia",
providerOptions: {},
disableInjectedProvider: false,
});
connectWallet().then(() => {
getDaoTreasuryBalance(),
getTokenBalance(),
getNoOfProposals(),
getDaoContractOwner();
});
}
}, [walletConnected]);
useEffect(() => {
if (selectedTab === "View Proposals") {
fetchAllProposals();
}
}, [selectedTab]);
// display the appropriate tabs whenver possible
function renderTabs() {
if (selectedTab === "Create Proposal") {
return renderCreateProposalsTab();
} else if (selectedTab === "View Proposals") {
return renderViewProposalsTab();
}
return null;
}
// render a create proposal tabs
function renderCreateProposalsTab() {
if (loading) {
return (
<div className={styles.description}>Waiting for transaction...</div>
);
} else if (tokenBalance === 0) {
return (
<div className={styles.description}>
You do not own any GD Tokens. <br />
{/* Bold text */}
<b>You cannot create or vote on proposals!!!</b>
</div>
);
} else {
return (
<div>
<label>Enter Title: </label>
<input
className={styles.input}
placeholder="Title"
type="string"
onChange={(e) => setTitle(e.target.value)}
/>
<br />
<label>Enter Description: </label>
<input
className={styles.input}
placeholder="description"
type="string"
onChange={(e) => setDescription(e.target.value)}
/>
<br />
<button className={styles.button3} onClick={createProposal}>
Create
</button>
<br />
</div>
);
}
}
// render a view proposals tab
function renderViewProposalsTab() {
if (loading) {
return (
<div className={styles.description}>Waiting for transaction...</div>
);
} else if (proposals.length === 0) {
return (
<div className={styles.description}>
<b>No proposals have been created yet!</b>
</div>
);
} else {
return (
<div className={styles.Cards}>
{proposals.map((p, index) => (
<div key={index} className={styles.proposalCard}>
<p>Proposal ID: {p.id.toString()}</p>
<p>Title: {p.title}</p>
<p>Description: {p.description}</p>
<p>Deadline: {p.deadline.toLocaleString()}</p>
<p>YES votes: {p.yesVotes}</p>
<p>NO Votes: {p.noVotes}</p>
<p>Executed?: {p.executed.toString()}</p>
{p.deadline.getTime() > Date.now() && !p.executed ? (
<div className={styles.flex}>
<button
className={styles.button2}
onClick={() => voteOnProposal(p.id, "YES")}
>
Vote YES
</button>
<button
className={styles.button2}
onClick={() => voteOnProposal(p.id, "NO")}
>
Vote NO
</button>
</div>
) : p.deadline.getTime() < Date.now() && !p.executed ? (
<div className={styles.flex}>
<button
className={styles.button2}
onClick={() => executeProposal(p.id)}
>
Execute Proposal {p.yesVotes > p.noVotes ? "(YES)" : "(NO)"}
</button>
</div>
) : (
<div className={styles.description}>
<b>Proposal Executed</b>
</div>
)}
</div>
))}
</div>
);
}
}
return (
<div className={styles.Top}>
<Head>
<title>Noire DAO</title>
<meta name="description" content="CryptoDevs DAO" />
<link rel="icon" href="/favicon.ico" />
</Head>
<header>
<div className={styles.header}>
<button
className={`${styles.radiobox} ${
walletConnected ? styles.green : ""
}`}
>
.
</button>
<Link href="/">
<button className={styles.HeaderButton}>HOME</button>
</Link>
<Link href="/joinUs">
<button className={styles.HeaderButton}>JOIN US</button>
</Link>
<Link href="/view">
<button className={styles.HeaderButton}>CONTACT US</button>
</Link>
</div>
</header>
<div className={styles.main}>
<div className={styles.middleMain}>
<div className={styles.innerMain}>
<h1 className={styles.title}>Welcome to Noire 3.0</h1>
<div className={styles.description}>Welcome to the future</div>
<div className={styles.description}>
Your GD Token Balance:{" "}
<b className={styles.innerValue}>{tokenBalance}</b>
<br />
Treasury Balance:{" "}
<b className={styles.innerValue}>
{formatEther(daoTreasury)} ETH
</b>
<br />
Total Number of Proposals:{" "}
<b className={styles.innerValue}>{noOfProposals}</b>
</div>
</div>
<div className={styles.flex}>
<button
className={styles.button}
onClick={() => setSelectedTab("Create Proposal")}
>
Create Proposal
</button>
<button
className={styles.button}
onClick={() => setSelectedTab("View Proposals")}
>
View Proposals
</button>
</div>
{renderTabs()}
{/* display withdraw button if the address is owner */}
{owner ? (
<div>
{loading ? (
<button className={styles.button}>Loading...</button>
) : (
<button className={styles.button} onClick={withdrawETHFromDao}>
Withdraw DAO ETH
</button>
)}
</div>
) : (
""
)}
</div>
<div className={styles.imgDiv}>
{/* <img className={styles.image} src="/0.svg" /> */}
</div>
</div>
<footer className={styles.footer}>
<div className={styles.innerFooter}>
{/* <h2 className={styles.footerText1}>NOIRE</h2> */}
<h3 className={styles.footerText1}>
Copyright © 2023 Noire, Inc.
</h3>
<h4 className={styles.footerText1}>
Legal Stuff | Privacy Policy | Security | Website Accessibility
</h4>
</div>
</footer>
</div>
);
}
export default Home; |
//
// Alerts.swift
// Gazelle
//
// Created by Angela Li Montez on 5/15/23.
//
import Foundation
import UIKit
extension UIViewController {
func showLoginAlert(description: String?) {
let alertController = UIAlertController(title: "Unable to Log in", message: description ?? "Unknown error", preferredStyle: .alert)
let action = UIAlertAction(title: "OK", style: .default)
alertController.addAction(action)
present(alertController, animated: true)
}
func showSignUpAlert(description: String?) {
let alertController = UIAlertController(title: "Unable to Sign Up", message: description ?? "Unknown error", preferredStyle: .alert)
let action = UIAlertAction(title: "OK", style: .default)
alertController.addAction(action)
present(alertController, animated: true)
}
func showMissingFieldsAlert() {
let alertController = UIAlertController(title: "Required Fields Missing", message: "All fields marked with an asterisk must be completed.", preferredStyle: .alert)
let action = UIAlertAction(title: "OK", style: .default)
alertController.addAction(action)
present(alertController, animated: true)
}
func showSucessAlert() {
let alertController = UIAlertController(title: "Success!", message: "Your request has been processed!", preferredStyle: .alert)
let action = UIAlertAction(title: "OK", style: .default)
alertController.addAction(action)
present(alertController, animated: true)
}
func showFailureAlert(description: String?) {
let alertController = UIAlertController(title: "Failure! Unable to process request", message: description ?? "Unknown error", preferredStyle: .alert)
let action = UIAlertAction(title: "OK", style: .default)
alertController.addAction(action)
present(alertController, animated: true)
}
func displayError(_ error: Error?) {
guard let error = error as NSError? else { return }
let alertController = UIAlertController(title: "Oops...", message: error.description, preferredStyle: .alert)
let action = UIAlertAction(title: "OK", style: .default)
alertController.addAction(action)
present(alertController, animated: true)
}
} |
import { Collapse, List, ListItemButton, ListItemIcon, ListItemText, Typography } from "@mui/material";
import { useState } from "react";
import ExpandLessOutlinedIcon from '@mui/icons-material/ExpandLessOutlined';
import ExpandMoreOutlinedIcon from '@mui/icons-material/ExpandMoreOutlined';
import { SidebarItem } from "./SidebarItem";
import ColorConfigs from "styling/ColorConfigs";
import { Navpath } from "@models/Navpath";
import { observer } from "mobx-react-lite";
type Props = {
item: Navpath;
sidebarOpen: boolean;
}
export const SidebarItemCollapse: React.FC<Props> = observer((props: Props) => {
const [open, setOpen] = useState(false);
return (
<>
<ListItemButton
onClick={() => setOpen(!open)}
sx={{
"&: hover": {
backgroundColor: ColorConfigs.sidebar.hoverBg
},
paddingY: "12px",
paddingX: "24px",
justifyContent: props.sidebarOpen ? "flex-start" : "center"
}}
>
<ListItemIcon sx={{
color: ColorConfigs.sidebar.color,
justifyContent: props.sidebarOpen ? "flex-start" : "center",
}}>
{props.item.icon && props.item.icon}
</ListItemIcon>
<ListItemText
disableTypography
primary={
<Typography>
{props.item.title}
</Typography>
}
/>
{open ? <ExpandLessOutlinedIcon /> : <ExpandMoreOutlinedIcon />}
</ListItemButton>
<Collapse in={open} timeout="auto">
<List>
{props.item.child && props.item.child.map((navpath, index) => (
navpath.child ? (
<SidebarItemCollapse item={navpath} sidebarOpen={props.sidebarOpen} key={index} />
) : (
<SidebarItem item={navpath} sidebarOpen={props.sidebarOpen} key={index} />
)
))}
</List>
</Collapse>
</>
);
}); |
package Entites;
import java.util.HashSet;
import java.util.Set;
import jakarta.persistence.Column;
import jakarta.persistence.DiscriminatorColumn;
import jakarta.persistence.Entity;
import jakarta.persistence.GeneratedValue;
import jakarta.persistence.GenerationType;
import jakarta.persistence.Id;
import jakarta.persistence.Inheritance;
import jakarta.persistence.InheritanceType;
import jakarta.persistence.JoinColumn;
import jakarta.persistence.JoinTable;
import jakarta.persistence.ManyToMany;
import jakarta.persistence.OneToMany;
/**
*
*/
@Entity
@Inheritance(strategy = InheritanceType.SINGLE_TABLE)
@DiscriminatorColumn(name="TYPE")
public class AbstractCompte {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
@Column(name="ID")
private int id;
private String numero;
private double solde;
@OneToMany(mappedBy = "compte")
private Set<Operation> operations = new HashSet<>();
@ManyToMany
@JoinTable(name="CPT_CLI",
joinColumns=@JoinColumn(name="ID_CPT", referencedColumnName = "ID"),
inverseJoinColumns = @JoinColumn(name="ID_CLI", referencedColumnName = "ID")
)
private Set<Client> clients;
@Override
public String toString() {
return "Compte [numero=" + numero + ", solde=" + solde + ", clients=" + clients + "]";
}
/** Getter pour id
* @return the id
*/
public int getId() {
return id;
}
/** Setter pour id
* @param id
*/
public void setId(int id) {
this.id = id;
}
/** Getter pour numero
* @return the numero
*/
public String getNumero() {
return numero;
}
/** Setter pour numero
* @param numero
*/
public void setNumero(String numero) {
this.numero = numero;
}
/** Getter pour solde
* @return the solde
*/
public double getSolde() {
return solde;
}
/** Setter pour solde
* @param solde
*/
public void setSolde(double solde) {
this.solde = solde;
}
/** Getter pour clients
* @return the clients
*/
public Set<Client> getClients() {
return clients;
}
/** Setter pour clients
* @param clients
*/
public void setClients(Set<Client> clients) {
this.clients = clients;
}
/** Getter pour operations
* @return the operations
*/
public Set<Operation> getOperations() {
return operations;
}
/** Setter pour operations
* @param operations
*/
public void setOperations(Set<Operation> operations) {
this.operations = operations;
}
/**
* @param type
*/
public void setType(String type) {
}
} |
"use strict";
var __awaiter = (this && this.__awaiter) || function (thisArg, _arguments, P, generator) {
function adopt(value) { return value instanceof P ? value : new P(function (resolve) { resolve(value); }); }
return new (P || (P = Promise))(function (resolve, reject) {
function fulfilled(value) { try { step(generator.next(value)); } catch (e) { reject(e); } }
function rejected(value) { try { step(generator["throw"](value)); } catch (e) { reject(e); } }
function step(result) { result.done ? resolve(result.value) : adopt(result.value).then(fulfilled, rejected); }
step((generator = generator.apply(thisArg, _arguments || [])).next());
});
};
Object.defineProperty(exports, "__esModule", { value: true });
exports.doCompletionResolve = exports.doCompletion = void 0;
const vscode_languageserver_1 = require("vscode-languageserver");
const yaml_1 = require("yaml");
const types_1 = require("yaml/types");
const ansible_1 = require("../utils/ansible");
const docsFormatter_1 = require("../utils/docsFormatter");
const misc_1 = require("../utils/misc");
const yaml_2 = require("../utils/yaml");
const priorityMap = {
nameKeyword: 1,
moduleName: 2,
redirectedModuleName: 3,
keyword: 4,
// options
requiredOption: 1,
option: 2,
aliasOption: 3,
};
function doCompletion(document, position, context) {
return __awaiter(this, void 0, void 0, function* () {
let preparedText = document.getText();
const offset = document.offsetAt(position);
// HACK: We need to insert a dummy mapping, so that the YAML parser can properly recognize the scope.
// This is particularly important when parser has nothing more than
// indentation to determine the scope of the current line. `_:` is ok here,
// since we expect to work on a Pair level
preparedText = misc_1.insert(preparedText, offset, '_:');
const yamlDocs = yaml_1.parseAllDocuments(preparedText);
// We need inclusive matching, since cursor position is the position of the character right after it
// NOTE: Might no longer be required due to the hack above
const path = yaml_2.getPathAt(document, position, yamlDocs, true);
if (path) {
const node = path[path.length - 1];
if (node) {
const docsLibrary = yield context.docsLibrary;
const isPlay = yaml_2.isPlayParam(path);
if (isPlay) {
return getKeywordCompletion(document, position, path, ansible_1.playKeywords);
}
if (yaml_2.isBlockParam(path)) {
return getKeywordCompletion(document, position, path, ansible_1.blockKeywords);
}
if (yaml_2.isRoleParam(path)) {
return getKeywordCompletion(document, position, path, ansible_1.roleKeywords);
}
if (yaml_2.isTaskParam(path)) {
// offer basic task keywords
const completionItems = getKeywordCompletion(document, position, path, ansible_1.taskKeywords);
if (isPlay === undefined) {
// this can still turn into a play, so we should offer those keywords too
completionItems.push(...getKeywordCompletion(document, position, path, ansible_1.playWithoutTaskKeywords));
}
// incidentally, the hack mentioned above prevents finding a module in
// case the cursor is on it
const module = yield yaml_2.findProvidedModule(path, document, docsLibrary);
if (!module) {
// offer the 'block' keyword (as it is not one of taskKeywords)
completionItems.push(...getKeywordCompletion(document, position, path, new Map([['block', ansible_1.blockKeywords.get('block')]])));
const inlineCollections = yaml_2.getDeclaredCollections(path);
const cursorAtEndOfLine = atEndOfLine(document, position);
let textEdit;
const nodeRange = getNodeRange(node, document);
if (nodeRange) {
textEdit = {
range: nodeRange,
newText: '', // placeholder
};
}
// offer modules
const moduleCompletionItems = [...docsLibrary.moduleFqcns].map((moduleFqcn) => {
var _a;
let priority, kind;
if ((_a = docsLibrary.getModuleRoute(moduleFqcn)) === null || _a === void 0 ? void 0 : _a.redirect) {
priority = priorityMap.redirectedModuleName;
kind = vscode_languageserver_1.CompletionItemKind.Reference;
}
else {
priority = priorityMap.moduleName;
kind = vscode_languageserver_1.CompletionItemKind.Class;
}
const [namespace, collection, name] = moduleFqcn.split('.');
return {
label: name,
kind: kind,
detail: `${namespace}.${collection}`,
sortText: `${priority}_${name}`,
filterText: `${name} ${moduleFqcn}`,
data: {
documentUri: document.uri,
moduleFqcn: moduleFqcn,
inlineCollections: inlineCollections,
atEndOfLine: cursorAtEndOfLine,
},
textEdit: textEdit,
};
});
completionItems.push(...moduleCompletionItems);
}
return completionItems;
}
// Finally, check if we're looking for module options
// In that case, the module name is a key of a map
const parentKeyPath = new yaml_2.AncestryBuilder(path)
.parentOfKey()
.parent(types_1.YAMLMap)
.getKeyPath();
if (parentKeyPath && yaml_2.isTaskParam(parentKeyPath)) {
const parentKeyNode = parentKeyPath[parentKeyPath.length - 1];
if (parentKeyNode instanceof types_1.Scalar) {
let module;
if (parentKeyNode.value === 'args') {
module = yield yaml_2.findProvidedModule(parentKeyPath, document, docsLibrary);
}
else {
[module] = yield docsLibrary.findModule(parentKeyNode.value, parentKeyPath, document.uri);
}
if (module && module.documentation) {
const moduleOptions = module.documentation.options;
const optionMap = new yaml_2.AncestryBuilder(parentKeyPath).parent(types_1.Pair).get().value;
// find options that have been already provided by the user
const providedOptions = new Set(yaml_2.getYamlMapKeys(optionMap));
const remainingOptions = [...moduleOptions.entries()].filter(([, specs]) => !providedOptions.has(specs.name));
const nodeRange = getNodeRange(node, document);
return remainingOptions
.map(([option, specs]) => {
return {
name: option,
specs: specs,
};
})
.map((option, index) => {
// translate option documentation to CompletionItem
const details = docsFormatter_1.getDetails(option.specs);
let priority;
if (isAlias(option)) {
priority = priorityMap.aliasOption;
}
else if (option.specs.required) {
priority = priorityMap.requiredOption;
}
else {
priority = priorityMap.option;
}
const completionItem = {
label: option.name,
detail: details,
// using index preserves order from the specification
// except when overridden by the priority
sortText: priority.toString() + index.toString().padStart(3),
kind: isAlias(option)
? vscode_languageserver_1.CompletionItemKind.Reference
: vscode_languageserver_1.CompletionItemKind.Property,
documentation: docsFormatter_1.formatOption(option.specs),
insertText: atEndOfLine(document, position)
? `${option.name}:`
: undefined,
};
const insertText = atEndOfLine(document, position)
? `${option.name}:`
: option.name;
if (nodeRange) {
completionItem.textEdit = {
range: nodeRange,
newText: insertText,
};
}
else {
completionItem.insertText = insertText;
}
return completionItem;
});
}
}
}
}
}
return null;
});
}
exports.doCompletion = doCompletion;
function getKeywordCompletion(document, position, path, keywords) {
const parameterMap = new yaml_2.AncestryBuilder(path)
.parent(types_1.YAMLMap)
.get();
// find options that have been already provided by the user
const providedParams = new Set(yaml_2.getYamlMapKeys(parameterMap));
const remainingParams = [...keywords.entries()].filter(([keyword]) => !providedParams.has(keyword));
const nodeRange = getNodeRange(path[path.length - 1], document);
return remainingParams.map(([keyword, description]) => {
const priority = keyword === 'name' ? priorityMap.nameKeyword : priorityMap.keyword;
const completionItem = {
label: keyword,
kind: vscode_languageserver_1.CompletionItemKind.Property,
sortText: `${priority}_${keyword}`,
documentation: description,
};
const insertText = atEndOfLine(document, position)
? `${keyword}:`
: keyword;
if (nodeRange) {
completionItem.textEdit = {
range: nodeRange,
newText: insertText,
};
}
else {
completionItem.insertText = insertText;
}
return completionItem;
});
}
/**
* Returns an LSP formatted range compensating for the `_:` hack, provided that
* the node has range information and is a string scalar.
*/
function getNodeRange(node, document) {
if (node.range && node instanceof types_1.Scalar && typeof node.value === 'string') {
const start = node.range[0];
let end = node.range[1];
// compensate for `_:`
if (node.value.includes('_:')) {
end -= 2;
}
else {
// colon, being at the end of the line, was excluded from the node
end -= 1;
}
return misc_1.toLspRange([start, end], document);
}
}
function doCompletionResolve(completionItem, context) {
var _a, _b, _c;
return __awaiter(this, void 0, void 0, function* () {
if (((_a = completionItem.data) === null || _a === void 0 ? void 0 : _a.moduleFqcn) && ((_b = completionItem.data) === null || _b === void 0 ? void 0 : _b.documentUri)) {
// resolve completion for a module
const docsLibrary = yield context.docsLibrary;
const [module] = yield docsLibrary.findModule(completionItem.data.moduleFqcn);
if (module && module.documentation) {
const [namespace, collection, name] = completionItem.data.moduleFqcn.split('.');
let useFqcn = (yield context.documentSettings.get(completionItem.data.documentUri)).ansible.useFullyQualifiedCollectionNames;
if (!useFqcn) {
// determine if the short name can really be used
const declaredCollections = ((_c = completionItem.data) === null || _c === void 0 ? void 0 : _c.inlineCollections) || [];
declaredCollections.push('ansible.builtin');
const metadata = yield context.documentMetadata.get(completionItem.data.documentUri);
if (metadata) {
declaredCollections.push(...metadata.collections);
}
const canUseShortName = declaredCollections.some((c) => c === `${namespace}.${collection}`);
if (!canUseShortName) {
// not an Ansible built-in module, and not part of the declared
// collections
useFqcn = true;
}
}
const insertName = useFqcn ? completionItem.data.moduleFqcn : name;
const insertText = completionItem.data.atEndOfLine
? `${insertName}:`
: insertName;
if (completionItem.textEdit) {
completionItem.textEdit.newText = insertText;
}
else {
completionItem.insertText = insertText;
}
completionItem.documentation = docsFormatter_1.formatModule(module.documentation, docsLibrary.getModuleRoute(completionItem.data.moduleFqcn));
}
}
return completionItem;
});
}
exports.doCompletionResolve = doCompletionResolve;
function isAlias(option) {
return option.name !== option.specs.name;
}
function atEndOfLine(document, position) {
const charAfterCursor = `${document.getText()}\n`[document.offsetAt(position)];
return charAfterCursor === '\n' || charAfterCursor === '\r';
}
//# sourceMappingURL=completionProvider.js.map |
import 'dart:convert';
import 'package:cloud_firestore/cloud_firestore.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:get/get.dart';
import 'package:quiz_app/firebase_ref/loading_status.dart';
import 'package:quiz_app/firebase_ref/reference.dart';
import 'package:quiz_app/modles/question_paper_model.dart';
//controller
class DataUploader extends GetxController {
@override
//This method used for ready the data in firebase;
void onReady() {
uploadData();
super.onReady();
}
final loadingStatus = LoadingStatus.loading.obs; //loadingStatus is obs
//This method used for upload the data in firebase;
Future<void> uploadData() async {
loadingStatus.value = LoadingStatus.loading; //0
final fireStore = FirebaseFirestore.instance;
final manifestContent = await DefaultAssetBundle.of(Get.context!)
.loadString("AssetManifest.json");
final Map<String, dynamic> manifestMap = json.decode(manifestContent);
//load json file and print paths
final papersInAssets = manifestMap.keys
.where((path) =>
path.startsWith("assets/DB/paper") && path.contains(".json"))
.toList();
List<QuestionPaperModel> questionPapers = [];
for (var paper in papersInAssets) {
String stringPaperContent = await rootBundle.loadString(paper);
questionPapers
.add(QuestionPaperModel.fromJson(json.decode(stringPaperContent)));
}
//print('Items number ${questionPapers.length}');
var batch = fireStore.batch();
for (var paper in questionPapers) {
batch.set(questionPaperRF.doc(paper.id), {
"title": paper.title,
"image_url": paper.imageUrl,
"description": paper.description,
"time_seconds": paper.timeSeconds,
"questions_count": paper.questions == null ? 0 : paper.questions!.length
});
for(var questions in paper.questions!){
final questionPath = questionRF(paperId: paper.id, questionId: questions.id);
batch.set(questionPath, {
"question": questions.question,
"correct_answer": questions.correctAnswer
});
for(var answer in questions.answers){
batch.set(questionPath.collection("answers").doc(answer.identifier), {
"identifier": answer.identifier,
"answer": answer.answer
});
}
}
}
await batch.commit();
loadingStatus.value = LoadingStatus.completed;
}
} |
"use client";
import { zodResolver } from "@hookform/resolvers/zod";
import { useForm, type DefaultValues } from "react-hook-form";
import * as z from "zod";
import { trpc } from "@/app/_trpc/client";
import { cn } from "@/lib/utils";
import { CalendarIcon } from "@radix-ui/react-icons";
import { format } from "date-fns";
import { Button } from "../ui/button";
import { Calendar } from "../ui/calendar";
import {
Form,
FormControl,
FormDescription,
FormField,
FormItem,
FormLabel,
FormMessage,
} from "../ui/form";
import { Input } from "../ui/input";
import { Popover, PopoverContent, PopoverTrigger } from "../ui/popover";
import {
Select,
SelectContent,
SelectItem,
SelectTrigger,
SelectValue,
} from "../ui/select";
import { useState } from "react";
const addTripSchema = z.object({
startLocation: z.string().min(1, {
message: "Start Location is required.",
}),
endLocation: z.string().min(1, {
message: "End Location is required.",
}),
milage: z
.string()
.min(1, {
message: "Milage is required.",
})
.refine((num) => !isNaN(Number(num)) && Number(num) > 0, {
message: "Milage is not valid",
}),
fuelConsumed: z
.string()
.min(1, {
message: "Fuel consumed is required.",
})
.refine((num) => !isNaN(Number(num)) && Number(num) > 0, {
message: "Fuel consumed is not valid",
}),
employeeId: z.string().min(1, {
message: "Driver is required.",
}),
vin: z.string().min(1, {
message: "Vehicle is required.",
}),
});
type TAddTripSchema = z.infer<typeof addTripSchema>;
const addTripDefaultValues: DefaultValues<TAddTripSchema> = {
startLocation: "",
endLocation: "",
employeeId: "",
vin: "",
};
type AddTripFormProps = (
| {
formType: "add";
}
| {
formType: "edit";
vin: string;
}
) & {
hideDialog: () => void;
};
type Options = {
value: string;
label: string;
};
export default function AddTripForm(props: AddTripFormProps) {
const [driverOptions, setDriverOptions] = useState<Options[]>([]);
const [vehiclesOptions, setVehiclesOptions] = useState<Options[]>([]);
const form = useForm<TAddTripSchema>({
resolver: zodResolver(addTripSchema),
mode: "onChange",
defaultValues: addTripDefaultValues,
});
const utils = trpc.useContext();
const { data: drivers } = trpc.getDrivers.useQuery(undefined, {
select: (data) => {
return data
.filter((driver) => driver.status === "AVAILABLE")
.map((driver) => ({
value: driver.employeeId,
label: `${driver.firstName} ${driver.lastName}`,
}));
},
onSuccess: (data) => {
setDriverOptions(data);
},
});
const { data: vehicles } = trpc.getVehicles.useQuery(undefined, {
select: (data) => {
return data
.filter((driver) => driver.vehicleStatus === "AVAILABLE")
.map((vehicle) => ({
value: vehicle.vin,
label: `${vehicle.plate} (${vehicle.vehicleType})`,
}));
},
onSuccess: (data) => {
setVehiclesOptions(data);
},
});
const { mutate: addTrip } = trpc.addTrip.useMutation();
const onSubmit = (data: TAddTripSchema) => {
if (props.formType === "add") {
addTrip(
{
startLocation: data.startLocation,
endLocation: data.endLocation,
mileage: Number(data.milage),
fuelConsumed: Number(data.fuelConsumed),
employeeId: data.employeeId,
vin: data.vin,
},
{
onSettled: () => {
utils.getTrips.invalidate();
},
}
);
}
props.hideDialog();
};
return (
<Form {...form}>
<form onSubmit={form.handleSubmit(onSubmit)} className="space-y-8">
<FormField
control={form.control}
name="startLocation"
render={({ field }) => (
<FormItem>
<FormLabel>Start Location</FormLabel>
<FormControl>
<Input {...field} />
</FormControl>
<FormMessage />
</FormItem>
)}
/>
<FormField
control={form.control}
name="endLocation"
render={({ field }) => (
<FormItem>
<FormLabel>End Location</FormLabel>
<FormControl>
<Input {...field} />
</FormControl>
<FormMessage />
</FormItem>
)}
/>
<FormField
control={form.control}
name="milage"
render={({ field }) => (
<FormItem>
<FormLabel>Milage</FormLabel>
<FormControl>
<Input {...field} />
</FormControl>
<FormDescription>
Add the millage in km(Kilometer).
</FormDescription>
<FormMessage />
</FormItem>
)}
/>
<FormField
control={form.control}
name="fuelConsumed"
render={({ field }) => (
<FormItem>
<FormLabel>Fuel Consumed</FormLabel>
<FormControl>
<Input {...field} />
</FormControl>
<FormDescription>Add consumed fuel in l(Liter).</FormDescription>
<FormMessage />
</FormItem>
)}
/>
<FormField
control={form.control}
name="employeeId"
render={({ field }) => (
<FormItem>
<FormLabel>Driver</FormLabel>
<Select onValueChange={field.onChange} {...field}>
<FormControl>
<SelectTrigger>
<SelectValue placeholder="Select driver" />
</SelectTrigger>
</FormControl>
<SelectContent>
{driverOptions.map((driverOption) => (
<SelectItem
key={driverOption.value}
value={driverOption.value}
>
{driverOption.label}
</SelectItem>
))}
</SelectContent>
</Select>
<FormMessage />
</FormItem>
)}
/>
<FormField
control={form.control}
name="vin"
render={({ field }) => (
<FormItem>
<FormLabel>Vehicle</FormLabel>
<Select onValueChange={field.onChange} {...field}>
<FormControl>
<SelectTrigger>
<SelectValue placeholder="Select vehicle" />
</SelectTrigger>
</FormControl>
<SelectContent>
{vehiclesOptions.map((vehicleOption) => (
<SelectItem
key={vehicleOption.value}
value={vehicleOption.value}
>
{vehicleOption.label}
</SelectItem>
))}
</SelectContent>
</Select>
<FormMessage />
</FormItem>
)}
/>
<div className="flex justify-end gap-x-2">
<Button variant="outline" type="button" onClick={props.hideDialog}>
Cancel
</Button>
<Button type="submit">
{props.formType === "add" ? "Add" : "Update"}
</Button>
</div>
</form>
</Form>
);
} |
#pragma once
#include <SFML/Graphics.hpp>
using namespace sf;
class Player
{
private:
const float START_SPEED = 200;
const float START_HEALTH = 100;
//player position
Vector2f m_pos;
//player sprite
Sprite m_sprite;
//player texture
Texture m_texture;
//screen resolution
Vector2f m_resolution;
//arena size
IntRect m_arena;
//tile size
int m_tile;
//direction of movement
bool m_up;
bool m_down;
bool m_left;
bool m_right;
//current health and max health
int m_health;
int m_maxHealth;
//when was the player last hit
Time m_lastHit;
//speed in pixels per second
float m_speed;
public:
Player();
void spawn(IntRect arena, Vector2f resolution, int tileSize);
//void resetPlayerStats();
//handles player being hit
bool hit(Time timeHit);
//when was player last hit
Time getLastHitTime();
//where is player
FloatRect getPosition();
//get centre of player
Vector2f getCentre();
//player angle
float getRotation();
//send copy of sprite to main
Sprite getSprite();
void moveLeft();
void moveRight();
void moveUp();
void moveDown();
void stopLeft();
void stopRight();
void stopUp();
void stopDown();
void update(float elapsedTime, Vector2i mousePos);
//increases player speed
void upgradeSpeed();
//increases player health
void upgradeHealth();
//Increases max health player can have
void increaseHealth(int amount);
//currenty health
int getHealth();
}; |
# Related Applications
The `prefer_related_applications` member is a boolean value that specifies that applications listed in `related_applications` should be preferred over the web application.
If the `prefer_related_applications` member is set to `true`, the user agent might suggest installing one of the related applications instead of this web application.
{% code title="/config/packages/pwa.yaml" lineNumbers="true" %}
```yaml
pwa:
manifest:
prefer_related_applications: true
related_applications:
- platform: "play"
url: "https://play.google.com/store/apps/details?id=com.example.app1"
id: "com.example.app1"
- platform: "itunes"
url: "https://itunes.apple.com/app/example-app1/id123456789"
- platform: "windows"
url: "https://apps.microsoft.com/store/detail/example-app1/id123456789"
```
{% endcode %}
Currently known possible values for the platform member are as follows;
* `"chrome_web_store"`: [Google Chrome Web Store](https://chrome.google.com/webstore).
* `"play"`: [Google Play Store](https://play.google.com/).
* `"chromeos_play"`: [Chrome OS Play](https://support.google.com/googleplay/answer/7021273).
* `"webapp"`: [Web apps](https://www.w3.org/TR/appmanifest/).
* `"windows"`: [Windows Store](https://www.microsoft.com/store/apps).
* `"f-droid"`: [F-droid](https://f-droid.org/).
* `"amazon"`: [Amazon App Store](https://www.amazon.com/gp/browse.html?node=2350149011). |
package Section;
use 5.006;
use strict;
use warnings;
=head1 NAME
Section
=head1 VERSION
Version 0.04
=cut
our $VERSION = '0.04';
=head1 SYNOPSIS
Section Object
use Section;
my $section = Section->new(
raw_data => "TITLE: An odd event
[1429.123.0457] Nowhere
Al looked again, and he was there.",
has_header => 1,
number => 1,
);
=head1 SUBROUTINES/METHODS
=head2 new
new takes a hash of data, to include: the section number ('number') if relevant,
and the raw_data from the file.
It uses the raw_data, and a "has_header" flag to create the section header.
The section's title is set by the first data line beginning with "TITLE:".
=cut
sub new {
my ($class, %data) = @_;
my $self = {
_file_name => $data{file_name},
_has_header => $data{has_header} || 0,
_header => undef,
_headless_data => undef,
_number => $data{number},
_raw_data => $data{raw_data},
_report => undef,
_title => undef,
};
bless $self, $class;
$self->_write_headless_data();
$self->_write_report();
return $self;
}
=head2 avg_sentence_length
Returns the average sentence length.
=cut
sub avg_sentence_length { $_[0]->{_report}->avg_sentence_length };
=head2 avg_word_length
Returns the average word length.
=cut
sub avg_word_length {
my ( $self ) = @_;
return $self->{_report}->avg_word_length;
}
=head2 file_name
Returns the file name for the section
=cut
sub file_name {
my ($self) = @_;
return $self->{_file_name}
}
=head2 grade_level
Returns the Flesch-Kincaid Grade Level score per: https://en.wikipedia.org/wiki/Flesch%E2%80%93Kincaid_readability_tests
=cut
sub grade_level {
my ($self) = @_;
my $f = sprintf("%0.2f", $self->{_report}->grade_level());
return $f;
}
=head2 header
Returns the section header, if appropriate, or undef.
=cut
sub header {
my ($self) = @_;
return undef unless $self->{_has_header};
my (@lines) = split(/\n/, $self->raw_data() );
foreach my $line (@lines) {
next if $line =~ m/TITLE:/;
chomp($line);
$line =~ s/^\s*//;
next unless length($line); # Skips extra blank lines at start.
$self->{_header} = $line;
last;
}
return $self->{_header};
}
=head2 headless_data
Returns the raw_data, minus any header or title.
=cut
sub headless_data { return $_[0]->{_headless_data}; }
=head2 number
Returns the section number.
=cut
sub number { $_[0]->{_number} };
=head2 raw_data
Returns the raw_data from the file.
=cut
sub raw_data { $_[0]->{_raw_data} };
=head2 sentence_count
Returns the number of sentences.
=cut
sub sentence_count { $_[0]->{_report}->sentence_count };
=head2 title
Returns the title.
=cut
sub title {
my ($self) = @_;
my (@lines) = split(/\n/, $self->raw_data() );
foreach my $line (@lines) {
if ( $line =~ m/TITLE:/ ) {
chomp($line);
$line =~ s/^\s*TITLE:\s*//;
$self->{_title} = $line;
last;
}
}
return $self->{_title};
}
=head2 word_count
Returns the word count of the section, minus title or header.
=cut
sub word_count { return $_[0]->{_report}->word_count; }
=head2 word_list
Returns a hash of the words used, in lowercase, with the usage count of that word as the value.
=cut
sub word_list {
my $self = shift;
my %word_list = $self->{_report}->sorted_word_list();
return %word_list;
}
=head2 write_report
Writes the report file.
=cut
sub write_report {
my $self = shift;
my $string = "File: " . $self->file_name() . "\n";
$string .= "Grade Level: " . $self->grade_level() . "\n";
$string .= "Word Frequency List:\n";
my %word_list = $self->{_report}->sorted_word_list();
my @unsorted_keys = ( keys %word_list );
my @sorted_keys = reverse ( sort { $a <=> $b } @unsorted_keys );
my $max_keys = 25;
foreach my $count ( @sorted_keys ){
$string .= " $count ";
foreach my $word ( @{$word_list{$count}} ){
$string .= " $word";
}
$string .= "\n";
$max_keys -= 1;
last unless $max_keys;
}
return $string;
}
=head2 _write_report
Writes the report data.
=cut
sub _write_report {
my ($self) = @_;
my $text = $self->headless_data;
use Report;
$self->{_report} = Report->new( string => $self->headless_data() );
}
=head2 _write_headless_data
Writes the headless data.
=cut
sub _write_headless_data {
my ($self) = @_;
my $data;
($data = $self->raw_data) =~ s/^TITLE:.*\n//;
$data =~ s/^\s*//;
# TODO: Not sure this covers multiple empty blank lines.
$data =~ s/^.*\n// if $self->{_has_header};
$data =~ s/^\s*(.*)\s*$/$1/;
$self->{_headless_data} = $data;
}
=head1 AUTHOR
Leam Hall, C<< <leamhall at gmail.com> >>
=head1 BUGS
Please report any bugs or feature requests to L<https://github.com/LeamHall/bookbot/issues>.
=head1 SUPPORT
You can find documentation for this module with the perldoc command.
perldoc lib/Section
You can also look for information at:
=over 4
=item * GitHub Project Page
L<https://github.com/LeamHall/bookbot>
=back
=head1 ACKNOWLEDGEMENTS
Besides Larry Wall, you can blame the folks on Freenode#perl for this stuff existing.
=head1 LICENSE AND COPYRIGHT
This software is Copyright (c) 2021 by Leam Hall.
This is free software, licensed under:
The Artistic License 2.0 (GPL Compatible)
=cut
1; # End of Section |
import {
View,
Image,
Pressable,
Text,
StyleSheet,
ScrollView,
} from 'react-native';
import { LinearGradient } from 'expo-linear-gradient';
import { MaterialIcons } from '@expo/vector-icons';
import { useNavigation } from '@react-navigation/native';
import { NativeStackNavigationProp } from '@react-navigation/native-stack';
import { users } from '../data/users';
import { stories } from '../data/stories';
const Stories = () => {
const navigation = useNavigation<NativeStackNavigationProp<any>>();
return (
<View style={styles.container}>
<ScrollView
horizontal={true}
bounces={false}
>
<Pressable
style={styles.addStory}
>
<>
<Image
style={styles.image}
source={require('../assets/portrait.jpg')}
/>
<Text style={[styles.profileNickname, { marginTop: 3 }]}>Your story</Text>
<MaterialIcons
name="add-circle"
size={20}
color="#0095F6"
style={{
position: 'absolute',
backgroundColor: 'white',
borderRadius: 24 / 2,
width: 20,
height: 20,
right: 3,
bottom: 3,
borderColor: 'black',
borderWidth: 4,
}}
/>
</>
</Pressable>
{stories.map((story, index) => (
<Pressable
style={styles.story}
key={index}
>
<Pressable
style={({ pressed }) => [
pressed && {
transform: [{
scale: 0.94,
}],
}
]}
onPress={() => {
navigation.navigate('StoryScreen',
{
nick: story.user,
story: story.story,
profilePicture: story.profilePicture,
})
}}
>
<LinearGradient
colors={['#CA1D7E', '#E35157', '#F2703F']}
start={{ x: 0.0, y: 1.0 }} end={{ x: 1.0, y: 1.0 }}
style={{ height: 70, width: 70, alignItems: 'center', justifyContent: 'center', borderRadius: 67 / 2 }}>
<Image
style={styles.image}
source={{ uri: story.profilePicture }}
/>
</LinearGradient>
</Pressable>
<Text style={styles.profileNickname}>{story.user}</Text>
</Pressable>
))}
</ScrollView>
</View >
);
}
export default Stories;
const styles = StyleSheet.create({
container: {
paddingVertical: 10,
borderBottomWidth: 0.2,
borderBottomColor: 'gray',
},
story: {
justifyContent: 'center',
alignItems: 'center',
marginLeft: 15,
},
image: {
width: 67,
height: 67,
borderRadius: 67 / 2,
padding: 10,
alignSelf: 'center',
borderColor: 'black',
borderWidth: 3,
},
profileNickname: {
color: 'white',
textAlign: 'center',
fontSize: 12,
},
addStory: {
width: 65,
height: 65,
borderRadius: 65 / 2,
marginLeft: 10,
}
}); |
# s2_change
Input sentinel 2 imagery and detect change
Change Detect Solo Project Workflow
Justin Fowler
last update 05/31/2024
1.access_sentinel_data dir: search_for_gran.py and download_seninel.py
search_for_gran.py:
input s2 gran, and exports to .csv all the s2 files that were in parameters
parameters: start_date, end_date,gran,productType,data_collection,cloudcover (set to 10)
manually select scenes:
look through csv's and select ID,Name of scenes wanted
Select as many scenes as needed
can check Copernicus browser to see thumbnail of scenes
save csv as {gran}_select.csv
Improvements: csv's probably only need to include ID and Name when created in search_for_gran.py
download_seninel.py:
given {gran}_select.csv, download all scenes to tile directory
currently can only download 3 at a time, have to rerun.
Improvements: add a wait function after 3 have been downloaded, so don't have to rerun to get all
2. safe_dir_reorg.py
Unzip .SAFE directories
Create date directories for where data will be stored
For L2A data creates MSIL2A directory, L1C data creates MSIL1C directory
Copies .jp2 bands 2,3,4,8,11,12 and SCL to MSIL2A
Copies .jp2 bands 2,3,4,8,11,12,8a,10 to MSIL1C
Deletes unzip'd .SAFE directories
Improvements:
Lot's of looping, could search for specific files more efficiently
3. download_glo30.py
Given tile name, uses sentinel_2_index_shapefile.shp to download GLO 30 dem from aws
Downloads 1x1's and mosaic's them (with buffer) to sentinel 2 grid
outputs:
a. [gran]_glo30_buf.tif
Improvements:
Currently not using DEM, add terrain shadow calculation further downstream
Came across a tile that covers 6 1x1's... so annoying
4. stack_vrt.py
Takes MSIL2A directory and stacks bands 2,3,4,8,11,12 to 1,2,3,4,5,6
Also uses SCL to mask out cloud mask and cloud shadow, set's nodata to -9999
Outputs:
a. [tile]_[date]_6band.vrt
b. [tile]_[date]_6band_masked.tif
stack_vrt_v2.py
Set's up files to be in {tile}\{month}\{date} dir's
To be immediately ready for mosaic_indiv_bands.py
4B. mosaic_images:
reorg_date_to_month.py
mosaic_indiv_bands.py
mosaic_month.py
Unmasked clouds are a real pain
Plan:
Download 1 scene per week (could do more but so much downloading...)
after all the scenes are masked, mosaic all scenes in a month
take the lowest value, missed clouds would be super high values theoretically
potentially will miss change, but it should be caught the next month so no biggie
Move all date dirs into yyyymm dirs, then go through and mosaic them based of yyyymm dirs
5.normalize_dif.py and create_indicies.py
normalize_dif.py
Creates functions
norm_dif: create normalized differences, like ndvi.
Clamps over |100|
assumes -9999 as nodata
msavi_calc: creates msavi
Clamps over |100|
assumes -9999
Improvements:
set up class correctly, with self as first input parameter
make nodata an input parameter
create_indicies
Uses function in normalize_dif.py
Loops through date directories, find's '_6band_masked.tif' and creates:
Outputs:
a.[tile]_[date]_ndvi.tif
b.[tile]_[date]_ndwi.tif
c.[tile]_[date]_ndbi.tif
d.[tile]_[date]_ndsi.tif
e.[tile]_[date]_msavi.tif
5B. Need to take ndwi's and mask out the masked.tif's again... maybe this should be done before...
can be part of masked process, do it with stack_vrt.py.
if part of mask, won't get water change...
maybe, just use ndwi time series to mask out for stats in
6. stack_time.py and stack_bands_time.py
stack_time.py:
takes date indicies(5.a->e) and stacks them in a time seires vrt
Output:
a.time_series/[tile]_ndvi_stack.vrt
b.time_series/[tile]_ndwi_stack.vrt
c.time_series/[tile]_ndbi_stack.vrt
d.time_series/[tile]_ndsi_stack.vrt
e.time_series/[tile]_msavi_stack.vrt
stack_bands_time.py:
takes date 6band_masked.tif's (4.b) and stacks them in a time seires vrt
Output:
a.time_series/[tile]_b1_stack.tif
b.time_series/[tile]_b2_stack.tif
c.time_series/[tile]_b3_stack.tif
d.time_series/[tile]_b4_stack.tif
e.time_series/[tile]_b5_stack.tif
f.time_series/[tile]_b6_stack.tif
Improvements:
Unsure if need 2 seperate scripts, or if need indicies time series at all
7. time_series_v2.py
Takes stats for time_series stacks
uses chips so doesnt destroy RAM
Outputs:
a. time_stats/[tile]_[timeseries]_mean.tif
b. time_stats/[tile]_[timeseries]_median.tif
c. time_stats/[tile]_[timeseries]_range.tif
d. time_stats/[tile]_[timeseries]_std.tif
8. temporal_slope_v3.py
take time_series and run linear regression on them, in order to determine temporal slope
Uses 512x512 chips to process so doesn't destroy RAM
numpy math to get linear regression slope
v3 now includes filter for outliers in stack. Things like missed clouds will be removed.
9. std_tempslope.py
create script to threshold based on standard deviation of temporal slope file
add water/ocean mask to get rid of std being skewed?
for water mask, use focal filters (check Geoprocessing with Python book)
10. step_time.py
get date of change
11. For now, just taking Blue band, will have to come up with better, change algorthim/rules later
added mean_filter_3x3.py
does a 3x3 uniform filter to smooth out the tempslope_std and date_num
Helps with edges of buildings type things, also just makes everything look cleaner.
a. vector_attrib/b1_change_layers.py
combine's standard deviation of temp slope and step filter date
Where std > |5|, keep the step filter date
inputs:
f'{gran}_b{bnum}_stacktempslope_std.tif' #standard deviation layer
f'{gran}_b{bnum}_date_num.tif' #step filter detect layer
output:
f'{gran}_b{bnum}_ch_tmp.tif'#tmp till goes through sieve
Missing some change in T18SUJ becaues the standard deviation isn't high enough.
new script: vector_attrib/b1_change_layers_v2.py
adding in another flat threshold for temporal slope, > |75|
will also include "confidence measure in vector layer, using tempslope and tempslope_std
inputs:
f'{gran}_b{bnum}_stacktempslope_std.tif' #standard deviation layer
f'{gran}_b{bnum}_date_num.tif' #step filter detect layer
f'{gran}_b{bnum}_stacktempslope.tif' #tempslope layer
output:
f'{gran}_b{bnum}_ch_tmp.tif'#tmp till goes through sieve
b. vector_attrib/ch_layer_sieve.py
Remove single pixels (seems to only remove single pixels surrounded by 0s)
input: f'{gran}_b{bnum}_ch_tmp.tif
output: f'{gran}_b{bnum}_ch.tif'
Improvements:
play around more with gdal_sieve.py, figure out how to remove all single pixels
c. vector_attrib/vectorize.py
Use gdal.Polygonize to export raster to vector
input: f'{gran}_b{bnum}_ch.tif'
output: f'{gran}_b{bnum}_ch_tmp.shp'
Looks like a bug? seeing a date=10, when the max should be 9...
12. Add attribution
vec_attrib1.py
uses rasterstats, zonal stats which takes forever
vec_attrib_test2.py, uses centriod, point is much faster, but centriod might not be value inside poly
slope_std, float
veg_mean, veg or non veg
veg_std, low/high variation
water_std, low/high variation
vec_attrib_v3.py
slope_std, float
veg_mean, veg or non veg
veg_std, low/high variation
water_std, low/high variation
conf, high, med, and low. Using both tempslope and tempslope_std
Future Attributes, layers not yet scripted
max ndvi post change (veg regrowth)
shadow likely hood
brightness increase/decrease (high albedo buildings) |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.