text
stringlengths 2
104M
| meta
dict |
---|---|
# Node.js - Aula 06 - Exercício
**user:** [carloshenriqueribeiro](https://github.com/carloshenriqueribeiro)
**autor:** Carlos Henrique Ribeiro
## Crie um Schema com cada tipo explicado, inserindo tanto um objeto correto, como um objeto que desencadeie erros de validação padrão, criar especificamente:
* 1.1. para String: `enum`, `match`, `maxlength` e `minlength`
* 1.2. para Number: `max` e `min`
**Schema Correto**
```js
'use strict';
const _schema = {
validate_match: { type: String, match: /^a/i }
, validate_enum: { type: String, enum: ['oi', 'tudo', 'bem', 'sim', 'nao'] }
, validate_maxlength: { type: String, maxlength: 15 }
, validate_minlength: { type: String, minlength: 5 }
, validate_max: { type: Number, max: 5 }
, validate_min: { type: Number, min: 3 }
}
, testSchema = new Schema(_schema)
, TestModel = mongoose.model('testValidate', testSchema)
;
let dataModel = {
validate_match: 'Aloca'
, validate_enum: 'bem'
, validate_maxlength: 'eh'
, validate_minlength: 'nois que voa bruxao'
, validate_max: 3
, validate_min: 10
}
, testing = new TestModel(dataModel)
;
testing.save(function(err, data){
if(err) return console.log('Error: ', err);
console.log('Passou nas validações! ', data);
});
// Output do terminal
Passou nas validações! { _id: 56ba956cc043d716eee48366,
validate_min: 10,
validate_max: 3,
validate_minlength: 'nois que voa bruxao',
validate_maxlength: 'eh',
validate_enum: 'bem',
validate_match: 'Aloca',
__v: 0 }
```
**Schema Incorreto**
```js
{ [ValidationError: testValidate validation failed]
message: 'testValidate validation failed',
name: 'ValidationError',
errors:
{ validate_min:
{ [ValidatorError: Path `validate_min` (1) is less than minimum allowed value (3).]
properties: [Object],
message: 'Path `validate_min` (1) is less than minimum allowed value (3).',
name: 'ValidatorError',
kind: 'min',
path: 'validate_min',
value: 1 },
validate_max:
{ [ValidatorError: Path `validate_max` (10) is more than maximum allowed value (5).]
properties: [Object],
message: 'Path `validate_max` (10) is more than maximum allowed value (5).',
name: 'ValidatorError',
kind: 'max',
path: 'validate_max',
value: 10 },
validate_minlength:
{ [ValidatorError: Path `validate_minlength` (`a`) is shorter than the minimum allowed length (2).]
properties: [Object],
message: 'Path `validate_minlength` (`a`) is shorter than the minimum allowed length (2).',
name: 'ValidatorError',
kind: 'minlength',
path: 'validate_minlength',
value: 'a' },
validate_maxlength:
{ [ValidatorError: Path `validate_maxlength` (`eh nois que voa`) is longer than the maximum allowed length (2).]
properties: [Object],
message: 'Path `validate_maxlength` (`eh nois que voa`) is longer than the maximum allowed length (2).',
name: 'ValidatorError',
kind: 'maxlength',
path: 'validate_maxlength',
value: 'eh nois que voa' },
validate_enum:
{ [ValidatorError: `incorreto` is not a valid enum value for path `validate_enum`.]
properties: [Object],
message: '`incorreto` is not a valid enum value for path `validate_enum`.',
name: 'ValidatorError',
kind: 'enum',
path: 'validate_enum',
value: 'incorreto' },
validate_match:
{ [ValidatorError: Path `validate_match` is invalid (bbbb).]
properties: [Object],
message: 'Path `validate_match` is invalid (bbbb).',
name: 'ValidatorError',
kind: 'regexp',
path: 'validate_match',
value: 'bbbb' } } }
```
## Cadastre 3 pokemons **de uma só vez**:
```js
const _schema = {
name: String
}
, testSchema = new Schema(_schema)
, TestModel = mongoose.model('testInsercao', testSchema)
;
let dataModel = [
{
name: 'Poke-01'
},
{
name: 'Poke-02'
},
{
name: 'Poke-03'
}
];
TestModel.create(dataModel, function(err, data){
if(err) return console.log('Error: ', err);
console.log('Inseriu todos pokemons! :D ', data);
});
// Output do terminal \\
Inseriu todos pokemons! :D [ { _id: 56bb44b415f642bdf1182962, name: 'Poke-01', __v: 0 },
{ _id: 56bb44b415f642bdf1182963, name: 'Poke-02', __v: 0 },
{ _id: 56bb44b415f642bdf1182964, name: 'Poke-03', __v: 0 } ]
```
## Busque **todos** os Pokemons com `attack > 50` e `height > 0.5`:
```js
const _schema = {
name: String
}
, pokemonSchema = new Schema(_schema)
, PokemonModel = mongoose.model('Pokemon', pokemonSchema)
;
function callback(err, data){
if(err) return console.log('Error: ', err);
console.log('Resultado: ', data);
}
PokemonModel.find({}).where({ $and:[ { attack: { $gt: 50 }}, { height: { $gt: 0.5}} ]}).exec(callback);
// Output do terminal \\
Resultado: [ { moves: [ 'Brasas', 'Encarar' ],
height: 0.6,
attack: 52,
type: 'fire',
description: 'Esse é o cão chupando manga de fofinho',
name: 'Charmander',
_id: 566a25407d0b654cdf58b763 } ]
```
## Altere, **inserindo**, o Pokemon `Nerdmon` com `attack` igual a 49 e com os valores dos outros campos a sua escolha.
```js
const _schema = {
name: String
, attack: Number
, defense: Number
, attacks: Array
}
, pokemonSchema = new Schema(_schema)
, PokemonModel = mongoose.model('Pokemon', pokemonSchema)
;
let query = {
name: /nerdmon/i
}
, mod = {
$setOnInsert: {
name: 'Nerdemon'
, attack: 49
, defense: 444
, attacks: ['Raio Virginador', 'Friend Zone']
}
}
, options = {
upsert: true
}
;
function callback(err, data){
if(err) return console.log('Error: ', err);
console.log('Resultado: ', data);
}
PokemonModel.update(query, mod, options, callback);
// Output do terminal \\
Resultado: { ok: 1,
nModified: 0,
n: 1,
upserted: [ { index: 0, _id: 56bb52ca2df027a2c53e7b8e } ] }
{
"_id": ObjectId("56bb52ca2df027a2c53e7b8e"),
"name": "Nerdemon",
"attack": 49,
"defense": 444,
"attacks": [
"Raio Virginador",
"Friend Zone"
]
}
```
## Remova **todos** os Pokemons com `attack` **acima de 50**.
```js
const _schema = {
attack: Number
}
, pokemonSchema = new Schema(_schema)
, PokemonModel = mongoose.model('Pokemon', pokemonSchema)
;
let query = {
attack: { $gt: 50 }
};
function callback(err, data){
if(err) return console.log('Error: ', err);
console.log('Removeu: ', data);
}
PokemonModel.remove(query, callback);
```
**Resposta do terminal**
```js
Removeu: { result: { ok: 1, n: 2 },
connection:
EventEmitter {
domain: null,
_events:
{ close: [Object],
error: [Object],
timeout: [Object],
parseError: [Object],
connect: [Function] },
_eventsCount: 5,
_maxListeners: undefined,
options:
{ socketOptions: {},
auto_reconnect: true,
host: 'localhost',
port: 27017,
cursorFactory: [Object],
reconnect: true,
emitError: true,
size: 5,
disconnectHandler: [Object],
bson: {},
messageHandler: [Function],
wireProtocolHandler: [Object] },
id: 2,
logger: { className: 'Connection' },
bson: {},
tag: undefined,
messageHandler: [Function],
maxBsonMessageSize: 67108864,
port: 27017,
host: 'localhost',
keepAlive: true,
keepAliveInitialDelay: 0,
noDelay: true,
connectionTimeout: 0,
socketTimeout: 0,
destroyed: false,
domainSocket: false,
singleBufferSerializtion: true,
serializationFunction: 'toBinUnified',
ca: null,
cert: null,
key: null,
passphrase: null,
ssl: false,
rejectUnauthorized: false,
checkServerIdentity: true,
responseOptions: { promoteLongs: true },
flushing: false,
queue: [],
connection:
Socket {
_connecting: false,
_hadError: false,
_handle: [Object],
_parent: null,
_host: 'localhost',
_readableState: [Object],
readable: true,
domain: null,
_events: [Object],
_eventsCount: 8,
_maxListeners: undefined,
_writableState: [Object],
writable: true,
allowHalfOpen: false,
destroyed: false,
bytesRead: 56,
_bytesDispatched: 175,
_sockname: null,
_pendingData: null,
_pendingEncoding: '',
_idleNext: null,
_idlePrev: null,
_idleTimeout: -1,
read: [Function],
_consuming: true },
writeStream: null,
buffer: null,
sizeOfMessage: 0,
bytesRead: 0,
stubBuffer: null } }
```
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 06 - Exercício
**user:** [DouglasHennrich](https://github.com/DouglasHennrich)
**autor:** Douglas Hennrich
## Crie um Schema com cada tipo explicado, inserindo tanto um objeto correto, como um objeto que desencadeie erros de validação padrão, criar especificamente:
* 1.1. para String: `enum`, `match`, `maxlength` e `minlength`
* 1.2. para Number: `max` e `min`
**Schema Correto**
```js
'use strict';
const _schema = {
validate_match: { type: String, match: /^a/i }
, validate_enum: { type: String, enum: ['oi', 'tudo', 'bem', 'sim', 'nao'] }
, validate_maxlength: { type: String, maxlength: 2 }
, validate_minlength: { type: String, minlength: 2 }
, validate_max: { type: Number, max: 5 }
, validate_min: { type: Number, min: 3 }
}
, testSchema = new Schema(_schema)
, TestModel = mongoose.model('testValidate', testSchema)
;
let dataModel = {
validate_match: 'Aloca'
, validate_enum: 'bem'
, validate_maxlength: 'eh'
, validate_minlength: 'nois que voa bruxao'
, validate_max: 3
, validate_min: 10
}
, testing = new TestModel(dataModel)
;
testing.save(function(err, data){
if(err) return console.log('Error: ', err);
console.log('Passou nas validações! :D ', data);
});
// Output do terminal \\
Passou nas validações! :D { _id: 56ba956cc043d716eee48366,
validate_min: 10,
validate_max: 3,
validate_minlength: 'nois que voa bruxao',
validate_maxlength: 'eh',
validate_enum: 'bem',
validate_match: 'Aloca',
__v: 0 }
```
**Schema Incorreto**
```js
{ [ValidationError: testValidate validation failed]
message: 'testValidate validation failed',
name: 'ValidationError',
errors:
{ validate_min:
{ [ValidatorError: Path `validate_min` (1) is less than minimum allowed value (3).]
properties: [Object],
message: 'Path `validate_min` (1) is less than minimum allowed value (3).',
name: 'ValidatorError',
kind: 'min',
path: 'validate_min',
value: 1 },
validate_max:
{ [ValidatorError: Path `validate_max` (10) is more than maximum allowed value (5).]
properties: [Object],
message: 'Path `validate_max` (10) is more than maximum allowed value (5).',
name: 'ValidatorError',
kind: 'max',
path: 'validate_max',
value: 10 },
validate_minlength:
{ [ValidatorError: Path `validate_minlength` (`a`) is shorter than the minimum allowed length (2).]
properties: [Object],
message: 'Path `validate_minlength` (`a`) is shorter than the minimum allowed length (2).',
name: 'ValidatorError',
kind: 'minlength',
path: 'validate_minlength',
value: 'a' },
validate_maxlength:
{ [ValidatorError: Path `validate_maxlength` (`eh nois que voa`) is longer than the maximum allowed length (2).]
properties: [Object],
message: 'Path `validate_maxlength` (`eh nois que voa`) is longer than the maximum allowed length (2).',
name: 'ValidatorError',
kind: 'maxlength',
path: 'validate_maxlength',
value: 'eh nois que voa' },
validate_enum:
{ [ValidatorError: `incorreto` is not a valid enum value for path `validate_enum`.]
properties: [Object],
message: '`incorreto` is not a valid enum value for path `validate_enum`.',
name: 'ValidatorError',
kind: 'enum',
path: 'validate_enum',
value: 'incorreto' },
validate_match:
{ [ValidatorError: Path `validate_match` is invalid (bbbb).]
properties: [Object],
message: 'Path `validate_match` is invalid (bbbb).',
name: 'ValidatorError',
kind: 'regexp',
path: 'validate_match',
value: 'bbbb' } } }
```
## Cadastre 3 pokemons **de uma só vez**:
```js
const _schema = {
name: String
}
, testSchema = new Schema(_schema)
, TestModel = mongoose.model('testInsercao', testSchema)
;
let dataModel = [
{
name: 'Poke-01'
},
{
name: 'Poke-02'
},
{
name: 'Poke-03'
}
];
TestModel.create(dataModel, function(err, data){
if(err) return console.log('Error: ', err);
console.log('Inseriu todos pokemons! :D ', data);
});
// Output do terminal \\
Inseriu todos pokemons! :D [ { _id: 56bb44b415f642bdf1182962, name: 'Poke-01', __v: 0 },
{ _id: 56bb44b415f642bdf1182963, name: 'Poke-02', __v: 0 },
{ _id: 56bb44b415f642bdf1182964, name: 'Poke-03', __v: 0 } ]
```
## Busque **todos** os Pokemons com `attack > 50` e `height > 0.5`:
```js
const _schema = {
name: String
}
, pokemonSchema = new Schema(_schema)
, PokemonModel = mongoose.model('Pokemon', pokemonSchema)
;
function callback(err, data){
if(err) return console.log('Error: ', err);
console.log('Resultado: ', data);
}
PokemonModel.find({}).where({ $and:[ { attack: { $gt: 50 }}, { height: { $gt: 0.5}} ]}).exec(callback);
// Output do terminal \\
Resultado: [ { moves: [ 'Brasas', 'Encarar' ],
height: 0.6,
attack: 52,
type: 'fire',
description: 'Esse é o cão chupando manga de fofinho',
name: 'Charmander',
_id: 566a25407d0b654cdf58b763 } ]
```
## Altere, **inserindo**, o Pokemon `Nerdmon` com `attack` igual a 49 e com os valores dos outros campos a sua escolha.
```js
const _schema = {
name: String
, attack: Number
, defense: Number
, attacks: Array
}
, pokemonSchema = new Schema(_schema)
, PokemonModel = mongoose.model('Pokemon', pokemonSchema)
;
let query = {
name: /nerdmon/i
}
, mod = {
$setOnInsert: {
name: 'Nerdemon'
, attack: 49
, defense: 444
, attacks: ['Raio Virginador', 'Friend Zone']
}
}
, options = {
upsert: true
}
;
function callback(err, data){
if(err) return console.log('Error: ', err);
console.log('Resultado: ', data);
}
PokemonModel.update(query, mod, options, callback);
// Output do terminal \\
Resultado: { ok: 1,
nModified: 0,
n: 1,
upserted: [ { index: 0, _id: 56bb52ca2df027a2c53e7b8e } ] }
{
"_id": ObjectId("56bb52ca2df027a2c53e7b8e"),
"name": "Nerdemon",
"attack": 49,
"defense": 444,
"attacks": [
"Raio Virginador",
"Friend Zone"
]
}
```
## Remova **todos** os Pokemons com `attack` **acima de 50**.
```js
const _schema = {
attack: Number
}
, pokemonSchema = new Schema(_schema)
, PokemonModel = mongoose.model('Pokemon', pokemonSchema)
;
let query = {
attack: { $gt: 50 }
};
function callback(err, data){
if(err) return console.log('Error: ', err);
console.log('Removeu: ', data);
}
PokemonModel.remove(query, callback);
```
**Resposta do terminal**
```js
Removeu: { result: { ok: 1, n: 2 },
connection:
EventEmitter {
domain: null,
_events:
{ close: [Object],
error: [Object],
timeout: [Object],
parseError: [Object],
connect: [Function] },
_eventsCount: 5,
_maxListeners: undefined,
options:
{ socketOptions: {},
auto_reconnect: true,
host: 'localhost',
port: 27017,
cursorFactory: [Object],
reconnect: true,
emitError: true,
size: 5,
disconnectHandler: [Object],
bson: {},
messageHandler: [Function],
wireProtocolHandler: [Object] },
id: 2,
logger: { className: 'Connection' },
bson: {},
tag: undefined,
messageHandler: [Function],
maxBsonMessageSize: 67108864,
port: 27017,
host: 'localhost',
keepAlive: true,
keepAliveInitialDelay: 0,
noDelay: true,
connectionTimeout: 0,
socketTimeout: 0,
destroyed: false,
domainSocket: false,
singleBufferSerializtion: true,
serializationFunction: 'toBinUnified',
ca: null,
cert: null,
key: null,
passphrase: null,
ssl: false,
rejectUnauthorized: false,
checkServerIdentity: true,
responseOptions: { promoteLongs: true },
flushing: false,
queue: [],
connection:
Socket {
_connecting: false,
_hadError: false,
_handle: [Object],
_parent: null,
_host: 'localhost',
_readableState: [Object],
readable: true,
domain: null,
_events: [Object],
_eventsCount: 8,
_maxListeners: undefined,
_writableState: [Object],
writable: true,
allowHalfOpen: false,
destroyed: false,
bytesRead: 56,
_bytesDispatched: 175,
_sockname: null,
_pendingData: null,
_pendingEncoding: '',
_idleNext: null,
_idlePrev: null,
_idleTimeout: -1,
read: [Function],
_consuming: true },
writeStream: null,
buffer: null,
sizeOfMessage: 0,
bytesRead: 0,
stubBuffer: null } }
```
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 06 - Exercício
**user**: [victorvoid](https://github.com/victorvoid)
**autor**: Victor Igor Gomes Martins
**date**: 1458069633190
## Crie um Schema com cada tipo explicado, inserindo tanto um objeto correto, como um objeto que desencadeie erros de validação padrão, criar especificamente:
1. para String: **enum**, **match**, **matchlength** e **minlength**
2. para Number: **max** e **min**
```js
/* COM O OBJETO DE DADOS CORRETO */
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
nome: {
type: String,
maxlength: 30,
minlength: 2
},
idade: {
type: Number,
min: 18,
max: 60
},
corFavorita: {
type: String,
enum: ['laranja', 'vermelho', 'azul'],
required: true
},
solteiro: Boolean,
attacks: Schema.Types.Mixed,
nomeFamiliares: [String],
curriculo: {type: String, match: /\.doc$/},
dtCadastrado: {type: Date, default: Date.now},
}
const pessoaSchema = new Schema(_schema);
/* simbora inserir */
const dados = {
nome: 'Victor Igor',
idade: 20,
corFavorita: 'azul',
solteiro: 1,
attacks:
[
{ nome: 'Socão',
poder: 100
},
{
nome: 'Vuadora',
poder: 99
}
],
nomeFamiliares: ['Mateus', 'Jorge', 'Maria'],
curriculo: 'curriculo.doc'
}
let Model = mongoose.model('testes', pessoaSchema);
let pes = new Model(dados);
pes.save(function(err, data){
if (err) return console.log('Ocorreu um erro =( '+err);
console.log('Cadastrado com sucesso!!! =D');
});
/* COM OBJETO DE DATA INVALIDO: */
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
nome: {
type: String,
maxlength: 30,
minlength: 2
},
idade: {
type: Number,
min: 18,
max: 60
},
corFavorita: {
type: String,
enum: ['laranja', 'vermelho', 'azul'],
required: true
},
solteiro: Boolean,
attacks: Schema.Types.Mixed,
nomeFamiliares: [String],
curriculo: {type: String, match: /\.doc$/},
dtCadastrado: {type: Date, default: Date.now},
}
const pessoaSchema = new Schema(_schema);
/* simbora inserir */
const dados = {
nome: 'A',
idade: 70,
corFavorita: 'azul',
solteiro: 1,
attacks:
[
{ nome: 'Socão',
poder: 100
},
{
nome: 'Vuadora',
poder: 99
}
],
nomeFamiliares: ['Mateus', 'Jorge', 'Maria'],
curriculo: 'curriculo.doc'
}
let Model = mongoose.model('testes', pessoaSchema);
let pes = new Model(dados);
pes.save(function(err, data){
if (err) return console.log('Ocorreu um erro =( '+err);
console.log('Cadastrado com sucesso!!! =D');
});
/*out:
node app.js
Ocorreu um erro =( ValidationError: Path `idade` (70) is more than maximum allowed value (60)., Path `nome` (`A`) is shorter than the minimum allowed length (2).
Connected by mongodb://localhost/be-mean-instagram
Mongoose open
*/
```
## Cadastre 3 pokemons **de uma só vez**:
```js
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
name: String,
description: String,
type: {
type: String,
enum: [
"Normal",
"Ghost",
"Dragon",
"Electric",
"Ground",
"Fighting",
"Poison",
"Psychic",
"Bug",
"Grass",
"Steel",
"Dark",
"Flying",
"Fire",
"Water",
"Ice",
"Fairy",
"Rock"
]
},
attack: {
type: Number,
max: 1000,
min: 0.1
},
defense: {
type: Number,
max: 1000,
min: 0.1
},
height: {
type: Number,
max: 10,
min: 0.1
}
}
let pokemonsSchema = new Schema (_schema);
/*inserindo 3 pokemons de uma só vez */
const data =
[
{
name: "bulbasaur",
description: "dragaozinho de merda",
type: "Fire",
attack: 30,
defense: 30,
height: 3.07
},
{
name: "Pikachu",
description: "fofo até de mais *--*",
type: "Electric",
attack: 30,
defense: 30,
height: 3.07
},
{
name: "Celebi",
description: "bixo feio",
type: "Eletric",
attack: 50,
defense: 40,
height: 2
}
];
let Model = mongoose.model('pokemons', pokemonsSchema);
/* Salvando todos os pokemons de dentro do array data: */
var quantidade = 0;
data.forEach(function(doc){
let poke = new Model(doc); //criando model para cada um
poke.save(function(err){
quantidade++;
if (err){
console.log('Ocorreu um erro =( --> ' + err);
}
if(quantidade == data.length){
console.log('Salvo com sucesso *---*');
}
});
});
/*out:
node app.js
Connected by mongodb://localhost/be-mean-instagram
Mongoose open
Salvo com sucesso *---*
*/
```
## Busque **todos** os Pokemons com `attack > 50` e `height > 0.5`:
```js
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
name: String
}
const pokemonSchema = new Schema(_schema);
const PokemonModel = mongoose.model('pokemons', pokemonSchema);
const query = {$and:[{attack:{$gt: 50}},{height: {$gt: 0.5}}]}
const fields = {name: 1, _id: 0}
PokemonModel.find(query, fields, (err, data)=>{
if (err)
return console.log('Ocorreu um erro: '+err);
if (data.length <= 0)
return console.log('Nenhum pokemon encontrado');
return console.log(data);
});
module.exports = PokemonModel;
/*
out:
node app.js
Connected by mongodb://localhost/be-mean-instagram
Mongoose open
Nenhum pokemon encontrado
*/
```
## Altere, **inserindo**, o Pokemon `Nerdmon` com `attack` igual a 49 e com os valores dos outros campos a sua escolha.
```
'use strict'
const mongoose = require('mongoose'),
Schema = mongoose.Schema;
const _schema = {
name: String,
description: String,
type: String,
attack: {
type: Number,
min: 5,
max: 190
},
defence: {
type: Number,
min: 5,
max: 230
},
height: Number
};
const query = {name: 'Nerdmon'};
const mod = {
$setOnInsert: {
name: 'Nerdmon',
description: 'Faz nada',
type: 'Fire',
attack: 49,
defense: 10,
height: 2
}
}
const option = { upsert: true };
const pokemonSchema = new Schema(_schema);
const Model = mongoose.model('pokemons',pokemonSchema);
Model.update(query, mod, option,(err, data)=>{
if (err) return console.log('ERRO: '+err);
return console.log('--> ' + data);
});
```
## Remova **todos** os Pokemons com `attack` **acima de 50**.
```
'use strict'
const mongoose = require('mongoose'),
Schema = mongoose.Schema;
const _schema = {
name: String,
description: String,
type: String,
attack: {
type: Number,
min: 5,
max: 190
},
defence: {
type: Number,
min: 5,
max: 230
},
height: Number
};
const query = {
attack: {$gt: 50}
}
const pokemonSchema = new Schema(_schema);
const pokemonModel = mongoose.model('pokemons', pokemonSchema);
pokemonModel.remove(query, (err, data) => {
if (err) return console.log('Ocorreu um erro: '+err);
return console.log('Removeu com sucesso: '+data);
});
/*
OUT:
node app.js
Connected by mongodb://localhost/be-mean-instagram
Mongoose open
Removeu com sucesso: {"ok":1,"n":0}
*/
``` | {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# NodeJS - Aula 06 - Exercício
**user**: [wellerson010](https://github.com/wellerson010)
**autor**: Wellerson Roberto
**date**: 17/03/2016 21:28:08
# 1) Crie um Schema com cada tipo especificado, inserindo tanto um objeto correto, como um objeto que desencadeie erros na validação padrão, criar especificamente:
- para String: **enum**, **match**, **maxlength**, **minlength**
- para Number: **max** e **min**
Objeto correto:
```
'use strict';
const dbUri = 'mongodb://127.0.0.1/pokemon';
const mongoose = require('mongoose');
mongoose.connect(dbUri);
var Schema = mongoose.Schema;
var pokemonSchema = new Schema({
name: { type: String, maxlength: 10, minlength: 4, enum: ['Pikachu', 'Beedril', 'Beedril2'], match: /^[a-z]+$/i },
attack: {type: Number, max: 100, min: 0},
created: {type: Date, default: Date.now},
isDead: Boolean,
moves: [String],
children: Schema.Types.Mixed,
id: Schema.Types.ObjectId
});
var model = mongoose.model('pokemon', pokemonSchema);
var pokeModel = new model({
name: 'Pikachu',
attack: 50
});
pokeModel.save(pokeModel, function (err, data) {
if (err) console.log(err);
console.log(data);
});
```
Agora os erros de validação...
**erro de max e maxlength**
```
{ [ValidationError: Pokemon validation failed]
message: 'Pokemon validation failed',
name: 'ValidationError',
errors:
{ attack:
{ [ValidatorError: Path `attack` (101) is more than maximum allowed value (100).]
properties: [Object],
message: 'Path `attack` (101) is more than maximum allowed value (100).',
name: 'ValidatorError',
kind: 'max',
path: 'attack',
value: 101 },
name:
{ [ValidatorError: Path `name` (`Pikachuzzzzzzz`) is longer than the maximum allowed length (10).]
properties: [Object],
message: 'Path `name` (`Pikachuzzzzzzz`) is longer than the maximum allowed length (10).',
name: 'ValidatorError',
kind: 'maxlength',
path: 'name',
value: 'Pikachuzzzzzzz' } } }
undefined
```
**erro de min e minlength**
```
{ [ValidationError: Pokemon validation failed]
message: 'Pokemon validation failed',
name: 'ValidationError',
errors:
{ attack:
{ [ValidatorError: Path `attack` (-20) is less than minimum allowed value (0).]
properties: [Object],
message: 'Path `attack` (-20) is less than minimum allowed value (0).',
name: 'ValidatorError',
kind: 'min',
path: 'attack',
value: -20 },
name:
{ [ValidatorError: Path `name` (`c`) is shorter than the minimum allowed length (4).]
properties: [Object],
message: 'Path `name` (`c`) is shorter than the minimum allowed length (4).',
name: 'ValidatorError',
kind: 'minlength',
path: 'name',
value: 'c' } } }
undefined
```
**erro de enum**
```
{ [ValidationError: Pokemon validation failed]
message: 'Pokemon validation failed',
name: 'ValidationError',
errors:
{ attack:
{ [ValidatorError: Path `attack` (-20) is less than minimum allowed value (0).]
properties: [Object],
message: 'Path `attack` (-20) is less than minimum allowed value (0).',
name: 'ValidatorError',
kind: 'min',
path: 'attack',
value: -20 },
name:
{ [ValidatorError: `csdsss` is not a valid enum value for path `name`.]
properties: [Object],
message: '`csdsss` is not a valid enum value for path `name`.',
name: 'ValidatorError',
kind: 'enum',
path: 'name',
value: 'csdsss' } } }
undefined
```
**erro de match**
```
{ [ValidationError: pokemon validation failed]
message: 'pokemon validation failed',
name: 'ValidationError',
errors:
{ name:
{ [ValidatorError: Path `name` is invalid (Beedril2).]
properties: [Object],
message: 'Path `name` is invalid (Beedril2).',
name: 'ValidatorError',
kind: 'regexp',
path: 'name',
value: 'Beedril2' } } }
undefined
```
# 2) Cadastre 3 Pokemons de uma só vez:
```
var Schema = mongoose.Schema;
var pokemonSchema = new Schema({
name: String,
attack: Number,
created: {type: Date, default: Date.now},
isDead: Boolean,
moves: [String],
children: Schema.Types.Mixed,
id: Schema.Types.ObjectId
});
var model = mongoose.model('pokemon', pokemonSchema);
var pokeOne = new model({
name: 'Master Fuck',
attack: 101
});
var pokeTwo = new model({
name: 'Pikamole',
attack: 19
});
var pokeFour = new model({
name: 'BigAss',
attack: 69
});
var pokemons = [pokeOne, pokeTwo, pokeFour];
model.create(pokemons, function (err, data) {
if (err) console.log(err);
console.log(data);
});
```
# 3) Busque todos os pokemons com attack > 50 e height > 0.5
```
var model = mongoose.model('pokemon', pokemonSchema);
var query = { attack: { $gt: 50 }, height: {$gt: 50}}
model.find(query, function (err, data) {
if (err) console.log(err);
console.log(data);
})
```
# 4) Altere, inserindo, o Pokemon Nerdmon com attack = 49 e com os valores de outro campo de sua escolha.
```
var model = mongoose.model('pokemon', pokemonSchema);
var query = { attack: 49, name: 'Nerdmon' };
model.update(query, query, { upsert: true }, function (err, data) {
if (err) console.log(err);
console.log(data);
})
```
# 5) Remova todos os Pokemons com attack > 50.
```
var model = mongoose.model('pokemon', pokemonSchema);
var query = { attack: {$gt: 50} };
model.remove(query, function (err, data) {
if (err) console.log(err);
console.log(data);
})
```
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 06 - Exercício
**user:** [vitorcapretz](https://github.com/vitorcapretz)
**autor:** Vitor Capretz
**date:** 1466382355764
## 1. Crie um Schema com cada tipo explicado, inserindo tanto um objeto correto, como um objeto que desencadeie erros de validação padrão, criar especificamente:
### 1.1 Para String: `enum`, `match`, `maxlength` e `minlength`
### 1.2 Para Number: `max` e `min`
* Código
```js
'use strict';
const mongoose = require('mongoose');
const dbURI = 'mongodb://localhost/be-mean-pokemons';
mongoose.connect(dbURI);
const Schema = mongoose.Schema;
const _schema = {
address: {type: String, match: /^av.*\,\s[0-9]+$/i},
state: {type: String, enum: ['SP', 'RJ', 'MG', 'RS', 'AM']},
course: {type: String, minlength: 5},
type: {type: String, maxlength: 10},
age: {type: Number, max: 99},
course_hours: {type: Number, min: 10}
};
const data = {
address: 'Avenida 9 de julho, 8872',
state: 'SP',
course: 'Be MEAN',
type: 'student',
age: 20,
course_hours: 15
};
const validationSchema = new Schema(_schema);
const validationModel = mongoose.model('Validation', validationSchema);
const val = new validationModel(data);
val.save((err, data) => {
if(err) return console.log('ERRO: ', err);
console.log('inseriu: ', data);
});
module.exports = validationSchema;
```
* Retorno sem erros
```
inseriu: { _id: 57673c54d4e6f1a92646cb0a,
course_hours: 15,
age: 20,
type: 'student',
course: 'Be MEAN',
state: 'SP',
address: 'Avenida 9 de julho, 8872',
__v: 0 }
```
* Retorno com erros
```
ERRO: { ValidationError: Validation validation failed
at MongooseError.ValidationError (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/error/validation.js:22:11)
at model.Document.invalidate (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/document.js:1399:32)
at /var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/document.js:1275:17
at validate (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:701:7)
at /var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:732:9
at Array.forEach (native)
at SchemaNumber.SchemaType.doValidate (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:706:19)
at /var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/document.js:1273:9
at _combinedTickCallback (internal/process/next_tick.js:67:7)
at process._tickCallback (internal/process/next_tick.js:98:9)
at Function.Module.runMain (module.js:577:11)
at startup (node.js:160:18)
at node.js:456:3
message: 'Validation validation failed',
name: 'ValidationError',
errors:
{ course_hours:
{ ValidatorError: Path `course_hours` (2) is less than minimum allowed value (10).
at MongooseError.ValidatorError (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/error/validator.js:24:11)
at validate (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:700:13)
at /var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:732:9
at Array.forEach (native)
at SchemaNumber.SchemaType.doValidate (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:706:19)
at /var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/document.js:1273:9
at _combinedTickCallback (internal/process/next_tick.js:67:7)
at process._tickCallback (internal/process/next_tick.js:98:9)
at Function.Module.runMain (module.js:577:11)
at startup (node.js:160:18)
at node.js:456:3
message: 'Path `course_hours` (2) is less than minimum allowed value (10).',
name: 'ValidatorError',
properties: [Object],
kind: 'min',
path: 'course_hours',
value: 2 },
age:
{ ValidatorError: Path `age` (450) is more than maximum allowed value (99).
at MongooseError.ValidatorError (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/error/validator.js:24:11)
at validate (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:700:13)
at /var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:732:9
at Array.forEach (native)
at SchemaNumber.SchemaType.doValidate (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:706:19)
at /var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/document.js:1273:9
at _combinedTickCallback (internal/process/next_tick.js:67:7)
at process._tickCallback (internal/process/next_tick.js:98:9)
at Function.Module.runMain (module.js:577:11)
at startup (node.js:160:18)
at node.js:456:3
message: 'Path `age` (450) is more than maximum allowed value (99).',
name: 'ValidatorError',
properties: [Object],
kind: 'max',
path: 'age',
value: 450 },
type:
{ ValidatorError: Path `type` (`student_teacher`) is longer than the maximum allowed length (10).
at MongooseError.ValidatorError (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/error/validator.js:24:11)
at validate (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:700:13)
at /var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:732:9
at Array.forEach (native)
at SchemaString.SchemaType.doValidate (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:706:19)
at /var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/document.js:1273:9
at _combinedTickCallback (internal/process/next_tick.js:67:7)
at process._tickCallback (internal/process/next_tick.js:98:9)
at Function.Module.runMain (module.js:577:11)
at startup (node.js:160:18)
at node.js:456:3
message: 'Path `type` (`student_teacher`) is longer than the maximum allowed length (10).',
name: 'ValidatorError',
properties: [Object],
kind: 'maxlength',
path: 'type',
value: 'student_teacher' },
course:
{ ValidatorError: Path `course` (`MEAN`) is shorter than the minimum allowed length (5).
at MongooseError.ValidatorError (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/error/validator.js:24:11)
at validate (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:700:13)
at /var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:732:9
at Array.forEach (native)
at SchemaString.SchemaType.doValidate (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:706:19)
at /var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/document.js:1273:9
at _combinedTickCallback (internal/process/next_tick.js:67:7)
at process._tickCallback (internal/process/next_tick.js:98:9)
at Function.Module.runMain (module.js:577:11)
at startup (node.js:160:18)
at node.js:456:3
message: 'Path `course` (`MEAN`) is shorter than the minimum allowed length (5).',
name: 'ValidatorError',
properties: [Object],
kind: 'minlength',
path: 'course',
value: 'MEAN' },
state:
{ ValidatorError: `PR` is not a valid enum value for path `state`.
at MongooseError.ValidatorError (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/error/validator.js:24:11)
at validate (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:700:13)
at /var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:732:9
at Array.forEach (native)
at SchemaString.SchemaType.doValidate (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:706:19)
at /var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/document.js:1273:9
at _combinedTickCallback (internal/process/next_tick.js:67:7)
at process._tickCallback (internal/process/next_tick.js:98:9)
at Function.Module.runMain (module.js:577:11)
at startup (node.js:160:18)
at node.js:456:3
message: '`PR` is not a valid enum value for path `state`.',
name: 'ValidatorError',
properties: [Object],
kind: 'enum',
path: 'state',
value: 'PR' },
address:
{ ValidatorError: Path `address` is invalid (9 de julho, 8872).
at MongooseError.ValidatorError (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/error/validator.js:24:11)
at validate (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:700:13)
at /var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:732:9
at Array.forEach (native)
at SchemaString.SchemaType.doValidate (/var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:706:19)
at /var/www/html/workshop-be-mean/nodejs/mongoose-pokemons/node_modules/mongoose/lib/document.js:1273:9
at _combinedTickCallback (internal/process/next_tick.js:67:7)
at process._tickCallback (internal/process/next_tick.js:98:9)
at Function.Module.runMain (module.js:577:11)
at startup (node.js:160:18)
at node.js:456:3
message: 'Path `address` is invalid (9 de julho, 8872).',
name: 'ValidatorError',
properties: [Object],
kind: 'regexp',
path: 'address',
value: '9 de julho, 8872' } } }
```
## 2. Cadastre 3 pokemóns de uma só vez:
* Código
```js
'use strict';
const mongoose = require('mongoose');
const dbURI = 'mongodb://localhost/be-mean-pokemons';
mongoose.connect(dbURI);
const Schema = mongoose.Schema;
const _schema = {
name: String,
descripion: String,
type: String,
attack: Number,
defense: Number,
height: Number
};
const pokemonSchema = new Schema(_schema);
const pokeModel = mongoose.model('Pokemon', pokemonSchema);
const data = [
{
name: 'Spearow',
descripion: "Pombo sem doença",
type: 'normal',
attack: 60,
defense: 30,
height: 0.3
},
{
name: 'Oddish',
descripion: "Beterraba?",
type: 'grass',
attack: 50,
defense: 55,
height: 0.51
},
{
name: 'Psyduck',
descripion: "quase que perry o ornitorrinco",
type: 'water',
attack: 52,
defense: 48,
height: 0.79
}
];
pokeModel.create(data, (err, data) => {
if(err) return console.log('ERRO: ', err);
console.log('inseriu: ', data);
});
module.exports = pokemonSchema;
```
* Retorno
```
inseriu: [ { _id: 57673f314ea09c7a296db64c,
height: 0.3,
defense: 30,
attack: 60,
type: 'normal',
descripion: 'Pombo sem doença',
name: 'Spearow',
__v: 0 },
{ _id: 57673f314ea09c7a296db64d,
height: 0.51,
defense: 55,
attack: 50,
type: 'grass',
descripion: 'Beterraba?',
name: 'Oddish',
__v: 0 },
{ _id: 57673f314ea09c7a296db64e,
height: 0.79,
defense: 48,
attack: 52,
type: 'water',
descripion: 'quase que perry o ornitorrinco',
name: 'Psyduck',
__v: 0 } ]
```
## 3. Busque todos os Pokemons com `attack` > 50 e `height` > 0.5
* Código
```js
'use strict';
const mongoose = require('mongoose');
const dbURI = 'mongodb://localhost/be-mean-pokemons';
mongoose.connect(dbURI);
const Schema = mongoose.Schema;
const _schema = {
name: String,
descripion: String,
type: String,
attack: Number,
defense: Number,
height: Number
};
const pokemonSchema = new Schema(_schema);
const pokeModel = mongoose.model('Pokemon', pokemonSchema);
const query = {$and: [
{height: {$gt: 0.5}},
{attack: {$gt: 50}}
]};
const callback = (err, data) => {
if(err) return consol.log('erro:', err);
console.log('busca: ', data);
};
pokeModel.find(query, callback);
module.exports = pokemonSchema;
```
* Retorno
```
busca: [ { height: 0.6,
defense: 43,
attack: 52,
type: 'Fogo',
description: 'o que vira o charizard (fodelao)',
name: 'Charmander',
_id: 57672ddd23aa7f10362224f7 },
{ __v: 0,
height: 0.79,
defense: 48,
attack: 52,
type: 'water',
descripion: 'quase que perry o ornitorrinco',
name: 'Psyduck',
_id: 57673f314ea09c7a296db64e } ]
```
## 4. Altere, inserindo, o Pokemon `Nerdmon` com `attack` igual a 49 e com os valores dos outros campos a sua escolha.
* Código
```js
'use strict';
const mongoose = require('mongoose');
const dbURI = 'mongodb://localhost/be-mean-pokemons';
mongoose.connect(dbURI);
const Schema = mongoose.Schema;
const _schema = {
name: String,
descripion: String,
type: String,
attack: Number,
defense: Number,
height: Number
};
const pokemonSchema = new Schema(_schema);
const pokeModel = mongoose.model('Pokemon', pokemonSchema);
const query = {name: /Nerdmon/i};
const mod = {
$setOnInsert: {
name: 'Nerdmon',
attack: 49,
defense: 70,
height: 1.6
}
};
const options = {upsert: true};
pokeModel.update(query, mod, options, (err, data) => {
if(err) return console.log('ERRO: ', err);
console.log('alterou: ', data);
});
module.exports = pokemonSchema;
```
* Retorno
```
alterou: { ok: 1,
nModified: 0,
n: 1,
upserted: [ { index: 0, _id: 576765cdb8050d654c17a2b5 } ] }
```
## 5. Remova todos os Pokemons com `attack` acima de 50.
* Código
```js
'use strict';
const mongoose = require('mongoose');
const dbURI = 'mongodb://localhost/be-mean-pokemons';
mongoose.connect(dbURI);
const Schema = mongoose.Schema;
const _schema = {
name: String,
descripion: String,
type: String,
attack: Number,
defense: Number,
height: Number
};
const pokemonSchema = new Schema(_schema);
const pokeModel = mongoose.model('Pokemon', pokemonSchema);
const query = {attack: {$gt: 50}};
pokeModel.remove(query, (err, data) => {
if(err) return console.log('ERRO: ', err);
console.log('removeu: ', data);
});
module.exports = pokemonSchema;
```
* Retorno
```
removeu: { result: { ok: 1, n: 4 },
connection:
EventEmitter {
domain: null,
_events:
{ close: [Object],
error: [Object],
timeout: [Object],
parseError: [Object],
connect: [Function] },
_eventsCount: 5,
_maxListeners: undefined,
options:
{ socketOptions: {},
auto_reconnect: true,
host: 'localhost',
port: 27017,
cursorFactory: [Object],
reconnect: true,
emitError: true,
size: 5,
disconnectHandler: [Object],
bson: {},
messageHandler: [Function],
wireProtocolHandler: {} },
id: 0,
logger: { className: 'Connection' },
bson: {},
tag: undefined,
messageHandler: [Function],
maxBsonMessageSize: 67108864,
port: 27017,
host: 'localhost',
keepAlive: true,
keepAliveInitialDelay: 0,
noDelay: true,
connectionTimeout: 0,
socketTimeout: 0,
destroyed: false,
domainSocket: false,
singleBufferSerializtion: true,
serializationFunction: 'toBinUnified',
ca: null,
cert: null,
key: null,
passphrase: null,
ssl: false,
rejectUnauthorized: false,
checkServerIdentity: true,
responseOptions: { promoteLongs: true },
flushing: false,
queue: [],
connection:
Socket {
connecting: false,
_hadError: false,
_handle: [Object],
_parent: null,
_host: 'localhost',
_readableState: [Object],
readable: true,
domain: null,
_events: [Object],
_eventsCount: 8,
_maxListeners: undefined,
_writableState: [Object],
writable: true,
allowHalfOpen: false,
destroyed: false,
_bytesDispatched: 230,
_sockname: null,
_pendingData: null,
_pendingEncoding: '',
server: null,
_server: null,
_idleNext: null,
_idlePrev: null,
_idleTimeout: -1,
read: [Function],
_consuming: true },
writeStream: null,
hashedName: '29bafad3b32b11dc7ce934204952515ea5984b3c',
buffer: null,
sizeOfMessage: 0,
bytesRead: 0,
stubBuffer: null } }
```
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 06 - Exercício
**user:** [xereda](http://github.com/xereda)
**autor:** Jackson Ricardo Schroeder
**data:** 1465935383915
## Crie um Schema com cada tipo explicado, inserindo tanto um objeto correto, como um objeto que desencadeie erros de validação padrão, criar especificamente:
* 1.1. para String: `enum`, `match`, `maxlength` e `minlength`
* 1.2. para Number: `max` e `min`
### Definção do _schema_ com validações
```js
// validacao do formato de email - validate
const validateEmail = function(email) {
let re = /^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/;
return re.test(email);
};
// regex para verificar se a imagem possui extensao .jpg (validacao match)
const matchFoto = [ /\.jpg$/, "A imagem não possui extensão .jpg ({VALUE})" ];
// regex para verificar se foi informado apenas numeros no telefone (validacao match)
const matchTelefone = [ /^[0-9]*$/, "Informe apenas números para o telefone" ];
const Schema = mongoose.Schema;
// definacao do esquema com as validacoes
const _schema = {
nome: { type: String, minlength: 5, maxlength: 40, required: "O nome é obrigatório" },
idade: { type: Number, min: 10, max: 110 },
operadora: { type: String, enum: ["claro", "vivo", "gvt", "telefonica", "tim"] },
telefone: { type: String, match: matchTelefone, minlength: 8, maxlength: 12, required: "Telefone é obrigatório" },
foto: { type: String, match: matchFoto },
email: { type: String, required: "Email é obrigatório", validate: [validateEmail, "Informe um e-mai válido!"] }
};
```
### Script completo para simular a inserção de um objeto de forma _INCORRETA_
```js
"use strict";
const mongoose = require("mongoose");
const dbURI = "mongodb://localhost/agenda";
mongoose.connect(dbURI);
const validateEmail = function(email) {
let re = /^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/;
return re.test(email);
};
const matchFoto = [ /\.jpg$/, "A imagem não possui extensão .jpg ({VALUE})" ];
const matchTelefone = [ /^[0-9]*$/, "Informe apenas números para o telefone" ];
const Schema = mongoose.Schema;
const _schema = {
nome: { type: String, minlength: 5, maxlength: 40, required: "O nome é obrigatório" },
idade: { type: Number, min: 10, max: 110 },
operadora: { type: String, enum: ["claro", "vivo", "gvt", "telefonica", "tim"] },
telefone: { type: String, match: matchTelefone, minlength: 8, maxlength: 12, required: "Telefone é obrigatório" },
foto: { type: String, match: matchFoto },
email: { type: String, required: "Email é obrigatório", validate: [validateEmail, "Informe um e-mai válido!"] }
};
const ContatoSchema = new Schema(_schema);
const Contatos = mongoose.model("contatos", ContatoSchema);
const dados = {
nome: "Fulano da Silva",
idade: 77,
operadora: "vivo",
telefone: "4799999999",
foto: "imagem.gif",
email: "fulano_da_silva@gmail.com"
};
Contatos.create(dados, (err, data) => {
if (err) return console.log("ERRO: ", err);
console.log("Salvou: ", data);
});
```
#### Retorno - _ERRO_
```
ERRO: { ValidationError: contatos validation failed
at MongooseError.ValidationError (/Users/xereda/Sites/bemean/nodejs/aula06/mongoose-pokemons/node_modules/mongoose/lib/error/validation.js:22:11)
at model.Document.invalidate (/Users/xereda/Sites/bemean/nodejs/aula06/mongoose-pokemons/node_modules/mongoose/lib/document.js:1399:32)
at /Users/xereda/Sites/bemean/nodejs/aula06/mongoose-pokemons/node_modules/mongoose/lib/document.js:1275:17
at validate (/Users/xereda/Sites/bemean/nodejs/aula06/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:701:7)
at /Users/xereda/Sites/bemean/nodejs/aula06/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:732:9
at Array.forEach (native)
at SchemaString.SchemaType.doValidate (/Users/xereda/Sites/bemean/nodejs/aula06/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:706:19)
at /Users/xereda/Sites/bemean/nodejs/aula06/mongoose-pokemons/node_modules/mongoose/lib/document.js:1273:9
at _combinedTickCallback (internal/process/next_tick.js:67:7)
at process._tickCallback (internal/process/next_tick.js:98:9)
at Function.Module.runMain (module.js:577:11)
at startup (node.js:160:18)
at node.js:449:3
message: 'contatos validation failed',
name: 'ValidationError',
errors:
{ foto:
{ ValidatorError: A imagem não possui extensão .jpg (imagem.gif)
at MongooseError.ValidatorError (/Users/xereda/Sites/bemean/nodejs/aula06/mongoose-pokemons/node_modules/mongoose/lib/error/validator.js:24:11)
at validate (/Users/xereda/Sites/bemean/nodejs/aula06/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:700:13)
at /Users/xereda/Sites/bemean/nodejs/aula06/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:732:9
at Array.forEach (native)
at SchemaString.SchemaType.doValidate (/Users/xereda/Sites/bemean/nodejs/aula06/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:706:19)
at /Users/xereda/Sites/bemean/nodejs/aula06/mongoose-pokemons/node_modules/mongoose/lib/document.js:1273:9
at _combinedTickCallback (internal/process/next_tick.js:67:7)
at process._tickCallback (internal/process/next_tick.js:98:9)
at Function.Module.runMain (module.js:577:11)
at startup (node.js:160:18)
at node.js:449:3
message: 'A imagem não possui extensão .jpg (imagem.gif)',
name: 'ValidatorError',
properties: [Object],
kind: 'regexp',
path: 'foto',
value: 'imagem.gif' } } }
```
### Script completo para simular a inserção de um objeto de forma _CORRETA_
```js
"use strict";
const mongoose = require("mongoose");
const dbURI = "mongodb://localhost/agenda";
mongoose.connect(dbURI);
const validateEmail = function(email) {
let re = /^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/;
return re.test(email);
};
const matchFoto = [ /\.jpg$/, "A imagem não possui extensão .jpg ({VALUE})" ];
const matchTelefone = [ /^[0-9]*$/, "Informe apenas números para o telefone" ];
const Schema = mongoose.Schema;
const _schema = {
nome: { type: String, minlength: 5, maxlength: 40, required: "O nome é obrigatório" },
idade: { type: Number, min: 10, max: 110 },
operadora: { type: String, enum: ["claro", "vivo", "gvt", "telefonica", "tim"] },
telefone: { type: String, match: matchTelefone, minlength: 8, maxlength: 12, required: "Telefone é obrigatório" },
foto: { type: String, match: matchFoto },
email: { type: String, required: "Email é obrigatório", validate: [validateEmail, "Informe um e-mai válido!"] }
};
const ContatoSchema = new Schema(_schema);
const Contatos = mongoose.model("contatos", ContatoSchema);
const dados = {
nome: "Fulano da Silva",
idade: 77,
operadora: "vivo",
telefone: "4799999999",
foto: "imagem.jpg",
email: "fulano_da_silva@gmail.com"
};
Contatos.create(dados, (err, data) => {
if (err) return console.log("ERRO: ", err);
console.log("Salvou: ", data);
});
```
### Retorno do script acima
```js
Salvou: { _id: 5760ded5dcce1fd533e829bb,
email: 'fulano_da_silva@gmail.com',
foto: 'imagem.jpg',
telefone: '4799999999',
operadora: 'vivo',
idade: 77,
nome: 'Fulano da Silva',
__v: 0 }
```
## Cadastre 3 pokemons **de uma só vez**:
```js
"use strict";
const mongoose = require("mongoose");
const dbURI = "mongodb://localhost/be-mean-instagram";
mongoose.connect(dbURI);
const Schema = mongoose.Schema;
const _schema = {
"attack": Number,
"created": Date,
"defense": Number,
"height": String,
"name": String,
"speed": Number,
"types": [String]
};
const PokemonSchema = new Schema(_schema);
const Pokemon = mongoose.model("pokemons", PokemonSchema);
const data = [
{
"attack": 77,
"created": new Date(),
"defense": 77,
"height": "77",
"name": "Pokemon Custom 01",
"speed": 77,
"types": ["water"]
},
{
"attack": 88,
"created": new Date(),
"defense": 88,
"height": "88",
"name": "Pokemon Custom 02",
"speed": 88,
"types": ["water"]
},
{
"attack": 99,
"created": new Date(),
"defense": 99,
"height": "99",
"name": "Pokemon Custom 03",
"speed": 99,
"types": ["water"]
}
];
Pokemon.create(data, (err, data) => {
if (err) return console.log("ERRO: ", err);
console.log("Criou os pokemons: ", data);
});
```
### Resultado:
```js
db.pokemons.find({ name: /pokemon custom /i });
{
"_id": ObjectId("5760d231a42f06db31ca1071"),
"attack": 77,
"created": ISODate("2016-06-15T03:57:37.756Z"),
"defense": 77,
"height": "77",
"name": "Pokemon Custom 01",
"speed": 77,
"types": [
"water"
],
"__v": 0
}
{
"_id": ObjectId("5760d231a42f06db31ca1072"),
"attack": 88,
"created": ISODate("2016-06-15T03:57:37.756Z"),
"defense": 88,
"height": "88",
"name": "Pokemon Custom 02",
"speed": 88,
"types": [
"water"
],
"__v": 0
}
{
"_id": ObjectId("5760d231a42f06db31ca1073"),
"attack": 99,
"created": ISODate("2016-06-15T03:57:37.756Z"),
"defense": 99,
"height": "99",
"name": "Pokemon Custom 03",
"speed": 99,
"types": [
"water"
],
"__v": 0
}
Fetched 3 record(s) in 3ms
```
## Busque **todos** os Pokemons com `attack > 180` e `speed > 70`:
### Teste no console do MongoDB
```
// todos os pokemons
db.pokemons.count();
613
// definicao do filtro
var query = { attack: { $gt:180 }, speed: { $gt: 70 } };
// retorna a quantidade apos aplicacao do filtro
db.pokemons.count(query);
2
```
### Teste com o nodeJS - _SCRIPT_
```js
"use strict";
const mongoose = require("mongoose");
const dbURI = "mongodb://localhost/be-mean-instagram";
mongoose.connect(dbURI);
const Schema = mongoose.Schema;
const _schema = {};
const pokemonSchema = new Schema(_schema);
const PokemonModel = mongoose.model("pokemons", pokemonSchema);
const query = {attack: { $gt: 180 }, speed: { $gt: 70 } };
PokemonModel.find(query, (err, data) => {
if (err) return console.log("ERRO: ", err);
return console.log("Buscou: ", data);
});
```
### Teste com o nodeJS - _RESULTADO_
```js
[nodemon] starting `node aula06_03.js`
Buscou: [ { types: [ 'fighting', 'psychic' ],
speed: 130,
name: 'Mewtwo-mega-x',
hp: 106,
height: '0',
defense: 100,
created: '2013-11-03T15:05:42.554794',
attack: 190,
_id: 564b1de525337263280d06a2 },
{ types: [ 'fighting', 'bug' ],
speed: 75,
name: 'Heracross-mega',
hp: 80,
height: '0',
defense: 115,
created: '2013-11-03T15:05:42.561123',
attack: 185,
_id: 564b1de525337263280d06a6 } ]
```
## Altere, **inserindo**, o Pokemon `Nerdmon` com `attack` igual a 49 e com os valores dos outros campos a sua escolha.
### Confirmando no console do MongoDB que não há o Nerdmon já inserido
```
var query = { name: /Nerdmon/ };
db.pokemons.find(query);
Fetched 0 record(s) in 1ms
```
### Script
```js
"use strict";
const mongoose = require("mongoose");
const dbURI = "mongodb://localhost/be-mean-instagram";
mongoose.connect(dbURI);
const Schema = mongoose.Schema;
const _schema = {
"attack": Number,
"created": Date,
"defense": Number,
"height": String,
"name": String,
"speed": Number,
"types": [String]
};
const PokemonSchema = new Schema(_schema);
const Pokemon = mongoose.model("pokemons", PokemonSchema);
const query = { name: /Nerdmon/ };
const mod = {
$setOnInsert: {
"name": "Nerdmon",
"attack": 49,
"created": new Date(),
"defense": 80,
"height": "56",
"speed": 99,
"types": ["water", "flash", "ghost"] }
};
const options = {upsert: true};
Pokemon.update(query, mod, options, (err, data) => {
if (err) return console.log("ERRO: ", err);
console.log("Criou o Nerdmon com upsert: ", data);
});
```
### Resultado da execução do script no nodeJS
```
Criou o Nerdmon com upsert: { ok: 1,
nModified: 0,
n: 1,
upserted: [ { index: 0, _id: 5760db14ec918dcbc3f73da7 } ] }
```
### Consulta no console do MongoDB:
```js
var query = { name: /Nerdmon/ };
db.pokemons.find(query);
{
"_id": ObjectId("5760db14ec918dcbc3f73da7"),
"name": "Nerdmon",
"attack": 49,
"created": ISODate("2016-06-15T04:35:32.133Z"),
"defense": 80,
"height": "56",
"speed": 99,
"types": [
"water",
"flash",
"ghost"
]
}
Fetched 1 record(s) in 2ms
```
## Remova **todos** os Pokemons com `attack` **acima de 50**.
### Verifica no console do MongoDB a quantidade total de pokemons com attack acima de 50 antes da exclusão
```
var query = { attack: { $gt: 50 } };
db.pokemons.count(query);
465
```
### Script
```js
"use strict";
const mongoose = require("mongoose");
const dbURI = "mongodb://localhost/be-mean-instagram";
mongoose.connect(dbURI);
const Schema = mongoose.Schema;
const _schema = {};
const PokemonSchema = new Schema(_schema);
const Pokemon = mongoose.model("pokemons", PokemonSchema);
const query = { attack: { $gt: 50 } };
Pokemon.remove(query, (err, data) => {
if (err) return console.log("ERRO: ", err);
console.log("Removeu os pokemons com ataque acima de 50: ", data);
});
```
### Resultado
```
Removeu os pokemons com ataque acima de 50: { result: { ok: 1, n: 465 },
connection:
EventEmitter {
domain: null,
_events:
{ close: [Object],
error: [Object],
timeout: [Object],
parseError: [Object],
connect: [Function] },
_eventsCount: 5,
_maxListeners: undefined,
options:
{ socketOptions: {},
auto_reconnect: true,
host: 'localhost',
port: 27017,
cursorFactory: [Object],
reconnect: true,
emitError: true,
size: 5,
disconnectHandler: [Object],
bson: {},
messageHandler: [Function],
wireProtocolHandler: [Object] },
id: 0,
logger: { className: 'Connection' },
bson: {},
tag: undefined,
messageHandler: [Function],
maxBsonMessageSize: 67108864,
port: 27017,
host: 'localhost',
keepAlive: true,
keepAliveInitialDelay: 0,
noDelay: true,
connectionTimeout: 0,
socketTimeout: 0,
destroyed: false,
domainSocket: false,
singleBufferSerializtion: true,
serializationFunction: 'toBinUnified',
ca: null,
cert: null,
key: null,
passphrase: null,
ssl: false,
rejectUnauthorized: false,
checkServerIdentity: true,
responseOptions: { promoteLongs: true },
flushing: false,
queue: [],
connection:
Socket {
connecting: false,
_hadError: false,
_handle: [Object],
_parent: null,
_host: 'localhost',
_readableState: [Object],
readable: true,
domain: null,
_events: [Object],
_eventsCount: 8,
_maxListeners: undefined,
_writableState: [Object],
writable: true,
allowHalfOpen: false,
destroyed: false,
_bytesDispatched: 231,
_sockname: null,
_pendingData: null,
_pendingEncoding: '',
server: null,
_server: null,
_idleNext: null,
_idlePrev: null,
_idleTimeout: -1,
read: [Function],
_consuming: true },
writeStream: null,
hashedName: '29bafad3b32b11dc7ce934204952515ea5984b3c',
buffer: null,
sizeOfMessage: 0,
bytesRead: 0,
stubBuffer: null } }
```
### Verifica no console do MongoDB se realmente os pokemons foram removidos
```
var query = { attack: { $gt: 50 } };
db.pokemons.count(query);
0
```
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 06 - Exercício
**user:** [FranciscoValerio](https://github.com/FranciscoValerio)
**autor:** Francisco Henrique Ruiz Valério
## Crie um Schema com cada tipo explicado, inserindo tanto um objeto correto, como um objeto que desencadeie erros de validação padrão, criar especificamente:
**Retorno da inserção de um objeto correto:**
```
Inseriu: { created_at: Wed Jan 27 2016 20:14:56 GMT-0200 (Horário brasileiro de verão),
type: [ 5691d60743056d6e1566274e ],
tags: [ 'schema', 'nodejs', 'be-mean' ],
_id: 56a941602a0af6000383ea8d,
attacks:
[ { name: 'Soco alto', power: 6500, order: 1, active: 1 },
{ name: 'Soco baixo', power: 3400, order: 2, active: 0 } ],
active: true,
image_bin:
Binary {
_bsontype: 'Binary',
sub_type: 0,
position: 32,
buffer: <Buffer e3 b0 c4 42 98 fc 1c 14 9a fb f4 c8 99 6f b9 24 27 ae 41 e4 64 9b 93 4c a4 95 99 1b 78 52 b8 55> },
attack: 15,
pokebola: 'open',
name: 'SchemaMon',
__v: 0 }
```
* 1.1. para String: `enum`, `match`, `maxlength` e `minlength`
**enum | match | maxlenght:**
```
ERRO: { [ValidationError: pokemons validation failed]
message: 'pokemons validation failed',
name: 'ValidationError',
errors:
{ pokebola:
{ [ValidatorError: `cabo` is not a valid enum value for path `pokebola`.]
properties: [Object],
message: '`cabo` is not a valid enum value for path `pokebola`.',
name: 'ValidatorError',
kind: 'enum',
path: 'pokebola',
value: 'cabo' },
name:
{ [ValidatorError: Path `name` is invalid (vishMonMaisDeDez).]
properties: [Object],
message: 'Path `name` is invalid (vishMonMaisDeDez).',
name: 'ValidatorError',
kind: 'regexp',
path: 'name',
value: 'vishMonMaisDeDez'
}
}
}
```
**minlength:**
```
ERRO: { [ValidationError: pokemons validation failed]
message: 'pokemons validation failed',
name: 'ValidationError',
errors:
{ name:
{ [ValidatorError: Path `name` is invalid (vi).]
properties: [Object],
message: 'Path `name` is invalid (vi).',
name: 'ValidatorError',
kind: 'regexp',
path: 'name',
value: 'vi'
}
}
}
```
* 1.2. para Number: `max` e `min`
**min:**
```
ERRO: { [ValidationError: pokemons validation failed]
message: 'pokemons validation failed',
name: 'ValidationError',
errors:
{ attack:
{ [ValidatorError: Path `attack` (1) is less than minimum allowed value (2).]
properties: [Object],
message: 'Path `attack` (1) is less than minimum allowed value (2).',
name: 'ValidatorError',
kind: 'min',
path: 'attack',
value: 1 },
name:
{ [ValidatorError: Path `name` is invalid (vishMon).]
properties: [Object],
message: 'Path `name` is invalid (vishMon).',
name: 'ValidatorError',
kind: 'regexp',
path: 'name',
value: 'vishMon'
}
}
}
```
**max:**
```
ERRO: { [ValidationError: pokemons validation failed]
message: 'pokemons validation failed',
name: 'ValidationError',
errors:
{ attack:
{ [ValidatorError: Path `attack` (1000000) is more than maximum allowed value (9999).]
properties: [Object],
message: 'Path `attack` (1000000) is more than maximum allowed value (9999).',
name: 'ValidatorError',
kind: 'max',
path: 'attack',
value: 1000000 },
name:
{ [ValidatorError: Path `name` is invalid (vishMon).]
properties: [Object],
message: 'Path `name` is invalid (vishMon).',
name: 'ValidatorError',
kind: 'regexp',
path: 'name',
value: 'vishMon'
}
}
}
```
**Código completo com o objeto correto:**
```JS
const crypto = require('crypto');
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
var states = 'opening open closing closed'.split(' ')
const _schema = {
name: { type: String, maxlength: 10, minlength: 3 },
pokebola: { type: String, enum: states },
attack: { type: Number, min: 2, max: 9999 },
created_at: { type: Date, default: Date.now },
image_bin: Buffer,
active: Boolean,
attacks: Schema.Types.Mixed,
type: [{type: Schema.Types.ObjectId, ref: 'types'}],
tags: [String]
}
const mixed = [
{ name: 'Soco alto',
power: 6500,
order: 1,
active: 1,
},
{ name: 'Soco baixo',
power: 3400,
order: 2,
active: 0,
}
];
const buffer = new Buffer(crypto.createHash('sha256').update(new Buffer(0)).digest('binary'), 'binary');
const data = { name: "SchemaMon",
pokebola: 'open',
attack: 15,
image_bin: buffer,
active: 1,
attacks: mixed,
type: '5691d60743056d6e1566274e',
tags: ['schema', 'nodejs', 'be-mean'],
};
const pokemonSchema = new Schema(_schema);
const PokemonModel = mongoose.model('pokemons', pokemonSchema);
var pokemon = new PokemonModel(data);
pokemon.save( function (err, data){
if(err) return console.log('ERRO: ', err);
return console.log('Inseriu: ', data);
});
module.exports = pokemonSchema;
```
## Cadastre 3 pokemons **de uma só vez**:
**Código:**
```JS
var Mongoose = require('Mongoose');
var db = Mongoose.connection;
db.on('error', console.error);
db.once('open', function() {
console.log('Conectado.')
var pokemonSchema = new Mongoose.Schema({
name: String,
attack: Number,
created_at: { type: Date, default: Date.now },
image_bin: Buffer,
active: Boolean,
tags: [String],
hasCreditCookie: Boolean
});
pokemonSchema.statics.findAllWithCreditCookies = function(callback) {
return this.find({ hasCreditCookie: true }, callback);
};
var Pokemon = Mongoose.model('Pokemon', pokemonSchema);
for (var i =1; i<=3; i++){
var pokemon = new Pokemon({
name: 'Pokemon ' + i,
attack: 22 + i,
hasCreditCookie: true
});
pokemon.save(function(err, pokemon) {
if (err) return console.error(err);
console.log('Salvo: ' + pokemon);
});
}
setTimeout(function(){
Pokemon.findAllWithCreditCookies(function(err, pokemons) {
if (err) return console.error(err);
console.log('Buscado: ' + pokemons);
});
}, 1000);
});
Mongoose.connect('mongodb://localhost/be-mean-instagram');
```
**Retorno:**
```
Salvo: { created_at: Wed Jan 27 2016 21:33:43 GMT-0200 (Horário brasileiro de verão),
tags: [],
_id: 56a953d7cdb6f5d0126dbada,
hasCreditCookie: true,
attack: 23,
name: 'Pokemon 1',
__v: 0 }
Salvo: { created_at: Wed Jan 27 2016 21:33:43 GMT-0200 (Horário brasileiro de verão),
tags: [],
_id: 56a953d7cdb6f5d0126dbadb,
hasCreditCookie: true,
attack: 24,
name: 'Pokemon 2',
__v: 0 }
Salvo: { created_at: Wed Jan 27 2016 21:33:43 GMT-0200 (Horário brasileiro de verão),
tags: [],
_id: 56a953d7cdb6f5d0126dbadc,
hasCreditCookie: true,
attack: 25,
name: 'Pokemon 3',
__v: 0 }
Buscado: { created_at: Wed Jan 27 2016 21:32:16 GMT-0200 (Horário brasileiro de verão),
tags: [],
__v: 0,
hasCreditCookie: true,
attack: 23,
name: 'Pokemon 1',
_id: 56a9538058e17770032a1b5b },{ created_at: Wed Jan 27 2016 21:32:16 GMT-0200 (Horário brasileiro de verão),
tags: [],
__v: 0,
hasCreditCookie: true,
attack: 24,
name: 'Pokemon 2',
_id: 56a9538058e17770032a1b5c },{ created_at: Wed Jan 27 2016 21:32:16 GMT-0200 (Horário brasileiro de verão),
tags: [],
__v: 0,
hasCreditCookie: true,
attack: 25,
name: 'Pokemon 3',
_id: 56a9538058e17770032a1b5d },{ created_at: Wed Jan 27 2016 21:32:43 GMT-0200 (Horário brasileiro de verão),
```
## Busque **todos** os Pokemons com `attack > 50` e `height > 0.5`:
**Código:**
```JS
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
name: String
}
const pokemonSchema = new Schema(_schema);
const PokemonModel = mongoose.model('pokemons', pokemonSchema);
const query = { $and: [ { attack: { $gt: 50 }}, { height: { $gt: 0.5 }} ] };
PokemonModel.find(query, function (err, data){
if(err) return console.log('ERRO: ', err);
return console.log('Buscou: ', data);
});
module.exports = pokemonSchema;
```
**Retorno:**
```
Buscou: [ { moves: [ 'investida', 'lança-chamas', 'run', 'esquiva', 'desvio' ],
active: false,
height: 0.6,
attack: 52,
type: 'fogo',
description: 'Esse é o cão chupando manda de fofinho',
name: 'Charmander',
_id: 56429adb65048c35cec000c7 } ]
```
## Altere, **inserindo**, o Pokemon `Nerdmon` com `attack` igual a 49 e com os valores dos outros campos a sua escolha.
**Código:**
```JS
const crypto = require('crypto');
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
var states = 'opening open closing closed'.split(' ')
const _schema = {
name: { type: String, maxlength: 10, minlength: 3 },
pokebola: { type: String, enum: states },
attack: { type: Number, min: 2, max: 9999 },
created_at: { type: Date, default: Date.now },
image_bin: Buffer,
active: Boolean,
attacks: Schema.Types.Mixed,
type: [{type: Schema.Types.ObjectId, ref: 'types'}],
tags: [String]
}
const mixed = [
{ name: 'Chuve alto',
power: 10500,
order: 1,
active: 1,
},
{ name: 'Chuve baixo',
power: 1000,
order: 2,
active: 0,
}
];
const buffer = new Buffer(crypto.createHash('sha256').update(new Buffer(0)).digest('binary'), 'binary');
const data = { name: "Nerdmon",
pokebola: 'closed',
attack: 49,
image_bin: buffer,
active: 1,
attacks: mixed,
type: '5691d60743056d6e1566274e',
tags: ['schema', 'nodejs', 'be-mean'],
};
const pokemonSchema = new Schema(_schema);
const PokemonModel = mongoose.model('pokemons', pokemonSchema);
var pokemon = new PokemonModel(data);
pokemon.save( function (err, data){
if(err) return console.log('ERRO: ', err);
return console.log('Inseriu: ', data);
});
module.exports = pokemonSchema;
```
**Retorno:**
```
Inseriu: { created_at: Wed Jan 27 2016 20:41:22 GMT-0200 (Horário brasileiro de verão),
type: [ 5691d60743056d6e1566274e ],
tags: [ 'schema', 'nodejs', 'be-mean' ],
_id: 56a94792f0fcdc8c17ee94d5,
attacks:
[ { name: 'Chuve alto', power: 10500, order: 1, active: 1 },
{ name: 'Chuve baixo', power: 1000, order: 2, active: 0 } ],
active: true,
image_bin:
Binary {
_bsontype: 'Binary',
sub_type: 0,
position: 32,
buffer: <Buffer e3 b0 c4 42 98 fc 1c 14 9a fb f4 c8 99 6f b9 24 27 ae 41 e4 64 9b 93 4c a4 95 99 1b 78 52 b8 55> },
attack: 49,
pokebola: 'closed',
name: 'Nerdmon',
__v: 0 }
```
## Remova **todos** os Pokemons com `attack` **acima de 50**.
**Código:**
```JS
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
var states = 'opening open closing closed'.split(' ')
const _schema = {
name: { type: String, match: /^S/, maxlength: 10, minlength: 3 },
pokebola: { type: String, enum: states },
attack: { type: Number, min: 2, max: 9999 },
created_at: { type: Date, default: Date.now },
image_bin: Buffer,
active: Boolean,
attacks: Schema.Types.Mixed,
type: [{type: Schema.Types.ObjectId, ref: 'types'}],
tags: [String]
}
const PokemonSchema = new Schema(_schema);
const Pokemon = mongoose.model('pokemons', PokemonSchema);
const query = { attack: { $gt: 50 } };
Pokemon.remove(query, function (err, data) {
if (err) return console.log('ERRO: ', err);
return console.log('Deletou:', data);
});
```
**Retorno:**
```
Deletou: { result: { ok: 1, n: 2 },
connection:
EventEmitter {
domain: null,
_events:
{ close: [Object],
error: [Object],
timeout: [Object],
parseError: [Object],
connect: [Function] },
_eventsCount: 5,
_maxListeners: undefined,
options:
{ socketOptions: {},
auto_reconnect: true,
host: 'localhost',
port: 27017,
cursorFactory: [Object],
reconnect: true,
emitError: true,
size: 5,
disconnectHandler: [Object],
bson: {},
messageHandler: [Function],
wireProtocolHandler: {} },
id: 2,
logger: { className: 'Connection' },
bson: {},
tag: undefined,
messageHandler: [Function],
maxBsonMessageSize: 67108864,
port: 27017,
host: 'localhost',
keepAlive: true,
keepAliveInitialDelay: 0,
noDelay: true,
connectionTimeout: 0,
socketTimeout: 0,
destroyed: false,
domainSocket: false,
singleBufferSerializtion: true,
serializationFunction: 'toBinUnified',
ca: null,
cert: null,
key: null,
passphrase: null,
ssl: false,
rejectUnauthorized: false,
checkServerIdentity: true,
responseOptions: { promoteLongs: true },
flushing: false,
queue: [],
connection:
Socket {
_connecting: false,
_hadError: false,
_handle: [Object],
_parent: null,
_host: 'localhost',
_readableState: [Object],
readable: true,
domain: null,
_events: [Object],
_eventsCount: 8,
_maxListeners: undefined,
_writableState: [Object],
writable: true,
allowHalfOpen: false,
destroyed: false,
bytesRead: 56,
_bytesDispatched: 176,
_sockname: null,
_pendingData: null,
_pendingEncoding: '',
_idleNext: null,
_idlePrev: null,
_idleTimeout: -1,
read: [Function],
_consuming: true },
writeStream: null,
buffer: null,
sizeOfMessage: 0,
bytesRead: 0,
stubBuffer: null } }
```
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 06 - Exercício
**User:** ronal2do
**Author:** Ronaldo Lima
**Date:** 1468073529260
##### [Exercício-01](#crie-um-schema-com-cada-tipo-explicado-inserindo-tanto-um-objeto-correto-como-um-objeto-que-desencadeie-erros-de-valida%C3%A7%C3%A3o-padr%C3%A3o-criar-especificamente)
##### [Exercício-02](#cadastre-3-pokemons-de-uma-s%C3%B3-vez)
##### [Exercício-03](#busque-todos-os-pokemons-com-attack--120-e-speed--70)
##### [Exercício-04](#altere-inserindo-o-pokemon-nerdmon-com-attack-igual-a-49-e-com-os-valores-dos-outros-campos-a-sua-escolha)
##### [Exercício-05](#remova-todos-os-pokemons-com-attack-acima-de-50)
## Crie um Schema com cada tipo explicado, inserindo tanto um objeto correto, como um objeto que desencadeie erros de validação padrão, criar especificamente:
* Number: possui os validadores de `max` e `min`
* String: possui os validadores de `enum`, `match`, `maxlength` e `minlength`
```js
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
name: {type: String, match: /^./i }
, age: {type: Number, min: 15}
, gender: {type: String, enum: ['M', 'F']}
, city: {type: String, maxlength: 10}
, state: {type: String, minlength: 2}
}
// Criação do Schema
const pokemonSchema = new Schema(_schema);
const data = {
name: '2D Lima',
age: 28,
gender: 'M',
city: 'Pallet',
state: 'PL'
}
const Model = mongoose.model('pokemons', pokemonSchema);
const poke = new Model(data);
poke.save(function (err, data) {
if (err) return console.log('ERRO: ', err);
console.log('Inseriu: ', data)
})
module.exports = pokemonSchema;
```
#### Saída
```
Inseriu: { __v: 0,
name: '2D Lima',
age: 28,
gender: 'M',
city: 'Pallet',
state: 'PL',
_id: 5781ce4a2f3a11730f5f80df }
```
### Dados com falha de validação.
```js
const data = {
name: '2D Lima',
age: 10,
gender: 'M',
city: 'Pallet',
state: 'P'
}
```
### Saída
```js
ERRO: { [ValidationError: pokemons validation failed]
message: 'pokemons validation failed',
name: 'ValidationError',
errors:
{ state:
{ [ValidatorError: Path `state` (`P`) is shorter than the minimum allowed length (2).]
message: 'Path `state` (`P`) is shorter than the minimum allowed length (2).',
name: 'ValidatorError',
properties: [Object],
kind: 'minlength',
path: 'state',
value: 'P' },
age:
{ [ValidatorError: Path `age` (10) is less than minimum allowed value (15).]
message: 'Path `age` (10) is less than minimum allowed value (15).',
name: 'ValidatorError',
properties: [Object],
kind: 'min',
path: 'age',
value: 10 } } }
```
## Cadastre 3 pokemons **de uma só vez**:
```js
"use strict";
const mongoose = require("mongoose");
const dbURI = "mongodb://localhost/pokemons";
mongoose.connect(dbURI);
const Schema = mongoose.Schema;
const _schema = {
attack: Number,
created: Date,
defense: Number,
height: String,
name: String,
speed: Number,
types: [String]
};
const PokemonSchema = new Schema(_schema);
const Pokemon = mongoose.model("pokemons", PokemonSchema);
const data = [
{
"attack": 80,
"created": new Date(),
"defense": 90,
"height": "14",
"name": "Walrein",
"speed": 65,
"types": ["water", "ice"]
},
{
"attack": 107,
"created": new Date(),
"defense": 122,
"height": "0",
"name": "Chesnaught",
"speed": 64,
"types": ["fighting", "grass"]
},
{
"attack": 45,
"created": new Date(),
"defense": 40,
"height": "99",
"name": "Fennekin",
"speed": 60,
"types": ["fire"]
}
];
Pokemon.create(data, (err, data) => {
if (err) return console.log("ERRO: ", err);
console.log("Pokémons cadastrados: ", data);
});
```
### Resultado:
```
Pokémons cadastrados: [ { __v: 0,
attack: 80,
created: Sun Jul 10 2016 00:33:34 GMT-0300 (BRT),
defense: 90,
height: '14',
name: 'Walrein',
speed: 65,
_id: 5781c20e87d241940ef3d09e,
types: [ 'water', 'ice' ] },
{ __v: 0,
attack: 107,
created: Sun Jul 10 2016 00:33:34 GMT-0300 (BRT),
defense: 122,
height: '0',
name: 'Chesnaught',
speed: 64,
_id: 5781c20e87d241940ef3d09f,
types: [ 'fighting', 'grass' ] },
{ __v: 0,
attack: 45,
created: Sun Jul 10 2016 00:33:34 GMT-0300 (BRT),
defense: 40,
height: '99',
name: 'Fennekin',
speed: 60,
_id: 5781c20e87d241940ef3d0a0,
types: [ 'fire' ] } ]
```
## Busque **todos** os Pokemons com `attack > 120` e `speed > 70`:
### Mongo
```
MacBook-Pro-de-Ronaldo(mongod-3.2.7) pokemons> db.pokemons.count();
49
MacBook-Pro-de-Ronaldo(mongod-3.2.7) pokemons> var query = { attack: { $gt:120 }, speed: { $gt: 70 } };
MacBook-Pro-de-Ronaldo(mongod-3.2.7) pokemons> db.pokemons.count(query);
3
```
### Node
```js
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {};
const pokemonSchema = new Schema(_schema);
const PokemonModel = mongoose.model("pokemons", pokemonSchema);
const query = {attack: { $gt: 120 }, speed: { $gt: 70 } };
PokemonModel.find(query, (err, data) => {
if (err) return console.log("ERRO: ", err);
return console.log("Resultado para a busca: ", data);
});
```
### Saída
```js
Resultado para a busca: [ { _id: 5781c34fb9b95aa10ebed6d8,
attack: 121,
created: Sun Jul 10 2016 00:38:55 GMT-0300 (BRT),
defense: 90,
height: '14',
name: 'Teste Exercício',
speed: 71,
types: [ 'water', 'ice' ],
__v: 0 },
{ _id: 5781c35cd25798a50e7410d6,
attack: 121,
created: Sun Jul 10 2016 00:39:08 GMT-0300 (BRT),
defense: 90,
height: '14',
name: 'Teste Exercício',
speed: 71,
types: [ 'water', 'ice', 'WTF' ],
__v: 0 },
{ _id: 5781c364472d1aa90eba07f6,
attack: 121,
created: Sun Jul 10 2016 00:39:16 GMT-0300 (BRT),
defense: 90,
height: '14',
name: 'Teste Exercício',
speed: 71,
types: [ 'water', 'ice', 'WTF' ],
__v: 0 } ]
```
## Altere, **inserindo**, o Pokemon `Nerdmon` com `attack` igual a 49 e com os valores dos outros campos a sua escolha.
### Confirmando no console do MongoDB que não há o Nerdmon já inserido
```js
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
attack: Number,
created: Date,
defense: Number,
height: String,
name: String,
speed: Number,
types: [String]
};
const pokemonSchema = new Schema(_schema);
const pokeModel = mongoose.model('Pokemon', pokemonSchema);
const query = {name: /Nerdmon/i};
const mod = {
$setOnInsert: {
attack: 49,
created: new Date(),
defense: 90,
height: "14",
name: "Nerdmon",
speed: 71,
types: ["water", "ice", "WTF"]
}
};
const options = {upsert: true};
pokeModel.update(query, mod, options, (err, data) => {
if(err) return console.log('ERRO: ', err);
console.log('Alterou: ', data);
});
module.exports = pokemonSchema;
```
### Saída
```
Alterou: { ok: 1,
nModified: 0,
n: 1,
upserted: [ { index: 0, _id: 5781c8efebecb288fb05923b } ] }
```
## Remova **todos** os Pokemons com `attack` **acima de 50**.
### Verifica no console do MongoDB a quantidade total de pokemons com attack acima de 50 antes da exclusão
```js
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
};
const pokemonSchema = new Schema(_schema);
const pokeModel = mongoose.model('Pokemon', pokemonSchema);
const query = {attack: {$gt: 50}};
pokeModel.remove(query, (err, data) => {
if(err) return console.log('ERRO: ', err);
console.log('Removendo: ', data);
});
module.exports = pokemonSchema;
```
### Saída
```
Removendo: { result: { ok: 1, n: 25 },
connection:
EventEmitter {
domain: null,
_events:
{ close: [Object],
error: [Object],
timeout: [Object],
parseError: [Object],
connect: [Function] },
_eventsCount: 5,
_maxListeners: undefined,
options:
{ socketOptions: {},
auto_reconnect: true,
host: 'localhost',
port: 27017,
cursorFactory: [Object],
reconnect: true,
emitError: true,
size: 5,
disconnectHandler: [Object],
bson: {},
messageHandler: [Function],
wireProtocolHandler: [Object] },
id: 0,
logger: { className: 'Connection' },
bson: {},
tag: undefined,
messageHandler: [Function],
maxBsonMessageSize: 67108864,
port: 27017,
host: 'localhost',
keepAlive: true,
keepAliveInitialDelay: 0,
noDelay: true,
connectionTimeout: 0,
socketTimeout: 0,
destroyed: false,
domainSocket: false,
singleBufferSerializtion: true,
serializationFunction: 'toBinUnified',
ca: null,
cert: null,
key: null,
passphrase: null,
ssl: false,
rejectUnauthorized: false,
checkServerIdentity: true,
responseOptions: { promoteLongs: true },
flushing: false,
queue: [],
connection:
Socket {
_connecting: false,
_hadError: false,
_handle: [Object],
_parent: null,
_host: 'localhost',
_readableState: [Object],
readable: true,
domain: null,
_events: [Object],
_eventsCount: 8,
_maxListeners: undefined,
_writableState: [Object],
writable: true,
allowHalfOpen: false,
destroyed: false,
bytesRead: 250,
_bytesDispatched: 222,
_sockname: null,
_pendingData: null,
_pendingEncoding: '',
server: null,
_server: null,
_idleNext: null,
_idlePrev: null,
_idleTimeout: -1,
read: [Function],
_consuming: true },
writeStream: null,
hashedName: '29bafad3b32b11dc7ce934204952515ea5984b3c',
buffer: null,
sizeOfMessage: 0,
bytesRead: 0,
stubBuffer: null } }
``` | {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 06 - Exercício
**user:** [Diego Ferreira](https://github.com/tuchedsf)
**autor:** Diego Ferreira
## 1- Crie um Schema com cada tipo explicado, inserindo tanto um objeto correto, como um objeto que desencadeie erros de validação padrão, criar especificamente:
* 1.1. para String: `enum`, `match`, `maxlength` e `minlength`
* 1.2. para Number: `max` e `min`
```js
'use strict';
const mongoose = required('mongoose');
const _schema = {
name : {type : String, required: true} ,
description: {type: String, minlength : 5, maxlength : 50},
attack: {type: Number, min: 40, max: 95},
defense : {type : Number, match: /\d/g },
height : Number,
cor: {type: String, enum: ['Red', 'Blue', 'Green'], required: true},
created_at: { type: Date, default: Date.now }
}
const PokemonSchema = new mongoose.Schema(_schema);
const PokemonModel = mongoose.model('pokemons', PokemonSchema);
//cadastro dados corretos
const dadosCorretos = {
name : "pokebola",
description: "tipo de pokemon que guarda outros pokemons",
attack: 40,
defense : 100,
height : 0.5,
cor: "Red"
}
const pokemon = new PokemonModel(dadosCorretos);
pokemon.save(function(err,data){
if (err) return console.log("Erro de validação: " + err);
return console.log("Dados ok! Validção OK: " + data);
});
```
// Saida Console
```
Dados ok! Validção OK: { created_at: Tue May 03 2016 21:56:53 GMT-0300 (BRT),
_id: 572948d5d4c92cc2d56ef17f,
cor: 'Red',
height: 5,
defense: 100,
attack: 40,
description: 'tipo de pokemon que guarda outros pokemons',
name: 'pokebola',
__v: 0 }
```
```js
//cadastro dados errados
const dadosErrados = {
name : "",
description: "",
attack: 20,
defense : "grande",
height : 0.5,
cor: "Yellow"
}
const pokemon = new PokemonModel(dadosErrados);
pokemon.save(function(err,data){
if (err) return console.log("Erro de validação: " + err);
return console.log("Dados ok! Validção OK: " + data);
});
```
// Saida Console:
```
Erro de validação:
ValidationError:
CastError: Cast to Number failed for value "grande" at path "defense",
`Yellow` is not a valid enum value for path `cor`.,
Path `attack` (20) is less than minimum allowed value (40).,
Path `description` (``) is shorter than the minimum allowed length (5).,
Path `name` is required.
```
## 2- Cadastre 3 pokemons **de uma só vez**:
```js
'use strict';
const mongoose = required('mongoose');
const _schema = {
name : {type : String, required: true} ,
description: {type: String, minlength : 5, maxlength : 50},
attack: {type: Number, min: 40, max: 95},
defense : {type : Number, match: /\d/g },
height : Number,
cor: {type: String, enum: ['Red', 'Blue', 'Green'], required: true},
created_at: { type: Date, default: Date.now }
}
const PokemonSchema = new mongoose.Schema(_schema);
const PokemonModel = mongoose.model('pokemons', PokemonSchema);
const array = [
{
name : "Yoytubemon",
description: "engana os pokes projetando videos na tela",
attack: 95,
defense : 20,
height : 1,
cor: "Red"
},
{
name : "Gramosauro",
description: "Pokemon especialista em grama",
attack: 40,
defense : 30,
height : 0.4,
cor: "Green"
},
{
name : "SublimeTextmon",
description: "Pokemon especialista em grama",
attack: 82,
defense : 60,
height : 0.5,
cor: "Blue"
}
]
PokemonModel.create(array, function(err,data){
if (err) return console.log("Erro: " + err);
return console.log("Dados: " + data);
});
```
// Saida Console:
```
Dados: { created_at: Tue May 03 2016 22:09:51 GMT-0300 (BRT),
_id: 57294bdfb6de032bd6811515,
cor: 'Red',
height: 1,
defense: 20,
attack: 95,
description: 'engana os pokes projetando videos na tela',
name: 'Yoytubemon',
__v: 0 },{ created_at: Tue May 03 2016 22:09:51 GMT-0300 (BRT),
_id: 57294bdfb6de032bd6811516,
cor: 'Green',
height: 0.4,
defense: 30,
attack: 40,
description: 'Pokemon especialista em grama',
name: 'Gramosauro',
__v: 0 },{ created_at: Tue May 03 2016 22:09:51 GMT-0300 (BRT),
_id: 57294bdfb6de032bd6811517,
cor: 'Blue',
height: 0.5,
defense: 60,
attack: 82,
description: 'Pokemon especialista em grama',
name: 'SublimeTextmon',
__v: 0 }
```
## 3- Busque **todos** os Pokemons com `attack > 50` e `height > 0.5`:
```js
'use strict';
const mongoose = require('mongoose');
const _schema = {
name : {type : String, required: true} ,
description: {type: String, minlength : 5, maxlength : 50},
attack: {type: Number, min: 40, max: 95},
defense : {type : Number, match: /\d/g },
height : Number,
cor: {type: String, enum: ['Red', 'Blue', 'Green'], required: true},
created_at: { type: Date, default: Date.now }
}
const PokemonSchema = new mongoose.Schema(_schema);
const PokemonModel = mongoose.model('pokemons', PokemonSchema);
const query = { $and:[ { attack: { $gt: 50 } }, { height: { $gt: 0.5 } } ] }
PokemonModel.find(query,function(err,data){
if (err) return console.log("Erro " + err);
return console.log("Dados " + data);
});
```
// Saida Console:
```
Dados { created_at: Tue May 03 2016 22:09:51 GMT-0300 (BRT),
__v: 0,
cor: 'Red',
height: 1,
defense: 20,
attack: 95,
description: 'engana os pokes projetando videos na tela',
name: 'Yoytubemon',
_id: 57294bdfb6de032bd6811515 }
```
## 4 - Altere, **inserindo**, o Pokemon `Nerdmon` com `attack` igual a 49 e com os valores dos outros campos a sua escolha.
```js
'use strict';
const mongoose = require('mongoose');
const _schema = {
name : {type : String, required: true} ,
description: {type: String, minlength : 5, maxlength : 50},
attack: {type: Number, min: 40, max: 95},
defense : {type : Number, match: /\d/g },
height : Number,
cor: {type: String, enum: ['Red', 'Blue', 'Green'], required: true},
created_at: { type: Date, default: Date.now }
}
const PokemonSchema = new mongoose.Schema(_schema);
const PokemonModel = mongoose.model('pokemons', PokemonSchema);
const query = {name: /nerdmon/i}
const mod = {$setOnInsert: {
name : "Nerdmon",
description: "Pokemon que altera inserindo",
attack: 40,
defense : 40,
height : 0.7,
cor: "Blue"
}}
const options = {upsert: true}
PokemonModel.update(query,mod,options,function(err, data){
if (err) return console.log("Erro " + err);
return console.log("Alterou: " + data);
});
```
// Saida Console:
```
Alterou: { ok: 1,
nModified: 0,
n: 1,
upserted: [ { index: 0, _id: 57294fe05daea5abb1cb1c4f } ] }
db.pokemons.find({name:/nerdmon/i})
{
"_id": ObjectId("57294fe05daea5abb1cb1c4f"),
"name": "Nerdmon",
"description": "Pokemon que altera inserindo",
"attack": 40,
"defense": 40,
"height": 0.7,
"cor": "Blue"
}
Fetched 1 record(s) in 50ms
```
## 5 - Remova **todos** os Pokemons com `attack` **acima de 50**.
```js
const mongoose = require('mongoose');
const _schema = {
name : {type : String, required: true} ,
description: {type: String, minlength : 5, maxlength : 50},
attack: {type: Number, min: 40, max: 95},
defense : {type : Number, match: /\d/g },
height : Number,
cor: {type: String, enum: ['Red', 'Blue', 'Green'], required: true},
created_at: { type: Date, default: Date.now }
}
const PokemonSchema = new mongoose.Schema(_schema);
const PokemonModel = mongoose.model('pokemons', PokemonSchema);
const query = {attack : {$gt : 50}}
PokemonModel.remove(query,function(err,data){
if (err) return console.log("Erro " + err);
return console.log("Dados " + data);
});
```
// Saida Console:
```
Dados {"ok":1,"n":2}
```
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 06 - Exercício
**user:** https://github.com/paulosilva92
**autor:** Paulo Roberto da Silva
**date:** Sat Mar 12 2016 22:40:30 GMT-0300 (BRT)
## 1. Crie um schema com cada tipo explicado, inserindo tanto um objeto correto, como um objeto que desencadeie erro de validação padrão, criar especificamente:
### 1.1 para String: enum, match, maxlength e minlength
### 1.2 para Number: max e min
##### Inserção correta
```js
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const schemaObj = {
string : {
type : String,
enum : ['string', 'não string'],
match : [/string/i, 'Não foi encontrada a palavra string 1'],
maxlength : [7, 'String muito grande'],
minlength: [2, 'String muito curta']
},
boolean : {
type : Boolean
},
number : {
type : Number,
max : [10, 'Numero muito grande'],
min : [2, 'Numero muito pequeno']
},
array : {
type : [String]
},
date : {
type : Date
},
objectID : {
type : Schema.Types.ObjectId
},
mixed : {
type : Schema.Types.Mixed
}
};
const schemaTest = new Schema(schemaObj);
const Correto = {
string : 'string',
boolean : true,
number : 4,
array : ['Paulo', 'Silva'],
date : Date.now(),
objectID : ['56e4d08d45228b2a7019d969'],
mixed : [{name : 'obj1'}, {name : 'obj2'}]
};
const Model = mongoose.model('model',schemaTest);
const modelTeste = new Model(Correto);
modelTeste.save((err, data)=>{
if(err) return console.log('Erro ',err);
console.log('Foi ',data);
});
```
##### Resultado
```js
Foi { array: [ 'Paulo', 'Silva' ],
_id: 56e4da30cfbc315979eaeed2,
mixed: [ { name: 'obj1' }, { name: 'obj2' } ],
objectID: 56e4d08d45228b2a7019d969,
date: Sun Mar 13 2016 00:10:40 GMT-0300 (BRT),
number: 4,
boolean: true,
string: 'string',
__v: 0 }
```
##### Inserção com erros
```js
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const schemaObj = {
string : {
type : String,
enum : ['string', 'não string'],
match : [/string/i, 'Não foi encontrada a palavra string 1'],
maxlength : [7, 'String muito grande'],
minlength: [2, 'String muito curta']
},
boolean : {
type : Boolean
},
number : {
type : Number,
max : [10, 'Numero muito grande'],
min : [2, 'Numero muito pequeno']
},
array : {
type : [String]
},
date : {
type : Date
},
objectID : {
type : Schema.Types.ObjectId
},
mixed : {
type : Schema.Types.Mixed
}
};
const schemaTest = new Schema(schemaObj);
const Incorreto = {
string : 'stringnumero2',
boolean : true,
number : 12,
array : ['Paulo', 'Silva'],
date : Date.now(),
objectID : ['56e4d08d45228b2a7019d969'],
mixed : [{name : 'obj1'}, {name : 'obj2'}]
};
const Model = mongoose.model('model',schemaTest);
const modelTeste = new Model(Incorreto);
modelTeste.save((err, data)=>{
if(err) return console.log('Erro ',err);
console.log('Foi ',data);
});
```
##### Resultado
```js
Erro { [ValidationError: model validation failed]
message: 'model validation failed',
name: 'ValidationError',
errors:
{ number:
{ [ValidatorError: Numero muito grande]
properties: [Object],
message: 'Numero muito grande',
name: 'ValidatorError',
kind: 'max',
path: 'number',
value: 12 },
string:
{ [ValidatorError: `stringnumero2` is not a valid enum value for path `string`.]
properties: [Object],
message: '`stringnumero2` is not a valid enum value for path `string`.',
name: 'ValidatorError',
kind: 'enum',
path: 'string',
value: 'stringnumero2' } } }
```
## Cadastre 3 pokemons de uma vez só
```js
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/be-mean-instagram');
const Schema = mongoose.Schema;
const _schema = {
attack: Number,
created: {type : Date, default : Date.now},
defense: Number,
height: Number,
hp: Number,
name: String,
speed: Number,
types: [String]
}
const pokemonSchema = new Schema(_schema);
const data = [
{
"attack": 52,
"created": "2013-11-03T15:05:41.271082",
"defense": 43,
"height": "6",
"hp": 39,
"name": "Charmander",
"speed": 65,
"types": [
"fire"
]
},
{
"attack": 64,
"created": "2013-11-03T15:05:41.273462",
"defense": 58,
"height": "11",
"hp": 58,
"name": "Charmeleon",
"speed": 80,
"types": [
"fire"
]
},
{
"attack": 56,
"created": "2013-11-03T15:05:41.305777",
"defense": 35,
"height": "3",
"hp": 30,
"name": "Rattata",
"speed": 72,
"types": [
"normal"
]
}
]
const pokemonModel = mongoose.model('pokemons', pokemonSchema);
pokemonModel.create(data,function (err, data) {
if (err) return console.log('ERRO: ', err);
console.log('Inseriu: ', data)
});
```
##### Resultado
```js
Inseriu: [ { created: Sun Nov 03 2013 13:05:41 GMT-0200 (BRST),
types: [ 'fire' ],
_id: 56e4e7e2c8f021a80345a462,
speed: 65,
name: 'Charmander',
hp: 39,
height: 6,
defense: 43,
attack: 52,
__v: 0 },
{ created: Sun Nov 03 2013 13:05:41 GMT-0200 (BRST),
types: [ 'fire' ],
_id: 56e4e7e2c8f021a80345a463,
speed: 80,
name: 'Charmeleon',
hp: 58,
height: 11,
defense: 58,
attack: 64,
__v: 0 },
{ created: Sun Nov 03 2013 13:05:41 GMT-0200 (BRST),
types: [ 'normal' ],
_id: 56e4e7e2c8f021a80345a464,
speed: 72,
name: 'Rattata',
hp: 30,
height: 3,
defense: 35,
attack: 56,
__v: 0 } ]
```
## Busque todos os pokemons com attack > 50 e height > 0.5
```js
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/be-mean-instagram');
const Schema = mongoose.Schema;
const _schema = {
attack: Number,
created: {type : Date, default : Date.now},
defense: Number,
height: Number,
hp: Number,
name: String,
speed: Number,
types: [String]
}
const pokemonSchema = new Schema(_schema);
const pokemonModel = mongoose.model('pokemons', pokemonSchema);
const query = {$and : [{attack : {$gt : 50}}, {height : {$gt : 0.5}} ] }
pokemonModel.find(query, (err,data)=>{
if (err) return console.log('ERRO: ', err);
console.log('Listou: ', data)
});
```
##### Resultado
```js
Listou: [ { created: Sun Nov 03 2013 13:05:41 GMT-0200 (BRST),
types: [ 'fire' ],
__v: 0,
speed: 65,
name: 'Charmander',
hp: 39,
height: 6,
defense: 43,
attack: 52,
_id: 56e4e7e2c8f021a80345a462 },
{ created: Sun Nov 03 2013 13:05:41 GMT-0200 (BRST),
types: [ 'fire' ],
__v: 0,
speed: 80,
name: 'Charmeleon',
hp: 58,
height: 11,
defense: 58,
attack: 64,
_id: 56e4e7e2c8f021a80345a463 },
{ created: Sun Nov 03 2013 13:05:41 GMT-0200 (BRST),
types: [ 'normal' ],
__v: 0,
speed: 72,
name: 'Rattata',
hp: 30,
height: 3,
defense: 35,
attack: 56,
_id: 56e4e7e2c8f021a80345a464 } ]
```
## Altere, inserindo, o pokemon Nerdmon com attack = 49 e com os valores dos outros campos a sua escolha
```js
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/be-mean-instagram');
const Schema = mongoose.Schema;
const _schema = {
attack: Number,
created: {type : Date, default : Date.now},
defense: Number,
height: Number,
hp: Number,
name: String,
speed: Number,
types: [String]
}
const pokemonSchema = new Schema(_schema);
const pokemonModel = mongoose.model('pokemons', pokemonSchema);
const query = {name : /nerdmon/i, attack : 49 };
const nerdmon = {
name: 'Nerdmon',
attack : 49,
defense : 35,
height : 10,
hp : 200,
speed : 300,
types : ['fire']
};
const options = { upsert : true };
pokemonModel.update(query,nerdmon, options, (err,data)=>{
if (err) return console.log('ERRO: ', err);
console.log('Atualizou ou Inseriu: ', data);
});
```
##### Procurando no mongo pelo id do nerdmon inserido
```js
> db.pokemons.find({_id: ObjectId('56e4edea660f144f23c1ad6c')}).pretty()
{
"_id" : ObjectId("56e4edea660f144f23c1ad6c"),
"attack" : 49,
"types" : [
"fire"
],
"speed" : 300,
"hp" : 200,
"height" : 10,
"defense" : 35,
"name" : "Nerdmon"
}
```
## Remova todos os pokemons com attack > 50
```js
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/be-mean-instagram');
const Schema = mongoose.Schema;
const _schema = {
attack: Number,
created: {type : Date, default : Date.now},
defense: Number,
height: Number,
hp: Number,
name: String,
speed: Number,
types: [String]
}
const pokemonSchema = new Schema(_schema);
const pokemonModel = mongoose.model('pokemons', pokemonSchema);
const query = { attack : {$gt : 50} };
pokemonModel.remove(query, (err, data)=>{
if (err) return console.log('ERRO: ', err);
console.log('Apagou: ', data)
});
```
##### Resultado
```js
Apagou: { result: { ok: 1, n: 3 },
``` | {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# NodeJS - Aula 06 - Exercício
**Autor**: Igor luíz
**Github**: [Halfeld](https://github.com/Halfeld)
**Data**: 1458017385252
## Crie um Schema com cada tipo explicado, inserindo tanto um objeto correto, como um objeto que desenvadeie erros de validação padrão, crir especificamente:
1. Para string: `enum`, `match`, `maxlength` e `minlength`
2. Para number: `max` e `min`
#### Dando objeto correto
```js
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
pessoa: {type: String, match: /^[a-z]/i },
sexo: {type: String, enum: ['Masculino', 'Feminino'] },
senha: {type: String, maxlength: 20, minlength: 6, match: /^\w./ },
idade: {type: Number, max: 115 },
idiomas: {type: Number, min: 1}
};
const pessoaSchema = new Schema(_schema);
const PessoaModel = mongoose.model('pessoas', pessoaSchema);
const dados = {
pessoa: 'Igor Luíz',
sexo: 'Masculino',
senha: 'helloworld',
idade: 17,
idiomas: 2
};
const pessoa1 = new PessoaModel(dados);
pessoa1.save((err, data) => {
if (err) return console.log('ERROR: ', err);
console.log('Inseriu: ', data);
});
module.exports = pessoaSchema;
// Saída do terminal
Mongoose esta conectado em: mongodb://localhost/teste
Mongoose esta com a conexão aberta
Inseriu: { _id: 56e780414fad665c02a5272b,
idiomas: 2,
idade: 17,
senha: 'helloworld',
sexo: 'Masculino',
pessoa: 'Igor Luíz',
__v: 0 }
```
#### Dando objeto com erro na validação
```js
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
pessoa: {type: String, match: /^[a-z]/i },
sexo: {type: String, enum: ['Masculino', 'Feminino'] },
senha: {type: String, maxlength: 20, minlength: 6, match: /^\w./ },
idade: {type: Number, max: 115 },
idiomas: {type: Number, min: 1}
};
const pessoaSchema = new Schema(_schema);
const PessoaModel = mongoose.model('pessoas', pessoaSchema);
const dados = {
pessoa: '7Igor Luíz', // Nome começando com número
sexo: 'alien', // Sem ser uma opção
senha: 'hello', // Menos de 6 caracteres
idade: 200, // Idade maior que o limite
idiomas: 0 // Sem idioma
};
const pessoa1 = new PessoaModel(dados);
pessoa1.save((err, data) => {
if (err) return console.log('ERROR: ', err);
console.log('Inseriu: ', data);
});
module.exports = pessoaSchema;
// Saída do terminal
ERROR: { [ValidationError: pessoas validation failed]
message: 'pessoas validation failed',
name: 'ValidationError',
errors:
{ idiomas:
{ [ValidatorError: Path `idiomas` (0) is less than minimum allowed value (1).]
properties: [Object],
message: 'Path `idiomas` (0) is less than minimum allowed value (1).',
name: 'ValidatorError',
kind: 'min',
path: 'idiomas',
value: 0 },
idade:
{ [ValidatorError: Path `idade` (200) is more than maximum allowed value (115).]
properties: [Object],
message: 'Path `idade` (200) is more than maximum allowed value (115).',
name: 'ValidatorError',
kind: 'max',
path: 'idade',
value: 200 },
senha:
{ [ValidatorError: Path `senha` (`hello`) is shorter than the minimum allowed length (6).]
properties: [Object],
message: 'Path `senha` (`hello`) is shorter than the minimum allowed length (6).',
name: 'ValidatorError',
kind: 'minlength',
path: 'senha',
value: 'hello' },
sexo:
{ [ValidatorError: `alien` is not a valid enum value for path `sexo`.]
properties: [Object],
message: '`alien` is not a valid enum value for path `sexo`.',
name: 'ValidatorError',
kind: 'enum',
path: 'sexo',
value: 'alien' },
pessoa:
{ [ValidatorError: Path `pessoa` is invalid (7Igor Luíz).]
properties: [Object],
message: 'Path `pessoa` is invalid (7Igor Luíz).',
name: 'ValidatorError',
kind: 'regexp',
path: 'pessoa',
value: '7Igor Luíz' } } }
Mongoose esta conectado em: mongodb://localhost/teste
Mongoose esta com a conexão aberta
```
## Cadrastre 3 pokemons de uma só vez. (pesquisar)
```js
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
name: String,
type: String
};
const poke = [
{ name: 'pokemon_1', type: 'tipo_1' },
{ name: 'pokemon_2', type: 'tipo_2' },
{ name: 'pokemon_3', type: 'tipo_3' }
];
const pokeSchema = new Schema(_schema);
const PokeModel = mongoose.model('intopoke', pokeSchema);
PokeModel.create(poke, (err, data) => {
if (err) return console.log('ERROR: ', err);
console.log('Inseriu isso aí: ', data);
});
// Saída do terminal
Mongoose esta conectado em: mongodb://localhost/teste
Mongoose esta com a conexão aberta
Inseriu isso aí: [ { _id: 56e78793ac90f4920c99e130,
type: 'tipo_1',
name: 'pokemon_1',
__v: 0 },
{ _id: 56e78793ac90f4920c99e131,
type: 'tipo_2',
name: 'pokemon_2',
__v: 0 },
{ _id: 56e78793ac90f4920c99e132,
type: 'tipo_3',
name: 'pokemon_3',
__v: 0 } ]
```
## Busque todos os Pokemons com `attack > 50` e `height > 0.5`
```js
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
name: String,
description: String,
type: String,
attack: Number,
height: Number,
defense: Number,
active: Boolean,
moves: Array
};
const mainSchema = new Schema(_schema);
const FindModel = mongoose.model('pokemons', mainSchema);
const mod = {$and: [ {attack: {$gt: 50}} , {height: {$gt: 0.5}} ]}
FindModel.find(mod, (err, data) => {
if(err) return console.log('ERROR: ', err);
console.log('Retornou: ', data);
});
// saída do terminal
Mongoose esta conectado em: mongodb://localhost/be-mean-pokemons
Mongoose esta com a conexão aberta
Retornou: [ { moves: [ 'investida', 'desvio' ],
active: false,
type: 'agua',
height: 1.6,
defense: 60,
attack: 60,
description: 'pokemon \'Vem monstro!\'',
name: 'Blastoise',
_id: 56b1390d21f82f17b072ce50 } ]
```
## Altere, **inserindo**, o pokemon Nerdmon com `attach = 49` e com os valores dos outros campos a sua escolha
```js
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
name: String,
description: String,
type: String,
attack: Number,
height: Number,
defense: Number,
active: Boolean,
moves: Array
};
const pokeSchema = new Schema(_schema);
const PokeModel = mongoose.model('pokemons', pokeSchema);
let query = {name: "Nerdmon"};
let mod = {
$setOnInsert: {
name: "Nerdmon",
attack: 49,
active: true,
type: "Lokão"
}
};
let opts = { upsert: true }
PokeModel.update(query, mod, opts, (err, data) => {
if (err) return console.log('ERROR: ', err);
console.log('Inseriu: ', data);
});
// Saída do terminal
Mongoose esta conectado em: mongodb://localhost/be-mean-pokemons
Mongoose esta com a conexão aberta
Inseriu: { ok: 1,
nModified: 0,
n: 1,
upserted: [ { index: 0, _id: 56e7915c3e3f3f320fdf296c } ] }
// Verificando se inseriu no mongo
fedora(mongod-3.0.9) be-mean-pokemons> db.pokemons.find({name: /nerdmon/i})
{
"_id": ObjectId("56e7915c3e3f3f320fdf296c"),
"name": "Nerdmon",
"attack": 49,
"active": true,
"type": "Lokão"
}
Fetched 1 record(s) in 1ms
```
## Remova todos os Pokemons com `attack > 40`
> Coloquei 40 para não remover todos os pokemons, o 40 é a média no meu caso
```js
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
attack: Number
};
const querySchema = new Schema(_schema);
const RemovePoke = mongoose.model('pokemons', querySchema);
RemovePoke.remove({ attack: {$gt: 50} }, (err, data) => {
if(err) return console.log('ERROR: ', err);
console.log('Removeu', data);
});
// Saída do terminal
Mongoose esta conectado em: mongodb://localhost/be-mean-pokemons
Mongoose esta com a conexão aberta
Removeu { result: { ok: 1, n: 3 },
connection:
EventEmitter {
domain: null,
_events:
{ close: [Object],
error: [Object],
timeout: [Object],
parseError: [Object],
connect: [Function] },
_eventsCount: 5,
_maxListeners: undefined,
options:
{ socketOptions: {},
auto_reconnect: true,
host: 'localhost',
port: 27017,
cursorFactory: [Object],
reconnect: true,
emitError: true,
size: 5,
disconnectHandler: [Object],
bson: {},
messageHandler: [Function],
wireProtocolHandler: {} },
id: 0,
logger: { className: 'Connection' },
bson: {},
tag: undefined,
messageHandler: [Function],
maxBsonMessageSize: 67108864,
port: 27017,
host: 'localhost',
keepAlive: true,
keepAliveInitialDelay: 0,
noDelay: true,
connectionTimeout: 0,
socketTimeout: 0,
destroyed: false,
domainSocket: false,
singleBufferSerializtion: true,
serializationFunction: 'toBinUnified',
ca: null,
cert: null,
key: null,
passphrase: null,
ssl: false,
rejectUnauthorized: false,
checkServerIdentity: true,
responseOptions: { promoteLongs: true },
flushing: false,
queue: [],
connection:
Socket {
_connecting: false,
_hadError: false,
_handle: [Object],
_parent: null,
_host: 'localhost',
_readableState: [Object],
readable: true,
domain: null,
_events: [Object],
_eventsCount: 8,
_maxListeners: undefined,
_writableState: [Object],
writable: true,
allowHalfOpen: false,
destroyed: false,
bytesRead: 250,
_bytesDispatched: 230,
_sockname: null,
_pendingData: null,
_pendingEncoding: '',
_idleNext: null,
_idlePrev: null,
_idleTimeout: -1,
read: [Function],
_consuming: true },
writeStream: null,
hashedName: '29bafad3b32b11dc7ce934204952515ea5984b3c',
buffer: null,
sizeOfMessage: 0,
bytesRead: 0,
stubBuffer: null } }
```
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 06 - Exercício
**User:** [gkal19](https://github.com/gkal19)
**Autor:** Gabriel Kalani
**Data:** 1465408594
## Índice
##### [Exercício-01](#exercicio-01)
* [Schema Correto](#schema-correto)
* [Schema Incorreto](#schema-incorreto)
<br>
##### [Exercício-02](#exercicio-02)
##### [Exercício-03](#exercicio-03)
##### [Exercício-04](#exercicio-04)
##### [Exercício-05](#exercicio-05)
##### [Resumo da Aula](#resumo-da-aula)
<br>
> A organização de códigos utilizadas nas aulas de Node.js utilizando `app.js` e `config.js` será usada nos exercícios daqui em diante.
## Resumo da Aula
=============
Ao termino dessas aulas pude chegar a concluir que:
<br>
Utilizado na programação com NodeJS, o Mongoose é quem será o responsável por gerenciar schemas do nosso banco de dados. É uma ferramenta do MongoDB para modelagem de objetos projetados para trabalhar em um ambiente assíncrono. Para definir uma conexão é necessário utilizaro `mongoose.connect` e caso para a criação de conexões adicionais é simples utilizando o `mongoose.createConnection`.
```js
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/meu-banco-de-dados');
```
E ele possue 4 tipos de eventos:
- error: quando acontece um erro
- connected: quando conectamos com o MongoDb
- open: quando a conexão foi aberta com o MongoDb
- disconnected: quando o MongoDb é desconectado da nossa aplicação
```js
mongoose.connection.on('connected', () => {
console.log(`Mongoose default connection open to ${dbURI}`);
});
mongoose.connection.on('error',err => {
console.log(`Mongoose default connection error: ${err}`);
});
mongoose.connection.on('disconnected', () => {
console.log('Mongoose default connection disconnected');
});
mongoose.connection.on('open', () => {
console.log('Mongoose default connection is open');
});
```
Por último para fechar a conexão usaremos o `process.on` com o evento `SIGINIT`
```js
process.on('SIGINT', () => {
mongoose.connection.close(() => {
console.log('Mongoose default connection disconnected through app termination');
process.exit(0);
});
});
```
## Exercicio-01
=============
> Crie um schema com cada tipo explicado inserindo tanto um objeto correto como um objeto que desencadeie erros de validação padrão criar especificamente:
* 1.1. para String: `enum`, `match`, `maxlength` e `minlength`
* 1.2. para Number: `max` e `min`
## Schema-correto
```js
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const pokemonSchema = new Schema({
name: {type: String, match: /^(\D)*$/g},
password: {type: String, minlength: 8},
description: {type: String, maxlength: 20},
type: {type: String, enum: ['Water', 'Fire', 'Stone', 'Bug', 'People']},
attack: {type: Number, min: 30},
defense: {type: Number, max: 70},
hasSpecial: {type: Boolean, required: true},
skills: [String],
created_at: {type: Date, default: Date.now},
relatedTo: [{type: Schema.Types.ObjectId, ref: 'pokemons'}]
});
const PokemonModel = mongoose.model('pokemons', pokemonSchema);
let poke = new PokemonModel({
name: 'Suissa',
password: 'gatinhoslindosefos',
description: 'Pokemon do KCT',
type: 'People',
attack: 100,
defense: 45,
hasSpecial: true,
skills: ['js','ensinar', 'fumar']
});
poke.validate((err, data) => {
if (err) return console.log(err);
console.log('Objeto validado com sucesso');
});
```
Resultado:
```bash
{}
Objeto validado com sucesso
Mongoose default connection connected to mongodb://localhost/be-mean-instagram
Mongoose default connection is open
```
## Schema-Incorreto
```js
let poke = new PokemonModel({
name: 'Suissa',
password: 'gatinhoslindosefos',
description: 'um pokémon que ama seus amigos e, bla bla bla bla',
type: 'Adorador de Gatos',
attack: 10,
defense: 100,
skills: true
});
```
Resultado:
```js
{}
{ [ValidationError: pokemons validation failed]
message: 'pokemons validation failed',
name: 'ValidationError',
errors:
{ hasSpecial:
{ [ValidatorError: Path `hasSpecial` is required.]
message: 'Path `hasSpecial` is required.',
name: 'ValidatorError',
properties: [Object],
kind: 'required',
path: 'hasSpecial',
value: undefined },
defense:
{ [ValidatorError: Path `defense` (100) is more than maximum allowed value (70).]
message: 'Path `defense` (100) is more than maximum allowed value (70).',
name: 'ValidatorError',
properties: [Object],
kind: 'max',
path: 'defense',
value: 100 },
attack:
{ [ValidatorError: Path `attack` (10) is less than minimum allowed value (30).]
message: 'Path `attack` (10) is less than minimum allowed value (30).',
name: 'ValidatorError',
properties: [Object],
kind: 'min',
path: 'attack',
value: 10 },
type:
{ [ValidatorError: `Adorador de Gatos` is not a valid enum value for path `type`.]
message: '`Adorador de Gatos` is not a valid enum value for path `type`.',
name: 'ValidatorError',
properties: [Object],
kind: 'enum',
path: 'type',
value: 'Adorador de Gatos' },
description:
{ [ValidatorError: Path `description` (`um pokémon que ama seus amigos e, bla bla bla bla`) is longer than the maximum allowed length (20).]
message: 'Path `description` (`um pokémon que ama seus amigos e, bla bla bla bla`) is longer than the maximum allowed length (20).',
name: 'ValidatorError',
properties: [Object],
kind: 'maxlength',
path: 'description',
value: 'um pokémon que ama seus amigos e, bla bla bla bla' } } }
Mongoose default connection connected to mongodb://localhost/be-mean-instagram
Mongoose default connection is open
```
## Exercicio-02
=============
> Cadastre 3 pokemons **de uma só vez**:
```js
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const pokemonSchema = new Schema({
name: String,
attack: Number,
defense: Number,
hp: Number,
speed: Number,
height: String,
types: [String],
created: {type: Date, default: Date.now}
});
const PokemonModel = mongoose.model('pokemons', pokemonSchema);
let pokes = [
new PokemonModel({
name: 'Suissa corretor de imoveis',
attack: 60,
defense: 50,
hp: 200,
speed: 30,
height: '1',
types: ['corretor', 'imoveis']
}),
new PokemonModel({
name: 'Suissa filosofo',
attack: 100,
defense: 30,
hp: 100,
speed: 50,
height: '0.7',
types: ['filosofo','loucao']
}),
new PokemonModel({
name: 'Suissa faz nada',
attack: 47,
defense: 33,
hp: 50,
speed: 30,
height: '3',
types: ['vagabundo','preguicoso']
}),
];
PokemonModel.create(pokes, (err, data) => {
if (err) return console.log('Erro detectado: ', err);
console.log('Inserido: ', data);
});
module.export = PokemonModel;
```
Resultado:
```shell
Mongoose default connection connected to mongodb://localhost/be-mean-instagram
Mongoose default connection is open
Inserido: [ { types: [ 'corretor', 'imoveis' ],
created: Wed Jun 08 2016 19:59:10 GMT+0000 (UTC),
name: 'Suissa corretor de imoveis',
attack: 60,
defense: 50,
hp: 200,
speed: 30,
height: '1',
_id: 5758790e5fe2e3730dbeeb16,
__v: 0 },
{ types: [ 'filosofo', 'loucao' ],
created: Wed Jun 08 2016 19:59:10 GMT+0000 (UTC),
name: 'Suissa filosofo',
attack: 100,
defense: 30,
hp: 100,
speed: 50,
height: '0.7',
_id: 5758790e5fe2e3730dbeeb17,
__v: 0 },
{ types: [ 'vagabundo', 'preguicoso' ],
created: Wed Jun 08 2016 19:59:10 GMT+0000 (UTC),
name: 'Suissa faz nada',
attack: 47,
defense: 33,
hp: 50,
speed: 30,
height: '3',
_id: 5758790e5fe2e3730dbeeb18,
__v: 0 } ]
```
## Exercicio-03
=============
> Busque **todos** os Pokemons com `attack > 50` e `height > 0.5`:
```js
let query = {$and: [{attack: {$gt: 50}}, {height: {$gt: 0.5}}]};
PokemonModel.find(query, (err, data) => {
if (err) return console.log('Erro detectado: ', err);
console.log('Foi retornado: ', data.length);
});
module.export = PokemonModel;
```
NOTA: Foi retornado 4 pokémons
## Exercicio-04
=============
> Altere, **inserindo**, o Pokemon `Nerdmon` com `attack` igual a 49 e com os valores dos outros campos a sua escolha.
```js
let query = {name: 'Nerdmon'};
let options = {upsert: true};
let mod = {
name: 'Nerdmon',
attack: 49,
defense: 45,
hp: 100,
speed: 30,
height: '4',
types: ['nerd', 'foda'],
};
```
Resultado:
```shell
{ ok: 1,
nModified: 0,
n: 1,
upserted: [ { index: 0, _id: 575879db3a2ac61c40d0c87e } ] }
```
## Exercicio-05
=============
> Remova **todos** os Pokemons com `attack` **acima de 50**.
```js
PokemonModel.remove({attack: {$gt: 50}}, (err, data) => {
if (err) return console.log('ERROR: ', err);
console.log(data);
});
```
Resultado:
````shell
Mongoose default connection connected to mongodb://localhost/be-mean-instagram
Mongoose default connection is open
{ result: { ok: 1, n: 11 },
connection:
EventEmitter {
domain: null,
_events:
{ close: [Object],
error: [Object],
timeout: [Object],
parseError: [Object],
connect: [Function] },
_eventsCount: 5,
_maxListeners: undefined,
options:
{ socketOptions: {},
auto_reconnect: true,
host: 'localhost',
port: 27017,
cursorFactory: [Object],
reconnect: true,
emitError: true,
size: 5,
disconnectHandler: [Object],
bson: {},
messageHandler: [Function],
wireProtocolHandler: {} },
id: 0,
logger: { className: 'Connection' },
bson: {},
tag: undefined,
messageHandler: [Function],
maxBsonMessageSize: 67108864,
port: 27017,
host: 'localhost',
keepAlive: true,
keepAliveInitialDelay: 0,
noDelay: true,
connectionTimeout: 0,
socketTimeout: 0,
destroyed: false,
domainSocket: false,
singleBufferSerializtion: true,
serializationFunction: 'toBinUnified',
ca: null,
cert: null,
key: null,
passphrase: null,
ssl: false,
rejectUnauthorized: false,
checkServerIdentity: true,
responseOptions: { promoteLongs: true },
flushing: false,
queue: [],
connection:
Socket {
_connecting: false,
_hadError: false,
_handle: [Object],
_parent: null,
_host: 'localhost',
_readableState: [Object],
readable: true,
domain: null,
_events: [Object],
_eventsCount: 8,
_maxListeners: undefined,
_writableState: [Object],
writable: true,
allowHalfOpen: false,
destroyed: false,
bytesRead: 250,
_bytesDispatched: 231,
_sockname: null,
_pendingData: null,
_pendingEncoding: '',
_idleNext: null,
_idlePrev: null,
_idleTimeout: -1,
read: [Function],
_consuming: true },
writeStream: null,
hashedName: '29bafad3b32b11dc7ce934204952515ea5984b3c',
buffer: null,
sizeOfMessage: 0,
bytesRead: 0,
stubBuffer: null } }
``` | {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 06 - Exercício
**user:** [felipelopesrita](https://github.com/felipelopesrita)
**autor:** Felipe José Lopes Rita
## Crie um Schema com cada tipo explicado, inserindo tanto um objeto correto, como um objeto que desencadeie erros de validação padrão, criar especificamente:
* 1.1. para String: `enum`, `match`, `maxlength` e `minlength`
**Schema**
```js
const _schema =
{ user_name: { type:String, match: /^\w/, maxlength: 15, minlength:2 }
, status: { type: String, enum: _states }
}
const userSchema = new Schema(_schema);
```
**Objeto correto**
```js
var userModel = mongoose.model('users', userSchema);
var user = new userModel(
{ user_name: 'felipe'
, status: 'online'
});
user.save( (err, result)=> {
if( err ) throw err;
console.log(result)
} );
/*
Mongoose default connection connected to mongodb://localhost/be-mean-instagram
Mongoose default connection is open
{ _id: 56e1b5c439bc29cc59722070,
status: 'online',
user_name: 'felipe',
__v: 0 }
*/
```
**Objeto com erros**
```js
var user = new userModel(
{ user_name: '@'
, status: 'block'
});
user.save( (err, result)=> {
if( err ) console.log(err);
console.log(result)
} );
/*
{ [ValidationError: users validation failed]
message: 'users validation failed',
name: 'ValidationError',
errors:
{ status:
{ [ValidatorError: `block` is not a valid enum value for path `status`.]
properties: [Object],
message: '`block` is not a valid enum value for path `status`.',
name: 'ValidatorError',
kind: 'enum',
path: 'status',
value: 'block' },
user_name:
{ [ValidatorError: Path `user_name` is invalid (@).]
properties: [Object],
message: 'Path `user_name` is invalid (@).',
name: 'ValidatorError',
kind: 'regexp',
path: 'user_name',
value: '@' } } }
*/
```
* 1.2. para Number: `max` e `min`
**Schema**
```js
const _schema =
{ name: String
, quantity: { type:Number, max: 50, min: 0 }
}
const productSchema = new Schema(_schema);
```
**Objeto correto**
```js
var produto = new productModel(
{ name: 'Macarrão'
, quantity: 20
});
produto.save( (err, result)=> {
if( err ) console.log(err);
console.log(result)
} );
/*
{ _id: 56e1b8e51d75859d5f70883f,
quantity: 20,
name: 'Macarrão',
__v: 0 }
*/
```
**Objeto com erro**
```js
var produto = new productModel(
{ name: 'Macarrão'
, quantity: 53
});
produto.save( (err, result)=> {
if( err ) console.log(err);
console.log(result)
} );
/*
{ [ValidationError: products validation failed]
message: 'products validation failed',
name: 'ValidationError',
errors:
{ quantity:
{ [ValidatorError: Path `quantity` (53) is more than maximum allowed value (50).]
properties: [Object],
message: 'Path `quantity` (53) is more than maximum allowed value (50).',
name: 'ValidatorError',
kind: 'max',
path: 'quantity',
value: 53 } } }
*/
```
## Cadastre 3 pokemons **de uma só vez**:
```js
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema =
{ name: String
, description: String
, type: String
, attack: Number
, defense: Number
, height: Number
}
const pokemonSchema = new Schema(_schema);
const pokemonModel = mongoose.model('pokemons', pokemonSchema);
const pokeData = [ { name: 'Blastoise' }, { name: 'Mudkip' }, { name: 'Lucario' } ];
pokemonModel.insertMany(pokeData, (err, result)=>{
if(err) console.log(err);
console.log(result);
});
/*
Mongoose default connection connected to mongodb://localhost/be-mean-instagram
Mongoose default connection is open
[ { _id: 56e1bd8ad3635ad56626018f, name: 'Blastoise' },
{ _id: 56e1bd8ad3635ad566260190, name: 'Mudkip' },
{ _id: 56e1bd8ad3635ad566260191, name: 'Lucario' } ]
*/
```
## Busque **todos** os Pokemons com `attack > 50` e `height > 0.5`:
```js
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema =
{ name: String
, description: String
, type: String
, attack: Number
, defense: Number
, height: Number
}
const pokemonSchema = new Schema(_schema);
const pokemonModel = mongoose.model('pokemons', pokemonSchema);
var query = { attack: {$gt: 50}, height: {$gt: 0.5} };
pokemonModel.find(query, (err, data)=> {
if(err) console.log(err);
console.log(data);
});
/*
Mongoose default connection connected to mongodb://localhost/be-mean-instagram
Mongoose default connection is open
[ { moves: [ 'desvio', 'Investida' ],
height: 1.7,
defense: 78,
attack: 84,
description: 'Pokemon mais pica das galáxias',
name: 'Charizard',
_id: 56b4237765cd05bf6bff3324 },
{ moves: [ 'desvio', 'Investida' ],
height: 0.7,
defense: 40,
attack: 65,
description: 'Raposa preta (deve ser do capeta) foda e fofa',
name: 'Zorua',
_id: 56b4237765cd05bf6bff3326 },
{ defense: 43,
moves: [ 'Investida', 'Multiplicar', 'desvio' ],
height: 0.6,
attack: 52,
type: 'fogo',
description: 'Esse é o cão chupando manga de fofinho',
name: 'Charmander',
_id: 56bb4baa43e8eae26e3d71cc } ]
*/
```
## Altere, **inserindo**, o Pokemon `Nerdmon` com `attack` igual a 49 e com os valores dos outros campos a sua escolha.
```js
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema =
{ name: String
, description: String
, type: String
, attack: Number
, defense: Number
, height: Number
}
const pokemonSchema = new Schema(_schema);
const pokemonModel = mongoose.model('pokemons', pokemonSchema);
var query = { name: 'Nerdmon' }
, mod =
{ name: 'Nerdmon'
, description: 'Pokemon criado no exercício'
, type: 'fire'
, attack: 49
, defense: 80
, height: 1.80
}
, opt = { upsert: true };
pokemonModel.update(query, mod, opt, (err, data)=> {
if(err) console.log(err);
console.log(data);
});
/*
Mongoose default connection connected to mongodb://localhost/be-mean-instagram
Mongoose default connection is open
{ ok: 1,
nModified: 0,
n: 1,
upserted: [ { index: 0, _id: 56e8af7e024ad117a164b501 } ] }
*/
```
## Remova **todos** os Pokemons com `attack` **acima de 50**.
```js
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema =
{ name: String
, description: String
, type: String
, attack: Number
, defense: Number
, height: Number
}
const pokemonSchema = new Schema(_schema);
const pokemonModel = mongoose.model('pokemons', pokemonSchema);
var query = { attack: {$gt: 50} };
pokemonModel.remove(query, (err, data)=> {
if(err) console.log(err);
console.log(data.resul);
});
/*
Mongoose default connection connected to mongodb://localhost/be-mean-instagram
Mongoose default connection is open
{ ok: 1, n: 5 }
*/
```
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 06 - Exercícios
**User:** [angelorubin](https://github.com/angelorubin)
**Autor:** Angelo Rogério Rubin
**Date:** 1453886160
## Crie um Schema com cada tipo explicado, inserindo tanto um objeto correto, como um objeto que desencadeie erros de validação padrão, criar especificamente:
* para string: enum, match, maxlenght e minlenght
* para Number: min e max
### Código com erros de validação:
/*
* Testing validation of various Schema Types
*/
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
let weekdays = ['monday', 'tuesday', 'wednesday', 'thursday',
'friday'];
// Schema validations
let _schema = new Schema({
vString: { type: String },
vStringMatch : {type: String, match: /^(mon|tue|wed|thu|fri)day$/i},
vStringEnum: { type: String, enum: weekdays },
vStringMinMaxLength: { type: String, minlength: 1, maxlength: 8 },
vNumber: { type: Number, min: 13, max: 19 },
vBoolean: { type: Boolean },
vDate: { type: Date },
vObjectId: Schema.Types.ObjectId,
vMixed: Schema.Types.Mixed
});
let pokeModel = mongoose.model('Pokemons', _schema);
let poke = new pokeModel;
// Validating with errors
poke.vString = {};
poke.vStringMatch = 'ang';
poke.vStringEnum = false;
poke.vStringMinMaxLength = 'dilmassauro';
poke.vNumber = 'string';
poke.vBoolean = 'string';
poke.vDate = {};
poke.vObjectId = 'only objectId here';
poke.save( (err, data) => {
if (err) {
return console.error(err);
}
console.log('Data entered in the database:' + data);
});
### Resultados com erros de validação:
PS C:\Projetos\mongoose-pokemons> node app
{ [ValidationError: Pokemons validation failed]
message: 'Pokemons validation failed',
name: 'ValidationError',
errors:
{ vString:
{ [CastError: Cast to String failed for value "[object Object]" at path "vString"]
message: 'Cast to String failed for value "[object Object]" at path "vString"',
name: 'CastError',
kind: 'String',
value: {},
path: 'vString',
reason: undefined },
vNumber:
{ [CastError: Cast to Number failed for value "string" at path "vNumber"]
message: 'Cast to Number failed for value "string" at path "vNumber"',
name: 'CastError',
kind: 'Number',
value: 'string',
path: 'vNumber',
reason: undefined },
vDate:
{ [CastError: Cast to Date failed for value "[object Object]" at path "vDate"]
message: 'Cast to Date failed for value "[object Object]" at path "vDate"',
name: 'CastError',
kind: 'Date',
value: {},
path: 'vDate',
reason: undefined },
vObjectId:
{ [CastError: Cast to ObjectID failed for value "only objectId here" at path "vObjectId"]
message: 'Cast to ObjectID failed for value "only objectId here" at path "vObjectId"',
name: 'CastError',
kind: 'ObjectID',
value: 'only objectId here',
path: 'vObjectId',
reason: undefined },
vStringMatch:
{ [ValidatorError: Path `vStringMatch` is invalid (ang).]
properties: [Object],
message: 'Path `vStringMatch` is invalid (ang).',
name: 'ValidatorError',
kind: 'regexp',
path: 'vStringMatch',
value: 'ang' },
vStringEnum:
{ [ValidatorError: `false` is not a valid enum value for path `vStringEnum`.]
properties: [Object],
message: '`false` is not a valid enum value for path `vStringEnum`.',
name: 'ValidatorError',
kind: 'enum',
path: 'vStringEnum',
value: 'false' },
vStringMinMaxLength:
{ [ValidatorError: Path `vStringMinMaxLength` (`dilmassauro`) is longer than the maximum allowed length (8).]
properties: [Object],
message: 'Path `vStringMinMaxLength` (`dilmassauro`) is longer than the maximum allowed length (8).',
name: 'ValidatorError',
kind: 'maxlength',
path: 'vStringMinMaxLength',
value: 'dilmassauro' } } }
Connected: mongodb://127.0.0.1:27017/be-mean-pokemons
Mongoose default connection is open
### Código sem erros de validação:
/*
* Testing validation of various Schema Types
*/
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
let weekdays = ['monday', 'tuesday', 'wednesday', 'thursday',
'friday'];
// Schema validations
let _schema = new Schema({
vString: { type: String },
vStringMatch : {type: String, match: /^(mon|tue|wed|thu|fri)day$/i},
vStringEnum: { type: String, enum: weekdays },
vStringMinMaxLength: { type: String, minlength: 1, maxlength: 8 },
vNumber: { type: Number, min: 13, max: 19 },
vBoolean: { type: Boolean },
vDate: { type: Date },
vObjectId: Schema.Types.ObjectId,
vMixed: Schema.Types.Mixed
});
let pokeModel = mongoose.model('Pokemons', _schema);
let poke = new pokeModel;
// Validating without errors
poke.vString = 'angelomon';
poke.vStringMatch = 'monday';
poke.vStringEnum = 'monday';
poke.vStringMinMaxLength = 'angelo';
poke.vNumber = 18;
poke.vBoolean = 1;
poke.vDate = '2016-01-25 14:42:00';
poke.vObjectId = '56a61ea0ebd4076c1f656eb3';
poke.vMixed = 1.7;
// Validating with errors
/*
poke.vString = {};
poke.vStringMatch = 'ang';
poke.vStringEnum = false;
poke.vStringMinMaxLength = 'dilmassauro';
poke.vNumber = 'string';
poke.vBoolean = 'string';
poke.vDate = {};
poke.vObjectId = 'only objectId here';
*/
poke.save( (err, data) => {
if (err) {
return console.error(err);
}
console.log('Os dados foram salvos:' + data);
});
### Resultado sem erros de validação:
PS C:\Projetos\mongoose-pokemons> node app
Connected: mongodb://127.0.0.1:27017/be-mean-pokemons
Mongoose default connection is open
Data entered in the database:{
_id: 56a65ef5a1628740190dc3a2,
vString: 'angelomon',
vStringMatch: 'monday',
vStringEnum: 'monday',
vStringMinMaxLength: 'angelo',
vNumber: 18,
vBoolean: true,
vDate: Mon Jan 25 2016 14:42:00 GMT-0200 (Horário brasileiro de verão),
vObjectId: 56a61ea0ebd4076c1f656eb3,
vMixed: 1.7,
__v: 0
}
## Cadastre 3 pokemons de uma só vez. (pesquisar).
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
// Schema validations
const pokeSchema = new Schema({
"attack" : Number,
"created" : Date,
"defense" : Number,
"height" : Number,
"hp" : Number,
"name" : String,
"speed" : Number,
"types" : Array
});
const pokeModel = mongoose.model('pokemons', pokeSchema);
const pokemons = [
{
"attack" : 80,
"created" : Date.now(),
"defense" : 100,
"height" : 10,
"hp" : 40,
"name" : 'Angelomon',
"speed" : 200,
"types" : ['earth','fire']
},
{
"attack" : 70,
"created" : Date.now(),
"defense" : 90,
"height" : 9,
"hp" : 30,
"name" : 'Toscomon',
"speed" : 100,
"types" : ['fire']
},
{
"attack" : 30,
"created" : Date.now(),
"defense" : 30,
"height" : 5,
"hp" : 20,
"name" : 'Dilmamon',
"speed" : 20,
"types" : ['water']
}
];
pokeModel.create(pokemons, (err, data) => {
if (err) {
console.log(err);
}
console.log('(' + data.length + ') pokemons foram inseridos no banco de dados com sucesso.');
});
### Resultado
PS C:\Projetos\mongoose-pokemons> node app
Connected: mongodb://127.0.0.1:27017/be-mean-pokemons
Mongoose default connection is open
(3) pokemons foram inseridos no banco de dados com sucesso.
## Busque todos os Pokemons com attack > 50 e height > 0.5.
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
name: String
}
const pokemonSchema = new Schema(_schema);
const PokemonModel = mongoose.model('Pokemon', pokemonSchema);
const query = {
$and: [
{
attack: { $gt: 50 },
height: { $gt: 0.5 }
}
]
};
PokemonModel.find(query, (err, data) => {
if (err) {
return console.log('ERRO: ', err);
}
return console.log('FOUND:', data);
});
### Resultado:
PS C:\Projetos\mongoose-pokemons> node app
Connected: mongodb://127.0.0.1:27017/be-mean-pokemons
Mongoose default connection is open
FOUND: [
{
__v: 0,
types: [ 'earth', 'fire' ],
speed: 200,
name: 'Angelomon',
hp: 40,
height: 10,
defense: 100,
created: Tue Jan 26 2016 16:34:01 GMT-0200 (Horário brasileiro de verão),
attack: 80,
_id: 56a7bc19ec645064182251e5
},
{
__v: 0,
types: [ 'fire' ],
speed: 100,
name: 'Toscomon',
hp: 30,
height: 9,
defense: 90,
created: Tue Jan 26 2016 16:34:01 GMT-0200 (Horário brasileiro de verão),
attack: 70,
_id: 56a7bc19ec645064182251e6
}
]
## Altere, inserindo, o Pokemon Nerdmon com attack = 49 e com os valores dos outros campos a sua escolha.
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
"attack" : Number,
"created" : Date,
"defense" : Number,
"height" : Number,
"hp" : Number,
"name" : String,
"speed" : Number,
"types" : Array
};
const pokemonSchema = new Schema(_schema);
const pokemonModel = mongoose.model('Pokemon', pokemonSchema);
const query = { name: 'Angelomon Pro' };
const update = {
"attack" : 49,
"created" : Date.now(),
"defense" : 200,
"height" : 15,
"hp" : 80,
"name" : 'Nerdmon',
"speed" : 300,
"types" : ['earth','fire','water']
};
pokemonModel.findOneAndUpdate(query, update, (err, data) => {
if(err){
return console.log(err);
}
console.log('O documento foi alterado com sucesso.');
});
### Resultado:
PS C:\Projetos\mongoose-pokemons> node app
Connected: mongodb://127.0.0.1:27017/be-mean-pokemons
Mongoose default connection is open
O documento foi alterado com sucesso.
## Remova todos os Pokemons com attack > 50.
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
// Schema validations
let _schema = new Schema({
attack: Number
});
let pokeModel = mongoose.model('Pokemons', _schema);
const conditions = { attack: { $gt: 50 } };
pokeModel.remove(conditions, (err) => {
if(err) {
console.log(err);
}
console.log('Pokemon(s) removido(s) com sucesso.');
});
### Resultado:
PS C:\Projetos\mongoose-pokemons> node app
Connected: mongodb://127.0.0.1:27017/be-mean-pokemons
Mongoose default connection is open
Pokemon(s) removido(s) com sucesso.
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 06 - Exercício
**user:** charles7421(https://github.com/charles7421)
**autor:** Charles de Freitas Garcia
## Crie um Schema com cada tipo explicado, inserindo tanto um objeto correto, como um objeto que desencadeie erros de validação padrão, criar especificamente:
```javascript
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
name: {
type: String,
enum: ['Charles', 'Ana Paula', 'João Lucas', ]
},
idade: {
type: Number,
min: [18, 'Somente maiores de 18 anos'],
max: [60, 'Somente menores de 60 anos']
},
cpf: {
type: String,
match: /([0-9]{2}[\.]?[0-9]{3}[\.]?[0-9]{3}[\/]?[0-9]{4}[-]?[0-9]{2})|([0-9]{3}[\.]?[0-9]{3}[\.]?[0-9]{3}[-]?[0-9]{2})/g
},
cep: {
type: String,
minlength: 8,
maxlength: 9
}
}
const testeSchema = new Schema(_schema);
const TesteModel = mongoose.model('teste', testeSchema);
const data = {
name: null,
idade: null,
cpf: null,
cep: null
};
const Charles = new TesteModel(data);
Charles.save(function (err, data) {
if (err) return console.log('ERRO: ', err);
return console.log('Inseriu: ', data);
})
module.exports = TesteModel;
```
* 1.1. para String: `enum`, `match`, `maxlength` e `minlength`
* **Dados ok!**
```javascript
const data = {
name: 'Charles',
idade: 27,
cpf: '08145391571',
cep: '38700-000'
}
Inseriu: { _id: 570853584ae985531647fda8,
cep: '38700-000',
cpf: '08145391571',
idade: 27,
name: 'Charles',
__v: 0 }
```
* **Nome fora do Array "enum"**
```javascript
const data = {
name: 'Israel',
idade: 27,
cpf: '08145391571',
cep: '38700-000'
}
ERRO: { [ValidationError: teste validation failed]
message: 'teste validation failed',
name: 'ValidationError',
errors:
{ name:
{ [ValidatorError: `Israel` is not a valid enum value for path `name`.]
message: '`Israel` is not a valid enum value for path `name`.',
name: 'ValidatorError',
properties: [Object],
kind: 'enum',
path: 'name',
value: 'Israel' } } }
```
* **CPF não batendo com a RegEx imposta no "match"**
```javascript
const data = {
name: 'Ana Paula',
idade: 27,
cpf: '081-453-915.71',
cep: '38700-000'
}
ERRO: { [ValidationError: teste validation failed]
message: 'teste validation failed',
name: 'ValidationError',
errors:
{ cpf:
{ [ValidatorError: Path `cpf` is invalid (087-549-036-02).]
message: 'Path `cpf` is invalid (087-549-036-02).',
name: 'ValidatorError',
properties: [Object],
kind: 'regexp',
path: 'cpf',
value: '087-549-036-02' } } }
```
* **CEP com mais caracteres do que o definido no maxlength**
```javascript
const data = {
name: 'Ana Paula',
idade: 27,
cpf: '08145391571',
cep: '38700--000'
}
ERRO: { [ValidationError: teste validation failed]
message: 'teste validation failed',
name: 'ValidationError',
errors:
{ cep:
{ [ValidatorError: Path `cep` (`38700--000`) is longer than the maximum allowed length (9).]
message: 'Path `cep` (`38700--000`) is longer than the maximum allowed length (9).',
name: 'ValidatorError',
properties: [Object],
kind: 'maxlength',
path: 'cep',
value: '38700--000' } } }
```
* **CEP com menos caracteres do que o definido no minlength**
```javascript
const data = {
name: 'Ana Paula',
idade: 27,
cpf: '08145391571',
cep: '3870000'
}
ERRO: { [ValidationError: teste validation failed]
message: 'teste validation failed',
name: 'ValidationError',
errors:
{ cep:
{ [ValidatorError: Path `cep` (`3870000`) is shorter than the minimum allowed length (8).]
message: 'Path `cep` (`3870000`) is shorter than the minimum allowed length (8).',
name: 'ValidatorError',
properties: [Object],
kind: 'minlength',
path: 'cep',
value: '3870000' } } }
```
* 1.2. para Number: `max` e `min`
* **Idade menor do que o definido no "min"**
```javascript
const data = {
name: 'Ana Paula',
idade: 17,
cpf: '08145391571',
cep: '38700000'
}
ERRO: { [ValidationError: teste validation failed]
message: 'teste validation failed',
name: 'ValidationError',
errors:
{ idade:
{ [ValidatorError: Somente maiores de 18 anos]
message: 'Somente maiores de 18 anos',
name: 'ValidatorError',
properties: [Object],
kind: 'min',
path: 'idade',
value: 17 } } }
```
* **Idade maior do que o definido no "max"**
```javascript
const data = {
name: 'João Lucas',
idade: 61,
cpf: '08145391571',
cep: '38700000'
}
ERRO: { [ValidationError: teste validation failed]
message: 'teste validation failed',
name: 'ValidationError',
errors:
{ idade:
{ [ValidatorError: Somente menores de 60 anos]
message: 'Somente menores de 60 anos',
name: 'ValidatorError',
properties: [Object],
kind: 'max',
path: 'idade',
value: 61 } } }
```
## Cadastre 3 pokemons **de uma só vez**:
```javascript
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
attack: Number,
created: { type: Date, default: Date.now },
defense: Number,
height: String,
hp: Number,
name: String,
speed: Number,
types: [String]
}
const PokemonSchema = new Schema(_schema);
const PokemonModel = mongoose.model('poke', PokemonSchema);
const data = {
attack: 55,
defense: 40,
height: "2",
hp: 100,
name: "Poke01",
speed: 45,
types: ["bug", "poison"]
}
const data2 = {
attack: 35,
defense: 68,
height: "4",
hp: 100,
name: "Poke02",
speed: 45,
types: ["ice", "water"]
}
const data3 = {
attack: 70,
defense: 10,
height: "4",
hp: 100,
name: "Poke03",
speed: 10,
types: ["normal", "flying"]
}
var array = [data, data2, data3];
const Charles = new PokemonModel(array);
PokemonModel.create(array, function (err, data) {
if (err) return console.log('ERRO: ', err);
return console.log('Inseriu: ', data);
})
module.exports = PokemonModel;
```
## Busque **todos** os Pokemons com `attack > 50` e `height > 0.5`:
```javascript
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
name: String
}
const pokemonSchema = new Schema(_schema);
const PokemonModel = mongoose.model('Pokemon', pokemonSchema);
const query = { $and: [ { attack: {$gt: 50} }, { height: {$gt: 0.5} } ] };
PokemonModel.find(query, function (err, data) {
if (err) return console.log('ERRO: ', err);
return console.log('Buscou:', data);
})
Buscou: []
```
## Altere, **inserindo**, o Pokemon `Nerdmon` com `attack` igual a 49 e com os valores dos outros campos a sua escolha.
```javascript
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
attack: Number,
created: { type: Date, default: Date.now },
defense: Number,
height: String,
hp: Number,
name: String,
speed: Number,
types: [String]
}
const PokemonSchema = new Schema(_schema);
const Pokemon = mongoose.model('Pokemon', PokemonSchema);
const query = {name: "Nerdmon"};
const mod = {
attack: 49,
defense: 33,
height: "2",
hp: 100,
name: "Smaug",
speed: 23,
types: ["dragon"]
};
var options = {upsert: true};
Pokemon.update(query, mod, options, function (err, data) {
if (err) return console.log('ERRO: ', err);
return console.log('Alterou: ', data);
});
Alterou: { ok: 1,
nModified: 0,
n: 1,
upserted: [ { index: 0, _id: 57086055363fa7f2af51e135 } ] }
```
## Remova **todos** os Pokemons com `attack` **acima de 50**.
```javascript
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const _schema = {
attack: Number,
created: { type: Date, default: Date.now },
defense: Number,
height: String,
hp: Number,
name: String,
speed: Number,
types: [String]
}
const PokemonSchema = new Schema(_schema);
const Pokemon = mongoose.model('Pokemon', PokemonSchema);
const query = { attack: {$gt: 50} };
Pokemon.remove(query, function (err, data) {
if (err) return console.log('ERRO: ', err);
return console.log('Deletou: ', data);
});
DELETOU: { result: { ok: 1, n: 462 },
connection:
EventEmitter {
domain: null,
_events:
{ close: [Object],
error: [Object],
timeout: [Object],
parseError: [Object],
connect: [Function] },
_eventsCount: 5,
_maxListeners: undefined,
options:
{ socketOptions: {},
auto_reconnect: true,
host: 'localhost',
port: 27017,
cursorFactory: [Object],
reconnect: true,
emitError: true,
size: 5,
disconnectHandler: [Object],
bson: {},
messageHandler: [Function],
wireProtocolHandler: {} },
id: 0,
logger: { className: 'Connection' },
bson: {},
tag: undefined,
messageHandler: [Function],
maxBsonMessageSize: 67108864,
port: 27017,
host: 'localhost',
keepAlive: true,
keepAliveInitialDelay: 0,
noDelay: true,
connectionTimeout: 0,
socketTimeout: 0,
destroyed: false,
domainSocket: false,
singleBufferSerializtion: true,
serializationFunction: 'toBinUnified',
ca: null,
cert: null,
key: null,
passphrase: null,
ssl: false,
rejectUnauthorized: false,
checkServerIdentity: true,
responseOptions: { promoteLongs: true },
flushing: false,
queue: [],
connection:
Socket {
_connecting: false,
_hadError: false,
_handle: [Object],
_parent: null,
_host: 'localhost',
_readableState: [Object],
readable: true,
domain: null,
_events: [Object],
_eventsCount: 8,
_maxListeners: undefined,
_writableState: [Object],
writable: true,
allowHalfOpen: false,
destroyed: false,
bytesRead: 250,
_bytesDispatched: 231,
_sockname: null,
_pendingData: null,
_pendingEncoding: '',
server: null,
_server: null,
_idleNext: null,
_idlePrev: null,
_idleTimeout: -1,
read: [Function],
_consuming: true },
writeStream: null,
hashedName: '29bafad3b32b11dc7ce934204952515ea5984b3c',
buffer: null,
sizeOfMessage: 0,
bytesRead: 0,
stubBuffer: null } }
```
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 06 - Exercício
**user:** [fauker](http://github.com/fauker)
**autor:** LUCAS DA SILVA MOREIRA
## Crie um Schema com cada tipo explicado, inserindo tanto um objeto correto, como um objeto que desencadeie erros de validação padrão, criar especificamente:
* 1.1. para String: `enum`, `match`, `maxlength` e `minlength`
* 1.2. para Number: `max` e `min`
**Objeto com erros de validação**:
```
require('./config');
var mongoose = require('mongoose');
var Schema = mongoose.Schema;
var _schema = {
nome: {type: String, minlength: 5},
sobrenome: {type: String, maxlength: 5},
tecnologias: {type: String, enum: ['Node.js', 'MongoDB', 'Express', 'Angular.js']},
telefone: {type: String, match: /^[1-9]/},
idade: {type: Number, max: 2},
amigos: {type: Number, min: 5}
};
var pessoaSchema = new Schema(_schema);
var PessoaModel = mongoose.model('Pessoa', pessoaSchema);
var dataModel = {
nome: 'José',
sobrenome: 'Moreira',
tecnologias: 'php',
telefone: 'a3333-3333',
idade: 10,
amigos: 2
};
var Jose = new PessoaModel(dataModel);
Jose.save(function(err, data) {
if (err) return console.log('DEU PAU: ' + err);
return console.log('Salvou a pessoa: ' + data);
});
//resultado
DEU PAU: ValidationError: Path `amigos` (2) is less than minimum allowed value (5)., Path `idade` (10) is more than maximum allowed value (2)., Path `telefone` is invalid (a3333-3333)., `php` is not a valid enum value for path `tecnologias`., Path `sobrenome` (`Moreira`) is longer than the maximum allowed length (5)., Path `nome` (`José`) is shorter than the minimum allowed length (5).
```
**Inserindo um objeto correto**:
```
require('./config');
var mongoose = require('mongoose');
var Schema = mongoose.Schema;
var _schema = {
nome: {type: String, minlength: 5},
sobrenome: {type: String, maxlength: 5},
tecnologias: {type: String, enum: ['Node.js', 'MongoDB', 'Express', 'Angular.js']},
telefone: {type: String, match: /^[1-9]/},
idade: {type: Number, max: 2},
amigos: {type: Number, min: 5}
};
var pessoaSchema = new Schema(_schema);
var PessoaModel = mongoose.model('Pessoa', pessoaSchema);
var dataModel = {
nome: 'Francisco',
sobrenome: 'Silva',
tecnologias: 'Node.js',
telefone: '3333-3333',
idade: 1,
amigos: 6
};
var Jose = new PessoaModel(dataModel);
Jose.save(function(err, data) {
if (err) return console.log('DEU PAU: ' + err);
return console.log('Salvou a pessoa: ' + data);
});
//resultado
Salvou a pessoa: { _id: 5729623d0c29593454bd65f7,
amigos: 6,
idade: 1,
telefone: '3333-3333',
tecnologias: 'Node.js',
sobrenome: 'Silva',
nome: 'Francisco',
__v: 0 }
```
## Cadastre 3 pokemons **de uma só vez**:
```
require('./config');
var mongoose = require('mongoose');
var Schema = mongoose.Schema;
var _schema = {
name: String,
description: String,
type: String,
attack: Number,
defense: Number,
height: Number
};
var pokemonSchema = new Schema(_schema);
var PokemonModel = mongoose.model('Pokemon', pokemonSchema);
var dataModel = [{
name: 'LucasMon',
description: 'Um pokemon muito bolado',
type: 'fogo',
attack: 100,
defense: 50,
height: 1
}, {
name: 'AmigoMon',
description: 'Um brother',
type: 'agua',
attack: 200,
defense: 10,
height: 0.4
}, {
name: 'NodeMon',
description: 'Sinixtro',
type: 'fogo',
attack: 50,
defense: 50,
height: 20
}];
PokemonModel.create(dataModel, function(err, data) {
if (err) return console.log(err);
console.log('Pokemons Inseridos: ', data);
});
```
Resultado:
```
Pokemons Inseridos: [ { _id: 572974fec3c044ae57196a32,
height: 1,
defense: 50,
attack: 100,
type: 'fogo',
description: 'Um pokemon muito bolado',
name: 'LucasMon',
__v: 0 },
{ _id: 572974fec3c044ae57196a33,
height: 0.4,
defense: 10,
attack: 200,
type: 'agua',
description: 'Um brother',
name: 'AmigoMon',
__v: 0 },
{ _id: 572974fec3c044ae57196a34,
height: 20,
defense: 50,
attack: 50,
type: 'fogo',
description: 'Sinixtro',
name: 'NodeMon',
__v: 0 }]
```
## Busque **todos** os Pokemons com `attack > 50` e `height > 0.5`:
```
require('./config');
var mongoose = require('mongoose');
var Schema = mongoose.Schema;
var _schema = {
name: String,
description: String,
type: String,
attack: Number,
defense: Number,
height: Number
};
var pokemonSchema = new Schema(_schema);
var PokemonModel = mongoose.model('Pokemon', pokemonSchema);
var query = {$and: [{attack: {$gt: 50}}, {height: {$gt: 0.5}}]};
PokemonModel.find(query, function (err, data) {
if (err) return console.log('ERRO: ', err);
return console.log('Buscou:', data);
});
```
Resultado:
```
Buscou: [ { __v: 0,
height: 1,
defense: 50,
attack: 100,
type: 'fogo',
description: 'Um pokemon muito bolado',
name: 'LucasMon',
_id: 572974fec3c044ae57196a32 } ]
lucasmore
```
## Altere, **inserindo**, o Pokemon `Nerdmon` com `attack` igual a 49 e com os valores dos outros campos a sua escolha.
Resultado:
```
require('./config');
var mongoose = require('mongoose');
var Schema = mongoose.Schema;
var _schema = {
name: String,
description: String,
type: String,
attack: Number,
defense: Number,
height: Number
};
var pokemonSchema = new Schema(_schema);
var PokemonModel = mongoose.model('Pokemon', pokemonSchema);
var query = {name: 'Nerdmon'};
var mod = {
$setOnInsert: {
name: 'Nerdmon',
description: 'Pokemon mt nerd',
type: 'fogo',
attack: 49,
defense: 50,
height: 100
}
};
var options = {upsert: true};
PokemonModel.update(query, mod, options, function(err, data) {
if (err) return console.log(err);
console.log('Pokemon Inserido com Upsert: ', data);
});
```
```
Pokemon Inserido com Upsert: { ok: 1,
nModified: 0,
n: 1,
upserted: [ { index: 0, _id: 57297a013b4cb9a31a868f50 } ] }
```
Consulta no MongoDB:
```
var query = {name: 'Nerdmon'}
db.pokemons.find(query)
{
"_id": ObjectId("57297a013b4cb9a31a868f50"),
"name": "Nerdmon",
"description": "Pokemon mt nerd",
"type": "fogo",
"attack": 49,
"defense": 50,
"height": 100
}
```
## Remova **todos** os Pokemons com `attack` **acima de 50**.
```
require('./config');
var mongoose = require('mongoose');
var Schema = mongoose.Schema;
var _schema = {
name: String,
description: String,
type: String,
attack: Number,
defense: Number,
height: Number
};
var pokemonSchema = new Schema(_schema);
var PokemonModel = mongoose.model('Pokemon', pokemonSchema);
var query = {attack: {$gt: 50}};
PokemonModel.remove(query, function(err, data) {
if (err) return console.log(err);
console.log('Pokemon Excluídos: ', data);
});
```
Resultado:
```
Pokemon Excluídos: { result: { ok: 1, n: 2 },
connection:
EventEmitter {
domain: null,
_events:
{ close: [Object],
error: [Object],
timeout: [Object],
parseError: [Object],
connect: [Function] },
_eventsCount: 5,
_maxListeners: undefined,
options:
{ socketOptions: {},
auto_reconnect: true,
host: 'localhost',
port: 27017,
cursorFactory: [Object],
reconnect: true,
emitError: true,
size: 5,
disconnectHandler: [Object],
bson: {},
messageHandler: [Function],
wireProtocolHandler: [Object] },
id: 0,
logger: { className: 'Connection' },
bson: {},
tag: undefined,
messageHandler: [Function],
maxBsonMessageSize: 67108864,
port: 27017,
host: 'localhost',
keepAlive: true,
keepAliveInitialDelay: 0,
noDelay: true,
connectionTimeout: 0,
socketTimeout: 0,
destroyed: false,
domainSocket: false,
singleBufferSerializtion: true,
serializationFunction: 'toBinUnified',
ca: null,
cert: null,
key: null,
passphrase: null,
ssl: false,
rejectUnauthorized: false,
checkServerIdentity: true,
responseOptions: { promoteLongs: true },
flushing: false,
queue: [],
connection:
Socket {
_connecting: false,
_hadError: false,
_handle: [Object],
_parent: null,
_host: 'localhost',
_readableState: [Object],
readable: true,
domain: null,
_events: [Object],
_eventsCount: 8,
_maxListeners: undefined,
_writableState: [Object],
writable: true,
allowHalfOpen: false,
destroyed: false,
bytesRead: 250,
_bytesDispatched: 231,
_sockname: null,
_pendingData: null,
_pendingEncoding: '',
_idleNext: null,
_idlePrev: null,
_idleTimeout: -1,
read: [Function],
_consuming: true },
writeStream: null,
hashedName: '29bafad3b32b11dc7ce934204952515ea5984b3c',
buffer: null,
sizeOfMessage: 0,
bytesRead: 0,
stubBuffer: null } }
```
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 06 - Exercício
**user:** [ednilsonamaral](https://github.com/ednilsonamaral)
**autor:** Ednilson Amaral
## Crie um Schema com cada tipo explicado, inserindo tanto um objeto correto, como um objeto que desencadeie erros de validação padrão, criar especificamente:
* 1.1. para String: `enum`, `match`, `maxlength` e `minlength`
* 1.2. para Number: `max` e `min`
No banco *be-mean-instagram* estou pedindo para criar uma nova *collection*, chamada `alunos`.
No JS abaixo, eu inseri na *collection* um objeto correto.
```js
require('./config.js');
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const aluno = {
//o match aceita regex, no caso abaixo, o nome pode ser case insensitive e começar com qualquer caractere
nome: {type: String, match: /^./i },
//max serve para setar o campo para que seja inserido um número máximo, no caso no máximo até 30, se não dá erro
idade: {type: Number, max: 30},
//o enum irá setar o sexo do cara, Masculino ou Feminino
sexo: {type: String, enum: ['M', 'F']},
//a ideia desse campo é que o aluno tenho no mínimo 5 aulas, caso contrário dá erro
aulas: {type: Number, min: 5},
//o nome da cidade deve conter no máximo 10 caracteres
cidade: {type: String, maxlength: 10},
//o nome do estado deve conter no mínimo 2 caracteres
estado: {type: String, minlength: 2}
}
const alunoSchema = new Schema(aluno);
const AlunoModel = mongoose.model('alunos', alunoSchema);
const data = (
{
nome: 'Ednilson',
idade: 24,
sexo: 'M',
aulas: 16,
cidade: 'Itapeva',
estado: 'SP'
}
);
const Ednilson = new AlunoModel(data);
Ednilson.save(function (err, data) {
if (err) return console.log('Erro: ', err);
console.log('Inserido o aluno: ', data);
});
```
A saída no *nodemon* foi a seguinte:
```
Inserido o aluno: { _id: 56ac40f7dd143b141efb4685,
estado: 'SP',
cidade: 'Itapeva',
aulas: 16,
sexo: 'M',
idade: 24,
nome: 'Ednilson',
__v: 0 }
```
Agora, no JS abaixo, é o objeto com erros de validação padrão:
```js
require('./config.js');
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const aluno = {
//o match aceita regex, no caso abaixo, o nome pode ser case insensitive e começar com qualquer caractere
nome: {type: String, match: /^./i },
//max serve para setar o campo para que seja inserido um número máximo, no caso no máximo até 30, se não dá erro
idade: {type: Number, max: 30},
//o enum irá setar o sexo do cara, Masculino ou Feminino
sexo: {type: String, enum: ['M', 'F']},
//a ideia desse campo é que o aluno tenho no mínimo 5 aulas, caso contrário dá erro
aulas: {type: Number, min: 5},
//o nome da cidade deve conter no máximo 10 caracteres
cidade: {type: String, maxlength: 10},
//o nome do estado deve conter no mínimo 2 caracteres
estado: {type: String, minlength: 2}
}
const alunoSchema = new Schema(aluno);
const AlunoModel = mongoose.model('alunos', alunoSchema);
const data = (
{
nome: 'Aline',
idade: 31,
sexo: 'J',
aulas: 3,
cidade: 'São Carlos',
estado: 'SP'
}
);
const Ednilson = new AlunoModel(data);
Ednilson.save(function (err, data) {
if (err) return console.log('Erro: ', err);
console.log('Inserido o(a) aluno(a): ', data);
});
```
E a saída no *nodemon* foi a seguinte:
```
Erro: { [ValidationError: alunos validation failed]
message: 'alunos validation failed',
name: 'ValidationError',
errors:
{ aulas:
{ [ValidatorError: Path `aulas` (3) is less than minimum allowed value (5).]
properties: [Object],
message: 'Path `aulas` (3) is less than minimum allowed value (5).',
name: 'ValidatorError',
kind: 'min',
path: 'aulas',
value: 3 },
sexo:
{ [ValidatorError: `J` is not a valid enum value for path `sexo`.]
properties: [Object],
message: '`J` is not a valid enum value for path `sexo`.',
name: 'ValidatorError',
kind: 'enum',
path: 'sexo',
value: 'J' },
idade:
{ [ValidatorError: Path `idade` (31) is more than maximum allowed value (30).]
properties: [Object],
message: 'Path `idade` (31) is more than maximum allowed value (30).',
name: 'ValidatorError',
kind: 'max',
path: 'idade',
value: 31 } } }
```
## Cadastre 3 pokemons **de uma só vez**.
Arquivo JS:
```js
require('./config.js');
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const poke = {
name: String,
description: String,
type: String,
attack: Number,
defense: Number,
height: Number
}
const PokemonSchema = new Schema(poke);
const PokemonModel = mongoose.model('pokemons', PokemonSchema);
const data = [
{
name: "Bowlmon",
description: "O pokemon mais viciado em NFL que qualquer extraterreste da Terra",
type: "nflmon",
attack: 98,
defense: 97,
height: 2.1
},
{
name: "Elimon",
description: "O caçula",
type: "nflmon",
attack: 83,
defense: 79,
height: 1.9
},
{
name: "Ricemon",
description: "O cabeludo pirado",
type: "nflmon",
attack: 91,
defense: 85,
height: 2
}
];
PokemonModel.create(data, function (err, data) {
if (err) return console.log('Erro: ', err);
console.log('Inserido(s): ', data);
});
```
Executando `node app.js` temos:
```
ednilson@EDNILSON-NB:/var/www/mongoose-pokemons$ node app.js
Mongoose default connection open to mongodb://localhost/be-mean-pokemons
Mongoose default connection is open
Inserido(s): [ { _id: 56aeb4a5aad359a813657598,
height: 2.1,
defense: 97,
attack: 98,
type: 'nflmon',
description: 'O pokemon mais viciado em NFL que qualquer extraterreste da Terra',
name: 'Bowlmon',
__v: 0 },
{ _id: 56aeb4a5aad359a813657599,
height: 1.9,
defense: 79,
attack: 83,
type: 'nflmon',
description: 'O caçula',
name: 'Elimon',
__v: 0 },
{ _id: 56aeb4a5aad359a81365759a,
height: 2,
defense: 85,
attack: 91,
type: 'nflmon',
description: 'O cabeludo pirado',
name: 'Ricemon',
__v: 0 } ]
```
Buscando pelo `type: 'nflmon'` pelo MongoDB, resultamos em:
```
be-mean-pokemons> var query = {type: /nflmon/i}
be-mean-pokemons> db.pokemons.find(query)
{
"_id": ObjectId("56aeb4a5aad359a813657598"),
"name": "Bowlmon",
"description": "O pokemon mais viciado em NFL que qualquer extraterreste da Terra",
"type": "nflmon",
"attack": 98,
"defense": 97,
"height": 2.1,
"__v": 0
}
{
"_id": ObjectId("56aeb4a5aad359a813657599"),
"name": "Elimon",
"description": "O caçula",
"type": "nflmon",
"attack": 83,
"defense": 79,
"height": 1.9,
"__v": 0
}
{
"_id": ObjectId("56aeb4a5aad359a81365759a"),
"name": "Ricemon",
"description": "O cabeludo pirado",
"type": "nflmon",
"attack": 91,
"defense": 85,
"height": 2,
"__v": 0
}
Fetched 3 record(s) in 4ms
```
## Busque **todos** os Pokemons com `attack > 50` e `height > 0.5`.
Arquivo JS:
```js
require('./config.js');
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const poke = {
name: String,
description: String,
type: String,
attack: Number,
defense: Number,
height: Number
}
const PokemonSchema = new Schema(poke);
const PokemonModel = mongoose.model('pokemons', PokemonSchema);
const data = {$and: [{attack: {$gt: 50}}, {height: {$gt: 0.5}}]};
PokemonModel.find(data, function (err, data) {
if (err) return console.log('Erro: ', err);
console.log('Buscou: ', data);
});
module.exports = PokemonModel;
```
Executando o `node app.js` temos:
```
ednilson@EDNILSON-NB:/var/www/mongoose-pokemons$ node app.js
Mongoose default connection open to mongodb://localhost/be-mean-pokemons
Mongoose default connection is open
Buscou: [ { moves: [ 'investida', 'desvio' ],
active: false,
height: 1.6,
defense: 60,
attack: 165,
type: 'rocha',
description: 'Pode levar qualquer cascudo na cabeça que não vai sentir porra nenhuma',
name: 'Rampardos',
_id: 5644da2d329b6e6a8376bd42 },
{ moves: [ 'investida', 'desvio' ],
active: false,
height: 2,
defense: 100,
attack: 160,
type: 'normal',
description: 'Pense em um cara preguiçoso, é esse pokebola aí',
name: 'Slaking',
_id: 5644da44329b6e6a8376bd43 },
{ moves: [ 'investida', 'desvio' ],
active: false,
height: 1.8,
defense: 90,
attack: 147,
type: 'dragão',
description: 'Esse é dócil viu, mas se baixar a pomba gira nele, fica muito agressivo',
name: 'Haxorus',
_id: 5644da4e329b6e6a8376bd44 },
{ moves: [ 'investida', 'desvio' ],
active: false,
height: 2.4,
defense: 130,
attack: 140,
type: 'rocha',
description: 'Esse resiste até a erupções',
name: 'Rhyperior',
_id: 5644da5a329b6e6a8376bd45 },
{ moves: [ 'investida', 'desvio' ],
active: false,
height: 1.6,
defense: 130,
attack: 135,
type: 'psíquico',
description: 'Esse não tem Windows como SO',
name: 'Metagross',
_id: 5644da63329b6e6a8376bd46 },
{ moves: [ 'engole fogo', 'assopra veneno', 'desvio' ],
active: false,
height: 0.6,
attack: 52,
type: 'fogo',
description: 'Esse é o cão chupando manga de fofinho',
name: 'Charmander',
_id: 564cff04f9025dedb2553205 },
{ __v: 0,
height: 2.1,
defense: 97,
attack: 98,
type: 'nflmon',
description: 'O pokemon mais viciado em NFL que qualquer extraterreste da Terra',
name: 'Bowlmon',
_id: 56aeb4a5aad359a813657598 },
{ __v: 0,
height: 1.9,
defense: 79,
attack: 83,
type: 'nflmon',
description: 'O caçula',
name: 'Elimon',
_id: 56aeb4a5aad359a813657599 },
{ __v: 0,
height: 2,
defense: 85,
attack: 91,
type: 'nflmon',
description: 'O cabeludo pirado',
name: 'Ricemon',
_id: 56aeb4a5aad359a81365759a } ]
```
Para tirar as dúvidas, busquei também pelo MongoDB:
```
be-mean-pokemons> var query = {$and: [{attack: {$gt: 50}}, {height: {$gt: 0.5}}]}
be-mean-pokemons> db.pokemons.find(query)
{
"_id": ObjectId("5644da2d329b6e6a8376bd42"),
"name": "Rampardos",
"description": "Pode levar qualquer cascudo na cabeça que não vai sentir porra nenhuma",
"type": "rocha",
"attack": 165,
"defense": 60,
"height": 1.6,
"active": false,
"moves": [
"investida",
"desvio"
]
}
{
"_id": ObjectId("5644da44329b6e6a8376bd43"),
"name": "Slaking",
"description": "Pense em um cara preguiçoso, é esse pokebola aí",
"type": "normal",
"attack": 160,
"defense": 100,
"height": 2,
"active": false,
"moves": [
"investida",
"desvio"
]
}
{
"_id": ObjectId("5644da4e329b6e6a8376bd44"),
"name": "Haxorus",
"description": "Esse é dócil viu, mas se baixar a pomba gira nele, fica muito agressivo",
"type": "dragão",
"attack": 147,
"defense": 90,
"height": 1.8,
"active": false,
"moves": [
"investida",
"desvio"
]
}
{
"_id": ObjectId("5644da5a329b6e6a8376bd45"),
"name": "Rhyperior",
"description": "Esse resiste até a erupções",
"type": "rocha",
"attack": 140,
"defense": 130,
"height": 2.4,
"active": false,
"moves": [
"investida",
"desvio"
]
}
{
"_id": ObjectId("5644da63329b6e6a8376bd46"),
"name": "Metagross",
"description": "Esse não tem Windows como SO",
"type": "psíquico",
"attack": 135,
"defense": 130,
"height": 1.6,
"active": false,
"moves": [
"investida",
"desvio"
]
}
{
"_id": ObjectId("564cff04f9025dedb2553205"),
"name": "Charmander",
"description": "Esse é o cão chupando manga de fofinho",
"type": "fogo",
"attack": 52,
"height": 0.6,
"active": false,
"moves": [
"engole fogo",
"assopra veneno",
"desvio"
]
}
{
"_id": ObjectId("56aeb4a5aad359a813657598"),
"name": "Bowlmon",
"description": "O pokemon mais viciado em NFL que qualquer extraterreste da Terra",
"type": "nflmon",
"attack": 98,
"defense": 97,
"height": 2.1,
"__v": 0
}
{
"_id": ObjectId("56aeb4a5aad359a813657599"),
"name": "Elimon",
"description": "O caçula",
"type": "nflmon",
"attack": 83,
"defense": 79,
"height": 1.9,
"__v": 0
}
{
"_id": ObjectId("56aeb4a5aad359a81365759a"),
"name": "Ricemon",
"description": "O cabeludo pirado",
"type": "nflmon",
"attack": 91,
"defense": 85,
"height": 2,
"__v": 0
}
Fetched 9 record(s) in 10ms
```
## Altere, **inserindo**, o Pokemon `Nerdmon` com `attack` igual a 49 e com os valores dos outros campos a sua escolha
O arquivo JS ficou da seguinte maneira:
```js
require('./config.js');
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const poke = {
name: String,
description: String,
type: String,
attack: Number,
defense: Number,
height: Number
}
const PokemonSchema = new Schema(poke);
const PokemonModel = mongoose.model('pokemons', PokemonSchema);
const insere = {
name: "Nerdmon",
description: "O pokemon mais nerd que já existiu, que existe ou que existirá",
type: "nerd",
attack: 999,
defense: 888,
height: 1.8
};
const Model = new PokemonModel(insere);
Model.save(insere, function (err, insere) {
if (err) return console.log('Erro: ', err);
console.log('Inserido: ', insere);
});
const alteraQuery = {name: /nerdmon/i};
const alteraMod = {attack: 49};
PokemonModel.update(alteraQuery, alteraMod, function (err, data){
if (err) return console.log('Erro: ', err);
console.log('Alterado: ', data);
});
```
Ao executar `node app.js` temos:
```
ednilson@EDNILSON-NB:/var/www/mongoose-pokemons$ node app.js
Mongoose default connection open to mongodb://localhost/be-mean-pokemons
Mongoose default connection is open
Inserido: { _id: 56aeb9d04bb106b114749436,
height: 1.8,
defense: 888,
attack: 999,
type: 'nerd',
description: 'O pokemon mais nerd que já existiu, que existe ou que existirá',
name: 'Nerdmon',
__v: 0 }
Alterado: { ok: 1, nModified: 1, n: 1 }
```
E, por via das dúvidas, consultando no MongoDB, temos:
```
be-mean-pokemons> var query = {name: /nerdmon/i}
be-mean-pokemons> db.pokemons.find(query)
{
"_id": ObjectId("56aeb9d04bb106b114749436"),
"name": "Nerdmon",
"description": "O pokemon mais nerd que já existiu, que existe ou que existirá",
"type": "nerd",
"attack": 49,
"defense": 888,
"height": 1.8,
"__v": 0
}
Fetched 1 record(s) in 3ms
```
## Remova **todos** os Pokemons com `attack` **acima de 50**
Arquivo JS:
```js
require('./config.js');
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const poke = {
name: String,
description: String,
type: String,
attack: Number,
defense: Number,
height: Number
}
const PokemonSchema = new Schema(poke);
const PokemonModel = mongoose.model('pokemons', PokemonSchema);
const query = {attack: {$gt: 50}};
PokemonModel.remove(query, function (err, query) {
if (err) return console.log('Erro: ', err);
console.log('Removeu: ', query);
});
```
Executando `node app.js` temos:
```
ednilson@EDNILSON-NB:/var/www/mongoose-pokemons$ node app.js
Mongoose default connection open to mongodb://localhost/be-mean-pokemons
Mongoose default connection is open
Removeu: { result: { ok: 1, n: 0 },
connection:
EventEmitter {
domain: null,
_events:
{ close: [Object],
error: [Object],
timeout: [Object],
parseError: [Object],
connect: [Function] },
_eventsCount: 5,
_maxListeners: undefined,
options:
{ socketOptions: {},
auto_reconnect: true,
host: 'localhost',
port: 27017,
cursorFactory: [Object],
reconnect: true,
emitError: true,
size: 5,
disconnectHandler: [Object],
bson: {},
messageHandler: [Function],
wireProtocolHandler: [Object] },
id: 2,
logger: { className: 'Connection' },
bson: {},
tag: undefined,
messageHandler: [Function],
maxBsonMessageSize: 67108864,
port: 27017,
host: 'localhost',
keepAlive: true,
keepAliveInitialDelay: 0,
noDelay: true,
connectionTimeout: 0,
socketTimeout: 0,
destroyed: false,
domainSocket: false,
singleBufferSerializtion: true,
serializationFunction: 'toBinUnified',
ca: null,
cert: null,
key: null,
passphrase: null,
ssl: false,
rejectUnauthorized: false,
checkServerIdentity: true,
responseOptions: { promoteLongs: true },
flushing: false,
queue: [],
connection:
Socket {
_connecting: false,
_hadError: false,
_handle: [Object],
_parent: null,
_host: 'localhost',
_readableState: [Object],
readable: true,
domain: null,
_events: [Object],
_eventsCount: 8,
_maxListeners: undefined,
_writableState: [Object],
writable: true,
allowHalfOpen: false,
destroyed: false,
bytesRead: 56,
_bytesDispatched: 175,
_sockname: null,
_pendingData: null,
_pendingEncoding: '',
_idleNext: null,
_idlePrev: null,
_idleTimeout: -1,
read: [Function],
_consuming: true },
writeStream: null,
buffer: null,
sizeOfMessage: 0,
bytesRead: 0,
stubBuffer: null } }
^CMongoose default connection disconnected
Mongoose default connection disconnected through app termination
```
Por via das dúvidas, no MongoDB, antes de executar o `app.js` busquei quantos tinhas segundo a condição do exercício. Após isso, obtive o número total de objetos salvos e então o *count* após executar o `app.js`.
```
be-mean-pokemons> var query = {attack: {$gt: 50}}
be-mean-pokemons> db.pokemons.count(query)
10
be-mean-pokemons> db.pokemons.count()
15
be-mean-pokemons> db.pokemons.count()
5
``` | {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 06 - Exercício
autor: Bruno Lima da Silva
## 1. Crie um Schema com cada tipo explicado, inserindo tanto um objeto correto, como um objeto que desencadeie erros de validação padrão, criar especificamente:
### -1.1 para String: enum, match, maxlength e minlength
String enum:
```js
const mongoose = require ('mongoose');
const Schema = mongoose.Schema;
const _schema = {
n: {
type: String,
enum: 'Valid'
}
};
const dataSchema = new Schema (_schema);
const data = { n: 'invalid' };
const model = mongoose.model ('pokemons', dataSchema);
const value = new model (data);
value.save ( (err, data) => {
if (err) return console.log ('ERRO:', err);
return console.log ('Inseriu:', data);
});
```
Saída
```
message: 'pokemons validation failed',
name: 'ValidationError',
errors:
{ n:
{ ValidatorError: `invalid` is not a valid enum value for path `n`.
at MongooseError.ValidatorError (/home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/error/validator.js:24:11)
at validate (/home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:704:13)
at /home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:742:9
at Array.forEach (native)
at SchemaString.SchemaType.doValidate (/home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:710:19)
at /home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/document.js:1354:9
at _combinedTickCallback (internal/process/next_tick.js:67:7)
at process._tickCallback (internal/process/next_tick.js:98:9)
at Module.runMain (module.js:606:11)
at run (bootstrap_node.js:394:7)
at startup (bootstrap_node.js:149:9)
at bootstrap_node.js:509:3
message: '`invalid` is not a valid enum value for path `n`.',
name: 'ValidatorError',
properties: [Object],
kind: 'enum',
path: 'n',
value: 'invalid' } } }
```
String match:
```js
const mongoose = require ('mongoose');
const Schema = mongoose.Schema;
const _schema = {
n: {
type: String,
match: /^a/
}
};
const dataSchema = new Schema (_schema);
const data = { n: 'Invalid' };
const model = mongoose.model ('pokemons', dataSchema);
const value = new model (data);
value.save ( (err, data) => {
if (err) return console.log ('ERRO:', err);
return console.log ('Inseriu:', data);
});
```
Saída
```
message: 'pokemons validation failed',
name: 'ValidationError',
errors:
{ n:
{ ValidatorError: Path `n` is invalid (Invalid).
at MongooseError.ValidatorError (/home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/error/validator.js:24:11)
at validate (/home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:704:13)
at /home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:742:9
at Array.forEach (native)
at SchemaString.SchemaType.doValidate (/home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:710:19)
at /home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/document.js:1354:9
at _combinedTickCallback (internal/process/next_tick.js:67:7)
at process._tickCallback (internal/process/next_tick.js:98:9)
at Module.runMain (module.js:606:11)
at run (bootstrap_node.js:394:7)
at startup (bootstrap_node.js:149:9)
at bootstrap_node.js:509:3
message: 'Path `n` is invalid (Invalid).',
name: 'ValidatorError',
properties: [Object],
kind: 'regexp',
path: 'n',
value: 'Invalid' } } }
```
String maxlength:
```js
const mongoose = require ('mongoose');
const Schema = mongoose.Schema;
const _schema = {
n: {
type: String,
maxlength: 5
}
};
const dataSchema = new Schema (_schema);
const data = { n: 'Bruno Lima da Silva' };
const model = mongoose.model ('pokemons', dataSchema);
const value = new model (data);
value.save ( (err, data) => {
if (err) return console.log ('ERRO:', err);
return console.log ('Inseriu:', data);
});
```
Saída
```
message: 'pokemons validation failed',
name: 'ValidationError',
errors:
{ n:
{ ValidatorError: Path `n` (`Bruno Lima da Silva`) is longer than the maximum allowed length (5).
at MongooseError.ValidatorError (/home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/error/validator.js:24:11)
at validate (/home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:704:13)
at /home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:742:9
at Array.forEach (native)
at SchemaString.SchemaType.doValidate (/home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:710:19)
at /home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/document.js:1354:9
at _combinedTickCallback (internal/process/next_tick.js:67:7)
at process._tickCallback (internal/process/next_tick.js:98:9)
at Module.runMain (module.js:606:11)
at run (bootstrap_node.js:394:7)
at startup (bootstrap_node.js:149:9)
at bootstrap_node.js:509:3
message: 'Path `n` (`Bruno Lima da Silva`) is longer than the maximum allowed length (5).',
name: 'ValidatorError',
properties: [Object],
kind: 'maxlength',
path: 'n',
value: 'Bruno Lima da Silva' } } }
```
String minlength:
```js
const mongoose = require ('mongoose');
const Schema = mongoose.Schema;
const _schema = {
n: {
type: String,
minlength: 8
}
};
const dataSchema = new Schema (_schema);
const data = { n: 'Bruno' };
const model = mongoose.model ('pokemons', dataSchema);
const value = new model (data);
value.save ( (err, data) => {
if (err) return console.log ('ERRO:', err);
return console.log ('Inseriu:', data);
});
```
Saída
```
message: 'pokemons validation failed',
name: 'ValidationError',
errors:
{ n:
{ ValidatorError: Path `n` (`Bruno`) is shorter than the minimum allowed length (8).
at MongooseError.ValidatorError (/home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/error/validator.js:24:11)
at validate (/home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:704:13)
at /home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:742:9
at Array.forEach (native)
at SchemaString.SchemaType.doValidate (/home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:710:19)
at /home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/document.js:1354:9
at _combinedTickCallback (internal/process/next_tick.js:67:7)
at process._tickCallback (internal/process/next_tick.js:98:9)
at Module.runMain (module.js:606:11)
at run (bootstrap_node.js:394:7)
at startup (bootstrap_node.js:149:9)
at bootstrap_node.js:509:3
message: 'Path `n` (`Bruno`) is shorter than the minimum allowed length (8).',
name: 'ValidatorError',
properties: [Object],
kind: 'minlength',
path: 'n',
value: 'Bruno' } } }
```
### -1.2 para Number:max e min
Number max:
```js
const mongoose = require ('mongoose');
const Schema = mongoose.Schema;
const _schema = {
n: {
type: Number,
max: 10
}
};
const dataSchema = new Schema (_schema);
const data = { n: 11 };
const model = mongoose.model ('pokemons', dataSchema);
const value = new model (data);
value.save ( (err, data) => {
if (err) return console.log ('ERRO:', err);
return console.log ('Inseriu:', data);
});
```
Saída
```
message: 'pokemons validation failed',
name: 'ValidationError',
errors:
{ n:
{ ValidatorError: Path `n` (11) is more than maximum allowed value (10).
at MongooseError.ValidatorError (/home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/error/validator.js:24:11)
at validate (/home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:704:13)
at /home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:742:9
at Array.forEach (native)
at SchemaNumber.SchemaType.doValidate (/home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:710:19)
at /home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/document.js:1354:9
at _combinedTickCallback (internal/process/next_tick.js:67:7)
at process._tickCallback (internal/process/next_tick.js:98:9)
at Module.runMain (module.js:606:11)
at run (bootstrap_node.js:394:7)
at startup (bootstrap_node.js:149:9)
at bootstrap_node.js:509:3
message: 'Path `n` (11) is more than maximum allowed value (10).',
name: 'ValidatorError',
properties: [Object],
kind: 'max',
path: 'n',
value: 11 } } }
```
Number min:
```js
const mongoose = require ('mongoose');
const Schema = mongoose.Schema;
const _schema = {
n: {
type: Number,
min: 7
}
};
const dataSchema = new Schema (_schema);
const data = { n: 5 };
const model = mongoose.model ('pokemons', dataSchema);
const value = new model (data);
value.save ( (err, data) => {
if (err) return console.log ('ERRO:', err);
return console.log ('Inseriu:', data);
});
```
Saída
```
message: 'pokemons validation failed',
name: 'ValidationError',
errors:
{ n:
{ ValidatorError: Path `n` (5) is less than minimum allowed value (7).
at MongooseError.ValidatorError (/home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/error/validator.js:24:11)
at validate (/home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:704:13)
at /home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:742:9
at Array.forEach (native)
at SchemaNumber.SchemaType.doValidate (/home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/schematype.js:710:19)
at /home/fsociety/be-mean-instagram/nodeJS/mongoose-pokemons/node_modules/mongoose/lib/document.js:1354:9
at _combinedTickCallback (internal/process/next_tick.js:67:7)
at process._tickCallback (internal/process/next_tick.js:98:9)
at Module.runMain (module.js:606:11)
at run (bootstrap_node.js:394:7)
at startup (bootstrap_node.js:149:9)
at bootstrap_node.js:509:3
message: 'Path `n` (5) is less than minimum allowed value (7).',
name: 'ValidatorError',
properties: [Object],
kind: 'min',
path: 'n',
value: 5 } } }
```
## 2. Cadastre 3 pokemons de uma só vez. (pesquisar)
```js
const mongoose = require ('mongoose');
const dbURI = 'mongodb://localhost/be-mean-instagram';
mongoose.connect (dbURI);
const Schema = mongoose.Schema;
const _schema = {
name: String
};
const pokemonSchema = new Schema (_schema);
const PokemonModel = mongoose.model ('pokemons', pokemonSchema);
const poke = [
{
name: 'blsdotrocks'
},
{
name: 'Karoline'
},
{
name: 'Suissa'
}
];
PokemonModel.create (poke, (err, poke) => {
if (err) return console.log ('ERRO:', err);
return console.log ('Inseriu:', poke);
});
```
Saída
```
➜ mongoose-pokemons node schema09.js
Inseriu: [ { __v: 0, name: 'blsdotrocks', _id: 582ce6bf5db3ae2c9f1faf3e },
{ __v: 0, name: 'Karoline', _id: 582ce6bf5db3ae2c9f1faf3f },
{ __v: 0, name: 'Suissa', _id: 582ce6bf5db3ae2c9f1faf40 } ]
```
## 3. Busque todos os Pokemons com attack > 50 e height > 0.5.
```js
const mongoose = require ('mongoose');
const dbURI = 'mongodb://localhost/be-mean-instagram';
mongoose.connect (dbURI);
const Schema = mongoose.Schema;
const _schema = {};
const pokemonSchema = new Schema (_schema);
const PokemonModel = mongoose.model ('pokemon', pokemonSchema);
const query = {attack: {$gt: 50}, height: {$gt: "0.5"}};
PokemonModel.find (query, (err, data) => {
if (err) return console.log ('ERRO:', err);
return console.log ('Buscou:', data);
});
```
## 4. Altere, inserindo, o Pokemon Nerdmon com attack = 49 e com os valores dos outros campos a sua escolha.
```js
const mongoose = require ('mongoose');
const dbURI = 'mongodb://localhost/be-mean-instagram';
mongoose.connect (dbURI);
const Schema = mongoose.Schema;
const _schema = {
name: String,
created: {type: Date, default: Date.now},
type: [String],
attack: Number,
defense: Number,
height: String,
hp: Number,
Speed: Number
};
const pokemonSchema = new Schema(_schema);
const PokemonModel = mongoose.model ('pokemons', pokemonSchema);
const query = {
name: 'Nerdmon',
attack: 49
};
PokemonModel.update (query, (err, data) => {
if (err) return console.log ('ERRO:', err);
return console.log ('Inseriu:', data);
});
```
Saída
```
Inseriu: { ok: 1, nModified: 1, n: 1 }
```
## 5. Remova todos os Pokemons com attack > 50.
```js
const mongoose = require ('mongoose');
const dbURI = 'mongodb://localhost/be-mean-instagram';
mongoose.connect (dbURI);
const Schema = mongoose.Schema;
const _schema = {
name: String,
created: {type: Date, default: Date.now},
type: [String],
attack: Number,
defense: Number,
height: String,
hp: Number,
Speed: Number
};
const pokemonSchema = new Schema (_schema);
const PokemonModel = mongoose.model ('pokemons', pokemonSchema);
const query = {attack: {$gt: 50}};
PokemonModel.remove (query, (err, data) => {
if (err) return console.log ('ERRO', err);
return console.log ('Delete', data);
});
```
Saída
```
result: { ok: 1, n: 461 },
```
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 06 - Exercício
**user:** [filipe1309](https://github.com/filipe1309)
**autor:** Filipe Leuch Bonfim
## Crie um Schema com cada tipo explicado, inserindo tanto um objeto correto, como um objeto que desencadeie erros de validação padrão, criar especificamente:
* 1.1. para String: `enum`, `match`, `maxlength` e `minlength`
* 1.2. para Number: `max` e `min`
```js
const mongoose = require('mongoose');
// Conexão com o mongo
const dbURI = 'mongodb://localhost/be-mean-instagram';
mongoose.connect(dbURI);
const Schema = mongoose.Schema;
// Definição do Schema
const meanArr = 'mongodb expressjs angularjs nodejs'.split(' ');
const _schema = {
stringEnum: { type: String, enum: meanArr }
, stringMatch: { type: String, match: /node((\.js)|js)?/i }
, stringMinLength: { type: String, minlength: 5 }
, stringMaxLength: { type: String, maxlength: 9 }
, numMin: { type: Number, min: 12 }
, numMax: { type: Number, max: 14 }
, dateValue: { type: Date }
, bufferValue: Buffer
, booleanValue: Boolean
, mixedValue: Schema.Types.Mixed
, objectValue: Schema.Types.ObjectId
, arrayValue: [String]
};
const class06Ex1Schema = new Schema(_schema);
const class06Ex1Model = mongoose.model('class06', class06Ex1Schema);
var class06Ex1Object = new class06Ex1Model;
// Inserções válidas
class06Ex1Object.stringEnum = 'nodejs';
class06Ex1Object.stringMatch = 'nodejs';
class06Ex1Object.stringMinLength = '1234567';
class06Ex1Object.stringMaxLength = '1234567';
class06Ex1Object.numMin = 13;
class06Ex1Object.numMax = 13;
class06Ex1Object.dateValue = Date.now();
class06Ex1Object.bufferValue = new Buffer(0);
class06Ex1Object.booleanValue = 1;
class06Ex1Object.mixedValue = 'Mean Stack';
class06Ex1Object.objectValue = new mongoose.Types.ObjectId;
class06Ex1Object.arrayValue.push('Inseriu!!!!');
// Inserções inválidas
class06Ex1Object.stringEnum = 'php';
class06Ex1Object.stringMatch = 'dog';
class06Ex1Object.stringMinLength = '1234';
class06Ex1Object.stringMaxLength = '1234567890';
class06Ex1Object.numMin = 11;
class06Ex1Object.numMax = 15;
class06Ex1Object.dateValue = '11/11/11';
class06Ex1Object.bufferValue = 'buf';
class06Ex1Object.booleanValue = {};
class06Ex1Object.mixedValue = undefined;
class06Ex1Object.objectValue = 'objeto';
class06Ex1Object.arrayValue.push(1);
class06Ex1Object.save(function (err, data) {
if(err) return console.log(err);
return console.log('Sucesso: ', data);
});
```
#### Saída
```js
// Inserções válidas
Sucesso: { arrayValue: [ 'Inseriu!!!!' ],
_id: 56e2338885ca37d42959fc1c,
stringEnum: 'nodejs',
stringMatch: 'nodejs',
stringMinLength: '1234567',
stringMaxLength: '1234567',
numMin: 13,
numMax: 13,
dateValue: Thu Mar 10 2016 23:55:04 GMT-0300 (BRT),
bufferValue:
Binary {
_bsontype: 'Binary',
sub_type: 0,
position: 0,
buffer: <Buffer > },
booleanValue: true,
mixedValue: 'Mean Stack',
objectValue: 56e2338885ca37d42959fc1d,
__v: 0 }
// Inserções inválidas
{ [ValidationError: class06 validation failed]
message: 'class06 validation failed',
name: 'ValidationError',
errors:
{ objectValue:
{ [CastError: Cast to ObjectID failed for value "objeto" at path "objectValue"]
message: 'Cast to ObjectID failed for value "objeto" at path "objectValue"',
name: 'CastError',
kind: 'ObjectID',
value: 'objeto',
path: 'objectValue',
reason: undefined },
stringEnum:
{ [ValidatorError: `php` is not a valid enum value for path `stringEnum`.]
properties: [Object],
message: '`php` is not a valid enum value for path `stringEnum`.',
name: 'ValidatorError',
kind: 'enum',
path: 'stringEnum',
value: 'php' },
stringMatch:
{ [ValidatorError: Path `stringMatch` is invalid (dog).]
properties: [Object],
message: 'Path `stringMatch` is invalid (dog).',
name: 'ValidatorError',
kind: 'regexp',
path: 'stringMatch',
value: 'dog' },
stringMinLength:
{ [ValidatorError: Path `stringMinLength` (`1234`) is shorter than the minimum allowed length (5).]
properties: [Object],
message: 'Path `stringMinLength` (`1234`) is shorter than the minimum allowed length (5).',
name: 'ValidatorError',
kind: 'minlength',
path: 'stringMinLength',
value: '1234' },
stringMaxLength:
{ [ValidatorError: Path `stringMaxLength` (`1234567890`) is longer than the maximum allowed length (9).]
properties: [Object],
message: 'Path `stringMaxLength` (`1234567890`) is longer than the maximum allowed length (9).',
name: 'ValidatorError',
kind: 'maxlength',
path: 'stringMaxLength',
value: '1234567890' },
numMin:
{ [ValidatorError: Path `numMin` (11) is less than minimum allowed value (12).]
properties: [Object],
message: 'Path `numMin` (11) is less than minimum allowed value (12).',
name: 'ValidatorError',
kind: 'min',
path: 'numMin',
value: 11 },
numMax:
{ [ValidatorError: Path `numMax` (15) is more than maximum allowed value (14).]
properties: [Object],
message: 'Path `numMax` (15) is more than maximum allowed value (14).',
name: 'ValidatorError',
kind: 'max',
path: 'numMax',
value: 15 }
}
}
```
## Cadastre 3 pokemons **de uma só vez**:
```js
const mongoose = require('mongoose');
// Conexão com o mongo
const dbURI = 'mongodb://localhost/be-mean-instagram';
mongoose.connect(dbURI);
const Schema = mongoose.Schema;
// Definição do Schema
const _schema = {
attack: Number
, created: { type: Date, default: Date.now }
, defense: Number
, height: Number
, hp: Number
, name: String
, speed: Number
, types: [String]
};
const pokemonsSchema = new Schema(_schema);
var pokemonsModel = mongoose.model('pokemons', pokemonsSchema);
var pokemon1 = {
name: 'BeboMon'
, attack: 51
, defense: 51
, height: 12.1
}
var pokemon2 = {
name: 'CodeMon'
, attack: 42
, defense: 31
, height: 6.18
}
var pokemon3 = {
name: 'SonoMon'
, attack: 10
, defense: 09
, height: 08
}
var novosPokemons = [pokemon1, pokemon2, pokemon3];
pokemonsModel.insertMany(novosPokemons, function(error, docs) {
if(error) return console.log(error);
return console.log('Sucesso: ', docs);
});
```
#### Saída
```js
Sucesso: [ { created: Fri Mar 11 2016 00:38:28 GMT-0300 (BRT),
types: [],
_id: 56e23db4bd5799c830b1b07a,
height: 12.1,
defense: 51,
attack: 51,
name: 'BeboMon' },
{ created: Fri Mar 11 2016 00:38:28 GMT-0300 (BRT),
types: [],
_id: 56e23db4bd5799c830b1b07b,
height: 6.18,
defense: 31,
attack: 42,
name: 'CodeMon' },
{ created: Fri Mar 11 2016 00:38:28 GMT-0300 (BRT),
types: [],
_id: 56e23db4bd5799c830b1b07c,
height: 8,
defense: 9,
attack: 10,
name: 'SonoMon' } ]
```
## Busque **todos** os Pokemons com `attack > 50` e `height > 0.5`:
```js
const mongoose = require('mongoose');
// Conexão com o mongo
const dbURI = 'mongodb://localhost/be-mean-instagram';
mongoose.connect(dbURI);
const Schema = mongoose.Schema;
// Definição do Schema
const _schema = {
attack: Number
, created: { type: Date, default: Date.now }
, defense: Number
, height: Number
, hp: Number
, name: String
, speed: Number
, types: [String]
};
const pokemonsSchema = new Schema(_schema);
const pokemonsModel = mongoose.model('pokemons', pokemonsSchema);
var query = {$and: [{attack: {$gt: 50}}, {height: {$gt: 0.5}}]}
pokemonsModel.find(query, function (err, data) {
if (err) return console.log('ERRO: ', err);
return console.log('Buscou: ', data);
});
```
#### Saída
```js
Buscou: [ { created: Fri Mar 11 2016 00:38:28 GMT-0300 (BRT),
types: [],
height: 12.1,
defense: 51,
attack: 51,
name: 'BeboMon',
_id: 56e23db4bd5799c830b1b07a }
]
```
## Altere, **inserindo**, o Pokemon `Nerdmon` com `attack` igual a 49 e com os valores dos outros campos a sua escolha.
```js
const mongoose = require('mongoose');
// Conexão com o mongo
const dbURI = 'mongodb://localhost/be-mean-instagram';
mongoose.connect(dbURI);
const Schema = mongoose.Schema;
// Definição do Schema
const _schema = {
attack: Number
, created: { type: Date, default: Date.now }
, defense: Number
, height: Number
, hp: Number
, name: String
, speed: Number
, types: [String]
};
const pokemonsSchema = new Schema(_schema);
const PokemonsModel = mongoose.model('pokemons', pokemonsSchema);
var nerdMon = {
name: 'Nerdmon'
, attack: 49
, defense: 51
}
var nerdMonObj = new PokemonsModel(nerdMon);
nerdMonObj.save(function (err, data) {
if (err) return console.log('ERRO: ', err);
console.log('Sucesso: ', data)
});
```
#### Saída
```js
Sucesso: { created: Fri Mar 11 2016 01:13:49 GMT-0300 (BRT),
types: [],
_id: 56e245fd5e430205354d4a4e,
defense: 51,
attack: 49,
name: 'Nerdmon',
__v: 0 }
```
## Remova **todos** os Pokemons com `attack` **acima de 50**.
```js
const mongoose = require('mongoose');
// Conexão com o mongo
const dbURI = 'mongodb://localhost/be-mean-instagram';
mongoose.connect(dbURI);
const Schema = mongoose.Schema;
// Definição do Schema
const _schema = {
attack: Number
, created: { type: Date, default: Date.now }
, defense: Number
, height: Number
, hp: Number
, name: String
, speed: Number
, types: [String]
};
const PokemonsSchema = new Schema(_schema);
const PokemonsModel = mongoose.model('pokemons', PokemonsSchema);
var query = {attack: {$gt: 50} }
PokemonsModel.remove(query, function (err, data) {
if (err) return console.log('ERRO: ', err);
return console.log('Excluiu: ', data);
});
```
#### Saída
```js
Excluiu: { result: { ok: 1, n: 463 },
connection:
EventEmitter {
domain: null,
_events:
{ close: [Object],
error: [Object],
timeout: [Object],
parseError: [Object],
connect: [Function] },
_eventsCount: 5,
_maxListeners: undefined,
options:
{ socketOptions: {},
auto_reconnect: true,
host: 'localhost',
port: 27017,
cursorFactory: [Object],
reconnect: true,
emitError: true,
size: 5,
disconnectHandler: [Object],
bson: {},
messageHandler: [Function],
wireProtocolHandler: [Object] },
id: 0,
logger: { className: 'Connection' },
bson: {},
tag: undefined,
messageHandler: [Function],
maxBsonMessageSize: 67108864,
port: 27017,
host: 'localhost',
keepAlive: true,
keepAliveInitialDelay: 0,
noDelay: true,
connectionTimeout: 0,
socketTimeout: 0,
destroyed: false,
domainSocket: false,
singleBufferSerializtion: true,
serializationFunction: 'toBinUnified',
ca: null,
cert: null,
key: null,
passphrase: null,
ssl: false,
rejectUnauthorized: false,
checkServerIdentity: true,
responseOptions: { promoteLongs: true },
flushing: false,
queue: [],
connection:
Socket {
_connecting: false,
_hadError: false,
_handle: [Object],
_parent: null,
_host: 'localhost',
_readableState: [Object],
readable: true,
domain: null,
_events: [Object],
_eventsCount: 8,
_maxListeners: undefined,
_writableState: [Object],
writable: true,
allowHalfOpen: false,
destroyed: false,
bytesRead: 250,
_bytesDispatched: 231,
_sockname: null,
_pendingData: null,
_pendingEncoding: '',
_idleNext: null,
_idlePrev: null,
_idleTimeout: -1,
read: [Function],
_consuming: true },
writeStream: null,
hashedName: '29bafad3b32b11dc7ce934204952515ea5984b3c',
buffer: null,
sizeOfMessage: 0,
bytesRead: 0,
stubBuffer: null } }
```
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 12 - Exercício
**user:** [tuchedsf](https://github.com/tuchedsf)
**autor:** Diego Ferreira
**data:** 1467668568530
O quark que utilizei para este exercício foi a validacão do isbn-13, utilizado para livros deste 2007, podendo ajudar quem deseja construir alguma aplicação que catalogue livros ou bibliotecas.
Fonte: https://pt.wikipedia.org/wiki/International_Standard_Book_Number#ISBN-13
>A edição de 2005 do manual oficial do International ISBN Agency,[10] que abrange os ISBNs emitidos a partir de Janeiro de 2007, descreve como é efectuado o calculo, do dígito de verificação, do ISBN de 13 dígitos. O cálculo do dígito de verificação do ISBN-13 começa com os primeiros 12 dígitos dos 13, multiplicando alternadamente por 1 ou 3 , da esquerda para a direita, em seguida, estes produtos são somados módulo 10 para dar um valor que varia de 0 a 9, que subtraído a 10, que deixa um resultado de 1 a 10. Um zero (0) substitui 0 dez (10), garantindo assim, que o resultado da verificação não é maior que um dígito.
Link para o repositório: https://github.com/Webschool-io/Node-Atomic-Design_QUARKS/pull/10
Pasta: isISBN-13
Arquivos:
isISBN-13.js
```js
'use strict';
module.exports = (value) => {
const arrayValues = value.toString().split("").map(Number);
//console.log(arrayValues);
let digCalculado = 0;
//Se isbn nao tiver 13 posiçoes já pode ser considerado inválido
if (arrayValues.length != 13 ) return false;
/*
1 passo:
da posicao 1 a 12 do array deve-se multiplicar da seguinte forma:
indice impar multiplica-se por 1
indice par multiplica-se por 3
*/
arrayValues.forEach(function (element,index){
if (index != arrayValues.length -1) {
//console.log("index: " + index + " element: " + element);
if(index % 2 === 0){
digCalculado = digCalculado + element;
}else {
digCalculado = digCalculado + (element * 3);
}
}
});
/* passo2:
- e feito o mod 10 sobre a soma calculada e este valor subtraido de 10.
- feito isso o resultado mod 10 deve ser igual ao elemento verificador a ultima posiçao do array
*/
digCalculado = (10 - (digCalculado % 10)) % 10;
/* passo3:
comparacao se o digito calculado é igual ao passado no array
*/
if (digCalculado === arrayValues[arrayValues.length -1]) return true;
return false;
}
```
isISBN-13.test.js
```js
'use strict';
const describes = [
{type: true
, message:"ISBN válido"
, values: [9780306406157, 9788525423498, 9788581303079]
}
, {type: false
, message:"ISBN inválido"
, values: [1111111111111, 123, 2367463526234, 9780306406156]
}
];
//chama a classe generica de testes informando qual o arquivo do teste, e quais os testes a serem feitos.
const test = require('./module.tests.is.js')('isISBN-13', describes);
```
module.tests.is.js
```js
'use strict';
const expect = require('chai').expect;
module.exports = (testName, describes) => {
const test = (values, msg, valueToTest) => {
values.forEach((element, index) =>{
it(msg + " "+ element + " ", () =>{
expect(require('./'+ testName)(element)).to.equal(valueToTest);
});
});
}
describe (testName, ()=> {
describes.forEach((element, index) => {
describe(element.message, () => {
test(element.values,element.message, element.type)
});
});
});
};
```
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 12 - Exercício
**User:** [gkal19](https://github.com/gkal19)
**Autor:** Gabriel Kalani
**Data:** 1468533500436
[MEU QUARK](https://github.com/Webschool-io/Node-Atomic-Design_QUARKS/pull/11)
> O que é um Quark?
Como dizia o Sábio Sennin Jean Carlo Nascimento JavaScript Da Silva (eita), é nada mais é que a menor parte/módulo/função utilizada pelo Átomo.
> Quero saber mais...
Então vê a aulas aqui rsrs
[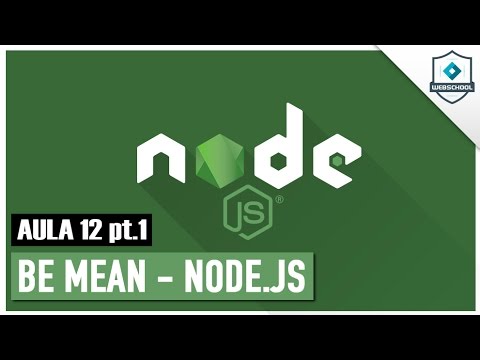](http://www.youtube.com/watch?v=2lml_4kOhgU "Be MEAN: Node.JS (AULA 12 pt.1/3) Quarks")
Falou!!
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 09 - Exercício
**user:** [tuchedsf](https://github.com/tuchedsf)
**autor:** Diego Ferreira
## Parte 1
###1) Faça uma lista de todas as funções que o modulo assert possui e coloque no md.
- assert -> Sinônimo de assert.ok, apenas atesta a existência da variável se é verdadeira.
- assert.deepEqual(atual, esperado, mensagem) -> testa se o valor atual é igual ao valor esperado, e valores primitivos são testados com comparação simples "==".
- assert.deepStrictEqual(atual, esperado, mensagem) -> idêntico ao deepEqual. A única diferença que aqui os valores primitivos são testados com "===", ou seja, além do "valor" também consideram o tipo do valor.
- assert.doesNotThrow(block, error, message) -> verifica se a função passada em "block" não retorna nenhum erro.
- assert.equal(atual, esperado, mensagem) -> verifica se o valor passada como atual é igual ao esperado e em caso de objetos se o objeto atual é igual ao esperado.
- assert.fail(atual, esperado, mensagem, operador) -> O operador serve para separar o elemento atual do esperado, em caso em caso de erro e se a mensagem não estiver preenchida. Caso a mensagem esteja preenchida é exibida a mensagem.
- assert.ifError(value) -> Testa se o valor não é um valor falso e dispara o throws se for verdadeiro.
- assert.notDeepEqual(atual, esperado, mensagem) -> Testa qualquer diferença entre os objetos tanto valor quanto tipo.
- assert.notDeepStrictEqual(atual, esperado, mensagem ) -> Testa se o valor do objeto atual é diferente do esperado porém leva em consideração o tipo de objeto.
- assert.notEqual(atual, esperado, mensagem) -> Testa se o valor do atual é diferente do esperado, leva em consideração o tipo de objeto.
- assert.notStrictEqual(atual, esperado, mensagem) -> Testa se o valor do atual é diferente do esperado, não leva em consideração o tipo de objeto.
- assert.ok(valor, mensagem) -> testa se o valor é verdadeiro.
- assert.strictEqual(atual, esperado, mensagem) -> testa se o valor e tipo do objeto são iguais.
- assert.throws(função[erro], mensagem) -> testa erros em funções.
###2) Modifique todos os testes que estão usando assert.equal para assert.deepStrictEqual(valor, result, "comentario") faça todos passarem, coloque os testes no md. ( primeira parte da aula)
Alteração assert add.spec.js
```js
'use strict';
const assert = require("assert");
const add = require("./assert_add");
//assert.equal(3, add(1,2));
//assert.equal(8, add(1,2,5));
assert.deepStrictEqual(3, add(1,2), "Resultado da soma diferente");
assert.deepStrictEqual(8, add(1,2,5), "Resultado da soma diferente");
```
Resultado:
```
Falha:
MacBook-Air-Diego:9tdd diego$ mocha assert_add_spec.js
assert.js:89
throw new assert.AssertionError({
^
AssertionError: Resultado da soma diferente
Correção:
➜ test mocha assert_add_spec.js
2 passing (4ms)
```
babystep.js
```js
'use strict';
const assert = require('assert');
const log = require('./log');
//assert.equal('Hello World', log('Hello World'));
//assert.equal('final do hello world', log('final do hello world'));
assert.deepStrictEqual('Hello World', log('Hello World'));
assert.deepStrictEqual('final do hello world', log('final do hello world'));
```
Resultado:
```
Erro:
➜ test mocha log_spec.js
assert.js:89
throw new assert.AssertionError({
^
AssertionError: 'Hell World' == 'Hello World'
Correção:
➜ test mocha log_spec.js
2 passing (4ms)
```
###3) Na calculadora do CHAI crie mais 3 testes, um para divisão, outro para multiplicação e 1 para raiz quadrada, lembrando que não deve-se aceitar divisão por zero.
calc.js
```js
'use strict';
const Calc = {
sum : (number1, number2) =>{
return number1 + number2;
}
, subtract : (number1, number2) => {
return number1 - number2;
}
, multiply : (number1, number2) => {
return number1 * number2;
}
, divide : (number1, number2) => {
return number1 / number2;
}
, square : (number) => {
return Math.sqrt(number);
}
};
module.exports = Calc;
```
chai_calc.js
```js
'use strict';
const Calc = require('../calc.js');
const expect = require('chai').expect;
describe("Calc", ()=> {
describe("calc sum off two numbers", () =>{
it("the function return the sum of two numbers", () => {
const sum = Calc.sum(5,5);
expect(sum).to.be.a('number');
expect(sum).to.equal(10);
expect(Calc).to.have.property('sum');
});
});
describe("calc sub off two numbers", () =>{
it("the function return the subtract of two numbers", () => {
const subtract = Calc.subtract(5,5);
expect(subtract).to.be.a('number');
expect(subtract).to.equal(0);
expect(Calc).to.have.property('subtract');
});
});
describe("calc multiply two numbers", () =>{
it("the function return the multiply of two numbers", () => {
const multiply = Calc.multiply(5,5);
expect(multiply).to.be.a('number');
expect(multiply).to.equal(25);
expect(Calc).to.have.property('multiply');
});
});
describe("calc divide two numbers", () =>{
const number1 = 5;
const number2 = 1;
it("the divisor great then 0", () => {
expect(number2).to.be.a('number');
expect(number2).not.to.be.NaN;
expect(number2).to.be.at.least(1);
});
it("the function return the divide of two numbers", () => {
const divide = Calc.divide(5,5);
expect(divide).to.be.a('number');
expect(divide).to.equal(1);
expect(Calc).to.have.property('divide');
});
});
describe("calc square two numbers", () =>{
it("the function return the square of two numbers", () => {
const square = Calc.square(9);
expect(square).to.be.a('number');
expect(square).to.equal(3);
expect(Calc).to.have.property('square');
});
});
});
```
Resultado após declaração dos testes sem implementação
```
mocha chai_ex_01.js
Calc
calc sum off two numbers
✓ the function return the sum of two numbers
calc sub off two numbers
✓ the function return the subtract of two numbers
calc multiply two numbers
✓ the function return the multiply of two numbers
calc divide two numbers
1) the divisor great then 0
2) the function return the divide of two numbers
calc square two numbers
3) the function return the square of two numbers
3 passing (27ms)
3 failing
1) Calc calc divide two numbers the divisor great then 0:
AssertionError: expected 0 to be at least 1
at Context.<anonymous> (chai_ex_01.js:43:29)
2) Calc calc divide two numbers the function return the divide of two numbers:
TypeError: Calc.divide is not a function
at Context.<anonymous> (chai_ex_01.js:47:24)
3) Calc calc square two numbers the function return the square of two numbers:
TypeError: Calc.square is not a function
at Context.<anonymous> (chai_ex_01.js:56:24)
```
Resultado apos implementação dos métodos:
```
mocha chai_ex_01.js
Calc
calc sum off two numbers
✓ the function return the sum of two numbers
calc sub off two numbers
✓ the function return the subtract of two numbers
calc multiply two numbers
✓ the function return the multiply of two numbers
calc divide two numbers
✓ the divisor great then 0
✓ the function return the divide of two numbers
calc square two numbers
✓ the function return the square of two numbers
6 passing (21ms)
```
###4) Use o expect para testar a exceção da divisão por zero. (complemento do exercício 3)
Feito no exercício antetior, abaixo apenas o teste criado para barrar divisão por 0.
```
describe("calc divide two numbers", () =>{
const number1 = 5;
const number2 = 0;
it("the divisor great then 0", () => {
expect(number2).to.be.a('number');
expect(number2).not.to.be.NaN;
expect(number2).to.be.at.least(1);
});
```
##Parte 02
###1) Use o exemplo de testes do getter e setter e crie um teste para o method. use o exemplo: (https://github.com/Webschool-io/be-mean-instagram/blob/master/Apostila%2Fmodule-nodejs%2Fsrc%2Fmongoose-atomic%2Fschema-method.js)
schema.js
```js
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
mongoose.connect('mongodb://localhost/be-mean-instagram');
const _schema = {
name: String
, description: String
, type: String
, attack: Number
, defense: Number
, height: Number
};
const PokemonSchema = new Schema(_schema);
PokemonSchema.methods.findSimilarType = function (cb) {
return this.model('Pokemon').find({ type: this.type }, cb);
};
const Pokemon = mongoose.model('Pokemon', PokemonSchema);
module.exports = Pokemon;
```
schema-method-test.js
```js
'use strict';
const Pokemon = require('../schema-method');
const chai = require('chai');
const expect = chai.expect;
const util = require('util');
describe('Pokemon methods test', ()=>{
const Poke = { name: 'Teste', type: 'besta' };
describe("validate find similar type", ()=> {
it('lista apenas pokemons types iguais', (done) => {
let pokeBusca = new Pokemon({name: 'Besta 2', type: 'besta'});
pokeBusca.findSimilarType((err,data) => {
expect(err).to.not.exist;
expect(data).to.be.instanceof(Array);
data.forEach((pokemon) => expect(pokemon).to.have.property('type').and.equal('besta'));
done();
});
});
});
});
```
Resultado:
```
mocha schema-method-test.js
Pokemon methods test
validate find similar type
✓ lista apenas timos iguais de pokemon
1 passing (60ms)
```
###2) Use o exemplo de testes do getter e setter, o static, use o exemplo: https://github.com/Webschool-io/be-mean-instagram/blob/master/Apostila%2Fmodule-nodejs%2Fsrc%2Fmongoose-atomic%2Fschema-static.js
schema-static.js
```js
'use strict';
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
mongoose.connect('mongodb://localhost/be-mean-instagram');
const _schema = {
name: String
, description: String
, type: String
, attack: Number
, defense: Number
, height: Number
};
const PokemonSchema = new Schema(_schema);
PokemonSchema.statics.search = function (name, cb) {
return this.where('name', new RegExp(name, 'i')).exec(cb);
};
const Pokemon = mongoose.model('Pokemon', PokemonSchema);
module.exports = Pokemon;
```
schema-static-test.js
```js
'use strict';
const Pokemon = require('../schema-static');
const chai = require('chai');
const expect = chai.expect;
const util = require('util');
describe('Pokemon static test', ()=>{
describe("validate find nomes iguais", ()=> {
it('lista pokemons de mesmo nome', (done) => {
Pokemon.search('pikachu', function (err, data) {
expect(err).to.not.exist;
expect(data).to.be.instanceof(Array);
data.forEach((pokemon) => expect(pokemon).to.have.property('name').and.equal('Pikachu'));
done();
});
});
});
});
```
Resultado:
```
mocha schema-static-test.js
Pokemon static test
validate find nomes iguais
✓ lista pokemons de mesmo nome
1 passing (57ms)
```
###3) No controller ainda falta dois métodos para testar, o update e o delete, crie-os e faça os testes, no mesmo arquivo que contem os testes create e retrive.
controller-poke-test.js
```js
'use strict';
const util = require('util');
const chai = require('chai');
const expect = chai.expect;
const pokeController = require('../controller-pokemon');
const Pokemon = require('../schema-static');
describe("Controller User teste", () => {
const poke = {
name: 'Novo Poke', type: 'besta', attack: 28, defense: 42, height: 0.87
};
describe( "Create new pokemon", () => {
it('expect a new pokemon has created', (done) => {
pokeController.create(poke, (err,data) => {
expect(err).to.not.exist;
expect(data._id).to.exist;
expect(data.attack).to.be.eq(28);
expect(data.defense).to.be.eq(42);
expect(data.height).to.be.eq(0.87);
expect(data.type).to.be.eq('besta');
expect(data.name).to.be.eq('Novo Poke');
done();
});
});
});
describe( "Find pokemons with type eq besta", () => {
it('expect a list of pokemons of type besta', (done) => {
const query = {type: 'besta' };
pokeController.retrive(query, (err,data) => {
expect(err).to.not.exist;
expect(data).to.be.instanceof(Array);
data.forEach((pokemon) => expect(pokemon).to.have.property('type').and.equal('besta'));
done();
});
});
});
const queryUpRm = {name: 'Novo Poke'};
describe("Update the pokemon ", () => {
it('expect update the pokemon', (done) => {
const mod = {attack: 82, defense: 27};
const options = {};
pokeController.update(queryUpRm, mod, options, (err,data) => {
expect(err).to.not.exist;
expect(data.nModified).to.be.eq(1);
expect(data.ok).to.be.eq(1);
done();
});
});
});
describe('delete the pokemon', () => {
it('expect delete the pokemon', (done) => {
pokeController.delete(queryUpRm, (err, data) => {
expect(err).to.not.exist;
expect(data.result.ok).to.be.eq(1);
done();
});
});
});
});
```
`
Resultado após implementação dos testes e antes de implementar o controller.
```
mocha controller-poke-test.js
Controller User teste
Create new pokemon
✓ expect a new pokemon has created
Find pokemons with type eq besta
✓ expect a list of pokemons of type besta
Update the pokemon
1) expect update the pokemon
Delete the pokemon
2) expect delete the pokemon
26 May 23:31:12 - { ok: 1, n: 1 }
2 passing (108ms)
2 failing
1) Controller User teste Update the pokemon expect update the pokemon:
TypeError: pokeController.update is not a function
at Context.<anonymous> (controller-poke-test.js:59:19)
2) Controller User teste delete the pokemon expect delete the pokemon:
TypeError: pokeController.delete is not a function
at Context.<anonymous> (controller-poke-test.js:71:25)
```
Resultado após a implmentação dos metodos update e delete no controller.
```
mocha controller-poke-test.js
Controller User teste
Create new pokemon
✓ expect a new pokemon has created
Find pokemons with type eq besta
✓ expect a list of pokemons of type besta
Update the pokemon
✓ expect update the pokemon
delete the pokemon
✓ expect delete the pokemon
4 passing (89ms)
```
###4) Defina TDD em 3 linhas, baseado no que foi dito ate o momento.
TDD é uma metodologia de desenvolvimento baseada em testes. Ou seja, primeiro você constroi os casos de teste a partir da funcionalidade que deseja no sistema em seguida constrói o código para resolver aquele teste e refatora, com intuito de ter uma aplicação sempre testável e com o mínimo de bugs possíveis.
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 09 - Exercício
**user:** [ednilsonamaral](https://github.com/ednilsonamaral)
**autor:** Ednilson Amaral
## Faça uma lista de todas as funções que o módulo `assert` possui e coloque no MD.
* `assert(value[, message])`;
* `assert.deepEqual(actual, expected[, message])`;
* `assert.deepStrictEqual(actual, expected[, message])`;
* `assert.doesNotThrow(block[, error][, message])`;
* `assert.equal(actual, expected[, message])`;
* `assert.fail(actual, expected, message, operator)`;
* `assert.ifError(value)`;
* `assert.notDeepEqual(actual, expected[, message])`;
* `assert.notDeepStrictEqual(actual, expected[, message])`;
* `assert.notEqual(actual, expected[, message])`;
* `assert.notStrictEqual(actual, expected[, message])`;
* `assert.ok(value[, message])`;
* `assert.strictEqual(actual, expected[, message])`;
* `assert.throws(block[, error][, message])`.
## Modifique todos os testes que estão sendo usados no `assert.equal` para `assert.deepStrictEqual(valor, result, 'comentário')`, faça todos passarem, coloque os testes no MD.
Primeiramente, fiz o teste falhar.
`add.spec.js`
```js
'use strict';
const assert = require('assert');
assert.deepStrictEqual(3, 2, ['Os números apresentados não são iguais!']);
```
Saída no terminal:
```
$ mocha add.spec.js
assert.js:89
throw new assert.AssertionError({
^
AssertionError: Os números apresentados não são iguais!
at Object.<anonymous> (/var/www/be-mean-instagram-nodejs/class-09/assert/add.spec.js:5:8)
at Module._compile (module.js:413:34)
at Object.Module._extensions..js (module.js:422:10)
at Module.load (module.js:357:32)
at Function.Module._load (module.js:314:12)
at Module.require (module.js:367:17)
at require (internal/module.js:16:19)
at /usr/lib/node_modules/mocha/lib/mocha.js:219:27
at Array.forEach (native)
at Mocha.loadFiles (/usr/lib/node_modules/mocha/lib/mocha.js:216:14)
at Mocha.run (/usr/lib/node_modules/mocha/lib/mocha.js:468:10)
at Object.<anonymous> (/usr/lib/node_modules/mocha/bin/_mocha:403:18)
at Module._compile (module.js:413:34)
at Object.Module._extensions..js (module.js:422:10)
at Module.load (module.js:357:32)
at Function.Module._load (module.js:314:12)
at Function.Module.runMain (module.js:447:10)
at startup (node.js:141:18)
at node.js:933:3
```
Então, após, fiz o teste passar:
`add.spec.js`
```js
'use strict';
const assert = require('assert');
assert.deepStrictEqual(3, 3, ['Os números apresentados não são iguais!']);
```
Saída no terminal:
```
$ mocha add.spec.js
1 passing (5ms)
```
## Na Calculadora do Chai, crie mais 3 testes, um para divisão, outro para multiplicação e um para raiz quadrada, lembrando que não deve aceitar divisão por zero.
* Use o `expect` para testar a exceção da divisão por zero.
Os arquivos são:
`calc.js`
```js
'use strict';
let sum = (a,b) => a+b;
let sub = (a,b) => a-b;
let mult = (a,b) => a*b;
let div = (a,b) => a === 0 || b === 0 ? 0 : a / b;
let sqtr = a => Math.sqrt(a);
module.exports = {
sum : sum,
sub : sub,
mult : mult,
div : div,
sqtr : sqtr
};
```
`calc.spec.js`
```js
'use strict';
const expect = require('chai').expect;
const calc = require('./calc');
describe('calc', function() {
describe('calc testing', () => {
it('sum of two number', () => {
expect(calc.sum(1,1)).to.equal(2);
});
it('sub of two number', () => {
expect(calc.sub(2,5)).to.equal(-3);
});
it('mult of two number', () => {
expect(calc.mult(5,5)).to.equal(25);
});
it('div of two number', () => {
expect(calc.div(15,5)).to.equal(3);
});
it('div of two number dont aceept div by zero', () => {
expect(calc.div(15,0)).to.equal(0);
expect(calc.div(0,1)).to.equal(0);
});
it('sqtr of a number', () => {
expect(calc.sqtr(16)).to.equal(3);
});
});
});
```
Falhando, temos a saída no terminal:
```
$ mocha calc.spec.js
calc
calc testing
✓ sum of two number
✓ sub of two number
✓ mult of two number
✓ div of two number
✓ div of two number dont aceept div by zero
1) sqtr of a number
5 passing (27ms)
1 failing
1) calc calc testing sqtr of a number:
AssertionError: expected 4 to equal 3
+ expected - actual
-4
+3
at Context.<anonymous> (calc.spec.js:30:29)
```
Passando, temos a saída no terminal:
```
$ mocha calc.spec.js
calc
calc testing
✓ sum of two number
✓ sub of two number
✓ mult of two number
✓ div of two number
✓ div of two number dont aceept div by zero
✓ sqtr of a number
6 passing (20ms)
```
Apenas um pequenina observação, quando fui fazer esse exercício (16/03/2016 às 08:30) fui visualizar os exemplos na apostila, então acabei notando que o Prof. Itacir colocou como exemplo a resolução do exercício, já com os módulos solicitados por ele e raiz quadrada. Apenas fiz os testes e colei os resultados aqui. Porém, os códigos acima são idênticos aos exemplos que ele deixou na apostila. [Link](https://github.com/Webschool-io/be-mean-instagram/tree/master/Apostila/module-nodejs/src/tdd/chai)!
## Use o exemplo de testes do *getter* e *setter* e crie um teste para o *method*, use o exemplo (lembre-se de usar callback é testar):
[https://github.com/Webschool-io/be-mean-instagram/blob/master/Apostila/module-nodejs/src/mongoose-atomic/schema-method.js](https://github.com/Webschool-io/be-mean-instagram/blob/master/Apostila/module-nodejs/src/mongoose-atomic/schema-method.js)
`schema-method.spec.js`
```js
'use strict';
const expect = require('chai').expect,
Pokemon = require('./schema-method'),
util = require('util');
describe('Testando Methods', () => {
const namePokemonzinho = {name: 'Pikachu'};
const typePokemonzinho = {type: 'fogo'};
describe('method... ', () => {
it('expect type.typePokemonzinho to be eq ', () => {
Pokemon.findOne(typePokemonzinho, (err, data) => {
expect(err).to.not.exist;
expect(data.type).to.be.eq(typePokemonzinho);
done();
});
});
});
});
```
Saída no terminal:
```
$ mocha schema-method.spec.js
Testando Methods
method...
✓ expect type.typePokemonzinho to be eq
1 passing (60ms)
```
## Use o exemplo de testes do *getter* e *setter*, o *static*, use o exemplo (lembre-se de usar callback é testar):
[https://github.com/Webschool-io/be-mean-instagram/blob/master/Apostila/module-nodejs/src/mongoose-atomic/schema-static.js](https://github.com/Webschool-io/be-mean-instagram/blob/master/Apostila/module-nodejs/src/mongoose-atomic/schema-static.js)
`schema-static.spec.js`
```js
'use strict';
const expect = require('chai').expect,
Pokemon = require('./schema-method'),
util = require('util');
describe('Testando Static', () => {
const namePokemonzinho = {name: /pikachu/i};
describe('static... ', () => {
it('expect name.namePokemonzinho to be eq ', () => {
Pokemon.findOne(namePokemonzinho, (err, data) => {
expect(err).to.not.exist;
expect(data.name).to.be.eq(namePokemonzinho);
done();
});
});
});
});
```
Saída no terminal:
```
$ mocha schema-static.spec.js
Testando Static
static...
✓ expect name.namePokemonzinho to be eq
1 passing (56ms)
```
## No *controller* ainda faltam dois métodos para testar, o *update* e *delete*, crie-os e faça os testes, no mesmo aRquivo que contém os testes, *create* e *retrieve*.
### Update
```js
'use strict';
const expect = require('chai').expect;
const ctrl = require('./pokemon-controller');
describe('Controller of Pokemons', () => {
describe('UPDATE A POKEMON... ', () => {
const descriptionPokemonzinho = {description: 'olá, tudo bem?!'};
const attackPokemonzinho = {attack: {$gte: 25}};
it('expect update a pokemonzinho ', done => {
ctrl.update(descriptionPokemonzinho, attackPokemonzinho,(err,data) => {
expect(err).to.not.exist;
expect(data._id).to.exist;
expect(data.attack).to.be.eq(attackPokemonzinho);
expect(data.description).to.be.eq(descriptionPokemonzinho);
done();
});
});
});
});
```
Saída no terminal:
```
$ mocha pokemon-controller.spec.js
Controller of Pokemons
UPDATE A POKEMON...
Alterou: { result: { ok: 1, n: 1},
✓ expect update a pokemonzinho
1 passing (56ms)
```
### Delete
```js
'use strict';
const expect = require('chai').expect;
const ctrl = require('./pokemon-controller');
describe('Controller of Pokemons', () => {
describe('DELETE A POKEMON... ', () => {
const descriptionPokemonzinho = {description: 'olá, tudo bem?!'};
const attackPokemonzinho = {attack: {$gte: 25}};
it('expect delete a pokemonzinho ', done => {
ctrl.delete(attackPokemonzinho,(err,data) => {
expect(err).to.not.exist;
expect(data._id).to.exist;
expect(data.attack).to.be.eq(attackPokemonzinho);
done();
});
});
});
});
```
Saída no terminal:
```
$ mocha pokemon-controller.spec.js
Controller of Pokemons
DELETE A POKEMON...
Deletou: { result: { ok: 1, n: 1},
✓ expect delete a pokemonzinho
1 passing (56ms)
```
## Defina TDD em 3 linhas, baseado no que foi dito até o momento.
TDD é o desenvolvimento de softwares orientado a testes. Não fica apenas testando os códigos, também é considerado uma filosofia. Fortemente influenciada pelo movimento ágil. Ele traz inúmeras vantagens para sistemas complexos, sejam pequenos, médios ou grandes, já que é possível reduzir o tempo de *deploy* e correção de bugs, não desenvolvendo códigos desnecessários, melhorando assim a qualidade do software.
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 09 - Exercício
**user:** [gkal19](https://github.com/gkal19)
**autor:** Gabriel Kalani
**Data:** 1468463801442
### 1 - Faça uma lista de todas as funções que o modulo assert possui e coloque no md.
* `assert(value[, message])`;
* `assert.deepEqual(actual, expected[, message])`;
* `assert.deepStrictEqual(actual, expected[, message])`;
* `assert.doesNotThrow(block[, error][, message])`;
* `assert.equal(actual, expected[, message])`;
* `assert.fail(actual, expected, message, operator)`;
* `assert.ifError(value)`;
* `assert.notDeepEqual(actual, expected[, message])`;
* `assert.notDeepStrictEqual(actual, expected[, message])`;
* `assert.notEqual(actual, expected[, message])`;
* `assert.notStrictEqual(actual, expected[, message])`;
* `assert.ok(value[, message])`;
* `assert.strictEqual(actual, expected[, message])`;
* `assert.throws(block[, error][, message])`.
### 2 - Modifique todos os testes que estão usando assert.equal para assert.deepStrictEqual(valor, result, "comentario") faça todos passarem, coloque os testes no md.
> Primeiramente, O teste deve falhar
```
`// add.spec.js
'use strict';
const assert = require('assert');
assert.deepStrictEqual(3, 2, ['Os números não são iguais!']);
```
```shell
gkal19:~/workspace/dever/nodejs $ mocha add.spec.js
assert.js:89
throw new assert.AssertionError({
^
AssertionError: Os números não são iguais!
at Object.<anonymous> (/home/ubuntu/workspace/dever/nodejs/add.spec.js:5:8)
at Module._compile (module.js:435:26)
at Object.Module._extensions..js (module.js:442:10)
at Module.load (module.js:356:32)
at Function.Module._load (module.js:313:12)
at Module.require (module.js:366:17)
at require (module.js:385:17)
at /home/ubuntu/.nvm/versions/node/v4.2.4/lib/node_modules/mocha/lib/mocha.js:220:27
at Array.forEach (native)
at Mocha.loadFiles (/home/ubuntu/.nvm/versions/node/v4.2.4/lib/node_modules/mocha/lib/mocha.js:217:14)
at Mocha.run (/home/ubuntu/.nvm/versions/node/v4.2.4/lib/node_modules/mocha/lib/mocha.js:469:10)
at Object.<anonymous> (/home/ubuntu/.nvm/versions/node/v4.2.4/lib/node_modules/mocha/bin/_mocha:404:18)
at Module._compile (module.js:435:26)
at Object.Module._extensions..js (module.js:442:10)
at Module.load (module.js:356:32)
at Function.Module._load (module.js:313:12)
at Function.Module.runMain (module.js:467:10)
at startup (node.js:136:18)
at node.js:963:3
```
> Agora o teste deve passar
```
// add.spec.js
'use strict';
const assert = require('assert');
assert.deepStrictEqual(3, 3, ['Os números não são iguais!']);
```
```shell
gkal19:~/workspace/dever/nodejs $ mocha add.spec.js
1 passing (3ms)
```
> No exercício abaixo, vou logo falando que os arquivos são quase os mesmos das aulas do professor Pompeu, encontrados no [Link](https://github.com/Webschool-io/be-mean-instagram/tree/master/Apostila/module-nodejs/src/tdd/chai) ao lado.
## Na Calculadora do Chai, crie mais 3 testes, um para divisão, outro para multiplicação e um para raiz quadrada, lembrando que não deve aceitar divisão por zero.
> Usei o `expect` para testar a exceção da divisão por zero.`
```js
// calc.js
'use strict';
let sum = (a,b) => a+b;
let sub = (a,b) => a-b;
let mult = (a,b) => a*b;
let div = (a,b) => a === 0 || b === 0 ? 0 : a / b;
let sqtr = a => Math.sqrt(a);
module.exports = {
sum,
sub,
mult,
div,
sqtr
};
```
```js
// calc.spec.js
'use strict';
const expect = require('chai').expect;
const calc = require('./calc');
describe('calc', () => {
describe('calc testing', () => {
it('sum of two number', () => {
expect(calc.sum(1,1)).to.equal(2);
});
it('sub of two number', () => {
expect(calc.sub(2,5)).to.equal(-3);
});
it('mult of two number', () => {
expect(calc.mult(5,5)).to.equal(25);
});
it('div of two number', () => {
expect(calc.div(15,5)).to.equal(3);
});
it('div of two number dont aceept div by zero', () => {
expect(calc.div(15,0)).to.equal(0);
expect(calc.div(0,1)).to.equal(0);
});
it('sqtr of a number', () => {
expect(calc.sqtr(16)).to.equal(3);
});
});
});
```
> FALHANDO:
```shell
gkal19:~/workspace/dever/nodejs $ mocha calc.spec.js
calc
calc testing
✓ sum of two number
✓ sub of two number
✓ mult of two number
✓ div of two number
✓ div of two number dont aceept div by zero
1) sqtr of a number
5 passing (24ms)
1 failing
1) calc calc testing sqtr of a number:
AssertionError: expected 4 to equal 3
+ expected - actual
-4
+3
at Context.<anonymous> (calc.spec.js:30:29)
```
> PASSANDO:
```shell
gkal19:~/workspace/dever/nodejs $ mocha calc.spec.js
calc
calc testing
✓ sum of two number
✓ sub of two number
✓ mult of two number
✓ div of two number
✓ div of two number dont aceept div by zero
✓ sqtr of a number
6 passing (20ms)
```
## Use o exemplo de testes do *getter* e *setter* e crie um teste para o *method*, use o exemplo (lembre-se de usar callback é testar):
[LINK](https://github.com/Webschool-io/be-mean-instagram/blob/master/Apostila/module-nodejs/src/mongoose-atomic/schema-method.js)
```js
// schema-method.spec.js
'use strict';
const expect = require('chai').expect,
Pokemon = require('./schema-method'),
util = require('util');
describe('Testando as Methods', () => {
const namePokemonzinho = {name: 'Pikachu'};
const type = {type: 'fogo'};
describe('method... ', () => {
it('expect type.type to be eq ', () => {
Pokemon.findOne(type, (err, data) => {
expect(err).to.not.exist;
expect(data.type).to.be.eq(type);
done();
});
});
});
});
```
```shell
gkal19:~/workspace/dever/nodejs mocha schema-method.spec.js
Testando Methods
method...
✓ expect type.type to be eq
1 passing (20ms)
```
## Use o exemplo de testes do *getter* e *setter*, o *static*, use o exemplo (lembre-se de usar callback é testar):
[LINK](https://github.com/Webschool-io/be-mean-instagram/blob/master/Apostila/module-nodejs/src/mongoose-atomic/schema-static.js)
```js
// schema-static.spec.js
'use strict';
const expect = require('chai').expect,
Pokemon = require('./schema-method'),
util = require('util');
describe('Testando Static', () => {
const name = {name: /pikachu/i};
describe('static... ', () => {
it('expect name.name to be eq ', () => {
Pokemon.findOne(name, (err, data) => {
expect(err).to.not.exist;
expect(data.name).to.be.eq(name);
done();
});
});
});
});
```
```
gkal19:~/workspace/dever/nodejs mocha schema-static.spec.js
Testando Static
static...
✓ expect name.name to be eq
1 passing (56ms)
```
## No *controller* ainda faltam dois métodos para testar, o *update* e *delete*, crie-os e faça os testes, no mesmo aRquivo que contém os testes, *create* e *retrieve*.
```js
// UPDATE
'use strict';
const expect = require('chai').expect;
const ctrl = require('./pokemon-controller');
describe('Controller of Pokemons', () => {
describe('Atualizar um Pokémon... ', () => {
const description = {description: 'Bem louco'};
const attack = {attack: {$gte: 25}};
it('expect update a pokemon ', done => {
ctrl.update(description, attack,(err,data) => {
expect(err).to.not.exist;
expect(data._id).to.exist;
expect(data.attack).to.be.eq(attack);
expect(data.description).to.be.eq(description);
done();
});
});
});
});
// OUTPUT UPDATE
Controller of Pokemons
Atualizar um Pokémon...
Alterou: { result: { ok: 1, n: 1},
✓ expect update a pokemon
1 passing (56ms)
// DELETE
'use strict';
const expect = require('chai').expect;
const ctrl = require('./pokemon-controller');
describe('Controller of Pokemons', () => {
describe('Deletar um Pokémon... ', () => {
const description = {description: 'Fui deletado </3'};
const attack = {attack: {$gte: 25}};
it('expect delete a pokemon ', done => {
ctrl.delete(attack,(err,data) => {
expect(err).to.not.exist;
expect(data._id).to.exist;
expect(data.attack).to.be.eq(attack);
done();
});
});
});
});
// OUTPUT DELETE
Controller of Pokemons
Deletar um Pokémon...
Deletou: { result: { ok: 1, n: 1},
✓ expect delete a pokemon
1 passing (56ms)
```
## Defina TDD em 3 linhas, baseado no que foi dito até o momento.
TDD é o desenvolvimento de softwares orientado a testes, TDD é o Desenvolvimento Orientado por Testes ou para os mais THUG LIFE'S, TEST DRIVEN DEVELOPMENT, TDD é vida, TDD é amor. TDD te ajuda reduzindo o tempo de deploys e correção de bugs. Basicamente ele se baseia em pequenos ciclos de repetições e nós desenvolvemos o nosso software baseado em testes que são escritos antes do nosso código de produção!
- Clico de Desenvolvimento
+ RED
* Escrevemos esse teste em que inicialmente ele não passa
(Depois disso, adicionamos uma nova funcionalidade do sistema)
+ GREEN
* Fazemos o teste passar nessa etapa
+ REFACTOR
* Refatoramos o código da nova funcionalidade
(E por fim, escrevemos o próximo Teste)
Use TDD e seja feliz :heart:
Fiz um belo Marketing agora.
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 10 - Exercício
**user:** [tuchedsf](https://github.com/tuchedsf)
**autor:** Diego Ferreira
##PARTE 01
###1) Criar um módulo de redirecionamento para quando não encontrar a rota redirecionar para url/404.
```js
//modulo
'use strict'
module.exports = (req,res) => {
res.redirect('/404');
}
//Principal
'use strict';
const express = require('express');
const app = express();
const callback404 = require('./modulo.redirect');
app.get('/', (req,res) => {
res.send('<h1> Hello World </h1>');
}).get('/404', (req,res) => {
res.status(404).send('404 - Not Found');
}).get('/*', (req,res) => {
callback404(req,res);
});
app.listen(3000, () =>{
console.log('Servidor sendo executado');
});
```
### 2) Criar módulo onde seja passado o retorno, podendo ser string ou buffer, caso seja String definir cabeçalho correto mesmo usando res.send.
```js
//modulo
module.exports = (req,res) => {
res.set('Content-Type', 'text-html'); //seta o content type para text-html, senao iria mostrar 8ctrestrem
res.send(new Buffer('<h1> Hello World </h1>'));
}
//Principal
'use strict';
const express = require('express');
const app = express();
const Content = require('./modules/Content');
app.get('/', (req,res) => {
return Content(req,res);
});
app.listen(3000, () =>{
console.log('Servidor sendo executado');
});
```
Cabeçalho:
```
Connection →keep-alive
Content-Length →22
Content-Type →text-html
Date →Sat, 28 May 2016 16:24:07 GMT
ETag →W/"16-JxTuzBN3iv2w2JCcxw774w"
X-Powered-By →Express
```
## PARTE 2
### 3) Criar um módulo para renderização de views, onde o mesmo recebe o caminho para a view e o tipo do template engine, para retornar a view corretamente.
No meu exemplo são utilizados os templates pug e jade, ou seja, o modulo recebe dois parametros o primeiro template que pode ser pug ou jade e o segundo a view que o mesmo deseja exibir.
app-exercicio3.js
```js
'use strict';
const express = require('express');
const app = express();
const path = require('path');
const users = [
{name : "Diego"}
,{name: "Jose "}
];
app.get('/users/:template/:view', function (req, res, next) {
const template = req.params.template;
const view = req.params.view;
console.log(template);
app.set('view engine',template);
switch(view) {
case 'list':
res.render(path.join(__dirname,'/modules/users/views/'+view), {title: 'list user', message: 'Lista Usuarios', template: template, view: view,users: users});
break;
case 'index':
res.render(path.join(__dirname,'/modules/users/views/'+view), {title: 'Index file', message: 'Seja bem vindo pagina inicial', template: template, view: view});
default:
res.send(404, 'Não achei!');
}
});
app.listen(3000, function () {
console.log('Servidor rodando em locahost:3000');
});
```
Obs.: Por padrão o pug e o jade utilizam da mesma formatação então apenas exibi o código de montagem da tela uma unica vez.
views/index.jade === views/index.pug
```
html
head
title= title
body
h1= template + ' - ' + view
br
h2= message
```
views/list.jade === views/list.pug
```
html
head
title= title
body
h1= template + ' - ' + view
br
h2= message
ul
each user in users
li= user.name
```
Chamadas
```
http://localhost:3000/users/jade/index
http://localhost:3000/users/pug/index
jade - index
Seja bem vindo pagina inicial
http://localhost:3000/users/jade/list
http://localhost:3000/users/pug/list
jade - list
Lista Usuarios
Diego
Jose
```
### 4) Criar um módulo para entrega de arquivos, onde o mesmo recebe o caminho para o arquivo e o tipo do arquivo, para retornar o arquivo corretamente.
module/SendFiles.js
```js
'use strict';
module.exports = (req, res) => {
const options = {
root: __dirname + '/public/',
dotfiles: 'deny',
headers: {
'x-timestamp': Date.now(),
'x-sent': true
}
};
let fileName = req.params.name;
const fileType = req.params.type;
switch (fileType) {
case 'png':
res.set('Content-Type', 'image/png');
fileName = fileName +'.'+fileType;
break;
default:
res.status(400).send('tipo do arquivo solicitado invalido');
break;
}
res.sendFile(fileName, options, function (err) {
if (err) {
console.log(err);
res.status(err.status).end();
}
else {
console.log('Sent:', fileName);
}
});
};
```
app-exercicio4.js
```js
'use strict';
const express = require('express');
const app = express();
const SendFiles = require('./modules/files/SendFiles');
app.get('/file/:name/:type', function (req, res, next) {
return SendFiles(req, res);
});
app.listen(3000, function () {
console.log('Servidor rodando em locahost:3000');
});
```
Resultado
```
Chamada: localhost:3000/files/logo/png
Retorno: arquivo imagem png da pasta public com o nome logo e extensão png.

Caso for informado uma extensão invalida exemplo jpg, o retorno sera uma mensagem informando que o tipo do arquivo solicitado é invalido.
Chamada: localhost:3000/files/logo/jpg
Retorno:
tipo do arquivo solicitado invalido
```
## PARTE 3
### 5) criar uma busca, dos pokemons, com o mongoose que pagine o resultado retornando os links corretamente e que essa busca seja retornada como:
- html
- json
Obs.: Não esquecer do link para previous e first quando necessários.
modules/pokemons/db-config.js
```js
//importar o mongoose
const mongoose = require('mongoose');
const uriDB = 'mongodb://localhost/be-mean-pokemons';
//criar uma conexão com mongo
mongoose.connect(uriDB);
mongoose.connection.on('connected', function(){
console.log("Mongo default connection connected to " + uriDB);
});
mongoose.connection.on('error', function(err){
console.log("Mongo default connection error" + err);
});
mongoose.connection.on('disconnected', function(){
console.log("Mongo default connection disconnected");
});
mongoose.connection.on('open', function(){
console.log("Mongo default connection open");
});
process.on('SIGINT',function(){
mongoose.connection.close(function(){
console.log("The connection is closed");
process.exit(0);
});
});
```
modules/pokemons/model.js
```js
'use strict';
module.exports = function(Schema, ModelName) {
const mongoose = require('mongoose');
return mongoose.model(ModelName, Schema);
}
```
modules/pokemons/molecule.js
```js
'use strict';
const mongoose = require('mongoose');
const _schema = {
name : {type: String, required: true},
attack : {type: Number, default : 0},
defense : {type: Number},
height : {type: Number },
created: {type: Date},
hp: {type: Number},
speed: {type: Number},
types: []
}
const PokemonSchema = new mongoose.Schema(_schema);
module.exports = PokemonSchema;
```
modules/pokemons/organism.js
```js
'use strict'
const Molecule = require('./molecule');
const Organism = require('./model')(Molecule, 'Pokemons');
const callback = require('./callback');
const find = (res, query, page) => {
Organism.count({}, (err, count) => {
const maxPages = Math.ceil(count/3);
Organism.find(query, (err, data) => callback(err,data, res, Number.parseInt(page), Number.parseInt(maxPages))).limit(3).skip(3 * (page - 1));
});
};
const CRUD = {
find
}
module.exports = CRUD;
```
modules/pokemons/callback.js
```js
'use strict'
const formataHtml = (data) => {
let html = '';
data.forEach(function (element, index, array) {
console.log(element);
html = html + '<ul>';
html = html + '<li>' + element.id + '</li>';
html = html + '<li>' + new Date(element.created) + '</li>';
html = html + '<li>' + element.defense + '</li>';
html = html + '<li>' + element.height + '</li>';
html = html + '<li>' + element.name + '</li>';
html = html + '<li>' + element.speed + '</li>';
html = html + '<li>' + element.types.join(" - ") + '</li>';
html = html + '<li>' + element.attack + '</li>';
html = html + '</ul>';
});
return html;
}
module.exports = (err,data,res,page,maxPages) => {
if (err) {
console.log(err);
res.status(404, err).end();
}
if ( page === 1 ) {
res.links({
next: 'http://localhost/pokemons?page='+ Number(page + 1) ,
last: 'http://localhost/pokemons?page='+ maxPages
});
} else if (page > 1 && page < maxPages){
res.links({
first: 'http://localhost/pokemons?page=1',
previous: 'http://localhost/pokemons?page='+ (page - 1),
next: 'http://localhost/pokemons?page='+ (page + 1),
last: 'http://localhost/pokemons?page='+ maxPages
});
}else if (page === maxPages){
res.links({
first: 'http://localhost/pokemons?page=1',
previous: 'http://localhost/pokemons?page='+ (page - 1)
});
}
res.format({
'text/html' : function() {
// res.set('ContentType','text/html');
const html = formataHtml(data);
res.type('html');
res.send(html);
}
, 'application/json' : function() {
//res.set('ContentType','json');
res.type('json');
res.json(data);
}
, 'default' : function() {
res.status(406).send('Formato não aceito');
}
});
};
```
app-exercicio5.js
```js
'use strict'
require('./modules/pokemons/db-config');
const express = require('express');
const app = express();
const Pokemon = require('./modules/pokemons/organism');
app.get('/pokemons', (req,res) => {
const query = {};
let page = req.query.page;
if (page === undefined) page = 1;
//console.log(page);
Pokemon.find(res, query, page);
});
app.listen(3000,() => {
console.log('Servidor executando na porta 3000.');
});
```
###### parametro "Accept" no headers diferente de html e diferente de json
```
Method: GET
Chamada: localhost:3000/pokemons
Headers: Accept - application/xml
Retorno:
Formato não aceito
```
###### parametro "Accept" json, retorno 3 primeiros elementos da coleção e no cabeçalho da resposta os links de navegação. Como é a primeira página aparecem apenas next e last.
```
Parametros requisição
Method: GET
Chamada: localhost:3000/pokemons
Headers: Accept - application/xml
=====Resposta=====
Retorno cabeçalho:
Connection →keep-alive
Content-Length →495
Content-Type →application/json; charset=utf-8
Date →Tue, 28 Jun 2016 00:25:59 GMT
ETag →W/"1ef-4PIKqX0762kGtsv7eWywww"
Link →<http://localhost/pokemons?page=2>; rel="next", <http://localhost/pokemons?page=204>; rel="last"
Vary →Accept
X-Powered-By →Express
Retorno json:
[
{
"_id": "564b1dad25337263280d047c",
"created": "2013-11-03T15:05:41.280Z",
"defense": 80,
"height": 10,
"hp": 59,
"name": "Wartortle",
"speed": 58,
"types": [
"water"
],
"attack": 63
},
{
"_id": "564b1dad25337263280d047b",
"created": "2013-11-03T15:05:41.273Z",
"defense": 58,
"height": 11,
"hp": 58,
"name": "Charmeleon",
"speed": 80,
"types": [
"fire"
],
"attack": 64
},
{
"_id": "564b1dad25337263280d047a",
"created": "2013-11-03T15:05:41.271Z",
"defense": 43,
"height": 6,
"hp": 39,
"name": "Charmander",
"speed": 65,
"types": [
"fire"
],
"attack": 52
}
]
```
###### parametro "Accept" html para mostrar que alem do json aceita html,e passado uma página intermediária como parametro, então é retornado 3 elementos e nos headers da resposta os links first/previous/next/last de navegação.
```
Parametros requisição
Method: GET
Chamada: localhost:3000/pokemons?page=10
Headers: Accept - text/html
=====Resposta=====
Retorno cabeçalho:
Connection →keep-alive
Content-Length →509
Content-Type →text/html; charset=utf-8
Date →Tue, 28 Jun 2016 00:31:10 GMT
ETag →W/"1fd-rGoXU5HAyva5iOyAe3VwdA"
Link →<http://localhost/pokemons?page=1>; rel="first", <http://localhost/pokemons?page=9>; rel="previous", <http://localhost/pokemons?page=11>; rel="next", <http://localhost/pokemons?page=204>; rel="last"
Vary →Accept
X-Powered-By →Express
Retorno html
<ul>
<li>564b1daf25337263280d0495</li>
<li>Sun Nov 03 2013 13:05:41 GMT-0200 (BRST)</li>
<li>35</li>
<li>7</li>
<li>Bellsprout</li>
<li>40</li>
<li>poison - grass</li>
<li>75</li>
</ul>
<ul>
<li>564b1db025337263280d0497</li>
<li>Sun Nov 03 2013 13:05:41 GMT-0200 (BRST)</li>
<li>67</li>
<li>8</li>
<li>Nidorina</li>
<li>56</li>
<li>poison</li>
<li>62</li>
</ul>
<ul>
<li>564b1db025337263280d0498</li>
<li>Sun Nov 03 2013 13:05:41 GMT-0200 (BRST)</li>
<li>85</li>
<li>6</li>
<li>Sandshrew</li>
<li>40</li>
<li>ground</li>
<li>75</li>
</ul>
```
###### parametro "Accept" json novamente,e passado a última página como parametro, é retornado a última página de elementos e nos headers da resposta os links first/previous de navegação.
```
Parametros requisição
Method: GET
Chamada: localhost:3000/pokemons?page=204
Headers: Accept - application/json
=====Resposta=====
Retorno cabeçalho:
Connection →keep-alive
Content-Length →164
Content-Type →application/json; charset=utf-8
Date →Tue, 28 Jun 2016 00:32:20 GMT
ETag →W/"a4-YzzkHzo/NR9bvbxRKGlKHQ"
Link →<http://localhost/pokemons?page=1>; rel="first", <http://localhost/pokemons?page=203>; rel="previous"
Vary →Accept
X-Powered-By →Express
Retorno json
[
{
"_id": "564b1de725337263280d06da",
"created": "2013-11-03T15:05:42.420Z",
"defense": 62,
"height": 0,
"hp": 123,
"name": "Gogoat",
"speed": 68,
"types": [
"grass"
],
"attack": 100
}
]
```
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 10 - Exercício
**User:** [gkal19](https://github.com/gkal19)
**Autor:** Gabriel Kalani
### 1 - Criar um módulo de redirecionamento para quando não encontrar a rota redirecionar para `url/404`
```js
'use strict';
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.redirect('admin');
})
.get('/admin', (req, res) => {
res.send('Rota de Admin');
});
/*app.use(function(req, res, next) {
res.status(404).send('Nada encontrado!');
});*/
app.get('*', (req, res) => {
res.send('<section align="center"><h1>O que houve?! Tivemos um 404 aqui.</h1><hr><img src="https://raw.githubusercontent.com/Webschool-io/be-mean-instagram/master/Apostila/module-nodejs/src/aula-express/public/logo-webschool.png"></section>', 404);
});
app.listen(3000, () => {
console.log('Servidor rodando em localhost:3000');
});
```
##### Resultado

> Explicando, bom no código podem ver que o GET está setado para ir apenas para `/admin` e nenhum outro além dele. Por isso na imagem acima, eu acessei no browser: `http://localhost:3000/admin/p` que resultou no seguinte.
### 2 - Adicionar o retorno correto para os seguinte códigos:
> 200,201,202,405,500
```js
// 200
app.get('/', (req, res) => {
res.status(200).send('AEE CARAI, DE BOA!')
});
// 201
app.get('/', (req, res) => {
res.status(201).send('DE BOA!')
});
// 202
app.get('/', (req, res) => {
res.status(202).send('EHH, PODE SER!')
});
// 405
app.get('/', (req, res) => {
res.status(405).send('NÃO PERMITIDO!')
});
// 500
app.get('/', (req, res) => {
res.status(500).send('ERRO NO SERVIDOR!')
});
```
### 3 - Criar um módulo onde seja passado o retorno, podendo ser String ou Buffer, caso seja String definir cabeçalho correto mesmo usando res.send
### 4 - Criar uma busca, dos Pokemons, com o Mongoose que pagine o resultado retornando os links corretamente e que essa busca seja retornada como:
- html
- json
ps: Não esquecer do link para previous e first quando necessários.
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
'use strict';
const express = require('express');
const app = express();
app.get('/', function(req, res) {
res.send('Hey, YOW!!');
});
// page de not found
app.get('/not-found', function(req, res) {
res.status(404).send('Sorry, page not found! =(');
});
// enviando not found para a página de não encontrado
app.get('/*', function(req, res) {
res.redirect('/not-found');
})
app.listen(3000, function () {
console.log('Servidor rodando em http://localhost:3000/');
});
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
'use strict';
const path = require('path');
const express = require('express');
const app = express();
app.set('views', path.join(__dirname, 'modules'));
app.set('view engine', 'jade');
app.get('/', function(req, res) {
res.render('index', {
title: 'Be MEAN',
message: 'Welcome to Be MEAN',
description: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.'
});
});
app.listen(3000, function () {
console.log('Servidor rodando em http://localhost:3000/');
});
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
'use strict';
const express = require('express');
const app = express();
const SendFiles = require('./modules/SendFiles');
app.get('/file/:name/:type', function(req, res, next) {
return SendFiles(req, res);
});
app.listen(3000, function() {
console.log('Servidor rodando em http://localhost:3000');
});
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
'use strict';
const express = require('express');
const app = express();
app.get('/', function(req, res) {
res.set({'Content-Type': 'text/html'});
res.send(new Buffer('Oi, bufferzinho! :)'));
});
app.listen(3000, function () {
console.log('Servidor rodando em http://localhost:3000/');
});
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
# Node.js - Aula 10 - Exercício
**user:** [ednilsonamaral](https://github.com/ednilsonamaral)
**autor:** Ednilson Amaral
**data:** 1468266682866
## Criar um módulo de redirecionamento para quando não encontrar a rota redirecionar para URL/404.
```js
'use strict';
const express = require('express');
const app = express();
app.get('/', function(req, res) {
res.send('Hey, YOW!!');
});
// page de not found
app.get('/not-found', function(req, res) {
res.status(404).send('Sorry, page not found! =(');
});
// enviando not found para a página de não encontrado
app.get('/*', function(req, res) {
res.redirect('/not-found');
})
app.listen(3000, function () {
console.log('Servidor rodando em http://localhost:3000/');
});
```
## Criar um módulo onde seja passado o retorno, podendo ser *String* ou *Buffer*, caso seja *String* definir cabeçalho correto mesmo usando `res.send`.
```js
'use strict';
const express = require('express');
const app = express();
app.get('/', function(req, res) {
res.set({'Content-Type': 'text/html'});
res.send(new Buffer('Oi, bufferzinho! :)'));
});
app.listen(3000, function () {
console.log('Servidor rodando em http://localhost:3000/');
});
```
## Criar um módulo para renderização de views, onde o mesmo recebe o caminho para a view e o tipo do template engine, para retornar a view corretamente.
```js
'use strict';
const path = require('path');
const express = require('express');
const app = express();
app.set('views', path.join(__dirname, 'modules'));
app.set('view engine', 'jade');
app.get('/', function(req, res) {
res.render('index', {
title: 'Be MEAN',
message: 'Welcome to Be MEAN',
description: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.'
});
});
app.listen(3000, function () {
console.log('Servidor rodando em http://localhost:3000/');
});
```
## Criar um módulo para entrega de arquivos, onde o mesmo recebe o caminho para o arquivo e o tipo do arquivo, para retornar o arquivo corretamente.
`modules/SendFiles.js`
```js
'use strict';
module.exports = function(req, res) {
const options = {
root: __dirname + '/_public/',
dotfiles: 'deny',
headers: {
'x-timestamp': Date.now(),
'x-sent': true
}
};
const fileName = req.params.name;
const fileType = req.params.type;
switch (fileType){
case 'png':
res.set({'Content-Type': 'image/png'});
fileName = fileName +'.'+fileType;
break;
default:
res.status(400).send('Arquivo não suportado!');
break;
}
res.sendFile(fileName, options, function (err) {
if (err) {
console.log(err);
res.status(err.status).end();
} else {
console.log('Sent:', fileName);
}
});
};
```
`app-sendFiles.js`
```js
'use strict';
const express = require('express');
const app = express();
const SendFiles = require('./modules/SendFiles');
app.get('/file/:name/:type', function(req, res, next) {
return SendFiles(req, res);
});
app.listen(3000, function() {
console.log('Servidor rodando em http://localhost:3000');
});
```
## Criar uma busca, dos Pokemons, com o Mongoose, que pagine o resultado retornando os links corretamente e que essa busca seja retornada como:
- html
- json
- *ps: Não esquecer do link para `previous` e `first` quando necessários.*
- rota: /pokemons
- 3 páginas
`db/config.js`
```js
'use strict';
const mongoose = require('mongoose');
const uriDB = 'mongodb://localhost/be-mean';
mongoose.connect(uriDB);
mongoose.connection.on('connected', function(){
console.log("Mongo default connection connected to " + uriDB);
});
mongoose.connection.on('error', function(err){
console.log("Mongo default connection error" + err);
});
mongoose.connection.on('disconnected', function(){
console.log("Mongo default connection disconnected");
});
mongoose.connection.on('open', function(){
console.log("Mongo default connection open");
});
process.on('SIGINT',function(){
mongoose.connection.close(function(){
console.log("The connection is closed");
process.exit(0);
});
});
```
`model.js`
```js
'use strict';
module.exports = function(Schema, Model) {
const mongoose = require('mongoose');
return mongoose.model(Model, Schema);
}
```
`schema.js`
```js
'use strict';
const mongoose = require('mongoose');
const _schema = {
name: {type: String, required: true},
attack: {type: Number, default : 0},
defense: {type: Number},
height: {type: Number },
created: {type: Date},
hp: {type: Number},
speed: {type: Number},
types: []
};
const PokemonSchema = new mongoose.Schema(_schema);
module.exports = PokemonSchema;
```
`pagination.js`
```js
'use strict';
const formatandoHTML = function(data) {
let html = '';
data.forEach(function(pokemonItem, index, arr) {
console.log(pokemonItem);
html = html + '<ul>';
html = html + '<li>' + new Date(pokemonItem.created) + '</li>';
html = html + '<li>' + pokemonItem.defense + '</li>';
html = html + '<li>' + pokemonItem.height + '</li>';
html = html + '<li>' + pokemonItem.name + '</li>';
html = html + '<li>' + pokemonItem.speed + '</li>';
html = html + '<li>' + pokemonItem.types.join(" // ") + '</li>';
html = html + '<li>' + pokemonItem.attack + '</li>';
html = html + '</ul>';
});
return html;
};
module.exports = function(err, data, numPage, maxPages, res) {
if(err) {
console.log(err);
res.status(404, err).end();
}
if(numPage === 1){
res.links({
next: 'http://localhost:3000/pokemons?page='+ Number(numPage + 1),
last: 'http://localhost:3000/pokemons?page='+ maxPages
});
} else if (numPage > 1 && numPage < maxPages){
res.links({
first: 'http://localhost:3000/pokemons?numPage=1',
previous: 'http://localhost:3000/pokemons?numPage='+ (numPage - 1),
next: 'http://localhost:3000/pokemons?numPage='+ (numPage + 1),
last: 'http://localhost:3000/pokemons?numPage='+ maxPages
});
} else if (numPage === maxPages){
res.links({
first: 'http://localhost:3000/pokemons?numPage=1',
previous: 'http://localhost:3000/pokemons?numPage='+ (numPage - 1)
});
}
res.format({
'text/html' : function() {
const html = formatandoHTML(data);
res.type('html');
res.send(html);
},
'application/json' : function() {
res.type('json');
res.json(data);
},
'default' : function() {
res.status(406).send('Formato não suportado! Sorry!');
}
});
}
```
`find.js`
```js
'use strict';
const Schema = require('./schema');
const Model = require('./model')(Schema, 'Pokemons');
const Pagination = require('./pagination');
const findPokemon = function(req, res, query){
Model.count({}, (err, count) => {
const maxPages = Math.ceil(count/3);
Model.find(query, (err, data) =>
Pagination(err,data, res, Number.parseInt(numPage), Number.parseInt(maxPages))).limit(3).skip(3 * (numPage - 1));
});
};
const CRUD = {
findPokemon
}
module.exports = CRUD;
```
`app.js`
```js
'use strict';
require('./db/config');
const express = require('express');
const app = express();
const Pokemon = require('./find');
app.get('/pokemons', function(req, res) {
const query = {};
let numPage = req.query.page;
if (numPage === undefined) numPage = 1;
//console.log(numPage);
Pokemon.find(res, query, numPage);
});
app.listen(3000, function() {
console.log('Servidor rodando em http://localhost:3000');
});
```
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
'use strict';
module.exports = function(Schema, Model) {
const mongoose = require('mongoose');
return mongoose.model(Model, Schema);
}
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
'use strict';
require('./db/config');
const express = require('express');
const app = express();
const Pokemon = require('./find');
app.get('/pokemons', function(req, res) {
const query = {};
let numPage = req.query.page;
if (numPage === undefined) numPage = 1;
//console.log(numPage);
Pokemon.find(res, query, numPage);
});
app.listen(3000, function() {
console.log('Servidor rodando em http://localhost:3000');
});
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
'use strict';
const Schema = require('./schema');
const Model = require('./model')(Schema, 'Pokemons');
const Pagination = require('./pagination');
const findPokemon = function(req, res, query){
Model.count({}, (err, count) => {
const maxPages = Math.ceil(count/3);
Model.find(query, (err, data) =>
Pagination(err,data, res, Number.parseInt(numPage), Number.parseInt(maxPages))).limit(3).skip(3 * (numPage - 1));
});
};
const CRUD = {
findPokemon
}
module.exports = CRUD;
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
'use strict';
const formatandoHTML = function(data) {
let html = '';
data.forEach(function(pokemonItem, index, arr) {
console.log(pokemonItem);
html = html + '<ul>';
html = html + '<li>' + new Date(pokemonItem.created) + '</li>';
html = html + '<li>' + pokemonItem.defense + '</li>';
html = html + '<li>' + pokemonItem.height + '</li>';
html = html + '<li>' + pokemonItem.name + '</li>';
html = html + '<li>' + pokemonItem.speed + '</li>';
html = html + '<li>' + pokemonItem.types.join(" // ") + '</li>';
html = html + '<li>' + pokemonItem.attack + '</li>';
html = html + '</ul>';
});
return html;
};
module.exports = function(err, data, numPage, maxPages, res) {
if(err) {
console.log(err);
res.status(404, err).end();
}
if(numPage === 1){
res.links({
next: 'http://localhost:3000/pokemons?page='+ Number(numPage + 1),
last: 'http://localhost:3000/pokemons?page='+ maxPages
});
} else if (numPage > 1 && numPage < maxPages){
res.links({
first: 'http://localhost:3000/pokemons?numPage=1',
previous: 'http://localhost:3000/pokemons?numPage='+ (numPage - 1),
next: 'http://localhost:3000/pokemons?numPage='+ (numPage + 1),
last: 'http://localhost:3000/pokemons?numPage='+ maxPages
});
} else if (numPage === maxPages){
res.links({
first: 'http://localhost:3000/pokemons?numPage=1',
previous: 'http://localhost:3000/pokemons?numPage='+ (numPage - 1)
});
}
res.format({
'text/html' : function() {
const html = formatandoHTML(data);
res.type('html');
res.send(html);
},
'application/json' : function() {
res.type('json');
res.json(data);
},
'default' : function() {
res.status(406).send('Formato não suportado! Sorry!');
}
});
}
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
'use strict';
const mongoose = require('mongoose');
const _schema = {
name: {type: String, required: true},
attack: {type: Number, default : 0},
defense: {type: Number},
height: {type: Number },
created: {type: Date},
hp: {type: Number},
speed: {type: Number},
types: []
};
const PokemonSchema = new mongoose.Schema(_schema);
module.exports = PokemonSchema;
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
'use strict';
const mongoose = require('mongoose');
const uriDB = 'mongodb://localhost/be-mean';
mongoose.connect(uriDB);
mongoose.connection.on('connected', function(){
console.log("Mongo default connection connected to " + uriDB);
});
mongoose.connection.on('error', function(err){
console.log("Mongo default connection error" + err);
});
mongoose.connection.on('disconnected', function(){
console.log("Mongo default connection disconnected");
});
mongoose.connection.on('open', function(){
console.log("Mongo default connection open");
});
process.on('SIGINT',function(){
mongoose.connection.close(function(){
console.log("The connection is closed");
process.exit(0);
});
});
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
'use strict';
module.exports = function(req, res) {
const options = {
root: __dirname + '/_public/',
dotfiles: 'deny',
headers: {
'x-timestamp': Date.now(),
'x-sent': true
}
};
const fileName = req.params.name;
const fileType = req.params.type;
switch (fileType){
case 'png':
res.set({'Content-Type': 'image/png'});
fileName = fileName +'.'+fileType;
break;
default:
res.status(400).send('Arquivo não suportado!');
break;
}
res.sendFile(fileName, options, function (err) {
if (err) {
console.log(err);
res.status(err.status).end();
} else {
console.log('Sent:', fileName);
}
});
};
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
html
head
title= title
body
h1= message
p= description
| {
"repo_name": "Webschool-io/be-mean-instagram-nodejs-exercises",
"stars": "33",
"repo_language": "JavaScript",
"file_name": "index.jade",
"mime_type": "text/plain"
} |
<!DOCTYPE html>
<html lang="en">
<head>
<link
href="mermaid.min.css"
rel="stylesheet"
/>
<style>
body {
background-color: #0d1117;
font-family: -apple-system,BlinkMacSystemFont,"Segoe UI","Noto Sans",Helvetica,Arial,sans-serif,"Apple Color Emoji","Segoe UI Emoji";
}
a:link {
color: #58A6FF;
background-color: transparent;
text-decoration: none;
}
a:visited {
color: #58A6FF;
background-color: transparent;
text-decoration: none;
}
a:hover {
color: #58A6FF;
background-color: transparent;
text-decoration: underline;
}
a:active {
color: #58A6FF;
background-color: transparent;
text-decoration: underline;
}
.gridjs-pagination {
color: #c9d1d9;
}
input.gridjs-input {
background-color: rgb(40 44 50);
border: 0px;
color: #c9d1d9;
}
.gridjs-pagination .gridjs-pages button {
background-color: #0f131a;
border: 1px solid #242e3b;
color: #c9d1d9;
}
.gridjs-pagination .gridjs-pages button:last-child {
border-right: 1px solid #242e3b;
}
.gridjs-pagination .gridjs-pages button:disabled, .gridjs-pagination .gridjs-pages button:hover:disabled, .gridjs-pagination .gridjs-pages button[disabled] {
background-color: #0f131a;
}
.gridjs-pagination .gridjs-pages button:disabled, .gridjs-pagination .gridjs-pages button:hover:enabled, .gridjs-pagination .gridjs-pages button[disabled] {
background-color: #0d1117;
}
.gridjs-pagination .gridjs-pages button.gridjs-currentPage {
background-color: #19212c;
}
.gridjs-pagination .gridjs-pages button.gridjs-spread {
background-color: #0d1117;
}
.gridjs-tbody, td.gridjs-td {
background-color: transparent !important;
}
tr:nth-child(even) {
background-color: #0f131a
}
tr:nth-child(odd) {
background-color: #0d1117
}
.gridjs-pagination .gridjs-summary {
color: #8B949E;
}
</style>
<title>AI Creator Archive</title>
</head>
<body>
<div id="wrapper"></div>
<script src="https://unpkg.com/gridjs/dist/gridjs.umd.js"></script>
<script src="index.js"></script>
</body>
</html>
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
new gridjs.Grid({
sort: true,
resizable: true,
search: true,
pagination: true,
pagination: {
limit: 7,
summary: true
},
fixedHeader: true,
height: '780px',
columns: [{
id: 'creator_username',
name: 'username',
}, {
id: 'model_name',
name: 'model',
}, {
id: 'model_version',
name: 'version',
}, {
id: 'links',
name: 'links',
formatter: (cells) => gridjs.html(`<a href='${cells[0]}'>${cells[0].split('/').pop().split('.').pop()}</a>`),
}, {
id: 'tokens',
name: 'tokens',
width : '300px',
formatter: (cells) => gridjs.html(`<small>${cells}</small>`),
}, {
id: 'vae',
name: 'vae',
formatter: (cells) => cells[0] ? gridjs.html(`<a href='${cells[0]}'>vae</a>`) : '',
}, {
id: 'online',
name: 'online',
formatter: (cells) => gridjs.html(`<a href='${cells[0]}'>colab</a>`),
}, {
id: 'images',
name: 'images',
width : '350px',
formatter: (cells) => gridjs.html(`<img src='https://via.placeholder.com/64'> <img src='https://via.placeholder.com/64'> <img src='https://via.placeholder.com/64'> <img src='https://via.placeholder.com/64'>`),
}, {
id: 'info',
name: 'info',
formatter: (cell) => gridjs.html(`<a href='${cell}'>info</a>`),
}, {
id: 'type',
name: 'type',
}],
server: {
url: 'https://aica.pages.dev/all.json',
then: data => data.map(creator =>
[creator.creator_username, creator.model_name, creator.model_version,creator.links,creator.tokens,creator.vae,creator.online,creator.images,creator.info,creator.type]
)
},
style: {
header : {
},
container : {
},
table: {
color: '#c9d1d9',
border: '0px',
},
th: {
'background-color': '#161B22',
color: '#8B949E',
border: '0px',
},
td: {
'background-color': 'transparent !important',
border: '0px solid rgb(40 44 50)',
},
footer : {
'background-color': '#161B22',
border: '0px',
}
},
}).render(document.getElementById("wrapper"));
var div = document.getElementsByClassName('gridjs-head')[0];
const divInside = document.createElement('div');
divInside.style.textAlign = "right";
divInside.style.color = "#c9d1d9";
divInside.style.paddingTop = "6px";
const json = document.createElement('a');
const jsonSpan = document.createElement('span');
jsonSpan.textContent = ' ' + String.fromCodePoint(128223) + ' ';
json.href = "https://aica.pages.dev/all.json";
json.textContent = 'json';
divInside.appendChild(jsonSpan);
divInside.appendChild(json);
const repo = document.createElement('a');
const repoSpan = document.createElement('span');
repoSpan.textContent = ' ' + String.fromCodePoint(128451) + ' ';
repo.href = "https://github.com/camenduru/ai-creator-archive";
repo.textContent = 'github';
divInside.appendChild(repoSpan);
divInside.appendChild(repo);
const colab = document.createElement('a');
const colabSpan = document.createElement('span');
colabSpan.textContent = ' ' + String.fromCodePoint(129426) + ' ';
colab.href = "https://github.com/camenduru/stable-diffusion-webui-colab";
colab.textContent = 'colab';
divInside.appendChild(colabSpan);
divInside.appendChild(colab);
const cTwitter = document.createElement('a');
const cTwitterSpan = document.createElement('span');
cTwitterSpan.textContent = ' ' + String.fromCodePoint(128035) + ' ';
cTwitter.href = "https://twitter.com/camenduru";
cTwitter.textContent = '@camenduru';
divInside.appendChild(cTwitterSpan);
divInside.appendChild(cTwitter);
div.appendChild(divInside); | {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
```
.
└───creators
├───22h
│ └───vintedois_diffusion
│ └───010
├───852wa
│ └───8528_diffusion
│ └───100
├───aipicasso
│ └───cool_japan_diffusion
│ └───210
├───alexds9
│ └───babes
│ └───100
├───amethyst_vera
│ └───simp_maker_3k1
│ └───100
├───andite
│ ├───anything
│ │ └───400
│ └───yohan_diffusion
│ └───100
├───astralite_heart
│ └───pony_diffusion
│ └───200
├───aybeeceedee
│ └───knollingcase
│ └───100
├───basunat
│ └───cinematic_diffusion
│ └───100
├───best_jammer
│ └───hasdx
│ └───400
├───cafeai
│ └───cafe_instagram_sd_1_5
│ └───600
├───chilon249
│ └───yiffy_mix
│ └───100
├───claudfuen
│ └───photorealistic_fuen
│ └───100
├───comp_vis
│ └───stable_diffusion_original
│ └───140
├───conflictx
│ └───complex_lineart
│ └───100
├───coreco
│ └───seek_art_mega
│ └───100
├───dallinmackay
│ └───van_gogh_diffusion
│ └───200
├───dg_spitzer
│ └───cyberpunk_anime_diffusion
│ └───100
├───doubleyobro
│ └───yiffy_e18
│ └───100
├───dreamlike_art
│ ├───dreamlike_diffusion
│ │ └───100
│ └───dreamlike_photoreal
│ └───200
├───duc_haiten
│ └───duc_haiten_aIart
│ └───011
├───eimiss
│ └───eimis_anime_diffusion
│ └───100
├───envvi
│ └───inkpunk_diffusion
│ └───100
├───fiacr
│ └───comics_blend
│ └───100
├───fictiverse
│ └───stable_diffusion_paper_cut_model
│ └───100
├───gsdf
│ └───counterfeit
│ └───200
├───hakurei
│ └───waifu_diffusion
│ ├───130
│ └───140
├───hassanblend
│ └───hassanblend
│ └───140
├───hesw23168
│ └───sd_elysium_model
│ └───100
├───inzamam567
│ └───any_gape
│ └───100
├───its_jay_qz
│ └───synthwave_punk
│ └───200
├───josephus_cheung
│ ├───a_certainty
│ │ └───100
│ ├───a_certain_model
│ │ └───100
│ └───a_certain_thing
│ └───100
├───mattgroy
│ └───dream_like_sam_kuvshinov
│ └───200
├───mehjourney_closed_ai
│ └───open_anime_journey
│ └───100
├───n75242
│ └───monstermash_anyv3
│ └───100
├───nitrosocke
│ ├───arcane_diffusion
│ │ └───300
│ └───mo_di_diffusion
│ └───100
├───ogkalu
│ ├───comic_diffusion
│ │ └───200
│ └───illustration_diffusion
│ └───100
├───plasmo
│ ├───food_crit
│ │ └───100
│ └───woolitize
│ └───100
├───prompthero
│ ├───midjourney_diffusion
│ │ └───400
│ └───openjourney
│ └───200
├───public_prompts
│ └───all_in_one_pixel_model
│ └───100
├───ray_hell
│ └───popup_book_diffusion
│ └───100
├───riffusion
│ └───riffusion_model
│ └───100
├───runwayml
│ ├───stable_diffusion
│ │ └───150
│ └───stable_diffusion_inpainting
│ └───100
├───sandro_halpo
│ └───sam_does_art
│ └───300
├───sentet1c
│ └───rando_mix3
│ └───100
├───stabilityai
│ ├───stable_diffusion
│ │ ├───200
│ │ └───210
│ ├───stable_diffusion_base
│ │ ├───200
│ │ └───210
│ ├───stable_diffusion_depth
│ │ └───200
│ └───stable_diffusion_inpainting
│ └───200
├───thecollector
│ └───sam_does_sexy_blend
│ └───100
├───unknown
│ ├───anything
│ │ └───300
│ ├───elldreths_lucid_mix
│ │ └───100
│ ├───f222
│ │ └───100
│ ├───grapefruit
│ │ └───100
│ ├───iz
│ │ └───100
│ ├───protogen
│ │ └───220
│ ├───rbm
│ │ └───100
│ └───urp
│ └───100
├───warrior_mama777
│ └───orange_mixs
│ └───100
└───wavymulder
└───analog_diffusion
└───100
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
526_mix_webui_colab
7th_layer_webui_colab
8528_diffusion_webui_colab
a_certain_model_webui_colab
a_certain_thing_webui_colab
a_certainty_webui_colab
abyss_orange_mix_2_webui_colab
abyss_orange_mix_3_webui_colab
alfamix_webui_colab
all_in_one_pixel_model_webui_colab
analog_diffusion_webui_colab
anylora_webui_colab
anything_3_webui_colab
anything_4_webui_colab
anything_gape_webui_colab
arcane_diffusion_3_webui_colab
babes_blend_webui_colab
berry_mix_1_5_webui_colab
cafe_instagram_v6_webui_colab
cetus_mix_v3_1_webui_colab
cheese_daddys_landscapes_mix_webui_colab
chillout_mix_webui_colab
cinematic_diffusion_webui_colab
color_bomb_mix_webui_colab
comic_diffusion_v2_webui_colab
comics_blend_webui_colab
complex_lineart_webui_colab
cool_japan_diffusion_2_1_webui_colab
counterfeit_v2_0_webui_colab
cyberpunk_anime_diffusion_webui_colab
dark_sushi_mix_mix_webui_colab
defmix_v2_webui_colab
deliberate_webui_colab
dosmix_webui_colab
dpepmkmp_webui_colab
dream_like_sam_kuvshino_webui_colab
dreamlike_diffusion_1_webui_colab
dreamlike_photoreal_2_webui_colab
dreamshaper_3_32_webui_colab
duchaitenaiart_v1_1_webui_colab
eimis_anime_diffusion_1_webui_colab
elldreths_lucid_mix_v10_webui_colab
elysium_anime_2_webui_colab
experience_webui_colab
f222_webui_colab
faetastic_v1_webui_colab
fantasy_world_v1_webui_colab
food_crit_webui_colab
grapefruit_webui_colab
graphic_art_webui_colab
hasdx_webui_colab
hassan_blend_1_4_webui_colab
illustration_diffusion_webui_colab
inkpunk_webui_colab
izumi_v1_webui_colab
knollingcase_webui_colab
liberty_webui_colab
lucky_strike_mix_webui_colab
magical_mix_v1_webui_colab
magical_mix_v2_webui_colab
mare_coloris_webui_colab
meina_mix_v8_webui_colab
midjourney_v4_diffusion_webui_colab
mo_di_diffusion_webui_colab
monstermash_any_3_webui_colab
mousey_mix_webui_colab
neverending_dream_webui_colab
nostalgia_clear_webui_colab
open_anime_journey_webui_colab
openjourney_v2_diffusion_webui_colab
openjourney_v4_webui_colab
padoru_v1_webui_colab
papercut_diffusion_webui_colab
pastel_boys_2d_webui_colab
pastel_mix_stylized_anime_webui_colab
pegasus_9_mix_webui_colab
perfect_world_v2_webui_colab
photorealistic_fuen_v1_webui_colab
pony_diffusion_2_webui_colab
pony_diffusion_v3_webui_colab
pony_v4_webui_colab
popup_book_diffusion_webui_colab
protogen_nova_webui_colab
protogen_v2_2_webui_colab
protogen_x3_4_webui_colab
pvc_v3_webui_colab
rando_mix_3_webui_colab
realbiter_v1_0_webui_colab
realistic_vision_v13_webui_colab
realistic_vision_v20_webui_colab
refslave_v2_webui_colab
rev_animated_v1_1_webui_colab
rmada_merge_v5_webui_colab
rmadart_v5_webui_colab
robo_diffusion_webui_colab
rpg_v4_webui_colab
samdoesart_diffusion_webui_colab
samdoessexy_blend_webui_colab
seek_art_mega_v1_webui_colab
seekart_mega_webui_colab
simpmaker_3k_webui_colab
stable_diffusion_1_5_webui_colab
stable_diffusion_inpainting_webui_colab
stable_diffusion_v2_1_base_webui_colab
stable_diffusion_v2_1_webui_colab
stable_diffusion_v2_base_webui_colab
stable_diffusion_v2_depth_webui_colab
stable_diffusion_v2_inpainting_webui_colab
stable_diffusion_v2_webui_colab
stable_diffusion_webui_colab
stablydiffuseds_aesthetic_mix_webui_colab
sukiyaki_mix_v1_webui_colab
synthwave_punk_v2_webui_colab
uber_realistic_merge_webui_colab
uber_realistic_v1_webui_colab
van_gogh_diffusion_webui_colab
vinte_protogen_mix_v_1_0_webui_colab
vintedois_diffusion_0_1_webui_colab
voxo_webui_colab
waifu_diffusion_v1_4_webui_colab
waifu_diffusion_v1_5_b2_webui_colab
waifu_diffusion_v1_5_webui_colab
waifu_diffusion_webui_colab
woolitize_webui_colab
yiffy_e18_webui_colab
yiffy_mix_webui_colab
yohan_diffusion_webui_colab
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
mo_di_diffusion_webui_colab 1080129500274245744
vinte_protogen_mix_v_1_0_webui_colab 1080132398781575249
waifu_diffusion_v1_5_b2_webui_colab 1084979718358581310
stablydiffuseds_aesthetic_mix_webui_colab 1084979612800532561
graphic_art_webui_colab 1084979552809390202
pvc_v3_webui_colab 1084979506370068511
magical_mix_v1_webui_colab 1084979421561225216
voxo_webui_colab 1084979382910722198
padoru_v1_webui_colab 1084979343165497455
realistic_vision_v13_webui_colab 1084979258084036658
liberty_webui_colab 1084978412449435719
sukiyaki_mix_v1_webui_colab 1084978296300785846
alfamix_webui_colab 1084978268471570572
mare_coloris_webui_colab 1084978193582268449
defmix_v2_webui_colab 1084978124464337037
perfect_world_v2_webui_colab 1084978094504415374
refslave_v2_webui_colab 1084977996877799445
uber_realistic_v1_webui_colab 1080131357151342693
stable_diffusion_inpainting_webui_colab 1080128693445333003
stable_diffusion_1_5_webui_colab 1080129446108999700
riffusion_v1_webui_colab 1080131014803849316
waifu_diffusion_webui_colab 1080128404529094779
stable_diffusion_webui_colab 1080128346282807396
cafe_instagram_v6_webui_colab 1080131169376542850
vintedois_diffusion_0_1_webui_colab 1080130677518893068
cinematic_diffusion_webui_colab 1080131208354209902
all_in_one_pixel_model_webui_colab 1080131135079718942
illustration_diffusion_webui_colab 1080130898294476810
synthwave_punk_v2_webui_colab 1080130861443334234
8528_diffusion_webui_colab 1080131632238973010
popup_book_diffusion_webui_colab 1080157291489284146
chillout_mix_webui_colab 1080132425121792000
food_crit_webui_colab 1080131252029501440
openjourney_v2_diffusion_webui_colab 1080130644094496768
waifu_diffusion_v1_4_webui_colab 1080131045032218624
dream_like_sam_kuvshino_webui_colab 1080131599846350969
abyss_orange_mix_2_webui_colab 1080131688723660880
dreamlike_photoreal_2_webui_colab 1080131718624854058
cool_japan_diffusion_2_1_webui_colab 1080131786685816933
a_certain_model_webui_colab 1080131985701347338
a_certain_thing_webui_colab 1080132011580194896
pix2pix_webui_colab 1080132066676592682
protogen_nova_webui_colab 1080132153448337468
protogen_x3_4_webui_colab 1080132256229761025
neverending_dream_webui_colab 1080132340967276666
deliberate_webui_colab 1080132367886323832
pastel_mix_stylized_anime_webui_colab 1080132454318362654
comics_blend_webui_colab 1080131565423693844
woolitize_webui_colab 1080129883839139910
mousey_mix_webui_colab 1080132554667077683
illuminati_v1_0_webui_colab 1080132498975105137
seek_art_mega_v1_webui_colab 1080130939881013298
yohan_diffusion_webui_colab 1080130612255527044
eimis_anime_diffusion_1_webui_colab 1080130422073204757
comic_diffusion_v2_webui_colab 1080130785421574144
pony_diffusion_2_webui_colab 1080130350036045884
hassan_blend_1_4_webui_colab 1080130284932051034
complex_lineart_webui_colab 1080130827058434119
analog_diffusion_webui_colab 1080130538855223376
van_gogh_diffusion_webui_colab 1080130502817763408
dreamlike_diffusion_1_webui_colab 1080130468923572424
knollingcase_webui_colab 1080130718602104862
inkpunk_webui_colab 1080129920904204288
samdoesart_diffusion_webui_colab 1080129807389577266
arcane_diffusion_3_webui_colab 1080129552090660905
cyberpunk_anime_diffusion_webui_colab 1080129636559753336
midjourney_v4_diffusion_webui_colab 1080129702393565345
papercut_diffusion_webui_colab 1080129755841560596
illuminati_v1_1_webui_colab 1080132633478041760
dpepmkmp_webui_colab 1080132588498337923
color_bomb_mix_webui_colab 1080132529090220083
abyss_orange_mix_3_webui_colab 1080132313511370914
dreamshaper_3_32_webui_colab 1080132287972245625
control_net_webui_colab 1080132217918984202
waifu_diffusion_v1_5_webui_colab 1080132126013411328
rpg_v4_webui_colab 1080132097626362006
7th_layer_webui_colab 1080132038008524850
a_certainty_webui_colab 1080131961835753524
open_anime_journey_webui_colab 1080131929740939355
grapefruit_webui_colab 1080131900976398386
counterfeit_v2_0_webui_colab 1080131874346762360
anything_4_webui_colab 1080131842046447626
anything_gape_webui_colab 1080131813881683978
photorealistic_fuen_v1_webui_colab 1080131747724922932
hasdx_webui_colab 1080131659652935701
samdoessexy_blend_webui_colab 1080131516165791754
babes_blend_webui_colab 1080131446729093120
yiffy_mix_webui_colab 1080131418543357963
izumi_v1_webui_colab 1080131390261178378
duchaitenaiart_v1_1_webui_colab 1080131316927955037
simpmaker_3k_webui_colab 1080131284594077726
elldreths_lucid_mix_v10_webui_colab 1080131098618646548
protogen_v2_2_webui_colab 1080131071355662397
monstermash_any_3_webui_colab 1080130573621800992
elysium_anime_2_webui_colab 1080130383510777896
yiffy_e18_webui_colab 1080130319220486166
stable_diffusion_v2_inpainting_webui_colab 1080130243379089479
stable_diffusion_v2_depth_webui_colab 1080130213188468738
stable_diffusion_v2_base_webui_colab 1080130182184194078
stable_diffusion_v2_webui_colab 1080130150672384102
stable_diffusion_v2_1_base_webui_colab 1080130120758595744
stable_diffusion_v2_1_webui_colab 1080130090173734922
berry_mix_1_5_webui_colab 1080130044237713408
f222_webui_colab 1080130010117058600
rando_mix_3_webui_colab 1080129971240050718
anything_3_webui_colab 1080129845834567730 | {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
🐣 Please follow me for new updates https://twitter.com/camenduru <br />
🔥 Please join our discord server https://discord.gg/k5BwmmvJJU <br />
🥳 Please join my patreon community https://patreon.com/camenduru <br />
## 🚦WIP🚦
## JSON & Preview
JSON: https://aica.pages.dev/all.json <br />
Preview Grid: https://aica.vercel.app <br />
Preview Table: https://aica.pages.dev
## Motivation and Action
Maybe someday, your favorite AI company will force you to make your stable diffusion models private. It's time to copy and paste them to other cloud providers to ensure they can't be taken down. This repo will begin with 105 models from the [stable-diffusion-webui-colab](https://github.com/camenduru/stable-diffusion-webui-colab) project and will provide a simple JSON file for the data inside. It will continue to grow.
## What is the AI Creator Archive
An AI Creator Archive is a repository of information about the individuals and teams who create and develop artificial intelligence models.
The archive can include information such as the creators' notable contributions to the field of AI.
It also include information about the AI models they have developed.
The purpose of an AI Creator Archive is to provide transparency and recognition for the creators of AI models.
It is also useful for people who are interested in learning about the history and development of AI and the people behind it.
## Contributing to the AI Creator Archive
Just open an issue with your `username`, `model name`, `model version`, `model token(s)`, `model direct link` or `Google Drive link`, `4 images with PNG info`, and if you want, an `info.txt`
## Image Formats
Images should not contain any nudity. Remember how GitHub deleted the web UI without warning.
## Automation Design
All files and the Colab notebook will be created with the server, the Colab will be published on this link: https://github.com/camenduru/stable-diffusion-webui-colab
### Folder Structure
```
.
├── ...
├── creator_username # username format if UseR_Nam3 should be user_nam3
│ └── model_name # model name format if OrangeCocoaMix should be orange_cocoa_mix
│ └── model_version # model version format if v0.1.0 should be 0_1_0
│ ├── 1.png # 4 images should be generated for each version
│ ├── 2.png # using the corresponding model version
│ ├── 3.png # the PNG info should be included within the images
│ ├── 4.png # all images will auto regenerated with the PNG info and overwrite these four images
│ ├── links.txt # check links.txt format
│ ├── tokens.txt # check tokens.txt format
│ ├── online.txt # check online.txt format
│ ├── images.txt # check images.txt format
│ ├── type.txt # check type.txt format
│ ├── vae.txt # check vae.txt format
│ └── info.txt # check info.txt format
├── all.json
└── ...
```
#### links.txt format
```
Models should be PyTorch safetensors format if not please convert with this https://github.com/camenduru/converter-colab
Each line must contain one direct link to the model, not the model page. The magnet should contain only the model.
├── https://huggingface.co/ckpt/stable-diffusion-2/resolve/main/768-v-ema.ckpt
├── https://civitai.com/api/download/models/0000?type=Pruned%20Model&format=SafeTensor
└── magnet:?xt=urn:btih:000000&dn=model&tr=udp://tracker.com&tr=udp://tracker.org/announce
```
#### tokens.txt format
```
Each line must contain one token.
├── analog style
└── dreamlikeart style
```
#### online.txt format
```
Online service links, such as Google Colab
Each line must contain one link.
├── https://colab.research.google.com/github/camenduru/stable-diffusion-webui-colab/blob/main/stable/stable_diffusion_webui_colab.ipynb
```
#### images.txt format
```
4 images should be generated for each version using the corresponding model version
The PNG info should be included within the images
All images will auto regenerated with the PNG info and overwrite these four images
Each line must contain one image url.
├── https://aica.pages.dev/creators/basunat/cinematic_diffusion/1_0_0/1.png
├── https://aica.pages.dev/creators/basunat/cinematic_diffusion/1_0_0/2.png
├── https://aica.pages.dev/creators/basunat/cinematic_diffusion/1_0_0/3.png
└── https://aica.pages.dev/creators/basunat/cinematic_diffusion/1_0_0/4.png
```
#### type.txt format
```
fine_tune, dreambooth, textual_inversion, hypernetwork, aesthetic_gradient, lora, blend
```
#### vae.txt format
```
VAE link for the model, name should be same_name_with_model.vae.pt
Each line must contain one link.
├── https://huggingface.co/ckpt/sd-vae-ft-mse-original/resolve/main/vae-ft-mse-840000-ema-pruned.ckpt
```
#### info.txt format
```
info.txt file will follow the markdown guide, with a file size of 10KB, do whatever you want. maybe example prompts.
```
#### all.json
```
all.json contains all of the data inside the files links.txt, tokens.txt, online.txt, info.txt, and image links.
[
{
"creator_username": "22h",
"images": [
"https://aica.pages.dev/creators/22h/vintedois_diffusion/0_1_0/1.png",
"https://aica.pages.dev/creators/22h/vintedois_diffusion/0_1_0/2.png",
"https://aica.pages.dev/creators/22h/vintedois_diffusion/0_1_0/3.png",
"https://aica.pages.dev/creators/22h/vintedois_diffusion/0_1_0/4.png"
],
"info": "https://aica.pages.dev/creators/22h/vintedois_diffusion/0_1_0/info.txt",
"links": [
"https://huggingface.co/ckpt/vintedois-diffusion-v0-1/resolve/main/vintedois_0_1.ckpt"
],
"model_name": "vintedois_diffusion",
"model_version": "0_1_0",
"online": [
"https://colab.research.google.com/github/camenduru/stable-diffusion-webui-colab/blob/main/stable/vintedois_diffusion_0_1_webui_colab.ipynb"
],
"tokens": [
"estilovintedois"
],
"type": [
"dreambooth"
],
"vae": []
},
{
"creator_username": "852wa",
"images": [
"https://aica.pages.dev/creators/852wa/8528_diffusion/1_0_0/1.png",
"https://aica.pages.dev/creators/852wa/8528_diffusion/1_0_0/2.png",
"https://aica.pages.dev/creators/852wa/8528_diffusion/1_0_0/3.png",
"https://aica.pages.dev/creators/852wa/8528_diffusion/1_0_0/4.png"
],
"info": "https://aica.pages.dev/creators/852wa/8528_diffusion/1_0_0/info.txt",
"links": [
"https://huggingface.co/ckpt/8528-diffusion/resolve/main/8528d-final.ckpt"
],
"model_name": "8528_diffusion",
"model_version": "1_0_0",
"online": [
"https://colab.research.google.com/github/camenduru/stable-diffusion-webui-colab/blob/main/stable/8528_diffusion_webui_colab.ipynb"
],
"tokens": [],
"type": [
"fine_tune"
],
"vae": []
}
]
```
## Archive PyTorch Backup
All PyTorch safetensors and ckpt models in this archive will be stored at this link: https://huggingface.co/ckpt and the link will be written in the 'models links.txt' file.
If we can find more cloud storage sponsors, we will create multiple backups, including backups of backups and backups of backups of backups of backups of ... <br />
We will also publish reports on the relationships between each model and its parent models, as well as the mixture percentages.
## Archive Flax Diffusers Lib Backup
All Flax Diffusers Lib safetensors and msgpack weights in this archive will be stored at this link: https://huggingface.co/flax and the link will be written in the 'models flax-links.txt' file.
## Archive PyTorch Diffusers Lib Backup
All PyTorch Diffusers Lib safetensors and bin weights in this archive will be stored at this link: https://huggingface.co/flax `pytorch` branch and the link will be written in the 'models pt-links.txt' file.
## Archive Core ML Backup
All Core ML Models in this archive will be stored at this link: https://huggingface.co/core-ml and the link will be written in the 'models coreml-links.txt' file.
## Archive Embed Backup
Textual Inversion, LoRA models will be stored at this link: https://huggingface.co/embed (Another archival project will be announced soon)
## Archive TensorRT Backup
TensorRT models will be stored at this link: https://huggingface.co/trrt (Another archival project will be announced soon)
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
# These are supported funding model platforms
github: # Replace with up to 4 GitHub Sponsors-enabled usernames e.g., [user1, user2]
patreon: camenduru
open_collective: # Replace with a single Open Collective username
ko_fi: camenduru
tidelift: # Replace with a single Tidelift platform-name/package-name e.g., npm/babel
community_bridge: # Replace with a single Community Bridge project-name e.g., cloud-foundry
liberapay: # Replace with a single Liberapay username
issuehunt: # Replace with a single IssueHunt username
otechie: # Replace with a single Otechie username
lfx_crowdfunding: # Replace with a single LFX Crowdfunding project-name e.g., cloud-foundry
custom: # Replace with up to 4 custom sponsorship URLs e.g., ['link1', 'link2']
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/vsukiyaki/sukiyaki_mix/1_0_0/1.png
https://aica.pages.dev/creators/vsukiyaki/sukiyaki_mix/1_0_0/2.png
https://aica.pages.dev/creators/vsukiyaki/sukiyaki_mix/1_0_0/3.png
https://aica.pages.dev/creators/vsukiyaki/sukiyaki_mix/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/inzamam567/any_gape/1_0_0/1.png
https://aica.pages.dev/creators/inzamam567/any_gape/1_0_0/2.png
https://aica.pages.dev/creators/inzamam567/any_gape/1_0_0/3.png
https://aica.pages.dev/creators/inzamam567/any_gape/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/jawgboi/padoru/1_0_0/1.png
https://aica.pages.dev/creators/jawgboi/padoru/1_0_0/2.png
https://aica.pages.dev/creators/jawgboi/padoru/1_0_0/3.png
https://aica.pages.dev/creators/jawgboi/padoru/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/claudfuen/photorealistic_fuen/1_0_0/1.png
https://aica.pages.dev/creators/claudfuen/photorealistic_fuen/1_0_0/2.png
https://aica.pages.dev/creators/claudfuen/photorealistic_fuen/1_0_0/3.png
https://aica.pages.dev/creators/claudfuen/photorealistic_fuen/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/civitai/chilloutmix/1_0_0/1.png
https://aica.pages.dev/creators/civitai/chilloutmix/1_0_0/2.png
https://aica.pages.dev/creators/civitai/chilloutmix/1_0_0/3.png
https://aica.pages.dev/creators/civitai/chilloutmix/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/fiacr/comics_blend/1_0_0/1.png
https://aica.pages.dev/creators/fiacr/comics_blend/1_0_0/2.png
https://aica.pages.dev/creators/fiacr/comics_blend/1_0_0/3.png
https://aica.pages.dev/creators/fiacr/comics_blend/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
ComplexLA style
nvinkpunk
marioalberti artstyle
ghibli style | {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/illuminati_ai/illuminati/1_1_0/1.png
https://aica.pages.dev/creators/illuminati_ai/illuminati/1_1_0/2.png
https://aica.pages.dev/creators/illuminati_ai/illuminati/1_1_0/3.png
https://aica.pages.dev/creators/illuminati_ai/illuminati/1_1_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/fictiverse/stable_diffusion_paper_cut_model/1_0_0/1.png
https://aica.pages.dev/creators/fictiverse/stable_diffusion_paper_cut_model/1_0_0/2.png
https://aica.pages.dev/creators/fictiverse/stable_diffusion_paper_cut_model/1_0_0/3.png
https://aica.pages.dev/creators/fictiverse/stable_diffusion_paper_cut_model/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/22h/vintedois_diffusion/0_1_0/1.png
https://aica.pages.dev/creators/22h/vintedois_diffusion/0_1_0/2.png
https://aica.pages.dev/creators/22h/vintedois_diffusion/0_1_0/3.png
https://aica.pages.dev/creators/22h/vintedois_diffusion/0_1_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/shatalin_art/alfamix/1_0_0/1.png
https://aica.pages.dev/creators/shatalin_art/alfamix/1_0_0/2.png
https://aica.pages.dev/creators/shatalin_art/alfamix/1_0_0/3.png
https://aica.pages.dev/creators/shatalin_art/alfamix/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/mekabu/magical_mix/1_0_0/1.png
https://aica.pages.dev/creators/mekabu/magical_mix/1_0_0/2.png
https://aica.pages.dev/creators/mekabu/magical_mix/1_0_0/3.png
https://aica.pages.dev/creators/mekabu/magical_mix/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/public_prompts/all_in_one_pixel_model/1_0_0/1.png
https://aica.pages.dev/creators/public_prompts/all_in_one_pixel_model/1_0_0/2.png
https://aica.pages.dev/creators/public_prompts/all_in_one_pixel_model/1_0_0/3.png
https://aica.pages.dev/creators/public_prompts/all_in_one_pixel_model/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
pixelsprite
16bitscene | {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/defpoint/defmix/2_0_0/1.png
https://aica.pages.dev/creators/defpoint/defmix/2_0_0/2.png
https://aica.pages.dev/creators/defpoint/defmix/2_0_0/3.png
https://aica.pages.dev/creators/defpoint/defmix/2_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/stablydiffused/stablydiffuseds_aesthetic_mix/1_0_0/1.png
https://aica.pages.dev/creators/stablydiffused/stablydiffuseds_aesthetic_mix/1_0_0/2.png
https://aica.pages.dev/creators/stablydiffused/stablydiffuseds_aesthetic_mix/1_0_0/3.png
https://aica.pages.dev/creators/stablydiffused/stablydiffuseds_aesthetic_mix/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/mattgroy/dream_like_sam_kuvshinov/2_0_0/1.png
https://aica.pages.dev/creators/mattgroy/dream_like_sam_kuvshinov/2_0_0/2.png
https://aica.pages.dev/creators/mattgroy/dream_like_sam_kuvshinov/2_0_0/3.png
https://aica.pages.dev/creators/mattgroy/dream_like_sam_kuvshinov/2_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/darkstorm2150/protogen_nova/1_0_0/1.png
https://aica.pages.dev/creators/darkstorm2150/protogen_nova/1_0_0/2.png
https://aica.pages.dev/creators/darkstorm2150/protogen_nova/1_0_0/3.png
https://aica.pages.dev/creators/darkstorm2150/protogen_nova/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/darkstorm2150/protogen_x/3_4_0/1.png
https://aica.pages.dev/creators/darkstorm2150/protogen_x/3_4_0/2.png
https://aica.pages.dev/creators/darkstorm2150/protogen_x/3_4_0/3.png
https://aica.pages.dev/creators/darkstorm2150/protogen_x/3_4_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://huggingface.co/ckpt/SD-Elysium-Model/resolve/main/Elysium_Anime_V2.ckpt
https://huggingface.co/ckpt/SD-Elysium-Model/resolve/main/Elysium_Anime_V3.safetensors | {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/hesw23168/sd_elysium_model/1_0_0/1.png
https://aica.pages.dev/creators/hesw23168/sd_elysium_model/1_0_0/2.png
https://aica.pages.dev/creators/hesw23168/sd_elysium_model/1_0_0/3.png
https://aica.pages.dev/creators/hesw23168/sd_elysium_model/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/852wa/8528_diffusion/1_0_0/1.png
https://aica.pages.dev/creators/852wa/8528_diffusion/1_0_0/2.png
https://aica.pages.dev/creators/852wa/8528_diffusion/1_0_0/3.png
https://aica.pages.dev/creators/852wa/8528_diffusion/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/coreco/seek_art_mega/1_0_0/1.png
https://aica.pages.dev/creators/coreco/seek_art_mega/1_0_0/2.png
https://aica.pages.dev/creators/coreco/seek_art_mega/1_0_0/3.png
https://aica.pages.dev/creators/coreco/seek_art_mega/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/dallinmackay/van_gogh_diffusion/2_0_0/1.png
https://aica.pages.dev/creators/dallinmackay/van_gogh_diffusion/2_0_0/2.png
https://aica.pages.dev/creators/dallinmackay/van_gogh_diffusion/2_0_0/3.png
https://aica.pages.dev/creators/dallinmackay/van_gogh_diffusion/2_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/bloodsuga/perfect_world/2_0_0/1.png
https://aica.pages.dev/creators/bloodsuga/perfect_world/2_0_0/2.png
https://aica.pages.dev/creators/bloodsuga/perfect_world/2_0_0/3.png
https://aica.pages.dev/creators/bloodsuga/perfect_world/2_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/thecollector/sam_does_sexy_blend/1_0_0/1.png
https://aica.pages.dev/creators/thecollector/sam_does_sexy_blend/1_0_0/2.png
https://aica.pages.dev/creators/thecollector/sam_does_sexy_blend/1_0_0/3.png
https://aica.pages.dev/creators/thecollector/sam_does_sexy_blend/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/hakurei/waifu_diffusion/1_4_0/1.png
https://aica.pages.dev/creators/hakurei/waifu_diffusion/1_4_0/2.png
https://aica.pages.dev/creators/hakurei/waifu_diffusion/1_4_0/3.png
https://aica.pages.dev/creators/hakurei/waifu_diffusion/1_4_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/hakurei/waifu_diffusion/1_3_0/1.png
https://aica.pages.dev/creators/hakurei/waifu_diffusion/1_3_0/2.png
https://aica.pages.dev/creators/hakurei/waifu_diffusion/1_3_0/3.png
https://aica.pages.dev/creators/hakurei/waifu_diffusion/1_3_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/dg_spitzer/cyberpunk_anime_diffusion/1_0_0/1.png
https://aica.pages.dev/creators/dg_spitzer/cyberpunk_anime_diffusion/1_0_0/2.png
https://aica.pages.dev/creators/dg_spitzer/cyberpunk_anime_diffusion/1_0_0/3.png
https://aica.pages.dev/creators/dg_spitzer/cyberpunk_anime_diffusion/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/riffusion/riffusion_model/1_0_0/1.png
https://aica.pages.dev/creators/riffusion/riffusion_model/1_0_0/2.png
https://aica.pages.dev/creators/riffusion/riffusion_model/1_0_0/3.png
https://aica.pages.dev/creators/riffusion/riffusion_model/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/conflictx/complex_lineart/1_0_0/1.png
https://aica.pages.dev/creators/conflictx/complex_lineart/1_0_0/2.png
https://aica.pages.dev/creators/conflictx/complex_lineart/1_0_0/3.png
https://aica.pages.dev/creators/conflictx/complex_lineart/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/p1atdev/pvc/3_0_0/1.png
https://aica.pages.dev/creators/p1atdev/pvc/3_0_0/2.png
https://aica.pages.dev/creators/p1atdev/pvc/3_0_0/3.png
https://aica.pages.dev/creators/p1atdev/pvc/3_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://huggingface.co/ckpt/DucHaitenAIart_v1.1a/resolve/main/DucHaitenAIart_v1.1a_emaonly.ckpt
https://huggingface.co/ckpt/DucHaitenAIart_v2.0/resolve/main/DucHaitenAIart_v2.0-emaonly.safetensors
https://huggingface.co/DucHaiten/DucHaitenDarkside/resolve/main/DucHaitenDarkside_v1.0.safetensors | {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/duc_haiten/duc_haiten_ai_art/0_1_1/1.png
https://aica.pages.dev/creators/duc_haiten/duc_haiten_ai_art/0_1_1/2.png
https://aica.pages.dev/creators/duc_haiten/duc_haiten_ai_art/0_1_1/3.png
https://aica.pages.dev/creators/duc_haiten/duc_haiten_ai_art/0_1_1/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/aine_captain/liberty/1_0_0/1.png
https://aica.pages.dev/creators/aine_captain/liberty/1_0_0/2.png
https://aica.pages.dev/creators/aine_captain/liberty/1_0_0/3.png
https://aica.pages.dev/creators/aine_captain/liberty/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/ratkovm8640/dream_like_sam_kuvshino/1_0_0/1.png
https://aica.pages.dev/creators/ratkovm8640/dream_like_sam_kuvshino/1_0_0/2.png
https://aica.pages.dev/creators/ratkovm8640/dream_like_sam_kuvshino/1_0_0/3.png
https://aica.pages.dev/creators/ratkovm8640/dream_like_sam_kuvshino/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/best_jammer/hasdx/4_0_0/1.png
https://aica.pages.dev/creators/best_jammer/hasdx/4_0_0/2.png
https://aica.pages.dev/creators/best_jammer/hasdx/4_0_0/3.png
https://aica.pages.dev/creators/best_jammer/hasdx/4_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/aybeeceedee/knollingcase/1_0_0/1.png
https://aica.pages.dev/creators/aybeeceedee/knollingcase/1_0_0/2.png
https://aica.pages.dev/creators/aybeeceedee/knollingcase/1_0_0/3.png
https://aica.pages.dev/creators/aybeeceedee/knollingcase/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/waifu_diffusion/waifu_diffusion/1_5_0/1.png
https://aica.pages.dev/creators/waifu_diffusion/waifu_diffusion/1_5_0/2.png
https://aica.pages.dev/creators/waifu_diffusion/waifu_diffusion/1_5_0/3.png
https://aica.pages.dev/creators/waifu_diffusion/waifu_diffusion/1_5_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/waifu_diffusion/waifu_diffusion/1_5_2/1.png
https://aica.pages.dev/creators/waifu_diffusion/waifu_diffusion/1_5_2/2.png
https://aica.pages.dev/creators/waifu_diffusion/waifu_diffusion/1_5_2/3.png
https://aica.pages.dev/creators/waifu_diffusion/waifu_diffusion/1_5_2/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/eimiss/eimis_anime_diffusion/1_0_0/1.png
https://aica.pages.dev/creators/eimiss/eimis_anime_diffusion/1_0_0/2.png
https://aica.pages.dev/creators/eimiss/eimis_anime_diffusion/1_0_0/3.png
https://aica.pages.dev/creators/eimiss/eimis_anime_diffusion/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/agra_fl/refslave/2_0_0/1.png
https://aica.pages.dev/creators/agra_fl/refslave/2_0_0/2.png
https://aica.pages.dev/creators/agra_fl/refslave/2_0_0/3.png
https://aica.pages.dev/creators/agra_fl/refslave/2_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/nitrosocke/mo_di_diffusion/1_0_0/1.png
https://aica.pages.dev/creators/nitrosocke/mo_di_diffusion/1_0_0/2.png
https://aica.pages.dev/creators/nitrosocke/mo_di_diffusion/1_0_0/3.png
https://aica.pages.dev/creators/nitrosocke/mo_di_diffusion/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/nitrosocke/arcane_diffusion/3_0_0/1.png
https://aica.pages.dev/creators/nitrosocke/arcane_diffusion/3_0_0/2.png
https://aica.pages.dev/creators/nitrosocke/arcane_diffusion/3_0_0/3.png
https://aica.pages.dev/creators/nitrosocke/arcane_diffusion/3_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/lykon/neverending_dream/1_0_0/1.png
https://aica.pages.dev/creators/lykon/neverending_dream/1_0_0/2.png
https://aica.pages.dev/creators/lykon/neverending_dream/1_0_0/3.png
https://aica.pages.dev/creators/lykon/neverending_dream/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://huggingface.co/ckpt/monstermash_anyv3/resolve/main/monstermash4%2Banyv3.safetensors
https://huggingface.co/ckpt/monstermash_anyv3/resolve/main/monstermash6%2Banyv3.safetensors | {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/n75242/monstermash_anyv3/1_0_0/1.png
https://aica.pages.dev/creators/n75242/monstermash_anyv3/1_0_0/2.png
https://aica.pages.dev/creators/n75242/monstermash_anyv3/1_0_0/3.png
https://aica.pages.dev/creators/n75242/monstermash_anyv3/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/basunat/cinematic_diffusion/1_0_0/1.png
https://aica.pages.dev/creators/basunat/cinematic_diffusion/1_0_0/2.png
https://aica.pages.dev/creators/basunat/cinematic_diffusion/1_0_0/3.png
https://aica.pages.dev/creators/basunat/cinematic_diffusion/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/runwayml/stable_diffusion/1_5_0/1.png
https://aica.pages.dev/creators/runwayml/stable_diffusion/1_5_0/2.png
https://aica.pages.dev/creators/runwayml/stable_diffusion/1_5_0/3.png
https://aica.pages.dev/creators/runwayml/stable_diffusion/1_5_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/runwayml/stable_diffusion_inpainting/1_0_0/1.png
https://aica.pages.dev/creators/runwayml/stable_diffusion_inpainting/1_0_0/2.png
https://aica.pages.dev/creators/runwayml/stable_diffusion_inpainting/1_0_0/3.png
https://aica.pages.dev/creators/runwayml/stable_diffusion_inpainting/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/xpuc_t/deliberate/1_0_0/1.png
https://aica.pages.dev/creators/xpuc_t/deliberate/1_0_0/2.png
https://aica.pages.dev/creators/xpuc_t/deliberate/1_0_0/3.png
https://aica.pages.dev/creators/xpuc_t/deliberate/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://huggingface.co/ckpt/yohan-diffusion/resolve/main/yohan-diffusion.ckpt
https://huggingface.co/ckpt/yohan-diffusion/resolve/main/Cocoa.ckpt | {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://aica.pages.dev/creators/andite/yohan_diffusion/1_0_0/1.png
https://aica.pages.dev/creators/andite/yohan_diffusion/1_0_0/2.png
https://aica.pages.dev/creators/andite/yohan_diffusion/1_0_0/3.png
https://aica.pages.dev/creators/andite/yohan_diffusion/1_0_0/4.png
| {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |
https://huggingface.co/ckpt/anything-v4.0/resolve/main/anything-v4.0-pruned.ckpt
https://huggingface.co/ckpt/anything-v4.0/resolve/main/anything-v4.5-pruned.ckpt | {
"repo_name": "camenduru/ai-creator-archive",
"stars": "27",
"repo_language": "Jupyter Notebook",
"file_name": "images.txt",
"mime_type": "text/plain"
} |