file_path
stringlengths 21
202
| content
stringlengths 12
1.02M
| size
int64 12
1.02M
| lang
stringclasses 9
values | avg_line_length
float64 3.33
100
| max_line_length
int64 10
993
| alphanum_fraction
float64 0.27
0.93
|
---|---|---|---|---|---|---|
renanmb/Omniverse_legged_robotics/URDF-Descriptions/Mini_pupper/QuadrupedRobot-mini_pupper/PS4Joystick/PS4Joystick.py |
import sys
import time
import subprocess
import math
from threading import Thread
from collections import OrderedDict, deque
from ds4drv.actions import ActionRegistry
from ds4drv.backends import BluetoothBackend, HidrawBackend
from ds4drv.config import load_options
from ds4drv.daemon import Daemon
from ds4drv.eventloop import EventLoop
from ds4drv.exceptions import BackendError
from ds4drv.action import ReportAction
from ds4drv.__main__ import create_controller_thread
class ActionShim(ReportAction):
""" intercepts the joystick report"""
def __init__(self, *args, **kwargs):
super(ActionShim, self).__init__(*args, **kwargs)
self.timer = self.create_timer(0.02, self.intercept)
self.values = None
self.timestamps = deque(range(10), maxlen=10)
def enable(self):
self.timer.start()
def disable(self):
self.timer.stop()
self.values = None
def load_options(self, options):
pass
def deadzones(self,values):
deadzone = 0.14
if math.sqrt( values['left_analog_x'] ** 2 + values['left_analog_y'] ** 2) < deadzone:
values['left_analog_y'] = 0.0
values['left_analog_x'] = 0.0
if math.sqrt( values['right_analog_x'] ** 2 + values['right_analog_y'] ** 2) < deadzone:
values['right_analog_y'] = 0.0
values['right_analog_x'] = 0.0
return values
def intercept(self, report):
new_out = OrderedDict()
for key in report.__slots__:
value = getattr(report, key)
new_out[key] = value
for key in ["left_analog_x", "left_analog_y",
"right_analog_x", "right_analog_y",
"l2_analog", "r2_analog"]:
new_out[key] = 2*( new_out[key]/255 ) - 1
new_out = self.deadzones(new_out)
self.timestamps.append(new_out['timestamp'])
if len(set(self.timestamps)) <= 1:
self.values = None
else:
self.values = new_out
return True
class Joystick:
def __init__(self):
self.thread = None
options = load_options()
if options.hidraw:
raise ValueError("HID mode not supported")
backend = HidrawBackend(Daemon.logger)
else:
subprocess.run(["hciconfig", "hciX", "up"])
backend = BluetoothBackend(Daemon.logger)
backend.setup()
self.thread = create_controller_thread(1, options.controllers[0])
self.thread.controller.setup_device(next(backend.devices))
self.shim = ActionShim(self.thread.controller)
self.thread.controller.actions.append(self.shim)
self.shim.enable()
self._color = (None, None, None)
self._rumble = (None, None)
self._flash = (None, None)
# ensure we get a value before returning
while self.shim.values is None:
pass
def close(self):
if self.thread is None:
return
self.thread.controller.exit("Cleaning up...")
self.thread.controller.loop.stop()
def __del__(self):
self.close()
@staticmethod
def map(val, in_min, in_max, out_min, out_max):
""" helper static method that helps with rescaling """
in_span = in_max - in_min
out_span = out_max - out_min
value_scaled = float(val - in_min) / float(in_span)
value_mapped = (value_scaled * out_span) + out_min
if value_mapped < out_min:
value_mapped = out_min
if value_mapped > out_max:
value_mapped = out_max
return value_mapped
def get_input(self):
""" returns ordered dict with state of all inputs """
if self.thread.controller.error:
raise IOError("Encountered error with controller")
if self.shim.values is None:
raise TimeoutError("Joystick hasn't updated values in last 200ms")
return self.shim.values
def led_color(self, red=0, green=0, blue=0):
""" set RGB color in range 0-255"""
color = (int(red),int(green),int(blue))
if( self._color == color ):
return
self._color = color
self.thread.controller.device.set_led( *self._color )
def rumble(self, small=0, big=0):
""" rumble in range 0-255 """
rumble = (int(small),int(big))
if( self._rumble == rumble ):
return
self._rumble = rumble
self.thread.controller.device.rumble( *self._rumble )
def led_flash(self, on=0, off=0):
""" flash led: on and off times in range 0 - 255 """
flash = (int(on),int(off))
if( self._flash == flash ):
return
self._flash = flash
if( self._flash == (0,0) ):
self.thread.controller.device.stop_led_flash()
else:
self.thread.controller.device.start_led_flash( *self._flash )
if __name__ == "__main__":
j = Joystick()
while 1:
for key, value in j.get_input().items():
print(key,value)
print()
time.sleep(0.1)
| 5,123 | Python | 28.28 | 96 | 0.579934 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/Mini_pupper/QuadrupedRobot-mini_pupper/PS4Joystick/mac_joystick.py | import os
import pygame
from UDPComms import Publisher
os.environ["SDL_VIDEODRIVER"] = "dummy"
drive_pub = Publisher(8830)
arm_pub = Publisher(8410)
pygame.display.init()
pygame.joystick.init()
# wait until joystick is connected
while 1:
try:
pygame.joystick.Joystick(0).init()
break
except pygame.error:
pygame.time.wait(500)
# Prints the joystick's name
JoyName = pygame.joystick.Joystick(0).get_name()
print("Name of the joystick:")
print(JoyName)
# Gets the number of axes
JoyAx = pygame.joystick.Joystick(0).get_numaxes()
print("Number of axis:")
print(JoyAx)
while True:
pygame.event.pump()
forward = (pygame.joystick.Joystick(0).get_axis(3))
twist = (pygame.joystick.Joystick(0).get_axis(2))
on = (pygame.joystick.Joystick(0).get_button(5))
if on:
print({'f':-150*forward,'t':-80*twist})
drive_pub.send({'f':-150*forward,'t':-80*twist})
else:
drive_pub.send({'f':0,'t':0})
pygame.time.wait(100)
| 994 | Python | 22.139534 | 56 | 0.662978 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/Mini_pupper/QuadrupedRobot-mini_pupper/PS4Joystick/setup.py | #!/usr/bin/env python
from distutils.core import setup
setup(name='PS4Joystick',
version='2.0',
py_modules=['PS4Joystick'],
description='Interfaces with a PS4 joystick over Bluetooth',
author='Michal Adamkiewicz',
author_email='mikadam@stanford.edu',
url='https://github.com/stanfordroboticsclub/JoystickUDP',
)
| 356 | Python | 24.499998 | 66 | 0.679775 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/Mini_pupper/QuadrupedRobot-mini_pupper/PS4Joystick/local_or_remote.py | import os
import RPi.GPIO as GPIO
import time
GPIO.setmode(GPIO.BCM) # Broadcom pin-numbering scheme
GPIO.setup(21, GPIO.IN, pull_up_down=GPIO.PUD_UP)
while 1:
if not GPIO.input(21):
print("eanbling joystick")
os.system("sudo systemctl start ds4drv")
os.system("sudo systemctl start joystick")
#os.system("screen sudo python3 /home/pi/RoverCommand/joystick.py")
else:
os.system("sudo systemctl stop ds4drv")
os.system("sudo systemctl stop joystick")
print("not eanbling joystick")
time.sleep(5)
| 566 | Python | 27.349999 | 75 | 0.673145 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/Mini_pupper/QuadrupedRobot-mini_pupper/PS4Joystick/README.md | # PS4Joystick
Code allowing the use of a DualShock (PS4 Joystick) over Bluetooth on Linux. Over USB comming soon. `mac_joystick.py` shows how to emulate something similar on macOS, but without the fancy features.
Note: We have updated from the previous version so make sure you disable ds4drv from running automatically at startup as they will conflict.
This method still requires [ds4drv](https://github.com/chrippa/ds4drv) however it doesn't run it as a separate service and then separately pull joystick data using Pygame. Instead it imports ds4drv directly which gives us much more control over the joystick behaviour. Specifically:
- It will only pair to one joystick allowing us to run multiple robots at a time
- Allows for launching joystick code via systemd at boot using `sudo systemctl enable joystick`
- Can change joystick colors and using rumble directly from Python (can also access the touchpad and IMU!)
- Is a much nicer interface than using Pygame, as the axes are actually named as opposed to arbitrarly numbered! The axis directions are consistant with Pygame.
- Doesn't need $DISPLAY hacks to run on headless devices
### Usage
Take a look at `rover_example.py` as it demonstrates most features.
To implement this functionality to a new repository (say [PupperCommand](https://github.com/stanfordroboticsclub/PupperCommand)) you can just call `from PS4Joystick import Joystick` anywhere once you've installed the module. Replicate `joystick.service` in that repository.
### Install
``` sudo bash install.sh ```
### macOS
Sadly ds4drv doesn't work on Macs. But you can get some of the functionality by installing Pygame with `sudo pip3 install Pygame`. Take a look in `mac_joystick.py` for an example. Note this only works over USB (plug the controller in using a micro usb cable) and the mapping is different than using Pygame with ds4drv | 1,873 | Markdown | 65.928569 | 317 | 0.789642 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/Mini_pupper/QuadrupedRobot-mini_pupper/PS4Joystick/rover_example.py | from UDPComms import Publisher
from PS4Joystick import Joystick
import time
from enum import Enum
drive_pub = Publisher(8830)
arm_pub = Publisher(8410)
j=Joystick()
MODES = Enum('MODES', 'SAFE DRIVE ARM')
mode = MODES.SAFE
while True:
values = j.get_input()
if( values['button_ps'] ):
if values['dpad_up']:
mode = MODES.DRIVE
j.led_color(red=255)
elif values['dpad_right']:
mode = MODES.ARM
j.led_color(blue=255)
elif values['dpad_down']:
mode = MODES.SAFE
j.led_color(green=255)
# overwrite when swiching modes to prevent phantom motions
values['dpad_down'] = 0
values['dpad_up'] = 0
values['dpad_right'] = 0
values['dpad_left'] = 0
if mode == MODES.DRIVE:
forward_left = - values['left_analog_y']
forward_right = - values['right_analog_y']
twist = values['right_analog_x']
on_right = values['button_r1']
on_left = values['button_l1']
l_trigger = values['l2_analog']
if on_left or on_right:
if on_right:
forward = forward_right
else:
forward = forward_left
slow = 150
fast = 500
max_speed = (fast+slow)/2 + l_trigger*(fast-slow)/2
out = {'f':(max_speed*forward),'t':-150*twist}
drive_pub.send(out)
print(out)
else:
drive_pub.send({'f':0,'t':0})
elif mode == MODES.ARM:
r_forward = - values['right_analog_y']
r_side = values['right_analog_x']
l_forward = - values['left_analog_y']
l_side = values['left_analog_x']
r_shoulder = values['button_r1']
l_shoulder = values['button_l1']
r_trigger = values['r2_analog']
l_trigger = values['l2_analog']
square = values['button_square']
cross = values['button_cross']
circle = values['button_circle']
triangle = values['button_triangle']
PS = values['button_ps']
# hat directions could be reversed from previous version
hat = [ values["dpad_up"] - values["dpad_down"],
values["dpad_right"] - values["dpad_left"] ]
reset = (PS == 1) and (triangle == 1)
reset_dock = (PS==1) and (square ==1)
target_vel = {"x": l_side,
"y": l_forward,
"z": (r_trigger - l_trigger)/2,
"yaw": r_side,
"pitch": r_forward,
"roll": (r_shoulder - l_shoulder),
"grip": cross - square,
"hat": hat,
"reset": reset,
"resetdock":reset_dock,
"trueXYZ": circle,
"dock": triangle}
print(target_vel)
arm_pub.send(target_vel)
elif mode == MODES.SAFE:
# random stuff to demo color features
triangle = values['button_triangle']
square = values['button_square']
j.rumble(small = 255*triangle, big = 255*square)
r2 = values['r2_analog']
r2 = j.map( r2, -1, 1, 0 ,255)
j.led_color( green = 255, blue = r2)
else:
pass
time.sleep(0.1)
| 3,285 | Python | 26.847457 | 66 | 0.506849 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/Mini_pupper/stanford_pupper_Chandykunju Alex/package.xml | <package format="2">
<name>standford_pupper_description</name>
<version>1.0.0</version>
<description>
<p>URDF Description package for standford_pupper</p>
<p>This package contains configuration data, 3D models and launch files
for standford_pupper robot</p>
</description>
<author>Chandy Alex</author>
<maintainer email="chandyalex92@gmail.com" />
<license>BSD</license>
<buildtool_depend>catkin</buildtool_depend>
<depend>roslaunch</depend>
<depend>robot_state_publisher</depend>
<depend>rviz</depend>
<depend>joint_state_publisher_gui</depend>
<depend>gazebo</depend>
<export>
<architecture_independent />
</export>
</package> | 670 | XML | 30.952379 | 75 | 0.722388 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/Mini_pupper/stanford_pupper_Chandykunju Alex/README.md |
# Stanford Pupper Robot Description (URDF)
## Overview
This package contains a simplified robot description (URDF) of the [Stanford Pupper](https://stanfordstudentrobotics.org/pupper) developed by [Stanford Robotics club](https://github.com/stanfordroboticsclub/StanfordQuadruped).
## License
This software is released under a [BSD 3-Clause license](LICENSE).
| 369 | Markdown | 23.666665 | 226 | 0.783198 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/CODE_OF_CONDUCT.md | # Contributor Covenant Code of Conduct
## Our Pledge
In the interest of fostering an open and welcoming environment, we as
contributors and maintainers pledge to making participation in our project and
our community a harassment-free experience for everyone, regardless of age, body
size, disability, ethnicity, sex characteristics, gender identity and expression,
level of experience, education, socio-economic status, nationality, personal
appearance, race, religion, or sexual identity and orientation.
## Our Standards
Examples of behavior that contributes to creating a positive environment
include:
* Using welcoming and inclusive language
* Being respectful of differing viewpoints and experiences
* Gracefully accepting constructive criticism
* Focusing on what is best for the community
* Showing empathy towards other community members
Examples of unacceptable behavior by participants include:
* The use of sexualized language or imagery and unwelcome sexual attention or
advances
* Trolling, insulting/derogatory comments, and personal or political attacks
* Public or private harassment
* Publishing others' private information, such as a physical or electronic
address, without explicit permission
* Other conduct which could reasonably be considered inappropriate in a
professional setting
## Our Responsibilities
Project maintainers are responsible for clarifying the standards of acceptable
behavior and are expected to take appropriate and fair corrective action in
response to any instances of unacceptable behavior.
Project maintainers have the right and responsibility to remove, edit, or
reject comments, commits, code, wiki edits, issues, and other contributions
that are not aligned to this Code of Conduct, or to ban temporarily or
permanently any contributor for other behaviors that they deem inappropriate,
threatening, offensive, or harmful.
## Scope
This Code of Conduct applies both within project spaces and in public spaces
when an individual is representing the project or its community. Examples of
representing a project or community include using an official project e-mail
address, posting via an official social media account, or acting as an appointed
representative at an online or offline event. Representation of a project may be
further defined and clarified by project maintainers.
## Enforcement
Instances of abusive, harassing, or otherwise unacceptable behavior may be
reported by contacting the project team at mauricerahme2020@u.northwestern.edu. All
complaints will be reviewed and investigated and will result in a response that
is deemed necessary and appropriate to the circumstances. The project team is
obligated to maintain confidentiality with regard to the reporter of an incident.
Further details of specific enforcement policies may be posted separately.
Project maintainers who do not follow or enforce the Code of Conduct in good
faith may face temporary or permanent repercussions as determined by other
members of the project's leadership.
## Attribution
This Code of Conduct is adapted from the [Contributor Covenant][homepage], version 1.4,
available at https://www.contributor-covenant.org/version/1/4/code-of-conduct.html
[homepage]: https://www.contributor-covenant.org
For answers to common questions about this code of conduct, see
https://www.contributor-covenant.org/faq
| 3,367 | Markdown | 42.740259 | 87 | 0.822097 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/CONTRIBUTING.md | # Contributing
When contributing to this repository, please first discuss the change you wish to make via issue,
[email](mauricerahme2020@u.northwestern.edu), or any other direct method with me. For those of you on the [Spot Micro Slack](https://spotmicroai-inviter.herokuapp.com/), contact me there (Maurice Rahme). Please be sure to follow the code of conduct.
## Examples of Contributions
1. New platform support (e.g. Gazebo, MuJoCo).
2. RL Agent addition (e.g. DDPG, PPO).
3. Simulation improvement (e.g. motor response in Pybullet)
4. Sensing capabilities in simulation (e.g. LIDAR, Camera)
If in doubt, please talk to me, and I'll let you know if your idea will be helpful to the project. Some things are better served as issues, such as:
## Examples of Issues
1. Bugs.
2. Feature Requests.
3. Clarifications.
4. Platform support (e.g. something is not working in your specific environment).
## Pull Request Process
1. Fork this repository.
2. Create a new branch titled `your-username`
3. Once your changes are ready, please send me a link to your branch so that I can try it out (there will be unit tests in the future).
4. If I approve your change, you may merge to `spot` and submit a PR.
| 1,207 | Markdown | 43.740739 | 249 | 0.755592 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/readthedocs.yml | # .readthedocs.yml
# Read the Docs configuration file
# See https://docs.readthedocs.io/en/stable/config-file/v2.html for details
# Required
version: 2
# Build documentation in the docs/ directory with Sphinx
sphinx:
configuration: docs/conf.py
# Build documentation with MkDocs
#mkdocs:
# configuration: mkdocs.yml
# Optionally build your docs in additional formats such as PDF
formats:
- pdf
# Optionally set the version of Python and requirements required to build your docs
python:
version: 3.7
install:
- requirements: docs/requirements.txt | 563 | YAML | 22.499999 | 83 | 0.765542 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/toc.md |
Table of Contents
=================
* [Spot Mini Mini OpenAI Gym Environment](#spot-mini-mini-openai-gym-environment)
* [Motivation](#motivation)
* [Kinematics:](#kinematics)
* [Reinforcement Learning](#reinforcement-learning)
* [Stability on Difficult Terrain](#stability-on-difficult-terrain)
* [Drift Correction](#drift-correction)
* [Gait:](#gait)
* [How To Run](#how-to-run)
* [Dependencies](#dependencies)
* [Joystick Control with ROS](#joystick-control-with-ros)
* [Testing Environment (Non-Joystick)](#testing-environment-non-joystick)
* [Reinforcement Learning Agent Evaluation](#reinforcement-learning-agent-evaluation)
* [Using Different Terrain](#using-different-terrain)
Created by [gh-md-toc](https://github.com/ekalinin/github-markdown-toc)
| 834 | Markdown | 40.749998 | 91 | 0.676259 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/README.md | Note: development for this project was haulted in November 2020 to respect my NDA with my employer.
## Spot Mini Mini OpenAI Gym Environment
[](https://github.com/moribots/spot_mini_mini/releases)
[](https://spot-mini-mini.readthedocs.io/en/latest/?badge=latest)
[](https://github.com/moribots/spot_mini_mini/graphs/commit-activity)
[](https://github.com/moribots/spot_mini_mini/pulls)
[](https://github.com/moribots)
[](https://github.com/moribots/spot_mini_mini/blob/spot/LICENSE)
<!--  -->
<p float="left">
<img src="spot_real/media/spot_hello.gif" width="670" />
</p>
**Featured in [Robotics Weekly](https://weeklyrobotics.com/weekly-robotics-98) and [Mithi's Robotics Coursework](https://github.com/mithi/robotics-coursework/#hands-on-and-blogs)!**
## Motivation
As part of the [Spot Micro](https://spotmicroai.readthedocs.io/en/latest/) community, I saw the need for a reliable and versatile simulator for those who wanted to try things out without risking damage to their robots. To that end, I developed my own in Pybullet which can also be used as a Gym environment for Reinforcement Learning tasks.
<p float="left">
<img src="spot_bullet/media/spot_new_demo.gif" width="335" />
<img src="spot_real/media/full_control.gif" width="335" />
</p>
You'll notice that there are gifs of the original `SpotMicro` as well a new version designed for added real world fidelity. The default branch simulates the new version, but you can work with `SpotMicro` in the [spotmicroai](https://github.com/moribots/spot_mini_mini/tree/spotmicroai) branch of this repo. The new version also has a more reliable URDF, with more accurate inertial calculations.
If you don't need a Gym environment, that's okay too! `env_tester.py` works without RL or Gym, it is designed to accept any gait implementation, and provides a GUI for testing it out! In my case, I've implemented a 12-point Bezier gait.
<!--
<p float="left">
<img src="spot_real/media/spot_demo.gif" width="670" />
</p>
-->
**Read the [docs](https://spot-mini-mini.readthedocs.io/en/latest/index.html)!**
Table of Contents
-----------------
* [Motivation](#motivation)
* [Kinematics](#kinematics)
* [D^2 Gait Modulation with Bezier Curves](#d2-gait-modulation-with-bezier-curves)
* [Training](#Training)
* [Real World Validation](#real-world-validation)
* [Gait](#gait)
* [How To Run](#how-to-run)
* [Dependencies](#dependencies)
* [Joystick Control with ROS](#joystick-control-with-ros)
* [Testing Environment (Non-Joystick)](#testing-environment-non-joystick)
* [Reinforcement Learning Agent Training](#reinforcement-learning-agent-training)
* [Reinforcement Learning Agent Evaluation](#reinforcement-learning-agent-evaluation)
* [Using Different Terrain](#using-different-terrain)
* [Hardware](https://github.com/moribots/spot_mini_mini/tree/spot/spot_real)
* [Assembly & Calibration](https://github.com/moribots/spot_mini_mini/tree/spot/spot_real/Calibration.md)
* [Citing Spot Mini Mini](#citing-spot-mini-mini)
* [Credits](#credits)
### Kinematics
Body manipulation with [leg IK](https://www.researchgate.net/publication/320307716_Inverse_Kinematic_Analysis_Of_A_Quadruped_Robot) and [body IK](https://moribots.github.io/project/spot-mini-mini) descriptions.
<img src="spot_bullet/media/spot_rpy.gif" alt="SIK" width="500"/>
<img src="spot_real/media/rpy.gif" alt="SRIK" width="500"/>
### D^2 Gait Modulation with Bezier Curves
I'm using this platform to validate a novel Reinforcement Learning method for locomotion by myself and my co-authors Matthew L. Elwin, Ian Abraham, and Todd D. Murphey. Instead of learning a gait from scratch, we propose using an existing scheme as a baseline over which we optimize via training. The method is called `D^2 Gait Modulation with Bezier Curves`. To learn more, visit our [website](https://sites.google.com/view/drgmbc)
<p float="left">
<img src="spot_real/media/V_descent.gif" width="335" />
<img src="spot_real/media/A_descent.gif" width="335" />
</p>
#### Training
During training, simple Proportional controller was employed to deliver yaw correction as would be the case if the robot were teleoperated or able to localize itself. For increased policy robustness, the terrain, link masses and foot frictions are randomized on each environment reset.
Here, the action space is 14-dimensional, consisting of `Clearance Height` (1), `Body Height` (1), and `Foot XYZ Residual` modulations (12). `Clearance Height` is treated through an exponential filter (`alpha = 0.7`), but all other actions are processed directly. These results were trained with only 149 epochs.
Before training, the robot falls almost immediately:

After training, the robot successfully navigates the terrain:

What's even better, is that the same agent `#149` is able to adapt to unseen commands, making high-level system integration straightforward. Here it is being teleoperated using `Forward`, `Lateral`, and `Yaw` commands.

Here's an example of the new URDF being teleoperated with a trained agent on 2x higher terrain:

#### Real World Validation
Here are some experimental results where the agent is on the right.
<p float="left">
<img src="spot_real/media/V2_3.gif" width="335" />
<img src="spot_real/media/T2_1.gif" width="335" />
</p>
### Gait
Open-Loop Gait using 12-Point Bezier Curves based on [MIT Cheetah Paper](https://dspace.mit.edu/handle/1721.1/98270) with [modifications](https://spot-mini-mini.readthedocs.io/en/latest/source/spotmicro.GaitGenerator.html#spotmicro.GaitGenerator.Bezier.BezierGait.GetPhase) for low step velocity discontinuity.
Forward and Lateral Motion:

Yaw logic based on [4-wheel steering car](http://www.inase.org/library/2014/santorini/bypaper/ROBCIRC/ROBCIRC-54.pdf):

## How To Run
### Dependencies
* ROS Melodic
* Gazebo
* Pytorch
* Pybullet
* Gym
* OpenCV
* Scipy
* Numpy
### Joystick Control with ROS
First, you're going to need a joystick (okay, not really, but it's more fun if you have one).
**Setting Up The Joystick:**
* Get Number (you will see something like jsX): `ls /dev/input/`
* Make available to ROS: `sudo chmod a+rw /dev/input/jsX`
* Make sure `<param name="dev" type="string" value="/dev/input/jsX"/>` matches your setup in the launchfile
Then simply: `roslaunch mini_ros spot_move.launch`
You can ignore this msg: `[ERROR] [1591631380.406690714]: Couldn't open joystick force feedback!` It just means your controller is missing some functionality, but this package doesn't use it.
**Controls:**
Assuming you have a Logitech Gamepad F310:
`A`: switch between stepping and RPY
`X`: E-STOP (engage and disengage)
**Stepping Mode**:
* `Right Stick Up/Down`: Step Length
* `Right Stick Left/Right`: Lateral Fraction
* `Left Stick Up/Down`: Robot Height
* `Left Stick Left/Right`: Yaw Rate
* `Arrow Pad Up/Down` (DISCRETE): Step Height
* `Arrow Pad Left/Right` (DISCRETE): Step Depth
* `Bottom Right/Left Bumpers`: Step Velocity (modulate)
* `Top Right/Left Bumpers`: reset all to default
**Viewing Mode**:
* `Right Stick Up/Down`: Pitch
* `Right Stick Left/Right`: Roll
* `Left Stick Up/Down`: Robot Height
* `Left Stick Left/Right`: Yaw
Changing `Step Velocity` while moving forward:

Changing `Step Length` while moving forward:

Yaw In Place: Slightly push the `Right Stick` forward while pushing the `Left Stick` maximally in either direction:

### Testing Environment (Non-Joystick)
If you don't have a joystick, go to `spot_bullet/src` and do `./env_tester.py`. A Pybullet sim will open up for you with the same controls you would have on the joystick, except each is on its own scrollbar. You may also use the following optional arguments:
```
-h, --help show this help message and exit
-hf, --HeightField Use HeightField
-r, --DebugRack Put Spot on an Elevated Rack
-p, --DebugPath Draw Spot's Foot Path
-ay, --AutoYaw Automatically Adjust Spot's Yaw
-ar, --AutoReset Automatically Reset Environment When Spot Falls
```
### Reinforcement Learning Agent Training
Go to `spot_bullet/src` and do `./spot_ars.py`. Models will be saved every `9th` episode to `spot_bullet/models/`. I will add some more arguments in the future to give you finer control of the heightfield mesh from the command line.
### Reinforcement Learning Agent Evaluation
Go to `spot_bullet/src` and do `./spot_ars_eval.py`. You may also use the following optional arguments. Note that if you don't use the `-a` argument, no agent will be loaded, so you will be using the open-loop policy. For example, if you enter `149` after `-a`, you will see the first successful policy, but if you enter `2229`, you will see a much more aggressive policy.
```
-h, --help show this help message and exit
-hf, --HeightField Use HeightField
-r, --DebugRack Put Spot on an Elevated Rack
-p, --DebugPath Draw Spot's Foot Path
-gui, --GUI Control The Robot Yourself With a GUI
-a, --AgentNum Agent Number To Load (followed by number)
```
### Using Different Terrain
Navigate to `spotmicro/heightfield.py` and take a look at `useProgrammatic` and `useTerrainFromPNG` (you can play around with the mesh scales for each) to experiment with different terrains. Make sure that the `spotBezierEnv` instance has `height_field=True` in `env_tester.py` and `spot_pybullet_interface` depending on whether you're using the joystick/ROS version. The same goes for the RL environments. Note: these were adapted from the [pybullet](https://github.com/bulletphysics/bullet3/blob/master/examples/pybullet/examples/heightfield.py) source code.
`useTerrainFromPNG`

`useProgrammatic`

With this terrain type, I programmed in a randomizer that triggers upon reset. This, along with the body randomizer from `Pybullet's Minitaur` increases your RL Policy's robustness.

## Citing Spot Mini Mini
```
@software{spotminimini2020github,
author = {Maurice Rahme and Ian Abraham and Matthew Elwin and Todd Murphey},
title = {SpotMiniMini: Pybullet Gym Environment for Gait Modulation with Bezier Curves},
url = {https://github.com/moribots/spot_mini_mini},
version = {2.1.0},
year = {2020},
}
```
## Credits
* Original Spot Design and CAD files: [Spot Micro AI Community](https://spotmicroai.readthedocs.io/en/latest/)
* Collaborator on `OpenQuadruped` design, including mechanical parts, custom PCB, and Teensy interface: [Adham Elarabawy](https://github.com/adham-elarabawy/OpenQuadruped)
* OpenAI Gym and Heightfield Interface: [Minitaur Environment](https://github.com/bulletphysics/bullet3/blob/master/examples/pybullet/gym/pybullet_envs/bullet/minitaur.py)
* Deprecated URDF for earlier development: [Rex Gym](https://github.com/nicrusso7/rex-gym)
| 12,090 | Markdown | 47.558233 | 560 | 0.750538 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/spot_ars.py | #!/usr/bin/env python
import numpy as np
import matplotlib.pyplot as plt
import sys
sys.path.append('../../')
from spotmicro.util.gui import GUI
from spotmicro.GymEnvs.spot_bezier_env import spotBezierEnv
from spotmicro.Kinematics.SpotKinematics import SpotModel
from spotmicro.GaitGenerator.Bezier import BezierGait
from spotmicro.OpenLoopSM.SpotOL import BezierStepper
from spotmicro.spot_env_randomizer import SpotEnvRandomizer
import time
from ars_lib.ars import ARSAgent, Normalizer, Policy, ParallelWorker
# Multiprocessing package for python
# Parallelization improvements based on:
# https://github.com/bulletphysics/bullet3/blob/master/examples/pybullet/gym/pybullet_envs/ARS/ars.py
import multiprocessing as mp
from multiprocessing import Pipe
import os
import argparse
# ARGUMENTS
descr = "Spot Mini Mini ARS Agent Trainer."
parser = argparse.ArgumentParser(description=descr)
parser.add_argument("-hf",
"--HeightField",
help="Use HeightField",
action='store_true')
parser.add_argument("-nc",
"--NoContactSensing",
help="Disable Contact Sensing",
action='store_true')
parser.add_argument("-dr",
"--DontRandomize",
help="Do NOT Randomize State and Environment.",
action='store_true')
parser.add_argument("-s", "--Seed", help="Seed (Default: 0).")
ARGS = parser.parse_args()
# Messages for Pipe
_RESET = 1
_CLOSE = 2
_EXPLORE = 3
def main():
""" The main() function. """
# Hold mp pipes
mp.freeze_support()
print("STARTING SPOT TRAINING ENV")
seed = 0
if ARGS.Seed:
seed = int(ARGS.Seed)
print("SEED: {}".format(seed))
max_timesteps = 4e6
eval_freq = 1e1
save_model = True
file_name = "spot_ars_"
if ARGS.HeightField:
height_field = True
else:
height_field = False
if ARGS.NoContactSensing:
contacts = False
else:
contacts = True
if ARGS.DontRandomize:
env_randomizer = None
rand_name = "norand_"
else:
env_randomizer = SpotEnvRandomizer()
rand_name = "rand_"
# Find abs path to this file
my_path = os.path.abspath(os.path.dirname(__file__))
results_path = os.path.join(my_path, "../results")
if contacts:
models_path = os.path.join(my_path, "../models/contact")
else:
models_path = os.path.join(my_path, "../models/no_contact")
if not os.path.exists(results_path):
os.makedirs(results_path)
if not os.path.exists(models_path):
os.makedirs(models_path)
env = spotBezierEnv(render=False,
on_rack=False,
height_field=height_field,
draw_foot_path=False,
contacts=contacts,
env_randomizer=env_randomizer)
# Set seeds
env.seed(seed)
np.random.seed(seed)
state_dim = env.observation_space.shape[0]
print("STATE DIM: {}".format(state_dim))
action_dim = env.action_space.shape[0]
print("ACTION DIM: {}".format(action_dim))
max_action = float(env.action_space.high[0])
env.reset()
g_u_i = GUI(env.spot.quadruped)
spot = SpotModel()
T_bf = spot.WorldToFoot
bz_step = BezierStepper(dt=env._time_step)
bzg = BezierGait(dt=env._time_step)
# Initialize Normalizer
normalizer = Normalizer(state_dim)
# Initialize Policy
policy = Policy(state_dim, action_dim, seed=seed)
# Initialize Agent with normalizer, policy and gym env
agent = ARSAgent(normalizer, policy, env, bz_step, bzg, spot)
agent_num = 0
if os.path.exists(models_path + "/" + file_name + str(agent_num) +
"_policy"):
print("Loading Existing agent")
agent.load(models_path + "/" + file_name + str(agent_num))
env.reset(agent.desired_velocity, agent.desired_rate)
episode_reward = 0
episode_timesteps = 0
episode_num = 0
# Create mp pipes
num_processes = policy.num_deltas
processes = []
childPipes = []
parentPipes = []
# Store mp pipes
for pr in range(num_processes):
parentPipe, childPipe = Pipe()
parentPipes.append(parentPipe)
childPipes.append(childPipe)
# Start multiprocessing
# Start multiprocessing
for proc_num in range(num_processes):
p = mp.Process(target=ParallelWorker,
args=(childPipes[proc_num], env, state_dim))
p.start()
processes.append(p)
print("STARTED SPOT TRAINING ENV")
t = 0
while t < (int(max_timesteps)):
# Maximum timesteps per rollout
episode_reward, episode_timesteps = agent.train_parallel(parentPipes)
t += episode_timesteps
# episode_reward = agent.train()
# +1 to account for 0 indexing.
# +0 on ep_timesteps since it will increment +1 even if done=True
print(
"Total T: {} Episode Num: {} Episode T: {} Reward: {:.2f} REWARD PER STEP: {:.2f}"
.format(t + 1, episode_num, episode_timesteps, episode_reward,
episode_reward / float(episode_timesteps)))
# Store Results (concat)
if episode_num == 0:
res = np.array(
[[episode_reward, episode_reward / float(episode_timesteps)]])
else:
new_res = np.array(
[[episode_reward, episode_reward / float(episode_timesteps)]])
res = np.concatenate((res, new_res))
# Also Save Results So Far (Overwrite)
# Results contain 2D numpy array of total reward for each ep
# and reward per timestep for each ep
np.save(
results_path + "/" + str(file_name) + rand_name + "seed" +
str(seed), res)
# Evaluate episode
if (episode_num + 1) % eval_freq == 0:
if save_model:
agent.save(models_path + "/" + str(file_name) +
str(episode_num))
episode_num += 1
# Close pipes and hence envs
for parentPipe in parentPipes:
parentPipe.send([_CLOSE, "pay2"])
for p in processes:
p.join()
if __name__ == '__main__':
main()
| 6,299 | Python | 28.166667 | 101 | 0.600572 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/env_tester.py | #!/usr/bin/env python
import numpy as np
import matplotlib.pyplot as plt
import copy
import sys
sys.path.append('../../')
from spotmicro.GymEnvs.spot_bezier_env import spotBezierEnv
from spotmicro.util.gui import GUI
from spotmicro.Kinematics.SpotKinematics import SpotModel
from spotmicro.Kinematics.LieAlgebra import RPY
from spotmicro.GaitGenerator.Bezier import BezierGait
from spotmicro.spot_env_randomizer import SpotEnvRandomizer
# TESTING
from spotmicro.OpenLoopSM.SpotOL import BezierStepper
import time
import os
import argparse
# ARGUMENTS
descr = "Spot Mini Mini Environment Tester (No Joystick)."
parser = argparse.ArgumentParser(description=descr)
parser.add_argument("-hf",
"--HeightField",
help="Use HeightField",
action='store_true')
parser.add_argument("-r",
"--DebugRack",
help="Put Spot on an Elevated Rack",
action='store_true')
parser.add_argument("-p",
"--DebugPath",
help="Draw Spot's Foot Path",
action='store_true')
parser.add_argument("-ay",
"--AutoYaw",
help="Automatically Adjust Spot's Yaw",
action='store_true')
parser.add_argument("-ar",
"--AutoReset",
help="Automatically Reset Environment When Spot Falls",
action='store_true')
parser.add_argument("-dr",
"--DontRandomize",
help="Do NOT Randomize State and Environment.",
action='store_true')
ARGS = parser.parse_args()
def main():
""" The main() function. """
print("STARTING SPOT TEST ENV")
seed = 0
max_timesteps = 4e6
# Find abs path to this file
my_path = os.path.abspath(os.path.dirname(__file__))
results_path = os.path.join(my_path, "../results")
models_path = os.path.join(my_path, "../models")
if not os.path.exists(results_path):
os.makedirs(results_path)
if not os.path.exists(models_path):
os.makedirs(models_path)
if ARGS.DebugRack:
on_rack = True
else:
on_rack = False
if ARGS.DebugPath:
draw_foot_path = True
else:
draw_foot_path = False
if ARGS.HeightField:
height_field = True
else:
height_field = False
if ARGS.DontRandomize:
env_randomizer = None
else:
env_randomizer = SpotEnvRandomizer()
env = spotBezierEnv(render=True,
on_rack=on_rack,
height_field=height_field,
draw_foot_path=draw_foot_path,
env_randomizer=env_randomizer)
# Set seeds
env.seed(seed)
np.random.seed(seed)
state_dim = env.observation_space.shape[0]
print("STATE DIM: {}".format(state_dim))
action_dim = env.action_space.shape[0]
print("ACTION DIM: {}".format(action_dim))
max_action = float(env.action_space.high[0])
state = env.reset()
g_u_i = GUI(env.spot.quadruped)
spot = SpotModel()
T_bf0 = spot.WorldToFoot
T_bf = copy.deepcopy(T_bf0)
bzg = BezierGait(dt=env._time_step)
bz_step = BezierStepper(dt=env._time_step, mode=0)
action = env.action_space.sample()
FL_phases = []
FR_phases = []
BL_phases = []
BR_phases = []
FL_Elbow = []
yaw = 0.0
print("STARTED SPOT TEST ENV")
t = 0
while t < (int(max_timesteps)):
bz_step.ramp_up()
pos, orn, StepLength, LateralFraction, YawRate, StepVelocity, ClearanceHeight, PenetrationDepth = bz_step.StateMachine(
)
pos, orn, StepLength, LateralFraction, YawRate, StepVelocity, ClearanceHeight, PenetrationDepth, SwingPeriod = g_u_i.UserInput(
)
# Update Swing Period
bzg.Tswing = SwingPeriod
yaw = env.return_yaw()
P_yaw = 5.0
if ARGS.AutoYaw:
YawRate += -yaw * P_yaw
# print("YAW RATE: {}".format(YawRate))
# TEMP
bz_step.StepLength = StepLength
bz_step.LateralFraction = LateralFraction
bz_step.YawRate = YawRate
bz_step.StepVelocity = StepVelocity
contacts = state[-4:]
FL_phases.append(env.spot.LegPhases[0])
FR_phases.append(env.spot.LegPhases[1])
BL_phases.append(env.spot.LegPhases[2])
BR_phases.append(env.spot.LegPhases[3])
# Get Desired Foot Poses
T_bf = bzg.GenerateTrajectory(StepLength, LateralFraction, YawRate,
StepVelocity, T_bf0, T_bf,
ClearanceHeight, PenetrationDepth,
contacts)
joint_angles = spot.IK(orn, pos, T_bf)
FL_Elbow.append(np.degrees(joint_angles[0][-1]))
# for i, (key, Tbf_in) in enumerate(T_bf.items()):
# print("{}: \t Angle: {}".format(key, np.degrees(joint_angles[i])))
# print("-------------------------")
env.pass_joint_angles(joint_angles.reshape(-1))
# Get External Observations
env.spot.GetExternalObservations(bzg, bz_step)
# Step
state, reward, done, _ = env.step(action)
# print("IMU Roll: {}".format(state[0]))
# print("IMU Pitch: {}".format(state[1]))
# print("IMU GX: {}".format(state[2]))
# print("IMU GY: {}".format(state[3]))
# print("IMU GZ: {}".format(state[4]))
# print("IMU AX: {}".format(state[5]))
# print("IMU AY: {}".format(state[6]))
# print("IMU AZ: {}".format(state[7]))
# print("-------------------------")
if done:
print("DONE")
if ARGS.AutoReset:
env.reset()
# plt.plot()
# # plt.plot(FL_phases, label="FL")
# # plt.plot(FR_phases, label="FR")
# # plt.plot(BL_phases, label="BL")
# # plt.plot(BR_phases, label="BR")
# plt.plot(FL_Elbow, label="FL ELbow (Deg)")
# plt.xlabel("dt")
# plt.ylabel("value")
# plt.title("Leg Phases")
# plt.legend()
# plt.show()
# time.sleep(1.0)
t += 1
env.close()
print(joint_angles)
if __name__ == '__main__':
main()
| 6,407 | Python | 27.864865 | 135 | 0.547214 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/spot_ars_eval.py | #!/usr/bin/env python
import numpy as np
import sys
sys.path.append('../../')
from ars_lib.ars import ARSAgent, Normalizer, Policy
from spotmicro.util.gui import GUI
from spotmicro.Kinematics.SpotKinematics import SpotModel
from spotmicro.GaitGenerator.Bezier import BezierGait
from spotmicro.OpenLoopSM.SpotOL import BezierStepper
from spotmicro.GymEnvs.spot_bezier_env import spotBezierEnv
from spotmicro.spot_env_randomizer import SpotEnvRandomizer
import matplotlib.pyplot as plt
import seaborn as sns
sns.set()
import os
import argparse
# ARGUMENTS
descr = "Spot Mini Mini ARS Agent Evaluator."
parser = argparse.ArgumentParser(description=descr)
parser.add_argument("-hf",
"--HeightField",
help="Use HeightField",
action='store_true')
parser.add_argument("-nr",
"--DontRender",
help="Don't Render environment",
action='store_true')
parser.add_argument("-r",
"--DebugRack",
help="Put Spot on an Elevated Rack",
action='store_true')
parser.add_argument("-p",
"--DebugPath",
help="Draw Spot's Foot Path",
action='store_true')
parser.add_argument("-gui",
"--GUI",
help="Control The Robot Yourself With a GUI",
action='store_true')
parser.add_argument("-nc",
"--NoContactSensing",
help="Disable Contact Sensing",
action='store_true')
parser.add_argument("-a", "--AgentNum", help="Agent Number To Load")
parser.add_argument("-dr",
"--DontRandomize",
help="Do NOT Randomize State and Environment.",
action='store_true')
parser.add_argument("-pp",
"--PlotPolicy",
help="Plot Policy Output after each Episode.",
action='store_true')
parser.add_argument("-ta",
"--TrueAction",
help="Plot Action as seen by the Robot.",
action='store_true')
parser.add_argument(
"-save",
"--SaveData",
help="Save the Policy Output to a .npy file in the results folder.",
action='store_true')
parser.add_argument("-s", "--Seed", help="Seed (Default: 0).")
ARGS = parser.parse_args()
def main():
""" The main() function. """
print("STARTING MINITAUR ARS")
# TRAINING PARAMETERS
# env_name = "MinitaurBulletEnv-v0"
seed = 0
if ARGS.Seed:
seed = ARGS.Seed
max_timesteps = 4e6
file_name = "spot_ars_"
if ARGS.DebugRack:
on_rack = True
else:
on_rack = False
if ARGS.DebugPath:
draw_foot_path = True
else:
draw_foot_path = False
if ARGS.HeightField:
height_field = True
else:
height_field = False
if ARGS.NoContactSensing:
contacts = False
else:
contacts = True
if ARGS.DontRender:
render = False
else:
render = True
if ARGS.DontRandomize:
env_randomizer = None
else:
env_randomizer = SpotEnvRandomizer()
# Find abs path to this file
my_path = os.path.abspath(os.path.dirname(__file__))
results_path = os.path.join(my_path, "../results")
if contacts:
models_path = os.path.join(my_path, "../models/contact")
else:
models_path = os.path.join(my_path, "../models/no_contact")
if not os.path.exists(results_path):
os.makedirs(results_path)
if not os.path.exists(models_path):
os.makedirs(models_path)
env = spotBezierEnv(render=render,
on_rack=on_rack,
height_field=height_field,
draw_foot_path=draw_foot_path,
contacts=contacts,
env_randomizer=env_randomizer)
# Set seeds
env.seed(seed)
np.random.seed(seed)
state_dim = env.observation_space.shape[0]
print("STATE DIM: {}".format(state_dim))
action_dim = env.action_space.shape[0]
print("ACTION DIM: {}".format(action_dim))
max_action = float(env.action_space.high[0])
env.reset()
spot = SpotModel()
bz_step = BezierStepper(dt=env._time_step)
bzg = BezierGait(dt=env._time_step)
# Initialize Normalizer
normalizer = Normalizer(state_dim)
# Initialize Policy
policy = Policy(state_dim, action_dim)
# to GUI or not to GUI
if ARGS.GUI:
gui = True
else:
gui = False
# Initialize Agent with normalizer, policy and gym env
agent = ARSAgent(normalizer, policy, env, bz_step, bzg, spot, gui)
agent_num = 0
if ARGS.AgentNum:
agent_num = ARGS.AgentNum
if os.path.exists(models_path + "/" + file_name + str(agent_num) +
"_policy"):
print("Loading Existing agent")
agent.load(models_path + "/" + file_name + str(agent_num))
agent.policy.episode_steps = np.inf
policy = agent.policy
env.reset()
episode_reward = 0
episode_timesteps = 0
episode_num = 0
print("STARTED MINITAUR TEST SCRIPT")
t = 0
while t < (int(max_timesteps)):
episode_reward, episode_timesteps = agent.deployTG()
t += episode_timesteps
# episode_reward = agent.train()
# +1 to account for 0 indexing.
# +0 on ep_timesteps since it will increment +1 even if done=True
print("Total T: {} Episode Num: {} Episode T: {} Reward: {}".format(
t, episode_num, episode_timesteps, episode_reward))
episode_num += 1
# Plot Policy Output
if ARGS.PlotPolicy or ARGS.TrueAction or ARGS.SaveData:
if ARGS.TrueAction:
action_name = "robot_act"
action = np.array(agent.true_action_history)
else:
action_name = "agent_act"
action = np.array(agent.action_history)
if ARGS.SaveData:
if height_field:
terrain_name = "rough_"
else:
terrain_name = "flat_"
np.save(
results_path + "/" + "policy_out_" + terrain_name + action_name, action)
print("SAVED DATA")
ClearHeight_act = action[:, 0]
BodyHeight_act = action[:, 1]
Residuals_act = action[:, 2:]
plt.plot(ClearHeight_act,
label='Clearance Height Mod',
color='black')
plt.plot(BodyHeight_act,
label='Body Height Mod',
color='darkviolet')
# FL
plt.plot(Residuals_act[:, 0],
label='Residual: FL (x)',
color='limegreen')
plt.plot(Residuals_act[:, 1],
label='Residual: FL (y)',
color='lime')
plt.plot(Residuals_act[:, 2],
label='Residual: FL (z)',
color='green')
# FR
plt.plot(Residuals_act[:, 3],
label='Residual: FR (x)',
color='lightskyblue')
plt.plot(Residuals_act[:, 4],
label='Residual: FR (y)',
color='dodgerblue')
plt.plot(Residuals_act[:, 5],
label='Residual: FR (z)',
color='blue')
# BL
plt.plot(Residuals_act[:, 6],
label='Residual: BL (x)',
color='firebrick')
plt.plot(Residuals_act[:, 7],
label='Residual: BL (y)',
color='crimson')
plt.plot(Residuals_act[:, 8],
label='Residual: BL (z)',
color='red')
# BR
plt.plot(Residuals_act[:, 9],
label='Residual: BR (x)',
color='gold')
plt.plot(Residuals_act[:, 10],
label='Residual: BR (y)',
color='orange')
plt.plot(Residuals_act[:, 11],
label='Residual: BR (z)',
color='coral')
plt.xlabel("Epoch Iteration")
plt.ylabel("Action Value")
plt.title("Policy Output")
plt.legend()
plt.show()
env.close()
if __name__ == '__main__':
main()
| 8,583 | Python | 29.119298 | 96 | 0.518467 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/old_eval_scripts/sac_eval.py | #!/usr/bin/env python
import numpy as np
from sac_lib import SoftActorCritic, NormalizedActions, ReplayBuffer, PolicyNetwork
from mini_bullet.minitaur_gym_env import MinitaurBulletEnv
import gym
import torch
import os
import time
def main():
""" The main() function. """
print("STARTING MINITAUR TD3")
# TRAINING PARAMETERS
# env_name = "MinitaurBulletEnv-v0"
seed = 0
max_timesteps = 4e6
file_name = "mini_td3_"
# Find abs path to this file
my_path = os.path.abspath(os.path.dirname(__file__))
results_path = os.path.join(my_path, "../results")
models_path = os.path.join(my_path, "../models")
if not os.path.exists(results_path):
os.makedirs(results_path)
if not os.path.exists(models_path):
os.makedirs(models_path)
env = NormalizedActions(MinitaurBulletEnv(render=True))
# Set seeds
env.seed(seed)
torch.manual_seed(seed)
np.random.seed(seed)
state_dim = env.observation_space.shape[0]
print("STATE DIM: {}".format(state_dim))
action_dim = env.action_space.shape[0]
print("ACTION DIM: {}".format(action_dim))
max_action = float(env.action_space.high[0])
print("RECORDED MAX ACTION: {}".format(max_action))
hidden_dim = 256
policy = PolicyNetwork(state_dim, action_dim, hidden_dim)
replay_buffer_size = 1000000
replay_buffer = ReplayBuffer(replay_buffer_size)
sac = SoftActorCritic(policy=policy,
state_dim=state_dim,
action_dim=action_dim,
replay_buffer=replay_buffer)
policy_num = 2239999
if os.path.exists(models_path + "/" + file_name + str(policy_num) +
"_critic"):
print("Loading Existing Policy")
sac.load(models_path + "/" + file_name + str(policy_num))
policy = sac.policy_net
# Evaluate untrained policy and init list for storage
evaluations = []
state = env.reset()
done = False
episode_reward = 0
episode_timesteps = 0
episode_num = 0
print("STARTED MINITAUR TEST SCRIPT")
for t in range(int(max_timesteps)):
episode_timesteps += 1
# Deterministic Policy Action
action = np.clip(policy.get_action(np.array(state)),
-max_action, max_action)
# rospy.logdebug("Selected Acton: {}".format(action))
# Perform action
next_state, reward, done, _ = env.step(action)
state = next_state
episode_reward += reward
# print("DT REWARD: {}".format(reward))
if done:
# +1 to account for 0 indexing.
# +0 on ep_timesteps since it will increment +1 even if done=True
print(
"Total T: {} Episode Num: {} Episode T: {} Reward: {}".format(
t + 1, episode_num, episode_timesteps, episode_reward))
# Reset environment
state, done = env.reset(), False
evaluations.append(episode_reward)
episode_reward = 0
episode_timesteps = 0
episode_num += 1
if __name__ == '__main__':
main()
| 3,153 | Python | 26.911504 | 83 | 0.595623 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/old_eval_scripts/ars_eval.py | #!/usr/bin/env python
import numpy as np
from ars_lib.ars import ARSAgent, Normalizer, Policy, ParallelWorker
from mini_bullet.minitaur_gym_env import MinitaurBulletEnv
import torch
import os
def main():
""" The main() function. """
print("STARTING MINITAUR ARS")
# TRAINING PARAMETERS
# env_name = "MinitaurBulletEnv-v0"
seed = 0
max_timesteps = 4e6
file_name = "mini_ars_"
# Find abs path to this file
my_path = os.path.abspath(os.path.dirname(__file__))
results_path = os.path.join(my_path, "../results")
models_path = os.path.join(my_path, "../models")
if not os.path.exists(results_path):
os.makedirs(results_path)
if not os.path.exists(models_path):
os.makedirs(models_path)
env = MinitaurBulletEnv(render=True)
# Set seeds
env.seed(seed)
torch.manual_seed(seed)
np.random.seed(seed)
state_dim = env.observation_space.shape[0]
print("STATE DIM: {}".format(state_dim))
action_dim = env.action_space.shape[0]
print("ACTION DIM: {}".format(action_dim))
max_action = float(env.action_space.high[0])
print("RECORDED MAX ACTION: {}".format(max_action))
# Initialize Normalizer
normalizer = Normalizer(state_dim)
# Initialize Policy
policy = Policy(state_dim, action_dim)
# Initialize Agent with normalizer, policy and gym env
agent = ARSAgent(normalizer, policy, env)
agent_num = raw_input("Policy Number: ")
if os.path.exists(models_path + "/" + file_name + str(agent_num) +
"_policy"):
print("Loading Existing agent")
agent.load(models_path + "/" + file_name + str(agent_num))
agent.policy.episode_steps = 3000
policy = agent.policy
# Evaluate untrained agent and init list for storage
evaluations = []
env.reset()
episode_reward = 0
episode_timesteps = 0
episode_num = 0
print("STARTED MINITAUR TEST SCRIPT")
t = 0
while t < (int(max_timesteps)):
# Maximum timesteps per rollout
t += policy.episode_steps
episode_timesteps += 1
episode_reward = agent.deploy()
# episode_reward = agent.train()
# +1 to account for 0 indexing.
# +0 on ep_timesteps since it will increment +1 even if done=True
print("Total T: {} Episode Num: {} Episode T: {} Reward: {}".format(
t, episode_num, policy.episode_steps, episode_reward))
# Reset environment
evaluations.append(episode_reward)
episode_reward = 0
episode_timesteps = 0
episode_num += 1
env.close()
if __name__ == '__main__':
main()
| 2,662 | Python | 25.63 | 76 | 0.622089 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/old_eval_scripts/spot_sac_eval.py | #!/usr/bin/env python
import numpy as np
from sac_lib import SoftActorCritic, NormalizedActions, ReplayBuffer, PolicyNetwork
import copy
from gym import spaces
import sys
sys.path.append('../../')
from spotmicro.GymEnvs.spot_bezier_env import spotBezierEnv
from spotmicro.Kinematics.SpotKinematics import SpotModel
from spotmicro.GaitGenerator.Bezier import BezierGait
# TESTING
from spotmicro.OpenLoopSM.SpotOL import BezierStepper
import time
import torch
import os
def main():
""" The main() function. """
print("STARTING SPOT SAC")
# TRAINING PARAMETERS
seed = 0
max_timesteps = 4e6
batch_size = 256
eval_freq = 1e4
save_model = True
file_name = "spot_sac_"
# Find abs path to this file
my_path = os.path.abspath(os.path.dirname(__file__))
results_path = os.path.join(my_path, "../results")
models_path = os.path.join(my_path, "../models")
if not os.path.exists(results_path):
os.makedirs(results_path)
if not os.path.exists(models_path):
os.makedirs(models_path)
env = spotBezierEnv(render=True,
on_rack=False,
height_field=False,
draw_foot_path=False)
env = NormalizedActions(env)
# Set seeds
env.seed(seed)
torch.manual_seed(seed)
np.random.seed(seed)
state_dim = env.observation_space.shape[0]
print("STATE DIM: {}".format(state_dim))
action_dim = env.action_space.shape[0]
print("ACTION DIM: {}".format(action_dim))
max_action = float(env.action_space.high[0])
print("RECORDED MAX ACTION: {}".format(max_action))
hidden_dim = 256
policy = PolicyNetwork(state_dim, action_dim, hidden_dim)
replay_buffer_size = 1000000
replay_buffer = ReplayBuffer(replay_buffer_size)
sac = SoftActorCritic(policy=policy,
state_dim=state_dim,
action_dim=action_dim,
replay_buffer=replay_buffer)
policy_num = raw_input("Policy Number: ")
if os.path.exists(models_path + "/" + file_name + str(policy_num) +
"_policy_net"):
print("Loading Existing Policy")
sac.load(models_path + "/" + file_name + str(policy_num))
policy = sac.policy_net
# Evaluate untrained policy and init list for storage
evaluations = []
state = env.reset()
done = False
episode_reward = 0
episode_timesteps = 0
episode_num = 0
max_t_per_ep = 5000
# State Machine for Random Controller Commands
bz_step = BezierStepper(dt=0.01, mode=0)
# Bezier Gait Generator
bzg = BezierGait(dt=0.01)
# Spot Model
spot = SpotModel()
T_bf0 = spot.WorldToFoot
T_bf = copy.deepcopy(T_bf0)
BaseClearanceHeight = bz_step.ClearanceHeight
BasePenetrationDepth = bz_step.PenetrationDepth
print("STARTED SPOT SAC")
for t in range(int(max_timesteps)):
contacts = state[-4:]
t += 1
episode_timesteps += 1
pos, orn, StepLength, LateralFraction, YawRate, StepVelocity, ClearanceHeight, PenetrationDepth = bz_step.StateMachine(
)
env.spot.GetExternalObservations(bzg, bz_step)
# Read UPDATED state based on controls and phase
state = env.return_state()
action = sac.policy_net.get_action(state)
# Bezier params specced by action
CD_SCALE = 0.002
SLV_SCALE = 0.01
StepLength += action[0] * CD_SCALE
StepVelocity += action[1] * SLV_SCALE
LateralFraction += action[2] * SLV_SCALE
YawRate = action[3]
ClearanceHeight += action[4] * CD_SCALE
PenetrationDepth += action[5] * CD_SCALE
# CLIP EVERYTHING
StepLength = np.clip(StepLength, bz_step.StepLength_LIMITS[0],
bz_step.StepLength_LIMITS[1])
StepVelocity = np.clip(StepVelocity, bz_step.StepVelocity_LIMITS[0],
bz_step.StepVelocity_LIMITS[1])
LateralFraction = np.clip(LateralFraction,
bz_step.LateralFraction_LIMITS[0],
bz_step.LateralFraction_LIMITS[1])
YawRate = np.clip(YawRate, bz_step.YawRate_LIMITS[0],
bz_step.YawRate_LIMITS[1])
ClearanceHeight = np.clip(ClearanceHeight,
bz_step.ClearanceHeight_LIMITS[0],
bz_step.ClearanceHeight_LIMITS[1])
PenetrationDepth = np.clip(PenetrationDepth,
bz_step.PenetrationDepth_LIMITS[0],
bz_step.PenetrationDepth_LIMITS[1])
contacts = state[-4:]
# Get Desired Foot Poses
T_bf = bzg.GenerateTrajectory(StepLength, LateralFraction, YawRate,
StepVelocity, T_bf0, T_bf,
ClearanceHeight, PenetrationDepth,
contacts)
# Add DELTA to XYZ Foot Poses
RESIDUALS_SCALE = 0.05
# T_bf["FL"][3, :3] += action[6:9] * RESIDUALS_SCALE
# T_bf["FR"][3, :3] += action[9:12] * RESIDUALS_SCALE
# T_bf["BL"][3, :3] += action[12:15] * RESIDUALS_SCALE
# T_bf["BR"][3, :3] += action[15:18] * RESIDUALS_SCALE
T_bf["FL"][3, 2] += action[6] * RESIDUALS_SCALE
T_bf["FR"][3, 2] += action[7] * RESIDUALS_SCALE
T_bf["BL"][3, 2] += action[8] * RESIDUALS_SCALE
T_bf["BR"][3, 2] += action[9] * RESIDUALS_SCALE
joint_angles = spot.IK(orn, pos, T_bf)
# Pass Joint Angles
env.pass_joint_angles(joint_angles.reshape(-1))
# Perform action
state, reward, done, _ = env.step(action)
episode_reward += reward
# print("DT REWARD: {}".format(reward))
if done:
# +1 to account for 0 indexing.
# +0 on ep_timesteps since it will increment +1 even if done=True
print(
"Total T: {} Episode Num: {} Episode T: {} Reward: {}".format(
t + 1, episode_num, episode_timesteps, episode_reward))
# Reset environment
state, done = env.reset(), False
evaluations.append(episode_reward)
episode_reward = 0
episode_timesteps = 0
episode_num += 1
env.close()
if __name__ == '__main__':
main() | 6,449 | Python | 31.089552 | 127 | 0.575748 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/old_eval_scripts/tg_eval.py | #!/usr/bin/env python
import numpy as np
import matplotlib.pyplot as plt
from ars_lib.ars import ARSAgent, Normalizer, Policy, ParallelWorker
from mini_bullet.minitaur_gym_env import MinitaurBulletEnv
from tg_lib.tg_policy import TGPolicy
import time
import torch
import os
def main():
""" The main() function. """
print("STARTING MINITAUR ARS")
# TRAINING PARAMETERS
# env_name = "MinitaurBulletEnv-v0"
seed = 0
max_timesteps = 1e6
file_name = "mini_tg_ars_"
# Find abs path to this file
my_path = os.path.abspath(os.path.dirname(__file__))
results_path = os.path.join(my_path, "../results")
models_path = os.path.join(my_path, "../models")
if not os.path.exists(results_path):
os.makedirs(results_path)
if not os.path.exists(models_path):
os.makedirs(models_path)
env = MinitaurBulletEnv(render=True, on_rack=False)
dt = env._time_step
# TRAJECTORY GENERATOR
movetype = "walk"
# movetype = "trot"
# movetype = "bound"
# movetype = "pace"
# movetype = "pronk"
TG = TGPolicy(movetype=movetype,
center_swing=0.0,
amplitude_extension=0.2,
amplitude_lift=0.4)
TG_state_dim = len(TG.get_TG_state())
TG_action_dim = 5 # f_tg, Beta, alpha_tg, h_tg, intensity
state_dim = env.observation_space.shape[0] + TG_state_dim
print("STATE DIM: {}".format(state_dim))
action_dim = env.action_space.shape[0] + TG_action_dim
print("ACTION DIM: {}".format(action_dim))
max_action = float(env.action_space.high[0])
print("RECORDED MAX ACTION: {}".format(max_action))
# Initialize Normalizer
normalizer = Normalizer(state_dim)
# Initialize Policy
policy = Policy(state_dim, action_dim)
# Initialize Agent with normalizer, policy and gym env
agent = ARSAgent(normalizer, policy, env, TGP=TG)
agent_num = raw_input("Policy Number: ")
if os.path.exists(models_path + "/" + file_name + str(agent_num) +
"_policy"):
print("Loading Existing agent")
agent.load(models_path + "/" + file_name + str(agent_num))
agent.policy.episode_steps = 1000
policy = agent.policy
# Set seeds
env.seed(seed)
torch.manual_seed(seed)
np.random.seed(seed)
env.reset()
episode_reward = 0
episode_timesteps = 0
episode_num = 0
print("STARTED MINITAUR TEST SCRIPT")
# Just to store correct action space
action = env.action_space.sample()
# Record extends for plot
# LF_ext = []
# LB_ext = []
# RF_ext = []
# RB_ext = []
LF_tp = []
LB_tp = []
RF_tp = []
RB_tp = []
t = 0
while t < (int(max_timesteps)):
action[:] = 0.0
# # Get Action from TG [no policies here]
# action = TG.get_utg(action, alpha_tg, h_tg, intensity,
# env.minitaur.num_motors)
# LF_ext.append(action[env.minitaur.num_motors / 2])
# LB_ext.append(action[1 + env.minitaur.num_motors / 2])
# RF_ext.append(action[2 + env.minitaur.num_motors / 2])
# RB_ext.append(action[3 + env.minitaur.num_motors / 2])
# # Perform action
# next_state, reward, done, _ = env.step(action)
obs = agent.TGP.get_TG_state()
# LF_tp.append(obs[0])
# LB_tp.append(obs[1])
# RF_tp.append(obs[2])
# RB_tp.append(obs[3])
# # Increment phase
# TG.increment(dt, f_tg, Beta)
# # time.sleep(1.0)
# t += 1
# Maximum timesteps per rollout
t += policy.episode_steps
episode_timesteps += 1
episode_reward = agent.deployTG()
# episode_reward = agent.train()
# +1 to account for 0 indexing.
# +0 on ep_timesteps since it will increment +1 even if done=True
print("Total T: {} Episode Num: {} Episode T: {} Reward: {}".format(
t, episode_num, policy.episode_steps, episode_reward))
# Reset environment
episode_reward = 0
episode_timesteps = 0
episode_num += 1
plt.plot(0)
plt.plot(LF_tp, label="LF")
plt.plot(LB_tp, label="LB")
plt.plot(RF_tp, label="RF")
plt.plot(RB_tp, label="RB")
plt.xlabel("t")
plt.ylabel("EXT")
plt.title("Leg Extensions")
plt.legend()
plt.show()
env.close()
if __name__ == '__main__':
main()
| 4,424 | Python | 25.981707 | 76 | 0.583635 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/td3_lib/td3.py | #!/usr/bin/env python
import copy
import pickle
import numpy as np
import torch
import torch.nn as nn
import torch.nn.functional as F
import gym
import os
# Twin Delayed Deterministic Policy Gradient
# Algorithm Steps
# 1. Initiailize Networks
class Actor(nn.Module):
"""Initialize parameters and build model.
An nn.Module contains layers, and a method
forward(input)that returns the output.
Weights (learnable params) are inherently defined here.
Args:
state_dim (int): Dimension of each state
action_dim (int): Dimension of each action
max_action (float): highest action to take
Return:
action output of network with tanh activation
"""
def __init__(self, state_dim, action_dim, max_action):
# Super calls the nn.Module Constructor
super(Actor, self).__init__()
# input layer
self.fc1 = nn.Linear(state_dim, 256)
# hidden layer
self.fc2 = nn.Linear(256, 256)
# output layer
self.fc3 = nn.Linear(256, action_dim)
# wrap from -max to +max
self.max_action = max_action
def forward(self, state):
# You just have to define the forward function,
# and the backward function (where gradients are computed)
# is automatically defined for you using autograd.
# Learnable params can be accessed using Actor.parameters
# Here, we create the tensor architecture
# state into layer 1
a = F.relu(self.fc1(state))
# layer 1 output into layer 2
a = F.relu(self.fc2(a))
# layer 2 output into layer 3 into tanh activation
return self.max_action * torch.tanh(self.fc3(a))
class Critic(nn.Module):
"""Initialize parameters and build model.
Args:
state_dim (int): Dimension of each state
action_dim (int): Dimension of each action
Return:
value output of network
"""
def __init__(self, state_dim, action_dim):
# Super calls the nn.Module Constructor
super(Critic, self).__init__()
# Q1 architecture
self.fc1 = nn.Linear(state_dim + action_dim, 256)
self.fc2 = nn.Linear(256, 256)
self.fc3 = nn.Linear(256, 1)
# Q2 architecture
self.fc4 = nn.Linear(state_dim + action_dim, 256)
self.fc5 = nn.Linear(256, 256)
self.fc6 = nn.Linear(256, 1)
def forward(self, state, action):
# concatenate state and actions by adding rows
# to form 1D input layer
sa = torch.cat([state, action], 1)
# s,a into input layer into relu activation
q1 = F.relu(self.fc1(sa))
# l1 output into l2 into relu activation
q1 = F.relu(self.fc2(q1))
# l2 output into l3
q1 = self.fc3(q1)
# s,a into input layer into relu activation
q2 = F.relu(self.fc4(sa))
# l4 output into l5 into relu activation
q2 = F.relu(self.fc5(q2))
# l5 output into l6
q2 = self.fc6(q2)
return q1, q2
def Q1(self, state, action):
# Return Q1 for gradient Ascent on Actor
# Note that only Q1 is used for Actor Update
# concatenate state and actions by adding rows
# to form 1D input layer
sa = torch.cat([state, action], 1)
# s,a into input layer into relu activation
q1 = F.relu(self.fc1(sa))
# l1 output into l2 into relu activation
q1 = F.relu(self.fc2(q1))
# l2 output into l3
q1 = self.fc3(q1)
return q1
# https://github.com/openai/baselines/blob/master/baselines/deepq/replay_buffer.py
# Expects tuples of (state, next_state, action, reward, done)
class ReplayBuffer(object):
"""Buffer to store tuples of experience replay"""
def __init__(self, max_size=1000000):
"""
Args:
max_size (int): total amount of tuples to store
"""
self.storage = []
self.max_size = max_size
self.ptr = 0
my_path = os.path.abspath(os.path.dirname(__file__))
self.buffer_path = os.path.join(my_path, "../../replay_buffer")
def add(self, data):
"""Add experience tuples to buffer
Args:
data (tuple): experience replay tuple
"""
if len(self.storage) == self.max_size:
self.storage[int(self.ptr)] = data
self.ptr = (self.ptr + 1) % self.max_size
else:
self.storage.append(data)
def save(self, iterations):
if not os.path.exists(self.buffer_path):
os.makedirs(self.buffer_path)
with open(
self.buffer_path + '/' + 'replay_buffer_' + str(iterations) +
'.data', 'wb') as filehandle:
pickle.dump(self.storage, filehandle)
def load(self, iterations):
with open(
self.buffer_path + '/' + 'replay_buffer_' + str(iterations) +
'.data', 'rb') as filehandle:
self.storage = pickle.load(filehandle)
def sample(self, batch_size):
"""Samples a random amount of experiences from buffer of batch size
NOTE: We don't delete samples here, only overwrite when max_size
Args:
batch_size (int): size of sample
"""
device = torch.device("cuda:1" if torch.cuda.is_available() else "cpu")
ind = np.random.randint(0, len(self.storage), size=batch_size)
states, actions, next_states, rewards, dones = [], [], [], [], []
for i in ind:
s, a, s_, r, d = self.storage[i]
states.append(np.array(s, copy=False))
state = torch.FloatTensor(np.array(states)).to(device)
actions.append(np.array(a, copy=False))
action = torch.FloatTensor(np.array(actions)).to(device)
next_states.append(np.array(s_, copy=False))
next_state = torch.FloatTensor(np.array(next_states)).to(device)
rewards.append(np.array(r, copy=False))
reward = torch.FloatTensor(np.array(rewards).reshape(-1,
1)).to(device)
dones.append(np.array(d, copy=False))
not_done = torch.FloatTensor(
1. - (np.array(dones).reshape(-1, 1))).to(device)
return state, action, next_state, reward, not_done
class TD3Agent(object):
"""Agent class that handles the training of the networks and
provides outputs as actions
Args:
state_dim (int): state size
action_dim (int): action size
max_action (float): highest action to take
device (device): cuda or cpu to process tensors
env (env): gym environment to use
batch_size(int): batch size to sample from replay buffer
discount (float): discount factor
tau (float): soft update for main networks to target networks
"""
def __init__(self,
state_dim,
action_dim,
max_action,
discount=0.99,
tau=0.005,
policy_noise=0.2,
noise_clip=0.5,
policy_freq=2):
self.device = torch.device(
"cuda:1" if torch.cuda.is_available() else "cpu")
self.actor = Actor(state_dim, action_dim, max_action).to(self.device)
self.actor_target = copy.deepcopy(self.actor)
self.actor_optimizer = torch.optim.Adam(self.actor.parameters(),
lr=3e-4)
self.critic = Critic(state_dim, action_dim).to(self.device)
self.critic_target = copy.deepcopy(self.critic)
self.critic_optimizer = torch.optim.Adam(self.critic.parameters(),
lr=3e-4)
self.max_action = max_action
self.discount = discount
self.tau = tau
self.policy_noise = policy_noise
self.noise_clip = noise_clip
self.policy_freq = policy_freq
self.total_it = 0
def select_action(self, state):
"""Select an appropriate action from the agent policy
Args:
state (array): current state of environment
Returns:
action (float): action clipped within action range
"""
# Turn float value into a CUDA Float Tensor
state = torch.FloatTensor(state.reshape(1, -1)).to(self.device)
action = self.actor(state).cpu().data.numpy().flatten()
return action
def train(self, replay_buffer, batch_size=100):
"""Train and update actor and critic networks
Args:
replay_buffer (ReplayBuffer): buffer for experience replay
batch_size(int): batch size to sample from replay buffer\
Return:
actor_loss (float): loss from actor network
critic_loss (float): loss from critic network
"""
self.total_it += 1
# Sample replay buffer
state, action, next_state, reward, not_done = replay_buffer.sample(
batch_size)
with torch.no_grad():
"""
Autograd: if you set its attribute .requires_gras as True,
(DEFAULT)
it tracks all operations on it. When you finish your
computation, call .backward() to have all gradients computed
automatically. The gradient for this tensor is then accumulated
into the .grad attribute.
To prevent tracking history and using memory, wrap the code block
in "with torch.no_grad()". This is heplful when evaluating a model
as it may have trainable params with requires_grad=True (DEFAULT),
but for which we don't need the gradients.
Here, we don't want to track the acyclic graph's history
when getting our next action because we DON'T want to train
our actor in this step. We train our actor ONLY when we perform
the periodic policy update. Could have done .detach() at
target_Q = reward + not_done * self.discount * target_Q
for the same effect
"""
# Select action according to policy and add clipped noise
noise = (torch.randn_like(action) * self.policy_noise).clamp(
-self.noise_clip, self.noise_clip)
next_action = (self.actor_target(next_state) + noise).clamp(
-self.max_action, self.max_action)
# Compute the target Q value
target_Q1, target_Q2 = self.critic_target(next_state, next_action)
target_Q = torch.min(target_Q1, target_Q2)
target_Q = reward + not_done * self.discount * target_Q
# Get current Q estimates
current_Q1, current_Q2 = self.critic(state, action)
# Compute critic loss
# A loss function takes the (output, target) pair of inputs,
# and computes a value that estimates how far away the output
# is from the target.
critic_loss = F.mse_loss(current_Q1, target_Q) + F.mse_loss(
current_Q2, target_Q)
# Optimize the critic
# Zero the gradient buffers of all parameters
self.critic_optimizer.zero_grad()
# Backprops with random gradients
# When we call loss.backward(), the whole graph is differentiated
# w.r.t. the loss, and all Tensors in the graph that has
# requires_grad=True (DEFAULT) will have their .grad Tensor
# accumulated with the gradient.
critic_loss.backward()
# Does the update
self.critic_optimizer.step()
# Delayed policy updates
if self.total_it % self.policy_freq == 0:
# Compute actor losse
actor_loss = -self.critic.Q1(state, self.actor(state)).mean()
# Optimize the actor
# Zero the gradient buffers
self.actor_optimizer.zero_grad()
# Differentiate the whole graph wrt loss
actor_loss.backward()
# Does the update
self.actor_optimizer.step()
# Update target networks (Critic 1, Critic 2, Actor)
for param, target_param in zip(self.critic.parameters(),
self.critic_target.parameters()):
target_param.data.copy_(self.tau * param.data +
(1 - self.tau) * target_param.data)
for param, target_param in zip(self.actor.parameters(),
self.actor_target.parameters()):
target_param.data.copy_(self.tau * param.data +
(1 - self.tau) * target_param.data)
def save(self, filename):
torch.save(self.critic.state_dict(), filename + "_critic")
torch.save(self.critic_optimizer.state_dict(),
filename + "_critic_optimizer")
torch.save(self.actor.state_dict(), filename + "_actor")
torch.save(self.actor_optimizer.state_dict(),
filename + "_actor_optimizer")
def load(self, filename):
self.critic.load_state_dict(
torch.load(filename + "_critic", map_location=self.device))
self.critic_optimizer.load_state_dict(
torch.load(filename + "_critic_optimizer",
map_location=self.device))
self.actor.load_state_dict(
torch.load(filename + "_actor", map_location=self.device))
self.actor_optimizer.load_state_dict(
torch.load(filename + "_actor_optimizer",
map_location=self.device))
# Runs policy for X episodes and returns average reward
# A fixed seed is used for the eval environment
def evaluate_policy(policy, env_name, seed, eval_episodes=10, render=False):
"""run several episodes using the best agent policy
Args:
policy (agent): agent to evaluate
env (env): gym environment
eval_episodes (int): how many test episodes to run
render (bool): show training
Returns:
avg_reward (float): average reward over the number of evaluations
"""
eval_env = gym.make(env_name, render=render)
eval_env.seed(seed + 100)
avg_reward = 0.
for _ in range(eval_episodes):
state, done = eval_env.reset(), False
while not done:
# if render:
# eval_env.render()
# sleep(0.01)
action = policy.select_action(np.array(state))
state, reward, done, _ = eval_env.step(action)
avg_reward += reward
avg_reward /= eval_episodes
print("---------------------------------------")
print("Evaluation over {} episodes: {}".format(eval_episodes, avg_reward))
print("---------------------------------------")
if render:
eval_env.close()
return avg_reward
def trainer(env_name,
seed,
max_timesteps,
start_timesteps,
expl_noise,
batch_size,
eval_freq,
save_model,
file_name="best_avg"):
""" Test Script on stock OpenAI Gym Envs
"""
if not os.path.exists("../results"):
os.makedirs("../results")
if not os.path.exists("../models"):
os.makedirs("../models")
env = gym.make(env_name)
# Set seeds
env.seed(seed)
torch.manual_seed(seed)
np.random.seed(seed)
state_dim = env.observation_space.shape[0]
action_dim = env.action_space.shape[0]
max_action = float(env.action_space.high[0])
policy = TD3Agent(state_dim, action_dim, max_action)
replay_buffer = ReplayBuffer()
# Evaluate untrained policy and init list for storage
evaluations = [evaluate_policy(policy, env_name, seed, 1)]
state = env.reset()
done = False
episode_reward = 0
episode_timesteps = 0
episode_num = 0
for t in range(int(max_timesteps)):
episode_timesteps += 1
# Select action randomly or according to policy
# Random Action - no training yet, just storing in buffer
if t < start_timesteps:
action = env.action_space.sample()
else:
# According to policy + Exploraton Noise
action = (policy.select_action(np.array(state)) + np.random.normal(
0, max_action * expl_noise, size=action_dim)).clip(
-max_action, max_action)
# Perform action
next_state, reward, done, _ = env.step(action)
done_bool = float(
done) if episode_timesteps < env._max_episode_steps else 0
# Store data in replay buffer
replay_buffer.add((state, action, next_state, reward, done_bool))
state = next_state
episode_reward += reward
# Train agent after collecting sufficient data for buffer
if t >= start_timesteps:
policy.train(replay_buffer, batch_size)
if done:
# +1 to account for 0 indexing.
# +0 on ep_timesteps since it will increment +1 even if done=True
print(
"Total T: {} Episode Num: {} Episode T: {} Reward: {}".format(
t + 1, episode_num, episode_timesteps, episode_reward))
# Reset environment
state, done = env.reset(), False
episode_reward = 0
episode_timesteps = 0
episode_num += 1
# Evaluate episode
if (t + 1) % eval_freq == 0:
evaluations.append(evaluate_policy(policy, env_name, seed, 1))
np.save("../results/" + str(file_name) + str(t), evaluations)
if save_model:
policy.save("../models/" + str(file_name) + str(t))
if __name__ == "__main__":
""" The Main Function """
trainer("BipedalWalker-v2", 0, 1e6, 1e4, 0.1, 100, 15e3, True) | 18,049 | Python | 34.392157 | 82 | 0.57017 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/td3_lib/plot_reward.py | #!/usr/bin/env python
import matplotlib.pyplot as plt
import numpy as np
if __name__ == '__main__':
reward = np.load("../../results/plen_walk_gazebo_.npy")
plt.figure(0)
plt.autoscale(enable=True, axis='both', tight=None)
plt.title('Dominant Foot Trajectories - SS')
plt.ylabel('positon (mm)')
plt.xlabel('timestep')
plt.plot(reward, color='b', label="Reward")
plt.legend()
plt.show()
| 426 | Python | 21.473683 | 59 | 0.626761 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/ars_lib/ars.py | # from tg_lib.tg_policy import TGPolicy
import pickle
import numpy as np
from scipy.signal import butter, filtfilt
from spotmicro.GaitGenerator.Bezier import BezierGait
from spotmicro.OpenLoopSM.SpotOL import BezierStepper
from spotmicro.Kinematics.SpotKinematics import SpotModel
from spotmicro.Kinematics.LieAlgebra import TransToRp
import copy
from spotmicro.util.gui import GUI
np.random.seed(0)
# Multiprocessing package for python
# Parallelization improvements based on:
# https://github.com/bulletphysics/bullet3/blob/master/examples/pybullet/gym/pybullet_envs/ARS/ars.py
# Messages for Pipes
_RESET = 1
_CLOSE = 2
_EXPLORE = 3
_EXPLORE_TG = 4
# Params for TG
CD_SCALE = 0.05
SLV_SCALE = 0.05
RESIDUALS_SCALE = 0.015
Z_SCALE = 0.05
# Filter actions
alpha = 0.7
# Added this to avoid filtering residuals
# -1 for all
actions_to_filter = 14
# For auto yaw control
P_yaw = 5.0
# Cummulative timestep exponential reward
# cum_dt_exp = 1.1
cum_dt_exp = 0.0
def butter_lowpass_filter(data, cutoff, fs, order=2):
""" Pass two subsequent datapoints in here to be filtered
"""
nyq = 0.5 * fs # Nyquist Frequency
normal_cutoff = cutoff / nyq
# Get the filter coefficients
b, a = butter(order, normal_cutoff, btype='low', analog=False)
y = filtfilt(b, a, data)
return y
def ParallelWorker(childPipe, env, nb_states):
""" Function to deploy multiple ARS agents in parallel
"""
# nb_states = env.observation_space.shape[0]
# common normalizer
normalizer = Normalizer(nb_states)
max_action = float(env.action_space.high[0])
_ = env.reset()
n = 0
while True:
n += 1
try:
# Only block for short times to have keyboard exceptions be raised.
if not childPipe.poll(0.001):
continue
message, payload = childPipe.recv()
except (EOFError, KeyboardInterrupt):
break
if message == _RESET:
_ = env.reset()
childPipe.send(["reset ok"])
continue
if message == _EXPLORE:
# Payloads received by parent in ARSAgent.train()
# [0]: normalizer, [1]: policy, [2]: direction, [3]: delta
# we use local normalizer so no need for [0] (optional)
# normalizer = payload[0]
policy = payload[1]
direction = payload[2]
delta = payload[3]
desired_velocity = payload[4]
desired_rate = payload[5]
state = env.reset(desired_velocity, desired_rate)
sum_rewards = 0.0
timesteps = 0
done = False
while not done and timesteps < policy.episode_steps:
normalizer.observe(state)
# Normalize State
state = normalizer.normalize(state)
action = policy.evaluate(state, delta, direction)
# # Clip action between +-1 for execution in env
# for a in range(len(action)):
# action[a] = np.clip(action[a], -max_action, max_action)
state, reward, done, _ = env.step(action)
reward = max(min(reward, 1), -1)
sum_rewards += reward
timesteps += 1
childPipe.send([sum_rewards])
continue
if message == _EXPLORE_TG:
# Payloads received by parent in ARSAgent.train()
# [0]: normalizer, [1]: policy, [2]: direction, [3]: delta
# [4]: desired_velocity, [5]: desired_rate, [6]: Trajectory Gen
# we use local normalizer so no need for [0] (optional)
# normalizer = payload[0]
policy = payload[1]
direction = payload[2]
delta = payload[3]
desired_velocity = payload[4]
desired_rate = payload[5]
TGP = payload[6]
smach = payload[7]
spot = payload[8]
state = env.reset()
sum_rewards = 0.0
timesteps = 0
done = False
T_bf = copy.deepcopy(spot.WorldToFoot)
T_b0 = copy.deepcopy(spot.WorldToFoot)
action = env.action_space.sample()
action[:] = 0.0
old_act = action[:actions_to_filter]
# For auto yaw control
yaw = 0.0
while not done and timesteps < policy.episode_steps:
# smach.ramp_up()
pos, orn, StepLength, LateralFraction, YawRate, StepVelocity, ClearanceHeight, PenetrationDepth = smach.StateMachine(
)
env.spot.GetExternalObservations(TGP, smach)
# Read UPDATED state based on controls and phase
state = env.return_state()
normalizer.observe(state)
# NOTE: Don't normalize contacts - must stay 0/1
state = normalizer.normalize(state)
action = policy.evaluate(state, delta, direction)
contacts = state[-4:]
action = np.tanh(action)
# EXP FILTER
action[:actions_to_filter] = alpha * old_act + (
1.0 - alpha) * action[:actions_to_filter]
old_act = action[:actions_to_filter]
ClearanceHeight += action[0] * CD_SCALE
# CLIP EVERYTHING
StepLength = np.clip(StepLength, smach.StepLength_LIMITS[0],
smach.StepLength_LIMITS[1])
StepVelocity = np.clip(StepVelocity,
smach.StepVelocity_LIMITS[0],
smach.StepVelocity_LIMITS[1])
LateralFraction = np.clip(LateralFraction,
smach.LateralFraction_LIMITS[0],
smach.LateralFraction_LIMITS[1])
YawRate = np.clip(YawRate, smach.YawRate_LIMITS[0],
smach.YawRate_LIMITS[1])
ClearanceHeight = np.clip(ClearanceHeight,
smach.ClearanceHeight_LIMITS[0],
smach.ClearanceHeight_LIMITS[1])
PenetrationDepth = np.clip(PenetrationDepth,
smach.PenetrationDepth_LIMITS[0],
smach.PenetrationDepth_LIMITS[1])
# For auto yaw control
yaw = env.return_yaw()
YawRate += -yaw * P_yaw
# Get Desired Foot Poses
if timesteps > 20:
T_bf = TGP.GenerateTrajectory(StepLength, LateralFraction,
YawRate, StepVelocity, T_b0,
T_bf, ClearanceHeight,
PenetrationDepth, contacts)
else:
T_bf = TGP.GenerateTrajectory(0.0, 0.0, 0.0, 0.1, T_b0,
T_bf, ClearanceHeight,
PenetrationDepth, contacts)
action[:] = 0.0
action[2:] *= RESIDUALS_SCALE
# Add DELTA to XYZ Foot Poses
T_bf_copy = copy.deepcopy(T_bf)
T_bf_copy["FL"][:3, 3] += action[2:5]
T_bf_copy["FR"][:3, 3] += action[5:8]
T_bf_copy["BL"][:3, 3] += action[8:11]
T_bf_copy["BR"][:3, 3] += action[11:14]
# Adjust Body Height with action!
pos[2] += abs(action[1]) * Z_SCALE
joint_angles = spot.IK(orn, pos, T_bf_copy)
# Pass Joint Angles
env.pass_joint_angles(joint_angles.reshape(-1))
# Perform action
next_state, reward, done, _ = env.step(action)
sum_rewards += reward
timesteps += 1
# Divide reward by timesteps for normalized reward + add exponential surival reward
childPipe.send([(sum_rewards + timesteps**cum_dt_exp) / timesteps])
continue
if message == _CLOSE:
childPipe.send(["close ok"])
break
childPipe.close()
class Policy():
""" state --> action
"""
def __init__(
self,
state_dim,
action_dim,
# how much weights are changed each step
learning_rate=0.03,
# number of random expl_noise variations generated
# each step
# each one will be run for 2 epochs, + and -
num_deltas=16,
# used to update weights, sorted by highest rwrd
num_best_deltas=16,
# number of timesteps per episode per rollout
episode_steps=5000,
# weight of sampled exploration noise
expl_noise=0.05,
# for seed gen
seed=0):
# Tunable Hyperparameters
self.learning_rate = learning_rate
self.num_deltas = num_deltas
self.num_best_deltas = num_best_deltas
# there cannot be more best_deltas than there are deltas
assert self.num_best_deltas <= self.num_deltas
self.episode_steps = episode_steps
self.expl_noise = expl_noise
self.seed = seed
np.random.seed(seed)
self.state_dim = state_dim
self.action_dim = action_dim
# input/ouput matrix with weights set to zero
# this is the perception matrix (policy)
self.theta = np.zeros((action_dim, state_dim))
def evaluate(self, state, delta=None, direction=None):
""" state --> action
"""
# if direction is None, deployment mode: takes dot product
# to directly sample from (use) policy
if direction is None:
return self.theta.dot(state)
# otherwise, add (+-) directed expl_noise before taking dot product (policy)
# this is where the 2*num_deltas rollouts comes from
elif direction == "+":
return (self.theta + self.expl_noise * delta).dot(state)
elif direction == "-":
return (self.theta - self.expl_noise * delta).dot(state)
def sample_deltas(self):
""" generate array of random expl_noise matrices. Length of
array = num_deltas
matrix dimension: pxn where p=observation dim and
n=action dim
"""
deltas = []
# print("SHAPE THING with *: {}".format(*self.theta.shape))
# print("SHAPE THING NORMALLY: ({}, {})".format(self.theta.shape[0],
# self.theta.shape[1]))
# print("ACTUAL SHAPE: {}".format(self.theta.shape))
# print("SHAPE OF EXAMPLE DELTA WITH *: {}".format(
# np.random.randn(*self.theta.shape).shape))
# print("SHAPE OF EXAMPLE DELTA NOMRALLY: {}".format(
# np.random.randn(self.theta.shape[0], self.theta.shape[1]).shape))
for _ in range(self.num_deltas):
deltas.append(
np.random.randn(self.theta.shape[0], self.theta.shape[1]))
return deltas
def update(self, rollouts, std_dev_rewards):
""" Update policy weights (theta) based on rewards
from 2*num_deltas rollouts
"""
step = np.zeros(self.theta.shape)
for r_pos, r_neg, delta in rollouts:
# how much to deviate from policy
step += (r_pos - r_neg) * delta
self.theta += self.learning_rate / (self.num_best_deltas *
std_dev_rewards) * step
class Normalizer():
""" this ensures that the policy puts equal weight upon
each state component.
"""
# Normalizes the states
def __init__(self, state_dim):
""" Initialize state space (all zero)
"""
self.state = np.zeros(state_dim)
self.mean = np.zeros(state_dim)
self.mean_diff = np.zeros(state_dim)
self.var = np.zeros(state_dim)
def observe(self, x):
""" Compute running average and variance
clip variance >0 to avoid division by zero
"""
self.state += 1.0
last_mean = self.mean.copy()
# running avg
self.mean += (x - self.mean) / self.state
# used to compute variance
self.mean_diff += (x - last_mean) * (x - self.mean)
# variance
self.var = (self.mean_diff / self.state).clip(min=1e-2)
def normalize(self, states):
""" subtract mean state value from current state
and divide by standard deviation (sqrt(var))
to normalize
"""
state_mean = self.mean
state_std = np.sqrt(self.var)
return (states - state_mean) / state_std
class ARSAgent():
def __init__(self,
normalizer,
policy,
env,
smach=None,
TGP=None,
spot=None,
gui=False):
self.normalizer = normalizer
self.policy = policy
self.state_dim = self.policy.state_dim
self.action_dim = self.policy.action_dim
self.env = env
self.max_action = float(self.env.action_space.high[0])
self.successes = 0
self.phase = 0
self.desired_velocity = 0.5
self.desired_rate = 0.0
self.flip = 0
self.increment = 0
self.scaledown = True
self.type = "Stop"
self.smach = smach
if smach is not None:
self.BaseClearanceHeight = self.smach.ClearanceHeight
self.BasePenetrationDepth = self.smach.PenetrationDepth
self.TGP = TGP
self.spot = spot
if gui:
self.g_u_i = GUI(self.env.spot.quadruped)
else:
self.g_u_i = None
self.action_history = []
self.true_action_history = []
# Deploy Policy in one direction over one whole episode
# DO THIS ONCE PER ROLLOUT OR DURING DEPLOYMENT
def deploy(self, direction=None, delta=None):
state = self.env.reset(self.desired_velocity, self.desired_rate)
sum_rewards = 0.0
timesteps = 0
done = False
while not done and timesteps < self.policy.episode_steps:
# print("STATE: ", state)
# print("dt: {}".format(timesteps))
self.normalizer.observe(state)
# Normalize State
state = self.normalizer.normalize(state)
action = self.policy.evaluate(state, delta, direction)
# Clip action between +-1 for execution in env
for a in range(len(action)):
action[a] = np.clip(action[a], -self.max_action,
self.max_action)
# print("ACTION: ", action)
state, reward, done, _ = self.env.step(action)
# print("STATE: ", state)
# Clip reward between -1 and 1 to prevent outliers from
# distorting weights
reward = np.clip(reward, -self.max_action, self.max_action)
sum_rewards += reward
timesteps += 1
# Divide rewards by timesteps for reward-per-step + exp survive rwd
return (sum_rewards + timesteps**cum_dt_exp) / timesteps
# Deploy Policy in one direction over one whole episode
# DO THIS ONCE PER ROLLOUT OR DURING DEPLOYMENT
def deployTG(self, direction=None, delta=None):
state = self.env.reset()
sum_rewards = 0.0
timesteps = 0
done = False
# alpha = []
# h = []
# f = []
T_bf = copy.deepcopy(self.spot.WorldToFoot)
T_b0 = copy.deepcopy(self.spot.WorldToFoot)
self.action_history = []
self.true_action_history = []
action = self.env.action_space.sample()
action[:] = 0.0
old_act = action[:actions_to_filter]
# For auto yaw correction
yaw = 0.0
while not done and timesteps < self.policy.episode_steps:
# self.smach.ramp_up()
pos, orn, StepLength, LateralFraction, YawRate, StepVelocity, ClearanceHeight, PenetrationDepth = self.smach.StateMachine(
)
if self.g_u_i:
pos, orn, StepLength, LateralFraction, YawRate, StepVelocity, ClearanceHeight, PenetrationDepth, SwingPeriod = self.g_u_i.UserInput(
)
self.TGP.Tswing = SwingPeriod
self.env.spot.GetExternalObservations(self.TGP, self.smach)
# Read UPDATED state based on controls and phase
state = self.env.return_state()
self.normalizer.observe(state)
# Don't normalize contacts
state = self.normalizer.normalize(state)
action = self.policy.evaluate(state, delta, direction)
# Action History
self.action_history.append(np.tanh(action))
# Action History
true_action = copy.deepcopy(np.tanh(action))
true_action[0] *= CD_SCALE
true_action[1] = abs(true_action[1]) * Z_SCALE
true_action[2:] *= RESIDUALS_SCALE
self.true_action_history.append(true_action)
action = np.tanh(action)
# EXP FILTER
action[:actions_to_filter] = alpha * old_act + (
1.0 - alpha) * action[:actions_to_filter]
old_act = action[:actions_to_filter]
ClearanceHeight += action[0] * CD_SCALE
# CLIP EVERYTHING
StepLength = np.clip(StepLength, self.smach.StepLength_LIMITS[0],
self.smach.StepLength_LIMITS[1])
StepVelocity = np.clip(StepVelocity,
self.smach.StepVelocity_LIMITS[0],
self.smach.StepVelocity_LIMITS[1])
LateralFraction = np.clip(LateralFraction,
self.smach.LateralFraction_LIMITS[0],
self.smach.LateralFraction_LIMITS[1])
YawRate = np.clip(YawRate, self.smach.YawRate_LIMITS[0],
self.smach.YawRate_LIMITS[1])
ClearanceHeight = np.clip(ClearanceHeight,
self.smach.ClearanceHeight_LIMITS[0],
self.smach.ClearanceHeight_LIMITS[1])
PenetrationDepth = np.clip(PenetrationDepth,
self.smach.PenetrationDepth_LIMITS[0],
self.smach.PenetrationDepth_LIMITS[1])
contacts = copy.deepcopy(state[-4:])
# contacts = [0, 0, 0, 0]
# print("CONTACTS: {}".format(contacts))
yaw = self.env.return_yaw()
if not self.g_u_i:
YawRate += -yaw * P_yaw
# Get Desired Foot Poses
if timesteps > 20:
T_bf = self.TGP.GenerateTrajectory(StepLength, LateralFraction,
YawRate, StepVelocity, T_b0,
T_bf, ClearanceHeight,
PenetrationDepth, contacts)
else:
T_bf = self.TGP.GenerateTrajectory(0.0, 0.0, 0.0, 0.1, T_b0,
T_bf, ClearanceHeight,
PenetrationDepth, contacts)
action[:] = 0.0
action[2:] *= RESIDUALS_SCALE
# Add DELTA to XYZ Foot Poses
T_bf_copy = copy.deepcopy(T_bf)
T_bf_copy["FL"][:3, 3] += action[2:5]
T_bf_copy["FR"][:3, 3] += action[5:8]
T_bf_copy["BL"][:3, 3] += action[8:11]
T_bf_copy["BR"][:3, 3] += action[11:14]
# Adjust Height!
pos[2] += abs(action[1]) * Z_SCALE
joint_angles = self.spot.IK(orn, pos, T_bf_copy)
# Pass Joint Angles
self.env.pass_joint_angles(joint_angles.reshape(-1))
# Perform action
next_state, reward, done, _ = self.env.step(action)
sum_rewards += reward
timesteps += 1
self.TGP.reset()
self.smach.reshuffle()
self.smach.PenetrationDepth = self.BasePenetrationDepth
self.smach.ClearanceHeight = self.BaseClearanceHeight
return sum_rewards, timesteps
def returnPose(self):
return self.env.spot.GetBasePosition()
def train(self):
# Sample random expl_noise deltas
print("-------------------------------")
# print("Sampling Deltas")
deltas = self.policy.sample_deltas()
# Initialize +- reward list of size num_deltas
positive_rewards = [0] * self.policy.num_deltas
negative_rewards = [0] * self.policy.num_deltas
# Execute 2*num_deltas rollouts and store +- rewards
print("Deploying Rollouts")
for i in range(self.policy.num_deltas):
print("Rollout #{}".format(i + 1))
positive_rewards[i] = self.deploy(direction="+", delta=deltas[i])
negative_rewards[i] = self.deploy(direction="-", delta=deltas[i])
# Calculate std dev
std_dev_rewards = np.array(positive_rewards + negative_rewards).std()
# Order rollouts in decreasing list using cum reward as criterion
unsorted_rollouts = [(positive_rewards[i], negative_rewards[i],
deltas[i])
for i in range(self.policy.num_deltas)]
# When sorting, take the max between the reward for +- disturbance
sorted_rollouts = sorted(
unsorted_rollouts,
key=lambda x: max(unsorted_rollouts[0], unsorted_rollouts[1]),
reverse=True)
# Only take first best_num_deltas rollouts
rollouts = sorted_rollouts[:self.policy.num_best_deltas]
# Update Policy
self.policy.update(rollouts, std_dev_rewards)
# Execute Current Policy
eval_reward = self.deploy()
return eval_reward
def train_parallel(self, parentPipes):
""" Execute rollouts in parallel using multiprocessing library
based on: # https://github.com/bulletphysics/bullet3/blob/master/examples/pybullet/gym/pybullet_envs/ARS/ars.py
"""
# USE VANILLA OR TG POLICY
if self.TGP is None:
exploration = _EXPLORE
else:
exploration = _EXPLORE_TG
# Initializing the perturbations deltas and the positive/negative rewards
deltas = self.policy.sample_deltas()
# Initialize +- reward list of size num_deltas
positive_rewards = [0] * self.policy.num_deltas
negative_rewards = [0] * self.policy.num_deltas
smach = copy.deepcopy(self.smach)
smach.ClearanceHeight = self.BaseClearanceHeight
smach.PenetrationDepth = self.BasePenetrationDepth
smach.reshuffle()
if parentPipes:
for i in range(self.policy.num_deltas):
# Execute each rollout on a separate thread
parentPipe = parentPipes[i]
# NOTE: target for parentPipe specified in main_ars.py
# (target is ParallelWorker fcn defined up top)
parentPipe.send([
exploration,
[
self.normalizer, self.policy, "+", deltas[i],
self.desired_velocity, self.desired_rate, self.TGP,
smach, self.spot
]
])
for i in range(self.policy.num_deltas):
# Receive cummulative reward from each rollout
positive_rewards[i] = parentPipes[i].recv()[0]
for i in range(self.policy.num_deltas):
# Execute each rollout on a separate thread
parentPipe = parentPipes[i]
parentPipe.send([
exploration,
[
self.normalizer, self.policy, "-", deltas[i],
self.desired_velocity, self.desired_rate, self.TGP,
smach, self.spot
]
])
for i in range(self.policy.num_deltas):
# Receive cummulative reward from each rollout
negative_rewards[i] = parentPipes[i].recv()[0]
else:
raise ValueError(
"Select 'train' method if you are not using multiprocessing!")
# Calculate std dev
std_dev_rewards = np.array(positive_rewards + negative_rewards).std()
# Order rollouts in decreasing list using cum reward as criterion
# take max between reward for +- disturbance as that rollout's reward
# Store max between positive and negative reward as key for sort
scores = {
k: max(r_pos, r_neg)
for k, (
r_pos,
r_neg) in enumerate(zip(positive_rewards, negative_rewards))
}
indeces = sorted(scores.keys(), key=lambda x: scores[x],
reverse=True)[:self.policy.num_deltas]
# print("INDECES: ", indeces)
rollouts = [(positive_rewards[k], negative_rewards[k], deltas[k])
for k in indeces]
# Update Policy
self.policy.update(rollouts, std_dev_rewards)
# Execute Current Policy USING VANILLA OR TG
if self.TGP is None:
return self.deploy()
else:
return self.deployTG()
def save(self, filename):
""" Save the Policy
:param filename: the name of the file where the policy is saved
"""
with open(filename + '_policy', 'wb') as filehandle:
pickle.dump(self.policy.theta, filehandle)
def load(self, filename):
""" Load the Policy
:param filename: the name of the file where the policy is saved
"""
with open(filename + '_policy', 'rb') as filehandle:
self.policy.theta = pickle.load(filehandle)
| 26,330 | Python | 37.836283 | 148 | 0.536308 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/mini_bullet/terrain_env_randomizer.py | """Generates a random terrain at Minitaur gym environment reset."""
from __future__ import absolute_import
from __future__ import division
from __future__ import print_function
import random
import os, inspect
currentdir = os.path.dirname(
os.path.abspath(inspect.getfile(inspect.currentframe())))
parentdir = os.path.dirname(os.path.dirname(currentdir))
parentdir = os.path.dirname(os.path.dirname(parentdir))
os.sys.path.insert(0, parentdir)
import itertools
import math
import enum
import numpy as np
from pybullet_envs.minitaur.envs import env_randomizer_base
_GRID_LENGTH = 15
_GRID_WIDTH = 10
_MAX_SAMPLE_SIZE = 30
_MIN_BLOCK_DISTANCE = 0.7
_MAX_BLOCK_LENGTH = _MIN_BLOCK_DISTANCE
_MIN_BLOCK_LENGTH = _MAX_BLOCK_LENGTH / 2
_MAX_BLOCK_HEIGHT = 0.05
_MIN_BLOCK_HEIGHT = _MAX_BLOCK_HEIGHT / 2
class PoissonDisc2D(object):
"""Generates 2D points using Poisson disk sampling method.
Implements the algorithm described in:
http://www.cs.ubc.ca/~rbridson/docs/bridson-siggraph07-poissondisk.pdf
Unlike the uniform sampling method that creates small clusters of points,
Poisson disk method enforces the minimum distance between points and is more
suitable for generating a spatial distribution of non-overlapping objects.
"""
def __init__(self, grid_length, grid_width, min_radius, max_sample_size):
"""Initializes the algorithm.
Args:
grid_length: The length of the bounding square in which points are
sampled.
grid_width: The width of the bounding square in which points are
sampled.
min_radius: The minimum distance between any pair of points.
max_sample_size: The maximum number of sample points around a active site.
See details in the algorithm description.
"""
self._cell_length = min_radius / math.sqrt(2)
self._grid_length = grid_length
self._grid_width = grid_width
self._grid_size_x = int(grid_length / self._cell_length) + 1
self._grid_size_y = int(grid_width / self._cell_length) + 1
self._min_radius = min_radius
self._max_sample_size = max_sample_size
# Flattern the 2D grid as an 1D array. The grid is used for fast nearest
# point searching.
self._grid = [None] * self._grid_size_x * self._grid_size_y
# Generate the first sample point and set it as an active site.
first_sample = np.array(
np.random.random_sample(2)) * [grid_length, grid_width]
self._active_list = [first_sample]
# Also store the sample point in the grid.
self._grid[self._point_to_index_1d(first_sample)] = first_sample
def _point_to_index_1d(self, point):
"""Computes the index of a point in the grid array.
Args:
point: A 2D point described by its coordinates (x, y).
Returns:
The index of the point within the self._grid array.
"""
return self._index_2d_to_1d(self._point_to_index_2d(point))
def _point_to_index_2d(self, point):
"""Computes the 2D index (aka cell ID) of a point in the grid.
Args:
point: A 2D point (list) described by its coordinates (x, y).
Returns:
x_index: The x index of the cell the point belongs to.
y_index: The y index of the cell the point belongs to.
"""
x_index = int(point[0] / self._cell_length)
y_index = int(point[1] / self._cell_length)
return x_index, y_index
def _index_2d_to_1d(self, index2d):
"""Converts the 2D index to the 1D position in the grid array.
Args:
index2d: The 2D index of a point (aka the cell ID) in the grid.
Returns:
The 1D position of the cell within the self._grid array.
"""
return index2d[0] + index2d[1] * self._grid_size_x
def _is_in_grid(self, point):
"""Checks if the point is inside the grid boundary.
Args:
point: A 2D point (list) described by its coordinates (x, y).
Returns:
Whether the point is inside the grid.
"""
return (0 <= point[0] < self._grid_length) and (0 <= point[1] <
self._grid_width)
def _is_in_range(self, index2d):
"""Checks if the cell ID is within the grid.
Args:
index2d: The 2D index of a point (aka the cell ID) in the grid.
Returns:
Whether the cell (2D index) is inside the grid.
"""
return (0 <= index2d[0] < self._grid_size_x) and (0 <= index2d[1] <
self._grid_size_y)
def _is_close_to_existing_points(self, point):
"""Checks if the point is close to any already sampled (and stored) points.
Args:
point: A 2D point (list) described by its coordinates (x, y).
Returns:
True iff the distance of the point to any existing points is smaller than
the min_radius
"""
px, py = self._point_to_index_2d(point)
# Now we can check nearby cells for existing points
for neighbor_cell in itertools.product(xrange(px - 1, px + 2),
xrange(py - 1, py + 2)):
if not self._is_in_range(neighbor_cell):
continue
maybe_a_point = self._grid[self._index_2d_to_1d(neighbor_cell)]
if maybe_a_point is not None and np.linalg.norm(
maybe_a_point - point) < self._min_radius:
return True
return False
def sample(self):
"""Samples new points around some existing point.
Removes the sampling base point and also stores the new jksampled points if
they are far enough from all existing points.
"""
active_point = self._active_list.pop()
for _ in xrange(self._max_sample_size):
# Generate random points near the current active_point between the radius
random_radius = np.random.uniform(self._min_radius,
2 * self._min_radius)
random_angle = np.random.uniform(0, 2 * math.pi)
# The sampled 2D points near the active point
sample = random_radius * np.array(
[np.cos(random_angle),
np.sin(random_angle)]) + active_point
if not self._is_in_grid(sample):
continue
if self._is_close_to_existing_points(sample):
continue
self._active_list.append(sample)
self._grid[self._point_to_index_1d(sample)] = sample
def generate(self):
"""Generates the Poisson disc distribution of 2D points.
Although the while loop looks scary, the algorithm is in fact O(N), where N
is the number of cells within the grid. When we sample around a base point
(in some base cell), new points will not be pushed into the base cell
because of the minimum distance constraint. Once the current base point is
removed, all future searches cannot start from within the same base cell.
Returns:
All sampled points. The points are inside the quare [0, grid_length] x [0,
grid_width]
"""
while self._active_list:
self.sample()
all_sites = []
for p in self._grid:
if p is not None:
all_sites.append(p)
return all_sites
class TerrainType(enum.Enum):
"""The randomzied terrain types we can use in the gym env."""
RANDOM_BLOCKS = 1
TRIANGLE_MESH = 2
class MinitaurTerrainRandomizer(env_randomizer_base.EnvRandomizerBase):
"""Generates an uneven terrain in the gym env."""
def __init__(self,
terrain_type=TerrainType.TRIANGLE_MESH,
mesh_filename="terrain9735.obj",
mesh_scale=None):
"""Initializes the randomizer.
Args:
terrain_type: Whether to generate random blocks or load a triangle mesh.
mesh_filename: The mesh file to be used. The mesh will only be loaded if
terrain_type is set to TerrainType.TRIANGLE_MESH.
mesh_scale: the scaling factor for the triangles in the mesh file.
"""
self._terrain_type = terrain_type
self._mesh_filename = mesh_filename
self._mesh_scale = mesh_scale if mesh_scale else [1.0, 1.0, 0.3]
def randomize_env(self, env):
"""Generate a random terrain for the current env.
Args:
env: A minitaur gym environment.
"""
if self._terrain_type is TerrainType.TRIANGLE_MESH:
self._load_triangle_mesh(env)
if self._terrain_type is TerrainType.RANDOM_BLOCKS:
self._generate_convex_blocks(env)
def _load_triangle_mesh(self, env):
"""Represents the random terrain using a triangle mesh.
It is possible for Minitaur leg to stuck at the common edge of two triangle
pieces. To prevent this from happening, we recommend using hard contacts
(or high stiffness values) for Minitaur foot in sim.
Args:
env: A minitaur gym environment.
"""
env.pybullet_client.removeBody(env.ground_id)
terrain_collision_shape_id = env.pybullet_client.createCollisionShape(
shapeType=env.pybullet_client.GEOM_MESH,
fileName=self._mesh_filename,
flags=1,
meshScale=self._mesh_scale)
env.ground_id = env.pybullet_client.createMultiBody(
baseMass=0,
baseCollisionShapeIndex=terrain_collision_shape_id,
basePosition=[0, 0, 0])
def _generate_convex_blocks(self, env):
"""Adds random convex blocks to the flat ground.
We use the Possion disk algorithm to add some random blocks on the ground.
Possion disk algorithm sets the minimum distance between two sampling
points, thus voiding the clustering effect in uniform N-D distribution.
Args:
env: A minitaur gym environment.
"""
poisson_disc = PoissonDisc2D(_GRID_LENGTH, _GRID_WIDTH,
_MIN_BLOCK_DISTANCE, _MAX_SAMPLE_SIZE)
block_centers = poisson_disc.generate()
for center in block_centers:
# We want the blocks to be in front of the robot.
shifted_center = np.array(center) - [2, _GRID_WIDTH / 2]
# Do not place blocks near the point [0, 0], where the robot will start.
if abs(shifted_center[0]) < 1.0 and abs(shifted_center[1]) < 1.0:
continue
half_length = np.random.uniform(
_MIN_BLOCK_LENGTH, _MAX_BLOCK_LENGTH) / (2 * math.sqrt(2))
half_height = np.random.uniform(_MIN_BLOCK_HEIGHT,
_MAX_BLOCK_HEIGHT) / 2
box_id = env.pybullet_client.createCollisionShape(
env.pybullet_client.GEOM_BOX,
halfExtents=[half_length, half_length, half_height])
env.pybullet_client.createMultiBody(baseMass=0,
baseCollisionShapeIndex=box_id,
basePosition=[
shifted_center[0],
shifted_center[1],
half_height
])
def _generate_height_field(self, env):
random.seed(10)
env.pybullet_client.configureDebugVisualizer(
env.pybullet_client.COV_ENABLE_RENDERING, 0)
terrainShape = env.pybullet_client.createCollisionShape(
shapeType=env.pybullet_client.GEOM_HEIGHTFIELD,
meshScale=[.1, .1, 24],
fileName="heightmaps/wm_height_out.png")
textureId = env.pybullet_client.loadTexture("gimp_overlay_out.png")
terrain = env.pybullet_client.createMultiBody(0, terrainShape)
env.pybullet_client.changeVisualShape(terrain,
-1,
textureUniqueId=textureId)
env.pybullet_client.changeVisualShape(terrain,
-1,
rgbaColor=[1, 1, 1, 1]) | 12,192 | Python | 39.916107 | 85 | 0.602116 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/mini_bullet/env_randomizer_base.py | """Abstract base class for environment randomizer."""
import abc
class EnvRandomizerBase(object):
"""Abstract base class for environment randomizer.
An EnvRandomizer is called in environment.reset(). It will
randomize physical parameters of the objects in the simulation.
The physical parameters will be fixed for that episode and be
randomized again in the next environment.reset().
"""
__metaclass__ = abc.ABCMeta
@abc.abstractmethod
def randomize_env(self, env):
"""Randomize the simulated_objects in the environment.
Args:
env: The environment to be randomized.
"""
pass
| 622 | Python | 23.919999 | 65 | 0.726688 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/mini_bullet/minitaur.py | """This file implements the functionalities of a minitaur using pybullet.
"""
import copy
import math
import numpy as np
from . import motor
import os
INIT_POSITION = [0, 0, .2]
INIT_ORIENTATION = [0, 0, 0, 1]
KNEE_CONSTRAINT_POINT_RIGHT = [0, 0.005, 0.2]
KNEE_CONSTRAINT_POINT_LEFT = [0, 0.01, 0.2]
OVERHEAT_SHUTDOWN_TORQUE = 2.45
OVERHEAT_SHUTDOWN_TIME = 1.0
LEG_POSITION = ["front_left", "back_left", "front_right", "back_right"]
MOTOR_NAMES = [
"motor_front_leftL_joint", "motor_front_leftR_joint",
"motor_back_leftL_joint", "motor_back_leftR_joint",
"motor_front_rightL_joint", "motor_front_rightR_joint",
"motor_back_rightL_joint", "motor_back_rightR_joint"
]
LEG_LINK_ID = [2, 3, 5, 6, 8, 9, 11, 12, 15, 16, 18, 19, 21, 22, 24, 25]
MOTOR_LINK_ID = [1, 4, 7, 10, 14, 17, 20, 23]
FOOT_LINK_ID = [3, 6, 9, 12, 16, 19, 22, 25]
BASE_LINK_ID = -1
class Minitaur(object):
"""The minitaur class that simulates a quadruped robot from Ghost Robotics.
"""
def __init__(self,
pybullet_client,
urdf_root=os.path.join(os.path.dirname(__file__), "../data"),
time_step=0.01,
self_collision_enabled=False,
motor_velocity_limit=np.inf,
pd_control_enabled=False,
accurate_motor_model_enabled=False,
motor_kp=0.7,
motor_kd=0.02,
torque_control_enabled=False,
motor_overheat_protection=False,
on_rack=False,
kd_for_pd_controllers=0.3,
desired_velocity=0.5,
desired_rate=0.0):
"""Constructs a minitaur and reset it to the initial states.
Args:
pybullet_client: The instance of BulletClient to manage different
simulations.
urdf_root: The path to the urdf folder.
time_step: The time step of the simulation.
self_collision_enabled: Whether to enable self collision.
motor_velocity_limit: The upper limit of the motor velocity.
pd_control_enabled: Whether to use PD control for the motors.
accurate_motor_model_enabled: Whether to use the accurate DC motor model.
motor_kp: proportional gain for the accurate motor model
motor_kd: derivative gain for the acurate motor model
torque_control_enabled: Whether to use the torque control, if set to
False, pose control will be used.
motor_overheat_protection: Whether to shutdown the motor that has exerted
large torque (OVERHEAT_SHUTDOWN_TORQUE) for an extended amount of time
(OVERHEAT_SHUTDOWN_TIME). See ApplyAction() in minitaur.py for more
details.
on_rack: Whether to place the minitaur on rack. This is only used to debug
the walking gait. In this mode, the minitaur's base is hanged midair so
that its walking gait is clearer to visualize.
kd_for_pd_controllers: kd value for the pd controllers of the motors.
desired_velocity: additional observation space dimension for policy
desired_rate: additional observation space dimension for policy
"""
# used to calculate minitaur acceleration
self.prev_lin_twist = np.array([0, 0, 0])
self.prev_lin_acc = np.array([0, 0, 0])
self.num_motors = 8
self.num_legs = int(self.num_motors / 2)
self._pybullet_client = pybullet_client
self._urdf_root = urdf_root
self._self_collision_enabled = self_collision_enabled
self._motor_velocity_limit = motor_velocity_limit
self._pd_control_enabled = pd_control_enabled
self._motor_direction = [-1, -1, -1, -1, 1, 1, 1, 1]
self._observed_motor_torques = np.zeros(self.num_motors)
self._applied_motor_torques = np.zeros(self.num_motors)
self._max_force = 3.5
self._accurate_motor_model_enabled = accurate_motor_model_enabled
self._torque_control_enabled = torque_control_enabled
self._motor_overheat_protection = motor_overheat_protection
self._on_rack = on_rack
if self._accurate_motor_model_enabled:
self._kp = motor_kp
self._kd = motor_kd
self._motor_model = motor.MotorModel(
torque_control_enabled=self._torque_control_enabled,
kp=self._kp,
kd=self._kd)
elif self._pd_control_enabled:
self._kp = 8
self._kd = kd_for_pd_controllers
else:
self._kp = 1
self._kd = 1
self.time_step = time_step
self.desired_velocity = desired_velocity
self.desired_rate = desired_rate
self.Reset()
def _RecordMassInfoFromURDF(self):
self._base_mass_urdf = self._pybullet_client.getDynamicsInfo(
self.quadruped, BASE_LINK_ID)[0]
self._leg_masses_urdf = []
self._leg_masses_urdf.append(
self._pybullet_client.getDynamicsInfo(self.quadruped,
LEG_LINK_ID[0])[0])
self._leg_masses_urdf.append(
self._pybullet_client.getDynamicsInfo(self.quadruped,
MOTOR_LINK_ID[0])[0])
def _BuildJointNameToIdDict(self):
num_joints = self._pybullet_client.getNumJoints(self.quadruped)
self._joint_name_to_id = {}
for i in range(num_joints):
joint_info = self._pybullet_client.getJointInfo(self.quadruped, i)
self._joint_name_to_id[joint_info[1].decode(
"UTF-8")] = joint_info[0]
def _BuildMotorIdList(self):
self._motor_id_list = [
self._joint_name_to_id[motor_name] for motor_name in MOTOR_NAMES
]
def Reset(self, reload_urdf=True, desired_velocity=None, desired_rate=None):
"""Reset the minitaur to its initial states.
Args:
reload_urdf: Whether to reload the urdf file. If not, Reset() just place
the minitaur back to its starting position.
"""
# UPDATE DESIRED VELOCITY AND RATE STATES
if desired_velocity is not None:
self.desired_velocity = desired_velocity
if desired_rate is not None:
self.desired_rate = desired_rate
if reload_urdf:
if self._self_collision_enabled:
self.quadruped = self._pybullet_client.loadURDF(
"%s/quadruped/minitaur.urdf" % self._urdf_root,
INIT_POSITION,
flags=self._pybullet_client.URDF_USE_SELF_COLLISION)
else:
self.quadruped = self._pybullet_client.loadURDF(
"%s/quadruped/minitaur.urdf" % self._urdf_root,
INIT_POSITION)
self._BuildJointNameToIdDict()
self._BuildMotorIdList()
self._RecordMassInfoFromURDF()
self.ResetPose(add_constraint=True)
if self._on_rack:
self._pybullet_client.createConstraint(
self.quadruped, -1, -1, -1,
self._pybullet_client.JOINT_FIXED, [0, 0, 0], [0, 0, 0],
[0, 0, 1])
else:
self._pybullet_client.resetBasePositionAndOrientation(
self.quadruped, INIT_POSITION, INIT_ORIENTATION)
self._pybullet_client.resetBaseVelocity(self.quadruped, [0, 0, 0],
[0, 0, 0])
self.ResetPose(add_constraint=False)
self._overheat_counter = np.zeros(self.num_motors)
self._motor_enabled_list = [True] * self.num_motors\
def _SetMotorTorqueById(self, motor_id, torque):
self._pybullet_client.setJointMotorControl2(
bodyIndex=self.quadruped,
jointIndex=motor_id,
controlMode=self._pybullet_client.TORQUE_CONTROL,
force=torque)
def _SetDesiredMotorAngleById(self, motor_id, desired_angle):
self._pybullet_client.setJointMotorControl2(
bodyIndex=self.quadruped,
jointIndex=motor_id,
controlMode=self._pybullet_client.POSITION_CONTROL,
targetPosition=desired_angle,
positionGain=self._kp,
velocityGain=self._kd,
force=self._max_force)
def _SetDesiredMotorAngleByName(self, motor_name, desired_angle):
self._SetDesiredMotorAngleById(self._joint_name_to_id[motor_name],
desired_angle)
def ResetPose(self, add_constraint):
"""Reset the pose of the minitaur.
Args:
add_constraint: Whether to add a constraint at the joints of two feet.
"""
for i in range(self.num_legs):
self._ResetPoseForLeg(i, add_constraint)
def _ResetPoseForLeg(self, leg_id, add_constraint):
"""Reset the initial pose for the leg.
Args:
leg_id: It should be 0, 1, 2, or 3, which represents the leg at
front_left, back_left, front_right and back_right.
add_constraint: Whether to add a constraint at the joints of two feet.
"""
knee_friction_force = 0
half_pi = math.pi / 2.0
knee_angle = -2.1834
leg_position = LEG_POSITION[leg_id]
self._pybullet_client.resetJointState(
self.quadruped,
self._joint_name_to_id["motor_" + leg_position + "L_joint"],
self._motor_direction[2 * leg_id] * half_pi,
targetVelocity=0)
self._pybullet_client.resetJointState(
self.quadruped,
self._joint_name_to_id["knee_" + leg_position + "L_link"],
self._motor_direction[2 * leg_id] * knee_angle,
targetVelocity=0)
self._pybullet_client.resetJointState(
self.quadruped,
self._joint_name_to_id["motor_" + leg_position + "R_joint"],
self._motor_direction[2 * leg_id + 1] * half_pi,
targetVelocity=0)
self._pybullet_client.resetJointState(
self.quadruped,
self._joint_name_to_id["knee_" + leg_position + "R_link"],
self._motor_direction[2 * leg_id + 1] * knee_angle,
targetVelocity=0)
if add_constraint:
self._pybullet_client.createConstraint(
self.quadruped,
self._joint_name_to_id["knee_" + leg_position + "R_link"],
self.quadruped,
self._joint_name_to_id["knee_" + leg_position + "L_link"],
self._pybullet_client.JOINT_POINT2POINT, [0, 0, 0],
KNEE_CONSTRAINT_POINT_RIGHT, KNEE_CONSTRAINT_POINT_LEFT)
if self._accurate_motor_model_enabled or self._pd_control_enabled:
# Disable the default motor in pybullet.
self._pybullet_client.setJointMotorControl2(
bodyIndex=self.quadruped,
jointIndex=(self._joint_name_to_id["motor_" + leg_position +
"L_joint"]),
controlMode=self._pybullet_client.VELOCITY_CONTROL,
targetVelocity=0,
force=knee_friction_force)
self._pybullet_client.setJointMotorControl2(
bodyIndex=self.quadruped,
jointIndex=(self._joint_name_to_id["motor_" + leg_position +
"R_joint"]),
controlMode=self._pybullet_client.VELOCITY_CONTROL,
targetVelocity=0,
force=knee_friction_force)
else:
self._SetDesiredMotorAngleByName(
"motor_" + leg_position + "L_joint",
self._motor_direction[2 * leg_id] * half_pi)
self._SetDesiredMotorAngleByName(
"motor_" + leg_position + "R_joint",
self._motor_direction[2 * leg_id + 1] * half_pi)
self._pybullet_client.setJointMotorControl2(
bodyIndex=self.quadruped,
jointIndex=(self._joint_name_to_id["knee_" + leg_position +
"L_link"]),
controlMode=self._pybullet_client.VELOCITY_CONTROL,
targetVelocity=0,
force=knee_friction_force)
self._pybullet_client.setJointMotorControl2(
bodyIndex=self.quadruped,
jointIndex=(self._joint_name_to_id["knee_" + leg_position +
"R_link"]),
controlMode=self._pybullet_client.VELOCITY_CONTROL,
targetVelocity=0,
force=knee_friction_force)
def GetBasePosition(self):
"""Get the position of minitaur's base.
Returns:
The position of minitaur's base.
"""
position, _ = (self._pybullet_client.getBasePositionAndOrientation(
self.quadruped))
return position
def GetBaseOrientation(self):
"""Get the orientation of minitaur's base, represented as quaternion.
Returns:
The orientation of minitaur's base.
"""
_, orientation = (self._pybullet_client.getBasePositionAndOrientation(
self.quadruped))
return orientation
def GetBaseTwitst(self):
"""Get the Twist of minitaur's base.
Returns:
The Twist of the minitaur's base.
"""
return self._pybullet_client.getBaseVelocity(self.quadruped)
def GetActionDimension(self):
"""Get the length of the action list.
Returns:
The length of the action list.
"""
return self.num_motors
def GetObservationUpperBound(self):
"""Get the upper bound of the observation.
Returns:
The upper bound of an observation. See GetObservation() for the details
of each element of an observation.
NOTE: Changed just like GetObservation()
"""
upper_bound = np.array([0.0] * self.GetObservationDimension())
# roll, pitch
upper_bound[0:2] = np.pi / 2.0
# acc, rate in x,y,z
upper_bound[2:8] = np.inf
# 8:10 are velocity and rate bounds, min and max are +-10
# upper_bound[8:10] = 10
# NOTE: ORIGINAL BELOW
# upper_bound[10:10 + self.num_motors] = math.pi # Joint angle.
# upper_bound[self.num_motors + 10:2 * self.num_motors + 10] = (
# motor.MOTOR_SPEED_LIMIT) # Joint velocity.
# upper_bound[2 * self.num_motors + 10:3 * self.num_motors + 10] = (
# motor.OBSERVED_TORQUE_LIMIT) # Joint torque.
# upper_bound[3 *
# self.num_motors:] = 1.0 # Quaternion of base orientation.
# print("UPPER BOUND{}".format(upper_bound))
return upper_bound
def GetObservationLowerBound(self):
"""Get the lower bound of the observation."""
return -self.GetObservationUpperBound()
def GetObservationDimension(self):
"""Get the length of the observation list.
Returns:
The length of the observation list.
"""
return len(self.GetObservation())
def GetObservation(self):
"""Get the observations of minitaur.
It includes the angles, velocities, torques and the orientation of the base.
Returns:
The observation list. observation[0:8] are motor angles. observation[8:16]
are motor velocities, observation[16:24] are motor torques.
observation[24:28] is the orientation of the base, in quaternion form.
NOTE: DIVERGES FROM STOCK MINITAUR ENV. WILL LEAVE ORIGINAL COMMENTED
For my purpose, the observation space includes Roll and Pitch, as well as
acceleration and gyroscopic rate along the x,y,z axes. All of this
information can be collected from an onboard IMU. The reward function
will contain a hidden velocity reward (fwd, bwd) which cannot be measured
and so is not included. For spinning, the gyroscopic z rate will be used
as the (explicit) velocity reward.
This version operates without motor torques, angles and velocities. Erwin
Coumans' paper suggests a sparse observation space leads to higher reward
"""
observation = []
ori = self.GetBaseOrientation()
# Get roll and pitch
roll, pitch, _ = self._pybullet_client.getEulerFromQuaternion(
[ori[0], ori[1], ori[2], ori[3]])
# Get linear accelerations and angular rates
lt, ang_twist = self.GetBaseTwitst()
lin_twist = np.array([lt[0], lt[1], lt[2]])
# Get linear accelerations
lin_acc = self.prev_lin_twist - lin_twist
# if lin_acc.all() == 0.0:
# lin_acc = self.prev_lin_acc
self.prev_lin_acc = lin_acc
# print("LIN ACC: ", lin_acc)
self.prev_lin_twist = lin_twist
# order: roll, pitch, acc(x,y,z), gyro(x,y,z)
observation.append(roll)
observation.append(pitch)
observation.extend(lin_acc.tolist())
observation.extend(list(ang_twist))
# velocity and rate
# observation.append(self.desired_velocity)
# observation.append(self.desired_rate)
# NOTE: ORIGINAL BELOW
# observation.extend(self.GetMotorAngles().tolist())
# observation.extend(self.GetMotorVelocities().tolist())
# observation.extend(self.GetMotorTorques().tolist())
# observation.extend(list(self.GetBaseOrientation()))
return observation
def ApplyAction(self, motor_commands):
"""Set the desired motor angles to the motors of the minitaur.
The desired motor angles are clipped based on the maximum allowed velocity.
If the pd_control_enabled is True, a torque is calculated according to
the difference between current and desired joint angle, as well as the joint
velocity. This torque is exerted to the motor. For more information about
PD control, please refer to: https://en.wikipedia.org/wiki/PID_controller.
Args:
motor_commands: The eight desired motor angles.
"""
if self._motor_velocity_limit < np.inf:
current_motor_angle = self.GetMotorAngles()
motor_commands_max = (current_motor_angle +
self.time_step * self._motor_velocity_limit)
motor_commands_min = (current_motor_angle -
self.time_step * self._motor_velocity_limit)
motor_commands = np.clip(motor_commands, motor_commands_min,
motor_commands_max)
if self._accurate_motor_model_enabled or self._pd_control_enabled:
q = self.GetMotorAngles()
qdot = self.GetMotorVelocities()
if self._accurate_motor_model_enabled:
actual_torque, observed_torque = self._motor_model.convert_to_torque(
motor_commands, q, qdot)
if self._motor_overheat_protection:
for i in range(self.num_motors):
if abs(actual_torque[i]) > OVERHEAT_SHUTDOWN_TORQUE:
self._overheat_counter[i] += 1
else:
self._overheat_counter[i] = 0
if (self._overheat_counter[i] >
OVERHEAT_SHUTDOWN_TIME / self.time_step):
self._motor_enabled_list[i] = False
# The torque is already in the observation space because we use
# GetMotorAngles and GetMotorVelocities.
self._observed_motor_torques = observed_torque
# Transform into the motor space when applying the torque.
self._applied_motor_torque = np.multiply(
actual_torque, self._motor_direction)
for motor_id, motor_torque, motor_enabled in zip(
self._motor_id_list, self._applied_motor_torque,
self._motor_enabled_list):
if motor_enabled:
self._SetMotorTorqueById(motor_id, motor_torque)
else:
self._SetMotorTorqueById(motor_id, 0)
else:
torque_commands = -self._kp * (
q - motor_commands) - self._kd * qdot
# The torque is already in the observation space because we use
# GetMotorAngles and GetMotorVelocities.
self._observed_motor_torques = torque_commands
# Transform into the motor space when applying the torque.
self._applied_motor_torques = np.multiply(
self._observed_motor_torques, self._motor_direction)
for motor_id, motor_torque in zip(self._motor_id_list,
self._applied_motor_torques):
self._SetMotorTorqueById(motor_id, motor_torque)
else:
motor_commands_with_direction = np.multiply(
motor_commands, self._motor_direction)
for motor_id, motor_command_with_direction in zip(
self._motor_id_list, motor_commands_with_direction):
self._SetDesiredMotorAngleById(motor_id,
motor_command_with_direction)
def GetMotorAngles(self):
"""Get the eight motor angles at the current moment.
Returns:
Motor angles.
"""
motor_angles = [
self._pybullet_client.getJointState(self.quadruped, motor_id)[0]
for motor_id in self._motor_id_list
]
motor_angles = np.multiply(motor_angles, self._motor_direction)
return motor_angles
def GetMotorVelocities(self):
"""Get the velocity of all eight motors.
Returns:
Velocities of all eight motors.
"""
motor_velocities = [
self._pybullet_client.getJointState(self.quadruped, motor_id)[1]
for motor_id in self._motor_id_list
]
motor_velocities = np.multiply(motor_velocities, self._motor_direction)
return motor_velocities
def GetMotorTorques(self):
"""Get the amount of torques the motors are exerting.
Returns:
Motor torques of all eight motors.
"""
if self._accurate_motor_model_enabled or self._pd_control_enabled:
return self._observed_motor_torques
else:
motor_torques = [
self._pybullet_client.getJointState(self.quadruped,
motor_id)[3]
for motor_id in self._motor_id_list
]
motor_torques = np.multiply(motor_torques, self._motor_direction)
return motor_torques
def ConvertFromLegModel(self, actions):
"""Convert the actions that use leg model to the real motor actions.
Args:
actions: The theta, phi of the leg model.
actions are of form = [phi, phi, phi, phi, theta, theta, theta, theta]
where phi = swing and theta = extension
Returns:
The eight desired motor angles that can be used in ApplyActions().
"""
motor_angle = copy.deepcopy(actions)
scale_for_singularity = 1
offset_for_singularity = 1.5
half_num_motors = int(self.num_motors / 2)
quater_pi = math.pi / 4
for i in range(self.num_motors):
action_idx = i // 2
forward_backward_component = (
-scale_for_singularity * quater_pi *
(actions[action_idx + half_num_motors] +
offset_for_singularity))
extension_component = (-1)**i * quater_pi * actions[action_idx]
if i >= half_num_motors:
extension_component = -extension_component
motor_angle[i] = (math.pi + forward_backward_component +
extension_component)
return motor_angle
def GetBaseMassFromURDF(self):
"""Get the mass of the base from the URDF file."""
return self._base_mass_urdf
def GetLegMassesFromURDF(self):
"""Get the mass of the legs from the URDF file."""
return self._leg_masses_urdf
def SetBaseMass(self, base_mass):
self._pybullet_client.changeDynamics(self.quadruped,
BASE_LINK_ID,
mass=base_mass)
def SetLegMasses(self, leg_masses):
"""Set the mass of the legs.
A leg includes leg_link and motor. All four leg_links have the same mass,
which is leg_masses[0]. All four motors have the same mass, which is
leg_mass[1].
Args:
leg_masses: The leg masses. leg_masses[0] is the mass of the leg link.
leg_masses[1] is the mass of the motor.
"""
for link_id in LEG_LINK_ID:
self._pybullet_client.changeDynamics(self.quadruped,
link_id,
mass=leg_masses[0])
for link_id in MOTOR_LINK_ID:
self._pybullet_client.changeDynamics(self.quadruped,
link_id,
mass=leg_masses[1])
def SetFootFriction(self, foot_friction):
"""Set the lateral friction of the feet.
Args:
foot_friction: The lateral friction coefficient of the foot. This value is
shared by all four feet.
"""
for link_id in FOOT_LINK_ID:
self._pybullet_client.changeDynamics(self.quadruped,
link_id,
lateralFriction=foot_friction)
def SetBatteryVoltage(self, voltage):
if self._accurate_motor_model_enabled:
self._motor_model.set_voltage(voltage)
def SetMotorViscousDamping(self, viscous_damping):
if self._accurate_motor_model_enabled:
self._motor_model.set_viscous_damping(viscous_damping) | 25,949 | Python | 42.540268 | 85 | 0.582951 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/mini_bullet/minitaur_gym_env.py | """This file implements the gym environment of minitaur.
"""
import os
import inspect
import math
import time
import gym
from gym import spaces
from gym.utils import seeding
import numpy as np
import pybullet
import pybullet_utils.bullet_client as bc
from . import minitaur
import pybullet_data
from . import minitaur_env_randomizer
from pkg_resources import parse_version
from gym.envs.registration import register
currentdir = os.path.dirname(
os.path.abspath(inspect.getfile(inspect.currentframe())))
parentdir = os.path.dirname(os.path.dirname(currentdir))
os.sys.path.insert(0, parentdir)
NUM_SUBSTEPS = 5
NUM_MOTORS = 8
MOTOR_ANGLE_OBSERVATION_INDEX = 0
MOTOR_VELOCITY_OBSERVATION_INDEX = MOTOR_ANGLE_OBSERVATION_INDEX + NUM_MOTORS
MOTOR_TORQUE_OBSERVATION_INDEX = MOTOR_VELOCITY_OBSERVATION_INDEX + NUM_MOTORS
BASE_ORIENTATION_OBSERVATION_INDEX = MOTOR_TORQUE_OBSERVATION_INDEX + NUM_MOTORS
ACTION_EPS = 0.01
OBSERVATION_EPS = 0.01
RENDER_HEIGHT = 720
RENDER_WIDTH = 960
# Register as OpenAI Gym Environment
register(
id="MinitaurBulletEnv-v999",
entry_point='mini_bullet.minitaur_gym_env:MinitaurBulletEnv',
max_episode_steps=500,
)
class MinitaurBulletEnv(gym.Env):
"""The gym environment for the minitaur.
It simulates the locomotion of a minitaur, a quadruped robot. The state space
include the angles, velocities and torques for all the motors and the action
space is the desired motor angle for each motor. The reward function is based
on how far the minitaur walks in 1000 steps and penalizes the energy
expenditure.
"""
metadata = {
"render.modes": ["human", "rgb_array"],
"video.frames_per_second": 50
}
def __init__(
self,
urdf_root=pybullet_data.getDataPath(),
action_repeat=1,
# WEIGHTS
distance_weight=1.0,
rotation_weight=1.0,
energy_weight=0.0005,
shake_weight=0.005,
drift_weight=2.0,
rp_weight=0.1,
rate_weight=0.1,
distance_limit=float("inf"),
observation_noise_stdev=0.0,
self_collision_enabled=True,
motor_velocity_limit=np.inf,
pd_control_enabled=False, # not needed to be true if accurate motor model is enabled (has its own better PD)
leg_model_enabled=True,
accurate_motor_model_enabled=True,
motor_kp=0.7,
motor_kd=0.02,
torque_control_enabled=False,
motor_overheat_protection=True,
hard_reset=True,
on_rack=False,
render=False,
kd_for_pd_controllers=0.3,
env_randomizer=minitaur_env_randomizer.MinitaurEnvRandomizer(),
desired_velocity=0.5,
desired_rate=0.0,
lateral=False):
"""Initialize the minitaur gym environment.
Args:
urdf_root: The path to the urdf data folder.
action_repeat: The number of simulation steps before actions are applied.
distance_weight: The weight of the distance term in the reward.
energy_weight: The weight of the energy term in the reward.
shake_weight: The weight of the vertical shakiness term in the reward.
drift_weight: The weight of the sideways drift term in the reward.
distance_limit: The maximum distance to terminate the episode.
observation_noise_stdev: The standard deviation of observation noise.
self_collision_enabled: Whether to enable self collision in the sim.
motor_velocity_limit: The velocity limit of each motor.
pd_control_enabled: Whether to use PD controller for each motor.
leg_model_enabled: Whether to use a leg motor to reparameterize the action
space.
accurate_motor_model_enabled: Whether to use the accurate DC motor model.
motor_kp: proportional gain for the accurate motor model.
motor_kd: derivative gain for the accurate motor model.
torque_control_enabled: Whether to use the torque control, if set to
False, pose control will be used.
motor_overheat_protection: Whether to shutdown the motor that has exerted
large torque (OVERHEAT_SHUTDOWN_TORQUE) for an extended amount of time
(OVERHEAT_SHUTDOWN_TIME). See ApplyAction() in minitaur.py for more
details.
hard_reset: Whether to wipe the simulation and load everything when reset
is called. If set to false, reset just place the minitaur back to start
position and set its pose to initial configuration.
on_rack: Whether to place the minitaur on rack. This is only used to debug
the walking gait. In this mode, the minitaur's base is hanged midair so
that its walking gait is clearer to visualize.
render: Whether to render the simulation.
kd_for_pd_controllers: kd value for the pd controllers of the motors
env_randomizer: An EnvRandomizer to randomize the physical properties
during reset().
"""
self._time_step = 0.01
self._action_repeat = action_repeat
self._num_bullet_solver_iterations = 300
self._urdf_root = urdf_root
self._self_collision_enabled = self_collision_enabled
self._motor_velocity_limit = motor_velocity_limit
self._observation = []
self._env_step_counter = 0
self._is_render = render
self._last_base_position = [0, 0, 0]
self._distance_weight = distance_weight
self._rotation_weight = rotation_weight
self._energy_weight = energy_weight
self._drift_weight = drift_weight
self._shake_weight = shake_weight
self._rp_weight = rp_weight
self._rate_weight = rate_weight
self._distance_limit = distance_limit
self._observation_noise_stdev = observation_noise_stdev
self._action_bound = 1
self._pd_control_enabled = pd_control_enabled
self._leg_model_enabled = leg_model_enabled
self._accurate_motor_model_enabled = accurate_motor_model_enabled
self._motor_kp = motor_kp
self._motor_kd = motor_kd
self._torque_control_enabled = torque_control_enabled
self._motor_overheat_protection = motor_overheat_protection
self._on_rack = on_rack
self._cam_dist = 1.0
self._cam_yaw = 0
self._cam_pitch = -30
self._hard_reset = True
self._kd_for_pd_controllers = kd_for_pd_controllers
self._last_frame_time = 0.0
print("urdf_root=" + self._urdf_root)
self._env_randomizer = env_randomizer
# PD control needs smaller time step for stability.
if pd_control_enabled or accurate_motor_model_enabled:
self._time_step /= NUM_SUBSTEPS
self._num_bullet_solver_iterations /= NUM_SUBSTEPS
self._action_repeat *= NUM_SUBSTEPS
if self._is_render:
self._pybullet_client = bc.BulletClient(
connection_mode=pybullet.GUI)
else:
self._pybullet_client = bc.BulletClient()
self.seed()
np.random.seed(0)
self.desired_velocity = desired_velocity
self.desired_rate = desired_rate
# Change fwd/bwd reward to side-side reward
self.lateral = lateral
self.reset()
observation_high = (self.minitaur.GetObservationUpperBound() +
OBSERVATION_EPS)
observation_low = (self.minitaur.GetObservationLowerBound() -
OBSERVATION_EPS)
action_dim = 8
action_high = np.array([self._action_bound] * action_dim)
self.action_space = spaces.Box(-action_high,
action_high,
dtype=np.float32)
self.observation_space = spaces.Box(observation_low,
observation_high,
dtype=np.float32)
self.viewer = None
self._hard_reset = hard_reset # This assignment need to be after reset()
def set_env_randomizer(self, env_randomizer):
self._env_randomizer = env_randomizer
def configure(self, args):
self._args = args
def reset(self, desired_velocity=None, desired_rate=None):
if desired_velocity is not None:
self.desired_velocity = desired_velocity
if desired_rate is not None:
self.desired_rate = desired_rate
if self._hard_reset:
self._pybullet_client.resetSimulation()
self._pybullet_client.setPhysicsEngineParameter(
numSolverIterations=int(self._num_bullet_solver_iterations))
self._pybullet_client.setTimeStep(self._time_step)
plane = self._pybullet_client.loadURDF("%s/plane.urdf" %
self._urdf_root)
self._pybullet_client.changeVisualShape(plane,
-1,
rgbaColor=[1, 1, 1, 0.9])
self._pybullet_client.configureDebugVisualizer(
self._pybullet_client.COV_ENABLE_PLANAR_REFLECTION, 0)
self._pybullet_client.setGravity(0, 0, -9.81)
acc_motor = self._accurate_motor_model_enabled
motor_protect = self._motor_overheat_protection
self.minitaur = (minitaur.Minitaur(
pybullet_client=self._pybullet_client,
urdf_root=self._urdf_root,
time_step=self._time_step,
self_collision_enabled=self._self_collision_enabled,
motor_velocity_limit=self._motor_velocity_limit,
pd_control_enabled=self._pd_control_enabled,
accurate_motor_model_enabled=acc_motor,
motor_kp=self._motor_kp,
motor_kd=self._motor_kd,
torque_control_enabled=self._torque_control_enabled,
motor_overheat_protection=motor_protect,
on_rack=self._on_rack,
kd_for_pd_controllers=self._kd_for_pd_controllers,
desired_velocity=self.desired_velocity,
desired_rate=self.desired_rate))
else:
self.minitaur.Reset(reload_urdf=False,
desired_velocity=self.desired_velocity,
desired_rate=self.desired_rate)
if self._env_randomizer is not None:
self._env_randomizer.randomize_env(self)
self._env_step_counter = 0
self._last_base_position = [0, 0, 0]
self._objectives = []
self._pybullet_client.resetDebugVisualizerCamera(
self._cam_dist, self._cam_yaw, self._cam_pitch, [0, 0, 0])
if not self._torque_control_enabled:
for _ in range(100):
if self._pd_control_enabled or self._accurate_motor_model_enabled:
self.minitaur.ApplyAction([math.pi / 2] * 8)
self._pybullet_client.stepSimulation()
return self._noisy_observation()
def seed(self, seed=None):
self.np_random, seed = seeding.np_random(seed)
return [seed]
def _transform_action_to_motor_command(self, action):
if self._leg_model_enabled:
for i, action_component in enumerate(action):
if not (-self._action_bound - ACTION_EPS <= action_component <=
self._action_bound + ACTION_EPS):
raise ValueError("{}th action {} out of bounds.".format(
i, action_component))
action = self.minitaur.ConvertFromLegModel(action)
return action
def step(self, action):
"""Step forward the simulation, given the action.
Args:
action: A list of desired motor angles for eight motors.
Returns:
observations: The angles, velocities and torques of all motors.
reward: The reward for the current state-action pair.
done: Whether the episode has ended.
info: A dictionary that stores diagnostic information.
Raises:
ValueError: The action dimension is not the same as the number of motors.
ValueError: The magnitude of actions is out of bounds.
"""
if self._is_render:
# Sleep, otherwise the computation takes less time than real time,
# which will make the visualization like a fast-forward video.
time_spent = time.time() - self._last_frame_time
self._last_frame_time = time.time()
time_to_sleep = self._action_repeat * self._time_step - time_spent
if time_to_sleep > 0:
time.sleep(time_to_sleep)
base_pos = self.minitaur.GetBasePosition()
camInfo = self._pybullet_client.getDebugVisualizerCamera()
curTargetPos = camInfo[11]
distance = camInfo[10]
yaw = camInfo[8]
pitch = camInfo[9]
targetPos = [
0.95 * curTargetPos[0] + 0.05 * base_pos[0],
0.95 * curTargetPos[1] + 0.05 * base_pos[1], curTargetPos[2]
]
self._pybullet_client.resetDebugVisualizerCamera(
distance, yaw, pitch, base_pos)
action = self._transform_action_to_motor_command(action)
for _ in range(self._action_repeat):
self.minitaur.ApplyAction(action)
self._pybullet_client.stepSimulation()
self._env_step_counter += 1
reward = self._reward()
done = self._termination()
return np.array(self._noisy_observation()), reward, done, {}
def render(self, mode="rgb_array", close=False):
if mode != "rgb_array":
return np.array([])
base_pos = self.minitaur.GetBasePosition()
view_matrix = self._pybullet_client.computeViewMatrixFromYawPitchRoll(
cameraTargetPosition=base_pos,
distance=self._cam_dist,
yaw=self._cam_yaw,
pitch=self._cam_pitch,
roll=0,
upAxisIndex=2)
proj_matrix = self._pybullet_client.computeProjectionMatrixFOV(
fov=60,
aspect=float(RENDER_WIDTH) / RENDER_HEIGHT,
nearVal=0.1,
farVal=100.0)
(_, _, px, _, _) = self._pybullet_client.getCameraImage(
width=RENDER_WIDTH,
height=RENDER_HEIGHT,
viewMatrix=view_matrix,
projectionMatrix=proj_matrix,
renderer=pybullet.ER_BULLET_HARDWARE_OPENGL)
rgb_array = np.array(px)
rgb_array = rgb_array[:, :, :3]
return rgb_array
def get_minitaur_motor_angles(self):
"""Get the minitaur's motor angles.
Returns:
A numpy array of motor angles.
"""
return np.array(
self._observation[MOTOR_ANGLE_OBSERVATION_INDEX:
MOTOR_ANGLE_OBSERVATION_INDEX + NUM_MOTORS])
def get_minitaur_motor_velocities(self):
"""Get the minitaur's motor velocities.
Returns:
A numpy array of motor velocities.
"""
return np.array(
self._observation[MOTOR_VELOCITY_OBSERVATION_INDEX:
MOTOR_VELOCITY_OBSERVATION_INDEX + NUM_MOTORS])
def get_minitaur_motor_torques(self):
"""Get the minitaur's motor torques.
Returns:
A numpy array of motor torques.
"""
return np.array(
self._observation[MOTOR_TORQUE_OBSERVATION_INDEX:
MOTOR_TORQUE_OBSERVATION_INDEX + NUM_MOTORS])
def get_minitaur_base_orientation(self):
"""Get the minitaur's base orientation, represented by a quaternion.
Returns:
A numpy array of minitaur's orientation.
"""
return np.array(self._observation[BASE_ORIENTATION_OBSERVATION_INDEX:])
def is_fallen(self):
"""Decide whether the minitaur has fallen.
If the up directions between the base and the world is larger (the dot
product is smaller than 0.85) or the base is very low on the ground
(the height is smaller than 0.13 meter), the minitaur is considered fallen.
Returns:
Boolean value that indicates whether the minitaur has fallen.
"""
orientation = self.minitaur.GetBaseOrientation()
rot_mat = self._pybullet_client.getMatrixFromQuaternion(orientation)
local_up = rot_mat[6:]
pos = self.minitaur.GetBasePosition()
return (np.dot(np.asarray([0, 0, 1]), np.asarray(local_up)) < 0.85
or pos[2] < 0.10)
def _termination(self):
position = self.minitaur.GetBasePosition()
distance = math.sqrt(position[0]**2 + position[1]**2)
return self.is_fallen() or distance > self._distance_limit
def _reward(self):
""" NOTE: reward now consists of:
roll, pitch at desired 0
acc (y,z) = 0
FORWARD-BACKWARD: rate(x,y,z) = 0
--> HIDDEN REWARD: x(+-) velocity reference, not incl. in obs
SPIN: acc(x) = 0, rate(x,y) = 0, rate (z) = rate reference
Also include drift, energy vanilla rewards
"""
current_base_position = self.minitaur.GetBasePosition()
# get observation
obs = self._get_observation()
# forward_reward = current_base_position[0] - self._last_base_position[0]
# POSITIVE FOR FORWARD, NEGATIVE FOR BACKWARD | NOTE: HIDDEN
fwd_speed = self.minitaur.prev_lin_twist[0]
lat_speed = self.minitaur.prev_lin_twist[1]
# print("FORWARD SPEED: {} \t STATE SPEED: {}".format(
# fwd_speed, self.desired_velocity))
# self.desired_velocity = 0.4
# Modification for lateral/fwd rewards
# FORWARD
if not self.lateral:
# f(x)=-(x-desired))^(2)*((1/desired)^2)+1
# to make sure that at 0vel there is 0 reawrd.
# also squishes allowable tolerance
reward_max = 1.0
forward_reward = reward_max * np.exp(
-(fwd_speed - self.desired_velocity)**2 / (0.1))
# LATERAL
else:
reward_max = 1.0
forward_reward = reward_max * np.exp(
-(lat_speed - self.desired_rate)**2 / (0.1))
if forward_reward < 0.0:
forward_reward = 0.0
yaw_rate = obs[7]
if self.desired_rate != 0:
rot_reward = (-(yaw_rate - (self.desired_rate))**2) * (
(1.0 / self.desired_rate)**2) + 1.0
else:
rot_reward = (-(yaw_rate -
(self.desired_rate))**2) * ((1.0 / 0.1)**2) + 1.0
# Make sure that for forward-policy there is the appropriate rotation penalty
if self.desired_velocity != 0:
self._rotation_weight = self._rate_weight
rot_reward = -abs(obs[7])
elif self.desired_rate != 0:
forward_reward = 0.0
# penalty for nonzero roll, pitch
rp_reward = -(abs(obs[0]) + abs(obs[1]))
# print("ROLL: {} \t PITCH: {}".format(obs[0], obs[1]))
# penalty for nonzero acc(z)
shake_reward = -abs(obs[4])
# penalty for nonzero rate (x,y,z)
rate_reward = -(abs(obs[5]) + abs(obs[6]))
# drift_reward = -abs(current_base_position[1] -
# self._last_base_position[1])
# this penalizes absolute error, and does not penalize correction
# NOTE: for side-side, drift reward becomes in x instead
drift_reward = -abs(current_base_position[1])
# If Lateral, change drift reward
if self.lateral:
drift_reward = -abs(current_base_position[0])
# shake_reward = -abs(current_base_position[2] -
# self._last_base_position[2])
self._last_base_position = current_base_position
energy_reward = -np.abs(
np.dot(self.minitaur.GetMotorTorques(),
self.minitaur.GetMotorVelocities())) * self._time_step
reward = (self._distance_weight * forward_reward +
self._rotation_weight * rot_reward +
self._energy_weight * energy_reward +
self._drift_weight * drift_reward +
self._shake_weight * shake_reward +
self._rp_weight * rp_reward +
self._rate_weight * rate_reward)
self._objectives.append(
[forward_reward, energy_reward, drift_reward, shake_reward])
return reward
def get_objectives(self):
return self._objectives
def _get_observation(self):
self._observation = self.minitaur.GetObservation()
return self._observation
def _noisy_observation(self):
self._get_observation()
observation = np.array(self._observation)
if self._observation_noise_stdev > 0:
observation += (self.np_random.normal(
scale=self._observation_noise_stdev, size=observation.shape) *
self.minitaur.GetObservationUpperBound())
return observation
if parse_version(gym.__version__) < parse_version('0.9.6'):
_render = render
_reset = reset
_seed = seed
_step = step | 21,275 | Python | 40.554687 | 121 | 0.600188 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/mini_bullet/minitaur_env_randomizer.py | """Randomize the minitaur_gym_env when reset() is called."""
import numpy as np
from . import env_randomizer_base
# Relative range.
MINITAUR_BASE_MASS_ERROR_RANGE = (-0.2, 0.2) # 0.2 means 20%
MINITAUR_LEG_MASS_ERROR_RANGE = (-0.2, 0.2) # 0.2 means 20%
# Absolute range.
BATTERY_VOLTAGE_RANGE = (14.8, 16.8) # Unit: Volt
MOTOR_VISCOUS_DAMPING_RANGE = (0, 0.01) # Unit: N*m*s/rad (torque/angular vel)
MINITAUR_LEG_FRICTION = (0.8, 1.5) # Unit: dimensionless
class MinitaurEnvRandomizer(env_randomizer_base.EnvRandomizerBase):
"""A randomizer that change the minitaur_gym_env during every reset."""
def __init__(self,
minitaur_base_mass_err_range=MINITAUR_BASE_MASS_ERROR_RANGE,
minitaur_leg_mass_err_range=MINITAUR_LEG_MASS_ERROR_RANGE,
battery_voltage_range=BATTERY_VOLTAGE_RANGE,
motor_viscous_damping_range=MOTOR_VISCOUS_DAMPING_RANGE):
self._minitaur_base_mass_err_range = minitaur_base_mass_err_range
self._minitaur_leg_mass_err_range = minitaur_leg_mass_err_range
self._battery_voltage_range = battery_voltage_range
self._motor_viscous_damping_range = motor_viscous_damping_range
np.random.seed(0)
def randomize_env(self, env):
self._randomize_minitaur(env.minitaur)
def _randomize_minitaur(self, minitaur):
"""Randomize various physical properties of minitaur.
It randomizes the mass/inertia of the base, mass/inertia of the legs,
friction coefficient of the feet, the battery voltage and the motor damping
at each reset() of the environment.
Args:
minitaur: the Minitaur instance in minitaur_gym_env environment.
"""
base_mass = minitaur.GetBaseMassFromURDF()
randomized_base_mass = np.random.uniform(
base_mass * (1.0 + self._minitaur_base_mass_err_range[0]),
base_mass * (1.0 + self._minitaur_base_mass_err_range[1]))
minitaur.SetBaseMass(randomized_base_mass)
leg_masses = minitaur.GetLegMassesFromURDF()
leg_masses_lower_bound = np.array(leg_masses) * (
1.0 + self._minitaur_leg_mass_err_range[0])
leg_masses_upper_bound = np.array(leg_masses) * (
1.0 + self._minitaur_leg_mass_err_range[1])
randomized_leg_masses = [
np.random.uniform(leg_masses_lower_bound[i],
leg_masses_upper_bound[i])
for i in range(len(leg_masses))
]
minitaur.SetLegMasses(randomized_leg_masses)
randomized_battery_voltage = np.random.uniform(
BATTERY_VOLTAGE_RANGE[0], BATTERY_VOLTAGE_RANGE[1])
minitaur.SetBatteryVoltage(randomized_battery_voltage)
randomized_motor_damping = np.random.uniform(
MOTOR_VISCOUS_DAMPING_RANGE[0], MOTOR_VISCOUS_DAMPING_RANGE[1])
minitaur.SetMotorViscousDamping(randomized_motor_damping)
randomized_foot_friction = np.random.uniform(MINITAUR_LEG_FRICTION[0],
MINITAUR_LEG_FRICTION[1])
minitaur.SetFootFriction(randomized_foot_friction)
| 3,133 | Python | 43.771428 | 79 | 0.651452 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/mini_bullet/motor.py | """This file implements an accurate motor model."""
import numpy as np
VOLTAGE_CLIPPING = 50
OBSERVED_TORQUE_LIMIT = 5.7
MOTOR_VOLTAGE = 16.0
MOTOR_RESISTANCE = 0.186
MOTOR_TORQUE_CONSTANT = 0.0954
MOTOR_VISCOUS_DAMPING = 0
MOTOR_SPEED_LIMIT = MOTOR_VOLTAGE / (MOTOR_VISCOUS_DAMPING +
MOTOR_TORQUE_CONSTANT)
class MotorModel(object):
"""The accurate motor model, which is based on the physics of DC motors.
The motor model support two types of control: position control and torque
control. In position control mode, a desired motor angle is specified, and a
torque is computed based on the internal motor model. When the torque control
is specified, a pwm signal in the range of [-1.0, 1.0] is converted to the
torque.
The internal motor model takes the following factors into consideration:
pd gains, viscous friction, back-EMF voltage and current-torque profile.
"""
def __init__(self, torque_control_enabled=False, kp=1.2, kd=0):
self._torque_control_enabled = torque_control_enabled
self._kp = kp
self._kd = kd
self._resistance = MOTOR_RESISTANCE
self._voltage = MOTOR_VOLTAGE
self._torque_constant = MOTOR_TORQUE_CONSTANT
self._viscous_damping = MOTOR_VISCOUS_DAMPING
self._current_table = [0, 10, 20, 30, 40, 50, 60]
self._torque_table = [0, 1, 1.9, 2.45, 3.0, 3.25, 3.5]
def set_voltage(self, voltage):
self._voltage = voltage
def get_voltage(self):
return self._voltage
def set_viscous_damping(self, viscous_damping):
self._viscous_damping = viscous_damping
def get_viscous_dampling(self):
return self._viscous_damping
def convert_to_torque(self, motor_commands, current_motor_angle,
current_motor_velocity):
"""Convert the commands (position control or torque control) to torque.
Args:
motor_commands: The desired motor angle if the motor is in position
control mode. The pwm signal if the motor is in torque control mode.
current_motor_angle: The motor angle at the current time step.
current_motor_velocity: The motor velocity at the current time step.
Returns:
actual_torque: The torque that needs to be applied to the motor.
observed_torque: The torque observed by the sensor.
"""
if self._torque_control_enabled:
pwm = motor_commands
else:
pwm = (-self._kp * (current_motor_angle - motor_commands) -
self._kd * current_motor_velocity)
pwm = np.clip(pwm, -1.0, 1.0)
return self._convert_to_torque_from_pwm(pwm, current_motor_velocity)
def _convert_to_torque_from_pwm(self, pwm, current_motor_velocity):
"""Convert the pwm signal to torque.
Args:
pwm: The pulse width modulation.
current_motor_velocity: The motor velocity at the current time step.
Returns:
actual_torque: The torque that needs to be applied to the motor.
observed_torque: The torque observed by the sensor.
"""
observed_torque = np.clip(
self._torque_constant * (pwm * self._voltage / self._resistance),
-OBSERVED_TORQUE_LIMIT, OBSERVED_TORQUE_LIMIT)
# Net voltage is clipped at 50V by diodes on the motor controller.
voltage_net = np.clip(
pwm * self._voltage -
(self._torque_constant + self._viscous_damping) *
current_motor_velocity, -VOLTAGE_CLIPPING, VOLTAGE_CLIPPING)
current = voltage_net / self._resistance
current_sign = np.sign(current)
current_magnitude = np.absolute(current)
# Saturate torque based on empirical current relation.
actual_torque = np.interp(current_magnitude, self._current_table,
self._torque_table)
actual_torque = np.multiply(current_sign, actual_torque)
return actual_torque, observed_torque
| 3,985 | Python | 39.673469 | 79 | 0.652698 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/mini_bullet/heightfield.py | import pybullet as p
import pybullet_data as pd
import math
import time
textureId = -1
useProgrammatic = 0
useTerrainFromPNG = 1
useDeepLocoCSV = 2
updateHeightfield = False
heightfieldSource = useProgrammatic
import random
random.seed(10)
class HeightField():
def __init__(self):
self.hf_id = 0
def _generate_field(self, env, heightPerturbationRange=0.08):
env.pybullet_client.setAdditionalSearchPath(pd.getDataPath())
env.pybullet_client.configureDebugVisualizer(
env.pybullet_client.COV_ENABLE_RENDERING, 0)
heightPerturbationRange = heightPerturbationRange
if heightfieldSource == useProgrammatic:
numHeightfieldRows = 256
numHeightfieldColumns = 256
heightfieldData = [0] * numHeightfieldRows * numHeightfieldColumns
for j in range(int(numHeightfieldColumns / 2)):
for i in range(int(numHeightfieldRows / 2)):
height = random.uniform(0, heightPerturbationRange)
heightfieldData[2 * i +
2 * j * numHeightfieldRows] = height
heightfieldData[2 * i + 1 +
2 * j * numHeightfieldRows] = height
heightfieldData[2 * i +
(2 * j + 1) * numHeightfieldRows] = height
heightfieldData[2 * i + 1 +
(2 * j + 1) * numHeightfieldRows] = height
terrainShape = env.pybullet_client.createCollisionShape(
shapeType=env.pybullet_client.GEOM_HEIGHTFIELD,
meshScale=[.05, .05, 1],
heightfieldTextureScaling=(numHeightfieldRows - 1) / 2,
heightfieldData=heightfieldData,
numHeightfieldRows=numHeightfieldRows,
numHeightfieldColumns=numHeightfieldColumns)
terrain = env.pybullet_client.createMultiBody(
0, terrainShape)
env.pybullet_client.resetBasePositionAndOrientation(
terrain, [0, 0, 0], [0, 0, 0, 1])
if heightfieldSource == useDeepLocoCSV:
terrainShape = env.pybullet_client.createCollisionShape(
shapeType=env.pybullet_client.GEOM_HEIGHTFIELD,
meshScale=[.5, .5, 2.5],
fileName="heightmaps/ground0.txt",
heightfieldTextureScaling=128)
terrain = env.pybullet_client.createMultiBody(
0, terrainShape)
env.pybullet_client.resetBasePositionAndOrientation(
terrain, [0, 0, 0], [0, 0, 0, 1])
if heightfieldSource == useTerrainFromPNG:
terrainShape = env.pybullet_client.createCollisionShape(
shapeType=env.pybullet_client.GEOM_HEIGHTFIELD,
meshScale=[.05, .05, 5],
fileName="heightmaps/wm_height_out.png")
textureId = env.pybullet_client.loadTexture(
"heightmaps/gimp_overlay_out.png")
terrain = env.pybullet_client.createMultiBody(
0, terrainShape)
env.pybullet_client.changeVisualShape(terrain,
-1,
textureUniqueId=textureId)
self.hf_id = terrainShape
print("TERRAIN SHAPE: {}".format(terrainShape))
env.pybullet_client.changeVisualShape(terrain,
-1,
rgbaColor=[1, 1, 1, 1])
env.pybullet_client.configureDebugVisualizer(
env.pybullet_client.COV_ENABLE_RENDERING, 1)
| 3,706 | Python | 40.188888 | 78 | 0.565839 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/sac_lib/softQnetwork.py | import torch
import torch.nn as nn
import torch.optim as optim
import torch.nn.functional as F
from torch.distributions import Normal
class SoftQNetwork(nn.Module):
def __init__(self, num_inputs, num_actions, hidden_size, init_w=3e-3):
super(SoftQNetwork, self).__init__()
self.q1 = nn.Sequential(
nn.Linear(num_inputs + num_actions, hidden_size), nn.ReLU(),
nn.Linear(hidden_size, hidden_size), nn.ReLU(),
nn.Linear(hidden_size, 1)
)
self.q2 = nn.Sequential(
nn.Linear(num_inputs + num_actions, hidden_size), nn.ReLU(),
nn.Linear(hidden_size, hidden_size), nn.ReLU(),
nn.Linear(hidden_size, 1)
)
def forward(self, state, action):
state_action = torch.cat([state, action], 1)
return self.q1(state_action), self.q2(state_action)
| 866 | Python | 35.124999 | 74 | 0.615473 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/sac_lib/policynetwork.py | import numpy as np
import torch
import torch.nn as nn
import torch.optim as optim
import torch.nn.functional as F
from torch.distributions import Normal
device = torch.device("cuda:1" if torch.cuda.is_available() else "cpu")
class PolicyNetwork(nn.Module):
def __init__(self,
num_inputs,
num_actions,
hidden_size,
init_w=3e-3,
log_std_min=-20,
log_std_max=2):
super(PolicyNetwork, self).__init__()
self.log_std_min = log_std_min
self.log_std_max = log_std_max
self.linear1 = nn.Linear(num_inputs, hidden_size)
self.linear2 = nn.Linear(hidden_size, hidden_size)
self.mean_linear = nn.Linear(hidden_size, num_actions)
self.mean_linear.weight.data.uniform_(-init_w, init_w)
self.mean_linear.bias.data.uniform_(-init_w, init_w)
self.log_std_linear1 = nn.Linear(hidden_size, hidden_size)
self.log_std_linear2 = nn.Linear(hidden_size, num_actions)
self.log_std_linear2.weight.data.uniform_(-init_w, init_w)
self.log_std_linear2.bias.data.uniform_(-init_w, init_w)
# self.log_std_linear.weight.data.zero_()
# self.log_std_linear.bias.data.zero_()
def forward(self, state):
x = F.relu(self.linear1(state))
x = F.relu(self.linear2(x))
mean = self.mean_linear(x)
log_std = self.log_std_linear2(F.relu(self.log_std_linear1(x)))
log_std = torch.clamp(log_std, self.log_std_min, self.log_std_max)
return mean, log_std
def evaluate(self, state, epsilon=1e-6):
mean, log_std = self.forward(state)
std = log_std.exp()
normal = Normal(mean, std)
z = normal.rsample()
action = torch.tanh(z)
log_prob = normal.log_prob(z) - torch.log(1 - action.pow(2) + epsilon)
log_prob = log_prob.sum(-1, keepdim=True)
return action, log_prob, z, mean, log_std
def get_action(self, state):
state = torch.FloatTensor(state).unsqueeze(0).to(device)
mean, log_std = self.forward(state)
std = log_std.exp()
normal = Normal(mean, std)
z = normal.sample()
action = torch.tanh(z)
action = action.detach().cpu().numpy()
return action[0]
| 2,319 | Python | 31.222222 | 78 | 0.593359 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/sac_lib/replay_buffer.py | import numpy as np
import random
class ReplayBuffer:
def __init__(self, capacity):
self.capacity = capacity
self.buffer = []
self.position = 0
def push(self, state, action, reward, next_state, done):
if len(self.buffer) < self.capacity:
self.buffer.append(None)
self.buffer[self.position] = (state, action, reward, next_state, done)
self.position = (self.position + 1) % self.capacity
def sample(self, batch_size):
batch = random.sample(self.buffer, batch_size)
state, action, reward, next_state, done = map(np.stack, zip(*batch))
return state, action, reward, next_state, done
def __len__(self):
return len(self.buffer)
| 733 | Python | 30.913042 | 78 | 0.619372 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/sac_lib/__init__.py | from .sac import SoftActorCritic
from .policynetwork import PolicyNetwork
from .replay_buffer import ReplayBuffer
from .normalized_actions import NormalizedActions
| 164 | Python | 31.999994 | 49 | 0.865854 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/sac_lib/normalized_actions.py | import gym
import numpy as np
class NormalizedActions(gym.ActionWrapper):
def action(self, action):
low_bound = self.action_space.low
upper_bound = self.action_space.high
action = low_bound + (action + 1.0) * 0.5 * (upper_bound - low_bound)
action = np.clip(action, low_bound, upper_bound)
return action
def reverse_action(self, action):
low_bound = self.action_space.low
upper_bound = self.action_space.high
action = 2 * (action - low_bound) / (upper_bound - low_bound) - 1
action = np.clip(action, low_bound, upper_bound)
return actions
| 638 | Python | 26.782608 | 77 | 0.62069 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/sac_lib/sac.py | import numpy as np
import torch
import torch.nn as nn
import torch.optim as optim
import torch.nn.functional as F
from torch.distributions import Normal
# alg specific imports
from .softQnetwork import SoftQNetwork
from .valuenetwork import ValueNetwork
class SoftActorCritic(object):
def __init__(self,
policy,
state_dim,
action_dim,
replay_buffer,
hidden_dim=256,
soft_q_lr=3e-3,
policy_lr=3e-3,
device=torch.device(
"cuda:1" if torch.cuda.is_available() else "cpu")):
self.device = device
# set up the networks
self.policy_net = policy.to(device)
self.soft_q_net = SoftQNetwork(state_dim, action_dim,
hidden_dim).to(device)
self.target_soft_q_net = SoftQNetwork(state_dim, action_dim,
hidden_dim).to(device)
# ent coeff
self.target_entropy = -action_dim
self.log_ent_coef = torch.FloatTensor(np.log(np.array([.1
]))).to(device)
self.log_ent_coef.requires_grad = True
# copy the target params over
for target_param, param in zip(self.target_soft_q_net.parameters(),
self.soft_q_net.parameters()):
target_param.data.copy_(param.data)
# set the losses
self.soft_q_criterion = nn.MSELoss()
# set the optimizers
self.soft_q_optimizer = optim.Adam(self.soft_q_net.parameters(),
lr=soft_q_lr)
self.policy_optimizer = optim.Adam(self.policy_net.parameters(),
lr=policy_lr)
self.ent_coef_optimizer = optim.Adam([self.log_ent_coef], lr=3e-3)
# reference the replay buffer
self.replay_buffer = replay_buffer
self.log = {'entropy_loss': [], 'q_value_loss': [], 'policy_loss': []}
def soft_q_update(self, batch_size, gamma=0.99, soft_tau=0.01):
state, action, reward, next_state, done = self.replay_buffer.sample(
batch_size)
state = torch.FloatTensor(state).to(self.device)
next_state = torch.FloatTensor(next_state).to(self.device)
action = torch.FloatTensor(action).to(self.device)
reward = torch.FloatTensor(reward).unsqueeze(1).to(self.device)
done = torch.FloatTensor(np.float32(done)).unsqueeze(1).to(self.device)
ent_coef = torch.exp(self.log_ent_coef.detach())
new_action, log_prob, z, mean, log_std = self.policy_net.evaluate(
next_state)
target_q1_value, target_q2_value = self.target_soft_q_net(
next_state, new_action)
target_value = reward + (1 - done) * gamma * (
torch.min(target_q2_value, target_q2_value) - ent_coef * log_prob)
expected_q1_value, expected_q2_value = self.soft_q_net(state, action)
q_value_loss = self.soft_q_criterion(expected_q1_value, target_value.detach()) \
+ self.soft_q_criterion(expected_q2_value, target_value.detach())
self.soft_q_optimizer.zero_grad()
q_value_loss.backward()
self.soft_q_optimizer.step()
new_action, log_prob, z, mean, log_std = self.policy_net.evaluate(
state)
expected_new_q1_value, expected_new_q2_value = self.soft_q_net(
state, new_action)
expected_new_q_value = torch.min(expected_new_q1_value,
expected_new_q2_value)
policy_loss = (ent_coef * log_prob - expected_new_q_value).mean()
self.policy_optimizer.zero_grad()
policy_loss.backward()
self.policy_optimizer.step()
self.ent_coef_optimizer.zero_grad()
ent_loss = torch.mean(
torch.exp(self.log_ent_coef) *
(-log_prob - self.target_entropy).detach())
ent_loss.backward()
self.ent_coef_optimizer.step()
for target_param, param in zip(self.target_soft_q_net.parameters(),
self.soft_q_net.parameters()):
target_param.data.copy_(target_param.data * (1.0 - soft_tau) +
param.data * soft_tau)
self.log['q_value_loss'].append(q_value_loss.item())
self.log['entropy_loss'].append(ent_loss.item())
self.log['policy_loss'].append(policy_loss.item())
def save(self, filename):
torch.save(self.policy_net.state_dict(), filename + "_policy_net")
torch.save(self.policy_optimizer.state_dict(),
filename + "_policy_optimizer")
torch.save(self.soft_q_net.state_dict(), filename + "_soft_q_net")
torch.save(self.soft_q_optimizer.state_dict(),
filename + "_soft_q_optimizer")
def load(self, filename):
self.policy_net.load_state_dict(
torch.load(filename + "_policy_net", map_location=self.device))
self.policy_optimizer.load_state_dict(
torch.load(filename + "_policy_optimizer",
map_location=self.device))
# self.soft_q_net.load_state_dict(
# torch.load(filename + "_soft_q_net", map_location=self.device))
# self.soft_q_optimizer.load_state_dict(
# torch.load(filename + "_soft_q_optimizer",
# map_location=self.device))
# self.target_soft_q_net = copy.deepcopy(self.soft_q_net)
| 5,614 | Python | 40.286764 | 93 | 0.563591 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/sac_lib/valuenetwork.py | import torch
import torch.nn as nn
import torch.optim as optim
import torch.nn.functional as F
from torch.distributions import Normal
class ValueNetwork(nn.Module):
def __init__(self, state_dim, hidden_dim, init_w=3e-3):
super(ValueNetwork, self).__init__()
self.linear1 = nn.Linear(state_dim, hidden_dim)
self.linear2 = nn.Linear(hidden_dim, hidden_dim)
self.linear3 = nn.Linear(hidden_dim, 1)
self.linear3.weight.data.uniform_(-init_w, init_w)
self.linear3.bias.data.uniform_(-init_w, init_w)
def forward(self, state):
x = F.relu(self.linear1(state))
x = F.relu(self.linear2(x))
x = self.linear3(x)
return x
| 726 | Python | 30.608694 | 59 | 0.62259 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/tg_lib/traj_gen.py | import numpy as np
PHASE_PERIOD = 2.0 * np.pi
class CyclicIntegrator():
def __init__(self, dphi_leg=0.0):
# Phase starts with offset
self.tprime = PHASE_PERIOD * dphi_leg
def progress_tprime(self, dt, f_tg, swing_stance_speed_ratio):
""" swing_stance_speed_ratio is Beta in the paper
set by policy at each step, but default is 1/3
delta_period is just dt
This moves the phase based on delta
(which is one parameter * delta_time_step).
The speed of the phase depends on swing vs stance phase
(phase > np.pi or phase < np.pi) which has different speeds.
"""
time_mult = dt * f_tg
stance_speed_coef = (swing_stance_speed_ratio +
1) * 0.5 / swing_stance_speed_ratio
swing_speed_coef = (swing_stance_speed_ratio + 1) * 0.5
if self.tprime < PHASE_PERIOD / 2.0: # Swing
delta_phase_multiplier = stance_speed_coef * PHASE_PERIOD
self.tprime += np.fmod(time_mult * delta_phase_multiplier,
PHASE_PERIOD)
else: # Stance
delta_phase_multiplier = swing_speed_coef * PHASE_PERIOD
self.tprime += np.fmod(time_mult * delta_phase_multiplier,
PHASE_PERIOD)
self.tprime = np.fmod(self.tprime, PHASE_PERIOD)
class TrajectoryGenerator():
def __init__(self,
center_swing=0.0,
amplitude_extension=0.2,
amplitude_lift=0.4,
dphi_leg=0.0):
# Cyclic Integrator
self.CI = CyclicIntegrator(dphi_leg)
self.center_swing = center_swing
self.amplitude_extension = amplitude_extension
self.amplitude_lift = amplitude_lift
def get_state_based_on_phase(self):
return np.array([(np.cos(self.CI.tprime) + 1) / 2.0,
(np.sin(self.CI.tprime) + 1) / 2.0])
def get_swing_extend_based_on_phase(self,
amplitude_swing=0.0,
center_extension=0.0,
intensity=1.0,
theta=0.0):
""" Eqn 2 in paper appendix
Cs: center_swing
Ae: amplitude_extension
theta: extention difference between end of swing and stance (good for climbing)
POLICY PARAMS:
h_tg = center_extension --> walking height (+short/-tall)
alpha_th = amplitude_swing --> swing fwd (-) or bwd (+)
"""
# Set amplitude_extension
amplitude_extension = self.amplitude_extension
if self.CI.tprime > PHASE_PERIOD / 2.0:
amplitude_extension = self.amplitude_lift
# E(t)
extend = center_extension + (amplitude_extension * np.sin(
self.CI.tprime)) * intensity + theta * np.cos(self.CI.tprime)
# S(t)
swing = self.center_swing + amplitude_swing * np.cos(
self.CI.tprime) * intensity
return swing, extend
| 3,133 | Python | 38.175 | 91 | 0.5391 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/tg_lib/tg_policy.py | import numpy as np
from tg_lib.traj_gen import TrajectoryGenerator
class TGPolicy():
""" state --> action
"""
def __init__(
self,
movetype="walk",
# offset for leg swing.
# mostly useless except walking up/down hill
# Might be good to make it a policy param
center_swing=0.0,
# push legs towards body
amplitude_extension=0.0,
# push legs away from body
amplitude_lift=0.0,
):
""" movetype decides which type of
TG we are training for
OPTIONS:
walk: one leg at a time LF, LB, RF, RB
trot: LF|RB together followed by
bound
pace
pronk
"""
# Trajectory Generators
self.TG_dict = {}
movetype_dict = {
"walk": [0, 0.25, 0.5, 0.75], # ORDER: RF | LF | RB | LB
"trot": [0, 0.5, 0.5, 0], # ORDER: LF + RB | LB + RF
"bound": [0, 0.5, 0, 0.5], # ORDER: LF + LB | RF + RB
"pace": [0, 0, 0.5, 0.5], # ORDER: LF + RF | LB + RB
"pronk": [0, 0, 0, 0] # LF + LB + RF + RB
}
TG_LF = TrajectoryGenerator(center_swing, amplitude_extension,
amplitude_lift, movetype_dict[movetype][0])
TG_LB = TrajectoryGenerator(center_swing, amplitude_extension,
amplitude_lift, movetype_dict[movetype][1])
TG_RF = TrajectoryGenerator(center_swing, amplitude_extension,
amplitude_lift, movetype_dict[movetype][2])
TG_RB = TrajectoryGenerator(center_swing, amplitude_extension,
amplitude_lift, movetype_dict[movetype][3])
self.TG_dict["LF"] = TG_LF
self.TG_dict["LB"] = TG_LB
self.TG_dict["RF"] = TG_RF
self.TG_dict["RB"] = TG_RB
def increment(self, dt, f_tg, Beta):
# Increment phase
for (key, tg) in self.TG_dict.items():
tg.CI.progress_tprime(dt, f_tg, Beta)
def get_TG_state(self):
# NOTE: MAYBE RETURN ONLY tprime for TG1 since that's
# the 'master' phase
# We get two observations per TG
# obs = np.array([])
# for i, (key, tg) in enumerate(self.TG_dict.items()):
# obs = np.append(obs, tg.get_state_based_on_phase[0])
# obs = np.append(obs, tg.get_state_based_on_phase[1])
# return obs
# OR just return phase, not sure why sin and cos is relevant...
# obs = np.array([])
# for i, (key, tg) in enumerate(self.TG_dict.items()):
# obs = np.append(obs, tg.CI.tprime)
# Return MASTER phase
obs = self.TG_dict["LF"].get_state_based_on_phase()
return obs
def get_utg(self, action, alpha_tg, h_tg, intensity, num_motors,
theta=0.0):
""" INPUTS:
action: residuals for each motor
from Policy
alpha_tg: swing amplitude from Policy
h_tg: center extension from Policy
ie walking height
num_motors: number of motors on minitaur
OUTPUTS:
action: residuals + TG
"""
# Get Action from TG [no policies here]
half_num_motors = int(num_motors / 2)
for i, (key, tg) in enumerate(self.TG_dict.items()):
action_idx = i
swing, extend = tg.get_swing_extend_based_on_phase(
alpha_tg, h_tg, intensity, theta)
# NOTE: ADDING to residuals
action[action_idx] += swing
action[action_idx + half_num_motors] += extend
return action
| 3,806 | Python | 35.257143 | 79 | 0.505255 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/debug_scripts/loader.py | #!/usr/bin/env python
import pybullet as p
import time
import pybullet_data
import numpy as np
import sys
sys.path.append('../../../')
from spotmicro.util import pybullet_data as pd
physicsClient = p.connect(p.GUI) # or p.DIRECT for non-graphical version
p.setAdditionalSearchPath(pybullet_data.getDataPath()) # optionally
p.setGravity(0, 0, -9.81)
# p.setTimeStep(1./240.) # slow, accurate
p.setRealTimeSimulation(0) # we want to be faster than real time :)
planeId = p.loadURDF("plane.urdf")
StartPos = [0, 0, 0.3]
StartOrientation = p.getQuaternionFromEuler([0, 0, 0])
p.resetDebugVisualizerCamera(cameraDistance=0.8,
cameraYaw=45,
cameraPitch=-30,
cameraTargetPosition=[0, 0, 0])
boxId = p.loadURDF(pd.getDataPath() + "/assets/urdf/spot.urdf",
StartPos,
StartOrientation,
flags=p.URDF_USE_SELF_COLLISION_EXCLUDE_PARENT)
numj = p.getNumJoints(boxId)
numb = p.getNumBodies()
Pos, Orn = p.getBasePositionAndOrientation(boxId)
print(Pos, Orn)
print("Number of joints {}".format(numj))
print("Number of links {}".format(numb))
joint = []
movingJoints = [
6,
7,
8, # FL
10,
11,
12, # FR
15,
16,
17, # BL
19,
20,
21 # BR
]
maxVelocity = 100
mode = p.POSITION_CONTROL
p.setJointMotorControlArray(bodyUniqueId=boxId,
jointIndices=movingJoints,
controlMode=p.POSITION_CONTROL,
targetPositions=np.zeros(12),
targetVelocities=np.zeros(12),
forces=np.ones(12) * np.inf)
counter = 0
angle1 = -np.pi / 2.0
angle2 = 0.0
angle = angle1
for i in range(100000000):
counter += 1
if counter % 1000 == 0:
p.setJointMotorControlArray(
bodyUniqueId=boxId,
jointIndices=[8, 12, 17, 21], # FWrists
controlMode=p.POSITION_CONTROL,
targetPositions=np.ones(4) * angle,
targetVelocities=np.zeros(4),
forces=np.ones(4) * 0.15)
counter = 0
if angle == angle1:
angle = angle2
else:
angle = angle1
p.stepSimulation()
p.disconnect() | 2,296 | Python | 26.674698 | 73 | 0.578397 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/old_training_scripts/mini_td3.py | #!/usr/bin/env python
import numpy as np
from td3_lib.td3 import ReplayBuffer, TD3Agent, evaluate_policy
from mini_bullet.minitaur_gym_env import MinitaurBulletEnv
import torch
import os
def main():
""" The main() function. """
print("STARTING MINITAUR TD3")
# TRAINING PARAMETERS
# env_name = "MinitaurBulletEnv-v0"
seed = 0
max_timesteps = 4e6
start_timesteps = 1e4 # 1e3 for testing purposes, use 1e4 for real
expl_noise = 0.1
batch_size = 100
eval_freq = 1e4
save_model = True
file_name = "mini_td3_"
# Find abs path to this file
my_path = os.path.abspath(os.path.dirname(__file__))
results_path = os.path.join(my_path, "../results")
models_path = os.path.join(my_path, "../models")
if not os.path.exists(results_path):
os.makedirs(results_path)
if not os.path.exists(models_path):
os.makedirs(models_path)
env = MinitaurBulletEnv(render=False)
# Set seeds
env.seed(seed)
torch.manual_seed(seed)
np.random.seed(seed)
state_dim = env.observation_space.shape[0]
print("STATE DIM: {}".format(state_dim))
action_dim = env.action_space.shape[0]
print("ACTION DIM: {}".format(action_dim))
max_action = float(env.action_space.high[0])
print("RECORDED MAX ACTION: {}".format(max_action))
policy = TD3Agent(state_dim, action_dim, max_action)
policy_num = 0
if os.path.exists(models_path + "/" + file_name +
str(policy_num) + "_critic"):
print("Loading Existing Policy")
policy.load(models_path + "/" + file_name + str(policy_num))
replay_buffer = ReplayBuffer()
# Optionally load existing policy, replace 9999 with num
buffer_number = 0 # BY DEFAULT WILL LOAD NOTHING, CHANGE THIS
if os.path.exists(replay_buffer.buffer_path + "/" + "replay_buffer_" +
str(buffer_number) + '.data'):
print("Loading Replay Buffer " + str(buffer_number))
replay_buffer.load(buffer_number)
# print(replay_buffer.storage)
# Evaluate untrained policy and init list for storage
evaluations = []
state = env.reset()
done = False
episode_reward = 0
episode_timesteps = 0
episode_num = 0
print("STARTED MINITAUR TD3")
for t in range(int(max_timesteps)):
episode_timesteps += 1
# Select action randomly or according to policy
# Random Action - no training yet, just storing in buffer
if t < start_timesteps:
action = env.action_space.sample()
# rospy.logdebug("Sampled Action")
else:
# According to policy + Exploraton Noise
# print("POLICY Action")
""" Note we clip at +-0.99.... because Gazebo
has problems executing actions at the
position limit (breaks model)
"""
action = np.clip(
(policy.select_action(np.array(state)) + np.random.normal(
0, max_action * expl_noise, size=action_dim)),
-max_action, max_action)
# rospy.logdebug("Selected Acton: {}".format(action))
# Perform action
next_state, reward, done, _ = env.step(action)
done_bool = float(done)
# Store data in replay buffer
replay_buffer.add((state, action, next_state, reward, done_bool))
state = next_state
episode_reward += reward
# Train agent after collecting sufficient data for buffer
if t >= start_timesteps:
policy.train(replay_buffer, batch_size)
if done:
# +1 to account for 0 indexing.
# +0 on ep_timesteps since it will increment +1 even if done=True
print(
"Total T: {} Episode Num: {} Episode T: {} Reward: {}".format(
t + 1, episode_num, episode_timesteps, episode_reward))
# Reset environment
state, done = env.reset(), False
evaluations.append(episode_reward)
episode_reward = 0
episode_timesteps = 0
episode_num += 1
# Evaluate episode
if (t + 1) % eval_freq == 0:
# evaluate_policy(policy, env_name, seed,
np.save(results_path + "/" + str(file_name), evaluations)
if save_model:
policy.save(models_path + "/" + str(file_name) + str(t))
# replay_buffer.save(t)
env.close()
if __name__ == '__main__':
main() | 4,520 | Python | 30.615384 | 78 | 0.584735 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/src/old_training_scripts/mini_ars.py | #!/usr/bin/env python
import numpy as np
from ars_lib.ars import ARSAgent, Normalizer, Policy, ParallelWorker
from mini_bullet.minitaur_gym_env import MinitaurBulletEnv
import torch
import os
# Multiprocessing package for python
# Parallelization improvements based on:
# https://github.com/bulletphysics/bullet3/blob/master/examples/pybullet/gym/pybullet_envs/ARS/ars.py
import multiprocessing as mp
from multiprocessing import Pipe
# Messages for Pipe
_RESET = 1
_CLOSE = 2
_EXPLORE = 3
def main():
""" The main() function. """
# Hold mp pipes
mp.freeze_support()
print("STARTING MINITAUR ARS")
# TRAINING PARAMETERS
# env_name = "MinitaurBulletEnv-v0"
seed = 0
max_timesteps = 4e6
eval_freq = 1e1
save_model = True
file_name = "mini_ars_"
# Find abs path to this file
my_path = os.path.abspath(os.path.dirname(__file__))
results_path = os.path.join(my_path, "../results")
models_path = os.path.join(my_path, "../models")
if not os.path.exists(results_path):
os.makedirs(results_path)
if not os.path.exists(models_path):
os.makedirs(models_path)
env = MinitaurBulletEnv(render=False)
# Set seeds
env.seed(seed)
torch.manual_seed(seed)
np.random.seed(seed)
state_dim = env.observation_space.shape[0]
print("STATE DIM: {}".format(state_dim))
action_dim = env.action_space.shape[0]
print("ACTION DIM: {}".format(action_dim))
max_action = float(env.action_space.high[0])
print("RECORDED MAX ACTION: {}".format(max_action))
# Initialize Normalizer
normalizer = Normalizer(state_dim)
# Initialize Policy
policy = Policy(state_dim, action_dim)
# Initialize Agent with normalizer, policy and gym env
agent = ARSAgent(normalizer, policy, env)
agent_num = 0
if os.path.exists(models_path + "/" + file_name + str(agent_num) +
"_policy"):
print("Loading Existing agent")
agent.load(models_path + "/" + file_name + str(agent_num))
# Evaluate untrained agent and init list for storage
evaluations = []
env.reset(agent.desired_velocity, agent.desired_rate)
episode_reward = 0
episode_timesteps = 0
episode_num = 0
# MULTIPROCESSING
# Create mp pipes
num_processes = policy.num_deltas
processes = []
childPipes = []
parentPipes = []
# Store mp pipes
for pr in range(num_processes):
parentPipe, childPipe = Pipe()
parentPipes.append(parentPipe)
childPipes.append(childPipe)
# Start multiprocessing
for proc_num in range(num_processes):
p = mp.Process(target=ParallelWorker, args=(childPipes[proc_num], env))
p.start()
processes.append(p)
print("STARTED MINITAUR ARS")
t = 0
while t < (int(max_timesteps)):
# Maximum timesteps per rollout
t += policy.episode_steps
episode_timesteps += 1
episode_reward = agent.train_parallel(parentPipes)
# episode_reward = agent.train()
# +1 to account for 0 indexing.
# +0 on ep_timesteps since it will increment +1 even if done=True
print("Total T: {} Episode Num: {} Episode T: {} Reward: {}, >400: {}".
format(t, episode_num, policy.episode_steps, episode_reward,
agent.successes))
# Reset environment
evaluations.append(episode_reward)
episode_reward = 0
episode_timesteps = 0
# Evaluate episode
if (episode_num + 1) % eval_freq == 0:
# evaluate_agent(agent, env_name, seed,
np.save(results_path + "/" + str(file_name), evaluations)
if save_model:
agent.save(models_path + "/" + str(file_name) +
str(episode_num))
# replay_buffer.save(t)
episode_num += 1
# Close pipes and hence envs
for parentPipe in parentPipes:
parentPipe.send([_CLOSE, "pay2"])
for p in processes:
p.join()
if __name__ == '__main__':
main()
| 4,077 | Python | 26.186666 | 101 | 0.619328 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/paper/GMBC_data_collector.py | #!/usr/bin/env python
import numpy as np
import sys
sys.path.append('../../')
from ars_lib.ars import ARSAgent, Normalizer, Policy
from spotmicro.util.gui import GUI
from spotmicro.Kinematics.SpotKinematics import SpotModel
from spotmicro.GaitGenerator.Bezier import BezierGait
from spotmicro.OpenLoopSM.SpotOL import BezierStepper
from spotmicro.GymEnvs.spot_bezier_env import spotBezierEnv
from spotmicro.spot_env_randomizer import SpotEnvRandomizer
import os
import argparse
import pickle
import matplotlib.pyplot as plt
import pandas as pd
import seaborn as sns
sns.set()
# ARGUMENTS
descr = "Spot Mini Mini ARS Agent Evaluator."
parser = argparse.ArgumentParser(description=descr)
parser.add_argument("-hf",
"--HeightField",
help="Use HeightField",
action='store_true')
parser.add_argument("-a", "--AgentNum", help="Agent Number To Load")
parser.add_argument("-nep",
"--NumberOfEpisodes",
help="Number of Episodes to Collect Data For")
parser.add_argument("-dr",
"--DontRandomize",
help="Do NOT Randomize State and Environment.",
action='store_true')
parser.add_argument("-nc",
"--NoContactSensing",
help="Disable Contact Sensing",
action='store_true')
parser.add_argument("-s",
"--Seed",
help="Seed (Default: 0).")
ARGS = parser.parse_args()
def main():
""" The main() function. """
print("STARTING MINITAUR ARS")
# TRAINING PARAMETERS
# env_name = "MinitaurBulletEnv-v0"
seed = 0
if ARGS.Seed:
seed = ARGS.Seed
max_episodes = 1000
if ARGS.NumberOfEpisodes:
max_episodes = ARGS.NumberOfEpisodes
if ARGS.HeightField:
height_field = True
else:
height_field = False
if ARGS.NoContactSensing:
contacts = False
else:
contacts = True
if ARGS.DontRandomize:
env_randomizer = None
else:
env_randomizer = SpotEnvRandomizer()
file_name = "spot_ars_"
# Find abs path to this file
my_path = os.path.abspath(os.path.dirname(__file__))
results_path = os.path.join(my_path, "../results")
if contacts:
models_path = os.path.join(my_path, "../models/contact")
else:
models_path = os.path.join(my_path, "../models/no_contact")
if not os.path.exists(results_path):
os.makedirs(results_path)
if not os.path.exists(models_path):
os.makedirs(models_path)
if ARGS.HeightField:
height_field = True
else:
height_field = False
env = spotBezierEnv(render=False,
on_rack=False,
height_field=height_field,
draw_foot_path=False,
contacts=contacts,
env_randomizer=env_randomizer)
# Set seeds
env.seed(seed)
np.random.seed(seed)
state_dim = env.observation_space.shape[0]
print("STATE DIM: {}".format(state_dim))
action_dim = env.action_space.shape[0]
print("ACTION DIM: {}".format(action_dim))
max_action = float(env.action_space.high[0])
env.reset()
spot = SpotModel()
bz_step = BezierStepper(dt=env._time_step)
bzg = BezierGait(dt=env._time_step)
# Initialize Normalizer
normalizer = Normalizer(state_dim)
# Initialize Policy
policy = Policy(state_dim, action_dim, episode_steps=np.inf)
# Initialize Agent with normalizer, policy and gym env
agent = ARSAgent(normalizer, policy, env, bz_step, bzg, spot, False)
use_agent = False
agent_num = 0
if ARGS.AgentNum:
agent_num = ARGS.AgentNum
use_agent = True
if os.path.exists(models_path + "/" + file_name + str(agent_num) +
"_policy"):
print("Loading Existing agent")
agent.load(models_path + "/" + file_name + str(agent_num))
agent.policy.episode_steps = 50000
policy = agent.policy
env.reset()
episode_reward = 0
episode_timesteps = 0
episode_num = 0
print("STARTED MINITAUR TEST SCRIPT")
# Used to create gaussian distribution of survival distance
surv_pos = []
# Store results
if use_agent:
# Store _agent
agt = "agent_" + str(agent_num)
else:
# Store _vanilla
agt = "vanilla"
while episode_num < (int(max_episodes)):
episode_reward, episode_timesteps = agent.deployTG()
# We only care about x/y pos
travelled_pos = list(agent.returnPose())
# NOTE: FORMAT: X, Y, TIMESTEPS -
# tells us if robobt was just stuck forever. didn't actually fall.
travelled_pos[-1] = episode_timesteps
episode_num += 1
# Store dt and frequency for prob distribution
surv_pos.append(travelled_pos)
print("Episode Num: {} Episode T: {} Reward: {}".format(
episode_num, episode_timesteps, episode_reward))
print("Survival Pos: {}".format(surv_pos[-1]))
# Save/Overwrite each time
with open(
results_path + "/" + str(file_name) + agt + '_survival_' +
str(max_episodes), 'wb') as filehandle:
pickle.dump(surv_pos, filehandle)
env.close()
print("---------------------------------------")
if __name__ == '__main__':
main()
| 5,460 | Python | 27.442708 | 74 | 0.59359 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_bullet/paper/GMBC_data_plotter.py | #!/usr/bin/env python
import numpy as np
import sys
import os
import argparse
import pickle
import matplotlib.pyplot as plt
import pandas as pd
import seaborn as sns
import copy
from scipy.stats import norm
sns.set()
# ARGUMENTS
descr = "Spot Mini Mini ARS Agent Evaluator."
parser = argparse.ArgumentParser(description=descr)
parser.add_argument("-nep",
"--NumberOfEpisodes",
help="Number of Episodes to Plot Data For")
parser.add_argument("-maw",
"--MovingAverageWindow",
help="Moving Average Window for Plotting (Default: 50)")
parser.add_argument("-surv",
"--Survival",
help="Plot Survival Curve",
action='store_true')
parser.add_argument("-tr",
"--TrainingData",
help="Plot Training Curve",
action='store_true')
parser.add_argument("-tot",
"--TotalReward",
help="Show Total Reward instead of Reward Per Timestep",
action='store_true')
parser.add_argument("-ar",
"--RandAgentNum",
help="Randomized Agent Number To Load")
parser.add_argument("-anor",
"--NoRandAgentNum",
help="Non-Randomized Agent Number To Load")
parser.add_argument("-raw",
"--Raw",
help="Plot Raw Data in addition to Moving Averaged Data",
action='store_true')
parser.add_argument(
"-s",
"--Seed",
help="Seed [UP TO, e.g. 0 | 0, 1 | 0, 1, 2 ...] (Default: 0).")
parser.add_argument("-pout",
"--PolicyOut",
help="Plot Policy Output Data",
action='store_true')
parser.add_argument("-rough",
"--Rough",
help="Plot Policy Output Data for Rough Terrain",
action='store_true')
parser.add_argument(
"-tru",
"--TrueAct",
help="Plot the Agent Action instead of what the robot sees",
action='store_true')
ARGS = parser.parse_args()
MA_WINDOW = 50
if ARGS.MovingAverageWindow:
MA_WINDOW = int(ARGS.MovingAverageWindow)
def moving_average(a, n=MA_WINDOW):
MA = np.cumsum(a, dtype=float)
MA[n:] = MA[n:] - MA[:-n]
return MA[n - 1:] / n
def extract_data_bounds(min=0, max=5, dist_data=None, dt_data=None):
""" 3 bounds: lower, mid, highest
"""
if dist_data is not None:
# Get Survival Data, dt
# Lowest Bound: x <= max
bound = np.array([0])
if min == 0:
less_max_cond = dist_data <= max
bound = np.where(less_max_cond)
else:
# Highest Bound: min <= x
if max == np.inf:
gtr_min_cond = dist_data >= min
bound = np.where(gtr_min_cond)
# Mid Bound: min < x < max
else:
less_max_gtr_min_cond = np.logical_and(dist_data > min,
dist_data < max)
bound = np.where(less_max_gtr_min_cond)
if dt_data is not None:
dt_bounded = dt_data[bound]
num_surv = np.array(np.where(dt_bounded == 50000))[0].shape[0]
else:
num_surv = None
return dist_data[bound], num_surv
else:
return None
def main():
""" The main() function. """
file_name = "spot_ars_"
seed = 0
if ARGS.Seed:
seed = ARGS.Seed
# Find abs path to this file
my_path = os.path.abspath(os.path.dirname(__file__))
results_path = os.path.join(my_path, "../results")
if not os.path.exists(results_path):
os.makedirs(results_path)
vanilla_surv = np.random.randn(1000)
agent_surv = np.random.randn(1000)
nep = 1000
if ARGS.NumberOfEpisodes:
nep = ARGS.NumberOfEpisodes
if ARGS.TrainingData:
training = True
else:
training = False
if ARGS.Survival:
surv = True
else:
surv = False
if ARGS.PolicyOut or ARGS.Rough or ARGS.TrueAct:
pout = True
else:
pout = False
if not pout and not surv and not training:
print(
"Please Select which Data you would like to plot (-pout | -surv | -tr)"
)
rand_agt = 579
norand_agt = 569
if ARGS.RandAgentNum:
rand_agt = ARGS.RandAgentNum
if ARGS.NoRandAgentNum:
norand_agt = ARGS.NoRandAgentNum
if surv:
# Vanilla Data
if os.path.exists(results_path + "/" + file_name + "vanilla" +
'_survival_{}'.format(nep)):
with open(
results_path + "/" + file_name + "vanilla" +
'_survival_{}'.format(nep), 'rb') as filehandle:
vanilla_surv = np.array(pickle.load(filehandle))
# Rand Agent Data
if os.path.exists(results_path + "/" + file_name +
"agent_{}".format(rand_agt) +
'_survival_{}'.format(nep)):
with open(
results_path + "/" + file_name +
"agent_{}".format(rand_agt) + '_survival_{}'.format(nep),
'rb') as filehandle:
d2gmbc_surv = np.array(pickle.load(filehandle))
# NoRand Agent Data
if os.path.exists(results_path + "/" + file_name +
"agent_{}".format(norand_agt) +
'_survival_{}'.format(nep)):
with open(
results_path + "/" + file_name +
"agent_{}".format(norand_agt) + '_survival_{}'.format(nep),
'rb') as filehandle:
gmbc_surv = np.array(pickle.load(filehandle))
# print(gmbc_surv[:, 0])
# Extract useful values
vanilla_surv_x = vanilla_surv[:1000, 0]
d2gmbc_surv_x = d2gmbc_surv[:, 0]
gmbc_surv_x = gmbc_surv[:, 0]
# convert the lists to series
data = {
'Open Loop': vanilla_surv_x,
'GMBC': d2gmbc_surv_x,
'D^2-GMBC': gmbc_surv_x
}
colors = ['r', 'g', 'b']
# get dataframe
df = pd.DataFrame(data)
print(df)
# get dataframe2
# Extract useful values
vanilla_surv_dt = vanilla_surv[:1000, -1]
d2gmbc_surv_dt = d2gmbc_surv[:, -1]
gmbc_surv_dt = gmbc_surv[:, -1]
# convert the lists to series
data2 = {
'Open Loop': vanilla_surv_dt,
'GMBC': d2gmbc_surv_dt,
'D^2-GMBC': gmbc_surv_dt
}
df2 = pd.DataFrame(data2)
# Plot
for i, col in enumerate(df.columns):
sns.distplot(df[[col]], color=colors[i])
plt.legend(labels=['D^2-GMBC', 'GMBC', 'Open Loop'])
plt.xlabel("Forward Survived Distance (m)")
plt.ylabel("Kernel Density Estimate")
plt.show()
# Print AVG and STDEV
norand_avg = np.average(copy.deepcopy(gmbc_surv_x))
norand_std = np.std(copy.deepcopy(gmbc_surv_x))
rand_avg = np.average(copy.deepcopy(d2gmbc_surv_x))
rand_std = np.std(copy.deepcopy(d2gmbc_surv_x))
vanilla_avg = np.average(copy.deepcopy(vanilla_surv_x))
vanilla_std = np.std(copy.deepcopy(vanilla_surv_x))
print("Open Loop: AVG [{}] | STD [{}] | AMOUNT [{}]".format(
vanilla_avg, vanilla_std, gmbc_surv_x.shape[0]))
print("D^2-GMBC: AVG [{}] | STD [{}] AMOUNT [{}]".format(
rand_avg, rand_std, d2gmbc_surv_x.shape[0]))
print("GMBC: AVG [{}] | STD [{}] AMOUNT [{}]".format(
norand_avg, norand_std, vanilla_surv_x.shape[0]))
# collect data
gmbc_surv_x_less_5, gmbc_surv_num_less_5 = extract_data_bounds(
0, 5, gmbc_surv_x, gmbc_surv_dt)
d2gmbc_surv_x_less_5, d2gmbc_surv_num_less_5 = extract_data_bounds(
0, 5, d2gmbc_surv_x, d2gmbc_surv_dt)
vanilla_surv_x_less_5, vanilla_surv_num_less_5 = extract_data_bounds(
0, 5, vanilla_surv_x, vanilla_surv_dt)
# <=5
# Make sure all arrays filled
if gmbc_surv_x_less_5.size == 0:
gmbc_surv_x_less_5 = np.array([0])
if d2gmbc_surv_x_less_5.size == 0:
d2gmbc_surv_x_less_5 = np.array([0])
if vanilla_surv_x_less_5.size == 0:
vanilla_surv_x_less_5 = np.array([0])
norand_avg = np.average(gmbc_surv_x_less_5)
norand_std = np.std(gmbc_surv_x_less_5)
rand_avg = np.average(d2gmbc_surv_x_less_5)
rand_std = np.std(d2gmbc_surv_x_less_5)
vanilla_avg = np.average(vanilla_surv_x_less_5)
vanilla_std = np.std(vanilla_surv_x_less_5)
print("<= 5m")
print(
"Open Loop: AVG [{}] | STD [{}] | AMOUNT DEAD [{}] | AMOUNT ALIVE [{}]"
.format(vanilla_avg, vanilla_std,
vanilla_surv_x_less_5.shape[0] - vanilla_surv_num_less_5,
vanilla_surv_num_less_5))
print(
"D^2-GMBC: AVG [{}] | STD [{}] AMOUNT DEAD [{}] | AMOUNT ALIVE [{}]"
.format(rand_avg, rand_std,
d2gmbc_surv_x_less_5.shape[0] - d2gmbc_surv_num_less_5,
d2gmbc_surv_num_less_5))
print("GMBC: AVG [{}] | STD [{}] AMOUNT DEAD [{}] | AMOUNT ALIVE [{}]".
format(norand_avg, norand_std,
gmbc_surv_x_less_5.shape[0] - gmbc_surv_num_less_5,
gmbc_surv_num_less_5))
# collect data
gmbc_surv_x_gtr_5, gmbc_surv_num_gtr_5 = extract_data_bounds(
5, 90, gmbc_surv_x, gmbc_surv_dt)
d2gmbc_surv_x_gtr_5, d2gmbc_surv_num_gtr_5 = extract_data_bounds(
5, 90, d2gmbc_surv_x, d2gmbc_surv_dt)
vanilla_surv_x_gtr_5, vanilla_surv_num_gtr_5 = extract_data_bounds(
5, 90, vanilla_surv_x, vanilla_surv_dt)
# >5 <90
# Make sure all arrays filled
if gmbc_surv_x_gtr_5.size == 0:
gmbc_surv_x_gtr_5 = np.array([0])
if d2gmbc_surv_x_gtr_5.size == 0:
d2gmbc_surv_x_gtr_5 = np.array([0])
if vanilla_surv_x_gtr_5.size == 0:
vanilla_surv_x_gtr_5 = np.array([0])
norand_avg = np.average(gmbc_surv_x_gtr_5)
norand_std = np.std(gmbc_surv_x_gtr_5)
rand_avg = np.average(d2gmbc_surv_x_gtr_5)
rand_std = np.std(d2gmbc_surv_x_gtr_5)
vanilla_avg = np.average(vanilla_surv_x_gtr_5)
vanilla_std = np.std(vanilla_surv_x_gtr_5)
print("> 5m and <90m")
print(
"Open Loop: AVG [{}] | STD [{}] | AMOUNT DEAD [{}] | AMOUNT ALIVE [{}]"
.format(vanilla_avg, vanilla_std,
vanilla_surv_x_gtr_5.shape[0] - vanilla_surv_num_gtr_5,
vanilla_surv_num_gtr_5))
print(
"D^2-GMBC: AVG [{}] | STD [{}] AMOUNT DEAD [{}] | AMOUNT ALIVE [{}]"
.format(rand_avg, rand_std,
d2gmbc_surv_x_gtr_5.shape[0] - d2gmbc_surv_num_gtr_5,
d2gmbc_surv_num_gtr_5))
print("GMBC: AVG [{}] | STD [{}] AMOUNT DEAD [{}] | AMOUNT ALIVE [{}]".
format(norand_avg, norand_std,
gmbc_surv_x_gtr_5.shape[0] - gmbc_surv_num_gtr_5,
gmbc_surv_num_gtr_5))
# collect data
gmbc_surv_x_gtr_90, gmbc_surv_num_gtr_90 = extract_data_bounds(
90, np.inf, gmbc_surv_x, gmbc_surv_dt)
d2gmbc_surv_x_gtr_90, d2gmbc_surv_num_gtr_90 = extract_data_bounds(
90, np.inf, d2gmbc_surv_x, d2gmbc_surv_dt)
vanilla_surv_x_gtr_90, vanilla_surv_num_gtr_90 = extract_data_bounds(
90, np.inf, vanilla_surv_x, vanilla_surv_dt)
# >90
# Make sure all arrays filled
if gmbc_surv_x_gtr_90.size == 0:
gmbc_surv_x_gtr_90 = np.array([0])
if d2gmbc_surv_x_gtr_90.size == 0:
d2gmbc_surv_x_gtr_90 = np.array([0])
if vanilla_surv_x_gtr_90.size == 0:
vanilla_surv_x_gtr_90 = np.array([0])
norand_avg = np.average(gmbc_surv_x_gtr_90)
norand_std = np.std(gmbc_surv_x_gtr_90)
rand_avg = np.average(d2gmbc_surv_x_gtr_90)
rand_std = np.std(d2gmbc_surv_x_gtr_90)
vanilla_avg = np.average(vanilla_surv_x_gtr_90)
vanilla_std = np.std(vanilla_surv_x_gtr_90)
print(">= 90m")
print(
"Open Loop: AVG [{}] | STD [{}] | AAMOUNT DEAD [{}] | AMOUNT ALIVE [{}]"
.format(vanilla_avg, vanilla_std,
vanilla_surv_x_gtr_90.shape[0] - vanilla_surv_num_gtr_90,
vanilla_surv_num_gtr_90))
print(
"D^2-GMBC: AVG [{}] | STD [{}] AMOUNT DEAD [{}] | AMOUNT ALIVE [{}]"
.format(rand_avg, rand_std,
d2gmbc_surv_x_gtr_90.shape[0] - d2gmbc_surv_num_gtr_90,
d2gmbc_surv_num_gtr_90))
print("GMBC: AVG [{}] | STD [{}] AMOUNT DEAD [{}] | AMOUNT ALIVE [{}]".
format(norand_avg, norand_std,
gmbc_surv_x_gtr_90.shape[0] - gmbc_surv_num_gtr_90,
gmbc_surv_num_gtr_90))
# Save to excel
df.to_excel(results_path + "/SurvDist.xlsx", index=False)
df2.to_excel(results_path + "/SurvDT.xlsx", index=False)
elif training:
rand_data_list = []
norand_data_list = []
rand_shortest_length = np.inf
norand_shortest_length = np.inf
for i in range(int(seed) + 1):
# Training Data Plotter
rand_data_temp = np.load(results_path + "/spot_ars_rand_" +
"seed" + str(i) + ".npy")
norand_data_temp = np.load(results_path + "/spot_ars_norand_" +
"seed" + str(i) + ".npy")
rand_shortest_length = min(
np.shape(rand_data_temp[:, 1])[0], rand_shortest_length)
norand_shortest_length = min(
np.shape(norand_data_temp[:, 1])[0], norand_shortest_length)
rand_data_list.append(rand_data_temp)
norand_data_list.append(norand_data_temp)
tot_rand_data = []
tot_norand_data = []
norm_rand_data = []
norm_norand_data = []
for i in range(int(seed) + 1):
tot_rand_data.append(
moving_average(rand_data_list[i][:rand_shortest_length, 0]))
tot_norand_data.append(
moving_average(
norand_data_list[i][:norand_shortest_length, 0]))
norm_rand_data.append(
moving_average(rand_data_list[i][:rand_shortest_length, 1]))
norm_norand_data.append(
moving_average(
norand_data_list[i][:norand_shortest_length, 1]))
tot_rand_data = np.array(tot_rand_data)
tot_norand_data = np.array(tot_norand_data)
norm_rand_data = np.array(norm_rand_data)
norm_norand_data = np.array(norm_norand_data)
# column-wise
axis = 0
# MEAN
tot_rand_mean = tot_rand_data.mean(axis=axis)
tot_norand_mean = tot_norand_data.mean(axis=axis)
norm_rand_mean = norm_rand_data.mean(axis=axis)
norm_norand_mean = norm_norand_data.mean(axis=axis)
# STD
tot_rand_std = tot_rand_data.std(axis=axis)
tot_norand_std = tot_norand_data.std(axis=axis)
norm_rand_std = norm_rand_data.std(axis=axis)
norm_norand_std = norm_norand_data.std(axis=axis)
aranged_rand = np.arange(np.shape(tot_rand_mean)[0])
aranged_norand = np.arange(np.shape(tot_norand_mean)[0])
if ARGS.TotalReward:
if ARGS.Raw:
plt.plot(rand_data_list[0][:, 0],
label="Randomized (Total Reward)",
color='g')
plt.plot(norand_data_list[0][:, 0],
label="Non-Randomized (Total Reward)",
color='r')
plt.plot(aranged_norand,
tot_norand_mean,
label="MA: Non-Randomized (Total Reward)",
color='r')
plt.fill_between(aranged_norand,
tot_norand_mean - tot_norand_std,
tot_norand_mean + tot_norand_std,
color='r',
alpha=0.2)
plt.plot(aranged_rand,
tot_rand_mean,
label="MA: Randomized (Total Reward)",
color='g')
plt.fill_between(aranged_rand,
tot_rand_mean - tot_rand_std,
tot_rand_mean + tot_rand_std,
color='g',
alpha=0.2)
else:
if ARGS.Raw:
plt.plot(rand_data_list[0][:, 1],
label="Randomized (Reward/dt)",
color='g')
plt.plot(norand_data_list[0][:, 1],
label="Non-Randomized (Reward/dt)",
color='r')
plt.plot(aranged_norand,
norm_norand_mean,
label="MA: Non-Randomized (Reward/dt)",
color='r')
plt.fill_between(aranged_norand,
norm_norand_mean - norm_norand_std,
norm_norand_mean + norm_norand_std,
color='r',
alpha=0.2)
plt.plot(aranged_rand,
norm_rand_mean,
label="MA: Randomized (Reward/dt)",
color='g')
plt.fill_between(aranged_rand,
norm_rand_mean - norm_rand_std,
norm_rand_mean + norm_rand_std,
color='g',
alpha=0.2)
plt.xlabel("Epoch #")
plt.ylabel("Reward")
plt.title(
"Training Performance with {} seed samples".format(int(seed) + 1))
plt.legend()
plt.show()
elif pout:
if ARGS.Rough:
terrain_name = "rough_"
else:
terrain_name = "flat_"
if ARGS.TrueAct:
action_name = "agent_act"
else:
action_name = "robot_act"
action = np.load(results_path + "/" + "policy_out_" + terrain_name +
action_name + ".npy")
ClearHeight_act = action[:, 0]
BodyHeight_act = action[:, 1]
Residuals_act = action[:, 2:]
plt.plot(ClearHeight_act, label='Clearance Height Mod', color='black')
plt.plot(BodyHeight_act, label='Body Height Mod', color='darkviolet')
# FL
plt.plot(Residuals_act[:, 0],
label='Residual: FL (x)',
color='limegreen')
plt.plot(Residuals_act[:, 1], label='Residual: FL (y)', color='lime')
plt.plot(Residuals_act[:, 2], label='Residual: FL (z)', color='green')
# FR
plt.plot(Residuals_act[:, 3],
label='Residual: FR (x)',
color='lightskyblue')
plt.plot(Residuals_act[:, 4],
label='Residual: FR (y)',
color='dodgerblue')
plt.plot(Residuals_act[:, 5], label='Residual: FR (z)', color='blue')
# BL
plt.plot(Residuals_act[:, 6],
label='Residual: BL (x)',
color='firebrick')
plt.plot(Residuals_act[:, 7],
label='Residual: BL (y)',
color='crimson')
plt.plot(Residuals_act[:, 8], label='Residual: BL (z)', color='red')
# BR
plt.plot(Residuals_act[:, 9], label='Residual: BR (x)', color='gold')
plt.plot(Residuals_act[:, 10],
label='Residual: BR (y)',
color='orange')
plt.plot(Residuals_act[:, 11], label='Residual: BR (z)', color='coral')
plt.xlabel("Epoch Iteration")
plt.ylabel("Action Value")
plt.title("Policy Output")
plt.legend()
plt.show()
if __name__ == '__main__':
main()
| 20,353 | Python | 36.484346 | 84 | 0.5014 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Calibration.md | # Spot Mini Mini - The Real Deal
### Assembly Instructions
I'm in the middle of moving so it's difficult for me to get detailed images/instructions. For now, please consult the [CAD Model](https://cad.onshape.com/documents/9d0f96878c54300abf1157ac/w/c9cdf8daa98d8a0d7d50c8d3/e/fa0d7caf0ed2ef46834ecc24), which should be straightforward to look at and intuit.
During assembly, make sure the motors are powered and that you select `NOMINAL_PWM` mode in the [main.cpp](https://github.com/moribots/spot_mini_mini/blob/spot/spot_real/Control/Teensy/SpotMiniMini/src/main.cpp) file that runs on the `Teensy`. **NOTE:** Be sure to consult `nominal_servo_pwm()` to input your correct servo angle and pwm ranges for a successful calibration. For example, I am using `270` degree motors with `500` min and `2500` max PWM as shown below.


When the assembly in this mode is finished, the robot should have its legs extended and perpendicular to the body, like this:

#### Motor Plug In Order
```
M01 front left shoulder
M02 front left elbow
M03 front left wrist
M04 front right shoulder
M05 front right elbow
M06 front right wrist
M07 back left shoulder
M08 back left elbow
M09 back left wrist
M10 back right shoulder
M11 back right elbow
M12 back right wrist
```
#### Recommended Build Order
* Main Body
* Legs
* Inner Hips
* Outer Hips
* Covers
#### Main Body
* press-fit M3 nuts onto both sides of the main electronics plate, and M2 nuts onto the bottom of the adapter plate.
* Fasten the IMU onto the middle of the electronics plate. Regardless of your make/model, calibrate it to make sure your inertial axes follow the right hand rule.
* Fasten the adapter plate onto the main plate using 16mm M3 bolts.
* Fasten the battery to the bottom of the main plate using the battery holder and some M3 bolts.
* Fasten the Raspberry Pi and Spot Mini Mini boards onto the adapter plate using 8mm M2 bolts.
* Do your wiring now to avoid a hassle later. **TODO: Wiring Instructions - (simple enough to figure out in the meantime by following [The Diagram](https://easyeda.com/adhamelarabawy/PowerDistributionBoard))**.
#### Legs
* Fasten two of your motors into each shoulder joint.
* Fasten a disc servo horn onto the upper leg.
* Fasten the upper leg onto the shoulder motor (the one that does not have an opposing nub) through the disc horn. The upper leg should be perpendicular to the shoulder.
* Squeeze in an M3 nut onto the floor of the inner upper leg and an M5 nut onto the idler adjustor. Then, fit a bearing onto the idler using an 8mm M5 bolt.
* Push the idler onto the floor of the inner upper leg. While you're here, press-fit an M5 nut onto the bottom of the upper leg.
* Fasten a disc servo horn onto the belt pulley.
* Fasten the assembled pulley onto each leg's third motor. Place a bearing inside the pulley once this is done.
* After placing a belt around the motor's pulley, press-fit the pulley onto the upper leg.
* Press-fit two bearings into either side of the lower leg pulley.
* Slot the belt around the lower leg (try to keep it parallel to the upper leg) and secure it through the upper leg with a 30mm M5 bolt. This should be easy if your idler is untentioned.
* Tension your idler.
* The leg should be fully extended, with the upper and lower leg being parallel to each other and perpendicular to the shoulder.
* Once you're happy with this, fasten the servo cover onto the protruding motor, press-fit the bearing, and fasten the support bridge between the bearing and the shoulder joint.
#### Inner Hips
* Fasten two disc servo horns into the rear inner hip.
* Fasten the rear left and right legs to the rear inner hip, making sure the legs are parallel to the side of the body.
* Fasten the rear inner hip to both side chassis brackets.
* Slot the finished Main Body assembly into the chassis bracket lips. Secure with nuts and bolts.
* Fasten the front inner hip to both side chassis brackets. The main body should now be fully secured.
* Press-fit two bearings into the front inner hip.
#### Outer Hips
* Fasten two disc servo horns into the front outer hip.
* Fasten the front left and right legs to the front outer hip, making sure the legs are parallel to the side of the body.
* After slotting M3 nuts into the front inner hip, secure the front outer hip assembly (with the legs) using 16mm M3 bolts. Note that the nubs on the shoulder joints should fit into the bearings.
* Press-fit two bearings into the rear outer hip.
* After slotting M3 nuts into the rear inner hip, secure the rear outer hip assembly (with the legs) using 16mm M3 bolts. Note that the nubs on the shoulder joints should fit into the bearings.
### Motor Calibration Modes and Method
After turning on Spot's power switch, and `ssh`-ing into the Raspberry Pi (assuming you've done all the standard ROS stuff: `source devel/setup.bash` and `catkin_make`), do: `roslaunch mini_ros spot_calibrate.launch`. This will establish the serial connection between the Pi and the Teensy, and you should be able to give Spot's joints some PWM commands.
* After launching the calibration node, use `rosservice call /servo_calibrator <TAB> <TAB>` (the double `TAB` auto-completes the format) on each joint `0-11` and give it a few different PWM commands (carefully) to inspect its behavior.
* Once you are familiar with the joint, hone in on a PWM command that sends it to `two` known and measurable positions (`0` and `90` degrees works great - for the wrists, `165` degrees is also an option).
* Record the PWM value and corresponding position for each joint in the `Initialize()` method for the joints in `main.cpp` in the following order: `[PWM0, PWM1, ANG0, ANG1]`. Your motors will inevitably have non-linearities so it is imperative to perform these steps for each joint and to find two reference points that minimize the presence of non-linearities.
Here is an example for two joint calibrations I did:

Within [main.cpp](https://github.com/moribots/spot_mini_mini/blob/spot/spot_real/Control/Teensy/SpotMiniMini/src/main.cpp) (runs on Teensy), you can select the following modes to **verify** your calibration. Spending extra time here goes a long way.
* `STRAIGHT_LEGS`: Spot will start by lying down, and then extend its legs straight after a few seconds.


* `LIEDOWN`: Spot will stay lying down.


* `PERPENDICULAR_LEGS`: Spot will start by lying down, and then make its upper leg perpendicular to its shoulder, and its lower leg perpendicular to its upper leg. **NOTE: Make sure Spot is on a stand during this mode as it will fall over!**


* `RUN`: Spot will start by lying down, and raise itself to its normal stance once all sensors/communications are ready. This is the default mode.

Thank you [Vincent](https://github.com/elpimous) for your feedback regarding this guide's clarity! | 7,179 | Markdown | 61.434782 | 467 | 0.766681 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/README.md | # Spot Mini Mini - The Real Deal
## Software
### Teensy Low Level Speed Controller: `Teensy`
Custom Serialized ROS messages have been built using `ROSSerial` for ROS Melodic. These allow the Teensy to communicate with the Raspberry Pi safely and efficiently. The Teensy firmware is split into multiple header files for Servo Motor Operations, Inverse Kinematics, IMU and Contact Sensor readings, as well as the ROSSerial Interface. Becuase of this split, I have opted to use `PlatformIO` for compilation instead of the Arduino IDE.
The `RPi` directory has some testing scripts which you will most likely not use.
#### Installation Instructions:
* Install `PlatformIO`.
* Install `Ubuntu 18.04` and `ROS Melodic` on Raspberry Pi and well as `ROSSerial`.
* Navigate to [firmware](https://github.com/moribots/spot_mini_mini/tree/spot/spot_real/Control/Teensy/SpotMiniMini) directory and run `platformio run -t upload` while connected to Teensy 4.0.
#### Launch Instructions:
After turning on Spot's power switch, and `ssh`-ing into the Raspberry Pi (assuming you've done all the standard ROS stuff: `source devel/setup.bash` and `catkin_make`), do: `roslaunch mini_ros spot_real.launch`. This will establish the serial connection between the Pi and the Teensy, and you should be able to give Spot joystick commands.
## Hardware
### Power Distribution Board: `PDB`
[Adham Elarabawy](https://github.com/adham-elarabawy/OpenQuadruped/blob/master/README.md) and I designed this Power Distribution Board for the [Spot Micro](https://spotmicroai.readthedocs.io/en/latest/). There are two available versions, one that supports a single power supply (mine - available in the `PDB` directory), and one that supports dual power supplies (his). The second version exists because users who do not use HV servos will need UBECs to buck the 2S Lipo's voltage down to whatever their motors require. In general, these UBECs come with limited current support, which means that two of them are requrired.
This power distribution board has a `1.5mm Track Width` to support up to `6A` at a `10C` temperature increase (conservative estimate). There are also copper grounding planes on both sides of the board to help with heat dissipation, and parallel tracks for the power lines are provided for the same reason. The PDB also includes shunt capactiors for each servo motor to smooth out the power input. You are free to select your own capaciors as recommendations range from `100uF` to `470uF` depending on the motors. Make sure you use electrolytic capacitors. Check out the [EasyEDA Project](https://easyeda.com/adhamelarabawy/PowerDistributionBoard)!

This board interfaces with a sensor array (used for foot sensors on this project) and contains two I2C terminals and a regulated 5V power rail. At the center of the board is a Teensy 4.0 which communicates with a Raspberry Pi over Serial to control the 12 servo motors and read the analogue sensors. The Teensy allows for motor speed control, but if you don't need this, it defaults at 100deg/sec (you can change this). The Gerber files for the single supply version are in this directory.
### STEP/STL Files for new design:
Together with [Adham Elarabawy](https://github.com/adham-elarabawy/OpenQuadruped), I have a completed a total mechanical redesign of SpotMicro. We call it `OpenQuadruped`! Check out the [STEP](https://cad.onshape.com/documents/9d0f96878c54300abf1157ac/w/c9cdf8daa98d8a0d7d50c8d3/e/fa0d7caf0ed2ef46834ecc24) files here!
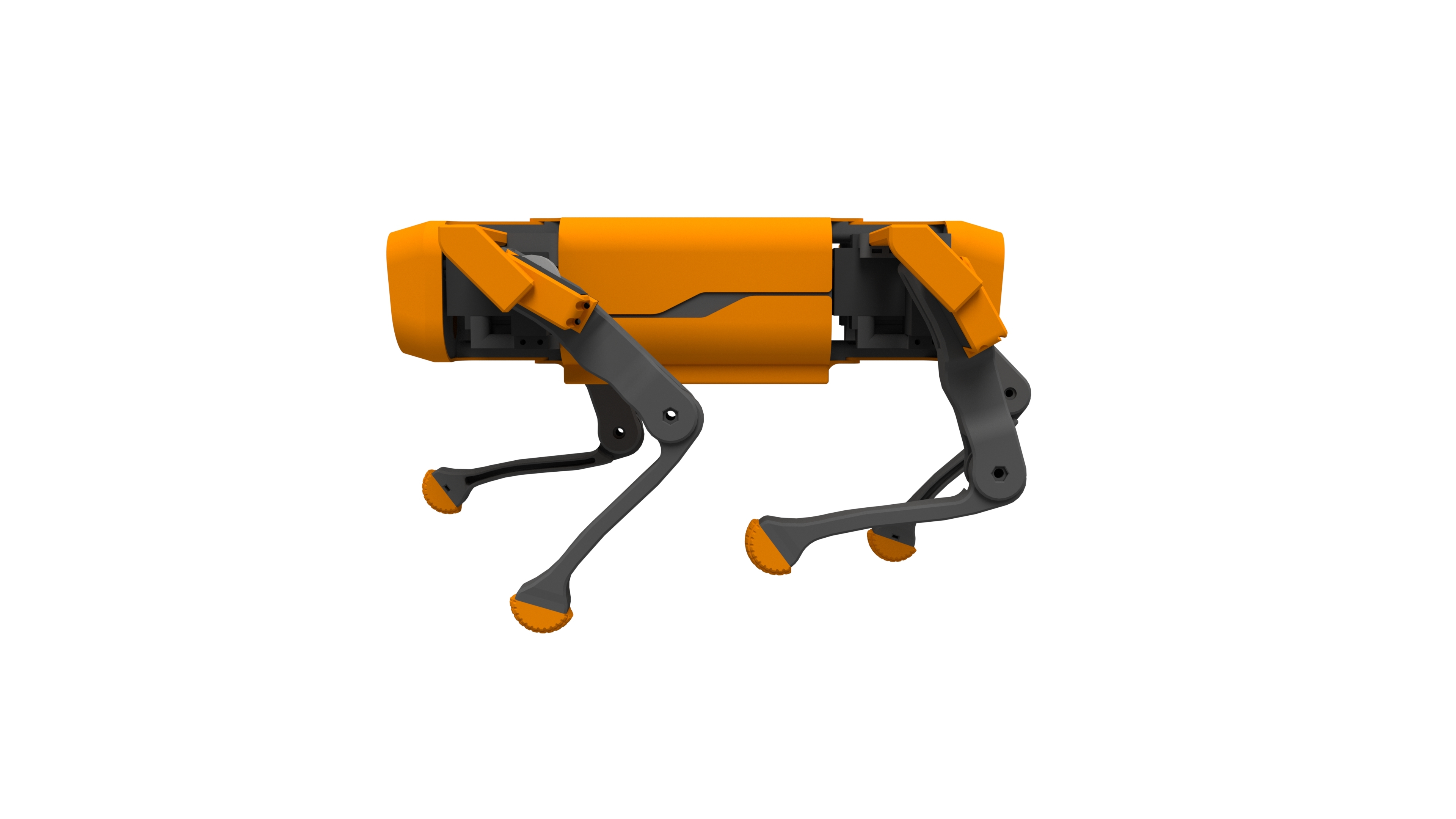
Main improvements:
* Shortened the body by 40mm while making more room for our electronics with adapter plates.
* Moved all the servos to the hip to save 60g on the lower legs, which are now actuated using belt-drives.
* Added support bridge on hip joint for added longevity.
* Added flush slots for hall effect sensors on the feet.
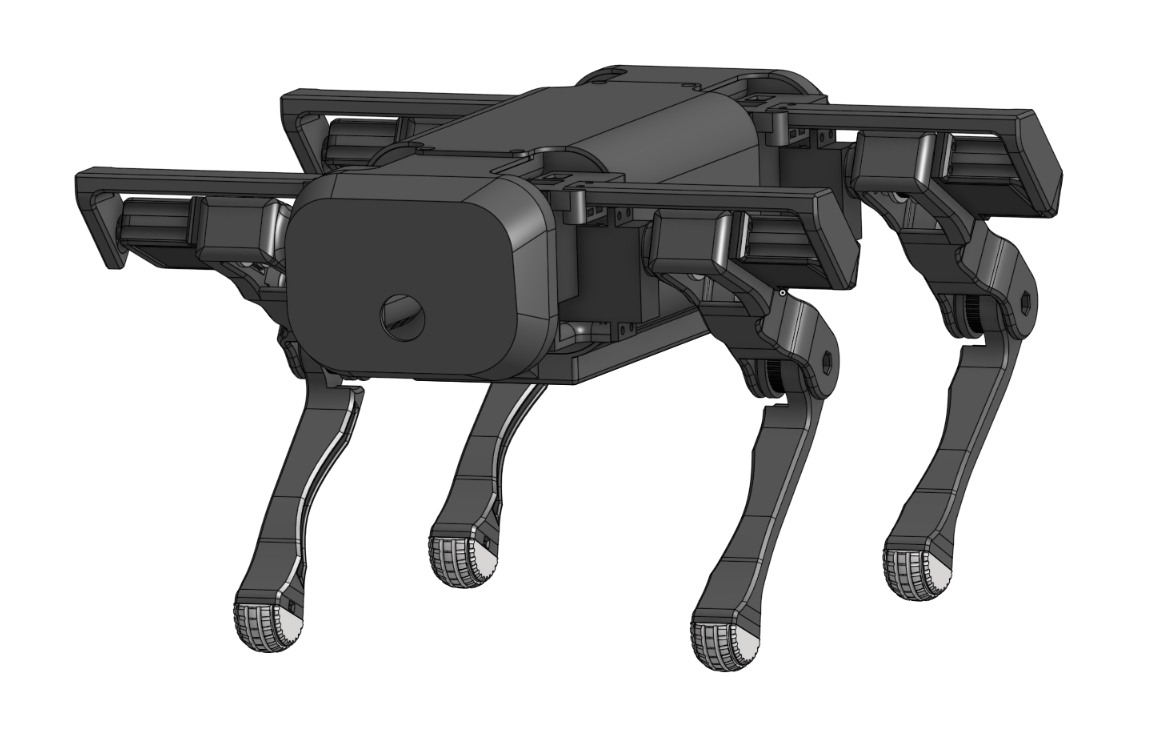
### Bill of Materials
See most recent [BOM](https://docs.google.com/spreadsheets/d/1Z4y59K8bY3r_442I70xe564zAFuP0pVIFEJ6bNZaCi0/edit?usp=sharing)!

Note that the actual cost of this project is reflected in the first group of items totalling `590 USD`. For users such as myself who did not own any hobbyist components before this project, I have included an expanded list of required purchases.
### Assembly & Calibration
Please consult  for assembly and calibration instructions. | 4,673 | Markdown | 75.62295 | 647 | 0.787717 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/PDB/README.md | ## Spot Micro Power Distribution Board
You should be able to upload this zip file into any PCB fulfiller to order yours. Make sure you select `2oz` copper, this is crucial. | 172 | Markdown | 85.499957 | 133 | 0.784884 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/src/main.cpp | /// \file
/// \brief Teensy Main.
#include <Arduino.h>
#include <SpotServo.hpp>
#include <ContactSensor.hpp>
#include <Kinematics.hpp>
#include <Utilities.hpp>
#include <IMU.hpp>
#include <Servo.h>
#include <ROSSerial.hpp>
#define DEBUGSERIAL Serial
// NOTE: IMPORTANT - CALIBRATION VS RUN PARAMS
/* Instructions:
- When assembling your servos, use NOMINAL_PWM mode, and be sure to enter the
appropriate degree and pwm range too.
- Use servo_calibration.launch on your Ubuntu machine running ROS Melodic and
hence the servo_calibrator service to send PWM values to each joint, and then
enter the resultant values in the Initialize() method of the SpotServo class.
- STRAIGHT_LEGS, LIEDOWN, and PERPENDICULAR_LEGS are used to validate your calibraton.
- RUN is used when you are finished calibrating, and are ready to run normal operations.
*/
enum MODE {NOMINAL_PWM, STRAIGHT_LEGS, LIEDOWN, PERPENDICULAR_LEGS, RUN};
MODE spot_mode = RUN;
bool ESTOPPED = false;
int viewing_speed = 400; // doesn't really mean anything, theoretically deg/sec
int walking_speed = 1500; // doesn't really mean anything, theoretically deg/sec
double last_estop = millis();
static unsigned long prev_publish_time;
const int ledPin = 13;
SpotServo FL_Shoulder, FL_Elbow, FL_Wrist, FR_Shoulder, FR_Elbow, FR_Wrist, BL_Shoulder, BL_Elbow, BL_Wrist, BR_Shoulder, BR_Elbow, BR_Wrist;
ContactSensor FL_sensor, FR_sensor, BL_sensor, BR_sensor;
SpotServo * Shoulders[4] = {&FL_Shoulder, &FR_Shoulder, &BL_Shoulder, &BR_Shoulder};
SpotServo * Elbows[4] = {&FL_Elbow, &FR_Elbow, &BL_Elbow, &BR_Elbow};
SpotServo * Wrists[4] = {&FL_Wrist, &FR_Wrist, &BL_Wrist, &BR_Wrist};
SpotServo * AllServos[12] = {&FL_Shoulder, &FL_Elbow, &FL_Wrist, &FR_Shoulder, &FR_Elbow, &FR_Wrist, &BL_Shoulder, &BL_Elbow, &BL_Wrist, &BR_Shoulder, &BR_Elbow, &BR_Wrist};
Utilities util;
Kinematics ik;
IMU imu_sensor;
LegType fl_leg = FL;
LegType fr_leg = FR;
LegType bl_leg = BL;
LegType br_leg = BR;
LegJoints FL_ = LegJoints(fl_leg);
LegJoints FR_ = LegJoints(fr_leg);
LegJoints BL_ = LegJoints(bl_leg);
LegJoints BR_ = LegJoints(br_leg);
ros_srl::ROSSerial ros_serial;
void update_servos()
{
// ShoulderS
FL_Shoulder.update_clk();
FR_Shoulder.update_clk();
BL_Shoulder.update_clk();
BR_Shoulder.update_clk();
// Elbow
FL_Elbow.update_clk();
FR_Elbow.update_clk();
BL_Elbow.update_clk();
BR_Elbow.update_clk();
// WRIST
FL_Wrist.update_clk();
FR_Wrist.update_clk();
BL_Wrist.update_clk();
BR_Wrist.update_clk();
}
void command_servos(const LegJoints & legjoint, const bool & step_or_view = true)
{
int leg = -1;
if (legjoint.legtype == FL)
{
leg = 0;
} else if (legjoint.legtype == FR)
{
leg = 1;
} else if (legjoint.legtype == BL)
{
leg = 2;
} else if (legjoint.legtype == BR)
{
leg = 3;
}
double shoulder_home = (*Shoulders[leg]).return_home();
double elbow_home = (*Elbows[leg]).return_home();
double wrist_home = (*Wrists[leg]).return_home();
double Shoulder_angle = util.angleConversion(legjoint.shoulder, shoulder_home, legjoint.legtype, Shoulder);
double Elbow_angle = legjoint.elbow;
double Wrist_angle = legjoint.wrist;
double s_dist = abs(Shoulder_angle - (*Shoulders[leg]).GetPoseEstimate());
double e_dist = abs(Elbow_angle - (*Elbows[leg]).GetPoseEstimate());
double w_dist = abs(Wrist_angle - (*Wrists[leg]).GetPoseEstimate());
double scaling_factor = util.max(s_dist, e_dist, w_dist);
s_dist /= scaling_factor;
e_dist /= scaling_factor;
w_dist /= scaling_factor;
double s_speed = 0.0;
double e_speed = 0.0;
double w_speed = 0.0;
if (step_or_view)
{
s_speed = viewing_speed * s_dist;
s_speed = max(s_speed, viewing_speed / 10.0);
e_speed = viewing_speed * e_dist;
e_speed = max(e_speed, viewing_speed / 10.0);
w_speed = viewing_speed * w_dist;
w_speed = max(w_speed, viewing_speed / 10.0);
} else
{
s_speed = walking_speed * s_dist;
e_speed = walking_speed * e_dist;
w_speed = walking_speed * w_dist;
}
(*Shoulders[leg]).SetGoal(Shoulder_angle, s_speed, step_or_view);
(*Elbows[leg]).SetGoal(Elbow_angle, e_speed, step_or_view);
(*Wrists[leg]).SetGoal(Wrist_angle, w_speed, step_or_view);
}
void update_sensors()
{
FL_sensor.update_clk();
FR_sensor.update_clk();
BL_sensor.update_clk();
BR_sensor.update_clk();
}
void set_stance(const double & f_shoulder_stance = 0.0, const double & f_elbow_stance = 0.0, const double & f_wrist_stance = 0.0,
const double & r_shoulder_stance = 0.0, const double & r_elbow_stance = 0.0, const double & r_wrist_stance = 0.0)
{
// Legs
FL_.FillLegJoint(f_shoulder_stance, f_elbow_stance, f_wrist_stance);
FR_.FillLegJoint(f_shoulder_stance, f_elbow_stance, f_wrist_stance);
BL_.FillLegJoint(r_shoulder_stance, r_elbow_stance, r_wrist_stance);
BR_.FillLegJoint(r_shoulder_stance, r_elbow_stance, r_wrist_stance);
command_servos(FL_);
command_servos(FR_);
command_servos(BL_);
command_servos(BR_);
// Loop until goal reached - check BR Wrist (last one)
while (!BR_Wrist.GoalReached())
{
update_servos();
}
}
// Set servo pwm values to nominal assuming 500~2500 range and 270 degree servos.
void nominal_servo_pwm(const double & servo_range = 270, const int & min_pwm = 500, const int & max_pwm = 2500)
{
// Attach motors for assembly
// Shoulders
FL_Shoulder.AssemblyInit(2, min_pwm, max_pwm);
FR_Shoulder.AssemblyInit(5, min_pwm, max_pwm);
BL_Shoulder.AssemblyInit(8, min_pwm, max_pwm);
BR_Shoulder.AssemblyInit(11, min_pwm, max_pwm);
//Elbows
FL_Elbow.AssemblyInit(3, min_pwm, max_pwm);
FR_Elbow.AssemblyInit(6, min_pwm, max_pwm);
BL_Elbow.AssemblyInit(9, min_pwm, max_pwm);
BR_Elbow.AssemblyInit(12, min_pwm, max_pwm);
//Wrists
FL_Wrist.AssemblyInit(4, min_pwm, max_pwm);
FR_Wrist.AssemblyInit(7, min_pwm, max_pwm);
BL_Wrist.AssemblyInit(10, min_pwm, max_pwm);
BR_Wrist.AssemblyInit(13, min_pwm, max_pwm);
// halfway for shoulder and elbow
int halfway_pulse = round(0.5 * (max_pwm - min_pwm) + min_pwm); // 1500
int shoulder_pulse = halfway_pulse; // 1500
int elbow_pulse = halfway_pulse; // 1500
// wrists need roughly 180
int remaining_range = round(((180.0 - (servo_range / 2.0)) / double(servo_range)) * (max_pwm - min_pwm));
int left_wrist_pulse = halfway_pulse - remaining_range; // 1167
int right_wrist_pulse = halfway_pulse + remaining_range; // 1833
//FL
AllServos[0]->writePulse(shoulder_pulse);
AllServos[1]->writePulse(elbow_pulse);
AllServos[2]->writePulse(left_wrist_pulse);
//FR
AllServos[3]->writePulse(shoulder_pulse);
AllServos[4]->writePulse(elbow_pulse);
AllServos[5]->writePulse(right_wrist_pulse);
//BL
AllServos[6]->writePulse(shoulder_pulse);
AllServos[7]->writePulse(elbow_pulse);
AllServos[8]->writePulse(left_wrist_pulse);
//BR
AllServos[9]->writePulse(shoulder_pulse);
AllServos[10]->writePulse(elbow_pulse);
AllServos[11]->writePulse(right_wrist_pulse);
}
void run_sequence()
{
// Move to Crouching Stance
delay(2000);
double f_shoulder_stance = 0.0;
double f_elbow_stance = 36.13;
double f_wrist_stance = -75.84;
double r_shoulder_stance = 0.0;
double r_elbow_stance = 36.13;
double r_wrist_stance = -75.84;
set_stance(f_shoulder_stance, f_elbow_stance, f_wrist_stance, r_shoulder_stance, r_elbow_stance, r_wrist_stance);
}
void straight_calibration_sequence()
{
set_stance();
}
void lie_calibration_sequence()
{
// Move to Extended stance
delay(2000);
double shoulder_stance = 0.0;
double elbow_stance = 90.0;
double wrist_stance = -170.3;
set_stance(shoulder_stance, elbow_stance, wrist_stance, shoulder_stance, elbow_stance, wrist_stance);
}
void perpendicular_calibration_sequence()
{
// Move to Extended stance
delay(2000);
set_stance();
// Move to Extended stance
delay(10000);
double shoulder_stance = 0.0;
double elbow_stance = 90.0;
double wrist_stance = -90.0;
set_stance(shoulder_stance, elbow_stance, wrist_stance, shoulder_stance, elbow_stance, wrist_stance);
}
// THIS ONLY RUNS ONCE
void setup()
{
Serial.begin(9600);
// NOTE: See top of file for spot_mode explanation:
// ONLY USE THIS MODE DURING ASSEMBLY
if (spot_mode == NOMINAL_PWM)
{
// Neutral Setting
nominal_servo_pwm();
// Prevent Servo Updates
ESTOPPED = true;
} else
{
// IK - unused
ik.Initialize(0.04, 0.1, 0.1);
// SERVOS: Pin, StandAngle, HomeAngle, Offset, LegType, JointType, min_pwm, max_pwm, min_pwm_angle, max_pwm_angle
// Shoulders
double shoulder_liedown = 0.0;
FL_Shoulder.Initialize(2, 135 + shoulder_liedown, 135, -7.25, FL, Shoulder, 500, 2400); // 0 | 0: STRAIGHT | 90: OUT | -90 IN
FR_Shoulder.Initialize(5, 135 - shoulder_liedown, 135, -5.5, FR, Shoulder, 500, 2400); // 1 | 0: STRAIGHT | 90: IN | -90 OUT
BL_Shoulder.Initialize(8, 135 + shoulder_liedown, 135, 5.75, BL, Shoulder, 500, 2400); // 2 | 0: STRAIGHT | 90: OUT | -90 IN
BR_Shoulder.Initialize(11, 135 - shoulder_liedown, 135, -4.0, BR, Shoulder, 500, 2400); // 3 | 0: STRAIGHT | 90: IN | -90 OUT
//Elbows
double elbow_liedown = 90.0;
FL_Elbow.Initialize(3, elbow_liedown, 0, 0.0, FL, Elbow, 1410, 2062, 0.0, 90.0); // 4 | 0: STRAIGHT | 90: BACK
FR_Elbow.Initialize(6, elbow_liedown, 0, 0.0, FR, Elbow, 1408, 733, 0.0, 90.0); // 5 | 0: STRAIGHT | 90: BACK
BL_Elbow.Initialize(9, elbow_liedown, 0, 0.0, BL, Elbow, 1460, 2095, 0.0, 90.0); // 6 | 0: STRAIGHT | 90: BACK
BR_Elbow.Initialize(12, elbow_liedown, 0, 0.0, BR, Elbow, 1505, 850, 0.0, 90.0); // 7 | 0: STRAIGHT | 90: BACK
//Wrists
double wrist_liedown = -160.0;
FL_Wrist.Initialize(4, wrist_liedown, 0, 0.0, FL, Wrist, 1755, 2320, -90.0, -165.0); // 8 | 0: STRAIGHT | -90: FORWARD
FR_Wrist.Initialize(7, wrist_liedown, 0, 0.0, FR, Wrist, 1805, 1150, 0.0, -90.0); // 9 | 0: STRAIGHT | -90: FORWARD
BL_Wrist.Initialize(10, wrist_liedown, 0, 0.0, BL, Wrist, 1100, 1733, 0.0, -90.0); // 10 | 0: STRAIGHT | -90: FORWARD
BR_Wrist.Initialize(13, wrist_liedown, 0, 0.0, BR, Wrist, 1788, 1153, 0.0, -90.0); // 11 | 0: STRAIGHT | -90: FORWARD
// Contact Sensors
FL_sensor.Initialize(A9, 17);
FR_sensor.Initialize(A8, 16);
BL_sensor.Initialize(A7, 15);
BR_sensor.Initialize(A6, 14);
// IMU
imu_sensor.Initialize();
// NOTE: See top of file for spot_mode explanation:
if (spot_mode == STRAIGHT_LEGS)
{
straight_calibration_sequence();
} else if (spot_mode == LIEDOWN)
{
lie_calibration_sequence();
} else if (spot_mode == PERPENDICULAR_LEGS)
{
perpendicular_calibration_sequence();
} else
{
run_sequence();
}
}
last_estop = millis();
prev_publish_time = micros();
}
// THIS LOOPS FOREVER
void loop()
{
// CHECK SENSORS AND SEND INFO
// CONTACT
// 1'000'000 / 20'000 = 50hz
if ((micros() - prev_publish_time) >= 20000)
{
ros_serial.publishContacts(FL_sensor.isTriggered(), FR_sensor.isTriggered(), BL_sensor.isTriggered(), BR_sensor.isTriggered());
//IMU
if (imu_sensor.available())
{
imu::Vector<3> eul = imu_sensor.GetEuler();
imu::Vector<3> acc = imu_sensor.GetAcc();
imu::Vector<3> gyro = imu_sensor.GetGyro();
ros_serial.publishIMU(eul, acc, gyro);
}
prev_publish_time = micros();
}
// Only allow controller commands if not E-STOPPED
// Direct pulse commands from calibration still allowed.
if(!ESTOPPED)
{
update_servos();
}
// Update Sensors
update_sensors();
// Command Servos
if (ros_serial.jointsInputIsActive())
{
bool step_or_view = false;
ros_serial.returnJoints(FL_, FR_, BL_, BR_, step_or_view);
command_servos(FL_, step_or_view);
command_servos(FR_, step_or_view);
command_servos(BL_, step_or_view);
command_servos(BR_, step_or_view);
} else if (ros_serial.jointsPulseIsActive())
{
int servo_num = ros_serial.returnServoNum();
int pulse = ros_serial.returnPulse();
if (servo_num > -1 and servo_num < 12)
{
AllServos[servo_num]->writePulse(pulse);
}
ros_serial.resetPulseTopic();
}
// Update ROS Node (spinOnce etc...)
ros_serial.run();
}
| 12,395 | C++ | 30.622449 | 173 | 0.653651 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/Kinematics/Kinematics.cpp | #include "Kinematics.hpp"
void Kinematics::Initialize(const double & shoulder_length_, const double & elbow_length_, const double & wrist_length_)
{
shoulder_length = shoulder_length_;
elbow_length = elbow_length_;
wrist_length = wrist_length_;
}
double Kinematics::GetDomain(const double & x, const double & y, const double & z)
{
double D = (pow(y, 2) + pow(-z, 2) - pow(shoulder_length, 2) +
pow(-x, 2) - pow(elbow_length, 2) - pow(wrist_length, 2)) / (
2.0 * wrist_length * elbow_length);
if (D > 1.0)
{
D = 1.0;
} if (D < -1.0)
{
D = -1.0;
}
return D;
}
void Kinematics::RightIK(const double & x, const double & y, const double & z, const double & D, double (& angles) [3])
{
double wrist_angle = atan2(-sqrt(1.0 - pow(D, 2)), D);
double sqrt_component = pow(y, 2) + pow(-z, 2) - pow(shoulder_length, 2);
if (sqrt_component < 0.0)
{
sqrt_component = 0.0;
}
double shoulder_angle = -atan2(z, y) - atan2(
sqrt(sqrt_component), -shoulder_length);
double elbow_angle = atan2(-x, sqrt(sqrt_component)) - atan2(
wrist_length * sin(wrist_angle),
elbow_length + wrist_length * cos(wrist_angle));
angles[0] = shoulder_angle;
angles[1] = -elbow_angle;
angles[2] = -wrist_angle;
}
void Kinematics::LeftIK(const double & x, const double & y, const double & z, const double & D, double (& angles) [3])
{
double wrist_angle = atan2(-sqrt(1.0 - pow(D, 2)), D);
double sqrt_component = pow(y, 2) + pow(-z, 2) - pow(shoulder_length, 2);
if (sqrt_component < 0.0)
{
sqrt_component = 0.0;
}
double shoulder_angle = -atan2(z, y) - atan2(
sqrt(sqrt_component), shoulder_length);
double elbow_angle = atan2(-x, sqrt(sqrt_component)) - atan2(
wrist_length * sin(wrist_angle),
elbow_length + wrist_length * cos(wrist_angle));
angles[0] = shoulder_angle;
angles[1] = -elbow_angle;
angles[2] = -wrist_angle;
}
void Kinematics::GetJointAngles(const double & x, const double & y, const double & z, const LegQuadrant & legquad, double (& angles) [3])
{
double D = GetDomain(x, y, z);
if (legquad == Right)
{
RightIK(x, y, z, D, angles);
} else
{
LeftIK(x, y, z, D, angles);
}
}
| 2,273 | C++ | 28.921052 | 137 | 0.597448 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/Kinematics/Kinematics.hpp | #ifndef KINEMATICS_INCLUDE_GUARD_HPP
#define KINEMATICS_INCLUDE_GUARD_HPP
/// \file
/// \brief Leg Kinematics Library.
#include <Arduino.h>
enum LegQuadrant {Right, Left};
class Kinematics {
private:
double shoulder_length = 0.0;
double elbow_length = 0.0;
double wrist_length = 0.0;
public:
// using default constructor
/// \brief Initialize parameters
/// \param shoulder_length_: length of shoulder link
/// \param elbow_length_: length of elbow link
/// \param wrist_length_: length of wrist link
/// \param leg_type_: right or left legs
void Initialize(const double & shoulder_length_, const double & elbow_length_, const double & wrist_length_);
/// \brief Calculates the leg's Domain and caps it in case of a breach
/// \param x: x coordinate of Hip To Foot Vector
/// \param y: y coordinate of Hip To Foot Vector
/// \param z: z coordinate of Hip To Foot Vector
/// \returns: Leg Domain D
double GetDomain(const double & x, const double & y, const double & z);
/// \brief Right Leg Inverse Kinematics Solver
/// \param x: x coordinate of Hip To Foot Vector
/// \param y: y coordinate of Hip To Foot Vector
/// \param z: z coordinate of Hip To Foot Vector
/// \param D: the leg domain
/// \param angles: array to populate with IK angles
/// \returns: pointer to beginning of array containing joint angles for this leg
void RightIK(const double & x, const double & y, const double & z, const double & D, double (& angles) [3]);
/// \brief Left Leg Inverse Kinematics Solver
/// \param x: x coordinate of Hip To Foot Vector
/// \param y: y coordinate of Hip To Foot Vector
/// \param z: z coordinate of Hip To Foot Vector
/// \param D: the leg domain
/// \param angles: array to populate with IK angles
/// \returns: pointer to beginning of array containing joint angles for this leg
void LeftIK(const double & x, const double & y, const double & z, const double & D, double (& angles) [3]);
/// \brief Retrives Joint Angles using a Hip To Foot Vector (x, y, z)
/// \param x: x coordinate of Hip To Foot Vector
/// \param y: y coordinate of Hip To Foot Vector
/// \param z: z coordinate of Hip To Foot Vector
/// \param legquad: Leg quadrant (left or right)
/// \param angles: array to populate with IK angles
/// \returns: pointer to beginning of array containing joint angles for this leg
void GetJointAngles(const double & x, const double & y, const double & z, const LegQuadrant & legquad, double (& angles) [3]);
};
#endif | 2,552 | C++ | 41.549999 | 128 | 0.681426 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/Utilities/Utilities.cpp | #include "Utilities.hpp"
#include <Arduino.h>
double Utilities::toDegrees(double radianVal) {
return radianVal * 57296 / 1000.0;
}
double Utilities::max(double a0, double a1, double a2) {
if(a0 >= a1 && a0 >= a2) {
return a0;
}
if(a1 >= a0 && a1 >= a2) {
return a1;
}
if(a2 >= a1 && a2 >= a0) {
return a2;
}
}
double Utilities::angleConversion(double angle, double home_angle, LegType legtype, JointType joint_type) {
double mod_angle = home_angle;
if (joint_type == Shoulder)
{
mod_angle = home_angle - angle;
} else if (joint_type == Elbow)
{
if (legtype == FR or legtype == BR)
{
mod_angle = home_angle - angle;
} else
// FL or BL
{
mod_angle = home_angle + angle;
}
} else if (joint_type == Wrist)
{
if (legtype == FR or legtype == BR)
{
mod_angle = home_angle + angle;
} else
// FL or BL
{
mod_angle = home_angle - angle;
}
}
return mod_angle;
}
| 977 | C++ | 18.17647 | 107 | 0.564995 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/Utilities/Utilities.hpp | #ifndef UTILITIES_INCLUDE_GUARD_HPP
#define UTILITIES_INCLUDE_GUARD_HPP
#include "SpotServo.hpp"
/// \file
/// \brief Utilities Library.
class Utilities {
public:
double angleConversion(double angle, double home_angle, LegType legtype, JointType joint_type);
double toDegrees(double radianVal);
double max(double a0, double a1, double a2);
};
#endif
| 359 | C++ | 24.714284 | 97 | 0.754875 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/IMU/IMU2.cpp | // #include "IMU2.hpp"
// void IMU2::Initialize()
// {
// if (!lsm.begin())
// {
// Serial.println("Oops ... unable to initialize the LSM9DS1. Check your wiring!");
// ok = false;
// } else
// {
// // 1.) Set the accelerometer range
// lsm.setupAccel(lsm.LSM9DS1_ACCELRANGE_2G);
// //lsm.setupAccel(lsm.LSM9DS1_ACCELRANGE_4G);
// //lsm.setupAccel(lsm.LSM9DS1_ACCELRANGE_8G);
// //lsm.setupAccel(lsm.LSM9DS1_ACCELRANGE_16G);
// // 2.) Set the magnetometer sensitivity
// lsm.setupMag(lsm.LSM9DS1_MAGGAIN_4GAUSS);
// //lsm.setupMag(lsm.LSM9DS1_MAGGAIN_8GAUSS);
// //lsm.setupMag(lsm.LSM9DS1_MAGGAIN_12GAUSS);
// //lsm.setupMag(lsm.LSM9DS1_MAGGAIN_16GAUSS);
// // 3.) Setup the gyroscope
// lsm.setupGyro(lsm.LSM9DS1_GYROSCALE_245DPS);
// //lsm.setupGyro(lsm.LSM9DS1_GYROSCALE_500DPS);
// //lsm.setupGyro(lsm.LSM9DS1_GYROSCALE_2000DPS);
// }
// }
// bool IMU2::available()
// {
// return ok;
// }
| 941 | C++ | 22.549999 | 84 | 0.639745 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/IMU/IMU2.hpp | // #ifndef IMU2_INCLUDE_GUARD_HPP
// #define IMU2_INCLUDE_GUARD_HPP
// /// \file
// /// \brief IMU Library.
// #include <Arduino.h>
// #include <Wire.h>
// #include <SPI.h>
// #include <Adafruit_LSM9DS1.h>
// #include <Adafruit_Sensor.h> // not used in this demo but required!
// #define LSM9DS1_SCK A5
// #define LSM9DS1_MISO 12
// #define LSM9DS1_MOSI A4
// #define LSM9DS1_XGCS 6
// #define LSM9DS1_MCS 5
// class IMU2 {
// private:
// bool ok = true;
// public:
// Adafruit_LSM9DS1 lsm = Adafruit_LSM9DS1();
// void Initialize();
// bool available();
// };
// #endif | 581 | C++ | 21.384615 | 71 | 0.633391 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/IMU/IMU.hpp | #ifndef IMU_INCLUDE_GUARD_HPP
#define IMU_INCLUDE_GUARD_HPP
/// \file
/// \brief IMU Library.
#include <Arduino.h>
#include <Wire.h>
#include <Adafruit_Sensor.h>
#include <Adafruit_BNO055.h>
#include <utility/imumaths.h>
#include <EEPROM.h>
class IMU {
private:
Adafruit_BNO055 bno = Adafruit_BNO055(55);
bool ok = true;
public:
void displaySensorDetails(void);
void displaySensorStatus(void);
void displayCalStatus(void);
void displaySensorOffsets(const adafruit_bno055_offsets_t &calibData);
void Initialize();
bool available();
imu::Vector<3> GetEuler();
imu::Quaternion GetQuat();
imu::Vector<3> GetAcc();
imu::Vector<3> GetGyro();
};
#endif | 663 | C++ | 21.896551 | 71 | 0.72549 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/IMU/IMU.cpp | #include "IMU.hpp"
#include <Arduino.h>
/* Set the delay between fresh samples */
#define BNO055_SAMPLERATE_DELAY_MS (100)
/**************************************************************************/
/*
Displays some basic information on this sensor from the unified
sensor API sensor_t type (see Adafruit_Sensor for more information)
*/
/**************************************************************************/
void IMU::displaySensorDetails(void)
{
sensor_t sensor;
bno.getSensor(&sensor);
Serial.println("------------------------------------");
Serial.print("Sensor: "); Serial.println(sensor.name);
Serial.print("Driver Ver: "); Serial.println(sensor.version);
Serial.print("Unique ID: "); Serial.println(sensor.sensor_id);
Serial.print("Max Value: "); Serial.print(sensor.max_value); Serial.println(" xxx");
Serial.print("Min Value: "); Serial.print(sensor.min_value); Serial.println(" xxx");
Serial.print("Resolution: "); Serial.print(sensor.resolution); Serial.println(" xxx");
Serial.println("------------------------------------");
Serial.println("");
delay(500);
}
/**************************************************************************/
/*
Display some basic info about the sensor status
*/
/**************************************************************************/
void IMU::displaySensorStatus(void)
{
/* Get the system status values (mostly for debugging purposes) */
uint8_t system_status, self_test_results, system_error;
system_status = self_test_results = system_error = 0;
bno.getSystemStatus(&system_status, &self_test_results, &system_error);
/* Display the results in the Serial Monitor */
Serial.println("");
Serial.print("System Status: 0x");
Serial.println(system_status, HEX);
Serial.print("Self Test: 0x");
Serial.println(self_test_results, HEX);
Serial.print("System Error: 0x");
Serial.println(system_error, HEX);
Serial.println("");
delay(500);
}
/**************************************************************************/
/*
Display sensor calibration status
*/
/**************************************************************************/
void IMU::displayCalStatus(void)
{
/* Get the four calibration values (0..3) */
/* Any sensor data reporting 0 should be ignored, */
/* 3 means 'fully calibrated" */
uint8_t system, gyro, accel, mag;
system = gyro = accel = mag = 0;
bno.getCalibration(&system, &gyro, &accel, &mag);
/* The data should be ignored until the system calibration is > 0 */
Serial.print("\t");
if (!system)
{
Serial.print("! ");
}
/* Display the individual values */
Serial.print("Sys:");
Serial.print(system, DEC);
Serial.print(" G:");
Serial.print(gyro, DEC);
Serial.print(" A:");
Serial.print(accel, DEC);
Serial.print(" M:");
Serial.print(mag, DEC);
}
/**************************************************************************/
/*
Display the raw calibration offset and radius data
*/
/**************************************************************************/
void IMU::displaySensorOffsets(const adafruit_bno055_offsets_t &calibData)
{
Serial.print("Accelerometer: ");
Serial.print(calibData.accel_offset_x); Serial.print(" ");
Serial.print(calibData.accel_offset_y); Serial.print(" ");
Serial.print(calibData.accel_offset_z); Serial.print(" ");
Serial.print("\nGyro: ");
Serial.print(calibData.gyro_offset_x); Serial.print(" ");
Serial.print(calibData.gyro_offset_y); Serial.print(" ");
Serial.print(calibData.gyro_offset_z); Serial.print(" ");
Serial.print("\nMag: ");
Serial.print(calibData.mag_offset_x); Serial.print(" ");
Serial.print(calibData.mag_offset_y); Serial.print(" ");
Serial.print(calibData.mag_offset_z); Serial.print(" ");
Serial.print("\nAccel Radius: ");
Serial.print(calibData.accel_radius);
Serial.print("\nMag Radius: ");
Serial.print(calibData.mag_radius);
}
void IMU::Initialize()
{
if(!bno.begin())
{
/* There was a problem detecting the BNO055 ... check your connections */
Serial.print("Ooops, no BNO055 detected ... Check your wiring or I2C ADDR!\n");
ok = false;
} else
{
int eeAddress = 0;
long bnoID;
bool foundCalib = false;
EEPROM.get(eeAddress, bnoID);
adafruit_bno055_offsets_t calibrationData;
sensor_t sensor;
/*
* Look for the sensor's unique ID at the beginning oF EEPROM.
* This isn't foolproof, but it's better than nothing.
*/
bno.getSensor(&sensor);
if (bnoID != sensor.sensor_id)
{
Serial.println("\nNo Calibration Data for this sensor exists in EEPROM");
delay(500);
}
else
{
Serial.println("\nFound Calibration for this sensor in EEPROM.");
eeAddress += sizeof(long);
EEPROM.get(eeAddress, calibrationData);
displaySensorOffsets(calibrationData);
Serial.println("\n\nRestoring Calibration data to the BNO055...");
bno.setSensorOffsets(calibrationData);
Serial.println("\n\nCalibration data loaded into BNO055");
foundCalib = true;
}
delay(1000);
/* Display some basic information on this sensor */
displaySensorDetails();
/* Optional: Display current status */
displaySensorStatus();
/* Crystal must be configured AFTER loading calibration data into BNO055. */
bno.setExtCrystalUse(true);
sensors_event_t event;
bno.getEvent(&event);
/* always recal the mag as It goes out of calibration very often */
if (foundCalib){
// NOTE: UNCOMMENT IF PLANNING TO USE MAGNEMOMETER
// Serial.println("Move sensor slightly to calibrate magnetometers");
// while (!bno.isFullyCalibrated())
// {
// bno.getEvent(&event);
// delay(BNO055_SAMPLERATE_DELAY_MS);
// }
Serial.println("Loading Calibration...");
}
else
{
Serial.println("Please Calibrate Sensor: ");
while (!bno.isFullyCalibrated())
{
bno.getEvent(&event);
Serial.print("X: ");
Serial.print(event.orientation.x, 4);
Serial.print("\tY: ");
Serial.print(event.orientation.y, 4);
Serial.print("\tZ: ");
Serial.print(event.orientation.z, 4);
/* Optional: Display calibration status */
displayCalStatus();
/* New line for the next sample */
Serial.println("");
/* Wait the specified delay before requesting new data */
delay(BNO055_SAMPLERATE_DELAY_MS);
}
}
Serial.println("\nFully calibrated!");
Serial.println("--------------------------------");
Serial.println("Calibration Results: ");
adafruit_bno055_offsets_t newCalib;
bno.getSensorOffsets(newCalib);
displaySensorOffsets(newCalib);
Serial.println("\n\nStoring calibration data to EEPROM...");
eeAddress = 0;
bno.getSensor(&sensor);
bnoID = sensor.sensor_id;
EEPROM.put(eeAddress, bnoID);
eeAddress += sizeof(long);
EEPROM.put(eeAddress, newCalib);
Serial.println("Data stored to EEPROM.");
Serial.println("\n--------------------------------\n");
// REMAP AXIS. IMPORTANT
/** Remap settings **/
// typedef enum {
// REMAP_CONFIG_P0 = 0x21,
// REMAP_CONFIG_P1 = 0x24, // default
// REMAP_CONFIG_P2 = 0x24,
// REMAP_CONFIG_P3 = 0x21,
// REMAP_CONFIG_P4 = 0x24,
// REMAP_CONFIG_P5 = 0x21,
// REMAP_CONFIG_P6 = 0x21,
// REMAP_CONFIG_P7 = 0x24
// } adafruit_bno055_axis_remap_config_t;
// * Remap Signs *
// typedef enum {
// REMAP_SIGN_P0 = 0x04,
// REMAP_SIGN_P1 = 0x00, // default
// REMAP_SIGN_P2 = 0x06,
// REMAP_SIGN_P3 = 0x02,
// REMAP_SIGN_P4 = 0x03,
// REMAP_SIGN_P5 = 0x01,
// REMAP_SIGN_P6 = 0x07,
// REMAP_SIGN_P7 = 0x05
// } adafruit_bno055_axis_remap_sign_t;
Adafruit_BNO055::adafruit_bno055_axis_remap_config_t r;
// NOTE: with this we just swap roll and pitch from eul.x/z
r = Adafruit_BNO055::REMAP_CONFIG_P7;
bno.setAxisRemap(r);
// bno.setAxisSign(0x00);
delay(500);
}
// delay(1000);
bno.setExtCrystalUse(true);
}
bool IMU::available()
{
return ok;
}
// NOTE: not really useful, use GetQuat()
imu::Vector<3> IMU::GetEuler()
{
// return bno.getVector(Adafruit_BNO055::VECTOR_EULER);
imu::Quaternion quat = GetQuat();
// Normalize
quat.normalize();
// convert quat to eul
imu::Vector<3> eul = quat.toEuler();
return eul;
}
imu::Quaternion IMU::GetQuat()
{
return bno.getQuat();
}
imu::Vector<3> IMU::GetAcc()
{
return bno.getVector(Adafruit_BNO055::VECTOR_ACCELEROMETER);
}
imu::Vector<3> IMU::GetGyro()
{
return bno.getVector(Adafruit_BNO055::VECTOR_GYROSCOPE);
}
| 8,968 | C++ | 29.097315 | 92 | 0.571811 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ROSSerial/ROSSerial.hpp | #ifndef ROSSERIAL_HPP
#define ROSSERIAL_HPP
#define USE_TEENSY_HW_SERIAL
#include <ros.h>
#include <ros/time.h>
#include <Arduino.h>
#include <mini_ros/ContactData.h>
#include <mini_ros/IMUdata.h>
#include <mini_ros/JointAngles.h>
#include <mini_ros/JointPulse.h>
#include <SpotServo.hpp>
#include <IMU.hpp>
struct LegJoints
{
LegType legtype;
double shoulder = 0.0;
double elbow = 0.0;
double wrist = 0.0;
LegJoints(const LegType & legtype_)
{
legtype = legtype_;
}
void FillLegJoint(const double & s, const double & e, const double & w)
{
shoulder = s;
elbow = e;
wrist = w;
}
};
namespace ros_srl
{
class ROSSerial
{
// Node Handle
ros::NodeHandle nh_;
// Joint Angle Subscriber
ros::Subscriber<mini_ros::JointAngles, ROSSerial> ja_sub_;
ros::Subscriber<mini_ros::JointPulse, ROSSerial> jp_sub_;
// joint msg timer
unsigned long prev_joints_time_;
unsigned long prev_resetter_time_;
// joint cmd msg flag
bool joints_cmd_active_ = false;
// joint pulse msg flag
bool joints_pulse_active_ = false;
int joint_num = -1;
int pulse = 1250;
// move type
// False is step, True is view
bool step_or_view = false;
// joint msgs
LegType fl_leg = FL;
LegType fr_leg = FR;
LegType bl_leg = BL;
LegType br_leg = BR;
LegJoints FL_ = LegJoints(fl_leg);
LegJoints FR_ = LegJoints(fr_leg);
LegJoints BL_ = LegJoints(bl_leg);
LegJoints BR_ = LegJoints(br_leg);
// IMU msg and publisher
mini_ros::IMUdata imu_msg_;
ros::Publisher imu_pub_;
// Contact msg and publisher
mini_ros::ContactData contact_msg_;
ros::Publisher contact_pub_;
void JointCommandCallback(const mini_ros::JointAngles& ja_msg)
{
prev_joints_time_ = micros();
FL_.FillLegJoint(ja_msg.fls, ja_msg.fle, ja_msg.flw);
FR_.FillLegJoint(ja_msg.frs, ja_msg.fre, ja_msg.frw);
BL_.FillLegJoint(ja_msg.bls, ja_msg.ble, ja_msg.blw);
BR_.FillLegJoint(ja_msg.brs, ja_msg.bre, ja_msg.brw);
step_or_view = ja_msg.step_or_view;
// flag
joints_cmd_active_ = true;
}
void JointPulseCallback(const mini_ros::JointPulse& jp_msg)
{
joint_num = jp_msg.servo_num;
pulse = jp_msg.servo_pulse;
// flag
joints_pulse_active_ = true;
// disable joint cmd flag
joints_cmd_active_ = false;
}
public:
ROSSerial():
ja_sub_("spot/joints", &ROSSerial::JointCommandCallback, this),
jp_sub_("spot/pulse", &ROSSerial::JointPulseCallback, this),
imu_pub_("spot/imu", &imu_msg_),
contact_pub_("spot/contact", &contact_msg_)
{
nh_.initNode();
nh_.getHardware()->setBaud(500000);
nh_.subscribe(ja_sub_);
nh_.subscribe(jp_sub_);
nh_.advertise(imu_pub_);
nh_.advertise(contact_pub_);
nh_.loginfo("SPOT MINI MINI ROS CLIENT CONNECTED");
}
void returnJoints(LegJoints & FL_ref, LegJoints & FR_ref, LegJoints & BL_ref, LegJoints & BR_ref, bool & step_or_view_)
{
FL_ref = FL_;
FR_ref = FR_;
BL_ref = BL_;
BR_ref = BR_;
step_or_view_ = step_or_view;
}
bool jointsInputIsActive()
{
return joints_cmd_active_;
}
bool jointsPulseIsActive()
{
return joints_pulse_active_;
}
int returnPulse()
{
return pulse;
}
int returnServoNum()
{
return joint_num;
}
void resetPulseTopic()
{
joints_pulse_active_ = false;
pulse = 1250;
joint_num = -1;
}
void run()
{
// timeout
unsigned long now = micros();
if((now - prev_joints_time_) > 1000000)
{
joints_cmd_active_ = false;
}
nh_.spinOnce();
}
void publishContacts(const bool & FLC, const bool & FRC, const bool & BLC, const bool & BRC)
{
contact_msg_.FL = FLC;
contact_msg_.FR = FRC;
contact_msg_.BL = BLC;
contact_msg_.BR = BRC;
contact_pub_.publish(&contact_msg_);
}
void publishIMU(imu::Vector<3> eul, imu::Vector<3> acc, imu::Vector<3> gyro)
{
// NOTE: switching eul.x and eul.x because Bosch is weird..
imu_msg_.roll = eul.z();
imu_msg_.pitch = eul.y();
imu_msg_.acc_x = acc.x();
imu_msg_.acc_y = acc.y();
imu_msg_.acc_z = acc.z();
imu_msg_.gyro_x = gyro.x();
imu_msg_.gyro_y = gyro.y();
imu_msg_.gyro_z = gyro.z();
imu_pub_.publish(&imu_msg_);
}
};
}
#endif | 5,489 | C++ | 26.86802 | 132 | 0.482237 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/time.cpp | /*
* Software License Agreement (BSD License)
*
* Copyright (c) 2011, Willow Garage, Inc.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials provided
* with the distribution.
* * Neither the name of Willow Garage, Inc. nor the names of its
* contributors may be used to endorse or promote prducts derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
* FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
* COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
* BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
* LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN
* ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
#include "ros/time.h"
namespace ros
{
void normalizeSecNSec(uint32_t& sec, uint32_t& nsec)
{
uint32_t nsec_part = nsec % 1000000000UL;
uint32_t sec_part = nsec / 1000000000UL;
sec += sec_part;
nsec = nsec_part;
}
Time& Time::fromNSec(int32_t t)
{
sec = t / 1000000000;
nsec = t % 1000000000;
normalizeSecNSec(sec, nsec);
return *this;
}
Time& Time::operator +=(const Duration &rhs)
{
sec += rhs.sec;
nsec += rhs.nsec;
normalizeSecNSec(sec, nsec);
return *this;
}
Time& Time::operator -=(const Duration &rhs)
{
sec += -rhs.sec;
nsec += -rhs.nsec;
normalizeSecNSec(sec, nsec);
return *this;
}
}
| 2,271 | C++ | 31 | 71 | 0.725672 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/ArduinoTcpHardware.h | /*
* Software License Agreement (BSD License)
*
* Copyright (c) 2011, Willow Garage, Inc.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials provided
* with the distribution.
* * Neither the name of Willow Garage, Inc. nor the names of its
* contributors may be used to endorse or promote prducts derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
* FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
* COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
* BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
* LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN
* ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
#ifndef ROS_ARDUINO_TCP_HARDWARE_H_
#define ROS_ARDUINO_TCP_HARDWARE_H_
#include <Arduino.h>
#if defined(ESP8266)
#include <ESP8266WiFi.h>
#else
#include <SPI.h>
#include <Ethernet.h>
#endif
class ArduinoHardware {
public:
ArduinoHardware()
{
}
void setConnection(IPAddress &server, int port = 11411)
{
server_ = server;
serverPort_ = port;
}
IPAddress getLocalIP()
{
#if defined(ESP8266)
return tcp_.localIP();
#else
return Ethernet.localIP();
#endif
}
void init()
{
tcp_.connect(server_, serverPort_);
}
int read(){
if (tcp_.connected())
{
return tcp_.read();
}
else
{
tcp_.connect(server_, serverPort_);
}
return -1;
}
void write(const uint8_t* data, int length)
{
tcp_.write(data, length);
}
unsigned long time()
{
return millis();
}
protected:
#if defined(ESP8266)
WiFiClient tcp_;
#else
EthernetClient tcp_;
#endif
IPAddress server_;
uint16_t serverPort_ = 11411;
};
#endif | 2,655 | C | 24.538461 | 71 | 0.702825 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/ArduinoHardware.h | /*
* Software License Agreement (BSD License)
*
* Copyright (c) 2011, Willow Garage, Inc.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials provided
* with the distribution.
* * Neither the name of Willow Garage, Inc. nor the names of its
* contributors may be used to endorse or promote prducts derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
* FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
* COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
* BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
* LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN
* ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
#ifndef ROS_ARDUINO_HARDWARE_H_
#define ROS_ARDUINO_HARDWARE_H_
#if ARDUINO>=100
#include <Arduino.h> // Arduino 1.0
#else
#include <WProgram.h> // Arduino 0022
#endif
#if defined(__MK20DX128__) || defined(__MK20DX256__) || defined(__MK64FX512__) || defined(__MK66FX1M0__) || defined(__MKL26Z64__) || defined(__IMXRT1062__)
#if defined(USE_TEENSY_HW_SERIAL)
#define SERIAL_CLASS HardwareSerial // Teensy HW Serial
#else
#include <usb_serial.h> // Teensy 3.0 and 3.1
#define SERIAL_CLASS usb_serial_class
#endif
#elif defined(_SAM3XA_)
#include <UARTClass.h> // Arduino Due
#define SERIAL_CLASS UARTClass
#elif defined(USE_USBCON)
// Arduino Leonardo USB Serial Port
#define SERIAL_CLASS Serial_
#elif (defined(__STM32F1__) and !(defined(USE_STM32_HW_SERIAL))) or defined(SPARK)
// Stm32duino Maple mini USB Serial Port
#define SERIAL_CLASS USBSerial
#else
#include <HardwareSerial.h> // Arduino AVR
#define SERIAL_CLASS HardwareSerial
#endif
class ArduinoHardware {
public:
ArduinoHardware(SERIAL_CLASS* io , long baud= 500000){
iostream = io;
baud_ = baud;
}
ArduinoHardware()
{
#if defined(USBCON) and !(defined(USE_USBCON))
/* Leonardo support */
iostream = &Serial1;
#elif defined(USE_TEENSY_HW_SERIAL) or defined(USE_STM32_HW_SERIAL)
iostream = &Serial1;
#else
iostream = &Serial;
#endif
baud_ = 500000;
}
ArduinoHardware(ArduinoHardware& h){
this->iostream = h.iostream;
this->baud_ = h.baud_;
}
void setBaud(long baud){
this->baud_= baud;
}
int getBaud(){return baud_;}
void init(){
#if defined(USE_USBCON)
// Startup delay as a fail-safe to upload a new sketch
delay(3000);
#endif
iostream->begin(baud_);
}
int read(){return iostream->read();};
void write(uint8_t* data, int length){
for(int i=0; i<length; i++)
iostream->write(data[i]);
}
unsigned long time(){return millis();}
protected:
SERIAL_CLASS* iostream;
long baud_;
};
#endif
| 3,716 | C | 30.769231 | 155 | 0.690797 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/move_base_msgs/MoveBaseActionGoal.h | #ifndef _ROS_move_base_msgs_MoveBaseActionGoal_h
#define _ROS_move_base_msgs_MoveBaseActionGoal_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "std_msgs/Header.h"
#include "actionlib_msgs/GoalID.h"
#include "move_base_msgs/MoveBaseGoal.h"
namespace move_base_msgs
{
class MoveBaseActionGoal : public ros::Msg
{
public:
typedef std_msgs::Header _header_type;
_header_type header;
typedef actionlib_msgs::GoalID _goal_id_type;
_goal_id_type goal_id;
typedef move_base_msgs::MoveBaseGoal _goal_type;
_goal_type goal;
MoveBaseActionGoal():
header(),
goal_id(),
goal()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->header.serialize(outbuffer + offset);
offset += this->goal_id.serialize(outbuffer + offset);
offset += this->goal.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->header.deserialize(inbuffer + offset);
offset += this->goal_id.deserialize(inbuffer + offset);
offset += this->goal.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "move_base_msgs/MoveBaseActionGoal"; };
const char * getMD5(){ return "660d6895a1b9a16dce51fbdd9a64a56b"; };
};
}
#endif
| 1,439 | C | 24.263157 | 74 | 0.653231 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/move_base_msgs/MoveBaseGoal.h | #ifndef _ROS_move_base_msgs_MoveBaseGoal_h
#define _ROS_move_base_msgs_MoveBaseGoal_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "geometry_msgs/PoseStamped.h"
namespace move_base_msgs
{
class MoveBaseGoal : public ros::Msg
{
public:
typedef geometry_msgs::PoseStamped _target_pose_type;
_target_pose_type target_pose;
MoveBaseGoal():
target_pose()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->target_pose.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->target_pose.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "move_base_msgs/MoveBaseGoal"; };
const char * getMD5(){ return "257d089627d7eb7136c24d3593d05a16"; };
};
}
#endif
| 953 | C | 20.2 | 72 | 0.654774 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/move_base_msgs/MoveBaseAction.h | #ifndef _ROS_move_base_msgs_MoveBaseAction_h
#define _ROS_move_base_msgs_MoveBaseAction_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "move_base_msgs/MoveBaseActionGoal.h"
#include "move_base_msgs/MoveBaseActionResult.h"
#include "move_base_msgs/MoveBaseActionFeedback.h"
namespace move_base_msgs
{
class MoveBaseAction : public ros::Msg
{
public:
typedef move_base_msgs::MoveBaseActionGoal _action_goal_type;
_action_goal_type action_goal;
typedef move_base_msgs::MoveBaseActionResult _action_result_type;
_action_result_type action_result;
typedef move_base_msgs::MoveBaseActionFeedback _action_feedback_type;
_action_feedback_type action_feedback;
MoveBaseAction():
action_goal(),
action_result(),
action_feedback()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->action_goal.serialize(outbuffer + offset);
offset += this->action_result.serialize(outbuffer + offset);
offset += this->action_feedback.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->action_goal.deserialize(inbuffer + offset);
offset += this->action_result.deserialize(inbuffer + offset);
offset += this->action_feedback.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "move_base_msgs/MoveBaseAction"; };
const char * getMD5(){ return "70b6aca7c7f7746d8d1609ad94c80bb8"; };
};
}
#endif
| 1,635 | C | 27.701754 | 75 | 0.685015 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/move_base_msgs/MoveBaseResult.h | #ifndef _ROS_move_base_msgs_MoveBaseResult_h
#define _ROS_move_base_msgs_MoveBaseResult_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
namespace move_base_msgs
{
class MoveBaseResult : public ros::Msg
{
public:
MoveBaseResult()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
return offset;
}
const char * getType(){ return "move_base_msgs/MoveBaseResult"; };
const char * getMD5(){ return "d41d8cd98f00b204e9800998ecf8427e"; };
};
}
#endif
| 675 | C | 16.333333 | 72 | 0.645926 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/move_base_msgs/MoveBaseFeedback.h | #ifndef _ROS_move_base_msgs_MoveBaseFeedback_h
#define _ROS_move_base_msgs_MoveBaseFeedback_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "geometry_msgs/PoseStamped.h"
namespace move_base_msgs
{
class MoveBaseFeedback : public ros::Msg
{
public:
typedef geometry_msgs::PoseStamped _base_position_type;
_base_position_type base_position;
MoveBaseFeedback():
base_position()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->base_position.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->base_position.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "move_base_msgs/MoveBaseFeedback"; };
const char * getMD5(){ return "3fb824c456a757373a226f6d08071bf0"; };
};
}
#endif
| 985 | C | 20.911111 | 72 | 0.66599 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/move_base_msgs/MoveBaseActionResult.h | #ifndef _ROS_move_base_msgs_MoveBaseActionResult_h
#define _ROS_move_base_msgs_MoveBaseActionResult_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "std_msgs/Header.h"
#include "actionlib_msgs/GoalStatus.h"
#include "move_base_msgs/MoveBaseResult.h"
namespace move_base_msgs
{
class MoveBaseActionResult : public ros::Msg
{
public:
typedef std_msgs::Header _header_type;
_header_type header;
typedef actionlib_msgs::GoalStatus _status_type;
_status_type status;
typedef move_base_msgs::MoveBaseResult _result_type;
_result_type result;
MoveBaseActionResult():
header(),
status(),
result()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->header.serialize(outbuffer + offset);
offset += this->status.serialize(outbuffer + offset);
offset += this->result.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->header.deserialize(inbuffer + offset);
offset += this->status.deserialize(inbuffer + offset);
offset += this->result.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "move_base_msgs/MoveBaseActionResult"; };
const char * getMD5(){ return "1eb06eeff08fa7ea874431638cb52332"; };
};
}
#endif
| 1,467 | C | 24.754386 | 76 | 0.66394 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/object_recognition_msgs/ObjectRecognitionAction.h | #ifndef _ROS_object_recognition_msgs_ObjectRecognitionAction_h
#define _ROS_object_recognition_msgs_ObjectRecognitionAction_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "object_recognition_msgs/ObjectRecognitionActionGoal.h"
#include "object_recognition_msgs/ObjectRecognitionActionResult.h"
#include "object_recognition_msgs/ObjectRecognitionActionFeedback.h"
namespace object_recognition_msgs
{
class ObjectRecognitionAction : public ros::Msg
{
public:
typedef object_recognition_msgs::ObjectRecognitionActionGoal _action_goal_type;
_action_goal_type action_goal;
typedef object_recognition_msgs::ObjectRecognitionActionResult _action_result_type;
_action_result_type action_result;
typedef object_recognition_msgs::ObjectRecognitionActionFeedback _action_feedback_type;
_action_feedback_type action_feedback;
ObjectRecognitionAction():
action_goal(),
action_result(),
action_feedback()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->action_goal.serialize(outbuffer + offset);
offset += this->action_result.serialize(outbuffer + offset);
offset += this->action_feedback.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->action_goal.deserialize(inbuffer + offset);
offset += this->action_result.deserialize(inbuffer + offset);
offset += this->action_feedback.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "object_recognition_msgs/ObjectRecognitionAction"; };
const char * getMD5(){ return "7d8979a0cf97e5078553ee3efee047d2"; };
};
}
#endif
| 1,824 | C | 31.017543 | 93 | 0.717654 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/object_recognition_msgs/ObjectRecognitionGoal.h | #ifndef _ROS_object_recognition_msgs_ObjectRecognitionGoal_h
#define _ROS_object_recognition_msgs_ObjectRecognitionGoal_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
namespace object_recognition_msgs
{
class ObjectRecognitionGoal : public ros::Msg
{
public:
typedef bool _use_roi_type;
_use_roi_type use_roi;
uint32_t filter_limits_length;
typedef float _filter_limits_type;
_filter_limits_type st_filter_limits;
_filter_limits_type * filter_limits;
ObjectRecognitionGoal():
use_roi(0),
filter_limits_length(0), filter_limits(NULL)
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
union {
bool real;
uint8_t base;
} u_use_roi;
u_use_roi.real = this->use_roi;
*(outbuffer + offset + 0) = (u_use_roi.base >> (8 * 0)) & 0xFF;
offset += sizeof(this->use_roi);
*(outbuffer + offset + 0) = (this->filter_limits_length >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (this->filter_limits_length >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (this->filter_limits_length >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (this->filter_limits_length >> (8 * 3)) & 0xFF;
offset += sizeof(this->filter_limits_length);
for( uint32_t i = 0; i < filter_limits_length; i++){
union {
float real;
uint32_t base;
} u_filter_limitsi;
u_filter_limitsi.real = this->filter_limits[i];
*(outbuffer + offset + 0) = (u_filter_limitsi.base >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (u_filter_limitsi.base >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (u_filter_limitsi.base >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (u_filter_limitsi.base >> (8 * 3)) & 0xFF;
offset += sizeof(this->filter_limits[i]);
}
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
union {
bool real;
uint8_t base;
} u_use_roi;
u_use_roi.base = 0;
u_use_roi.base |= ((uint8_t) (*(inbuffer + offset + 0))) << (8 * 0);
this->use_roi = u_use_roi.real;
offset += sizeof(this->use_roi);
uint32_t filter_limits_lengthT = ((uint32_t) (*(inbuffer + offset)));
filter_limits_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1);
filter_limits_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2);
filter_limits_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3);
offset += sizeof(this->filter_limits_length);
if(filter_limits_lengthT > filter_limits_length)
this->filter_limits = (float*)realloc(this->filter_limits, filter_limits_lengthT * sizeof(float));
filter_limits_length = filter_limits_lengthT;
for( uint32_t i = 0; i < filter_limits_length; i++){
union {
float real;
uint32_t base;
} u_st_filter_limits;
u_st_filter_limits.base = 0;
u_st_filter_limits.base |= ((uint32_t) (*(inbuffer + offset + 0))) << (8 * 0);
u_st_filter_limits.base |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1);
u_st_filter_limits.base |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2);
u_st_filter_limits.base |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3);
this->st_filter_limits = u_st_filter_limits.real;
offset += sizeof(this->st_filter_limits);
memcpy( &(this->filter_limits[i]), &(this->st_filter_limits), sizeof(float));
}
return offset;
}
const char * getType(){ return "object_recognition_msgs/ObjectRecognitionGoal"; };
const char * getMD5(){ return "49bea2f03a1bba0ad05926e01e3525fa"; };
};
}
#endif
| 3,769 | C | 36.326732 | 106 | 0.57575 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/object_recognition_msgs/ObjectType.h | #ifndef _ROS_object_recognition_msgs_ObjectType_h
#define _ROS_object_recognition_msgs_ObjectType_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
namespace object_recognition_msgs
{
class ObjectType : public ros::Msg
{
public:
typedef const char* _key_type;
_key_type key;
typedef const char* _db_type;
_db_type db;
ObjectType():
key(""),
db("")
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
uint32_t length_key = strlen(this->key);
varToArr(outbuffer + offset, length_key);
offset += 4;
memcpy(outbuffer + offset, this->key, length_key);
offset += length_key;
uint32_t length_db = strlen(this->db);
varToArr(outbuffer + offset, length_db);
offset += 4;
memcpy(outbuffer + offset, this->db, length_db);
offset += length_db;
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
uint32_t length_key;
arrToVar(length_key, (inbuffer + offset));
offset += 4;
for(unsigned int k= offset; k< offset+length_key; ++k){
inbuffer[k-1]=inbuffer[k];
}
inbuffer[offset+length_key-1]=0;
this->key = (char *)(inbuffer + offset-1);
offset += length_key;
uint32_t length_db;
arrToVar(length_db, (inbuffer + offset));
offset += 4;
for(unsigned int k= offset; k< offset+length_db; ++k){
inbuffer[k-1]=inbuffer[k];
}
inbuffer[offset+length_db-1]=0;
this->db = (char *)(inbuffer + offset-1);
offset += length_db;
return offset;
}
const char * getType(){ return "object_recognition_msgs/ObjectType"; };
const char * getMD5(){ return "ac757ec5be1998b0167e7efcda79e3cf"; };
};
}
#endif
| 1,855 | C | 24.424657 | 75 | 0.59407 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/object_recognition_msgs/RecognizedObjectArray.h | #ifndef _ROS_object_recognition_msgs_RecognizedObjectArray_h
#define _ROS_object_recognition_msgs_RecognizedObjectArray_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "std_msgs/Header.h"
#include "object_recognition_msgs/RecognizedObject.h"
namespace object_recognition_msgs
{
class RecognizedObjectArray : public ros::Msg
{
public:
typedef std_msgs::Header _header_type;
_header_type header;
uint32_t objects_length;
typedef object_recognition_msgs::RecognizedObject _objects_type;
_objects_type st_objects;
_objects_type * objects;
uint32_t cooccurrence_length;
typedef float _cooccurrence_type;
_cooccurrence_type st_cooccurrence;
_cooccurrence_type * cooccurrence;
RecognizedObjectArray():
header(),
objects_length(0), objects(NULL),
cooccurrence_length(0), cooccurrence(NULL)
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->header.serialize(outbuffer + offset);
*(outbuffer + offset + 0) = (this->objects_length >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (this->objects_length >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (this->objects_length >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (this->objects_length >> (8 * 3)) & 0xFF;
offset += sizeof(this->objects_length);
for( uint32_t i = 0; i < objects_length; i++){
offset += this->objects[i].serialize(outbuffer + offset);
}
*(outbuffer + offset + 0) = (this->cooccurrence_length >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (this->cooccurrence_length >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (this->cooccurrence_length >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (this->cooccurrence_length >> (8 * 3)) & 0xFF;
offset += sizeof(this->cooccurrence_length);
for( uint32_t i = 0; i < cooccurrence_length; i++){
union {
float real;
uint32_t base;
} u_cooccurrencei;
u_cooccurrencei.real = this->cooccurrence[i];
*(outbuffer + offset + 0) = (u_cooccurrencei.base >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (u_cooccurrencei.base >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (u_cooccurrencei.base >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (u_cooccurrencei.base >> (8 * 3)) & 0xFF;
offset += sizeof(this->cooccurrence[i]);
}
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->header.deserialize(inbuffer + offset);
uint32_t objects_lengthT = ((uint32_t) (*(inbuffer + offset)));
objects_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1);
objects_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2);
objects_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3);
offset += sizeof(this->objects_length);
if(objects_lengthT > objects_length)
this->objects = (object_recognition_msgs::RecognizedObject*)realloc(this->objects, objects_lengthT * sizeof(object_recognition_msgs::RecognizedObject));
objects_length = objects_lengthT;
for( uint32_t i = 0; i < objects_length; i++){
offset += this->st_objects.deserialize(inbuffer + offset);
memcpy( &(this->objects[i]), &(this->st_objects), sizeof(object_recognition_msgs::RecognizedObject));
}
uint32_t cooccurrence_lengthT = ((uint32_t) (*(inbuffer + offset)));
cooccurrence_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1);
cooccurrence_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2);
cooccurrence_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3);
offset += sizeof(this->cooccurrence_length);
if(cooccurrence_lengthT > cooccurrence_length)
this->cooccurrence = (float*)realloc(this->cooccurrence, cooccurrence_lengthT * sizeof(float));
cooccurrence_length = cooccurrence_lengthT;
for( uint32_t i = 0; i < cooccurrence_length; i++){
union {
float real;
uint32_t base;
} u_st_cooccurrence;
u_st_cooccurrence.base = 0;
u_st_cooccurrence.base |= ((uint32_t) (*(inbuffer + offset + 0))) << (8 * 0);
u_st_cooccurrence.base |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1);
u_st_cooccurrence.base |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2);
u_st_cooccurrence.base |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3);
this->st_cooccurrence = u_st_cooccurrence.real;
offset += sizeof(this->st_cooccurrence);
memcpy( &(this->cooccurrence[i]), &(this->st_cooccurrence), sizeof(float));
}
return offset;
}
const char * getType(){ return "object_recognition_msgs/RecognizedObjectArray"; };
const char * getMD5(){ return "bad6b1546b9ebcabb49fb3b858d78964"; };
};
}
#endif
| 4,972 | C | 42.243478 | 160 | 0.60358 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/object_recognition_msgs/Table.h | #ifndef _ROS_object_recognition_msgs_Table_h
#define _ROS_object_recognition_msgs_Table_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "std_msgs/Header.h"
#include "geometry_msgs/Pose.h"
#include "geometry_msgs/Point.h"
namespace object_recognition_msgs
{
class Table : public ros::Msg
{
public:
typedef std_msgs::Header _header_type;
_header_type header;
typedef geometry_msgs::Pose _pose_type;
_pose_type pose;
uint32_t convex_hull_length;
typedef geometry_msgs::Point _convex_hull_type;
_convex_hull_type st_convex_hull;
_convex_hull_type * convex_hull;
Table():
header(),
pose(),
convex_hull_length(0), convex_hull(NULL)
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->header.serialize(outbuffer + offset);
offset += this->pose.serialize(outbuffer + offset);
*(outbuffer + offset + 0) = (this->convex_hull_length >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (this->convex_hull_length >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (this->convex_hull_length >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (this->convex_hull_length >> (8 * 3)) & 0xFF;
offset += sizeof(this->convex_hull_length);
for( uint32_t i = 0; i < convex_hull_length; i++){
offset += this->convex_hull[i].serialize(outbuffer + offset);
}
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->header.deserialize(inbuffer + offset);
offset += this->pose.deserialize(inbuffer + offset);
uint32_t convex_hull_lengthT = ((uint32_t) (*(inbuffer + offset)));
convex_hull_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1);
convex_hull_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2);
convex_hull_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3);
offset += sizeof(this->convex_hull_length);
if(convex_hull_lengthT > convex_hull_length)
this->convex_hull = (geometry_msgs::Point*)realloc(this->convex_hull, convex_hull_lengthT * sizeof(geometry_msgs::Point));
convex_hull_length = convex_hull_lengthT;
for( uint32_t i = 0; i < convex_hull_length; i++){
offset += this->st_convex_hull.deserialize(inbuffer + offset);
memcpy( &(this->convex_hull[i]), &(this->st_convex_hull), sizeof(geometry_msgs::Point));
}
return offset;
}
const char * getType(){ return "object_recognition_msgs/Table"; };
const char * getMD5(){ return "39efebc7d51e44bd2d72f2df6c7823a2"; };
};
}
#endif
| 2,720 | C | 34.337662 | 130 | 0.613235 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/object_recognition_msgs/TableArray.h | #ifndef _ROS_object_recognition_msgs_TableArray_h
#define _ROS_object_recognition_msgs_TableArray_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "std_msgs/Header.h"
#include "object_recognition_msgs/Table.h"
namespace object_recognition_msgs
{
class TableArray : public ros::Msg
{
public:
typedef std_msgs::Header _header_type;
_header_type header;
uint32_t tables_length;
typedef object_recognition_msgs::Table _tables_type;
_tables_type st_tables;
_tables_type * tables;
TableArray():
header(),
tables_length(0), tables(NULL)
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->header.serialize(outbuffer + offset);
*(outbuffer + offset + 0) = (this->tables_length >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (this->tables_length >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (this->tables_length >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (this->tables_length >> (8 * 3)) & 0xFF;
offset += sizeof(this->tables_length);
for( uint32_t i = 0; i < tables_length; i++){
offset += this->tables[i].serialize(outbuffer + offset);
}
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->header.deserialize(inbuffer + offset);
uint32_t tables_lengthT = ((uint32_t) (*(inbuffer + offset)));
tables_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1);
tables_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2);
tables_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3);
offset += sizeof(this->tables_length);
if(tables_lengthT > tables_length)
this->tables = (object_recognition_msgs::Table*)realloc(this->tables, tables_lengthT * sizeof(object_recognition_msgs::Table));
tables_length = tables_lengthT;
for( uint32_t i = 0; i < tables_length; i++){
offset += this->st_tables.deserialize(inbuffer + offset);
memcpy( &(this->tables[i]), &(this->st_tables), sizeof(object_recognition_msgs::Table));
}
return offset;
}
const char * getType(){ return "object_recognition_msgs/TableArray"; };
const char * getMD5(){ return "d1c853e5acd0ed273eb6682dc01ab428"; };
};
}
#endif
| 2,408 | C | 32.929577 | 135 | 0.608804 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/object_recognition_msgs/ObjectRecognitionResult.h | #ifndef _ROS_object_recognition_msgs_ObjectRecognitionResult_h
#define _ROS_object_recognition_msgs_ObjectRecognitionResult_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "object_recognition_msgs/RecognizedObjectArray.h"
namespace object_recognition_msgs
{
class ObjectRecognitionResult : public ros::Msg
{
public:
typedef object_recognition_msgs::RecognizedObjectArray _recognized_objects_type;
_recognized_objects_type recognized_objects;
ObjectRecognitionResult():
recognized_objects()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->recognized_objects.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->recognized_objects.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "object_recognition_msgs/ObjectRecognitionResult"; };
const char * getMD5(){ return "868e41288f9f8636e2b6c51f1af6aa9c"; };
};
}
#endif
| 1,126 | C | 24.044444 | 88 | 0.706039 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/object_recognition_msgs/RecognizedObject.h | #ifndef _ROS_object_recognition_msgs_RecognizedObject_h
#define _ROS_object_recognition_msgs_RecognizedObject_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "std_msgs/Header.h"
#include "object_recognition_msgs/ObjectType.h"
#include "sensor_msgs/PointCloud2.h"
#include "shape_msgs/Mesh.h"
#include "geometry_msgs/Point.h"
#include "geometry_msgs/PoseWithCovarianceStamped.h"
namespace object_recognition_msgs
{
class RecognizedObject : public ros::Msg
{
public:
typedef std_msgs::Header _header_type;
_header_type header;
typedef object_recognition_msgs::ObjectType _type_type;
_type_type type;
typedef float _confidence_type;
_confidence_type confidence;
uint32_t point_clouds_length;
typedef sensor_msgs::PointCloud2 _point_clouds_type;
_point_clouds_type st_point_clouds;
_point_clouds_type * point_clouds;
typedef shape_msgs::Mesh _bounding_mesh_type;
_bounding_mesh_type bounding_mesh;
uint32_t bounding_contours_length;
typedef geometry_msgs::Point _bounding_contours_type;
_bounding_contours_type st_bounding_contours;
_bounding_contours_type * bounding_contours;
typedef geometry_msgs::PoseWithCovarianceStamped _pose_type;
_pose_type pose;
RecognizedObject():
header(),
type(),
confidence(0),
point_clouds_length(0), point_clouds(NULL),
bounding_mesh(),
bounding_contours_length(0), bounding_contours(NULL),
pose()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->header.serialize(outbuffer + offset);
offset += this->type.serialize(outbuffer + offset);
union {
float real;
uint32_t base;
} u_confidence;
u_confidence.real = this->confidence;
*(outbuffer + offset + 0) = (u_confidence.base >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (u_confidence.base >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (u_confidence.base >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (u_confidence.base >> (8 * 3)) & 0xFF;
offset += sizeof(this->confidence);
*(outbuffer + offset + 0) = (this->point_clouds_length >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (this->point_clouds_length >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (this->point_clouds_length >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (this->point_clouds_length >> (8 * 3)) & 0xFF;
offset += sizeof(this->point_clouds_length);
for( uint32_t i = 0; i < point_clouds_length; i++){
offset += this->point_clouds[i].serialize(outbuffer + offset);
}
offset += this->bounding_mesh.serialize(outbuffer + offset);
*(outbuffer + offset + 0) = (this->bounding_contours_length >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (this->bounding_contours_length >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (this->bounding_contours_length >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (this->bounding_contours_length >> (8 * 3)) & 0xFF;
offset += sizeof(this->bounding_contours_length);
for( uint32_t i = 0; i < bounding_contours_length; i++){
offset += this->bounding_contours[i].serialize(outbuffer + offset);
}
offset += this->pose.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->header.deserialize(inbuffer + offset);
offset += this->type.deserialize(inbuffer + offset);
union {
float real;
uint32_t base;
} u_confidence;
u_confidence.base = 0;
u_confidence.base |= ((uint32_t) (*(inbuffer + offset + 0))) << (8 * 0);
u_confidence.base |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1);
u_confidence.base |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2);
u_confidence.base |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3);
this->confidence = u_confidence.real;
offset += sizeof(this->confidence);
uint32_t point_clouds_lengthT = ((uint32_t) (*(inbuffer + offset)));
point_clouds_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1);
point_clouds_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2);
point_clouds_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3);
offset += sizeof(this->point_clouds_length);
if(point_clouds_lengthT > point_clouds_length)
this->point_clouds = (sensor_msgs::PointCloud2*)realloc(this->point_clouds, point_clouds_lengthT * sizeof(sensor_msgs::PointCloud2));
point_clouds_length = point_clouds_lengthT;
for( uint32_t i = 0; i < point_clouds_length; i++){
offset += this->st_point_clouds.deserialize(inbuffer + offset);
memcpy( &(this->point_clouds[i]), &(this->st_point_clouds), sizeof(sensor_msgs::PointCloud2));
}
offset += this->bounding_mesh.deserialize(inbuffer + offset);
uint32_t bounding_contours_lengthT = ((uint32_t) (*(inbuffer + offset)));
bounding_contours_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1);
bounding_contours_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2);
bounding_contours_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3);
offset += sizeof(this->bounding_contours_length);
if(bounding_contours_lengthT > bounding_contours_length)
this->bounding_contours = (geometry_msgs::Point*)realloc(this->bounding_contours, bounding_contours_lengthT * sizeof(geometry_msgs::Point));
bounding_contours_length = bounding_contours_lengthT;
for( uint32_t i = 0; i < bounding_contours_length; i++){
offset += this->st_bounding_contours.deserialize(inbuffer + offset);
memcpy( &(this->bounding_contours[i]), &(this->st_bounding_contours), sizeof(geometry_msgs::Point));
}
offset += this->pose.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "object_recognition_msgs/RecognizedObject"; };
const char * getMD5(){ return "f92c4cb29ba11f26c5f7219de97e900d"; };
};
}
#endif
| 6,243 | C | 43.920863 | 148 | 0.620215 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/object_recognition_msgs/ObjectRecognitionFeedback.h | #ifndef _ROS_object_recognition_msgs_ObjectRecognitionFeedback_h
#define _ROS_object_recognition_msgs_ObjectRecognitionFeedback_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
namespace object_recognition_msgs
{
class ObjectRecognitionFeedback : public ros::Msg
{
public:
ObjectRecognitionFeedback()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
return offset;
}
const char * getType(){ return "object_recognition_msgs/ObjectRecognitionFeedback"; };
const char * getMD5(){ return "d41d8cd98f00b204e9800998ecf8427e"; };
};
}
#endif
| 766 | C | 18.666666 | 90 | 0.68799 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/object_recognition_msgs/GetObjectInformation.h | #ifndef _ROS_SERVICE_GetObjectInformation_h
#define _ROS_SERVICE_GetObjectInformation_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "object_recognition_msgs/ObjectType.h"
#include "object_recognition_msgs/ObjectInformation.h"
namespace object_recognition_msgs
{
static const char GETOBJECTINFORMATION[] = "object_recognition_msgs/GetObjectInformation";
class GetObjectInformationRequest : public ros::Msg
{
public:
typedef object_recognition_msgs::ObjectType _type_type;
_type_type type;
GetObjectInformationRequest():
type()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->type.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->type.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return GETOBJECTINFORMATION; };
const char * getMD5(){ return "0d72b69e80da0fe473b0bdcdd7a28d4d"; };
};
class GetObjectInformationResponse : public ros::Msg
{
public:
typedef object_recognition_msgs::ObjectInformation _information_type;
_information_type information;
GetObjectInformationResponse():
information()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->information.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->information.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return GETOBJECTINFORMATION; };
const char * getMD5(){ return "a62c5d1c41e250373b3e8e912a13a9cb"; };
};
class GetObjectInformation {
public:
typedef GetObjectInformationRequest Request;
typedef GetObjectInformationResponse Response;
};
}
#endif
| 2,003 | C | 23.144578 | 90 | 0.685971 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/object_recognition_msgs/ObjectInformation.h | #ifndef _ROS_object_recognition_msgs_ObjectInformation_h
#define _ROS_object_recognition_msgs_ObjectInformation_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "shape_msgs/Mesh.h"
#include "sensor_msgs/PointCloud2.h"
namespace object_recognition_msgs
{
class ObjectInformation : public ros::Msg
{
public:
typedef const char* _name_type;
_name_type name;
typedef shape_msgs::Mesh _ground_truth_mesh_type;
_ground_truth_mesh_type ground_truth_mesh;
typedef sensor_msgs::PointCloud2 _ground_truth_point_cloud_type;
_ground_truth_point_cloud_type ground_truth_point_cloud;
ObjectInformation():
name(""),
ground_truth_mesh(),
ground_truth_point_cloud()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
uint32_t length_name = strlen(this->name);
varToArr(outbuffer + offset, length_name);
offset += 4;
memcpy(outbuffer + offset, this->name, length_name);
offset += length_name;
offset += this->ground_truth_mesh.serialize(outbuffer + offset);
offset += this->ground_truth_point_cloud.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
uint32_t length_name;
arrToVar(length_name, (inbuffer + offset));
offset += 4;
for(unsigned int k= offset; k< offset+length_name; ++k){
inbuffer[k-1]=inbuffer[k];
}
inbuffer[offset+length_name-1]=0;
this->name = (char *)(inbuffer + offset-1);
offset += length_name;
offset += this->ground_truth_mesh.deserialize(inbuffer + offset);
offset += this->ground_truth_point_cloud.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "object_recognition_msgs/ObjectInformation"; };
const char * getMD5(){ return "921ec39f51c7b927902059cf3300ecde"; };
};
}
#endif
| 1,998 | C | 28.397058 | 82 | 0.652653 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/roscpp_tutorials/TwoInts.h | #ifndef _ROS_SERVICE_TwoInts_h
#define _ROS_SERVICE_TwoInts_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
namespace roscpp_tutorials
{
static const char TWOINTS[] = "roscpp_tutorials/TwoInts";
class TwoIntsRequest : public ros::Msg
{
public:
typedef int64_t _a_type;
_a_type a;
typedef int64_t _b_type;
_b_type b;
TwoIntsRequest():
a(0),
b(0)
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
union {
int64_t real;
uint64_t base;
} u_a;
u_a.real = this->a;
*(outbuffer + offset + 0) = (u_a.base >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (u_a.base >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (u_a.base >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (u_a.base >> (8 * 3)) & 0xFF;
*(outbuffer + offset + 4) = (u_a.base >> (8 * 4)) & 0xFF;
*(outbuffer + offset + 5) = (u_a.base >> (8 * 5)) & 0xFF;
*(outbuffer + offset + 6) = (u_a.base >> (8 * 6)) & 0xFF;
*(outbuffer + offset + 7) = (u_a.base >> (8 * 7)) & 0xFF;
offset += sizeof(this->a);
union {
int64_t real;
uint64_t base;
} u_b;
u_b.real = this->b;
*(outbuffer + offset + 0) = (u_b.base >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (u_b.base >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (u_b.base >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (u_b.base >> (8 * 3)) & 0xFF;
*(outbuffer + offset + 4) = (u_b.base >> (8 * 4)) & 0xFF;
*(outbuffer + offset + 5) = (u_b.base >> (8 * 5)) & 0xFF;
*(outbuffer + offset + 6) = (u_b.base >> (8 * 6)) & 0xFF;
*(outbuffer + offset + 7) = (u_b.base >> (8 * 7)) & 0xFF;
offset += sizeof(this->b);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
union {
int64_t real;
uint64_t base;
} u_a;
u_a.base = 0;
u_a.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0);
u_a.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1);
u_a.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2);
u_a.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3);
u_a.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4);
u_a.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5);
u_a.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6);
u_a.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7);
this->a = u_a.real;
offset += sizeof(this->a);
union {
int64_t real;
uint64_t base;
} u_b;
u_b.base = 0;
u_b.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0);
u_b.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1);
u_b.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2);
u_b.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3);
u_b.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4);
u_b.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5);
u_b.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6);
u_b.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7);
this->b = u_b.real;
offset += sizeof(this->b);
return offset;
}
const char * getType(){ return TWOINTS; };
const char * getMD5(){ return "36d09b846be0b371c5f190354dd3153e"; };
};
class TwoIntsResponse : public ros::Msg
{
public:
typedef int64_t _sum_type;
_sum_type sum;
TwoIntsResponse():
sum(0)
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
union {
int64_t real;
uint64_t base;
} u_sum;
u_sum.real = this->sum;
*(outbuffer + offset + 0) = (u_sum.base >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (u_sum.base >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (u_sum.base >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (u_sum.base >> (8 * 3)) & 0xFF;
*(outbuffer + offset + 4) = (u_sum.base >> (8 * 4)) & 0xFF;
*(outbuffer + offset + 5) = (u_sum.base >> (8 * 5)) & 0xFF;
*(outbuffer + offset + 6) = (u_sum.base >> (8 * 6)) & 0xFF;
*(outbuffer + offset + 7) = (u_sum.base >> (8 * 7)) & 0xFF;
offset += sizeof(this->sum);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
union {
int64_t real;
uint64_t base;
} u_sum;
u_sum.base = 0;
u_sum.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0);
u_sum.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1);
u_sum.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2);
u_sum.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3);
u_sum.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4);
u_sum.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5);
u_sum.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6);
u_sum.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7);
this->sum = u_sum.real;
offset += sizeof(this->sum);
return offset;
}
const char * getType(){ return TWOINTS; };
const char * getMD5(){ return "b88405221c77b1878a3cbbfff53428d7"; };
};
class TwoInts {
public:
typedef TwoIntsRequest Request;
typedef TwoIntsResponse Response;
};
}
#endif
| 5,536 | C | 32.155688 | 72 | 0.481575 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/geometry_msgs/PointStamped.h | #ifndef _ROS_geometry_msgs_PointStamped_h
#define _ROS_geometry_msgs_PointStamped_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "std_msgs/Header.h"
#include "geometry_msgs/Point.h"
namespace geometry_msgs
{
class PointStamped : public ros::Msg
{
public:
typedef std_msgs::Header _header_type;
_header_type header;
typedef geometry_msgs::Point _point_type;
_point_type point;
PointStamped():
header(),
point()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->header.serialize(outbuffer + offset);
offset += this->point.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->header.deserialize(inbuffer + offset);
offset += this->point.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "geometry_msgs/PointStamped"; };
const char * getMD5(){ return "c63aecb41bfdfd6b7e1fac37c7cbe7bf"; };
};
}
#endif
| 1,139 | C | 21.352941 | 72 | 0.646181 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/geometry_msgs/Quaternion.h | #ifndef _ROS_geometry_msgs_Quaternion_h
#define _ROS_geometry_msgs_Quaternion_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
namespace geometry_msgs
{
class Quaternion : public ros::Msg
{
public:
typedef double _x_type;
_x_type x;
typedef double _y_type;
_y_type y;
typedef double _z_type;
_z_type z;
typedef double _w_type;
_w_type w;
Quaternion():
x(0),
y(0),
z(0),
w(0)
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
union {
double real;
uint64_t base;
} u_x;
u_x.real = this->x;
*(outbuffer + offset + 0) = (u_x.base >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (u_x.base >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (u_x.base >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (u_x.base >> (8 * 3)) & 0xFF;
*(outbuffer + offset + 4) = (u_x.base >> (8 * 4)) & 0xFF;
*(outbuffer + offset + 5) = (u_x.base >> (8 * 5)) & 0xFF;
*(outbuffer + offset + 6) = (u_x.base >> (8 * 6)) & 0xFF;
*(outbuffer + offset + 7) = (u_x.base >> (8 * 7)) & 0xFF;
offset += sizeof(this->x);
union {
double real;
uint64_t base;
} u_y;
u_y.real = this->y;
*(outbuffer + offset + 0) = (u_y.base >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (u_y.base >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (u_y.base >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (u_y.base >> (8 * 3)) & 0xFF;
*(outbuffer + offset + 4) = (u_y.base >> (8 * 4)) & 0xFF;
*(outbuffer + offset + 5) = (u_y.base >> (8 * 5)) & 0xFF;
*(outbuffer + offset + 6) = (u_y.base >> (8 * 6)) & 0xFF;
*(outbuffer + offset + 7) = (u_y.base >> (8 * 7)) & 0xFF;
offset += sizeof(this->y);
union {
double real;
uint64_t base;
} u_z;
u_z.real = this->z;
*(outbuffer + offset + 0) = (u_z.base >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (u_z.base >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (u_z.base >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (u_z.base >> (8 * 3)) & 0xFF;
*(outbuffer + offset + 4) = (u_z.base >> (8 * 4)) & 0xFF;
*(outbuffer + offset + 5) = (u_z.base >> (8 * 5)) & 0xFF;
*(outbuffer + offset + 6) = (u_z.base >> (8 * 6)) & 0xFF;
*(outbuffer + offset + 7) = (u_z.base >> (8 * 7)) & 0xFF;
offset += sizeof(this->z);
union {
double real;
uint64_t base;
} u_w;
u_w.real = this->w;
*(outbuffer + offset + 0) = (u_w.base >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (u_w.base >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (u_w.base >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (u_w.base >> (8 * 3)) & 0xFF;
*(outbuffer + offset + 4) = (u_w.base >> (8 * 4)) & 0xFF;
*(outbuffer + offset + 5) = (u_w.base >> (8 * 5)) & 0xFF;
*(outbuffer + offset + 6) = (u_w.base >> (8 * 6)) & 0xFF;
*(outbuffer + offset + 7) = (u_w.base >> (8 * 7)) & 0xFF;
offset += sizeof(this->w);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
union {
double real;
uint64_t base;
} u_x;
u_x.base = 0;
u_x.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0);
u_x.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1);
u_x.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2);
u_x.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3);
u_x.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4);
u_x.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5);
u_x.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6);
u_x.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7);
this->x = u_x.real;
offset += sizeof(this->x);
union {
double real;
uint64_t base;
} u_y;
u_y.base = 0;
u_y.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0);
u_y.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1);
u_y.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2);
u_y.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3);
u_y.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4);
u_y.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5);
u_y.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6);
u_y.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7);
this->y = u_y.real;
offset += sizeof(this->y);
union {
double real;
uint64_t base;
} u_z;
u_z.base = 0;
u_z.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0);
u_z.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1);
u_z.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2);
u_z.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3);
u_z.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4);
u_z.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5);
u_z.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6);
u_z.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7);
this->z = u_z.real;
offset += sizeof(this->z);
union {
double real;
uint64_t base;
} u_w;
u_w.base = 0;
u_w.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0);
u_w.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1);
u_w.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2);
u_w.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3);
u_w.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4);
u_w.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5);
u_w.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6);
u_w.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7);
this->w = u_w.real;
offset += sizeof(this->w);
return offset;
}
const char * getType(){ return "geometry_msgs/Quaternion"; };
const char * getMD5(){ return "a779879fadf0160734f906b8c19c7004"; };
};
}
#endif
| 6,295 | C | 36.700599 | 72 | 0.453058 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/geometry_msgs/Accel.h | #ifndef _ROS_geometry_msgs_Accel_h
#define _ROS_geometry_msgs_Accel_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "geometry_msgs/Vector3.h"
namespace geometry_msgs
{
class Accel : public ros::Msg
{
public:
typedef geometry_msgs::Vector3 _linear_type;
_linear_type linear;
typedef geometry_msgs::Vector3 _angular_type;
_angular_type angular;
Accel():
linear(),
angular()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->linear.serialize(outbuffer + offset);
offset += this->angular.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->linear.deserialize(inbuffer + offset);
offset += this->angular.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "geometry_msgs/Accel"; };
const char * getMD5(){ return "9f195f881246fdfa2798d1d3eebca84a"; };
};
}
#endif
| 1,097 | C | 20.96 | 72 | 0.639927 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/geometry_msgs/PoseWithCovariance.h | #ifndef _ROS_geometry_msgs_PoseWithCovariance_h
#define _ROS_geometry_msgs_PoseWithCovariance_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "geometry_msgs/Pose.h"
namespace geometry_msgs
{
class PoseWithCovariance : public ros::Msg
{
public:
typedef geometry_msgs::Pose _pose_type;
_pose_type pose;
double covariance[36];
PoseWithCovariance():
pose(),
covariance()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->pose.serialize(outbuffer + offset);
for( uint32_t i = 0; i < 36; i++){
union {
double real;
uint64_t base;
} u_covariancei;
u_covariancei.real = this->covariance[i];
*(outbuffer + offset + 0) = (u_covariancei.base >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (u_covariancei.base >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (u_covariancei.base >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (u_covariancei.base >> (8 * 3)) & 0xFF;
*(outbuffer + offset + 4) = (u_covariancei.base >> (8 * 4)) & 0xFF;
*(outbuffer + offset + 5) = (u_covariancei.base >> (8 * 5)) & 0xFF;
*(outbuffer + offset + 6) = (u_covariancei.base >> (8 * 6)) & 0xFF;
*(outbuffer + offset + 7) = (u_covariancei.base >> (8 * 7)) & 0xFF;
offset += sizeof(this->covariance[i]);
}
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->pose.deserialize(inbuffer + offset);
for( uint32_t i = 0; i < 36; i++){
union {
double real;
uint64_t base;
} u_covariancei;
u_covariancei.base = 0;
u_covariancei.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0);
u_covariancei.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1);
u_covariancei.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2);
u_covariancei.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3);
u_covariancei.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4);
u_covariancei.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5);
u_covariancei.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6);
u_covariancei.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7);
this->covariance[i] = u_covariancei.real;
offset += sizeof(this->covariance[i]);
}
return offset;
}
const char * getType(){ return "geometry_msgs/PoseWithCovariance"; };
const char * getMD5(){ return "c23e848cf1b7533a8d7c259073a97e6f"; };
};
}
#endif
| 2,680 | C | 32.5125 | 79 | 0.558582 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/geometry_msgs/PoseArray.h | #ifndef _ROS_geometry_msgs_PoseArray_h
#define _ROS_geometry_msgs_PoseArray_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "std_msgs/Header.h"
#include "geometry_msgs/Pose.h"
namespace geometry_msgs
{
class PoseArray : public ros::Msg
{
public:
typedef std_msgs::Header _header_type;
_header_type header;
uint32_t poses_length;
typedef geometry_msgs::Pose _poses_type;
_poses_type st_poses;
_poses_type * poses;
PoseArray():
header(),
poses_length(0), poses(NULL)
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->header.serialize(outbuffer + offset);
*(outbuffer + offset + 0) = (this->poses_length >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (this->poses_length >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (this->poses_length >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (this->poses_length >> (8 * 3)) & 0xFF;
offset += sizeof(this->poses_length);
for( uint32_t i = 0; i < poses_length; i++){
offset += this->poses[i].serialize(outbuffer + offset);
}
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->header.deserialize(inbuffer + offset);
uint32_t poses_lengthT = ((uint32_t) (*(inbuffer + offset)));
poses_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1);
poses_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2);
poses_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3);
offset += sizeof(this->poses_length);
if(poses_lengthT > poses_length)
this->poses = (geometry_msgs::Pose*)realloc(this->poses, poses_lengthT * sizeof(geometry_msgs::Pose));
poses_length = poses_lengthT;
for( uint32_t i = 0; i < poses_length; i++){
offset += this->st_poses.deserialize(inbuffer + offset);
memcpy( &(this->poses[i]), &(this->st_poses), sizeof(geometry_msgs::Pose));
}
return offset;
}
const char * getType(){ return "geometry_msgs/PoseArray"; };
const char * getMD5(){ return "916c28c5764443f268b296bb671b9d97"; };
};
}
#endif
| 2,277 | C | 31.084507 | 110 | 0.59025 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/geometry_msgs/Wrench.h | #ifndef _ROS_geometry_msgs_Wrench_h
#define _ROS_geometry_msgs_Wrench_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "geometry_msgs/Vector3.h"
namespace geometry_msgs
{
class Wrench : public ros::Msg
{
public:
typedef geometry_msgs::Vector3 _force_type;
_force_type force;
typedef geometry_msgs::Vector3 _torque_type;
_torque_type torque;
Wrench():
force(),
torque()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->force.serialize(outbuffer + offset);
offset += this->torque.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->force.deserialize(inbuffer + offset);
offset += this->torque.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "geometry_msgs/Wrench"; };
const char * getMD5(){ return "4f539cf138b23283b520fd271b567936"; };
};
}
#endif
| 1,090 | C | 20.82 | 72 | 0.637615 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/geometry_msgs/TwistWithCovarianceStamped.h | #ifndef _ROS_geometry_msgs_TwistWithCovarianceStamped_h
#define _ROS_geometry_msgs_TwistWithCovarianceStamped_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "std_msgs/Header.h"
#include "geometry_msgs/TwistWithCovariance.h"
namespace geometry_msgs
{
class TwistWithCovarianceStamped : public ros::Msg
{
public:
typedef std_msgs::Header _header_type;
_header_type header;
typedef geometry_msgs::TwistWithCovariance _twist_type;
_twist_type twist;
TwistWithCovarianceStamped():
header(),
twist()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->header.serialize(outbuffer + offset);
offset += this->twist.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->header.deserialize(inbuffer + offset);
offset += this->twist.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "geometry_msgs/TwistWithCovarianceStamped"; };
const char * getMD5(){ return "8927a1a12fb2607ceea095b2dc440a96"; };
};
}
#endif
| 1,237 | C | 23.274509 | 81 | 0.674212 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/geometry_msgs/Polygon.h | #ifndef _ROS_geometry_msgs_Polygon_h
#define _ROS_geometry_msgs_Polygon_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "geometry_msgs/Point32.h"
namespace geometry_msgs
{
class Polygon : public ros::Msg
{
public:
uint32_t points_length;
typedef geometry_msgs::Point32 _points_type;
_points_type st_points;
_points_type * points;
Polygon():
points_length(0), points(NULL)
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
*(outbuffer + offset + 0) = (this->points_length >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (this->points_length >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (this->points_length >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (this->points_length >> (8 * 3)) & 0xFF;
offset += sizeof(this->points_length);
for( uint32_t i = 0; i < points_length; i++){
offset += this->points[i].serialize(outbuffer + offset);
}
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
uint32_t points_lengthT = ((uint32_t) (*(inbuffer + offset)));
points_lengthT |= ((uint32_t) (*(inbuffer + offset + 1))) << (8 * 1);
points_lengthT |= ((uint32_t) (*(inbuffer + offset + 2))) << (8 * 2);
points_lengthT |= ((uint32_t) (*(inbuffer + offset + 3))) << (8 * 3);
offset += sizeof(this->points_length);
if(points_lengthT > points_length)
this->points = (geometry_msgs::Point32*)realloc(this->points, points_lengthT * sizeof(geometry_msgs::Point32));
points_length = points_lengthT;
for( uint32_t i = 0; i < points_length; i++){
offset += this->st_points.deserialize(inbuffer + offset);
memcpy( &(this->points[i]), &(this->st_points), sizeof(geometry_msgs::Point32));
}
return offset;
}
const char * getType(){ return "geometry_msgs/Polygon"; };
const char * getMD5(){ return "cd60a26494a087f577976f0329fa120e"; };
};
}
#endif
| 2,075 | C | 30.938461 | 119 | 0.590843 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/geometry_msgs/WrenchStamped.h | #ifndef _ROS_geometry_msgs_WrenchStamped_h
#define _ROS_geometry_msgs_WrenchStamped_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "std_msgs/Header.h"
#include "geometry_msgs/Wrench.h"
namespace geometry_msgs
{
class WrenchStamped : public ros::Msg
{
public:
typedef std_msgs::Header _header_type;
_header_type header;
typedef geometry_msgs::Wrench _wrench_type;
_wrench_type wrench;
WrenchStamped():
header(),
wrench()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->header.serialize(outbuffer + offset);
offset += this->wrench.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->header.deserialize(inbuffer + offset);
offset += this->wrench.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "geometry_msgs/WrenchStamped"; };
const char * getMD5(){ return "d78d3cb249ce23087ade7e7d0c40cfa7"; };
};
}
#endif
| 1,152 | C | 21.607843 | 72 | 0.650174 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/geometry_msgs/PoseStamped.h | #ifndef _ROS_geometry_msgs_PoseStamped_h
#define _ROS_geometry_msgs_PoseStamped_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "std_msgs/Header.h"
#include "geometry_msgs/Pose.h"
namespace geometry_msgs
{
class PoseStamped : public ros::Msg
{
public:
typedef std_msgs::Header _header_type;
_header_type header;
typedef geometry_msgs::Pose _pose_type;
_pose_type pose;
PoseStamped():
header(),
pose()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->header.serialize(outbuffer + offset);
offset += this->pose.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->header.deserialize(inbuffer + offset);
offset += this->pose.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "geometry_msgs/PoseStamped"; };
const char * getMD5(){ return "d3812c3cbc69362b77dc0b19b345f8f5"; };
};
}
#endif
| 1,126 | C | 21.098039 | 72 | 0.642096 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/geometry_msgs/AccelWithCovariance.h | #ifndef _ROS_geometry_msgs_AccelWithCovariance_h
#define _ROS_geometry_msgs_AccelWithCovariance_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "geometry_msgs/Accel.h"
namespace geometry_msgs
{
class AccelWithCovariance : public ros::Msg
{
public:
typedef geometry_msgs::Accel _accel_type;
_accel_type accel;
double covariance[36];
AccelWithCovariance():
accel(),
covariance()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->accel.serialize(outbuffer + offset);
for( uint32_t i = 0; i < 36; i++){
union {
double real;
uint64_t base;
} u_covariancei;
u_covariancei.real = this->covariance[i];
*(outbuffer + offset + 0) = (u_covariancei.base >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (u_covariancei.base >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (u_covariancei.base >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (u_covariancei.base >> (8 * 3)) & 0xFF;
*(outbuffer + offset + 4) = (u_covariancei.base >> (8 * 4)) & 0xFF;
*(outbuffer + offset + 5) = (u_covariancei.base >> (8 * 5)) & 0xFF;
*(outbuffer + offset + 6) = (u_covariancei.base >> (8 * 6)) & 0xFF;
*(outbuffer + offset + 7) = (u_covariancei.base >> (8 * 7)) & 0xFF;
offset += sizeof(this->covariance[i]);
}
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->accel.deserialize(inbuffer + offset);
for( uint32_t i = 0; i < 36; i++){
union {
double real;
uint64_t base;
} u_covariancei;
u_covariancei.base = 0;
u_covariancei.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0);
u_covariancei.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1);
u_covariancei.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2);
u_covariancei.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3);
u_covariancei.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4);
u_covariancei.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5);
u_covariancei.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6);
u_covariancei.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7);
this->covariance[i] = u_covariancei.real;
offset += sizeof(this->covariance[i]);
}
return offset;
}
const char * getType(){ return "geometry_msgs/AccelWithCovariance"; };
const char * getMD5(){ return "ad5a718d699c6be72a02b8d6a139f334"; };
};
}
#endif
| 2,693 | C | 32.675 | 79 | 0.560713 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/geometry_msgs/Transform.h | #ifndef _ROS_geometry_msgs_Transform_h
#define _ROS_geometry_msgs_Transform_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "geometry_msgs/Vector3.h"
#include "geometry_msgs/Quaternion.h"
namespace geometry_msgs
{
class Transform : public ros::Msg
{
public:
typedef geometry_msgs::Vector3 _translation_type;
_translation_type translation;
typedef geometry_msgs::Quaternion _rotation_type;
_rotation_type rotation;
Transform():
translation(),
rotation()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->translation.serialize(outbuffer + offset);
offset += this->rotation.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->translation.deserialize(inbuffer + offset);
offset += this->rotation.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "geometry_msgs/Transform"; };
const char * getMD5(){ return "ac9eff44abf714214112b05d54a3cf9b"; };
};
}
#endif
| 1,194 | C | 22.431372 | 72 | 0.662479 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/geometry_msgs/AccelWithCovarianceStamped.h | #ifndef _ROS_geometry_msgs_AccelWithCovarianceStamped_h
#define _ROS_geometry_msgs_AccelWithCovarianceStamped_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "std_msgs/Header.h"
#include "geometry_msgs/AccelWithCovariance.h"
namespace geometry_msgs
{
class AccelWithCovarianceStamped : public ros::Msg
{
public:
typedef std_msgs::Header _header_type;
_header_type header;
typedef geometry_msgs::AccelWithCovariance _accel_type;
_accel_type accel;
AccelWithCovarianceStamped():
header(),
accel()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->header.serialize(outbuffer + offset);
offset += this->accel.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->header.deserialize(inbuffer + offset);
offset += this->accel.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "geometry_msgs/AccelWithCovarianceStamped"; };
const char * getMD5(){ return "96adb295225031ec8d57fb4251b0a886"; };
};
}
#endif
| 1,237 | C | 23.274509 | 81 | 0.674212 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/geometry_msgs/Pose.h | #ifndef _ROS_geometry_msgs_Pose_h
#define _ROS_geometry_msgs_Pose_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "geometry_msgs/Point.h"
#include "geometry_msgs/Quaternion.h"
namespace geometry_msgs
{
class Pose : public ros::Msg
{
public:
typedef geometry_msgs::Point _position_type;
_position_type position;
typedef geometry_msgs::Quaternion _orientation_type;
_orientation_type orientation;
Pose():
position(),
orientation()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->position.serialize(outbuffer + offset);
offset += this->orientation.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->position.deserialize(inbuffer + offset);
offset += this->orientation.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "geometry_msgs/Pose"; };
const char * getMD5(){ return "e45d45a5a1ce597b249e23fb30fc871f"; };
};
}
#endif
| 1,165 | C | 21.862745 | 72 | 0.654077 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/geometry_msgs/PoseWithCovarianceStamped.h | #ifndef _ROS_geometry_msgs_PoseWithCovarianceStamped_h
#define _ROS_geometry_msgs_PoseWithCovarianceStamped_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "std_msgs/Header.h"
#include "geometry_msgs/PoseWithCovariance.h"
namespace geometry_msgs
{
class PoseWithCovarianceStamped : public ros::Msg
{
public:
typedef std_msgs::Header _header_type;
_header_type header;
typedef geometry_msgs::PoseWithCovariance _pose_type;
_pose_type pose;
PoseWithCovarianceStamped():
header(),
pose()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->header.serialize(outbuffer + offset);
offset += this->pose.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->header.deserialize(inbuffer + offset);
offset += this->pose.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "geometry_msgs/PoseWithCovarianceStamped"; };
const char * getMD5(){ return "953b798c0f514ff060a53a3498ce6246"; };
};
}
#endif
| 1,224 | C | 23.019607 | 80 | 0.670752 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/geometry_msgs/Vector3Stamped.h | #ifndef _ROS_geometry_msgs_Vector3Stamped_h
#define _ROS_geometry_msgs_Vector3Stamped_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "std_msgs/Header.h"
#include "geometry_msgs/Vector3.h"
namespace geometry_msgs
{
class Vector3Stamped : public ros::Msg
{
public:
typedef std_msgs::Header _header_type;
_header_type header;
typedef geometry_msgs::Vector3 _vector_type;
_vector_type vector;
Vector3Stamped():
header(),
vector()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->header.serialize(outbuffer + offset);
offset += this->vector.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->header.deserialize(inbuffer + offset);
offset += this->vector.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "geometry_msgs/Vector3Stamped"; };
const char * getMD5(){ return "7b324c7325e683bf02a9b14b01090ec7"; };
};
}
#endif
| 1,159 | C | 21.745098 | 72 | 0.652286 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/geometry_msgs/TransformStamped.h | #ifndef _ROS_geometry_msgs_TransformStamped_h
#define _ROS_geometry_msgs_TransformStamped_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "std_msgs/Header.h"
#include "geometry_msgs/Transform.h"
namespace geometry_msgs
{
class TransformStamped : public ros::Msg
{
public:
typedef std_msgs::Header _header_type;
_header_type header;
typedef const char* _child_frame_id_type;
_child_frame_id_type child_frame_id;
typedef geometry_msgs::Transform _transform_type;
_transform_type transform;
TransformStamped():
header(),
child_frame_id(""),
transform()
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
offset += this->header.serialize(outbuffer + offset);
uint32_t length_child_frame_id = strlen(this->child_frame_id);
varToArr(outbuffer + offset, length_child_frame_id);
offset += 4;
memcpy(outbuffer + offset, this->child_frame_id, length_child_frame_id);
offset += length_child_frame_id;
offset += this->transform.serialize(outbuffer + offset);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
offset += this->header.deserialize(inbuffer + offset);
uint32_t length_child_frame_id;
arrToVar(length_child_frame_id, (inbuffer + offset));
offset += 4;
for(unsigned int k= offset; k< offset+length_child_frame_id; ++k){
inbuffer[k-1]=inbuffer[k];
}
inbuffer[offset+length_child_frame_id-1]=0;
this->child_frame_id = (char *)(inbuffer + offset-1);
offset += length_child_frame_id;
offset += this->transform.deserialize(inbuffer + offset);
return offset;
}
const char * getType(){ return "geometry_msgs/TransformStamped"; };
const char * getMD5(){ return "b5764a33bfeb3588febc2682852579b0"; };
};
}
#endif
| 1,957 | C | 27.794117 | 78 | 0.646398 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/geometry_msgs/Inertia.h | #ifndef _ROS_geometry_msgs_Inertia_h
#define _ROS_geometry_msgs_Inertia_h
#include <stdint.h>
#include <string.h>
#include <stdlib.h>
#include "ros/msg.h"
#include "geometry_msgs/Vector3.h"
namespace geometry_msgs
{
class Inertia : public ros::Msg
{
public:
typedef double _m_type;
_m_type m;
typedef geometry_msgs::Vector3 _com_type;
_com_type com;
typedef double _ixx_type;
_ixx_type ixx;
typedef double _ixy_type;
_ixy_type ixy;
typedef double _ixz_type;
_ixz_type ixz;
typedef double _iyy_type;
_iyy_type iyy;
typedef double _iyz_type;
_iyz_type iyz;
typedef double _izz_type;
_izz_type izz;
Inertia():
m(0),
com(),
ixx(0),
ixy(0),
ixz(0),
iyy(0),
iyz(0),
izz(0)
{
}
virtual int serialize(unsigned char *outbuffer) const
{
int offset = 0;
union {
double real;
uint64_t base;
} u_m;
u_m.real = this->m;
*(outbuffer + offset + 0) = (u_m.base >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (u_m.base >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (u_m.base >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (u_m.base >> (8 * 3)) & 0xFF;
*(outbuffer + offset + 4) = (u_m.base >> (8 * 4)) & 0xFF;
*(outbuffer + offset + 5) = (u_m.base >> (8 * 5)) & 0xFF;
*(outbuffer + offset + 6) = (u_m.base >> (8 * 6)) & 0xFF;
*(outbuffer + offset + 7) = (u_m.base >> (8 * 7)) & 0xFF;
offset += sizeof(this->m);
offset += this->com.serialize(outbuffer + offset);
union {
double real;
uint64_t base;
} u_ixx;
u_ixx.real = this->ixx;
*(outbuffer + offset + 0) = (u_ixx.base >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (u_ixx.base >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (u_ixx.base >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (u_ixx.base >> (8 * 3)) & 0xFF;
*(outbuffer + offset + 4) = (u_ixx.base >> (8 * 4)) & 0xFF;
*(outbuffer + offset + 5) = (u_ixx.base >> (8 * 5)) & 0xFF;
*(outbuffer + offset + 6) = (u_ixx.base >> (8 * 6)) & 0xFF;
*(outbuffer + offset + 7) = (u_ixx.base >> (8 * 7)) & 0xFF;
offset += sizeof(this->ixx);
union {
double real;
uint64_t base;
} u_ixy;
u_ixy.real = this->ixy;
*(outbuffer + offset + 0) = (u_ixy.base >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (u_ixy.base >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (u_ixy.base >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (u_ixy.base >> (8 * 3)) & 0xFF;
*(outbuffer + offset + 4) = (u_ixy.base >> (8 * 4)) & 0xFF;
*(outbuffer + offset + 5) = (u_ixy.base >> (8 * 5)) & 0xFF;
*(outbuffer + offset + 6) = (u_ixy.base >> (8 * 6)) & 0xFF;
*(outbuffer + offset + 7) = (u_ixy.base >> (8 * 7)) & 0xFF;
offset += sizeof(this->ixy);
union {
double real;
uint64_t base;
} u_ixz;
u_ixz.real = this->ixz;
*(outbuffer + offset + 0) = (u_ixz.base >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (u_ixz.base >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (u_ixz.base >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (u_ixz.base >> (8 * 3)) & 0xFF;
*(outbuffer + offset + 4) = (u_ixz.base >> (8 * 4)) & 0xFF;
*(outbuffer + offset + 5) = (u_ixz.base >> (8 * 5)) & 0xFF;
*(outbuffer + offset + 6) = (u_ixz.base >> (8 * 6)) & 0xFF;
*(outbuffer + offset + 7) = (u_ixz.base >> (8 * 7)) & 0xFF;
offset += sizeof(this->ixz);
union {
double real;
uint64_t base;
} u_iyy;
u_iyy.real = this->iyy;
*(outbuffer + offset + 0) = (u_iyy.base >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (u_iyy.base >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (u_iyy.base >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (u_iyy.base >> (8 * 3)) & 0xFF;
*(outbuffer + offset + 4) = (u_iyy.base >> (8 * 4)) & 0xFF;
*(outbuffer + offset + 5) = (u_iyy.base >> (8 * 5)) & 0xFF;
*(outbuffer + offset + 6) = (u_iyy.base >> (8 * 6)) & 0xFF;
*(outbuffer + offset + 7) = (u_iyy.base >> (8 * 7)) & 0xFF;
offset += sizeof(this->iyy);
union {
double real;
uint64_t base;
} u_iyz;
u_iyz.real = this->iyz;
*(outbuffer + offset + 0) = (u_iyz.base >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (u_iyz.base >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (u_iyz.base >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (u_iyz.base >> (8 * 3)) & 0xFF;
*(outbuffer + offset + 4) = (u_iyz.base >> (8 * 4)) & 0xFF;
*(outbuffer + offset + 5) = (u_iyz.base >> (8 * 5)) & 0xFF;
*(outbuffer + offset + 6) = (u_iyz.base >> (8 * 6)) & 0xFF;
*(outbuffer + offset + 7) = (u_iyz.base >> (8 * 7)) & 0xFF;
offset += sizeof(this->iyz);
union {
double real;
uint64_t base;
} u_izz;
u_izz.real = this->izz;
*(outbuffer + offset + 0) = (u_izz.base >> (8 * 0)) & 0xFF;
*(outbuffer + offset + 1) = (u_izz.base >> (8 * 1)) & 0xFF;
*(outbuffer + offset + 2) = (u_izz.base >> (8 * 2)) & 0xFF;
*(outbuffer + offset + 3) = (u_izz.base >> (8 * 3)) & 0xFF;
*(outbuffer + offset + 4) = (u_izz.base >> (8 * 4)) & 0xFF;
*(outbuffer + offset + 5) = (u_izz.base >> (8 * 5)) & 0xFF;
*(outbuffer + offset + 6) = (u_izz.base >> (8 * 6)) & 0xFF;
*(outbuffer + offset + 7) = (u_izz.base >> (8 * 7)) & 0xFF;
offset += sizeof(this->izz);
return offset;
}
virtual int deserialize(unsigned char *inbuffer)
{
int offset = 0;
union {
double real;
uint64_t base;
} u_m;
u_m.base = 0;
u_m.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0);
u_m.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1);
u_m.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2);
u_m.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3);
u_m.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4);
u_m.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5);
u_m.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6);
u_m.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7);
this->m = u_m.real;
offset += sizeof(this->m);
offset += this->com.deserialize(inbuffer + offset);
union {
double real;
uint64_t base;
} u_ixx;
u_ixx.base = 0;
u_ixx.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0);
u_ixx.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1);
u_ixx.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2);
u_ixx.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3);
u_ixx.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4);
u_ixx.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5);
u_ixx.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6);
u_ixx.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7);
this->ixx = u_ixx.real;
offset += sizeof(this->ixx);
union {
double real;
uint64_t base;
} u_ixy;
u_ixy.base = 0;
u_ixy.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0);
u_ixy.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1);
u_ixy.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2);
u_ixy.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3);
u_ixy.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4);
u_ixy.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5);
u_ixy.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6);
u_ixy.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7);
this->ixy = u_ixy.real;
offset += sizeof(this->ixy);
union {
double real;
uint64_t base;
} u_ixz;
u_ixz.base = 0;
u_ixz.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0);
u_ixz.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1);
u_ixz.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2);
u_ixz.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3);
u_ixz.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4);
u_ixz.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5);
u_ixz.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6);
u_ixz.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7);
this->ixz = u_ixz.real;
offset += sizeof(this->ixz);
union {
double real;
uint64_t base;
} u_iyy;
u_iyy.base = 0;
u_iyy.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0);
u_iyy.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1);
u_iyy.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2);
u_iyy.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3);
u_iyy.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4);
u_iyy.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5);
u_iyy.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6);
u_iyy.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7);
this->iyy = u_iyy.real;
offset += sizeof(this->iyy);
union {
double real;
uint64_t base;
} u_iyz;
u_iyz.base = 0;
u_iyz.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0);
u_iyz.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1);
u_iyz.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2);
u_iyz.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3);
u_iyz.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4);
u_iyz.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5);
u_iyz.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6);
u_iyz.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7);
this->iyz = u_iyz.real;
offset += sizeof(this->iyz);
union {
double real;
uint64_t base;
} u_izz;
u_izz.base = 0;
u_izz.base |= ((uint64_t) (*(inbuffer + offset + 0))) << (8 * 0);
u_izz.base |= ((uint64_t) (*(inbuffer + offset + 1))) << (8 * 1);
u_izz.base |= ((uint64_t) (*(inbuffer + offset + 2))) << (8 * 2);
u_izz.base |= ((uint64_t) (*(inbuffer + offset + 3))) << (8 * 3);
u_izz.base |= ((uint64_t) (*(inbuffer + offset + 4))) << (8 * 4);
u_izz.base |= ((uint64_t) (*(inbuffer + offset + 5))) << (8 * 5);
u_izz.base |= ((uint64_t) (*(inbuffer + offset + 6))) << (8 * 6);
u_izz.base |= ((uint64_t) (*(inbuffer + offset + 7))) << (8 * 7);
this->izz = u_izz.real;
offset += sizeof(this->izz);
return offset;
}
const char * getType(){ return "geometry_msgs/Inertia"; };
const char * getMD5(){ return "1d26e4bb6c83ff141c5cf0d883c2b0fe"; };
};
}
#endif
| 11,093 | C | 40.241636 | 72 | 0.465158 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/ros/service_client.h | /*
* Software License Agreement (BSD License)
*
* Copyright (c) 2011, Willow Garage, Inc.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials provided
* with the distribution.
* * Neither the name of Willow Garage, Inc. nor the names of its
* contributors may be used to endorse or promote prducts derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
* FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
* COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
* BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
* LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN
* ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
#ifndef _ROS_SERVICE_CLIENT_H_
#define _ROS_SERVICE_CLIENT_H_
#include "rosserial_msgs/TopicInfo.h"
#include "ros/publisher.h"
#include "ros/subscriber.h"
namespace ros
{
template<typename MReq , typename MRes>
class ServiceClient : public Subscriber_
{
public:
ServiceClient(const char* topic_name) :
pub(topic_name, &req, rosserial_msgs::TopicInfo::ID_SERVICE_CLIENT + rosserial_msgs::TopicInfo::ID_PUBLISHER)
{
this->topic_ = topic_name;
this->waiting = true;
}
virtual void call(const MReq & request, MRes & response)
{
if (!pub.nh_->connected()) return;
ret = &response;
waiting = true;
pub.publish(&request);
while (waiting && pub.nh_->connected())
if (pub.nh_->spinOnce() < 0) break;
}
// these refer to the subscriber
virtual void callback(unsigned char *data)
{
ret->deserialize(data);
waiting = false;
}
virtual const char * getMsgType()
{
return this->resp.getType();
}
virtual const char * getMsgMD5()
{
return this->resp.getMD5();
}
virtual int getEndpointType()
{
return rosserial_msgs::TopicInfo::ID_SERVICE_CLIENT + rosserial_msgs::TopicInfo::ID_SUBSCRIBER;
}
MReq req;
MRes resp;
MRes * ret;
bool waiting;
Publisher pub;
};
}
#endif
| 2,907 | C | 29.291666 | 113 | 0.714482 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/ros/subscriber.h | /*
* Software License Agreement (BSD License)
*
* Copyright (c) 2011, Willow Garage, Inc.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials provided
* with the distribution.
* * Neither the name of Willow Garage, Inc. nor the names of its
* contributors may be used to endorse or promote prducts derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
* FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
* COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
* BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
* LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN
* ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
#ifndef ROS_SUBSCRIBER_H_
#define ROS_SUBSCRIBER_H_
#include "rosserial_msgs/TopicInfo.h"
namespace ros
{
/* Base class for objects subscribers. */
class Subscriber_
{
public:
virtual void callback(unsigned char *data) = 0;
virtual int getEndpointType() = 0;
// id_ is set by NodeHandle when we advertise
int id_;
virtual const char * getMsgType() = 0;
virtual const char * getMsgMD5() = 0;
const char * topic_;
};
/* Bound function subscriber. */
template<typename MsgT, typename ObjT = void>
class Subscriber: public Subscriber_
{
public:
typedef void(ObjT::*CallbackT)(const MsgT&);
MsgT msg;
Subscriber(const char * topic_name, CallbackT cb, ObjT* obj, int endpoint = rosserial_msgs::TopicInfo::ID_SUBSCRIBER) :
cb_(cb),
obj_(obj),
endpoint_(endpoint)
{
topic_ = topic_name;
};
virtual void callback(unsigned char* data)
{
msg.deserialize(data);
(obj_->*cb_)(msg);
}
virtual const char * getMsgType()
{
return this->msg.getType();
}
virtual const char * getMsgMD5()
{
return this->msg.getMD5();
}
virtual int getEndpointType()
{
return endpoint_;
}
private:
CallbackT cb_;
ObjT* obj_;
int endpoint_;
};
/* Standalone function subscriber. */
template<typename MsgT>
class Subscriber<MsgT, void>: public Subscriber_
{
public:
typedef void(*CallbackT)(const MsgT&);
MsgT msg;
Subscriber(const char * topic_name, CallbackT cb, int endpoint = rosserial_msgs::TopicInfo::ID_SUBSCRIBER) :
cb_(cb),
endpoint_(endpoint)
{
topic_ = topic_name;
};
virtual void callback(unsigned char* data)
{
msg.deserialize(data);
this->cb_(msg);
}
virtual const char * getMsgType()
{
return this->msg.getType();
}
virtual const char * getMsgMD5()
{
return this->msg.getMD5();
}
virtual int getEndpointType()
{
return endpoint_;
}
private:
CallbackT cb_;
int endpoint_;
};
}
#endif
| 3,579 | C | 24.390071 | 121 | 0.700754 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/ros/node_handle.h | /*
* Software License Agreement (BSD License)
*
* Copyright (c) 2011, Willow Garage, Inc.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials provided
* with the distribution.
* * Neither the name of Willow Garage, Inc. nor the names of its
* contributors may be used to endorse or promote prducts derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
* FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
* COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
* BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
* LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN
* ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
#ifndef ROS_NODE_HANDLE_H_
#define ROS_NODE_HANDLE_H_
#include <stdint.h>
#include "std_msgs/Time.h"
#include "rosserial_msgs/TopicInfo.h"
#include "rosserial_msgs/Log.h"
#include "rosserial_msgs/RequestParam.h"
#include "ros/msg.h"
namespace ros
{
class NodeHandleBase_
{
public:
virtual int publish(int id, const Msg* msg) = 0;
virtual int spinOnce() = 0;
virtual bool connected() = 0;
};
}
#include "ros/publisher.h"
#include "ros/subscriber.h"
#include "ros/service_server.h"
#include "ros/service_client.h"
namespace ros
{
const int SPIN_OK = 0;
const int SPIN_ERR = -1;
const int SPIN_TIMEOUT = -2;
const uint8_t SYNC_SECONDS = 5;
const uint8_t MODE_FIRST_FF = 0;
/*
* The second sync byte is a protocol version. It's value is 0xff for the first
* version of the rosserial protocol (used up to hydro), 0xfe for the second version
* (introduced in hydro), 0xfd for the next, and so on. Its purpose is to enable
* detection of mismatched protocol versions (e.g. hydro rosserial_python with groovy
* rosserial_arduino. It must be changed in both this file and in
* rosserial_python/src/rosserial_python/SerialClient.py
*/
const uint8_t MODE_PROTOCOL_VER = 1;
const uint8_t PROTOCOL_VER1 = 0xff; // through groovy
const uint8_t PROTOCOL_VER2 = 0xfe; // in hydro
const uint8_t PROTOCOL_VER = PROTOCOL_VER2;
const uint8_t MODE_SIZE_L = 2;
const uint8_t MODE_SIZE_H = 3;
const uint8_t MODE_SIZE_CHECKSUM = 4; // checksum for msg size received from size L and H
const uint8_t MODE_TOPIC_L = 5; // waiting for topic id
const uint8_t MODE_TOPIC_H = 6;
const uint8_t MODE_MESSAGE = 7;
const uint8_t MODE_MSG_CHECKSUM = 8; // checksum for msg and topic id
const uint8_t SERIAL_MSG_TIMEOUT = 20; // 20 milliseconds to recieve all of message data
using rosserial_msgs::TopicInfo;
/* Node Handle */
template<class Hardware,
int MAX_SUBSCRIBERS = 25,
int MAX_PUBLISHERS = 25,
int INPUT_SIZE = 512,
int OUTPUT_SIZE = 512>
class NodeHandle_ : public NodeHandleBase_
{
protected:
Hardware hardware_;
/* time used for syncing */
uint32_t rt_time;
/* used for computing current time */
uint32_t sec_offset, nsec_offset;
/* Spinonce maximum work timeout */
uint32_t spin_timeout_;
uint8_t message_in[INPUT_SIZE];
uint8_t message_out[OUTPUT_SIZE];
Publisher * publishers[MAX_PUBLISHERS];
Subscriber_ * subscribers[MAX_SUBSCRIBERS];
/*
* Setup Functions
*/
public:
NodeHandle_() : configured_(false)
{
for (unsigned int i = 0; i < MAX_PUBLISHERS; i++)
publishers[i] = 0;
for (unsigned int i = 0; i < MAX_SUBSCRIBERS; i++)
subscribers[i] = 0;
for (unsigned int i = 0; i < INPUT_SIZE; i++)
message_in[i] = 0;
for (unsigned int i = 0; i < OUTPUT_SIZE; i++)
message_out[i] = 0;
req_param_resp.ints_length = 0;
req_param_resp.ints = NULL;
req_param_resp.floats_length = 0;
req_param_resp.floats = NULL;
req_param_resp.ints_length = 0;
req_param_resp.ints = NULL;
spin_timeout_ = 0;
}
Hardware* getHardware()
{
return &hardware_;
}
/* Start serial, initialize buffers */
void initNode()
{
hardware_.init();
mode_ = 0;
bytes_ = 0;
index_ = 0;
topic_ = 0;
};
/* Start a named port, which may be network server IP, initialize buffers */
void initNode(char *portName)
{
hardware_.init(portName);
mode_ = 0;
bytes_ = 0;
index_ = 0;
topic_ = 0;
};
/**
* @brief Sets the maximum time in millisconds that spinOnce() can work.
* This will not effect the processing of the buffer, as spinOnce processes
* one byte at a time. It simply sets the maximum time that one call can
* process for. You can choose to clear the buffer if that is beneficial if
* SPIN_TIMEOUT is returned from spinOnce().
* @param timeout The timeout in milliseconds that spinOnce will function.
*/
void setSpinTimeout(const uint32_t& timeout)
{
spin_timeout_ = timeout;
}
protected:
//State machine variables for spinOnce
int mode_;
int bytes_;
int topic_;
int index_;
int checksum_;
bool configured_;
/* used for syncing the time */
uint32_t last_sync_time;
uint32_t last_sync_receive_time;
uint32_t last_msg_timeout_time;
public:
/* This function goes in your loop() function, it handles
* serial input and callbacks for subscribers.
*/
virtual int spinOnce()
{
/* restart if timed out */
uint32_t c_time = hardware_.time();
if ((c_time - last_sync_receive_time) > (SYNC_SECONDS * 2200))
{
configured_ = false;
}
/* reset if message has timed out */
if (mode_ != MODE_FIRST_FF)
{
if (c_time > last_msg_timeout_time)
{
mode_ = MODE_FIRST_FF;
}
}
/* while available buffer, read data */
while (true)
{
// If a timeout has been specified, check how long spinOnce has been running.
if (spin_timeout_ > 0)
{
// If the maximum processing timeout has been exceeded, exit with error.
// The next spinOnce can continue where it left off, or optionally
// based on the application in use, the hardware buffer could be flushed
// and start fresh.
if ((hardware_.time() - c_time) > spin_timeout_)
{
// Exit the spin, processing timeout exceeded.
return SPIN_TIMEOUT;
}
}
int data = hardware_.read();
if (data < 0)
break;
checksum_ += data;
if (mode_ == MODE_MESSAGE) /* message data being recieved */
{
message_in[index_++] = data;
bytes_--;
if (bytes_ == 0) /* is message complete? if so, checksum */
mode_ = MODE_MSG_CHECKSUM;
}
else if (mode_ == MODE_FIRST_FF)
{
if (data == 0xff)
{
mode_++;
last_msg_timeout_time = c_time + SERIAL_MSG_TIMEOUT;
}
else if (hardware_.time() - c_time > (SYNC_SECONDS * 1000))
{
/* We have been stuck in spinOnce too long, return error */
configured_ = false;
return SPIN_TIMEOUT;
}
}
else if (mode_ == MODE_PROTOCOL_VER)
{
if (data == PROTOCOL_VER)
{
mode_++;
}
else
{
mode_ = MODE_FIRST_FF;
if (configured_ == false)
requestSyncTime(); /* send a msg back showing our protocol version */
}
}
else if (mode_ == MODE_SIZE_L) /* bottom half of message size */
{
bytes_ = data;
index_ = 0;
mode_++;
checksum_ = data; /* first byte for calculating size checksum */
}
else if (mode_ == MODE_SIZE_H) /* top half of message size */
{
bytes_ += data << 8;
mode_++;
}
else if (mode_ == MODE_SIZE_CHECKSUM)
{
if ((checksum_ % 256) == 255)
mode_++;
else
mode_ = MODE_FIRST_FF; /* Abandon the frame if the msg len is wrong */
}
else if (mode_ == MODE_TOPIC_L) /* bottom half of topic id */
{
topic_ = data;
mode_++;
checksum_ = data; /* first byte included in checksum */
}
else if (mode_ == MODE_TOPIC_H) /* top half of topic id */
{
topic_ += data << 8;
mode_ = MODE_MESSAGE;
if (bytes_ == 0)
mode_ = MODE_MSG_CHECKSUM;
}
else if (mode_ == MODE_MSG_CHECKSUM) /* do checksum */
{
mode_ = MODE_FIRST_FF;
if ((checksum_ % 256) == 255)
{
if (topic_ == TopicInfo::ID_PUBLISHER)
{
requestSyncTime();
negotiateTopics();
last_sync_time = c_time;
last_sync_receive_time = c_time;
return SPIN_ERR;
}
else if (topic_ == TopicInfo::ID_TIME)
{
syncTime(message_in);
}
else if (topic_ == TopicInfo::ID_PARAMETER_REQUEST)
{
req_param_resp.deserialize(message_in);
param_recieved = true;
}
else if (topic_ == TopicInfo::ID_TX_STOP)
{
configured_ = false;
}
else
{
if (subscribers[topic_ - 100])
subscribers[topic_ - 100]->callback(message_in);
}
}
}
}
/* occasionally sync time */
if (configured_ && ((c_time - last_sync_time) > (SYNC_SECONDS * 500)))
{
requestSyncTime();
last_sync_time = c_time;
}
return SPIN_OK;
}
/* Are we connected to the PC? */
virtual bool connected()
{
return configured_;
};
/********************************************************************
* Time functions
*/
void requestSyncTime()
{
std_msgs::Time t;
publish(TopicInfo::ID_TIME, &t);
rt_time = hardware_.time();
}
void syncTime(uint8_t * data)
{
std_msgs::Time t;
uint32_t offset = hardware_.time() - rt_time;
t.deserialize(data);
t.data.sec += offset / 1000;
t.data.nsec += (offset % 1000) * 1000000UL;
this->setNow(t.data);
last_sync_receive_time = hardware_.time();
}
Time now()
{
uint32_t ms = hardware_.time();
Time current_time;
current_time.sec = ms / 1000 + sec_offset;
current_time.nsec = (ms % 1000) * 1000000UL + nsec_offset;
normalizeSecNSec(current_time.sec, current_time.nsec);
return current_time;
}
void setNow(Time & new_now)
{
uint32_t ms = hardware_.time();
sec_offset = new_now.sec - ms / 1000 - 1;
nsec_offset = new_now.nsec - (ms % 1000) * 1000000UL + 1000000000UL;
normalizeSecNSec(sec_offset, nsec_offset);
}
/********************************************************************
* Topic Management
*/
/* Register a new publisher */
bool advertise(Publisher & p)
{
for (int i = 0; i < MAX_PUBLISHERS; i++)
{
if (publishers[i] == 0) // empty slot
{
publishers[i] = &p;
p.id_ = i + 100 + MAX_SUBSCRIBERS;
p.nh_ = this;
return true;
}
}
return false;
}
/* Register a new subscriber */
template<typename SubscriberT>
bool subscribe(SubscriberT& s)
{
for (int i = 0; i < MAX_SUBSCRIBERS; i++)
{
if (subscribers[i] == 0) // empty slot
{
subscribers[i] = static_cast<Subscriber_*>(&s);
s.id_ = i + 100;
return true;
}
}
return false;
}
/* Register a new Service Server */
template<typename MReq, typename MRes, typename ObjT>
bool advertiseService(ServiceServer<MReq, MRes, ObjT>& srv)
{
bool v = advertise(srv.pub);
for (int i = 0; i < MAX_SUBSCRIBERS; i++)
{
if (subscribers[i] == 0) // empty slot
{
subscribers[i] = static_cast<Subscriber_*>(&srv);
srv.id_ = i + 100;
return v;
}
}
return false;
}
/* Register a new Service Client */
template<typename MReq, typename MRes>
bool serviceClient(ServiceClient<MReq, MRes>& srv)
{
bool v = advertise(srv.pub);
for (int i = 0; i < MAX_SUBSCRIBERS; i++)
{
if (subscribers[i] == 0) // empty slot
{
subscribers[i] = static_cast<Subscriber_*>(&srv);
srv.id_ = i + 100;
return v;
}
}
return false;
}
void negotiateTopics()
{
rosserial_msgs::TopicInfo ti;
int i;
for (i = 0; i < MAX_PUBLISHERS; i++)
{
if (publishers[i] != 0) // non-empty slot
{
ti.topic_id = publishers[i]->id_;
ti.topic_name = (char *) publishers[i]->topic_;
ti.message_type = (char *) publishers[i]->msg_->getType();
ti.md5sum = (char *) publishers[i]->msg_->getMD5();
ti.buffer_size = OUTPUT_SIZE;
publish(publishers[i]->getEndpointType(), &ti);
}
}
for (i = 0; i < MAX_SUBSCRIBERS; i++)
{
if (subscribers[i] != 0) // non-empty slot
{
ti.topic_id = subscribers[i]->id_;
ti.topic_name = (char *) subscribers[i]->topic_;
ti.message_type = (char *) subscribers[i]->getMsgType();
ti.md5sum = (char *) subscribers[i]->getMsgMD5();
ti.buffer_size = INPUT_SIZE;
publish(subscribers[i]->getEndpointType(), &ti);
}
}
configured_ = true;
}
virtual int publish(int id, const Msg * msg)
{
if (id >= 100 && !configured_)
return 0;
/* serialize message */
int l = msg->serialize(message_out + 7);
/* setup the header */
message_out[0] = 0xff;
message_out[1] = PROTOCOL_VER;
message_out[2] = (uint8_t)((uint16_t)l & 255);
message_out[3] = (uint8_t)((uint16_t)l >> 8);
message_out[4] = 255 - ((message_out[2] + message_out[3]) % 256);
message_out[5] = (uint8_t)((int16_t)id & 255);
message_out[6] = (uint8_t)((int16_t)id >> 8);
/* calculate checksum */
int chk = 0;
for (int i = 5; i < l + 7; i++)
chk += message_out[i];
l += 7;
message_out[l++] = 255 - (chk % 256);
if (l <= OUTPUT_SIZE)
{
hardware_.write(message_out, l);
return l;
}
else
{
logerror("Message from device dropped: message larger than buffer.");
return -1;
}
}
/********************************************************************
* Logging
*/
private:
void log(char byte, const char * msg)
{
rosserial_msgs::Log l;
l.level = byte;
l.msg = (char*)msg;
publish(rosserial_msgs::TopicInfo::ID_LOG, &l);
}
public:
void logdebug(const char* msg)
{
log(rosserial_msgs::Log::ROSDEBUG, msg);
}
void loginfo(const char * msg)
{
log(rosserial_msgs::Log::INFO, msg);
}
void logwarn(const char *msg)
{
log(rosserial_msgs::Log::WARN, msg);
}
void logerror(const char*msg)
{
log(rosserial_msgs::Log::ERROR, msg);
}
void logfatal(const char*msg)
{
log(rosserial_msgs::Log::FATAL, msg);
}
/********************************************************************
* Parameters
*/
private:
bool param_recieved;
rosserial_msgs::RequestParamResponse req_param_resp;
bool requestParam(const char * name, int time_out = 1000)
{
param_recieved = false;
rosserial_msgs::RequestParamRequest req;
req.name = (char*)name;
publish(TopicInfo::ID_PARAMETER_REQUEST, &req);
uint32_t end_time = hardware_.time() + time_out;
while (!param_recieved)
{
spinOnce();
if (hardware_.time() > end_time)
{
logwarn("Failed to get param: timeout expired");
return false;
}
}
return true;
}
public:
bool getParam(const char* name, int* param, int length = 1, int timeout = 1000)
{
if (requestParam(name, timeout))
{
if (length == req_param_resp.ints_length)
{
//copy it over
for (int i = 0; i < length; i++)
param[i] = req_param_resp.ints[i];
return true;
}
else
{
logwarn("Failed to get param: length mismatch");
}
}
return false;
}
bool getParam(const char* name, float* param, int length = 1, int timeout = 1000)
{
if (requestParam(name, timeout))
{
if (length == req_param_resp.floats_length)
{
//copy it over
for (int i = 0; i < length; i++)
param[i] = req_param_resp.floats[i];
return true;
}
else
{
logwarn("Failed to get param: length mismatch");
}
}
return false;
}
bool getParam(const char* name, char** param, int length = 1, int timeout = 1000)
{
if (requestParam(name, timeout))
{
if (length == req_param_resp.strings_length)
{
//copy it over
for (int i = 0; i < length; i++)
strcpy(param[i], req_param_resp.strings[i]);
return true;
}
else
{
logwarn("Failed to get param: length mismatch");
}
}
return false;
}
bool getParam(const char* name, bool* param, int length = 1, int timeout = 1000)
{
if (requestParam(name, timeout))
{
if (length == req_param_resp.ints_length)
{
//copy it over
for (int i = 0; i < length; i++)
param[i] = req_param_resp.ints[i];
return true;
}
else
{
logwarn("Failed to get param: length mismatch");
}
}
return false;
}
};
}
#endif
| 18,164 | C | 25.441048 | 93 | 0.568212 |
renanmb/Omniverse_legged_robotics/URDF-Descriptions/OpenQuadruped/OpenQuadruped-spot_mini_mini-spot/spot_real/Control/Teensy/SpotMiniMini/lib/ros_lib/ros/msg.h | /*
* Software License Agreement (BSD License)
*
* Copyright (c) 2011, Willow Garage, Inc.
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above
* copyright notice, this list of conditions and the following
* disclaimer in the documentation and/or other materials provided
* with the distribution.
* * Neither the name of Willow Garage, Inc. nor the names of its
* contributors may be used to endorse or promote prducts derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS
* FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE
* COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING,
* BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
* LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN
* ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
#ifndef _ROS_MSG_H_
#define _ROS_MSG_H_
#include <stdint.h>
#include <stddef.h>
namespace ros
{
/* Base Message Type */
class Msg
{
public:
virtual int serialize(unsigned char *outbuffer) const = 0;
virtual int deserialize(unsigned char *data) = 0;
virtual const char * getType() = 0;
virtual const char * getMD5() = 0;
/**
* @brief This tricky function handles promoting a 32bit float to a 64bit
* double, so that AVR can publish messages containing float64
* fields, despite AVV having no native support for double.
*
* @param[out] outbuffer pointer for buffer to serialize to.
* @param[in] f value to serialize.
*
* @return number of bytes to advance the buffer pointer.
*
*/
static int serializeAvrFloat64(unsigned char* outbuffer, const float f)
{
const int32_t* val = (int32_t*) &f;
int32_t exp = ((*val >> 23) & 255);
if (exp != 0)
{
exp += 1023 - 127;
}
int32_t sig = *val;
*(outbuffer++) = 0;
*(outbuffer++) = 0;
*(outbuffer++) = 0;
*(outbuffer++) = (sig << 5) & 0xff;
*(outbuffer++) = (sig >> 3) & 0xff;
*(outbuffer++) = (sig >> 11) & 0xff;
*(outbuffer++) = ((exp << 4) & 0xF0) | ((sig >> 19) & 0x0F);
*(outbuffer++) = (exp >> 4) & 0x7F;
// Mark negative bit as necessary.
if (f < 0)
{
*(outbuffer - 1) |= 0x80;
}
return 8;
}
/**
* @brief This tricky function handles demoting a 64bit double to a
* 32bit float, so that AVR can understand messages containing
* float64 fields, despite AVR having no native support for double.
*
* @param[in] inbuffer pointer for buffer to deserialize from.
* @param[out] f pointer to place the deserialized value in.
*
* @return number of bytes to advance the buffer pointer.
*/
static int deserializeAvrFloat64(const unsigned char* inbuffer, float* f)
{
uint32_t* val = (uint32_t*)f;
inbuffer += 3;
// Copy truncated mantissa.
*val = ((uint32_t)(*(inbuffer++)) >> 5 & 0x07);
*val |= ((uint32_t)(*(inbuffer++)) & 0xff) << 3;
*val |= ((uint32_t)(*(inbuffer++)) & 0xff) << 11;
*val |= ((uint32_t)(*inbuffer) & 0x0f) << 19;
// Copy truncated exponent.
uint32_t exp = ((uint32_t)(*(inbuffer++)) & 0xf0) >> 4;
exp |= ((uint32_t)(*inbuffer) & 0x7f) << 4;
if (exp != 0)
{
*val |= ((exp) - 1023 + 127) << 23;
}
// Copy negative sign.
*val |= ((uint32_t)(*(inbuffer++)) & 0x80) << 24;
return 8;
}
// Copy data from variable into a byte array
template<typename A, typename V>
static void varToArr(A arr, const V var)
{
for (size_t i = 0; i < sizeof(V); i++)
arr[i] = (var >> (8 * i));
}
// Copy data from a byte array into variable
template<typename V, typename A>
static void arrToVar(V& var, const A arr)
{
var = 0;
for (size_t i = 0; i < sizeof(V); i++)
var |= (arr[i] << (8 * i));
}
};
} // namespace ros
#endif
| 4,620 | C | 30.013423 | 76 | 0.635281 |