file_path
stringlengths 21
202
| content
stringlengths 12
1.02M
| size
int64 12
1.02M
| lang
stringclasses 9
values | avg_line_length
float64 3.33
100
| max_line_length
int64 10
993
| alphanum_fraction
float64 0.27
0.93
|
---|---|---|---|---|---|---|
arhix52/Strelka/src/HdStrelka/Camera.cpp | #include "Camera.h"
#include <pxr/imaging/hd/sceneDelegate.h>
#include <pxr/base/gf/vec4d.h>
#include <pxr/base/gf/camera.h>
#include <cmath>
#include <glm/glm.hpp>
#include <glm/gtc/matrix_transform.hpp>
#include <glm/gtc/quaternion.hpp>
#include <glm/gtc/type_ptr.hpp>
#include <glm/gtx/compatibility.hpp>
#include <glm/gtx/matrix_decompose.hpp>
PXR_NAMESPACE_OPEN_SCOPE
HdStrelkaCamera::HdStrelkaCamera(const SdfPath& id, oka::Scene& scene) : HdCamera(id), mScene(scene), m_vfov(M_PI_2)
{
const std::string& name = id.GetString();
oka::Camera okaCamera;
okaCamera.name = name;
mCameraIndex = mScene.addCamera(okaCamera);
}
HdStrelkaCamera::~HdStrelkaCamera()
{
}
float HdStrelkaCamera::GetVFov() const
{
return m_vfov;
}
uint32_t HdStrelkaCamera::GetCameraIndex() const
{
return mCameraIndex;
}
void HdStrelkaCamera::Sync(HdSceneDelegate* sceneDelegate, HdRenderParam* renderParam, HdDirtyBits* dirtyBits)
{
HdDirtyBits dirtyBitsCopy = *dirtyBits;
HdCamera::Sync(sceneDelegate, renderParam, &dirtyBitsCopy);
if (*dirtyBits & DirtyBits::DirtyParams)
{
// See https://wiki.panotools.org/Field_of_View
float aperture = _verticalAperture * GfCamera::APERTURE_UNIT;
float focalLength = _focalLength * GfCamera::FOCAL_LENGTH_UNIT;
float vfov = 2.0f * std::atan(aperture / (2.0f * focalLength));
m_vfov = vfov;
oka::Camera cam = _ConstructStrelkaCamera();
mScene.updateCamera(cam, mCameraIndex);
}
*dirtyBits = DirtyBits::Clean;
}
HdDirtyBits HdStrelkaCamera::GetInitialDirtyBitsMask() const
{
return DirtyBits::DirtyParams | DirtyBits::DirtyTransform;
}
oka::Camera HdStrelkaCamera::_ConstructStrelkaCamera()
{
oka::Camera strelkaCamera;
GfMatrix4d perspMatrix = ComputeProjectionMatrix();
GfMatrix4d absInvViewMatrix = GetTransform();
GfMatrix4d relViewMatrix = absInvViewMatrix; //*m_rootMatrix;
glm::float4x4 xform;
for (int i = 0; i < 4; ++i)
{
for (int j = 0; j < 4; ++j)
{
xform[i][j] = (float)relViewMatrix[i][j];
}
}
glm::float4x4 persp;
for (int i = 0; i < 4; ++i)
{
for (int j = 0; j < 4; ++j)
{
persp[i][j] = (float)perspMatrix[i][j];
}
}
{
glm::vec3 scale;
glm::quat rotation;
glm::vec3 translation;
glm::vec3 skew;
glm::vec4 perspective;
glm::decompose(xform, scale, rotation, translation, skew, perspective);
rotation = glm::conjugate(rotation);
strelkaCamera.position = translation * scale;
strelkaCamera.mOrientation = rotation;
}
strelkaCamera.matrices.perspective = persp;
strelkaCamera.matrices.invPerspective = glm::inverse(persp);
strelkaCamera.fov = glm::degrees(GetVFov());
const std::string& name = GetId().GetString();
strelkaCamera.name = name;
return strelkaCamera;
}
PXR_NAMESPACE_CLOSE_SCOPE
| 2,967 | C++ | 26.229358 | 116 | 0.658915 |
arhix52/Strelka/src/HdStrelka/RenderPass.cpp | #include "RenderPass.h"
#include "Camera.h"
#include "Instancer.h"
#include "Material.h"
#include "Mesh.h"
#include "BasisCurves.h"
#include "Light.h"
#include "RenderBuffer.h"
#include "Tokens.h"
#include <pxr/base/gf/matrix3d.h>
#include <pxr/base/gf/quatd.h>
#include <pxr/imaging/hd/renderDelegate.h>
#include <pxr/imaging/hd/renderPassState.h>
#include <pxr/imaging/hd/rprim.h>
#include <pxr/imaging/hd/basisCurves.h>
#include <log.h>
#include <glm/glm.hpp>
#include <glm/gtc/matrix_transform.hpp>
#include <glm/gtc/type_ptr.hpp>
#include <glm/gtx/compatibility.hpp>
PXR_NAMESPACE_OPEN_SCOPE
HdStrelkaRenderPass::HdStrelkaRenderPass(HdRenderIndex* index,
const HdRprimCollection& collection,
const HdRenderSettingsMap& settings,
oka::Render* renderer,
oka::Scene* scene)
: HdRenderPass(index, collection),
m_settings(settings),
m_isConverged(false),
m_lastSceneStateVersion(UINT32_MAX),
m_lastRenderSettingsVersion(UINT32_MAX),
mRenderer(renderer),
mScene(scene)
{
}
HdStrelkaRenderPass::~HdStrelkaRenderPass()
{
}
bool HdStrelkaRenderPass::IsConverged() const
{
return m_isConverged;
}
// valid range of coordinates [-1; 1]
uint32_t packNormal(const glm::float3& normal)
{
uint32_t packed = (uint32_t)((normal.x + 1.0f) / 2.0f * 511.99999f);
packed += (uint32_t)((normal.y + 1.0f) / 2.0f * 511.99999f) << 10;
packed += (uint32_t)((normal.z + 1.0f) / 2.0f * 511.99999f) << 20;
return packed;
}
// valid range of coordinates [-10; 10]
uint32_t packUV(const glm::float2& uv)
{
int32_t packed = (uint32_t)((uv.x + 10.0f) / 20.0f * 16383.99999f);
packed += (uint32_t)((uv.y + 10.0f) / 20.0f * 16383.99999f) << 16;
return packed;
}
void HdStrelkaRenderPass::_BakeMeshInstance(const HdStrelkaMesh* mesh, GfMatrix4d transform, uint32_t materialIndex)
{
const GfMatrix4d normalMatrix = transform.GetInverse().GetTranspose();
const std::vector<GfVec3f>& meshPoints = mesh->GetPoints();
const std::vector<GfVec3f>& meshNormals = mesh->GetNormals();
const std::vector<GfVec3f>& meshTangents = mesh->GetTangents();
const std::vector<GfVec3i>& meshFaces = mesh->GetFaces();
const std::vector<GfVec2f>& meshUVs = mesh->GetUVs();
TF_VERIFY(meshPoints.size() == meshNormals.size());
const size_t vertexCount = meshPoints.size();
std::vector<oka::Scene::Vertex> vertices(vertexCount);
std::vector<uint32_t> indices(meshFaces.size() * 3);
for (size_t j = 0; j < meshFaces.size(); ++j)
{
const GfVec3i& vertexIndices = meshFaces[j];
indices[j * 3 + 0] = vertexIndices[0];
indices[j * 3 + 1] = vertexIndices[1];
indices[j * 3 + 2] = vertexIndices[2];
}
for (size_t j = 0; j < vertexCount; ++j)
{
const GfVec3f& point = meshPoints[j];
const GfVec3f& normal = meshNormals[j];
const GfVec3f& tangent = meshTangents[j];
oka::Scene::Vertex& vertex = vertices[j];
vertex.pos[0] = point[0];
vertex.pos[1] = point[1];
vertex.pos[2] = point[2];
const glm::float3 glmNormal = glm::float3(normal[0], normal[1], normal[2]);
vertex.normal = packNormal(glmNormal);
const glm::float3 glmTangent = glm::float3(tangent[0], tangent[1], tangent[2]);
vertex.tangent = packNormal(glmTangent);
// Texture coord
if (!meshUVs.empty())
{
const GfVec2f& uv = meshUVs[j];
const glm::float2 glmUV = glm::float2(uv[0], 1.0f - uv[1]); // Flip v coordinate
vertex.uv = packUV(glmUV);
}
}
glm::float4x4 glmTransform;
for (int i = 0; i < 4; ++i)
{
for (int j = 0; j < 4; ++j)
{
glmTransform[i][j] = (float)transform[i][j];
}
}
uint32_t meshId = mScene->createMesh(vertices, indices);
assert(meshId != -1);
uint32_t instId = mScene->createInstance(oka::Instance::Type::eMesh, meshId, materialIndex, glmTransform);
assert(instId != -1);
}
void HdStrelkaRenderPass::_BakeMeshes(HdRenderIndex* renderIndex, GfMatrix4d rootTransform)
{
TfHashMap<SdfPath, uint32_t, SdfPath::Hash> materialMapping;
materialMapping[SdfPath::EmptyPath()] = 0;
auto getOrCreateMaterial = [&](const SdfPath& materialId) {
uint32_t materialIndex = 0;
if (materialMapping.find(materialId) != materialMapping.end())
{
materialIndex = materialMapping[materialId];
}
else
{
HdSprim* sprim = renderIndex->GetSprim(HdPrimTypeTokens->material, materialId);
if (!sprim)
{
STRELKA_ERROR("Cannot retrive material!");
return 0u;
}
HdStrelkaMaterial* material = dynamic_cast<HdStrelkaMaterial*>(sprim);
if (material->isMdl())
{
const std::string& fileUri = material->getFileUri();
const std::string& name = material->getSubIdentifier();
oka::Scene::MaterialDescription materialDesc;
materialDesc.file = fileUri;
materialDesc.name = name;
materialDesc.type = oka::Scene::MaterialDescription::Type::eMdl;
materialDesc.params = material->getParams();
materialIndex = mScene->addMaterial(materialDesc);
}
else
{
const std::string& code = material->GetStrelkaMaterial();
const std::string& name = material->getSubIdentifier();
oka::Scene::MaterialDescription materialDesc;
materialDesc.name = name;
materialDesc.code = code;
materialDesc.type = oka::Scene::MaterialDescription::Type::eMaterialX;
materialDesc.params = material->getParams();
materialIndex = mScene->addMaterial(materialDesc);
}
materialMapping[materialId] = materialIndex;
}
return materialIndex;
};
for (const auto& rprimId : renderIndex->GetRprimIds())
{
const HdRprim* rprim = renderIndex->GetRprim(rprimId);
if (dynamic_cast<const HdMesh*>(rprim))
{
const HdStrelkaMesh* mesh = dynamic_cast<const HdStrelkaMesh*>(rprim);
if (!mesh->IsVisible())
{
// TODO: add UI/setting control here
continue;
}
const TfToken renderTag = mesh->GetRenderTag();
if ((renderTag != "geometry") && (renderTag != "render"))
{
// skip all proxy meshes
continue;
}
VtMatrix4dArray transforms;
const SdfPath& instancerId = mesh->GetInstancerId();
if (instancerId.IsEmpty())
{
transforms.resize(1);
transforms[0] = GfMatrix4d(1.0);
}
else
{
HdInstancer* boxedInstancer = renderIndex->GetInstancer(instancerId);
HdStrelkaInstancer* instancer = dynamic_cast<HdStrelkaInstancer*>(boxedInstancer);
const SdfPath& meshId = mesh->GetId();
transforms = instancer->ComputeInstanceTransforms(meshId);
}
const SdfPath& materialId = mesh->GetMaterialId();
const std::string& materialName = materialId.GetString();
STRELKA_INFO("Hydra: Mesh: {0} \t Material: {1}", mesh->getName(), materialName.c_str());
uint32_t materialIndex = 0;
if (materialId.IsEmpty())
{
GfVec3f color(1.0f);
if (mesh->HasColor())
{
color = mesh->GetColor();
}
// materialName += "_color";
const std::string& fileUri = "default.mdl";
const std::string& name = "default_material";
oka::Scene::MaterialDescription material;
material.file = fileUri;
material.name = name;
material.type = oka::Scene::MaterialDescription::Type::eMdl;
material.color = glm::float3(color[0], color[1], color[2]);
material.hasColor = true;
oka::MaterialManager::Param colorParam = {};
colorParam.name = "diffuse_color";
colorParam.type = oka::MaterialManager::Param::Type::eFloat3;
colorParam.value.resize(sizeof(float) * 3);
memcpy(colorParam.value.data(), glm::value_ptr(material.color), sizeof(float) * 3);
material.params.push_back(colorParam);
materialIndex = mScene->addMaterial(material);
}
else
{
materialIndex = getOrCreateMaterial(materialId);
}
const GfMatrix4d& prototypeTransform = mesh->GetPrototypeTransform();
for (size_t i = 0; i < transforms.size(); i++)
{
const GfMatrix4d transform = prototypeTransform * transforms[i]; // *rootTransform;
// GfMatrix4d transform = GfMatrix4d(1.0);
_BakeMeshInstance(mesh, transform, materialIndex);
}
}
else if (dynamic_cast<const HdBasisCurves*>(rprim))
{
const HdStrelkaBasisCurves* curve = dynamic_cast<const HdStrelkaBasisCurves*>(rprim);
const std::vector<glm::float3>& points = curve->GetPoints();
const std::vector<float>& widths = curve->GetWidths();
const std::vector<uint32_t>& vertexCounts = curve->GetVertexCounts();
const SdfPath& materialId = curve->GetMaterialId();
const std::string& materialName = materialId.GetString();
STRELKA_INFO("Hydra: Curve: {0} \t Material: {1}", curve->getName(), materialName.c_str());
const uint32_t materialIndex = getOrCreateMaterial(materialId);
const GfMatrix4d& prototypeTransform = curve->GetPrototypeTransform();
glm::float4x4 glmTransform;
for (int i = 0; i < 4; ++i)
{
for (int j = 0; j < 4; ++j)
{
glmTransform[i][j] = (float)prototypeTransform[i][j];
}
}
uint32_t curveId = mScene->createCurve(oka::Curve::Type::eCubic, vertexCounts, points, widths);
mScene->createInstance(oka::Instance::Type::eCurve, curveId, materialIndex, glmTransform, -1);
}
}
STRELKA_INFO("Meshes: {}", mScene->getMeshes().size());
STRELKA_INFO("Instances: {}", mScene->getInstances().size());
STRELKA_INFO("Materials: {}", mScene->getMaterials().size());
STRELKA_INFO("Curves: {}", mScene->getCurves().size());
}
void HdStrelkaRenderPass::_Execute(const HdRenderPassStateSharedPtr& renderPassState, const TfTokenVector& renderTags)
{
TF_UNUSED(renderTags);
HD_TRACE_FUNCTION();
HF_MALLOC_TAG_FUNCTION();
m_isConverged = false;
const auto* camera = dynamic_cast<const HdStrelkaCamera*>(renderPassState->GetCamera());
if (!camera)
{
return;
}
const HdRenderPassAovBindingVector& aovBindings = renderPassState->GetAovBindings();
if (aovBindings.empty())
{
return;
}
const HdRenderPassAovBinding* colorAovBinding = nullptr;
for (const HdRenderPassAovBinding& aovBinding : aovBindings)
{
if (aovBinding.aovName != HdAovTokens->color)
{
HdStrelkaRenderBuffer* renderBuffer = dynamic_cast<HdStrelkaRenderBuffer*>(aovBinding.renderBuffer);
renderBuffer->SetConverged(true);
continue;
}
colorAovBinding = &aovBinding;
}
if (!colorAovBinding)
{
return;
}
HdRenderIndex* renderIndex = GetRenderIndex();
HdChangeTracker& changeTracker = renderIndex->GetChangeTracker();
HdRenderDelegate* renderDelegate = renderIndex->GetRenderDelegate();
HdStrelkaRenderBuffer* renderBuffer = dynamic_cast<HdStrelkaRenderBuffer*>(colorAovBinding->renderBuffer);
uint32_t sceneStateVersion = changeTracker.GetSceneStateVersion();
uint32_t renderSettingsStateVersion = renderDelegate->GetRenderSettingsVersion();
bool sceneChanged = (sceneStateVersion != m_lastSceneStateVersion);
bool renderSettingsChanged = (renderSettingsStateVersion != m_lastRenderSettingsVersion);
// if (!sceneChanged && !renderSettingsChanged)
//{
// renderBuffer->SetConverged(true);
// return;
//}
oka::Buffer* outputImage = renderBuffer->GetResource(false).UncheckedGet<oka::Buffer*>();
renderBuffer->SetConverged(false);
m_lastSceneStateVersion = sceneStateVersion;
m_lastRenderSettingsVersion = renderSettingsStateVersion;
// Transform scene into camera space to increase floating point precision.
GfMatrix4d viewMatrix = camera->GetTransform().GetInverse();
static int counter = 0;
if (counter == 0)
{
++counter;
_BakeMeshes(renderIndex, viewMatrix);
m_rootMatrix = viewMatrix;
mRenderer->setScene(mScene);
const uint32_t camIndex = camera->GetCameraIndex();
// mRenderer->setActiveCameraIndex(camIndex);
oka::Scene::UniformLightDesc desc{};
desc.color = glm::float3(1.0f);
desc.height = 0.4f;
desc.width = 0.4f;
desc.position = glm::float3(0, 1.1, 0.67);
desc.orientation = glm::float3(179.68, 29.77, -89.97);
desc.intensity = 160.0f;
static const TfTokenVector lightTypes = { HdPrimTypeTokens->domeLight, HdPrimTypeTokens->simpleLight,
HdPrimTypeTokens->sphereLight, HdPrimTypeTokens->rectLight,
HdPrimTypeTokens->diskLight, HdPrimTypeTokens->cylinderLight,
HdPrimTypeTokens->distantLight };
size_t count = 0;
// TF_FOR_ALL(it, lightTypes)
{
// TODO: refactor this to more generic code, templates?
if (renderIndex->IsSprimTypeSupported(HdPrimTypeTokens->rectLight))
{
SdfPathVector sprimPaths =
renderIndex->GetSprimSubtree(HdPrimTypeTokens->rectLight, SdfPath::AbsoluteRootPath());
for (int lightIdx = 0; lightIdx < sprimPaths.size(); ++lightIdx)
{
HdSprim* sprim = renderIndex->GetSprim(HdPrimTypeTokens->rectLight, sprimPaths[lightIdx]);
HdStrelkaLight* light = dynamic_cast<HdStrelkaLight*>(sprim);
mScene->createLight(light->getLightDesc());
}
}
if (renderIndex->IsSprimTypeSupported(HdPrimTypeTokens->diskLight))
{
SdfPathVector sprimPaths =
renderIndex->GetSprimSubtree(HdPrimTypeTokens->diskLight, SdfPath::AbsoluteRootPath());
for (int lightIdx = 0; lightIdx < sprimPaths.size(); ++lightIdx)
{
HdSprim* sprim = renderIndex->GetSprim(HdPrimTypeTokens->diskLight, sprimPaths[lightIdx]);
HdStrelkaLight* light = dynamic_cast<HdStrelkaLight*>(sprim);
mScene->createLight(light->getLightDesc());
}
}
if (renderIndex->IsSprimTypeSupported(HdPrimTypeTokens->sphereLight))
{
SdfPathVector sprimPaths =
renderIndex->GetSprimSubtree(HdPrimTypeTokens->sphereLight, SdfPath::AbsoluteRootPath());
for (int lightIdx = 0; lightIdx < sprimPaths.size(); ++lightIdx)
{
HdSprim* sprim = renderIndex->GetSprim(HdPrimTypeTokens->sphereLight, sprimPaths[lightIdx]);
HdStrelkaLight* light = dynamic_cast<HdStrelkaLight*>(sprim);
mScene->createLight(light->getLightDesc());
}
}
if (renderIndex->IsSprimTypeSupported(HdPrimTypeTokens->distantLight))
{
SdfPathVector sprimPaths =
renderIndex->GetSprimSubtree(HdPrimTypeTokens->distantLight, SdfPath::AbsoluteRootPath());
for (int lightIdx = 0; lightIdx < sprimPaths.size(); ++lightIdx)
{
HdSprim* sprim = renderIndex->GetSprim(HdPrimTypeTokens->distantLight, sprimPaths[lightIdx]);
HdStrelkaLight* light = dynamic_cast<HdStrelkaLight*>(sprim);
mScene->createLight(light->getLightDesc());
}
}
}
}
// mScene.createLight(desc);
float* img_data = (float*)renderBuffer->Map();
mRenderer->render(outputImage);
renderBuffer->Unmap();
// renderBuffer->SetConverged(true);
m_isConverged = true;
}
PXR_NAMESPACE_CLOSE_SCOPE
| 17,099 | C++ | 37 | 118 | 0.587578 |
arhix52/Strelka/src/HdStrelka/MaterialNetworkTranslator.h | // Copyright (C) 2021 Pablo Delgado Krämer
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program. If not, see <https://www.gnu.org/licenses/>.
#pragma once
#include <pxr/usd/sdf/path.h>
#include <string>
#include <MaterialXCore/Document.h>
#include <memory>
PXR_NAMESPACE_OPEN_SCOPE
struct HdMaterialNetwork2;
class MaterialNetworkTranslator
{
public:
MaterialNetworkTranslator(const std::string& mtlxLibPath);
std::string ParseNetwork(const SdfPath& id, const HdMaterialNetwork2& network) const;
static bool ParseMdlNetwork(const HdMaterialNetwork2& network, std::string& fileUri, std::string& subIdentifier);
private:
MaterialX::DocumentPtr CreateMaterialXDocumentFromNetwork(const SdfPath& id, const HdMaterialNetwork2& network) const;
void patchMaterialNetwork(HdMaterialNetwork2& network) const;
private:
MaterialX::DocumentPtr m_nodeLib;
};
PXR_NAMESPACE_CLOSE_SCOPE
| 1,568 | C | 32.382978 | 122 | 0.714286 |
arhix52/Strelka/src/HdStrelka/Mesh.cpp | #include "Mesh.h"
#include <pxr/imaging/hd/instancer.h>
#include <pxr/imaging/hd/meshUtil.h>
#include <pxr/imaging/hd/smoothNormals.h>
#include <pxr/imaging/hd/vertexAdjacency.h>
#include <log.h>
PXR_NAMESPACE_OPEN_SCOPE
// clang-format off
TF_DEFINE_PRIVATE_TOKENS(_tokens,
(st)
);
// clang-format on
HdStrelkaMesh::HdStrelkaMesh(const SdfPath& id, oka::Scene* scene)
: HdMesh(id), mPrototypeTransform(1.0), mColor(0.0, 0.0, 0.0), mHasColor(false), mScene(scene)
{
}
HdStrelkaMesh::~HdStrelkaMesh() = default;
void HdStrelkaMesh::Sync(HdSceneDelegate* sceneDelegate,
HdRenderParam* renderParam,
HdDirtyBits* dirtyBits,
const TfToken& reprToken)
{
TF_UNUSED(renderParam);
TF_UNUSED(reprToken);
HD_TRACE_FUNCTION();
HF_MALLOC_TAG_FUNCTION();
HdRenderIndex& renderIndex = sceneDelegate->GetRenderIndex();
if ((*dirtyBits & HdChangeTracker::DirtyInstancer) | (*dirtyBits & HdChangeTracker::DirtyInstanceIndex))
{
HdDirtyBits dirtyBitsCopy = *dirtyBits;
_UpdateInstancer(sceneDelegate, &dirtyBitsCopy);
const SdfPath& instancerId = GetInstancerId();
HdInstancer::_SyncInstancerAndParents(renderIndex, instancerId);
}
const SdfPath& id = GetId();
mName = id.GetText();
if (*dirtyBits & HdChangeTracker::DirtyMaterialId)
{
const SdfPath& materialId = sceneDelegate->GetMaterialId(id);
SetMaterialId(materialId);
}
if (*dirtyBits & HdChangeTracker::DirtyTransform)
{
mPrototypeTransform = sceneDelegate->GetTransform(id);
}
const bool updateGeometry = (*dirtyBits & HdChangeTracker::DirtyPoints) |
(*dirtyBits & HdChangeTracker::DirtyNormals) |
(*dirtyBits & HdChangeTracker::DirtyTopology);
*dirtyBits = HdChangeTracker::Clean;
if (!updateGeometry)
{
return;
}
mFaces.clear();
mPoints.clear();
mNormals.clear();
_UpdateGeometry(sceneDelegate);
}
// valid range of coordinates [-1; 1]
static uint32_t packNormal(const glm::float3& normal)
{
uint32_t packed = (uint32_t)((normal.x + 1.0f) / 2.0f * 511.99999f);
packed += (uint32_t)((normal.y + 1.0f) / 2.0f * 511.99999f) << 10;
packed += (uint32_t)((normal.z + 1.0f) / 2.0f * 511.99999f) << 20;
return packed;
}
void HdStrelkaMesh::_ConvertMesh()
{
const std::vector<GfVec3f>& meshPoints = GetPoints();
const std::vector<GfVec3f>& meshNormals = GetNormals();
const std::vector<GfVec3i>& meshFaces = GetFaces();
TF_VERIFY(meshPoints.size() == meshNormals.size());
const size_t vertexCount = meshPoints.size();
std::vector<oka::Scene::Vertex> vertices(vertexCount);
std::vector<uint32_t> indices(meshFaces.size() * 3);
for (size_t j = 0; j < meshFaces.size(); ++j)
{
const GfVec3i& vertexIndices = meshFaces[j];
indices[j * 3 + 0] = vertexIndices[0];
indices[j * 3 + 1] = vertexIndices[1];
indices[j * 3 + 2] = vertexIndices[2];
}
for (size_t j = 0; j < vertexCount; ++j)
{
const GfVec3f& point = meshPoints[j];
const GfVec3f& normal = meshNormals[j];
oka::Scene::Vertex& vertex = vertices[j];
vertex.pos[0] = point[0];
vertex.pos[1] = point[1];
vertex.pos[2] = point[2];
const glm::float3 glmNormal = glm::float3(normal[0], normal[1], normal[2]);
vertex.normal = packNormal(glmNormal);
}
mStrelkaMeshId = mScene->createMesh(vertices, indices);
assert(mStrelkaMeshId != -1);
}
void HdStrelkaMesh::_UpdateGeometry(HdSceneDelegate* sceneDelegate)
{
const HdMeshTopology& topology = GetMeshTopology(sceneDelegate);
const SdfPath& id = GetId();
const HdMeshUtil meshUtil(&topology, id);
VtVec3iArray indices;
VtIntArray primitiveParams;
meshUtil.ComputeTriangleIndices(&indices, &primitiveParams);
VtVec3fArray points;
VtVec3fArray normals;
VtVec2fArray uvs;
bool indexedNormals;
bool indexedUVs;
_PullPrimvars(sceneDelegate, points, normals, uvs, indexedNormals, indexedUVs, mColor, mHasColor);
const bool hasUVs = !uvs.empty();
for (int i = 0; i < indices.size(); i++)
{
GfVec3i newFaceIndices(i * 3 + 0, i * 3 + 1, i * 3 + 2);
mFaces.push_back(newFaceIndices);
const GfVec3i& faceIndices = indices[i];
mPoints.push_back(points[faceIndices[0]]);
mPoints.push_back(points[faceIndices[1]]);
mPoints.push_back(points[faceIndices[2]]);
auto computeTangent = [](const GfVec3f& normal) {
GfVec3f c1 = GfCross(normal, GfVec3f(1.0f, 0.0f, 0.0f));
GfVec3f c2 = GfCross(normal, GfVec3f(0.0f, 1.0f, 0.0f));
GfVec3f tangent;
if (c1.GetLengthSq() > c2.GetLengthSq())
{
tangent = c1;
}
else
{
tangent = c2;
}
GfNormalize(&tangent);
return tangent;
};
mNormals.push_back(normals[indexedNormals ? faceIndices[0] : newFaceIndices[0]]);
mTangents.push_back(computeTangent(normals[indexedNormals ? faceIndices[0] : newFaceIndices[0]]));
mNormals.push_back(normals[indexedNormals ? faceIndices[1] : newFaceIndices[1]]);
mTangents.push_back(computeTangent(normals[indexedNormals ? faceIndices[1] : newFaceIndices[1]]));
mNormals.push_back(normals[indexedNormals ? faceIndices[2] : newFaceIndices[2]]);
mTangents.push_back(computeTangent(normals[indexedNormals ? faceIndices[2] : newFaceIndices[2]]));
if (hasUVs)
{
mUvs.push_back(uvs[indexedUVs ? faceIndices[0] : newFaceIndices[0]]);
mUvs.push_back(uvs[indexedUVs ? faceIndices[1] : newFaceIndices[1]]);
mUvs.push_back(uvs[indexedUVs ? faceIndices[2] : newFaceIndices[2]]);
}
}
}
bool HdStrelkaMesh::_FindPrimvar(HdSceneDelegate* sceneDelegate,
const TfToken& primvarName,
HdInterpolation& interpolation) const
{
const HdInterpolation interpolations[] = {
HdInterpolation::HdInterpolationVertex, HdInterpolation::HdInterpolationFaceVarying,
HdInterpolation::HdInterpolationConstant, HdInterpolation::HdInterpolationUniform,
HdInterpolation::HdInterpolationVarying, HdInterpolation::HdInterpolationInstance
};
for (const HdInterpolation& currInteroplation : interpolations)
{
const auto& primvarDescs = GetPrimvarDescriptors(sceneDelegate, currInteroplation);
for (const HdPrimvarDescriptor& primvar : primvarDescs)
{
if (primvar.name == primvarName)
{
interpolation = currInteroplation;
return true;
}
}
}
return false;
}
void HdStrelkaMesh::_PullPrimvars(HdSceneDelegate* sceneDelegate,
VtVec3fArray& points,
VtVec3fArray& normals,
VtVec2fArray& uvs,
bool& indexedNormals,
bool& indexedUVs,
GfVec3f& color,
bool& hasColor) const
{
const SdfPath& id = GetId();
// Handle points.
HdInterpolation pointInterpolation;
const bool foundPoints = _FindPrimvar(sceneDelegate, HdTokens->points, pointInterpolation);
if (!foundPoints)
{
TF_RUNTIME_ERROR("Points primvar not found!");
return;
}
else if (pointInterpolation != HdInterpolation::HdInterpolationVertex)
{
TF_RUNTIME_ERROR("Points primvar is not vertex-interpolated!");
return;
}
const VtValue boxedPoints = sceneDelegate->Get(id, HdTokens->points);
points = boxedPoints.Get<VtVec3fArray>();
// Handle color.
HdInterpolation colorInterpolation;
const bool foundColor = _FindPrimvar(sceneDelegate, HdTokens->displayColor, colorInterpolation);
if (foundColor && colorInterpolation == HdInterpolation::HdInterpolationConstant)
{
const VtValue boxedColors = sceneDelegate->Get(id, HdTokens->displayColor);
const VtVec3fArray& colors = boxedColors.Get<VtVec3fArray>();
color = colors[0];
hasColor = true;
}
const HdMeshTopology topology = GetMeshTopology(sceneDelegate);
// Handle normals.
HdInterpolation normalInterpolation;
const bool foundNormals = _FindPrimvar(sceneDelegate, HdTokens->normals, normalInterpolation);
if (foundNormals && normalInterpolation == HdInterpolation::HdInterpolationVertex)
{
const VtValue boxedNormals = sceneDelegate->Get(id, HdTokens->normals);
normals = boxedNormals.Get<VtVec3fArray>();
indexedNormals = true;
}
if (foundNormals && normalInterpolation == HdInterpolation::HdInterpolationFaceVarying)
{
const VtValue boxedFvNormals = sceneDelegate->Get(id, HdTokens->normals);
const VtVec3fArray& fvNormals = boxedFvNormals.Get<VtVec3fArray>();
const HdMeshUtil meshUtil(&topology, id);
VtValue boxedTriangulatedNormals;
if (!meshUtil.ComputeTriangulatedFaceVaryingPrimvar(
fvNormals.cdata(), fvNormals.size(), HdTypeFloatVec3, &boxedTriangulatedNormals))
{
TF_CODING_ERROR("Unable to triangulate face-varying normals of %s", id.GetText());
}
normals = boxedTriangulatedNormals.Get<VtVec3fArray>();
indexedNormals = false;
}
else
{
Hd_VertexAdjacency adjacency;
adjacency.BuildAdjacencyTable(&topology);
normals = Hd_SmoothNormals::ComputeSmoothNormals(&adjacency, points.size(), points.cdata());
indexedNormals = true;
}
// Handle texture coords
HdInterpolation textureCoordInterpolation;
const bool foundTextureCoord = _FindPrimvar(sceneDelegate, _tokens->st, textureCoordInterpolation);
if (foundTextureCoord && textureCoordInterpolation == HdInterpolationVertex)
{
uvs = sceneDelegate->Get(id, _tokens->st).Get<VtVec2fArray>();
indexedUVs = true;
}
if (foundTextureCoord && textureCoordInterpolation == HdInterpolation::HdInterpolationFaceVarying)
{
const VtValue boxedUVs = sceneDelegate->Get(id, _tokens->st);
const VtVec2fArray& fvUVs = boxedUVs.Get<VtVec2fArray>();
const HdMeshUtil meshUtil(&topology, id);
VtValue boxedTriangulatedUVS;
if (!meshUtil.ComputeTriangulatedFaceVaryingPrimvar(
fvUVs.cdata(), fvUVs.size(), HdTypeFloatVec2, &boxedTriangulatedUVS))
{
TF_CODING_ERROR("Unable to triangulate face-varying UVs of %s", id.GetText());
}
uvs = boxedTriangulatedUVS.Get<VtVec2fArray>();
indexedUVs = false;
}
}
const TfTokenVector& HdStrelkaMesh::GetBuiltinPrimvarNames() const
{
return BUILTIN_PRIMVAR_NAMES;
}
const std::vector<GfVec3f>& HdStrelkaMesh::GetPoints() const
{
return mPoints;
}
const std::vector<GfVec3f>& HdStrelkaMesh::GetNormals() const
{
return mNormals;
}
const std::vector<GfVec3f>& HdStrelkaMesh::GetTangents() const
{
return mTangents;
}
const std::vector<GfVec3i>& HdStrelkaMesh::GetFaces() const
{
return mFaces;
}
const std::vector<GfVec2f>& HdStrelkaMesh::GetUVs() const
{
return mUvs;
}
const GfMatrix4d& HdStrelkaMesh::GetPrototypeTransform() const
{
return mPrototypeTransform;
}
const GfVec3f& HdStrelkaMesh::GetColor() const
{
return mColor;
}
bool HdStrelkaMesh::HasColor() const
{
return mHasColor;
}
const char* HdStrelkaMesh::getName() const
{
return mName.c_str();
}
HdDirtyBits HdStrelkaMesh::GetInitialDirtyBitsMask() const
{
return HdChangeTracker::DirtyPoints | HdChangeTracker::DirtyNormals | HdChangeTracker::DirtyTopology |
HdChangeTracker::DirtyInstancer | HdChangeTracker::DirtyInstanceIndex | HdChangeTracker::DirtyTransform |
HdChangeTracker::DirtyMaterialId | HdChangeTracker::DirtyPrimvar;
}
HdDirtyBits HdStrelkaMesh::_PropagateDirtyBits(HdDirtyBits bits) const
{
return bits;
}
void HdStrelkaMesh::_InitRepr(const TfToken& reprName, HdDirtyBits* dirtyBits)
{
TF_UNUSED(reprName);
TF_UNUSED(dirtyBits);
}
PXR_NAMESPACE_CLOSE_SCOPE
| 12,415 | C++ | 32.109333 | 116 | 0.648731 |
arhix52/Strelka/src/HdStrelka/Instancer.h | // Copyright (C) 2021 Pablo Delgado Krämer
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program. If not, see <https://www.gnu.org/licenses/>.
#pragma once
#include <pxr/imaging/hd/instancer.h>
PXR_NAMESPACE_OPEN_SCOPE
class HdStrelkaInstancer final : public HdInstancer
{
public:
HdStrelkaInstancer(HdSceneDelegate* delegate,
const SdfPath& id);
~HdStrelkaInstancer() override;
public:
VtMatrix4dArray ComputeInstanceTransforms(const SdfPath& prototypeId);
void Sync(HdSceneDelegate* sceneDelegate,
HdRenderParam* renderParam,
HdDirtyBits* dirtyBits) override;
private:
TfHashMap<TfToken, VtValue, TfToken::HashFunctor> m_primvarMap;
};
PXR_NAMESPACE_CLOSE_SCOPE
| 1,403 | C | 32.428571 | 106 | 0.676408 |
arhix52/Strelka/src/HdStrelka/Light.h | #pragma once
#include "pxr/pxr.h"
#include <pxr/imaging/hd/light.h>
#include <pxr/imaging/hd/sceneDelegate.h>
#include <scene/scene.h>
PXR_NAMESPACE_OPEN_SCOPE
class HdStrelkaLight final : public HdLight
{
public:
HF_MALLOC_TAG_NEW("new HdStrelkaLight");
HdStrelkaLight(const SdfPath& id, TfToken const& lightType);
~HdStrelkaLight() override;
public:
void Sync(HdSceneDelegate* delegate,
HdRenderParam* renderParam,
HdDirtyBits* dirtyBits) override;
HdDirtyBits GetInitialDirtyBitsMask() const override;
oka::Scene::UniformLightDesc getLightDesc();
private:
TfToken mLightType;
oka::Scene::UniformLightDesc mLightDesc;
};
PXR_NAMESPACE_CLOSE_SCOPE
| 720 | C | 19.599999 | 64 | 0.719444 |
arhix52/Strelka/src/HdStrelka/MdlParserPlugin.h | // Copyright (C) 2021 Pablo Delgado Krämer
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program. If not, see <https://www.gnu.org/licenses/>.
#pragma once
#include <pxr/usd/ndr/parserPlugin.h>
PXR_NAMESPACE_OPEN_SCOPE
class HdStrelkaMdlParserPlugin final : public NdrParserPlugin
{
public:
NdrNodeUniquePtr Parse(const NdrNodeDiscoveryResult& discoveryResult) override;
const NdrTokenVec& GetDiscoveryTypes() const override;
const TfToken& GetSourceType() const override;
};
PXR_NAMESPACE_CLOSE_SCOPE
| 1,174 | C | 34.60606 | 106 | 0.695911 |
arhix52/Strelka/src/HdStrelka/MaterialNetworkTranslator.cpp | // Copyright (C) 2021 Pablo Delgado Krämer
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program. If not, see <https://www.gnu.org/licenses/>.
#include "MaterialNetworkTranslator.h"
#include <pxr/imaging/hd/material.h>
#include <pxr/usd/sdr/registry.h>
#include <pxr/imaging/hdMtlx/hdMtlx.h>
#include <pxr/imaging/hd/tokens.h>
#include <MaterialXCore/Document.h>
#include <MaterialXCore/Library.h>
#include <MaterialXCore/Material.h>
#include <MaterialXCore/Definition.h>
#include <MaterialXFormat/File.h>
#include <MaterialXFormat/Util.h>
#include "Tokens.h"
using std::string;
namespace mx = MaterialX;
PXR_NAMESPACE_OPEN_SCOPE
// clang-format off
TF_DEFINE_PRIVATE_TOKENS(
_tokens,
// USD node types
(UsdPreviewSurface)
(UsdUVTexture)
(UsdTransform2d)
(UsdPrimvarReader_float)
(UsdPrimvarReader_float2)
(UsdPrimvarReader_float3)
(UsdPrimvarReader_float4)
(UsdPrimvarReader_int)
(UsdPrimvarReader_string)
(UsdPrimvarReader_normal)
(UsdPrimvarReader_point)
(UsdPrimvarReader_vector)
(UsdPrimvarReader_matrix)
// MaterialX USD node type equivalents
(ND_UsdPreviewSurface_surfaceshader)
(ND_UsdUVTexture)
(ND_UsdPrimvarReader_integer)
(ND_UsdPrimvarReader_boolean)
(ND_UsdPrimvarReader_string)
(ND_UsdPrimvarReader_float)
(ND_UsdPrimvarReader_vector2)
(ND_UsdPrimvarReader_vector3)
(ND_UsdPrimvarReader_vector4)
(ND_UsdTransform2d)
(ND_UsdPrimvarReader_matrix44)
(mdl)
(subIdentifier)
(ND_convert_color3_vector3)
(diffuse_color_constant)
(normal)
(rgb)
(in)
(out)
);
// clang-format on
bool _ConvertNodesToMaterialXNodes(const HdMaterialNetwork2& network, HdMaterialNetwork2& mtlxNetwork)
{
mtlxNetwork = network;
for (auto& node : mtlxNetwork.nodes)
{
TfToken& nodeTypeId = node.second.nodeTypeId;
SdrRegistry& sdrRegistry = SdrRegistry::GetInstance();
if (sdrRegistry.GetShaderNodeByIdentifierAndType(nodeTypeId, HdStrelkaDiscoveryTypes->mtlx))
{
continue;
}
if (nodeTypeId == _tokens->UsdPreviewSurface)
{
nodeTypeId = _tokens->ND_UsdPreviewSurface_surfaceshader;
}
else if (nodeTypeId == _tokens->UsdUVTexture)
{
nodeTypeId = _tokens->ND_UsdUVTexture;
}
else if (nodeTypeId == _tokens->UsdTransform2d)
{
nodeTypeId = _tokens->ND_UsdTransform2d;
}
else if (nodeTypeId == _tokens->UsdPrimvarReader_float)
{
nodeTypeId = _tokens->ND_UsdPrimvarReader_float;
}
else if (nodeTypeId == _tokens->UsdPrimvarReader_float2)
{
nodeTypeId = _tokens->ND_UsdPrimvarReader_vector2;
}
else if (nodeTypeId == _tokens->UsdPrimvarReader_float3)
{
nodeTypeId = _tokens->ND_UsdPrimvarReader_vector3;
}
else if (nodeTypeId == _tokens->UsdPrimvarReader_float4)
{
nodeTypeId = _tokens->ND_UsdPrimvarReader_vector4;
}
else if (nodeTypeId == _tokens->UsdPrimvarReader_int)
{
nodeTypeId = _tokens->ND_UsdPrimvarReader_integer;
}
else if (nodeTypeId == _tokens->UsdPrimvarReader_string)
{
nodeTypeId = _tokens->ND_UsdPrimvarReader_string;
}
else if (nodeTypeId == _tokens->UsdPrimvarReader_normal)
{
nodeTypeId = _tokens->ND_UsdPrimvarReader_vector3;
}
else if (nodeTypeId == _tokens->UsdPrimvarReader_point)
{
nodeTypeId = _tokens->ND_UsdPrimvarReader_vector3;
}
else if (nodeTypeId == _tokens->UsdPrimvarReader_vector)
{
nodeTypeId = _tokens->ND_UsdPrimvarReader_vector3;
}
else if (nodeTypeId == _tokens->UsdPrimvarReader_matrix)
{
nodeTypeId = _tokens->ND_UsdPrimvarReader_matrix44;
}
else
{
TF_WARN("Unable to translate material node of type %s to MaterialX counterpart", nodeTypeId.GetText());
return false;
}
}
return true;
}
bool GetMaterialNetworkSurfaceTerminal(const HdMaterialNetwork2& network2,
HdMaterialNode2& surfaceTerminal,
SdfPath& terminalPath)
{
const auto& connectionIt = network2.terminals.find(HdMaterialTerminalTokens->surface);
if (connectionIt == network2.terminals.end())
{
return false;
}
const HdMaterialConnection2& connection = connectionIt->second;
terminalPath = connection.upstreamNode;
const auto& nodeIt = network2.nodes.find(terminalPath);
if (nodeIt == network2.nodes.end())
{
return false;
}
surfaceTerminal = nodeIt->second;
return true;
}
MaterialNetworkTranslator::MaterialNetworkTranslator(const std::string& mtlxLibPath)
{
m_nodeLib = mx::createDocument();
const mx::FilePathVec libFolders; // All directories if left empty.
const mx::FileSearchPath folderSearchPath(mtlxLibPath);
mx::loadLibraries(libFolders, folderSearchPath, m_nodeLib);
}
std::string MaterialNetworkTranslator::ParseNetwork(const SdfPath& id, const HdMaterialNetwork2& network) const
{
HdMaterialNetwork2 mtlxNetwork;
if (!_ConvertNodesToMaterialXNodes(network, mtlxNetwork))
{
return "";
}
patchMaterialNetwork(mtlxNetwork);
const mx::DocumentPtr doc = CreateMaterialXDocumentFromNetwork(id, mtlxNetwork);
if (!doc)
{
return "";
}
mx::string docStr = mx::writeToXmlString(doc);
return docStr;
}
bool MaterialNetworkTranslator::ParseMdlNetwork(const HdMaterialNetwork2& network,
std::string& fileUri,
std::string& subIdentifier)
{
if (network.nodes.size() == 1)
{
const HdMaterialNode2& node = network.nodes.begin()->second;
SdrRegistry& sdrRegistry = SdrRegistry::GetInstance();
SdrShaderNodeConstPtr sdrNode = sdrRegistry.GetShaderNodeByIdentifier(node.nodeTypeId);
if ((sdrNode == nullptr) || sdrNode->GetContext() != _tokens->mdl)
{
return false;
}
const NdrTokenMap& metadata = sdrNode->GetMetadata();
const auto& subIdentifierIt = metadata.find(_tokens->subIdentifier);
TF_DEV_AXIOM(subIdentifierIt != metadata.end());
subIdentifier = (*subIdentifierIt).second;
fileUri = sdrNode->GetResolvedImplementationURI();
return true;
}
TF_RUNTIME_ERROR("Unsupported multi-node MDL material!");
return false;
}
mx::DocumentPtr MaterialNetworkTranslator::CreateMaterialXDocumentFromNetwork(const SdfPath& id,
const HdMaterialNetwork2& network) const
{
HdMaterialNode2 surfaceTerminal;
SdfPath terminalPath;
if (!GetMaterialNetworkSurfaceTerminal(network, surfaceTerminal, terminalPath))
{
TF_WARN("Unable to find surface terminal for material network");
return nullptr;
}
HdMtlxTexturePrimvarData mxHdData;
return HdMtlxCreateMtlxDocumentFromHdNetwork(network, surfaceTerminal, terminalPath, id, m_nodeLib, &mxHdData);
}
void MaterialNetworkTranslator::patchMaterialNetwork(HdMaterialNetwork2& network) const
{
for (auto& pathNodePair : network.nodes)
{
HdMaterialNode2& node = pathNodePair.second;
if (node.nodeTypeId != _tokens->ND_UsdPreviewSurface_surfaceshader)
{
continue;
}
auto& inputs = node.inputConnections;
const auto patchColor3Vector3InputConnection = [&inputs, &network](const TfToken& inputName) {
auto inputIt = inputs.find(inputName);
if (inputIt == inputs.end())
{
return;
}
auto& connections = inputIt->second;
for (HdMaterialConnection2& connection : connections)
{
if (connection.upstreamOutputName != _tokens->rgb)
{
continue;
}
SdfPath upstreamNodePath = connection.upstreamNode;
SdfPath convertNodePath = upstreamNodePath;
for (int i = 0; network.nodes.count(convertNodePath) > 0; i++)
{
const std::string convertNodeName = "convert" + std::to_string(i);
convertNodePath = upstreamNodePath.AppendElementString(convertNodeName);
}
HdMaterialNode2 convertNode;
convertNode.nodeTypeId = _tokens->ND_convert_color3_vector3;
convertNode.inputConnections[_tokens->in] = { { upstreamNodePath, _tokens->rgb } };
network.nodes[convertNodePath] = convertNode;
connection.upstreamNode = convertNodePath;
connection.upstreamOutputName = _tokens->out;
}
};
patchColor3Vector3InputConnection(_tokens->normal);
}
}
PXR_NAMESPACE_CLOSE_SCOPE
| 9,749 | C++ | 30.758958 | 118 | 0.634014 |
arhix52/Strelka/src/HdStrelka/Mesh.h | #pragma once
#include <pxr/pxr.h>
#include <pxr/imaging/hd/mesh.h>
#include <scene/scene.h>
#include <pxr/base/gf/vec2f.h>
PXR_NAMESPACE_OPEN_SCOPE
class HdStrelkaMesh final : public HdMesh
{
public:
HF_MALLOC_TAG_NEW("new HdStrelkaMesh");
HdStrelkaMesh(const SdfPath& id, oka::Scene* scene);
~HdStrelkaMesh() override;
void Sync(HdSceneDelegate* delegate,
HdRenderParam* renderParam,
HdDirtyBits* dirtyBits,
const TfToken& reprToken) override;
HdDirtyBits GetInitialDirtyBitsMask() const override;
const TfTokenVector& GetBuiltinPrimvarNames() const override;
const std::vector<GfVec3f>& GetPoints() const;
const std::vector<GfVec3f>& GetNormals() const;
const std::vector<GfVec3f>& GetTangents() const;
const std::vector<GfVec3i>& GetFaces() const;
const std::vector<GfVec2f>& GetUVs() const;
const GfMatrix4d& GetPrototypeTransform() const;
const GfVec3f& GetColor() const;
bool HasColor() const;
const char* getName() const;
protected:
HdDirtyBits _PropagateDirtyBits(HdDirtyBits bits) const override;
void _InitRepr(const TfToken& reprName, HdDirtyBits* dirtyBits) override;
private:
void _ConvertMesh();
void _UpdateGeometry(HdSceneDelegate* sceneDelegate);
bool _FindPrimvar(HdSceneDelegate* sceneDelegate, const TfToken& primvarName, HdInterpolation& interpolation) const;
void _PullPrimvars(HdSceneDelegate* sceneDelegate,
VtVec3fArray& points,
VtVec3fArray& normals,
VtVec2fArray& uvs,
bool& indexedNormals,
bool& indexedUVs,
GfVec3f& color,
bool& hasColor) const;
const TfTokenVector BUILTIN_PRIMVAR_NAMES = { HdTokens->points, HdTokens->normals };
GfMatrix4d mPrototypeTransform;
std::vector<GfVec3f> mPoints;
std::vector<GfVec3f> mNormals;
std::vector<GfVec3f> mTangents;
std::vector<GfVec2f> mUvs;
std::vector<GfVec3i> mFaces;
GfVec3f mColor;
bool mHasColor;
oka::Scene* mScene;
std::string mName;
uint32_t mStrelkaMeshId;
};
PXR_NAMESPACE_CLOSE_SCOPE
| 2,224 | C | 26.8125 | 120 | 0.665018 |
arhix52/Strelka/src/HdStrelka/RenderBuffer.h | #pragma once
#include <pxr/imaging/hd/renderBuffer.h>
#include <pxr/pxr.h>
#include <render/common.h>
#include <render/buffer.h>
PXR_NAMESPACE_OPEN_SCOPE
class HdStrelkaRenderBuffer final : public HdRenderBuffer
{
public:
HdStrelkaRenderBuffer(const SdfPath& id, oka::SharedContext* ctx);
~HdStrelkaRenderBuffer() override;
public:
bool Allocate(const GfVec3i& dimensions, HdFormat format, bool multiSamples) override;
public:
unsigned int GetWidth() const override;
unsigned int GetHeight() const override;
unsigned int GetDepth() const override;
HdFormat GetFormat() const override;
bool IsMultiSampled() const override;
VtValue GetResource(bool multiSampled) const override;
public:
bool IsConverged() const override;
void SetConverged(bool converged);
public:
void* Map() override;
bool IsMapped() const override;
void Unmap() override;
void Resolve() override;
protected:
void _Deallocate() override;
private:
// oka::Image* mResult = nullptr;
oka::SharedContext* mCtx = nullptr;
oka::Buffer* mResult = nullptr;
void* m_bufferMem = nullptr;
uint32_t m_width;
uint32_t m_height;
HdFormat m_format;
bool m_isMultiSampled;
bool m_isMapped;
bool m_isConverged;
};
PXR_NAMESPACE_CLOSE_SCOPE
| 1,317 | C | 18.969697 | 90 | 0.709188 |
arhix52/Strelka/src/HdStrelka/RenderPass.h | #pragma once
#include <pxr/imaging/hd/renderDelegate.h>
#include <pxr/imaging/hd/renderPass.h>
#include <pxr/pxr.h>
#include <scene/camera.h>
#include <scene/scene.h>
#include <render/render.h>
PXR_NAMESPACE_OPEN_SCOPE
class HdStrelkaCamera;
class HdStrelkaMesh;
class HdStrelkaRenderPass final : public HdRenderPass
{
public:
HdStrelkaRenderPass(HdRenderIndex* index,
const HdRprimCollection& collection,
const HdRenderSettingsMap& settings,
oka::Render* renderer,
oka::Scene* scene);
~HdStrelkaRenderPass() override;
bool IsConverged() const override;
protected:
void _Execute(const HdRenderPassStateSharedPtr& renderPassState,
const TfTokenVector& renderTags) override;
private:
void _BakeMeshInstance(const HdStrelkaMesh* mesh,
GfMatrix4d transform,
uint32_t materialIndex);
void _BakeMeshes(HdRenderIndex* renderIndex,
GfMatrix4d rootTransform);
const HdRenderSettingsMap& m_settings;
bool m_isConverged;
uint32_t m_lastSceneStateVersion;
uint32_t m_lastRenderSettingsVersion;
GfMatrix4d m_rootMatrix;
oka::Scene* mScene;
// ptr to global render
oka::Render* mRenderer;
};
PXR_NAMESPACE_CLOSE_SCOPE
| 1,350 | C | 24.490566 | 68 | 0.664444 |
arhix52/Strelka/src/HdStrelka/RendererPlugin.cpp | #include "RendererPlugin.h"
#include "RenderDelegate.h"
#include <pxr/imaging/hd/rendererPluginRegistry.h>
#include <pxr/base/plug/plugin.h>
#include "pxr/base/plug/thisPlugin.h"
#include <log.h>
PXR_NAMESPACE_OPEN_SCOPE
TF_REGISTRY_FUNCTION(TfType)
{
HdRendererPluginRegistry::Define<HdStrelkaRendererPlugin>();
}
HdStrelkaRendererPlugin::HdStrelkaRendererPlugin()
{
const PlugPluginPtr plugin = PLUG_THIS_PLUGIN;
const std::string& resourcePath = plugin->GetResourcePath();
STRELKA_INFO("Resource path: {}", resourcePath.c_str());
const std::string shaderPath = resourcePath + "/shaders";
const std::string mtlxmdlPath = resourcePath + "/mtlxmdl";
const std::string mtlxlibPath = resourcePath + "/mtlxlib";
// m_translator = std::make_unique<MaterialNetworkTranslator>(mtlxlibPath);
const char* envUSDPath = std::getenv("USD_DIR");
if (!envUSDPath)
{
STRELKA_FATAL("Please, set USD_DIR variable\n");
assert(0);
m_isSupported = false;
}
else
{
const std::string USDPath(envUSDPath);
m_translator = std::make_unique<MaterialNetworkTranslator>(USDPath + "/libraries");
m_isSupported = true;
}
}
HdStrelkaRendererPlugin::~HdStrelkaRendererPlugin()
{
}
HdRenderDelegate* HdStrelkaRendererPlugin::CreateRenderDelegate()
{
HdRenderSettingsMap settingsMap = {};
return new HdStrelkaRenderDelegate(settingsMap, *m_translator);
}
HdRenderDelegate* HdStrelkaRendererPlugin::CreateRenderDelegate(const HdRenderSettingsMap& settingsMap)
{
return new HdStrelkaRenderDelegate(settingsMap, *m_translator);
}
void HdStrelkaRendererPlugin::DeleteRenderDelegate(HdRenderDelegate* renderDelegate)
{
delete renderDelegate;
}
bool HdStrelkaRendererPlugin::IsSupported(bool gpuEnabled) const
{
return m_isSupported;
}
PXR_NAMESPACE_CLOSE_SCOPE
| 1,865 | C++ | 25.657142 | 103 | 0.730831 |
arhix52/Strelka/src/materialmanager/mdlMaterialCompiler.cpp | // Copyright (C) 2021 Pablo Delgado Krämer
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program. If not, see <https://www.gnu.org/licenses/>.
#include "mdlMaterialCompiler.h"
#include <mi/mdl_sdk.h>
#include <atomic>
#include <cassert>
#include <iostream>
namespace oka
{
std::string _makeModuleName(const std::string& identifier)
{
return "::" + identifier;
}
MdlMaterialCompiler::MdlMaterialCompiler(MdlRuntime& runtime)
{
mLogger = mi::base::Handle<MdlLogger>(runtime.getLogger());
mDatabase = mi::base::Handle<mi::neuraylib::IDatabase>(runtime.getDatabase());
mTransaction = mi::base::Handle<mi::neuraylib::ITransaction>(runtime.getTransaction());
mFactory = mi::base::Handle<mi::neuraylib::IMdl_factory>(runtime.getFactory());
mImpExpApi = mi::base::Handle<mi::neuraylib::IMdl_impexp_api>(runtime.getImpExpApi());
}
bool MdlMaterialCompiler::createModule(const std::string& identifier,
std::string& moduleName)
{
mi::base::Handle<mi::neuraylib::IMdl_execution_context> context(mFactory->create_execution_context());
moduleName = _makeModuleName(identifier);
mi::Sint32 result = mImpExpApi->load_module(mTransaction.get(), moduleName.c_str(), context.get());
mLogger->flushContextMessages(context.get());
return result == 0 || result == 1;
}
bool MdlMaterialCompiler::createMaterialInstace(const char* moduleName, const char* identifier, mi::base::Handle<mi::neuraylib::IFunction_call>& matInstance)
{
mi::base::Handle<mi::neuraylib::IMdl_execution_context> context(mFactory->create_execution_context());
mi::base::Handle<const mi::IString> moduleDbName(mFactory->get_db_module_name(moduleName));
mi::base::Handle<const mi::neuraylib::IModule> module(mTransaction->access<mi::neuraylib::IModule>(moduleDbName->get_c_str()));
assert(module);
std::string materialDbName = std::string(moduleDbName->get_c_str()) + "::" + identifier;
mi::base::Handle<const mi::IArray> funcs(module->get_function_overloads(materialDbName.c_str(), (const mi::neuraylib::IExpression_list*)nullptr));
if (funcs->get_length() == 0)
{
std::string errorMsg = std::string("Material with identifier ") + identifier + " not found in MDL module\n";
mLogger->message(mi::base::MESSAGE_SEVERITY_ERROR, errorMsg.c_str());
return false;
}
if (funcs->get_length() > 1)
{
std::string errorMsg = std::string("Ambigious material identifier ") + identifier + " for MDL module\n";
mLogger->message(mi::base::MESSAGE_SEVERITY_ERROR, errorMsg.c_str());
return false;
}
mi::base::Handle<const mi::IString> exactMaterialDbName(funcs->get_element<mi::IString>(0));
// get material definition from database
mi::base::Handle<const mi::neuraylib::IFunction_definition> matDefinition(mTransaction->access<mi::neuraylib::IFunction_definition>(exactMaterialDbName->get_c_str()));
if (!matDefinition)
{
return false;
}
mi::Sint32 result;
// instantiate material with default parameters and store in database
matInstance = matDefinition->create_function_call(nullptr, &result);
if (result != 0 || !matInstance)
{
return false;
}
return true;
}
bool MdlMaterialCompiler::compileMaterial(mi::base::Handle<mi::neuraylib::IFunction_call>& instance, mi::base::Handle<mi::neuraylib::ICompiled_material>& compiledMaterial)
{
if (!instance)
{
// TODO: log error
return false;
}
mi::base::Handle<mi::neuraylib::IMdl_execution_context> context(mFactory->create_execution_context());
// performance optimizations available only in class compilation mode
// (all parameters are folded in instance mode)
context->set_option("fold_all_bool_parameters", true);
// context->set_option("fold_all_enum_parameters", true);
context->set_option("ignore_noinline", true);
context->set_option("fold_ternary_on_df", true);
auto flags = mi::neuraylib::IMaterial_instance::CLASS_COMPILATION;
// auto flags = mi::neuraylib::IMaterial_instance::DEFAULT_OPTIONS;
mi::base::Handle<mi::neuraylib::IMaterial_instance> material_instance2(
instance->get_interface<mi::neuraylib::IMaterial_instance>());
compiledMaterial = mi::base::Handle<mi::neuraylib::ICompiled_material>(material_instance2->create_compiled_material(flags, context.get()));
mLogger->flushContextMessages(context.get());
return true;
}
mi::base::Handle<mi::neuraylib::IMdl_factory>& MdlMaterialCompiler::getFactory()
{
return mFactory;
}
mi::base::Handle<mi::neuraylib::ITransaction>& MdlMaterialCompiler::getTransaction()
{
return mTransaction;
}
} // namespace oka
| 5,372 | C++ | 40.976562 | 171 | 0.687826 |
arhix52/Strelka/src/materialmanager/mdlNeurayLoader.cpp | // Copyright (C) 2021 Pablo Delgado Krämer
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program. If not, see <https://www.gnu.org/licenses/>.
#include "mdlNeurayLoader.h"
#include <mi/mdl_sdk.h>
#ifdef MI_PLATFORM_WINDOWS
# include <mi/base/miwindows.h>
#else
# include <dlfcn.h>
#endif
#include "iostream"
#include <string>
namespace oka
{
MdlNeurayLoader::MdlNeurayLoader()
: mDsoHandle(nullptr), mNeuray(nullptr)
{
}
MdlNeurayLoader::~MdlNeurayLoader()
{
if (mNeuray)
{
mNeuray->shutdown();
mNeuray.reset();
}
if (mDsoHandle)
{
unloadDso();
}
}
bool MdlNeurayLoader::init(const char* resourcePath, const char* imagePluginPath)
{
if (!loadDso(resourcePath))
{
return false;
}
if (!loadNeuray())
{
return false;
}
if (!loadPlugin(imagePluginPath))
{
return false;
}
return mNeuray->start() == 0;
}
mi::base::Handle<mi::neuraylib::INeuray> MdlNeurayLoader::getNeuray() const
{
return mNeuray;
}
bool MdlNeurayLoader::loadPlugin(const char* imagePluginPath)
{
// init plugin for texture support
mi::base::Handle<mi::neuraylib::INeuray> neuray(getNeuray());
mi::base::Handle<mi::neuraylib::IPlugin_configuration> configPl(neuray->get_api_component<mi::neuraylib::IPlugin_configuration>());
if (configPl->load_plugin_library(imagePluginPath)) // This function can only be called before the MDL SDK has been started.
{
std::cout << "Plugin file path not found, translation not possible" << std::endl;
return false;
}
return true;
}
bool MdlNeurayLoader::loadDso(const char* resourcePath)
{
std::string dsoFilename = std::string(resourcePath) + std::string("/libmdl_sdk" MI_BASE_DLL_FILE_EXT);
#ifdef MI_PLATFORM_WINDOWS
HMODULE handle = LoadLibraryA(dsoFilename.c_str());
if (!handle)
{
LPTSTR buffer = NULL;
LPCTSTR message = TEXT("unknown failure");
DWORD error_code = GetLastError();
if (FormatMessage(FORMAT_MESSAGE_ALLOCATE_BUFFER | FORMAT_MESSAGE_FROM_SYSTEM |
FORMAT_MESSAGE_IGNORE_INSERTS,
0, error_code,
MAKELANGID(LANG_NEUTRAL, SUBLANG_DEFAULT), (LPTSTR)&buffer, 0, 0))
{
message = buffer;
}
fprintf(stderr, "Failed to load library (%u): %s", error_code, message);
if (buffer)
{
LocalFree(buffer);
}
return false;
}
#else
void* handle = dlopen(dsoFilename.c_str(), RTLD_LAZY);
if (!handle)
{
fprintf(stderr, "%s\n", dlerror());
return false;
}
#endif
mDsoHandle = handle;
return true;
}
bool MdlNeurayLoader::loadNeuray()
{
#ifdef MI_PLATFORM_WINDOWS
void* symbol = GetProcAddress(reinterpret_cast<HMODULE>(mDsoHandle), "mi_factory");
if (!symbol)
{
LPTSTR buffer = NULL;
LPCTSTR message = TEXT("unknown failure");
DWORD error_code = GetLastError();
if (FormatMessage(FORMAT_MESSAGE_ALLOCATE_BUFFER | FORMAT_MESSAGE_FROM_SYSTEM |
FORMAT_MESSAGE_IGNORE_INSERTS,
0, error_code,
MAKELANGID(LANG_NEUTRAL, SUBLANG_DEFAULT), (LPTSTR)&buffer, 0, 0))
{
message = buffer;
}
fprintf(stderr, "GetProcAddress error (%u): %s", error_code, message);
if (buffer)
{
LocalFree(buffer);
}
return false;
}
#else
void* symbol = dlsym(mDsoHandle, "mi_factory");
if (!symbol)
{
fprintf(stderr, "%s\n", dlerror());
return false;
}
#endif
mNeuray = mi::base::Handle<mi::neuraylib::INeuray>(mi::neuraylib::mi_factory<mi::neuraylib::INeuray>(symbol));
if (mNeuray.is_valid_interface())
{
return true;
}
mi::base::Handle<mi::neuraylib::IVersion> version(mi::neuraylib::mi_factory<mi::neuraylib::IVersion>(symbol));
if (!version)
{
fprintf(stderr, "Error: Incompatible library.\n");
}
else
{
fprintf(stderr, "Error: Library version %s does not match header version %s.\n", version->get_product_version(), MI_NEURAYLIB_PRODUCT_VERSION_STRING);
}
return false;
}
void MdlNeurayLoader::unloadDso()
{
#ifdef MI_PLATFORM_WINDOWS
if (FreeLibrary(reinterpret_cast<HMODULE>(mDsoHandle)))
{
return;
}
LPTSTR buffer = 0;
LPCTSTR message = TEXT("unknown failure");
DWORD error_code = GetLastError();
if (FormatMessage(FORMAT_MESSAGE_ALLOCATE_BUFFER | FORMAT_MESSAGE_FROM_SYSTEM |
FORMAT_MESSAGE_IGNORE_INSERTS,
0, error_code,
MAKELANGID(LANG_NEUTRAL, SUBLANG_DEFAULT), (LPTSTR)&buffer, 0, 0))
{
message = buffer;
}
fprintf(stderr, "Failed to unload library (%u): %s", error_code, message);
if (buffer)
{
LocalFree(buffer);
}
#else
if (dlclose(mDsoHandle) != 0)
{
printf("%s\n", dlerror());
}
#endif
}
} // namespace oka
| 5,771 | C++ | 27.019417 | 158 | 0.601109 |
arhix52/Strelka/src/materialmanager/materialmanager.cpp | #include "materialmanager.h"
#include "materials.h"
#include <mi/mdl_sdk.h>
#include "mdlPtxCodeGen.h"
#include "mdlLogger.h"
#include "mdlMaterialCompiler.h"
#include "mdlNeurayLoader.h"
#include "mdlRuntime.h"
#include "mtlxMdlCodeGen.h"
#include <filesystem>
#include <fstream>
#include <iostream>
#include <unordered_map>
#include <log.h>
namespace fs = std::filesystem;
namespace oka
{
struct MaterialManager::Module
{
std::string moduleName;
std::string identifier;
};
struct MaterialManager::MaterialInstance
{
mi::base::Handle<mi::neuraylib::IFunction_call> instance;
};
struct MaterialManager::CompiledMaterial
{
mi::base::Handle<mi::neuraylib::ICompiled_material> compiledMaterial;
std::string name;
};
struct MaterialManager::TargetCode
{
struct InternalMaterial
{
mi::base::Handle<const mi::neuraylib::ITarget_code> targetCode;
std::string codePtx;
CompiledMaterial* compiledMaterial;
mi::base::Uuid hash;
uint32_t hash32;
uint32_t functionNum;
bool isUnique = false;
mi::base::Handle<mi::neuraylib::ITarget_argument_block> arg_block;
mi::Size argument_block_layout_index = -1;
uint32_t arg_block_offset = -1; // offset for arg block in argBlockData
uint32_t ro_offset = -1;
};
std::vector<InternalMaterial> internalMaterials;
std::unordered_map<uint32_t, uint32_t> uidToInternalIndex;
std::unordered_map<CompiledMaterial*, uint32_t> ptrToInternalIndex;
std::vector<Mdl_resource_info> resourceInfo;
std::vector<oka::MdlPtxCodeGen::InternalMaterialInfo> internalsInfo;
std::vector<const mi::neuraylib::ICompiled_material*> compiledMaterials;
bool isInitialized = false;
// MDL data for GPU buffers
std::vector<MdlMaterial> mdlMaterials;
std::vector<uint8_t> argBlockData;
std::vector<uint8_t> roData;
};
struct MaterialManager::TextureDescription
{
std::string dbName;
mi::base::Handle<const mi::neuraylib::IType_texture> textureType;
};
class Resource_callback : public mi::base::Interface_implement<mi::neuraylib::ITarget_resource_callback>
{
public:
/// Constructor.
Resource_callback(mi::base::Handle<mi::neuraylib::ITransaction>& transaction,
const mi::base::Handle<const mi::neuraylib::ITarget_code>& target_code)
: m_transaction(transaction), m_target_code(target_code)
{
}
/// Destructor.
virtual ~Resource_callback() = default;
/// Returns a resource index for the given resource value usable by the target code
/// resource handler for the corresponding resource type.
///
/// \param resource the resource value
///
/// \returns a resource index or 0 if no resource index can be returned
mi::Uint32 get_resource_index(mi::neuraylib::IValue_resource const* resource) override
{
// check whether we already know the resource index
auto it = m_resource_cache.find(resource);
if (it != m_resource_cache.end())
return it->second;
const char* filePath = resource->get_file_path();
// handle resources already known by the target code
mi::Uint32 res_idx = m_target_code->get_known_resource_index(m_transaction.get(), resource);
if (res_idx > 0)
{
// only accept body resources
switch (resource->get_kind())
{
case mi::neuraylib::IValue::VK_TEXTURE:
if (res_idx < m_target_code->get_texture_count())
return res_idx;
break;
case mi::neuraylib::IValue::VK_LIGHT_PROFILE:
if (res_idx < m_target_code->get_light_profile_count())
return res_idx;
break;
case mi::neuraylib::IValue::VK_BSDF_MEASUREMENT:
if (res_idx < m_target_code->get_bsdf_measurement_count())
return res_idx;
break;
default:
return 0u; // invalid kind
}
}
// invalid (or empty) resource
const char* name = resource->get_value();
if (!name)
{
return 0;
}
switch (resource->get_kind())
{
case mi::neuraylib::IValue::VK_TEXTURE: {
mi::base::Handle<mi::neuraylib::IValue_texture const> val_texture(
resource->get_interface<mi::neuraylib::IValue_texture const>());
if (!val_texture)
return 0u; // unknown resource
mi::base::Handle<const mi::neuraylib::IType_texture> texture_type(val_texture->get_type());
mi::neuraylib::ITarget_code::Texture_shape shape =
mi::neuraylib::ITarget_code::Texture_shape(texture_type->get_shape());
// m_compile_result.textures.emplace_back(resource->get_value(), shape);
// res_idx = m_compile_result.textures.size() - 1;
break;
}
case mi::neuraylib::IValue::VK_LIGHT_PROFILE:
// m_compile_result.light_profiles.emplace_back(resource->get_value());
// res_idx = m_compile_result.light_profiles.size() - 1;
break;
case mi::neuraylib::IValue::VK_BSDF_MEASUREMENT:
// m_compile_result.bsdf_measurements.emplace_back(resource->get_value());
// res_idx = m_compile_result.bsdf_measurements.size() - 1;
break;
default:
return 0u; // invalid kind
}
m_resource_cache[resource] = res_idx;
return res_idx;
}
/// Returns a string identifier for the given string value usable by the target code.
///
/// The value 0 is always the "not known string".
///
/// \param s the string value
mi::Uint32 get_string_index(mi::neuraylib::IValue_string const* s) override
{
char const* str_val = s->get_value();
if (str_val == nullptr)
return 0u;
for (mi::Size i = 0, n = m_target_code->get_string_constant_count(); i < n; ++i)
{
if (strcmp(m_target_code->get_string_constant(i), str_val) == 0)
return mi::Uint32(i);
}
// string not known by code
return 0u;
}
private:
mi::base::Handle<mi::neuraylib::ITransaction> m_transaction;
mi::base::Handle<const mi::neuraylib::ITarget_code> m_target_code;
std::unordered_map<mi::neuraylib::IValue_resource const*, mi::Uint32> m_resource_cache;
};
class MaterialManager::Context
{
public:
Context()
{
configurePaths();
};
~Context(){};
// paths is array of resource pathes + mdl path
bool addMdlSearchPath(const char* paths[], uint32_t numPaths)
{
mRuntime = std::make_unique<oka::MdlRuntime>();
if (!mRuntime->init(paths, numPaths, mPathso.c_str(), mImagePluginPath.c_str()))
{
return false;
}
mMtlxCodeGen = std::make_unique<oka::MtlxMdlCodeGen>(mtlxLibPath.generic_string().c_str(), mRuntime.get());
mMatCompiler = std::make_unique<oka::MdlMaterialCompiler>(*mRuntime);
mTransaction = mi::base::Handle<mi::neuraylib::ITransaction>(mRuntime->getTransaction());
mNeuray = mRuntime->getNeuray();
mCodeGen = std::make_unique<MdlPtxCodeGen>();
mCodeGen->init(*mRuntime);
std::vector<char> data;
{
const fs::path cwdPath = fs::current_path();
const fs::path precompiledPath = cwdPath / "optix/shaders/OptixRender_radiance_closest_hit.bc";
std::ifstream file(precompiledPath.c_str(), std::ios::in | std::ios::binary);
if (!file.is_open())
{
STRELKA_FATAL("Cannot open precompiled closest hit file.\n");
return false;
}
file.seekg(0, std::ios::end);
data.resize(file.tellg());
file.seekg(0, std::ios::beg);
file.read(data.data(), data.size());
}
if (!mCodeGen->setOptionBinary("llvm_renderer_module", data.data(), data.size()))
{
STRELKA_ERROR("Unable to set binary options!");
return false;
}
return true;
}
Module* createMtlxModule(const char* mtlSrc)
{
assert(mMtlxCodeGen);
std::unique_ptr<Module> module = std::make_unique<Module>();
bool res = mMtlxCodeGen->translate(mtlSrc, mMdlSrc, module->identifier);
if (res)
{
std::string mtlxFile = "./data/materials/mtlx/" + module->identifier + ".mtlx";
std::string mdlFile = "./data/materials/mtlx/" + module->identifier + ".mdl";
auto dumpToFile = [](const std::string& fileName, const std::string& content) {
std::ofstream material(fileName.c_str());
if (!material.is_open())
{
STRELKA_ERROR("can not create file: {}", fileName.c_str());
return;
}
material << content;
material.close();
return;
};
dumpToFile(mtlxFile, mtlSrc);
dumpToFile(mdlFile, mMdlSrc);
if (!mMatCompiler->createModule(module->identifier, module->moduleName))
{
STRELKA_ERROR("failed to create MDL module: {} identifier: {}", module->moduleName.c_str(),
module->identifier.c_str());
return nullptr;
}
return module.release();
}
else
{
STRELKA_ERROR("failed to translate MaterialX -> MDL");
return nullptr;
}
};
Module* createModule(const char* file)
{
std::unique_ptr<Module> module = std::make_unique<Module>();
const fs::path materialFile = file;
module->identifier = materialFile.stem().string();
if (!mMatCompiler->createModule(module->identifier, module->moduleName))
{
return nullptr;
}
return module.release();
};
void destroyModule(Module* module)
{
assert(module);
delete module;
};
MaterialInstance* createMaterialInstance(MaterialManager::Module* module, const char* materialName)
{
assert(module);
assert(materialName);
std::unique_ptr<MaterialInstance> material = std::make_unique<MaterialInstance>();
if (strcmp(materialName, "") == 0) // mtlx
{
materialName = module->identifier.c_str();
}
if (!mMatCompiler->createMaterialInstace(module->moduleName.c_str(), materialName, material->instance))
{
return nullptr;
}
return material.release();
}
void destroyMaterialInstance(MaterialInstance* matInst)
{
assert(matInst);
delete matInst;
}
void dumpParams(const TargetCode* targetCode, uint32_t materialIdx, CompiledMaterial* material)
{
assert(targetCode);
assert(targetCode->isInitialized);
assert(material);
const uint32_t internalIndex = materialIdx;// targetCode->ptrToInternalIndex.at(material);
const mi::Size argLayoutIndex = targetCode->internalMaterials[internalIndex].argument_block_layout_index;
mi::base::Handle<const mi::neuraylib::ITarget_value_layout> arg_layout(
targetCode->internalMaterials[internalIndex].targetCode->get_argument_block_layout(argLayoutIndex));
if (targetCode->internalMaterials[internalIndex].arg_block == nullptr)
{
STRELKA_ERROR("Material : {} has no arg block!\n", material->name.c_str());
return;
}
char* baseArgBlockData = targetCode->internalMaterials[internalIndex].arg_block->get_data();
const mi::Size argBlockOffset = targetCode->internalMaterials[internalIndex].arg_block_offset;
const mi::Size paramCount = material->compiledMaterial->get_parameter_count();
STRELKA_DEBUG("Material name: {0}\t param count: {1}", material->name.c_str(), paramCount);
for (int pi = 0; pi < paramCount; ++pi)
{
const char* name = material->compiledMaterial->get_parameter_name(pi);
STRELKA_DEBUG("# {0}: Param name: {1}", pi, name);
mi::neuraylib::Target_value_layout_state valLayoutState = arg_layout->get_nested_state(pi);
mi::neuraylib::IValue::Kind kind;
mi::Size arg_size;
const mi::Size offsetInArgBlock = arg_layout->get_layout(kind, arg_size, valLayoutState);
STRELKA_DEBUG("\t offset = {0} \t size = {1}", offsetInArgBlock, arg_size);
// char* data = baseArgBlockData + offsetInArgBlock;
const uint8_t* data = targetCode->argBlockData.data() + argBlockOffset + offsetInArgBlock;
switch (kind)
{
case mi::neuraylib::IValue::Kind::VK_FLOAT: {
float val = *((float*)data);
STRELKA_DEBUG("\t type float");
STRELKA_DEBUG("\t val = {}", val);
break;
}
case mi::neuraylib::IValue::Kind::VK_INT: {
int val = *((int*)data);
STRELKA_DEBUG("\t type int");
STRELKA_DEBUG("\t val = {}", val);
break;
}
case mi::neuraylib::IValue::Kind::VK_BOOL: {
bool val = *((bool*)data);
STRELKA_DEBUG("\t type bool");
STRELKA_DEBUG("\t val = {}", val);
break;
}
case mi::neuraylib::IValue::Kind::VK_COLOR: {
float* val = (float*)data;
STRELKA_DEBUG("\t type color");
STRELKA_DEBUG("\t val = ({}, {}, {})", val[0], val[1], val[2]);
break;
}
case mi::neuraylib::IValue::Kind::VK_TEXTURE: {
STRELKA_DEBUG("\t type texture");
int val = *((int*)data);
STRELKA_DEBUG("\t val = {}", val);
break;
}
default:
break;
}
// uint8_t* dst = targetCode->argBlockData.data() + argBlockOffset + offsetInArgBlock;
// memcpy(dst, param.value.data(), param.value.size());
// isParamFound = true;
}
}
bool setParam(TargetCode* targetCode, uint32_t materialIdx, CompiledMaterial* material, const Param& param)
{
assert(targetCode);
assert(targetCode->isInitialized);
assert(material);
bool isParamFound = false;
for (int pi = 0; pi < material->compiledMaterial->get_parameter_count(); ++pi)
{
if (!strcmp(material->compiledMaterial->get_parameter_name(pi), param.name.c_str()))
{
const uint32_t internalIndex = materialIdx; //targetCode->ptrToInternalIndex[material];
const mi::Size argLayoutIndex = targetCode->internalMaterials[internalIndex].argument_block_layout_index;
mi::base::Handle<const mi::neuraylib::ITarget_value_layout> arg_layout(
targetCode->internalMaterials[internalIndex].targetCode->get_argument_block_layout(argLayoutIndex));
mi::neuraylib::Target_value_layout_state valLayoutState = arg_layout->get_nested_state(pi);
mi::neuraylib::IValue::Kind kind;
mi::Size arg_size;
const mi::Size offsetInArgBlock = arg_layout->get_layout(kind, arg_size, valLayoutState);
assert(arg_size == param.value.size()); // TODO: should match, otherwise error
const mi::Size argBlockOffset = targetCode->internalMaterials[internalIndex].arg_block_offset;
uint8_t* dst = targetCode->argBlockData.data() + argBlockOffset + offsetInArgBlock;
memcpy(dst, param.value.data(), param.value.size());
isParamFound = true;
break;
}
}
return isParamFound;
}
TextureDescription* createTextureDescription(const char* name, const char* gammaMode)
{
assert(name);
float gamma = 1.0;
const char* texGamma = "";
if (strcmp(gammaMode, "srgb") == 0)
{
gamma = 2.2f;
texGamma = "_srgb";
}
else if (strcmp(gammaMode, "linear") == 0)
{
gamma = 1.0f;
texGamma = "_linear";
}
std::string textureDbName = std::string(name) + std::string(texGamma);
mi::base::Handle<const mi::neuraylib::ITexture> texAccess = mi::base::Handle<const mi::neuraylib::ITexture>(
mMatCompiler->getTransaction()->access<mi::neuraylib::ITexture>(textureDbName.c_str()));
// check if it is in DB
if (!texAccess.is_valid_interface())
{
// Load it
mi::base::Handle<mi::neuraylib::ITexture> tex(
mMatCompiler->getTransaction()->create<mi::neuraylib::ITexture>("Texture"));
mi::base::Handle<mi::neuraylib::IImage> image(
mMatCompiler->getTransaction()->create<mi::neuraylib::IImage>("Image"));
if (image->reset_file(name) != 0)
{
// TODO: report error could not find texture!
return nullptr;
}
std::string imageName = textureDbName + std::string("_image");
mMatCompiler->getTransaction()->store(image.get(), imageName.c_str());
tex->set_image(imageName.c_str());
tex->set_gamma(gamma);
mMatCompiler->getTransaction()->store(tex.get(), textureDbName.c_str());
}
mi::base::Handle<mi::neuraylib::IType_factory> typeFactory(
mMatCompiler->getFactory()->create_type_factory(mMatCompiler->getTransaction().get()));
// TODO: texture could be 1D, 3D
mi::base::Handle<const mi::neuraylib::IType_texture> textureType(
typeFactory->create_texture(mi::neuraylib::IType_texture::TS_2D));
TextureDescription* texDesc = new TextureDescription;
texDesc->dbName = textureDbName;
texDesc->textureType = textureType;
return texDesc;
}
const char* getTextureDbName(TextureDescription* texDesc)
{
return texDesc->dbName.c_str();
}
CompiledMaterial* compileMaterial(MaterialInstance* matInstance)
{
assert(matInstance);
std::unique_ptr<CompiledMaterial> material = std::make_unique<CompiledMaterial>();
if (!mMatCompiler->compileMaterial(matInstance->instance, material->compiledMaterial))
{
return nullptr;
}
material->name = matInstance->instance->get_function_definition();
return material.release();
};
void destroyCompiledMaterial(CompiledMaterial* materials)
{
assert(materials);
delete materials;
}
const char* getName(CompiledMaterial* compMaterial)
{
return compMaterial->name.c_str();
}
TargetCode* generateTargetCode(CompiledMaterial** materials, const uint32_t numMaterials)
{
TargetCode* targetCode = new TargetCode;
std::unordered_map<uint32_t, uint32_t> uidToFunctionNum;
std::vector<TargetCode::InternalMaterial>& internalMaterials = targetCode->internalMaterials;
internalMaterials.resize(numMaterials);
std::vector<const mi::neuraylib::ICompiled_material*> materialsToCompile;
for (int i = 0; i < numMaterials; ++i)
{
internalMaterials[i].compiledMaterial = materials[i];
internalMaterials[i].hash = materials[i]->compiledMaterial->get_hash();
internalMaterials[i].hash32 = uuid_hash32(internalMaterials[i].hash);
uint32_t functionNum = -1;
bool isUnique = false;
if (uidToFunctionNum.find(internalMaterials[i].hash32) != uidToFunctionNum.end())
{
functionNum = uidToFunctionNum[internalMaterials[i].hash32];
}
else
{
functionNum = uidToFunctionNum.size();
uidToFunctionNum[internalMaterials[i].hash32] = functionNum;
isUnique = true;
materialsToCompile.push_back(internalMaterials[i].compiledMaterial->compiledMaterial.get());
// log output
STRELKA_DEBUG("Material to compile: {}", getName(internalMaterials[i].compiledMaterial));
}
internalMaterials[i].isUnique = isUnique;
internalMaterials[i].functionNum = functionNum;
targetCode->uidToInternalIndex[internalMaterials[i].hash32] = i;
targetCode->ptrToInternalIndex[materials[i]] = i;
}
const uint32_t numMaterialsToCompile = materialsToCompile.size();
STRELKA_INFO("Num Materials to compile: {}", numMaterialsToCompile);
std::vector<oka::MaterialManager::TargetCode::InternalMaterial> compiledInternalMaterials(numMaterialsToCompile);
for (int i = 0; i < numMaterialsToCompile; ++i)
{
oka::MdlPtxCodeGen::InternalMaterialInfo internalsInfo;
compiledInternalMaterials[i].targetCode =
mCodeGen->translate(materialsToCompile[i], compiledInternalMaterials[i].codePtx, internalsInfo);
compiledInternalMaterials[i].argument_block_layout_index = internalsInfo.argument_block_index;
}
targetCode->mdlMaterials.resize(numMaterials);
for (uint32_t i = 0; i < numMaterials; ++i)
{
const uint32_t compiledOrder = internalMaterials[i].functionNum;
assert(compiledOrder < numMaterialsToCompile);
internalMaterials[i].argument_block_layout_index = compiledInternalMaterials[compiledOrder].argument_block_layout_index;
internalMaterials[i].targetCode = compiledInternalMaterials[compiledOrder].targetCode;
internalMaterials[i].codePtx = compiledInternalMaterials[compiledOrder].codePtx;
targetCode->mdlMaterials[i].functionId = compiledOrder;
}
targetCode->argBlockData = loadArgBlocks(targetCode);
targetCode->roData = loadROData(targetCode);
// uint32_t texCount = targetCode->targetCode->get_texture_count();
// if (texCount > 0)
// {
// targetCode->resourceInfo.resize(texCount);
// for (uint32_t i = 0; i < texCount; ++i)
// {
// targetCode->resourceInfo[i].gpu_resource_array_start = i;
// const char* texUrl = targetCode->targetCode->get_texture_url(i);
// const int texBody = targetCode->targetCode->get_body_texture_count();
// const char* texName = getTextureName(targetCode, i);
// // const float* data = getTextureData(targetCode, i);
// // uint32_t width = getTextureWidth(targetCode, i);
// // uint32_t height = getTextureHeight(targetCode, i);
// // const char* type = getTextureType(targetCode, i);
// printf("Material texture name: %s\n", texName);
// }
// }
// else
//{
// targetCode->resourceInfo.resize(1);
// }
targetCode->isInitialized = true;
return targetCode;
};
int registerResource(TargetCode* targetCode, int index)
{
Mdl_resource_info ri{ 0 };
ri.gpu_resource_array_start = index;
targetCode->resourceInfo.push_back(ri);
return (int)targetCode->resourceInfo.size(); // resource id 0 is reserved for invalid, but we store resources
// from 0 internally
}
const char* getShaderCode(const TargetCode* targetCode, uint32_t materialIdx)
{
const uint32_t compiledOrder = targetCode->internalMaterials[materialIdx].functionNum;
return targetCode->internalMaterials[compiledOrder].codePtx.c_str();
}
uint32_t getArgBlockOffset(const TargetCode* targetCode, uint32_t materialId)
{
return targetCode->internalMaterials[materialId].arg_block_offset;
}
uint32_t getReadOnlyBlockOffset(const TargetCode* targetCode, uint32_t materialId)
{
return targetCode->internalMaterials[materialId].ro_offset;
}
uint32_t getReadOnlyBlockSize(const TargetCode* shaderCode)
{
return shaderCode->roData.size();
}
const uint8_t* getReadOnlyBlockData(const TargetCode* targetCode)
{
return targetCode->roData.data();
}
uint32_t getArgBufferSize(const TargetCode* shaderCode)
{
return shaderCode->argBlockData.size();
}
const uint8_t* getArgBufferData(const TargetCode* targetCode)
{
return targetCode->argBlockData.data();
}
uint32_t getResourceInfoSize(const TargetCode* targetCode)
{
return targetCode->resourceInfo.size() * sizeof(Mdl_resource_info);
}
const uint8_t* getResourceInfoData(const TargetCode* targetCode)
{
return reinterpret_cast<const uint8_t*>(targetCode->resourceInfo.data());
}
uint32_t getMdlMaterialSize(const TargetCode* targetCode)
{
return targetCode->mdlMaterials.size() * sizeof(MdlMaterial);
}
const uint8_t* getMdlMaterialData(const TargetCode* targetCode)
{
return reinterpret_cast<const uint8_t*>(targetCode->mdlMaterials.data());
}
uint32_t getTextureCount(const TargetCode* targetCode, uint32_t materialId)
{
return targetCode->internalMaterials[materialId].targetCode->get_texture_count();
}
const char* getTextureName(const TargetCode* targetCode, uint32_t materialId, uint32_t index)
{
assert(index); // index == 0 is invalid
return targetCode->internalMaterials[materialId].targetCode->get_texture(index);
}
const float* getTextureData(const TargetCode* targetCode, uint32_t materialId, uint32_t index)
{
// assert(index); // index == 0 is invalid
assert(mTransaction);
auto cachedCanvas = m_indexToCanvas.find(index);
if (cachedCanvas == m_indexToCanvas.end())
{
mi::base::Handle<mi::neuraylib::IImage_api> image_api(mNeuray->get_api_component<mi::neuraylib::IImage_api>());
mi::base::Handle<const mi::neuraylib::ITexture> texture(mTransaction->access<mi::neuraylib::ITexture>(
targetCode->internalMaterials[materialId].targetCode->get_texture(index)));
mi::base::Handle<const mi::neuraylib::IImage> image(
mTransaction->access<mi::neuraylib::IImage>(texture->get_image()));
mi::base::Handle<const mi::neuraylib::ICanvas> canvas(image->get_canvas(0, 0, 0));
char const* image_type = image->get_type(0, 0);
// if (canvas->get_tiles_size_x() != 1 || canvas->get_tiles_size_y() != 1)
// {
// mLogger->message(mi::base::MESSAGE_SEVERITY_ERROR, "The example does not support tiled images!");
// return nullptr;
// }
if (texture->get_effective_gamma(0, 0) != 1.0f)
{
// Copy/convert to float4 canvas and adjust gamma from "effective gamma" to 1.
mi::base::Handle<mi::neuraylib::ICanvas> gamma_canvas(image_api->convert(canvas.get(), "Color"));
gamma_canvas->set_gamma(texture->get_effective_gamma(0, 0));
image_api->adjust_gamma(gamma_canvas.get(), 1.0f);
canvas = gamma_canvas;
}
else if (strcmp(image_type, "Color") != 0 && strcmp(image_type, "Float32<4>") != 0)
{
// Convert to expected format
canvas = image_api->convert(canvas.get(), "Color");
}
m_indexToCanvas[index] = canvas;
cachedCanvas = m_indexToCanvas.find(index);
}
mi::Float32 const* data = nullptr;
mi::neuraylib::ITarget_code::Texture_shape texture_shape =
targetCode->internalMaterials[materialId].targetCode->get_texture_shape(index);
if (texture_shape == mi::neuraylib::ITarget_code::Texture_shape_2d)
{
mi::base::Handle<const mi::neuraylib::ITile> tile(cachedCanvas->second->get_tile());
data = static_cast<mi::Float32 const*>(tile->get_data());
}
return data;
}
const char* getTextureType(const TargetCode* targetCode, uint32_t materialId, uint32_t index)
{
// assert(index); // index == 0 is invalid
mi::base::Handle<const mi::neuraylib::ITexture> texture(mTransaction->access<mi::neuraylib::ITexture>(
targetCode->internalMaterials[materialId].targetCode->get_texture(index)));
mi::base::Handle<const mi::neuraylib::IImage> image(
mTransaction->access<mi::neuraylib::IImage>(texture->get_image()));
char const* imageType = image->get_type(0, 0);
return imageType;
}
uint32_t getTextureWidth(const TargetCode* targetCode, uint32_t materialId, uint32_t index)
{
// assert(index); // index == 0 is invalid
mi::base::Handle<const mi::neuraylib::ITexture> texture(mTransaction->access<mi::neuraylib::ITexture>(
targetCode->internalMaterials[materialId].targetCode->get_texture(index)));
mi::base::Handle<const mi::neuraylib::IImage> image(
mTransaction->access<mi::neuraylib::IImage>(texture->get_image()));
mi::base::Handle<const mi::neuraylib::ICanvas> canvas(image->get_canvas(0, 0, 0));
mi::Uint32 texWidth = canvas->get_resolution_x();
return texWidth;
}
uint32_t getTextureHeight(const TargetCode* targetCode, uint32_t materialId, uint32_t index)
{
// assert(index); // index == 0 is invalid
mi::base::Handle<const mi::neuraylib::ITexture> texture(mTransaction->access<mi::neuraylib::ITexture>(
targetCode->internalMaterials[materialId].targetCode->get_texture(index)));
mi::base::Handle<const mi::neuraylib::IImage> image(
mTransaction->access<mi::neuraylib::IImage>(texture->get_image()));
mi::base::Handle<const mi::neuraylib::ICanvas> canvas(image->get_canvas(0, 0, 0));
mi::Uint32 texHeight = canvas->get_resolution_y();
return texHeight;
}
uint32_t getTextureBytesPerTexel(const TargetCode* targetCode, uint32_t materialId, uint32_t index)
{
// assert(index); // index == 0 is invalid
mi::base::Handle<mi::neuraylib::IImage_api> image_api(mNeuray->get_api_component<mi::neuraylib::IImage_api>());
mi::base::Handle<const mi::neuraylib::ITexture> texture(mTransaction->access<mi::neuraylib::ITexture>(
targetCode->internalMaterials[materialId].targetCode->get_texture(index)));
mi::base::Handle<const mi::neuraylib::IImage> image(
mTransaction->access<mi::neuraylib::IImage>(texture->get_image()));
char const* imageType = image->get_type(0, 0);
int cpp = image_api->get_components_per_pixel(imageType);
int bpc = image_api->get_bytes_per_component(imageType);
int bpp = cpp * bpc;
return bpp;
}
private:
std::string mImagePluginPath;
std::string mPathso;
std::string mMdlSrc;
fs::path mtlxLibPath;
std::unordered_map<uint32_t, mi::base::Handle<const mi::neuraylib::ICanvas>> m_indexToCanvas;
void configurePaths()
{
using namespace std;
const fs::path cwd = fs::current_path();
#ifdef MI_PLATFORM_WINDOWS
mPathso = cwd.string();
mImagePluginPath = cwd.string() + "/nv_openimageio.dll";
#else
mPathso = cwd.string();
mImagePluginPath = cwd.string() + "/nv_openimageio.so";
#endif
}
std::unique_ptr<oka::MdlPtxCodeGen> mCodeGen = nullptr;
std::unique_ptr<oka::MdlMaterialCompiler> mMatCompiler = nullptr;
std::unique_ptr<oka::MdlRuntime> mRuntime = nullptr;
std::unique_ptr<oka::MtlxMdlCodeGen> mMtlxCodeGen = nullptr;
mi::base::Handle<mi::neuraylib::ITransaction> mTransaction;
mi::base::Handle<oka::MdlLogger> mLogger;
mi::base::Handle<mi::neuraylib::INeuray> mNeuray;
std::vector<uint8_t> loadArgBlocks(TargetCode* targetCode);
std::vector<uint8_t> loadROData(TargetCode* targetCode);
};
MaterialManager::MaterialManager()
{
mContext = std::make_unique<Context>();
}
MaterialManager::~MaterialManager()
{
mContext.reset(nullptr);
};
bool MaterialManager::addMdlSearchPath(const char* paths[], uint32_t numPaths)
{
return mContext->addMdlSearchPath(paths, numPaths);
}
MaterialManager::Module* MaterialManager::createModule(const char* file)
{
return mContext->createModule(file);
}
MaterialManager::Module* MaterialManager::createMtlxModule(const char* file)
{
return mContext->createMtlxModule(file);
}
void MaterialManager::destroyModule(MaterialManager::Module* module)
{
return mContext->destroyModule(module);
}
MaterialManager::MaterialInstance* MaterialManager::createMaterialInstance(MaterialManager::Module* module,
const char* materialName)
{
return mContext->createMaterialInstance(module, materialName);
}
void MaterialManager::destroyMaterialInstance(MaterialManager::MaterialInstance* matInst)
{
return mContext->destroyMaterialInstance(matInst);
}
MaterialManager::TextureDescription* MaterialManager::createTextureDescription(const char* name, const char* gamma)
{
return mContext->createTextureDescription(name, gamma);
}
const char* MaterialManager::getTextureDbName(TextureDescription* texDesc)
{
return mContext->getTextureDbName(texDesc);
}
void MaterialManager::dumpParams(const TargetCode* targetCode, uint32_t materialIdx, CompiledMaterial* material)
{
return mContext->dumpParams(targetCode, materialIdx, material);
}
bool MaterialManager::setParam(TargetCode* targetCode, uint32_t materialIdx, CompiledMaterial* material, const Param& param)
{
return mContext->setParam(targetCode, materialIdx, material, param);
}
MaterialManager::CompiledMaterial* MaterialManager::compileMaterial(MaterialManager::MaterialInstance* matInstance)
{
return mContext->compileMaterial(matInstance);
}
void MaterialManager::destroyCompiledMaterial(MaterialManager::CompiledMaterial* material)
{
return mContext->destroyCompiledMaterial(material);
}
const char* MaterialManager::getName(CompiledMaterial* compMaterial)
{
return mContext->getName(compMaterial);
}
MaterialManager::TargetCode* MaterialManager::generateTargetCode(CompiledMaterial** materials, uint32_t numMaterials)
{
return mContext->generateTargetCode(materials, numMaterials);
}
const char* MaterialManager::getShaderCode(const TargetCode* targetCode, uint32_t materialId) // get shader code
{
return mContext->getShaderCode(targetCode, materialId);
}
uint32_t MaterialManager::getReadOnlyBlockSize(const TargetCode* targetCode)
{
return mContext->getReadOnlyBlockSize(targetCode);
}
const uint8_t* MaterialManager::getReadOnlyBlockData(const TargetCode* targetCode)
{
return mContext->getReadOnlyBlockData(targetCode);
}
uint32_t MaterialManager::getArgBufferSize(const TargetCode* targetCode)
{
return mContext->getArgBufferSize(targetCode);
}
const uint8_t* MaterialManager::getArgBufferData(const TargetCode* targetCode)
{
return mContext->getArgBufferData(targetCode);
}
uint32_t MaterialManager::getArgBlockOffset(const TargetCode* targetCode, uint32_t materialId)
{
return mContext->getArgBlockOffset(targetCode, materialId);
}
uint32_t MaterialManager::getReadOnlyOffset(const TargetCode* targetCode, uint32_t materialId)
{
return mContext->getArgBlockOffset(targetCode, materialId);
}
uint32_t MaterialManager::getResourceInfoSize(const TargetCode* targetCode)
{
return mContext->getResourceInfoSize(targetCode);
}
const uint8_t* MaterialManager::getResourceInfoData(const TargetCode* targetCode)
{
return mContext->getResourceInfoData(targetCode);
}
int MaterialManager::registerResource(TargetCode* targetCode, int index)
{
return mContext->registerResource(targetCode, index);
}
uint32_t MaterialManager::getMdlMaterialSize(const TargetCode* targetCode)
{
return mContext->getMdlMaterialSize(targetCode);
}
const uint8_t* MaterialManager::getMdlMaterialData(const TargetCode* targetCode)
{
return mContext->getMdlMaterialData(targetCode);
}
uint32_t MaterialManager::getTextureCount(const TargetCode* targetCode, uint32_t materialId)
{
return mContext->getTextureCount(targetCode, materialId);
}
const char* MaterialManager::getTextureName(const TargetCode* targetCode, uint32_t materialId, uint32_t index)
{
return mContext->getTextureName(targetCode, materialId, index);
}
const float* MaterialManager::getTextureData(const TargetCode* targetCode, uint32_t materialId, uint32_t index)
{
return mContext->getTextureData(targetCode, materialId, index);
}
const char* MaterialManager::getTextureType(const TargetCode* targetCode, uint32_t materialId, uint32_t index)
{
return mContext->getTextureType(targetCode, materialId, index);
}
uint32_t MaterialManager::getTextureWidth(const TargetCode* targetCode, uint32_t materialId, uint32_t index)
{
return mContext->getTextureWidth(targetCode, materialId, index);
}
uint32_t MaterialManager::getTextureHeight(const TargetCode* targetCode, uint32_t materialId, uint32_t index)
{
return mContext->getTextureHeight(targetCode, materialId, index);
}
uint32_t MaterialManager::getTextureBytesPerTexel(const TargetCode* targetCode, uint32_t materialId, uint32_t index)
{
return mContext->getTextureBytesPerTexel(targetCode, materialId, index);
}
inline size_t round_to_power_of_two(size_t value, size_t power_of_two_factor)
{
return (value + (power_of_two_factor - 1)) & ~(power_of_two_factor - 1);
}
std::vector<uint8_t> MaterialManager::Context::loadArgBlocks(TargetCode* targetCode)
{
std::vector<uint8_t> res;
for (int i = 0; i < targetCode->internalMaterials.size(); ++i)
{
mi::base::Handle<const mi::neuraylib::ITarget_code>& mdlTargetCode = targetCode->internalMaterials[i].targetCode;
mi::base::Handle<Resource_callback> callback(new Resource_callback(mTransaction, mdlTargetCode));
// const uint32_t compiledIndex = targetCode->internalMaterials[i].functionNum;
const mi::Size argLayoutIndex = targetCode->internalMaterials[i].argument_block_layout_index;
const mi::Size layoutCount = mdlTargetCode->get_argument_layout_count();
if (argLayoutIndex != static_cast<mi::Size>(-1) && argLayoutIndex < layoutCount)
{
mi::neuraylib::ICompiled_material* compiledMaterial =
targetCode->internalMaterials[i].compiledMaterial->compiledMaterial.get();
targetCode->internalMaterials[i].arg_block =
mdlTargetCode->create_argument_block(argLayoutIndex, compiledMaterial, callback.get());
if (!targetCode->internalMaterials[i].arg_block)
{
std::cerr << ("Failed to create material argument block: ") << std::endl;
res.resize(4);
return res;
}
// create a buffer to provide those parameters to the shader
// align to 4 bytes and pow of two
const size_t buffer_size = round_to_power_of_two(targetCode->internalMaterials[i].arg_block->get_size(), 4);
std::vector<uint8_t> argBlockData = std::vector<uint8_t>(buffer_size, 0);
memcpy(argBlockData.data(), targetCode->internalMaterials[i].arg_block->get_data(),
targetCode->internalMaterials[i].arg_block->get_size());
// set offset in common arg block buffer
targetCode->internalMaterials[i].arg_block_offset = (uint32_t)res.size();
targetCode->mdlMaterials[i].arg_block_offset = (int)res.size();
res.insert(res.end(), argBlockData.begin(), argBlockData.end());
}
}
if (res.empty())
{
res.resize(4);
}
return res;
}
std::vector<uint8_t> MaterialManager::Context::loadROData(TargetCode* targetCode)
{
std::vector<uint8_t> roData;
for (int i = 0; i < targetCode->internalMaterials.size(); ++i)
{
const size_t segCount = targetCode->internalMaterials[i].targetCode->get_ro_data_segment_count();
targetCode->internalMaterials[i].ro_offset = roData.size();
for (size_t s = 0; s < segCount; ++s)
{
const char* data = targetCode->internalMaterials[i].targetCode->get_ro_data_segment_data(s);
size_t dataSize = targetCode->internalMaterials[i].targetCode->get_ro_data_segment_size(s);
const char* name = targetCode->internalMaterials[i].targetCode->get_ro_data_segment_name(s);
std::cerr << "MDL segment [" << s << "] name : " << name << std::endl;
if (dataSize != 0)
{
roData.insert(roData.end(), data, data + dataSize);
}
}
}
if (roData.empty())
{
roData.resize(4);
}
return roData;
}
} // namespace oka
| 41,296 | C++ | 37.096863 | 132 | 0.627809 |
arhix52/Strelka/src/materialmanager/mdlPtxCodeGen.cpp | #include "mdlPtxCodeGen.h"
#include "materials.h"
#include <mi/mdl_sdk.h>
#include <cassert>
#include <sstream>
#include <log.h>
namespace oka
{
const char* SCATTERING_FUNC_NAME = "mdl_bsdf_scattering";
const char* EMISSION_FUNC_NAME = "mdl_edf_emission";
const char* EMISSION_INTENSITY_FUNC_NAME = "mdl_edf_emission_intensity";
const char* MATERIAL_STATE_NAME = "Shading_state_material";
bool MdlPtxCodeGen::init(MdlRuntime& runtime)
{
mi::base::Handle<mi::neuraylib::IMdl_backend_api> backendApi(runtime.getBackendApi());
mBackend = mi::base::Handle<mi::neuraylib::IMdl_backend>(
backendApi->get_backend(mi::neuraylib::IMdl_backend_api::MB_CUDA_PTX));
if (!mBackend.is_valid_interface())
{
mLogger->message(mi::base::MESSAGE_SEVERITY_FATAL, "CUDA backend not supported by MDL runtime");
return false;
}
// 75 - Turing
// 86 - Ampere
// 89 - Ada
if (mBackend->set_option("sm_version", "75") != 0)
{
mLogger->message(mi::base::MESSAGE_SEVERITY_FATAL, "ERROR: Setting PTX option sm_version failed");
return false;
}
if (mBackend->set_option("num_texture_spaces", "2") != 0)
{
mLogger->message(mi::base::MESSAGE_SEVERITY_FATAL, "ERROR: Setting PTX option num_texture_spaces failed");
return false;
}
if (mBackend->set_option("num_texture_results", "16") != 0)
{
mLogger->message(mi::base::MESSAGE_SEVERITY_FATAL, "ERROR: Setting PTX option num_texture_results failed");
return false;
}
if (mBackend->set_option("tex_lookup_call_mode", "direct_call") != 0)
{
mLogger->message(mi::base::MESSAGE_SEVERITY_FATAL, "ERROR: Setting PTX option tex_lookup_call_mode failed");
return false;
}
mLogger = mi::base::Handle<MdlLogger>(runtime.getLogger());
mLoader = std::move(runtime.mLoader);
mi::base::Handle<mi::neuraylib::IMdl_factory> factory(runtime.getFactory());
mContext = mi::base::Handle<mi::neuraylib::IMdl_execution_context>(factory->create_execution_context());
mDatabase = mi::base::Handle<mi::neuraylib::IDatabase>(runtime.getDatabase());
mTransaction = mi::base::Handle<mi::neuraylib::ITransaction>(runtime.getTransaction());
return true;
}
mi::base::Handle<const mi::neuraylib::ITarget_code> MdlPtxCodeGen::translate(
const mi::neuraylib::ICompiled_material* material, std::string& ptxSrc, InternalMaterialInfo& internalsInfo)
{
assert(material);
mi::base::Handle<mi::neuraylib::ILink_unit> linkUnit(mBackend->create_link_unit(mTransaction.get(), mContext.get()));
mLogger->flushContextMessages(mContext.get());
if (!linkUnit)
{
throw "Failed to create link unit";
}
mi::Size argBlockIndex;
if (!appendMaterialToLinkUnit(0, material, linkUnit.get(), argBlockIndex))
{
throw "Failed to append material to the link unit";
}
internalsInfo.argument_block_index = argBlockIndex;
mi::base::Handle<const mi::neuraylib::ITarget_code> targetCode(
mBackend->translate_link_unit(linkUnit.get(), mContext.get()));
mLogger->flushContextMessages(mContext.get());
if (!targetCode)
{
throw "No target code";
}
ptxSrc = targetCode->get_code();
return targetCode;
}
mi::base::Handle<const mi::neuraylib::ITarget_code> MdlPtxCodeGen::translate(
const std::vector<const mi::neuraylib::ICompiled_material*>& materials,
std::string& ptxSrc,
std::vector<InternalMaterialInfo>& internalsInfo)
{
mi::base::Handle<mi::neuraylib::ILink_unit> linkUnit(mBackend->create_link_unit(mTransaction.get(), mContext.get()));
mLogger->flushContextMessages(mContext.get());
if (!linkUnit)
{
throw "Failed to create link unit";
}
uint32_t materialCount = materials.size();
internalsInfo.resize(materialCount);
mi::Size argBlockIndex;
for (uint32_t i = 0; i < materialCount; i++)
{
const mi::neuraylib::ICompiled_material* material = materials.at(i);
assert(material);
if (!appendMaterialToLinkUnit(i, material, linkUnit.get(), argBlockIndex))
{
throw "Failed to append material to the link unit";
}
internalsInfo[i].argument_block_index = argBlockIndex;
}
mi::base::Handle<const mi::neuraylib::ITarget_code> targetCode(
mBackend->translate_link_unit(linkUnit.get(), mContext.get()));
mLogger->flushContextMessages(mContext.get());
if (!targetCode)
{
throw "No target code";
}
ptxSrc = targetCode->get_code();
return targetCode;
}
bool MdlPtxCodeGen::appendMaterialToLinkUnit(uint32_t idx,
const mi::neuraylib::ICompiled_material* compiledMaterial,
mi::neuraylib::ILink_unit* linkUnit,
mi::Size& argBlockIndex)
{
std::string idxStr = std::to_string(idx);
auto scatteringFuncName = std::string("mdlcode");
auto emissionFuncName = std::string(EMISSION_FUNC_NAME) + "_" + idxStr;
auto emissionIntensityFuncName = std::string(EMISSION_INTENSITY_FUNC_NAME) + "_" + idxStr;
// Here we need to detect if current material for hair, if so, replace name in function description
mi::base::Handle<mi::neuraylib::IExpression const> hairExpr(compiledMaterial->lookup_sub_expression("hair"));
bool isHair = false;
if (hairExpr != nullptr)
{
if (hairExpr->get_kind() != mi::neuraylib::IExpression::EK_CONSTANT)
{
isHair = true;
}
}
std::vector<mi::neuraylib::Target_function_description> genFunctions;
genFunctions.push_back(mi::neuraylib::Target_function_description( isHair ? "hair" : "surface.scattering", scatteringFuncName.c_str()));
genFunctions.push_back(
mi::neuraylib::Target_function_description("surface.emission.emission", emissionFuncName.c_str()));
genFunctions.push_back(
mi::neuraylib::Target_function_description("surface.emission.intensity", emissionIntensityFuncName.c_str()));
mi::Sint32 result =
linkUnit->add_material(compiledMaterial, genFunctions.data(), genFunctions.size(), mContext.get());
mLogger->flushContextMessages(mContext.get());
if (result == 0)
{
argBlockIndex = genFunctions[0].argument_block_index;
}
return result == 0;
}
bool MdlPtxCodeGen::setOptionBinary(const char* name, const char* data, size_t size)
{
if (mBackend->set_option_binary(name, data, size) != 0)
{
return false;
}
// limit functions for which PTX code is generated to the entry functions
if (mBackend->set_option("visible_functions", "__closesthit__radiance") != 0)
{
STRELKA_ERROR("Setting PTX option visible_functions failed");
return false;
}
return true;
}
} // namespace oka
| 6,873 | C++ | 35.178947 | 140 | 0.657064 |
arhix52/Strelka/src/materialmanager/mtlxMdlCodeGen.cpp | // Copyright (C) 2021 Pablo Delgado Krämer
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program. If not, see <https://www.gnu.org/licenses/>.
#include "mtlxMdlCodeGen.h"
#include <MaterialXCore/Definition.h>
#include <MaterialXCore/Document.h>
#include <MaterialXCore/Library.h>
#include <MaterialXCore/Material.h>
#include <MaterialXFormat/File.h>
#include <MaterialXFormat/Util.h>
#include <MaterialXGenMdl/MdlShaderGenerator.h>
#include <MaterialXGenShader/DefaultColorManagementSystem.h>
#include <MaterialXGenShader/GenContext.h>
#include <MaterialXGenShader/GenOptions.h>
#include <MaterialXGenShader/Library.h>
#include <MaterialXGenShader/Shader.h>
#include <MaterialXGenShader/Util.h>
#include <unordered_set>
#include <log.h>
namespace mx = MaterialX;
namespace oka
{
class MdlStringResolver;
using MdlStringResolverPtr = std::shared_ptr<MdlStringResolver>;
// Original source from https://github.com/NVIDIA/MDL-SDK/blob/190249748ddfe75b133b9da9028cc6272928c1b5/examples/mdl_sdk/dxr/mdl_d3d12/materialx/mdl_generator.cpp#L53
class MdlStringResolver : public mx::StringResolver
{
public:
/// Create a new string resolver.
static MdlStringResolverPtr create()
{
return MdlStringResolverPtr(new MdlStringResolver());
}
~MdlStringResolver() = default;
void initialize(mx::DocumentPtr document, mi::neuraylib::IMdl_configuration* config)
{
// remove duplicates and keep order by using a set
auto less = [](const mx::FilePath& lhs, const mx::FilePath& rhs) { return lhs.asString() < rhs.asString(); };
std::set<mx::FilePath, decltype(less)> mtlx_paths(less);
m_mtlx_document_paths.clear();
m_mdl_search_paths.clear();
// use the source search paths as base
mx::FilePath p = mx::FilePath(document->getSourceUri()).getParentPath().getNormalized();
mtlx_paths.insert(p);
m_mtlx_document_paths.append(p);
for (auto sp : mx::getSourceSearchPath(document))
{
sp = sp.getNormalized();
if(sp.exists() && mtlx_paths.insert(sp).second)
m_mtlx_document_paths.append(sp);
}
// add all search paths known to MDL
for (size_t i = 0, n = config->get_mdl_paths_length(); i < n; i++)
{
mi::base::Handle<const mi::IString> sp_istring(config->get_mdl_path(i));
p = mx::FilePath(sp_istring->get_c_str()).getNormalized();
if (p.exists() && mtlx_paths.insert(p).second)
m_mtlx_document_paths.append(p);
// keep a list of MDL search paths for resource resolution
m_mdl_search_paths.append(p);
}
}
std::string resolve(const std::string& str, const std::string& type) const override
{
mx::FilePath normalizedPath = mx::FilePath(str).getNormalized();
// in case the path is absolute we need to find a proper search path to put the file in
if (normalizedPath.isAbsolute())
{
// find the highest priority search path that is a prefix of the resource path
for (const auto& sp : m_mdl_search_paths)
{
if (sp.size() > normalizedPath.size())
continue;
bool isParent = true;
for (size_t i = 0; i < sp.size(); ++i)
{
if (sp[i] != normalizedPath[i])
{
isParent = false;
break;
}
}
if (!isParent)
continue;
// found a search path that is a prefix of the resource
std::string resource_path =
normalizedPath.asString(mx::FilePath::FormatPosix).substr(
sp.asString(mx::FilePath::FormatPosix).size());
if (resource_path[0] != '/')
resource_path = "/" + resource_path;
return resource_path;
}
}
STRELKA_ERROR("MaterialX resource can not be accessed through an MDL search path. \n Dropping the resource from the Material. Resource Path: {} ", normalizedPath.asString());
// drop the resource by returning the empty string.
// alternatively, the resource could be copied into an MDL search path,
// maybe even only temporary.
return "";
}
// Get the MaterialX paths used to load the current document as well the current MDL search
// paths in order to resolve resources by the MaterialX SDK.
const mx::FileSearchPath& get_search_paths() const { return m_mtlx_document_paths; }
private:
// List of paths from which MaterialX can locate resources.
// This includes the document folder and the search paths used to load the document.
mx::FileSearchPath m_mtlx_document_paths;
// List of MDL search paths from which we can locate resources.
// This is only a subset of the MaterialX document paths and needs to be extended by using the
// `--mdl_path` option when starting the application if needed.
mx::FileSearchPath m_mdl_search_paths;
};
MtlxMdlCodeGen::MtlxMdlCodeGen(const char* mtlxlibPath, MdlRuntime* mdlRuntime)
: mMtlxlibPath(mtlxlibPath),
mMdlRuntime(mdlRuntime)
{
// Init shadergen.
mShaderGen = mx::MdlShaderGenerator::create();
std::string target = mShaderGen->getTarget();
// MaterialX libs.
mStdLib = mx::createDocument();
mx::FilePathVec libFolders;
mx::loadLibraries(libFolders, mMtlxlibPath, mStdLib);
// Color management.
mx::DefaultColorManagementSystemPtr colorSystem = mx::DefaultColorManagementSystem::create(target);
colorSystem->loadLibrary(mStdLib);
mShaderGen->setColorManagementSystem(colorSystem);
// Unit management.
mx::UnitSystemPtr unitSystem = mx::UnitSystem::create(target);
unitSystem->loadLibrary(mStdLib);
mx::UnitConverterRegistryPtr unitRegistry = mx::UnitConverterRegistry::create();
mx::UnitTypeDefPtr distanceTypeDef = mStdLib->getUnitTypeDef("distance");
unitRegistry->addUnitConverter(distanceTypeDef, mx::LinearUnitConverter::create(distanceTypeDef));
mx::UnitTypeDefPtr angleTypeDef = mStdLib->getUnitTypeDef("angle");
unitRegistry->addUnitConverter(angleTypeDef, mx::LinearUnitConverter::create(angleTypeDef));
unitSystem->setUnitConverterRegistry(unitRegistry);
mShaderGen->setUnitSystem(unitSystem);
}
mx::TypedElementPtr _FindSurfaceShaderElement(mx::DocumentPtr doc)
{
// Find renderable element.
std::vector<mx::TypedElementPtr> renderableElements;
mx::findRenderableElements(doc, renderableElements);
if (renderableElements.size() != 1)
{
return nullptr;
}
// Extract surface shader node.
mx::TypedElementPtr renderableElement = renderableElements.at(0);
mx::NodePtr node = renderableElement->asA<mx::Node>();
if (node && node->getType() == mx::MATERIAL_TYPE_STRING)
{
auto shaderNodes = mx::getShaderNodes(node, mx::SURFACE_SHADER_TYPE_STRING);
if (!shaderNodes.empty())
{
renderableElement = *shaderNodes.begin();
}
}
mx::ElementPtr surfaceElement = doc->getDescendant(renderableElement->getNamePath());
if (!surfaceElement)
{
return nullptr;
}
return surfaceElement->asA<mx::TypedElement>();
}
bool MtlxMdlCodeGen::translate(const char* mtlxSrc, std::string& mdlSrc, std::string& subIdentifier)
{
// Don't cache the context because it is thread-local.
mx::GenContext context(mShaderGen);
context.registerSourceCodeSearchPath(mMtlxlibPath);
mx::GenOptions& contextOptions = context.getOptions();
contextOptions.targetDistanceUnit = "meter";
mx::ShaderPtr shader = nullptr;
try
{
mx::DocumentPtr doc = mx::createDocument();
doc->importLibrary(mStdLib);
mx::readFromXmlString(doc, mtlxSrc); // originally from string
auto custom_resolver = MdlStringResolver::create();
custom_resolver->initialize(doc, mMdlRuntime->getConfig().get());
mx::flattenFilenames(doc, custom_resolver->get_search_paths(), custom_resolver);
mx::TypedElementPtr element = _FindSurfaceShaderElement(doc);
if (!element)
{
return false;
}
subIdentifier = element->getName();
shader = mShaderGen->generate(subIdentifier, element, context);
}
catch (const std::exception& ex)
{
STRELKA_ERROR("Exception generating MDL code: {}", ex.what());
}
if (!shader)
{
return false;
}
mx::ShaderStage pixelStage = shader->getStage(mx::Stage::PIXEL);
mdlSrc = pixelStage.getSourceCode();
return true;
}
} // namespace oka
| 9,457 | C++ | 35.517374 | 182 | 0.64936 |
arhix52/Strelka/src/materialmanager/mdlRuntime.cpp | // Copyright (C) 2021 Pablo Delgado Krämer
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program. If not, see <https://www.gnu.org/licenses/>.
#include "mdlRuntime.h"
#include <mi/mdl_sdk.h>
#include <vector>
namespace oka
{
MdlRuntime::MdlRuntime()
{
}
MdlRuntime::~MdlRuntime()
{
if (mTransaction)
{
mTransaction->commit();
}
}
bool MdlRuntime::init(const char* paths[], uint32_t numPaths, const char* neurayPath, const char* imagePluginPath)
{
mLoader = std::make_unique<MdlNeurayLoader>();
if (!mLoader->init(neurayPath, imagePluginPath))
{
return false;
}
mi::base::Handle<mi::neuraylib::INeuray> neuray(mLoader->getNeuray());
mConfig = neuray->get_api_component<mi::neuraylib::IMdl_configuration>();
mi::base::Handle<mi::neuraylib::ILogging_configuration> logging_conf(
neuray->get_api_component<mi::neuraylib::ILogging_configuration>());
mLogger = mi::base::Handle<MdlLogger>(new MdlLogger());
logging_conf->set_receiving_logger(mLogger.get());
for (uint32_t i = 0; i < numPaths; i++)
{
if (mConfig->add_mdl_path(paths[i]) != 0 || mConfig->add_resource_path(paths[i]) != 0)
{
mLogger->message(mi::base::MESSAGE_SEVERITY_FATAL, "MDL file path not found, translation not possible");
return false;
}
}
mDatabase = mi::base::Handle<mi::neuraylib::IDatabase>(neuray->get_api_component<mi::neuraylib::IDatabase>());
mi::base::Handle<mi::neuraylib::IScope> scope(mDatabase->get_global_scope());
mTransaction = mi::base::Handle<mi::neuraylib::ITransaction>(scope->create_transaction());
mFactory = mi::base::Handle<mi::neuraylib::IMdl_factory>(neuray->get_api_component<mi::neuraylib::IMdl_factory>());
mImpExpApi = mi::base::Handle<mi::neuraylib::IMdl_impexp_api>(neuray->get_api_component<mi::neuraylib::IMdl_impexp_api>());
mBackendApi = mi::base::Handle<mi::neuraylib::IMdl_backend_api>(neuray->get_api_component<mi::neuraylib::IMdl_backend_api>());
return true;
}
mi::base::Handle<mi::neuraylib::IMdl_configuration> MdlRuntime::getConfig()
{
return mConfig;
}
mi::base::Handle<mi::neuraylib::INeuray> MdlRuntime::getNeuray()
{
return mLoader->getNeuray();
}
mi::base::Handle<MdlLogger> MdlRuntime::getLogger()
{
return mLogger;
}
mi::base::Handle<mi::neuraylib::IDatabase> MdlRuntime::getDatabase()
{
return mDatabase;
}
mi::base::Handle<mi::neuraylib::ITransaction> MdlRuntime::getTransaction()
{
return mTransaction;
}
mi::base::Handle<mi::neuraylib::IMdl_factory> MdlRuntime::getFactory()
{
return mFactory;
}
mi::base::Handle<mi::neuraylib::IMdl_impexp_api> MdlRuntime::getImpExpApi()
{
return mImpExpApi;
}
mi::base::Handle<mi::neuraylib::IMdl_backend_api> MdlRuntime::getBackendApi()
{
return mBackendApi;
}
} // namespace oka
| 3,511 | C++ | 30.639639 | 130 | 0.668755 |
arhix52/Strelka/src/materialmanager/mdlLogger.cpp | // Copyright (C) 2021 Pablo Delgado Krämer
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program. If not, see <https://www.gnu.org/licenses/>.
#include "mdlLogger.h"
#include <log.h>
namespace oka
{
const char* _miMessageSeverityToCStr(mi::base::Message_severity severity)
{
switch (severity)
{
case mi::base::MESSAGE_SEVERITY_FATAL:
return "fatal";
case mi::base::MESSAGE_SEVERITY_ERROR:
return "error";
case mi::base::MESSAGE_SEVERITY_WARNING:
return "warning";
case mi::base::MESSAGE_SEVERITY_INFO:
return "info";
case mi::base::MESSAGE_SEVERITY_VERBOSE:
return "verbose";
case mi::base::MESSAGE_SEVERITY_DEBUG:
return "debug";
default:
break;
}
return "";
}
const char* _miMessageKindToCStr(mi::neuraylib::IMessage::Kind kind)
{
switch (kind)
{
case mi::neuraylib::IMessage::MSG_INTEGRATION:
return "MDL SDK";
case mi::neuraylib::IMessage::MSG_IMP_EXP:
return "Importer/Exporter";
case mi::neuraylib::IMessage::MSG_COMILER_BACKEND:
return "Compiler Backend";
case mi::neuraylib::IMessage::MSG_COMILER_CORE:
return "Compiler Core";
case mi::neuraylib::IMessage::MSG_COMPILER_ARCHIVE_TOOL:
return "Compiler Archive Tool";
case mi::neuraylib::IMessage::MSG_COMPILER_DAG:
return "Compiler DAG generator";
default:
break;
}
return "";
}
void MdlLogger::message(mi::base::Message_severity level,
const char* moduleCategory,
const mi::base::Message_details& details,
const char* message)
{
#ifdef NDEBUG
const mi::base::Message_severity minLogLevel = mi::base::MESSAGE_SEVERITY_WARNING;
#else
const mi::base::Message_severity minLogLevel = mi::base::MESSAGE_SEVERITY_VERBOSE;
#endif
if (level <= minLogLevel)
{
// const char* s_severity = _miMessageSeverityToCStr(level);
// FILE* os = (level <= mi::base::MESSAGE_SEVERITY_ERROR) ? stderr : stdout;
// fprintf(os, "[%s] (%s) %s\n", s_severity, moduleCategory, message);
switch (level)
{
case mi::base::MESSAGE_SEVERITY_FATAL:
STRELKA_FATAL("MDL: ({0}) {1}", moduleCategory, message);
break;
case mi::base::MESSAGE_SEVERITY_ERROR:
STRELKA_ERROR("MDL: ({0}) {1}", moduleCategory, message);
break;
case mi::base::MESSAGE_SEVERITY_WARNING:
STRELKA_WARNING("MDL: ({0}) {1}", moduleCategory, message);
break;
case mi::base::MESSAGE_SEVERITY_INFO:
STRELKA_INFO("MDL: ({0}) {1}", moduleCategory, message);
break;
case mi::base::MESSAGE_SEVERITY_VERBOSE:
STRELKA_TRACE("MDL: ({0}) {1}", moduleCategory, message);
break;
case mi::base::MESSAGE_SEVERITY_DEBUG:
STRELKA_DEBUG("MDL: ({0}) {1}", moduleCategory, message);
break;
default:
break;
}
}
}
void MdlLogger::message(mi::base::Message_severity level, const char* moduleCategory, const char* message)
{
this->message(level, moduleCategory, mi::base::Message_details{}, message);
}
void MdlLogger::message(mi::base::Message_severity level, const char* message)
{
const char* MODULE_CATEGORY = "shadergen";
this->message(level, MODULE_CATEGORY, message);
}
void MdlLogger::flushContextMessages(mi::neuraylib::IMdl_execution_context* context)
{
for (mi::Size i = 0, n = context->get_messages_count(); i < n; ++i)
{
mi::base::Handle<const mi::neuraylib::IMessage> message(context->get_message(i));
const char* s_msg = message->get_string();
const char* s_kind = _miMessageKindToCStr(message->get_kind());
this->message(message->get_severity(), s_kind, s_msg);
}
context->clear_messages();
}
} // namespace oka
| 4,575 | C++ | 34.2 | 106 | 0.615519 |
arhix52/Strelka/src/render/render.cpp | #include "render.h"
#ifdef __APPLE__
# include "metal/MetalRender.h"
#else
# include "optix/OptixRender.h"
#endif
using namespace oka;
Render* RenderFactory::createRender(const RenderType type)
{
#ifdef __APPLE__
if (type == RenderType::eMetal)
{
return new MetalRender();
}
// unsupported
return nullptr;
#else
if (type == RenderType::eOptiX)
{
return new OptiXRender();
}
return nullptr;
#endif
}
| 459 | C++ | 16.037036 | 58 | 0.627451 |
arhix52/Strelka/src/render/optix/RandomSampler.h | #pragma once
#include <stdint.h>
// source:
// https://github.com/mmp/pbrt-v4/blob/5acc5e46cf4b5c3382babd6a3b93b87f54d79b0a/src/pbrt/util/float.h#L46C1-L47C1
static constexpr float FloatOneMinusEpsilon = 0x1.fffffep-1;
__device__ const unsigned int primeNumbers[32] = {
2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53,
59, 61, 67, 71, 73, 79, 83, 89, 97, 101, 103, 107, 109, 113, 127, 131,
};
enum struct SampleDimension : uint32_t
{
ePixelX,
ePixelY,
eLightId,
eLightPointX,
eLightPointY,
eBSDF0,
eBSDF1,
eBSDF2,
eBSDF3,
eRussianRoulette,
eNUM_DIMENSIONS
};
struct SamplerState
{
uint32_t seed;
uint32_t sampleIdx;
uint32_t depth;
};
#define MAX_BOUNCES 128
// Based on: https://www.reedbeta.com/blog/hash-functions-for-gpu-rendering/
__device__ inline unsigned int pcg_hash(unsigned int seed)
{
unsigned int state = seed * 747796405u + 2891336453u;
unsigned int word = ((state >> ((state >> 28u) + 4u)) ^ state) * 277803737u;
return (word >> 22u) ^ word;
}
// __device__ inline unsigned hash_combine(unsigned a, unsigned b)
// {
// return a ^ (b + 0x9e3779b9 + (a << 6) + (a >> 2));
// }
__device__ __inline__ uint32_t hash_combine(uint32_t seed, uint32_t v)
{
return seed ^ (v + (seed << 6) + (seed >> 2));
}
__device__ inline unsigned int hash_with(unsigned int seed, unsigned int hash)
{
// Wang hash
seed = (seed ^ 61u) ^ hash;
seed += seed << 3;
seed ^= seed >> 4;
seed *= 0x27d4eb2du;
return seed;
}
// Generate random unsigned int in [0, 2^24)
static __host__ __device__ __inline__ unsigned int lcg(unsigned int& prev)
{
const unsigned int LCG_A = 1664525u;
const unsigned int LCG_C = 1013904223u;
prev = (LCG_A * prev + LCG_C);
return prev & 0x00FFFFFF;
}
// jenkins hash
static __device__ unsigned int jenkins_hash(unsigned int a)
{
a = (a + 0x7ED55D16) + (a << 12);
a = (a ^ 0xC761C23C) ^ (a >> 19);
a = (a + 0x165667B1) + (a << 5);
a = (a + 0xD3A2646C) ^ (a << 9);
a = (a + 0xFD7046C5) + (a << 3);
a = (a ^ 0xB55A4F09) ^ (a >> 16);
return a;
}
__device__ __inline__ uint32_t hash(uint32_t x)
{
// finalizer from murmurhash3
x ^= x >> 16;
x *= 0x85ebca6bu;
x ^= x >> 13;
x *= 0xc2b2ae35u;
x ^= x >> 16;
return x;
}
static __device__ float halton(uint32_t index, uint32_t base)
{
const float s = 1.0f / float(base);
unsigned int i = index;
float result = 0.0f;
float f = s;
while (i)
{
const unsigned int digit = i % base;
result += f * float(digit);
i = (i - digit) / base;
f *= s;
}
return clamp(result, 0.0f, FloatOneMinusEpsilon);
}
// source https://fgiesen.wordpress.com/2009/12/13/decoding-morton-codes/
// "Insert" a 0 bit after each of the 16 low bits of x
__device__ __inline__ uint32_t Part1By1(uint32_t x)
{
x &= 0x0000ffff; // x = ---- ---- ---- ---- fedc ba98 7654 3210
x = (x ^ (x << 8)) & 0x00ff00ff; // x = ---- ---- fedc ba98 ---- ---- 7654 3210
x = (x ^ (x << 4)) & 0x0f0f0f0f; // x = ---- fedc ---- ba98 ---- 7654 ---- 3210
x = (x ^ (x << 2)) & 0x33333333; // x = --fe --dc --ba --98 --76 --54 --32 --10
x = (x ^ (x << 1)) & 0x55555555; // x = -f-e -d-c -b-a -9-8 -7-6 -5-4 -3-2 -1-0
return x;
}
__device__ __inline__ uint32_t EncodeMorton2(uint32_t x, uint32_t y)
{
return (Part1By1(y) << 1) + Part1By1(x);
}
static __device__ SamplerState initSampler(uint32_t pixelX, uint32_t pixelY, uint32_t linearPixelIndex, uint32_t pixelSampleIndex, uint32_t maxSampleCount, uint32_t seed)
{
SamplerState sampler{};
sampler.seed = seed;
// sampler.sampleIdx = pixelSampleIndex + linearPixelIndex * maxSampleCount;
sampler.sampleIdx = EncodeMorton2(pixelX, pixelY) * maxSampleCount + pixelSampleIndex;
return sampler;
}
__device__ const uint32_t sb_matrix[5][32] = {
0x80000000, 0x40000000, 0x20000000, 0x10000000, 0x08000000, 0x04000000, 0x02000000, 0x01000000,
0x00800000, 0x00400000, 0x00200000, 0x00100000, 0x00080000, 0x00040000, 0x00020000, 0x00010000,
0x00008000, 0x00004000, 0x00002000, 0x00001000, 0x00000800, 0x00000400, 0x00000200, 0x00000100,
0x00000080, 0x00000040, 0x00000020, 0x00000010, 0x00000008, 0x00000004, 0x00000002, 0x00000001,
0x80000000, 0xc0000000, 0xa0000000, 0xf0000000, 0x88000000, 0xcc000000, 0xaa000000, 0xff000000,
0x80800000, 0xc0c00000, 0xa0a00000, 0xf0f00000, 0x88880000, 0xcccc0000, 0xaaaa0000, 0xffff0000,
0x80008000, 0xc000c000, 0xa000a000, 0xf000f000, 0x88008800, 0xcc00cc00, 0xaa00aa00, 0xff00ff00,
0x80808080, 0xc0c0c0c0, 0xa0a0a0a0, 0xf0f0f0f0, 0x88888888, 0xcccccccc, 0xaaaaaaaa, 0xffffffff,
0x80000000, 0xc0000000, 0x60000000, 0x90000000, 0xe8000000, 0x5c000000, 0x8e000000, 0xc5000000,
0x68800000, 0x9cc00000, 0xee600000, 0x55900000, 0x80680000, 0xc09c0000, 0x60ee0000, 0x90550000,
0xe8808000, 0x5cc0c000, 0x8e606000, 0xc5909000, 0x6868e800, 0x9c9c5c00, 0xeeee8e00, 0x5555c500,
0x8000e880, 0xc0005cc0, 0x60008e60, 0x9000c590, 0xe8006868, 0x5c009c9c, 0x8e00eeee, 0xc5005555,
0x80000000, 0xc0000000, 0x20000000, 0x50000000, 0xf8000000, 0x74000000, 0xa2000000, 0x93000000,
0xd8800000, 0x25400000, 0x59e00000, 0xe6d00000, 0x78080000, 0xb40c0000, 0x82020000, 0xc3050000,
0x208f8000, 0x51474000, 0xfbea2000, 0x75d93000, 0xa0858800, 0x914e5400, 0xdbe79e00, 0x25db6d00,
0x58800080, 0xe54000c0, 0x79e00020, 0xb6d00050, 0x800800f8, 0xc00c0074, 0x200200a2, 0x50050093,
0x80000000, 0x40000000, 0x20000000, 0xb0000000, 0xf8000000, 0xdc000000, 0x7a000000, 0x9d000000,
0x5a800000, 0x2fc00000, 0xa1600000, 0xf0b00000, 0xda880000, 0x6fc40000, 0x81620000, 0x40bb0000,
0x22878000, 0xb3c9c000, 0xfb65a000, 0xddb2d000, 0x78022800, 0x9c0b3c00, 0x5a0fb600, 0x2d0ddb00,
0xa2878080, 0xf3c9c040, 0xdb65a020, 0x6db2d0b0, 0x800228f8, 0x400b3cdc, 0x200fb67a, 0xb00ddb9d,
};
__device__ __inline__ uint32_t sobol_uint(uint32_t index, uint32_t dim)
{
uint32_t X = 0;
for (int bit = 0; bit < 32; bit++)
{
int mask = (index >> bit) & 1;
X ^= mask * sb_matrix[dim][bit];
}
return X;
}
__device__ __inline__ float sobol(uint32_t index, uint32_t dim)
{
return min(sobol_uint(index, dim) * 0x1p-32f, FloatOneMinusEpsilon);
}
__device__ __inline__ uint32_t laine_karras_permutation(uint32_t value, uint32_t seed)
{
value += seed;
value ^= value * 0x6c50b47cu;
value ^= value * 0xb82f1e52u;
value ^= value * 0xc7afe638u;
value ^= value * 0x8d22f6e6u;
return value;
}
__device__ __inline__ uint32_t ReverseBits(uint32_t value)
{
#ifdef __CUDACC__
return __brev(value);
#else
value = (((value & 0xaaaaaaaa) >> 1) | ((value & 0x55555555) << 1));
value = (((value & 0xcccccccc) >> 2) | ((value & 0x33333333) << 2));
value = (((value & 0xf0f0f0f0) >> 4) | ((value & 0x0f0f0f0f) << 4));
value = (((value & 0xff00ff00) >> 8) | ((value & 0x00ff00ff) << 8));
return ((value >> 16) | (value << 16));
#endif
}
__device__ __inline__ uint32_t nested_uniform_scramble(uint32_t value, uint32_t seed)
{
value = ReverseBits(value);
value = laine_karras_permutation(value, seed);
value = ReverseBits(value);
return value;
}
__device__ __inline__ float sobol_scramble(uint32_t index, uint32_t dim, uint32_t seed)
{
seed = hash(seed);
index = nested_uniform_scramble(index, seed);
uint32_t result = nested_uniform_scramble(sobol_uint(index, dim), hash_combine(seed, dim));
return min(result * 0x1p-32f, FloatOneMinusEpsilon);
}
template <SampleDimension Dim>
__device__ __inline__ float random(SamplerState& state)
{
const uint32_t dimension = (uint32_t(Dim) + state.depth * uint32_t(SampleDimension::eNUM_DIMENSIONS)) % 5;
return sobol_scramble(state.sampleIdx, dimension, state.seed + state.depth);
}
| 7,854 | C | 33.603524 | 170 | 0.650751 |
arhix52/Strelka/src/render/optix/OptixBuffer.h | #pragma once
#include "buffer.h"
#include <stdint.h>
#include <vector>
namespace oka
{
class OptixBuffer : public Buffer
{
public:
OptixBuffer(void* devicePtr, BufferFormat format, uint32_t width, uint32_t height);
virtual ~OptixBuffer();
void resize(uint32_t width, uint32_t height) override;
void* map() override;
void unmap() override;
void* getNativePtr()
{
return mDeviceData;
}
protected:
void* mDeviceData = nullptr;
uint32_t mDeviceIndex = 0;
};
} // namespace oka
| 529 | C | 15.5625 | 87 | 0.667297 |
arhix52/Strelka/src/render/optix/OptixRenderParams.h | #pragma once
#include <optix_types.h>
#include <vector_types.h>
#include <sutil/Matrix.h>
#include "RandomSampler.h"
#include "Lights.h"
#define GEOMETRY_MASK_TRIANGLE 1
#define GEOMETRY_MASK_CURVE 2
#define GEOMETRY_MASK_LIGHT 4
#define GEOMETRY_MASK_GEOMETRY (GEOMETRY_MASK_TRIANGLE | GEOMETRY_MASK_CURVE)
#define RAY_MASK_PRIMARY (GEOMETRY_MASK_GEOMETRY | GEOMETRY_MASK_LIGHT)
#define RAY_MASK_SHADOW GEOMETRY_MASK_GEOMETRY
#define RAY_MASK_SECONDARY GEOMETRY_MASK_GEOMETRY
struct Vertex
{
float3 position;
uint32_t tangent;
uint32_t normal;
uint32_t uv;
float pad0;
float pad1;
};
struct SceneData
{
Vertex* vb;
uint32_t* ib;
UniformLight* lights;
uint32_t numLights;
};
struct Params
{
uint32_t subframe_index;
uint32_t samples_per_launch;
uint32_t maxSampleCount;
float4* image;
float4* accum;
float4* diffuse;
uint16_t* diffuseCounter;
float4* specular;
uint16_t* specularCounter;
uint32_t image_width;
uint32_t image_height;
uint32_t max_depth;
uint32_t rectLightSamplingMethod;
float3 exposure;
float clipToView[16];
float viewToWorld[16];
OptixTraversableHandle handle;
SceneData scene;
bool enableAccumulation;
// developers settings:
uint32_t debug;
float shadowRayTmin;
float materialRayTmin;
};
enum class EventType: uint8_t
{
eUndef,
eAbsorb,
eDiffuse,
eSpecular,
eLast,
};
struct PerRayData
{
SamplerState sampler;
uint32_t linearPixelIndex;
uint32_t sampleIndex;
uint32_t depth; // bounce
float3 radiance;
float3 throughput;
float3 origin;
float3 dir;
bool inside;
bool specularBounce;
float lastBsdfPdf;
EventType firstEventType;
};
enum RayType
{
RAY_TYPE_RADIANCE = 0,
RAY_TYPE_OCCLUSION = 1,
RAY_TYPE_COUNT
};
struct RayGenData
{
// No data needed
};
struct MissData
{
float3 bg_color;
};
struct HitGroupData
{
int32_t indexOffset;
int32_t indexCount;
int32_t vertexOffset;
int32_t lightId; // only for lights. -1 for others
CUdeviceptr argData;
CUdeviceptr roData;
CUdeviceptr resHandler;
float4 world_to_object[4] = {};
float4 object_to_world[4] = {};
};
| 2,254 | C | 17.185484 | 77 | 0.681012 |
arhix52/Strelka/src/render/optix/texture_support_cuda.h | /******************************************************************************
* Copyright 2022 NVIDIA Corporation. All rights reserved.
*****************************************************************************/
// examples/mdl_sdk/shared/texture_support_cuda.h
//
// This file contains the implementations and the vtables of the texture access functions.
#ifndef TEXTURE_SUPPORT_CUDA_H
#define TEXTURE_SUPPORT_CUDA_H
#include <cuda.h>
#include <cuda_runtime.h>
#include <math.h>
#include <mi/neuraylib/target_code_types.h>
#define USE_SMOOTHERSTEP_FILTER
#ifndef M_PI
#define M_PI 3.14159265358979323846
#endif
#define M_ONE_OVER_PI 0.318309886183790671538
typedef mi::neuraylib::tct_deriv_float tct_deriv_float;
typedef mi::neuraylib::tct_deriv_float2 tct_deriv_float2;
typedef mi::neuraylib::tct_deriv_arr_float_2 tct_deriv_arr_float_2;
typedef mi::neuraylib::tct_deriv_arr_float_3 tct_deriv_arr_float_3;
typedef mi::neuraylib::tct_deriv_arr_float_4 tct_deriv_arr_float_4;
typedef mi::neuraylib::Shading_state_material_with_derivs Shading_state_material_with_derivs;
typedef mi::neuraylib::Shading_state_material Shading_state_material;
typedef mi::neuraylib::Texture_handler_base Texture_handler_base;
typedef mi::neuraylib::Tex_wrap_mode Tex_wrap_mode;
typedef mi::neuraylib::Mbsdf_part Mbsdf_part;
// Custom structure representing an MDL texture, containing filtered and unfiltered CUDA texture
// objects and the size of the texture.
struct Texture
{
explicit Texture()
: filtered_object(0)
, unfiltered_object(0)
, size(make_uint3(0, 0, 0))
, inv_size(make_float3(0.0f, 0.0f, 0.0f))
{}
explicit Texture(
cudaTextureObject_t filtered_object,
cudaTextureObject_t unfiltered_object,
uint3 size)
: filtered_object(filtered_object)
, unfiltered_object(unfiltered_object)
, size(size)
, inv_size(make_float3(1.0f / size.x, 1.0f / size.y, 1.0f / size.z))
{}
cudaTextureObject_t filtered_object; // uses filter mode cudaFilterModeLinear
cudaTextureObject_t unfiltered_object; // uses filter mode cudaFilterModePoint
uint3 size; // size of the texture, needed for texel access
float3 inv_size; // the inverse values of the size of the texture
};
// Custom structure representing an MDL BSDF measurement.
struct Mbsdf
{
unsigned has_data[2]; // true if there is a measurement for this part
cudaTextureObject_t eval_data[2]; // uses filter mode cudaFilterModeLinear
float max_albedo[2]; // max albedo used to limit the multiplier
float* sample_data[2]; // CDFs for sampling a BSDF measurement
float* albedo_data[2]; // max albedo for each theta (isotropic)
uint2 angular_resolution[2]; // size of the dataset, needed for texel access
float2 inv_angular_resolution[2]; // the inverse values of the size of the dataset
unsigned num_channels[2]; // number of color channels (1 or 3)
};
// Structure representing a Light Profile
struct Lightprofile
{
explicit Lightprofile()
: angular_resolution(make_uint2(0, 0))
, inv_angular_resolution(make_float2(0.0f, 0.0f))
, theta_phi_start(make_float2(0.0f, 0.0f))
, theta_phi_delta(make_float2(0.0f, 0.0f))
, theta_phi_inv_delta(make_float2(0.0f, 0.0f))
, candela_multiplier(0.0f)
, total_power(0.0f)
, eval_data(0)
{
}
uint2 angular_resolution; // angular resolution of the grid
float2 inv_angular_resolution; // inverse angular resolution of the grid
float2 theta_phi_start; // start of the grid
float2 theta_phi_delta; // angular step size
float2 theta_phi_inv_delta; // inverse step size
float candela_multiplier; // factor to rescale the normalized data
float total_power;
cudaTextureObject_t eval_data; // normalized data sampled on grid
float* cdf_data; // CDFs for sampling a light profile
};
// The texture handler structure required by the MDL SDK with custom additional fields.
struct Texture_handler : Texture_handler_base {
// additional data for the texture access functions can be provided here
size_t num_textures; // the number of textures used by the material
// (without the invalid texture)
Texture const *textures; // the textures used by the material
// (without the invalid texture)
size_t num_mbsdfs; // the number of mbsdfs used by the material
// (without the invalid mbsdf)
Mbsdf const *mbsdfs; // the mbsdfs used by the material
// (without the invalid mbsdf)
size_t num_lightprofiles; // number of elements in the lightprofiles field
// (without the invalid light profile)
Lightprofile const *lightprofiles; // a device pointer to a list of mbsdfs objects, if used
// (without the invalid light profile)
};
// The texture handler structure required by the MDL SDK with custom additional fields.
struct Texture_handler_deriv : mi::neuraylib::Texture_handler_deriv_base {
// additional data for the texture access functions can be provided here
size_t num_textures; // the number of textures used by the material
// (without the invalid texture)
Texture const *textures; // the textures used by the material
// (without the invalid texture)
size_t num_mbsdfs; // the number of mbsdfs used by the material
// (without the invalid texture)
Mbsdf const *mbsdfs; // the mbsdfs used by the material
// (without the invalid texture)
size_t num_lightprofiles; // number of elements in the lightprofiles field
// (without the invalid light profile)
Lightprofile const *lightprofiles; // a device pointer to a list of mbsdfs objects, if used
// (without the invalid light profile)
};
#if defined(__CUDACC__)
// Stores a float4 in a float[4] array.
__device__ inline void store_result4(float res[4], const float4 &v)
{
res[0] = v.x;
res[1] = v.y;
res[2] = v.z;
res[3] = v.w;
}
// Stores a float in all elements of a float[4] array.
__device__ inline void store_result4(float res[4], float s)
{
res[0] = res[1] = res[2] = res[3] = s;
}
// Stores the given float values in a float[4] array.
__device__ inline void store_result4(
float res[4], float v0, float v1, float v2, float v3)
{
res[0] = v0;
res[1] = v1;
res[2] = v2;
res[3] = v3;
}
// Stores a float3 in a float[3] array.
__device__ inline void store_result3(float res[3], float3 const&v)
{
res[0] = v.x;
res[1] = v.y;
res[2] = v.z;
}
// Stores a float4 in a float[3] array, ignoring v.w.
__device__ inline void store_result3(float res[3], const float4 &v)
{
res[0] = v.x;
res[1] = v.y;
res[2] = v.z;
}
// Stores a float in all elements of a float[3] array.
__device__ inline void store_result3(float res[3], float s)
{
res[0] = res[1] = res[2] = s;
}
// Stores the given float values in a float[3] array.
__device__ inline void store_result3(float res[3], float v0, float v1, float v2)
{
res[0] = v0;
res[1] = v1;
res[2] = v2;
}
// Stores the luminance if a given float[3] in a float.
__device__ inline void store_result1(float* res, float3 const& v)
{
// store luminance
*res = 0.212671 * v.x + 0.715160 * v.y + 0.072169 * v.z;
}
// Stores the luminance if a given float[3] in a float.
__device__ inline void store_result1(float* res, float v0, float v1, float v2)
{
// store luminance
*res = 0.212671 * v0 + 0.715160 * v1 + 0.072169 * v2;
}
// Stores a given float in a float
__device__ inline void store_result1(float* res, float s)
{
*res = s;
}
// ------------------------------------------------------------------------------------------------
// Textures
// ------------------------------------------------------------------------------------------------
// Applies wrapping and cropping to the given coordinate.
// Note: This macro returns if wrap mode is clip and the coordinate is out of range.
#define WRAP_AND_CROP_OR_RETURN_BLACK(val, inv_dim, wrap_mode, crop_vals, store_res_func) \
do { \
if ( (wrap_mode) == mi::neuraylib::TEX_WRAP_REPEAT && \
(crop_vals)[0] == 0.0f && (crop_vals)[1] == 1.0f ) { \
/* Do nothing, use texture sampler default behavior */ \
} \
else \
{ \
if ( (wrap_mode) == mi::neuraylib::TEX_WRAP_REPEAT ) \
val = val - floorf(val); \
else { \
if ( (wrap_mode) == mi::neuraylib::TEX_WRAP_CLIP && (val < 0.0f || val >= 1.0f) ) { \
store_res_func(result, 0.0f); \
return; \
} \
else if ( (wrap_mode) == mi::neuraylib::TEX_WRAP_MIRRORED_REPEAT ) { \
float floored_val = floorf(val); \
if ( (int(floored_val) & 1) != 0 ) \
val = 1.0f - (val - floored_val); \
else \
val = val - floored_val; \
} \
float inv_hdim = 0.5f * (inv_dim); \
val = fminf(fmaxf(val, inv_hdim), 1.f - inv_hdim); \
} \
val = val * ((crop_vals)[1] - (crop_vals)[0]) + (crop_vals)[0]; \
} \
} while ( 0 )
#ifdef USE_SMOOTHERSTEP_FILTER
// Modify texture coordinates to get better texture filtering,
// see http://www.iquilezles.org/www/articles/texture/texture.htm
#define APPLY_SMOOTHERSTEP_FILTER() \
do { \
u = u * tex.size.x + 0.5f; \
v = v * tex.size.y + 0.5f; \
\
float u_i = floorf(u), v_i = floorf(v); \
float u_f = u - u_i; \
float v_f = v - v_i; \
u_f = u_f * u_f * u_f * (u_f * (u_f * 6.f - 15.f) + 10.f); \
v_f = v_f * v_f * v_f * (v_f * (v_f * 6.f - 15.f) + 10.f); \
u = u_i + u_f; \
v = v_i + v_f; \
\
u = (u - 0.5f) * tex.inv_size.x; \
v = (v - 0.5f) * tex.inv_size.y; \
} while ( 0 )
#else
#define APPLY_SMOOTHERSTEP_FILTER()
#endif
// Implementation of tex::lookup_float4() for a texture_2d texture.
extern "C" __device__ void tex_lookup_float4_2d(
float result[4],
Texture_handler_base const *self_base,
unsigned texture_idx,
float const coord[2],
Tex_wrap_mode const wrap_u,
Tex_wrap_mode const wrap_v,
float const crop_u[2],
float const crop_v[2],
float /*frame*/)
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if ( texture_idx == 0 || texture_idx - 1 >= self->num_textures ) {
// invalid texture returns zero
store_result4(result, 0.0f);
return;
}
Texture const &tex = self->textures[texture_idx - 1];
float u = coord[0], v = coord[1];
WRAP_AND_CROP_OR_RETURN_BLACK(u, tex.inv_size.x, wrap_u, crop_u, store_result4);
WRAP_AND_CROP_OR_RETURN_BLACK(v, tex.inv_size.y, wrap_v, crop_v, store_result4);
APPLY_SMOOTHERSTEP_FILTER();
store_result4(result, tex2D<float4>(tex.filtered_object, u, v));
}
// Implementation of tex::lookup_float4() for a texture_2d texture.
extern "C" __device__ void tex_lookup_deriv_float4_2d(
float result[4],
Texture_handler_base const *self_base,
unsigned texture_idx,
tct_deriv_float2 const *coord,
Tex_wrap_mode const wrap_u,
Tex_wrap_mode const wrap_v,
float const crop_u[2],
float const crop_v[2],
float /*frame*/)
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if ( texture_idx == 0 || texture_idx - 1 >= self->num_textures ) {
// invalid texture returns zero
store_result4(result, 0.0f);
return;
}
Texture const &tex = self->textures[texture_idx - 1];
float u = coord->val.x, v = coord->val.y;
WRAP_AND_CROP_OR_RETURN_BLACK(u, tex.inv_size.x, wrap_u, crop_u, store_result4);
WRAP_AND_CROP_OR_RETURN_BLACK(v, tex.inv_size.y, wrap_v, crop_v, store_result4);
APPLY_SMOOTHERSTEP_FILTER();
store_result4(result, tex2DGrad<float4>(tex.filtered_object, u, v, coord->dx, coord->dy));
}
// Implementation of tex::lookup_float3() for a texture_2d texture.
extern "C" __device__ void tex_lookup_float3_2d(
float result[3],
Texture_handler_base const *self_base,
unsigned texture_idx,
float const coord[2],
Tex_wrap_mode const wrap_u,
Tex_wrap_mode const wrap_v,
float const crop_u[2],
float const crop_v[2],
float /*frame*/)
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if ( texture_idx == 0 || texture_idx - 1 >= self->num_textures ) {
// invalid texture returns zero
store_result3(result, 0.0f);
return;
}
Texture const &tex = self->textures[texture_idx - 1];
float u = coord[0], v = coord[1];
WRAP_AND_CROP_OR_RETURN_BLACK(u, tex.inv_size.x, wrap_u, crop_u, store_result3);
WRAP_AND_CROP_OR_RETURN_BLACK(v, tex.inv_size.y, wrap_v, crop_v, store_result3);
APPLY_SMOOTHERSTEP_FILTER();
store_result3(result, tex2D<float4>(tex.filtered_object, u, v));
}
// Implementation of tex::lookup_float3() for a texture_2d texture.
extern "C" __device__ void tex_lookup_deriv_float3_2d(
float result[3],
Texture_handler_base const *self_base,
unsigned texture_idx,
tct_deriv_float2 const *coord,
Tex_wrap_mode const wrap_u,
Tex_wrap_mode const wrap_v,
float const crop_u[2],
float const crop_v[2],
float /*frame*/)
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if ( texture_idx == 0 || texture_idx - 1 >= self->num_textures ) {
// invalid texture returns zero
store_result3(result, 0.0f);
return;
}
Texture const &tex = self->textures[texture_idx - 1];
float u = coord->val.x, v = coord->val.y;
WRAP_AND_CROP_OR_RETURN_BLACK(u, tex.inv_size.x, wrap_u, crop_u, store_result3);
WRAP_AND_CROP_OR_RETURN_BLACK(v, tex.inv_size.y, wrap_v, crop_v, store_result3);
APPLY_SMOOTHERSTEP_FILTER();
store_result3(result, tex2DGrad<float4>(tex.filtered_object, u, v, coord->dx, coord->dy));
}
// Implementation of tex::texel_float4() for a texture_2d texture.
// Note: uvtile and/or animated textures are not supported
extern "C" __device__ void tex_texel_float4_2d(
float result[4],
Texture_handler_base const *self_base,
unsigned texture_idx,
int const coord[2],
int const /*uv_tile*/[2],
float /*frame*/)
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if ( texture_idx == 0 || texture_idx - 1 >= self->num_textures ) {
// invalid texture returns zero
store_result4(result, 0.0f);
return;
}
Texture const &tex = self->textures[texture_idx - 1];
store_result4(result, tex2D<float4>(
tex.unfiltered_object,
float(coord[0]) * tex.inv_size.x,
float(coord[1]) * tex.inv_size.y));
}
// Implementation of tex::lookup_float4() for a texture_3d texture.
extern "C" __device__ void tex_lookup_float4_3d(
float result[4],
Texture_handler_base const *self_base,
unsigned texture_idx,
float const coord[3],
Tex_wrap_mode wrap_u,
Tex_wrap_mode wrap_v,
Tex_wrap_mode wrap_w,
float const crop_u[2],
float const crop_v[2],
float const crop_w[2],
float /*frame*/)
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if ( texture_idx == 0 || texture_idx - 1 >= self->num_textures ) {
// invalid texture returns zero
store_result4(result, 0.0f);
return;
}
Texture const &tex = self->textures[texture_idx - 1];
float u = coord[0], v = coord[1], w = coord[2];
WRAP_AND_CROP_OR_RETURN_BLACK(u, tex.inv_size.x, wrap_u, crop_u, store_result4);
WRAP_AND_CROP_OR_RETURN_BLACK(v, tex.inv_size.y, wrap_v, crop_v, store_result4);
WRAP_AND_CROP_OR_RETURN_BLACK(w, tex.inv_size.z, wrap_w, crop_w, store_result4);
store_result4(result, tex3D<float4>(tex.filtered_object, u, v, w));
}
// Implementation of tex::lookup_float3() for a texture_3d texture.
extern "C" __device__ void tex_lookup_float3_3d(
float result[3],
Texture_handler_base const *self_base,
unsigned texture_idx,
float const coord[3],
Tex_wrap_mode wrap_u,
Tex_wrap_mode wrap_v,
Tex_wrap_mode wrap_w,
float const crop_u[2],
float const crop_v[2],
float const crop_w[2],
float /*frame*/)
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if ( texture_idx == 0 || texture_idx - 1 >= self->num_textures ) {
// invalid texture returns zero
store_result3(result, 0.0f);
return;
}
Texture const &tex = self->textures[texture_idx - 1];
float u = coord[0], v = coord[1], w = coord[2];
WRAP_AND_CROP_OR_RETURN_BLACK(u, tex.inv_size.x, wrap_u, crop_u, store_result3);
WRAP_AND_CROP_OR_RETURN_BLACK(v, tex.inv_size.y, wrap_v, crop_v, store_result3);
WRAP_AND_CROP_OR_RETURN_BLACK(w, tex.inv_size.z, wrap_w, crop_w, store_result3);
store_result3(result, tex3D<float4>(tex.filtered_object, u, v, w));
}
// Implementation of tex::texel_float4() for a texture_3d texture.
extern "C" __device__ void tex_texel_float4_3d(
float result[4],
Texture_handler_base const *self_base,
unsigned texture_idx,
const int coord[3],
float /*frame*/)
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if ( texture_idx == 0 || texture_idx - 1 >= self->num_textures ) {
// invalid texture returns zero
store_result4(result, 0.0f);
return;
}
Texture const &tex = self->textures[texture_idx - 1];
store_result4(result, tex3D<float4>(
tex.unfiltered_object,
float(coord[0]) * tex.inv_size.x,
float(coord[1]) * tex.inv_size.y,
float(coord[2]) * tex.inv_size.z));
}
// Implementation of tex::lookup_float4() for a texture_cube texture.
extern "C" __device__ void tex_lookup_float4_cube(
float result[4],
Texture_handler_base const *self_base,
unsigned texture_idx,
float const coord[3])
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if ( texture_idx == 0 || texture_idx - 1 >= self->num_textures ) {
// invalid texture returns zero
store_result4(result, 0.0f);
return;
}
Texture const &tex = self->textures[texture_idx - 1];
store_result4(result, texCubemap<float4>(tex.filtered_object, coord[0], coord[1], coord[2]));
}
// Implementation of tex::lookup_float3() for a texture_cube texture.
extern "C" __device__ void tex_lookup_float3_cube(
float result[3],
Texture_handler_base const *self_base,
unsigned texture_idx,
float const coord[3])
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if ( texture_idx == 0 || texture_idx - 1 >= self->num_textures ) {
// invalid texture returns zero
store_result3(result, 0.0f);
return;
}
Texture const &tex = self->textures[texture_idx - 1];
store_result3(result, texCubemap<float4>(tex.filtered_object, coord[0], coord[1], coord[2]));
}
// Implementation of resolution_2d function needed by generated code.
// Note: uvtile and/or animated textures are not supported
extern "C" __device__ void tex_resolution_2d(
int result[2],
Texture_handler_base const *self_base,
unsigned texture_idx,
int const /*uv_tile*/[2],
float /*frame*/)
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if ( texture_idx == 0 || texture_idx - 1 >= self->num_textures ) {
// invalid texture returns zero
result[0] = 0;
result[1] = 0;
return;
}
Texture const &tex = self->textures[texture_idx - 1];
result[0] = tex.size.x;
result[1] = tex.size.y;
}
// Implementation of resolution_3d function needed by generated code.
extern "C" __device__ void tex_resolution_3d(
int result[3],
Texture_handler_base const *self_base,
unsigned texture_idx,
float /*frame*/)
{
Texture_handler const* self = static_cast<Texture_handler const*>(self_base);
if (texture_idx == 0 || texture_idx - 1 >= self->num_textures) {
// invalid texture returns zero
result[0] = 0;
result[1] = 0;
result[2] = 0;
}
Texture const& tex = self->textures[texture_idx - 1];
result[0] = tex.size.x;
result[1] = tex.size.y;
result[2] = tex.size.z;
}
// Implementation of texture_isvalid().
extern "C" __device__ bool tex_texture_isvalid(
Texture_handler_base const *self_base,
unsigned texture_idx)
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
return texture_idx != 0 && texture_idx - 1 < self->num_textures;
}
// Implementation of frame function needed by generated code.
extern "C" __device__ void tex_frame(
int result[2],
Texture_handler_base const *self_base,
unsigned texture_idx)
{
Texture_handler const* self = static_cast<Texture_handler const*>(self_base);
if (texture_idx == 0 || texture_idx - 1 >= self->num_textures) {
// invalid texture returns zero
result[0] = 0;
result[1] = 0;
}
// Texture const& tex = self->textures[texture_idx - 1];
result[0] = 0;
result[1] = 0;
}
// ------------------------------------------------------------------------------------------------
// Light Profiles
// ------------------------------------------------------------------------------------------------
// Implementation of light_profile_power() for a light profile.
extern "C" __device__ float df_light_profile_power(
Texture_handler_base const *self_base,
unsigned light_profile_idx)
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if (light_profile_idx == 0 || light_profile_idx - 1 >= self->num_lightprofiles)
return 0.0f; // invalid light profile returns zero
const Lightprofile& lp = self->lightprofiles[light_profile_idx - 1];
return lp.total_power;
}
// Implementation of light_profile_maximum() for a light profile.
extern "C" __device__ float df_light_profile_maximum(
Texture_handler_base const *self_base,
unsigned light_profile_idx)
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if (light_profile_idx == 0 || light_profile_idx - 1 >= self->num_lightprofiles)
return 0.0f; // invalid light profile returns zero
const Lightprofile& lp = self->lightprofiles[light_profile_idx - 1];
return lp.candela_multiplier;
}
// Implementation of light_profile_isvalid() for a light profile.
extern "C" __device__ bool df_light_profile_isvalid(
Texture_handler_base const *self_base,
unsigned light_profile_idx)
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
return light_profile_idx != 0 && light_profile_idx - 1 < self->num_lightprofiles;
}
// binary search through CDF
__device__ inline unsigned sample_cdf(
const float* cdf,
unsigned cdf_size,
float xi)
{
unsigned li = 0;
unsigned ri = cdf_size - 1;
unsigned m = (li + ri) / 2;
while (ri > li)
{
if (xi < cdf[m])
ri = m;
else
li = m + 1;
m = (li + ri) / 2;
}
return m;
}
// Implementation of df::light_profile_evaluate() for a light profile.
extern "C" __device__ float df_light_profile_evaluate(
Texture_handler_base const *self_base,
unsigned light_profile_idx,
float const theta_phi[2])
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if (light_profile_idx == 0 || light_profile_idx - 1 >= self->num_lightprofiles)
return 0.0f; // invalid light profile returns zero
const Lightprofile& lp = self->lightprofiles[light_profile_idx - 1];
// map theta to 0..1 range
float u = (theta_phi[0] - lp.theta_phi_start.x) *
lp.theta_phi_inv_delta.x * lp.inv_angular_resolution.x;
// converting input phi from -pi..pi to 0..2pi
float phi = (theta_phi[1] > 0.0f) ? theta_phi[1] : (float(2.0 * M_PI) + theta_phi[1]);
// floorf wraps phi range into 0..2pi
phi = phi - lp.theta_phi_start.y -
floorf((phi - lp.theta_phi_start.y) * float(0.5 / M_PI)) * float(2.0 * M_PI);
// (phi < 0.0f) is no problem, this is handle by the (black) border
// since it implies lp.theta_phi_start.y > 0 (and we really have "no data" below that)
float v = phi * lp.theta_phi_inv_delta.y * lp.inv_angular_resolution.y;
// half pixel offset
// see https://docs.nvidia.com/cuda/cuda-c-programming-guide/index.html#linear-filtering
u += 0.5f * lp.inv_angular_resolution.x;
v += 0.5f * lp.inv_angular_resolution.y;
// wrap_mode: border black would be an alternative (but it produces artifacts at low res)
if (u < 0.0f || u > 1.0f || v < 0.0f || v > 1.0f) return 0.0f;
return tex2D<float>(lp.eval_data, u, v) * lp.candela_multiplier;
}
// Implementation of df::light_profile_sample() for a light profile.
extern "C" __device__ void df_light_profile_sample(
float result[3], // output: theta, phi, pdf
Texture_handler_base const *self_base,
unsigned light_profile_idx,
float const xi[3]) // uniform random values
{
result[0] = -1.0f; // negative theta means no emission
result[1] = -1.0f;
result[2] = 0.0f;
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if (light_profile_idx == 0 || light_profile_idx - 1 >= self->num_lightprofiles)
return; // invalid light profile returns zero
const Lightprofile& lp = self->lightprofiles[light_profile_idx - 1];
uint2 res = lp.angular_resolution;
// sample theta_out
//-------------------------------------------
float xi0 = xi[0];
const float* cdf_data_theta = lp.cdf_data; // CDF theta
unsigned idx_theta = sample_cdf(cdf_data_theta, res.x - 1, xi0); // binary search
float prob_theta = cdf_data_theta[idx_theta];
if (idx_theta > 0)
{
const float tmp = cdf_data_theta[idx_theta - 1];
prob_theta -= tmp;
xi0 -= tmp;
}
xi0 /= prob_theta; // rescale for re-usage
// sample phi_out
//-------------------------------------------
float xi1 = xi[1];
const float* cdf_data_phi = cdf_data_theta + (res.x - 1) // CDF theta block
+ (idx_theta * (res.y - 1)); // selected CDF for phi
const unsigned idx_phi = sample_cdf(cdf_data_phi, res.y - 1, xi1); // binary search
float prob_phi = cdf_data_phi[idx_phi];
if (idx_phi > 0)
{
const float tmp = cdf_data_phi[idx_phi - 1];
prob_phi -= tmp;
xi1 -= tmp;
}
xi1 /= prob_phi; // rescale for re-usage
// compute theta and phi
//-------------------------------------------
// sample uniformly within the patch (grid cell)
const float2 start = lp.theta_phi_start;
const float2 delta = lp.theta_phi_delta;
const float cos_theta_0 = cosf(start.x + float(idx_theta) * delta.x);
const float cos_theta_1 = cosf(start.x + float(idx_theta + 1u) * delta.x);
// n = \int_{\theta_0}^{\theta_1} \sin{\theta} \delta \theta
// = 1 / (\cos{\theta_0} - \cos{\theta_1})
//
// \xi = n * \int_{\theta_0}^{\theta_1} \sin{\theta} \delta \theta
// => \cos{\theta} = (1 - \xi) \cos{\theta_0} + \xi \cos{\theta_1}
const float cos_theta = (1.0f - xi1) * cos_theta_0 + xi1 * cos_theta_1;
result[0] = acosf(cos_theta);
result[1] = start.y + (float(idx_phi) + xi0) * delta.y;
// align phi
if (result[1] > float(2.0 * M_PI)) result[1] -= float(2.0 * M_PI); // wrap
if (result[1] > float(1.0 * M_PI)) result[1] = float(-2.0 * M_PI) + result[1]; // to [-pi, pi]
// compute pdf
//-------------------------------------------
result[2] = prob_theta * prob_phi / (delta.y * (cos_theta_0 - cos_theta_1));
}
// Implementation of df::light_profile_pdf() for a light profile.
extern "C" __device__ float df_light_profile_pdf(
Texture_handler_base const *self_base,
unsigned light_profile_idx,
float const theta_phi[2])
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if (light_profile_idx == 0 || light_profile_idx - 1 >= self->num_lightprofiles)
return 0.0f; // invalid light profile returns zero
const Lightprofile& lp = self->lightprofiles[light_profile_idx - 1];
// CDF data
const uint2 res = lp.angular_resolution;
const float* cdf_data_theta = lp.cdf_data;
// map theta to 0..1 range
const float theta = theta_phi[0] - lp.theta_phi_start.x;
const int idx_theta = int(theta * lp.theta_phi_inv_delta.x);
// converting input phi from -pi..pi to 0..2pi
float phi = (theta_phi[1] > 0.0f) ? theta_phi[1] : (float(2.0 * M_PI) + theta_phi[1]);
// floorf wraps phi range into 0..2pi
phi = phi - lp.theta_phi_start.y -
floorf((phi - lp.theta_phi_start.y) * float(0.5 / M_PI)) * float(2.0 * M_PI);
// (phi < 0.0f) is no problem, this is handle by the (black) border
// since it implies lp.theta_phi_start.y > 0 (and we really have "no data" below that)
const int idx_phi = int(phi * lp.theta_phi_inv_delta.y);
// wrap_mode: border black would be an alternative (but it produces artifacts at low res)
if (idx_theta < 0 || idx_theta > (res.x - 2) || idx_phi < 0 || idx_phi >(res.x - 2))
return 0.0f;
// get probability for theta
//-------------------------------------------
float prob_theta = cdf_data_theta[idx_theta];
if (idx_theta > 0)
{
const float tmp = cdf_data_theta[idx_theta - 1];
prob_theta -= tmp;
}
// get probability for phi
//-------------------------------------------
const float* cdf_data_phi = cdf_data_theta
+ (res.x - 1) // CDF theta block
+ (idx_theta * (res.y - 1)); // selected CDF for phi
float prob_phi = cdf_data_phi[idx_phi];
if (idx_phi > 0)
{
const float tmp = cdf_data_phi[idx_phi - 1];
prob_phi -= tmp;
}
// compute probability to select a position in the sphere patch
const float2 start = lp.theta_phi_start;
const float2 delta = lp.theta_phi_delta;
const float cos_theta_0 = cos(start.x + float(idx_theta) * delta.x);
const float cos_theta_1 = cos(start.x + float(idx_theta + 1u) * delta.x);
return prob_theta * prob_phi / (delta.y * (cos_theta_0 - cos_theta_1));
}
// ------------------------------------------------------------------------------------------------
// BSDF Measurements
// ------------------------------------------------------------------------------------------------
// Implementation of bsdf_measurement_isvalid() for an MBSDF.
extern "C" __device__ bool df_bsdf_measurement_isvalid(
Texture_handler_base const *self_base,
unsigned bsdf_measurement_index)
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
return bsdf_measurement_index != 0 && bsdf_measurement_index - 1 < self->num_mbsdfs;
}
// Implementation of df::bsdf_measurement_resolution() function needed by generated code,
// which retrieves the angular and chromatic resolution of the given MBSDF.
// The returned triple consists of: number of equi-spaced steps of theta_i and theta_o,
// number of equi-spaced steps of phi, and number of color channels (1 or 3).
extern "C" __device__ void df_bsdf_measurement_resolution(
unsigned result[3],
Texture_handler_base const *self_base,
unsigned bsdf_measurement_index,
Mbsdf_part part)
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if (bsdf_measurement_index == 0 || bsdf_measurement_index - 1 >= self->num_mbsdfs)
{
// invalid MBSDF returns zero
result[0] = 0;
result[1] = 0;
result[2] = 0;
return;
}
Mbsdf const &bm = self->mbsdfs[bsdf_measurement_index - 1];
const unsigned part_index = static_cast<unsigned>(part);
// check for the part
if (bm.has_data[part_index] == 0)
{
result[0] = 0;
result[1] = 0;
result[2] = 0;
return;
}
// pass out the information
result[0] = bm.angular_resolution[part_index].x;
result[1] = bm.angular_resolution[part_index].y;
result[2] = bm.num_channels[part_index];
}
__device__ inline float3 bsdf_compute_uvw(const float theta_phi_in[2],
const float theta_phi_out[2])
{
// assuming each phi is between -pi and pi
float u = theta_phi_out[1] - theta_phi_in[1];
if (u < 0.0) u += float(2.0 * M_PI);
if (u > float(1.0 * M_PI)) u = float(2.0 * M_PI) - u;
u *= M_ONE_OVER_PI;
const float v = theta_phi_out[0] * float(2.0 / M_PI);
const float w = theta_phi_in[0] * float(2.0 / M_PI);
return make_float3(u, v, w);
}
template<typename T>
__device__ inline T bsdf_measurement_lookup(const cudaTextureObject_t& eval_volume,
const float theta_phi_in[2],
const float theta_phi_out[2])
{
// 3D volume on the GPU (phi_delta x theta_out x theta_in)
const float3 uvw = bsdf_compute_uvw(theta_phi_in, theta_phi_out);
return tex3D<T>(eval_volume, uvw.x, uvw.y, uvw.z);
}
// Implementation of df::bsdf_measurement_evaluate() for an MBSDF.
extern "C" __device__ void df_bsdf_measurement_evaluate(
float result[3],
Texture_handler_base const *self_base,
unsigned bsdf_measurement_index,
float const theta_phi_in[2],
float const theta_phi_out[2],
Mbsdf_part part)
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if (bsdf_measurement_index == 0 || bsdf_measurement_index - 1 >= self->num_mbsdfs)
{
// invalid MBSDF returns zero
store_result3(result, 0.0f);
return;
}
const Mbsdf& bm = self->mbsdfs[bsdf_measurement_index - 1];
const unsigned part_index = static_cast<unsigned>(part);
// check for the parta
if (bm.has_data[part_index] == 0)
{
store_result3(result, 0.0f);
return;
}
// handle channels
if (bm.num_channels[part_index] == 3)
{
const float4 sample = bsdf_measurement_lookup<float4>(
bm.eval_data[part_index], theta_phi_in, theta_phi_out);
store_result3(result, sample.x, sample.y, sample.z);
}
else
{
const float sample = bsdf_measurement_lookup<float>(
bm.eval_data[part_index], theta_phi_in, theta_phi_out);
store_result3(result, sample);
}
}
// Implementation of df::bsdf_measurement_sample() for an MBSDF.
extern "C" __device__ void df_bsdf_measurement_sample(
float result[3], // output: theta, phi, pdf
Texture_handler_base const *self_base,
unsigned bsdf_measurement_index,
float const theta_phi_out[2],
float const xi[3], // uniform random values
Mbsdf_part part)
{
result[0] = -1.0f; // negative theta means absorption
result[1] = -1.0f;
result[2] = 0.0f;
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if (bsdf_measurement_index == 0 || bsdf_measurement_index - 1 >= self->num_mbsdfs)
return; // invalid MBSDFs returns zero
const Mbsdf& bm = self->mbsdfs[bsdf_measurement_index - 1];
unsigned part_index = static_cast<unsigned>(part);
if (bm.has_data[part_index] == 0)
return; // check for the part
// CDF data
uint2 res = bm.angular_resolution[part_index];
const float* sample_data = bm.sample_data[part_index];
// compute the theta_in index (flipping input and output, BSDFs are symmetric)
unsigned idx_theta_in = unsigned(theta_phi_out[0] * M_ONE_OVER_PI * 2.0f * float(res.x));
idx_theta_in = min(idx_theta_in, res.x - 1);
// sample theta_out
//-------------------------------------------
float xi0 = xi[0];
const float* cdf_theta = sample_data + idx_theta_in * res.x;
unsigned idx_theta_out = sample_cdf(cdf_theta, res.x, xi0); // binary search
float prob_theta = cdf_theta[idx_theta_out];
if (idx_theta_out > 0)
{
const float tmp = cdf_theta[idx_theta_out - 1];
prob_theta -= tmp;
xi0 -= tmp;
}
xi0 /= prob_theta; // rescale for re-usage
// sample phi_out
//-------------------------------------------
float xi1 = xi[1];
const float* cdf_phi = sample_data +
(res.x * res.x) + // CDF theta block
(idx_theta_in * res.x + idx_theta_out) * res.y; // selected CDF phi
// select which half-circle to choose with probability 0.5
const bool flip = (xi1 > 0.5f);
if (flip)
xi1 = 1.0f - xi1;
xi1 *= 2.0f;
unsigned idx_phi_out = sample_cdf(cdf_phi, res.y, xi1); // binary search
float prob_phi = cdf_phi[idx_phi_out];
if (idx_phi_out > 0)
{
const float tmp = cdf_phi[idx_phi_out - 1];
prob_phi -= tmp;
xi1 -= tmp;
}
xi1 /= prob_phi; // rescale for re-usage
// compute theta and phi out
//-------------------------------------------
const float2 inv_res = bm.inv_angular_resolution[part_index];
const float s_theta = float(0.5 * M_PI) * inv_res.x;
const float s_phi = float(1.0 * M_PI) * inv_res.y;
const float cos_theta_0 = cosf(float(idx_theta_out) * s_theta);
const float cos_theta_1 = cosf(float(idx_theta_out + 1u) * s_theta);
const float cos_theta = cos_theta_0 * (1.0f - xi1) + cos_theta_1 * xi1;
result[0] = acosf(cos_theta);
result[1] = (float(idx_phi_out) + xi0) * s_phi;
if (flip)
result[1] = float(2.0 * M_PI) - result[1]; // phi \in [0, 2pi]
// align phi
result[1] += (theta_phi_out[1] > 0) ? theta_phi_out[1] : (float(2.0 * M_PI) + theta_phi_out[1]);
if (result[1] > float(2.0 * M_PI)) result[1] -= float(2.0 * M_PI);
if (result[1] > float(1.0 * M_PI)) result[1] = float(-2.0 * M_PI) + result[1]; // to [-pi, pi]
// compute pdf
//-------------------------------------------
result[2] = prob_theta * prob_phi * 0.5f
/ (s_phi * (cos_theta_0 - cos_theta_1));
}
// Implementation of df::bsdf_measurement_pdf() for an MBSDF.
extern "C" __device__ float df_bsdf_measurement_pdf(
Texture_handler_base const *self_base,
unsigned bsdf_measurement_index,
float const theta_phi_in[2],
float const theta_phi_out[2],
Mbsdf_part part)
{
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if (bsdf_measurement_index == 0 || bsdf_measurement_index - 1 >= self->num_mbsdfs)
return 0.0f; // invalid MBSDF returns zero
const Mbsdf& bm = self->mbsdfs[bsdf_measurement_index - 1];
unsigned part_index = static_cast<unsigned>(part);
// check for the part
if (bm.has_data[part_index] == 0)
return 0.0f;
// CDF data and resolution
const float* sample_data = bm.sample_data[part_index];
uint2 res = bm.angular_resolution[part_index];
// compute indices in the CDF data
float3 uvw = bsdf_compute_uvw(theta_phi_in, theta_phi_out); // phi_delta, theta_out, theta_in
unsigned idx_theta_in = unsigned(theta_phi_in[0] * M_ONE_OVER_PI * 2.0f * float(res.x));
unsigned idx_theta_out = unsigned(theta_phi_out[0] * M_ONE_OVER_PI * 2.0f * float(res.x));
unsigned idx_phi_out = unsigned(uvw.x * float(res.y));
idx_theta_in = min(idx_theta_in, res.x - 1);
idx_theta_out = min(idx_theta_out, res.x - 1);
idx_phi_out = min(idx_phi_out, res.y - 1);
// get probability to select theta_out
const float* cdf_theta = sample_data + idx_theta_in * res.x;
float prob_theta = cdf_theta[idx_theta_out];
if (idx_theta_out > 0)
{
const float tmp = cdf_theta[idx_theta_out - 1];
prob_theta -= tmp;
}
// get probability to select phi_out
const float* cdf_phi = sample_data +
(res.x * res.x) + // CDF theta block
(idx_theta_in * res.x + idx_theta_out) * res.y; // selected CDF phi
float prob_phi = cdf_phi[idx_phi_out];
if (idx_phi_out > 0)
{
const float tmp = cdf_phi[idx_phi_out - 1];
prob_phi -= tmp;
}
// compute probability to select a position in the sphere patch
float2 inv_res = bm.inv_angular_resolution[part_index];
const float s_theta = float(0.5 * M_PI) * inv_res.x;
const float s_phi = float(1.0 * M_PI) * inv_res.y;
const float cos_theta_0 = cosf(float(idx_theta_out) * s_theta);
const float cos_theta_1 = cosf(float(idx_theta_out + 1u) * s_theta);
return prob_theta * prob_phi * 0.5f
/ (s_phi * (cos_theta_0 - cos_theta_1));
}
__device__ inline void df_bsdf_measurement_albedo(
float result[2], // output: max (in case of color) albedo
// for the selected direction ([0]) and
// global ([1])
Texture_handler const *self,
unsigned bsdf_measurement_index,
float const theta_phi[2],
Mbsdf_part part)
{
const Mbsdf& bm = self->mbsdfs[bsdf_measurement_index - 1];
const unsigned part_index = static_cast<unsigned>(part);
// check for the part
if (bm.has_data[part_index] == 0)
return;
const uint2 res = bm.angular_resolution[part_index];
unsigned idx_theta = unsigned(theta_phi[0] * float(2.0 / M_PI) * float(res.x));
idx_theta = min(idx_theta, res.x - 1u);
result[0] = bm.albedo_data[part_index][idx_theta];
result[1] = bm.max_albedo[part_index];
}
// Implementation of df::bsdf_measurement_albedos() for an MBSDF.
extern "C" __device__ void df_bsdf_measurement_albedos(
float result[4], // output: [0] albedo refl. for theta_phi
// [1] max albedo refl. global
// [2] albedo trans. for theta_phi
// [3] max albedo trans. global
Texture_handler_base const *self_base,
unsigned bsdf_measurement_index,
float const theta_phi[2])
{
result[0] = 0.0f;
result[1] = 0.0f;
result[2] = 0.0f;
result[3] = 0.0f;
Texture_handler const *self = static_cast<Texture_handler const *>(self_base);
if (bsdf_measurement_index == 0 || bsdf_measurement_index - 1 >= self->num_mbsdfs)
return; // invalid MBSDF returns zero
df_bsdf_measurement_albedo(
&result[0],
self,
bsdf_measurement_index,
theta_phi,
mi::neuraylib::MBSDF_DATA_REFLECTION);
df_bsdf_measurement_albedo(
&result[2],
self,
bsdf_measurement_index,
theta_phi,
mi::neuraylib::MBSDF_DATA_TRANSMISSION);
}
// ------------------------------------------------------------------------------------------------
// Normal adaption (dummy functions)
//
// Can be enabled via backend option "use_renderer_adapt_normal".
// ------------------------------------------------------------------------------------------------
#ifndef TEX_SUPPORT_NO_DUMMY_ADAPTNORMAL
// Implementation of adapt_normal().
extern "C" __device__ void adapt_normal(
float result[3],
Texture_handler_base const *self_base,
Shading_state_material *state,
float const normal[3])
{
// just return original normal
result[0] = normal[0];
result[1] = normal[1];
result[2] = normal[2];
}
#endif // TEX_SUPPORT_NO_DUMMY_ADAPTNORMAL
// ------------------------------------------------------------------------------------------------
// Scene data (dummy functions)
// ------------------------------------------------------------------------------------------------
#ifndef TEX_SUPPORT_NO_DUMMY_SCENEDATA
// Implementation of scene_data_isvalid().
extern "C" __device__ bool scene_data_isvalid(
Texture_handler_base const *self_base,
Shading_state_material *state,
unsigned scene_data_id)
{
return false;
}
// Implementation of scene_data_lookup_float4().
extern "C" __device__ void scene_data_lookup_float4(
float result[4],
Texture_handler_base const *self_base,
Shading_state_material *state,
unsigned scene_data_id,
float const default_value[4],
bool uniform_lookup)
{
// just return default value
result[0] = default_value[0];
result[1] = default_value[1];
result[2] = default_value[2];
result[3] = default_value[3];
}
// Implementation of scene_data_lookup_float3().
extern "C" __device__ void scene_data_lookup_float3(
float result[3],
Texture_handler_base const *self_base,
Shading_state_material *state,
unsigned scene_data_id,
float const default_value[3],
bool uniform_lookup)
{
// just return default value
result[0] = default_value[0];
result[1] = default_value[1];
result[2] = default_value[2];
}
// Implementation of scene_data_lookup_color().
extern "C" __device__ void scene_data_lookup_color(
float result[3],
Texture_handler_base const *self_base,
Shading_state_material *state,
unsigned scene_data_id,
float const default_value[3],
bool uniform_lookup)
{
// just return default value
result[0] = default_value[0];
result[1] = default_value[1];
result[2] = default_value[2];
}
// Implementation of scene_data_lookup_float2().
extern "C" __device__ void scene_data_lookup_float2(
float result[2],
Texture_handler_base const *self_base,
Shading_state_material *state,
unsigned scene_data_id,
float const default_value[2],
bool uniform_lookup)
{
// just return default value
result[0] = default_value[0];
result[1] = default_value[1];
}
// Implementation of scene_data_lookup_float().
extern "C" __device__ float scene_data_lookup_float(
Texture_handler_base const *self_base,
Shading_state_material *state,
unsigned scene_data_id,
float const default_value,
bool uniform_lookup)
{
// just return default value
return default_value;
}
// Implementation of scene_data_lookup_int4().
extern "C" __device__ void scene_data_lookup_int4(
int result[4],
Texture_handler_base const *self_base,
Shading_state_material *state,
unsigned scene_data_id,
int const default_value[4],
bool uniform_lookup)
{
// just return default value
result[0] = default_value[0];
result[1] = default_value[1];
result[2] = default_value[2];
result[3] = default_value[3];
}
// Implementation of scene_data_lookup_int3().
extern "C" __device__ void scene_data_lookup_int3(
int result[3],
Texture_handler_base const *self_base,
Shading_state_material *state,
unsigned scene_data_id,
int const default_value[3],
bool uniform_lookup)
{
// just return default value
result[0] = default_value[0];
result[1] = default_value[1];
result[2] = default_value[2];
}
// Implementation of scene_data_lookup_int2().
extern "C" __device__ void scene_data_lookup_int2(
int result[2],
Texture_handler_base const *self_base,
Shading_state_material *state,
unsigned scene_data_id,
int const default_value[2],
bool uniform_lookup)
{
// just return default value
result[0] = default_value[0];
result[1] = default_value[1];
}
// Implementation of scene_data_lookup_int().
extern "C" __device__ int scene_data_lookup_int(
Texture_handler_base const *self_base,
Shading_state_material *state,
unsigned scene_data_id,
int default_value,
bool uniform_lookup)
{
// just return default value
return default_value;
}
// Implementation of scene_data_lookup_float4() with derivatives.
extern "C" __device__ void scene_data_lookup_deriv_float4(
tct_deriv_arr_float_4 *result,
Texture_handler_base const *self_base,
Shading_state_material_with_derivs *state,
unsigned scene_data_id,
tct_deriv_arr_float_4 const *default_value,
bool uniform_lookup)
{
// just return default value
*result = *default_value;
}
// Implementation of scene_data_lookup_float3() with derivatives.
extern "C" __device__ void scene_data_lookup_deriv_float3(
tct_deriv_arr_float_3 *result,
Texture_handler_base const *self_base,
Shading_state_material_with_derivs *state,
unsigned scene_data_id,
tct_deriv_arr_float_3 const *default_value,
bool uniform_lookup)
{
// just return default value
*result = *default_value;
}
// Implementation of scene_data_lookup_color() with derivatives.
extern "C" __device__ void scene_data_lookup_deriv_color(
tct_deriv_arr_float_3 *result,
Texture_handler_base const *self_base,
Shading_state_material_with_derivs *state,
unsigned scene_data_id,
tct_deriv_arr_float_3 const *default_value,
bool uniform_lookup)
{
// just return default value
*result = *default_value;
}
// Implementation of scene_data_lookup_float2() with derivatives.
extern "C" __device__ void scene_data_lookup_deriv_float2(
tct_deriv_arr_float_2 *result,
Texture_handler_base const *self_base,
Shading_state_material_with_derivs *state,
unsigned scene_data_id,
tct_deriv_arr_float_2 const *default_value,
bool uniform_lookup)
{
// just return default value
*result = *default_value;
}
// Implementation of scene_data_lookup_float() with derivatives.
extern "C" __device__ void scene_data_lookup_deriv_float(
tct_deriv_float *result,
Texture_handler_base const *self_base,
Shading_state_material_with_derivs *state,
unsigned scene_data_id,
tct_deriv_float const *default_value,
bool uniform_lookup)
{
// just return default value
*result = *default_value;
}
#endif // TEX_SUPPORT_NO_DUMMY_SCENEDATA
// ------------------------------------------------------------------------------------------------
// Vtables
// ------------------------------------------------------------------------------------------------
#ifndef TEX_SUPPORT_NO_VTABLES
// The vtable containing all texture access handlers required by the generated code
// in "vtable" mode.
__device__ mi::neuraylib::Texture_handler_vtable tex_vtable = {
tex_lookup_float4_2d,
tex_lookup_float3_2d,
tex_texel_float4_2d,
tex_lookup_float4_3d,
tex_lookup_float3_3d,
tex_texel_float4_3d,
tex_lookup_float4_cube,
tex_lookup_float3_cube,
tex_resolution_2d,
tex_resolution_3d,
tex_texture_isvalid,
tex_frame,
df_light_profile_power,
df_light_profile_maximum,
df_light_profile_isvalid,
df_light_profile_evaluate,
df_light_profile_sample,
df_light_profile_pdf,
df_bsdf_measurement_isvalid,
df_bsdf_measurement_resolution,
df_bsdf_measurement_evaluate,
df_bsdf_measurement_sample,
df_bsdf_measurement_pdf,
df_bsdf_measurement_albedos,
adapt_normal,
scene_data_isvalid,
scene_data_lookup_float,
scene_data_lookup_float2,
scene_data_lookup_float3,
scene_data_lookup_float4,
scene_data_lookup_int,
scene_data_lookup_int2,
scene_data_lookup_int3,
scene_data_lookup_int4,
scene_data_lookup_color,
};
// The vtable containing all texture access handlers required by the generated code
// in "vtable" mode with derivatives.
__device__ mi::neuraylib::Texture_handler_deriv_vtable tex_deriv_vtable = {
tex_lookup_deriv_float4_2d,
tex_lookup_deriv_float3_2d,
tex_texel_float4_2d,
tex_lookup_float4_3d,
tex_lookup_float3_3d,
tex_texel_float4_3d,
tex_lookup_float4_cube,
tex_lookup_float3_cube,
tex_resolution_2d,
tex_resolution_3d,
tex_texture_isvalid,
tex_frame,
df_light_profile_power,
df_light_profile_maximum,
df_light_profile_isvalid,
df_light_profile_evaluate,
df_light_profile_sample,
df_light_profile_pdf,
df_bsdf_measurement_isvalid,
df_bsdf_measurement_resolution,
df_bsdf_measurement_evaluate,
df_bsdf_measurement_sample,
df_bsdf_measurement_pdf,
df_bsdf_measurement_albedos,
adapt_normal,
scene_data_isvalid,
scene_data_lookup_float,
scene_data_lookup_float2,
scene_data_lookup_float3,
scene_data_lookup_float4,
scene_data_lookup_int,
scene_data_lookup_int2,
scene_data_lookup_int3,
scene_data_lookup_int4,
scene_data_lookup_color,
scene_data_lookup_deriv_float,
scene_data_lookup_deriv_float2,
scene_data_lookup_deriv_float3,
scene_data_lookup_deriv_float4,
scene_data_lookup_deriv_color,
};
#endif // TEX_SUPPORT_NO_VTABLES
#endif // __CUDACC__
#endif // TEXTURE_SUPPORT_CUDA_H
| 60,296 | C | 37.602433 | 100 | 0.532191 |
arhix52/Strelka/src/render/optix/OptixRender.h | #pragma once
#include "render.h"
#include <optix.h>
#include "OptixRenderParams.h"
#include <iostream>
#include <iomanip>
#include <scene/scene.h>
#include "common.h"
#include "buffer.h"
#include <materialmanager.h>
struct Texture;
namespace oka
{
enum RayType
{
RAY_TYPE_RADIANCE = 0,
RAY_TYPE_OCCLUSION = 1,
RAY_TYPE_COUNT
};
struct PathTracerState
{
OptixDeviceContext context = 0;
OptixTraversableHandle ias_handle;
CUdeviceptr d_instances = 0;
OptixTraversableHandle gas_handle = 0; // Traversable handle for triangle AS
CUdeviceptr d_gas_output_buffer = 0; // Triangle AS memory
CUdeviceptr d_vertices = 0;
OptixModuleCompileOptions module_compile_options = {};
OptixModule ptx_module = 0;
OptixPipelineCompileOptions pipeline_compile_options = {};
OptixPipeline pipeline = 0;
OptixModule m_catromCurveModule = 0;
OptixProgramGroup raygen_prog_group = 0;
OptixProgramGroup radiance_miss_group = 0;
OptixProgramGroup occlusion_miss_group = 0;
OptixProgramGroup radiance_default_hit_group = 0;
std::vector<OptixProgramGroup> radiance_hit_groups;
OptixProgramGroup occlusion_hit_group = 0;
OptixProgramGroup light_hit_group = 0;
CUstream stream = 0;
Params params = {};
Params prevParams = {};
CUdeviceptr d_params = 0;
OptixShaderBindingTable sbt = {};
};
class OptiXRender : public Render
{
private:
struct Mesh
{
OptixTraversableHandle gas_handle = 0;
CUdeviceptr d_gas_output_buffer = 0;
};
struct Curve
{
OptixTraversableHandle gas_handle = 0;
CUdeviceptr d_gas_output_buffer = 0;
};
struct Instance
{
OptixInstance instance;
};
// optix material
struct Material
{
OptixProgramGroup programGroup;
CUdeviceptr d_argData = 0;
size_t d_argDataSize = 0;
CUdeviceptr d_roData = 0;
size_t d_roSize = 0;
CUdeviceptr d_textureHandler;
};
struct View
{
oka::Camera::Matrices mCamMatrices;
};
View mPrevView;
PathTracerState mState;
bool mEnableValidation;
Mesh* createMesh(const oka::Mesh& mesh);
Curve* createCurve(const oka::Curve& curve);
bool compactAccel(CUdeviceptr& buffer, OptixTraversableHandle& handle, CUdeviceptr result, size_t outputSizeInBytes);
std::vector<Mesh*> mOptixMeshes;
std::vector<Curve*> mOptixCurves;
CUdeviceptr d_vb = 0;
CUdeviceptr d_ib = 0;
CUdeviceptr d_lights = 0;
CUdeviceptr d_points = 0;
CUdeviceptr d_widths = 0;
CUdeviceptr d_materialRoData = 0;
CUdeviceptr d_materialArgData = 0;
CUdeviceptr d_texturesHandler = 0;
CUdeviceptr d_texturesData = 0;
CUdeviceptr d_param = 0;
void createVertexBuffer();
void createIndexBuffer();
// curve utils
void createPointsBuffer();
void createWidthsBuffer();
void createLightBuffer();
Texture loadTextureFromFile(const std::string& fileName);
bool createOptixMaterials();
Material& getMaterial(int id);
MaterialManager mMaterialManager;
std::vector<Material> mMaterials;
void updatePathtracerParams(const uint32_t width, const uint32_t height);
public:
OptiXRender(/* args */);
~OptiXRender();
void init() override;
void render(Buffer* output_buffer) override;
Buffer* createBuffer(const BufferDesc& desc) override;
void createContext();
void createAccelerationStructure();
void createModule();
void createProgramGroups();
void createPipeline();
void createSbt();
OptixProgramGroup createRadianceClosestHitProgramGroup(PathTracerState& state,
char const* module_code,
size_t module_size);
};
} // namespace oka
| 3,873 | C | 22.621951 | 121 | 0.661245 |
arhix52/Strelka/src/render/optix/OptixBuffer.cpp | #include "OptixBuffer.h"
#include <cuda.h>
#include <cuda_runtime_api.h>
using namespace oka;
oka::OptixBuffer::OptixBuffer(void* devicePtr, BufferFormat format, uint32_t width, uint32_t height)
{
mDeviceData = devicePtr;
mFormat = format;
mWidth = width;
mHeight = height;
}
oka::OptixBuffer::~OptixBuffer()
{
// TODO:
if (mDeviceData)
{
cudaFree(mDeviceData);
}
}
void oka::OptixBuffer::resize(uint32_t width, uint32_t height)
{
if (mDeviceData)
{
cudaFree(mDeviceData);
}
mWidth = width;
mHeight = height;
const size_t bufferSize = mWidth * mHeight * getElementSize();
cudaMalloc(reinterpret_cast<void**>(&mDeviceData), bufferSize);
}
void* oka::OptixBuffer::map()
{
const size_t bufferSize = mWidth * mHeight * getElementSize();
mHostData.resize(bufferSize);
cudaMemcpy(static_cast<void*>(mHostData.data()), mDeviceData, bufferSize, cudaMemcpyDeviceToHost);
return nullptr;
}
void oka::OptixBuffer::unmap()
{
}
| 1,014 | C++ | 20.145833 | 102 | 0.670611 |
arhix52/Strelka/src/render/optix/OptixRender.cpp | #include "OptixRender.h"
#include "OptixBuffer.h"
#include <optix_function_table_definition.h>
#include <optix_stubs.h>
#include <optix_stack_size.h>
#include <glm/glm.hpp>
#include <glm/mat4x3.hpp>
#include <glm/gtx/compatibility.hpp>
#include <glm/gtc/type_ptr.hpp>
#include <glm/gtx/matrix_major_storage.hpp>
#include <glm/ext/matrix_relational.hpp>
#define STB_IMAGE_STATIC
#define STB_IMAGE_IMPLEMENTATION
#include <stb_image.h>
#include <vector_types.h>
#include <vector_functions.h>
#include <sutil/vec_math_adv.h>
#include "texture_support_cuda.h"
#include <filesystem>
#include <array>
#include <string>
#include <sstream>
#include <fstream>
#include <log.h>
#include "postprocessing/Tonemappers.h"
#include "Camera.h"
static void context_log_cb(unsigned int level, const char* tag, const char* message, void* /*cbdata */)
{
switch (level)
{
case 1:
STRELKA_FATAL("OptiX [{0}]: {1}", tag, message);
break;
case 2:
STRELKA_ERROR("OptiX [{0}]: {1}", tag, message);
break;
case 3:
STRELKA_WARNING("OptiX [{0}]: {1}", tag, message);
break;
case 4:
STRELKA_INFO("OptiX [{0}]: {1}", tag, message);
break;
default:
break;
}
}
static inline void optixCheck(OptixResult res, const char* call, const char* file, unsigned int line)
{
if (res != OPTIX_SUCCESS)
{
STRELKA_ERROR("OptiX call {0} failed: {1}:{2}", call, file, line);
assert(0);
}
}
static inline void optixCheckLog(OptixResult res,
const char* log,
size_t sizeof_log,
size_t sizeof_log_returned,
const char* call,
const char* file,
unsigned int line)
{
if (res != OPTIX_SUCCESS)
{
STRELKA_FATAL("OptiX call {0} failed: {1}:{2} : {3}", call, file, line, log);
assert(0);
}
}
inline void cudaCheck(cudaError_t error, const char* call, const char* file, unsigned int line)
{
if (error != cudaSuccess)
{
STRELKA_FATAL("CUDA call ({0}) failed with error: {1} {2}:{3}", call, cudaGetErrorString(error), file, line);
assert(0);
}
}
inline void cudaSyncCheck(const char* file, unsigned int line)
{
cudaDeviceSynchronize();
cudaError_t error = cudaGetLastError();
if (error != cudaSuccess)
{
STRELKA_FATAL("CUDA error on synchronize with error {0} , {1}:{2}", cudaGetErrorString(error), file, line);
assert(0);
}
}
//------------------------------------------------------------------------------
//
// OptiX error-checking
//
//------------------------------------------------------------------------------
#define OPTIX_CHECK(call) optixCheck(call, #call, __FILE__, __LINE__)
#define OPTIX_CHECK_LOG(call) optixCheckLog(call, log, sizeof(log), sizeof_log, #call, __FILE__, __LINE__)
#define CUDA_CHECK(call) cudaCheck(call, #call, __FILE__, __LINE__)
#define CUDA_SYNC_CHECK() cudaSyncCheck(__FILE__, __LINE__)
using namespace oka;
namespace fs = std::filesystem;
template <typename T>
struct SbtRecord
{
__align__(OPTIX_SBT_RECORD_ALIGNMENT) char header[OPTIX_SBT_RECORD_HEADER_SIZE];
T data;
};
typedef SbtRecord<RayGenData> RayGenSbtRecord;
typedef SbtRecord<MissData> MissSbtRecord;
typedef SbtRecord<HitGroupData> HitGroupSbtRecord;
void configureCamera(::Camera& cam, const uint32_t width, const uint32_t height)
{
cam.setEye({ 0.0f, 0.0f, 2.0f });
cam.setLookat({ 0.0f, 0.0f, 0.0f });
cam.setUp({ 0.0f, 1.0f, 3.0f });
cam.setFovY(45.0f);
cam.setAspectRatio((float)width / (float)height);
}
static bool readSourceFile(std::string& str, const fs::path& filename)
{
// Try to open file
std::ifstream file(filename.c_str(), std::ios::binary);
if (file.good())
{
// Found usable source file
std::vector<unsigned char> buffer = std::vector<unsigned char>(std::istreambuf_iterator<char>(file), {});
str.assign(buffer.begin(), buffer.end());
return true;
}
return false;
}
OptiXRender::OptiXRender(/* args */)
{
}
OptiXRender::~OptiXRender()
{
}
void OptiXRender::createContext()
{
// Initialize CUDA
CUDA_CHECK(cudaFree(0));
CUstream stream;
CUDA_CHECK(cudaStreamCreate(&stream));
mState.stream = stream;
OptixDeviceContext context;
CUcontext cu_ctx = 0; // zero means take the current context
OPTIX_CHECK(optixInit());
OptixDeviceContextOptions options = {};
options.logCallbackFunction = &context_log_cb;
options.logCallbackLevel = 4;
if (mEnableValidation)
{
options.validationMode = OPTIX_DEVICE_CONTEXT_VALIDATION_MODE_ALL;
}
else
{
options.validationMode = OPTIX_DEVICE_CONTEXT_VALIDATION_MODE_OFF;
}
OPTIX_CHECK(optixDeviceContextCreate(cu_ctx, &options, &context));
mState.context = context;
}
bool OptiXRender::compactAccel(CUdeviceptr& buffer,
OptixTraversableHandle& handle,
CUdeviceptr result,
size_t outputSizeInBytes)
{
bool flag = false;
size_t compacted_size;
CUDA_CHECK(cudaMemcpy(&compacted_size, (void*)result, sizeof(size_t), cudaMemcpyDeviceToHost));
CUdeviceptr compactedOutputBuffer;
if (compacted_size < outputSizeInBytes)
{
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&compactedOutputBuffer), compacted_size));
// use handle as input and output
OPTIX_CHECK(optixAccelCompact(mState.context, 0, handle, compactedOutputBuffer, compacted_size, &handle));
CUDA_CHECK(cudaFree(reinterpret_cast<void*>(buffer)));
buffer = compactedOutputBuffer;
flag = true;
}
return flag;
}
OptiXRender::Curve* OptiXRender::createCurve(const oka::Curve& curve)
{
Curve* rcurve = new Curve();
OptixAccelBuildOptions accel_options = {};
accel_options.buildFlags = OPTIX_BUILD_FLAG_ALLOW_COMPACTION | OPTIX_BUILD_FLAG_ALLOW_RANDOM_VERTEX_ACCESS |
OPTIX_BUILD_FLAG_PREFER_FAST_TRACE;
accel_options.operation = OPTIX_BUILD_OPERATION_BUILD;
const uint32_t pointsCount = mScene->getCurvesPoint().size(); // total points count in points buffer
const int degree = 3;
// each oka::Curves could contains many curves
const uint32_t numCurves = curve.mVertexCountsCount;
std::vector<int> segmentIndices;
uint32_t offsetInsideCurveArray = 0;
for (int curveIndex = 0; curveIndex < numCurves; ++curveIndex)
{
const std::vector<uint32_t>& vertexCounts = mScene->getCurvesVertexCounts();
const uint32_t numControlPoints = vertexCounts[curve.mVertexCountsStart + curveIndex];
const int segmentsCount = numControlPoints - degree;
for (int i = 0; i < segmentsCount; ++i)
{
int index = curve.mPointsStart + offsetInsideCurveArray + i;
segmentIndices.push_back(index);
}
offsetInsideCurveArray += numControlPoints;
}
const size_t segmentIndicesSize = sizeof(int) * segmentIndices.size();
CUdeviceptr d_segmentIndices = 0;
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&d_segmentIndices), segmentIndicesSize));
CUDA_CHECK(cudaMemcpy(
reinterpret_cast<void*>(d_segmentIndices), segmentIndices.data(), segmentIndicesSize, cudaMemcpyHostToDevice));
// Curve build input.
OptixBuildInput curve_input = {};
curve_input.type = OPTIX_BUILD_INPUT_TYPE_CURVES;
switch (degree)
{
case 1:
curve_input.curveArray.curveType = OPTIX_PRIMITIVE_TYPE_ROUND_LINEAR;
break;
case 2:
curve_input.curveArray.curveType = OPTIX_PRIMITIVE_TYPE_ROUND_QUADRATIC_BSPLINE;
break;
case 3:
curve_input.curveArray.curveType = OPTIX_PRIMITIVE_TYPE_ROUND_CUBIC_BSPLINE;
break;
}
curve_input.curveArray.numPrimitives = segmentIndices.size();
curve_input.curveArray.vertexBuffers = &d_points;
curve_input.curveArray.numVertices = pointsCount;
curve_input.curveArray.vertexStrideInBytes = sizeof(glm::float3);
curve_input.curveArray.widthBuffers = &d_widths;
curve_input.curveArray.widthStrideInBytes = sizeof(float);
curve_input.curveArray.normalBuffers = 0;
curve_input.curveArray.normalStrideInBytes = 0;
curve_input.curveArray.indexBuffer = d_segmentIndices;
curve_input.curveArray.indexStrideInBytes = sizeof(int);
curve_input.curveArray.flag = OPTIX_GEOMETRY_FLAG_NONE;
curve_input.curveArray.primitiveIndexOffset = 0;
// curve_input.curveArray.endcapFlags = OPTIX_CURVE_ENDCAP_ON;
OptixAccelBufferSizes gas_buffer_sizes;
OPTIX_CHECK(optixAccelComputeMemoryUsage(mState.context, &accel_options, &curve_input,
1, // Number of build inputs
&gas_buffer_sizes));
CUdeviceptr d_temp_buffer_gas;
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&d_temp_buffer_gas), gas_buffer_sizes.tempSizeInBytes));
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&rcurve->d_gas_output_buffer), gas_buffer_sizes.outputSizeInBytes));
CUdeviceptr compactedSizeBuffer;
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>((&compactedSizeBuffer)), sizeof(uint64_t)));
OptixAccelEmitDesc property = {};
property.type = OPTIX_PROPERTY_TYPE_COMPACTED_SIZE;
property.result = compactedSizeBuffer;
OPTIX_CHECK(optixAccelBuild(mState.context, 0, // CUDA stream
&accel_options, &curve_input,
1, // num build inputs
d_temp_buffer_gas, gas_buffer_sizes.tempSizeInBytes, rcurve->d_gas_output_buffer,
gas_buffer_sizes.outputSizeInBytes, &rcurve->gas_handle,
&property, // emitted property list
1)); // num emitted properties
compactAccel(rcurve->d_gas_output_buffer, rcurve->gas_handle, property.result, gas_buffer_sizes.outputSizeInBytes);
// We can now free the scratch space buffer used during build and the vertex
// inputs, since they are not needed by our trivial shading method
CUDA_CHECK(cudaFree(reinterpret_cast<void*>(d_temp_buffer_gas)));
CUDA_CHECK(cudaFree(reinterpret_cast<void*>(d_segmentIndices)));
return rcurve;
}
OptiXRender::Mesh* OptiXRender::createMesh(const oka::Mesh& mesh)
{
OptixTraversableHandle gas_handle;
CUdeviceptr d_gas_output_buffer;
{
// Use default options for simplicity. In a real use case we would want to
// enable compaction, etc
OptixAccelBuildOptions accel_options = {};
accel_options.buildFlags = OPTIX_BUILD_FLAG_ALLOW_COMPACTION | OPTIX_BUILD_FLAG_PREFER_FAST_TRACE;
accel_options.operation = OPTIX_BUILD_OPERATION_BUILD;
// std::vector<oka::Scene::Vertex>& vertices = mScene->getVertices();
// std::vector<uint32_t>& indices = mScene->getIndices();
const CUdeviceptr verticesDataStart = d_vb + mesh.mVbOffset * sizeof(oka::Scene::Vertex);
CUdeviceptr indicesDataStart = d_ib + mesh.mIndex * sizeof(uint32_t);
// Our build input is a simple list of non-indexed triangle vertices
const uint32_t triangle_input_flags[1] = { OPTIX_GEOMETRY_FLAG_NONE };
OptixBuildInput triangle_input = {};
triangle_input.type = OPTIX_BUILD_INPUT_TYPE_TRIANGLES;
triangle_input.triangleArray.vertexFormat = OPTIX_VERTEX_FORMAT_FLOAT3;
triangle_input.triangleArray.numVertices = mesh.mVertexCount;
triangle_input.triangleArray.vertexBuffers = &verticesDataStart;
triangle_input.triangleArray.vertexStrideInBytes = sizeof(oka::Scene::Vertex);
triangle_input.triangleArray.indexBuffer = indicesDataStart;
triangle_input.triangleArray.indexFormat = OptixIndicesFormat::OPTIX_INDICES_FORMAT_UNSIGNED_INT3;
triangle_input.triangleArray.indexStrideInBytes = sizeof(uint32_t) * 3;
triangle_input.triangleArray.numIndexTriplets = mesh.mCount / 3;
// triangle_input.triangleArray.
triangle_input.triangleArray.flags = triangle_input_flags;
triangle_input.triangleArray.numSbtRecords = 1;
OptixAccelBufferSizes gas_buffer_sizes;
OPTIX_CHECK(optixAccelComputeMemoryUsage(mState.context, &accel_options, &triangle_input,
1, // Number of build inputs
&gas_buffer_sizes));
CUdeviceptr d_temp_buffer_gas;
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&d_temp_buffer_gas), gas_buffer_sizes.tempSizeInBytes));
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&d_gas_output_buffer), gas_buffer_sizes.outputSizeInBytes));
CUdeviceptr compactedSizeBuffer;
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>((&compactedSizeBuffer)), sizeof(uint64_t)));
OptixAccelEmitDesc property = {};
property.type = OPTIX_PROPERTY_TYPE_COMPACTED_SIZE;
property.result = compactedSizeBuffer;
OPTIX_CHECK(optixAccelBuild(mState.context,
0, // CUDA stream
&accel_options, &triangle_input,
1, // num build inputs
d_temp_buffer_gas, gas_buffer_sizes.tempSizeInBytes, d_gas_output_buffer,
gas_buffer_sizes.outputSizeInBytes, &gas_handle,
&property, // emitted property list
1 // num emitted properties
));
compactAccel(d_gas_output_buffer, gas_handle, property.result, gas_buffer_sizes.outputSizeInBytes);
CUDA_CHECK(cudaFree(reinterpret_cast<void*>(d_temp_buffer_gas)));
CUDA_CHECK(cudaFree(reinterpret_cast<void*>(compactedSizeBuffer)));
}
Mesh* rmesh = new Mesh();
rmesh->d_gas_output_buffer = d_gas_output_buffer;
rmesh->gas_handle = gas_handle;
return rmesh;
}
void OptiXRender::createAccelerationStructure()
{
const std::vector<oka::Mesh>& meshes = mScene->getMeshes();
const std::vector<oka::Curve>& curves = mScene->getCurves();
const std::vector<oka::Instance>& instances = mScene->getInstances();
if (meshes.empty() && curves.empty())
{
return;
}
// TODO: add proper clear and free resources
mOptixMeshes.clear();
for (int i = 0; i < meshes.size(); ++i)
{
Mesh* m = createMesh(meshes[i]);
mOptixMeshes.push_back(m);
}
mOptixCurves.clear();
for (int i = 0; i < curves.size(); ++i)
{
Curve* c = createCurve(curves[i]);
mOptixCurves.push_back(c);
}
std::vector<OptixInstance> optixInstances;
glm::mat3x4 identity = glm::identity<glm::mat3x4>();
for (int i = 0; i < instances.size(); ++i)
{
OptixInstance oi = {};
const oka::Instance& curr = instances[i];
if (curr.type == oka::Instance::Type::eMesh)
{
oi.traversableHandle = mOptixMeshes[curr.mMeshId]->gas_handle;
oi.visibilityMask = GEOMETRY_MASK_TRIANGLE;
}
else if (curr.type == oka::Instance::Type::eCurve)
{
oi.traversableHandle = mOptixCurves[curr.mCurveId]->gas_handle;
oi.visibilityMask = GEOMETRY_MASK_CURVE;
}
else if (curr.type == oka::Instance::Type::eLight)
{
oi.traversableHandle = mOptixMeshes[curr.mMeshId]->gas_handle;
oi.visibilityMask = GEOMETRY_MASK_LIGHT;
}
else
{
assert(0);
}
// fill common instance data
memcpy(oi.transform, glm::value_ptr(glm::float3x4(glm::rowMajor4(curr.transform))), sizeof(float) * 12);
oi.sbtOffset = static_cast<unsigned int>(i * RAY_TYPE_COUNT);
optixInstances.push_back(oi);
}
size_t instances_size_in_bytes = sizeof(OptixInstance) * optixInstances.size();
CUDA_CHECK(cudaMalloc((void**)&mState.d_instances, instances_size_in_bytes));
CUDA_CHECK(
cudaMemcpy((void*)mState.d_instances, optixInstances.data(), instances_size_in_bytes, cudaMemcpyHostToDevice));
OptixBuildInput ias_instance_input = {};
ias_instance_input.type = OPTIX_BUILD_INPUT_TYPE_INSTANCES;
ias_instance_input.instanceArray.instances = mState.d_instances;
ias_instance_input.instanceArray.numInstances = static_cast<int>(optixInstances.size());
OptixAccelBuildOptions ias_accel_options = {};
ias_accel_options.buildFlags = OPTIX_BUILD_FLAG_ALLOW_COMPACTION | OPTIX_BUILD_FLAG_PREFER_FAST_TRACE;
ias_accel_options.motionOptions.numKeys = 1;
ias_accel_options.operation = OPTIX_BUILD_OPERATION_BUILD;
OptixAccelBufferSizes ias_buffer_sizes;
OPTIX_CHECK(
optixAccelComputeMemoryUsage(mState.context, &ias_accel_options, &ias_instance_input, 1, &ias_buffer_sizes));
// non-compacted output
CUdeviceptr d_buffer_temp_output_ias_and_compacted_size;
auto roundUp = [](size_t x, size_t y) { return ((x + y - 1) / y) * y; };
size_t compactedSizeOffset = roundUp(ias_buffer_sizes.outputSizeInBytes, 8ull);
CUDA_CHECK(
cudaMalloc(reinterpret_cast<void**>(&d_buffer_temp_output_ias_and_compacted_size), compactedSizeOffset + 8));
CUdeviceptr d_ias_temp_buffer;
// bool needIASTempBuffer = ias_buffer_sizes.tempSizeInBytes > state.temp_buffer_size;
// if( needIASTempBuffer )
{
CUDA_CHECK(cudaMalloc((void**)&d_ias_temp_buffer, ias_buffer_sizes.tempSizeInBytes));
}
// else
// {
// d_ias_temp_buffer = state.d_temp_buffer;
// }
CUdeviceptr compactedSizeBuffer;
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>((&compactedSizeBuffer)), sizeof(uint64_t)));
OptixAccelEmitDesc property = {};
property.type = OPTIX_PROPERTY_TYPE_COMPACTED_SIZE;
property.result = compactedSizeBuffer;
OPTIX_CHECK(optixAccelBuild(mState.context, 0, &ias_accel_options, &ias_instance_input, 1, d_ias_temp_buffer,
ias_buffer_sizes.tempSizeInBytes, d_buffer_temp_output_ias_and_compacted_size,
ias_buffer_sizes.outputSizeInBytes, &mState.ias_handle, &property, 1));
compactAccel(d_buffer_temp_output_ias_and_compacted_size, mState.ias_handle, property.result,
ias_buffer_sizes.outputSizeInBytes);
CUDA_CHECK(cudaFree(reinterpret_cast<void*>(compactedSizeBuffer)));
CUDA_CHECK(cudaFree(reinterpret_cast<void*>(d_ias_temp_buffer)));
}
void OptiXRender::createModule()
{
OptixModule module = nullptr;
OptixPipelineCompileOptions pipeline_compile_options = {};
OptixModuleCompileOptions module_compile_options = {};
{
if (mEnableValidation)
{
module_compile_options.optLevel = OPTIX_COMPILE_OPTIMIZATION_LEVEL_0;
module_compile_options.debugLevel = OPTIX_COMPILE_DEBUG_LEVEL_FULL;
}
else
{
module_compile_options.optLevel = OPTIX_COMPILE_OPTIMIZATION_DEFAULT;
module_compile_options.debugLevel = OPTIX_COMPILE_DEBUG_LEVEL_NONE;
}
pipeline_compile_options.usesMotionBlur = false;
pipeline_compile_options.traversableGraphFlags = OPTIX_TRAVERSABLE_GRAPH_FLAG_ALLOW_SINGLE_LEVEL_INSTANCING;
pipeline_compile_options.numPayloadValues = 2;
pipeline_compile_options.numAttributeValues = 2;
if (mEnableValidation) // Enables debug exceptions during optix launches. This may incur
// significant performance cost and should only be done during
// development.
pipeline_compile_options.exceptionFlags =
OPTIX_EXCEPTION_FLAG_USER | OPTIX_EXCEPTION_FLAG_TRACE_DEPTH | OPTIX_EXCEPTION_FLAG_STACK_OVERFLOW;
else
{
pipeline_compile_options.exceptionFlags = OPTIX_EXCEPTION_FLAG_NONE;
}
pipeline_compile_options.pipelineLaunchParamsVariableName = "params";
pipeline_compile_options.usesPrimitiveTypeFlags =
OPTIX_PRIMITIVE_TYPE_FLAGS_TRIANGLE | OPTIX_PRIMITIVE_TYPE_FLAGS_ROUND_CUBIC_BSPLINE;
size_t inputSize = 0;
std::string optixSource;
const fs::path cwdPath = fs::current_path();
const fs::path precompiledOptixPath = cwdPath / "optix/render_generated_OptixRender.cu.optixir";
readSourceFile(optixSource, precompiledOptixPath);
const char* input = optixSource.c_str();
inputSize = optixSource.size();
char log[2048]; // For error reporting from OptiX creation functions
size_t sizeof_log = sizeof(log);
OPTIX_CHECK_LOG(optixModuleCreate(mState.context, &module_compile_options, &pipeline_compile_options,
input, inputSize, log, &sizeof_log, &module));
}
mState.ptx_module = module;
mState.pipeline_compile_options = pipeline_compile_options;
mState.module_compile_options = module_compile_options;
// hair modules
OptixBuiltinISOptions builtinISOptions = {};
builtinISOptions.buildFlags = OPTIX_BUILD_FLAG_NONE;
// builtinISOptions.curveEndcapFlags = OPTIX_CURVE_ENDCAP_ON;
builtinISOptions.builtinISModuleType = OPTIX_PRIMITIVE_TYPE_ROUND_CUBIC_BSPLINE;
OPTIX_CHECK(optixBuiltinISModuleGet(mState.context, &mState.module_compile_options, &mState.pipeline_compile_options,
&builtinISOptions, &mState.m_catromCurveModule));
}
OptixProgramGroup OptiXRender::createRadianceClosestHitProgramGroup(PathTracerState& state,
char const* module_code,
size_t module_size)
{
char log[2048];
size_t sizeof_log = sizeof(log);
OptixModule mat_module = nullptr;
OPTIX_CHECK_LOG(optixModuleCreate(state.context, &state.module_compile_options, &state.pipeline_compile_options,
module_code, module_size, log, &sizeof_log, &mat_module));
OptixProgramGroupOptions program_group_options = {};
OptixProgramGroupDesc hit_prog_group_desc = {};
hit_prog_group_desc.kind = OPTIX_PROGRAM_GROUP_KIND_HITGROUP;
hit_prog_group_desc.hitgroup.moduleCH = mat_module;
hit_prog_group_desc.hitgroup.entryFunctionNameCH = "__closesthit__radiance";
hit_prog_group_desc.hitgroup.moduleIS = mState.m_catromCurveModule;
hit_prog_group_desc.hitgroup.entryFunctionNameIS = 0; // automatically supplied for built-in module
sizeof_log = sizeof(log);
OptixProgramGroup ch_hit_group = nullptr;
OPTIX_CHECK_LOG(optixProgramGroupCreate(state.context, &hit_prog_group_desc,
/*numProgramGroups=*/1, &program_group_options, log, &sizeof_log,
&ch_hit_group));
return ch_hit_group;
}
void OptiXRender::createProgramGroups()
{
OptixProgramGroupOptions program_group_options = {}; // Initialize to zeros
OptixProgramGroupDesc raygen_prog_group_desc = {}; //
raygen_prog_group_desc.kind = OPTIX_PROGRAM_GROUP_KIND_RAYGEN;
raygen_prog_group_desc.raygen.module = mState.ptx_module;
raygen_prog_group_desc.raygen.entryFunctionName = "__raygen__rg";
char log[2048]; // For error reporting from OptiX creation functions
size_t sizeof_log = sizeof(log);
OPTIX_CHECK_LOG(optixProgramGroupCreate(mState.context, &raygen_prog_group_desc,
1, // num program groups
&program_group_options, log, &sizeof_log, &mState.raygen_prog_group));
OptixProgramGroupDesc miss_prog_group_desc = {};
miss_prog_group_desc.kind = OPTIX_PROGRAM_GROUP_KIND_MISS;
miss_prog_group_desc.miss.module = mState.ptx_module;
miss_prog_group_desc.miss.entryFunctionName = "__miss__ms";
sizeof_log = sizeof(log);
OPTIX_CHECK_LOG(optixProgramGroupCreate(mState.context, &miss_prog_group_desc,
1, // num program groups
&program_group_options, log, &sizeof_log, &mState.radiance_miss_group));
memset(&miss_prog_group_desc, 0, sizeof(OptixProgramGroupDesc));
miss_prog_group_desc.kind = OPTIX_PROGRAM_GROUP_KIND_MISS;
miss_prog_group_desc.miss.module = nullptr; // NULL miss program for occlusion rays
miss_prog_group_desc.miss.entryFunctionName = nullptr;
sizeof_log = sizeof(log);
OPTIX_CHECK_LOG(optixProgramGroupCreate(mState.context, &miss_prog_group_desc,
1, // num program groups
&program_group_options, log, &sizeof_log, &mState.occlusion_miss_group));
OptixProgramGroupDesc hit_prog_group_desc = {};
hit_prog_group_desc.kind = OPTIX_PROGRAM_GROUP_KIND_HITGROUP;
hit_prog_group_desc.hitgroup.moduleCH = mState.ptx_module;
hit_prog_group_desc.hitgroup.entryFunctionNameCH = "__closesthit__ch";
sizeof_log = sizeof(log);
OptixProgramGroup radiance_hit_group;
OPTIX_CHECK_LOG(optixProgramGroupCreate(mState.context, &hit_prog_group_desc,
1, // num program groups
&program_group_options, log, &sizeof_log, &radiance_hit_group));
mState.radiance_default_hit_group = radiance_hit_group;
OptixProgramGroupDesc light_hit_prog_group_desc = {};
light_hit_prog_group_desc.kind = OPTIX_PROGRAM_GROUP_KIND_HITGROUP;
light_hit_prog_group_desc.hitgroup.moduleCH = mState.ptx_module;
light_hit_prog_group_desc.hitgroup.entryFunctionNameCH = "__closesthit__light";
sizeof_log = sizeof(log);
OptixProgramGroup light_hit_group;
OPTIX_CHECK_LOG(optixProgramGroupCreate(mState.context, &light_hit_prog_group_desc,
1, // num program groups
&program_group_options, log, &sizeof_log, &light_hit_group));
mState.light_hit_group = light_hit_group;
memset(&hit_prog_group_desc, 0, sizeof(OptixProgramGroupDesc));
hit_prog_group_desc.kind = OPTIX_PROGRAM_GROUP_KIND_HITGROUP;
hit_prog_group_desc.hitgroup.moduleCH = mState.ptx_module;
hit_prog_group_desc.hitgroup.entryFunctionNameCH = "__closesthit__occlusion";
hit_prog_group_desc.hitgroup.moduleIS = mState.m_catromCurveModule;
hit_prog_group_desc.hitgroup.entryFunctionNameIS = 0; // automatically supplied for built-in module
sizeof_log = sizeof(log);
OPTIX_CHECK(optixProgramGroupCreate(mState.context, &hit_prog_group_desc,
1, // num program groups
&program_group_options, log, &sizeof_log, &mState.occlusion_hit_group));
}
void OptiXRender::createPipeline()
{
OptixPipeline pipeline = nullptr;
{
const uint32_t max_trace_depth = 2;
std::vector<OptixProgramGroup> program_groups = {};
program_groups.push_back(mState.raygen_prog_group);
program_groups.push_back(mState.radiance_miss_group);
program_groups.push_back(mState.radiance_default_hit_group);
program_groups.push_back(mState.occlusion_miss_group);
program_groups.push_back(mState.occlusion_hit_group);
program_groups.push_back(mState.light_hit_group);
for (auto& m : mMaterials)
{
program_groups.push_back(m.programGroup);
}
OptixPipelineLinkOptions pipeline_link_options = {};
pipeline_link_options.maxTraceDepth = max_trace_depth;
// if (mEnableValidation)
// {
// pipeline_link_options.debugLevel = OPTIX_COMPILE_DEBUG_LEVEL_FULL;
// }
// else
// {
// pipeline_link_options.debugLevel = OPTIX_COMPILE_DEBUG_LEVEL_NONE;
// }
char log[2048]; // For error reporting from OptiX creation functions
size_t sizeof_log = sizeof(log);
OPTIX_CHECK_LOG(optixPipelineCreate(mState.context, &mState.pipeline_compile_options, &pipeline_link_options,
program_groups.data(), program_groups.size(), log, &sizeof_log, &pipeline));
OptixStackSizes stack_sizes = {};
for (auto& prog_group : program_groups)
{
OPTIX_CHECK(optixUtilAccumulateStackSizes(prog_group, &stack_sizes, pipeline));
}
uint32_t direct_callable_stack_size_from_traversal;
uint32_t direct_callable_stack_size_from_state;
uint32_t continuation_stack_size;
OPTIX_CHECK(optixUtilComputeStackSizes(&stack_sizes, max_trace_depth,
0, // maxCCDepth
0, // maxDCDepth
&direct_callable_stack_size_from_traversal,
&direct_callable_stack_size_from_state, &continuation_stack_size));
OPTIX_CHECK(optixPipelineSetStackSize(pipeline, direct_callable_stack_size_from_traversal,
direct_callable_stack_size_from_state, continuation_stack_size,
2 // maxTraversableDepth
));
}
mState.pipeline = pipeline;
}
void OptiXRender::createSbt()
{
OptixShaderBindingTable sbt = {};
{
CUdeviceptr raygen_record;
const size_t raygen_record_size = sizeof(RayGenSbtRecord);
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&raygen_record), raygen_record_size));
RayGenSbtRecord rg_sbt;
OPTIX_CHECK(optixSbtRecordPackHeader(mState.raygen_prog_group, &rg_sbt));
CUDA_CHECK(
cudaMemcpy(reinterpret_cast<void*>(raygen_record), &rg_sbt, raygen_record_size, cudaMemcpyHostToDevice));
CUdeviceptr miss_record;
const uint32_t miss_record_count = RAY_TYPE_COUNT;
size_t miss_record_size = sizeof(MissSbtRecord) * miss_record_count;
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&miss_record), miss_record_size));
std::vector<MissSbtRecord> missGroupDataCpu(miss_record_count);
MissSbtRecord& ms_sbt = missGroupDataCpu[RAY_TYPE_RADIANCE];
// ms_sbt.data.bg_color = { 0.5f, 0.5f, 0.5f };
ms_sbt.data.bg_color = { 0.0f, 0.0f, 0.0f };
OPTIX_CHECK(optixSbtRecordPackHeader(mState.radiance_miss_group, &ms_sbt));
MissSbtRecord& ms_sbt_occlusion = missGroupDataCpu[RAY_TYPE_OCCLUSION];
ms_sbt_occlusion.data = { 0.0f, 0.0f, 0.0f };
OPTIX_CHECK(optixSbtRecordPackHeader(mState.occlusion_miss_group, &ms_sbt_occlusion));
CUDA_CHECK(cudaMemcpy(
reinterpret_cast<void*>(miss_record), missGroupDataCpu.data(), miss_record_size, cudaMemcpyHostToDevice));
uint32_t hitGroupRecordCount = RAY_TYPE_COUNT;
CUdeviceptr hitgroup_record = 0;
size_t hitgroup_record_size = hitGroupRecordCount * sizeof(HitGroupSbtRecord);
const std::vector<oka::Instance>& instances = mScene->getInstances();
std::vector<HitGroupSbtRecord> hitGroupDataCpu(hitGroupRecordCount); // default sbt record
if (!instances.empty())
{
const std::vector<oka::Mesh>& meshes = mScene->getMeshes();
hitGroupRecordCount = instances.size() * RAY_TYPE_COUNT;
hitgroup_record_size = sizeof(HitGroupSbtRecord) * hitGroupRecordCount;
hitGroupDataCpu.resize(hitGroupRecordCount);
for (int i = 0; i < instances.size(); ++i)
{
const oka::Instance& instance = instances[i];
int hg_index = i * RAY_TYPE_COUNT + RAY_TYPE_RADIANCE;
HitGroupSbtRecord& hg_sbt = hitGroupDataCpu[hg_index];
// replace unknown material on default
const int materialIdx = instance.mMaterialId == -1 ? 0 : instance.mMaterialId;
const Material& mat = getMaterial(materialIdx);
if (instance.type == oka::Instance::Type::eLight)
{
hg_sbt.data.lightId = instance.mLightId;
const OptixProgramGroup& lightPg = mState.light_hit_group;
OPTIX_CHECK(optixSbtRecordPackHeader(lightPg, &hg_sbt));
}
else
{
const OptixProgramGroup& hitMaterial = mat.programGroup;
OPTIX_CHECK(optixSbtRecordPackHeader(hitMaterial, &hg_sbt));
}
// write all needed data for instances
hg_sbt.data.argData = mat.d_argData;
hg_sbt.data.roData = mat.d_roData;
hg_sbt.data.resHandler = mat.d_textureHandler;
if (instance.type == oka::Instance::Type::eMesh)
{
const oka::Mesh& mesh = meshes[instance.mMeshId];
hg_sbt.data.indexCount = mesh.mCount;
hg_sbt.data.indexOffset = mesh.mIndex;
hg_sbt.data.vertexOffset = mesh.mVbOffset;
hg_sbt.data.lightId = -1;
}
memcpy(hg_sbt.data.object_to_world, glm::value_ptr(glm::float4x4(glm::rowMajor4(instance.transform))),
sizeof(float4) * 4);
glm::mat4 world_to_object = glm::inverse(instance.transform);
memcpy(hg_sbt.data.world_to_object, glm::value_ptr(glm::float4x4(glm::rowMajor4(world_to_object))),
sizeof(float4) * 4);
// write data for visibility ray
hg_index = i * RAY_TYPE_COUNT + RAY_TYPE_OCCLUSION;
HitGroupSbtRecord& hg_sbt_occlusion = hitGroupDataCpu[hg_index];
OPTIX_CHECK(optixSbtRecordPackHeader(mState.occlusion_hit_group, &hg_sbt_occlusion));
}
}
else
{
// stub record
HitGroupSbtRecord& hg_sbt = hitGroupDataCpu[RAY_TYPE_RADIANCE];
OPTIX_CHECK(optixSbtRecordPackHeader(mState.radiance_default_hit_group, &hg_sbt));
HitGroupSbtRecord& hg_sbt_occlusion = hitGroupDataCpu[RAY_TYPE_OCCLUSION];
OPTIX_CHECK(optixSbtRecordPackHeader(mState.occlusion_hit_group, &hg_sbt_occlusion));
}
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&hitgroup_record), hitgroup_record_size));
CUDA_CHECK(cudaMemcpy(reinterpret_cast<void*>(hitgroup_record), hitGroupDataCpu.data(), hitgroup_record_size,
cudaMemcpyHostToDevice));
sbt.raygenRecord = raygen_record;
sbt.missRecordBase = miss_record;
sbt.missRecordStrideInBytes = sizeof(MissSbtRecord);
sbt.missRecordCount = RAY_TYPE_COUNT;
sbt.hitgroupRecordBase = hitgroup_record;
sbt.hitgroupRecordStrideInBytes = sizeof(HitGroupSbtRecord);
sbt.hitgroupRecordCount = hitGroupRecordCount;
}
mState.sbt = sbt;
}
void OptiXRender::updatePathtracerParams(const uint32_t width, const uint32_t height)
{
bool needRealloc = false;
if (mState.params.image_width != width || mState.params.image_height != height)
{
// new dimensions!
needRealloc = true;
// reset rendering
getSharedContext().mSubframeIndex = 0;
}
mState.params.image_width = width;
mState.params.image_height = height;
if (needRealloc)
{
getSharedContext().mSettingsManager->setAs<bool>("render/pt/isResized", true);
if (mState.params.accum)
{
CUDA_CHECK(cudaFree((void*)mState.params.accum));
}
if (mState.params.diffuse)
{
CUDA_CHECK(cudaFree((void*)mState.params.diffuse));
}
if (mState.params.specular)
{
CUDA_CHECK(cudaFree((void*)mState.params.specular));
}
if (mState.d_params)
{
CUDA_CHECK(cudaFree((void*)mState.d_params));
}
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&mState.d_params), sizeof(Params)));
const size_t frameSize = mState.params.image_width * mState.params.image_height;
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&mState.params.accum), frameSize * sizeof(float4)));
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&mState.params.diffuse), frameSize * sizeof(float4)));
CUDA_CHECK(cudaMemset(mState.params.diffuse, 0, frameSize * sizeof(float4)));
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&mState.params.diffuseCounter), frameSize * sizeof(uint16_t)));
CUDA_CHECK(cudaMemset(mState.params.diffuseCounter, 0, frameSize * sizeof(uint16_t)));
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&mState.params.specular), frameSize * sizeof(float4)));
CUDA_CHECK(cudaMemset(mState.params.specular, 0, frameSize * sizeof(float4)));
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&mState.params.specularCounter), frameSize * sizeof(uint16_t)));
CUDA_CHECK(cudaMemset(mState.params.specularCounter, 0, frameSize * sizeof(uint16_t)));
}
}
void OptiXRender::render(Buffer* output)
{
if (getSharedContext().mFrameNumber == 0)
{
createOptixMaterials();
createPipeline();
createVertexBuffer();
createIndexBuffer();
// upload all curve data
createPointsBuffer();
createWidthsBuffer();
createAccelerationStructure();
createSbt();
createLightBuffer();
}
const uint32_t width = output->width();
const uint32_t height = output->height();
updatePathtracerParams(width, height);
oka::Camera& camera = mScene->getCamera(0);
camera.updateAspectRatio(width / (float)height);
camera.updateViewMatrix();
View currView = {};
currView.mCamMatrices = camera.matrices;
if (glm::any(glm::notEqual(currView.mCamMatrices.perspective, mPrevView.mCamMatrices.perspective)) ||
glm::any(glm::notEqual(currView.mCamMatrices.view, mPrevView.mCamMatrices.view)))
{
// need reset
getSharedContext().mSubframeIndex = 0;
}
SettingsManager& settings = *getSharedContext().mSettingsManager;
bool settingsChanged = false;
static uint32_t rectLightSamplingMethodPrev = 0;
const uint32_t rectLightSamplingMethod = settings.getAs<uint32_t>("render/pt/rectLightSamplingMethod");
settingsChanged = (rectLightSamplingMethodPrev != rectLightSamplingMethod);
rectLightSamplingMethodPrev = rectLightSamplingMethod;
static bool enableAccumulationPrev = 0;
bool enableAccumulation = settings.getAs<bool>("render/pt/enableAcc");
settingsChanged |= (enableAccumulationPrev != enableAccumulation);
enableAccumulationPrev = enableAccumulation;
static uint32_t sspTotalPrev = 0;
const uint32_t sspTotal = settings.getAs<uint32_t>("render/pt/sppTotal");
settingsChanged |= (sspTotalPrev > sspTotal); // reset only if new spp less than already accumulated
sspTotalPrev = sspTotal;
const float gamma = settings.getAs<float>("render/post/gamma");
const ToneMapperType tonemapperType = (ToneMapperType)settings.getAs<uint32_t>("render/pt/tonemapperType");
if (settingsChanged)
{
getSharedContext().mSubframeIndex = 0;
}
Params& params = mState.params;
params.scene.vb = (Vertex*)d_vb;
params.scene.ib = (uint32_t*)d_ib;
params.scene.lights = (UniformLight*)d_lights;
params.scene.numLights = mScene->getLights().size();
params.image = (float4*)((OptixBuffer*)output)->getNativePtr();
params.samples_per_launch = settings.getAs<uint32_t>("render/pt/spp");
params.handle = mState.ias_handle;
params.max_depth = settings.getAs<uint32_t>("render/pt/depth");
params.rectLightSamplingMethod = settings.getAs<uint32_t>("render/pt/rectLightSamplingMethod");
params.enableAccumulation = settings.getAs<bool>("render/pt/enableAcc");
params.debug = settings.getAs<uint32_t>("render/pt/debug");
params.shadowRayTmin = settings.getAs<float>("render/pt/dev/shadowRayTmin");
params.materialRayTmin = settings.getAs<float>("render/pt/dev/materialRayTmin");
memcpy(params.viewToWorld, glm::value_ptr(glm::transpose(glm::inverse(camera.matrices.view))), sizeof(params.viewToWorld));
memcpy(params.clipToView, glm::value_ptr(glm::transpose(camera.matrices.invPerspective)), sizeof(params.clipToView));
params.subframe_index = getSharedContext().mSubframeIndex;
// Photometric Units from iray documentation
// Controls the sensitivity of the “camera film” and is expressed as an index; the ISO number of the film, also
// known as “film speed.” The higher this value, the greater the exposure. If this is set to a non-zero value,
// “Photographic” mode is enabled. If this is set to 0, “Arbitrary” mode is enabled, and all color scaling is then
// strictly defined by the value of cm^2 Factor.
float filmIso = settings.getAs<float>("render/post/tonemapper/filmIso");
// The candela per meter square factor
float cm2_factor = settings.getAs<float>("render/post/tonemapper/cm2_factor");
// The fractional aperture number; e.g., 11 means aperture “f/11.” It adjusts the size of the opening of the “camera
// iris” and is expressed as a ratio. The higher this value, the lower the exposure.
float fStop = settings.getAs<float>("render/post/tonemapper/fStop");
// Controls the duration, in fractions of a second, that the “shutter” is open; e.g., the value 100 means that the
// “shutter” is open for 1/100th of a second. The higher this value, the greater the exposure
float shutterSpeed = settings.getAs<float>("render/post/tonemapper/shutterSpeed");
// Specifies the main color temperature of the light sources; the color that will be mapped to “white” on output,
// e.g., an incoming color of this hue/saturation will be mapped to grayscale, but its intensity will remain
// unchanged. This is similar to white balance controls on digital cameras.
float3 whitePoint { 1.0f, 1.0f, 1.0f };
float3 exposureValue = all(whitePoint) ? 1.0f / whitePoint : make_float3(1.0f);
const float lum = dot(exposureValue, make_float3(0.299f, 0.587f, 0.114f));
if (filmIso > 0.0f)
{
// See https://www.nayuki.io/page/the-photographic-exposure-equation
exposureValue *= cm2_factor * filmIso / (shutterSpeed * fStop * fStop) / 100.0f;
}
else
{
exposureValue *= cm2_factor;
}
exposureValue /= lum;
params.exposure = exposureValue;
const uint32_t totalSpp = settings.getAs<uint32_t>("render/pt/sppTotal");
const uint32_t samplesPerLaunch = settings.getAs<uint32_t>("render/pt/spp");
const int32_t leftSpp = totalSpp - getSharedContext().mSubframeIndex;
// if accumulation is off then launch selected samples per pixel
uint32_t samplesThisLaunch =
enableAccumulation ? std::min((int32_t)samplesPerLaunch, leftSpp) : samplesPerLaunch;
if (params.debug == 1)
{
samplesThisLaunch = 1;
enableAccumulation = false;
}
params.samples_per_launch = samplesThisLaunch;
params.enableAccumulation = enableAccumulation;
params.maxSampleCount = totalSpp;
CUDA_CHECK(cudaMemcpy(reinterpret_cast<void*>(mState.d_params), ¶ms, sizeof(params), cudaMemcpyHostToDevice));
if (samplesThisLaunch != 0)
{
OPTIX_CHECK(optixLaunch(
mState.pipeline, mState.stream, mState.d_params, sizeof(Params), &mState.sbt, width, height, /*depth=*/1));
CUDA_SYNC_CHECK();
if (enableAccumulation)
{
getSharedContext().mSubframeIndex += samplesThisLaunch;
}
else
{
getSharedContext().mSubframeIndex = 0;
}
}
else
{
// just copy latest accumulated raw radiance to destination
if (params.debug == 0)
{
CUDA_CHECK(cudaMemcpy(params.image, params.accum,
mState.params.image_width * mState.params.image_height * sizeof(float4),
cudaMemcpyDeviceToDevice));
}
if (params.debug == 2)
{
CUDA_CHECK(cudaMemcpy(params.image, params.diffuse,
mState.params.image_width * mState.params.image_height * sizeof(float4),
cudaMemcpyDeviceToDevice));
}
if (params.debug == 3)
{
CUDA_CHECK(cudaMemcpy(params.image, params.specular,
mState.params.image_width * mState.params.image_height * sizeof(float4),
cudaMemcpyDeviceToDevice));
}
}
if (params.debug != 1)
{
// do not run post processing for debug output
tonemap(tonemapperType, exposureValue, gamma, params.image, width, height);
}
output->unmap();
getSharedContext().mFrameNumber++;
mPrevView = currView;
mState.prevParams = mState.params;
}
void OptiXRender::init()
{
// TODO: move USD_DIR to settings
const char* envUSDPath = std::getenv("USD_DIR");
mEnableValidation = getSharedContext().mSettingsManager->getAs<bool>("render/enableValidation");
fs::path usdMdlLibPath;
if (envUSDPath)
{
usdMdlLibPath = (fs::path(envUSDPath) / fs::path("libraries/mdl/")).make_preferred();
}
const fs::path cwdPath = fs::current_path();
STRELKA_DEBUG("cwdPath: {}", cwdPath.string().c_str());
const fs::path mtlxPath = (cwdPath / fs::path("data/materials/mtlx")).make_preferred();
STRELKA_DEBUG("mtlxPath: {}", mtlxPath.string().c_str());
const fs::path mdlPath = (cwdPath / fs::path("data/materials/mdl")).make_preferred();
const std::string usdMdlLibPathStr = usdMdlLibPath.string();
const std::string mtlxPathStr = mtlxPath.string().c_str();
const std::string mdlPathStr = mdlPath.string().c_str();
const char* paths[] = { usdMdlLibPathStr.c_str(), mtlxPathStr.c_str(), mdlPathStr.c_str() };
bool res = mMaterialManager.addMdlSearchPath(paths, sizeof(paths) / sizeof(char*));
if (!res)
{
STRELKA_FATAL("Wrong mdl paths configuration!");
assert(0);
return;
}
// default material
{
oka::Scene::MaterialDescription defaultMaterial{};
defaultMaterial.file = "default.mdl";
defaultMaterial.name = "default_material";
defaultMaterial.type = oka::Scene::MaterialDescription::Type::eMdl;
mScene->addMaterial(defaultMaterial);
}
createContext();
// createAccelerationStructure();
createModule();
createProgramGroups();
createPipeline();
// createSbt();
}
Buffer* OptiXRender::createBuffer(const BufferDesc& desc)
{
const size_t size = desc.height * desc.width * Buffer::getElementSize(desc.format);
assert(size != 0);
void* devicePtr = nullptr;
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&devicePtr), size));
OptixBuffer* res = new OptixBuffer(devicePtr, desc.format, desc.width, desc.height);
return res;
}
void OptiXRender::createPointsBuffer()
{
const std::vector<glm::float3>& points = mScene->getCurvesPoint();
std::vector<float3> data(points.size());
for (int i = 0; i < points.size(); ++i)
{
data[i] = make_float3(points[i].x, points[i].y, points[i].z);
}
const size_t size = data.size() * sizeof(float3);
if (d_points)
{
CUDA_CHECK(cudaFree(reinterpret_cast<void*>(d_points)));
}
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&d_points), size));
CUDA_CHECK(cudaMemcpy(reinterpret_cast<void*>(d_points), data.data(), size, cudaMemcpyHostToDevice));
}
void OptiXRender::createWidthsBuffer()
{
const std::vector<float>& data = mScene->getCurvesWidths();
const size_t size = data.size() * sizeof(float);
if (d_widths)
{
CUDA_CHECK(cudaFree(reinterpret_cast<void*>(d_widths)));
}
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&d_widths), size));
CUDA_CHECK(cudaMemcpy(reinterpret_cast<void*>(d_widths), data.data(), size, cudaMemcpyHostToDevice));
}
void OptiXRender::createVertexBuffer()
{
const std::vector<oka::Scene::Vertex>& vertices = mScene->getVertices();
const size_t vbsize = vertices.size() * sizeof(oka::Scene::Vertex);
if (d_vb)
{
CUDA_CHECK(cudaFree(reinterpret_cast<void*>(d_vb)));
}
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&d_vb), vbsize));
CUDA_CHECK(cudaMemcpy(reinterpret_cast<void*>(d_vb), vertices.data(), vbsize, cudaMemcpyHostToDevice));
}
void OptiXRender::createIndexBuffer()
{
const std::vector<uint32_t>& indices = mScene->getIndices();
const size_t ibsize = indices.size() * sizeof(uint32_t);
if (d_ib)
{
CUDA_CHECK(cudaFree(reinterpret_cast<void*>(d_ib)));
}
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&d_ib), ibsize));
CUDA_CHECK(cudaMemcpy(reinterpret_cast<void*>(d_ib), indices.data(), ibsize, cudaMemcpyHostToDevice));
}
void OptiXRender::createLightBuffer()
{
const std::vector<Scene::Light>& lightDescs = mScene->getLights();
const size_t lightBufferSize = lightDescs.size() * sizeof(Scene::Light);
if (d_lights)
{
CUDA_CHECK(cudaFree(reinterpret_cast<void*>(d_lights)));
}
if (lightBufferSize)
{
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&d_lights), lightBufferSize));
CUDA_CHECK(
cudaMemcpy(reinterpret_cast<void*>(d_lights), lightDescs.data(), lightBufferSize, cudaMemcpyHostToDevice));
}
}
Texture OptiXRender::loadTextureFromFile(const std::string& fileName)
{
int texWidth, texHeight, texChannels;
stbi_uc* data = stbi_load(fileName.c_str(), &texWidth, &texHeight, &texChannels, STBI_rgb_alpha);
if (!data)
{
STRELKA_ERROR("Unable to load texture from file: {}", fileName.c_str());
return Texture();
}
// convert to float4 format
// TODO: add compression here:
// std::vector<float> floatData(texWidth * texHeight * 4);
// for (int i = 0; i < texHeight; ++i)
// {
// for (int j = 0; j < texWidth; ++j)
// {
// const size_t linearPixelIndex = (i * texWidth + j) * 4;
// auto remapToFloat = [](const unsigned char v)
// {
// return float(v) / 255.0f;
// };
// floatData[linearPixelIndex + 0] = remapToFloat(data[linearPixelIndex + 0]);
// floatData[linearPixelIndex + 1] = remapToFloat(data[linearPixelIndex + 1]);
// floatData[linearPixelIndex + 2] = remapToFloat(data[linearPixelIndex + 2]);
// floatData[linearPixelIndex + 3] = remapToFloat(data[linearPixelIndex + 3]);
// }
// }
const void* dataPtr = data;
cudaChannelFormatDesc channel_desc = cudaCreateChannelDesc<uchar4>();
cudaResourceDesc res_desc{};
memset(&res_desc, 0, sizeof(res_desc));
cudaArray_t device_tex_array;
CUDA_CHECK(cudaMallocArray(&device_tex_array, &channel_desc, texWidth, texHeight));
CUDA_CHECK(cudaMemcpy2DToArray(device_tex_array, 0, 0, dataPtr, texWidth * sizeof(char) * 4,
texWidth * sizeof(char) * 4, texHeight, cudaMemcpyHostToDevice));
res_desc.resType = cudaResourceTypeArray;
res_desc.res.array.array = device_tex_array;
// Create filtered texture object
cudaTextureDesc tex_desc;
memset(&tex_desc, 0, sizeof(tex_desc));
cudaTextureAddressMode addr_mode = cudaAddressModeWrap;
tex_desc.addressMode[0] = addr_mode;
tex_desc.addressMode[1] = addr_mode;
tex_desc.addressMode[2] = addr_mode;
tex_desc.filterMode = cudaFilterModeLinear;
tex_desc.readMode = cudaReadModeNormalizedFloat;
tex_desc.normalizedCoords = 1;
if (res_desc.resType == cudaResourceTypeMipmappedArray)
{
tex_desc.mipmapFilterMode = cudaFilterModeLinear;
tex_desc.maxAnisotropy = 16;
tex_desc.minMipmapLevelClamp = 0.f;
tex_desc.maxMipmapLevelClamp = 1000.f; // default value in OpenGL
}
cudaTextureObject_t tex_obj = 0;
CUDA_CHECK(cudaCreateTextureObject(&tex_obj, &res_desc, &tex_desc, nullptr));
// Create unfiltered texture object if necessary (cube textures have no texel functions)
cudaTextureObject_t tex_obj_unfilt = 0;
// if (texture_shape != mi::neuraylib::ITarget_code::Texture_shape_cube)
{
// Use a black border for access outside of the texture
tex_desc.addressMode[0] = cudaAddressModeBorder;
tex_desc.addressMode[1] = cudaAddressModeBorder;
tex_desc.addressMode[2] = cudaAddressModeBorder;
tex_desc.filterMode = cudaFilterModePoint;
CUDA_CHECK(cudaCreateTextureObject(&tex_obj_unfilt, &res_desc, &tex_desc, nullptr));
}
return Texture(tex_obj, tex_obj_unfilt, make_uint3(texWidth, texHeight, 1));
}
bool OptiXRender::createOptixMaterials()
{
std::unordered_map<std::string, MaterialManager::Module*> mNameToModule;
std::unordered_map<std::string, MaterialManager::MaterialInstance*> mNameToInstance;
std::unordered_map<std::string, MaterialManager::CompiledMaterial*> mNameToCompiled;
std::unordered_map<std::string, MaterialManager::TargetCode*> mNameToTargetCode;
std::vector<MaterialManager::CompiledMaterial*> compiledMaterials;
MaterialManager::TargetCode* targetCode;
std::vector<Scene::MaterialDescription>& matDescs = mScene->getMaterials();
for (uint32_t i = 0; i < matDescs.size(); ++i)
{
oka::Scene::MaterialDescription& currMatDesc = matDescs[i];
if (currMatDesc.type == oka::Scene::MaterialDescription::Type::eMdl)
{
if (auto it = mNameToCompiled.find(currMatDesc.name); it != mNameToCompiled.end())
{
compiledMaterials.emplace_back(it->second);
continue;
}
MaterialManager::Module* mdlModule = nullptr;
if (mNameToModule.find(currMatDesc.file) != mNameToModule.end())
{
mdlModule = mNameToModule[currMatDesc.file];
}
else
{
mdlModule = mMaterialManager.createModule(currMatDesc.file.c_str());
if (mdlModule == nullptr)
{
STRELKA_ERROR("Unable to load MDL file: {}, Force replaced to default.mdl", currMatDesc.file.c_str());
mdlModule = mNameToModule["default.mdl"];
}
mNameToModule[currMatDesc.file] = mdlModule;
}
assert(mdlModule);
MaterialManager::MaterialInstance* materialInst = nullptr;
if (mNameToInstance.find(currMatDesc.name) != mNameToInstance.end())
{
materialInst = mNameToInstance[currMatDesc.name];
}
else
{
materialInst = mMaterialManager.createMaterialInstance(mdlModule, currMatDesc.name.c_str());
mNameToInstance[currMatDesc.name] = materialInst;
}
assert(materialInst);
MaterialManager::CompiledMaterial* materialComp = nullptr;
{
materialComp = mMaterialManager.compileMaterial(materialInst);
mNameToCompiled[currMatDesc.name] = materialComp;
}
assert(materialComp);
compiledMaterials.push_back(materialComp);
}
else
{
MaterialManager::Module* mdlModule = mMaterialManager.createMtlxModule(currMatDesc.code.c_str());
assert(mdlModule);
MaterialManager::MaterialInstance* materialInst = mMaterialManager.createMaterialInstance(mdlModule, "");
assert(materialInst);
MaterialManager::CompiledMaterial* materialComp = mMaterialManager.compileMaterial(materialInst);
assert(materialComp);
compiledMaterials.push_back(materialComp);
// MaterialManager::TargetCode* mdlTargetCode = mMaterialManager.generateTargetCode(&materialComp, 1);
// assert(mdlTargetCode);
// mNameToTargetCode[currMatDesc.name] = mdlTargetCode;
// targetCodes.push_back(mdlTargetCode);
}
}
targetCode = mMaterialManager.generateTargetCode(compiledMaterials.data(), compiledMaterials.size());
std::vector<Texture> materialTextures;
const auto searchPath = getSharedContext().mSettingsManager->getAs<std::string>("resource/searchPath");
fs::path resourcePath = fs::path(getSharedContext().mSettingsManager->getAs<std::string>("resource/searchPath"));
for (uint32_t i = 0; i < matDescs.size(); ++i)
{
for (const auto& param : matDescs[i].params)
{
bool res = false;
if (param.type == MaterialManager::Param::Type::eTexture)
{
std::string texPath(param.value.size(), 0);
memcpy(texPath.data(), param.value.data(), param.value.size());
// int texId = getTexManager()->loadTextureMdl(texPath);
fs::path fullTextureFilePath = resourcePath / texPath;
::Texture tex = loadTextureFromFile(fullTextureFilePath.string());
materialTextures.push_back(tex);
int texId = 0;
int resId = mMaterialManager.registerResource(targetCode, texId);
assert(resId > 0);
MaterialManager::Param newParam;
newParam.name = param.name;
newParam.type = MaterialManager::Param::Type::eInt;
newParam.value.resize(sizeof(resId));
memcpy(newParam.value.data(), &resId, sizeof(resId));
res = mMaterialManager.setParam(targetCode, i, compiledMaterials[i], newParam);
}
else
{
res = mMaterialManager.setParam(targetCode, i, compiledMaterials[i], param);
}
if (!res)
{
STRELKA_ERROR(
"Unable to set parameter: {0} for material: {1}", param.name.c_str(), matDescs[i].name.c_str());
// assert(0);
}
}
mMaterialManager.dumpParams(targetCode, i, compiledMaterials[i]);
}
const uint8_t* argData = mMaterialManager.getArgBufferData(targetCode);
const size_t argDataSize = mMaterialManager.getArgBufferSize(targetCode);
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&d_materialArgData), argDataSize));
CUDA_CHECK(cudaMemcpy((void*)d_materialArgData, argData, argDataSize, cudaMemcpyHostToDevice));
const uint8_t* roData = mMaterialManager.getReadOnlyBlockData(targetCode);
const size_t roDataSize = mMaterialManager.getReadOnlyBlockSize(targetCode);
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&d_materialRoData), roDataSize));
CUDA_CHECK(cudaMemcpy((void*)d_materialRoData, roData, roDataSize, cudaMemcpyHostToDevice));
const size_t texturesBuffSize = sizeof(Texture) * materialTextures.size();
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&d_texturesData), texturesBuffSize));
CUDA_CHECK(cudaMemcpy((void*)d_texturesData, materialTextures.data(), texturesBuffSize, cudaMemcpyHostToDevice));
Texture_handler resourceHandler;
resourceHandler.num_textures = materialTextures.size();
resourceHandler.textures = (const Texture*)d_texturesData;
CUDA_CHECK(cudaMalloc(reinterpret_cast<void**>(&d_texturesHandler), sizeof(Texture_handler)));
CUDA_CHECK(cudaMemcpy((void*)d_texturesHandler, &resourceHandler, sizeof(Texture_handler), cudaMemcpyHostToDevice));
std::unordered_map<MaterialManager::CompiledMaterial*, OptixProgramGroup> compiledToOptixPG;
for (int i = 0; i < compiledMaterials.size(); ++i)
{
if (compiledToOptixPG.find(compiledMaterials[i]) == compiledToOptixPG.end())
{
const char* codeData = mMaterialManager.getShaderCode(targetCode, i);
assert(codeData);
const size_t codeSize = strlen(codeData);
assert(codeSize);
OptixProgramGroup pg = createRadianceClosestHitProgramGroup(mState, codeData, codeSize);
compiledToOptixPG[compiledMaterials[i]] = pg;
}
Material optixMaterial;
optixMaterial.programGroup = compiledToOptixPG[compiledMaterials[i]];
optixMaterial.d_argData = d_materialArgData + mMaterialManager.getArgBlockOffset(targetCode, i);
optixMaterial.d_argDataSize = argDataSize;
optixMaterial.d_roData = d_materialRoData + mMaterialManager.getReadOnlyOffset(targetCode, i);
optixMaterial.d_roSize = roDataSize;
optixMaterial.d_textureHandler = d_texturesHandler;
mMaterials.push_back(optixMaterial);
}
return true;
}
OptiXRender::Material& OptiXRender::getMaterial(int id)
{
assert(id < mMaterials.size());
return mMaterials[id];
}
| 61,289 | C++ | 41.5625 | 127 | 0.640066 |
arhix52/Strelka/src/render/optix/postprocessing/Utils.h | #pragma once
#include <sutil/vec_math.h>
// utility function for accumulation and HDR <=> LDR
__device__ __inline__ float3 tonemap(float3 color, const float3 exposure)
{
color *= exposure;
return color / (color + 1.0f);
}
__device__ __inline__ float3 inverseTonemap(const float3 color, const float3 exposure)
{
return color / (exposure - color * exposure);
}
| 373 | C | 23.933332 | 86 | 0.683646 |
arhix52/Strelka/src/render/optix/postprocessing/Tonemappers.h | #pragma once
#include <sutil/vec_math.h>
#include <stdint.h>
enum class ToneMapperType : uint32_t
{
eNone = 0,
eReinhard,
eACES,
eFilmic,
};
extern "C" void tonemap(const ToneMapperType type,
const float3 exposure,
const float gamma,
float4* image,
const uint32_t width,
const uint32_t height);
| 433 | C | 21.842104 | 50 | 0.512702 |
arhix52/Strelka/src/render/metal/MetalBuffer.h | #pragma once
#include "buffer.h"
#include <Metal/Metal.hpp>
namespace oka
{
class MetalBuffer : public Buffer
{
public:
MetalBuffer(MTL::Buffer* buff, BufferFormat format, uint32_t width, uint32_t height);
~MetalBuffer() override;
void resize(uint32_t width, uint32_t height) override;
void* map() override;
void unmap() override;
MTL::Buffer* getNativePtr()
{
return mBuffer;
}
void* getHostPointer() override
{
return map();
}
size_t getHostDataSize() override
{
return mBuffer->length();
}
protected:
MTL::Buffer* mBuffer;
};
} // namespace oka | 640 | C | 15.868421 | 89 | 0.63125 |
arhix52/Strelka/src/render/metal/MetalBuffer.cpp |
#include "MetalBuffer.h"
using namespace oka;
oka::MetalBuffer::MetalBuffer(MTL::Buffer* buff, BufferFormat format, uint32_t width, uint32_t height) : mBuffer(buff)
{
mFormat = format;
mWidth = width;
mHeight = height;
}
oka::MetalBuffer::~MetalBuffer()
{
mBuffer->release();
}
void oka::MetalBuffer::resize(uint32_t width, uint32_t height)
{
}
void* oka::MetalBuffer::map()
{
return mBuffer->contents();
}
void oka::MetalBuffer::unmap()
{
}
| 470 | C++ | 14.193548 | 118 | 0.676596 |
arhix52/Strelka/src/render/metal/MetalRender.cpp | #define NS_PRIVATE_IMPLEMENTATION
#define MTL_PRIVATE_IMPLEMENTATION
#define MTK_PRIVATE_IMPLEMENTATION
#include <Metal/Metal.hpp>
#include "MetalRender.h"
#include "MetalBuffer.h"
#include <cassert>
#include <filesystem>
#include <glm/glm.hpp>
#include <glm/mat4x3.hpp>
#include <glm/gtx/compatibility.hpp>
#include <glm/gtc/type_ptr.hpp>
#include <glm/gtx/matrix_major_storage.hpp>
#include <glm/ext/matrix_relational.hpp>
#define STB_IMAGE_STATIC
#define STB_IMAGE_IMPLEMENTATION
#include <stb_image.h>
#include <log.h>
#include <simd/simd.h>
#include "shaders/ShaderTypes.h"
using namespace oka;
namespace fs = std::filesystem;
MetalRender::MetalRender(/* args */) = default;
MetalRender::~MetalRender() = default;
void MetalRender::init()
{
mDevice = MTL::CreateSystemDefaultDevice();
mCommandQueue = mDevice->newCommandQueue();
buildComputePipeline();
buildTonemapperPipeline();
}
MTL::Texture* MetalRender::loadTextureFromFile(const std::string& fileName)
{
int texWidth = 0;
int texHeight = 0;
int texChannels = 0;
stbi_uc* data = stbi_load(fileName.c_str(), &texWidth, &texHeight, &texChannels, STBI_rgb_alpha);
if (data == nullptr)
{
STRELKA_ERROR("Unable to load texture from file: {}", fileName.c_str());
return nullptr;
}
MTL::TextureDescriptor* pTextureDesc = MTL::TextureDescriptor::alloc()->init();
pTextureDesc->setWidth(texWidth);
pTextureDesc->setHeight(texHeight);
pTextureDesc->setPixelFormat(MTL::PixelFormatRGBA8Unorm);
pTextureDesc->setTextureType(MTL::TextureType2D);
pTextureDesc->setStorageMode(MTL::StorageModeManaged);
pTextureDesc->setUsage(MTL::ResourceUsageSample | MTL::ResourceUsageRead);
MTL::Texture* pTexture = mDevice->newTexture(pTextureDesc);
const MTL::Region region = MTL::Region::Make3D(0, 0, 0, texWidth, texHeight, 1);
pTexture->replaceRegion(region, 0, data, 4ull * texWidth);
pTextureDesc->release();
return pTexture;
}
void MetalRender::createMetalMaterials()
{
using simd::float3;
const std::vector<Scene::MaterialDescription>& matDescs = mScene->getMaterials();
std::vector<Material> gpuMaterials;
const fs::path resourcePath = getSharedContext().mSettingsManager->getAs<std::string>("resource/searchPath");
for (const Scene::MaterialDescription& currMatDesc : matDescs)
{
Material material = {};
material.diffuse = { 1.0f, 1.0f, 1.0f };
for (const auto& param : currMatDesc.params)
{
if (param.name == "diffuse_color" || param.name == "diffuseColor" || param.name == "diffuse_color_constant")
{
memcpy(&material.diffuse, param.value.data(), sizeof(float) * 3);
}
if (param.type == MaterialManager::Param::Type::eTexture)
{
std::string texPath(param.value.size(), 0);
memcpy(texPath.data(), param.value.data(), param.value.size());
const fs::path fullTextureFilePath = resourcePath / texPath;
if (param.name == "diffuse_texture")
{
MTL::Texture* diffuseTex = loadTextureFromFile(fullTextureFilePath.string());
mMaterialTextures.push_back(diffuseTex);
material.diffuseTexture = diffuseTex->gpuResourceID();
}
if (param.name == "normalmap_texture")
{
MTL::Texture* normalTex = loadTextureFromFile(fullTextureFilePath.string());
mMaterialTextures.push_back(normalTex);
material.normalTexture = normalTex->gpuResourceID();
}
}
}
gpuMaterials.push_back(material);
}
const size_t materialsDataSize = sizeof(Material) * gpuMaterials.size();
mMaterialBuffer = mDevice->newBuffer(materialsDataSize, MTL::ResourceStorageModeManaged);
memcpy(mMaterialBuffer->contents(), gpuMaterials.data(), materialsDataSize);
mMaterialBuffer->didModifyRange(NS::Range::Make(0, mMaterialBuffer->length()));
}
void MetalRender::render(Buffer* output)
{
using simd::float3;
using simd::float4;
using simd::float4x4;
NS::AutoreleasePool* pPool = NS::AutoreleasePool::alloc()->init();
if (getSharedContext().mFrameNumber == 0)
{
buildBuffers();
createMetalMaterials();
createAccelerationStructures();
// create accum buffer, we don't need cpu access, make it device only
mAccumulationBuffer = mDevice->newBuffer(
output->width() * output->height() * output->getElementSize(), MTL::ResourceStorageModePrivate);
}
mFrameIndex = (mFrameIndex + 1) % kMaxFramesInFlight;
// Update camera state:
const uint32_t width = output->width();
const uint32_t height = output->height();
oka::Camera& camera = mScene->getCamera(1);
camera.updateAspectRatio(width / (float)height);
camera.updateViewMatrix();
View currView = {};
currView.mCamMatrices = camera.matrices;
if (glm::any(glm::notEqual(currView.mCamMatrices.perspective, mPrevView.mCamMatrices.perspective)) ||
glm::any(glm::notEqual(currView.mCamMatrices.view, mPrevView.mCamMatrices.view)))
{
// need reset
getSharedContext().mSubframeIndex = 0;
}
SettingsManager& settings = *getSharedContext().mSettingsManager;
MTL::Buffer* pUniformBuffer = mUniformBuffers[mFrameIndex];
MTL::Buffer* pUniformTMBuffer = mUniformTMBuffers[mFrameIndex];
Uniforms* pUniformData = reinterpret_cast<Uniforms*>(pUniformBuffer->contents());
UniformsTonemap* pUniformTonemap = reinterpret_cast<UniformsTonemap*>(pUniformTMBuffer->contents());
pUniformData->frameIndex = mFrameIndex;
pUniformData->subframeIndex = getSharedContext().mSubframeIndex;
pUniformData->height = height;
pUniformData->width = width;
pUniformData->numLights = mScene->getLightsDesc().size();
pUniformData->samples_per_launch = settings.getAs<uint32_t>("render/pt/spp");
pUniformData->enableAccumulation = (uint32_t)settings.getAs<bool>("render/pt/enableAcc");
pUniformData->missColor = float3(0.0f);
pUniformData->maxDepth = settings.getAs<uint32_t>("render/pt/depth");
pUniformData->debug = settings.getAs<uint32_t>("render/pt/debug");
pUniformTonemap->width = width;
pUniformTonemap->height = height;
pUniformTonemap->tonemapperType = settings.getAs<uint32_t>("render/pt/tonemapperType");
pUniformTonemap->gamma = settings.getAs<float>("render/post/gamma");
bool settingsChanged = false;
static uint32_t rectLightSamplingMethodPrev = 0;
pUniformData->rectLightSamplingMethod = settings.getAs<uint32_t>("render/pt/rectLightSamplingMethod");
settingsChanged = (rectLightSamplingMethodPrev != pUniformData->rectLightSamplingMethod);
rectLightSamplingMethodPrev = pUniformData->rectLightSamplingMethod;
static bool enableAccumulationPrev = false;
const bool enableAccumulation = settings.getAs<bool>("render/pt/enableAcc");
settingsChanged |= (enableAccumulationPrev != enableAccumulation);
enableAccumulationPrev = enableAccumulation;
static uint32_t sspTotalPrev = 0;
const uint32_t sspTotal = settings.getAs<uint32_t>("render/pt/sppTotal");
settingsChanged |= (sspTotalPrev > sspTotal); // reset only if new spp less than already accumulated
sspTotalPrev = sspTotal;
if (settingsChanged)
{
getSharedContext().mSubframeIndex = 0;
}
glm::float4x4 invView = glm::inverse(camera.matrices.view);
for (int column = 0; column < 4; column++)
{
for (int row = 0; row < 4; row++)
{
pUniformData->viewToWorld.columns[column][row] = invView[column][row];
}
}
for (int column = 0; column < 4; column++)
{
for (int row = 0; row < 4; row++)
{
pUniformData->clipToView.columns[column][row] = camera.matrices.invPerspective[column][row];
}
}
pUniformData->subframeIndex = getSharedContext().mSubframeIndex;
// Photometric Units from iray documentation
// Controls the sensitivity of the “camera film” and is expressed as an index; the ISO number of the film, also
// known as “film speed.” The higher this value, the greater the exposure. If this is set to a non-zero value,
// “Photographic” mode is enabled. If this is set to 0, “Arbitrary” mode is enabled, and all color scaling is then
// strictly defined by the value of cm^2 Factor.
float filmIso = settings.getAs<float>("render/post/tonemapper/filmIso");
// The candela per meter square factor
float cm2_factor = settings.getAs<float>("render/post/tonemapper/cm2_factor");
// The fractional aperture number; e.g., 11 means aperture “f/11.” It adjusts the size of the opening of the “camera
// iris” and is expressed as a ratio. The higher this value, the lower the exposure.
float fStop = settings.getAs<float>("render/post/tonemapper/fStop");
// Controls the duration, in fractions of a second, that the “shutter” is open; e.g., the value 100 means that the
// “shutter” is open for 1/100th of a second. The higher this value, the greater the exposure
float shutterSpeed = settings.getAs<float>("render/post/tonemapper/shutterSpeed");
// Specifies the main color temperature of the light sources; the color that will be mapped to “white” on output,
// e.g., an incoming color of this hue/saturation will be mapped to grayscale, but its intensity will remain
// unchanged. This is similar to white balance controls on digital cameras.
float3 whitePoint{ 1.0f, 1.0f, 1.0f };
auto all = [](float3 v) { return v.x > 0.0f && v.y > 0.0f && v.z > 0.0f; };
float3 exposureValue = all(whitePoint) ? 1.0f / whitePoint : float3(1.0f);
const float lum = simd::dot(exposureValue, float3{ 0.299f, 0.587f, 0.114f });
if (filmIso > 0.0f)
{
// See https://www.nayuki.io/page/the-photographic-exposure-equation
exposureValue *= cm2_factor * filmIso / (shutterSpeed * fStop * fStop) / 100.0f;
}
else
{
exposureValue *= cm2_factor;
}
exposureValue /= lum;
pUniformTonemap->exposureValue = exposureValue;
pUniformData->exposureValue = exposureValue; // need for proper accumulation
const uint32_t totalSpp = settings.getAs<uint32_t>("render/pt/sppTotal");
const uint32_t samplesPerLaunch = settings.getAs<uint32_t>("render/pt/spp");
const int32_t leftSpp = totalSpp - getSharedContext().mSubframeIndex;
// if accumulation is off then launch selected samples per pixel
const uint32_t samplesThisLaunch =
enableAccumulation ? std::min((int32_t)samplesPerLaunch, leftSpp) : samplesPerLaunch;
if (samplesThisLaunch != 0)
{
pUniformData->samples_per_launch = samplesThisLaunch;
pUniformBuffer->didModifyRange(NS::Range::Make(0, sizeof(Uniforms)));
pUniformTMBuffer->didModifyRange(NS::Range::Make(0, sizeof(UniformsTonemap)));
MTL::CommandBuffer* pCmd = mCommandQueue->commandBuffer();
MTL::ComputeCommandEncoder* pComputeEncoder = pCmd->computeCommandEncoder();
pComputeEncoder->useResource(mMaterialBuffer, MTL::ResourceUsageRead);
pComputeEncoder->useResource(mLightBuffer, MTL::ResourceUsageRead);
pComputeEncoder->useResource(mInstanceAccelerationStructure, MTL::ResourceUsageRead);
for (const MTL::AccelerationStructure* primitiveAccel : mPrimitiveAccelerationStructures)
{
pComputeEncoder->useResource(primitiveAccel, MTL::ResourceUsageRead);
}
for (auto& materialTexture : mMaterialTextures)
{
pComputeEncoder->useResource(materialTexture, MTL::ResourceUsageRead);
}
pComputeEncoder->useResource(((MetalBuffer*)output)->getNativePtr(), MTL::ResourceUsageWrite);
pComputeEncoder->setComputePipelineState(mPathTracingPSO);
pComputeEncoder->setBuffer(pUniformBuffer, 0, 0);
pComputeEncoder->setBuffer(mInstanceBuffer, 0, 1);
pComputeEncoder->setAccelerationStructure(mInstanceAccelerationStructure, 2);
pComputeEncoder->setBuffer(mLightBuffer, 0, 3);
pComputeEncoder->setBuffer(mMaterialBuffer, 0, 4);
// Output
pComputeEncoder->setBuffer(((MetalBuffer*)output)->getNativePtr(), 0, 5);
pComputeEncoder->setBuffer(mAccumulationBuffer, 0, 6);
{
const MTL::Size gridSize = MTL::Size(width, height, 1);
const NS::UInteger threadGroupSize = mPathTracingPSO->maxTotalThreadsPerThreadgroup();
const MTL::Size threadgroupSize(threadGroupSize, 1, 1);
pComputeEncoder->dispatchThreads(gridSize, threadgroupSize);
}
// Disable tonemapping for debug output
if (pUniformData->debug == 0)
{
pComputeEncoder->setComputePipelineState(mTonemapperPSO);
pComputeEncoder->useResource(
((MetalBuffer*)output)->getNativePtr(), MTL::ResourceUsageRead | MTL::ResourceUsageWrite);
pComputeEncoder->setBuffer(pUniformTMBuffer, 0, 0);
pComputeEncoder->setBuffer(((MetalBuffer*)output)->getNativePtr(), 0, 1);
{
const MTL::Size gridSize = MTL::Size(width, height, 1);
const NS::UInteger threadGroupSize = mTonemapperPSO->maxTotalThreadsPerThreadgroup();
const MTL::Size threadgroupSize(threadGroupSize, 1, 1);
pComputeEncoder->dispatchThreads(gridSize, threadgroupSize);
}
}
pComputeEncoder->endEncoding();
pCmd->commit();
pCmd->waitUntilCompleted();
if (enableAccumulation)
{
getSharedContext().mSubframeIndex += samplesThisLaunch;
}
else
{
getSharedContext().mSubframeIndex = 0;
}
}
else
{
MTL::CommandBuffer* pCmd = mCommandQueue->commandBuffer();
MTL::BlitCommandEncoder* pBlitEncoder = pCmd->blitCommandEncoder();
pBlitEncoder->copyFromBuffer(
mAccumulationBuffer, 0, ((MetalBuffer*)output)->getNativePtr(), 0, width * height * sizeof(float4));
pBlitEncoder->endEncoding();
// Disable tonemapping for debug output
if (pUniformData->debug == 0)
{
MTL::ComputeCommandEncoder* pComputeEncoder = pCmd->computeCommandEncoder();
pComputeEncoder->setComputePipelineState(mTonemapperPSO);
pComputeEncoder->useResource(
((MetalBuffer*)output)->getNativePtr(), MTL::ResourceUsageRead | MTL::ResourceUsageWrite);
pComputeEncoder->setBuffer(pUniformTMBuffer, 0, 0);
pComputeEncoder->setBuffer(((MetalBuffer*)output)->getNativePtr(), 0, 1);
{
const MTL::Size gridSize = MTL::Size(width, height, 1);
const NS::UInteger threadGroupSize = mTonemapperPSO->maxTotalThreadsPerThreadgroup();
const MTL::Size threadgroupSize(threadGroupSize, 1, 1);
pComputeEncoder->dispatchThreads(gridSize, threadgroupSize);
}
pComputeEncoder->endEncoding();
}
pCmd->commit();
pCmd->waitUntilCompleted();
}
pPool->release();
mPrevView = currView;
getSharedContext().mFrameNumber++;
}
Buffer* MetalRender::createBuffer(const BufferDesc& desc)
{
assert(mDevice);
const size_t size = desc.height * desc.width * Buffer::getElementSize(desc.format);
assert(size != 0);
MTL::Buffer* buff = mDevice->newBuffer(size, MTL::ResourceStorageModeManaged);
assert(buff);
MetalBuffer* res = new MetalBuffer(buff, desc.format, desc.width, desc.height);
assert(res);
return res;
}
void MetalRender::buildComputePipeline()
{
NS::Error* pError = nullptr;
MTL::Library* pComputeLibrary =
mDevice->newLibrary(NS::String::string("./metal/shaders/pathtrace.metallib", NS::UTF8StringEncoding), &pError);
if (!pComputeLibrary)
{
STRELKA_FATAL("{}", pError->localizedDescription()->utf8String());
assert(false);
}
MTL::Function* pPathTraceFn =
pComputeLibrary->newFunction(NS::String::string("raytracingKernel", NS::UTF8StringEncoding));
mPathTracingPSO = mDevice->newComputePipelineState(pPathTraceFn, &pError);
if (!mPathTracingPSO)
{
STRELKA_FATAL("{}", pError->localizedDescription()->utf8String());
assert(false);
}
pPathTraceFn->release();
pComputeLibrary->release();
}
void MetalRender::buildTonemapperPipeline()
{
NS::Error* pError = nullptr;
MTL::Library* pComputeLibrary =
mDevice->newLibrary(NS::String::string("./metal/shaders/tonemapper.metallib", NS::UTF8StringEncoding), &pError);
if (!pComputeLibrary)
{
STRELKA_FATAL("{}", pError->localizedDescription()->utf8String());
assert(false);
}
MTL::Function* pTonemapperFn =
pComputeLibrary->newFunction(NS::String::string("toneMappingComputeShader", NS::UTF8StringEncoding));
mTonemapperPSO = mDevice->newComputePipelineState(pTonemapperFn, &pError);
if (!mTonemapperPSO)
{
STRELKA_FATAL("{}", pError->localizedDescription()->utf8String());
assert(false);
}
pTonemapperFn->release();
pComputeLibrary->release();
}
void MetalRender::buildBuffers()
{
const std::vector<Scene::Vertex>& vertices = mScene->getVertices();
const std::vector<uint32_t>& indices = mScene->getIndices();
const std::vector<Scene::Light>& lightDescs = mScene->getLights();
static_assert(sizeof(Scene::Light) == sizeof(UniformLight));
const size_t lightBufferSize = sizeof(Scene::Light) * lightDescs.size();
const size_t vertexDataSize = sizeof(Scene::Vertex) * vertices.size();
const size_t indexDataSize = sizeof(uint32_t) * indices.size();
MTL::Buffer* pLightBuffer = mDevice->newBuffer(lightBufferSize, MTL::ResourceStorageModeManaged);
MTL::Buffer* pVertexBuffer = mDevice->newBuffer(vertexDataSize, MTL::ResourceStorageModeManaged);
MTL::Buffer* pIndexBuffer = mDevice->newBuffer(indexDataSize, MTL::ResourceStorageModeManaged);
mLightBuffer = pLightBuffer;
mVertexBuffer = pVertexBuffer;
mIndexBuffer = pIndexBuffer;
memcpy(mLightBuffer->contents(), lightDescs.data(), lightBufferSize);
memcpy(mVertexBuffer->contents(), vertices.data(), vertexDataSize);
memcpy(mIndexBuffer->contents(), indices.data(), indexDataSize);
mLightBuffer->didModifyRange(NS::Range::Make(0, mLightBuffer->length()));
mVertexBuffer->didModifyRange(NS::Range::Make(0, mVertexBuffer->length()));
mIndexBuffer->didModifyRange(NS::Range::Make(0, mIndexBuffer->length()));
for (MTL::Buffer*& uniformBuffer : mUniformBuffers)
{
uniformBuffer = mDevice->newBuffer(sizeof(Uniforms), MTL::ResourceStorageModeManaged);
}
for (MTL::Buffer*& uniformBuffer : mUniformTMBuffers)
{
uniformBuffer = mDevice->newBuffer(sizeof(UniformsTonemap), MTL::ResourceStorageModeManaged);
}
}
MTL::AccelerationStructure* MetalRender::createAccelerationStructure(MTL::AccelerationStructureDescriptor* descriptor)
{
NS::AutoreleasePool* pPool = NS::AutoreleasePool::alloc()->init();
// Query for the sizes needed to store and build the acceleration structure.
const MTL::AccelerationStructureSizes accelSizes = mDevice->accelerationStructureSizes(descriptor);
// Allocate an acceleration structure large enough for this descriptor. This doesn't actually
// build the acceleration structure, it just allocates memory.
MTL::AccelerationStructure* accelerationStructure =
mDevice->newAccelerationStructure(accelSizes.accelerationStructureSize);
// Allocate scratch space Metal uses to build the acceleration structure.
// Use MTLResourceStorageModePrivate for best performance because the sample
// doesn't need access to the buffer's contents.
MTL::Buffer* scratchBuffer = mDevice->newBuffer(accelSizes.buildScratchBufferSize, MTL::ResourceStorageModePrivate);
// Create a command buffer to perform the acceleration structure build.
MTL::CommandBuffer* commandBuffer = mCommandQueue->commandBuffer();
// Create an acceleration structure command encoder.
MTL::AccelerationStructureCommandEncoder* commandEncoder = commandBuffer->accelerationStructureCommandEncoder();
// Allocate a buffer for Metal to write the compacted accelerated structure's size into.
MTL::Buffer* compactedSizeBuffer = mDevice->newBuffer(sizeof(uint32_t), MTL::ResourceStorageModeShared);
// Schedule the actual acceleration structure build.
commandEncoder->buildAccelerationStructure(accelerationStructure, descriptor, scratchBuffer, 0UL);
// Compute and write the compacted acceleration structure size into the buffer. You
// need to already have a built accelerated structure because Metal determines the compacted
// size based on the final size of the acceleration structure. Compacting an acceleration
// structure can potentially reclaim significant amounts of memory because Metal must
// create the initial structure using a conservative approach.
commandEncoder->writeCompactedAccelerationStructureSize(accelerationStructure, compactedSizeBuffer, 0UL);
// End encoding and commit the command buffer so the GPU can start building the
// acceleration structure.
commandEncoder->endEncoding();
commandBuffer->commit();
// The sample waits for Metal to finish executing the command buffer so that it can
// read back the compacted size.
// Note: Don't wait for Metal to finish executing the command buffer if you aren't compacting
// the acceleration structure because doing so requires CPU/GPU synchronization. You don't have
// to compact acceleration structures, but it's helpful when creating large static acceleration
// structures, such as static scene geometry. Avoid compacting acceleration structures that
// you rebuild every frame because the synchronization cost may be significant.
commandBuffer->waitUntilCompleted();
const uint32_t compactedSize = *(uint32_t*)compactedSizeBuffer->contents();
// commandBuffer->release();
// commandEncoder->release();
// Allocate a smaller acceleration structure based on the returned size.
MTL::AccelerationStructure* compactedAccelerationStructure = mDevice->newAccelerationStructure(compactedSize);
// Create another command buffer and encoder.
commandBuffer = mCommandQueue->commandBuffer();
commandEncoder = commandBuffer->accelerationStructureCommandEncoder();
// Encode the command to copy and compact the acceleration structure into the
// smaller acceleration structure.
commandEncoder->copyAndCompactAccelerationStructure(accelerationStructure, compactedAccelerationStructure);
// End encoding and commit the command buffer. You don't need to wait for Metal to finish
// executing this command buffer as long as you synchronize any ray-intersection work
// to run after this command buffer completes. The sample relies on Metal's default
// dependency tracking on resources to automatically synchronize access to the new
// compacted acceleration structure.
commandEncoder->endEncoding();
commandBuffer->commit();
// commandEncoder->release();
// commandBuffer->release();
accelerationStructure->release();
scratchBuffer->release();
compactedSizeBuffer->release();
pPool->release();
return compactedAccelerationStructure->retain();
}
constexpr simd_float4x4 makeIdentity()
{
using simd::float4;
return (simd_float4x4){ (float4){ 1.f, 0.f, 0.f, 0.f }, (float4){ 0.f, 1.f, 0.f, 0.f },
(float4){ 0.f, 0.f, 1.f, 0.f }, (float4){ 0.f, 0.f, 0.f, 1.f } };
}
MetalRender::Mesh* MetalRender::createMesh(const oka::Mesh& mesh)
{
MetalRender::Mesh* result = new MetalRender::Mesh();
const uint32_t triangleCount = mesh.mCount / 3;
const std::vector<Scene::Vertex>& vertices = mScene->getVertices();
const std::vector<uint32_t>& indices = mScene->getIndices();
std::vector<Triangle> triangleData(triangleCount);
for (int i = 0; i < triangleCount; ++i)
{
Triangle& curr = triangleData[i];
const uint32_t i0 = indices[mesh.mIndex + i * 3 + 0];
const uint32_t i1 = indices[mesh.mIndex + i * 3 + 1];
const uint32_t i2 = indices[mesh.mIndex + i * 3 + 2];
// Positions
using simd::float3;
curr.positions[0] = { vertices[mesh.mVbOffset + i0].pos.x, vertices[mesh.mVbOffset + i0].pos.y,
vertices[mesh.mVbOffset + i0].pos.z };
curr.positions[1] = { vertices[mesh.mVbOffset + i1].pos.x, vertices[mesh.mVbOffset + i1].pos.y,
vertices[mesh.mVbOffset + i1].pos.z };
curr.positions[2] = { vertices[mesh.mVbOffset + i2].pos.x, vertices[mesh.mVbOffset + i2].pos.y,
vertices[mesh.mVbOffset + i2].pos.z };
// Normals
curr.normals[0] = vertices[mesh.mVbOffset + i0].normal;
curr.normals[1] = vertices[mesh.mVbOffset + i1].normal;
curr.normals[2] = vertices[mesh.mVbOffset + i2].normal;
// Tangents
curr.tangent[0] = vertices[mesh.mVbOffset + i0].tangent;
curr.tangent[1] = vertices[mesh.mVbOffset + i1].tangent;
curr.tangent[2] = vertices[mesh.mVbOffset + i2].tangent;
// UVs
curr.uv[0] = vertices[mesh.mVbOffset + i0].uv;
curr.uv[1] = vertices[mesh.mVbOffset + i1].uv;
curr.uv[2] = vertices[mesh.mVbOffset + i2].uv;
}
MTL::Buffer* perPrimitiveBuffer =
mDevice->newBuffer(triangleData.size() * sizeof(Triangle), MTL::ResourceStorageModeManaged);
memcpy(perPrimitiveBuffer->contents(), triangleData.data(), sizeof(Triangle) * triangleData.size());
perPrimitiveBuffer->didModifyRange(NS::Range(0, perPrimitiveBuffer->length()));
MTL::AccelerationStructureTriangleGeometryDescriptor* geomDescriptor =
MTL::AccelerationStructureTriangleGeometryDescriptor::alloc()->init();
geomDescriptor->setVertexBuffer(mVertexBuffer);
geomDescriptor->setVertexBufferOffset(mesh.mVbOffset * sizeof(Scene::Vertex));
geomDescriptor->setVertexStride(sizeof(Scene::Vertex));
geomDescriptor->setIndexBuffer(mIndexBuffer);
geomDescriptor->setIndexBufferOffset(mesh.mIndex * sizeof(uint32_t));
geomDescriptor->setIndexType(MTL::IndexTypeUInt32);
geomDescriptor->setTriangleCount(triangleCount);
// Setup per primitive data
geomDescriptor->setPrimitiveDataBuffer(perPrimitiveBuffer);
geomDescriptor->setPrimitiveDataBufferOffset(0);
geomDescriptor->setPrimitiveDataElementSize(sizeof(Triangle));
geomDescriptor->setPrimitiveDataStride(sizeof(Triangle));
const NS::Array* geomDescriptors = NS::Array::array((const NS::Object* const*)&geomDescriptor, 1UL);
MTL::PrimitiveAccelerationStructureDescriptor* primDescriptor =
MTL::PrimitiveAccelerationStructureDescriptor::alloc()->init();
primDescriptor->setGeometryDescriptors(geomDescriptors);
result->mGas = createAccelerationStructure(primDescriptor);
primDescriptor->release();
geomDescriptor->release();
perPrimitiveBuffer->release();
return result;
}
void MetalRender::createAccelerationStructures()
{
NS::AutoreleasePool* pPool = NS::AutoreleasePool::alloc()->init();
const std::vector<oka::Mesh>& meshes = mScene->getMeshes();
const std::vector<oka::Curve>& curves = mScene->getCurves();
const std::vector<oka::Instance>& instances = mScene->getInstances();
if (meshes.empty() && curves.empty())
{
return;
}
for (const oka::Mesh& currMesh : meshes)
{
MetalRender::Mesh* metalMesh = createMesh(currMesh);
mMetalMeshes.push_back(metalMesh);
mPrimitiveAccelerationStructures.push_back(metalMesh->mGas);
}
mInstanceBuffer = mDevice->newBuffer(
sizeof(MTL::AccelerationStructureUserIDInstanceDescriptor) * instances.size(), MTL::ResourceStorageModeManaged);
MTL::AccelerationStructureUserIDInstanceDescriptor* instanceDescriptors =
(MTL::AccelerationStructureUserIDInstanceDescriptor*)mInstanceBuffer->contents();
for (int i = 0; i < instances.size(); ++i)
{
const Instance& curr = instances[i];
instanceDescriptors[i].accelerationStructureIndex = curr.mMeshId;
instanceDescriptors[i].options = MTL::AccelerationStructureInstanceOptionOpaque;
instanceDescriptors[i].intersectionFunctionTableOffset = 0;
instanceDescriptors[i].userID = curr.type == Instance::Type::eLight ? curr.mLightId : curr.mMaterialId;
instanceDescriptors[i].mask = curr.type == Instance::Type::eLight ? GEOMETRY_MASK_LIGHT : GEOMETRY_MASK_TRIANGLE;
for (int column = 0; column < 4; column++)
{
for (int row = 0; row < 3; row++)
{
instanceDescriptors[i].transformationMatrix.columns[column][row] = curr.transform[column][row];
}
}
}
mInstanceBuffer->didModifyRange(NS::Range::Make(0, mInstanceBuffer->length()));
const NS::Array* instancedAccelerationStructures = NS::Array::array(
(const NS::Object* const*)mPrimitiveAccelerationStructures.data(), mPrimitiveAccelerationStructures.size());
MTL::InstanceAccelerationStructureDescriptor* accelDescriptor =
MTL::InstanceAccelerationStructureDescriptor::descriptor();
accelDescriptor->setInstancedAccelerationStructures(instancedAccelerationStructures);
accelDescriptor->setInstanceCount(instances.size());
accelDescriptor->setInstanceDescriptorBuffer(mInstanceBuffer);
accelDescriptor->setInstanceDescriptorType(MTL::AccelerationStructureInstanceDescriptorTypeUserID);
mInstanceAccelerationStructure = createAccelerationStructure(accelDescriptor);
pPool->release();
}
| 30,059 | C++ | 43.932735 | 121 | 0.69144 |
arhix52/Strelka/src/render/metal/MetalRender.h | #pragma once
#include "render.h"
#include <Metal/Metal.hpp>
#include <MetalKit/MetalKit.hpp>
namespace oka
{
static constexpr size_t kMaxFramesInFlight = 3;
class MetalRender : public Render
{
public:
MetalRender(/* args */);
~MetalRender() override;
void init() override;
void render(Buffer* output) override;
Buffer* createBuffer(const BufferDesc& desc) override;
void* getNativeDevicePtr() override
{
return mDevice;
}
private:
struct Mesh
{
MTL::AccelerationStructure* mGas;
};
Mesh* createMesh(const oka::Mesh& mesh);
struct View
{
oka::Camera::Matrices mCamMatrices;
};
View mPrevView;
MTL::Device* mDevice;
MTL::CommandQueue* mCommandQueue;
MTL::Library* mShaderLibrary;
MTL::ComputePipelineState* mPathTracingPSO;
MTL::ComputePipelineState* mTonemapperPSO;
MTL::Buffer* mAccumulationBuffer;
MTL::Buffer* mLightBuffer;
MTL::Buffer* mVertexBuffer;
MTL::Buffer* mUniformBuffers[kMaxFramesInFlight];
MTL::Buffer* mUniformTMBuffers[kMaxFramesInFlight];
MTL::Buffer* mIndexBuffer;
uint32_t mTriangleCount;
std::vector<MetalRender::Mesh*> mMetalMeshes;
std::vector<MTL::AccelerationStructure*> mPrimitiveAccelerationStructures;
MTL::AccelerationStructure* mInstanceAccelerationStructure;
MTL::Buffer* mInstanceBuffer;
MTL::Buffer* mMaterialBuffer;
std::vector<MTL::Texture*> mMaterialTextures;
uint32_t mFrameIndex;
void buildComputePipeline();
void buildTonemapperPipeline();
void buildBuffers();
MTL::Texture* loadTextureFromFile(const std::string& fileName);
void createMetalMaterials();
MTL::AccelerationStructure* createAccelerationStructure(MTL::AccelerationStructureDescriptor* descriptor);
void createAccelerationStructures();
};
} // namespace oka
| 1,873 | C | 24.324324 | 110 | 0.710091 |
arhix52/Strelka/src/render/metal/shaders/random.h | #pragma once
#include <simd/simd.h>
using namespace metal;
float uintToFloat(uint x)
{
return as_type<float>(0x3f800000 | (x >> 9)) - 1.f;
}
enum class SampleDimension : uint32_t
{
ePixelX,
ePixelY,
eLightId,
eLightPointX,
eLightPointY,
eBSDF0,
eBSDF1,
eBSDF2,
eBSDF3,
eRussianRoulette,
eNUM_DIMENSIONS
};
struct SamplerState
{
uint32_t seed;
uint32_t sampleIdx;
uint32_t depth;
};
#define MAX_BOUNCES 128
// Based on: https://www.reedbeta.com/blog/hash-functions-for-gpu-rendering/
inline unsigned pcg_hash(unsigned seed) {
unsigned state = seed * 747796405u + 2891336453u;
unsigned word = ((state >> ((state >> 28u) + 4u)) ^ state) * 277803737u;
return (word >> 22u) ^ word;
}
inline unsigned hash_with(unsigned seed, unsigned hash) {
// Wang hash
seed = (seed ^ 61) ^ hash;
seed += seed << 3;
seed ^= seed >> 4;
seed *= 0x27d4eb2d;
return seed;
}
// jenkins hash
unsigned int hash(unsigned int a)
{
a = (a + 0x7ED55D16) + (a << 12);
a = (a ^ 0xC761C23C) ^ (a >> 19);
a = (a + 0x165667B1) + (a << 5);
a = (a + 0xD3A2646C) ^ (a << 9);
a = (a + 0xFD7046C5) + (a << 3);
a = (a ^ 0xB55A4F09) ^ (a >> 16);
return a;
}
uint32_t hash2(uint32_t x, uint32_t seed)
{
x ^= x * 0x3d20adea;
x += seed;
x *= (seed >> 16) | 1;
x ^= x * 0x05526c56;
x ^= x * 0x53a22864;
return x;
}
uint jenkinsHash(uint x)
{
x += x << 10;
x ^= x >> 6;
x += x << 3;
x ^= x >> 11;
x += x << 15;
return x;
}
constant unsigned int primeNumbers[32] =
{
2, 3, 5, 7, 11, 13, 17, 19, 23, 29,
31, 37, 41, 43, 47, 53, 59, 61, 67, 71,
73, 79, 83, 89, 97, 101, 103, 107, 109, 113,
127, 131
};
float halton(uint32_t index, uint32_t base)
{
const float s = 1.0f / float(base);
unsigned int i = index;
float result = 0.0f;
float f = s;
while (i)
{
const unsigned int digit = i % base;
result += f * float(digit);
i = (i - digit) / base;
f *= s;
}
return clamp(result, 0.0f, 1.0f - 1e-6f); // TODO: 1minusEps
}
static SamplerState initSampler(uint32_t linearPixelIndex, uint32_t pixelSampleIndex, uint32_t seed)
{
SamplerState sampler {};
sampler.seed = hash(linearPixelIndex); //^ 0x736caf6fu;
sampler.sampleIdx = pixelSampleIndex;
sampler.depth = 0;
return sampler;
}
template <SampleDimension Dim>
static float random(thread SamplerState& state)
{
const uint32_t dimension = uint32_t(Dim) + state.depth * uint32_t(SampleDimension::eNUM_DIMENSIONS);
const uint32_t base = primeNumbers[dimension & 31u];
return halton(state.seed + state.sampleIdx, base);
}
uint xorshift(thread uint& rngState)
{
rngState ^= rngState << 13;
rngState ^= rngState >> 17;
rngState ^= rngState << 5;
return rngState;
}
template<unsigned int N>
static __inline__ unsigned int tea( unsigned int val0, unsigned int val1 )
{
unsigned int v0 = val0;
unsigned int v1 = val1;
unsigned int s0 = 0;
for( unsigned int n = 0; n < N; n++ )
{
s0 += 0x9e3779b9;
v0 += ((v1<<4)+0xa341316c)^(v1+s0)^((v1>>5)+0xc8013ea4);
v1 += ((v0<<4)+0xad90777d)^(v0+s0)^((v0>>5)+0x7e95761e);
}
return v0;
}
// Generate random unsigned int in [0, 2^24)
static __inline__ unsigned int lcg(thread unsigned int &prev)
{
const unsigned int LCG_A = 1664525u;
const unsigned int LCG_C = 1013904223u;
prev = (LCG_A * prev + LCG_C);
return prev & 0x00FFFFFF;
}
static __inline__ unsigned int lcg2(thread unsigned int &prev)
{
prev = (prev*8121 + 28411) % 134456;
return prev;
}
// Generate random float in [0, 1)
static __inline__ float rnd(thread unsigned int &prev)
{
return ((float) lcg(prev) / (float) 0x01000000);
}
static __inline__ unsigned int rot_seed( unsigned int seed, unsigned int frame )
{
return seed ^ frame;
}
// Implementetion from Ray Tracing gems
// https://github.com/boksajak/referencePT/blob/master/shaders/PathTracer.hlsl
uint initRNG(uint2 pixelCoords, uint2 resolution, uint frameNumber)
{
uint t = dot(float2(pixelCoords), float2(1, resolution.x));
uint seed = t ^ jenkinsHash(frameNumber);
// uint seed = dot(pixelCoords, uint2(1, resolution.x)) ^ jenkinsHash(frameNumber);
return jenkinsHash(seed);
}
uint owen_scramble_rev(uint x, uint seed)
{
x ^= x * 0x3d20adea;
x += seed;
x *= (seed >> 16) | 1;
x ^= x * 0x05526c56;
x ^= x * 0x53a22864;
return x;
}
| 4,402 | C | 21.695876 | 104 | 0.62199 |
arhix52/Strelka/src/render/metal/shaders/lights.h | #pragma once
#include <metal_stdlib>
#include <simd/simd.h>
#include "ShaderTypes.h"
using namespace metal;
struct LightSampleData
{
float3 pointOnLight;
float pdf;
float3 normal;
float area;
float3 L;
float distToLight;
};
__inline__ float misWeightBalance(const float a, const float b)
{
return 1.0f / ( 1.0f + (b / a) );
}
static float calcLightArea(device const UniformLight& l)
{
float area = 0.0f;
if (l.type == 0) // rectangle area
{
float3 e1 = float3(l.points[1]) - float3(l.points[0]);
float3 e2 = float3(l.points[3]) - float3(l.points[0]);
area = length(cross(e1, e2));
}
else if (l.type == 1) // disc area
{
area = l.points[0].x * l.points[0].x * M_PI_F; // pi * radius^2
}
else if (l.type == 2) // sphere area
{
area = l.points[0].x * l.points[0].x * 4.0f * M_PI_F; // 4 * pi * radius^2
}
return area;
}
static float3 calcLightNormal(device const UniformLight& l, thread const float3& hitPoint)
{
float3 norm = float3(0.0f);
if (l.type == 0)
{
float3 e1 = float3(l.points[1]) - float3(l.points[0]);
float3 e2 = float3(l.points[3]) - float3(l.points[0]);
norm = -normalize(cross(e1, e2));
}
else if (l.type == 1)
{
norm = float3(l.normal);
}
else if (l.type == 2)
{
norm = normalize(hitPoint - float3(l.points[1]));
}
return norm;
}
static void fillLightData(device const UniformLight& l, thread const float3& hitPoint, thread LightSampleData& lightSampleData)
{
lightSampleData.area = calcLightArea(l);
lightSampleData.normal = calcLightNormal(l, hitPoint);
const float3 toLight = lightSampleData.pointOnLight - hitPoint;
const float lenToLight = length(toLight);
lightSampleData.L = toLight / lenToLight;
lightSampleData.distToLight = lenToLight;
}
struct SphQuad
{
float3 o, x, y, z;
float z0, z0sq;
float x0, y0, y0sq; // rectangle coords in ’R’
float x1, y1, y1sq;
float b0, b1, b0sq, k;
float S;
};
// Precomputation of constants for the spherical rectangle Q.
static SphQuad init(device const UniformLight& l, const float3 o)
{
SphQuad squad;
float3 ex = float3(l.points[1]) - float3(l.points[0]);
float3 ey = float3(l.points[3]) - float3(l.points[0]);
float3 s = float3(l.points[0]);
float exl = length(ex);
float eyl = length(ey);
squad.o = o;
squad.x = ex / exl;
squad.y = ey / eyl;
squad.z = cross(squad.x, squad.y);
// compute rectangle coords in local reference system
float3 d = s - o;
squad.z0 = dot(d, squad.z);
// flip ’z’ to make it point against ’Q’
if (squad.z0 > 0.0f)
{
squad.z *= -1.0f;
squad.z0 *= -1.0f;
}
squad.z0sq = squad.z0 * squad.z0;
squad.x0 = dot(d, squad.x);
squad.y0 = dot(d, squad.y);
squad.x1 = squad.x0 + exl;
squad.y1 = squad.y0 + eyl;
squad.y0sq = squad.y0 * squad.y0;
squad.y1sq = squad.y1 * squad.y1;
// create vectors to four vertices
float3 v00 = { squad.x0, squad.y0, squad.z0 };
float3 v01 = { squad.x0, squad.y1, squad.z0 };
float3 v10 = { squad.x1, squad.y0, squad.z0 };
float3 v11 = { squad.x1, squad.y1, squad.z0 };
// compute normals to edges
float3 n0 = normalize(cross(v00, v10));
float3 n1 = normalize(cross(v10, v11));
float3 n2 = normalize(cross(v11, v01));
float3 n3 = normalize(cross(v01, v00));
// compute internal angles (gamma_i)
float g0 = fast::acos(-dot(n0, n1));
float g1 = fast::acos(-dot(n1, n2));
float g2 = fast::acos(-dot(n2, n3));
float g3 = fast::acos(-dot(n3, n0));
// compute predefined constants
squad.b0 = n0.z;
squad.b1 = n2.z;
squad.b0sq = squad.b0 * squad.b0;
const float twoPi = 2.0f * M_PI_F;
squad.k = twoPi - g2 - g3;
// compute solid angle from internal angles
squad.S = g0 + g1 - squad.k;
return squad;
}
static float3 SphQuadSample(const SphQuad squad, const float2 uv)
{
float u = uv.x;
float v = uv.y;
// 1. compute cu
float au = u * squad.S + squad.k;
float fu = (cos(au) * squad.b0 - squad.b1) / sin(au);
float cu = 1 / sqrt(fu * fu + squad.b0sq) * (fu > 0 ? 1 : -1);
cu = clamp(cu, -1.0f, 1.0f); // avoid NaNs
// 2. compute xu
float xu = -(cu * squad.z0) / sqrt(1 - cu * cu);
xu = clamp(xu, squad.x0, squad.x1); // avoid Infs
// 3. compute yv
float d = sqrt(xu * xu + squad.z0sq);
float h0 = squad.y0 / sqrt(d * d + squad.y0sq);
float h1 = squad.y1 / sqrt(d * d + squad.y1sq);
float hv = h0 + v * (h1 - h0);
float hv2 = hv * hv;
float eps = 1e-6f;
float yv = (hv2 < 1.0f - eps) ? (hv * d) / sqrt(1.0f - hv2) : squad.y1;
// 4. transform (xu, yv, z0) to world coords
return (squad.o + xu * squad.x + yv * squad.y + squad.z0 * squad.z);
}
static LightSampleData SampleRectLight(device const UniformLight& l, thread const float2& u, thread const float3& hitPoint)
{
LightSampleData lightSampleData;
float3 e1 = float3(l.points[1]) - float3(l.points[0]);
float3 e2 = float3(l.points[3]) - float3(l.points[0]);
// https://www.arnoldrenderer.com/research/egsr2013_spherical_rectangle.pdf
SphQuad quad = init(l, hitPoint);
if (quad.S <= 0.0f)
{
lightSampleData.pdf = 0.0f;
lightSampleData.pointOnLight = float3(l.points[0]) + e1 * u.x + e2 * u.y;
fillLightData(l, hitPoint, lightSampleData);
return lightSampleData;
}
if (quad.S < 1e-3f)
{
// light too small, use uniform
lightSampleData.pointOnLight = float3(l.points[0]) + e1 * u.x + e2 * u.y;
fillLightData(l, hitPoint, lightSampleData);
lightSampleData.pdf = lightSampleData.distToLight * lightSampleData.distToLight /
(dot(-lightSampleData.L, lightSampleData.normal) * lightSampleData.area);
return lightSampleData;
}
lightSampleData.pointOnLight = SphQuadSample(quad, u);
fillLightData(l, hitPoint, lightSampleData);
lightSampleData.pdf = max(0.0f, 1.0f / quad.S);
return lightSampleData;
}
static __inline__ LightSampleData SampleRectLightUniform(device const UniformLight& l, thread const float2& u, thread const float3& hitPoint)
{
LightSampleData lightSampleData;
// uniform sampling
float3 e1 = float3(l.points[1]) - float3(l.points[0]);
float3 e2 = float3(l.points[3]) - float3(l.points[0]);
lightSampleData.pointOnLight = float3(l.points[0]) + e1 * u.x + e2 * u.y;
fillLightData(l, hitPoint, lightSampleData);
lightSampleData.pdf = lightSampleData.distToLight * lightSampleData.distToLight /
(dot(-lightSampleData.L, lightSampleData.normal) * lightSampleData.area);
return lightSampleData;
}
static __inline__ float getRectLightPdf(device const UniformLight& l, const float3 lightHitPoint, const float3 surfaceHitPoint)
{
LightSampleData lightSampleData {};
lightSampleData.pointOnLight = lightHitPoint;
fillLightData(l, surfaceHitPoint, lightSampleData);
lightSampleData.pdf = lightSampleData.distToLight * lightSampleData.distToLight /
(dot(-lightSampleData.L, lightSampleData.normal) * lightSampleData.area);
return lightSampleData.pdf;
}
static void createCoordinateSystem(thread const float3& N, thread float3& Nt, thread float3& Nb) {
if (fabs(N.x) > fabs(N.y)) {
float invLen = 1.0f / sqrt(N.x * N.x + N.z * N.z);
Nt = float3(-N.z * invLen, 0.0f, N.x * invLen);
} else {
float invLen = 1.0f / sqrt(N.y * N.y + N.z * N.z);
Nt = float3(0.0f, N.z * invLen, -N.y * invLen);
}
Nb = cross(N, Nt);
}
static __inline__ float getDirectLightPdf(float angle)
{
return 1.0f / (2.0f * M_PI_F * (1.0f - cos(angle)));
}
static __inline__ float getSphereLightPdf()
{
return 1.0f / (4.0f * M_PI_F);
}
static float3 SampleCone(float2 uv, float angle, float3 direction, thread float& pdf) {
float phi = 2.0 * M_PI_F * uv.x;
float cosTheta = 1.0 - uv.y * (1.0 - cos(angle));
// Convert spherical coordinates to 3D direction
float sinTheta = sqrt(1.0 - cosTheta * cosTheta);
float3 u, v;
createCoordinateSystem(direction, u, v);
float3 sampledDir = normalize(cos(phi) * sinTheta * u + sin(phi) * sinTheta * v + cosTheta * direction);
// Calculate the PDF for the sampled direction
pdf = getDirectLightPdf(angle);
return sampledDir;
}
static __inline__ LightSampleData SampleDistantLight(device const UniformLight& l, const float2 u, const float3 hitPoint)
{
LightSampleData lightSampleData;
float pdf = 0.0f;
float3 coneSample = SampleCone(u, l.halfAngle, -float3(l.normal), pdf);
lightSampleData.area = 0.0f;
lightSampleData.distToLight = 1e9;
lightSampleData.L = coneSample;
lightSampleData.normal = float3(l.normal);
lightSampleData.pdf = pdf;
lightSampleData.pointOnLight = coneSample;
return lightSampleData;
}
static __inline__ LightSampleData SampleSphereLight(device const UniformLight& l, const float2 u, const float3 hitPoint)
{
LightSampleData lightSampleData;
// Generate a random direction on the sphere using solid angle sampling
float cosTheta = 1.0f - 2.0f * u.x; // cosTheta is uniformly distributed between [-1, 1]
float sinTheta = sqrt(1.0f - cosTheta * cosTheta);
float phi = 2.0f * M_PI_F * u.y; // phi is uniformly distributed between [0, 2*pi]
const float radius = l.points[0].x;
// Convert spherical coordinates to Cartesian coordinates
float3 sphereDirection = float3(sinTheta * cos(phi), sinTheta * sin(phi), cosTheta);
// Scale the direction by the radius of the sphere and move it to the light position
float3 lightPoint = float3(l.points[1]) + radius * sphereDirection;
// Calculate the direction from the hit point to the sampled point on the light
lightSampleData.L = normalize(lightPoint - hitPoint);
// Calculate the distance to the light
lightSampleData.distToLight = length(lightPoint - hitPoint);
lightSampleData.area = 0.0f;
lightSampleData.normal = sphereDirection;
lightSampleData.pdf = 1.0f / (4.0f * M_PI_F);
lightSampleData.pointOnLight = lightPoint;
return lightSampleData;
}
static __inline__ float getLightPdf(device const UniformLight& l, const float3 lightHitPoint, const float3 surfaceHitPoint)
{
switch (l.type)
{
case 0:
// Rect
return getRectLightPdf(l, lightHitPoint, surfaceHitPoint);
break;
case 2:
// sphere
return getSphereLightPdf();
break;
case 3:
// Distant
return getDirectLightPdf(l.halfAngle);
break;
default:
break;
}
return 0.0f;
}
| 10,860 | C | 30.75731 | 141 | 0.630295 |
arhix52/Strelka/src/render/metal/shaders/tonemappers.h | #pragma once
#include <simd/simd.h>
using namespace metal;
enum class ToneMapperType : uint32_t
{
eNone = 0,
eReinhard,
eACES,
eFilmic,
};
// https://github.com/TheRealMJP/BakingLab/blob/master/BakingLab/ACES.hlsl
// sRGB => XYZ => D65_2_D60 => AP1 => RRT_SAT
static constant float3x3 ACESInputMat =
{
{0.59719, 0.35458, 0.04823},
{0.07600, 0.90834, 0.01566},
{0.02840, 0.13383, 0.83777}
};
// ODT_SAT => XYZ => D60_2_D65 => sRGB
static constant float3x3 ACESOutputMat =
{
{ 1.60475, -0.53108, -0.07367},
{-0.10208, 1.10813, -0.00605},
{-0.00327, -0.07276, 1.07602}
};
float3 RRTAndODTFit(float3 v)
{
float3 a = v * (v + 0.0245786f) - 0.000090537f;
float3 b = v * (0.983729f * v + 0.4329510f) + 0.238081f;
return a / b;
}
float3 ACESFitted(float3 color)
{
color = transpose(ACESInputMat) * color;
// Apply RRT and ODT
color = RRTAndODTFit(color);
color = transpose(ACESOutputMat) * color;
// Clamp to [0, 1]
color = saturate(color);
return color;
}
// https://knarkowicz.wordpress.com/2016/01/06/aces-filmic-tone-mapping-curve/
float3 ACESFilm(float3 x)
{
float a = 2.51f;
float b = 0.03f;
float c = 2.43f;
float d = 0.59f;
float e = 0.14f;
return saturate((x*(a*x+b))/(x*(c*x+d)+e));
}
// original implementation https://github.com/NVIDIAGameWorks/Falcor/blob/5236495554f57a734cc815522d95ae9a7dfe458a/Source/RenderPasses/ToneMapper/ToneMapping.ps.slang
float calcLuminance(float3 color)
{
return dot(color, float3(0.299, 0.587, 0.114));
}
float3 reinhard(float3 color)
{
float luminance = calcLuminance(color);
// float reinhard = luminance / (luminance + 1);
return color / (luminance + 1.0f);
}
float gammaFloat(const float c, const float gamma)
{
if (isnan(c))
{
return 0.0f;
}
if (c > 1.0f)
{
return 1.0f;
}
else if (c < 0.0f)
{
return 0.0f;
}
else if (c < 0.0031308f)
{
return 12.92f * c;
}
else
{
return 1.055f * pow(c, 1.0f / gamma) - 0.055f;
}
}
float3 srgbGamma(const float3 color, const float gamma)
{
return float3(gammaFloat(color.r, gamma), gammaFloat(color.g, gamma), gammaFloat(color.b, gamma));
}
// utility function for accumulation and HDR <=> LDR
float3 tonemap(float3 color, const float3 exposure)
{
color *= exposure;
return color / (color + 1.0f);
}
float3 inverseTonemap(const float3 color, const float3 exposure)
{
return color / (exposure - color * exposure);
}
| 2,540 | C | 21.289473 | 166 | 0.626378 |
arhix52/Strelka/src/render/metal/shaders/ShaderTypes.h | #ifndef ShaderTypes_h
#define ShaderTypes_h
#include <simd/simd.h>
#ifndef __METAL_VERSION__
#ifdef __cplusplus
#include <Metal/MTLTypes.hpp>
#endif
#endif
#define GEOMETRY_MASK_TRIANGLE 1
#define GEOMETRY_MASK_CURVE 2
#define GEOMETRY_MASK_LIGHT 4
#define GEOMETRY_MASK_GEOMETRY (GEOMETRY_MASK_TRIANGLE | GEOMETRY_MASK_CURVE)
#define RAY_MASK_PRIMARY (GEOMETRY_MASK_GEOMETRY | GEOMETRY_MASK_LIGHT)
#define RAY_MASK_SHADOW GEOMETRY_MASK_GEOMETRY
#define RAY_MASK_SECONDARY GEOMETRY_MASK_GEOMETRY
#ifndef __METAL_VERSION__
struct packed_float3
{
# ifdef __cplusplus
packed_float3() = default;
packed_float3(vector_float3 v) : x(v.x), y(v.y), z(v.z)
{
}
# endif
float x;
float y;
float z;
};
#endif
enum class DebugMode : uint32_t
{
eNone = 0,
eNormal,
};
struct Vertex
{
vector_float3 pos;
uint32_t tangent;
uint32_t normal;
uint32_t uv;
float pad0;
float pad1;
};
struct Uniforms
{
simd::float4x4 viewToWorld;
simd::float4x4 clipToView;
vector_float3 missColor;
uint32_t width;
uint32_t height;
uint32_t frameIndex;
uint32_t subframeIndex;
uint32_t numLights;
uint32_t enableAccumulation;
uint32_t samples_per_launch;
uint32_t maxDepth;
uint32_t rectLightSamplingMethod;
uint32_t tonemapperType; // 0 - "None", "Reinhard", "ACES", "Filmic"
float gamma; // 0 - off
vector_float3 exposureValue;
uint32_t debug;
};
struct UniformsTonemap
{
uint32_t width;
uint32_t height;
uint32_t tonemapperType; // 0 - "None", "Reinhard", "ACES", "Filmic"
float gamma; // 0 - off
vector_float3 exposureValue;
};
struct Triangle
{
vector_float3 positions[3];
uint32_t normals[3];
uint32_t tangent[3];
uint32_t uv[3];
};
// GPU side structure
struct UniformLight
{
vector_float4 points[4];
vector_float4 color;
vector_float4 normal;
int type;
float halfAngle;
float pad0;
float pad1;
};
struct Material
{
simd::float3 diffuse;
#ifdef __METAL_VERSION__
texture2d<float> diffuseTexture;
texture2d<float> normalTexture;
#else
MTL::ResourceID diffuseTexture; // uint64_t - alignment 8
MTL::ResourceID normalTexture;
#endif
};
#endif
| 2,255 | C | 17.491803 | 77 | 0.668736 |
arhix52/Strelka/src/settings/settings.cpp | #include "settings/settings.h"
namespace oka
{
} // namespace oka
| 68 | C++ | 8.857142 | 30 | 0.705882 |
arhix52/Strelka/src/app/main.cpp | #include <display/Display.h>
#include <render/common.h>
#include <render/buffer.h>
#include <render/Render.h>
#include <sceneloader/gltfloader.h>
#include <log.h>
#include <logmanager.h>
#include <cxxopts.hpp>
#include <filesystem>
class CameraController : public oka::InputHandler
{
oka::Camera mCam;
glm::quat mOrientation;
glm::float3 mPosition;
glm::float3 mWorldUp;
glm::float3 mWorldForward;
float rotationSpeed = 0.025f;
float movementSpeed = 1.0f;
double pitch = 0.0;
double yaw = 0.0;
double max_pitch_rate = 5;
double max_yaw_rate = 5;
public:
virtual ~CameraController() = default;
struct
{
bool left = false;
bool right = false;
bool up = false;
bool down = false;
bool forward = false;
bool back = false;
} keys;
struct MouseButtons
{
bool left = false;
bool right = false;
bool middle = false;
} mouseButtons;
glm::float2 mMousePos;
// // local cameras axis
// glm::float3 getFront() const
// {
// return mOrientation.Transform(GfVec3d(0.0, 0.0, -1.0));
// }
// glm::float3 getUp() const
// {
// return mOrientation.Transform(GfVec3d(0.0, 1.0, 0.0));
// }
// glm::float3 getRight() const
// {
// return mOrientation.Transform(GfVec3d(1.0, 0.0, 0.0));
// }
// // global camera axis depending on scene settings
// GfVec3d getWorldUp() const
// {
// return mWorldUp;
// }
// GfVec3d getWorldForward() const
// {
// return mWorldForward;
// }
bool moving()
{
return keys.left || keys.right || keys.up || keys.down || keys.forward || keys.back || mouseButtons.right ||
mouseButtons.left || mouseButtons.middle;
}
void update(double deltaTime, float speed)
{
mCam.rotationSpeed = rotationSpeed;
mCam.movementSpeed = speed;
mCam.update(deltaTime);
// movementSpeed = speed;
// if (moving())
// {
// const float moveSpeed = deltaTime * movementSpeed;
// if (keys.up)
// mPosition += getWorldUp() * moveSpeed;
// if (keys.down)
// mPosition -= getWorldUp() * moveSpeed;
// if (keys.left)
// mPosition -= getRight() * moveSpeed;
// if (keys.right)
// mPosition += getRight() * moveSpeed;
// if (keys.forward)
// mPosition += getFront() * moveSpeed;
// if (keys.back)
// mPosition -= getFront() * moveSpeed;
// updateViewMatrix();
// }
}
// void rotate(double rightAngle, double upAngle)
// {
// GfRotation a(getRight(), upAngle * rotationSpeed);
// GfRotation b(getWorldUp(), rightAngle * rotationSpeed);
// GfRotation c = a * b;
// GfQuatd cq = c.GetQuat();
// cq.Normalize();
// mOrientation = cq * mOrientation;
// mOrientation.Normalize();
// updateViewMatrix();
// }
// void translate(GfVec3d delta)
// {
// // mPosition += mOrientation.Transform(delta);
// // updateViewMatrix();
// mCam.translate()
// }
void updateViewMatrix()
{
// GfMatrix4d view(1.0);
// view.SetRotateOnly(mOrientation);
// view.SetTranslateOnly(mPosition);
// mGfCam.SetTransform(view);
mCam.updateViewMatrix();
}
oka::Camera& getCamera()
{
return mCam;
}
CameraController(oka::Camera& cam, bool isYup)
{
if (isYup)
{
cam.setWorldUp(glm::float3(0.0, 1.0, 0.0));
cam.setWorldForward(glm::float3(0.0, 0.0, -1.0));
}
else
{
cam.setWorldUp(glm::float3(0.0, 0.0, 1.0));
cam.setWorldForward(glm::float3(0.0, 1.0, 0.0));
}
mCam = cam;
// GfMatrix4d xform = mGfCam.GetTransform();
// xform.Orthonormalize();
// mOrientation = xform.ExtractRotationQuat();
// mOrientation.Normalize();
// mPosition = xform.ExtractTranslation();
}
void keyCallback(int key, [[maybe_unused]] int scancode, int action, [[maybe_unused]] int mods) override
{
const bool keyState = ((GLFW_REPEAT == action) || (GLFW_PRESS == action)) ? true : false;
switch (key)
{
case GLFW_KEY_W: {
mCam.keys.forward = keyState;
break;
}
case GLFW_KEY_S: {
mCam.keys.back = keyState;
break;
}
case GLFW_KEY_A: {
mCam.keys.left = keyState;
break;
}
case GLFW_KEY_D: {
mCam.keys.right = keyState;
break;
}
case GLFW_KEY_Q: {
mCam.keys.up = keyState;
break;
}
case GLFW_KEY_E: {
mCam.keys.down = keyState;
break;
}
default:
break;
}
}
void mouseButtonCallback(int button, int action, [[maybe_unused]] int mods) override
{
if (button == GLFW_MOUSE_BUTTON_RIGHT)
{
if (action == GLFW_PRESS)
{
mCam.mouseButtons.right = true;
}
else if (action == GLFW_RELEASE)
{
mCam.mouseButtons.right = false;
}
}
else if (button == GLFW_MOUSE_BUTTON_LEFT)
{
if (action == GLFW_PRESS)
{
mCam.mouseButtons.left = true;
}
else if (action == GLFW_RELEASE)
{
mCam.mouseButtons.left = false;
}
}
}
void handleMouseMoveCallback([[maybe_unused]] double xpos, [[maybe_unused]] double ypos) override
{
const float dx = mCam.mousePos[0] - xpos;
const float dy = mCam.mousePos[1] - ypos;
// ImGuiIO& io = ImGui::GetIO();
// bool handled = io.WantCaptureMouse;
// if (handled)
//{
// camera.mousePos = glm::vec2((float)xpos, (float)ypos);
// return;
//}
if (mCam.mouseButtons.right)
{
mCam.rotate(-dx, -dy);
}
if (mCam.mouseButtons.left)
{
mCam.translate(glm::float3(-0.0, 0.0, -dy * .005 * movementSpeed));
}
if (mCam.mouseButtons.middle)
{
mCam.translate(glm::float3(-dx * 0.01, -dy * 0.01, 0.0f));
}
mCam.mousePos[0] = xpos;
mCam.mousePos[1] = ypos;
}
};
int main(int argc, const char* argv[])
{
const oka::Logmanager loggerManager;
cxxopts::Options options("Strelka -s <Scene path>", "commands");
// clang-format off
options.add_options()
("s, scene", "scene path", cxxopts::value<std::string>()->default_value(""))
("i, iteration", "Iteration to capture", cxxopts::value<int32_t>()->default_value("-1"))
("h, help", "Print usage")("t, spp_total", "spp total", cxxopts::value<int32_t>()->default_value("64"))
("f, spp_subframe", "spp subframe", cxxopts::value<int32_t>()->default_value("1"))
("c, need_screenshot", "Screenshot after spp total", cxxopts::value<bool>()->default_value("false"))
("v, validation", "Enable Validation", cxxopts::value<bool>()->default_value("false"));
// clang-format on
options.parse_positional({ "s" });
auto result = options.parse(argc, argv);
if (result.count("help"))
{
std::cout << options.help() << std::endl;
return 0;
}
// check params
const std::string sceneFile(result["s"].as<std::string>());
if (sceneFile.empty())
{
STRELKA_FATAL("Specify scene file name");
return 1;
}
if (!std::filesystem::exists(sceneFile))
{
STRELKA_FATAL("Specified scene file: {} doesn't exist", sceneFile.c_str());
return -1;
}
const std::filesystem::path sceneFilePath = { sceneFile.c_str() };
const std::string resourceSearchPath = sceneFilePath.parent_path().string();
STRELKA_DEBUG("Resource search path {}", resourceSearchPath);
auto* ctx = new oka::SharedContext();
// Set up rendering context.
uint32_t imageWidth = 1024;
uint32_t imageHeight = 768;
ctx->mSettingsManager = new oka::SettingsManager();
ctx->mSettingsManager->setAs<uint32_t>("render/width", imageWidth);
ctx->mSettingsManager->setAs<uint32_t>("render/height", imageHeight);
ctx->mSettingsManager->setAs<uint32_t>("render/pt/depth", 4);
ctx->mSettingsManager->setAs<uint32_t>("render/pt/sppTotal", result["t"].as<int32_t>());
ctx->mSettingsManager->setAs<uint32_t>("render/pt/spp", result["f"].as<int32_t>());
ctx->mSettingsManager->setAs<uint32_t>("render/pt/iteration", 0);
ctx->mSettingsManager->setAs<uint32_t>("render/pt/stratifiedSamplingType", 0); // 0 - none, 1 - random, 2 -
// stratified sampling, 3 - optimized
// stratified sampling
ctx->mSettingsManager->setAs<uint32_t>("render/pt/tonemapperType", 0); // 0 - reinhard, 1 - aces, 2 - filmic
ctx->mSettingsManager->setAs<uint32_t>("render/pt/debug", 0); // 0 - none, 1 - normals
ctx->mSettingsManager->setAs<float>("render/cameraSpeed", 1.0f);
ctx->mSettingsManager->setAs<float>("render/pt/upscaleFactor", 0.5f);
ctx->mSettingsManager->setAs<bool>("render/pt/enableUpscale", true);
ctx->mSettingsManager->setAs<bool>("render/pt/enableAcc", true);
ctx->mSettingsManager->setAs<bool>("render/pt/enableTonemap", true);
ctx->mSettingsManager->setAs<bool>("render/pt/isResized", false);
ctx->mSettingsManager->setAs<bool>("render/pt/needScreenshot", false);
ctx->mSettingsManager->setAs<bool>("render/pt/screenshotSPP", result["c"].as<bool>());
ctx->mSettingsManager->setAs<uint32_t>("render/pt/rectLightSamplingMethod", 0);
ctx->mSettingsManager->setAs<bool>("render/enableValidation", result["v"].as<bool>());
ctx->mSettingsManager->setAs<std::string>("resource/searchPath", resourceSearchPath);
// Postprocessing settings:
ctx->mSettingsManager->setAs<float>("render/post/tonemapper/filmIso", 100.0f);
ctx->mSettingsManager->setAs<float>("render/post/tonemapper/cm2_factor", 1.0f);
ctx->mSettingsManager->setAs<float>("render/post/tonemapper/fStop", 4.0f);
ctx->mSettingsManager->setAs<float>("render/post/tonemapper/shutterSpeed", 100.0f);
ctx->mSettingsManager->setAs<float>("render/post/gamma", 2.4f); // 0.0f - off
// Dev settings:
ctx->mSettingsManager->setAs<float>("render/pt/dev/shadowRayTmin", 0.0f); // offset to avoid self-collision in light
// sampling
ctx->mSettingsManager->setAs<float>("render/pt/dev/materialRayTmin", 0.0f); // offset to avoid self-collision in
oka::Display* display = oka::DisplayFactory::createDisplay();
display->init(imageWidth, imageHeight, ctx);
oka::RenderType type = oka::RenderType::eOptiX;
oka::Render* render = oka::RenderFactory::createRender(type);
oka::Scene scene;
oka::GltfLoader sceneLoader;
sceneLoader.loadGltf(sceneFilePath.string(), scene);
CameraController cameraController(scene.getCamera(0), true);
oka::Camera camera;
camera.name = "Main";
camera.fov = 45;
camera.position = glm::vec3(0, 0, -10);
camera.mOrientation = glm::quat(glm::vec3(0,0,0));
camera.updateViewMatrix();
scene.addCamera(camera);
render->setScene(&scene);
render->setSharedContext(ctx);
render->init();
ctx->mRender = render;
oka::BufferDesc desc{};
desc.format = oka::BufferFormat::FLOAT4;
desc.width = imageWidth;
desc.height = imageHeight;
oka::Buffer* outputBuffer = ctx->mRender->createBuffer(desc);
display->setInputHandler(&cameraController);
while (!display->windowShouldClose())
{
auto start = std::chrono::high_resolution_clock::now();
display->pollEvents();
static auto prevTime = std::chrono::high_resolution_clock::now();
auto currentTime = std::chrono::high_resolution_clock::now();
const double deltaTime = std::chrono::duration<double, std::milli>(currentTime - prevTime).count() / 1000.0;
const auto cameraSpeed = ctx->mSettingsManager->getAs<float>("render/cameraSpeed");
cameraController.update(deltaTime, cameraSpeed);
prevTime = currentTime;
scene.updateCamera(cameraController.getCamera(), 0);
display->onBeginFrame();
render->render(outputBuffer);
outputBuffer->map();
oka::ImageBuffer outputImage;
outputImage.data = outputBuffer->getHostPointer();
outputImage.dataSize = outputBuffer->getHostDataSize();
outputImage.height = outputBuffer->height();
outputImage.width = outputBuffer->width();
outputImage.pixel_format = oka::BufferFormat::FLOAT4;
display->drawFrame(outputImage); // blit rendered image to swapchain
display->drawUI(); // render ui to swapchain image in window resolution
display->onEndFrame(); // submit command buffer and present
outputBuffer->unmap();
const uint32_t currentSpp = ctx->mSubframeIndex;
auto finish = std::chrono::high_resolution_clock::now();
const double frameTime = std::chrono::duration<double, std::milli>(finish - start).count();
display->setWindowTitle((std::string("Strelka") + " [" + std::to_string(frameTime) + " ms]" + " [" +
std::to_string(currentSpp) + " spp]")
.c_str()); }
display->destroy();
return 0;
}
| 13,969 | C++ | 33.408867 | 120 | 0.573198 |
arhix52/Strelka/src/hdRunner/SimpleRenderTask.h | #pragma once
#include <pxr/pxr.h>
#include <pxr/imaging/hd/task.h>
#include <pxr/imaging/hd/renderPass.h>
#include <pxr/imaging/hd/renderPassState.h>
PXR_NAMESPACE_OPEN_SCOPE
class SimpleRenderTask final : public HdTask
{
public:
SimpleRenderTask(const HdRenderPassSharedPtr& renderPass,
const HdRenderPassStateSharedPtr& renderPassState,
const TfTokenVector& renderTags);
void Sync(HdSceneDelegate* sceneDelegate,
HdTaskContext* taskContext,
HdDirtyBits* dirtyBits) override;
void Prepare(HdTaskContext* taskContext,
HdRenderIndex* renderIndex) override;
void Execute(HdTaskContext* taskContext) override;
const TfTokenVector& GetRenderTags() const override;
private:
HdRenderPassSharedPtr m_renderPass;
HdRenderPassStateSharedPtr m_renderPassState;
TfTokenVector m_renderTags;
};
PXR_NAMESPACE_CLOSE_SCOPE
| 936 | C | 25.771428 | 71 | 0.719017 |
arhix52/Strelka/src/hdRunner/SimpleRenderTask.cpp | #include "SimpleRenderTask.h"
PXR_NAMESPACE_OPEN_SCOPE
SimpleRenderTask::SimpleRenderTask(const HdRenderPassSharedPtr& renderPass,
const HdRenderPassStateSharedPtr& renderPassState,
const TfTokenVector& renderTags)
: HdTask(SdfPath::EmptyPath()), m_renderPass(renderPass), m_renderPassState(renderPassState), m_renderTags(renderTags)
{
}
void SimpleRenderTask::Sync(HdSceneDelegate* sceneDelegate,
HdTaskContext* taskContext,
HdDirtyBits* dirtyBits)
{
TF_UNUSED(sceneDelegate);
TF_UNUSED(taskContext);
m_renderPass->Sync();
*dirtyBits = HdChangeTracker::Clean;
}
void SimpleRenderTask::Prepare(HdTaskContext* taskContext,
HdRenderIndex* renderIndex)
{
TF_UNUSED(taskContext);
const HdResourceRegistrySharedPtr& resourceRegistry = renderIndex->GetResourceRegistry();
m_renderPassState->Prepare(resourceRegistry);
//m_renderPass->Prepare(m_renderTags);
}
void SimpleRenderTask::Execute(HdTaskContext* taskContext)
{
TF_UNUSED(taskContext);
m_renderPass->Execute(m_renderPassState, m_renderTags);
}
const TfTokenVector& SimpleRenderTask::GetRenderTags() const
{
return m_renderTags;
}
PXR_NAMESPACE_CLOSE_SCOPE
| 1,325 | C++ | 26.624999 | 122 | 0.685283 |
arhix52/Strelka/src/hdRunner/main.cpp | #include "SimpleRenderTask.h"
#include <pxr/base/gf/gamma.h>
#include <pxr/base/gf/rotation.h>
#include <pxr/base/tf/stopwatch.h>
#include <pxr/imaging/hd/camera.h>
#include <pxr/imaging/hd/engine.h>
#include <pxr/imaging/hd/pluginRenderDelegateUniqueHandle.h>
#include <pxr/imaging/hd/renderBuffer.h>
#include <pxr/imaging/hd/renderDelegate.h>
#include <pxr/imaging/hd/renderIndex.h>
#include <pxr/imaging/hd/renderPass.h>
#include <pxr/imaging/hd/renderPassState.h>
#include <pxr/imaging/hd/rendererPlugin.h>
#include <pxr/imaging/hd/rendererPluginRegistry.h>
#include <pxr/imaging/hf/pluginDesc.h>
#include <pxr/imaging/hgi/hgi.h>
#include <pxr/imaging/hgi/tokens.h>
#include <pxr/imaging/hio/image.h>
#include <pxr/imaging/hio/imageRegistry.h>
#include <pxr/imaging/hio/types.h>
#include <pxr/pxr.h>
#include <pxr/usd/ar/resolver.h>
#include <pxr/usd/usd/stage.h>
#include <pxr/usd/usdGeom/camera.h>
#include <pxr/usd/usdGeom/metrics.h>
#include <pxr/usdImaging/usdImaging/delegate.h>
#include <render/common.h>
#include <render/buffer.h>
#include <display/Display.h>
#include <log.h>
#include <logmanager.h>
#include <algorithm>
#include <cxxopts.hpp>
#include <iostream>
#include <filesystem>
// #include <cuda_runtime.h>
PXR_NAMESPACE_USING_DIRECTIVE
TF_DEFINE_PRIVATE_TOKENS(_AppTokens, (HdStrelkaDriver)(HdStrelkaRendererPlugin));
HdRendererPluginHandle GetHdStrelkaPlugin()
{
HdRendererPluginRegistry& registry = HdRendererPluginRegistry::GetInstance();
const TfToken& pluginId = _AppTokens->HdStrelkaRendererPlugin;
HdRendererPluginHandle plugin = registry.GetOrCreateRendererPlugin(pluginId);
return plugin;
}
HdCamera* FindCamera(UsdStageRefPtr& stage, HdRenderIndex* renderIndex, SdfPath& cameraPath)
{
UsdPrimRange primRange = stage->TraverseAll();
for (auto prim = primRange.cbegin(); prim != primRange.cend(); prim++)
{
if (!prim->IsA<UsdGeomCamera>())
{
continue;
}
cameraPath = prim->GetPath();
break;
}
auto* camera = (HdCamera*)dynamic_cast<HdCamera*>(renderIndex->GetSprim(HdTokens->camera, cameraPath));
return camera;
}
std::vector<std::pair<HdCamera*, SdfPath>> FindAllCameras(UsdStageRefPtr& stage, HdRenderIndex* renderIndex)
{
UsdPrimRange primRange = stage->TraverseAll();
HdCamera* camera{};
SdfPath cameraPath{};
std::vector<std::pair<HdCamera*, SdfPath>> cameras{};
for (auto prim = primRange.cbegin(); prim != primRange.cend(); prim++)
{
if (!prim->IsA<UsdGeomCamera>())
{
continue;
}
cameraPath = prim->GetPath();
camera = (HdCamera*)dynamic_cast<HdCamera*>(renderIndex->GetSprim(HdTokens->camera, cameraPath));
cameras.emplace_back(camera, cameraPath);
}
return cameras;
}
class CameraController : public oka::InputHandler
{
GfCamera mGfCam;
GfQuatd mOrientation;
GfVec3d mPosition;
GfVec3d mWorldUp;
GfVec3d mWorldForward;
float rotationSpeed = 0.025f;
float movementSpeed = 1.0f;
double pitch = 0.0;
double yaw = 0.0;
double max_pitch_rate = 5;
double max_yaw_rate = 5;
public:
virtual ~CameraController() = default;
struct
{
bool left = false;
bool right = false;
bool up = false;
bool down = false;
bool forward = false;
bool back = false;
} keys;
struct MouseButtons
{
bool left = false;
bool right = false;
bool middle = false;
} mouseButtons;
GfVec2d mMousePos;
// local cameras axis
GfVec3d getFront() const
{
return mOrientation.Transform(GfVec3d(0.0, 0.0, -1.0));
}
GfVec3d getUp() const
{
return mOrientation.Transform(GfVec3d(0.0, 1.0, 0.0));
}
GfVec3d getRight() const
{
return mOrientation.Transform(GfVec3d(1.0, 0.0, 0.0));
}
// global camera axis depending on scene settings
GfVec3d getWorldUp() const
{
return mWorldUp;
}
GfVec3d getWorldForward() const
{
return mWorldForward;
}
bool moving()
{
return keys.left || keys.right || keys.up || keys.down || keys.forward || keys.back || mouseButtons.right ||
mouseButtons.left || mouseButtons.middle;
}
void update(double deltaTime, float speed)
{
movementSpeed = speed;
if (moving())
{
const float moveSpeed = deltaTime * movementSpeed;
if (keys.up)
mPosition += getWorldUp() * moveSpeed;
if (keys.down)
mPosition -= getWorldUp() * moveSpeed;
if (keys.left)
mPosition -= getRight() * moveSpeed;
if (keys.right)
mPosition += getRight() * moveSpeed;
if (keys.forward)
mPosition += getFront() * moveSpeed;
if (keys.back)
mPosition -= getFront() * moveSpeed;
updateViewMatrix();
}
}
void rotate(double rightAngle, double upAngle)
{
GfRotation a(getRight(), upAngle * rotationSpeed);
GfRotation b(getWorldUp(), rightAngle * rotationSpeed);
GfRotation c = a * b;
GfQuatd cq = c.GetQuat();
cq.Normalize();
mOrientation = cq * mOrientation;
mOrientation.Normalize();
updateViewMatrix();
}
void translate(GfVec3d delta)
{
mPosition += mOrientation.Transform(delta);
updateViewMatrix();
}
void updateViewMatrix()
{
GfMatrix4d view(1.0);
view.SetRotateOnly(mOrientation);
view.SetTranslateOnly(mPosition);
mGfCam.SetTransform(view);
}
GfCamera& getCamera()
{
return mGfCam;
}
CameraController(UsdGeomCamera& cam, bool isYup)
{
if (isYup)
{
mWorldUp = GfVec3d(0.0, 1.0, 0.0);
mWorldForward = GfVec3d(0.0, 0.0, -1.0);
}
else
{
mWorldUp = GfVec3d(0.0, 0.0, 1.0);
mWorldForward = GfVec3d(0.0, 1.0, 0.0);
}
mGfCam = cam.GetCamera(0.0);
GfMatrix4d xform = mGfCam.GetTransform();
xform.Orthonormalize();
mOrientation = xform.ExtractRotationQuat();
mOrientation.Normalize();
mPosition = xform.ExtractTranslation();
}
void keyCallback(int key, [[maybe_unused]] int scancode, int action, [[maybe_unused]] int mods) override
{
const bool keyState = ((GLFW_REPEAT == action) || (GLFW_PRESS == action)) ? true : false;
switch (key)
{
case GLFW_KEY_W: {
keys.forward = keyState;
break;
}
case GLFW_KEY_S: {
keys.back = keyState;
break;
}
case GLFW_KEY_A: {
keys.left = keyState;
break;
}
case GLFW_KEY_D: {
keys.right = keyState;
break;
}
case GLFW_KEY_Q: {
keys.up = keyState;
break;
}
case GLFW_KEY_E: {
keys.down = keyState;
break;
}
default:
break;
}
}
void mouseButtonCallback(int button, int action, [[maybe_unused]] int mods) override
{
if (button == GLFW_MOUSE_BUTTON_RIGHT)
{
if (action == GLFW_PRESS)
{
mouseButtons.right = true;
}
else if (action == GLFW_RELEASE)
{
mouseButtons.right = false;
}
}
else if (button == GLFW_MOUSE_BUTTON_LEFT)
{
if (action == GLFW_PRESS)
{
mouseButtons.left = true;
}
else if (action == GLFW_RELEASE)
{
mouseButtons.left = false;
}
}
}
void handleMouseMoveCallback([[maybe_unused]] double xpos, [[maybe_unused]] double ypos) override
{
const float dx = mMousePos[0] - xpos;
const float dy = mMousePos[1] - ypos;
// ImGuiIO& io = ImGui::GetIO();
// bool handled = io.WantCaptureMouse;
// if (handled)
//{
// camera.mousePos = glm::vec2((float)xpos, (float)ypos);
// return;
//}
if (mouseButtons.right)
{
rotate(dx, dy);
}
if (mouseButtons.left)
{
translate(GfVec3d(-0.0, 0.0, -dy * .005 * movementSpeed));
}
if (mouseButtons.middle)
{
translate(GfVec3d(-dx * 0.01, -dy * 0.01, 0.0f));
}
mMousePos[0] = xpos;
mMousePos[1] = ypos;
}
};
class RenderSurfaceController : public oka::ResizeHandler
{
uint32_t imageWidth = 800;
uint32_t imageHeight = 600;
oka::SettingsManager* mSettingsManager = nullptr;
std::array<HdRenderBuffer*, 3> mRenderBuffers;
bool mDirty[3] = { true };
bool mInUse[3] = { false };
public:
RenderSurfaceController(oka::SettingsManager* settingsManager, std::array<HdRenderBuffer*, 3>& renderBuffers)
: mSettingsManager(settingsManager), mRenderBuffers(renderBuffers)
{
imageWidth = mSettingsManager->getAs<uint32_t>("render/width");
imageHeight = mSettingsManager->getAs<uint32_t>("render/height");
}
void framebufferResize(int newWidth, int newHeight)
{
assert(mSettingsManager);
mSettingsManager->setAs<uint32_t>("render/width", newWidth);
mSettingsManager->setAs<uint32_t>("render/height", newHeight);
imageWidth = newWidth;
imageHeight = newHeight;
for (bool& i : mDirty)
{
i = true;
}
// reallocateBuffers();
}
bool isDirty(uint32_t index)
{
return mDirty[index];
}
void acquire(uint32_t index)
{
mInUse[index] = true;
}
void release(uint32_t index)
{
mInUse[index] = false;
}
HdRenderBuffer* getRenderBuffer(uint32_t index)
{
assert(index < 3);
if (mDirty[index] && !mInUse[index])
{
mRenderBuffers[index]->Allocate(GfVec3i(imageWidth, imageHeight, 1), HdFormatFloat32Vec4, false);
mDirty[index] = false;
}
return mRenderBuffers[index];
}
};
void setDefaultCamera(UsdGeomCamera& cam)
{
// y - up, camera looks at (0, 0, 0)
std::vector<float> r0 = { 0, -1, 0, 0 };
std::vector<float> r1 = { 0, 0, 1, 0 };
std::vector<float> r2 = { -1, 0, 0, 0 };
std::vector<float> r3 = { 0, 0, 0, 1 };
GfMatrix4d xform(r0, r1, r2, r3);
GfCamera mGfCam{};
mGfCam.SetTransform(xform);
GfRange1f clippingRange = GfRange1f{ 0.1, 1000 };
mGfCam.SetClippingRange(clippingRange);
mGfCam.SetVerticalAperture(20.25);
mGfCam.SetVerticalApertureOffset(0);
mGfCam.SetHorizontalAperture(36);
mGfCam.SetHorizontalApertureOffset(0);
mGfCam.SetFocalLength(50);
GfCamera::Projection projection = GfCamera::Projection::Perspective;
mGfCam.SetProjection(projection);
cam.SetFromCamera(mGfCam, 0.0);
}
bool saveScreenshot(std::string& outputFilePath, unsigned char* mappedMem, uint32_t imageWidth, uint32_t imageHeight)
{
TF_VERIFY(mappedMem != nullptr);
int pixelCount = imageWidth * imageHeight;
// Write image to file.
TfStopwatch timerWrite;
timerWrite.Start();
HioImageSharedPtr image = HioImage::OpenForWriting(outputFilePath);
if (!image)
{
STRELKA_ERROR("Unable to open output file for writing!");
return false;
}
HioImage::StorageSpec storage;
storage.width = (int)imageWidth;
storage.height = (int)imageHeight;
storage.depth = (int)1;
storage.format = HioFormat::HioFormatFloat32Vec4;
storage.flipped = true;
storage.data = mappedMem;
VtDictionary metadata;
image->Write(storage, metadata);
timerWrite.Stop();
STRELKA_INFO("Wrote image {}", timerWrite.GetSeconds());
return true;
}
int main(int argc, const char* argv[])
{
const oka::Logmanager loggerManager;
// config. options
cxxopts::Options options("Strelka -s <USD Scene path>", "commands");
// clang-format off
options.add_options()
("s, scene", "scene path", cxxopts::value<std::string>()->default_value(""))
("i, iteration", "Iteration to capture", cxxopts::value<int32_t>()->default_value("-1"))
("h, help", "Print usage")("t, spp_total", "spp total", cxxopts::value<int32_t>()->default_value("64"))
("f, spp_subframe", "spp subframe", cxxopts::value<int32_t>()->default_value("1"))
("c, need_screenshot", "Screenshot after spp total", cxxopts::value<bool>()->default_value("false"))
("v, validation", "Enable Validation", cxxopts::value<bool>()->default_value("false"));
// clang-format on
options.parse_positional({ "s" });
auto result = options.parse(argc, argv);
if (result.count("help"))
{
std::cout << options.help() << std::endl;
return 0;
}
// check params
const std::string usdFile(result["s"].as<std::string>());
if (usdFile.empty())
{
STRELKA_FATAL("Specify usd file name");
return 1;
}
if (!std::filesystem::exists(usdFile))
{
STRELKA_FATAL("Specified usd file: {} doesn't exist", usdFile.c_str());
return -1;
}
const std::filesystem::path usdFilePath = { usdFile.c_str() };
const std::string resourceSearchPath = usdFilePath.parent_path().string();
STRELKA_DEBUG("Resource path {}", resourceSearchPath);
const int32_t iterationToCapture(result["i"].as<int32_t>());
// Init plugin.
const HdRendererPluginHandle pluginHandle = GetHdStrelkaPlugin();
if (!pluginHandle)
{
STRELKA_FATAL("HdStrelka plugin not found!");
return EXIT_FAILURE;
}
if (!pluginHandle->IsSupported())
{
STRELKA_FATAL("HdStrelka plugin is not supported!");
return EXIT_FAILURE;
}
HdDriverVector drivers;
// Set up rendering context.
uint32_t imageWidth = 1024;
uint32_t imageHeight = 768;
auto* ctx = new oka::SharedContext(); // &display.getSharedContext();
ctx->mSettingsManager = new oka::SettingsManager();
ctx->mSettingsManager->setAs<uint32_t>("render/width", imageWidth);
ctx->mSettingsManager->setAs<uint32_t>("render/height", imageHeight);
ctx->mSettingsManager->setAs<uint32_t>("render/pt/depth", 4);
ctx->mSettingsManager->setAs<uint32_t>("render/pt/sppTotal", result["t"].as<int32_t>());
ctx->mSettingsManager->setAs<uint32_t>("render/pt/spp", result["f"].as<int32_t>());
ctx->mSettingsManager->setAs<uint32_t>("render/pt/iteration", 0);
ctx->mSettingsManager->setAs<uint32_t>("render/pt/stratifiedSamplingType", 0); // 0 - none, 1 - random, 2 -
// stratified sampling, 3 - optimized
// stratified sampling
ctx->mSettingsManager->setAs<uint32_t>("render/pt/tonemapperType", 0); // 0 - reinhard, 1 - aces, 2 - filmic
ctx->mSettingsManager->setAs<uint32_t>("render/pt/debug", 0); // 0 - none, 1 - normals
ctx->mSettingsManager->setAs<float>("render/cameraSpeed", 1.0f);
ctx->mSettingsManager->setAs<float>("render/pt/upscaleFactor", 0.5f);
ctx->mSettingsManager->setAs<bool>("render/pt/enableUpscale", true);
ctx->mSettingsManager->setAs<bool>("render/pt/enableAcc", true);
ctx->mSettingsManager->setAs<bool>("render/pt/enableTonemap", true);
ctx->mSettingsManager->setAs<bool>("render/pt/isResized", false);
ctx->mSettingsManager->setAs<bool>("render/pt/needScreenshot", false);
ctx->mSettingsManager->setAs<bool>("render/pt/screenshotSPP", result["c"].as<bool>());
ctx->mSettingsManager->setAs<uint32_t>("render/pt/rectLightSamplingMethod", 0);
ctx->mSettingsManager->setAs<bool>("render/enableValidation", result["v"].as<bool>());
ctx->mSettingsManager->setAs<std::string>("resource/searchPath", resourceSearchPath);
// Postprocessing settings:
ctx->mSettingsManager->setAs<float>("render/post/tonemapper/filmIso", 100.0f);
ctx->mSettingsManager->setAs<float>("render/post/tonemapper/cm2_factor", 1.0f);
ctx->mSettingsManager->setAs<float>("render/post/tonemapper/fStop", 4.0f);
ctx->mSettingsManager->setAs<float>("render/post/tonemapper/shutterSpeed", 100.0f);
ctx->mSettingsManager->setAs<float>("render/post/gamma", 2.4f); // 0.0f - off
// Dev settings:
ctx->mSettingsManager->setAs<float>("render/pt/dev/shadowRayTmin", 0.0f); // offset to avoid self-collision in light
// sampling
ctx->mSettingsManager->setAs<float>("render/pt/dev/materialRayTmin", 0.0f); // offset to avoid self-collision in
// bsdf sampling
HdDriver driver;
driver.name = _AppTokens->HdStrelkaDriver;
driver.driver = VtValue(ctx);
drivers.push_back(&driver);
HdRenderDelegate* renderDelegate = pluginHandle->CreateRenderDelegate();
TF_VERIFY(renderDelegate);
renderDelegate->SetDrivers(drivers);
oka::Display* display = oka::DisplayFactory::createDisplay();
display->init(imageWidth, imageHeight, ctx);
// Handle cmdline args.
// Load scene.
TfStopwatch timerLoad;
timerLoad.Start();
// ArGetResolver().ConfigureResolverForAsset(settings.sceneFilePath);
const std::string& usdPath = usdFile;
UsdStageRefPtr stage = UsdStage::Open(usdPath);
timerLoad.Stop();
if (!stage)
{
STRELKA_FATAL("Unable to open USD stage file.");
return EXIT_FAILURE;
}
STRELKA_INFO("USD scene loaded {}", timerLoad.GetSeconds());
// Print the up-axis
const TfToken upAxis = UsdGeomGetStageUpAxis(stage);
STRELKA_INFO("Stage up-axis: {}", (std::string)upAxis);
// Print the stage's linear units, or "meters per unit"
STRELKA_INFO("Meters per unit: {}", UsdGeomGetStageMetersPerUnit(stage));
HdRenderIndex* renderIndex = HdRenderIndex::New(renderDelegate, HdDriverVector());
TF_VERIFY(renderIndex);
UsdImagingDelegate sceneDelegate(renderIndex, SdfPath::AbsoluteRootPath());
sceneDelegate.Populate(stage->GetPseudoRoot());
sceneDelegate.SetTime(0);
sceneDelegate.SetRefineLevelFallback(4);
const double meterPerUnit = UsdGeomGetStageMetersPerUnit(stage);
// Init camera from scene
SdfPath cameraPath = SdfPath::EmptyPath();
HdCamera* camera = FindCamera(stage, renderIndex, cameraPath);
UsdGeomCamera cam;
if (camera)
{
cam = UsdGeomCamera::Get(stage, cameraPath);
}
else
{
// Init default camera
cameraPath = SdfPath("/defaultCamera");
cam = UsdGeomCamera::Define(stage, cameraPath);
setDefaultCamera(cam);
}
CameraController cameraController(cam, upAxis == UsdGeomTokens->y);
// std::vector<std::pair<HdCamera*, SdfPath>> cameras = FindAllCameras(stage, renderIndex);
std::array<HdRenderBuffer*, 3> renderBuffers{};
for (int i = 0; i < 3; ++i)
{
renderBuffers[i] = (HdRenderBuffer*)renderDelegate->CreateFallbackBprim(HdPrimTypeTokens->renderBuffer);
renderBuffers[i]->Allocate(GfVec3i(imageWidth, imageHeight, 1), HdFormatFloat32Vec4, false);
}
CameraUtilFraming framing;
framing.dataWindow = GfRect2i(GfVec2i(0, 0), GfVec2i(imageWidth, imageHeight));
framing.displayWindow = GfRange2f(GfVec2f(0.0f, 0.0f), GfVec2f((float)imageWidth, (float)imageHeight));
framing.pixelAspectRatio = 1.0f;
const std::optional<CameraUtilConformWindowPolicy> overrideWindowPolicy(CameraUtilFit);
// TODO: add UI control here
TfTokenVector renderTags{ HdRenderTagTokens->geometry, HdRenderTagTokens->render };
HdRprimCollection renderCollection(HdTokens->geometry, HdReprSelector(HdReprTokens->refined));
HdRenderPassSharedPtr renderPass = renderDelegate->CreateRenderPass(renderIndex, renderCollection);
std::shared_ptr<HdRenderPassState> renderPassState[3];
std::shared_ptr<SimpleRenderTask> renderTasks[3];
for (int i = 0; i < 3; ++i)
{
renderPassState[i] = std::make_shared<HdRenderPassState>();
renderPassState[i]->SetCamera(camera);
renderPassState[i]->SetFraming(framing);
renderPassState[i]->SetOverrideWindowPolicy(overrideWindowPolicy);
HdRenderPassAovBindingVector aovBindings(1);
aovBindings[0].aovName = HdAovTokens->color;
aovBindings[0].renderBuffer = renderBuffers[i];
renderPassState[i]->SetAovBindings(aovBindings);
renderTasks[i] = std::make_shared<SimpleRenderTask>(renderPass, renderPassState[i], renderTags);
}
// Perform rendering.
TfStopwatch timerRender;
timerRender.Start();
HdEngine engine;
display->setInputHandler(&cameraController);
RenderSurfaceController surfaceController(ctx->mSettingsManager, renderBuffers);
display->setResizeHandler(&surfaceController);
uint64_t frameCount = 0;
while (!display->windowShouldClose())
{
auto start = std::chrono::high_resolution_clock::now();
HdTaskSharedPtrVector tasks;
const uint32_t versionId = frameCount % oka::MAX_FRAMES_IN_FLIGHT;
// relocation?
surfaceController.acquire(versionId);
if (surfaceController.isDirty(versionId))
{
HdRenderPassAovBindingVector aovBindings(1);
aovBindings[0].aovName = HdAovTokens->color;
surfaceController.release(versionId);
aovBindings[0].renderBuffer = surfaceController.getRenderBuffer(versionId);
surfaceController.acquire(versionId);
renderPassState[versionId]->SetAovBindings(aovBindings);
renderTasks[versionId] =
std::make_shared<SimpleRenderTask>(renderPass, renderPassState[versionId], renderTags);
}
tasks.push_back(renderTasks[versionId]);
sceneDelegate.SetTime(1.0f);
display->pollEvents();
static auto prevTime = std::chrono::high_resolution_clock::now();
auto currentTime = std::chrono::high_resolution_clock::now();
const double deltaTime = std::chrono::duration<double, std::milli>(currentTime - prevTime).count() / 1000.0;
const auto cameraSpeed = ctx->mSettingsManager->getAs<float>("render/cameraSpeed");
cameraController.update(deltaTime, cameraSpeed);
prevTime = currentTime;
cam.SetFromCamera(cameraController.getCamera(), 0.0);
display->onBeginFrame();
engine.Execute(renderIndex, &tasks); // main path tracing rendering in fixed render resolution
auto* outputBuffer =
surfaceController.getRenderBuffer(versionId)->GetResource(false).UncheckedGet<oka::Buffer*>();
oka::ImageBuffer outputImage;
// upload to host
outputBuffer->map();
outputImage.data = outputBuffer->getHostPointer();
outputImage.dataSize = outputBuffer->getHostDataSize();
outputImage.height = outputBuffer->height();
outputImage.width = outputBuffer->width();
outputImage.pixel_format = outputBuffer->getFormat();
const auto totalSpp = ctx->mSettingsManager->getAs<uint32_t>("render/pt/sppTotal");
const uint32_t currentSpp = ctx->mSubframeIndex;
bool needScreenshot = ctx->mSettingsManager->getAs<bool>("render/pt/needScreenshot");
if (ctx->mSettingsManager->getAs<bool>("render/pt/screenshotSPP") && (currentSpp == totalSpp))
{
needScreenshot = true;
// need to store screen only once
ctx->mSettingsManager->setAs<bool>("render/pt/screenshotSPP", false);
}
if (needScreenshot)
{
const std::size_t foundSlash = usdPath.find_last_of("/\\");
const std::size_t foundDot = usdPath.find_last_of('.');
std::string fileName = usdPath.substr(0, foundDot);
fileName = fileName.substr(foundSlash + 1);
auto generateName = [&](const uint32_t attempt) {
std::string outputFilePath = fileName + "_" + std::to_string(currentSpp) + "i_" +
std::to_string(ctx->mSettingsManager->getAs<uint32_t>("render/pt/depth")) +
"d_" + std::to_string(totalSpp) + "spp_" + std::to_string(attempt) + ".png";
return outputFilePath;
};
uint32_t attempt = 0;
std::string outputFilePath;
do
{
outputFilePath = generateName(attempt++);
} while (std::filesystem::exists(std::filesystem::path(outputFilePath.c_str())));
unsigned char* mappedMem = (unsigned char*)outputImage.data;
if (saveScreenshot(outputFilePath, mappedMem, outputImage.width, outputImage.height))
{
ctx->mSettingsManager->setAs<bool>("render/pt/needScreenshot", false);
}
}
display->drawFrame(outputImage); // blit rendered image to swapchain
display->drawUI(); // render ui to swapchain image in window resolution
display->onEndFrame(); // submit command buffer and present
auto finish = std::chrono::high_resolution_clock::now();
const double frameTime = std::chrono::duration<double, std::milli>(finish - start).count();
surfaceController.release(versionId);
display->setWindowTitle((std::string("Strelka") + " [" + std::to_string(frameTime) + " ms]" + " [" +
std::to_string(currentSpp) + " spp]")
.c_str());
++frameCount;
}
// renderBuffer->Resolve();
// TF_VERIFY(renderBuffer->IsConverged());
timerRender.Stop();
display->destroy();
STRELKA_INFO("Rendering finished {}", timerRender.GetSeconds());
for (int i = 0; i < 3; ++i)
{
renderDelegate->DestroyBprim(renderBuffers[i]);
}
return EXIT_SUCCESS;
}
| 26,275 | C++ | 32.687179 | 121 | 0.61785 |
arhix52/Strelka/src/scene/camera.cpp | #include "camera.h"
#include <glm/gtx/quaternion.hpp>
#include <glm-wrapper.hpp>
namespace oka
{
void Camera::updateViewMatrix()
{
const glm::float4x4 rotM{ mOrientation };
const glm::float4x4 transM = glm::translate(glm::float4x4(1.0f), -position);
if (type == CameraType::firstperson)
{
matrices.view = rotM * transM;
}
else
{
matrices.view = transM * rotM;
}
updated = true;
}
glm::float3 Camera::getFront() const
{
return glm::conjugate(mOrientation) * glm::float3(0.0f, 0.0f, -1.0f);
}
glm::float3 Camera::getUp() const
{
return glm::conjugate(mOrientation) * glm::float3(0.0f, 1.0f, 0.0f);
}
glm::float3 Camera::getRight() const
{
return glm::conjugate(mOrientation) * glm::float3(1.0f, 0.0f, 0.0f);
}
bool Camera::moving() const
{
return keys.left || keys.right || keys.up || keys.down || keys.forward || keys.back || mouseButtons.right || mouseButtons.left || mouseButtons.middle;
}
float Camera::getNearClip() const
{
return znear;
}
float Camera::getFarClip() const
{
return zfar;
}
void Camera::setFov(float fov)
{
this->fov = fov;
}
// original implementation: https://vincent-p.github.io/notes/20201216234910-the_projection_matrix_in_vulkan/
glm::float4x4 perspective(float fov, float aspect_ratio, float n, float f, glm::float4x4* inverse)
{
const float focal_length = 1.0f / std::tan(glm::radians(fov) / 2.0f);
const float x = focal_length / aspect_ratio;
const float y = focal_length;
const float A = n / (f - n);
const float B = f * A;
//glm::float4x4 projection = glm::perspective(fov, aspect_ratio, n, f);
//if (inverse)
//{
// *inverse = glm::inverse(projection);
//}
glm::float4x4 projection({
x,
0.0f,
0.0f,
0.0f,
0.0f,
y,
0.0f,
0.0f,
0.0f,
0.0f,
A,
B,
0.0f,
0.0f,
-1.0f,
0.0f,
});
if (inverse)
{
*inverse = glm::transpose(glm::float4x4({ // glm inverse
1 / x,
0.0f,
0.0f,
0.0f,
0.0f,
1 / y,
0.0f,
0.0f,
0.0f,
0.0f,
0.0f,
-1.0f,
0.0f,
0.0f,
1 / B,
A / B,
}));
}
return glm::transpose(projection);
}
void Camera::setPerspective(float _fov, float _aspect, float _znear, float _zfar)
{
fov = _fov;
znear = _znear;
zfar = _zfar;
// swap near and far plane for reverse z
matrices.perspective = perspective(fov, _aspect, zfar, znear, &matrices.invPerspective);
}
void Camera::setWorldUp(const glm::float3 up)
{
mWorldUp = up;
}
glm::float3 Camera::getWorldUp()
{
return mWorldUp;
}
void Camera::setWorldForward(const glm::float3 forward)
{
mWorldForward = forward;
}
glm::float3 Camera::getWorldForward()
{
return mWorldForward;
}
glm::float4x4& Camera::getPerspective()
{
return matrices.perspective;
}
glm::float4x4 Camera::getView()
{
return matrices.view;
}
void Camera::updateAspectRatio(float _aspect)
{
setPerspective(fov, _aspect, znear, zfar);
}
void Camera::setPosition(glm::float3 _position)
{
position = _position;
updateViewMatrix();
}
glm::float3 Camera::getPosition()
{
return position;
}
void Camera::setRotation(glm::quat rotation)
{
mOrientation = rotation;
updateViewMatrix();
}
void Camera::rotate(float rightAngle, float upAngle)
{
const glm::quat a = glm::angleAxis(glm::radians(upAngle) * rotationSpeed, glm::float3(1.0f, 0.0f, 0.0f));
const glm::quat b = glm::angleAxis(glm::radians(rightAngle) * rotationSpeed, glm::float3(0.0f, 1.0f, 0.0f));
// const glm::quat a = glm::angleAxis(glm::radians(upAngle) * rotationSpeed, getRight());
// const glm::quat b = glm::angleAxis(glm::radians(rightAngle) * rotationSpeed, getWorldUp());
// auto c = a * b;
// c = glm::normalize(c);
mOrientation = glm::normalize(a * mOrientation * b);
// mOrientation = glm::normalize(c * mOrientation);
updateViewMatrix();
}
glm::quat Camera::getOrientation()
{
return mOrientation;
}
void Camera::setTranslation(glm::float3 translation)
{
position = translation;
updateViewMatrix();
}
void Camera::translate(glm::float3 delta)
{
position += glm::conjugate(mOrientation) * delta;
updateViewMatrix();
}
void Camera::update(float deltaTime)
{
updated = false;
if (type == CameraType::firstperson)
{
if (moving())
{
float moveSpeed = deltaTime * movementSpeed;
if (keys.up)
position += getWorldUp() * moveSpeed;
if (keys.down)
position -= getWorldUp() * moveSpeed;
if (keys.left)
position -= getRight() * moveSpeed;
if (keys.right)
position += getRight() * moveSpeed;
if (keys.forward)
position += getFront() * moveSpeed;
if (keys.back)
position -= getFront() * moveSpeed;
updateViewMatrix();
}
}
}
} // namespace oka
| 5,209 | C++ | 20.618257 | 154 | 0.582261 |
arhix52/Strelka/src/scene/scene.cpp | #include "scene.h"
#include <glm/gtc/quaternion.hpp>
#include <glm/gtc/type_ptr.hpp>
#include <glm/gtx/norm.hpp>
#include <algorithm>
#include <array>
#include <filesystem>
#include <map>
#include <utility>
namespace fs = std::filesystem;
namespace oka
{
uint32_t Scene::createMesh(const std::vector<Vertex>& vb, const std::vector<uint32_t>& ib)
{
std::scoped_lock lock(mMeshMutex);
Mesh* mesh = nullptr;
uint32_t meshId = -1;
if (mDelMesh.empty())
{
meshId = mMeshes.size(); // add mesh to storage
mMeshes.push_back({});
mesh = &mMeshes.back();
}
else
{
meshId = mDelMesh.top(); // get index from stack
mDelMesh.pop(); // del taken index from stack
mesh = &mMeshes[meshId];
}
mesh->mIndex = mIndices.size(); // Index of 1st index in index buffer
mesh->mCount = ib.size(); // amount of indices in mesh
mesh->mVbOffset = mVertices.size();
mesh->mVertexCount = vb.size();
// const uint32_t ibOffset = mVertices.size(); // adjust indices for global index buffer
// for (int i = 0; i < ib.size(); ++i)
// {
// mIndices.push_back(ibOffset + ib[i]);
// }
mIndices.insert(mIndices.end(), ib.begin(), ib.end());
mVertices.insert(mVertices.end(), vb.begin(), vb.end()); // copy vertices
return meshId;
}
uint32_t Scene::createInstance(const Instance::Type type,
const uint32_t geomId,
const uint32_t materialId,
const glm::mat4& transform,
const uint32_t lightId)
{
std::scoped_lock lock(mInstanceMutex);
Instance* inst = nullptr;
uint32_t instId = -1;
if (mDelInstances.empty())
{
instId = mInstances.size(); // add instance to storage
mInstances.push_back({});
inst = &mInstances.back();
}
else
{
instId = mDelInstances.top(); // get index from stack
mDelInstances.pop(); // del taken index from stack
inst = &mInstances[instId];
}
inst->type = type;
if (inst->type == Instance::Type::eMesh || inst->type == Instance::Type::eLight)
{
inst->mMeshId = geomId;
}
else if (inst->type == Instance::Type::eCurve)
{
inst->mCurveId = geomId;
}
inst->mMaterialId = materialId;
inst->transform = transform;
inst->mLightId = lightId;
mOpaqueInstances.push_back(instId);
return instId;
}
uint32_t Scene::addMaterial(const MaterialDescription& material)
{
// TODO: fix here
uint32_t res = mMaterialsDescs.size();
mMaterialsDescs.push_back(material);
return res;
}
std::string Scene::getSceneFileName()
{
fs::path p(modelPath);
return p.filename().string();
};
std::string Scene::getSceneDir()
{
fs::path p(modelPath);
return p.parent_path().string();
};
// valid range of coordinates [-1; 1]
uint32_t packNormals(const glm::float3& normal)
{
uint32_t packed = (uint32_t)((normal.x + 1.0f) / 2.0f * 511.99999f);
packed += (uint32_t)((normal.y + 1.0f) / 2.0f * 511.99999f) << 10;
packed += (uint32_t)((normal.z + 1.0f) / 2.0f * 511.99999f) << 20;
return packed;
}
uint32_t Scene::createRectLightMesh()
{
if (mRectLightMeshId != -1)
{
return mRectLightMeshId;
}
std::vector<Scene::Vertex> vb;
Scene::Vertex v1, v2, v3, v4;
v1.pos = glm::float4(0.5f, 0.5f, 0.0f, 1.0f); // top right 0
v2.pos = glm::float4(-0.5f, 0.5f, 0.0f, 1.0f); // top left 1
v3.pos = glm::float4(-0.5f, -0.5f, 0.0f, 1.0f); // bottom left 2
v4.pos = glm::float4(0.5f, -0.5f, 0.0f, 1.0f); // bottom right 3
glm::float3 normal = glm::float3(0.f, 0.f, 1.f);
v1.normal = v2.normal = v3.normal = v4.normal = packNormals(normal);
std::vector<uint32_t> ib = { 0, 1, 2, 2, 3, 0 };
vb.push_back(v1);
vb.push_back(v2);
vb.push_back(v3);
vb.push_back(v4);
uint32_t meshId = createMesh(vb, ib);
assert(meshId != -1);
return meshId;
}
uint32_t Scene::createSphereLightMesh()
{
if (mSphereLightMeshId != -1)
{
return mSphereLightMeshId;
}
std::vector<Scene::Vertex> vertices;
std::vector<uint32_t> indices;
const int segments = 16;
const int rings = 16;
const float radius = 1.0f;
// Generate vertices and normals
for (int i = 0; i <= rings; ++i)
{
float theta = static_cast<float>(i) * static_cast<float>(M_PI) / static_cast<float>(rings);
float sinTheta = sin(theta);
float cosTheta = cos(theta);
for (int j = 0; j <= segments; ++j)
{
float phi = static_cast<float>(j) * 2.0f * static_cast<float>(M_PI) / static_cast<float>(segments);
float sinPhi = sin(phi);
float cosPhi = cos(phi);
float x = cosPhi * sinTheta;
float y = cosTheta;
float z = sinPhi * sinTheta;
glm::float3 pos = { radius * x, radius * y, radius * z };
glm::float3 normal = { x, y, z };
vertices.push_back(Scene::Vertex{ pos, 0, packNormals(normal) });
}
}
// Generate indices
for (int i = 0; i < rings; ++i)
{
for (int j = 0; j < segments; ++j)
{
int p0 = i * (segments + 1) + j;
int p1 = p0 + 1;
int p2 = (i + 1) * (segments + 1) + j;
int p3 = p2 + 1;
indices.push_back(p0);
indices.push_back(p1);
indices.push_back(p2);
indices.push_back(p2);
indices.push_back(p1);
indices.push_back(p3);
}
}
const uint32_t meshId = createMesh(vertices, indices);
assert(meshId != -1);
return meshId;
}
uint32_t Scene::createDiscLightMesh()
{
if (mDiskLightMeshId != -1)
{
return mDiskLightMeshId;
}
std::vector<Scene::Vertex> vertices;
std::vector<uint32_t> indices;
Scene::Vertex v1, v2;
v1.pos = glm::float4(0.f, 0.f, 0.f, 1.f);
v2.pos = glm::float4(1.0f, 0.f, 0.f, 1.f);
glm::float3 normal = glm::float3(0.f, 0.f, 1.f);
v1.normal = v2.normal = packNormals(normal);
vertices.push_back(v1); // central point
vertices.push_back(v2); // first point
const float diskRadius = 1.0f; // param
const float step = 2.0f * M_PI / 16;
float angle = 0;
for (int i = 0; i < 16; ++i)
{
indices.push_back(0); // each triangle have central point
indices.push_back(vertices.size() - 1); // prev vertex
angle += step;
const float x = cos(angle) * diskRadius;
const float y = sin(angle) * diskRadius;
Scene::Vertex v;
v.pos = glm::float4(x, y, 0.0f, 1.0f);
v.normal = packNormals(normal);
vertices.push_back(v);
indices.push_back(vertices.size() - 1); // added vertex
}
uint32_t meshId = createMesh(vertices, indices);
assert(meshId != -1);
return meshId;
}
void Scene::updateAnimation(const float time)
{
if (mAnimations.empty())
{
return;
}
auto& animation = mAnimations[0];
for (auto& channel : animation.channels)
{
assert(channel.node < mNodes.size());
auto& sampler = animation.samplers[channel.samplerIndex];
if (sampler.inputs.size() > sampler.outputsVec4.size())
{
continue;
}
for (size_t i = 0; i < sampler.inputs.size() - 1; i++)
{
if ((time >= sampler.inputs[i]) && (time <= sampler.inputs[i + 1]))
{
float u = std::max(0.0f, time - sampler.inputs[i]) / (sampler.inputs[i + 1] - sampler.inputs[i]);
if (u <= 1.0f)
{
switch (channel.path)
{
case AnimationChannel::PathType::TRANSLATION: {
glm::vec4 trans = glm::mix(sampler.outputsVec4[i], sampler.outputsVec4[i + 1], u);
mNodes[channel.node].translation = glm::float3(trans);
break;
}
case AnimationChannel::PathType::SCALE: {
glm::vec4 scale = glm::mix(sampler.outputsVec4[i], sampler.outputsVec4[i + 1], u);
mNodes[channel.node].scale = glm::float3(scale);
break;
}
case AnimationChannel::PathType::ROTATION: {
float floatRotation[4] = { (float)sampler.outputsVec4[i][3], (float)sampler.outputsVec4[i][0],
(float)sampler.outputsVec4[i][1], (float)sampler.outputsVec4[i][2] };
float floatRotation1[4] = { (float)sampler.outputsVec4[i + 1][3],
(float)sampler.outputsVec4[i + 1][0],
(float)sampler.outputsVec4[i + 1][1],
(float)sampler.outputsVec4[i + 1][2] };
glm::quat q1 = glm::make_quat(floatRotation);
glm::quat q2 = glm::make_quat(floatRotation1);
mNodes[channel.node].rotation = glm::normalize(glm::slerp(q1, q2, u));
break;
}
}
}
}
}
}
mCameras[0].matrices.view = getTransform(mCameras[0].node);
}
uint32_t Scene::createLight(const UniformLightDesc& desc)
{
uint32_t lightId = (uint32_t)mLights.size();
Light l;
mLights.push_back(l);
mLightDesc.push_back(desc);
updateLight(lightId, desc);
// TODO: only for rect light
// Lazy init light mesh
glm::float4x4 scaleMatrix = glm::float4x4(0.f);
uint32_t currentLightMeshId = 0;
if (desc.type == 0)
{
mRectLightMeshId = createRectLightMesh();
currentLightMeshId = mRectLightMeshId;
scaleMatrix = glm::scale(glm::float4x4(1.0f), glm::float3(desc.width, desc.height, 1.0f));
}
else if (desc.type == 1)
{
mDiskLightMeshId = createDiscLightMesh();
currentLightMeshId = mDiskLightMeshId;
scaleMatrix = glm::scale(glm::float4x4(1.0f), glm::float3(desc.radius, desc.radius, desc.radius));
}
else if (desc.type == 2)
{
mSphereLightMeshId = createSphereLightMesh();
currentLightMeshId = mSphereLightMeshId;
scaleMatrix = glm::scale(glm::float4x4(1.0f), glm::float3(desc.radius, desc.radius, desc.radius));
}
else if (desc.type == 3)
{
// distant light
currentLightMeshId = 0; // empty
scaleMatrix = glm::scale(glm::float4x4(1.0f), glm::float3(desc.radius, desc.radius, desc.radius));
}
const glm::float4x4 transform = desc.useXform ? desc.xform * scaleMatrix : getTransform(desc);
uint32_t instId = createInstance(Instance::Type::eLight, currentLightMeshId, (uint32_t)-1, transform, lightId);
assert(instId != -1);
mLightIdToInstanceId[lightId] = instId;
return lightId;
}
void Scene::updateLight(const uint32_t lightId, const UniformLightDesc& desc)
{
const float intensityPerPoint = desc.intensity; // light intensity
// transform to GPU light
// Rect Light
if (desc.type == 0)
{
const glm::float4x4 scaleMatrix = glm::scale(glm::float4x4(1.0f), glm::float3(desc.width, desc.height, 1.0f));
const glm::float4x4 localTransform = desc.useXform ? desc.xform * scaleMatrix : getTransform(desc);
mLights[lightId].points[0] = localTransform * glm::float4(0.5f, 0.5f, 0.0f, 1.0f);
mLights[lightId].points[1] = localTransform * glm::float4(-0.5f, 0.5f, 0.0f, 1.0f);
mLights[lightId].points[2] = localTransform * glm::float4(-0.5f, -0.5f, 0.0f, 1.0f);
mLights[lightId].points[3] = localTransform * glm::float4(0.5f, -0.5f, 0.0f, 1.0f);
mLights[lightId].type = 0;
}
else if (desc.type == 1)
{
// Disk Light
const glm::float4x4 scaleMatrix =
glm::scale(glm::float4x4(1.0f), glm::float3(desc.radius, desc.radius, desc.radius));
const glm::float4x4 localTransform = desc.useXform ? desc.xform * scaleMatrix : getTransform(desc);
mLights[lightId].points[0] = glm::float4(desc.radius, 0.f, 0.f, 0.f); // save radius
mLights[lightId].points[1] = localTransform * glm::float4(0.f, 0.f, 0.f, 1.f); // save O
mLights[lightId].points[2] = localTransform * glm::float4(1.f, 0.f, 0.f, 0.f); // OXws
mLights[lightId].points[3] = localTransform * glm::float4(0.f, 1.f, 0.f, 0.f); // OYws
glm::float4 normal = localTransform * glm::float4(0, 0, 1.f, 0.0f);
mLights[lightId].normal = normal;
mLights[lightId].type = 1;
}
else if (desc.type == 2)
{
// Sphere Light
const glm::float4x4 scaleMatrix = glm::scale(glm::float4x4(1.0f), glm::float3(1.0f, 1.0f, 1.0f));
const glm::float4x4 localTransform = desc.useXform ? scaleMatrix * desc.xform : getTransform(desc);
mLights[lightId].points[0] = glm::float4(desc.radius, 0.f, 0.f, 0.f); // save radius
mLights[lightId].points[1] = localTransform * glm::float4(0.f, 0.f, 0.f, 1.f); // save O
mLights[lightId].type = 2;
}
else if (desc.type == 3)
{
// distant light https://openusd.org/release/api/class_usd_lux_distant_light.html
mLights[lightId].type = 3;
mLights[lightId].halfAngle = desc.halfAngle;
const glm::float4x4 scaleMatrix = glm::float4x4(1.0f);
const glm::float4x4 localTransform = desc.useXform ? desc.xform * scaleMatrix : getTransform(desc);
mLights[lightId].normal = glm::normalize(localTransform * glm::float4(0.0f, 0.0f, -1.0f, 0.0f)); // -Z
}
mLights[lightId].color = glm::float4(desc.color, 1.0f) * intensityPerPoint;
}
void Scene::removeInstance(const uint32_t instId)
{
mDelInstances.push(instId); // marked as removed
}
void Scene::removeMesh(const uint32_t meshId)
{
mDelMesh.push(meshId); // marked as removed
}
void Scene::removeMaterial(const uint32_t materialId)
{
mDelMaterial.push(materialId); // marked as removed
}
std::vector<uint32_t>& Scene::getOpaqueInstancesToRender(const glm::float3& camPos)
{
return mOpaqueInstances;
}
std::vector<uint32_t>& Scene::getTransparentInstancesToRender(const glm::float3& camPos)
{
return mTransparentInstances;
}
std::set<uint32_t> Scene::getDirtyInstances()
{
return this->mDirtyInstances;
}
bool Scene::getFrMod()
{
return this->FrMod;
}
void Scene::updateInstanceTransform(uint32_t instId, glm::float4x4 newTransform)
{
Instance& inst = mInstances[instId];
inst.transform = newTransform;
mDirtyInstances.insert(instId);
}
void Scene::beginFrame()
{
FrMod = true;
mDirtyInstances.clear();
}
void Scene::endFrame()
{
FrMod = false;
}
uint32_t Scene::createCurve(const Curve::Type type,
const std::vector<uint32_t>& vertexCounts,
const std::vector<glm::float3>& points,
const std::vector<float>& widths)
{
Curve c = {};
c.mPointsStart = mCurvePoints.size();
c.mPointsCount = points.size();
mCurvePoints.insert(mCurvePoints.end(), points.begin(), points.end());
c.mVertexCountsStart = mCurveVertexCounts.size();
c.mVertexCountsCount = vertexCounts.size();
mCurveVertexCounts.insert(mCurveVertexCounts.end(), vertexCounts.begin(), vertexCounts.end());
if (!widths.empty())
{
c.mWidthsCount = widths.size();
c.mWidthsStart = mCurveWidths.size();
mCurveWidths.insert(mCurveWidths.end(), widths.begin(), widths.end());
}
else
{
c.mWidthsCount = -1;
c.mWidthsStart = -1;
}
uint32_t res = mCurves.size();
mCurves.push_back(c);
return res;
}
} // namespace oka
| 15,925 | C++ | 31.108871 | 120 | 0.576641 |
arhix52/Strelka/src/log/logmanager.cpp | #include "logmanager.h"
#include <spdlog/spdlog.h>
#include <spdlog/cfg/env.h>
#include <spdlog/sinks/stdout_color_sinks.h>
#include <spdlog/sinks/basic_file_sink.h>
#include <memory>
#include <vector>
oka::Logmanager::Logmanager()
{
initialize();
}
oka::Logmanager::~Logmanager()
{
shutdown();
}
void oka::Logmanager::initialize()
{
auto logger = spdlog::get("Strelka");
if (!logger)
{
spdlog::cfg::load_env_levels();
auto consolesink = std::make_shared<spdlog::sinks::stdout_color_sink_mt>();
auto fileSink = std::make_shared<spdlog::sinks::basic_file_sink_mt>("strelka.log");
std::vector<spdlog::sink_ptr> sinks = { consolesink, fileSink };
logger = std::make_shared<spdlog::logger>("Strelka", sinks.begin(), sinks.end());
// TODO: env var doesn't work on linux
logger->set_level(spdlog::level::trace);
logger->flush_on(spdlog::level::trace);
#if defined(WIN32)
logger->set_level(spdlog::level::trace);
logger->flush_on(spdlog::level::trace);
#endif
spdlog::register_logger(logger);
}
}
void oka::Logmanager::shutdown()
{
spdlog::shutdown();
}
| 1,170 | C++ | 23.914893 | 91 | 0.637607 |
arhix52/Strelka/src/sceneloader/gltfloader.cpp | #include "gltfloader.h"
#include "camera.h"
#define STB_IMAGE_IMPLEMENTATION
#define STB_IMAGE_WRITE_IMPLEMENTATION
#define TINYGLTF_IMPLEMENTATION
#include "tiny_gltf.h"
#include <glm/glm.hpp>
#include <glm/gtc/matrix_transform.hpp>
#include <glm/gtc/quaternion.hpp>
#include <glm/gtc/type_ptr.hpp>
#include <glm/gtx/compatibility.hpp>
#include <glm/gtx/matrix_decompose.hpp>
#include <iostream>
#include <log/log.h>
namespace fs = std::filesystem;
#include "nlohmann/json.hpp"
using json = nlohmann::json;
namespace oka
{
// valid range of coordinates [-10; 10]
uint32_t packUV(const glm::float2& uv)
{
int32_t packed = (uint32_t)((uv.x + 10.0f) / 20.0f * 16383.99999f);
packed += (uint32_t)((uv.y + 10.0f) / 20.0f * 16383.99999f) << 16;
return packed;
}
// valid range of coordinates [-1; 1]
uint32_t packNormal(const glm::float3& normal)
{
uint32_t packed = (uint32_t)((normal.x + 1.0f) / 2.0f * 511.99999f);
packed += (uint32_t)((normal.y + 1.0f) / 2.0f * 511.99999f) << 10;
packed += (uint32_t)((normal.z + 1.0f) / 2.0f * 511.99999f) << 20;
return packed;
}
// valid range of coordinates [-10; 10]
uint32_t packTangent(const glm::float3& tangent)
{
uint32_t packed = (uint32_t)((tangent.x + 10.0f) / 20.0f * 511.99999f);
packed += (uint32_t)((tangent.y + 10.0f) / 20.0f * 511.99999f) << 10;
packed += (uint32_t)((tangent.z + 10.0f) / 20.0f * 511.99999f) << 20;
return packed;
}
glm::float2 unpackUV(uint32_t val)
{
glm::float2 uv;
uv.y = ((val & 0xffff0000) >> 16) / 16383.99999f * 10.0f - 5.0f;
uv.x = (val & 0x0000ffff) / 16383.99999f * 10.0f - 5.0f;
return uv;
}
void computeTangent(std::vector<Scene::Vertex>& vertices,
const std::vector<uint32_t>& indices)
{
const size_t lastIndex = indices.size();
Scene::Vertex& v0 = vertices[indices[lastIndex - 3]];
Scene::Vertex& v1 = vertices[indices[lastIndex - 2]];
Scene::Vertex& v2 = vertices[indices[lastIndex - 1]];
glm::float2 uv0 = unpackUV(v0.uv);
glm::float2 uv1 = unpackUV(v1.uv);
glm::float2 uv2 = unpackUV(v2.uv);
glm::float3 deltaPos1 = v1.pos - v0.pos;
glm::float3 deltaPos2 = v2.pos - v0.pos;
glm::vec2 deltaUV1 = uv1 - uv0;
glm::vec2 deltaUV2 = uv2 - uv0;
glm::vec3 tangent{ 0.0f, 0.0f, 1.0f };
const float d = deltaUV1.x * deltaUV2.y - deltaUV1.y * deltaUV2.x;
if (abs(d) > 1e-6)
{
float r = 1.0f / d;
tangent = (deltaPos1 * deltaUV2.y - deltaPos2 * deltaUV1.y) * r;
}
glm::uint32_t packedTangent = packTangent(tangent);
v0.tangent = packedTangent;
v1.tangent = packedTangent;
v2.tangent = packedTangent;
}
void processPrimitive(const tinygltf::Model& model, oka::Scene& scene, const tinygltf::Primitive& primitive, const glm::float4x4& transform, const float globalScale)
{
using namespace std;
assert(primitive.attributes.find("POSITION") != primitive.attributes.end());
const tinygltf::Accessor& positionAccessor = model.accessors[primitive.attributes.find("POSITION")->second];
const tinygltf::BufferView& positionView = model.bufferViews[positionAccessor.bufferView];
const float* positionData = reinterpret_cast<const float*>(&model.buffers[positionView.buffer].data[positionAccessor.byteOffset + positionView.byteOffset]);
assert(positionData != nullptr);
const uint32_t vertexCount = static_cast<uint32_t>(positionAccessor.count);
assert(vertexCount != 0);
const int byteStride = positionAccessor.ByteStride(positionView);
assert(byteStride > 0); // -1 means invalid glTF
int posStride = byteStride / sizeof(float);
// Normals
const float* normalsData = nullptr;
int normalStride = 0;
if (primitive.attributes.find("NORMAL") != primitive.attributes.end())
{
const tinygltf::Accessor& normalAccessor = model.accessors[primitive.attributes.find("NORMAL")->second];
const tinygltf::BufferView& normView = model.bufferViews[normalAccessor.bufferView];
normalsData = reinterpret_cast<const float*>(&(model.buffers[normView.buffer].data[normalAccessor.byteOffset + normView.byteOffset]));
assert(normalsData != nullptr);
normalStride = normalAccessor.ByteStride(normView) / sizeof(float);
assert(normalStride > 0);
}
// UVs
const float* texCoord0Data = nullptr;
int texCoord0Stride = 0;
if (primitive.attributes.find("TEXCOORD_0") != primitive.attributes.end())
{
const tinygltf::Accessor& uvAccessor = model.accessors[primitive.attributes.find("TEXCOORD_0")->second];
const tinygltf::BufferView& uvView = model.bufferViews[uvAccessor.bufferView];
texCoord0Data = reinterpret_cast<const float*>(&(model.buffers[uvView.buffer].data[uvAccessor.byteOffset + uvView.byteOffset]));
texCoord0Stride = uvAccessor.ByteStride(uvView) / sizeof(float);
}
int matId = primitive.material;
if (matId == -1)
{
matId = 0; // TODO: should be index of default material
}
glm::float3 sum = glm::float3(0.0f, 0.0f, 0.0f);
std::vector<oka::Scene::Vertex> vertices;
vertices.reserve(vertexCount);
for (uint32_t v = 0; v < vertexCount; ++v)
{
oka::Scene::Vertex vertex{};
vertex.pos = glm::make_vec3(&positionData[v * posStride]) * globalScale;
vertex.normal = packNormal(glm::normalize(glm::vec3(normalsData ? glm::make_vec3(&normalsData[v * normalStride]) : glm::vec3(0.0f))));
vertex.uv = packUV(texCoord0Data ? glm::make_vec2(&texCoord0Data[v * texCoord0Stride]) : glm::vec3(0.0f));
vertices.push_back(vertex);
sum += vertex.pos;
}
const glm::float3 massCenter = sum / (float)vertexCount;
uint32_t indexCount = 0;
std::vector<uint32_t> indices;
const bool hasIndices = (primitive.indices != -1);
assert(hasIndices); // currently support only this mode
if (hasIndices)
{
const tinygltf::Accessor& accessor = model.accessors[primitive.indices > -1 ? primitive.indices : 0];
const tinygltf::BufferView& bufferView = model.bufferViews[accessor.bufferView];
const tinygltf::Buffer& buffer = model.buffers[bufferView.buffer];
indexCount = static_cast<uint32_t>(accessor.count);
assert(indexCount != 0 && (indexCount % 3 == 0));
const void* dataPtr = &(buffer.data[accessor.byteOffset + bufferView.byteOffset]);
indices.reserve(indexCount);
switch (accessor.componentType)
{
case TINYGLTF_PARAMETER_TYPE_UNSIGNED_INT: {
const uint32_t* buf = static_cast<const uint32_t*>(dataPtr);
for (size_t index = 0; index < indexCount; index++)
{
indices.push_back(buf[index]);
}
computeTangent(vertices, indices);
break;
}
case TINYGLTF_PARAMETER_TYPE_UNSIGNED_SHORT: {
const uint16_t* buf = static_cast<const uint16_t*>(dataPtr);
for (size_t index = 0; index < indexCount; index++)
{
indices.push_back(buf[index]);
}
computeTangent(vertices, indices);
break;
}
case TINYGLTF_PARAMETER_TYPE_UNSIGNED_BYTE: {
const uint8_t* buf = static_cast<const uint8_t*>(dataPtr);
for (size_t index = 0; index < indexCount; index++)
{
indices.push_back(buf[index]);
}
computeTangent(vertices, indices);
break;
}
default:
std::cerr << "Index component type " << accessor.componentType << " not supported!" << std::endl;
return;
}
}
uint32_t meshId = scene.createMesh(vertices, indices);
assert(meshId != -1);
uint32_t instId = scene.createInstance(Instance::Type::eMesh, meshId, matId, transform);
assert(instId != -1);
}
void processMesh(const tinygltf::Model& model, oka::Scene& scene, const tinygltf::Mesh& mesh, const glm::float4x4& transform, const float globalScale)
{
using namespace std;
cout << "Mesh name: " << mesh.name << endl;
cout << "Primitive count: " << mesh.primitives.size() << endl;
for (size_t i = 0; i < mesh.primitives.size(); ++i)
{
processPrimitive(model, scene, mesh.primitives[i], transform, globalScale);
}
}
glm::float4x4 getTransform(const tinygltf::Node& node, const float globalScale)
{
if (node.matrix.empty())
{
glm::float3 scale{ 1.0f };
if (!node.scale.empty())
{
scale = glm::float3((float)node.scale[0], (float)node.scale[1], (float)node.scale[2]);
// check that scale is uniform, otherwise we have to support it in shader
// assert(scale.x == scale.y && scale.y == scale.z);
}
glm::quat rotation = glm::quat(1.0f, 0.0f, 0.0f, 0.0f);
if (!node.rotation.empty())
{
const float floatRotation[4] = {
(float)node.rotation[3],
(float)node.rotation[0],
(float)node.rotation[1],
(float)node.rotation[2],
};
rotation = glm::make_quat(floatRotation);
}
glm::float3 translation{ 0.0f };
if (!node.translation.empty())
{
translation = glm::float3((float)node.translation[0], (float)node.translation[1], (float)node.translation[2]);
translation *= globalScale;
}
const glm::float4x4 translationMatrix = glm::translate(glm::float4x4(1.0f), translation);
const glm::float4x4 rotationMatrix{ rotation };
const glm::float4x4 scaleMatrix = glm::scale(glm::float4x4(1.0f), scale);
const glm::float4x4 localTransform = translationMatrix * rotationMatrix * scaleMatrix;
return localTransform;
}
else
{
glm::float4x4 localTransform = glm::make_mat4(node.matrix.data());
return localTransform;
}
}
void processNode(const tinygltf::Model& model, oka::Scene& scene, const tinygltf::Node& node, const uint32_t currentNodeId, const glm::float4x4& baseTransform, const float globalScale)
{
using namespace std;
cout << "Node name: " << node.name << endl;
const glm::float4x4 localTransform = getTransform(node, globalScale);
const glm::float4x4 globalTransform = baseTransform * localTransform;
if (node.mesh != -1) // mesh exist
{
const tinygltf::Mesh& mesh = model.meshes[node.mesh];
processMesh(model, scene, mesh, globalTransform, globalScale);
}
else if (node.camera != -1) // camera node
{
glm::vec3 scale;
glm::quat rotation;
glm::vec3 translation;
glm::vec3 skew;
glm::vec4 perspective;
glm::decompose(globalTransform, scale, rotation, translation, skew, perspective);
rotation = glm::conjugate(rotation);
scene.getCamera(node.camera).node = currentNodeId;
scene.getCamera(node.camera).position = translation * scale;
scene.getCamera(node.camera).mOrientation = rotation;
scene.getCamera(node.camera).updateViewMatrix();
}
for (int i = 0; i < node.children.size(); ++i)
{
scene.mNodes[node.children[i]].parent = currentNodeId;
processNode(model, scene, model.nodes[node.children[i]], node.children[i], globalTransform, globalScale);
}
}
oka::Scene::MaterialDescription convertToOmniPBR(const tinygltf::Model& model, const tinygltf::Material& material)
{
const std::string& fileUri = "OmniPBR.mdl";
const std::string& name = "OmniPBR";
oka::Scene::MaterialDescription materialDesc{};
materialDesc.file = fileUri;
materialDesc.name = name;
materialDesc.type = oka::Scene::MaterialDescription::Type::eMdl;
materialDesc.color =
glm::float3(material.pbrMetallicRoughness.baseColorFactor[0], material.pbrMetallicRoughness.baseColorFactor[1],
material.pbrMetallicRoughness.baseColorFactor[2]);
materialDesc.hasColor = true;
oka::MaterialManager::Param colorParam = {};
colorParam.name = "diffuse_color_constant";
colorParam.type = oka::MaterialManager::Param::Type::eFloat3;
colorParam.value.resize(sizeof(float) * 3);
memcpy(colorParam.value.data(), glm::value_ptr(materialDesc.color), sizeof(float) * 3);
materialDesc.params.push_back(colorParam);
auto addFloat = [&](float value, const char* materialParamName) {
oka::MaterialManager::Param param{};
param.name = materialParamName;
param.type = oka::MaterialManager::Param::Type::eFloat;
param.value.resize(sizeof(float));
*((float*)param.value.data()) = value;
materialDesc.params.push_back(param);
};
addFloat((float)material.pbrMetallicRoughness.roughnessFactor, "reflection_roughness_constant");
addFloat((float)material.pbrMetallicRoughness.metallicFactor, "metallic_constant");
auto addTexture = [&](int texId, const char* materialParamName) {
const auto imageId = model.textures[texId].source;
const auto textureUri = model.images[imageId].uri;
oka::MaterialManager::Param paramTexture{};
paramTexture.name = materialParamName;
paramTexture.type = oka::MaterialManager::Param::Type::eTexture;
paramTexture.value.resize(textureUri.size());
memcpy(paramTexture.value.data(), textureUri.data(), textureUri.size());
materialDesc.params.push_back(paramTexture);
};
auto texId = material.pbrMetallicRoughness.baseColorTexture.index;
if (texId >= 0)
{
addTexture(texId, "diffuse_texture");
}
auto normalTexId = material.normalTexture.index;
if (normalTexId >= 0)
{
addTexture(normalTexId, "normalmap_texture");
}
return materialDesc;
}
oka::Scene::MaterialDescription convertToOmniGlass(const tinygltf::Model& model, const tinygltf::Material& material)
{
oka::Scene::MaterialDescription materialDesc{};
materialDesc.file = "OmniGlass.mdl";
materialDesc.name = "OmniGlass";
materialDesc.type = oka::Scene::MaterialDescription::Type::eMdl;
oka::MaterialManager::Param param{};
param.name = "enable_opacity";
param.type = oka::MaterialManager::Param::Type::eBool;
param.value.resize(sizeof(bool));
*((bool*)param.value.data()) = true;
materialDesc.params.push_back(param);
// materialDesc.color =
// glm::float3(material.pbrMetallicRoughness.baseColorFactor[0], material.pbrMetallicRoughness.baseColorFactor[1],
// material.pbrMetallicRoughness.baseColorFactor[2]);
// oka::MaterialManager::Param colorParam = {};
// colorParam.name = "glass_color";
// colorParam.type = oka::MaterialManager::Param::Type::eFloat3;
// colorParam.value.resize(sizeof(float) * 3);
// memcpy(colorParam.value.data(), glm::value_ptr(materialDesc.color), sizeof(float) * 3);
// materialDesc.params.push_back(colorParam);
auto addBool = [&](bool value, const char* materialParamName) {
oka::MaterialManager::Param param{};
param.name = materialParamName;
param.type = oka::MaterialManager::Param::Type::eBool;
param.value.resize(sizeof(float));
*((bool*)param.value.data()) = value;
materialDesc.params.push_back(param);
};
addBool(false, "thin_walled");
auto addFloat = [&](float value, const char* materialParamName) {
oka::MaterialManager::Param param{};
param.name = materialParamName;
param.type = oka::MaterialManager::Param::Type::eFloat;
param.value.resize(sizeof(float));
*((float*)param.value.data()) = value;
materialDesc.params.push_back(param);
};
addFloat((float)material.pbrMetallicRoughness.roughnessFactor, "frosting_roughness");
return materialDesc;
}
void loadMaterials(const tinygltf::Model& model, oka::Scene& scene)
{
for (const tinygltf::Material& material : model.materials)
{
if (material.alphaMode == "OPAQUE")
{
scene.addMaterial(convertToOmniPBR(model, material));
}
else
{
scene.addMaterial(convertToOmniGlass(model, material));
}
}
}
void loadCameras(const tinygltf::Model& model, oka::Scene& scene)
{
for (uint32_t i = 0; i < model.cameras.size(); ++i)
{
const tinygltf::Camera& cameraGltf = model.cameras[i];
if (strcmp(cameraGltf.type.c_str(), "perspective") == 0)
{
oka::Camera camera;
camera.fov = cameraGltf.perspective.yfov * (180.0f / 3.1415926f);
camera.znear = cameraGltf.perspective.znear;
camera.zfar = cameraGltf.perspective.zfar;
camera.name = cameraGltf.name;
scene.addCamera(camera);
}
else
{
// not supported
}
}
if (scene.getCameraCount() == 0)
{
// add default camera
Camera camera;
camera.updateViewMatrix();
scene.addCamera(camera);
}
}
void loadAnimation(const tinygltf::Model& model, oka::Scene& scene)
{
std::vector<oka::Scene::Animation> animations;
using namespace std;
for (const tinygltf::Animation& animation : model.animations)
{
oka::Scene::Animation anim{};
cout << "Animation name: " << animation.name << endl;
for (const tinygltf::AnimationSampler& sampler : animation.samplers)
{
oka::Scene::AnimationSampler samp{};
{
const tinygltf::Accessor& accessor = model.accessors[sampler.input];
const tinygltf::BufferView& bufferView = model.bufferViews[accessor.bufferView];
const tinygltf::Buffer& buffer = model.buffers[bufferView.buffer];
assert(accessor.componentType == TINYGLTF_COMPONENT_TYPE_FLOAT);
const void* dataPtr = &buffer.data[accessor.byteOffset + bufferView.byteOffset];
const float* buf = static_cast<const float*>(dataPtr);
for (size_t index = 0; index < accessor.count; index++)
{
samp.inputs.push_back(buf[index]);
}
for (auto input : samp.inputs)
{
if (input < anim.start)
{
anim.start = input;
};
if (input > anim.end)
{
anim.end = input;
}
}
}
{
const tinygltf::Accessor& accessor = model.accessors[sampler.output];
const tinygltf::BufferView& bufferView = model.bufferViews[accessor.bufferView];
const tinygltf::Buffer& buffer = model.buffers[bufferView.buffer];
assert(accessor.componentType == TINYGLTF_COMPONENT_TYPE_FLOAT);
const void* dataPtr = &buffer.data[accessor.byteOffset + bufferView.byteOffset];
switch (accessor.type)
{
case TINYGLTF_TYPE_VEC3: {
const glm::vec3* buf = static_cast<const glm::vec3*>(dataPtr);
for (size_t index = 0; index < accessor.count; index++)
{
samp.outputsVec4.push_back(glm::vec4(buf[index], 0.0f));
}
break;
}
case TINYGLTF_TYPE_VEC4: {
const glm::vec4* buf = static_cast<const glm::vec4*>(dataPtr);
for (size_t index = 0; index < accessor.count; index++)
{
samp.outputsVec4.push_back(buf[index]);
}
break;
}
default: {
std::cout << "unknown type" << std::endl;
break;
}
}
anim.samplers.push_back(samp);
}
}
for (const tinygltf::AnimationChannel& channel : animation.channels)
{
oka::Scene::AnimationChannel chan{};
if (channel.target_path == "rotation")
{
chan.path = oka::Scene::AnimationChannel::PathType::ROTATION;
}
if (channel.target_path == "translation")
{
chan.path = oka::Scene::AnimationChannel::PathType::TRANSLATION;
}
if (channel.target_path == "scale")
{
chan.path = oka::Scene::AnimationChannel::PathType::SCALE;
}
if (channel.target_path == "weights")
{
std::cout << "weights not yet supported, skipping channel" << std::endl;
continue;
}
chan.samplerIndex = channel.sampler;
chan.node = channel.target_node;
if (chan.node < 0)
{
std::cout << "skipping channel" << std::endl;
continue;
}
anim.channels.push_back(chan);
}
animations.push_back(anim);
}
scene.mAnimations = animations;
}
void loadNodes(const tinygltf::Model& model, oka::Scene& scene, const float globalScale = 1.0f)
{
for (const auto& node : model.nodes)
{
oka::Scene::Node n{};
n.name = node.name;
n.children = node.children;
glm::float3 scale{ 1.0f };
if (!node.scale.empty())
{
scale = glm::float3((float)node.scale[0], (float)node.scale[1], (float)node.scale[2]);
// check that scale is uniform, otherwise we have to support it in shader
// assert(scale.x == scale.y && scale.y == scale.z);
}
n.scale = scale;
//glm::quat rotation = glm::quat(1.0f, 0.0f, 0.0f, 0.0f);
glm::quat rotation = glm::quat_cast(glm::float4x4(1.0f));
if (!node.rotation.empty())
{
const float floatRotation[4] = {
(float)node.rotation[3],
(float)node.rotation[0],
(float)node.rotation[1],
(float)node.rotation[2],
};
rotation = glm::make_quat(floatRotation);
}
n.rotation = rotation;
glm::float3 translation{ 0.0f };
if (!node.translation.empty())
{
translation = glm::float3((float)node.translation[0], (float)node.translation[1], (float)node.translation[2]);
translation *= globalScale;
}
n.translation = translation;
scene.mNodes.push_back(n);
}
}
oka::Scene::UniformLightDesc parseFromJson(const json& light)
{
oka::Scene::UniformLightDesc desc{};
const auto position = light["position"];
desc.position = glm::float3(position[0], position[1], position[2]);
const auto orientation = light["orientation"];
desc.orientation = glm::float3(orientation[0], orientation[1], orientation[2]);
desc.width = float(light["width"]);
desc.height = light["height"];
const auto color = light["color"];
desc.color = glm::float3(color[0], color[1], color[2]);
desc.intensity = float(light["intensity"]);
desc.useXform = false;
desc.type = 0;
return desc;
}
bool loadLightsFromJson(const std::string& modelPath, oka::Scene& scene)
{
std::string fileName = modelPath.substr(0, modelPath.rfind('.')); // w/o extension
std::string jsonPath = fileName + "_light" + ".json";
if (fs::exists(jsonPath))
{
STRELKA_INFO("Found light file, loading lights from it");
std::ifstream i(jsonPath);
json light;
i >> light;
for (const auto& light : light["lights"])
{
Scene::UniformLightDesc desc = parseFromJson(light);
scene.createLight(desc);
}
return true;
}
return false;
}
bool GltfLoader::loadGltf(const std::string& modelPath, oka::Scene& scene)
{
if (modelPath.empty())
{
return false;
}
using namespace std;
tinygltf::Model model;
tinygltf::TinyGLTF gltf_ctx;
std::string err;
std::string warn;
bool res = gltf_ctx.LoadASCIIFromFile(&model, &err, &warn, modelPath.c_str());
if (!res)
{
STRELKA_ERROR("Unable to load file: {}", modelPath);
return res;
}
int sceneId = model.defaultScene;
loadMaterials(model, scene);
if (loadLightsFromJson(modelPath, scene) == false)
{
STRELKA_WARNING("No light is scene, adding default distant light");
oka::Scene::UniformLightDesc lightDesc {};
// lightDesc.xform = glm::mat4(1.0f);
// lightDesc.useXform = true;
lightDesc.useXform = false;
lightDesc.position = glm::float3(0.0f, 0.0f, 0.0f);
lightDesc.orientation = glm::float3(-45.0f, 15.0f, 0.0f);
lightDesc.type = 3; // distant light
lightDesc.halfAngle = 10.0f * 0.5f * (M_PI / 180.0f);
lightDesc.intensity = 100000;
lightDesc.color = glm::float3(1.0);
scene.createLight(lightDesc);
}
loadCameras(model, scene);
const float globalScale = 1.0f;
loadNodes(model, scene, globalScale);
for (int i = 0; i < model.scenes[sceneId].nodes.size(); ++i)
{
const int rootNodeIdx = model.scenes[sceneId].nodes[i];
processNode(model, scene, model.nodes[rootNodeIdx], rootNodeIdx, glm::float4x4(1.0f), globalScale);
}
loadAnimation(model, scene);
return res;
}
} // namespace oka
| 25,554 | C++ | 35.982634 | 184 | 0.604406 |
arhix52/Strelka/src/display/DisplayFactory.cpp | #ifdef __APPLE__
#include "metal/glfwdisplay.h"
#else
#include "opengl/glfwdisplay.h"
#endif
using namespace oka;
Display* DisplayFactory::createDisplay()
{
return new glfwdisplay();
}
| 191 | C++ | 13.76923 | 40 | 0.727749 |
arhix52/Strelka/src/display/Display.cpp | #include "Display.h"
#include "imgui.h"
#include "imgui_impl_glfw.h"
using namespace oka;
void Display::framebufferResizeCallback(GLFWwindow* window, int width, int height)
{
assert(window);
if (width == 0 || height == 0)
{
return;
}
auto app = reinterpret_cast<Display*>(glfwGetWindowUserPointer(window));
// app->framebufferResized = true;
ResizeHandler* handler = app->getResizeHandler();
if (handler)
{
handler->framebufferResize(width, height);
}
}
void Display::keyCallback(GLFWwindow* window,
[[maybe_unused]] int key,
[[maybe_unused]] int scancode,
[[maybe_unused]] int action,
[[maybe_unused]] int mods)
{
assert(window);
auto app = reinterpret_cast<Display*>(glfwGetWindowUserPointer(window));
InputHandler* handler = app->getInputHandler();
assert(handler);
handler->keyCallback(key, scancode, action, mods);
}
void Display::mouseButtonCallback(GLFWwindow* window,
[[maybe_unused]] int button,
[[maybe_unused]] int action,
[[maybe_unused]] int mods)
{
assert(window);
auto app = reinterpret_cast<Display*>(glfwGetWindowUserPointer(window));
InputHandler* handler = app->getInputHandler();
if (handler)
{
handler->mouseButtonCallback(button, action, mods);
}
}
void Display::handleMouseMoveCallback(GLFWwindow* window, [[maybe_unused]] double xpos, [[maybe_unused]] double ypos)
{
assert(window);
auto app = reinterpret_cast<Display*>(glfwGetWindowUserPointer(window));
InputHandler* handler = app->getInputHandler();
if (handler)
{
handler->handleMouseMoveCallback(xpos, ypos);
}
}
void Display::scrollCallback(GLFWwindow* window, [[maybe_unused]] double xoffset, [[maybe_unused]] double yoffset)
{
assert(window);
}
void Display::drawUI()
{
ImGui_ImplGlfw_NewFrame();
ImGui::NewFrame();
ImGuiIO& io = ImGui::GetIO();
const char* debugItems[] = { "None", "Normals", "Diffuse AOV", "Specular AOV" };
static int currentDebugItemId = 0;
/*
bool openFD = false;
static uint32_t showPropertiesId = -1;
static uint32_t lightId = -1;
static bool isLight = false;
static bool openInspector = false;
const char* stratifiedSamplingItems[] = { "None", "Random", "Stratified", "Optimized" };
static int currentSamplingItemId = 1;
*/
ImGui::Begin("Menu:"); // begin window
if (ImGui::BeginCombo("Debug view", debugItems[currentDebugItemId]))
{
for (int n = 0; n < IM_ARRAYSIZE(debugItems); n++)
{
bool is_selected = (currentDebugItemId == n);
if (ImGui::Selectable(debugItems[n], is_selected))
{
currentDebugItemId = n;
}
if (is_selected)
{
ImGui::SetItemDefaultFocus();
}
}
ImGui::EndCombo();
}
mCtx->mSettingsManager->setAs<uint32_t>("render/pt/debug", currentDebugItemId);
if (ImGui::TreeNode("Path Tracer"))
{
const char* rectlightSamplingMethodItems[] = { "Uniform", "Advanced" };
static int currentRectlightSamplingMethodItemId = 0;
if (ImGui::BeginCombo("Rect Light Sampling", rectlightSamplingMethodItems[currentRectlightSamplingMethodItemId]))
{
for (int n = 0; n < IM_ARRAYSIZE(rectlightSamplingMethodItems); n++)
{
bool is_selected = (currentRectlightSamplingMethodItemId == n);
if (ImGui::Selectable(rectlightSamplingMethodItems[n], is_selected))
{
currentRectlightSamplingMethodItemId = n;
}
if (is_selected)
{
ImGui::SetItemDefaultFocus();
}
}
ImGui::EndCombo();
}
mCtx->mSettingsManager->setAs<uint32_t>(
"render/pt/rectLightSamplingMethod", currentRectlightSamplingMethodItemId);
uint32_t maxDepth = mCtx->mSettingsManager->getAs<uint32_t>("render/pt/depth");
ImGui::SliderInt("Max Depth", (int*)&maxDepth, 1, 16);
mCtx->mSettingsManager->setAs<uint32_t>("render/pt/depth", maxDepth);
uint32_t sppTotal = mCtx->mSettingsManager->getAs<uint32_t>("render/pt/sppTotal");
ImGui::SliderInt("SPP Total", (int*)&sppTotal, 1, 10000);
mCtx->mSettingsManager->setAs<uint32_t>("render/pt/sppTotal", sppTotal);
uint32_t sppSubframe = mCtx->mSettingsManager->getAs<uint32_t>("render/pt/spp");
ImGui::SliderInt("SPP Subframe", (int*)&sppSubframe, 1, 32);
mCtx->mSettingsManager->setAs<uint32_t>("render/pt/spp", sppSubframe);
bool enableAccumulation = mCtx->mSettingsManager->getAs<bool>("render/pt/enableAcc");
ImGui::Checkbox("Enable Path Tracer Acc", &enableAccumulation);
mCtx->mSettingsManager->setAs<bool>("render/pt/enableAcc", enableAccumulation);
/*
if (ImGui::BeginCombo("Stratified Sampling", stratifiedSamplingItems[currentSamplingItemId]))
{
for (int n = 0; n < IM_ARRAYSIZE(stratifiedSamplingItems); n++)
{
bool is_selected = (currentSamplingItemId == n);
if (ImGui::Selectable(stratifiedSamplingItems[n], is_selected))
{
currentSamplingItemId = n;
}
if (is_selected)
{
ImGui::SetItemDefaultFocus();
}
}
ImGui::EndCombo();
}
mCtx->mSettingsManager->setAs<uint32_t>("render/pt/stratifiedSamplingType", currentSamplingItemId);
*/
ImGui::TreePop();
}
if (ImGui::Button("Capture Screen"))
{
mCtx->mSettingsManager->setAs<bool>("render/pt/needScreenshot", true);
}
float cameraSpeed = mCtx->mSettingsManager->getAs<float>("render/cameraSpeed");
ImGui::InputFloat("Camera Speed", (float*)&cameraSpeed, 0.5);
mCtx->mSettingsManager->setAs<float>("render/cameraSpeed", cameraSpeed);
const char* tonemapItems[] = { "None", "Reinhard", "ACES", "Filmic" };
static int currentTonemapItemId = 1;
if (ImGui::BeginCombo("Tonemap", tonemapItems[currentTonemapItemId]))
{
for (int n = 0; n < IM_ARRAYSIZE(tonemapItems); n++)
{
bool is_selected = (currentTonemapItemId == n);
if (ImGui::Selectable(tonemapItems[n], is_selected))
{
currentTonemapItemId = n;
}
if (is_selected)
{
ImGui::SetItemDefaultFocus();
}
}
ImGui::EndCombo();
}
mCtx->mSettingsManager->setAs<uint32_t>("render/pt/tonemapperType", currentTonemapItemId);
float gamma = mCtx->mSettingsManager->getAs<float>("render/post/gamma");
ImGui::InputFloat("Gamma", (float*)&gamma, 0.5);
mCtx->mSettingsManager->setAs<float>("render/post/gamma", gamma);
float materialRayTmin = mCtx->mSettingsManager->getAs<float>("render/pt/dev/materialRayTmin");
ImGui::InputFloat("Material ray T min", (float*)&materialRayTmin, 0.1);
mCtx->mSettingsManager->setAs<float>("render/pt/dev/materialRayTmin", materialRayTmin);
float shadowRayTmin = mCtx->mSettingsManager->getAs<float>("render/pt/dev/shadowRayTmin");
ImGui::InputFloat("Shadow ray T min", (float*)&shadowRayTmin, 0.1);
mCtx->mSettingsManager->setAs<float>("render/pt/dev/shadowRayTmin", shadowRayTmin);
/*
bool enableUpscale = mCtx->mSettingsManager->getAs<bool>("render/pt/enableUpscale");
ImGui::Checkbox("Enable Upscale", &enableUpscale);
mCtx->mSettingsManager->setAs<bool>("render/pt/enableUpscale", enableUpscale);
float upscaleFactor = 0.0f;
if (enableUpscale)
{
upscaleFactor = 0.5f;
}
else
{
upscaleFactor = 1.0f;
}
mCtx->mSettingsManager->setAs<float>("render/pt/upscaleFactor", upscaleFactor);
if (ImGui::Button("Capture Screen"))
{
mCtx->mSettingsManager->setAs<bool>("render/pt/needScreenshot", true);
}
// bool isRecreate = ImGui::Button("Recreate BVH");
// renderConfig.recreateBVH = isRecreate ? true : false;
*/
ImGui::End(); // end window
// Rendering
ImGui::Render();
}
| 8,486 | C++ | 34.3625 | 121 | 0.603936 |
arhix52/Strelka/src/display/metal/metalcpphelp.cpp | #define NS_PRIVATE_IMPLEMENTATION
#define MTL_PRIVATE_IMPLEMENTATION
// #define MTK_PRIVATE_IMPLEMENTATION
#define CA_PRIVATE_IMPLEMENTATION
#include <Metal/Metal.hpp>
#include <QuartzCore/QuartzCore.hpp>
| 205 | C++ | 28.428567 | 37 | 0.819512 |
arhix52/Strelka/src/display/metal/glfwdisplay.h | #pragma once
#define GLFW_INCLUDE_NONE
#define GLFW_EXPOSE_NATIVE_COCOA
#include "Display.h"
#include <Metal/Metal.hpp>
#include <QuartzCore/QuartzCore.hpp>
namespace oka
{
class glfwdisplay : public Display
{
public:
glfwdisplay()
{
}
virtual ~glfwdisplay()
{
}
virtual void init(int width, int height, oka::SharedContext* ctx) override;
virtual void destroy() override;
virtual void onBeginFrame() override;
virtual void onEndFrame() override;
virtual void drawFrame(ImageBuffer& result) override;
virtual void drawUI() override;
private:
static constexpr size_t kMaxFramesInFlight = 3;
MTL::Device* _pDevice;
MTL::CommandQueue* _pCommandQueue;
MTL::Library* _pShaderLibrary;
MTL::RenderPipelineState* _pPSO;
MTL::Texture* mTexture;
uint32_t mTexWidth = 32;
uint32_t mTexHeight = 32;
dispatch_semaphore_t _semaphore;
CA::MetalLayer* layer;
MTL::RenderPassDescriptor *renderPassDescriptor;
MTL::Texture* buildTexture(uint32_t width, uint32_t heigth);
void buildShaders();
MTL::CommandBuffer* commandBuffer;
MTL::RenderCommandEncoder* renderEncoder;
CA::MetalDrawable* drawable;
};
} // namespace oka
| 1,226 | C | 22.596153 | 79 | 0.700653 |
arhix52/Strelka/src/display/opengl/glfwdisplay.h | #pragma once
#include <glad/glad.h>
#include "Display.h"
namespace oka
{
class glfwdisplay : public Display
{
private:
/* data */
GLuint m_render_tex = 0u;
GLuint m_program = 0u;
GLint m_render_tex_uniform_loc = -1;
GLuint m_quad_vertex_buffer = 0;
GLuint m_dislpayPbo = 0;
static const std::string s_vert_source;
static const std::string s_frag_source;
public:
glfwdisplay(/* args */);
virtual ~glfwdisplay();
public:
virtual void init(int width, int height, oka::SharedContext* ctx) override;
void destroy();
void onBeginFrame();
void onEndFrame();
void drawFrame(ImageBuffer& result);
void drawUI();
void display(const int32_t screen_res_x,
const int32_t screen_res_y,
const int32_t framebuf_res_x,
const int32_t framebuf_res_y,
const uint32_t pbo) const;
};
} // namespace oka
| 927 | C | 20.090909 | 79 | 0.618123 |
arhix52/Strelka/src/display/opengl/glfwdisplay.cpp | #include "glfwdisplay.h"
#include "imgui.h"
#include "imgui_impl_glfw.h"
#include "imgui_impl_opengl3.h"
#define GL_SILENCE_DEPRECATION
#include <sstream>
using namespace oka;
inline const char* getGLErrorString(GLenum error)
{
switch (error)
{
case GL_NO_ERROR:
return "No error";
case GL_INVALID_ENUM:
return "Invalid enum";
case GL_INVALID_VALUE:
return "Invalid value";
case GL_INVALID_OPERATION:
return "Invalid operation";
// case GL_STACK_OVERFLOW: return "Stack overflow";
// case GL_STACK_UNDERFLOW: return "Stack underflow";
case GL_OUT_OF_MEMORY:
return "Out of memory";
// case GL_TABLE_TOO_LARGE: return "Table too large";
default:
return "Unknown GL error";
}
}
inline void glCheck(const char* call, const char* file, unsigned int line)
{
GLenum err = glGetError();
if (err != GL_NO_ERROR)
{
std::stringstream ss;
ss << "GL error " << getGLErrorString(err) << " at " << file << "(" << line << "): " << call << '\n';
std::cerr << ss.str() << std::endl;
// throw Exception(ss.str().c_str());
assert(0);
}
}
#define GL_CHECK(call) \
do \
{ \
call; \
glCheck(#call, __FILE__, __LINE__); \
} while (false)
const std::string glfwdisplay::s_vert_source = R"(
#version 330 core
layout(location = 0) in vec3 vertexPosition_modelspace;
out vec2 UV;
void main()
{
gl_Position = vec4(vertexPosition_modelspace,1);
UV = (vec2( vertexPosition_modelspace.x, vertexPosition_modelspace.y )+vec2(1,1))/2.0;
}
)";
const std::string glfwdisplay::s_frag_source = R"(
#version 330 core
in vec2 UV;
out vec3 color;
uniform sampler2D render_tex;
uniform bool correct_gamma;
void main()
{
color = texture( render_tex, UV ).xyz;
}
)";
GLuint createGLShader(const std::string& source, GLuint shader_type)
{
GLuint shader = glCreateShader(shader_type);
{
const GLchar* source_data = reinterpret_cast<const GLchar*>(source.data());
glShaderSource(shader, 1, &source_data, nullptr);
glCompileShader(shader);
GLint is_compiled = 0;
glGetShaderiv(shader, GL_COMPILE_STATUS, &is_compiled);
if (is_compiled == GL_FALSE)
{
GLint max_length = 0;
glGetShaderiv(shader, GL_INFO_LOG_LENGTH, &max_length);
std::string info_log(max_length, '\0');
GLchar* info_log_data = reinterpret_cast<GLchar*>(&info_log[0]);
glGetShaderInfoLog(shader, max_length, nullptr, info_log_data);
glDeleteShader(shader);
std::cerr << "Compilation of shader failed: " << info_log << std::endl;
return 0;
}
}
// GL_CHECK_ERRORS();
return shader;
}
GLuint createGLProgram(const std::string& vert_source, const std::string& frag_source)
{
GLuint vert_shader = createGLShader(vert_source, GL_VERTEX_SHADER);
if (vert_shader == 0)
return 0;
GLuint frag_shader = createGLShader(frag_source, GL_FRAGMENT_SHADER);
if (frag_shader == 0)
{
glDeleteShader(vert_shader);
return 0;
}
GLuint program = glCreateProgram();
glAttachShader(program, vert_shader);
glAttachShader(program, frag_shader);
glLinkProgram(program);
GLint is_linked = 0;
glGetProgramiv(program, GL_LINK_STATUS, &is_linked);
if (is_linked == GL_FALSE)
{
GLint max_length = 0;
glGetProgramiv(program, GL_INFO_LOG_LENGTH, &max_length);
std::string info_log(max_length, '\0');
GLchar* info_log_data = reinterpret_cast<GLchar*>(&info_log[0]);
glGetProgramInfoLog(program, max_length, nullptr, info_log_data);
std::cerr << "Linking of program failed: " << info_log << std::endl;
glDeleteProgram(program);
glDeleteShader(vert_shader);
glDeleteShader(frag_shader);
return 0;
}
glDetachShader(program, vert_shader);
glDetachShader(program, frag_shader);
// GL_CHECK_ERRORS();
return program;
}
GLint getGLUniformLocation(GLuint program, const std::string& name)
{
GLint loc = glGetUniformLocation(program, name.c_str());
return loc;
}
glfwdisplay::glfwdisplay(/* args */)
{
}
glfwdisplay::~glfwdisplay()
{
}
void glfwdisplay::init(int width, int height, oka::SharedContext* ctx)
{
mWindowWidth = width;
mWindowHeight = height;
mCtx = ctx;
glfwInit();
const char* glsl_version = "#version 130";
glfwWindowHint(GLFW_CONTEXT_VERSION_MAJOR, 3);
glfwWindowHint(GLFW_CONTEXT_VERSION_MINOR, 3);
glfwWindowHint(GLFW_OPENGL_FORWARD_COMPAT, GL_TRUE);
glfwWindowHint(GLFW_OPENGL_PROFILE, GLFW_OPENGL_CORE_PROFILE);
mWindow = glfwCreateWindow(mWindowWidth, mWindowHeight, "Strelka", nullptr, nullptr);
glfwSetWindowUserPointer(mWindow, this);
glfwSetFramebufferSizeCallback(mWindow, framebufferResizeCallback);
glfwSetKeyCallback(mWindow, keyCallback);
glfwSetMouseButtonCallback(mWindow, mouseButtonCallback);
glfwSetCursorPosCallback(mWindow, handleMouseMoveCallback);
glfwSetScrollCallback(mWindow, scrollCallback);
glfwMakeContextCurrent(mWindow);
if (!gladLoadGLLoader((GLADloadproc)glfwGetProcAddress))
{
std::cout << "Failed to initialize GLAD" << std::endl;
assert(0);
}
GLuint m_vertex_array;
GL_CHECK(glGenVertexArrays(1, &m_vertex_array));
GL_CHECK(glBindVertexArray(m_vertex_array));
m_program = createGLProgram(s_vert_source, s_frag_source);
m_render_tex_uniform_loc = getGLUniformLocation(m_program, "render_tex");
GL_CHECK(glGenTextures(1, &m_render_tex));
GL_CHECK(glBindTexture(GL_TEXTURE_2D, m_render_tex));
GL_CHECK(glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_NEAREST));
GL_CHECK(glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_NEAREST));
GL_CHECK(glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE));
GL_CHECK(glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE));
static const GLfloat g_quad_vertex_buffer_data[] = {
-1.0f, -1.0f, 0.0f, 1.0f, -1.0f, 0.0f, -1.0f, 1.0f, 0.0f,
-1.0f, 1.0f, 0.0f, 1.0f, -1.0f, 0.0f, 1.0f, 1.0f, 0.0f,
};
GL_CHECK(glGenBuffers(1, &m_quad_vertex_buffer));
GL_CHECK(glBindBuffer(GL_ARRAY_BUFFER, m_quad_vertex_buffer));
GL_CHECK(glBufferData(GL_ARRAY_BUFFER, sizeof(g_quad_vertex_buffer_data), g_quad_vertex_buffer_data, GL_STATIC_DRAW));
// GL_CHECK_ERRORS();
// Setup Dear ImGui context
IMGUI_CHECKVERSION();
ImGui::CreateContext();
ImGuiIO& io = ImGui::GetIO();
(void)io;
// io.ConfigFlags |= ImGuiConfigFlags_NavEnableKeyboard; // Enable Keyboard Controls
// io.ConfigFlags |= ImGuiConfigFlags_NavEnableGamepad; // Enable Gamepad Controls
// Setup Dear ImGui style
ImGui::StyleColorsDark();
// ImGui::StyleColorsLight();
// Setup Platform/Renderer backends
ImGui_ImplGlfw_InitForOpenGL(mWindow, true);
ImGui_ImplOpenGL3_Init(glsl_version);
}
void glfwdisplay::display(const int32_t screen_res_x,
const int32_t screen_res_y,
const int32_t framebuf_res_x,
const int32_t framebuf_res_y,
const uint32_t pbo) const
{
GL_CHECK(glBindFramebuffer(GL_FRAMEBUFFER, 0));
GL_CHECK(glViewport(0, 0, framebuf_res_x, framebuf_res_y));
GL_CHECK(glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT));
GL_CHECK(glUseProgram(m_program));
// Bind our texture in Texture Unit 0
GL_CHECK(glActiveTexture(GL_TEXTURE0));
GL_CHECK(glBindTexture(GL_TEXTURE_2D, m_render_tex));
GL_CHECK(glBindBuffer(GL_PIXEL_UNPACK_BUFFER, pbo));
GL_CHECK(glPixelStorei(GL_UNPACK_ALIGNMENT, 4)); // TODO!!!!!!
size_t elmt_size = 4 * sizeof(float); // pixelFormatSize(m_image_format);
if (elmt_size % 8 == 0)
glPixelStorei(GL_UNPACK_ALIGNMENT, 8);
else if (elmt_size % 4 == 0)
glPixelStorei(GL_UNPACK_ALIGNMENT, 4);
else if (elmt_size % 2 == 0)
glPixelStorei(GL_UNPACK_ALIGNMENT, 2);
else
glPixelStorei(GL_UNPACK_ALIGNMENT, 1);
bool convertToSrgb = true;
// if (m_image_format == BufferImageFormat::UNSIGNED_BYTE4)
// {
// // input is assumed to be in srgb since it is only 1 byte per channel in
// // size
// glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA8, screen_res_x, screen_res_y, 0, GL_RGBA, GL_UNSIGNED_BYTE, nullptr);
// convertToSrgb = false;
// }
// else if (m_image_format == BufferImageFormat::FLOAT3)
// glTexImage2D(GL_TEXTURE_2D, 0, GL_RGB32F, screen_res_x, screen_res_y, 0, GL_RGB, GL_FLOAT, nullptr);
// else if (m_image_format == BufferImageFormat::FLOAT4)
glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA32F, screen_res_x, screen_res_y, 0, GL_RGBA, GL_FLOAT, nullptr);
convertToSrgb = false;
// else
// throw Exception("Unknown buffer format");
GL_CHECK(glBindBuffer(GL_PIXEL_UNPACK_BUFFER, 0));
GL_CHECK(glUniform1i(m_render_tex_uniform_loc, 0));
// 1st attribute buffer : vertices
GL_CHECK(glEnableVertexAttribArray(0));
GL_CHECK(glBindBuffer(GL_ARRAY_BUFFER, m_quad_vertex_buffer));
GL_CHECK(glVertexAttribPointer(0, // attribute 0. No particular reason for 0,
// but must match the layout in the shader.
3, // size
GL_FLOAT, // type
GL_FALSE, // normalized?
0, // stride
(void*)0 // array buffer offset
));
if (convertToSrgb)
GL_CHECK(glEnable(GL_FRAMEBUFFER_SRGB));
else
GL_CHECK(glDisable(GL_FRAMEBUFFER_SRGB));
// Draw the triangles !
GL_CHECK(glDrawArrays(GL_TRIANGLES, 0,
6)); // 2*3 indices starting at 0 -> 2 triangles
GL_CHECK(glDisableVertexAttribArray(0));
GL_CHECK(glDisable(GL_FRAMEBUFFER_SRGB));
// GL_CHECK_ERRORS();
}
void glfwdisplay::drawFrame(ImageBuffer& result)
{
glClear(GL_COLOR_BUFFER_BIT);
int framebuf_res_x = 0, framebuf_res_y = 0;
glfwGetFramebufferSize(mWindow, &framebuf_res_x, &framebuf_res_y);
if (m_dislpayPbo == 0)
{
GL_CHECK(glGenBuffers(1, &m_dislpayPbo));
}
GL_CHECK(glBindBuffer(GL_ARRAY_BUFFER, m_dislpayPbo));
GL_CHECK(glBufferData(GL_ARRAY_BUFFER, result.dataSize, result.data, GL_STREAM_DRAW));
GL_CHECK(glBindBuffer(GL_ARRAY_BUFFER, 0));
display(result.width, result.height, framebuf_res_x, framebuf_res_y, m_dislpayPbo);
}
void glfwdisplay::destroy()
{
}
void glfwdisplay::onBeginFrame()
{
}
void glfwdisplay::onEndFrame()
{
glfwSwapBuffers(mWindow);
}
void glfwdisplay::drawUI()
{
ImGui_ImplOpenGL3_NewFrame();
Display::drawUI();
int display_w, display_h;
glfwGetFramebufferSize(mWindow, &display_w, &display_h);
glViewport(0, 0, display_w, display_h);
ImGui_ImplOpenGL3_RenderDrawData(ImGui::GetDrawData());
}
| 11,703 | C++ | 31.065753 | 122 | 0.604375 |
arhix52/Strelka/include/HdStrelka/RendererPlugin.h | #pragma once
#include <pxr/imaging/hd/rendererPlugin.h>
#include <memory>
#include <log/logmanager.h>
#include "MaterialNetworkTranslator.h"
PXR_NAMESPACE_OPEN_SCOPE
class HdStrelkaRendererPlugin final : public HdRendererPlugin
{
public:
HdStrelkaRendererPlugin();
~HdStrelkaRendererPlugin() override;
public:
HdRenderDelegate* CreateRenderDelegate() override;
HdRenderDelegate* CreateRenderDelegate(const HdRenderSettingsMap& settingsMap) override;
void DeleteRenderDelegate(HdRenderDelegate* renderDelegate) override;
bool IsSupported(bool gpuEnabled = true) const override;
private:
oka::Logmanager mLoggerManager;
std::unique_ptr<MaterialNetworkTranslator> m_translator;
bool m_isSupported;
};
PXR_NAMESPACE_CLOSE_SCOPE
| 772 | C | 21.085714 | 92 | 0.782383 |
arhix52/Strelka/include/materialmanager/mdlLogger.h | // Copyright (C) 2021 Pablo Delgado Krämer
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program. If not, see <https://www.gnu.org/licenses/>.
#pragma once
#include <mi/base/interface_implement.h>
#include <mi/base/ilogger.h>
#include <mi/neuraylib/imdl_execution_context.h>
namespace oka
{
class MdlLogger : public mi::base::Interface_implement<mi::base::ILogger>
{
public:
void message(mi::base::Message_severity level,
const char* moduleCategory,
const mi::base::Message_details& details,
const char* message) override;
void message(mi::base::Message_severity level,
const char* moduleCategory,
const char* message) override;
void message(mi::base::Message_severity level,
const char* message);
void flushContextMessages(mi::neuraylib::IMdl_execution_context* context);
};
}
| 1,561 | C | 36.190475 | 106 | 0.647662 |
arhix52/Strelka/include/materialmanager/materialmanager.h | #pragma once
#include <memory>
#include <stdint.h>
#include <string>
#include <vector>
namespace oka
{
class MaterialManager
{
class Context;
std::unique_ptr<Context> mContext;
public:
struct Module;
struct MaterialInstance;
struct CompiledMaterial;
struct TargetCode;
struct TextureDescription;
bool addMdlSearchPath(const char* paths[], uint32_t numPaths);
Module* createModule(const char* file);
Module* createMtlxModule(const char* file);
void destroyModule(Module* module);
MaterialInstance* createMaterialInstance(Module* module, const char* materialName);
void destroyMaterialInstance(MaterialInstance* material);
struct Param
{
enum class Type : uint32_t
{
eFloat = 0,
eInt,
eBool,
eFloat2,
eFloat3,
eFloat4,
eTexture
};
Type type;
std::string name;
std::vector<uint8_t> value;
};
void dumpParams(const TargetCode* targetCode, uint32_t materialIdx, CompiledMaterial* material);
bool setParam(TargetCode* targetCode, uint32_t materialIdx, CompiledMaterial* material, const Param& param);
TextureDescription* createTextureDescription(const char* name, const char* gamma);
const char* getTextureDbName(TextureDescription* texDesc);
CompiledMaterial* compileMaterial(MaterialInstance* matInstance);
void destroyCompiledMaterial(CompiledMaterial* compMaterial);
const char* getName(CompiledMaterial* compMaterial);
TargetCode* generateTargetCode(CompiledMaterial** materials, const uint32_t numMaterials);
const char* getShaderCode(const TargetCode* targetCode, uint32_t materialId);
uint32_t getReadOnlyBlockSize(const TargetCode* targetCode);
const uint8_t* getReadOnlyBlockData(const TargetCode* targetCode);
uint32_t getArgBufferSize(const TargetCode* targetCode);
const uint8_t* getArgBufferData(const TargetCode* targetCode);
uint32_t getResourceInfoSize(const TargetCode* targetCode);
const uint8_t* getResourceInfoData(const TargetCode* targetCode);
int registerResource(TargetCode* targetCode, int index);
uint32_t getMdlMaterialSize(const TargetCode* targetCode);
const uint8_t* getMdlMaterialData(const TargetCode* targetCode);
uint32_t getArgBlockOffset(const TargetCode* targetCode, uint32_t materialId);
uint32_t getReadOnlyOffset(const TargetCode* targetCode, uint32_t materialId);
uint32_t getTextureCount(const TargetCode* targetCode, uint32_t materialId);
const char* getTextureName(const TargetCode* targetCode, uint32_t materialId, uint32_t index);
const float* getTextureData(const TargetCode* targetCode, uint32_t materialId, uint32_t index);
const char* getTextureType(const TargetCode* targetCode, uint32_t materialId, uint32_t index);
uint32_t getTextureWidth(const TargetCode* targetCode, uint32_t materialId, uint32_t index);
uint32_t getTextureHeight(const TargetCode* targetCode, uint32_t materialId, uint32_t index);
uint32_t getTextureBytesPerTexel(const TargetCode* targetCode, uint32_t materialId, uint32_t index);
MaterialManager();
~MaterialManager();
};
} // namespace oka
| 3,244 | C | 34.65934 | 112 | 0.733046 |
arhix52/Strelka/include/materialmanager/mdlPtxCodeGen.h | #pragma once
#include "mdlLogger.h"
#include "mdlRuntime.h"
#include <MaterialXCore/Document.h>
#include <MaterialXFormat/File.h>
#include <MaterialXGenShader/ShaderGenerator.h>
#include <mi/mdl_sdk.h>
namespace oka
{
class MdlPtxCodeGen
{
public:
explicit MdlPtxCodeGen(){};
bool init(MdlRuntime& runtime);
struct InternalMaterialInfo
{
mi::Size argument_block_index;
};
bool setOptionBinary(const char* name, const char* data, size_t size);
mi::base::Handle<const mi::neuraylib::ITarget_code> translate(
const mi::neuraylib::ICompiled_material* material,
std::string& ptxSrc,
InternalMaterialInfo& internalsInfo);
mi::base::Handle<const mi::neuraylib::ITarget_code> translate(
const std::vector<const mi::neuraylib::ICompiled_material*>& materials,
std::string& ptxSrc,
std::vector<InternalMaterialInfo>& internalsInfo);
private:
bool appendMaterialToLinkUnit(uint32_t idx,
const mi::neuraylib::ICompiled_material* compiledMaterial,
mi::neuraylib::ILink_unit* linkUnit,
mi::Size& argBlockIndex);
std::unique_ptr<MdlNeurayLoader> mLoader;
mi::base::Handle<MdlLogger> mLogger;
mi::base::Handle<mi::neuraylib::IMdl_backend> mBackend;
mi::base::Handle<mi::neuraylib::IDatabase> mDatabase;
mi::base::Handle<mi::neuraylib::ITransaction> mTransaction;
mi::base::Handle<mi::neuraylib::IMdl_execution_context> mContext;
};
} // namespace oka
| 1,564 | C | 29.096153 | 92 | 0.663683 |
arhix52/Strelka/include/materialmanager/mdlNeurayLoader.h | // Copyright (C) 2021 Pablo Delgado Krämer
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program. If not, see <https://www.gnu.org/licenses/>.
#pragma once
#include <mi/base/handle.h>
#include <mi/neuraylib/ineuray.h>
namespace oka
{
class MdlNeurayLoader
{
public:
MdlNeurayLoader();
~MdlNeurayLoader();
public:
bool init(const char* resourcePath, const char* imagePluginPath);
mi::base::Handle<mi::neuraylib::INeuray> getNeuray() const;
private:
bool loadDso(const char* resourcePath);
bool loadNeuray();
bool loadPlugin(const char* imagePluginPath);
void unloadDso();
private:
void* mDsoHandle;
mi::base::Handle<mi::neuraylib::INeuray> mNeuray;
};
}
| 1,359 | C | 29.222222 | 106 | 0.668138 |
arhix52/Strelka/include/materialmanager/mdlMaterialCompiler.h | // Copyright (C) 2021 Pablo Delgado Krämer
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program. If not, see <https://www.gnu.org/licenses/>.
#pragma once
#include <string>
#include <mi/base/handle.h>
#include <mi/neuraylib/icompiled_material.h>
#include <mi/neuraylib/idatabase.h>
#include <mi/neuraylib/itransaction.h>
#include <mi/neuraylib/imaterial_instance.h>
#include <mi/neuraylib/imdl_impexp_api.h>
#include <mi/neuraylib/imdl_execution_context.h>
#include <mi/neuraylib/imdl_factory.h>
#include "mdlRuntime.h"
namespace oka
{
class MdlMaterialCompiler
{
public:
MdlMaterialCompiler(MdlRuntime& runtime);
public:
bool createModule(const std::string& identifier,
std::string& moduleName);
bool createMaterialInstace(const char* moduleName, const char* identifier,
mi::base::Handle<mi::neuraylib::IFunction_call>& matInstance);
bool compileMaterial(mi::base::Handle<mi::neuraylib::IFunction_call>& instance,
mi::base::Handle<mi::neuraylib::ICompiled_material>& compiledMaterial);
mi::base::Handle<mi::neuraylib::IMdl_factory>& getFactory();
mi::base::Handle<mi::neuraylib::ITransaction>& getTransaction();
private:
mi::base::Handle<MdlLogger> mLogger;
mi::base::Handle<mi::neuraylib::IDatabase> mDatabase;
mi::base::Handle<mi::neuraylib::ITransaction> mTransaction;
mi::base::Handle<mi::neuraylib::IMdl_factory> mFactory;
mi::base::Handle<mi::neuraylib::IMdl_impexp_api> mImpExpApi;
};
}
| 2,165 | C | 35.711864 | 106 | 0.687298 |
arhix52/Strelka/include/materialmanager/mdlRuntime.h | // Copyright (C) 2021 Pablo Delgado Krämer
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program. If not, see <https://www.gnu.org/licenses/>.
#pragma once
#include "mdlLogger.h"
#include "mdlNeurayLoader.h"
#include <mi/base/handle.h>
#include <mi/neuraylib/idatabase.h>
#include <mi/neuraylib/itransaction.h>
#include <mi/neuraylib/imdl_backend_api.h>
#include <mi/neuraylib/imdl_impexp_api.h>
#include <mi/neuraylib/imdl_factory.h>
#include <mi/mdl_sdk.h>
#include <memory>
#include <vector>
namespace oka
{
class MdlRuntime
{
public:
MdlRuntime();
~MdlRuntime();
public:
bool init(const char* paths[], uint32_t numPaths, const char* neurayPath, const char* imagePluginPath);
mi::base::Handle<MdlLogger> getLogger();
mi::base::Handle<mi::neuraylib::IDatabase> getDatabase();
mi::base::Handle<mi::neuraylib::ITransaction> getTransaction();
mi::base::Handle<mi::neuraylib::IMdl_factory> getFactory();
mi::base::Handle<mi::neuraylib::IMdl_impexp_api> getImpExpApi();
mi::base::Handle<mi::neuraylib::IMdl_backend_api> getBackendApi();
mi::base::Handle<mi::neuraylib::INeuray> getNeuray();
mi::base::Handle<mi::neuraylib::IMdl_configuration> getConfig();
std::unique_ptr<MdlNeurayLoader> mLoader;
private:
mi::base::Handle<MdlLogger> mLogger;
mi::base::Handle<mi::neuraylib::IDatabase> mDatabase;
mi::base::Handle<mi::neuraylib::ITransaction> mTransaction;
mi::base::Handle<mi::neuraylib::IMdl_factory> mFactory;
mi::base::Handle<mi::neuraylib::IMdl_backend_api> mBackendApi;
mi::base::Handle<mi::neuraylib::IMdl_impexp_api> mImpExpApi;
mi::base::Handle<mi::neuraylib::IMdl_configuration> mConfig;
};
} // namespace oka
| 2,369 | C | 34.373134 | 107 | 0.68721 |
arhix52/Strelka/include/materialmanager/mtlxMdlCodeGen.h | // Copyright (C) 2021 Pablo Delgado Krämer
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program. If not, see <https://www.gnu.org/licenses/>.
#pragma once
#include <stdint.h>
#include <string>
#include <MaterialXCore/Document.h>
#include <MaterialXFormat/File.h>
#include <MaterialXGenShader/ShaderGenerator.h>
#include "mdlRuntime.h"
namespace oka
{
class MtlxMdlCodeGen
{
public:
explicit MtlxMdlCodeGen(const char* mtlxlibPath, MdlRuntime* mdlRuntime);
public:
bool translate(const char* mtlxSrc, std::string& mdlSrc, std::string& subIdentifier);
private:
const MaterialX::FileSearchPath mMtlxlibPath;
MaterialX::DocumentPtr mStdLib;
MaterialX::ShaderGeneratorPtr mShaderGen;
MdlRuntime* mMdlRuntime;
};
}
| 1,400 | C | 30.840908 | 106 | 0.695714 |
arhix52/Strelka/include/render/buffer.h | #pragma once
#include "common.h"
#include <stdint.h>
#include <vector>
namespace oka
{
enum class BufferFormat : char
{
UNSIGNED_BYTE4,
FLOAT4,
FLOAT3
};
struct BufferDesc
{
uint32_t width;
uint32_t height;
BufferFormat format;
};
class Buffer
{
public:
virtual ~Buffer() = default;
virtual void resize(uint32_t width, uint32_t height) = 0;
virtual void* map() = 0;
virtual void unmap() = 0;
uint32_t width() const
{
return mWidth;
}
uint32_t height() const
{
return mHeight;
}
// Get output buffer
virtual void* getHostPointer()
{
return mHostData.data();
}
virtual size_t getHostDataSize()
{
return mHostData.size();
}
static size_t getElementSize(BufferFormat format)
{
switch (format)
{
case BufferFormat::FLOAT4:
return 4 * sizeof(float);
break;
case BufferFormat::FLOAT3:
return 3 * sizeof(float);
break;
case BufferFormat::UNSIGNED_BYTE4:
return 4 * sizeof(char);
break;
default:
break;
}
assert(0);
return 0;
}
size_t getElementSize() const
{
return Buffer::getElementSize(mFormat);
}
BufferFormat getFormat() const
{
return mFormat;
}
protected:
uint32_t mWidth = 0u;
uint32_t mHeight = 0u;
BufferFormat mFormat;
std::vector<char> mHostData;
};
struct ImageBuffer
{
void* data = nullptr;
size_t dataSize = 0;
unsigned int width = 0;
unsigned int height = 0;
BufferFormat pixel_format;
};
} // namespace oka
| 1,699 | C | 16 | 61 | 0.567393 |
arhix52/Strelka/include/render/Lights.h | #pragma once
#include <vector_types.h>
#include <sutil/vec_math.h>
struct UniformLight
{
float4 points[4];
float4 color;
float4 normal;
int type;
float halfAngle;
float pad0;
float pad1;
};
struct LightSampleData
{
float3 pointOnLight;
float pdf;
float3 normal;
float area;
float3 L;
float distToLight;
};
__forceinline__ __device__ float misWeightBalance(const float a, const float b)
{
return 1.0f / ( 1.0f + (b / a) );
}
static __inline__ __device__ float calcLightArea(const UniformLight& l)
{
float area = 0.0f;
if (l.type == 0) // rectangle area
{
float3 e1 = make_float3(l.points[1]) - make_float3(l.points[0]);
float3 e2 = make_float3(l.points[3]) - make_float3(l.points[0]);
area = length(cross(e1, e2));
}
else if (l.type == 1) // disc area
{
area = M_PIf * l.points[0].x * l.points[0].x; // pi * radius^2
}
else if (l.type == 2) // sphere area
{
area = 4.0f * M_PIf * l.points[0].x * l.points[0].x; // 4 * pi * radius^2
}
return area;
}
static __inline__ __device__ float3 calcLightNormal(const UniformLight& l, const float3 hitPoint)
{
float3 norm = make_float3(0.0f);
if (l.type == 0)
{
float3 e1 = make_float3(l.points[1]) - make_float3(l.points[0]);
float3 e2 = make_float3(l.points[3]) - make_float3(l.points[0]);
norm = -normalize(cross(e1, e2));
}
else if (l.type == 1)
{
norm = make_float3(l.normal);
}
else if (l.type == 2)
{
norm = normalize(hitPoint - make_float3(l.points[1]));
}
return norm;
}
static __inline__ __device__ void fillLightData(const UniformLight& l, const float3 hitPoint, LightSampleData& lightSampleData)
{
lightSampleData.area = calcLightArea(l);
lightSampleData.normal = calcLightNormal(l, hitPoint);
const float3 toLight = lightSampleData.pointOnLight - hitPoint;
const float lenToLight = length(toLight);
lightSampleData.L = toLight / lenToLight;
lightSampleData.distToLight = lenToLight;
}
struct SphQuad
{
float3 o, x, y, z;
float z0, z0sq;
float x0, y0, y0sq; // rectangle coords in ’R’
float x1, y1, y1sq;
float b0, b1, b0sq, k;
float S;
};
// Precomputation of constants for the spherical rectangle Q.
static __device__ SphQuad init(const UniformLight& l, const float3 o)
{
SphQuad squad;
float3 ex = make_float3(l.points[1]) - make_float3(l.points[0]);
float3 ey = make_float3(l.points[3]) - make_float3(l.points[0]);
float3 s = make_float3(l.points[0]);
float exl = length(ex);
float eyl = length(ey);
squad.o = o;
squad.x = ex / exl;
squad.y = ey / eyl;
squad.z = cross(squad.x, squad.y);
// compute rectangle coords in local reference system
float3 d = s - o;
squad.z0 = dot(d, squad.z);
// flip ’z’ to make it point against ’Q’
if (squad.z0 > 0)
{
squad.z *= -1;
squad.z0 *= -1;
}
squad.z0sq = squad.z0 * squad.z0;
squad.x0 = dot(d, squad.x);
squad.y0 = dot(d, squad.y);
squad.x1 = squad.x0 + exl;
squad.y1 = squad.y0 + eyl;
squad.y0sq = squad.y0 * squad.y0;
squad.y1sq = squad.y1 * squad.y1;
// create vectors to four vertices
float3 v00 = { squad.x0, squad.y0, squad.z0 };
float3 v01 = { squad.x0, squad.y1, squad.z0 };
float3 v10 = { squad.x1, squad.y0, squad.z0 };
float3 v11 = { squad.x1, squad.y1, squad.z0 };
// compute normals to edges
float3 n0 = normalize(cross(v00, v10));
float3 n1 = normalize(cross(v10, v11));
float3 n2 = normalize(cross(v11, v01));
float3 n3 = normalize(cross(v01, v00));
// compute internal angles (gamma_i)
float g0 = acos(-dot(n0, n1));
float g1 = acos(-dot(n1, n2));
float g2 = acos(-dot(n2, n3));
float g3 = acos(-dot(n3, n0));
// compute predefined constants
squad.b0 = n0.z;
squad.b1 = n2.z;
squad.b0sq = squad.b0 * squad.b0;
squad.k = 2.0f * M_PIf - g2 - g3;
// compute solid angle from internal angles
squad.S = g0 + g1 - squad.k;
return squad;
}
static __device__ float3 SphQuadSample(const SphQuad& squad, const float2 uv)
{
float u = uv.x;
float v = uv.y;
// 1. compute cu
float au = u * squad.S + squad.k;
float fu = (cosf(au) * squad.b0 - squad.b1) / sinf(au);
float cu = 1.0f / sqrtf(fu * fu + squad.b0sq) * (fu > 0.0f ? 1.0f : -1.0f);
cu = clamp(cu, -1.0f, 1.0f); // avoid NaNs
// 2. compute xu
float xu = -(cu * squad.z0) / sqrtf(1.0f - cu * cu);
xu = clamp(xu, squad.x0, squad.x1); // avoid Infs
// 3. compute yv
float d = sqrtf(xu * xu + squad.z0sq);
float h0 = squad.y0 / sqrtf(d * d + squad.y0sq);
float h1 = squad.y1 / sqrtf(d * d + squad.y1sq);
float hv = h0 + v * (h1 - h0);
float hv2 = hv * hv;
float eps = 1e-5f;
float yv = (hv < 1.0f - eps) ? (hv * d) / sqrtf(1 - hv2) : squad.y1;
// 4. transform (xu, yv, z0) to world coords
return (squad.o + xu * squad.x + yv * squad.y + squad.z0 * squad.z);
}
static __inline__ __device__ float getLightPdf(const UniformLight& l, const float3 hitPoint)
{
SphQuad quad = init(l, hitPoint);
if (quad.S <= 0.0f)
{
return 0.0f;
}
return 1.0f / quad.S;
}
static __inline__ __device__ float getRectLightPdf(const UniformLight& l, const float3 lightHitPoint, const float3 surfaceHitPoint)
{
LightSampleData lightSampleData {};
lightSampleData.pointOnLight = lightHitPoint;
fillLightData(l, surfaceHitPoint, lightSampleData);
lightSampleData.pdf = lightSampleData.distToLight * lightSampleData.distToLight /
(dot(-lightSampleData.L, lightSampleData.normal) * lightSampleData.area);
return lightSampleData.pdf;
}
static __inline__ __device__ float getDirectLightPdf(float angle)
{
return 1.0f / (2.0f * M_PIf * (1.0f - cos(angle)));
}
static __inline__ __device__ float getSphereLightPdf()
{
return 1.0f / (4.0f * M_PIf);
}
static __inline__ __device__ float getLightPdf(const UniformLight& l,
const float3 lightHitPoint,
const float3 surfaceHitPoint)
{
switch (l.type)
{
case 0:
// Rect
return getRectLightPdf(l, lightHitPoint, surfaceHitPoint);
break;
case 2:
// sphere
return getSphereLightPdf();
break;
case 3:
// Distant
return getDirectLightPdf(l.halfAngle);
break;
default:
break;
}
return 0.0f;
}
static __inline__ __device__ LightSampleData SampleRectLight(const UniformLight& l, const float2 u, const float3 hitPoint)
{
LightSampleData lightSampleData;
float3 e1 = make_float3(l.points[1]) - make_float3(l.points[0]);
float3 e2 = make_float3(l.points[3]) - make_float3(l.points[0]);
// lightSampleData.pointOnLight = make_float3(l.points[0]) + e1 * u.x + e2 * u.y;
// https://www.arnoldrenderer.com/research/egsr2013_spherical_rectangle.pdf
SphQuad quad = init(l, hitPoint);
if (quad.S <= 0.0f)
{
lightSampleData.pdf = 0.0f;
lightSampleData.pointOnLight = make_float3(l.points[0]) + e1 * u.x + e2 * u.y;
fillLightData(l, hitPoint, lightSampleData);
return lightSampleData;
}
if (quad.S < 1e-3f)
{
// just use uniform, because rectangle too small
lightSampleData.pointOnLight = make_float3(l.points[0]) + e1 * u.x + e2 * u.y;
fillLightData(l, hitPoint, lightSampleData);
lightSampleData.pdf = lightSampleData.distToLight * lightSampleData.distToLight /
(-dot(lightSampleData.L, lightSampleData.normal) * lightSampleData.area);
return lightSampleData;
}
lightSampleData.pointOnLight = SphQuadSample(quad, u);
fillLightData(l, hitPoint, lightSampleData);
lightSampleData.pdf = 1.0f / quad.S;
return lightSampleData;
}
static __inline__ __device__ LightSampleData SampleRectLightUniform(const UniformLight& l, const float2 u, const float3 hitPoint)
{
LightSampleData lightSampleData;
// // uniform sampling
float3 e1 = make_float3(l.points[1]) - make_float3(l.points[0]);
float3 e2 = make_float3(l.points[3]) - make_float3(l.points[0]);
lightSampleData.pointOnLight = make_float3(l.points[0]) + e1 * u.x + e2 * u.y;
fillLightData(l, hitPoint, lightSampleData);
// here is conversion from are to solid angle: dist2 / cos
lightSampleData.pdf = lightSampleData.distToLight * lightSampleData.distToLight /
(-dot(lightSampleData.L, lightSampleData.normal) * lightSampleData.area);
return lightSampleData;
}
static __device__ void createCoordinateSystem(const float3& N, float3& Nt, float3& Nb) {
if (fabs(N.x) > fabs(N.y)) {
float invLen = 1.0f / sqrt(N.x * N.x + N.z * N.z);
Nt = make_float3(-N.z * invLen, 0.0f, N.x * invLen);
} else {
float invLen = 1.0f / sqrt(N.y * N.y + N.z * N.z);
Nt = make_float3(0.0f, N.z * invLen, -N.y * invLen);
}
Nb = cross(N, Nt);
}
static __device__ float3 SampleCone(float2 uv, float angle, float3 direction, float& pdf) {
float phi = 2.0 * M_PIf * uv.x;
float cosTheta = 1.0 - uv.y * (1.0 - cos(angle));
// Convert spherical coordinates to 3D direction
float sinTheta = sqrt(1.0 - cosTheta * cosTheta);
float3 u, v;
createCoordinateSystem(direction, u, v);
float3 sampledDir = normalize(cos(phi) * sinTheta * u + sin(phi) * sinTheta * v + cosTheta * direction);
// Calculate the PDF for the sampled direction
pdf = 1.0 / (2.0 * M_PIf * (1.0 - cos(angle)));
return sampledDir;
}
static __inline__ __device__ LightSampleData SampleDistantLight(const UniformLight& l, const float2 u, const float3 hitPoint)
{
LightSampleData lightSampleData;
float pdf = 0.0f;
float3 coneSample = SampleCone(u, l.halfAngle, -make_float3(l.normal), pdf);
lightSampleData.area = 0.0f;
lightSampleData.distToLight = 1e9;
lightSampleData.L = coneSample;
lightSampleData.normal = make_float3(l.normal);
lightSampleData.pdf = pdf;
lightSampleData.pointOnLight = coneSample;
return lightSampleData;
}
static __inline__ __device__ LightSampleData SampleSphereLight(const UniformLight& l, const float2 u, const float3 hitPoint)
{
LightSampleData lightSampleData;
// Generate a random direction on the sphere using solid angle sampling
float cosTheta = 1.0f - 2.0f * u.x; // cosTheta is uniformly distributed between [-1, 1]
float sinTheta = sqrt(1.0f - cosTheta * cosTheta);
float phi = 2.0f * M_PIf * u.y; // phi is uniformly distributed between [0, 2*pi]
const float radius = l.points[0].x;
// Convert spherical coordinates to Cartesian coordinates
float3 sphereDirection = make_float3(sinTheta * cos(phi), sinTheta * sin(phi), cosTheta);
// Scale the direction by the radius of the sphere and move it to the light position
float3 lightPoint = make_float3(l.points[1]) + radius * sphereDirection;
// Calculate the direction from the hit point to the sampled point on the light
lightSampleData.L = normalize(lightPoint - hitPoint);
// Calculate the distance to the light
lightSampleData.distToLight = length(lightPoint - hitPoint);
lightSampleData.area = 0.0f;
lightSampleData.normal = sphereDirection;
lightSampleData.pdf = 1.0f / (4.0f * M_PIf);
lightSampleData.pointOnLight = lightPoint;
return lightSampleData;
}
| 11,611 | C | 30.988981 | 131 | 0.623374 |
arhix52/Strelka/include/render/Camera.h | #pragma once
#include <glm/glm.hpp>
#include <glm/gtx/compatibility.hpp>
class Camera
{
public:
Camera()
: m_eye(glm::float3(1.0f)),
m_lookat(glm::float3(0.0f)),
m_up(glm::float3(0.0f, 1.0f, 0.0f)),
m_fovY(35.0f),
m_aspectRatio(1.0f)
{
}
Camera(const glm::float3& eye, const glm::float3& lookat, const glm::float3& up, float fovY, float aspectRatio)
: m_eye(eye), m_lookat(lookat), m_up(up), m_fovY(fovY), m_aspectRatio(aspectRatio)
{
}
glm::float3 direction() const
{
return glm::normalize(m_lookat - m_eye);
}
void setDirection(const glm::float3& dir)
{
m_lookat = m_eye + glm::length(m_lookat - m_eye) * dir;
}
const glm::float3& eye() const
{
return m_eye;
}
void setEye(const glm::float3& val)
{
m_eye = val;
}
const glm::float3& lookat() const
{
return m_lookat;
}
void setLookat(const glm::float3& val)
{
m_lookat = val;
}
const glm::float3& up() const
{
return m_up;
}
void setUp(const glm::float3& val)
{
m_up = val;
}
const float& fovY() const
{
return m_fovY;
}
void setFovY(const float& val)
{
m_fovY = val;
}
const float& aspectRatio() const
{
return m_aspectRatio;
}
void setAspectRatio(const float& val)
{
m_aspectRatio = val;
}
// UVW forms an orthogonal, but not orthonormal basis!
void UVWFrame(glm::float3& U, glm::float3& V, glm::float3& W) const
{
W = m_lookat - m_eye; // Do not normalize W -- it implies focal length
float wlen = glm::length(W);
U = glm::normalize(glm::cross(W, m_up));
V = glm::normalize(glm::cross(U, W));
float vlen = wlen * tanf(0.5f * m_fovY * M_PI / 180.0f);
V *= vlen;
float ulen = vlen * m_aspectRatio;
U *= ulen;
}
private:
glm::float3 m_eye;
glm::float3 m_lookat;
glm::float3 m_up;
float m_fovY;
float m_aspectRatio;
};
| 2,098 | C | 21.329787 | 115 | 0.534795 |
arhix52/Strelka/include/render/render.h | #pragma once
#include "common.h"
#include "buffer.h"
#include <scene/scene.h>
namespace oka
{
enum class RenderType : int
{
eOptiX = 0,
eMetal,
eCompute,
};
/**
* Render interface
*/
class Render
{
public:
virtual ~Render() = default;
virtual void init() = 0;
virtual void render(Buffer* output) = 0;
virtual Buffer* createBuffer(const BufferDesc& desc) = 0;
virtual void* getNativeDevicePtr()
{
return nullptr;
}
void setSharedContext(SharedContext* ctx)
{
mSharedCtx = ctx;
}
SharedContext& getSharedContext()
{
return *mSharedCtx;
}
void setScene(Scene* scene)
{
mScene = scene;
}
Scene* getScene()
{
return mScene;
}
protected:
SharedContext* mSharedCtx = nullptr;
oka::Scene* mScene = nullptr;
};
class RenderFactory
{
public:
static Render* createRender(RenderType type);
};
} // namespace oka
| 954 | C | 13.692307 | 61 | 0.610063 |
arhix52/Strelka/include/render/common.h | #pragma once
#include <glm/glm.hpp>
#include <glm/gtx/compatibility.hpp>
#include <settings/settings.h>
namespace oka
{
static constexpr int MAX_FRAMES_IN_FLIGHT = 3;
#ifdef __APPLE__
struct float3;
struct float4;
#else
using Float3 = glm::float3;
using Float4 = glm::float4;
#endif
class Render;
struct SharedContext
{
size_t mFrameNumber = 0;
size_t mSubframeIndex = 0;
SettingsManager* mSettingsManager = nullptr;
Render* mRender = nullptr;
};
enum class Result : uint32_t
{
eOk,
eFail,
eOutOfMemory,
};
} // namespace oka
| 562 | C | 13.815789 | 48 | 0.69395 |
arhix52/Strelka/include/render/materials.h | #pragma once
#ifdef __cplusplus
# define GLM_FORCE_SILENT_WARNINGS
# define GLM_LANG_STL11_FORCED
# define GLM_ENABLE_EXPERIMENTAL
# define GLM_FORCE_CTOR_INIT
# define GLM_FORCE_RADIANS
# define GLM_FORCE_DEPTH_ZERO_TO_ONE
# include <glm/glm.hpp>
# include <glm/gtx/compatibility.hpp>
# define float4 glm::float4
# define float3 glm::float3
# define uint glm::uint
#endif
struct MdlMaterial
{
int arg_block_offset = 0; // in bytes
int ro_data_segment_offset = 0; // in bytes
int functionId = 0;
int pad1;
};
/// Information passed to GPU for mapping id requested in the runtime functions to buffer
/// views of the corresponding type.
struct Mdl_resource_info
{
// index into the tex2d, tex3d, ... buffers, depending on the type requested
uint gpu_resource_array_start;
uint pad0;
uint pad1;
uint pad2;
};
#ifdef __cplusplus
# undef float4
# undef float3
# undef uint
#endif
| 958 | C | 22.390243 | 89 | 0.679541 |
arhix52/Strelka/include/settings/settings.h | #pragma once
#include <iostream>
#include <string>
#include <unordered_map>
#include <cassert>
namespace oka
{
class SettingsManager
{
private:
/* data */
std::unordered_map<std::string, std::string> mMap;
void isNameValid(const char* name)
{
if (mMap.find(name) == mMap.end())
{
std::cerr << "The setting " << name << " does not exist" << std::endl;
assert(0);
}
}
public:
SettingsManager(/* args */) = default;
~SettingsManager() = default;
template <typename T>
void setAs(const char* name, const T& value)
{
// mMap[name] = std::to_string(value);
mMap[name] = toString(value);
}
template <typename T>
T getAs(const char* name)
{
isNameValid(name);
return convertValue<T>(mMap[name]);
}
private:
template <typename T>
T convertValue(const std::string& value)
{
// Default implementation for non-specialized types
return T{};
}
static std::string toString(const std::string& value)
{
return value;
}
template <typename T>
std::string toString(const T& value)
{
// Default implementation for non-specialized types
return std::to_string(value);
}
};
template<>
inline void SettingsManager::setAs(const char* name, const std::string& value)
{
mMap[name] = value;
}
template <>
inline bool SettingsManager::convertValue(const std::string& value)
{
return static_cast<bool>(atoi(value.c_str()));
}
template <>
inline float SettingsManager::convertValue(const std::string& value)
{
return static_cast<float>(atof(value.c_str()));
}
template <>
inline uint32_t SettingsManager::convertValue(const std::string& value)
{
return static_cast<uint32_t>(atoi(value.c_str()));
}
template <>
inline std::string SettingsManager::convertValue(const std::string& value)
{
return value;
}
template <>
inline std::string SettingsManager::toString(const std::string& value)
{
return value;
}
} // namespace oka
| 2,057 | C | 19.17647 | 82 | 0.628585 |
arhix52/Strelka/include/scene/camera.h | #pragma once
#define GLM_FORCE_SILENT_WARNINGS
#define GLM_FORCE_RADIANS
#define GLM_FORCE_DEPTH_ZERO_TO_ONE
#include <glm/glm.hpp>
#include <glm/gtc/matrix_transform.hpp>
#include <glm/gtc/quaternion.hpp>
#include <glm/gtx/compatibility.hpp>
#include <string>
namespace oka
{
class Camera
{
public:
std::string name = "Default camera";
int node = -1;
enum class CameraType : uint32_t
{
lookat,
firstperson
};
CameraType type = CameraType::firstperson;
float fov = 45.0f;
float znear = 0.1f, zfar = 1000.0f;
// View dir -Z
glm::quat mOrientation = { 1.0f, 0.0f, 0.0f, 0.0f };
glm::float3 position = { 0.0f, 0.0f, 10.0f };
glm::float3 mWorldUp = {0.0, 1.0, 0.0};
glm::float3 mWorldForward = {0.0, 0.0, -1.0};
glm::quat getOrientation();
float rotationSpeed = 0.025f;
float movementSpeed = 5.0f;
bool updated = false;
bool isDirty = true;
struct MouseButtons
{
bool left = false;
bool right = false;
bool middle = false;
} mouseButtons;
glm::float2 mousePos;
struct Matrices
{
glm::float4x4 perspective;
glm::float4x4 invPerspective;
glm::float4x4 view;
};
Matrices matrices;
void updateViewMatrix();
struct
{
bool left = false;
bool right = false;
bool up = false;
bool down = false;
bool forward = false;
bool back = false;
} keys;
glm::float3 getFront() const;
glm::float3 getUp() const;
glm::float3 getRight() const;
bool moving() const;
float getNearClip() const;
float getFarClip() const;
void setFov(float fov);
void setPerspective(float fov, float aspect, float znear, float zfar);
void setWorldUp(const glm::float3 up);
glm::float3 getWorldUp();
void setWorldForward(const glm::float3 forward);
glm::float3 getWorldForward();
glm::float4x4& getPerspective();
glm::float4x4 getView();
void updateAspectRatio(float aspect);
void setPosition(glm::float3 position);
glm::float3 getPosition();
void setRotation(glm::quat rotation);
void rotate(float, float);
void setTranslation(glm::float3 translation);
void translate(glm::float3 delta);
void update(float deltaTime);
};
} // namespace oka
| 2,328 | C | 23.010309 | 74 | 0.629725 |
arhix52/Strelka/include/scene/scene.h | #pragma once
#include "camera.h"
#include "glm-wrapper.hpp"
// #include "materials.h"
// #undef float4
// #undef float3
#include <cstdint>
#include <mutex>
#include <set>
#include <stack>
#include <unordered_map>
#include <vector>
#include <mutex>
#include <materialmanager/materialmanager.h>
namespace oka
{
struct Mesh
{
uint32_t mIndex; // Index of 1st index in index buffer
uint32_t mCount; // amount of indices in mesh
uint32_t mVbOffset; // start in vb
uint32_t mVertexCount; // number of vertices in mesh
};
struct Curve
{
enum class Type : uint8_t
{
eLinear,
eCubic,
};
uint32_t mVertexCountsStart;
uint32_t mVertexCountsCount;
uint32_t mPointsStart;
uint32_t mPointsCount;
uint32_t mWidthsStart;
uint32_t mWidthsCount;
};
struct Instance
{
glm::mat4 transform;
enum class Type : uint8_t
{
eMesh,
eLight,
eCurve
} type;
union
{
uint32_t mMeshId;
uint32_t mCurveId;
};
uint32_t mMaterialId = 0;
uint32_t mLightId = (uint32_t)-1;
};
class Scene
{
public:
struct MaterialDescription
{
enum class Type
{
eMdl,
eMaterialX
} type;
std::string code;
std::string file;
std::string name;
bool hasColor = false;
glm::float3 color;
std::vector<MaterialManager::Param> params;
};
struct Vertex
{
glm::float3 pos;
uint32_t tangent;
uint32_t normal;
uint32_t uv;
float pad0;
float pad1;
};
struct Node
{
std::string name;
glm::float3 translation;
glm::float3 scale;
glm::quat rotation;
int parent = -1;
std::vector<int> children;
};
std::vector<Node> mNodes;
enum class AnimationState : uint32_t
{
eStop,
ePlay,
eScroll,
};
AnimationState mAnimState = AnimationState::eStop;
struct AnimationSampler
{
enum class InterpolationType
{
LINEAR,
STEP,
CUBICSPLINE
};
InterpolationType interpolation;
std::vector<float> inputs;
std::vector<glm::float4> outputsVec4;
};
struct AnimationChannel
{
enum class PathType
{
TRANSLATION,
ROTATION,
SCALE
};
PathType path;
int node;
uint32_t samplerIndex;
};
struct Animation
{
std::string name;
std::vector<AnimationSampler> samplers;
std::vector<AnimationChannel> channels;
float start = std::numeric_limits<float>::max();
float end = std::numeric_limits<float>::min();
};
std::vector<Animation> mAnimations;
// GPU side structure
struct Light
{
glm::float4 points[4];
glm::float4 color = glm::float4(1.0f);
glm::float4 normal;
int type;
float halfAngle;
float pad0;
float pad1;
};
// CPU side structure
struct UniformLightDesc
{
int32_t type;
glm::float4x4 xform{ 1.0 };
glm::float3 position; // world position
glm::float3 orientation; // euler angles in degrees
bool useXform;
// OX - axis of light or normal
glm::float3 color;
float intensity;
// rectangle light
float width; // OY
float height; // OZ
// disc/sphere light
float radius;
// distant light
float halfAngle;
};
std::vector<UniformLightDesc> mLightDesc;
enum class DebugView : uint32_t
{
eNone = 0,
eNormals = 1,
eShadows = 2,
eLTC = 3,
eMotion = 4,
eCustomDebug = 5,
ePTDebug = 11
};
DebugView mDebugViewSettings = DebugView::eNone;
bool transparentMode = true;
bool opaqueMode = true;
glm::float4 mLightPosition{ 10.0, 10.0, 10.0, 1.0 };
std::mutex mMeshMutex;
std::mutex mInstanceMutex;
std::vector<Vertex> mVertices;
std::vector<uint32_t> mIndices;
std::vector<glm::float3> mCurvePoints;
std::vector<float> mCurveWidths;
std::vector<uint32_t> mCurveVertexCounts;
std::string modelPath;
std::string getSceneFileName();
std::string getSceneDir();
std::vector<Mesh> mMeshes;
std::vector<Curve> mCurves;
std::vector<Instance> mInstances;
std::vector<Light> mLights;
std::vector<uint32_t> mTransparentInstances;
std::vector<uint32_t> mOpaqueInstances;
Scene() = default;
~Scene() = default;
std::unordered_map<uint32_t, uint32_t> mLightIdToInstanceId{};
std::unordered_map<int32_t, std::string> mTexIdToTexName{};
std::vector<Vertex>& getVertices()
{
return mVertices;
}
std::vector<uint32_t>& getIndices()
{
return mIndices;
}
std::vector<MaterialDescription>& getMaterials()
{
return mMaterialsDescs;
}
std::vector<Light>& getLights()
{
return mLights;
}
std::vector<UniformLightDesc>& getLightsDesc()
{
return mLightDesc;
}
uint32_t findCameraByName(const std::string& name)
{
std::scoped_lock lock(mCameraMutex);
if (mNameToCamera.find(name) != mNameToCamera.end())
{
return mNameToCamera[name];
}
return (uint32_t)-1;
}
uint32_t addCamera(Camera& camera)
{
std::scoped_lock lock(mCameraMutex);
mCameras.push_back(camera);
// store camera index
mNameToCamera[camera.name] = (uint32_t)mCameras.size() - 1;
return (uint32_t)mCameras.size() - 1;
}
void updateCamera(Camera& camera, uint32_t index)
{
assert(index < mCameras.size());
std::scoped_lock lock(mCameraMutex);
mCameras[index] = camera;
}
Camera& getCamera(uint32_t index)
{
assert(index < mCameras.size());
std::scoped_lock lock(mCameraMutex);
return mCameras[index];
}
const std::vector<Camera>& getCameras()
{
std::scoped_lock lock(mCameraMutex);
return mCameras;
}
size_t getCameraCount()
{
std::scoped_lock lock(mCameraMutex);
return mCameras.size();
}
const std::vector<Instance>& getInstances() const
{
return mInstances;
}
const std::vector<Mesh>& getMeshes() const
{
return mMeshes;
}
const std::vector<Curve>& getCurves() const
{
return mCurves;
}
const std::vector<glm::float3>& getCurvesPoint() const
{
return mCurvePoints;
}
const std::vector<float>& getCurvesWidths() const
{
return mCurveWidths;
}
const std::vector<uint32_t>& getCurvesVertexCounts() const
{
return mCurveVertexCounts;
}
void updateCamerasParams(int width, int height)
{
for (Camera& camera : mCameras)
{
camera.updateAspectRatio((float)width / height);
}
}
glm::float4x4 getTransform(const Scene::UniformLightDesc& desc)
{
const glm::float4x4 translationMatrix = glm::translate(glm::float4x4(1.0f), desc.position);
glm::quat rotation = glm::quat(glm::radians(desc.orientation)); // to quaternion
const glm::float4x4 rotationMatrix{ rotation };
glm::float3 scale = { desc.width, desc.height, 1.0f };
const glm::float4x4 scaleMatrix = glm::scale(glm::float4x4(1.0f), scale);
const glm::float4x4 localTransform = translationMatrix * rotationMatrix * scaleMatrix;
return localTransform;
}
glm::float4x4 getTransformFromRoot(int nodeIdx)
{
std::stack<glm::float4x4> xforms;
while (nodeIdx != -1)
{
const Node& n = mNodes[nodeIdx];
glm::float4x4 xform = glm::translate(glm::float4x4(1.0f), n.translation) * glm::float4x4(n.rotation) *
glm::scale(glm::float4x4(1.0f), n.scale);
xforms.push(xform);
nodeIdx = n.parent;
}
glm::float4x4 xform = glm::float4x4(1.0);
while (!xforms.empty())
{
xform = xform * xforms.top();
xforms.pop();
}
return xform;
}
glm::float4x4 getTransform(int nodeIdx)
{
glm::float4x4 xform = glm::float4x4(1.0);
while (nodeIdx != -1)
{
const Node& n = mNodes[nodeIdx];
xform = glm::translate(glm::float4x4(1.0f), n.translation) * glm::float4x4(n.rotation) *
glm::scale(glm::float4x4(1.0f), n.scale) * xform;
nodeIdx = n.parent;
}
return xform;
}
glm::float4x4 getCameraTransform(int nodeIdx)
{
int child = mNodes[nodeIdx].children[0];
return getTransform(child);
}
void updateAnimation(const float dt);
void updateLight(uint32_t lightId, const UniformLightDesc& desc);
/// <summary>
/// Create Mesh geometry
/// </summary>
/// <param name="vb">Vertices</param>
/// <param name="ib">Indices</param>
/// <returns>Mesh id in scene</returns>
uint32_t createMesh(const std::vector<Vertex>& vb, const std::vector<uint32_t>& ib);
/// <summary>
/// Creates Instance
/// </summary>
/// <param name="meshId">valid mesh id</param>
/// <param name="materialId">valid material id</param>
/// <param name="transform">transform</param>
/// <returns>Instance id in scene</returns>
uint32_t createInstance(const Instance::Type type,
const uint32_t geomId,
const uint32_t materialId,
const glm::mat4& transform,
const uint32_t lightId = (uint32_t)-1);
uint32_t addMaterial(const MaterialDescription& material);
uint32_t createCurve(const Curve::Type type,
const std::vector<uint32_t>& vertexCounts,
const std::vector<glm::float3>& points,
const std::vector<float>& widths);
uint32_t createLight(const UniformLightDesc& desc);
/// <summary>
/// Removes instance/mesh/material
/// </summary>
/// <param name="meshId">valid mesh id</param>
/// <param name="materialId">valid material id</param>
/// <param name="instId">valid instance id</param>
/// <returns>Nothing</returns>
void removeInstance(uint32_t instId);
void removeMesh(uint32_t meshId);
void removeMaterial(uint32_t materialId);
std::vector<uint32_t>& getOpaqueInstancesToRender(const glm::float3& camPos);
std::vector<uint32_t>& getTransparentInstancesToRender(const glm::float3& camPos);
/// <summary>
/// Get set of DirtyInstances
/// </summary>
/// <returns>Set of instances</returns>
std::set<uint32_t> getDirtyInstances();
/// <summary>
/// Get Frame mode (bool)
/// </summary>
/// <returns>Bool</returns>
bool getFrMod();
/// <summary>
/// Updates Instance matrix(transform)
/// </summary>
/// <param name="instId">valid instance id</param>
/// <param name="newTransform">new transformation matrix</param>
/// <returns>Nothing</returns>
void updateInstanceTransform(uint32_t instId, glm::float4x4 newTransform);
/// <summary>
/// Changes status of scene and cleans up mDirty* sets
/// </summary>
/// <returns>Nothing</returns>
void beginFrame();
/// <summary>
/// Changes status of scene
/// </summary>
/// <returns>Nothing</returns>
void endFrame();
private:
std::vector<Camera> mCameras;
std::unordered_map<std::string, uint32_t> mNameToCamera;
std::mutex mCameraMutex;
std::stack<uint32_t> mDelInstances;
std::stack<uint32_t> mDelMesh;
std::stack<uint32_t> mDelMaterial;
std::vector<MaterialDescription> mMaterialsDescs;
uint32_t createRectLightMesh();
uint32_t createDiscLightMesh();
uint32_t createSphereLightMesh();
bool FrMod{};
std::set<uint32_t> mDirtyInstances;
uint32_t mRectLightMeshId = (uint32_t)-1;
uint32_t mDiskLightMeshId = (uint32_t)-1;
uint32_t mSphereLightMeshId = (uint32_t)-1;
};
} // namespace oka
| 12,324 | C | 24.256147 | 114 | 0.586498 |
arhix52/Strelka/include/scene/glm-wrapper.hpp | #pragma once
#define GLM_FORCE_SILENT_WARNINGS
#define GLM_LANG_STL11_FORCED
#define GLM_ENABLE_EXPERIMENTAL
#define GLM_FORCE_CTOR_INIT
#define GLM_FORCE_RADIANS
#define GLM_FORCE_DEPTH_ZERO_TO_ONE
#include <glm/vec2.hpp>
#include <glm/vec3.hpp>
#include <glm/vec4.hpp>
#include <glm/mat4x4.hpp>
#include <glm/gtx/hash.hpp>
#include <glm/gtc/matrix_transform.hpp>
#include <glm/gtx/compatibility.hpp>
| 403 | C++ | 24.249998 | 39 | 0.769231 |
arhix52/Strelka/include/log/logmanager.h | #pragma once
#include <spdlog/spdlog.h>
namespace oka
{
class Logmanager
{
public:
Logmanager(/* args */);
~Logmanager();
void initialize();
void shutdown();
};
} // namespace oka
| 200 | C | 10.166666 | 27 | 0.625 |
arhix52/Strelka/include/log/log.h | #pragma once
#include <spdlog/spdlog.h>
#define STRELKA_DEFAULT_LOGGER_NAME "Strelka"
#ifndef RELEASE
#define STRELKA_TRACE(...) if (spdlog::get(STRELKA_DEFAULT_LOGGER_NAME) != nullptr) {spdlog::get(STRELKA_DEFAULT_LOGGER_NAME)->trace(__VA_ARGS__);}
#define STRELKA_DEBUG(...) if (spdlog::get(STRELKA_DEFAULT_LOGGER_NAME) != nullptr) {spdlog::get(STRELKA_DEFAULT_LOGGER_NAME)->debug(__VA_ARGS__);}
#define STRELKA_INFO(...) if (spdlog::get(STRELKA_DEFAULT_LOGGER_NAME) != nullptr) {spdlog::get(STRELKA_DEFAULT_LOGGER_NAME)->info(__VA_ARGS__);}
#define STRELKA_WARNING(...) if (spdlog::get(STRELKA_DEFAULT_LOGGER_NAME) != nullptr) {spdlog::get(STRELKA_DEFAULT_LOGGER_NAME)->warn(__VA_ARGS__);}
#define STRELKA_ERROR(...) if (spdlog::get(STRELKA_DEFAULT_LOGGER_NAME) != nullptr) {spdlog::get(STRELKA_DEFAULT_LOGGER_NAME)->error(__VA_ARGS__);}
#define STRELKA_FATAL(...) if (spdlog::get(STRELKA_DEFAULT_LOGGER_NAME) != nullptr) {spdlog::get(STRELKA_DEFAULT_LOGGER_NAME)->critical(__VA_ARGS__);}
#else
define STRELKA_TRACE(...) void(0);
define STRELKA_DEBUG(...) void(0);
define STRELKA_INFO(...) void(0);
define STRELKA_WARNING(...) void(0);
define STRELKA_ERROR(...) void(0);
define STRELKA_FATAL(...) void(0);
#endif
| 1,219 | C | 54.454543 | 150 | 0.700574 |
arhix52/Strelka/include/sceneloader/gltfloader.h | #pragma once
#include <scene/scene.h>
#include <string>
#include <vector>
namespace oka
{
class GltfLoader
{
private:
public:
explicit GltfLoader(){}
bool loadGltf(const std::string& modelPath, Scene& mScene);
void computeTangent(std::vector<Scene::Vertex>& _vertices,
const std::vector<uint32_t>& _indices) const;
};
} // namespace oka
| 380 | C | 14.874999 | 69 | 0.655263 |
arhix52/Strelka/include/display/Display.h | #pragma once
#include <render/common.h>
#include <render/buffer.h>
#include <GLFW/glfw3.h>
namespace oka
{
class InputHandler
{
public:
virtual void keyCallback(int key, [[maybe_unused]] int scancode, int action, [[maybe_unused]] int mods) = 0;
virtual void mouseButtonCallback(int button, int action, [[maybe_unused]] int mods) = 0;
virtual void handleMouseMoveCallback([[maybe_unused]] double xpos, [[maybe_unused]] double ypos) = 0;
};
class ResizeHandler
{
public:
virtual void framebufferResize(int newWidth, int newHeight) = 0;
};
class Display
{
public:
Display()
{
}
virtual ~Display()
{
}
virtual void init(int width, int height, oka::SharedContext* ctx) = 0;
virtual void destroy() = 0;
void setWindowTitle(const char* title)
{
glfwSetWindowTitle(mWindow, title);
}
void setInputHandler(InputHandler* handler)
{
mInputHandler = handler;
}
InputHandler* getInputHandler()
{
return mInputHandler;
}
void setResizeHandler(ResizeHandler* handler)
{
mResizeHandler = handler;
}
ResizeHandler* getResizeHandler()
{
return mResizeHandler;
}
bool windowShouldClose()
{
return glfwWindowShouldClose(mWindow);
}
void pollEvents()
{
glfwPollEvents();
}
virtual void onBeginFrame() = 0;
virtual void onEndFrame() = 0;
virtual void drawFrame(ImageBuffer& result) = 0;
virtual void drawUI();
protected:
static void framebufferResizeCallback(GLFWwindow* window, int width, int height);
static void keyCallback(
GLFWwindow* window, int key, [[maybe_unused]] int scancode, int action, [[maybe_unused]] int mods);
static void mouseButtonCallback(GLFWwindow* window, int button, int action, int mods);
static void handleMouseMoveCallback(GLFWwindow* window, double xpos, double ypos);
static void scrollCallback(GLFWwindow* window, double xoffset, double yoffset);
int mWindowWidth = 800;
int mWindowHeight = 600;
InputHandler* mInputHandler = nullptr;
ResizeHandler* mResizeHandler = nullptr;
oka::SharedContext* mCtx = nullptr;
GLFWwindow* mWindow;
};
class DisplayFactory
{
public:
static Display* createDisplay();
};
} // namespace oka
| 2,313 | C | 21.25 | 112 | 0.671855 |
arhix52/Strelka/cuda/random.h | //
// Copyright (c) 2021, NVIDIA CORPORATION. All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
// * Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
// * Redistributions in binary form must reproduce the above copyright
// notice, this list of conditions and the following disclaimer in the
// documentation and/or other materials provided with the distribution.
// * Neither the name of NVIDIA CORPORATION nor the names of its
// contributors may be used to endorse or promote products derived
// from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ``AS IS'' AND ANY
// EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
// IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
// PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
// CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
// EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
// PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
// PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY
// OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
// (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
// OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
//
#pragma once
template<unsigned int N>
static __host__ __device__ __inline__ unsigned int tea( unsigned int val0, unsigned int val1 )
{
unsigned int v0 = val0;
unsigned int v1 = val1;
unsigned int s0 = 0;
for( unsigned int n = 0; n < N; n++ )
{
s0 += 0x9e3779b9;
v0 += ((v1<<4)+0xa341316c)^(v1+s0)^((v1>>5)+0xc8013ea4);
v1 += ((v0<<4)+0xad90777d)^(v0+s0)^((v0>>5)+0x7e95761e);
}
return v0;
}
// Generate random unsigned int in [0, 2^24)
static __host__ __device__ __inline__ unsigned int lcg(unsigned int &prev)
{
const unsigned int LCG_A = 1664525u;
const unsigned int LCG_C = 1013904223u;
prev = (LCG_A * prev + LCG_C);
return prev & 0x00FFFFFF;
}
static __host__ __device__ __inline__ unsigned int lcg2(unsigned int &prev)
{
prev = (prev*8121 + 28411) % 134456;
return prev;
}
// Generate random float in [0, 1)
static __host__ __device__ __inline__ float rnd(unsigned int &prev)
{
return ((float) lcg(prev) / (float) 0x01000000);
}
static __host__ __device__ __inline__ unsigned int rot_seed( unsigned int seed, unsigned int frame )
{
return seed ^ frame;
}
| 2,678 | C | 35.69863 | 100 | 0.707244 |
arhix52/Strelka/cuda/curve.h | //
// Copyright (c) 2021, NVIDIA CORPORATION. All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
// * Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
// * Redistributions in binary form must reproduce the above copyright
// notice, this list of conditions and the following disclaimer in the
// documentation and/or other materials provided with the distribution.
// * Neither the name of NVIDIA CORPORATION nor the names of its
// contributors may be used to endorse or promote products derived
// from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ``AS IS'' AND ANY
// EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
// IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
// PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
// CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
// EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
// PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
// PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY
// OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
// (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
// OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
//
#pragma once
#include <optix.h>
#include <sutil/vec_math.h>
#include <vector_types.h>
//
// First order polynomial interpolator
//
struct LinearInterpolator
{
__device__ __forceinline__ LinearInterpolator() {}
__device__ __forceinline__ void initialize( const float4* q )
{
p[0] = q[0];
p[1] = q[1] - q[0];
}
__device__ __forceinline__ float4 position4( float u ) const
{
return p[0] + u * p[1]; // Horner scheme
}
__device__ __forceinline__ float3 position3( float u ) const
{
return make_float3( position4( u ) );
}
__device__ __forceinline__ float radius( const float& u ) const
{
return position4( u ).w;
}
__device__ __forceinline__ float4 velocity4( float u ) const
{
return p[1];
}
__device__ __forceinline__ float3 velocity3( float u ) const
{
return make_float3( velocity4( u ) );
}
__device__ __forceinline__ float derivative_of_radius( float u ) const
{
return velocity4( u ).w;
}
__device__ __forceinline__ float3 acceleration3( float u ) const { return make_float3( 0.f ); }
__device__ __forceinline__ float4 acceleration4( float u ) const { return make_float4( 0.f ); }
float4 p[2];
};
//
// Second order polynomial interpolator
//
struct QuadraticInterpolator
{
__device__ __forceinline__ QuadraticInterpolator() {}
__device__ __forceinline__ void initializeFromBSpline( const float4* q )
{
// Bspline-to-Poly = Matrix([[1/2, -1, 1/2],
// [-1, 1, 0],
// [1/2, 1/2, 0]])
p[0] = ( q[0] - 2.0f * q[1] + q[2] ) / 2.0f;
p[1] = ( -2.0f * q[0] + 2.0f * q[1] ) / 2.0f;
p[2] = ( q[0] + q[1] ) / 2.0f;
}
__device__ __forceinline__ void export2BSpline( float4 bs[3] ) const
{
// inverse of initializeFromBSpline
// Bspline-to-Poly = Matrix([[1/2, -1, 1/2],
// [-1, 1, 0],
// [1/2, 1/2, 0]])
// invert to get:
// Poly-to-Bspline = Matrix([[0, -1/2, 1],
// [0, 1/2, 1],
// [2, 3/2, 1]])
bs[0] = p[0] - p[1] / 2;
bs[1] = p[0] + p[1] / 2;
bs[2] = p[0] + 1.5f * p[1] + 2 * p[2];
}
__device__ __forceinline__ float4 position4( float u ) const
{
return ( p[0] * u + p[1] ) * u + p[2]; // Horner scheme
}
__device__ __forceinline__ float3 position3( float u ) const
{
return make_float3( position4( u ) );
}
__device__ __forceinline__ float radius( float u ) const
{
return position4( u ).w;
}
__device__ __forceinline__ float4 velocity4( float u ) const
{
return 2.0f * p[0] * u + p[1];
}
__device__ __forceinline__ float3 velocity3( float u ) const
{
return make_float3( velocity4( u ) );
}
__device__ __forceinline__ float derivative_of_radius( float u ) const
{
return velocity4( u ).w;
}
__device__ __forceinline__ float4 acceleration4( float u ) const
{
return 2.0f * p[0];
}
__device__ __forceinline__ float3 acceleration3( float u ) const
{
return make_float3( acceleration4( u ) );
}
float4 p[3];
};
//
// Third order polynomial interpolator
//
// Storing {p0, p1, p2, p3} for evaluation:
// P(u) = p0 * u^3 + p1 * u^2 + p2 * u + p3
//
struct CubicInterpolator
{
__device__ __forceinline__ CubicInterpolator() {}
// TODO: Initialize from polynomial weights. Check that sample doesn't rely on
// legacy behavior.
// __device__ __forceinline__ CubicBSplineSegment( const float4* q ) { initializeFromBSpline( q ); }
__device__ __forceinline__ void initializeFromBSpline( const float4* q )
{
// Bspline-to-Poly = Matrix([[-1/6, 1/2, -1/2, 1/6],
// [ 1/2, -1, 1/2, 0],
// [-1/2, 0, 1/2, 0],
// [ 1/6, 2/3, 1/6, 0]])
p[0] = ( q[0] * ( -1.0f ) + q[1] * ( 3.0f ) + q[2] * ( -3.0f ) + q[3] ) / 6.0f;
p[1] = ( q[0] * ( 3.0f ) + q[1] * ( -6.0f ) + q[2] * ( 3.0f ) ) / 6.0f;
p[2] = ( q[0] * ( -3.0f ) + q[2] * ( 3.0f ) ) / 6.0f;
p[3] = ( q[0] * ( 1.0f ) + q[1] * ( 4.0f ) + q[2] * ( 1.0f ) ) / 6.0f;
}
__device__ __forceinline__ void export2BSpline( float4 bs[4] ) const
{
// inverse of initializeFromBSpline
// Bspline-to-Poly = Matrix([[-1/6, 1/2, -1/2, 1/6],
// [ 1/2, -1, 1/2, 0],
// [-1/2, 0, 1/2, 0],
// [ 1/6, 2/3, 1/6, 0]])
// invert to get:
// Poly-to-Bspline = Matrix([[0, 2/3, -1, 1],
// [0, -1/3, 0, 1],
// [0, 2/3, 1, 1],
// [6, 11/3, 2, 1]])
bs[0] = ( p[1] * ( 2.0f ) + p[2] * ( -1.0f ) + p[3] ) / 3.0f;
bs[1] = ( p[1] * ( -1.0f ) + p[3] ) / 3.0f;
bs[2] = ( p[1] * ( 2.0f ) + p[2] * ( 1.0f ) + p[3] ) / 3.0f;
bs[3] = ( p[0] + p[1] * ( 11.0f ) + p[2] * ( 2.0f ) + p[3] ) / 3.0f;
}
__device__ __forceinline__ void initializeFromCatrom(const float4* q)
{
// Catrom-to-Poly = Matrix([[-1/2, 3/2, -3/2, 1/2],
// [1, -5/2, 2, -1/2],
// [-1/2, 0, 1/2, 0],
// [0, 1, 0, 0]])
p[0] = ( -1.0f * q[0] + ( 3.0f ) * q[1] + ( -3.0f ) * q[2] + ( 1.0f ) * q[3] ) / 2.0f;
p[1] = ( 2.0f * q[0] + ( -5.0f ) * q[1] + ( 4.0f ) * q[2] + ( -1.0f ) * q[3] ) / 2.0f;
p[2] = ( -1.0f * q[0] + ( 1.0f ) * q[2] ) / 2.0f;
p[3] = ( ( 2.0f ) * q[1] ) / 2.0f;
}
__device__ __forceinline__ void export2Catrom(float4 cr[4]) const
{
// Catrom-to-Poly = Matrix([[-1/2, 3/2, -3/2, 1/2],
// [1, -5/2, 2, -1/2],
// [-1/2, 0, 1/2, 0],
// [0, 1, 0, 0]])
// invert to get:
// Poly-to-Catrom = Matrix([[1, 1, -1, 1],
// [0, 0, 0, 1],
// [1, 1, 1, 1],
// [6, 4, 2, 1]])
cr[0] = ( p[0] * 6.f/6.f ) - ( p[1] * 5.f/6.f ) + ( p[2] * 2.f/6.f ) + ( p[3] * 1.f/6.f );
cr[1] = ( p[0] * 6.f/6.f ) ;
cr[2] = ( p[0] * 6.f/6.f ) + ( p[1] * 1.f/6.f ) + ( p[2] * 2.f/6.f ) + ( p[3] * 1.f/6.f );
cr[3] = ( p[0] * 6.f/6.f ) + ( p[3] * 6.f/6.f );
}
__device__ __forceinline__ float4 position4( float u ) const
{
return ( ( ( p[0] * u ) + p[1] ) * u + p[2] ) * u + p[3]; // Horner scheme
}
__device__ __forceinline__ float3 position3( float u ) const
{
// rely on compiler and inlining for dead code removal
return make_float3( position4( u ) );
}
__device__ __forceinline__ float radius( float u ) const
{
return position4( u ).w;
}
__device__ __forceinline__ float4 velocity4( float u ) const
{
// adjust u to avoid problems with tripple knots.
if( u == 0 )
u = 0.000001f;
if( u == 1 )
u = 0.999999f;
return ( ( 3.0f * p[0] * u ) + 2.0f * p[1] ) * u + p[2];
}
__device__ __forceinline__ float3 velocity3( float u ) const
{
return make_float3( velocity4( u ) );
}
__device__ __forceinline__ float derivative_of_radius( float u ) const
{
return velocity4( u ).w;
}
__device__ __forceinline__ float4 acceleration4( float u ) const
{
return 6.0f * p[0] * u + 2.0f * p[1]; // Horner scheme
}
__device__ __forceinline__ float3 acceleration3( float u ) const
{
return make_float3( acceleration4( u ) );
}
float4 p[4];
};
// Compute curve primitive surface normal in object space.
//
// Template parameters:
// CurveType - A B-Spline evaluator class.
// type - 0 ~ cylindrical approximation (correct if radius' == 0)
// 1 ~ conic approximation (correct if curve'' == 0)
// other ~ the bona fide surface normal
//
// Parameters:
// bc - A B-Spline evaluator object.
// u - segment parameter of hit-point.
// ps - hit-point on curve's surface in object space; usually
// computed like this.
// float3 ps = ray_orig + t_hit * ray_dir;
// the resulting point is slightly offset away from the
// surface. For this reason (Warning!) ps gets modified by this
// method, projecting it onto the surface
// in case it is not already on it. (See also inline
// comments.)
//
template <typename CurveType, int type = 2>
__device__ __forceinline__ float3 surfaceNormal( const CurveType& bc, float u, float3& ps )
{
float3 normal;
if( u == 0.0f )
{
normal = -bc.velocity3( 0 ); // special handling for flat endcaps
}
else if( u == 1.0f )
{
normal = bc.velocity3( 1 ); // special handling for flat endcaps
}
else
{
// ps is a point that is near the curve's offset surface,
// usually ray.origin + ray.direction * rayt.
// We will push it exactly to the surface by projecting it to the plane(p,d).
// The function derivation:
// we (implicitly) transform the curve into coordinate system
// {p, o1 = normalize(ps - p), o2 = normalize(curve'(t)), o3 = o1 x o2} in which
// curve'(t) = (0, length(d), 0); ps = (r, 0, 0);
float4 p4 = bc.position4( u );
float3 p = make_float3( p4 );
float r = p4.w; // == length(ps - p) if ps is already on the surface
float4 d4 = bc.velocity4( u );
float3 d = make_float3( d4 );
float dr = d4.w;
float dd = dot( d, d );
float3 o1 = ps - p; // dot(modified_o1, d) == 0 by design:
o1 -= ( dot( o1, d ) / dd ) * d; // first, project ps to the plane(p,d)
o1 *= r / length( o1 ); // and then drop it to the surface
ps = p + o1; // fine-tuning the hit point
if( type == 0 )
{
normal = o1; // cylindrical approximation
}
else
{
if( type != 1 )
{
dd -= dot( bc.acceleration3( u ), o1 );
}
normal = dd * o1 - ( dr * r ) * d;
}
}
return normalize( normal );
}
template <int type = 1>
__device__ __forceinline__ float3 surfaceNormal( const LinearInterpolator& bc, float u, float3& ps )
{
float3 normal;
if( u == 0.0f )
{
normal = ps - ( float3 & )( bc.p[0] ); // special handling for round endcaps
}
else if( u >= 1.0f )
{
// reconstruct second control point (Note: the interpolator pre-transforms
// the control-points to speed up repeated evaluation.
const float3 p1 = ( float3 & ) (bc.p[1] ) + ( float3 & )( bc.p[0] );
normal = ps - p1; // special handling for round endcaps
}
else
{
// ps is a point that is near the curve's offset surface,
// usually ray.origin + ray.direction * rayt.
// We will push it exactly to the surface by projecting it to the plane(p,d).
// The function derivation:
// we (implicitly) transform the curve into coordinate system
// {p, o1 = normalize(ps - p), o2 = normalize(curve'(t)), o3 = o1 x o2} in which
// curve'(t) = (0, length(d), 0); ps = (r, 0, 0);
float4 p4 = bc.position4( u );
float3 p = make_float3( p4 );
float r = p4.w; // == length(ps - p) if ps is already on the surface
float4 d4 = bc.velocity4( u );
float3 d = make_float3( d4 );
float dr = d4.w;
float dd = dot( d, d );
float3 o1 = ps - p; // dot(modified_o1, d) == 0 by design:
o1 -= ( dot( o1, d ) / dd ) * d; // first, project ps to the plane(p,d)
o1 *= r / length( o1 ); // and then drop it to the surface
ps = p + o1; // fine-tuning the hit point
if( type == 0 )
{
normal = o1; // cylindrical approximation
}
else
{
normal = dd * o1 - ( dr * r ) * d;
}
}
return normalize( normal );
}
// Compute curve primitive tangent in object space.
//
// Template parameters:
// CurveType - A B-Spline evaluator class.
//
// Parameters:
// bc - A B-Spline evaluator object.
// u - segment parameter of tangent location on curve.
//
template <typename CurveType>
__device__ __forceinline__ float3 curveTangent( const CurveType& bc, float u )
{
float3 tangent = bc.velocity3( u );
return normalize( tangent );
}
| 14,969 | C | 34.813397 | 104 | 0.48567 |
arhix52/Strelka/cuda/helpers.h | //
// Copyright (c) 2021, NVIDIA CORPORATION. All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
// * Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
// * Redistributions in binary form must reproduce the above copyright
// notice, this list of conditions and the following disclaimer in the
// documentation and/or other materials provided with the distribution.
// * Neither the name of NVIDIA CORPORATION nor the names of its
// contributors may be used to endorse or promote products derived
// from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ``AS IS'' AND ANY
// EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
// IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
// PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
// CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
// EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
// PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
// PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY
// OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
// (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
// OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
//
#pragma once
#include <vector_types.h>
#include <sutil/vec_math.h>
__forceinline__ __device__ float3 toSRGB( const float3& c )
{
float invGamma = 1.0f / 2.4f;
float3 powed = make_float3( powf( c.x, invGamma ), powf( c.y, invGamma ), powf( c.z, invGamma ) );
return make_float3(
c.x < 0.0031308f ? 12.92f * c.x : 1.055f * powed.x - 0.055f,
c.y < 0.0031308f ? 12.92f * c.y : 1.055f * powed.y - 0.055f,
c.z < 0.0031308f ? 12.92f * c.z : 1.055f * powed.z - 0.055f );
}
//__forceinline__ __device__ float dequantizeUnsigned8Bits( const unsigned char i )
//{
// enum { N = (1 << 8) - 1 };
// return min((float)i / (float)N), 1.f)
//}
__forceinline__ __device__ unsigned char quantizeUnsigned8Bits( float x )
{
x = clamp( x, 0.0f, 1.0f );
enum { N = (1 << 8) - 1, Np1 = (1 << 8) };
return (unsigned char)min((unsigned int)(x * (float)Np1), (unsigned int)N);
}
__forceinline__ __device__ uchar4 make_color( const float3& c )
{
// first apply gamma, then convert to unsigned char
float3 srgb = toSRGB( clamp( c, 0.0f, 1.0f ) );
return make_uchar4( quantizeUnsigned8Bits( srgb.x ), quantizeUnsigned8Bits( srgb.y ), quantizeUnsigned8Bits( srgb.z ), 255u );
}
__forceinline__ __device__ uchar4 make_color( const float4& c )
{
return make_color( make_float3( c.x, c.y, c.z ) );
}
| 2,911 | C | 42.462686 | 130 | 0.687736 |
arhix52/Strelka/tests/tests_main.cpp | #define DOCTEST_CONFIG_IMPLEMENT_WITH_MAIN
#include <doctest/doctest.h>
// This is all that is needed to compile a test-runner executable.
// More tests can be added here, or in a new tests/*.cpp file.
| 203 | C++ | 32.999995 | 66 | 0.748768 |
arhix52/Strelka/tests/materialmanager/test_materialmanager.cpp | #include <materialmanager/materialmanager.h>
// #include <render/render.h>
#include <doctest/doctest.h>
#include <filesystem>
#include <fstream>
#include <iostream>
#include <cassert>
using namespace oka;
namespace fs = std::filesystem;
TEST_CASE("mtlx to hlsl code gen test")
{
// static const char* DEFAULT_MTLX_DOC_1 =
// "<?xml version=\"1.0\"?>"
// "<materialx version=\"1.38\" colorspace=\"lin_rec709\">"
// " <UsdPreviewSurface name=\"SR_Invalid\" type=\"surfaceshader\">"
// " <input name=\"diffuseColor\" type=\"color3\" value=\"1.0, 0.0, 1.0\" />"
// " <input name=\"roughness\" type=\"float\" value=\"1.0\" />"
// " </UsdPreviewSurface>"
// " <surfacematerial name=\"invalid\" type=\"material\">"
// " <input name=\"surfaceshader\" type=\"surfaceshader\" nodename=\"SR_Invalid\" />"
// " </surfacematerial>"
// "</materialx>";
static const char* DEFAULT_MTLX_DOC_1 =
"<?xml version=\"1.0\"?>"
"<materialx version=\"1.38\" colorspace=\"lin_rec709\">"
" <UsdPreviewSurface name=\"SR_default\" type=\"surfaceshader\">"
" <input name=\"diffuseColor\" type=\"color3\" value=\"0.18, 0.18, 0.18\" />"
" <input name=\"emissiveColor\" type=\"color3\" value=\"0, 0, 0\" />"
" <input name=\"useSpecularWorkflow\" type=\"integer\" value=\"0\" />"
" <input name=\"specularColor\" type=\"color3\" value=\"0, 0, 0\" />"
" <input name=\"metallic\" type=\"float\" value=\"0\" />"
" <input name=\"roughness\" type=\"float\" value=\"0.5\" />"
" <input name=\"clearcoat\" type=\"float\" value=\"0\" />"
" <input name=\"clearcoatRoughness\" type=\"float\" value=\"0.01\" />"
" <input name=\"opacity\" type=\"float\" value=\"1\" />"
" <input name=\"opacityThreshold\" type=\"float\" value=\"0\" />"
" <input name=\"ior\" type=\"float\" value=\"1.5\" />"
" <input name=\"normal\" type=\"vector3\" value=\"0, 0, 1\" />"
" <input name=\"displacement\" type=\"float\" value=\"0\" />"
" <input name=\"occlusion\" type=\"float\" value=\"1\" />"
" </UsdPreviewSurface>"
" <surfacematerial name=\"USD_Default\" type=\"material\">"
" <input name=\"surfaceshader\" type=\"surfaceshader\" nodename=\"SR_default\" />"
" </surfacematerial>"
"</materialx>";
static const char* DEFAULT_MTLX_DOC_2 =
"<?xml version=\"1.0\"?>"
"<materialx version=\"1.38\" colorspace=\"lin_rec709\">"
" <UsdPreviewSurface name=\"SR_Invalid\" type=\"surfaceshader\">"
" <input name=\"diffuseColor\" type=\"color3\" value=\"0.0, 0.0, 1.0\" />"
" <input name=\"roughness\" type=\"float\" value=\"1.0\" />"
" </UsdPreviewSurface>"
" <surfacematerial name=\"invalid\" type=\"material\">"
" <input name=\"surfaceshader\" type=\"surfaceshader\" nodename=\"SR_Invalid\" />"
" </surfacematerial>"
"</materialx>";
using namespace std;
const fs::path cwd = fs::current_path();
cout << cwd.c_str() << endl;
std::string mdlFile = "material.mdl";
std::ofstream mdlMaterial(mdlFile.c_str());
MaterialManager* matMngr = new MaterialManager();
CHECK(matMngr);
const char* envUSDPath = std::getenv("USD_DIR");
if (!envUSDPath)
{
printf("Please, set USD_DIR variable\n");
assert(0);
}
const std::string usdMdlLibPath = std::string(envUSDPath) + "\\libraries\\mdl\\";
const char* paths[] = { usdMdlLibPath.c_str(), "./data/materials/mtlx/" };
bool res = matMngr->addMdlSearchPath(paths, 2);
CHECK(res);
MaterialManager::Module* mdlModule = matMngr->createMtlxModule(DEFAULT_MTLX_DOC_1);
assert(mdlModule);
MaterialManager::MaterialInstance* materialInst = matMngr->createMaterialInstance(mdlModule, "");
assert(materialInst);
MaterialManager::CompiledMaterial* materialComp = matMngr->compileMaterial(materialInst);
assert(materialComp);
MaterialManager::Module* mdlModule2 = matMngr->createMtlxModule(DEFAULT_MTLX_DOC_2);
assert(mdlModule2);
MaterialManager::MaterialInstance* materialInst2 = matMngr->createMaterialInstance(mdlModule2, "");
assert(materialInst2);
MaterialManager::CompiledMaterial* materialComp2 = matMngr->compileMaterial(materialInst2);
assert(materialComp2);
MaterialManager::CompiledMaterial* materials[2] = { materialComp, materialComp2 };
// std::vector<MaterialManager::CompiledMaterial*> materials;
// materials.push_back(materialComp);
// materials.push_back(materialComp2);
const MaterialManager::TargetCode* code = matMngr->generateTargetCode(materials, 2);
CHECK(code);
const char* hlsl = matMngr->getShaderCode(code, 0);
std::string shaderFile = "test_material_output.hlsl";
std::ofstream out(shaderFile.c_str());
out << hlsl << std::endl;
out.close();
delete matMngr;
}
TEST_CASE("MDL OmniPBR")
{
using namespace std;
const fs::path cwd = fs::current_path();
cout << cwd.c_str() << endl;
std::string mdlFile = "C:\\work\\StrelkaOptix\\build\\data\\materials\\mtlx\\OmniPBR.mdl";
// std::ofstream mdlMaterial(mdlFile.c_str());
MaterialManager* matMngr = new MaterialManager();
CHECK(matMngr);
const char* envUSDPath = std::getenv("USD_DIR");
if (!envUSDPath)
{
printf("Please, set USD_DIR variable\n");
assert(0);
}
const std::string usdMdlLibPath = std::string(envUSDPath) + "\\libraries\\mdl\\";
const char* paths[] = { usdMdlLibPath.c_str(), "./data/materials/mtlx/",
"C:\\work\\StrelkaOptix\\build\\data\\materials\\mtlx\\",
"C:\\work\\Strelka\\misc\\test_data\\mdl" };
bool res = matMngr->addMdlSearchPath(paths, 4);
CHECK(res);
MaterialManager::Module* currModule = matMngr->createModule(mdlFile.c_str());
CHECK(currModule);
MaterialManager::MaterialInstance* materialInst1 = matMngr->createMaterialInstance(currModule, "OmniPBR");
CHECK(materialInst1);
MaterialManager::CompiledMaterial* materialComp1 = matMngr->compileMaterial(materialInst1);
CHECK(materialComp1);
MaterialManager::CompiledMaterial* materials[1] = { materialComp1 };
const MaterialManager::TargetCode* targetCode = matMngr->generateTargetCode(materials, 1);
CHECK(targetCode);
const char* ptx = matMngr->getShaderCode(targetCode, 0);
string ptxFileName = cwd.string() + "/output.ptx";
ofstream outPtxFile(ptxFileName.c_str());
outPtxFile << ptx << endl;
outPtxFile.close();
delete matMngr;
}
// TEST_CASE("mdl to hlsl code gen test")
// {
// using namespace std;
// const fs::path cwd = fs::current_path();
// cout << cwd.c_str() << endl;
// std::string ptFile = cwd.string() + "/output.ptx";
// std::ofstream outHLSLShaderFile(ptFile.c_str());
// // Render r;
// // r.HEIGHT = 600;
// // r.WIDTH = 800;
// // r.initWindow();
// // r.initVulkan();
// MaterialManager* matMngr = new MaterialManager();
// CHECK(matMngr);
// const char* envUSDPath = std::getenv("USD_DIR");
// if (!envUSDPath)
// {
// printf("Please, set USD_DIR variable\n");
// assert(0);
// }
// const std::string usdMdlLibPath = std::string(envUSDPath) + "\\libraries\\mdl\\";
// const char* paths[] = { usdMdlLibPath.c_str(), "./data/materials/mtlx/" };
// bool res = matMngr->addMdlSearchPath(paths, 2);
// CHECK(res);
// MaterialManager::Module* defaultModule = matMngr->createModule("default.mdl");
// CHECK(defaultModule);
// MaterialManager::MaterialInstance* materialInst0 = matMngr->createMaterialInstance(defaultModule, "default_material");
// CHECK(materialInst0);
// MaterialManager::CompiledMaterial* materialComp0 = matMngr->compileMaterial(materialInst0);
// CHECK(materialComp0);
// MaterialManager::CompiledMaterial* materialComp00 = matMngr->compileMaterial(materialInst0);
// CHECK(materialComp00);
// MaterialManager::CompiledMaterial* materialComp000 = matMngr->compileMaterial(materialInst0);
// CHECK(materialComp000);
// MaterialManager::Module* currModule = matMngr->createModule("OmniGlass.mdl");
// CHECK(currModule);
// MaterialManager::MaterialInstance* materialInst1 = matMngr->createMaterialInstance(currModule, "OmniGlass");
// CHECK(materialInst1);
// MaterialManager::CompiledMaterial* materialComp1 = matMngr->compileMaterial(materialInst1);
// CHECK(materialComp1);
// MaterialManager::CompiledMaterial* materialsDefault[1] = { materialComp0 };
// // const MaterialManager::TargetCode* codeDefault = matMngr->generateTargetCode(materialsDefault, 1);
// // CHECK(codeDefault);
// MaterialManager::CompiledMaterial* materials[4] = { materialComp0, materialComp00, materialComp1, materialComp000 };
// const MaterialManager::TargetCode* code = matMngr->generateTargetCode(materials, 4);
// CHECK(code);
// const char* hlsl = matMngr->getShaderCode(code);
// // std::cout << hlsl << std::endl;
// uint32_t size = matMngr->getArgBufferSize(code);
// CHECK(size != 0);
// size = matMngr->getArgBufferSize(code);
// CHECK(size != 0);
// size = matMngr->getResourceInfoSize(code);
// CHECK(size != 0);
// matMngr->dumpParams(code, materialComp1);
// // nevk::TextureManager* mTexManager = new nevk::TextureManager(r.getDevice(), r.getPhysicalDevice(),
// // r.getResManager());
// uint32_t texSize = matMngr->getTextureCount(code);
// // CHECK(texSize == 8);
// // for (uint32_t i = 0; i < texSize; ++i)
// // {
// // const float* data = matMngr->getTextureData(code, i);
// // uint32_t width = matMngr->getTextureWidth(code, i);
// // uint32_t height = matMngr->getTextureHeight(code, i);
// // const char* type = matMngr->getTextureType(code, i);
// // std::string name = matMngr->getTextureName(code, i);
// // // mTexManager->loadTextureMdl(data, width, height, type, name);
// // }
// // CHECK(mTexManager->textures.size() == 7);
// // CHECK(mTexManager->textures[0].texWidth == 512);
// // CHECK(mTexManager->textures[0].texHeight == 512);
// delete matMngr;
// }
// TEST_CASE("mtlx to mdl code gen test")
// {
// using namespace std;
// const fs::path cwd = fs::current_path();
// cout << cwd.c_str() << endl;
// std::string mdlFile = "material.mdl";
// std::ofstream mdlMaterial(mdlFile.c_str());
// std::string ptFile = cwd.string() + "/shaders/newPT.hlsl";
// std::ofstream outHLSLShaderFile(ptFile.c_str());
// Render r;
// r.HEIGHT = 600;
// r.WIDTH = 800;
// r.initWindow();
// r.initVulkan();
// MaterialManager* matMngr = new MaterialManager();
// CHECK(matMngr);
// const char* path[2] = { "misc/test_data/mdl/", "misc/test_data/mtlx" }; // todo: configure paths
// bool res = matMngr->addMdlSearchPath(path, 2);
// CHECK(res);
// std::string file = "misc/test_data/mtlx/standard_surface_wood_tiled.mtlx";
// std::unique_ptr<MaterialManager::Module> currModule = matMngr->createMtlxModule(file.c_str());
// CHECK(currModule);
// std::unique_ptr<MaterialManager::MaterialInstance> materialInst1 =
// matMngr->createMaterialInstance(currModule.get(), ""); CHECK(materialInst1);
// std::unique_ptr<MaterialManager::CompiledMaterial> materialComp1 = matMngr->compileMaterial(materialInst1.get());
// CHECK(materialComp1);
// std::vector<std::unique_ptr<MaterialManager::CompiledMaterial>> materials;
// materials.push_back(std::move(materialComp1));
// const MaterialManager::TargetCode* code = matMngr->generateTargetCode(materials);
// CHECK(code);
// const char* hlsl = matMngr->getShaderCode(code);
// //std::cout << hlsl << std::endl;
// uint32_t size = matMngr->getArgBufferSize(code);
// CHECK(size != 0);
// size = matMngr->getArgBufferSize(code);
// CHECK(size != 0);
// size = matMngr->getResourceInfoSize(code);
// CHECK(size != 0);
// nevk::TextureManager* mTexManager = new nevk::TextureManager(r.getDevice(), r.getPhysicalDevice(),
// r.getResManager()); uint32_t texSize = matMngr->getTextureCount(code); for (uint32_t i = 1; i < texSize; ++i)
// {
// const float* data = matMngr->getTextureData(code, i);
// uint32_t width = matMngr->getTextureWidth(code, i);
// uint32_t height = matMngr->getTextureHeight(code, i);
// const char* type = matMngr->getTextureType(code, i);
// std::string name = matMngr->getTextureName(code, i);
// if (data != NULL) // todo: for bsdf_text data is NULL in COMPILATION_CLASS. in default class there is no
// bsdf_tex
// {
// mTexManager->loadTextureMdl(data, width, height, type, name);
// }
// }
// CHECK(mTexManager->textures.size() == 2);
// CHECK(mTexManager->textures[0].texWidth == 512);
// CHECK(mTexManager->textures[0].texHeight == 512);
// }
| 13,259 | C++ | 40.698113 | 125 | 0.621465 |
arhix52/Strelka/sutil/Quaternion.h | //
// Copyright (c) 2021, NVIDIA CORPORATION. All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
// * Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
// * Redistributions in binary form must reproduce the above copyright
// notice, this list of conditions and the following disclaimer in the
// documentation and/or other materials provided with the distribution.
// * Neither the name of NVIDIA CORPORATION nor the names of its
// contributors may be used to endorse or promote products derived
// from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ``AS IS'' AND ANY
// EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
// IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
// PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
// CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
// EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
// PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
// PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY
// OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
// (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
// OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
//
#pragma once
#include <sutil/Matrix.h>
//------------------------------------------------------------------------------
//
// Quaternion class
//
//------------------------------------------------------------------------------
namespace sutil
{
class Quaternion
{
public:
Quaternion()
{ q[0] = q[1] = q[2] = q[3] = 0.0; }
Quaternion( float w, float x, float y, float z )
{ q[0] = w; q[1] = x; q[2] = y; q[3] = z; }
Quaternion( const float3& from, const float3& to );
Quaternion( const Quaternion& a )
{ q[0] = a[0]; q[1] = a[1]; q[2] = a[2]; q[3] = a[3]; }
Quaternion ( float angle, const float3& axis );
// getters and setters
void setW(float _w) { q[0] = _w; }
void setX(float _x) { q[1] = _x; }
void setY(float _y) { q[2] = _y; }
void setZ(float _z) { q[3] = _z; }
float w() const { return q[0]; }
float x() const { return q[1]; }
float y() const { return q[2]; }
float z() const { return q[3]; }
Quaternion& operator-=(const Quaternion& r)
{ q[0] -= r[0]; q[1] -= r[1]; q[2] -= r[2]; q[3] -= r[3]; return *this; }
Quaternion& operator+=(const Quaternion& r)
{ q[0] += r[0]; q[1] += r[1]; q[2] += r[2]; q[3] += r[3]; return *this; }
Quaternion& operator*=(const Quaternion& r);
Quaternion& operator/=(const float a);
Quaternion conjugate()
{ return Quaternion( q[0], -q[1], -q[2], -q[3] ); }
void rotation( float& angle, float3& axis ) const;
void rotation( float& angle, float& x, float& y, float& z ) const;
Matrix4x4 rotationMatrix() const;
float& operator[](int i) { return q[i]; }
float operator[](int i)const { return q[i]; }
// l2 norm
float norm() const
{ return sqrtf(q[0]*q[0] + q[1]*q[1] + q[2]*q[2] + q[3]*q[3]); }
float normalize();
private:
float q[4];
};
inline Quaternion::Quaternion( const float3& from, const float3& to )
{
const float3 c = cross( from, to );
q[0] = dot(from, to);
q[1] = c.x;
q[2] = c.y;
q[3] = c.z;
}
inline Quaternion::Quaternion( float angle, const float3& axis )
{
const float n = length( axis );
const float inverse = 1.0f/n;
const float3 naxis = axis*inverse;
const float s = sinf(angle/2.0f);
q[0] = naxis.x*s*inverse;
q[1] = naxis.y*s*inverse;
q[2] = naxis.z*s*inverse;
q[3] = cosf(angle/2.0f);
}
inline Quaternion& Quaternion::operator*=(const Quaternion& r)
{
float w = q[0]*r[0] - q[1]*r[1] - q[2]*r[2] - q[3]*r[3];
float x = q[0]*r[1] + q[1]*r[0] + q[2]*r[3] - q[3]*r[2];
float y = q[0]*r[2] + q[2]*r[0] + q[3]*r[1] - q[1]*r[3];
float z = q[0]*r[3] + q[3]*r[0] + q[1]*r[2] - q[2]*r[1];
q[0] = w;
q[1] = x;
q[2] = y;
q[3] = z;
return *this;
}
inline Quaternion& Quaternion::operator/=(const float a)
{
float inverse = 1.0f/a;
q[0] *= inverse;
q[1] *= inverse;
q[2] *= inverse;
q[3] *= inverse;
return *this;
}
inline void Quaternion::rotation( float& angle, float3& axis ) const
{
Quaternion n = *this;
n.normalize();
axis.x = n[1];
axis.y = n[2];
axis.z = n[3];
angle = 2.0f * acosf(n[0]);
}
inline void Quaternion::rotation(
float& angle,
float& x,
float& y,
float& z
) const
{
Quaternion n = *this;
n.normalize();
x = n[1];
y = n[2];
z = n[3];
angle = 2.0f * acosf(n[0]);
}
inline float Quaternion::normalize()
{
float n = norm();
float inverse = 1.0f/n;
q[0] *= inverse;
q[1] *= inverse;
q[2] *= inverse;
q[3] *= inverse;
return n;
}
inline Quaternion operator*(const float a, const Quaternion &r)
{ return Quaternion(a*r[0], a*r[1], a*r[2], a*r[3]); }
inline Quaternion operator*(const Quaternion &r, const float a)
{ return Quaternion(a*r[0], a*r[1], a*r[2], a*r[3]); }
inline Quaternion operator/(const Quaternion &r, const float a)
{
float inverse = 1.0f/a;
return Quaternion( r[0]*inverse, r[1]*inverse, r[2]*inverse, r[3]*inverse);
}
inline Quaternion operator/(const float a, const Quaternion &r)
{
float inverse = 1.0f/a;
return Quaternion( r[0]*inverse, r[1]*inverse, r[2]*inverse, r[3]*inverse);
}
inline Quaternion operator-(const Quaternion& l, const Quaternion& r)
{ return Quaternion(l[0]-r[0], l[1]-r[1], l[2]-r[2], l[3]-r[3]); }
inline bool operator==(const Quaternion& l, const Quaternion& r)
{ return ( l[0] == r[0] && l[1] == r[1] && l[2] == r[2] && l[3] == r[3] ); }
inline bool operator!=(const Quaternion& l, const Quaternion& r)
{ return !(l == r); }
inline Quaternion operator+(const Quaternion& l, const Quaternion& r)
{ return Quaternion(l[0]+r[0], l[1]+r[1], l[2]+r[2], l[3]+r[3]); }
inline Quaternion operator*(const Quaternion& l, const Quaternion& r)
{
float w = l[0]*r[0] - l[1]*r[1] - l[2]*r[2] - l[3]*r[3];
float x = l[0]*r[1] + l[1]*r[0] + l[2]*r[3] - l[3]*r[2];
float y = l[0]*r[2] + l[2]*r[0] + l[3]*r[1] - l[1]*r[3];
float z = l[0]*r[3] + l[3]*r[0] + l[1]*r[2] - l[2]*r[1];
return Quaternion( w, x, y, z );
}
inline float dot( const Quaternion& l, const Quaternion& r )
{
return l.w()*r.w() + l.x()*r.x() + l.y()*r.y() + l.z()*r.z();
}
inline Matrix4x4 Quaternion::rotationMatrix() const
{
Matrix4x4 m;
const float qw = q[0];
const float qx = q[1];
const float qy = q[2];
const float qz = q[3];
m[0*4+0] = 1.0f - 2.0f*qy*qy - 2.0f*qz*qz;
m[0*4+1] = 2.0f*qx*qy - 2.0f*qz*qw;
m[0*4+2] = 2.0f*qx*qz + 2.0f*qy*qw;
m[0*4+3] = 0.0f;
m[1*4+0] = 2.0f*qx*qy + 2.0f*qz*qw;
m[1*4+1] = 1.0f - 2.0f*qx*qx - 2.0f*qz*qz;
m[1*4+2] = 2.0f*qy*qz - 2.0f*qx*qw;
m[1*4+3] = 0.0f;
m[2*4+0] = 2.0f*qx*qz - 2.0f*qy*qw;
m[2*4+1] = 2.0f*qy*qz + 2.0f*qx*qw;
m[2*4+2] = 1.0f - 2.0f*qx*qx - 2.0f*qy*qy;
m[2*4+3] = 0.0f;
m[3*4+0] = 0.0f;
m[3*4+1] = 0.0f;
m[3*4+2] = 0.0f;
m[3*4+3] = 1.0f;
return m;
}
} // end namespace sutil
| 7,605 | C | 26.963235 | 80 | 0.56121 |
arhix52/Strelka/sutil/Matrix.h | //
// Copyright (c) 2021, NVIDIA CORPORATION. All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
// * Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
// * Redistributions in binary form must reproduce the above copyright
// notice, this list of conditions and the following disclaimer in the
// documentation and/or other materials provided with the distribution.
// * Neither the name of NVIDIA CORPORATION nor the names of its
// contributors may be used to endorse or promote products derived
// from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ``AS IS'' AND ANY
// EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
// IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
// PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
// CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
// EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
// PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
// PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY
// OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
// (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
// OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
//
#pragma once
#include <sutil/sutilapi.h>
#include <sutil/Preprocessor.h>
#include <sutil/vec_math.h>
#if !defined(__CUDACC_RTC__)
#include <cmath>
#include <initializer_list>
#endif
#define RT_MATRIX_ACCESS(m,i,j) m[i*N+j]
#define RT_MAT_DECL template <unsigned int M, unsigned int N>
namespace sutil
{
template <int DIM> struct VectorDim { };
template <> struct VectorDim<2> { typedef float2 VectorType; };
template <> struct VectorDim<3> { typedef float3 VectorType; };
template <> struct VectorDim<4> { typedef float4 VectorType; };
template <unsigned int M, unsigned int N> class Matrix;
template <unsigned int M> SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<M,M>& operator*=(Matrix<M,M>& m1, const Matrix<M,M>& m2);
RT_MAT_DECL SUTIL_INLINE SUTIL_HOSTDEVICE bool operator==(const Matrix<M,N>& m1, const Matrix<M,N>& m2);
RT_MAT_DECL SUTIL_INLINE SUTIL_HOSTDEVICE bool operator!=(const Matrix<M,N>& m1, const Matrix<M,N>& m2);
RT_MAT_DECL SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<M,N>& operator-=(Matrix<M,N>& m1, const Matrix<M,N>& m2);
RT_MAT_DECL SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<M,N>& operator+=(Matrix<M,N>& m1, const Matrix<M,N>& m2);
RT_MAT_DECL SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<M,N>& operator*=(Matrix<M,N>& m1, float f);
RT_MAT_DECL SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<M,N>&operator/=(Matrix<M,N>& m1, float f);
RT_MAT_DECL SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<M,N> operator-(const Matrix<M,N>& m1, const Matrix<M,N>& m2);
RT_MAT_DECL SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<M,N> operator+(const Matrix<M,N>& m1, const Matrix<M,N>& m2);
RT_MAT_DECL SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<M,N> operator/(const Matrix<M,N>& m, float f);
RT_MAT_DECL SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<M,N> operator*(const Matrix<M,N>& m, float f);
RT_MAT_DECL SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<M,N> operator*(float f, const Matrix<M,N>& m);
RT_MAT_DECL SUTIL_INLINE SUTIL_HOSTDEVICE typename Matrix<M,N>::floatM operator*(const Matrix<M,N>& m, const typename Matrix<M,N>::floatN& v );
RT_MAT_DECL SUTIL_INLINE SUTIL_HOSTDEVICE typename Matrix<M,N>::floatN operator*(const typename Matrix<M,N>::floatM& v, const Matrix<M,N>& m);
template<unsigned int M, unsigned int N, unsigned int R> SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<M,R> operator*(const Matrix<M,N>& m1, const Matrix<N,R>& m2);
// Partial specializations to make matrix vector multiplication more efficient
template <unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE float2 operator*(const Matrix<2,N>& m, const typename Matrix<2,N>::floatN& vec );
template <unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE float3 operator*(const Matrix<3,N>& m, const typename Matrix<3,N>::floatN& vec );
SUTIL_INLINE SUTIL_HOSTDEVICE float3 operator*(const Matrix<3,4>& m, const float4& vec );
template <unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE float4 operator*(const Matrix<4,N>& m, const typename Matrix<4,N>::floatN& vec );
SUTIL_INLINE SUTIL_HOSTDEVICE float4 operator*(const Matrix<4,4>& m, const float4& vec );
/**
* @brief A matrix with M rows and N columns
*
* @ingroup CUDACTypes
*
* <B>Description</B>
*
* @ref Matrix provides a utility class for small-dimension floating-point
* matrices, such as transformation matrices. @ref Matrix may also be useful
* in other computation and can be used in both host and device code.
* Typedefs are provided for 2x2 through 4x4 matrices.
*
*/
template <unsigned int M, unsigned int N>
class Matrix
{
public:
typedef typename VectorDim<N>::VectorType floatN; /// A row of the matrix
typedef typename VectorDim<M>::VectorType floatM; /// A column of the matrix
/** Create an uninitialized matrix */
SUTIL_HOSTDEVICE Matrix();
/** Create a matrix from the specified float array */
SUTIL_HOSTDEVICE explicit Matrix( const float data[M*N] ) { for(unsigned int i = 0; i < M*N; ++i) m_data[i] = data[i]; }
/** Copy the matrix */
SUTIL_HOSTDEVICE Matrix( const Matrix& m );
SUTIL_HOSTDEVICE Matrix( const std::initializer_list<float>& list );
/** Assignment operator */
SUTIL_HOSTDEVICE Matrix& operator=( const Matrix& b );
/** Access the specified element 0..N*M-1 */
SUTIL_HOSTDEVICE float operator[]( unsigned int i )const { return m_data[i]; }
/** Access the specified element 0..N*M-1 */
SUTIL_HOSTDEVICE float& operator[]( unsigned int i ) { return m_data[i]; }
/** Access the specified row 0..M. Returns float, float2, float3 or float4 depending on the matrix size */
SUTIL_HOSTDEVICE floatN getRow( unsigned int m )const;
/** Access the specified column 0..N. Returns float, float2, float3 or float4 depending on the matrix size */
SUTIL_HOSTDEVICE floatM getCol( unsigned int n )const;
/** Returns a pointer to the internal data array. The data array is stored in row-major order. */
SUTIL_HOSTDEVICE float* getData();
/** Returns a const pointer to the internal data array. The data array is stored in row-major order. */
SUTIL_HOSTDEVICE const float* getData()const;
/** Assign the specified row 0..M. Takes a float, float2, float3 or float4 depending on the matrix size */
SUTIL_HOSTDEVICE void setRow( unsigned int m, const floatN &r );
/** Assign the specified column 0..N. Takes a float, float2, float3 or float4 depending on the matrix size */
SUTIL_HOSTDEVICE void setCol( unsigned int n, const floatM &c );
/** Returns the transpose of the matrix */
SUTIL_HOSTDEVICE Matrix<N,M> transpose() const;
/** Returns the inverse of the matrix */
SUTIL_HOSTDEVICE Matrix<4,4> inverse() const;
/** Returns the determinant of the matrix */
SUTIL_HOSTDEVICE float det() const;
/** Returns a rotation matrix */
SUTIL_HOSTDEVICE static Matrix<4,4> rotate(const float radians, const float3& axis);
/** Returns a translation matrix */
SUTIL_HOSTDEVICE static Matrix<4,4> translate(const float3& vec);
/** Returns a scale matrix */
SUTIL_HOSTDEVICE static Matrix<4,4> scale(const float3& vec);
/** Creates a matrix from an ONB and center point */
SUTIL_HOSTDEVICE static Matrix<4,4> fromBasis( const float3& u, const float3& v, const float3& w, const float3& c );
/** Returns the identity matrix */
SUTIL_HOSTDEVICE static Matrix<3,4> affineIdentity();
SUTIL_HOSTDEVICE static Matrix<N,N> identity();
/** Ordered comparison operator so that the matrix can be used in an STL container */
SUTIL_HOSTDEVICE bool operator<( const Matrix<M, N>& rhs ) const;
private:
/** The data array is stored in row-major order */
float m_data[M*N];
};
template<unsigned int M, unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<M,N>::Matrix()
{
}
template<unsigned int M, unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<M,N>::Matrix( const Matrix<M,N>& m )
{
for(unsigned int i = 0; i < M*N; ++i)
m_data[i] = m[i];
}
template<unsigned int M, unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<M,N>::Matrix( const std::initializer_list<float>& list )
{
int i = 0;
for( auto it = list.begin(); it != list.end(); ++it )
m_data[ i++ ] = *it;
}
template<unsigned int M, unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<M,N>& Matrix<M,N>::operator=( const Matrix& b )
{
for(unsigned int i = 0; i < M*N; ++i)
m_data[i] = b[i];
return *this;
}
/*
template<unsigned int M, unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE float Matrix<M,N>::operator[]( unsigned int i )const
{
return m_data[i];
}
template<unsigned int M, unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE float& Matrix<M,N>::operator[]( unsigned int i )
{
return m_data[i];
}
*/
template<unsigned int M, unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE typename Matrix<M,N>::floatN Matrix<M,N>::getRow( unsigned int m )const
{
typename Matrix<M,N>::floatN temp;
float* v = reinterpret_cast<float*>( &temp );
const float* row = &( m_data[m*N] );
for(unsigned int i = 0; i < N; ++i)
v[i] = row[i];
return temp;
}
template<unsigned int M, unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE typename Matrix<M,N>::floatM Matrix<M,N>::getCol( unsigned int n )const
{
typename Matrix<M,N>::floatM temp;
float* v = reinterpret_cast<float*>( &temp );
for ( unsigned int i = 0; i < M; ++i )
v[i] = RT_MATRIX_ACCESS( m_data, i, n );
return temp;
}
template<unsigned int M, unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE float* Matrix<M,N>::getData()
{
return m_data;
}
template<unsigned int M, unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE const float* Matrix<M,N>::getData() const
{
return m_data;
}
template<unsigned int M, unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE void Matrix<M,N>::setRow( unsigned int m, const typename Matrix<M,N>::floatN &r )
{
const float* v = reinterpret_cast<const float*>( &r );
float* row = &( m_data[m*N] );
for(unsigned int i = 0; i < N; ++i)
row[i] = v[i];
}
template<unsigned int M, unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE void Matrix<M,N>::setCol( unsigned int n, const typename Matrix<M,N>::floatM &c )
{
const float* v = reinterpret_cast<const float*>( &c );
for ( unsigned int i = 0; i < M; ++i )
RT_MATRIX_ACCESS( m_data, i, n ) = v[i];
}
// Compare two matrices using exact float comparison
template<unsigned int M, unsigned int N>
SUTIL_HOSTDEVICE bool operator==(const Matrix<M,N>& m1, const Matrix<M,N>& m2)
{
for ( unsigned int i = 0; i < M*N; ++i )
if ( m1[i] != m2[i] ) return false;
return true;
}
template<unsigned int M, unsigned int N>
SUTIL_HOSTDEVICE bool operator!=(const Matrix<M,N>& m1, const Matrix<M,N>& m2)
{
for ( unsigned int i = 0; i < M*N; ++i )
if ( m1[i] != m2[i] ) return true;
return false;
}
// Subtract two matrices of the same size.
template<unsigned int M, unsigned int N>
SUTIL_HOSTDEVICE Matrix<M,N> operator-(const Matrix<M,N>& m1, const Matrix<M,N>& m2)
{
Matrix<M,N> temp( m1 );
temp -= m2;
return temp;
}
// Subtract two matrices of the same size.
template<unsigned int M, unsigned int N>
SUTIL_HOSTDEVICE Matrix<M,N>& operator-=(Matrix<M,N>& m1, const Matrix<M,N>& m2)
{
for ( unsigned int i = 0; i < M*N; ++i )
m1[i] -= m2[i];
return m1;
}
// Add two matrices of the same size.
template<unsigned int M, unsigned int N>
SUTIL_HOSTDEVICE Matrix<M,N> operator+(const Matrix<M,N>& m1, const Matrix<M,N>& m2)
{
Matrix<M,N> temp( m1 );
temp += m2;
return temp;
}
// Add two matrices of the same size.
template<unsigned int M, unsigned int N>
SUTIL_HOSTDEVICE Matrix<M,N>& operator+=(Matrix<M,N>& m1, const Matrix<M,N>& m2)
{
for ( unsigned int i = 0; i < M*N; ++i )
m1[i] += m2[i];
return m1;
}
// Multiply two compatible matrices.
template<unsigned int M, unsigned int N, unsigned int R>
SUTIL_HOSTDEVICE Matrix<M,R> operator*( const Matrix<M,N>& m1, const Matrix<N,R>& m2)
{
Matrix<M,R> temp;
for ( unsigned int i = 0; i < M; ++i ) {
for ( unsigned int j = 0; j < R; ++j ) {
float sum = 0.0f;
for ( unsigned int k = 0; k < N; ++k ) {
float ik = m1[ i*N+k ];
float kj = m2[ k*R+j ];
sum += ik * kj;
}
temp[i*R+j] = sum;
}
}
return temp;
}
// Multiply two compatible matrices.
template<unsigned int M>
SUTIL_HOSTDEVICE Matrix<M,M>& operator*=(Matrix<M,M>& m1, const Matrix<M,M>& m2)
{
m1 = m1*m2;
return m1;
}
// Multiply matrix by vector
template<unsigned int M, unsigned int N>
SUTIL_HOSTDEVICE typename Matrix<M,N>::floatM operator*(const Matrix<M,N>& m, const typename Matrix<M,N>::floatN& vec )
{
typename Matrix<M,N>::floatM temp;
float* t = reinterpret_cast<float*>( &temp );
const float* v = reinterpret_cast<const float*>( &vec );
for (unsigned int i = 0; i < M; ++i) {
float sum = 0.0f;
for (unsigned int j = 0; j < N; ++j) {
sum += RT_MATRIX_ACCESS( m, i, j ) * v[j];
}
t[i] = sum;
}
return temp;
}
// Multiply matrix2xN by floatN
template<unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE float2 operator*(const Matrix<2,N>& m, const typename Matrix<2,N>::floatN& vec )
{
float2 temp = { 0.0f, 0.0f };
const float* v = reinterpret_cast<const float*>( &vec );
int index = 0;
for (unsigned int j = 0; j < N; ++j)
temp.x += m[index++] * v[j];
for (unsigned int j = 0; j < N; ++j)
temp.y += m[index++] * v[j];
return temp;
}
// Multiply matrix3xN by floatN
template<unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE float3 operator*(const Matrix<3,N>& m, const typename Matrix<3,N>::floatN& vec )
{
float3 temp = { 0.0f, 0.0f, 0.0f };
const float* v = reinterpret_cast<const float*>( &vec );
int index = 0;
for (unsigned int j = 0; j < N; ++j)
temp.x += m[index++] * v[j];
for (unsigned int j = 0; j < N; ++j)
temp.y += m[index++] * v[j];
for (unsigned int j = 0; j < N; ++j)
temp.z += m[index++] * v[j];
return temp;
}
// Multiply matrix4xN by floatN
template<unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE float4 operator*(const Matrix<4,N>& m, const typename Matrix<4,N>::floatN& vec )
{
float4 temp = { 0.0f, 0.0f, 0.0f, 0.0f };
const float* v = reinterpret_cast<const float*>( &vec );
int index = 0;
for (unsigned int j = 0; j < N; ++j)
temp.x += m[index++] * v[j];
for (unsigned int j = 0; j < N; ++j)
temp.y += m[index++] * v[j];
for (unsigned int j = 0; j < N; ++j)
temp.z += m[index++] * v[j];
for (unsigned int j = 0; j < N; ++j)
temp.w += m[index++] * v[j];
return temp;
}
// Multiply matrix4x4 by float4
SUTIL_INLINE SUTIL_HOSTDEVICE float3 operator*(const Matrix<3,4>& m, const float4& vec )
{
float3 temp;
temp.x = m[ 0] * vec.x +
m[ 1] * vec.y +
m[ 2] * vec.z +
m[ 3] * vec.w;
temp.y = m[ 4] * vec.x +
m[ 5] * vec.y +
m[ 6] * vec.z +
m[ 7] * vec.w;
temp.z = m[ 8] * vec.x +
m[ 9] * vec.y +
m[10] * vec.z +
m[11] * vec.w;
return temp;
}
// Multiply matrix4x4 by float4
SUTIL_INLINE SUTIL_HOSTDEVICE float4 operator*(const Matrix<4,4>& m, const float4& vec )
{
float4 temp;
temp.x = m[ 0] * vec.x +
m[ 1] * vec.y +
m[ 2] * vec.z +
m[ 3] * vec.w;
temp.y = m[ 4] * vec.x +
m[ 5] * vec.y +
m[ 6] * vec.z +
m[ 7] * vec.w;
temp.z = m[ 8] * vec.x +
m[ 9] * vec.y +
m[10] * vec.z +
m[11] * vec.w;
temp.w = m[12] * vec.x +
m[13] * vec.y +
m[14] * vec.z +
m[15] * vec.w;
return temp;
}
// Multiply vector by matrix
template<unsigned int M, unsigned int N>
SUTIL_HOSTDEVICE typename Matrix<M,N>::floatN operator*(const typename Matrix<M,N>::floatM& vec, const Matrix<M,N>& m)
{
typename Matrix<M,N>::floatN temp;
float* t = reinterpret_cast<float*>( &temp );
const float* v = reinterpret_cast<const float*>( &vec);
for (unsigned int i = 0; i < N; ++i) {
float sum = 0.0f;
for (unsigned int j = 0; j < M; ++j) {
sum += v[j] * RT_MATRIX_ACCESS( m, j, i ) ;
}
t[i] = sum;
}
return temp;
}
// Multply matrix by a scalar.
template<unsigned int M, unsigned int N>
SUTIL_HOSTDEVICE Matrix<M,N> operator*(const Matrix<M,N>& m, float f)
{
Matrix<M,N> temp( m );
temp *= f;
return temp;
}
// Multply matrix by a scalar.
template<unsigned int M, unsigned int N>
SUTIL_HOSTDEVICE Matrix<M,N>& operator*=(Matrix<M,N>& m, float f)
{
for ( unsigned int i = 0; i < M*N; ++i )
m[i] *= f;
return m;
}
// Multply matrix by a scalar.
template<unsigned int M, unsigned int N>
SUTIL_HOSTDEVICE Matrix<M,N> operator*(float f, const Matrix<M,N>& m)
{
Matrix<M,N> temp;
for ( unsigned int i = 0; i < M*N; ++i )
temp[i] = m[i]*f;
return temp;
}
// Divide matrix by a scalar.
template<unsigned int M, unsigned int N>
SUTIL_HOSTDEVICE Matrix<M,N> operator/(const Matrix<M,N>& m, float f)
{
Matrix<M,N> temp( m );
temp /= f;
return temp;
}
// Divide matrix by a scalar.
template<unsigned int M, unsigned int N>
SUTIL_HOSTDEVICE Matrix<M,N>& operator/=(Matrix<M,N>& m, float f)
{
float inv_f = 1.0f / f;
for ( unsigned int i = 0; i < M*N; ++i )
m[i] *= inv_f;
return m;
}
// Returns the transpose of the matrix.
template<unsigned int M, unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<N,M> Matrix<M,N>::transpose() const
{
Matrix<N,M> ret;
for( unsigned int row = 0; row < M; ++row )
for( unsigned int col = 0; col < N; ++col )
ret[col*M+row] = m_data[row*N+col];
return ret;
}
// Returns the determinant of the matrix.
template<>
SUTIL_INLINE SUTIL_HOSTDEVICE float Matrix<3,3>::det() const
{
const float* m = m_data;
float d = m[0]*m[4]*m[8] + m[1]*m[5]*m[6] + m[2]*m[3]*m[7]
- m[0]*m[5]*m[7] - m[1]*m[3]*m[8] - m[2]*m[4]*m[6];
return d;
}
// Returns the determinant of the matrix.
template<>
SUTIL_INLINE SUTIL_HOSTDEVICE float Matrix<4,4>::det() const
{
const float* m = m_data;
float d =
m[0]*m[5]*m[10]*m[15]-
m[0]*m[5]*m[11]*m[14]+m[0]*m[9]*m[14]*m[7]-
m[0]*m[9]*m[6]*m[15]+m[0]*m[13]*m[6]*m[11]-
m[0]*m[13]*m[10]*m[7]-m[4]*m[1]*m[10]*m[15]+m[4]*m[1]*m[11]*m[14]-
m[4]*m[9]*m[14]*m[3]+m[4]*m[9]*m[2]*m[15]-
m[4]*m[13]*m[2]*m[11]+m[4]*m[13]*m[10]*m[3]+m[8]*m[1]*m[6]*m[15]-
m[8]*m[1]*m[14]*m[7]+m[8]*m[5]*m[14]*m[3]-
m[8]*m[5]*m[2]*m[15]+m[8]*m[13]*m[2]*m[7]-
m[8]*m[13]*m[6]*m[3]-
m[12]*m[1]*m[6]*m[11]+m[12]*m[1]*m[10]*m[7]-
m[12]*m[5]*m[10]*m[3]+m[12]*m[5]*m[2]*m[11]-
m[12]*m[9]*m[2]*m[7]+m[12]*m[9]*m[6]*m[3];
return d;
}
// Returns the inverse of the matrix.
template<>
SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<4,4> Matrix<4,4>::inverse() const
{
Matrix<4,4> dst;
const float* m = m_data;
const float d = 1.0f / det();
dst[0] = d * (m[5] * (m[10] * m[15] - m[14] * m[11]) + m[9] * (m[14] * m[7] - m[6] * m[15]) + m[13] * (m[6] * m[11] - m[10] * m[7]));
dst[4] = d * (m[6] * (m[8] * m[15] - m[12] * m[11]) + m[10] * (m[12] * m[7] - m[4] * m[15]) + m[14] * (m[4] * m[11] - m[8] * m[7]));
dst[8] = d * (m[7] * (m[8] * m[13] - m[12] * m[9]) + m[11] * (m[12] * m[5] - m[4] * m[13]) + m[15] * (m[4] * m[9] - m[8] * m[5]));
dst[12] = d * (m[4] * (m[13] * m[10] - m[9] * m[14]) + m[8] * (m[5] * m[14] - m[13] * m[6]) + m[12] * (m[9] * m[6] - m[5] * m[10]));
dst[1] = d * (m[9] * (m[2] * m[15] - m[14] * m[3]) + m[13] * (m[10] * m[3] - m[2] * m[11]) + m[1] * (m[14] * m[11] - m[10] * m[15]));
dst[5] = d * (m[10] * (m[0] * m[15] - m[12] * m[3]) + m[14] * (m[8] * m[3] - m[0] * m[11]) + m[2] * (m[12] * m[11] - m[8] * m[15]));
dst[9] = d * (m[11] * (m[0] * m[13] - m[12] * m[1]) + m[15] * (m[8] * m[1] - m[0] * m[9]) + m[3] * (m[12] * m[9] - m[8] * m[13]));
dst[13] = d * (m[8] * (m[13] * m[2] - m[1] * m[14]) + m[12] * (m[1] * m[10] - m[9] * m[2]) + m[0] * (m[9] * m[14] - m[13] * m[10]));
dst[2] = d * (m[13] * (m[2] * m[7] - m[6] * m[3]) + m[1] * (m[6] * m[15] - m[14] * m[7]) + m[5] * (m[14] * m[3] - m[2] * m[15]));
dst[6] = d * (m[14] * (m[0] * m[7] - m[4] * m[3]) + m[2] * (m[4] * m[15] - m[12] * m[7]) + m[6] * (m[12] * m[3] - m[0] * m[15]));
dst[10] = d * (m[15] * (m[0] * m[5] - m[4] * m[1]) + m[3] * (m[4] * m[13] - m[12] * m[5]) + m[7] * (m[12] * m[1] - m[0] * m[13]));
dst[14] = d * (m[12] * (m[5] * m[2] - m[1] * m[6]) + m[0] * (m[13] * m[6] - m[5] * m[14]) + m[4] * (m[1] * m[14] - m[13] * m[2]));
dst[3] = d * (m[1] * (m[10] * m[7] - m[6] * m[11]) + m[5] * (m[2] * m[11] - m[10] * m[3]) + m[9] * (m[6] * m[3] - m[2] * m[7]));
dst[7] = d * (m[2] * (m[8] * m[7] - m[4] * m[11]) + m[6] * (m[0] * m[11] - m[8] * m[3]) + m[10] * (m[4] * m[3] - m[0] * m[7]));
dst[11] = d * (m[3] * (m[8] * m[5] - m[4] * m[9]) + m[7] * (m[0] * m[9] - m[8] * m[1]) + m[11] * (m[4] * m[1] - m[0] * m[5]));
dst[15] = d * (m[0] * (m[5] * m[10] - m[9] * m[6]) + m[4] * (m[9] * m[2] - m[1] * m[10]) + m[8] * (m[1] * m[6] - m[5] * m[2]));
return dst;
}
// Returns a rotation matrix.
// This is a static member.
template<>
SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<4,4> Matrix<4,4>::rotate(const float radians, const float3& axis)
{
Matrix<4,4> Mat = Matrix<4,4>::identity();
float *m = Mat.getData();
// NOTE: Element 0,1 is wrong in Foley and Van Dam, Pg 227!
float sintheta=sinf(radians);
float costheta=cosf(radians);
float ux=axis.x;
float uy=axis.y;
float uz=axis.z;
m[0*4+0]=ux*ux+costheta*(1-ux*ux);
m[0*4+1]=ux*uy*(1-costheta)-uz*sintheta;
m[0*4+2]=uz*ux*(1-costheta)+uy*sintheta;
m[0*4+3]=0;
m[1*4+0]=ux*uy*(1-costheta)+uz*sintheta;
m[1*4+1]=uy*uy+costheta*(1-uy*uy);
m[1*4+2]=uy*uz*(1-costheta)-ux*sintheta;
m[1*4+3]=0;
m[2*4+0]=uz*ux*(1-costheta)-uy*sintheta;
m[2*4+1]=uy*uz*(1-costheta)+ux*sintheta;
m[2*4+2]=uz*uz+costheta*(1-uz*uz);
m[2*4+3]=0;
m[3*4+0]=0;
m[3*4+1]=0;
m[3*4+2]=0;
m[3*4+3]=1;
return Matrix<4,4>( m );
}
// Returns a translation matrix.
// This is a static member.
template<>
SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<4,4> Matrix<4,4>::translate(const float3& vec)
{
Matrix<4,4> Mat = Matrix<4,4>::identity();
float *m = Mat.getData();
m[3] = vec.x;
m[7] = vec.y;
m[11]= vec.z;
return Matrix<4,4>( m );
}
// Returns a scale matrix.
// This is a static member.
template<>
SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<4,4> Matrix<4,4>::scale(const float3& vec)
{
Matrix<4,4> Mat = Matrix<4,4>::identity();
float *m = Mat.getData();
m[0] = vec.x;
m[5] = vec.y;
m[10]= vec.z;
return Matrix<4,4>( m );
}
// This is a static member.
template<>
SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<4,4> Matrix<4,4>::fromBasis( const float3& u, const float3& v, const float3& w, const float3& c )
{
float m[16];
m[ 0] = u.x;
m[ 1] = v.x;
m[ 2] = w.x;
m[ 3] = c.x;
m[ 4] = u.y;
m[ 5] = v.y;
m[ 6] = w.y;
m[ 7] = c.y;
m[ 8] = u.z;
m[ 9] = v.z;
m[10] = w.z;
m[11] = c.z;
m[12] = 0.0f;
m[13] = 0.0f;
m[14] = 0.0f;
m[15] = 1.0f;
return Matrix<4,4>( m );
}
template<>
SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<3,4> Matrix<3,4>::affineIdentity()
{
Matrix<3,4> m;
m.m_data[ 0] = 1.0f;
m.m_data[ 1] = 0.0f;
m.m_data[ 2] = 0.0f;
m.m_data[ 3] = 0.0f;
m.m_data[ 4] = 0.0f;
m.m_data[ 5] = 1.0f;
m.m_data[ 6] = 0.0f;
m.m_data[ 7] = 0.0f;
m.m_data[ 8] = 0.0f;
m.m_data[ 9] = 0.0f;
m.m_data[10] = 1.0f;
m.m_data[11] = 0.0f;
return m;
}
// Returns the identity matrix.
// This is a static member.
template<unsigned int M, unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<N,N> Matrix<M,N>::identity()
{
float temp[N*N];
for(unsigned int i = 0; i < N*N; ++i)
temp[i] = 0;
for( unsigned int i = 0; i < N; ++i )
RT_MATRIX_ACCESS( temp,i,i ) = 1.0f;
return Matrix<N,N>( temp );
}
// Ordered comparison operator so that the matrix can be used in an STL container.
template<unsigned int M, unsigned int N>
SUTIL_INLINE SUTIL_HOSTDEVICE bool Matrix<M,N>::operator<( const Matrix<M, N>& rhs ) const
{
for( unsigned int i = 0; i < N*M; ++i ) {
if( m_data[i] < rhs[i] )
return true;
else if( m_data[i] > rhs[i] )
return false;
}
return false;
}
typedef Matrix<2, 2> Matrix2x2;
typedef Matrix<2, 3> Matrix2x3;
typedef Matrix<2, 4> Matrix2x4;
typedef Matrix<3, 2> Matrix3x2;
typedef Matrix<3, 3> Matrix3x3;
typedef Matrix<3, 4> Matrix3x4;
typedef Matrix<4, 2> Matrix4x2;
typedef Matrix<4, 3> Matrix4x3;
typedef Matrix<4, 4> Matrix4x4;
SUTIL_INLINE SUTIL_HOSTDEVICE Matrix<3,3> make_matrix3x3(const Matrix<4,4> &matrix)
{
Matrix<3,3> Mat;
float *m = Mat.getData();
const float *m4x4 = matrix.getData();
m[0*3+0]=m4x4[0*4+0];
m[0*3+1]=m4x4[0*4+1];
m[0*3+2]=m4x4[0*4+2];
m[1*3+0]=m4x4[1*4+0];
m[1*3+1]=m4x4[1*4+1];
m[1*3+2]=m4x4[1*4+2];
m[2*3+0]=m4x4[2*4+0];
m[2*3+1]=m4x4[2*4+1];
m[2*3+2]=m4x4[2*4+2];
return Mat;
}
} // end namespace sutil
#undef RT_MATRIX_ACCESS
#undef RT_MAT_DECL
| 26,645 | C | 31.855734 | 158 | 0.576994 |
arhix52/Strelka/sutil/vec_math.h | //
// Copyright (c) 2021, NVIDIA CORPORATION. All rights reserved.
//
// Redistribution and use in source and binary forms, with or without
// modification, are permitted provided that the following conditions
// are met:
// * Redistributions of source code must retain the above copyright
// notice, this list of conditions and the following disclaimer.
// * Redistributions in binary form must reproduce the above copyright
// notice, this list of conditions and the following disclaimer in the
// documentation and/or other materials provided with the distribution.
// * Neither the name of NVIDIA CORPORATION nor the names of its
// contributors may be used to endorse or promote products derived
// from this software without specific prior written permission.
//
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS ``AS IS'' AND ANY
// EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
// IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
// PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
// CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
// EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
// PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
// PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY
// OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
// (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
// OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
//
#pragma once
#include <sutil/Preprocessor.h>
#include <vector_functions.h>
#include <vector_types.h>
#if !defined(__CUDACC_RTC__)
#include <cmath>
#include <cstdlib>
#endif
/* scalar functions used in vector functions */
#ifndef M_PIf
#define M_PIf 3.14159265358979323846f
#endif
#ifndef M_PI_2f
#define M_PI_2f 1.57079632679489661923f
#endif
#ifndef M_1_PIf
#define M_1_PIf 0.318309886183790671538f
#endif
#if !defined(__CUDACC__)
SUTIL_INLINE SUTIL_HOSTDEVICE int max(int a, int b)
{
return a > b ? a : b;
}
SUTIL_INLINE SUTIL_HOSTDEVICE int min(int a, int b)
{
return a < b ? a : b;
}
SUTIL_INLINE SUTIL_HOSTDEVICE long long max(long long a, long long b)
{
return a > b ? a : b;
}
SUTIL_INLINE SUTIL_HOSTDEVICE long long min(long long a, long long b)
{
return a < b ? a : b;
}
SUTIL_INLINE SUTIL_HOSTDEVICE unsigned int max(unsigned int a, unsigned int b)
{
return a > b ? a : b;
}
SUTIL_INLINE SUTIL_HOSTDEVICE unsigned int min(unsigned int a, unsigned int b)
{
return a < b ? a : b;
}
SUTIL_INLINE SUTIL_HOSTDEVICE unsigned long long max(unsigned long long a, unsigned long long b)
{
return a > b ? a : b;
}
SUTIL_INLINE SUTIL_HOSTDEVICE unsigned long long min(unsigned long long a, unsigned long long b)
{
return a < b ? a : b;
}
SUTIL_INLINE SUTIL_HOSTDEVICE float min(const float a, const float b)
{
return a < b ? a : b;
}
/** lerp */
SUTIL_INLINE SUTIL_HOSTDEVICE float lerp(const float a, const float b, const float t)
{
return a + t*(b-a);
}
/** bilerp */
SUTIL_INLINE SUTIL_HOSTDEVICE float bilerp(const float x00, const float x10, const float x01, const float x11,
const float u, const float v)
{
return lerp( lerp( x00, x10, u ), lerp( x01, x11, u ), v );
}
template <typename IntegerType>
SUTIL_INLINE SUTIL_HOSTDEVICE IntegerType roundUp(IntegerType x, IntegerType y)
{
return ( ( x + y - 1 ) / y ) * y;
}
#endif
/** clamp */
SUTIL_INLINE SUTIL_HOSTDEVICE float clamp( const float f, const float a, const float b )
{
return fmaxf( a, fminf( f, b ) );
}
/* float2 functions */
/******************************************************************************/
/** additional constructors
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float2 make_float2(const float s)
{
return make_float2(s, s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float2 make_float2(const int2& a)
{
return make_float2(float(a.x), float(a.y));
}
SUTIL_INLINE SUTIL_HOSTDEVICE float2 make_float2(const uint2& a)
{
return make_float2(float(a.x), float(a.y));
}
/** @} */
/** negate */
SUTIL_INLINE SUTIL_HOSTDEVICE float2 operator-(const float2& a)
{
return make_float2(-a.x, -a.y);
}
/** min
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float2 fminf(const float2& a, const float2& b)
{
return make_float2(fminf(a.x,b.x), fminf(a.y,b.y));
}
SUTIL_INLINE SUTIL_HOSTDEVICE float fminf(const float2& a)
{
return fminf(a.x, a.y);
}
/** @} */
/** max
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float2 fmaxf(const float2& a, const float2& b)
{
return make_float2(fmaxf(a.x,b.x), fmaxf(a.y,b.y));
}
SUTIL_INLINE SUTIL_HOSTDEVICE float fmaxf(const float2& a)
{
return fmaxf(a.x, a.y);
}
/** @} */
/** add
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float2 operator+(const float2& a, const float2& b)
{
return make_float2(a.x + b.x, a.y + b.y);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float2 operator+(const float2& a, const float b)
{
return make_float2(a.x + b, a.y + b);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float2 operator+(const float a, const float2& b)
{
return make_float2(a + b.x, a + b.y);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator+=(float2& a, const float2& b)
{
a.x += b.x; a.y += b.y;
}
/** @} */
/** subtract
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float2 operator-(const float2& a, const float2& b)
{
return make_float2(a.x - b.x, a.y - b.y);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float2 operator-(const float2& a, const float b)
{
return make_float2(a.x - b, a.y - b);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float2 operator-(const float a, const float2& b)
{
return make_float2(a - b.x, a - b.y);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator-=(float2& a, const float2& b)
{
a.x -= b.x; a.y -= b.y;
}
/** @} */
/** multiply
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float2 operator*(const float2& a, const float2& b)
{
return make_float2(a.x * b.x, a.y * b.y);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float2 operator*(const float2& a, const float s)
{
return make_float2(a.x * s, a.y * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float2 operator*(const float s, const float2& a)
{
return make_float2(a.x * s, a.y * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator*=(float2& a, const float2& s)
{
a.x *= s.x; a.y *= s.y;
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator*=(float2& a, const float s)
{
a.x *= s; a.y *= s;
}
/** @} */
/** divide
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float2 operator/(const float2& a, const float2& b)
{
return make_float2(a.x / b.x, a.y / b.y);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float2 operator/(const float2& a, const float s)
{
float inv = 1.0f / s;
return a * inv;
}
SUTIL_INLINE SUTIL_HOSTDEVICE float2 operator/(const float s, const float2& a)
{
return make_float2( s/a.x, s/a.y );
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator/=(float2& a, const float s)
{
float inv = 1.0f / s;
a *= inv;
}
/** @} */
/** lerp */
SUTIL_INLINE SUTIL_HOSTDEVICE float2 lerp(const float2& a, const float2& b, const float t)
{
return a + t*(b-a);
}
/** bilerp */
SUTIL_INLINE SUTIL_HOSTDEVICE float2 bilerp(const float2& x00, const float2& x10, const float2& x01, const float2& x11,
const float u, const float v)
{
return lerp( lerp( x00, x10, u ), lerp( x01, x11, u ), v );
}
/** clamp
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float2 clamp(const float2& v, const float a, const float b)
{
return make_float2(clamp(v.x, a, b), clamp(v.y, a, b));
}
SUTIL_INLINE SUTIL_HOSTDEVICE float2 clamp(const float2& v, const float2& a, const float2& b)
{
return make_float2(clamp(v.x, a.x, b.x), clamp(v.y, a.y, b.y));
}
/** @} */
/** dot product */
SUTIL_INLINE SUTIL_HOSTDEVICE float dot(const float2& a, const float2& b)
{
return a.x * b.x + a.y * b.y;
}
/** length */
SUTIL_INLINE SUTIL_HOSTDEVICE float length(const float2& v)
{
return sqrtf(dot(v, v));
}
/** normalize */
SUTIL_INLINE SUTIL_HOSTDEVICE float2 normalize(const float2& v)
{
float invLen = 1.0f / sqrtf(dot(v, v));
return v * invLen;
}
/** floor */
SUTIL_INLINE SUTIL_HOSTDEVICE float2 floor(const float2& v)
{
return make_float2(::floorf(v.x), ::floorf(v.y));
}
/** reflect */
SUTIL_INLINE SUTIL_HOSTDEVICE float2 reflect(const float2& i, const float2& n)
{
return i - 2.0f * n * dot(n,i);
}
/** Faceforward
* Returns N if dot(i, nref) > 0; else -N;
* Typical usage is N = faceforward(N, -ray.dir, N);
* Note that this is opposite of what faceforward does in Cg and GLSL */
SUTIL_INLINE SUTIL_HOSTDEVICE float2 faceforward(const float2& n, const float2& i, const float2& nref)
{
return n * copysignf( 1.0f, dot(i, nref) );
}
/** exp */
SUTIL_INLINE SUTIL_HOSTDEVICE float2 expf(const float2& v)
{
return make_float2(::expf(v.x), ::expf(v.y));
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE float getByIndex(const float2& v, int i)
{
return ((float*)(&v))[i];
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE void setByIndex(float2& v, int i, float x)
{
((float*)(&v))[i] = x;
}
/* float3 functions */
/******************************************************************************/
/** additional constructors
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float3 make_float3(const float s)
{
return make_float3(s, s, s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float3 make_float3(const float2& a)
{
return make_float3(a.x, a.y, 0.0f);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float3 make_float3(const int3& a)
{
return make_float3(float(a.x), float(a.y), float(a.z));
}
SUTIL_INLINE SUTIL_HOSTDEVICE float3 make_float3(const uint3& a)
{
return make_float3(float(a.x), float(a.y), float(a.z));
}
/** @} */
/** negate */
SUTIL_INLINE SUTIL_HOSTDEVICE float3 operator-(const float3& a)
{
return make_float3(-a.x, -a.y, -a.z);
}
/** min
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float3 fminf(const float3& a, const float3& b)
{
return make_float3(fminf(a.x,b.x), fminf(a.y,b.y), fminf(a.z,b.z));
}
SUTIL_INLINE SUTIL_HOSTDEVICE float fminf(const float3& a)
{
return fminf(fminf(a.x, a.y), a.z);
}
/** @} */
/** max
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float3 fmaxf(const float3& a, const float3& b)
{
return make_float3(fmaxf(a.x,b.x), fmaxf(a.y,b.y), fmaxf(a.z,b.z));
}
SUTIL_INLINE SUTIL_HOSTDEVICE float fmaxf(const float3& a)
{
return fmaxf(fmaxf(a.x, a.y), a.z);
}
/** @} */
/** add
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float3 operator+(const float3& a, const float3& b)
{
return make_float3(a.x + b.x, a.y + b.y, a.z + b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float3 operator+(const float3& a, const float b)
{
return make_float3(a.x + b, a.y + b, a.z + b);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float3 operator+(const float a, const float3& b)
{
return make_float3(a + b.x, a + b.y, a + b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator+=(float3& a, const float3& b)
{
a.x += b.x; a.y += b.y; a.z += b.z;
}
/** @} */
/** subtract
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float3 operator-(const float3& a, const float3& b)
{
return make_float3(a.x - b.x, a.y - b.y, a.z - b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float3 operator-(const float3& a, const float b)
{
return make_float3(a.x - b, a.y - b, a.z - b);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float3 operator-(const float a, const float3& b)
{
return make_float3(a - b.x, a - b.y, a - b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator-=(float3& a, const float3& b)
{
a.x -= b.x; a.y -= b.y; a.z -= b.z;
}
/** @} */
/** multiply
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float3 operator*(const float3& a, const float3& b)
{
return make_float3(a.x * b.x, a.y * b.y, a.z * b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float3 operator*(const float3& a, const float s)
{
return make_float3(a.x * s, a.y * s, a.z * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float3 operator*(const float s, const float3& a)
{
return make_float3(a.x * s, a.y * s, a.z * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator*=(float3& a, const float3& s)
{
a.x *= s.x; a.y *= s.y; a.z *= s.z;
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator*=(float3& a, const float s)
{
a.x *= s; a.y *= s; a.z *= s;
}
/** @} */
/** divide
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float3 operator/(const float3& a, const float3& b)
{
return make_float3(a.x / b.x, a.y / b.y, a.z / b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float3 operator/(const float3& a, const float s)
{
float inv = 1.0f / s;
return a * inv;
}
SUTIL_INLINE SUTIL_HOSTDEVICE float3 operator/(const float s, const float3& a)
{
return make_float3( s/a.x, s/a.y, s/a.z );
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator/=(float3& a, const float s)
{
float inv = 1.0f / s;
a *= inv;
}
/** @} */
/** lerp */
SUTIL_INLINE SUTIL_HOSTDEVICE float3 lerp(const float3& a, const float3& b, const float t)
{
return a + t*(b-a);
}
/** bilerp */
SUTIL_INLINE SUTIL_HOSTDEVICE float3 bilerp(const float3& x00, const float3& x10, const float3& x01, const float3& x11,
const float u, const float v)
{
return lerp( lerp( x00, x10, u ), lerp( x01, x11, u ), v );
}
/** clamp
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float3 clamp(const float3& v, const float a, const float b)
{
return make_float3(clamp(v.x, a, b), clamp(v.y, a, b), clamp(v.z, a, b));
}
SUTIL_INLINE SUTIL_HOSTDEVICE float3 clamp(const float3& v, const float3& a, const float3& b)
{
return make_float3(clamp(v.x, a.x, b.x), clamp(v.y, a.y, b.y), clamp(v.z, a.z, b.z));
}
/** @} */
/** dot product */
SUTIL_INLINE SUTIL_HOSTDEVICE float dot(const float3& a, const float3& b)
{
return a.x * b.x + a.y * b.y + a.z * b.z;
}
/** cross product */
SUTIL_INLINE SUTIL_HOSTDEVICE float3 cross(const float3& a, const float3& b)
{
return make_float3(a.y*b.z - a.z*b.y, a.z*b.x - a.x*b.z, a.x*b.y - a.y*b.x);
}
/** length */
SUTIL_INLINE SUTIL_HOSTDEVICE float length(const float3& v)
{
return sqrtf(dot(v, v));
}
/** normalize */
SUTIL_INLINE SUTIL_HOSTDEVICE float3 normalize(const float3& v)
{
float invLen = 1.0f / sqrtf(dot(v, v));
return v * invLen;
}
/** floor */
SUTIL_INLINE SUTIL_HOSTDEVICE float3 floor(const float3& v)
{
return make_float3(::floorf(v.x), ::floorf(v.y), ::floorf(v.z));
}
/** reflect */
SUTIL_INLINE SUTIL_HOSTDEVICE float3 reflect(const float3& i, const float3& n)
{
return i - 2.0f * n * dot(n,i);
}
/** Faceforward
* Returns N if dot(i, nref) > 0; else -N;
* Typical usage is N = faceforward(N, -ray.dir, N);
* Note that this is opposite of what faceforward does in Cg and GLSL */
SUTIL_INLINE SUTIL_HOSTDEVICE float3 faceforward(const float3& n, const float3& i, const float3& nref)
{
return n * copysignf( 1.0f, dot(i, nref) );
}
/** exp */
SUTIL_INLINE SUTIL_HOSTDEVICE float3 expf(const float3& v)
{
return make_float3(::expf(v.x), ::expf(v.y), ::expf(v.z));
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE float getByIndex(const float3& v, int i)
{
return ((float*)(&v))[i];
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE void setByIndex(float3& v, int i, float x)
{
((float*)(&v))[i] = x;
}
/* float4 functions */
/******************************************************************************/
/** additional constructors
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float4 make_float4(const float s)
{
return make_float4(s, s, s, s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float4 make_float4(const float3& a)
{
return make_float4(a.x, a.y, a.z, 0.0f);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float4 make_float4(const int4& a)
{
return make_float4(float(a.x), float(a.y), float(a.z), float(a.w));
}
SUTIL_INLINE SUTIL_HOSTDEVICE float4 make_float4(const uint4& a)
{
return make_float4(float(a.x), float(a.y), float(a.z), float(a.w));
}
/** @} */
/** negate */
SUTIL_INLINE SUTIL_HOSTDEVICE float4 operator-(const float4& a)
{
return make_float4(-a.x, -a.y, -a.z, -a.w);
}
/** min
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float4 fminf(const float4& a, const float4& b)
{
return make_float4(fminf(a.x,b.x), fminf(a.y,b.y), fminf(a.z,b.z), fminf(a.w,b.w));
}
SUTIL_INLINE SUTIL_HOSTDEVICE float fminf(const float4& a)
{
return fminf(fminf(a.x, a.y), fminf(a.z, a.w));
}
/** @} */
/** max
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float4 fmaxf(const float4& a, const float4& b)
{
return make_float4(fmaxf(a.x,b.x), fmaxf(a.y,b.y), fmaxf(a.z,b.z), fmaxf(a.w,b.w));
}
SUTIL_INLINE SUTIL_HOSTDEVICE float fmaxf(const float4& a)
{
return fmaxf(fmaxf(a.x, a.y), fmaxf(a.z, a.w));
}
/** @} */
/** add
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float4 operator+(const float4& a, const float4& b)
{
return make_float4(a.x + b.x, a.y + b.y, a.z + b.z, a.w + b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float4 operator+(const float4& a, const float b)
{
return make_float4(a.x + b, a.y + b, a.z + b, a.w + b);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float4 operator+(const float a, const float4& b)
{
return make_float4(a + b.x, a + b.y, a + b.z, a + b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator+=(float4& a, const float4& b)
{
a.x += b.x; a.y += b.y; a.z += b.z; a.w += b.w;
}
/** @} */
/** subtract
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float4 operator-(const float4& a, const float4& b)
{
return make_float4(a.x - b.x, a.y - b.y, a.z - b.z, a.w - b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float4 operator-(const float4& a, const float b)
{
return make_float4(a.x - b, a.y - b, a.z - b, a.w - b);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float4 operator-(const float a, const float4& b)
{
return make_float4(a - b.x, a - b.y, a - b.z, a - b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator-=(float4& a, const float4& b)
{
a.x -= b.x; a.y -= b.y; a.z -= b.z; a.w -= b.w;
}
/** @} */
/** multiply
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float4 operator*(const float4& a, const float4& s)
{
return make_float4(a.x * s.x, a.y * s.y, a.z * s.z, a.w * s.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float4 operator*(const float4& a, const float s)
{
return make_float4(a.x * s, a.y * s, a.z * s, a.w * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float4 operator*(const float s, const float4& a)
{
return make_float4(a.x * s, a.y * s, a.z * s, a.w * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator*=(float4& a, const float4& s)
{
a.x *= s.x; a.y *= s.y; a.z *= s.z; a.w *= s.w;
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator*=(float4& a, const float s)
{
a.x *= s; a.y *= s; a.z *= s; a.w *= s;
}
/** @} */
/** divide
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float4 operator/(const float4& a, const float4& b)
{
return make_float4(a.x / b.x, a.y / b.y, a.z / b.z, a.w / b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float4 operator/(const float4& a, const float s)
{
float inv = 1.0f / s;
return a * inv;
}
SUTIL_INLINE SUTIL_HOSTDEVICE float4 operator/(const float s, const float4& a)
{
return make_float4( s/a.x, s/a.y, s/a.z, s/a.w );
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator/=(float4& a, const float s)
{
float inv = 1.0f / s;
a *= inv;
}
/** @} */
/** lerp */
SUTIL_INLINE SUTIL_HOSTDEVICE float4 lerp(const float4& a, const float4& b, const float t)
{
return a + t*(b-a);
}
/** bilerp */
SUTIL_INLINE SUTIL_HOSTDEVICE float4 bilerp(const float4& x00, const float4& x10, const float4& x01, const float4& x11,
const float u, const float v)
{
return lerp( lerp( x00, x10, u ), lerp( x01, x11, u ), v );
}
/** clamp
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float4 clamp(const float4& v, const float a, const float b)
{
return make_float4(clamp(v.x, a, b), clamp(v.y, a, b), clamp(v.z, a, b), clamp(v.w, a, b));
}
SUTIL_INLINE SUTIL_HOSTDEVICE float4 clamp(const float4& v, const float4& a, const float4& b)
{
return make_float4(clamp(v.x, a.x, b.x), clamp(v.y, a.y, b.y), clamp(v.z, a.z, b.z), clamp(v.w, a.w, b.w));
}
/** @} */
/** dot product */
SUTIL_INLINE SUTIL_HOSTDEVICE float dot(const float4& a, const float4& b)
{
return a.x * b.x + a.y * b.y + a.z * b.z + a.w * b.w;
}
/** length */
SUTIL_INLINE SUTIL_HOSTDEVICE float length(const float4& r)
{
return sqrtf(dot(r, r));
}
/** normalize */
SUTIL_INLINE SUTIL_HOSTDEVICE float4 normalize(const float4& v)
{
float invLen = 1.0f / sqrtf(dot(v, v));
return v * invLen;
}
/** floor */
SUTIL_INLINE SUTIL_HOSTDEVICE float4 floor(const float4& v)
{
return make_float4(::floorf(v.x), ::floorf(v.y), ::floorf(v.z), ::floorf(v.w));
}
/** reflect */
SUTIL_INLINE SUTIL_HOSTDEVICE float4 reflect(const float4& i, const float4& n)
{
return i - 2.0f * n * dot(n,i);
}
/**
* Faceforward
* Returns N if dot(i, nref) > 0; else -N;
* Typical usage is N = faceforward(N, -ray.dir, N);
* Note that this is opposite of what faceforward does in Cg and GLSL
*/
SUTIL_INLINE SUTIL_HOSTDEVICE float4 faceforward(const float4& n, const float4& i, const float4& nref)
{
return n * copysignf( 1.0f, dot(i, nref) );
}
/** exp */
SUTIL_INLINE SUTIL_HOSTDEVICE float4 expf(const float4& v)
{
return make_float4(::expf(v.x), ::expf(v.y), ::expf(v.z), ::expf(v.w));
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE float getByIndex(const float4& v, int i)
{
return ((float*)(&v))[i];
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE void setByIndex(float4& v, int i, float x)
{
((float*)(&v))[i] = x;
}
/* int functions */
/******************************************************************************/
/** clamp */
SUTIL_INLINE SUTIL_HOSTDEVICE int clamp(const int f, const int a, const int b)
{
return max(a, min(f, b));
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE int getByIndex(const int1& v, int i)
{
return ((int*)(&v))[i];
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE void setByIndex(int1& v, int i, int x)
{
((int*)(&v))[i] = x;
}
/* int2 functions */
/******************************************************************************/
/** additional constructors
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE int2 make_int2(const int s)
{
return make_int2(s, s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE int2 make_int2(const float2& a)
{
return make_int2(int(a.x), int(a.y));
}
/** @} */
/** negate */
SUTIL_INLINE SUTIL_HOSTDEVICE int2 operator-(const int2& a)
{
return make_int2(-a.x, -a.y);
}
/** min */
SUTIL_INLINE SUTIL_HOSTDEVICE int2 min(const int2& a, const int2& b)
{
return make_int2(min(a.x,b.x), min(a.y,b.y));
}
/** max */
SUTIL_INLINE SUTIL_HOSTDEVICE int2 max(const int2& a, const int2& b)
{
return make_int2(max(a.x,b.x), max(a.y,b.y));
}
/** add
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE int2 operator+(const int2& a, const int2& b)
{
return make_int2(a.x + b.x, a.y + b.y);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator+=(int2& a, const int2& b)
{
a.x += b.x; a.y += b.y;
}
/** @} */
/** subtract
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE int2 operator-(const int2& a, const int2& b)
{
return make_int2(a.x - b.x, a.y - b.y);
}
SUTIL_INLINE SUTIL_HOSTDEVICE int2 operator-(const int2& a, const int b)
{
return make_int2(a.x - b, a.y - b);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator-=(int2& a, const int2& b)
{
a.x -= b.x; a.y -= b.y;
}
/** @} */
/** multiply
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE int2 operator*(const int2& a, const int2& b)
{
return make_int2(a.x * b.x, a.y * b.y);
}
SUTIL_INLINE SUTIL_HOSTDEVICE int2 operator*(const int2& a, const int s)
{
return make_int2(a.x * s, a.y * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE int2 operator*(const int s, const int2& a)
{
return make_int2(a.x * s, a.y * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator*=(int2& a, const int s)
{
a.x *= s; a.y *= s;
}
/** @} */
/** clamp
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE int2 clamp(const int2& v, const int a, const int b)
{
return make_int2(clamp(v.x, a, b), clamp(v.y, a, b));
}
SUTIL_INLINE SUTIL_HOSTDEVICE int2 clamp(const int2& v, const int2& a, const int2& b)
{
return make_int2(clamp(v.x, a.x, b.x), clamp(v.y, a.y, b.y));
}
/** @} */
/** equality
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator==(const int2& a, const int2& b)
{
return a.x == b.x && a.y == b.y;
}
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator!=(const int2& a, const int2& b)
{
return a.x != b.x || a.y != b.y;
}
/** @} */
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE int getByIndex(const int2& v, int i)
{
return ((int*)(&v))[i];
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE void setByIndex(int2& v, int i, int x)
{
((int*)(&v))[i] = x;
}
/* int3 functions */
/******************************************************************************/
/** additional constructors
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE int3 make_int3(const int s)
{
return make_int3(s, s, s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE int3 make_int3(const float3& a)
{
return make_int3(int(a.x), int(a.y), int(a.z));
}
/** @} */
/** negate */
SUTIL_INLINE SUTIL_HOSTDEVICE int3 operator-(const int3& a)
{
return make_int3(-a.x, -a.y, -a.z);
}
/** min */
SUTIL_INLINE SUTIL_HOSTDEVICE int3 min(const int3& a, const int3& b)
{
return make_int3(min(a.x,b.x), min(a.y,b.y), min(a.z,b.z));
}
/** max */
SUTIL_INLINE SUTIL_HOSTDEVICE int3 max(const int3& a, const int3& b)
{
return make_int3(max(a.x,b.x), max(a.y,b.y), max(a.z,b.z));
}
/** add
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE int3 operator+(const int3& a, const int3& b)
{
return make_int3(a.x + b.x, a.y + b.y, a.z + b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator+=(int3& a, const int3& b)
{
a.x += b.x; a.y += b.y; a.z += b.z;
}
/** @} */
/** subtract
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE int3 operator-(const int3& a, const int3& b)
{
return make_int3(a.x - b.x, a.y - b.y, a.z - b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator-=(int3& a, const int3& b)
{
a.x -= b.x; a.y -= b.y; a.z -= b.z;
}
/** @} */
/** multiply
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE int3 operator*(const int3& a, const int3& b)
{
return make_int3(a.x * b.x, a.y * b.y, a.z * b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE int3 operator*(const int3& a, const int s)
{
return make_int3(a.x * s, a.y * s, a.z * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE int3 operator*(const int s, const int3& a)
{
return make_int3(a.x * s, a.y * s, a.z * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator*=(int3& a, const int s)
{
a.x *= s; a.y *= s; a.z *= s;
}
/** @} */
/** divide
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE int3 operator/(const int3& a, const int3& b)
{
return make_int3(a.x / b.x, a.y / b.y, a.z / b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE int3 operator/(const int3& a, const int s)
{
return make_int3(a.x / s, a.y / s, a.z / s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE int3 operator/(const int s, const int3& a)
{
return make_int3(s /a.x, s / a.y, s / a.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator/=(int3& a, const int s)
{
a.x /= s; a.y /= s; a.z /= s;
}
/** @} */
/** clamp
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE int3 clamp(const int3& v, const int a, const int b)
{
return make_int3(clamp(v.x, a, b), clamp(v.y, a, b), clamp(v.z, a, b));
}
SUTIL_INLINE SUTIL_HOSTDEVICE int3 clamp(const int3& v, const int3& a, const int3& b)
{
return make_int3(clamp(v.x, a.x, b.x), clamp(v.y, a.y, b.y), clamp(v.z, a.z, b.z));
}
/** @} */
/** equality
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator==(const int3& a, const int3& b)
{
return a.x == b.x && a.y == b.y && a.z == b.z;
}
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator!=(const int3& a, const int3& b)
{
return a.x != b.x || a.y != b.y || a.z != b.z;
}
/** @} */
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE int getByIndex(const int3& v, int i)
{
return ((int*)(&v))[i];
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE void setByIndex(int3& v, int i, int x)
{
((int*)(&v))[i] = x;
}
/* int4 functions */
/******************************************************************************/
/** additional constructors
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE int4 make_int4(const int s)
{
return make_int4(s, s, s, s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE int4 make_int4(const float4& a)
{
return make_int4((int)a.x, (int)a.y, (int)a.z, (int)a.w);
}
/** @} */
/** negate */
SUTIL_INLINE SUTIL_HOSTDEVICE int4 operator-(const int4& a)
{
return make_int4(-a.x, -a.y, -a.z, -a.w);
}
/** min */
SUTIL_INLINE SUTIL_HOSTDEVICE int4 min(const int4& a, const int4& b)
{
return make_int4(min(a.x,b.x), min(a.y,b.y), min(a.z,b.z), min(a.w,b.w));
}
/** max */
SUTIL_INLINE SUTIL_HOSTDEVICE int4 max(const int4& a, const int4& b)
{
return make_int4(max(a.x,b.x), max(a.y,b.y), max(a.z,b.z), max(a.w,b.w));
}
/** add
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE int4 operator+(const int4& a, const int4& b)
{
return make_int4(a.x + b.x, a.y + b.y, a.z + b.z, a.w + b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator+=(int4& a, const int4& b)
{
a.x += b.x; a.y += b.y; a.z += b.z; a.w += b.w;
}
/** @} */
/** subtract
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE int4 operator-(const int4& a, const int4& b)
{
return make_int4(a.x - b.x, a.y - b.y, a.z - b.z, a.w - b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator-=(int4& a, const int4& b)
{
a.x -= b.x; a.y -= b.y; a.z -= b.z; a.w -= b.w;
}
/** @} */
/** multiply
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE int4 operator*(const int4& a, const int4& b)
{
return make_int4(a.x * b.x, a.y * b.y, a.z * b.z, a.w * b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE int4 operator*(const int4& a, const int s)
{
return make_int4(a.x * s, a.y * s, a.z * s, a.w * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE int4 operator*(const int s, const int4& a)
{
return make_int4(a.x * s, a.y * s, a.z * s, a.w * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator*=(int4& a, const int s)
{
a.x *= s; a.y *= s; a.z *= s; a.w *= s;
}
/** @} */
/** divide
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE int4 operator/(const int4& a, const int4& b)
{
return make_int4(a.x / b.x, a.y / b.y, a.z / b.z, a.w / b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE int4 operator/(const int4& a, const int s)
{
return make_int4(a.x / s, a.y / s, a.z / s, a.w / s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE int4 operator/(const int s, const int4& a)
{
return make_int4(s / a.x, s / a.y, s / a.z, s / a.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator/=(int4& a, const int s)
{
a.x /= s; a.y /= s; a.z /= s; a.w /= s;
}
/** @} */
/** clamp
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE int4 clamp(const int4& v, const int a, const int b)
{
return make_int4(clamp(v.x, a, b), clamp(v.y, a, b), clamp(v.z, a, b), clamp(v.w, a, b));
}
SUTIL_INLINE SUTIL_HOSTDEVICE int4 clamp(const int4& v, const int4& a, const int4& b)
{
return make_int4(clamp(v.x, a.x, b.x), clamp(v.y, a.y, b.y), clamp(v.z, a.z, b.z), clamp(v.w, a.w, b.w));
}
/** @} */
/** equality
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator==(const int4& a, const int4& b)
{
return a.x == b.x && a.y == b.y && a.z == b.z && a.w == b.w;
}
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator!=(const int4& a, const int4& b)
{
return a.x != b.x || a.y != b.y || a.z != b.z || a.w != b.w;
}
/** @} */
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE int getByIndex(const int4& v, int i)
{
return ((int*)(&v))[i];
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE void setByIndex(int4& v, int i, int x)
{
((int*)(&v))[i] = x;
}
/* uint functions */
/******************************************************************************/
/** clamp */
SUTIL_INLINE SUTIL_HOSTDEVICE unsigned int clamp(const unsigned int f, const unsigned int a, const unsigned int b)
{
return max(a, min(f, b));
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE unsigned int getByIndex(const uint1& v, unsigned int i)
{
return ((unsigned int*)(&v))[i];
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE void setByIndex(uint1& v, int i, unsigned int x)
{
((unsigned int*)(&v))[i] = x;
}
/* uint2 functions */
/******************************************************************************/
/** additional constructors
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE uint2 make_uint2(const unsigned int s)
{
return make_uint2(s, s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE uint2 make_uint2(const float2& a)
{
return make_uint2((unsigned int)a.x, (unsigned int)a.y);
}
/** @} */
/** min */
SUTIL_INLINE SUTIL_HOSTDEVICE uint2 min(const uint2& a, const uint2& b)
{
return make_uint2(min(a.x,b.x), min(a.y,b.y));
}
/** max */
SUTIL_INLINE SUTIL_HOSTDEVICE uint2 max(const uint2& a, const uint2& b)
{
return make_uint2(max(a.x,b.x), max(a.y,b.y));
}
/** add
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE uint2 operator+(const uint2& a, const uint2& b)
{
return make_uint2(a.x + b.x, a.y + b.y);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator+=(uint2& a, const uint2& b)
{
a.x += b.x; a.y += b.y;
}
/** @} */
/** subtract
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE uint2 operator-(const uint2& a, const uint2& b)
{
return make_uint2(a.x - b.x, a.y - b.y);
}
SUTIL_INLINE SUTIL_HOSTDEVICE uint2 operator-(const uint2& a, const unsigned int b)
{
return make_uint2(a.x - b, a.y - b);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator-=(uint2& a, const uint2& b)
{
a.x -= b.x; a.y -= b.y;
}
/** @} */
/** multiply
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE uint2 operator*(const uint2& a, const uint2& b)
{
return make_uint2(a.x * b.x, a.y * b.y);
}
SUTIL_INLINE SUTIL_HOSTDEVICE uint2 operator*(const uint2& a, const unsigned int s)
{
return make_uint2(a.x * s, a.y * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE uint2 operator*(const unsigned int s, const uint2& a)
{
return make_uint2(a.x * s, a.y * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator*=(uint2& a, const unsigned int s)
{
a.x *= s; a.y *= s;
}
/** @} */
/** clamp
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE uint2 clamp(const uint2& v, const unsigned int a, const unsigned int b)
{
return make_uint2(clamp(v.x, a, b), clamp(v.y, a, b));
}
SUTIL_INLINE SUTIL_HOSTDEVICE uint2 clamp(const uint2& v, const uint2& a, const uint2& b)
{
return make_uint2(clamp(v.x, a.x, b.x), clamp(v.y, a.y, b.y));
}
/** @} */
/** equality
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator==(const uint2& a, const uint2& b)
{
return a.x == b.x && a.y == b.y;
}
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator!=(const uint2& a, const uint2& b)
{
return a.x != b.x || a.y != b.y;
}
/** @} */
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE unsigned int getByIndex(const uint2& v, unsigned int i)
{
return ((unsigned int*)(&v))[i];
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE void setByIndex(uint2& v, int i, unsigned int x)
{
((unsigned int*)(&v))[i] = x;
}
/* uint3 functions */
/******************************************************************************/
/** additional constructors
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE uint3 make_uint3(const unsigned int s)
{
return make_uint3(s, s, s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE uint3 make_uint3(const float3& a)
{
return make_uint3((unsigned int)a.x, (unsigned int)a.y, (unsigned int)a.z);
}
/** @} */
/** min */
SUTIL_INLINE SUTIL_HOSTDEVICE uint3 min(const uint3& a, const uint3& b)
{
return make_uint3(min(a.x,b.x), min(a.y,b.y), min(a.z,b.z));
}
/** max */
SUTIL_INLINE SUTIL_HOSTDEVICE uint3 max(const uint3& a, const uint3& b)
{
return make_uint3(max(a.x,b.x), max(a.y,b.y), max(a.z,b.z));
}
/** add
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE uint3 operator+(const uint3& a, const uint3& b)
{
return make_uint3(a.x + b.x, a.y + b.y, a.z + b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator+=(uint3& a, const uint3& b)
{
a.x += b.x; a.y += b.y; a.z += b.z;
}
/** @} */
/** subtract
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE uint3 operator-(const uint3& a, const uint3& b)
{
return make_uint3(a.x - b.x, a.y - b.y, a.z - b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator-=(uint3& a, const uint3& b)
{
a.x -= b.x; a.y -= b.y; a.z -= b.z;
}
/** @} */
/** multiply
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE uint3 operator*(const uint3& a, const uint3& b)
{
return make_uint3(a.x * b.x, a.y * b.y, a.z * b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE uint3 operator*(const uint3& a, const unsigned int s)
{
return make_uint3(a.x * s, a.y * s, a.z * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE uint3 operator*(const unsigned int s, const uint3& a)
{
return make_uint3(a.x * s, a.y * s, a.z * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator*=(uint3& a, const unsigned int s)
{
a.x *= s; a.y *= s; a.z *= s;
}
/** @} */
/** divide
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE uint3 operator/(const uint3& a, const uint3& b)
{
return make_uint3(a.x / b.x, a.y / b.y, a.z / b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE uint3 operator/(const uint3& a, const unsigned int s)
{
return make_uint3(a.x / s, a.y / s, a.z / s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE uint3 operator/(const unsigned int s, const uint3& a)
{
return make_uint3(s / a.x, s / a.y, s / a.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator/=(uint3& a, const unsigned int s)
{
a.x /= s; a.y /= s; a.z /= s;
}
/** @} */
/** clamp
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE uint3 clamp(const uint3& v, const unsigned int a, const unsigned int b)
{
return make_uint3(clamp(v.x, a, b), clamp(v.y, a, b), clamp(v.z, a, b));
}
SUTIL_INLINE SUTIL_HOSTDEVICE uint3 clamp(const uint3& v, const uint3& a, const uint3& b)
{
return make_uint3(clamp(v.x, a.x, b.x), clamp(v.y, a.y, b.y), clamp(v.z, a.z, b.z));
}
/** @} */
/** equality
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator==(const uint3& a, const uint3& b)
{
return a.x == b.x && a.y == b.y && a.z == b.z;
}
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator!=(const uint3& a, const uint3& b)
{
return a.x != b.x || a.y != b.y || a.z != b.z;
}
/** @} */
/** If used on the device, this could place the the 'v' in local memory
*/
SUTIL_INLINE SUTIL_HOSTDEVICE unsigned int getByIndex(const uint3& v, unsigned int i)
{
return ((unsigned int*)(&v))[i];
}
/** If used on the device, this could place the the 'v' in local memory
*/
SUTIL_INLINE SUTIL_HOSTDEVICE void setByIndex(uint3& v, int i, unsigned int x)
{
((unsigned int*)(&v))[i] = x;
}
/* uint4 functions */
/******************************************************************************/
/** additional constructors
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 make_uint4(const unsigned int s)
{
return make_uint4(s, s, s, s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 make_uint4(const float4& a)
{
return make_uint4((unsigned int)a.x, (unsigned int)a.y, (unsigned int)a.z, (unsigned int)a.w);
}
/** @} */
/** min
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 min(const uint4& a, const uint4& b)
{
return make_uint4(min(a.x,b.x), min(a.y,b.y), min(a.z,b.z), min(a.w,b.w));
}
/** @} */
/** max
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 max(const uint4& a, const uint4& b)
{
return make_uint4(max(a.x,b.x), max(a.y,b.y), max(a.z,b.z), max(a.w,b.w));
}
/** @} */
/** add
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 operator+(const uint4& a, const uint4& b)
{
return make_uint4(a.x + b.x, a.y + b.y, a.z + b.z, a.w + b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator+=(uint4& a, const uint4& b)
{
a.x += b.x; a.y += b.y; a.z += b.z; a.w += b.w;
}
/** @} */
/** subtract
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 operator-(const uint4& a, const uint4& b)
{
return make_uint4(a.x - b.x, a.y - b.y, a.z - b.z, a.w - b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator-=(uint4& a, const uint4& b)
{
a.x -= b.x; a.y -= b.y; a.z -= b.z; a.w -= b.w;
}
/** @} */
/** multiply
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 operator*(const uint4& a, const uint4& b)
{
return make_uint4(a.x * b.x, a.y * b.y, a.z * b.z, a.w * b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 operator*(const uint4& a, const unsigned int s)
{
return make_uint4(a.x * s, a.y * s, a.z * s, a.w * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 operator*(const unsigned int s, const uint4& a)
{
return make_uint4(a.x * s, a.y * s, a.z * s, a.w * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator*=(uint4& a, const unsigned int s)
{
a.x *= s; a.y *= s; a.z *= s; a.w *= s;
}
/** @} */
/** divide
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 operator/(const uint4& a, const uint4& b)
{
return make_uint4(a.x / b.x, a.y / b.y, a.z / b.z, a.w / b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 operator/(const uint4& a, const unsigned int s)
{
return make_uint4(a.x / s, a.y / s, a.z / s, a.w / s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 operator/(const unsigned int s, const uint4& a)
{
return make_uint4(s / a.x, s / a.y, s / a.z, s / a.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator/=(uint4& a, const unsigned int s)
{
a.x /= s; a.y /= s; a.z /= s; a.w /= s;
}
/** @} */
/** clamp
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 clamp(const uint4& v, const unsigned int a, const unsigned int b)
{
return make_uint4(clamp(v.x, a, b), clamp(v.y, a, b), clamp(v.z, a, b), clamp(v.w, a, b));
}
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 clamp(const uint4& v, const uint4& a, const uint4& b)
{
return make_uint4(clamp(v.x, a.x, b.x), clamp(v.y, a.y, b.y), clamp(v.z, a.z, b.z), clamp(v.w, a.w, b.w));
}
/** @} */
/** equality
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator==(const uint4& a, const uint4& b)
{
return a.x == b.x && a.y == b.y && a.z == b.z && a.w == b.w;
}
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator!=(const uint4& a, const uint4& b)
{
return a.x != b.x || a.y != b.y || a.z != b.z || a.w != b.w;
}
/** @} */
/** If used on the device, this could place the the 'v' in local memory
*/
SUTIL_INLINE SUTIL_HOSTDEVICE unsigned int getByIndex(const uint4& v, unsigned int i)
{
return ((unsigned int*)(&v))[i];
}
/** If used on the device, this could place the the 'v' in local memory
*/
SUTIL_INLINE SUTIL_HOSTDEVICE void setByIndex(uint4& v, int i, unsigned int x)
{
((unsigned int*)(&v))[i] = x;
}
/* long long functions */
/******************************************************************************/
/** clamp */
SUTIL_INLINE SUTIL_HOSTDEVICE long long clamp(const long long f, const long long a, const long long b)
{
return max(a, min(f, b));
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE long long getByIndex(const longlong1& v, int i)
{
return ((long long*)(&v))[i];
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE void setByIndex(longlong1& v, int i, long long x)
{
((long long*)(&v))[i] = x;
}
/* longlong2 functions */
/******************************************************************************/
/** additional constructors
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE longlong2 make_longlong2(const long long s)
{
return make_longlong2(s, s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE longlong2 make_longlong2(const float2& a)
{
return make_longlong2(int(a.x), int(a.y));
}
/** @} */
/** negate */
SUTIL_INLINE SUTIL_HOSTDEVICE longlong2 operator-(const longlong2& a)
{
return make_longlong2(-a.x, -a.y);
}
/** min */
SUTIL_INLINE SUTIL_HOSTDEVICE longlong2 min(const longlong2& a, const longlong2& b)
{
return make_longlong2(min(a.x, b.x), min(a.y, b.y));
}
/** max */
SUTIL_INLINE SUTIL_HOSTDEVICE longlong2 max(const longlong2& a, const longlong2& b)
{
return make_longlong2(max(a.x, b.x), max(a.y, b.y));
}
/** add
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE longlong2 operator+(const longlong2& a, const longlong2& b)
{
return make_longlong2(a.x + b.x, a.y + b.y);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator+=(longlong2& a, const longlong2& b)
{
a.x += b.x; a.y += b.y;
}
/** @} */
/** subtract
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE longlong2 operator-(const longlong2& a, const longlong2& b)
{
return make_longlong2(a.x - b.x, a.y - b.y);
}
SUTIL_INLINE SUTIL_HOSTDEVICE longlong2 operator-(const longlong2& a, const long long b)
{
return make_longlong2(a.x - b, a.y - b);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator-=(longlong2& a, const longlong2& b)
{
a.x -= b.x; a.y -= b.y;
}
/** @} */
/** multiply
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE longlong2 operator*(const longlong2& a, const longlong2& b)
{
return make_longlong2(a.x * b.x, a.y * b.y);
}
SUTIL_INLINE SUTIL_HOSTDEVICE longlong2 operator*(const longlong2& a, const long long s)
{
return make_longlong2(a.x * s, a.y * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE longlong2 operator*(const long long s, const longlong2& a)
{
return make_longlong2(a.x * s, a.y * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator*=(longlong2& a, const long long s)
{
a.x *= s; a.y *= s;
}
/** @} */
/** clamp
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE longlong2 clamp(const longlong2& v, const long long a, const long long b)
{
return make_longlong2(clamp(v.x, a, b), clamp(v.y, a, b));
}
SUTIL_INLINE SUTIL_HOSTDEVICE longlong2 clamp(const longlong2& v, const longlong2& a, const longlong2& b)
{
return make_longlong2(clamp(v.x, a.x, b.x), clamp(v.y, a.y, b.y));
}
/** @} */
/** equality
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator==(const longlong2& a, const longlong2& b)
{
return a.x == b.x && a.y == b.y;
}
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator!=(const longlong2& a, const longlong2& b)
{
return a.x != b.x || a.y != b.y;
}
/** @} */
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE long long getByIndex(const longlong2& v, int i)
{
return ((long long*)(&v))[i];
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE void setByIndex(longlong2& v, int i, long long x)
{
((long long*)(&v))[i] = x;
}
/* longlong3 functions */
/******************************************************************************/
/** additional constructors
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE longlong3 make_longlong3(const long long s)
{
return make_longlong3(s, s, s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE longlong3 make_longlong3(const float3& a)
{
return make_longlong3( (long long)a.x, (long long)a.y, (long long)a.z);
}
/** @} */
/** negate */
SUTIL_INLINE SUTIL_HOSTDEVICE longlong3 operator-(const longlong3& a)
{
return make_longlong3(-a.x, -a.y, -a.z);
}
/** min */
SUTIL_INLINE SUTIL_HOSTDEVICE longlong3 min(const longlong3& a, const longlong3& b)
{
return make_longlong3(min(a.x, b.x), min(a.y, b.y), min(a.z, b.z));
}
/** max */
SUTIL_INLINE SUTIL_HOSTDEVICE longlong3 max(const longlong3& a, const longlong3& b)
{
return make_longlong3(max(a.x, b.x), max(a.y, b.y), max(a.z, b.z));
}
/** add
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE longlong3 operator+(const longlong3& a, const longlong3& b)
{
return make_longlong3(a.x + b.x, a.y + b.y, a.z + b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator+=(longlong3& a, const longlong3& b)
{
a.x += b.x; a.y += b.y; a.z += b.z;
}
/** @} */
/** subtract
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE longlong3 operator-(const longlong3& a, const longlong3& b)
{
return make_longlong3(a.x - b.x, a.y - b.y, a.z - b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator-=(longlong3& a, const longlong3& b)
{
a.x -= b.x; a.y -= b.y; a.z -= b.z;
}
/** @} */
/** multiply
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE longlong3 operator*(const longlong3& a, const longlong3& b)
{
return make_longlong3(a.x * b.x, a.y * b.y, a.z * b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE longlong3 operator*(const longlong3& a, const long long s)
{
return make_longlong3(a.x * s, a.y * s, a.z * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE longlong3 operator*(const long long s, const longlong3& a)
{
return make_longlong3(a.x * s, a.y * s, a.z * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator*=(longlong3& a, const long long s)
{
a.x *= s; a.y *= s; a.z *= s;
}
/** @} */
/** divide
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE longlong3 operator/(const longlong3& a, const longlong3& b)
{
return make_longlong3(a.x / b.x, a.y / b.y, a.z / b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE longlong3 operator/(const longlong3& a, const long long s)
{
return make_longlong3(a.x / s, a.y / s, a.z / s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE longlong3 operator/(const long long s, const longlong3& a)
{
return make_longlong3(s /a.x, s / a.y, s / a.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator/=(longlong3& a, const long long s)
{
a.x /= s; a.y /= s; a.z /= s;
}
/** @} */
/** clamp
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE longlong3 clamp(const longlong3& v, const long long a, const long long b)
{
return make_longlong3(clamp(v.x, a, b), clamp(v.y, a, b), clamp(v.z, a, b));
}
SUTIL_INLINE SUTIL_HOSTDEVICE longlong3 clamp(const longlong3& v, const longlong3& a, const longlong3& b)
{
return make_longlong3(clamp(v.x, a.x, b.x), clamp(v.y, a.y, b.y), clamp(v.z, a.z, b.z));
}
/** @} */
/** equality
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator==(const longlong3& a, const longlong3& b)
{
return a.x == b.x && a.y == b.y && a.z == b.z;
}
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator!=(const longlong3& a, const longlong3& b)
{
return a.x != b.x || a.y != b.y || a.z != b.z;
}
/** @} */
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE long long getByIndex(const longlong3& v, int i)
{
return ((long long*)(&v))[i];
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE void setByIndex(longlong3& v, int i, int x)
{
((long long*)(&v))[i] = x;
}
/* longlong4 functions */
/******************************************************************************/
/** additional constructors
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 make_longlong4(const long long s)
{
return make_longlong4(s, s, s, s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 make_longlong4(const float4& a)
{
return make_longlong4((long long)a.x, (long long)a.y, (long long)a.z, (long long)a.w);
}
/** @} */
/** negate */
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 operator-(const longlong4& a)
{
return make_longlong4(-a.x, -a.y, -a.z, -a.w);
}
/** min */
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 min(const longlong4& a, const longlong4& b)
{
return make_longlong4(min(a.x, b.x), min(a.y, b.y), min(a.z, b.z), min(a.w, b.w));
}
/** max */
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 max(const longlong4& a, const longlong4& b)
{
return make_longlong4(max(a.x, b.x), max(a.y, b.y), max(a.z, b.z), max(a.w, b.w));
}
/** add
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 operator+(const longlong4& a, const longlong4& b)
{
return make_longlong4(a.x + b.x, a.y + b.y, a.z + b.z, a.w + b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator+=(longlong4& a, const longlong4& b)
{
a.x += b.x; a.y += b.y; a.z += b.z; a.w += b.w;
}
/** @} */
/** subtract
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 operator-(const longlong4& a, const longlong4& b)
{
return make_longlong4(a.x - b.x, a.y - b.y, a.z - b.z, a.w - b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator-=(longlong4& a, const longlong4& b)
{
a.x -= b.x; a.y -= b.y; a.z -= b.z; a.w -= b.w;
}
/** @} */
/** multiply
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 operator*(const longlong4& a, const longlong4& b)
{
return make_longlong4(a.x * b.x, a.y * b.y, a.z * b.z, a.w * b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 operator*(const longlong4& a, const long long s)
{
return make_longlong4(a.x * s, a.y * s, a.z * s, a.w * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 operator*(const long long s, const longlong4& a)
{
return make_longlong4(a.x * s, a.y * s, a.z * s, a.w * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator*=(longlong4& a, const long long s)
{
a.x *= s; a.y *= s; a.z *= s; a.w *= s;
}
/** @} */
/** divide
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 operator/(const longlong4& a, const longlong4& b)
{
return make_longlong4(a.x / b.x, a.y / b.y, a.z / b.z, a.w / b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 operator/(const longlong4& a, const long long s)
{
return make_longlong4(a.x / s, a.y / s, a.z / s, a.w / s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 operator/(const long long s, const longlong4& a)
{
return make_longlong4(s / a.x, s / a.y, s / a.z, s / a.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator/=(longlong4& a, const long long s)
{
a.x /= s; a.y /= s; a.z /= s; a.w /= s;
}
/** @} */
/** clamp
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 clamp(const longlong4& v, const long long a, const long long b)
{
return make_longlong4(clamp(v.x, a, b), clamp(v.y, a, b), clamp(v.z, a, b), clamp(v.w, a, b));
}
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 clamp(const longlong4& v, const longlong4& a, const longlong4& b)
{
return make_longlong4(clamp(v.x, a.x, b.x), clamp(v.y, a.y, b.y), clamp(v.z, a.z, b.z), clamp(v.w, a.w, b.w));
}
/** @} */
/** equality
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator==(const longlong4& a, const longlong4& b)
{
return a.x == b.x && a.y == b.y && a.z == b.z && a.w == b.w;
}
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator!=(const longlong4& a, const longlong4& b)
{
return a.x != b.x || a.y != b.y || a.z != b.z || a.w != b.w;
}
/** @} */
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE long long getByIndex(const longlong4& v, int i)
{
return ((long long*)(&v))[i];
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE void setByIndex(longlong4& v, int i, long long x)
{
((long long*)(&v))[i] = x;
}
/* ulonglong functions */
/******************************************************************************/
/** clamp */
SUTIL_INLINE SUTIL_HOSTDEVICE unsigned long long clamp(const unsigned long long f, const unsigned long long a, const unsigned long long b)
{
return max(a, min(f, b));
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE unsigned long long getByIndex(const ulonglong1& v, unsigned int i)
{
return ((unsigned long long*)(&v))[i];
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE void setByIndex(ulonglong1& v, int i, unsigned long long x)
{
((unsigned long long*)(&v))[i] = x;
}
/* ulonglong2 functions */
/******************************************************************************/
/** additional constructors
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong2 make_ulonglong2(const unsigned long long s)
{
return make_ulonglong2(s, s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong2 make_ulonglong2(const float2& a)
{
return make_ulonglong2((unsigned long long)a.x, (unsigned long long)a.y);
}
/** @} */
/** min */
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong2 min(const ulonglong2& a, const ulonglong2& b)
{
return make_ulonglong2(min(a.x, b.x), min(a.y, b.y));
}
/** max */
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong2 max(const ulonglong2& a, const ulonglong2& b)
{
return make_ulonglong2(max(a.x, b.x), max(a.y, b.y));
}
/** add
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong2 operator+(const ulonglong2& a, const ulonglong2& b)
{
return make_ulonglong2(a.x + b.x, a.y + b.y);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator+=(ulonglong2& a, const ulonglong2& b)
{
a.x += b.x; a.y += b.y;
}
/** @} */
/** subtract
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong2 operator-(const ulonglong2& a, const ulonglong2& b)
{
return make_ulonglong2(a.x - b.x, a.y - b.y);
}
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong2 operator-(const ulonglong2& a, const unsigned long long b)
{
return make_ulonglong2(a.x - b, a.y - b);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator-=(ulonglong2& a, const ulonglong2& b)
{
a.x -= b.x; a.y -= b.y;
}
/** @} */
/** multiply
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong2 operator*(const ulonglong2& a, const ulonglong2& b)
{
return make_ulonglong2(a.x * b.x, a.y * b.y);
}
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong2 operator*(const ulonglong2& a, const unsigned long long s)
{
return make_ulonglong2(a.x * s, a.y * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong2 operator*(const unsigned long long s, const ulonglong2& a)
{
return make_ulonglong2(a.x * s, a.y * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator*=(ulonglong2& a, const unsigned long long s)
{
a.x *= s; a.y *= s;
}
/** @} */
/** clamp
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong2 clamp(const ulonglong2& v, const unsigned long long a, const unsigned long long b)
{
return make_ulonglong2(clamp(v.x, a, b), clamp(v.y, a, b));
}
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong2 clamp(const ulonglong2& v, const ulonglong2& a, const ulonglong2& b)
{
return make_ulonglong2(clamp(v.x, a.x, b.x), clamp(v.y, a.y, b.y));
}
/** @} */
/** equality
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator==(const ulonglong2& a, const ulonglong2& b)
{
return a.x == b.x && a.y == b.y;
}
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator!=(const ulonglong2& a, const ulonglong2& b)
{
return a.x != b.x || a.y != b.y;
}
/** @} */
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE unsigned long long getByIndex(const ulonglong2& v, unsigned int i)
{
return ((unsigned long long*)(&v))[i];
}
/** If used on the device, this could place the the 'v' in local memory */
SUTIL_INLINE SUTIL_HOSTDEVICE void setByIndex(ulonglong2& v, int i, unsigned long long x)
{
((unsigned long long*)(&v))[i] = x;
}
/* ulonglong3 functions */
/******************************************************************************/
/** additional constructors
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong3 make_ulonglong3(const unsigned long long s)
{
return make_ulonglong3(s, s, s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong3 make_ulonglong3(const float3& a)
{
return make_ulonglong3((unsigned long long)a.x, (unsigned long long)a.y, (unsigned long long)a.z);
}
/** @} */
/** min */
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong3 min(const ulonglong3& a, const ulonglong3& b)
{
return make_ulonglong3(min(a.x, b.x), min(a.y, b.y), min(a.z, b.z));
}
/** max */
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong3 max(const ulonglong3& a, const ulonglong3& b)
{
return make_ulonglong3(max(a.x, b.x), max(a.y, b.y), max(a.z, b.z));
}
/** add
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong3 operator+(const ulonglong3& a, const ulonglong3& b)
{
return make_ulonglong3(a.x + b.x, a.y + b.y, a.z + b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator+=(ulonglong3& a, const ulonglong3& b)
{
a.x += b.x; a.y += b.y; a.z += b.z;
}
/** @} */
/** subtract
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong3 operator-(const ulonglong3& a, const ulonglong3& b)
{
return make_ulonglong3(a.x - b.x, a.y - b.y, a.z - b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator-=(ulonglong3& a, const ulonglong3& b)
{
a.x -= b.x; a.y -= b.y; a.z -= b.z;
}
/** @} */
/** multiply
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong3 operator*(const ulonglong3& a, const ulonglong3& b)
{
return make_ulonglong3(a.x * b.x, a.y * b.y, a.z * b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong3 operator*(const ulonglong3& a, const unsigned long long s)
{
return make_ulonglong3(a.x * s, a.y * s, a.z * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong3 operator*(const unsigned long long s, const ulonglong3& a)
{
return make_ulonglong3(a.x * s, a.y * s, a.z * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator*=(ulonglong3& a, const unsigned long long s)
{
a.x *= s; a.y *= s; a.z *= s;
}
/** @} */
/** divide
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong3 operator/(const ulonglong3& a, const ulonglong3& b)
{
return make_ulonglong3(a.x / b.x, a.y / b.y, a.z / b.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong3 operator/(const ulonglong3& a, const unsigned long long s)
{
return make_ulonglong3(a.x / s, a.y / s, a.z / s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong3 operator/(const unsigned long long s, const ulonglong3& a)
{
return make_ulonglong3(s / a.x, s / a.y, s / a.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator/=(ulonglong3& a, const unsigned long long s)
{
a.x /= s; a.y /= s; a.z /= s;
}
/** @} */
/** clamp
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong3 clamp(const ulonglong3& v, const unsigned long long a, const unsigned long long b)
{
return make_ulonglong3(clamp(v.x, a, b), clamp(v.y, a, b), clamp(v.z, a, b));
}
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong3 clamp(const ulonglong3& v, const ulonglong3& a, const ulonglong3& b)
{
return make_ulonglong3(clamp(v.x, a.x, b.x), clamp(v.y, a.y, b.y), clamp(v.z, a.z, b.z));
}
/** @} */
/** equality
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator==(const ulonglong3& a, const ulonglong3& b)
{
return a.x == b.x && a.y == b.y && a.z == b.z;
}
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator!=(const ulonglong3& a, const ulonglong3& b)
{
return a.x != b.x || a.y != b.y || a.z != b.z;
}
/** @} */
/** If used on the device, this could place the the 'v' in local memory
*/
SUTIL_INLINE SUTIL_HOSTDEVICE unsigned long long getByIndex(const ulonglong3& v, unsigned int i)
{
return ((unsigned long long*)(&v))[i];
}
/** If used on the device, this could place the the 'v' in local memory
*/
SUTIL_INLINE SUTIL_HOSTDEVICE void setByIndex(ulonglong3& v, int i, unsigned long long x)
{
((unsigned long long*)(&v))[i] = x;
}
/* ulonglong4 functions */
/******************************************************************************/
/** additional constructors
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 make_ulonglong4(const unsigned long long s)
{
return make_ulonglong4(s, s, s, s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 make_ulonglong4(const float4& a)
{
return make_ulonglong4((unsigned long long)a.x, (unsigned long long)a.y, (unsigned long long)a.z, (unsigned long long)a.w);
}
/** @} */
/** min
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 min(const ulonglong4& a, const ulonglong4& b)
{
return make_ulonglong4(min(a.x, b.x), min(a.y, b.y), min(a.z, b.z), min(a.w, b.w));
}
/** @} */
/** max
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 max(const ulonglong4& a, const ulonglong4& b)
{
return make_ulonglong4(max(a.x, b.x), max(a.y, b.y), max(a.z, b.z), max(a.w, b.w));
}
/** @} */
/** add
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 operator+(const ulonglong4& a, const ulonglong4& b)
{
return make_ulonglong4(a.x + b.x, a.y + b.y, a.z + b.z, a.w + b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator+=(ulonglong4& a, const ulonglong4& b)
{
a.x += b.x; a.y += b.y; a.z += b.z; a.w += b.w;
}
/** @} */
/** subtract
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 operator-(const ulonglong4& a, const ulonglong4& b)
{
return make_ulonglong4(a.x - b.x, a.y - b.y, a.z - b.z, a.w - b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator-=(ulonglong4& a, const ulonglong4& b)
{
a.x -= b.x; a.y -= b.y; a.z -= b.z; a.w -= b.w;
}
/** @} */
/** multiply
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 operator*(const ulonglong4& a, const ulonglong4& b)
{
return make_ulonglong4(a.x * b.x, a.y * b.y, a.z * b.z, a.w * b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 operator*(const ulonglong4& a, const unsigned long long s)
{
return make_ulonglong4(a.x * s, a.y * s, a.z * s, a.w * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 operator*(const unsigned long long s, const ulonglong4& a)
{
return make_ulonglong4(a.x * s, a.y * s, a.z * s, a.w * s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator*=(ulonglong4& a, const unsigned long long s)
{
a.x *= s; a.y *= s; a.z *= s; a.w *= s;
}
/** @} */
/** divide
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 operator/(const ulonglong4& a, const ulonglong4& b)
{
return make_ulonglong4(a.x / b.x, a.y / b.y, a.z / b.z, a.w / b.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 operator/(const ulonglong4& a, const unsigned long long s)
{
return make_ulonglong4(a.x / s, a.y / s, a.z / s, a.w / s);
}
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 operator/(const unsigned long long s, const ulonglong4& a)
{
return make_ulonglong4(s / a.x, s / a.y, s / a.z, s / a.w);
}
SUTIL_INLINE SUTIL_HOSTDEVICE void operator/=(ulonglong4& a, const unsigned long long s)
{
a.x /= s; a.y /= s; a.z /= s; a.w /= s;
}
/** @} */
/** clamp
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 clamp(const ulonglong4& v, const unsigned long long a, const unsigned long long b)
{
return make_ulonglong4(clamp(v.x, a, b), clamp(v.y, a, b), clamp(v.z, a, b), clamp(v.w, a, b));
}
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 clamp(const ulonglong4& v, const ulonglong4& a, const ulonglong4& b)
{
return make_ulonglong4(clamp(v.x, a.x, b.x), clamp(v.y, a.y, b.y), clamp(v.z, a.z, b.z), clamp(v.w, a.w, b.w));
}
/** @} */
/** equality
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator==(const ulonglong4& a, const ulonglong4& b)
{
return a.x == b.x && a.y == b.y && a.z == b.z && a.w == b.w;
}
SUTIL_INLINE SUTIL_HOSTDEVICE bool operator!=(const ulonglong4& a, const ulonglong4& b)
{
return a.x != b.x || a.y != b.y || a.z != b.z || a.w != b.w;
}
/** @} */
/** If used on the device, this could place the the 'v' in local memory
*/
SUTIL_INLINE SUTIL_HOSTDEVICE unsigned long long getByIndex(const ulonglong4& v, unsigned int i)
{
return ((unsigned long long*)(&v))[i];
}
/** If used on the device, this could place the the 'v' in local memory
*/
SUTIL_INLINE SUTIL_HOSTDEVICE void setByIndex(ulonglong4& v, int i, unsigned long long x)
{
((unsigned long long*)(&v))[i] = x;
}
/******************************************************************************/
/** Narrowing functions
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE int2 make_int2(const int3& v0) { return make_int2( v0.x, v0.y ); }
SUTIL_INLINE SUTIL_HOSTDEVICE int2 make_int2(const int4& v0) { return make_int2( v0.x, v0.y ); }
SUTIL_INLINE SUTIL_HOSTDEVICE int3 make_int3(const int4& v0) { return make_int3( v0.x, v0.y, v0.z ); }
SUTIL_INLINE SUTIL_HOSTDEVICE uint2 make_uint2(const uint3& v0) { return make_uint2( v0.x, v0.y ); }
SUTIL_INLINE SUTIL_HOSTDEVICE uint2 make_uint2(const uint4& v0) { return make_uint2( v0.x, v0.y ); }
SUTIL_INLINE SUTIL_HOSTDEVICE uint3 make_uint3(const uint4& v0) { return make_uint3( v0.x, v0.y, v0.z ); }
SUTIL_INLINE SUTIL_HOSTDEVICE longlong2 make_longlong2(const longlong3& v0) { return make_longlong2( v0.x, v0.y ); }
SUTIL_INLINE SUTIL_HOSTDEVICE longlong2 make_longlong2(const longlong4& v0) { return make_longlong2( v0.x, v0.y ); }
SUTIL_INLINE SUTIL_HOSTDEVICE longlong3 make_longlong3(const longlong4& v0) { return make_longlong3( v0.x, v0.y, v0.z ); }
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong2 make_ulonglong2(const ulonglong3& v0) { return make_ulonglong2( v0.x, v0.y ); }
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong2 make_ulonglong2(const ulonglong4& v0) { return make_ulonglong2( v0.x, v0.y ); }
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong3 make_ulonglong3(const ulonglong4& v0) { return make_ulonglong3( v0.x, v0.y, v0.z ); }
SUTIL_INLINE SUTIL_HOSTDEVICE float2 make_float2(const float3& v0) { return make_float2( v0.x, v0.y ); }
SUTIL_INLINE SUTIL_HOSTDEVICE float2 make_float2(const float4& v0) { return make_float2( v0.x, v0.y ); }
SUTIL_INLINE SUTIL_HOSTDEVICE float3 make_float3(const float4& v0) { return make_float3( v0.x, v0.y, v0.z ); }
/** @} */
/** Assemble functions from smaller vectors
* @{
*/
SUTIL_INLINE SUTIL_HOSTDEVICE int3 make_int3(const int v0, const int2& v1) { return make_int3( v0, v1.x, v1.y ); }
SUTIL_INLINE SUTIL_HOSTDEVICE int3 make_int3(const int2& v0, const int v1) { return make_int3( v0.x, v0.y, v1 ); }
SUTIL_INLINE SUTIL_HOSTDEVICE int4 make_int4(const int v0, const int v1, const int2& v2) { return make_int4( v0, v1, v2.x, v2.y ); }
SUTIL_INLINE SUTIL_HOSTDEVICE int4 make_int4(const int v0, const int2& v1, const int v2) { return make_int4( v0, v1.x, v1.y, v2 ); }
SUTIL_INLINE SUTIL_HOSTDEVICE int4 make_int4(const int2& v0, const int v1, const int v2) { return make_int4( v0.x, v0.y, v1, v2 ); }
SUTIL_INLINE SUTIL_HOSTDEVICE int4 make_int4(const int v0, const int3& v1) { return make_int4( v0, v1.x, v1.y, v1.z ); }
SUTIL_INLINE SUTIL_HOSTDEVICE int4 make_int4(const int3& v0, const int v1) { return make_int4( v0.x, v0.y, v0.z, v1 ); }
SUTIL_INLINE SUTIL_HOSTDEVICE int4 make_int4(const int2& v0, const int2& v1) { return make_int4( v0.x, v0.y, v1.x, v1.y ); }
SUTIL_INLINE SUTIL_HOSTDEVICE uint3 make_uint3(const unsigned int v0, const uint2& v1) { return make_uint3( v0, v1.x, v1.y ); }
SUTIL_INLINE SUTIL_HOSTDEVICE uint3 make_uint3(const uint2& v0, const unsigned int v1) { return make_uint3( v0.x, v0.y, v1 ); }
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 make_uint4(const unsigned int v0, const unsigned int v1, const uint2& v2) { return make_uint4( v0, v1, v2.x, v2.y ); }
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 make_uint4(const unsigned int v0, const uint2& v1, const unsigned int v2) { return make_uint4( v0, v1.x, v1.y, v2 ); }
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 make_uint4(const uint2& v0, const unsigned int v1, const unsigned int v2) { return make_uint4( v0.x, v0.y, v1, v2 ); }
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 make_uint4(const unsigned int v0, const uint3& v1) { return make_uint4( v0, v1.x, v1.y, v1.z ); }
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 make_uint4(const uint3& v0, const unsigned int v1) { return make_uint4( v0.x, v0.y, v0.z, v1 ); }
SUTIL_INLINE SUTIL_HOSTDEVICE uint4 make_uint4(const uint2& v0, const uint2& v1) { return make_uint4( v0.x, v0.y, v1.x, v1.y ); }
SUTIL_INLINE SUTIL_HOSTDEVICE longlong3 make_longlong3(const long long v0, const longlong2& v1) { return make_longlong3(v0, v1.x, v1.y); }
SUTIL_INLINE SUTIL_HOSTDEVICE longlong3 make_longlong3(const longlong2& v0, const long long v1) { return make_longlong3(v0.x, v0.y, v1); }
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 make_longlong4(const long long v0, const long long v1, const longlong2& v2) { return make_longlong4(v0, v1, v2.x, v2.y); }
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 make_longlong4(const long long v0, const longlong2& v1, const long long v2) { return make_longlong4(v0, v1.x, v1.y, v2); }
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 make_longlong4(const longlong2& v0, const long long v1, const long long v2) { return make_longlong4(v0.x, v0.y, v1, v2); }
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 make_longlong4(const long long v0, const longlong3& v1) { return make_longlong4(v0, v1.x, v1.y, v1.z); }
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 make_longlong4(const longlong3& v0, const long long v1) { return make_longlong4(v0.x, v0.y, v0.z, v1); }
SUTIL_INLINE SUTIL_HOSTDEVICE longlong4 make_longlong4(const longlong2& v0, const longlong2& v1) { return make_longlong4(v0.x, v0.y, v1.x, v1.y); }
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong3 make_ulonglong3(const unsigned long long v0, const ulonglong2& v1) { return make_ulonglong3(v0, v1.x, v1.y); }
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong3 make_ulonglong3(const ulonglong2& v0, const unsigned long long v1) { return make_ulonglong3(v0.x, v0.y, v1); }
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 make_ulonglong4(const unsigned long long v0, const unsigned long long v1, const ulonglong2& v2) { return make_ulonglong4(v0, v1, v2.x, v2.y); }
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 make_ulonglong4(const unsigned long long v0, const ulonglong2& v1, const unsigned long long v2) { return make_ulonglong4(v0, v1.x, v1.y, v2); }
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 make_ulonglong4(const ulonglong2& v0, const unsigned long long v1, const unsigned long long v2) { return make_ulonglong4(v0.x, v0.y, v1, v2); }
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 make_ulonglong4(const unsigned long long v0, const ulonglong3& v1) { return make_ulonglong4(v0, v1.x, v1.y, v1.z); }
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 make_ulonglong4(const ulonglong3& v0, const unsigned long long v1) { return make_ulonglong4(v0.x, v0.y, v0.z, v1); }
SUTIL_INLINE SUTIL_HOSTDEVICE ulonglong4 make_ulonglong4(const ulonglong2& v0, const ulonglong2& v1) { return make_ulonglong4(v0.x, v0.y, v1.x, v1.y); }
SUTIL_INLINE SUTIL_HOSTDEVICE float3 make_float3(const float2& v0, const float v1) { return make_float3(v0.x, v0.y, v1); }
SUTIL_INLINE SUTIL_HOSTDEVICE float3 make_float3(const float v0, const float2& v1) { return make_float3( v0, v1.x, v1.y ); }
SUTIL_INLINE SUTIL_HOSTDEVICE float4 make_float4(const float v0, const float v1, const float2& v2) { return make_float4( v0, v1, v2.x, v2.y ); }
SUTIL_INLINE SUTIL_HOSTDEVICE float4 make_float4(const float v0, const float2& v1, const float v2) { return make_float4( v0, v1.x, v1.y, v2 ); }
SUTIL_INLINE SUTIL_HOSTDEVICE float4 make_float4(const float2& v0, const float v1, const float v2) { return make_float4( v0.x, v0.y, v1, v2 ); }
SUTIL_INLINE SUTIL_HOSTDEVICE float4 make_float4(const float v0, const float3& v1) { return make_float4( v0, v1.x, v1.y, v1.z ); }
SUTIL_INLINE SUTIL_HOSTDEVICE float4 make_float4(const float3& v0, const float v1) { return make_float4( v0.x, v0.y, v0.z, v1 ); }
SUTIL_INLINE SUTIL_HOSTDEVICE float4 make_float4(const float2& v0, const float2& v1) { return make_float4( v0.x, v0.y, v1.x, v1.y ); }
/** @} */
| 73,688 | C | 27.104119 | 184 | 0.631785 |
arhix52/Strelka/sutil/vec_math_adv.h | #pragma once
#include "sutil/vec_math.h"
#include <sutil/Preprocessor.h>
#include <vector_functions.h>
#include <vector_types.h>
#if !defined(__CUDACC_RTC__)
#include <cmath>
#include <cstdlib>
#endif
// SUTIL_INLINE SUTIL_HOSTDEVICE float clamp( const float f, const float a, const float b )
// {
// return fmaxf( a, fminf( f, b ) );
// }
// SUTIL_INLINE SUTIL_HOSTDEVICE float4 make_float4(const float3& a, float w)
// {
// return make_float4(a.x, a.y, a.z, w);
// }
SUTIL_INLINE SUTIL_HOSTDEVICE float3 saturate(const float3 v)
{
float3 r = v;
r.x = clamp(r.x, 0.0f, 1.0f);
r.y = clamp(r.y, 0.0f, 1.0f);
r.z = clamp(r.z, 0.0f, 1.0f);
return r;
}
SUTIL_INLINE SUTIL_HOSTDEVICE float saturate(const float v)
{
return clamp(v, 0.0f, 1.0f);
}
SUTIL_INLINE SUTIL_HOSTDEVICE bool all(const float3 v)
{
return v.x != 0.0f && v.y != 0.0f && v.z != 0.0f;
}
SUTIL_INLINE SUTIL_HOSTDEVICE bool any(const float3 v)
{
return v.x != 0.0f || v.y != 0.0f || v.z != 0.0f;
}
SUTIL_INLINE SUTIL_HOSTDEVICE bool isnan(const float3 v)
{
return isnan(v.x) || isnan(v.y) || isnan(v.z);
}
SUTIL_INLINE SUTIL_HOSTDEVICE float3 powf(const float3 v, const float p)
{
float3 r = v;
r.x = powf(r.x, p);
r.y = powf(r.y, p);
r.z = powf(r.z, p);
return r;
}
| 1,303 | C | 20.032258 | 91 | 0.617038 |
cadop/HumanGenerator/README.md | # Overview
ovmh (ov_makehuman) is a MakeHuman extension for Nvidia Omniverse. This project relies on the makehuman project from https://github.com/makehumancommunity/makehuman.
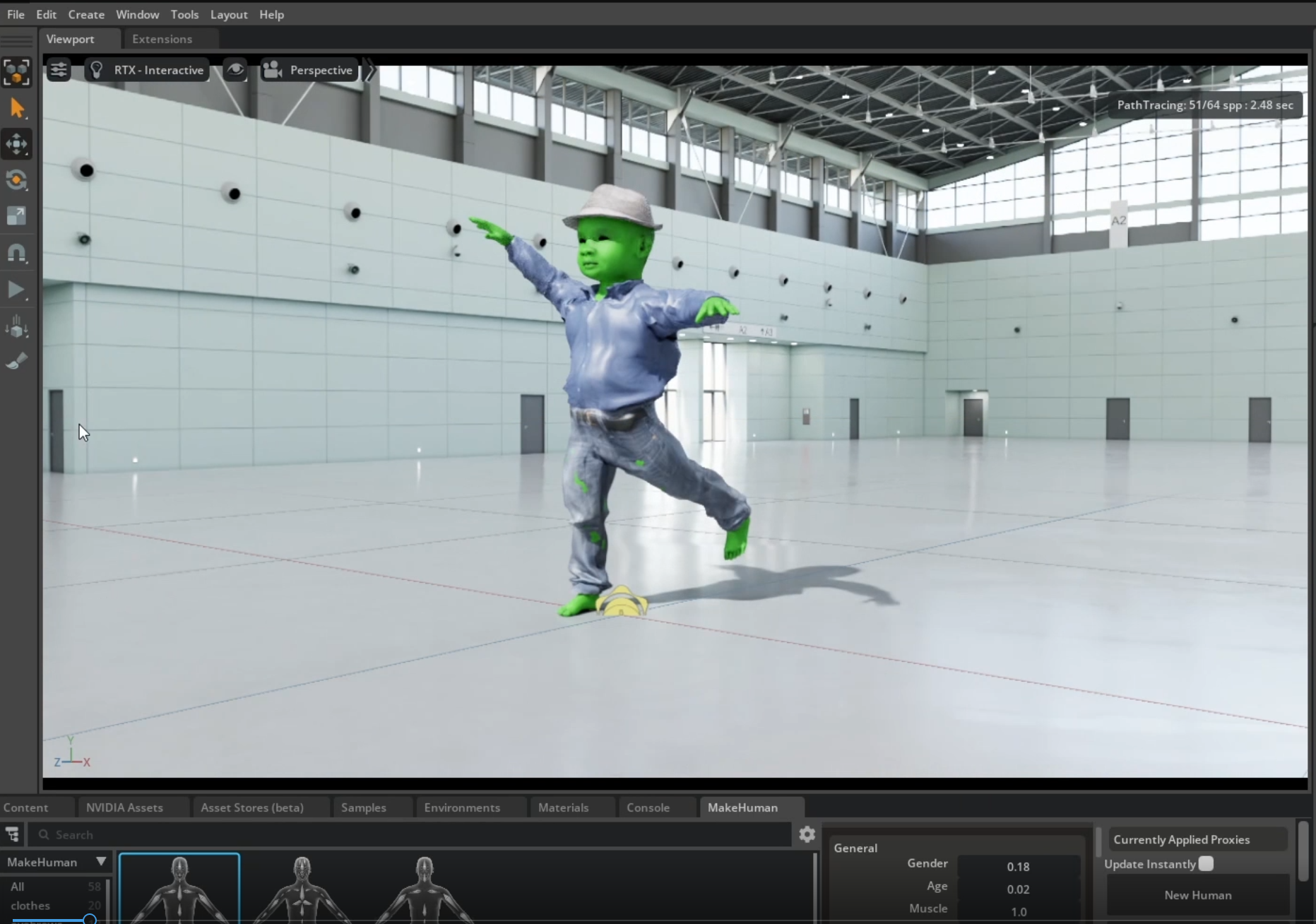
# Getting Started
The easiest way to get started is using Omniverse Create. Navigate to the Extensions window and click on "Community". Search for `makehuman` and you should see our extension show up.
The extension may take a few minutes to install as it will download makehuman and install it in the Omniverse's local Python instance.
For use, check out the walkthrough video
[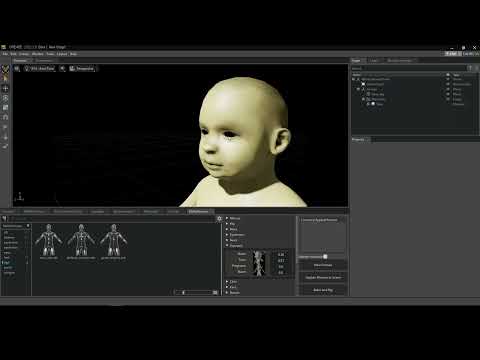](https://www.youtube.com/watch?v=8GD3ld1Ep7c)
## License
*Our license restrictions are due to the AGPL of MakeHuman. In line with the statements from MakeHuman, the targets and resulting characters are CC0, meaning you can use whatever you create for free, without restrictions. It is only the codebase that is AGPL.
| 1,073 | Markdown | 43.749998 | 259 | 0.788444 |
cadop/HumanGenerator/exts/siborg.create.human/API_EXAMPLE.py | # Human Generator API Example
# Author: Joshua Grebler | SiBORG Lab | 2023
# Description: This is an example of how to use the Human Generator API to create human models in NVIDIA Omniverse.
# The siborg.create.human extension must be installed and enabled for this to work.
# The script generates 10 humans, placing them throughout the stage. Random modifiers and clothing are applied to each.
import siborg.create.human as hg
from siborg.create.human.shared import data_path
import omni.usd
import random
# Get the stage
context = omni.usd.get_context()
stage = context.get_stage()
# Make a single Human to start with
human = hg.Human()
human.add_to_scene()
# Apply a modifier by name (you can find the names of all the available modifiers
# by using the `get_modifier_names()` method)
height = human.get_modifier_by_name("macrodetails-height/Height")
human.set_modifier_value(height, 1)
# Update the human in the scene
human.update_in_scene(human.prim_path)
# Gather some default clothing items (additional clothing can be downloaded from the extension UI)
clothes = ["nvidia_Casual/nvidia_casual.mhclo", "omni_casual/omni_casual.mhclo", "siborg_casual/siborg_casual.mhclo"]
# Convert the clothing names to their full paths.
clothes = [data_path(f"clothes/{c}") for c in clothes]
# Create 20 humans, placing them randomly throughout the scene, and applying random modifier values
for _ in range(10):
h = hg.Human()
h.add_to_scene()
# Apply a random translation and Y rotation to the human prim
translateOp = h.prim.AddTranslateOp()
translateOp.Set((random.uniform(-50, 50), 0, random.uniform(-50, 50)))
rotateOp = h.prim.AddRotateXYZOp()
rotateOp.Set((0, random.uniform(0, 360), 0))
# Apply a random value to the last 9 modifiers in the list.
# These modifiers are macros that affect the overall shape of the human more than any individual modifier.
# Get the last 9 modifiers
modifiers = h.get_modifiers()[-9:]
# Apply a random value to each modifier. Use the modifier's min/max values to ensure the value is within range.
for m in modifiers:
h.set_modifier_value(m, random.uniform(m.getMin(), m.getMax()))
# Update the human in the scene
h.update_in_scene(h.prim_path)
# Add a random clothing item to the human
h.add_item(random.choice(clothes))
| 2,343 | Python | 36.806451 | 119 | 0.733248 |
cadop/HumanGenerator/exts/siborg.create.human/siborg/create/human/human.py | from typing import Tuple, List, Dict, Union
from .mhcaller import MHCaller
import numpy as np
import omni.kit
import omni.usd
from pxr import Sdf, Usd, UsdGeom, UsdSkel
from .shared import sanitize, data_path
from .skeleton import Skeleton
from module3d import Object3D
from pxr import Usd, UsdGeom, UsdPhysics, UsdShade, Sdf, Gf, Tf, UsdSkel, Vt
import carb
from .materials import get_mesh_texture, create_material, bind_material
class Human:
"""Class representing a human in the scene. This class is used to add a human to the scene,
and to update the human in the scene. The class also contains functions to add and remove
proxies (clothing, etc.) and apply modifiers, as well as a skeleton.
Attributes
----------
name : str
Name of the human
prim : UsdSkel.Root
Reference to the usd prim for the skelroot representing the human in the stage. Can be changed using set_prim()
prim_path : str
Path to the human prim
scale : float
Scale factor for the human. Defaults to 10 (Omniverse provided humans are 10 times larger than makehuman)
skeleton : Makehuman.Skeleton
Skeleton object for the human
usd_skel : UsdSkel.Skeleton
Skeleton object for the human in the USD stage. Imported from the skeleton object.
objects : List[Object3D]
List of objects attached to the human. Fetched from the makehuman app
mh_meshes : List[Object3D]
List of meshes attached to the human. Fetched from the makehuman app
"""
def __init__(self, name='human', **kwargs):
"""Constructs an instance of Human.
Parameters
----------
name : str
Name of the human. Defaults to 'human'
"""
self.name = name
# Reference to the usd prim for the skelroot representing the human in the stage
self.prim = None
# Provide a scale factor (Omniverse provided humans are 10 times larger than makehuman)
self.scale = 10
# Create a skeleton object for the human
self.skeleton = Skeleton(self.scale)
# usd_skel is none until the human is added to the stage
self.usd_skel = None
# Set the human in makehuman to default values
MHCaller.reset_human()
def reset(self):
"""Resets the human in makehuman and adds a new skeleton to the human"""
# Reset the human in makehuman
MHCaller.reset_human()
# Re-add the skeleton to the human
self.skeleton = Skeleton(self.scale)
def delete_proxies(self):
"""Deletes the prims corresponding to proxies attached to the human"""
# Delete any child prims corresponding to proxies
if self.prim:
# Get the children of the human prim and delete them all at once
proxy_prims = [child.GetPath() for child in self.prim.GetChildren() if child.GetCustomDataByKey("Proxy_path:")]
omni.kit.commands.execute("DeletePrims", paths=proxy_prims)
@property
def prim_path(self):
"""Path to the human prim"""
if self.prim:
return self.prim.GetPath().pathString
else:
return None
@property
def objects(self):
"""List of objects attached to the human. Fetched from the makehuman app"""
return MHCaller.objects
@property
def mh_meshes(self):
"""List of meshes attached to the human. Fetched from the makehuman app"""
return MHCaller.meshes
def add_to_scene(self):
"""Adds the human to the scene. Creates a prim for the human with custom attributes
to hold modifiers and proxies. Also creates a prim for each proxy and attaches it to
the human prim.
Returns
-------
str
Path to the human prim"""
# Get the current stage
stage = omni.usd.get_context().get_stage()
root_path = "/"
# Get default prim.
default_prim = stage.GetDefaultPrim()
if default_prim.IsValid():
# Set the rootpath under the stage's default prim, if the default prim is valid
root_path = default_prim.GetPath().pathString
# Create a path for the next available prim
prim_path = omni.usd.get_stage_next_free_path(stage, root_path + "/" + self.name, False)
# Create a prim for the human
# Prim should be a SkelRoot so we can rig the human with a skeleton later
self.prim = UsdSkel.Root.Define(stage, prim_path)
# Write the properties of the human to the prim
self.write_properties(prim_path, stage)
# Get the objects of the human from mhcaller
objects = MHCaller.objects
# Get the human object from the list of objects
human = objects[0]
# Determine the offset for the human from the ground
offset = -1 * human.getJointPosition("ground")
# Import makehuman objects into the scene
mesh_paths = self.import_meshes(prim_path, stage, offset = offset)
# Add the skeleton to the scene
self.usd_skel= self.skeleton.add_to_stage(stage, prim_path, offset = offset)
# Create bindings between meshes and the skeleton. Returns a list of
# bindings the length of the number of meshes
bindings = self.setup_bindings(mesh_paths, stage, self.usd_skel)
# Setup weights for corresponding mh_meshes (which hold the data) and
# bindings (which link USD_meshes to the skeleton)
self.setup_weights(self.mh_meshes, bindings, self.skeleton.joint_names, self.skeleton.joint_paths)
self.setup_materials(self.mh_meshes, mesh_paths, root_path, stage)
# Explicitly setup material for human skin
texture_path = data_path("skins/textures/skin.png")
skin = create_material(texture_path, "Skin", root_path, stage)
# Bind the skin material to the first prim in the list (the human)
bind_material(mesh_paths[0], skin, stage)
Human._set_scale(self.prim.GetPrim(), self.scale)
return self.prim
def update_in_scene(self, prim_path: str):
"""Updates the human in the scene. Writes the properties of the human to the
human prim and imports the human and proxy meshes. This is called when the
human is updated
Parameters
----------
prim_path : str
Path to the human prim (prim type is SkelRoot)
"""
usd_context = omni.usd.get_context()
stage = usd_context.get_stage()
prim = stage.GetPrimAtPath(prim_path)
prim = stage.GetPrimAtPath(prim_path)
if prim and stage:
print(prim.GetPath().pathString)
prim_kind = prim.GetTypeName()
# Check if the prim is a SkelRoot and a human
if prim_kind == "SkelRoot" and prim.GetCustomDataByKey("human"):
# Get default prim.
default_prim = stage.GetDefaultPrim()
if default_prim.IsValid():
# Set the rootpath under the stage's default prim, if the default prim is valid
root_path = default_prim.GetPath().pathString
else:
root_path = "/"
# Write the properties of the human to the prim
self.write_properties(prim_path, stage)
# Get the objects of the human from mhcaller
objects = MHCaller.objects
# Get the human object from the list of objects
human = objects[0]
# Determine the offset for the human from the ground
offset = -1 * human.getJointPosition("ground")
# Import makehuman objects into the scene
mesh_paths = self.import_meshes(prim_path, stage, offset = offset)
# Update the skeleton values and insert it into the stage
self.usd_skel = self.skeleton.update_in_scene(stage, prim_path, offset = offset)
# Create bindings between meshes and the skeleton. Returns a list of
# bindings the length of the number of meshes
bindings = self.setup_bindings(mesh_paths, stage, self.usd_skel)
# Setup weights for corresponding mh_meshes (which hold the data) and
# bindings (which link USD_meshes to the skeleton)
self.setup_weights(self.mh_meshes, bindings, self.skeleton.joint_names, self.skeleton.joint_paths)
self.setup_materials(self.mh_meshes, mesh_paths, root_path, stage)
# Explicitly setup material for human skin
texture_path = data_path("skins/textures/skin.png")
skin = create_material(texture_path, "Skin", root_path, stage)
# Bind the skin material to the first prim in the list (the human)
bind_material(mesh_paths[0], skin, stage)
else:
carb.log_warn("The selected prim must be a human!")
else:
carb.log_warn("Can't update human. No prim selected!")
def import_meshes(self, prim_path: str, stage: Usd.Stage, offset: List[float] = [0, 0, 0]):
"""Imports the meshes of the human into the scene. This is called when the human is
added to the scene, and when the human is updated. This function creates mesh prims
for both the human and its proxies, and attaches them to the human prim. If a mesh already
exists in the scene, its values are updated instead of creating a new mesh.
Parameters
----------
prim_path : str
Path to the human prim
stage : Usd.Stage
Stage to write to
offset : List[float], optional
Offset to move the mesh relative to the prim origin, by default [0, 0, 0]
Returns
-------
paths : array of: Sdf.Path
Usd Sdf paths to geometry prims in the scene
"""
# Get the objects of the human from mhcaller
objects = MHCaller.objects
# Get the meshes of the human and its proxies
meshes = [o.mesh for o in objects]
usd_mesh_paths = []
for mesh in meshes:
# Number of vertices per face
nPerFace = mesh.vertsPerFaceForExport
# Lists to hold pruned lists of vertex and UV indices
newvertindices = []
newuvindices = []
# Array of coordinates organized [[x1,y1,z1],[x2,y2,z2]...]
# Adding the given offset moves the mesh relative to the prim origin
coords = mesh.getCoords() + offset
for fn, fv in enumerate(mesh.fvert):
if not mesh.face_mask[fn]:
continue
# only include <nPerFace> verts for each face, and order them
# consecutively
newvertindices += [(fv[n]) for n in range(nPerFace)]
fuv = mesh.fuvs[fn]
# build an array of (u,v)s for each face
newuvindices += [(fuv[n]) for n in range(nPerFace)]
# Type conversion
newvertindices = np.array(newvertindices)
# Create mesh prim at appropriate path. Does not yet hold any data
name = sanitize(mesh.name)
usd_mesh_path = prim_path + "/" + name
usd_mesh_paths.append(usd_mesh_path)
# Check to see if the mesh prim already exists
prim = stage.GetPrimAtPath(usd_mesh_path)
if prim.IsValid():
# omni.kit.commands.execute("DeletePrims", paths=[usd_mesh_path])
point_attr = prim.GetAttribute('points')
point_attr.Set(coords)
face_count = prim.GetAttribute('faceVertexCounts')
nface = [nPerFace] * int(len(newvertindices) / nPerFace)
face_count.Set(nface)
face_idx = prim.GetAttribute('faceVertexIndices')
face_idx.Set(newvertindices)
normals_attr = prim.GetAttribute('normals')
normals_attr.Set(mesh.getNormals())
meshGeom = UsdGeom.Mesh(prim)
# If it doesn't exist, make it. This will run the first time a human is created and
# whenever a new proxy is added
else:
# First determine if the mesh is a proxy
p = mesh.object.proxy
if p:
# Determine if the mesh is a clothes proxy or a proxymesh. If not, then
# an existing proxy of this type already exists, and we must overwrite it
type = p.type if p.type else "proxymeshes"
if not (type == "clothes" or type == "proxymeshes"):
for child in self.prim.GetChildren():
child_type = child.GetCustomDataByKey("Proxy_type:")
if child_type == type:
# If the child prim has the same type as the proxy, delete it
omni.kit.commands.execute("DeletePrims", paths=[child.GetPath()])
break
meshGeom = UsdGeom.Mesh.Define(stage, usd_mesh_path)
prim = meshGeom.GetPrim()
# Set vertices. This is a list of tuples for ALL vertices in an unassociated
# cloud. Faces are built based on indices of this list.
# Example: 3 explicitly defined vertices:
# meshGeom.CreatePointsAttr([(-10, 0, -10), (-10, 0, 10), (10, 0, 10)]
meshGeom.CreatePointsAttr(coords)
# Set face vertex count. This is an array where each element is the number
# of consecutive vertex indices to include in each face definition, as
# indices are given as a single flat list. The length of this list is the
# same as the number of faces
# Example: 4 faces with 4 vertices each
# meshGeom.CreateFaceVertexCountsAttr([4, 4, 4, 4])
nface = [nPerFace] * int(len(newvertindices) / nPerFace)
meshGeom.CreateFaceVertexCountsAttr(nface)
# Set face vertex indices.
# Example: one face with 4 vertices defined by 4 indices.
# meshGeom.CreateFaceVertexIndicesAttr([0, 1, 2, 3])
meshGeom.CreateFaceVertexIndicesAttr(newvertindices)
# Set vertex normals. Normals are represented as a list of tuples each of
# which is a vector indicating the direction a point is facing. This is later
# Used to calculate face normals
# Example: Normals for 3 vertices
# meshGeom.CreateNormalsAttr([(0, 1, 0), (0, 1, 0), (0, 1, 0), (0, 1,
# 0)])
meshGeom.CreateNormalsAttr(mesh.getNormals())
meshGeom.SetNormalsInterpolation("vertex")
# If the mesh is a proxy, write the proxy path to the mesh prim
if mesh.object.proxy:
p = mesh.object.proxy
type = p.type if p.type else "proxymeshes"
prim.SetCustomDataByKey("Proxy_path:", p.file)
prim.SetCustomDataByKey("Proxy_type:", type)
prim.SetCustomDataByKey("Proxy_name:", p.name)
# Set vertex uvs. UVs are represented as a list of tuples, each of which is a 2D
# coordinate. UV's are used to map textures to the surface of 3D geometry
# Example: texture coordinates for 3 vertices
# texCoords.Set([(0, 1), (0, 0), (1, 0)])
texCoords = meshGeom.CreatePrimvar(
"st", Sdf.ValueTypeNames.TexCoord2fArray, UsdGeom.Tokens.faceVarying
)
texCoords.Set(mesh.getUVs(newuvindices))
# # Subdivision is set to none. The mesh is as imported and not further refined
meshGeom.CreateSubdivisionSchemeAttr().Set("none")
# ConvertPath strings to USD Sdf paths. TODO change to map() for performance
paths = [Sdf.Path(mesh_path) for mesh_path in usd_mesh_paths]
return paths
def get_written_modifiers(self) -> Union[Dict[str, float], None]:
"""List of modifier names and values written to the human prim.
MAY BE STALE IF THE HUMAN HAS BEEN UPDATED IN MAKEHUMAN AND THE CHANGES HAVE NOT BEEN WRITTEN TO THE PRIM.
Returns
-------
Dict[str, float]
Dictionary of modifier names and values. Keys are modifier names, values are modifier values"""
return self.prim.GetCustomDataByKey("Modifiers") if self.prim else None
def get_changed_modifiers(self):
"""List of modifiers which have been changed in makehuman. Fetched from the human in makehuman.
MAY NOT MATCH `get_written_modifiers()` IF CHANGES HAVE NOT BEEN WRITTEN TO THE PRIM."""
return MHCaller.modifiers
def get_modifiers(self):
"""Retrieve the list of all modifiers available to the human, whether or not their values have changed."""
return MHCaller.default_modifiers
def set_modifier_value(self, modifier, value: float):
"""Sets the value of a modifier in makehuman. Validates the value before setting it.
Returns true if the value was set, false otherwise.
Parameters
----------
modifier : makehuman.humanmodifier.Modifier
Modifier to change
value : float
Value to set the modifier to
"""
# Get the range of the modifier
val_min = modifier.getMin()
val_max = modifier.getMax()
# Check if the value is within the range of the modifier
if value >= val_min and value <= val_max:
# Set the value of the modifier
modifier.setValue(value)
return True
else:
carb.log_warn(f"Value must be between {str(val_min)} and {str(val_max)}")
return False
def get_modifier_by_name(self, name: str):
"""Gets a modifier from the list of modifiers attached to the human by name
Parameters
----------
name : str
Name of the modifier to get
Returns
-------
makehuman.modifiers.Modifier
Modifier with the given name
"""
return MHCaller.human.getModifier(name)
def get_modifier_names(self):
return MHCaller.human.getModifierNames()
def write_properties(self, prim_path: str, stage: Usd.Stage):
"""Writes the properties of the human to the human prim. This includes modifiers and
proxies. This is called when the human is added to the scene, and when the human is
updated
Parameters
----------
prim_path : str
Path to the human prim
stage : Usd.Stage
Stage to write to
"""
prim = stage.GetPrimAtPath(prim_path)
# Add custom data to the prim by key, designating the prim is a human
prim.SetCustomDataByKey("human", True)
# Get the modifiers of the human in mhcaller
modifiers = MHCaller.modifiers
for m in modifiers:
# Add the modifier to the prim as custom data by key. For modifiers,
# the format is "group/modifer:value"
prim.SetCustomDataByKey("Modifiers:" + m.fullName, m.getValue())
# NOTE We are not currently using proxies in the USD export. Proxy data is stored
# in their respective mesh prims, so that deleting proxy prims will also remove the
# proxies. The following code is left here for reference.
# Get the proxies of the human in mhcaller
# proxies = MHCaller.proxies
# for p in proxies:
# # Add the proxy to the prim as custom data by key under "Proxies".
# # Proxy type should be "proxymeshes" if type cannot be determined from the
# # proxy.type property.
# type = p.type if p.type else "proxymeshes"
# # Only "proxymeshes" and "clothes" should be subdictionaries of "Proxies"
# if type == "clothes" or type == "proxymeshes":
# prim.SetCustomDataByKey("Proxies:" + type + ":" + p.name, p.file)
# # Other proxy types should be added as a key to the prim with their
# # type as the key and the path as the value
# else:
# prim.SetCustomDataByKey("Proxies:" + type, p.file)
def set_prim(self, usd_prim : Usd.Prim):
"""Updates the human based on the given prim's attributes
Parameters
----------
usd_prim : Usd.Prim
Prim from which to update the human model."""
self.prim = usd_prim
# Get the data from the prim
humandata = self.prim.GetCustomData()
# Get the list of modifiers from the prim
modifiers = humandata.get("Modifiers")
for m, v in modifiers.items():
MHCaller.human.getModifier(m).setValue(v, skipDependencies=False)
# Gather proxies from the prim children
proxies = []
for child in self.prim.GetChildren():
if child.GetTypeName() == "Mesh" and child.GetCustomDataByKey("Proxy_path:"):
proxies.append(child)
# Clear the makehuman proxies
MHCaller.clear_proxies()
# # Make sure the proxy list is not empty
if proxies:
for p in proxies:
type = p.GetCustomDataByKey("Proxy_type:")
path = p.GetCustomDataByKey("Proxy_path:")
# name = p.GetCustomDataByKey("Proxy_name:")
MHCaller.add_proxy(path, type)
# Update the human in MHCaller
MHCaller.human.applyAllTargets()
def setup_weights(self, mh_meshes: List['Object3D'], bindings: List[UsdSkel.BindingAPI], joint_names: List[str], joint_paths: List[str]):
"""Apply weights to USD meshes using data from makehuman. USD meshes,
bindings and skeleton must already be in the active scene
Parameters
----------
mh_meshes : list of `Object3D`
Makehuman meshes which store weight data
bindings : list of `UsdSkel.BindingAPI`
USD bindings between meshes and skeleton
joint_names : list of str
Unique, plaintext names of all joints in the skeleton in USD
(breadth-first) order.
joint_paths : list of str
List of the full usd path to each joint corresponding to the skeleton to bind to
"""
# Generate bone weights for all meshes up front so they can be reused for all
rawWeights = MHCaller.human.getVertexWeights(
MHCaller.human.getSkeleton()
) # Basemesh weights
for mesh in self.mh_meshes:
if mesh.object.proxy:
# Transfer weights to proxy
parentWeights = mesh.object.proxy.getVertexWeights(
rawWeights, MHCaller.human.getSkeleton()
)
else:
parentWeights = rawWeights
# Transfer weights to face/vert masked and/or subdivided mesh
weights = mesh.getVertexWeights(parentWeights)
# Attach these vertexWeights to the mesh to pass them around the
# exporter easier, the cloned mesh is discarded afterwards, anyway
# if this is the same person, just skip updating weights
mesh.vertexWeights = weights
# Iterate through corresponding meshes and bindings
for mh_mesh, binding in zip(mh_meshes, bindings):
# Calculate vertex weights
indices, weights = self.calculate_influences(mh_mesh, joint_names)
# Type conversion to native ints and floats from numpy
indices = list(map(int, indices))
weights = list(map(float, weights))
# Type conversion to USD
indices = Vt.IntArray(indices)
weights = Vt.FloatArray(weights)
# The number of weights to apply to each vertex, taken directly from
# MakeHuman data
elementSize = int(mh_mesh.vertexWeights._nWeights)
# weight_data = list(mh_mesh.vertexWeights.data) TODO remove
# We might not need to normalize. Makehuman weights are automatically
# normalized when loaded, see:
# http://www.makehumancommunity.org/wiki/Technical_notes_on_MakeHuman
UsdSkel.NormalizeWeights(weights, elementSize)
UsdSkel.SortInfluences(indices, weights, elementSize)
# Assign indices to binding
indices_attribute = binding.CreateJointIndicesPrimvar(
constant=False, elementSize=elementSize
)
joint_attr = binding.GetPrim().GetAttribute('skel:joints')
joint_attr.Set(joint_paths)
indices_attribute.Set(indices)
# Assign weights to binding
weights_attribute = binding.CreateJointWeightsPrimvar(
constant=False, elementSize=elementSize
)
weights_attribute.Set(weights)
def calculate_influences(self, mh_mesh: Object3D, joint_names: List[str]):
"""Build arrays of joint indices and corresponding weights for each vertex.
Joints are in USD (breadth-first) order.
Parameters
----------
mh_mesh : Object3D
Makehuman-format mesh. Contains weight and vertex data.
joint_names : list of str
Unique, plaintext names of all joints in the skeleton in USD
(breadth-first) order.
Returns
-------
indices : list of int
Flat list of joint indices for each vertex
weights : list of float
Flat list of weights corresponding to joint indices
"""
# The maximum number of weights a vertex might have
max_influences = mh_mesh.vertexWeights._nWeights
# Named joints corresponding to vertices and weights ie.
# {"joint",([indices],[weights])}
influence_joints = mh_mesh.vertexWeights.data
num_verts = mh_mesh.getVertexCount(excludeMaskedVerts=False)
# all skeleton joints in USD order
binding_joints = joint_names
# Corresponding arrays of joint indices and weights of length num_verts.
# Allots the maximum number of weights for every vertex, and pads any
# remaining weights with 0's, per USD spec, see:
# https://graphics.pixar.com/usd/dev/api/_usd_skel__schemas.html#UsdSkel_BindingAPI
# "If a point has fewer influences than are needed for other points, the
# unused array elements of that point should be filled with 0, both for
# joint indices and for weights."
indices = np.zeros((num_verts, max_influences))
weights = np.zeros((num_verts, max_influences))
# Keep track of the number of joint influences on each vertex
influence_counts = np.zeros(num_verts, dtype=int)
for joint, joint_data in influence_joints.items():
# get the index of the joint in our USD-ordered list of all joints
joint_index = binding_joints.index(joint)
for vert_index, weight in zip(*joint_data):
# Use influence_count to keep from overwriting existing influences
influence_count = influence_counts[vert_index]
# Add the joint index to our vertex array
indices[vert_index][influence_count] = joint_index
# Add the weight to the same vertex
weights[vert_index][influence_count] = weight
# Add to the influence count for this vertex
influence_counts[vert_index] += 1
# Check for any unweighted verts (this is a test routine)
# for i, d in enumerate(indices): if np.all((d == 0)): print(i)
# Flatten arrays to one dimensional lists
indices = indices.flatten()
weights = weights.flatten()
return indices, weights
def setup_bindings(self, paths: List[Sdf.Path], stage: Usd.Stage, skeleton: UsdSkel.Skeleton):
"""Setup bindings between meshes in the USD scene and the skeleton
Parameters
----------
paths : List of Sdf.Path
USD Sdf paths to each mesh prim
stage : Usd.Stage
The USD stage where the prims can be found
skeleton : UsdSkel.Skeleton
The USD skeleton to apply bindings to
Returns
-------
array of: UsdSkel.BindingAPI
Array of bindings between each mesh and the skeleton, in "path" order
"""
bindings = []
# TODO rename "mesh" to "path"
for mesh in paths:
# Get the prim in the stage
prim = stage.GetPrimAtPath(mesh)
attrs = prim.GetAttribute('primvars:skel:jointWeights')
# Check if joint weights have already been applied
if attrs.IsValid():
prim_path = prim.GetPath()
sdf_path = Sdf.Path(prim_path)
binding = UsdSkel.BindingAPI.Get(stage, sdf_path)
# relationships = prim.GetRelationships()
# 'material:binding' , 'proxyPrim', 'skel:animationSource','skel:blendShapeTargets','skel:skeleton'
# get_binding.GetSkeletonRel()
else:
# Create a binding applied to the prim
binding = UsdSkel.BindingAPI.Apply(prim)
# Create a relationship between the binding and the skeleton
binding.CreateSkeletonRel().SetTargets([skeleton.GetPath()])
# Add the binding to the list to return
bindings.append(binding)
return bindings
def setup_materials(self, mh_meshes: List['Object3D'], meshes: List[Sdf.Path], root: str, stage: Usd.Stage):
"""Fetches materials from Makehuman meshes and applies them to their corresponding
Usd mesh prims in the stage.
Parameters
----------
mh_meshes : List['Object3D']
List of makehuman meshes
meshes : List[Sdf.Path]
Paths to Usd meshes in the stage
root : str
The root path under which to create new prims
stage : Usd.Stage
Usd stage in which to create materials, and which contains the meshes
to which to apply materials
"""
for mh_mesh, mesh in zip(self.mh_meshes, meshes):
# Get a texture path and name from the makehuman mesh
texture, name = get_mesh_texture(mh_mesh)
if texture:
# If we can get a texture from the makehuman mesh, create a material
# from it and bind it to the corresponding USD mesh in the stage
material = create_material(texture, name, root, stage)
bind_material(mesh, material, stage)
def add_item(self, path: str):
"""Add a new asset to the human. Propagates changes to the Makehuman app
and then upates the stage with the new asset. If the asset is a proxy,
targets will not be applied. If the asset is a skeleton, targets must
be applied.
Parameters
----------
path : str
Path to an asset on disk
"""
# Check if human has a prim
if self.prim:
# Add an item through the MakeHuman instance and update the widget view
MHCaller.add_item(path)
self.update_in_scene(self.prim.GetPath().pathString)
else:
carb.log_warn("Can't add asset. No human prim selected!")
@staticmethod
def _set_scale(prim : Usd.Prim, scale : float):
"""Set scale of a prim.
Parameters
----------
prim : Usd.Prim
The prim to scale.
scale : float
The scale to apply."""
if prim == None:
return
# Uniform scale.
sV = Gf.Vec3f(scale, scale, scale)
scale = prim.GetAttribute("xformOp:scale").Get()
if scale != None:
prim.GetAttribute("xformOp:scale").Set(Gf.Vec3f(sV))
else:
# xformOpOrder is also updated.
xformAPI = UsdGeom.XformCommonAPI(prim)
xformAPI.SetScale(Gf.Vec3f(sV)) | 32,659 | Python | 40.030151 | 141 | 0.598212 |
cadop/HumanGenerator/exts/siborg.create.human/siborg/create/human/styles.py | # Stylesheet for parameter panels
panel_style = {
"Rectangle::group_rect": {
"background_color": 0xFF313333,
"border_radius": 5,
"margin": 5,
},
"VStack::contents": {
"margin": 10,
},
}
# Stylesheet for sliderentry widgets
sliderentry_style = {
"Label::label_param": {
"margin_width": 10,
},
}
# stylesheet for collapseable frame widgets, used for each modifier category
frame_style = {
"CollapsableFrame": {
"background_color": 0xFF1F2123,
},
}
# stylesheet for main UI window
window_style = {
"Rectangle::splitter": {"background_color": 0xFF454545},
"Rectangle::splitter:hovered": {"background_color": 0xFFFFCA83},
}
# Stylesheet for buttons
button_style = {
"Button:disabled": {
"background_color": 0xFF424242,
},
"Button:disabled.Label": {
"color": 0xFF848484,
},
}
| 894 | Python | 20.309523 | 76 | 0.608501 |
cadop/HumanGenerator/exts/siborg.create.human/siborg/create/human/mhcaller.py | from typing import TypeVar, Union
import warnings
import io
import makehuman
from pathlib import Path
# Makehuman loads most modules by manipulating the system path, so we have to
# run this before we can run the rest of our makehuman imports
makehuman.set_sys_path()
import human
import animation
import bvh
import files3d
import mh
from core import G
from mhmain import MHApplication
from shared import wavefront
import humanmodifier, skeleton
import proxy, gui3d, events3d, targets
from getpath import findFile
import numpy as np
import carb
from .shared import data_path
class classproperty:
"""Class property decorator. Allows us to define a property on a class
method rather than an instance method."""
def __init__(cls, fget):
cls.fget = fget
def __get__(cls, obj, owner):
return cls.fget(owner)
class MHCaller:
"""A singleton wrapper around the Makehuman app. Lets us use Makehuman functions without
launching the whole application. Also holds all data about the state of our Human
and available modifiers/assets, and allows us to create new humans without creating a new
instance of MHApp.
Attributes
----------
G : Globals
Makehuman global object. Stores globals needed by Makehuman internally
human : Human
Makehuman Human object. Encapsulates all human data (parameters, available)
modifiers, skeletons, meshes, assets, etc) and functions.
"""
G = G
human = None
def __init__(cls):
"""Constructs an instance of MHCaller. This involves setting up the
needed components to use makehuman modules independent of the GUI.
This includes app globals (G) and the human object."""
cls._config_mhapp()
cls.init_human()
def __new__(cls):
"""Singleton pattern. Only one instance of MHCaller can exist at a time."""
if not hasattr(cls, 'instance'):
cls.instance = super(MHCaller, cls).__new__(cls)
return cls.instance
@classmethod
def _config_mhapp(cls):
"""Declare and initialize the makehuman app, and move along if we
encounter any errors (omniverse sometimes fails to purge the app
singleton on extension reload, which can throw an error. This just means
the app already exists)
"""
try:
cls.G.app = MHApplication()
except:
return
cls.human_mapper = {}
@classmethod
def reset_human(cls):
"""Resets the human object to its initial state. This involves setting the
human's name to its default, resetting all modifications, and resetting all
proxies. Does not reset the skeleton. Also flags the human as having been
reset so that the new name can be created when adding to the Usd stage.
"""
cls.human.resetMeshValues()
# Subdivide the human mesh. This also means that any proxies added to the human are subdivided
cls.human.setSubdivided(True)
# Restore eyes
# cls.add_proxy(data_path("eyes/high-poly/high-poly.mhpxy"), "eyes")
# Reset skeleton to the game skeleton
cls.human.setSkeleton(cls.game_skel)
# Reset the human to tpose
cls.set_tpose()
# HACK Set the age to itcls to force an update of targets, otherwise humans
# are created with the MH base mesh, see:
# http://static.makehumancommunity.org/makehuman/docs/professional_mesh_topology.html
cls.human.setAge(cls.human.getAge())
@classmethod
def init_human(cls):
"""Initialize the human and set some required files from disk. This
includes the skeleton and any proxies (hair, clothes, accessories etc.)
The weights from the base skeleton must be transfered to the chosen
skeleton or else there will be unweighted verts on the meshes.
"""
cls.human = human.Human(files3d.loadMesh(mh.getSysDataPath("3dobjs/base.obj"), maxFaces=5))
# set the makehuman instance human so that features (eg skeletons) can
# access it globally
cls.G.app.selectedHuman = cls.human
humanmodifier.loadModifiers(mh.getSysDataPath("modifiers/modeling_modifiers.json"), cls.human)
# Add eyes
# cls.add_proxy(data_path("eyes/high-poly/high-poly.mhpxy"), "eyes")
cls.base_skel = skeleton.load(
mh.getSysDataPath("rigs/default.mhskel"),
cls.human.meshData,
)
# Load the game developer skeleton
# The root of this skeleton is at the origin which is better for animation
# retargeting
cls.game_skel = skeleton.load(data_path("rigs/game_engine.mhskel"), cls.human.meshData)
# Build joint weights on our chosen skeleton, derived from the base
# skeleton
cls.game_skel.autoBuildWeightReferences(cls.base_skel)
# Set the base skeleton
cls.human.setBaseSkeleton(cls.base_skel)
# Set the game skeleton
cls.human.setSkeleton(cls.game_skel)
@classproperty
def objects(cls):
"""List of objects attached to the human.
Returns
-------
list of: guiCommon.Object
All 3D objects included in the human. This includes the human
itcls, as well as any proxies
"""
# Make sure proxies are up-to-date
cls.update()
return cls.human.getObjects()
@classproperty
def meshes(cls):
"""All of the meshes of all of the objects attached to a human. This
includes the mesh of the human itcls as well as the meshes of all proxies
(clothing, hair, musculature, eyes, etc.)"""
return [o.mesh for o in cls.objects]
@classproperty
def modifiers(cls):
"""List of modifers attached to the human. These are all macros as well as any
individual modifiers which have changed.
Returns
-------
list of: humanmodifier.Modifier
The macros and changed modifiers included in the human
"""
return [m for m in cls.human.modifiers if m.getValue() or m.isMacro()]
@classproperty
def default_modifiers(cls):
"""List of all the loaded modifiers, whether or not their default values have been changed.
-------
list of: humanmodifier.Modifier
The macros and changed modifiers included in the human
"""
return cls.human.modifiers
@classproperty
def proxies(cls):
"""List of proxies attached to the human.
Returns
-------
list of: proxy.Proxy
All proxies included in the human
"""
return cls.human.getProxies()
@classmethod
def update(cls):
"""Propagate changes to meshes and proxies"""
# For every mesh object except for the human (first object), update the
# mesh and corresponding proxy
# See https://github.com/makehumancommunity/makehuman/search?q=adaptproxytohuman
for obj in cls.human.getObjects()[1:]:
mesh = obj.getSeedMesh()
pxy = obj.getProxy()
# Update the proxy and fit to posed human
# args are (mesh, fit_to_posed = false) by default
pxy.update(mesh, True)
# Update the mesh
mesh.update()
@classmethod
def add_proxy(cls, proxypath : str, proxy_type : str = None):
"""Load a proxy (hair, nails, clothes, etc.) and apply it to the human
Parameters
----------
proxypath : str
Path to the proxy file on disk
proxy_type: str, optional
Proxy type, None by default
Can be automatically determined using path names, but otherwise
must be defined (this is a limitation of how makehuman handles
proxies)
"""
# Derived from work by @tomtom92 at the MH-Community forums
# See: http://www.makehumancommunity.org/forum/viewtopic.php?f=9&t=17182&sid=7c2e6843275d8c6c6e70288bc0a27ae9
# Get proxy type if none is given
if proxy_type is None:
proxy_type = cls.guess_proxy_type(proxypath)
# Load the proxy
pxy = proxy.loadProxy(cls.human, proxypath, type=proxy_type)
# Get the mesh and Object3D object from the proxy applied to the human
mesh, obj = pxy.loadMeshAndObject(cls.human)
# TODO is this next line needed?
mesh.setPickable(True)
# TODO Can this next line be deleted? The app isn't running
gui3d.app.addObject(obj)
# Fit the proxy mesh to the human
mesh2 = obj.getSeedMesh()
fit_to_posed = True
pxy.update(mesh2, fit_to_posed)
mesh2.update()
# Set the object to be subdivided if the human is subdivided
obj.setSubdivided(cls.human.isSubdivided())
# Set/add proxy based on type
if proxy_type == "eyes":
cls.human.setEyesProxy(pxy)
elif proxy_type == "clothes":
cls.human.addClothesProxy(pxy)
elif proxy_type == "eyebrows":
cls.human.setEyebrowsProxy(pxy)
elif proxy_type == "eyelashes":
cls.human.setEyelashesProxy(pxy)
elif proxy_type == "hair":
cls.human.setHairProxy(pxy)
else:
# Body proxies (musculature, etc)
cls.human.setProxy(pxy)
vertsMask = np.ones(cls.human.meshData.getVertexCount(), dtype=bool)
proxyVertMask = proxy.transferVertexMaskToProxy(vertsMask, pxy)
# Apply accumulated mask from previous layers on this proxy
obj.changeVertexMask(proxyVertMask)
# Delete masked vertices
# TODO add toggle for this feature in UI
# verts = np.argwhere(pxy.deleteVerts)[..., 0]
# vertsMask[verts] = False
# cls.human.changeVertexMask(vertsMask)
Proxy = TypeVar("Proxy")
@classmethod
def remove_proxy(cls, proxy: Proxy):
"""Removes a proxy from the human. Executes a particular method for removal
based on proxy type.
Parameters
----------
proxy : proxy.Proxy
The Makehuman proxy to remove from the human
"""
proxy_type = proxy.type.lower()
# Use MakeHuman internal methods to remove proxy based on type
if proxy_type == "eyes":
cls.human.setEyesProxy(None)
elif proxy_type == "clothes":
cls.human.removeClothesProxy(proxy.uuid)
elif proxy_type == "eyebrows":
cls.human.setEyebrowsProxy(None)
elif proxy_type == "eyelashes":
cls.human.setEyelashesProxy(None)
elif proxy_type == "hair":
cls.human.setHairProxy(None)
else:
# Body proxies (musculature, etc)
cls.human.setProxy(None)
@classmethod
def clear_proxies(cls):
"""Removes all proxies from the human"""
for pxy in cls.proxies:
cls.remove_proxy(pxy)
Skeleton = TypeVar("Skeleton")
@classmethod
def remove_item(cls, item : Union[Skeleton, Proxy]):
"""Removes a Makehuman asset from the human. Assets include Skeletons
as well as proxies. Determines removal method based on asset object type.
Parameters
----------
item : Union[Skeleton,Proxy]
Makehuman skeleton or proxy to remove from the human
"""
if isinstance(item, proxy.Proxy):
cls.remove_proxy(item)
else:
return
@classmethod
def add_item(cls, path : str):
"""Add a Makehuman asset (skeleton or proxy) to the human.
Parameters
----------
path : str
Path to the asset on disk
"""
if "mhpxy" in path or "mhclo" in path:
cls.add_proxy(path)
elif "mhskel" in path:
cls.set_skel(path)
@classmethod
def set_skel(cls, path : str):
"""Change the skeleton applied to the human. Loads a skeleton from disk.
The skeleton position can be used to drive the human position in the scene.
Parameters
----------
path : str
The path to the skeleton to load from disk
"""
# Load skeleton from path
skel = skeleton.load(path, cls.human.meshData)
# Build skeleton weights based on base skeleton
skel.autoBuildWeightReferences(cls.base_skel)
# Set the skeleton and update the human
cls.human.setSkeleton(skel)
cls.human.applyAllTargets()
# Return the skeleton object
return skel
@classmethod
def guess_proxy_type(cls, path : str):
"""Guesses a proxy's type based on the path from which it is loaded.
Parameters
----------
path : str
The path to the proxy on disk
Returns
-------
Union[str,None]
The proxy type, or none if the type could not be determined
"""
proxy_types = ("eyes", "clothes", "eyebrows", "eyelashes", "hair")
for type in proxy_types:
if type in path:
return type
return None
@classmethod
def set_tpose(cls):
"""Sets the human to the T-Pose"""
# Load the T-Pose BVH file
filepath = data_path('poses\\tpose.bvh')
bvh_file = bvh.load(filepath, convertFromZUp="auto")
# Create an animation track from the BVH file
anim = bvh_file.createAnimationTrack(cls.human.getBaseSkeleton())
# Add the animation to the human
cls.human.addAnimation(anim)
# Set the active animation to the T-Pose
cls.human.setActiveAnimation(anim.name)
# Refresh the human pose
cls.human.refreshPose()
return
# Create an instance of MHCaller when imported
MHCaller()
| 13,875 | Python | 34.218274 | 118 | 0.622198 |
cadop/HumanGenerator/exts/siborg.create.human/siborg/create/human/extension.py | import omni.ext
import omni.ui as ui
import carb
import carb.events
import omni
from functools import partial
import asyncio
import omni.usd
from pxr import Usd
from typing import Union
from .window import MHWindow, WINDOW_TITLE, MENU_PATH
class MakeHumanExtension(omni.ext.IExt):
# ext_id is current extension id. It can be used with extension manager to query additional information, like where
# this extension is located on filesystem.
def on_startup(self, ext_id):
# subscribe to stage events
# see https://github.com/mtw75/kit_customdata_view
_usd_context = omni.usd.get_context()
self._selection = _usd_context.get_selection()
self._human_selection_event = carb.events.type_from_string("siborg.create.human.human_selected")
# subscribe to stage events
self._events = _usd_context.get_stage_event_stream()
self._stage_event_sub = self._events.create_subscription_to_push(
self._on_stage_event,
name='human seletion changed',
)
# get message bus event stream so we can push events to the message bus
self._bus = omni.kit.app.get_app().get_message_bus_event_stream()
ui.Workspace.set_show_window_fn(WINDOW_TITLE, partial(self.show_window, None))
# create a menu item to open the window
editor_menu = omni.kit.ui.get_editor_menu()
if editor_menu:
self._menu = editor_menu.add_item(
MENU_PATH, self.show_window, toggle=True, value=True
)
# show the window
ui.Workspace.show_window(WINDOW_TITLE)
print("[siborg.create.human] HumanGeneratorExtension startup")
def on_shutdown(self):
self._menu = None
if self._window:
self._window.destroy()
self._window = None
# Deregister the function that shows the window from omni.ui
ui.Workspace.set_show_window_fn(WINDOW_TITLE, None)
async def _destroy_window_async(self):
# wait one frame, this is due to the one frame defer
# in Window::_moveToMainOSWindow()
await omni.kit.app.get_app().next_update_async()
if self._window:
self._window.destroy()
self._window = None
def visibility_changed(self, visible):
# Called when window closed by user
editor_menu = omni.kit.ui.get_editor_menu()
# Update the menu item to reflect the window state
if editor_menu:
editor_menu.set_value(MENU_PATH, visible)
if not visible:
# Destroy the window, since we are creating new window
# in show_window
asyncio.ensure_future(self._destroy_window_async())
def show_window(self, menu, value):
"""Handles showing and hiding the window"""
if value:
self._window = MHWindow(WINDOW_TITLE)
# # Dock window wherever the "Content" tab is found (bottom panel by default)
self._window.deferred_dock_in("Content", ui.DockPolicy.CURRENT_WINDOW_IS_ACTIVE)
self._window.set_visibility_changed_fn(self.visibility_changed)
elif self._window:
self._window.visible = False
def _on_stage_event(self, event):
"""Handles stage events. This is where we get notified when the user selects/deselects a prim in the viewport."""
if event.type == int(omni.usd.StageEventType.SELECTION_CHANGED):
# Get the current selection
selection = self._selection.get_selected_prim_paths()
# Check if the selection is empty
if not selection:
# Push an event to the message bus with "None" as a payload
# This event will be picked up by the window and used to update the UI
carb.log_warn("Human deselected")
self._bus.push(self._human_selection_event, payload={"prim_path": None})
else:
# Get the stage
_usd_context = omni.usd.get_context()
stage = _usd_context.get_stage()
if selection and stage:
if len(selection) > 0:
path = selection[-1]
print(path)
prim = stage.GetPrimAtPath(path)
prim = self._get_typed_parent(prim, "SkelRoot")
# If the selection is a human, push an event to the event stream with the prim as a payload
# This event will be picked up by the window and used to update the UI
if prim and prim.GetCustomDataByKey("human"):
# carb.log_warn("Human selected")
path = prim.GetPath().pathString
self._bus.push(self._human_selection_event, payload={"prim_path": path})
else:
# carb.log_warn("Human deselected")
self._bus.push(self._human_selection_event, payload={"prim_path": None})
def _get_typed_parent(self, prim: Union[Usd.Prim, None], type_name: str, level: int = 5):
"""Returns the first parent of the given prim with the given type name. If no parent is found, returns None.
Parameters:
-----------
prim : Usd.Prim or None
The prim to search from. If None, returns None.
type_name : str
The parent type name to search for
level : int
The maximum number of levels to traverse. Defaults to 5.
Returns:
--------
Usd.Prim
The first parent of the given prim with the given type name. If no match is found, returns None.
"""
if (not prim) or level == 0:
return None
elif prim and prim.GetTypeName() == type_name:
return prim
else:
return self._get_typed_parent(prim.GetParent(), type_name, level - 1)
| 6,003 | Python | 40.986014 | 121 | 0.590038 |
cadop/HumanGenerator/exts/siborg.create.human/siborg/create/human/skeleton.py | from pxr import Usd, Gf, UsdSkel
from typing import List
import numpy as np
from .shared import sanitize
from .mhcaller import skeleton as mhskel
from .mhcaller import MHCaller
class Bone:
"""Bone which constitutes skeletons to be imported using the HumanGenerator
extension. Has a parent and children, transforms in space, and named joints
at the head and tail.
Attributes
----------
name : str
Human-readable bone name.
"""
def __init__(self, skel: 'Skeleton', name: str, parent: str, head: str, tail: str) -> None:
"""Create a Bone instance
Parameters
----------
skel : Skeleton
Skeleton to which the bone belongs
name : str
Name of the bone
parent : str
Name of the parent bone. This is the bone "above" and is one level closer to
the root of the skeleton
head : str
Name of the head joint
tail : str
Name of the tail joint
"""
self._mh_bone = mhskel.Bone(skel, name, parent, head, tail)
self.name = name
self.skeleton = skel
self.headJoint = head
self.tailJoint = tail
def getRelativeMatrix(self, offset: List[float] = [0, 0, 0]) -> np.ndarray:
"""_summary_
Parameters
----------
offset : List[float], optional
Geometric translation to apply, by default [0, 0, 0]
Returns
-------
np.ndarray
_description_
"""
return self._mh_bone.getRelativeMatrix(offset)
def getRestMatrix(self, offset: List[float] = [0, 0, 0]) -> np.ndarray:
"""_summary_
Parameters
----------
offset : List[float], optional
Geometric translation to apply, by default [0, 0, 0]
Returns
-------
np.ndarray
_description_
"""
return self._mh_bone.getRestMatrix(offset)
def getBindMatrix(self, offset: List[float] = [0, 0, 0]) -> np.ndarray:
"""_summary_
Parameters
----------
offset : List[float], optional
Geometric translation to apply, by default [0, 0, 0]
Returns
-------
np.ndarray
_description_
"""
return self._mh_bone.getBindMatrix(offset)[1]
class Skeleton:
"""Skeleton which can be imported using the HumanGenerator extension. Provides
root bone(s), which have a tree of children that can be traversed to get the data
for the entire rig.
Attributes
----------
name : str
Name of the skeleton rig, by default "Skeleton"
roots : list of Bone
Root bones. Bones which have children that can be traversed to constitute the
entire skeleton.
joint_paths : list of: str
Paths to joints in the stage hierarchy that are used as joint indices
joint_names : list of: str
List of joint names in USD (breadth-first traversal) order. It is
important that joints be ordered this way so that their indices can be
used for skinning / weighting.
"""
def __init__(self, name="Skeleton") -> None:
"""Create a skeleton instance
Parameters
----------
name : str, optional
Name of the skeleton, by default "Skeleton"
"""
# Set the skeleton to the makehuman default
_mh_skeleton = MHCaller.human.getSkeleton()
self._rel_transforms = []
self._bind_transforms = []
self.roots = _mh_skeleton.roots
self.joint_paths = []
self.joint_names = []
self.name = name
def addBone(self, name: str, parent: str, head: str, tail: str) -> Bone:
"""Add a new bone to the Skeleton
Parameters
----------
name : str
Name of the new bone
parent : str
Name of the parent bone under which to put the new bone
head : str
Name of the joint at the head of the new bone
tail : str
Name of the joint at the tail of the new bone
Returns
-------
Bone
The bone which has been added to the skeleton
"""
_bone = Bone(self, name, parent, head, tail)
# HACK Bone() creates a new Bone for _mh_bone by default. How can we
# avoid doing this twice without revealing it to the user?
_bone._mh_bone = self._mh_skeleton.addBone(name, parent, head, tail)
return _bone
def add_to_stage(self, stage: Usd.Stage, skel_root_path: str, offset: List[float] = [0, 0, 0], new_root_bone: bool = False):
"""Adds the skeleton to the USD stage
Parameters
----------
stage : Usd.Stage
Stage in which to create skeleton prims
skelroot_path : str
Path to the human root prim in the stage
offset : List[float], optional
Geometric translation to apply, by default [0, 0, 0]
new_root_bone : bool, optional
Whether or not to prepend a new root at the origin, by default False
"""
root_bone = self.roots[0]
if new_root_bone:
root_bone = self.prepend_root(root_bone)
self.setup_skeleton(root_bone, offset=offset)
skeleton_path = skel_root_path + "/Skeleton"
usdSkel = UsdSkel.Skeleton.Define(stage, skeleton_path)
# add joints to skeleton by path
attribute = usdSkel.GetJointsAttr()
# exclude root
attribute.Set(self.joint_paths)
# Add bind transforms to skeleton
usdSkel.CreateBindTransformsAttr(self._bind_transforms)
# setup rest transforms in joint-local space
usdSkel.CreateRestTransformsAttr(self._rel_transforms)
return usdSkel
def prepend_root(self, oldRoot: Bone, newroot_name: str = "RootJoint", offset: List[float] = [0, 0, 0]) -> Bone:
"""Adds a new root bone to the head of a skeleton, ahead of the existing root bone.
Parameters
----------
oldRoot : Bone
The original MakeHuman root bone
newroot_name : str, optional
The name for the new root bone, by default "RootJoint"
offset : List[float], optional
Geometric translation to apply, by default [0, 0, 0]
Returns
-------
newRoot : Bone
The new root bone of the Skeleton
"""
# make a "super-root" bone, parent to the root, with identity transforms so
# we can abide by Lina Halper's animation retargeting guidelines:
# https://docs.omniverse.nvidia.com/prod_extensions/prod_extensions/ext_animation-retargeting.html
newRoot = self.addBone(
newroot_name, None, "newRoot_head", oldRoot.tailJoint)
oldRoot.parent = newRoot
newRoot.headPos -= offset
newRoot.build()
newRoot.children.append(oldRoot)
return newRoot
def _process_bone(self, bone: Bone, path: str, offset: List[float] = [0, 0, 0]) -> None:
"""Get the name, path, relative transform, and bind transform of a joint
and add its values to the lists of stored values
Parameters
----------
bone : Bone
The bone to process for Usd
path : str
Path to the parent of this bone
offset : List[float], optional
Geometric translation to apply, by default [0, 0, 0]
"""
# sanitize the name for USD paths
name = sanitize(bone.name)
path += name
self.joint_paths.append(path)
# store original name for later joint weighting
self.joint_names.append(bone.name)
# Get matrix for joint transform relative to its parent. Move to offset
# to match mesh transform in scene
relxform = bone.getRelativeMatrix(offsetVect=offset)
# Transpose the matrix as USD stores transforms in row-major format
relxform = relxform.transpose()
# Convert type for USD and store
relative_transform = Gf.Matrix4d(relxform.tolist())
self._rel_transforms.append(relative_transform)
# Get matrix which represents a joints transform in its binding position
# for binding to a mesh. Move to offset to match mesh transform.
# getBindMatrix() returns a tuple of the bind matrix and the bindinv
# matrix. Since omniverse uses row-major format, we can just use the
# already transposed bind matrix.
bxform = bone.getBindMatrix(offsetVect=offset)
# Convert type for USD and store
bind_transform = Gf.Matrix4d(bxform[1].tolist())
# bind_transform = Gf.Matrix4d().SetIdentity() TODO remove
self._bind_transforms.append(bind_transform)
def setup_skeleton(self, bone: Bone, offset: List[float] = [0, 0, 0]) -> None:
"""Traverse the imported skeleton and get the data for each bone for
adding to the stage
Parameters
----------
bone : Bone
The root bone at which to start traversing the imported skeleton.
offset : List[float], optional
Geometric translation to apply, by default [0, 0, 0]
"""
# Setup a breadth-first search of our skeleton as a tree
# Use the new root of the imported skeleton as the root bone of our tree
visited = [] # List to keep track of visited bones.
queue = [] # Initialize a queue
path_queue = [] # Keep track of paths in a parallel queue
visited.append(bone)
queue.append(bone)
name = sanitize(bone.name)
path_queue.append(name + "/")
# joints are relative to the root, so we don't prepend a path for the root
self._process_bone(bone, "", offset=offset)
# Traverse skeleton (breadth-first) and store joint data
while queue:
v = queue.pop(0)
path = path_queue.pop(0)
for neighbor in v.children:
if neighbor not in visited:
visited.append(neighbor)
queue.append(neighbor)
name = sanitize(neighbor.name)
path_queue.append(path + name + "/")
self._process_bone(neighbor, path, offset)
def update_in_scene(self, stage: Usd.Stage, skel_root_path: str, offset: List[float] = [0, 0, 0]):
"""Resets the skeleton values in the stage, updates the skeleton from makehuman.
Parameters
----------
stage : Usd.Stage
The stage in which to update the skeleton
skel_root_path : str
The path to the skeleton root in the stage
offset : List[float], optional
Geometric translation to apply, by default [0, 0, 0]
Returns
-------
UsdSkel.Skeleton
The updated skeleton in USD
"""
# Get the skeleton from makehuman
_mh_skeleton = MHCaller.human.getSkeleton()
# Clear out any existing data
self._rel_transforms = []
self._bind_transforms = []
self.joint_paths = []
self.joint_names = []
# Get the root bone(s) of the skeleton
self.roots = _mh_skeleton.roots
# Overwrite the skeleton in the stage with the new skeleton
return self.add_to_stage(stage, skel_root_path, offset)
| 11,468 | Python | 33.032641 | 128 | 0.586327 |
cadop/HumanGenerator/exts/siborg.create.human/siborg/create/human/__init__.py | from .extension import *
from .human import Human | 49 | Python | 23.999988 | 24 | 0.795918 |
cadop/HumanGenerator/exts/siborg.create.human/siborg/create/human/materials.py | from typing import List
from module3d import Object3D
from pxr import Usd, UsdGeom, UsdShade, Sdf
def get_mesh_texture(mh_mesh: Object3D):
"""Gets mesh diffuse texture from a Makehuman mesh object
Parameters
----------
mh_mesh : Object3D
A Makehuman mesh object. Contains path to bound material/textures
Returns
-------
Tuple (str,str)
Returns the path to a texture on disk, and a name for the texture
Returns (None, None) if no texture exists
"""
# TODO return additional maps (AO, roughness, normals, etc)
material = mh_mesh.material
texture = material.diffuseTexture
name = material.name
if texture:
return texture, name
else:
return (None, None)
def create_material(diffuse_image_path: str, name: str, root_path: str, stage: Usd.Stage):
"""Create OmniPBR Material with specified diffuse texture
Parameters
----------
diffuse_image_path : str
Path to diffuse texture on disk
name : str
Material name
root_path : str
Root path under which to place material scope
stage : Usd.Stage
USD stage into which to add the material
Returns
-------
UsdShade.Material
Material with diffuse texture applied
"""
materialScopePath = root_path + "/Materials"
# Check for a scope in which to keep materials. If it doesn't exist, make
# one
scopePrim = stage.GetPrimAtPath(materialScopePath)
if scopePrim.IsValid() is False:
UsdGeom.Scope.Define(stage, materialScopePath)
# Create material (omniPBR).
materialPath = materialScopePath + "/" + name
material = UsdShade.Material.Define(stage, materialPath)
# Store shaders inside their respective material path
shaderPath = materialPath + "/Shader"
# Create shader
shader = UsdShade.Shader.Define(stage, shaderPath)
# Use OmniPBR as a source to define our shader
shader.SetSourceAsset("OmniPBR.mdl", "mdl")
shader.GetPrim().CreateAttribute(
"info:mdl:sourceAsset:subIdentifier",
Sdf.ValueTypeNames.Token,
False,
Sdf.VariabilityUniform,
).Set("OmniPBR")
# Set Diffuse texture.
diffTexIn = shader.CreateInput("diffuse_texture", Sdf.ValueTypeNames.Asset)
diffTexIn.Set(diffuse_image_path)
diffTexIn.GetAttr().SetColorSpace("sRGB")
# Set Diffuse value. TODO make default color NVIDIA Green
# diffTintIn = shader.CreateInput("diffuse_tint", Sdf.ValueTypeNames.Color3f)
# diffTintIn.Set((0.9, 0.9, 0.9))
# Connect Material to Shader.
mdlOutput = material.CreateSurfaceOutput("mdl")
mdlOutput.ConnectToSource(shader, "out")
return material
def bind_material(mesh_path: Sdf.Path, material: UsdShade.Material, stage: Usd.Stage):
"""Bind a material to a mesh
Parameters
----------
mesh_path : Sdf.Path
The USD formatted path to a mesh prim
material : UsdShade.Material
USD material object
stage : Usd.Stage
Stage in which to find mesh prim
"""
# Get the mesh prim
meshPrim = stage.GetPrimAtPath(mesh_path)
# Bind the mesh
UsdShade.MaterialBindingAPI(meshPrim).Bind(material) | 3,210 | Python | 29.292453 | 90 | 0.669782 |
cadop/HumanGenerator/exts/siborg.create.human/siborg/create/human/ext_ui.py | import omni.ui as ui
from typing import List, TypeVar, Union, Callable
from dataclasses import dataclass, field
from . import styles
from .mhcaller import MHCaller
from pxr import Usd
import os
import inspect
import makehuman
import targets
from siborg.create.human.shared import data_path
class SliderEntry:
"""Custom UI element that encapsulates a labeled slider and field
Attributes
----------
label : str
Label to display for slider/field
model : ui.SimpleFloatModel
Model to publish changes to
fn : object
Function to run when updating the human after changes are made
image: str
Path on disk to an image to display
step : float
Division between values for the slider
min : float
Minimum value
max : float
Maximum value
default : float
Default parameter value
"""
def __init__(
self,
label: str,
model: ui.SimpleFloatModel,
fn: object,
image: str = None,
step: float = 0.01,
min: float = None,
max: float = None,
default: float = 0,
):
"""Constructs an instance of SliderEntry
Parameters
----------
label : str
Label to display for slider/field
model : ui.SimpleFloatModel
Model to publish changes to
fn : object
Function to run when changes are made
image: str, optional
Path on disk to an image to display. By default None
step : float, optional
Division between values for the slider, by default 0.01
min : float, optional
Minimum value, by default None
max : float, optional
Maximum value, by default None
default : float, optional
Default parameter value, by default 0
"""
self.label = label
self.model = model
self.fn = fn
self.step = step
self.min = min
self.max = max
self.default = default
self.image = image
self._build_widget()
def _build_widget(self):
"""Construct the UI elements"""
with ui.HStack(height=0, style=styles.sliderentry_style):
# If an image is available, display it
if self.image:
ui.Image(self.image, height=75, style={"border_radius": 5})
# Stack the label and slider on top of each other
with ui.VStack(spacing = 5):
ui.Label(
self.label,
height=15,
alignment=ui.Alignment.CENTER,
name="label_param",
)
# create a floatdrag (can be used as a slider or an entry field) to
# input parameter values
self.drag = ui.FloatDrag(model=self.model, step=self.step)
# Limit drag values to within min and max if provided
if self.min is not None:
self.drag.min = self.min
if self.max is not None:
self.drag.max = self.max
@dataclass
class Param:
"""Dataclass to store SliderEntry parameter data
Attributes
----------
name: str
The name of the parameter. Used for labeling.
full_name: str
The full name of the parameter. Used for referencing
fn: object
The method to execute when making changes to the parameter
image: str, optional
The path to the image to use for labeling. By default None
min: float, optional
The minimum allowed value of the parameter. By default 0
max: float
The maximum allowed value of the parameter. By default 1
default: float
The default value of the parameter. By default 0.5
value : ui.SimpleFloatModel
The model to track the current value of the parameter. By default None
"""
name: str
full_name: str
fn: object
image: str = None
min: float = 0
max: float = 1
default: float = 0.5
value: ui.SimpleFloatModel = None
class SliderEntryPanelModel:
"""Provides a model for referencing SliderEntryPanel data. References models
for each individual SliderEntry widget in the SliderEntryPanel widget.
Attributes
----------
params : list of `Param`
List of parameter objects. Each contains a float model to track the current value
toggle : ui.SimpleBoolModel
Tracks whether or not the human should update immediately when changes are made
instant_update : Callable
A function to call when instant update is toggled
subscriptions : list of `Subscription`
List of event subscriptions triggered by editing a SliderEntry
"""
def __init__(self, params: List[Param], toggle: ui.SimpleBoolModel = None, instant_update: Callable = None):
"""Constructs an instance of SliderEntryPanelModel and instantiates models
to hold parameter data for individual SliderEntries
Parameters
----------
params : list of `Param`
A list of parameter objects, each of which contains the data to create
a SliderEntry widget and a model to track the current value
toggle : ui.SimpleBoolModel, optional
Tracks whether or not the human should update immediately when changes are made, by default None
instant_update : Callable
A function to call when instant update is toggled
"""
self.params = []
"""Param objects corresponding to each SliderEntry widget"""
self.changed_params = []
"""Params o SliderEntry widgets that have been changed"""
self.toggle = toggle
self.instant_update = instant_update
self.subscriptions = []
"""List of event subscriptions triggered by editing a SliderEntry"""
for p in params:
self.add_param(p)
def add_param(self, param: Param):
"""Adds a parameter to the SliderEntryPanelModel. Subscribes to the parameter's model
to check for editing changes
Parameters
----------
param : Param
The Parameter object from which to create the subscription
"""
# Create a model to track the current value of the parameter. Set the value to the default
param.value = ui.SimpleFloatModel(param.default)
# Add the parameter to the list of parameters
self.params.append(param)
# Subscribe to changes in parameter editing
self.subscriptions.append(
param.value.subscribe_end_edit_fn(
lambda m: self._sanitize_and_run(param))
)
def reset(self):
"""Resets the values of each floatmodel to parameter default for UI reset
"""
for param in self.params:
param.value.set_value(param.default)
def _sanitize_and_run(self, param: Param):
"""Make sure that values are within an acceptable range and then add the parameter to the
list of changed parameters
Parameters
----------
param : Param
Parameter object which contains acceptable value bounds and
references the function to run
"""
m = param.value
# Get the value from the slider model
getval = m.get_value_as_float
# Set the value to the min or max if it goes under or over respectively
if getval() < param.min:
m.set_value(param.min)
if getval() > param.max:
m.set_value(param.max)
# Check if the parameter is already in the list of changed parameters. If so, remove it.
# Then, add the parameter to the list of changed parameters
if param in self.changed_params:
self.changed_params.remove(param)
self.changed_params.append(param)
# If instant update is toggled on, add the changes to the stage instantly
if self.toggle.get_value_as_bool():
# Apply the changes
self.apply_changes()
# Run the instant update function
self.instant_update()
def apply_changes(self):
"""Apply the changes made to the parameters. Runs the function associated with each
parameter using the value from the widget
"""
for param in self.changed_params:
param.fn(param.value.get_value_as_float())
# Clear the list of changed parameters
self.changed_params = []
def destroy(self):
"""Destroys the instance of SliderEntryPanelModel. Deletes event
subscriptions. Important for preventing zombie-UI and unintended behavior
when the extension is reloaded.
"""
self.subscriptions = None
class SliderEntryPanel:
"""A UI widget providing a labeled group of slider entries
Attributes
----------
model : SliderEntryPanelModel
Model to hold parameters for each slider
label : str
Display title for the group. Can be none if no title is desired.
"""
def __init__(self, model: SliderEntryPanelModel, label: str = None):
"""
Parameters
----------
model : SliderEntryPanelModel
Model to hold parameters
label : str, Optional
Display title for the group, by default None
"""
self.label = label
self.model = model
self._build_widget()
def _build_widget(self):
"""Construct the UI elements"""
# Layer widgets on top of a rectangle to create a group frame
with ui.ZStack(style=styles.panel_style, height=0):
ui.Rectangle(name="group_rect")
with ui.VStack(name="contents", spacing = 8):
# If the panel has a label, show it
if self.label:
ui.Label(self.label, height=0)
# Create a slider entry for each parameter
for param in self.model.params:
SliderEntry(
param.name,
param.value,
param.fn,
image=param.image,
min=param.min,
max=param.max,
default=param.default,
)
def destroy(self):
"""Destroys the instance of SliderEntryPanel. Executes the destructor of
the SliderEntryPanel's SliderEntryPanelModel instance.
"""
self.model.destroy()
class ParamPanelModel(ui.AbstractItemModel):
def __init__(self, toggle: ui.SimpleBoolModel, **kwargs):
"""Constructs an instance of ParamPanelModel, which stores data for a ParamPanel.
Parameters
----------
toggle : ui.SimpleBoolModel
Model to track whether changes should be instant
"""
super().__init__(**kwargs)
# model to track whether changes should be instant
self.toggle = toggle
# Reference to models for each modifier/parameter. The models store modifier
# data for reference in the UI, and track the values of the sliders
self.models = []
class ParamPanel(ui.Frame):
"""UI Widget for displaying and modifying human parameters
Attributes
----------
model : ParamPanelModel
Stores data for the panel
toggle : ui.SimpleBoolModel
Model to track whether changes should be instant
models : list of SliderEntryPanelModel
Models for each group of parameter sliders
"""
def __init__(self, model: ParamPanelModel, instant_update : Callable = None, **kwargs):
"""Constructs an instance of ParamPanel. Panel contains a scrollable list of collapseable groups. These include
a group of macros (which affect multiple modifiers simultaneously), as well as groups of modifiers for
different body parts. Each modifier can be adjusted using a slider or doubleclicking to enter values directly.
Values are restricted based on the limits of a particular modifier.
Parameters
----------
model: ParamPanelModel
Stores data for the panel. Contains a toggle model to track whether changes should be instant
instant_update : Callable
Function to call when a parameter is changed (if instant update is toggle on)
"""
# Subclassing ui.Frame allows us to use styling on the whole widget
super().__init__(**kwargs)
self.model = model
self.toggle = model.toggle
# If no instant update function is passed, use a dummy function and do nothing
self.instant_update = instant_update if instant_update else lambda *args: None
self.models = model.models
self.set_build_fn(self._build_widget)
def _build_widget(self):
"""Build widget UI
"""
Modifier = TypeVar('Modifier')
def modifier_param(m: Modifier):
"""Generate a parameter data object from a human modifier,
Parameters
----------
m : Modifier
Makehuman Human modifier object. Represents a set of targets to apply to the human when modifying
Returns
-------
Param
Parameter data object holding all the modifier data needed to build UI elements
"""
# print(m.name)
# Guess a suitable title from the modifier name
tlabel = m.name.split("-")
if "|" in tlabel[len(tlabel) - 1]:
tlabel = tlabel[:-1]
if len(tlabel) > 1 and tlabel[0] == m.groupName:
label = tlabel[1:]
else:
label = tlabel
label = " ".join([word.capitalize() for word in label])
# Guess a suitable image path from modifier name
tlabel = m.name.replace("|", "-").split("-")
image = modifier_image(("%s.png" % "-".join(tlabel)).lower())
# Store modifier info in dataclass for building UI elements
return Param(
label,
m.fullName,
m.updateValue,
image=image,
min=m.getMin(),
max=m.getMax(),
default=m.getDefaultValue(),
)
def group_params(group: str):
"""Creates a list of parameters for all the modifiers in the given group
Parameters
----------
group : str
The name name of a modifier group
Returns
-------
List of Param
A list of all the parameters built from modifiers in the group
"""
params = [modifier_param(m)
for m in MHCaller.human.getModifiersByGroup(group)]
return params
def build_macro_frame():
"""Builds UI widget for the group of macro modifiers (which affect multiple individual modifiers
simultaneously). This includes:
+ Gender
+ Age
+ Muscle
+ Weight
+ Height
+ Proportions
Parameters that affect how much the human resembles a particular racial group:
+ African
+ Asian
+ Caucasian
"""
# Shorten human reference for convenience
human = MHCaller.human
# Explicitly create parameters for panel of macros (general modifiers that
# affect a group of targets). Otherwise these look bad. Creates a nice
# panel to have open by default
macro_params = (
Param("Gender", "macrodetails/Gender", human.setGender),
Param("Age", "macrodetails/Age", human.setAge),
Param("Muscle", "macrodetails-universal/Muscle", human.setMuscle),
Param("Weight", "macrodetails-universal/Weight", human.setWeight),
Param("Height", "macrodetails-height/Height", human.setHeight),
Param("Proportions", "macrodetails-proportions/BodyProportions", human.setBodyProportions),
)
# Create a model for storing macro parameter data
macro_model = SliderEntryPanelModel(macro_params, self.toggle, self.instant_update)
# Separate set of race parameters to also be included in the Macros group
# TODO make race parameters automatically normalize in UI
race_params = (
Param("African", "macrodetails/African", human.setAfrican),
Param("Asian", "macrodetails/Asian", human.setAsian),
Param("Caucasian", "macrodetails/Caucasian", human.setCaucasian),
)
# Create a model for storing race parameter data
race_model = SliderEntryPanelModel(race_params, self.toggle, self.instant_update)
self.models.append(macro_model)
self.models.append(race_model)
# Create category widget for macros
with ui.CollapsableFrame("Macros", style=styles.frame_style, height=0, collapsed=True):
with ui.VStack():
# Create panels for macros and race
self.panels = (
SliderEntryPanel(macro_model, label="General"),
SliderEntryPanel(race_model, label="Race"),
)
# The scrollable list of modifiers
with ui.ScrollingFrame():
with ui.VStack():
# Add the macros frame first
build_macro_frame()
# Create a set of all modifier groups that include macros
macrogroups = [
g for g in MHCaller.human.modifierGroups if "macrodetails" in g]
macrogroups = set(macrogroups)
# Remove macro groups from list of modifier groups as we have already
# included them explicitly
allgroups = set(
MHCaller.human.modifierGroups).difference(macrogroups)
for group in allgroups:
# Create a collapseable frame for each modifier group
with ui.CollapsableFrame(group.capitalize(), style=styles.frame_style, collapsed=True):
# Model to hold panel parameters
model = SliderEntryPanelModel(
group_params(group), self.toggle,self.instant_update)
self.models.append(model)
# Create panel of slider entries for modifier group
SliderEntryPanel(model)
def reset(self):
"""Reset every SliderEntryPanel to set UI values to defaults
"""
for model in self.models:
model.reset()
def load_values(self, human_prim: Usd.Prim):
"""Load values from the human prim into the UI. Specifically, this function
loads the values of the modifiers from the prim and updates any which
have changed.
Parameters
----------
HumanPrim : Usd.Prim
The USD prim representing the human
"""
# Make the prim exists
if not human_prim.IsValid():
return
# Reset the UI to defaults
self.reset()
# Get the data from the prim
humandata = human_prim.GetCustomData()
modifiers = humandata.get("Modifiers")
# Set any changed values in the models
for SliderEntryPanelModel in self.models:
for param in SliderEntryPanelModel.params:
if param.full_name in modifiers:
param.value.set_value(modifiers[param.full_name])
def update_models(self):
"""Update all models"""
for model in self.models:
model.apply_changes()
def destroy(self):
"""Destroys the ParamPanel instance as well as the models attached to each group of parameters
"""
super().destroy()
for model in self.models:
model.destroy()
class NoSelectionNotification:
"""
When no human selected, show notification.
"""
def __init__(self):
self._container = ui.ZStack()
with self._container:
ui.Rectangle()
with ui.VStack(spacing=10):
ui.Spacer(height=10)
with ui.HStack(height=0):
ui.Spacer()
ui.ImageWithProvider(
data_path('human_icon.png'),
width=192,
height=192,
fill_policy=ui.IwpFillPolicy.IWP_PRESERVE_ASPECT_FIT
)
ui.Spacer()
self._message_label = ui.Label(
"No human is current selected.",
height=0,
alignment=ui.Alignment.CENTER
)
self._suggestion_label = ui.Label(
"Select a human prim to see its properties here.",
height=0,
alignment=ui.Alignment.CENTER
)
@property
def visible(self) -> bool:
return self._container.visible
@visible.setter
def visible(self, value) -> None:
self._container.visible = value
def set_message(self, message: str) -> None:
messages = message.split("\n")
self._message_label.text = messages[0]
self._suggestion_label.text = messages[1]
def modifier_image(name : str):
"""Guess the path to a modifier's corresponding image on disk based on the name
of the modifier. Useful for building UI for list of modifiers.
Parameters
----------
name : str
Name of the modifier
Returns
-------
str
The path to the image on disk
"""
if name is None:
# If no modifier name is provided, we can't guess the file name
return None
name = name.lower()
# Return the modifier path based on the modifier name
# TODO determine if images can be loaded from the Makehuman module stored in
# site-packages so we don't have to include the data twice
return os.path.join(os.path.dirname(inspect.getfile(makehuman)),targets.getTargets().images.get(name, name))
| 22,469 | Python | 35.359223 | 119 | 0.582936 |
cadop/HumanGenerator/exts/siborg.create.human/siborg/create/human/shared.py | from pathlib import Path
import os
# Shared methods that are useful to several modules
def data_path(path):
"""Returns the absolute path of a path given relative to "exts/<omni.ext>/data"
Parameters
----------
path : str
Relative path
Returns
-------
str
Absolute path
"""
# Uses an absolute path, and then works its way up the folder directory to find the data folder
data = os.path.join(str(Path(__file__).parents[3]), "data", path)
return data
def sanitize(s: str):
"""Sanitize strings for use a prim names. Strips and replaces illegal
characters.
Parameters
----------
s : str
Input string
Returns
-------
s : str
Primpath-safe output string
"""
# List of illegal characters
# TODO create more comprehensive list
# TODO switch from blacklisting illegal characters to whitelisting valid ones
illegal = (".", "-")
for c in illegal:
# Replace illegal characters with underscores
s = s.replace(c, "_")
return s
| 1,072 | Python | 21.829787 | 99 | 0.613806 |
cadop/HumanGenerator/exts/siborg.create.human/siborg/create/human/window.py | from .ext_ui import ParamPanelModel, ParamPanel, NoSelectionNotification
from .browser import MHAssetBrowserModel, AssetBrowserFrame
from .human import Human
from .mhcaller import MHCaller
from .styles import window_style, button_style
import omni.ui as ui
import omni.kit.ui
import omni
import carb
WINDOW_TITLE = "Human Generator"
MENU_PATH = f"Window/{WINDOW_TITLE}"
class MHWindow(ui.Window):
"""
Main UI window. Contains all UI widgets. Extends omni.ui.Window.
Attributes
-----------
panel : HumanPanel
A widget that includes panels for modifiers, listing/removing applied
proxies, and executing human creation and updates
browser: AssetBrowserFrame
A browser for MakeHuman assets, including clothing, hair, and skeleton rigs.
"""
def __init__(self, title):
"""Constructs an instance of MHWindow
Parameters
----------
menu_path : str
The path to the menu item that opens the window
"""
super().__init__(title)
# Holds the state of the realtime toggle
self.toggle_model = ui.SimpleBoolModel()
# Holds the state of the parameter list
self.param_model = ParamPanelModel(self.toggle_model)
# Keep track of the human
self._human = Human()
# A model to hold browser data
self.browser_model = MHAssetBrowserModel(
self._human,
filter_file_suffixes=["mhpxy", "mhskel", "mhclo"],
timeout=carb.settings.get_settings().get(
"/exts/siborg.create.human.browser.asset/data/timeout"
),
)
# Subscribe to selection events on the message bus
bus = omni.kit.app.get_app().get_message_bus_event_stream()
selection_event = carb.events.type_from_string("siborg.create.human.human_selected")
self._selection_sub = bus.create_subscription_to_push_by_type(selection_event, self._on_selection_changed)
self.frame.set_build_fn(self._build_ui)
def _build_ui(self):
spacer_width = 3
with self.frame:
# Widgets are built starting on the left
with ui.HStack(style=window_style):
# Widget to show if no human is selected
self.no_selection_notification = NoSelectionNotification()
self.property_panel = ui.HStack(visible=False)
with self.property_panel:
with ui.ZStack(width=0):
# Draggable splitter
with ui.Placer(offset_x=self.frame.computed_content_width/1.8, draggable=True, drag_axis=ui.Axis.X):
ui.Rectangle(width=spacer_width, name="splitter")
with ui.HStack():
# Left-most panel is a browser for MakeHuman assets. It includes
# a reference to the list of applied proxies so that an update
# can be triggered when new assets are added
self.browser = AssetBrowserFrame(self.browser_model)
ui.Spacer(width=spacer_width)
with ui.HStack():
with ui.VStack():
self.param_panel = ParamPanel(self.param_model,self.update_human)
with ui.HStack(height=0):
# Toggle whether changes should propagate instantly
ui.ToolButton(text = "Update Instantly", model = self.toggle_model)
with ui.VStack(width = 100, style=button_style):
# Creates a new human in scene and resets modifiers and assets
ui.Button(
"New Human",
clicked_fn=self.new_human,
)
# Updates current human in omniverse scene
self.update_button = ui.Button(
"Update Human",
clicked_fn=self.update_human,
enabled=False,
)
# Resets modifiers and assets on selected human
self.reset_button = ui.Button(
"Reset Human",
clicked_fn=self.reset_human,
enabled=False,
)
def _on_selection_changed(self, event):
"""Callback for human selection events
Parameters
----------
event : carb.events.Event
The event that was pushed to the event stream. Contains payload data with
the selected prim path, or "None" if no human is selected
"""
# Get the stage
stage = omni.usd.get_context().get_stage()
prim_path = event.payload["prim_path"]
# If a valid human prim is selected,
if not prim_path or not stage.GetPrimAtPath(prim_path):
# Hide the property panel
self.property_panel.visible = False
# Show the no selection notification
self.no_selection_notification.visible = True
# Deactivate the update and reset buttons
self.update_button.enabled = False
self.reset_button.enabled = False
else:
# Show the property panel
self.property_panel.visible = True
# Hide the no selection notification
self.no_selection_notification.visible = False
# Activate the update and reset buttons
self.update_button.enabled = True
self.reset_button.enabled = True
# Get the prim from the path in the event payload
prim = stage.GetPrimAtPath(prim_path)
# Update the human in MHCaller
self._human.set_prim(prim)
# Update the list of applied modifiers
self.param_panel.load_values(prim)
def new_human(self):
"""Creates a new human in the scene and selects it"""
# Reset the human class
self._human.reset()
# Create a new human
self._human.prim = self._human.add_to_scene()
# Get selection.
selection = omni.usd.get_context().get_selection()
# Select the new human.
selection.set_selected_prim_paths([self._human.prim_path], True)
def update_human(self):
"""Updates the current human in the scene"""
# Collect changed values from the parameter panel
self.param_panel.update_models()
# Update the human in the scene
self._human.update_in_scene(self._human.prim_path)
def reset_human(self):
"""Resets the current human in the scene"""
# Reset the human
self._human.reset()
# Delete the proxy prims
self._human.delete_proxies()
# Update the human in the scene and reset parameter widgets
self.update_human()
def destroy(self):
"""Called when the window is destroyed. Unsuscribes from human selection events"""
self._selection_sub.unsubscribe()
self._selection_sub = None
super().destroy()
| 7,214 | Python | 36 | 124 | 0.570834 |
cadop/HumanGenerator/exts/siborg.create.human/siborg/create/human/browser/delegate.py | import carb
from omni.kit.browser.folder.core.models.folder_browser_item import FileDetailItem
import omni.ui as ui
import omni.kit.app
from omni.kit.browser.core import get_legacy_viewport_interface
from omni.kit.browser.folder.core import FolderDetailDelegate
from .model import MHAssetBrowserModel, AssetDetailItem
from ..mhcaller import MHCaller
import asyncio
from pathlib import Path
from typing import Optional
# TODO remove unused imports
# TODO remove
CURRENT_PATH = Path(__file__).parent
ICON_PATH = CURRENT_PATH.parent.parent.parent.parent.joinpath("icons")
class AssetDetailDelegate(FolderDetailDelegate):
"""Delegate to show Makehuman asset item in detail view and execute drag-and-
drop and doubleclick behavior.
Attributes
----------
model : MHAssetBrowserModel
Model that stores AssetBrowser data
"""
def __init__(self, model: MHAssetBrowserModel):
"""Constructs an instance of AssetDetailDelegate, which handles
execution of functions
Parameters
----------
model : MHAssetBrowserModel
Makehuman asset browser model
"""
super().__init__(model=model)
# Reference to the browser asset model
self.model = model
# Reference to the human
self._human = model.human
self._settings = carb.settings.get_settings()
# The context menu that opens on right_click
self._context_menu: Optional[ui.Menu] = None
self._action_item: Optional[AssetDetailItem] = None
self._viewport = None
self._drop_helper = None
def destroy(self):
"""Destructor for AssetDetailDelegate. Removes references and destroys superclass."""
self._viewport = None
self._drop_helper = None
super().destroy()
def get_thumbnail(self, item : AssetDetailItem) -> str:
"""Get the thumbnail for an asset
Parameters
----------
item : AssetDetailItem
The item in the browser for which we are getting a thumbnail
Returns
-------
str
Path to the thumbnail image
"""
return item.thumbnail
def on_drag(self, item: AssetDetailItem) -> str:
"""Displays a translucent UI widget when an asset is dragged
Parameters
----------
item : AssetDetailItem
The item being dragged
Returns
-------
str
The path on disk of the item being dragged (passed to whatever widget
accepts the drop)
"""
thumbnail = self.get_thumbnail(item)
icon_size = 128
with ui.VStack(width=icon_size):
if thumbnail:
ui.Spacer(height=2)
with ui.HStack():
ui.Spacer()
ui.ImageWithProvider(
thumbnail, width=icon_size, height=icon_size
)
ui.Spacer()
ui.Label(
item.name,
word_wrap=False,
elided_text=True,
skip_draw_when_clipped=True,
alignment=ui.Alignment.TOP,
style_type_name_override="GridView.Item",
)
# Return the path of the item being dragged so it can be accessed by
# the widget on which it is dropped
return item.url
def on_double_click(self, item: FileDetailItem):
"""Method to execute when an asset is doubleclicked. Adds the asset to the
human.
Parameters
----------
item : FileDetailItem
The item that has been doubleclicked
"""
self._human.add_item(item.url)
| 3,725 | Python | 30.05 | 93 | 0.595973 |
cadop/HumanGenerator/exts/siborg.create.human/siborg/create/human/browser/downloader.py | from typing import Callable
import carb
import aiohttp
import omni.client
import os, zipfile
class Downloader:
"""Downloads and unzips remote files and tracks download status/progress"""
def __init__(self, log_fn : Callable[[float], None]) -> None:
"""Construct an instance of Downloader. Assigns the logging function and sets initial is_downloading status
Parameters
----------
log_fn : Callable[[float], None]
Function to which to pass progress. Recieves a proportion that represents the amount downloaded
"""
self._is_downloading = False
self._log_fn = log_fn
async def download(self, url : str, dest_url : str) -> None:
"""Download a given url to disk and unzip it
Parameters
----------
url : str
Remote URL to fetch
dest_url : str
Local path at which to write and then unzip the downloaded files
Returns
-------
dict of str, Union[omni.client.Result, str]
Error message and location on disk
"""
ret_value = {"url": None}
async with aiohttp.ClientSession() as session:
self._is_downloading = True
content = bytearray()
# Download content from the given url
downloaded = 0
async with session.get(url) as response:
size = int(response.headers.get("content-length", 0))
if size > 0:
async for chunk in response.content.iter_chunked(1024 * 512):
content.extend(chunk)
downloaded += len(chunk)
if self._log_fn:
self._log_fn(float(downloaded) / size)
else:
if self._log_fn:
self._log_fn(0)
content = await response.read()
if self._log_fn:
self._log_fn(1)
if response.ok:
# Write to destination
filename = os.path.basename(url.split("?")[0])
dest_url = f"{dest_url}/{filename}"
(result, list_entry) = await omni.client.stat_async(dest_url)
ret_value["status"] = await omni.client.write_file_async(dest_url, content)
ret_value["url"] = dest_url
if ret_value["status"] == omni.client.Result.OK:
# TODO handle file already exists
pass
z = zipfile.ZipFile(dest_url, 'r')
z.extractall(os.path.dirname(dest_url))
else:
carb.log_error(f"[access denied: {url}")
ret_value["status"] = omni.client.Result.ERROR_ACCESS_DENIED
self._is_downloading = False
return ret_value
def not_downloading(self):
return not self._is_downloading | 2,935 | Python | 37.12987 | 115 | 0.529131 |
cadop/HumanGenerator/exts/siborg.create.human/siborg/create/human/browser/__init__.py | from omni.kit.browser.folder.core import FolderBrowserWidget
from .delegate import AssetDetailDelegate
from .model import MHAssetBrowserModel
from .options_menu import FolderOptionsMenu
import omni.ui as ui
class AssetBrowserFrame:
"""A widget to browse and select Makehuman assets
Attributes
----------
mhcaller : MHCaller
Wrapper object for Makehuman functions
"""
def __init__(self, model: MHAssetBrowserModel, **kwargs):
"""Constructs an instance of AssetBrowserFrame. This is a browser that
displays available Makehuman assets (skeletons/rigs, proxies) and allows
a user to apply them to the human.
Parameters
----------
model : MHAssetBrowserModel
A model to hold browser data
"""
self.model = model
self.build_widget()
def build_widget(self):
"""Build UI widget"""
# The delegate to execute browser actions
self._delegate = AssetDetailDelegate(self.model)
# Drop down menu to hold options
self._options_menu = FolderOptionsMenu()
with ui.VStack():
self._widget = FolderBrowserWidget(
self.model, detail_delegate=self._delegate, options_menu=self._options_menu)
ui.Separator(height=2)
# Progress bar to show download progress (initially hidden)
self._progress_bar = ui.ProgressBar(height=20, visible=False)
self._options_menu.bind_progress_bar(self._progress_bar)
| 1,520 | Python | 34.372092 | 92 | 0.653289 |
cadop/HumanGenerator/exts/siborg.create.human/siborg/create/human/browser/model.py | import os
from typing import List, Union
import carb.settings
import omni.kit.commands
from siborg.create.human.mhcaller import MHCaller
import omni.usd
from omni.kit.browser.core import DetailItem
from omni.kit.browser.folder.core import (
FolderBrowserModel,
FileDetailItem,
BrowserFile,
)
from siborg.create.human.shared import data_path
from ..human import Human
class AssetDetailItem(FileDetailItem):
"""Represents Makehuman asset detail item
"""
def __init__(self, file: BrowserFile):
"""Constructs an instance of AssetDetailItem
Parameters
----------
file : BrowserFile
BrowserFile object from which to create detail item
"""
dirs = file.url.split("/")
name = dirs[-1]
super().__init__(name, file.url, file, file.thumbnail)
class MHAssetBrowserModel(FolderBrowserModel):
"""Represents Makehuman asset browser model
"""
def __init__(self, human: Human, *args, **kwargs):
"""Constructs an instance of MHAssetBrowserModel
Parameters
----------
human : Human
The human to which to add assets
"""
self.human = human
super().__init__(
*args,
show_category_subfolders=True,
hide_file_without_thumbnails=False,
**kwargs,
)
# Add the data path as the root folder from which to build a collection
super().append_root_folder(data_path(""), name="MakeHuman")
def create_detail_item(
self, file: BrowserFile
) -> Union[FileDetailItem, List[FileDetailItem]]:
"""Create detail item(s) from a file.
A file may include multiple detail items.
Overwrite parent function to add thumbnails.
Parameters
----------
file : BrowserFile
File object to create detail item(s)
Returns
-------
Union[FileDetailItem, List[FileDetailItem]]
FileDetailItem or list of items created from file
"""
dirs = file.url.split("/")
name = dirs[-1]
# Get the file name without the extension
filename_noext = os.path.splitext(file.url)[0]
thumb = filename_noext + ".thumb"
thumb_png = filename_noext + ".png"
# If there is already a PNG, get it. If not, rename the thumb file to a PNG
# (They are the same format just with different extensions). This lets us
# use Makehuman's asset thumbnails
if os.path.exists(thumb_png):
thumb = thumb_png
elif os.path.exists(thumb):
os.rename(thumb, thumb_png)
thumb = thumb_png
else:
thumb = None
return FileDetailItem(name, file.url, file, thumb)
| 2,789 | Python | 28.0625 | 83 | 0.603801 |
cadop/HumanGenerator/exts/siborg.create.human/siborg/create/human/browser/options_menu.py | from omni.kit.browser.core import OptionMenuDescription, OptionsMenu
from omni.kit.browser.folder.core.models.folder_browser_item import FolderCollectionItem
import carb
import asyncio
from ..shared import data_path
from .downloader import Downloader
import omni.ui as ui
class FolderOptionsMenu(OptionsMenu):
"""
Represent options menu used in material browser.
"""
def __init__(self):
super().__init__()
# Progress bar widget to show download progress
self._progress_bar : ui.ProgressBar = None
self.downloader = Downloader(self.progress_fn,)
self._download_menu_desc = OptionMenuDescription(
"Download Assets",
clicked_fn=self._on_download_assets,
get_text_fn=self._get_menu_item_text,
enabled_fn=self.downloader.not_downloading
)
self.append_menu_item(self._download_menu_desc)
def destroy(self) -> None:
super().destroy()
def progress_fn(self, proportion: float):
carb.log_info(f"Download is {int(proportion * 100)}% done")
if self._progress_bar:
self._progress_bar.model.set_value(proportion)
def _get_menu_item_text(self) -> str:
# Show download state if download starts
if self.downloader._is_downloading:
return "Download In Progress"
return "Download Assets"
def bind_progress_bar(self, progress_bar):
self._progress_bar = progress_bar
def _on_download_assets(self):
# Show progress bar
if self._progress_bar:
self._progress_bar.visible = True
loop = asyncio.get_event_loop()
asyncio.run_coroutine_threadsafe(self._download(), loop)
def _is_remove_collection_enabled(self) -> None:
'''Don't allow removing the default collection'''
if self._browser_widget is not None:
return self._browser_widget.collection_index >= 1
else:
return False
def _on_remove_collection(self) -> None:
if self._browser_widget is None or self._browser_widget.collection_index < 0:
return
else:
browser_model = self._browser_widget.model
collection_items = browser_model.get_collection_items()
if browser_model.remove_collection(collection_items[self._browser_widget.collection_index]):
# Update collection combobox and default none selected
browser_model._item_changed(None)
self._browser_widget.collection_index -= 1
def _hide_progress_bar(self):
if self._progress_bar:
self._progress_bar.visible = False
async def _download(self):
# Makehuman system assets
url = "http://files.makehumancommunity.org/asset_packs/makehuman_system_assets/makehuman_system_assets_cc0.zip"
# Smaller zip for testing
# url = "https://download.tuxfamily.org/makehuman/asset_packs/shirts03/shirts03_ccby.zip"
dest_url = data_path("")
await self.downloader.download(url, dest_url)
self._hide_progress_bar()
self.refresh_collection()
def refresh_collection(self):
collection_item: FolderCollectionItem = self._browser_widget.collection_selection
if collection_item:
folder = collection_item.folder
folder._timeout = 10
asyncio.ensure_future(folder.start_traverse())
| 3,422 | Python | 37.033333 | 119 | 0.643776 |