text
stringlengths 2
104M
| meta
dict |
---|---|
/*
==============================================================================
FractionalGrid.h
Created: 24 Mar 2019 1:58:51pm
Author: Vincenzo
Wrapper class for setting preset data in a ValueTree.
The general idea is you can load up to 128 modes into a preset, and this will allow you to
maniuplate the mode slots and keeps track of which mode slots are loaded,
with mode selectors 0 and 1 being the main ones (0 = input, 1 = output).
A custom mode has it's own slot and there can only be one at a time. To take advantage of
mode slot features, the custom mode must be saved to file and loaded into a slot.
Slot numbers and slot indicies do not need to match, and the slot indicies are only
used internally
==============================================================================
*/
#pragma once
#include "../JuceLibraryCode/JuceHeader.h"
#include "../PluginIDs.h"
#include "../CommonFunctions.h"
#include "../Constants.h"
#include "Mode.h"
class SvkPreset
{
protected:
ValueTree parentNode;
ValueTree thePropertiesNode;
ValueTree theModeSlots;
ValueTree theModeSelectors;
ValueTree theCustomMode;
ValueTree theKeyboardNode;
ValueTree theMidiSettingsNode;
ValueTree theMappingsNode;
public:
SvkPreset();
SvkPreset(const ValueTree presetNodeIn);
SvkPreset(const SvkPreset& presetToCopy);
~SvkPreset();
/*
Returns the parent node of the preset
Will sort mode slots by slot number if called with true.
*/
ValueTree getPresetNode(bool sortModeSlots = false);
/*
Returns the keyboard node of the preset
*/
ValueTree getKeyboardNode();
/*
Returns the midi settings node of the preset
*/
ValueTree getMidiSettingsNode();
/*
Returns the mappings node of the preset
*/
ValueTree getMappingsNode();
/*
Recreate a preset from a ValueTree
*/
bool restoreFromNode(ValueTree presetNodeIn, bool createCopy = false); // TODO: Revise default parameter
/*
Returns array of slot numbers in use
*/
Array<int> getSlotNumbersInUse() const;
/*
Returns the mode selector that's currently in view
*/
int getModeSelectorViewed() const;
/*
Returns true if given slot number is being used
*/
bool isSlotNumberInUse(int modeSlotNumberIn) const;
/*
Returns the amount of modes loaded into slots
*/
int getModeSlotsSize() const;
/*
Returns the slot number of the mode loaded by the given selector
*/
int getSlotNumberBySelector(int selectorNumIn) const;
/*
Returns the slot number of the mode loaded by selector 0 (input keyboard)
*/
int getMode1SlotNumber() const;
/*
Returns the slot number of the mode loaded by selector 1 (output keyboard)
*/
int getMode2SlotNumber() const;
/*
Returns the mode slot number of the mode selector currently viewed
*/
int getModeViewedSlotNumber() const;
/*
Return the root note of the given mode selector
*/
int getModeSelectorRootNote(int modeSelectorNum) const;
/*
Returns the mode slots node
*/
ValueTree getModeSlots();
/*
Returns the mode with the given slot number (which may be different from the index it's in)
*/
ValueTree getModeInSlot(int slotNum);
/*
Returns the mode loaded in the given mode selector
*/
ValueTree getModeBySelector(int selectorNumIn);
/*
Returns the mode loaded in selector 0 (input keyboard)
*/
ValueTree getMode1();
/*
Returns the mode loaded in selector 1 (output keyboard)
*/
ValueTree getMode2();
/*
Returns the mode currently being viewed
*/
ValueTree getModeViewed();
/*
Returns the current custom mode
*/
ValueTree getCustomMode();
/*
Finds the slot index of a given slot number, or returns -1 if not found
*/
int getSlotNumberIndex(int slotNumIn);
/*
Sets the slot number used by the given selector, and returns the slot index
If slot number doesn't exist, nothing will happen and this will return -1
*/
int setModeSelectorSlotNum(int selectorNumIn, int slotNumIn);
void setMode1SlotNumber(int slotNumIn);
void setMode2SlotNumber(int slotNumIn);
/*
Sets the midi root note used by the mode selector
*/
void setModeSelectorRootNote(int modeSelectorNumIn, int rootNoteIn);
/*
Loads the given mode into the given slot number, and returns the slot index
*/
int setModeSlot(ValueTree modeNodeIn, int slotNumber);
/*
Puts the given mode in the smallest available free slot and returns the slot number
*/
int addModeSlot(ValueTree modeNodeIn);
/*
Sets the custom mode and returns a reference to the mode node
*/
ValueTree setCustomMode(ValueTree customModeNodeIn, bool createCopy = false);
/*
Empties the given mode slot and retunrs the slot index it was in
*/
int removeModeSlot(int slotNumberIn);
/*
Replaces mode slots 1 & 2 with standard tuning, and removes other modes
Also resets selectors 0 and 1 to respective mode slots
*/
void resetModeSlots();
/*
Returns a readable version of the parent node
*/
String toString();
static bool isValidPresetNode(ValueTree presetNodeIn);
static SvkPreset getDefaultPreset();
private:
int getNextFreeSlotNumber();
private:
Array<int> slotNumbersInUse;
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
FractionalGrid.cpp
Created: 22 Apr 2019 12:05:22pm
Author: Vincenzo
==============================================================================
*/
#include "FractionalGrid.h"
FractionalGrid::FractionalGrid(float columsIn, float rowsIn)
{
columns = columsIn;
rows = rowsIn;
bounds = Rectangle<int>();
}
FractionalGrid::~FractionalGrid() {}
Rectangle<int> FractionalGrid::setBounds(int x, int y, int w, int h)
{
bounds = Rectangle<int>(x, y, w, h);
updateGrid();
return bounds;
}
Rectangle<int> FractionalGrid::setBounds(Rectangle<int> rectIn)
{
bounds = rectIn;
updateGrid();
return bounds;
}
Rectangle<int> FractionalGrid::getBounds()
{
return bounds;
}
void FractionalGrid::scaleToColumnWidth(float columnWidthIn)
{
float factor = columnWidthIn / getColumnWidth();
bounds = bounds.withWidth((getColumnWidth() * factor + columnGap) * columns);
bounds = bounds.withHeight((getRowHeight() * factor + rowGap) * rows);
updateGrid();
}
void FractionalGrid::scaleToRowHeight(float rowHeightIn)
{
float factor = rowHeightIn / getRowHeight();
bounds = bounds.withWidth((getColumnWidth() * factor + columnGap) * columns);
bounds = bounds.withHeight((getRowHeight() * factor + rowGap) * rows);
updateGrid();
}
void FractionalGrid::scaleToProportion(float proportion, float rowSizeIn)
{
bounds = bounds.withWidth((rowSizeIn * proportion + columnGap) * columns);
bounds = bounds.withHeight((rowSizeIn + rowGap) * rows);
updateGrid();
}
void FractionalGrid::updateGrid()
{
colFraction = 1.0 / columns;
rowFraction = 1.0 / rows;
colSize = (bounds.getWidth() + columns * columnGap) / columns;
rowSize = (bounds.getHeight() + rows * rowGap) / rows;
}
float FractionalGrid::getColumnWidth()
{
return colSize;
}
float FractionalGrid::getRowHeight()
{
return rowSize;
}
float FractionalGrid::getColumns()
{
return columns;
}
float FractionalGrid::getRows()
{
return rows;
}
void FractionalGrid::setColumnGap(int colGapIn)
{
columnGap = colGapIn;
updateGrid();
}
void FractionalGrid::setRowGap(int rowGapIn)
{
rowGap = rowGapIn;
updateGrid();
}
int FractionalGrid::getColumnGap()
{
return columnGap;
}
int FractionalGrid::getRowGap()
{
return rowGap;
}
Point<int> FractionalGrid::place_component(Component* item, int col, int row)
{
if (bounds.getX() == 0 || bounds.getY() == 0)
throw "Cannot have 0 size";
Point<int> pt = Point<int>((int)(col * (colSize + columnGap)), (int)(row * (rowSize + rowGap)));
item->setTopLeftPosition(pt);
return pt;
}
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
MappingHelper.h
Created: 21 Aug 2019 4:38:52pm
Author: Vincenzo Sicurella
==============================================================================
*/
#pragma once
#include "MappingEditorBase.h"
class MappingHelper : public MappingEditor
{
public:
MappingHelper(NoteMap mappingIn = NoteMap());
~MappingHelper();
//================================================================
// MappingEditor implementation
NoteMap getCurrentNoteMapping() const override;
void mapMidiNoteToKey(int midiNoteIn, int keyNumberOut) override;
void resetMapping(NoteMap mappingIn = NoteMap(), bool sendMessage = true) override;
//================================================================
bool isWaitingForKeyInput();
int getVirtualKeyToMap();
bool isMappingAllPeriods();
void prepareKeyToMap(int virtualKeyClicked, bool allPeriodsIn);
void mapPreparedKeyToNote(int midiNoteTriggered, bool mapAllPeriods, Mode* modeFrom = nullptr, Mode* modeTo = nullptr);
void cancelKeyMap();
Array<int> getKeyInAllPeriods(int keyNumber, Mode* modeToReference);
private:
NoteMap noteMapping;
int virtualKeyToMap = -1;
bool allPeriods = false;
bool waitingForKeyInput = false;
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
OwnedHashMap.h
Created: 17 Nov 2019 4:50:00pm
Author: Vincenzo Sicurella
==============================================================================
*/
#pragma once
#include "../../JuceLibraryCode/JuceHeader.h"
template <typename KeyType,
typename ValueType,
class HFunctionType = DefaultHashFunctions,
class CriticalSectionToUse = DummyCriticalSection>
class OwnedHashMap : public OwnedArray<ValueType, CriticalSectionToUse>,
public HashMap<KeyType, ValueType*, HFunctionType, CriticalSectionToUse>
{
public:
typedef OwnedArray<ValueType> TheArray;
typedef HashMap<KeyType, ValueType*, HFunctionType, CriticalSectionToUse> TheHash;
OwnedHashMap(int numberOfSlots=101, HFunctionType hashFunction=HFunctionType())
: TheArray(), TheHash(numberOfSlots, hashFunction)
{
}
~OwnedHashMap()
{
this->TheHash::clear();
this->TheArray::clear();
}
TheArray& getOwnedArray()
{
return dynamic_cast<TheArray&>(*this);
}
TheHash& getHashMap()
{
return dynamic_cast<TheHash&>(*this);
}
int getSize()
{
return this->TheArray::size();
}
ValueType* stash(KeyType keyIn, ValueType* valueIn)
{
ValueType* valueOut = valueIn;
this->add(valueIn);
this->TheHash::set(keyIn, valueOut);
return valueOut;
}
ValueType* grab(const KeyType& keyIn) const
{
return this->TheHash::operator[](keyIn);
}
void toss(KeyType keyIn)
{
this->TheArray::removeObject(grab(keyIn));
this->TheHash::remove(keyIn);
}
static void test()
{
// keep the "fun" in "function"
OwnedHashMap<String, RangedAudioParameter> parameters;
Identifier normal("NormalcyAmount");
parameters.stash(normal.toString(), new AudioParameterFloat(normal.toString(), "Sounds good", 0.0f, 100.0f, 50.0f));
Identifier weird("WeirdnessAmount");
parameters.stash(weird.toString(), new AudioParameterFloat(weird.toString(), "What??", 0.0f, 100.0f, 50.0f));
parameters.grab(weird.toString())->setValue(100.0f);
parameters.grab(normal.toString())->setValue(0.0f);
parameters.toss(normal.toString());
}
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
MappingEditorBase.h
Created: 18 Oct 2020 8:32:13pm
Author: Vincenzo
==============================================================================
*/
#pragma once
#include "NoteMap.h"
class MappingEditor
{
public:
MappingEditor() {};
virtual NoteMap getCurrentNoteMapping() const = 0;
virtual void mapMidiNoteToKey(int midiNoteIn, int keyNumberOut) = 0;
virtual void resetMapping(NoteMap mappingIn, bool sendMessage) = 0;
public:
class Listener
{
public:
virtual void keyMappingStatusChanged(int keyNumber, bool preparedToMap) {};
virtual void keyMapConfirmed(int keyNumber, int midiNote) {};
virtual void mappingEditorChanged(NoteMap& newMapping) {};
};
void addListener(Listener* listenerIn) { listeners.add(listenerIn); }
void removeListener(Listener* listenerIn) { listeners.remove(listenerIn); }
void removeAllListeners() { listeners.clear(); }
protected:
ListenerList<Listener> listeners;
}; | {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
FractionalGrid.h
Created: 24 Mar 2019 1:58:51pm
Author: Vincenzo
==============================================================================
*/
#include "../JuceLibraryCode/JuceHeader.h"
#pragma once
class FractionalGrid
{
protected:
// Parameters
float columns;
float rows;
Rectangle<int> bounds;
// Properties
double colFraction;
double rowFraction;
float colSize;
float rowSize;
int columnGap = 1;
int rowGap = 1;
// Methods
void updateGrid();
public:
FractionalGrid(float columsIn, float rowsIn);
~FractionalGrid();
Rectangle<int> setBounds(int x, int y, int w, int h);
Rectangle<int> setBounds(Rectangle<int> rectIn);
Rectangle<int> getBounds();
void scaleToColumnWidth(float columnWidthIn);
void scaleToRowHeight(float rowHeightIn);
void scaleToProportion(float proportion, float factor);
float getColumns();
float getRows();
float getColumnWidth();
float getRowHeight();
int getColumnGap();
int getRowGap();
void setColumnGap(int colGapIn);
void setRowGap(int rowGapIn);
/* WARNING: mutates Component's position*/
Point<int> place_component(Component* item, int col, int row);
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
MidiFilter.h
Created: 14 May 2019 10:25:01pm
Author: Vincenzo
==============================================================================
*/
#pragma once
#include "../JuceLibraryCode/JuceHeader.h"
#include "NoteMap.h"
class MidiFilter
{
std::unique_ptr<NoteMap> midiNoteMapping;
Point<int> noteRange;
public:
MidiFilter();
MidiFilter(Array<int> mapIn);
MidiFilter(NoteMap mapIn);
int getNoteRemapped(int midiNoteIn) const;
int getNoteMidi(int remappedNoteIn) const;
Array<int> getRemappedNotes();
Point<int> getNoteRange() const;
NoteMap* getNoteMap();
int setNote(int noteIn, int noteOut);
void setNoteRange(int lowestMidiNote, int highestMidiNote);
NoteMap* setNoteMap(Array<int> mapToCopy);
NoteMap* setNoteMap(NoteMap mapToCopy);
int size() const;
String toString();
static Array<int> getStandardMap();
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
MidiInputFilter.cpp
Created: 14 May 2019 10:25:01pm
Author: Vincenzo
==============================================================================
*/
#include "MidiFilter.h"
MidiFilter::MidiFilter()
{
noteRange = Point<int>(-1, 127);
midiNoteMapping = std::make_unique<NoteMap>(128, true);
}
MidiFilter::MidiFilter(Array<int> mapIn)
{
noteRange = Point<int>(-1, 127);
setNoteMap(mapIn);
}
MidiFilter::MidiFilter(NoteMap mapIn)
{
noteRange = Point<int>(-1, 127);
setNoteMap(mapIn.getValues());
}
NoteMap* MidiFilter::setNoteMap(Array<int> mapToCopy)
{
midiNoteMapping = std::make_unique<NoteMap>(mapToCopy);
return midiNoteMapping.get();
}
NoteMap* MidiFilter::setNoteMap(NoteMap mapToCopy)
{
midiNoteMapping = std::make_unique<NoteMap>(mapToCopy);
return midiNoteMapping.get();
}
int MidiFilter::getNoteRemapped(int midiNoteIn) const
{
int midiNoteOut = jlimit(noteRange.x, noteRange.y, midiNoteIn);
if (midiNoteMapping.get())
midiNoteOut = midiNoteMapping->getValue(midiNoteOut);
return midiNoteOut;//jlimit(noteRange.x, noteRange.y, midiNoteOut);
}
int MidiFilter::getNoteMidi(int remappedNoteIn) const
{
int remappedNote = jlimit(noteRange.x, noteRange.y, remappedNoteIn);
int noteOut = -1;
if (midiNoteMapping.get())
noteOut = midiNoteMapping->getKey(remappedNote);
return jlimit(noteRange.x, noteRange.y, noteOut);
}
Point<int> MidiFilter::getNoteRange() const
{
return noteRange;
}
int MidiFilter::setNote(int noteIn, int noteOut)
{
if (midiNoteMapping.get())
midiNoteMapping->setValue(noteIn, noteOut);
return noteOut;
}
void MidiFilter::setNoteRange(int lowestMidiNote, int highestMidiNote)
{
noteRange = Point<int>(lowestMidiNote, highestMidiNote);
}
NoteMap* MidiFilter::getNoteMap()
{
return midiNoteMapping.get();
}
Array<int> MidiFilter::getRemappedNotes()
{
return midiNoteMapping->getValues();
}
int MidiFilter::size() const
{
int sizeOut = 0;
if (midiNoteMapping.get())
sizeOut = midiNoteMapping->getSize();
return sizeOut;
}
String MidiFilter::toString()
{
String out;
for (int i = 0; i < midiNoteMapping->getSize(); i++)
{
out << "Midi Note In " << i << " --> " << midiNoteMapping->getValue(i) << "\t Midi Note Out " << i << " <-- " << midiNoteMapping->getKey(i) << "\n";
}
return out;
}
Array<int> MidiFilter::getStandardMap()
{
Array <int> mapOut;
mapOut.resize(128);
for (int i = 0; i < 128; i++)
mapOut.set(i, i);
return mapOut;
}
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
ModeMapper.h
Created: 30 May 2019 8:02:42pm
Author: Vincenzo
TODO: Lots of cleaning up, simplifying, and generalizing
==============================================================================
*/
#pragma once
#include "../../JuceLibraryCode/JuceHeader.h"
#include "../PluginModes.h"
#include "Mode.h"
#include "NoteMap.h"
class ModeMapper
{
ValueTree mappingNode;
int mappingStyle = 1;
int mapByOrderNum1 = 0;
int mapByOrderNum2 = 0;
int mapByOrderOffset1 = 0;
int mapByOrderOffset2 = 0;
NoteMap previousOrderMap;
public:
ModeMapper();
ModeMapper(ValueTree modeMappingNodeIn);
ValueTree getMappingNode();
int getMode1OrderNum() const;
int getMode2OrderNum() const;
int getMode1OrderOffset() const;
int getMode2OrderOffset() const;
void setMappingStyle(int mapTypeIn);
void setMapOrdersParameters(int order1, int order2, int offset1, int offset2);
void setMode1OrderNum(int orderNumber);
void setMode2OrderNum(int orderNumber);
void setMode1OrderOffset(int orderOffset);
void setMode2OrderOffset(int orderOffset);
void setPreviousOrderNoteMap(NoteMap prevNoteMapIn);
NoteMap map(const Mode& mode1, const Mode& mode2, NoteMap prevMap = NoteMap());
// Returns certain type of mapping based off of passed in parameters
NoteMap map(const Mode& mode1, const Mode& mode2, int mapStyleIn = -1, int order1=0, int order2=0, int offset1=0, int offset2=0,
NoteMap prevMap = NoteMap());
Array<int> getSelectedPeriodMap(const Mode& mode1, const Mode& mode2) const;
//
static NoteMap mapFull(const Mode& mode1, const Mode& mode2, Array<int> degreeMapIn = Array<int>());
// Creates a mapping of mode1 onto mode2 via aligning key orders (white vs black vs colored)
static NoteMap mapByOrder(const Mode& mode1, const Mode& mode2, int mode1Order=0, int mode2Order=0, int mode1Offset=0, int mode2Offset=0, NoteMap prevMap=NoteMap());
//
static NoteMap mapToMode1Period(const Mode& mapFrom, const Mode& mapTo, Array<int> degreeMapIn=Array<int>());
//
static NoteMap mapToMode1Scale(const Mode& mode1, const Mode& mode2, int mode2Order=0);
// Maps standard keyboard layout (white keys) to new mode (white keys)
static NoteMap stdMidiToMode(const Mode& modeMapped, int rootNoteStd = 60);
// A basic one-period mode-based mapping
static Array<int> getModeToModePeriodMap(const Mode& mode1, const Mode& mode2);
// A basic one-period scale-based mapping, dependent on output mode's subscale being the same size as input mode's full scale
static Array<int> getScaleToModePeriodMap(const Mode& mode1, const Mode& mode2);
//
static Array<int> degreeMapFullMode(const Mode& mode1, const Mode& mode2);
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
ModeLayout.cpp
Created: 19 Apr 2019 11:15:14pm
Author: Vincenzo
==============================================================================
*/
#include "Mode.h"
Mode::Mode()
{
stepsString = "1";
family = "undefined";
rootNote = 60;
steps = parseIntStringToArray(stepsString);
ordersDefault = unfoldStepsToOrders(steps);
scaleSize = 1;
modeSize = 1;
name = getDescription();
updateProperties();
updateNode(true);
}
Mode::Mode(String stepsIn, String familyIn, int rootNoteIn, String nameIn, String infoIn)
{
stepsString = stepsIn;
family = familyIn;
rootNote = rootNoteIn;
info = infoIn;
steps = parseIntStringToArray(stepsIn);
ordersDefault = unfoldStepsToOrders(steps);
scaleSize = ordersDefault.size();
modeSize = steps.size();
offset = getOffset() * -1;
if (nameIn == "")
name = getDescription();
else
name = nameIn;
updateProperties();
updateNode(true);
}
Mode::Mode(Array<int> stepsIn, String familyIn, int rootNoteIn, String nameIn, String infoIn)
{
stepsString = intArrayToString(stepsIn);
family = familyIn;
rootNote = rootNoteIn;
info = infoIn;
steps = stepsIn;
ordersDefault = unfoldStepsToOrders(steps);
scaleSize = ordersDefault.size();
modeSize = steps.size();
offset = getOffset() * -1;
if (nameIn == "")
name = getDescription();
else
name = nameIn;
updateProperties();
updateNode(true);
}
Mode::Mode(ValueTree modeNodeIn, bool copyNode)
: Mode()
{
if (isValidMode(modeNodeIn))
{
if (copyNode)
modeNode = modeNodeIn.createCopy();
else
modeNode = modeNodeIn;
stepsString = modeNode[IDs::stepString];
steps = parseIntStringToArray(stepsString);
ordersDefault = unfoldStepsToOrders(steps);
scaleSize = modeNode[IDs::scaleSize];
if (scaleSize == 0)
scaleSize = ordersDefault.size();
modeSize = modeNode[IDs::modeSize];
if (modeSize == 0)
modeSize = steps.size();
family = modeNode[IDs::family];
if (family == "")
family = "undefined";
info = modeNode[IDs::modeInfo];
name = modeNode[IDs::modeName];
if (name == "")
name = getDescription();
rootNote = modeNode[IDs::rootMidiNote];
if (rootNote < 0 || rootNote > 127)
rootNote = 60;
updateProperties();
updateNode();
}
}
Mode::~Mode() {}
void Mode::updateNode(bool initializeNode)
{
if (initializeNode)
modeNode = ValueTree(IDs::modePresetNode);
modeNode.setProperty(IDs::modeName, name, nullptr);
modeNode.setProperty(IDs::scaleSize, scaleSize, nullptr);
modeNode.setProperty(IDs::modeSize, modeSize, nullptr);
modeNode.setProperty(IDs::stepString, stepsString, nullptr);
modeNode.setProperty(IDs::family, family, nullptr);
modeNode.setProperty(IDs::modeInfo, info, nullptr);
modeNode.setProperty(IDs::factoryPreset, false, nullptr);
modeNode.setProperty(IDs::rootMidiNote, rootNote, nullptr);
}
void Mode::restoreNode(ValueTree nodeIn, bool useNodeRoot)
{
if (isValidMode(nodeIn))
{
modeNode = nodeIn;
stepsString = modeNode[IDs::stepString];
steps = parseIntStringToArray(stepsString);
ordersDefault = unfoldStepsToOrders(steps);
scaleSize = modeNode[IDs::scaleSize];
if (scaleSize == 0)
scaleSize = ordersDefault.size();
modeSize = modeNode[IDs::modeSize];
if (modeSize == 0)
modeSize = steps.size();
family = modeNode[IDs::family];
if (family == "")
family = "undefined";
info = modeNode[IDs::modeInfo];
name = modeNode[IDs::modeName];
if (name == "")
name = getDescription();
if (useNodeRoot)
rootNote = modeNode[IDs::rootMidiNote];
if (rootNote < 0 || rootNote > 127)
rootNote = 60;
updateProperties();
updateNode();
}
}
bool Mode::isValidMode(ValueTree nodeIn)
{
String steps = nodeIn.getProperty(IDs::stepString).toString();
Array<int> stepsArray = Mode::parseIntStringToArray(steps);
int length = stepsArray.size();
int numDegrees = sumUpToIndex(stepsArray, length);
return length > 0 && numDegrees <= 128;
}
ValueTree Mode::createNode(String stepsIn, String familyIn, String nameIn, String infoIn, int rootNoteIn, bool factoryPreset)
{
ValueTree modeNodeOut = ValueTree(IDs::modePresetNode);
Array<int> steps = parseIntStringToArray(stepsIn);
Array<int> orders = unfoldStepsToOrders(steps);
Array<int> mosClass = intervalAmounts(steps);
if (rootNoteIn < 0 || rootNoteIn > 127)
rootNoteIn = 60;
modeNodeOut.setProperty(IDs::scaleSize, orders.size(), nullptr);
modeNodeOut.setProperty(IDs::modeSize, steps.size(), nullptr);
modeNodeOut.setProperty(IDs::stepString, stepsIn, nullptr);
modeNodeOut.setProperty(IDs::family, familyIn, nullptr);
modeNodeOut.setProperty(IDs::modeInfo, infoIn, nullptr);
modeNodeOut.setProperty(IDs::rootMidiNote, rootNoteIn, nullptr);
modeNodeOut.setProperty(IDs::factoryPreset, factoryPreset, nullptr);
if (nameIn == "")
{
String modeName = familyIn + "[" + String(steps.size()) + "] " + String(orders.size());
modeNodeOut.setProperty(IDs::modeName, modeName, nullptr);
}
else
modeNodeOut.setProperty(IDs::modeName, nameIn, nullptr);
return modeNodeOut;
}
ValueTree Mode::createNode(Array<int> stepsIn, String familyIn, String nameIn, String infoIn, int rootNoteIn, bool factoryPreset)
{
ValueTree modeNodeOut = ValueTree(IDs::modePresetNode);
Array<int> orders = unfoldStepsToOrders(stepsIn);
Array<int> mosClass = intervalAmounts(stepsIn);
String stepsStr = intArrayToString(stepsIn);
if (rootNoteIn < 0 || rootNoteIn > 127)
rootNoteIn = 60;
modeNodeOut.setProperty(IDs::scaleSize, orders.size(), nullptr);
modeNodeOut.setProperty(IDs::modeSize, stepsIn.size(), nullptr);
modeNodeOut.setProperty(IDs::stepString, stepsStr, nullptr);
modeNodeOut.setProperty(IDs::family, familyIn, nullptr);
modeNodeOut.setProperty(IDs::modeInfo, infoIn, nullptr);
modeNodeOut.setProperty(IDs::rootMidiNote, rootNoteIn, nullptr);
modeNodeOut.setProperty(IDs::factoryPreset, factoryPreset, nullptr);
if (nameIn == "")
{
String modeName = familyIn + "[" + String(stepsStr.length()) + "] " + String(orders.size());
modeNodeOut.setProperty(IDs::modeName, modeName, nullptr);
}
else
modeNodeOut.setProperty(IDs::modeName, nameIn, nullptr);
return modeNodeOut;
}
void Mode::updateProperties()
{
offset = -getOffset();
fixedOffset = (rootNote / scaleSize) * scaleSize + rootNote % scaleSize;
orders = repeatArray(ordersDefault, 128, offset);
modeDegrees = ordersToModalDegrees(orders);
scaleDegrees = generateScaleDegrees(scaleSize, offset);
mosClass = intervalAmounts(steps);
keyboardOrdersSizes = intervalAmounts(orders);
stepsOfOrders = repeatIndicies(foldOrdersToSteps(orders));
}
void Mode::setFamily(String familyIn)
{
family = familyIn;
modeNode.setProperty(IDs::family, family, nullptr);
}
void Mode::setName(String nameIn)
{
name = nameIn;
modeNode.setProperty(IDs::modeName, name, nullptr);
}
void Mode::setInfo(String infoIn)
{
info = infoIn;
modeNode.setProperty(IDs::modeInfo, infoIn, nullptr);
}
void Mode::setRootNote(int rootNoteIn)
{
if (rootNote != rootNoteIn)
{
rootNote = rootNoteIn;
updateProperties();
}
modeNode.setProperty(IDs::rootMidiNote, rootNote, nullptr);
}
void Mode::rotate(int rotateAmt)
{
int amt = totalModulus(rotateAmt, modeSize);
Array<int> newSteps;
for (int i = 0; i < steps.size(); i++)
newSteps.add(steps[(amt + i) % steps.size()]);
steps = newSteps;
stepsString = intArrayToString(steps);
ValueTree degreeColors = modeNode.getChildWithName(IDs::pianoKeyColorsNode).getChildWithName(IDs::pianoKeyColorsDegree);
for (auto degreeNode : degreeColors)
{
int newDeg = totalModulus((int)degreeNode[IDs::pianoKeyColorsDegree] - rotateAmt, scaleSize);
degreeNode.setProperty(IDs::pianoKeyColorsDegree, newDeg, nullptr);
}
updateProperties();
}
void Mode::addTag(String tagIn)
{
tags.add(tagIn);
}
int Mode::removeTag(String tagIn)
{
int index = tags.indexOf(tagIn);
tags.remove(index);
return index;
}
int Mode::getRootNote() const
{
return rootNote;
}
int Mode::getOffset() const
{
return totalModulus(rootNote, scaleSize);
}
int Mode::getScaleSize() const
{
return scaleSize;
}
int Mode::getModeSize() const
{
return modeSize;
}
Array<int> Mode::getIntervalSizeCount() const
{
return intervalAmounts(steps);
}
Array<int> Mode::getStepsOfOrders() const
{
return stepsOfOrders;
}
int Mode::getNoteStep(int noteNumberIn) const
{
return stepsOfOrders[noteNumberIn];
}
String Mode::getFamily() const
{
return family;
}
String Mode::getName() const
{
return name;
}
String Mode::getInfo() const
{
return info;
}
StringArray Mode::getTags() const
{
return tags;
}
Array<int> Mode::getSteps(int rotationIn) const
{
if (rotationIn == 0)
return steps;
else
{
Array<int> rotatedSteps;
rotationIn = totalModulus(rotationIn, modeSize);
for (int i = 0; i < modeSize; i++)
rotatedSteps.add(steps[(i + rotationIn) % modeSize]);
return rotatedSteps;
}
}
String Mode::getStepsString(int rotationIn) const
{
if (rotationIn == 0)
return stepsString;
else
{
// lol feelin lazy about this boring function
Array<int> stepsRotated = getSteps(rotationIn);
return intArrayToString(stepsRotated);
}
}
Array<int>* Mode::getKeyboardOrdersSizes()
{
return &keyboardOrdersSizes;
}
int Mode::getKeyboardOrdersSize(int ordersIn) const
{
int o = ordersIn % getMaxStep();
return keyboardOrdersSizes[o];
}
Array<int> Mode::getOrdersDefault() const
{
return ordersDefault;
}
Array<int> Mode::getOrders() const
{
return orders;
}
int Mode::getOrder(int noteNumberIn) const
{
return orders[noteNumberIn];
}
Array<float> Mode::getModalDegrees() const
{
return modeDegrees;
}
Array<int> Mode::getScaleDegrees() const
{
return scaleDegrees;
}
int Mode::getScaleDegree(int midiNoteIn) const
{
return totalModulus(midiNoteIn - rootNote, scaleSize);
}
float Mode::getModeDegree(int midiNoteIn) const
{
return modeDegrees[midiNoteIn % 128];
}
int Mode::getFixedDegree(int midiNoteIn) const
{
return midiNoteIn - fixedOffset;
}
int Mode::fixedDegreeToNoteNumber(int fixedDegreeIn) const
{
return fixedDegreeIn + fixedOffset;
}
Point<int> Mode::getPeriodsAndDegree(int midiNoteIn) const
{
return Point<int>((midiNoteIn - rootNote) / scaleSize, (midiNoteIn - rootNote) % scaleSize);
}
int Mode::getMidiNote(int scaleDegreeIn) const
{
int periods = scaleDegreeIn / scaleSize;
int offsetDeg = totalModulus(scaleDegreeIn - rootNote, scaleSize);
return periods * scaleSize + offsetDeg;
}
int Mode::getMidiNote(int periodIn, int scaleDegreeIn) const
{
scaleDegreeIn = totalModulus(scaleDegreeIn - rootNote, scaleSize);
return periodIn * scaleSize + scaleDegreeIn;
}
int Mode::getMaxStep() const
{
int step = 1;
for (int i = 0; i < steps.size(); i++)
{
if (steps[i] > step)
step = steps[i];
}
return step;
}
Array<int> Mode::getNotesOfOrder(int order) const
{
Array<int> notesOut;
for (int i = 0; i < orders.size(); i++)
{
if (orders.getUnchecked(i) == order)
notesOut.add(i);
}
return notesOut;
}
Array<int> Mode::getMOSClass() const
{
return mosClass;
}
String Mode::getDescription() const
{
return family + "[" + String(modeSize) + "] " + String(scaleSize);
}
String Mode::getScaleDescription() const
{
return String(scaleSize) + " " + getDescription().dropLastCharacters(2);
}
String Mode::getModeDescription() const
{
return String(modeSize) + " " + family + " " + String(scaleSize);
}
int Mode::indexOfTag(String tagNameIn)
{
return tags.indexOf(tagNameIn);
}
int Mode::isSimilarTo(Mode* modeToCompare) const
{
Array<int> mode1Steps = steps;
Array<int> mode2Steps = modeToCompare->getSteps();
int rotations = 0;
if (mode1Steps.size() != mode2Steps.size())
return -1;
while (mode1Steps != mode2Steps || rotations < mode1Steps.size())
{
mode2Steps.move(0, mode2Steps.size());
}
return (rotations % (mode1Steps.size() + 1)) - 1;
}
Array<Array<int>> Mode::getNotesOrders()
{
Array<Array<int>> notesOut;
notesOut.resize(getMaxStep());
for (int i = 0; i < getMaxStep(); i++)
{
notesOut.getReference(i).addArray(getNotesOfOrder(i));
}
return notesOut;
}
Array<Array<int>> Mode::getNotesInScaleDegrees()
{
Array<Array<int>> notesOut;
notesOut.resize(getScaleSize());
return notesOut;
}
Array<Array<int>> Mode::getNotesInModalDegrees()
{
Array<Array<int>> notesOut;
notesOut.resize(getModeSize());
return notesOut;
}
Array<int> Mode::parseIntStringToArray(String stepsIn)
{
Array<int> stepsOut;
std::string theSteps = stepsIn.toStdString();
char c;
int step;
int digits;
int i = 0;
while (i < stepsIn.length())
{
digits = 0;
c = stepsIn[i];
while ((c >= 48 && c < 58) && (i + digits) < stepsIn.length())
{
digits++;
c = stepsIn[i + digits];
}
if (digits > 0)
{
step = stepsIn.substring(i, i + digits).getIntValue();
stepsOut.add(step);
i += digits + 1;
}
else
i++;
}
stepsOut.minimiseStorageOverheads();
return stepsOut;
}
Array<int> Mode::repeatIndicies(Array<int> arrayToRepeat, Array<int> repeatMask)
{
Array<int> stepsOut;
if (repeatMask.size() == 0)
repeatMask = arrayToRepeat;
for (int i = 0; i < arrayToRepeat.size(); i++)
{
for (int s = 0; s < repeatMask[i % repeatMask.size()]; s++)
stepsOut.add(arrayToRepeat[i]);
}
stepsOut.minimiseStorageOverheads();
return stepsOut;
}
String Mode::intArrayToString(Array<int> stepsIn)
{
String out;
for (int i = 0; i < stepsIn.size(); i++)
{
out += String(stepsIn[i]);
if (i < stepsIn.size() - 1)
out += ' ';
}
return out;
}
Array<int> Mode::unfoldStepsToOrders(Array<int> stepsIn)
{
Array<int> ordersOut;
for (int i = 0; i < stepsIn.size(); i++)
{
for (int j = 0; j < stepsIn[i]; j++)
ordersOut.add(j);
}
ordersOut.minimiseStorageOverheads();
return ordersOut;
}
// needs revision to allow for notes to start in the middle of a step
Array<int> Mode::repeatArray(Array<int> ordersIn, int sizeIn, int offsetIn)
{
Array<int> ordersOut;
int period = ordersIn.size();
int off = totalModulus(offsetIn, ordersIn.size());
int index;
for (int i = 0; i < sizeIn; i++)
{
index = (off + i) % period;
// adjust first few notes if it starts in the middle of a whole step
if (i == 0 && ordersIn[index] != 0)
{
int localOffset = ordersIn[index];
while (ordersIn[index] != 0)
{
ordersOut.add(ordersIn[index] - localOffset);
i++;
index = (off + i) % period;
}
}
ordersOut.add(ordersIn[index]);
}
jassert(ordersOut.size() == 128);
ordersOut.minimiseStorageOverheads();
return ordersOut;
}
Array<int> Mode::foldOrdersToSteps(Array<int> layoutIn)
{
Array<int> stepsOut;
int i = 0;
int j = 0;
int step = 0;
while (i < layoutIn.size())
{
if (layoutIn[i] == 0 || i == 0)
{
j = i + 1;
step = 1;
while (layoutIn[j] != 0 && j < layoutIn.size())
{
j++;
step++;
}
}
i = j;
stepsOut.add(step);
}
stepsOut.minimiseStorageOverheads();
return stepsOut;
}
Array<float> Mode::ordersToModalDegrees(Array<int> ordersIn)
{
Array<float> degreesOut;
float deg = -1;
int stepSize = 0;
int i = 0;
while (degreesOut.size() < ordersIn.size())
{
stepSize++;
if ((i + stepSize) == (ordersIn.size() - 1) ||
ordersIn[i + stepSize] == 0)
{
deg++;
for (int j = 0; j < stepSize; j++)
degreesOut.add(deg + (j / (float) stepSize));
i += stepSize;
stepSize = 0;
}
}
degreesOut.minimiseStorageOverheads();
return degreesOut;
}
Array<int> Mode::generateScaleDegrees(int scaleSize, int offset)
{
Array<int> degreesOut;
for (int i = 0; i < scaleSize; i++)
{
degreesOut.add(totalModulus(i - offset, scaleSize));
}
degreesOut.minimiseStorageOverheads();
return degreesOut;
}
Array<int> Mode::intervalAmounts(Array<int> stepsIn)
{
HashMap<int, int> intervalCount;
Array<int> stepTypes;
for (auto step : stepsIn)
{
if (intervalCount.contains(step))
intervalCount.set(step, intervalCount[step] + 1);
else
{
intervalCount.set(step, 1);
stepTypes.add(step);
}
}
stepTypes.sort();
Array<int> intervalAmountsOut;
for (int i = stepTypes.size() - 1; i >= 0; i--)
{
intervalAmountsOut.add(intervalCount[stepTypes[i]]);
}
return intervalAmountsOut;
}
Array<int> Mode::sumArray(Array<int> stepsIn, int offsetIn, bool includePeriod)
{
Array<int> sumsOut;
int sum = -offsetIn;
sumsOut.add(sum);
for (int i = 1; i < stepsIn.size(); i++)
{
sum += stepsIn[i - 1];
sumsOut.add(sum);
}
if (includePeriod)
sumsOut.add(sum + stepsIn[stepsIn.size() - 1]);
//DBGArray(sumsOut, "Sums Out");
sumsOut.minimiseStorageOverheads();
return sumsOut;
}
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
ModeMapper.cpp
Created: 30 May 2019 8:02:42pm
Author: Vincenzo
==============================================================================
*/
#include "ModeMapper.h"
ModeMapper::ModeMapper()
{
mappingNode = ValueTree(IDs::midiMapNode);
setMappingStyle(0);
setMapOrdersParameters(0, 0, 0, 0);
}
ModeMapper::ModeMapper(ValueTree modeMappingNodeIn)
{
mappingNode = modeMappingNodeIn;
mappingStyle = mappingNode[IDs::autoMappingStyle];
mapByOrderNum1 = mappingNode[IDs::mode1OrderMapping];
mapByOrderNum2 = mappingNode[IDs::mode2OrderMapping];
mapByOrderOffset1 = mappingNode[IDs::mode1OrderOffsetMapping];
mapByOrderOffset2 = mappingNode[IDs::mode2OrderOffsetMapping];
}
ValueTree ModeMapper::getMappingNode()
{
return mappingNode;
}
int ModeMapper::getMode1OrderNum() const
{
return mapByOrderNum1;
}
int ModeMapper::getMode2OrderNum() const
{
return mapByOrderNum2;
}
int ModeMapper::getMode1OrderOffset() const
{
return mapByOrderOffset1;
}
int ModeMapper::getMode2OrderOffset() const
{
return mapByOrderOffset2;
}
void ModeMapper::setMappingStyle(int mapTypeIn)
{
mappingStyle = mapTypeIn;
mappingNode.setProperty(IDs::autoMappingStyle, mapTypeIn, nullptr);
}
void ModeMapper::setMapOrdersParameters(int order1, int order2, int offset1, int offset2)
{
setMode1OrderNum(order1);
setMode2OrderNum(order2);
setMode1OrderOffset(offset1);
setMode2OrderOffset(offset2);
}
void ModeMapper::setMode1OrderNum(int orderNumber)
{
mapByOrderNum1 = orderNumber;
mappingNode.setProperty(IDs::mode1OrderMapping, mapByOrderNum1, nullptr);
}
void ModeMapper::setMode2OrderNum(int orderNumber)
{
mapByOrderNum2 = orderNumber;
mappingNode.setProperty(IDs::mode2OrderMapping, mapByOrderNum2, nullptr);
}
void ModeMapper::setMode1OrderOffset(int orderOffset)
{
mapByOrderOffset1 = orderOffset;
mappingNode.setProperty(IDs::mode1OrderOffsetMapping, mapByOrderOffset1, nullptr);
}
void ModeMapper::setMode2OrderOffset(int orderOffset)
{
mapByOrderOffset2 = orderOffset;
mappingNode.setProperty(IDs::mode2OrderOffsetMapping, mapByOrderOffset2, nullptr);
}
void ModeMapper::setPreviousOrderNoteMap(NoteMap prevNoteMapIn)
{
previousOrderMap = prevNoteMapIn;
}
NoteMap ModeMapper::map(const Mode& mode1, const Mode& mode2, NoteMap prevMap)
{
return map(mode1, mode2, mappingStyle, mapByOrderNum1, mapByOrderNum2, mapByOrderOffset1, mapByOrderOffset2, prevMap);
}
NoteMap ModeMapper::map(const Mode& mode1, const Mode& mode2, int mapStyleIn, int order1, int order2, int offset1, int offset2,
NoteMap prevMap)
{
NoteMap mapOut;
if (mapStyleIn < 0)
mapStyleIn = mappingStyle;
else
setMappingStyle(mapStyleIn);
mappingNode.setProperty(IDs::autoMappingStyle, mappingStyle, nullptr);
switch (mappingStyle)
{
case ModeToScale:
mapOut = mapToMode1Scale(mode1, mode2);
break;
case ModeByOrder:
setMapOrdersParameters(order1, order2, offset1, offset2);
previousOrderMap = prevMap;
mapOut = mapByOrder(mode1, mode2, mapByOrderNum1, mapByOrderNum2, mapByOrderOffset1, mapByOrderOffset2, previousOrderMap);
break;
default:
mapOut = mapFull(mode1, mode2);
break;
}
return mapOut;
}
Array<int> ModeMapper::getSelectedPeriodMap(const Mode& mode1, const Mode& mode2) const
{
Array<int> mapOut;
NoteMap fullMap;
switch (mappingStyle)
{
case ModeToScale:
if (mode1.getScaleSize() == mode2.getModeSize())
mapOut = getScaleToModePeriodMap(mode1, mode2);
else // TODO: improve
mapOut = mapFull(mode1, mode2).getValues();
break;
case ModeByOrder: // TODO: improve
mapOut = mapFull(mode1, mode2, previousOrderMap.getValues()).getValues();
break;
default: // ModeToMode
if (mode1.getModeSize() == mode2.getModeSize())
mapOut = getModeToModePeriodMap(mode1, mode2);
else
mapOut = degreeMapFullMode(mode1, mode2);
break;
}
return mapOut;
}
NoteMap ModeMapper::mapFull(const Mode& mode1, const Mode& mode2, Array<int> degreeMapIn)
{
if (degreeMapIn.size() != mode1.getOrders().size())
degreeMapIn = degreeMapFullMode(mode1, mode2);
else
degreeMapIn = getModeToModePeriodMap(mode1, mode2);
NoteMap mappingOut;
int midiNote;
for (int m = 0; m < mappingOut.getSize(); m++)
{
midiNote = degreeMapIn[m];
if (midiNote >= 0)
{
mappingOut.setValue(m, midiNote);
}
else
{
mappingOut.setValue(m, -1);
}
}
//DBG("Root note from mode 1 is " + String(mode1.getRootNote()) +
// " and it produces the note " + String(mappingOut.getValue(mode1.getRootNote())) +
// ". Root note from mode 2 is " + String(mode2.getRootNote()));
return mappingOut;
}
NoteMap ModeMapper::mapByOrder(const Mode& mode1, const Mode& mode2, int mode1Order, int mode2Order, int mode1Offset, int mode2Offset, NoteMap prevMap)
{
mode1Order = jlimit(0, mode1.getMaxStep() - 1, mode1Order);
mode2Order = jlimit(0, mode2.getMaxStep() - 1, mode2Order);
Array<int> midiNotesFrom = mode1.getNotesOfOrder(mode1Order);
Array<int> midiNotesTo = mode2.getNotesOfOrder(mode2Order);
mode1Offset = jlimit(0, midiNotesFrom.size() - 1, mode1Offset);
mode2Offset = jlimit(0, midiNotesTo.size() - 1, mode2Offset);
int rootNoteFrom = mode1.getRootNote();
int rootNoteTo = mode2.getRootNote();
int rootIndexFrom = mode1.getNotesOfOrder().indexOf(rootNoteFrom);
int rootIndexTo = mode2.getNotesOfOrder().indexOf(rootNoteTo);
int rootIndexOffset = rootIndexTo - rootIndexFrom + mode2Offset - mode1Offset;
int mode1Index, mode2Index;
int noteFrom, noteTo;
for (int m = 0; m < midiNotesFrom.size(); m++)
{
// might make more sense to not have a mode1Offset
mode1Index = totalModulus(m + mode1Offset, midiNotesFrom.size());
mode2Index = totalModulus(m + rootIndexOffset + mode2Offset, midiNotesTo.size());
noteFrom = midiNotesFrom[mode1Index];
noteTo = midiNotesTo[mode2Index];
if (noteFrom > 0)
prevMap.setValue(noteFrom, noteTo);
}
return prevMap;
}
NoteMap ModeMapper::mapToMode1Period(const Mode& mode1, const Mode& mode2, Array<int> degreeMapIn)
{
// ensure degree map is the same size as Mode1 Period
if (degreeMapIn.size() != mode1.getScaleSize())
degreeMapIn = getModeToModePeriodMap(mode1, mode2);
int rootNoteFrom = mode1.getRootNote();
int rootNoteTo = mode2.getRootNote();
int rootNoteOffset = rootNoteTo - rootNoteFrom;
int degreeOffset = rootNoteTo - (ceil(rootNoteTo / (float) mode2.getScaleSize())) * degreeMapIn.size();
int midiOffset = (int)(ceil(rootNoteTo / (float) mode2.getScaleSize())) * mode2.getScaleSize() - rootNoteTo + rootNoteOffset;
//DBG("Degree Offset is " + String(degreeOffset));
//DBG("Midi Offset is " + String(midiOffset));
NoteMap mappingOut;
int mapIndex;
int periods;
int midiNote;
for (int m = 0; m < mappingOut.getSize(); m++)
{
mapIndex = m - degreeOffset;
if (mapIndex >= 0)
{
periods = mapIndex / degreeMapIn.size();
midiNote = degreeMapIn[mapIndex % degreeMapIn.size()] + periods * mode2.getScaleSize() - midiOffset;
}
else
midiNote = -1;
mappingOut.setValue(m, midiNote);
//if (m == rootNoteFrom)
// DBG("Root Note From (" + String(rootNoteFrom) + ") produces note " + String(mappingOut.getValue(rootNoteFrom)) + " and comapre that to the Root note to (" + String(rootNoteTo) + ")");
}
return mappingOut;
}
NoteMap ModeMapper::mapToMode1Scale(const Mode& mode1, const Mode& mode2, int mode2Order)
{
Array<int> mode2ModalNotes = mode2.getNotesOfOrder(mode2Order);
int mode1RootIndex = mode1.getRootNote();
int mode2RootIndex = mode2ModalNotes.indexOf(mode2.getRootNote());
int rootIndexOffset = mode2RootIndex - mode1RootIndex;
NoteMap mappingOut;
int degToAdd;
int mode2ModeIndex = 0;
for (int m = 0; m < mode1.getOrders().size(); m++)
{
mode2ModeIndex = m + rootIndexOffset;
if (mode2ModeIndex < 0)
{
degToAdd = mode2ModeIndex;
}
else if (mode2ModeIndex >= mode2ModalNotes.size())
{
degToAdd = 128;
}
else
{
degToAdd = mode2ModalNotes[mode2ModeIndex];
}
mappingOut.setValue(m, degToAdd);
//if (m == mode1.getRootNote())
// DBG("Root Note From (" + String(mode1.getRootNote()) + ") produces note " + String(mappingOut.getValue(mode1.getRootNote())) + " and comapre that to the Root note to (" + String(mode2.getRootNote()) + ")");
}
return mappingOut;
}
NoteMap ModeMapper::stdMidiToMode(const Mode& modeMapped, int rootNoteStd)
{
Mode meantone7_12(STD_TUNING_MODE_NODE);
if (modeMapped.getModeSize() == meantone7_12.getModeSize())
return mapToMode1Period(meantone7_12, modeMapped);
else
return mapFull(meantone7_12, modeMapped);
}
Array<int> ModeMapper::getModeToModePeriodMap(const Mode& mode1, const Mode& mode2)
{
Array<int> degreeMapOut;
Array<int> mode1Steps = mode1.getSteps();
Array<int> mode2Steps = mode2.getSteps();
int mode1ModeSize = mode1.getModeSize();
int mode2ModeSize = mode2.getModeSize();
int mode2ScaleIndex = 0;
int degToAdd;
int degSub;
float stepFraction;
float mode1Step;
float mode2Step;
for (int m1 = 0; m1 < mode2ModeSize; m1++)
{
mode1Step = mode1Steps[m1 % mode1ModeSize];
mode2Step = mode2Steps[m1];
degreeMapOut.add(mode2ScaleIndex);
for (int d1 = 1; d1 < mode1Step; d1++)
{
stepFraction = d1 / mode1Step;
// round up to the next mode degree
degSub = mode2Step;
// find closest degree
for (int d2 = 1; d2 < mode2Step; d2++)
{
if (abs(d2 / mode2Step - stepFraction) < abs(degSub / mode2Step - stepFraction))
degSub = d2;
}
// resulting degree is the sum of previous steps, plus the next closest sub degree within the modal step
degToAdd = mode2ScaleIndex + degSub;
degreeMapOut.add(degToAdd);
}
mode2ScaleIndex += mode2Step;
}
//DBGArray(degreeMapOut, "Mode1 -> Mode2 Scale Degrees");
return degreeMapOut;
}
Array<int> ModeMapper::getScaleToModePeriodMap(const Mode& mode1, const Mode& mode2)
{
Array<int> degreeMapOut;
Array<int> mode2Steps = mode2.getSteps();
int mode2ScaleIndex = 0;
for (int i = 0; i < mode2Steps.size(); i++)
{
degreeMapOut.add(mode2ScaleIndex);
mode2ScaleIndex += mode2Steps[i];
}
//DBGArray(degreeMapOut, "Mode1 -> Mode2 Scale Degrees");
return degreeMapOut;
}
Array<int> ModeMapper::degreeMapFullMode(const Mode& mode1, const Mode& mode2)
{
Array<int> degreeMapOut;
//DBG("Mode1 Root: " + String(mode1.getRootNote()) + "\tMode2Root: " + String(mode2.getRootNote()));
Array<int> mode1Steps = Mode::foldOrdersToSteps(mode1.getOrders());
Array<int> mode2Steps = Mode::foldOrdersToSteps(mode2.getOrders());
Array<int> mode1MidiNotes = Mode::sumArray(mode1Steps, 0, true);
Array<int> mode2MidiNotes = Mode::sumArray(mode2Steps, 0, true);
int mode1RootIndex = mode1MidiNotes.indexOf(mode1.getRootNote());
int mode2RootIndex = mode2MidiNotes.indexOf(mode2.getRootNote());
int rootIndexOffset = mode2RootIndex - mode1RootIndex;
//DBG("Mode1 Root Index: " + String(mode1RootIndex) + "\tMode2Root: " + String(mode2RootIndex));
//DBGArray(mode2MidiNotes, "Mode2 Midi Notes");
int mode2ScaleIndex = 0;
if (rootIndexOffset > 0)
{
mode2ScaleIndex = sumUpToIndex(mode2Steps, rootIndexOffset);
}
int degToAdd, degSub;
float stepFraction;
float mode1Step, mode2Step;
int mode2ModeIndex = 0;
for (int m = 0; m < mode1Steps.size(); m++)
{
mode2ModeIndex = m + rootIndexOffset;
// this is assuming that the order will always start at 0
mode1Step = mode1Steps[m];
if (mode2ModeIndex < 0)
{
mode2Step = 0;
degToAdd = mode2ModeIndex;
}
else if (mode2ModeIndex >= mode2Steps.size())
{
mode2Step = 0;
degToAdd = 128;
}
else
{
mode2Step = mode2Steps[mode2ModeIndex];
degToAdd = mode2ScaleIndex;
}
degreeMapOut.add(degToAdd);
for (int d1 = 1; d1 < mode1Step; d1++)
{
stepFraction = d1 / mode1Step;
// round up to the next mode degree
degSub = mode2Step;
// find closest degree
for (int d2 = 1; d2 < mode2Step; d2++)
{
if (abs(d2 / mode2Step - stepFraction) < abs(degSub / mode2Step - stepFraction))
degSub = d2;
}
// resulting degree is the sum of previous steps, plus the next closest sub degree within the modal step
degToAdd = mode2ScaleIndex + degSub;
if (mode2ModeIndex < 0)
degreeMapOut.add(mode2ModeIndex);
else
degreeMapOut.add(degToAdd);
}
mode2ScaleIndex += mode2Step;
}
return degreeMapOut;
}
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
ModeLayout.h
Created: 31 Mar 2019 2:16:09pm
Author: Vincenzo
==============================================================================
*/
#pragma once
#include "../PluginIDs.h"
#include "../CommonFunctions.h"
/*
A class for representing the layout of a virtualKeyboard
based on a given mode. The mode can be applied to different scales.
*/
class Mode
{
String name;
int scaleSize;
int modeSize;
String family;
String info;
StringArray tags;
int rootNote = 60;
int offset;
int fixedOffset;
String stepsString;
Array<int> steps;
Array<int> ordersDefault;
Array<int> mosClass;
Array<int> orders;
Array<int> scaleDegrees;
Array<float> modeDegrees;
// Keyboard Convenience
Array<int> stepsOfOrders; // each index is the step which the note is associated with
Array<int> keyboardOrdersSizes; // amount of keys in each key order groupings
public:
ValueTree modeNode;
Mode();
Mode(String stepsIn, String familyIn="undefined", int rootNoteIn=60, String nameIn="", String infoIn="");
Mode(Array<int> stepsIn, String familyIn="undefined", int rootNoteIn=60, String nameIn="", String infoIn="");
Mode(ValueTree modeNodeIn, bool copyNode=false);
~Mode();
void updateNode(bool initializeNode=false);
void restoreNode(ValueTree nodeIn, bool useNodeRoot=true);
static bool isValidMode(ValueTree nodeIn);
static ValueTree createNode(String stepsIn, String familyIn = "undefined", String nameIn = "", String infoIn="", int rootNoteIn=60, bool factoryPreset = false);
static ValueTree createNode(Array<int> stepsIn, String familyIn = "undefined", String nameIn = "", String infoIn="", int rootNoteIn=60, bool factoryPreset = false);
/*
Sets temperament family name.
*/
void setFamily(String familyIn);
/*
Sets custom name of the mode.
*/
void setName(String nameIn);
/*
Sets info regarding the mode.
*/
void setInfo(String infoIn);
/*
Recalculates mode properties based on parameter change
*/
void updateProperties();
/*
Sets the offset of the mode and updates parameters so that the
offset replaces the current visualization of the mode
*/
void setRootNote(int rootNoteIn);
/*
Rotates the scale by moving the index of the first interval
by the rotation amount given.
*/
void rotate(int rotateAmt);
void addTag(String tagIn);
int removeTag(String tagIn);
int getRootNote() const;
int getOffset() const;
int getScaleSize() const;
int getModeSize() const;
Array<int> getIntervalSizeCount() const;
String getFamily() const;
String getName() const;
String getInfo() const;
StringArray getTags() const;
Array<int>* getKeyboardOrdersSizes();
int getKeyboardOrdersSize(int ordersIn) const;
Array<int> getStepsOfOrders() const;
int getNoteStep(int noteNumberIn) const;
Array<int> getSteps(int rotationIn=0) const;
String getStepsString(int rotationIn=0) const;
Array<int> getOrdersDefault() const;
Array<int> getOrders() const;
int getOrder(int noteNumberIn) const;
Array<float> getModalDegrees() const;
Array<int> getScaleDegrees() const;
int getScaleDegree(int midiNoteIn) const;
float getModeDegree(int midiNoteIn) const;
int getFixedDegree(int midiNoteIn) const;
int fixedDegreeToNoteNumber(int fixedDegreeIn) const;
Point<int> getPeriodsAndDegree(int midiNoteIn) const;
int getMidiNote(int scaleDegreeIn) const;
int getMidiNote(int periodIn, int scaleDegreeIn) const;
int getMaxStep() const;
Array<int> getMOSClass() const;
String getDescription() const;
String getScaleDescription() const;
String getModeDescription() const;
Array<int> getNotesOfOrder(int order = 0) const;
int indexOfTag(String tagNameIn);
/*
Returns -1 if modes are different, otherwise returns the number of rotations
needed to make the modes equivalent.
*/
int isSimilarTo(Mode* modeToCompare) const;
/*
Returns a table of note numbers (with current root) organized by key order.
*/
Array<Array<int>> getNotesOrders();
/*
Returns a table of note numbers (with current root) organized by scale degree.
*/
Array<Array<int>> getNotesInScaleDegrees();
/*
Returns a table of note numbers (with current root) organized by modal degree.
*/
Array<Array<int>> getNotesInModalDegrees();
/*
Simply parses a string reprsenting step sizes and returns a vector
*/
static Array<int> parseIntStringToArray(String stepsIn);
/*
Takes in step vector like {2, 2 , 1 , 2 , 2 , 2 , 1}
Returns a string like "2 2 1 2 2 2 1"
*/
static String intArrayToString(Array<int> stepsIn);
/*
Takes in a vector like {2, 2, 1, 2, 2, 2, 1} and a mask like {0, 0, 2, 0, 0, 0, 2}
Returns a vector of each index repeated by the mask {2, 2, 1, 1, 2, 2, 2, 1, 1}
If no mask supplied, then it uses it's own magnitudes.
*/
static Array<int> repeatIndicies(Array<int> arrayToRepeat, Array<int> repeatMask=Array<int>());
/*
Takes in step vector like {2, 2, 1, 2, 2, 2, 1}
Returns a vector of key orders {0, 1, 0, 1, 0, 0, 1,...}
*/
static Array<int> unfoldStepsToOrders(Array<int> stepsIn);
/*
Takes in array of a scale layout of note orders (1:1) {0, 1, 0, 1 , 0, 0, 1,...}
and returns scale step size layout "2, 2, 1, 2,..."
*/
static Array<int> foldOrdersToSteps(Array<int> layoutIn);
/*
Takes in a period-size vector, a new vector size, and an offset
Returns a vector at new size using original vector's period for repetition with the given offset
*/
// needs revision to allow for notes to start in the middle of a step
static Array<int> repeatArray(Array<int> ordersIn, int sizeIn, int offsetIn=0);
/*
Takes in array of a scale layout of note orders (1:1) {0, 1, 0, 1 , 0, 0, 1,...}
and returns scale step size layout "0, 0.5, 1, 1.5,..."
*/
static Array<float> ordersToModalDegrees(Array<int> ordersIn);
/*
Simply creates an array of scale degrees based off of scale size and offset
*/
static Array<int> generateScaleDegrees(int scaleSize, int offset = 0);
/*
Takes in a vector like {2, 2, 1, 2, 2, 2, 1}
Returns a vector of sizes large->small like {5, 2}
*/
static Array<int> intervalAmounts(Array<int> stepsIn);
/*
Takes in a vector like {2, 2, 1, 2, 2, 2, 1}
And returns a sum of the previous indicies at each index like {0, 2, 4, 5, 7, 9, 11}
*/
static Array<int> sumArray(Array<int> stepsIn, int offsetIn = 0, bool includePeriod = false);
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
NoteMap.cpp
Created: 25 May 2019 10:34:43pm
Author: Vincenzo
==============================================================================
*/
#include "NoteMap.h"
NoteMap::NoteMap()
: NoteMap(128, true, -1)
{
}
NoteMap::NoteMap(int sizeIn, bool useIdentity, int nullValIn)
{
if (sizeIn > 0)
size = sizeIn;
else
size = 128;
keys.clear();
values.clear();
nullVal = nullValIn;
int defaultVal = nullVal;
for (int i = 0; i < size; i++)
{
if (useIdentity)
defaultVal = i;
keys.add(defaultVal);
values.add(defaultVal);
}
}
NoteMap::NoteMap(Array<int> valuesIn, int nullValIn)
{
nullVal = nullValIn;
setValues(valuesIn);
}
NoteMap::NoteMap(ValueTree noteMappingNode)
: NoteMap(noteMappingNode[IDs::mappingSize], true, -1)
{
for (auto child : noteMappingNode)
{
if (child.hasType(IDs::noteMapNode))
{
int key = child[IDs::mappingMidiNoteIn];
if (key < size)
setValue(key, child[IDs::pianoKeyNumber]);
}
}
}
NoteMap::NoteMap(const NoteMap& mapToCopy)
{
size = mapToCopy.size;
nullVal = mapToCopy.nullVal;
keys.clear();
values.clear();
for (int i = 0; i < size; i++)
{
keys.add(mapToCopy.keys.getUnchecked(i));
values.add(mapToCopy.values.getUnchecked(i));
}
}
ValueTree NoteMap::getAsValueTree(Identifier parentNodeId, bool includeIdentities) const
{
ValueTree node(parentNodeId);
node.setProperty(IDs::mappingSize, size, nullptr);
for (int i = 0; i < size; i++)
{
if (includeIdentities || values[i] != i)
{
ValueTree value(IDs::noteMapNode);
value.setProperty(IDs::mappingMidiNoteIn, i, nullptr);
value.setProperty(IDs::pianoKeyNumber, values[i], nullptr);
node.appendChild(value, nullptr);
}
}
return node;
}
void NoteMap::setValue(int keyNum, int valIn)
{
int oldVal = values.getUnchecked(keyNum);
if (oldVal >= 0)
keys.set(oldVal, nullVal);
values.set(keyNum, valIn);
if (valIn >= 0)
keys.set(valIn, keyNum);
}
void NoteMap::setValues(Array<int> valuesIn)
{
size = valuesIn.size();
values.clear();
keys.clear();
values.resize(size);
keys.resize(size);
for (int i = 0; i < size; i++)
{
setValue(i, valuesIn[i]);
}
}
int* NoteMap::setNullVal(int nullValIn)
{
nullVal = nullValIn;
return &nullVal;
}
int NoteMap::getSize()
{
return size;
}
int NoteMap::getKey(int valIn)
{
return keys.getUnchecked(valIn);
}
int NoteMap::getValue(int keyNum)
{
return values.getUnchecked(keyNum);
}
const Array<int>& NoteMap::getKeys() const
{
return keys;
}
const Array<int>& NoteMap::getValues() const
{
return values;
}
int NoteMap::getNullVal()
{
return nullVal;
}
String NoteMap::toString()
{
String toStr = "";
for (int i = 0; i < size; i++)
{
toStr += String(i) + "\t: " + String(values.getUnchecked(i)) + "\n";
}
return toStr;
}
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
This file was auto-generated!
It contains the basic framework code for a JUCE plugin editor.
==============================================================================
*/
#pragma once
#include <JuceHeader.h>
#include "../PluginProcessor.h"
#include "Components/PluginControlComponent.h"
class SvkPluginEditor : public AudioProcessorEditor, public SvkPluginState::Listener, protected ValueTree::Listener
{
public:
SvkPluginEditor (SvkAudioProcessor&);
~SvkPluginEditor();
void paint (Graphics&) override;
void resized() override;
void presetLoaded(ValueTree presetNodeIn) override;
protected:
void valueTreePropertyChanged(ValueTree&, const Identifier&) override;
private:
SvkAudioProcessor& processor;
SvkPluginState* pluginState;
ValueTree pluginEditorNode;
std::unique_ptr<PluginControlComponent> controlComponent;
int defaultMinWidth = 750;
int defaultMinHeight = 172;
int defaultMaxWidth = 10e4;
int defaultMaxHeight = 10e4;
TooltipWindow tooltip;
JUCE_DECLARE_NON_COPYABLE_WITH_LEAK_DETECTOR (SvkPluginEditor)
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
This file was auto-generated!
It contains the basic framework code for a JUCE plugin editor.
==============================================================================
*/
#include "PluginEditor.h"
#include "../PluginProcessor.h"
using namespace VirtualKeyboard;
//==============================================================================
SvkPluginEditor::SvkPluginEditor(SvkAudioProcessor& p)
: AudioProcessorEditor(&p), processor(p)
{
setName("SuperVirtualKeyboard");
setResizable(true, true);
setBroughtToFrontOnMouseClick(true);
setMouseClickGrabsKeyboardFocus(true);
addMouseListener(this, true);
pluginState = processor.getPluginState();
pluginEditorNode = pluginState->getPluginEditorNode();
// Intialization
if (!pluginEditorNode.isValid())
{
pluginEditorNode = ValueTree(IDs::pluginEditorNode);
pluginEditorNode.setProperty(IDs::windowBoundsW, 1000, nullptr);
pluginEditorNode.setProperty(IDs::windowBoundsH, 210, nullptr);
pluginEditorNode.setProperty(IDs::viewportPosition, 60, nullptr);
pluginEditorNode.setProperty(IDs::settingsOpen, false, nullptr);
pluginState->pluginStateNode.appendChild(pluginEditorNode, nullptr);
}
controlComponent.reset(new PluginControlComponent(pluginState));
addAndMakeVisible(controlComponent.get());
setSize(controlComponent->getWidth(), controlComponent->getHeight());
setResizeLimits(defaultMinWidth, defaultMinHeight, defaultMaxWidth, defaultMaxHeight);
if (pluginEditorNode[IDs::settingsOpen])
controlComponent->showSettingsDialog();
pluginState->addListener(this);
pluginEditorNode.addListener(this);
}
SvkPluginEditor::~SvkPluginEditor()
{
pluginEditorNode.removeListener(this);
pluginState->removeListener(this);
controlComponent = nullptr;
}
//==============================================================================
void SvkPluginEditor::paint(Graphics& g)
{
g.fillAll(Colour(0xff323e44));
}
void SvkPluginEditor::resized()
{
controlComponent->setBounds(0, 0, getWidth(), getHeight());
}
void SvkPluginEditor::presetLoaded(ValueTree presetNodeIn)
{
if (pluginEditorNode.isValid())
pluginEditorNode.removeListener(this);
pluginEditorNode = pluginState->getPluginEditorNode();
controlComponent->loadPresetNode(presetNodeIn);
if (pluginEditorNode[IDs::settingsOpen])
controlComponent->showSettingsDialog();
pluginEditorNode.addListener(this);
}
//==============================================================================
void SvkPluginEditor::valueTreePropertyChanged(ValueTree& parent, const Identifier& property)
{
if (parent == pluginEditorNode && property == IDs::windowBoundsH)
{
Viewport* viewport = controlComponent->getViewport();
VirtualKeyboard::Keyboard* keyboard = controlComponent->getKeyboard();
int viewportHeight = viewport->getHeight() - viewport->getScrollBarThickness();
int keyboardWidth = keyboard->getPianoWidth(viewportHeight);
// Not sure if this is a great solution, but should keep mins < maxes.
if (viewportHeight > 1 && keyboardWidth > 1)
{
setResizeLimits(
defaultMinWidth,
jmax(defaultMinHeight, getHeight() - viewportHeight + 100 + 1),
jmax(defaultMaxWidth, controlComponent->getViewport()->getX() * 2 + keyboardWidth),
defaultMaxHeight
);
}
}
}
// Would like to turn these command descriptions into tooltips...or something
//void SvkPluginEditor::getCommandInfo(CommandID commandID, ApplicationCommandInfo &result)
//{
// switch (commandID)
// {
// case IDs::CommandIDs::savePresetToFile:
// result.setInfo("Save Preset", "Save your custom layout to a file.", "Preset", 0);
// break;
// case IDs::CommandIDs::saveMode:
// result.setInfo("Save Mode", "Save the currently viewed mode.", "Preset", 0);
// break;
// case IDs::CommandIDs::showSaveMenu:
// result.setInfo("Show Saving Options", "Save current mode or whole preset.", "Preset", 0);
// break;
// case IDs::CommandIDs::loadPreset:
// result.setInfo("Load Preset", "Load a custom layout from a file.", "Preset", 0);
// break;
// case IDs::CommandIDs::loadMode:
// result.setInfo("Load Mode", "Load only the mode of a preset.", "Preset", 0);
// break;
// case IDs::CommandIDs::showLoadMenu:
// result.setInfo("Show Loading Options", "Load a mode or whole preset.", "Preset", 0);
// break;
// case IDs::CommandIDs::exportReaperMap:
// result.setInfo("Export for Reaper", "Exports the current preset to a MIDI Note Name text file for use in Reaper's piano roll.", "Preset", 0);
// break;
// case IDs::CommandIDs::exportAbletonMap:
// result.setInfo("Export for Ableton", "Exports the mode mapping to a MIDI file for to use in Ableton's piano roll for folding.", "Preset", 0);
// break;
// case IDs::CommandIDs::showExportMenu:
// result.setInfo("Show Export Options", "Shows different ways you can export a mode or preset.", "Preset", 0);
// break;
// case IDs::CommandIDs::showSettingsDialog:
// result.setInfo("Show Settings Dialog", "Change default directories", "Settings", 0);
// break;
// case IDs::CommandIDs::commitCustomScale:
// result.setInfo("Commit custom scale", "Registers the entered scale steps as the current custom scale.", "Preset", 0);
// break;
// case IDs::CommandIDs::setMode1:
// result.setInfo("Set Mode 1", "Loads the mode into the Mode 1 slot.", "Preset", 0);
// break;
// case IDs::CommandIDs::setMode2:
// result.setInfo("Set Mode 2", "Loads the mode into the Mode 2 slot.", "Preset", 0);
// break;
// case IDs::CommandIDs::setMode1RootNote:
// result.setInfo("Set Mode 1 Root", "Applies the selected root note for Mode 1.", "Preset", 0);
// break;
// case IDs::CommandIDs::setMode2RootNote:
// result.setInfo("Set Mode 2 Root", "Applies the selected root note for Mode 2.", "Preset", 0);
// break;
// case IDs::CommandIDs::setModeViewed:
// result.setInfo("Set Mode Viewed", "Shows the mode slot on the keyboard.", "Keyboard", 0);
// break;
// case IDs::CommandIDs::showModeInfo:
// result.setInfo("Show Mode Info", "Shows information regarding the selected Mode.", "Mode", 0);
// break;
// case IDs::CommandIDs::setMappingStyle:
// result.setInfo("Mapping Style", "Choose a mapping style for remapping MIDI notes.", "Midi", 0);
// break;
// case IDs::CommandIDs::showMapOrderEdit:
// result.setInfo("Edit Mappings by Order", "Choose how to map modes with the order mapping method.", "Preset", 0);
// break;
// case IDs::CommandIDs::applyMapping:
// result.setInfo("Apply Mapping", "Map incoming MIDI notes to Mode 2 with the selected mapping style.", "Midi", 0);
// break;
// case IDs::CommandIDs::setMappingMode:
// result.setInfo("Auto Map to Scale", "Remap Midi notes when scale changes", "Midi", 0);
// break;
// case IDs::CommandIDs::beginColorEditing:
// result.setInfo("Change Keyboard Colors", "Allows you to change the default colors for the rows of keys.", "Keyboard", 0);
// break;
//case IDs::CommandIDs::setPeriodShift:
// result.setInfo("Shift by Mode 2 Period", "Shift the outgoing MIDI notes by the selected number of Mode 2 periods.", "Midi", 0);
// break;
//case IDs::CommandIDs::setMidiChannelOut:
// result.setInfo("Set MIDI Channel Out", "Set the outgoing MIDI Notes to the selected MIDI Channel.", "Midi", 0);
// break;
//case IDs::CommandIDs::showMidiNoteNumbers:
// result.setInfo("Show Midi Note Numbers", "Shows incoming MIDI notes on Mode 1 and outgoing MIDI Notes on Mode 2.", "Keyboard", 0);
// break;
//case IDs::CommandIDs::setKeyStyle:
// result.setInfo("Set Key Style", "Sets the selected style for drawing overlapping degrees between mode degrees.", "Keyboard", 0);
// break;
//case IDs::CommandIDs::setHighlightStyle:
// result.setInfo("Set Highlight Style", "Sets the selected style for drawing triggered notes.", "Keyboard", 0);
// break;
// default:
// break;
// }
//} | {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
SvkUiPanel.h
Created: 26 Dec 2019 3:54:15pm
Author: Vincenzo Sicurella
A base class for a UI Panel in SuperVirtualKeyboard, which currently just requires
a helper function to be defined for connecting parameters to the AudioProcessor.
==============================================================================
*/
#pragma once
#include "../../JuceLibraryCode/JuceHeader.h"
//typedef AudioProcessorValueTreeState::ButtonAttachment ButtonAttachment;
//typedef AudioProcessorValueTreeState::ComboBoxAttachment ComboBoxAttachment;
//typedef AudioProcessorValueTreeState::SliderAttachment SliderAttachment;
class SvkUiPanel : public Component
{
protected:
//AudioProcessorValueTreeState& processorTree;
public:
SvkUiPanel(/*AudioProcessorValueTreeState& processorTreeIn*/)
// : processorTree(processorTreeIn)
{};
virtual ~SvkUiPanel() = 0;
//virtual void connectToProcessor() = 0;
virtual void paint(Graphics& g) override;
virtual void resized() override;
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
VectorResources.h
Created: 10 Nov 2019 10:50:29pm
Author: Vincenzo Sicurella
==============================================================================
*/
#pragma once
#include "../../JuceLibraryCode/JuceHeader.h"
static void drawSaveIcon(Graphics& g, Rectangle<int> bounds, Colour backgroundFill=Colour(),
Colour outLineColor=Colours::lightgrey)
{
g.setColour(backgroundFill);
g.fillRoundedRectangle(bounds.toFloat(), 3);
g.setColour(outLineColor);
g.drawRoundedRectangle(bounds.toFloat(), 3, 1);
g.setColour(Colours::whitesmoke);
int boundsSize = bounds.getWidth();
float imgSize = boundsSize * 7.0f / 8.0f;
float thickness = imgSize / 32.0f;
Rectangle<float> boundsReduced = bounds.reduced(bounds.getWidth() - imgSize).toFloat();
Path outline = Path();
outline.addLineSegment(Line<float>(boundsReduced.getTopLeft().toFloat(), boundsReduced.getBottomLeft().toFloat()), thickness);
outline.addLineSegment(Line<float>(boundsReduced.getBottomLeft().toFloat(), boundsReduced.getBottomRight().toFloat()), thickness);
float corner = imgSize / 6.0f;
Point<float> cornerBottom = boundsReduced.getTopRight().withY( boundsReduced.getTopRight().getY() + corner);
Point<float> cornerLeft = boundsReduced.getTopRight().withX( boundsReduced.getTopRight().getX() - corner);
outline.addLineSegment(Line<float>(boundsReduced.getBottomRight().toFloat(), cornerBottom), thickness);
outline.addLineSegment(Line<float>(cornerBottom, cornerLeft), thickness);
outline.addLineSegment(Line<float>(cornerLeft, boundsReduced.getTopLeft().toFloat()), thickness);
outline = outline.createPathWithRoundedCorners(2.0f);
g.fillPath(outline);
g.drawRect(boundsReduced.reduced(corner, 0).withTrimmedBottom(boundsReduced.getHeight() * 2.0f/3.0f));
float lineWidth = bounds.getWidth() * 1.0f / 8.0f;
float lineX1 = boundsReduced.getTopLeft().getX() + lineWidth;
float lineX2 = boundsReduced.getTopRight().getX() - lineWidth;
float height = boundsReduced.getHeight();
g.drawHorizontalLine(boundsReduced.getTopLeft().getY() + height * 0.6f, lineX1, lineX2);
g.drawHorizontalLine(boundsReduced.getTopLeft().getY() + height * 0.7f, lineX1, lineX2);
g.drawHorizontalLine(boundsReduced.getTopLeft().getY() + height * 0.8f, lineX1, lineX2);
}
static void drawLoadIcon(Graphics& g, Rectangle<int> bounds, Colour backgroundFill=Colour(),
Colour outLineColor=Colours::whitesmoke)
{
g.setColour(backgroundFill);
g.fillRoundedRectangle(bounds.toFloat(), 3);
g.setColour(outLineColor);
g.drawRoundedRectangle(bounds.toFloat(), 3, 1);
g.setColour(Colours::whitesmoke);
int boundsSize = bounds.getWidth();
float imgSize = boundsSize * 7.0f / 8.0f;
float thickness = imgSize / 32.0f;
float tabHeight = imgSize / 9.0f;
float tabWidth = imgSize / 3.0f;
Rectangle<float> boundsReduced = bounds.reduced(bounds.getWidth() - imgSize).toFloat();
boundsReduced.reduce(0, imgSize/20);
Path outline = Path();
outline.addLineSegment(Line<float>(boundsReduced.getTopLeft(), boundsReduced.getBottomLeft()), thickness);
outline.addLineSegment(Line<float>(boundsReduced.getBottomLeft(), boundsReduced.getBottomRight()), thickness);
Point<float> tabCornerBottomRight = boundsReduced.getTopRight().translated(0, tabHeight);
Point<float> tabCornerBottomLeft = boundsReduced.getTopLeft().translated(tabWidth*1.15, tabHeight);
Point<float> tabCornerTop = boundsReduced.getTopLeft().translated(tabWidth, 0);
outline.addLineSegment(Line<float>(boundsReduced.getBottomRight(), tabCornerBottomRight), thickness);
outline.addLineSegment(Line<float>(tabCornerBottomRight, tabCornerBottomLeft), thickness);
outline.addLineSegment(Line<float>(tabCornerBottomLeft, tabCornerTop), thickness);
outline.addLineSegment(Line<float>(tabCornerTop, boundsReduced.getTopLeft()), thickness);
outline = outline.createPathWithRoundedCorners(2.0f);
g.fillPath(outline);
}
static void drawSettingsIcon(Graphics& g, Rectangle<int> bounds, Colour backgroundFill=Colour(),
Colour outLineColor=Colours::whitesmoke)
{
g.setColour(backgroundFill);
g.fillRoundedRectangle(bounds.toFloat(), 3);
g.setColour(outLineColor);
g.drawRoundedRectangle(bounds.toFloat(), 3, 1);
g.setColour(Colours::whitesmoke);
int boundsSize = bounds.getWidth();
float imgSize = boundsSize * 7.0f / 8.0f;
float thickness = imgSize / 36.0f;
Rectangle<float> boundsReduced = bounds.reduced(bounds.getWidth() - imgSize).toFloat();
Path gear = Path();
int n = 7;
float radiusBody = imgSize * 3.0f / 11.0f;
float radiusSpoke = radiusBody * 12.0f/8.0f;
Point<float> bodyRight;
Point<float> bodyLeft;
Point<float> spokeRight;
Point<float> spokeLeft;
Point<float> nextSpoke;
Point<float> center = bounds.getCentre().toFloat();
float r = 0;
float rStep = 6.28318530718f / n;
float spokeDif = rStep * 4.0f/19.0f;
float bodDif = spokeDif * 5.0f / 3.0f;
float rStepNext;
for (int i = 0; i < n; i++)
{
r = rStep * i;
bodyRight = Point<float>(cosf(r-bodDif) * radiusBody + center.getX(), sinf(r-bodDif) * radiusBody + center.getY());
spokeRight = Point<float>(cosf(r-spokeDif) * radiusSpoke + center.getX(), sinf(r-spokeDif) * radiusSpoke + center.getY());
bodyLeft = Point<float>(cosf(r+bodDif) * radiusBody + center.getX(), sinf(r+bodDif) * radiusBody + center.getY());
spokeLeft = Point<float>(cosf(r+spokeDif) * radiusSpoke + center.getX(), sinf(r+spokeDif) * radiusSpoke + center.getY());
rStepNext = rStep * (i + 1);
nextSpoke = Point<float>(cosf(rStepNext-bodDif) * radiusBody + center.getX(), sinf(rStepNext-bodDif) * radiusBody + center.getY());
gear.addLineSegment(Line<float>(bodyRight, spokeRight), thickness);
gear.addLineSegment(Line<float>(spokeRight, spokeLeft), thickness);
gear.addLineSegment(Line<float>(spokeLeft, bodyLeft), thickness);
gear.addLineSegment(Line<float>(bodyLeft, nextSpoke), thickness);
}
gear = gear.createPathWithRoundedCorners(thickness);
g.fillPath(gear);
float cR = radiusBody / 2.0f;
g.drawEllipse(center.getX() - cR, center.getY() - cR, cR * 2, cR * 2, thickness);
}
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
ColourLibraryComponent.cpp
Created: 18 Sep 2020 9:56:16pm
Author: Vincenzo
==============================================================================
*/
#include <JuceHeader.h>
#include "ColourLibraryComponent.h"
//==============================================================================
ColourLibraryComponent::ColourLibraryComponent(StringArray rowNamesIn, Array<Array<Colour>*> rowColoursIn, bool showLabelsIn, bool onlyOpaqueIn)
: rowColours(rowColoursIn), showLabels(showLabelsIn), onlyOpaque(onlyOpaqueIn)
{
for (auto rowName : rowNamesIn)
{
Label* label = new Label(rowName + "_Label", rowName);
rowLabels.add(label);
addAndMakeVisible(label);
}
if (rowColoursIn.size() > 0)
setupFlex();
setColour(ColourLibraryComponent::ColourIds::backgroundColourId, Colour());
setColour(ColourLibraryComponent::ColourIds::outLineColourId, Colour());
setColour(ColourLibraryComponent::ColourIds::rowBackgroundColourId, Colour());
setColour(ColourLibraryComponent::ColourIds::rowOutlineColourId, Colour());
}
ColourLibraryComponent::~ColourLibraryComponent()
{
}
void ColourLibraryComponent::paint (juce::Graphics& g)
{
g.fillAll(findColour(ColourLibraryComponent::ColourIds::backgroundColourId));
g.setColour(findColour(ColourLibraryComponent::ColourIds::outLineColourId));
g.drawRect(getLocalBounds());
for (int i = 0; i < flexBox.items.size() / 2; i++)
{
Component* label = flexBox.items.getReference(i * 2).associatedComponent;
FlexItem& item = flexBox.items.getReference(i * 2 + 1);
Rectangle<int> rowRect = { label->getX(), label->getY(), jmax(label->getWidth(), (int)item.width), label->getHeight() + (int)item.height };
g.setColour(findColour(ColourLibraryComponent::ColourIds::rowBackgroundColourId));
g.fillRect(rowRect);
g.setColour(findColour(ColourLibraryComponent::ColourIds::rowOutlineColourId));
g.drawRect(rowRect);
}
}
void ColourLibraryComponent::resized()
{
for (int i = 0; i < flexBox.items.size(); i++)
{
int maxWidth = getWidth();
if (maxSwatchColumns > 0)
maxWidth = 25 * maxSwatchColumns + 8; // default width plus margins
flexBox.items.getReference(i).width = maxWidth;
flexBox.items.getReference(i).height = 24;
}
flexBox.performLayout(getLocalBounds());
}
void ColourLibraryComponent::showSwatchLabels(bool showLabelsIn)
{
showLabels = showLabelsIn;
}
void ColourLibraryComponent::onlyShowOpaque(bool onlyOpaqueIn)
{
if ((int)onlyOpaque + (int)onlyOpaqueIn == 1)
{
onlyOpaque = onlyOpaqueIn;
setupFlex();
return;
}
onlyOpaque = onlyOpaqueIn;
}
void ColourLibraryComponent::setNewRows(Array<Array<Colour>*> arraysToControl)
{
rowColours = arraysToControl;
refreshSwatches();
}
void ColourLibraryComponent::refreshSwatches()
{
swatches.clear();
for (int r = 0; r < rowBoxes.size(); r++)
{
FlexBox& rowBox = rowBoxes.getReference(r);
Array<Colour>* colorRow = rowColours.getReference(r);
rowBox.items.clear();
for (int i = 0; i < colorRow->size(); i++)
{
Colour& c = colorRow->getReference(i);
if (onlyOpaque && c.getAlpha() < 0xff)
continue;
PaintSwatch* swatch = new PaintSwatch(*colorRow, i, String(i + 1), showLabels);
addAndMakeVisible(swatch);
swatches.add(swatch);
FlexItem swatchItem(20, 20, *swatch);
swatchItem.margin = FlexItem::Margin(2);
rowBox.items.add(swatchItem);
}
}
resized();
}
void ColourLibraryComponent::forceSwatchColumns(int maxNumColumns)
{
maxSwatchColumns = maxNumColumns;
}
void ColourLibraryComponent::setupFlex()
{
flexBox = FlexBox(
FlexBox::Direction::column,
FlexBox::Wrap::noWrap,
FlexBox::AlignContent::flexStart,
FlexBox::AlignItems::stretch,
FlexBox::JustifyContent::flexStart
);
rowBoxes.clear();
for (int r = 0; r < rowLabels.size(); r++)
{
rowBoxes.add(FlexBox(
FlexBox::Direction::row,
FlexBox::Wrap::wrap,
FlexBox::AlignContent::spaceAround,
FlexBox::AlignItems::stretch,
FlexBox::JustifyContent::flexStart
));
Label* row = rowLabels[r];
FlexItem labelAsItem;
labelAsItem.associatedComponent = row;
labelAsItem.minHeight = 24;
labelAsItem.minWidth = Font().getStringWidth(row->getText());
flexBox.items.add(labelAsItem);
FlexItem boxAsItem;
boxAsItem.associatedFlexBox = &rowBoxes.getReference(r);
boxAsItem.alignSelf = FlexItem::AlignSelf::stretch;
boxAsItem.margin = FlexItem::Margin(2, 0, 2, 8);
flexBox.items.add(boxAsItem);
}
refreshSwatches();
} | {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
NoteMapEditor.cpp
Created: 18 Oct 2020 5:13:12pm
Author: Vincenzo
==============================================================================
*/
#include "NoteMapEditor.h"
NoteMapEditor::NoteMapEditor(NoteMap noteMapIn)
{
header = &table.getHeader();
header->addColumn("Note In", ColumnType::MidiNoteIn,font.getStringWidth("128"), font.getStringWidth("Note In"));
header->addColumn("Key", ColumnType::KeyNumber, font.getStringWidth("128"), font.getStringWidth("Key"));
header->addColumn("Note Out", ColumnType::MidiNoteOut, font.getStringWidth("128"), font.getStringWidth("Note Out"));
header->addColumn("+/-", ColumnType::ToggleMapping, font.getStringWidth("+/-"), font.getStringWidth("+/-"));
header->setStretchToFitActive(true);
table.setColour(ListBox::outlineColourId, Colours::darkgrey);
table.setOutlineThickness(1);
addAndMakeVisible(table);
resetMapping(noteMapIn, false);
}
NoteMapEditor::~NoteMapEditor()
{
}
void NoteMapEditor::resized()
{
table.setBoundsInset(BorderSize<int>(1));
header->resizeAllColumnsToFit(table.getWidth() - table.getVerticalScrollBar().getWidth());
}
//=============================================================================
int NoteMapEditor::getNumRows()
{
return currentNoteMapNode.getNumChildren() + 1;
}
void NoteMapEditor::paintRowBackground(Graphics& g, int rowNumber, int w, int h, bool rowIsSelected)
{
g.setColour(rowNumber % 2 == 0 ? Colours::grey : Colours::lightgrey);
g.fillAll();
if (rowNumber == currentNoteMapNode.getNumChildren())
{
g.setColour(Colours::black);
g.drawText(newMappingTrans, 0, 0, w, h, Justification::left);
}
}
void NoteMapEditor::paintCell(Graphics& g, int rowNumber, int columnId, int w, int h, bool isSelected)
{
}
Component* NoteMapEditor::refreshComponentForCell(int rowNumber, int columnId, bool isRowSelected, Component* existingComponentToUpdate)
{
if (columnId < ColumnType::ToggleMapping)
{
if (rowNumber < currentNoteMapNode.getNumChildren())
{
ValueTree node = currentNoteMapNode.getChild(rowNumber);
var value;
switch (columnId)
{
case ColumnType::MidiNoteIn:
if (node.hasProperty(IDs::mappingMidiNoteIn))
value = node[IDs::mappingMidiNoteIn];
else
value = noneTrans;
break;
case ColumnType::KeyNumber:
if (node.hasProperty(IDs::pianoKeyNumber))
value = node[IDs::pianoKeyNumber];
else
value = noneTrans;
break;
case ColumnType::MidiNoteOut:
// TODO: note out mapping implementation
if (node.hasProperty(IDs::pianoKeyNumber))
value = node[IDs::pianoKeyNumber];
else
value = noneTrans;
break;
}
if ((int)value < 0)
value = belowThreshTrans;
else if ((int)value > 127)
value = aboveThreshTrans;
if (value.isVoid())
value = 0;
auto* valueLabel = static_cast<TableLabel*> (existingComponentToUpdate);
if (valueLabel == nullptr)
{
valueLabel = new TableLabel();
valueLabel->setColour(Label::ColourIds::textColourId, Colours::black);
valueLabel->setColour(Label::ColourIds::outlineColourId, Colours::black);
valueLabel->setJustificationType(Justification::centred);
// TODO: note out mapping implementation
if (columnId == ColumnType::KeyNumber || (columnId != ColumnType::MidiNoteOut && value == noneTrans))
{
valueLabel->setEditable(true);
valueLabel->addListener(this);
}
}
valueLabel->setRowNumber(rowNumber);
valueLabel->setColumnId(columnId);
valueLabel->setText(value.toString(), dontSendNotification);
return valueLabel;
}
else
{
// don't understand why I have to do this
delete existingComponentToUpdate;
}
}
// Add / Delete Column
else
{
auto* button = static_cast<TableButton*>(existingComponentToUpdate);
if (existingComponentToUpdate == nullptr)
{
button = new TableButton();
button->addListener(this);
}
button->setRowNumber(rowNumber);
button->setColumnId(columnId);
if (rowNumber < currentNoteMapNode.getNumChildren())
button->setButtonText(deleteTrans);
else
button->setButtonText("+");
return button;
}
return nullptr;
}
int NoteMapEditor::getColumnAutoSizeWidth(int columnId)
{
switch (ColumnType(columnId))
{
case ColumnType::ToggleMapping:
return font.getStringWidth("+");
default:
return font.getStringWidth("128");
}
}
String NoteMapEditor::getCellTooltip(int rowNumber, int columnId)
{
return String();
}
void NoteMapEditor::deleteKeyPressed(int lastRowSelected)
{
}
void NoteMapEditor::returnKeyPressed(int lastRowSelected)
{
}
//=============================================================================
NoteMap NoteMapEditor::getCurrentNoteMapping() const
{
return currentNoteMap;
}
void NoteMapEditor::mapMidiNoteToKey(int midiNoteIn, int keyNumberOut)
{
currentNoteMap.setValue(midiNoteIn, keyNumberOut);
listeners.call(&MappingEditor::Listener::mappingEditorChanged, currentNoteMap);
resetMapping(currentNoteMap, false);
}
void NoteMapEditor::resetMapping(NoteMap mappingIn, bool sendMessage)
{
currentNoteMap = mappingIn;
currentNoteMapNode = currentNoteMap.getAsValueTree(IDs::midiInputRemap);
if (sendMessage)
listeners.call(&MappingEditor::Listener::mappingEditorChanged, currentNoteMap);
table.updateContent();
}
//=============================================================================
void NoteMapEditor::editorShown(Label* label, TextEditor& editor)
{
TableLabel* tl = static_cast<TableLabel*>(label);
ValueTree mapNode = currentNoteMapNode.getChild(tl->getRowNumber());
editor.setInputRestrictions(4, "-0123456789");
switch (tl->getColumnId())
{
case ColumnType::KeyNumber:
editor.setText(mapNode[IDs::pianoKeyNumber]);
break;
// TODO: implement actual midi channel out
case ColumnType::MidiChannelOut:
editor.setText(mapNode[IDs::pianoKeyNumber]);
break;
}
}
void NoteMapEditor::labelTextChanged(Label* label)
{
TableLabel* tl = static_cast<TableLabel*>(label);
switch (tl->getColumnId())
{
case ColumnType::KeyNumber:
if (label->getText().length() > 0)
{
int newKeyNum = label->getText().getIntValue();
if (newKeyNum < 0)
label->setText(belowThreshTrans, dontSendNotification);
else if (newKeyNum > 127)
label->setText(aboveThreshTrans, dontSendNotification);
else
editMapping(tl->getRowNumber(), newKeyNum, false);
}
else
{
label->setText(noneTrans, dontSendNotification);
currentNoteMapNode.getChild(tl->getRowNumber()).removeProperty(IDs::pianoKeyNumber, nullptr);
}
listeners.call(&MappingEditor::Listener::mappingEditorChanged, currentNoteMap);
break;
case ColumnType::MidiNoteIn:
if (tl->getText().length() > 0)
{
int newNoteIn = tl->getText().getIntValue();
int keyMappedToNote = currentNoteMap.getValue(newNoteIn);
// Midi Note In mapping already exists
if (keyMappedToNote != newNoteIn)
{
AlertWindow::showMessageBox(
AlertWindow::AlertIconType::WarningIcon,
mappingErrorTrans,
getAlreadyMappedMessage(newNoteIn, keyMappedToNote),
okTrans
);
label->setText(noneTrans, dontSendNotification);
}
else
{
currentNoteMapNode.getChild(tl->getRowNumber()).setProperty(IDs::mappingMidiNoteIn, newNoteIn, nullptr);
}
}
else
{
label->setText(noneTrans, dontSendNotification);
currentNoteMapNode.getChild(tl->getRowNumber()).removeProperty(IDs::mappingMidiNoteIn, nullptr);
}
break;
}
}
void NoteMapEditor::buttonClicked(Button* button)
{
TableButton* tb = static_cast<TableButton*>(button);
int rowNum = tb->getRowNumber();
// Delete mapping
if (rowNum < currentNoteMapNode.getNumChildren())
{
removeMapping(rowNum);
}
// Add new mapping
else
{
addNewMapping();
}
}
//=============================================================================
void NoteMapEditor::addNewMapping()
{
currentNoteMapNode.appendChild(ValueTree(IDs::noteMapNode), nullptr);
table.updateContent();
}
void NoteMapEditor::editMapping(int rowNumber, int keyNumberOut, bool sendMappingChangedMessage)
{
currentNoteMapNode.getChild(rowNumber).setProperty(IDs::pianoKeyNumber, keyNumberOut, nullptr);
currentNoteMap = NoteMap(currentNoteMapNode);
resetMapping(currentNoteMap, sendMappingChangedMessage);
}
void NoteMapEditor::removeMapping(int rowNumber)
{
currentNoteMapNode.removeChild(rowNumber, nullptr);
currentNoteMap = NoteMap(currentNoteMapNode);
resetMapping(currentNoteMap);
}
String NoteMapEditor::getAlreadyMappedMessage(int noteIn, int keyNumOut)
{
return "Note " + String(noteIn) + " is already mapped to key " + String(keyNumOut) + ". Edit key number instead.";
} | {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
PresetLibraryBox.h
Created: 27 May 2019 12:02:43pm
Author: Vincenzo
==============================================================================
*/
#pragma once
#include "../../../JuceLibraryCode/JuceHeader.h"
class ReferencedComboBox : public ComboBox
{
PopupMenu rootMenu;
JUCE_DECLARE_NON_COPYABLE_WITH_LEAK_DETECTOR(ReferencedComboBox)
public:
ReferencedComboBox(const String& nameIn={}, const PopupMenu* menuIn=nullptr);
~ReferencedComboBox();
void setMenu(const PopupMenu& menuIn);
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
NoteMapEditor.h
Created: 18 Oct 2020 5:13:12pm
Author: Vincenzo
A component for viewing and editing note mappings.
==============================================================================
*/
#pragma once
#include <JuceHeader.h>
#include "../../Structures/MappingEditorBase.h"
#include "../../CommonFunctions.h"
// Table Helpers
class TableComponent
{
int rowNumber;
int columnId;
public:
void setRowNumber(int rowNumberIn)
{
rowNumber = rowNumberIn;
}
void setColumnId(int columnIdIn)
{
columnId = columnIdIn;
}
int getRowNumber() const
{
return rowNumber;
}
int getColumnId() const
{
return columnId;
}
};
class TableLabel : public TableComponent, public Label {};
class TableButton : public TableComponent, public TextButton {};
class TableSlider : public TableComponent, public Slider {};
class NoteMapEditor : public Component,
public TableListBoxModel,
public MappingEditor,
private Button::Listener,
private Label::Listener
{
public:
enum ColumnType
{
MidiNoteIn = 1,
MidiChannelIn,
KeyNumber,
MidiNoteOut,
MidiChannelOut,
ToggleMapping
};
public:
NoteMapEditor(NoteMap noteMapIn);
~NoteMapEditor();
void resized() override;
//=============================================================================
// TableListBoxModel implementation
int getNumRows() override;
void paintRowBackground(Graphics&, int rowNumber, int w, int h, bool rowIsSelected) override;
void paintCell(Graphics&, int rowNumber, int columnId, int w, int h, bool isSelected) override;
Component* refreshComponentForCell(int rowNumber, int columnId, bool isRowSelected, Component*) override;
int getColumnAutoSizeWidth(int columnId) override;
String getCellTooltip(int rowNumber, int columnId) override;
void deleteKeyPressed(int lastRowSelected) override;
void returnKeyPressed(int lastRowSelected) override;
//=============================================================================
// MappingEditor implementation
NoteMap getCurrentNoteMapping() const override;
void mapMidiNoteToKey(int midiNoteIn, int keyNumberOut) override;
void resetMapping(NoteMap mappingIn = NoteMap(), bool sendMessage = true) override;
//=============================================================================
// Label::Listener implementation
void editorShown(Label*, TextEditor&) override;
void labelTextChanged(Label*) override;
// Button::Listener implementation
void buttonClicked(Button*) override;
//=============================================================================
void addNewMapping();
// TODO: add midi note out & channel editing
void editMapping(int rowNumber, int keyNumOut, bool sendMappingChangedMessage = true);
void removeMapping(int rowNumber);
private:
String getAlreadyMappedMessage(int noteIn, int keyNumOut);
private:
NoteMap currentNoteMap;
ValueTree currentNoteMapNode;
Font font = Font(11, Font::plain);
TableListBox table = { {}, this };
TableHeaderComponent* header;
String newMappingTrans = translate(" Add new mapping");
String belowThreshTrans = translate("Too low");
String aboveThreshTrans = translate("Too high");
String mappingErrorTrans = translate("Mapping Error");
String noneTrans = translate("None");
String deleteTrans = translate("Delete");
String okTrans = translate("Ok");
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
FocussedComponent.h
Created: 17 Sep 2020 6:31:58pm
Author: Vincenzo
==============================================================================
*/
#pragma once
#include <JuceHeader.h>
class FocussedComponent;
class FocusableComponent
{
public:
FocusableComponent(FocussedComponent* focusserIn = nullptr, std::function<void(var)> callbackIn = {})
: currentFocusser(focusserIn)
{
}
virtual void setNewFocusser(FocussedComponent* newFocusserIn)
{
currentFocusser = newFocusserIn;
}
bool isFocussedOn() const
{
return currentFocusser != nullptr;
}
virtual void performFocusFunction(var dataIn)
{
focusFunctionCallback(dataIn);
}
protected:
FocussedComponent* currentFocusser;
std::function<void(var)> focusFunctionCallback;
};
class FocussedComponent
{
public:
FocussedComponent(Component* initialComponentToFocus = nullptr, std::function<void(Component*, var)> focusserCallbackIn = {})
: currentFocus(initialComponentToFocus), focusserCallback(focusserCallbackIn)
{
}
virtual void setComponentToFocusOn(Component* componentPtr)
{
FocusableComponent* focusable = dynamic_cast<FocusableComponent*>(currentFocus);
if (focusable)
focusable->setNewFocusser(nullptr);
currentFocus = componentPtr;
focusable = dynamic_cast<FocusableComponent*>(currentFocus);
if (focusable)
focusable->setNewFocusser(this);
}
void setFocusserCallback(std::function<void(Component*, var)> focusserCallbackIn)
{
focusserCallback = focusserCallbackIn;
}
protected:
Component* currentFocus;
std::function<void(Component*, var)> focusserCallback;
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
LabelledComponent.h
Created: 6 Sep 2020 4:43:16pm
Author: Vincenzo
A container for a component that allows a offsetLabel to be attached a certain way.
==============================================================================
*/
#pragma once
#include <JuceHeader.h>
class LabelledComponent : public Component
{
public:
enum LabelPosition
{
LeftOfComponent = 0,
TopOfComponent,
RightOfComponent,
BottomOfComponent
};
enum LabelJustification
{
LeftJustify = 0,
CentredJustify,
RightJustify
};
public:
LabelledComponent(
Component& componentIn,
String labelTextIn,
bool ownsComponent = true,
LabelPosition positionIn = LabelPosition::LeftOfComponent,
LabelJustification justificationIn = LabelJustification::LeftJustify,
Font fontIn = Font()
) :
componentReference (componentIn),
deleteComponent (ownsComponent),
position (positionIn),
justification (justificationIn),
font (fontIn)
{
(Component*)&componentReference;
if (deleteComponent)
{
component.reset(&componentReference);
}
addAndMakeVisible(&componentReference);
label.reset(new Label(componentReference.getName() + "_Label"));
label->setText(labelTextIn, dontSendNotification);
label->setFont(font);
label->setJustificationType(Justification::verticallyCentred);
addAndMakeVisible(label.get());
setLabelSize(font.getStringWidth(label->getText()) + 8, 24);
// Default Size
component->setSize(225, 24);
}
~LabelledComponent()
{
if (deleteComponent)
component = nullptr;
label = nullptr;
}
void resized() override
{
//if ((expectedWidth > 0 && expectedHeight > 0)
// && (expectedWidth != getWidth() || expectedHeight != getHeight())
// )
//{
// float widthRatio = getWidth() / expectedWidth;
// float heightRatio = getHeight() / expectedHeight;
// componentReference.setSize(componentReference.getWidth() * widthRatio, componentReference.getHeight() * heightRatio);
// offsetLabel->setSize(offsetLabel->getWidth() * widthRatio, offsetLabel->getHeight() * heightRatio);
//}
int labelX = 0, labelY = 0, compX = 0, compY = 0;
if (position % 2 == 0)
{
if (position == LabelPosition::LeftOfComponent)
compX = label->getWidth();
else
labelX = componentReference.getWidth();
}
else
{
if (position == LabelPosition::TopOfComponent)
compY = label->getHeight();
else
labelY = componentReference.getHeight();
if (justification == LabelJustification::CentredJustify)
labelX = (getWidth() - label->getWidth()) / 2;
else if (justification == LabelJustification::RightJustify)
labelX = getWidth() - label->getWidth();
}
label->setTopLeftPosition(labelX, labelY);
componentReference.setTopLeftPosition(compX, compY);
}
void paintOverChildren(Graphics& g) override
{
//g.setColour(Colours::red);
//g.drawRect(getLocalBounds(), 1);
}
void setComponentSize(int widthIn, int heightIn)
{
componentReference.setSize(widthIn, heightIn);
if (position % 2 == 0)
{
expectedWidth = labelWidth + componentReference.getWidth();
expectedHeight = jmax(labelHeight, componentReference.getHeight());
}
else
{
expectedWidth = jmax(labelWidth, componentReference.getWidth());
expectedHeight = labelHeight + componentReference.getHeight();
}
setSize(expectedWidth, expectedHeight);
}
void setLabelSize(int widthIn, int heightIn)
{
labelWidth = widthIn;
labelHeight = heightIn;
label->setSize(labelWidth, labelHeight);
setComponentSize(componentReference.getWidth(), componentReference.getHeight());
}
void setLabelPosition(LabelPosition positionIn)
{
position = positionIn;
setComponentSize(componentReference.getWidth(), componentReference.getHeight());
}
void setLabelJustification(LabelJustification justificationIn)
{
justification = justificationIn;
resized();
}
Component* get()
{
return &componentReference;
}
Label* getLabel() const
{
return label.get();
}
String getLabelText() const
{
return label->getText();
}
bool hasOwnership() const
{
return deleteComponent;
}
LabelPosition getLabelPosition() const
{
return position;
}
public:
template <class ComponentType>
static ComponentType* getComponentPointer(LabelledComponent* labelledComponentIn)
{
return dynamic_cast<ComponentType*>(labelledComponentIn->get());
}
private:
std::unique_ptr<Component> component;
Component& componentReference;
bool deleteComponent;
LabelPosition position;
LabelJustification justification;
Font font;
float expectedWidth, expectedHeight;
int labelWidth, labelHeight;
std::unique_ptr<Label> label;
}; | {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
PresetLibraryBox.cpp
Created: 27 May 2019 12:02:43pm
Author: Vincenzo
==============================================================================
*/
#include "ReferencedComboBox.h"
ReferencedComboBox::ReferencedComboBox(const String& nameIn, const PopupMenu* menuIn)
: ComboBox(nameIn), rootMenu(PopupMenu())
{
if (menuIn)
setMenu(*menuIn);
}
ReferencedComboBox::~ReferencedComboBox()
{
}
void ReferencedComboBox::setMenu(const PopupMenu& menuIn)
{
rootMenu = menuIn;
PopupMenu& currentMenu = *getRootMenu();
currentMenu = rootMenu;
}
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
ColourLibraryComponent.h
Created: 18 Sep 2020 9:56:16pm
Author: Vincenzo
==============================================================================
*/
#pragma once
#include <JuceHeader.h>
#include "FocussedComponent.h"
//==============================================================================
/*
*/
class PaintSwatch : public Component, public FocusableComponent
{
public:
PaintSwatch(Array<Colour>& parentPaletteIn, int indexIn, String swatchLabelIn = "", bool showLabelIn = false, std::function<void(var)> callback = {})
: parentPalette(parentPaletteIn), index(indexIn), swatchLabel(swatchLabelIn), showLabel(showLabelIn), FocusableComponent(nullptr, callback)
{
setName("Swatch_" + swatchLabelIn);
};
~PaintSwatch() {};
void paint(Graphics& g) override
{
g.fillAll(getCurrentColour());
if (getCurrentColour().isTransparent())
{
g.setColour(Colours::darkgrey.darker());
g.drawRect(getLocalBounds());
}
g.setColour(getCurrentColour().contrasting());
if (showLabel)
{
g.drawFittedText(swatchLabel, getLocalBounds(), Justification::centred, 1, 0.1f);
}
if (isFocussedOn())
{
g.drawRect(getLocalBounds(), 2.0f);
}
}
void setNewFocusser(FocussedComponent* focusserIn) override
{
FocusableComponent::setNewFocusser(focusserIn);
repaint();
}
void setLabelText(String labelTextIn)
{
swatchLabel = labelTextIn;
}
void performFocusFunction(var dataIn) override
{
parentPalette.set(index, Colour::fromString(dataIn.toString()));
repaint();
}
Colour getCurrentColour() const
{
return parentPalette[index];
}
private:
Array<Colour>& parentPalette;
const int index;
String swatchLabel;
bool showLabel;
};
//==============================================================================
/*
*/
class ColourLibraryComponent : public juce::Component
{
public:
enum ColourIds
{
backgroundColourId = 0x00010100,
rowBackgroundColourId = 0x00010101,
outLineColourId = 0x00010200,
rowOutlineColourId = 0x00010201
};
public:
ColourLibraryComponent(StringArray rowNamesIn, Array<Array<Colour>*> arraysToControl = {}, bool showLabelsIn = false, bool onlyOpaqueIn = false);
~ColourLibraryComponent() override;
void paint(juce::Graphics&) override;
void resized() override;
void showSwatchLabels(bool toShowLabels);
void onlyShowOpaque(bool onlyOpaque);
void setNewRows(Array<Array<Colour>*> arraysToControl);
void refreshSwatches();
void forceSwatchColumns(int maxNumColumns);
private:
void setupFlex();
private:
Array<Array<Colour>*> rowColours;
FlexBox flexBox;
Array<FlexBox> rowBoxes;
OwnedArray<Label> rowLabels;
OwnedArray<PaintSwatch> swatches;
bool showLabels;
bool onlyOpaque;
int maxSwatchColumns = 0;
JUCE_DECLARE_NON_COPYABLE_WITH_LEAK_DETECTOR(ColourLibraryComponent)
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
PluginControlComponent.h
Created: 8 Jul 2019
Author: Vincenzo Sicurella
==============================================================================
*/
#pragma once
#include "../JuceLibraryCode/JuceHeader.h"
#include "../../PluginState.h"
#include "../VectorResources.h"
#include "ReferencedComboBox.h"
#include "VirtualKeyboard/KeyboardComponent.h"
#include "VirtualKeyboard/KeyboardViewport.h"
#include "../../Structures/MappingHelper.h"
#include "Settings/SettingsContainer.h"
#include "../Dialogs/ModeInfoDialog.h"
#include "../Dialogs/MapByOrderDialog.h"
#include "../Dialogs/ExportKbmDialog.h"
#include "../../File IO/ReaperWriter.h"
#include "../../File IO/AbletonMidiWriter.h"
#include "../../File IO/KbmWriter.h"
class PluginControlComponent : public Component,
public TextEditor::Listener,
public ComboBox::Listener,
public Slider::Listener,
public Button::Listener,
public ScrollBar::Listener,
public ChangeListener,
private SvkPluginState::Listener,
private SettingsContainer::Listener,
private MappingHelper::Listener,
private Timer
{
public:
enum SaveMenuOptions
{
SaveMode = 1,
SavePreset,
ExportKBM,
ExportReaper,
ExportAbleton
};
enum LoadMenuOptions
{
LoadMode = 1,
LoadPreset,
LoadKBM
};
public:
//==============================================================================
PluginControlComponent (SvkPluginState* pluginStateIn);
~PluginControlComponent();
//==============================================================================
void paint(Graphics& g) override;
void resized() override;
void mouseDown(const MouseEvent& e) override;
void comboBoxChanged(ComboBox* comboBoxThatHasChanged) override;
void sliderValueChanged(Slider* sliderThatWasMoved) override;
void buttonClicked(Button* buttonThatWasClicked) override;
void textEditorTextChanged(TextEditor& textEditor) override {};
void textEditorEscapeKeyPressed(TextEditor& textEditor) override {};
void textEditorReturnKeyPressed(TextEditor& textEditor) override;
void textEditorFocusLost(TextEditor& textEditor) override {};
void scrollBarMoved(ScrollBar* bar, double newRangeStart) override;
void changeListenerCallback(ChangeBroadcaster* source) override;
void timerCallback() override;
//==============================================================================
void resyncPresetNode(ValueTree presetNode) override;
void modeViewedChanged(Mode* modeIn, int selectorNumber, int slotNumber) override;
void mappingModeChanged(int mappingModeId) override;
void inputMappingChanged(NoteMap& inputNoteMap) override;
void customModeChanged(Mode* newCustomMode) override;
void modeInfoChanged(Mode* modeEdited) override;
void settingsTabChanged(int tabIndex, const String& tabName, SvkSettingsPanel* panelChangedTo) override;
void keyMappingStatusChanged(int keyNumber, bool preparedToMap) override;
void keyMapConfirmed(int keyNumber, int midiNote) override;
void mappingEditorChanged(NoteMap&) override;
//==============================================================================
String getScaleEntryText();
void setScaleEntryText(String textIn, NotificationType notify = NotificationType::dontSendNotification);
VirtualKeyboard::Keyboard* getKeyboard();
TextEditor* getScaleTextEditor();
Viewport* getViewport();
ComboBox* getMappingStyleBox();
ReferencedComboBox* getMode1Box();
ReferencedComboBox* getMode2Box();
void updateModeBoxMenus();
ImageButton* getSettingsButton();
TextButton* getModeInfoButton();
//==============================================================================
/*
Sets controls to plugin state
*/
void loadPresetNode(ValueTree presetNodeIn);
/*
Updates UI to the new mode
*/
void onModeViewedChange(Mode* modeViewed);
int getModeSelectorViewed();
void setMapModeBoxId(int mappingModeId, NotificationType notify = NotificationType::sendNotification);
void setMappingStyleId(int idIn, NotificationType notify = NotificationType::sendNotification);
void setMode1Root(int rootIn, NotificationType notify = NotificationType::dontSendNotification);
void setMode2Root(int rootIn, NotificationType notify = NotificationType::dontSendNotification);
void submitCustomScale();
void updateRootNoteLabels();
//==============================================================================
void showModeInfo();
void showMapOrderEditDialog();
void showSettingsDialog();
void hideSettings();
void beginColorEditing();
void endColorEditing();
void beginManualMapping();
//==============================================================================
bool browseForModeToOpen();
bool browseForPresetToOpen();
bool exportModeViewedForReaper();
bool exportModeViewedForAbleton();
void exportKbmMapping();
private:
SvkPluginState* pluginState;
SvkPresetManager* presetManager;
std::unique_ptr<Image> saveIcon;
std::unique_ptr<Image> openIcon;
std::unique_ptr<Image> settingsIcon;
std::unique_ptr<PopupMenu> saveMenu;
Array<PopupMenu::Item> saveMenuItems;
std::unique_ptr<PopupMenu> loadMenu;
Array<PopupMenu::Item> loadMenuItems;
MappingHelper* manualMappingHelper;
std::unique_ptr<SettingsContainer> settingsContainer;
ScrollBar* viewportScrollBar;
ModeInfoDialog* modeInfo;
MapByOrderDialog* mapByOrderDialog;
Array<Component*> mappingComponents;
bool settingsPanelOpen = false;
bool isColorEditing = false;
bool inMappingMode = false;
bool mappingSettingsOpen = false;
String noKeySelectedTrans = "No key selected.";
String waitingForTrans = "Waiting for input to map to key ";
String inputModeTooltip = "The input scale that represents your physical keyboard or DAW piano roll.";
String outputModeTooltip = "The output scale that you want to play or reference.";
String mapModeTooltip =
"Maps MIDI note input to notes in the selected scale (Mode 2).\n"
"Auto Map: automatically maps notes based on Mode 1 and Mode 2.\n"
"Manual: create a custom mapping by clicking notes on the virtual keyboard.";
String mapStyleTooltip =
"The way notes are mapped from the input to output.\n"
"Mode To Mode: natural notes from Mode 1 are mapped to natural Notes in Mode 2.\n"
"Scale To Mode: each note from Mode 1 is mapped to natural notes in Mode 2.\n"
"by Layer: Notes from a layer in Mode 1 are mapped to a layer in Mode 2.";
float centerKeyPos = 60.5f;
int defaultHeight = 210;
int barHeight = 24;
int gap = 8;
//==============================================================================
std::unique_ptr<TextEditor> scaleTextBox;
std::unique_ptr<ReferencedComboBox> mode1Box;
std::unique_ptr<ReferencedComboBox> mode2Box;
std::unique_ptr<Slider> mode1RootSld;
std::unique_ptr<Slider> mode2RootSld;
std::unique_ptr<TextButton> scaleEntryBtn;
std::unique_ptr<TextButton> modeInfoButton;
std::unique_ptr<ToggleButton> mode1ViewBtn;
std::unique_ptr<ToggleButton> mode2ViewBtn;
std::unique_ptr<Label> mode1RootLbl;
std::unique_ptr<Label> mode2RootLbl;
std::unique_ptr<ComboBox> mapStyleBox;
std::unique_ptr<Label> mapStyleLbl;
std::unique_ptr<TextButton> mapOrderEditBtn;
std::unique_ptr<ComboBox> mapModeBox;
std::unique_ptr<TextButton> mapApplyBtn;
std::unique_ptr<Label> mapManualTip;
std::unique_ptr<Label> mapManualStatus;
std::unique_ptr<TextButton> mapManualCancel;
std::unique_ptr<TextButton> mapManualResetBtn;
std::unique_ptr<TextButton> mapCopyToManualBtn;
std::unique_ptr<VirtualKeyboard::Keyboard> keyboard;
std::unique_ptr<KeyboardViewport> keyboardViewport;
std::unique_ptr<ImageButton> saveButton;
std::unique_ptr<ImageButton> openButton;
std::unique_ptr<ImageButton> settingsButton;
//==============================================================================
JUCE_DECLARE_NON_COPYABLE_WITH_LEAK_DETECTOR (PluginControlComponent)
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
PluginControlComponent.cpp
Created: 8 Jul 2019
Author: Vincenzo Sicurella
==============================================================================
*/
#include "PluginControlComponent.h"
PluginControlComponent::PluginControlComponent(SvkPluginState* pluginStateIn)
: pluginState(pluginStateIn)
{
pluginState->addListener(this);
presetManager = pluginState->getPresetManager();
scaleTextBox.reset(new TextEditor("Scale Text Box"));
addAndMakeVisible(scaleTextBox.get());
scaleTextBox->setMultiLine(false);
scaleTextBox->setReturnKeyStartsNewLine(false);
scaleTextBox->setReadOnly(false);
scaleTextBox->setScrollbarsShown(true);
scaleTextBox->setCaretVisible(true);
scaleTextBox->setPopupMenuEnabled(true);
scaleTextBox->setText(String());
mode1Box.reset(new ReferencedComboBox("Mode1 Box"));
addAndMakeVisible(mode1Box.get());
mode1Box->setEditableText(false);
mode1Box->setJustificationType(Justification::centredLeft);
mode1Box->setTextWhenNothingSelected(String());
mode1Box->setTextWhenNoChoicesAvailable(TRANS("(no choices)"));
mode1Box->setTooltip(inputModeTooltip);
mode1Box->addListener(this);
mode2Box.reset(new ReferencedComboBox("Mode2 Box"));
addAndMakeVisible(mode2Box.get());
mode2Box->setEditableText(false);
mode2Box->setJustificationType(Justification::centredLeft);
mode2Box->setTextWhenNothingSelected(String());
mode2Box->setTextWhenNoChoicesAvailable(TRANS("(no choices)"));
mode2Box->setTooltip(outputModeTooltip);
mode2Box->addListener(this);
mode1RootSld.reset(new Slider("Mode1 Root Slider"));
addAndMakeVisible(mode1RootSld.get());
mode1RootSld->setRange(0, 127, 1);
mode1RootSld->setSliderStyle(Slider::IncDecButtons);
mode1RootSld->setTextBoxStyle(Slider::TextBoxLeft, false, 40, 20);
mode1RootSld->addListener(this);
mode2RootSld.reset(new Slider("mode2RootSld"));
addAndMakeVisible(mode2RootSld.get());
mode2RootSld->setRange(0, 127, 1);
mode2RootSld->setSliderStyle(Slider::IncDecButtons);
mode2RootSld->setTextBoxStyle(Slider::TextBoxLeft, false, 40, 20);
mode2RootSld->addListener(this);
scaleEntryBtn.reset(new TextButton("Scale Entry Button"));
addAndMakeVisible(scaleEntryBtn.get());
scaleEntryBtn->setButtonText(TRANS("OK"));
scaleEntryBtn->addListener(this);
modeInfoButton.reset(new TextButton("Mode Info Button"));
addAndMakeVisible(modeInfoButton.get());
modeInfoButton->setButtonText(TRANS("i"));
modeInfoButton->addListener(this);
mode1ViewBtn.reset(new ToggleButton("Mode1 View Button"));
addChildComponent(mode1ViewBtn.get());
mode1ViewBtn->setButtonText(String());
mode1ViewBtn->setRadioGroupId(10);
mode1ViewBtn->addListener(this);
mode1ViewBtn->setToggleState(true, dontSendNotification);
mode2ViewBtn.reset(new ToggleButton("Mode2 View Button"));
addChildComponent(mode2ViewBtn.get());
mode2ViewBtn->setButtonText(String());
mode2ViewBtn->setRadioGroupId(10);
mode2ViewBtn->addListener(this);
mode1RootLbl.reset(new Label("Mode1 Root Label",
TRANS("C4")));
addAndMakeVisible(mode1RootLbl.get());
mode1RootLbl->setFont(Font(15.00f, Font::plain).withTypefaceStyle("Regular"));
mode1RootLbl->setJustificationType(Justification::centredLeft);
mode1RootLbl->setEditable(false, false, false);
mode1RootLbl->setColour(TextEditor::textColourId, Colours::black);
mode1RootLbl->setColour(TextEditor::backgroundColourId, Colour(0x00000000));
mode2RootLbl.reset(new Label("Mode 2 Root Label",
TRANS("F5")));
addAndMakeVisible(mode2RootLbl.get());
mode2RootLbl->setFont(Font(15.00f, Font::plain).withTypefaceStyle("Regular"));
mode2RootLbl->setJustificationType(Justification::centredLeft);
mode2RootLbl->setEditable(false, false, false);
mode2RootLbl->setColour(TextEditor::textColourId, Colours::black);
mode2RootLbl->setColour(TextEditor::backgroundColourId, Colour(0x00000000));
mapStyleBox.reset(new ComboBox("Mapping Style Box"));
addAndMakeVisible(mapStyleBox.get());
mapStyleBox->setEditableText(false);
mapStyleBox->setJustificationType(Justification::centredLeft);
mapStyleBox->setTextWhenNothingSelected(TRANS("Mode To Mode"));
mapStyleBox->setTextWhenNoChoicesAvailable(TRANS("(no choices)"));
mapStyleBox->addItem(TRANS("Mode To Mode"), 1);
mapStyleBox->addItem(TRANS("Scale To Mode"), 2);
mapStyleBox->addItem(TRANS("By Key Layers"), 3);
mapStyleBox->setTooltip(mapStyleTooltip);
mapStyleBox->addListener(this);
mapStyleLbl.reset(new Label("Mapping Style Label",
TRANS("Mapping Style:")));
addAndMakeVisible(mapStyleLbl.get());
mapStyleLbl->setFont(Font(15.00f, Font::plain).withTypefaceStyle("Regular"));
mapStyleLbl->setJustificationType(Justification::centredLeft);
mapStyleLbl->setEditable(false, false, false);
mapStyleLbl->setColour(TextEditor::textColourId, Colours::black);
mapStyleLbl->setColour(TextEditor::backgroundColourId, Colour(0x00000000));
mapOrderEditBtn.reset(new TextButton("Map Order Edit Button", TRANS("Set layer mappings")));
addChildComponent(mapOrderEditBtn.get());
mapOrderEditBtn->setButtonText(TRANS("Layers"));
mapOrderEditBtn->addListener(this);
mapModeBox.reset(new ComboBox("Mapping Mode Box"));
addAndMakeVisible(mapModeBox.get());
mapModeBox->setEditableText(false);
mapModeBox->setJustificationType(Justification::centredLeft);
mapModeBox->setTextWhenNothingSelected(TRANS("Mapping Off"));
mapModeBox->setTextWhenNoChoicesAvailable(TRANS("Mapping Off"));
mapModeBox->addItem(TRANS("Mapping Off"), 1);
mapModeBox->addItem(TRANS("Auto Map"), 2);
mapModeBox->addItem(TRANS("Manual Map"), 3);
mapModeBox->setTooltip(mapModeTooltip);
mapModeBox->addListener(this);
mapApplyBtn.reset(new TextButton("Apply Layer Map Button"));
addChildComponent(mapApplyBtn.get());
mapApplyBtn->setButtonText(TRANS("Apply"));
mapApplyBtn->addListener(this);
mapCopyToManualBtn.reset(new TextButton("Copy Mapping to Manual Button", TRANS("Edit this mapping in Manual mode.")));
addChildComponent(mapCopyToManualBtn.get());
mapCopyToManualBtn->setButtonText(TRANS("Edit"));
mapCopyToManualBtn->setSize(40, barHeight);
mapCopyToManualBtn->addListener(this);
mapManualTip.reset(new Label("Manual Mapping Tip", TRANS("Right-click output key, then trigger input MIDI note.")));
addChildComponent(mapManualTip.get());
mapManualStatus.reset(new Label("Manual Mapping Status", noKeySelectedTrans));
addChildComponent(mapManualStatus.get());
mapManualCancel.reset(new TextButton("Manual Mapping Cancel", TRANS("Cancel key mapping.")));
addChildComponent(mapManualCancel.get());
mapManualCancel->setButtonText(juce::CharPointer_UTF8("\xc3\x97"));
mapManualCancel->addListener(this);
mapManualCancel->setSize(barHeight * 0.75f, barHeight * 0.75f);
mapManualResetBtn.reset(new TextButton("Reset Manual Mapping Button", TRANS("Reset mapping.")));
addChildComponent(mapManualResetBtn.get());
mapManualResetBtn->setButtonText(TRANS("Reset"));
mapManualResetBtn->setSize(48, barHeight);
mapManualResetBtn->addListener(this);
keyboard.reset(new VirtualKeyboard::Keyboard());
keyboard->addListener(pluginState->getMidiProcessor());
keyboardViewport.reset(new KeyboardViewport(keyboard.get(), "Keyboard Viewport"));
addAndMakeVisible(keyboardViewport.get());
viewportScrollBar = &keyboardViewport->getHorizontalScrollBar();
saveButton.reset(new ImageButton("Save Button"));
addAndMakeVisible(saveButton.get());
saveButton->setButtonText(TRANS("Save"));
saveButton->addListener(this);
saveButton->setImages(false, true, true,
Image(), 1.000f, Colour(0x00000000),
Image(), 1.000f, Colour(0x00000000),
Image(), 1.000f, Colour(0x00000000));
saveButton->setSize(28, 28);
openButton.reset(new ImageButton("Open Button"));
addAndMakeVisible(openButton.get());
openButton->setButtonText(TRANS("Open"));
openButton->addListener(this);
openButton->setImages(false, true, true,
Image(), 1.000f, Colour(0x00000000),
Image(), 1.000f, Colour(0x00000000),
Image(), 1.000f, Colour(0x00000000));
openButton->setSize(28, 28);
settingsButton.reset(new ImageButton("Settings Button"));
addAndMakeVisible(settingsButton.get());
settingsButton->setButtonText(TRANS("Settings"));
settingsButton->addListener(this);
settingsButton->setImages(false, true, true,
Image(), 1.000f, Colour(0x00000000),
Image(), 1.000f, Colour(0x00000000),
Image(), 1.000f, Colour(0x00000000));
settingsButton->setSize(28, 28);
saveIcon.reset(new Image(Image::PixelFormat::RGB, saveButton->getWidth(), saveButton->getHeight(), true));
openIcon.reset(new Image(Image::PixelFormat::RGB, openButton->getWidth(), openButton->getHeight(), true));
saveButton->setImages(true, true, true, *saveIcon.get(), 0.0f, Colour(), *saveIcon.get(), 0.0f, Colours::white.withAlpha(0.25f), *saveIcon.get(), 0.0f, Colours::white.withAlpha(0.5f));
openButton->setImages(true, true, true, *openIcon.get(), 0.0f, Colour(), *openIcon.get(), 0.0f, Colours::white.withAlpha(0.25f), *openIcon.get(), 0.0f, Colours::white.withAlpha(0.5f));
scaleTextBox->addListener(this);
scaleTextBox->setInputRestrictions(0, " 0123456789");
//keyboardViewport->setScrollingMode(3);
// allows for implementing mouseDown() to update the menus
mode1Box->setInterceptsMouseClicks(false, false);
mode2Box->setInterceptsMouseClicks(false, false);
saveMenu.reset(new PopupMenu());
saveMenuItems =
{
PopupMenu::Item("Save Mode").setID(SaveMenuOptions::SaveMode).setAction([&]() { pluginState->saveModeViewedToFile(); }),
PopupMenu::Item("Save Preset").setID(SaveMenuOptions::SavePreset).setAction([&]() { pluginState->savePresetToFile(); }),
PopupMenu::Item("Export Mapping as KBM").setID(SaveMenuOptions::ExportKBM).setAction([&]() { exportKbmMapping(); }),
PopupMenu::Item("Export for Reaper Note Names").setID(SaveMenuOptions::ExportReaper).setAction([&]() { exportModeViewedForReaper(); }),
PopupMenu::Item("Export for Ableton Folding").setID(SaveMenuOptions::ExportAbleton).setAction([&]() { exportModeViewedForAbleton(); })
};
for (auto item : saveMenuItems)
saveMenu->addItem(item);
loadMenu.reset(new PopupMenu());
loadMenuItems =
{
PopupMenu::Item("Load Mode").setID(LoadMenuOptions::LoadMode).setAction([&]() { browseForModeToOpen(); }),
PopupMenu::Item("Load Preset").setID(LoadMenuOptions::LoadPreset).setAction([&]() { browseForPresetToOpen(); })
};
for (auto item : loadMenuItems)
loadMenu->addItem(item);
ValueTree pluginWindowNode = pluginState->getPluginEditorNode();
int recallWidth = pluginWindowNode[IDs::windowBoundsW];
int recallHeight = pluginWindowNode[IDs::windowBoundsH];
centerKeyPos = pluginWindowNode[IDs::viewportPosition];
setSize(
recallWidth > 0 ? recallWidth : 1000,
recallHeight > 0 ? recallHeight : defaultHeight
);
settingsIcon.reset(new Image(Image::PixelFormat::RGB, settingsButton->getWidth(), settingsButton->getHeight(), true));
settingsButton->setImages(true, true, true, *settingsIcon.get(), 0.0f, Colour(), *settingsIcon.get(), 0.0f, Colours::white.withAlpha(0.25f), *settingsIcon.get(), 0.0f, Colours::white.withAlpha(0.5f));
settingsButton->setClickingTogglesState(true);
mappingComponents =
{
mapStyleLbl.get(),
mapStyleBox.get(),
mapOrderEditBtn.get(),
mode1RootLbl.get(),
mode1RootSld.get(),
mode1Box.get(),
mode1ViewBtn.get(),
mode2ViewBtn.get(),
mapCopyToManualBtn.get()
};
loadPresetNode(pluginState->getPresetNode());
viewportScrollBar->addListener(this);
// Update keyboard to show external midi input
#if (!JUCE_ANDROID && !JUCE_IOS)
startTimerHz(30);
#endif
}
PluginControlComponent::~PluginControlComponent()
{
pluginState->removeListener(this);
if (settingsPanelOpen)
{
pluginState->removeListener(settingsContainer.get());
settingsContainer = nullptr;
}
scaleTextBox = nullptr;
mode1Box = nullptr;
mode2Box = nullptr;
mode1RootSld = nullptr;
mode2RootSld = nullptr;
scaleEntryBtn = nullptr;
modeInfoButton = nullptr;
mode1ViewBtn = nullptr;
mode2ViewBtn = nullptr;
mode1RootLbl = nullptr;
mode2RootLbl = nullptr;
mapStyleBox = nullptr;
mapStyleLbl = nullptr;
mapManualTip = nullptr;
mapManualStatus = nullptr;
mapOrderEditBtn = nullptr;
mapManualCancel = nullptr;
mapModeBox = nullptr;
mapApplyBtn = nullptr;
keyboard = nullptr;
keyboardViewport = nullptr;
saveButton = nullptr;
openButton = nullptr;
settingsButton = nullptr;
}
//==============================================================================
void PluginControlComponent::loadPresetNode(ValueTree presetNodeIn)
{
if (presetNodeIn.hasType(IDs::presetNode) && (float)presetNodeIn[IDs::pluginPresetVersion] == SVK_PRESET_VERSION)
{
ValueTree presetNode = presetNodeIn;
ValueTree properties = presetNode.getChildWithName(IDs::presetProperties);
if (properties.isValid())
{
mode2ViewBtn->setToggleState((int)properties[IDs::modeSelectorViewed], dontSendNotification);
}
ValueTree keyboardSettings = presetNode.getChildWithName(IDs::pianoNode);
if (keyboardSettings.isValid())
{
keyboard->restoreNode(keyboardSettings);
}
ValueTree mapping = presetNode.getChildWithName(IDs::midiMapNode);
if (mapping.isValid())
{
setMapModeBoxId(mapping[IDs::mappingMode]);
setMappingStyleId(mapping[IDs::autoMappingStyle]);
}
ValueTree modeSelectors = presetNode.getChildWithName(IDs::modeSelectorsNode);
if (modeSelectors.isValid())
{
for (int num = 0; num < modeSelectors.getNumChildren(); num++)
{
ValueTree selector = modeSelectors.getChild(num);
Mode* mode = presetManager->getModeBySelector(num);
// TODO: generalize
if (num == 0)
{
mode1RootSld->setValue(selector[IDs::modeSelectorRootNote], dontSendNotification);
mode1Box->setText(mode->getName(), dontSendNotification);
}
else if (num == 1)
{
mode2RootSld->setValue(selector[IDs::modeSelectorRootNote], dontSendNotification);
mode2Box->setText(mode->getName(), dontSendNotification);
}
updateRootNoteLabels();
if (num == (int)presetNode.getChildWithName(IDs::presetProperties)[IDs::modeSelectorViewed])
{
modeViewedChanged(mode, num, 0 /*unused*/);
}
}
}
}
}
void PluginControlComponent::onModeViewedChange(Mode* modeViewed)
{
setScaleEntryText(modeViewed->getStepsString());
keyboard->applyMode(modeViewed, true);
resized();
}
void PluginControlComponent::paint (Graphics& g)
{
g.fillAll (Colour (0xff323e44));
Colour buttonFill = getLookAndFeel().findColour(TextButton::ColourIds::buttonColourId);
Colour buttonOutline = getLookAndFeel().findColour(TextEditor::ColourIds::outlineColourId);
drawSaveIcon(g, saveButton->getBoundsInParent(), buttonFill, buttonOutline);
drawLoadIcon(g, openButton->getBoundsInParent(), buttonFill, buttonOutline);
drawSettingsIcon(g, settingsButton->getBoundsInParent(), buttonFill, buttonOutline);
}
void PluginControlComponent::resized()
{
int basicHeight = getHeight();
if (settingsPanelOpen)
{
basicHeight -= defaultHeight;
settingsContainer->setBounds(0, basicHeight, getWidth(), defaultHeight);
settingsContainer->resized();
}
scaleTextBox->setSize(proportionOfWidth(0.2f), barHeight);
scaleTextBox->setCentrePosition(proportionOfWidth(0.5f), barHeight / 2 + gap);
scaleEntryBtn->setBounds(scaleTextBox->getRight() + 8, scaleTextBox->getY(), 31, 24);
modeInfoButton->setBounds(scaleTextBox->getX() - 32, scaleTextBox->getY(), 24, 24);
saveButton->setSize(barHeight, barHeight);
saveButton->setTopLeftPosition(gap, scaleTextBox->getY());
openButton->setSize(barHeight, barHeight);
openButton->setTopLeftPosition(saveButton->getRight() + gap, saveButton->getY());
settingsButton->setSize(barHeight, barHeight);
settingsButton->setTopLeftPosition(openButton->getRight() + gap, saveButton->getY());
mapModeBox->setTopLeftPosition(settingsButton->getRight() + gap, gap);
mapModeBox->setSize(jmin(proportionOfWidth(0.15f), modeInfoButton->getX() - mapModeBox->getX() - gap), barHeight);
mode1ViewBtn->setBounds(getWidth() - 32, gap, 32, barHeight);
mode2ViewBtn->setBounds(getWidth() - 32, mode1ViewBtn->getBottom() + gap, 32, barHeight);
int keyboardY = barHeight + gap * 2;
if (inMappingMode)
{
mode1Box->setSize(proportionOfWidth(0.15f), barHeight);
mode1Box->setTopLeftPosition(mode1ViewBtn->getX() - mode1Box->getWidth() - gap / 2, gap);
mode2Box->setSize(proportionOfWidth(0.15f), barHeight);
mode2Box->setTopLeftPosition(mode1Box->getX(), mode2ViewBtn->getY());
mapStyleLbl->setTopLeftPosition(gap / 2, scaleTextBox->getBottom() + gap);
mapStyleLbl->setSize(mapModeBox->getX() - mapStyleLbl->getX(), barHeight);
mapStyleBox->setBounds(mapStyleLbl->getRight(), mapStyleLbl->getY(), mapModeBox->getWidth(), barHeight);
mapManualTip->setBounds(mapStyleLbl->getX(), mapStyleLbl->getY(), mapManualTip->getFont().getStringWidth(mapManualTip->getText()), barHeight);
mapManualStatus->setBounds(mapManualTip->getRight(), mapManualTip->getY(), mapManualStatus->getFont().getStringWidth(mapManualStatus->getText()) + 8, barHeight);
mapOrderEditBtn->setBounds(mapStyleBox->getRight() + gap, mapStyleBox->getY(), 55, barHeight);
mapApplyBtn->setBounds(mapOrderEditBtn->getRight() + gap, mapOrderEditBtn->getY(), 55, barHeight);
if (mapApplyBtn->isVisible())
mapCopyToManualBtn->setTopLeftPosition(mapApplyBtn->getRight() + gap, mapApplyBtn->getY());
else
mapCopyToManualBtn->setTopLeftPosition(mapOrderEditBtn->getPosition());
mapManualCancel->setTopLeftPosition(mapManualStatus->getRight() + gap / 2, mapManualStatus->getY() + mapManualCancel->getHeight() / 4);
if (mapModeBox->getSelectedId() == 3)
{
if (mapManualCancel->isVisible())
mapManualResetBtn->setTopLeftPosition(mapManualCancel->getRight() + gap, mapManualStatus->getY());
else
mapManualResetBtn->setTopLeftPosition(mapManualStatus->getRight() + gap, mapManualStatus->getY());
}
keyboardY = (keyboardY * 2) - gap;
}
else
{
mode2Box->setSize(proportionOfWidth(0.15f) + mode2ViewBtn->getWidth(), barHeight);
mode2Box->setTopLeftPosition(getWidth() - mode2Box->getWidth() - gap, gap);
}
mode1RootSld->setBounds(mode1Box->getX() - 80 - gap, mode1Box->getY(), 80, barHeight);
mode2RootSld->setBounds(mode2Box->getX() - 80 - gap, mode2Box->getY(), 80, barHeight);
mode1RootLbl->setBounds(mode1RootSld->getX() - 32, mode1Box->getY(), 32, barHeight);
mode2RootLbl->setBounds(mode2RootSld->getX() - 32, mode2Box->getY(), 32, barHeight);
viewportScrollBar->removeListener(this);
int scrollBarThickness = basicHeight / 28;
int keyboardViewWidth = getWidth() - gap * 2;
int keyboardViewHeight = jmax(basicHeight - keyboardY - gap, 1);
keyboardViewport->setBounds(gap, keyboardY, keyboardViewWidth, keyboardViewHeight);
keyboardViewport->setScrollBarThickness(scrollBarThickness);
keyboardViewport->resizeKeyboard();
keyboardViewport->centerOnKey((int)centerKeyPos);
if (keyboard->getWidth() < keyboardViewWidth)
{
keyboardViewport->setSize(keyboard->getWidth(), keyboardViewHeight);
keyboardViewport->setTopLeftPosition((getWidth() - keyboard->getWidth()) / 2, keyboardY);
}
viewportScrollBar->addListener(this);
pluginState->getPluginEditorNode().setProperty(IDs::windowBoundsW, getWidth(), nullptr);
pluginState->getPluginEditorNode().setProperty(IDs::windowBoundsH, basicHeight, nullptr);
}
void PluginControlComponent::mouseDown(const MouseEvent& e)
{
if (mode1Box->isVisible() && mode1Box->getBounds().contains(e.getPosition()))
{
String display = mode1Box->getText();
presetManager->requestModeMenu(mode1Box->getRootMenu());
mode1Box->setText(display, dontSendNotification);
mode1Box->showPopup();
}
if (mode2Box->isVisible() && mode2Box->getBounds().contains(e.getPosition()))
{
String display = mode2Box->getText();
presetManager->requestModeMenu(mode2Box->getRootMenu());
mode2Box->setText(display, dontSendNotification);
mode2Box->showPopup();
}
}
void PluginControlComponent::comboBoxChanged (ComboBox* comboBoxThatHasChanged)
{
if (comboBoxThatHasChanged == mode1Box.get())
{
pluginState->handleModeSelection(0, mode1Box->getSelectedId());
}
else if (comboBoxThatHasChanged == mode2Box.get())
{
pluginState->handleModeSelection(1, mode2Box->getSelectedId());
}
else if (comboBoxThatHasChanged == mapModeBox.get())
{
setMapModeBoxId(mapModeBox->getSelectedId());
}
else if (comboBoxThatHasChanged == mapStyleBox.get())
{
setMappingStyleId(mapStyleBox->getSelectedId());
}
}
void PluginControlComponent::sliderValueChanged (Slider* sliderThatWasMoved)
{
if (sliderThatWasMoved == mode1RootSld.get())
{
pluginState->setModeSelectorRoot(0, mode1RootSld->getValue());
updateRootNoteLabels();
}
else if (sliderThatWasMoved == mode2RootSld.get())
{
pluginState->setModeSelectorRoot(1, mode2RootSld->getValue());
updateRootNoteLabels();
}
}
void PluginControlComponent::buttonClicked (Button* buttonThatWasClicked)
{
if (buttonThatWasClicked == scaleEntryBtn.get())
{
submitCustomScale();
}
else if (buttonThatWasClicked == modeInfoButton.get())
{
showModeInfo();
}
else if (buttonThatWasClicked == mode1ViewBtn.get() || buttonThatWasClicked == mode2ViewBtn.get())
{
pluginState->setModeSelectorViewed(mode2ViewBtn->getToggleState());
scaleTextBox->setText(pluginState->getModeViewed()->getStepsString(), false);
}
else if (buttonThatWasClicked == mapCopyToManualBtn.get())
{
NoteMap autoMap = *pluginState->getMidiInputFilterMap();
mapModeBox->setSelectedId(3, sendNotificationSync);
manualMappingHelper->resetMapping(autoMap);
}
else if (buttonThatWasClicked == mapOrderEditBtn.get())
{
showMapOrderEditDialog();
}
else if (buttonThatWasClicked == mapApplyBtn.get())
{
pluginState->doAutoMapping();
}
else if (buttonThatWasClicked == saveButton.get())
{
saveMenu->showAt(saveButton.get());
}
else if (buttonThatWasClicked == openButton.get())
{
loadMenu->showAt(openButton.get());
}
else if (buttonThatWasClicked == settingsButton.get())
{
showSettingsDialog();
}
else if (buttonThatWasClicked == mapManualCancel.get())
{
manualMappingHelper->cancelKeyMap();
}
else if (buttonThatWasClicked == mapManualResetBtn.get())
{
manualMappingHelper->resetMapping();
keyMappingStatusChanged(-1, false);
}
}
void PluginControlComponent::textEditorReturnKeyPressed(TextEditor& textEditor)
{
submitCustomScale();
}
void PluginControlComponent::scrollBarMoved(ScrollBar* bar, double newRangeStart)
{
centerKeyPos = keyboardViewport->getCenterKeyProportion();
pluginState->getPluginEditorNode().setProperty(IDs::viewportPosition, centerKeyPos, nullptr);
}
void PluginControlComponent::changeListenerCallback(ChangeBroadcaster* source)
{
// Mode Info Changed
if (source == modeInfo)
{
pluginState->commitModeInfo();
onModeViewedChange(pluginState->getModeViewed());
}
}
void PluginControlComponent::timerCallback()
{
keyboard->repaint();
}
//==============================================================================
void PluginControlComponent::resyncPresetNode(ValueTree presetNode)
{
keyboard->restoreNode(presetNode.getChildWithName(IDs::pianoNode), false);
}
void PluginControlComponent::modeViewedChanged(Mode* modeIn, int selectorNumber, int slotNumber)
{
MidiKeyboardState* displayState = selectorNumber == 0
? pluginState->getMidiProcessor()->getOriginalKeyboardState()
: pluginState->getMidiProcessor()->getRemappedKeyboardState();
keyboard->displayKeyboardState(displayState);
onModeViewedChange(modeIn);
}
void PluginControlComponent::mappingModeChanged(int mappingModeId)
{
inMappingMode = mappingModeId > 1;
for (auto c : mappingComponents)
{
c->setVisible(inMappingMode);
c->setEnabled(inMappingMode);
}
if (inMappingMode)
{
// this is a fallback
if (mappingModeId == 2)
mapStyleBox->setSelectedId(pluginState->getMappingNode()[IDs::autoMappingStyle], dontSendNotification);
if (mappingModeId == 2 && mapStyleBox->getSelectedId() == 3)
{
mapOrderEditBtn->setVisible(true);
mapApplyBtn->setVisible(true);
}
else
{
mapOrderEditBtn->setVisible(false);
mapApplyBtn->setVisible(false);
}
mode2ViewBtn->setToggleState(true, sendNotification);
toggleMenuItemEnablement(*saveMenu, SaveMenuOptions::ExportKBM, true);
}
else
{
toggleMenuItemEnablement(*saveMenu, SaveMenuOptions::ExportKBM, false);
}
if (mappingModeId == 3)
{
beginManualMapping();
}
else
{
mapManualTip->setVisible(false);
mapManualStatus->setVisible(false);
mapManualCancel->setVisible(false);
mapManualResetBtn->setVisible(false);
keyboard->setUIMode(VirtualKeyboard::UIMode::playMode);
mapManualStatus->setText(noKeySelectedTrans, sendNotification);
}
resized();
}
void PluginControlComponent::inputMappingChanged(NoteMap& inputNoteMap)
{
keyboard->setInputNoteMap(inputNoteMap);
}
void PluginControlComponent::customModeChanged(Mode* newCustomMode)
{
// This is a hack and should be handled better
String customModeName = newCustomMode->getName();
if (getModeSelectorViewed() == 1)
mode2Box->setText(customModeName, dontSendNotification);
else
mode1Box->setText(customModeName, dontSendNotification);
}
void PluginControlComponent::modeInfoChanged(Mode* modeEdited)
{
if (getModeSelectorViewed() == 1)
mode2Box->setText(modeEdited->getName(), dontSendNotification);
else
mode1Box->setText(modeEdited->getName(), dontSendNotification);
}
void PluginControlComponent::settingsTabChanged(int tabIndex, const String& tabName, SvkSettingsPanel* panelChangedTo)
{
DBG("Settings changed to " + tabName + ", tab: " + String(tabIndex));
if (panelChangedTo->getName() == "ColourSettingsPanel")
{
// TODO clean up
beginColorEditing();
}
else if (isColorEditing)
{
endColorEditing();
}
if (tabName == "Mapping")
{
mappingSettingsOpen = true;
static_cast<MappingSettingsPanel*>(panelChangedTo)->registerEditorListener(this);
}
else if (mappingSettingsOpen)
{
mappingSettingsOpen = false;
// MappingSettingsPanel removes listeners from NoteMapEditor
}
pluginState->getPluginEditorNode().setProperty(IDs::settingsTabName, tabName, nullptr);
}
void PluginControlComponent::keyMappingStatusChanged(int keyNumber, bool preparedToMap)
{
if (preparedToMap)
{
mapManualStatus->setText(waitingForTrans + String(keyNumber), sendNotification);
mapManualCancel->setVisible(true);
}
else
{
mapManualStatus->setText(noKeySelectedTrans, sendNotification);
mapManualCancel->setVisible(false);
}
mapManualStatus->setSize(mapManualStatus->getFont().getStringWidth(mapManualStatus->getText()) + 8, mapManualStatus->getHeight());
resized();
}
void PluginControlComponent::keyMapConfirmed(int keyNumber, int midiNote)
{
mapManualStatus->setText("Key " + String(keyNumber) + " mapped to MIDI Note " + String(midiNote), sendNotification);
mapManualCancel->setVisible(false);
resized();
}
void PluginControlComponent::mappingEditorChanged(NoteMap& newMapping)
{
DBG("MAPPING CHANGED");
pluginState->setMidiInputMap(newMapping, mapModeBox->getSelectedId() == 3);
}
//==============================================================================
VirtualKeyboard::Keyboard* PluginControlComponent::getKeyboard()
{
return keyboard.get();
}
Viewport* PluginControlComponent::getViewport()
{
return keyboardViewport.get();
}
TextEditor* PluginControlComponent::getScaleTextEditor()
{
return scaleTextBox.get();
}
ComboBox* PluginControlComponent::getMappingStyleBox()
{
return mapStyleBox.get();
}
String PluginControlComponent::getScaleEntryText()
{
return scaleTextBox->getText();
}
void PluginControlComponent::setScaleEntryText(String textIn, NotificationType notify)
{
scaleTextBox->setText(textIn, notify == sendNotification);
}
ReferencedComboBox* PluginControlComponent::getMode1Box()
{
return mode1Box.get();
}
ReferencedComboBox* PluginControlComponent::getMode2Box()
{
return mode2Box.get();
}
void PluginControlComponent::updateModeBoxMenus()
{
}
ImageButton* PluginControlComponent::getSettingsButton()
{
return settingsButton.get();
}
TextButton* PluginControlComponent::getModeInfoButton()
{
return modeInfoButton.get();
}
int PluginControlComponent::getModeSelectorViewed()
{
return mode2ViewBtn->getToggleState();
}
void PluginControlComponent::setMapModeBoxId(int mappingModeId, NotificationType notify)
{
mapModeBox->setSelectedId(mappingModeId, dontSendNotification);
if (notify != dontSendNotification)
pluginState->setMapMode(mapModeBox->getSelectedId());
}
void PluginControlComponent::setMappingStyleId(int idIn, NotificationType notify)
{
mapStyleBox->setSelectedId(idIn, dontSendNotification);
if (idIn == 3 && mapModeBox->getSelectedId() == 2)
{
mapOrderEditBtn->setVisible(true);
mapApplyBtn->setVisible(true);
}
else
{
mapOrderEditBtn->setVisible(false);
mapApplyBtn->setVisible(false);
}
if (notify != dontSendNotification)
pluginState->setAutoMapStyle(mapStyleBox->getSelectedId());
resized();
}
void PluginControlComponent::submitCustomScale()
{
pluginState->setModeCustom(scaleTextBox->getText());
}
void PluginControlComponent::updateRootNoteLabels()
{
mode1RootLbl->setText(MidiMessage::getMidiNoteName(mode1RootSld->getValue(), true, true, 4), dontSendNotification);
mode2RootLbl->setText(MidiMessage::getMidiNoteName(mode2RootSld->getValue(), true, true, 4), dontSendNotification);
}
//==============================================================================
void PluginControlComponent::showModeInfo()
{
modeInfo = new ModeInfoDialog(pluginState->getModeViewed());
modeInfo->addChangeListener(this);
CallOutBox::launchAsynchronously(std::unique_ptr<Component>(modeInfo), scaleTextBox->getScreenBounds(), nullptr);
}
void PluginControlComponent::showMapOrderEditDialog()
{
mapByOrderDialog = new MapByOrderDialog(pluginState->getModeMapper(), pluginState->getMode1(), pluginState->getMode2());
CallOutBox::launchAsynchronously(std::unique_ptr<Component>(mapByOrderDialog), mapStyleBox->getScreenBounds(), nullptr);
}
void PluginControlComponent::showSettingsDialog()
{
if (!settingsPanelOpen)
{
settingsContainer.reset(new SettingsContainer(pluginState));
settingsContainer->setKeyboardPointer(keyboard.get());
settingsContainer->addListener(this);
pluginState->addListener(settingsContainer.get());
settingsPanelOpen = true;
pluginState->getPluginEditorNode().setProperty(IDs::settingsOpen, true, nullptr);
settingsButton->setToggleState(true, dontSendNotification);
addAndMakeVisible(settingsContainer.get());
getParentComponent()->setSize(getWidth(), getHeight() + defaultHeight);
int setToTab = 0;
String lastTab = pluginState->getPluginEditorNode()[IDs::settingsTabName].toString();
DBG("Last tab: " + lastTab);
if (lastTab.length() > 0)
setToTab = settingsContainer->getTabNames().indexOf(lastTab);
settingsContainer->setCurrentTabIndex(setToTab, true);
}
else
{
hideSettings();
}
}
void PluginControlComponent::hideSettings()
{
settingsContainer->removeListener(this);
settingsContainer->setVisible(false);
pluginState->removeListener(settingsContainer.get());
settingsContainer = nullptr;
settingsPanelOpen = false;
pluginState->getPluginEditorNode().setProperty(IDs::settingsOpen, false, nullptr);
if (isColorEditing)
endColorEditing();
getParentComponent()->setSize(getWidth(), getHeight() - defaultHeight);
}
void PluginControlComponent::beginColorEditing()
{
keyboard->setUIMode(VirtualKeyboard::UIMode::editMode);
isColorEditing = true;
keyMappingStatusChanged(-1, false);
mapModeBox->setEnabled(false);
mapStyleBox->setEnabled(false);
mapManualResetBtn->setEnabled(false);
mapOrderEditBtn->setEnabled(false);
mapApplyBtn->setEnabled(false);
mapCopyToManualBtn->setEnabled(false);
}
void PluginControlComponent::endColorEditing()
{
keyboard->setUIMode(VirtualKeyboard::UIMode::playMode);
isColorEditing = false;
mapModeBox->setEnabled(true);
mapStyleBox->setEnabled(true);
mapManualResetBtn->setEnabled(true);
mapOrderEditBtn->setEnabled(true);
mapApplyBtn->setEnabled(true);
mapCopyToManualBtn->setEnabled(true);
// Restore previous UI state
pluginState->setMapMode(mapModeBox->getSelectedId());
}
void PluginControlComponent::beginManualMapping()
{
mapManualStatus->setText(noKeySelectedTrans, sendNotification);
mapStyleBox->setVisible(false);
mapStyleLbl->setVisible(false);
mapCopyToManualBtn->setVisible(false);
mapManualTip->setVisible(true);
mapManualStatus->setVisible(true);
mapManualResetBtn->setVisible(true);
manualMappingHelper = pluginState->getManualMappingHelper();
manualMappingHelper->addListener(this);
keyboard->setUIMode(VirtualKeyboard::UIMode::mapMode);
// Selects the key to map a MIDI note to
keyboard->setMappingHelper(manualMappingHelper);
}
void PluginControlComponent::setMode1Root(int rootIn, NotificationType notify)
{
mode1RootSld->setValue(rootIn, notify);
mode1RootLbl->setText(MidiMessage::getMidiNoteName(rootIn, true, true, 4), dontSendNotification);
}
void PluginControlComponent::setMode2Root(int rootIn, NotificationType notify)
{
mode2RootSld->setValue(rootIn, notify);
mode2RootLbl->setText(MidiMessage::getMidiNoteName(rootIn, true, true, 4), dontSendNotification);
}
bool PluginControlComponent::browseForModeToOpen()
{
ValueTree modeNode = presetManager->nodeFromFile("Open Mode", "*.svk", pluginState->getPluginSettings()->getModePath());
if (Mode::isValidMode(modeNode))
{
presetManager->addSlotAndSetSelection(getModeSelectorViewed(), modeNode);
onModeViewedChange(pluginState->getModeViewed());
return true;
}
return false;
}
bool PluginControlComponent::browseForPresetToOpen()
{
ValueTree presetLoaded = presetManager->presetFromFile(pluginState->getPluginSettings()->getPresetPath());
pluginState->recallState(presetLoaded);
return true;
}
bool PluginControlComponent::exportModeViewedForReaper()
{
ReaperWriter rpp = ReaperWriter(pluginState->getModeViewed());
return rpp.write();
}
bool PluginControlComponent::exportModeViewedForAbleton()
{
AbletonMidiWriter amw(*pluginState->getModeViewed());
return amw.write();
}
void PluginControlComponent::exportKbmMapping()
{
DialogWindow::LaunchOptions options;
options.dialogTitle = "Export KBM file";
options.escapeKeyTriggersCloseButton = true;
options.componentToCentreAround = this;
options.content = OptionalScopedPointer<Component>(new ExportKbmDialog(
pluginState->getMappingNode(),
*pluginState->getMode1(), *pluginState->getMode2(), *pluginState->getModeMapper()), true
);
options.content->setSize(316, 112);
if (JUCEApplication::isStandaloneApp())
options.runModal();
else
options.launchAsync();
} | {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
DirectoryBrowserComponent.h
Created: 5 Sep 2020 9:59:39pm
Author: Vincenzo
Simple combination of a text editor and button to trigger browsing for a
directory and then displaying the full directory path.
==============================================================================
*/
#pragma once
#include <JuceHeader.h>
class DirectoryBrowserComponent : public Component, public Button::Listener
{
public:
DirectoryBrowserComponent(const String dialogBoxTitle, const File& directoryIn = File())
: chooser(dialogBoxTitle, directoryIn)
{
setName(dialogBoxTitle);
editor.reset(new TextEditor(dialogBoxTitle + "Editor"));
editor->setMultiLine(false, false);
editor->setReadOnly(true);
addAndMakeVisible(editor.get());
openButton.reset(new TextButton(dialogBoxTitle + "Button", "Choose directory for " + dialogBoxTitle));
openButton->setButtonText("...");
openButton->setConnectedEdges(Button::ConnectedEdgeFlags::ConnectedOnLeft);
addAndMakeVisible(openButton.get());
openButton->addListener(this);
}
~DirectoryBrowserComponent()
{
editor = nullptr;
openButton = nullptr;
}
void resized() override
{
openButton->setSize(getHeight(), getHeight());
openButton->setTopRightPosition(getWidth() - 1, 0);
editor->setBounds(0, 0, getWidth() - getHeight(), getHeight());
}
void buttonClicked(Button* buttonThatWasClicked) override
{
if (chooser.browseForDirectory())
{
editor->setText(chooser.getResult().getFullPathName(), false);
listeners.call(&DirectoryBrowserComponent::Listener::directoryChanged, this, chooser.getResult());
}
}
void setText(String textIn)
{
editor->setText(textIn);
}
class Listener
{
public:
Listener() {};
virtual ~Listener() {};
virtual void directoryChanged(DirectoryBrowserComponent* source, File directorySelected) = 0;
};
void addListener(DirectoryBrowserComponent::Listener* listenerIn)
{
listeners.add(listenerIn);
}
void removeListener(DirectoryBrowserComponent::Listener* listenerIn)
{
listeners.remove(listenerIn);
}
protected:
ListenerList<DirectoryBrowserComponent::Listener> listeners;
private:
FileChooser chooser;
std::unique_ptr<TextEditor> editor;
std::unique_ptr<TextButton> openButton;
std::function<void(const File&)> directorySetCallback;
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
MappingSettingsPanel.h
Created: 7 Oct 2020 10:00:18pm
Author: Vincenzo
==============================================================================
*/
#pragma once
#include "SvkSettingsPanel.h"
#include "../NoteMapEditor.h"
class MappingSettingsPanel : public SvkSettingsPanel,
public SvkPluginState::Listener,
public MappingEditor::Listener
{
public:
MappingSettingsPanel(SvkPluginState* pluginStateIn);
~MappingSettingsPanel();
void visibilityChanged() override;
void mappingModeChanged(int mappingModeId) override;
void inputMappingChanged(NoteMap&) override;
void mappingEditorChanged(NoteMap&) override;
void registerEditorListener(MappingEditor::Listener* listenerIn);
private:
Label* currentMappingLabel;
NoteMapEditor* noteMapEditor;
}; | {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
SettingsContainer.h
Created: 13 Nov 2019 7:13:39pm
Author: Vincenzo Sicurella
==============================================================================
*/
#pragma once
#include "../../../PluginState.h"
#include "GeneralSettingsPanel.h"
#include "MidiSettingsPanel.h"
#include "KeyboardSettingsPanel.h"
#include "MappingSettingsPanel.h"
#include "ColourSettingsPanel.h"
//#include "ControlSettingsPanel.h"
#include "DebugSettingsPanel.h"
//==============================================================================
/*
*/
class SettingsContainer : public TabbedComponent, public SvkPluginState::Listener
{
public:
SettingsContainer(SvkPluginState* pluginStateIn)
: TabbedComponent(TabbedButtonBar::Orientation::TabsAtTop),
pluginState(pluginStateIn)
{
tabColour = Colour(0xff323e44);
backgroundColour = tabColour.darker();
textColour = tabColour.contrasting();
setColour(backgroundColourId, backgroundColour);
//view.reset(new Viewport("SettingsViewport"));
//view->setScrollOnDragEnabled(true);
//view->setScrollBarsShown(true, false);
//addAndMakeVisible(view.get());
for (auto panelName : panelNames)
{
if (panelName == "General")
panels.add(new GeneralSettingsPanel(pluginState));
else if (panelName == "Midi")
panels.add(new MidiSettingsPanel(pluginState));
else if (panelName == "Keyboard")
panels.add(new KeyboardSettingsPanel(pluginState));
else if (panelName == "Mapping")
panels.add(new MappingSettingsPanel(pluginState));
else if (panelName == "Colors")
panels.add(new ColourSettingsPanel(pluginState));
else if (panelName == "Debug")
panels.add(new DebugSettingsPanel(pluginState));
}
for (int i = 0; i < panels.size(); i++)
{
addTab(panelNames[i], tabColour, panels[i], true);
}
setSize(100, 100);
}
~SettingsContainer()
{
view = nullptr;
}
SvkSettingsPanel* getComponentViewed()
{
return componentViewed;
}
void currentTabChanged (int newCurrentTabIndex, const String &newCurrentTabName) override
{
componentViewed = panels.getUnchecked(newCurrentTabIndex);
componentViewed->resized();
listeners.call(&SettingsContainer::Listener::settingsTabChanged,
newCurrentTabIndex,
newCurrentTabName,
componentViewed
);
}
void setKeyboardPointer(VirtualKeyboard::Keyboard* keyboardPtrIn)
{
for (int i = 0; i < panelNames.size(); i++)
{
if (keyboardPanels.contains(panelNames[i]))
panels[i]->setKeyboardPointer(keyboardPtrIn);
}
}
void modeViewedChanged(Mode* modeIn, int selectorNumber, int slotNumber) override
{
for (auto p : panels)
{
if (p->getName() == "ColourSettingsPanel")
p->refreshPanel();
}
}
public:
class Listener
{
public:
virtual void settingsTabChanged(int newTabIndex, const String& tabName, SvkSettingsPanel* panelChangedTo) = 0;
};
void addListener(SettingsContainer::Listener* listenerIn) { listeners.add(listenerIn); }
void removeListener(SettingsContainer::Listener* listenerIn) { listeners.remove(listenerIn); }
protected:
ListenerList<Listener> listeners;
private:
SvkPluginState* pluginState;
Array<SvkSettingsPanel*> panels;
std::unique_ptr<Viewport> view;
SvkSettingsPanel* componentViewed = nullptr;
int defaultControlHeight = 50;
Colour backgroundColour;
Colour tabColour;
Colour textColour;
StringArray panelNames =
{
"General"
, "Midi"
, "Keyboard"
, "Mapping"
, "Colors"
//#if JUCE_DEBUG
// , "Debug"
//#endif
};
StringArray keyboardPanels = { "Keyboard", "Colors", "Debug" };
JUCE_DECLARE_NON_COPYABLE_WITH_LEAK_DETECTOR (SettingsContainer)
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
ColourSettingsPanel.cpp
Created: 13 Sep 2020 2:41:09pm
Author: Vincenzo
==============================================================================
*/
#include "ColourSettingsPanel.h"
ColourSettingsPanel::ColourSettingsPanel(SvkPluginState* stateIn)
: SvkSettingsPanel("ColourSettingsPanel", stateIn,
{
"",
"Choose settings then click keys to paint:",
"Select a box to adjust the color:",
""
},
{
IDs::pianoKeyColor,
IDs::pianoKeyColorsDegree,
IDs::pianoKeyColorsLayer,
IDs::pianoKeyColorsIndividual,
IDs::pianoKeyColorReset,
Identifier("KeyColourLibrary"),
Identifier("NoteOnColourLibrary")
},
{
SvkControlProperties(ControlTypeNames::GenericControl, "Color Selector"),
SvkControlProperties(ControlTypeNames::ToggleControl, "Paint by Scale Degree", false, 1),
SvkControlProperties(ControlTypeNames::ToggleControl, "Paint by Layer (hold Shift)", false, 1),
SvkControlProperties(ControlTypeNames::ToggleControl, "Paint by Individual Key (hold Ctrl)", false, 1),
SvkControlProperties(ControlTypeNames::ToggleControl, "Reset Color to Default (Right-click)", false, 1),
SvkControlProperties(ControlTypeNames::GenericControl, "Key Colors", false, 2),
SvkControlProperties(ControlTypeNames::GenericControl, "Note On Colors", false, 3)
},
FlexBox(), {}, { FlexItem() }
)
{
// Set up Color Selector and replace generic control
colourSelector = new FocussedColourSelector();
addAndMakeVisible(colourSelector);
controls.set(0, colourSelector, true);
getSectionFlexBox(0)->items.getReference(0).associatedComponent = colourSelector;
degreePaintButton = static_cast<TextButton*>(idToControl[IDs::pianoKeyColorsDegree]);
degreePaintButton->setRadioGroupId(paintTypeRadioGroup, dontSendNotification);
degreePaintButton->setToggleState(true, dontSendNotification);
degreePaintButton->addListener(this);
layerPaintButton = static_cast<TextButton*>(idToControl[IDs::pianoKeyColorsLayer]);
layerPaintButton->setRadioGroupId(paintTypeRadioGroup, dontSendNotification);
layerPaintButton->addListener(this);
keyPaintButton = static_cast<TextButton*>(idToControl[IDs::pianoKeyColorsIndividual]);
keyPaintButton->setRadioGroupId(paintTypeRadioGroup, dontSendNotification);
keyPaintButton->addListener(this);
resetColourToggle = static_cast<TextButton*>(idToControl[IDs::pianoKeyColorReset]);
resetColourToggle->addListener(this);
flexParent.items.getReference(numSections - 1).margin = FlexItem::Margin(0, 5, 0, 0);
addMouseListener(this, true);
setSize(100, 100);
}
ColourSettingsPanel::~ColourSettingsPanel()
{
colourSelector = nullptr;
if (virtualKeyboard)
virtualKeyboard->removeMouseListener(this);
}
void ColourSettingsPanel::resized()
{
getSectionFlexBox(0)->items.getReference(0).height = getHeight();
SvkSettingsPanel::resized();
}
void ColourSettingsPanel::buttonClicked(Button* buttonThatWasClicked)
{
if (buttonThatWasClicked == degreePaintButton)
{
virtualKeyboard->getProperties().set(IDs::pianoKeyPaintType, 0);
}
else if (buttonThatWasClicked == layerPaintButton)
{
virtualKeyboard->getProperties().set(IDs::pianoKeyPaintType, 1);
}
else if (buttonThatWasClicked == keyPaintButton)
{
virtualKeyboard->getProperties().set(IDs::pianoKeyPaintType, 2);
}
if (buttonThatWasClicked == resetColourToggle)
{
virtualKeyboard->getProperties().set(IDs::pianoKeyColorReset, buttonThatWasClicked->getToggleState());
userClickedReset = buttonThatWasClicked->getToggleState();
}
}
void ColourSettingsPanel::modifierKeysChanged(const ModifierKeys& modifiers)
{
checkModifiers(modifiers);
}
void ColourSettingsPanel::mouseMove(const MouseEvent& event)
{
if (!virtualKeyboard->isMouseOver())
checkModifiers(event.mods);
}
void ColourSettingsPanel::mouseDown(const MouseEvent& event)
{
if (event.mods.isRightButtonDown())
{
resetColourToggle->setToggleState(true, dontSendNotification);
userClickedReset = false;
}
// TODO: allow one click on virtual keyboard to set focus before painting?
if (event.eventComponent->getParentComponent() == virtualKeyboard)
{
colourSelector->setComponentToFocusOn(virtualKeyboard);
// TODO: better messaging for VirtualKeyboard changes
virtualKeyboard->updateKeyColors(false);
keyColourLibrary->refreshSwatches();
}
else if (colourSelector->isMouseOver() || event.eventComponent->getParentComponent() == colourSelector)
return;
else if (dynamic_cast<FocusableComponent*>(event.eventComponent))
{
colourSelector->setComponentToFocusOn(event.eventComponent);
// TODO: find out why this isn't being done in ColourSelector focusser callback
virtualKeyboard->getProperties().set(IDs::colorSelected, colourSelector->getCurrentColour().toString());
}
else
colourSelector->setComponentToFocusOn(nullptr);
}
void ColourSettingsPanel::mouseUp(const MouseEvent& event)
{
if (!userClickedReset && resetColourToggle->getToggleState())
resetColourToggle->setToggleState(false, dontSendNotification);
}
void ColourSettingsPanel::setKeyboardPointer(VirtualKeyboard::Keyboard* keyboardPointer)
{
virtualKeyboard = keyboardPointer;
Colour init = Colour::fromString(virtualKeyboard->getProperties()[IDs::colorSelected].toString());
if (init.isTransparent())
init = virtualKeyboard->getKeyLayerColor(0);
colourSelector->setCurrentColour(init, dontSendNotification);
// fill key color swatches
keyColourLibrary = new ColourLibraryComponent(
{ "Key Layer Colors:",
"Scale Degree Colors:",
"Individual Key Colors:" },
{ virtualKeyboard->getKeyLayerColours(),
virtualKeyboard->getKeyDegreeColours(),
virtualKeyboard->getKeyIndividualColours() }
, true, true
);
keyColourLibrary->setName("KeyColourLibrary");
keyColourLibrary->setColour(ColourLibraryComponent::ColourIds::backgroundColourId, Colour(Colour(0xff323e44).brighter(0.125f)));
controls.set(5, keyColourLibrary, true);
addAndMakeVisible(keyColourLibrary);
getSectionFlexBox(2)->items.getReference(1).associatedComponent = keyColourLibrary;
// fill note on color swatches
noteOnColourLibrary = new ColourLibraryComponent({ "Midi Channel Note On Colors:" }, { virtualKeyboard->getKeyNoteOnColours() }, true);
noteOnColourLibrary->setName("NoteOnColourLibrary");
noteOnColourLibrary->setColour(ColourLibraryComponent::ColourIds::backgroundColourId, Colour(Colour(0xff323e44).brighter(0.125f)));
noteOnColourLibrary->forceSwatchColumns(8);
controls.set(6, noteOnColourLibrary, true);
addAndMakeVisible(noteOnColourLibrary);
getSectionFlexBox(3)->items.getReference(0).associatedComponent = noteOnColourLibrary;
// callback for setting color in keyboard
colourSelector->setFocusserCallback([&](Component* c, var dataIn)
{
if (dynamic_cast<PaintSwatch*>(c))
virtualKeyboard->updateKeyColors();
else
virtualKeyboard->getProperties().set(IDs::colorSelected, dataIn);
});
virtualKeyboard->addMouseListener(this, true);
}
void ColourSettingsPanel::refreshPanel()
{
if (keyColourLibrary)
keyColourLibrary->refreshSwatches();
}
ColourSelector* ColourSettingsPanel::getColourSelector()
{
return colourSelector;
}
void ColourSettingsPanel::checkModifiers(const ModifierKeys& modifiers)
{
bool valueChanged = true;
if (modifiers.isCtrlDown() && !ctrlHeld)
ctrlHeld = true;
else if (ctrlHeld && !modifiers.isCtrlDown())
ctrlHeld = false;
else if (modifiers.isShiftDown() && !shiftHeld)
shiftHeld = true;
else if (shiftHeld && !modifiers.isShiftDown())
shiftHeld = false;
else
valueChanged = false;
if (valueChanged)
{
if (ctrlHeld)
keyPaintButton->setToggleState(true, sendNotification);
else if (shiftHeld)
layerPaintButton->setToggleState(true, sendNotification);
else
degreePaintButton->setToggleState(true, sendNotification);
}
} | {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
MidiSettingsPanel.cpp
Created: 13 Nov 2019 7:09:59pm
Author: Vincenzo Sicurella
==============================================================================
*/
#include "MidiSettingsPanel.h"
MidiSettingsPanel::MidiSettingsPanel(SvkPluginState* pluginStateIn)
: SvkSettingsPanel("MidiSettingsPanel", pluginStateIn,
{
"Filters",
"Devices"
},
{
IDs::periodShift,
IDs::transposeAmt,
IDs::keyboardMidiChannel,
IDs::midiInputName,
IDs::midiOutputName
},
{
SvkControlProperties(ControlTypeNames::SliderControl, "Period Shift", true, 0, 0, -10, 10),
SvkControlProperties(ControlTypeNames::SliderControl, "Transpose", true, 0, 0, -128, 128),
SvkControlProperties(ControlTypeNames::SliderControl, "MIDI Channel Out", true, 0, 1, 1, 16),
SvkControlProperties(ControlTypeNames::MenuControl, "Midi Input Device", true, 1),
SvkControlProperties(ControlTypeNames::MenuControl, "Midi Output Device", true, 1)
}
)
{
Array<Identifier> labelledControlIDs =
{
IDs::periodShift,
IDs::transposeAmt,
IDs::keyboardMidiChannel,
IDs::midiInputName,
IDs::midiOutputName
};
for (auto id : labelledControlIDs)
{
idToLabelledControl.set(id, static_cast<LabelledComponent*>(idToControl[id]));
}
midiProcessor = pluginState->getMidiProcessor();
idToLabelledControl[IDs::periodShift]->setComponentSize(100, 24);
periodShiftSlider = LabelledComponent::getComponentPointer<Slider>(idToLabelledControl[IDs::periodShift]);
periodShiftSlider->setSliderStyle(Slider::SliderStyle::IncDecButtons);
periodShiftSlider->setTextBoxStyle(Slider::TextBoxLeft, false, 40, 24);
periodShiftSlider->setValue(midiProcessor->getPeriodShift());
periodShiftSlider->addListener(this);
idToLabelledControl[IDs::transposeAmt]->setComponentSize(100, 24);
transposeSlider = LabelledComponent::getComponentPointer<Slider>(idToLabelledControl[IDs::transposeAmt]);
transposeSlider->setSliderStyle(Slider::SliderStyle::IncDecButtons);
transposeSlider->setTextBoxStyle(Slider::TextBoxLeft, false, 40, 24);
transposeSlider->setValue(midiProcessor->getTransposeAmt());
transposeSlider->addListener(this);
idToLabelledControl[IDs::keyboardMidiChannel]->setComponentSize(100, 24);
midiChannelSlider = LabelledComponent::getComponentPointer<Slider>(idToLabelledControl[IDs::keyboardMidiChannel]);
midiChannelSlider->setSliderStyle(Slider::SliderStyle::IncDecButtons);
midiChannelSlider->setTextBoxStyle(Slider::TextBoxLeft, false, 40, 24);
midiChannelSlider->setValue(midiProcessor->getMidiChannelOut());
midiChannelSlider->addListener(this);
// Setup device settings if on standalone version, or hide
if (JUCEApplication::isStandaloneApp())
{
inputBoxLabelled = static_cast<LabelledComponent*>(idToControl[IDs::midiInputName]);
inputBoxLabelled->setComponentSize(320, 24);
inputBox = LabelledComponent::getComponentPointer<ComboBox>(inputBoxLabelled);
inputBox->addListener(this);
outputBoxLabelled = static_cast<LabelledComponent*>(idToControl[IDs::midiOutputName]);
outputBoxLabelled->setComponentSize(320, 24);
outputBox = LabelledComponent::getComponentPointer<ComboBox>(outputBoxLabelled);
outputBox->addListener(this);
}
else
{
controls.removeObject(idToControl[IDs::midiInputName], true);
controls.removeObject(idToControl[IDs::midiOutputName], true);
sectionHeaderLabels.remove(1);
flexParent.items.remove(1);
}
}
MidiSettingsPanel::~MidiSettingsPanel()
{
}
void MidiSettingsPanel::visibilityChanged()
{
if (isVisible() && JUCEApplication::isStandaloneApp())
{
refreshDevices();
startTimer(1000);
}
else
{
stopTimer();
}
}
void MidiSettingsPanel::sliderValueChanged(Slider* sliderThatChanged)
{
if (sliderThatChanged == periodShiftSlider)
{
midiProcessor->setPeriodShift(sliderThatChanged->getValue());
}
else if (sliderThatChanged == transposeSlider)
{
midiProcessor->setTransposeAmt(sliderThatChanged->getValue());
}
else if (sliderThatChanged == periodShiftSlider)
{
midiProcessor->setMidiChannelOut(sliderThatChanged->getValue());
}
}
void MidiSettingsPanel::comboBoxChanged(ComboBox* comboBoxThatChanged)
{
// Midi Input Changed
if (inputBox == comboBoxThatChanged)
{
MidiDeviceInfo& device = availableIns.getReference(inputBox->getSelectedId() - 1);
DBG("Midi Input Selected: " + device.name);
pluginState->getMidiProcessor()->setMidiInput(device.identifier);
}
// Midi Output Changed
else if (outputBox == comboBoxThatChanged)
{
MidiDeviceInfo& device = availableOuts.getReference(outputBox->getSelectedId() - 1);
DBG("Midi Output Selected: " + device.name);
pluginState->getMidiProcessor()->setMidiOutput(device.identifier);
}
}
void MidiSettingsPanel::timerCallback()
{
refreshDevices();
}
void MidiSettingsPanel::refreshDevices()
{
Array<MidiDeviceInfo> inputDevices = MidiInput::getAvailableDevices();
if (availableIns != inputDevices && !inputBox->isPopupActive())
{
availableIns = inputDevices;
inputBox->clear(dontSendNotification);
inputBox->setText(pluginState->getMidiProcessor()->getInputName(), dontSendNotification);
int i = 1;
for (auto device : availableIns)
{
inputBox->addItem(device.name, i++);
}
}
Array<MidiDeviceInfo> outputDevices = MidiOutput::getAvailableDevices();
if (availableOuts != outputDevices && !outputBox->isPopupActive())
{
availableOuts = outputDevices;
outputBox->clear(dontSendNotification);
outputBox->setText(pluginState->getMidiProcessor()->getOutputName(), dontSendNotification);
int i = 1;
for (auto device : availableOuts)
{
outputBox->addItem(device.name, i++);
}
}
} | {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
MappingSettingsPanel.cpp
Created: 7 Oct 2020 10:00:18pm
Author: Vincenzo
==============================================================================
*/
#include "MappingSettingsPanel.h"
MappingSettingsPanel::MappingSettingsPanel(SvkPluginState* pluginStateIn)
: SvkSettingsPanel(
"MappingSettings", pluginStateIn,
{
"Current Mapping",
""
//"Advanced Mapping"
},
{
IDs::noteMapNode,
Identifier("Message")
},
{
SvkControlProperties(ControlTypeNames::GenericControl, "Current Mapping", false, 0),
SvkControlProperties(ControlTypeNames::GenericControl, "New features message", false, 1)
//SvkControlProperties(ControlTypeNames::GenericControl, "Advanced Mapping Note", false, 1)
}
)
{
currentMappingLabel = static_cast<Label*>(getSectionFlexBox(0)->items.getReference(0).associatedComponent);
noteMapEditor = new NoteMapEditor(*pluginState->getMidiInputFilterMap());
controls.set(0, noteMapEditor, true);
addAndMakeVisible(noteMapEditor);
getSectionFlexBox(0)->items.getReference(1).associatedComponent = noteMapEditor;
mappingModeChanged(pluginState->getMappingMode());
pluginState->addListener(this);
Label* msg = new Label("Message", "Currently, you can only edit the key number. More mapping features on the way! :)");
controls.set(1, msg, true);
addAndMakeVisible(msg);
getSectionFlexBox(1)->items.getReference(0).associatedComponent = msg;
flexParent.items.getReference(0).maxWidth = 400;
}
MappingSettingsPanel::~MappingSettingsPanel()
{
pluginState->removeListener(this);
}
void MappingSettingsPanel::visibilityChanged()
{
if (!isVisible())
{
noteMapEditor->removeAllListeners();
}
}
void MappingSettingsPanel::mappingModeChanged(int mappingModeId)
{
// Mapping off
if (mappingModeId == 1)
{
noteMapEditor->setEnabled(false);
currentMappingLabel->setText("Current Mapping (No mapping active)", dontSendNotification);
noteMapEditor->resetMapping(pluginState->getMidiProcessor()->getManualNoteMap(), false);
}
// Auto Map
else if (mappingModeId == 2)
{
noteMapEditor->setEnabled(false);
currentMappingLabel->setText("Current Mapping (Auto Map / Read-only)", dontSendNotification);
}
// Manual Map
else if (mappingModeId == 3)
{
noteMapEditor->setEnabled(true);
currentMappingLabel->setText("Current Mapping", dontSendNotification);
}
}
void MappingSettingsPanel::inputMappingChanged(NoteMap& newNoteMap)
{
noteMapEditor->resetMapping(newNoteMap, false);
}
void MappingSettingsPanel::mappingEditorChanged(NoteMap& newNoteMap)
{
pluginState->setMidiInputMap(newNoteMap, true, false);
}
void MappingSettingsPanel::registerEditorListener(MappingEditor::Listener* listenerIn)
{
noteMapEditor->addListener(listenerIn);
} | {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
GeneralSettingsPanel.h
Created: 3 Sep 2020 9:11:33pm
Author: Vincenzo Sicurella
==============================================================================
*/
#pragma once
#include "SvkSettingsPanel.h"
class GeneralSettingsPanel : public SvkSettingsPanel
{
public:
GeneralSettingsPanel(SvkPluginState*);
~GeneralSettingsPanel() override;
void directoryChanged(DirectoryBrowserComponent*, File) override;
private:
LabelledComponent* presetLabel;
LabelledComponent* modeLabel;
LabelledComponent* settingsLabel;
DirectoryBrowserComponent* presetDirectoryBrowser;
DirectoryBrowserComponent* modeDirectoryBrowser;
DirectoryBrowserComponent* settingsDirectoryBrowser;
JUCE_DECLARE_NON_COPYABLE_WITH_LEAK_DETECTOR (GeneralSettingsPanel)
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
KeyboardSettingsPanel.cpp
Created: 13 Nov 2019 7:09:31pm
Author: Vincenzo Sicurella
==============================================================================
*/
#include "KeyboardSettingsPanel.h"
KeyboardSettingsPanel::KeyboardSettingsPanel(SvkPluginState* pluginStateIn)
: SvkSettingsPanel("KeyboardSettingsPanel", pluginStateIn,
{
"Keyboard settings"
},
{
IDs::keyboardKeysStyle,
IDs::keyboardHighlightStyle,
IDs::pianoWHRatio,
IDs::pianoKeyShowNumber
},
{
SvkControlProperties(ControlTypeNames::MenuControl, "Key Layout Style", true),
SvkControlProperties(ControlTypeNames::MenuControl, "Key Highlight Style", true),
SvkControlProperties(ControlTypeNames::SliderControl, "Key Proportion", true),
SvkControlProperties(ControlTypeNames::ToggleControl, "Show Key Numbers")
}
)
{
layoutLabel = static_cast<LabelledComponent*>(controls[0]);
layoutLabel->setComponentSize(136, controlMinHeight);
keyLayoutBox = LabelledComponent::getComponentPointer<ComboBox>(layoutLabel);
keyLayoutBox->addListener(this);
keyLayoutBox->setEditableText(false);
keyLayoutBox->setJustificationType(Justification::centredLeft);
keyLayoutBox->setTextWhenNothingSelected(String());
keyLayoutBox->setTextWhenNoChoicesAvailable(TRANS("(no choices)"));
keyLayoutBox->addItem(TRANS("Nested Right"), 1);
keyLayoutBox->addItem(TRANS("Nested Center"), 2);
keyLayoutBox->addItem(TRANS("Flat"), 3);
keyLayoutBox->addItem(TRANS("Adjacent"), 4);
highlightLabel = static_cast<LabelledComponent*>(controls[1]);
highlightLabel->setComponentSize(96, controlMinHeight);
keyHighlightBox = LabelledComponent::getComponentPointer<ComboBox>(highlightLabel);
keyHighlightBox->addListener(this);
keyHighlightBox->setEditableText(false);
keyHighlightBox->setJustificationType(Justification::centredLeft);
keyHighlightBox->setTextWhenNothingSelected(String());
keyHighlightBox->setTextWhenNoChoicesAvailable(TRANS("(no choices)"));
keyHighlightBox->addItem(TRANS("Full Key"), 1);
keyHighlightBox->addItem(TRANS("Inside"), 2);
keyHighlightBox->addItem(TRANS("Border"), 3);
keyHighlightBox->addItem(TRANS("Circles"), 4);
keyHighlightBox->addItem(TRANS("Squares"), 5);
keyHighlightBox->addListener(this);
ratioLabel = static_cast<LabelledComponent*>(controls[2]);
ratioLabel->setComponentSize(225, controlMinHeight);
flexSections.getReference(0).items.getReference(2).minWidth = ratioLabel->getWidth();
keyRatioSlider = LabelledComponent::getComponentPointer<Slider>(ratioLabel);
keyRatioSlider->setRange(0.01, 1, 0.01);
keyRatioSlider->addListener(this);
showNoteNumbers = static_cast<TextButton*>(controls[3]);
showNoteNumbers->setSize(Font().getStringWidth(showNoteNumbers->getButtonText()) + 5, controlMinHeight);
showNoteNumbers->addListener(this);
}
void KeyboardSettingsPanel::comboBoxChanged(ComboBox* boxThatChanged)
{
if (virtualKeyboard)
{
if (boxThatChanged == keyLayoutBox)
{
virtualKeyboard->setKeyStyle(boxThatChanged->getSelectedId());
virtualKeyboard->resized();
}
else if (boxThatChanged == keyHighlightBox)
{
virtualKeyboard->setHighlightStyle(boxThatChanged->getSelectedId());
}
}
}
void KeyboardSettingsPanel::sliderValueChanged(Slider* sliderChanged)
{
if (virtualKeyboard)
{
if (sliderChanged == keyRatioSlider)
{
virtualKeyboard->setKeySizeRatio(sliderChanged->getValue(), false);
getParentComponent()->getParentComponent()->resized();
}
}
}
void KeyboardSettingsPanel::buttonClicked(Button* clickedButton)
{
if (virtualKeyboard)
{
if (clickedButton == showNoteNumbers)
{
virtualKeyboard->setShowNoteNumbers(clickedButton->getToggleState());
}
}
}
void KeyboardSettingsPanel::setKeyboardPointer(VirtualKeyboard::Keyboard* keyboardPointer)
{
virtualKeyboard = keyboardPointer;
keyLayoutBox->setSelectedId(virtualKeyboard->getKeyPlacementStyle(), dontSendNotification);
keyHighlightBox->setSelectedId(virtualKeyboard->getHighlightStyle(), dontSendNotification);
keyRatioSlider->setValue(virtualKeyboard->getKeySizeRatio(), dontSendNotification);
showNoteNumbers->setToggleState(virtualKeyboard->isShowingNoteNumbers(), dontSendNotification);
} | {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
ModeSettingsPanel.h
Created: 26 Sep 2020 1:59:11pm
Author: Vincenzo
==============================================================================
*/
#pragma once
#include "SvkSettingsPanel.h"
class ModeSettingsPanel : public SvkSettingsPanel
{
public:
ModeSettingsPanel(SvkPluginState*);
~ModeSettingsPanel();
}; | {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
GeneralSettingsPanel.cpp
Created: 13 Nov 2019 7:11:33pm
Author: Vincenzo Sicurella
==============================================================================
*/
#include "GeneralSettingsPanel.h"
GeneralSettingsPanel::GeneralSettingsPanel(SvkPluginState* pluginStateIn)
: SvkSettingsPanel("GeneralSettingsPanel", pluginStateIn, { "File Directories" },
{
IDs::presetDirectory,
IDs::modeDirectory,
IDs::settingsDirectory
},
{
SvkControlProperties(ControlTypeNames::DirectoryControl, "Preset Directory", true),
SvkControlProperties(ControlTypeNames::DirectoryControl, "Mode Directory", true),
SvkControlProperties(ControlTypeNames::DirectoryControl, "Settings Directory", true)
},
FlexBox(), {}
)
{
presetLabel = static_cast<LabelledComponent*>(idToControl[IDs::presetDirectory]);
presetLabel->setComponentSize(320, 24);
presetDirectoryBrowser = LabelledComponent::getComponentPointer<DirectoryBrowserComponent>(presetLabel);
presetDirectoryBrowser->setText(pluginState->getPluginSettings()->getPresetPath());
presetDirectoryBrowser->addListener(this);
modeLabel = static_cast<LabelledComponent*>(idToControl[IDs::modeDirectory]);
modeLabel->setComponentSize(320, 24);
modeDirectoryBrowser = LabelledComponent::getComponentPointer<DirectoryBrowserComponent>(modeLabel);
modeDirectoryBrowser->setText(pluginState->getPluginSettings()->getModePath());
modeDirectoryBrowser->addListener(this);
settingsLabel = static_cast<LabelledComponent*>(idToControl[IDs::settingsDirectory]);
settingsLabel->setComponentSize(320, 24);
settingsDirectoryBrowser = LabelledComponent::getComponentPointer<DirectoryBrowserComponent>(settingsLabel);
settingsDirectoryBrowser->setText(pluginState->getPluginSettings()->getSettingsPath());
settingsDirectoryBrowser->addListener(this);
setSize(100, 100);
}
GeneralSettingsPanel::~GeneralSettingsPanel()
{
}
void GeneralSettingsPanel::directoryChanged(DirectoryBrowserComponent* browser, File directorySelected)
{
if (browser == presetLabel->get())
{
pluginState->getPluginSettings()->setPresetDirectory(directorySelected);
}
else if (browser == modeLabel->get())
{
pluginState->getPluginSettings()->setModeDirectory(directorySelected);
}
else if (browser == settingsLabel->get())
{
pluginState->getPluginSettings()->setSettingsDirectory(directorySelected);
}
} | {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
KeyboardSettingsPanel.h
Created: 13 Nov 2019 7:09:31pm
Author: Vincenzo Sicurella
==============================================================================
*/
#pragma once
#include "SvkSettingsPanel.h"
class KeyboardSettingsPanel : public SvkSettingsPanel
{
public:
KeyboardSettingsPanel(SvkPluginState*);
void comboBoxChanged(ComboBox*) override;
void sliderValueChanged(Slider*) override;
void buttonClicked(Button*) override;
void setKeyboardPointer(VirtualKeyboard::Keyboard*) override;
private:
LabelledComponent* layoutLabel;
LabelledComponent* highlightLabel;
LabelledComponent* ratioLabel;
ComboBox* keyLayoutBox;
ComboBox* keyHighlightBox;
Slider* keyRatioSlider;
TextButton* showNoteNumbers;
JUCE_DECLARE_NON_COPYABLE_WITH_LEAK_DETECTOR (KeyboardSettingsPanel)
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
ControlSettingsPanel.cpp
Created: 13 Nov 2019 7:11:08pm
Author: Vincenzo Sicurella
==============================================================================
*/
#include "ControlSettingsPanel.h"
ControlSettingsPanel::ControlSettingsPanel(AudioProcessorValueTreeState& processorTreeIn)
: SvkUiPanel(processorTreeIn)
{
}
ControlSettingsPanel::~ControlSettingsPanel()
{
}
void ControlSettingsPanel::connectToProcessor()
{
}
void ControlSettingsPanel::paint(Graphics& g)
{
}
void ControlSettingsPanel::resized()
{
}
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
DebugSettingsPanel.h
Created: 14 Nov 2019 11:46:31pm
Author: Vincenzo Sicurella
==============================================================================
*/
#pragma once
#include "SvkSettingsPanel.h"
class DebugSettingsPanel : public SvkSettingsPanel, public Timer
{
public:
DebugSettingsPanel(SvkPluginState*);
~DebugSettingsPanel();
//void paint(Graphics& g) override;
//void resized() override;
void timerCallback() override;
private:
SvkPluginState* pluginState;
JUCE_DECLARE_NON_COPYABLE_WITH_LEAK_DETECTOR(DebugSettingsPanel)
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
DebugSettingsPanel.cpp
Created: 14 Nov 2019 11:46:31pm
Author: Vincenzo Sicurella
==============================================================================
*/
#include "DebugSettingsPanel.h"
DebugSettingsPanel::DebugSettingsPanel(SvkPluginState* pluginStateIn)
: SvkSettingsPanel("DebugSettingsPanel", pluginState, { "" }, {}, {}),
pluginState(pluginStateIn)
{
setSize(100, 100);
}
DebugSettingsPanel::~DebugSettingsPanel()
{
}
//
//void DebugSettingsPanel::connectToProcessor()
//{
// Label* lbl;
// Component* c;
// Slider* s;
// String paramName;
// RangedAudioParameter* param;
// AudioParameterInt* api;
//
// for (int i = 0; i < paramIDs->size(); i++)
// {
// paramName = paramIDs->getUnchecked(i);
// param = processorTree.getParameter(paramName);
//
// lbl = labels.add(new Label("Lbl" + paramName, param->name));
// lbl->setColour(Label::textColourId, Colours::black);
// addAndMakeVisible(lbl);
//
// const NormalisableRange<float>& range = param->getNormalisableRange();
// s = new Slider("Sld" + paramName);
// c = controls.add(s);
//
// s->setSliderStyle(Slider::LinearHorizontal);
//
// api = dynamic_cast<AudioParameterInt*>(param);
// if (api)
// {
// s->setNormalisableRange(NormalisableRange<double>(range.start, range.end, 1));
// s->setSliderStyle(Slider::IncDecButtons);
// s->setValue(api->get(), dontSendNotification);
// }
// else
// {
// s->setNormalisableRange(NormalisableRange<double>(range.start, range.end, 0.01f));
// s->setValue(param->getValue(), dontSendNotification);
// }
//
// s->setScrollWheelEnabled(false);
// s->setTextBoxStyle (Slider::TextBoxLeft, false, 60, 20);
// s->setColour(Slider::textBoxTextColourId, Colours::black);
// s->addListener (this);
//
// sliderAttachments.add(new SliderAttachment(processorTree, paramName, *s));
// addAndMakeVisible(s);
// }
//}
//
//void DebugSettingsPanel::paint(Graphics& g)
//{
// g.setColour(Colours::palegreen);
// g.fillAll();
//
// if (controlIdentifiers.size() == 0)
// {
// g.setColour(Colours::black);
// g.drawText("No parameters", getWidth()/2, getHeight()/2,
// getWidth(), getHeight(), Justification::centred);
// }
//}
//
//void DebugSettingsPanel::sliderValueChanged(Slider *slider)
//{
// String paramName = slider->getName().substring(3);
// RangedAudioParameter* param = processorTree.getParameter(paramName);
// AudioParameterInt* api;
//
// if (paramName == IDs::pianoWHRatio.toString())
// {
// //param->setValue(slider->getValue());
// //pluginState->getKeyboard()->setKeySizeRatio(param->getValue());
// }
//
// else if (paramName == IDs::keyboardNumRows.toString())
// {
// //api = dynamic_cast<AudioParameterInt*>(param);
// //*api = slider->getValue();
// //pluginState->getKeyboard()->setNumRows(api->get());
// }
//
//}
//
void DebugSettingsPanel::timerCallback()
{
} | {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
MidiSettingsPanel.h
Created: 13 Nov 2019 7:09:59pm
Author: Vincenzo Sicurella
==============================================================================
*/
#pragma once
#include "SvkSettingsPanel.h"
class MidiSettingsPanel : public SvkSettingsPanel, public Timer
{
public:
MidiSettingsPanel(SvkPluginState*);
~MidiSettingsPanel();
void visibilityChanged() override;
void sliderValueChanged(Slider* sliderThatChanged) override;
void comboBoxChanged(ComboBox* comboBoxThatChanged) override;
void timerCallback() override;
void refreshDevices();
private:
HashMap<Identifier, LabelledComponent*, IDasStringHash> idToLabelledControl;
LabelledComponent* inputBoxLabelled;
LabelledComponent* outputBoxLabelled;
ComboBox* inputBox;
ComboBox* outputBox;
Slider* periodShiftSlider;
Slider* transposeSlider;
Slider* midiChannelSlider;
Array<MidiDeviceInfo> availableIns;
Array<MidiDeviceInfo> availableOuts;
SvkMidiProcessor* midiProcessor;
JUCE_DECLARE_NON_COPYABLE_WITH_LEAK_DETECTOR (MidiSettingsPanel)
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
ControlSettingsPanel.h
Created: 13 Nov 2019 7:11:08pm
Author: Vincenzo Sicurella
==============================================================================
*/
#pragma once
#include "../../../PluginState.h"
class ControlSettingsPanel : public Component
{
public:
ControlSettingsPanel(AudioProcessorValueTreeState& processorTreeIn);
~ControlSettingsPanel();
//void connectToProcessor() override;
void paint(Graphics& g) override;
void resized() override;
private:
SvkPluginState* pluginState;
OwnedArray<ButtonAttachment> buttonAttachments;
OwnedArray<ComboBoxAttachment> comboBoxAttachments;
OwnedArray<SliderAttachment> sliderAttachments;
JUCE_DECLARE_NON_COPYABLE_WITH_LEAK_DETECTOR (ControlSettingsPanel)
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
SvkSettingsPanel.h
Created: 5 Sep 2020 4:08:42pm
Author: Vincenzo
==============================================================================
*/
#pragma once
#include "../../../PluginState.h"
#include "../DirectoryBrowserComponent.h"
#include "../LabelledComponent.h"
#include "../VirtualKeyboard/KeyboardComponent.h"
#define defaultSectionFlexBox FlexBox(FlexBox::Direction::column, FlexBox::Wrap::wrap, FlexBox::AlignContent::stretch, FlexBox::AlignItems::flexStart, FlexBox::JustifyContent::spaceAround)
#define defaultSectionFlexItem FlexItem(250, 24).withFlex(1.0f).withMargin(FlexItem::Margin(5, 0, 5, 5))
#define defaultSectionAsFlexItem FlexItem(100, 100).withFlex(1.0f);
class SvkSettingsPanel : public Component,
protected Slider::Listener,
protected Button::Listener,
protected ComboBox::Listener,
protected DirectoryBrowserComponent::Listener
{
public:
enum ControlTypeNames
{
SliderControl = 0,
ToggleControl,
MenuControl,
DirectoryControl,
GenericControl
};
struct SvkControlProperties
{
int controlType;
String controlName;
bool controlLabelled;
int sectionNum;
var defaultValue;
var minValue;
var maxValue;
var increment;
SvkControlProperties(
int typeIn = 1, String nameIn = "", bool labelled = false, int sectionNumIn = 0,
var defaultIn = var(), var minIn = 0, var maxIn = 1, var incrementIn = 1
) :
controlType(typeIn), controlName(nameIn), controlLabelled(labelled), sectionNum(sectionNumIn),
defaultValue(defaultIn), minValue(minIn), maxValue(maxIn), increment(incrementIn)
{};
};
public:
SvkSettingsPanel(
String panelName,
SvkPluginState* pluginStateIn,
StringArray sectionNamesIn,
Array<Identifier> controlIdsIn,
Array<SvkControlProperties> controlTypesIn,
FlexBox flexParentStyle = FlexBox(),
Array<FlexBox> sectionBoxStyle = Array<FlexBox>(),
Array<FlexItem> sectionItemsStyle = Array<FlexItem>()
) :
pluginState(pluginStateIn),
sectionNames(sectionNamesIn),
controlIdentifiers(controlIdsIn),
controlTypes(controlTypesIn),
flexParent(flexParentStyle)
{
setName(panelName);
numSections = sectionNames.size();
flexParent.items.resize(numSections);
FlexItem sectionDefault = defaultSectionAsFlexItem;
flexParent.items.fill(sectionDefault);
// Combine given and default FlexBox styles
flexSections.addArray(sectionBoxStyle);
while (flexSections.size() < numSections)
flexSections.add(defaultSectionFlexBox);
// Combine given and default FlexItem styles
sectionsDefaultFlexItems.addArray(sectionItemsStyle);
while (sectionsDefaultFlexItems.size() < numSections)
sectionsDefaultFlexItems.add(defaultSectionFlexItem);
setupSections();
setupControls();
}
virtual ~SvkSettingsPanel()
{
}
virtual void paint(Graphics& g) override
{
//g.fillAll(Colours::darkgrey);
//g.setColour(Colours::blueviolet);
//float x = 0;
//for (int i = 0; i < flexParent.items.size(); i++)
//{
// FlexItem& item = flexParent.items.getReference(i);
// Rectangle<float> rect = { x, 0, item.width, item.height };
// x += item.width;
// g.fillRoundedRectangle(rect.reduced(3, 3), 3.0f);
//}
g.setColour(Colour(0xff323e44).darker());
for (auto label : sectionHeaderLabels)
{
g.fillRoundedRectangle(label->getBounds().reduced(2.0f, 0).toFloat(), 5.0f);
}
}
void resized() override
{
flexParent.performLayout(getLocalBounds());
}
virtual void setKeyboardPointer(VirtualKeyboard::Keyboard* keyboardPointer)
{
virtualKeyboard = keyboardPointer;
}
virtual void refreshPanel() {};
//=============================================================================================================
virtual void sliderValueChanged(Slider*) override {};
virtual void buttonClicked(Button*) override {};
virtual void comboBoxChanged(ComboBox*) override {};
virtual void directoryChanged(DirectoryBrowserComponent*, File) override {};
protected:
FlexBox* getSectionFlexBox(int sectionNum)
{
return flexParent.items.getReference(jmin(sectionNum, numSections - 1)).associatedFlexBox;
}
FlexItem* getControlFlexItem(int controlNum)
{
FlexBox* sectionBox = getSectionFlexBox(controlTypes[controlNum].sectionNum);
for (int i = 0; i < sectionBox->items.size(); i++)
{
FlexItem* item = §ionBox->items.getReference(i);
if (item->associatedComponent == controls[controlNum])
return item;
}
return nullptr;
}
bool setControlFlexItem(int controlNum, FlexItem flexItemIn)
{
FlexBox* sectionBox = getSectionFlexBox(controlTypes[controlNum].sectionNum);
for (int i = 0; i < sectionBox->items.size(); i++)
{
if (sectionBox->items.getReference(i).associatedComponent == controls[controlNum])
{
sectionBox->items.set(i, flexItemIn);
return true;
}
}
return false;
}
private:
void setupSections()
{
// Associate section flexboxes with parent FlexItems and add section headers
for (int i = 0; i < numSections; i++)
{
FlexBox& section = flexSections.getReference(i);
flexParent.items.getReference(i).associatedFlexBox = §ion;
String header = sectionNames[i];
if (header.length() > 0)
{
Label* l = sectionHeaderLabels.add(new Label(getName() + "_Header" + String(i), header));
addAndMakeVisible(l);
section.items.add(FlexItem(225, 24, *l));
}
}
}
void setupControls()
{
int numControls = jmin(controlIdentifiers.size(), controlTypes.size());
for (int i = 0; i < numControls; i++)
{
Identifier id = controlIdentifiers[i];
SvkControlProperties properties = controlTypes[i];
Slider* slider = nullptr;
Component* control = nullptr;
switch (properties.controlType)
{
case ControlTypeNames::SliderControl:
slider = new Slider(properties.controlName);
slider->setRange(properties.minValue, properties.maxValue, properties.increment);
slider->setValue(properties.defaultValue, dontSendNotification);
control = slider;
break;
case ControlTypeNames::ToggleControl:
control = new ToggleButton(properties.controlName);
break;
case ControlTypeNames::MenuControl:
control = new ComboBox(properties.controlName);
break;
case ControlTypeNames::DirectoryControl:
control = new DirectoryBrowserComponent(properties.controlName);
break;
default:
control = new Component(properties.controlName);
}
if (control)
{
if (properties.controlLabelled)
{
control = new LabelledComponent(*control, properties.controlName + ":");
}
controls.add(control);
int sectionNum = jmin(properties.sectionNum, numSections - 1);
FlexBox& section = flexSections.getReference(sectionNum);
FlexItem item = sectionsDefaultFlexItems[sectionNum];
item.associatedComponent = control;
section.items.add(item);
addAndMakeVisible(control);
idToControl.set(id, control);
}
}
}
protected:
SvkPluginState* pluginState;
StringArray sectionNames;
int numSections;
Array<Identifier> controlIdentifiers;
Array<SvkControlProperties> controlTypes;
OwnedArray<Label> sectionHeaderLabels;
Array<FlexBox> flexSections;
FlexBox flexParent;
Array<FlexItem> sectionsDefaultFlexItems;
OwnedArray<Component> controls;
HashMap<Component*, Label*> controlLabels;
HashMap<Identifier, Component*, IDasStringHash> idToControl;
int controlMinWidth = 250;
int controlMinHeight = 24;
VirtualKeyboard::Keyboard* virtualKeyboard = nullptr;
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
ColourSettingsPanel.h
Created: 13 Sep 2020 2:41:09pm
Author: Vincenzo
==============================================================================
*/
#pragma once
#include "SvkSettingsPanel.h"
#include "../ColourLibraryComponent.h"
class FocussedColourSelector : public FocussedComponent, public ColourSelector, private ChangeListener
{
public:
FocussedColourSelector(Component* componentToFocusOn = nullptr)
: FocussedComponent(componentToFocusOn), ColourSelector(
ColourSelector::ColourSelectorOptions::showColourAtTop
+ ColourSelector::ColourSelectorOptions::editableColour
+ ColourSelector::ColourSelectorOptions::showColourspace
, 4, 0)
{
setName("FocussedColourSelector");
// Called when colour changes
addChangeListener(this);
};
~FocussedColourSelector() {};
void setComponentToFocusOn(Component* componentPtr) override
{
FocussedComponent::setComponentToFocusOn(componentPtr);
PaintSwatch* swatch = dynamic_cast<PaintSwatch*>(componentPtr);
if (swatch)
setCurrentColour(swatch->getCurrentColour());
}
private:
void changeListenerCallback(ChangeBroadcaster* source) override
{
FocusableComponent* focussed = dynamic_cast<FocusableComponent*>(currentFocus);
if (focussed)
focussed->performFocusFunction(getCurrentColour().toString());
focusserCallback(currentFocus, getCurrentColour().toString());
}
};
class ColourSettingsPanel : public SvkSettingsPanel
{
public:
ColourSettingsPanel(SvkPluginState*);
~ColourSettingsPanel() override;
void resized() override;
void buttonClicked(Button*) override;
void modifierKeysChanged(const ModifierKeys&) override;
void mouseMove(const MouseEvent& event) override;
void mouseDown(const MouseEvent& event) override;
void mouseUp(const MouseEvent& event) override;
void setKeyboardPointer(VirtualKeyboard::Keyboard* keyboardPointer) override;
void refreshPanel() override;
ColourSelector* getColourSelector();
private:
void checkModifiers(const ModifierKeys& keys);
private:
FocussedColourSelector* colourSelector;
ColourLibraryComponent* keyColourLibrary;
ColourLibraryComponent* noteOnColourLibrary;
const int paintTypeRadioGroup = 100;
TextButton* degreePaintButton;
TextButton* layerPaintButton;
TextButton* keyPaintButton;
TextButton* resetColourToggle;
bool userClickedReset = false;
bool ctrlHeld = false;
bool shiftHeld = false;
bool altOptionHeld = false;
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
ModeSettingsPanel.cpp
Created: 26 Sep 2020 1:59:11pm
Author: Vincenzo
==============================================================================
*/
#include "ModeSettingsPanel.h"
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
KeyboardController.cpp
Created: 1 Sep 2019 8:22:03pm
Author: Vincenzo
==============================================================================
*/
#include "KeyboardController.h"
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
KeyboardController.h
Created: 1 Sep 2019 8:22:03pm
Author: Vincenzo
Will be used for higher level keyboard controlling.
==============================================================================
*/
#pragma once
#include "../../../../JuceLibraryCode/JuceHeader.h"
#include "KeyboardComponent.h"
#include "../../../PluginIDs.h"
namespace VirtualKeyboard
{
class SvkController : public MidiKeyboardStateListener
{
public:
SvkController(Keyboard* keyboardIn);
~SvkController();
ValueTree getNode();
void restoreNode(ValueTree nodeIn);
Keyboard* getKeyboard();
void setInputSource(MidiKeyboardState* sourceIn);
void setInputFilteredSource(MidiKeyboardState* sourceIn);
// Keyboard Actions
void triggerNoteOn(int midiChannel, int midiNote, float velocity);
void triggerNoteOff(int midiChannel, int midiNote);
void allNotesOff();
// Interfacing
void setMidiChannelOut(int midiChannelOutIn);
void setNoteColor(int midiNoteIn, Colour colorIn);
void setDegreeColor(int degreeIn, Colour colorIn);
void setChannelTriggeredColor(int midiChannelIn, Colour colorIn);
void handleNoteOn(MidiKeyboardState* source, int midiChannel, int midiNote, float velocity) override;
void handleNoteOff(MidiKeyboardState* source, int midiChannel, int midiNote, float velocity) override;
private:
Keyboard* keyboard;
MidiKeyboardState* input;
MidiKeyboardState* inputFiltered;
Array<Key> keyProperties;
ValueTree keyNodes;
bool showNoteNumbers = false;
bool showNoteNames = false;
bool showInput = true;
bool showInputFiltered = false;
Array<Colour> colorsDegrees;
Array<Point<int>> offsetsByOrder;
Array<Point<int>> offsetsByDegree;
Array<Point<float>> ratiosByOrder;
Array<Point<float>> ratiosByDegree;
};
}; | {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
KeyboardViewPort.h
Created: 31 Oct 2019 2:41:37pm
Author: Vincenzo Sicurella
==============================================================================
*/
#pragma once
#include "../../../../JuceLibraryCode/JuceHeader.h"
#include "KeyboardComponent.h"
using namespace VirtualKeyboard;
class KeyboardViewport : public Viewport,
private Button::Listener
{
public:
KeyboardViewport(VirtualKeyboard::Keyboard* keyboardIn, const String& nameIn = String(), int scrollingModeIn = 1, int scrollingStyleIn = 1);
void viewedComponentChanged(Component*) override;
void visibleAreaChanged(const Rectangle<int>&) override;
int getStepSmall();
int getStepLarge();
int getButtonWidth();
bool isShowingButtons();
float getCenterKeyProportion() const;
void setButtonWidth(int widthIn);
void setScrollingMode(int modeIdIn);
void setScrollingStyle(int styleIdIn);
void stepSmallForward();
void stepSmallBackward();
void stepLargeForward();
void stepLargeBackward();
void centerOnKey(int keyNumberIn);
void centerOnKey(float keyNumberProportionIn);
void resizeKeyboard();
void resized() override;
void buttonClicked(Button* button) override;
enum ScrollingMode
{
NoScrolling = 0,
Smooth,
Stepped,
SmoothWithButtons
};
enum ScrollingStyle
{
Hidden = 0,
Bar,
KeyboardView,
Dots
};
private:
// returns altitude
int drawAngleBracket(Graphics& g, bool isRightPointing, int sideLength, int xCenter, int yCenter, float thickness = 1.0f);
void redrawButtons(int heightIn);
private:
int stepSmall = 0;
int stepLarge = 0;
int buttonWidth = 16;
int scrollingModeSelected = 1;
int scrollingStyleSelected = 1;
std::unique_ptr<ImageButton> stepLeftSmall;
std::unique_ptr<ImageButton> stepLeftLarge;
std::unique_ptr<ImageButton> stepRightSmall;
std::unique_ptr<ImageButton> stepRightLarge;
Keyboard* keyboard;
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
ViewPianoComponent.h
Created: 14 Mar 2019 4:50:31pm
Author: Vincenzo
A keyboard where keys can be rearranged and other stylization options.
==============================================================================
*/
#pragma once
#include "../../../../JuceLibraryCode/JuceHeader.h"
#include "../../../CommonFunctions.h"
#include "../../../Constants.h"
#include "../../../PluginIDs.h"
#include "../../../Midi/MidiProcessor.h"
#include "../../../Structures/Mode.h"
#include "../../../Structures/MappingHelper.h"
#include "VirtualKeyboardKey.h"
#include "VirtualKeyboardGrid.h"
#include "VirtualKeyboardGraphics.h"
#include <iostream>
//==============================================================================
/*
*/
namespace VirtualKeyboard
{
class Keyboard :
public Component,
public MidiKeyboardState,
public MidiKeyboardStateListener
// private Timer
{
public:
//===============================================================================================
Keyboard();
Keyboard(ValueTree keyboardNodeIn, Mode* modeIn=nullptr, NoteMap* inputFilterMapIn = nullptr);
~Keyboard();
//===============================================================================================
void restoreNode(ValueTree keyboardNodeIn, bool reinitializeKeys = true, bool resetIfInvalid=false);
void reset();
void initializeKeys(int size=128);
//===============================================================================================
void paint(juce::Graphics& g) override;
void paintOverChildren(juce::Graphics& g) override;
void resized() override;
//===============================================================================================
void mouseMove(const MouseEvent& e) override;
void mouseExit(const MouseEvent& e) override;
void mouseDown(const MouseEvent& e) override;
void mouseDrag(const MouseEvent& e) override;
void mouseUp(const MouseEvent& e) override;
//===============================================================================================
bool keyStateChanged(bool isKeyDown) override;
bool keyPressed(const KeyPress& key) override;
void modifierKeysChanged(const ModifierKeys& modifiers) override;
//===============================================================================================
// void timerCallback() override;
//===============================================================================================
/*
Returns Keyboard settings node
*/
ValueTree getNode();
/*
Returns a struct with the given key's data.
*/
Key* getKey(int keyNumIn);
/*
Returns a value tree with the given key's data.
*/
ValueTree getKeyNode(int keyNumIn);
/*
Returns an array of pointers to the keys corresponding to the given order.
*/
Array<Key*> getKeysByOrder(int orderIn);
/*
Returns an array of pointers to the keys corresponding to the given order.
*/
Array<Key*> getKeysByScaleDegree(int degreeIn);
/*
Returns an array of pointers to the keys corresponding to the given order.
*/
Array<Key*> getKeysByModalDegree(int degreeIn);
/*
Returns the number of the last key clicked by the user.
*/
int getLastKeyClicked();
/*
Returns the area of the given key relative to the top left corner of the full keyboard.
*/
Rectangle<int> getKeyArea(int midiNoteIn);
/*
Returns the area of the given key relative to the top left corner of the viewport.
*/
Rectangle<int> getKeyAreaRelative(int midiNoteIn);
/*
Returns the key width in pixels.
*/
int getKeyWidth();
/*
Returns the key height in pixels.
*/
int getKeyHeight();
/*
Returns the piano width from given height
*/
int getPianoWidth(int heightIn);
/*
Returns the current proportion of key width to height.
*/
float getKeySizeRatio();
/*
Returns the current size of the keys within the given order.
*/
Point<int> getKeyOrderSize(int orderIn);
/*
Returns the current size of the keys within the given scale degree.
*/
Point<int> getKeyDegreeSize(int degreeIn);
/*
Returns the key which holds the given point relative to the top left corner of the component.
*/
Key* getKeyFromPosition(Point<int> posIn);
/*
Returns the key which holds the given MouseEvent point relative to the top left corner of the component.
*/
Key* getKeyFromPositionMouseEvent(const MouseEvent& e);
/*
Returns the velocity for a given key and point relative to the key's top left corner
*/
float getKeyVelocity(Key* keyIn, Point<int> posIn);
/*
Returns the Midi Channel the keyboard is sending output on.
*/
int getMidiChannelOut() const;
/*
Returns the current mode size
*/
int getModeSize() const;
//===============================================================================================
/*
Returns the UI mode of which the keyboard is in.
*/
int getUIMode();
/*
Returns the orientation of which the keyboard is in.
*/
int getOrientation();
/*
Returns the style of which the keys are nested.
*/
int getKeyPlacementStyle();
/*
Returns whether or not note numbers are showing
*/
bool isShowingNoteNumbers();
/*
Returns whether or not filtered note numbers are showing
*/
bool isShowingFilteredNumbers();
/*
Returns whether or not note names are showing
*/
bool isShowingNoteNames();
/*
Returns the style of which the keys get highlighted.
*/
int getHighlightStyle();
/*
Returns the style of note velocity.
*/
int getVelocityStyle();
/*
Returns the value of velocity if in fixed mode.
*/
float getVelocityFixed();
/*
Returns whether or not Midi input is being scaled to the selected velocity style
*/
bool isInputVelocityScaled();
/*
Returns the scrolling style selected
*/
int getScrollingStyle();
//===============================================================================================
/*
Rearranges the keys to fit the current mode, without changing other key data.
*/
void applyMode(Mode* modeIn, bool resize = false);
/*
Applies the key data to the keyboard so that it matches the data passed in.
*/
void applyKeyData(ValueTree keyDataTreeIn);
/*
Allows the keyboard to listen the filtered midi input
*/
void displayKeyboardState(MidiKeyboardState* keyboardStateIn);
//===============================================================================================
/*
Sets the keyboards UI mode.
*/
void setUIMode(int uiModeIn);
/*
Sets the orientation of the keyboard.
*/
void setOrientation(int orientationIn);
/*
Sets the amount of rows to split the keyboard into
*/
void setNumRows(int numRowsIn);
/*
Sets the style of which the keys are nested in.
*/
void setKeyStyle(int placementIn);
/*
Set the way the keys are highlighted
*/
void setHighlightStyle(int styleIn);
/*
Sets the velocity behavior of the keyboard
*/
void setVelocityBehavior(int behaviorNumIn, bool scaleInputVelocity = false);
/*
Sets the scrolling style of the keyboard.
*/
void setScrollingStyle(int scrollingStyleIn);
/*
Set the Midi Channel that the keyboard outputs on clicks.
*/
void setMidiChannelOut(int midiChannelOutIn);
/*
Set the fixed output velocity
*/
void setVelocityFixed(float velocityIn);
/*
Sets whether or not midi input velocity should be scaled
*/
void setInputVelocityScaled(bool shouldBeScaled);
/*
Set whether note numbers are showing
*/
void setShowNoteNumbers(bool shouldShowNumbers);
/*
Set whether filtered note numbers are showing
*/
void setShowFilteredNumbers(bool shouldShowNumbers);
/*
Set whether pitch names are shown
*/
void setShowNoteLabels(bool shouldShowPitchNames);
// might want to restructure these so this is not necessary
void setLastKeyClicked(int keyNumIn);
/*
Set the Input NoteMap being used.
*/
void setInputNoteMap(NoteMap& noteMapIn);
//===============================================================================================
/*
Adds a reference to a mapping helper for manual mapping
*/
void setMappingHelper(MappingHelper*);
/*
Hightlights the given key, or removes highlight if false is passed in.
*/
void highlightKey(int keyNumberIn, bool highlightOn = true);
//===============================================================================================
/*
Returns a pointer to the individual key colors
*/
Array<Colour>* getKeyIndividualColours();
/*
Returns the color of the given key.
*/
Colour getKeyColor(int keyNumIn);
/*
Returns a pointer to the key layer colors
*/
Array<Colour>* getKeyLayerColours();
/*
Returns the current key layer of the given order.
*/
Colour getKeyLayerColor(int orderIn);
/*
Returns a pointer to the key scale degree colors
*/
Array<Colour>* getKeyDegreeColours();
/*
Returns the current key color of the given scale degree.
*/
Colour getKeyDegreeColor(int degIn);
/*
Returns a pointer to the note on colors by midi channel
*/
Array<Colour>* getKeyNoteOnColours();
//===============================================================================================
/*
Sets the display color of all the keys
*/
void updateKeyColors(bool writeToNode = true);
/*
Resets the color of all the keys in the given order to the current order color.
*/
void resetLayerColors();
/*
Resets the color of all the keys of the scale degree to the current order color.
*/
void resetDegreeColors();
/*
Reset all keys to the current colors of their orders.
*/
void resetKeyColors();
//===============================================================================================
/*
Sets the color of all keys of the given scale degree
*/
void setKeyDegreeColor(int scaleDegreeIn, Colour colourIn);
/*
Sets the color of all keys of the given layer
*/
void setKeyLayerColor(int layerNumIn, Colour colourIn);
/*
Sets the color of the given key
*/
void setKeyColor(int keyNumIn, Colour colourIn);
//===============================================================================================
/*
Sets the proportion of the keys' width to height. Default is 0.25.
*/
void setKeySizeRatio(float keySizeRatioIn, bool resizeSelf = true);
/*
Sets the width of the keys to a given size and conforms the keyboard to the current proportions.
*/
void setKeyWidthSize(int widthSizeIn);
/*
Sets a scaling factor for different orders of keys.
*/
void setKeyOrderSizeScalar(float scalarIn);
//===============================================================================================
/*
Turns all notes off with the selected midi channel
*/
void allNotesOff();
/*
Turns all notes off except for the last one clicked.
*/
void isolateLastNote();
/*
Will resend a Note On message for all notes currently on.
*/
void retriggerNotes();
/*
Will trigger a midi message for the key number given
*/
void triggerKey(int keyNumberIn, bool doNoteOn = true, float velocity = 1);
/*
Will trigger a midi messages for the key numbers given with the given velocity.
*/
void triggerKeys(Array<int> keyNumbers, bool doNoteOn = true, float velocity = 1);
/*
Returns the order of which all held notes are a part of.
Values less than 0 mean the notes are a part of different orders
*/
int getOrderOfNotesOn();
/*
Transposes the given key (if on) to a certain amount of modal steps.
*/
int transposeKeyModally(int keyNumIn, int stepsIn);
/*
Transposes the given key (if on) to a certain amount of scale degrees.
*/
int transposeKeyChromatically(int keyNumIn, int degreesIn);
/*
Transposes the keys on to a certain amount of modal steps.
If needsSameOrder is true, this will only work if all keys are in the same order.
If it is false, then the keys in higher orders will be transposed the same amount of degrees
of the modal steps of the lowest note if useLastClickedRoot is false, or the last clicked note
if that is true.
*/
void transposeKeysOnModally(int modalStepsIn, bool needsSameOrder=true, bool useLastClickedRoot=false);
/*
Transposes the keys on to a certain amount of scale degrees.
*/
void transposeKeysOnChromatically(int modalStepsIn);
//===============================================================================================
void handleNoteOn(MidiKeyboardState* source, int midiChannel, int midiNote, float velocity) override;
void handleNoteOff(MidiKeyboardState* source, int midiChannel, int midiNote, float velocity) override;
//===============================================================================================
private:
// Functionality
UndoManager* undo;
KeyboardPositioner keyPositioner;
MidiKeyboardState* externalKeyboardToDisplay = nullptr;
NoteMap noteMapOnDisplay;
bool hasDirtyKeys = true;
MidiBuffer buffer;
MappingHelper* manualMappingHelper;
Array<Key*> highlightedKeys;
//Array<Path> keyboardKeysPath;
// Parameters
int uiModeSelected = 0;
int orientationSelected = 0;
int keyPlacementSelected = 1;
int highlightSelected = 1;
int velocitySelected = 1;
int scrollingSelected = 1;
int numRows = 1;
bool showNoteLabels = false;
bool showNoteNumbers = false;
bool showFilteredNoteNums = false;
int midiChannelOut = 1;
float velocityFixed = 1;
bool scaleMidiInputVelocity = false;
float keySizeRatio = 0.25f;
// Data
ValueTree pianoNode;
ValueTree keyTreeNode;
OwnedArray<Key> keys;
Array<Colour> keyColorsOrders;
Array<Colour> keyColorsDegrees;
Array<Colour> keyColorsIndividual;
Array<Colour> keyOnColorsByChannel;
Mode* mode;
Mode modeDefault = Mode(STD_TUNING_MODE_NODE, true);
Array<Colour> colorsDefaultOrders = {
Colours::white,
Colours::black,
Colours::crimson.withSaturation(1.0f),
Colours::cornflowerblue.withSaturation(0.8f),
Colours::mediumseagreen.withSaturation(0.9f),
Colours::gold.withBrightness(0.75f),
Colours::mediumpurple,
Colours::orangered,
Colours::saddlebrown
};
// Properties
int keyWidth = 50;
int keyHeight = 200;
float layerKeysWidthRatio = 0.8f;
float layerKeysHeightRatio = 0.55f;
int lastKeyOver = 0;
int lastKeyClicked = 0;
int numOrder0Keys = 128;
int pianoWidth;
int minWindowHeight;
int modeSize;
Array<Array<int>> keysOrder;
Array<Array<int>> keysScaleDegree;
Array<Array<int>> keysModalDegree;
Array<int> keysOn;
Array<int> keysByMouseTouch;
// Locks
bool rightMouseHeld = false;
bool shiftHeld = false;
bool altHeld = false;
bool ctrlHeld = false;
bool upHeld = false;
bool downHeld = false;
bool leftHeld = false;
bool rightHeld = false;
bool spaceHeld = false;
bool displayIsReady = false;
JUCE_DECLARE_NON_COPYABLE_WITH_LEAK_DETECTOR(Keyboard)
};
}
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
KeyboardViewPort.cpp
Created: 31 Oct 2019 2:41:37pm
Author: Vincenzo Sicurella
==============================================================================
*/
#include "KeyboardViewport.h"
KeyboardViewport::KeyboardViewport(VirtualKeyboard::Keyboard* keyboardIn, const String& nameIn, int scrollingModeIn, int scrollingStyleIn)
: Viewport(nameIn)
{
stepRightLarge.reset(new ImageButton());
stepRightLarge->addListener(this);
addChildComponent(stepRightLarge.get());
stepRightSmall.reset(new ImageButton());
stepRightSmall->addListener(this);
addChildComponent(stepRightSmall.get());
stepLeftLarge.reset(new ImageButton());
stepLeftLarge->addListener(this);
addChildComponent(stepLeftLarge.get());
stepLeftSmall.reset(new ImageButton());
stepLeftSmall->addListener(this);
addChildComponent(stepLeftSmall.get());
setScrollingMode(scrollingModeIn);
setScrollingStyle(scrollingStyleIn);
keyboard = keyboardIn;
setViewedComponent(keyboard);
}
void KeyboardViewport::viewedComponentChanged(Component* newComponent)
{
keyboard = dynamic_cast<Keyboard*>(newComponent);
resizeKeyboard();
}
void KeyboardViewport::visibleAreaChanged(const Rectangle<int>&)
{
if (keyboard->getHeight() > getMaximumVisibleHeight())
{
resizeKeyboard();
}
}
int KeyboardViewport::getStepSmall()
{
return stepSmall;
}
int KeyboardViewport::getStepLarge()
{
return stepLarge;
}
int KeyboardViewport::getButtonWidth()
{
if (isShowingButtons())
return buttonWidth;
return 0;
}
bool KeyboardViewport::isShowingButtons()
{
return scrollingModeSelected > 1;
}
float KeyboardViewport::getCenterKeyProportion() const
{
int center = getViewPositionX() + getMaximumVisibleWidth() / 2;
Key* key = keyboard->getKeyFromPosition({ center, 0 });
if (key)
return key->keyNumber + (center % key->getWidth()) / (float)key->getWidth();
return -1;
}
void KeyboardViewport::setButtonWidth(int widthIn)
{
buttonWidth = widthIn;
}
void KeyboardViewport::setScrollingMode(int modeIdIn)
{
scrollingModeSelected = modeIdIn;
if (scrollingModeSelected > 1)
{
stepRightLarge->setVisible(true);
stepRightSmall->setVisible(true);
stepLeftLarge->setVisible(true);
stepLeftSmall->setVisible(true);
}
else
{
stepRightLarge->setVisible(false);
stepRightSmall->setVisible(false);
stepLeftLarge->setVisible(false);
stepLeftSmall->setVisible(false);
}
}
void KeyboardViewport::setScrollingStyle(int styleIdIn)
{
scrollingStyleSelected = styleIdIn;
// do stuff
}
void KeyboardViewport::stepSmallForward()
{
Viewport::setViewPosition(getViewPositionX() + stepSmall, getViewPositionY());
}
void KeyboardViewport::stepSmallBackward()
{
Viewport::setViewPosition(getViewPositionX() - stepSmall, getViewPositionY());
}
void KeyboardViewport::stepLargeForward()
{
Viewport::setViewPosition(getViewPositionX() + stepLarge, getViewPositionY());
}
void KeyboardViewport::stepLargeBackward()
{
Viewport::setViewPosition(getViewPositionX() - stepLarge, getViewPositionY());
}
void KeyboardViewport::centerOnKey(int keyNumberIn)
{
Key* key = keyboard->getKey(keyNumberIn);
if (key)
{
int position = key->getBoundsInParent().getX() - getMaximumVisibleWidth() / 2;
Viewport::setViewPosition(position, getViewPositionY());
}
}
void KeyboardViewport::centerOnKey(float keyNumberProportionIn)
{
Key* key = keyboard->getKey((int)keyNumberProportionIn);
if (key)
{
int proportion = (keyNumberProportionIn - (int)keyNumberProportionIn) * key->getWidth();
int position = key->getBoundsInParent().getX() + proportion - getMaximumVisibleWidth() / 2;
Viewport::setViewPosition(position, getViewPositionY());
}
}
int KeyboardViewport::drawAngleBracket(Graphics& g, bool isRightPointing, int sideLength, int xCenter, int yCenter, float thickness)
{
float halfSide = sideLength / 2.0f;
float altitude = sqrtf(powf(sideLength, 2.0f) - powf(halfSide, 2.0f));
float halfAltitude = altitude / 2.0f;
int x1 = xCenter - halfAltitude;
int x2 = xCenter + halfAltitude;
int y1 = yCenter + halfSide;
int y2 = yCenter - halfSide;
if (isRightPointing)
{
g.drawLine(Line<float>(x1, y1, x2, yCenter), thickness);
g.drawLine(Line<float>(x1, y2, x2, yCenter), thickness);
}
else
{
g.drawLine(Line<float>(x2, y1, x1, yCenter), thickness);
g.drawLine(Line<float>(x2, y2, x1, yCenter), thickness);
}
return altitude;
}
void KeyboardViewport::redrawButtons(int heightIn)
{
Image singleBracketImage = Image(Image::PixelFormat::ARGB, buttonWidth, heightIn, false);
Image doubleBracketImage = singleBracketImage.createCopy();
Image singleBracketFlipped = singleBracketImage.createCopy();
Image doubleBracketFlipped = singleBracketImage.createCopy();
Colour buttonColour = Colours::steelblue.darker().withSaturation(0.33f);
Colour bracketColour = Colours::black;
Colour mouseOverColor = Colours::lightgrey.withAlpha(0.33f);
Colour mouseClickColor = Colours::darkgrey.withAlpha(0.75f);
int sideLength = round(buttonWidth * 0.33f);
int altitude;
int xCenter = buttonWidth / 2;
int yCenter = getHeight() / 4;
int doubleSeparation = 3;
Graphics g1(singleBracketImage);
g1.setColour(buttonColour);
g1.fillAll();
g1.setColour(bracketColour);
altitude = drawAngleBracket(g1, true, sideLength, xCenter, yCenter, 1);
Graphics g2(doubleBracketImage);
g2.setColour(buttonColour);
g2.fillAll();
g2.setColour(bracketColour);
drawAngleBracket(g2, true, sideLength, xCenter - doubleSeparation, yCenter, 1);
drawAngleBracket(g2, true, sideLength, xCenter + doubleSeparation, yCenter, 1);
Graphics g3(singleBracketFlipped);
g3.setColour(buttonColour);
g3.fillAll();
g3.setColour(bracketColour);
altitude = drawAngleBracket(g3, false, sideLength, xCenter, yCenter, 1);
Graphics g4(doubleBracketFlipped);
g4.setColour(buttonColour);
g4.fillAll();
g4.setColour(bracketColour);
drawAngleBracket(g4, false, sideLength, xCenter - doubleSeparation, yCenter, 1);
drawAngleBracket(g4, false, sideLength, xCenter + doubleSeparation, yCenter, 1);
stepLeftSmall->setImages(false, false, false,
singleBracketImage, 1.0f, Colours::transparentBlack,
singleBracketImage, 1.0f, mouseOverColor,
singleBracketImage, 1.0f, mouseClickColor);
stepLeftLarge->setImages(false, false, false,
doubleBracketImage, 1.0f, Colours::transparentBlack,
doubleBracketImage, 1.0f, mouseOverColor,
doubleBracketImage, 1.0f, mouseClickColor);
stepRightSmall->setImages(false, false, false,
singleBracketFlipped, 1.0f, Colours::transparentBlack,
singleBracketFlipped, 1.0f, mouseOverColor,
singleBracketFlipped, 1.0f, mouseClickColor);
stepRightLarge->setImages(false, false, false,
doubleBracketFlipped, 1.0f, Colours::transparentBlack,
doubleBracketFlipped, 1.0f, mouseOverColor,
doubleBracketFlipped, 1.0f, mouseClickColor);
}
void KeyboardViewport::resizeKeyboard()
{
int heightWithScrollbar = getHeight() - getScrollBarThickness();
keyboard->setBounds(0, 0, keyboard->getPianoWidth(heightWithScrollbar), heightWithScrollbar);
}
void KeyboardViewport::resized()
{
Viewport::resized();
stepSmall = keyboard->getWidth() / keyboard->getKeysByOrder(0).size();
stepLarge = stepSmall * keyboard->getModeSize();
// draw step buttons
if (scrollingModeSelected > 1)
{
int halfHeight = round(getMaximumVisibleHeight() / 2.0f);
redrawButtons(halfHeight);
stepRightLarge->setBounds(0, 0, buttonWidth, halfHeight);
stepRightSmall->setBounds(0, halfHeight, buttonWidth, halfHeight);
stepLeftLarge->setBounds(getWidth() - buttonWidth, 0, buttonWidth, halfHeight);
stepLeftSmall->setBounds(getWidth() - buttonWidth, halfHeight, buttonWidth, halfHeight);
}
}
void KeyboardViewport::buttonClicked(Button* button)
{
if (button == stepRightLarge.get())
{
stepLargeBackward();
}
else if (button == stepRightSmall.get())
{
stepSmallBackward();
}
else if (button == stepLeftLarge.get())
{
stepLargeForward();
}
else if (button == stepLeftSmall.get())
{
stepSmallForward();
}
}
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
VirtualKeyboardGraphics.cpp
Created: 25 Jul 2020 1:49:41am
Author: Vincenzo
==============================================================================
*/
#include "VirtualKeyboardGraphics.h"
VirtualKeyboard::KeyGraphics::KeyGraphics(const Mode& modeIn, int baseKeyHeightIn, int baseKeyWidthIn, float heightLayerMultIn, float widthLayerMultIn)
: mode(modeIn),
baseKeyHeight(baseKeyHeightIn),
baseKeyWidth(baseKeyWidthIn),
heightLayerMult(heightLayerMultIn),
widthLayerMult(widthLayerMultIn)
{
}
void VirtualKeyboard::KeyGraphics::setBaseKeyWidth(int keyWidthIn)
{
baseKeyWidth = keyWidthIn;
}
void VirtualKeyboard::KeyGraphics::setBaseKeyHeight(int keyHeightIn)
{
baseKeyHeight = keyHeightIn;
}
void VirtualKeyboard::KeyGraphics::setWidthMultiplier(float widthMultIn)
{
widthLayerMult = widthMultIn;
}
void VirtualKeyboard::KeyGraphics::setHeightMultiplier(float heightMultIn)
{
heightLayerMult = heightMultIn;
}
void VirtualKeyboard::KeyGraphics::setKeyPlacementStyle(VirtualKeyboard::KeyPlacementType placementTypeIn)
{
keyPlacement = placementTypeIn;
}
Array<Path> VirtualKeyboard::KeyGraphics::getKeyboardOctave(int scaleDegreeOffset)
{
Array<Path> octavePathOut;
int scaleIndex = mode.getRootNote() + scaleDegreeOffset;
for (auto step : mode.getSteps())
{
for (int i = 0; i < step; i++)
{
octavePathOut.add(getKey(scaleIndex++));
}
}
return octavePathOut;
}
Array<Path> VirtualKeyboard::KeyGraphics::getFullKeyboard()
{
Array<Path> keyboardPathOut;
//Array<Path> keyboardPeriod = getKeyboardOctave();
//Array<Path> keyboardBegin = getKeyboardOctave(-(mode.getRootNote() * 2));
//Array<Path> keyboardEnd = getKeyboardOctave(128 - mode.getRootNote() - mode.getScaleSize());
//keyboardPathOut.addArray(keyboardBegin, mode.getRootNote() & mode.getScaleSize());
//
//for (int period = 1; period < 128 / mode.getScaleSize(); period++)
//{
// keyboardPathOut.addArray(keyboardPeriod);
//}
//int endStart = (128 - mode.getRootNote() % mode.getScaleSize());
//keyboardPathOut.addArray(keyboardEnd, endStart, mode.getScaleSize() - endStart);
for (int i = 0; i < 128; i++)
keyboardPathOut.add(getKey(i));
return keyboardPathOut;
}
Path VirtualKeyboard::KeyGraphics::getKey(int keyNumber)
{
int order = mode.getOrders()[keyNumber];
int step = mode.getStepsOfOrders()[keyNumber];
int prevOrder = mode.getOrders()[keyNumber - 1];
int prevStep = mode.getStepsOfOrders()[keyNumber - 1];
int nextOrder = mode.getOrders()[keyNumber + 1];
int nextStep = mode.getStepsOfOrders()[keyNumber + 1];
// get key style
int keyShape = 0;
if (order == 0)
{
// has left notch
if (prevStep > 1)
{
keyShape += VirtualKeyboard::NotchPrevious;
}
// has right notch
if (nextStep > 1)
{
keyShape += VirtualKeyboard::NotchNext;
}
}
// Not a top layer key
else if (order < step - 1)
{
if (keyPlacement == VirtualKeyboard::nestedCenter)
keyShape = VirtualKeyboard::nestedCenter;
else
keyShape += VirtualKeyboard::NotchNext;
}
else
{
// Key is a rectangle
}
float keyWidth = baseKeyWidth - (order * baseKeyWidth * widthLayerMult);
float keyHeight = baseKeyHeight - (order * baseKeyHeight * heightLayerMult);
Array<Point<float>> keyPathPoints;
keyPathPoints.add({ 0, keyHeight });
keyPathPoints.add({ keyWidth, keyHeight });
if (keyShape & VirtualKeyboard::NotchPrevious != 0)
{
float notchWidth = (baseKeyWidth - (prevOrder * baseKeyWidth * widthLayerMult)) / 2.0f;
float prevKeyHeight = baseKeyHeight - (prevOrder * baseKeyHeight * heightLayerMult);
keyPathPoints.insert(0, { 0, prevKeyHeight });
keyPathPoints.insert(0, { notchWidth, prevKeyHeight });
keyPathPoints.insert(0, { notchWidth, 0 });
}
else
{
keyPathPoints.insert(0, { 0, 0 });
}
if (keyShape & VirtualKeyboard::NotchNext != 0)
{
float notchX = keyWidth - (baseKeyWidth - (nextOrder * baseKeyWidth * widthLayerMult)) / 2.0f;
float nextKeyHeight = baseKeyHeight - (nextOrder * baseKeyHeight * heightLayerMult);
keyPathPoints.add({ keyWidth, nextKeyHeight });
keyPathPoints.add({ notchX, nextKeyHeight });
keyPathPoints.add({ notchX, 0 });
}
else
{
keyPathPoints.add({ keyWidth, 0 });
}
if (keyShape == VirtualKeyboard::NotchCenter)
{
float nextKeyWidth = (baseKeyWidth - (nextOrder * baseKeyWidth * widthLayerMult)) / 2.0f;
float notchX0 = (keyWidth - nextKeyWidth) / 2;
float notchX1 = keyWidth - notchX0;
float nextKeyHeight = baseKeyHeight - (nextOrder * baseKeyHeight * heightLayerMult);
keyPathPoints.insert(0, { 0, 0 });
keyPathPoints.insert(0, { notchX0, 0 });
keyPathPoints.insert(0, { notchX0, nextKeyHeight });
keyPathPoints.add({ keyWidth, 0 });
keyPathPoints.add({ notchX1, 0 });
keyPathPoints.add({ notchX1, nextKeyHeight });
}
// Build Path
Path keyPath;
keyPath.startNewSubPath(keyPathPoints.removeAndReturn(0));
for (auto point : keyPathPoints)
{
keyPath.lineTo(point);
}
keyPath.closeSubPath();
return keyPath;
} | {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
VirtualKeyboardKey.cpp
Created: 17 Apr 2019 2:11:53pm
Author: Vincenzo
==============================================================================
*/
#include "VirtualKeyboardKey.h"
using namespace VirtualKeyboard;
Key::Key()
{
setName("Key");
node = ValueTree(IDs::pianoKeyNode);
setDisplayColor(Colours::transparentBlack);
}
Key::Key(int keyNumIn)
: Key()
{
keyNumber = keyNumIn;
}
Key::Key(int keyNumIn, int orderIn, int scaleDegreeIn, int modeDegreeIn, int stepIn,
String pitchNameIn, int widthModIn, int heightModIn, int xOff, int yOff,
bool showNoteNumIn, bool showNoteNameIn, Colour colorIn)
: Key()
{
keyNumber = keyNumIn;
order = orderIn;
scaleDegree = scaleDegreeIn;
modeDegree = modeDegreeIn;
step = stepIn;
pitchName = pitchNameIn;
widthMod = widthModIn;
heightMod = heightModIn;
xOffset = xOff;
yOffset = yOff;
showNoteNumber = showNoteNumIn;
showNoteLabel = showNoteNameIn;
color = colorIn;
}
Key::Key(ValueTree keyNodeIn)
: Key()
{
if (keyNodeIn.hasType(IDs::pianoKeyNode))
{
applyParameters(keyNodeIn);
node = keyNodeIn;
}
}
Key::Key(const Key& keyToCopy)
: Key(keyToCopy.keyNumber, keyToCopy.order, keyToCopy.scaleDegree, keyToCopy.modeDegree, keyToCopy.step,
keyToCopy.pitchName, keyToCopy.widthMod, keyToCopy.heightMod, keyToCopy.xOffset, keyToCopy.yOffset,
keyToCopy.showNoteNumber, keyToCopy.showNoteLabel, keyToCopy.color)
{
node = keyToCopy.node.createCopy();
}
void Key::setPath(Path keyPathIn)
{
keyPath = keyPathIn;
}
void Key::applyParameters(ValueTree nodeIn)
{
if (nodeIn.hasType(IDs::pianoKeyNode))
{
Identifier id;
for (int i = 0; i < nodeIn.getNumProperties(); i++)
{
id = nodeIn.getPropertyName(i);
if (id == IDs::pianoKeyNumber)
keyNumber = nodeIn.getProperty(id);
else if (id == IDs::pianoKeyWidthMod)
widthMod = nodeIn.getProperty(id);
else if (id == IDs::pianoKeyHeightMod)
heightMod = nodeIn.getProperty(id);
else if (id == IDs::pianoKeyXOffset)
xOffset = nodeIn.getProperty(id);
else if (id == IDs::pianoKeyYOffset)
yOffset = nodeIn.getProperty(id);
else if (id == IDs::pianoKeyShowNumber)
showNoteNumber = nodeIn.getProperty(id);
else if (id == IDs::keyboardShowsNoteLabels)
showNoteLabel = nodeIn.getProperty(id);
else if (id == IDs::pianoKeyColorsNode)
color = Colour::fromString(nodeIn.getProperty(id).toString());
}
node.copyPropertiesAndChildrenFrom(nodeIn, nullptr);
}
}
Colour Key::getDisplayColor() const
{
return color;
}
void Key::paint(Graphics& g)
{
g.fillAll(color);
Colour colorToUse = color;
if (isClicked || isMouseOver() || exPressed)
{
float contrast = 0;
if (isClicked)
contrast = 0.7f;
else if (isMouseOver())
contrast = 0.25f;
if (exPressed)
colorToUse = exInputColor;
else
colorToUse = color.contrasting(contrast);
if (colorToUse.getPerceivedBrightness() < 0.6667f)
g.setColour(colorToUse.contrasting(0.333f));
g.setColour(colorToUse);
// Full or Inside
if (highlightStyleId < 3)
{
Rectangle<int> bounds = getLocalBounds();
if (highlightStyleId == 2)
{
int margin = sqrt(getWidth() * getHeight()) * 0.07;
bounds.reduce(margin, margin);
}
g.fillRect(bounds);
}
// Border
else if (highlightStyleId == 3)
{
float margin = sqrt(getWidth() * getHeight()) * 0.07f;
g.drawRect(getLocalBounds(), margin);
}
else
{
float margin = sqrt(getWidth() * getHeight()) * 0.05f;
float boundsWidth = getWidth() - margin * 2;
Rectangle<float> shapeBounds = { margin, getHeight() - margin - boundsWidth, boundsWidth, boundsWidth };
// Circle
if (highlightStyleId == 4)
g.fillEllipse(shapeBounds);
// Square
else
g.fillRect(shapeBounds);
}
}
if (order == 0)
{
if (colorToUse.getPerceivedBrightness() < 0.6667f)
g.setColour(colorToUse.contrasting(0.333f));
else
g.setColour(Colours::black);
Rectangle<int> outline = getLocalBounds();
g.drawRect(outline, 1.0f);
}
if (showNoteNumber)
{
g.setColour(colorToUse.contrasting());
String numTxt = String(keyNumber);
Rectangle<int> txtArea = getLocalBounds();
txtArea.removeFromBottom(6);
g.drawText(numTxt, txtArea, Justification::centredBottom);
}
isDirty = false;
}
void Key::setDisplayColor(Colour colorIn)
{
color = colorIn;
node.setProperty(IDs::pianoKeyColorsNode, color.toString(), nullptr);
repaint();
}
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
VirtualKeyboardGraphics.h
Created: 25 Jul 2020 1:49:41am
Author: Vincenzo
==============================================================================
*/
#pragma once
#include <JuceHeader.h>
#include "../../../Structures/Mode.h"
#include "../../../PluginModes.h"
namespace VirtualKeyboard
{
class KeyGraphics
{
public:
KeyGraphics(const Mode& modeIn, int baseKeyHeightIn, int baseKeyWidthIn, float heightLayerMultIn, float widthLayerMultIn);
void setBaseKeyWidth(int keyWidthIn);
void setBaseKeyHeight(int keyHeightIn);
void setWidthMultiplier(float widthMultIn);
void setHeightMultiplier(float heightMultIn);
void setKeyPlacementStyle(VirtualKeyboard::KeyPlacementType placementTypeIn);
Array<Path> getKeyboardOctave(int scaleDegreeOffset = 0);
Array<Path> getFullKeyboard();
Path getKey(int keyNumber);
private:
const Mode& mode;
int baseKeyHeight;
int baseKeyWidth;
float heightLayerMult;
float widthLayerMult;
VirtualKeyboard::KeyPlacementType keyPlacement = VirtualKeyboard::nestedRight;
};
} | {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
VirtualKeyboardGrid.h
Created: 17 Apr 2019 2:12:23pm
Author: Vincenzo
==============================================================================
*/
#pragma once
#include "../../../PluginModes.h"
#include "../../../Structures/FractionalGrid.h"
#include "../../../Structures/Mode.h"
#include "VirtualKeyboardKey.h"
namespace VirtualKeyboard
{
class KeyboardPositioner
{
Component* parent;
int numModeKeys;
int keyGap = 0;
int keyPlacement = 1;
float baseWidthRatio = 0.8f;
float baseHeightRatio = 0.55f;
float keyWidth = 0;
public:
KeyboardPositioner(Component* parentIn, int numModeKeysIn = 128);
~KeyboardPositioner() {};
void parentResized();
float getKeyWidth() const;
int getKeyGap() const;
void setNumModeKeys(int numKeysIn);
void setKeyGap(int keyGapIn);
void setKeyPlacement(int keyPlacementTypeIn);
void setLayerKeysWidthRatio(float widthRatioIn);
void setLayerKeysHeightRatio(float widthRatioIn);
void resizeKey(Key& key);
void placeKey(Key& key);
};
}
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
VirtualKeyboardKey.h
Created: 17 Apr 2019 2:11:53pm
Author: Vincenzo
Struct for holding virtual keyboard key data.
==============================================================================
*/
#pragma once
#include "../JuceLibraryCode/JuceHeader.h"
#include "../../../PluginIDs.h"
#include "../../../PluginModes.h"
namespace VirtualKeyboard
{
class Key : public Component
{
public:
Key();
Key(int keyNumIn);
Key(int keyNumIn, int orderIn, int scaleDegreeIn, int modeDegreeIn, int stepIn,
String pitchNameIn = "", int widthModIn = 0, int heightModIn = 0, int xOff = 0, int yOff = 0,
bool showNoteNumIn = false, bool showNoteNameIn = false, Colour colorIn = Colours::transparentBlack);
Key(ValueTree keyNodeIn);
Key(const Key& keyToCopy);
Colour getDisplayColor() const;
void setDisplayColor(Colour colorIn);
void applyParameters(ValueTree nodeIn);
void setPath(Path keyPathIn);
void paint(juce::Graphics& g) override;
ValueTree node;
Path keyPath;
// Properties
int keyNumber = 0;
int order = 0; // front most keys are 0, "black" keys are 1, etc
int scaleDegree = 0; // corresponds to chromatic degree in the period
float modeDegree = 0; // fractional degree. in Meantone[7] in 12edo set in C, F# would be 3.5
int step = 0; // the interval step that this note is a part of
float velocity = 0; // recorded when key is clicked
bool isClicked = false; // clicked by mouse
bool exPressed = false; // pressed by midi input
Colour exInputColor; // for midi controller input, will use this color if it's opaque
bool isDirty = true;
// Parameters
String pitchName = "";
float widthMod = -1;
float heightMod = -1;
float xOffset = 0;
float yOffset = 0;
bool showNoteNumber = false;
bool showNoteLabel = false;
float velocityFixed = 1;
Colour color = Colours::transparentBlack;
int highlightStyleId = HighlightStyle::full;
};
}
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
VirtualKeyboard.h
Created: 14 Mar 2019 4:50:31pm
Author: Vincenzo
==============================================================================
*/
#include "../../../../JuceLibraryCode/JuceHeader.h"
#include "KeyboardComponent.h"
using namespace VirtualKeyboard;
Keyboard::Keyboard()
: keyPositioner(this)
{
setName("VirtualKeyboard");
addMouseListener(this, true);
setWantsKeyboardFocus(true);
initializeKeys();
reset();
setSize(1000, 250);
setOpaque(true);
}
Keyboard::Keyboard(ValueTree keyboardNodeIn, Mode* modeIn, NoteMap* inputFilterMapIn)
: keyPositioner(this)
{
setName("VirtualKeyboard");
addMouseListener(this, true);
setWantsKeyboardFocus(true);
initializeKeys(); // todo: load keyboard size
restoreNode(keyboardNodeIn, true, true);
if (modeIn)
mode = modeIn;
applyMode(mode);
setSize(1000, 250);
setOpaque(true);
}
Keyboard::~Keyboard()
{
if (externalKeyboardToDisplay)
externalKeyboardToDisplay->removeListener(this);
}
//===============================================================================================
void Keyboard::restoreNode(ValueTree pianoNodeIn, bool reinitializeKeys, bool resetIfInvalid)
{
if (pianoNodeIn.hasType(IDs::pianoNode))
{
pianoNode = pianoNodeIn;
DBG("RESTORING KEYBOARD NODE: " + pianoNode.toXmlString());
showNoteNumbers = pianoNode[IDs::pianoKeysShowNoteNumbers];
orientationSelected = pianoNode[IDs::keyboardOrientation];
keyPlacementSelected = pianoNode[IDs::keyboardKeysStyle];
highlightSelected = pianoNode[IDs::keyboardHighlightStyle];
lastKeyClicked = pianoNode[IDs::pianoLastKeyClicked];
keySizeRatio = (float) pianoNode[IDs::pianoWHRatio];
keyPositioner.setKeyPlacement(keyPlacementSelected);
// safeguard
if (keySizeRatio < 0.01)
keySizeRatio = 0.25f;
if (reinitializeKeys)
{
// TODO: keyboard key editing
pianoNode.removeChild(pianoNode.getChildWithName(IDs::pianoKeyTreeNode), nullptr);
// unpack key data
//keyTreeNode = pianoNode.getOrCreateChildWithName(IDs::pianoKeyTreeNode, nullptr);
//if (keyTreeNode.getNumChildren() == keys.size())
//{
// for (auto key : keys)
// key->applyParameters(keyTreeNode.getChild(key->keyNumber));
//}
//else
//{
// keyTreeNode.removeAllChildren(nullptr);
initializeKeys();
//for (auto key : keys)
//{
// keyTreeNode.addChild(key->node, key->keyNumber, nullptr);
//}
//}
}
}
else
{
DBG("Invalid Piano Node.");
if (resetIfInvalid)
{
reset();
DBG("Keyboard is reset.");
}
}
}
void Keyboard::reset()
{
pianoNode = ValueTree(IDs::pianoNode);
setUIMode(UIMode::playMode);
setOrientation(Orientation::horizontal);
setKeyStyle(KeyPlacementType::nestedRight);
setHighlightStyle(HighlightStyle::full);
setVelocityBehavior(VelocityStyle::linear);
setScrollingStyle(ScrollingStyle::smooth);
setShowNoteLabels(false);
setShowNoteNumbers(false);
setShowFilteredNumbers(false);
setMidiChannelOut(1);
setVelocityFixed(1);
setInputVelocityScaled(false);
setKeySizeRatio(0.25f);
setKeyOrderSizeScalar(1);
keyOnColorsByChannel.resize(16);
keyOnColorsByChannel.fill(Colour());
mode = &modeDefault;
applyMode(mode);
}
void Keyboard::initializeKeys(int size)
{
keys.clear();
for (int i = 0; i < size; i++)
{
Key* key = keys.add(new Key(i));
addAndMakeVisible(key);
key->showNoteNumber = showNoteNumbers; // hacky ?
key->highlightStyleId = highlightSelected;
}
keyColorsIndividual.resize(keys.size());
keysOn = Array<int>();
keysByMouseTouch = Array<int>();
}
//===============================================================================================
void Keyboard::paint(juce::Graphics& g)
{
g.fillAll(getLookAndFeel().findColour(ResizableWindow::backgroundColourId));
}
void Keyboard::paintOverChildren(juce::Graphics& g)
{
g.setColour(Colours::black);
g.drawRect(getLocalBounds(), 1);
}
void Keyboard::resized()
{
keyPositioner.parentResized();
keyWidth = keyPositioner.getKeyWidth();
keyHeight = getHeight();
keyPositioner.setLayerKeysWidthRatio(layerKeysWidthRatio);
keyPositioner.setLayerKeysHeightRatio(layerKeysHeightRatio);
for (int i = 0; i < keys.size(); i++)
{
Key* key = keys[i];
keyPositioner.resizeKey(*key);
}
float keyOrder1Width = keyWidth * layerKeysWidthRatio;
float adjacentStyleStepWidth;
// Place Keys
Array<int>& modeKeys = keysOrder.getReference(0);
for (int i = 0; i < numOrder0Keys; i++)
{
Key& modeKey = *keys[modeKeys[i]];
int keyX = (keyWidth + keyPositioner.getKeyGap() - 1) * i;
modeKey.setTopLeftPosition(keyX, 0);
if (modeKey.step > 1)
{
adjacentStyleStepWidth = keyOrder1Width / (modeKey.step - 1);
for (int s = 1; s < modeKey.step; s++)
{
Key* stepKey = keys[modeKey.keyNumber + s];
if (s > 1 && keyPlacementSelected == KeyPlacementType::nestedRight)
{
keyX = modeKey.getX() + keyWidth + (keys[modeKey.keyNumber + 1]->getWidth() / 2) - stepKey->getWidth();
}
else if (stepKey->step > 2 && keyPlacementSelected == KeyPlacementType::adjacent)
{
keyX = modeKey.getX() + keyWidth - (keyOrder1Width / 2) + (stepKey->order - 1) * adjacentStyleStepWidth;
}
else
{
keyX = modeKey.getX() + keyWidth - stepKey->getWidth() / 2;
}
stepKey->setTopLeftPosition(keyX, 0);
}
}
}
}
//===============================================================================================
void Keyboard::mouseMove(const MouseEvent& e)
{
Key* key = getKeyFromPositionMouseEvent(e);
if (uiModeSelected != UIMode::editMode)
{
if (key)
{
lastKeyOver = key->keyNumber;
}
}
else
{
if (key)
{
lastKeyOver = key->keyNumber;
setMouseCursor(MouseCursor::CrosshairCursor);
}
else
{
setMouseCursor(MouseCursor::NormalCursor);
}
}
}
void Keyboard::mouseExit(const MouseEvent& e)
{
if (uiModeSelected != UIMode::editMode)
{
int touchIndex = e.source.getIndex();
if (keysByMouseTouch[touchIndex] > 0)
{
Key* key = keys[keysByMouseTouch[touchIndex]];
if (!e.source.isDragging())
{
triggerKey(key->keyNumber, false);
keysByMouseTouch.set(touchIndex, -1);
}
}
Key* key = keys[lastKeyOver];
key->repaint();
}
}
void Keyboard::mouseDown(const MouseEvent& e)
{
Key* key = getKeyFromPositionMouseEvent(e);
bool triggerNote = false;
if (uiModeSelected == UIMode::editMode)
{
if (key)
{
if (e.mods.isRightButtonDown() || getProperties()[IDs::pianoKeyColorReset])
{
if (e.mods.isShiftDown())
keyColorsOrders.set(key->order, Colour());
else if (e.mods.isCtrlDown())
keyColorsIndividual.set(key->keyNumber, Colour());
else
{
if (keyColorsIndividual[key->keyNumber].isOpaque())
keyColorsIndividual.set(key->keyNumber, Colour());
keyColorsDegrees.set(key->scaleDegree, Colour());
}
}
else if (e.mods.isCtrlDown() || (int)getProperties()[IDs::pianoKeyPaintType] == 2)
{
keyColorsIndividual.set(key->keyNumber, Colour::fromString(getProperties()[IDs::colorSelected].toString()));
}
else if (e.mods.isShiftDown() || (int)getProperties()[IDs::pianoKeyPaintType] == 1)
{
if (getKeyDegreeColor(key->scaleDegree).isOpaque())
keyColorsDegrees.set(key->scaleDegree, Colour());
else if (getKeyColor(key->keyNumber).isOpaque())
keyColorsIndividual.set(key->keyNumber, Colour());
keyColorsOrders.set(key->order, Colour::fromString(getProperties()[IDs::colorSelected].toString()));
}
else
{
if (keyColorsIndividual[key->keyNumber].isOpaque())
keyColorsIndividual.set(key->keyNumber, Colour());
keyColorsDegrees.set(key->scaleDegree, Colour::fromString(getProperties()[IDs::colorSelected].toString()));
}
updateKeyColors();
}
}
else if (uiModeSelected == UIMode::mapMode)
{
if (key)
{
if (e.mods.isRightButtonDown())
{
bool allPeriods = true;
if (e.mods.isCtrlDown())
allPeriods = false;
manualMappingHelper->prepareKeyToMap(key->keyNumber, false);
highlightKey(key->keyNumber);
DBG("Preparing to map key: " + String(key->keyNumber));
}
else
{
triggerNote = true;
}
}
if (!key || !isMouseOver(true))
{
highlightKey(manualMappingHelper->getVirtualKeyToMap(), false);
manualMappingHelper->cancelKeyMap();
}
}
if (uiModeSelected == UIMode::playMode || triggerNote)
{
if (key)
{
if (e.mods.isShiftDown() && !e.mods.isAltDown() && key->isClicked)
{
// note off
lastKeyClicked = key->keyNumber;
triggerKey(key->keyNumber, false);
}
else
{
// need to implement a velocity class
triggerKey(key->keyNumber);
lastKeyClicked = key->keyNumber;
keysByMouseTouch.set(e.source.getIndex(), key->keyNumber);
if (e.mods.isAltDown())
{
isolateLastNote();
}
}
}
}
}
void Keyboard::mouseDrag(const MouseEvent& e)
{
if (uiModeSelected != UIMode::editMode && !e.mods.isRightButtonDown())
{
Key* key = getKeyFromPositionMouseEvent(e);
if (key)
{
//bool alreadyDown = false;
int touchIndex = e.source.getIndex();
int keyIndex = keysByMouseTouch[touchIndex];
if (key->keyNumber != keyIndex)
{
if (!e.mods.isShiftDown())
triggerKey(keyIndex, false);
triggerKey(key->keyNumber);
lastKeyClicked = key->keyNumber;
keysByMouseTouch.set(touchIndex, key->keyNumber);
}
}
}
}
void Keyboard::mouseUp(const MouseEvent& e)
{
if (uiModeSelected != UIMode::editMode)
{
int touchIndex = e.source.getIndex();
int keyIndex = keysByMouseTouch[touchIndex];
if (keyIndex > 0)
{
Key* key = keys[keyIndex];
if (key)// && mappingHelper->getVirtualKeyToMap() != key->keyNumber)
{
keysByMouseTouch.set(touchIndex, -1);
if (!shiftHeld)
{
triggerKey(key->keyNumber, false);
}
}
}
}
}
//===============================================================================================
bool Keyboard::keyStateChanged(bool isKeyDown)
{
if (!KeyPress::isKeyCurrentlyDown(KeyPress::upKey) && upHeld)
{
upHeld = false;
return true;
}
if (!KeyPress::isKeyCurrentlyDown(KeyPress::downKey) && downHeld)
{
downHeld = false;
return true;
}
if (!KeyPress::isKeyCurrentlyDown(KeyPress::leftKey) && leftHeld)
{
leftHeld = false;
return true;
}
if (!KeyPress::isKeyCurrentlyDown(KeyPress::rightKey) && rightHeld)
{
rightHeld = false;
return true;
}
if (!KeyPress::isKeyCurrentlyDown(KeyPress::spaceKey) && spaceHeld)
{
spaceHeld = false;
return true;
}
return false;
}
bool Keyboard::keyPressed(const KeyPress& key)
{
if (KeyPress::isKeyCurrentlyDown(KeyPress::upKey) && !upHeld)
{
upHeld = true;
if (uiModeSelected != UIMode::editMode)
{
if (shiftHeld && keysOn.size() > 0)
{
transposeKeysOnChromatically(1);
repaint();
}
}
return true;
}
if (KeyPress::isKeyCurrentlyDown(KeyPress::downKey) && !downHeld)
{
downHeld = true;
if (uiModeSelected != UIMode::editMode)
{
if (shiftHeld && keysOn.size() > 0)
{
transposeKeysOnChromatically(-1);
repaint();
}
}
return true;
}
if (KeyPress::isKeyCurrentlyDown(KeyPress::leftKey) && !leftHeld)
{
leftHeld = true;
if (uiModeSelected != UIMode::editMode)
{
if (shiftHeld && keysOn.size() > 0)
{
transposeKeysOnModally(-1);
}
}
return true;
}
if (KeyPress::isKeyCurrentlyDown(KeyPress::rightKey) && !rightHeld)
{
rightHeld = true;
if (uiModeSelected != UIMode::editMode)
{
if (shiftHeld && keysOn.size() > 0)
{
transposeKeysOnModally(1);
}
}
return true;
}
if (KeyPress::isKeyCurrentlyDown(KeyPress::spaceKey) && !spaceHeld)
{
spaceHeld = true;
if (uiModeSelected != UIMode::editMode)
{
if (shiftHeld && keysOn.size() > 0)
{
retriggerNotes();
}
}
return true;
}
return false;
}
void Keyboard::modifierKeysChanged(const ModifierKeys& modifiers)
{
if (!rightMouseHeld && modifiers.isRightButtonDown())
{
rightMouseHeld = true;
}
if (rightMouseHeld && !modifiers.isRightButtonDown())
{
rightMouseHeld = false;
}
if (!shiftHeld && modifiers.isShiftDown())
{
shiftHeld = true;
}
if (shiftHeld && !modifiers.isShiftDown())
{
shiftHeld = false;
if (uiModeSelected != UIMode::editMode)
{
isolateLastNote();
Key* key = keys[lastKeyClicked];
if (!(key->isMouseOver() && key->isMouseButtonDownAnywhere()))
triggerKey(lastKeyClicked, false);
}
}
if (!altHeld && modifiers.isAltDown())
{
altHeld = true;
if (uiModeSelected != UIMode::editMode)
{
isolateLastNote();
repaint();
}
}
else if (altHeld && !modifiers.isAltDown())
{
altHeld = false;
}
if (!ctrlHeld && modifiers.isCtrlDown())
{
ctrlHeld = true;
}
else if (ctrlHeld && !modifiers.isCtrlDown())
{
ctrlHeld = false;
}
}
//===============================================================================================
//void Keyboard::timerCallback()
//{
//if (hasDirtyKeys)
//{
// for (auto key : *keys.get())
// {
// if (key->isDirty)
// {
// key->repaint();
// }
// }
//}
//hasDirtyKeys = false;
//}
//===============================================================================================
ValueTree Keyboard::getNode()
{
return pianoNode;
}
Key* Keyboard::getKey(int keyNumIn)
{
return keys[keyNumIn];
}
ValueTree Keyboard::getKeyNode(int keyNumIn)
{
return keys[keyNumIn]->node;
}
Array<Key*> Keyboard::getKeysByOrder(int orderIn)
{
Array<Key*> keysOut;
Array<int> orderArray = keysOrder[orderIn];
for (int i = 0; i < orderArray.size(); i++)
{
keysOut.add(keys[orderArray[i]]);
}
return keysOut;
}
Array<Key*> Keyboard::getKeysByScaleDegree(int degreeIn)
{
Array<Key*> keysOut;
Array<int> degreeArray = keysScaleDegree[degreeIn];
for (int i = 0; i < degreeArray.size(); i++)
{
keysOut.add(keys[degreeArray[i]]);
}
return keysOut;
}
Array<Key*> Keyboard::getKeysByModalDegree(int degreeIn)
{
Array<Key*> keysOut;
Array<int> degreeArray = keysModalDegree[degreeIn];
for (int i = 0; i < degreeArray.size(); i++)
{
keysOut.add(keys[degreeArray[i]]);
}
return keysOut;
}
int Keyboard::getLastKeyClicked()
{
return lastKeyClicked;
}
Rectangle<int> Keyboard::getKeyArea(int midiNoteIn)
{
// TODO
return Rectangle<int>();
}
Rectangle<int> Keyboard::getKeyAreaRelative(int midiNoteIn)
{
// TODO
return Rectangle<int>();
}
int Keyboard::getKeyWidth()
{
return keyWidth;
}
int Keyboard::getKeyHeight()
{
return keyHeight;
}
int Keyboard::getPianoWidth(int heightIn)
{
return numOrder0Keys * (heightIn * keySizeRatio + keyPositioner.getKeyGap() - 1);
}
float Keyboard::getKeySizeRatio()
{
return keySizeRatio;
}
Point<int> Keyboard::getKeyOrderSize(int orderIn)
{
//TODO (use the scalar)
return Point<int>();
}
Key* Keyboard::getKeyFromPosition(Point<int> posIn)
{
Key* keyOut = nullptr;
Component* c = getComponentAt(posIn);
if (c != nullptr && c->getName().startsWith("Key"))
keyOut = dynamic_cast<Key*>(c);
return keyOut;
}
Key* Keyboard::getKeyFromPositionMouseEvent(const MouseEvent& e)
{
Point<int> ep = Point<int>(e.getScreenX() - getScreenX(), e.getPosition().getY());
return getKeyFromPosition(ep);
}
float Keyboard::getKeyVelocity(Key* keyIn, Point<int> posIn)
{
//TODO
return velocityFixed;
}
int Keyboard::getMidiChannelOut() const
{
return midiChannelOut;
}
int Keyboard::getModeSize() const
{
return mode->getModeSize();
}
//===============================================================================================
int Keyboard::getUIMode()
{
return uiModeSelected;
}
int Keyboard::getOrientation()
{
return orientationSelected;
}
int Keyboard::getKeyPlacementStyle()
{
return keyPlacementSelected;
}
bool Keyboard::isShowingNoteNumbers()
{
return showNoteNumbers;
}
bool Keyboard::isShowingFilteredNumbers()
{
return showFilteredNoteNums;
}
bool Keyboard::isShowingNoteNames()
{
return showNoteLabels;
}
int Keyboard::getHighlightStyle()
{
return highlightSelected;
}
int Keyboard::getVelocityStyle()
{
return velocitySelected;
}
float Keyboard::getVelocityFixed()
{
return velocityFixed;
}
bool Keyboard::isInputVelocityScaled()
{
return scaleMidiInputVelocity;
}
int Keyboard::getScrollingStyle()
{
return scrollingSelected;
}
//===============================================================================================
void Keyboard::applyMode(Mode* modeIn, bool resize)
{
mode = modeIn;
if (mode == nullptr)
mode = &modeDefault;
keysOrder.clear();
keysOrder.resize(mode->getMaxStep());
int period = 0;
for (int i = 0; i < keys.size(); i++)
{
Key* key = keys[i];
period = i / mode->getScaleSize();
key->order = mode->getOrder(i);
key->scaleDegree = mode->getScaleDegree(i);
key->modeDegree = mode->getModeDegree(i);
key->step = mode->getNoteStep(i);
keysOrder.getReference(key->order).add(key->keyNumber);
}
numOrder0Keys = keysOrder.getReference(0).size();
keyPositioner.setNumModeKeys(numOrder0Keys);
keysScaleDegree = mode->getNotesInScaleDegrees();
keysModalDegree = mode->getNotesInModalDegrees();
// add from back to front
for (int o = 0; o < keysOrder.size(); o++)
{
Array<int>& orderArray = keysOrder.getReference(o);
for (int keyNum = 0; keyNum < orderArray.size(); keyNum++)
{
Key* key = keys[(orderArray.getReference(keyNum))];
//addAndMakeVisible(key);
key->toFront(false);
}
}
keyColorsOrders.resize(mode->getMaxStep());
keyColorsDegrees.resize(mode->getScaleSize());
// unpack color data
ValueTree keyColorsNode = mode->modeNode.getOrCreateChildWithName(IDs::pianoKeyColorsNode, nullptr);
keyColorsOrders.fill(Colour());
for (auto layerColor : keyColorsNode.getChildWithName(IDs::pianoKeyColorsLayer))
{
keyColorsOrders.set(
layerColor[IDs::pianoKeyColorsLayer],
Colour::fromString(layerColor[IDs::pianoKeyColor].toString())
);
}
keyColorsDegrees.fill(Colour());
for (auto degreeColor : keyColorsNode.getChildWithName(IDs::pianoKeyColorsDegree))
{
keyColorsDegrees.set(
degreeColor[IDs::pianoKeyColorsDegree],
Colour::fromString(degreeColor[IDs::pianoKeyColor].toString())
);
}
keyColorsIndividual.fill(Colour());
for (auto keyColor : keyColorsNode.getChildWithName(IDs::pianoKeyColorsIndividual))
{
keyColorsIndividual.set(
mode->fixedDegreeToNoteNumber(keyColor[IDs::pianoKeyColorsIndividual]),
Colour::fromString(keyColor[IDs::pianoKeyColor].toString())
);
}
keyOnColorsByChannel.fill(Colour());
for (auto noteOnColor : keyColorsNode.getChildWithName(IDs::pianoKeyColorsNoteOn))
{
keyOnColorsByChannel.set(
noteOnColor[IDs::pianoKeyColorsNoteOn],
Colour::fromString(noteOnColor[IDs::pianoKeyColor].toString())
);
}
updateKeyColors();
if (resize)
resized();
else
hasDirtyKeys = true;
}
void Keyboard::applyKeyData(ValueTree keyDataTreeIn)
{
ValueTree keyNode;
int numKeys = jmin(keys.size(), keyDataTreeIn.getNumChildren());
for (int i = 0; i < numKeys; i++)
{
Key* key = keys[i];
key->applyParameters(keyDataTreeIn.getChild(i));
}
}
void Keyboard::displayKeyboardState(MidiKeyboardState* keyboardStateIn)
{
if (externalKeyboardToDisplay)
externalKeyboardToDisplay->removeListener(this);
externalKeyboardToDisplay = keyboardStateIn;
if (externalKeyboardToDisplay)
externalKeyboardToDisplay->addListener(this);
}
//===============================================================================================
void Keyboard::setUIMode(int uiModeIn)
{
if (uiModeSelected == UIMode::editMode && uiModeIn != uiModeSelected)
updateKeyColors();
uiModeSelected = uiModeIn;
pianoNode.setProperty(IDs::pianoUIMode, uiModeSelected, nullptr);
}
void Keyboard::setOrientation(int orientationIn)
{
orientationSelected = orientationIn;
pianoNode.setProperty(IDs::keyboardOrientation, orientationSelected, nullptr);
// do stuff
}
void Keyboard::setNumRows(int numRowsIn)
{
numRows = jlimit(1, 16, numRowsIn);
pianoNode.setProperty(IDs::keyboardNumRows, numRows, nullptr);
applyMode(mode);
}
void Keyboard::setKeyStyle(int placementIn)
{
keyPlacementSelected = placementIn;
keyPositioner.setKeyPlacement(keyPlacementSelected);
pianoNode.setProperty(IDs::keyboardKeysStyle, keyPlacementSelected, nullptr);
}
void Keyboard::setHighlightStyle(int styleIn)
{
highlightSelected = styleIn;
pianoNode.setProperty(IDs::keyboardHighlightStyle, highlightSelected, nullptr);
for (auto key : keys)
{
key->highlightStyleId = highlightSelected;
key->repaint();
}
}
void Keyboard::setVelocityBehavior(int behaviorNumIn, bool scaleInputVelocity)
{
velocitySelected = behaviorNumIn;
pianoNode.setProperty(IDs::pianoVelocityBehavior, velocitySelected, nullptr);
scaleMidiInputVelocity = scaleInputVelocity;
pianoNode.setProperty(IDs::pianoVelocityScaleInput, scaleMidiInputVelocity, nullptr);
}
void Keyboard::setScrollingStyle(int scrollingStyleIn)
{
scrollingSelected = scrollingStyleIn;
pianoNode.setProperty(IDs::keyboardScrollingStyle, scrollingSelected, nullptr);
// do stuff?
}
void Keyboard::setMidiChannelOut(int midiChannelOutIn)
{
midiChannelOut = jlimit(1, 16, midiChannelOutIn);
pianoNode.setProperty(IDs::keyboardMidiChannel, midiChannelOut, nullptr);
}
void Keyboard::setVelocityFixed(float velocityIn)
{
velocityFixed = velocityIn;
pianoNode.setProperty(IDs::pianoVelocityValue, velocityFixed, nullptr);
}
void Keyboard::setInputVelocityScaled(bool shouldBeScaled)
{
scaleMidiInputVelocity = shouldBeScaled;
pianoNode.setProperty(IDs::pianoVelocityScaleInput, scaleMidiInputVelocity, nullptr);
}
void Keyboard::setShowNoteNumbers(bool shouldShowNumbers)
{
showNoteNumbers = shouldShowNumbers;
pianoNode.setProperty(IDs::pianoKeysShowNoteNumbers, showNoteNumbers, nullptr);
for (int i = 0; i < keys.size(); i++)
{
Key* key = keys[i];
key->showNoteNumber = shouldShowNumbers;
key->repaint();
}
}
void Keyboard::setShowFilteredNumbers(bool shouldShowNumbers)
{
showFilteredNoteNums = shouldShowNumbers;
pianoNode.setProperty(IDs::pianoKeysShowFilteredNotes, showFilteredNoteNums, nullptr);
}
void Keyboard::setShowNoteLabels(bool shouldShowPitchNames)
{
showNoteLabels = shouldShowPitchNames;
pianoNode.setProperty(IDs::keyboardShowsNoteLabels, showNoteLabels, nullptr);
}
void Keyboard::setLastKeyClicked(int keyNumIn)
{
lastKeyClicked = keyNumIn;
pianoNode.setProperty(IDs::pianoLastKeyClicked, lastKeyClicked, nullptr);
}
void Keyboard::setInputNoteMap(NoteMap& noteMapIn)
{
noteMapOnDisplay = noteMapIn;
}
//===============================================================================================
void Keyboard::setMappingHelper(MappingHelper* mappingHelperIn)
{
manualMappingHelper = mappingHelperIn;
}
void Keyboard::highlightKey(int keyNumberIn, bool highlightOn)
{
if (keyNumberIn < 0 || keyNumberIn >= keys.size())
return;
if (highlightOn)
highlightedKeys.add(keys[keyNumberIn]);
else
highlightedKeys.removeAllInstancesOf(keys[keyNumberIn]);
}
//===============================================================================================
Array<Colour>* Keyboard::getKeyIndividualColours()
{
return &keyColorsIndividual;
}
Colour Keyboard::getKeyColor(int keyNumIn)
{
Colour c;
if (keyNumIn >= 0 && keyNumIn < keys.size())
{
Key* key = keys[keyNumIn];
if (keyColorsIndividual[key->keyNumber].isOpaque()) // need to fix this for switching modes
c = keyColorsIndividual[key->keyNumber];
else if (keyColorsDegrees[key->scaleDegree].isOpaque())
c = keyColorsDegrees[key->scaleDegree];
else if (keyColorsOrders[key->order].isOpaque())
c = keyColorsOrders[key->order];
else
c = colorsDefaultOrders[key->order];
}
return c;
}
Array<Colour>* Keyboard::getKeyLayerColours()
{
return &keyColorsOrders;
}
Colour Keyboard::getKeyLayerColor(int orderIn)
{
Colour c;
if (keyColorsOrders[orderIn].isOpaque())
c = keyColorsOrders[orderIn];
else
c = colorsDefaultOrders[orderIn];
return c;
}
Array<Colour>* Keyboard::getKeyDegreeColours()
{
return &keyColorsDegrees;
}
Colour Keyboard::getKeyDegreeColor(int degIn)
{
Colour c;
if (keyColorsDegrees[degIn].isOpaque())
c = keyColorsDegrees[degIn];
else // this deg->order conversion is probably wrong
c = colorsDefaultOrders[mode->getOrder(degIn)];
return c;
}
Array<Colour>* Keyboard::getKeyNoteOnColours()
{
return &keyOnColorsByChannel;
}
void Keyboard::updateKeyColors(bool writeToNode)
{
for (int i = 0; i < keys.size(); i++)
{
Key* key = keys[i];
key->setDisplayColor(getKeyColor(i));
}
if (writeToNode)
{
ValueTree keyColorsNode = mode->modeNode.getOrCreateChildWithName(IDs::pianoKeyColorsNode, nullptr);
ValueTree layersColorNode = ValueTree(IDs::pianoKeyColorsLayer);
for (int i = 0; i < keyColorsOrders.size(); i++)
{
if (keyColorsOrders[i].isOpaque())
{
ValueTree node(IDs::pianoKeyColor);
node.setProperty(IDs::pianoKeyColorsLayer, i, nullptr);
node.setProperty(IDs::pianoKeyColor, keyColorsOrders[i].toString(), nullptr);
layersColorNode.appendChild(node, nullptr);
}
}
keyColorsNode.getOrCreateChildWithName(IDs::pianoKeyColorsLayer, nullptr).copyPropertiesAndChildrenFrom(layersColorNode, nullptr);
ValueTree degreesColorNode = ValueTree(IDs::pianoKeyColorsDegree);
for (int i = 0; i < keyColorsDegrees.size(); i++)
{
if (keyColorsDegrees[i].isOpaque())
{
ValueTree node(IDs::pianoKeyColor);
node.setProperty(IDs::pianoKeyColorsDegree, i, nullptr);
node.setProperty(IDs::pianoKeyColor, keyColorsDegrees[i].toString(), nullptr);
degreesColorNode.appendChild(node, nullptr);
}
}
keyColorsNode.getOrCreateChildWithName(IDs::pianoKeyColorsDegree, nullptr).copyPropertiesAndChildrenFrom(degreesColorNode, nullptr);
ValueTree individualColorNode = ValueTree(IDs::pianoKeyColorsIndividual);
for (int i = 0; i < keyColorsIndividual.size(); i++)
{
if (keyColorsIndividual[i].isOpaque())
{
ValueTree node(IDs::pianoKeyColor);
node.setProperty(IDs::pianoKeyColorsIndividual, mode->getFixedDegree(i), nullptr);
node.setProperty(IDs::pianoKeyColor, keyColorsIndividual[i].toString(), nullptr);
individualColorNode.appendChild(node, nullptr);
}
}
keyColorsNode.getOrCreateChildWithName(IDs::pianoKeyColorsIndividual, nullptr).copyPropertiesAndChildrenFrom(individualColorNode, nullptr);
ValueTree keyOnColorNode = ValueTree(IDs::pianoKeyColorsNoteOn);
for (int i = 0; i < 16; i++)
{
if (keyOnColorsByChannel[i].isOpaque())
{
ValueTree node(IDs::pianoKeyColor);
node.setProperty(IDs::pianoKeyColorsNoteOn, i, nullptr);
node.setProperty(IDs::pianoKeyColor, keyOnColorsByChannel[i].toString(), nullptr);
keyOnColorNode.appendChild(node, nullptr);
}
}
keyColorsNode.getOrCreateChildWithName(IDs::pianoKeyColorsNoteOn, nullptr).copyPropertiesAndChildrenFrom(keyOnColorNode, nullptr);
}
}
void Keyboard::resetLayerColors()
{
keyColorsOrders.fill(Colour());
}
void Keyboard::resetDegreeColors()
{
keyColorsDegrees.fill(Colour());
}
void Keyboard::resetKeyColors()
{
resetLayerColors();
resetDegreeColors();
keyColorsIndividual.fill(Colour());
updateKeyColors();
}
//===============================================================================================
void Keyboard::setKeyDegreeColor(int scaleDegreeIn, Colour colourIn)
{
int degree = totalModulus(scaleDegreeIn, mode->getScaleSize());
keyColorsDegrees.set(degree, colourIn);
}
void Keyboard::setKeyLayerColor(int layerNumIn, Colour colourIn)
{
int layer = totalModulus(layerNumIn, mode->getMaxStep());
keyColorsOrders.set(layer, colourIn);
}
void Keyboard::setKeyColor(int keyNumIn, Colour colourIn)
{
int keyNum = totalModulus(keyNumIn, keys.size());
keyColorsIndividual.set(keyNum, colourIn);
}
//===============================================================================================
void Keyboard::setKeySizeRatio(float keySizeRatioIn, bool resizeSelf)
{
keySizeRatio = keySizeRatioIn;
pianoNode.setProperty(IDs::pianoWHRatio, keySizeRatio, nullptr);
if (resizeSelf)
setSize(getPianoWidth(getHeight()), getHeight());
}
void Keyboard::setKeyWidthSize(int widthSizeIn)
{
// do stuff
}
void Keyboard::setKeyOrderSizeScalar(float scalarIn)
{
// do stuff
}
//===============================================================================================
void Keyboard::allNotesOff()
{
MidiKeyboardState::allNotesOff(midiChannelOut);
for (int i = 0; i < keys.size(); i++)
{
triggerKey(i, false);
}
}
void Keyboard::isolateLastNote()
{
if (lastKeyClicked >= 0 && lastKeyClicked < 128)
{
for (int i = 0; i < keys.size(); i++)
{
if (i != lastKeyClicked)
triggerKey(i, false);
}
}
}
void Keyboard::retriggerNotes()
{
Array<int> retrigger = Array<int>(keysOn);
triggerKeys(retrigger, false, 0);
triggerKeys(retrigger, true, 0.7f);
}
void Keyboard::triggerKey(int keyNumberIn, bool doNoteOn, float velocity)
{
if (keyNumberIn < 0 || keyNumberIn >= keys.size())
return;
Key* key = keys[keyNumberIn];
if (doNoteOn)
{
noteOn(midiChannelOut, keyNumberIn, velocity);
key->isClicked = true;
keysOn.addIfNotAlreadyThere(keyNumberIn);
}
else
{
noteOff(midiChannelOut, keyNumberIn, 0);
key->isClicked = false;
keysOn.removeAllInstancesOf(keyNumberIn);
}
key->repaint();
}
void Keyboard::triggerKeys(Array<int> keyNumbers, bool doNoteOn, float velocity)
{
for (auto keyNum : keyNumbers)
{
triggerKey(keyNum, doNoteOn, velocity);
}
}
int Keyboard::getOrderOfNotesOn()
{
float orderSum = 0;
int orderDetected;
for (int i = 0; i < keysOn.size(); i++)
{
Key* key = keys[keysOn[i]];
orderSum += (key->order + 1);
}
orderDetected = orderSum / keysOn.size();
if (orderDetected == (int)orderDetected)
orderDetected -= 1;
else
orderDetected = -1;
return orderDetected;
}
int Keyboard::transposeKeyModally(int keyNumIn, int stepsIn)
{
if (keyNumIn < 0 || keyNumIn >= keys.size())
return -1;
Key* key = keys[keyNumIn];
Array<int> orderArray = keysOrder[key->order];
int newKey = -1;
for (int i = 0; i < orderArray.size(); i++)
{
if (key->keyNumber == orderArray.getUnchecked(i))
{
newKey = i;
break;
}
}
newKey += stepsIn;
if (newKey >= 0 && newKey < orderArray.size())
return orderArray[newKey];
else
return -1;
}
int Keyboard::transposeKeyChromatically(int keyNumIn, int stepsIn)
{
int newKey = keyNumIn + stepsIn;
if (newKey >= 0 && newKey <= keys.size())
return newKey;
else
return -1;
}
void Keyboard::transposeKeysOnModally(int modalStepsIn, bool needsSameOrder, bool useLastClickedRoot)
{
int keyRoot;
int rootOrder;
int newKey;
Array<int> oldKeys = Array<int>(keysOn);
Array<int> newKeys;
if (needsSameOrder)
{
rootOrder = keys[keysOn[0]]->order;
if (rootOrder != getOrderOfNotesOn())
return;
for (int i = 0; i < oldKeys.size(); i++)
{
newKey = transposeKeyModally(oldKeys[i], modalStepsIn);
if (newKey > -1)
newKeys.add(newKey);
}
}
else
{
int stepsToUse; // TODO: implement this so that the chord quality is retained
if (useLastClickedRoot)
{
keyRoot = lastKeyClicked;
}
else // find lowest key
{
keyRoot = keys.size();
for (int i = 0; i < keysOn.size(); i++)
{
if (keysOn[i] < keyRoot)
keyRoot = keysOn[i];
}
}
rootOrder = keys[keyRoot]->order;
for (int i = 0; i < oldKeys.size(); i++)
{
newKey = transposeKeyModally(oldKeys[i], modalStepsIn);
if (newKey > -1)
newKeys.add(newKey);
}
}
// TODO: implement velocities further
lastKeyClicked = transposeKeyModally(lastKeyClicked, modalStepsIn);
triggerKeys(oldKeys, false);
triggerKeys(newKeys);
}
void Keyboard::transposeKeysOnChromatically(int stepsIn)
{
Array<int> oldKeys = Array<int>(keysOn);
Array<int> newKeys;
int keyNum;
int newKey;
float velocity;
for (int i = 0; i < oldKeys.size(); i++)
{
keyNum = oldKeys.getUnchecked(i);
Key* key = keys[keyNum];
velocity = key->velocity;
newKey = transposeKeyChromatically(keyNum, stepsIn);
if (newKey > -1)
newKeys.add(newKey);
}
// TODO: implement velocities further
lastKeyClicked = transposeKeyChromatically(lastKeyClicked, stepsIn);
triggerKeys(oldKeys, false);
triggerKeys(newKeys);
}
//===============================================================================================
void Keyboard::handleNoteOn(MidiKeyboardState* source, int midiChannel, int midiNote, float velocity)
{
int keyTriggered = midiNote;
Key* key = keys[keyTriggered];
key->exPressed = true;
Colour keyOnColour = keyOnColorsByChannel[midiChannel];
if (!keyOnColour.isOpaque())
keyOnColour = key->color.contrasting(0.75f);
key->exInputColor = keyOnColour;
key->isDirty = true;
hasDirtyKeys = true;
}
void Keyboard::handleNoteOff(MidiKeyboardState* source, int midiChannel, int midiNote, float velocity)
{
int keyTriggered = midiNote;
Key* key = keys[keyTriggered];
key->exPressed = false;
key->isDirty = true;
hasDirtyKeys = true;
}
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
VirtualKeyboardGrid.cpp
Created: 17 Apr 2019 2:12:23pm
Author: Vincenzo
==============================================================================
*/
#include "VirtualKeyboardGrid.h"
using namespace VirtualKeyboard;
KeyboardPositioner::KeyboardPositioner(Component* parentIn, int numModeKeysIn)
: parent(parentIn)
{
setNumModeKeys(numModeKeysIn);
}
void KeyboardPositioner::parentResized()
{
keyWidth = (parent->getWidth() - keyGap) / numModeKeys;
}
float KeyboardPositioner::getKeyWidth() const
{
return keyWidth;
}
int KeyboardPositioner::getKeyGap() const
{
return keyGap;
}
void KeyboardPositioner::setNumModeKeys(int numKeysIn)
{
numModeKeys = numKeysIn;
parentResized();
}
void KeyboardPositioner::setKeyGap(int keyGapIn)
{
keyGap = keyGapIn;
parentResized();
}
void KeyboardPositioner::setKeyPlacement(int keyPlacementTypeIn)
{
keyPlacement = keyPlacementTypeIn;
}
void KeyboardPositioner::setLayerKeysWidthRatio(float widthRatioIn)
{
baseWidthRatio = widthRatioIn;
}
void KeyboardPositioner::setLayerKeysHeightRatio(float heightRatioIn)
{
baseHeightRatio = heightRatioIn;
}
void KeyboardPositioner::resizeKey(Key& key)
{
float spread = 4; // TODO make setter
float height, width;
if (key.order == 0)
{
height = 1;
width = 1;
}
else
{
float stepHeight = baseHeightRatio + (key.step - 2) / 100.0f * spread;
width = baseWidthRatio;
switch (keyPlacement)
{
case(KeyPlacementType::flat):
{
height = stepHeight - stepHeight * (key.order - 1) / (float)key.step * 1.125f;
break;
}
case(KeyPlacementType::adjacent):
{
height = stepHeight;
width /= (key.step - 1) * 0.9f; // mode keys of step 1 will not be in this else condition
if (key.step == 2)
width *= 0.75f;
break;
}
default: // nested keys
{
height = stepHeight - stepHeight * (key.order - 1) / (key.step * 1.08f);
width -= (key.order - 1) * 0.1f;
break;
}
}
}
key.setSize(width * keyWidth, height * parent->getHeight());
}
void KeyboardPositioner::placeKey(Key& key)
{
}
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
ModeInfoDialog.h
Vincenzo Sicurella
==============================================================================
*/
#pragma once
#include "../../../JuceLibraryCode/JuceHeader.h"
#include "../../CommonFunctions.h"
#include "../../Structures/Mode.h"
class ModeInfoDialog : public Component,
public ChangeBroadcaster,
public TextEditor::Listener,
public Button::Listener,
public Slider::Listener
{
public:
//==============================================================================
ModeInfoDialog (Mode* modeIn);
~ModeInfoDialog();
//==============================================================================
void commitMode();
void textEditorTextChanged(TextEditor& textEditor) override;
void textEditorEscapeKeyPressed(TextEditor& textEditor) override {};
void textEditorReturnKeyPressed(TextEditor& textEditor) override {};
void textEditorFocusLost(TextEditor& textEditor) override {};
void paint (Graphics& g) override;
void resized() override {};
void buttonClicked (Button* buttonThatWasClicked) override;
void sliderValueChanged (Slider* sliderThatWasMoved) override;
private:
Mode* modeOriginal;
Mode modeWorking;
ValueTree modeNode;
int lastRotateAmt = 0;
//==============================================================================
std::unique_ptr<TextEditor> familyBox;
std::unique_ptr<TextEditor> stepsBox;
std::unique_ptr<TextEditor> infoBox;
std::unique_ptr<Label> familyNameLbl;
std::unique_ptr<Label> stepsLbl;
std::unique_ptr<Label> intervalSizesLbl;
std::unique_ptr<Label> nameLabel;
std::unique_ptr<Label> modeSizeLbl;
std::unique_ptr<Label> scaleSizeLbl;
std::unique_ptr<ToggleButton> defaultNameBtn;
std::unique_ptr<Label> infoLbl;
std::unique_ptr<TextEditor> nameBox;
std::unique_ptr<TextButton> saveButton;
std::unique_ptr<Slider> rotateSld;
std::unique_ptr<Label> modeRotateLbl;
std::unique_ptr<Label> scaleSizeReadout;
std::unique_ptr<Label> modeSizeReadout;
std::unique_ptr<Label> intervalSizeReadout;
std::unique_ptr<TextButton> closeButton;
//==============================================================================
JUCE_DECLARE_NON_COPYABLE_WITH_LEAK_DETECTOR (ModeInfoDialog)
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
ModeInfoDialog.cpp
Vincenzo Sicurella
==============================================================================
*/
#include "ModeInfoDialog.h"
//==============================================================================
ModeInfoDialog::ModeInfoDialog (Mode* modeIn)
: modeOriginal(modeIn), modeWorking(Mode(modeIn->modeNode)), modeNode(modeWorking.modeNode)
{
familyBox.reset (new TextEditor ("Family Box"));
addAndMakeVisible (familyBox.get());
familyBox->setMultiLine (false);
familyBox->setReturnKeyStartsNewLine (false);
familyBox->setReadOnly (false);
familyBox->setScrollbarsShown (true);
familyBox->setCaretVisible (true);
familyBox->setPopupMenuEnabled (true);
familyBox->setText (TRANS("Meantone"));
familyBox->setBounds (8, 168, 192, 24);
stepsBox.reset (new TextEditor ("Steps Box"));
addAndMakeVisible (stepsBox.get());
stepsBox->setMultiLine (false);
stepsBox->setReturnKeyStartsNewLine (false);
stepsBox->setReadOnly (true);
stepsBox->setScrollbarsShown (true);
stepsBox->setCaretVisible (false);
stepsBox->setPopupMenuEnabled (true);
stepsBox->setText (TRANS("2 2 1 2 2 2 1"));
stepsBox->setBounds (8, 40, 192, 24);
infoBox.reset (new TextEditor ("Info Box"));
addAndMakeVisible (infoBox.get());
infoBox->setMultiLine (true);
infoBox->setReturnKeyStartsNewLine (false);
infoBox->setReadOnly (false);
infoBox->setScrollbarsShown (true);
infoBox->setCaretVisible (true);
infoBox->setPopupMenuEnabled (true);
infoBox->setText (TRANS("Ever hear of it?"));
infoBox->setBounds (8, 336, 320, 128);
familyNameLbl.reset (new Label ("Family Name Label",
TRANS("Temperament Family:\n")));
addAndMakeVisible (familyNameLbl.get());
familyNameLbl->setFont (Font (15.00f, Font::plain).withTypefaceStyle ("Regular"));
familyNameLbl->setJustificationType (Justification::centredLeft);
familyNameLbl->setEditable (false, false, false);
familyNameLbl->setColour (TextEditor::textColourId, Colours::black);
familyNameLbl->setColour (TextEditor::backgroundColourId, Colour (0x00000000));
familyNameLbl->setBounds (8, 136, 150, 24);
stepsLbl.reset (new Label ("Steps Label",
TRANS("Steps:\n")));
addAndMakeVisible (stepsLbl.get());
stepsLbl->setFont (Font (15.00f, Font::plain).withTypefaceStyle ("Regular"));
stepsLbl->setJustificationType (Justification::centredLeft);
stepsLbl->setEditable (false, false, false);
stepsLbl->setColour (TextEditor::textColourId, Colours::black);
stepsLbl->setColour (TextEditor::backgroundColourId, Colour (0x00000000));
stepsLbl->setBounds (8, 8, 56, 24);
intervalSizesLbl.reset (new Label ("Interval Sizes Label",
TRANS("Interval Sizes:")));
addAndMakeVisible (intervalSizesLbl.get());
intervalSizesLbl->setFont (Font (15.00f, Font::plain).withTypefaceStyle ("Regular"));
intervalSizesLbl->setJustificationType (Justification::centredLeft);
intervalSizesLbl->setEditable (false, false, false);
intervalSizesLbl->setColour (TextEditor::textColourId, Colours::black);
intervalSizesLbl->setColour (TextEditor::backgroundColourId, Colour (0x00000000));
intervalSizesLbl->setBounds (8, 272, 95, 24);
nameLabel.reset (new Label ("Name Label",
TRANS("Mode Name:")));
addAndMakeVisible (nameLabel.get());
nameLabel->setFont (Font (15.00f, Font::plain).withTypefaceStyle ("Regular"));
nameLabel->setJustificationType (Justification::centredLeft);
nameLabel->setEditable (false, false, false);
nameLabel->setColour (TextEditor::textColourId, Colours::black);
nameLabel->setColour (TextEditor::backgroundColourId, Colour (0x00000000));
nameLabel->setBounds (9, 73, 96, 24);
modeSizeLbl.reset (new Label ("Mode Size Label",
TRANS("Mode Size:")));
addAndMakeVisible (modeSizeLbl.get());
modeSizeLbl->setFont (Font (15.00f, Font::plain).withTypefaceStyle ("Regular"));
modeSizeLbl->setJustificationType (Justification::centredLeft);
modeSizeLbl->setEditable (false, false, false);
modeSizeLbl->setColour (TextEditor::textColourId, Colours::black);
modeSizeLbl->setColour (TextEditor::backgroundColourId, Colour (0x00000000));
modeSizeLbl->setBounds (8, 240, 80, 24);
scaleSizeLbl.reset (new Label ("Scale Size Label",
TRANS("Scale Size:")));
addAndMakeVisible (scaleSizeLbl.get());
scaleSizeLbl->setFont (Font (15.00f, Font::plain).withTypefaceStyle ("Regular"));
scaleSizeLbl->setJustificationType (Justification::centredLeft);
scaleSizeLbl->setEditable (false, false, false);
scaleSizeLbl->setColour (TextEditor::textColourId, Colours::black);
scaleSizeLbl->setColour (TextEditor::backgroundColourId, Colour (0x00000000));
scaleSizeLbl->setBounds (8, 208, 71, 24);
defaultNameBtn.reset (new ToggleButton ("Default Name Button"));
addAndMakeVisible (defaultNameBtn.get());
defaultNameBtn->setButtonText (TRANS("Use Default"));
defaultNameBtn->addListener (this);
defaultNameBtn->setToggleState (true, dontSendNotification);
defaultNameBtn->setBounds (217, 105, 111, 24);
infoLbl.reset (new Label ("Info Label",
TRANS("Info:")));
addAndMakeVisible (infoLbl.get());
infoLbl->setFont (Font (15.00f, Font::plain).withTypefaceStyle ("Regular"));
infoLbl->setJustificationType (Justification::centredLeft);
infoLbl->setEditable (false, false, false);
infoLbl->setColour (TextEditor::textColourId, Colours::black);
infoLbl->setColour (TextEditor::backgroundColourId, Colour (0x00000000));
infoLbl->setBounds (8, 304, 40, 24);
nameBox.reset (new TextEditor ("Name Box"));
addAndMakeVisible (nameBox.get());
nameBox->setMultiLine (false);
nameBox->setReturnKeyStartsNewLine (false);
nameBox->setReadOnly (false);
nameBox->setScrollbarsShown (true);
nameBox->setCaretVisible (true);
nameBox->setPopupMenuEnabled (true);
nameBox->setText (TRANS("Meantone[7] 12"));
nameBox->setBounds (9, 105, 192, 24);
saveButton.reset (new TextButton ("Save Button"));
addAndMakeVisible (saveButton.get());
saveButton->setButtonText (TRANS("Save"));
saveButton->addListener (this);
saveButton->setBounds (32, 488, 104, 24);
rotateSld.reset (new Slider ("Rotate Slider"));
addAndMakeVisible (rotateSld.get());
rotateSld->setRange (-3, 4, 1);
rotateSld->setSliderStyle (Slider::IncDecButtons);
rotateSld->setTextBoxStyle (Slider::TextBoxLeft, false, 48, 20);
rotateSld->addListener (this);
rotateSld->setBounds (216, 40, 96, 24);
modeRotateLbl.reset (new Label ("Mode Rotate Label",
TRANS("Shift Mode:")));
addAndMakeVisible (modeRotateLbl.get());
modeRotateLbl->setFont (Font (15.00f, Font::plain).withTypefaceStyle ("Regular"));
modeRotateLbl->setJustificationType (Justification::centredLeft);
modeRotateLbl->setEditable (false, false, false);
modeRotateLbl->setColour (TextEditor::textColourId, Colours::black);
modeRotateLbl->setColour (TextEditor::backgroundColourId, Colour (0x00000000));
modeRotateLbl->setBounds (216, 8, 103, 24);
scaleSizeReadout.reset (new Label ("Scale Size Readout",
TRANS("12\n")));
addAndMakeVisible (scaleSizeReadout.get());
scaleSizeReadout->setFont (Font (15.00f, Font::plain).withTypefaceStyle ("Regular"));
scaleSizeReadout->setJustificationType (Justification::centredLeft);
scaleSizeReadout->setEditable (false, false, false);
scaleSizeReadout->setColour (TextEditor::textColourId, Colours::black);
scaleSizeReadout->setColour (TextEditor::backgroundColourId, Colour (0x00000000));
scaleSizeReadout->setBounds (88, 208, 31, 24);
modeSizeReadout.reset (new Label ("Mode Size Readout",
TRANS("7")));
addAndMakeVisible (modeSizeReadout.get());
modeSizeReadout->setFont (Font (15.00f, Font::plain).withTypefaceStyle ("Regular"));
modeSizeReadout->setJustificationType (Justification::centredLeft);
modeSizeReadout->setEditable (false, false, false);
modeSizeReadout->setColour (TextEditor::textColourId, Colours::black);
modeSizeReadout->setColour (TextEditor::backgroundColourId, Colour (0x00000000));
modeSizeReadout->setBounds (88, 240, 103, 24);
intervalSizeReadout.reset (new Label ("Interval Size Readout",
TRANS("7, 5")));
addAndMakeVisible (intervalSizeReadout.get());
intervalSizeReadout->setFont (Font (15.00f, Font::plain).withTypefaceStyle ("Regular"));
intervalSizeReadout->setJustificationType (Justification::centredLeft);
intervalSizeReadout->setEditable (false, false, false);
intervalSizeReadout->setColour (TextEditor::textColourId, Colours::black);
intervalSizeReadout->setColour (TextEditor::backgroundColourId, Colour (0x00000000));
intervalSizeReadout->setBounds (104, 272, 143, 24);
closeButton.reset (new TextButton ("closeButton"));
addAndMakeVisible (closeButton.get());
closeButton->setButtonText (TRANS("Close"));
closeButton->addListener (this);
closeButton->setBounds (192, 488, 104, 24);
rotateSld->setIncDecButtonsMode(Slider::IncDecButtonMode::incDecButtonsDraggable_Horizontal);
familyBox->addListener(this);
stepsBox->setText(modeWorking.getStepsString());
familyBox->setText(modeWorking.getFamily());
nameBox->setText(modeWorking.getName());
nameBox->setEnabled(false);
defaultNameBtn->setClickingTogglesState(true);
if (modeWorking.getName() != modeWorking.getDescription())
defaultNameBtn->setToggleState(false, sendNotification);
scaleSizeReadout->setText(String(modeWorking.getScaleSize()), dontSendNotification);
modeSizeReadout->setText(String(modeWorking.getModeSize()), dontSendNotification);
intervalSizeReadout->setText(arrayToString(modeWorking.getIntervalSizeCount()), dontSendNotification);
infoBox->setText(modeWorking.getInfo());
if (modeWorking.getModeSize() > 1)
rotateSld->setRange(-(modeWorking.getModeSize() - 1), modeWorking.getModeSize() - 1, 1);
else
rotateSld->setEnabled(false);
setSize (340, 525);
}
ModeInfoDialog::~ModeInfoDialog()
{
removeAllChangeListeners();
familyBox = nullptr;
stepsBox = nullptr;
infoBox = nullptr;
familyNameLbl = nullptr;
stepsLbl = nullptr;
intervalSizesLbl = nullptr;
nameLabel = nullptr;
modeSizeLbl = nullptr;
scaleSizeLbl = nullptr;
defaultNameBtn = nullptr;
infoLbl = nullptr;
nameBox = nullptr;
saveButton = nullptr;
rotateSld = nullptr;
modeRotateLbl = nullptr;
scaleSizeReadout = nullptr;
modeSizeReadout = nullptr;
intervalSizeReadout = nullptr;
closeButton = nullptr;
}
//==============================================================================
void ModeInfoDialog::paint (Graphics& g)
{
g.fillAll (Colour (0xff323e44));
}
void ModeInfoDialog::buttonClicked (Button* buttonThatWasClicked)
{
if (buttonThatWasClicked == defaultNameBtn.get())
{
if (defaultNameBtn->getToggleState())
{
nameBox->setEnabled(false);
modeWorking.setFamily(familyBox->getText());
modeNode.setProperty(IDs::family, familyBox->getText(), nullptr);
nameBox->setText(modeWorking.getDescription());
}
else
{
nameBox->setEnabled(true);
}
}
else if (buttonThatWasClicked == saveButton.get())
{
commitMode();
sendChangeMessage();
getParentComponent()->exitModalState(0);
}
else if (buttonThatWasClicked == closeButton.get())
{
getParentComponent()->exitModalState(0);
}
}
void ModeInfoDialog::sliderValueChanged (Slider* sliderThatWasMoved)
{
if (sliderThatWasMoved == rotateSld.get())
{
modeWorking.rotate(lastRotateAmt - sliderThatWasMoved->getValue());
lastRotateAmt = sliderThatWasMoved->getValue();
modeNode.setProperty(IDs::stepString, modeWorking.getStepsString(), nullptr);
stepsBox->setText(modeWorking.getStepsString());
}
}
void ModeInfoDialog::textEditorTextChanged(TextEditor& textEditor)
{
if (textEditor.getName() == familyBox->getName())
{
if (defaultNameBtn->getToggleState())
{
modeWorking.setFamily(familyBox->getText());
nameBox->setText(modeWorking.getDescription());
}
}
}
void ModeInfoDialog::commitMode()
{
modeNode.setProperty(IDs::modeName, nameBox->getText(), nullptr);
modeNode.setProperty(IDs::family, familyBox->getText(), nullptr);
modeNode.setProperty(IDs::modeInfo, infoBox->getText(), nullptr);
modeOriginal->restoreNode(modeNode);
}
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
ExportKbmDialog.h
Created: 24 Oct 2020 4:47:57pm
Author: Vincenzo
==============================================================================
*/
#pragma once
#include <JuceHeader.h>
#include "../Components/LabelledComponent.h"
#include "../../File IO/KbmWriter.h"
//==============================================================================
/*
*/
class ExportKbmDialog : public Component
{
public:
ExportKbmDialog(ValueTree mappingNodeIn, const Mode& modeInput, const Mode& modeOutput, const ModeMapper& mapperIn)
: mappingNode(mappingNodeIn), inputMode(modeInput), outputMode(modeOutput), mapper(mapperIn)
{
if (!mappingNode.isValid())
mappingNode = ValueTree(IDs::midiMapNode);
refNoteSld = new Slider(Slider::SliderStyle::IncDecButtons, Slider::TextBoxLeft);
refNoteSld->setRange(0, 127, 1);
refNoteSld->setTextBoxStyle(Slider::TextBoxLeft, false, 48, 24);
refNoteSldLabel.reset(new LabelledComponent(*refNoteSld, "Tuning Reference MIDI Note:"));
addAndMakeVisible(refNoteSldLabel.get());
refFreqEditor = new TextEditor("ReferenceFreqEditor");
refFreqEditor->setInputRestrictions(0, "123456890.");
refFreqEditLabel.reset(new LabelledComponent(*refFreqEditor, "Tuning Reference Frequency:"));
addAndMakeVisible(refFreqEditLabel.get());
autoFreqButton.reset(new TextButton("AutoFreqButton", "Use a standard tuning frequency based on selected MIDI note."));
autoFreqButton->setButtonText("Auto");
autoFreqButton->onClick = [&]() { refFreqEditor->setText(String(getStdFrequency(refNoteSld->getValue()))); };
addAndMakeVisible(autoFreqButton.get());
saveButton.reset(new TextButton("SaveButton"));
saveButton->setButtonText("Save");
saveButton->onClick = [&]() { ExportKbmDialog::saveAndClose(); };
addAndMakeVisible(saveButton.get());
cancelButton.reset(new TextButton("CancelButton"));
cancelButton->setButtonText("Cancel");
cancelButton->onClick = [&]() { delete getParentComponent(); };
addAndMakeVisible(cancelButton.get());
if (mappingNode.hasProperty(IDs::tuningRootNote))
refNoteSld->setValue((int)mappingNode[IDs::tuningRootNote]);
else
refNoteSld->setValue(69);
if (mappingNode.hasProperty(IDs::tuningRootFreq))
refFreqEditor->setText(mappingNode[IDs::tuningRootFreq].toString());
else
refFreqEditor->setText("440.0");
}
~ExportKbmDialog() override
{
}
void paint (juce::Graphics& g) override
{
g.fillAll (getLookAndFeel().findColour (juce::ResizableWindow::backgroundColourId)); // clear the background
g.setColour (juce::Colours::grey);
g.drawRect (getLocalBounds(), 1); // draw an outline around the component
}
void resized() override
{
refNoteSldLabel->setComponentSize(88, 24);
refNoteSldLabel->setTopLeftPosition(0, 8);
refFreqEditLabel->setComponentSize(80, 24);
refFreqEditLabel->setTopLeftPosition(0, refNoteSldLabel->getBottom() + 8);
autoFreqButton->setBounds(refFreqEditLabel->getRight() + 4, refFreqEditLabel->getY(), 44, 24);
double unit = proportionOfWidth(1 / 17.0f);
saveButton->setBounds(unit * 2, getHeight() - 40, unit * 5, 36);
cancelButton->setBounds(unit * 10, saveButton->getY(), unit * 5, 36);
}
void saveAndClose()
{
bool success = false;
File initialDirectory;
if (mappingNode.hasProperty(IDs::kbmFileLocation) && File::isAbsolutePath(mappingNode[IDs::kbmFileLocation].toString()))
initialDirectory = File(mappingNode[IDs::kbmFileLocation]);
FileChooser chooser("Save KBM file...", initialDirectory, "*.kbm");
if (chooser.browseForFileToSave(true))
{
KbmWriter kbm = KbmWriter::fromModes(
&inputMode, &outputMode, mapper, 0, 127, -1,
refNoteSld->getValue(), refFreqEditor->getText().getDoubleValue()
);
if (kbm.writeTo(chooser.getResult()))
{
success = true;
mappingNode.setProperty(IDs::tuningRootNote, refNoteSld->getValue(), nullptr);
mappingNode.setProperty(IDs::tuningRootFreq, refFreqEditor->getText().getDoubleValue(), nullptr);
mappingNode.setProperty(IDs::kbmFileLocation, chooser.getResult().getParentDirectory().getFullPathName(), nullptr);
}
}
if (!success)
{
AlertWindow::showMessageBox(AlertWindow::AlertIconType::WarningIcon, "File Write Error", "Could not write .kbm file", "Ok", this);
}
delete getParentComponent();
}
private:
ValueTree mappingNode;
const Mode& inputMode;
const Mode& outputMode;
const ModeMapper& mapper;
std::unique_ptr<LabelledComponent> refNoteSldLabel;
Slider* refNoteSld;
std::unique_ptr<LabelledComponent> refFreqEditLabel;
TextEditor* refFreqEditor;
std::unique_ptr<TextButton> autoFreqButton;
std::unique_ptr<TextButton> saveButton;
std::unique_ptr<TextButton> cancelButton;
JUCE_DECLARE_NON_COPYABLE_WITH_LEAK_DETECTOR (ExportKbmDialog)
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
Created with Projucer version: 5.4.4
------------------------------------------------------------------------------
The Projucer is part of the JUCE library.
Copyright (c) 2017 - ROLI Ltd.
==============================================================================
*/
#pragma once
#include "../../../JuceLibraryCode/JuceHeader.h"
#include "../../CommonFunctions.h"
#include "../../Structures/ModeMapper.h"
#include "../Components/ReferencedComboBox.h"
//==============================================================================
class MapByOrderDialog : public Component,
public ComboBox::Listener,
public Slider::Listener
{
public:
//==============================================================================
MapByOrderDialog (ModeMapper* modeMapperIn, Mode* mode1In, Mode* mode2In);
~MapByOrderDialog();
//==============================================================================
void paint (Graphics& g) override;
void resized() override;
void comboBoxChanged (ComboBox* comboBoxThatHasChanged) override;
void sliderValueChanged (Slider* sliderThatWasMoved) override;
private:
ModeMapper* modeMapper;
Mode* mode1;
Mode* mode2;
int boxW = 10;
int boxH = 8;
//==============================================================================
std::unique_ptr<ComboBox> mode1OrderBox;
std::unique_ptr<ComboBox> mode2OrderBox;
std::unique_ptr<Label> orderSelectionLbl;
std::unique_ptr<Slider> orderOffsetSld1;
std::unique_ptr<Slider> orderOffsetSld2;
std::unique_ptr<Label> offsetLabel;
std::unique_ptr<Label> inputLabel;
std::unique_ptr<Label> outputLabel;
//==============================================================================
JUCE_DECLARE_NON_COPYABLE_WITH_LEAK_DETECTOR (MapByOrderDialog)
};
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
/*
==============================================================================
Created with Projucer version: 5.4.4
------------------------------------------------------------------------------
The Projucer is part of the JUCE library.
Copyright (c) 2017 - ROLI Ltd.
==============================================================================
*/
#include "MapByOrderDialog.h"
//==============================================================================
MapByOrderDialog::MapByOrderDialog (ModeMapper* modeMapperIn, Mode* mode1In, Mode* mode2In)
: modeMapper(modeMapperIn)
{
mode1OrderBox.reset (new ComboBox ("Mode 1 Order Box"));
addAndMakeVisible (mode1OrderBox.get());
mode1OrderBox->setEditableText (false);
mode1OrderBox->setJustificationType (Justification::centredLeft);
mode1OrderBox->setTextWhenNothingSelected (String());
mode1OrderBox->setTextWhenNoChoicesAvailable (TRANS("(no choices)"));
mode1OrderBox->addListener (this);
mode1OrderBox->setBounds (76, 25, 63, 24);
mode2OrderBox.reset (new ComboBox ("Mode 2 Order Box"));
addAndMakeVisible (mode2OrderBox.get());
mode2OrderBox->setEditableText (false);
mode2OrderBox->setJustificationType (Justification::centredLeft);
mode2OrderBox->setTextWhenNothingSelected (String());
mode2OrderBox->setTextWhenNoChoicesAvailable (TRANS("(no choices)"));
mode2OrderBox->addListener (this);
mode2OrderBox->setBounds (156, 25, 63, 24);
orderSelectionLbl.reset (new Label ("Order Label",
TRANS("Layer:")));
addAndMakeVisible (orderSelectionLbl.get());
orderSelectionLbl->setFont (Font (15.00f, Font::plain).withTypefaceStyle ("Regular"));
orderSelectionLbl->setJustificationType (Justification::centredLeft);
orderSelectionLbl->setEditable (false, false, false);
orderSelectionLbl->setColour (TextEditor::textColourId, Colours::black);
orderSelectionLbl->setColour (TextEditor::backgroundColourId, Colour (0x00000000));
orderSelectionLbl->setBounds (20, 24, 48, 24);
orderOffsetSld1.reset (new Slider ("Order Offset Slider 1"));
addAndMakeVisible (orderOffsetSld1.get());
orderOffsetSld1->setRange (0, 10, 1);
orderOffsetSld1->setSliderStyle (Slider::IncDecButtons);
orderOffsetSld1->setTextBoxStyle (Slider::TextBoxAbove, false, 80, 30);
orderOffsetSld1->addListener (this);
orderOffsetSld1->setBounds (76, 66, 64, 56);
orderOffsetSld2.reset (new Slider ("Order Offset Slider 2"));
addAndMakeVisible (orderOffsetSld2.get());
orderOffsetSld2->setRange (0, 10, 1);
orderOffsetSld2->setSliderStyle (Slider::IncDecButtons);
orderOffsetSld2->setTextBoxStyle (Slider::TextBoxAbove, false, 80, 30);
orderOffsetSld2->addListener (this);
orderOffsetSld2->setBounds (156, 66, 64, 56);
offsetLabel.reset (new Label ("new label",
TRANS("Degree\n"
"Offsets:")));
addAndMakeVisible (offsetLabel.get());
offsetLabel->setFont (Font (15.00f, Font::plain).withTypefaceStyle ("Regular"));
offsetLabel->setJustificationType (Justification::centredLeft);
offsetLabel->setEditable (false, false, false);
offsetLabel->setColour (TextEditor::textColourId, Colours::black);
offsetLabel->setColour (TextEditor::backgroundColourId, Colour (0x00000000));
offsetLabel->setBounds (12, 58, 64, 40);
inputLabel.reset(new Label("Input Label", TRANS("In")));
addAndMakeVisible(inputLabel.get());
inputLabel->setJustificationType(Justification::centred);
inputLabel->setBounds(mode1OrderBox->getX(), mode1OrderBox->getY() - 28, mode1OrderBox->getWidth(), 30);
outputLabel.reset(new Label("Output Label", TRANS("Out")));
addAndMakeVisible(outputLabel.get());
outputLabel->setJustificationType(Justification::centred);
outputLabel->setBounds(mode2OrderBox->getX(), mode2OrderBox->getY() - 28, mode2OrderBox->getWidth(), 30);
mode1 = mode1In;
mode2 = mode2In;
mode1OrderBox->clear();
mode2OrderBox->clear();
for (int i = 0; i < mode1->getMaxStep(); i++)
mode1OrderBox->addItem(String(i), i+1);
for (int i = 0; i < mode2->getMaxStep(); i++)
mode2OrderBox->addItem(String(i), i+1);
mode1OrderBox->setSelectedId(modeMapper->getMode1OrderNum() + 1, dontSendNotification);
mode2OrderBox->setSelectedId(modeMapper->getMode2OrderNum() + 1, dontSendNotification);
orderOffsetSld1->setValue(modeMapper->getMode1OrderOffset(), dontSendNotification);
orderOffsetSld2->setValue(modeMapper->getMode2OrderOffset(), dontSendNotification);
setSize (250, 130);
}
MapByOrderDialog::~MapByOrderDialog()
{
mode1OrderBox = nullptr;
mode2OrderBox = nullptr;
orderSelectionLbl = nullptr;
orderOffsetSld1 = nullptr;
orderOffsetSld2 = nullptr;
offsetLabel = nullptr;
}
//==============================================================================
void MapByOrderDialog::paint (Graphics& g)
{
g.fillAll (Colour (0xff323e44));
}
void MapByOrderDialog::resized()
{
}
void MapByOrderDialog::comboBoxChanged (ComboBox* comboBoxThatHasChanged)
{
if (comboBoxThatHasChanged == mode1OrderBox.get())
{
modeMapper->setMode1OrderNum(mode1OrderBox->getSelectedId()-1);
}
else if (comboBoxThatHasChanged == mode2OrderBox.get())
{
modeMapper->setMode2OrderNum(mode2OrderBox->getSelectedId()-1);
}
}
void MapByOrderDialog::sliderValueChanged (Slider* sliderThatWasMoved)
{
if (sliderThatWasMoved == orderOffsetSld1.get())
{
modeMapper->setMode1OrderOffset(orderOffsetSld1->getValue());
}
else if (sliderThatWasMoved == orderOffsetSld2.get())
{
modeMapper->setMode2OrderOffset(orderOffsetSld2->getValue());
}
}
| {
"repo_name": "vsicurella/SuperVirtualKeyboard",
"stars": "38",
"repo_language": "C++",
"file_name": "MapByOrderDialog.cpp",
"mime_type": "text/x-c"
} |
Creative Commons Legal Code
CC0 1.0 Universal
CREATIVE COMMONS CORPORATION IS NOT A LAW FIRM AND DOES NOT PROVIDE
LEGAL SERVICES. DISTRIBUTION OF THIS DOCUMENT DOES NOT CREATE AN
ATTORNEY-CLIENT RELATIONSHIP. CREATIVE COMMONS PROVIDES THIS
INFORMATION ON AN "AS-IS" BASIS. CREATIVE COMMONS MAKES NO WARRANTIES
REGARDING THE USE OF THIS DOCUMENT OR THE INFORMATION OR WORKS
PROVIDED HEREUNDER, AND DISCLAIMS LIABILITY FOR DAMAGES RESULTING FROM
THE USE OF THIS DOCUMENT OR THE INFORMATION OR WORKS PROVIDED
HEREUNDER.
Statement of Purpose
The laws of most jurisdictions throughout the world automatically confer
exclusive Copyright and Related Rights (defined below) upon the creator
and subsequent owner(s) (each and all, an "owner") of an original work of
authorship and/or a database (each, a "Work").
Certain owners wish to permanently relinquish those rights to a Work for
the purpose of contributing to a commons of creative, cultural and
scientific works ("Commons") that the public can reliably and without fear
of later claims of infringement build upon, modify, incorporate in other
works, reuse and redistribute as freely as possible in any form whatsoever
and for any purposes, including without limitation commercial purposes.
These owners may contribute to the Commons to promote the ideal of a free
culture and the further production of creative, cultural and scientific
works, or to gain reputation or greater distribution for their Work in
part through the use and efforts of others.
For these and/or other purposes and motivations, and without any
expectation of additional consideration or compensation, the person
associating CC0 with a Work (the "Affirmer"), to the extent that he or she
is an owner of Copyright and Related Rights in the Work, voluntarily
elects to apply CC0 to the Work and publicly distribute the Work under its
terms, with knowledge of his or her Copyright and Related Rights in the
Work and the meaning and intended legal effect of CC0 on those rights.
1. Copyright and Related Rights. A Work made available under CC0 may be
protected by copyright and related or neighboring rights ("Copyright and
Related Rights"). Copyright and Related Rights include, but are not
limited to, the following:
i. the right to reproduce, adapt, distribute, perform, display,
communicate, and translate a Work;
ii. moral rights retained by the original author(s) and/or performer(s);
iii. publicity and privacy rights pertaining to a person's image or
likeness depicted in a Work;
iv. rights protecting against unfair competition in regards to a Work,
subject to the limitations in paragraph 4(a), below;
v. rights protecting the extraction, dissemination, use and reuse of data
in a Work;
vi. database rights (such as those arising under Directive 96/9/EC of the
European Parliament and of the Council of 11 March 1996 on the legal
protection of databases, and under any national implementation
thereof, including any amended or successor version of such
directive); and
vii. other similar, equivalent or corresponding rights throughout the
world based on applicable law or treaty, and any national
implementations thereof.
2. Waiver. To the greatest extent permitted by, but not in contravention
of, applicable law, Affirmer hereby overtly, fully, permanently,
irrevocably and unconditionally waives, abandons, and surrenders all of
Affirmer's Copyright and Related Rights and associated claims and causes
of action, whether now known or unknown (including existing as well as
future claims and causes of action), in the Work (i) in all territories
worldwide, (ii) for the maximum duration provided by applicable law or
treaty (including future time extensions), (iii) in any current or future
medium and for any number of copies, and (iv) for any purpose whatsoever,
including without limitation commercial, advertising or promotional
purposes (the "Waiver"). Affirmer makes the Waiver for the benefit of each
member of the public at large and to the detriment of Affirmer's heirs and
successors, fully intending that such Waiver shall not be subject to
revocation, rescission, cancellation, termination, or any other legal or
equitable action to disrupt the quiet enjoyment of the Work by the public
as contemplated by Affirmer's express Statement of Purpose.
3. Public License Fallback. Should any part of the Waiver for any reason
be judged legally invalid or ineffective under applicable law, then the
Waiver shall be preserved to the maximum extent permitted taking into
account Affirmer's express Statement of Purpose. In addition, to the
extent the Waiver is so judged Affirmer hereby grants to each affected
person a royalty-free, non transferable, non sublicensable, non exclusive,
irrevocable and unconditional license to exercise Affirmer's Copyright and
Related Rights in the Work (i) in all territories worldwide, (ii) for the
maximum duration provided by applicable law or treaty (including future
time extensions), (iii) in any current or future medium and for any number
of copies, and (iv) for any purpose whatsoever, including without
limitation commercial, advertising or promotional purposes (the
"License"). The License shall be deemed effective as of the date CC0 was
applied by Affirmer to the Work. Should any part of the License for any
reason be judged legally invalid or ineffective under applicable law, such
partial invalidity or ineffectiveness shall not invalidate the remainder
of the License, and in such case Affirmer hereby affirms that he or she
will not (i) exercise any of his or her remaining Copyright and Related
Rights in the Work or (ii) assert any associated claims and causes of
action with respect to the Work, in either case contrary to Affirmer's
express Statement of Purpose.
4. Limitations and Disclaimers.
a. No trademark or patent rights held by Affirmer are waived, abandoned,
surrendered, licensed or otherwise affected by this document.
b. Affirmer offers the Work as-is and makes no representations or
warranties of any kind concerning the Work, express, implied,
statutory or otherwise, including without limitation warranties of
title, merchantability, fitness for a particular purpose, non
infringement, or the absence of latent or other defects, accuracy, or
the present or absence of errors, whether or not discoverable, all to
the greatest extent permissible under applicable law.
c. Affirmer disclaims responsibility for clearing rights of other persons
that may apply to the Work or any use thereof, including without
limitation any person's Copyright and Related Rights in the Work.
Further, Affirmer disclaims responsibility for obtaining any necessary
consents, permissions or other rights required for any use of the
Work.
d. Affirmer understands and acknowledges that Creative Commons is not a
party to this document and has no duty or obligation with respect to
this CC0 or use of the Work.
| {
"repo_name": "kdeldycke/awesome-iam",
"stars": "1284",
"repo_language": "None",
"file_name": "autofix.yaml",
"mime_type": "text/plain"
} |
<!--lint disable awesome-heading-->
<p align="center">
<a href="https://github.com/kdeldycke/awesome-iam#readme">
<img src="https://raw.githubusercontent.com/kdeldycke/awesome-iam/main/assets/awesome-iam-header.jpg" alt="Awesome IAM">
</a>
</p>
<p align="center">
<a href="https://github.com/kdeldycke/awesome-iam#readme" hreflang="en"><img src="https://img.shields.io/badge/lang-English-blue?style=flat-square" lang="en" alt="English"></a>
<a href="https://github.com/kdeldycke/awesome-iam/blob/main/readme.zh.md" hreflang="zh"><img src="https://img.shields.io/badge/lang-汉语-blue?style=flat-square" lang="zh" alt="汉语"></a>
</p>
<p align="center">
<sup>This list is <a href="#sponsor-def">sponsored<sup id="sponsor-ref">[0]</sup></a> by:</sup><br>
</p>
<p align="center">
<a href="https://www.descope.com/?utm_source=awesome-iam&utm_medium=referral&utm_campaign=awesome-iam-oss-sponsorship">
<picture>
<source media="(prefers-color-scheme: dark)" srcset="https://raw.githubusercontent.com/kdeldycke/awesome-iam/main/assets/descope-logo-dark-background.svg">
<source media="(prefers-color-scheme: light)" srcset="https://raw.githubusercontent.com/kdeldycke/awesome-iam/main/assets/descope-logo-light-background.svg">
<img width="300" src="https://raw.githubusercontent.com/kdeldycke/awesome-iam/main/assets/descope-logo-light-background.svg">
</picture>
<br/>
<strong>Drag and drop your auth.</strong><br/>
Add authentication, user management, and authorization to your app with a few lines of code.
</a>
<!-- Comment this sponsorship call-to-action if there is a sponsor logo to increase its impact. -->
<!--
<a href="https://github.com/sponsors/kdeldycke">
<strong>Yᴏᴜʀ Iᴅᴇɴᴛɪᴛʏ & Aᴜᴛʜᴇɴᴛɪᴄᴀᴛɪᴏɴ Pʀᴏᴅᴜᴄᴛ ʜᴇʀᴇ!</strong>
<br/>
<sup>Add a link to your company or project here: back me up via a GitHub sponsorship.</sup>
</a>
-->
</p>
---
<p align="center">
<i>Trusting is hard. Knowing who to trust, even harder.</i><br>
— Maria V. Snyder<sup id="intro-quote-ref"><a href="#intro-quote-def">[1]</a></sup>
</p>
<!--lint disable double-link-->
[IAM](https://en.wikipedia.org/wiki/Identity_management) stands for Identity and Access Management. It is a complex domain which covers **user accounts, authentication, authorization, roles, permissions and privacy**. It is an essential pillar of the cloud stack, where users, products and security meets. The [other pillar being billing & payments 💰](https://github.com/kdeldycke/awesome-billing/).
This curated [](https://github.com/sindresorhus/awesome) list expose all the technologies, protocols and jargon of the domain in a comprehensive and actionable manner.
<!--lint enable double-link-->
## Contents
<!-- mdformat-toc start --slug=github --no-anchors --maxlevel=6 --minlevel=2 -->
- [Overview](#overview)
- [Security](#security)
- [Account Management](#account-management)
- [Cryptography](#cryptography)
- [Identifiers](#identifiers)
- [Zero-trust Network](#zero-trust-network)
- [Authentication](#authentication)
- [Password-based](#password-based)
- [Password-less](#password-less)
- [Security Key](#security-key)
- [Multi-Factor](#multi-factor)
- [SMS-based](#sms-based)
- [Public-Key Infrastructure (PKI)](#public-key-infrastructure-pki)
- [JWT](#jwt)
- [OAuth2 & OpenID](#oauth2--openid)
- [SAML](#saml)
- [Authorization](#authorization)
- [Policy models](#policy-models)
- [Open-source policy frameworks](#open-source-policy-frameworks)
- [AWS policy tools](#aws-policy-tools)
- [Macaroons](#macaroons)
- [Secret Management](#secret-management)
- [Hardware Security Module (HSM)](#hardware-security-module-hsm)
- [Trust & Safety](#trust--safety)
- [User Identity](#user-identity)
- [Fraud](#fraud)
- [Moderation](#moderation)
- [Threat Intelligence](#threat-intelligence)
- [Captcha](#captcha)
- [Blocklists](#blocklists)
- [Hostnames and Subdomains](#hostnames-and-subdomains)
- [Emails](#emails)
- [Reserved IDs](#reserved-ids)
- [Profanity](#profanity)
- [Privacy](#privacy)
- [Anonymization](#anonymization)
- [GDPR](#gdpr)
- [UX/UI](#uxui)
- [Competitive Analysis](#competitive-analysis)
- [History](#history)
<!-- mdformat-toc end -->
## Overview
<img align="right" width="50%" src="./assets/cloud-software-stack-iam.jpg"/>
In a Stanford class providing an [overview of cloud computing](http://web.stanford.edu/class/cs349d/docs/L01_overview.pdf), the software architecture of the platform is described as in the right diagram →
Here we set out the big picture: definition and strategic importance of the domain, its place in the larger ecosystem, plus some critical features.
- [The EnterpriseReady SaaS Feature Guides](https://www.enterpriseready.io) - The majority of the features making B2B users happy will be implemented by the IAM perimeter.
- [IAM is hard. It's really hard.](https://twitter.com/kmcquade3/status/1291801858676228098) - “Overly permissive AWS IAM policies that allowed `s3:GetObject` to `*` (all) resources”, led to \$80 million fine for Capital One. The only reason why you can't overlook IAM as a business owner.
- [IAM Is The Real Cloud Lock-In](https://forrestbrazeal.com/2019/02/18/cloud-irregular-iam-is-the-real-cloud-lock-in/) - A little *click-baity*, but author admit that “It depends on how much you trust them to 1. Stay in business; 2. Not jack up your prices; 3. Not deprecate services out from under you; 4. Provide more value to you in business acceleration than they take away in flexibility.”
## Security
Security is one of the most central pillar of IAM foundations. Here are some broad concepts.
- [Enterprise Information Security](https://infosec.mozilla.org) - Mozilla's security and access guidelines.
- [Mitigating Cloud Vulnerabilities](https://media.defense.gov/2020/Jan/22/2002237484/-1/-1/0/CSI-MITIGATING-CLOUD-VULNERABILITIES_20200121.PDF) - “This document divides cloud vulnerabilities into four classes (misconfiguration, poor access control, shared tenancy vulnerabilities, and supply chain vulnerabilities)”.
- [Cartography](https://github.com/lyft/cartography) - A Neo4J-based tool to map out dependencies and relationships between services and resources. Supports AWS, GCP, GSuite, Okta and GitHub.
- [Open guide to AWS Security and IAM](https://github.com/open-guides/og-aws#security-and-iam)
## Account Management
The foundation of IAM: the definition and life-cycle of users, groups, roles and permissions.
- [As a user, I want…](https://mobile.twitter.com/oktopushup/status/1030457418206068736) - A meta-critic of account management, in which features expected by the business clash with real user needs, in the form of user stories written by a fictional project manager.
- [Things end users care about but programmers don't](https://instadeq.com/blog/posts/things-end-users-care-about-but-programmers-dont/) - In the same spirit as above, but broader: all the little things we overlook as developers but users really care about. In the top of that list lies account-centric features, diverse integration and import/export tools. I.e. all the enterprise customers needs to cover.
- [Separate the account, user and login/auth details](https://news.ycombinator.com/item?id=21151830) - Sound advice to lay down the foundation of a future-proof IAM API.
- [Identity Beyond Usernames](https://lord.io/blog/2020/usernames/) - On the concept of usernames as identifiers, and the complexities introduced when unicode characters meets uniqueness requirements.
- [Kratos](https://github.com/ory/kratos) - User login, user registration, 2FA and profile management.
- [Conjur](https://github.com/cyberark/conjur) - Automatically secures secrets used by privileged users and machine identities.
- [SuperTokens](https://github.com/supertokens/supertokens-core) - Open-source project for login and session management which supports passwordless, social login, email and phone logins.
- [UserFrosting](https://github.com/userfrosting/UserFrosting) - Modern PHP user login and management framework.
## Cryptography
The whole authentication stack is based on cryptography primitives. This can't be overlooked.
- [Cryptographic Right Answers](https://latacora.micro.blog/2018/04/03/cryptographic-right-answers.html) - An up to date set of recommendations for developers who are not cryptography engineers. There's even a [shorter summary](https://news.ycombinator.com/item?id=16749140) available.
- [Real World Crypto Symposium](https://rwc.iacr.org) - Aims to bring together cryptography researchers with developers, focusing on uses in real-world environments such as the Internet, the cloud, and embedded devices.
- [An Overview of Cryptography](https://www.garykessler.net/library/crypto.html) - “This paper has two major purposes. The first is to define some of the terms and concepts behind basic cryptographic methods, and to offer a way to compare the myriad cryptographic schemes in use today. The second is to provide some real examples of cryptography in use today.”
- [Papers we love: Cryptography](https://github.com/papers-we-love/papers-we-love/blob/master/cryptography/README.md) - Foundational papers of cryptography.
- [Lifetimes of cryptographic hash functions](http://valerieaurora.org/hash.html) - “If you are using compare-by-hash to generate addresses for data that can be supplied by malicious users, you should have a plan to migrate to a new hash every few years”.
### Identifiers
Tokens, primary keys, UUIDs, … Whatever the end use, you'll have to generate these numbers with some randomness and uniqueness properties.
- [Security Recommendations for Any Device that Depends on Randomly-Generated Numbers](https://www.av8n.com/computer/htm/secure-random.htm) - “The phrase ‘random number generator’ should be parsed as follows: It is a random generator of numbers. It is not a generator of random numbers.”
- [RFC #4122: UUID - Security Considerations](https://www.rfc-editor.org/rfc/rfc4122#section-6) - “Do not assume that UUIDs are hard to guess; they should not be used as security capabilities (identifiers whose mere possession grants access)”. UUIDs are designed to be unique, not to be random or unpredictable: do not use UUIDs as a secret.
- [Awesome Identifiers](https://adileo.github.io/awesome-identifiers/) - A benchmark of all identifier formats.
- [Awesome GUID](https://github.com/secretGeek/AwesomeGUID) - Funny take on the global aspect of unique identifiers.
## Zero-trust Network
Zero trust network security operates under the principle “never trust, always verify”.
- [BeyondCorp: A New Approach to Enterprise Security](https://www.usenix.org/system/files/login/articles/login_dec14_02_ward.pdf) - Quick overview of Google's Zero-trust Network initiative.
- [What is BeyondCorp? What is Identity-Aware Proxy?](https://medium.com/google-cloud/what-is-beyondcorp-what-is-identity-aware-proxy-de525d9b3f90) - More companies add extra layers of VPNs, firewalls, restrictions and constraints, resulting in a terrible experience and a slight security gain. There's a better way.
- [oathkeeper](https://github.com/ory/oathkeeper) - Identity & Access Proxy and Access Control Decision API that authenticates, authorizes, and mutates incoming HTTP requests. Inspired by the BeyondCorp / Zero Trust white paper.
- [transcend](https://github.com/cogolabs/transcend) - BeyondCorp-inspired Access Proxy server.
- [Pomerium](https://github.com/pomerium/pomerium) - An identity-aware proxy that enables secure access to internal applications.
## Authentication
Protocols and technologies to verify that you are who you pretend to be.
- [API Tokens: A Tedious Survey](https://fly.io/blog/api-tokens-a-tedious-survey/) - An overview and comparison of all token-based authentication schemes for end-user APIs.
- [A Child's Garden of Inter-Service Authentication Schemes](https://web.archive.org/web/20200507173734/https://latacora.micro.blog/a-childs-garden/) - In the same spirit as above, but this time at the service level.
- [Scaling backend authentication at Facebook](https://www.youtube.com/watch?v=kY-Bkv3qxMc) - How-to in a nutshell: 1. Small root of trust; 2. TLS isn't enough; 3. Certificate-based tokens; 4. Crypto Auth Tokens (CATs). See the [slides](https://rwc.iacr.org/2018/Slides/Lewi.pdf) for more details.
### Password-based
- [The new NIST password guidance](https://pciguru.wordpress.com/2019/03/11/the-new-nist-password-guidance/) - A summary of [NIST Special Publication 800-63B](https://pages.nist.gov/800-63-3/sp800-63b.html) covering new password complexity guidelines.
- [Password Storage Cheat Sheet](https://cheatsheetseries.owasp.org/cheatsheets/Password_Storage_Cheat_Sheet.html) - The only way to slow down offline attacks is by carefully choosing hash algorithms that are as resource intensive as possible.
- [Password expiration is dead](https://techcrunch.com/2019/06/02/password-expiration-is-dead-long-live-your-passwords/) - Recent scientific research calls into question the value of many long-standing password-security practices such as password expiration policies, and points instead to better alternatives such as enforcing banned-password lists and MFA.
- [Practical Recommendations for Stronger, More Usable Passwords](http://www.andrew.cmu.edu/user/nicolasc/publications/Tan-CCS20.pdf) - This study recommend the association of: blocklist checks against commonly leaked passwords, password policies without character-class requirements, minimum-strength policies.
- [Banks, Arbitrary Password Restrictions and Why They Don't Matter](https://www.troyhunt.com/banks-arbitrary-password-restrictions-and-why-they-dont-matter/) - “Arbitrary low limits on length and character composition are bad. They look bad, they lead to negative speculation about security posture and they break tools like password managers.”
- [Dumb Password Rules](https://github.com/dumb-password-rules/dumb-password-rules) - Shaming sites with dumb password rules.
- [Plain Text Offenders](https://plaintextoffenders.com/about/) - Public shaming of websites storing passwords in plain text.
- [Password Manager Resources](https://github.com/apple/password-manager-resources) - A collection of password rules, change URLs and quirks by sites.
- [A Well-Known URL for Changing Passwords](https://github.com/WICG/change-password-url) - Specification defining site resource for password updates.
- [How to change the hashing scheme of already hashed user's passwords](https://news.ycombinator.com/item?id=20109360) - Good news: you're not stuck with a legacy password saving scheme. Here is a trick to transparently upgrade to stronger hashing algorithm.
### Password-less
- [An argument for passwordless](https://web.archive.org/web/20190515230752/https://biarity.gitlab.io/2018/02/23/passwordless/) - Passwords are not the be-all and end-all of user authentication. This article tries to tell you why.
- [Magic Links – Are they Actually Outdated?](https://zitadel.com/blog/magic-links) - What are magic links, their origin, pros and cons.
- [WebAuthn guide](https://webauthn.guide) - A very accessible guide to WebAuthn, a standard allowing “servers to register and authenticate users using public key cryptography instead of a password”, supported by all major browsers.
### Security Key
- [Webauthn and security keys](https://www.imperialviolet.org/2018/03/27/webauthn.html) - Describe how authentication works with security keys, details the protocols, and how they articulates with WebAuthn. Key takeaway: “There is no way to create a U2F key with webauthn however. (…) So complete the transition to webauthn of your login process first, then transition registration.”
- [Getting started with security keys](https://paulstamatiou.com/getting-started-with-security-keys/) - A practical guide to stay safe online and prevent phishing with FIDO2, WebAuthn and security keys.
- [Solo](https://github.com/solokeys/solo) - Open security key supporting FIDO2 & U2F over USB + NFC.
- [OpenSK](https://github.com/google/OpenSK) - Open-source implementation for security keys written in Rust that supports both FIDO U2F and FIDO2 standards.
- [YubiKey Guide](https://github.com/drduh/YubiKey-Guide) - Guide to using YubiKey as a SmartCard for storing GPG encryption, signing and authentication keys, which can also be used for SSH. Many of the principles in this document are applicable to other smart card devices.
- [YubiKey at Datadog](https://github.com/DataDog/yubikey) - Guide to setup Yubikey, U2F, GPG, git, SSH, Keybase, VMware Fusion and Docker Content Trust.
### Multi-Factor
- [Breaking Password Dependencies: Challenges in the Final Mile at Microsoft](https://www.youtube.com/watch?v=B_mhJO2qHlQ) - The primary source of account hacks is password spraying (on legacy auth like SMTP, IMAP, POP, etc.), second is replay attack. Takeaway: password are insecure, use and enforce MFA.
- [Beyond Passwords: 2FA, U2F and Google Advanced Protection](https://www.troyhunt.com/beyond-passwords-2fa-u2f-and-google-advanced-protection/) - An excellent walk-trough over all these technologies.
- [A Comparative Long-Term Study of Fallback Authentication](https://maximiliangolla.com/files/2019/papers/usec2019-30-wip-fallback-long-term-study-finalv5.pdf) - Key take-away: “schemes based on email and SMS are more usable. Mechanisms based on designated trustees and personal knowledge questions, on the other hand, fall short, both in terms of convenience and efficiency.”
- [Secrets, Lies, and Account Recovery: Lessons from the Use of Personal Knowledge Questions at Google](https://static.googleusercontent.com/media/research.google.com/en/us/pubs/archive/43783.pdf) - “Our analysis confirms that secret questions generally offer a security level that is far lower than user-chosen passwords. (…) Surprisingly, we found that a significant cause of this insecurity is that users often don't answer truthfully. (…) On the usability side, we show that secret answers have surprisingly poor memorability”.
- [How effective is basic account hygiene at preventing hijacking](https://security.googleblog.com/2019/05/new-research-how-effective-is-basic.html) - Google security team's data shows 2FA blocks 100% of automated bot hacks.
- [Your Pa\$\$word doesn't matter](https://techcommunity.microsoft.com/t5/Azure-Active-Directory-Identity/Your-Pa-word-doesn-t-matter/ba-p/731984) - Same conclusion as above from Microsoft: “Based on our studies, your account is more than 99.9% less likely to be compromised if you use MFA.”
- [Attacking Google Authenticator](https://unix-ninja.com/p/attacking_google_authenticator) - Probably on the verge of paranoia, but might be a reason to rate limit 2FA validation attempts.
- [Compromising online accounts by cracking voicemail systems](https://www.martinvigo.com/voicemailcracker/) - Or why you should not rely on automated phone calls as a method to reach the user and reset passwords, 2FA or for any kind of verification. Not unlike SMS-based 2FA, it is currently insecure and can be compromised by the way of its weakest link: voicemail systems.
- [Getting 2FA Right in 2019](https://blog.trailofbits.com/2019/06/20/getting-2fa-right-in-2019/) - On the UX aspects of 2FA.
- [2FA is missing a key feature](https://syslog.ravelin.com/2fa-is-missing-a-key-feature-c781c3861db) - “When my 2FA code is entered incorrectly I'd like to know about it”.
- [SMS Multifactor Authentication in Antarctica](https://brr.fyi/posts/sms-mfa) - Doesn't work because there are no cell phone towers at stations in Antarctica.
- [Authelia](https://github.com/authelia/authelia) - Open-source authentication and authorization server providing two-factor authentication and single sign-on (SSO) for your applications via a web portal.
- [Kanidm](https://github.com/kanidm/kanidm) - Simple, secure and fast identity management platform.
### SMS-based
TL;DR: don't. For details, see articles below.
- [SMS 2FA auth is deprecated by NIST](https://techcrunch.com/2016/07/25/nist-declares-the-age-of-sms-based-2-factor-authentication-over/) - NIST has said that 2FA via SMS is bad and awful since 2016.
- [SMS: The most popular and least secure 2FA method](https://www.allthingsauth.com/2018/02/27/sms-the-most-popular-and-least-secure-2fa-method/)
- [Is SMS 2FA Secure? No.](https://www.issms2fasecure.com) - Definitive research project demonstrating successful attempts at SIM swapping.
- [Hackers Hit Twitter C.E.O. Jack Dorsey in a 'SIM Swap.' You're at Risk, Too.](https://www.nytimes.com/2019/09/05/technology/sim-swap-jack-dorsey-hack.html)
- [AT&T rep handed control of his cellphone account to a hacker](https://www.theregister.co.uk/2017/07/10/att_falls_for_hacker_tricks/)
- [The Most Expensive Lesson Of My Life: Details of SIM port hack](https://medium.com/coinmonks/the-most-expensive-lesson-of-my-life-details-of-sim-port-hack-35de11517124)
- [SIM swap horror story](https://www.zdnet.com/article/sim-swap-horror-story-ive-lost-decades-of-data-and-google-wont-lift-a-finger/)
- [AWS is on its way to deprecate SMS-based 2FA](https://aws.amazon.com/iam/details/mfa/) - “We encourage you to use MFA through a U2F security key, hardware device, or virtual (software-based) MFA device. You can continue using this feature until January 31, 2019.”
### Public-Key Infrastructure (PKI)
Certificate-based authentication.
- [PKI for busy people](https://gist.github.com/hoffa/5a939fd0f3bcd2a6a0e4754cb2cf3f1b) - Quick overview of the important stuff.
- [Everything you should know about certificates and PKI but are too afraid to ask](https://smallstep.com/blog/everything-pki.html) - PKI lets you define a system cryptographically. It's universal and vendor neutral.
- [`lemur`](https://github.com/Netflix/lemur) - Acts as a broker between CAs and environments, providing a central portal for developers to issue TLS certificates with 'sane' defaults.
- [CFSSL](https://github.com/cloudflare/cfssl) - A swiss army knife for PKI/TLS by CloudFlare. Command line tool and an HTTP API server for signing, verifying, and bundling TLS certificates.
- [JA3](https://github.com/salesforce/ja3) - Method for creating SSL/TLS client fingerprints that should be easy to produce on any platform and can be easily shared for threat intelligence.
### JWT
[JSON Web Token](https://en.wikipedia.org/wiki/JSON_Web_Token) is a bearer's token.
- [Introduction to JSON Web Tokens](https://jwt.io/introduction/) - Get up to speed on JWT with this article.
- [Learn how to use JWT for Authentication](https://github.com/dwyl/learn-json-web-tokens) - Learn how to use JWT to secure your web app.
- [Using JSON Web Tokens as API Keys](https://auth0.com/blog/using-json-web-tokens-as-api-keys/) - Compared to API keys, JWTs offers granular security, homogenous auth architecture, decentralized issuance, OAuth2 compliance, debuggability, expiration control, device management.
- [Managing a Secure JSON Web Token Implementation](https://cursorblog.com/managing-a-secure-json-web-token-implementation/) - JWT has all sorts of flexibility that make it hard to use well.
- [Hardcoded secrets, unverified tokens, and other common JWT mistakes](https://r2c.dev/blog/2020/hardcoded-secrets-unverified-tokens-and-other-common-jwt-mistakes/) - A good recap of all JWT pitfalls.
- [Adding JSON Web Token API Keys to a DenyList](https://auth0.com/blog/denylist-json-web-token-api-keys/) - On token invalidation.
- [Stop using JWT for sessions](http://cryto.net/~joepie91/blog/2016/06/13/stop-using-jwt-for-sessions/) - And [why your "solution" doesn't work](http://cryto.net/%7Ejoepie91/blog/2016/06/19/stop-using-jwt-for-sessions-part-2-why-your-solution-doesnt-work/), because [stateless JWT tokens cannot be invalidated or updated](https://news.ycombinator.com/item?id=18354141). They will introduce either size issues or security issues depending on where you store them. Stateful JWT tokens are functionally the same as session cookies, but without the battle-tested and well-reviewed implementations or client support.
- [JWT, JWS and JWE for Not So Dummies!](https://medium.facilelogin.com/jwt-jws-and-jwe-for-not-so-dummies-b63310d201a3) - A signed JWT is known as a JWS (JSON Web Signature). In fact a JWT does not exist itself — either it has to be a JWS or a JWE (JSON Web Encryption). Its like an abstract class — the JWS and JWE are the concrete implementations.
- [JOSE is a Bad Standard That Everyone Should Avoid](https://paragonie.com/blog/2017/03/jwt-json-web-tokens-is-bad-standard-that-everyone-should-avoid) - The standards are either completely broken or complex minefields hard to navigate.
- [JWT.io](https://jwt.io) - Allows you to decode, verify and generate JWT.
- [`loginsrv`](https://github.com/tarent/loginsrv) - Standalone minimalistic login server providing a JWT login for multiple login backends (htpasswd, OSIAM, user/password, HTTP basic authentication, OAuth2: GitHub, Google, Bitbucket, Facebook, Gitlab).
- [jwtXploiter](https://github.com/DontPanicO/jwtXploiter) - A tool to test security of json web token.
### OAuth2 & OpenID
[OAuth 2.0](https://en.wikipedia.org/wiki/OAuth#OAuth_2.0) is a *delegated authorization* framework. [OpenID Connect (OIDC)](https://en.wikipedia.org/wiki/OpenID_Connect) is an *authentication* layer on top of it.
The old *OpenID* is dead; the new *OpenID Connect* is very much not-dead.
- [The problem with OAuth for Authentication](http://www.thread-safe.com/2012/01/problem-with-oauth-for-authentication.html) - “The problem is that OAuth 2.0 is a Delegated Authorization protocol, and not a Authentication protocol.” 10 years after, this article is still the best explanation on [why use OpenID Connect instead of plain OAuth2](https://security.stackexchange.com/a/260519)?
- [An Illustrated Guide to OAuth and OpenID Connect](https://developer.okta.com/blog/2019/10/21/illustrated-guide-to-oauth-and-oidc) - Explain how these standards work using simplified illustrations.
- [OAuth 2 Simplified](https://aaronparecki.com/oauth-2-simplified/) - A reference article describing the protocol in simplified format to help developers and service providers implement it.
- [OAuth 2.0 and OpenID Connect (in plain English)](https://www.youtube.com/watch?v=996OiexHze0) - Starts with an historical context on how these standards came to be, clears up the innacuracies in the vocabulary, then details the protocols and its pitfalls to make it less intimidating.
- [Everything You Need to Know About OAuth (2.0)](https://gravitational.com/blog/everything-you-need-to-know-about-oauth/) - A good overview with a practical case study on how Teleport, an open-source remote access tool, allows users to log in through GitHub SSO.
- [OAuth in one picture](https://mobile.twitter.com/kamranahmedse/status/1276994010423361540) - A nice summary card.
- [How to Implement a Secure Central Authentication Service in Six Steps](https://engineering.shopify.com/blogs/engineering/implement-secure-central-authentication-service-six-steps) - Got multiple legacy systems to merge with their own login methods and accounts? Here is how to merge all that mess by the way of OIDC.
- [Open-Sourcing BuzzFeed's SSO Experience](https://increment.com/security/open-sourcing-buzzfeeds-single-sign-on-process/) - OAuth2-friendly adaptation of the Central Authentication Service (CAS) protocol. You'll find there good OAuth user flow diagrams.
- [OAuth 2.0 Security Best Current Practice](https://tools.ietf.org/html/draft-ietf-oauth-security-topics-16) - “Updates and extends the OAuth 2.0 Security Threat Model to incorporate practical experiences gathered since OAuth 2.0 was published and covers new threats relevant due to the broader application”.
- [Hidden OAuth attack vectors](https://portswigger.net/web-security/oauth) - How to identify and exploit some of the key vulnerabilities found in OAuth 2.0 authentication mechanisms.
- [PKCE Explained](https://www.loginradius.com/blog/engineering/pkce/) - “PKCE is used to provide one more security layer to the authorization code flow in OAuth and OpenID Connect.”
- [Hydra](https://gethydra.sh) - Open-source OIDC & OAuth2 Server.
- [Keycloak](https://www.keycloak.org) - Open-source Identity and Access Management. Supports OIDC, OAuth 2 and SAML 2, LDAP and AD directories, password policies.
- [Casdoor](https://github.com/casbin/casdoor) - A UI-first centralized authentication / Single-Sign-On (SSO) platform based. Supports OIDC and OAuth 2, social logins, user management, 2FA based on Email and SMS.
- [authentik](https://goauthentik.io/?#correctness) - Open-source Identity Provider similar to Keycloak.
- [ZITADEL](https://github.com/zitadel/zitadel) - An Open-Source solution built with Go and Angular to manage all your systems, users and service accounts together with their roles and external identities. ZITADEL provides you with OIDC, OAuth 2.0, login & register flows, passwordless and MFA authentication. All this is built on top of eventsourcing in combination with CQRS to provide a great audit trail.
- [a12n-server](https://github.com/curveball/a12n-server) - A simple authentication system which only implements the relevant parts of the OAuth2 standards.
- [Logto](https://github.com/logto-io/logto) - Build the sign-in, auth, and user identity with this OIDC-based identity service.
- [The Decline of OpenID](https://penguindreams.org/blog/the-decline-of-openid/) - OpenID is being replaced in the public web to a mix of OAuth 1, OAuth 2 or other proprietary SSO protocols.
- [Why Mastercard Doesn't Use OAuth 2.0](https://developer.mastercard.com/blog/why-mastercard-doesnt-use-oauth-20) - “They did this to provide message-level integrity. OAuth 2 switched to transport-level confidentiality/integrity.” (which TLS provides) ([source](https://news.ycombinator.com/item?id=17486165)).
- [OAuth 2.0 and the Road to Hell](https://gist.github.com/nckroy/dd2d4dfc86f7d13045ad715377b6a48f) - The resignation letter from the lead author and editor of the Oauth 2.0 specification.
### SAML
Security Assertion Markup Language (SAML) 2.0 is a means to exchange authorization and authentication between services, like OAuth/OpenID protocols above.
Typical SAML identity provider is an institution or a big corporation's internal SSO, while the typical OIDC/OAuth provider is a tech company that runs a data silo.
- [SAML vs. OAuth](https://web.archive.org/web/20230327071347/https://www.cloudflare.com/learning/access-management/what-is-oauth/) - “OAuth is a protocol for authorization: it ensures Bob goes to the right parking lot. In contrast, SAML is a protocol for authentication, or allowing Bob to get past the guardhouse.”
- [The Difference Between SAML 2.0 and OAuth 2.0](https://www.ubisecure.com/uncategorized/difference-between-saml-and-oauth/) - “Even though SAML was actually designed to be widely applicable, its contemporary usage is typically shifted towards enterprise SSO scenarios. On the other hand, OAuth was designed for use with applications on the Internet, especially for delegated authorisation.”
- [What's the Difference Between OAuth, OpenID Connect, and SAML?](https://www.okta.com/identity-101/whats-the-difference-between-oauth-openid-connect-and-saml/) - Identity is hard. Another take on the different protocol is always welcome to help makes sense of it all.
- [How SAML 2.0 Authentication Works](https://gravitational.com/blog/how-saml-authentication-works/) - Overview of the how and why of SSO and SAML.
- [Web Single Sign-On, the SAML 2.0 perspective](https://blog.theodo.com/2019/06/web-single-sign-on-the-saml-2-0-perspective/) - Another naive explanation of SAML workflow in the context of corporate SSO implementation.
- [The Beer Drinker's Guide to SAML](https://duo.com/blog/the-beer-drinkers-guide-to-saml) - SAML is arcane at times. A another analogy might helps get more sense out of it.
- [SAML is insecure by design](https://joonas.fi/2021/08/saml-is-insecure-by-design/) - Not only weird, SAML is also insecure by design, as it relies on signatures based on XML canonicalization, not XML byte stream. Which means you can exploit XML parser/encoder differences.
- [The Difficulties of SAML Single Logout](https://wiki.shibboleth.net/confluence/display/CONCEPT/SLOIssues) - On the technical and UX issues of single logout implementations.
- [The SSO Wall of Shame](https://sso.tax) - A documented rant on the exessive pricing practiced by SaaS providers to activate SSO on their product. The author's point is, as a core security feature, SSO should be reasonnably priced and not part of an exclusive tier.
## Authorization
Now we know you are you. But are you allowed to do what you want to do?
Policy specification is the science, enforcement is the art.
### Policy models
As a concept, access control policies can be designed to follow very different archetypes, from classic [Access Control Lists](https://en.wikipedia.org/wiki/Access-control_list) to [Role Based Access Control](https://en.wikipedia.org/wiki/Role-based_access_control). In this section we explore lots of different patterns and architectures.
- [Why Authorization is Hard](https://www.osohq.com/post/why-authorization-is-hard) - Because it needs multiple tradeoffs on Enforcement which is required in so many places, on Decision architecture to split business logic from authorization logic, and on Modeling to balance power and complexity.
- [The never-ending product requirements of user authorization](https://alexolivier.me/posts/the-never-ending-product-requirements-of-user-authorization) - How a simple authorization model based on roles is not enough and gets complicated fast due to product packaging, data locality, enterprise organizations and compliance.
- [RBAC like it was meant to be](https://tailscale.com/blog/rbac-like-it-was-meant-to-be/) - How we got from DAC (unix permissions, secret URL), to MAC (DRM, MFA, 2FA, SELinux), to RBAC. Details how the latter allows for better modeling of policies, ACLs, users and groups.
- [The Case for Granular Permissions](https://cerbos.dev/blog/the-case-for-granular-permissions) - Discuss the limitations of RBAC and how ABAC (Attribute-Based Access Control) addresses them.
- [In Search For a Perfect Access Control System](https://goteleport.com/blog/access-controls/) - The historical origins of authorization schemes. Hints at the future of sharing, trust and delegation between different teams and organizations.
- [AWS IAM Roles, a tale of unnecessary complexity](https://infosec.rodeo/posts/thoughts-on-aws-iam/) - The history of fast-growing AWS explains how the current sheme came to be, and how it compares to GCP's resource hierarchy.
- [Semantic-based Automated Reasoning for AWS Access Policies using SMT](https://d1.awsstatic.com/Security/pdfs/Semantic_Based_Automated_Reasoning_for_AWS_Access_Policies_Using_SMT.pdf) - Zelkova is how AWS does it. This system perform symbolic analysis of IAM policies, and solve the reachability of resources according user's rights and access constraints. Also see the higher-level [introduction given at re:inforce 2019](https://youtu.be/x6wsTFnU3eY?t=2111).
- [Zanzibar: Google's Consistent, Global Authorization System](https://ai.google/research/pubs/pub48190) - Scales to trillions of access control lists and millions of authorization requests per second to support services used by billions of people. It has maintained 95th-percentile latency of less than 10 milliseconds and availability of greater than 99.999% over 3 years of production use. [Other bits not in the paper](https://twitter.com/LeaKissner/status/1136626971566149633). [Zanzibar Academy](https://zanzibar.academy/) is a site dedicated to explaining how Zanzibar works.
- [SpiceDB](https://github.com/authzed/spicedb) - An open source database system for managing security-critical application permissions inspired by Zanzibar.
- Description of an [authz system that is built around labeled security and RBAC concepts](https://news.ycombinator.com/item?id=20136831).
### Open-source policy frameworks
Collection of open-source projects if you're looking to roll your own policy implementation.
- [Keto](https://github.com/ory/keto) - Policy decision point. It uses a set of access control policies, similar to AWS policies, in order to determine whether a subject is authorized to perform a certain action on a resource.
- [Ladon](https://github.com/ory/ladon) - Access control library, inspired by AWS.
- [Athenz](https://github.com/yahoo/athenz) - Set of services and libraries supporting service authentication and role-based authorization (RBAC) for provisioning and configuration.
- [Casbin](https://github.com/casbin/casbin) - Open-source access control library for Golang projects.
- [Open Policy Agent](https://github.com/open-policy-agent/opa) - An open-source general-purpose decision engine to create and enforce attribute-based access control (ABAC) policies.
- [Topaz](https://github.com/aserto-dev/topaz) - An open-source project which combines the policy-as-code and decision logging of OPA with a Zanzibar-modeled directory.
- [Open Policy Administration Layer](https://github.com/permitio/opal) - Open Source administration layer for OPA, detecting changes to both policy and policy data in realtime and pushing live updates to OPA agents. OPAL brings open-policy up to the speed needed by live applications.
- [Gubernator](https://github.com/mailgun/gubernator) - High performance rate-limiting micro-service and library.
- [Biscuit](https://www.clever-cloud.com/blog/engineering/2021/04/12/introduction-to-biscuit/) - Biscuit merge concepts from cookies, JWTs, macaroons and Open Policy Agent. “It provide a logic language based on Datalog to write authorization policies. It can store data, like JWT, or small conditions like Macaroons, but it is also able to represent more complex rules like role-based access control, delegation, hierarchies.”
- [Oso](https://github.com/osohq/oso) - A batteries-included library for building authorization in your application.
- [Cerbos](https://github.com/cerbos/cerbos) - An authorization endpoint to write context-aware access control policies.
### AWS policy tools
Tools and resources exclusively targetting the [AWS IAM policies](http://docs.aws.amazon.com/IAM/latest/UserGuide/access_policies.html) ecosystem.
- [Become an AWS IAM Policy Ninja](https://www.youtube.com/watch?v=y7-fAT3z8Lo) - “In my nearly 5 years at Amazon, I carve out a little time each day, each week to look through the forums, customer tickets to try to find out where people are having trouble.”
- [Cloudsplaining](https://github.com/salesforce/cloudsplaining) - Security assessment tool that identifies violations of least privilege and generates a risk-prioritized report.
- [Policy Sentry](https://github.com/salesforce/policy_sentry) - Writing security-conscious IAM Policies by hand can be very tedious and inefficient. Policy Sentry helps users to create least-privilege policies in a matter of seconds.
- [Aardvark and Repokid](https://netflixtechblog.com/introducing-aardvark-and-repokid-53b081bf3a7e) - Netflix tools to enforce least privilege on AWS. The idea is that the default policy on new things is deny all, and then it monitors cloudtrail for privilege failures and reconfigures IAM to allow the smallest possible privilege to get rid of that deny message.
- [Principal Mapper](https://github.com/nccgroup/PMapper) - Quickly evaluates permissions.
- [PolicyUniverse](https://github.com/Netflix-Skunkworks/policyuniverse) - Parse and process AWS policies, statements, ARNs, and wildcards.
- [IAM Floyd](https://github.com/udondan/iam-floyd) - AWS IAM policy statement generator with fluent interface. Helps with creating type safe IAM policies and writing more restrictive/secure statements by offering conditions and ARN generation via IntelliSense. Available for Node.js, Python, .Net and Java.
- [ConsoleMe](https://github.com/Netflix/consoleme) - A self-service tool for AWS that provides end-users and administrators credentials and console access to the onboarded accounts based on their authorization level of managing permissions across multiple accounts, while encouraging least-privilege permissions.
- [IAMbic](https://github.com/noqdev/iambic) - GitOps for IAM. The Terraform of Cloud IAM. IAMbic is a multi-cloud identity and access management (IAM) control plane that centralizes and simplifies cloud access and permissions. It maintains an eventually consistent, human-readable, bi-directional representation of IAM in version control.
### Macaroons
A clever curiosity to distribute and delegate authorization.
- [Google's Macaroons in Five Minutes or Less](https://blog.bren2010.io/blog/googles-macaroons) - If I'm given a Macaroon that authorizes me to perform some action(s) under certain restrictions, I can non-interactively build a second Macaroon with stricter restrictions that I can then give to you.
- [Macaroons: Cookies with Contextual Caveats for Decentralized Authorization in the Cloud](https://ai.google/research/pubs/pub41892) - Google's original paper.
- [Google paper's author compares Macaroons and JWTs](https://news.ycombinator.com/item?id=14294463) - As a consumer/verifier of macaroons, they allow you (through third-party caveats) to defer some authorization decisions to someone else. JWTs don't.
## Secret Management
Architectures, software and hardware allowing the storage and usage of secrets to allow for authentication and authorization, while maintaining the chain of trust.
- [Secret at Scale at Netflix](https://www.youtube.com/watch?v=K0EOPddWpsE) - Solution based on blind signatures. See the [slides](https://rwc.iacr.org/2018/Slides/Mehta.pdf).
- [High Availability in Google's Internal KMS](https://www.youtube.com/watch?v=5T_c-lqgjso) - Not GCP's KMS, but the one at the core of their infrastructure. See the [slides](https://rwc.iacr.org/2018/Slides/Kanagala.pdf).
- [`vault`](https://www.vaultproject.io) - Secure, store and tightly control access to tokens, passwords, certificates, encryption keys.
- [`sops`](https://github.com/mozilla/sops) - Encrypts the values of YAML and JSON files, not the keys.
- [`gitleaks`](https://github.com/zricethezav/gitleaks) - Audit git repos for secrets.
- [`truffleHog`](https://github.com/dxa4481/truffleHog) - Searches through git repositories for high entropy strings and secrets, digging deep into commit history.
- [Keywhiz](https://square.github.io/keywhiz/) - A system for managing and distributing secrets, which can fit well with a service oriented architecture (SOA).
- [`roca`](https://github.com/crocs-muni/roca) - Python module to check for weak RSA moduli in various key formats.
### Hardware Security Module (HSM)
HSMs are physical devices guaranteeing security of secret management at the hardware level.
- [HSM: What they are and why it's likely that you've (indirectly) used one today](https://rwc.iacr.org/2015/Slides/RWC-2015-Hampton.pdf) - Really basic overview of HSM usages.
- [Tidbits on AWS Cloud HSM hardware](https://news.ycombinator.com/item?id=16759383) - AWS CloudHSM Classic is backed by SafeNet's Luna HSM, current CloudHSM rely on Cavium's Nitrox, which allows for partitionable "virtual HSMs".
- [CrypTech](https://cryptech.is) - An open hardware HSM.
- [Keystone](https://keystone-enclave.org) - Open-source project for building trusted execution environments (TEE) with secure hardware enclaves, based on the RISC-V architecture.
- [Project Oak](https://github.com/project-oak/oak) - A specification and a reference implementation for the secure transfer, storage and processing of data.
- [Everybody be cool, this is a robbery!](https://www.sstic.org/2019/presentation/hsm/) - A case study of vulnerability and exploitability of a HSM (in French, sorry).
## Trust & Safety
Once you've got a significant user base, it is called a community. You'll then be responsible to protect it: the customer, people, the company, the business, and facilitate all interactions and transactions happening therein.
A critical intermediation complex driven by a policy and constraint by local laws, the Trust & Safety department is likely embodied by a cross-functional team of 24/7 operators and systems of highly advanced moderation and administration tools. You can see it as an extension of customer support services, specialized in edge-cases like manual identity checks, moderation of harmful content, stopping harassment, handling of warrants and copyright claims, data sequestration and other credit card disputes.
- [Trust and safety 101](https://www.csoonline.com/article/3206127/trust-and-safety-101.html) - A great introduction on the domain and its responsabilities.
- [What the Heck is Trust and Safety?](https://www.linkedin.com/pulse/what-heck-trust-safety-kenny-shi) - A couple of real use-case to demonstrate the role of a TnS team.
<!--lint disable double-link-->
- [Awesome List of Billing and Payments: Fraud links](https://github.com/kdeldycke/awesome-billing#fraud) - Section dedicated to fraud management for billing and payment, from our sister repository.
<!--lint enable double-link-->
### User Identity
Most businesses do not collect customer's identity to create user profiles to sell to third party, no. But you still have to: local laws require to keep track of contract relationships under the large [Know You Customer (KYC)](https://en.wikipedia.org/wiki/Know_your_customer) banner.
- [The Laws of Identity](https://www.identityblog.com/stories/2005/05/13/TheLawsOfIdentity.pdf) - Is this paper aims at identity metasystem, its laws still provides great insights at smaller scale, especially the first law: to always allow user control and ask for consent to earn trust.
- [How Uber Got Lost](https://www.nytimes.com/2019/08/23/business/how-uber-got-lost.html) - “To limit "friction" Uber allowed riders to sign up without requiring them to provide identity beyond an email — easily faked — or a phone number. (…) Vehicles were stolen and burned; drivers were assaulted, robbed and occasionally murdered. The company stuck with the low-friction sign-up system, even as violence increased.”
- [A Comparison of Personal Name Matching: Techniques and Practical Issues](http://users.cecs.anu.edu.au/~Peter.Christen/publications/tr-cs-06-02.pdf) - Customer name matching has lots of application, from account deduplication to fraud monitoring.
- [Statistically Likely Usernames](https://github.com/insidetrust/statistically-likely-usernames) - Wordlists for creating statistically likely usernames for use in username-enumeration, simulated password-attacks and other security testing tasks.
- [Facebook Dangerous Individuals and Organizations List](https://theintercept.com/document/2021/10/12/facebook-dangerous-individuals-and-organizations-list-reproduced-snapshot/) - Some groups and content are illegal in some juridictions. This is an example of a blocklist.
- [Ballerine](https://github.com/ballerine-io/ballerine) - An open-source infrastructure for user identity and risk management.
- [Sherlock](https://github.com/sherlock-project/sherlock) - Hunt down social media accounts by username across social networks.
### Fraud
As an online service provider, you're exposed to fraud, crime and abuses. You'll be surprised by how much people gets clever when it comes to money. Expect any bug or discrepancies in your workflow to be exploited for financial gain.
- [After Car2Go eased its background checks, 75 of its vehicles were stolen in one day.](https://web.archive.org/web/20230526073109/https://www.bloomberg.com/news/articles/2019-07-11/mercedes-thieves-showed-just-how-vulnerable-car-sharing-can-be) - Why background check are sometimes necessary.
- [Investigation into the Unusual Signups](https://openstreetmap.lu/MWGGlobalLogicReport20181226.pdf) - A really detailed analysis of suspicious contributor signups on OpenStreetMap. This beautiful and high-level report demonstrating an orchestrated and directed campaign might serve as a template for fraud reports.
- [MIDAS: Detecting Microcluster Anomalies in Edge Streams](https://github.com/bhatiasiddharth/MIDAS) - A proposed method to “detects microcluster anomalies, or suddenly arriving groups of suspiciously similar edges, in edge streams, using constant time and memory.”
- [Gephi](https://github.com/gephi/gephi) - Open-source platform for visualizing and manipulating large graphs.
### Moderation
Any online communities, not only those related to gaming and social networks, requires their operator to invest a lot of resource and energy to moderate it.
- [Still Logged In: What AR and VR Can Learn from MMOs](https://youtu.be/kgw8RLHv1j4?t=534) - “If you host an online community, where people can harm another person: you are on the hook. And if you can't afford to be on the hook, don't host an online community”.
- [You either die an MVP or live long enough to build content moderation](https://mux.com/blog/you-either-die-an-mvp-or-live-long-enough-to-build-content-moderation/) - “You can think about the solution space for this problem by considering three dimensions: cost, accuracy and speed. And two approaches: human review and machine review. Humans are great in one of these dimensions: accuracy. The downside is that humans are expensive and slow. Machines, or robots, are great at the other two dimensions: cost and speed - they're much cheaper and faster. But the goal is to find a robot solution that is also sufficiently accurate for your needs.”
- [Keep out the bad apples: How to moderate a marketplace](https://www.twosided.io/p/keep-out-the-bad-apples-how-to-moderate) - “With great power comes great responsibility. Some of my tips and tricks to make your marketplace a safer place.”
- [The despair and darkness of people will get to you](https://restofworld.org/2020/facebook-international-content-moderators/) - Moderation of huge social networks is performed by an army of outsourced subcontractors. These people are exposed to the worst and generally ends up with PTSD.
- [The Cleaners](https://thoughtmaybe.com/the-cleaners/) - A documentary on these teams of underpaid people removing posts and deleting accounts.
### Threat Intelligence
How to detect, unmask and classify offensive online activities. Most of the time these are monitored by security, networking and/or infrastructure engineering teams. Still, these are good resources for T&S and IAM people, who might be called upon for additional expertise for analysis and handling of threats.
- [Awesome Threat Intelligence](https://github.com/hslatman/awesome-threat-intelligence) - “A concise definition of Threat Intelligence: evidence-based knowledge, including context, mechanisms, indicators, implications and actionable advice, about an existing or emerging menace or hazard to assets that can be used to inform decisions regarding the subject's response to that menace or hazard.”
- [SpiderFoot](https://github.com/smicallef/spiderfoot) - An open source intelligence (OSINT) automation tool. It integrates with just about every data source available and uses a range of methods for data analysis, making that data easy to navigate.
- [Standards related to Threat Intelligence](https://www.threat-intelligence.eu/standards/) - Open standards, tools and methodologies to support threat intelligence analysis.
- [MISP taxonomies and classification](https://www.misp-project.org/taxonomies.html) - Tags to organize information on “threat intelligence including cyber security indicators, financial fraud or counter-terrorism information.”
- [Browser Fingerprinting: A survey](https://arxiv.org/pdf/1905.01051.pdf) - Fingerprints can be used as a source of signals to identify bots and fraudsters.
- [The challenges of file formats](https://speakerdeck.com/ange/the-challenges-of-file-formats) - At one point you will let users upload files in your system. Here is a [corpus of suspicious media files](https://github.com/corkami/pocs) that can be leveraged by scammers =to bypass security or fool users.
- [SecLists](https://github.com/danielmiessler/SecLists) - Collection of multiple types of lists used during security assessments, collected in one place. List types include usernames, passwords, URLs, sensitive data patterns, fuzzing payloads, web shells, and many more.
- [PhishingKitTracker](https://github.com/neonprimetime/PhishingKitTracker) - CSV database of email addresses used by threat actor in phishing kits.
- [PhoneInfoga](https://github.com/sundowndev/PhoneInfoga) - Tools to scan phone numbers using only free resources. The goal is to first gather standard information such as country, area, carrier and line type on any international phone numbers with a very good accuracy. Then search for footprints on search engines to try to find the VoIP provider or identify the owner.
- [Confusable Homoglyphs](https://github.com/vhf/confusable_homoglyphs) - Homoglyphs is a common phishing trick.
### Captcha
Another line of defense against spammers.
- [Awesome Captcha](https://github.com/ZYSzys/awesome-captcha) - Reference all open-source captcha libraries, integration, alternatives and cracking tools.
- [reCaptcha](https://www.google.com/recaptcha) - reCaptcha is still an effective, economical and quick solution when your company can't afford to have a dedicated team to fight bots and spammers at internet scale.
- [You (probably) don't need ReCAPTCHA](https://web.archive.org/web/20190611190134/https://kevv.net/you-probably-dont-need-recaptcha/) - Starts with a rant on how the service is a privacy nightmare and is tedious UI-wise, then list alternatives.
- [Anti-captcha](https://anti-captcha.com) - Captchas solving service.
## Blocklists
The first mechanical line of defense against abuses consist in plain and simple deny-listing. This is the low-hanging fruit of fraud fighting, but you'll be surprised how they're still effective.
- [Bloom Filter](https://en.wikipedia.org/wiki/Bloom_filter) - Perfect for this use-case, as bloom filters are designed to quickly check if an element is not in a (large) set. Variations of bloom filters exist for specific data types.
- [How Radix trees made blocking IPs 5000 times faster](https://blog.sqreen.com/demystifying-radix-trees/) - Radix trees might come handy to speed-up IP blocklists.
### Hostnames and Subdomains
Useful to identified clients, catch and block swarms of bots, and limit effects of dDOS.
- [`hosts`](https://github.com/StevenBlack/hosts) - Consolidates reputable hosts files, and merges them into a unified hosts file with duplicates removed.
- [`nextdns/metadata`](https://github.com/nextdns/metadata) - Extensive collection of list for security, privacy and parental control.
- [The Public Suffix List](https://publicsuffix.org) - Mozilla's registry of public suffixes, under which Internet users can (or historically could) directly register names.
- [Country IP Blocks](https://github.com/herrbischoff/country-ip-blocks) - CIDR country-level IP data, straight from the Regional Internet Registries, updated hourly.
- [Certificate Transparency Subdomains](https://github.com/internetwache/CT_subdomains) - An hourly updated list of subdomains gathered from certificate transparency logs.
- Subdomain denylists: [#1](https://gist.github.com/artgon/5366868), [#2](https://github.com/sandeepshetty/subdomain-blacklist/blob/master/subdomain-blacklist.txt), [#3](https://github.com/nccgroup/typofinder/blob/master/TypoMagic/datasources/subdomains.txt), [#4](https://www.quora.com/How-do-sites-prevent-vanity-URLs-from-colliding-with-future-features).
- [`common-domain-prefix-suffix-list.tsv`](https://gist.github.com/erikig/826f49442929e9ecfab6d7c481870700) - Top-5000 most common domain prefix/suffix list.
- [`hosts-blocklists`](https://github.com/notracking/hosts-blocklists) - No more ads, tracking and other virtual garbage.
- [`xkeyscorerules100.txt`](https://gist.github.com/sehrgut/324626fa370f044dbca7) - NSA's [XKeyscore](https://en.wikipedia.org/wiki/XKeyscore) matching rules for TOR and other anonymity preserving tools.
- [`pyisp`](https://github.com/ActivisionGameScience/pyisp) - IP to ISP lookup library (includes ASN).
- [AMF site blocklist](https://www.amf-france.org/Epargne-Info-Service/Proteger-son-epargne/Listes-noires) - Official French denylist of money-related fraud sites.
### Emails
- [Burner email providers](https://github.com/wesbos/burner-email-providers) - A list of temporary email providers. And its [derivative Python module](https://github.com/martenson/disposable-email-domains).
- [MailChecker](https://github.com/FGRibreau/mailchecker) - Cross-language temporary (disposable/throwaway) email detection library.
- [Temporary Email Address Domains](https://gist.github.com/adamloving/4401361) - A list of domains for disposable and temporary email addresses. Useful for filtering your email list to increase open rates (sending email to these domains likely will not be opened).
- [`gman`](https://github.com/benbalter/gman) - “A ruby gem to check if the owner of a given email address or website is working for THE MAN (a.k.a verifies government domains).” Good resource to hunt for potential government customers in your user base.
- [`Swot`](https://github.com/leereilly/swot) - In the same spirit as above, but this time to flag academic users.
### Reserved IDs
- [General List of Reserved Words](https://gist.github.com/stuartpb/5710271) - This is a general list of words you may want to consider reserving, in a system where users can pick any name.
- [Hostnames and usernames to reserve](https://ldpreload.com/blog/names-to-reserve) - List of all the names that should be restricted from registration in automated systems.
### Profanity
- [List of Dirty, Naughty, Obscene, and Otherwise Bad Words](https://github.com/LDNOOBW/List-of-Dirty-Naughty-Obscene-and-Otherwise-Bad-Words) - Profanity blocklist from Shutterstock.
- [`profanity-check`](https://github.com/vzhou842/profanity-check) - Uses a linear SVM model trained on 200k human-labeled samples of clean and profane text strings.
## Privacy
As the guardian of user's data, the IAM stack is deeply bounded by the respect of privacy.
- [Privacy Enhancing Technologies Decision Tree](https://www.private-ai.com/wp-content/uploads/2021/10/PETs-Decision-Tree.pdf) - A flowchart to select the right tool depending on data type and context.
- [Paper we love: Privacy](https://github.com/papers-we-love/papers-we-love/tree/master/privacy) - A collection of scientific studies of schemes providing privacy by design.
- [IRMA Authentication](https://news.ycombinator.com/item?id=20144240) - Open-source app and protocol that offers privacy-friendly attribute based authentication and signing using [Camenisch and Lysyanskaya's Idemix](https://privacybydesign.foundation/publications/).
- [Have I been Pwned?](https://haveibeenpwned.com) - Data breach index.
- [Automated security testing for Software Developers](https://fahrplan.events.ccc.de/camp/2019/Fahrplan/system/event_attachments/attachments/000/003/798/original/security_cccamp.pdf) - Most privacy breaches were allowed by known vulnerabilities in third-party dependencies. Here is how to detect them by the way of CI/CD.
- [Email marketing regulations around the world](https://github.com/threeheartsdigital/email-marketing-regulations) - As the world becomes increasingly connected, the email marketing regulation landscape becomes more and more complex.
- [World's Biggest Data Breaches & Hacks](https://www.informationisbeautiful.net/visualizations/worlds-biggest-data-breaches-hacks/) - Don't be the next company leaking your customer's data.
### Anonymization
As a central repository of user data, the IAM stack stakeholders have to prevent any leakage of business and customer data. To allow for internal analytics, anonymization is required.
- [The False Allure of Hashing for Anonymization](https://gravitational.com/blog/hashing-for-anonymization/) - Hashing is not sufficient for anonymization no. But still it is good enough for pseudonymization (which is allowed by the GDPR).
- [Four cents to deanonymize: Companies reverse hashed email addresses](https://freedom-to-tinker.com/2018/04/09/four-cents-to-deanonymize-companies-reverse-hashed-email-addresses/) - “Hashed email addresses can be easily reversed and linked to an individual”.
- [Why differential privacy is awesome](https://desfontain.es/privacy/differential-privacy-awesomeness.html) - Explain the intuition behind [differential privacy](https://en.wikipedia.org/wiki/Differential_privacy), a theoretical framework which allow sharing of aggregated data without compromising confidentiality. See follow-up articles with [more details](https://desfontain.es/privacy/differential-privacy-in-more-detail.html) and [practical aspects](https://desfontain.es/privacy/differential-privacy-in-practice.html).
- [k-anonymity: an introduction](https://www.privitar.com/listing/k-anonymity-an-introduction) - An alternative anonymity privacy model.
- [Presidio](https://github.com/microsoft/presidio) - Context aware, pluggable and customizable data protection and PII data anonymization service for text and images.
- [Diffix: High-Utility Database Anonymization](https://aircloak.com/wp-content/uploads/apf17-aspen.pdf) - Diffix try to provide anonymization, avoid pseudonymization and preserve data quality. [Written in Elixir at Aircloak](https://elixirforum.com/t/aircloak-anonymized-analitycs/10930), it acts as an SQL proxy between the analyst and an unmodified live database.
### GDPR
The well-known European privacy framework
- [GDPR Tracker](https://gdpr.eu) - Europe's reference site.
- [GDPR Developer Guide](https://github.com/LINCnil/GDPR-Developer-Guide) - Best practices for developers.
- [GDPR – A Practical guide for Developers](https://techblog.bozho.net/gdpr-practical-guide-developers/) - A one-page summary of the above.
- [GDPR Tracker](https://gdprtracker.io) - Track the GDPR compliance of cloud services and subprocessors.
- [GDPR documents](https://github.com/good-lly/gdpr-documents) - Templates for personal use to have companies comply with "Data Access" requests.
- [Dark Patterns after the GDPR](https://arxiv.org/pdf/2001.02479.pdf) - This paper demonstrates that, because of the lack of GDPR law enforcements, dark patterns and implied consent are ubiquitous.
- [GDPR Enforcement Tracker](http://enforcementtracker.com) - List of GDPR fines and penalties.
## UX/UI
As stakeholder of the IAM stack, you're going to implement in the backend the majority of the primitives required to build-up the sign-up tunnel and user onboarding. This is the first impression customers will get from your product, and can't be overlooked: you'll have to carefully design it with front-end experts. Here is a couple of guides to help you polish that experience.
- [The 2020 State of SaaS Product Onboarding](https://userpilot.com/saas-product-onboarding/) - Covers all the important facets of user onboarding.
- [User Onboarding Teardowns](https://www.useronboard.com/user-onboarding-teardowns/) - A huge list of deconstructed first-time user signups.
- [Discover UI Design Decisions Of Leading Companies](https://goodui.org/leaks/) - From Leaked Screenshots & A/B Tests.
- [Conversion Optimization](https://www.nickkolenda.com/conversion-optimization-psychology/#cro-tactic11) - A collection of tactics to increase the chance of users finishing the account creation funnel.
- [Trello User Onboarding](https://growth.design/case-studies/trello-user-onboarding/) - A detailed case study, nicely presented, on how to improve user onboarding.
- [11 Tips for Better Signup / Login UX](https://learnui.design/blog/tips-signup-login-ux.html) - Some basic tips on the login form.
- [Don't get clever with login forms](http://bradfrost.com/blog/post/dont-get-clever-with-login-forms/) - Create login forms that are simple, linkable, predictable, and play nicely with password managers.
- [Why are the username and password on two different pages?](https://www.twilio.com/blog/why-username-and-password-on-two-different-pages) - To support both SSO and password-based login. Now if breaking the login funnel in 2 steps is too infuriating to users, solve this as Dropbox does: [an AJAX request when you enter your username](https://news.ycombinator.com/item?id=19174355).
- [HTML attributes to improve your users' two factor authentication experience](https://www.twilio.com/blog/html-attributes-two-factor-authentication-autocomplete) - “In this post we will look at the humble `<input>` element and the HTML attributes that will help speed up our users' two factor authentication experience”.
- [Remove password masking](http://passwordmasking.com) - Summarizes the results from an academic study investigating the impact removing password masking has on consumer trust.
- [For anybody who thinks "I could build that in a weekend," this is how Slack decides to send a notification](https://twitter.com/ProductHunt/status/979912670970249221) - Notifications are hard. Really hard.
## Competitive Analysis
Keep track on the activity of open-source projects and companies operating in the domain.
- [Best-of Digital Identity](https://github.com/jruizaranguren/best-of-digital-identity) - Ranking, popularity and activity status of open-source digital identity projects.
- [AWS Security, Identity & Compliance announcements](https://aws.amazon.com/about-aws/whats-new/security_identity_and_compliance/) - The source of all new features added to the IAM perimeter.
- [GCP IAM release notes](https://cloud.google.com/iam/docs/release-notes) - Also of note: [Identity](https://cloud.google.com/identity/docs/release-notes), [Identity Platform](https://cloud.google.com/identity-platform/docs/release-notes), [Resource Manager](https://cloud.google.com/resource-manager/docs/release-notes), [Key Management Service/HSM](https://cloud.google.com/kms/docs/release-notes), [Access Context Manager](https://cloud.google.com/access-context-manager/docs/release-notes), [Identity-Aware Proxy](https://cloud.google.com/iap/docs/release-notes), [Data Loss Prevention](https://cloud.google.com/dlp/docs/release-notes) and [Security Scanner](https://cloud.google.com/security-scanner/docs/release-notes).
- [Unofficial Weekly Google Cloud Platform newsletter](https://www.gcpweekly.com) - Relevant keywords: [`IAM`](https://www.gcpweekly.com/gcp-resources/tag/iam/) and [`Security`](https://www.gcpweekly.com/gcp-resources/tag/security/).
- [DigitalOcean Accounts changelog](http://docs.digitalocean.com/release-notes/accounts/) - All the latest accounts updates on DO.
- [163 AWS services explained in one line each](https://adayinthelifeof.nl/2020/05/20/aws.html#discovering-aws) - Help makes sense of their huge service catalog. In the same spirit: [AWS in simple terms](https://netrixllc.com/blog/aws-services-in-simple-terms/) & [AWS In Plain English](https://expeditedsecurity.com/aws-in-plain-english/).
- [Google Cloud Developer's Cheat Sheet](https://github.com/gregsramblings/google-cloud-4-words#the-google-cloud-developers-cheat-sheet) - Describe all GCP products in 4 words or less.
## History
- [cryptoanarchy.wiki](https://cryptoanarchy.wiki) - Cypherpunks overlaps with security. This wiki compiles information about the movement, its history and the people/events of note.
## Contributing
Your contributions are always welcome! Please take a look at the [contribution guidelines](.github/contributing.md) first.
## Footnotes
The [header image](https://github.com/kdeldycke/awesome-iam/blob/main/assets/awesome-iam-header.jpg) is based on a modified [photo](https://unsplash.com/photos/2LowviVHZ-E) by [Ben Sweet](https://unsplash.com/@benjaminsweet).
<!--lint disable no-undefined-references-->
<a name="sponsor-def">\[0\]</a>: You can <a href="https://github.com/sponsors/kdeldycke">add your Identity & Authentication product in the list of sponsors via a GitHub sponsorship</a>. [\[↑\]](#sponsor-ref)
<a name="intro-quote-def">\[1\]</a>: [*Poison Study*](https://www.amazon.com/dp/0778324338?&linkCode=ll1&tag=kevideld-20&linkId=0b92c3d92371bd53daca5457bdad327e&language=en_US&ref_=as_li_ss_tl) (Mira, 2007). [\[↑\]](#intro-quote-ref)
| {
"repo_name": "kdeldycke/awesome-iam",
"stars": "1284",
"repo_language": "None",
"file_name": "autofix.yaml",
"mime_type": "text/plain"
} |
<!--lint disable awesome-heading-->
<p align="center">
<a href="https://github.com/kdeldycke/awesome-iam#readme">
<img src="https://raw.githubusercontent.com/kdeldycke/awesome-iam/main/assets/awesome-iam-header.jpg" alt="Awesome IAM">
</a>
</p>
<p align="center">
<a href="https://github.com/kdeldycke/awesome-iam#readme" hreflang="en"><img src="https://img.shields.io/badge/lang-English-blue?style=flat-square" lang="en" alt="English"></a>
<a href="https://github.com/kdeldycke/awesome-iam/blob/main/readme.zh.md" hreflang="zh"><img src="https://img.shields.io/badge/lang-汉语-blue?style=flat-square" lang="zh" alt="汉语"></a>
</p>
<p align="center">
<sup><a href="#sponsor-def">此列表由以下机构赞助<sup id="sponsor-ref">[0]</sup></a>:</sup><br>
</p>
<p align="center">
<a href="https://www.descope.com/?utm_source=awesome-iam&utm_medium=referral&utm_campaign=awesome-iam-oss-sponsorship">
<picture>
<source media="(prefers-color-scheme: dark)" srcset="https://raw.githubusercontent.com/kdeldycke/awesome-iam/main/assets/descope-logo-dark-background.svg">
<source media="(prefers-color-scheme: light)" srcset="https://raw.githubusercontent.com/kdeldycke/awesome-iam/main/assets/descope-logo-light-background.svg">
<img width="300" src="https://raw.githubusercontent.com/kdeldycke/awesome-iam/main/assets/descope-logo-light-background.svg">
</picture>
<br/>
<strong>拖放您的身份验证。</strong><br/>
使用几行代码向您的应用程序添加身份验证、用户管理和授权。
</a>
<!-- Comment this sponsorship call-to-action if there is a sponsor logo to increase its impact. -->
<!--
<a href="https://github.com/sponsors/kdeldycke">
<strong>Yᴏᴜʀ Iᴅᴇɴᴛɪᴛʏ & Aᴜᴛʜᴇɴᴛɪᴄᴀᴛɪᴏɴ Pʀᴏᴅᴜᴄᴛ ʜᴇʀᴇ!</strong>
<br/>
<sup>Add a link to your company or project here: back me up via a GitHub sponsorship.</sup>
</a>
-->
</p>
---
<p align="center">
<i>Trusting is hard. Knowing who to trust, even harder.(信任是困难的。知道该信任谁,更难。)</i><br>
— Maria V. Snyder<sup id="intro-quote-ref"><a href="#intro-quote-def">[1]</a></sup>
</p>
<!--lint disable double-link-->
[IAM](https://zh.wikipedia.org/wiki/身份管理) 代表身份和访问管理。 它是一个复杂的域,涵盖**用户帐户、身份验证、授权、角色、权限和隐私**。 它是云服务平台的重要支柱,是用户、产品和安全的交汇点。[另一个支柱是账单和支付 💰](https://github.com/kdeldycke/awesome-billing/).
这个精选清单 [](https://github.com/sindresorhus/awesome) 以全面且可操作的方式公开该领域的所有技术、协议和行话。
<!--lint enable double-link-->
## Contents
<!-- mdformat-toc start --slug=github --no-anchors --maxlevel=6 --minlevel=2 -->
- [概述](#概述)
- [安全](#安全)
- [账户管理](#账户管理)
- [密码学](#密码学)
- [标识符](#标识符)
- [零信任网络](#零信任网络)
- [认证](#认证)
- [基于密码](#基于密码)
- [无密码](#无密码)
- [安全密钥](#安全密钥)
- [多因素](#多因素)
- [基于短信](#基于短信)
- [公钥基础设施](#公钥基础设施)
- [JWT](#jwt)
- [OAuth2 & OpenID](#oauth2--openid)
- [SAML](#saml)
- [授权](#授权)
- [策略模型](#策略模型)
- [开源策略框架](#开源策略框架)
- [AWS 策略工具](#AWS-策略工具)
- [Macaroons](#macaroons)
- [秘密管理](#秘密管理)
- [硬件安全模块 (HSM)](#硬件安全模块-hsm)
- [信任与安全](#信任与安全)
- [用户身份](#用户身份)
- [欺诈](#欺诈)
- [Moderation](#moderation)
- [威胁情报](#威胁情报)
- [验证码](#验证码)
- [黑名单](#黑名单)
- [主机名和子域](#主机名和子域)
- [邮件](#邮件)
- [保留的 ID](#保留的-ID)
- [诽谤](#诽谤)
- [隐私](#隐私)
- [匿名化](#匿名化)
- [GDPR](#gdpr)
- [UX/UI](#uxui)
- [竞争分析](#竞争分析)
- [历史](#历史)
<!-- mdformat-toc end -->
## 概述
<img align="right" width="50%" src="./assets/cloud-software-stack-iam.jpg"/>
在[云计算概述](http://web.stanford.edu/class/cs349d/docs/L01_overview.pdf)的斯坦福课程中,提供的平台的软件架构如右图所示 →
在这里,我们列出了全局:域的定义和战略重要性、它在更大的生态系统中的位置,以及一些关键特性。
- [EnterpriseReady SaaS 功能指南](https://www.enterpriseready.io) - 大多数让 B2B 用户满意的功能将由 IAM 外围实现。
- [IAM 很难,真的很难](https://twitter.com/kmcquade3/status/1291801858676228098) - “过于宽松的 AWS IAM 策略允许 `s3:GetObject` 访问 `*`(所有)资源”,导致 Capital One 被罚款 8000 万美元。这是作为企业主不能忽视 IAM 的唯一原因。
- [IAM 是真正的云锁定](https://forrestbrazeal.com/2019/02/18/cloud-irregular-iam-is-the-real-cloud-lock-in/) - 虽然是小小的 *点击诱饵*,但作者承认“这取决于您对他们的信任程度 1. 继续经营; 2. 不抬高价格; 3. 不贬低您下属的服务; 4. 在业务加速方面为您提供的价值多于他们在灵活性方面带来的价值。
## 安全
安全性是 IAM 基金会最核心的支柱之一。 这里有一些广泛的概念。
- [企业信息安全](https://infosec.mozilla.org) - Mozilla 的安全和访问指南。
- [缓解云漏洞](https://media.defense.gov/2020/Jan/22/2002237484/-1/-1/0/CSI-MITIGATING-CLOUD-VULNERABILITIES_20200121.PDF) - “本文档将云漏洞分为四类(配置错误、访问控制不当、共享租户漏洞和供应链漏洞)”。
- [Cartography](https://github.com/lyft/cartography) - 一种基于 Neo4J 的工具,用于映射服务和资源之间的依赖关系和关系。 支持 AWS、GCP、GSuite、Okta 和 GitHub。
- [AWS 安全性和 IAM 开放指南](https://github.com/open-guides/og-aws#security-and-iam)
## 账户管理
IAM 的基础:用户、组、角色和权限的定义和生命周期。
- [作为一个用户,我想要…](https://mobile.twitter.com/oktopushup/status/1030457418206068736) - 客户管理的元评论家,其中业务预期的功能与真实用户需求发生冲突,以虚构项目经理编写的用户故事的形式出现。
- [终端用户关心但程序员不关心的事情](https://instadeq.com/blog/posts/things-end-users-care-about-but-programmers-dont/) - 与上述精神相同,但范围更广:所有我们作为开发者而忽视但用户真正关心的小事。在这个列表的顶部是以账户为中心的功能,多样化的集成和导入/导出工具。也就是所有企业客户需要涵盖的内容。
- [将账户、用户和登录/授权细节分开](https://news.ycombinator.com/item?id=21151830) - 为面向未来的 IAM API 奠定基础的合理建议。
- [超越用户名的身份](https://lord.io/blog/2020/usernames/) - 关于用户名作为标识符的概念,以及当 unicode 字符满足唯一性要求时引入的复杂问题。
- [Kratos](https://github.com/ory/kratos) -用户登录、用户注册、2FA 和个人资料管理。
- [Conjur](https://github.com/cyberark/conjur) - 自动保护特权用户和机器身份所使用的秘密信息。
- [SuperTokens](https://github.com/supertokens/supertokens-core) - 用于登录和会话管理的开源项目,支持无密码、社交登录、电子邮件和电话登录。
- [UserFrosting](https://github.com/userfrosting/UserFrosting) - 现代PHP用户登录和管理框架。
## 密码学
整个认证技术栈是基于密码学原理的。这一点不能被忽视。
- [密码学的正确答案](https://latacora.micro.blog/2018/04/03/cryptographic-right-answers.html) - 为非密码学工程师的开发人员提供的一组最新建议。甚至还有[更短的摘要](https://news.ycombinator.com/item?id=16749140)可用。
- [真实世界的密码研讨会](https://rwc.iacr.org) - 旨在将密码学研究人员与开发人员聚集在一起,专注于在互联网、云和嵌入式设备等现实环境中的使用。
- [密码学概述](https://www.garykessler.net/library/crypto.html) - “这篇论文有两个主要目的。 首先是定义基本密码方法背后的一些术语和概念,并提供一种方法来比较当今使用的无数密码方案。 第二个是提供一些当今使用的密码学的真实例子。”
- [我们喜欢的论文:密码学](https://github.com/papers-we-love/papers-we-love/blob/master/cryptography/README.md) - 密码学基础论文。
- [加密哈希函数的生命周期](http://valerieaurora.org/hash.html) - "如果你使用逐个哈希值比较来生成可由恶意用户提供的数据的地址,你应该有一个计划,每隔几年就迁移到一个新的哈希值"。
### 标识符
令牌、主键、UUID......无论最终用途是什么,你都必须生成这些具有一定随机性和唯一性的数字。
- [对任何依赖随机生成的号码的设备的安全建议](https://www.av8n.com/computer/htm/secure-random.htm) - "'随机数生成器'这一短语应作如下解析。它是一个数字的随机发生器。它不是一个随机数的生成器"。
- [RFC #4122: UUID - 安全方面的考虑](https://www.rfc-editor.org/rfc/rfc4122#section-6) - "不要认为UUID难以猜测;它们不应该被用作安全能力(仅仅拥有它就能授予访问权的标识)"。UUIDs被设计成唯一的,而不是随机的或不可预测的:不要把UUIDs作为一个秘密。
- [Awesome Identifiers](https://adileo.github.io/awesome-identifiers/) - 所有标识符格式的一个基准。
- [Awesome GUID](https://github.com/secretGeek/AwesomeGUID) - 对唯一标识的全局性方面做的有趣讨论。
## 零信任网络
零信任网络安全的运作原则是 "永不信任,永远验证"。
- [BeyondCorp:企业安全的新方法](https://www.usenix.org/system/files/login/articles/login_dec14_02_ward.pdf) - 简要概述谷歌的零信任网络方案。
- [什么是 BeyondCorp? 什么是身份感知代理?](https://medium.com/google-cloud/what-is-beyondcorp-what-is-identity-aware-proxy-de525d9b3f90) - 越来越多的公司添加了额外的 VPN、防火墙、限制和限制层,导致糟糕的体验和轻微的安全增益。是存在更好的方法。
- [oathkeeper](https://github.com/ory/oathkeeper) - 身份与访问代理和访问控制决策API,对进入的HTTP请求进行认证、授权和变异。受BeyondCorp / Zero Trust白皮书的启发。
- [transcend](https://github.com/cogolabs/transcend) - BeyondCorp 启发的访问代理服务器。
- [Pomerium](https://github.com/pomerium/pomerium) - 一种身份感知代理,支持对内部应用程序的安全访问。
## 认证
用于确认你是相应的人的协议和技术。
- [API Tokens: A Tedious Survey](https://fly.io/blog/api-tokens-a-tedious-survey/) - 对终端用户 API 的所有基于令牌的认证方案进行概述和比较。
- [服务间认证方案的儿童花园](https://web.archive.org/web/20200507173734/https://latacora.micro.blog/a-childs-garden/) - 与上述精神相同,但这次是在服务层面。
- [在 Facebook 扩展后端身份验证](https://www.youtube.com/watch?v=kY-Bkv3qxMc) - 简而言之,如何做:1.小的信任根;2.TLS 是不够的;3.基于证书的令牌;4.加密认证令牌(CATs)。更多细节见[幻灯片](https://rwc.iacr.org/2018/Slides/Lewi.pdf)。
### 基于密码
- [新的 NIST 密码指南](https://pciguru.wordpress.com/2019/03/11/the-new-nist-password-guidance/) - [NIST Special Publication 800-63B](https://pages.nist.gov/800-63-3/sp800-63b.html) 的摘要,涵盖了新的密码复杂性指南。
- [密码存储备忘](https://cheatsheetseries.owasp.org/cheatsheets/Password_Storage_Cheat_Sheet.html) - 减缓离线攻击的唯一方法是谨慎地选择尽可能密集资源的哈希算法。
- [密码过期作废](https://techcrunch.com/2019/06/02/password-expiration-is-dead-long-live-your-passwords/) - 最近的科学研究对许多长期存在的密码安全实践(例如密码过期策略)的价值提出质疑,并指出更好的替代方案,例如执行禁止密码列表和 MFA。
- [更强大、更实用的密码的实用建议](http://www.andrew.cmu.edu/user/nicolasc/publications/Tan-CCS20.pdf) - 本研究建议关联如下几个方法:针对常见泄露密码的黑名单检查、无字符类要求的密码策略、最小强度策略。
- [银行、任意的密码限制以及为什么它们并不重要](https://www.troyhunt.com/banks-arbitrary-password-restrictions-and-why-they-dont-matter/) - “对长度和字符组成的任意低限制是不好的。 它们看起来很糟糕,会导致对安全状况的负面猜测,并且会破坏密码管理器等工具。”
- [愚蠢的密码规则](https://github.com/dumb-password-rules/dumb-password-rules) - 使用愚蠢的密码规则的糟糕网站。
- [普通文本罪犯](https://plaintextoffenders.com/about/) - 公开羞辱以纯文本存储密码的网站。
- [密码管理器资源](https://github.com/apple/password-manager-resources) - 一个按网站分类的密码规则、更改URL和怪癖的集合。
- [更改密码的著名网址](https://github.com/WICG/change-password-url) - 定义密码更新的网站资源的规范。
- [如何改变已经散列的用户密码的散列方案](https://news.ycombinator.com/item?id=20109360) - 好消息是:你并没有被困在一个传统的密码保存方案中。这里有一个技巧,可以透明地升级到更强大的散列算法。
### 无密码
- [无密码的争论](https://web.archive.org/web/20190515230752/https://biarity.gitlab.io/2018/02/23/passwordless/) - 密码不是用户身份验证的全部和最终结果。这篇文章试图告诉你为什么。
- [神奇的链接 - 它们实际上已经过时了吗?](https://zitadel.com/blog/magic-links) - 什么是神奇的链接,它们的起源,优点和缺点。
- [WebAuthn 指南](https://webauthn.guide) - 这是一份非常容易理解的WebAuthn指南,该标准允许 "服务器使用公钥加密技术而不是密码来注册和验证用户",所有主要浏览器都支持。
### 安全密钥
- [Webauthn 和安全密钥](https://www.imperialviolet.org/2018/03/27/webauthn.html) - 描述身份验证如何使用安全密钥,详细说明协议,以及它们如何与 WebAuthn 结合。 要点:“但是,无法使用 webauthn 创建 U2F 密钥。 (...) 所以先完成登录过程到 webauthn 的过渡,然后再过渡注册。”
- [开始使用安全密钥](https://paulstamatiou.com/getting-started-with-security-keys/) - 使用 FIDO2、WebAuthn 和安全密钥保持在线安全和防止网络钓鱼的实用指南。
- [Solo](https://github.com/solokeys/solo) - 通过 USB + NFC 打开支持 FIDO2 和 U2F 的安全密钥。
- [OpenSK](https://github.com/google/OpenSK) - 用 Rust 编写的安全密钥的开源实现,支持 FIDO U2F 和 FIDO2 标准。
- [YubiKey 指南](https://github.com/drduh/YubiKey-Guide) - 使用 YubiKey 作为存储 GPG 加密、签名和身份验证密钥的智能卡的指南,它也可以用于 SSH。 本文档中的许多原则适用于其他智能卡设备。
- [YubiKey at Datadog](https://github.com/DataDog/yubikey) - Yubikey、U2F、GPG、git、SSH、Keybase、VMware Fusion 和 Docker Content Trust 设置指南。
### 多因素
- [打破密码的依赖性。微软最后一公里的挑战](https://www.youtube.com/watch?v=B_mhJO2qHlQ) - 帐户黑客攻击的主要来源是密码喷洒(在 SMTP、IMAP、POP 等传统身份验证上),其次是重放攻击。 要点:密码不安全,使用并执行 MFA。
- [超越密码:2FA、U2F 和 Google 高级保护](https://www.troyhunt.com/beyond-passwords-2fa-u2f-and-google-advanced-protection/) - 全面了解所有这些技术。
- [回溯认证的长期比较研究](https://maximiliangolla.com/files/2019/papers/usec2019-30-wip-fallback-long-term-study-finalv5.pdf) - 要点:“基于电子邮件和短信的方案更有用。 另一方面,基于指定受托人和个人知识问题的机制在便利性和效率方面都存在不足。”
- [秘密、谎言和帐户恢复:谷歌使用个人知识问题的经验教训](https://static.googleusercontent.com/media/research.google.com/en/us/pubs/archive/43783.pdf) - "我们的分析证实,秘密问题通常提供的安全级别远远低于用户选择的密码。(......)令人惊讶的是,我们发现造成这种不安全的一个重要原因是用户经常不如实回答。(......)在可用性方面,我们表明秘密答案的记忆性出奇的差"。
- [基本的账户卫生在防止劫持方面的效果如何](https://security.googleblog.com/2019/05/new-research-how-effective-is-basic.html) - 谷歌安全团队的数据显示,2FA 阻止了100%的自动机器人黑客。
- [你的 Pa\$\$word 无关紧要](https://techcommunity.microsoft.com/t5/Azure-Active-Directory-Identity/Your-Pa-word-doesn-t-matter/ba-p/731984) - 与上述 Microsoft 的结论相同:“根据我们的研究,如果您使用 MFA,您的帐户被盗用的可能性会降低 99.9% 以上。”
- [攻击 Google 身份验证器](https://unix-ninja.com/p/attacking_google_authenticator) - 可能处于偏执狂的边缘,但可能是限制 2FA 验证尝试的原因。
- [通过破解语音邮件系统来破坏在线帐户](https://www.martinvigo.com/voicemailcracker/) - 或者说,为什么你不应该依靠自动电话作为联系用户和重置密码、2FA 或进行任何形式的验证的方法。与基于短信的 2FA 不一样,它目前是不安全的,可以通过其最薄弱的环节:语音邮件系统的方式进行破坏。
- [2019 年正确对待 2FA](https://blog.trailofbits.com/2019/06/20/getting-2fa-right-in-2019/) - 关于 2FA 的用户体验方面。
- [2FA 缺少一个关键功能](https://syslog.ravelin.com/2fa-is-missing-a-key-feature-c781c3861db) - “当我的 2FA 代码输入错误时,我想知道这件事”。
- [南极洲的 SMS 多因素认证](https://brr.fyi/posts/sms-mfa) - 不起作用,因为在南极洲的站点没有手机信号塔。
- [Authelia](https://github.com/authelia/authelia) - 开源认证和授权服务器,通过网络门户为你的应用程序提供双因素认证和单点登录(SSO)。
- [Kanidm](https://github.com/kanidm/kanidm) - 简单、安全、快速的身份管理平台。
### 基于短信
太长了,细节详情见下面的文章
- [短信 2FA 认证已被 NIST 废止](https://techcrunch.com/2016/07/25/nist-declares-the-age-of-sms-based-2-factor-authentication-over/) - NIST 表示,自2016年以来,通过短信进行的 2FA 是糟糕的、可怕的。
- [SMS:最流行但最不安全的 2FA 方法](https://www.allthingsauth.com/2018/02/27/sms-the-most-popular-and-least-secure-2fa-method/)
- [SMS 2FA 安全吗? 不。](https://www.issms2fasecure.com) - 权威研究项目展示了 SIM 交换的成功尝试。
- [黑客攻击 Twitter CEO 杰克·多尔西 (Jack Dorsey) 在“SIM 交换”中。 你也有危险。](https://www.nytimes.com/2019/09/05/technology/sim-swap-jack-dorsey-hack.html)
- [美国电话电报公司代表将其手机账户的控制权交给黑客](https://www.theregister.co.uk/2017/07/10/att_falls_for_hacker_tricks/)
- [我一生中最昂贵的一课:SIM 端口黑客攻击的详细信息](https://medium.com/coinmonks/the-most-expensive-lesson-of-my-life-details-of-sim-port-hack-35de11517124)
- [SIM 卡交换恐怖故事](https://www.zdnet.com/article/sim-swap-horror-story-ive-lost-decades-of-data-and-google-wont-lift-a-finger/)
- [AWS 正在逐步弃用基于 SMS 的 2FA](https://aws.amazon.com/iam/details/mfa/) - “我们鼓励您通过 U2F 安全密钥、硬件设备或虚拟(基于软件的)MFA 设备使用 MFA。 您可以在 2019 年 1 月 31 日之前继续使用此功能。”
### 公钥基础设施
基于证书的身份验证。
- [忙碌者的 PKI](https://gist.github.com/hoffa/5a939fd0f3bcd2a6a0e4754cb2cf3f1b) - 重要内容的快速概述。
- [关于证书和 PKI 你应该知道但不敢问的一切](https://smallstep.com/blog/everything-pki.html) - PKI 让你以加密方式定义一个系统。它是通用的,并且是供应商中立的。
- [`lemur`](https://github.com/Netflix/lemur) - 充当 CA 和环境之间的代理,为开发人员提供中央门户以颁发具有“正常”默认值的 TLS 证书。
- [CFSSL](https://github.com/cloudflare/cfssl) - CloudFlare 的 PKI/TLS 瑞士军刀。 用于签署、验证和捆绑 TLS 证书的命令行工具和 HTTP API 服务器。
- [JA3](https://github.com/salesforce/ja3) - 创建 SSL/TLS 客户端指纹的方法,应该可以在任何平台上轻松生成,并且可以轻松共享以获取威胁情报。
### JWT
[JSON 网络令牌](https://en.wikipedia.org/wiki/JSON_Web_Token) 是不记名的令牌。
- [JSON Web Token 简介](https://jwt.io/introduction/) - 通过本文快速了解 JWT。
- [了解如何使用 JWT 进行身份验证](https://github.com/dwyl/learn-json-web-tokens) - 了解如何使用 JWT 来保护您的 Web 应用程序。
- [使用 JSON Web 令牌作为 API 密钥](https://auth0.com/blog/using-json-web-tokens-as-api-keys/) - 与 API 密钥相比,JWT 提供了细粒度的安全性、同质身份验证架构、去中心化发布、OAuth2 合规性、可调试性、过期控制、设备管理。
- [管理一个安全的 JSON 网络令牌实现](https://cursorblog.com/managing-a-secure-json-web-token-implementation/) - JWT有各种各样的灵活性,使它很难用好。
- [硬编码的密钥、未经验证的令牌和其他常见的 JWT 错误](https://r2c.dev/blog/2020/hardcoded-secrets-unverified-tokens-and-other-common-jwt-mistakes/) - 对所有 JWT 的陷阱进行了很好的总结。
- [将 JSON 网络令牌 API 密钥添加到拒绝列表中](https://auth0.com/blog/denylist-json-web-token-api-keys/) - 在令牌失效时。
- [停止对会话使用 JWT](http://cryto.net/~joepie91/blog/2016/06/13/stop-using-jwt-for-sessions/) - 以及[为什么你的 "解决方案 "不起作用](http://cryto.net/%7Ejoepie91/blog/2016/06/19/stop-using-jwt-for-sessions-part-2-why-your-solution-doesnt-work/),因为[无状态的JWT令牌不能被废止或更新](https://news.ycombinator.com/item?id=18354141)。它们会引入大小问题或安全问题,这取决于你将它们存储在哪里。有状态的 JWT 令牌在功能上与会话 cookie 相同,但没有经过实战检验和充分审查的实现或客户端支持。
- [JWT、JWS 和 JWE 是为不那么愚蠢的人准备的!](https://medium.facilelogin.com/jwt-jws-and-jwe-for-not-so-dummies-b63310d201a3) - 经过签名的 JWT 被称为 JWS(JSON Web Signature)。事实上,JWT 本身并不存在--它必须是一个JWS 或 JWE(JSON Web Encryption)。它就像一个抽象类,JWS 和 JWE 是具体的实现。
- [JOSE 是每个人都应该避免的坏标准](https://paragonie.com/blog/2017/03/jwt-json-web-tokens-is-bad-standard-that-everyone-should-avoid) - 这些标准要么是完全破碎的,要么是难以驾驭的复杂雷区。
- [JWT.io](https://jwt.io) - 允许你解码、验证和生成JWT。
- [`loginsrv`](https://github.com/tarent/loginsrv) - 独立的简约登录服务器,为多个登录后端(htpasswd、OSIAM、用户/密码、HTTP 基本身份验证、OAuth2:GitHub、Google、Bitbucket、Facebook、Gitlab)提供 JWT 登录。
- [jwtXploiter](https://github.com/DontPanicO/jwtXploiter) - 一个测试 json web token 安全性的工具。
### OAuth2 & OpenID
[OAuth 2.0](https://zh.wikipedia.org/wiki/开放授权#OAuth_2.0) 是一个*委托授权*框架。 [OpenID Connect (OIDC)](https://en.wikipedia.org/wiki/OpenID_Connect) 是其之上的*身份验证*层。
旧的 *OpenID* 已死; 新的 *OpenID Connect* 还没有死。
- [OAuth 身份验证的问题](http://www.thread-safe.com/2012/01/problem-with-oauth-for-authentication.html) - “问题是 OAuth 2.0 是委托授权 协议,而不是身份验证协议。” 10年后,这篇文章仍然是关于[为什么使用OpenID Connect而不是普通OAuth2](https://security.stackexchange.com/a/260519)的最好解释?
- [OAuth 和 OpenID Connect 图解指南](https://developer.okta.com/blog/2019/10/21/illustrated-guide-to-oauth-and-oidc) - 使用简化的插图解释这些标准的工作原理。
- [OAuth 2 简化版](https://aaronparecki.com/oauth-2-simplified/) - 以简化格式描述协议的参考文章,以帮助开发人员和服务提供商实施它。
- [OAuth 2.0 和 OpenID 连接(通俗易懂)](https://www.youtube.com/watch?v=996OiexHze0) - 首先介绍了这些标准是如何形成的历史背景,澄清了词汇中的不正确之处,然后详细介绍了协议及其陷阱,使其不那么令人生畏。
- [关于 OAuth (2.0) 你需要知道的一切](https://gravitational.com/blog/everything-you-need-to-know-about-oauth/) - 很好的概述和实际案例研究,介绍了开源远程访问工具 Teleport 如何允许用户通过 GitHub SSO 登录。
- [一张图看懂 OAuth](https://mobile.twitter.com/kamranahmedse/status/1276994010423361540) - 一张漂亮的总结卡。
- [如何通过六个步骤实现安全的中央认证服务](https://engineering.shopify.com/blogs/engineering/implement-secure-central-authentication-service-six-steps) - 有多个遗留系统要与它们自己的登录方式和账户合并?这里是如何通过 OIDC 的方式来合并所有这些混乱的系统。
- [开源 BuzzFeed 的 SSO 体验](https://increment.com/security/open-sourcing-buzzfeeds-single-sign-on-process/) - 中央认证服务 (CAS) 协议的 OAuth2 友好改编。 您会在那里找到很好的 OAuth 用户流程图。
- [OAuth 2.0 安全的当前最佳实践](https://tools.ietf.org/html/draft-ietf-oauth-security-topics-16) - "更新和扩展了 OAuth 2.0 的安全威胁模型,以纳入自 OAuth 2.0 发布以来收集的实际经验,并涵盖了由于更广泛的应用而产生的相关新威胁"。
- [隐藏的 OAuth 攻击载体](https://portswigger.net/web-security/oauth) - 如何识别和利用 OAuth 2.0 认证机制中发现的一些关键漏洞。
- [PKCE 的解释](https://www.loginradius.com/blog/engineering/pkce/) - "PKCE 用于为 OAuth 和 OpenID Connect 中的授权代码流提供多一个安全层。"
- [Hydra](https://gethydra.sh) - 开源的 OIDC 和 OAuth2 服务器。
- [Keycloak](https://www.keycloak.org) - 开源的身份和访问管理。支持 OIDC、OAuth 2和SAML 2、LDAP 和 AD 目录、密码策略。
- [Casdoor](https://github.com/casbin/casdoor) - 基于 UI 优先的集中式身份验证/单点登录 (SSO) 平台。 支持 OIDC 和 OAuth 2、社交登录、用户管理、基于电子邮件和短信的 2FA。
- [authentik](https://goauthentik.io/?#correctness) - 类似于 Keycloak 的开源身份提供者。
- [ZITADEL](https://github.com/zitadel/zitadel) - 使用 Go 和 Angular 构建的开源解决方案,用于管理您的所有系统、用户和服务帐户及其角色和外部身份。 ZITADEL 为您提供 OIDC、OAuth 2.0、登录和注册流程、无密码和 MFA 身份验证。 所有这一切都建立在事件溯源之上,并结合 CQRS 来提供出色的审计跟踪。
- [a12n-server](https://github.com/curveball/a12n-server) - 一个简单的身份验证系统,仅实现 OAuth2 标准的相关部分。
- [Logto](https://github.com/logto-io/logto) - 使用此基于 OIDC 的身份服务构建登录、身份验证和用户身份。
- [OpenID 的衰落D](https://penguindreams.org/blog/the-decline-of-openid/) - OpenID 在公共网络中被替换为 OAuth 1、OAuth 2 或其他专有 SSO 协议的混合体。
- [为什么 Mastercard 不使用 OAuth 2.0](https://developer.mastercard.com/blog/why-mastercard-doesnt-use-oauth-20) - "他们这样做是为了提供消息级的完整性。OAuth 2改成了传输级的保密性/完整性。" (由 TLS 提供这个定性) ([来源](https://news.ycombinator.com/item?id=17486165)).
- [OAuth 2.0 and the Road to Hell](https://gist.github.com/nckroy/dd2d4dfc86f7d13045ad715377b6a48f) - Oauth 2.0 規範的主要作者和編輯的辭職信。
### SAML
安全断言标记语言 (SAML) 2.0 是一种在服务之间交换授权和身份验证的方法,例如上面的 OAuth/OpenID 协议。
典型的 SAML 身份提供商是机构或大公司的内部 SSO,而典型的 OIDC/OAuth 提供商是运行数据孤岛的科技公司。
- [SAML vs. OAuth](https://web.archive.org/web/20230327071347/https://www.cloudflare.com/learning/access-management/what-is-oauth/) - “OAuth 是一种授权协议:它确保 Bob 前往正确的停车场。 相比之下,SAML 是一种用于身份验证的协议,或者允许 Bob 通过警卫室。”
- [SAML 2.0 和 OAuth 2.0 的区别](https://www.ubisecure.com/uncategorized/difference-between-saml-and-oauth/) - “尽管 SAML 实际上被设计为具有广泛的适用性,但其当代用途通常转向企业 SSO 场景。 另一方面,OAuth 被设计用于 Internet 上的应用程序,尤其是委托授权。”
- [OAuth、OpenID Connect 和 SAML 之间有什么区别?](https://www.okta.com/identity-101/whats-the-difference-between-oauth-openid-connect-and-saml/) - 身份是困难的。我们总是欢迎对不同协议的另一种看法,以帮助理解这一切。
- [SAML 2.0 认证如何工作](https://gravitational.com/blog/how-saml-authentication-works/) - 概述 SSO 和 SAML 的方式和原因。
- [Web 单点登录,SAML 2.0 视角](https://blog.theodo.com/2019/06/web-single-sign-on-the-saml-2-0-perspective/) - 在公司 SSO 实施的上下文中对 SAML 工作流的另一种简要解释。
- [SAML 啤酒饮用者指南](https://duo.com/blog/the-beer-drinkers-guide-to-saml) - SAML 有时很神秘。 另一个类比可能有助于从中获得更多意义。
- [SAML 在设计上是不安全的](https://joonas.fi/2021/08/saml-is-insecure-by-design/) - 不仅奇怪,SAML 在设计上也不安全,因为它依赖于基于 XML 规范化的签名,而不是 XML 字节流。 这意味着您可以利用 XML 解析器/编码器的差异。
- [SAML 单点退出的难点 ](https://wiki.shibboleth.net/confluence/display/CONCEPT/SLOIssues) - 关于单点注销实施的技术和用户体验问题。
- [SSO的耻辱墙](https://sso.tax) - 对 SaaS 提供商为在其产品上激活 SSO 而实行的过高定价进行了有记录的咆哮。 作者的观点是,作为核心安全功能,SSO 应该合理定价,而不是排他性层的一部分。
## 授权
现在我们知道你就是你。 但是你可以做你想做的事吗?
策略规范是科学,执行是艺术。
### 策略模型
作为一个概念,访问控制策略可以设计为遵循非常不同的原型,从经典的[访问控制列表](https://en.wikipedia.org/wiki/Access-control_list)到[基于角色的访问控制](https://zh.wikipedia.org/wiki/以角色為基礎的存取控制)。 在本节中,我们将探索许多不同的模式和架构。
- [为什么授权很难](https://www.osohq.com/post/why-authorization-is-hard) - 因为它需要在很多地方需要的执行、决策架构上进行多重权衡以将业务逻辑与授权逻辑分开,以及在建模上平衡功率和复杂性。
- [用户授权的永无止境的产品要求](https://alexolivier.me/posts/the-never-ending-product-requirements-of-user-authorization) - 基于角色的简单授权模型是如何不够的,并且由于产品包装、数据定位、企业组织和合规性而迅速变得复杂。
- [拟采用的 RBAC 方式](https://tailscale.com/blog/rbac-like-it-was-meant-to-be/) -我们如何从 DAC(unix 权限、秘密 URL)到 MAC(DRM、MFA、2FA、SELinux),再到 RBAC。 详细说明后者如何允许更好地建模策略、ACL、用户和组。
- [细粒度权限的案例](https://cerbos.dev/blog/the-case-for-granular-permissions) - 讨论 RBAC 的局限性以及 ABAC(基于属性的访问控制)如何解决这些问题。
- [寻找完美的访问控制系统](https://goteleport.com/blog/access-controls/) - 授权计划的历史渊源。暗示了不同团队和组织之间共享、信任和授权的未来。
- [AWS IAM 角色,一个不必要的复杂故事](https://infosec.rodeo/posts/thoughts-on-aws-iam/) - 快速增长的 AWS 的历史解释了当前方案是如何形成的,以及它与 GCP 资源层次结构的比较。
- [使用 SMT 的 AWS 访问策略的基于语义的自动推理](https://d1.awsstatic.com/Security/pdfs/Semantic_Based_Automated_Reasoning_for_AWS_Access_Policies_Using_SMT.pdf) - Zelkova 是 AWS 的做法。 该系统对IAM策略进行符号分析,根据用户权限和访问约束解决资源可达性问题。 另请参阅更高级别的 [在 re:inforce 2019 上给出的介绍](https://youtu.be/x6wsTFnU3eY?t=2111)。
- [Zanzibar:谷歌一致的全球授权系统](https://ai.google/research/pubs/pub48190) - 可扩展到每秒数万亿个访问控制列表和数百万个授权请求,以支持数十亿人使用的服务。 在 3 年的生产使用中,它一直保持低于 10 毫秒的 95% 延迟和高于 99.999% 的可用性。 [论文中没有的其他内容](https://twitter.com/LeaKissner/status/1136626971566149633)。 [Zanzibar Academy](https://zanzibar.academy/) 是一个致力于解释 Zanzibar 运作方式的网站。
- [SpiceDB](https://github.com/authzed/spicedb) - 一个开源数据库系统,用于管理受 Zanzibar 启发的安全关键应用程序权限。
- [围绕标记的安全性和 RBAC 概念构建的 authz 系统](https://news.ycombinator.com/item?id=20136831) 的描述。
### 开源策略框架
如果你想推出你自己的策略实施,收集的开源项目。
- [Keto](https://github.com/ory/keto) - 策略决定点。 它使用一组访问控制策略,类似于 AWS 策略,以确定主体是否有权对资源执行特定操作。
- [Ladon](https://github.com/ory/ladon) - 受 AWS 启发的访问控制库。
- [Athenz](https://github.com/yahoo/athenz) - 支持服务身份验证和基于角色的授权 (RBAC) 的服务和库集,用于部署和配置。
- [Casbin](https://github.com/casbin/casbin) - Golang 项目的开源访问控制库。
- [Open Policy Agent](https://github.com/open-policy-agent/opa) - 一个开源通用决策引擎,用于创建和实施基于属性的访问控制 (ABAC) 策略。
- [Topaz](https://github.com/aserto-dev/topaz) - 一个开源项目,它将 OPA 的策略即代码和决策日志记录与 Zanzibar 模型目录相结合。
- [Open Policy Administration Layer](https://github.com/permitio/opal) - OPA 的开源管理层,实时检测政策和政策数据的变化,并将实时更新推送给 OPA 代理。 OPAL 使开放策略达到实时应用程序所需的速度。
- [Gubernator](https://github.com/mailgun/gubernator) - 高性能限速微服务和库。
- [Biscuit](https://www.clever-cloud.com/blog/engineering/2021/04/12/introduction-to-biscuit/) - Biscuit 合并了来自 cookies、JWTs、macaroons 和 Open Policy Agent 的概念。 “它提供了一种基于 Datalog 的逻辑语言来编写授权策略。 它可以存储数据,如 JWT,或像 Macaroons 这样的小条件,但它也能够表示更复杂的规则,如基于角色的访问控制、委托、层次结构。”
- [Oso](https://github.com/osohq/oso) - 一个包含电池的库,用于在您的应用程序中构建授权。
- [Cerbos](https://github.com/cerbos/cerbos) - 用于编写上下文感知访问控制策略的授权端点。
### AWS 策略工具
专门针对 [AWS IAM 策略](http://docs.aws.amazon.com/IAM/latest/UserGuide/access_policies.html) 生态系统的工具和资源。
- [成为 AWS IAM 策略忍者](https://www.youtube.com/watch?v=y7-fAT3z8Lo) - “在亚马逊工作近 5 年的时间里,我每天、每周都会抽出一点时间浏览论坛、客户工单,试图找出人们遇到问题的地方。”
- [Cloudsplaining](https://github.com/salesforce/cloudsplaining) - 安全评估工具,可识别违反最小特权的行为并生成风险优先报告。
- [Policy Sentry](https://github.com/salesforce/policy_sentry) - 手动编写具有安全意识的 IAM 策略可能非常乏味且效率低下。 Policy Sentry 可帮助用户在几秒钟内创建最低权限策略。
- [Aardvark and Repokid](https://netflixtechblog.com/introducing-aardvark-and-repokid-53b081bf3a7e) - 用于在 AWS 上实施最低权限的 Netflix 工具。 这个想法是,对新事物的默认策略是全部拒绝,然后它会监控 cloudtrail 是否存在权限故障,并重新配置 IAM 以允许尽可能小的权限来摆脱该拒绝消息。
- [Principal Mapper](https://github.com/nccgroup/PMapper) - 快速评估权限。
- [PolicyUniverse](https://github.com/Netflix-Skunkworks/policyuniverse) - 解析和处理 AWS 策略、语句、ARN 和通配符。
- [IAM Floyd](https://github.com/udondan/iam-floyd) - 具有流畅界面的 AWS IAM 策略语句生成器。 通过 IntelliSense 提供条件和 ARN 生成,帮助创建类型安全的 IAM 策略并编写更具限制性/安全的语句。 适用于 Node.js、Python、.Net 和 Java。
- [ConsoleMe](https://github.com/Netflix/consoleme) - 一种适用于 AWS 的自助服务工具,它根据跨多个账户管理权限的授权级别为最终用户和管理员提供登录账户的凭据和控制台访问权限,同时鼓励使用最低权限。
- [IAMbic](https://github.com/noqdev/iambic) - 適用於 IAM 的 GitOps。 Cloud IAM 的 Terraform。 IAMbic 是一個多雲身份和訪問管理 (IAM) 控制平面,可集中和簡化雲訪問和權限。 它在版本控制中維護 IAM 的最終一致、人類可讀的雙向表示。
### Macaroons
分配和委托授权的巧妙好奇。
- [五分钟或更短时间内完成 Google 的 Macaroon](https://blog.bren2010.io/blog/googles-macaroons) - 如果给我一个授权我在某些限制下执行某些操作的 Macaroon,我可以非交互地构建第二个具有更严格限制的 Macaroon,然后我可以给你。
- [Macaroons: 为云中的分散式授权提供带有上下文警告的Cookies](https://ai.google/research/pubs/pub41892) - 谷歌的原始论文。
- [Google 论文的作者比较了 Macaroons 和 JWT](https://news.ycombinator.com/item?id=14294463) - 作为 Macaroons 的消费者/验证者,它们允许您(通过第三方警告)将某些授权决定推迟给其他人,JWT 没有。
## 秘密管理
允许存储和使用秘密的架构、软件和硬件允许进行身份验证和授权,同时维护信任链。
- [Netflix 的大规模秘密](https://www.youtube.com/watch?v=K0EOPddWpsE) - 基于盲签名的解决方案。 见[幻灯片](https://rwc.iacr.org/2018/Slides/Mehta.pdf).
- [Google 内部 KMS 中的高可用性](https://www.youtube.com/watch?v=5T_c-lqgjso) - 不是 GCP 的 KMS,而是其基础架构的核心。 见[幻灯片](https://rwc.iacr.org/2018/Slides/Kanagala.pdf).
- [`vault`](https://www.vaultproject.io) - 保护、存储和严格控制对令牌、密码、证书、加密密钥的访问。
- [`sops`](https://github.com/mozilla/sops) - 加密 YAML 和 JSON 文件的值,而不是密钥。
- [`gitleaks`](https://github.com/zricethezav/gitleaks) - 审计 git repos 的秘密。
- [`truffleHog`](https://github.com/dxa4481/truffleHog) - 在 git 存储库中搜索高熵字符串和秘密,深入挖掘提交历史。
- [Keywhiz](https://square.github.io/keywhiz/) - 一种用于管理和分发机密的系统,可以很好地适应面向服务的体系结构 (SOA)。
- [`roca`](https://github.com/crocs-muni/roca) - 用于检查各种密钥格式的弱 RSA 模块的 Python 模块。
### 硬件安全模块 (HSM)
HSM 是在硬件层面保证秘密管理安全的物理设备。
- [HSM:它们是什么以及为什么您今天可能(间接)使用过它们](https://rwc.iacr.org/2015/Slides/RWC-2015-Hampton.pdf) - HSM 用法的真正基本概述。
- [AWS Cloud HSM 硬件花絮](https://news.ycombinator.com/item?id=16759383) - AWS CloudHSM Classic 由 SafeNet 的 Luna HSM 提供支持,当前的 CloudHSM 依赖于 Cavium 的 Nitrox,它允许分区的“虚拟 HSM”。
- [CrypTech](https://cryptech.is) - 一个开放的硬件 HSM。
- [Keystone](https://keystone-enclave.org) - 用于基于 RISC-V 架构构建具有安全硬件飞地的可信执行环境 (TEE) 的开源项目。
- [Project Oak](https://github.com/project-oak/oak) - 数据安全传输、存储和处理的规范和参考实现。
- [大家冷静点,这是抢劫!](https://www.sstic.org/2019/presentation/hsm/) - HSM 漏洞和可利用性的案例研究(这一篇文章是法语 😅)。
## 信任与安全
一旦你有了一个重要的用户群,它就被称为一个社区。然后你将负责保护它:客户、人们、公司、企业,并促进其中发生的所有互动和交易。
信任与安全部门是一个受政策驱动和当地法律约束的关键中介机构,可能由一个由 24/7 运营商和高度先进的调节和管理工具系统组成的跨职能团队体现。 您可以将其视为客户支持服务的延伸,专门处理边缘案例,例如手动身份检查、有害内容的审核、停止骚扰、处理授权和版权索赔、数据封存和其他信用卡纠纷。
- [信任与安全 101](https://www.csoonline.com/article/3206127/trust-and-safety-101.html) - 关于域及其职责的精彩介绍。
- [信任和安全到底是什么?](https://www.linkedin.com/pulse/what-heck-trust-safety-kenny-shi) - 几个真实的用例来展示 TnS 团队的作用。
<!--lint disable double-link-->
- [账单和付款清单的备忘列表:欺诈链接](https://github.com/kdeldycke/awesome-billing#fraud) - 专门用于计费和支付欺诈管理的部分,来自我们的姊妹 Git 仓库。
<!--lint enable double-link-->
### 用户身份
大多数企业不会收集客户的身份信息来创建用户档案以出售给第三方,不会。但你仍然必须这样做:当地法律要求在 ["了解你的客户" (Know You Customer KYC)] 的大旗下跟踪合同关系。
- [身份法则](https://www.identityblog.com/stories/2005/05/13/TheLawsOfIdentity.pdf) - 虽然本文的目标是身份元系统,但它的法则在较小的范围内仍然提供了很好的见解,特别是第一条法则:总是允许用户控制并征求同意以赢得信任。
- [Uber 是如何迷路的](https://www.nytimes.com/2019/08/23/business/how-uber-got-lost.html) - “为了限制‘摩擦’,Uber 允许乘客在注册时无需提供电子邮件(很容易伪造)或电话号码以外的身份信息。 (...) 车辆被盗并被烧毁; 司机遭到殴打、抢劫,有时甚至被谋杀。 该公司坚持使用低摩擦注册系统,即使暴力事件有所增加。”
- [个人姓名匹配的比较:技术和实际问题](http://users.cecs.anu.edu.au/~Peter.Christen/publications/tr-cs-06-02.pdf) - 客户姓名匹配有很多应用,从重复数据删除到欺诈监控。
- [统计学上可能的用户名](https://github.com/insidetrust/statistically-likely-usernames) - 用于创建统计学上可能的用户名的词表,以用于用户名枚举、模拟密码攻击和其他安全测试任务。
- [Facebook 上的危险个人和组织名单](https://theintercept.com/document/2021/10/12/facebook-dangerous-individuals-and-organizations-list-reproduced-snapshot/) - 一些团体和内容在一些司法管辖区是非法的。这是一个封锁名单的例子。
- [Ballerine](https://github.com/ballerine-io/ballerine) - 一个用于用户身份和风险管理的开源基础设施。
- [Sherlock](https://github.com/sherlock-project/sherlock) - 在社交网络中按用户名猎取社交媒体账户。
### 欺诈
作为一个在线服务提供商,你面临着欺诈、犯罪和滥用的风险。你会惊讶于人们在涉及到金钱时的聪明程度。预计你的工作流程中的任何错误或差异都会被利用来获取经济利益。
- [在 Car2Go 放宽背景调查后,其75辆车在一天内被盗。](https://web.archive.org/web/20230526073109/https://www.bloomberg.com/news/articles/2019-07-11/mercedes-thieves-showed-just-how-vulnerable-car-sharing-can-be) - 为什么背景调查有时是必要的。
- [调查异常注册](https://openstreetmap.lu/MWGGlobalLogicReport20181226.pdf) - 对 OpenStreetMap 上可疑贡献者注册的详细分析。 这份精美而高层次的报告展示了一场精心策划和定向的活动,可以作为欺诈报告的模板。
- [MIDAS:检测边缘流中的微集群异常](https://github.com/bhatiasiddharth/MIDAS) - 一种提议方法“使用恒定时间和内存检测边缘流中的微簇异常,或突然到达的可疑相似边缘组。”
- [Gephi](https://github.com/gephi/gephi) - 用于可视化和操作大型图形的开源平台。
### Moderation
任何在线社区,不仅是与游戏和社交网络相关的社区,都需要其运营商投入大量资源和精力来对其进行管理。
- [仍在登录了。AR 和 VR 可以从 MMO 中学习什么?](https://youtu.be/kgw8RLHv1j4?t=534) - “如果你主持一个在线社区,在那里人们可以伤害另一个人:你就上钩了。 如果你负担不起被骗的后果,就不要主持在线社区”。
- [你要么死于 MVP,要么活到足够长的时间来建立内容节制。](https://mux.com/blog/you-either-die-an-mvp-or-live-long-enough-to-build-content-moderation/) - "你可以通过考虑三个维度来思考这个问题的解决空间:成本、准确性和速度。还有两种方法:人类审查和机器审查。人类在其中一个维度上很出色:准确性。缺点是,人类的成本高,速度慢。机器,或称机器人,在另外两个方面很出色:成本和速度--它们要便宜得多,速度也快。但是,目标是要找到一个机器人解决方案,同时对你的需求有足够的准确性"。
- [把坏苹果拒之门外:如何调节市场](https://www.twosided.io/p/keep-out-the-bad-apples-how-to-moderate) - “拥有权利的同时也被赋予了重大的责任。 我的一些提示和技巧可以让你的市场变得更安全。”
- [人们的绝望和黑暗会影响到你](https://restofworld.org/2020/facebook-international-content-moderators/) - 大量的外包分包商负责管理庞大的社交网络。 这些人暴露在最坏的情况下,通常最终会患上创伤后应激障碍。
- [The Cleaners](https://thoughtmaybe.com/the-cleaners/) - 一部关于这些薪酬过低的团队删除帖子和删除帐户的纪录片。
### 威胁情报
如何检测、解密和分类攻击性的在线活动。大多数时候,这些都是由安全、网络和/或基础设施工程团队监控的。不过,这些都是技术与服务和 IAM 人员的良好资源,他们可能会被要求提供额外的专业知识来分析和处理威胁。
- [很棒的威胁情报](https://github.com/hslatman/awesome-threat-intelligence) - "威胁情报的简明定义:基于证据的知识,包括背景、机制、指标、影响和可操作的建议,涉及对资产的现有或新出现的威胁或危险,可用于为主体应对该威胁或危险的决策提供信息。"
- [SpiderFoot](https://github.com/smicallef/spiderfoot) - 一个开源的情报(OSINT)自动化工具。它与几乎所有可用的数据源集成,并使用一系列的方法进行数据分析,使这些数据易于浏览。
- [与威胁情报有关的标准](https://www.threat-intelligence.eu/standards/) - 支持威胁情报分析的开放标准、工具和方法。
- [MISP 分类法和分类](https://www.misp-project.org/taxonomies.html) - 组织有关“威胁情报,包括网络安全指标、金融欺诈或反恐信息”的信息的标签。
- [浏览器指纹识别:调查](https://arxiv.org/pdf/1905.01051.pdf) - 指纹可作为识别机器人和欺诈者的信号来源。
- [文件格式的挑战](https://speakerdeck.com/ange/the-challenges-of-file-formats) - 在某个时候,你会让用户在你的系统中上传文件。这里有一个[可疑媒体文件的语料库](https://github.com/corkami/pocs),可以被骗子利用来绕过安全或愚弄用户。
- [SecLists](https://github.com/danielmiessler/SecLists) - 收集安全评估期间使用的多种类型的列表,收集在一个地方。列表类型包括用户名、密码、URL、敏感数据模式、模糊处理有效载荷、网络外壳等等。
- [PhishingKitTracker](https://github.com/neonprimetime/PhishingKitTracker) - 威胁行为者在网络钓鱼工具包中使用的电子邮件地址的 CSV 数据库。
- [PhoneInfoga](https://github.com/sundowndev/PhoneInfoga) - 扫描电话号码的工具,只使用免费资源。目标是首先收集标准信息,如国家、地区、运营商和任何国际电话号码的线路类型,并有非常好的准确性。然后在搜索引擎上搜索足迹,试图找到网络电话供应商或确定其所有者。
- [易混淆的同音字](https://github.com/vhf/confusable_homoglyphs) - 同音字是一种常见的网络钓鱼伎俩。
### 验证码
对付垃圾邮件的另一道防线。
- [Awesome Captcha](https://github.com/ZYSzys/awesome-captcha) - 参考所有开源的验证码库、集成、替代品和破解工具。
- [reCaptcha](https://www.google.com/recaptcha) - 当你的公司没有能力拥有一个专门的团队在互联网规模上打击机器人和垃圾邮件的时候,reCaptcha 仍然是一个有效、经济和快速的解决方案。
- [你(可能)不需要ReCAPTCHA](https://web.archive.org/web/20190611190134/https://kevv.net/you-probably-dont-need-recaptcha/) - 开始时咆哮说该服务是一个隐私的噩梦,在用户界面上也很乏味,然后列出替代方案。
- [Anti-captcha](https://anti-captcha.com) - 验证码的解决服务。
## 黑名单
防止滥用的第一道机械防线包括简单明了的拒绝列表。这是打击欺诈行为的低垂果实,但你会惊讶地发现它们仍然有效。
- [Bloom Filter](https://zh.wikipedia.org/wiki/布隆过滤器) - 非常适合这种用例,因为布隆过滤器旨在快速检查元素是否不在(大)集合中。 特定数据类型存在布隆过滤器的变体。
- [Radix 树如何使阻断 IP 的速度提高5000倍](https://blog.sqreen.com/demystifying-radix-trees/) -Radix 树可能对加快 IP 封锁名单的速度很有帮助。
### 主机名和子域
有助于识别客户,捕捉和阻止机器人群,并限制 dDOS 的影响。
- [`hosts`](https://github.com/StevenBlack/hosts) - 合并有信誉的主机文件,并将它们合并成一个统一的主机文件,删除重复的部分。
- [`nextdns/metadata`](https://github.com/nextdns/metadata) - 广泛收集安全、隐私和家长控制的清单。
- [公共后缀列表](https://publicsuffix.org) - Mozilla的公共后缀注册处,互联网用户可以(或在历史上可以)直接注册名字。
- [国家IP区块](https://github.com/herrbischoff/country-ip-blocks) - CIDR 国家层面的 IP 数据,直接来自区域互联网注册中心,每小时更新一次。
- [证书透明化子域](https://github.com/internetwache/CT_subdomains) - 每小时更新一次从证书透明度日志中收集的子域列表。
- 子域否认列表: [#1](https://gist.github.com/artgon/5366868), [#2](https://github.com/sandeepshetty/subdomain-blacklist/blob/master/subdomain-blacklist.txt), [#3](https://github.com/nccgroup/typofinder/blob/master/TypoMagic/datasources/subdomains.txt), [#4](https://www.quora.com/How-do-sites-prevent-vanity-URLs-from-colliding-with-future-features).
- [`common-domain-prefix-suffix-list.tsv`](https://gist.github.com/erikig/826f49442929e9ecfab6d7c481870700) - 前5000个最常见的域名前缀/后缀列表。
- [`hosts-blocklists`](https://github.com/notracking/hosts-blocklists) -没有更多的广告、跟踪和其他虚拟垃圾。
- [`xkeyscorerules100.txt`](https://gist.github.com/sehrgut/324626fa370f044dbca7) - NSA 的 [XKeyscore](https://zh.wikipedia.org/wiki/XKeyscore) 对TOR和其他匿名保存工具的匹配规则。
- [`pyisp`](https://github.com/ActivisionGameScience/pyisp) - IP 到 ISP 的查询库(包括 ASN)。
- [AMF网站封锁名单](https://www.amf-france.org/Epargne-Info-Service/Proteger-son-epargne/Listes-noires) - 法国官方否认与金钱有关的欺诈网站名单。
### 邮件
- [烧录机电子邮件供应商](https://github.com/wesbos/burner-email-providers) - 一个临时电子邮件提供商的列表。以及其[衍生的Python模块](https://github.com/martenson/disposable-email-domains).
- [MailChecker](https://github.com/FGRibreau/mailchecker) - 跨语言的临时(一次性/抛弃式)电子邮件检测库。
- [临时电子邮件地址域名](https://gist.github.com/adamloving/4401361) - 一次性和临时电子邮件地址的域名列表。用于过滤你的电子邮件列表,以提高打开率(向这些域名发送电子邮件可能不会被打开)。
- [`gman`](https://github.com/benbalter/gman) - "一个红宝石,用于检查一个给定的电子邮件地址或网站的所有者是否在为政府工作(又称验证政府域)。" 在你的用户群中寻找潜在的政府客户的良好资源。
- [`Swot`](https://github.com/leereilly/swot) - 与上述精神相同,但这次是为了标记学术用户。
### 保留的 ID
- [保留字的总清单](https://gist.github.com/stuartpb/5710271) - 这是你可能要考虑保留的一个一般的单词列表,在一个系统中,用户可以挑选任何名字。
- [要保留的主机名和用户名](https://ldpreload.com/blog/names-to-reserve) - 所有应限制在自动系统中注册的名字的清单。
### 诽谤
- [肮脏、顽皮、淫秽和其他坏词列表](https://github.com/LDNOOBW/List-of-Dirty-Naughty-Obscene-and-Otherwise-Bad-Words) - 来自 Shutterstock 的诽谤黑名单。
- [`profanity-check`](https://github.com/vzhou842/profanity-check) - 使用在 200k 人类标记的干净和亵渎文本字符串样本上训练的线性 SVM 模型。
## 隐私
作为用户数据的守护者,IAM 技术栈中深受隐私尊重的约束。
- [隐私增强技术决策树](https://www.private-ai.com/wp-content/uploads/2021/10/PETs-Decision-Tree.pdf) - 根据数据类型和上下文选择正确工具的流程图。
- [我们喜欢的论文:隐私](https://github.com/papers-we-love/papers-we-love/tree/master/privacy) - 通过设计提供隐私的方案的科学研究集合。
- [IRMA 认证](https://news.ycombinator.com/item?id=20144240) - 使用 [Camenisch 和 Lysyanskaya 的 Idemix](https://privacybydesign.foundation/publications/) 提供隐私友好的基于属性的身份验证和签名的开源应用程序和协议。
- [我被骗了吗?](https://haveibeenpwned.com) - 数据泄露指数。
- [软件开发人员的自动化安全测试](https://fahrplan.events.ccc.de/camp/2019/Fahrplan/system/event_attachments/attachments/000/003/798/original/security_cccamp.pdf) -第三方依赖项中的已知漏洞允许大多数隐私泄露。 下面介绍如何通过 CI/CD 的方式检测它们。
- [世界各地的电子邮件营销法规](https://github.com/threeheartsdigital/email-marketing-regulations) - 随着世界的联系越来越紧密,电子邮件营销的监管情况也变得越来越复杂。
- [世界上最大的数据泄露和黑客攻击事件](https://www.informationisbeautiful.net/visualizations/worlds-biggest-data-breaches-hacks/) - 不要成为下一个泄露客户数据的公司。
### 匿名化
作为用户数据的中央存储库,IAM 技术栈的相关人员必须防止任何业务和客户数据的泄漏。为了允许内部分析,需要进行匿名化。
- [哈希法用于匿名的虚假诱惑](https://gravitational.com/blog/hashing-for-anonymization/) - Hashing 不足以实现匿名化。但对于假名化(GDPR允许的)来说,它仍然足够好。
- [四分钱去掉匿名:公司反向散列的电子邮件地址](https://freedom-to-tinker.com/2018/04/09/four-cents-to-deanonymize-companies-reverse-hashed-email-addresses/) - "哈希的电子邮件地址可以很容易地被逆转,并与个人联系起来"。
- [为什么差异化的隐私是了不起的](https://desfontain.es/privacy/differential-privacy-awesomeness.html) - 解释[差异隐私](https://zh.wikipedia.org/wiki/差分隐私)背后的直觉,这是一个理论框架,允许在不影响保密性的情况下共享聚合数据。参见后续文章[更多细节](https://desfontain.es/privacy/differential-privacy-in-more-detail.html)和[实践方面](https://desfontain.es/privacy/differential-privacy-in-practice.html)。
- [K-匿名性:简介](https://www.privitar.com/listing/k-anonymity-an-introduction) - 一个替代性的匿名隐私模型。
- [Presidio](https://github.com/microsoft/presidio) - 语境感知、可插拔和可定制的数据保护和PII数据匿名化服务,用于文本和图像。
- [Diffix:高实用性数据库匿名化](https://aircloak.com/wp-content/uploads/apf17-aspen.pdf) - Diffix 试图提供匿名化,避免假名化并保持数据质量。[在Aircloak 用 Elixir 编写](https://elixirforum.com/t/aircloak-anonymized-analitycs/10930),它作为分析师和未修改的实时数据库之间的一个SQL代理。
### GDPR
众所周知的欧洲隐私框架
- [GDPR Tracker](https://gdpr.eu) - 欧洲的参考网站。
- [GDPR 开发指南](https://github.com/LINCnil/GDPR-Developer-Guide) - 开发者的最佳实践。
- [GDPR – 开发人员的实用指南](https://techblog.bozho.net/gdpr-practical-guide-developers/) - 上述内容的一页摘要。
- [GDPR Tracker](https://gdprtracker.io) - 追踪云服务和子处理者的GDPR合规性。
- [GDPR 文档](https://github.com/good-lly/gdpr-documents) - 供个人使用的模板,让公司遵守 "数据访问 "要求。
- [GDPR 之后的黑暗模式](https://arxiv.org/pdf/2001.02479.pdf) - 本文表明,由于缺乏 GDPR 法律的执行,黑暗模式和默示同意无处不在。
- [GDPR 执行情况跟踪](http://enforcementtracker.com) - GDPR的罚款和处罚清单。
## UX/UI
作为 IAM 技术栈的利益相关者,你将在后端实现建立注册通道和用户入职所需的大部分原语。这是客户对你的产品的第一印象,不能被忽视:你必须和前端专家一起精心设计。这里有几个指南可以帮助你打磨这种体验。
- [2020 年 SaaS 产品入职的状况](https://userpilot.com/saas-product-onboarding/) - 涵盖了用户入职的所有重要方面。
- [用户入职拆解](https://www.useronboard.com/user-onboarding-teardowns/) - 一个巨大的被解构的首次用户注册的列表。
- [发现领先公司的 UI 设计决策](https://goodui.org/leaks/) - 从泄露的截图和 A/B 测试。
- [转换优化](https://www.nickkolenda.com/conversion-optimization-psychology/#cro-tactic11) - 一组战术,以增加用户完成账户创建漏斗的机会。
- [Trello 用户入职培训](https://growth.design/case-studies/trello-user-onboarding/) - 一个详细的案例研究,很好地介绍了如何改善用户的入职。
- [改善注册/登录用户体验的11个技巧](https://learnui.design/blog/tips-signup-login-ux.html) - 关于登录表格的一些基本提示。
- [不要在登录表格上耍小聪明](http://bradfrost.com/blog/post/dont-get-clever-with-login-forms/) - 创建简单、可链接、可预测的登录表格,并与密码管理器很好地配合。
- [为什么用户名和密码在两个不同的页面上?](https://www.twilio.com/blog/why-username-and-password-on-two-different-pages) - 要同时支持SSO和基于密码的登录。现在,如果将登录漏斗分成两步,对用户来说太令人生气了,可以像Dropbox那样解决这个问题:[当你输入用户名时,会有一个AJAX请求](https://news.ycombinator.com/item?id=19174355).
- [用 HTML 属性来改善你的用户的双因素认证体验](https://www.twilio.com/blog/html-attributes-two-factor-authentication-autocomplete) - "在这篇文章中,我们将看看不起眼的 `<input>` 元素和 HTML 属性,这将有助于加快我们用户的双因素认证体验"。
- [移除密码掩码](http://passwordmasking.com) - 总结了一项学术研究的结果,该研究调查了去除密码掩码对消费者信任的影响。
- [对于那些认为 "我可以在一个周末建立 "的人,Slack 是这样决定发送通知的](https://twitter.com/ProductHunt/status/979912670970249221) - 通知是困难的。真的很难。
## 竞争分析
一堆资源,以跟踪所有在该领域经营的公司的现状和进展。
- [AWS 安全、身份与合规公告](https://aws.amazon.com/about-aws/whats-new/security_identity_and_compliance/) - 所有添加到 IAM 周边的新功能的来源。
- [GCP IAM 发布说明](https://cloud.google.com/iam/docs/release-notes) - Also of note: [身份](https://cloud.google.com/identity/docs/release-notes), [身份平台](https://cloud.google.com/identity-platform/docs/release-notes), [资源管理](https://cloud.google.com/resource-manager/docs/release-notes), [密钥服务/HSM](https://cloud.google.com/kms/docs/release-notes), [访问环境管理器](https://cloud.google.com/access-context-manager/docs/release-notes), [身份感知代理](https://cloud.google.com/iap/docs/release-notes), [数据丢失预防](https://cloud.google.com/dlp/docs/release-notes) and [安全扫描器](https://cloud.google.com/security-scanner/docs/release-notes).
- [非官方的谷歌云平台周报](https://www.gcpweekly.com) - Relevant keywords: [`IAM`](https://www.gcpweekly.com/gcp-resources/tag/iam/) and [`安全`](https://www.gcpweekly.com/gcp-resources/tag/security/).
- [DigitalOcean 账户变化日志](http://docs.digitalocean.com/release-notes/accounts/) - 关于 DO 的所有最新账户更新。
- [163 项 AWS 服务各用一行解释](https://adayinthelifeof.nl/2020/05/20/aws.html#discovering-aws) -帮助使他们巨大的服务目录变得有意义。本着同样的精神:[AWS 的简单术语](https://netrixllc.com/blog/aws-services-in-simple-terms/) & [通俗易懂的 AWS](https://expeditedsecurity.com/aws-in-plain-english/).
- [谷歌云开发者的小抄](https://github.com/gregsramblings/google-cloud-4-words#the-google-cloud-developers-cheat-sheet) - 用4个字或更少描述所有 GCP 产品。
## 历史
- [cryptoanarchy.wiki](https://cryptoanarchy.wiki) - Cypherpunks 与安全重合。这个维基汇编了有关该运动、其历史和值得注意的人/事件的信息。
## 贡献
我们永远欢迎你的贡献! 请先看一下[贡献指南](.github/contributing.md)。
## Footnotes
[标题图片](https://github.com/kdeldycke/awesome-iam/blob/main/assets/awesome-iam-header.jpg) 是基于[Ben Sweet](https://unsplash.com/@benjaminsweet).的[照片](https://unsplash.com/photos/2LowviVHZ-E)修改的。
<!--lint disable no-undefined-references-->
<a name="sponsor-def">\[0\]</a>: <a href="https://github.com/sponsors/kdeldycke">您可以通过 GitHub 赞助将您的身份和身份验证产品添加到赞助商列表中</a>。 [\[↑\]](#sponsor-ref)
<a name="intro-quote-def">\[1\]</a>: [*Poison Study*](https://www.amazon.com/dp/0778324338?&linkCode=ll1&tag=kevideld-20&linkId=0b92c3d92371bd53daca5457bdad327e&language=en_US&ref_=as_li_ss_tl) (Mira, 2007). [\[↑\]](#intro-quote-ref)
| {
"repo_name": "kdeldycke/awesome-iam",
"stars": "1284",
"repo_language": "None",
"file_name": "autofix.yaml",
"mime_type": "text/plain"
} |
# Contributor Covenant Code of Conduct
## Our Pledge
In the interest of fostering an open and welcoming environment, we as
contributors and maintainers pledge to making participation in our project and
our community a harassment-free experience for everyone, regardless of age, body
size, disability, ethnicity, gender identity and expression, level of experience,
nationality, personal appearance, race, religion, or sexual identity and
orientation.
## Our Standards
Examples of behavior that contributes to creating a positive environment
include:
- Using welcoming and inclusive language
- Being respectful of differing viewpoints and experiences
- Gracefully accepting constructive criticism
- Focusing on what is best for the community
- Showing empathy towards other community members
Examples of unacceptable behavior by participants include:
- The use of sexualized language or imagery and unwelcome sexual attention or
advances
- Trolling, insulting/derogatory comments, and personal or political attacks
- Public or private harassment
- Publishing others' private information, such as a physical or electronic
address, without explicit permission
- Other conduct which could reasonably be considered inappropriate in a
professional setting
## Our Responsibilities
Project maintainers are responsible for clarifying the standards of acceptable
behavior and are expected to take appropriate and fair corrective action in
response to any instances of unacceptable behavior.
Project maintainers have the right and responsibility to remove, edit, or
reject comments, commits, code, wiki edits, issues, and other contributions
that are not aligned to this Code of Conduct, or to ban temporarily or
permanently any contributor for other behaviors that they deem inappropriate,
threatening, offensive, or harmful.
## Scope
This Code of Conduct applies both within project spaces and in public spaces
when an individual is representing the project or its community. Examples of
representing a project or community include using an official project e-mail
address, posting via an official social media account, or acting as an appointed
representative at an online or offline event. Representation of a project may be
further defined and clarified by project maintainers.
## Enforcement
Instances of abusive, harassing, or otherwise unacceptable behavior may be
reported by contacting the project team at kevin@deldycke.com. All
complaints will be reviewed and investigated and will result in a response that
is deemed necessary and appropriate to the circumstances. The project team is
obligated to maintain confidentiality with regard to the reporter of an incident.
Further details of specific enforcement policies may be posted separately.
Project maintainers who do not follow or enforce the Code of Conduct in good
faith may face temporary or permanent repercussions as determined by other
members of the project's leadership.
## Attribution
This Code of Conduct is adapted from the [Contributor Covenant][homepage], version 1.4,
available at [https://contributor-covenant.org/version/1/4][version]
[homepage]: https://contributor-covenant.org
[version]: https://contributor-covenant.org/version/1/4/
| {
"repo_name": "kdeldycke/awesome-iam",
"stars": "1284",
"repo_language": "None",
"file_name": "autofix.yaml",
"mime_type": "text/plain"
} |
---
github: kdeldycke
| {
"repo_name": "kdeldycke/awesome-iam",
"stars": "1284",
"repo_language": "None",
"file_name": "autofix.yaml",
"mime_type": "text/plain"
} |
# https://docs.github.com/en/github/administering-a-repository
# /configuration-options-for-dependency-updates
---
version: 2
updates:
- package-ecosystem: "github-actions"
directory: "/"
schedule:
interval: "daily"
labels:
- "📦 dependencies"
assignees:
- "kdeldycke"
| {
"repo_name": "kdeldycke/awesome-iam",
"stars": "1284",
"repo_language": "None",
"file_name": "autofix.yaml",
"mime_type": "text/plain"
} |
# Contributing
Your contributions are always welcome! Here are some guidelines.
## Status
This repository has reached an equilibrium state. We are past its accumulation phase, and in the middle of the curation process. Meaning we're more into refining its concepts, smooth the progression and carefully evaluating the addition of new content.
## Pull-requests and issues
- Search past and current issues and pull-requests for previous suggestions before making a new one, as yours may be a duplicate or a work in progress.
- Only one list item per commit.
- Only one commit per pull-request. Always squash commits after applying changes.
- Check your spelling and grammar.
- Add the reason why the linked resource is awesome. And what it adds to existing content.
## Linting
Have your pull-request pass the [official Awesome List's linter](https://github.com/sindresorhus/awesome-lint).
No extra work is required here as it is [already integrated by the way of GitHub actions](https://github.com/kdeldycke/awesome-iam/tree/main/.github/workflows).
To run the linter locally, do:
```shell-session
$ npm i npx
$ npx awesome-lint
```
## Formatting
Additional rules not covered by `awesome-lint`, to keep the content clean and tidy.
If one of these rule conflict with the linter, the linter's rule should takes precedence. Apply it.
### General content
- Remove any trailing whitespaces.
- Use spaces, no tabs, for indention.
- Apostrophes should be using the single ASCII mark: `'`.
- Try to quote the original content as-is to summarize the point of the linked content.
- If a straight quote doesn't cut it, feel free to paraphrase both the item's title and description. Remember, this is curation: we are increasing the value of the original content by aggregation and categorization, yes, but also by smart editorializing. As long as you respect the spirit of the original content it's OK.
### Sections
- Sections are not in the alphabetical order to provide a progression, from general to specific topics.
- Section might feature one paragraph introduction and a figure (graph, drawing, photo).
### Item title
- URL must use HTTPs protocol if available.
- No `“` and `”` curved quotation marks. This is reserved for original content quotation in descriptions.
- To quote, use either the single or double variations: `'` and `"`. Keep them properly balanced.
### Item description
- Try to provide an actionable TL;DR as a description, quoting the original text if it stands by itself.
- [Removes `TL;DR:` prefix in description](https://github.com/kdeldycke/awesome-iam/commit/537cbfd8beaca18d44a0962e107a6db9171a0345). Every description is a short summary anyway.
- Quotes should be properly delimited with the `“` and `”` curved quotation marks.
- You can reduce the original text by using an ellipsis in parenthesis `(…)`.
- For quoting inside a quote, use single or double `'` and `"` ASCII marks. Keep them properly balanced.
- To serialize a list into a description, use the following format:
> This is my own description. Here is a list quoted from the original content about **“a random subject: 1. Blah blah blah; 2. Blah blah blah? 3. Blah blah blah.”** This makes sense right?
This provides visual anchor points that help readability and quick content scanning.
- You can skip some items from the original list and renumber it.
- You shouldn't have to re-order it though.
- An additional link in the description is allowed. This must be limited to some rare cases. Like pointing to a bigger concept, an acronym definition, or reference material (book, biography, …).
### CLI helpers
One-liners to fix-up some common formatting mistakes. Use with great caution and always double-check and edit the results.
- Replaces star list item markers by dashes:
```shell-session
$ sed -i 's/^* /- /g' ./README.md
```
- Replaces typographic quotes with ASCII ones:
```shell-session
$ sed -i "s/‘/\'/g" ./readme.md
$ sed -i "s/’/\'/g" ./readme.md
```
- Forces quotes to end with a dot:
```shell-session
$ sed -i 's/`$/`\./g' ./readme.md
```
| {
"repo_name": "kdeldycke/awesome-iam",
"stars": "1284",
"repo_language": "None",
"file_name": "autofix.yaml",
"mime_type": "text/plain"
} |
### Motivation
<!--
Explain the motivation for adding this link to the current collection. What's special about it?
Note: you can skip this section if you're proposing something as trivial as fixing a typo.
-->
This new link is special because...
### Affiliation
<!-- Please indicate how you are associated with the new proposed content: -->
- [ ] I am the author of the article or project
- [ ] I am working for/with the company which is publishing the article or project
- [ ] I'm just a rando who stumbled upon this via social networks
### Self checks
- [ ] I have [read the Code of Conduct](https://github.com/kdeldycke/awesome-iam/blob/main/.github/code-of-conduct.md)
- [ ] I applied all rules from the [Contributing guide](https://github.com/kdeldycke/awesome-iam/blob/main/.github/contributing.md)
- [ ] I have checked there is no other [Issues](https://github.com/kdeldycke/awesome-iam/issues) or [Pull Requests](https://github.com/kdeldycke/awesome-iam/pulls) covering the same topic to open
| {
"repo_name": "kdeldycke/awesome-iam",
"stars": "1284",
"repo_language": "None",
"file_name": "autofix.yaml",
"mime_type": "text/plain"
} |
name: Add new link
description: You would like to add a new link to the collection
labels: ["🆕 new link"]
body:
- type: input
id: link
attributes:
label: URL of the article or project
validations:
required: true
- type: textarea
id: motivation
attributes:
label: Motivation
description: >
Explain the motivation for adding this link to the current collection. What's special about it?
placeholder: |
This new link is special because...
validations:
required: true
- type: checkboxes
id: affiliation
attributes:
label: Affiliation
description: >
Please indicate how you are associated with the new proposed content.
options:
- label: "I am the author of the article or project"
- label: "I am working for/with the company which is publishing the article or project"
- label: "I'm just a rando who stumbled upon this via social networks"
validations:
required: true
- type: checkboxes
id: self-checks
attributes:
label: Self checks
options:
- label: >
I have [read the Code of
Conduct](https://github.com/kdeldycke/awesome-iam/blob/main/.github/code-of-conduct.md)
- label: >
I applied all rules from the [Contributing
guide](https://github.com/kdeldycke/awesome-iam/blob/main/.github/contributing.md)
- label: >
I have checked there is no other [Issues](https://github.com/kdeldycke/awesome-iam/issues) or [Pull
Requests](https://github.com/kdeldycke/awesome-iam/pulls) covering the same topic to open
validations:
required: true | {
"repo_name": "kdeldycke/awesome-iam",
"stars": "1284",
"repo_language": "None",
"file_name": "autofix.yaml",
"mime_type": "text/plain"
} |
---
name: Label sponsors
"on":
pull_request:
types:
- opened
issues:
types:
- opened
jobs:
label-sponsors:
uses: kdeldycke/workflows/.github/workflows/label-sponsors.yaml@v2.17.4 | {
"repo_name": "kdeldycke/awesome-iam",
"stars": "1284",
"repo_language": "None",
"file_name": "autofix.yaml",
"mime_type": "text/plain"
} |
---
name: Autolock
"on":
schedule:
# Run weekly, every Monday at 8:43.
- cron: "43 8 * * 1"
jobs:
autolock:
uses: kdeldycke/workflows/.github/workflows/autolock.yaml@v2.17.4
| {
"repo_name": "kdeldycke/awesome-iam",
"stars": "1284",
"repo_language": "None",
"file_name": "autofix.yaml",
"mime_type": "text/plain"
} |
---
name: Lint
"on":
push:
jobs:
lint:
uses: kdeldycke/workflows/.github/workflows/lint.yaml@v2.17.4 | {
"repo_name": "kdeldycke/awesome-iam",
"stars": "1284",
"repo_language": "None",
"file_name": "autofix.yaml",
"mime_type": "text/plain"
} |
---
name: Labels
"on":
push:
branches:
- main
jobs:
labels:
uses: kdeldycke/workflows/.github/workflows/labels.yaml@v2.17.4 | {
"repo_name": "kdeldycke/awesome-iam",
"stars": "1284",
"repo_language": "None",
"file_name": "autofix.yaml",
"mime_type": "text/plain"
} |
---
name: Docs
"on":
push:
# Only targets main branch to avoid amplification effects of auto-fixing
# the exact same stuff in multiple non-rebased branches.
branches:
- main
jobs:
docs:
uses: kdeldycke/workflows/.github/workflows/docs.yaml@v2.17.4 | {
"repo_name": "kdeldycke/awesome-iam",
"stars": "1284",
"repo_language": "None",
"file_name": "autofix.yaml",
"mime_type": "text/plain"
} |
---
name: Autofix
"on":
push:
# Only targets main branch to avoid amplification effects of auto-fixing
# the exact same stuff in multiple non-rebased branches.
branches:
- main
jobs:
autofix:
uses: kdeldycke/workflows/.github/workflows/autofix.yaml@v2.17.4 | {
"repo_name": "kdeldycke/awesome-iam",
"stars": "1284",
"repo_language": "None",
"file_name": "autofix.yaml",
"mime_type": "text/plain"
} |
[cfi-derived-cast]
fun:*ObjectWrap*
| {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
<!-- Generated by documentation.js. Update this documentation by updating the source code. -->
### Table of Contents
* [hello][1]
* [Examples][2]
* [helloAsync][3]
* [Parameters][4]
* [Examples][5]
* [helloPromise][6]
* [Parameters][7]
* [Examples][8]
* [HelloObject][9]
* [Examples][10]
* [hello][11]
* [Examples][12]
* [HelloObjectAsync][13]
* [Examples][14]
* [helloAsync][15]
* [Parameters][16]
* [Examples][17]
## hello
This is a synchronous standalone function that logs a string.
### Examples
```javascript
const { hello } = require('@mapbox/node-cpp-skel');
const check = hello();
console.log(check); // => "hello world"
```
Returns **[string][18]**
## helloAsync
This is an asynchronous standalone function that logs a string.
### Parameters
* `args` **[Object][19]** different ways to alter the string
* `args.louder` **[boolean][20]** adds exclamation points to the string
* `args.buffer` **[boolean][20]** returns value as a node buffer rather than a string
* `callback` **[Function][21]** from whence the hello comes, returns a string
### Examples
```javascript
const { helloAsync } = require('@mapbox/node-cpp-skel');
helloAsync({ louder: true }, function(err, result) {
if (err) throw err;
console.log(result); // => "...threads are busy async bees...hello
world!!!!"
});
```
Returns **[string][18]**
## helloPromise
This is a function that returns a promise. It multiplies a string N times.
### Parameters
* `options` **[Object][19]?** different ways to alter the string
* `options.phrase` **[string][18]** the string to multiply (optional, default `hello`)
* `options.multiply` **[Number][22]** duplicate the string this number of times (optional, default `1`)
### Examples
```javascript
const { helloPromise } = require('@mapbox/node-cpp-skel');
const result = await helloAsync({ phrase: 'Howdy', multiply: 3 });
console.log(result); // HowdyHowdyHowdy
```
Returns **[Promise][23]**
## HelloObject
Synchronous class, called HelloObject
### Examples
```javascript
const { HelloObject } = require('@mapbox/node-cpp-skel');
const Obj = new HelloObject('greg');
```
### hello
Say hello
#### Examples
```javascript
const x = Obj.hello();
console.log(x); // => '...initialized an object...hello greg'
```
Returns **[String][18]**
## HelloObjectAsync
Asynchronous class, called HelloObjectAsync
### Examples
```javascript
const { HelloObjectAsync } = require('@mapbox/node-cpp-skel');
const Obj = new module.HelloObjectAsync('greg');
```
### helloAsync
Say hello while doing expensive work in threads
#### Parameters
* `args` **[Object][19]** different ways to alter the string
* `args.louder` **[boolean][20]** adds exclamation points to the string
* `args.buffer` **[buffer][24]** returns object as a node buffer rather then string
* `callback` **[Function][21]** from whence the hello comes, returns a string
#### Examples
```javascript
const { HelloObjectAsync } = require('@mapbox/node-cpp-skel');
const Obj = new HelloObjectAsync('greg');
Obj.helloAsync({ louder: true }, function(err, result) {
if (err) throw err;
console.log(result); // => '...threads are busy async bees...hello greg!!!'
});
```
Returns **[String][18]**
[1]: #hello
[2]: #examples
[3]: #helloasync
[4]: #parameters
[5]: #examples-1
[6]: #hellopromise
[7]: #parameters-1
[8]: #examples-2
[9]: #helloobject
[10]: #examples-3
[11]: #hello-1
[12]: #examples-4
[13]: #helloobjectasync
[14]: #examples-5
[15]: #helloasync-1
[16]: #parameters-2
[17]: #examples-6
[18]: https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/String
[19]: https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Object
[20]: https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Boolean
[21]: https://developer.mozilla.org/docs/Web/JavaScript/Reference/Statements/function
[22]: https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Number
[23]: https://developer.mozilla.org/docs/Web/JavaScript/Reference/Global_Objects/Promise
[24]: https://nodejs.org/api/buffer.html
| {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
# Code of conduct
Everyone is invited to participate in Mapbox’s open source projects and public discussions: we want to create a welcoming and friendly environment. Harassment of participants or other unethical and unprofessional behavior will not be tolerated in our spaces. The [Contributor Covenant](http://contributor-covenant.org) applies to all projects under the Mapbox organization and we ask that you please read [the full text](http://contributor-covenant.org/version/1/2/0/).
You can learn more about our open source philosophy on [mapbox.com](https://www.mapbox.com/about/open/). | {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
# 2/21/2022
* Add `helloPromise` function example using [Napi::Promise](https://github.com/nodejs/node-addon-api/blob/c54aeef5fd37d3304e61af189672f9f61d403e6f/doc/promises.md)
* Move all JSDoc to lib/index.js since versions >=5 do not support the `--polyglot` flag and give docs a little refresh
# 1/9/2018
* Add memory stats option to bench tests
# 1/4/2018
* Add doc note about remote vs local coverage using `LCOV`
# 11/17/2017
* Add liftoff script, setup docs, and more contributing details per https://github.com/mapbox/node-cpp-skel/pull/87
# 10/31/2017
* Add [sanitzer flag doc](https://github.com/mapbox/node-cpp-skel/pull/84)
* Add [sanitizer script](hhttps://github.com/mapbox/node-cpp-skel/pull/85) and enable [leak sanitizer](https://github.com/mapbox/node-cpp-skel/commit/725601e4c7df6cb8477a128f018fb064a9f6f9aa)
*
# 10/20/2017
* Add [code of conduct](https://github.com/mapbox/node-cpp-skel/pull/82)
* Add [CC0 license](https://github.com/mapbox/node-cpp-skel/pull/82)
* Point to [cpp glossary](https://github.com/mapbox/node-cpp-skel/pull/83)
# 10/12/2017
* Update compiler flags per best practices per https://github.com/mapbox/cpp/issues/37
- https://github.com/mapbox/node-cpp-skel/pull/80
- https://github.com/mapbox/node-cpp-skel/pull/78
- https://github.com/mapbox/node-cpp-skel/pull/77
# 09/10/2017
* [Sanitize update](https://github.com/mapbox/node-cpp-skel/pull/74)
# 08/24/2017
* Clang tidy updates
- https://github.com/mapbox/node-cpp-skel/pull/68
- https://github.com/mapbox/node-cpp-skel/issues/65
- https://github.com/mapbox/node-cpp-skel/pull/64
- https://github.com/mapbox/node-cpp-skel/pull/66
# 08/15/2017
* Add [bench scripts](https://github.com/mapbox/node-cpp-skel/pull/61) for async examples
# 08/09/2017
* Add comments about "new" allocation
# 08/08/2017
* Add [clang-format](https://github.com/mapbox/node-cpp-skel/pull/56)
# 08/04/2017
* Use Nan's safer and high performance `Nan::Utf8String` when accepting string args per https://github.com/mapbox/node-cpp-skel/pull/55
# 08/3/2017
* Reorganize [documentation](https://github.com/mapbox/node-cpp-skel/pull/53)
# 07/21/2017
* Add [object_async example](https://github.com/mapbox/node-cpp-skel/pull/52)
# 07/11/2017
* Add [build docs](https://github.com/mapbox/node-cpp-skel/pull/51)
* It begins | {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
# Contributing
Thanks for getting involved and contributing to the skel :tada: Below are a few things to setup when submitting a PR.
## Code comments
If adding new code, be sure to include relevant code comments. Code comments are a great way for others to learn from your code. This is especially true within the skeleton, since it is made for learning.
## Update Documentation
Be sure to update any documentation relevant to your change. This includes updating the [CHANGELOG.md](https://github.com/mapbox/node-cpp-skel/blob/master/CHANGELOG.md).
## [Code Formatting](/docs/extended-tour.md#clang-tools)
We use [this script](/scripts/clang-format.sh#L20) to install a consistent version of [`clang-format`](https://clang.llvm.org/docs/ClangFormat.html) to format the code base. The format is automatically checked via a Travis CI build as well. Run the following script locally to ensure formatting is ready to merge:
make format
We also use [`clang-tidy`](https://clang.llvm.org/extra/clang-tidy/) as a C++ linter. Run the following command to lint and ensure your code is ready to merge:
make tidy
These commands are set from within [the Makefile](./Makefile). | {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
{
'target_defaults': {
'default_configuration': 'Release',
'cflags_cc' : [
'-std=c++14',
# The assumption is that projects based on node-cpp-skel will also
# depend on mason packages. Currently (this will change in future mason versions)
# mason packages default to being built/linked with the CXX11_ABI=0.
# So we need to link this way too. This allows source
# compiling your module on any ubuntu version, even the latest
# where the CXX11_ABI default has flipped to 1
# More details at https://github.com/mapbox/mason/issues/319
'-D_GLIBCXX_USE_CXX11_ABI=0'
],
'cflags_cc!': ['-std=gnu++0x','-std=gnu++1y', '-fno-rtti', '-fno-exceptions'],
'configurations': {
'Debug': {
'defines!': [
'NDEBUG'
],
'cflags_cc!': [
'-O3',
'-Os',
'-DNDEBUG'
],
'xcode_settings': {
'OTHER_CPLUSPLUSFLAGS!': [
'-O3',
'-Os',
'-DDEBUG'
],
'GCC_OPTIMIZATION_LEVEL': '0',
'GCC_GENERATE_DEBUGGING_SYMBOLS': 'YES'
}
},
'Release': {
'defines': [
'NDEBUG'
],
'cflags': [
'-flto'
],
'ldflags': [
'-flto',
'-fuse-ld=<(module_root_dir)/mason_packages/.link/bin/ld'
],
'xcode_settings': {
'OTHER_CPLUSPLUSFLAGS!': [
'-Os',
'-O2'
],
'OTHER_LDFLAGS':[ '-flto' ],
'OTHER_CPLUSPLUSFLAGS': [ '-flto' ],
'GCC_OPTIMIZATION_LEVEL': '3',
'GCC_GENERATE_DEBUGGING_SYMBOLS': 'NO',
'DEAD_CODE_STRIPPING': 'YES',
'GCC_INLINES_ARE_PRIVATE_EXTERN': 'YES'
}
}
}
}
}
| {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
ignore:
- "test" | {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
[headers]
protozero=1.6.8
[compiled]
clang++=10.0.0
clang-tidy=10.0.0
clang-format=10.0.0
llvm-cov=10.0.0
binutils=2.35
| {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
# This file inherits default targets for Node addons, see https://github.com/nodejs/node-gyp/blob/master/addon.gypi
{
# https://github.com/springmeyer/gyp/blob/master/test/make_global_settings/wrapper/wrapper.gyp
'make_global_settings': [
['CXX', '<(module_root_dir)/mason_packages/.link/bin/clang++'],
['CC', '<(module_root_dir)/mason_packages/.link/bin/clang'],
['LINK', '<(module_root_dir)/mason_packages/.link/bin/clang++'],
['AR', '<(module_root_dir)/mason_packages/.link/bin/llvm-ar'],
['NM', '<(module_root_dir)/mason_packages/.link/bin/llvm-nm']
],
'includes': [ 'common.gypi' ], # brings in a default set of options that are inherited from gyp
'variables': { # custom variables we use specific to this file
'error_on_warnings%':'true', # can be overriden by a command line variable because of the % sign using "WERROR" (defined in Makefile)
# Use this variable to silence warnings from mason dependencies and from NAN
# It's a variable to make easy to pass to
# cflags (linux) and xcode (mac)
'system_includes': [
"-isystem <!@(node -p \"require('node-addon-api').include.slice(1,-1)\")",
"-isystem <(module_root_dir)/mason_packages/.link/include/"
],
# Flags we pass to the compiler to ensure the compiler
# warns us about potentially buggy or dangerous code
'compiler_checks': [
'-Wall',
'-Wextra',
'-Weffc++',
'-Wconversion',
'-pedantic-errors',
'-Wconversion',
'-Wshadow',
'-Wfloat-equal',
'-Wuninitialized',
'-Wunreachable-code',
'-Wold-style-cast',
'-Wno-error=unused-variable'
]
},
# `targets` is a list of targets for gyp to run.
# Different types of targets:
# - [executable](https://github.com/mapbox/cpp/blob/master/glossary.md#executable)
# - [loadable_module](https://github.com/mapbox/cpp/blob/master/glossary.md#loadable-module)
# - [static library](https://github.com/mapbox/cpp/blob/master/glossary.md#static-library)
# - [shared library](https://github.com/mapbox/cpp/blob/master/glossary.md#shared-library)
# - none: a trick to tell gyp not to run the compiler for a given target.
'targets': [
{
'target_name': 'action_before_build',
'type': 'none',
'hard_dependency': 1,
'actions': [
{
'action_name': 'install_deps',
'inputs': ['./node_modules/.bin/mason-js'],
'outputs': ['./mason_packages'],
'action': ['./node_modules/.bin/mason-js', 'install']
},
{
'action_name': 'link_deps',
'inputs': ['./node_modules/.bin/mason-js'],
'outputs': ['./mason_packages/.link'],
'action': ['./node_modules/.bin/mason-js', 'link']
}
]
},
{
# module_name and module_path are both variables passed by node-pre-gyp from package.json
'target_name': '<(module_name)', # sets the name of the binary file
'product_dir': '<(module_path)', # controls where the node binary file gets copied to (./lib/binding/module.node)
'type': 'loadable_module',
'dependencies': [ 'action_before_build' ],
# "make" only watches files specified here, and will sometimes cache these files after the first compile.
# This cache can sometimes cause confusing errors when removing/renaming/adding new files.
# Running "make clean" helps to prevent this "mysterious error by cache" scenario
# This also is where the benefits of using a "glob" come into play...
# See: https://github.com/mapbox/node-cpp-skel/pull/44#discussion_r122050205
'sources': [
'./src/module.cpp',
'./src/standalone/hello.cpp',
'./src/standalone_async/hello_async.cpp',
'./src/standalone_promise/hello_promise.cpp',
'./src/object_sync/hello.cpp',
'./src/object_async/hello_async.cpp'
],
'ldflags': [
'-Wl,-z,now',
],
'conditions': [
['error_on_warnings == "true"', {
'cflags_cc' : [ '-Werror' ],
'xcode_settings': {
'OTHER_CPLUSPLUSFLAGS': [ '-Werror' ]
}
}]
],
'cflags_cc': [
'<@(system_includes)',
'<@(compiler_checks)'
],
'xcode_settings': {
'OTHER_LDFLAGS':[
'-Wl,-bind_at_load'
],
'OTHER_CPLUSPLUSFLAGS': [
'<@(system_includes)',
'<@(compiler_checks)'
],
'GCC_ENABLE_CPP_RTTI': 'YES',
'GCC_ENABLE_CPP_EXCEPTIONS': 'YES',
'MACOSX_DEPLOYMENT_TARGET':'10.8',
'CLANG_CXX_LIBRARY': 'libc++',
'CLANG_CXX_LANGUAGE_STANDARD':'c++14',
'GCC_VERSION': 'com.apple.compilers.llvm.clang.1_0'
}
}
]
}
| {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
# This Makefile serves a few purposes:
#
# 1. It provides an interface to iterate quickly while developing the C++ code in src/
# by typing `make` or `make debug`. To make iteration as fast as possible it calls out
# directly to underlying build tools and skips running steps that appear to have already
# been run (determined by the presence of a known file or directory). What `make` does is
# the same as running `npm install --build-from-source` except that it is faster when
# run a second time because it will skip re-running expensive steps.
# Note: in rare cases (like if you hit `crtl-c` during an install) you might end up with
# build deps only partially installed. In this case you should run `make distclean` to fully
# restore your repo to is starting state and then running `make` again should start from
# scratch, fixing any inconsistencies.
#
# 2. It provides a few commands that call out to external scripts like `make coverage` or
# `make tidy`. These scripts can be called directly but this Makefile provides a more uniform
# interface to call them.
#
# To learn more about the build system see https://github.com/mapbox/node-cpp-skel/blob/master/docs/extended-tour.md#builds
# Whether to turn compiler warnings into errors
export WERROR ?= true
# the default target. This line means that
# just typing `make` will call `make release`
default: release
node_modules/node-addon-api:
npm install --ignore-scripts
mason_packages/headers: node_modules/node-addon-api
node_modules/.bin/mason-js install
mason_packages/.link/include: mason_packages/headers
node_modules/.bin/mason-js link
build-deps: mason_packages/.link/include
release: build-deps
V=1 ./node_modules/.bin/node-pre-gyp configure build --error_on_warnings=$(WERROR) --loglevel=error
@echo "run 'make clean' for full rebuild"
debug: mason_packages/.link/include
V=1 ./node_modules/.bin/node-pre-gyp configure build --error_on_warnings=$(WERROR) --loglevel=error --debug
@echo "run 'make clean' for full rebuild"
coverage: build-deps
./scripts/coverage.sh
tidy: build-deps
./scripts/clang-tidy.sh
format: build-deps
./scripts/clang-format.sh
sanitize: build-deps
./scripts/sanitize.sh
clean:
rm -rf lib/binding
rm -rf build
# remove remains from running 'make coverage'
rm -f *.profraw
rm -f *.profdata
@echo "run 'make distclean' to also clear node_modules, mason_packages, and .mason directories"
distclean: clean
rm -rf node_modules
rm -rf mason_packages
# variable used in the `xcode` target below
MODULE_NAME := $(shell node -e "console.log(require('./package.json').binary.module_name)")
xcode: node_modules
./node_modules/.bin/node-pre-gyp configure -- -f xcode
@# If you need more targets, e.g. to run other npm scripts, duplicate the last line and change NPM_ARGUMENT
SCHEME_NAME="$(MODULE_NAME)" SCHEME_TYPE=library BLUEPRINT_NAME=$(MODULE_NAME) BUILDABLE_NAME=$(MODULE_NAME).node scripts/create_scheme.sh
SCHEME_NAME="npm test" SCHEME_TYPE=node BLUEPRINT_NAME=$(MODULE_NAME) BUILDABLE_NAME=$(MODULE_NAME).node NODE_ARGUMENT="`npm bin tape`/tape test/*.test.js" scripts/create_scheme.sh
open build/binding.xcodeproj
testpack:
rm -f ./*tgz
npm pack
tar -ztvf *tgz
docs:
npm run docs
test:
npm test
.PHONY: test docs
| {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
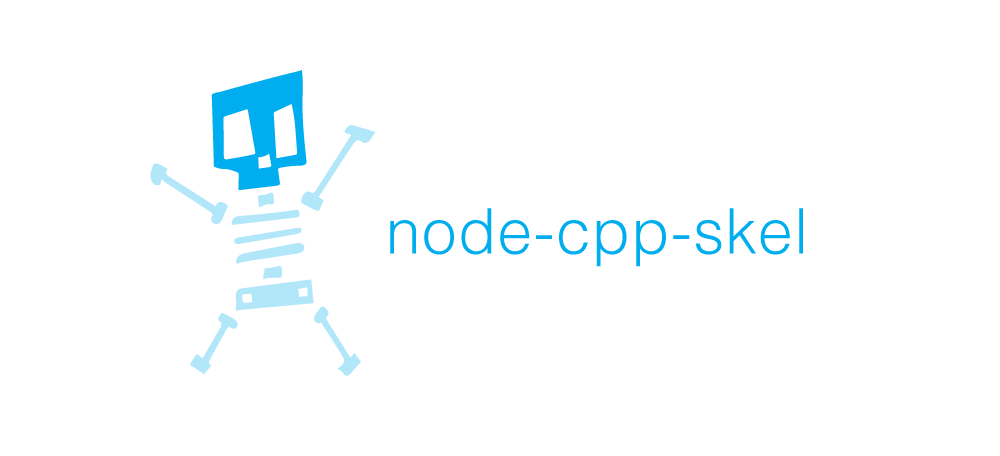
[](https://travis-ci.com/mapbox/node-cpp-skel)
[](https://codecov.io/gh/mapbox/node-cpp-skel)
A skeleton for building a C++ addon for Node.js. This is a small, helper repository that generates simple `HelloWorld` Javascript example constructors. The examples have a number of methods to demonstrate different ways to use the Node C+ API for building particular types of functionality (i.e. asynchronous functions). Use this repo as both a template for your own code as well as a learning tool if you're just starting to develop Node/C++ Addons.
**Why port C++ to Node.js?**. That's a great question! C++ is a high performance language that allows you to execute operations without clogging up the event loop. Node.js is single-threaded, which blocks execution. Even in highly optimized javascript code it may be impossible to improve performance. Passing heavy operations into C++ and subsequently into C++ workers can greatly improve the overall runtime of the code. Porting C++ code to Node.js is also referred to as creating an ["Addon"](https://github.com/mapbox/cpp/blob/master/node-cpp.md).
More examples of how to port C++ libraries to node can be found at [nodejs.org/api/addons.html](https://nodejs.org/api/addons.html).
# What's in the box? :package:
This repository itself can be cloned and edited to your needs. The skeleton prepares a C++ port to Node.js and provides the following for quick development:
* **Tests**: created with [Tape](https://github.com/substack/tape) in the `test/` directory. Travis CI file is prepared to build and test your project on every push.
* **Documentation**: use this README as a template and customize for your own project. Also, this skeleton uses [documentation.js](http://documentation.js.org/) to generate API documentation from JSDOC comments in the `.cpp` files. Docs are located in `API.md`.
* **[Benchmarking](./docs/benchmarking.md)**: Easily test the performance of your code using the built-in benchmark tests provided in this skeleton.
* **Build system**: [node-pre-gyp](https://github.com/mapbox/node-pre-gyp) generates binaries with the proper system architecture flags
* **[Publishing](./docs/publishing-binaries.md)**: Structured as a node module with a `package.json` that can be deployed to NPM's registry.
* **Learning resources**: Read the detailed inline comments within the example code to learn exactly what is happening behind the scenes. Also, check out the [extended tour](./docs/extended-tour.md) to learn more about Node/C++ Addon development, builds, Xcode, and more details about the configuration of this skeleton.
# Installation
Each `make` command is specified in [`Makefile`](./Makefile)
```bash
git clone git@github.com:mapbox/node-cpp-skel.git
cd node-cpp-skel
# Build binaries. This looks to see if there were changes in the C++ code. This does not reinstall deps.
make
# Run tests
make test
# Cleans your current builds and removes potential cache
make clean
# Build binaries in Debug mode (https://github.com/mapbox/cpp/blob/master/glossary.md#debug-build)
make debug
# Cleans everything, including the things you download from the network in order to compile (ex: npm packages).
# This is useful if you want to nuke everything and start from scratch.
# For example, it's super useful for making sure everything works for Travis, production, someone else's machine, etc
make distclean
# This skel uses documentation.js to auto-generate API docs.
# If you'd like to generate docs for your code, you'll need to install documentation.js,
# and then add your subdirectory to the docs command in package.json
npm install -g documentation@4.0.0
npm run docs
```
NOTE: we are pinned to `documentation@4.0.0` because 5.x removed C++ support: https://github.com/documentationjs/documentation/blob/master/CHANGELOG.md#500-2017-07-27
### Customizing the compiler toolchain
By default we use `clang++` via [mason](https://github.com/mapbox/mason). The reason we do this is:
- We want to run the latest and greatest compiler version, to catch the most bugs, provide the best developer experience, and trigger the most helpful warnings
- We use clang-format to format the code and each version of clang-format formats code slightly differently. To avoid friction around this (and ensure all devs format the code the same) we default to using the same version of clang++ via mason.
- We want to support [LTO](https://github.com/mapbox/cpp/blob/master/glossary.md#link-time-optimization) in the builds, which is difficult to do on linux unless you control the toolchain tightly.
The version of the clang++ binary (and related tools) is controlled by the [`mason-versions.ini`](./mason-versions.ini), and uses `mason-js` uses to install the toolchain.
All that said, it is still absolutely possible and encouraged to compile your module with another compiler toolchain. In fact we hope that modules based on node-cpp-skel do this!
To customize the toolchain you can override the defaults by setting these environment variables: CXX, CC, LINK, AR, NM. For example to use g++-6 you could do:
```bash
export CXX="g++-6"
export CC="gcc-6"
export LINK="g++-6"
export AR="ar"
export NM="nm"
make
```
These environment variables will override the compiler toolchain defaults in `make_global_settings` in the [`binding.gyp`](./binding.gyp).
### Warnings as errors
By default the build errors on compiler warnings. To disable this do:
```
WERROR=false make
```
### Sanitizers
You can run the [sanitizers](https://github.com/mapbox/cpp/blob/master/glossary.md#sanitizers), to catch additional bugs, by doing:
```shell
make sanitize
```
The sanitizers [are part of the compiler](https://github.com/mapbox/cpp/blob/master/glossary.md#sanitizers) and are also run in a specific job on Travis.
# Add Custom Code
Depending on your usecase, there are a variety of ways to start using this skeleton for your project.
### Setup new project
Easily use this skeleton as a starting off point for a _new_ custom project:
```
# Clone node-cpp-skel locally
git clone git@github.com:mapbox/node-cpp-skel.git
cd node-cpp-skel/
# Create your new repo on GitHub and have the remote repo url handy for liftoff
# Then run the liftoff script from within your local node-cpp-skel root directory.
#
# This will:
# - prompt you for the new name of your project and the new remote repo url
# - automatically create a new directory for your new project repo
# - create a new branch called "node-cpp-skel-port" within your new repo directory
# - add, commit, and push that branch to your new repo
./scripts/liftoff.sh
```
### Add your code
Once your project has ported node-cpp-skel, follow these steps to integrate your own code:
- Create a dir in `./src` to hold your custom code. See the example code within `/src` for reference.
- Add your new method or class to `./src/module.cpp`, and `#include` it at the top
- Add your new file-to-be-compiled to the list of target sources in `./binding.gyp`
- Run `make` and see what surprises await on your new journey :boat:
### Adding dependencies
With updated versions of npm, a `package-lock.json` file is created, which is now included in node-cpp-skel. See [`npm-and-package-lock.md`](./docs/npm-and-package-lock.md) for more info on how to interact with this file and how to add new dependencies.
### Interactive Debugging
* [Debugging with VS Code](./docs/debugging-with-vs-code.md)
# Code coverage
Code coverage is critical for knowing how well your tests actually test all your code. To see code coverage you can view current results online at [](https://codecov.io/gh/mapbox/node-cpp-skel) or you can build in a customized way and display coverage locally like:
```
make coverage
```
**Note**
Use [`// LCOV_EXCL_START` and `// LCOV_EXCL_STOP`](https://github.com/mapbox/vtvalidate/blob/master/src/vtvalidate.cpp#L70-L73) to ignore from [codecov](https://codecov.io/gh/mapbox/node-cpp-skel) _remotely_. However, this won't ignore when running coverage _locally_.
For more details about what `make coverage` is doing under the hood see https://github.com/mapbox/cpp#code-coverage.
# Contributing and License
Contributors are welcome! :sparkles: This repo exists as a place to gather C++/Node Addon knowledge that will benefit the larger community. Please contribute your knowledge if you'd like.
Node-cpp-skel is licensed under [CC0](https://creativecommons.org/share-your-work/public-domain/cc0/). Attribution is not required, but definitely welcome! If your project uses this skeleton, please add the node-cpp-skel badge to your readme so that others can learn about the resource.
[](https://github.com/mapbox/node-cpp-skel)
To include the badge, paste this into your README.md file:
```
[](https://github.com/mapbox/node-cpp-skel)
```
See [CONTRIBUTING](CONTRIBUTING.md) and [LICENSE](LICENSE.md) for more info.
| {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
# Extended Tour
Welcome to the extended tour of node-cpp-skel. This documentation is especially handy if you're just starting out with building [Node Addon-ons](https://github.com/mapbox/cpp/blob/master/node-cpp.md) or writing C++ :tada:
## Table of Contents:
- [Walkthrough of example code](extended-tour.md#walkthrough-example-code)
- [What does "build" mean?](extended-tour.md#what-does-build-mean)
- [Software build components](extended-tour.md#software-build-components)
- [Configuration files](extended-tour.md#configuration-files)
- [Autogenerated files](extended-tour.md#autogenerated-files)
- [Overall flow](extended-tour.md#overall-flow)
- [Clang Tools](extended-tour.md#clang-tools)
- [Xcode](extended-tour.md#xcode)
# Walkthrough example code
This skeleton includes a few examples of how you might design your application, including standalone functions and creating Objects/Classes. Both the synchronous and asynchronous versions of each are included to give an iterative example of what the progression from sync to async looks like. Let's run through reasons why you'd design your code in these ways:
1. [Standalone function](../API.md#hello-1)
2. [Standalone asynchronous function](../API.md#helloasync-1)
3. [Object/Class](../API.md#helloobject)
4. [Asynchronous Object/Class](../API.md#helloobjectasync)
### When would you use a standalone function?
A standalone function is a function that exists at the top level of the module scope rather than as a member of an object that is instantiated. So if your module is `wonderful`, a standalone function would be called like `wonderful.callme()`.
A standalone function makes sense when the only data needed by the function can be easily passed as arguments. When it is not easy or clean to pass data as arguments then you should consider encapsulation, for example, exposing a function as a member of an object. One of the benefits of creating a standalone function is that it can help [separate concerns](https://en.wikipedia.org/wiki/Separation_of_concerns), which is mainly a stylistic design decision.
Example:
- [vtinfo](https://github.com/mapbox/vtinfo/blob/master/src/vtinfo.cpp): A synchronous standalone function that is simply getting info about a single vector tile buffer.
### When would you use an object/class?
Create an object/class when you need to do some kind of data preprocessing before going into the thread pool. It's best to write your code so that the preprocessing happens _once_ as a separate operation, then continues through to the thread pool after the preprocessing is finished and the object is ready. Examples:
- [node-mapnik](https://github.com/mapnik/node-mapnik/blob/fe80ce5d79c0e90cfbb5a2b992bf0ae2b8f88198/src/mapnik_map.hpp#L20): we create a Map object once, then use the object multiple times for rendering each vector tile.
- [node-addon-examples](https://github.com/nodejs/node-addon-examples/tree/master/6_object_wrap/node-addon-api) for another example of what an object-focused Addon looks like.
Objects/Classes are useful when you start doing more complex operations and need to consider performance more heavily, when performance constraints start to matter. Use classes to compute the value of something once, rather than every time you call a function.
One step further is using an asynchronous object or class, which enables you to pass data into the threadpool. The aim is to process data in the threadpool in the most efficient way possible. [Efficiency](https://github.com/mapbox/cpp/blob/master/glossary.md#efficiency) and high performance are the main goals of the `HelloObjectAsync` example in this skel. The `HelloObjectAsync` example demonstrates high load, when _many_ objects in _many_ threads are being allocated. This scenario is where reducing unnecessary allocations really pays off, and is also why this example uses "move semantics" for even better performance.
- **Move semantics**: move semantics avoid data being copied (allocating memory), to limit unnecessary memory allocation, and are most useful when working with big data.
Other thoughts related to move semantics:
- Always best to use move semantics instead of passing by reference, espeically with objects like [`std::string`](http://shaharmike.com/cpp/std-string/), which can be expensive.
- Relatedly, best to use [`std::unique_ptr`](http://en.cppreference.com/w/cpp/memory/unique_ptr) instead of using `std::shared_ptr` because `std::unique_ptr` is non-copyable. So you're forced to avoid copying, which is a good practice.
# Builds
### What does "build" mean?
When you "build" your module, you are compiling and linking your C++ [source code](https://github.com/mapbox/cpp/blob/master/glossary.md#source-code) to produce a binary file that allows Node.js to load and run your module. Once loaded your C++ module can be used as if it were a Javascript module. A large list of tools come together to make this possible. In the below section we'll describe:
- [Software build components](extended-tour.md##software-build-components)
- Listing of [configuration files](extended-tour.md#configuration-files) for the build
- Listing of [autogenerated files](extended-tour.md#autogenerated-files) created when you run a build
- Overall [build flow](extended-tour.md#build-flow) of what happens when you run a build
### Software build components
The primary components involved in the build
#### node-pre-gyp
node-pre-gyp is a javascript command line tool used in this project as a [front end](https://github.com/mapbox/cpp/blob/master/glossary.md#front-end) to [node-gyp](#node-gyp) to either compile your code or install it from binaries.
It is installed as a dependency in the [package.json](../package.json).
Learn more about node-pre-gyp [here](https://github.com/mapbox/node-pre-gyp)
#### node-gyp
node-gyp is a javascript command line tool used in this project as a [front end](https://github.com/mapbox/cpp/blob/master/glossary.md#front-end) to [gyp](#gyp).
node-gyp is bundled inside [npm](#npm) and does not need to be installed separately. Although, if installed in [package.json](../package.json), that version will be used by [node-pre-gyp](#node-pre-gyp).
Learn more about node-gyp [here](https://github.com/nodejs/node-gyp)
#### gyp
gyp is a python command line tool used in this project as a [front end](https://github.com/mapbox/cpp/blob/master/glossary.md#front-end) to [make](#make).
Learn more about gyp [here](https://github.com/mapbox/cpp/blob/master/glossary.md#gyp)
#### make
make is a command line tool, written in C, that is installed by default on most unix systems. It is used in this project in two ways:
- We provide a `Makefile` that acts as a simple entry point for developers wanting to source compile node-pre-gyp
- When [node-gyp](#node-gyp) is run, it generates a set of `Makefile`s automatically which are used to call out to the [compiler](#compiler) and [linker](#linker) to assemble your binary C++ module.
Learn more about make [here](https://github.com/mapbox/cpp/blob/master/glossary.md#make)
#### compiler
The command line program able to compile C++ source code, in this case `clang++`.
Learn more about what a compiler is [here](https://github.com/mapbox/cpp/blob/master/glossary.md#compiler)
#### linker
The command line program able to link C++ source code, in this case also `clang++`, which acts as a [front end](https://github.com/mapbox/cpp/blob/master/glossary.md#front-end) to the [system linker](https://github.com/mapbox/cpp/blob/master/glossary.md#linker)
### Configuration files
Files you will find inside this repo and their purpose. For more info look inside each file for detailed comments.
- [Makefile](../Makefile) - entry point to building from source. This is invoked when you type `make` in the root directory. By default the `default` target is run which maps to the `release` target. See the comments inside the Makefile for more detail.
- [binding.gyp](../binding.gyp) - JSON configuration file for [node-gyp](#node-gyp). Must be named `binding.gyp` and present in the root directory so that `npm` detects it. Will be passed to [gyp](#gyp) by [node-gyp](#node-gyp). Because [gyp](#gyp) is python and has less strict JSON parsing rules, code comments with `#` are allowed (this would not be the case if parsed with node.js).
- [common.gypi](../common.gypi) - "gypi" stands for gyp include file. This is referenced by the [binding.gyp](../binding.gyp)
- [package.json](../package.json) - configuration file for npm. But it also contains a custom `binary` object that is the configuration for [node-pre-gyp](#node-pre-gyp).
- [lib/index.js](../lib/index.js) - entry point for the javascript module. Referenced in the `main` property in the [package.json](../package.json). This is the file that is run when the module is loaded by `require` from another module.
- [scripts/setup.sh](../scripts/setup.sh) - script used to 1) install [Mason](https://github.com/mapbox/cpp/blob/master/glossary.md#mason) and [clang++](https://github.com/mapbox/cpp/blob/master/glossary.md#clang-1) and 2) create a `local.env` that can be sourced in `bash` in order to set up [Mason](https://github.com/mapbox/cpp/blob/master/glossary.md#mason) and [clang++](https://github.com/mapbox/cpp/blob/master/glossary.md#clang-1) on your PATH.
- [scripts/install_deps.sh](../scripts/install_deps.sh) - script that invokes [Mason](https://github.com/mapbox/cpp/blob/master/glossary.md#mason) to install mason packages
- [scripts/publish.sh](../scripts/publish.sh) - script to publish the C++ binary module with [node-pre-gyp](#node-pre-gyp). Designed to be run on [travisci.org](https://travis-ci.org/)
- [.travis.yml](../.travis.yml) - configuration for this module on [travisci.org](https://travis-ci.org/). Used to test the code and publish binaries for various node versions and compiler options.
Note: the `binding.gyp` file also inherits from two files that you will not find inside the node-cpp-skel repo. These are gyp include files that come from node core and node-gyp and are invoked when [`node-gyp configure` is called](https://github.com/nodejs/node-gyp/blob/a8ba5288cb25d4edcafdc75d0cd59b474b7225e8/lib/configure.js#L279-L280):
- https://github.com/nodejs/node/blob/v4.x/common.gypi
- https://github.com/nodejs/node-gyp/blob/master/addon.gypi
### Autogenerated files
Files you will notice are created when you build from source by running `make`:
- `build/` - a directory created by [node-gyp](#node-gyp) to hold a variety of autogenerated Makefiles, gyp files, and binary outputs.
- `build/Release/` - directory created to hold binary files for a `Release` build. A `Release` build is the default build when you run `make`. For more info on release builds see [this definition](https://github.com/mapbox/cpp/blob/master/glossary.md#release-build)
- `build/Release/module.node` - The C++ binary module, for a `Release build, in the form of a [loadable module](https://github.com/mapbox/cpp/blob/master/glossary.md#loadable-module). This file was ultimately created by the [linker](#linker) and ended up at this path thanks to [node-gyp](#node-gyp).
- `lib/binding/module.node` - the final resting place for the C++ binary module. Copied to this location from either `build/Release/module.node` or `build/Debug/module.node`. This location is configured via the `module_path` variable in the [package.json](../package.json) and is used by [node-pre-gyp](#node-pre-gyp) when assembling a package to publish remotely (to allow users to install via binaries).
- `build/Release/obj.target/module/src/module.o`: the [object file](https://github.com/mapbox/cpp/blob/master/glossary.md#object-file) that corresponds to the [`src/module.cpp`](../src/module.cpp).
- `build/Release/obj.target/module/standalone/hello.o`: the [object file](https://github.com/mapbox/cpp/blob/master/glossary.md#object-file) that corresponds to the [`src/standalone/hello.cpp`](../src/standalone/hello.cpp).
- `build/Debug/` - directory created to hold binary files for a `Debug` build. A `Debug` build is trigged when you run `make debug`. For more info on debug builds see [this definition](https://github.com/mapbox/cpp/blob/master/glossary.md#debug-build)
- `build/Debug/module.node` - The C++ binary module, for a `Debug build, in the form of a [loadable module](https://github.com/mapbox/cpp/blob/master/glossary.md#loadable-module). This file was ultimately created by the [linker](#linker) and ended up at this path thanks to [node-gyp](#node-gyp).
### Overall flow
The overall flow in terms of software components is:
```
make -> node-pre-gyp -> node-gyp -> gyp -> make -> compiler/linker
```
The overall flow, including operations, is:
```
Developer (you) runs 'make'
-> make reads Makefile
-> Makefile has custom line that calls out to 'node-pre-gyp configure build'
-> node-pre-gyp passes variables in the package.json along to 'node-gyp rebuild'
-> node-gyp finds the `binding.gyp` and passes it to gyp along with other .gyp includes (addon.gypi and a common.gypi from node core)
-> gyp loads the `binding.gyp` and the `common.gypi` and generates Makefiles inside `build/` directory
-> node-gyp runs make in the `build/` directory
-> make runs all the targets in the makefiles generated by gyp in the `build/` directory
-> these makefiles run the `install_deps.sh` and invoke the compiler and linker for all sources to compile
-> `install_deps.sh` puts mason packages in `/mason_packages` directory
-> compiler outputs object files in `build/`
-> linker outputs the loadable module in `build/`
-> make copies the 'module.node` from `build/Release` to `lib/binding`
```
Then the module is ready to use. What happens when it is used is:
```
User of your module runs 'npm install'
-> npm fetches your module
-> npm notices an 'install' target that calls out to node-pre-gyp
-> node-pre-gyp downloads the C++ binary from remote url (as specified in the node-pre-gyp config in the package.json)
-> node-pre-gyp places the C++ binary at the ['module_path']('../lib/binding/module.node')
-> the index.js reads '../lib/binding/module.node'
```
This binary file `../lib/binding/module.node` is what `require()` points to within Node.js.
# Clang Tools
This skeleton uses two clang/llvm tools for automated formatting and static fixes.
Each of these tools run within their own [Travis jobs](https://github.com/mapbox/node-cpp-skel/blob/master/.travis.yml). You can disable these Travis jobs if you'd like, by commenting them out in `.travis.yml`. This may be necessary if you're porting this skel into an already-existing project that triggers tons of clang-tidy errors, and you'd like to work through them gradually while still actively iterating on other parts of your code.
### [clang-format](https://clang.llvm.org/docs/ClangFormat.html)
Automates coding style enforcement, for example whitespace, tabbing, wrapping.
To run
```
make format
```
- Set format preferences in [`.clang-format`](https://github.com/mapbox/node-cpp-skel/blob/master/.clang-format)
- Installed and run via [`/scripts/clang-format.sh`](https://github.com/mapbox/node-cpp-skel/blob/master/scripts/clang-format.sh)
### [clang-tidy](https://clang.llvm.org/extra/clang-tidy/)
Lint framework that can do very powerful checks, for example bugs that can be deduced via [static analysis](https://github.com/mapbox/cpp/blob/master/glossary.md#static-analysis), unneeded copies, inefficient for-loops, and improved readability. In many cases, it can fix and modernize your code.
To run
```
make tidy
```
- The clang-tidy tool has a large set of checks and when one fails, the error message contains the shorthand for the check name. See llvm's docs for details about what failed check is referring to. For example, see ["performance-unnecessary-copy-initialization"](https://clang.llvm.org/extra/clang-tidy/checks/performance-unnecessary-copy-initialization.html).
- Set tidy preferences in [`.clang-tidy`](https://github.com/mapbox/node-cpp-skel/blob/master/.clang-tidy). When you run clang-tidy, you'll see output of a number of specific checks that tidy runs. You can add/remove any of these checks via the `.clang-tidy` file, configure these checks, and specify the files you'd like to run these checks against. The skel runs clang-tidy against everything within the `/src` directory.
- This skel runs clang-tidy with the `-fix` option, which will automatically apply fixes to your code. Though some warnings will need to be fixed manually.
- Installed and run via [`/scripts/clang-tidy.sh`](https://github.com/mapbox/node-cpp-skel/blob/master/scripts/clang-tidy.sh)
- `NOLINT`: In the case that clang-tidy throws for code that you either want to keep as-is or can't control, you can [use `NOLINT`](https://github.com/mapbox/node-cpp-skel/blob/87c8d51f2d7d05ac44f3ea7a607f60e73a2af9c8/src/module.cpp#L43-L46) to silent clang-tidy.
- Since clang-tidy must compile the code to do its checks, skel [generates](https://github.com/mapbox/node-cpp-skel/blob/master/scripts/generate_compile_commands.py) a JSON file that tells clang-tidy which files to run against and what compile command to run. This newly generated file, `/build/compile_command.json`, is created when running `make tidy`. See [clang's JSON compilation format docs](https://clang.llvm.org/docs/JSONCompilationDatabase.html) for more info.
# Xcode

If you're developing on macOS and have Xcode installed, you can also type `make xcode` to generate and open an Xcode project. In the dropdown, choose `npm test` to run the npm tests. You can also add more targets by adding the appropriate lines in `Makefile`, and rerunning `make xcode`. If you are modifying `binding.gyp`, e.g. by adding more source files, make sure to rerun `make xcode` so that Xcode knows about the new source files.
| {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
# Workflow
So your code is compiling, tested, benched, and ready to be shared. How to get it into the wild? This document will go through what's next.
Generally speaking, the workflow for a node-addon isn't much different than publishing a regular Javascript node module. The main difference is an added step and a bit more configuration in order to handle the binary. If the concept of a binary file is new, give [this doc a gander](https://github.com/mapbox/node-cpp-skel/blob/dbc48924b3e30bba903e6b9220b0cdf2854f717f/docs/extended-tour.md#builds).
The typical workflow for a regular node module may look something like this:
1. merge to master
2. git tag
3. npm publish (Now it's ready to be npm installed)
The workflow for a node add-on looks very similar:
1. merge to master
2. git tag
3. publish binaries
4. npm publish
Let's talk generally about the relationship between the third and fourth steps. Since your code is in C++, any projects that `npm install` your module as a dependency will need the C++ code precompiled so that Node can use your module in Javascript world. Before publishing your module to npm, you will publish your binaries by putting them on s3 (**Note**: you will likely publish multiple binaries, one for each Node version and various operating systems). This s3 location is reflected in your module's [package.json file](https://github.com/mapbox/node-cpp-skel/blob/dbc48924b3e30bba903e6b9220b0cdf2854f717f/package.json#L35). Your package.json file is also redefining [the install command](https://github.com/mapbox/node-cpp-skel/blob/dbc48924b3e30bba903e6b9220b0cdf2854f717f/package.json#L14), by running node-pre-gyp instead.
[Node-pre-gyp](https://github.com/mapbox/node-pre-gyp) is responsible for installing the binary by pulling the relevant binary from s3 and placing it in the specified and expected location defined by your module's [main index.js file](https://github.com/mapbox/node-cpp-skel/blob/dbc48924b3e30bba903e6b9220b0cdf2854f717f/lib/index.js#L3). So when a project runs `require()` on your module, they are directly accessing the binary. In a bit more detail, node-pre-gyp will detect [what version of Node is being used and which operating system](https://github.com/mapbox/node-cpp-skel/blob/dbc48924b3e30bba903e6b9220b0cdf2854f717f/package.json#L37), then go to s3 to retrieve the binary that matches.
Continue reading below to learn how to publish your binaries to s3 so they're ready to be installed.
# Publishing Binaries
It's a good idea to publish pre-built binaries of your module if you want others to be able to easily install it on their system without needing to install a compiler like g++ or clang++. Node-pre-gyp does a lot of the heavy lifting for us (like detecting which system you are building on and deploying to s3) but you'll need a few things configured to get started.
#### 1) In the `package.json`, update the `"binary"` field to the appropriate s3 bucket `host`.
For Mapbox staff we recommend using a host setting of `"host": "https://mapbox-node-binary.s3.amazonaws.com",` which will publish to `s3://mapbox-node-binary/<your module name>`.
Note: for namespaced modules the path will end up being `s3://mapbox-node-binary/@org/<your module name>`.
#### 2) Copy the ci.template.js
Copy the `ci.template.js` from this repo into your repo and place it at `./cloudformation/ci.template.js`
#### 3) Install deps for validating and managing cloudformation templates
```bash
npm install -g @mapbox/cfn-config # deploying stacks
npm install -g @mapbox/cloudfriend # validating and building templates
```
#### 4) Create a user with permissions to upload to `s3://<bucket name>/<your module name>/`
First configure your AWS creds. You will need to set at least the `AWS_ACCESS_KEY_ID` and `AWS_SECRET_ACCESS_KEY` keys. And also `AWS_SESSION_TOKEN` if you are using 2-factor auth.
Then run:
```bash
validate-template cloudformation/ci.template.js
build-template cloudformation/ci.template.js > cloudformation/ci.template
```
Next we will actually create the user. But first let's discuss what happens here. In addition to creating the user we also write details about the user to a separate bucket (for east auditing purposes). In the below command we:
- Create a `ci-binary-publish` user
- Using the `cloudformation/ci.template`
- And ask to save our stack configuration to the `cfn-config` bucket. If you are outside of Mapbox, see [you will need to pass a different bucket and also a `--template-bucket` option](https://github.com/mapbox/cfn-config#prerequisites)
Now, run the command to create the user:
```
cfn-config create ci-binary-publish cloudformation/ci.template -c cfn-configs
```
It will prompt you, choose:
- New configuration
- Ready to create the stack? Y
It will fail if the stack already exists. In this case you can recreate a new user by deleting the stack by running `./node_modules/.bin/cfn-config delete ci-binary-publish cloudformation/ci.template` and then creating a new one.
#### 5) Get the user keys
After the create step succeeds you will have a new user. You now need to get get the users `AccessKeyId` and `SecretAccessKey`.
You can do this in two ways: 1) finding the keys through the AWS console, or 2) using cfn-config to show the stack information
**Tokens via cfn-config**
Run the command `cfn-config info ci-binary-publish` and you'll see a JSON output with `AccessKeyId` and `SecretAccessKey`.
**Tokens via the AWS console**
- Go to https://console.aws.amazon.com/cloudformation/home?region=us-east-1#/stacks?filter=active&tab=outputs
- Search for `ci-binary-publish`. You should see a stack called `<your module name>-ci-binary-publish`
- Click the checkbox beside your `<your module name>-ci-binary-publish` stack
- Click the `Output` tab to access the `AccessKeyId` and `SecretAccessKey` for this new user.
#### 6) Add the keys to the travis
**Adding to travis UI settings**
- Go to https://travis-ci.org/<your user or org>/<your module>/settings
- Scroll to the bottom and find the `Environment Variables` section
- Add a variable called `AWS_ACCESS_KEY_ID` and put the value of the `AccessKeyId` in it
- CRITICAL: Choose `OFF` for `Display value in build log` to ensure the variables are not shown in the logs
- Click `Add`
- Add a variable called `AWS_SECRET_ACCESS_KEY` and put the value of the `SecretAccessKey` in it
- CRITICAL: Choose `OFF` for `Display value in build log` to ensure the variables are not shown in the logs
- Click `Add`
#### 7) All done!
Now that you have generated keys for a user that can publish to s3 and provided these keys to travis in a secure way, you should be able to publish binaries. But this should be done in an automated way. See the next section below for how to do that with travis.ci.
**Publishing on Travis CI**
This project includes a `script/publish.sh` command that builds binaries and publishes them to s3. This script checks your commit message for either `[publish binary]` or `[republish binary]` in order to begin publishing. This allows you to publish binaries according to the version specified in your `package.json` like this:
```
git commit -m 'releasing 0.1.0 [publish binary]'
```
Republishing a binary overrides the current version and must be specified with `[republish binary]`.
**Adding new operating systems and node versions**
The `.travis.yml` file uses the `matrix` to set up each individual job, which specifies the operating system, node version, and other environment variables for running the scripts. To add more operating systems and node versions to the binaries you publish, add another job to the matrix like this:
```yaml
- os: {operating system}
env: NODE="{your node version}" TARGET="Release"
install: *setup
script: *test
after_script: *publish
```
### Dev releases
You may want to test your module works correctly, in downstream dependencies, before formally publishing. To do this we recommend you:
1. Create a branch of your node c++ module
2. Modify the `version` string in your `package.json` like:
```diff
diff --git a/package.json b/package.json
index e00b7b5..22f7cd9 100644
--- a/package.json
+++ b/package.json
@@ -1,6 +1,6 @@
{
"name": "@mapbox/node-cpp-skel",
- "version": "0.1.0",
+ "version": "0.1.0-alpha",
"description": "Skeleton for bindings to C++ libraries for Node.js using NAN",
"url": "http://github.com/mapbox/node-cpp-skel",
"main": "./lib/index.js",
```
3. Publishing C++ binaries by pushing a commit with `[publish binary]` per https://github.com/mapbox/node-cpp-skel/blob/master/docs/publishing-binaries.md#7-all-done
4. **Option A)** Require your module in downstream applications like:
```js
"your-module": "https://github.com/<your-org>/<your-module>/tarball/<your-branch>",
```
If you're publishing from a private repo, generate a dev release and then reference the url in the appropriate `package.json` file. For example, `zip` the repo, put to S3, and then reference the S3 url in `package.json`.
**Option B)** Issue a npm dev release after the binary is published.
Run `npm publish --tag dev` and then require your module in downstream applications like:
```js
"your-module": "0.1.0-alpha",
```
For npm dev releases, it’s good to use the `--tag <something>` to avoid publishing to the latest tag. If you run `npm publish` then `0.1.0-alpha` is what anyone running `npm install <your module> --save` will receive.
#### Before `npm publish`
Before publishing to npm, you can ensure the final packaged tarball will include what you expect. For instance, you want to avoid a large accidental file being packaged by npm and make sure the package contains all needed dependencies.
Take a peek at what npm will publish by running:
```
make
make testpack
```
This will create a tarball locally and print every file included. A couple basic checks:
- make sure `.mason` is not included
- make sure `node-pre-gyp` directory is included, because it is responsible for knowing where to grab the binary from s3.
**Hot tips** :hot_pepper:
- Node and npm versions can have differing `npm pack` and `npm publish` behaviour, so be mindful of what your environment is using
- You can use the resulting tarball locally and install it within another local repo to make sure it works:
```
npm install <path to tarball>
```
| {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
# Benchmarking
This project includes [bench tests](https://github.com/mapbox/node-cpp-skel/tree/master/bench) you can use to experiment with and measure performance. These bench tests hit the functions that [simulate expensive work being done in the threadpool](https://github.com/mapbox/node-cpp-skel/blob/master/src/object_async/hello_async.cpp#L121-L122). This is intended to model realworld use cases where you, as the developer, have expensive computation you'd like to dispatch to worker threads. Adapt these tests to your custom code and monitor the performance of your code.
We've included two bench tests for the async examples, demonstrating the affects of concurrency and threads within a process. For example, you can run:
```
node bench/hello_async.bench.js --iterations 50 --concurrency 10 --mem
```
This will run a bunch of calls to the module's `helloAsync()` function. You can control two things:
- iterations: number of times to call `helloAsync()`
- concurrency: max number of threads the test can utilize, by setting `UV_THREADPOOL_SIZE`. When running the bench script, you can see this number of threads reflected in your [Activity Monitor](https://github.com/springmeyer/profiling-guide#activity-monitorapp-on-os-x)/[htop window](https://hisham.hm/htop/).
- `--mem`: Optional arg to show memory stats per [`process.memoryUsage()`](https://nodejs.org/api/process.html#process_process_memoryusage)
### Ideal Benchmarks
**Ideally, you want your workers to run your code ~99% of the time and never idle.** This reflects a healthy node c++ addon and what you would expect to see when you've picked a good problem to solve with node.
The bench tests and async functions that come with `node-cpp-skel` out of the box demonstrate this behaviour:
- An async function that is CPU intensive and takes a while to finish (expensive creation and querying of a `std::map` and string comparisons).
- Worker threads are busy doing a lot of work, and the main loop is relatively idle. Depending on how many threads (concurrency) you enable, you may see your CPU% sky-rocket and your cores max out. Yeaahhh!!!
- If you bump up `--iterations` to 500 and [profile in Activity Monitor.app](https://github.com/springmeyer/profiling-guide#activity-monitorapp-on-os-x), you'll see the main loop is idle as expected since the threads are doing all the work. You'll also see the threads busy doing work in AsyncHelloWorker roughly 99% of the time :tada:
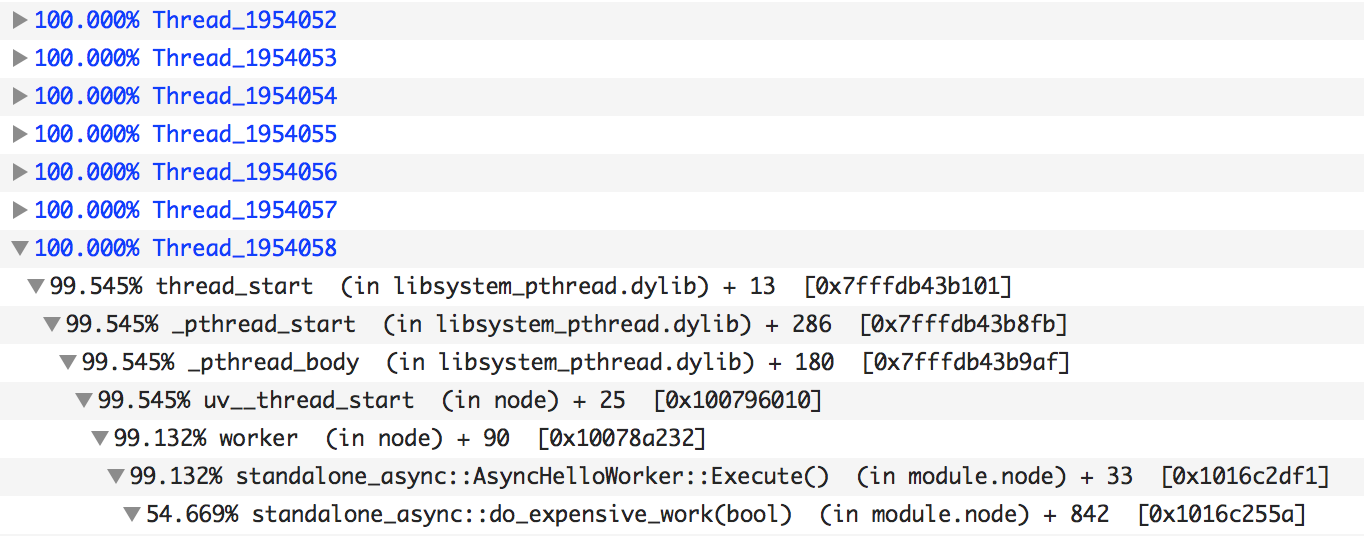 | {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
## Using npm, package.json, and package-lock.json
With updated versions of npm, there is now such thing as a `package-lock.json` file, which is now included in node-cpp-skel. Read this doc for more info, also discussion at https://github.com/mapbox/node-cpp-skel/issues/124.
`package.json` defines the dependencies required by any given library. It often defines a range of acceptable version of those dependencies, for example:
```
"dependencies": {
"eslint": "^4.3.0"
}
```
... means that any version of eslint >4.3.0 and <5 is acceptable.
`package-lock.json` defines _explicit versions of dependencies that should be used_. This is useful, because by using `package-lock.json` you can be confident that the version of your dependencies deployed in production matches exactly with the versions you install in your local testing environment.
Running `npm install` in a folder that contains a `package.json` file will create a `package-lock.json` file if one does not exist. **You should commit this file**.
If a `package-lock.json` file exists in the repository, but some of the versions of dependencies are out-of-sync with what's specified in `package.json` then running `npm install` will update the `package-lock.json` file. This may happen if you manually edited a version of a dependency in `package.json`, which you should not do (see below).
If you are creating a PR, you should notice if that PR contains changes to `package-lock.json`, and you should be able to explain in the PR why those changes are being made.
To take full advantage of the explicit dependency versions specified in `package-lock.json`, don't use `npm install`. Instead, run
```
npm ci
```
Note: this `ci` requires at least npm >= v5.7.1 (https://blog.npmjs.org/post/171556855892/introducing-npm-ci-for-faster-more-reliable).
This will remove your existing `node_modules` folder, and then install the exact versions defined by `package-lock.json`. It will **never** make changes to `package.json` or `package-lock.json`, unlike `npm install`.
### Avoid editing package.json directly
If you need to change the range of acceptable versions for a dependency:
```
npm install --save eslint@^4.4.8
```
If you want to upgrade a dependency to the most recent version specified by the existing range in `package.json`;
```
npm update eslint
```
If you want to remove a dependency:
```
npm remove eslint
```
| {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
# How to debug C++ with VS Code
VS Code is a great source code editor for developing code in many languages. This document is to tell you how to debug a node C++ addon with VS Code.
### 1. Setting up VS Code debug
First open the node C++ addon folder in VS Code by choosing `File > Open`.
Then to start debugging there are two ways:
#### Option 1
Press `Cmd + Shift + P` to open the command bar, type open `open launch.json`, and then choose `C++`.
Note: the first time you do this you will need to `install` the C++ Extension and reload the app.
#### Option 2
Click the debug button on the right sidebar, click the `⚙` button on the right top, and then choose `C++`.

In the `launch.json` you can see some template, like this:
```json
{
"version": "0.2.0",
"configurations": [
{
"name": "(lldb) Launch",
"type": "cppdbg",
"request": "launch",
"program": "enter program name, for example ${workspaceFolder}/a.out",
"args": [],
"stopAtEntry": false,
"cwd": "${workspaceFolder}",
"environment": [],
"externalConsole": true,
"MIMode": "lldb"
}
]
}
```
`launch.json` defines which program you want to run after you click the run debug button. Let's say we want run `node test/vtshaver.test.js`. In this case we need to change the configurations, put `program` to node's absolute path (you can use `which node` to find the path of current version of node), and change the `args` to `${workspaceFolder}/test/vtshaver.test.js`.
Additional we want build C++ everytime before we start debug, so we add `preLaunchTask` into the config:
```
"preLaunchTask": "npm: build:dev",
```
Now the config file could look like this:
```json
{
"version": "0.2.0",
"configurations": [
{
"name": "(lldb) Launch",
"type": "cppdbg",
"request": "launch",
"preLaunchTask": "npm: build:dev",
"program": "/Users/mapbox-mofei/.nvm/versions/node/v8.11.3/bin/node",
"args": ["${workspaceFolder}/test/vtshaver.test.js"],
"stopAtEntry": false,
"cwd": "${workspaceFolder}",
"environment": [],
"externalConsole": true,
"MIMode": "lldb"
}
]
}
```
Now you can open any C++ files and click the left side of the line number to add a breakpoint, then go to the debug button on the sidebar, click the run button on the top.

Now everything is done! Debug is Done! The program will stop at the breakpoint, you can use your mouse to explore the variable. If you want your program to continue past the breakpoint, you can navigate the program using the top control box.

| {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
#pragma once
#include <chrono>
#include <string>
#include <thread>
#include <vector>
namespace detail {
// simulate CPU intensive task
inline std::unique_ptr<std::vector<char>> do_expensive_work(std::string const& name, bool louder)
{
std::this_thread::sleep_for(std::chrono::milliseconds(100));
std::string str = "...threads are busy async bees...hello " + name;
std::unique_ptr<std::vector<char>> result = std::make_unique<std::vector<char>>(str.begin(), str.end());
if (louder)
{
result->push_back('!');
result->push_back('!');
result->push_back('!');
result->push_back('!');
}
return result;
}
} // namespace detail
| {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
#pragma once
#include <memory>
#include <napi.h>
#include <string>
namespace utils {
/*
* This is an internal function used to return callback error messages instead of
* throwing errors.
* Usage:
*
* return CallbackError(env, "error message", callback);
*
*/
inline Napi::Value CallbackError(Napi::Env env, std::string const& message, Napi::Function const& func)
{
Napi::Object obj = Napi::Object::New(env);
obj.Set("message", message);
return func.Call({obj});
}
} // namespace utils
namespace gsl {
template <typename T>
using owner = T;
} // namespace gsl
// ^^^ type alias required for clang-tidy (cppcoreguidelines-owning-memory)
| {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
#include "object_async/hello_async.hpp"
#include "object_sync/hello.hpp"
#include "standalone/hello.hpp"
#include "standalone_async/hello_async.hpp"
#include "standalone_promise/hello_promise.hpp"
#include <napi.h>
// #include "your_code.hpp"
Napi::Object init(Napi::Env env, Napi::Object exports)
{
// expose hello method
exports.Set(Napi::String::New(env, "hello"), Napi::Function::New(env, standalone::hello));
// expose helloAsync method
exports.Set(Napi::String::New(env, "helloAsync"), Napi::Function::New(env, standalone_async::helloAsync));
// expose helloPromise method
exports.Set(Napi::String::New(env, "helloPromise"), Napi::Function::New(env, standalone_promise::helloPromise));
// expose HelloObject class
object_sync::HelloObject::Init(env, exports);
// expose HelloObjectAsync class
object_async::HelloObjectAsync::Init(env, exports);
return exports;
/**
* You may have noticed there are multiple "hello" functions as part of this
* module.
* They are exposed a bit differently.
* 1) standalone::hello // exposed above
* 2) HelloObject.hello // exposed in object_sync/hello.cpp as part of
* HelloObject
*/
// add more methods/classes below that youd like to use in Javascript world
// then create a directory in /src with a .cpp and a .hpp file.
// Include your .hpp file at the top of this file.
}
// Initialize the module (we only do this once)
// Mark this NOLINT to avoid the clang-tidy checks
// NODE_GYP_MODULE_NAME is the name of our module as defined in 'target_name'
// variable in the 'binding.gyp', which is passed along as a compiler define
NODE_API_MODULE(NODE_GYP_MODULE_NAME, init) // NOLINT
| {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
#include "hello_async.hpp"
#include "../cpu_intensive_task.hpp"
#include "../module_utils.hpp"
#include <exception>
#include <iostream>
#include <map>
#include <memory>
#include <stdexcept>
namespace standalone_async {
// This is the worker running asynchronously and calling a user-provided
// callback when done.
// Consider storing all C++ objects you need by value or by shared_ptr to keep
// them alive until done.
// Napi::AsyncWorker docs:
// https://github.com/nodejs/node-addon-api/blob/master/doc/async_worker.md
struct AsyncHelloWorker : Napi::AsyncWorker
{
using Base = Napi::AsyncWorker;
// ctor
AsyncHelloWorker(bool louder, bool buffer, Napi::Function const& cb)
: Base(cb),
louder_(louder),
buffer_(buffer) {}
// The Execute() function is getting called when the worker starts to run.
// - You only have access to member variables stored in this worker.
// - You do not have access to Javascript v8 objects here.
void Execute() override
{
// The try/catch is critical here: if code was added that could throw an
// unhandled error INSIDE the threadpool, it would be disasterous
try
{
result_ = detail::do_expensive_work("world", louder_);
}
catch (std::exception const& e)
{
SetError(e.what());
}
}
// The OnOK() is getting called when Execute() successfully
// completed.
// - In case Execute() invoked SetErrorMessage("") this function is not
// getting called.
// - You have access to Javascript v8 objects again
// - You have to translate from C++ member variables to Javascript v8 objects
// - Finally, you call the user's callback with your results
void OnOK() final
{
if (!Callback().IsEmpty() && result_)
{
if (buffer_)
{
char* data = result_->data();
std::size_t size = result_->size();
auto buffer = Napi::Buffer<char>::New(
Env(),
data,
size,
[](Napi::Env /*unused*/, char* /*unused*/, gsl::owner<std::vector<char>*> v) {
delete v;
},
result_.release());
Callback().Call({Env().Null(), buffer});
}
else
{
Callback().Call({Env().Null(), Napi::String::New(Env(), result_->data(), result_->size())});
}
}
}
std::unique_ptr<std::vector<char>> result_ = nullptr;
const bool louder_;
const bool buffer_;
};
// helloAsync is a "standalone function" because it's not a class.
// If this function was not defined within a namespace ("standalone_async"
// specified above), it would be in the global scope.
Napi::Value helloAsync(Napi::CallbackInfo const& info)
{
bool louder = false;
bool buffer = false;
Napi::Env env = info.Env();
// Check second argument, should be a 'callback' function.
if (!info[1].IsFunction())
{
Napi::TypeError::New(env, "second arg 'callback' must be a function").ThrowAsJavaScriptException();
return env.Null();
}
Napi::Function callback = info[1].As<Napi::Function>();
// Check first argument, should be an 'options' object
if (!info[0].IsObject())
{
return utils::CallbackError(env, "first arg 'options' must be an object", callback);
}
Napi::Object options = info[0].As<Napi::Object>();
// Check options object for the "louder" property, which should be a boolean
// value
if (options.Has(Napi::String::New(env, "louder")))
{
Napi::Value louder_val = options.Get(Napi::String::New(env, "louder"));
if (!louder_val.IsBoolean())
{
return utils::CallbackError(env, "option 'louder' must be a boolean", callback);
}
louder = louder_val.As<Napi::Boolean>().Value();
}
// Check options object for the "buffer" property, which should be a boolean value
if (options.Has(Napi::String::New(env, "buffer")))
{
Napi::Value buffer_val = options.Get(Napi::String::New(env, "buffer"));
if (!buffer_val.IsBoolean())
{
return utils::CallbackError(env, "option 'buffer' must be a boolean", callback);
}
buffer = buffer_val.As<Napi::Boolean>().Value();
}
// Creates a worker instance and queues it to run asynchronously, invoking the
// callback when done.
// - Napi::AsyncWorker takes a pointer to a Napi::FunctionReference and deletes the
// pointer automatically.
// - Napi::AsyncQueueWorker takes a pointer to a Napi::AsyncWorker and deletes
// the pointer automatically.
auto* worker = new AsyncHelloWorker{louder, buffer, callback}; // NOLINT
worker->Queue();
return env.Undefined(); // NOLINT
}
} // namespace standalone_async
| {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
#pragma once
#include <napi.h>
namespace standalone_async {
// hello, custom sync method
// method's logic lives in hello.cpp
Napi::Value helloAsync(Napi::CallbackInfo const& info);
} // namespace standalone_async
| {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |
#pragma once
#include <napi.h>
namespace standalone {
// hello, custom sync method
Napi::Value hello(Napi::CallbackInfo const& info);
} // namespace standalone
| {
"repo_name": "mapbox/node-cpp-skel",
"stars": "70",
"repo_language": "C++",
"file_name": "ci.template.js",
"mime_type": "text/plain"
} |