output_description
stringlengths 15
956
| submission_id
stringlengths 10
10
| status
stringclasses 3
values | problem_id
stringlengths 6
6
| input_description
stringlengths 9
2.55k
| attempt
stringlengths 1
13.7k
| problem_description
stringlengths 7
5.24k
| samples
stringlengths 2
2.72k
|
---|---|---|---|---|---|---|---|
Print the minimum possible total cost incurred.
* * * | s304762708 | Wrong Answer | p03173 | Input is given from Standard Input in the following format:
N
a_1 a_2 \ldots a_N | """
sum a_i から
DPじゃなくて貪欲に小さい方からくっつけるのじゃだめ?
"""
from heapq import heapify, heappop, heappush, heappushpop
class PriorityQueue:
def __init__(self, heap):
"""
heap ... list
"""
self.heap = heap
heapify(self.heap)
def push(self, item):
heappush(self.heap, item)
def pop(self):
return heappop(self.heap)
def pushpop(self, item):
return heappushpop(self.heap, item)
def __call__(self):
return self.heap
def __len__(self):
return len(self.heap)
import sys
sys.setrecursionlimit(1 << 25)
readline = sys.stdin.buffer.readline
read = sys.stdin.readline # 文字列読み込む時はこっち
ra = range
enu = enumerate
def exit(*argv, **kwarg):
print(*argv, **kwarg)
sys.exit()
def mina(*argv, sub=1):
return list(map(lambda x: x - sub, argv))
# 受け渡されたすべての要素からsubだけ引く.リストを*をつけて展開しておくこと
def a_int():
return int(readline())
def ints():
return list(map(int, readline().split()))
N = a_int()
A = ints()
pq = PriorityQueue(A)
ans = 0
while len(pq) > 1:
a = pq.pop()
b = pq.pop()
s = a + b
ans += s
pq.push(s)
print(ans)
| Statement
There are N slimes lining up in a row. Initially, the i-th slime from the left
has a size of a_i.
Taro is trying to combine all the slimes into a larger slime. He will perform
the following operation repeatedly until there is only one slime:
* Choose two adjacent slimes, and combine them into a new slime. The new slime has a size of x + y, where x and y are the sizes of the slimes before combining them. Here, a cost of x + y is incurred. The positional relationship of the slimes does not change while combining slimes.
Find the minimum possible total cost incurred. | [{"input": "4\n 10 20 30 40", "output": "190\n \n\nTaro should do as follows (slimes being combined are shown in bold):\n\n * (**10** , **20** , 30, 40) \u2192 (**30** , 30, 40)\n * (**30** , **30** , 40) \u2192 (**60** , 40)\n * (**60** , **40**) \u2192 (**100**)\n\n* * *"}, {"input": "5\n 10 10 10 10 10", "output": "120\n \n\nTaro should do, for example, as follows:\n\n * (**10** , **10** , 10, 10, 10) \u2192 (**20** , 10, 10, 10)\n * (20, **10** , **10** , 10) \u2192 (20, **20** , 10)\n * (20, **20** , **10**) \u2192 (20, **30**)\n * (**20** , **30**) \u2192 (**50**)\n\n* * *"}, {"input": "3\n 1000000000 1000000000 1000000000", "output": "5000000000\n \n\nThe answer may not fit into a 32-bit integer type.\n\n* * *"}, {"input": "6\n 7 6 8 6 1 1", "output": "68\n \n\nTaro should do, for example, as follows:\n\n * (7, 6, 8, 6, **1** , **1**) \u2192 (7, 6, 8, 6, **2**)\n * (7, 6, 8, **6** , **2**) \u2192 (7, 6, 8, **8**)\n * (**7** , **6** , 8, 8) \u2192 (**13** , 8, 8)\n * (13, **8** , **8**) \u2192 (13, **16**)\n * (**13** , **16**) \u2192 (**29**)"}] |
Print the minimum possible total cost incurred.
* * * | s649686933 | Wrong Answer | p03173 | Input is given from Standard Input in the following format:
N
a_1 a_2 \ldots a_N | from bisect import bisect_left as bl
from bisect import bisect_right as br
from heapq import heappush, heappop, heapify
import math
from collections import *
from functools import reduce, cmp_to_key
import sys
input = sys.stdin.readline
from itertools import accumulate
from functools import lru_cache
M = mod = 998244353
def factors(n):
return sorted(
set(
reduce(
list.__add__,
([i, n // i] for i in range(1, int(n**0.5) + 1) if n % i == 0),
)
)
)
def inv_mod(n):
return pow(n, mod - 2, mod)
def li():
return [int(i) for i in input().rstrip("\n").split()]
def st():
return input().rstrip("\n")
def val():
return int(input().rstrip("\n"))
def li2():
return [i for i in input().rstrip("\n")]
def li3():
return [int(i) for i in input().rstrip("\n")]
n = val()
l = li()
# @lru_cache(None)
def dp(l):
# l = list(l)
ans = float("inf")
n = len(l)
if n == 1:
return 0
ind = 0
# print(l)
for i in range(1, n):
curr = l[i] + l[i - 1]
if curr < ans:
ans = curr
ind = i - 1
return ans + dp(l[:ind] + [ans] + l[ind + 2 :])
print(dp(l))
| Statement
There are N slimes lining up in a row. Initially, the i-th slime from the left
has a size of a_i.
Taro is trying to combine all the slimes into a larger slime. He will perform
the following operation repeatedly until there is only one slime:
* Choose two adjacent slimes, and combine them into a new slime. The new slime has a size of x + y, where x and y are the sizes of the slimes before combining them. Here, a cost of x + y is incurred. The positional relationship of the slimes does not change while combining slimes.
Find the minimum possible total cost incurred. | [{"input": "4\n 10 20 30 40", "output": "190\n \n\nTaro should do as follows (slimes being combined are shown in bold):\n\n * (**10** , **20** , 30, 40) \u2192 (**30** , 30, 40)\n * (**30** , **30** , 40) \u2192 (**60** , 40)\n * (**60** , **40**) \u2192 (**100**)\n\n* * *"}, {"input": "5\n 10 10 10 10 10", "output": "120\n \n\nTaro should do, for example, as follows:\n\n * (**10** , **10** , 10, 10, 10) \u2192 (**20** , 10, 10, 10)\n * (20, **10** , **10** , 10) \u2192 (20, **20** , 10)\n * (20, **20** , **10**) \u2192 (20, **30**)\n * (**20** , **30**) \u2192 (**50**)\n\n* * *"}, {"input": "3\n 1000000000 1000000000 1000000000", "output": "5000000000\n \n\nThe answer may not fit into a 32-bit integer type.\n\n* * *"}, {"input": "6\n 7 6 8 6 1 1", "output": "68\n \n\nTaro should do, for example, as follows:\n\n * (7, 6, 8, 6, **1** , **1**) \u2192 (7, 6, 8, 6, **2**)\n * (7, 6, 8, **6** , **2**) \u2192 (7, 6, 8, **8**)\n * (**7** , **6** , 8, 8) \u2192 (**13** , 8, 8)\n * (13, **8** , **8**) \u2192 (13, **16**)\n * (**13** , **16**) \u2192 (**29**)"}] |
Print the minimum possible total cost incurred.
* * * | s883490983 | Wrong Answer | p03173 | Input is given from Standard Input in the following format:
N
a_1 a_2 \ldots a_N | n = int(input())
arr = list(map(int, input().split()))
dp = [-1] * n
for i in range(n):
dp[i] = -(i + 1)
ct = n - 1
ans = 0
while ct:
mini = 999999999999999999999
ind = -1
for i in range(n - 1):
if (dp[i] != dp[i + 1]) and arr[i] + arr[i + 1] < mini:
mini = arr[i] + arr[i + 1]
ind = i
arr[ind] = mini
arr[ind + 1] = mini
j = ind
while j >= 1 and dp[j] == dp[j - 1]:
dp[j] = ct
arr[j] = mini
j -= 1
if j == 0:
dp[0] = ct
arr[0] = mini
if j == ind:
dp[j] = ct
j = ind + 1
while j < n - 1 and dp[j] == dp[j + 1]:
arr[j] = mini
dp[j] = ct
j += 1
if j == n - 1:
dp[j] = ct
arr[j] = mini
if j == ind + 1:
dp[j] = ct
# for j in range(ind+2,n):
# if dp[j]<0:
# break
# arr[j]=mini
# dp[j]=ct
# for j in range(ind-1,-1,-1):
# if dp[j]<0:
# break
# arr[j]=mini
# dp[j]=ct
ans += mini
ct -= 1
print(ans)
| Statement
There are N slimes lining up in a row. Initially, the i-th slime from the left
has a size of a_i.
Taro is trying to combine all the slimes into a larger slime. He will perform
the following operation repeatedly until there is only one slime:
* Choose two adjacent slimes, and combine them into a new slime. The new slime has a size of x + y, where x and y are the sizes of the slimes before combining them. Here, a cost of x + y is incurred. The positional relationship of the slimes does not change while combining slimes.
Find the minimum possible total cost incurred. | [{"input": "4\n 10 20 30 40", "output": "190\n \n\nTaro should do as follows (slimes being combined are shown in bold):\n\n * (**10** , **20** , 30, 40) \u2192 (**30** , 30, 40)\n * (**30** , **30** , 40) \u2192 (**60** , 40)\n * (**60** , **40**) \u2192 (**100**)\n\n* * *"}, {"input": "5\n 10 10 10 10 10", "output": "120\n \n\nTaro should do, for example, as follows:\n\n * (**10** , **10** , 10, 10, 10) \u2192 (**20** , 10, 10, 10)\n * (20, **10** , **10** , 10) \u2192 (20, **20** , 10)\n * (20, **20** , **10**) \u2192 (20, **30**)\n * (**20** , **30**) \u2192 (**50**)\n\n* * *"}, {"input": "3\n 1000000000 1000000000 1000000000", "output": "5000000000\n \n\nThe answer may not fit into a 32-bit integer type.\n\n* * *"}, {"input": "6\n 7 6 8 6 1 1", "output": "68\n \n\nTaro should do, for example, as follows:\n\n * (7, 6, 8, 6, **1** , **1**) \u2192 (7, 6, 8, 6, **2**)\n * (7, 6, 8, **6** , **2**) \u2192 (7, 6, 8, **8**)\n * (**7** , **6** , 8, 8) \u2192 (**13** , 8, 8)\n * (13, **8** , **8**) \u2192 (13, **16**)\n * (**13** , **16**) \u2192 (**29**)"}] |
Print the minimum possible total cost incurred.
* * * | s443944818 | Accepted | p03173 | Input is given from Standard Input in the following format:
N
a_1 a_2 \ldots a_N | # dp[i][j] denotes answer for subarray from i to j
n = int(input())
l = input().split()
li = [int(i) for i in l]
dp = [[0 for i in range(n)] for i in range(n)]
pref = [0 for i in range(n)]
sumi = 0
for i in range(n):
sumi += li[i]
pref[i] = sumi
for i in range(n):
dp[i][i] = 0
for gap in range(1, n):
for i in range(n - gap):
left = i
right = i + gap
mina = []
if left == 0:
curr = pref[right]
else:
curr = pref[right] - pref[left - 1]
for j in range(left, right):
mina.append(dp[left][j] + dp[j + 1][right] + curr)
dp[left][right] = min(mina)
print(dp[0][n - 1])
| Statement
There are N slimes lining up in a row. Initially, the i-th slime from the left
has a size of a_i.
Taro is trying to combine all the slimes into a larger slime. He will perform
the following operation repeatedly until there is only one slime:
* Choose two adjacent slimes, and combine them into a new slime. The new slime has a size of x + y, where x and y are the sizes of the slimes before combining them. Here, a cost of x + y is incurred. The positional relationship of the slimes does not change while combining slimes.
Find the minimum possible total cost incurred. | [{"input": "4\n 10 20 30 40", "output": "190\n \n\nTaro should do as follows (slimes being combined are shown in bold):\n\n * (**10** , **20** , 30, 40) \u2192 (**30** , 30, 40)\n * (**30** , **30** , 40) \u2192 (**60** , 40)\n * (**60** , **40**) \u2192 (**100**)\n\n* * *"}, {"input": "5\n 10 10 10 10 10", "output": "120\n \n\nTaro should do, for example, as follows:\n\n * (**10** , **10** , 10, 10, 10) \u2192 (**20** , 10, 10, 10)\n * (20, **10** , **10** , 10) \u2192 (20, **20** , 10)\n * (20, **20** , **10**) \u2192 (20, **30**)\n * (**20** , **30**) \u2192 (**50**)\n\n* * *"}, {"input": "3\n 1000000000 1000000000 1000000000", "output": "5000000000\n \n\nThe answer may not fit into a 32-bit integer type.\n\n* * *"}, {"input": "6\n 7 6 8 6 1 1", "output": "68\n \n\nTaro should do, for example, as follows:\n\n * (7, 6, 8, 6, **1** , **1**) \u2192 (7, 6, 8, 6, **2**)\n * (7, 6, 8, **6** , **2**) \u2192 (7, 6, 8, **8**)\n * (**7** , **6** , 8, 8) \u2192 (**13** , 8, 8)\n * (13, **8** , **8**) \u2192 (13, **16**)\n * (**13** , **16**) \u2192 (**29**)"}] |
Print the minimum possible total cost incurred.
* * * | s235065342 | Wrong Answer | p03173 | Input is given from Standard Input in the following format:
N
a_1 a_2 \ldots a_N | n = int(input())
a = [int(x) for x in input().strip().split()]
c = [[None for i in range(n)] for j in range(n)]
s = [[0 for i in range(n)] for j in range(n)]
for i in range(n):
c[i][i] = 0
s[i][i] = a[i]
for i in range(n):
for j in range(i + 1, n):
s[i][j] = s[i][j - 1] + a[j]
def solve(i, j):
if c[i][j] is not None:
return c[i][j]
mn = 10**10
for k in range(i, j):
mn = min(mn, solve(i, k) + solve(k + 1, j) + s[i][k] + s[k + 1][j])
c[i][j] = mn
return c[i][j]
# print(s)
print(solve(0, n - 1))
# print(c)
| Statement
There are N slimes lining up in a row. Initially, the i-th slime from the left
has a size of a_i.
Taro is trying to combine all the slimes into a larger slime. He will perform
the following operation repeatedly until there is only one slime:
* Choose two adjacent slimes, and combine them into a new slime. The new slime has a size of x + y, where x and y are the sizes of the slimes before combining them. Here, a cost of x + y is incurred. The positional relationship of the slimes does not change while combining slimes.
Find the minimum possible total cost incurred. | [{"input": "4\n 10 20 30 40", "output": "190\n \n\nTaro should do as follows (slimes being combined are shown in bold):\n\n * (**10** , **20** , 30, 40) \u2192 (**30** , 30, 40)\n * (**30** , **30** , 40) \u2192 (**60** , 40)\n * (**60** , **40**) \u2192 (**100**)\n\n* * *"}, {"input": "5\n 10 10 10 10 10", "output": "120\n \n\nTaro should do, for example, as follows:\n\n * (**10** , **10** , 10, 10, 10) \u2192 (**20** , 10, 10, 10)\n * (20, **10** , **10** , 10) \u2192 (20, **20** , 10)\n * (20, **20** , **10**) \u2192 (20, **30**)\n * (**20** , **30**) \u2192 (**50**)\n\n* * *"}, {"input": "3\n 1000000000 1000000000 1000000000", "output": "5000000000\n \n\nThe answer may not fit into a 32-bit integer type.\n\n* * *"}, {"input": "6\n 7 6 8 6 1 1", "output": "68\n \n\nTaro should do, for example, as follows:\n\n * (7, 6, 8, 6, **1** , **1**) \u2192 (7, 6, 8, 6, **2**)\n * (7, 6, 8, **6** , **2**) \u2192 (7, 6, 8, **8**)\n * (**7** , **6** , 8, 8) \u2192 (**13** , 8, 8)\n * (13, **8** , **8**) \u2192 (13, **16**)\n * (**13** , **16**) \u2192 (**29**)"}] |
Print the number of cells whose density value is 0 in first line.
Print the maximum value of density in the second line. | s363613860 | Accepted | p00026 | x1,y1,s1
x2,y2,s2
:
:
(xi, yi) represents the position of the i-th drop and si denotes its size. The
number of drops is less than or equal to 50. | if __name__ == "__main__":
DI = [[0 for _ in range(10)] for _ in range(10)]
while True:
try:
x, y, s = map(int, input().split(","))
if s == 0:
break
if s == 1:
# 自身
DI[x][y] += 1
# up
if y >= 1:
DI[x][y - 1] += 1
# down
if y <= 8:
DI[x][y + 1] += 1
# left
if x >= 1:
DI[x - 1][y] += 1
# right
if x <= 8:
DI[x + 1][y] += 1
elif s == 2:
# 自身
DI[x][y] += 1
# up
if y >= 1:
DI[x][y - 1] += 1
# up left
if x >= 1 and y >= 1:
DI[x - 1][y - 1] += 1
# up right
if x <= 8 and y >= 1:
DI[x + 1][y - 1] += 1
# down
if y <= 8:
DI[x][y + 1] += 1
# down left
if x >= 1 and y <= 8:
DI[x - 1][y + 1] += 1
# down right
if x <= 8 and y <= 8:
DI[x + 1][y + 1] += 1
# left
if x >= 1:
DI[x - 1][y] += 1
# right
if x <= 8:
DI[x + 1][y] += 1
else:
# 自身
DI[x][y] += 1
# up
if y >= 1:
DI[x][y - 1] += 1
# up left
if x >= 1 and y >= 1:
DI[x - 1][y - 1] += 1
# up right
if x <= 8 and y >= 1:
DI[x + 1][y - 1] += 1
# up +1
if y >= 2:
DI[x][y - 2] += 1
# down
if y <= 8:
DI[x][y + 1] += 1
# down left
if x >= 1 and y <= 8:
DI[x - 1][y + 1] += 1
# down right
if x <= 8 and y <= 8:
DI[x + 1][y + 1] += 1
# down + 1
if y <= 7:
DI[x][y + 2] += 1
# left
if x >= 1:
DI[x - 1][y] += 1
# left + 1
if x >= 2:
DI[x - 2][y] += 1
# right
if x <= 8:
DI[x + 1][y] += 1
# right + 1
if x <= 7:
DI[x + 2][y] += 1
except EOFError:
break
# for j in DI:
# print(j)
cnt = 0
maxcnt = 0
for k in DI:
cnt += k.count(0)
if maxcnt < max(k):
maxcnt = max(k)
print(cnt)
print(maxcnt)
| Dropping Ink
As shown in the following figure, there is a paper consisting of a grid
structure where each cell is indicated by (x, y) coordinate system.
We are going to put drops of ink on the paper. A drop comes in three different
sizes: Large, Medium, and Small. From the point of fall, the ink sinks into
surrounding cells as shown in the figure depending on its size. In the figure,
a star denotes the point of fall and a circle denotes the surrounding cells.
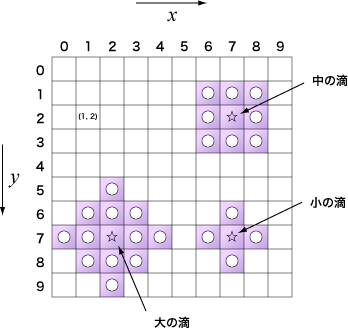
Originally, the paper is white that means for each cell the value of density
is 0. The value of density is increased by 1 when the ink sinks into the
corresponding cells. For example, if we put a drop of Small ink at (1, 2) and
a drop of Medium ink at (3, 2), the ink will sink as shown in the following
figure (left side):
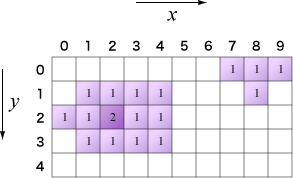
In the figure, density values of empty cells are 0. The ink sinking into out
of the paper should be ignored as shown in the figure (top side). We can put
several drops of ink at the same point.
Your task is to write a program which reads a sequence of points of fall (x,
y) with its size (Small = 1, Medium = 2, Large = 3), and prints the number of
cells whose density value is 0. The program must also print the maximum value
of density.
You may assume that the paper always consists of 10 × 10, and 0 ≤ x < 10, 0 ≤
y < 10\. | [{"input": ",5,3\n 3,6,1\n 3,4,2\n 4,5,2\n 3,6,3\n 2,4,1", "output": "5"}] |
Print the number of cells whose density value is 0 in first line.
Print the maximum value of density in the second line. | s246112465 | Accepted | p00026 | x1,y1,s1
x2,y2,s2
:
:
(xi, yi) represents the position of the i-th drop and si denotes its size. The
number of drops is less than or equal to 50. | class Paper:
def __init__(self, x, y):
self.A = [[0 for i in range(x)] for j in range(y)]
self.size = (x, y)
def drop(self, x, y, s):
if s == 1:
if y + 1 <= self.size[1] - 1:
self.A[y + 1][x] += 1
for i in range(max(0, x - 1), 1 + min(self.size[0] - 1, x + 1)):
self.A[y][i] += 1
if y - 1 >= 0:
self.A[y - 1][x] += 1
if s == 2:
if y + 1 <= self.size[1] - 1:
for i in range(max(0, x - 1), 1 + min(self.size[0] - 1, x + 1)):
self.A[y + 1][i] += 1
for i in range(max(0, x - 1), 1 + min(self.size[0] - 1, x + 1)):
self.A[y][i] += 1
if y - 1 >= 0:
for i in range(max(0, x - 1), 1 + min(self.size[0] - 1, x + 1)):
self.A[y - 1][i] += 1
if s == 3:
if y + 2 <= self.size[1] - 1:
self.A[y + 2][x] += 1
if y + 1 <= self.size[1] - 1:
for i in range(max(0, x - 1), 1 + min(self.size[0] - 1, x + 1)):
self.A[y + 1][i] += 1
for i in range(max(0, x - 2), 1 + min(self.size[0] - 1, x + 2)):
self.A[y][i] += 1
if y - 1 >= 0:
for i in range(max(0, x - 1), 1 + min(self.size[0] - 1, x + 1)):
self.A[y - 1][i] += 1
if y - 2 >= 0:
self.A[y - 2][x] += 1
if __name__ == "__main__":
import sys
p = Paper(10, 10)
L = sys.stdin.readlines()
for l in L:
x, y, s = map(int, l.split(","))
p.drop(x, y, s)
# for i in range(10):
# print(p.A[i])
# print()
temp = []
for y in p.A:
temp.extend(y)
A1 = temp.count(0)
A2 = max(temp)
print(A1)
print(A2)
| Dropping Ink
As shown in the following figure, there is a paper consisting of a grid
structure where each cell is indicated by (x, y) coordinate system.
We are going to put drops of ink on the paper. A drop comes in three different
sizes: Large, Medium, and Small. From the point of fall, the ink sinks into
surrounding cells as shown in the figure depending on its size. In the figure,
a star denotes the point of fall and a circle denotes the surrounding cells.
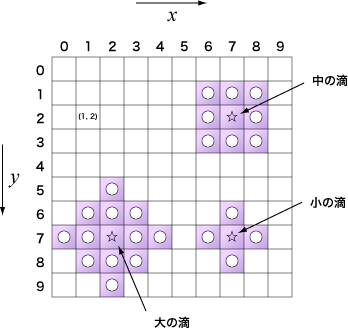
Originally, the paper is white that means for each cell the value of density
is 0. The value of density is increased by 1 when the ink sinks into the
corresponding cells. For example, if we put a drop of Small ink at (1, 2) and
a drop of Medium ink at (3, 2), the ink will sink as shown in the following
figure (left side):
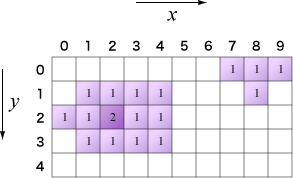
In the figure, density values of empty cells are 0. The ink sinking into out
of the paper should be ignored as shown in the figure (top side). We can put
several drops of ink at the same point.
Your task is to write a program which reads a sequence of points of fall (x,
y) with its size (Small = 1, Medium = 2, Large = 3), and prints the number of
cells whose density value is 0. The program must also print the maximum value
of density.
You may assume that the paper always consists of 10 × 10, and 0 ≤ x < 10, 0 ≤
y < 10\. | [{"input": ",5,3\n 3,6,1\n 3,4,2\n 4,5,2\n 3,6,3\n 2,4,1", "output": "5"}] |
Print the maximum possible number of happy people after at most K operations.
* * * | s239228944 | Accepted | p02918 | Input is given from Standard Input in the following format:
N K
S | n, s = open(0)
n, k = map(int, n.split())
print(n - max(sum(i != j for i, j in zip(s, s[1:])) - 2 * k, 1))
| Statement
There are N people standing in a queue from west to east.
Given is a string S of length N representing the directions of the people. The
i-th person from the west is facing west if the i-th character of S is `L`,
and east if that character of S is `R`.
A person is happy if the person in front of him/her is facing the same
direction. If no person is standing in front of a person, however, he/she is
not happy.
You can perform the following operation any number of times between 0 and K
(inclusive):
Operation: Choose integers l and r such that 1 \leq l \leq r \leq N, and
rotate by 180 degrees the part of the queue: the l-th, (l+1)-th, ..., r-th
persons. That is, for each i = 0, 1, ..., r-l, the (l + i)-th person from the
west will stand the (r - i)-th from the west after the operation, facing east
if he/she is facing west now, and vice versa.
What is the maximum possible number of happy people you can have? | [{"input": "6 1\n LRLRRL", "output": "3\n \n\nIf we choose (l, r) = (2, 5), we have `LLLRLL`, where the 2-nd, 3-rd, and 6-th\npersons from the west are happy.\n\n* * *"}, {"input": "13 3\n LRRLRLRRLRLLR", "output": "9\n \n\n* * *"}, {"input": "10 1\n LLLLLRRRRR", "output": "9\n \n\n* * *"}, {"input": "9 2\n RRRLRLRLL", "output": "7"}] |
Print the maximum possible number of happy people after at most K operations.
* * * | s017883745 | Runtime Error | p02918 | Input is given from Standard Input in the following format:
N K
S | N = int(input())
B = list(map(int, input().split()))
cnt = B[0] + B[N - 2]
for i in range(1, N - 1):
cnt += min(B[i - 1], B[i])
print(cnt)
| Statement
There are N people standing in a queue from west to east.
Given is a string S of length N representing the directions of the people. The
i-th person from the west is facing west if the i-th character of S is `L`,
and east if that character of S is `R`.
A person is happy if the person in front of him/her is facing the same
direction. If no person is standing in front of a person, however, he/she is
not happy.
You can perform the following operation any number of times between 0 and K
(inclusive):
Operation: Choose integers l and r such that 1 \leq l \leq r \leq N, and
rotate by 180 degrees the part of the queue: the l-th, (l+1)-th, ..., r-th
persons. That is, for each i = 0, 1, ..., r-l, the (l + i)-th person from the
west will stand the (r - i)-th from the west after the operation, facing east
if he/she is facing west now, and vice versa.
What is the maximum possible number of happy people you can have? | [{"input": "6 1\n LRLRRL", "output": "3\n \n\nIf we choose (l, r) = (2, 5), we have `LLLRLL`, where the 2-nd, 3-rd, and 6-th\npersons from the west are happy.\n\n* * *"}, {"input": "13 3\n LRRLRLRRLRLLR", "output": "9\n \n\n* * *"}, {"input": "10 1\n LLLLLRRRRR", "output": "9\n \n\n* * *"}, {"input": "9 2\n RRRLRLRLL", "output": "7"}] |
Print the maximum possible number of happy people after at most K operations.
* * * | s682488995 | Accepted | p02918 | Input is given from Standard Input in the following format:
N K
S | ## 幸福度が変わるかどうかは操作Kによって変更されたl, r番目の人間だけなので、
## 左右l,r番目の人間が幸福であるかどうかを見てゆく
## for(n)回のループでまず「既に 1 + x, 任意の r が幸福であるかどうか」を確認して、
## 幸福度2, 1の順でそのl,rをK回覚える
n, k = map(int, input().split())
humans = list(input())
k_count = 0
last_r = n ## 最後に操作したr(番目)
dev_null = 0
## ポイントを計算
happy_point = 0
for x in range(n - 1):
if humans[x] == humans[x + 1]:
happy_point += 1
print(min(n - 1, happy_point + 2 * k))
| Statement
There are N people standing in a queue from west to east.
Given is a string S of length N representing the directions of the people. The
i-th person from the west is facing west if the i-th character of S is `L`,
and east if that character of S is `R`.
A person is happy if the person in front of him/her is facing the same
direction. If no person is standing in front of a person, however, he/she is
not happy.
You can perform the following operation any number of times between 0 and K
(inclusive):
Operation: Choose integers l and r such that 1 \leq l \leq r \leq N, and
rotate by 180 degrees the part of the queue: the l-th, (l+1)-th, ..., r-th
persons. That is, for each i = 0, 1, ..., r-l, the (l + i)-th person from the
west will stand the (r - i)-th from the west after the operation, facing east
if he/she is facing west now, and vice versa.
What is the maximum possible number of happy people you can have? | [{"input": "6 1\n LRLRRL", "output": "3\n \n\nIf we choose (l, r) = (2, 5), we have `LLLRLL`, where the 2-nd, 3-rd, and 6-th\npersons from the west are happy.\n\n* * *"}, {"input": "13 3\n LRRLRLRRLRLLR", "output": "9\n \n\n* * *"}, {"input": "10 1\n LLLLLRRRRR", "output": "9\n \n\n* * *"}, {"input": "9 2\n RRRLRLRLL", "output": "7"}] |
Print the maximum possible number of happy people after at most K operations.
* * * | s250037718 | Wrong Answer | p02918 | Input is given from Standard Input in the following format:
N K
S | n, k = map(int, input().split())
s = input()
comp = []
bef = s[0]
temp = 0
# if s[0] != "L":
# comp.append(0)
#
# if s[-1] != "L":
# comp.append(0)
for c in s:
if c == bef:
temp += 1
else:
comp.append(temp)
temp = 1
bef = c
comp.append(temp)
# print(comp)
#
# n = len(comp)
#
# leng = k*2 +1
#
# if leng >= n:
# print("over")
# print(sum(comp))
# else:
# step = (n-leng)//2
# now = 0
# ans = 0
# for i in range(leng):
# now += comp[i]
# ans = now
# print(ans)
# for i in range(step):
# now -= (comp[i*2]+comp[i*2+1])
# now += (comp[i*2+2]+comp[i*2+3])
# if now > ans:
# ans = now
# print(ans)
#
# print(ans)
init = 0
n = len(comp)
for c in comp:
init += c - 1
inside = 0
if n % 2 == 0:
inside = n // 2 - 1
else:
inside = n // 2
outside = 0
if n % 2 == 0:
outside = n // 2 - 1
else:
outside = 0
tot = inside + outside
if k >= tot:
print(sum(comp) - 1)
elif k > inside:
print(init + 2 * inside + outside)
else:
print(init + 2 * k)
| Statement
There are N people standing in a queue from west to east.
Given is a string S of length N representing the directions of the people. The
i-th person from the west is facing west if the i-th character of S is `L`,
and east if that character of S is `R`.
A person is happy if the person in front of him/her is facing the same
direction. If no person is standing in front of a person, however, he/she is
not happy.
You can perform the following operation any number of times between 0 and K
(inclusive):
Operation: Choose integers l and r such that 1 \leq l \leq r \leq N, and
rotate by 180 degrees the part of the queue: the l-th, (l+1)-th, ..., r-th
persons. That is, for each i = 0, 1, ..., r-l, the (l + i)-th person from the
west will stand the (r - i)-th from the west after the operation, facing east
if he/she is facing west now, and vice versa.
What is the maximum possible number of happy people you can have? | [{"input": "6 1\n LRLRRL", "output": "3\n \n\nIf we choose (l, r) = (2, 5), we have `LLLRLL`, where the 2-nd, 3-rd, and 6-th\npersons from the west are happy.\n\n* * *"}, {"input": "13 3\n LRRLRLRRLRLLR", "output": "9\n \n\n* * *"}, {"input": "10 1\n LLLLLRRRRR", "output": "9\n \n\n* * *"}, {"input": "9 2\n RRRLRLRLL", "output": "7"}] |
Print the maximum possible number of happy people after at most K operations.
* * * | s238385894 | Wrong Answer | p02918 | Input is given from Standard Input in the following format:
N K
S | N, K = map(int, input().split())
S = str(input())
def happy_now(s):
number1 = 0
number2 = 0
number3 = 0
if s[0] == "L":
number1 += 1
number2 += 1
if s[len(s) - 1] == "R":
number1 += 1
number2 += 1
for i in range(len(s) - 1):
if s[i] == "R" and s[i + 1] == "L":
number1 += 2
number3 += 1
return [len(s) - number1, number2, number3]
happy_people = happy_now(S)[0]
unhappy_edge_place = happy_now(S)[1]
unhappy_not_edge_place = happy_now(S)[2]
# print(happy_people,unhappy_edge_place,unhappy_not_edge_place)
ans = 0
if unhappy_not_edge_place - 1 >= K:
ans = happy_people + 2 * K
else:
ans = happy_people + 2 * max(unhappy_not_edge_place - 1, 0)
if K - (unhappy_not_edge_place - 1) >= 3:
ans += 1 + unhappy_edge_place
elif K - (unhappy_not_edge_place - 1) == 2:
ans += min(1 + unhappy_edge_place, 2)
elif K - (unhappy_not_edge_place) == 1:
ans += min(1 + unhappy_edge_place, 1)
print(min(ans, len(S) - 1))
| Statement
There are N people standing in a queue from west to east.
Given is a string S of length N representing the directions of the people. The
i-th person from the west is facing west if the i-th character of S is `L`,
and east if that character of S is `R`.
A person is happy if the person in front of him/her is facing the same
direction. If no person is standing in front of a person, however, he/she is
not happy.
You can perform the following operation any number of times between 0 and K
(inclusive):
Operation: Choose integers l and r such that 1 \leq l \leq r \leq N, and
rotate by 180 degrees the part of the queue: the l-th, (l+1)-th, ..., r-th
persons. That is, for each i = 0, 1, ..., r-l, the (l + i)-th person from the
west will stand the (r - i)-th from the west after the operation, facing east
if he/she is facing west now, and vice versa.
What is the maximum possible number of happy people you can have? | [{"input": "6 1\n LRLRRL", "output": "3\n \n\nIf we choose (l, r) = (2, 5), we have `LLLRLL`, where the 2-nd, 3-rd, and 6-th\npersons from the west are happy.\n\n* * *"}, {"input": "13 3\n LRRLRLRRLRLLR", "output": "9\n \n\n* * *"}, {"input": "10 1\n LLLLLRRRRR", "output": "9\n \n\n* * *"}, {"input": "9 2\n RRRLRLRLL", "output": "7"}] |
Print the maximum possible number of happy people after at most K operations.
* * * | s181944059 | Wrong Answer | p02918 | Input is given from Standard Input in the following format:
N K
S | N, K = map(int, input().split())
S = input()
left_ac = [0]
right_ac = [0]
prev = ""
for i in range(N):
s = S[i]
if s == "L":
if i - 1 >= 0:
if S[i - 1] == "L":
left_ac.append(left_ac[-1] + 1)
else:
left_ac.append(left_ac[-1])
else:
left_ac.append(left_ac[-1])
right_ac.append(right_ac[-1])
else:
if i + 1 < N:
if S[i + 1] == "R":
right_ac.append(right_ac[-1] + 1)
else:
right_ac.append(right_ac[-1])
else:
right_ac.append(right_ac[-1])
left_ac.append(left_ac[-1])
left_ac.pop(0)
right_ac.pop(0)
# print(left_ac)
# print(right_ac)
left = 0
right = 0
ans = right_ac[-1] + left_ac[-1]
remain = K
while left < N:
s = S[left]
while remain > 0 and right < N:
if S[right] != s:
if (right + 1 < N and S[right + 1] == s) or (
right == N - 1 and S[N - 1] != s
):
remain -= 1
right += 1
if s == "L":
# print(s, right - left, left, right)
tmp = right - left
if right == N:
tmp -= 1
if right < N - 1:
tmp += right_ac[-1] - right_ac[right + 1]
tmp += left_ac[-1] - left_ac[right + 1]
if left > 0:
tmp += left_ac[left - 1] - left_ac[0]
tmp += right_ac[left - 1] - right_ac[0]
ans = max(ans, tmp)
else:
# print(s, right - left, left, right)
tmp = right - left
if right == N:
tmp -= 1
if right < N - 1:
tmp += right_ac[-1] - right_ac[right + 1]
tmp += left_ac[-1] - left_ac[right + 1]
if left > 0:
tmp += left_ac[left - 1] - left_ac[0]
tmp += right_ac[left - 1] - right_ac[0]
ans = max(ans, tmp)
if right == left:
right += 1
if left + 1 < N and S[left + 1] != s:
remain += 1
left += 1
print(ans)
| Statement
There are N people standing in a queue from west to east.
Given is a string S of length N representing the directions of the people. The
i-th person from the west is facing west if the i-th character of S is `L`,
and east if that character of S is `R`.
A person is happy if the person in front of him/her is facing the same
direction. If no person is standing in front of a person, however, he/she is
not happy.
You can perform the following operation any number of times between 0 and K
(inclusive):
Operation: Choose integers l and r such that 1 \leq l \leq r \leq N, and
rotate by 180 degrees the part of the queue: the l-th, (l+1)-th, ..., r-th
persons. That is, for each i = 0, 1, ..., r-l, the (l + i)-th person from the
west will stand the (r - i)-th from the west after the operation, facing east
if he/she is facing west now, and vice versa.
What is the maximum possible number of happy people you can have? | [{"input": "6 1\n LRLRRL", "output": "3\n \n\nIf we choose (l, r) = (2, 5), we have `LLLRLL`, where the 2-nd, 3-rd, and 6-th\npersons from the west are happy.\n\n* * *"}, {"input": "13 3\n LRRLRLRRLRLLR", "output": "9\n \n\n* * *"}, {"input": "10 1\n LLLLLRRRRR", "output": "9\n \n\n* * *"}, {"input": "9 2\n RRRLRLRLL", "output": "7"}] |
Print the maximum possible number of happy people after at most K operations.
* * * | s764840140 | Runtime Error | p02918 | Input is given from Standard Input in the following format:
N K
S | N, K = map(int, input().split())
S = input()
if N == 1:
print(0)
exit()
# 左端をLにする場合
now = ""
num_substr = 0
ex = ""
stack = []
for i in range(N):
if (ex == "L" or ex == "") and S[i] == "R":
start = i
if ex == "R" and S[i] == "L":
end = i - 1
stack.append((start, end))
num_substr += 1
ex = S[i]
# 左からひっくり返す
if num_substr == 0:
print(N - 1)
exit()
tmp = min(K, num_substr, N)
num = stack[tmp - 1][1]
ss = "L" * (num + 1) + S[num + 1 :]
ll = num
for i in range(num + 1, N):
if i == 0:
if ss[i + 1] == "R" and ss[i] == "R":
ll += 1
elif i == N - 1:
if ss[i - 1] == "L" and ss[i] == "L":
ll += 1
else:
if (ss[i - 1] == "L" and ss[i] == "L") or (ss[i + 1] == "R" and ss[i] == "R"):
ll += 1
# 右からひっくり返す
if num_substr == 0:
print(N - 1)
exit()
tmp = min(K, num_substr, N)
num = stack[len(stack) - tmp][0]
ss = S[: (N - 1 - num)] + "L" * (N - 1 - num)
lr = N - 1 - num
for i in range(num):
if i == 0:
if ss[i + 1] == "R" and ss[i] == "R":
lr += 1
elif i == N - 1:
if ss[i - 1] == "L" and ss[i] == "L":
lr += 1
else:
if (ss[i - 1] == "L" and ss[i] == "L") or (ss[i + 1] == "R" and ss[i] == "R"):
lr += 1
######################################################################
# 左端をRにする場合
now = ""
num_substr = 0
ex = ""
stack = []
for i in range(N):
if (ex == "R" or ex == "") and S[i] == "L":
start = i
if ex == "L" and S[i] == "R":
end = i - 1
stack.append((start, end))
num_substr += 1
ex = S[i]
# 左からひっくり返す
if num_substr == 0:
print(N - 1)
exit()
tmp = min(K, num_substr, N)
num = stack[tmp - 1][1]
ss = "R" * (num + 1) + S[num + 1 :]
rl = num
# print(ll, tmp)
for i in range(num + 1, N):
if i == 0:
if ss[i + 1] == "R" and ss[i] == "R":
rl += 1
elif i == N - 1:
if ss[i - 1] == "L" and ss[i] == "L":
rl += 1
else:
if (ss[i - 1] == "L" and ss[i] == "L") or (ss[i + 1] == "R" and ss[i] == "R"):
rl += 1
# 右からひっくり返す
if num_substr == 0:
print(N - 1)
exit()
tmp = min(K, num_substr, N)
num = stack[len(stack) - tmp][0]
ss = S[: (N - 1 - num)] + "R" * (N - 1 - num)
rr = N - 1 - num
# print(ll, tmp)
for i in range(num):
if i == 0:
if ss[i + 1] == "R" and ss[i] == "R":
rr += 1
elif i == N - 1:
if ss[i - 1] == "L" and ss[i] == "L":
rr += 1
else:
if (ss[i - 1] == "L" and ss[i] == "L") or (ss[i + 1] == "R" and ss[i] == "R"):
rr += 1
print(max([ll, lr, rl, rr]))
| Statement
There are N people standing in a queue from west to east.
Given is a string S of length N representing the directions of the people. The
i-th person from the west is facing west if the i-th character of S is `L`,
and east if that character of S is `R`.
A person is happy if the person in front of him/her is facing the same
direction. If no person is standing in front of a person, however, he/she is
not happy.
You can perform the following operation any number of times between 0 and K
(inclusive):
Operation: Choose integers l and r such that 1 \leq l \leq r \leq N, and
rotate by 180 degrees the part of the queue: the l-th, (l+1)-th, ..., r-th
persons. That is, for each i = 0, 1, ..., r-l, the (l + i)-th person from the
west will stand the (r - i)-th from the west after the operation, facing east
if he/she is facing west now, and vice versa.
What is the maximum possible number of happy people you can have? | [{"input": "6 1\n LRLRRL", "output": "3\n \n\nIf we choose (l, r) = (2, 5), we have `LLLRLL`, where the 2-nd, 3-rd, and 6-th\npersons from the west are happy.\n\n* * *"}, {"input": "13 3\n LRRLRLRRLRLLR", "output": "9\n \n\n* * *"}, {"input": "10 1\n LLLLLRRRRR", "output": "9\n \n\n* * *"}, {"input": "9 2\n RRRLRLRLL", "output": "7"}] |
Print the maximum possible number of happy people after at most K operations.
* * * | s153039751 | Runtime Error | p02918 | Input is given from Standard Input in the following format:
N K
S | def d(s, type):
first_index = None
last_index = None
for i in range(0, n - 1):
if first_index == None:
if s[i] != type:
first_index = i
if s[len(s) - 1] != type:
last_index = len(s) - 1
break
if s[i] != type and s[i + 1] == type:
last_index = i
if last_index == None and first_index != None:
last_index = len(s) - 1
return first_index, last_index
def find(n, k, s):
f1, l1 = d(s, "R")
f2, l2 = d(s, "L")
valid1 = f1 is not None and l1 is not None
valid2 = f2 is not None and l2 is not None
if not valid2:
return f1, l1
if not valid1:
return f2, l2
if (l2 - f2) < (l1 - f1):
return f2, l2
else:
return f1, l1
def o(s):
if s == "L":
return "R"
else:
return "L"
def turn(list, first_index, last_index):
for i in range(last_index - first_index):
l = first_index + i
r = last_index - i
if l > r:
break
org_l = list[l]
org_r = list[r]
list[l] = o(org_r)
list[r] = o(org_l)
def calc(list):
r = 0
for i in range(len(list)):
if list[i] == "R" and i < len(list) - 1:
if list[i] == list[i + 1]:
r += 1
if list[i] == "L" and i >= 1:
if list[i] == list[i - 1]:
r += 1
return r
n, k = list(map(int, input().split()))
s = list(input())
for _ in range(k):
first_index, last_index = find(n, k, s)
turn(s, first_index, last_index)
print(calc(s))
| Statement
There are N people standing in a queue from west to east.
Given is a string S of length N representing the directions of the people. The
i-th person from the west is facing west if the i-th character of S is `L`,
and east if that character of S is `R`.
A person is happy if the person in front of him/her is facing the same
direction. If no person is standing in front of a person, however, he/she is
not happy.
You can perform the following operation any number of times between 0 and K
(inclusive):
Operation: Choose integers l and r such that 1 \leq l \leq r \leq N, and
rotate by 180 degrees the part of the queue: the l-th, (l+1)-th, ..., r-th
persons. That is, for each i = 0, 1, ..., r-l, the (l + i)-th person from the
west will stand the (r - i)-th from the west after the operation, facing east
if he/she is facing west now, and vice versa.
What is the maximum possible number of happy people you can have? | [{"input": "6 1\n LRLRRL", "output": "3\n \n\nIf we choose (l, r) = (2, 5), we have `LLLRLL`, where the 2-nd, 3-rd, and 6-th\npersons from the west are happy.\n\n* * *"}, {"input": "13 3\n LRRLRLRRLRLLR", "output": "9\n \n\n* * *"}, {"input": "10 1\n LLLLLRRRRR", "output": "9\n \n\n* * *"}, {"input": "9 2\n RRRLRLRLL", "output": "7"}] |
Print the maximum possible number of happy people after at most K operations.
* * * | s612563440 | Runtime Error | p02918 | Input is given from Standard Input in the following format:
N K
S | N, K = map(int, input().split())
S = input()
largest = 0
largest_left_index = -1
sum_list = list()
count = 0
for i in range(N):
if S[i] == "L":
if count <= 0:
count = count - 1
else:
sum_list.append(count)
count = -1
elif S[i] == "R":
if count >= 0:
count = count + 1
else:
sum_list.append(-count)
count = 1
else:
sum_list.append(count) if count > 0 else sum_list.append(-count)
length = len(sum_list)
current_max = 0
current_max_index = 0
for j in range(length):
current_sum = sum_list[j]
if j < length - 1:
current_sum = current_sum + sum_list[j + 1]
if j < length - 2:
current_sum = current_sum + sum_list[j + 2]
if current_sum > current_max:
current_max = current_sum
current_max_index = j
if current_max_index < length - 2:
sum_list.pop(current_max_index + 2)
if current_max_index < length - 1:
sum_list.pop(current_max_index + 1)
sum_list.pop(current_max_index)
sum_list.insert(current_max_index, current_max)
for i in range(K - 1):
left = sum_list[current_max_index]
if current_max_index > 1:
left = left + sum_list[current_max_index - 1]
if current_max_index > 2:
left = left + sum_list[current_max_index - 2]
right = sum_list[current_max_index]
if current_max_index < len(sum_list) - 1:
right = right + sum_list[current_max_index + 1]
if current_max_index < len(sum_list) - 2:
right = right + sum_list[current_max_index + 2]
if left >= right:
sum_list.pop(current_max_index)
sum_list.insert(current_max_index, left)
if current_max_index > 1:
sum_list.pop(current_max_index - 1)
if current_max_index > 2:
sum_list.pop(current_max_index - 2)
else:
length = len(sum_list)
if current_max_index < length - 2:
sum_list.pop(current_max_index + 2)
if current_max_index < length - 1:
sum_list.pop(current_max_index + 1)
sum_list.pop(current_max_index)
sum_list.insert(current_max_index, right)
res = 0
for i in range(len(sum_list)):
res = res + sum_list[i] - 1
print(res)
| Statement
There are N people standing in a queue from west to east.
Given is a string S of length N representing the directions of the people. The
i-th person from the west is facing west if the i-th character of S is `L`,
and east if that character of S is `R`.
A person is happy if the person in front of him/her is facing the same
direction. If no person is standing in front of a person, however, he/she is
not happy.
You can perform the following operation any number of times between 0 and K
(inclusive):
Operation: Choose integers l and r such that 1 \leq l \leq r \leq N, and
rotate by 180 degrees the part of the queue: the l-th, (l+1)-th, ..., r-th
persons. That is, for each i = 0, 1, ..., r-l, the (l + i)-th person from the
west will stand the (r - i)-th from the west after the operation, facing east
if he/she is facing west now, and vice versa.
What is the maximum possible number of happy people you can have? | [{"input": "6 1\n LRLRRL", "output": "3\n \n\nIf we choose (l, r) = (2, 5), we have `LLLRLL`, where the 2-nd, 3-rd, and 6-th\npersons from the west are happy.\n\n* * *"}, {"input": "13 3\n LRRLRLRRLRLLR", "output": "9\n \n\n* * *"}, {"input": "10 1\n LLLLLRRRRR", "output": "9\n \n\n* * *"}, {"input": "9 2\n RRRLRLRLL", "output": "7"}] |
Print the maximum possible number of happy people after at most K operations.
* * * | s211701525 | Wrong Answer | p02918 | Input is given from Standard Input in the following format:
N K
S | print("a")
| Statement
There are N people standing in a queue from west to east.
Given is a string S of length N representing the directions of the people. The
i-th person from the west is facing west if the i-th character of S is `L`,
and east if that character of S is `R`.
A person is happy if the person in front of him/her is facing the same
direction. If no person is standing in front of a person, however, he/she is
not happy.
You can perform the following operation any number of times between 0 and K
(inclusive):
Operation: Choose integers l and r such that 1 \leq l \leq r \leq N, and
rotate by 180 degrees the part of the queue: the l-th, (l+1)-th, ..., r-th
persons. That is, for each i = 0, 1, ..., r-l, the (l + i)-th person from the
west will stand the (r - i)-th from the west after the operation, facing east
if he/she is facing west now, and vice versa.
What is the maximum possible number of happy people you can have? | [{"input": "6 1\n LRLRRL", "output": "3\n \n\nIf we choose (l, r) = (2, 5), we have `LLLRLL`, where the 2-nd, 3-rd, and 6-th\npersons from the west are happy.\n\n* * *"}, {"input": "13 3\n LRRLRLRRLRLLR", "output": "9\n \n\n* * *"}, {"input": "10 1\n LLLLLRRRRR", "output": "9\n \n\n* * *"}, {"input": "9 2\n RRRLRLRLL", "output": "7"}] |
Print the maximum possible number of happy people after at most K operations.
* * * | s757240358 | Accepted | p02918 | Input is given from Standard Input in the following format:
N K
S | import re
from collections import deque
N, K = list(map(int, input().split()))
S = input()
token = re.findall(r"(L+|R+)", S)
def flip(s):
if s[0] == "R":
return "L" * len(s)
else:
return "R" * len(s)
even = False
flipped_token = deque([])
flip_count = 0
for s in token:
if flip_count >= K:
flipped_token.append(s)
else:
if even:
s = flip(s)
flip_count += 1
flipped_token.append(s)
even = False
else:
flipped_token.append(s)
even = True
flipped_S = "".join(flipped_token)
token = re.findall(r"(L+|R+)", flipped_S)
happy_count = 0
for s in token:
l = len(s)
happy_count += l - 1
print(happy_count)
| Statement
There are N people standing in a queue from west to east.
Given is a string S of length N representing the directions of the people. The
i-th person from the west is facing west if the i-th character of S is `L`,
and east if that character of S is `R`.
A person is happy if the person in front of him/her is facing the same
direction. If no person is standing in front of a person, however, he/she is
not happy.
You can perform the following operation any number of times between 0 and K
(inclusive):
Operation: Choose integers l and r such that 1 \leq l \leq r \leq N, and
rotate by 180 degrees the part of the queue: the l-th, (l+1)-th, ..., r-th
persons. That is, for each i = 0, 1, ..., r-l, the (l + i)-th person from the
west will stand the (r - i)-th from the west after the operation, facing east
if he/she is facing west now, and vice versa.
What is the maximum possible number of happy people you can have? | [{"input": "6 1\n LRLRRL", "output": "3\n \n\nIf we choose (l, r) = (2, 5), we have `LLLRLL`, where the 2-nd, 3-rd, and 6-th\npersons from the west are happy.\n\n* * *"}, {"input": "13 3\n LRRLRLRRLRLLR", "output": "9\n \n\n* * *"}, {"input": "10 1\n LLLLLRRRRR", "output": "9\n \n\n* * *"}, {"input": "9 2\n RRRLRLRLL", "output": "7"}] |
Print the maximum possible number of happy people after at most K operations.
* * * | s731352176 | Runtime Error | p02918 | Input is given from Standard Input in the following format:
N K
S | n, k = map(int, input().split())
s = input()
s = list(s)
print(s)
before = s[1]
flag = False
place = -1
end = -1
cnt = 0
for i in range(1, n - 1):
if s[i] != before:
flag = True
place = i
if flag:
print(s, i)
if i + 1 == n - 1:
if s[place] == s[i + 1]:
end = i + 1
else:
end = i
print(s, i, end, "end")
if s[place] == "L":
if end == i:
s[place:end] = "R"
else:
s[place:end] = "R"
# s[end-1] ='R'
else:
if end == i:
s[place:end] = "L"
else:
s[place:end] = "L"
# s[end-1] = 'L'
print(s, "end2")
cnt += 1
if cnt == k:
break
flag = False
before = s[i]
continue
if s[i] != s[i + 1]:
print(s)
if s[place] == "L":
s[place : i + 1] = "R"
else:
s[place : i + 1] = "L"
cnt += 1
print(s)
if cnt == k:
break
flag = False
before = s[i]
continue
before = s[i]
print(s)
if cnt != k:
if s[0] != s[1]:
s[0] = s[1]
if k >= cnt + 2:
if s[n - 1] != s[n - 2]:
s[n - 1] = s[n - 2]
seqCnt = 0
ans = 0
print(s)
for i in range(n - 1):
print(i, seqCnt)
if s[i] == s[i + 1]:
seqCnt += 1
if s[i] != s[i + 1] or i == n - 2:
print(ans, seqCnt, i)
if seqCnt >= 1:
ans += seqCnt
seqCnt = 0
print(ans)
| Statement
There are N people standing in a queue from west to east.
Given is a string S of length N representing the directions of the people. The
i-th person from the west is facing west if the i-th character of S is `L`,
and east if that character of S is `R`.
A person is happy if the person in front of him/her is facing the same
direction. If no person is standing in front of a person, however, he/she is
not happy.
You can perform the following operation any number of times between 0 and K
(inclusive):
Operation: Choose integers l and r such that 1 \leq l \leq r \leq N, and
rotate by 180 degrees the part of the queue: the l-th, (l+1)-th, ..., r-th
persons. That is, for each i = 0, 1, ..., r-l, the (l + i)-th person from the
west will stand the (r - i)-th from the west after the operation, facing east
if he/she is facing west now, and vice versa.
What is the maximum possible number of happy people you can have? | [{"input": "6 1\n LRLRRL", "output": "3\n \n\nIf we choose (l, r) = (2, 5), we have `LLLRLL`, where the 2-nd, 3-rd, and 6-th\npersons from the west are happy.\n\n* * *"}, {"input": "13 3\n LRRLRLRRLRLLR", "output": "9\n \n\n* * *"}, {"input": "10 1\n LLLLLRRRRR", "output": "9\n \n\n* * *"}, {"input": "9 2\n RRRLRLRLL", "output": "7"}] |
Print the maximum possible number of happy people after at most K operations.
* * * | s354531147 | Wrong Answer | p02918 | Input is given from Standard Input in the following format:
N K
S | import sys
sys.setrecursionlimit(10**9)
input = sys.stdin.readline
inpl = lambda: list(map(int, input().split()))
def get_sequence(arr):
if len(arr) == 0:
return [], []
elements = []
nums = []
n = 1
prev = arr[0]
for c in arr[1:]:
if c == prev:
n += 1
else:
elements.append(prev)
nums.append(n)
prev = c
n = 1
elements.append(prev)
nums.append(n)
return elements, nums
N, K = inpl()
S = input().rstrip("\n")
elements, nums = get_sequence(S)
if elements[0] == "R":
gap = len(elements) // 2
if len(elements) % 2:
boundary = 1
else:
boundary = 0
else:
gap = (len(elements) - 1) // 2
if len(elements) % 2:
boundary = 1
else:
boundary = 2
if K <= gap:
gap -= K
elif K <= gap + boundary:
gap = 0
boundary = boundary + gap - K
else:
gap = boundary = 0
print(N - gap * 2 - boundary)
| Statement
There are N people standing in a queue from west to east.
Given is a string S of length N representing the directions of the people. The
i-th person from the west is facing west if the i-th character of S is `L`,
and east if that character of S is `R`.
A person is happy if the person in front of him/her is facing the same
direction. If no person is standing in front of a person, however, he/she is
not happy.
You can perform the following operation any number of times between 0 and K
(inclusive):
Operation: Choose integers l and r such that 1 \leq l \leq r \leq N, and
rotate by 180 degrees the part of the queue: the l-th, (l+1)-th, ..., r-th
persons. That is, for each i = 0, 1, ..., r-l, the (l + i)-th person from the
west will stand the (r - i)-th from the west after the operation, facing east
if he/she is facing west now, and vice versa.
What is the maximum possible number of happy people you can have? | [{"input": "6 1\n LRLRRL", "output": "3\n \n\nIf we choose (l, r) = (2, 5), we have `LLLRLL`, where the 2-nd, 3-rd, and 6-th\npersons from the west are happy.\n\n* * *"}, {"input": "13 3\n LRRLRLRRLRLLR", "output": "9\n \n\n* * *"}, {"input": "10 1\n LLLLLRRRRR", "output": "9\n \n\n* * *"}, {"input": "9 2\n RRRLRLRLL", "output": "7"}] |
Print the maximum possible number of happy people after at most K operations.
* * * | s511775035 | Wrong Answer | p02918 | Input is given from Standard Input in the following format:
N K
S | #!/usr/bin/env python3
# -*- coding: utf-8 -*-
import sys
import re
N, K = tuple(map(int, sys.stdin.readline().rstrip().split()))
s = sys.stdin.readline().rstrip()
# print(N,K)
# print(s)
def rev_comp(s):
tmp = s.translate(str.maketrans("LR", "RL"))
return tmp[::-1]
def score(s):
sc = 0
for i in range(len(s) - 1):
sl = s[i : i + 2]
if sl == "RR" or sl == "LL":
sc += 1
return sc
lr = re.compile("LR.*RL")
rl = re.compile("RL.*LR")
lrl = re.compile("LRL")
rlr = re.compile("RLR")
r_l = re.compile("R+L+")
l_r = re.compile("L+R+")
ronly = re.compile("R+")
lonly = re.compile("L+")
flag = True
while K > 0 and flag:
flag = False
m = re.search(lrl, s)
while m and K > 0:
start = m.start()
end = m.end()
s = s[:start] + "LLL" + s[end:]
flag = True
K = K - 1
m = re.search(lrl, s)
m = re.search(rlr, s)
while m and K > 0:
start = m.start()
end = m.end()
s = s[:start] + "RRR" + s[end:]
flag = True
K = K - 1
m = re.search(rlr, s)
m = re.search(lr, s)
while m and K > 0:
start = m.start()
end = m.end()
print(start, end)
s = s[: start + 1] + rev_comp(s[start + 1 : end - 1]) + s[end - 1 :]
flag = True
K = K - 1
m = re.search(lr, s)
m = re.search(rl, s)
while m and K > 0:
start = m.start()
end = m.end()
print(start, end)
s = s[: start + 1] + rev_comp(s[start + 1 : end - 1]) + s[end - 1 :]
flag = True
K = K - 1
m = re.search(rl, s)
flag = True
while K > 0 and flag:
flag = False
m = re.search(r_l, s)
while m and K > 0:
end = re.search(ronly, s).end()
s = rev_comp(s[:end]) + s[end:]
flag = True
K = K - 1
m = re.search(r_l, s)
m = re.search(l_r, s)
while m and K > 0:
end = re.search(lonly, s).end()
s = rev_comp(s[:end]) + s[end:]
flag = True
K = K - 1
m = re.search(r_l, s)
# print(s)
print(score(s))
| Statement
There are N people standing in a queue from west to east.
Given is a string S of length N representing the directions of the people. The
i-th person from the west is facing west if the i-th character of S is `L`,
and east if that character of S is `R`.
A person is happy if the person in front of him/her is facing the same
direction. If no person is standing in front of a person, however, he/she is
not happy.
You can perform the following operation any number of times between 0 and K
(inclusive):
Operation: Choose integers l and r such that 1 \leq l \leq r \leq N, and
rotate by 180 degrees the part of the queue: the l-th, (l+1)-th, ..., r-th
persons. That is, for each i = 0, 1, ..., r-l, the (l + i)-th person from the
west will stand the (r - i)-th from the west after the operation, facing east
if he/she is facing west now, and vice versa.
What is the maximum possible number of happy people you can have? | [{"input": "6 1\n LRLRRL", "output": "3\n \n\nIf we choose (l, r) = (2, 5), we have `LLLRLL`, where the 2-nd, 3-rd, and 6-th\npersons from the west are happy.\n\n* * *"}, {"input": "13 3\n LRRLRLRRLRLLR", "output": "9\n \n\n* * *"}, {"input": "10 1\n LLLLLRRRRR", "output": "9\n \n\n* * *"}, {"input": "9 2\n RRRLRLRLL", "output": "7"}] |
Print the maximum possible number of happy people after at most K operations.
* * * | s049123915 | Wrong Answer | p02918 | Input is given from Standard Input in the following format:
N K
S | N, K = map(int, input().split())
S = input()
same_L_cnt = 0
up_cnt = 0
same_cnt = 0
before = ""
buf = []
for i in range(N):
now_S = S[i]
if now_S == "L":
now_S = 1
else:
now_S = 0
# 前回と同じか確認
if now_S == before:
# 同じならカウントアップ
same_cnt += 1
# L R R ..... R Lが出現したか確認する
buf.append(now_S)
# print('------ buffer ------')
# pprint(buf)
# print('--------------------')
if buf[0] != 1:
buf = []
elif len(buf) == 2 and buf[1] == 1:
buf = [1]
elif len(buf) < 3:
pass
else:
t = buf[1 : len(buf) - 2]
if sum(t) != 0:
buf = []
elif buf[len(buf) - 1] == 1:
up_cnt += 1
buf = [1]
else:
pass
before = now_S
# print(up_cnt)
# print(same_cnt)
up_flg = 0
if sum(buf) == 1:
up_flg = 1
pt = same_cnt
rev_cnt = min(K, up_cnt)
remain_cnt = K - rev_cnt
extra = 0
if up_flg and remain_cnt > 0:
extra = 1
print(same_cnt + rev_cnt * 2 + extra)
| Statement
There are N people standing in a queue from west to east.
Given is a string S of length N representing the directions of the people. The
i-th person from the west is facing west if the i-th character of S is `L`,
and east if that character of S is `R`.
A person is happy if the person in front of him/her is facing the same
direction. If no person is standing in front of a person, however, he/she is
not happy.
You can perform the following operation any number of times between 0 and K
(inclusive):
Operation: Choose integers l and r such that 1 \leq l \leq r \leq N, and
rotate by 180 degrees the part of the queue: the l-th, (l+1)-th, ..., r-th
persons. That is, for each i = 0, 1, ..., r-l, the (l + i)-th person from the
west will stand the (r - i)-th from the west after the operation, facing east
if he/she is facing west now, and vice versa.
What is the maximum possible number of happy people you can have? | [{"input": "6 1\n LRLRRL", "output": "3\n \n\nIf we choose (l, r) = (2, 5), we have `LLLRLL`, where the 2-nd, 3-rd, and 6-th\npersons from the west are happy.\n\n* * *"}, {"input": "13 3\n LRRLRLRRLRLLR", "output": "9\n \n\n* * *"}, {"input": "10 1\n LLLLLRRRRR", "output": "9\n \n\n* * *"}, {"input": "9 2\n RRRLRLRLL", "output": "7"}] |
Print the maximum possible number of happy people after at most K operations.
* * * | s422677123 | Runtime Error | p02918 | Input is given from Standard Input in the following format:
N K
S | ## 幸福度が変わるかどうかは操作Kによって変更されたl, r番目の人間だけなので、
## 左右l,r番目の人間が幸福であるかどうかを見てゆく
## for(n)回のループでまず「既に 1 + x, 任意の r が幸福であるかどうか」を確認して、
## 幸福度2, 1の順でそのl,rをK回覚える
n, k = map(int, input().split())
humans = list(input())
k_count = 0
last_r = n ## 最後に操作したr(番目)
dev_null = 0
for x in range(n - 1):
if x == last_r:
break ## すべての可能性を確認した
if x == 0:
continue
l = r = "n"
if k_count == k:
break ## 既にK回試行したのでbreak
if humans[x] == "L" and humans[x - 1] == "L":
continue
elif humans[x] == "R" and humans[x - 1] == "R" and humans[x + 1] == "R":
continue
else:
## 不幸なので、lを確定
l = x + 1 ## 不幸な人
if l == last_r - 1:
break ## 前に操作したrとlが等しい
## rを探す(x + 1からlast_rまで)
for y in reversed(range(l, last_r)): ## l, l+1, last_r - 1
if y == n - 1 and humans[x] == "R":
## 不幸なので、rを確定
r = y
last_r = r
k_count += 1
break
elif humans[y] == "L" and humans[y - 1] == "L":
dev_null += 1 ## 既に同じ方向を向いてる
elif humans[y] == "R" and humans[y + 1] == "R":
dev_null += 1 ## 既に同じ方向を向いてる
else:
## 不幸なので、rを確定
r = y
last_r = r
k_count += 1
break
if l != "n" and r != "n":
## ともに値があるなら操作Kを実行
for z in range(l, r + 1):
if humans[z - 1] == "L":
humans[z - 1] = "R"
else:
humans[z - 1] = "L"
## ポイントを計算
happy_point = 0
for x in range(n):
if x == 0 and humans[x] == "L":
## do nothing
dev_null += 1
elif x == n - 1 and humans[x] == "R":
## do nothing
dev_null += 1
else:
if humans[x] == "L" and humans[x - 1] == "L":
happy_point += 1
elif humans[x] == "R" and humans[x + 1] == "R":
happy_point += 1
print(happy_point)
| Statement
There are N people standing in a queue from west to east.
Given is a string S of length N representing the directions of the people. The
i-th person from the west is facing west if the i-th character of S is `L`,
and east if that character of S is `R`.
A person is happy if the person in front of him/her is facing the same
direction. If no person is standing in front of a person, however, he/she is
not happy.
You can perform the following operation any number of times between 0 and K
(inclusive):
Operation: Choose integers l and r such that 1 \leq l \leq r \leq N, and
rotate by 180 degrees the part of the queue: the l-th, (l+1)-th, ..., r-th
persons. That is, for each i = 0, 1, ..., r-l, the (l + i)-th person from the
west will stand the (r - i)-th from the west after the operation, facing east
if he/she is facing west now, and vice versa.
What is the maximum possible number of happy people you can have? | [{"input": "6 1\n LRLRRL", "output": "3\n \n\nIf we choose (l, r) = (2, 5), we have `LLLRLL`, where the 2-nd, 3-rd, and 6-th\npersons from the west are happy.\n\n* * *"}, {"input": "13 3\n LRRLRLRRLRLLR", "output": "9\n \n\n* * *"}, {"input": "10 1\n LLLLLRRRRR", "output": "9\n \n\n* * *"}, {"input": "9 2\n RRRLRLRLL", "output": "7"}] |
For each dataset, print the sum of the outputs of all query operations in one
line. | s062456607 | Runtime Error | p01288 | The input consists of multiple datasets. Each dataset has the following
format:
The first line of the input contains two integers _N_ and _Q_ , which denotes
the number of nodes in the tree _T_ and the number of operations,
respectively. These numbers meet the following conditions: 1 ≤ _N_ ≤ 100000
and 1 ≤ _Q_ ≤ 100000\.
The following _N_ \- 1 lines describe the configuration of the tree _T_. Each
line contains a single integer _p i_ (_i_ = 2, ... , _N_), which represents
the index of the parent of _i_ -th node.
The next _Q_ lines contain operations in order. Each operation is formatted as
"M v" or "Q v", where _v_ is the index of a node.
The last dataset is followed by a line containing two zeros. This line is not
a part of any dataset and should not be processed. | def solve():
import sys
file_input = sys.stdin
def marked_ancestor(v):
if v == ancestor[v]:
return v
else:
ancestor[v] = marked_ancestor(parent[v])
return ancestor[v]
def line_ans(line):
v = int(line[2:])
if line[0] == 'M':
ancestor[v] = v
return 0
else:
return marked_ancestor(v)
while True:
N, Q = map(int, file_input.readline().split())
if N == 0:
break
parent = [None, None]
parent += [int(file_input.readline()) for _ in range(N - 1)]
ancestor = [1] * (N + 1)
ans = (line_ans(file_input.readline()) for _ in range(Q))
print(sum(ans))
solve()
| F: Marked Ancestor
You are given a tree _T_ that consists of _N_ nodes. Each node is numbered
from 1 to _N_ , and node 1 is always the root node of _T_. Consider the
following two operations on _T_ :
* M v: (Mark) Mark node v.
* Q v: (Query) Print the index of the nearest marked ancestor of node v which is nearest to it. Initially, only the root node is marked. Note that a node is an ancestor of itself.
Your job is to write a program that performs a sequence of these operations on
a given tree and calculates the value that each Q operation will print. To
avoid too large output file, your program is requested to print the sum of the
outputs of all query operations. Note that the judges confirmed that it is
possible to calculate every output of query operations in a given sequence. | [{"input": "3\n 1\n 1\n 2\n 3\n 3\n Q 5\n M 3\n Q 5\n 0 0", "output": "4"}] |
Print the number of different kinds of colors of the paint cans.
* * * | s219988484 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | N = map(int, input().split())
print(len(set(N)))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s673965203 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | s = list(input().split())
print(len(set(s)) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s524592591 | Wrong Answer | p03962 | The input is given from Standard Input in the following format:
a b c | print(1)
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s289576398 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | 3 3 33 | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s811625741 | Wrong Answer | p03962 | The input is given from Standard Input in the following format:
a b c | print(len(set(input())) - 1)
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s741267308 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | #
# abc046 a
#
import sys
from io import StringIO
import unittest
class TestClass(unittest.TestCase):
def assertIO(self, input, output):
stdout, stdin = sys.stdout, sys.stdin
sys.stdout, sys.stdin = StringIO(), StringIO(input)
resolve()
sys.stdout.seek(0)
out = sys.stdout.read()[:-1]
sys.stdout, sys.stdin = stdout, stdin
self.assertEqual(out, output)
def test_入力例_1(self):
input = """3 1 4"""
output = """3"""
self.assertIO(input, output)
def test_入力例_2(self):
input = """3 3 33"""
output = """2"""
self.assertIO(input, output)
def resolve():
a, b, c = map(int, input().split())
ans = 0
if a == b == c:
print("1")
elif a == b or b == c or c == a:
print("2")
else:
print("3")
if __name__ == "__main__":
# unittest.main()
resolve()
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s300003209 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | a,b,c=map(int,input().split())
if a=b and b=c:
print(1)
elif a=b and a!=c:
print(2)
elif a!=b and b=c:
print(2)
elif a=c and a!=b:
print(2)
else:
print(3) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s300318023 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | def iroha():
a, b, c = map(int, input().split())
if a == b and b == c and a == c:
print(1)
elif a != b and b != c and a != c:
print(3)
elif else:
print(2)
if __name__ == "__main__":
iroha()
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s799515345 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | #入力
lst = input().split()
#整数値化
for i in range(len(lst)):
lst[i] = int(lst[i])
#ソート
lst.sort()
#出力
if lst[0] == lst[2]
print(3)
elif lst[0] == lst[1] or lst[1] == lst[2]:
print(2)
else:
print(1) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s751415062 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | #入力
lst = input().spilit()
#整数値化
for i in range(len(lst)):
lst[i] = int(lst[i])
#ソート
lst.sort()
#出力
if lst[0] == lst[2]
print(3)
elif lst[0] == lst[1] or lst[1] == lst[2]:
print(2)
else:
print(1) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s358381965 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | # # -*- coding: utf-8 -*-
a_b_c = set(map(int, input().split()))
print(len(a_b_c))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s335308251 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | co = list(map(int, input().split()))
cos = set(co)
# print(cos)
print(len(cos))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s537211053 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | a, b, c = map(int, input().split(" "))
ink = [a, b, c]
print(len(set(ink)))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s294333037 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | paint_list = input().split()
print(len(set(paint_list)))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s087892474 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | print(len(list(set(list(map(lambda n: int(n), input().split(" ")))))))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s473257821 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | al = list(map(int, input().split()))
print(len(set(al)))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s682568593 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | p_list = [int(x) for x in input().split()]
print(len(set(p_list)))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s886813828 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | x = list(map(int, input().split()))
print(len(set(x)) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s689347793 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | print(len(list(set(map(int, input().split())))))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s859681691 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | penkis = input().split()
print(len(set(penkis)))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s900886112 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | print(len({int(x) for x in input().split()}))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s775784418 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | w = set(map(int, input().split()))
print(len(w))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s229162007 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | colors = len(set(input().split()))
print(colors)
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s878896347 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | print(len(set([int(i) in input().split()])))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s822735909 | Wrong Answer | p03962 | The input is given from Standard Input in the following format:
a b c | s = set(input().split())
len(s)
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s598523680 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | icase = 46
if icase == 46:
a = list(map(int, input().split()))
icnt = 1
if a[0] != a[1]:
icnt = icnt + 1
if a[0] != a[2] and a[1] != a[2]:
icnt = icnt + 1
print(icnt)
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s213607888 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | n = int(input())
t = 1
a = 1
for i in range(n):
T, A = map(int, input().split())
k = max(1, ~-t // T + 1, ~-a // A + 1)
t = k * T
a = k * A
print(t + a)
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s990698213 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | シカのAtCoDeerくんはペンキをこれまでに3つ買いました。おととい買ったペンキの色は a , 昨日買ったペンキの色は b , 今日買ったペンキの色は c です。各ペンキの色は1以上100以下の整数で表されます。 AtCoDeerくんはわすれんぼうなため、同じ色のペンキを買ってしまっていることがあります。AtCoDeerくんが買ったペンキの色の種類の個数を教えてあげてください。
a,b,c=map(int, input().split())
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s628852019 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | s = list(input().split())
print(len(set(s)) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s523380146 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | a=list(map(int,input().split()))
ans=3
for i in range(0,2):
for j in range(i+1,3):
if a[i]==a[j]:
ans -= 1
else:
j += 1
print(ans)
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s743839355 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | a,b,c = map(int,input().split())
if a=b and b=c:
print(1)
elif (a=b or b=c or a=c):
print(2)
else:
print(3)
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s532790974 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | a, b, c = map(int, input().split())
m = set([a, b, c])
print(len(m) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s759601180 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | lst = input().split()
print(len(list(set(lst))))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s604932184 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | print(len(set([i for i in input().split()])))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s417500269 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | print(len(a=set(input())) - 1)
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s016094278 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | print(len(list(set(int, input().split()))))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s963223891 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | print(list(set(int(input().split()))))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s610180585 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | print(len(set(input().split())) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s588514433 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | li ~ list(map(int,input().split()))
cnt = 3
if li[0] == li[1]:
cnt -= 1
if li[0] == li[2]:
cnt -= 1
if li[1] == li[2]:
cnt -= 1
if li[0] == li[1] and li[1] == li[2]:
cnt = 1
print(cnt) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s563930528 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | color = []
color.append(int(input(),range(3)))
x = count(color[0])
y = count(color[1])
if x == 1 and y == 1:
print("3")
else if x == 1 and y == 2:
print("2")
else if x == 2:
print("2")
else:
print("1") | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s886306501 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | a, b, c = map(int, input().split())
if a == b and b == c:
print(1)
elif a == b and b != c or b == c and a != b or a==c and a!=b
print(2)
else:
print(3) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s211545926 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | nums = list(map(int, input().split()))
n = 1
if nums[0] != nums[1]:
n += 1
if nums[1] != nums[2] and nums[0] != nums[2]:
n += 1
print(n)
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s375861187 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | colors = [int(i) for i in input().split()]
s_colors = set(colors)
print(len(s_colors))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s842462619 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | N = input().split()
d = dict.fromkeys(N, 0)
for n in N:
d[n] += 1
print(sum(d.values())
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s193195906 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | x = len(set(list(map(int, input().split()))))
print(x)
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s064003096 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | A, B C = set(map(int.input().split))
print(len(A, B, C)) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s396653115 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | i = lambda: int(input())
print(len(set([i(), i(), i()])))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s295670819 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | List = input().split()
print(len(list(set(List))))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s595466453 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | print(len(list(set(list(input().split())))))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s347681923 | Wrong Answer | p03962 | The input is given from Standard Input in the following format:
a b c | print(set([int(i) for i in input().split()]))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s309233067 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | a = set(list(input.split()))
print(len(list(a)))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s830026198 | Wrong Answer | p03962 | The input is given from Standard Input in the following format:
a b c | print(set(list(map(int, input().split()))))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s318979092 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | c = list(input().split())
print(len(set(c)) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s463139966 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | c = list(input().split())
print(len(set(c)) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s231342406 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | print(len(set(map(input().split()))))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s122319226 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | print(len(set([input().split()])))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s307239541 | Wrong Answer | p03962 | The input is given from Standard Input in the following format:
a b c | S = input().split()
print(set(S))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s664110656 | Wrong Answer | p03962 | The input is given from Standard Input in the following format:
a b c | color = input().split()
len(set(color))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s675166602 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | print)(len(set(list(map(int,input().split())))) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s807160580 | Wrong Answer | p03962 | The input is given from Standard Input in the following format:
a b c | print(len(set([i for i in input()])))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s775668339 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | print(len(set(map(int, input().split()))) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s606740594 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | print(len(set(input().split())) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s818166119 | Wrong Answer | p03962 | The input is given from Standard Input in the following format:
a b c | print(len(set(input())))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s969836592 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | s = input().split()
print(len(set(s)) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s101352337 | Wrong Answer | p03962 | The input is given from Standard Input in the following format:
a b c | print(len(set(list(input()))))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s179269555 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | a,b,c = map(int,input().split())
if a != b != c:
print(3)
if a == b and a != c and b != c:
print(2)
if b == c and b != a and c != a:
print(2)
iif a == c and a != b and c != b:
print(2)
if a == b == c:
print(1) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s581265018 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | l = list(map(int, input().split()))
s = 3
for i in range(3):
for j in range(i + 1, 3):
if l[i] == l[j]:
s = s - 1
if l[0] = l[1] and l[1] = l[2]:
s = s + 1
print(s) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s501605468 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | ls = list()
lt = list()
la = list()
LS = list()
LT = list()
LA = list()
N = int(input())
for i in range(1, N + 1):
T, A = map(int, input().split())
X = T + A
ls.append(X)
lt.append(T)
la.append(A)
LS.append(X)
LT.append(T)
LA.append(A)
print(lt, la, LT, LA)
for j in range(1, N):
while ls[j] < ls[j - 1]:
ls[j] += LS[j]
while lt[j] < lt[j - 1] or la[j] < la[j - 1]:
lt[j] += LT[j]
la[j] += LA[j]
print(lt[j], la[j], LT[j], LA[j])
print(lt[N - 1] + la[N - 1])
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s335338828 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | a, b, c = map(int, input().split())
if a == b:
if a == c:
print(1)
else: print(2)
elif a == c:
print(2)
else: print(3)
elif b == c:
print(2)
else: print(3) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s547461197 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | a=input().split()
#a=int(input())
#b=int(input())
#c=int(input())
#d=int(input())
if a[0]==a[1]==a[2]:
print(1)
elif a[0]!=a[1]! and a[1]!=a[2] and a[0]!=a[2]:
print(3)
else:
print(2)
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s811089537 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | a = input().split()
num = 3
if a[0] == a[1]:
num = num - 1
if a[0] == a[2]:
num = num - 1:
elif a[0] == a[2]:
num = num - 1
elif a[1] == a[2]:
num = num - 1
print(num) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s746768898 | Wrong Answer | p03962 | The input is given from Standard Input in the following format:
a b c | lis = list(map(int, input().split()))
unique_list = []
for i in range(len(lis)):
if lis[i] in unique_list or lis[i] in lis[:i] + lis[i + 1 :]:
continue
unique_list.append(lis[i])
print(len(unique_list))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s934403296 | Accepted | p03962 | The input is given from Standard Input in the following format:
a b c | i_list = list(map(int, input().split()))
i_set = set(i_list)
print(len(i_set))
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s294098424 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | a,b,c=list(map(int,input().split()))
print("3" if a=b=c else "2" if a=b or a=c or b=c else "3") | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s884995291 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | l = list(map(int,input()split()))
ans = list(dict.fromkeys(l))
print(len(ans)+1) | Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |
Print the number of different kinds of colors of the paint cans.
* * * | s275554448 | Runtime Error | p03962 | The input is given from Standard Input in the following format:
a b c | nums = list(map(int, input().split()))
n = 1
if nums[0] != nums[1]:
n++
if nums[1] != nums[2] and nums[0] != nums[2]:
n++
print(n)
| Statement
AtCoDeer the deer recently bought three paint cans. The color of the one he
bought two days ago is a, the color of the one he bought yesterday is b, and
the color of the one he bought today is c. Here, the color of each paint can
is represented by an integer between 1 and 100, inclusive.
Since he is forgetful, he might have bought more than one paint can in the
same color. Count the number of different kinds of colors of these paint cans
and tell him. | [{"input": "3 1 4", "output": "3\n \n\nThree different colors: 1, 3, and 4.\n\n* * *"}, {"input": "3 3 33", "output": "2\n \n\nTwo different colors: 3 and 33."}] |