entities
listlengths 1
2.22k
| max_stars_repo_path
stringlengths 6
98
| max_stars_repo_name
stringlengths 9
55
| max_stars_count
int64 0
8.27k
| content
stringlengths 93
965k
| id
stringlengths 2
5
| new_content
stringlengths 85
964k
| modified
bool 1
class | references
stringlengths 98
970k
| language
stringclasses 1
value |
---|---|---|---|---|---|---|---|---|---|
[
{
"context": " getDeviceList \n , clientId =\n \"cfe2208fcad346cda755e6f65a7c171e\"\n , scope =\n [\"user-read-playback-state",
"end": 1599,
"score": 0.9840475917,
"start": 1567,
"tag": "KEY",
"value": "cfe2208fcad346cda755e6f65a7c171e"
}
] | src/Main.elm | superechnik/SpotifyConnectionVerifier | 0 | {-
the majority of the auth code in this file
was pulled off the oath2 package demo
github with little alteration
-}
port module Main exposing (main)
import Base64.Encode as Base64
import Browser exposing (Document, application)
import Browser.Navigation as Navigation exposing (Key)
import Delay exposing (TimeUnit(..), after)
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (..)
import Http
import Json.Decode as Json
import OAuth
import OAuth.Implicit as OAuth
import Url exposing (Protocol(..), Url)
import Json.Decode exposing (Decoder)
import Json.Decode exposing (map7)
import Helpers exposing (..)
type alias FlagObject =
Maybe (List Int)
main : Program FlagObject Model Msg
main =
application
{ init =
Maybe.map convertBytes >> init
, update =
update
, subscriptions =
always <| randomBytes GotRandomBytes
, onUrlRequest =
always NoOp
, onUrlChange =
always NoOp
, view =
view
{ title = "SpotifyConnectionVerifier"
, btnClass = class "CTA__secondary--FVl7a"
}
}
configuration : Configuration
configuration =
{ authorizationEndpoint =
{ defaultHttpsUrl | host = "accounts.spotify.com", path = "/authorize" }
, accountPlayerEndpoint =
{ defaultHttpsUrl | host = "api.spotify.com", path = "/v1/me/player/devices" }
, accountPlayerDecoder =
getDeviceList
, clientId =
"cfe2208fcad346cda755e6f65a7c171e"
, scope =
["user-read-playback-state"]
}
--decoder for spotify devices
decodeDeviceList : Decoder AccountPlayer
decodeDeviceList =
Json.map7
AccountPlayer
(Json.Decode.at ["id"] Json.string)
(Json.Decode.at ["is_active"] Json.bool)
(Json.Decode.at ["is_private_session"] Json.bool)
(Json.Decode.at ["is_restricted"] Json.bool)
(Json.Decode.at ["name"] Json.string)
(Json.Decode.at ["type"] Json.string)
(Json.Decode.at ["volume_percent"] Json.int)
getDeviceList : Decoder (List AccountPlayer)
getDeviceList =
Json.Decode.at ["devices"] (Json.Decode.list decodeDeviceList)
--
-- Model
--
type alias Model =
{ redirectUri : Url
, flow : Flow
}
type Flow
= Idle
| Authorized OAuth.Token
| Done (List AccountPlayer)
| Errored Error
type Error
= ErrStateMismatch
| ErrAuthorization OAuth.AuthorizationError
| ErrHTTPGetAccountPlayerInfo
type alias UserInfo =
{ name : String}
type alias AccountPlayer =
{id: String
,is_active: Bool
,is_private_session: Bool
,is_restricted: Bool
,name: String
,playerType: String
,volume_percent: Int
}
type alias Configuration =
{ authorizationEndpoint : Url
, accountPlayerEndpoint : Url
, accountPlayerDecoder : Json.Decoder (List AccountPlayer)
, clientId : String
, scope : List String
}
init : Maybe { state : String } -> Url -> Key -> ( Model, Cmd Msg )
init mflags origin navigationKey =
let
redirectUri =
{ origin | query = Nothing, fragment = Nothing }
clearUrl =
Navigation.replaceUrl navigationKey (Url.toString redirectUri)
in
case OAuth.parseToken origin of
OAuth.Empty ->
( { flow = Idle, redirectUri = redirectUri }
, Cmd.none
)
OAuth.Success { token, state } ->
case mflags of
Nothing ->
( { flow = Errored ErrStateMismatch, redirectUri = redirectUri }
, clearUrl
)
Just flags ->
if state /= Just flags.state then
( { flow = Errored ErrStateMismatch, redirectUri = redirectUri }
, clearUrl
)
else
( { flow = Authorized token, redirectUri = redirectUri }
, Cmd.batch
[ after 0 Millisecond AccountPlayerInfoRequested
, clearUrl
]
)
OAuth.Error error ->
( { flow = Errored <| ErrAuthorization error, redirectUri = redirectUri }
, clearUrl
)
--
-- Msg
--
type Msg
= NoOp
| SignInRequested
| GotRandomBytes (List Int)
| AccountPlayerInfoRequested
| GotAccountPlayerInfo (Result Http.Error (List AccountPlayer))
| SignOutRequested
getAccountPlayers : Configuration -> OAuth.Token -> Cmd Msg
getAccountPlayers {accountPlayerDecoder, accountPlayerEndpoint} token =
Http.request
{ method = "GET"
, body = Http.emptyBody
, headers = OAuth.useToken token []
, url = Url.toString accountPlayerEndpoint
, expect = Http.expectJson GotAccountPlayerInfo accountPlayerDecoder
, timeout = Nothing
, tracker = Nothing
}
port genRandomBytes : Int -> Cmd msg
port randomBytes : (List Int -> msg) -> Sub msg
--
-- Update
--
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case ( model.flow, msg ) of
( Idle, SignInRequested ) ->
signInRequested model
( Idle, GotRandomBytes bytes ) ->
gotRandomBytes model bytes
( Authorized token, AccountPlayerInfoRequested) ->
accountPlayerInfoRequested model token
( Authorized _, GotAccountPlayerInfo accountPlayerResponse) ->
gotAccountPlayerInfo model accountPlayerResponse
( Done _, SignOutRequested ) ->
signOutRequested model
_ ->
noOp model
noOp : Model -> ( Model, Cmd Msg )
noOp model =
( model, Cmd.none )
signInRequested : Model -> ( Model, Cmd Msg )
signInRequested model =
( { model | flow = Idle }
, genRandomBytes 16
)
gotRandomBytes : Model -> List Int -> ( Model, Cmd Msg )
gotRandomBytes model bytes =
let
{ state } =
convertBytes bytes
authorization =
{ clientId = configuration.clientId
, redirectUri = model.redirectUri
, scope = configuration.scope
, state = Just state
, url = configuration.authorizationEndpoint
}
in
( { model | flow = Idle }
, authorization
|> OAuth.makeAuthorizationUrl
|> Url.toString
|> Navigation.load
)
accountPlayerInfoRequested : Model -> OAuth.Token -> (Model, Cmd Msg)
accountPlayerInfoRequested model token =
( {model | flow = Authorized token}
, getAccountPlayers configuration token
)
gotAccountPlayerInfo : Model -> Result Http.Error (List AccountPlayer) -> (Model, Cmd Msg)
gotAccountPlayerInfo model accountPlayerResponse =
case accountPlayerResponse of
Err _ ->
(
{ model | flow = Errored ErrHTTPGetAccountPlayerInfo }
, Cmd.none
)
Ok accountPlayer ->
({ model | flow = Done accountPlayer }
, Cmd.none
)
signOutRequested : Model -> ( Model, Cmd Msg )
signOutRequested model =
( { model | flow = Idle }
, Navigation.load (Url.toString model.redirectUri)
)
--
-- View
--
type alias ViewConfiguration msg =
{ title : String
, btnClass : Attribute msg
}
view : ViewConfiguration Msg -> Model -> Document Msg
view ({ title } as config) model =
{ title = title
, body = viewBody config model
}
viewBody : ViewConfiguration Msg -> Model -> List (Html Msg)
viewBody config model =
[ div [ class "flex", class "flex-column", class "flex-space-around"] <|
case model.flow of
Idle ->
div [ class "flex" ]
[ viewAuthorizationStep False
, viewStepSeparator False
]
:: viewIdle config
Authorized _ ->
div [ class "flex" ]
[ viewAuthorizationStep True
, viewStepSeparator True
]
:: viewAuthorized
Done accountPlayerInfo ->
div [ class "flex"]
[ viewAuthorizationStep True
, viewStepSeparator True
, viewGetAccountPlayerInfoStep True
]
:: viewAccountPlayerInfo config accountPlayerInfo
Errored err ->
div [ class "flex" ]
[ viewErroredStep
]
:: viewErrored err
]
viewIdle : ViewConfiguration Msg -> List (Html Msg)
viewIdle { btnClass } =
[ button
[ onClick SignInRequested, btnClass ]
[ text "Sign in" ]
]
viewAuthorized : List (Html Msg)
viewAuthorized =
[ span [] [ text "Getting info..." ]
]
viewAccountPlayerInfo : ViewConfiguration Msg -> (List AccountPlayer) -> List (Html Msg)
viewAccountPlayerInfo {btnClass } ls =
[ div [class "flex", class "flex-column"]
[ul []
(List.map (\l -> li [] [(text l.id),(text ", "),(text l.name),(text ", "),(text (boolToString l.is_active)) ]) ls)
, div []
[ button
[ onClick SignOutRequested, btnClass ]
[ text "Sign out" ]
]
]
]
viewErrored : Error -> List (Html Msg)
viewErrored error =
[ span [ class "span-error" ] [ viewError error ] ]
viewError : Error -> Html Msg
viewError e =
text <|
case e of
ErrStateMismatch ->
"'state' doesn't match, the request has likely been forged by an adversary!"
ErrAuthorization error ->
oauthErrorToString { error = error.error, errorDescription = error.errorDescription }
ErrHTTPGetAccountPlayerInfo ->
"Unable to retrive account player info: HTTP request failed."
viewAuthorizationStep : Bool -> Html Msg
viewAuthorizationStep isActive =
viewStep isActive ( "Authorization", style "left" "-110%" )
viewGetAccountPlayerInfoStep : Bool -> Html Msg
viewGetAccountPlayerInfoStep isActive =
viewStep isActive ("Get Account Device Info", style "left" "-135%")
viewErroredStep : Html Msg
viewErroredStep =
div
[ class "step", class "step-errored" ]
[ span [ style "left" "-50%" ] [ text "Errored" ] ]
viewStep : Bool -> ( String, Attribute Msg ) -> Html Msg
viewStep isActive ( step, position ) =
let
stepClass =
class "step"
:: (if isActive then
[ class "step-active" ]
else
[]
)
in
div stepClass [ span [ position ] [ text step ] ]
viewStepSeparator : Bool -> Html Msg
viewStepSeparator isActive =
let
stepClass =
class "step-separator"
:: (if isActive then
[ class "step-active" ]
else
[]
)
in
span stepClass [] | 34135 | {-
the majority of the auth code in this file
was pulled off the oath2 package demo
github with little alteration
-}
port module Main exposing (main)
import Base64.Encode as Base64
import Browser exposing (Document, application)
import Browser.Navigation as Navigation exposing (Key)
import Delay exposing (TimeUnit(..), after)
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (..)
import Http
import Json.Decode as Json
import OAuth
import OAuth.Implicit as OAuth
import Url exposing (Protocol(..), Url)
import Json.Decode exposing (Decoder)
import Json.Decode exposing (map7)
import Helpers exposing (..)
type alias FlagObject =
Maybe (List Int)
main : Program FlagObject Model Msg
main =
application
{ init =
Maybe.map convertBytes >> init
, update =
update
, subscriptions =
always <| randomBytes GotRandomBytes
, onUrlRequest =
always NoOp
, onUrlChange =
always NoOp
, view =
view
{ title = "SpotifyConnectionVerifier"
, btnClass = class "CTA__secondary--FVl7a"
}
}
configuration : Configuration
configuration =
{ authorizationEndpoint =
{ defaultHttpsUrl | host = "accounts.spotify.com", path = "/authorize" }
, accountPlayerEndpoint =
{ defaultHttpsUrl | host = "api.spotify.com", path = "/v1/me/player/devices" }
, accountPlayerDecoder =
getDeviceList
, clientId =
"<KEY>"
, scope =
["user-read-playback-state"]
}
--decoder for spotify devices
decodeDeviceList : Decoder AccountPlayer
decodeDeviceList =
Json.map7
AccountPlayer
(Json.Decode.at ["id"] Json.string)
(Json.Decode.at ["is_active"] Json.bool)
(Json.Decode.at ["is_private_session"] Json.bool)
(Json.Decode.at ["is_restricted"] Json.bool)
(Json.Decode.at ["name"] Json.string)
(Json.Decode.at ["type"] Json.string)
(Json.Decode.at ["volume_percent"] Json.int)
getDeviceList : Decoder (List AccountPlayer)
getDeviceList =
Json.Decode.at ["devices"] (Json.Decode.list decodeDeviceList)
--
-- Model
--
type alias Model =
{ redirectUri : Url
, flow : Flow
}
type Flow
= Idle
| Authorized OAuth.Token
| Done (List AccountPlayer)
| Errored Error
type Error
= ErrStateMismatch
| ErrAuthorization OAuth.AuthorizationError
| ErrHTTPGetAccountPlayerInfo
type alias UserInfo =
{ name : String}
type alias AccountPlayer =
{id: String
,is_active: Bool
,is_private_session: Bool
,is_restricted: Bool
,name: String
,playerType: String
,volume_percent: Int
}
type alias Configuration =
{ authorizationEndpoint : Url
, accountPlayerEndpoint : Url
, accountPlayerDecoder : Json.Decoder (List AccountPlayer)
, clientId : String
, scope : List String
}
init : Maybe { state : String } -> Url -> Key -> ( Model, Cmd Msg )
init mflags origin navigationKey =
let
redirectUri =
{ origin | query = Nothing, fragment = Nothing }
clearUrl =
Navigation.replaceUrl navigationKey (Url.toString redirectUri)
in
case OAuth.parseToken origin of
OAuth.Empty ->
( { flow = Idle, redirectUri = redirectUri }
, Cmd.none
)
OAuth.Success { token, state } ->
case mflags of
Nothing ->
( { flow = Errored ErrStateMismatch, redirectUri = redirectUri }
, clearUrl
)
Just flags ->
if state /= Just flags.state then
( { flow = Errored ErrStateMismatch, redirectUri = redirectUri }
, clearUrl
)
else
( { flow = Authorized token, redirectUri = redirectUri }
, Cmd.batch
[ after 0 Millisecond AccountPlayerInfoRequested
, clearUrl
]
)
OAuth.Error error ->
( { flow = Errored <| ErrAuthorization error, redirectUri = redirectUri }
, clearUrl
)
--
-- Msg
--
type Msg
= NoOp
| SignInRequested
| GotRandomBytes (List Int)
| AccountPlayerInfoRequested
| GotAccountPlayerInfo (Result Http.Error (List AccountPlayer))
| SignOutRequested
getAccountPlayers : Configuration -> OAuth.Token -> Cmd Msg
getAccountPlayers {accountPlayerDecoder, accountPlayerEndpoint} token =
Http.request
{ method = "GET"
, body = Http.emptyBody
, headers = OAuth.useToken token []
, url = Url.toString accountPlayerEndpoint
, expect = Http.expectJson GotAccountPlayerInfo accountPlayerDecoder
, timeout = Nothing
, tracker = Nothing
}
port genRandomBytes : Int -> Cmd msg
port randomBytes : (List Int -> msg) -> Sub msg
--
-- Update
--
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case ( model.flow, msg ) of
( Idle, SignInRequested ) ->
signInRequested model
( Idle, GotRandomBytes bytes ) ->
gotRandomBytes model bytes
( Authorized token, AccountPlayerInfoRequested) ->
accountPlayerInfoRequested model token
( Authorized _, GotAccountPlayerInfo accountPlayerResponse) ->
gotAccountPlayerInfo model accountPlayerResponse
( Done _, SignOutRequested ) ->
signOutRequested model
_ ->
noOp model
noOp : Model -> ( Model, Cmd Msg )
noOp model =
( model, Cmd.none )
signInRequested : Model -> ( Model, Cmd Msg )
signInRequested model =
( { model | flow = Idle }
, genRandomBytes 16
)
gotRandomBytes : Model -> List Int -> ( Model, Cmd Msg )
gotRandomBytes model bytes =
let
{ state } =
convertBytes bytes
authorization =
{ clientId = configuration.clientId
, redirectUri = model.redirectUri
, scope = configuration.scope
, state = Just state
, url = configuration.authorizationEndpoint
}
in
( { model | flow = Idle }
, authorization
|> OAuth.makeAuthorizationUrl
|> Url.toString
|> Navigation.load
)
accountPlayerInfoRequested : Model -> OAuth.Token -> (Model, Cmd Msg)
accountPlayerInfoRequested model token =
( {model | flow = Authorized token}
, getAccountPlayers configuration token
)
gotAccountPlayerInfo : Model -> Result Http.Error (List AccountPlayer) -> (Model, Cmd Msg)
gotAccountPlayerInfo model accountPlayerResponse =
case accountPlayerResponse of
Err _ ->
(
{ model | flow = Errored ErrHTTPGetAccountPlayerInfo }
, Cmd.none
)
Ok accountPlayer ->
({ model | flow = Done accountPlayer }
, Cmd.none
)
signOutRequested : Model -> ( Model, Cmd Msg )
signOutRequested model =
( { model | flow = Idle }
, Navigation.load (Url.toString model.redirectUri)
)
--
-- View
--
type alias ViewConfiguration msg =
{ title : String
, btnClass : Attribute msg
}
view : ViewConfiguration Msg -> Model -> Document Msg
view ({ title } as config) model =
{ title = title
, body = viewBody config model
}
viewBody : ViewConfiguration Msg -> Model -> List (Html Msg)
viewBody config model =
[ div [ class "flex", class "flex-column", class "flex-space-around"] <|
case model.flow of
Idle ->
div [ class "flex" ]
[ viewAuthorizationStep False
, viewStepSeparator False
]
:: viewIdle config
Authorized _ ->
div [ class "flex" ]
[ viewAuthorizationStep True
, viewStepSeparator True
]
:: viewAuthorized
Done accountPlayerInfo ->
div [ class "flex"]
[ viewAuthorizationStep True
, viewStepSeparator True
, viewGetAccountPlayerInfoStep True
]
:: viewAccountPlayerInfo config accountPlayerInfo
Errored err ->
div [ class "flex" ]
[ viewErroredStep
]
:: viewErrored err
]
viewIdle : ViewConfiguration Msg -> List (Html Msg)
viewIdle { btnClass } =
[ button
[ onClick SignInRequested, btnClass ]
[ text "Sign in" ]
]
viewAuthorized : List (Html Msg)
viewAuthorized =
[ span [] [ text "Getting info..." ]
]
viewAccountPlayerInfo : ViewConfiguration Msg -> (List AccountPlayer) -> List (Html Msg)
viewAccountPlayerInfo {btnClass } ls =
[ div [class "flex", class "flex-column"]
[ul []
(List.map (\l -> li [] [(text l.id),(text ", "),(text l.name),(text ", "),(text (boolToString l.is_active)) ]) ls)
, div []
[ button
[ onClick SignOutRequested, btnClass ]
[ text "Sign out" ]
]
]
]
viewErrored : Error -> List (Html Msg)
viewErrored error =
[ span [ class "span-error" ] [ viewError error ] ]
viewError : Error -> Html Msg
viewError e =
text <|
case e of
ErrStateMismatch ->
"'state' doesn't match, the request has likely been forged by an adversary!"
ErrAuthorization error ->
oauthErrorToString { error = error.error, errorDescription = error.errorDescription }
ErrHTTPGetAccountPlayerInfo ->
"Unable to retrive account player info: HTTP request failed."
viewAuthorizationStep : Bool -> Html Msg
viewAuthorizationStep isActive =
viewStep isActive ( "Authorization", style "left" "-110%" )
viewGetAccountPlayerInfoStep : Bool -> Html Msg
viewGetAccountPlayerInfoStep isActive =
viewStep isActive ("Get Account Device Info", style "left" "-135%")
viewErroredStep : Html Msg
viewErroredStep =
div
[ class "step", class "step-errored" ]
[ span [ style "left" "-50%" ] [ text "Errored" ] ]
viewStep : Bool -> ( String, Attribute Msg ) -> Html Msg
viewStep isActive ( step, position ) =
let
stepClass =
class "step"
:: (if isActive then
[ class "step-active" ]
else
[]
)
in
div stepClass [ span [ position ] [ text step ] ]
viewStepSeparator : Bool -> Html Msg
viewStepSeparator isActive =
let
stepClass =
class "step-separator"
:: (if isActive then
[ class "step-active" ]
else
[]
)
in
span stepClass [] | true | {-
the majority of the auth code in this file
was pulled off the oath2 package demo
github with little alteration
-}
port module Main exposing (main)
import Base64.Encode as Base64
import Browser exposing (Document, application)
import Browser.Navigation as Navigation exposing (Key)
import Delay exposing (TimeUnit(..), after)
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (..)
import Http
import Json.Decode as Json
import OAuth
import OAuth.Implicit as OAuth
import Url exposing (Protocol(..), Url)
import Json.Decode exposing (Decoder)
import Json.Decode exposing (map7)
import Helpers exposing (..)
type alias FlagObject =
Maybe (List Int)
main : Program FlagObject Model Msg
main =
application
{ init =
Maybe.map convertBytes >> init
, update =
update
, subscriptions =
always <| randomBytes GotRandomBytes
, onUrlRequest =
always NoOp
, onUrlChange =
always NoOp
, view =
view
{ title = "SpotifyConnectionVerifier"
, btnClass = class "CTA__secondary--FVl7a"
}
}
configuration : Configuration
configuration =
{ authorizationEndpoint =
{ defaultHttpsUrl | host = "accounts.spotify.com", path = "/authorize" }
, accountPlayerEndpoint =
{ defaultHttpsUrl | host = "api.spotify.com", path = "/v1/me/player/devices" }
, accountPlayerDecoder =
getDeviceList
, clientId =
"PI:KEY:<KEY>END_PI"
, scope =
["user-read-playback-state"]
}
--decoder for spotify devices
decodeDeviceList : Decoder AccountPlayer
decodeDeviceList =
Json.map7
AccountPlayer
(Json.Decode.at ["id"] Json.string)
(Json.Decode.at ["is_active"] Json.bool)
(Json.Decode.at ["is_private_session"] Json.bool)
(Json.Decode.at ["is_restricted"] Json.bool)
(Json.Decode.at ["name"] Json.string)
(Json.Decode.at ["type"] Json.string)
(Json.Decode.at ["volume_percent"] Json.int)
getDeviceList : Decoder (List AccountPlayer)
getDeviceList =
Json.Decode.at ["devices"] (Json.Decode.list decodeDeviceList)
--
-- Model
--
type alias Model =
{ redirectUri : Url
, flow : Flow
}
type Flow
= Idle
| Authorized OAuth.Token
| Done (List AccountPlayer)
| Errored Error
type Error
= ErrStateMismatch
| ErrAuthorization OAuth.AuthorizationError
| ErrHTTPGetAccountPlayerInfo
type alias UserInfo =
{ name : String}
type alias AccountPlayer =
{id: String
,is_active: Bool
,is_private_session: Bool
,is_restricted: Bool
,name: String
,playerType: String
,volume_percent: Int
}
type alias Configuration =
{ authorizationEndpoint : Url
, accountPlayerEndpoint : Url
, accountPlayerDecoder : Json.Decoder (List AccountPlayer)
, clientId : String
, scope : List String
}
init : Maybe { state : String } -> Url -> Key -> ( Model, Cmd Msg )
init mflags origin navigationKey =
let
redirectUri =
{ origin | query = Nothing, fragment = Nothing }
clearUrl =
Navigation.replaceUrl navigationKey (Url.toString redirectUri)
in
case OAuth.parseToken origin of
OAuth.Empty ->
( { flow = Idle, redirectUri = redirectUri }
, Cmd.none
)
OAuth.Success { token, state } ->
case mflags of
Nothing ->
( { flow = Errored ErrStateMismatch, redirectUri = redirectUri }
, clearUrl
)
Just flags ->
if state /= Just flags.state then
( { flow = Errored ErrStateMismatch, redirectUri = redirectUri }
, clearUrl
)
else
( { flow = Authorized token, redirectUri = redirectUri }
, Cmd.batch
[ after 0 Millisecond AccountPlayerInfoRequested
, clearUrl
]
)
OAuth.Error error ->
( { flow = Errored <| ErrAuthorization error, redirectUri = redirectUri }
, clearUrl
)
--
-- Msg
--
type Msg
= NoOp
| SignInRequested
| GotRandomBytes (List Int)
| AccountPlayerInfoRequested
| GotAccountPlayerInfo (Result Http.Error (List AccountPlayer))
| SignOutRequested
getAccountPlayers : Configuration -> OAuth.Token -> Cmd Msg
getAccountPlayers {accountPlayerDecoder, accountPlayerEndpoint} token =
Http.request
{ method = "GET"
, body = Http.emptyBody
, headers = OAuth.useToken token []
, url = Url.toString accountPlayerEndpoint
, expect = Http.expectJson GotAccountPlayerInfo accountPlayerDecoder
, timeout = Nothing
, tracker = Nothing
}
port genRandomBytes : Int -> Cmd msg
port randomBytes : (List Int -> msg) -> Sub msg
--
-- Update
--
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case ( model.flow, msg ) of
( Idle, SignInRequested ) ->
signInRequested model
( Idle, GotRandomBytes bytes ) ->
gotRandomBytes model bytes
( Authorized token, AccountPlayerInfoRequested) ->
accountPlayerInfoRequested model token
( Authorized _, GotAccountPlayerInfo accountPlayerResponse) ->
gotAccountPlayerInfo model accountPlayerResponse
( Done _, SignOutRequested ) ->
signOutRequested model
_ ->
noOp model
noOp : Model -> ( Model, Cmd Msg )
noOp model =
( model, Cmd.none )
signInRequested : Model -> ( Model, Cmd Msg )
signInRequested model =
( { model | flow = Idle }
, genRandomBytes 16
)
gotRandomBytes : Model -> List Int -> ( Model, Cmd Msg )
gotRandomBytes model bytes =
let
{ state } =
convertBytes bytes
authorization =
{ clientId = configuration.clientId
, redirectUri = model.redirectUri
, scope = configuration.scope
, state = Just state
, url = configuration.authorizationEndpoint
}
in
( { model | flow = Idle }
, authorization
|> OAuth.makeAuthorizationUrl
|> Url.toString
|> Navigation.load
)
accountPlayerInfoRequested : Model -> OAuth.Token -> (Model, Cmd Msg)
accountPlayerInfoRequested model token =
( {model | flow = Authorized token}
, getAccountPlayers configuration token
)
gotAccountPlayerInfo : Model -> Result Http.Error (List AccountPlayer) -> (Model, Cmd Msg)
gotAccountPlayerInfo model accountPlayerResponse =
case accountPlayerResponse of
Err _ ->
(
{ model | flow = Errored ErrHTTPGetAccountPlayerInfo }
, Cmd.none
)
Ok accountPlayer ->
({ model | flow = Done accountPlayer }
, Cmd.none
)
signOutRequested : Model -> ( Model, Cmd Msg )
signOutRequested model =
( { model | flow = Idle }
, Navigation.load (Url.toString model.redirectUri)
)
--
-- View
--
type alias ViewConfiguration msg =
{ title : String
, btnClass : Attribute msg
}
view : ViewConfiguration Msg -> Model -> Document Msg
view ({ title } as config) model =
{ title = title
, body = viewBody config model
}
viewBody : ViewConfiguration Msg -> Model -> List (Html Msg)
viewBody config model =
[ div [ class "flex", class "flex-column", class "flex-space-around"] <|
case model.flow of
Idle ->
div [ class "flex" ]
[ viewAuthorizationStep False
, viewStepSeparator False
]
:: viewIdle config
Authorized _ ->
div [ class "flex" ]
[ viewAuthorizationStep True
, viewStepSeparator True
]
:: viewAuthorized
Done accountPlayerInfo ->
div [ class "flex"]
[ viewAuthorizationStep True
, viewStepSeparator True
, viewGetAccountPlayerInfoStep True
]
:: viewAccountPlayerInfo config accountPlayerInfo
Errored err ->
div [ class "flex" ]
[ viewErroredStep
]
:: viewErrored err
]
viewIdle : ViewConfiguration Msg -> List (Html Msg)
viewIdle { btnClass } =
[ button
[ onClick SignInRequested, btnClass ]
[ text "Sign in" ]
]
viewAuthorized : List (Html Msg)
viewAuthorized =
[ span [] [ text "Getting info..." ]
]
viewAccountPlayerInfo : ViewConfiguration Msg -> (List AccountPlayer) -> List (Html Msg)
viewAccountPlayerInfo {btnClass } ls =
[ div [class "flex", class "flex-column"]
[ul []
(List.map (\l -> li [] [(text l.id),(text ", "),(text l.name),(text ", "),(text (boolToString l.is_active)) ]) ls)
, div []
[ button
[ onClick SignOutRequested, btnClass ]
[ text "Sign out" ]
]
]
]
viewErrored : Error -> List (Html Msg)
viewErrored error =
[ span [ class "span-error" ] [ viewError error ] ]
viewError : Error -> Html Msg
viewError e =
text <|
case e of
ErrStateMismatch ->
"'state' doesn't match, the request has likely been forged by an adversary!"
ErrAuthorization error ->
oauthErrorToString { error = error.error, errorDescription = error.errorDescription }
ErrHTTPGetAccountPlayerInfo ->
"Unable to retrive account player info: HTTP request failed."
viewAuthorizationStep : Bool -> Html Msg
viewAuthorizationStep isActive =
viewStep isActive ( "Authorization", style "left" "-110%" )
viewGetAccountPlayerInfoStep : Bool -> Html Msg
viewGetAccountPlayerInfoStep isActive =
viewStep isActive ("Get Account Device Info", style "left" "-135%")
viewErroredStep : Html Msg
viewErroredStep =
div
[ class "step", class "step-errored" ]
[ span [ style "left" "-50%" ] [ text "Errored" ] ]
viewStep : Bool -> ( String, Attribute Msg ) -> Html Msg
viewStep isActive ( step, position ) =
let
stepClass =
class "step"
:: (if isActive then
[ class "step-active" ]
else
[]
)
in
div stepClass [ span [ position ] [ text step ] ]
viewStepSeparator : Bool -> Html Msg
viewStepSeparator isActive =
let
stepClass =
class "step-separator"
:: (if isActive then
[ class "step-active" ]
else
[]
)
in
span stepClass [] | elm |
[
{
"context": "\"\n , \"areolas,7667\"\n , \"ariane,2084\"\n , \"arielrebel,13053\"\n , \"asaakira,8006\"\n , \"AsianCumsluts",
"end": 836,
"score": 0.9745720625,
"start": 826,
"tag": "USERNAME",
"value": "arielrebel"
},
{
"context": "6\"\n , \"damngoodinterracial,14987\"\n , \"DanielleSharp,5570\"\n , \"DarkAngels,36217\"\n , \"DarkHumor,4",
"end": 4830,
"score": 0.6173806787,
"start": 4823,
"tag": "USERNAME",
"value": "leSharp"
},
{
"context": "liciousTraps,5631\"\n , \"DesiBoners,6455\"\n , \"DiamondJackson,841\"\n , \"DirtyFamilyPhotos,13784\"\n , \"dirty",
"end": 5083,
"score": 0.7945931554,
"start": 5069,
"tag": "NAME",
"value": "DiamondJackson"
},
{
"context": "als,32993\"\n , \"dirtypenpals,74555\"\n , \"dirtyr4r,40526\"\n , \"dirtysmall,136317\"\n , \"DirtySna",
"end": 5189,
"score": 0.5460226536,
"start": 5187,
"tag": "USERNAME",
"value": "r4"
},
{
"context": "penpals,74555\"\n , \"dirtyr4r,40526\"\n , \"dirtysmall,136317\"\n , \"DirtySnapchat,31502\"\n , \"disney",
"end": 5215,
"score": 0.5305256248,
"start": 5210,
"tag": "USERNAME",
"value": "small"
},
{
"context": "ysmall,136317\"\n , \"DirtySnapchat,31502\"\n , \"disneyporn,9475\"\n , \"distension,40247\"\n , \"doppelbangh",
"end": 5269,
"score": 0.6504744291,
"start": 5259,
"tag": "USERNAME",
"value": "disneyporn"
},
{
"context": "neyporn,9475\"\n , \"distension,40247\"\n , \"doppelbangher,26184\"\n , \"dota2smut,6949\"\n , \"doublepenetr",
"end": 5321,
"score": 0.6127782464,
"start": 5312,
"tag": "USERNAME",
"value": "elbangher"
},
{
"context": "ion,40247\"\n , \"doppelbangher,26184\"\n , \"dota2smut,6949\"\n , \"doublepenetration,8700\"\n , \"dou",
"end": 5343,
"score": 0.5421367884,
"start": 5340,
"tag": "USERNAME",
"value": "2sm"
},
{
"context": " , \"DownBra,9851\"\n , \"dreamjobs,13943\"\n , \"drunkencookery,12918\"\n , \"DSLs,9883\"\n , \"DumpsterSluts,202",
"end": 5498,
"score": 0.8725283146,
"start": 5485,
"tag": "USERNAME",
"value": "runkencookery"
},
{
"context": " \"DSLs,9883\"\n , \"DumpsterSluts,20270\"\n , \"dykesgonewild,34039\"\n , \"easterneuropeangirls,12899\"\n , \"",
"end": 5572,
"score": 0.7405854464,
"start": 5561,
"tag": "USERNAME",
"value": "kesgonewild"
},
{
"context": "\n , \"ecchigifs,2582\"\n , \"eFukt,5453\"\n , \"Eliza_cs,2615\"\n , \"emmaglover,4749\"\n , \"EngorgedV",
"end": 5709,
"score": 0.9279262424,
"start": 5704,
"tag": "NAME",
"value": "Eliza"
},
{
"context": " , \"gfur,7117\"\n , \"ghostnipples,4787\"\n , \"GiannaMichaels,16702\"\n , \"gifcest,1567\"\n , \"gifsgonewild,5",
"end": 7418,
"score": 0.9250621796,
"start": 7406,
"tag": "NAME",
"value": "annaMichaels"
},
{
"context": "suNights,1975\"\n , \"KateeOwen,26976\"\n , \"kati3kat,7846\"\n , \"kinderyiff,2342\"\n , \"kinksters",
"end": 10332,
"score": 0.568959713,
"start": 10331,
"tag": "USERNAME",
"value": "3"
},
{
"context": "Leave,18542\"\n , \"MakeUpFetish,11899\"\n , \"MalenaMorgan,10088\"\n , \"manass,6408\"\n , \"mangonewild,187",
"end": 11105,
"score": 0.6582416892,
"start": 11096,
"tag": "NAME",
"value": "enaMorgan"
},
{
"context": "enus,21951\"\n , \"mycleavage,17212\"\n , \"Naruto_Hentai,8227\"\n , \"naturaltitties,18749\"\n , \"N",
"end": 11749,
"score": 0.5024742484,
"start": 11749,
"tag": "USERNAME",
"value": ""
},
{
"context": "us,21951\"\n , \"mycleavage,17212\"\n , \"Naruto_Hentai,8227\"\n , \"naturaltitties,18749\"\n , \"Nekom",
"end": 11754,
"score": 0.5521839261,
"start": 11751,
"tag": "NAME",
"value": "ent"
},
{
"context": "orn,25106\"\n , \"OldSchoolCoolNSFW,28864\"\n , \"omgbeckylookathiscock,27781\"\n , \"OnHerKnees,44792\"\n , \"OnOff,1843",
"end": 12828,
"score": 0.9985902309,
"start": 12807,
"tag": "USERNAME",
"value": "omgbeckylookathiscock"
},
{
"context": "trangers,24537\"\n , \"slutwife,9641\"\n , \"smalldickporn,524\"\n , \"smashbros34,4666\"\n , \"snapchat_sl",
"end": 15613,
"score": 0.5681618452,
"start": 15606,
"tag": "USERNAME",
"value": "dickpor"
},
{
"context": " \"Upskirt,71682\"\n , \"usedpanties,9873\"\n , \"Vaganny,486\"\n , \"vagina,19014\"\n , \"vikingsgonew",
"end": 17616,
"score": 0.6545283794,
"start": 17614,
"tag": "NAME",
"value": "ag"
}
] | src/elm/Nsfw.elm | Dobiasd/RedditTimeMachine | 21 | module Nsfw (..) where
nsfwRaw : List String
nsfwRaw =
[ "18_19,24484"
, "60fpsporn,148203"
, "aa_cups,23506"
, "AbbyWinters,12577"
, "ABDL,5852"
, "AbusePorn2,14554"
, "accidentalnudity,27933"
, "adorableporn,14666"
, "adultgifs,29753"
, "after_the_shot,14139"
, "AgedBeauty,17686"
, "AgeplayPenPals,3997"
, "ahegao,10317"
, "alteredbuttholes,4324"
, "altgonewild,66176"
, "Amateur,244751"
, "AmateurArchives,87045"
, "amateurcumsluts,45204"
, "AmateurPorn,13364"
, "AmateurWifes,10962"
, "amazingtits,2490"
, "AnaCheri,2180"
, "anal,81903"
, "AnalGW,48267"
, "AnimeBooty,3344"
, "AnimeFeet,1310"
, "animeplot,14694"
, "Anjelica_Ebbi,32289"
, "ArabPorn,9546"
, "areolas,7667"
, "ariane,2084"
, "arielrebel,13053"
, "asaakira,8006"
, "AsianCumsluts,12412"
, "AsianFetish,9068"
, "AsianHotties,118250"
, "AsianNSFW,44501"
, "AsianPorn,33780"
, "Asianpornin15seconds,6637"
, "AsiansGoneWild,132028"
, "AskRedditAfterDark,21313"
, "ass,212858"
, "asshole,45189"
, "AssholeBehindThong,26892"
, "assholegonewild,19089"
, "assinthong,48561"
, "asslick,19197"
, "asstastic,123590"
, "AthleticGirls,33227"
, "AvatarPorn,13156"
, "avocadosgonewild,24228"
, "awwyea,3000"
, "BadDragon,5626"
, "Balls,4385"
, "ballsdeepandcumming,4990"
, "BBCSluts,9657"
, "BBW,22720"
, "BBWGW,23910"
, "B_Cups,13776"
, "bdsm,58817"
, "BDSMcommunity,44370"
, "BDSMGW,39917"
, "BDSMpersonals,15920"
, "BeautifulTitsAndAss,13457"
, "beef_flaps,12633"
, "before_after_cumsluts,30887"
, "Bestiality,6711"
, "bestofblowjobs,6505"
, "bestofboobies,9856"
, "bestofcollege,32777"
, "bestofcumsluts,13574"
, "bestoflingerie,3900"
, "bestofworldstar,43773"
, "Beth_Lily,6372"
, "BHMGoneWild,5031"
, "bigasses,38039"
, "bigboobs,15021"
, "BigBoobsGonewild,30051"
, "BigBoobsGW,63596"
, "bigclit,7054"
, "bigonewild,9797"
, "Bigtitssmalltits,13541"
, "bikinibridge,20064"
, "bikinis,40786"
, "bimbofetish,41761"
, "bimbofication,7362"
, "blackchickswhitedicks,21792"
, "BlackCrimeMatters,1536"
, "Blonde,30956"
, "blowbang,9638"
, "BlowJob,11002"
, "blowjob_eyes,8850"
, "BlowjobGifs,26677"
, "Blowjobs,119089"
, "blowjobsandwich,25966"
, "bodyperfection,20335"
, "bodyshots,24452"
, "boltedontits,61128"
, "Bondage,67688"
, "BonerMaterial,64112"
, "boobbounce,55981"
, "boobgifs,21473"
, "Boobies,219679"
, "boobs,44694"
, "booty,28469"
, "BorednIgnored,30986"
, "Bottomless_Vixens,44832"
, "Brazzers_Network,3069"
, "BreastEnvy,32075"
, "BreastExpansion,9933"
, "breastsucking,3878"
, "Breeding,17945"
, "broslikeus,19536"
, "brunette,30380"
, "BubbleButts,21270"
, "bubbling,9960"
, "Bulges,9188"
, "BurningLibrary,35"
, "burstingout,85792"
, "bustyasians,20150"
, "bustybabes,22596"
, "Bustyfit,12946"
, "BustyNats,3729"
, "BustyNaturals,8845"
, "BustyPetite,233193"
, "butterface,7741"
, "buttplug,45727"
, "ButtsAndBareFeet,12313"
, "buttsex,13970"
, "CamelToeGirls,13152"
, "CamSluts,20545"
, "camwhores,57858"
, "CandidFashionPolice,65014"
, "cardsagainsthumanity,25841"
, "cat_girls,21561"
, "CelebFakes,25971"
, "celebnsfw,139023"
, "CelebOops,8986"
, "CelebrityButts,20657"
, "CelebrityNipples,19472"
, "CelebrityPussy,37756"
, "celebsnaked,29058"
, "celebsunleashed,9458"
, "cfnm,23842"
, "CharlotteMcKinney,3941"
, "chastity,7850"
, "cheatingwives,6914"
, "CheekyBottoms,10873"
, "chesthairporn,7507"
, "chickflixxx,35961"
, "choking,7219"
, "ChristianGirls,45343"
, "chubby,36225"
, "cinnamonmermaids,1223"
, "cleavage,37843"
, "ClopClop,16429"
, "cocaine,6289"
, "cock,8378"
, "CockOutline,3647"
, "collared,32214"
, "CollegeAmateurs,49920"
, "collegesluts,99495"
, "comics18_story,14893"
, "ConfusedBoners,39957"
, "consentacles,4110"
, "cougars,16995"
, "couplesgonewild,11583"
, "creampie,20395"
, "creampiegifs,19352"
, "creampies,60796"
, "crouch,3451"
, "Cuckold,43103"
, "cuckoldcaptions,6454"
, "cumcoveredfucking,42377"
, "cumfetish,60077"
, "CumHaters,31134"
, "cumontongue,6670"
, "cumshots,16021"
, "cumsluts,203095"
, "CumSwallowing,11310"
, "curvy,119160"
, "cutecunts,6710"
, "CuteGuyButts,8870"
, "CuteModeSlutMode,46138"
, "DadsGoneWild,496"
, "damngoodinterracial,14987"
, "DanielleSharp,5570"
, "DarkAngels,36217"
, "DarkHumor,4633"
, "DarkNetMarkets,80737"
, "datgap,93167"
, "datgrip,11198"
, "deepthroat,40451"
, "DegradingHoles,5571"
, "DeliciousTraps,5631"
, "DesiBoners,6455"
, "DiamondJackson,841"
, "DirtyFamilyPhotos,13784"
, "dirtykikpals,32993"
, "dirtypenpals,74555"
, "dirtyr4r,40526"
, "dirtysmall,136317"
, "DirtySnapchat,31502"
, "disneyporn,9475"
, "distension,40247"
, "doppelbangher,26184"
, "dota2smut,6949"
, "doublepenetration,8700"
, "doujinshi,21636"
, "downblouse,45814"
, "DownBra,9851"
, "dreamjobs,13943"
, "drunkencookery,12918"
, "DSLs,9883"
, "DumpsterSluts,20270"
, "dykesgonewild,34039"
, "easterneuropeangirls,12899"
, "Ebony,28317"
, "ecchi,37105"
, "ecchigifs,2582"
, "eFukt,5453"
, "Eliza_cs,2615"
, "emmaglover,4749"
, "EngorgedVeinyBreasts,15262"
, "EntExchange,6696"
, "ErectElephantDicks,16"
, "eroticauthors,5308"
, "ExhibitionistSex,23485"
, "exotic_oasis,9619"
, "exposedinpublic,18077"
, "extreme_gifs,3581"
, "Exxxtras,45153"
, "facedownassup,86307"
, "FaceFuck,16794"
, "facesitting,39903"
, "FacialFun,39472"
, "Facials,15022"
, "FapFap,6102"
, "faponher,6893"
, "FearMe,33613"
, "feet,24237"
, "FemBoys,10116"
, "Femdom,24830"
, "FestivalSluts,72778"
, "FiftyFifty,288727"
, "FilthyGirls,20789"
, "Fisting,16730"
, "fit2phat,1325"
, "fitdrawngirls,2895"
, "fitgirls,57889"
, "FlashingGirls,42831"
, "flatchests,7015"
, "flexi,13923"
, "FMN,6707"
, "forcedorgasms,33891"
, "foreskin,9331"
, "freeuse,30250"
, "Fretton,2849"
, "fuckyeahcollegesluts,11251"
, "fuckyeahdrunksluts,18154"
, "fuckyeahfitgirls,4683"
, "fuckyeahsluttybimbos,4773"
, "fuckyeahsofuckable,6058"
, "funsized,28628"
, "FunWithFriends,25949"
, "futanari,36809"
, "fuxtaposition,25951"
, "fyeahsexyteens,10676"
, "gag_spit,11724"
, "gangbang,22923"
, "GarterBelts,12460"
, "gaybears,9790"
, "GaybrosGoneWild,30015"
, "GayCreampie,835"
, "gaycumsluts,10079"
, "GayDaddiesPics,5837"
, "GayGifs,15200"
, "gayincest,5344"
, "GayKink,6469"
, "gaymersgonewild,18877"
, "gaynsfw,14604"
, "gayporn,15475"
, "gaypornhunters,9155"
, "gaystoriesgonewild,10478"
, "Gemplugs,3831"
, "gettingherselfoff,61269"
, "gfur,7117"
, "ghostnipples,4787"
, "GiannaMichaels,16702"
, "gifcest,1567"
, "gifsgonewild,52697"
, "Gif_source,149"
, "gilf,12717"
, "ginger,140484"
, "GirlsCuddling,7441"
, "girlsdoingnerdythings,27814"
, "girlsdoporn,9926"
, "GirlsFinishingTheJob,171255"
, "girlsflashing,11572"
, "GirlsHumpingThings,29849"
, "girlsinleggings,24975"
, "GirlsinSchoolUniforms,31825"
, "GirlsinStripedSocks,32691"
, "GirlsInTheBathroom,389"
, "girlsinyogapants,171734"
, "girlskissing,34111"
, "girlswhoride,12617"
, "GirlswithGlasses,121113"
, "GirlswithNeonHair,46301"
, "Gloryholes,11188"
, "GoneErotic,9821"
, "GoneInsane,19856"
, "GoneMild,65200"
, "Gonewild18,1274"
, "gonewild30plus,13852"
, "gonewild,843110"
, "gonewildaudio,78812"
, "GoneWildCD,16785"
, "gonewildchubby,5193"
, "gonewildcolor,53520"
, "gonewildcouples,39524"
, "gonewildcurvy,168173"
, "GoneWildHairy,22057"
, "GoneWildPlus,92479"
, "GoneWildScrubs,20356"
, "GoneWildSmiles,39168"
, "gonewildstories,96304"
, "GoneWildTrans,18419"
, "GoneWildTube,102715"
, "gonwild,21212"
, "grool,79211"
, "GroupOfNudeGirls,41008"
, "GushingGirls,15189"
, "GuysFromBehind,3672"
, "GWABackstage,1210"
, "GWbanned,11034"
, "gwcosplay,11527"
, "GWCouples,107728"
, "gwcumsluts,63864"
, "GWNerdy,78086"
, "gwpublic,27365"
, "hadom,3829"
, "HairyArmpits,3435"
, "HairyPussy,22641"
, "handbra,12802"
, "HappyEmbarrassedGirls,121185"
, "hardanal,9938"
, "HardBoltOns,8857"
, "havoc_bot,6681"
, "hentai,95242"
, "hentaibondage,14956"
, "HENTAI_GIF,27931"
, "HerBush,4007"
, "highheelsNSFW,16805"
, "HighMileageHoles,9416"
, "HighResNSFW,83488"
, "hipcleavage,13387"
, "holdmyturban,13832"
, "holdthemoan,223742"
, "homegrowntits,42553"
, "HomemadePorn,9947"
, "homemadexxx,122095"
, "Hotchickswithtattoos,120592"
, "Hotwife,61092"
, "hugeboobs,77828"
, "Hugeboobshardcore,21026"
, "HugeDickTinyChick,29890"
, "hugefutanari,4664"
, "hugenaturals,20316"
, "HungryButts,16331"
, "Hyiff,2296"
, "iateacrayon,6315"
, "Ifyouhadtopickone,14070"
, "ImaginaryBoners,10338"
, "ImGoingToHellForThis,503147"
, "incest,55424"
, "IncestComics,18632"
, "Incest_Gifs,3856"
, "IncestPorn,27977"
, "IndianBabes,48961"
, "IndianPorn,9680"
, "IndiansGoneWild,24650"
, "IndianTeens,12059"
, "Innie,43412"
, "insertions,32142"
, "IntenseBDSM,6422"
, "iWantToFuckHer,47799"
, "JapanesePorn2,19840"
, "JavDownloadCenter,15046"
, "Jewdank,20970"
, "JiggleFuck,21003"
, "jilling,76929"
, "jobuds,7193"
, "joi,18674"
, "juicyasians,80772"
, "JustOneBoob,12369"
, "KanMusuNights,1975"
, "KateeOwen,26976"
, "kati3kat,7846"
, "kinderyiff,2342"
, "kinksters_gone_wild,15872"
, "KinkyStuff,3431"
, "kpopfap,27478"
, "LabiaGW,31435"
, "lactation,14580"
, "ladybonersgw,73296"
, "latinas,43997"
, "LatinasGW,16088"
, "LegalCollegeGirls,1681"
, "LegalTeens,185026"
, "LegalTeensXXX,30374"
, "legendarylootz,6324"
, "leggingsgonewild,24557"
, "legs,40997"
, "legsup,20993"
, "legwrap,23286"
, "leotards,27721"
, "lesbians,83608"
, "lesserknownpornstars,8051"
, "lewdgestures,4194"
, "lineups,12259"
, "lingerie,52073"
, "LipsThatGrip,102947"
, "littlespace,8004"
, "lolgrindr,10393"
, "lovegaymale,14054"
, "LoveToWatchYouLeave,18542"
, "MakeUpFetish,11899"
, "MalenaMorgan,10088"
, "manass,6408"
, "mangonewild,18777"
, "MassiveCock,36277"
, "MasterOfAnal,17320"
, "MastersOfAnal,362"
, "maturewomen,10179"
, "MCBourbonnais,2616"
, "mellisaclarke,6928"
, "MensHighJinx,5700"
, "MiddleEasternHotties,16949"
, "milf,189385"
, "Milfie,10295"
, "Milfinstockings,3494"
, "milf_nowandforever,6478"
, "MilitaryGoneWild,28966"
, "MistressPorn,3998"
, "mmgirls,29077"
, "monster_cocks,8243"
, "MonsterGirl,15410"
, "Mooning,18263"
, "MorbidReality,199435"
, "Morphs,4714"
, "MoundofVenus,21951"
, "mycleavage,17212"
, "Naruto_Hentai,8227"
, "naturaltitties,18749"
, "Nekomimi,9066"
, "Nipples,31889"
, "nipslip,21758"
, "normalnudes,19985"
, "notgayporn,3849"
, "NotSafeForNature,33964"
, "NowYouReallySeeMe,12900"
, "nsfw2,85112"
, "NSFW411,41427"
, "nsfw,619457"
, "Nsfw_Amateurs,30250"
, "nsfwasmr,17370"
, "NSFWBarista,11093"
, "nsfwcelebgifs,14493"
, "nsfwcosplay,92462"
, "NSFWCostumes,29258"
, "NSFWfashion,9646"
, "NSFWFunny,120163"
, "nsfwgif,11381"
, "NSFW_GIF,444769"
, "nsfw_gifs,275755"
, "nsfwhardcore,149726"
, "NSFW_HTML5,84757"
, "NSFWIAMA,17481"
, "NSFW_Japan,48017"
, "NSFW_Korea,21277"
, "NSFW_nospam,36611"
, "nsfwoutfits,80662"
, "NSFW_Plowcam,16101"
, "NSFW_Snapchat,46100"
, "nsfwsports,15357"
, "nsfw_videos,54269"
, "NSFW_Wallpapers,27968"
, "nsfw_wtf,41894"
, "Objects,28620"
, "oculusnsfw,8319"
, "O_Faces,88301"
, "OffensiveSpeech,2068"
, "Oilporn,25106"
, "OldSchoolCoolNSFW,28864"
, "omgbeckylookathiscock,27781"
, "OnHerKnees,44792"
, "OnOff,184350"
, "OpenShirt,10388"
, "outercourse,27863"
, "Page3Glamour,28383"
, "painal,32954"
, "palegirls,100724"
, "paleskin,7065"
, "pantsu,17621"
, "Pantyfetish,28625"
, "pantyhose,10234"
, "passionx,59476"
, "pawg,48293"
, "Pee,20091"
, "peegonewild,15600"
, "Pegging,34258"
, "pelfie,19023"
, "penis,28641"
, "petite,21158"
, "PetiteGirls,11221"
, "PetiteGoneWild,138254"
, "photoplunder,48664"
, "PiercedNSFW,19428"
, "Playboy,25672"
, "PlayfulGirls,5082"
, "PokePorn,28191"
, "pokies,39944"
, "porn,61231"
, "porn_gifs,65373"
, "pornID,25944"
, "porninfifteenseconds,69115"
, "porn_irl,1387"
, "PornStars,18744"
, "pornvids,144272"
, "preggo,6602"
, "PreggoPorn,29370"
, "prematurecumshots,5118"
, "Presenting,18893"
, "primes,16123"
, "pronebone,11836"
, "PublicBoys,10645"
, "PublicFlashing,40754"
, "Puffies,15400"
, "PunkGirls,18050"
, "pussy,90627"
, "pussyjobs,13757"
, "PussyJuices,11737"
, "PussyMound,18778"
, "quiver,42504"
, "RandomActsOfBlowJob,57817"
, "RandomActsOfMuffDive,26518"
, "randomsexiness,108173"
, "RateMyNudeBody,15430"
, "RealGirls,500214"
, "realmoms,9517"
, "rearpussy,68313"
, "RedditorCum,6165"
, "reddtube,1483"
, "redheads,130037"
, "RemyLaCroix,22780"
, "RepressedGoneWild,26693"
, "ResearchMarkets,3461"
, "RollaGoneWild,196"
, "RopesandRoundThings,524"
, "RugsOnly,14065"
, "RuinedOrgasms,25075"
, "rule34,163083"
, "rule34celebs,6481"
, "rule34_comics,45072"
, "rule34feet,3842"
, "Rule34LoL,25018"
, "russiangirls,8482"
, "RWBYNSFW,3191"
, "Saggy,13386"
, "SaraJUnderwood,11930"
, "scissoring,26368"
, "sea_girls,8563"
, "seethru,29190"
, "selfservice,8018"
, "sex_comics,35364"
, "SexiestPetites,18782"
, "SexInFrontOfOthers,32286"
, "Sexsells,11753"
, "SexShows,9930"
, "SexToys,11036"
, "Sexy,31638"
, "SexyButNotPorn,43830"
, "SexyFrex,48227"
, "SexyGirlsInBoots,17162"
, "sexygirlsinjeans,22536"
, "sexytgirls,7684"
, "SexyTummies,17663"
, "Shadman,3095"
, "Sharktits,3507"
, "SheLikesItRough,46307"
, "Shemales,18524"
, "ShinyPorn,33412"
, "shorthairchicks,32275"
, "sideboob,12252"
, "simps,38791"
, "Singlets,5067"
, "Sissies,18319"
, "sissyhypno,12887"
, "Sissyperfection,7430"
, "skinnytail,25088"
, "skivvies,10936"
, "slightcellulite,3263"
, "slimgirls,14166"
, "SluttyHalloween,22066"
, "SluttyStrangers,24537"
, "slutwife,9641"
, "smalldickporn,524"
, "smashbros34,4666"
, "snapchat_sluts,26970"
, "snapleaks,20340"
, "SocialMediaSluts,11252"
, "sodomy,12505"
, "softies,6895"
, "SoFuckable,14879"
, "Spanking,13720"
, "spreadeagle,14882"
, "SpreadEm,20109"
, "spreading,13811"
, "squirting,40828"
, "ssbbw,3072"
, "Stacked,74848"
, "Steroidsourcetalk,7141"
, "stockings,53328"
, "StomachDownFeetUp,7113"
, "StraightGirlsPlaying,64462"
, "straponlesbians,2754"
, "StretchingIt,9820"
, "StruggleFucking,35561"
, "StrugglePorn,11899"
, "Stuffers,3886"
, "suicidegirls,87512"
, "Sukebei,8178"
, "superheroporn,4904"
, "sweatermeat,28465"
, "Swingersgw,17068"
, "TallGoneWild,22606"
, "tanlines,31041"
, "TBoIRule34,1089"
, "Tgifs,12920"
, "Tgirls,42572"
, "thefullbush,8242"
, "TheHangingBoobs,25255"
, "TheHottestBabes,1734"
, "theratio,18115"
, "TheRedFox,22052"
, "TheUnderboob,30281"
, "TheUnderbun,32463"
, "thick,92258"
, "ThickChixxx,17944"
, "thickloads,32225"
, "ThickThighs,13548"
, "thighhighs,26000"
, "thinspo,38863"
, "Throatfucking,9389"
, "throatpies,31332"
, "tightdresses,99184"
, "TightsAndTightClothes,3033"
, "TightShorts,30016"
, "tight_shorts,34247"
, "TinyAsianTits,7369"
, "TinyTits,103655"
, "tipofmypenis,69706"
, "titfuck,23529"
, "tits,20395"
, "Titties,14990"
, "TittyDrop,97809"
, "torpedotits,18064"
, "TotallyStraight,23505"
, "transformation,4418"
, "translucent_porn,14520"
, "transporn,1341"
, "traps,38877"
, "trashyboners,39484"
, "TrashyPorn,6319"
, "treesgonewild,70558"
, "TributeMe,31704"
, "twerking,12030"
, "TwinGuys,559"
, "twinks,14936"
, "Unashamed,86525"
, "UncutPorn,9592"
, "UnderTail,1255"
, "UnderwearGW,36741"
, "upherbutt,13848"
, "Upskirt,71682"
, "usedpanties,9873"
, "Vaganny,486"
, "vagina,19014"
, "vikingsgonewild,6364"
, "VintageBabes,21387"
, "voluptuous,59581"
, "Vore,3307"
, "watchitforthecat,7063"
, "WatchItForThePlot,140279"
, "watchpeopledie,92815"
, "watersports,9380"
, "WeAllGoWild,6888"
, "WeddingRingsShowing,20896"
, "WeddingsGoneWild,26648"
, "WesternHentai,22661"
, "wet,29784"
, "WhipItOut,2715"
, "whooties,15565"
, "whynotasource,12838"
, "wifesharing,71509"
, "wildchicks,19547"
, "wincest,29725"
, "WomenOfColor,41357"
, "womenofcolorXXX,17855"
, "WomenOfColour,26912"
, "workgonewild,82309"
, "WorkIt,9982"
, "Workoutgonewild,23199"
, "WouldYouFuckMyWife,37655"
, "WtSSTaDaMiT,91151"
, "XChangePill,4260"
, "xray,17712"
, "xsmallgirls,36517"
, "Xsome,28602"
, "xxxcaptions,12808"
, "yaoi,7060"
, "YAYamateurs,29424"
, "yiff,27964"
, "yiffcomics,7957"
, "YogaPants,43138"
, "youngporn,16792"
, "youtubetitties,34514"
, "yuri,9468"
]
| 36289 | module Nsfw (..) where
nsfwRaw : List String
nsfwRaw =
[ "18_19,24484"
, "60fpsporn,148203"
, "aa_cups,23506"
, "AbbyWinters,12577"
, "ABDL,5852"
, "AbusePorn2,14554"
, "accidentalnudity,27933"
, "adorableporn,14666"
, "adultgifs,29753"
, "after_the_shot,14139"
, "AgedBeauty,17686"
, "AgeplayPenPals,3997"
, "ahegao,10317"
, "alteredbuttholes,4324"
, "altgonewild,66176"
, "Amateur,244751"
, "AmateurArchives,87045"
, "amateurcumsluts,45204"
, "AmateurPorn,13364"
, "AmateurWifes,10962"
, "amazingtits,2490"
, "AnaCheri,2180"
, "anal,81903"
, "AnalGW,48267"
, "AnimeBooty,3344"
, "AnimeFeet,1310"
, "animeplot,14694"
, "Anjelica_Ebbi,32289"
, "ArabPorn,9546"
, "areolas,7667"
, "ariane,2084"
, "arielrebel,13053"
, "asaakira,8006"
, "AsianCumsluts,12412"
, "AsianFetish,9068"
, "AsianHotties,118250"
, "AsianNSFW,44501"
, "AsianPorn,33780"
, "Asianpornin15seconds,6637"
, "AsiansGoneWild,132028"
, "AskRedditAfterDark,21313"
, "ass,212858"
, "asshole,45189"
, "AssholeBehindThong,26892"
, "assholegonewild,19089"
, "assinthong,48561"
, "asslick,19197"
, "asstastic,123590"
, "AthleticGirls,33227"
, "AvatarPorn,13156"
, "avocadosgonewild,24228"
, "awwyea,3000"
, "BadDragon,5626"
, "Balls,4385"
, "ballsdeepandcumming,4990"
, "BBCSluts,9657"
, "BBW,22720"
, "BBWGW,23910"
, "B_Cups,13776"
, "bdsm,58817"
, "BDSMcommunity,44370"
, "BDSMGW,39917"
, "BDSMpersonals,15920"
, "BeautifulTitsAndAss,13457"
, "beef_flaps,12633"
, "before_after_cumsluts,30887"
, "Bestiality,6711"
, "bestofblowjobs,6505"
, "bestofboobies,9856"
, "bestofcollege,32777"
, "bestofcumsluts,13574"
, "bestoflingerie,3900"
, "bestofworldstar,43773"
, "Beth_Lily,6372"
, "BHMGoneWild,5031"
, "bigasses,38039"
, "bigboobs,15021"
, "BigBoobsGonewild,30051"
, "BigBoobsGW,63596"
, "bigclit,7054"
, "bigonewild,9797"
, "Bigtitssmalltits,13541"
, "bikinibridge,20064"
, "bikinis,40786"
, "bimbofetish,41761"
, "bimbofication,7362"
, "blackchickswhitedicks,21792"
, "BlackCrimeMatters,1536"
, "Blonde,30956"
, "blowbang,9638"
, "BlowJob,11002"
, "blowjob_eyes,8850"
, "BlowjobGifs,26677"
, "Blowjobs,119089"
, "blowjobsandwich,25966"
, "bodyperfection,20335"
, "bodyshots,24452"
, "boltedontits,61128"
, "Bondage,67688"
, "BonerMaterial,64112"
, "boobbounce,55981"
, "boobgifs,21473"
, "Boobies,219679"
, "boobs,44694"
, "booty,28469"
, "BorednIgnored,30986"
, "Bottomless_Vixens,44832"
, "Brazzers_Network,3069"
, "BreastEnvy,32075"
, "BreastExpansion,9933"
, "breastsucking,3878"
, "Breeding,17945"
, "broslikeus,19536"
, "brunette,30380"
, "BubbleButts,21270"
, "bubbling,9960"
, "Bulges,9188"
, "BurningLibrary,35"
, "burstingout,85792"
, "bustyasians,20150"
, "bustybabes,22596"
, "Bustyfit,12946"
, "BustyNats,3729"
, "BustyNaturals,8845"
, "BustyPetite,233193"
, "butterface,7741"
, "buttplug,45727"
, "ButtsAndBareFeet,12313"
, "buttsex,13970"
, "CamelToeGirls,13152"
, "CamSluts,20545"
, "camwhores,57858"
, "CandidFashionPolice,65014"
, "cardsagainsthumanity,25841"
, "cat_girls,21561"
, "CelebFakes,25971"
, "celebnsfw,139023"
, "CelebOops,8986"
, "CelebrityButts,20657"
, "CelebrityNipples,19472"
, "CelebrityPussy,37756"
, "celebsnaked,29058"
, "celebsunleashed,9458"
, "cfnm,23842"
, "CharlotteMcKinney,3941"
, "chastity,7850"
, "cheatingwives,6914"
, "CheekyBottoms,10873"
, "chesthairporn,7507"
, "chickflixxx,35961"
, "choking,7219"
, "ChristianGirls,45343"
, "chubby,36225"
, "cinnamonmermaids,1223"
, "cleavage,37843"
, "ClopClop,16429"
, "cocaine,6289"
, "cock,8378"
, "CockOutline,3647"
, "collared,32214"
, "CollegeAmateurs,49920"
, "collegesluts,99495"
, "comics18_story,14893"
, "ConfusedBoners,39957"
, "consentacles,4110"
, "cougars,16995"
, "couplesgonewild,11583"
, "creampie,20395"
, "creampiegifs,19352"
, "creampies,60796"
, "crouch,3451"
, "Cuckold,43103"
, "cuckoldcaptions,6454"
, "cumcoveredfucking,42377"
, "cumfetish,60077"
, "CumHaters,31134"
, "cumontongue,6670"
, "cumshots,16021"
, "cumsluts,203095"
, "CumSwallowing,11310"
, "curvy,119160"
, "cutecunts,6710"
, "CuteGuyButts,8870"
, "CuteModeSlutMode,46138"
, "DadsGoneWild,496"
, "damngoodinterracial,14987"
, "DanielleSharp,5570"
, "DarkAngels,36217"
, "DarkHumor,4633"
, "DarkNetMarkets,80737"
, "datgap,93167"
, "datgrip,11198"
, "deepthroat,40451"
, "DegradingHoles,5571"
, "DeliciousTraps,5631"
, "DesiBoners,6455"
, "<NAME>,841"
, "DirtyFamilyPhotos,13784"
, "dirtykikpals,32993"
, "dirtypenpals,74555"
, "dirtyr4r,40526"
, "dirtysmall,136317"
, "DirtySnapchat,31502"
, "disneyporn,9475"
, "distension,40247"
, "doppelbangher,26184"
, "dota2smut,6949"
, "doublepenetration,8700"
, "doujinshi,21636"
, "downblouse,45814"
, "DownBra,9851"
, "dreamjobs,13943"
, "drunkencookery,12918"
, "DSLs,9883"
, "DumpsterSluts,20270"
, "dykesgonewild,34039"
, "easterneuropeangirls,12899"
, "Ebony,28317"
, "ecchi,37105"
, "ecchigifs,2582"
, "eFukt,5453"
, "<NAME>_cs,2615"
, "emmaglover,4749"
, "EngorgedVeinyBreasts,15262"
, "EntExchange,6696"
, "ErectElephantDicks,16"
, "eroticauthors,5308"
, "ExhibitionistSex,23485"
, "exotic_oasis,9619"
, "exposedinpublic,18077"
, "extreme_gifs,3581"
, "Exxxtras,45153"
, "facedownassup,86307"
, "FaceFuck,16794"
, "facesitting,39903"
, "FacialFun,39472"
, "Facials,15022"
, "FapFap,6102"
, "faponher,6893"
, "FearMe,33613"
, "feet,24237"
, "FemBoys,10116"
, "Femdom,24830"
, "FestivalSluts,72778"
, "FiftyFifty,288727"
, "FilthyGirls,20789"
, "Fisting,16730"
, "fit2phat,1325"
, "fitdrawngirls,2895"
, "fitgirls,57889"
, "FlashingGirls,42831"
, "flatchests,7015"
, "flexi,13923"
, "FMN,6707"
, "forcedorgasms,33891"
, "foreskin,9331"
, "freeuse,30250"
, "Fretton,2849"
, "fuckyeahcollegesluts,11251"
, "fuckyeahdrunksluts,18154"
, "fuckyeahfitgirls,4683"
, "fuckyeahsluttybimbos,4773"
, "fuckyeahsofuckable,6058"
, "funsized,28628"
, "FunWithFriends,25949"
, "futanari,36809"
, "fuxtaposition,25951"
, "fyeahsexyteens,10676"
, "gag_spit,11724"
, "gangbang,22923"
, "GarterBelts,12460"
, "gaybears,9790"
, "GaybrosGoneWild,30015"
, "GayCreampie,835"
, "gaycumsluts,10079"
, "GayDaddiesPics,5837"
, "GayGifs,15200"
, "gayincest,5344"
, "GayKink,6469"
, "gaymersgonewild,18877"
, "gaynsfw,14604"
, "gayporn,15475"
, "gaypornhunters,9155"
, "gaystoriesgonewild,10478"
, "Gemplugs,3831"
, "gettingherselfoff,61269"
, "gfur,7117"
, "ghostnipples,4787"
, "Gi<NAME>,16702"
, "gifcest,1567"
, "gifsgonewild,52697"
, "Gif_source,149"
, "gilf,12717"
, "ginger,140484"
, "GirlsCuddling,7441"
, "girlsdoingnerdythings,27814"
, "girlsdoporn,9926"
, "GirlsFinishingTheJob,171255"
, "girlsflashing,11572"
, "GirlsHumpingThings,29849"
, "girlsinleggings,24975"
, "GirlsinSchoolUniforms,31825"
, "GirlsinStripedSocks,32691"
, "GirlsInTheBathroom,389"
, "girlsinyogapants,171734"
, "girlskissing,34111"
, "girlswhoride,12617"
, "GirlswithGlasses,121113"
, "GirlswithNeonHair,46301"
, "Gloryholes,11188"
, "GoneErotic,9821"
, "GoneInsane,19856"
, "GoneMild,65200"
, "Gonewild18,1274"
, "gonewild30plus,13852"
, "gonewild,843110"
, "gonewildaudio,78812"
, "GoneWildCD,16785"
, "gonewildchubby,5193"
, "gonewildcolor,53520"
, "gonewildcouples,39524"
, "gonewildcurvy,168173"
, "GoneWildHairy,22057"
, "GoneWildPlus,92479"
, "GoneWildScrubs,20356"
, "GoneWildSmiles,39168"
, "gonewildstories,96304"
, "GoneWildTrans,18419"
, "GoneWildTube,102715"
, "gonwild,21212"
, "grool,79211"
, "GroupOfNudeGirls,41008"
, "GushingGirls,15189"
, "GuysFromBehind,3672"
, "GWABackstage,1210"
, "GWbanned,11034"
, "gwcosplay,11527"
, "GWCouples,107728"
, "gwcumsluts,63864"
, "GWNerdy,78086"
, "gwpublic,27365"
, "hadom,3829"
, "HairyArmpits,3435"
, "HairyPussy,22641"
, "handbra,12802"
, "HappyEmbarrassedGirls,121185"
, "hardanal,9938"
, "HardBoltOns,8857"
, "havoc_bot,6681"
, "hentai,95242"
, "hentaibondage,14956"
, "HENTAI_GIF,27931"
, "HerBush,4007"
, "highheelsNSFW,16805"
, "HighMileageHoles,9416"
, "HighResNSFW,83488"
, "hipcleavage,13387"
, "holdmyturban,13832"
, "holdthemoan,223742"
, "homegrowntits,42553"
, "HomemadePorn,9947"
, "homemadexxx,122095"
, "Hotchickswithtattoos,120592"
, "Hotwife,61092"
, "hugeboobs,77828"
, "Hugeboobshardcore,21026"
, "HugeDickTinyChick,29890"
, "hugefutanari,4664"
, "hugenaturals,20316"
, "HungryButts,16331"
, "Hyiff,2296"
, "iateacrayon,6315"
, "Ifyouhadtopickone,14070"
, "ImaginaryBoners,10338"
, "ImGoingToHellForThis,503147"
, "incest,55424"
, "IncestComics,18632"
, "Incest_Gifs,3856"
, "IncestPorn,27977"
, "IndianBabes,48961"
, "IndianPorn,9680"
, "IndiansGoneWild,24650"
, "IndianTeens,12059"
, "Innie,43412"
, "insertions,32142"
, "IntenseBDSM,6422"
, "iWantToFuckHer,47799"
, "JapanesePorn2,19840"
, "JavDownloadCenter,15046"
, "Jewdank,20970"
, "JiggleFuck,21003"
, "jilling,76929"
, "jobuds,7193"
, "joi,18674"
, "juicyasians,80772"
, "JustOneBoob,12369"
, "KanMusuNights,1975"
, "KateeOwen,26976"
, "kati3kat,7846"
, "kinderyiff,2342"
, "kinksters_gone_wild,15872"
, "KinkyStuff,3431"
, "kpopfap,27478"
, "LabiaGW,31435"
, "lactation,14580"
, "ladybonersgw,73296"
, "latinas,43997"
, "LatinasGW,16088"
, "LegalCollegeGirls,1681"
, "LegalTeens,185026"
, "LegalTeensXXX,30374"
, "legendarylootz,6324"
, "leggingsgonewild,24557"
, "legs,40997"
, "legsup,20993"
, "legwrap,23286"
, "leotards,27721"
, "lesbians,83608"
, "lesserknownpornstars,8051"
, "lewdgestures,4194"
, "lineups,12259"
, "lingerie,52073"
, "LipsThatGrip,102947"
, "littlespace,8004"
, "lolgrindr,10393"
, "lovegaymale,14054"
, "LoveToWatchYouLeave,18542"
, "MakeUpFetish,11899"
, "Mal<NAME>,10088"
, "manass,6408"
, "mangonewild,18777"
, "MassiveCock,36277"
, "MasterOfAnal,17320"
, "MastersOfAnal,362"
, "maturewomen,10179"
, "MCBourbonnais,2616"
, "mellisaclarke,6928"
, "MensHighJinx,5700"
, "MiddleEasternHotties,16949"
, "milf,189385"
, "Milfie,10295"
, "Milfinstockings,3494"
, "milf_nowandforever,6478"
, "MilitaryGoneWild,28966"
, "MistressPorn,3998"
, "mmgirls,29077"
, "monster_cocks,8243"
, "MonsterGirl,15410"
, "Mooning,18263"
, "MorbidReality,199435"
, "Morphs,4714"
, "MoundofVenus,21951"
, "mycleavage,17212"
, "Naruto_H<NAME>ai,8227"
, "naturaltitties,18749"
, "Nekomimi,9066"
, "Nipples,31889"
, "nipslip,21758"
, "normalnudes,19985"
, "notgayporn,3849"
, "NotSafeForNature,33964"
, "NowYouReallySeeMe,12900"
, "nsfw2,85112"
, "NSFW411,41427"
, "nsfw,619457"
, "Nsfw_Amateurs,30250"
, "nsfwasmr,17370"
, "NSFWBarista,11093"
, "nsfwcelebgifs,14493"
, "nsfwcosplay,92462"
, "NSFWCostumes,29258"
, "NSFWfashion,9646"
, "NSFWFunny,120163"
, "nsfwgif,11381"
, "NSFW_GIF,444769"
, "nsfw_gifs,275755"
, "nsfwhardcore,149726"
, "NSFW_HTML5,84757"
, "NSFWIAMA,17481"
, "NSFW_Japan,48017"
, "NSFW_Korea,21277"
, "NSFW_nospam,36611"
, "nsfwoutfits,80662"
, "NSFW_Plowcam,16101"
, "NSFW_Snapchat,46100"
, "nsfwsports,15357"
, "nsfw_videos,54269"
, "NSFW_Wallpapers,27968"
, "nsfw_wtf,41894"
, "Objects,28620"
, "oculusnsfw,8319"
, "O_Faces,88301"
, "OffensiveSpeech,2068"
, "Oilporn,25106"
, "OldSchoolCoolNSFW,28864"
, "omgbeckylookathiscock,27781"
, "OnHerKnees,44792"
, "OnOff,184350"
, "OpenShirt,10388"
, "outercourse,27863"
, "Page3Glamour,28383"
, "painal,32954"
, "palegirls,100724"
, "paleskin,7065"
, "pantsu,17621"
, "Pantyfetish,28625"
, "pantyhose,10234"
, "passionx,59476"
, "pawg,48293"
, "Pee,20091"
, "peegonewild,15600"
, "Pegging,34258"
, "pelfie,19023"
, "penis,28641"
, "petite,21158"
, "PetiteGirls,11221"
, "PetiteGoneWild,138254"
, "photoplunder,48664"
, "PiercedNSFW,19428"
, "Playboy,25672"
, "PlayfulGirls,5082"
, "PokePorn,28191"
, "pokies,39944"
, "porn,61231"
, "porn_gifs,65373"
, "pornID,25944"
, "porninfifteenseconds,69115"
, "porn_irl,1387"
, "PornStars,18744"
, "pornvids,144272"
, "preggo,6602"
, "PreggoPorn,29370"
, "prematurecumshots,5118"
, "Presenting,18893"
, "primes,16123"
, "pronebone,11836"
, "PublicBoys,10645"
, "PublicFlashing,40754"
, "Puffies,15400"
, "PunkGirls,18050"
, "pussy,90627"
, "pussyjobs,13757"
, "PussyJuices,11737"
, "PussyMound,18778"
, "quiver,42504"
, "RandomActsOfBlowJob,57817"
, "RandomActsOfMuffDive,26518"
, "randomsexiness,108173"
, "RateMyNudeBody,15430"
, "RealGirls,500214"
, "realmoms,9517"
, "rearpussy,68313"
, "RedditorCum,6165"
, "reddtube,1483"
, "redheads,130037"
, "RemyLaCroix,22780"
, "RepressedGoneWild,26693"
, "ResearchMarkets,3461"
, "RollaGoneWild,196"
, "RopesandRoundThings,524"
, "RugsOnly,14065"
, "RuinedOrgasms,25075"
, "rule34,163083"
, "rule34celebs,6481"
, "rule34_comics,45072"
, "rule34feet,3842"
, "Rule34LoL,25018"
, "russiangirls,8482"
, "RWBYNSFW,3191"
, "Saggy,13386"
, "SaraJUnderwood,11930"
, "scissoring,26368"
, "sea_girls,8563"
, "seethru,29190"
, "selfservice,8018"
, "sex_comics,35364"
, "SexiestPetites,18782"
, "SexInFrontOfOthers,32286"
, "Sexsells,11753"
, "SexShows,9930"
, "SexToys,11036"
, "Sexy,31638"
, "SexyButNotPorn,43830"
, "SexyFrex,48227"
, "SexyGirlsInBoots,17162"
, "sexygirlsinjeans,22536"
, "sexytgirls,7684"
, "SexyTummies,17663"
, "Shadman,3095"
, "Sharktits,3507"
, "SheLikesItRough,46307"
, "Shemales,18524"
, "ShinyPorn,33412"
, "shorthairchicks,32275"
, "sideboob,12252"
, "simps,38791"
, "Singlets,5067"
, "Sissies,18319"
, "sissyhypno,12887"
, "Sissyperfection,7430"
, "skinnytail,25088"
, "skivvies,10936"
, "slightcellulite,3263"
, "slimgirls,14166"
, "SluttyHalloween,22066"
, "SluttyStrangers,24537"
, "slutwife,9641"
, "smalldickporn,524"
, "smashbros34,4666"
, "snapchat_sluts,26970"
, "snapleaks,20340"
, "SocialMediaSluts,11252"
, "sodomy,12505"
, "softies,6895"
, "SoFuckable,14879"
, "Spanking,13720"
, "spreadeagle,14882"
, "SpreadEm,20109"
, "spreading,13811"
, "squirting,40828"
, "ssbbw,3072"
, "Stacked,74848"
, "Steroidsourcetalk,7141"
, "stockings,53328"
, "StomachDownFeetUp,7113"
, "StraightGirlsPlaying,64462"
, "straponlesbians,2754"
, "StretchingIt,9820"
, "StruggleFucking,35561"
, "StrugglePorn,11899"
, "Stuffers,3886"
, "suicidegirls,87512"
, "Sukebei,8178"
, "superheroporn,4904"
, "sweatermeat,28465"
, "Swingersgw,17068"
, "TallGoneWild,22606"
, "tanlines,31041"
, "TBoIRule34,1089"
, "Tgifs,12920"
, "Tgirls,42572"
, "thefullbush,8242"
, "TheHangingBoobs,25255"
, "TheHottestBabes,1734"
, "theratio,18115"
, "TheRedFox,22052"
, "TheUnderboob,30281"
, "TheUnderbun,32463"
, "thick,92258"
, "ThickChixxx,17944"
, "thickloads,32225"
, "ThickThighs,13548"
, "thighhighs,26000"
, "thinspo,38863"
, "Throatfucking,9389"
, "throatpies,31332"
, "tightdresses,99184"
, "TightsAndTightClothes,3033"
, "TightShorts,30016"
, "tight_shorts,34247"
, "TinyAsianTits,7369"
, "TinyTits,103655"
, "tipofmypenis,69706"
, "titfuck,23529"
, "tits,20395"
, "Titties,14990"
, "TittyDrop,97809"
, "torpedotits,18064"
, "TotallyStraight,23505"
, "transformation,4418"
, "translucent_porn,14520"
, "transporn,1341"
, "traps,38877"
, "trashyboners,39484"
, "TrashyPorn,6319"
, "treesgonewild,70558"
, "TributeMe,31704"
, "twerking,12030"
, "TwinGuys,559"
, "twinks,14936"
, "Unashamed,86525"
, "UncutPorn,9592"
, "UnderTail,1255"
, "UnderwearGW,36741"
, "upherbutt,13848"
, "Upskirt,71682"
, "usedpanties,9873"
, "V<NAME>anny,486"
, "vagina,19014"
, "vikingsgonewild,6364"
, "VintageBabes,21387"
, "voluptuous,59581"
, "Vore,3307"
, "watchitforthecat,7063"
, "WatchItForThePlot,140279"
, "watchpeopledie,92815"
, "watersports,9380"
, "WeAllGoWild,6888"
, "WeddingRingsShowing,20896"
, "WeddingsGoneWild,26648"
, "WesternHentai,22661"
, "wet,29784"
, "WhipItOut,2715"
, "whooties,15565"
, "whynotasource,12838"
, "wifesharing,71509"
, "wildchicks,19547"
, "wincest,29725"
, "WomenOfColor,41357"
, "womenofcolorXXX,17855"
, "WomenOfColour,26912"
, "workgonewild,82309"
, "WorkIt,9982"
, "Workoutgonewild,23199"
, "WouldYouFuckMyWife,37655"
, "WtSSTaDaMiT,91151"
, "XChangePill,4260"
, "xray,17712"
, "xsmallgirls,36517"
, "Xsome,28602"
, "xxxcaptions,12808"
, "yaoi,7060"
, "YAYamateurs,29424"
, "yiff,27964"
, "yiffcomics,7957"
, "YogaPants,43138"
, "youngporn,16792"
, "youtubetitties,34514"
, "yuri,9468"
]
| true | module Nsfw (..) where
nsfwRaw : List String
nsfwRaw =
[ "18_19,24484"
, "60fpsporn,148203"
, "aa_cups,23506"
, "AbbyWinters,12577"
, "ABDL,5852"
, "AbusePorn2,14554"
, "accidentalnudity,27933"
, "adorableporn,14666"
, "adultgifs,29753"
, "after_the_shot,14139"
, "AgedBeauty,17686"
, "AgeplayPenPals,3997"
, "ahegao,10317"
, "alteredbuttholes,4324"
, "altgonewild,66176"
, "Amateur,244751"
, "AmateurArchives,87045"
, "amateurcumsluts,45204"
, "AmateurPorn,13364"
, "AmateurWifes,10962"
, "amazingtits,2490"
, "AnaCheri,2180"
, "anal,81903"
, "AnalGW,48267"
, "AnimeBooty,3344"
, "AnimeFeet,1310"
, "animeplot,14694"
, "Anjelica_Ebbi,32289"
, "ArabPorn,9546"
, "areolas,7667"
, "ariane,2084"
, "arielrebel,13053"
, "asaakira,8006"
, "AsianCumsluts,12412"
, "AsianFetish,9068"
, "AsianHotties,118250"
, "AsianNSFW,44501"
, "AsianPorn,33780"
, "Asianpornin15seconds,6637"
, "AsiansGoneWild,132028"
, "AskRedditAfterDark,21313"
, "ass,212858"
, "asshole,45189"
, "AssholeBehindThong,26892"
, "assholegonewild,19089"
, "assinthong,48561"
, "asslick,19197"
, "asstastic,123590"
, "AthleticGirls,33227"
, "AvatarPorn,13156"
, "avocadosgonewild,24228"
, "awwyea,3000"
, "BadDragon,5626"
, "Balls,4385"
, "ballsdeepandcumming,4990"
, "BBCSluts,9657"
, "BBW,22720"
, "BBWGW,23910"
, "B_Cups,13776"
, "bdsm,58817"
, "BDSMcommunity,44370"
, "BDSMGW,39917"
, "BDSMpersonals,15920"
, "BeautifulTitsAndAss,13457"
, "beef_flaps,12633"
, "before_after_cumsluts,30887"
, "Bestiality,6711"
, "bestofblowjobs,6505"
, "bestofboobies,9856"
, "bestofcollege,32777"
, "bestofcumsluts,13574"
, "bestoflingerie,3900"
, "bestofworldstar,43773"
, "Beth_Lily,6372"
, "BHMGoneWild,5031"
, "bigasses,38039"
, "bigboobs,15021"
, "BigBoobsGonewild,30051"
, "BigBoobsGW,63596"
, "bigclit,7054"
, "bigonewild,9797"
, "Bigtitssmalltits,13541"
, "bikinibridge,20064"
, "bikinis,40786"
, "bimbofetish,41761"
, "bimbofication,7362"
, "blackchickswhitedicks,21792"
, "BlackCrimeMatters,1536"
, "Blonde,30956"
, "blowbang,9638"
, "BlowJob,11002"
, "blowjob_eyes,8850"
, "BlowjobGifs,26677"
, "Blowjobs,119089"
, "blowjobsandwich,25966"
, "bodyperfection,20335"
, "bodyshots,24452"
, "boltedontits,61128"
, "Bondage,67688"
, "BonerMaterial,64112"
, "boobbounce,55981"
, "boobgifs,21473"
, "Boobies,219679"
, "boobs,44694"
, "booty,28469"
, "BorednIgnored,30986"
, "Bottomless_Vixens,44832"
, "Brazzers_Network,3069"
, "BreastEnvy,32075"
, "BreastExpansion,9933"
, "breastsucking,3878"
, "Breeding,17945"
, "broslikeus,19536"
, "brunette,30380"
, "BubbleButts,21270"
, "bubbling,9960"
, "Bulges,9188"
, "BurningLibrary,35"
, "burstingout,85792"
, "bustyasians,20150"
, "bustybabes,22596"
, "Bustyfit,12946"
, "BustyNats,3729"
, "BustyNaturals,8845"
, "BustyPetite,233193"
, "butterface,7741"
, "buttplug,45727"
, "ButtsAndBareFeet,12313"
, "buttsex,13970"
, "CamelToeGirls,13152"
, "CamSluts,20545"
, "camwhores,57858"
, "CandidFashionPolice,65014"
, "cardsagainsthumanity,25841"
, "cat_girls,21561"
, "CelebFakes,25971"
, "celebnsfw,139023"
, "CelebOops,8986"
, "CelebrityButts,20657"
, "CelebrityNipples,19472"
, "CelebrityPussy,37756"
, "celebsnaked,29058"
, "celebsunleashed,9458"
, "cfnm,23842"
, "CharlotteMcKinney,3941"
, "chastity,7850"
, "cheatingwives,6914"
, "CheekyBottoms,10873"
, "chesthairporn,7507"
, "chickflixxx,35961"
, "choking,7219"
, "ChristianGirls,45343"
, "chubby,36225"
, "cinnamonmermaids,1223"
, "cleavage,37843"
, "ClopClop,16429"
, "cocaine,6289"
, "cock,8378"
, "CockOutline,3647"
, "collared,32214"
, "CollegeAmateurs,49920"
, "collegesluts,99495"
, "comics18_story,14893"
, "ConfusedBoners,39957"
, "consentacles,4110"
, "cougars,16995"
, "couplesgonewild,11583"
, "creampie,20395"
, "creampiegifs,19352"
, "creampies,60796"
, "crouch,3451"
, "Cuckold,43103"
, "cuckoldcaptions,6454"
, "cumcoveredfucking,42377"
, "cumfetish,60077"
, "CumHaters,31134"
, "cumontongue,6670"
, "cumshots,16021"
, "cumsluts,203095"
, "CumSwallowing,11310"
, "curvy,119160"
, "cutecunts,6710"
, "CuteGuyButts,8870"
, "CuteModeSlutMode,46138"
, "DadsGoneWild,496"
, "damngoodinterracial,14987"
, "DanielleSharp,5570"
, "DarkAngels,36217"
, "DarkHumor,4633"
, "DarkNetMarkets,80737"
, "datgap,93167"
, "datgrip,11198"
, "deepthroat,40451"
, "DegradingHoles,5571"
, "DeliciousTraps,5631"
, "DesiBoners,6455"
, "PI:NAME:<NAME>END_PI,841"
, "DirtyFamilyPhotos,13784"
, "dirtykikpals,32993"
, "dirtypenpals,74555"
, "dirtyr4r,40526"
, "dirtysmall,136317"
, "DirtySnapchat,31502"
, "disneyporn,9475"
, "distension,40247"
, "doppelbangher,26184"
, "dota2smut,6949"
, "doublepenetration,8700"
, "doujinshi,21636"
, "downblouse,45814"
, "DownBra,9851"
, "dreamjobs,13943"
, "drunkencookery,12918"
, "DSLs,9883"
, "DumpsterSluts,20270"
, "dykesgonewild,34039"
, "easterneuropeangirls,12899"
, "Ebony,28317"
, "ecchi,37105"
, "ecchigifs,2582"
, "eFukt,5453"
, "PI:NAME:<NAME>END_PI_cs,2615"
, "emmaglover,4749"
, "EngorgedVeinyBreasts,15262"
, "EntExchange,6696"
, "ErectElephantDicks,16"
, "eroticauthors,5308"
, "ExhibitionistSex,23485"
, "exotic_oasis,9619"
, "exposedinpublic,18077"
, "extreme_gifs,3581"
, "Exxxtras,45153"
, "facedownassup,86307"
, "FaceFuck,16794"
, "facesitting,39903"
, "FacialFun,39472"
, "Facials,15022"
, "FapFap,6102"
, "faponher,6893"
, "FearMe,33613"
, "feet,24237"
, "FemBoys,10116"
, "Femdom,24830"
, "FestivalSluts,72778"
, "FiftyFifty,288727"
, "FilthyGirls,20789"
, "Fisting,16730"
, "fit2phat,1325"
, "fitdrawngirls,2895"
, "fitgirls,57889"
, "FlashingGirls,42831"
, "flatchests,7015"
, "flexi,13923"
, "FMN,6707"
, "forcedorgasms,33891"
, "foreskin,9331"
, "freeuse,30250"
, "Fretton,2849"
, "fuckyeahcollegesluts,11251"
, "fuckyeahdrunksluts,18154"
, "fuckyeahfitgirls,4683"
, "fuckyeahsluttybimbos,4773"
, "fuckyeahsofuckable,6058"
, "funsized,28628"
, "FunWithFriends,25949"
, "futanari,36809"
, "fuxtaposition,25951"
, "fyeahsexyteens,10676"
, "gag_spit,11724"
, "gangbang,22923"
, "GarterBelts,12460"
, "gaybears,9790"
, "GaybrosGoneWild,30015"
, "GayCreampie,835"
, "gaycumsluts,10079"
, "GayDaddiesPics,5837"
, "GayGifs,15200"
, "gayincest,5344"
, "GayKink,6469"
, "gaymersgonewild,18877"
, "gaynsfw,14604"
, "gayporn,15475"
, "gaypornhunters,9155"
, "gaystoriesgonewild,10478"
, "Gemplugs,3831"
, "gettingherselfoff,61269"
, "gfur,7117"
, "ghostnipples,4787"
, "GiPI:NAME:<NAME>END_PI,16702"
, "gifcest,1567"
, "gifsgonewild,52697"
, "Gif_source,149"
, "gilf,12717"
, "ginger,140484"
, "GirlsCuddling,7441"
, "girlsdoingnerdythings,27814"
, "girlsdoporn,9926"
, "GirlsFinishingTheJob,171255"
, "girlsflashing,11572"
, "GirlsHumpingThings,29849"
, "girlsinleggings,24975"
, "GirlsinSchoolUniforms,31825"
, "GirlsinStripedSocks,32691"
, "GirlsInTheBathroom,389"
, "girlsinyogapants,171734"
, "girlskissing,34111"
, "girlswhoride,12617"
, "GirlswithGlasses,121113"
, "GirlswithNeonHair,46301"
, "Gloryholes,11188"
, "GoneErotic,9821"
, "GoneInsane,19856"
, "GoneMild,65200"
, "Gonewild18,1274"
, "gonewild30plus,13852"
, "gonewild,843110"
, "gonewildaudio,78812"
, "GoneWildCD,16785"
, "gonewildchubby,5193"
, "gonewildcolor,53520"
, "gonewildcouples,39524"
, "gonewildcurvy,168173"
, "GoneWildHairy,22057"
, "GoneWildPlus,92479"
, "GoneWildScrubs,20356"
, "GoneWildSmiles,39168"
, "gonewildstories,96304"
, "GoneWildTrans,18419"
, "GoneWildTube,102715"
, "gonwild,21212"
, "grool,79211"
, "GroupOfNudeGirls,41008"
, "GushingGirls,15189"
, "GuysFromBehind,3672"
, "GWABackstage,1210"
, "GWbanned,11034"
, "gwcosplay,11527"
, "GWCouples,107728"
, "gwcumsluts,63864"
, "GWNerdy,78086"
, "gwpublic,27365"
, "hadom,3829"
, "HairyArmpits,3435"
, "HairyPussy,22641"
, "handbra,12802"
, "HappyEmbarrassedGirls,121185"
, "hardanal,9938"
, "HardBoltOns,8857"
, "havoc_bot,6681"
, "hentai,95242"
, "hentaibondage,14956"
, "HENTAI_GIF,27931"
, "HerBush,4007"
, "highheelsNSFW,16805"
, "HighMileageHoles,9416"
, "HighResNSFW,83488"
, "hipcleavage,13387"
, "holdmyturban,13832"
, "holdthemoan,223742"
, "homegrowntits,42553"
, "HomemadePorn,9947"
, "homemadexxx,122095"
, "Hotchickswithtattoos,120592"
, "Hotwife,61092"
, "hugeboobs,77828"
, "Hugeboobshardcore,21026"
, "HugeDickTinyChick,29890"
, "hugefutanari,4664"
, "hugenaturals,20316"
, "HungryButts,16331"
, "Hyiff,2296"
, "iateacrayon,6315"
, "Ifyouhadtopickone,14070"
, "ImaginaryBoners,10338"
, "ImGoingToHellForThis,503147"
, "incest,55424"
, "IncestComics,18632"
, "Incest_Gifs,3856"
, "IncestPorn,27977"
, "IndianBabes,48961"
, "IndianPorn,9680"
, "IndiansGoneWild,24650"
, "IndianTeens,12059"
, "Innie,43412"
, "insertions,32142"
, "IntenseBDSM,6422"
, "iWantToFuckHer,47799"
, "JapanesePorn2,19840"
, "JavDownloadCenter,15046"
, "Jewdank,20970"
, "JiggleFuck,21003"
, "jilling,76929"
, "jobuds,7193"
, "joi,18674"
, "juicyasians,80772"
, "JustOneBoob,12369"
, "KanMusuNights,1975"
, "KateeOwen,26976"
, "kati3kat,7846"
, "kinderyiff,2342"
, "kinksters_gone_wild,15872"
, "KinkyStuff,3431"
, "kpopfap,27478"
, "LabiaGW,31435"
, "lactation,14580"
, "ladybonersgw,73296"
, "latinas,43997"
, "LatinasGW,16088"
, "LegalCollegeGirls,1681"
, "LegalTeens,185026"
, "LegalTeensXXX,30374"
, "legendarylootz,6324"
, "leggingsgonewild,24557"
, "legs,40997"
, "legsup,20993"
, "legwrap,23286"
, "leotards,27721"
, "lesbians,83608"
, "lesserknownpornstars,8051"
, "lewdgestures,4194"
, "lineups,12259"
, "lingerie,52073"
, "LipsThatGrip,102947"
, "littlespace,8004"
, "lolgrindr,10393"
, "lovegaymale,14054"
, "LoveToWatchYouLeave,18542"
, "MakeUpFetish,11899"
, "MalPI:NAME:<NAME>END_PI,10088"
, "manass,6408"
, "mangonewild,18777"
, "MassiveCock,36277"
, "MasterOfAnal,17320"
, "MastersOfAnal,362"
, "maturewomen,10179"
, "MCBourbonnais,2616"
, "mellisaclarke,6928"
, "MensHighJinx,5700"
, "MiddleEasternHotties,16949"
, "milf,189385"
, "Milfie,10295"
, "Milfinstockings,3494"
, "milf_nowandforever,6478"
, "MilitaryGoneWild,28966"
, "MistressPorn,3998"
, "mmgirls,29077"
, "monster_cocks,8243"
, "MonsterGirl,15410"
, "Mooning,18263"
, "MorbidReality,199435"
, "Morphs,4714"
, "MoundofVenus,21951"
, "mycleavage,17212"
, "Naruto_HPI:NAME:<NAME>END_PIai,8227"
, "naturaltitties,18749"
, "Nekomimi,9066"
, "Nipples,31889"
, "nipslip,21758"
, "normalnudes,19985"
, "notgayporn,3849"
, "NotSafeForNature,33964"
, "NowYouReallySeeMe,12900"
, "nsfw2,85112"
, "NSFW411,41427"
, "nsfw,619457"
, "Nsfw_Amateurs,30250"
, "nsfwasmr,17370"
, "NSFWBarista,11093"
, "nsfwcelebgifs,14493"
, "nsfwcosplay,92462"
, "NSFWCostumes,29258"
, "NSFWfashion,9646"
, "NSFWFunny,120163"
, "nsfwgif,11381"
, "NSFW_GIF,444769"
, "nsfw_gifs,275755"
, "nsfwhardcore,149726"
, "NSFW_HTML5,84757"
, "NSFWIAMA,17481"
, "NSFW_Japan,48017"
, "NSFW_Korea,21277"
, "NSFW_nospam,36611"
, "nsfwoutfits,80662"
, "NSFW_Plowcam,16101"
, "NSFW_Snapchat,46100"
, "nsfwsports,15357"
, "nsfw_videos,54269"
, "NSFW_Wallpapers,27968"
, "nsfw_wtf,41894"
, "Objects,28620"
, "oculusnsfw,8319"
, "O_Faces,88301"
, "OffensiveSpeech,2068"
, "Oilporn,25106"
, "OldSchoolCoolNSFW,28864"
, "omgbeckylookathiscock,27781"
, "OnHerKnees,44792"
, "OnOff,184350"
, "OpenShirt,10388"
, "outercourse,27863"
, "Page3Glamour,28383"
, "painal,32954"
, "palegirls,100724"
, "paleskin,7065"
, "pantsu,17621"
, "Pantyfetish,28625"
, "pantyhose,10234"
, "passionx,59476"
, "pawg,48293"
, "Pee,20091"
, "peegonewild,15600"
, "Pegging,34258"
, "pelfie,19023"
, "penis,28641"
, "petite,21158"
, "PetiteGirls,11221"
, "PetiteGoneWild,138254"
, "photoplunder,48664"
, "PiercedNSFW,19428"
, "Playboy,25672"
, "PlayfulGirls,5082"
, "PokePorn,28191"
, "pokies,39944"
, "porn,61231"
, "porn_gifs,65373"
, "pornID,25944"
, "porninfifteenseconds,69115"
, "porn_irl,1387"
, "PornStars,18744"
, "pornvids,144272"
, "preggo,6602"
, "PreggoPorn,29370"
, "prematurecumshots,5118"
, "Presenting,18893"
, "primes,16123"
, "pronebone,11836"
, "PublicBoys,10645"
, "PublicFlashing,40754"
, "Puffies,15400"
, "PunkGirls,18050"
, "pussy,90627"
, "pussyjobs,13757"
, "PussyJuices,11737"
, "PussyMound,18778"
, "quiver,42504"
, "RandomActsOfBlowJob,57817"
, "RandomActsOfMuffDive,26518"
, "randomsexiness,108173"
, "RateMyNudeBody,15430"
, "RealGirls,500214"
, "realmoms,9517"
, "rearpussy,68313"
, "RedditorCum,6165"
, "reddtube,1483"
, "redheads,130037"
, "RemyLaCroix,22780"
, "RepressedGoneWild,26693"
, "ResearchMarkets,3461"
, "RollaGoneWild,196"
, "RopesandRoundThings,524"
, "RugsOnly,14065"
, "RuinedOrgasms,25075"
, "rule34,163083"
, "rule34celebs,6481"
, "rule34_comics,45072"
, "rule34feet,3842"
, "Rule34LoL,25018"
, "russiangirls,8482"
, "RWBYNSFW,3191"
, "Saggy,13386"
, "SaraJUnderwood,11930"
, "scissoring,26368"
, "sea_girls,8563"
, "seethru,29190"
, "selfservice,8018"
, "sex_comics,35364"
, "SexiestPetites,18782"
, "SexInFrontOfOthers,32286"
, "Sexsells,11753"
, "SexShows,9930"
, "SexToys,11036"
, "Sexy,31638"
, "SexyButNotPorn,43830"
, "SexyFrex,48227"
, "SexyGirlsInBoots,17162"
, "sexygirlsinjeans,22536"
, "sexytgirls,7684"
, "SexyTummies,17663"
, "Shadman,3095"
, "Sharktits,3507"
, "SheLikesItRough,46307"
, "Shemales,18524"
, "ShinyPorn,33412"
, "shorthairchicks,32275"
, "sideboob,12252"
, "simps,38791"
, "Singlets,5067"
, "Sissies,18319"
, "sissyhypno,12887"
, "Sissyperfection,7430"
, "skinnytail,25088"
, "skivvies,10936"
, "slightcellulite,3263"
, "slimgirls,14166"
, "SluttyHalloween,22066"
, "SluttyStrangers,24537"
, "slutwife,9641"
, "smalldickporn,524"
, "smashbros34,4666"
, "snapchat_sluts,26970"
, "snapleaks,20340"
, "SocialMediaSluts,11252"
, "sodomy,12505"
, "softies,6895"
, "SoFuckable,14879"
, "Spanking,13720"
, "spreadeagle,14882"
, "SpreadEm,20109"
, "spreading,13811"
, "squirting,40828"
, "ssbbw,3072"
, "Stacked,74848"
, "Steroidsourcetalk,7141"
, "stockings,53328"
, "StomachDownFeetUp,7113"
, "StraightGirlsPlaying,64462"
, "straponlesbians,2754"
, "StretchingIt,9820"
, "StruggleFucking,35561"
, "StrugglePorn,11899"
, "Stuffers,3886"
, "suicidegirls,87512"
, "Sukebei,8178"
, "superheroporn,4904"
, "sweatermeat,28465"
, "Swingersgw,17068"
, "TallGoneWild,22606"
, "tanlines,31041"
, "TBoIRule34,1089"
, "Tgifs,12920"
, "Tgirls,42572"
, "thefullbush,8242"
, "TheHangingBoobs,25255"
, "TheHottestBabes,1734"
, "theratio,18115"
, "TheRedFox,22052"
, "TheUnderboob,30281"
, "TheUnderbun,32463"
, "thick,92258"
, "ThickChixxx,17944"
, "thickloads,32225"
, "ThickThighs,13548"
, "thighhighs,26000"
, "thinspo,38863"
, "Throatfucking,9389"
, "throatpies,31332"
, "tightdresses,99184"
, "TightsAndTightClothes,3033"
, "TightShorts,30016"
, "tight_shorts,34247"
, "TinyAsianTits,7369"
, "TinyTits,103655"
, "tipofmypenis,69706"
, "titfuck,23529"
, "tits,20395"
, "Titties,14990"
, "TittyDrop,97809"
, "torpedotits,18064"
, "TotallyStraight,23505"
, "transformation,4418"
, "translucent_porn,14520"
, "transporn,1341"
, "traps,38877"
, "trashyboners,39484"
, "TrashyPorn,6319"
, "treesgonewild,70558"
, "TributeMe,31704"
, "twerking,12030"
, "TwinGuys,559"
, "twinks,14936"
, "Unashamed,86525"
, "UncutPorn,9592"
, "UnderTail,1255"
, "UnderwearGW,36741"
, "upherbutt,13848"
, "Upskirt,71682"
, "usedpanties,9873"
, "VPI:NAME:<NAME>END_PIanny,486"
, "vagina,19014"
, "vikingsgonewild,6364"
, "VintageBabes,21387"
, "voluptuous,59581"
, "Vore,3307"
, "watchitforthecat,7063"
, "WatchItForThePlot,140279"
, "watchpeopledie,92815"
, "watersports,9380"
, "WeAllGoWild,6888"
, "WeddingRingsShowing,20896"
, "WeddingsGoneWild,26648"
, "WesternHentai,22661"
, "wet,29784"
, "WhipItOut,2715"
, "whooties,15565"
, "whynotasource,12838"
, "wifesharing,71509"
, "wildchicks,19547"
, "wincest,29725"
, "WomenOfColor,41357"
, "womenofcolorXXX,17855"
, "WomenOfColour,26912"
, "workgonewild,82309"
, "WorkIt,9982"
, "Workoutgonewild,23199"
, "WouldYouFuckMyWife,37655"
, "WtSSTaDaMiT,91151"
, "XChangePill,4260"
, "xray,17712"
, "xsmallgirls,36517"
, "Xsome,28602"
, "xxxcaptions,12808"
, "yaoi,7060"
, "YAYamateurs,29424"
, "yiff,27964"
, "yiffcomics,7957"
, "YogaPants,43138"
, "youngporn,16792"
, "youtubetitties,34514"
, "yuri,9468"
]
| elm |
[
{
"context": "{--\n\nCopyright (c) 2016, William Whitacre\nAll rights reserved.\n\nRedistribution and use in s",
"end": 41,
"score": 0.9998480678,
"start": 25,
"tag": "NAME",
"value": "William Whitacre"
}
] | src/Scaffold/Machine.elm | williamwhitacre/scaffold | 8 | {--
Copyright (c) 2016, William Whitacre
All rights reserved.
Redistribution and use in source and binary forms, with or without modification, are permitted
provided that the following conditions are met:
1. Redistributions of source code must retain the above copyright notice, this list of conditions
and the following disclaimer.
2. Redistributions in binary form must reproduce the above copyright notice, this list of conditions
and the following disclaimer in the documentation and/or other materials provided with the
distribution.
3. Neither the name of the copyright holder nor the names of its contributors may be used to endorse
or promote products derived from this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR
IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND
FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR
CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER
IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF
THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
--}
module Scaffold.Machine
(Machine,
machine,
getModel, replaceModel,
dispatching, dispatchingAs,
presenting, presentingAs,
staging, stagingAs,
updating, integrate,
maybeGetModel, maybeReplaceModel,
maybeDispatching, maybeDispatchingAs,
maybePresenting, maybePresentingAs,
maybeStaging, maybeStagingAs,
maybeUpdating, maybeIntegrate,
machineResource,
resourceGetModel,
resourceReplaceModel,
resourceDispatching,
resourceDispatchingAs,
resourceIntegrate,
resourceUpdating,
resourceStaging,
resourceStagingAs,
resourcePresenting,
resourcePresentingAs
)
where
{-| This module builds a more usable state machine snapshot with the Elm Architecture in mind on
top of the Gigan App.
# Definition
@docs Machine
# Constructor
@docs machine
# Get and Set the Model
@docs getModel, replaceModel
# Using Machines
@docs dispatching, dispatchingAs, presenting, presentingAs, staging, stagingAs, updating, integrate
# Get and Set the Model of Maybe Machines
@docs maybeGetModel, maybeReplaceModel
# Optional Machines Using Maybe
@docs maybeDispatching, maybeDispatchingAs, maybePresenting, maybePresentingAs, maybeStaging, maybeStagingAs, maybeUpdating, maybeIntegrate
# Resource Dependant Machines
@docs machineResource, resourceGetModel, resourceReplaceModel, resourceDispatching, resourceDispatchingAs, resourceIntegrate, resourceUpdating, resourceStaging, resourceStagingAs, resourcePresenting, resourcePresentingAs
-}
import Scaffold.App exposing (..)
import Scaffold.Resource as Resource exposing (Resource)
import Set exposing (Set)
import Dict exposing (Dict)
import Time exposing (Time)
{-| A Machine is an instantaneous description of some model associated with an ProgramInput (see App)
that specifies how it should be updated, staged and presented. -}
type alias Machine a b c bad =
{ input : ProgramInput a b c bad
, state : ProgramSnapshot a b bad
}
{-| Create a machine from an ProgramInput -}
machine : ProgramInput a b c bad -> Machine a b c bad
machine input =
{ input = input
, state = programSnapshot input.model0 (dispatchTasks [])
}
{-| Get the model of a machine. -}
getModel : Machine a b c bad -> b
getModel machine' =
machine'.state.model'
{-| Create a machine with a different model from a replacement model and an original machine. -}
replaceModel : b -> Machine a b c bad -> Machine a b c bad
replaceModel model' machine' =
let machine'state = machine'.state in
{ machine' | state = { machine'state | model' = model' } }
-- FLAT STEM OPERATORS
{-| Dispatch the tasks of a Machine. This function is in the depreciated family of `dispatch'*`
functions. Use `dispatching*` and `integrate` instead. -}
dispatch' : Machine a b c bad -> (Machine a b c bad, TaskDispatchment bad a)
dispatch' machine' =
programSnapshotDispatch machine'.state
|> \result -> ({ machine' | state = fst result }, snd result)
{-| Get the currently waiting dispatchment. -}
dispatching : Machine a b c bad -> TaskDispatchment bad a
dispatching =
dispatch' >> snd
{-| Dispatch the tasks of a Machine using some transducer to translate the action list. This function
is in the depreciated family of `dispatch'*` functions. Use `dispatching*` and
`integrate` instead. -}
dispatchAs' : (List a -> List a') -> Machine a b c bad -> (Machine a b c bad, TaskDispatchment bad a')
dispatchAs' xdcr machine' =
dispatch' machine'
|> \(machine'', dispatchment) -> dispatchment
|> promoteDispatchment xdcr
|> \dispatchment' -> (machine'', dispatchment')
{-| Get the currently waiting task dispatchment, but apply some action type transformation. -}
dispatchingAs : (List a -> List a') -> Machine a b c bad -> TaskDispatchment bad a'
dispatchingAs xdcr =
dispatchAs' xdcr >> snd
{-| Remove the currently waiting task dispatchment. This should be done only after retrieving any
possibly waiting dispatchment. -}
integrate : Machine a b c bad -> Machine a b c bad
integrate =
dispatch' >> fst
{-| Run the update function from the configured ProgramInput of the Machine on the Machine's current model to produce a new model and dispatch any asynchronous tasks desired.
This takes a list of actions, the current time, and the Machine. -}
updating : List a -> Time -> Machine a b c bad -> Machine a b c bad
updating actions now machine' =
{ machine' | state = programSnapshotUpdate machine'.input actions now machine'.state }
{-| Run the update function from the configured ProgramInput of the Machine on the Machine's current model to produce a new model and dispatch any asynchronous tasks desired.
This takes an address for actions to be sent to, the current time, and the Machine. -}
staging : Signal.Address (List a) -> Time -> Machine a b c bad -> Machine a b c bad
staging address now machine' =
{ machine' | state = programSnapshotStage machine'.input address now machine'.state }
{-| Same as staging, but with a transformation to apply to action lists before they are sent to the address. -}
stagingAs : (List a -> List a') -> Signal.Address (List a') -> Time -> Machine a b c bad -> Machine a b c bad
stagingAs xdcr address =
staging (Signal.forwardTo address xdcr)
{-| Run the present function from the configured ProgramInput of the Machine on the Machine's current model to produce a ViewOutput, which includes the an element of the Machine's view type, and a TaskDispatchment for any desired asynchronous tasks.
This takes an address for actions to be sent to, the current time, and the Machine. -}
presenting : Signal.Address (List a) -> Time -> Machine a b c bad -> ViewOutput a c bad
presenting address now machine' =
programSnapshotPresent machine'.input address now machine'.state
{-| Same as presenting, but with a transformation to apply to action lists before they are sent to the address. -}
presentingAs : (List a -> List a') -> Signal.Address (List a') -> Time -> Machine a b c bad -> ViewOutput a' c bad
presentingAs xdcr address now machine' =
presenting (Signal.forwardTo address xdcr) now machine'
|> \view' -> { view' | dispatchment = promoteDispatchment xdcr view'.dispatchment }
{--
OPTIONAL MACHINES USING MAYBE
--}
{-| Create a possible machine from possible model given a partial program definition (without the
model). -}
maybeMachine : (b -> ProgramInput a b c bad) -> Maybe b -> Maybe (Machine a b c bad)
maybeMachine modelInput maybeModel =
Maybe.map (modelInput >> machine >> Just) maybeModel
|> Maybe.withDefault Nothing
{-| -}
maybeGetModel : Maybe (Machine a b c bad) -> Maybe b
maybeGetModel =
Maybe.map (.state >> .model')
{-| -}
maybeReplaceModel : b -> Maybe (Machine a b c bad) -> Maybe (Machine a b c bad)
maybeReplaceModel model' =
Maybe.map (replaceModel model')
{-| -}
maybeDispatch : Maybe (Machine a b c bad) -> Maybe (Machine a b c bad, TaskDispatchment bad a)
maybeDispatch =
Maybe.map dispatch'
{-| -}
maybeDispatching : Maybe (Machine a b c bad) -> TaskDispatchment bad a
maybeDispatching machine' =
Maybe.map dispatching machine'
|> Maybe.withDefault (dispatchTasks [])
-- variant of dispatch' that accepts a transducer with which to promote actions.
{-| -}
maybeDispatchAs : (List a -> List a') -> Maybe (Machine a b c bad) -> Maybe (Machine a b c bad, TaskDispatchment bad a')
maybeDispatchAs xdcr =
Maybe.map (dispatchAs' xdcr)
{-| -}
maybeDispatchingAs : (List a -> List a') -> Maybe (Machine a b c bad) -> TaskDispatchment bad a'
maybeDispatchingAs xdcr machine' =
Maybe.map (dispatchingAs xdcr) machine'
|> Maybe.withDefault (dispatchTasks [])
{-| -}
maybeIntegrate : Maybe (Machine a b c bad) -> Maybe (Machine a b c bad)
maybeIntegrate =
Maybe.map integrate
{-| -}
maybeUpdating : List a -> Time -> Maybe (Machine a b c bad) -> Maybe (Machine a b c bad)
maybeUpdating actions now =
Maybe.map (updating actions now)
{-| -}
maybeStaging : Signal.Address (List a) -> Time -> Maybe (Machine a b c bad) -> Maybe (Machine a b c bad)
maybeStaging address now =
Maybe.map (staging address now)
{-| -}
maybeStagingAs : (List a -> List a') -> Signal.Address (List a') -> Time -> Maybe (Machine a b c bad) -> Maybe (Machine a b c bad)
maybeStagingAs xdcr address now =
Maybe.map (stagingAs xdcr address now)
{-| -}
maybePresenting : Signal.Address (List a) -> Time -> Maybe (Machine a b c bad) -> Maybe (ViewOutput a c bad)
maybePresenting address now =
Maybe.map (presenting address now)
{-| -}
maybePresentingAs : (List a -> List a') -> Signal.Address (List a') -> Time -> Maybe (Machine a b c bad) -> Maybe (ViewOutput a' c bad)
maybePresentingAs xdcr address now =
Maybe.map (presentingAs xdcr address now)
{--
RESOURCE DEPENDANT MACHINES USING RESOURCE
--}
{-| Create a machine resource from a model resource given a partial program definition (without the
model). -}
machineResource : (b -> ProgramInput a b c bad) -> Resource euser b -> Resource euser (Machine a b c bad)
machineResource modelInput modelResource =
Resource.toProgram modelInput modelResource
|> Resource.therefore machine
{-| -}
resourceGetModel : Resource euser (Machine a b c bad) -> Resource euser b
resourceGetModel =
Resource.therefore (.state >> .model')
{-| -}
resourceReplaceModel : b -> Resource euser (Machine a b c bad) -> Resource euser (Machine a b c bad)
resourceReplaceModel model' =
Resource.therefore (replaceModel model')
{-| -}
resourceDispatching : Resource euser (Machine a b c bad) -> TaskDispatchment bad a
resourceDispatching machine' =
Resource.therefore dispatching machine'
|> Resource.otherwise (dispatchTasks [])
{-| -}
resourceDispatchingAs : (List a -> List a') -> Resource euser (Machine a b c bad) -> TaskDispatchment bad a'
resourceDispatchingAs xdcr machine' =
Resource.therefore (dispatchingAs xdcr) machine'
|> Resource.otherwise (dispatchTasks [])
{-| -}
resourceIntegrate : Resource euser (Machine a b c bad) -> Resource euser (Machine a b c bad)
resourceIntegrate =
Resource.therefore integrate
{-| -}
resourceUpdating : List a -> Time -> Resource euser (Machine a b c bad) -> Resource euser (Machine a b c bad)
resourceUpdating actions now =
Resource.therefore (updating actions now)
{-| -}
resourceStaging : Signal.Address (List a) -> Time -> Resource euser (Machine a b c bad) -> Resource euser (Machine a b c bad)
resourceStaging address now =
Resource.therefore (staging address now)
{-| -}
resourceStagingAs : (List a -> List a') -> Signal.Address (List a') -> Time -> Resource euser (Machine a b c bad) -> Resource euser (Machine a b c bad)
resourceStagingAs xdcr address now =
Resource.therefore (stagingAs xdcr address now)
{-| -}
resourcePresenting : Signal.Address (List a) -> Time -> Resource euser (Machine a b c bad) -> Resource euser (ViewOutput a c bad)
resourcePresenting address now =
Resource.therefore (presenting address now)
{-| -}
resourcePresentingAs : (List a -> List a') -> Signal.Address (List a') -> Time -> Resource euser (Machine a b c bad) -> Resource euser (ViewOutput a' c bad)
resourcePresentingAs xdcr address now =
Resource.therefore (presentingAs xdcr address now)
| 41960 | {--
Copyright (c) 2016, <NAME>
All rights reserved.
Redistribution and use in source and binary forms, with or without modification, are permitted
provided that the following conditions are met:
1. Redistributions of source code must retain the above copyright notice, this list of conditions
and the following disclaimer.
2. Redistributions in binary form must reproduce the above copyright notice, this list of conditions
and the following disclaimer in the documentation and/or other materials provided with the
distribution.
3. Neither the name of the copyright holder nor the names of its contributors may be used to endorse
or promote products derived from this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR
IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND
FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR
CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER
IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF
THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
--}
module Scaffold.Machine
(Machine,
machine,
getModel, replaceModel,
dispatching, dispatchingAs,
presenting, presentingAs,
staging, stagingAs,
updating, integrate,
maybeGetModel, maybeReplaceModel,
maybeDispatching, maybeDispatchingAs,
maybePresenting, maybePresentingAs,
maybeStaging, maybeStagingAs,
maybeUpdating, maybeIntegrate,
machineResource,
resourceGetModel,
resourceReplaceModel,
resourceDispatching,
resourceDispatchingAs,
resourceIntegrate,
resourceUpdating,
resourceStaging,
resourceStagingAs,
resourcePresenting,
resourcePresentingAs
)
where
{-| This module builds a more usable state machine snapshot with the Elm Architecture in mind on
top of the Gigan App.
# Definition
@docs Machine
# Constructor
@docs machine
# Get and Set the Model
@docs getModel, replaceModel
# Using Machines
@docs dispatching, dispatchingAs, presenting, presentingAs, staging, stagingAs, updating, integrate
# Get and Set the Model of Maybe Machines
@docs maybeGetModel, maybeReplaceModel
# Optional Machines Using Maybe
@docs maybeDispatching, maybeDispatchingAs, maybePresenting, maybePresentingAs, maybeStaging, maybeStagingAs, maybeUpdating, maybeIntegrate
# Resource Dependant Machines
@docs machineResource, resourceGetModel, resourceReplaceModel, resourceDispatching, resourceDispatchingAs, resourceIntegrate, resourceUpdating, resourceStaging, resourceStagingAs, resourcePresenting, resourcePresentingAs
-}
import Scaffold.App exposing (..)
import Scaffold.Resource as Resource exposing (Resource)
import Set exposing (Set)
import Dict exposing (Dict)
import Time exposing (Time)
{-| A Machine is an instantaneous description of some model associated with an ProgramInput (see App)
that specifies how it should be updated, staged and presented. -}
type alias Machine a b c bad =
{ input : ProgramInput a b c bad
, state : ProgramSnapshot a b bad
}
{-| Create a machine from an ProgramInput -}
machine : ProgramInput a b c bad -> Machine a b c bad
machine input =
{ input = input
, state = programSnapshot input.model0 (dispatchTasks [])
}
{-| Get the model of a machine. -}
getModel : Machine a b c bad -> b
getModel machine' =
machine'.state.model'
{-| Create a machine with a different model from a replacement model and an original machine. -}
replaceModel : b -> Machine a b c bad -> Machine a b c bad
replaceModel model' machine' =
let machine'state = machine'.state in
{ machine' | state = { machine'state | model' = model' } }
-- FLAT STEM OPERATORS
{-| Dispatch the tasks of a Machine. This function is in the depreciated family of `dispatch'*`
functions. Use `dispatching*` and `integrate` instead. -}
dispatch' : Machine a b c bad -> (Machine a b c bad, TaskDispatchment bad a)
dispatch' machine' =
programSnapshotDispatch machine'.state
|> \result -> ({ machine' | state = fst result }, snd result)
{-| Get the currently waiting dispatchment. -}
dispatching : Machine a b c bad -> TaskDispatchment bad a
dispatching =
dispatch' >> snd
{-| Dispatch the tasks of a Machine using some transducer to translate the action list. This function
is in the depreciated family of `dispatch'*` functions. Use `dispatching*` and
`integrate` instead. -}
dispatchAs' : (List a -> List a') -> Machine a b c bad -> (Machine a b c bad, TaskDispatchment bad a')
dispatchAs' xdcr machine' =
dispatch' machine'
|> \(machine'', dispatchment) -> dispatchment
|> promoteDispatchment xdcr
|> \dispatchment' -> (machine'', dispatchment')
{-| Get the currently waiting task dispatchment, but apply some action type transformation. -}
dispatchingAs : (List a -> List a') -> Machine a b c bad -> TaskDispatchment bad a'
dispatchingAs xdcr =
dispatchAs' xdcr >> snd
{-| Remove the currently waiting task dispatchment. This should be done only after retrieving any
possibly waiting dispatchment. -}
integrate : Machine a b c bad -> Machine a b c bad
integrate =
dispatch' >> fst
{-| Run the update function from the configured ProgramInput of the Machine on the Machine's current model to produce a new model and dispatch any asynchronous tasks desired.
This takes a list of actions, the current time, and the Machine. -}
updating : List a -> Time -> Machine a b c bad -> Machine a b c bad
updating actions now machine' =
{ machine' | state = programSnapshotUpdate machine'.input actions now machine'.state }
{-| Run the update function from the configured ProgramInput of the Machine on the Machine's current model to produce a new model and dispatch any asynchronous tasks desired.
This takes an address for actions to be sent to, the current time, and the Machine. -}
staging : Signal.Address (List a) -> Time -> Machine a b c bad -> Machine a b c bad
staging address now machine' =
{ machine' | state = programSnapshotStage machine'.input address now machine'.state }
{-| Same as staging, but with a transformation to apply to action lists before they are sent to the address. -}
stagingAs : (List a -> List a') -> Signal.Address (List a') -> Time -> Machine a b c bad -> Machine a b c bad
stagingAs xdcr address =
staging (Signal.forwardTo address xdcr)
{-| Run the present function from the configured ProgramInput of the Machine on the Machine's current model to produce a ViewOutput, which includes the an element of the Machine's view type, and a TaskDispatchment for any desired asynchronous tasks.
This takes an address for actions to be sent to, the current time, and the Machine. -}
presenting : Signal.Address (List a) -> Time -> Machine a b c bad -> ViewOutput a c bad
presenting address now machine' =
programSnapshotPresent machine'.input address now machine'.state
{-| Same as presenting, but with a transformation to apply to action lists before they are sent to the address. -}
presentingAs : (List a -> List a') -> Signal.Address (List a') -> Time -> Machine a b c bad -> ViewOutput a' c bad
presentingAs xdcr address now machine' =
presenting (Signal.forwardTo address xdcr) now machine'
|> \view' -> { view' | dispatchment = promoteDispatchment xdcr view'.dispatchment }
{--
OPTIONAL MACHINES USING MAYBE
--}
{-| Create a possible machine from possible model given a partial program definition (without the
model). -}
maybeMachine : (b -> ProgramInput a b c bad) -> Maybe b -> Maybe (Machine a b c bad)
maybeMachine modelInput maybeModel =
Maybe.map (modelInput >> machine >> Just) maybeModel
|> Maybe.withDefault Nothing
{-| -}
maybeGetModel : Maybe (Machine a b c bad) -> Maybe b
maybeGetModel =
Maybe.map (.state >> .model')
{-| -}
maybeReplaceModel : b -> Maybe (Machine a b c bad) -> Maybe (Machine a b c bad)
maybeReplaceModel model' =
Maybe.map (replaceModel model')
{-| -}
maybeDispatch : Maybe (Machine a b c bad) -> Maybe (Machine a b c bad, TaskDispatchment bad a)
maybeDispatch =
Maybe.map dispatch'
{-| -}
maybeDispatching : Maybe (Machine a b c bad) -> TaskDispatchment bad a
maybeDispatching machine' =
Maybe.map dispatching machine'
|> Maybe.withDefault (dispatchTasks [])
-- variant of dispatch' that accepts a transducer with which to promote actions.
{-| -}
maybeDispatchAs : (List a -> List a') -> Maybe (Machine a b c bad) -> Maybe (Machine a b c bad, TaskDispatchment bad a')
maybeDispatchAs xdcr =
Maybe.map (dispatchAs' xdcr)
{-| -}
maybeDispatchingAs : (List a -> List a') -> Maybe (Machine a b c bad) -> TaskDispatchment bad a'
maybeDispatchingAs xdcr machine' =
Maybe.map (dispatchingAs xdcr) machine'
|> Maybe.withDefault (dispatchTasks [])
{-| -}
maybeIntegrate : Maybe (Machine a b c bad) -> Maybe (Machine a b c bad)
maybeIntegrate =
Maybe.map integrate
{-| -}
maybeUpdating : List a -> Time -> Maybe (Machine a b c bad) -> Maybe (Machine a b c bad)
maybeUpdating actions now =
Maybe.map (updating actions now)
{-| -}
maybeStaging : Signal.Address (List a) -> Time -> Maybe (Machine a b c bad) -> Maybe (Machine a b c bad)
maybeStaging address now =
Maybe.map (staging address now)
{-| -}
maybeStagingAs : (List a -> List a') -> Signal.Address (List a') -> Time -> Maybe (Machine a b c bad) -> Maybe (Machine a b c bad)
maybeStagingAs xdcr address now =
Maybe.map (stagingAs xdcr address now)
{-| -}
maybePresenting : Signal.Address (List a) -> Time -> Maybe (Machine a b c bad) -> Maybe (ViewOutput a c bad)
maybePresenting address now =
Maybe.map (presenting address now)
{-| -}
maybePresentingAs : (List a -> List a') -> Signal.Address (List a') -> Time -> Maybe (Machine a b c bad) -> Maybe (ViewOutput a' c bad)
maybePresentingAs xdcr address now =
Maybe.map (presentingAs xdcr address now)
{--
RESOURCE DEPENDANT MACHINES USING RESOURCE
--}
{-| Create a machine resource from a model resource given a partial program definition (without the
model). -}
machineResource : (b -> ProgramInput a b c bad) -> Resource euser b -> Resource euser (Machine a b c bad)
machineResource modelInput modelResource =
Resource.toProgram modelInput modelResource
|> Resource.therefore machine
{-| -}
resourceGetModel : Resource euser (Machine a b c bad) -> Resource euser b
resourceGetModel =
Resource.therefore (.state >> .model')
{-| -}
resourceReplaceModel : b -> Resource euser (Machine a b c bad) -> Resource euser (Machine a b c bad)
resourceReplaceModel model' =
Resource.therefore (replaceModel model')
{-| -}
resourceDispatching : Resource euser (Machine a b c bad) -> TaskDispatchment bad a
resourceDispatching machine' =
Resource.therefore dispatching machine'
|> Resource.otherwise (dispatchTasks [])
{-| -}
resourceDispatchingAs : (List a -> List a') -> Resource euser (Machine a b c bad) -> TaskDispatchment bad a'
resourceDispatchingAs xdcr machine' =
Resource.therefore (dispatchingAs xdcr) machine'
|> Resource.otherwise (dispatchTasks [])
{-| -}
resourceIntegrate : Resource euser (Machine a b c bad) -> Resource euser (Machine a b c bad)
resourceIntegrate =
Resource.therefore integrate
{-| -}
resourceUpdating : List a -> Time -> Resource euser (Machine a b c bad) -> Resource euser (Machine a b c bad)
resourceUpdating actions now =
Resource.therefore (updating actions now)
{-| -}
resourceStaging : Signal.Address (List a) -> Time -> Resource euser (Machine a b c bad) -> Resource euser (Machine a b c bad)
resourceStaging address now =
Resource.therefore (staging address now)
{-| -}
resourceStagingAs : (List a -> List a') -> Signal.Address (List a') -> Time -> Resource euser (Machine a b c bad) -> Resource euser (Machine a b c bad)
resourceStagingAs xdcr address now =
Resource.therefore (stagingAs xdcr address now)
{-| -}
resourcePresenting : Signal.Address (List a) -> Time -> Resource euser (Machine a b c bad) -> Resource euser (ViewOutput a c bad)
resourcePresenting address now =
Resource.therefore (presenting address now)
{-| -}
resourcePresentingAs : (List a -> List a') -> Signal.Address (List a') -> Time -> Resource euser (Machine a b c bad) -> Resource euser (ViewOutput a' c bad)
resourcePresentingAs xdcr address now =
Resource.therefore (presentingAs xdcr address now)
| true | {--
Copyright (c) 2016, PI:NAME:<NAME>END_PI
All rights reserved.
Redistribution and use in source and binary forms, with or without modification, are permitted
provided that the following conditions are met:
1. Redistributions of source code must retain the above copyright notice, this list of conditions
and the following disclaimer.
2. Redistributions in binary form must reproduce the above copyright notice, this list of conditions
and the following disclaimer in the documentation and/or other materials provided with the
distribution.
3. Neither the name of the copyright holder nor the names of its contributors may be used to endorse
or promote products derived from this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR
IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND
FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR
CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER
IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF
THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
--}
module Scaffold.Machine
(Machine,
machine,
getModel, replaceModel,
dispatching, dispatchingAs,
presenting, presentingAs,
staging, stagingAs,
updating, integrate,
maybeGetModel, maybeReplaceModel,
maybeDispatching, maybeDispatchingAs,
maybePresenting, maybePresentingAs,
maybeStaging, maybeStagingAs,
maybeUpdating, maybeIntegrate,
machineResource,
resourceGetModel,
resourceReplaceModel,
resourceDispatching,
resourceDispatchingAs,
resourceIntegrate,
resourceUpdating,
resourceStaging,
resourceStagingAs,
resourcePresenting,
resourcePresentingAs
)
where
{-| This module builds a more usable state machine snapshot with the Elm Architecture in mind on
top of the Gigan App.
# Definition
@docs Machine
# Constructor
@docs machine
# Get and Set the Model
@docs getModel, replaceModel
# Using Machines
@docs dispatching, dispatchingAs, presenting, presentingAs, staging, stagingAs, updating, integrate
# Get and Set the Model of Maybe Machines
@docs maybeGetModel, maybeReplaceModel
# Optional Machines Using Maybe
@docs maybeDispatching, maybeDispatchingAs, maybePresenting, maybePresentingAs, maybeStaging, maybeStagingAs, maybeUpdating, maybeIntegrate
# Resource Dependant Machines
@docs machineResource, resourceGetModel, resourceReplaceModel, resourceDispatching, resourceDispatchingAs, resourceIntegrate, resourceUpdating, resourceStaging, resourceStagingAs, resourcePresenting, resourcePresentingAs
-}
import Scaffold.App exposing (..)
import Scaffold.Resource as Resource exposing (Resource)
import Set exposing (Set)
import Dict exposing (Dict)
import Time exposing (Time)
{-| A Machine is an instantaneous description of some model associated with an ProgramInput (see App)
that specifies how it should be updated, staged and presented. -}
type alias Machine a b c bad =
{ input : ProgramInput a b c bad
, state : ProgramSnapshot a b bad
}
{-| Create a machine from an ProgramInput -}
machine : ProgramInput a b c bad -> Machine a b c bad
machine input =
{ input = input
, state = programSnapshot input.model0 (dispatchTasks [])
}
{-| Get the model of a machine. -}
getModel : Machine a b c bad -> b
getModel machine' =
machine'.state.model'
{-| Create a machine with a different model from a replacement model and an original machine. -}
replaceModel : b -> Machine a b c bad -> Machine a b c bad
replaceModel model' machine' =
let machine'state = machine'.state in
{ machine' | state = { machine'state | model' = model' } }
-- FLAT STEM OPERATORS
{-| Dispatch the tasks of a Machine. This function is in the depreciated family of `dispatch'*`
functions. Use `dispatching*` and `integrate` instead. -}
dispatch' : Machine a b c bad -> (Machine a b c bad, TaskDispatchment bad a)
dispatch' machine' =
programSnapshotDispatch machine'.state
|> \result -> ({ machine' | state = fst result }, snd result)
{-| Get the currently waiting dispatchment. -}
dispatching : Machine a b c bad -> TaskDispatchment bad a
dispatching =
dispatch' >> snd
{-| Dispatch the tasks of a Machine using some transducer to translate the action list. This function
is in the depreciated family of `dispatch'*` functions. Use `dispatching*` and
`integrate` instead. -}
dispatchAs' : (List a -> List a') -> Machine a b c bad -> (Machine a b c bad, TaskDispatchment bad a')
dispatchAs' xdcr machine' =
dispatch' machine'
|> \(machine'', dispatchment) -> dispatchment
|> promoteDispatchment xdcr
|> \dispatchment' -> (machine'', dispatchment')
{-| Get the currently waiting task dispatchment, but apply some action type transformation. -}
dispatchingAs : (List a -> List a') -> Machine a b c bad -> TaskDispatchment bad a'
dispatchingAs xdcr =
dispatchAs' xdcr >> snd
{-| Remove the currently waiting task dispatchment. This should be done only after retrieving any
possibly waiting dispatchment. -}
integrate : Machine a b c bad -> Machine a b c bad
integrate =
dispatch' >> fst
{-| Run the update function from the configured ProgramInput of the Machine on the Machine's current model to produce a new model and dispatch any asynchronous tasks desired.
This takes a list of actions, the current time, and the Machine. -}
updating : List a -> Time -> Machine a b c bad -> Machine a b c bad
updating actions now machine' =
{ machine' | state = programSnapshotUpdate machine'.input actions now machine'.state }
{-| Run the update function from the configured ProgramInput of the Machine on the Machine's current model to produce a new model and dispatch any asynchronous tasks desired.
This takes an address for actions to be sent to, the current time, and the Machine. -}
staging : Signal.Address (List a) -> Time -> Machine a b c bad -> Machine a b c bad
staging address now machine' =
{ machine' | state = programSnapshotStage machine'.input address now machine'.state }
{-| Same as staging, but with a transformation to apply to action lists before they are sent to the address. -}
stagingAs : (List a -> List a') -> Signal.Address (List a') -> Time -> Machine a b c bad -> Machine a b c bad
stagingAs xdcr address =
staging (Signal.forwardTo address xdcr)
{-| Run the present function from the configured ProgramInput of the Machine on the Machine's current model to produce a ViewOutput, which includes the an element of the Machine's view type, and a TaskDispatchment for any desired asynchronous tasks.
This takes an address for actions to be sent to, the current time, and the Machine. -}
presenting : Signal.Address (List a) -> Time -> Machine a b c bad -> ViewOutput a c bad
presenting address now machine' =
programSnapshotPresent machine'.input address now machine'.state
{-| Same as presenting, but with a transformation to apply to action lists before they are sent to the address. -}
presentingAs : (List a -> List a') -> Signal.Address (List a') -> Time -> Machine a b c bad -> ViewOutput a' c bad
presentingAs xdcr address now machine' =
presenting (Signal.forwardTo address xdcr) now machine'
|> \view' -> { view' | dispatchment = promoteDispatchment xdcr view'.dispatchment }
{--
OPTIONAL MACHINES USING MAYBE
--}
{-| Create a possible machine from possible model given a partial program definition (without the
model). -}
maybeMachine : (b -> ProgramInput a b c bad) -> Maybe b -> Maybe (Machine a b c bad)
maybeMachine modelInput maybeModel =
Maybe.map (modelInput >> machine >> Just) maybeModel
|> Maybe.withDefault Nothing
{-| -}
maybeGetModel : Maybe (Machine a b c bad) -> Maybe b
maybeGetModel =
Maybe.map (.state >> .model')
{-| -}
maybeReplaceModel : b -> Maybe (Machine a b c bad) -> Maybe (Machine a b c bad)
maybeReplaceModel model' =
Maybe.map (replaceModel model')
{-| -}
maybeDispatch : Maybe (Machine a b c bad) -> Maybe (Machine a b c bad, TaskDispatchment bad a)
maybeDispatch =
Maybe.map dispatch'
{-| -}
maybeDispatching : Maybe (Machine a b c bad) -> TaskDispatchment bad a
maybeDispatching machine' =
Maybe.map dispatching machine'
|> Maybe.withDefault (dispatchTasks [])
-- variant of dispatch' that accepts a transducer with which to promote actions.
{-| -}
maybeDispatchAs : (List a -> List a') -> Maybe (Machine a b c bad) -> Maybe (Machine a b c bad, TaskDispatchment bad a')
maybeDispatchAs xdcr =
Maybe.map (dispatchAs' xdcr)
{-| -}
maybeDispatchingAs : (List a -> List a') -> Maybe (Machine a b c bad) -> TaskDispatchment bad a'
maybeDispatchingAs xdcr machine' =
Maybe.map (dispatchingAs xdcr) machine'
|> Maybe.withDefault (dispatchTasks [])
{-| -}
maybeIntegrate : Maybe (Machine a b c bad) -> Maybe (Machine a b c bad)
maybeIntegrate =
Maybe.map integrate
{-| -}
maybeUpdating : List a -> Time -> Maybe (Machine a b c bad) -> Maybe (Machine a b c bad)
maybeUpdating actions now =
Maybe.map (updating actions now)
{-| -}
maybeStaging : Signal.Address (List a) -> Time -> Maybe (Machine a b c bad) -> Maybe (Machine a b c bad)
maybeStaging address now =
Maybe.map (staging address now)
{-| -}
maybeStagingAs : (List a -> List a') -> Signal.Address (List a') -> Time -> Maybe (Machine a b c bad) -> Maybe (Machine a b c bad)
maybeStagingAs xdcr address now =
Maybe.map (stagingAs xdcr address now)
{-| -}
maybePresenting : Signal.Address (List a) -> Time -> Maybe (Machine a b c bad) -> Maybe (ViewOutput a c bad)
maybePresenting address now =
Maybe.map (presenting address now)
{-| -}
maybePresentingAs : (List a -> List a') -> Signal.Address (List a') -> Time -> Maybe (Machine a b c bad) -> Maybe (ViewOutput a' c bad)
maybePresentingAs xdcr address now =
Maybe.map (presentingAs xdcr address now)
{--
RESOURCE DEPENDANT MACHINES USING RESOURCE
--}
{-| Create a machine resource from a model resource given a partial program definition (without the
model). -}
machineResource : (b -> ProgramInput a b c bad) -> Resource euser b -> Resource euser (Machine a b c bad)
machineResource modelInput modelResource =
Resource.toProgram modelInput modelResource
|> Resource.therefore machine
{-| -}
resourceGetModel : Resource euser (Machine a b c bad) -> Resource euser b
resourceGetModel =
Resource.therefore (.state >> .model')
{-| -}
resourceReplaceModel : b -> Resource euser (Machine a b c bad) -> Resource euser (Machine a b c bad)
resourceReplaceModel model' =
Resource.therefore (replaceModel model')
{-| -}
resourceDispatching : Resource euser (Machine a b c bad) -> TaskDispatchment bad a
resourceDispatching machine' =
Resource.therefore dispatching machine'
|> Resource.otherwise (dispatchTasks [])
{-| -}
resourceDispatchingAs : (List a -> List a') -> Resource euser (Machine a b c bad) -> TaskDispatchment bad a'
resourceDispatchingAs xdcr machine' =
Resource.therefore (dispatchingAs xdcr) machine'
|> Resource.otherwise (dispatchTasks [])
{-| -}
resourceIntegrate : Resource euser (Machine a b c bad) -> Resource euser (Machine a b c bad)
resourceIntegrate =
Resource.therefore integrate
{-| -}
resourceUpdating : List a -> Time -> Resource euser (Machine a b c bad) -> Resource euser (Machine a b c bad)
resourceUpdating actions now =
Resource.therefore (updating actions now)
{-| -}
resourceStaging : Signal.Address (List a) -> Time -> Resource euser (Machine a b c bad) -> Resource euser (Machine a b c bad)
resourceStaging address now =
Resource.therefore (staging address now)
{-| -}
resourceStagingAs : (List a -> List a') -> Signal.Address (List a') -> Time -> Resource euser (Machine a b c bad) -> Resource euser (Machine a b c bad)
resourceStagingAs xdcr address now =
Resource.therefore (stagingAs xdcr address now)
{-| -}
resourcePresenting : Signal.Address (List a) -> Time -> Resource euser (Machine a b c bad) -> Resource euser (ViewOutput a c bad)
resourcePresenting address now =
Resource.therefore (presenting address now)
{-| -}
resourcePresentingAs : (List a -> List a') -> Signal.Address (List a') -> Time -> Resource euser (Machine a b c bad) -> Resource euser (ViewOutput a' c bad)
resourcePresentingAs xdcr address now =
Resource.therefore (presentingAs xdcr address now)
| elm |
[
{
"context": "exposing (..)\n\nbaseUrl : String\nbaseUrl = \"http://13.115.226.214:9999/api/trades?\"",
"end": 75,
"score": 0.9482345581,
"start": 61,
"tag": "IP_ADDRESS",
"value": "13.115.226.214"
}
] | src/Api.elm | sovmedcare/farm-trans | 15 | module Api exposing (..)
baseUrl : String
baseUrl = "http://13.115.226.214:9999/api/trades?" | 14744 | module Api exposing (..)
baseUrl : String
baseUrl = "http://172.16.17.32:9999/api/trades?" | true | module Api exposing (..)
baseUrl : String
baseUrl = "http://PI:IP_ADDRESS:172.16.17.32END_PI:9999/api/trades?" | elm |
[
{
"context": " [ h1 []\n [ text \"Helmtris\" ]\n , text <| \"GAME OVER with scor",
"end": 8285,
"score": 0.9818140268,
"start": 8277,
"tag": "NAME",
"value": "Helmtris"
},
{
"context": " [ h1 []\n [ text \"Helmtris\" ]\n , div\n [ cl",
"end": 8633,
"score": 0.9705299139,
"start": 8625,
"tag": "NAME",
"value": "Helmtris"
},
{
"context": " [ h1 []\n [ text \"Helmtris\" ]\n , viewPlayField game.grid <| N",
"end": 9085,
"score": 0.9097798467,
"start": 9077,
"tag": "NAME",
"value": "Helmtris"
},
{
"context": " [ h1 []\n [ text \"Helmtris\" ]\n , viewPlayField (Grid.empty pl",
"end": 9383,
"score": 0.9079140425,
"start": 9375,
"tag": "NAME",
"value": "Helmtris"
}
] | src/Main.elm | hallski/helmtris | 0 | module Main exposing (main)
import Grid
import Block
import AnimationFrame as AF
import Char exposing (KeyCode)
import Collage
import Color exposing (..)
import Element
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick, on, keyCode)
import Keyboard as KB
import Random
import Time
import Transform exposing (..)
defaultTimeToUpdate : Time.Time
defaultTimeToUpdate =
450 * Time.millisecond
boostedTimeToUpdate : Time.Time
boostedTimeToUpdate =
50 * Time.millisecond
playFieldSize : { cols : Int, rows : Int }
playFieldSize =
{ cols = 10, rows = 20 }
rowsBetweenLevels : Int
rowsBetweenLevels =
10
type alias Score =
Int
type alias Game =
{ grid : Grid.Grid
, activeBlock : Block.Block
, nextBlock : Maybe Block.Block
, score : Score
, timeToUpdate : Time.Time
, boost : Bool
, level : Int
, rowsToNewLevel : Int
}
type GameState
= Initial
| Playing Game
| Paused Game
| GameOver Score
type alias Model =
{ state : GameState
}
init : ( Model, Cmd Msg )
init =
Model Initial ! []
-- Update
type Msg
= Tick Time.Time
| TogglePlay
| Left
| Right
| Rotate
| Boost Bool
| Reset
| InitialBlocks ( Block.Block, Block.Block )
| NextBlock Block.Block
| NoOp -- Needed by handleKey
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case ( msg, model.state ) of
( Tick diff, Playing game ) ->
let
( updatedGameState, cmd ) =
updateGame game diff
in
{ model | state = updatedGameState } ! [ cmd ]
( TogglePlay, _ ) ->
togglePlaying model
( Left, Playing game ) ->
playingNoCmd model <| modifyActiveBlock (Block.moveOn -1) game
( Right, Playing game ) ->
playingNoCmd model <| modifyActiveBlock (Block.moveOn 1) game
( Rotate, Playing game ) ->
playingNoCmd model <| modifyActiveBlock Block.rotateOn game
( Boost onOff, Playing game ) ->
playingNoCmd model <| setBoost onOff game
( Reset, _ ) ->
init
( InitialBlocks blocks, Initial ) ->
startPlaying model blocks ! []
( NextBlock block, Playing game ) ->
playingNoCmd model <| nextBlockSpawned game block
_ ->
model ! []
playingNoCmd : Model -> Game -> ( Model, Cmd Msg )
playingNoCmd model game =
{ model | state = Playing game } ! []
spawnNextBlock : Cmd Msg
spawnNextBlock =
Random.generate NextBlock Block.getRandom
spawnInitialBlocks : Cmd Msg
spawnInitialBlocks =
Random.pair Block.getRandom Block.getRandom
|> Random.generate InitialBlocks
togglePlaying : Model -> ( Model, Cmd Msg )
togglePlaying model =
case model.state of
Playing game ->
{ model | state = Paused game } ! []
Paused game ->
{ model | state = Playing game } ! []
Initial ->
model ! [ spawnInitialBlocks ]
GameOver _ ->
{ model | state = Initial } ! [ spawnInitialBlocks ]
startPlaying : Model -> ( Block.Block, Block.Block ) -> Model
startPlaying model ( block, nextBlock ) =
let
grid =
Grid.empty playFieldSize.cols playFieldSize.rows
game =
Game grid block (Just nextBlock) 0 defaultTimeToUpdate False 1 rowsBetweenLevels
in
{ model | state = Playing game }
nextBlockSpawned : Game -> Block.Block -> Game
nextBlockSpawned game block =
{ game | nextBlock = Just block }
setBoost : Bool -> Game -> Game
setBoost onOff game =
{ game | boost = onOff }
modifyActiveBlock : Block.BlockManipulation -> Game -> Game
modifyActiveBlock fn game =
case fn game.grid game.activeBlock of
Ok block ->
{ game | activeBlock = block }
_ ->
game
copyBlockToGrid : Game -> Game
copyBlockToGrid game =
{ game | grid = Block.copyOntoGrid game.activeBlock game.grid }
calculateScore : Int -> Int -> Int
calculateScore removedLines oldScore =
case removedLines of
0 ->
oldScore
n ->
calculateScore (n - 1) (oldScore + n * 10)
removeFullRows : Game -> ( Int, Game )
removeFullRows game =
let
( removed, grid ) =
Grid.removeFullRows game.grid
score =
calculateScore removed game.score
in
( removed, { game | grid = grid, score = score } )
updateLevel : ( Int, Game ) -> Game
updateLevel ( removedRows, game ) =
let
rowsUntilNextLevel =
game.rowsToNewLevel - removedRows
( level, rows ) =
if rowsUntilNextLevel <= 0 then
( game.level + 1, rowsBetweenLevels + rowsUntilNextLevel )
else
( game.level, rowsUntilNextLevel )
in
{ game | level = level, rowsToNewLevel = rows }
attemptNextBlock : Game -> ( GameState, Cmd Msg )
attemptNextBlock game =
case game.nextBlock of
Just nextBlock ->
if Block.detectCollisionInGrid nextBlock game.grid then
GameOver game.score ! []
else
Playing
{ game
| activeBlock = nextBlock
, nextBlock = Nothing
}
! [ spawnNextBlock ]
Nothing ->
Playing game ! []
landBlock : Game -> ( GameState, Cmd Msg )
landBlock game =
copyBlockToGrid game
|> removeFullRows
|> updateLevel
|> attemptNextBlock
resetTimeToNextUpdate : Game -> Game
resetTimeToNextUpdate game =
let
timeToUpdate =
if game.boost then
boostedTimeToUpdate
else
defaultTimeToUpdate - (toFloat game.level) * 50
in
{ game | timeToUpdate = timeToUpdate }
updateGame : Game -> Time.Time -> ( GameState, Cmd Msg )
updateGame game diff =
let
timeToUpdate =
game.timeToUpdate - diff
in
if timeToUpdate < 0 then
advanceGame <| resetTimeToNextUpdate game
else
Playing { game | timeToUpdate = timeToUpdate } ! []
advanceGame : Game -> ( GameState, Cmd Msg )
advanceGame game =
case Block.moveYOn 1 game.grid game.activeBlock of
Ok block ->
Playing { game | activeBlock = block } ! []
_ ->
landBlock game
-- Subscriptions
subscriptions : Model -> Sub Msg
subscriptions model =
case model.state of
Playing _ ->
Sub.batch
[ AF.diffs Tick
, KB.downs handleDownKey
, KB.ups handleUpKey
]
_ ->
Sub.none
handleDownKey : KeyCode -> Msg
handleDownKey code =
case code of
-- WASD
65 ->
Left
68 ->
Right
87 ->
Rotate
83 ->
Boost True
-- Arrow keys
37 ->
Left
38 ->
Rotate
39 ->
Right
40 ->
Boost True
_ ->
NoOp
handleUpKey : KeyCode -> Msg
handleUpKey code =
case code of
-- WASD
83 ->
Boost False
-- Arrow keys
40 ->
Boost False
_ ->
NoOp
-- View
{--
Simplify the rendering code by applying a transformation on all forms drawn.
Since Collage in elm-graphics puts origin in the center with Y growing upwards and draws all
forms anchored to the center as well, flip the Y axis and translate origin to be half a cellSize
in from the top left.
--}
canvasTranslation : Int -> Int -> Transform.Transform
canvasTranslation width height =
let
startX =
-(toFloat <| width - Grid.cellSize) / 2
startY =
-(toFloat <| height - Grid.cellSize) / 2
in
Transform.multiply (Transform.scaleY -1) (Transform.translation startX startY)
view : Model -> Html Msg
view model =
case model.state of
GameOver score ->
div []
[ h1 []
[ text "Helmtris" ]
, text <| "GAME OVER with score: " ++ (toString score)
, viewPlayField (Grid.empty playFieldSize.cols playFieldSize.rows) Nothing
, button [ onClick Reset ] [ text "Reset" ]
]
Playing game ->
div []
[ h1 []
[ text "Helmtris" ]
, div
[ class "game-container" ]
[ viewPlayField game.grid <| Just game.activeBlock
, viewGameInfo game
]
, button [ onClick TogglePlay ] [ text "Pause" ]
, button [ onClick Reset ] [ text "Reset" ]
]
Paused game ->
div []
[ h1 []
[ text "Helmtris" ]
, viewPlayField game.grid <| Nothing
, button [ onClick TogglePlay ] [ text "Play" ]
, button [ onClick Reset ] [ text "Reset" ]
]
Initial ->
div []
[ h1 []
[ text "Helmtris" ]
, viewPlayField (Grid.empty playFieldSize.cols playFieldSize.rows) Nothing
, button [ onClick TogglePlay ] [ text "Play" ]
, button [ onClick Reset ] [ text "Reset" ]
]
viewGameInfo : Game -> Html Msg
viewGameInfo game =
case game.nextBlock of
Just block ->
let
( width, height ) =
Block.dimensions block
transformation =
canvasTranslation width height
forms =
[ Block.renderPreview block ]
preview =
Collage.collage width
height
[ Collage.groupTransform transformation forms ]
|> Element.toHtml
in
div [ class "preview" ]
[ h2 [] [ text <| "Score: " ++ toString game.score ]
, h2 [] [ text <| "Level: " ++ toString game.level ]
, h2 [] [ text "Next block:" ]
, preview
]
Nothing ->
div [] []
viewPlayField : Grid.Grid -> Maybe Block.Block -> Html Msg
viewPlayField grid maybeBlock =
let
forms =
case maybeBlock of
Just block ->
[ Grid.render 0 0 grid
, Block.render block
]
Nothing ->
[ Grid.render 0 0 grid ]
( gridWidth, gridHeight ) =
Grid.dimensions grid
transformation =
canvasTranslation gridWidth gridHeight
in
Collage.collage gridWidth
gridHeight
[ Collage.groupTransform transformation forms ]
|> Element.color gray
|> Element.toHtml
-- Main
main : Program Never Model Msg
main =
Html.program
{ init = init
, view = view
, update = update
, subscriptions = subscriptions
}
| 33099 | module Main exposing (main)
import Grid
import Block
import AnimationFrame as AF
import Char exposing (KeyCode)
import Collage
import Color exposing (..)
import Element
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick, on, keyCode)
import Keyboard as KB
import Random
import Time
import Transform exposing (..)
defaultTimeToUpdate : Time.Time
defaultTimeToUpdate =
450 * Time.millisecond
boostedTimeToUpdate : Time.Time
boostedTimeToUpdate =
50 * Time.millisecond
playFieldSize : { cols : Int, rows : Int }
playFieldSize =
{ cols = 10, rows = 20 }
rowsBetweenLevels : Int
rowsBetweenLevels =
10
type alias Score =
Int
type alias Game =
{ grid : Grid.Grid
, activeBlock : Block.Block
, nextBlock : Maybe Block.Block
, score : Score
, timeToUpdate : Time.Time
, boost : Bool
, level : Int
, rowsToNewLevel : Int
}
type GameState
= Initial
| Playing Game
| Paused Game
| GameOver Score
type alias Model =
{ state : GameState
}
init : ( Model, Cmd Msg )
init =
Model Initial ! []
-- Update
type Msg
= Tick Time.Time
| TogglePlay
| Left
| Right
| Rotate
| Boost Bool
| Reset
| InitialBlocks ( Block.Block, Block.Block )
| NextBlock Block.Block
| NoOp -- Needed by handleKey
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case ( msg, model.state ) of
( Tick diff, Playing game ) ->
let
( updatedGameState, cmd ) =
updateGame game diff
in
{ model | state = updatedGameState } ! [ cmd ]
( TogglePlay, _ ) ->
togglePlaying model
( Left, Playing game ) ->
playingNoCmd model <| modifyActiveBlock (Block.moveOn -1) game
( Right, Playing game ) ->
playingNoCmd model <| modifyActiveBlock (Block.moveOn 1) game
( Rotate, Playing game ) ->
playingNoCmd model <| modifyActiveBlock Block.rotateOn game
( Boost onOff, Playing game ) ->
playingNoCmd model <| setBoost onOff game
( Reset, _ ) ->
init
( InitialBlocks blocks, Initial ) ->
startPlaying model blocks ! []
( NextBlock block, Playing game ) ->
playingNoCmd model <| nextBlockSpawned game block
_ ->
model ! []
playingNoCmd : Model -> Game -> ( Model, Cmd Msg )
playingNoCmd model game =
{ model | state = Playing game } ! []
spawnNextBlock : Cmd Msg
spawnNextBlock =
Random.generate NextBlock Block.getRandom
spawnInitialBlocks : Cmd Msg
spawnInitialBlocks =
Random.pair Block.getRandom Block.getRandom
|> Random.generate InitialBlocks
togglePlaying : Model -> ( Model, Cmd Msg )
togglePlaying model =
case model.state of
Playing game ->
{ model | state = Paused game } ! []
Paused game ->
{ model | state = Playing game } ! []
Initial ->
model ! [ spawnInitialBlocks ]
GameOver _ ->
{ model | state = Initial } ! [ spawnInitialBlocks ]
startPlaying : Model -> ( Block.Block, Block.Block ) -> Model
startPlaying model ( block, nextBlock ) =
let
grid =
Grid.empty playFieldSize.cols playFieldSize.rows
game =
Game grid block (Just nextBlock) 0 defaultTimeToUpdate False 1 rowsBetweenLevels
in
{ model | state = Playing game }
nextBlockSpawned : Game -> Block.Block -> Game
nextBlockSpawned game block =
{ game | nextBlock = Just block }
setBoost : Bool -> Game -> Game
setBoost onOff game =
{ game | boost = onOff }
modifyActiveBlock : Block.BlockManipulation -> Game -> Game
modifyActiveBlock fn game =
case fn game.grid game.activeBlock of
Ok block ->
{ game | activeBlock = block }
_ ->
game
copyBlockToGrid : Game -> Game
copyBlockToGrid game =
{ game | grid = Block.copyOntoGrid game.activeBlock game.grid }
calculateScore : Int -> Int -> Int
calculateScore removedLines oldScore =
case removedLines of
0 ->
oldScore
n ->
calculateScore (n - 1) (oldScore + n * 10)
removeFullRows : Game -> ( Int, Game )
removeFullRows game =
let
( removed, grid ) =
Grid.removeFullRows game.grid
score =
calculateScore removed game.score
in
( removed, { game | grid = grid, score = score } )
updateLevel : ( Int, Game ) -> Game
updateLevel ( removedRows, game ) =
let
rowsUntilNextLevel =
game.rowsToNewLevel - removedRows
( level, rows ) =
if rowsUntilNextLevel <= 0 then
( game.level + 1, rowsBetweenLevels + rowsUntilNextLevel )
else
( game.level, rowsUntilNextLevel )
in
{ game | level = level, rowsToNewLevel = rows }
attemptNextBlock : Game -> ( GameState, Cmd Msg )
attemptNextBlock game =
case game.nextBlock of
Just nextBlock ->
if Block.detectCollisionInGrid nextBlock game.grid then
GameOver game.score ! []
else
Playing
{ game
| activeBlock = nextBlock
, nextBlock = Nothing
}
! [ spawnNextBlock ]
Nothing ->
Playing game ! []
landBlock : Game -> ( GameState, Cmd Msg )
landBlock game =
copyBlockToGrid game
|> removeFullRows
|> updateLevel
|> attemptNextBlock
resetTimeToNextUpdate : Game -> Game
resetTimeToNextUpdate game =
let
timeToUpdate =
if game.boost then
boostedTimeToUpdate
else
defaultTimeToUpdate - (toFloat game.level) * 50
in
{ game | timeToUpdate = timeToUpdate }
updateGame : Game -> Time.Time -> ( GameState, Cmd Msg )
updateGame game diff =
let
timeToUpdate =
game.timeToUpdate - diff
in
if timeToUpdate < 0 then
advanceGame <| resetTimeToNextUpdate game
else
Playing { game | timeToUpdate = timeToUpdate } ! []
advanceGame : Game -> ( GameState, Cmd Msg )
advanceGame game =
case Block.moveYOn 1 game.grid game.activeBlock of
Ok block ->
Playing { game | activeBlock = block } ! []
_ ->
landBlock game
-- Subscriptions
subscriptions : Model -> Sub Msg
subscriptions model =
case model.state of
Playing _ ->
Sub.batch
[ AF.diffs Tick
, KB.downs handleDownKey
, KB.ups handleUpKey
]
_ ->
Sub.none
handleDownKey : KeyCode -> Msg
handleDownKey code =
case code of
-- WASD
65 ->
Left
68 ->
Right
87 ->
Rotate
83 ->
Boost True
-- Arrow keys
37 ->
Left
38 ->
Rotate
39 ->
Right
40 ->
Boost True
_ ->
NoOp
handleUpKey : KeyCode -> Msg
handleUpKey code =
case code of
-- WASD
83 ->
Boost False
-- Arrow keys
40 ->
Boost False
_ ->
NoOp
-- View
{--
Simplify the rendering code by applying a transformation on all forms drawn.
Since Collage in elm-graphics puts origin in the center with Y growing upwards and draws all
forms anchored to the center as well, flip the Y axis and translate origin to be half a cellSize
in from the top left.
--}
canvasTranslation : Int -> Int -> Transform.Transform
canvasTranslation width height =
let
startX =
-(toFloat <| width - Grid.cellSize) / 2
startY =
-(toFloat <| height - Grid.cellSize) / 2
in
Transform.multiply (Transform.scaleY -1) (Transform.translation startX startY)
view : Model -> Html Msg
view model =
case model.state of
GameOver score ->
div []
[ h1 []
[ text "<NAME>" ]
, text <| "GAME OVER with score: " ++ (toString score)
, viewPlayField (Grid.empty playFieldSize.cols playFieldSize.rows) Nothing
, button [ onClick Reset ] [ text "Reset" ]
]
Playing game ->
div []
[ h1 []
[ text "<NAME>" ]
, div
[ class "game-container" ]
[ viewPlayField game.grid <| Just game.activeBlock
, viewGameInfo game
]
, button [ onClick TogglePlay ] [ text "Pause" ]
, button [ onClick Reset ] [ text "Reset" ]
]
Paused game ->
div []
[ h1 []
[ text "<NAME>" ]
, viewPlayField game.grid <| Nothing
, button [ onClick TogglePlay ] [ text "Play" ]
, button [ onClick Reset ] [ text "Reset" ]
]
Initial ->
div []
[ h1 []
[ text "<NAME>" ]
, viewPlayField (Grid.empty playFieldSize.cols playFieldSize.rows) Nothing
, button [ onClick TogglePlay ] [ text "Play" ]
, button [ onClick Reset ] [ text "Reset" ]
]
viewGameInfo : Game -> Html Msg
viewGameInfo game =
case game.nextBlock of
Just block ->
let
( width, height ) =
Block.dimensions block
transformation =
canvasTranslation width height
forms =
[ Block.renderPreview block ]
preview =
Collage.collage width
height
[ Collage.groupTransform transformation forms ]
|> Element.toHtml
in
div [ class "preview" ]
[ h2 [] [ text <| "Score: " ++ toString game.score ]
, h2 [] [ text <| "Level: " ++ toString game.level ]
, h2 [] [ text "Next block:" ]
, preview
]
Nothing ->
div [] []
viewPlayField : Grid.Grid -> Maybe Block.Block -> Html Msg
viewPlayField grid maybeBlock =
let
forms =
case maybeBlock of
Just block ->
[ Grid.render 0 0 grid
, Block.render block
]
Nothing ->
[ Grid.render 0 0 grid ]
( gridWidth, gridHeight ) =
Grid.dimensions grid
transformation =
canvasTranslation gridWidth gridHeight
in
Collage.collage gridWidth
gridHeight
[ Collage.groupTransform transformation forms ]
|> Element.color gray
|> Element.toHtml
-- Main
main : Program Never Model Msg
main =
Html.program
{ init = init
, view = view
, update = update
, subscriptions = subscriptions
}
| true | module Main exposing (main)
import Grid
import Block
import AnimationFrame as AF
import Char exposing (KeyCode)
import Collage
import Color exposing (..)
import Element
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick, on, keyCode)
import Keyboard as KB
import Random
import Time
import Transform exposing (..)
defaultTimeToUpdate : Time.Time
defaultTimeToUpdate =
450 * Time.millisecond
boostedTimeToUpdate : Time.Time
boostedTimeToUpdate =
50 * Time.millisecond
playFieldSize : { cols : Int, rows : Int }
playFieldSize =
{ cols = 10, rows = 20 }
rowsBetweenLevels : Int
rowsBetweenLevels =
10
type alias Score =
Int
type alias Game =
{ grid : Grid.Grid
, activeBlock : Block.Block
, nextBlock : Maybe Block.Block
, score : Score
, timeToUpdate : Time.Time
, boost : Bool
, level : Int
, rowsToNewLevel : Int
}
type GameState
= Initial
| Playing Game
| Paused Game
| GameOver Score
type alias Model =
{ state : GameState
}
init : ( Model, Cmd Msg )
init =
Model Initial ! []
-- Update
type Msg
= Tick Time.Time
| TogglePlay
| Left
| Right
| Rotate
| Boost Bool
| Reset
| InitialBlocks ( Block.Block, Block.Block )
| NextBlock Block.Block
| NoOp -- Needed by handleKey
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case ( msg, model.state ) of
( Tick diff, Playing game ) ->
let
( updatedGameState, cmd ) =
updateGame game diff
in
{ model | state = updatedGameState } ! [ cmd ]
( TogglePlay, _ ) ->
togglePlaying model
( Left, Playing game ) ->
playingNoCmd model <| modifyActiveBlock (Block.moveOn -1) game
( Right, Playing game ) ->
playingNoCmd model <| modifyActiveBlock (Block.moveOn 1) game
( Rotate, Playing game ) ->
playingNoCmd model <| modifyActiveBlock Block.rotateOn game
( Boost onOff, Playing game ) ->
playingNoCmd model <| setBoost onOff game
( Reset, _ ) ->
init
( InitialBlocks blocks, Initial ) ->
startPlaying model blocks ! []
( NextBlock block, Playing game ) ->
playingNoCmd model <| nextBlockSpawned game block
_ ->
model ! []
playingNoCmd : Model -> Game -> ( Model, Cmd Msg )
playingNoCmd model game =
{ model | state = Playing game } ! []
spawnNextBlock : Cmd Msg
spawnNextBlock =
Random.generate NextBlock Block.getRandom
spawnInitialBlocks : Cmd Msg
spawnInitialBlocks =
Random.pair Block.getRandom Block.getRandom
|> Random.generate InitialBlocks
togglePlaying : Model -> ( Model, Cmd Msg )
togglePlaying model =
case model.state of
Playing game ->
{ model | state = Paused game } ! []
Paused game ->
{ model | state = Playing game } ! []
Initial ->
model ! [ spawnInitialBlocks ]
GameOver _ ->
{ model | state = Initial } ! [ spawnInitialBlocks ]
startPlaying : Model -> ( Block.Block, Block.Block ) -> Model
startPlaying model ( block, nextBlock ) =
let
grid =
Grid.empty playFieldSize.cols playFieldSize.rows
game =
Game grid block (Just nextBlock) 0 defaultTimeToUpdate False 1 rowsBetweenLevels
in
{ model | state = Playing game }
nextBlockSpawned : Game -> Block.Block -> Game
nextBlockSpawned game block =
{ game | nextBlock = Just block }
setBoost : Bool -> Game -> Game
setBoost onOff game =
{ game | boost = onOff }
modifyActiveBlock : Block.BlockManipulation -> Game -> Game
modifyActiveBlock fn game =
case fn game.grid game.activeBlock of
Ok block ->
{ game | activeBlock = block }
_ ->
game
copyBlockToGrid : Game -> Game
copyBlockToGrid game =
{ game | grid = Block.copyOntoGrid game.activeBlock game.grid }
calculateScore : Int -> Int -> Int
calculateScore removedLines oldScore =
case removedLines of
0 ->
oldScore
n ->
calculateScore (n - 1) (oldScore + n * 10)
removeFullRows : Game -> ( Int, Game )
removeFullRows game =
let
( removed, grid ) =
Grid.removeFullRows game.grid
score =
calculateScore removed game.score
in
( removed, { game | grid = grid, score = score } )
updateLevel : ( Int, Game ) -> Game
updateLevel ( removedRows, game ) =
let
rowsUntilNextLevel =
game.rowsToNewLevel - removedRows
( level, rows ) =
if rowsUntilNextLevel <= 0 then
( game.level + 1, rowsBetweenLevels + rowsUntilNextLevel )
else
( game.level, rowsUntilNextLevel )
in
{ game | level = level, rowsToNewLevel = rows }
attemptNextBlock : Game -> ( GameState, Cmd Msg )
attemptNextBlock game =
case game.nextBlock of
Just nextBlock ->
if Block.detectCollisionInGrid nextBlock game.grid then
GameOver game.score ! []
else
Playing
{ game
| activeBlock = nextBlock
, nextBlock = Nothing
}
! [ spawnNextBlock ]
Nothing ->
Playing game ! []
landBlock : Game -> ( GameState, Cmd Msg )
landBlock game =
copyBlockToGrid game
|> removeFullRows
|> updateLevel
|> attemptNextBlock
resetTimeToNextUpdate : Game -> Game
resetTimeToNextUpdate game =
let
timeToUpdate =
if game.boost then
boostedTimeToUpdate
else
defaultTimeToUpdate - (toFloat game.level) * 50
in
{ game | timeToUpdate = timeToUpdate }
updateGame : Game -> Time.Time -> ( GameState, Cmd Msg )
updateGame game diff =
let
timeToUpdate =
game.timeToUpdate - diff
in
if timeToUpdate < 0 then
advanceGame <| resetTimeToNextUpdate game
else
Playing { game | timeToUpdate = timeToUpdate } ! []
advanceGame : Game -> ( GameState, Cmd Msg )
advanceGame game =
case Block.moveYOn 1 game.grid game.activeBlock of
Ok block ->
Playing { game | activeBlock = block } ! []
_ ->
landBlock game
-- Subscriptions
subscriptions : Model -> Sub Msg
subscriptions model =
case model.state of
Playing _ ->
Sub.batch
[ AF.diffs Tick
, KB.downs handleDownKey
, KB.ups handleUpKey
]
_ ->
Sub.none
handleDownKey : KeyCode -> Msg
handleDownKey code =
case code of
-- WASD
65 ->
Left
68 ->
Right
87 ->
Rotate
83 ->
Boost True
-- Arrow keys
37 ->
Left
38 ->
Rotate
39 ->
Right
40 ->
Boost True
_ ->
NoOp
handleUpKey : KeyCode -> Msg
handleUpKey code =
case code of
-- WASD
83 ->
Boost False
-- Arrow keys
40 ->
Boost False
_ ->
NoOp
-- View
{--
Simplify the rendering code by applying a transformation on all forms drawn.
Since Collage in elm-graphics puts origin in the center with Y growing upwards and draws all
forms anchored to the center as well, flip the Y axis and translate origin to be half a cellSize
in from the top left.
--}
canvasTranslation : Int -> Int -> Transform.Transform
canvasTranslation width height =
let
startX =
-(toFloat <| width - Grid.cellSize) / 2
startY =
-(toFloat <| height - Grid.cellSize) / 2
in
Transform.multiply (Transform.scaleY -1) (Transform.translation startX startY)
view : Model -> Html Msg
view model =
case model.state of
GameOver score ->
div []
[ h1 []
[ text "PI:NAME:<NAME>END_PI" ]
, text <| "GAME OVER with score: " ++ (toString score)
, viewPlayField (Grid.empty playFieldSize.cols playFieldSize.rows) Nothing
, button [ onClick Reset ] [ text "Reset" ]
]
Playing game ->
div []
[ h1 []
[ text "PI:NAME:<NAME>END_PI" ]
, div
[ class "game-container" ]
[ viewPlayField game.grid <| Just game.activeBlock
, viewGameInfo game
]
, button [ onClick TogglePlay ] [ text "Pause" ]
, button [ onClick Reset ] [ text "Reset" ]
]
Paused game ->
div []
[ h1 []
[ text "PI:NAME:<NAME>END_PI" ]
, viewPlayField game.grid <| Nothing
, button [ onClick TogglePlay ] [ text "Play" ]
, button [ onClick Reset ] [ text "Reset" ]
]
Initial ->
div []
[ h1 []
[ text "PI:NAME:<NAME>END_PI" ]
, viewPlayField (Grid.empty playFieldSize.cols playFieldSize.rows) Nothing
, button [ onClick TogglePlay ] [ text "Play" ]
, button [ onClick Reset ] [ text "Reset" ]
]
viewGameInfo : Game -> Html Msg
viewGameInfo game =
case game.nextBlock of
Just block ->
let
( width, height ) =
Block.dimensions block
transformation =
canvasTranslation width height
forms =
[ Block.renderPreview block ]
preview =
Collage.collage width
height
[ Collage.groupTransform transformation forms ]
|> Element.toHtml
in
div [ class "preview" ]
[ h2 [] [ text <| "Score: " ++ toString game.score ]
, h2 [] [ text <| "Level: " ++ toString game.level ]
, h2 [] [ text "Next block:" ]
, preview
]
Nothing ->
div [] []
viewPlayField : Grid.Grid -> Maybe Block.Block -> Html Msg
viewPlayField grid maybeBlock =
let
forms =
case maybeBlock of
Just block ->
[ Grid.render 0 0 grid
, Block.render block
]
Nothing ->
[ Grid.render 0 0 grid ]
( gridWidth, gridHeight ) =
Grid.dimensions grid
transformation =
canvasTranslation gridWidth gridHeight
in
Collage.collage gridWidth
gridHeight
[ Collage.groupTransform transformation forms ]
|> Element.color gray
|> Element.toHtml
-- Main
main : Program Never Model Msg
main =
Html.program
{ init = init
, view = view
, update = update
, subscriptions = subscriptions
}
| elm |
[
{
"context": " Maybe String\n , email : Email\n , password : Password\n , plan : Plan\n }\n\n\nnameField =\n Form.te",
"end": 1528,
"score": 0.9957033992,
"start": 1520,
"tag": "PASSWORD",
"value": "Password"
},
{
"context": " { label = \"Email\"\n , placeholder = \"you@example.com\"\n , htmlAttributes = []\n }\n",
"end": 2280,
"score": 0.9999206662,
"start": 2265,
"tag": "EMAIL",
"value": "you@example.com"
}
] | assets/elm/DemoApp/src/DemoElmApp.elm | rolojf/base3 | 0 | port module DemoElmApp exposing (..)
import Browser
import Css.Global as Global
import Email
import Form exposing (..)
import Form.View
import Html.Styled exposing (..)
import Html.Styled.Attributes exposing (..)
import Html.Styled.Events exposing (on, onClick, onInput)
import Json.Decode as D
import TW.Utilities as TW
-- MAIN
main : Program () Model Msg
main =
Browser.element
{ init = init
, view = view >> Html.Styled.toUnstyled
, update = update
, subscriptions = subscriptions
}
-- PORTS
port sendMessage : String -> Cmd msg
port messageReceiver : (Int -> msg) -> Sub msg
-- MODEL
type alias Model =
{ draft : String
, messages : Int
, forma : Form.View.Model InputValues
}
init : () -> ( Model, Cmd Msg )
init flags =
( { draft = ""
, messages = 0
, forma =
Form.View.idle
{ name = ""
, email = ""
, password = ""
, repeatPassword = ""
, plan = "Pro"
, agreedToTerms = False
}
}
, Cmd.none
)
type Plan
= Basic
| Pro
| Enterprise
type Email
= Email String
type Password
= Password String
type alias InputValues =
{ name : String
, email : String
, password : String
, repeatPassword : String
, plan : String
, agreedToTerms : Bool
}
type alias UserDetails =
{ name : Maybe String
, email : Email
, password : Password
, plan : Plan
}
nameField =
Form.textField
{ parser = Ok
, value = .name
, error = always Nothing
, update = \value values -> { values | name = value }
, attributes =
{ label = "Name"
, placeholder = "Your name"
, htmlAttributes = []
}
}
parseEmail correo =
if Email.isValid correo then
Ok <| Email correo
else
Err "Invalid email"
emailField =
Form.emailField
{ parser = parseEmail
, value = .email
, error = always Nothing
, update = \value values -> { values | email = value }
, attributes =
{ label = "Email"
, placeholder = "you@example.com"
, htmlAttributes = []
}
}
parsePassword s =
if String.length s >= 6 then
Ok <| Password s
else
Err "Password must be at least 6 characters"
passwordField =
Form.passwordField
{ parser = parsePassword
, value = .password
, error = always Nothing
, update = \value values -> { values | password = value }
, attributes =
{ label = "Password"
, placeholder = "Your password"
, htmlAttributes = []
}
}
repeatPasswordField =
Form.meta
(\values ->
Form.passwordField
{ parser =
\value ->
if value == values.password then
Ok ()
else
Err "The passwords must match"
, value = .repeatPassword
, error = always Nothing
, update =
\newValue values_ ->
{ values_ | repeatPassword = newValue }
, attributes =
{ label = "Repeat password"
, placeholder = "Repeat password"
, htmlAttributes = []
}
}
)
parsePlan s =
case s of
"Basic" ->
Ok Basic
"Pro" ->
Ok Pro
"Enterprise" ->
Ok Enterprise
_ ->
Err "Invalid plan"
planSelector =
Form.selectField
{ parser = parsePlan
, value = .plan
, error = always Nothing
, update =
\value values ->
{ values | plan = value }
, attributes =
{ label = "Choose a plan"
, placeholder = "Choose a plan"
, htmlAttributes = []
, options =
[ ( "Basic", "Basic" )
, ( "Pro", "Pro" )
, ( "Enterprise", "Enterprise" )
]
}
}
termsCheckbox =
Form.checkboxField
{ parser =
\value ->
if value then
Ok ()
else
Err "You must accept the terms"
, value = .agreedToTerms
, error = always Nothing
, update =
\value values ->
{ values | agreedToTerms = value }
, attributes =
{ label = "I agree to terms and conditions"
, htmlAttributes = []
}
}
form : Form InputValues UserDetails
form =
Form.succeed
(\name email password plan _ ->
UserDetails name email password plan
)
|> Form.append (Form.optional nameField)
|> Form.append emailField
|> Form.append
(Form.succeed (\password _ -> password)
|> Form.append passwordField
|> Form.append repeatPasswordField
|> Form.group
)
|> Form.append planSelector
|> Form.append termsCheckbox
-- UPDATE
type Msg
= DraftChanged String
| Send
| Recv Int
| FormChanged (Form.View.Model InputValues)
| Signup UserDetails
-- Use the `sendMessage` port when someone presses ENTER or clicks
-- the "Send" button. Check out index.html to see the corresponding
-- JS where this is piped into a WebSocket.
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
DraftChanged borrador ->
( { model | draft = borrador }
, Cmd.none
)
Send ->
( { model | draft = "" }
, sendMessage model.draft
)
Recv mensaje ->
( { model | messages = mensaje }
, Cmd.none
)
FormChanged newForm ->
( { model | forma = newForm }
, Cmd.none
)
Signup _ ->
( model
, Cmd.none
)
-- SUBSCRIPTIONS
-- Subscribe to the `messageReceiver` port to hear about messages coming in
-- from JS. C
--
subscriptions : Model -> Sub Msg
subscriptions _ =
messageReceiver Recv
-- VIEW
view : Model -> Html Msg
view model =
div []
[ h1 [] [ text "Echo Chat" ]
, p
[ css
[ TW.text_4xl
, TW.font_semibold
, TW.text_indigo_400
]
]
[ text <| String.fromInt model.messages ]
, input
[ type_ "text"
, placeholder "Draft"
, onInput DraftChanged
, on "keydown" (ifIsEnter Send)
, value model.draft
]
[]
, button [ onClick Send ] [ text "Send" ]
, Global.global
[ Global.class "elm-form-label"
[ TW.text_blue_400
, TW.text_sm
]
]
, Html.Styled.fromUnstyled <|
Form.View.asHtml
{ onChange = FormChanged
, action = "Sign up"
, loading = "Signing up"
, validation = Form.View.ValidateOnSubmit
}
(Form.map Signup form)
model.forma
, h1
[]
[ text "PRIMERO TITULO" ]
]
-- DETECT ENTER
ifIsEnter : msg -> D.Decoder msg
ifIsEnter msg =
D.field "key" D.string
|> D.andThen
(\key ->
if key == "Enter" then
D.succeed msg
else
D.fail "some other key"
)
| 32374 | port module DemoElmApp exposing (..)
import Browser
import Css.Global as Global
import Email
import Form exposing (..)
import Form.View
import Html.Styled exposing (..)
import Html.Styled.Attributes exposing (..)
import Html.Styled.Events exposing (on, onClick, onInput)
import Json.Decode as D
import TW.Utilities as TW
-- MAIN
main : Program () Model Msg
main =
Browser.element
{ init = init
, view = view >> Html.Styled.toUnstyled
, update = update
, subscriptions = subscriptions
}
-- PORTS
port sendMessage : String -> Cmd msg
port messageReceiver : (Int -> msg) -> Sub msg
-- MODEL
type alias Model =
{ draft : String
, messages : Int
, forma : Form.View.Model InputValues
}
init : () -> ( Model, Cmd Msg )
init flags =
( { draft = ""
, messages = 0
, forma =
Form.View.idle
{ name = ""
, email = ""
, password = ""
, repeatPassword = ""
, plan = "Pro"
, agreedToTerms = False
}
}
, Cmd.none
)
type Plan
= Basic
| Pro
| Enterprise
type Email
= Email String
type Password
= Password String
type alias InputValues =
{ name : String
, email : String
, password : String
, repeatPassword : String
, plan : String
, agreedToTerms : Bool
}
type alias UserDetails =
{ name : Maybe String
, email : Email
, password : <PASSWORD>
, plan : Plan
}
nameField =
Form.textField
{ parser = Ok
, value = .name
, error = always Nothing
, update = \value values -> { values | name = value }
, attributes =
{ label = "Name"
, placeholder = "Your name"
, htmlAttributes = []
}
}
parseEmail correo =
if Email.isValid correo then
Ok <| Email correo
else
Err "Invalid email"
emailField =
Form.emailField
{ parser = parseEmail
, value = .email
, error = always Nothing
, update = \value values -> { values | email = value }
, attributes =
{ label = "Email"
, placeholder = "<EMAIL>"
, htmlAttributes = []
}
}
parsePassword s =
if String.length s >= 6 then
Ok <| Password s
else
Err "Password must be at least 6 characters"
passwordField =
Form.passwordField
{ parser = parsePassword
, value = .password
, error = always Nothing
, update = \value values -> { values | password = value }
, attributes =
{ label = "Password"
, placeholder = "Your password"
, htmlAttributes = []
}
}
repeatPasswordField =
Form.meta
(\values ->
Form.passwordField
{ parser =
\value ->
if value == values.password then
Ok ()
else
Err "The passwords must match"
, value = .repeatPassword
, error = always Nothing
, update =
\newValue values_ ->
{ values_ | repeatPassword = newValue }
, attributes =
{ label = "Repeat password"
, placeholder = "Repeat password"
, htmlAttributes = []
}
}
)
parsePlan s =
case s of
"Basic" ->
Ok Basic
"Pro" ->
Ok Pro
"Enterprise" ->
Ok Enterprise
_ ->
Err "Invalid plan"
planSelector =
Form.selectField
{ parser = parsePlan
, value = .plan
, error = always Nothing
, update =
\value values ->
{ values | plan = value }
, attributes =
{ label = "Choose a plan"
, placeholder = "Choose a plan"
, htmlAttributes = []
, options =
[ ( "Basic", "Basic" )
, ( "Pro", "Pro" )
, ( "Enterprise", "Enterprise" )
]
}
}
termsCheckbox =
Form.checkboxField
{ parser =
\value ->
if value then
Ok ()
else
Err "You must accept the terms"
, value = .agreedToTerms
, error = always Nothing
, update =
\value values ->
{ values | agreedToTerms = value }
, attributes =
{ label = "I agree to terms and conditions"
, htmlAttributes = []
}
}
form : Form InputValues UserDetails
form =
Form.succeed
(\name email password plan _ ->
UserDetails name email password plan
)
|> Form.append (Form.optional nameField)
|> Form.append emailField
|> Form.append
(Form.succeed (\password _ -> password)
|> Form.append passwordField
|> Form.append repeatPasswordField
|> Form.group
)
|> Form.append planSelector
|> Form.append termsCheckbox
-- UPDATE
type Msg
= DraftChanged String
| Send
| Recv Int
| FormChanged (Form.View.Model InputValues)
| Signup UserDetails
-- Use the `sendMessage` port when someone presses ENTER or clicks
-- the "Send" button. Check out index.html to see the corresponding
-- JS where this is piped into a WebSocket.
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
DraftChanged borrador ->
( { model | draft = borrador }
, Cmd.none
)
Send ->
( { model | draft = "" }
, sendMessage model.draft
)
Recv mensaje ->
( { model | messages = mensaje }
, Cmd.none
)
FormChanged newForm ->
( { model | forma = newForm }
, Cmd.none
)
Signup _ ->
( model
, Cmd.none
)
-- SUBSCRIPTIONS
-- Subscribe to the `messageReceiver` port to hear about messages coming in
-- from JS. C
--
subscriptions : Model -> Sub Msg
subscriptions _ =
messageReceiver Recv
-- VIEW
view : Model -> Html Msg
view model =
div []
[ h1 [] [ text "Echo Chat" ]
, p
[ css
[ TW.text_4xl
, TW.font_semibold
, TW.text_indigo_400
]
]
[ text <| String.fromInt model.messages ]
, input
[ type_ "text"
, placeholder "Draft"
, onInput DraftChanged
, on "keydown" (ifIsEnter Send)
, value model.draft
]
[]
, button [ onClick Send ] [ text "Send" ]
, Global.global
[ Global.class "elm-form-label"
[ TW.text_blue_400
, TW.text_sm
]
]
, Html.Styled.fromUnstyled <|
Form.View.asHtml
{ onChange = FormChanged
, action = "Sign up"
, loading = "Signing up"
, validation = Form.View.ValidateOnSubmit
}
(Form.map Signup form)
model.forma
, h1
[]
[ text "PRIMERO TITULO" ]
]
-- DETECT ENTER
ifIsEnter : msg -> D.Decoder msg
ifIsEnter msg =
D.field "key" D.string
|> D.andThen
(\key ->
if key == "Enter" then
D.succeed msg
else
D.fail "some other key"
)
| true | port module DemoElmApp exposing (..)
import Browser
import Css.Global as Global
import Email
import Form exposing (..)
import Form.View
import Html.Styled exposing (..)
import Html.Styled.Attributes exposing (..)
import Html.Styled.Events exposing (on, onClick, onInput)
import Json.Decode as D
import TW.Utilities as TW
-- MAIN
main : Program () Model Msg
main =
Browser.element
{ init = init
, view = view >> Html.Styled.toUnstyled
, update = update
, subscriptions = subscriptions
}
-- PORTS
port sendMessage : String -> Cmd msg
port messageReceiver : (Int -> msg) -> Sub msg
-- MODEL
type alias Model =
{ draft : String
, messages : Int
, forma : Form.View.Model InputValues
}
init : () -> ( Model, Cmd Msg )
init flags =
( { draft = ""
, messages = 0
, forma =
Form.View.idle
{ name = ""
, email = ""
, password = ""
, repeatPassword = ""
, plan = "Pro"
, agreedToTerms = False
}
}
, Cmd.none
)
type Plan
= Basic
| Pro
| Enterprise
type Email
= Email String
type Password
= Password String
type alias InputValues =
{ name : String
, email : String
, password : String
, repeatPassword : String
, plan : String
, agreedToTerms : Bool
}
type alias UserDetails =
{ name : Maybe String
, email : Email
, password : PI:PASSWORD:<PASSWORD>END_PI
, plan : Plan
}
nameField =
Form.textField
{ parser = Ok
, value = .name
, error = always Nothing
, update = \value values -> { values | name = value }
, attributes =
{ label = "Name"
, placeholder = "Your name"
, htmlAttributes = []
}
}
parseEmail correo =
if Email.isValid correo then
Ok <| Email correo
else
Err "Invalid email"
emailField =
Form.emailField
{ parser = parseEmail
, value = .email
, error = always Nothing
, update = \value values -> { values | email = value }
, attributes =
{ label = "Email"
, placeholder = "PI:EMAIL:<EMAIL>END_PI"
, htmlAttributes = []
}
}
parsePassword s =
if String.length s >= 6 then
Ok <| Password s
else
Err "Password must be at least 6 characters"
passwordField =
Form.passwordField
{ parser = parsePassword
, value = .password
, error = always Nothing
, update = \value values -> { values | password = value }
, attributes =
{ label = "Password"
, placeholder = "Your password"
, htmlAttributes = []
}
}
repeatPasswordField =
Form.meta
(\values ->
Form.passwordField
{ parser =
\value ->
if value == values.password then
Ok ()
else
Err "The passwords must match"
, value = .repeatPassword
, error = always Nothing
, update =
\newValue values_ ->
{ values_ | repeatPassword = newValue }
, attributes =
{ label = "Repeat password"
, placeholder = "Repeat password"
, htmlAttributes = []
}
}
)
parsePlan s =
case s of
"Basic" ->
Ok Basic
"Pro" ->
Ok Pro
"Enterprise" ->
Ok Enterprise
_ ->
Err "Invalid plan"
planSelector =
Form.selectField
{ parser = parsePlan
, value = .plan
, error = always Nothing
, update =
\value values ->
{ values | plan = value }
, attributes =
{ label = "Choose a plan"
, placeholder = "Choose a plan"
, htmlAttributes = []
, options =
[ ( "Basic", "Basic" )
, ( "Pro", "Pro" )
, ( "Enterprise", "Enterprise" )
]
}
}
termsCheckbox =
Form.checkboxField
{ parser =
\value ->
if value then
Ok ()
else
Err "You must accept the terms"
, value = .agreedToTerms
, error = always Nothing
, update =
\value values ->
{ values | agreedToTerms = value }
, attributes =
{ label = "I agree to terms and conditions"
, htmlAttributes = []
}
}
form : Form InputValues UserDetails
form =
Form.succeed
(\name email password plan _ ->
UserDetails name email password plan
)
|> Form.append (Form.optional nameField)
|> Form.append emailField
|> Form.append
(Form.succeed (\password _ -> password)
|> Form.append passwordField
|> Form.append repeatPasswordField
|> Form.group
)
|> Form.append planSelector
|> Form.append termsCheckbox
-- UPDATE
type Msg
= DraftChanged String
| Send
| Recv Int
| FormChanged (Form.View.Model InputValues)
| Signup UserDetails
-- Use the `sendMessage` port when someone presses ENTER or clicks
-- the "Send" button. Check out index.html to see the corresponding
-- JS where this is piped into a WebSocket.
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
DraftChanged borrador ->
( { model | draft = borrador }
, Cmd.none
)
Send ->
( { model | draft = "" }
, sendMessage model.draft
)
Recv mensaje ->
( { model | messages = mensaje }
, Cmd.none
)
FormChanged newForm ->
( { model | forma = newForm }
, Cmd.none
)
Signup _ ->
( model
, Cmd.none
)
-- SUBSCRIPTIONS
-- Subscribe to the `messageReceiver` port to hear about messages coming in
-- from JS. C
--
subscriptions : Model -> Sub Msg
subscriptions _ =
messageReceiver Recv
-- VIEW
view : Model -> Html Msg
view model =
div []
[ h1 [] [ text "Echo Chat" ]
, p
[ css
[ TW.text_4xl
, TW.font_semibold
, TW.text_indigo_400
]
]
[ text <| String.fromInt model.messages ]
, input
[ type_ "text"
, placeholder "Draft"
, onInput DraftChanged
, on "keydown" (ifIsEnter Send)
, value model.draft
]
[]
, button [ onClick Send ] [ text "Send" ]
, Global.global
[ Global.class "elm-form-label"
[ TW.text_blue_400
, TW.text_sm
]
]
, Html.Styled.fromUnstyled <|
Form.View.asHtml
{ onChange = FormChanged
, action = "Sign up"
, loading = "Signing up"
, validation = Form.View.ValidateOnSubmit
}
(Form.map Signup form)
model.forma
, h1
[]
[ text "PRIMERO TITULO" ]
]
-- DETECT ENTER
ifIsEnter : msg -> D.Decoder msg
ifIsEnter msg =
D.field "key" D.string
|> D.andThen
(\key ->
if key == "Enter" then
D.succeed msg
else
D.fail "some other key"
)
| elm |
[
{
"context": "ord password ->\n ( { model | password = password }, Cmd.none )\n\n SetConfirmPassword passwor",
"end": 2336,
"score": 0.9988494515,
"start": 2328,
"tag": "PASSWORD",
"value": "password"
},
{
"context": "sword ->\n ( { model | confirmPassword = password }, Cmd.none )\n\n SetRegistrationType t ->\n ",
"end": 2441,
"score": 0.9986771345,
"start": 2433,
"tag": "PASSWORD",
"value": "password"
},
{
"context": " [ class \"my-2\"\n , placeholder \"Confirm Password\"\n , onInput SetConfirmPassword\n ",
"end": 8760,
"score": 0.9620594978,
"start": 8744,
"tag": "PASSWORD",
"value": "Confirm Password"
}
] | frontend/app/src/Page/Register.elm | ThreeMinuteLearning/3ml | 1 | module Page.Register exposing (Model, Msg, init, update, view)
import Api
import Components
import Data.Session exposing (Session, authorization)
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick, onInput, onSubmit)
import Http
import Route
import SHA1
import Util exposing (defaultHttpErrorMsg)
import Validate exposing (Validator, fromErrors, ifBlank, ifInvalidEmail, validate)
import Views.Form as Form
type alias Model =
{ errors : List Error
, email : String
, registrationType : Maybe RegistrationType
, code : Maybe String
, schoolName : String
, teacherName : String
, password : String
, confirmPassword : String
, status : Status
}
type PwnedCount
= Zero
| One
| Pwned Int
| Error
type Status
= NotSent
| AwaitingPwnedResponse
| AwaitingResponse
| Completed
type RegistrationType
= WithCode
| NewSchool
type Msg
= SubmitForm
| SetEmail String
| SetCode String
| SetSchoolName String
| SetRegistrationType RegistrationType
| SetTeacherName String
| SetPassword String
| SetConfirmPassword String
| RegisterResponse (Result Http.Error Api.NoContent)
| PwnedResults (Result Http.Error String)
init : Model
init =
{ errors = []
, email = ""
, registrationType = Nothing
, code = Nothing
, schoolName = ""
, teacherName = ""
, password = ""
, confirmPassword = ""
, status = NotSent
}
update : Session -> Msg -> Model -> ( Model, Cmd Msg )
update session msg model =
case msg of
SubmitForm ->
case validate validator model of
Err errors ->
( { model | errors = errors }, Cmd.none )
_ ->
( { model | status = AwaitingPwnedResponse, errors = [] }, getPwnedMatches model.password )
SetEmail email ->
( { model | email = email }, Cmd.none )
SetCode code ->
( { model | code = Just code, schoolName = code }, Cmd.none )
SetSchoolName name ->
( { model | schoolName = name }, Cmd.none )
SetTeacherName name ->
( { model | teacherName = name }, Cmd.none )
SetPassword password ->
( { model | password = password }, Cmd.none )
SetConfirmPassword password ->
( { model | confirmPassword = password }, Cmd.none )
SetRegistrationType t ->
( { model | registrationType = Just t }, Cmd.none )
RegisterResponse (Err error) ->
let
errorMessages =
case error of
Http.BadStatus { status } ->
case status.code of
409 ->
[ "Account already registered" ]
403 ->
[ "The registration code was not found or may have expired" ]
_ ->
[ "There was an error during registration" ]
_ ->
[ defaultHttpErrorMsg error ]
in
( { model | status = NotSent, errors = List.map (\errorMessage -> ( Form, errorMessage )) errorMessages }, Cmd.none )
RegisterResponse (Ok _) ->
( { model | status = Completed }, Cmd.none )
PwnedResults (Err _) ->
sendForm model session
PwnedResults (Ok matches) ->
let
pwnedCount =
pwnedCountFromResponse model.password matches
errors =
validatePwned pwnedCount
in
if errors == [] then
sendForm model session
else
( { model | errors = errors, status = NotSent }, Cmd.none )
sendForm : Model -> Session -> ( Model, Cmd Msg )
sendForm model session =
( { model | status = AwaitingResponse, errors = [] }
, Api.postAccountRegister (authorization session) (Api.Registration model.email model.code model.schoolName model.teacherName model.password)
|> Http.send RegisterResponse
)
view : Model -> { title : String, content : Html Msg }
view model =
{ title = "Register with 3ml"
, content =
div [ class "max-w-xl mx-auto flex flex-col" ]
[ h1 [ class "text-xs-center text-gray-600" ] [ text (heading model.registrationType) ]
, p [ class "my-2 text-lg leading-normal" ]
[ text (blurb model.registrationType)
]
, p [ class "mb-4" ]
[ a [ Route.href Route.Login, class "text-blue-500 hover:text-blue-700" ]
[ text "Have an account already?" ]
]
, Form.viewErrors model.errors
, if model.registrationType == Nothing then
viewRegistrationOptions
else if model.status == Completed then
div [ class "text-lg" ]
[ p [] [ text "Registration complete." ]
, p []
[ case model.registrationType of
Just WithCode ->
text "Your school admin should let you know when they have activated your account."
_ ->
text "Your account should be activated within an hour."
]
, p [] [ text "You can then sign in using your email and password." ]
]
else
viewForm model
]
}
heading : Maybe RegistrationType -> String
heading rt =
case rt of
Nothing ->
"Sign up"
Just NewSchool ->
"Register a new school"
Just WithCode ->
"Register as a teacher in an existing school"
blurb : Maybe RegistrationType -> String
blurb rt =
case rt of
Nothing ->
"""
You can either register a new school or, if your school is already using 3ml, as a teacher in an existing school.
"""
Just WithCode ->
"""To register for an existing school, a 3ml admin at your school should have given
you a registration code. Please enter it in the form below. If you don't have a code, please
ask the teacher who registered your school for one. The code is only valid for a short time, so you
should complete your registration right away.
"""
Just NewSchool ->
"""
Registering will create a new school in the 3ml system for which you will be the administrator.
You will be able to create accounts for the children in your school to use 3ml and also
accounts for other teachers. You will be responsible for obtaining consent for your students
to use the system, for controlling the data that they enter and for maintaining the accounts
for your school.
"""
viewRegistrationOptions : Html Msg
viewRegistrationOptions =
div [ class "flex justify-around" ]
[ Components.btn [ id "register-school", class "mr-2", type_ "button", onClick (SetRegistrationType NewSchool) ] [ text "Register a new school" ]
, Components.btn [ id "register-teacher", type_ "button", onClick (SetRegistrationType WithCode) ] [ text "Register as a teacher in an existing school" ]
]
viewForm : Model -> Html Msg
viewForm model =
Html.form [ class "flex flex-col", onSubmit SubmitForm ]
[ case model.registrationType of
Just WithCode ->
Form.input
[ class ""
, placeholder "Registration code"
, onInput SetCode
, maxlength 100
]
[]
_ ->
Form.input
[ class ""
, placeholder "School name"
, onInput SetSchoolName
, maxlength 100
]
[]
, Form.input
[ class "my-2"
, placeholder "Your name"
, onInput SetTeacherName
, maxlength 100
]
[]
, Form.input
[ class "mb-2"
, placeholder "Email"
, onInput SetEmail
, maxlength 50
]
[]
, Form.password
[ class ""
, placeholder "Choose a password"
, onInput SetPassword
, maxlength 100
, type_ "password"
]
[]
, Form.password
[ class "my-2"
, placeholder "Confirm Password"
, onInput SetConfirmPassword
, maxlength 100
]
[]
, Components.btn [ class "mt-1", disabled (model.status /= NotSent) ]
[ text "Sign up" ]
]
-- VALIDATION --
type Field
= Form
| SchoolName
| TeacherName
| Email
| Password
type alias Error =
( Field, String )
validator : Validator Error Model
validator =
Validate.all
[ ifBlank .schoolName ( SchoolName, "school name can't be blank." )
, ifInvalidEmail .email (\_ -> ( Email, "please enter a valid email." ))
, ifBlank .teacherName ( TeacherName, "name can't be blank." )
, fromErrors validatePassword
]
validatePassword : Model -> List Error
validatePassword { password, confirmPassword } =
case password of
"" ->
[ ( Password, "password can't be blank" ) ]
_ ->
if password /= confirmPassword then
[ ( Form, "the passwords must be the same" ) ]
else if String.length password < 8 then
[ ( Password, "password must be at least 8 characters" ) ]
else
[]
validatePwned : PwnedCount -> List Error
validatePwned pwned =
case pwned of
Zero ->
[]
One ->
[ ( Password, "Insecure password (found in a compromised database)!" ) ]
Pwned n ->
[ ( Password, "Insecure password (found " ++ String.fromInt n ++ " times in compromised databases)!" ) ]
Error ->
[]
sha1 : String -> String
sha1 s =
SHA1.fromString s
|> SHA1.toHex
|> String.toUpper
pwnedCountFromResponse : String -> String -> PwnedCount
pwnedCountFromResponse password response =
let
suffix =
sha1 password |> String.dropLeft 5
match =
String.lines response
|> List.filter (String.startsWith suffix)
|> List.head
|> Maybe.map (String.dropLeft 36)
|> Maybe.andThen String.toInt
in
case match of
Nothing ->
Zero
Just 1 ->
One
Just count ->
Pwned count
getPwnedMatches : String -> Cmd Msg
getPwnedMatches password =
Http.send PwnedResults <|
Http.getString ("https://api.pwnedpasswords.com/range/" ++ String.left 5 (sha1 password))
| 349 | module Page.Register exposing (Model, Msg, init, update, view)
import Api
import Components
import Data.Session exposing (Session, authorization)
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick, onInput, onSubmit)
import Http
import Route
import SHA1
import Util exposing (defaultHttpErrorMsg)
import Validate exposing (Validator, fromErrors, ifBlank, ifInvalidEmail, validate)
import Views.Form as Form
type alias Model =
{ errors : List Error
, email : String
, registrationType : Maybe RegistrationType
, code : Maybe String
, schoolName : String
, teacherName : String
, password : String
, confirmPassword : String
, status : Status
}
type PwnedCount
= Zero
| One
| Pwned Int
| Error
type Status
= NotSent
| AwaitingPwnedResponse
| AwaitingResponse
| Completed
type RegistrationType
= WithCode
| NewSchool
type Msg
= SubmitForm
| SetEmail String
| SetCode String
| SetSchoolName String
| SetRegistrationType RegistrationType
| SetTeacherName String
| SetPassword String
| SetConfirmPassword String
| RegisterResponse (Result Http.Error Api.NoContent)
| PwnedResults (Result Http.Error String)
init : Model
init =
{ errors = []
, email = ""
, registrationType = Nothing
, code = Nothing
, schoolName = ""
, teacherName = ""
, password = ""
, confirmPassword = ""
, status = NotSent
}
update : Session -> Msg -> Model -> ( Model, Cmd Msg )
update session msg model =
case msg of
SubmitForm ->
case validate validator model of
Err errors ->
( { model | errors = errors }, Cmd.none )
_ ->
( { model | status = AwaitingPwnedResponse, errors = [] }, getPwnedMatches model.password )
SetEmail email ->
( { model | email = email }, Cmd.none )
SetCode code ->
( { model | code = Just code, schoolName = code }, Cmd.none )
SetSchoolName name ->
( { model | schoolName = name }, Cmd.none )
SetTeacherName name ->
( { model | teacherName = name }, Cmd.none )
SetPassword password ->
( { model | password = <PASSWORD> }, Cmd.none )
SetConfirmPassword password ->
( { model | confirmPassword = <PASSWORD> }, Cmd.none )
SetRegistrationType t ->
( { model | registrationType = Just t }, Cmd.none )
RegisterResponse (Err error) ->
let
errorMessages =
case error of
Http.BadStatus { status } ->
case status.code of
409 ->
[ "Account already registered" ]
403 ->
[ "The registration code was not found or may have expired" ]
_ ->
[ "There was an error during registration" ]
_ ->
[ defaultHttpErrorMsg error ]
in
( { model | status = NotSent, errors = List.map (\errorMessage -> ( Form, errorMessage )) errorMessages }, Cmd.none )
RegisterResponse (Ok _) ->
( { model | status = Completed }, Cmd.none )
PwnedResults (Err _) ->
sendForm model session
PwnedResults (Ok matches) ->
let
pwnedCount =
pwnedCountFromResponse model.password matches
errors =
validatePwned pwnedCount
in
if errors == [] then
sendForm model session
else
( { model | errors = errors, status = NotSent }, Cmd.none )
sendForm : Model -> Session -> ( Model, Cmd Msg )
sendForm model session =
( { model | status = AwaitingResponse, errors = [] }
, Api.postAccountRegister (authorization session) (Api.Registration model.email model.code model.schoolName model.teacherName model.password)
|> Http.send RegisterResponse
)
view : Model -> { title : String, content : Html Msg }
view model =
{ title = "Register with 3ml"
, content =
div [ class "max-w-xl mx-auto flex flex-col" ]
[ h1 [ class "text-xs-center text-gray-600" ] [ text (heading model.registrationType) ]
, p [ class "my-2 text-lg leading-normal" ]
[ text (blurb model.registrationType)
]
, p [ class "mb-4" ]
[ a [ Route.href Route.Login, class "text-blue-500 hover:text-blue-700" ]
[ text "Have an account already?" ]
]
, Form.viewErrors model.errors
, if model.registrationType == Nothing then
viewRegistrationOptions
else if model.status == Completed then
div [ class "text-lg" ]
[ p [] [ text "Registration complete." ]
, p []
[ case model.registrationType of
Just WithCode ->
text "Your school admin should let you know when they have activated your account."
_ ->
text "Your account should be activated within an hour."
]
, p [] [ text "You can then sign in using your email and password." ]
]
else
viewForm model
]
}
heading : Maybe RegistrationType -> String
heading rt =
case rt of
Nothing ->
"Sign up"
Just NewSchool ->
"Register a new school"
Just WithCode ->
"Register as a teacher in an existing school"
blurb : Maybe RegistrationType -> String
blurb rt =
case rt of
Nothing ->
"""
You can either register a new school or, if your school is already using 3ml, as a teacher in an existing school.
"""
Just WithCode ->
"""To register for an existing school, a 3ml admin at your school should have given
you a registration code. Please enter it in the form below. If you don't have a code, please
ask the teacher who registered your school for one. The code is only valid for a short time, so you
should complete your registration right away.
"""
Just NewSchool ->
"""
Registering will create a new school in the 3ml system for which you will be the administrator.
You will be able to create accounts for the children in your school to use 3ml and also
accounts for other teachers. You will be responsible for obtaining consent for your students
to use the system, for controlling the data that they enter and for maintaining the accounts
for your school.
"""
viewRegistrationOptions : Html Msg
viewRegistrationOptions =
div [ class "flex justify-around" ]
[ Components.btn [ id "register-school", class "mr-2", type_ "button", onClick (SetRegistrationType NewSchool) ] [ text "Register a new school" ]
, Components.btn [ id "register-teacher", type_ "button", onClick (SetRegistrationType WithCode) ] [ text "Register as a teacher in an existing school" ]
]
viewForm : Model -> Html Msg
viewForm model =
Html.form [ class "flex flex-col", onSubmit SubmitForm ]
[ case model.registrationType of
Just WithCode ->
Form.input
[ class ""
, placeholder "Registration code"
, onInput SetCode
, maxlength 100
]
[]
_ ->
Form.input
[ class ""
, placeholder "School name"
, onInput SetSchoolName
, maxlength 100
]
[]
, Form.input
[ class "my-2"
, placeholder "Your name"
, onInput SetTeacherName
, maxlength 100
]
[]
, Form.input
[ class "mb-2"
, placeholder "Email"
, onInput SetEmail
, maxlength 50
]
[]
, Form.password
[ class ""
, placeholder "Choose a password"
, onInput SetPassword
, maxlength 100
, type_ "password"
]
[]
, Form.password
[ class "my-2"
, placeholder "<PASSWORD>"
, onInput SetConfirmPassword
, maxlength 100
]
[]
, Components.btn [ class "mt-1", disabled (model.status /= NotSent) ]
[ text "Sign up" ]
]
-- VALIDATION --
type Field
= Form
| SchoolName
| TeacherName
| Email
| Password
type alias Error =
( Field, String )
validator : Validator Error Model
validator =
Validate.all
[ ifBlank .schoolName ( SchoolName, "school name can't be blank." )
, ifInvalidEmail .email (\_ -> ( Email, "please enter a valid email." ))
, ifBlank .teacherName ( TeacherName, "name can't be blank." )
, fromErrors validatePassword
]
validatePassword : Model -> List Error
validatePassword { password, confirmPassword } =
case password of
"" ->
[ ( Password, "password can't be blank" ) ]
_ ->
if password /= confirmPassword then
[ ( Form, "the passwords must be the same" ) ]
else if String.length password < 8 then
[ ( Password, "password must be at least 8 characters" ) ]
else
[]
validatePwned : PwnedCount -> List Error
validatePwned pwned =
case pwned of
Zero ->
[]
One ->
[ ( Password, "Insecure password (found in a compromised database)!" ) ]
Pwned n ->
[ ( Password, "Insecure password (found " ++ String.fromInt n ++ " times in compromised databases)!" ) ]
Error ->
[]
sha1 : String -> String
sha1 s =
SHA1.fromString s
|> SHA1.toHex
|> String.toUpper
pwnedCountFromResponse : String -> String -> PwnedCount
pwnedCountFromResponse password response =
let
suffix =
sha1 password |> String.dropLeft 5
match =
String.lines response
|> List.filter (String.startsWith suffix)
|> List.head
|> Maybe.map (String.dropLeft 36)
|> Maybe.andThen String.toInt
in
case match of
Nothing ->
Zero
Just 1 ->
One
Just count ->
Pwned count
getPwnedMatches : String -> Cmd Msg
getPwnedMatches password =
Http.send PwnedResults <|
Http.getString ("https://api.pwnedpasswords.com/range/" ++ String.left 5 (sha1 password))
| true | module Page.Register exposing (Model, Msg, init, update, view)
import Api
import Components
import Data.Session exposing (Session, authorization)
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick, onInput, onSubmit)
import Http
import Route
import SHA1
import Util exposing (defaultHttpErrorMsg)
import Validate exposing (Validator, fromErrors, ifBlank, ifInvalidEmail, validate)
import Views.Form as Form
type alias Model =
{ errors : List Error
, email : String
, registrationType : Maybe RegistrationType
, code : Maybe String
, schoolName : String
, teacherName : String
, password : String
, confirmPassword : String
, status : Status
}
type PwnedCount
= Zero
| One
| Pwned Int
| Error
type Status
= NotSent
| AwaitingPwnedResponse
| AwaitingResponse
| Completed
type RegistrationType
= WithCode
| NewSchool
type Msg
= SubmitForm
| SetEmail String
| SetCode String
| SetSchoolName String
| SetRegistrationType RegistrationType
| SetTeacherName String
| SetPassword String
| SetConfirmPassword String
| RegisterResponse (Result Http.Error Api.NoContent)
| PwnedResults (Result Http.Error String)
init : Model
init =
{ errors = []
, email = ""
, registrationType = Nothing
, code = Nothing
, schoolName = ""
, teacherName = ""
, password = ""
, confirmPassword = ""
, status = NotSent
}
update : Session -> Msg -> Model -> ( Model, Cmd Msg )
update session msg model =
case msg of
SubmitForm ->
case validate validator model of
Err errors ->
( { model | errors = errors }, Cmd.none )
_ ->
( { model | status = AwaitingPwnedResponse, errors = [] }, getPwnedMatches model.password )
SetEmail email ->
( { model | email = email }, Cmd.none )
SetCode code ->
( { model | code = Just code, schoolName = code }, Cmd.none )
SetSchoolName name ->
( { model | schoolName = name }, Cmd.none )
SetTeacherName name ->
( { model | teacherName = name }, Cmd.none )
SetPassword password ->
( { model | password = PI:PASSWORD:<PASSWORD>END_PI }, Cmd.none )
SetConfirmPassword password ->
( { model | confirmPassword = PI:PASSWORD:<PASSWORD>END_PI }, Cmd.none )
SetRegistrationType t ->
( { model | registrationType = Just t }, Cmd.none )
RegisterResponse (Err error) ->
let
errorMessages =
case error of
Http.BadStatus { status } ->
case status.code of
409 ->
[ "Account already registered" ]
403 ->
[ "The registration code was not found or may have expired" ]
_ ->
[ "There was an error during registration" ]
_ ->
[ defaultHttpErrorMsg error ]
in
( { model | status = NotSent, errors = List.map (\errorMessage -> ( Form, errorMessage )) errorMessages }, Cmd.none )
RegisterResponse (Ok _) ->
( { model | status = Completed }, Cmd.none )
PwnedResults (Err _) ->
sendForm model session
PwnedResults (Ok matches) ->
let
pwnedCount =
pwnedCountFromResponse model.password matches
errors =
validatePwned pwnedCount
in
if errors == [] then
sendForm model session
else
( { model | errors = errors, status = NotSent }, Cmd.none )
sendForm : Model -> Session -> ( Model, Cmd Msg )
sendForm model session =
( { model | status = AwaitingResponse, errors = [] }
, Api.postAccountRegister (authorization session) (Api.Registration model.email model.code model.schoolName model.teacherName model.password)
|> Http.send RegisterResponse
)
view : Model -> { title : String, content : Html Msg }
view model =
{ title = "Register with 3ml"
, content =
div [ class "max-w-xl mx-auto flex flex-col" ]
[ h1 [ class "text-xs-center text-gray-600" ] [ text (heading model.registrationType) ]
, p [ class "my-2 text-lg leading-normal" ]
[ text (blurb model.registrationType)
]
, p [ class "mb-4" ]
[ a [ Route.href Route.Login, class "text-blue-500 hover:text-blue-700" ]
[ text "Have an account already?" ]
]
, Form.viewErrors model.errors
, if model.registrationType == Nothing then
viewRegistrationOptions
else if model.status == Completed then
div [ class "text-lg" ]
[ p [] [ text "Registration complete." ]
, p []
[ case model.registrationType of
Just WithCode ->
text "Your school admin should let you know when they have activated your account."
_ ->
text "Your account should be activated within an hour."
]
, p [] [ text "You can then sign in using your email and password." ]
]
else
viewForm model
]
}
heading : Maybe RegistrationType -> String
heading rt =
case rt of
Nothing ->
"Sign up"
Just NewSchool ->
"Register a new school"
Just WithCode ->
"Register as a teacher in an existing school"
blurb : Maybe RegistrationType -> String
blurb rt =
case rt of
Nothing ->
"""
You can either register a new school or, if your school is already using 3ml, as a teacher in an existing school.
"""
Just WithCode ->
"""To register for an existing school, a 3ml admin at your school should have given
you a registration code. Please enter it in the form below. If you don't have a code, please
ask the teacher who registered your school for one. The code is only valid for a short time, so you
should complete your registration right away.
"""
Just NewSchool ->
"""
Registering will create a new school in the 3ml system for which you will be the administrator.
You will be able to create accounts for the children in your school to use 3ml and also
accounts for other teachers. You will be responsible for obtaining consent for your students
to use the system, for controlling the data that they enter and for maintaining the accounts
for your school.
"""
viewRegistrationOptions : Html Msg
viewRegistrationOptions =
div [ class "flex justify-around" ]
[ Components.btn [ id "register-school", class "mr-2", type_ "button", onClick (SetRegistrationType NewSchool) ] [ text "Register a new school" ]
, Components.btn [ id "register-teacher", type_ "button", onClick (SetRegistrationType WithCode) ] [ text "Register as a teacher in an existing school" ]
]
viewForm : Model -> Html Msg
viewForm model =
Html.form [ class "flex flex-col", onSubmit SubmitForm ]
[ case model.registrationType of
Just WithCode ->
Form.input
[ class ""
, placeholder "Registration code"
, onInput SetCode
, maxlength 100
]
[]
_ ->
Form.input
[ class ""
, placeholder "School name"
, onInput SetSchoolName
, maxlength 100
]
[]
, Form.input
[ class "my-2"
, placeholder "Your name"
, onInput SetTeacherName
, maxlength 100
]
[]
, Form.input
[ class "mb-2"
, placeholder "Email"
, onInput SetEmail
, maxlength 50
]
[]
, Form.password
[ class ""
, placeholder "Choose a password"
, onInput SetPassword
, maxlength 100
, type_ "password"
]
[]
, Form.password
[ class "my-2"
, placeholder "PI:PASSWORD:<PASSWORD>END_PI"
, onInput SetConfirmPassword
, maxlength 100
]
[]
, Components.btn [ class "mt-1", disabled (model.status /= NotSent) ]
[ text "Sign up" ]
]
-- VALIDATION --
type Field
= Form
| SchoolName
| TeacherName
| Email
| Password
type alias Error =
( Field, String )
validator : Validator Error Model
validator =
Validate.all
[ ifBlank .schoolName ( SchoolName, "school name can't be blank." )
, ifInvalidEmail .email (\_ -> ( Email, "please enter a valid email." ))
, ifBlank .teacherName ( TeacherName, "name can't be blank." )
, fromErrors validatePassword
]
validatePassword : Model -> List Error
validatePassword { password, confirmPassword } =
case password of
"" ->
[ ( Password, "password can't be blank" ) ]
_ ->
if password /= confirmPassword then
[ ( Form, "the passwords must be the same" ) ]
else if String.length password < 8 then
[ ( Password, "password must be at least 8 characters" ) ]
else
[]
validatePwned : PwnedCount -> List Error
validatePwned pwned =
case pwned of
Zero ->
[]
One ->
[ ( Password, "Insecure password (found in a compromised database)!" ) ]
Pwned n ->
[ ( Password, "Insecure password (found " ++ String.fromInt n ++ " times in compromised databases)!" ) ]
Error ->
[]
sha1 : String -> String
sha1 s =
SHA1.fromString s
|> SHA1.toHex
|> String.toUpper
pwnedCountFromResponse : String -> String -> PwnedCount
pwnedCountFromResponse password response =
let
suffix =
sha1 password |> String.dropLeft 5
match =
String.lines response
|> List.filter (String.startsWith suffix)
|> List.head
|> Maybe.map (String.dropLeft 36)
|> Maybe.andThen String.toInt
in
case match of
Nothing ->
Zero
Just 1 ->
One
Just count ->
Pwned count
getPwnedMatches : String -> Cmd Msg
getPwnedMatches password =
Http.send PwnedResults <|
Http.getString ("https://api.pwnedpasswords.com/range/" ++ String.left 5 (sha1 password))
| elm |
[
{
"context": "-- Copyright (c) 2016, Richard Feldman\n-- All rights reserved.\n-- \n-- Redistribution and",
"end": 38,
"score": 0.9997396469,
"start": 23,
"tag": "NAME",
"value": "Richard Feldman"
}
] | Hex.elm | dwendelen/adventofcode_2017 | 0 | -- Copyright (c) 2016, Richard Feldman
-- All rights reserved.
--
-- Redistribution and use in source and binary forms, with or without
-- modification, are permitted provided that the following conditions are met:
--
-- * Redistributions of source code must retain the above copyright notice, this
-- list of conditions and the following disclaimer.
--
-- * Redistributions in binary form must reproduce the above copyright notice,
-- this list of conditions and the following disclaimer in the documentation
-- and/or other materials provided with the distribution.
--
-- * Neither the name of the copyright holder nor the names of its
-- contributors may be used to endorse or promote products derived from
-- this software without specific prior written permission.
--
-- THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
-- AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
-- IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
-- DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
-- FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
-- DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
-- SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
-- CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
-- OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
-- OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
module Hex exposing (fromString, toString)
{-| Convert to and from Hex strings.
@docs fromString, toString
-}
{-| Convert a hexdecimal string such as "abc94f" to a decimal integer.
Hex.fromString "a5" == Ok 165
Hex.fromString "hat" == Err "invalid hexadecimal string"
-}
fromString : String -> Result String Int
fromString str =
if String.isEmpty str then
Err "Empty strings are not valid hexadecimal strings."
else
let
result =
if String.startsWith "-" str then
let
list =
str
|> String.toList
|> List.tail
|> Maybe.withDefault []
in
fromStringHelp (List.length list - 1) list 0
|> Result.map negate
else
fromStringHelp (String.length str - 1) (String.toList str) 0
formatError err =
String.join " "
[ "\"" ++ str ++ "\""
, "is not a valid hexadecimal string because"
, err
]
in
Result.mapError formatError result
fromStringHelp : Int -> List Char -> Int -> Result String Int
fromStringHelp position chars accumulated =
case chars of
[] ->
Ok accumulated
char :: rest ->
-- NOTE: It's important to have this call `fromStringHelp` directly.
-- Previously this called a helper function, but that meant this
-- was not tail-call optimized; it did not compile to a `while` loop
-- the way it does now. See 240c3d5aa4f97463b924728935d2989621e9fd6b
case char of
'0' ->
fromStringHelp (position - 1) rest accumulated
'1' ->
fromStringHelp (position - 1) rest (accumulated + (16 ^ position))
'2' ->
fromStringHelp (position - 1) rest (accumulated + (2 * (16 ^ position)))
'3' ->
fromStringHelp (position - 1) rest (accumulated + (3 * (16 ^ position)))
'4' ->
fromStringHelp (position - 1) rest (accumulated + (4 * (16 ^ position)))
'5' ->
fromStringHelp (position - 1) rest (accumulated + (5 * (16 ^ position)))
'6' ->
fromStringHelp (position - 1) rest (accumulated + (6 * (16 ^ position)))
'7' ->
fromStringHelp (position - 1) rest (accumulated + (7 * (16 ^ position)))
'8' ->
fromStringHelp (position - 1) rest (accumulated + (8 * (16 ^ position)))
'9' ->
fromStringHelp (position - 1) rest (accumulated + (9 * (16 ^ position)))
'a' ->
fromStringHelp (position - 1) rest (accumulated + (10 * (16 ^ position)))
'b' ->
fromStringHelp (position - 1) rest (accumulated + (11 * (16 ^ position)))
'c' ->
fromStringHelp (position - 1) rest (accumulated + (12 * (16 ^ position)))
'd' ->
fromStringHelp (position - 1) rest (accumulated + (13 * (16 ^ position)))
'e' ->
fromStringHelp (position - 1) rest (accumulated + (14 * (16 ^ position)))
'f' ->
fromStringHelp (position - 1) rest (accumulated + (15 * (16 ^ position)))
nonHex ->
Err (String.fromChar nonHex ++ " is not a valid hexadecimal character.")
{-| Convert a decimal integer to a hexdecimal string such as `"abc94f"`.
Hex.toString 165 == "a5"
-}
toString : Int -> String
toString num =
String.fromList <|
if num < 0 then
'-' :: unsafePositiveToDigits [] (negate num)
else
unsafePositiveToDigits [] num
{-| ONLY EVER CALL THIS WITH POSITIVE INTEGERS!
-}
unsafePositiveToDigits : List Char -> Int -> List Char
unsafePositiveToDigits digits num =
if num < 16 then
unsafeToDigit num :: digits
else
unsafePositiveToDigits (unsafeToDigit (num % 16) :: digits) (num // 16)
{-| ONLY EVER CALL THIS WITH INTEGERS BETWEEN 0 and 15!
-}
unsafeToDigit : Int -> Char
unsafeToDigit num =
case num of
0 ->
'0'
1 ->
'1'
2 ->
'2'
3 ->
'3'
4 ->
'4'
5 ->
'5'
6 ->
'6'
7 ->
'7'
8 ->
'8'
9 ->
'9'
10 ->
'a'
11 ->
'b'
12 ->
'c'
13 ->
'd'
14 ->
'e'
15 ->
'f'
_ ->
Debug.crash ("Tried to convert " ++ toString num ++ " to hexadecimal.")
| 42316 | -- Copyright (c) 2016, <NAME>
-- All rights reserved.
--
-- Redistribution and use in source and binary forms, with or without
-- modification, are permitted provided that the following conditions are met:
--
-- * Redistributions of source code must retain the above copyright notice, this
-- list of conditions and the following disclaimer.
--
-- * Redistributions in binary form must reproduce the above copyright notice,
-- this list of conditions and the following disclaimer in the documentation
-- and/or other materials provided with the distribution.
--
-- * Neither the name of the copyright holder nor the names of its
-- contributors may be used to endorse or promote products derived from
-- this software without specific prior written permission.
--
-- THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
-- AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
-- IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
-- DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
-- FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
-- DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
-- SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
-- CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
-- OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
-- OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
module Hex exposing (fromString, toString)
{-| Convert to and from Hex strings.
@docs fromString, toString
-}
{-| Convert a hexdecimal string such as "abc94f" to a decimal integer.
Hex.fromString "a5" == Ok 165
Hex.fromString "hat" == Err "invalid hexadecimal string"
-}
fromString : String -> Result String Int
fromString str =
if String.isEmpty str then
Err "Empty strings are not valid hexadecimal strings."
else
let
result =
if String.startsWith "-" str then
let
list =
str
|> String.toList
|> List.tail
|> Maybe.withDefault []
in
fromStringHelp (List.length list - 1) list 0
|> Result.map negate
else
fromStringHelp (String.length str - 1) (String.toList str) 0
formatError err =
String.join " "
[ "\"" ++ str ++ "\""
, "is not a valid hexadecimal string because"
, err
]
in
Result.mapError formatError result
fromStringHelp : Int -> List Char -> Int -> Result String Int
fromStringHelp position chars accumulated =
case chars of
[] ->
Ok accumulated
char :: rest ->
-- NOTE: It's important to have this call `fromStringHelp` directly.
-- Previously this called a helper function, but that meant this
-- was not tail-call optimized; it did not compile to a `while` loop
-- the way it does now. See 240c3d5aa4f97463b924728935d2989621e9fd6b
case char of
'0' ->
fromStringHelp (position - 1) rest accumulated
'1' ->
fromStringHelp (position - 1) rest (accumulated + (16 ^ position))
'2' ->
fromStringHelp (position - 1) rest (accumulated + (2 * (16 ^ position)))
'3' ->
fromStringHelp (position - 1) rest (accumulated + (3 * (16 ^ position)))
'4' ->
fromStringHelp (position - 1) rest (accumulated + (4 * (16 ^ position)))
'5' ->
fromStringHelp (position - 1) rest (accumulated + (5 * (16 ^ position)))
'6' ->
fromStringHelp (position - 1) rest (accumulated + (6 * (16 ^ position)))
'7' ->
fromStringHelp (position - 1) rest (accumulated + (7 * (16 ^ position)))
'8' ->
fromStringHelp (position - 1) rest (accumulated + (8 * (16 ^ position)))
'9' ->
fromStringHelp (position - 1) rest (accumulated + (9 * (16 ^ position)))
'a' ->
fromStringHelp (position - 1) rest (accumulated + (10 * (16 ^ position)))
'b' ->
fromStringHelp (position - 1) rest (accumulated + (11 * (16 ^ position)))
'c' ->
fromStringHelp (position - 1) rest (accumulated + (12 * (16 ^ position)))
'd' ->
fromStringHelp (position - 1) rest (accumulated + (13 * (16 ^ position)))
'e' ->
fromStringHelp (position - 1) rest (accumulated + (14 * (16 ^ position)))
'f' ->
fromStringHelp (position - 1) rest (accumulated + (15 * (16 ^ position)))
nonHex ->
Err (String.fromChar nonHex ++ " is not a valid hexadecimal character.")
{-| Convert a decimal integer to a hexdecimal string such as `"abc94f"`.
Hex.toString 165 == "a5"
-}
toString : Int -> String
toString num =
String.fromList <|
if num < 0 then
'-' :: unsafePositiveToDigits [] (negate num)
else
unsafePositiveToDigits [] num
{-| ONLY EVER CALL THIS WITH POSITIVE INTEGERS!
-}
unsafePositiveToDigits : List Char -> Int -> List Char
unsafePositiveToDigits digits num =
if num < 16 then
unsafeToDigit num :: digits
else
unsafePositiveToDigits (unsafeToDigit (num % 16) :: digits) (num // 16)
{-| ONLY EVER CALL THIS WITH INTEGERS BETWEEN 0 and 15!
-}
unsafeToDigit : Int -> Char
unsafeToDigit num =
case num of
0 ->
'0'
1 ->
'1'
2 ->
'2'
3 ->
'3'
4 ->
'4'
5 ->
'5'
6 ->
'6'
7 ->
'7'
8 ->
'8'
9 ->
'9'
10 ->
'a'
11 ->
'b'
12 ->
'c'
13 ->
'd'
14 ->
'e'
15 ->
'f'
_ ->
Debug.crash ("Tried to convert " ++ toString num ++ " to hexadecimal.")
| true | -- Copyright (c) 2016, PI:NAME:<NAME>END_PI
-- All rights reserved.
--
-- Redistribution and use in source and binary forms, with or without
-- modification, are permitted provided that the following conditions are met:
--
-- * Redistributions of source code must retain the above copyright notice, this
-- list of conditions and the following disclaimer.
--
-- * Redistributions in binary form must reproduce the above copyright notice,
-- this list of conditions and the following disclaimer in the documentation
-- and/or other materials provided with the distribution.
--
-- * Neither the name of the copyright holder nor the names of its
-- contributors may be used to endorse or promote products derived from
-- this software without specific prior written permission.
--
-- THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
-- AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
-- IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
-- DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
-- FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
-- DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
-- SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
-- CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
-- OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
-- OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
module Hex exposing (fromString, toString)
{-| Convert to and from Hex strings.
@docs fromString, toString
-}
{-| Convert a hexdecimal string such as "abc94f" to a decimal integer.
Hex.fromString "a5" == Ok 165
Hex.fromString "hat" == Err "invalid hexadecimal string"
-}
fromString : String -> Result String Int
fromString str =
if String.isEmpty str then
Err "Empty strings are not valid hexadecimal strings."
else
let
result =
if String.startsWith "-" str then
let
list =
str
|> String.toList
|> List.tail
|> Maybe.withDefault []
in
fromStringHelp (List.length list - 1) list 0
|> Result.map negate
else
fromStringHelp (String.length str - 1) (String.toList str) 0
formatError err =
String.join " "
[ "\"" ++ str ++ "\""
, "is not a valid hexadecimal string because"
, err
]
in
Result.mapError formatError result
fromStringHelp : Int -> List Char -> Int -> Result String Int
fromStringHelp position chars accumulated =
case chars of
[] ->
Ok accumulated
char :: rest ->
-- NOTE: It's important to have this call `fromStringHelp` directly.
-- Previously this called a helper function, but that meant this
-- was not tail-call optimized; it did not compile to a `while` loop
-- the way it does now. See 240c3d5aa4f97463b924728935d2989621e9fd6b
case char of
'0' ->
fromStringHelp (position - 1) rest accumulated
'1' ->
fromStringHelp (position - 1) rest (accumulated + (16 ^ position))
'2' ->
fromStringHelp (position - 1) rest (accumulated + (2 * (16 ^ position)))
'3' ->
fromStringHelp (position - 1) rest (accumulated + (3 * (16 ^ position)))
'4' ->
fromStringHelp (position - 1) rest (accumulated + (4 * (16 ^ position)))
'5' ->
fromStringHelp (position - 1) rest (accumulated + (5 * (16 ^ position)))
'6' ->
fromStringHelp (position - 1) rest (accumulated + (6 * (16 ^ position)))
'7' ->
fromStringHelp (position - 1) rest (accumulated + (7 * (16 ^ position)))
'8' ->
fromStringHelp (position - 1) rest (accumulated + (8 * (16 ^ position)))
'9' ->
fromStringHelp (position - 1) rest (accumulated + (9 * (16 ^ position)))
'a' ->
fromStringHelp (position - 1) rest (accumulated + (10 * (16 ^ position)))
'b' ->
fromStringHelp (position - 1) rest (accumulated + (11 * (16 ^ position)))
'c' ->
fromStringHelp (position - 1) rest (accumulated + (12 * (16 ^ position)))
'd' ->
fromStringHelp (position - 1) rest (accumulated + (13 * (16 ^ position)))
'e' ->
fromStringHelp (position - 1) rest (accumulated + (14 * (16 ^ position)))
'f' ->
fromStringHelp (position - 1) rest (accumulated + (15 * (16 ^ position)))
nonHex ->
Err (String.fromChar nonHex ++ " is not a valid hexadecimal character.")
{-| Convert a decimal integer to a hexdecimal string such as `"abc94f"`.
Hex.toString 165 == "a5"
-}
toString : Int -> String
toString num =
String.fromList <|
if num < 0 then
'-' :: unsafePositiveToDigits [] (negate num)
else
unsafePositiveToDigits [] num
{-| ONLY EVER CALL THIS WITH POSITIVE INTEGERS!
-}
unsafePositiveToDigits : List Char -> Int -> List Char
unsafePositiveToDigits digits num =
if num < 16 then
unsafeToDigit num :: digits
else
unsafePositiveToDigits (unsafeToDigit (num % 16) :: digits) (num // 16)
{-| ONLY EVER CALL THIS WITH INTEGERS BETWEEN 0 and 15!
-}
unsafeToDigit : Int -> Char
unsafeToDigit num =
case num of
0 ->
'0'
1 ->
'1'
2 ->
'2'
3 ->
'3'
4 ->
'4'
5 ->
'5'
6 ->
'6'
7 ->
'7'
8 ->
'8'
9 ->
'9'
10 ->
'a'
11 ->
'b'
12 ->
'c'
13 ->
'd'
14 ->
'e'
15 ->
'f'
_ ->
Debug.crash ("Tried to convert " ++ toString num ++ " to hexadecimal.")
| elm |
[
{
"context": "e =\n let\n nodes =\n [ Node 0 \"Socks\"\n , Node 1 \"Undershorts\"\n ,",
"end": 2074,
"score": 0.9803011417,
"start": 2069,
"tag": "NAME",
"value": "Socks"
},
{
"context": " [ Node 0 \"Socks\"\n , Node 1 \"Undershorts\"\n , Node 2 \"Pants\"\n ]\n\n ",
"end": 2109,
"score": 0.9738795757,
"start": 2098,
"tag": "NAME",
"value": "Undershorts"
},
{
"context": " , Node 1 \"Undershorts\"\n , Node 2 \"Pants\"\n ]\n\n e from to =\n E",
"end": 2138,
"score": 0.9933710098,
"start": 2133,
"tag": "NAME",
"value": "Pants"
}
] | tests/Tests/Graph.elm | janwirth/graph | 59 | module Tests.Graph exposing (all)
import Expect
import Graph exposing (Edge, Graph, Node, NodeContext, NodeId)
import IntDict exposing (IntDict)
import Test exposing (..)
isJust : Maybe a -> Bool
isJust m =
case m of
Just _ ->
True
_ ->
False
isOk : Result m n -> Bool
isOk result =
case result of
Err _ ->
False
Ok _ ->
True
isErr : Result m n -> Bool
isErr result =
not (isOk result)
expectEqualComparing : (a -> b) -> a -> a -> Expect.Expectation
expectEqualComparing f a b =
Expect.equal (f a) (f b)
edgeTriples : Graph n e -> List ( NodeId, NodeId, e )
edgeTriples =
Graph.edges >> List.map (\e -> ( e.from, e.to, e.label ))
dressUp : Graph String ()
dressUp =
let
nodes =
[ Node 0 "Socks"
, Node 1 "Undershorts"
, Node 2 "Pants"
, Node 3 "Shoes"
, Node 4 "Watch"
, Node 5 "Shirt"
, Node 6 "Belt"
, Node 7 "Tie"
, Node 8 "Jacket"
]
e from to =
Edge from to ()
edges =
[ e 0 3 -- socks before shoes
, e 1 2 -- undershorts before pants
, e 1 3 -- undershorts before shoes
, e 2 3 -- pants before shoes
, e 2 6 -- pants before belt
, e 5 6 -- shirt before belt
, e 5 7 -- shirt before tie
, e 4 8 -- watch before jacket
, e 6 8 -- belt before jacket
, e 7 8 -- tie before jacket
]
in
Graph.fromNodesAndEdges nodes edges
simple : Graph String (Maybe String)
simple =
let
nodes =
[ Node 0 "first"
, Node 1 "second"
, Node 2 "third"
]
edges =
[ Edge 0 1 Nothing
, Edge 0 2 (Just "relationship")
]
in
Graph.fromNodesAndEdges nodes edges
dressUpWithCycle : Graph String ()
dressUpWithCycle =
let
nodes =
[ Node 0 "Socks"
, Node 1 "Undershorts"
, Node 2 "Pants"
]
e from to =
Edge from to ()
edges =
[ e 0 1
, e 1 2
, e 2 0
]
in
Graph.fromNodesAndEdges nodes edges
connectedComponents : Graph Char ()
connectedComponents =
let
nodes =
[ 'a', 'b', 'c', 'd', 'e', 'f', 'g', 'h' ]
edges =
[ ( 0, 1 )
, ( 1, 2 )
, ( 1, 4 )
, ( 1, 5 )
, ( 2, 3 )
, ( 2, 6 )
, ( 3, 2 )
, ( 3, 7 )
, ( 4, 0 )
, ( 4, 5 )
, ( 5, 6 )
, ( 6, 5 )
, ( 6, 7 )
]
in
Graph.fromNodeLabelsAndEdgePairs nodes edges
noNeighbors : Node String -> NodeContext String ()
noNeighbors node =
NodeContext node IntDict.empty IntDict.empty
isValidTopologicalOrderingOf : Graph n e -> List (NodeContext n e) -> Bool
isValidTopologicalOrderingOf graph ordering =
ordering
|> List.foldl
(\ctx maybeIds ->
maybeIds
|> Maybe.andThen
(\ids ->
if List.all (\i -> IntDict.member i ids) (IntDict.keys ctx.incoming) then
ids |> IntDict.insert ctx.node.id () |> Just
else
Nothing
)
)
(Just IntDict.empty)
|> isJust
|> (&&) (List.length ordering == Graph.size graph)
expectTopologicalOrderingOf : Graph String e -> List (NodeContext String e) -> Expect.Expectation
expectTopologicalOrderingOf graph ordering =
let
message =
String.join "\n"
[ "Expected a valid topological ordering of "
, " " ++ Graph.toString Just (always <| Just "") graph
, "but got"
, " " ++ Debug.toString ordering
]
in
Expect.true message (isValidTopologicalOrderingOf graph ordering)
all : Test
all =
let
emptyTests =
describe "empty"
[ test "has size 0" <| \() -> Expect.equal 0 (Graph.size Graph.empty)
, test "isEmpty" <| \() -> Expect.equal True (Graph.isEmpty Graph.empty)
]
memberTests =
describe "member"
[ test "True" <| \() -> Expect.equal True (Graph.member 0 dressUp)
, test "False" <| \() -> Expect.equal False (Graph.member 99 dressUp)
]
getTests =
describe "get"
[ test "id 0, the socks" <|
\() ->
Expect.equal
(Just "Socks")
(dressUp |> Graph.get 0 |> Maybe.map (.node >> .label))
, test "id 99, Nothing" <| \() -> Expect.equal Nothing (Graph.get 99 dressUp)
]
nodeIdRangeTests =
describe "nodeIdRange"
[ test "dressUp: [0, 8]" <|
\() ->
Expect.equal
(Just ( 0, 8 ))
(Graph.nodeIdRange dressUp)
, test "dressUp - 0: [1, 8]" <|
\() ->
Expect.equal
(Just ( 1, 8 ))
(dressUp |> Graph.remove 0 |> Graph.nodeIdRange)
, test "dressUp - 8: [0, 7]" <|
\() ->
Expect.equal
(Just ( 0, 7 ))
(dressUp |> Graph.remove 8 |> Graph.nodeIdRange)
]
listRepTests =
describe "list conversions"
[ test "nodeIds" <|
\() ->
Expect.equal
[ 0, 1, 2, 3, 4, 5, 6, 7, 8 ]
(dressUp |> Graph.nodeIds)
, test "nodes" <|
\() ->
Expect.equal
[ 0, 1, 2, 3, 4, 5, 6, 7, 8 ]
(dressUp |> Graph.nodes |> List.map .id)
, test "edges" <|
\() ->
Expect.equal
[ ( 0, 3 ), ( 1, 2 ), ( 1, 3 ), ( 2, 3 ), ( 2, 6 ), ( 4, 8 ), ( 5, 6 ), ( 5, 7 ), ( 6, 8 ), ( 7, 8 ) ]
(dressUp
|> Graph.edges
|> List.map (\e -> ( e.from, e.to ))
|> List.sort
)
]
insertTests =
describe "insert"
[ test "new node - size" <|
\() ->
Expect.equal
(dressUp |> Graph.size |> (+) 1)
(dressUp |> Graph.insert (noNeighbors (Node 99 "Ring")) |> Graph.size)
, test "new node - can get it" <|
\() ->
Expect.equal
(Just "Ring")
(dressUp
|> Graph.insert (noNeighbors (Node 99 "Ring"))
|> Graph.get 99
|> Maybe.map (.node >> .label)
)
, test "replace node - size" <|
\() ->
Expect.equal
(dressUp |> Graph.size)
(dressUp |> Graph.insert (noNeighbors (Node 0 "Ring")) |> Graph.size)
, test "replace node - can get it" <|
\() ->
Expect.equal
(Just "Ring")
(dressUp
|> Graph.insert (noNeighbors (Node 0 "Ring"))
|> Graph.get 0
|> Maybe.map (.node >> .label)
)
, test "replace node - replaces adjacency" <|
\() ->
Expect.equal
(Just True)
(dressUp
|> Graph.insert (noNeighbors (Node 0 "Ring"))
|> Graph.get 0
|> Maybe.map (\ctx -> IntDict.isEmpty ctx.incoming && IntDict.isEmpty ctx.outgoing)
)
]
removeTests =
describe "remove"
[ test "nonexistent node" <|
\() ->
Expect.equal
dressUp
(dressUp |> Graph.remove 99)
, test "existing node - size" <|
\() ->
Expect.equal
(dressUp |> Graph.size |> (\i -> (-) i 1))
(dressUp |> Graph.remove 0 |> Graph.size)
, test "existing node - can't get it" <|
\() ->
Expect.equal
Nothing
(dressUp |> Graph.remove 0 |> Graph.get 0)
]
updateTests =
describe "update"
[ test "remove outgoing edges" <|
\() ->
Expect.equal
(Just True)
(dressUp
|> Graph.update 0
-- "Shorts" has outgoing edges
(Maybe.map (\n -> { n | outgoing = IntDict.empty }))
|> Graph.get 0
|> Maybe.map (.outgoing >> IntDict.isEmpty)
)
]
inducedSubgraphTests =
describe "inducedSubgraph"
[ test "should not have any dangling edges" <|
\() ->
expectEqualComparing
(edgeTriples >> List.sortBy (\( f, t, _ ) -> ( f, t )))
(Graph.fromNodesAndEdges
[ Node 0 'a', Node 1 'b', Node 4 'e' ]
[ Edge 0 1 (), Edge 1 4 (), Edge 4 0 () ]
)
(Graph.inducedSubgraph [ 0, 1, 4 ] connectedComponents)
]
fromNodesAndEdgesTests =
describe "fromNodesAndEdges"
[ test "should not have any dangling edges" <|
\() ->
Expect.equal
[ Edge 0 0 () ]
(Graph.edges
(Graph.fromNodesAndEdges
[ Node 0 'a' ]
[ Edge 0 0 (), Edge 0 1 (), Edge 1 0 (), Edge 1 1 () ]
)
)
]
foldTests =
describe "fold"
[ test "sum up ids" <|
\() ->
Expect.equal
36
(dressUp
|> Graph.fold (\ctx -> (+) ctx.node.id) 0
)
]
mapTests =
describe "map*"
[ test "mapContexts over id is the id" <|
\() ->
Expect.equal
dressUp
(dressUp |> Graph.mapContexts identity)
, test "mapNodes over id is the id" <|
\() ->
Expect.equal
dressUp
(dressUp |> Graph.mapNodes identity)
, test "mapEdges over id is the id" <|
\() ->
Expect.equal
dressUp
(dressUp |> Graph.mapNodes identity)
-- This should be backed by more tests, but I'm not in the mood for that :/
]
toStringTests =
describe "toString"
[ test "works as expected" <|
\() ->
Expect.equal
(Graph.toString Just identity simple)
"Graph [Node 0 (first), Node 1 (second), Node 2 (third)] [Edge 0->2 (relationship), Edge 0->1]"
]
graphOpsTests =
describe "Graph ops"
[ test "symmetricClosure is symmetric" <|
\() ->
Expect.true
"expected all incoming edges to also be outgoing and vice versa"
(dressUp
|> Graph.symmetricClosure (\_ _ e _ -> e)
|> Graph.fold
(\ctx acc ->
ctx.incoming == ctx.outgoing && acc
)
True
)
, test "reverseEdges" <|
\() ->
Expect.equal
(dressUp
|> Graph.edges
|> List.map (\e -> ( e.from, e.to ))
|> List.sort
)
(dressUp
|> Graph.reverseEdges
|> Graph.edges
|> List.map (\e -> ( e.to, e.from ))
|> List.sort
)
]
checkAcyclicTests =
describe "checkAcyclicTests" <|
[ test "Ok for graph with no cycles" <|
\() ->
Expect.true
"Should return Ok"
(isOk (Graph.checkAcyclic dressUp))
, test "Err for cyclic graph" <|
\() ->
Expect.true
"Should return Err"
(isErr (Graph.checkAcyclic dressUpWithCycle))
, test "Err for connectedComponents" <|
\() ->
Expect.true
"Should return Err"
(isErr (Graph.checkAcyclic connectedComponents))
]
topologicalSortTests =
describe "topologicalSort"
[ test "valid topological ordering" <|
\() ->
case Graph.checkAcyclic dressUp of
Err e ->
Expect.fail
("dressUp should be acylic, but returned edge " ++ Debug.toString e)
Ok acyclic ->
acyclic
|> Graph.topologicalSort
|> expectTopologicalOrderingOf dressUp
, test "heightLevels" <|
\() ->
case Graph.checkAcyclic dressUp of
Err e ->
Expect.fail
("dressUp should be acylic, but returned edge " ++ Debug.toString e)
Ok acyclic ->
acyclic
|> Graph.heightLevels
|> List.concat
|> expectTopologicalOrderingOf dressUp
]
bfsTests =
describe "BFS"
[ test "breadth-first node order" <|
\() ->
Expect.equal
[ 0, 3, 1, 2, 6, 8, 4, 5, 7 ]
(dressUp
|> Graph.bfs (Graph.ignorePath (::)) []
|> List.map (.node >> .id)
|> List.reverse
)
]
graphWithLoop =
Graph.fromNodeLabelsAndEdgePairs [ 0 ] [ ( 0, 0 ) ]
sccTests =
let
result =
Graph.stronglyConnectedComponents connectedComponents
sg nodeIds =
connectedComponents
|> Graph.inducedSubgraph nodeIds
|> Graph.toString (Just << String.fromChar) (always <| Just "")
in
describe "Strongly connected components"
[ test "The input graph was acyclic" <|
\() ->
Expect.true
"Result should be Err"
(isErr result)
, test "The expected SCCs in order" <|
\() ->
Expect.equal
[ sg [ 0, 1, 4 ] -- "abe"
, sg [ 2, 3 ] -- "cd"
, sg [ 5, 6 ] -- "ef"
, sg [ 7 ] -- "h"
]
(case result of
Err components ->
List.map (Graph.toString (Just << String.fromChar) (always <| Just "")) components
Ok _ ->
[]
-- should never happen oO
)
, test "dressUp is acyclic" <|
\() ->
Expect.true
"Should be Ok"
(isOk (Graph.stronglyConnectedComponents dressUp))
, test "The input graph has loops" <|
\() ->
Expect.true
"Should be Err"
(isErr (Graph.stronglyConnectedComponents graphWithLoop))
]
unitTests =
describe "unit tests"
[ emptyTests
, memberTests
, getTests
, nodeIdRangeTests
, listRepTests
, insertTests
, removeTests
, updateTests
, inducedSubgraphTests
, fromNodesAndEdgesTests
, foldTests
, mapTests
, toStringTests
, graphOpsTests
, checkAcyclicTests
, topologicalSortTests
, bfsTests
, sccTests
]
examples =
describe "examples"
[ test "README - iWantToWearShoes" <|
\() ->
Expect.equal
[ "Pants", "Undershorts", "Socks", "Shoes" ]
iWantToWearShoes
, test "insert" <|
\() ->
Expect.true "Graph size wasn't 2" insertExample
, test "fold" <|
\() ->
Expect.true "The graph had a loop." foldExample
, test "mapContexts" <|
\() ->
Expect.true "Mapped edge flip should've reversed edges" mapContextsExample
]
in
describe "The Graph module"
[ unitTests
, examples
]
-- EXAMPLE SECTION
-- The code of the more complex examples is exercised here
-- This is from the README
iWantToWearShoes : List String
iWantToWearShoes =
Graph.guidedDfs
Graph.alongIncomingEdges
-- which edges to follow
(Graph.onDiscovery
(\ctx list ->
-- append node labels on finish
ctx.node.label :: list
)
)
[ 3
{- "Shoes" NodeId -}
]
-- start with the node labelled "Shoes"
[]
-- accumulate starting with the empty list
dressUp
-- traverse our dressUp graph from above
|> Tuple.first
-- ignores the untraversed rest of the graph
insertExample : Bool
insertExample =
let
graph1 =
Graph.fromNodesAndEdges [ Node 1 "1" ] []
newNode =
{ node = Node 2 "2"
, incoming = IntDict.singleton 1 () -- so there will be an edge from 1 to 2
, outgoing = IntDict.empty
}
graph2 =
Graph.insert newNode graph1
in
Graph.size graph2 == 2
foldExample : Bool
foldExample =
let
hasLoop ctx =
IntDict.member ctx.node.id ctx.incoming
graph =
Graph.fromNodesAndEdges [ Node 1 "1", Node 2 "2" ] [ Edge 1 2 "->" ]
-- The graph should not have any loop.
in
Graph.fold (\ctx acc -> acc || hasLoop ctx) False graph == False
mapContextsExample : Bool
mapContextsExample =
let
flipEdges ctx =
{ ctx | incoming = ctx.outgoing, outgoing = ctx.incoming }
graph =
Graph.fromNodesAndEdges [ Node 1 "1", Node 2 "2" ] [ Edge 1 2 "->" ]
in
Graph.reverseEdges graph == Graph.mapContexts flipEdges graph
symmetricClosureExample : Bool
symmetricClosureExample =
let
graph =
Graph.fromNodesAndEdges [ Node 1 "1", Node 2 "2" ] [ Edge 1 2 "->" ]
onlyUndirectedEdges ctx =
ctx.incoming == ctx.outgoing
merger from to outgoingLabel incomingLabel =
outgoingLabel
-- quite arbitrary, will not be called for the above graph
in
Graph.fold
(\ctx acc -> acc && onlyUndirectedEdges ctx)
True
(Graph.symmetricClosure merger graph)
== True
onDiscoveryExample : ()
-- Just let it compile
onDiscoveryExample =
let
dfsPostOrder : Graph n e -> List (NodeContext n e)
dfsPostOrder graph =
Graph.dfs (Graph.onDiscovery (::)) [] graph
in
dfsPostOrder Graph.empty |> (\_ -> ())
onFinishExample : ()
-- Just let it compile
onFinishExample =
let
dfsPreOrder : Graph n e -> List (NodeContext n e)
dfsPreOrder graph =
Graph.dfs (Graph.onFinish (::)) [] graph
in
dfsPreOrder Graph.empty |> (\_ -> ())
ignorePathExample : ()
-- Just let it compile
ignorePathExample =
let
bfsLevelOrder : Graph n e -> List (NodeContext n e)
bfsLevelOrder graph =
graph
|> Graph.bfs (Graph.ignorePath (::)) []
|> List.reverse
in
bfsLevelOrder Graph.empty |> (\_ -> ())
| 3580 | module Tests.Graph exposing (all)
import Expect
import Graph exposing (Edge, Graph, Node, NodeContext, NodeId)
import IntDict exposing (IntDict)
import Test exposing (..)
isJust : Maybe a -> Bool
isJust m =
case m of
Just _ ->
True
_ ->
False
isOk : Result m n -> Bool
isOk result =
case result of
Err _ ->
False
Ok _ ->
True
isErr : Result m n -> Bool
isErr result =
not (isOk result)
expectEqualComparing : (a -> b) -> a -> a -> Expect.Expectation
expectEqualComparing f a b =
Expect.equal (f a) (f b)
edgeTriples : Graph n e -> List ( NodeId, NodeId, e )
edgeTriples =
Graph.edges >> List.map (\e -> ( e.from, e.to, e.label ))
dressUp : Graph String ()
dressUp =
let
nodes =
[ Node 0 "Socks"
, Node 1 "Undershorts"
, Node 2 "Pants"
, Node 3 "Shoes"
, Node 4 "Watch"
, Node 5 "Shirt"
, Node 6 "Belt"
, Node 7 "Tie"
, Node 8 "Jacket"
]
e from to =
Edge from to ()
edges =
[ e 0 3 -- socks before shoes
, e 1 2 -- undershorts before pants
, e 1 3 -- undershorts before shoes
, e 2 3 -- pants before shoes
, e 2 6 -- pants before belt
, e 5 6 -- shirt before belt
, e 5 7 -- shirt before tie
, e 4 8 -- watch before jacket
, e 6 8 -- belt before jacket
, e 7 8 -- tie before jacket
]
in
Graph.fromNodesAndEdges nodes edges
simple : Graph String (Maybe String)
simple =
let
nodes =
[ Node 0 "first"
, Node 1 "second"
, Node 2 "third"
]
edges =
[ Edge 0 1 Nothing
, Edge 0 2 (Just "relationship")
]
in
Graph.fromNodesAndEdges nodes edges
dressUpWithCycle : Graph String ()
dressUpWithCycle =
let
nodes =
[ Node 0 "<NAME>"
, Node 1 "<NAME>"
, Node 2 "<NAME>"
]
e from to =
Edge from to ()
edges =
[ e 0 1
, e 1 2
, e 2 0
]
in
Graph.fromNodesAndEdges nodes edges
connectedComponents : Graph Char ()
connectedComponents =
let
nodes =
[ 'a', 'b', 'c', 'd', 'e', 'f', 'g', 'h' ]
edges =
[ ( 0, 1 )
, ( 1, 2 )
, ( 1, 4 )
, ( 1, 5 )
, ( 2, 3 )
, ( 2, 6 )
, ( 3, 2 )
, ( 3, 7 )
, ( 4, 0 )
, ( 4, 5 )
, ( 5, 6 )
, ( 6, 5 )
, ( 6, 7 )
]
in
Graph.fromNodeLabelsAndEdgePairs nodes edges
noNeighbors : Node String -> NodeContext String ()
noNeighbors node =
NodeContext node IntDict.empty IntDict.empty
isValidTopologicalOrderingOf : Graph n e -> List (NodeContext n e) -> Bool
isValidTopologicalOrderingOf graph ordering =
ordering
|> List.foldl
(\ctx maybeIds ->
maybeIds
|> Maybe.andThen
(\ids ->
if List.all (\i -> IntDict.member i ids) (IntDict.keys ctx.incoming) then
ids |> IntDict.insert ctx.node.id () |> Just
else
Nothing
)
)
(Just IntDict.empty)
|> isJust
|> (&&) (List.length ordering == Graph.size graph)
expectTopologicalOrderingOf : Graph String e -> List (NodeContext String e) -> Expect.Expectation
expectTopologicalOrderingOf graph ordering =
let
message =
String.join "\n"
[ "Expected a valid topological ordering of "
, " " ++ Graph.toString Just (always <| Just "") graph
, "but got"
, " " ++ Debug.toString ordering
]
in
Expect.true message (isValidTopologicalOrderingOf graph ordering)
all : Test
all =
let
emptyTests =
describe "empty"
[ test "has size 0" <| \() -> Expect.equal 0 (Graph.size Graph.empty)
, test "isEmpty" <| \() -> Expect.equal True (Graph.isEmpty Graph.empty)
]
memberTests =
describe "member"
[ test "True" <| \() -> Expect.equal True (Graph.member 0 dressUp)
, test "False" <| \() -> Expect.equal False (Graph.member 99 dressUp)
]
getTests =
describe "get"
[ test "id 0, the socks" <|
\() ->
Expect.equal
(Just "Socks")
(dressUp |> Graph.get 0 |> Maybe.map (.node >> .label))
, test "id 99, Nothing" <| \() -> Expect.equal Nothing (Graph.get 99 dressUp)
]
nodeIdRangeTests =
describe "nodeIdRange"
[ test "dressUp: [0, 8]" <|
\() ->
Expect.equal
(Just ( 0, 8 ))
(Graph.nodeIdRange dressUp)
, test "dressUp - 0: [1, 8]" <|
\() ->
Expect.equal
(Just ( 1, 8 ))
(dressUp |> Graph.remove 0 |> Graph.nodeIdRange)
, test "dressUp - 8: [0, 7]" <|
\() ->
Expect.equal
(Just ( 0, 7 ))
(dressUp |> Graph.remove 8 |> Graph.nodeIdRange)
]
listRepTests =
describe "list conversions"
[ test "nodeIds" <|
\() ->
Expect.equal
[ 0, 1, 2, 3, 4, 5, 6, 7, 8 ]
(dressUp |> Graph.nodeIds)
, test "nodes" <|
\() ->
Expect.equal
[ 0, 1, 2, 3, 4, 5, 6, 7, 8 ]
(dressUp |> Graph.nodes |> List.map .id)
, test "edges" <|
\() ->
Expect.equal
[ ( 0, 3 ), ( 1, 2 ), ( 1, 3 ), ( 2, 3 ), ( 2, 6 ), ( 4, 8 ), ( 5, 6 ), ( 5, 7 ), ( 6, 8 ), ( 7, 8 ) ]
(dressUp
|> Graph.edges
|> List.map (\e -> ( e.from, e.to ))
|> List.sort
)
]
insertTests =
describe "insert"
[ test "new node - size" <|
\() ->
Expect.equal
(dressUp |> Graph.size |> (+) 1)
(dressUp |> Graph.insert (noNeighbors (Node 99 "Ring")) |> Graph.size)
, test "new node - can get it" <|
\() ->
Expect.equal
(Just "Ring")
(dressUp
|> Graph.insert (noNeighbors (Node 99 "Ring"))
|> Graph.get 99
|> Maybe.map (.node >> .label)
)
, test "replace node - size" <|
\() ->
Expect.equal
(dressUp |> Graph.size)
(dressUp |> Graph.insert (noNeighbors (Node 0 "Ring")) |> Graph.size)
, test "replace node - can get it" <|
\() ->
Expect.equal
(Just "Ring")
(dressUp
|> Graph.insert (noNeighbors (Node 0 "Ring"))
|> Graph.get 0
|> Maybe.map (.node >> .label)
)
, test "replace node - replaces adjacency" <|
\() ->
Expect.equal
(Just True)
(dressUp
|> Graph.insert (noNeighbors (Node 0 "Ring"))
|> Graph.get 0
|> Maybe.map (\ctx -> IntDict.isEmpty ctx.incoming && IntDict.isEmpty ctx.outgoing)
)
]
removeTests =
describe "remove"
[ test "nonexistent node" <|
\() ->
Expect.equal
dressUp
(dressUp |> Graph.remove 99)
, test "existing node - size" <|
\() ->
Expect.equal
(dressUp |> Graph.size |> (\i -> (-) i 1))
(dressUp |> Graph.remove 0 |> Graph.size)
, test "existing node - can't get it" <|
\() ->
Expect.equal
Nothing
(dressUp |> Graph.remove 0 |> Graph.get 0)
]
updateTests =
describe "update"
[ test "remove outgoing edges" <|
\() ->
Expect.equal
(Just True)
(dressUp
|> Graph.update 0
-- "Shorts" has outgoing edges
(Maybe.map (\n -> { n | outgoing = IntDict.empty }))
|> Graph.get 0
|> Maybe.map (.outgoing >> IntDict.isEmpty)
)
]
inducedSubgraphTests =
describe "inducedSubgraph"
[ test "should not have any dangling edges" <|
\() ->
expectEqualComparing
(edgeTriples >> List.sortBy (\( f, t, _ ) -> ( f, t )))
(Graph.fromNodesAndEdges
[ Node 0 'a', Node 1 'b', Node 4 'e' ]
[ Edge 0 1 (), Edge 1 4 (), Edge 4 0 () ]
)
(Graph.inducedSubgraph [ 0, 1, 4 ] connectedComponents)
]
fromNodesAndEdgesTests =
describe "fromNodesAndEdges"
[ test "should not have any dangling edges" <|
\() ->
Expect.equal
[ Edge 0 0 () ]
(Graph.edges
(Graph.fromNodesAndEdges
[ Node 0 'a' ]
[ Edge 0 0 (), Edge 0 1 (), Edge 1 0 (), Edge 1 1 () ]
)
)
]
foldTests =
describe "fold"
[ test "sum up ids" <|
\() ->
Expect.equal
36
(dressUp
|> Graph.fold (\ctx -> (+) ctx.node.id) 0
)
]
mapTests =
describe "map*"
[ test "mapContexts over id is the id" <|
\() ->
Expect.equal
dressUp
(dressUp |> Graph.mapContexts identity)
, test "mapNodes over id is the id" <|
\() ->
Expect.equal
dressUp
(dressUp |> Graph.mapNodes identity)
, test "mapEdges over id is the id" <|
\() ->
Expect.equal
dressUp
(dressUp |> Graph.mapNodes identity)
-- This should be backed by more tests, but I'm not in the mood for that :/
]
toStringTests =
describe "toString"
[ test "works as expected" <|
\() ->
Expect.equal
(Graph.toString Just identity simple)
"Graph [Node 0 (first), Node 1 (second), Node 2 (third)] [Edge 0->2 (relationship), Edge 0->1]"
]
graphOpsTests =
describe "Graph ops"
[ test "symmetricClosure is symmetric" <|
\() ->
Expect.true
"expected all incoming edges to also be outgoing and vice versa"
(dressUp
|> Graph.symmetricClosure (\_ _ e _ -> e)
|> Graph.fold
(\ctx acc ->
ctx.incoming == ctx.outgoing && acc
)
True
)
, test "reverseEdges" <|
\() ->
Expect.equal
(dressUp
|> Graph.edges
|> List.map (\e -> ( e.from, e.to ))
|> List.sort
)
(dressUp
|> Graph.reverseEdges
|> Graph.edges
|> List.map (\e -> ( e.to, e.from ))
|> List.sort
)
]
checkAcyclicTests =
describe "checkAcyclicTests" <|
[ test "Ok for graph with no cycles" <|
\() ->
Expect.true
"Should return Ok"
(isOk (Graph.checkAcyclic dressUp))
, test "Err for cyclic graph" <|
\() ->
Expect.true
"Should return Err"
(isErr (Graph.checkAcyclic dressUpWithCycle))
, test "Err for connectedComponents" <|
\() ->
Expect.true
"Should return Err"
(isErr (Graph.checkAcyclic connectedComponents))
]
topologicalSortTests =
describe "topologicalSort"
[ test "valid topological ordering" <|
\() ->
case Graph.checkAcyclic dressUp of
Err e ->
Expect.fail
("dressUp should be acylic, but returned edge " ++ Debug.toString e)
Ok acyclic ->
acyclic
|> Graph.topologicalSort
|> expectTopologicalOrderingOf dressUp
, test "heightLevels" <|
\() ->
case Graph.checkAcyclic dressUp of
Err e ->
Expect.fail
("dressUp should be acylic, but returned edge " ++ Debug.toString e)
Ok acyclic ->
acyclic
|> Graph.heightLevels
|> List.concat
|> expectTopologicalOrderingOf dressUp
]
bfsTests =
describe "BFS"
[ test "breadth-first node order" <|
\() ->
Expect.equal
[ 0, 3, 1, 2, 6, 8, 4, 5, 7 ]
(dressUp
|> Graph.bfs (Graph.ignorePath (::)) []
|> List.map (.node >> .id)
|> List.reverse
)
]
graphWithLoop =
Graph.fromNodeLabelsAndEdgePairs [ 0 ] [ ( 0, 0 ) ]
sccTests =
let
result =
Graph.stronglyConnectedComponents connectedComponents
sg nodeIds =
connectedComponents
|> Graph.inducedSubgraph nodeIds
|> Graph.toString (Just << String.fromChar) (always <| Just "")
in
describe "Strongly connected components"
[ test "The input graph was acyclic" <|
\() ->
Expect.true
"Result should be Err"
(isErr result)
, test "The expected SCCs in order" <|
\() ->
Expect.equal
[ sg [ 0, 1, 4 ] -- "abe"
, sg [ 2, 3 ] -- "cd"
, sg [ 5, 6 ] -- "ef"
, sg [ 7 ] -- "h"
]
(case result of
Err components ->
List.map (Graph.toString (Just << String.fromChar) (always <| Just "")) components
Ok _ ->
[]
-- should never happen oO
)
, test "dressUp is acyclic" <|
\() ->
Expect.true
"Should be Ok"
(isOk (Graph.stronglyConnectedComponents dressUp))
, test "The input graph has loops" <|
\() ->
Expect.true
"Should be Err"
(isErr (Graph.stronglyConnectedComponents graphWithLoop))
]
unitTests =
describe "unit tests"
[ emptyTests
, memberTests
, getTests
, nodeIdRangeTests
, listRepTests
, insertTests
, removeTests
, updateTests
, inducedSubgraphTests
, fromNodesAndEdgesTests
, foldTests
, mapTests
, toStringTests
, graphOpsTests
, checkAcyclicTests
, topologicalSortTests
, bfsTests
, sccTests
]
examples =
describe "examples"
[ test "README - iWantToWearShoes" <|
\() ->
Expect.equal
[ "Pants", "Undershorts", "Socks", "Shoes" ]
iWantToWearShoes
, test "insert" <|
\() ->
Expect.true "Graph size wasn't 2" insertExample
, test "fold" <|
\() ->
Expect.true "The graph had a loop." foldExample
, test "mapContexts" <|
\() ->
Expect.true "Mapped edge flip should've reversed edges" mapContextsExample
]
in
describe "The Graph module"
[ unitTests
, examples
]
-- EXAMPLE SECTION
-- The code of the more complex examples is exercised here
-- This is from the README
iWantToWearShoes : List String
iWantToWearShoes =
Graph.guidedDfs
Graph.alongIncomingEdges
-- which edges to follow
(Graph.onDiscovery
(\ctx list ->
-- append node labels on finish
ctx.node.label :: list
)
)
[ 3
{- "Shoes" NodeId -}
]
-- start with the node labelled "Shoes"
[]
-- accumulate starting with the empty list
dressUp
-- traverse our dressUp graph from above
|> Tuple.first
-- ignores the untraversed rest of the graph
insertExample : Bool
insertExample =
let
graph1 =
Graph.fromNodesAndEdges [ Node 1 "1" ] []
newNode =
{ node = Node 2 "2"
, incoming = IntDict.singleton 1 () -- so there will be an edge from 1 to 2
, outgoing = IntDict.empty
}
graph2 =
Graph.insert newNode graph1
in
Graph.size graph2 == 2
foldExample : Bool
foldExample =
let
hasLoop ctx =
IntDict.member ctx.node.id ctx.incoming
graph =
Graph.fromNodesAndEdges [ Node 1 "1", Node 2 "2" ] [ Edge 1 2 "->" ]
-- The graph should not have any loop.
in
Graph.fold (\ctx acc -> acc || hasLoop ctx) False graph == False
mapContextsExample : Bool
mapContextsExample =
let
flipEdges ctx =
{ ctx | incoming = ctx.outgoing, outgoing = ctx.incoming }
graph =
Graph.fromNodesAndEdges [ Node 1 "1", Node 2 "2" ] [ Edge 1 2 "->" ]
in
Graph.reverseEdges graph == Graph.mapContexts flipEdges graph
symmetricClosureExample : Bool
symmetricClosureExample =
let
graph =
Graph.fromNodesAndEdges [ Node 1 "1", Node 2 "2" ] [ Edge 1 2 "->" ]
onlyUndirectedEdges ctx =
ctx.incoming == ctx.outgoing
merger from to outgoingLabel incomingLabel =
outgoingLabel
-- quite arbitrary, will not be called for the above graph
in
Graph.fold
(\ctx acc -> acc && onlyUndirectedEdges ctx)
True
(Graph.symmetricClosure merger graph)
== True
onDiscoveryExample : ()
-- Just let it compile
onDiscoveryExample =
let
dfsPostOrder : Graph n e -> List (NodeContext n e)
dfsPostOrder graph =
Graph.dfs (Graph.onDiscovery (::)) [] graph
in
dfsPostOrder Graph.empty |> (\_ -> ())
onFinishExample : ()
-- Just let it compile
onFinishExample =
let
dfsPreOrder : Graph n e -> List (NodeContext n e)
dfsPreOrder graph =
Graph.dfs (Graph.onFinish (::)) [] graph
in
dfsPreOrder Graph.empty |> (\_ -> ())
ignorePathExample : ()
-- Just let it compile
ignorePathExample =
let
bfsLevelOrder : Graph n e -> List (NodeContext n e)
bfsLevelOrder graph =
graph
|> Graph.bfs (Graph.ignorePath (::)) []
|> List.reverse
in
bfsLevelOrder Graph.empty |> (\_ -> ())
| true | module Tests.Graph exposing (all)
import Expect
import Graph exposing (Edge, Graph, Node, NodeContext, NodeId)
import IntDict exposing (IntDict)
import Test exposing (..)
isJust : Maybe a -> Bool
isJust m =
case m of
Just _ ->
True
_ ->
False
isOk : Result m n -> Bool
isOk result =
case result of
Err _ ->
False
Ok _ ->
True
isErr : Result m n -> Bool
isErr result =
not (isOk result)
expectEqualComparing : (a -> b) -> a -> a -> Expect.Expectation
expectEqualComparing f a b =
Expect.equal (f a) (f b)
edgeTriples : Graph n e -> List ( NodeId, NodeId, e )
edgeTriples =
Graph.edges >> List.map (\e -> ( e.from, e.to, e.label ))
dressUp : Graph String ()
dressUp =
let
nodes =
[ Node 0 "Socks"
, Node 1 "Undershorts"
, Node 2 "Pants"
, Node 3 "Shoes"
, Node 4 "Watch"
, Node 5 "Shirt"
, Node 6 "Belt"
, Node 7 "Tie"
, Node 8 "Jacket"
]
e from to =
Edge from to ()
edges =
[ e 0 3 -- socks before shoes
, e 1 2 -- undershorts before pants
, e 1 3 -- undershorts before shoes
, e 2 3 -- pants before shoes
, e 2 6 -- pants before belt
, e 5 6 -- shirt before belt
, e 5 7 -- shirt before tie
, e 4 8 -- watch before jacket
, e 6 8 -- belt before jacket
, e 7 8 -- tie before jacket
]
in
Graph.fromNodesAndEdges nodes edges
simple : Graph String (Maybe String)
simple =
let
nodes =
[ Node 0 "first"
, Node 1 "second"
, Node 2 "third"
]
edges =
[ Edge 0 1 Nothing
, Edge 0 2 (Just "relationship")
]
in
Graph.fromNodesAndEdges nodes edges
dressUpWithCycle : Graph String ()
dressUpWithCycle =
let
nodes =
[ Node 0 "PI:NAME:<NAME>END_PI"
, Node 1 "PI:NAME:<NAME>END_PI"
, Node 2 "PI:NAME:<NAME>END_PI"
]
e from to =
Edge from to ()
edges =
[ e 0 1
, e 1 2
, e 2 0
]
in
Graph.fromNodesAndEdges nodes edges
connectedComponents : Graph Char ()
connectedComponents =
let
nodes =
[ 'a', 'b', 'c', 'd', 'e', 'f', 'g', 'h' ]
edges =
[ ( 0, 1 )
, ( 1, 2 )
, ( 1, 4 )
, ( 1, 5 )
, ( 2, 3 )
, ( 2, 6 )
, ( 3, 2 )
, ( 3, 7 )
, ( 4, 0 )
, ( 4, 5 )
, ( 5, 6 )
, ( 6, 5 )
, ( 6, 7 )
]
in
Graph.fromNodeLabelsAndEdgePairs nodes edges
noNeighbors : Node String -> NodeContext String ()
noNeighbors node =
NodeContext node IntDict.empty IntDict.empty
isValidTopologicalOrderingOf : Graph n e -> List (NodeContext n e) -> Bool
isValidTopologicalOrderingOf graph ordering =
ordering
|> List.foldl
(\ctx maybeIds ->
maybeIds
|> Maybe.andThen
(\ids ->
if List.all (\i -> IntDict.member i ids) (IntDict.keys ctx.incoming) then
ids |> IntDict.insert ctx.node.id () |> Just
else
Nothing
)
)
(Just IntDict.empty)
|> isJust
|> (&&) (List.length ordering == Graph.size graph)
expectTopologicalOrderingOf : Graph String e -> List (NodeContext String e) -> Expect.Expectation
expectTopologicalOrderingOf graph ordering =
let
message =
String.join "\n"
[ "Expected a valid topological ordering of "
, " " ++ Graph.toString Just (always <| Just "") graph
, "but got"
, " " ++ Debug.toString ordering
]
in
Expect.true message (isValidTopologicalOrderingOf graph ordering)
all : Test
all =
let
emptyTests =
describe "empty"
[ test "has size 0" <| \() -> Expect.equal 0 (Graph.size Graph.empty)
, test "isEmpty" <| \() -> Expect.equal True (Graph.isEmpty Graph.empty)
]
memberTests =
describe "member"
[ test "True" <| \() -> Expect.equal True (Graph.member 0 dressUp)
, test "False" <| \() -> Expect.equal False (Graph.member 99 dressUp)
]
getTests =
describe "get"
[ test "id 0, the socks" <|
\() ->
Expect.equal
(Just "Socks")
(dressUp |> Graph.get 0 |> Maybe.map (.node >> .label))
, test "id 99, Nothing" <| \() -> Expect.equal Nothing (Graph.get 99 dressUp)
]
nodeIdRangeTests =
describe "nodeIdRange"
[ test "dressUp: [0, 8]" <|
\() ->
Expect.equal
(Just ( 0, 8 ))
(Graph.nodeIdRange dressUp)
, test "dressUp - 0: [1, 8]" <|
\() ->
Expect.equal
(Just ( 1, 8 ))
(dressUp |> Graph.remove 0 |> Graph.nodeIdRange)
, test "dressUp - 8: [0, 7]" <|
\() ->
Expect.equal
(Just ( 0, 7 ))
(dressUp |> Graph.remove 8 |> Graph.nodeIdRange)
]
listRepTests =
describe "list conversions"
[ test "nodeIds" <|
\() ->
Expect.equal
[ 0, 1, 2, 3, 4, 5, 6, 7, 8 ]
(dressUp |> Graph.nodeIds)
, test "nodes" <|
\() ->
Expect.equal
[ 0, 1, 2, 3, 4, 5, 6, 7, 8 ]
(dressUp |> Graph.nodes |> List.map .id)
, test "edges" <|
\() ->
Expect.equal
[ ( 0, 3 ), ( 1, 2 ), ( 1, 3 ), ( 2, 3 ), ( 2, 6 ), ( 4, 8 ), ( 5, 6 ), ( 5, 7 ), ( 6, 8 ), ( 7, 8 ) ]
(dressUp
|> Graph.edges
|> List.map (\e -> ( e.from, e.to ))
|> List.sort
)
]
insertTests =
describe "insert"
[ test "new node - size" <|
\() ->
Expect.equal
(dressUp |> Graph.size |> (+) 1)
(dressUp |> Graph.insert (noNeighbors (Node 99 "Ring")) |> Graph.size)
, test "new node - can get it" <|
\() ->
Expect.equal
(Just "Ring")
(dressUp
|> Graph.insert (noNeighbors (Node 99 "Ring"))
|> Graph.get 99
|> Maybe.map (.node >> .label)
)
, test "replace node - size" <|
\() ->
Expect.equal
(dressUp |> Graph.size)
(dressUp |> Graph.insert (noNeighbors (Node 0 "Ring")) |> Graph.size)
, test "replace node - can get it" <|
\() ->
Expect.equal
(Just "Ring")
(dressUp
|> Graph.insert (noNeighbors (Node 0 "Ring"))
|> Graph.get 0
|> Maybe.map (.node >> .label)
)
, test "replace node - replaces adjacency" <|
\() ->
Expect.equal
(Just True)
(dressUp
|> Graph.insert (noNeighbors (Node 0 "Ring"))
|> Graph.get 0
|> Maybe.map (\ctx -> IntDict.isEmpty ctx.incoming && IntDict.isEmpty ctx.outgoing)
)
]
removeTests =
describe "remove"
[ test "nonexistent node" <|
\() ->
Expect.equal
dressUp
(dressUp |> Graph.remove 99)
, test "existing node - size" <|
\() ->
Expect.equal
(dressUp |> Graph.size |> (\i -> (-) i 1))
(dressUp |> Graph.remove 0 |> Graph.size)
, test "existing node - can't get it" <|
\() ->
Expect.equal
Nothing
(dressUp |> Graph.remove 0 |> Graph.get 0)
]
updateTests =
describe "update"
[ test "remove outgoing edges" <|
\() ->
Expect.equal
(Just True)
(dressUp
|> Graph.update 0
-- "Shorts" has outgoing edges
(Maybe.map (\n -> { n | outgoing = IntDict.empty }))
|> Graph.get 0
|> Maybe.map (.outgoing >> IntDict.isEmpty)
)
]
inducedSubgraphTests =
describe "inducedSubgraph"
[ test "should not have any dangling edges" <|
\() ->
expectEqualComparing
(edgeTriples >> List.sortBy (\( f, t, _ ) -> ( f, t )))
(Graph.fromNodesAndEdges
[ Node 0 'a', Node 1 'b', Node 4 'e' ]
[ Edge 0 1 (), Edge 1 4 (), Edge 4 0 () ]
)
(Graph.inducedSubgraph [ 0, 1, 4 ] connectedComponents)
]
fromNodesAndEdgesTests =
describe "fromNodesAndEdges"
[ test "should not have any dangling edges" <|
\() ->
Expect.equal
[ Edge 0 0 () ]
(Graph.edges
(Graph.fromNodesAndEdges
[ Node 0 'a' ]
[ Edge 0 0 (), Edge 0 1 (), Edge 1 0 (), Edge 1 1 () ]
)
)
]
foldTests =
describe "fold"
[ test "sum up ids" <|
\() ->
Expect.equal
36
(dressUp
|> Graph.fold (\ctx -> (+) ctx.node.id) 0
)
]
mapTests =
describe "map*"
[ test "mapContexts over id is the id" <|
\() ->
Expect.equal
dressUp
(dressUp |> Graph.mapContexts identity)
, test "mapNodes over id is the id" <|
\() ->
Expect.equal
dressUp
(dressUp |> Graph.mapNodes identity)
, test "mapEdges over id is the id" <|
\() ->
Expect.equal
dressUp
(dressUp |> Graph.mapNodes identity)
-- This should be backed by more tests, but I'm not in the mood for that :/
]
toStringTests =
describe "toString"
[ test "works as expected" <|
\() ->
Expect.equal
(Graph.toString Just identity simple)
"Graph [Node 0 (first), Node 1 (second), Node 2 (third)] [Edge 0->2 (relationship), Edge 0->1]"
]
graphOpsTests =
describe "Graph ops"
[ test "symmetricClosure is symmetric" <|
\() ->
Expect.true
"expected all incoming edges to also be outgoing and vice versa"
(dressUp
|> Graph.symmetricClosure (\_ _ e _ -> e)
|> Graph.fold
(\ctx acc ->
ctx.incoming == ctx.outgoing && acc
)
True
)
, test "reverseEdges" <|
\() ->
Expect.equal
(dressUp
|> Graph.edges
|> List.map (\e -> ( e.from, e.to ))
|> List.sort
)
(dressUp
|> Graph.reverseEdges
|> Graph.edges
|> List.map (\e -> ( e.to, e.from ))
|> List.sort
)
]
checkAcyclicTests =
describe "checkAcyclicTests" <|
[ test "Ok for graph with no cycles" <|
\() ->
Expect.true
"Should return Ok"
(isOk (Graph.checkAcyclic dressUp))
, test "Err for cyclic graph" <|
\() ->
Expect.true
"Should return Err"
(isErr (Graph.checkAcyclic dressUpWithCycle))
, test "Err for connectedComponents" <|
\() ->
Expect.true
"Should return Err"
(isErr (Graph.checkAcyclic connectedComponents))
]
topologicalSortTests =
describe "topologicalSort"
[ test "valid topological ordering" <|
\() ->
case Graph.checkAcyclic dressUp of
Err e ->
Expect.fail
("dressUp should be acylic, but returned edge " ++ Debug.toString e)
Ok acyclic ->
acyclic
|> Graph.topologicalSort
|> expectTopologicalOrderingOf dressUp
, test "heightLevels" <|
\() ->
case Graph.checkAcyclic dressUp of
Err e ->
Expect.fail
("dressUp should be acylic, but returned edge " ++ Debug.toString e)
Ok acyclic ->
acyclic
|> Graph.heightLevels
|> List.concat
|> expectTopologicalOrderingOf dressUp
]
bfsTests =
describe "BFS"
[ test "breadth-first node order" <|
\() ->
Expect.equal
[ 0, 3, 1, 2, 6, 8, 4, 5, 7 ]
(dressUp
|> Graph.bfs (Graph.ignorePath (::)) []
|> List.map (.node >> .id)
|> List.reverse
)
]
graphWithLoop =
Graph.fromNodeLabelsAndEdgePairs [ 0 ] [ ( 0, 0 ) ]
sccTests =
let
result =
Graph.stronglyConnectedComponents connectedComponents
sg nodeIds =
connectedComponents
|> Graph.inducedSubgraph nodeIds
|> Graph.toString (Just << String.fromChar) (always <| Just "")
in
describe "Strongly connected components"
[ test "The input graph was acyclic" <|
\() ->
Expect.true
"Result should be Err"
(isErr result)
, test "The expected SCCs in order" <|
\() ->
Expect.equal
[ sg [ 0, 1, 4 ] -- "abe"
, sg [ 2, 3 ] -- "cd"
, sg [ 5, 6 ] -- "ef"
, sg [ 7 ] -- "h"
]
(case result of
Err components ->
List.map (Graph.toString (Just << String.fromChar) (always <| Just "")) components
Ok _ ->
[]
-- should never happen oO
)
, test "dressUp is acyclic" <|
\() ->
Expect.true
"Should be Ok"
(isOk (Graph.stronglyConnectedComponents dressUp))
, test "The input graph has loops" <|
\() ->
Expect.true
"Should be Err"
(isErr (Graph.stronglyConnectedComponents graphWithLoop))
]
unitTests =
describe "unit tests"
[ emptyTests
, memberTests
, getTests
, nodeIdRangeTests
, listRepTests
, insertTests
, removeTests
, updateTests
, inducedSubgraphTests
, fromNodesAndEdgesTests
, foldTests
, mapTests
, toStringTests
, graphOpsTests
, checkAcyclicTests
, topologicalSortTests
, bfsTests
, sccTests
]
examples =
describe "examples"
[ test "README - iWantToWearShoes" <|
\() ->
Expect.equal
[ "Pants", "Undershorts", "Socks", "Shoes" ]
iWantToWearShoes
, test "insert" <|
\() ->
Expect.true "Graph size wasn't 2" insertExample
, test "fold" <|
\() ->
Expect.true "The graph had a loop." foldExample
, test "mapContexts" <|
\() ->
Expect.true "Mapped edge flip should've reversed edges" mapContextsExample
]
in
describe "The Graph module"
[ unitTests
, examples
]
-- EXAMPLE SECTION
-- The code of the more complex examples is exercised here
-- This is from the README
iWantToWearShoes : List String
iWantToWearShoes =
Graph.guidedDfs
Graph.alongIncomingEdges
-- which edges to follow
(Graph.onDiscovery
(\ctx list ->
-- append node labels on finish
ctx.node.label :: list
)
)
[ 3
{- "Shoes" NodeId -}
]
-- start with the node labelled "Shoes"
[]
-- accumulate starting with the empty list
dressUp
-- traverse our dressUp graph from above
|> Tuple.first
-- ignores the untraversed rest of the graph
insertExample : Bool
insertExample =
let
graph1 =
Graph.fromNodesAndEdges [ Node 1 "1" ] []
newNode =
{ node = Node 2 "2"
, incoming = IntDict.singleton 1 () -- so there will be an edge from 1 to 2
, outgoing = IntDict.empty
}
graph2 =
Graph.insert newNode graph1
in
Graph.size graph2 == 2
foldExample : Bool
foldExample =
let
hasLoop ctx =
IntDict.member ctx.node.id ctx.incoming
graph =
Graph.fromNodesAndEdges [ Node 1 "1", Node 2 "2" ] [ Edge 1 2 "->" ]
-- The graph should not have any loop.
in
Graph.fold (\ctx acc -> acc || hasLoop ctx) False graph == False
mapContextsExample : Bool
mapContextsExample =
let
flipEdges ctx =
{ ctx | incoming = ctx.outgoing, outgoing = ctx.incoming }
graph =
Graph.fromNodesAndEdges [ Node 1 "1", Node 2 "2" ] [ Edge 1 2 "->" ]
in
Graph.reverseEdges graph == Graph.mapContexts flipEdges graph
symmetricClosureExample : Bool
symmetricClosureExample =
let
graph =
Graph.fromNodesAndEdges [ Node 1 "1", Node 2 "2" ] [ Edge 1 2 "->" ]
onlyUndirectedEdges ctx =
ctx.incoming == ctx.outgoing
merger from to outgoingLabel incomingLabel =
outgoingLabel
-- quite arbitrary, will not be called for the above graph
in
Graph.fold
(\ctx acc -> acc && onlyUndirectedEdges ctx)
True
(Graph.symmetricClosure merger graph)
== True
onDiscoveryExample : ()
-- Just let it compile
onDiscoveryExample =
let
dfsPostOrder : Graph n e -> List (NodeContext n e)
dfsPostOrder graph =
Graph.dfs (Graph.onDiscovery (::)) [] graph
in
dfsPostOrder Graph.empty |> (\_ -> ())
onFinishExample : ()
-- Just let it compile
onFinishExample =
let
dfsPreOrder : Graph n e -> List (NodeContext n e)
dfsPreOrder graph =
Graph.dfs (Graph.onFinish (::)) [] graph
in
dfsPreOrder Graph.empty |> (\_ -> ())
ignorePathExample : ()
-- Just let it compile
ignorePathExample =
let
bfsLevelOrder : Graph n e -> List (NodeContext n e)
bfsLevelOrder graph =
graph
|> Graph.bfs (Graph.ignorePath (::)) []
|> List.reverse
in
bfsLevelOrder Graph.empty |> (\_ -> ())
| elm |
[
{
"context": " Password password ->\n { model | password = password, submitState = False }\n\n PasswordAgain passwor",
"end": 830,
"score": 0.9823200107,
"start": 822,
"tag": "PASSWORD",
"value": "password"
}
] | examples/3-form.elm | scott-ad-riley/elm-tutorials | 0 | import Html exposing (..)
import Html.App as Html
import Html.Attributes exposing (..)
import Html.Events exposing (onInput, onClick)
import String exposing (toUpper, toLower, any, toInt)
import Char exposing (isDigit)
main =
Html.beginnerProgram
{ model = model
, view = view
, update = update
}
-- MODEL
type alias Model =
{ name : String
, password : String
, passwordAgain : String
, age : String
, submitState : Bool
}
model : Model
model =
Model "" "" "" "" False
-- UPDATE
type Msg
= Name String
| Password String
| PasswordAgain String
| Age String
| SubmitForm
update : Msg -> Model -> Model
update msg model =
case msg of
Name name ->
{ model | name = name, submitState = False }
Password password ->
{ model | password = password, submitState = False }
PasswordAgain password ->
{ model | passwordAgain = password, submitState = False }
Age age ->
{ model | age = age, submitState = False }
SubmitForm ->
{ model | submitState = True }
-- VIEW
view : Model -> Html Msg
view model =
div []
[ input [ type' "text", placeholder "Name", onInput Name ] []
, input [ type' "password", placeholder "Password", onInput Password ] []
, input [ type' "password", placeholder "Re-enter Password", onInput PasswordAgain ] []
, input [ type' "text", placeholder "Age", onInput Age ] []
, input [ type' "button", value "Submit", onClick SubmitForm ] []
, viewValidation model
]
viewValidation : Model -> Html msg
viewValidation model =
let
(color, message) =
if not model.submitState then
("", "")
else if String.length model.password < 8 then
("red", "Password is too short")
else if model.password /= model.passwordAgain then
("red", "Passwords do not match!")
else if (passwordInvalid model.password) then
("red", "Password must contain atleast one uppercase character, one lowercase character and one number")
else if (ageValid model.age) then
("red", "Age must be a number")
else
("green", "OK")
in
div [ style [("color", color)] ] [ text message ]
passwordInvalid : String -> Bool
passwordInvalid password =
(String.toUpper password) == password || (String.toLower password) == password || not (any isDigit password)
ageValid : String -> Bool
ageValid age =
case toInt age of
Err msg -> True
Ok val -> False | 26156 | import Html exposing (..)
import Html.App as Html
import Html.Attributes exposing (..)
import Html.Events exposing (onInput, onClick)
import String exposing (toUpper, toLower, any, toInt)
import Char exposing (isDigit)
main =
Html.beginnerProgram
{ model = model
, view = view
, update = update
}
-- MODEL
type alias Model =
{ name : String
, password : String
, passwordAgain : String
, age : String
, submitState : Bool
}
model : Model
model =
Model "" "" "" "" False
-- UPDATE
type Msg
= Name String
| Password String
| PasswordAgain String
| Age String
| SubmitForm
update : Msg -> Model -> Model
update msg model =
case msg of
Name name ->
{ model | name = name, submitState = False }
Password password ->
{ model | password = <PASSWORD>, submitState = False }
PasswordAgain password ->
{ model | passwordAgain = password, submitState = False }
Age age ->
{ model | age = age, submitState = False }
SubmitForm ->
{ model | submitState = True }
-- VIEW
view : Model -> Html Msg
view model =
div []
[ input [ type' "text", placeholder "Name", onInput Name ] []
, input [ type' "password", placeholder "Password", onInput Password ] []
, input [ type' "password", placeholder "Re-enter Password", onInput PasswordAgain ] []
, input [ type' "text", placeholder "Age", onInput Age ] []
, input [ type' "button", value "Submit", onClick SubmitForm ] []
, viewValidation model
]
viewValidation : Model -> Html msg
viewValidation model =
let
(color, message) =
if not model.submitState then
("", "")
else if String.length model.password < 8 then
("red", "Password is too short")
else if model.password /= model.passwordAgain then
("red", "Passwords do not match!")
else if (passwordInvalid model.password) then
("red", "Password must contain atleast one uppercase character, one lowercase character and one number")
else if (ageValid model.age) then
("red", "Age must be a number")
else
("green", "OK")
in
div [ style [("color", color)] ] [ text message ]
passwordInvalid : String -> Bool
passwordInvalid password =
(String.toUpper password) == password || (String.toLower password) == password || not (any isDigit password)
ageValid : String -> Bool
ageValid age =
case toInt age of
Err msg -> True
Ok val -> False | true | import Html exposing (..)
import Html.App as Html
import Html.Attributes exposing (..)
import Html.Events exposing (onInput, onClick)
import String exposing (toUpper, toLower, any, toInt)
import Char exposing (isDigit)
main =
Html.beginnerProgram
{ model = model
, view = view
, update = update
}
-- MODEL
type alias Model =
{ name : String
, password : String
, passwordAgain : String
, age : String
, submitState : Bool
}
model : Model
model =
Model "" "" "" "" False
-- UPDATE
type Msg
= Name String
| Password String
| PasswordAgain String
| Age String
| SubmitForm
update : Msg -> Model -> Model
update msg model =
case msg of
Name name ->
{ model | name = name, submitState = False }
Password password ->
{ model | password = PI:PASSWORD:<PASSWORD>END_PI, submitState = False }
PasswordAgain password ->
{ model | passwordAgain = password, submitState = False }
Age age ->
{ model | age = age, submitState = False }
SubmitForm ->
{ model | submitState = True }
-- VIEW
view : Model -> Html Msg
view model =
div []
[ input [ type' "text", placeholder "Name", onInput Name ] []
, input [ type' "password", placeholder "Password", onInput Password ] []
, input [ type' "password", placeholder "Re-enter Password", onInput PasswordAgain ] []
, input [ type' "text", placeholder "Age", onInput Age ] []
, input [ type' "button", value "Submit", onClick SubmitForm ] []
, viewValidation model
]
viewValidation : Model -> Html msg
viewValidation model =
let
(color, message) =
if not model.submitState then
("", "")
else if String.length model.password < 8 then
("red", "Password is too short")
else if model.password /= model.passwordAgain then
("red", "Passwords do not match!")
else if (passwordInvalid model.password) then
("red", "Password must contain atleast one uppercase character, one lowercase character and one number")
else if (ageValid model.age) then
("red", "Age must be a number")
else
("green", "OK")
in
div [ style [("color", color)] ] [ text message ]
passwordInvalid : String -> Bool
passwordInvalid password =
(String.toUpper password) == password || (String.toLower password) == password || not (any isDigit password)
ageValid : String -> Bool
ageValid age =
case toInt age of
Err msg -> True
Ok val -> False | elm |
[
{
"context": "d ->\n UpdateResult { model | password = password } globals Cmd.none\n\n Login ->\n ",
"end": 1226,
"score": 0.740205884,
"start": 1218,
"tag": "PASSWORD",
"value": "password"
},
{
"context": "ue\nmodelToJson model =\n JE.object\n [ ( \"username\", JE.string model.username )\n , ( \"passwor",
"end": 2740,
"score": 0.6771520376,
"start": 2732,
"tag": "USERNAME",
"value": "username"
},
{
"context": "\n [ textFieldWithLabel ChangeUsername \"Username\" \"Username\"\n , inputFieldWithLabel\n ",
"end": 3655,
"score": 0.9575333595,
"start": 3647,
"tag": "USERNAME",
"value": "Username"
},
{
"context": " [ textFieldWithLabel ChangeUsername \"Username\" \"Username\"\n , inputFieldWithLabel\n ",
"end": 3666,
"score": 0.9545494914,
"start": 3658,
"tag": "USERNAME",
"value": "Username"
},
{
"context": "= \"Password\"\n , placeholderText = \"Password\"\n , hasError = False\n ",
"end": 3972,
"score": 0.6675733924,
"start": 3964,
"tag": "PASSWORD",
"value": "Password"
}
] | src/Components/Login.elm | bnkrs/bnk-frontend | 1 | port module Components.Login exposing (..)
import Html exposing (..)
import Html.Attributes exposing (..)
import String
import Json.Decode as JD exposing ((:=))
import Json.Encode as JE
import HttpBuilder exposing (..)
import Maybe
import Task
import Navigation
import Globals
import Utils.HttpUtils exposing (httpErrorToString)
import Utils.HtmlUtils exposing (..)
-- MODEL
type alias Model =
{ username : String
, password : String
, httpError : Maybe (Error String)
}
initialModel : Model
initialModel =
{ username = ""
, password = ""
, httpError = Nothing
}
port saveToLocalstorage : Globals.Model -> Cmd msg
-- UPDATE
type Msg
= ChangeUsername String
| ChangePassword String
| Login
| LoginFailed (Error String)
| LoginSuccessful (Response String)
| NoOp
type alias UpdateResult =
{ model : Model
, globals : Globals.Model
, cmd : Cmd Msg
}
update : Msg -> Model -> Globals.Model -> UpdateResult
update msg model globals =
case msg of
ChangeUsername name ->
UpdateResult { model | username = name } globals Cmd.none
ChangePassword password ->
UpdateResult { model | password = password } globals Cmd.none
Login ->
UpdateResult model globals (login model globals)
LoginFailed error ->
UpdateResult { model | httpError = Just error, password = "" } globals Cmd.none
LoginSuccessful token ->
let
newGlobals =
{ globals | apiToken = token.data, username = model.username }
in
UpdateResult
{ model | password = "", httpError = Nothing }
newGlobals
(Cmd.batch [ saveToLocalstorage newGlobals, Navigation.newUrl "#home" ])
NoOp ->
UpdateResult model globals Cmd.none
login : Model -> Globals.Model -> Cmd Msg
login model global =
Task.perform
LoginFailed
LoginSuccessful
(doLogin model global)
doLogin : Model -> Globals.Model -> Task.Task (Error String) (Response String)
doLogin model global =
let
loginUrl =
global.endpoint ++ "/auth/getToken"
loginSuccessReader =
jsonReader ("token" := JD.string)
loginFailReader =
jsonReader (JD.at [ "error" ] ("message" := JD.string))
body =
modelToJson model
in
HttpBuilder.post loginUrl
|> withJsonBody body
|> withHeader "Content-Type" "application/json"
|> send loginSuccessReader loginFailReader
modelToJson : Model -> JE.Value
modelToJson model =
JE.object
[ ( "username", JE.string model.username )
, ( "password", JE.string model.password )
]
-- VIEW
view : Model -> Html Msg
view model =
div [ class "row" ]
[ div [ class "col-sm-3" ] []
, div [ class "col-sm-6" ]
[ errorView model
, formView model
, a [ href "#register", class "text-center lead" ] [ text "No account? Register Here!" ]
]
, div [ class "col-sm-3" ] []
]
errorView : Model -> Html Msg
errorView model =
case model.httpError of
Just error ->
Utils.HtmlUtils.errorView (httpErrorToString error)
Nothing ->
text ""
formView : Model -> Html Msg
formView model =
div [ class "panel panel-primary login-form" ]
[ div [ class "panel-heading" ] [ text "Login" ]
, div [ class "panel-body" ]
[ textFieldWithLabel ChangeUsername "Username" "Username"
, inputFieldWithLabel
{ inputHandler = ChangePassword
, focusHandler = Nothing
, enterHandler = Just ( Login, NoOp )
, inputType = "password"
, labelText = "Password"
, placeholderText = "Password"
, hasError = False
, inputValue = Just model.password
}
, primaryButton Login (isValid model) "Login"
]
]
isValid : Model -> Bool
isValid model =
(String.length model.username) > 0 && (String.length model.password > 6)
| 20793 | port module Components.Login exposing (..)
import Html exposing (..)
import Html.Attributes exposing (..)
import String
import Json.Decode as JD exposing ((:=))
import Json.Encode as JE
import HttpBuilder exposing (..)
import Maybe
import Task
import Navigation
import Globals
import Utils.HttpUtils exposing (httpErrorToString)
import Utils.HtmlUtils exposing (..)
-- MODEL
type alias Model =
{ username : String
, password : String
, httpError : Maybe (Error String)
}
initialModel : Model
initialModel =
{ username = ""
, password = ""
, httpError = Nothing
}
port saveToLocalstorage : Globals.Model -> Cmd msg
-- UPDATE
type Msg
= ChangeUsername String
| ChangePassword String
| Login
| LoginFailed (Error String)
| LoginSuccessful (Response String)
| NoOp
type alias UpdateResult =
{ model : Model
, globals : Globals.Model
, cmd : Cmd Msg
}
update : Msg -> Model -> Globals.Model -> UpdateResult
update msg model globals =
case msg of
ChangeUsername name ->
UpdateResult { model | username = name } globals Cmd.none
ChangePassword password ->
UpdateResult { model | password = <PASSWORD> } globals Cmd.none
Login ->
UpdateResult model globals (login model globals)
LoginFailed error ->
UpdateResult { model | httpError = Just error, password = "" } globals Cmd.none
LoginSuccessful token ->
let
newGlobals =
{ globals | apiToken = token.data, username = model.username }
in
UpdateResult
{ model | password = "", httpError = Nothing }
newGlobals
(Cmd.batch [ saveToLocalstorage newGlobals, Navigation.newUrl "#home" ])
NoOp ->
UpdateResult model globals Cmd.none
login : Model -> Globals.Model -> Cmd Msg
login model global =
Task.perform
LoginFailed
LoginSuccessful
(doLogin model global)
doLogin : Model -> Globals.Model -> Task.Task (Error String) (Response String)
doLogin model global =
let
loginUrl =
global.endpoint ++ "/auth/getToken"
loginSuccessReader =
jsonReader ("token" := JD.string)
loginFailReader =
jsonReader (JD.at [ "error" ] ("message" := JD.string))
body =
modelToJson model
in
HttpBuilder.post loginUrl
|> withJsonBody body
|> withHeader "Content-Type" "application/json"
|> send loginSuccessReader loginFailReader
modelToJson : Model -> JE.Value
modelToJson model =
JE.object
[ ( "username", JE.string model.username )
, ( "password", JE.string model.password )
]
-- VIEW
view : Model -> Html Msg
view model =
div [ class "row" ]
[ div [ class "col-sm-3" ] []
, div [ class "col-sm-6" ]
[ errorView model
, formView model
, a [ href "#register", class "text-center lead" ] [ text "No account? Register Here!" ]
]
, div [ class "col-sm-3" ] []
]
errorView : Model -> Html Msg
errorView model =
case model.httpError of
Just error ->
Utils.HtmlUtils.errorView (httpErrorToString error)
Nothing ->
text ""
formView : Model -> Html Msg
formView model =
div [ class "panel panel-primary login-form" ]
[ div [ class "panel-heading" ] [ text "Login" ]
, div [ class "panel-body" ]
[ textFieldWithLabel ChangeUsername "Username" "Username"
, inputFieldWithLabel
{ inputHandler = ChangePassword
, focusHandler = Nothing
, enterHandler = Just ( Login, NoOp )
, inputType = "password"
, labelText = "Password"
, placeholderText = "<PASSWORD>"
, hasError = False
, inputValue = Just model.password
}
, primaryButton Login (isValid model) "Login"
]
]
isValid : Model -> Bool
isValid model =
(String.length model.username) > 0 && (String.length model.password > 6)
| true | port module Components.Login exposing (..)
import Html exposing (..)
import Html.Attributes exposing (..)
import String
import Json.Decode as JD exposing ((:=))
import Json.Encode as JE
import HttpBuilder exposing (..)
import Maybe
import Task
import Navigation
import Globals
import Utils.HttpUtils exposing (httpErrorToString)
import Utils.HtmlUtils exposing (..)
-- MODEL
type alias Model =
{ username : String
, password : String
, httpError : Maybe (Error String)
}
initialModel : Model
initialModel =
{ username = ""
, password = ""
, httpError = Nothing
}
port saveToLocalstorage : Globals.Model -> Cmd msg
-- UPDATE
type Msg
= ChangeUsername String
| ChangePassword String
| Login
| LoginFailed (Error String)
| LoginSuccessful (Response String)
| NoOp
type alias UpdateResult =
{ model : Model
, globals : Globals.Model
, cmd : Cmd Msg
}
update : Msg -> Model -> Globals.Model -> UpdateResult
update msg model globals =
case msg of
ChangeUsername name ->
UpdateResult { model | username = name } globals Cmd.none
ChangePassword password ->
UpdateResult { model | password = PI:PASSWORD:<PASSWORD>END_PI } globals Cmd.none
Login ->
UpdateResult model globals (login model globals)
LoginFailed error ->
UpdateResult { model | httpError = Just error, password = "" } globals Cmd.none
LoginSuccessful token ->
let
newGlobals =
{ globals | apiToken = token.data, username = model.username }
in
UpdateResult
{ model | password = "", httpError = Nothing }
newGlobals
(Cmd.batch [ saveToLocalstorage newGlobals, Navigation.newUrl "#home" ])
NoOp ->
UpdateResult model globals Cmd.none
login : Model -> Globals.Model -> Cmd Msg
login model global =
Task.perform
LoginFailed
LoginSuccessful
(doLogin model global)
doLogin : Model -> Globals.Model -> Task.Task (Error String) (Response String)
doLogin model global =
let
loginUrl =
global.endpoint ++ "/auth/getToken"
loginSuccessReader =
jsonReader ("token" := JD.string)
loginFailReader =
jsonReader (JD.at [ "error" ] ("message" := JD.string))
body =
modelToJson model
in
HttpBuilder.post loginUrl
|> withJsonBody body
|> withHeader "Content-Type" "application/json"
|> send loginSuccessReader loginFailReader
modelToJson : Model -> JE.Value
modelToJson model =
JE.object
[ ( "username", JE.string model.username )
, ( "password", JE.string model.password )
]
-- VIEW
view : Model -> Html Msg
view model =
div [ class "row" ]
[ div [ class "col-sm-3" ] []
, div [ class "col-sm-6" ]
[ errorView model
, formView model
, a [ href "#register", class "text-center lead" ] [ text "No account? Register Here!" ]
]
, div [ class "col-sm-3" ] []
]
errorView : Model -> Html Msg
errorView model =
case model.httpError of
Just error ->
Utils.HtmlUtils.errorView (httpErrorToString error)
Nothing ->
text ""
formView : Model -> Html Msg
formView model =
div [ class "panel panel-primary login-form" ]
[ div [ class "panel-heading" ] [ text "Login" ]
, div [ class "panel-body" ]
[ textFieldWithLabel ChangeUsername "Username" "Username"
, inputFieldWithLabel
{ inputHandler = ChangePassword
, focusHandler = Nothing
, enterHandler = Just ( Login, NoOp )
, inputType = "password"
, labelText = "Password"
, placeholderText = "PI:PASSWORD:<PASSWORD>END_PI"
, hasError = False
, inputValue = Just model.password
}
, primaryButton Login (isValid model) "Login"
]
]
isValid : Model -> Bool
isValid model =
(String.length model.username) > 0 && (String.length model.password > 6)
| elm |
[
{
"context": "f all kinds (and I guess coffee too)\n\nAuthor(s):\n* Madison Scott-Clary - http://github.com/makyo\n\nLicense:\nMIT\n-}\n\nimpor",
"end": 131,
"score": 0.9998768568,
"start": 112,
"tag": "NAME",
"value": "Madison Scott-Clary"
},
{
"context": "hor(s):\n* Madison Scott-Clary - http://github.com/makyo\n\nLicense:\nMIT\n-}\n\nimport Html exposing (..)\nimpor",
"end": 157,
"score": 0.999473393,
"start": 152,
"tag": "USERNAME",
"value": "makyo"
}
] | SteepList.elm | makyo/steep.love | 0 | module SteepList where
{-| Steep.♡ - a steep timer for tea of all kinds (and I guess coffee too)
Author(s):
* Madison Scott-Clary - http://github.com/makyo
License:
MIT
-}
import Html exposing (..)
import Signal exposing (Address, Signal)
-- main : Signal Html
-- main =
-- Signal.map (view actions.address) session
main : Html
main =
em [] [ text "Coming soon: storing multiple sessions in localStorage!" ]
| 34535 | module SteepList where
{-| Steep.♡ - a steep timer for tea of all kinds (and I guess coffee too)
Author(s):
* <NAME> - http://github.com/makyo
License:
MIT
-}
import Html exposing (..)
import Signal exposing (Address, Signal)
-- main : Signal Html
-- main =
-- Signal.map (view actions.address) session
main : Html
main =
em [] [ text "Coming soon: storing multiple sessions in localStorage!" ]
| true | module SteepList where
{-| Steep.♡ - a steep timer for tea of all kinds (and I guess coffee too)
Author(s):
* PI:NAME:<NAME>END_PI - http://github.com/makyo
License:
MIT
-}
import Html exposing (..)
import Signal exposing (Address, Signal)
-- main : Signal Html
-- main =
-- Signal.map (view actions.address) session
main : Html
main =
em [] [ text "Coming soon: storing multiple sessions in localStorage!" ]
| elm |
[
{
"context": " Int Animal String\n animals =\n [ (Cat, \"Tom\"), (Mouse, \"Jerry\") ]\n |> fromList ani",
"end": 5907,
"score": 0.9996019006,
"start": 5904,
"tag": "NAME",
"value": "Tom"
},
{
"context": "ng\n animals =\n [ (Cat, \"Tom\"), (Mouse, \"Jerry\") ]\n |> fromList animalToInt\n\n get ",
"end": 5925,
"score": 0.9995942116,
"start": 5920,
"tag": "NAME",
"value": "Jerry"
},
{
"context": "ist animalToInt\n\n get Cat animals\n -> Just \"Tom\"\n\n get Mouse animals\n --> Just \"Jerry\"\n\n ",
"end": 6003,
"score": 0.999550581,
"start": 6000,
"tag": "NAME",
"value": "Tom"
},
{
"context": "> Just \"Tom\"\n\n get Mouse animals\n --> Just \"Jerry\"\n\n get Dog animals\n --> Nothing\n\n-}\nget : k",
"end": 6047,
"score": 0.9995827675,
"start": 6042,
"tag": "NAME",
"value": "Jerry"
},
{
"context": "ON object.\n\n import Json.Encode\n\n type Key = Foo | Bar\n\n toString : Key -> String\n toString ",
"end": 14033,
"score": 0.5877886415,
"start": 14030,
"tag": "KEY",
"value": "Foo"
},
{
"context": "ample =\n fromList personToString [(Person \"Jeve\" \"Sobs\", 9001), (Person \"Tim\" \"Berners-Lee\", 1234",
"end": 15224,
"score": 0.9994463921,
"start": 15220,
"tag": "NAME",
"value": "Jeve"
},
{
"context": "\n fromList personToString [(Person \"Jeve\" \"Sobs\", 9001), (Person \"Tim\" \"Berners-Lee\", 1234)]\n\n ",
"end": 15231,
"score": 0.9991819859,
"start": 15227,
"tag": "NAME",
"value": "Sobs"
},
{
"context": "nToString [(Person \"Jeve\" \"Sobs\", 9001), (Person \"Tim\" \"Berners-Lee\", 1234)]\n\n encodeList (\\k v -> E",
"end": 15253,
"score": 0.9989579916,
"start": 15250,
"tag": "NAME",
"value": "Tim"
},
{
"context": "ing [(Person \"Jeve\" \"Sobs\", 9001), (Person \"Tim\" \"Berners-Lee\", 1234)]\n\n encodeList (\\k v -> Encode.list ide",
"end": 15267,
"score": 0.9964020252,
"start": 15256,
"tag": "NAME",
"value": "Berners-Lee"
},
{
"context": " |> Encode.encode 0\n --> \"[[{\\\"first\\\":\\\"Jeve\\\",\\\"last\\\":\\\"Sobs\\\"},9001],[{\\\"first\\\":\\\"Tim\\\",\\\"",
"end": 15424,
"score": 0.9993127584,
"start": 15420,
"tag": "NAME",
"value": "Jeve"
},
{
"context": "e 0\n --> \"[[{\\\"first\\\":\\\"Jeve\\\",\\\"last\\\":\\\"Sobs\\\"},9001],[{\\\"first\\\":\\\"Tim\\\",\\\"last\\\":\\\"Berners-L",
"end": 15442,
"score": 0.9822134972,
"start": 15438,
"tag": "NAME",
"value": "Sobs"
},
{
"context": "\":\\\"Jeve\\\",\\\"last\\\":\\\"Sobs\\\"},9001],[{\\\"first\\\":\\\"Tim\\\",\\\"last\\\":\\\"Berners-Lee\\\"},1234]]\"\n\n-}\nencodeLis",
"end": 15469,
"score": 0.9871366024,
"start": 15466,
"tag": "NAME",
"value": "Tim"
},
{
"context": "\\\":\\\"Sobs\\\"},9001],[{\\\"first\\\":\\\"Tim\\\",\\\"last\\\":\\\"Berners-Lee\\\"},1234]]\"\n\n-}\nencodeList : (k -> v -> Encode.Val",
"end": 15494,
"score": 0.8232983947,
"start": 15483,
"tag": "NAME",
"value": "Berners-Lee"
},
{
"context": ".field \"last\" Decode.string)\n\n \"[[{\\\"first\\\":\\\"Jeve\\\",\\\"last\\\":\\\"Sobs\\\"},9001],[{\\\"first\\\":\\\"Tim\\\",\\\"",
"end": 16183,
"score": 0.9992086291,
"start": 16179,
"tag": "NAME",
"value": "Jeve"
},
{
"context": "de.string)\n\n \"[[{\\\"first\\\":\\\"Jeve\\\",\\\"last\\\":\\\"Sobs\\\"},9001],[{\\\"first\\\":\\\"Tim\\\",\\\"last\\\":\\\"Berners-L",
"end": 16201,
"score": 0.992266655,
"start": 16197,
"tag": "NAME",
"value": "Sobs"
},
{
"context": "\":\\\"Jeve\\\",\\\"last\\\":\\\"Sobs\\\"},9001],[{\\\"first\\\":\\\"Tim\\\",\\\"last\\\":\\\"Berners-Lee\\\"},1234]]\"\n |> De",
"end": 16228,
"score": 0.9952753782,
"start": 16225,
"tag": "NAME",
"value": "Tim"
},
{
"context": "\\\":\\\"Sobs\\\"},9001],[{\\\"first\\\":\\\"Tim\\\",\\\"last\\\":\\\"Berners-Lee\\\"},1234]]\"\n |> Decode.decodeString (decode",
"end": 16253,
"score": 0.9395899773,
"start": 16242,
"tag": "NAME",
"value": "Berners-Lee"
},
{
"context": " --> Ok (fromList personToString [(Person \"Jeve\" \"Sobs\", 9001), (Person \"Tim\" \"Berners-Lee\", 1234",
"end": 16461,
"score": 0.9994388223,
"start": 16457,
"tag": "NAME",
"value": "Jeve"
},
{
"context": " --> Ok (fromList personToString [(Person \"Jeve\" \"Sobs\", 9001), (Person \"Tim\" \"Berners-Lee\", 1234)])\n\n-}",
"end": 16468,
"score": 0.9992434978,
"start": 16464,
"tag": "NAME",
"value": "Sobs"
},
{
"context": "nToString [(Person \"Jeve\" \"Sobs\", 9001), (Person \"Tim\" \"Berners-Lee\", 1234)])\n\n-}\ndecodeList : (k -> co",
"end": 16490,
"score": 0.9994186163,
"start": 16487,
"tag": "NAME",
"value": "Tim"
},
{
"context": "ing [(Person \"Jeve\" \"Sobs\", 9001), (Person \"Tim\" \"Berners-Lee\", 1234)])\n\n-}\ndecodeList : (k -> comparable) -> D",
"end": 16504,
"score": 0.9978007674,
"start": 16493,
"tag": "NAME",
"value": "Berners-Lee"
},
{
"context": " Int Animal String\n animals =\n [ (Cat, \"Tom\"), (Mouse, \"Jerry\") ]\n |> fromList ani",
"end": 17646,
"score": 0.9962713718,
"start": 17643,
"tag": "NAME",
"value": "Tom"
},
{
"context": "ng\n animals =\n [ (Cat, \"Tom\"), (Mouse, \"Jerry\") ]\n |> fromList animalToInt\n\n isAC",
"end": 17664,
"score": 0.9931938052,
"start": 17659,
"tag": "NAME",
"value": "Jerry"
},
{
"context": " Int Animal String\n animals =\n [ (Cat, \"Tom\"), (Mouse, \"Jerry\") ]\n |> fromList ani",
"end": 18360,
"score": 0.9976517558,
"start": 18357,
"tag": "NAME",
"value": "Tom"
},
{
"context": "ng\n animals =\n [ (Cat, \"Tom\"), (Mouse, \"Jerry\") ]\n |> fromList animalToInt\n\n aris",
"end": 18378,
"score": 0.9931262732,
"start": 18373,
"tag": "NAME",
"value": "Jerry"
},
{
"context": "t Animal String\n aristocats =\n [ (Cat, \"Marie\") ]\n |> fromList animalToInt\n\n isAC",
"end": 18502,
"score": 0.9989669323,
"start": 18497,
"tag": "NAME",
"value": "Marie"
},
{
"context": " Int Animal String\n animals =\n [ (Cat, \"Tom\"), (Mouse, \"Jerry\") ]\n |> fromList ani",
"end": 19283,
"score": 0.9973566532,
"start": 19280,
"tag": "NAME",
"value": "Tom"
},
{
"context": "ng\n animals =\n [ (Cat, \"Tom\"), (Mouse, \"Jerry\") ]\n |> fromList animalToInt\n\n only",
"end": 19301,
"score": 0.999139607,
"start": 19296,
"tag": "NAME",
"value": "Jerry"
},
{
"context": " Int Animal String\n onlyTom =\n [ (Cat, \"Tom\") ]\n |> fromList animalToInt\n\n getC",
"end": 19417,
"score": 0.9950299263,
"start": 19414,
"tag": "NAME",
"value": "Tom"
}
] | src/Dict/Any.elm | turboMaCk/any-dict | 20 | module Dict.Any exposing
( AnyDict, equal
, empty, singleton, insert, update, remove, removeAll
, isEmpty, member, get, getKey, size, any, all
, keys, values, toList, fromList
, map, foldl, foldr, filter, partition, filterMap
, union, intersect, diff, merge, groupBy
, toDict
, decode, decode_, decodeList, encode, encodeList
)
{-| A dictionary mapping unique keys to values.
Similar and based on Dict but without restriction on comparable keys.
Insert, remove, and query operations all take O(log n) time.
# Converting Types to Comparable
When writing a function for conversion from the type you want to use for keys to comparable
it's very important to make sure every distinct member of type k produces different value in set o of comparables.
Take for instance those two examples:
We can use Bool as a key for our Dict (No matter how unpractical it might seem)
boolToInt : Bool -> Int
boolToInt bool =
case bool of
False -> 0
True -> 1
empty boolToInt
|> insert True "foo"
|> get True
--> Just "foo"
or Maybe String.
comparableKey : Maybe String -> (Int, String)
comparableKey maybe =
case maybe of
Nothing -> (0, "")
Just str -> (1, str)
empty comparableKey
|> insert (Just "foo") 42
|> get (Just "foo")
--> Just 42
Note that we give Int code to either constructor and in Case of Nothing we default to `""` (empty string).
There is still a difference between `Nothing` and `Just ""` (`Int` value in the pair is different).
In fact, you can "hardcode" any value as the second member of the pair
in case of nothing but empty string seems like a reasonable option for this case.
Generally, this is how I would implement `toComparable` function for most of your custom data types.
Have a look at the longest constructor,
Define tuple where the first key is int (number of the constructor)
and other are types within the constructor and you're good to go.
# Dictionaries
@docs AnyDict, equal
# Build
@docs empty, singleton, insert, update, remove, removeAll
# Query
@docs isEmpty, member, get, getKey, size, any, all
# Lists
@docs keys, values, toList, fromList
# Transform
@docs map, foldl, foldr, filter, partition, filterMap
# Combine
@docs union, intersect, diff, merge, groupBy
# Dict
@docs toDict
# Json
@docs decode, decode_, decodeList, encode, encodeList
-}
import Dict exposing (Dict)
import Json.Decode as Decode
import Json.Encode as Encode
{-| Be aware that AnyDict stores a function internally.
Use [`equal`](#equal) function to check equality of two `AnyDict`s.
Using `(==)` would result in runtime exception because `AnyDict` type
contains a function.
-}
type AnyDict comparable k v
= AnyDict
{ dict : Dict comparable ( k, v )
, toKey : k -> comparable
}
{-| Check equality of two `AnyDict`s
* returns `True` if AnyDicts are equal
* returns `False` if AnyDicts are not equal
-}
equal : AnyDict comparable k v -> AnyDict comparable k v -> Bool
equal (AnyDict r1) (AnyDict r2) =
r1.dict == r2.dict
-- Build
{-| Create an empty dictionary by suppling function used for comparing keys.
**Note that it's important to make sure every key is turned to different comparable.**
Otherwise keys would conflict and overwrite each other.
-}
empty : (k -> comparable) -> AnyDict comparable k v
empty toKey =
AnyDict
{ dict = Dict.empty
, toKey = toKey
}
{-| Create a dictionary with one key-value pair.
**Note that it's important to make sure every key is turned to different comparable.**
Otherwise keys would conflict and overwrite each other.
-}
singleton : k -> v -> (k -> comparable) -> AnyDict comparable k v
singleton k v f =
empty f
|> insert k v
{-| Insert a key-value pair into a dictionary. Replaces value when there is a collision.
-}
insert : k -> v -> AnyDict comparable k v -> AnyDict comparable k v
insert k v (AnyDict inner) =
AnyDict { inner | dict = Dict.insert (inner.toKey k) ( k, v ) inner.dict }
{-| Update the value of a dictionary for a specific key with a given function.
-}
update : k -> (Maybe v -> Maybe v) -> AnyDict comparable k v -> AnyDict comparable k v
update k f (AnyDict inner) =
let
updateDict =
Maybe.map (\b -> ( k, b )) << f << Maybe.map Tuple.second
in
AnyDict { inner | dict = Dict.update (inner.toKey k) updateDict inner.dict }
{-| Remove a key-value pair from a dictionary.
If the key is not found, no changes are made.
-}
remove : k -> AnyDict comparable k v -> AnyDict comparable k v
remove k (AnyDict inner) =
AnyDict { inner | dict = Dict.remove (inner.toKey k) inner.dict }
{-| Remove all entries from AnyDict.
Useful when you need to create new empty AnyDict using
same comparable function for key type.
-}
removeAll : AnyDict comparable k v -> AnyDict comparable k x
removeAll (AnyDict inner) =
AnyDict
{ toKey = inner.toKey
, dict = Dict.empty
}
-- Query
{-| Determine if a dictionary is empty.
isEmpty (empty identity)
--> True
singleton 1 "foo" identity
|> isEmpty
--> False
-}
isEmpty : AnyDict comparable k v -> Bool
isEmpty (AnyDict { dict }) =
Dict.isEmpty dict
{-| Determine if a key is in a dictionary.
-}
member : k -> AnyDict comparable k v -> Bool
member k (AnyDict { dict, toKey }) =
Dict.member (toKey k) dict
{-| Get the value associated with a key.
If the key is not found, return Nothing.
This is useful when you are not sure
if a key will be in the dictionary.
type Animal = Cat | Mouse | Dog
animalToInt : Animal -> Int
animalToInt animal =
case animal of
Cat -> 0
Mouse -> 1
Dog -> 2
animals : AnyDict Int Animal String
animals =
[ (Cat, "Tom"), (Mouse, "Jerry") ]
|> fromList animalToInt
get Cat animals
-> Just "Tom"
get Mouse animals
--> Just "Jerry"
get Dog animals
--> Nothing
-}
get : k -> AnyDict comparable k v -> Maybe v
get k (AnyDict { dict, toKey }) =
Dict.get (toKey k) dict
|> Maybe.map Tuple.second
{-| Get a key associated with key.
This is useful in case of `AnyDict` because
some parts of a key might not be used
for generating comparable.
This function allows quering `AnyDict` with old
key to obtain updated one in such cases.
-}
getKey : k -> AnyDict comparable k v -> Maybe k
getKey k (AnyDict { dict, toKey }) =
Dict.get (toKey k) dict
|> Maybe.map Tuple.first
{-| Determine the number of key-value pairs in the dictionary.
-}
size : AnyDict comparable k v -> Int
size (AnyDict { dict }) =
Dict.size dict
-- List
{-| Get all of the keys in a dictionary, sorted from lowest to highest.
-}
keys : AnyDict comparable k v -> List k
keys =
List.map Tuple.first << toList
{-| Get all of the values in a dictionary, in the order of their keys.
-}
values : AnyDict comparable k v -> List v
values =
List.map Tuple.second << toList
{-| Convert a dictionary into an association list of key-value pairs,
sorted by keys.
-}
toList : AnyDict comparable k v -> List ( k, v )
toList (AnyDict { dict }) =
Dict.values dict
{-| Convert an association list into a dictionary.
**Note that it's important to make sure every key is turned to different comparable.**
Otherwise keys would conflict and overwrite each other.
-}
fromList : (k -> comparable) -> List ( k, v ) -> AnyDict comparable k v
fromList f xs =
AnyDict
{ toKey = f
, dict = Dict.fromList <| List.map (\( k, v ) -> ( f k, ( k, v ) )) xs
}
-- Transform
{-| Apply a function to all values in a dictionary.
-}
map : (a -> b -> c) -> AnyDict comparable a b -> AnyDict comparable a c
map f (AnyDict { dict, toKey }) =
AnyDict
{ dict = Dict.map (\_ ( k, v ) -> ( k, f k v )) dict
, toKey = toKey
}
{-| Fold over the key-value pairs in a dictionary, in order from lowest key to highest key.
-}
foldl : (k -> v -> b -> b) -> b -> AnyDict comparable k v -> b
foldl f acc (AnyDict { dict }) =
Dict.foldl (\_ ( k, v ) -> f k v) acc dict
{-| Fold over the key-value pairs in a dictionary, in order from highest key to lowest key.
-}
foldr : (k -> v -> b -> b) -> b -> AnyDict comparable k v -> b
foldr f acc (AnyDict { dict }) =
Dict.foldr (\_ ( k, v ) -> f k v) acc dict
{-| Keep a key-value pair when it satisfies a predicate.
-}
filter : (k -> v -> Bool) -> AnyDict comparable k v -> AnyDict comparable k v
filter f (AnyDict inner) =
AnyDict { inner | dict = Dict.filter (\_ ( k, v ) -> f k v) inner.dict }
{-| Partition a dictionary according to a predicate.
The first dictionary contains all key-value pairs which satisfy the predicate,
and the second contains the rest.
-}
partition : (k -> v -> Bool) -> AnyDict comparable k v -> ( AnyDict comparable k v, AnyDict comparable k v )
partition f (AnyDict inner) =
let
( left, right ) =
Dict.partition (\_ ( k, v ) -> f k v) inner.dict
in
( AnyDict { inner | dict = left }
, AnyDict { inner | dict = right }
)
-- Combine
{-| Combine two dictionaries. If there is a collision, preference is given to the first dictionary.
-}
union : AnyDict comparable k v -> AnyDict comparable k v -> AnyDict comparable k v
union (AnyDict inner) (AnyDict { dict }) =
AnyDict { inner | dict = Dict.union inner.dict dict }
{-| Keep a key-value pair when its key appears in the second dictionary.
Preference is given to values in the first dictionary.
-}
intersect : AnyDict comparable k v -> AnyDict comparable k v -> AnyDict comparable k v
intersect (AnyDict inner) (AnyDict { dict }) =
AnyDict { inner | dict = Dict.intersect inner.dict dict }
{-| Keep a key-value pair when its key does not appear in the second dictionary.
-}
diff : AnyDict comparable k v -> AnyDict comparable k v -> AnyDict comparable k v
diff (AnyDict inner) (AnyDict { dict }) =
AnyDict { inner | dict = Dict.diff inner.dict dict }
{-| The most general way of combining two dictionaries.
You provide three accumulators for when a given key appears:
1. Only in the left dictionary.
2. In both dictionaries.
3. Only in the right dictionary.
Only in the left dictionary.
In both dictionaries.
Only in the right dictionary.
-}
merge :
(k -> a -> result -> result)
-> (k -> a -> b -> result -> result)
-> (k -> b -> result -> result)
-> AnyDict comparable k a
-> AnyDict comparable k b
-> result
-> result
merge f g h (AnyDict inner) (AnyDict { dict }) =
let
l fc _ ( k, v ) =
fc k v
in
Dict.merge (l f) (\_ ( k, a ) ( _, b ) -> g k a b) (l h) inner.dict dict
-- Dict
{-| Convert `AnyDict` to plain dictionary with comparable keys.
-}
toDict : AnyDict comparable k v -> Dict comparable v
toDict (AnyDict { dict }) =
Dict.map (always Tuple.second) dict
{-| Decode a JSON object into an `AnyDict`.
This encoder is limitted for cases where JSON representation
for a given type is an JSON Object. In JSON, object keys must be of type
`String`.
If you need to decode different representation into `AnyDict` value,
just use primitive `Decoder` types directly and map `AnyDict` constructors
over these.
import Json.Decode
type Key = Foo | Bar
fromString : String -> Key
fromString str =
case str of
"foo" -> Foo
_ -> Bar
toString : Key -> String
toString key =
case key of
Foo -> "foo"
Bar -> "bar"
type alias Data = AnyDict String Key Int
dataDecoder : Json.Decode.Decoder Data
dataDecoder =
decode (\str _ -> fromString str) toString Json.Decode.int
Json.Decode.decodeString dataDecoder "{\"foo\":1,\"bar\":2}"
|> Result.map toList
--> Ok [(Bar, 2), (Foo, 1)]
-}
decode : (String -> v -> k) -> (k -> comparable) -> Decode.Decoder v -> Decode.Decoder (AnyDict comparable k v)
decode fromStr toComparable valueD =
let
construct strK v acc =
insert (fromStr strK v) v acc
in
Decode.dict valueD
|> Decode.map (Dict.foldr construct (empty toComparable))
{-| Decode a JSON object into an `AnyDict`.
This variant of decode allows you to fail with error while parsing key from String.
In such case whole Dict decoding will fail.
import Json.Decode
type Key = Foo | Bar
fromString : String -> Result String Key
fromString str =
case str of
"foo" -> Ok Foo
"bar" -> Ok Bar
_ -> Err <| "Unknown key " ++ str
toString : Key -> String
toString key =
case key of
Foo -> "foo"
Bar -> "bar"
type alias Data = AnyDict String Key Int
dataDecoder : Json.Decode.Decoder Data
dataDecoder =
decode_ (\str _ -> fromString str) toString Json.Decode.int
Json.Decode.decodeString dataDecoder "{\"foo\":1,\"bar\":2}"
|> Result.map toList
--> Ok [(Bar, 2), (Foo, 1)]
Json.Decode.decodeString dataDecoder "{\"foo\":1,\"baz\":2}"
|> Result.map toList
--> Json.Decode.decodeString (Json.Decode.fail "Unknown key baz") "{}"
-}
decode_ : (String -> v -> Result String k) -> (k -> comparable) -> Decode.Decoder v -> Decode.Decoder (AnyDict comparable k v)
decode_ fromStr toComparable valueD =
let
construct strK v =
Result.andThen
(\acc ->
fromStr strK v
|> Result.map (\key -> insert key v acc)
)
in
Decode.dict valueD
|> Decode.map (Dict.foldr construct (Ok <| empty toComparable))
|> Decode.andThen
(\res ->
case res of
Ok val ->
Decode.succeed val
Err err ->
Decode.fail err
)
{-| Turn an `AnyDict` into a JSON object.
import Json.Encode
type Key = Foo | Bar
toString : Key -> String
toString key =
case key of
Foo -> "foo"
Bar -> "bar"
type alias Data = AnyDict String Key Int
encodeData : Data -> Json.Encode.Value
encodeData =
encode toString Json.Encode.int
fromList toString [(Foo, 1), (Bar, 2)]
|> encodeData
|> Json.Encode.encode 0
--> "{\"bar\":2,\"foo\":1}"
-}
encode : (k -> String) -> (v -> Encode.Value) -> AnyDict comparable k v -> Encode.Value
encode keyE valueE =
Encode.object
<< List.map (Tuple.mapBoth keyE valueE)
<< toList
{-| Turn an AnyDict into a JSON list of tuples. This is useful when you have more complex types as keys
import Json.Decode as Decode
import Json.Encode as Encode
type alias Person = {first : String, last : String}
type alias Age = Int
personToString : Person -> String
personToString {first, last} = first ++ last
personEncode : Person -> Encode.Value
personEncode {first, last} =
Encode.object [("first", (Encode.string first)), ("last", (Encode.string last))]
example : AnyDict String Person Age
example =
fromList personToString [(Person "Jeve" "Sobs", 9001), (Person "Tim" "Berners-Lee", 1234)]
encodeList (\k v -> Encode.list identity [ personEncode k, Encode.int v ]) example
|> Encode.encode 0
--> "[[{\"first\":\"Jeve\",\"last\":\"Sobs\"},9001],[{\"first\":\"Tim\",\"last\":\"Berners-Lee\"},1234]]"
-}
encodeList : (k -> v -> Encode.Value) -> AnyDict comparable k v -> Encode.Value
encodeList encodeF =
Encode.list (\( k, v ) -> encodeF k v) << toList
{-| Decode an AnyDict from a JSON list of tuples.
import Json.Decode as Decode
import Json.Encode as Encode
type alias Person = {first : String, last : String}
type alias Age = Int
personToString : Person -> String
personToString {first, last} = first ++ last
personDecode : Decode.Decoder Person
personDecode =
Decode.map2
Person
(Decode.field "first" Decode.string)
(Decode.field "last" Decode.string)
"[[{\"first\":\"Jeve\",\"last\":\"Sobs\"},9001],[{\"first\":\"Tim\",\"last\":\"Berners-Lee\"},1234]]"
|> Decode.decodeString (decodeList personToString (Decode.map2 Tuple.pair (Decode.index 0 personDecode) (Decode.index 1 Decode.int)))
--> Ok (fromList personToString [(Person "Jeve" "Sobs", 9001), (Person "Tim" "Berners-Lee", 1234)])
-}
decodeList : (k -> comparable) -> Decode.Decoder ( k, v ) -> Decode.Decoder (AnyDict comparable k v)
decodeList keyToComparable =
Decode.map (fromList keyToComparable) << Decode.list
{-| Takes a key-fn and a list.
Creates an `AnyDict` which maps the key to a list of matching elements.
-}
groupBy : (value -> key) -> (key -> comparable) -> List value -> AnyDict comparable key (List value)
groupBy toKey keyToComparable list =
List.foldr
(\x acc ->
update
(toKey x)
(\maybeValues ->
maybeValues
|> Maybe.map ((::) x)
|> Maybe.withDefault [ x ]
|> Just
)
acc
)
(empty keyToComparable)
list
{-| Find out if there is any instance of something in a Dictionary.
type Animal = Cat | Mouse | Dog
animalToInt : Animal -> Int
animalToInt animal =
case animal of
Cat -> 0
Mouse -> 1
Dog -> 2
animals : AnyDict Int Animal String
animals =
[ (Cat, "Tom"), (Mouse, "Jerry") ]
|> fromList animalToInt
isACat : Animal -> String -> Bool
isACat animal _ =
case animal of
Cat -> True
_ -> False
any isACat animals
--> True
-}
any : (k -> v -> Bool) -> AnyDict comparable k v -> Bool
any predicate dict =
foldl
(\k v acc -> acc || predicate k v)
False
dict
{-| Find if all key value paris in Dictionary match a predicate.
type Animal = Cat | Mouse | Dog
animalToInt : Animal -> Int
animalToInt animal =
case animal of
Cat -> 0
Mouse -> 1
Dog -> 2
animals : AnyDict Int Animal String
animals =
[ (Cat, "Tom"), (Mouse, "Jerry") ]
|> fromList animalToInt
aristocats : AnyDict Int Animal String
aristocats =
[ (Cat, "Marie") ]
|> fromList animalToInt
isACat : Animal -> String -> Bool
isACat animal _ =
case animal of
Cat -> True
_ -> False
all isACat animals
--> False
all isACat aristocats
--> True
-}
all : (k -> v -> Bool) -> AnyDict comparable k v -> Bool
all predicate dict =
foldl
(\k v acc -> acc && predicate k v)
True
dict
{-| Apply a function that may or may not succeed to all entries in a dictionary, but only keep the successes.
type Animal = Cat | Mouse | Dog
animalToInt : Animal -> Int
animalToInt animal =
case animal of
Cat -> 0
Mouse -> 1
Dog -> 2
animals : AnyDict Int Animal String
animals =
[ (Cat, "Tom"), (Mouse, "Jerry") ]
|> fromList animalToInt
onlyTom : AnyDict Int Animal String
onlyTom =
[ (Cat, "Tom") ]
|> fromList animalToInt
getCatName : Animal -> String -> Maybe String
getCatName animal name =
case animal of
Cat -> Just name
_ -> Nothing
filterMap getCatName animals == onlyTom
--> True
-}
filterMap : (k -> v1 -> Maybe v2) -> AnyDict comparable k v1 -> AnyDict comparable k v2
filterMap f dict =
foldl
(\k v acc ->
case f k v of
Just newVal ->
insert k newVal acc
Nothing ->
acc
)
(removeAll dict)
dict
| 41031 | module Dict.Any exposing
( AnyDict, equal
, empty, singleton, insert, update, remove, removeAll
, isEmpty, member, get, getKey, size, any, all
, keys, values, toList, fromList
, map, foldl, foldr, filter, partition, filterMap
, union, intersect, diff, merge, groupBy
, toDict
, decode, decode_, decodeList, encode, encodeList
)
{-| A dictionary mapping unique keys to values.
Similar and based on Dict but without restriction on comparable keys.
Insert, remove, and query operations all take O(log n) time.
# Converting Types to Comparable
When writing a function for conversion from the type you want to use for keys to comparable
it's very important to make sure every distinct member of type k produces different value in set o of comparables.
Take for instance those two examples:
We can use Bool as a key for our Dict (No matter how unpractical it might seem)
boolToInt : Bool -> Int
boolToInt bool =
case bool of
False -> 0
True -> 1
empty boolToInt
|> insert True "foo"
|> get True
--> Just "foo"
or Maybe String.
comparableKey : Maybe String -> (Int, String)
comparableKey maybe =
case maybe of
Nothing -> (0, "")
Just str -> (1, str)
empty comparableKey
|> insert (Just "foo") 42
|> get (Just "foo")
--> Just 42
Note that we give Int code to either constructor and in Case of Nothing we default to `""` (empty string).
There is still a difference between `Nothing` and `Just ""` (`Int` value in the pair is different).
In fact, you can "hardcode" any value as the second member of the pair
in case of nothing but empty string seems like a reasonable option for this case.
Generally, this is how I would implement `toComparable` function for most of your custom data types.
Have a look at the longest constructor,
Define tuple where the first key is int (number of the constructor)
and other are types within the constructor and you're good to go.
# Dictionaries
@docs AnyDict, equal
# Build
@docs empty, singleton, insert, update, remove, removeAll
# Query
@docs isEmpty, member, get, getKey, size, any, all
# Lists
@docs keys, values, toList, fromList
# Transform
@docs map, foldl, foldr, filter, partition, filterMap
# Combine
@docs union, intersect, diff, merge, groupBy
# Dict
@docs toDict
# Json
@docs decode, decode_, decodeList, encode, encodeList
-}
import Dict exposing (Dict)
import Json.Decode as Decode
import Json.Encode as Encode
{-| Be aware that AnyDict stores a function internally.
Use [`equal`](#equal) function to check equality of two `AnyDict`s.
Using `(==)` would result in runtime exception because `AnyDict` type
contains a function.
-}
type AnyDict comparable k v
= AnyDict
{ dict : Dict comparable ( k, v )
, toKey : k -> comparable
}
{-| Check equality of two `AnyDict`s
* returns `True` if AnyDicts are equal
* returns `False` if AnyDicts are not equal
-}
equal : AnyDict comparable k v -> AnyDict comparable k v -> Bool
equal (AnyDict r1) (AnyDict r2) =
r1.dict == r2.dict
-- Build
{-| Create an empty dictionary by suppling function used for comparing keys.
**Note that it's important to make sure every key is turned to different comparable.**
Otherwise keys would conflict and overwrite each other.
-}
empty : (k -> comparable) -> AnyDict comparable k v
empty toKey =
AnyDict
{ dict = Dict.empty
, toKey = toKey
}
{-| Create a dictionary with one key-value pair.
**Note that it's important to make sure every key is turned to different comparable.**
Otherwise keys would conflict and overwrite each other.
-}
singleton : k -> v -> (k -> comparable) -> AnyDict comparable k v
singleton k v f =
empty f
|> insert k v
{-| Insert a key-value pair into a dictionary. Replaces value when there is a collision.
-}
insert : k -> v -> AnyDict comparable k v -> AnyDict comparable k v
insert k v (AnyDict inner) =
AnyDict { inner | dict = Dict.insert (inner.toKey k) ( k, v ) inner.dict }
{-| Update the value of a dictionary for a specific key with a given function.
-}
update : k -> (Maybe v -> Maybe v) -> AnyDict comparable k v -> AnyDict comparable k v
update k f (AnyDict inner) =
let
updateDict =
Maybe.map (\b -> ( k, b )) << f << Maybe.map Tuple.second
in
AnyDict { inner | dict = Dict.update (inner.toKey k) updateDict inner.dict }
{-| Remove a key-value pair from a dictionary.
If the key is not found, no changes are made.
-}
remove : k -> AnyDict comparable k v -> AnyDict comparable k v
remove k (AnyDict inner) =
AnyDict { inner | dict = Dict.remove (inner.toKey k) inner.dict }
{-| Remove all entries from AnyDict.
Useful when you need to create new empty AnyDict using
same comparable function for key type.
-}
removeAll : AnyDict comparable k v -> AnyDict comparable k x
removeAll (AnyDict inner) =
AnyDict
{ toKey = inner.toKey
, dict = Dict.empty
}
-- Query
{-| Determine if a dictionary is empty.
isEmpty (empty identity)
--> True
singleton 1 "foo" identity
|> isEmpty
--> False
-}
isEmpty : AnyDict comparable k v -> Bool
isEmpty (AnyDict { dict }) =
Dict.isEmpty dict
{-| Determine if a key is in a dictionary.
-}
member : k -> AnyDict comparable k v -> Bool
member k (AnyDict { dict, toKey }) =
Dict.member (toKey k) dict
{-| Get the value associated with a key.
If the key is not found, return Nothing.
This is useful when you are not sure
if a key will be in the dictionary.
type Animal = Cat | Mouse | Dog
animalToInt : Animal -> Int
animalToInt animal =
case animal of
Cat -> 0
Mouse -> 1
Dog -> 2
animals : AnyDict Int Animal String
animals =
[ (Cat, "<NAME>"), (Mouse, "<NAME>") ]
|> fromList animalToInt
get Cat animals
-> Just "<NAME>"
get Mouse animals
--> Just "<NAME>"
get Dog animals
--> Nothing
-}
get : k -> AnyDict comparable k v -> Maybe v
get k (AnyDict { dict, toKey }) =
Dict.get (toKey k) dict
|> Maybe.map Tuple.second
{-| Get a key associated with key.
This is useful in case of `AnyDict` because
some parts of a key might not be used
for generating comparable.
This function allows quering `AnyDict` with old
key to obtain updated one in such cases.
-}
getKey : k -> AnyDict comparable k v -> Maybe k
getKey k (AnyDict { dict, toKey }) =
Dict.get (toKey k) dict
|> Maybe.map Tuple.first
{-| Determine the number of key-value pairs in the dictionary.
-}
size : AnyDict comparable k v -> Int
size (AnyDict { dict }) =
Dict.size dict
-- List
{-| Get all of the keys in a dictionary, sorted from lowest to highest.
-}
keys : AnyDict comparable k v -> List k
keys =
List.map Tuple.first << toList
{-| Get all of the values in a dictionary, in the order of their keys.
-}
values : AnyDict comparable k v -> List v
values =
List.map Tuple.second << toList
{-| Convert a dictionary into an association list of key-value pairs,
sorted by keys.
-}
toList : AnyDict comparable k v -> List ( k, v )
toList (AnyDict { dict }) =
Dict.values dict
{-| Convert an association list into a dictionary.
**Note that it's important to make sure every key is turned to different comparable.**
Otherwise keys would conflict and overwrite each other.
-}
fromList : (k -> comparable) -> List ( k, v ) -> AnyDict comparable k v
fromList f xs =
AnyDict
{ toKey = f
, dict = Dict.fromList <| List.map (\( k, v ) -> ( f k, ( k, v ) )) xs
}
-- Transform
{-| Apply a function to all values in a dictionary.
-}
map : (a -> b -> c) -> AnyDict comparable a b -> AnyDict comparable a c
map f (AnyDict { dict, toKey }) =
AnyDict
{ dict = Dict.map (\_ ( k, v ) -> ( k, f k v )) dict
, toKey = toKey
}
{-| Fold over the key-value pairs in a dictionary, in order from lowest key to highest key.
-}
foldl : (k -> v -> b -> b) -> b -> AnyDict comparable k v -> b
foldl f acc (AnyDict { dict }) =
Dict.foldl (\_ ( k, v ) -> f k v) acc dict
{-| Fold over the key-value pairs in a dictionary, in order from highest key to lowest key.
-}
foldr : (k -> v -> b -> b) -> b -> AnyDict comparable k v -> b
foldr f acc (AnyDict { dict }) =
Dict.foldr (\_ ( k, v ) -> f k v) acc dict
{-| Keep a key-value pair when it satisfies a predicate.
-}
filter : (k -> v -> Bool) -> AnyDict comparable k v -> AnyDict comparable k v
filter f (AnyDict inner) =
AnyDict { inner | dict = Dict.filter (\_ ( k, v ) -> f k v) inner.dict }
{-| Partition a dictionary according to a predicate.
The first dictionary contains all key-value pairs which satisfy the predicate,
and the second contains the rest.
-}
partition : (k -> v -> Bool) -> AnyDict comparable k v -> ( AnyDict comparable k v, AnyDict comparable k v )
partition f (AnyDict inner) =
let
( left, right ) =
Dict.partition (\_ ( k, v ) -> f k v) inner.dict
in
( AnyDict { inner | dict = left }
, AnyDict { inner | dict = right }
)
-- Combine
{-| Combine two dictionaries. If there is a collision, preference is given to the first dictionary.
-}
union : AnyDict comparable k v -> AnyDict comparable k v -> AnyDict comparable k v
union (AnyDict inner) (AnyDict { dict }) =
AnyDict { inner | dict = Dict.union inner.dict dict }
{-| Keep a key-value pair when its key appears in the second dictionary.
Preference is given to values in the first dictionary.
-}
intersect : AnyDict comparable k v -> AnyDict comparable k v -> AnyDict comparable k v
intersect (AnyDict inner) (AnyDict { dict }) =
AnyDict { inner | dict = Dict.intersect inner.dict dict }
{-| Keep a key-value pair when its key does not appear in the second dictionary.
-}
diff : AnyDict comparable k v -> AnyDict comparable k v -> AnyDict comparable k v
diff (AnyDict inner) (AnyDict { dict }) =
AnyDict { inner | dict = Dict.diff inner.dict dict }
{-| The most general way of combining two dictionaries.
You provide three accumulators for when a given key appears:
1. Only in the left dictionary.
2. In both dictionaries.
3. Only in the right dictionary.
Only in the left dictionary.
In both dictionaries.
Only in the right dictionary.
-}
merge :
(k -> a -> result -> result)
-> (k -> a -> b -> result -> result)
-> (k -> b -> result -> result)
-> AnyDict comparable k a
-> AnyDict comparable k b
-> result
-> result
merge f g h (AnyDict inner) (AnyDict { dict }) =
let
l fc _ ( k, v ) =
fc k v
in
Dict.merge (l f) (\_ ( k, a ) ( _, b ) -> g k a b) (l h) inner.dict dict
-- Dict
{-| Convert `AnyDict` to plain dictionary with comparable keys.
-}
toDict : AnyDict comparable k v -> Dict comparable v
toDict (AnyDict { dict }) =
Dict.map (always Tuple.second) dict
{-| Decode a JSON object into an `AnyDict`.
This encoder is limitted for cases where JSON representation
for a given type is an JSON Object. In JSON, object keys must be of type
`String`.
If you need to decode different representation into `AnyDict` value,
just use primitive `Decoder` types directly and map `AnyDict` constructors
over these.
import Json.Decode
type Key = Foo | Bar
fromString : String -> Key
fromString str =
case str of
"foo" -> Foo
_ -> Bar
toString : Key -> String
toString key =
case key of
Foo -> "foo"
Bar -> "bar"
type alias Data = AnyDict String Key Int
dataDecoder : Json.Decode.Decoder Data
dataDecoder =
decode (\str _ -> fromString str) toString Json.Decode.int
Json.Decode.decodeString dataDecoder "{\"foo\":1,\"bar\":2}"
|> Result.map toList
--> Ok [(Bar, 2), (Foo, 1)]
-}
decode : (String -> v -> k) -> (k -> comparable) -> Decode.Decoder v -> Decode.Decoder (AnyDict comparable k v)
decode fromStr toComparable valueD =
let
construct strK v acc =
insert (fromStr strK v) v acc
in
Decode.dict valueD
|> Decode.map (Dict.foldr construct (empty toComparable))
{-| Decode a JSON object into an `AnyDict`.
This variant of decode allows you to fail with error while parsing key from String.
In such case whole Dict decoding will fail.
import Json.Decode
type Key = Foo | Bar
fromString : String -> Result String Key
fromString str =
case str of
"foo" -> Ok Foo
"bar" -> Ok Bar
_ -> Err <| "Unknown key " ++ str
toString : Key -> String
toString key =
case key of
Foo -> "foo"
Bar -> "bar"
type alias Data = AnyDict String Key Int
dataDecoder : Json.Decode.Decoder Data
dataDecoder =
decode_ (\str _ -> fromString str) toString Json.Decode.int
Json.Decode.decodeString dataDecoder "{\"foo\":1,\"bar\":2}"
|> Result.map toList
--> Ok [(Bar, 2), (Foo, 1)]
Json.Decode.decodeString dataDecoder "{\"foo\":1,\"baz\":2}"
|> Result.map toList
--> Json.Decode.decodeString (Json.Decode.fail "Unknown key baz") "{}"
-}
decode_ : (String -> v -> Result String k) -> (k -> comparable) -> Decode.Decoder v -> Decode.Decoder (AnyDict comparable k v)
decode_ fromStr toComparable valueD =
let
construct strK v =
Result.andThen
(\acc ->
fromStr strK v
|> Result.map (\key -> insert key v acc)
)
in
Decode.dict valueD
|> Decode.map (Dict.foldr construct (Ok <| empty toComparable))
|> Decode.andThen
(\res ->
case res of
Ok val ->
Decode.succeed val
Err err ->
Decode.fail err
)
{-| Turn an `AnyDict` into a JSON object.
import Json.Encode
type Key = <KEY> | Bar
toString : Key -> String
toString key =
case key of
Foo -> "foo"
Bar -> "bar"
type alias Data = AnyDict String Key Int
encodeData : Data -> Json.Encode.Value
encodeData =
encode toString Json.Encode.int
fromList toString [(Foo, 1), (Bar, 2)]
|> encodeData
|> Json.Encode.encode 0
--> "{\"bar\":2,\"foo\":1}"
-}
encode : (k -> String) -> (v -> Encode.Value) -> AnyDict comparable k v -> Encode.Value
encode keyE valueE =
Encode.object
<< List.map (Tuple.mapBoth keyE valueE)
<< toList
{-| Turn an AnyDict into a JSON list of tuples. This is useful when you have more complex types as keys
import Json.Decode as Decode
import Json.Encode as Encode
type alias Person = {first : String, last : String}
type alias Age = Int
personToString : Person -> String
personToString {first, last} = first ++ last
personEncode : Person -> Encode.Value
personEncode {first, last} =
Encode.object [("first", (Encode.string first)), ("last", (Encode.string last))]
example : AnyDict String Person Age
example =
fromList personToString [(Person "<NAME>" "<NAME>", 9001), (Person "<NAME>" "<NAME>", 1234)]
encodeList (\k v -> Encode.list identity [ personEncode k, Encode.int v ]) example
|> Encode.encode 0
--> "[[{\"first\":\"<NAME>\",\"last\":\"<NAME>\"},9001],[{\"first\":\"<NAME>\",\"last\":\"<NAME>\"},1234]]"
-}
encodeList : (k -> v -> Encode.Value) -> AnyDict comparable k v -> Encode.Value
encodeList encodeF =
Encode.list (\( k, v ) -> encodeF k v) << toList
{-| Decode an AnyDict from a JSON list of tuples.
import Json.Decode as Decode
import Json.Encode as Encode
type alias Person = {first : String, last : String}
type alias Age = Int
personToString : Person -> String
personToString {first, last} = first ++ last
personDecode : Decode.Decoder Person
personDecode =
Decode.map2
Person
(Decode.field "first" Decode.string)
(Decode.field "last" Decode.string)
"[[{\"first\":\"<NAME>\",\"last\":\"<NAME>\"},9001],[{\"first\":\"<NAME>\",\"last\":\"<NAME>\"},1234]]"
|> Decode.decodeString (decodeList personToString (Decode.map2 Tuple.pair (Decode.index 0 personDecode) (Decode.index 1 Decode.int)))
--> Ok (fromList personToString [(Person "<NAME>" "<NAME>", 9001), (Person "<NAME>" "<NAME>", 1234)])
-}
decodeList : (k -> comparable) -> Decode.Decoder ( k, v ) -> Decode.Decoder (AnyDict comparable k v)
decodeList keyToComparable =
Decode.map (fromList keyToComparable) << Decode.list
{-| Takes a key-fn and a list.
Creates an `AnyDict` which maps the key to a list of matching elements.
-}
groupBy : (value -> key) -> (key -> comparable) -> List value -> AnyDict comparable key (List value)
groupBy toKey keyToComparable list =
List.foldr
(\x acc ->
update
(toKey x)
(\maybeValues ->
maybeValues
|> Maybe.map ((::) x)
|> Maybe.withDefault [ x ]
|> Just
)
acc
)
(empty keyToComparable)
list
{-| Find out if there is any instance of something in a Dictionary.
type Animal = Cat | Mouse | Dog
animalToInt : Animal -> Int
animalToInt animal =
case animal of
Cat -> 0
Mouse -> 1
Dog -> 2
animals : AnyDict Int Animal String
animals =
[ (Cat, "<NAME>"), (Mouse, "<NAME>") ]
|> fromList animalToInt
isACat : Animal -> String -> Bool
isACat animal _ =
case animal of
Cat -> True
_ -> False
any isACat animals
--> True
-}
any : (k -> v -> Bool) -> AnyDict comparable k v -> Bool
any predicate dict =
foldl
(\k v acc -> acc || predicate k v)
False
dict
{-| Find if all key value paris in Dictionary match a predicate.
type Animal = Cat | Mouse | Dog
animalToInt : Animal -> Int
animalToInt animal =
case animal of
Cat -> 0
Mouse -> 1
Dog -> 2
animals : AnyDict Int Animal String
animals =
[ (Cat, "<NAME>"), (Mouse, "<NAME>") ]
|> fromList animalToInt
aristocats : AnyDict Int Animal String
aristocats =
[ (Cat, "<NAME>") ]
|> fromList animalToInt
isACat : Animal -> String -> Bool
isACat animal _ =
case animal of
Cat -> True
_ -> False
all isACat animals
--> False
all isACat aristocats
--> True
-}
all : (k -> v -> Bool) -> AnyDict comparable k v -> Bool
all predicate dict =
foldl
(\k v acc -> acc && predicate k v)
True
dict
{-| Apply a function that may or may not succeed to all entries in a dictionary, but only keep the successes.
type Animal = Cat | Mouse | Dog
animalToInt : Animal -> Int
animalToInt animal =
case animal of
Cat -> 0
Mouse -> 1
Dog -> 2
animals : AnyDict Int Animal String
animals =
[ (Cat, "<NAME>"), (Mouse, "<NAME>") ]
|> fromList animalToInt
onlyTom : AnyDict Int Animal String
onlyTom =
[ (Cat, "<NAME>") ]
|> fromList animalToInt
getCatName : Animal -> String -> Maybe String
getCatName animal name =
case animal of
Cat -> Just name
_ -> Nothing
filterMap getCatName animals == onlyTom
--> True
-}
filterMap : (k -> v1 -> Maybe v2) -> AnyDict comparable k v1 -> AnyDict comparable k v2
filterMap f dict =
foldl
(\k v acc ->
case f k v of
Just newVal ->
insert k newVal acc
Nothing ->
acc
)
(removeAll dict)
dict
| true | module Dict.Any exposing
( AnyDict, equal
, empty, singleton, insert, update, remove, removeAll
, isEmpty, member, get, getKey, size, any, all
, keys, values, toList, fromList
, map, foldl, foldr, filter, partition, filterMap
, union, intersect, diff, merge, groupBy
, toDict
, decode, decode_, decodeList, encode, encodeList
)
{-| A dictionary mapping unique keys to values.
Similar and based on Dict but without restriction on comparable keys.
Insert, remove, and query operations all take O(log n) time.
# Converting Types to Comparable
When writing a function for conversion from the type you want to use for keys to comparable
it's very important to make sure every distinct member of type k produces different value in set o of comparables.
Take for instance those two examples:
We can use Bool as a key for our Dict (No matter how unpractical it might seem)
boolToInt : Bool -> Int
boolToInt bool =
case bool of
False -> 0
True -> 1
empty boolToInt
|> insert True "foo"
|> get True
--> Just "foo"
or Maybe String.
comparableKey : Maybe String -> (Int, String)
comparableKey maybe =
case maybe of
Nothing -> (0, "")
Just str -> (1, str)
empty comparableKey
|> insert (Just "foo") 42
|> get (Just "foo")
--> Just 42
Note that we give Int code to either constructor and in Case of Nothing we default to `""` (empty string).
There is still a difference between `Nothing` and `Just ""` (`Int` value in the pair is different).
In fact, you can "hardcode" any value as the second member of the pair
in case of nothing but empty string seems like a reasonable option for this case.
Generally, this is how I would implement `toComparable` function for most of your custom data types.
Have a look at the longest constructor,
Define tuple where the first key is int (number of the constructor)
and other are types within the constructor and you're good to go.
# Dictionaries
@docs AnyDict, equal
# Build
@docs empty, singleton, insert, update, remove, removeAll
# Query
@docs isEmpty, member, get, getKey, size, any, all
# Lists
@docs keys, values, toList, fromList
# Transform
@docs map, foldl, foldr, filter, partition, filterMap
# Combine
@docs union, intersect, diff, merge, groupBy
# Dict
@docs toDict
# Json
@docs decode, decode_, decodeList, encode, encodeList
-}
import Dict exposing (Dict)
import Json.Decode as Decode
import Json.Encode as Encode
{-| Be aware that AnyDict stores a function internally.
Use [`equal`](#equal) function to check equality of two `AnyDict`s.
Using `(==)` would result in runtime exception because `AnyDict` type
contains a function.
-}
type AnyDict comparable k v
= AnyDict
{ dict : Dict comparable ( k, v )
, toKey : k -> comparable
}
{-| Check equality of two `AnyDict`s
* returns `True` if AnyDicts are equal
* returns `False` if AnyDicts are not equal
-}
equal : AnyDict comparable k v -> AnyDict comparable k v -> Bool
equal (AnyDict r1) (AnyDict r2) =
r1.dict == r2.dict
-- Build
{-| Create an empty dictionary by suppling function used for comparing keys.
**Note that it's important to make sure every key is turned to different comparable.**
Otherwise keys would conflict and overwrite each other.
-}
empty : (k -> comparable) -> AnyDict comparable k v
empty toKey =
AnyDict
{ dict = Dict.empty
, toKey = toKey
}
{-| Create a dictionary with one key-value pair.
**Note that it's important to make sure every key is turned to different comparable.**
Otherwise keys would conflict and overwrite each other.
-}
singleton : k -> v -> (k -> comparable) -> AnyDict comparable k v
singleton k v f =
empty f
|> insert k v
{-| Insert a key-value pair into a dictionary. Replaces value when there is a collision.
-}
insert : k -> v -> AnyDict comparable k v -> AnyDict comparable k v
insert k v (AnyDict inner) =
AnyDict { inner | dict = Dict.insert (inner.toKey k) ( k, v ) inner.dict }
{-| Update the value of a dictionary for a specific key with a given function.
-}
update : k -> (Maybe v -> Maybe v) -> AnyDict comparable k v -> AnyDict comparable k v
update k f (AnyDict inner) =
let
updateDict =
Maybe.map (\b -> ( k, b )) << f << Maybe.map Tuple.second
in
AnyDict { inner | dict = Dict.update (inner.toKey k) updateDict inner.dict }
{-| Remove a key-value pair from a dictionary.
If the key is not found, no changes are made.
-}
remove : k -> AnyDict comparable k v -> AnyDict comparable k v
remove k (AnyDict inner) =
AnyDict { inner | dict = Dict.remove (inner.toKey k) inner.dict }
{-| Remove all entries from AnyDict.
Useful when you need to create new empty AnyDict using
same comparable function for key type.
-}
removeAll : AnyDict comparable k v -> AnyDict comparable k x
removeAll (AnyDict inner) =
AnyDict
{ toKey = inner.toKey
, dict = Dict.empty
}
-- Query
{-| Determine if a dictionary is empty.
isEmpty (empty identity)
--> True
singleton 1 "foo" identity
|> isEmpty
--> False
-}
isEmpty : AnyDict comparable k v -> Bool
isEmpty (AnyDict { dict }) =
Dict.isEmpty dict
{-| Determine if a key is in a dictionary.
-}
member : k -> AnyDict comparable k v -> Bool
member k (AnyDict { dict, toKey }) =
Dict.member (toKey k) dict
{-| Get the value associated with a key.
If the key is not found, return Nothing.
This is useful when you are not sure
if a key will be in the dictionary.
type Animal = Cat | Mouse | Dog
animalToInt : Animal -> Int
animalToInt animal =
case animal of
Cat -> 0
Mouse -> 1
Dog -> 2
animals : AnyDict Int Animal String
animals =
[ (Cat, "PI:NAME:<NAME>END_PI"), (Mouse, "PI:NAME:<NAME>END_PI") ]
|> fromList animalToInt
get Cat animals
-> Just "PI:NAME:<NAME>END_PI"
get Mouse animals
--> Just "PI:NAME:<NAME>END_PI"
get Dog animals
--> Nothing
-}
get : k -> AnyDict comparable k v -> Maybe v
get k (AnyDict { dict, toKey }) =
Dict.get (toKey k) dict
|> Maybe.map Tuple.second
{-| Get a key associated with key.
This is useful in case of `AnyDict` because
some parts of a key might not be used
for generating comparable.
This function allows quering `AnyDict` with old
key to obtain updated one in such cases.
-}
getKey : k -> AnyDict comparable k v -> Maybe k
getKey k (AnyDict { dict, toKey }) =
Dict.get (toKey k) dict
|> Maybe.map Tuple.first
{-| Determine the number of key-value pairs in the dictionary.
-}
size : AnyDict comparable k v -> Int
size (AnyDict { dict }) =
Dict.size dict
-- List
{-| Get all of the keys in a dictionary, sorted from lowest to highest.
-}
keys : AnyDict comparable k v -> List k
keys =
List.map Tuple.first << toList
{-| Get all of the values in a dictionary, in the order of their keys.
-}
values : AnyDict comparable k v -> List v
values =
List.map Tuple.second << toList
{-| Convert a dictionary into an association list of key-value pairs,
sorted by keys.
-}
toList : AnyDict comparable k v -> List ( k, v )
toList (AnyDict { dict }) =
Dict.values dict
{-| Convert an association list into a dictionary.
**Note that it's important to make sure every key is turned to different comparable.**
Otherwise keys would conflict and overwrite each other.
-}
fromList : (k -> comparable) -> List ( k, v ) -> AnyDict comparable k v
fromList f xs =
AnyDict
{ toKey = f
, dict = Dict.fromList <| List.map (\( k, v ) -> ( f k, ( k, v ) )) xs
}
-- Transform
{-| Apply a function to all values in a dictionary.
-}
map : (a -> b -> c) -> AnyDict comparable a b -> AnyDict comparable a c
map f (AnyDict { dict, toKey }) =
AnyDict
{ dict = Dict.map (\_ ( k, v ) -> ( k, f k v )) dict
, toKey = toKey
}
{-| Fold over the key-value pairs in a dictionary, in order from lowest key to highest key.
-}
foldl : (k -> v -> b -> b) -> b -> AnyDict comparable k v -> b
foldl f acc (AnyDict { dict }) =
Dict.foldl (\_ ( k, v ) -> f k v) acc dict
{-| Fold over the key-value pairs in a dictionary, in order from highest key to lowest key.
-}
foldr : (k -> v -> b -> b) -> b -> AnyDict comparable k v -> b
foldr f acc (AnyDict { dict }) =
Dict.foldr (\_ ( k, v ) -> f k v) acc dict
{-| Keep a key-value pair when it satisfies a predicate.
-}
filter : (k -> v -> Bool) -> AnyDict comparable k v -> AnyDict comparable k v
filter f (AnyDict inner) =
AnyDict { inner | dict = Dict.filter (\_ ( k, v ) -> f k v) inner.dict }
{-| Partition a dictionary according to a predicate.
The first dictionary contains all key-value pairs which satisfy the predicate,
and the second contains the rest.
-}
partition : (k -> v -> Bool) -> AnyDict comparable k v -> ( AnyDict comparable k v, AnyDict comparable k v )
partition f (AnyDict inner) =
let
( left, right ) =
Dict.partition (\_ ( k, v ) -> f k v) inner.dict
in
( AnyDict { inner | dict = left }
, AnyDict { inner | dict = right }
)
-- Combine
{-| Combine two dictionaries. If there is a collision, preference is given to the first dictionary.
-}
union : AnyDict comparable k v -> AnyDict comparable k v -> AnyDict comparable k v
union (AnyDict inner) (AnyDict { dict }) =
AnyDict { inner | dict = Dict.union inner.dict dict }
{-| Keep a key-value pair when its key appears in the second dictionary.
Preference is given to values in the first dictionary.
-}
intersect : AnyDict comparable k v -> AnyDict comparable k v -> AnyDict comparable k v
intersect (AnyDict inner) (AnyDict { dict }) =
AnyDict { inner | dict = Dict.intersect inner.dict dict }
{-| Keep a key-value pair when its key does not appear in the second dictionary.
-}
diff : AnyDict comparable k v -> AnyDict comparable k v -> AnyDict comparable k v
diff (AnyDict inner) (AnyDict { dict }) =
AnyDict { inner | dict = Dict.diff inner.dict dict }
{-| The most general way of combining two dictionaries.
You provide three accumulators for when a given key appears:
1. Only in the left dictionary.
2. In both dictionaries.
3. Only in the right dictionary.
Only in the left dictionary.
In both dictionaries.
Only in the right dictionary.
-}
merge :
(k -> a -> result -> result)
-> (k -> a -> b -> result -> result)
-> (k -> b -> result -> result)
-> AnyDict comparable k a
-> AnyDict comparable k b
-> result
-> result
merge f g h (AnyDict inner) (AnyDict { dict }) =
let
l fc _ ( k, v ) =
fc k v
in
Dict.merge (l f) (\_ ( k, a ) ( _, b ) -> g k a b) (l h) inner.dict dict
-- Dict
{-| Convert `AnyDict` to plain dictionary with comparable keys.
-}
toDict : AnyDict comparable k v -> Dict comparable v
toDict (AnyDict { dict }) =
Dict.map (always Tuple.second) dict
{-| Decode a JSON object into an `AnyDict`.
This encoder is limitted for cases where JSON representation
for a given type is an JSON Object. In JSON, object keys must be of type
`String`.
If you need to decode different representation into `AnyDict` value,
just use primitive `Decoder` types directly and map `AnyDict` constructors
over these.
import Json.Decode
type Key = Foo | Bar
fromString : String -> Key
fromString str =
case str of
"foo" -> Foo
_ -> Bar
toString : Key -> String
toString key =
case key of
Foo -> "foo"
Bar -> "bar"
type alias Data = AnyDict String Key Int
dataDecoder : Json.Decode.Decoder Data
dataDecoder =
decode (\str _ -> fromString str) toString Json.Decode.int
Json.Decode.decodeString dataDecoder "{\"foo\":1,\"bar\":2}"
|> Result.map toList
--> Ok [(Bar, 2), (Foo, 1)]
-}
decode : (String -> v -> k) -> (k -> comparable) -> Decode.Decoder v -> Decode.Decoder (AnyDict comparable k v)
decode fromStr toComparable valueD =
let
construct strK v acc =
insert (fromStr strK v) v acc
in
Decode.dict valueD
|> Decode.map (Dict.foldr construct (empty toComparable))
{-| Decode a JSON object into an `AnyDict`.
This variant of decode allows you to fail with error while parsing key from String.
In such case whole Dict decoding will fail.
import Json.Decode
type Key = Foo | Bar
fromString : String -> Result String Key
fromString str =
case str of
"foo" -> Ok Foo
"bar" -> Ok Bar
_ -> Err <| "Unknown key " ++ str
toString : Key -> String
toString key =
case key of
Foo -> "foo"
Bar -> "bar"
type alias Data = AnyDict String Key Int
dataDecoder : Json.Decode.Decoder Data
dataDecoder =
decode_ (\str _ -> fromString str) toString Json.Decode.int
Json.Decode.decodeString dataDecoder "{\"foo\":1,\"bar\":2}"
|> Result.map toList
--> Ok [(Bar, 2), (Foo, 1)]
Json.Decode.decodeString dataDecoder "{\"foo\":1,\"baz\":2}"
|> Result.map toList
--> Json.Decode.decodeString (Json.Decode.fail "Unknown key baz") "{}"
-}
decode_ : (String -> v -> Result String k) -> (k -> comparable) -> Decode.Decoder v -> Decode.Decoder (AnyDict comparable k v)
decode_ fromStr toComparable valueD =
let
construct strK v =
Result.andThen
(\acc ->
fromStr strK v
|> Result.map (\key -> insert key v acc)
)
in
Decode.dict valueD
|> Decode.map (Dict.foldr construct (Ok <| empty toComparable))
|> Decode.andThen
(\res ->
case res of
Ok val ->
Decode.succeed val
Err err ->
Decode.fail err
)
{-| Turn an `AnyDict` into a JSON object.
import Json.Encode
type Key = PI:KEY:<KEY>END_PI | Bar
toString : Key -> String
toString key =
case key of
Foo -> "foo"
Bar -> "bar"
type alias Data = AnyDict String Key Int
encodeData : Data -> Json.Encode.Value
encodeData =
encode toString Json.Encode.int
fromList toString [(Foo, 1), (Bar, 2)]
|> encodeData
|> Json.Encode.encode 0
--> "{\"bar\":2,\"foo\":1}"
-}
encode : (k -> String) -> (v -> Encode.Value) -> AnyDict comparable k v -> Encode.Value
encode keyE valueE =
Encode.object
<< List.map (Tuple.mapBoth keyE valueE)
<< toList
{-| Turn an AnyDict into a JSON list of tuples. This is useful when you have more complex types as keys
import Json.Decode as Decode
import Json.Encode as Encode
type alias Person = {first : String, last : String}
type alias Age = Int
personToString : Person -> String
personToString {first, last} = first ++ last
personEncode : Person -> Encode.Value
personEncode {first, last} =
Encode.object [("first", (Encode.string first)), ("last", (Encode.string last))]
example : AnyDict String Person Age
example =
fromList personToString [(Person "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI", 9001), (Person "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI", 1234)]
encodeList (\k v -> Encode.list identity [ personEncode k, Encode.int v ]) example
|> Encode.encode 0
--> "[[{\"first\":\"PI:NAME:<NAME>END_PI\",\"last\":\"PI:NAME:<NAME>END_PI\"},9001],[{\"first\":\"PI:NAME:<NAME>END_PI\",\"last\":\"PI:NAME:<NAME>END_PI\"},1234]]"
-}
encodeList : (k -> v -> Encode.Value) -> AnyDict comparable k v -> Encode.Value
encodeList encodeF =
Encode.list (\( k, v ) -> encodeF k v) << toList
{-| Decode an AnyDict from a JSON list of tuples.
import Json.Decode as Decode
import Json.Encode as Encode
type alias Person = {first : String, last : String}
type alias Age = Int
personToString : Person -> String
personToString {first, last} = first ++ last
personDecode : Decode.Decoder Person
personDecode =
Decode.map2
Person
(Decode.field "first" Decode.string)
(Decode.field "last" Decode.string)
"[[{\"first\":\"PI:NAME:<NAME>END_PI\",\"last\":\"PI:NAME:<NAME>END_PI\"},9001],[{\"first\":\"PI:NAME:<NAME>END_PI\",\"last\":\"PI:NAME:<NAME>END_PI\"},1234]]"
|> Decode.decodeString (decodeList personToString (Decode.map2 Tuple.pair (Decode.index 0 personDecode) (Decode.index 1 Decode.int)))
--> Ok (fromList personToString [(Person "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI", 9001), (Person "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI", 1234)])
-}
decodeList : (k -> comparable) -> Decode.Decoder ( k, v ) -> Decode.Decoder (AnyDict comparable k v)
decodeList keyToComparable =
Decode.map (fromList keyToComparable) << Decode.list
{-| Takes a key-fn and a list.
Creates an `AnyDict` which maps the key to a list of matching elements.
-}
groupBy : (value -> key) -> (key -> comparable) -> List value -> AnyDict comparable key (List value)
groupBy toKey keyToComparable list =
List.foldr
(\x acc ->
update
(toKey x)
(\maybeValues ->
maybeValues
|> Maybe.map ((::) x)
|> Maybe.withDefault [ x ]
|> Just
)
acc
)
(empty keyToComparable)
list
{-| Find out if there is any instance of something in a Dictionary.
type Animal = Cat | Mouse | Dog
animalToInt : Animal -> Int
animalToInt animal =
case animal of
Cat -> 0
Mouse -> 1
Dog -> 2
animals : AnyDict Int Animal String
animals =
[ (Cat, "PI:NAME:<NAME>END_PI"), (Mouse, "PI:NAME:<NAME>END_PI") ]
|> fromList animalToInt
isACat : Animal -> String -> Bool
isACat animal _ =
case animal of
Cat -> True
_ -> False
any isACat animals
--> True
-}
any : (k -> v -> Bool) -> AnyDict comparable k v -> Bool
any predicate dict =
foldl
(\k v acc -> acc || predicate k v)
False
dict
{-| Find if all key value paris in Dictionary match a predicate.
type Animal = Cat | Mouse | Dog
animalToInt : Animal -> Int
animalToInt animal =
case animal of
Cat -> 0
Mouse -> 1
Dog -> 2
animals : AnyDict Int Animal String
animals =
[ (Cat, "PI:NAME:<NAME>END_PI"), (Mouse, "PI:NAME:<NAME>END_PI") ]
|> fromList animalToInt
aristocats : AnyDict Int Animal String
aristocats =
[ (Cat, "PI:NAME:<NAME>END_PI") ]
|> fromList animalToInt
isACat : Animal -> String -> Bool
isACat animal _ =
case animal of
Cat -> True
_ -> False
all isACat animals
--> False
all isACat aristocats
--> True
-}
all : (k -> v -> Bool) -> AnyDict comparable k v -> Bool
all predicate dict =
foldl
(\k v acc -> acc && predicate k v)
True
dict
{-| Apply a function that may or may not succeed to all entries in a dictionary, but only keep the successes.
type Animal = Cat | Mouse | Dog
animalToInt : Animal -> Int
animalToInt animal =
case animal of
Cat -> 0
Mouse -> 1
Dog -> 2
animals : AnyDict Int Animal String
animals =
[ (Cat, "PI:NAME:<NAME>END_PI"), (Mouse, "PI:NAME:<NAME>END_PI") ]
|> fromList animalToInt
onlyTom : AnyDict Int Animal String
onlyTom =
[ (Cat, "PI:NAME:<NAME>END_PI") ]
|> fromList animalToInt
getCatName : Animal -> String -> Maybe String
getCatName animal name =
case animal of
Cat -> Just name
_ -> Nothing
filterMap getCatName animals == onlyTom
--> True
-}
filterMap : (k -> v1 -> Maybe v2) -> AnyDict comparable k v1 -> AnyDict comparable k v2
filterMap f dict =
foldl
(\k v acc ->
case f k v of
Just newVal ->
insert k newVal acc
Nothing ->
acc
)
(removeAll dict)
dict
| elm |
[
{
"context": " , autofocus True\n , placeholder \"Username\"\n ]\n []\n ",
"end": 2781,
"score": 0.9989962578,
"start": 2773,
"tag": "USERNAME",
"value": "Username"
},
{
"context": ", type_ \"password\"\n , placeholder \"Password\"\n ]\n []\n ",
"end": 2956,
"score": 0.9916075468,
"start": 2948,
"tag": "PASSWORD",
"value": "Password"
}
] | src/elm/Page/Login.elm | rl-king/tokonoma | 0 | module Page.Login exposing (Model, Msg, init, update, view)
import Browser.Navigation as Navigation
import Css exposing (..)
import Css.Breakpoint as Breakpoint
import Css.Global as Global exposing (global)
import Data.Auth as Auth exposing (Auth(..))
import Data.Request as Request
import Data.Resource as Resource exposing (Resource)
import Data.Session as Session
import Data.User exposing (User)
import Html.Styled exposing (..)
import Html.Styled.Attributes
exposing
( autofocus
, css
, disabled
, placeholder
, type_
)
import Html.Styled.Events exposing (on, onClick, onInput, onSubmit)
import Html.Styled.Keyed as Keyed
import Http
import Markdown
import Specification exposing (colors)
import Task
import Time
-- MODEL
type alias Model =
{ session : Session.Data
, redirectUrl : Maybe String
, username : String
, password : String
}
init : Session.Data -> Maybe String -> ( Model, Cmd msg )
init session redirectUrl =
let
redirect =
case Session.getAuth session of
Auth.Auth _ ->
Navigation.replaceUrl (Session.getNavKey session) "/"
_ ->
Cmd.none
in
( Model session redirectUrl "" ""
, redirect
)
-- UPDATE
type Msg
= OnUsernameInput String
| OnPasswordInput String
| PerformLogin
| GotPerformLogin (Result Http.Error User)
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
OnUsernameInput input ->
( { model | username = input }, Cmd.none )
OnPasswordInput input ->
( { model | password = input }, Cmd.none )
PerformLogin ->
( model
, Task.attempt GotPerformLogin <|
Request.postLogin model.username model.password
)
GotPerformLogin (Ok user) ->
let
url =
Maybe.withDefault "/" model.redirectUrl
in
( { model | session = Session.insertAuth (Auth user) model.session }
, Navigation.replaceUrl (Session.getNavKey model.session) url
)
GotPerformLogin (Err err) ->
( { model | session = Session.insertAuth Anonymous model.session }
, Cmd.none
)
-- VIEW
view : Model -> Html Msg
view model =
let
disable =
String.isEmpty model.password
|| String.isEmpty model.username
in
section [ css styling.login ]
[ h1 [] [ text "Tokonoma" ]
, Html.Styled.form [ onSubmit PerformLogin ]
[ input
[ onInput OnUsernameInput
, autofocus True
, placeholder "Username"
]
[]
, input
[ onInput OnPasswordInput
, type_ "password"
, placeholder "Password"
]
[]
, button [ disabled disable ] [ text "Login" ]
]
]
styling =
{ login =
[ backgroundColor colors.offwhite
, padding (rem 1.5)
, width (rem 25)
, margin2 (vh 25) auto
, Global.descendants
[ Global.h1 [ marginBottom (rem 2) ]
, Global.button
[ backgroundColor colors.black
, padding2 (rem 0.5) (rem 1)
, color colors.white
, width (pct 100)
, Css.disabled
[ color colors.greyLighter
, cursor notAllowed
]
]
]
]
}
| 51422 | module Page.Login exposing (Model, Msg, init, update, view)
import Browser.Navigation as Navigation
import Css exposing (..)
import Css.Breakpoint as Breakpoint
import Css.Global as Global exposing (global)
import Data.Auth as Auth exposing (Auth(..))
import Data.Request as Request
import Data.Resource as Resource exposing (Resource)
import Data.Session as Session
import Data.User exposing (User)
import Html.Styled exposing (..)
import Html.Styled.Attributes
exposing
( autofocus
, css
, disabled
, placeholder
, type_
)
import Html.Styled.Events exposing (on, onClick, onInput, onSubmit)
import Html.Styled.Keyed as Keyed
import Http
import Markdown
import Specification exposing (colors)
import Task
import Time
-- MODEL
type alias Model =
{ session : Session.Data
, redirectUrl : Maybe String
, username : String
, password : String
}
init : Session.Data -> Maybe String -> ( Model, Cmd msg )
init session redirectUrl =
let
redirect =
case Session.getAuth session of
Auth.Auth _ ->
Navigation.replaceUrl (Session.getNavKey session) "/"
_ ->
Cmd.none
in
( Model session redirectUrl "" ""
, redirect
)
-- UPDATE
type Msg
= OnUsernameInput String
| OnPasswordInput String
| PerformLogin
| GotPerformLogin (Result Http.Error User)
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
OnUsernameInput input ->
( { model | username = input }, Cmd.none )
OnPasswordInput input ->
( { model | password = input }, Cmd.none )
PerformLogin ->
( model
, Task.attempt GotPerformLogin <|
Request.postLogin model.username model.password
)
GotPerformLogin (Ok user) ->
let
url =
Maybe.withDefault "/" model.redirectUrl
in
( { model | session = Session.insertAuth (Auth user) model.session }
, Navigation.replaceUrl (Session.getNavKey model.session) url
)
GotPerformLogin (Err err) ->
( { model | session = Session.insertAuth Anonymous model.session }
, Cmd.none
)
-- VIEW
view : Model -> Html Msg
view model =
let
disable =
String.isEmpty model.password
|| String.isEmpty model.username
in
section [ css styling.login ]
[ h1 [] [ text "Tokonoma" ]
, Html.Styled.form [ onSubmit PerformLogin ]
[ input
[ onInput OnUsernameInput
, autofocus True
, placeholder "Username"
]
[]
, input
[ onInput OnPasswordInput
, type_ "password"
, placeholder "<PASSWORD>"
]
[]
, button [ disabled disable ] [ text "Login" ]
]
]
styling =
{ login =
[ backgroundColor colors.offwhite
, padding (rem 1.5)
, width (rem 25)
, margin2 (vh 25) auto
, Global.descendants
[ Global.h1 [ marginBottom (rem 2) ]
, Global.button
[ backgroundColor colors.black
, padding2 (rem 0.5) (rem 1)
, color colors.white
, width (pct 100)
, Css.disabled
[ color colors.greyLighter
, cursor notAllowed
]
]
]
]
}
| true | module Page.Login exposing (Model, Msg, init, update, view)
import Browser.Navigation as Navigation
import Css exposing (..)
import Css.Breakpoint as Breakpoint
import Css.Global as Global exposing (global)
import Data.Auth as Auth exposing (Auth(..))
import Data.Request as Request
import Data.Resource as Resource exposing (Resource)
import Data.Session as Session
import Data.User exposing (User)
import Html.Styled exposing (..)
import Html.Styled.Attributes
exposing
( autofocus
, css
, disabled
, placeholder
, type_
)
import Html.Styled.Events exposing (on, onClick, onInput, onSubmit)
import Html.Styled.Keyed as Keyed
import Http
import Markdown
import Specification exposing (colors)
import Task
import Time
-- MODEL
type alias Model =
{ session : Session.Data
, redirectUrl : Maybe String
, username : String
, password : String
}
init : Session.Data -> Maybe String -> ( Model, Cmd msg )
init session redirectUrl =
let
redirect =
case Session.getAuth session of
Auth.Auth _ ->
Navigation.replaceUrl (Session.getNavKey session) "/"
_ ->
Cmd.none
in
( Model session redirectUrl "" ""
, redirect
)
-- UPDATE
type Msg
= OnUsernameInput String
| OnPasswordInput String
| PerformLogin
| GotPerformLogin (Result Http.Error User)
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
OnUsernameInput input ->
( { model | username = input }, Cmd.none )
OnPasswordInput input ->
( { model | password = input }, Cmd.none )
PerformLogin ->
( model
, Task.attempt GotPerformLogin <|
Request.postLogin model.username model.password
)
GotPerformLogin (Ok user) ->
let
url =
Maybe.withDefault "/" model.redirectUrl
in
( { model | session = Session.insertAuth (Auth user) model.session }
, Navigation.replaceUrl (Session.getNavKey model.session) url
)
GotPerformLogin (Err err) ->
( { model | session = Session.insertAuth Anonymous model.session }
, Cmd.none
)
-- VIEW
view : Model -> Html Msg
view model =
let
disable =
String.isEmpty model.password
|| String.isEmpty model.username
in
section [ css styling.login ]
[ h1 [] [ text "Tokonoma" ]
, Html.Styled.form [ onSubmit PerformLogin ]
[ input
[ onInput OnUsernameInput
, autofocus True
, placeholder "Username"
]
[]
, input
[ onInput OnPasswordInput
, type_ "password"
, placeholder "PI:PASSWORD:<PASSWORD>END_PI"
]
[]
, button [ disabled disable ] [ text "Login" ]
]
]
styling =
{ login =
[ backgroundColor colors.offwhite
, padding (rem 1.5)
, width (rem 25)
, margin2 (vh 25) auto
, Global.descendants
[ Global.h1 [ marginBottom (rem 2) ]
, Global.button
[ backgroundColor colors.black
, padding2 (rem 0.5) (rem 1)
, color colors.white
, width (pct 100)
, Css.disabled
[ color colors.greyLighter
, cursor notAllowed
]
]
]
]
}
| elm |
[
{
"context": " Encode.object\n [ ( \"username\", Encode.string model.username )\n ",
"end": 617,
"score": 0.6707237363,
"start": 609,
"tag": "USERNAME",
"value": "username"
},
{
"context": "dated username ->\n { model | username = username } ! []\n\n PasswordUpdated password ->\n ",
"end": 2067,
"score": 0.9716808796,
"start": 2059,
"tag": "USERNAME",
"value": "username"
},
{
"context": "dated password ->\n { model | password = password } ! []\n",
"end": 2153,
"score": 0.93659693,
"start": 2145,
"tag": "PASSWORD",
"value": "password"
}
] | episode-038/web/static/elm/Main.elm | thaterikperson/elmseeds | 84 | module Main exposing (..)
import Http
import HttpBuilder
import Html
import Json.Decode as Decode
import Json.Encode as Encode
import Model exposing (..)
import Task
import View
import Window
main : Program Never Model Msg
main =
Html.program
{ init = ( initialModel, Cmd.none )
, update = update
, view = View.mainView
, subscriptions = (\_ -> Sub.none)
}
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
Signin ->
let
jsonBody =
Encode.object
[ ( "username", Encode.string model.username )
, ( "password", Encode.string model.password )
]
builderCmd =
HttpBuilder.post "http://localhost:4000/signin"
|> HttpBuilder.withHeader "X-CSRF-TOKEN" model.token
|> HttpBuilder.withJsonBody jsonBody
|> HttpBuilder.withQueryParams [ ( "timestamp", (toString model.timestamp) ) ]
|> HttpBuilder.withExpect (Http.expectJson Decode.int)
|> HttpBuilder.send SigninCompleted
saveCmd =
Http.request
{ method = "POST"
, headers = [ Http.header "X-CSRF-TOKEN" model.token ]
, url = "http://localhost:4000/signin?timestamp=" ++ (toString model.timestamp)
, body = Http.jsonBody jsonBody
, expect = Http.expectJson Decode.int
, timeout = Nothing
, withCredentials = False
}
|> Http.send SigninCompleted
in
model ! [ saveCmd, builderCmd ]
SigninCompleted (Ok data) ->
model ! []
SigninCompleted (Err error) ->
model ! []
UsernameUpdated username ->
{ model | username = username } ! []
PasswordUpdated password ->
{ model | password = password } ! []
| 60061 | module Main exposing (..)
import Http
import HttpBuilder
import Html
import Json.Decode as Decode
import Json.Encode as Encode
import Model exposing (..)
import Task
import View
import Window
main : Program Never Model Msg
main =
Html.program
{ init = ( initialModel, Cmd.none )
, update = update
, view = View.mainView
, subscriptions = (\_ -> Sub.none)
}
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
Signin ->
let
jsonBody =
Encode.object
[ ( "username", Encode.string model.username )
, ( "password", Encode.string model.password )
]
builderCmd =
HttpBuilder.post "http://localhost:4000/signin"
|> HttpBuilder.withHeader "X-CSRF-TOKEN" model.token
|> HttpBuilder.withJsonBody jsonBody
|> HttpBuilder.withQueryParams [ ( "timestamp", (toString model.timestamp) ) ]
|> HttpBuilder.withExpect (Http.expectJson Decode.int)
|> HttpBuilder.send SigninCompleted
saveCmd =
Http.request
{ method = "POST"
, headers = [ Http.header "X-CSRF-TOKEN" model.token ]
, url = "http://localhost:4000/signin?timestamp=" ++ (toString model.timestamp)
, body = Http.jsonBody jsonBody
, expect = Http.expectJson Decode.int
, timeout = Nothing
, withCredentials = False
}
|> Http.send SigninCompleted
in
model ! [ saveCmd, builderCmd ]
SigninCompleted (Ok data) ->
model ! []
SigninCompleted (Err error) ->
model ! []
UsernameUpdated username ->
{ model | username = username } ! []
PasswordUpdated password ->
{ model | password = <PASSWORD> } ! []
| true | module Main exposing (..)
import Http
import HttpBuilder
import Html
import Json.Decode as Decode
import Json.Encode as Encode
import Model exposing (..)
import Task
import View
import Window
main : Program Never Model Msg
main =
Html.program
{ init = ( initialModel, Cmd.none )
, update = update
, view = View.mainView
, subscriptions = (\_ -> Sub.none)
}
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
Signin ->
let
jsonBody =
Encode.object
[ ( "username", Encode.string model.username )
, ( "password", Encode.string model.password )
]
builderCmd =
HttpBuilder.post "http://localhost:4000/signin"
|> HttpBuilder.withHeader "X-CSRF-TOKEN" model.token
|> HttpBuilder.withJsonBody jsonBody
|> HttpBuilder.withQueryParams [ ( "timestamp", (toString model.timestamp) ) ]
|> HttpBuilder.withExpect (Http.expectJson Decode.int)
|> HttpBuilder.send SigninCompleted
saveCmd =
Http.request
{ method = "POST"
, headers = [ Http.header "X-CSRF-TOKEN" model.token ]
, url = "http://localhost:4000/signin?timestamp=" ++ (toString model.timestamp)
, body = Http.jsonBody jsonBody
, expect = Http.expectJson Decode.int
, timeout = Nothing
, withCredentials = False
}
|> Http.send SigninCompleted
in
model ! [ saveCmd, builderCmd ]
SigninCompleted (Ok data) ->
model ! []
SigninCompleted (Err error) ->
model ! []
UsernameUpdated username ->
{ model | username = username } ! []
PasswordUpdated password ->
{ model | password = PI:PASSWORD:<PASSWORD>END_PI } ! []
| elm |
[
{
"context": "{-\n Swaggy Jenkins\n Jenkins API clients generated from Swagger / O",
"end": 20,
"score": 0.999653995,
"start": 6,
"tag": "NAME",
"value": "Swaggy Jenkins"
},
{
"context": "cation\n\n OpenAPI spec version: 1.1.1\n Contact: blah@cliffano.com\n\n NOTE: This file is auto generated by the open",
"end": 153,
"score": 0.999912858,
"start": 136,
"tag": "EMAIL",
"value": "blah@cliffano.com"
},
{
"context": "d by the openapi-generator.\n https://github.com/openapitools/openapi-generator.git\n Do not edit this file ma",
"end": 252,
"score": 0.9996265769,
"start": 240,
"tag": "USERNAME",
"value": "openapitools"
}
] | clients/elm/generated/src/Data/Hudson.elm | PankTrue/swaggy-jenkins | 23 | {-
Swaggy Jenkins
Jenkins API clients generated from Swagger / Open API specification
OpenAPI spec version: 1.1.1
Contact: blah@cliffano.com
NOTE: This file is auto generated by the openapi-generator.
https://github.com/openapitools/openapi-generator.git
Do not edit this file manually.
-}
module Data.Hudson exposing (Hudson, hudsonDecoder, hudsonEncoder)
import Data.HudsonassignedLabels exposing (HudsonassignedLabels, hudsonassignedLabelsDecoder, hudsonassignedLabelsEncoder)
import Data.FreeStyleProject exposing (FreeStyleProject, freeStyleProjectDecoder, freeStyleProjectEncoder)
import Data.AllView exposing (AllView, allViewDecoder, allViewEncoder)
import Data.UnlabeledLoadStatistics exposing (UnlabeledLoadStatistics, unlabeledLoadStatisticsDecoder, unlabeledLoadStatisticsEncoder)
import Data.AllView exposing (AllView, allViewDecoder, allViewEncoder)
import Json.Decode as Decode exposing (Decoder)
import Json.Decode.Pipeline exposing (decode, optional, required)
import Json.Encode as Encode
import Maybe exposing (map, withDefault)
type alias Hudson =
{ class : Maybe String
, assignedLabels : Maybe (List HudsonassignedLabels)
, mode : Maybe String
, nodeDescription : Maybe String
, nodeName : Maybe String
, numExecutors : Maybe Int
, description : Maybe String
, jobs : Maybe (List FreeStyleProject)
, primaryView : Maybe AllView
, quietingDown : Maybe Bool
, slaveAgentPort : Maybe Int
, unlabeledLoad : Maybe UnlabeledLoadStatistics
, useCrumbs : Maybe Bool
, useSecurity : Maybe Bool
, views : Maybe (List AllView)
}
hudsonDecoder : Decoder Hudson
hudsonDecoder =
decode Hudson
|> optional "_class" (Decode.nullable Decode.string) Nothing
|> optional "assignedLabels" (Decode.nullable (Decode.list hudsonassignedLabelsDecoder)) Nothing
|> optional "mode" (Decode.nullable Decode.string) Nothing
|> optional "nodeDescription" (Decode.nullable Decode.string) Nothing
|> optional "nodeName" (Decode.nullable Decode.string) Nothing
|> optional "numExecutors" (Decode.nullable Decode.int) Nothing
|> optional "description" (Decode.nullable Decode.string) Nothing
|> optional "jobs" (Decode.nullable (Decode.list freeStyleProjectDecoder)) Nothing
|> optional "primaryView" (Decode.nullable allViewDecoder) Nothing
|> optional "quietingDown" (Decode.nullable Decode.bool) Nothing
|> optional "slaveAgentPort" (Decode.nullable Decode.int) Nothing
|> optional "unlabeledLoad" (Decode.nullable unlabeledLoadStatisticsDecoder) Nothing
|> optional "useCrumbs" (Decode.nullable Decode.bool) Nothing
|> optional "useSecurity" (Decode.nullable Decode.bool) Nothing
|> optional "views" (Decode.nullable (Decode.list allViewDecoder)) Nothing
hudsonEncoder : Hudson -> Encode.Value
hudsonEncoder model =
Encode.object
[ ( "_class", withDefault Encode.null (map Encode.string model.class) )
, ( "assignedLabels", withDefault Encode.null (map (Encode.list << List.map hudsonassignedLabelsEncoder) model.assignedLabels) )
, ( "mode", withDefault Encode.null (map Encode.string model.mode) )
, ( "nodeDescription", withDefault Encode.null (map Encode.string model.nodeDescription) )
, ( "nodeName", withDefault Encode.null (map Encode.string model.nodeName) )
, ( "numExecutors", withDefault Encode.null (map Encode.int model.numExecutors) )
, ( "description", withDefault Encode.null (map Encode.string model.description) )
, ( "jobs", withDefault Encode.null (map (Encode.list << List.map freeStyleProjectEncoder) model.jobs) )
, ( "primaryView", withDefault Encode.null (map allViewEncoder model.primaryView) )
, ( "quietingDown", withDefault Encode.null (map Encode.bool model.quietingDown) )
, ( "slaveAgentPort", withDefault Encode.null (map Encode.int model.slaveAgentPort) )
, ( "unlabeledLoad", withDefault Encode.null (map unlabeledLoadStatisticsEncoder model.unlabeledLoad) )
, ( "useCrumbs", withDefault Encode.null (map Encode.bool model.useCrumbs) )
, ( "useSecurity", withDefault Encode.null (map Encode.bool model.useSecurity) )
, ( "views", withDefault Encode.null (map (Encode.list << List.map allViewEncoder) model.views) )
]
| 14735 | {-
<NAME>
Jenkins API clients generated from Swagger / Open API specification
OpenAPI spec version: 1.1.1
Contact: <EMAIL>
NOTE: This file is auto generated by the openapi-generator.
https://github.com/openapitools/openapi-generator.git
Do not edit this file manually.
-}
module Data.Hudson exposing (Hudson, hudsonDecoder, hudsonEncoder)
import Data.HudsonassignedLabels exposing (HudsonassignedLabels, hudsonassignedLabelsDecoder, hudsonassignedLabelsEncoder)
import Data.FreeStyleProject exposing (FreeStyleProject, freeStyleProjectDecoder, freeStyleProjectEncoder)
import Data.AllView exposing (AllView, allViewDecoder, allViewEncoder)
import Data.UnlabeledLoadStatistics exposing (UnlabeledLoadStatistics, unlabeledLoadStatisticsDecoder, unlabeledLoadStatisticsEncoder)
import Data.AllView exposing (AllView, allViewDecoder, allViewEncoder)
import Json.Decode as Decode exposing (Decoder)
import Json.Decode.Pipeline exposing (decode, optional, required)
import Json.Encode as Encode
import Maybe exposing (map, withDefault)
type alias Hudson =
{ class : Maybe String
, assignedLabels : Maybe (List HudsonassignedLabels)
, mode : Maybe String
, nodeDescription : Maybe String
, nodeName : Maybe String
, numExecutors : Maybe Int
, description : Maybe String
, jobs : Maybe (List FreeStyleProject)
, primaryView : Maybe AllView
, quietingDown : Maybe Bool
, slaveAgentPort : Maybe Int
, unlabeledLoad : Maybe UnlabeledLoadStatistics
, useCrumbs : Maybe Bool
, useSecurity : Maybe Bool
, views : Maybe (List AllView)
}
hudsonDecoder : Decoder Hudson
hudsonDecoder =
decode Hudson
|> optional "_class" (Decode.nullable Decode.string) Nothing
|> optional "assignedLabels" (Decode.nullable (Decode.list hudsonassignedLabelsDecoder)) Nothing
|> optional "mode" (Decode.nullable Decode.string) Nothing
|> optional "nodeDescription" (Decode.nullable Decode.string) Nothing
|> optional "nodeName" (Decode.nullable Decode.string) Nothing
|> optional "numExecutors" (Decode.nullable Decode.int) Nothing
|> optional "description" (Decode.nullable Decode.string) Nothing
|> optional "jobs" (Decode.nullable (Decode.list freeStyleProjectDecoder)) Nothing
|> optional "primaryView" (Decode.nullable allViewDecoder) Nothing
|> optional "quietingDown" (Decode.nullable Decode.bool) Nothing
|> optional "slaveAgentPort" (Decode.nullable Decode.int) Nothing
|> optional "unlabeledLoad" (Decode.nullable unlabeledLoadStatisticsDecoder) Nothing
|> optional "useCrumbs" (Decode.nullable Decode.bool) Nothing
|> optional "useSecurity" (Decode.nullable Decode.bool) Nothing
|> optional "views" (Decode.nullable (Decode.list allViewDecoder)) Nothing
hudsonEncoder : Hudson -> Encode.Value
hudsonEncoder model =
Encode.object
[ ( "_class", withDefault Encode.null (map Encode.string model.class) )
, ( "assignedLabels", withDefault Encode.null (map (Encode.list << List.map hudsonassignedLabelsEncoder) model.assignedLabels) )
, ( "mode", withDefault Encode.null (map Encode.string model.mode) )
, ( "nodeDescription", withDefault Encode.null (map Encode.string model.nodeDescription) )
, ( "nodeName", withDefault Encode.null (map Encode.string model.nodeName) )
, ( "numExecutors", withDefault Encode.null (map Encode.int model.numExecutors) )
, ( "description", withDefault Encode.null (map Encode.string model.description) )
, ( "jobs", withDefault Encode.null (map (Encode.list << List.map freeStyleProjectEncoder) model.jobs) )
, ( "primaryView", withDefault Encode.null (map allViewEncoder model.primaryView) )
, ( "quietingDown", withDefault Encode.null (map Encode.bool model.quietingDown) )
, ( "slaveAgentPort", withDefault Encode.null (map Encode.int model.slaveAgentPort) )
, ( "unlabeledLoad", withDefault Encode.null (map unlabeledLoadStatisticsEncoder model.unlabeledLoad) )
, ( "useCrumbs", withDefault Encode.null (map Encode.bool model.useCrumbs) )
, ( "useSecurity", withDefault Encode.null (map Encode.bool model.useSecurity) )
, ( "views", withDefault Encode.null (map (Encode.list << List.map allViewEncoder) model.views) )
]
| true | {-
PI:NAME:<NAME>END_PI
Jenkins API clients generated from Swagger / Open API specification
OpenAPI spec version: 1.1.1
Contact: PI:EMAIL:<EMAIL>END_PI
NOTE: This file is auto generated by the openapi-generator.
https://github.com/openapitools/openapi-generator.git
Do not edit this file manually.
-}
module Data.Hudson exposing (Hudson, hudsonDecoder, hudsonEncoder)
import Data.HudsonassignedLabels exposing (HudsonassignedLabels, hudsonassignedLabelsDecoder, hudsonassignedLabelsEncoder)
import Data.FreeStyleProject exposing (FreeStyleProject, freeStyleProjectDecoder, freeStyleProjectEncoder)
import Data.AllView exposing (AllView, allViewDecoder, allViewEncoder)
import Data.UnlabeledLoadStatistics exposing (UnlabeledLoadStatistics, unlabeledLoadStatisticsDecoder, unlabeledLoadStatisticsEncoder)
import Data.AllView exposing (AllView, allViewDecoder, allViewEncoder)
import Json.Decode as Decode exposing (Decoder)
import Json.Decode.Pipeline exposing (decode, optional, required)
import Json.Encode as Encode
import Maybe exposing (map, withDefault)
type alias Hudson =
{ class : Maybe String
, assignedLabels : Maybe (List HudsonassignedLabels)
, mode : Maybe String
, nodeDescription : Maybe String
, nodeName : Maybe String
, numExecutors : Maybe Int
, description : Maybe String
, jobs : Maybe (List FreeStyleProject)
, primaryView : Maybe AllView
, quietingDown : Maybe Bool
, slaveAgentPort : Maybe Int
, unlabeledLoad : Maybe UnlabeledLoadStatistics
, useCrumbs : Maybe Bool
, useSecurity : Maybe Bool
, views : Maybe (List AllView)
}
hudsonDecoder : Decoder Hudson
hudsonDecoder =
decode Hudson
|> optional "_class" (Decode.nullable Decode.string) Nothing
|> optional "assignedLabels" (Decode.nullable (Decode.list hudsonassignedLabelsDecoder)) Nothing
|> optional "mode" (Decode.nullable Decode.string) Nothing
|> optional "nodeDescription" (Decode.nullable Decode.string) Nothing
|> optional "nodeName" (Decode.nullable Decode.string) Nothing
|> optional "numExecutors" (Decode.nullable Decode.int) Nothing
|> optional "description" (Decode.nullable Decode.string) Nothing
|> optional "jobs" (Decode.nullable (Decode.list freeStyleProjectDecoder)) Nothing
|> optional "primaryView" (Decode.nullable allViewDecoder) Nothing
|> optional "quietingDown" (Decode.nullable Decode.bool) Nothing
|> optional "slaveAgentPort" (Decode.nullable Decode.int) Nothing
|> optional "unlabeledLoad" (Decode.nullable unlabeledLoadStatisticsDecoder) Nothing
|> optional "useCrumbs" (Decode.nullable Decode.bool) Nothing
|> optional "useSecurity" (Decode.nullable Decode.bool) Nothing
|> optional "views" (Decode.nullable (Decode.list allViewDecoder)) Nothing
hudsonEncoder : Hudson -> Encode.Value
hudsonEncoder model =
Encode.object
[ ( "_class", withDefault Encode.null (map Encode.string model.class) )
, ( "assignedLabels", withDefault Encode.null (map (Encode.list << List.map hudsonassignedLabelsEncoder) model.assignedLabels) )
, ( "mode", withDefault Encode.null (map Encode.string model.mode) )
, ( "nodeDescription", withDefault Encode.null (map Encode.string model.nodeDescription) )
, ( "nodeName", withDefault Encode.null (map Encode.string model.nodeName) )
, ( "numExecutors", withDefault Encode.null (map Encode.int model.numExecutors) )
, ( "description", withDefault Encode.null (map Encode.string model.description) )
, ( "jobs", withDefault Encode.null (map (Encode.list << List.map freeStyleProjectEncoder) model.jobs) )
, ( "primaryView", withDefault Encode.null (map allViewEncoder model.primaryView) )
, ( "quietingDown", withDefault Encode.null (map Encode.bool model.quietingDown) )
, ( "slaveAgentPort", withDefault Encode.null (map Encode.int model.slaveAgentPort) )
, ( "unlabeledLoad", withDefault Encode.null (map unlabeledLoadStatisticsEncoder model.unlabeledLoad) )
, ( "useCrumbs", withDefault Encode.null (map Encode.bool model.useCrumbs) )
, ( "useSecurity", withDefault Encode.null (map Encode.bool model.useSecurity) )
, ( "views", withDefault Encode.null (map (Encode.list << List.map allViewEncoder) model.views) )
]
| elm |
[
{
"context": " ->\n { title = x.title\n , author = x.author\n , article = x.article\n , vote = x.",
"end": 7039,
"score": 0.7050427794,
"start": 7033,
"tag": "USERNAME",
"value": "author"
},
{
"context": "hen\n { title = x.title\n , author = x.author\n , article = x.article\n , vote = x.",
"end": 8536,
"score": 0.6023058891,
"start": 8530,
"tag": "USERNAME",
"value": "author"
},
{
"context": "\n [ a\n [ href \"https://github.com/HaroldLeo\" ]\n [ text \"Written by Hongji Liu\" ]\n ",
"end": 10932,
"score": 0.9995158911,
"start": 10923,
"tag": "USERNAME",
"value": "HaroldLeo"
},
{
"context": "thub.com/HaroldLeo\" ]\n [ text \"Written by Hongji Liu\" ]\n ]\n ]\n\nviewArticle : List String -",
"end": 10975,
"score": 0.9998182058,
"start": 10965,
"tag": "NAME",
"value": "Hongji Liu"
},
{
"context": " [ type_ \"text\"\n , placeholder \"who are you\"\n , autofocus True\n ",
"end": 14899,
"score": 0.786262989,
"start": 14888,
"tag": "NAME",
"value": "who are you"
}
] | src/TreeHole.elm | HaroldLeo/elm-tree-hole | 0 | module TreeHole exposing (..)
-- Add/modify imports if you'd like. ------------------------------------------
import Browser
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick, onInput)
import Http
import Json.Decode as Decode exposing (Decoder, field, succeed)
import Json.Encode as Encode
import Json.Decode.Pipeline as DecodePipeline
-- Main -----------------------------------------------------------------------
main : Program Flags Model Msg
main =
Browser.element
{ init = init
, view = view
, update = update
, subscriptions = \_ -> Sub.none
}
-- Model ----------------------------------------------------------------------
type PostState = Posting | None
type alias Comment =
{ postId : Int
, author : String
, content : String
, id : Int
}
type alias Post =
{ title : String
, author : String
, article : String
, vote : Int
, comments : List Comment
, clicked : Bool
, newCommentAuthor : String
, newCommentContent : String
, id : Int
}
type alias Model =
{ posts : List Post
, comments : List Comment
, newTitle : String
, newAuthor : String
, newArticle : String
, postState : PostState
, newComPostId : Int
}
type alias Flags = ()
init : Flags -> (Model, Cmd Msg)
init () =
(initModel, getPosts)
initModel : Model
initModel =
{ posts = []
, comments = []
, newTitle = ""
, newAuthor = ""
, newArticle = ""
, postState = None
, newComPostId = 0
}
-- Update ---------------------------------------------------------------------
type Msg = NewPost (Result Http.Error Post)
| Upload
| GetPost (Result Http.Error (List Post))
| NewTitle String
| NewAuthor String
| NewArticle String
| Upvote Int
| Downvote Int
| VoteUpdated (Result Http.Error Post)
| ChangePostState PostState
| ChangeCommentState Int Bool
| GetComment (Result Http.Error (List Comment))
| NewCommentAuthor String
| NewCommentContent String
| CommentUpdated (Result Http.Error Comment)
| SendComment Int
update : Msg -> Model -> (Model, Cmd Msg)
update msg model =
case msg of
Upload ->
(model, sendPost model)
NewPost (Ok score) ->
({ model | newTitle = ""
, newAuthor = ""
, newArticle = ""
, postState = None}, getPosts)
NewPost (Err error) ->
let
_ = Debug.log "Cannot post a new post" error
in
(model, Cmd.none)
GetPost (Ok lists) ->
({ model | posts = List.reverse (List.sortBy .id lists)}, Cmd.none)
GetPost (Err error) ->
let
_ = Debug.log "cannoot request posts" error
in
(model, Cmd.none)
NewTitle t ->
({ model | newTitle = t }, Cmd.none)
NewAuthor a ->
({ model | newAuthor = a }, Cmd.none)
NewArticle ar ->
({ model | newArticle = ar }, Cmd.none)
Upvote id ->
case (findPostById id model.posts) of
Just post -> (model, updatePost (upvote post))
Nothing -> (model, Cmd.none)
Downvote id ->
case (findPostById id model.posts) of
Just post -> (model, updatePost (downvote post))
Nothing -> (model, Cmd.none)
VoteUpdated _ ->
(model, getPosts)
ChangePostState state ->
({ model | postState = state}, Cmd.none)
ChangeCommentState id state ->
case state of
True ->
({ model | posts = updateState model.posts id state
, newComPostId = id}, getComments)
False ->
({ model | posts = updateState model.posts id state
, newComPostId = 0}, getComments)
GetComment (Ok lists) ->
({ model | comments = List.reverse (List.sortBy .postId lists)
, posts = distributor model.posts lists}, Cmd.none)
GetComment (Err error) ->
let
_ = Debug.log "cannoot request comments" error
in
(model, Cmd.none)
NewCommentAuthor author ->
({ model | posts = updateComAuthor model.posts model.newComPostId author }
, Cmd.none)
NewCommentContent content ->
({ model | posts =
updateComContent model.posts model.newComPostId content }
, Cmd.none)
CommentUpdated _ ->
(model, getComments)
SendComment id ->
case (findPostById id model.posts) of
Just post ->
({ model | posts = sendingCom model.posts model.newComPostId }
, sendComment post)
Nothing -> (model, Cmd.none)
-- Posts
postsURL : String
postsURL = "http://localhost:3000/posts/"
getPosts : Cmd Msg
getPosts =
(Decode.list postDecoder)
|> Http.get postsURL
|> Http.send GetPost
postDecoder : Decoder Post
postDecoder =
Decode.succeed Post
|> DecodePipeline.required "title" Decode.string
|> DecodePipeline.required "author" Decode.string
|> DecodePipeline.required "article" Decode.string
|> DecodePipeline.required "vote" Decode.int
|> DecodePipeline.hardcoded []
|> DecodePipeline.hardcoded False
|> DecodePipeline.hardcoded ""
|> DecodePipeline.hardcoded ""
|> DecodePipeline.required "id" Decode.int
encodePost : Model -> Encode.Value
encodePost model =
Encode.object
[ ("title", Encode.string model.newTitle)
, ("author", Encode.string model.newAuthor)
, ("article", Encode.string model.newArticle)
, ("vote", Encode.int 0)
]
postEncoder : Post -> Encode.Value
postEncoder post =
Encode.object
[ ("title", Encode.string post.title)
, ("author", Encode.string post.author)
, ("article", Encode.string post.article)
, ("vote", Encode.int post.vote)
]
sendPost : Model -> Cmd Msg
sendPost model =
let
body =
encodePost model
|> Http.jsonBody
request =
Http.post postsURL body postDecoder
in
Http.send NewPost request
-- Comments
commentsURL : String
commentsURL = "http://localhost:3000/comments"
getComments : Cmd Msg
getComments =
(Decode.list commentDecoder)
|> Http.get commentsURL
|> Http.send GetComment
commentDecoder : Decoder Comment
commentDecoder =
Decode.map4 Comment
(field "postId" Decode.int)
(field "author" Decode.string)
(field "content" Decode.string)
(field "id" Decode.int)
encodeComment : Post -> Encode.Value
encodeComment post =
Encode.object
[ ("postId", Encode.int post.id)
, ("author", Encode.string post.newCommentAuthor)
, ("content", Encode.string post.newCommentContent)
]
sendComment : Post -> Cmd Msg
sendComment post =
let
body =
encodeComment post
|> Http.jsonBody
request =
Http.post commentsURL body commentDecoder
in
Http.send CommentUpdated request
distributor : List Post -> List Comment -> List Post
distributor posts comments =
case posts of
[] -> []
x :: xs ->
{ title = x.title
, author = x.author
, article = x.article
, vote = x.vote
, comments = List.filter (\c -> c.postId == x.id) comments
, clicked = x.clicked
, newCommentAuthor = x.newCommentAuthor
, newCommentContent = x.newCommentContent
, id = x.id } :: (distributor xs comments)
updateState : List Post -> Int -> Bool -> List Post
updateState posts id state =
case posts of
[] -> []
x :: xs ->
if x.id == id then
{ title = x.title
, author = x.author
, article = x.article
, vote = x.vote
, comments = x.comments
, clicked = state
, newCommentAuthor = x.newCommentAuthor
, newCommentContent = x.newCommentContent
, id = x.id } :: xs
else x :: (updateState xs id state)
updateComAuthor : List Post -> Int -> String -> List Post
updateComAuthor posts id author =
case posts of
[] -> []
x :: xs ->
if x.id == id then
{ title = x.title
, author = x.author
, article = x.article
, vote = x.vote
, comments = x.comments
, clicked = x.clicked
, newCommentAuthor = author
, newCommentContent = x.newCommentContent
, id = x.id } :: xs
else x :: (updateComAuthor xs id author)
updateComContent : List Post -> Int -> String -> List Post
updateComContent posts id content =
case posts of
[] -> []
x :: xs ->
if x.id == id then
{ title = x.title
, author = x.author
, article = x.article
, vote = x.vote
, comments = x.comments
, clicked = x.clicked
, newCommentAuthor = x.newCommentAuthor
, newCommentContent = content
, id = x.id } :: xs
else x :: (updateComContent xs id content)
sendingCom : List Post -> Int -> List Post
sendingCom posts id =
case posts of
[] -> []
x :: xs ->
if x.id == id then
{ title = x.title
, author = x.author
, article = x.article
, vote = x.vote
, comments = x.comments
, clicked = x.clicked
, newCommentAuthor = ""
, newCommentContent = ""
, id = x.id } :: xs
else x :: (sendingCom xs id)
-- Upvotes/Downvotes
findPostById : Int -> List Post -> Maybe Post
findPostById id posts =
case posts
|> List.filter (\post -> post.id == id)
|> List.head of
Just post -> Just post
Nothing -> Nothing
upvote : Post -> Post
upvote post =
{ title = post.title
, author = post.author
, article = post.article
, vote = post.vote + 1
, comments = post.comments
, clicked = post.clicked
, newCommentAuthor = post.newCommentAuthor
, newCommentContent = post.newCommentContent
, id = post.id }
downvote : Post -> Post
downvote post =
{ title = post.title
, author = post.author
, article = post.article
, vote = post.vote - 1
, comments = post.comments
, clicked = post.clicked
, newCommentAuthor = post.newCommentAuthor
, newCommentContent = post.newCommentContent
, id = post.id }
updatePost : Post -> Cmd Msg
updatePost post =
let
body =
postEncoder post
|> Http.jsonBody
request =
Http.request
{ method = "PATCH"
, headers = []
, url = postsURL ++ (Debug.toString post.id)
, body = body
, expect = Http.expectJson postDecoder
, timeout = Nothing
, withCredentials = False
}
in
Http.send VoteUpdated request
-- View -----------------------------------------------------------------------
viewHeader : String -> Html Msg
viewHeader title =
header [ class "header" ]
[ h1 [] [ text title ]
, h4 [] [ text "...where you can put your secrets in *shh*" ]
]
viewFooter : Html Msg
viewFooter =
div []
[ footer []
[ a
[ href "https://github.com/HaroldLeo" ]
[ text "Written by Hongji Liu" ]
]
]
viewArticle : List String -> List (Html Msg)
viewArticle list =
case list of
[] -> []
first :: rest ->
(p [ class "article" ] [ text first ]) :: viewArticle rest
viewVote : Post -> List (Html Msg)
viewVote post =
[ div [ class "vote" ]
[ button [ class "upvote", onClick (Upvote post.id)]
[ text "⇧" ]
, h5 [ class "vote-num" ]
[ text (String.fromInt post.vote) ]
, button [ class "downvote", onClick (Downvote post.id)]
[ text "⇩" ]
]]
viewPost : Post -> Html Msg
viewPost post =
article [ class "post" ]
([ h2 [ class "title" ] [ text post.title ]
, h4 [ class "author" ] [ text post.author ]
] ++ viewArticle (String.split "\n" post.article)
++ viewVote post ++ viewCommentList post)
viewPostList : List Post -> Html Msg
viewPostList posts =
let
postList = List.map viewPost posts
in
ul [ class "posts" ] postList
viewComment : Comment -> Html Msg
viewComment comment =
article [ class "comment" ]
([ h5 [ class "author" ] [ text (comment.author ++ ":") ]]
++ viewArticle (String.split "\n" comment.content))
viewCommentList : Post -> List (Html Msg)
viewCommentList post =
let
commentList = List.map viewComment post.comments
in
case post.clicked of
True ->
[ div [ class "commenting" ]
[ button
[ class "comment-button"
, onClick (ChangeCommentState post.id False)
]
[ text "comments" ]
]
, div [ class "commenting" ]
[ input
[ type_ "text"
, placeholder "you, the name"
, autofocus True
, onInput NewCommentAuthor
, value post.newCommentAuthor
]
[]
]
, div [ class "commenting" ]
[ textarea
[ cols 10
, rows 5
, placeholder "any thoughts?"
, autofocus True
, onInput NewCommentContent
, value post.newCommentContent
]
[]
]
, div [ class "commenting" ]
[ button
[ class "comment-submit"
, onClick (SendComment post.id)
, disabled (emptyComment post)
]
[ text "don't regret" ]
]
] ++ [ ul [ class "comments" ] commentList ]
False ->
[div [ class "commenting"]
[button
[ class "comment-button"
, onClick (ChangeCommentState post.id True)
]
[ text "comments" ]
]
]
emptyComment : Post -> Bool
emptyComment post =
if post.newCommentAuthor == "" || post.newCommentContent == ""
then True
else
False
emptyPost : Model -> Bool
emptyPost model =
if model.newTitle == "" || model.newAuthor == "" || model.newArticle == ""
then True
else
False
viewPostInput : Model -> Html Msg
viewPostInput model =
case model.postState of
Posting ->
div [ class "posting" ]
[ div [ class "form-group" ]
[ button
[ class "post-button"
, onClick (ChangePostState None)
]
[ text "let go" ]
]
, div [ class "form-group" ]
[ input
[ type_ "text"
, placeholder "a bit of a summary"
, autofocus True
, onInput NewTitle
, value model.newTitle
, class "post-title"
]
[]
]
, div [ class "form-group" ]
[ input
[ type_ "text"
, placeholder "who are you"
, autofocus True
, onInput NewAuthor
, value model.newAuthor
, class "post-author"
]
[]
]
, div [ class "form-group" ]
[ textarea
[ cols 50
, rows 10
, placeholder "please elaborate"
, autofocus True
, onInput NewArticle
, value model.newArticle
, class "post-article"
]
[]
]
, div [ class "form-group" ]
[ button
[ class "submit-button"
, onClick Upload
, disabled (emptyPost model)
]
[ text "push it in" ]
]
]
None ->
div [ class "posting" ]
[ button
[ class "post-button"
, onClick (ChangePostState Posting)
]
[ text "a new post" ]
]
view : Model -> Html Msg
view model =
div [ id "container" ]
[ viewHeader "a giant hole of a mysterious tree"
, viewPostInput model
, viewPostList model.posts
, viewFooter
]
| 52694 | module TreeHole exposing (..)
-- Add/modify imports if you'd like. ------------------------------------------
import Browser
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick, onInput)
import Http
import Json.Decode as Decode exposing (Decoder, field, succeed)
import Json.Encode as Encode
import Json.Decode.Pipeline as DecodePipeline
-- Main -----------------------------------------------------------------------
main : Program Flags Model Msg
main =
Browser.element
{ init = init
, view = view
, update = update
, subscriptions = \_ -> Sub.none
}
-- Model ----------------------------------------------------------------------
type PostState = Posting | None
type alias Comment =
{ postId : Int
, author : String
, content : String
, id : Int
}
type alias Post =
{ title : String
, author : String
, article : String
, vote : Int
, comments : List Comment
, clicked : Bool
, newCommentAuthor : String
, newCommentContent : String
, id : Int
}
type alias Model =
{ posts : List Post
, comments : List Comment
, newTitle : String
, newAuthor : String
, newArticle : String
, postState : PostState
, newComPostId : Int
}
type alias Flags = ()
init : Flags -> (Model, Cmd Msg)
init () =
(initModel, getPosts)
initModel : Model
initModel =
{ posts = []
, comments = []
, newTitle = ""
, newAuthor = ""
, newArticle = ""
, postState = None
, newComPostId = 0
}
-- Update ---------------------------------------------------------------------
type Msg = NewPost (Result Http.Error Post)
| Upload
| GetPost (Result Http.Error (List Post))
| NewTitle String
| NewAuthor String
| NewArticle String
| Upvote Int
| Downvote Int
| VoteUpdated (Result Http.Error Post)
| ChangePostState PostState
| ChangeCommentState Int Bool
| GetComment (Result Http.Error (List Comment))
| NewCommentAuthor String
| NewCommentContent String
| CommentUpdated (Result Http.Error Comment)
| SendComment Int
update : Msg -> Model -> (Model, Cmd Msg)
update msg model =
case msg of
Upload ->
(model, sendPost model)
NewPost (Ok score) ->
({ model | newTitle = ""
, newAuthor = ""
, newArticle = ""
, postState = None}, getPosts)
NewPost (Err error) ->
let
_ = Debug.log "Cannot post a new post" error
in
(model, Cmd.none)
GetPost (Ok lists) ->
({ model | posts = List.reverse (List.sortBy .id lists)}, Cmd.none)
GetPost (Err error) ->
let
_ = Debug.log "cannoot request posts" error
in
(model, Cmd.none)
NewTitle t ->
({ model | newTitle = t }, Cmd.none)
NewAuthor a ->
({ model | newAuthor = a }, Cmd.none)
NewArticle ar ->
({ model | newArticle = ar }, Cmd.none)
Upvote id ->
case (findPostById id model.posts) of
Just post -> (model, updatePost (upvote post))
Nothing -> (model, Cmd.none)
Downvote id ->
case (findPostById id model.posts) of
Just post -> (model, updatePost (downvote post))
Nothing -> (model, Cmd.none)
VoteUpdated _ ->
(model, getPosts)
ChangePostState state ->
({ model | postState = state}, Cmd.none)
ChangeCommentState id state ->
case state of
True ->
({ model | posts = updateState model.posts id state
, newComPostId = id}, getComments)
False ->
({ model | posts = updateState model.posts id state
, newComPostId = 0}, getComments)
GetComment (Ok lists) ->
({ model | comments = List.reverse (List.sortBy .postId lists)
, posts = distributor model.posts lists}, Cmd.none)
GetComment (Err error) ->
let
_ = Debug.log "cannoot request comments" error
in
(model, Cmd.none)
NewCommentAuthor author ->
({ model | posts = updateComAuthor model.posts model.newComPostId author }
, Cmd.none)
NewCommentContent content ->
({ model | posts =
updateComContent model.posts model.newComPostId content }
, Cmd.none)
CommentUpdated _ ->
(model, getComments)
SendComment id ->
case (findPostById id model.posts) of
Just post ->
({ model | posts = sendingCom model.posts model.newComPostId }
, sendComment post)
Nothing -> (model, Cmd.none)
-- Posts
postsURL : String
postsURL = "http://localhost:3000/posts/"
getPosts : Cmd Msg
getPosts =
(Decode.list postDecoder)
|> Http.get postsURL
|> Http.send GetPost
postDecoder : Decoder Post
postDecoder =
Decode.succeed Post
|> DecodePipeline.required "title" Decode.string
|> DecodePipeline.required "author" Decode.string
|> DecodePipeline.required "article" Decode.string
|> DecodePipeline.required "vote" Decode.int
|> DecodePipeline.hardcoded []
|> DecodePipeline.hardcoded False
|> DecodePipeline.hardcoded ""
|> DecodePipeline.hardcoded ""
|> DecodePipeline.required "id" Decode.int
encodePost : Model -> Encode.Value
encodePost model =
Encode.object
[ ("title", Encode.string model.newTitle)
, ("author", Encode.string model.newAuthor)
, ("article", Encode.string model.newArticle)
, ("vote", Encode.int 0)
]
postEncoder : Post -> Encode.Value
postEncoder post =
Encode.object
[ ("title", Encode.string post.title)
, ("author", Encode.string post.author)
, ("article", Encode.string post.article)
, ("vote", Encode.int post.vote)
]
sendPost : Model -> Cmd Msg
sendPost model =
let
body =
encodePost model
|> Http.jsonBody
request =
Http.post postsURL body postDecoder
in
Http.send NewPost request
-- Comments
commentsURL : String
commentsURL = "http://localhost:3000/comments"
getComments : Cmd Msg
getComments =
(Decode.list commentDecoder)
|> Http.get commentsURL
|> Http.send GetComment
commentDecoder : Decoder Comment
commentDecoder =
Decode.map4 Comment
(field "postId" Decode.int)
(field "author" Decode.string)
(field "content" Decode.string)
(field "id" Decode.int)
encodeComment : Post -> Encode.Value
encodeComment post =
Encode.object
[ ("postId", Encode.int post.id)
, ("author", Encode.string post.newCommentAuthor)
, ("content", Encode.string post.newCommentContent)
]
sendComment : Post -> Cmd Msg
sendComment post =
let
body =
encodeComment post
|> Http.jsonBody
request =
Http.post commentsURL body commentDecoder
in
Http.send CommentUpdated request
distributor : List Post -> List Comment -> List Post
distributor posts comments =
case posts of
[] -> []
x :: xs ->
{ title = x.title
, author = x.author
, article = x.article
, vote = x.vote
, comments = List.filter (\c -> c.postId == x.id) comments
, clicked = x.clicked
, newCommentAuthor = x.newCommentAuthor
, newCommentContent = x.newCommentContent
, id = x.id } :: (distributor xs comments)
updateState : List Post -> Int -> Bool -> List Post
updateState posts id state =
case posts of
[] -> []
x :: xs ->
if x.id == id then
{ title = x.title
, author = x.author
, article = x.article
, vote = x.vote
, comments = x.comments
, clicked = state
, newCommentAuthor = x.newCommentAuthor
, newCommentContent = x.newCommentContent
, id = x.id } :: xs
else x :: (updateState xs id state)
updateComAuthor : List Post -> Int -> String -> List Post
updateComAuthor posts id author =
case posts of
[] -> []
x :: xs ->
if x.id == id then
{ title = x.title
, author = x.author
, article = x.article
, vote = x.vote
, comments = x.comments
, clicked = x.clicked
, newCommentAuthor = author
, newCommentContent = x.newCommentContent
, id = x.id } :: xs
else x :: (updateComAuthor xs id author)
updateComContent : List Post -> Int -> String -> List Post
updateComContent posts id content =
case posts of
[] -> []
x :: xs ->
if x.id == id then
{ title = x.title
, author = x.author
, article = x.article
, vote = x.vote
, comments = x.comments
, clicked = x.clicked
, newCommentAuthor = x.newCommentAuthor
, newCommentContent = content
, id = x.id } :: xs
else x :: (updateComContent xs id content)
sendingCom : List Post -> Int -> List Post
sendingCom posts id =
case posts of
[] -> []
x :: xs ->
if x.id == id then
{ title = x.title
, author = x.author
, article = x.article
, vote = x.vote
, comments = x.comments
, clicked = x.clicked
, newCommentAuthor = ""
, newCommentContent = ""
, id = x.id } :: xs
else x :: (sendingCom xs id)
-- Upvotes/Downvotes
findPostById : Int -> List Post -> Maybe Post
findPostById id posts =
case posts
|> List.filter (\post -> post.id == id)
|> List.head of
Just post -> Just post
Nothing -> Nothing
upvote : Post -> Post
upvote post =
{ title = post.title
, author = post.author
, article = post.article
, vote = post.vote + 1
, comments = post.comments
, clicked = post.clicked
, newCommentAuthor = post.newCommentAuthor
, newCommentContent = post.newCommentContent
, id = post.id }
downvote : Post -> Post
downvote post =
{ title = post.title
, author = post.author
, article = post.article
, vote = post.vote - 1
, comments = post.comments
, clicked = post.clicked
, newCommentAuthor = post.newCommentAuthor
, newCommentContent = post.newCommentContent
, id = post.id }
updatePost : Post -> Cmd Msg
updatePost post =
let
body =
postEncoder post
|> Http.jsonBody
request =
Http.request
{ method = "PATCH"
, headers = []
, url = postsURL ++ (Debug.toString post.id)
, body = body
, expect = Http.expectJson postDecoder
, timeout = Nothing
, withCredentials = False
}
in
Http.send VoteUpdated request
-- View -----------------------------------------------------------------------
viewHeader : String -> Html Msg
viewHeader title =
header [ class "header" ]
[ h1 [] [ text title ]
, h4 [] [ text "...where you can put your secrets in *shh*" ]
]
viewFooter : Html Msg
viewFooter =
div []
[ footer []
[ a
[ href "https://github.com/HaroldLeo" ]
[ text "Written by <NAME>" ]
]
]
viewArticle : List String -> List (Html Msg)
viewArticle list =
case list of
[] -> []
first :: rest ->
(p [ class "article" ] [ text first ]) :: viewArticle rest
viewVote : Post -> List (Html Msg)
viewVote post =
[ div [ class "vote" ]
[ button [ class "upvote", onClick (Upvote post.id)]
[ text "⇧" ]
, h5 [ class "vote-num" ]
[ text (String.fromInt post.vote) ]
, button [ class "downvote", onClick (Downvote post.id)]
[ text "⇩" ]
]]
viewPost : Post -> Html Msg
viewPost post =
article [ class "post" ]
([ h2 [ class "title" ] [ text post.title ]
, h4 [ class "author" ] [ text post.author ]
] ++ viewArticle (String.split "\n" post.article)
++ viewVote post ++ viewCommentList post)
viewPostList : List Post -> Html Msg
viewPostList posts =
let
postList = List.map viewPost posts
in
ul [ class "posts" ] postList
viewComment : Comment -> Html Msg
viewComment comment =
article [ class "comment" ]
([ h5 [ class "author" ] [ text (comment.author ++ ":") ]]
++ viewArticle (String.split "\n" comment.content))
viewCommentList : Post -> List (Html Msg)
viewCommentList post =
let
commentList = List.map viewComment post.comments
in
case post.clicked of
True ->
[ div [ class "commenting" ]
[ button
[ class "comment-button"
, onClick (ChangeCommentState post.id False)
]
[ text "comments" ]
]
, div [ class "commenting" ]
[ input
[ type_ "text"
, placeholder "you, the name"
, autofocus True
, onInput NewCommentAuthor
, value post.newCommentAuthor
]
[]
]
, div [ class "commenting" ]
[ textarea
[ cols 10
, rows 5
, placeholder "any thoughts?"
, autofocus True
, onInput NewCommentContent
, value post.newCommentContent
]
[]
]
, div [ class "commenting" ]
[ button
[ class "comment-submit"
, onClick (SendComment post.id)
, disabled (emptyComment post)
]
[ text "don't regret" ]
]
] ++ [ ul [ class "comments" ] commentList ]
False ->
[div [ class "commenting"]
[button
[ class "comment-button"
, onClick (ChangeCommentState post.id True)
]
[ text "comments" ]
]
]
emptyComment : Post -> Bool
emptyComment post =
if post.newCommentAuthor == "" || post.newCommentContent == ""
then True
else
False
emptyPost : Model -> Bool
emptyPost model =
if model.newTitle == "" || model.newAuthor == "" || model.newArticle == ""
then True
else
False
viewPostInput : Model -> Html Msg
viewPostInput model =
case model.postState of
Posting ->
div [ class "posting" ]
[ div [ class "form-group" ]
[ button
[ class "post-button"
, onClick (ChangePostState None)
]
[ text "let go" ]
]
, div [ class "form-group" ]
[ input
[ type_ "text"
, placeholder "a bit of a summary"
, autofocus True
, onInput NewTitle
, value model.newTitle
, class "post-title"
]
[]
]
, div [ class "form-group" ]
[ input
[ type_ "text"
, placeholder "<NAME>"
, autofocus True
, onInput NewAuthor
, value model.newAuthor
, class "post-author"
]
[]
]
, div [ class "form-group" ]
[ textarea
[ cols 50
, rows 10
, placeholder "please elaborate"
, autofocus True
, onInput NewArticle
, value model.newArticle
, class "post-article"
]
[]
]
, div [ class "form-group" ]
[ button
[ class "submit-button"
, onClick Upload
, disabled (emptyPost model)
]
[ text "push it in" ]
]
]
None ->
div [ class "posting" ]
[ button
[ class "post-button"
, onClick (ChangePostState Posting)
]
[ text "a new post" ]
]
view : Model -> Html Msg
view model =
div [ id "container" ]
[ viewHeader "a giant hole of a mysterious tree"
, viewPostInput model
, viewPostList model.posts
, viewFooter
]
| true | module TreeHole exposing (..)
-- Add/modify imports if you'd like. ------------------------------------------
import Browser
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick, onInput)
import Http
import Json.Decode as Decode exposing (Decoder, field, succeed)
import Json.Encode as Encode
import Json.Decode.Pipeline as DecodePipeline
-- Main -----------------------------------------------------------------------
main : Program Flags Model Msg
main =
Browser.element
{ init = init
, view = view
, update = update
, subscriptions = \_ -> Sub.none
}
-- Model ----------------------------------------------------------------------
type PostState = Posting | None
type alias Comment =
{ postId : Int
, author : String
, content : String
, id : Int
}
type alias Post =
{ title : String
, author : String
, article : String
, vote : Int
, comments : List Comment
, clicked : Bool
, newCommentAuthor : String
, newCommentContent : String
, id : Int
}
type alias Model =
{ posts : List Post
, comments : List Comment
, newTitle : String
, newAuthor : String
, newArticle : String
, postState : PostState
, newComPostId : Int
}
type alias Flags = ()
init : Flags -> (Model, Cmd Msg)
init () =
(initModel, getPosts)
initModel : Model
initModel =
{ posts = []
, comments = []
, newTitle = ""
, newAuthor = ""
, newArticle = ""
, postState = None
, newComPostId = 0
}
-- Update ---------------------------------------------------------------------
type Msg = NewPost (Result Http.Error Post)
| Upload
| GetPost (Result Http.Error (List Post))
| NewTitle String
| NewAuthor String
| NewArticle String
| Upvote Int
| Downvote Int
| VoteUpdated (Result Http.Error Post)
| ChangePostState PostState
| ChangeCommentState Int Bool
| GetComment (Result Http.Error (List Comment))
| NewCommentAuthor String
| NewCommentContent String
| CommentUpdated (Result Http.Error Comment)
| SendComment Int
update : Msg -> Model -> (Model, Cmd Msg)
update msg model =
case msg of
Upload ->
(model, sendPost model)
NewPost (Ok score) ->
({ model | newTitle = ""
, newAuthor = ""
, newArticle = ""
, postState = None}, getPosts)
NewPost (Err error) ->
let
_ = Debug.log "Cannot post a new post" error
in
(model, Cmd.none)
GetPost (Ok lists) ->
({ model | posts = List.reverse (List.sortBy .id lists)}, Cmd.none)
GetPost (Err error) ->
let
_ = Debug.log "cannoot request posts" error
in
(model, Cmd.none)
NewTitle t ->
({ model | newTitle = t }, Cmd.none)
NewAuthor a ->
({ model | newAuthor = a }, Cmd.none)
NewArticle ar ->
({ model | newArticle = ar }, Cmd.none)
Upvote id ->
case (findPostById id model.posts) of
Just post -> (model, updatePost (upvote post))
Nothing -> (model, Cmd.none)
Downvote id ->
case (findPostById id model.posts) of
Just post -> (model, updatePost (downvote post))
Nothing -> (model, Cmd.none)
VoteUpdated _ ->
(model, getPosts)
ChangePostState state ->
({ model | postState = state}, Cmd.none)
ChangeCommentState id state ->
case state of
True ->
({ model | posts = updateState model.posts id state
, newComPostId = id}, getComments)
False ->
({ model | posts = updateState model.posts id state
, newComPostId = 0}, getComments)
GetComment (Ok lists) ->
({ model | comments = List.reverse (List.sortBy .postId lists)
, posts = distributor model.posts lists}, Cmd.none)
GetComment (Err error) ->
let
_ = Debug.log "cannoot request comments" error
in
(model, Cmd.none)
NewCommentAuthor author ->
({ model | posts = updateComAuthor model.posts model.newComPostId author }
, Cmd.none)
NewCommentContent content ->
({ model | posts =
updateComContent model.posts model.newComPostId content }
, Cmd.none)
CommentUpdated _ ->
(model, getComments)
SendComment id ->
case (findPostById id model.posts) of
Just post ->
({ model | posts = sendingCom model.posts model.newComPostId }
, sendComment post)
Nothing -> (model, Cmd.none)
-- Posts
postsURL : String
postsURL = "http://localhost:3000/posts/"
getPosts : Cmd Msg
getPosts =
(Decode.list postDecoder)
|> Http.get postsURL
|> Http.send GetPost
postDecoder : Decoder Post
postDecoder =
Decode.succeed Post
|> DecodePipeline.required "title" Decode.string
|> DecodePipeline.required "author" Decode.string
|> DecodePipeline.required "article" Decode.string
|> DecodePipeline.required "vote" Decode.int
|> DecodePipeline.hardcoded []
|> DecodePipeline.hardcoded False
|> DecodePipeline.hardcoded ""
|> DecodePipeline.hardcoded ""
|> DecodePipeline.required "id" Decode.int
encodePost : Model -> Encode.Value
encodePost model =
Encode.object
[ ("title", Encode.string model.newTitle)
, ("author", Encode.string model.newAuthor)
, ("article", Encode.string model.newArticle)
, ("vote", Encode.int 0)
]
postEncoder : Post -> Encode.Value
postEncoder post =
Encode.object
[ ("title", Encode.string post.title)
, ("author", Encode.string post.author)
, ("article", Encode.string post.article)
, ("vote", Encode.int post.vote)
]
sendPost : Model -> Cmd Msg
sendPost model =
let
body =
encodePost model
|> Http.jsonBody
request =
Http.post postsURL body postDecoder
in
Http.send NewPost request
-- Comments
commentsURL : String
commentsURL = "http://localhost:3000/comments"
getComments : Cmd Msg
getComments =
(Decode.list commentDecoder)
|> Http.get commentsURL
|> Http.send GetComment
commentDecoder : Decoder Comment
commentDecoder =
Decode.map4 Comment
(field "postId" Decode.int)
(field "author" Decode.string)
(field "content" Decode.string)
(field "id" Decode.int)
encodeComment : Post -> Encode.Value
encodeComment post =
Encode.object
[ ("postId", Encode.int post.id)
, ("author", Encode.string post.newCommentAuthor)
, ("content", Encode.string post.newCommentContent)
]
sendComment : Post -> Cmd Msg
sendComment post =
let
body =
encodeComment post
|> Http.jsonBody
request =
Http.post commentsURL body commentDecoder
in
Http.send CommentUpdated request
distributor : List Post -> List Comment -> List Post
distributor posts comments =
case posts of
[] -> []
x :: xs ->
{ title = x.title
, author = x.author
, article = x.article
, vote = x.vote
, comments = List.filter (\c -> c.postId == x.id) comments
, clicked = x.clicked
, newCommentAuthor = x.newCommentAuthor
, newCommentContent = x.newCommentContent
, id = x.id } :: (distributor xs comments)
updateState : List Post -> Int -> Bool -> List Post
updateState posts id state =
case posts of
[] -> []
x :: xs ->
if x.id == id then
{ title = x.title
, author = x.author
, article = x.article
, vote = x.vote
, comments = x.comments
, clicked = state
, newCommentAuthor = x.newCommentAuthor
, newCommentContent = x.newCommentContent
, id = x.id } :: xs
else x :: (updateState xs id state)
updateComAuthor : List Post -> Int -> String -> List Post
updateComAuthor posts id author =
case posts of
[] -> []
x :: xs ->
if x.id == id then
{ title = x.title
, author = x.author
, article = x.article
, vote = x.vote
, comments = x.comments
, clicked = x.clicked
, newCommentAuthor = author
, newCommentContent = x.newCommentContent
, id = x.id } :: xs
else x :: (updateComAuthor xs id author)
updateComContent : List Post -> Int -> String -> List Post
updateComContent posts id content =
case posts of
[] -> []
x :: xs ->
if x.id == id then
{ title = x.title
, author = x.author
, article = x.article
, vote = x.vote
, comments = x.comments
, clicked = x.clicked
, newCommentAuthor = x.newCommentAuthor
, newCommentContent = content
, id = x.id } :: xs
else x :: (updateComContent xs id content)
sendingCom : List Post -> Int -> List Post
sendingCom posts id =
case posts of
[] -> []
x :: xs ->
if x.id == id then
{ title = x.title
, author = x.author
, article = x.article
, vote = x.vote
, comments = x.comments
, clicked = x.clicked
, newCommentAuthor = ""
, newCommentContent = ""
, id = x.id } :: xs
else x :: (sendingCom xs id)
-- Upvotes/Downvotes
findPostById : Int -> List Post -> Maybe Post
findPostById id posts =
case posts
|> List.filter (\post -> post.id == id)
|> List.head of
Just post -> Just post
Nothing -> Nothing
upvote : Post -> Post
upvote post =
{ title = post.title
, author = post.author
, article = post.article
, vote = post.vote + 1
, comments = post.comments
, clicked = post.clicked
, newCommentAuthor = post.newCommentAuthor
, newCommentContent = post.newCommentContent
, id = post.id }
downvote : Post -> Post
downvote post =
{ title = post.title
, author = post.author
, article = post.article
, vote = post.vote - 1
, comments = post.comments
, clicked = post.clicked
, newCommentAuthor = post.newCommentAuthor
, newCommentContent = post.newCommentContent
, id = post.id }
updatePost : Post -> Cmd Msg
updatePost post =
let
body =
postEncoder post
|> Http.jsonBody
request =
Http.request
{ method = "PATCH"
, headers = []
, url = postsURL ++ (Debug.toString post.id)
, body = body
, expect = Http.expectJson postDecoder
, timeout = Nothing
, withCredentials = False
}
in
Http.send VoteUpdated request
-- View -----------------------------------------------------------------------
viewHeader : String -> Html Msg
viewHeader title =
header [ class "header" ]
[ h1 [] [ text title ]
, h4 [] [ text "...where you can put your secrets in *shh*" ]
]
viewFooter : Html Msg
viewFooter =
div []
[ footer []
[ a
[ href "https://github.com/HaroldLeo" ]
[ text "Written by PI:NAME:<NAME>END_PI" ]
]
]
viewArticle : List String -> List (Html Msg)
viewArticle list =
case list of
[] -> []
first :: rest ->
(p [ class "article" ] [ text first ]) :: viewArticle rest
viewVote : Post -> List (Html Msg)
viewVote post =
[ div [ class "vote" ]
[ button [ class "upvote", onClick (Upvote post.id)]
[ text "⇧" ]
, h5 [ class "vote-num" ]
[ text (String.fromInt post.vote) ]
, button [ class "downvote", onClick (Downvote post.id)]
[ text "⇩" ]
]]
viewPost : Post -> Html Msg
viewPost post =
article [ class "post" ]
([ h2 [ class "title" ] [ text post.title ]
, h4 [ class "author" ] [ text post.author ]
] ++ viewArticle (String.split "\n" post.article)
++ viewVote post ++ viewCommentList post)
viewPostList : List Post -> Html Msg
viewPostList posts =
let
postList = List.map viewPost posts
in
ul [ class "posts" ] postList
viewComment : Comment -> Html Msg
viewComment comment =
article [ class "comment" ]
([ h5 [ class "author" ] [ text (comment.author ++ ":") ]]
++ viewArticle (String.split "\n" comment.content))
viewCommentList : Post -> List (Html Msg)
viewCommentList post =
let
commentList = List.map viewComment post.comments
in
case post.clicked of
True ->
[ div [ class "commenting" ]
[ button
[ class "comment-button"
, onClick (ChangeCommentState post.id False)
]
[ text "comments" ]
]
, div [ class "commenting" ]
[ input
[ type_ "text"
, placeholder "you, the name"
, autofocus True
, onInput NewCommentAuthor
, value post.newCommentAuthor
]
[]
]
, div [ class "commenting" ]
[ textarea
[ cols 10
, rows 5
, placeholder "any thoughts?"
, autofocus True
, onInput NewCommentContent
, value post.newCommentContent
]
[]
]
, div [ class "commenting" ]
[ button
[ class "comment-submit"
, onClick (SendComment post.id)
, disabled (emptyComment post)
]
[ text "don't regret" ]
]
] ++ [ ul [ class "comments" ] commentList ]
False ->
[div [ class "commenting"]
[button
[ class "comment-button"
, onClick (ChangeCommentState post.id True)
]
[ text "comments" ]
]
]
emptyComment : Post -> Bool
emptyComment post =
if post.newCommentAuthor == "" || post.newCommentContent == ""
then True
else
False
emptyPost : Model -> Bool
emptyPost model =
if model.newTitle == "" || model.newAuthor == "" || model.newArticle == ""
then True
else
False
viewPostInput : Model -> Html Msg
viewPostInput model =
case model.postState of
Posting ->
div [ class "posting" ]
[ div [ class "form-group" ]
[ button
[ class "post-button"
, onClick (ChangePostState None)
]
[ text "let go" ]
]
, div [ class "form-group" ]
[ input
[ type_ "text"
, placeholder "a bit of a summary"
, autofocus True
, onInput NewTitle
, value model.newTitle
, class "post-title"
]
[]
]
, div [ class "form-group" ]
[ input
[ type_ "text"
, placeholder "PI:NAME:<NAME>END_PI"
, autofocus True
, onInput NewAuthor
, value model.newAuthor
, class "post-author"
]
[]
]
, div [ class "form-group" ]
[ textarea
[ cols 50
, rows 10
, placeholder "please elaborate"
, autofocus True
, onInput NewArticle
, value model.newArticle
, class "post-article"
]
[]
]
, div [ class "form-group" ]
[ button
[ class "submit-button"
, onClick Upload
, disabled (emptyPost model)
]
[ text "push it in" ]
]
]
None ->
div [ class "posting" ]
[ button
[ class "post-button"
, onClick (ChangePostState Posting)
]
[ text "a new post" ]
]
view : Model -> Html Msg
view model =
div [ id "container" ]
[ viewHeader "a giant hole of a mysterious tree"
, viewPostInput model
, viewPostList model.posts
, viewFooter
]
| elm |
[
{
"context": "\"\n , sourceCode = Just \"https://github.com/material-components/material-components-web/tree/master/packages/mdc-",
"end": 1516,
"score": 0.9979378581,
"start": 1497,
"tag": "USERNAME",
"value": "material-components"
},
{
"context": "\" ]\n , listItemText [] [ text \"user1@example.com\" ]\n ]\n , listIt",
"end": 4366,
"score": 0.9999187589,
"start": 4349,
"tag": "EMAIL",
"value": "user1@example.com"
},
{
"context": "\" ]\n , listItemText [] [ text \"user2@example.com\" ]\n ]\n , listIt",
"end": 4997,
"score": 0.9999046326,
"start": 4980,
"tag": "EMAIL",
"value": "user2@example.com"
},
{
"context": " []\n [ text \"\"\"\n Dorothy lived in the midst of the great Kansas prai",
"end": 7219,
"score": 0.5807910562,
"start": 7218,
"tag": "NAME",
"value": "D"
},
{
"context": "he great Kansas prairies,\n with Uncle Henry, who was a farmer, and Aunt Em, who was\n ",
"end": 7311,
"score": 0.9210759401,
"start": 7300,
"tag": "NAME",
"value": "Uncle Henry"
},
{
"context": " with Uncle Henry, who was a farmer, and Aunt Em, who was\n the far",
"end": 7329,
"score": 0.9867438078,
"start": 7326,
"tag": "NAME",
"value": "mer"
},
{
"context": " with Uncle Henry, who was a farmer, and Aunt Em, who was\n the farmer's wife. T",
"end": 7342,
"score": 0.9974031448,
"start": 7335,
"tag": "NAME",
"value": "Aunt Em"
},
{
"context": "ur chairs, and the\n beds. Uncle Henry and Aunt Em had a big bed in one corner,\n ",
"end": 7778,
"score": 0.8370599747,
"start": 7773,
"tag": "NAME",
"value": "Henry"
},
{
"context": " and the\n beds. Uncle Henry and Aunt Em had a big bed in one corner,\n ",
"end": 7790,
"score": 0.9967027307,
"start": 7783,
"tag": "NAME",
"value": "Aunt Em"
},
{
"context": "d a big bed in one corner,\n and Dorothy a little bed in another corner. There was no\n ",
"end": 7851,
"score": 0.9958916903,
"start": 7844,
"tag": "NAME",
"value": "Dorothy"
},
{
"context": " [ text \"\"\"\n When Dorothy stood in the doorway and looked around, she\n ",
"end": 8468,
"score": 0.9757140279,
"start": 8461,
"tag": "NAME",
"value": "Dorothy"
},
{
"context": " [ text \"\"\"\n When Aunt Em came there to live she was a young, pretty\n ",
"end": 9399,
"score": 0.992069006,
"start": 9392,
"tag": "NAME",
"value": "Aunt Em"
},
{
"context": "nt, and never smiled now.\n When Dorothy, who was an orphan, first came to her, Aunt Em\n ",
"end": 9789,
"score": 0.9609669447,
"start": 9782,
"tag": "NAME",
"value": "Dorothy"
},
{
"context": "hen Dorothy, who was an orphan, first came to her, Aunt Em\n had been so startled by th",
"end": 9833,
"score": 0.8349391222,
"start": 9829,
"tag": "NAME",
"value": "Aunt"
},
{
"context": "orothy, who was an orphan, first came to her, Aunt Em\n had been so startled by the c",
"end": 9836,
"score": 0.6043308377,
"start": 9834,
"tag": "NAME",
"value": "Em"
},
{
"context": " scream and press her hand upon her heart whenever Dorothy's\n merry voice reached h",
"end": 9988,
"score": 0.5327763557,
"start": 9987,
"tag": "NAME",
"value": "D"
},
{
"context": " [ text \"\"\"\n Uncle Henry never laughed. He worked hard from morning till\n ",
"end": 10303,
"score": 0.5978714824,
"start": 10301,
"tag": "NAME",
"value": "ry"
},
{
"context": " [ text \"\"\"\n It was Toto that made Dorothy laugh, and saved her from\n ",
"end": 10676,
"score": 0.6177129149,
"start": 10675,
"tag": "NAME",
"value": "T"
},
{
"context": "text \"\"\"\n It was Toto that made Dorothy laugh, and saved her from\n ",
"end": 10691,
"score": 0.5224183798,
"start": 10690,
"tag": "NAME",
"value": "D"
},
{
"context": "his funny, wee nose. Toto played all day long, and Dorothy\n played with him, and lo",
"end": 11027,
"score": 0.608856082,
"start": 11026,
"tag": "NAME",
"value": "D"
},
{
"context": " Today, however, they were not playing. Uncle Henry sat upon\n the doorstep and loo",
"end": 11254,
"score": 0.5425236821,
"start": 11252,
"tag": "NAME",
"value": "ry"
},
{
"context": "ch was\n even grayer than usual. Dorothy stood in the door with Toto\n ",
"end": 11385,
"score": 0.6769313216,
"start": 11384,
"tag": "NAME",
"value": "D"
},
{
"context": " grayer than usual. Dorothy stood in the door with Toto\n in her arms, and looked at",
"end": 11416,
"score": 0.737336576,
"start": 11415,
"tag": "NAME",
"value": "T"
},
{
"context": " in her arms, and looked at the sky too. Aunt Em was washing\n the dishes.\n ",
"end": 11487,
"score": 0.7323492169,
"start": 11480,
"tag": "NAME",
"value": "Aunt Em"
},
{
"context": "ail of the wind, and\n Uncle Henry and Dorothy could see where the long grass\n ",
"end": 11732,
"score": 0.5199046135,
"start": 11730,
"tag": "NAME",
"value": "ry"
},
{
"context": " the wind, and\n Uncle Henry and Dorothy could see where the long grass\n ",
"end": 11740,
"score": 0.5831535459,
"start": 11737,
"tag": "NAME",
"value": "Dor"
}
] | demo/src/Demo/Dialog.elm | SwiftEngineer/material-components-web-elm | 1 | module Demo.Dialog exposing (Model, Msg(..), defaultModel, update, view)
import Demo.CatalogPage exposing (CatalogPage)
import Demo.Helper.ResourceLink as ResourceLink
import Html exposing (Html, text)
import Html.Attributes exposing (class, style)
import Html.Events
import Material.Button exposing (buttonConfig, textButton)
import Material.Dialog exposing (dialog, dialogConfig)
import Material.Icon exposing (icon, iconConfig)
import Material.List exposing (list, listConfig, listItem, listItemConfig, listItemGraphic, listItemText)
import Material.Radio exposing (radio, radioConfig)
import Material.Typography as Typography
type alias Model =
{ openDialog : Maybe String
}
defaultModel : Model
defaultModel =
{ openDialog = Nothing
}
type Msg
= Close
| Show String
update : Msg -> Model -> Model
update msg model =
case msg of
Close ->
{ model | openDialog = Nothing }
Show index ->
{ model | openDialog = Just index }
view : Model -> CatalogPage Msg
view model =
{ title = "Dialog"
, prelude = "Dialogs inform users about a specific task and may contain critical information, require decisions, or involve multiple tasks."
, resources =
{ materialDesignGuidelines = Just "https://material.io/go/design-dialogs"
, documentation = Just "https://package.elm-lang.org/packages/aforemny/material-components-web-elm/latest/Material-Dialog"
, sourceCode = Just "https://github.com/material-components/material-components-web/tree/master/packages/mdc-dialog"
}
, hero = heroDialog
, content =
[ textButton
{ buttonConfig | onClick = Just (Show "dialog-alert-dialog") }
"Alert"
, text " "
, textButton
{ buttonConfig | onClick = Just (Show "dialog-simple-dialog") }
"Simple"
, text " "
, textButton
{ buttonConfig | onClick = Just (Show "dialog-confirmation-dialog") }
"Confirmation"
, text " "
, textButton
{ buttonConfig | onClick = Just (Show "dialog-scrollable-dialog") }
"Scrollable"
, text " "
, alertDialog model
, simpleDialog model
, confirmationDialog model
, scrollableDialog model
]
}
heroDialog : List (Html Msg)
heroDialog =
[ Html.div
[ class "mdc-dialog mdc-dialog--open"
, style "position" "relative"
]
[ Html.div
[ class "mdc-dialog__surface" ]
[ Html.div [ class "mdc-dialog__title" ] [ text "Get this party started?" ]
, Html.div [ class "mdc-dialog__content" ]
[ text "Turn up the jams and have a good time." ]
, Html.div [ class "mdc-dialog__actions" ]
[ textButton buttonConfig "Decline"
, textButton buttonConfig "Accept"
]
]
]
]
alertDialog : Model -> Html Msg
alertDialog model =
dialog
{ dialogConfig
| open = model.openDialog == Just "dialog-alert-dialog"
, onClose = Just Close
}
{ title = Nothing
, content =
[ text "Discard draft?" ]
, actions =
[ textButton { buttonConfig | onClick = Just Close } "Cancel"
, textButton { buttonConfig | onClick = Just Close } "Discard"
]
}
simpleDialog : Model -> Html Msg
simpleDialog model =
dialog
{ dialogConfig
| open = model.openDialog == Just "dialog-simple-dialog"
, onClose = Just Close
}
{ title = Just "Select an account"
, content =
[ list { listConfig | avatarList = True }
[ listItem
{ listItemConfig
| additionalAttributes =
[ Html.Attributes.tabindex 0
, Html.Events.onClick Close
]
}
[ listItemGraphic
[ Html.Attributes.style "background-color" "rgba(0,0,0,.3)"
, Html.Attributes.style "color" "#fff"
]
[ icon iconConfig "person" ]
, listItemText [] [ text "user1@example.com" ]
]
, listItem
{ listItemConfig
| additionalAttributes =
[ Html.Attributes.tabindex 0
, Html.Events.onClick Close
]
}
[ listItemGraphic
[ Html.Attributes.style "background-color" "rgba(0,0,0,.3)"
, Html.Attributes.style "color" "#fff"
]
[ icon iconConfig "person" ]
, listItemText [] [ text "user2@example.com" ]
]
, listItem
{ listItemConfig
| additionalAttributes =
[ Html.Attributes.tabindex 0
, Html.Events.onClick Close
]
}
[ listItemGraphic
[ Html.Attributes.style "background-color" "rgba(0,0,0,.3)"
, Html.Attributes.style "color" "#fff"
]
[ icon iconConfig "add" ]
, listItemText [] [ text "Add account" ]
]
]
]
, actions = []
}
confirmationDialog : Model -> Html Msg
confirmationDialog model =
dialog
{ dialogConfig
| open = model.openDialog == Just "dialog-confirmation-dialog"
, onClose = Just Close
}
{ title = Just "Phone ringtone"
, content =
[ list { listConfig | avatarList = True }
[ listItem listItemConfig
[ listItemGraphic [] [ radio { radioConfig | checked = True } ]
, listItemText [] [ text "Never Gonna Give You Up" ]
]
, listItem listItemConfig
[ listItemGraphic [] [ radio radioConfig ]
, listItemText [] [ text "Hot Cross Buns" ]
]
, listItem listItemConfig
[ listItemGraphic [] [ radio radioConfig ]
, listItemText [] [ text "None" ]
]
]
]
, actions =
[ textButton { buttonConfig | onClick = Just Close } "Cancel"
, textButton { buttonConfig | onClick = Just Close } "OK"
]
}
scrollableDialog : Model -> Html Msg
scrollableDialog model =
dialog
{ dialogConfig
| open = model.openDialog == Just "dialog-scrollable-dialog"
, onClose = Just Close
}
{ title = Just "The Wonderful Wizard of Oz"
, content =
[ Html.p []
[ text """
Dorothy lived in the midst of the great Kansas prairies,
with Uncle Henry, who was a farmer, and Aunt Em, who was
the farmer's wife. Their house was small, for the lumber to
build it had to be carried by wagon many miles. There were
four walls, a floor and a roof, which made one room; and
this room contained a rusty looking cookstove, a cupboard
for the dishes, a table, three or four chairs, and the
beds. Uncle Henry and Aunt Em had a big bed in one corner,
and Dorothy a little bed in another corner. There was no
garret at all, and no cellar--except a small hole dug in
the ground, called a cyclone cellar, where the family could
go in case one of those great whirlwinds arose, mighty
enough to crush any building in its path. It was reached by
a trap door in the middle of the floor, from which a ladder
led down into the small, dark hole.
"""
]
, Html.p []
[ text """
When Dorothy stood in the doorway and looked around, she
could see nothing but the great gray prairie on every side.
Not a tree nor a house broke the broad sweep of flat
country that reached to the edge of the sky in all
directions. The sun had baked the plowed land into a gray
mass, with little cracks running through it. Even the grass
was not green, for the sun had burned the tops of the long
blades until they were the same gray color to be seen
everywhere. Once the house had been painted, but the sun
blistered the paint and the rains washed it away, and now
the house was as dull and gray as everything else.
"""
]
, Html.p []
[ text """
When Aunt Em came there to live she was a young, pretty
wife. The sun and wind had changed her, too. They had taken
the sparkle from her eyes and left them a sober gray; they
had taken the red from her cheeks and lips, and they were
gray also. She was thin and gaunt, and never smiled now.
When Dorothy, who was an orphan, first came to her, Aunt Em
had been so startled by the child's laughter that she would
scream and press her hand upon her heart whenever Dorothy's
merry voice reached her ears; and she still looked at the
little girl with wonder that she could find anything to
laugh at.
"""
]
, Html.p []
[ text """
Uncle Henry never laughed. He worked hard from morning till
night and did not know what joy was. He was gray also, from
his long beard to his rough boots, and he looked stern and
solemn, and rarely spoke.
"""
]
, Html.p []
[ text """
It was Toto that made Dorothy laugh, and saved her from
growing as gray as her other surroundings. Toto was not
gray; he was a little black dog, with long silky hair and
small black eyes that twinkled merrily on either side of
his funny, wee nose. Toto played all day long, and Dorothy
played with him, and loved him dearly.
"""
]
, Html.p []
[ text """
Today, however, they were not playing. Uncle Henry sat upon
the doorstep and looked anxiously at the sky, which was
even grayer than usual. Dorothy stood in the door with Toto
in her arms, and looked at the sky too. Aunt Em was washing
the dishes.
"""
]
, Html.p []
[ text """
From the far north they heard a low wail of the wind, and
Uncle Henry and Dorothy could see where the long grass
bowed in waves before the coming storm. There now came a
sharp whistling in the air from the south, and as they
turned their eyes that way they saw ripples in the grass
coming from that direction also.
"""
]
]
, actions =
[ textButton { buttonConfig | onClick = Just Close } "Decline"
, textButton { buttonConfig | onClick = Just Close } "Continue"
]
}
| 50054 | module Demo.Dialog exposing (Model, Msg(..), defaultModel, update, view)
import Demo.CatalogPage exposing (CatalogPage)
import Demo.Helper.ResourceLink as ResourceLink
import Html exposing (Html, text)
import Html.Attributes exposing (class, style)
import Html.Events
import Material.Button exposing (buttonConfig, textButton)
import Material.Dialog exposing (dialog, dialogConfig)
import Material.Icon exposing (icon, iconConfig)
import Material.List exposing (list, listConfig, listItem, listItemConfig, listItemGraphic, listItemText)
import Material.Radio exposing (radio, radioConfig)
import Material.Typography as Typography
type alias Model =
{ openDialog : Maybe String
}
defaultModel : Model
defaultModel =
{ openDialog = Nothing
}
type Msg
= Close
| Show String
update : Msg -> Model -> Model
update msg model =
case msg of
Close ->
{ model | openDialog = Nothing }
Show index ->
{ model | openDialog = Just index }
view : Model -> CatalogPage Msg
view model =
{ title = "Dialog"
, prelude = "Dialogs inform users about a specific task and may contain critical information, require decisions, or involve multiple tasks."
, resources =
{ materialDesignGuidelines = Just "https://material.io/go/design-dialogs"
, documentation = Just "https://package.elm-lang.org/packages/aforemny/material-components-web-elm/latest/Material-Dialog"
, sourceCode = Just "https://github.com/material-components/material-components-web/tree/master/packages/mdc-dialog"
}
, hero = heroDialog
, content =
[ textButton
{ buttonConfig | onClick = Just (Show "dialog-alert-dialog") }
"Alert"
, text " "
, textButton
{ buttonConfig | onClick = Just (Show "dialog-simple-dialog") }
"Simple"
, text " "
, textButton
{ buttonConfig | onClick = Just (Show "dialog-confirmation-dialog") }
"Confirmation"
, text " "
, textButton
{ buttonConfig | onClick = Just (Show "dialog-scrollable-dialog") }
"Scrollable"
, text " "
, alertDialog model
, simpleDialog model
, confirmationDialog model
, scrollableDialog model
]
}
heroDialog : List (Html Msg)
heroDialog =
[ Html.div
[ class "mdc-dialog mdc-dialog--open"
, style "position" "relative"
]
[ Html.div
[ class "mdc-dialog__surface" ]
[ Html.div [ class "mdc-dialog__title" ] [ text "Get this party started?" ]
, Html.div [ class "mdc-dialog__content" ]
[ text "Turn up the jams and have a good time." ]
, Html.div [ class "mdc-dialog__actions" ]
[ textButton buttonConfig "Decline"
, textButton buttonConfig "Accept"
]
]
]
]
alertDialog : Model -> Html Msg
alertDialog model =
dialog
{ dialogConfig
| open = model.openDialog == Just "dialog-alert-dialog"
, onClose = Just Close
}
{ title = Nothing
, content =
[ text "Discard draft?" ]
, actions =
[ textButton { buttonConfig | onClick = Just Close } "Cancel"
, textButton { buttonConfig | onClick = Just Close } "Discard"
]
}
simpleDialog : Model -> Html Msg
simpleDialog model =
dialog
{ dialogConfig
| open = model.openDialog == Just "dialog-simple-dialog"
, onClose = Just Close
}
{ title = Just "Select an account"
, content =
[ list { listConfig | avatarList = True }
[ listItem
{ listItemConfig
| additionalAttributes =
[ Html.Attributes.tabindex 0
, Html.Events.onClick Close
]
}
[ listItemGraphic
[ Html.Attributes.style "background-color" "rgba(0,0,0,.3)"
, Html.Attributes.style "color" "#fff"
]
[ icon iconConfig "person" ]
, listItemText [] [ text "<EMAIL>" ]
]
, listItem
{ listItemConfig
| additionalAttributes =
[ Html.Attributes.tabindex 0
, Html.Events.onClick Close
]
}
[ listItemGraphic
[ Html.Attributes.style "background-color" "rgba(0,0,0,.3)"
, Html.Attributes.style "color" "#fff"
]
[ icon iconConfig "person" ]
, listItemText [] [ text "<EMAIL>" ]
]
, listItem
{ listItemConfig
| additionalAttributes =
[ Html.Attributes.tabindex 0
, Html.Events.onClick Close
]
}
[ listItemGraphic
[ Html.Attributes.style "background-color" "rgba(0,0,0,.3)"
, Html.Attributes.style "color" "#fff"
]
[ icon iconConfig "add" ]
, listItemText [] [ text "Add account" ]
]
]
]
, actions = []
}
confirmationDialog : Model -> Html Msg
confirmationDialog model =
dialog
{ dialogConfig
| open = model.openDialog == Just "dialog-confirmation-dialog"
, onClose = Just Close
}
{ title = Just "Phone ringtone"
, content =
[ list { listConfig | avatarList = True }
[ listItem listItemConfig
[ listItemGraphic [] [ radio { radioConfig | checked = True } ]
, listItemText [] [ text "Never Gonna Give You Up" ]
]
, listItem listItemConfig
[ listItemGraphic [] [ radio radioConfig ]
, listItemText [] [ text "Hot Cross Buns" ]
]
, listItem listItemConfig
[ listItemGraphic [] [ radio radioConfig ]
, listItemText [] [ text "None" ]
]
]
]
, actions =
[ textButton { buttonConfig | onClick = Just Close } "Cancel"
, textButton { buttonConfig | onClick = Just Close } "OK"
]
}
scrollableDialog : Model -> Html Msg
scrollableDialog model =
dialog
{ dialogConfig
| open = model.openDialog == Just "dialog-scrollable-dialog"
, onClose = Just Close
}
{ title = Just "The Wonderful Wizard of Oz"
, content =
[ Html.p []
[ text """
<NAME>orothy lived in the midst of the great Kansas prairies,
with <NAME>, who was a far<NAME>, and <NAME>, who was
the farmer's wife. Their house was small, for the lumber to
build it had to be carried by wagon many miles. There were
four walls, a floor and a roof, which made one room; and
this room contained a rusty looking cookstove, a cupboard
for the dishes, a table, three or four chairs, and the
beds. Uncle <NAME> and <NAME> had a big bed in one corner,
and <NAME> a little bed in another corner. There was no
garret at all, and no cellar--except a small hole dug in
the ground, called a cyclone cellar, where the family could
go in case one of those great whirlwinds arose, mighty
enough to crush any building in its path. It was reached by
a trap door in the middle of the floor, from which a ladder
led down into the small, dark hole.
"""
]
, Html.p []
[ text """
When <NAME> stood in the doorway and looked around, she
could see nothing but the great gray prairie on every side.
Not a tree nor a house broke the broad sweep of flat
country that reached to the edge of the sky in all
directions. The sun had baked the plowed land into a gray
mass, with little cracks running through it. Even the grass
was not green, for the sun had burned the tops of the long
blades until they were the same gray color to be seen
everywhere. Once the house had been painted, but the sun
blistered the paint and the rains washed it away, and now
the house was as dull and gray as everything else.
"""
]
, Html.p []
[ text """
When <NAME> came there to live she was a young, pretty
wife. The sun and wind had changed her, too. They had taken
the sparkle from her eyes and left them a sober gray; they
had taken the red from her cheeks and lips, and they were
gray also. She was thin and gaunt, and never smiled now.
When <NAME>, who was an orphan, first came to her, <NAME> <NAME>
had been so startled by the child's laughter that she would
scream and press her hand upon her heart whenever <NAME>orothy's
merry voice reached her ears; and she still looked at the
little girl with wonder that she could find anything to
laugh at.
"""
]
, Html.p []
[ text """
Uncle Hen<NAME> never laughed. He worked hard from morning till
night and did not know what joy was. He was gray also, from
his long beard to his rough boots, and he looked stern and
solemn, and rarely spoke.
"""
]
, Html.p []
[ text """
It was <NAME>oto that made <NAME>orothy laugh, and saved her from
growing as gray as her other surroundings. Toto was not
gray; he was a little black dog, with long silky hair and
small black eyes that twinkled merrily on either side of
his funny, wee nose. Toto played all day long, and <NAME>orothy
played with him, and loved him dearly.
"""
]
, Html.p []
[ text """
Today, however, they were not playing. Uncle Hen<NAME> sat upon
the doorstep and looked anxiously at the sky, which was
even grayer than usual. <NAME>orothy stood in the door with <NAME>oto
in her arms, and looked at the sky too. <NAME> was washing
the dishes.
"""
]
, Html.p []
[ text """
From the far north they heard a low wail of the wind, and
Uncle Hen<NAME> and <NAME>othy could see where the long grass
bowed in waves before the coming storm. There now came a
sharp whistling in the air from the south, and as they
turned their eyes that way they saw ripples in the grass
coming from that direction also.
"""
]
]
, actions =
[ textButton { buttonConfig | onClick = Just Close } "Decline"
, textButton { buttonConfig | onClick = Just Close } "Continue"
]
}
| true | module Demo.Dialog exposing (Model, Msg(..), defaultModel, update, view)
import Demo.CatalogPage exposing (CatalogPage)
import Demo.Helper.ResourceLink as ResourceLink
import Html exposing (Html, text)
import Html.Attributes exposing (class, style)
import Html.Events
import Material.Button exposing (buttonConfig, textButton)
import Material.Dialog exposing (dialog, dialogConfig)
import Material.Icon exposing (icon, iconConfig)
import Material.List exposing (list, listConfig, listItem, listItemConfig, listItemGraphic, listItemText)
import Material.Radio exposing (radio, radioConfig)
import Material.Typography as Typography
type alias Model =
{ openDialog : Maybe String
}
defaultModel : Model
defaultModel =
{ openDialog = Nothing
}
type Msg
= Close
| Show String
update : Msg -> Model -> Model
update msg model =
case msg of
Close ->
{ model | openDialog = Nothing }
Show index ->
{ model | openDialog = Just index }
view : Model -> CatalogPage Msg
view model =
{ title = "Dialog"
, prelude = "Dialogs inform users about a specific task and may contain critical information, require decisions, or involve multiple tasks."
, resources =
{ materialDesignGuidelines = Just "https://material.io/go/design-dialogs"
, documentation = Just "https://package.elm-lang.org/packages/aforemny/material-components-web-elm/latest/Material-Dialog"
, sourceCode = Just "https://github.com/material-components/material-components-web/tree/master/packages/mdc-dialog"
}
, hero = heroDialog
, content =
[ textButton
{ buttonConfig | onClick = Just (Show "dialog-alert-dialog") }
"Alert"
, text " "
, textButton
{ buttonConfig | onClick = Just (Show "dialog-simple-dialog") }
"Simple"
, text " "
, textButton
{ buttonConfig | onClick = Just (Show "dialog-confirmation-dialog") }
"Confirmation"
, text " "
, textButton
{ buttonConfig | onClick = Just (Show "dialog-scrollable-dialog") }
"Scrollable"
, text " "
, alertDialog model
, simpleDialog model
, confirmationDialog model
, scrollableDialog model
]
}
heroDialog : List (Html Msg)
heroDialog =
[ Html.div
[ class "mdc-dialog mdc-dialog--open"
, style "position" "relative"
]
[ Html.div
[ class "mdc-dialog__surface" ]
[ Html.div [ class "mdc-dialog__title" ] [ text "Get this party started?" ]
, Html.div [ class "mdc-dialog__content" ]
[ text "Turn up the jams and have a good time." ]
, Html.div [ class "mdc-dialog__actions" ]
[ textButton buttonConfig "Decline"
, textButton buttonConfig "Accept"
]
]
]
]
alertDialog : Model -> Html Msg
alertDialog model =
dialog
{ dialogConfig
| open = model.openDialog == Just "dialog-alert-dialog"
, onClose = Just Close
}
{ title = Nothing
, content =
[ text "Discard draft?" ]
, actions =
[ textButton { buttonConfig | onClick = Just Close } "Cancel"
, textButton { buttonConfig | onClick = Just Close } "Discard"
]
}
simpleDialog : Model -> Html Msg
simpleDialog model =
dialog
{ dialogConfig
| open = model.openDialog == Just "dialog-simple-dialog"
, onClose = Just Close
}
{ title = Just "Select an account"
, content =
[ list { listConfig | avatarList = True }
[ listItem
{ listItemConfig
| additionalAttributes =
[ Html.Attributes.tabindex 0
, Html.Events.onClick Close
]
}
[ listItemGraphic
[ Html.Attributes.style "background-color" "rgba(0,0,0,.3)"
, Html.Attributes.style "color" "#fff"
]
[ icon iconConfig "person" ]
, listItemText [] [ text "PI:EMAIL:<EMAIL>END_PI" ]
]
, listItem
{ listItemConfig
| additionalAttributes =
[ Html.Attributes.tabindex 0
, Html.Events.onClick Close
]
}
[ listItemGraphic
[ Html.Attributes.style "background-color" "rgba(0,0,0,.3)"
, Html.Attributes.style "color" "#fff"
]
[ icon iconConfig "person" ]
, listItemText [] [ text "PI:EMAIL:<EMAIL>END_PI" ]
]
, listItem
{ listItemConfig
| additionalAttributes =
[ Html.Attributes.tabindex 0
, Html.Events.onClick Close
]
}
[ listItemGraphic
[ Html.Attributes.style "background-color" "rgba(0,0,0,.3)"
, Html.Attributes.style "color" "#fff"
]
[ icon iconConfig "add" ]
, listItemText [] [ text "Add account" ]
]
]
]
, actions = []
}
confirmationDialog : Model -> Html Msg
confirmationDialog model =
dialog
{ dialogConfig
| open = model.openDialog == Just "dialog-confirmation-dialog"
, onClose = Just Close
}
{ title = Just "Phone ringtone"
, content =
[ list { listConfig | avatarList = True }
[ listItem listItemConfig
[ listItemGraphic [] [ radio { radioConfig | checked = True } ]
, listItemText [] [ text "Never Gonna Give You Up" ]
]
, listItem listItemConfig
[ listItemGraphic [] [ radio radioConfig ]
, listItemText [] [ text "Hot Cross Buns" ]
]
, listItem listItemConfig
[ listItemGraphic [] [ radio radioConfig ]
, listItemText [] [ text "None" ]
]
]
]
, actions =
[ textButton { buttonConfig | onClick = Just Close } "Cancel"
, textButton { buttonConfig | onClick = Just Close } "OK"
]
}
scrollableDialog : Model -> Html Msg
scrollableDialog model =
dialog
{ dialogConfig
| open = model.openDialog == Just "dialog-scrollable-dialog"
, onClose = Just Close
}
{ title = Just "The Wonderful Wizard of Oz"
, content =
[ Html.p []
[ text """
PI:NAME:<NAME>END_PIorothy lived in the midst of the great Kansas prairies,
with PI:NAME:<NAME>END_PI, who was a farPI:NAME:<NAME>END_PI, and PI:NAME:<NAME>END_PI, who was
the farmer's wife. Their house was small, for the lumber to
build it had to be carried by wagon many miles. There were
four walls, a floor and a roof, which made one room; and
this room contained a rusty looking cookstove, a cupboard
for the dishes, a table, three or four chairs, and the
beds. Uncle PI:NAME:<NAME>END_PI and PI:NAME:<NAME>END_PI had a big bed in one corner,
and PI:NAME:<NAME>END_PI a little bed in another corner. There was no
garret at all, and no cellar--except a small hole dug in
the ground, called a cyclone cellar, where the family could
go in case one of those great whirlwinds arose, mighty
enough to crush any building in its path. It was reached by
a trap door in the middle of the floor, from which a ladder
led down into the small, dark hole.
"""
]
, Html.p []
[ text """
When PI:NAME:<NAME>END_PI stood in the doorway and looked around, she
could see nothing but the great gray prairie on every side.
Not a tree nor a house broke the broad sweep of flat
country that reached to the edge of the sky in all
directions. The sun had baked the plowed land into a gray
mass, with little cracks running through it. Even the grass
was not green, for the sun had burned the tops of the long
blades until they were the same gray color to be seen
everywhere. Once the house had been painted, but the sun
blistered the paint and the rains washed it away, and now
the house was as dull and gray as everything else.
"""
]
, Html.p []
[ text """
When PI:NAME:<NAME>END_PI came there to live she was a young, pretty
wife. The sun and wind had changed her, too. They had taken
the sparkle from her eyes and left them a sober gray; they
had taken the red from her cheeks and lips, and they were
gray also. She was thin and gaunt, and never smiled now.
When PI:NAME:<NAME>END_PI, who was an orphan, first came to her, PI:NAME:<NAME>END_PI PI:NAME:<NAME>END_PI
had been so startled by the child's laughter that she would
scream and press her hand upon her heart whenever PI:NAME:<NAME>END_PIorothy's
merry voice reached her ears; and she still looked at the
little girl with wonder that she could find anything to
laugh at.
"""
]
, Html.p []
[ text """
Uncle HenPI:NAME:<NAME>END_PI never laughed. He worked hard from morning till
night and did not know what joy was. He was gray also, from
his long beard to his rough boots, and he looked stern and
solemn, and rarely spoke.
"""
]
, Html.p []
[ text """
It was PI:NAME:<NAME>END_PIoto that made PI:NAME:<NAME>END_PIorothy laugh, and saved her from
growing as gray as her other surroundings. Toto was not
gray; he was a little black dog, with long silky hair and
small black eyes that twinkled merrily on either side of
his funny, wee nose. Toto played all day long, and PI:NAME:<NAME>END_PIorothy
played with him, and loved him dearly.
"""
]
, Html.p []
[ text """
Today, however, they were not playing. Uncle HenPI:NAME:<NAME>END_PI sat upon
the doorstep and looked anxiously at the sky, which was
even grayer than usual. PI:NAME:<NAME>END_PIorothy stood in the door with PI:NAME:<NAME>END_PIoto
in her arms, and looked at the sky too. PI:NAME:<NAME>END_PI was washing
the dishes.
"""
]
, Html.p []
[ text """
From the far north they heard a low wail of the wind, and
Uncle HenPI:NAME:<NAME>END_PI and PI:NAME:<NAME>END_PIothy could see where the long grass
bowed in waves before the coming storm. There now came a
sharp whistling in the air from the south, and as they
turned their eyes that way they saw ripples in the grass
coming from that direction also.
"""
]
]
, actions =
[ textButton { buttonConfig | onClick = Just Close } "Decline"
, textButton { buttonConfig | onClick = Just Close } "Continue"
]
}
| elm |
[
{
"context": "e version of the OpenAPI document: 1.0\n Contact: sainth@sainth.de\n\n NOTE: This file is auto generated by the open",
"end": 191,
"score": 0.9999152422,
"start": 175,
"tag": "EMAIL",
"value": "sainth@sainth.de"
},
{
"context": "d by the openapi-generator.\n https://github.com/openapitools/openapi-generator.git\n Do not edit this file ma",
"end": 290,
"score": 0.9996531606,
"start": 278,
"tag": "USERNAME",
"value": "openapitools"
}
] | elm/src/Data/Memory.elm | sainthDE/pgtune_frontend | 0 | {-
pgtune
A service to generate some optimized configuration parameters for PostgreSQL based on best practices.
The version of the OpenAPI document: 1.0
Contact: sainth@sainth.de
NOTE: This file is auto generated by the openapi-generator.
https://github.com/openapitools/openapi-generator.git
Do not edit this file manually.
-}
module Data.Memory exposing (Memory, decoder, encode)
import Data.SizeUnit as SizeUnit exposing (SizeUnit)
import Dict exposing (Dict)
import Json.Decode as Decode exposing (Decoder)
import Json.Decode.Pipeline exposing (optional, required)
import Json.Encode as Encode
type alias Memory =
{ memory : Int
, unit : SizeUnit
}
decoder : Decoder Memory
decoder =
Decode.succeed Memory
|> required "memory" Decode.int
|> required "unit" SizeUnit.decoder
encode : Memory -> Encode.Value
encode model =
Encode.object
[ ( "memory", Encode.int model.memory )
, ( "unit", SizeUnit.encode model.unit )
]
| 38703 | {-
pgtune
A service to generate some optimized configuration parameters for PostgreSQL based on best practices.
The version of the OpenAPI document: 1.0
Contact: <EMAIL>
NOTE: This file is auto generated by the openapi-generator.
https://github.com/openapitools/openapi-generator.git
Do not edit this file manually.
-}
module Data.Memory exposing (Memory, decoder, encode)
import Data.SizeUnit as SizeUnit exposing (SizeUnit)
import Dict exposing (Dict)
import Json.Decode as Decode exposing (Decoder)
import Json.Decode.Pipeline exposing (optional, required)
import Json.Encode as Encode
type alias Memory =
{ memory : Int
, unit : SizeUnit
}
decoder : Decoder Memory
decoder =
Decode.succeed Memory
|> required "memory" Decode.int
|> required "unit" SizeUnit.decoder
encode : Memory -> Encode.Value
encode model =
Encode.object
[ ( "memory", Encode.int model.memory )
, ( "unit", SizeUnit.encode model.unit )
]
| true | {-
pgtune
A service to generate some optimized configuration parameters for PostgreSQL based on best practices.
The version of the OpenAPI document: 1.0
Contact: PI:EMAIL:<EMAIL>END_PI
NOTE: This file is auto generated by the openapi-generator.
https://github.com/openapitools/openapi-generator.git
Do not edit this file manually.
-}
module Data.Memory exposing (Memory, decoder, encode)
import Data.SizeUnit as SizeUnit exposing (SizeUnit)
import Dict exposing (Dict)
import Json.Decode as Decode exposing (Decoder)
import Json.Decode.Pipeline exposing (optional, required)
import Json.Encode as Encode
type alias Memory =
{ memory : Int
, unit : SizeUnit
}
decoder : Decoder Memory
decoder =
Decode.succeed Memory
|> required "memory" Decode.int
|> required "unit" SizeUnit.decoder
encode : Memory -> Encode.Value
encode model =
Encode.object
[ ( "memory", Encode.int model.memory )
, ( "unit", SizeUnit.encode model.unit )
]
| elm |
[
{
"context": " Element.paddingXY 12 0 ]\n [ Element.text \"Carlos\"\n , viewMenu\n ]\n\n\nviewMenu : Elemen",
"end": 1373,
"score": 0.9940210581,
"start": 1367,
"tag": "NAME",
"value": "Carlos"
}
] | s09-mi-portafolio-l03-menu/src/Main.elm | csaltos/crear-sitios-web-con-elm | 0 | module Main exposing (..)
import Browser
import Browser.Navigation
import Element
import Element.Font
import Url
main : Program () Model Msg
main =
Browser.application
{ init = init
, view = view
, update = update
, subscriptions = subscriptions
, onUrlChange = onUrlChange
, onUrlRequest = onUrlRequest
}
onUrlRequest : Browser.UrlRequest -> Msg
onUrlRequest urlRequest =
MsgCambiarDePagina
onUrlChange : Url.Url -> Msg
onUrlChange url =
MsgCambiarDePagina
subscriptions : Model -> Sub Msg
subscriptions model =
Sub.none
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
MsgCambiarDePagina ->
( { model | titulo = "Nueva página" }
, Cmd.none
)
view : Model -> Browser.Document Msg
view model =
{ title = model.titulo
, body = [ Element.layout [] viewPage ]
}
viewPage : Element.Element Msg
viewPage =
Element.column
[ Element.padding 22
, Element.spacing 12
]
[ viewCabecera
, Element.text "Home"
, viewContenido
]
viewContenido : Element.Element msg
viewContenido =
Element.text "Esta es la página del home"
viewCabecera : Element.Element msg
viewCabecera =
Element.row [ Element.paddingXY 12 0 ]
[ Element.text "Carlos"
, viewMenu
]
viewMenu : Element.Element msg
viewMenu =
Element.row
[ Element.paddingXY 12 0
, Element.spacing 12
]
[ Element.link [ Element.Font.underline ]
{ url = "/ayuda"
, label = Element.text "Ayuda"
}
, Element.link [ Element.Font.underline ]
{ url = "/hoja-de-vida"
, label = Element.text "Hoja de vida"
}
]
init : flags -> Url.Url -> Browser.Navigation.Key -> ( Model, Cmd Msg )
init flags url navigationKey =
( initModel, Cmd.none )
initModel =
{ titulo = "Home page" }
type Msg
= MsgCambiarDePagina
type alias Model =
{ titulo : String
}
| 51355 | module Main exposing (..)
import Browser
import Browser.Navigation
import Element
import Element.Font
import Url
main : Program () Model Msg
main =
Browser.application
{ init = init
, view = view
, update = update
, subscriptions = subscriptions
, onUrlChange = onUrlChange
, onUrlRequest = onUrlRequest
}
onUrlRequest : Browser.UrlRequest -> Msg
onUrlRequest urlRequest =
MsgCambiarDePagina
onUrlChange : Url.Url -> Msg
onUrlChange url =
MsgCambiarDePagina
subscriptions : Model -> Sub Msg
subscriptions model =
Sub.none
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
MsgCambiarDePagina ->
( { model | titulo = "Nueva página" }
, Cmd.none
)
view : Model -> Browser.Document Msg
view model =
{ title = model.titulo
, body = [ Element.layout [] viewPage ]
}
viewPage : Element.Element Msg
viewPage =
Element.column
[ Element.padding 22
, Element.spacing 12
]
[ viewCabecera
, Element.text "Home"
, viewContenido
]
viewContenido : Element.Element msg
viewContenido =
Element.text "Esta es la página del home"
viewCabecera : Element.Element msg
viewCabecera =
Element.row [ Element.paddingXY 12 0 ]
[ Element.text "<NAME>"
, viewMenu
]
viewMenu : Element.Element msg
viewMenu =
Element.row
[ Element.paddingXY 12 0
, Element.spacing 12
]
[ Element.link [ Element.Font.underline ]
{ url = "/ayuda"
, label = Element.text "Ayuda"
}
, Element.link [ Element.Font.underline ]
{ url = "/hoja-de-vida"
, label = Element.text "Hoja de vida"
}
]
init : flags -> Url.Url -> Browser.Navigation.Key -> ( Model, Cmd Msg )
init flags url navigationKey =
( initModel, Cmd.none )
initModel =
{ titulo = "Home page" }
type Msg
= MsgCambiarDePagina
type alias Model =
{ titulo : String
}
| true | module Main exposing (..)
import Browser
import Browser.Navigation
import Element
import Element.Font
import Url
main : Program () Model Msg
main =
Browser.application
{ init = init
, view = view
, update = update
, subscriptions = subscriptions
, onUrlChange = onUrlChange
, onUrlRequest = onUrlRequest
}
onUrlRequest : Browser.UrlRequest -> Msg
onUrlRequest urlRequest =
MsgCambiarDePagina
onUrlChange : Url.Url -> Msg
onUrlChange url =
MsgCambiarDePagina
subscriptions : Model -> Sub Msg
subscriptions model =
Sub.none
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
MsgCambiarDePagina ->
( { model | titulo = "Nueva página" }
, Cmd.none
)
view : Model -> Browser.Document Msg
view model =
{ title = model.titulo
, body = [ Element.layout [] viewPage ]
}
viewPage : Element.Element Msg
viewPage =
Element.column
[ Element.padding 22
, Element.spacing 12
]
[ viewCabecera
, Element.text "Home"
, viewContenido
]
viewContenido : Element.Element msg
viewContenido =
Element.text "Esta es la página del home"
viewCabecera : Element.Element msg
viewCabecera =
Element.row [ Element.paddingXY 12 0 ]
[ Element.text "PI:NAME:<NAME>END_PI"
, viewMenu
]
viewMenu : Element.Element msg
viewMenu =
Element.row
[ Element.paddingXY 12 0
, Element.spacing 12
]
[ Element.link [ Element.Font.underline ]
{ url = "/ayuda"
, label = Element.text "Ayuda"
}
, Element.link [ Element.Font.underline ]
{ url = "/hoja-de-vida"
, label = Element.text "Hoja de vida"
}
]
init : flags -> Url.Url -> Browser.Navigation.Key -> ( Model, Cmd Msg )
init flags url navigationKey =
( initModel, Cmd.none )
initModel =
{ titulo = "Home page" }
type Msg
= MsgCambiarDePagina
type alias Model =
{ titulo : String
}
| elm |
[
{
"context": "\n\ndefaultModel : Model\ndefaultModel =\n { name = \"Cool Person\" }\n\n\nupdate : Msg -> Model -> ( Model, Cmd Msg )\n",
"end": 335,
"score": 0.9971963167,
"start": 324,
"tag": "NAME",
"value": "Cool Person"
},
{
"context": "e msg of\n DoClick ->\n ( { model | name = \"Awesome Person\" }, Cmd.none )\n DoSpecialClick modifier ->\n ",
"end": 473,
"score": 0.9966956973,
"start": 459,
"tag": "NAME",
"value": "Awesome Person"
}
] | tests/src/Elmer/TestApps/LazyTestApp.elm | jbwatkins/elmer | 44 | module Elmer.TestApps.LazyTestApp exposing (..)
import Html exposing (Html)
import Html.Attributes as Attr
import Html.Events as Events
import Html.Lazy exposing (lazy, lazy2, lazy3)
type alias Model =
{ name : String }
type Msg
= DoClick
| DoSpecialClick String
defaultModel : Model
defaultModel =
{ name = "Cool Person" }
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
DoClick ->
( { model | name = "Awesome Person" }, Cmd.none )
DoSpecialClick modifier ->
( { model | name = modifier ++ " " ++ model.name }, Cmd.none )
view : Model -> Html Msg
view model =
Html.div
[ Attr.id "root", Attr.class "styled no-events", Events.onClick DoClick ]
[ Html.div [ Attr.id "name-text" ]
[ lazy subView model ]
]
subView : Model -> Html Msg
subView model =
Html.div [ Attr.id "lazy-div" ]
[ Html.text <| "Some name: " ++ model.name
]
lazyView2 : Model -> Html Msg
lazyView2 model =
Html.div
[ Attr.id "root", Attr.class "styled no-events" ]
[ lazy2 subView2 model "bowling" ]
subView2 : Model -> String -> Html Msg
subView2 model activity =
Html.div [ Attr.id "lazy-div", Events.onClick <| DoSpecialClick "Happy" ]
[ Html.text <| model.name ++ " likes " ++ activity
]
lazyView3 : Model -> Html Msg
lazyView3 model =
Html.div
[ Attr.id "root", Attr.class "styled no-events" ]
[ lazy3 subView3 model "bowling" 5 ]
subView3 : Model -> String -> Int -> Html Msg
subView3 model activity times =
model.name ++ " likes " ++ activity ++ " " ++ (String.fromInt times) ++ " times a week!"
|> Html.text
| 3276 | module Elmer.TestApps.LazyTestApp exposing (..)
import Html exposing (Html)
import Html.Attributes as Attr
import Html.Events as Events
import Html.Lazy exposing (lazy, lazy2, lazy3)
type alias Model =
{ name : String }
type Msg
= DoClick
| DoSpecialClick String
defaultModel : Model
defaultModel =
{ name = "<NAME>" }
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
DoClick ->
( { model | name = "<NAME>" }, Cmd.none )
DoSpecialClick modifier ->
( { model | name = modifier ++ " " ++ model.name }, Cmd.none )
view : Model -> Html Msg
view model =
Html.div
[ Attr.id "root", Attr.class "styled no-events", Events.onClick DoClick ]
[ Html.div [ Attr.id "name-text" ]
[ lazy subView model ]
]
subView : Model -> Html Msg
subView model =
Html.div [ Attr.id "lazy-div" ]
[ Html.text <| "Some name: " ++ model.name
]
lazyView2 : Model -> Html Msg
lazyView2 model =
Html.div
[ Attr.id "root", Attr.class "styled no-events" ]
[ lazy2 subView2 model "bowling" ]
subView2 : Model -> String -> Html Msg
subView2 model activity =
Html.div [ Attr.id "lazy-div", Events.onClick <| DoSpecialClick "Happy" ]
[ Html.text <| model.name ++ " likes " ++ activity
]
lazyView3 : Model -> Html Msg
lazyView3 model =
Html.div
[ Attr.id "root", Attr.class "styled no-events" ]
[ lazy3 subView3 model "bowling" 5 ]
subView3 : Model -> String -> Int -> Html Msg
subView3 model activity times =
model.name ++ " likes " ++ activity ++ " " ++ (String.fromInt times) ++ " times a week!"
|> Html.text
| true | module Elmer.TestApps.LazyTestApp exposing (..)
import Html exposing (Html)
import Html.Attributes as Attr
import Html.Events as Events
import Html.Lazy exposing (lazy, lazy2, lazy3)
type alias Model =
{ name : String }
type Msg
= DoClick
| DoSpecialClick String
defaultModel : Model
defaultModel =
{ name = "PI:NAME:<NAME>END_PI" }
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
DoClick ->
( { model | name = "PI:NAME:<NAME>END_PI" }, Cmd.none )
DoSpecialClick modifier ->
( { model | name = modifier ++ " " ++ model.name }, Cmd.none )
view : Model -> Html Msg
view model =
Html.div
[ Attr.id "root", Attr.class "styled no-events", Events.onClick DoClick ]
[ Html.div [ Attr.id "name-text" ]
[ lazy subView model ]
]
subView : Model -> Html Msg
subView model =
Html.div [ Attr.id "lazy-div" ]
[ Html.text <| "Some name: " ++ model.name
]
lazyView2 : Model -> Html Msg
lazyView2 model =
Html.div
[ Attr.id "root", Attr.class "styled no-events" ]
[ lazy2 subView2 model "bowling" ]
subView2 : Model -> String -> Html Msg
subView2 model activity =
Html.div [ Attr.id "lazy-div", Events.onClick <| DoSpecialClick "Happy" ]
[ Html.text <| model.name ++ " likes " ++ activity
]
lazyView3 : Model -> Html Msg
lazyView3 model =
Html.div
[ Attr.id "root", Attr.class "styled no-events" ]
[ lazy3 subView3 model "bowling" 5 ]
subView3 : Model -> String -> Int -> Html Msg
subView3 model activity times =
model.name ++ " likes " ++ activity ++ " " ++ (String.fromInt times) ++ " times a week!"
|> Html.text
| elm |
[
{
"context": "x\n , title = \"Welcome to Self-Review, FirstName!\"\n , content = Html.text \"This is wh",
"end": 1708,
"score": 0.7732272744,
"start": 1699,
"tag": "NAME",
"value": "FirstName"
}
] | styleguide-app/Examples/SlideModal.elm | michaelglass/noredink-ui | 0 | module Examples.SlideModal exposing (Msg, State, example, init, update)
{-|
@docs Msg, State, example, init, update
-}
import Accessibility.Styled as Html exposing (Html, div, h3, p, text)
import Assets
import Css
import Html.Styled exposing (fromUnstyled)
import Html.Styled.Attributes exposing (css)
import ModuleExample exposing (Category(..), ModuleExample)
import Nri.Ui.Button.V8 as Button
import Nri.Ui.Colors.V1 as Colors
import Nri.Ui.SlideModal.V2 as SlideModal
import Svg exposing (..)
import Svg.Attributes exposing (..)
{-| -}
type Msg
= ModalMsg SlideModal.State
{-| -}
type alias State =
{ modal : SlideModal.State }
{-| -}
example : (Msg -> msg) -> State -> ModuleExample msg
example parentMessage state =
{ name = "Nri.Ui.SlideModal.V2"
, category = Modals
, content =
[ viewModal state.modal
, modalLaunchButton
]
|> List.map (Html.map parentMessage)
}
{-| -}
init : State
init =
{ modal = SlideModal.closed
}
{-| -}
update : Msg -> State -> ( State, Cmd Msg )
update msg state =
case msg of
ModalMsg modal ->
( { state | modal = modal }, Cmd.none )
-- INTERNAL
modalLaunchButton : Html Msg
modalLaunchButton =
Button.button
{ onClick = ModalMsg SlideModal.open
, size = Button.Small
, style = Button.Secondary
, width = Button.WidthUnbounded
}
{ label = "Launch Modal"
, state = Button.Enabled
, icon = Nothing
}
viewModal : SlideModal.State -> Html Msg
viewModal state =
SlideModal.view
{ panels =
[ { icon = grayBox
, title = "Welcome to Self-Review, FirstName!"
, content = Html.text "This is where the content goes!"
, buttonLabel = "Continue"
}
, { icon = fancyIcon
, title = "Here are the steps we’ll take:"
, content =
div [ css [ Css.height (Css.px 400) ] ]
[ Html.text "Sometimes the content may change height."
]
, buttonLabel = "Okay, keep going!"
}
, { icon = grayBox
, title = "As you revise, remember:"
, content = Html.text "Sometimes things may change back."
, buttonLabel = "Let's get to it!"
}
]
, height = Css.px 400
, parentMsg = ModalMsg
}
state
grayBox : Html msg
grayBox =
div
[ css
[ Css.backgroundColor Colors.gray45
, Css.height (Css.pct 100)
, Css.width (Css.pct 100)
]
]
[]
{-| -}
fancyIcon : Html msg
fancyIcon =
svg [ viewBox "0 0 100 100", version "1.1" ]
[ g
[ id "Page-1"
, stroke "none"
, strokeWidth "1"
, fill "none"
, fillRule "evenodd"
]
[ g
[ id "tree-3"
, fillRule "nonzero"
]
[ Svg.path
[ d "M44.2554458,76.2957186 C36.1962642,76.2957186 29.6386609,69.7388768 29.6386609,61.6796952 L29.6386609,55.8332857 C29.6386609,54.2174899 30.9468311,52.9100811 32.5618657,52.9100811 C34.1776615,52.9100811 35.4858318,54.2174899 35.4858318,55.8332857 L35.4858318,61.6796952 C35.4858318,66.5156609 39.4194799,70.4493092 44.2554458,70.4493092 C45.8712416,70.4493092 47.1786506,71.7567179 47.1786506,73.3725139 C47.1786506,74.9883097 45.8712416,76.2957186 44.2554458,76.2957186 L44.2554458,76.2957186 Z"
, id "Path"
, fill "#D55F05"
]
[]
, Svg.path
[ d "M61.7946739,70.4493092 L55.9482646,70.4493092 C54.3324686,70.4493092 53.0250599,69.1419004 53.0250599,67.5261044 C53.0250599,65.9103087 54.3324686,64.6028997 55.9482646,64.6028997 L61.7946739,64.6028997 C66.6298784,64.6028997 70.5642881,60.6692516 70.5642881,55.8332857 C70.5642881,54.2174899 71.8716969,52.9100811 73.4874928,52.9100811 C75.1032886,52.9100811 76.4106974,54.2174899 76.4106974,55.8332857 C76.4106974,63.8924673 69.8530942,70.4493092 61.7946739,70.4493092 L61.7946739,70.4493092 Z"
, id "Path"
, fill "#913F02"
]
[]
, Svg.path
[ d "M58.8714693,55.8332857 L58.8714693,95.7256259 L41.3322411,95.7256259 L41.3322411,55.8332857 C41.3322411,54.1961693 42.6175679,52.9100811 44.2554458,52.9100811 L55.9482646,52.9100811 C57.585381,52.9100811 58.8714693,54.1961693 58.8714693,55.8332857 L58.8714693,55.8332857 Z"
, id "Path"
, fill "#D97F4A"
]
[]
, Svg.path
[ d "M58.8714693,55.8332857 L58.8714693,95.7256259 L50.1018553,95.7256259 L50.1018553,52.9100811 L55.9482646,52.9100811 C57.585381,52.9100811 58.8714693,54.1961693 58.8714693,55.8332857 L58.8714693,55.8332857 Z"
, id "Path"
, fill "#D55F05"
]
[]
, Svg.path
[ d "M99.9326344,40.1063075 L99.9326344,40.164939 C98.9975744,50.2206412 90.0916609,58.1716972 79.5090354,58.7564904 L44.2554458,58.7564904 L49.9259604,51.7412561 C49.984592,51.7412561 50.0432236,51.6826243 50.1018553,51.6239928 C55.246208,49.4020832 58.8714693,44.2569686 58.8714693,38.2940576 C58.8714693,32.2725149 55.246208,27.1274003 50.1018553,24.9054908 C48.2888439,24.0876939 46.3014607,23.6780341 44.2554458,23.6780341 L26.7154562,23.6780341 C25.0783398,23.6780341 23.7922517,22.391946 23.7922517,20.7548294 C23.7922517,9.47090917 32.9715254,0.097465538 44.2554458,0.097465538 L67.6410834,0.097465538 C78.5739756,0.097465538 87.5773547,8.94474751 88.1035161,19.7611378 C95.7621755,23.4442691 100.633929,31.5118267 99.9326344,40.1063075 L99.9326344,40.1063075 Z"
, id "Path"
, fill "#9CDD05"
]
[]
, Svg.path
[ d "M99.9326344,40.1063075 L99.9326344,40.164939 C98.9975744,50.2206412 90.0916609,58.1716972 79.5090354,58.7564904 L50.1018553,58.7564904 L50.1018553,51.6239928 C55.246208,49.4020832 58.8714693,44.2569686 58.8714693,38.2940576 C58.8714693,32.2725149 55.246208,27.1274003 50.1018553,24.9054908 L50.1018553,0.097465538 L67.6410834,0.097465538 C78.5739756,0.097465538 87.5773547,8.94474751 88.1035161,19.7611378 C95.7621755,23.4442691 100.633929,31.5118267 99.9326344,40.1063075 L99.9326344,40.1063075 Z"
, id "Path"
, fill "#66BB00"
]
[]
, Svg.path
[ d "M50.1018553,18.7080531 C48.2309737,18.1240213 46.3014607,17.8316247 44.2554458,17.8316247 L20.8690469,17.8316247 C15.1985323,17.8316247 9.93615468,20.0535344 6.07789013,24.1455642 C2.16099382,28.2383552 -0.0220819872,33.6751048 0.211682965,39.4050125 C0.796476291,50.045508 10.5795797,58.7564904 21.8048683,58.7564904 L44.2554458,58.7564904 C46.3014607,58.7564904 48.2309737,58.4640938 50.1018553,57.8793006 C58.5204412,55.3657558 64.7178786,47.5312018 64.7178786,38.2940576 C64.7178786,29.0569135 58.5204412,21.2223595 50.1018553,18.7080531 Z"
, id "Path"
, fill "#C3EA21"
]
[]
, Svg.path
[ d "M64.7178786,38.2940576 C64.7178786,47.5312018 58.5204412,55.3657558 50.1018553,57.8793006 L50.1018553,18.7080531 C58.5204412,21.2223595 64.7178786,29.0569135 64.7178786,38.2940576 L64.7178786,38.2940576 Z"
, id "Path"
, fill "#9CDD05"
]
[]
, Svg.path
[ d "M88.1035161,38.2940576 C88.1035161,39.9083306 86.7945844,41.2172622 85.1803114,41.2172622 C83.5660384,41.2172622 82.2571069,39.9083306 82.2571069,38.2940576 C82.2571069,36.6797847 83.5660384,35.3708529 85.1803114,35.3708529 C86.7945844,35.3708529 88.1035161,36.6797847 88.1035161,38.2940576 L88.1035161,38.2940576 Z"
, id "Path"
, fill "#CD0000"
]
[]
, Svg.path
[ d "M76.4106974,20.7548294 C76.4106974,22.3691024 75.1017658,23.6780341 73.4874928,23.6780341 C71.8732199,23.6780341 70.5642881,22.3691024 70.5642881,20.7548294 C70.5642881,19.1405564 71.8732199,17.8316247 73.4874928,17.8316247 C75.1017658,17.8316247 76.4106974,19.1405564 76.4106974,20.7548294 L76.4106974,20.7548294 Z"
, id "Path"
, fill "#CD0000"
]
[]
, Svg.path
[ d "M53.0250599,38.2940576 C53.0250599,39.931174 51.7389717,41.2172622 50.1018553,41.2172622 C48.4639774,41.2172622 47.1786506,39.931174 47.1786506,38.2940576 C47.1786506,36.6569411 48.4639774,35.3708529 50.1018553,35.3708529 C51.7389717,35.3708529 53.0250599,36.6569411 53.0250599,38.2940576 Z"
, id "Path"
, fill "#FF637B"
]
[]
, Svg.path
[ d "M76.4106974,44.1404669 C76.4106974,45.7547399 75.1017658,47.0636717 73.4874928,47.0636717 C71.8732199,47.0636717 70.5642881,45.7547399 70.5642881,44.1404669 C70.5642881,42.5261939 71.8732199,41.2172622 73.4874928,41.2172622 C75.1017658,41.2172622 76.4106974,42.5261939 76.4106974,44.1404669 L76.4106974,44.1404669 Z"
, id "Path"
, fill "#CD0000"
]
[]
, Svg.path
[ d "M41.3322411,44.1404669 C41.3322411,45.7547399 40.0233093,47.0636717 38.4090364,47.0636717 C36.794002,47.0636717 35.4858318,45.7547399 35.4858318,44.1404669 C35.4858318,42.5261939 36.794002,41.2172622 38.4090364,41.2172622 C40.0233093,41.2172622 41.3322411,42.5261939 41.3322411,44.1404669 Z"
, id "Path"
, fill "#FF637B"
]
[]
, Svg.path
[ d "M23.7922517,44.1404669 C23.7922517,45.7547399 22.4840813,47.0636717 20.8690469,47.0636717 C19.2547739,47.0636717 17.9458422,45.7547399 17.9458422,44.1404669 C17.9458422,42.5261939 19.2547739,41.2172622 20.8690469,41.2172622 C22.4840813,41.2172622 23.7922517,42.5261939 23.7922517,44.1404669 Z"
, id "Path"
, fill "#FF637B"
]
[]
, Svg.path
[ d "M64.7178786,14.9084201 C64.7178786,16.5226931 63.4089469,17.8316247 61.7946739,17.8316247 C60.1796395,17.8316247 58.8714693,16.5226931 58.8714693,14.9084201 C58.8714693,13.2941471 60.1796395,11.9852154 61.7946739,11.9852154 C63.4089469,11.9852154 64.7178786,13.2941471 64.7178786,14.9084201 L64.7178786,14.9084201 Z"
, id "Path"
, fill "#CD0000"
]
[]
, Svg.path
[ d "M29.6386609,32.4476482 C29.6386609,34.0619211 28.3304908,35.3708529 26.7154562,35.3708529 C25.1011832,35.3708529 23.7922517,34.0619211 23.7922517,32.4476482 C23.7922517,30.8333752 25.1011832,29.5244436 26.7154562,29.5244436 C28.3304908,29.5244436 29.6386609,30.8333752 29.6386609,32.4476482 Z"
, id "Path"
, fill "#FF637B"
]
[]
, Svg.path
[ d "M50.1018553,41.2172622 L50.1018553,35.3708529 C51.7389717,35.3708529 53.0250599,36.6569411 53.0250599,38.2940576 C53.0250599,39.931174 51.7389717,41.2172622 50.1018553,41.2172622 Z"
, id "Path"
, fill "#FF001E"
]
[]
, Svg.path
[ d "M76.4106974,96.9530825 C76.4106974,98.5901989 75.1246092,99.8762872 73.4874928,99.8762872 L26.7154562,99.8762872 C25.0783398,99.8762872 23.7922517,98.5901989 23.7922517,96.9530825 C23.7922517,95.3159659 25.0783398,94.0298778 26.7154562,94.0298778 L73.4874928,94.0298778 C75.1246092,94.0298778 76.4106974,95.3159659 76.4106974,96.9530825 L76.4106974,96.9530825 Z"
, id "Path"
, fill "#C3EA21"
]
[]
, Svg.path
[ d "M76.4106974,96.9530825 C76.4106974,98.5901989 75.1246092,99.8762872 73.4874928,99.8762872 L50.1018553,99.8762872 L50.1018553,94.0298778 L73.4874928,94.0298778 C75.1246092,94.0298778 76.4106974,95.3159659 76.4106974,96.9530825 L76.4106974,96.9530825 Z"
, id "Path"
, fill "#9CDD05"
]
[]
]
]
]
|> fromUnstyled
| 45346 | module Examples.SlideModal exposing (Msg, State, example, init, update)
{-|
@docs Msg, State, example, init, update
-}
import Accessibility.Styled as Html exposing (Html, div, h3, p, text)
import Assets
import Css
import Html.Styled exposing (fromUnstyled)
import Html.Styled.Attributes exposing (css)
import ModuleExample exposing (Category(..), ModuleExample)
import Nri.Ui.Button.V8 as Button
import Nri.Ui.Colors.V1 as Colors
import Nri.Ui.SlideModal.V2 as SlideModal
import Svg exposing (..)
import Svg.Attributes exposing (..)
{-| -}
type Msg
= ModalMsg SlideModal.State
{-| -}
type alias State =
{ modal : SlideModal.State }
{-| -}
example : (Msg -> msg) -> State -> ModuleExample msg
example parentMessage state =
{ name = "Nri.Ui.SlideModal.V2"
, category = Modals
, content =
[ viewModal state.modal
, modalLaunchButton
]
|> List.map (Html.map parentMessage)
}
{-| -}
init : State
init =
{ modal = SlideModal.closed
}
{-| -}
update : Msg -> State -> ( State, Cmd Msg )
update msg state =
case msg of
ModalMsg modal ->
( { state | modal = modal }, Cmd.none )
-- INTERNAL
modalLaunchButton : Html Msg
modalLaunchButton =
Button.button
{ onClick = ModalMsg SlideModal.open
, size = Button.Small
, style = Button.Secondary
, width = Button.WidthUnbounded
}
{ label = "Launch Modal"
, state = Button.Enabled
, icon = Nothing
}
viewModal : SlideModal.State -> Html Msg
viewModal state =
SlideModal.view
{ panels =
[ { icon = grayBox
, title = "Welcome to Self-Review, <NAME>!"
, content = Html.text "This is where the content goes!"
, buttonLabel = "Continue"
}
, { icon = fancyIcon
, title = "Here are the steps we’ll take:"
, content =
div [ css [ Css.height (Css.px 400) ] ]
[ Html.text "Sometimes the content may change height."
]
, buttonLabel = "Okay, keep going!"
}
, { icon = grayBox
, title = "As you revise, remember:"
, content = Html.text "Sometimes things may change back."
, buttonLabel = "Let's get to it!"
}
]
, height = Css.px 400
, parentMsg = ModalMsg
}
state
grayBox : Html msg
grayBox =
div
[ css
[ Css.backgroundColor Colors.gray45
, Css.height (Css.pct 100)
, Css.width (Css.pct 100)
]
]
[]
{-| -}
fancyIcon : Html msg
fancyIcon =
svg [ viewBox "0 0 100 100", version "1.1" ]
[ g
[ id "Page-1"
, stroke "none"
, strokeWidth "1"
, fill "none"
, fillRule "evenodd"
]
[ g
[ id "tree-3"
, fillRule "nonzero"
]
[ Svg.path
[ d "M44.2554458,76.2957186 C36.1962642,76.2957186 29.6386609,69.7388768 29.6386609,61.6796952 L29.6386609,55.8332857 C29.6386609,54.2174899 30.9468311,52.9100811 32.5618657,52.9100811 C34.1776615,52.9100811 35.4858318,54.2174899 35.4858318,55.8332857 L35.4858318,61.6796952 C35.4858318,66.5156609 39.4194799,70.4493092 44.2554458,70.4493092 C45.8712416,70.4493092 47.1786506,71.7567179 47.1786506,73.3725139 C47.1786506,74.9883097 45.8712416,76.2957186 44.2554458,76.2957186 L44.2554458,76.2957186 Z"
, id "Path"
, fill "#D55F05"
]
[]
, Svg.path
[ d "M61.7946739,70.4493092 L55.9482646,70.4493092 C54.3324686,70.4493092 53.0250599,69.1419004 53.0250599,67.5261044 C53.0250599,65.9103087 54.3324686,64.6028997 55.9482646,64.6028997 L61.7946739,64.6028997 C66.6298784,64.6028997 70.5642881,60.6692516 70.5642881,55.8332857 C70.5642881,54.2174899 71.8716969,52.9100811 73.4874928,52.9100811 C75.1032886,52.9100811 76.4106974,54.2174899 76.4106974,55.8332857 C76.4106974,63.8924673 69.8530942,70.4493092 61.7946739,70.4493092 L61.7946739,70.4493092 Z"
, id "Path"
, fill "#913F02"
]
[]
, Svg.path
[ d "M58.8714693,55.8332857 L58.8714693,95.7256259 L41.3322411,95.7256259 L41.3322411,55.8332857 C41.3322411,54.1961693 42.6175679,52.9100811 44.2554458,52.9100811 L55.9482646,52.9100811 C57.585381,52.9100811 58.8714693,54.1961693 58.8714693,55.8332857 L58.8714693,55.8332857 Z"
, id "Path"
, fill "#D97F4A"
]
[]
, Svg.path
[ d "M58.8714693,55.8332857 L58.8714693,95.7256259 L50.1018553,95.7256259 L50.1018553,52.9100811 L55.9482646,52.9100811 C57.585381,52.9100811 58.8714693,54.1961693 58.8714693,55.8332857 L58.8714693,55.8332857 Z"
, id "Path"
, fill "#D55F05"
]
[]
, Svg.path
[ d "M99.9326344,40.1063075 L99.9326344,40.164939 C98.9975744,50.2206412 90.0916609,58.1716972 79.5090354,58.7564904 L44.2554458,58.7564904 L49.9259604,51.7412561 C49.984592,51.7412561 50.0432236,51.6826243 50.1018553,51.6239928 C55.246208,49.4020832 58.8714693,44.2569686 58.8714693,38.2940576 C58.8714693,32.2725149 55.246208,27.1274003 50.1018553,24.9054908 C48.2888439,24.0876939 46.3014607,23.6780341 44.2554458,23.6780341 L26.7154562,23.6780341 C25.0783398,23.6780341 23.7922517,22.391946 23.7922517,20.7548294 C23.7922517,9.47090917 32.9715254,0.097465538 44.2554458,0.097465538 L67.6410834,0.097465538 C78.5739756,0.097465538 87.5773547,8.94474751 88.1035161,19.7611378 C95.7621755,23.4442691 100.633929,31.5118267 99.9326344,40.1063075 L99.9326344,40.1063075 Z"
, id "Path"
, fill "#9CDD05"
]
[]
, Svg.path
[ d "M99.9326344,40.1063075 L99.9326344,40.164939 C98.9975744,50.2206412 90.0916609,58.1716972 79.5090354,58.7564904 L50.1018553,58.7564904 L50.1018553,51.6239928 C55.246208,49.4020832 58.8714693,44.2569686 58.8714693,38.2940576 C58.8714693,32.2725149 55.246208,27.1274003 50.1018553,24.9054908 L50.1018553,0.097465538 L67.6410834,0.097465538 C78.5739756,0.097465538 87.5773547,8.94474751 88.1035161,19.7611378 C95.7621755,23.4442691 100.633929,31.5118267 99.9326344,40.1063075 L99.9326344,40.1063075 Z"
, id "Path"
, fill "#66BB00"
]
[]
, Svg.path
[ d "M50.1018553,18.7080531 C48.2309737,18.1240213 46.3014607,17.8316247 44.2554458,17.8316247 L20.8690469,17.8316247 C15.1985323,17.8316247 9.93615468,20.0535344 6.07789013,24.1455642 C2.16099382,28.2383552 -0.0220819872,33.6751048 0.211682965,39.4050125 C0.796476291,50.045508 10.5795797,58.7564904 21.8048683,58.7564904 L44.2554458,58.7564904 C46.3014607,58.7564904 48.2309737,58.4640938 50.1018553,57.8793006 C58.5204412,55.3657558 64.7178786,47.5312018 64.7178786,38.2940576 C64.7178786,29.0569135 58.5204412,21.2223595 50.1018553,18.7080531 Z"
, id "Path"
, fill "#C3EA21"
]
[]
, Svg.path
[ d "M64.7178786,38.2940576 C64.7178786,47.5312018 58.5204412,55.3657558 50.1018553,57.8793006 L50.1018553,18.7080531 C58.5204412,21.2223595 64.7178786,29.0569135 64.7178786,38.2940576 L64.7178786,38.2940576 Z"
, id "Path"
, fill "#9CDD05"
]
[]
, Svg.path
[ d "M88.1035161,38.2940576 C88.1035161,39.9083306 86.7945844,41.2172622 85.1803114,41.2172622 C83.5660384,41.2172622 82.2571069,39.9083306 82.2571069,38.2940576 C82.2571069,36.6797847 83.5660384,35.3708529 85.1803114,35.3708529 C86.7945844,35.3708529 88.1035161,36.6797847 88.1035161,38.2940576 L88.1035161,38.2940576 Z"
, id "Path"
, fill "#CD0000"
]
[]
, Svg.path
[ d "M76.4106974,20.7548294 C76.4106974,22.3691024 75.1017658,23.6780341 73.4874928,23.6780341 C71.8732199,23.6780341 70.5642881,22.3691024 70.5642881,20.7548294 C70.5642881,19.1405564 71.8732199,17.8316247 73.4874928,17.8316247 C75.1017658,17.8316247 76.4106974,19.1405564 76.4106974,20.7548294 L76.4106974,20.7548294 Z"
, id "Path"
, fill "#CD0000"
]
[]
, Svg.path
[ d "M53.0250599,38.2940576 C53.0250599,39.931174 51.7389717,41.2172622 50.1018553,41.2172622 C48.4639774,41.2172622 47.1786506,39.931174 47.1786506,38.2940576 C47.1786506,36.6569411 48.4639774,35.3708529 50.1018553,35.3708529 C51.7389717,35.3708529 53.0250599,36.6569411 53.0250599,38.2940576 Z"
, id "Path"
, fill "#FF637B"
]
[]
, Svg.path
[ d "M76.4106974,44.1404669 C76.4106974,45.7547399 75.1017658,47.0636717 73.4874928,47.0636717 C71.8732199,47.0636717 70.5642881,45.7547399 70.5642881,44.1404669 C70.5642881,42.5261939 71.8732199,41.2172622 73.4874928,41.2172622 C75.1017658,41.2172622 76.4106974,42.5261939 76.4106974,44.1404669 L76.4106974,44.1404669 Z"
, id "Path"
, fill "#CD0000"
]
[]
, Svg.path
[ d "M41.3322411,44.1404669 C41.3322411,45.7547399 40.0233093,47.0636717 38.4090364,47.0636717 C36.794002,47.0636717 35.4858318,45.7547399 35.4858318,44.1404669 C35.4858318,42.5261939 36.794002,41.2172622 38.4090364,41.2172622 C40.0233093,41.2172622 41.3322411,42.5261939 41.3322411,44.1404669 Z"
, id "Path"
, fill "#FF637B"
]
[]
, Svg.path
[ d "M23.7922517,44.1404669 C23.7922517,45.7547399 22.4840813,47.0636717 20.8690469,47.0636717 C19.2547739,47.0636717 17.9458422,45.7547399 17.9458422,44.1404669 C17.9458422,42.5261939 19.2547739,41.2172622 20.8690469,41.2172622 C22.4840813,41.2172622 23.7922517,42.5261939 23.7922517,44.1404669 Z"
, id "Path"
, fill "#FF637B"
]
[]
, Svg.path
[ d "M64.7178786,14.9084201 C64.7178786,16.5226931 63.4089469,17.8316247 61.7946739,17.8316247 C60.1796395,17.8316247 58.8714693,16.5226931 58.8714693,14.9084201 C58.8714693,13.2941471 60.1796395,11.9852154 61.7946739,11.9852154 C63.4089469,11.9852154 64.7178786,13.2941471 64.7178786,14.9084201 L64.7178786,14.9084201 Z"
, id "Path"
, fill "#CD0000"
]
[]
, Svg.path
[ d "M29.6386609,32.4476482 C29.6386609,34.0619211 28.3304908,35.3708529 26.7154562,35.3708529 C25.1011832,35.3708529 23.7922517,34.0619211 23.7922517,32.4476482 C23.7922517,30.8333752 25.1011832,29.5244436 26.7154562,29.5244436 C28.3304908,29.5244436 29.6386609,30.8333752 29.6386609,32.4476482 Z"
, id "Path"
, fill "#FF637B"
]
[]
, Svg.path
[ d "M50.1018553,41.2172622 L50.1018553,35.3708529 C51.7389717,35.3708529 53.0250599,36.6569411 53.0250599,38.2940576 C53.0250599,39.931174 51.7389717,41.2172622 50.1018553,41.2172622 Z"
, id "Path"
, fill "#FF001E"
]
[]
, Svg.path
[ d "M76.4106974,96.9530825 C76.4106974,98.5901989 75.1246092,99.8762872 73.4874928,99.8762872 L26.7154562,99.8762872 C25.0783398,99.8762872 23.7922517,98.5901989 23.7922517,96.9530825 C23.7922517,95.3159659 25.0783398,94.0298778 26.7154562,94.0298778 L73.4874928,94.0298778 C75.1246092,94.0298778 76.4106974,95.3159659 76.4106974,96.9530825 L76.4106974,96.9530825 Z"
, id "Path"
, fill "#C3EA21"
]
[]
, Svg.path
[ d "M76.4106974,96.9530825 C76.4106974,98.5901989 75.1246092,99.8762872 73.4874928,99.8762872 L50.1018553,99.8762872 L50.1018553,94.0298778 L73.4874928,94.0298778 C75.1246092,94.0298778 76.4106974,95.3159659 76.4106974,96.9530825 L76.4106974,96.9530825 Z"
, id "Path"
, fill "#9CDD05"
]
[]
]
]
]
|> fromUnstyled
| true | module Examples.SlideModal exposing (Msg, State, example, init, update)
{-|
@docs Msg, State, example, init, update
-}
import Accessibility.Styled as Html exposing (Html, div, h3, p, text)
import Assets
import Css
import Html.Styled exposing (fromUnstyled)
import Html.Styled.Attributes exposing (css)
import ModuleExample exposing (Category(..), ModuleExample)
import Nri.Ui.Button.V8 as Button
import Nri.Ui.Colors.V1 as Colors
import Nri.Ui.SlideModal.V2 as SlideModal
import Svg exposing (..)
import Svg.Attributes exposing (..)
{-| -}
type Msg
= ModalMsg SlideModal.State
{-| -}
type alias State =
{ modal : SlideModal.State }
{-| -}
example : (Msg -> msg) -> State -> ModuleExample msg
example parentMessage state =
{ name = "Nri.Ui.SlideModal.V2"
, category = Modals
, content =
[ viewModal state.modal
, modalLaunchButton
]
|> List.map (Html.map parentMessage)
}
{-| -}
init : State
init =
{ modal = SlideModal.closed
}
{-| -}
update : Msg -> State -> ( State, Cmd Msg )
update msg state =
case msg of
ModalMsg modal ->
( { state | modal = modal }, Cmd.none )
-- INTERNAL
modalLaunchButton : Html Msg
modalLaunchButton =
Button.button
{ onClick = ModalMsg SlideModal.open
, size = Button.Small
, style = Button.Secondary
, width = Button.WidthUnbounded
}
{ label = "Launch Modal"
, state = Button.Enabled
, icon = Nothing
}
viewModal : SlideModal.State -> Html Msg
viewModal state =
SlideModal.view
{ panels =
[ { icon = grayBox
, title = "Welcome to Self-Review, PI:NAME:<NAME>END_PI!"
, content = Html.text "This is where the content goes!"
, buttonLabel = "Continue"
}
, { icon = fancyIcon
, title = "Here are the steps we’ll take:"
, content =
div [ css [ Css.height (Css.px 400) ] ]
[ Html.text "Sometimes the content may change height."
]
, buttonLabel = "Okay, keep going!"
}
, { icon = grayBox
, title = "As you revise, remember:"
, content = Html.text "Sometimes things may change back."
, buttonLabel = "Let's get to it!"
}
]
, height = Css.px 400
, parentMsg = ModalMsg
}
state
grayBox : Html msg
grayBox =
div
[ css
[ Css.backgroundColor Colors.gray45
, Css.height (Css.pct 100)
, Css.width (Css.pct 100)
]
]
[]
{-| -}
fancyIcon : Html msg
fancyIcon =
svg [ viewBox "0 0 100 100", version "1.1" ]
[ g
[ id "Page-1"
, stroke "none"
, strokeWidth "1"
, fill "none"
, fillRule "evenodd"
]
[ g
[ id "tree-3"
, fillRule "nonzero"
]
[ Svg.path
[ d "M44.2554458,76.2957186 C36.1962642,76.2957186 29.6386609,69.7388768 29.6386609,61.6796952 L29.6386609,55.8332857 C29.6386609,54.2174899 30.9468311,52.9100811 32.5618657,52.9100811 C34.1776615,52.9100811 35.4858318,54.2174899 35.4858318,55.8332857 L35.4858318,61.6796952 C35.4858318,66.5156609 39.4194799,70.4493092 44.2554458,70.4493092 C45.8712416,70.4493092 47.1786506,71.7567179 47.1786506,73.3725139 C47.1786506,74.9883097 45.8712416,76.2957186 44.2554458,76.2957186 L44.2554458,76.2957186 Z"
, id "Path"
, fill "#D55F05"
]
[]
, Svg.path
[ d "M61.7946739,70.4493092 L55.9482646,70.4493092 C54.3324686,70.4493092 53.0250599,69.1419004 53.0250599,67.5261044 C53.0250599,65.9103087 54.3324686,64.6028997 55.9482646,64.6028997 L61.7946739,64.6028997 C66.6298784,64.6028997 70.5642881,60.6692516 70.5642881,55.8332857 C70.5642881,54.2174899 71.8716969,52.9100811 73.4874928,52.9100811 C75.1032886,52.9100811 76.4106974,54.2174899 76.4106974,55.8332857 C76.4106974,63.8924673 69.8530942,70.4493092 61.7946739,70.4493092 L61.7946739,70.4493092 Z"
, id "Path"
, fill "#913F02"
]
[]
, Svg.path
[ d "M58.8714693,55.8332857 L58.8714693,95.7256259 L41.3322411,95.7256259 L41.3322411,55.8332857 C41.3322411,54.1961693 42.6175679,52.9100811 44.2554458,52.9100811 L55.9482646,52.9100811 C57.585381,52.9100811 58.8714693,54.1961693 58.8714693,55.8332857 L58.8714693,55.8332857 Z"
, id "Path"
, fill "#D97F4A"
]
[]
, Svg.path
[ d "M58.8714693,55.8332857 L58.8714693,95.7256259 L50.1018553,95.7256259 L50.1018553,52.9100811 L55.9482646,52.9100811 C57.585381,52.9100811 58.8714693,54.1961693 58.8714693,55.8332857 L58.8714693,55.8332857 Z"
, id "Path"
, fill "#D55F05"
]
[]
, Svg.path
[ d "M99.9326344,40.1063075 L99.9326344,40.164939 C98.9975744,50.2206412 90.0916609,58.1716972 79.5090354,58.7564904 L44.2554458,58.7564904 L49.9259604,51.7412561 C49.984592,51.7412561 50.0432236,51.6826243 50.1018553,51.6239928 C55.246208,49.4020832 58.8714693,44.2569686 58.8714693,38.2940576 C58.8714693,32.2725149 55.246208,27.1274003 50.1018553,24.9054908 C48.2888439,24.0876939 46.3014607,23.6780341 44.2554458,23.6780341 L26.7154562,23.6780341 C25.0783398,23.6780341 23.7922517,22.391946 23.7922517,20.7548294 C23.7922517,9.47090917 32.9715254,0.097465538 44.2554458,0.097465538 L67.6410834,0.097465538 C78.5739756,0.097465538 87.5773547,8.94474751 88.1035161,19.7611378 C95.7621755,23.4442691 100.633929,31.5118267 99.9326344,40.1063075 L99.9326344,40.1063075 Z"
, id "Path"
, fill "#9CDD05"
]
[]
, Svg.path
[ d "M99.9326344,40.1063075 L99.9326344,40.164939 C98.9975744,50.2206412 90.0916609,58.1716972 79.5090354,58.7564904 L50.1018553,58.7564904 L50.1018553,51.6239928 C55.246208,49.4020832 58.8714693,44.2569686 58.8714693,38.2940576 C58.8714693,32.2725149 55.246208,27.1274003 50.1018553,24.9054908 L50.1018553,0.097465538 L67.6410834,0.097465538 C78.5739756,0.097465538 87.5773547,8.94474751 88.1035161,19.7611378 C95.7621755,23.4442691 100.633929,31.5118267 99.9326344,40.1063075 L99.9326344,40.1063075 Z"
, id "Path"
, fill "#66BB00"
]
[]
, Svg.path
[ d "M50.1018553,18.7080531 C48.2309737,18.1240213 46.3014607,17.8316247 44.2554458,17.8316247 L20.8690469,17.8316247 C15.1985323,17.8316247 9.93615468,20.0535344 6.07789013,24.1455642 C2.16099382,28.2383552 -0.0220819872,33.6751048 0.211682965,39.4050125 C0.796476291,50.045508 10.5795797,58.7564904 21.8048683,58.7564904 L44.2554458,58.7564904 C46.3014607,58.7564904 48.2309737,58.4640938 50.1018553,57.8793006 C58.5204412,55.3657558 64.7178786,47.5312018 64.7178786,38.2940576 C64.7178786,29.0569135 58.5204412,21.2223595 50.1018553,18.7080531 Z"
, id "Path"
, fill "#C3EA21"
]
[]
, Svg.path
[ d "M64.7178786,38.2940576 C64.7178786,47.5312018 58.5204412,55.3657558 50.1018553,57.8793006 L50.1018553,18.7080531 C58.5204412,21.2223595 64.7178786,29.0569135 64.7178786,38.2940576 L64.7178786,38.2940576 Z"
, id "Path"
, fill "#9CDD05"
]
[]
, Svg.path
[ d "M88.1035161,38.2940576 C88.1035161,39.9083306 86.7945844,41.2172622 85.1803114,41.2172622 C83.5660384,41.2172622 82.2571069,39.9083306 82.2571069,38.2940576 C82.2571069,36.6797847 83.5660384,35.3708529 85.1803114,35.3708529 C86.7945844,35.3708529 88.1035161,36.6797847 88.1035161,38.2940576 L88.1035161,38.2940576 Z"
, id "Path"
, fill "#CD0000"
]
[]
, Svg.path
[ d "M76.4106974,20.7548294 C76.4106974,22.3691024 75.1017658,23.6780341 73.4874928,23.6780341 C71.8732199,23.6780341 70.5642881,22.3691024 70.5642881,20.7548294 C70.5642881,19.1405564 71.8732199,17.8316247 73.4874928,17.8316247 C75.1017658,17.8316247 76.4106974,19.1405564 76.4106974,20.7548294 L76.4106974,20.7548294 Z"
, id "Path"
, fill "#CD0000"
]
[]
, Svg.path
[ d "M53.0250599,38.2940576 C53.0250599,39.931174 51.7389717,41.2172622 50.1018553,41.2172622 C48.4639774,41.2172622 47.1786506,39.931174 47.1786506,38.2940576 C47.1786506,36.6569411 48.4639774,35.3708529 50.1018553,35.3708529 C51.7389717,35.3708529 53.0250599,36.6569411 53.0250599,38.2940576 Z"
, id "Path"
, fill "#FF637B"
]
[]
, Svg.path
[ d "M76.4106974,44.1404669 C76.4106974,45.7547399 75.1017658,47.0636717 73.4874928,47.0636717 C71.8732199,47.0636717 70.5642881,45.7547399 70.5642881,44.1404669 C70.5642881,42.5261939 71.8732199,41.2172622 73.4874928,41.2172622 C75.1017658,41.2172622 76.4106974,42.5261939 76.4106974,44.1404669 L76.4106974,44.1404669 Z"
, id "Path"
, fill "#CD0000"
]
[]
, Svg.path
[ d "M41.3322411,44.1404669 C41.3322411,45.7547399 40.0233093,47.0636717 38.4090364,47.0636717 C36.794002,47.0636717 35.4858318,45.7547399 35.4858318,44.1404669 C35.4858318,42.5261939 36.794002,41.2172622 38.4090364,41.2172622 C40.0233093,41.2172622 41.3322411,42.5261939 41.3322411,44.1404669 Z"
, id "Path"
, fill "#FF637B"
]
[]
, Svg.path
[ d "M23.7922517,44.1404669 C23.7922517,45.7547399 22.4840813,47.0636717 20.8690469,47.0636717 C19.2547739,47.0636717 17.9458422,45.7547399 17.9458422,44.1404669 C17.9458422,42.5261939 19.2547739,41.2172622 20.8690469,41.2172622 C22.4840813,41.2172622 23.7922517,42.5261939 23.7922517,44.1404669 Z"
, id "Path"
, fill "#FF637B"
]
[]
, Svg.path
[ d "M64.7178786,14.9084201 C64.7178786,16.5226931 63.4089469,17.8316247 61.7946739,17.8316247 C60.1796395,17.8316247 58.8714693,16.5226931 58.8714693,14.9084201 C58.8714693,13.2941471 60.1796395,11.9852154 61.7946739,11.9852154 C63.4089469,11.9852154 64.7178786,13.2941471 64.7178786,14.9084201 L64.7178786,14.9084201 Z"
, id "Path"
, fill "#CD0000"
]
[]
, Svg.path
[ d "M29.6386609,32.4476482 C29.6386609,34.0619211 28.3304908,35.3708529 26.7154562,35.3708529 C25.1011832,35.3708529 23.7922517,34.0619211 23.7922517,32.4476482 C23.7922517,30.8333752 25.1011832,29.5244436 26.7154562,29.5244436 C28.3304908,29.5244436 29.6386609,30.8333752 29.6386609,32.4476482 Z"
, id "Path"
, fill "#FF637B"
]
[]
, Svg.path
[ d "M50.1018553,41.2172622 L50.1018553,35.3708529 C51.7389717,35.3708529 53.0250599,36.6569411 53.0250599,38.2940576 C53.0250599,39.931174 51.7389717,41.2172622 50.1018553,41.2172622 Z"
, id "Path"
, fill "#FF001E"
]
[]
, Svg.path
[ d "M76.4106974,96.9530825 C76.4106974,98.5901989 75.1246092,99.8762872 73.4874928,99.8762872 L26.7154562,99.8762872 C25.0783398,99.8762872 23.7922517,98.5901989 23.7922517,96.9530825 C23.7922517,95.3159659 25.0783398,94.0298778 26.7154562,94.0298778 L73.4874928,94.0298778 C75.1246092,94.0298778 76.4106974,95.3159659 76.4106974,96.9530825 L76.4106974,96.9530825 Z"
, id "Path"
, fill "#C3EA21"
]
[]
, Svg.path
[ d "M76.4106974,96.9530825 C76.4106974,98.5901989 75.1246092,99.8762872 73.4874928,99.8762872 L50.1018553,99.8762872 L50.1018553,94.0298778 L73.4874928,94.0298778 C75.1246092,94.0298778 76.4106974,95.3159659 76.4106974,96.9530825 L76.4106974,96.9530825 Z"
, id "Path"
, fill "#9CDD05"
]
[]
]
]
]
|> fromUnstyled
| elm |
[
{
"context": "8482bea5493a0d7b44ccc4749b65\"\n , name = \"Né ici\"\n , duration = 274080\n }\n ",
"end": 1091,
"score": 0.9961437583,
"start": 1085,
"tag": "NAME",
"value": "Né ici"
}
] | examples/music-player/src/Model.elm | lisardo/elm-ui-explorer | 0 | module Model exposing (..)
type alias Model =
{ album : Album
, isPlaying : Bool
, selectedTrack : Maybe Track
}
type alias Album =
{ cover : String
, title : String
, playlist : PlayList
}
type alias PlayList =
List Track
type alias Track =
{ url : String
, name : String
, duration : Int
}
model : Model
model =
{ album = mockAlbum
, isPlaying = False
, selectedTrack = Nothing
}
mockAlbum : Album
mockAlbum =
{ cover = "https://i.scdn.co/image/d025af49bb84dbb26393f42ff9646f1c748c3035"
, title = "Menu Best Of"
, playlist =
[ { url = "https://p.scdn.co/mp3-preview/fb897f080a9c6765cc9178d48abcb4d6f81b472c"
, name = "Viens voir le docteur - Dirty moog mix"
, duration = 322213
}
, { url = "https://p.scdn.co/mp3-preview/887d0e4b4aa31b5c4ed971394dbc21573aaa4e8c"
, name = "Nirvana"
, duration = 338840
}
, { url = "https://p.scdn.co/mp3-preview/2aac3d146c048482bea5493a0d7b44ccc4749b65"
, name = "Né ici"
, duration = 274080
}
]
}
| 30602 | module Model exposing (..)
type alias Model =
{ album : Album
, isPlaying : Bool
, selectedTrack : Maybe Track
}
type alias Album =
{ cover : String
, title : String
, playlist : PlayList
}
type alias PlayList =
List Track
type alias Track =
{ url : String
, name : String
, duration : Int
}
model : Model
model =
{ album = mockAlbum
, isPlaying = False
, selectedTrack = Nothing
}
mockAlbum : Album
mockAlbum =
{ cover = "https://i.scdn.co/image/d025af49bb84dbb26393f42ff9646f1c748c3035"
, title = "Menu Best Of"
, playlist =
[ { url = "https://p.scdn.co/mp3-preview/fb897f080a9c6765cc9178d48abcb4d6f81b472c"
, name = "Viens voir le docteur - Dirty moog mix"
, duration = 322213
}
, { url = "https://p.scdn.co/mp3-preview/887d0e4b4aa31b5c4ed971394dbc21573aaa4e8c"
, name = "Nirvana"
, duration = 338840
}
, { url = "https://p.scdn.co/mp3-preview/2aac3d146c048482bea5493a0d7b44ccc4749b65"
, name = "<NAME>"
, duration = 274080
}
]
}
| true | module Model exposing (..)
type alias Model =
{ album : Album
, isPlaying : Bool
, selectedTrack : Maybe Track
}
type alias Album =
{ cover : String
, title : String
, playlist : PlayList
}
type alias PlayList =
List Track
type alias Track =
{ url : String
, name : String
, duration : Int
}
model : Model
model =
{ album = mockAlbum
, isPlaying = False
, selectedTrack = Nothing
}
mockAlbum : Album
mockAlbum =
{ cover = "https://i.scdn.co/image/d025af49bb84dbb26393f42ff9646f1c748c3035"
, title = "Menu Best Of"
, playlist =
[ { url = "https://p.scdn.co/mp3-preview/fb897f080a9c6765cc9178d48abcb4d6f81b472c"
, name = "Viens voir le docteur - Dirty moog mix"
, duration = 322213
}
, { url = "https://p.scdn.co/mp3-preview/887d0e4b4aa31b5c4ed971394dbc21573aaa4e8c"
, name = "Nirvana"
, duration = 338840
}
, { url = "https://p.scdn.co/mp3-preview/2aac3d146c048482bea5493a0d7b44ccc4749b65"
, name = "PI:NAME:<NAME>END_PI"
, duration = 274080
}
]
}
| elm |
[
{
"context": " (second argument, `target`).\n\n confirmEnding \"Bastian\" \"n\" == True\n confirmEnding \"He has to give me",
"end": 701,
"score": 0.998916328,
"start": 694,
"tag": "NAME",
"value": "Bastian"
},
{
"context": "<| assertEqual expected actual\n inputs = [ (\"Bastian\", \"n\", True)\n , (\"Connor\", \"n\", Fal",
"end": 1309,
"score": 0.9986441135,
"start": 1302,
"tag": "NAME",
"value": "Bastian"
},
{
"context": "uts = [ (\"Bastian\", \"n\", True)\n , (\"Connor\", \"n\", False)\n , (\"Walking on water",
"end": 1348,
"score": 0.9960696101,
"start": 1342,
"tag": "NAME",
"value": "Connor"
}
] | src/basic-algorithm-scripting/ConfirmEnding.elm | huggablemonad/fcc | 0 | module ConfirmEnding
( main, confirmEnding
) where
{-| # Confirm the Ending
------------------------
Check if a string (first argument, `str`) ends with the given target string
(second argument, `target`).
This is a certification challenge.
<https://www.freecodecamp.com/challenges/confirm-the-ending>
# Core functions
@docs main, confirmEnding
-}
import Graphics.Element exposing (Element)
import List
import String
import ElmTest exposing (..)
{-| Main entry point.
This just runs the tests.
-}
main : Element
main = elementRunner testSuite
{-| Return true if a string (first argument, `str`) ends with the given target
string (second argument, `target`).
confirmEnding "Bastian" "n" == True
confirmEnding "He has to give me a new name" "na" == False
-}
confirmEnding : String -> String -> Bool
confirmEnding string target = String.endsWith target string
{-| Test suite for `ConfirmEnding`. -}
testSuite : Test
testSuite = suite "ConfirmEnding" [testConfirmEnding]
{-| Test suite for `confirmEnding`. -}
testConfirmEnding : Test
testConfirmEnding =
let f (str, target, expected) =
let name = "input: " ++ toString str ++ " " ++ toString target
actual = confirmEnding str target
in test name <| assertEqual expected actual
inputs = [ ("Bastian", "n", True)
, ("Connor", "n", False)
, ("Walking on water and developing software from a specification are easy if both are frozen", "specification", False)
, ("He has to give me a new name", "name", True)
, ("He has to give me a new name", "me", True)
, ("He has to give me a new name", "na", False)
, ("If you want to save our world, you must hurry. We dont know how much longer we can withstand the nothing", "mountain", False)
]
tests = List.map f inputs
in suite "confirmEnding" tests
| 59119 | module ConfirmEnding
( main, confirmEnding
) where
{-| # Confirm the Ending
------------------------
Check if a string (first argument, `str`) ends with the given target string
(second argument, `target`).
This is a certification challenge.
<https://www.freecodecamp.com/challenges/confirm-the-ending>
# Core functions
@docs main, confirmEnding
-}
import Graphics.Element exposing (Element)
import List
import String
import ElmTest exposing (..)
{-| Main entry point.
This just runs the tests.
-}
main : Element
main = elementRunner testSuite
{-| Return true if a string (first argument, `str`) ends with the given target
string (second argument, `target`).
confirmEnding "<NAME>" "n" == True
confirmEnding "He has to give me a new name" "na" == False
-}
confirmEnding : String -> String -> Bool
confirmEnding string target = String.endsWith target string
{-| Test suite for `ConfirmEnding`. -}
testSuite : Test
testSuite = suite "ConfirmEnding" [testConfirmEnding]
{-| Test suite for `confirmEnding`. -}
testConfirmEnding : Test
testConfirmEnding =
let f (str, target, expected) =
let name = "input: " ++ toString str ++ " " ++ toString target
actual = confirmEnding str target
in test name <| assertEqual expected actual
inputs = [ ("<NAME>", "n", True)
, ("<NAME>", "n", False)
, ("Walking on water and developing software from a specification are easy if both are frozen", "specification", False)
, ("He has to give me a new name", "name", True)
, ("He has to give me a new name", "me", True)
, ("He has to give me a new name", "na", False)
, ("If you want to save our world, you must hurry. We dont know how much longer we can withstand the nothing", "mountain", False)
]
tests = List.map f inputs
in suite "confirmEnding" tests
| true | module ConfirmEnding
( main, confirmEnding
) where
{-| # Confirm the Ending
------------------------
Check if a string (first argument, `str`) ends with the given target string
(second argument, `target`).
This is a certification challenge.
<https://www.freecodecamp.com/challenges/confirm-the-ending>
# Core functions
@docs main, confirmEnding
-}
import Graphics.Element exposing (Element)
import List
import String
import ElmTest exposing (..)
{-| Main entry point.
This just runs the tests.
-}
main : Element
main = elementRunner testSuite
{-| Return true if a string (first argument, `str`) ends with the given target
string (second argument, `target`).
confirmEnding "PI:NAME:<NAME>END_PI" "n" == True
confirmEnding "He has to give me a new name" "na" == False
-}
confirmEnding : String -> String -> Bool
confirmEnding string target = String.endsWith target string
{-| Test suite for `ConfirmEnding`. -}
testSuite : Test
testSuite = suite "ConfirmEnding" [testConfirmEnding]
{-| Test suite for `confirmEnding`. -}
testConfirmEnding : Test
testConfirmEnding =
let f (str, target, expected) =
let name = "input: " ++ toString str ++ " " ++ toString target
actual = confirmEnding str target
in test name <| assertEqual expected actual
inputs = [ ("PI:NAME:<NAME>END_PI", "n", True)
, ("PI:NAME:<NAME>END_PI", "n", False)
, ("Walking on water and developing software from a specification are easy if both are frozen", "specification", False)
, ("He has to give me a new name", "name", True)
, ("He has to give me a new name", "me", True)
, ("He has to give me a new name", "na", False)
, ("If you want to save our world, you must hurry. We dont know how much longer we can withstand the nothing", "mountain", False)
]
tests = List.map f inputs
in suite "confirmEnding" tests
| elm |
[
{
"context": "oat\n , reply : String\n }\n\n-- Http\napikey = \"DZZnkt3h7o4Sd5q9NhFIRj3iAdZVMywA\"\nnewRequest : String -> String\nnewRequest query =",
"end": 1673,
"score": 0.9997575283,
"start": 1641,
"tag": "KEY",
"value": "DZZnkt3h7o4Sd5q9NhFIRj3iAdZVMywA"
}
] | src/Talk001.elm | kalz2q/elm-examples | 0 | module Main exposing (..)
import Browser
import Html exposing (Html, div, h1, a, text, input, button)
import Html.Attributes exposing (class, value, disabled)
import Html.Events exposing (onClick, onInput)
import Http
import Json.Decode as D exposing (Decoder)
main =
Browser.element
{ init = init
, view = view
, update = update
, subscriptions = \_ -> Sub.none
}
-- Update
type Msg
= Send
| Input String
| Recieve (Result Http.Error Talk)
update : Msg -> Model -> (Model, Cmd Msg)
update msg model =
case msg of
Input newInput ->
({model | input = newInput }, Cmd.none)
Send ->
( {model | input = "", userState = Waiting}
, Http.post
{ url = "https://api.a3rt.recruit-tech.co.jp/talk/v1/smalltalk"
, body = Http.stringBody "application/x-www-form-urlencoded" (newRequest model.input)
, expect = Http.expectJson Recieve talkDecoder
}
)
Recieve (Ok talk) ->
({model | userState = Loaded talk}, Cmd.none)
Recieve (Err e) ->
({model | userState = Failed e}, Cmd.none)
-- Model
type alias Model =
{ input : String
, userState : UserState
}
type UserState
= Init
| Waiting
| Loaded Talk
| Failed Http.Error
init : () -> (Model, Cmd Msg)
init () =
( {input = "", userState = Init }, Cmd.none )
-- Data
type alias Talk =
{ status : Int
, message : String
, results : (List Response)
}
type alias Response =
{ perplexity : Float
, reply : String
}
-- Http
apikey = "DZZnkt3h7o4Sd5q9NhFIRj3iAdZVMywA"
newRequest : String -> String
newRequest query =
["apikey=", apikey, "&query=", query] |> String.concat
-- Decoder
talkDecoder : Decoder (Talk)
talkDecoder =
D.map3 Talk
(D.field "status" D.int)
(D.field "message" D.string)
(D.field "results" (D.list responseDecoder))
responseDecoder : Decoder (Response)
responseDecoder =
D.map2 Response
(D.field "perplexity" D.float)
(D.field "reply" D.string)
-- Render
renderResponse : (List Response) -> Html Msg
renderResponse results =
let
toA = \result -> a [] [text result.reply]
in
div [] (List.map toA results)
-- View
view : Model -> Html Msg
view model =
div [ class "container"]
[ div [] [
h1 [] [ text "テキストを入力してネ"]
, input [ onInput Input, value model.input] []
, button
[ onClick Send
, disabled
((model.userState == Waiting)
|| String.isEmpty (String.trim model.input)
)
]
[text "Submit"]
]
, div [] [
case model.userState of
Init ->
text ""
Waiting ->
text "waiting..."
Loaded talk ->
renderResponse talk.results
Failed error ->
text (Debug.toString error)
]
] | 36518 | module Main exposing (..)
import Browser
import Html exposing (Html, div, h1, a, text, input, button)
import Html.Attributes exposing (class, value, disabled)
import Html.Events exposing (onClick, onInput)
import Http
import Json.Decode as D exposing (Decoder)
main =
Browser.element
{ init = init
, view = view
, update = update
, subscriptions = \_ -> Sub.none
}
-- Update
type Msg
= Send
| Input String
| Recieve (Result Http.Error Talk)
update : Msg -> Model -> (Model, Cmd Msg)
update msg model =
case msg of
Input newInput ->
({model | input = newInput }, Cmd.none)
Send ->
( {model | input = "", userState = Waiting}
, Http.post
{ url = "https://api.a3rt.recruit-tech.co.jp/talk/v1/smalltalk"
, body = Http.stringBody "application/x-www-form-urlencoded" (newRequest model.input)
, expect = Http.expectJson Recieve talkDecoder
}
)
Recieve (Ok talk) ->
({model | userState = Loaded talk}, Cmd.none)
Recieve (Err e) ->
({model | userState = Failed e}, Cmd.none)
-- Model
type alias Model =
{ input : String
, userState : UserState
}
type UserState
= Init
| Waiting
| Loaded Talk
| Failed Http.Error
init : () -> (Model, Cmd Msg)
init () =
( {input = "", userState = Init }, Cmd.none )
-- Data
type alias Talk =
{ status : Int
, message : String
, results : (List Response)
}
type alias Response =
{ perplexity : Float
, reply : String
}
-- Http
apikey = "<KEY>"
newRequest : String -> String
newRequest query =
["apikey=", apikey, "&query=", query] |> String.concat
-- Decoder
talkDecoder : Decoder (Talk)
talkDecoder =
D.map3 Talk
(D.field "status" D.int)
(D.field "message" D.string)
(D.field "results" (D.list responseDecoder))
responseDecoder : Decoder (Response)
responseDecoder =
D.map2 Response
(D.field "perplexity" D.float)
(D.field "reply" D.string)
-- Render
renderResponse : (List Response) -> Html Msg
renderResponse results =
let
toA = \result -> a [] [text result.reply]
in
div [] (List.map toA results)
-- View
view : Model -> Html Msg
view model =
div [ class "container"]
[ div [] [
h1 [] [ text "テキストを入力してネ"]
, input [ onInput Input, value model.input] []
, button
[ onClick Send
, disabled
((model.userState == Waiting)
|| String.isEmpty (String.trim model.input)
)
]
[text "Submit"]
]
, div [] [
case model.userState of
Init ->
text ""
Waiting ->
text "waiting..."
Loaded talk ->
renderResponse talk.results
Failed error ->
text (Debug.toString error)
]
] | true | module Main exposing (..)
import Browser
import Html exposing (Html, div, h1, a, text, input, button)
import Html.Attributes exposing (class, value, disabled)
import Html.Events exposing (onClick, onInput)
import Http
import Json.Decode as D exposing (Decoder)
main =
Browser.element
{ init = init
, view = view
, update = update
, subscriptions = \_ -> Sub.none
}
-- Update
type Msg
= Send
| Input String
| Recieve (Result Http.Error Talk)
update : Msg -> Model -> (Model, Cmd Msg)
update msg model =
case msg of
Input newInput ->
({model | input = newInput }, Cmd.none)
Send ->
( {model | input = "", userState = Waiting}
, Http.post
{ url = "https://api.a3rt.recruit-tech.co.jp/talk/v1/smalltalk"
, body = Http.stringBody "application/x-www-form-urlencoded" (newRequest model.input)
, expect = Http.expectJson Recieve talkDecoder
}
)
Recieve (Ok talk) ->
({model | userState = Loaded talk}, Cmd.none)
Recieve (Err e) ->
({model | userState = Failed e}, Cmd.none)
-- Model
type alias Model =
{ input : String
, userState : UserState
}
type UserState
= Init
| Waiting
| Loaded Talk
| Failed Http.Error
init : () -> (Model, Cmd Msg)
init () =
( {input = "", userState = Init }, Cmd.none )
-- Data
type alias Talk =
{ status : Int
, message : String
, results : (List Response)
}
type alias Response =
{ perplexity : Float
, reply : String
}
-- Http
apikey = "PI:KEY:<KEY>END_PI"
newRequest : String -> String
newRequest query =
["apikey=", apikey, "&query=", query] |> String.concat
-- Decoder
talkDecoder : Decoder (Talk)
talkDecoder =
D.map3 Talk
(D.field "status" D.int)
(D.field "message" D.string)
(D.field "results" (D.list responseDecoder))
responseDecoder : Decoder (Response)
responseDecoder =
D.map2 Response
(D.field "perplexity" D.float)
(D.field "reply" D.string)
-- Render
renderResponse : (List Response) -> Html Msg
renderResponse results =
let
toA = \result -> a [] [text result.reply]
in
div [] (List.map toA results)
-- View
view : Model -> Html Msg
view model =
div [ class "container"]
[ div [] [
h1 [] [ text "テキストを入力してネ"]
, input [ onInput Input, value model.input] []
, button
[ onClick Send
, disabled
((model.userState == Waiting)
|| String.isEmpty (String.trim model.input)
)
]
[text "Submit"]
]
, div [] [
case model.userState of
Init ->
text ""
Waiting ->
text "waiting..."
Loaded talk ->
renderResponse talk.results
Failed error ->
text (Debug.toString error)
]
] | elm |
[
{
"context": ") ->\n Expect.equal\n [\"Alice\", \"Bob\", \"Chuck\"]\n (pluck .name [\n",
"end": 7235,
"score": 0.9998080134,
"start": 7230,
"tag": "NAME",
"value": "Alice"
},
{
"context": " Expect.equal\n [\"Alice\", \"Bob\", \"Chuck\"]\n (pluck .name [\n ",
"end": 7242,
"score": 0.9998311996,
"start": 7239,
"tag": "NAME",
"value": "Bob"
},
{
"context": " Expect.equal\n [\"Alice\", \"Bob\", \"Chuck\"]\n (pluck .name [\n ",
"end": 7251,
"score": 0.9997984171,
"start": 7246,
"tag": "NAME",
"value": "Chuck"
},
{
"context": " (pluck .name [\n { name=\"Alice\", height=1.62 }\n , { name=\"Bob\",",
"end": 7316,
"score": 0.9998544455,
"start": 7311,
"tag": "NAME",
"value": "Alice"
},
{
"context": "Alice\", height=1.62 }\n , { name=\"Bob\", height=1.85 }\n , { name=\"Chuck",
"end": 7364,
"score": 0.9998577833,
"start": 7361,
"tag": "NAME",
"value": "Bob"
},
{
"context": "=\"Bob\", height=1.85 }\n , { name=\"Chuck\", height=1.76 }\n ])\n ]\n ",
"end": 7414,
"score": 0.999728322,
"start": 7409,
"tag": "NAME",
"value": "Chuck"
},
{
"context": " expectedValue = [\n {name = \"Alice\"},\n {name = \"Bob\"},\n ",
"end": 8220,
"score": 0.9998437166,
"start": 8215,
"tag": "NAME",
"value": "Alice"
},
{
"context": " {name = \"Alice\"},\n {name = \"Bob\"},\n {name = \"Steve\"}\n ",
"end": 8254,
"score": 0.999830246,
"start": 8251,
"tag": "NAME",
"value": "Bob"
},
{
"context": " {name = \"Bob\"},\n {name = \"Steve\"}\n ]\n actualValue =",
"end": 8290,
"score": 0.9994302392,
"start": 8285,
"tag": "NAME",
"value": "Steve"
},
{
"context": "\n [\n {name = \"Bob\"},\n {name = \"Steve\"},\n ",
"end": 8406,
"score": 0.9998250604,
"start": 8403,
"tag": "NAME",
"value": "Bob"
},
{
"context": " {name = \"Bob\"},\n {name = \"Steve\"},\n {name = \"Alice\"}\n ",
"end": 8444,
"score": 0.9994353056,
"start": 8439,
"tag": "NAME",
"value": "Steve"
},
{
"context": " {name = \"Steve\"},\n {name = \"Alice\"}\n ]\n in\n ",
"end": 8482,
"score": 0.9998514652,
"start": 8477,
"tag": "NAME",
"value": "Alice"
}
] | tests/ListTest.elm | antivanov/underscore-elm | 2 | module ListTest exposing (..)
import Underscore.List exposing (
map,
reduce,
reduceRight,
find,
filter,
whereDict,
whereProperty,
findWhereDict,
findWhereProperty,
reject,
every,
some,
contains,
pluckDict,
pluck,
min,
max,
sortBy,
groupBy,
indexBy,
partition,
first)
import Dict exposing (fromList)
import Test exposing (..)
import Expect
all : Test
all =
describe "Underscore.elm"
[ describe "map"
[ test "non-empty list" <|
\() ->
Expect.equal [2, 4, 6] (Underscore.List.map (\x -> 2 * x) [1, 2, 3])
, test "empty list" <|
\() ->
Expect.equal [] (Underscore.List.map (\x -> 2 * x) [])
]
, describe "reduce"
[ test "non-empty list" <|
\() ->
Expect.equal 6 (reduce (\s x -> s + x) 0 [1, 2, 3])
, test "empty list" <|
\() ->
Expect.equal 0 (reduce (\s x -> s + x) 0 [])
]
, describe "reduceRight"
[ test "non-empty list" <|
\() ->
Expect.equal "123" (reduceRight (\s x -> s ++ x) "" ["1", "2", "3"])
, test "empty list" <|
\() ->
Expect.equal "" (reduceRight (\s x -> s ++ x) "" [])
]
, describe "find"
[ test "finds first element satisfying the predicate" <|
\() ->
Expect.equal (Just 2) (find (\x -> x % 2 == 0) [1, 2, 3, 4])
, test "returns Nothing if no element is found" <|
\() ->
Expect.equal Nothing (find (\x -> x % 2 == 0) [1, 3, 5])
, test "returns Nothing if list is empty" <|
\() ->
Expect.equal Nothing (find (\x -> x % 2 == 0) [])
]
, describe "filter"
[ test "leaves only the elements satisfying the predicate" <|
\() ->
Expect.equal [2, 4] (Underscore.List.filter (\x -> x % 2 == 0) [1, 2, 3, 4])
, test "returns empty list if list is empty" <|
\() ->
Expect.equal [] (Underscore.List.filter (\x -> x % 2 == 0) [1, 3, 5])
]
, describe "whereDict"
[ test "leaves only the elements matching the provided dictionary" <|
\() ->
Expect.equal [Dict.fromList [(1, "1"), (2, "2")], Dict.fromList [(2, "2"), (3, "3")]] (whereDict
(Dict.fromList [(2, "2")])
[Dict.fromList [(1, "1"), (2, "2")], Dict.fromList [(2, "2"), (3, "3")], Dict.fromList [(3, "3"), (4, "4")]]
)
, test "returns empty list if list is empty" <|
\() ->
Expect.equal [] (whereDict (Dict.fromList [(2, "2")]) [])
]
, describe "whereProperty"
[ test "leaves only the elements matching the provided property value" <|
\() ->
let
expectedValue = [
{ city = "Helsinki", country = "Finland" }
, { city = "Turku", country = "Finland" }
]
actualValue = (whereProperty .country "Finland" [
{ city = "Helsinki", country = "Finland" }
, { city = "Turku", country = "Finland" }
, { city = "Tallinn", country = "Estonia" }
])
in
Expect.equal expectedValue actualValue
]
, describe "findWhereDict"
[ test "return first element if there are multiple matching elements" <|
\() ->
Expect.equal (Just (Dict.fromList [(1, "1"), (2, "2")])) (findWhereDict
(Dict.fromList [(2, "2")])
[Dict.fromList [(1, "1"), (2, "2")], Dict.fromList [(2, "2"), (3, "3")], Dict.fromList [(3, "3"), (4, "4")]]
)
, test "returns Nothing if there are no matching elements" <|
\() ->
Expect.equal Nothing (findWhereDict (Dict.fromList [(2, "2")]) [])
]
, describe "findWhereProperty"
[ test "returns first element if there are multiple matching elements" <|
\() ->
let
expectedValue = Just ({ city = "Helsinki", country = "Finland" })
actualValue = (findWhereProperty .country "Finland" [
{ city = "Helsinki", country = "Finland" }
, { city = "Turku", country = "Finland" }
, { city = "Tallinn", country = "Estonia" }
])
in
Expect.equal expectedValue actualValue
, test "returns Nothing if there are no matching elements" <|
\() ->
let
expectedValue = Nothing
actualValue = (findWhereProperty .country "Latvia" [
{ city = "Helsinki", country = "Finland" }
, { city = "Turku", country = "Finland" }
, { city = "Tallinn", country = "Estonia" }
])
in
Expect.equal expectedValue actualValue
]
, describe "reject"
[ test "rejects the elements matching the provided dictionary" <|
\() ->
Expect.equal [1, 3] (Underscore.List.reject (\x -> x % 2 == 0) [1, 2, 3, 4])
, test "returns empty list if list is empty" <|
\() ->
Expect.equal [] (Underscore.List.reject (\x -> x % 2 == 0) [])
]
, describe "every"
[ test "every element satisfies the predicate" <|
\() ->
Expect.equal True (Underscore.List.every (\x -> x % 2 == 0) [2, 4, 6])
, test "at least one element does not satisfy the predicate" <|
\() ->
Expect.equal False (Underscore.List.every (\x -> x % 2 == 0) [2, 3, 6])
]
, describe "some"
[ test "not a single element satisfies the predicate" <|
\() ->
Expect.equal False (Underscore.List.some (\x -> x % 2 == 0) [1, 3, 5])
, test "at least one element satisfies the predicate" <|
\() ->
Expect.equal True (Underscore.List.some (\x -> x % 2 == 0) [2, 3, 6])
]
, describe "contains"
[ test "list contains element" <|
\() ->
Expect.equal True (Underscore.List.contains 2 [1, 2, 3])
, test "list does not contain element" <|
\() ->
Expect.equal False (Underscore.List.contains 4 [1, 2, 3])
]
, describe "pluckDict"
[ test "list of dictionaries some of which contain key" <|
\() ->
Expect.equal
[Just "name1", Nothing, Just "name3"]
(pluckDict "name" [
Dict.fromList [("name", "name1"), ("email", "email1")],
Dict.fromList [("email", "email2")],
Dict.fromList [("name", "name3"), ("email", "email3")]
])
]
, describe "pluck"
[ test "list some of which contain property" <|
\() ->
Expect.equal
["Alice", "Bob", "Chuck"]
(pluck .name [
{ name="Alice", height=1.62 }
, { name="Bob", height=1.85 }
, { name="Chuck", height=1.76 }
])
]
, describe "min"
[ test "list with a minimum element" <|
\() ->
Expect.equal (Just 1) (Underscore.List.min [2, 1, 3])
, test "empty list" <|
\() ->
Expect.equal Nothing (Underscore.List.min [])
]
, describe "max"
[ test "list with a maximum element" <|
\() ->
Expect.equal (Just 3) (Underscore.List.max [2, 1, 3])
, test "empty list" <|
\() ->
Expect.equal Nothing (Underscore.List.max [])
]
, describe "sortBy"
[ test "sorts list by given property" <|
\() ->
let
expectedValue = [
{name = "Alice"},
{name = "Bob"},
{name = "Steve"}
]
actualValue = sortBy .name
[
{name = "Bob"},
{name = "Steve"},
{name = "Alice"}
]
in
Expect.equal expectedValue actualValue
]
, describe "groupBy"
[ test "groups list by given property" <|
\() ->
let
expectedValue = Dict.fromList
[
("even", [0, 2]),
("odd", [1, 3])
]
actualValue = groupBy (\x -> if x % 2 == 0 then "even" else "odd") [0, 1, 2, 3]
in
Expect.equal expectedValue actualValue
]
, describe "indexBy"
[ test "indexes list by given property" <|
\() ->
let
expectedValue = Dict.fromList [
("a", "abc"),
("b", "bca"),
("c", "cab")
]
actualValue = indexBy
(\x ->
let
maybeFirstSymbol = List.head <| String.toList x
in case maybeFirstSymbol of
Just firstSymbol -> (String.fromChar firstSymbol)
Nothing -> "?")
["abc", "bca", "cab"]
in
Expect.equal expectedValue actualValue
]
, describe "partition"
[ test "partitions list by given predicate" <|
\() ->
Expect.equal ([2, 4], [1, 3, 5]) (partition (\x -> x % 2 == 0) [1, 2, 3, 4, 5])
]
, describe "first"
[ test "takes first n elements if n less than list length" <|
\() ->
Expect.equal [1, 2, 3] (first 3 [1, 2, 3, 4, 5]),
test "takes all list elements if n greater than list length" <|
\() ->
Expect.equal [1, 2, 3] (first 5 [1, 2, 3]),
test "takes no elements if n is not positive" <|
\() ->
Expect.equal [] (first -3 [1, 2, 3])
]
] | 46024 | module ListTest exposing (..)
import Underscore.List exposing (
map,
reduce,
reduceRight,
find,
filter,
whereDict,
whereProperty,
findWhereDict,
findWhereProperty,
reject,
every,
some,
contains,
pluckDict,
pluck,
min,
max,
sortBy,
groupBy,
indexBy,
partition,
first)
import Dict exposing (fromList)
import Test exposing (..)
import Expect
all : Test
all =
describe "Underscore.elm"
[ describe "map"
[ test "non-empty list" <|
\() ->
Expect.equal [2, 4, 6] (Underscore.List.map (\x -> 2 * x) [1, 2, 3])
, test "empty list" <|
\() ->
Expect.equal [] (Underscore.List.map (\x -> 2 * x) [])
]
, describe "reduce"
[ test "non-empty list" <|
\() ->
Expect.equal 6 (reduce (\s x -> s + x) 0 [1, 2, 3])
, test "empty list" <|
\() ->
Expect.equal 0 (reduce (\s x -> s + x) 0 [])
]
, describe "reduceRight"
[ test "non-empty list" <|
\() ->
Expect.equal "123" (reduceRight (\s x -> s ++ x) "" ["1", "2", "3"])
, test "empty list" <|
\() ->
Expect.equal "" (reduceRight (\s x -> s ++ x) "" [])
]
, describe "find"
[ test "finds first element satisfying the predicate" <|
\() ->
Expect.equal (Just 2) (find (\x -> x % 2 == 0) [1, 2, 3, 4])
, test "returns Nothing if no element is found" <|
\() ->
Expect.equal Nothing (find (\x -> x % 2 == 0) [1, 3, 5])
, test "returns Nothing if list is empty" <|
\() ->
Expect.equal Nothing (find (\x -> x % 2 == 0) [])
]
, describe "filter"
[ test "leaves only the elements satisfying the predicate" <|
\() ->
Expect.equal [2, 4] (Underscore.List.filter (\x -> x % 2 == 0) [1, 2, 3, 4])
, test "returns empty list if list is empty" <|
\() ->
Expect.equal [] (Underscore.List.filter (\x -> x % 2 == 0) [1, 3, 5])
]
, describe "whereDict"
[ test "leaves only the elements matching the provided dictionary" <|
\() ->
Expect.equal [Dict.fromList [(1, "1"), (2, "2")], Dict.fromList [(2, "2"), (3, "3")]] (whereDict
(Dict.fromList [(2, "2")])
[Dict.fromList [(1, "1"), (2, "2")], Dict.fromList [(2, "2"), (3, "3")], Dict.fromList [(3, "3"), (4, "4")]]
)
, test "returns empty list if list is empty" <|
\() ->
Expect.equal [] (whereDict (Dict.fromList [(2, "2")]) [])
]
, describe "whereProperty"
[ test "leaves only the elements matching the provided property value" <|
\() ->
let
expectedValue = [
{ city = "Helsinki", country = "Finland" }
, { city = "Turku", country = "Finland" }
]
actualValue = (whereProperty .country "Finland" [
{ city = "Helsinki", country = "Finland" }
, { city = "Turku", country = "Finland" }
, { city = "Tallinn", country = "Estonia" }
])
in
Expect.equal expectedValue actualValue
]
, describe "findWhereDict"
[ test "return first element if there are multiple matching elements" <|
\() ->
Expect.equal (Just (Dict.fromList [(1, "1"), (2, "2")])) (findWhereDict
(Dict.fromList [(2, "2")])
[Dict.fromList [(1, "1"), (2, "2")], Dict.fromList [(2, "2"), (3, "3")], Dict.fromList [(3, "3"), (4, "4")]]
)
, test "returns Nothing if there are no matching elements" <|
\() ->
Expect.equal Nothing (findWhereDict (Dict.fromList [(2, "2")]) [])
]
, describe "findWhereProperty"
[ test "returns first element if there are multiple matching elements" <|
\() ->
let
expectedValue = Just ({ city = "Helsinki", country = "Finland" })
actualValue = (findWhereProperty .country "Finland" [
{ city = "Helsinki", country = "Finland" }
, { city = "Turku", country = "Finland" }
, { city = "Tallinn", country = "Estonia" }
])
in
Expect.equal expectedValue actualValue
, test "returns Nothing if there are no matching elements" <|
\() ->
let
expectedValue = Nothing
actualValue = (findWhereProperty .country "Latvia" [
{ city = "Helsinki", country = "Finland" }
, { city = "Turku", country = "Finland" }
, { city = "Tallinn", country = "Estonia" }
])
in
Expect.equal expectedValue actualValue
]
, describe "reject"
[ test "rejects the elements matching the provided dictionary" <|
\() ->
Expect.equal [1, 3] (Underscore.List.reject (\x -> x % 2 == 0) [1, 2, 3, 4])
, test "returns empty list if list is empty" <|
\() ->
Expect.equal [] (Underscore.List.reject (\x -> x % 2 == 0) [])
]
, describe "every"
[ test "every element satisfies the predicate" <|
\() ->
Expect.equal True (Underscore.List.every (\x -> x % 2 == 0) [2, 4, 6])
, test "at least one element does not satisfy the predicate" <|
\() ->
Expect.equal False (Underscore.List.every (\x -> x % 2 == 0) [2, 3, 6])
]
, describe "some"
[ test "not a single element satisfies the predicate" <|
\() ->
Expect.equal False (Underscore.List.some (\x -> x % 2 == 0) [1, 3, 5])
, test "at least one element satisfies the predicate" <|
\() ->
Expect.equal True (Underscore.List.some (\x -> x % 2 == 0) [2, 3, 6])
]
, describe "contains"
[ test "list contains element" <|
\() ->
Expect.equal True (Underscore.List.contains 2 [1, 2, 3])
, test "list does not contain element" <|
\() ->
Expect.equal False (Underscore.List.contains 4 [1, 2, 3])
]
, describe "pluckDict"
[ test "list of dictionaries some of which contain key" <|
\() ->
Expect.equal
[Just "name1", Nothing, Just "name3"]
(pluckDict "name" [
Dict.fromList [("name", "name1"), ("email", "email1")],
Dict.fromList [("email", "email2")],
Dict.fromList [("name", "name3"), ("email", "email3")]
])
]
, describe "pluck"
[ test "list some of which contain property" <|
\() ->
Expect.equal
["<NAME>", "<NAME>", "<NAME>"]
(pluck .name [
{ name="<NAME>", height=1.62 }
, { name="<NAME>", height=1.85 }
, { name="<NAME>", height=1.76 }
])
]
, describe "min"
[ test "list with a minimum element" <|
\() ->
Expect.equal (Just 1) (Underscore.List.min [2, 1, 3])
, test "empty list" <|
\() ->
Expect.equal Nothing (Underscore.List.min [])
]
, describe "max"
[ test "list with a maximum element" <|
\() ->
Expect.equal (Just 3) (Underscore.List.max [2, 1, 3])
, test "empty list" <|
\() ->
Expect.equal Nothing (Underscore.List.max [])
]
, describe "sortBy"
[ test "sorts list by given property" <|
\() ->
let
expectedValue = [
{name = "<NAME>"},
{name = "<NAME>"},
{name = "<NAME>"}
]
actualValue = sortBy .name
[
{name = "<NAME>"},
{name = "<NAME>"},
{name = "<NAME>"}
]
in
Expect.equal expectedValue actualValue
]
, describe "groupBy"
[ test "groups list by given property" <|
\() ->
let
expectedValue = Dict.fromList
[
("even", [0, 2]),
("odd", [1, 3])
]
actualValue = groupBy (\x -> if x % 2 == 0 then "even" else "odd") [0, 1, 2, 3]
in
Expect.equal expectedValue actualValue
]
, describe "indexBy"
[ test "indexes list by given property" <|
\() ->
let
expectedValue = Dict.fromList [
("a", "abc"),
("b", "bca"),
("c", "cab")
]
actualValue = indexBy
(\x ->
let
maybeFirstSymbol = List.head <| String.toList x
in case maybeFirstSymbol of
Just firstSymbol -> (String.fromChar firstSymbol)
Nothing -> "?")
["abc", "bca", "cab"]
in
Expect.equal expectedValue actualValue
]
, describe "partition"
[ test "partitions list by given predicate" <|
\() ->
Expect.equal ([2, 4], [1, 3, 5]) (partition (\x -> x % 2 == 0) [1, 2, 3, 4, 5])
]
, describe "first"
[ test "takes first n elements if n less than list length" <|
\() ->
Expect.equal [1, 2, 3] (first 3 [1, 2, 3, 4, 5]),
test "takes all list elements if n greater than list length" <|
\() ->
Expect.equal [1, 2, 3] (first 5 [1, 2, 3]),
test "takes no elements if n is not positive" <|
\() ->
Expect.equal [] (first -3 [1, 2, 3])
]
] | true | module ListTest exposing (..)
import Underscore.List exposing (
map,
reduce,
reduceRight,
find,
filter,
whereDict,
whereProperty,
findWhereDict,
findWhereProperty,
reject,
every,
some,
contains,
pluckDict,
pluck,
min,
max,
sortBy,
groupBy,
indexBy,
partition,
first)
import Dict exposing (fromList)
import Test exposing (..)
import Expect
all : Test
all =
describe "Underscore.elm"
[ describe "map"
[ test "non-empty list" <|
\() ->
Expect.equal [2, 4, 6] (Underscore.List.map (\x -> 2 * x) [1, 2, 3])
, test "empty list" <|
\() ->
Expect.equal [] (Underscore.List.map (\x -> 2 * x) [])
]
, describe "reduce"
[ test "non-empty list" <|
\() ->
Expect.equal 6 (reduce (\s x -> s + x) 0 [1, 2, 3])
, test "empty list" <|
\() ->
Expect.equal 0 (reduce (\s x -> s + x) 0 [])
]
, describe "reduceRight"
[ test "non-empty list" <|
\() ->
Expect.equal "123" (reduceRight (\s x -> s ++ x) "" ["1", "2", "3"])
, test "empty list" <|
\() ->
Expect.equal "" (reduceRight (\s x -> s ++ x) "" [])
]
, describe "find"
[ test "finds first element satisfying the predicate" <|
\() ->
Expect.equal (Just 2) (find (\x -> x % 2 == 0) [1, 2, 3, 4])
, test "returns Nothing if no element is found" <|
\() ->
Expect.equal Nothing (find (\x -> x % 2 == 0) [1, 3, 5])
, test "returns Nothing if list is empty" <|
\() ->
Expect.equal Nothing (find (\x -> x % 2 == 0) [])
]
, describe "filter"
[ test "leaves only the elements satisfying the predicate" <|
\() ->
Expect.equal [2, 4] (Underscore.List.filter (\x -> x % 2 == 0) [1, 2, 3, 4])
, test "returns empty list if list is empty" <|
\() ->
Expect.equal [] (Underscore.List.filter (\x -> x % 2 == 0) [1, 3, 5])
]
, describe "whereDict"
[ test "leaves only the elements matching the provided dictionary" <|
\() ->
Expect.equal [Dict.fromList [(1, "1"), (2, "2")], Dict.fromList [(2, "2"), (3, "3")]] (whereDict
(Dict.fromList [(2, "2")])
[Dict.fromList [(1, "1"), (2, "2")], Dict.fromList [(2, "2"), (3, "3")], Dict.fromList [(3, "3"), (4, "4")]]
)
, test "returns empty list if list is empty" <|
\() ->
Expect.equal [] (whereDict (Dict.fromList [(2, "2")]) [])
]
, describe "whereProperty"
[ test "leaves only the elements matching the provided property value" <|
\() ->
let
expectedValue = [
{ city = "Helsinki", country = "Finland" }
, { city = "Turku", country = "Finland" }
]
actualValue = (whereProperty .country "Finland" [
{ city = "Helsinki", country = "Finland" }
, { city = "Turku", country = "Finland" }
, { city = "Tallinn", country = "Estonia" }
])
in
Expect.equal expectedValue actualValue
]
, describe "findWhereDict"
[ test "return first element if there are multiple matching elements" <|
\() ->
Expect.equal (Just (Dict.fromList [(1, "1"), (2, "2")])) (findWhereDict
(Dict.fromList [(2, "2")])
[Dict.fromList [(1, "1"), (2, "2")], Dict.fromList [(2, "2"), (3, "3")], Dict.fromList [(3, "3"), (4, "4")]]
)
, test "returns Nothing if there are no matching elements" <|
\() ->
Expect.equal Nothing (findWhereDict (Dict.fromList [(2, "2")]) [])
]
, describe "findWhereProperty"
[ test "returns first element if there are multiple matching elements" <|
\() ->
let
expectedValue = Just ({ city = "Helsinki", country = "Finland" })
actualValue = (findWhereProperty .country "Finland" [
{ city = "Helsinki", country = "Finland" }
, { city = "Turku", country = "Finland" }
, { city = "Tallinn", country = "Estonia" }
])
in
Expect.equal expectedValue actualValue
, test "returns Nothing if there are no matching elements" <|
\() ->
let
expectedValue = Nothing
actualValue = (findWhereProperty .country "Latvia" [
{ city = "Helsinki", country = "Finland" }
, { city = "Turku", country = "Finland" }
, { city = "Tallinn", country = "Estonia" }
])
in
Expect.equal expectedValue actualValue
]
, describe "reject"
[ test "rejects the elements matching the provided dictionary" <|
\() ->
Expect.equal [1, 3] (Underscore.List.reject (\x -> x % 2 == 0) [1, 2, 3, 4])
, test "returns empty list if list is empty" <|
\() ->
Expect.equal [] (Underscore.List.reject (\x -> x % 2 == 0) [])
]
, describe "every"
[ test "every element satisfies the predicate" <|
\() ->
Expect.equal True (Underscore.List.every (\x -> x % 2 == 0) [2, 4, 6])
, test "at least one element does not satisfy the predicate" <|
\() ->
Expect.equal False (Underscore.List.every (\x -> x % 2 == 0) [2, 3, 6])
]
, describe "some"
[ test "not a single element satisfies the predicate" <|
\() ->
Expect.equal False (Underscore.List.some (\x -> x % 2 == 0) [1, 3, 5])
, test "at least one element satisfies the predicate" <|
\() ->
Expect.equal True (Underscore.List.some (\x -> x % 2 == 0) [2, 3, 6])
]
, describe "contains"
[ test "list contains element" <|
\() ->
Expect.equal True (Underscore.List.contains 2 [1, 2, 3])
, test "list does not contain element" <|
\() ->
Expect.equal False (Underscore.List.contains 4 [1, 2, 3])
]
, describe "pluckDict"
[ test "list of dictionaries some of which contain key" <|
\() ->
Expect.equal
[Just "name1", Nothing, Just "name3"]
(pluckDict "name" [
Dict.fromList [("name", "name1"), ("email", "email1")],
Dict.fromList [("email", "email2")],
Dict.fromList [("name", "name3"), ("email", "email3")]
])
]
, describe "pluck"
[ test "list some of which contain property" <|
\() ->
Expect.equal
["PI:NAME:<NAME>END_PI", "PI:NAME:<NAME>END_PI", "PI:NAME:<NAME>END_PI"]
(pluck .name [
{ name="PI:NAME:<NAME>END_PI", height=1.62 }
, { name="PI:NAME:<NAME>END_PI", height=1.85 }
, { name="PI:NAME:<NAME>END_PI", height=1.76 }
])
]
, describe "min"
[ test "list with a minimum element" <|
\() ->
Expect.equal (Just 1) (Underscore.List.min [2, 1, 3])
, test "empty list" <|
\() ->
Expect.equal Nothing (Underscore.List.min [])
]
, describe "max"
[ test "list with a maximum element" <|
\() ->
Expect.equal (Just 3) (Underscore.List.max [2, 1, 3])
, test "empty list" <|
\() ->
Expect.equal Nothing (Underscore.List.max [])
]
, describe "sortBy"
[ test "sorts list by given property" <|
\() ->
let
expectedValue = [
{name = "PI:NAME:<NAME>END_PI"},
{name = "PI:NAME:<NAME>END_PI"},
{name = "PI:NAME:<NAME>END_PI"}
]
actualValue = sortBy .name
[
{name = "PI:NAME:<NAME>END_PI"},
{name = "PI:NAME:<NAME>END_PI"},
{name = "PI:NAME:<NAME>END_PI"}
]
in
Expect.equal expectedValue actualValue
]
, describe "groupBy"
[ test "groups list by given property" <|
\() ->
let
expectedValue = Dict.fromList
[
("even", [0, 2]),
("odd", [1, 3])
]
actualValue = groupBy (\x -> if x % 2 == 0 then "even" else "odd") [0, 1, 2, 3]
in
Expect.equal expectedValue actualValue
]
, describe "indexBy"
[ test "indexes list by given property" <|
\() ->
let
expectedValue = Dict.fromList [
("a", "abc"),
("b", "bca"),
("c", "cab")
]
actualValue = indexBy
(\x ->
let
maybeFirstSymbol = List.head <| String.toList x
in case maybeFirstSymbol of
Just firstSymbol -> (String.fromChar firstSymbol)
Nothing -> "?")
["abc", "bca", "cab"]
in
Expect.equal expectedValue actualValue
]
, describe "partition"
[ test "partitions list by given predicate" <|
\() ->
Expect.equal ([2, 4], [1, 3, 5]) (partition (\x -> x % 2 == 0) [1, 2, 3, 4, 5])
]
, describe "first"
[ test "takes first n elements if n less than list length" <|
\() ->
Expect.equal [1, 2, 3] (first 3 [1, 2, 3, 4, 5]),
test "takes all list elements if n greater than list length" <|
\() ->
Expect.equal [1, 2, 3] (first 5 [1, 2, 3]),
test "takes no elements if n is not positive" <|
\() ->
Expect.equal [] (first -3 [1, 2, 3])
]
] | elm |
[
{
"context": "\n\n\ncreator : Creator\ncreator =\n { id = \"creator-1\"\n , links = Dict.fromList [ ( \"self\", \"http://",
"end": 1210,
"score": 0.851672411,
"start": 1209,
"tag": "USERNAME",
"value": "1"
},
{
"context": " \"http://url-to-creator/1\" ) ]\n , firstname = \"John\"\n , lastname = \"Doe\"\n }\n\n\ncomment1 : Commen",
"end": 1305,
"score": 0.9994282126,
"start": 1301,
"tag": "NAME",
"value": "John"
},
{
"context": "1\" ) ]\n , content = \"Comment 1\"\n , email = \"email@email.com\"\n }\n\n\ncomment2 : Comment\ncomment2 =\n { id =",
"end": 1519,
"score": 0.9998466372,
"start": 1504,
"tag": "EMAIL",
"value": "email@email.com"
},
{
"context": "2\" ) ]\n , content = \"Comment 2\"\n , email = \"email@email.com\"\n }\n\n\ncomment3 : Comment\ncomment3 =\n { id =",
"end": 1710,
"score": 0.9925261736,
"start": 1695,
"tag": "EMAIL",
"value": "email@email.com"
},
{
"context": "3\" ) ]\n , content = \"Comment 3\"\n , email = \"email@email.com\"\n }\n\n\ncommentDecoder : ResourceInfo -> Decoder",
"end": 1901,
"score": 0.9999201894,
"start": 1886,
"tag": "EMAIL",
"value": "email@email.com"
},
{
"context": " \"http://url-to-creator/1\" ) ]\n , firstname = \"Fake\"\n , lastname = \"Fake\"\n }\n\n\nbadCreatorDecode",
"end": 3382,
"score": 0.741296649,
"start": 3378,
"tag": "NAME",
"value": "Fake"
},
{
"context": "d { id = \"test\", links = Dict.empty, firstname = \"John\", lastname = \"Doe\" })\n (succeed [])\n\n\npost",
"end": 4424,
"score": 0.999370873,
"start": 4420,
"tag": "NAME",
"value": "John"
},
{
"context": "nks = Dict.empty, firstname = \"John\", lastname = \"Doe\" })\n (succeed [])\n\n\npostWithoutAttributesD",
"end": 4442,
"score": 0.9920120835,
"start": 4439,
"tag": "NAME",
"value": "Doe"
}
] | tests/JsonApi/Data/Posts.elm | ChristophP/jsonapi | 0 | module JsonApi.Data.Posts exposing (..)
import Dict exposing (Dict)
import Json.Decode exposing (Decoder, field, string, succeed, decodeString, map4, map6, oneOf)
import JsonApi.Decode as Decode
import JsonApi exposing (ResourceInfo)
type alias Post =
{ id : String
, links : Dict String String
, title : String
, content : String
, creator : Creator
, comments : List Comment
}
type alias Creator =
{ id : String
, links : Dict String String
, firstname : String
, lastname : String
}
type alias Comment =
{ id : String
, links : Dict String String
, content : String
, email : String
}
posts : List Post
posts =
[ postNoLink, post2 ]
postNoLink : Post
postNoLink =
{ id = "post-1"
, links = Dict.empty
, title = "Post no link"
, content = "Post content no link"
, creator = creator
, comments = [ comment1, comment2 ]
}
post2 : Post
post2 =
{ id = "post-2"
, links = Dict.fromList [ ( "self", "http://url-to-post/2" ) ]
, title = "Post 2"
, content = "Post content 2"
, creator = creator
, comments = [ comment3 ]
}
creator : Creator
creator =
{ id = "creator-1"
, links = Dict.fromList [ ( "self", "http://url-to-creator/1" ) ]
, firstname = "John"
, lastname = "Doe"
}
comment1 : Comment
comment1 =
{ id = "comment-1"
, links = Dict.fromList [ ( "self", "http://url-to-comment/1" ) ]
, content = "Comment 1"
, email = "email@email.com"
}
comment2 : Comment
comment2 =
{ id = "comment-2"
, links = Dict.fromList [ ( "self", "http://url-to-comment/2" ) ]
, content = "Comment 2"
, email = "email@email.com"
}
comment3 : Comment
comment3 =
{ id = "comment-3"
, links = Dict.fromList [ ( "self", "http://url-to-comment/3" ) ]
, content = "Comment 3"
, email = "email@email.com"
}
commentDecoder : ResourceInfo -> Decoder Comment
commentDecoder resourceInfo =
map4 Comment
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "content" string)
(field "email" string)
creatorDecoder : ResourceInfo -> Decoder Creator
creatorDecoder resourceInfo =
map4 Creator
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "firstname" string)
(field "lastname" string)
postDecoder : ResourceInfo -> Decoder Post
postDecoder resourceInfo =
map6 Post
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "title" string)
(field "content" string)
(Decode.relationship "creator" resourceInfo creatorDecoder)
(Decode.relationships "comments" resourceInfo commentDecoder)
postDecoderWithoutCreator : ResourceInfo -> Decoder Post
postDecoderWithoutCreator resourceInfo =
map6 Post
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "title" string)
(field "content" string)
(oneOf [ Decode.relationship "creator" resourceInfo creatorDecoder, succeed fakeUser ])
(Decode.relationships "comments" resourceInfo commentDecoder)
fakeUser : Creator
fakeUser =
{ id = "creator-1"
, links = Dict.fromList [ ( "self", "http://url-to-creator/1" ) ]
, firstname = "Fake"
, lastname = "Fake"
}
badCreatorDecoder : ResourceInfo -> Decoder Creator
badCreatorDecoder resourceInfo =
map4 Creator
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "bad" string)
(field "lastname" string)
postBadCreatorDecoder : ResourceInfo -> Decoder Post
postBadCreatorDecoder resourceInfo =
map6 Post
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "title" string)
(field "content" string)
(Decode.relationship "creator" resourceInfo badCreatorDecoder)
(Decode.relationships "comments" resourceInfo commentDecoder)
postWithoutRelationshipsDecoder : ResourceInfo -> Decoder Post
postWithoutRelationshipsDecoder resourceInfo =
map6 Post
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "title" string)
(field "content" string)
(succeed { id = "test", links = Dict.empty, firstname = "John", lastname = "Doe" })
(succeed [])
postWithoutAttributesDecoder : ResourceInfo -> Decoder Post
postWithoutAttributesDecoder resourceInfo =
map6 Post
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(succeed "fake title")
(succeed "fake content")
(Decode.relationship "creator" resourceInfo creatorDecoder)
(Decode.relationships "comments" resourceInfo commentDecoder)
badPostDecoder : ResourceInfo -> Decoder Post
badPostDecoder resourceInfo =
map6 Post
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "bad" string)
(field "content" string)
(Decode.relationship "creator" resourceInfo creatorDecoder)
(Decode.relationships "comments" resourceInfo commentDecoder)
| 7292 | module JsonApi.Data.Posts exposing (..)
import Dict exposing (Dict)
import Json.Decode exposing (Decoder, field, string, succeed, decodeString, map4, map6, oneOf)
import JsonApi.Decode as Decode
import JsonApi exposing (ResourceInfo)
type alias Post =
{ id : String
, links : Dict String String
, title : String
, content : String
, creator : Creator
, comments : List Comment
}
type alias Creator =
{ id : String
, links : Dict String String
, firstname : String
, lastname : String
}
type alias Comment =
{ id : String
, links : Dict String String
, content : String
, email : String
}
posts : List Post
posts =
[ postNoLink, post2 ]
postNoLink : Post
postNoLink =
{ id = "post-1"
, links = Dict.empty
, title = "Post no link"
, content = "Post content no link"
, creator = creator
, comments = [ comment1, comment2 ]
}
post2 : Post
post2 =
{ id = "post-2"
, links = Dict.fromList [ ( "self", "http://url-to-post/2" ) ]
, title = "Post 2"
, content = "Post content 2"
, creator = creator
, comments = [ comment3 ]
}
creator : Creator
creator =
{ id = "creator-1"
, links = Dict.fromList [ ( "self", "http://url-to-creator/1" ) ]
, firstname = "<NAME>"
, lastname = "Doe"
}
comment1 : Comment
comment1 =
{ id = "comment-1"
, links = Dict.fromList [ ( "self", "http://url-to-comment/1" ) ]
, content = "Comment 1"
, email = "<EMAIL>"
}
comment2 : Comment
comment2 =
{ id = "comment-2"
, links = Dict.fromList [ ( "self", "http://url-to-comment/2" ) ]
, content = "Comment 2"
, email = "<EMAIL>"
}
comment3 : Comment
comment3 =
{ id = "comment-3"
, links = Dict.fromList [ ( "self", "http://url-to-comment/3" ) ]
, content = "Comment 3"
, email = "<EMAIL>"
}
commentDecoder : ResourceInfo -> Decoder Comment
commentDecoder resourceInfo =
map4 Comment
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "content" string)
(field "email" string)
creatorDecoder : ResourceInfo -> Decoder Creator
creatorDecoder resourceInfo =
map4 Creator
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "firstname" string)
(field "lastname" string)
postDecoder : ResourceInfo -> Decoder Post
postDecoder resourceInfo =
map6 Post
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "title" string)
(field "content" string)
(Decode.relationship "creator" resourceInfo creatorDecoder)
(Decode.relationships "comments" resourceInfo commentDecoder)
postDecoderWithoutCreator : ResourceInfo -> Decoder Post
postDecoderWithoutCreator resourceInfo =
map6 Post
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "title" string)
(field "content" string)
(oneOf [ Decode.relationship "creator" resourceInfo creatorDecoder, succeed fakeUser ])
(Decode.relationships "comments" resourceInfo commentDecoder)
fakeUser : Creator
fakeUser =
{ id = "creator-1"
, links = Dict.fromList [ ( "self", "http://url-to-creator/1" ) ]
, firstname = "<NAME>"
, lastname = "Fake"
}
badCreatorDecoder : ResourceInfo -> Decoder Creator
badCreatorDecoder resourceInfo =
map4 Creator
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "bad" string)
(field "lastname" string)
postBadCreatorDecoder : ResourceInfo -> Decoder Post
postBadCreatorDecoder resourceInfo =
map6 Post
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "title" string)
(field "content" string)
(Decode.relationship "creator" resourceInfo badCreatorDecoder)
(Decode.relationships "comments" resourceInfo commentDecoder)
postWithoutRelationshipsDecoder : ResourceInfo -> Decoder Post
postWithoutRelationshipsDecoder resourceInfo =
map6 Post
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "title" string)
(field "content" string)
(succeed { id = "test", links = Dict.empty, firstname = "<NAME>", lastname = "<NAME>" })
(succeed [])
postWithoutAttributesDecoder : ResourceInfo -> Decoder Post
postWithoutAttributesDecoder resourceInfo =
map6 Post
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(succeed "fake title")
(succeed "fake content")
(Decode.relationship "creator" resourceInfo creatorDecoder)
(Decode.relationships "comments" resourceInfo commentDecoder)
badPostDecoder : ResourceInfo -> Decoder Post
badPostDecoder resourceInfo =
map6 Post
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "bad" string)
(field "content" string)
(Decode.relationship "creator" resourceInfo creatorDecoder)
(Decode.relationships "comments" resourceInfo commentDecoder)
| true | module JsonApi.Data.Posts exposing (..)
import Dict exposing (Dict)
import Json.Decode exposing (Decoder, field, string, succeed, decodeString, map4, map6, oneOf)
import JsonApi.Decode as Decode
import JsonApi exposing (ResourceInfo)
type alias Post =
{ id : String
, links : Dict String String
, title : String
, content : String
, creator : Creator
, comments : List Comment
}
type alias Creator =
{ id : String
, links : Dict String String
, firstname : String
, lastname : String
}
type alias Comment =
{ id : String
, links : Dict String String
, content : String
, email : String
}
posts : List Post
posts =
[ postNoLink, post2 ]
postNoLink : Post
postNoLink =
{ id = "post-1"
, links = Dict.empty
, title = "Post no link"
, content = "Post content no link"
, creator = creator
, comments = [ comment1, comment2 ]
}
post2 : Post
post2 =
{ id = "post-2"
, links = Dict.fromList [ ( "self", "http://url-to-post/2" ) ]
, title = "Post 2"
, content = "Post content 2"
, creator = creator
, comments = [ comment3 ]
}
creator : Creator
creator =
{ id = "creator-1"
, links = Dict.fromList [ ( "self", "http://url-to-creator/1" ) ]
, firstname = "PI:NAME:<NAME>END_PI"
, lastname = "Doe"
}
comment1 : Comment
comment1 =
{ id = "comment-1"
, links = Dict.fromList [ ( "self", "http://url-to-comment/1" ) ]
, content = "Comment 1"
, email = "PI:EMAIL:<EMAIL>END_PI"
}
comment2 : Comment
comment2 =
{ id = "comment-2"
, links = Dict.fromList [ ( "self", "http://url-to-comment/2" ) ]
, content = "Comment 2"
, email = "PI:EMAIL:<EMAIL>END_PI"
}
comment3 : Comment
comment3 =
{ id = "comment-3"
, links = Dict.fromList [ ( "self", "http://url-to-comment/3" ) ]
, content = "Comment 3"
, email = "PI:EMAIL:<EMAIL>END_PI"
}
commentDecoder : ResourceInfo -> Decoder Comment
commentDecoder resourceInfo =
map4 Comment
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "content" string)
(field "email" string)
creatorDecoder : ResourceInfo -> Decoder Creator
creatorDecoder resourceInfo =
map4 Creator
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "firstname" string)
(field "lastname" string)
postDecoder : ResourceInfo -> Decoder Post
postDecoder resourceInfo =
map6 Post
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "title" string)
(field "content" string)
(Decode.relationship "creator" resourceInfo creatorDecoder)
(Decode.relationships "comments" resourceInfo commentDecoder)
postDecoderWithoutCreator : ResourceInfo -> Decoder Post
postDecoderWithoutCreator resourceInfo =
map6 Post
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "title" string)
(field "content" string)
(oneOf [ Decode.relationship "creator" resourceInfo creatorDecoder, succeed fakeUser ])
(Decode.relationships "comments" resourceInfo commentDecoder)
fakeUser : Creator
fakeUser =
{ id = "creator-1"
, links = Dict.fromList [ ( "self", "http://url-to-creator/1" ) ]
, firstname = "PI:NAME:<NAME>END_PI"
, lastname = "Fake"
}
badCreatorDecoder : ResourceInfo -> Decoder Creator
badCreatorDecoder resourceInfo =
map4 Creator
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "bad" string)
(field "lastname" string)
postBadCreatorDecoder : ResourceInfo -> Decoder Post
postBadCreatorDecoder resourceInfo =
map6 Post
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "title" string)
(field "content" string)
(Decode.relationship "creator" resourceInfo badCreatorDecoder)
(Decode.relationships "comments" resourceInfo commentDecoder)
postWithoutRelationshipsDecoder : ResourceInfo -> Decoder Post
postWithoutRelationshipsDecoder resourceInfo =
map6 Post
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "title" string)
(field "content" string)
(succeed { id = "test", links = Dict.empty, firstname = "PI:NAME:<NAME>END_PI", lastname = "PI:NAME:<NAME>END_PI" })
(succeed [])
postWithoutAttributesDecoder : ResourceInfo -> Decoder Post
postWithoutAttributesDecoder resourceInfo =
map6 Post
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(succeed "fake title")
(succeed "fake content")
(Decode.relationship "creator" resourceInfo creatorDecoder)
(Decode.relationships "comments" resourceInfo commentDecoder)
badPostDecoder : ResourceInfo -> Decoder Post
badPostDecoder resourceInfo =
map6 Post
(succeed (JsonApi.id resourceInfo))
(succeed (JsonApi.links resourceInfo))
(field "bad" string)
(field "content" string)
(Decode.relationship "creator" resourceInfo creatorDecoder)
(Decode.relationships "comments" resourceInfo commentDecoder)
| elm |
[
{
"context": "\n { url = \"https://mailchi.mp/145ed6fd7df3/danmarcab\"\n , label =\n Icon.view\n ",
"end": 1752,
"score": 0.9789002538,
"start": 1743,
"tag": "USERNAME",
"value": "danmarcab"
},
{
"context": "ewTabLink []\n { url = \"https://twitter.com/danmarcab\"\n , label =\n Icon.view\n ",
"end": 2100,
"score": 0.9985332489,
"start": 2091,
"tag": "USERNAME",
"value": "danmarcab"
},
{
"context": "newTabLink []\n { url = \"https://github.com/danmarcab\"\n , label =\n Icon.view\n ",
"end": 2448,
"score": 0.9996643066,
"start": 2439,
"tag": "USERNAME",
"value": "danmarcab"
},
{
"context": " Element.newTabLink []\n { url = \"mailto:danmarcab+web@gmail.com\"\n , label =\n Icon.view\n ",
"end": 2795,
"score": 0.9998853207,
"start": 2772,
"tag": "EMAIL",
"value": "danmarcab+web@gmail.com"
},
{
"context": "k []\n { url = \"https://www.linkedin.com/in/daniel-mar%C3%ADn-cabillas-09b50254/\"\n , label =\n ",
"end": 3154,
"score": 0.9271771908,
"start": 3144,
"tag": "USERNAME",
"value": "daniel-mar"
},
{
"context": " = \"https://www.linkedin.com/in/daniel-mar%C3%ADn-cabillas-09b50254/\"\n , label =\n Ico",
"end": 3165,
"score": 0.9059643149,
"start": 3162,
"tag": "USERNAME",
"value": "cab"
},
{
"context": "www.linkedin.com/in/daniel-mar%C3%ADn-cabillas-09b50254/\"\n , label =\n Icon.view\n ",
"end": 3177,
"score": 0.5547302365,
"start": 3174,
"tag": "USERNAME",
"value": "502"
},
{
"context": "ize.sm ] <|\n Element.text \"© 2019 - present Daniel Marin Cabillas\"\n",
"end": 3582,
"score": 0.999877274,
"start": 3561,
"tag": "NAME",
"value": "Daniel Marin Cabillas"
}
] | src/Widget/Footer.elm | danmarcab/danmarcab.com | 2 | module Widget.Footer exposing (copyrightView, mailingListView, profilesView, view)
import Element exposing (Element)
import Element.Border as Border
import Element.Font as Font
import FeatherIcons as Icon
import ViewSettings exposing (ViewSettings)
import Widget.Icon as Icon
view : { viewSettings : ViewSettings } -> Element msg
view { viewSettings } =
Element.wrappedRow
[ Element.width Element.fill
, Element.spacingXY viewSettings.spacing.xl viewSettings.spacing.md
, Element.spaceEvenly
, Element.alignBottom
, Border.widthEach
{ top = 5
, right = 0
, bottom = 0
, left = 0
}
, Element.paddingEach
{ top = viewSettings.spacing.sm
, right = 0
, bottom = 0
, left = 0
}
]
[ mailingListView viewSettings
, profilesView viewSettings
, copyrightView viewSettings
]
mailingListView : ViewSettings -> Element msg
mailingListView viewSettings =
Element.row [ Font.size viewSettings.font.size.sm, Element.spacing viewSettings.spacing.md ]
[ Element.text "Subscribe to my mailing list:", mailListLink viewSettings ]
profilesView : ViewSettings -> Element msg
profilesView viewSettings =
Element.row [ Font.size viewSettings.font.size.sm, Element.spacing viewSettings.spacing.md ]
[ Element.text "Find me on:"
, emailLink viewSettings
, twitterLink viewSettings
, githubLink viewSettings
, linkedinLink viewSettings
]
mailListLink : ViewSettings -> Element msg
mailListLink viewSettings =
Element.newTabLink []
{ url = "https://mailchi.mp/145ed6fd7df3/danmarcab"
, label =
Icon.view
{ size = viewSettings.font.size.lg
, color = viewSettings.font.color.primary
}
Icon.mail
}
twitterLink : ViewSettings -> Element msg
twitterLink viewSettings =
Element.newTabLink []
{ url = "https://twitter.com/danmarcab"
, label =
Icon.view
{ size = viewSettings.font.size.lg
, color = viewSettings.font.color.primary
}
Icon.twitter
}
githubLink : ViewSettings -> Element msg
githubLink viewSettings =
Element.newTabLink []
{ url = "https://github.com/danmarcab"
, label =
Icon.view
{ size = viewSettings.font.size.lg
, color = viewSettings.font.color.primary
}
Icon.github
}
emailLink : ViewSettings -> Element msg
emailLink viewSettings =
Element.newTabLink []
{ url = "mailto:danmarcab+web@gmail.com"
, label =
Icon.view
{ size = viewSettings.font.size.lg
, color = viewSettings.font.color.primary
}
Icon.mail
}
linkedinLink : ViewSettings -> Element msg
linkedinLink viewSettings =
Element.newTabLink []
{ url = "https://www.linkedin.com/in/daniel-mar%C3%ADn-cabillas-09b50254/"
, label =
Icon.view
{ size = viewSettings.font.size.lg
, color = viewSettings.font.color.primary
}
Icon.linkedin
}
copyrightView : ViewSettings -> Element msg
copyrightView viewSettings =
Element.el [ Font.size viewSettings.font.size.sm ] <|
Element.text "© 2019 - present Daniel Marin Cabillas"
| 51266 | module Widget.Footer exposing (copyrightView, mailingListView, profilesView, view)
import Element exposing (Element)
import Element.Border as Border
import Element.Font as Font
import FeatherIcons as Icon
import ViewSettings exposing (ViewSettings)
import Widget.Icon as Icon
view : { viewSettings : ViewSettings } -> Element msg
view { viewSettings } =
Element.wrappedRow
[ Element.width Element.fill
, Element.spacingXY viewSettings.spacing.xl viewSettings.spacing.md
, Element.spaceEvenly
, Element.alignBottom
, Border.widthEach
{ top = 5
, right = 0
, bottom = 0
, left = 0
}
, Element.paddingEach
{ top = viewSettings.spacing.sm
, right = 0
, bottom = 0
, left = 0
}
]
[ mailingListView viewSettings
, profilesView viewSettings
, copyrightView viewSettings
]
mailingListView : ViewSettings -> Element msg
mailingListView viewSettings =
Element.row [ Font.size viewSettings.font.size.sm, Element.spacing viewSettings.spacing.md ]
[ Element.text "Subscribe to my mailing list:", mailListLink viewSettings ]
profilesView : ViewSettings -> Element msg
profilesView viewSettings =
Element.row [ Font.size viewSettings.font.size.sm, Element.spacing viewSettings.spacing.md ]
[ Element.text "Find me on:"
, emailLink viewSettings
, twitterLink viewSettings
, githubLink viewSettings
, linkedinLink viewSettings
]
mailListLink : ViewSettings -> Element msg
mailListLink viewSettings =
Element.newTabLink []
{ url = "https://mailchi.mp/145ed6fd7df3/danmarcab"
, label =
Icon.view
{ size = viewSettings.font.size.lg
, color = viewSettings.font.color.primary
}
Icon.mail
}
twitterLink : ViewSettings -> Element msg
twitterLink viewSettings =
Element.newTabLink []
{ url = "https://twitter.com/danmarcab"
, label =
Icon.view
{ size = viewSettings.font.size.lg
, color = viewSettings.font.color.primary
}
Icon.twitter
}
githubLink : ViewSettings -> Element msg
githubLink viewSettings =
Element.newTabLink []
{ url = "https://github.com/danmarcab"
, label =
Icon.view
{ size = viewSettings.font.size.lg
, color = viewSettings.font.color.primary
}
Icon.github
}
emailLink : ViewSettings -> Element msg
emailLink viewSettings =
Element.newTabLink []
{ url = "mailto:<EMAIL>"
, label =
Icon.view
{ size = viewSettings.font.size.lg
, color = viewSettings.font.color.primary
}
Icon.mail
}
linkedinLink : ViewSettings -> Element msg
linkedinLink viewSettings =
Element.newTabLink []
{ url = "https://www.linkedin.com/in/daniel-mar%C3%ADn-cabillas-09b50254/"
, label =
Icon.view
{ size = viewSettings.font.size.lg
, color = viewSettings.font.color.primary
}
Icon.linkedin
}
copyrightView : ViewSettings -> Element msg
copyrightView viewSettings =
Element.el [ Font.size viewSettings.font.size.sm ] <|
Element.text "© 2019 - present <NAME>"
| true | module Widget.Footer exposing (copyrightView, mailingListView, profilesView, view)
import Element exposing (Element)
import Element.Border as Border
import Element.Font as Font
import FeatherIcons as Icon
import ViewSettings exposing (ViewSettings)
import Widget.Icon as Icon
view : { viewSettings : ViewSettings } -> Element msg
view { viewSettings } =
Element.wrappedRow
[ Element.width Element.fill
, Element.spacingXY viewSettings.spacing.xl viewSettings.spacing.md
, Element.spaceEvenly
, Element.alignBottom
, Border.widthEach
{ top = 5
, right = 0
, bottom = 0
, left = 0
}
, Element.paddingEach
{ top = viewSettings.spacing.sm
, right = 0
, bottom = 0
, left = 0
}
]
[ mailingListView viewSettings
, profilesView viewSettings
, copyrightView viewSettings
]
mailingListView : ViewSettings -> Element msg
mailingListView viewSettings =
Element.row [ Font.size viewSettings.font.size.sm, Element.spacing viewSettings.spacing.md ]
[ Element.text "Subscribe to my mailing list:", mailListLink viewSettings ]
profilesView : ViewSettings -> Element msg
profilesView viewSettings =
Element.row [ Font.size viewSettings.font.size.sm, Element.spacing viewSettings.spacing.md ]
[ Element.text "Find me on:"
, emailLink viewSettings
, twitterLink viewSettings
, githubLink viewSettings
, linkedinLink viewSettings
]
mailListLink : ViewSettings -> Element msg
mailListLink viewSettings =
Element.newTabLink []
{ url = "https://mailchi.mp/145ed6fd7df3/danmarcab"
, label =
Icon.view
{ size = viewSettings.font.size.lg
, color = viewSettings.font.color.primary
}
Icon.mail
}
twitterLink : ViewSettings -> Element msg
twitterLink viewSettings =
Element.newTabLink []
{ url = "https://twitter.com/danmarcab"
, label =
Icon.view
{ size = viewSettings.font.size.lg
, color = viewSettings.font.color.primary
}
Icon.twitter
}
githubLink : ViewSettings -> Element msg
githubLink viewSettings =
Element.newTabLink []
{ url = "https://github.com/danmarcab"
, label =
Icon.view
{ size = viewSettings.font.size.lg
, color = viewSettings.font.color.primary
}
Icon.github
}
emailLink : ViewSettings -> Element msg
emailLink viewSettings =
Element.newTabLink []
{ url = "mailto:PI:EMAIL:<EMAIL>END_PI"
, label =
Icon.view
{ size = viewSettings.font.size.lg
, color = viewSettings.font.color.primary
}
Icon.mail
}
linkedinLink : ViewSettings -> Element msg
linkedinLink viewSettings =
Element.newTabLink []
{ url = "https://www.linkedin.com/in/daniel-mar%C3%ADn-cabillas-09b50254/"
, label =
Icon.view
{ size = viewSettings.font.size.lg
, color = viewSettings.font.color.primary
}
Icon.linkedin
}
copyrightView : ViewSettings -> Element msg
copyrightView viewSettings =
Element.el [ Font.size viewSettings.font.size.sm ] <|
Element.text "© 2019 - present PI:NAME:<NAME>END_PI"
| elm |
[
{
"context": " , H.input\n [ HA.placeholder \"Password\"\n , HA.type_ \"password\"\n ",
"end": 3043,
"score": 0.9321292639,
"start": 3035,
"tag": "PASSWORD",
"value": "Password"
}
] | frontend/src/Page/Login.elm | benkolera/dissidence | 2 | module Page.Login exposing (Model, Msg, init, subscriptions, update, view)
import Browser
import Browser.Navigation as Nav
import Generated.Api as BE
import Html as H
import Html.Attributes as HA
import Html.Attributes.Aria as HAA
import Html.Events as HE
import Http
import Page
import Ports exposing (putPlayerSession)
import RemoteData exposing (RemoteData)
import Route
import Session
import Utils exposing (disabledIfLoading, maybe, maybeToList, remoteDataError)
type Msg
= SetPlayerId String
| SetPassword String
| Submit
| HandleResp (Result Http.Error BE.Token)
type alias Model =
{ playerId : String
, password : String
, submission : RemoteData String Session.Player
}
type alias PageMsg =
Page.SubMsg Msg
init : Nav.Key -> Maybe Session.Player -> ( Model, Cmd PageMsg )
init key player =
( { playerId = ""
, password = ""
, submission = RemoteData.NotAsked
}
, Utils.maybe Cmd.none (always (Route.pushRoute key Route.Lobby)) player
)
subscriptions : Maybe Session.Player -> Model -> Sub PageMsg
subscriptions _ _ =
Ports.onPlayerSessionChange (Page.wrapParentMsg (Page.SetPlayer Route.Lobby))
update : Nav.Key -> Maybe Session.Player -> Msg -> Model -> ( Model, Cmd PageMsg )
update key player msg model =
case msg of
SetPlayerId pId ->
( { model | playerId = pId }, Cmd.none )
SetPassword p ->
( { model | password = p }, Cmd.none )
Submit ->
( { model | submission = RemoteData.Loading }
, BE.postApiLogin
{ dbPlayerId = model.playerId, dbPlayerPassword = model.password }
(Page.wrapChildMsg HandleResp)
)
HandleResp r ->
let
remoteData =
RemoteData.fromResult r
|> RemoteData.mapError Utils.httpErrorToStr
|> RemoteData.map (\t -> { playerId = model.playerId, token = t })
in
( { model | submission = remoteData }
, RemoteData.unwrap
Cmd.none
(\us -> Cmd.batch [ putPlayerSession (Just us) ])
remoteData
)
view : Maybe Session.Player -> Model -> Browser.Document Msg
view player model =
{ title = "Dissidence - Login"
, body =
[ H.div [ HA.class "login-box" ]
[ H.h1 [] [ H.text "Login" ]
, H.p [ HA.class "login-alternative" ]
[ H.text "Don't have a player id? "
, Route.routeLink Route.Register [ H.text "Register" ]
, H.text " instead!"
]
, H.form [ HE.onSubmit Submit ]
[ H.input
[ HA.placeholder "Player Id"
, HE.onInput SetPlayerId
, HA.value model.playerId
, HAA.ariaLabel "Player ID"
]
[]
, H.input
[ HA.placeholder "Password"
, HA.type_ "password"
, HE.onInput SetPassword
, HA.value model.password
, HAA.ariaLabel "Password"
]
[]
, H.ul [ HA.class "warn" ]
(Maybe.map (\em -> H.li [] [ H.text em ]) (remoteDataError model.submission)
|> maybeToList
)
, H.button
[ HA.class "btn primary", disabledIfLoading model.submission ]
[ H.text "Login" ]
]
]
]
}
| 52871 | module Page.Login exposing (Model, Msg, init, subscriptions, update, view)
import Browser
import Browser.Navigation as Nav
import Generated.Api as BE
import Html as H
import Html.Attributes as HA
import Html.Attributes.Aria as HAA
import Html.Events as HE
import Http
import Page
import Ports exposing (putPlayerSession)
import RemoteData exposing (RemoteData)
import Route
import Session
import Utils exposing (disabledIfLoading, maybe, maybeToList, remoteDataError)
type Msg
= SetPlayerId String
| SetPassword String
| Submit
| HandleResp (Result Http.Error BE.Token)
type alias Model =
{ playerId : String
, password : String
, submission : RemoteData String Session.Player
}
type alias PageMsg =
Page.SubMsg Msg
init : Nav.Key -> Maybe Session.Player -> ( Model, Cmd PageMsg )
init key player =
( { playerId = ""
, password = ""
, submission = RemoteData.NotAsked
}
, Utils.maybe Cmd.none (always (Route.pushRoute key Route.Lobby)) player
)
subscriptions : Maybe Session.Player -> Model -> Sub PageMsg
subscriptions _ _ =
Ports.onPlayerSessionChange (Page.wrapParentMsg (Page.SetPlayer Route.Lobby))
update : Nav.Key -> Maybe Session.Player -> Msg -> Model -> ( Model, Cmd PageMsg )
update key player msg model =
case msg of
SetPlayerId pId ->
( { model | playerId = pId }, Cmd.none )
SetPassword p ->
( { model | password = p }, Cmd.none )
Submit ->
( { model | submission = RemoteData.Loading }
, BE.postApiLogin
{ dbPlayerId = model.playerId, dbPlayerPassword = model.password }
(Page.wrapChildMsg HandleResp)
)
HandleResp r ->
let
remoteData =
RemoteData.fromResult r
|> RemoteData.mapError Utils.httpErrorToStr
|> RemoteData.map (\t -> { playerId = model.playerId, token = t })
in
( { model | submission = remoteData }
, RemoteData.unwrap
Cmd.none
(\us -> Cmd.batch [ putPlayerSession (Just us) ])
remoteData
)
view : Maybe Session.Player -> Model -> Browser.Document Msg
view player model =
{ title = "Dissidence - Login"
, body =
[ H.div [ HA.class "login-box" ]
[ H.h1 [] [ H.text "Login" ]
, H.p [ HA.class "login-alternative" ]
[ H.text "Don't have a player id? "
, Route.routeLink Route.Register [ H.text "Register" ]
, H.text " instead!"
]
, H.form [ HE.onSubmit Submit ]
[ H.input
[ HA.placeholder "Player Id"
, HE.onInput SetPlayerId
, HA.value model.playerId
, HAA.ariaLabel "Player ID"
]
[]
, H.input
[ HA.placeholder "<PASSWORD>"
, HA.type_ "password"
, HE.onInput SetPassword
, HA.value model.password
, HAA.ariaLabel "Password"
]
[]
, H.ul [ HA.class "warn" ]
(Maybe.map (\em -> H.li [] [ H.text em ]) (remoteDataError model.submission)
|> maybeToList
)
, H.button
[ HA.class "btn primary", disabledIfLoading model.submission ]
[ H.text "Login" ]
]
]
]
}
| true | module Page.Login exposing (Model, Msg, init, subscriptions, update, view)
import Browser
import Browser.Navigation as Nav
import Generated.Api as BE
import Html as H
import Html.Attributes as HA
import Html.Attributes.Aria as HAA
import Html.Events as HE
import Http
import Page
import Ports exposing (putPlayerSession)
import RemoteData exposing (RemoteData)
import Route
import Session
import Utils exposing (disabledIfLoading, maybe, maybeToList, remoteDataError)
type Msg
= SetPlayerId String
| SetPassword String
| Submit
| HandleResp (Result Http.Error BE.Token)
type alias Model =
{ playerId : String
, password : String
, submission : RemoteData String Session.Player
}
type alias PageMsg =
Page.SubMsg Msg
init : Nav.Key -> Maybe Session.Player -> ( Model, Cmd PageMsg )
init key player =
( { playerId = ""
, password = ""
, submission = RemoteData.NotAsked
}
, Utils.maybe Cmd.none (always (Route.pushRoute key Route.Lobby)) player
)
subscriptions : Maybe Session.Player -> Model -> Sub PageMsg
subscriptions _ _ =
Ports.onPlayerSessionChange (Page.wrapParentMsg (Page.SetPlayer Route.Lobby))
update : Nav.Key -> Maybe Session.Player -> Msg -> Model -> ( Model, Cmd PageMsg )
update key player msg model =
case msg of
SetPlayerId pId ->
( { model | playerId = pId }, Cmd.none )
SetPassword p ->
( { model | password = p }, Cmd.none )
Submit ->
( { model | submission = RemoteData.Loading }
, BE.postApiLogin
{ dbPlayerId = model.playerId, dbPlayerPassword = model.password }
(Page.wrapChildMsg HandleResp)
)
HandleResp r ->
let
remoteData =
RemoteData.fromResult r
|> RemoteData.mapError Utils.httpErrorToStr
|> RemoteData.map (\t -> { playerId = model.playerId, token = t })
in
( { model | submission = remoteData }
, RemoteData.unwrap
Cmd.none
(\us -> Cmd.batch [ putPlayerSession (Just us) ])
remoteData
)
view : Maybe Session.Player -> Model -> Browser.Document Msg
view player model =
{ title = "Dissidence - Login"
, body =
[ H.div [ HA.class "login-box" ]
[ H.h1 [] [ H.text "Login" ]
, H.p [ HA.class "login-alternative" ]
[ H.text "Don't have a player id? "
, Route.routeLink Route.Register [ H.text "Register" ]
, H.text " instead!"
]
, H.form [ HE.onSubmit Submit ]
[ H.input
[ HA.placeholder "Player Id"
, HE.onInput SetPlayerId
, HA.value model.playerId
, HAA.ariaLabel "Player ID"
]
[]
, H.input
[ HA.placeholder "PI:PASSWORD:<PASSWORD>END_PI"
, HA.type_ "password"
, HE.onInput SetPassword
, HA.value model.password
, HAA.ariaLabel "Password"
]
[]
, H.ul [ HA.class "warn" ]
(Maybe.map (\em -> H.li [] [ H.text em ]) (remoteDataError model.submission)
|> maybeToList
)
, H.button
[ HA.class "btn primary", disabledIfLoading model.submission ]
[ H.text "Login" ]
]
]
]
}
| elm |
[
{
"context": "ly as possible to the CommonMark spec.\nWrite me at jxxcarlson@gmail.com with comments and bug reports,\nor post an issue o",
"end": 1544,
"score": 0.9999132156,
"start": 1524,
"tag": "EMAIL",
"value": "jxxcarlson@gmail.com"
},
{
"context": " an issue on the [GitHub repo](https://github.com/jxxcarlson/elm-markdown).\n\nFor installation, see the notes\na",
"end": 1643,
"score": 0.9474871755,
"start": 1633,
"tag": "USERNAME",
"value": "jxxcarlson"
},
{
"context": "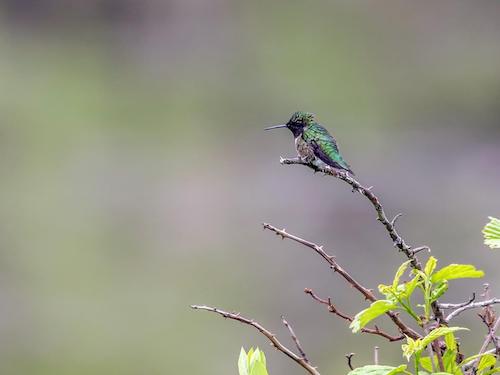\nHummingbird (Meditation)\n\nLink:",
"end": 1947,
"score": 0.7665268183,
"start": 1937,
"tag": "USERNAME",
"value": "jxxcarlson"
},
{
"context": "or the people, shall not perish from the earth.\n\n— Abraham Lincoln, *Gettysbug Address*\n\n## Poetry (Extension)\n\nPoet",
"end": 5974,
"score": 0.9998550415,
"start": 5959,
"tag": "NAME",
"value": "Abraham Lincoln"
}
] | Demos/simple/src/Placeholders.elm | elm-review-bot/elm-markdown | 27 | module Placeholders exposing (initialText, notes, testText)
testText : String
testText =
"""## Example
**Pythagoras** said: $a^2 + b^2 = c^2$.
An integral formula:
$$
\\int_{-\\infty}^\\infty e^{-x^2} dx = \\pi
$$
You should see two formulas above.
"""
initialText : String
initialText =
"""# A Pure Elm Markdown Parser
## Introduction
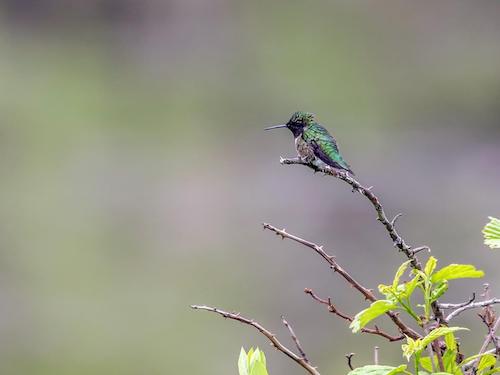 This project grew out of the need to have a pure Elm Markdown
parser-renderer that could also handle mathematical
text. Mathematical text is rendered by
MathJax 3, which has very large performance advantages
compared to its predecessors.
The Markdown used here offers three options: *Standard*,
*Extended* and *ExtendedMath*. The Extended option provides for strike-through
text, verbatim blocks, poetry blocks, and tables. ExtendedMath
handles
formulas written in TeX/LaTeX:
$$
\\int_{-\\infty}^\\infty e^{-x^2} dx = \\pi
$$
Note also that there is an automatically generated
active table of contents. It can be placed inside the document
at the top, to one side, as it is here, or it can be absent.
The [library](https://package.elm-lang.org/packages/jxxcarlson/elm-markdown/latest/)
with which this demo app is built is suitable for
light-weight writing tasks that require
mathematical notation — problem sets, short class notes, etc.
This project is a work in progress: more to to do make
it adhere as closely as possible to the CommonMark spec.
Write me at jxxcarlson@gmail.com with comments and bug reports,
or post an issue on the [GitHub repo](https://github.com/jxxcarlson/elm-markdown).
For installation, see the notes
at the end. This Markdown package is written in pure Elm.
It uses MathJax.js to render math formulas.
## Demo
Below we illustrate some typical Markdown elements: images, links, headings, etc.
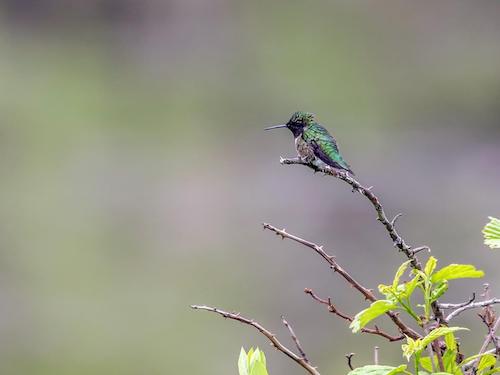
Hummingbird (Meditation)
Link: [New York Times](http://nytimes.com)
Text styles: **bold** *italic* ~~strike it out~~
## Inline Math
This is a test: $a^2 + b^2 = c^2$.
## Display Math
So is this:
$$
\\int_0^1 x^n dx = \\frac{1}{n+1}
$$
## Code
He said that `a := 0` is an initialization statement.
### Harmonic series:
```python
sum = 0
for n in range(1..100):
sum = sum + 1.0/n
sum
```
## SVG
@@svg
<svg width="100" height="100">
<circle cx="50" cy="50" r="40" stroke="blue" stroke-width="3" fill="cyan" />
</svg>
## Verbatim and Tables (Extensions)
A verbatim block begins and ends with four tick marks.
It is just like a code block, except that there is no
syntax highlighting. Verbatim blocks are an extension
of normal Markdown.
````
Verbatim text has many uses:
Element | Z
--------------------
Altium | 4/5
Brazilium | 7/5
Certium | 9/5
````
But better is to use Markdown tables:
| Element | Symbol | Z | A |
| Hydrogen | H | 1 | 1.008 |
| Helium | He | 2 | 4.0026 |
| Lithium | Li | 3 | 6.94 |
| Beryllium | Be | 4 | 9.0122 |
| Boron | B | 5 | 10.81 |
| Carbon | C | 6 | 12.011 |
| Nitrogen | N | 7 | 14.007 |
| Oxygen | O | 8 | 15.999 |
| Flourine | F | 9 | 18.998 |
| Neon | Ne | 10 | 20.180 |
## Lists
Indent by four spaces for each level. List items
are separated by blank lines.
- Solids
- Iron *(metal)*
- Iron disulfide (Pyrite): $FeS_2$, crystalline
- Iron(II) sulfed $FeS$, not stable, amorphous
- Selenium *(use for solar cells)*
- Liquids
- Alcohol *(careful!)*
- Water *(Ok to drink)*
## Numbered lists
### Problem Set 18
1. Compute the coefficient of $a^5b^2$ in $(a + b)^7$.
1. Do also: coefficient of $a^5b^5$ in $(a + 2b)^{10}$
2. Do also: coefficient of $a^7b^5$ in $(a - b)^{12}$
4. If $f'(2) = 0$, what can you say about the graph of $f$ at $x = 2$?
6. Suppose that in addition, $f''(2) > 0$. What else can say about the graph?
### Problem Set 19
4. Show that $u(x,t) = f(x - ct)$ is a solution to the equation $\\partial u(x,t)/\\partial x + c^{-1} \\partial u(x,t)/\\partial t = 0$.
3. State the wave equation and show that $u(x,t)$ as above is a solution to it.
2. In what direction does the wave defined by $u(x,t) = f(x - ct)$ move?
4. Find a solution of the wave equation that represents a pulse moving in the opposite direction.
## Quotations
Quotations are offset:
> Four score and seven years ago our fathers brought forth on this continent, a new nation, conceived in Liberty, and dedicated to the proposition that all men are created equal.
> Now we are engaged in a great civil war, testing whether that nation, or any nation so conceived and so dedicated, can long endure. We are met on a great battle-field of that war. We have come to dedicate a portion of that field, as a final resting place for those who here gave their lives that that nation might live. It is altogether fitting and proper that we should do this.
> But, in a larger sense, we can not dedicate—we can not consecrate—we can not hallow—this ground. The brave men, living and dead, who struggled here, have consecrated it, far above our poor power to add or detract. The world will little note, nor long remember what we say here, but it can never forget what they did here. It is for us the living, rather, to be dedicated here to the unfinished work which they who fought here have thus far so nobly advanced. It is rather for us to be here dedicated to the great task remaining before us—that from these honored dead we take increased devotion to that cause for which they gave the last full measure of devotion—that we here highly resolve that these dead shall not have died in vain—that this nation, under God, shall have a new birth of freedom—and that government of the people, by the people, for the people, shall not perish from the earth.
— Abraham Lincoln, *Gettysbug Address*
## Poetry (Extension)
Poetry blocks, an extension of normal Markdown,
begin with ">>"; line endings are respected.
>> Twas brillig, and the slithy toves
Did gyre and gimble in the wabe:
All mimsy were the borogoves,
And the mome raths outgrabe.
>> Beware the Jabberwock, my son!
The jaws that bite, the claws that catch!
Beware the Jubjub bird, and shun
The frumious Bandersnatch!
Etcetera!
___
NOTE: this Markdown implementation is an option for writing documents on [knode.io](https://knode.io).
Knode also offers MiniLaTeX, a web-friendly subset of TeX/LaTex. To see
how it works without a sign-in, please see [demo.minilatex.app](https://demo.minilatex.app).
___
## Installation
To compile, use
```elm
elm make --output=Main.js
```
Then open `index-katex.html` to run the app.
"""
notes : String
notes =
"""
# Notes
"""
| 44941 | module Placeholders exposing (initialText, notes, testText)
testText : String
testText =
"""## Example
**Pythagoras** said: $a^2 + b^2 = c^2$.
An integral formula:
$$
\\int_{-\\infty}^\\infty e^{-x^2} dx = \\pi
$$
You should see two formulas above.
"""
initialText : String
initialText =
"""# A Pure Elm Markdown Parser
## Introduction
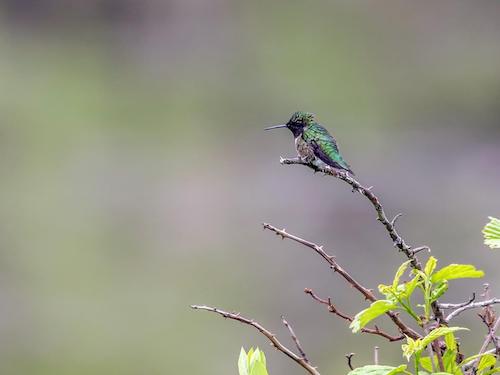 This project grew out of the need to have a pure Elm Markdown
parser-renderer that could also handle mathematical
text. Mathematical text is rendered by
MathJax 3, which has very large performance advantages
compared to its predecessors.
The Markdown used here offers three options: *Standard*,
*Extended* and *ExtendedMath*. The Extended option provides for strike-through
text, verbatim blocks, poetry blocks, and tables. ExtendedMath
handles
formulas written in TeX/LaTeX:
$$
\\int_{-\\infty}^\\infty e^{-x^2} dx = \\pi
$$
Note also that there is an automatically generated
active table of contents. It can be placed inside the document
at the top, to one side, as it is here, or it can be absent.
The [library](https://package.elm-lang.org/packages/jxxcarlson/elm-markdown/latest/)
with which this demo app is built is suitable for
light-weight writing tasks that require
mathematical notation — problem sets, short class notes, etc.
This project is a work in progress: more to to do make
it adhere as closely as possible to the CommonMark spec.
Write me at <EMAIL> with comments and bug reports,
or post an issue on the [GitHub repo](https://github.com/jxxcarlson/elm-markdown).
For installation, see the notes
at the end. This Markdown package is written in pure Elm.
It uses MathJax.js to render math formulas.
## Demo
Below we illustrate some typical Markdown elements: images, links, headings, etc.
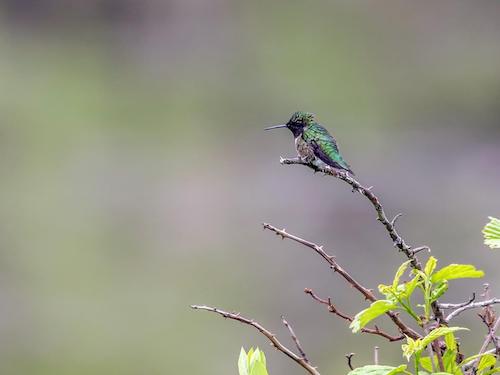
Hummingbird (Meditation)
Link: [New York Times](http://nytimes.com)
Text styles: **bold** *italic* ~~strike it out~~
## Inline Math
This is a test: $a^2 + b^2 = c^2$.
## Display Math
So is this:
$$
\\int_0^1 x^n dx = \\frac{1}{n+1}
$$
## Code
He said that `a := 0` is an initialization statement.
### Harmonic series:
```python
sum = 0
for n in range(1..100):
sum = sum + 1.0/n
sum
```
## SVG
@@svg
<svg width="100" height="100">
<circle cx="50" cy="50" r="40" stroke="blue" stroke-width="3" fill="cyan" />
</svg>
## Verbatim and Tables (Extensions)
A verbatim block begins and ends with four tick marks.
It is just like a code block, except that there is no
syntax highlighting. Verbatim blocks are an extension
of normal Markdown.
````
Verbatim text has many uses:
Element | Z
--------------------
Altium | 4/5
Brazilium | 7/5
Certium | 9/5
````
But better is to use Markdown tables:
| Element | Symbol | Z | A |
| Hydrogen | H | 1 | 1.008 |
| Helium | He | 2 | 4.0026 |
| Lithium | Li | 3 | 6.94 |
| Beryllium | Be | 4 | 9.0122 |
| Boron | B | 5 | 10.81 |
| Carbon | C | 6 | 12.011 |
| Nitrogen | N | 7 | 14.007 |
| Oxygen | O | 8 | 15.999 |
| Flourine | F | 9 | 18.998 |
| Neon | Ne | 10 | 20.180 |
## Lists
Indent by four spaces for each level. List items
are separated by blank lines.
- Solids
- Iron *(metal)*
- Iron disulfide (Pyrite): $FeS_2$, crystalline
- Iron(II) sulfed $FeS$, not stable, amorphous
- Selenium *(use for solar cells)*
- Liquids
- Alcohol *(careful!)*
- Water *(Ok to drink)*
## Numbered lists
### Problem Set 18
1. Compute the coefficient of $a^5b^2$ in $(a + b)^7$.
1. Do also: coefficient of $a^5b^5$ in $(a + 2b)^{10}$
2. Do also: coefficient of $a^7b^5$ in $(a - b)^{12}$
4. If $f'(2) = 0$, what can you say about the graph of $f$ at $x = 2$?
6. Suppose that in addition, $f''(2) > 0$. What else can say about the graph?
### Problem Set 19
4. Show that $u(x,t) = f(x - ct)$ is a solution to the equation $\\partial u(x,t)/\\partial x + c^{-1} \\partial u(x,t)/\\partial t = 0$.
3. State the wave equation and show that $u(x,t)$ as above is a solution to it.
2. In what direction does the wave defined by $u(x,t) = f(x - ct)$ move?
4. Find a solution of the wave equation that represents a pulse moving in the opposite direction.
## Quotations
Quotations are offset:
> Four score and seven years ago our fathers brought forth on this continent, a new nation, conceived in Liberty, and dedicated to the proposition that all men are created equal.
> Now we are engaged in a great civil war, testing whether that nation, or any nation so conceived and so dedicated, can long endure. We are met on a great battle-field of that war. We have come to dedicate a portion of that field, as a final resting place for those who here gave their lives that that nation might live. It is altogether fitting and proper that we should do this.
> But, in a larger sense, we can not dedicate—we can not consecrate—we can not hallow—this ground. The brave men, living and dead, who struggled here, have consecrated it, far above our poor power to add or detract. The world will little note, nor long remember what we say here, but it can never forget what they did here. It is for us the living, rather, to be dedicated here to the unfinished work which they who fought here have thus far so nobly advanced. It is rather for us to be here dedicated to the great task remaining before us—that from these honored dead we take increased devotion to that cause for which they gave the last full measure of devotion—that we here highly resolve that these dead shall not have died in vain—that this nation, under God, shall have a new birth of freedom—and that government of the people, by the people, for the people, shall not perish from the earth.
— <NAME>, *Gettysbug Address*
## Poetry (Extension)
Poetry blocks, an extension of normal Markdown,
begin with ">>"; line endings are respected.
>> Twas brillig, and the slithy toves
Did gyre and gimble in the wabe:
All mimsy were the borogoves,
And the mome raths outgrabe.
>> Beware the Jabberwock, my son!
The jaws that bite, the claws that catch!
Beware the Jubjub bird, and shun
The frumious Bandersnatch!
Etcetera!
___
NOTE: this Markdown implementation is an option for writing documents on [knode.io](https://knode.io).
Knode also offers MiniLaTeX, a web-friendly subset of TeX/LaTex. To see
how it works without a sign-in, please see [demo.minilatex.app](https://demo.minilatex.app).
___
## Installation
To compile, use
```elm
elm make --output=Main.js
```
Then open `index-katex.html` to run the app.
"""
notes : String
notes =
"""
# Notes
"""
| true | module Placeholders exposing (initialText, notes, testText)
testText : String
testText =
"""## Example
**Pythagoras** said: $a^2 + b^2 = c^2$.
An integral formula:
$$
\\int_{-\\infty}^\\infty e^{-x^2} dx = \\pi
$$
You should see two formulas above.
"""
initialText : String
initialText =
"""# A Pure Elm Markdown Parser
## Introduction
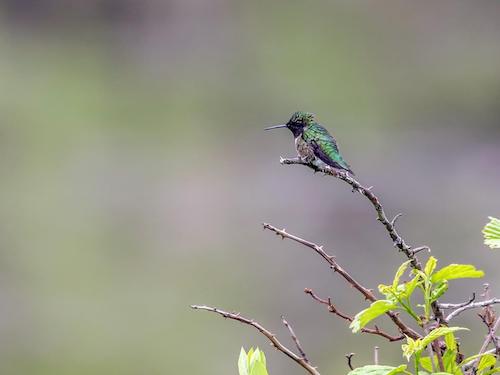 This project grew out of the need to have a pure Elm Markdown
parser-renderer that could also handle mathematical
text. Mathematical text is rendered by
MathJax 3, which has very large performance advantages
compared to its predecessors.
The Markdown used here offers three options: *Standard*,
*Extended* and *ExtendedMath*. The Extended option provides for strike-through
text, verbatim blocks, poetry blocks, and tables. ExtendedMath
handles
formulas written in TeX/LaTeX:
$$
\\int_{-\\infty}^\\infty e^{-x^2} dx = \\pi
$$
Note also that there is an automatically generated
active table of contents. It can be placed inside the document
at the top, to one side, as it is here, or it can be absent.
The [library](https://package.elm-lang.org/packages/jxxcarlson/elm-markdown/latest/)
with which this demo app is built is suitable for
light-weight writing tasks that require
mathematical notation — problem sets, short class notes, etc.
This project is a work in progress: more to to do make
it adhere as closely as possible to the CommonMark spec.
Write me at PI:EMAIL:<EMAIL>END_PI with comments and bug reports,
or post an issue on the [GitHub repo](https://github.com/jxxcarlson/elm-markdown).
For installation, see the notes
at the end. This Markdown package is written in pure Elm.
It uses MathJax.js to render math formulas.
## Demo
Below we illustrate some typical Markdown elements: images, links, headings, etc.
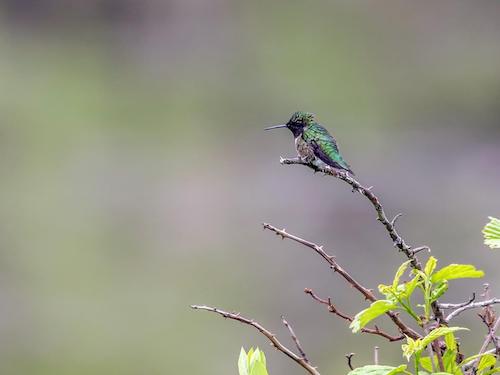
Hummingbird (Meditation)
Link: [New York Times](http://nytimes.com)
Text styles: **bold** *italic* ~~strike it out~~
## Inline Math
This is a test: $a^2 + b^2 = c^2$.
## Display Math
So is this:
$$
\\int_0^1 x^n dx = \\frac{1}{n+1}
$$
## Code
He said that `a := 0` is an initialization statement.
### Harmonic series:
```python
sum = 0
for n in range(1..100):
sum = sum + 1.0/n
sum
```
## SVG
@@svg
<svg width="100" height="100">
<circle cx="50" cy="50" r="40" stroke="blue" stroke-width="3" fill="cyan" />
</svg>
## Verbatim and Tables (Extensions)
A verbatim block begins and ends with four tick marks.
It is just like a code block, except that there is no
syntax highlighting. Verbatim blocks are an extension
of normal Markdown.
````
Verbatim text has many uses:
Element | Z
--------------------
Altium | 4/5
Brazilium | 7/5
Certium | 9/5
````
But better is to use Markdown tables:
| Element | Symbol | Z | A |
| Hydrogen | H | 1 | 1.008 |
| Helium | He | 2 | 4.0026 |
| Lithium | Li | 3 | 6.94 |
| Beryllium | Be | 4 | 9.0122 |
| Boron | B | 5 | 10.81 |
| Carbon | C | 6 | 12.011 |
| Nitrogen | N | 7 | 14.007 |
| Oxygen | O | 8 | 15.999 |
| Flourine | F | 9 | 18.998 |
| Neon | Ne | 10 | 20.180 |
## Lists
Indent by four spaces for each level. List items
are separated by blank lines.
- Solids
- Iron *(metal)*
- Iron disulfide (Pyrite): $FeS_2$, crystalline
- Iron(II) sulfed $FeS$, not stable, amorphous
- Selenium *(use for solar cells)*
- Liquids
- Alcohol *(careful!)*
- Water *(Ok to drink)*
## Numbered lists
### Problem Set 18
1. Compute the coefficient of $a^5b^2$ in $(a + b)^7$.
1. Do also: coefficient of $a^5b^5$ in $(a + 2b)^{10}$
2. Do also: coefficient of $a^7b^5$ in $(a - b)^{12}$
4. If $f'(2) = 0$, what can you say about the graph of $f$ at $x = 2$?
6. Suppose that in addition, $f''(2) > 0$. What else can say about the graph?
### Problem Set 19
4. Show that $u(x,t) = f(x - ct)$ is a solution to the equation $\\partial u(x,t)/\\partial x + c^{-1} \\partial u(x,t)/\\partial t = 0$.
3. State the wave equation and show that $u(x,t)$ as above is a solution to it.
2. In what direction does the wave defined by $u(x,t) = f(x - ct)$ move?
4. Find a solution of the wave equation that represents a pulse moving in the opposite direction.
## Quotations
Quotations are offset:
> Four score and seven years ago our fathers brought forth on this continent, a new nation, conceived in Liberty, and dedicated to the proposition that all men are created equal.
> Now we are engaged in a great civil war, testing whether that nation, or any nation so conceived and so dedicated, can long endure. We are met on a great battle-field of that war. We have come to dedicate a portion of that field, as a final resting place for those who here gave their lives that that nation might live. It is altogether fitting and proper that we should do this.
> But, in a larger sense, we can not dedicate—we can not consecrate—we can not hallow—this ground. The brave men, living and dead, who struggled here, have consecrated it, far above our poor power to add or detract. The world will little note, nor long remember what we say here, but it can never forget what they did here. It is for us the living, rather, to be dedicated here to the unfinished work which they who fought here have thus far so nobly advanced. It is rather for us to be here dedicated to the great task remaining before us—that from these honored dead we take increased devotion to that cause for which they gave the last full measure of devotion—that we here highly resolve that these dead shall not have died in vain—that this nation, under God, shall have a new birth of freedom—and that government of the people, by the people, for the people, shall not perish from the earth.
— PI:NAME:<NAME>END_PI, *Gettysbug Address*
## Poetry (Extension)
Poetry blocks, an extension of normal Markdown,
begin with ">>"; line endings are respected.
>> Twas brillig, and the slithy toves
Did gyre and gimble in the wabe:
All mimsy were the borogoves,
And the mome raths outgrabe.
>> Beware the Jabberwock, my son!
The jaws that bite, the claws that catch!
Beware the Jubjub bird, and shun
The frumious Bandersnatch!
Etcetera!
___
NOTE: this Markdown implementation is an option for writing documents on [knode.io](https://knode.io).
Knode also offers MiniLaTeX, a web-friendly subset of TeX/LaTex. To see
how it works without a sign-in, please see [demo.minilatex.app](https://demo.minilatex.app).
___
## Installation
To compile, use
```elm
elm make --output=Main.js
```
Then open `index-katex.html` to run the app.
"""
notes : String
notes =
"""
# Notes
"""
| elm |
[
{
"context": "-- Copyright 2018 Gregor Uhlenheuer\n--\n-- Licensed under the Apache License, Version ",
"end": 35,
"score": 0.9998822212,
"start": 18,
"tag": "NAME",
"value": "Gregor Uhlenheuer"
}
] | client/src/View/Planet.elm | kongo2002/ogonek | 1 | -- Copyright 2018 Gregor Uhlenheuer
--
-- Licensed under the Apache License, Version 2.0 (the "License");
-- you may not use this file except in compliance with the License.
-- You may obtain a copy of the License at
--
-- http://www.apache.org/licenses/LICENSE-2.0
--
-- Unless required by applicable law or agreed to in writing, software
-- distributed under the License is distributed on an "AS IS" BASIS,
-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-- See the License for the specific language governing permissions and
-- limitations under the License.
module View.Planet exposing (planet)
import Html exposing (..)
import Html.Attributes exposing (..)
import Types exposing (..)
import Utils exposing (capacityPercent, zonedIso8601)
import View.Utils exposing (..)
planet : ActivePlanet -> Model -> List (Html Msg)
planet active model =
let
planet0 =
active.planet
name =
planetName planet0
in
[ h2 [] [ text name ]
, div [ class "row" ]
[ ul [ id "planet-description", class "no-mobile nine columns" ]
[ li []
[ span [ class "description" ] [ text "Type: " ]
, span [ class "value" ] [ text <| Utils.planetToString planet0.planetType ]
]
, li []
[ span [ class "description" ] [ text "Size: " ]
, span [ class "value" ] [ text <| String.fromInt planet0.size ]
]
, li []
[ span [ class "description" ] [ text "Position: " ]
, span [ class "value" ] [ text <| coordStr planet0.position ]
]
]
, div [ class "u-pull-right three columns" ]
[ div [ class "u-full-width text-right" ]
[ planetImg planet0
]
]
]
]
-- vim: et sw=2 sts=2
| 22756 | -- Copyright 2018 <NAME>
--
-- Licensed under the Apache License, Version 2.0 (the "License");
-- you may not use this file except in compliance with the License.
-- You may obtain a copy of the License at
--
-- http://www.apache.org/licenses/LICENSE-2.0
--
-- Unless required by applicable law or agreed to in writing, software
-- distributed under the License is distributed on an "AS IS" BASIS,
-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-- See the License for the specific language governing permissions and
-- limitations under the License.
module View.Planet exposing (planet)
import Html exposing (..)
import Html.Attributes exposing (..)
import Types exposing (..)
import Utils exposing (capacityPercent, zonedIso8601)
import View.Utils exposing (..)
planet : ActivePlanet -> Model -> List (Html Msg)
planet active model =
let
planet0 =
active.planet
name =
planetName planet0
in
[ h2 [] [ text name ]
, div [ class "row" ]
[ ul [ id "planet-description", class "no-mobile nine columns" ]
[ li []
[ span [ class "description" ] [ text "Type: " ]
, span [ class "value" ] [ text <| Utils.planetToString planet0.planetType ]
]
, li []
[ span [ class "description" ] [ text "Size: " ]
, span [ class "value" ] [ text <| String.fromInt planet0.size ]
]
, li []
[ span [ class "description" ] [ text "Position: " ]
, span [ class "value" ] [ text <| coordStr planet0.position ]
]
]
, div [ class "u-pull-right three columns" ]
[ div [ class "u-full-width text-right" ]
[ planetImg planet0
]
]
]
]
-- vim: et sw=2 sts=2
| true | -- Copyright 2018 PI:NAME:<NAME>END_PI
--
-- Licensed under the Apache License, Version 2.0 (the "License");
-- you may not use this file except in compliance with the License.
-- You may obtain a copy of the License at
--
-- http://www.apache.org/licenses/LICENSE-2.0
--
-- Unless required by applicable law or agreed to in writing, software
-- distributed under the License is distributed on an "AS IS" BASIS,
-- WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
-- See the License for the specific language governing permissions and
-- limitations under the License.
module View.Planet exposing (planet)
import Html exposing (..)
import Html.Attributes exposing (..)
import Types exposing (..)
import Utils exposing (capacityPercent, zonedIso8601)
import View.Utils exposing (..)
planet : ActivePlanet -> Model -> List (Html Msg)
planet active model =
let
planet0 =
active.planet
name =
planetName planet0
in
[ h2 [] [ text name ]
, div [ class "row" ]
[ ul [ id "planet-description", class "no-mobile nine columns" ]
[ li []
[ span [ class "description" ] [ text "Type: " ]
, span [ class "value" ] [ text <| Utils.planetToString planet0.planetType ]
]
, li []
[ span [ class "description" ] [ text "Size: " ]
, span [ class "value" ] [ text <| String.fromInt planet0.size ]
]
, li []
[ span [ class "description" ] [ text "Position: " ]
, span [ class "value" ] [ text <| coordStr planet0.position ]
]
]
, div [ class "u-pull-right three columns" ]
[ div [ class "u-full-width text-right" ]
[ planetImg planet0
]
]
]
]
-- vim: et sw=2 sts=2
| elm |
[
{
"context": "\ninitialModel : Model\ninitialModel =\n { key = \"test1\"\n , value = \"\"\n , keyToGet = \"\"\n , value",
"end": 646,
"score": 0.9984562993,
"start": 641,
"tag": "KEY",
"value": "test1"
}
] | src/Main.elm | skipadu/elm-localstorage | 0 | port module Main exposing (main)
import Browser
import Html exposing (Html, button, div, h1, h2, input, label, p, text)
import Html.Attributes exposing (disabled, for, id, readonly, value)
import Html.Events exposing (onClick, onInput)
-- MAIN
main : Program () Model Msg
main =
Browser.element
{ init = init
, view = view
, update = update
, subscriptions = subscriptions
}
-- MODEL
type alias Model =
{ key : String
, value : String
, keyToGet : String
, valueGot : String
, keyToRemove : String
}
-- INIT
initialModel : Model
initialModel =
{ key = "test1"
, value = ""
, keyToGet = ""
, valueGot = ""
, keyToRemove = ""
}
init : () -> ( Model, Cmd Msg )
init _ =
( initialModel, Cmd.none )
-- UPDATE
type Msg
= SetValue String
| StoreItem
| SetKey String
| SetKeyToGet String
| GetItem String
| GotItem String
| SetKeyToRemove String
| RemoveItem
| Clear
type alias LocalStorageItem =
{ key : String
, value : String
}
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
SetValue newValue ->
( { model | value = newValue }, Cmd.none )
StoreItem ->
( model, setItem { key = model.key, value = model.value } )
SetKey newKey ->
( { model | key = newKey }, Cmd.none )
SetKeyToGet newKey ->
( { model | keyToGet = newKey }, Cmd.none )
GetItem key ->
( model, getItem key )
GotItem value ->
( { model | valueGot = value }, Cmd.none )
SetKeyToRemove key ->
( { model | keyToRemove = key }, Cmd.none )
RemoveItem ->
( model, removeItem model.keyToRemove )
Clear ->
( model, clear () )
-- VIEW
view : Model -> Html Msg
view model =
div []
[ h1 [] [ text "localStorage example" ]
, div []
[ h2 [] [ text "Set" ]
, div []
[ div []
[ label [ for "key-input" ] [ text "Item key" ]
, input [ id "key-input", onInput SetKey, value model.key ] []
]
, div []
[ label [ for "value-input" ] [ text "Item value" ]
, input [ id "value-input", onInput SetValue, value model.value ] []
]
, button [ onClick StoreItem ] [ text "Set" ]
]
]
, div []
[ h2 [] [ text "Get" ]
, div []
[ label [ for "key-to-get-input" ] [ text "Item key" ]
, input [ id "key-to-get-input", onInput SetKeyToGet ] []
, button
[ onClick (GetItem model.keyToGet)
, disabled (String.length (String.trim model.keyToGet) == 0)
]
[ text "Get" ]
]
, div []
[ label [ for "value-gotten-input" ] [ text " Value gotten" ]
, input [ id "value-gotten-input", readonly True, value model.valueGot ] []
]
]
, div []
[ h2 [] [ text "Remove" ]
, div []
[ label [ for "key-to-remove-input" ] [ text "Item key" ]
, input [ id "key-to-remove-input", onInput SetKeyToRemove ] []
, button
[ onClick RemoveItem
, disabled (String.length (String.trim model.keyToRemove) == 0)
]
[ text "Remove" ]
]
]
, div []
[ h2 [] [ text "Clear" ]
, div []
[ button
[ onClick Clear
]
[ text "Clear" ]
]
]
]
-- PORTS
port setItem : LocalStorageItem -> Cmd msg
port getItem : String -> Cmd msg
port gotItem : (String -> msg) -> Sub msg
port removeItem : String -> Cmd msg
port clear : () -> Cmd msg
-- port requestItem : (String -> msg) -> Sub msg
-- port arrivingItem : (String -> msg) -> Sub msg
-- SUBSCRIPTIONS
subscriptions : Model -> Sub Msg
subscriptions _ =
gotItem GotItem
| 3251 | port module Main exposing (main)
import Browser
import Html exposing (Html, button, div, h1, h2, input, label, p, text)
import Html.Attributes exposing (disabled, for, id, readonly, value)
import Html.Events exposing (onClick, onInput)
-- MAIN
main : Program () Model Msg
main =
Browser.element
{ init = init
, view = view
, update = update
, subscriptions = subscriptions
}
-- MODEL
type alias Model =
{ key : String
, value : String
, keyToGet : String
, valueGot : String
, keyToRemove : String
}
-- INIT
initialModel : Model
initialModel =
{ key = "<KEY>"
, value = ""
, keyToGet = ""
, valueGot = ""
, keyToRemove = ""
}
init : () -> ( Model, Cmd Msg )
init _ =
( initialModel, Cmd.none )
-- UPDATE
type Msg
= SetValue String
| StoreItem
| SetKey String
| SetKeyToGet String
| GetItem String
| GotItem String
| SetKeyToRemove String
| RemoveItem
| Clear
type alias LocalStorageItem =
{ key : String
, value : String
}
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
SetValue newValue ->
( { model | value = newValue }, Cmd.none )
StoreItem ->
( model, setItem { key = model.key, value = model.value } )
SetKey newKey ->
( { model | key = newKey }, Cmd.none )
SetKeyToGet newKey ->
( { model | keyToGet = newKey }, Cmd.none )
GetItem key ->
( model, getItem key )
GotItem value ->
( { model | valueGot = value }, Cmd.none )
SetKeyToRemove key ->
( { model | keyToRemove = key }, Cmd.none )
RemoveItem ->
( model, removeItem model.keyToRemove )
Clear ->
( model, clear () )
-- VIEW
view : Model -> Html Msg
view model =
div []
[ h1 [] [ text "localStorage example" ]
, div []
[ h2 [] [ text "Set" ]
, div []
[ div []
[ label [ for "key-input" ] [ text "Item key" ]
, input [ id "key-input", onInput SetKey, value model.key ] []
]
, div []
[ label [ for "value-input" ] [ text "Item value" ]
, input [ id "value-input", onInput SetValue, value model.value ] []
]
, button [ onClick StoreItem ] [ text "Set" ]
]
]
, div []
[ h2 [] [ text "Get" ]
, div []
[ label [ for "key-to-get-input" ] [ text "Item key" ]
, input [ id "key-to-get-input", onInput SetKeyToGet ] []
, button
[ onClick (GetItem model.keyToGet)
, disabled (String.length (String.trim model.keyToGet) == 0)
]
[ text "Get" ]
]
, div []
[ label [ for "value-gotten-input" ] [ text " Value gotten" ]
, input [ id "value-gotten-input", readonly True, value model.valueGot ] []
]
]
, div []
[ h2 [] [ text "Remove" ]
, div []
[ label [ for "key-to-remove-input" ] [ text "Item key" ]
, input [ id "key-to-remove-input", onInput SetKeyToRemove ] []
, button
[ onClick RemoveItem
, disabled (String.length (String.trim model.keyToRemove) == 0)
]
[ text "Remove" ]
]
]
, div []
[ h2 [] [ text "Clear" ]
, div []
[ button
[ onClick Clear
]
[ text "Clear" ]
]
]
]
-- PORTS
port setItem : LocalStorageItem -> Cmd msg
port getItem : String -> Cmd msg
port gotItem : (String -> msg) -> Sub msg
port removeItem : String -> Cmd msg
port clear : () -> Cmd msg
-- port requestItem : (String -> msg) -> Sub msg
-- port arrivingItem : (String -> msg) -> Sub msg
-- SUBSCRIPTIONS
subscriptions : Model -> Sub Msg
subscriptions _ =
gotItem GotItem
| true | port module Main exposing (main)
import Browser
import Html exposing (Html, button, div, h1, h2, input, label, p, text)
import Html.Attributes exposing (disabled, for, id, readonly, value)
import Html.Events exposing (onClick, onInput)
-- MAIN
main : Program () Model Msg
main =
Browser.element
{ init = init
, view = view
, update = update
, subscriptions = subscriptions
}
-- MODEL
type alias Model =
{ key : String
, value : String
, keyToGet : String
, valueGot : String
, keyToRemove : String
}
-- INIT
initialModel : Model
initialModel =
{ key = "PI:KEY:<KEY>END_PI"
, value = ""
, keyToGet = ""
, valueGot = ""
, keyToRemove = ""
}
init : () -> ( Model, Cmd Msg )
init _ =
( initialModel, Cmd.none )
-- UPDATE
type Msg
= SetValue String
| StoreItem
| SetKey String
| SetKeyToGet String
| GetItem String
| GotItem String
| SetKeyToRemove String
| RemoveItem
| Clear
type alias LocalStorageItem =
{ key : String
, value : String
}
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
SetValue newValue ->
( { model | value = newValue }, Cmd.none )
StoreItem ->
( model, setItem { key = model.key, value = model.value } )
SetKey newKey ->
( { model | key = newKey }, Cmd.none )
SetKeyToGet newKey ->
( { model | keyToGet = newKey }, Cmd.none )
GetItem key ->
( model, getItem key )
GotItem value ->
( { model | valueGot = value }, Cmd.none )
SetKeyToRemove key ->
( { model | keyToRemove = key }, Cmd.none )
RemoveItem ->
( model, removeItem model.keyToRemove )
Clear ->
( model, clear () )
-- VIEW
view : Model -> Html Msg
view model =
div []
[ h1 [] [ text "localStorage example" ]
, div []
[ h2 [] [ text "Set" ]
, div []
[ div []
[ label [ for "key-input" ] [ text "Item key" ]
, input [ id "key-input", onInput SetKey, value model.key ] []
]
, div []
[ label [ for "value-input" ] [ text "Item value" ]
, input [ id "value-input", onInput SetValue, value model.value ] []
]
, button [ onClick StoreItem ] [ text "Set" ]
]
]
, div []
[ h2 [] [ text "Get" ]
, div []
[ label [ for "key-to-get-input" ] [ text "Item key" ]
, input [ id "key-to-get-input", onInput SetKeyToGet ] []
, button
[ onClick (GetItem model.keyToGet)
, disabled (String.length (String.trim model.keyToGet) == 0)
]
[ text "Get" ]
]
, div []
[ label [ for "value-gotten-input" ] [ text " Value gotten" ]
, input [ id "value-gotten-input", readonly True, value model.valueGot ] []
]
]
, div []
[ h2 [] [ text "Remove" ]
, div []
[ label [ for "key-to-remove-input" ] [ text "Item key" ]
, input [ id "key-to-remove-input", onInput SetKeyToRemove ] []
, button
[ onClick RemoveItem
, disabled (String.length (String.trim model.keyToRemove) == 0)
]
[ text "Remove" ]
]
]
, div []
[ h2 [] [ text "Clear" ]
, div []
[ button
[ onClick Clear
]
[ text "Clear" ]
]
]
]
-- PORTS
port setItem : LocalStorageItem -> Cmd msg
port getItem : String -> Cmd msg
port gotItem : (String -> msg) -> Sub msg
port removeItem : String -> Cmd msg
port clear : () -> Cmd msg
-- port requestItem : (String -> msg) -> Sub msg
-- port arrivingItem : (String -> msg) -> Sub msg
-- SUBSCRIPTIONS
subscriptions : Model -> Sub Msg
subscriptions _ =
gotItem GotItem
| elm |
[
{
"context": " }\n\ninitialForm : Form\ninitialForm = { email = \"admin@mail.com\", password = \"admin\", passwordAgain = \"admin\", po",
"end": 972,
"score": 0.9999275208,
"start": 958,
"tag": "EMAIL",
"value": "admin@mail.com"
},
{
"context": "alForm = { email = \"admin@mail.com\", password = \"admin\", passwordAgain = \"admin\", postTitle = \"\", postBo",
"end": 992,
"score": 0.9995387793,
"start": 987,
"tag": "PASSWORD",
"value": "admin"
},
{
"context": "n@mail.com\", password = \"admin\", passwordAgain = \"admin\", postTitle = \"\", postBody = \"\" }\n\n",
"end": 1017,
"score": 0.9995198846,
"start": 1012,
"tag": "PASSWORD",
"value": "admin"
}
] | src/app/Models.elm | grrinchas/elm-blog | 8 | module Models exposing (..)
import Lorem
import RemoteData exposing (RemoteData(NotAsked), WebData)
import Routes exposing (Route(HomeRoute))
type alias User =
{ email : String
}
type alias Post =
{ id : String
, title : String
, body : String
}
type alias Form =
{ email : String
, password : String
, passwordAgain : String
, postTitle : String
, postBody : String
}
type alias Token =
{ accessToken : String
, idToken : String
, tokenType : String
, expiresIn : Int
}
type alias Model =
{ posts : WebData (List Post)
, form : Form
, route : Route
, user : WebData User
, token : WebData Token
, account : WebData ()
}
initialModel : Model
initialModel =
{ posts = NotAsked
, form = initialForm
, route = HomeRoute
, user = NotAsked
, token = NotAsked
, account = NotAsked
}
initialForm : Form
initialForm = { email = "admin@mail.com", password = "admin", passwordAgain = "admin", postTitle = "", postBody = "" }
| 35667 | module Models exposing (..)
import Lorem
import RemoteData exposing (RemoteData(NotAsked), WebData)
import Routes exposing (Route(HomeRoute))
type alias User =
{ email : String
}
type alias Post =
{ id : String
, title : String
, body : String
}
type alias Form =
{ email : String
, password : String
, passwordAgain : String
, postTitle : String
, postBody : String
}
type alias Token =
{ accessToken : String
, idToken : String
, tokenType : String
, expiresIn : Int
}
type alias Model =
{ posts : WebData (List Post)
, form : Form
, route : Route
, user : WebData User
, token : WebData Token
, account : WebData ()
}
initialModel : Model
initialModel =
{ posts = NotAsked
, form = initialForm
, route = HomeRoute
, user = NotAsked
, token = NotAsked
, account = NotAsked
}
initialForm : Form
initialForm = { email = "<EMAIL>", password = "<PASSWORD>", passwordAgain = "<PASSWORD>", postTitle = "", postBody = "" }
| true | module Models exposing (..)
import Lorem
import RemoteData exposing (RemoteData(NotAsked), WebData)
import Routes exposing (Route(HomeRoute))
type alias User =
{ email : String
}
type alias Post =
{ id : String
, title : String
, body : String
}
type alias Form =
{ email : String
, password : String
, passwordAgain : String
, postTitle : String
, postBody : String
}
type alias Token =
{ accessToken : String
, idToken : String
, tokenType : String
, expiresIn : Int
}
type alias Model =
{ posts : WebData (List Post)
, form : Form
, route : Route
, user : WebData User
, token : WebData Token
, account : WebData ()
}
initialModel : Model
initialModel =
{ posts = NotAsked
, form = initialForm
, route = HomeRoute
, user = NotAsked
, token = NotAsked
, account = NotAsked
}
initialForm : Form
initialForm = { email = "PI:EMAIL:<EMAIL>END_PI", password = "PI:PASSWORD:<PASSWORD>END_PI", passwordAgain = "PI:PASSWORD:<PASSWORD>END_PI", postTitle = "", postBody = "" }
| elm |
[
{
"context": "ed (NewItem workflowKind process { id = \"\", name = newItemName, description = \"\" } itemIndex))\n ,",
"end": 17661,
"score": 0.8843414187,
"start": 17658,
"tag": "NAME",
"value": "new"
}
] | src/frontend/src/ProcessDesigner.elm | chechulnikov/resolvent | 0 | module ProcessDesigner exposing (..)
import Controls
import Css exposing (..)
import Html.Styled exposing (..)
import Html.Styled.Attributes exposing (attribute, css)
import Html.Styled.Events exposing (..)
import List.Extra as List
import Utils exposing (boolToMaybe, boolToString, onStd, onWithoutDef, onWithoutProp, updateIfTrue)
type Msg
= Idle
| ToggleMode Mode
| TempIdRequested (Int -> Msg)
| NewProcess WorkflowKind Process Int
| NewItem WorkflowKind Process ProcessItem Int Int
| NewItemSlot WorkflowKind Process
| DragStart DraggingState
| DragEnd
| Drop WorkflowKind DropProcessItemTarget
| DragTargetOnDraggableArea Bool
| ToggleProcessItemSelection Process ProcessItem
type alias Model =
{ processes : List Process
, subWorkflow : Maybe SubWorkflowData
, mode : Mode
, draggingState : Maybe { state : DraggingState, hasTargeted : Bool}
}
type alias Workflow a =
{ a | processes : List Process }
type alias SubWorkflowData =
{ parentProcessItem : ProcessItem
, parentProcess : Process
, processes : List Process
}
type alias Process =
{ id : String
, name : String
, items : List (Maybe ProcessItem)
}
type alias ProcessItem =
{ id : String
, name : String
, description : String
}
type DraggingState
= DraggingProcessItemState
{ workflowKind : WorkflowKind
, process : Process
, item : ProcessItem
, itemIndex : Int
}
| DraggingEmptyItemState
{ workflowKind : WorkflowKind
, process : Process
, itemIndex : Int
}
| DraggingProcessState
{ workflowKind : WorkflowKind
, process : Process
}
type Mode
= ViewerMode
| EditorMode
type DropProcessItemTarget
= DropOnEmptySlot Process Int
| DropOnNewSlot Process
| DropInBin
| DropOnAnotherProcessItem Process ProcessItem Int
type DroppableAreaMode
= Normal
| ReadyToReceiveProcessItem
| ReadyToReceiveEmptyItem
| ReadyToReceiveProcess
type WorkflowKind
= MainWorkflow
| SubWorkflow
type ProcessItemState
= NormalProcessItemState
| ActiveProcessItemState
-- UPDATE
toggleMode : Mode -> Model -> Model
toggleMode mode model =
{ model | mode = mode }
updateWorkflow : WorkflowKind -> (Model-> Model) -> (SubWorkflowData -> SubWorkflowData) -> Model -> Model
updateWorkflow workflowType updateMainWorkflow updateSecondaryWorkflow model =
case workflowType of
MainWorkflow ->
updateMainWorkflow model
SubWorkflow ->
{ model | subWorkflow = model.subWorkflow |> Maybe.map updateSecondaryWorkflow }
newProcess : Int -> Process -> Workflow a -> Workflow a
newProcess tempId process workflow =
addProcess { process | id = String.fromInt tempId } workflow
addProcess : Process -> Workflow a -> Workflow a
addProcess process workflow =
{ workflow | processes = List.append workflow.processes [ process ] }
newItemToProcess : Int -> Int -> ProcessItem -> Process -> Workflow a -> Workflow a
newItemToProcess tempId itemIndex item process model =
addItemToProcess itemIndex { item | id = String.fromInt tempId } process model
addItemToProcess : Int -> ProcessItem -> Process -> Workflow a -> Workflow a
addItemToProcess itemIndex item process workflow =
let
update p =
{ p | items = p.items |> List.updateAt itemIndex (\_ -> Just item) }
updatedProcesses =
workflow.processes |> List.map (\p -> updateIfTrue update p (p.id == process.id))
in
{ workflow | processes = updatedProcesses }
removeItemFromProcess : Int -> Process -> Workflow a -> Workflow a
removeItemFromProcess itemIndex process workflow =
let
update p =
{ p | items = p.items |> List.updateAt itemIndex (\_ -> Nothing) }
updatedProcesses =
workflow.processes
|> List.map (\p -> updateIfTrue update p (p.id == process.id))
in
{ workflow | processes = updatedProcesses }
dropOn : DropProcessItemTarget -> Model -> Workflow a -> Workflow a
dropOn target model workflow =
model.draggingState
|> Maybe.map
(\{ state } ->
case state of
DraggingProcessItemState { workflowKind, process, item, itemIndex } ->
case target of
DropOnNewSlot targetProcess ->
workflow
|> addItemSlot targetProcess
|> addItemToProcess (List.length targetProcess.items) item targetProcess
|> removeItemFromProcess itemIndex process
DropInBin ->
removeItemFromProcess itemIndex process workflow
DropOnEmptySlot targetProcess targetItemIndex ->
workflow
|> addItemToProcess targetItemIndex item targetProcess
|> removeItemFromProcess itemIndex process
DropOnAnotherProcessItem targetProcess targetItem targetItemIndex ->
workflow
|> addItemToProcess itemIndex targetItem process
|> addItemToProcess targetItemIndex item targetProcess
DraggingEmptyItemState { process, itemIndex } ->
removeEmptySlot itemIndex process workflow
DraggingProcessState { process } ->
removeProcess process workflow
)
|> Maybe.withDefault workflow
addItemSlot : Process -> Workflow a -> Workflow a
addItemSlot process workflow =
let
update p =
{ p | items = List.append p.items [ Nothing ] }
updatedProcesses =
workflow.processes |> List.map (\p -> updateIfTrue update p (p.id == process.id))
in
{ workflow | processes = updatedProcesses }
removeEmptySlot : Int -> Process -> Workflow a -> Workflow a
removeEmptySlot itemIndex process workflow =
let
update p =
{ p | items = p.items |> List.removeAt itemIndex }
updatedProcesses =
workflow.processes
|> List.map (\p -> updateIfTrue update p (p.id == process.id))
in
{ workflow | processes = updatedProcesses }
removeProcess : Process -> Workflow a -> Workflow a
removeProcess process workflow =
{ workflow | processes = workflow.processes |> List.remove process }
setDraggingState : DraggingState -> Model -> Model
setDraggingState draggingState model =
{ model | draggingState = Just { state = draggingState, hasTargeted = False } }
hasDragTargeted : Model -> Bool
hasDragTargeted model =
model.draggingState
|> Maybe.map .hasTargeted
|> Maybe.withDefault False
clearDraggingState : Model -> Model
clearDraggingState model =
{ model | draggingState = Nothing }
toggleTargetingOfDraggingState : Bool -> Model -> Model
toggleTargetingOfDraggingState hasTargeted model =
{ model | draggingState = model.draggingState |> Maybe.map (\s -> { s | hasTargeted = hasTargeted}) }
toggleSubWorkflow : Process -> ProcessItem -> List Process -> Model -> Model
toggleSubWorkflow parentProcess parentProcessItem subProcesses model =
model.subWorkflow
|> Maybe.andThen
(\w -> boolToMaybe { model | subWorkflow = Nothing } (w.parentProcessItem == parentProcessItem))
|> Maybe.withDefault
{ model
| subWorkflow =
Just
{ parentProcessItem = parentProcessItem
, parentProcess = parentProcess
, processes = subProcesses
}
}
-- ATTRS
attrDraggable mode =
attribute "draggable" (boolToString (mode == EditorMode))
attrEditable mode =
attribute "contenteditable" (boolToString (mode == EditorMode))
-- VIEW
view : Model -> Html Msg
view model =
let
droppableAreaMode =
case model.draggingState of
Just { state } ->
case state of
DraggingProcessItemState _ ->
ReadyToReceiveProcessItem
DraggingEmptyItemState _ ->
ReadyToReceiveEmptyItem
DraggingProcessState _ ->
ReadyToReceiveProcess
Nothing ->
Normal
mode =
model.mode
toggleModeButton =
let
(label, newMode) =
case model.mode of
ViewerMode ->
("Edit", EditorMode)
EditorMode ->
("Back to viewer", ViewerMode)
in
Controls.viewToggle label newMode ToggleMode ((==) ViewerMode)
activeProcessItem =
model.subWorkflow
|> Maybe.map .parentProcessItem
addWorkflowControls workflowKind workflow ps =
mode == EditorMode
|> boolToMaybe
( List.append
ps
[ div
[ css [ displayFlex ] ]
[ viewAddProcessButton workflowKind workflow.processes ]
]
)
|> Maybe.withDefault ps
viewMainWorkflow =
model.processes
|> List.filter isUsefulProcess
|> List.map (viewProcess MainWorkflow mode droppableAreaMode activeProcessItem)
|> addWorkflowControls MainWorkflow model
|> List.append [ toggleModeButton ]
|> div
[ css
[ overflowX scroll
, whiteSpace noWrap
, height (vh 75)
]
]
viewSubWorkflow workflow =
workflow.processes
|> List.map (viewProcess SubWorkflow mode droppableAreaMode activeProcessItem)
|> addWorkflowControls SubWorkflow workflow
|> div
[ css
[ bottom (vh 0)
, right (rem 0)
, padding (rem 0.25)
, width (pct 100)
, height (vh 25)
, borderTop3 (rem 0.1) solid (rgb 4 4 4)
]
]
isUsefulProcess p =
(model.mode == EditorMode)
|| ((model.mode == ViewerMode) && (p.items /= [] && List.any ((/=) Nothing) p.items))
binWorkflow =
model.draggingState
|> Maybe.map .state
|> Maybe.map
( \state ->
case state of
DraggingProcessItemState { workflowKind } ->
workflowKind
DraggingEmptyItemState { workflowKind } ->
workflowKind
DraggingProcessState { workflowKind } ->
workflowKind
)
|> Maybe.withDefault MainWorkflow
in
div
[]
[ viewMainWorkflow
, model.subWorkflow
|> Maybe.map viewSubWorkflow
|> Maybe.withDefault (text "")
, viewBin binWorkflow droppableAreaMode
]
viewProcess : WorkflowKind -> Mode -> DroppableAreaMode -> Maybe ProcessItem -> Process -> Html Msg
viewProcess workflowKind mode droppableAreaMode activeProcessItem process =
let
getProcessItemState i =
activeProcessItem
|> Maybe.andThen (\x -> boolToMaybe ActiveProcessItemState (x == i))
|> Maybe.withDefault NormalProcessItemState
viewProcessItems =
let
items =
process.items
|> List.filter ((/=) Nothing >> (||) (mode == EditorMode))
|> List.indexedMap
( \itemIndex item ->
item
|> Maybe.map (\i -> viewProcessItem workflowKind (getProcessItemState i) mode process i)
|> Maybe.withDefault (viewEmptyItem workflowKind mode droppableAreaMode process itemIndex)
)
in
if mode == EditorMode then
List.append items [ viewNewSlotButton workflowKind droppableAreaMode process ]
else
items
in
div
[ css
[ displayFlex
, before
[ width (rem 2)
, minWidth (rem 2)
, margin (rem 0.25)
, backgroundColor (hex "#606dbc")
, property "content" "''"
]
, hover
[ backgroundColor (rgba 128 128 128 0.05)
]
, cursor (if mode == EditorMode then move else default)
]
, onWithoutProp "dragstart" (DragStart (DraggingProcessState { workflowKind = workflowKind, process = process }))
, onWithoutProp "dragend" DragEnd
, attrDraggable mode
]
viewProcessItems
viewProcessItem workflowKind state mode process processItem =
let
viewName =
div
[ css []
]
[ div
[ attrEditable mode
, css
[ focus [ outline none ]
, whiteSpace noWrap
]
]
[ text processItem.name ]
]
itemIndex =
process.items
|> List.elemIndex (Just processItem)
|> Maybe.withDefault 0 -- unattainable result
style =
case state of
NormalProcessItemState ->
Css.batch
[ border3 (rem 0.1) solid (rgb 4 4 4)
, backgroundColor (rgb 255 255 255)
]
ActiveProcessItemState ->
Css.batch
[ border3 (rem 0.1) solid (rgb 10 124 10)
, backgroundColor (rgb 216 255 211)
, color (rgb 10 124 10)
]
in
div
[ css
[ style
, margin (rem 0.5)
, padding (rem 0.25)
, minWidth (rem 10)
, height (rem 3)
, overflowX hidden
, hover
[ border3 (rem 0.1) solid (rgb 10 124 10)
, backgroundColor (rgb 216 255 211)
, color (rgb 10 124 10)
, cursor pointer
]
, active
[ border3 (rem 0.1) solid (rgb 26 171 26)
, backgroundColor (rgb 216 255 211)
, color (rgb 26 171 26)
, cursor pointer
]
]
, attrDraggable mode
, onWithoutProp
"dragstart"
(DragStart (DraggingProcessItemState { workflowKind = workflowKind, process = process, item = processItem, itemIndex = itemIndex }))
, onWithoutProp "dragend" DragEnd
, onWithoutProp "dragenter" (DragTargetOnDraggableArea True)
, onWithoutProp "dragleave" (DragTargetOnDraggableArea False)
, onWithoutProp "drop" (Drop workflowKind (DropOnAnotherProcessItem process processItem itemIndex))
, onWithoutDef "dragover" Idle
, onClick (ToggleProcessItemSelection process processItem)
]
[ viewName
]
viewEmptyItem workflowKind mode droppableAreaMode process itemIndex =
let
newItemName =
"New item " ++ (String.fromInt (itemIndex + 1))
droppableStyles =
case droppableAreaMode of
ReadyToReceiveProcessItem ->
Css.batch
[ border3 (rem 0.1) dashed (rgb 15 103 15)
, backgroundColor (rgb 216 255 211)
, color (rgb 13 110 13)
, opacity (num 0.5)
]
_ ->
Css.batch
[ border3 (rem 0.1) solid (rgb 255 255 255)
, opacity (num 0.25)
]
in
div
[ css
[ droppableStyles
, margin (rem 0.5)
, padding (rem 0.25)
, minWidth (rem 10)
, height (rem 3)
, hover
[ border3 (rem 0.1) solid (rgb 15 103 15)
, backgroundColor (rgb 216 255 211)
, color (rgb 13 110 13)
, cursor pointer
, opacity (num 0.5)
]
, active
[ border3 (rem 0.1) solid (rgb 26 171 26)
, backgroundColor (rgb 216 255 211)
, color (rgb 26 171 26)
, cursor pointer
, opacity (num 0.85)
]
]
, onClick (TempIdRequested (NewItem workflowKind process { id = "", name = newItemName, description = "" } itemIndex))
, onWithoutDef "dragover" Idle
, attrDraggable mode
, onStd "drop" (Drop workflowKind (DropOnEmptySlot process itemIndex))
, onWithoutProp
"dragstart"
(DragStart (DraggingEmptyItemState { workflowKind = workflowKind, process = process, itemIndex = itemIndex }))
, onWithoutProp "dragend" DragEnd
, onStd "dragenter" (DragTargetOnDraggableArea True)
, onStd "dragleave" (DragTargetOnDraggableArea False)
]
[ text "EMPTY" ]
viewNewSlotButton workflowKind droppableAreaMode process =
let
droppableStyles =
case droppableAreaMode of
ReadyToReceiveProcessItem ->
Css.batch
[ border3 (rem 0.1) dashed (rgb 15 103 15)
, backgroundColor (rgb 216 255 211)
, color (rgb 13 110 13)
, opacity (num 0.5)
]
_ ->
Css.batch
[ border3 (rem 0.1) dashed (rgb 4 4 4)
, opacity (num 0.25)
]
in
div
[ css
[ droppableStyles
, margin (rem 0.5)
, padding (rem 0.25)
, minWidth (rem 10)
, height (rem 3)
, hover
[ backgroundColor (rgb 216 255 211)
, color (rgb 13 110 13)
, cursor pointer
, opacity (num 0.5)
]
, active
[ border3 (rem 0.1) solid (rgb 26 171 26)
, backgroundColor (rgb 216 255 211)
, color (rgb 26 171 26)
, cursor pointer
, opacity (num 0.85)
]
]
, onClick (NewItemSlot workflowKind process)
, onWithoutDef "dragover" Idle
, onStd "drop" (Drop workflowKind (DropOnNewSlot process))
, onStd "dragenter" (DragTargetOnDraggableArea True)
, onStd "dragleave" (DragTargetOnDraggableArea False)
]
[ text "➕" ]
viewAddProcessButton : WorkflowKind -> List Process -> Html Msg
viewAddProcessButton workflowKind processes =
let
processesCount =
List.length processes
newProcessName =
"New item " ++ (String.fromInt (processesCount + 1))
in
div
[ css
[ border3 (rem 0.1) dashed (rgb 4 4 4)
, margin (rem 0.5)
, padding (rem 0.25)
, width (rem 20)
, height (rem 3)
, opacity (num 0.25)
, hover
[ border3 (rem 0.1) solid (rgb 15 103 15)
, backgroundColor (rgb 216 255 211)
, color (rgb 13 110 13)
, cursor pointer
, opacity (num 0.5)
]
, active
[ border3 (rem 0.1) solid (rgb 26 171 26)
, backgroundColor (rgb 216 255 211)
, color (rgb 26 171 26)
, cursor pointer
, opacity (num 0.85)
]
]
, onClick (TempIdRequested (NewProcess workflowKind (Process newProcessName newProcessName [])))
]
[ text "➕" ]
viewBin : WorkflowKind -> DroppableAreaMode -> Html Msg
viewBin workflowKind droppableAreaMode =
let
droppableStyles =
let
droppableReadyStyles =
Css.batch
[ border3 (rem 0.1) dashed (rgb 105 0 24)
, backgroundColor (rgb 255 211 216)
, color (rgb 105 0 24)
, opacity (num 0.5)
, display block
]
in
case droppableAreaMode of
Normal ->
Css.batch
[ border3 (rem 0.1) dashed (rgb 4 4 4)
, opacity (num 0.25)
, display none
]
_ ->
droppableReadyStyles
dropMsg =
case droppableAreaMode of
Normal ->
Idle
_ ->
Drop workflowKind DropInBin
in
div
[ css
[ droppableStyles
, position fixed
, bottom (vh 0)
, right (rem 0)
, margin (rem 0.5)
, padding (rem 0.25)
, width (vw 10)
, height (vh 10)
]
, onWithoutDef "dragover" Idle
, onStd "drop" dropMsg
, onStd "dragenter" (DragTargetOnDraggableArea True)
, onStd "dragleave" (DragTargetOnDraggableArea False)
]
[ text "🗑️" ]
| 36664 | module ProcessDesigner exposing (..)
import Controls
import Css exposing (..)
import Html.Styled exposing (..)
import Html.Styled.Attributes exposing (attribute, css)
import Html.Styled.Events exposing (..)
import List.Extra as List
import Utils exposing (boolToMaybe, boolToString, onStd, onWithoutDef, onWithoutProp, updateIfTrue)
type Msg
= Idle
| ToggleMode Mode
| TempIdRequested (Int -> Msg)
| NewProcess WorkflowKind Process Int
| NewItem WorkflowKind Process ProcessItem Int Int
| NewItemSlot WorkflowKind Process
| DragStart DraggingState
| DragEnd
| Drop WorkflowKind DropProcessItemTarget
| DragTargetOnDraggableArea Bool
| ToggleProcessItemSelection Process ProcessItem
type alias Model =
{ processes : List Process
, subWorkflow : Maybe SubWorkflowData
, mode : Mode
, draggingState : Maybe { state : DraggingState, hasTargeted : Bool}
}
type alias Workflow a =
{ a | processes : List Process }
type alias SubWorkflowData =
{ parentProcessItem : ProcessItem
, parentProcess : Process
, processes : List Process
}
type alias Process =
{ id : String
, name : String
, items : List (Maybe ProcessItem)
}
type alias ProcessItem =
{ id : String
, name : String
, description : String
}
type DraggingState
= DraggingProcessItemState
{ workflowKind : WorkflowKind
, process : Process
, item : ProcessItem
, itemIndex : Int
}
| DraggingEmptyItemState
{ workflowKind : WorkflowKind
, process : Process
, itemIndex : Int
}
| DraggingProcessState
{ workflowKind : WorkflowKind
, process : Process
}
type Mode
= ViewerMode
| EditorMode
type DropProcessItemTarget
= DropOnEmptySlot Process Int
| DropOnNewSlot Process
| DropInBin
| DropOnAnotherProcessItem Process ProcessItem Int
type DroppableAreaMode
= Normal
| ReadyToReceiveProcessItem
| ReadyToReceiveEmptyItem
| ReadyToReceiveProcess
type WorkflowKind
= MainWorkflow
| SubWorkflow
type ProcessItemState
= NormalProcessItemState
| ActiveProcessItemState
-- UPDATE
toggleMode : Mode -> Model -> Model
toggleMode mode model =
{ model | mode = mode }
updateWorkflow : WorkflowKind -> (Model-> Model) -> (SubWorkflowData -> SubWorkflowData) -> Model -> Model
updateWorkflow workflowType updateMainWorkflow updateSecondaryWorkflow model =
case workflowType of
MainWorkflow ->
updateMainWorkflow model
SubWorkflow ->
{ model | subWorkflow = model.subWorkflow |> Maybe.map updateSecondaryWorkflow }
newProcess : Int -> Process -> Workflow a -> Workflow a
newProcess tempId process workflow =
addProcess { process | id = String.fromInt tempId } workflow
addProcess : Process -> Workflow a -> Workflow a
addProcess process workflow =
{ workflow | processes = List.append workflow.processes [ process ] }
newItemToProcess : Int -> Int -> ProcessItem -> Process -> Workflow a -> Workflow a
newItemToProcess tempId itemIndex item process model =
addItemToProcess itemIndex { item | id = String.fromInt tempId } process model
addItemToProcess : Int -> ProcessItem -> Process -> Workflow a -> Workflow a
addItemToProcess itemIndex item process workflow =
let
update p =
{ p | items = p.items |> List.updateAt itemIndex (\_ -> Just item) }
updatedProcesses =
workflow.processes |> List.map (\p -> updateIfTrue update p (p.id == process.id))
in
{ workflow | processes = updatedProcesses }
removeItemFromProcess : Int -> Process -> Workflow a -> Workflow a
removeItemFromProcess itemIndex process workflow =
let
update p =
{ p | items = p.items |> List.updateAt itemIndex (\_ -> Nothing) }
updatedProcesses =
workflow.processes
|> List.map (\p -> updateIfTrue update p (p.id == process.id))
in
{ workflow | processes = updatedProcesses }
dropOn : DropProcessItemTarget -> Model -> Workflow a -> Workflow a
dropOn target model workflow =
model.draggingState
|> Maybe.map
(\{ state } ->
case state of
DraggingProcessItemState { workflowKind, process, item, itemIndex } ->
case target of
DropOnNewSlot targetProcess ->
workflow
|> addItemSlot targetProcess
|> addItemToProcess (List.length targetProcess.items) item targetProcess
|> removeItemFromProcess itemIndex process
DropInBin ->
removeItemFromProcess itemIndex process workflow
DropOnEmptySlot targetProcess targetItemIndex ->
workflow
|> addItemToProcess targetItemIndex item targetProcess
|> removeItemFromProcess itemIndex process
DropOnAnotherProcessItem targetProcess targetItem targetItemIndex ->
workflow
|> addItemToProcess itemIndex targetItem process
|> addItemToProcess targetItemIndex item targetProcess
DraggingEmptyItemState { process, itemIndex } ->
removeEmptySlot itemIndex process workflow
DraggingProcessState { process } ->
removeProcess process workflow
)
|> Maybe.withDefault workflow
addItemSlot : Process -> Workflow a -> Workflow a
addItemSlot process workflow =
let
update p =
{ p | items = List.append p.items [ Nothing ] }
updatedProcesses =
workflow.processes |> List.map (\p -> updateIfTrue update p (p.id == process.id))
in
{ workflow | processes = updatedProcesses }
removeEmptySlot : Int -> Process -> Workflow a -> Workflow a
removeEmptySlot itemIndex process workflow =
let
update p =
{ p | items = p.items |> List.removeAt itemIndex }
updatedProcesses =
workflow.processes
|> List.map (\p -> updateIfTrue update p (p.id == process.id))
in
{ workflow | processes = updatedProcesses }
removeProcess : Process -> Workflow a -> Workflow a
removeProcess process workflow =
{ workflow | processes = workflow.processes |> List.remove process }
setDraggingState : DraggingState -> Model -> Model
setDraggingState draggingState model =
{ model | draggingState = Just { state = draggingState, hasTargeted = False } }
hasDragTargeted : Model -> Bool
hasDragTargeted model =
model.draggingState
|> Maybe.map .hasTargeted
|> Maybe.withDefault False
clearDraggingState : Model -> Model
clearDraggingState model =
{ model | draggingState = Nothing }
toggleTargetingOfDraggingState : Bool -> Model -> Model
toggleTargetingOfDraggingState hasTargeted model =
{ model | draggingState = model.draggingState |> Maybe.map (\s -> { s | hasTargeted = hasTargeted}) }
toggleSubWorkflow : Process -> ProcessItem -> List Process -> Model -> Model
toggleSubWorkflow parentProcess parentProcessItem subProcesses model =
model.subWorkflow
|> Maybe.andThen
(\w -> boolToMaybe { model | subWorkflow = Nothing } (w.parentProcessItem == parentProcessItem))
|> Maybe.withDefault
{ model
| subWorkflow =
Just
{ parentProcessItem = parentProcessItem
, parentProcess = parentProcess
, processes = subProcesses
}
}
-- ATTRS
attrDraggable mode =
attribute "draggable" (boolToString (mode == EditorMode))
attrEditable mode =
attribute "contenteditable" (boolToString (mode == EditorMode))
-- VIEW
view : Model -> Html Msg
view model =
let
droppableAreaMode =
case model.draggingState of
Just { state } ->
case state of
DraggingProcessItemState _ ->
ReadyToReceiveProcessItem
DraggingEmptyItemState _ ->
ReadyToReceiveEmptyItem
DraggingProcessState _ ->
ReadyToReceiveProcess
Nothing ->
Normal
mode =
model.mode
toggleModeButton =
let
(label, newMode) =
case model.mode of
ViewerMode ->
("Edit", EditorMode)
EditorMode ->
("Back to viewer", ViewerMode)
in
Controls.viewToggle label newMode ToggleMode ((==) ViewerMode)
activeProcessItem =
model.subWorkflow
|> Maybe.map .parentProcessItem
addWorkflowControls workflowKind workflow ps =
mode == EditorMode
|> boolToMaybe
( List.append
ps
[ div
[ css [ displayFlex ] ]
[ viewAddProcessButton workflowKind workflow.processes ]
]
)
|> Maybe.withDefault ps
viewMainWorkflow =
model.processes
|> List.filter isUsefulProcess
|> List.map (viewProcess MainWorkflow mode droppableAreaMode activeProcessItem)
|> addWorkflowControls MainWorkflow model
|> List.append [ toggleModeButton ]
|> div
[ css
[ overflowX scroll
, whiteSpace noWrap
, height (vh 75)
]
]
viewSubWorkflow workflow =
workflow.processes
|> List.map (viewProcess SubWorkflow mode droppableAreaMode activeProcessItem)
|> addWorkflowControls SubWorkflow workflow
|> div
[ css
[ bottom (vh 0)
, right (rem 0)
, padding (rem 0.25)
, width (pct 100)
, height (vh 25)
, borderTop3 (rem 0.1) solid (rgb 4 4 4)
]
]
isUsefulProcess p =
(model.mode == EditorMode)
|| ((model.mode == ViewerMode) && (p.items /= [] && List.any ((/=) Nothing) p.items))
binWorkflow =
model.draggingState
|> Maybe.map .state
|> Maybe.map
( \state ->
case state of
DraggingProcessItemState { workflowKind } ->
workflowKind
DraggingEmptyItemState { workflowKind } ->
workflowKind
DraggingProcessState { workflowKind } ->
workflowKind
)
|> Maybe.withDefault MainWorkflow
in
div
[]
[ viewMainWorkflow
, model.subWorkflow
|> Maybe.map viewSubWorkflow
|> Maybe.withDefault (text "")
, viewBin binWorkflow droppableAreaMode
]
viewProcess : WorkflowKind -> Mode -> DroppableAreaMode -> Maybe ProcessItem -> Process -> Html Msg
viewProcess workflowKind mode droppableAreaMode activeProcessItem process =
let
getProcessItemState i =
activeProcessItem
|> Maybe.andThen (\x -> boolToMaybe ActiveProcessItemState (x == i))
|> Maybe.withDefault NormalProcessItemState
viewProcessItems =
let
items =
process.items
|> List.filter ((/=) Nothing >> (||) (mode == EditorMode))
|> List.indexedMap
( \itemIndex item ->
item
|> Maybe.map (\i -> viewProcessItem workflowKind (getProcessItemState i) mode process i)
|> Maybe.withDefault (viewEmptyItem workflowKind mode droppableAreaMode process itemIndex)
)
in
if mode == EditorMode then
List.append items [ viewNewSlotButton workflowKind droppableAreaMode process ]
else
items
in
div
[ css
[ displayFlex
, before
[ width (rem 2)
, minWidth (rem 2)
, margin (rem 0.25)
, backgroundColor (hex "#606dbc")
, property "content" "''"
]
, hover
[ backgroundColor (rgba 128 128 128 0.05)
]
, cursor (if mode == EditorMode then move else default)
]
, onWithoutProp "dragstart" (DragStart (DraggingProcessState { workflowKind = workflowKind, process = process }))
, onWithoutProp "dragend" DragEnd
, attrDraggable mode
]
viewProcessItems
viewProcessItem workflowKind state mode process processItem =
let
viewName =
div
[ css []
]
[ div
[ attrEditable mode
, css
[ focus [ outline none ]
, whiteSpace noWrap
]
]
[ text processItem.name ]
]
itemIndex =
process.items
|> List.elemIndex (Just processItem)
|> Maybe.withDefault 0 -- unattainable result
style =
case state of
NormalProcessItemState ->
Css.batch
[ border3 (rem 0.1) solid (rgb 4 4 4)
, backgroundColor (rgb 255 255 255)
]
ActiveProcessItemState ->
Css.batch
[ border3 (rem 0.1) solid (rgb 10 124 10)
, backgroundColor (rgb 216 255 211)
, color (rgb 10 124 10)
]
in
div
[ css
[ style
, margin (rem 0.5)
, padding (rem 0.25)
, minWidth (rem 10)
, height (rem 3)
, overflowX hidden
, hover
[ border3 (rem 0.1) solid (rgb 10 124 10)
, backgroundColor (rgb 216 255 211)
, color (rgb 10 124 10)
, cursor pointer
]
, active
[ border3 (rem 0.1) solid (rgb 26 171 26)
, backgroundColor (rgb 216 255 211)
, color (rgb 26 171 26)
, cursor pointer
]
]
, attrDraggable mode
, onWithoutProp
"dragstart"
(DragStart (DraggingProcessItemState { workflowKind = workflowKind, process = process, item = processItem, itemIndex = itemIndex }))
, onWithoutProp "dragend" DragEnd
, onWithoutProp "dragenter" (DragTargetOnDraggableArea True)
, onWithoutProp "dragleave" (DragTargetOnDraggableArea False)
, onWithoutProp "drop" (Drop workflowKind (DropOnAnotherProcessItem process processItem itemIndex))
, onWithoutDef "dragover" Idle
, onClick (ToggleProcessItemSelection process processItem)
]
[ viewName
]
viewEmptyItem workflowKind mode droppableAreaMode process itemIndex =
let
newItemName =
"New item " ++ (String.fromInt (itemIndex + 1))
droppableStyles =
case droppableAreaMode of
ReadyToReceiveProcessItem ->
Css.batch
[ border3 (rem 0.1) dashed (rgb 15 103 15)
, backgroundColor (rgb 216 255 211)
, color (rgb 13 110 13)
, opacity (num 0.5)
]
_ ->
Css.batch
[ border3 (rem 0.1) solid (rgb 255 255 255)
, opacity (num 0.25)
]
in
div
[ css
[ droppableStyles
, margin (rem 0.5)
, padding (rem 0.25)
, minWidth (rem 10)
, height (rem 3)
, hover
[ border3 (rem 0.1) solid (rgb 15 103 15)
, backgroundColor (rgb 216 255 211)
, color (rgb 13 110 13)
, cursor pointer
, opacity (num 0.5)
]
, active
[ border3 (rem 0.1) solid (rgb 26 171 26)
, backgroundColor (rgb 216 255 211)
, color (rgb 26 171 26)
, cursor pointer
, opacity (num 0.85)
]
]
, onClick (TempIdRequested (NewItem workflowKind process { id = "", name = <NAME>ItemName, description = "" } itemIndex))
, onWithoutDef "dragover" Idle
, attrDraggable mode
, onStd "drop" (Drop workflowKind (DropOnEmptySlot process itemIndex))
, onWithoutProp
"dragstart"
(DragStart (DraggingEmptyItemState { workflowKind = workflowKind, process = process, itemIndex = itemIndex }))
, onWithoutProp "dragend" DragEnd
, onStd "dragenter" (DragTargetOnDraggableArea True)
, onStd "dragleave" (DragTargetOnDraggableArea False)
]
[ text "EMPTY" ]
viewNewSlotButton workflowKind droppableAreaMode process =
let
droppableStyles =
case droppableAreaMode of
ReadyToReceiveProcessItem ->
Css.batch
[ border3 (rem 0.1) dashed (rgb 15 103 15)
, backgroundColor (rgb 216 255 211)
, color (rgb 13 110 13)
, opacity (num 0.5)
]
_ ->
Css.batch
[ border3 (rem 0.1) dashed (rgb 4 4 4)
, opacity (num 0.25)
]
in
div
[ css
[ droppableStyles
, margin (rem 0.5)
, padding (rem 0.25)
, minWidth (rem 10)
, height (rem 3)
, hover
[ backgroundColor (rgb 216 255 211)
, color (rgb 13 110 13)
, cursor pointer
, opacity (num 0.5)
]
, active
[ border3 (rem 0.1) solid (rgb 26 171 26)
, backgroundColor (rgb 216 255 211)
, color (rgb 26 171 26)
, cursor pointer
, opacity (num 0.85)
]
]
, onClick (NewItemSlot workflowKind process)
, onWithoutDef "dragover" Idle
, onStd "drop" (Drop workflowKind (DropOnNewSlot process))
, onStd "dragenter" (DragTargetOnDraggableArea True)
, onStd "dragleave" (DragTargetOnDraggableArea False)
]
[ text "➕" ]
viewAddProcessButton : WorkflowKind -> List Process -> Html Msg
viewAddProcessButton workflowKind processes =
let
processesCount =
List.length processes
newProcessName =
"New item " ++ (String.fromInt (processesCount + 1))
in
div
[ css
[ border3 (rem 0.1) dashed (rgb 4 4 4)
, margin (rem 0.5)
, padding (rem 0.25)
, width (rem 20)
, height (rem 3)
, opacity (num 0.25)
, hover
[ border3 (rem 0.1) solid (rgb 15 103 15)
, backgroundColor (rgb 216 255 211)
, color (rgb 13 110 13)
, cursor pointer
, opacity (num 0.5)
]
, active
[ border3 (rem 0.1) solid (rgb 26 171 26)
, backgroundColor (rgb 216 255 211)
, color (rgb 26 171 26)
, cursor pointer
, opacity (num 0.85)
]
]
, onClick (TempIdRequested (NewProcess workflowKind (Process newProcessName newProcessName [])))
]
[ text "➕" ]
viewBin : WorkflowKind -> DroppableAreaMode -> Html Msg
viewBin workflowKind droppableAreaMode =
let
droppableStyles =
let
droppableReadyStyles =
Css.batch
[ border3 (rem 0.1) dashed (rgb 105 0 24)
, backgroundColor (rgb 255 211 216)
, color (rgb 105 0 24)
, opacity (num 0.5)
, display block
]
in
case droppableAreaMode of
Normal ->
Css.batch
[ border3 (rem 0.1) dashed (rgb 4 4 4)
, opacity (num 0.25)
, display none
]
_ ->
droppableReadyStyles
dropMsg =
case droppableAreaMode of
Normal ->
Idle
_ ->
Drop workflowKind DropInBin
in
div
[ css
[ droppableStyles
, position fixed
, bottom (vh 0)
, right (rem 0)
, margin (rem 0.5)
, padding (rem 0.25)
, width (vw 10)
, height (vh 10)
]
, onWithoutDef "dragover" Idle
, onStd "drop" dropMsg
, onStd "dragenter" (DragTargetOnDraggableArea True)
, onStd "dragleave" (DragTargetOnDraggableArea False)
]
[ text "🗑️" ]
| true | module ProcessDesigner exposing (..)
import Controls
import Css exposing (..)
import Html.Styled exposing (..)
import Html.Styled.Attributes exposing (attribute, css)
import Html.Styled.Events exposing (..)
import List.Extra as List
import Utils exposing (boolToMaybe, boolToString, onStd, onWithoutDef, onWithoutProp, updateIfTrue)
type Msg
= Idle
| ToggleMode Mode
| TempIdRequested (Int -> Msg)
| NewProcess WorkflowKind Process Int
| NewItem WorkflowKind Process ProcessItem Int Int
| NewItemSlot WorkflowKind Process
| DragStart DraggingState
| DragEnd
| Drop WorkflowKind DropProcessItemTarget
| DragTargetOnDraggableArea Bool
| ToggleProcessItemSelection Process ProcessItem
type alias Model =
{ processes : List Process
, subWorkflow : Maybe SubWorkflowData
, mode : Mode
, draggingState : Maybe { state : DraggingState, hasTargeted : Bool}
}
type alias Workflow a =
{ a | processes : List Process }
type alias SubWorkflowData =
{ parentProcessItem : ProcessItem
, parentProcess : Process
, processes : List Process
}
type alias Process =
{ id : String
, name : String
, items : List (Maybe ProcessItem)
}
type alias ProcessItem =
{ id : String
, name : String
, description : String
}
type DraggingState
= DraggingProcessItemState
{ workflowKind : WorkflowKind
, process : Process
, item : ProcessItem
, itemIndex : Int
}
| DraggingEmptyItemState
{ workflowKind : WorkflowKind
, process : Process
, itemIndex : Int
}
| DraggingProcessState
{ workflowKind : WorkflowKind
, process : Process
}
type Mode
= ViewerMode
| EditorMode
type DropProcessItemTarget
= DropOnEmptySlot Process Int
| DropOnNewSlot Process
| DropInBin
| DropOnAnotherProcessItem Process ProcessItem Int
type DroppableAreaMode
= Normal
| ReadyToReceiveProcessItem
| ReadyToReceiveEmptyItem
| ReadyToReceiveProcess
type WorkflowKind
= MainWorkflow
| SubWorkflow
type ProcessItemState
= NormalProcessItemState
| ActiveProcessItemState
-- UPDATE
toggleMode : Mode -> Model -> Model
toggleMode mode model =
{ model | mode = mode }
updateWorkflow : WorkflowKind -> (Model-> Model) -> (SubWorkflowData -> SubWorkflowData) -> Model -> Model
updateWorkflow workflowType updateMainWorkflow updateSecondaryWorkflow model =
case workflowType of
MainWorkflow ->
updateMainWorkflow model
SubWorkflow ->
{ model | subWorkflow = model.subWorkflow |> Maybe.map updateSecondaryWorkflow }
newProcess : Int -> Process -> Workflow a -> Workflow a
newProcess tempId process workflow =
addProcess { process | id = String.fromInt tempId } workflow
addProcess : Process -> Workflow a -> Workflow a
addProcess process workflow =
{ workflow | processes = List.append workflow.processes [ process ] }
newItemToProcess : Int -> Int -> ProcessItem -> Process -> Workflow a -> Workflow a
newItemToProcess tempId itemIndex item process model =
addItemToProcess itemIndex { item | id = String.fromInt tempId } process model
addItemToProcess : Int -> ProcessItem -> Process -> Workflow a -> Workflow a
addItemToProcess itemIndex item process workflow =
let
update p =
{ p | items = p.items |> List.updateAt itemIndex (\_ -> Just item) }
updatedProcesses =
workflow.processes |> List.map (\p -> updateIfTrue update p (p.id == process.id))
in
{ workflow | processes = updatedProcesses }
removeItemFromProcess : Int -> Process -> Workflow a -> Workflow a
removeItemFromProcess itemIndex process workflow =
let
update p =
{ p | items = p.items |> List.updateAt itemIndex (\_ -> Nothing) }
updatedProcesses =
workflow.processes
|> List.map (\p -> updateIfTrue update p (p.id == process.id))
in
{ workflow | processes = updatedProcesses }
dropOn : DropProcessItemTarget -> Model -> Workflow a -> Workflow a
dropOn target model workflow =
model.draggingState
|> Maybe.map
(\{ state } ->
case state of
DraggingProcessItemState { workflowKind, process, item, itemIndex } ->
case target of
DropOnNewSlot targetProcess ->
workflow
|> addItemSlot targetProcess
|> addItemToProcess (List.length targetProcess.items) item targetProcess
|> removeItemFromProcess itemIndex process
DropInBin ->
removeItemFromProcess itemIndex process workflow
DropOnEmptySlot targetProcess targetItemIndex ->
workflow
|> addItemToProcess targetItemIndex item targetProcess
|> removeItemFromProcess itemIndex process
DropOnAnotherProcessItem targetProcess targetItem targetItemIndex ->
workflow
|> addItemToProcess itemIndex targetItem process
|> addItemToProcess targetItemIndex item targetProcess
DraggingEmptyItemState { process, itemIndex } ->
removeEmptySlot itemIndex process workflow
DraggingProcessState { process } ->
removeProcess process workflow
)
|> Maybe.withDefault workflow
addItemSlot : Process -> Workflow a -> Workflow a
addItemSlot process workflow =
let
update p =
{ p | items = List.append p.items [ Nothing ] }
updatedProcesses =
workflow.processes |> List.map (\p -> updateIfTrue update p (p.id == process.id))
in
{ workflow | processes = updatedProcesses }
removeEmptySlot : Int -> Process -> Workflow a -> Workflow a
removeEmptySlot itemIndex process workflow =
let
update p =
{ p | items = p.items |> List.removeAt itemIndex }
updatedProcesses =
workflow.processes
|> List.map (\p -> updateIfTrue update p (p.id == process.id))
in
{ workflow | processes = updatedProcesses }
removeProcess : Process -> Workflow a -> Workflow a
removeProcess process workflow =
{ workflow | processes = workflow.processes |> List.remove process }
setDraggingState : DraggingState -> Model -> Model
setDraggingState draggingState model =
{ model | draggingState = Just { state = draggingState, hasTargeted = False } }
hasDragTargeted : Model -> Bool
hasDragTargeted model =
model.draggingState
|> Maybe.map .hasTargeted
|> Maybe.withDefault False
clearDraggingState : Model -> Model
clearDraggingState model =
{ model | draggingState = Nothing }
toggleTargetingOfDraggingState : Bool -> Model -> Model
toggleTargetingOfDraggingState hasTargeted model =
{ model | draggingState = model.draggingState |> Maybe.map (\s -> { s | hasTargeted = hasTargeted}) }
toggleSubWorkflow : Process -> ProcessItem -> List Process -> Model -> Model
toggleSubWorkflow parentProcess parentProcessItem subProcesses model =
model.subWorkflow
|> Maybe.andThen
(\w -> boolToMaybe { model | subWorkflow = Nothing } (w.parentProcessItem == parentProcessItem))
|> Maybe.withDefault
{ model
| subWorkflow =
Just
{ parentProcessItem = parentProcessItem
, parentProcess = parentProcess
, processes = subProcesses
}
}
-- ATTRS
attrDraggable mode =
attribute "draggable" (boolToString (mode == EditorMode))
attrEditable mode =
attribute "contenteditable" (boolToString (mode == EditorMode))
-- VIEW
view : Model -> Html Msg
view model =
let
droppableAreaMode =
case model.draggingState of
Just { state } ->
case state of
DraggingProcessItemState _ ->
ReadyToReceiveProcessItem
DraggingEmptyItemState _ ->
ReadyToReceiveEmptyItem
DraggingProcessState _ ->
ReadyToReceiveProcess
Nothing ->
Normal
mode =
model.mode
toggleModeButton =
let
(label, newMode) =
case model.mode of
ViewerMode ->
("Edit", EditorMode)
EditorMode ->
("Back to viewer", ViewerMode)
in
Controls.viewToggle label newMode ToggleMode ((==) ViewerMode)
activeProcessItem =
model.subWorkflow
|> Maybe.map .parentProcessItem
addWorkflowControls workflowKind workflow ps =
mode == EditorMode
|> boolToMaybe
( List.append
ps
[ div
[ css [ displayFlex ] ]
[ viewAddProcessButton workflowKind workflow.processes ]
]
)
|> Maybe.withDefault ps
viewMainWorkflow =
model.processes
|> List.filter isUsefulProcess
|> List.map (viewProcess MainWorkflow mode droppableAreaMode activeProcessItem)
|> addWorkflowControls MainWorkflow model
|> List.append [ toggleModeButton ]
|> div
[ css
[ overflowX scroll
, whiteSpace noWrap
, height (vh 75)
]
]
viewSubWorkflow workflow =
workflow.processes
|> List.map (viewProcess SubWorkflow mode droppableAreaMode activeProcessItem)
|> addWorkflowControls SubWorkflow workflow
|> div
[ css
[ bottom (vh 0)
, right (rem 0)
, padding (rem 0.25)
, width (pct 100)
, height (vh 25)
, borderTop3 (rem 0.1) solid (rgb 4 4 4)
]
]
isUsefulProcess p =
(model.mode == EditorMode)
|| ((model.mode == ViewerMode) && (p.items /= [] && List.any ((/=) Nothing) p.items))
binWorkflow =
model.draggingState
|> Maybe.map .state
|> Maybe.map
( \state ->
case state of
DraggingProcessItemState { workflowKind } ->
workflowKind
DraggingEmptyItemState { workflowKind } ->
workflowKind
DraggingProcessState { workflowKind } ->
workflowKind
)
|> Maybe.withDefault MainWorkflow
in
div
[]
[ viewMainWorkflow
, model.subWorkflow
|> Maybe.map viewSubWorkflow
|> Maybe.withDefault (text "")
, viewBin binWorkflow droppableAreaMode
]
viewProcess : WorkflowKind -> Mode -> DroppableAreaMode -> Maybe ProcessItem -> Process -> Html Msg
viewProcess workflowKind mode droppableAreaMode activeProcessItem process =
let
getProcessItemState i =
activeProcessItem
|> Maybe.andThen (\x -> boolToMaybe ActiveProcessItemState (x == i))
|> Maybe.withDefault NormalProcessItemState
viewProcessItems =
let
items =
process.items
|> List.filter ((/=) Nothing >> (||) (mode == EditorMode))
|> List.indexedMap
( \itemIndex item ->
item
|> Maybe.map (\i -> viewProcessItem workflowKind (getProcessItemState i) mode process i)
|> Maybe.withDefault (viewEmptyItem workflowKind mode droppableAreaMode process itemIndex)
)
in
if mode == EditorMode then
List.append items [ viewNewSlotButton workflowKind droppableAreaMode process ]
else
items
in
div
[ css
[ displayFlex
, before
[ width (rem 2)
, minWidth (rem 2)
, margin (rem 0.25)
, backgroundColor (hex "#606dbc")
, property "content" "''"
]
, hover
[ backgroundColor (rgba 128 128 128 0.05)
]
, cursor (if mode == EditorMode then move else default)
]
, onWithoutProp "dragstart" (DragStart (DraggingProcessState { workflowKind = workflowKind, process = process }))
, onWithoutProp "dragend" DragEnd
, attrDraggable mode
]
viewProcessItems
viewProcessItem workflowKind state mode process processItem =
let
viewName =
div
[ css []
]
[ div
[ attrEditable mode
, css
[ focus [ outline none ]
, whiteSpace noWrap
]
]
[ text processItem.name ]
]
itemIndex =
process.items
|> List.elemIndex (Just processItem)
|> Maybe.withDefault 0 -- unattainable result
style =
case state of
NormalProcessItemState ->
Css.batch
[ border3 (rem 0.1) solid (rgb 4 4 4)
, backgroundColor (rgb 255 255 255)
]
ActiveProcessItemState ->
Css.batch
[ border3 (rem 0.1) solid (rgb 10 124 10)
, backgroundColor (rgb 216 255 211)
, color (rgb 10 124 10)
]
in
div
[ css
[ style
, margin (rem 0.5)
, padding (rem 0.25)
, minWidth (rem 10)
, height (rem 3)
, overflowX hidden
, hover
[ border3 (rem 0.1) solid (rgb 10 124 10)
, backgroundColor (rgb 216 255 211)
, color (rgb 10 124 10)
, cursor pointer
]
, active
[ border3 (rem 0.1) solid (rgb 26 171 26)
, backgroundColor (rgb 216 255 211)
, color (rgb 26 171 26)
, cursor pointer
]
]
, attrDraggable mode
, onWithoutProp
"dragstart"
(DragStart (DraggingProcessItemState { workflowKind = workflowKind, process = process, item = processItem, itemIndex = itemIndex }))
, onWithoutProp "dragend" DragEnd
, onWithoutProp "dragenter" (DragTargetOnDraggableArea True)
, onWithoutProp "dragleave" (DragTargetOnDraggableArea False)
, onWithoutProp "drop" (Drop workflowKind (DropOnAnotherProcessItem process processItem itemIndex))
, onWithoutDef "dragover" Idle
, onClick (ToggleProcessItemSelection process processItem)
]
[ viewName
]
viewEmptyItem workflowKind mode droppableAreaMode process itemIndex =
let
newItemName =
"New item " ++ (String.fromInt (itemIndex + 1))
droppableStyles =
case droppableAreaMode of
ReadyToReceiveProcessItem ->
Css.batch
[ border3 (rem 0.1) dashed (rgb 15 103 15)
, backgroundColor (rgb 216 255 211)
, color (rgb 13 110 13)
, opacity (num 0.5)
]
_ ->
Css.batch
[ border3 (rem 0.1) solid (rgb 255 255 255)
, opacity (num 0.25)
]
in
div
[ css
[ droppableStyles
, margin (rem 0.5)
, padding (rem 0.25)
, minWidth (rem 10)
, height (rem 3)
, hover
[ border3 (rem 0.1) solid (rgb 15 103 15)
, backgroundColor (rgb 216 255 211)
, color (rgb 13 110 13)
, cursor pointer
, opacity (num 0.5)
]
, active
[ border3 (rem 0.1) solid (rgb 26 171 26)
, backgroundColor (rgb 216 255 211)
, color (rgb 26 171 26)
, cursor pointer
, opacity (num 0.85)
]
]
, onClick (TempIdRequested (NewItem workflowKind process { id = "", name = PI:NAME:<NAME>END_PIItemName, description = "" } itemIndex))
, onWithoutDef "dragover" Idle
, attrDraggable mode
, onStd "drop" (Drop workflowKind (DropOnEmptySlot process itemIndex))
, onWithoutProp
"dragstart"
(DragStart (DraggingEmptyItemState { workflowKind = workflowKind, process = process, itemIndex = itemIndex }))
, onWithoutProp "dragend" DragEnd
, onStd "dragenter" (DragTargetOnDraggableArea True)
, onStd "dragleave" (DragTargetOnDraggableArea False)
]
[ text "EMPTY" ]
viewNewSlotButton workflowKind droppableAreaMode process =
let
droppableStyles =
case droppableAreaMode of
ReadyToReceiveProcessItem ->
Css.batch
[ border3 (rem 0.1) dashed (rgb 15 103 15)
, backgroundColor (rgb 216 255 211)
, color (rgb 13 110 13)
, opacity (num 0.5)
]
_ ->
Css.batch
[ border3 (rem 0.1) dashed (rgb 4 4 4)
, opacity (num 0.25)
]
in
div
[ css
[ droppableStyles
, margin (rem 0.5)
, padding (rem 0.25)
, minWidth (rem 10)
, height (rem 3)
, hover
[ backgroundColor (rgb 216 255 211)
, color (rgb 13 110 13)
, cursor pointer
, opacity (num 0.5)
]
, active
[ border3 (rem 0.1) solid (rgb 26 171 26)
, backgroundColor (rgb 216 255 211)
, color (rgb 26 171 26)
, cursor pointer
, opacity (num 0.85)
]
]
, onClick (NewItemSlot workflowKind process)
, onWithoutDef "dragover" Idle
, onStd "drop" (Drop workflowKind (DropOnNewSlot process))
, onStd "dragenter" (DragTargetOnDraggableArea True)
, onStd "dragleave" (DragTargetOnDraggableArea False)
]
[ text "➕" ]
viewAddProcessButton : WorkflowKind -> List Process -> Html Msg
viewAddProcessButton workflowKind processes =
let
processesCount =
List.length processes
newProcessName =
"New item " ++ (String.fromInt (processesCount + 1))
in
div
[ css
[ border3 (rem 0.1) dashed (rgb 4 4 4)
, margin (rem 0.5)
, padding (rem 0.25)
, width (rem 20)
, height (rem 3)
, opacity (num 0.25)
, hover
[ border3 (rem 0.1) solid (rgb 15 103 15)
, backgroundColor (rgb 216 255 211)
, color (rgb 13 110 13)
, cursor pointer
, opacity (num 0.5)
]
, active
[ border3 (rem 0.1) solid (rgb 26 171 26)
, backgroundColor (rgb 216 255 211)
, color (rgb 26 171 26)
, cursor pointer
, opacity (num 0.85)
]
]
, onClick (TempIdRequested (NewProcess workflowKind (Process newProcessName newProcessName [])))
]
[ text "➕" ]
viewBin : WorkflowKind -> DroppableAreaMode -> Html Msg
viewBin workflowKind droppableAreaMode =
let
droppableStyles =
let
droppableReadyStyles =
Css.batch
[ border3 (rem 0.1) dashed (rgb 105 0 24)
, backgroundColor (rgb 255 211 216)
, color (rgb 105 0 24)
, opacity (num 0.5)
, display block
]
in
case droppableAreaMode of
Normal ->
Css.batch
[ border3 (rem 0.1) dashed (rgb 4 4 4)
, opacity (num 0.25)
, display none
]
_ ->
droppableReadyStyles
dropMsg =
case droppableAreaMode of
Normal ->
Idle
_ ->
Drop workflowKind DropInBin
in
div
[ css
[ droppableStyles
, position fixed
, bottom (vh 0)
, right (rem 0)
, margin (rem 0.5)
, padding (rem 0.25)
, width (vw 10)
, height (vh 10)
]
, onWithoutDef "dragover" Idle
, onStd "drop" dropMsg
, onStd "dragenter" (DragTargetOnDraggableArea True)
, onStd "dragleave" (DragTargetOnDraggableArea False)
]
[ text "🗑️" ]
| elm |
[
{
"context": "nsecutiveItemsBy\n Tuple.second\n [ (\"Betty\", 1), (\"Tom\", 1), (\"Jane\", 2)] -- [(\"Betty\", 1), ",
"end": 2891,
"score": 0.9996955395,
"start": 2886,
"tag": "NAME",
"value": "Betty"
},
{
"context": "By\n Tuple.second\n [ (\"Betty\", 1), (\"Tom\", 1), (\"Jane\", 2)] -- [(\"Betty\", 1), (\"Jane\", 2)]",
"end": 2903,
"score": 0.9998113513,
"start": 2900,
"tag": "NAME",
"value": "Tom"
},
{
"context": "uple.second\n [ (\"Betty\", 1), (\"Tom\", 1), (\"Jane\", 2)] -- [(\"Betty\", 1), (\"Jane\", 2)]\n\n-}\ndeduplic",
"end": 2916,
"score": 0.999502182,
"start": 2912,
"tag": "NAME",
"value": "Jane"
},
{
"context": " [ (\"Betty\", 1), (\"Tom\", 1), (\"Jane\", 2)] -- [(\"Betty\", 1), (\"Jane\", 2)]\n\n-}\ndeduplicateConsecutiveItem",
"end": 2934,
"score": 0.9996693134,
"start": 2929,
"tag": "NAME",
"value": "Betty"
},
{
"context": " 1), (\"Tom\", 1), (\"Jane\", 2)] -- [(\"Betty\", 1), (\"Jane\", 2)]\n\n-}\ndeduplicateConsecutiveItemsBy : (a -> b",
"end": 2947,
"score": 0.9996161461,
"start": 2943,
"tag": "NAME",
"value": "Jane"
}
] | src/Ra.elm | JSuder-xx/elm-ra | 1 | module Ra exposing
( allPass, anyPass, both, either, complement, false, true
, lessThan, lessThanEqualTo, greaterThan, greaterThanEqualTo, equals
, adding, subtracting, dividedByInt, dividedByFloat, multiplying, negated
, ifElse, cond, condDefault, maybeWhen, when, unless, until
, converge, converge3, convergeList, curry, curry3, flip, fnContraMap, fnContraMap2, fnContraMap3, uncurry, uncurry3
, isMemberOf
, deduplicateConsecutiveItemsBy, partitionWhile
)
{-| Ra supports Pointfree style in Elm by providing various combinators to work with Predicates, Relations, Math, Functions, and Flow Control.
## Categories
### Predicate Combinators
Predicate combinators provide a huge readability win when you have named predicates.
@docs allPass, anyPass, both, either, complement, false, true
### Readable Chain and Composition Relations
Partial application of the native relation operators is not intuitive or readable because the order is reversed from what one might expect.
For example, `((>=) 21)` does not return True for numbers that are greater than or equal to 21 but rather returns True for numbers less than
21 because the 21 is applied on the left i.e. `n -> 21 >= n`.
By contrast, the `greaterThanEqualTo` function returns a test of numbers greater than or equal to the first number given. So `greaterThanEqualTo 21`
is `n -> n >= 21`.
@docs lessThan, lessThanEqualTo, greaterThan, greaterThanEqualTo, equals
### Readable Chain and Composition Math
@docs adding, subtracting, dividedByInt, dividedByFloat, multiplying, negated
### Flow Control
@docs ifElse, cond, condDefault, maybeWhen, when, unless, until
### Function Combinators
@docs converge, converge3, convergeList, curry, curry3, flip, fnContraMap, fnContraMap2, fnContraMap3, uncurry, uncurry3
### Dict
@docs isMemberOf
### List
@docs deduplicateConsecutiveItemsBy, partitionWhile
-}
import Dict
import List.Extra
-- ------------------------------------------------------------------------------
-- Dictionary
-- ------------------------------------------------------------------------------
{-| Dict.member with the arguments flipped in order to test the member of _many_ keys against a _single_ dictionary.
let
validPersonIds =
personIds
|> List.filter (isMemberOf idToPerson)
in
-- ...
-}
isMemberOf : Dict.Dict comparable v -> comparable -> Bool
isMemberOf =
flip Dict.member
-- ------------------------------------------------------------------------------
-- List
-- ------------------------------------------------------------------------------
{-| Deduplicate consecutive items in a list using a function to extract the element of interest.
deduplicateConsecutiveItemsBy identity [ 1, 1, 1, 2, 3, 3 ] -- [1, 2, 3]
deduplicateConsecutiveItemsBy
Tuple.second
[ ("Betty", 1), ("Tom", 1), ("Jane", 2)] -- [("Betty", 1), ("Jane", 2)]
-}
deduplicateConsecutiveItemsBy : (a -> b) -> List a -> List a
deduplicateConsecutiveItemsBy key =
List.Extra.groupWhile (fnContraMap2 key equals)
>> List.map Tuple.first
{-| Returns all of the items in the list that pass the given predicate up until the first item that fails in the first position
and the remaining items in the second.
partitionWhile (lessThanEqualTo 10) [1, 5, 10, 15, 10, 3]
-- ([1, 5, 10], [15, 10, 3])
partitionWhile (lessThanEqualTo 10) [11, 20, 30, 5, 3]
-- ([], [11, 20, 30, 5, 3])
partitionWhile (lessThanEqualTo 10) [1, 5, 10, 7]
-- ([1, 5, 10, 7], [])
-}
partitionWhile : (a -> Bool) -> List a -> ( List a, List a )
partitionWhile p list =
list
|> List.Extra.splitWhen (complement p)
|> Maybe.withDefault ( list, [] )
-- ------------------------------------------------------------------------------
-- Function
-- ------------------------------------------------------------------------------
{-| Flip the first and second arguments of a function.
- This is an incredibly useful function when needing to change the application of an existing function.
- **WARNING** However, it can also destroy the literate reading of code if overused and it is easy to overuse.
For example, the first argument to Dict.get is the key and the second is the dictionary.
What if we want to map over multiple keys? We would want to apply the dictionary first and get a function that accepts the key.
valuesForKeys : Dict comparable value -> List comparable -> List value
valuesForKeys =
flip Dict.get >> List.filterMap
More simply, consider the case of having a `(Dictionary k v)` of values but wanting to pass along a function `k -> Maybe v` to some provider
so that the provider doesn't couple to the Dictionary? This would be a good idea to Reduce Coupling and honor the Principle of Least Privilege.
someInterestingProvider : (Int -> Widget) -> String -> Maybe Widget
someInterestingProvider = Debug.todo "doesn't matter for the demonstration"
someConsumer : List Widget -> String -> Maybe Widget
someConsumer widgets =
someInterestingProvider (flip Dict.get (indexBy .id widgets))
-}
flip : (a -> b -> c) -> (b -> a -> c)
flip f a b =
f b a
{-| Converts a function from 2-tuple to normal curried function. This is mostly useful for reverting an uncurry.
-}
curry : (( a, b ) -> c) -> (a -> b -> c)
curry original =
\a -> \b -> original ( a, b )
{-| Converts a function from 3-tuple to normal curried function. This is mostly useful for reverting an uncurry.
-}
curry3 : (( a, b, c ) -> d) -> (a -> b -> c -> d)
curry3 original =
\a -> \b -> \c -> original ( a, b, c )
{-| Converts a normal Elm function to a function that accepts a 2-tuple. This can be useful if the output of some function happens to be
a tuple or a functor (List, Maybe, Result, etc.) of a tuple.
sums : List (number, number) -> List number
sums = List.map (uncurry adding)
sum : Maybe (number, number) -> Maybe number
sum = Maybe.map (uncurry adding)
-}
uncurry : (a -> b -> c) -> (( a, b ) -> c)
uncurry original =
\( a, b ) -> original a b
{-| Converts a standard Elm function to a function that accepts a 3-tuple.
-}
uncurry3 : (a -> b -> c -> d) -> (( a, b, c ) -> d)
uncurry3 original =
\( a, b, c ) -> original a b c
{-| This function can best be explained with examples as it is unusually useful when building data pipelines but has a tricky type signature.
Please do NOT be afraid of the type signature!
If you would like a function that transforms a Person to a String of their first and last name.
converge
String.append
( .firstName, .lastName >> String.append " " )
Or perhaps you are building a data pipeline
- where at some stage you have a `List Widget`
- and you have a function `extraWidgets: List Widget -> List Widget`
- and you would like to like to end up with a new list of widgets that contains both the original widgets and the extra widgets.
Then you might write
converge
List.append
(identity, extraWidgets)
-}
converge : (first -> second -> output) -> ( input -> first, input -> second ) -> input -> output
converge fn ( firstFn, secondFn ) input =
fn (firstFn input) (secondFn input)
{-| Converge for the case of a 3 argument function. See `converge` for examples.
-}
converge3 : (first -> second -> third -> output) -> ( input -> first, input -> second, input -> third ) -> input -> output
converge3 fn ( firstFn, secondFn, thirdFn ) input =
fn (firstFn input) (secondFn input) (thirdFn input)
{-| As with converge, convergeList is best explained with an example
convergeList
(String.join " ")
[ .firstName, .middleInitial, .lastName ]
Or to be more complete
nonEmptyStrings : List String -> List String
nonEmptyStrings = List.filter (complement String.isEmpty)
fullName : Person -> String
fullName =
convergeList
(nonEmptyStrings >> String.join " ")
[ .firstName, .middleInitial, .lastName ]
-}
convergeList : (List a -> output) -> List (input -> a) -> input -> output
convergeList reducer reducerInputs input =
reducerInputs |> List.map ((|>) input) |> reducer
{-| This is like `map` for functions but going the opposite direction (contra functor).
- map: (a -> b) -> F a -> F b
- contraMap: (a -> b) -> F b -> F a
OKAY. So that it probably a little or a lot confusing. How would you use this? Any time yout have a parent/child relation and a function that
will transform the child and you want to get back a function that transforms the parent.
addressesCount : Person -> Int
addressesCount = fnContraMap .addresses List.length
-}
fnContraMap : (a -> b) -> (b -> c) -> (a -> c)
fnContraMap ab original =
ab >> original
{-| Best described with an example. Let's say you have a parent/child relation, a function of 2 arguments that applies to the child type
and you would like to get back a function that works on the parent.
sameFavoriteColor : Person -> Person -> Bool
sameFavoriteColor = fnContraMap2 (.favorites >> .color) (==)
-}
fnContraMap2 : (a -> b) -> (b -> b -> c) -> (a -> a -> c)
fnContraMap2 ab original first second =
original (ab first) (ab second)
{-| Same as fnContraMap2 but for 3 argument functions.
-}
fnContraMap3 : (a -> b) -> (b -> b -> b -> c) -> (a -> a -> a -> c)
fnContraMap3 ab original first second third =
original (ab first) (ab second) (ab third)
-- ------------------------------------------------------------------------------
-- Predicate
-- ------------------------------------------------------------------------------
type alias Predicate a =
a -> Bool
{-| Return a new predicate that is true if both of the two predicates return true.
Note that this is short-circuited, meaning that the second predicate will not be invoked if the first returns false.
-}
both : Predicate a -> Predicate a -> Predicate a
both left right a =
left a && right a
{-| Takes a list of predicates and returns a predicate that returns true for a given list of arguments if every one of the provided predicates is satisfied by those arguments.
isAmazing : Person -> Bool
isAmazing = allPass [isKind, isCreative, isHardWorking]
-}
allPass : List (Predicate a) -> Predicate a
allPass preds a =
List.all ((|>) a) preds
{-| Takes a list of predicates and returns a predicate that returns true for a given list of arguments if at least one of the provided predicates is satisfied by those arguments.
isOkay : Person -> Bool
isOkay = anyPass [isKind, isCreative, isHardWorking]
-}
anyPass : List (Predicate a) -> Predicate a
anyPass preds a =
List.any ((|>) a) preds
{-| Return a new predicate that is true if either of the two predicates return true.
Note that this is short-circuited, meaning that the second predicate will not be invoked if the first returns true.
-}
either : Predicate a -> Predicate a -> Predicate a
either left right a =
left a || right a
{-| Invert (negate, complement) a predicate i.e if the predicate would have return True for some `a` it will now return False and vice versa.
-}
complement : Predicate a -> Predicate a
complement fn =
fn >> not
{-| A predicate that always returns true.
-}
true : Predicate a
true =
always True
{-| A predicate that always returns false.
-}
false : Predicate a
false =
always False
-- ------------------------------------------------------------------------------
-- Flow Control
-- ------------------------------------------------------------------------------
{-| Creates a function that will process either the whenTrue or the whenFalse function depending upon the result of the condition predicate.
ifElse
(.weightInLbs >> R.Relation.greaterThan 500)
(always "Sorry. You cannot ride the coaster.")
(.name >> String.append "Enjoy your ride ")
-}
ifElse : Predicate a -> (a -> b) -> (a -> b) -> (a -> b)
ifElse condition whenTrue whenFalse input =
if condition input then
whenTrue input
else
whenFalse input
{-| Returns a function which encapsulates if/else, if/else, ... logic given a list of (predicate, transformer) tuples.
- The condition pairs are processed in order
- and returns the transformation of the first predicate that passes
- If no conditions pass then returns Nothing.
-}
cond : List ( Predicate a, a -> b ) -> a -> Maybe b
cond cases input =
let
apply =
(|>) input
in
cases
|> List.Extra.find (Tuple.first >> apply)
|> Maybe.map (Tuple.second >> apply)
type alias Thunk a =
() -> a
{-| Same as `cond` but also provides a default option in the case that none of the conditions pass.
-}
condDefault : List ( Predicate a, a -> b ) -> Thunk b -> (a -> b)
condDefault cases defaultThunk a =
case cond cases a of
Just v ->
v
Nothing ->
defaultThunk ()
{-| Tests the final argument by passing it to the given predicate function.
- If the predicate is satisfied, the function will return the result of calling the whenTrueFn function with the same argument.
- If the predicate is not satisfied, the argument is returned as is.
-}
when : Predicate a -> (a -> a) -> (a -> a)
when condition transform a =
if condition a then
transform a
else
a
{-| Tests the final argument against the predicate and if true then performs the transformation.
This is super helpful when combined with List.filterMap. For example, let's say you wanted the names of just the cool people
allThePeople
|> List.filterMap (maybeWhen .isCool .fullName)
-}
maybeWhen : Predicate a -> (a -> b) -> a -> Maybe b
maybeWhen condition transform a =
if condition a then
a |> transform |> Just
else
Nothing
{-| Tests the final argument by passing it to the given predicate function.
- If the predicate is not satisfied, the function will return the result of calling the whenFalseFn function with the same argument.
- If the predicate is satisfied, the argument is returned as is.
-}
unless : Predicate a -> (a -> a) -> (a -> a)
unless whenFalse =
when (complement whenFalse)
{-| Takes a predicate, a transformation function, and an initial value, and returns a value of the same type as the initial value.
It does so by applying the transformation until the predicate is satisfied, at which point it returns the satisfactory value.
-}
until : Predicate a -> (a -> a) -> (a -> a)
until condition transform =
let
aux current =
if condition current then
current
else
aux (transform current)
in
aux
-- ------------------------------------------------------------------------------
-- Relation
-- ------------------------------------------------------------------------------
{-| Designed for use in pipelines or currying. Returns a predicate that tests if the value is less than the first value given.
[5, 10, 11, 20] |> List.map (lessThan 10)
-- [True, False, False, False]
-}
lessThan : comparable -> comparable -> Bool
lessThan a b =
b < a
{-| Designed for currying. Returns a predicate that tests if the value is less than or equal to the first value given.
[5, 10, 11, 20] |> List.map (lessThanEqualto 10)
-- [True, True, False, False]
-}
lessThanEqualTo : comparable -> Predicate comparable
lessThanEqualTo a b =
b <= a
{-| Designed for currying. Returns a predicate that tests if the value is greater than the first value given.
[5, 10, 11, 20] |> List.map (greaterThan 10)
-- [False, False, True, True]
-}
greaterThan : comparable -> Predicate comparable
greaterThan a b =
b > a
{-| Designed for currying. Returns a predicate that tests if the value is greater than or equal to the first value given.
[5, 10, 11, 20]
|> List.map (greaterThanEqualTo 10) -- [False, True, True, True]
-}
greaterThanEqualTo : comparable -> Predicate comparable
greaterThanEqualTo a b =
b >= a
{-| A natural language alias for `==` that also helps to avoid parenthesis when writing point-free flows
employees
|> List.filter (.employmentType >> equals Contractor)
-}
equals : a -> Predicate a
equals =
(==)
-- ------------------------------------------------------------------------------
-- Math
-- ------------------------------------------------------------------------------
{-| A literate alias for addition that is nice for building functional pipelines by avoiding parenthesis.
[5, 10, 15, 20] |> List.map (adding 5)
-- [10, 15, 20, 25]
-}
adding : number -> number -> number
adding a b =
a + b
{-| Subtraction alias designed for pipelines i.e. the order is swapped such that the subtrahend is the first argument.
[20, 30, 40]
|> List.map (subtracting 6)
-- [14, 24, 34]
-}
subtracting : number -> number -> number
subtracting subtrahend minuend =
minuend - subtrahend
{-| A natural language alias for \*.
-}
multiplying : number -> number -> number
multiplying =
(*)
{-| Division designed for pipelines i.e. the divisor is the first argument.
[20, 32, 40] |> List (divideByInt 4)
-- [5, 8, 10]
-}
dividedByInt : Int -> Int -> Int
dividedByInt divisor dividend =
dividend // divisor
{-| Division designed for pipelines i.e. the divisor is the first argument.
[20, 25, 26.25] |> List (divideByFloat 2.5)
-- [8.0, 10.0, 10.5]
-}
dividedByFloat : Float -> Float -> Float
dividedByFloat divisor dividend =
dividend / divisor
{-| Negate the given number.
-}
negated : number -> number
negated =
(*) -1
| 12514 | module Ra exposing
( allPass, anyPass, both, either, complement, false, true
, lessThan, lessThanEqualTo, greaterThan, greaterThanEqualTo, equals
, adding, subtracting, dividedByInt, dividedByFloat, multiplying, negated
, ifElse, cond, condDefault, maybeWhen, when, unless, until
, converge, converge3, convergeList, curry, curry3, flip, fnContraMap, fnContraMap2, fnContraMap3, uncurry, uncurry3
, isMemberOf
, deduplicateConsecutiveItemsBy, partitionWhile
)
{-| Ra supports Pointfree style in Elm by providing various combinators to work with Predicates, Relations, Math, Functions, and Flow Control.
## Categories
### Predicate Combinators
Predicate combinators provide a huge readability win when you have named predicates.
@docs allPass, anyPass, both, either, complement, false, true
### Readable Chain and Composition Relations
Partial application of the native relation operators is not intuitive or readable because the order is reversed from what one might expect.
For example, `((>=) 21)` does not return True for numbers that are greater than or equal to 21 but rather returns True for numbers less than
21 because the 21 is applied on the left i.e. `n -> 21 >= n`.
By contrast, the `greaterThanEqualTo` function returns a test of numbers greater than or equal to the first number given. So `greaterThanEqualTo 21`
is `n -> n >= 21`.
@docs lessThan, lessThanEqualTo, greaterThan, greaterThanEqualTo, equals
### Readable Chain and Composition Math
@docs adding, subtracting, dividedByInt, dividedByFloat, multiplying, negated
### Flow Control
@docs ifElse, cond, condDefault, maybeWhen, when, unless, until
### Function Combinators
@docs converge, converge3, convergeList, curry, curry3, flip, fnContraMap, fnContraMap2, fnContraMap3, uncurry, uncurry3
### Dict
@docs isMemberOf
### List
@docs deduplicateConsecutiveItemsBy, partitionWhile
-}
import Dict
import List.Extra
-- ------------------------------------------------------------------------------
-- Dictionary
-- ------------------------------------------------------------------------------
{-| Dict.member with the arguments flipped in order to test the member of _many_ keys against a _single_ dictionary.
let
validPersonIds =
personIds
|> List.filter (isMemberOf idToPerson)
in
-- ...
-}
isMemberOf : Dict.Dict comparable v -> comparable -> Bool
isMemberOf =
flip Dict.member
-- ------------------------------------------------------------------------------
-- List
-- ------------------------------------------------------------------------------
{-| Deduplicate consecutive items in a list using a function to extract the element of interest.
deduplicateConsecutiveItemsBy identity [ 1, 1, 1, 2, 3, 3 ] -- [1, 2, 3]
deduplicateConsecutiveItemsBy
Tuple.second
[ ("<NAME>", 1), ("<NAME>", 1), ("<NAME>", 2)] -- [("<NAME>", 1), ("<NAME>", 2)]
-}
deduplicateConsecutiveItemsBy : (a -> b) -> List a -> List a
deduplicateConsecutiveItemsBy key =
List.Extra.groupWhile (fnContraMap2 key equals)
>> List.map Tuple.first
{-| Returns all of the items in the list that pass the given predicate up until the first item that fails in the first position
and the remaining items in the second.
partitionWhile (lessThanEqualTo 10) [1, 5, 10, 15, 10, 3]
-- ([1, 5, 10], [15, 10, 3])
partitionWhile (lessThanEqualTo 10) [11, 20, 30, 5, 3]
-- ([], [11, 20, 30, 5, 3])
partitionWhile (lessThanEqualTo 10) [1, 5, 10, 7]
-- ([1, 5, 10, 7], [])
-}
partitionWhile : (a -> Bool) -> List a -> ( List a, List a )
partitionWhile p list =
list
|> List.Extra.splitWhen (complement p)
|> Maybe.withDefault ( list, [] )
-- ------------------------------------------------------------------------------
-- Function
-- ------------------------------------------------------------------------------
{-| Flip the first and second arguments of a function.
- This is an incredibly useful function when needing to change the application of an existing function.
- **WARNING** However, it can also destroy the literate reading of code if overused and it is easy to overuse.
For example, the first argument to Dict.get is the key and the second is the dictionary.
What if we want to map over multiple keys? We would want to apply the dictionary first and get a function that accepts the key.
valuesForKeys : Dict comparable value -> List comparable -> List value
valuesForKeys =
flip Dict.get >> List.filterMap
More simply, consider the case of having a `(Dictionary k v)` of values but wanting to pass along a function `k -> Maybe v` to some provider
so that the provider doesn't couple to the Dictionary? This would be a good idea to Reduce Coupling and honor the Principle of Least Privilege.
someInterestingProvider : (Int -> Widget) -> String -> Maybe Widget
someInterestingProvider = Debug.todo "doesn't matter for the demonstration"
someConsumer : List Widget -> String -> Maybe Widget
someConsumer widgets =
someInterestingProvider (flip Dict.get (indexBy .id widgets))
-}
flip : (a -> b -> c) -> (b -> a -> c)
flip f a b =
f b a
{-| Converts a function from 2-tuple to normal curried function. This is mostly useful for reverting an uncurry.
-}
curry : (( a, b ) -> c) -> (a -> b -> c)
curry original =
\a -> \b -> original ( a, b )
{-| Converts a function from 3-tuple to normal curried function. This is mostly useful for reverting an uncurry.
-}
curry3 : (( a, b, c ) -> d) -> (a -> b -> c -> d)
curry3 original =
\a -> \b -> \c -> original ( a, b, c )
{-| Converts a normal Elm function to a function that accepts a 2-tuple. This can be useful if the output of some function happens to be
a tuple or a functor (List, Maybe, Result, etc.) of a tuple.
sums : List (number, number) -> List number
sums = List.map (uncurry adding)
sum : Maybe (number, number) -> Maybe number
sum = Maybe.map (uncurry adding)
-}
uncurry : (a -> b -> c) -> (( a, b ) -> c)
uncurry original =
\( a, b ) -> original a b
{-| Converts a standard Elm function to a function that accepts a 3-tuple.
-}
uncurry3 : (a -> b -> c -> d) -> (( a, b, c ) -> d)
uncurry3 original =
\( a, b, c ) -> original a b c
{-| This function can best be explained with examples as it is unusually useful when building data pipelines but has a tricky type signature.
Please do NOT be afraid of the type signature!
If you would like a function that transforms a Person to a String of their first and last name.
converge
String.append
( .firstName, .lastName >> String.append " " )
Or perhaps you are building a data pipeline
- where at some stage you have a `List Widget`
- and you have a function `extraWidgets: List Widget -> List Widget`
- and you would like to like to end up with a new list of widgets that contains both the original widgets and the extra widgets.
Then you might write
converge
List.append
(identity, extraWidgets)
-}
converge : (first -> second -> output) -> ( input -> first, input -> second ) -> input -> output
converge fn ( firstFn, secondFn ) input =
fn (firstFn input) (secondFn input)
{-| Converge for the case of a 3 argument function. See `converge` for examples.
-}
converge3 : (first -> second -> third -> output) -> ( input -> first, input -> second, input -> third ) -> input -> output
converge3 fn ( firstFn, secondFn, thirdFn ) input =
fn (firstFn input) (secondFn input) (thirdFn input)
{-| As with converge, convergeList is best explained with an example
convergeList
(String.join " ")
[ .firstName, .middleInitial, .lastName ]
Or to be more complete
nonEmptyStrings : List String -> List String
nonEmptyStrings = List.filter (complement String.isEmpty)
fullName : Person -> String
fullName =
convergeList
(nonEmptyStrings >> String.join " ")
[ .firstName, .middleInitial, .lastName ]
-}
convergeList : (List a -> output) -> List (input -> a) -> input -> output
convergeList reducer reducerInputs input =
reducerInputs |> List.map ((|>) input) |> reducer
{-| This is like `map` for functions but going the opposite direction (contra functor).
- map: (a -> b) -> F a -> F b
- contraMap: (a -> b) -> F b -> F a
OKAY. So that it probably a little or a lot confusing. How would you use this? Any time yout have a parent/child relation and a function that
will transform the child and you want to get back a function that transforms the parent.
addressesCount : Person -> Int
addressesCount = fnContraMap .addresses List.length
-}
fnContraMap : (a -> b) -> (b -> c) -> (a -> c)
fnContraMap ab original =
ab >> original
{-| Best described with an example. Let's say you have a parent/child relation, a function of 2 arguments that applies to the child type
and you would like to get back a function that works on the parent.
sameFavoriteColor : Person -> Person -> Bool
sameFavoriteColor = fnContraMap2 (.favorites >> .color) (==)
-}
fnContraMap2 : (a -> b) -> (b -> b -> c) -> (a -> a -> c)
fnContraMap2 ab original first second =
original (ab first) (ab second)
{-| Same as fnContraMap2 but for 3 argument functions.
-}
fnContraMap3 : (a -> b) -> (b -> b -> b -> c) -> (a -> a -> a -> c)
fnContraMap3 ab original first second third =
original (ab first) (ab second) (ab third)
-- ------------------------------------------------------------------------------
-- Predicate
-- ------------------------------------------------------------------------------
type alias Predicate a =
a -> Bool
{-| Return a new predicate that is true if both of the two predicates return true.
Note that this is short-circuited, meaning that the second predicate will not be invoked if the first returns false.
-}
both : Predicate a -> Predicate a -> Predicate a
both left right a =
left a && right a
{-| Takes a list of predicates and returns a predicate that returns true for a given list of arguments if every one of the provided predicates is satisfied by those arguments.
isAmazing : Person -> Bool
isAmazing = allPass [isKind, isCreative, isHardWorking]
-}
allPass : List (Predicate a) -> Predicate a
allPass preds a =
List.all ((|>) a) preds
{-| Takes a list of predicates and returns a predicate that returns true for a given list of arguments if at least one of the provided predicates is satisfied by those arguments.
isOkay : Person -> Bool
isOkay = anyPass [isKind, isCreative, isHardWorking]
-}
anyPass : List (Predicate a) -> Predicate a
anyPass preds a =
List.any ((|>) a) preds
{-| Return a new predicate that is true if either of the two predicates return true.
Note that this is short-circuited, meaning that the second predicate will not be invoked if the first returns true.
-}
either : Predicate a -> Predicate a -> Predicate a
either left right a =
left a || right a
{-| Invert (negate, complement) a predicate i.e if the predicate would have return True for some `a` it will now return False and vice versa.
-}
complement : Predicate a -> Predicate a
complement fn =
fn >> not
{-| A predicate that always returns true.
-}
true : Predicate a
true =
always True
{-| A predicate that always returns false.
-}
false : Predicate a
false =
always False
-- ------------------------------------------------------------------------------
-- Flow Control
-- ------------------------------------------------------------------------------
{-| Creates a function that will process either the whenTrue or the whenFalse function depending upon the result of the condition predicate.
ifElse
(.weightInLbs >> R.Relation.greaterThan 500)
(always "Sorry. You cannot ride the coaster.")
(.name >> String.append "Enjoy your ride ")
-}
ifElse : Predicate a -> (a -> b) -> (a -> b) -> (a -> b)
ifElse condition whenTrue whenFalse input =
if condition input then
whenTrue input
else
whenFalse input
{-| Returns a function which encapsulates if/else, if/else, ... logic given a list of (predicate, transformer) tuples.
- The condition pairs are processed in order
- and returns the transformation of the first predicate that passes
- If no conditions pass then returns Nothing.
-}
cond : List ( Predicate a, a -> b ) -> a -> Maybe b
cond cases input =
let
apply =
(|>) input
in
cases
|> List.Extra.find (Tuple.first >> apply)
|> Maybe.map (Tuple.second >> apply)
type alias Thunk a =
() -> a
{-| Same as `cond` but also provides a default option in the case that none of the conditions pass.
-}
condDefault : List ( Predicate a, a -> b ) -> Thunk b -> (a -> b)
condDefault cases defaultThunk a =
case cond cases a of
Just v ->
v
Nothing ->
defaultThunk ()
{-| Tests the final argument by passing it to the given predicate function.
- If the predicate is satisfied, the function will return the result of calling the whenTrueFn function with the same argument.
- If the predicate is not satisfied, the argument is returned as is.
-}
when : Predicate a -> (a -> a) -> (a -> a)
when condition transform a =
if condition a then
transform a
else
a
{-| Tests the final argument against the predicate and if true then performs the transformation.
This is super helpful when combined with List.filterMap. For example, let's say you wanted the names of just the cool people
allThePeople
|> List.filterMap (maybeWhen .isCool .fullName)
-}
maybeWhen : Predicate a -> (a -> b) -> a -> Maybe b
maybeWhen condition transform a =
if condition a then
a |> transform |> Just
else
Nothing
{-| Tests the final argument by passing it to the given predicate function.
- If the predicate is not satisfied, the function will return the result of calling the whenFalseFn function with the same argument.
- If the predicate is satisfied, the argument is returned as is.
-}
unless : Predicate a -> (a -> a) -> (a -> a)
unless whenFalse =
when (complement whenFalse)
{-| Takes a predicate, a transformation function, and an initial value, and returns a value of the same type as the initial value.
It does so by applying the transformation until the predicate is satisfied, at which point it returns the satisfactory value.
-}
until : Predicate a -> (a -> a) -> (a -> a)
until condition transform =
let
aux current =
if condition current then
current
else
aux (transform current)
in
aux
-- ------------------------------------------------------------------------------
-- Relation
-- ------------------------------------------------------------------------------
{-| Designed for use in pipelines or currying. Returns a predicate that tests if the value is less than the first value given.
[5, 10, 11, 20] |> List.map (lessThan 10)
-- [True, False, False, False]
-}
lessThan : comparable -> comparable -> Bool
lessThan a b =
b < a
{-| Designed for currying. Returns a predicate that tests if the value is less than or equal to the first value given.
[5, 10, 11, 20] |> List.map (lessThanEqualto 10)
-- [True, True, False, False]
-}
lessThanEqualTo : comparable -> Predicate comparable
lessThanEqualTo a b =
b <= a
{-| Designed for currying. Returns a predicate that tests if the value is greater than the first value given.
[5, 10, 11, 20] |> List.map (greaterThan 10)
-- [False, False, True, True]
-}
greaterThan : comparable -> Predicate comparable
greaterThan a b =
b > a
{-| Designed for currying. Returns a predicate that tests if the value is greater than or equal to the first value given.
[5, 10, 11, 20]
|> List.map (greaterThanEqualTo 10) -- [False, True, True, True]
-}
greaterThanEqualTo : comparable -> Predicate comparable
greaterThanEqualTo a b =
b >= a
{-| A natural language alias for `==` that also helps to avoid parenthesis when writing point-free flows
employees
|> List.filter (.employmentType >> equals Contractor)
-}
equals : a -> Predicate a
equals =
(==)
-- ------------------------------------------------------------------------------
-- Math
-- ------------------------------------------------------------------------------
{-| A literate alias for addition that is nice for building functional pipelines by avoiding parenthesis.
[5, 10, 15, 20] |> List.map (adding 5)
-- [10, 15, 20, 25]
-}
adding : number -> number -> number
adding a b =
a + b
{-| Subtraction alias designed for pipelines i.e. the order is swapped such that the subtrahend is the first argument.
[20, 30, 40]
|> List.map (subtracting 6)
-- [14, 24, 34]
-}
subtracting : number -> number -> number
subtracting subtrahend minuend =
minuend - subtrahend
{-| A natural language alias for \*.
-}
multiplying : number -> number -> number
multiplying =
(*)
{-| Division designed for pipelines i.e. the divisor is the first argument.
[20, 32, 40] |> List (divideByInt 4)
-- [5, 8, 10]
-}
dividedByInt : Int -> Int -> Int
dividedByInt divisor dividend =
dividend // divisor
{-| Division designed for pipelines i.e. the divisor is the first argument.
[20, 25, 26.25] |> List (divideByFloat 2.5)
-- [8.0, 10.0, 10.5]
-}
dividedByFloat : Float -> Float -> Float
dividedByFloat divisor dividend =
dividend / divisor
{-| Negate the given number.
-}
negated : number -> number
negated =
(*) -1
| true | module Ra exposing
( allPass, anyPass, both, either, complement, false, true
, lessThan, lessThanEqualTo, greaterThan, greaterThanEqualTo, equals
, adding, subtracting, dividedByInt, dividedByFloat, multiplying, negated
, ifElse, cond, condDefault, maybeWhen, when, unless, until
, converge, converge3, convergeList, curry, curry3, flip, fnContraMap, fnContraMap2, fnContraMap3, uncurry, uncurry3
, isMemberOf
, deduplicateConsecutiveItemsBy, partitionWhile
)
{-| Ra supports Pointfree style in Elm by providing various combinators to work with Predicates, Relations, Math, Functions, and Flow Control.
## Categories
### Predicate Combinators
Predicate combinators provide a huge readability win when you have named predicates.
@docs allPass, anyPass, both, either, complement, false, true
### Readable Chain and Composition Relations
Partial application of the native relation operators is not intuitive or readable because the order is reversed from what one might expect.
For example, `((>=) 21)` does not return True for numbers that are greater than or equal to 21 but rather returns True for numbers less than
21 because the 21 is applied on the left i.e. `n -> 21 >= n`.
By contrast, the `greaterThanEqualTo` function returns a test of numbers greater than or equal to the first number given. So `greaterThanEqualTo 21`
is `n -> n >= 21`.
@docs lessThan, lessThanEqualTo, greaterThan, greaterThanEqualTo, equals
### Readable Chain and Composition Math
@docs adding, subtracting, dividedByInt, dividedByFloat, multiplying, negated
### Flow Control
@docs ifElse, cond, condDefault, maybeWhen, when, unless, until
### Function Combinators
@docs converge, converge3, convergeList, curry, curry3, flip, fnContraMap, fnContraMap2, fnContraMap3, uncurry, uncurry3
### Dict
@docs isMemberOf
### List
@docs deduplicateConsecutiveItemsBy, partitionWhile
-}
import Dict
import List.Extra
-- ------------------------------------------------------------------------------
-- Dictionary
-- ------------------------------------------------------------------------------
{-| Dict.member with the arguments flipped in order to test the member of _many_ keys against a _single_ dictionary.
let
validPersonIds =
personIds
|> List.filter (isMemberOf idToPerson)
in
-- ...
-}
isMemberOf : Dict.Dict comparable v -> comparable -> Bool
isMemberOf =
flip Dict.member
-- ------------------------------------------------------------------------------
-- List
-- ------------------------------------------------------------------------------
{-| Deduplicate consecutive items in a list using a function to extract the element of interest.
deduplicateConsecutiveItemsBy identity [ 1, 1, 1, 2, 3, 3 ] -- [1, 2, 3]
deduplicateConsecutiveItemsBy
Tuple.second
[ ("PI:NAME:<NAME>END_PI", 1), ("PI:NAME:<NAME>END_PI", 1), ("PI:NAME:<NAME>END_PI", 2)] -- [("PI:NAME:<NAME>END_PI", 1), ("PI:NAME:<NAME>END_PI", 2)]
-}
deduplicateConsecutiveItemsBy : (a -> b) -> List a -> List a
deduplicateConsecutiveItemsBy key =
List.Extra.groupWhile (fnContraMap2 key equals)
>> List.map Tuple.first
{-| Returns all of the items in the list that pass the given predicate up until the first item that fails in the first position
and the remaining items in the second.
partitionWhile (lessThanEqualTo 10) [1, 5, 10, 15, 10, 3]
-- ([1, 5, 10], [15, 10, 3])
partitionWhile (lessThanEqualTo 10) [11, 20, 30, 5, 3]
-- ([], [11, 20, 30, 5, 3])
partitionWhile (lessThanEqualTo 10) [1, 5, 10, 7]
-- ([1, 5, 10, 7], [])
-}
partitionWhile : (a -> Bool) -> List a -> ( List a, List a )
partitionWhile p list =
list
|> List.Extra.splitWhen (complement p)
|> Maybe.withDefault ( list, [] )
-- ------------------------------------------------------------------------------
-- Function
-- ------------------------------------------------------------------------------
{-| Flip the first and second arguments of a function.
- This is an incredibly useful function when needing to change the application of an existing function.
- **WARNING** However, it can also destroy the literate reading of code if overused and it is easy to overuse.
For example, the first argument to Dict.get is the key and the second is the dictionary.
What if we want to map over multiple keys? We would want to apply the dictionary first and get a function that accepts the key.
valuesForKeys : Dict comparable value -> List comparable -> List value
valuesForKeys =
flip Dict.get >> List.filterMap
More simply, consider the case of having a `(Dictionary k v)` of values but wanting to pass along a function `k -> Maybe v` to some provider
so that the provider doesn't couple to the Dictionary? This would be a good idea to Reduce Coupling and honor the Principle of Least Privilege.
someInterestingProvider : (Int -> Widget) -> String -> Maybe Widget
someInterestingProvider = Debug.todo "doesn't matter for the demonstration"
someConsumer : List Widget -> String -> Maybe Widget
someConsumer widgets =
someInterestingProvider (flip Dict.get (indexBy .id widgets))
-}
flip : (a -> b -> c) -> (b -> a -> c)
flip f a b =
f b a
{-| Converts a function from 2-tuple to normal curried function. This is mostly useful for reverting an uncurry.
-}
curry : (( a, b ) -> c) -> (a -> b -> c)
curry original =
\a -> \b -> original ( a, b )
{-| Converts a function from 3-tuple to normal curried function. This is mostly useful for reverting an uncurry.
-}
curry3 : (( a, b, c ) -> d) -> (a -> b -> c -> d)
curry3 original =
\a -> \b -> \c -> original ( a, b, c )
{-| Converts a normal Elm function to a function that accepts a 2-tuple. This can be useful if the output of some function happens to be
a tuple or a functor (List, Maybe, Result, etc.) of a tuple.
sums : List (number, number) -> List number
sums = List.map (uncurry adding)
sum : Maybe (number, number) -> Maybe number
sum = Maybe.map (uncurry adding)
-}
uncurry : (a -> b -> c) -> (( a, b ) -> c)
uncurry original =
\( a, b ) -> original a b
{-| Converts a standard Elm function to a function that accepts a 3-tuple.
-}
uncurry3 : (a -> b -> c -> d) -> (( a, b, c ) -> d)
uncurry3 original =
\( a, b, c ) -> original a b c
{-| This function can best be explained with examples as it is unusually useful when building data pipelines but has a tricky type signature.
Please do NOT be afraid of the type signature!
If you would like a function that transforms a Person to a String of their first and last name.
converge
String.append
( .firstName, .lastName >> String.append " " )
Or perhaps you are building a data pipeline
- where at some stage you have a `List Widget`
- and you have a function `extraWidgets: List Widget -> List Widget`
- and you would like to like to end up with a new list of widgets that contains both the original widgets and the extra widgets.
Then you might write
converge
List.append
(identity, extraWidgets)
-}
converge : (first -> second -> output) -> ( input -> first, input -> second ) -> input -> output
converge fn ( firstFn, secondFn ) input =
fn (firstFn input) (secondFn input)
{-| Converge for the case of a 3 argument function. See `converge` for examples.
-}
converge3 : (first -> second -> third -> output) -> ( input -> first, input -> second, input -> third ) -> input -> output
converge3 fn ( firstFn, secondFn, thirdFn ) input =
fn (firstFn input) (secondFn input) (thirdFn input)
{-| As with converge, convergeList is best explained with an example
convergeList
(String.join " ")
[ .firstName, .middleInitial, .lastName ]
Or to be more complete
nonEmptyStrings : List String -> List String
nonEmptyStrings = List.filter (complement String.isEmpty)
fullName : Person -> String
fullName =
convergeList
(nonEmptyStrings >> String.join " ")
[ .firstName, .middleInitial, .lastName ]
-}
convergeList : (List a -> output) -> List (input -> a) -> input -> output
convergeList reducer reducerInputs input =
reducerInputs |> List.map ((|>) input) |> reducer
{-| This is like `map` for functions but going the opposite direction (contra functor).
- map: (a -> b) -> F a -> F b
- contraMap: (a -> b) -> F b -> F a
OKAY. So that it probably a little or a lot confusing. How would you use this? Any time yout have a parent/child relation and a function that
will transform the child and you want to get back a function that transforms the parent.
addressesCount : Person -> Int
addressesCount = fnContraMap .addresses List.length
-}
fnContraMap : (a -> b) -> (b -> c) -> (a -> c)
fnContraMap ab original =
ab >> original
{-| Best described with an example. Let's say you have a parent/child relation, a function of 2 arguments that applies to the child type
and you would like to get back a function that works on the parent.
sameFavoriteColor : Person -> Person -> Bool
sameFavoriteColor = fnContraMap2 (.favorites >> .color) (==)
-}
fnContraMap2 : (a -> b) -> (b -> b -> c) -> (a -> a -> c)
fnContraMap2 ab original first second =
original (ab first) (ab second)
{-| Same as fnContraMap2 but for 3 argument functions.
-}
fnContraMap3 : (a -> b) -> (b -> b -> b -> c) -> (a -> a -> a -> c)
fnContraMap3 ab original first second third =
original (ab first) (ab second) (ab third)
-- ------------------------------------------------------------------------------
-- Predicate
-- ------------------------------------------------------------------------------
type alias Predicate a =
a -> Bool
{-| Return a new predicate that is true if both of the two predicates return true.
Note that this is short-circuited, meaning that the second predicate will not be invoked if the first returns false.
-}
both : Predicate a -> Predicate a -> Predicate a
both left right a =
left a && right a
{-| Takes a list of predicates and returns a predicate that returns true for a given list of arguments if every one of the provided predicates is satisfied by those arguments.
isAmazing : Person -> Bool
isAmazing = allPass [isKind, isCreative, isHardWorking]
-}
allPass : List (Predicate a) -> Predicate a
allPass preds a =
List.all ((|>) a) preds
{-| Takes a list of predicates and returns a predicate that returns true for a given list of arguments if at least one of the provided predicates is satisfied by those arguments.
isOkay : Person -> Bool
isOkay = anyPass [isKind, isCreative, isHardWorking]
-}
anyPass : List (Predicate a) -> Predicate a
anyPass preds a =
List.any ((|>) a) preds
{-| Return a new predicate that is true if either of the two predicates return true.
Note that this is short-circuited, meaning that the second predicate will not be invoked if the first returns true.
-}
either : Predicate a -> Predicate a -> Predicate a
either left right a =
left a || right a
{-| Invert (negate, complement) a predicate i.e if the predicate would have return True for some `a` it will now return False and vice versa.
-}
complement : Predicate a -> Predicate a
complement fn =
fn >> not
{-| A predicate that always returns true.
-}
true : Predicate a
true =
always True
{-| A predicate that always returns false.
-}
false : Predicate a
false =
always False
-- ------------------------------------------------------------------------------
-- Flow Control
-- ------------------------------------------------------------------------------
{-| Creates a function that will process either the whenTrue or the whenFalse function depending upon the result of the condition predicate.
ifElse
(.weightInLbs >> R.Relation.greaterThan 500)
(always "Sorry. You cannot ride the coaster.")
(.name >> String.append "Enjoy your ride ")
-}
ifElse : Predicate a -> (a -> b) -> (a -> b) -> (a -> b)
ifElse condition whenTrue whenFalse input =
if condition input then
whenTrue input
else
whenFalse input
{-| Returns a function which encapsulates if/else, if/else, ... logic given a list of (predicate, transformer) tuples.
- The condition pairs are processed in order
- and returns the transformation of the first predicate that passes
- If no conditions pass then returns Nothing.
-}
cond : List ( Predicate a, a -> b ) -> a -> Maybe b
cond cases input =
let
apply =
(|>) input
in
cases
|> List.Extra.find (Tuple.first >> apply)
|> Maybe.map (Tuple.second >> apply)
type alias Thunk a =
() -> a
{-| Same as `cond` but also provides a default option in the case that none of the conditions pass.
-}
condDefault : List ( Predicate a, a -> b ) -> Thunk b -> (a -> b)
condDefault cases defaultThunk a =
case cond cases a of
Just v ->
v
Nothing ->
defaultThunk ()
{-| Tests the final argument by passing it to the given predicate function.
- If the predicate is satisfied, the function will return the result of calling the whenTrueFn function with the same argument.
- If the predicate is not satisfied, the argument is returned as is.
-}
when : Predicate a -> (a -> a) -> (a -> a)
when condition transform a =
if condition a then
transform a
else
a
{-| Tests the final argument against the predicate and if true then performs the transformation.
This is super helpful when combined with List.filterMap. For example, let's say you wanted the names of just the cool people
allThePeople
|> List.filterMap (maybeWhen .isCool .fullName)
-}
maybeWhen : Predicate a -> (a -> b) -> a -> Maybe b
maybeWhen condition transform a =
if condition a then
a |> transform |> Just
else
Nothing
{-| Tests the final argument by passing it to the given predicate function.
- If the predicate is not satisfied, the function will return the result of calling the whenFalseFn function with the same argument.
- If the predicate is satisfied, the argument is returned as is.
-}
unless : Predicate a -> (a -> a) -> (a -> a)
unless whenFalse =
when (complement whenFalse)
{-| Takes a predicate, a transformation function, and an initial value, and returns a value of the same type as the initial value.
It does so by applying the transformation until the predicate is satisfied, at which point it returns the satisfactory value.
-}
until : Predicate a -> (a -> a) -> (a -> a)
until condition transform =
let
aux current =
if condition current then
current
else
aux (transform current)
in
aux
-- ------------------------------------------------------------------------------
-- Relation
-- ------------------------------------------------------------------------------
{-| Designed for use in pipelines or currying. Returns a predicate that tests if the value is less than the first value given.
[5, 10, 11, 20] |> List.map (lessThan 10)
-- [True, False, False, False]
-}
lessThan : comparable -> comparable -> Bool
lessThan a b =
b < a
{-| Designed for currying. Returns a predicate that tests if the value is less than or equal to the first value given.
[5, 10, 11, 20] |> List.map (lessThanEqualto 10)
-- [True, True, False, False]
-}
lessThanEqualTo : comparable -> Predicate comparable
lessThanEqualTo a b =
b <= a
{-| Designed for currying. Returns a predicate that tests if the value is greater than the first value given.
[5, 10, 11, 20] |> List.map (greaterThan 10)
-- [False, False, True, True]
-}
greaterThan : comparable -> Predicate comparable
greaterThan a b =
b > a
{-| Designed for currying. Returns a predicate that tests if the value is greater than or equal to the first value given.
[5, 10, 11, 20]
|> List.map (greaterThanEqualTo 10) -- [False, True, True, True]
-}
greaterThanEqualTo : comparable -> Predicate comparable
greaterThanEqualTo a b =
b >= a
{-| A natural language alias for `==` that also helps to avoid parenthesis when writing point-free flows
employees
|> List.filter (.employmentType >> equals Contractor)
-}
equals : a -> Predicate a
equals =
(==)
-- ------------------------------------------------------------------------------
-- Math
-- ------------------------------------------------------------------------------
{-| A literate alias for addition that is nice for building functional pipelines by avoiding parenthesis.
[5, 10, 15, 20] |> List.map (adding 5)
-- [10, 15, 20, 25]
-}
adding : number -> number -> number
adding a b =
a + b
{-| Subtraction alias designed for pipelines i.e. the order is swapped such that the subtrahend is the first argument.
[20, 30, 40]
|> List.map (subtracting 6)
-- [14, 24, 34]
-}
subtracting : number -> number -> number
subtracting subtrahend minuend =
minuend - subtrahend
{-| A natural language alias for \*.
-}
multiplying : number -> number -> number
multiplying =
(*)
{-| Division designed for pipelines i.e. the divisor is the first argument.
[20, 32, 40] |> List (divideByInt 4)
-- [5, 8, 10]
-}
dividedByInt : Int -> Int -> Int
dividedByInt divisor dividend =
dividend // divisor
{-| Division designed for pipelines i.e. the divisor is the first argument.
[20, 25, 26.25] |> List (divideByFloat 2.5)
-- [8.0, 10.0, 10.5]
-}
dividedByFloat : Float -> Float -> Float
dividedByFloat divisor dividend =
dividend / divisor
{-| Negate the given number.
-}
negated : number -> number
negated =
(*) -1
| elm |
[
{
"context": "\ninitialModel : Model\ninitialModel = \n { name = \"Anonymous\"\n , gameNumber = 1\n , entries = []\n , alertMes",
"end": 734,
"score": 0.5788097978,
"start": 725,
"tag": "NAME",
"value": "Anonymous"
}
] | Bingo.elm | desireco/elm_buzzword_bingo | 0 | module Bingo exposing (..)
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick, onInput)
import Random
import Http
import Json.Decode as Decode exposing (Decoder, field, succeed)
import Json.Encode as Encode
import ViewHelpers exposing (..)
import Entry
-- MODEL
type GameState = EnteringName | Playing
type alias Model =
{ name : PlayerName
, gameNumber : GameNumber
, entries : List Entry.Entry
, alertMessage : Maybe String
, nameInput : String
, gameState : GameState
}
type alias PlayerName = String
type alias GameNumber = Int
type alias Score =
{ id : Int
, name : String
, score : Int
}
initialModel : Model
initialModel =
{ name = "Anonymous"
, gameNumber = 1
, entries = []
, alertMessage = Nothing
, nameInput = ""
, gameState = EnteringName
}
-- UPDATE
type Msg
= NewGame
| Mark Int
| NewRandom Int
| NewEntries (Result Http.Error (List Entry.Entry))
| CloseAlert
| ShareScore
| NewScore (Result Http.Error Score)
| SetNameInput String
| SaveName
| CancelName
| ChangeGameState GameState
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
ChangeGameState state ->
( { model | gameState = state }, Cmd.none )
SaveName ->
if String.isEmpty model.nameInput then
( model, Cmd.none )
else
( { model | name = model.nameInput,
nameInput = "",
gameState = Playing }, Cmd.none )
CancelName ->
( { model | nameInput = "", gameState = Playing }, Cmd.none )
SetNameInput value ->
( { model | nameInput = value }, Cmd.none )
NewRandom randomNumber ->
( { model | gameNumber = randomNumber }, Cmd.none )
ShareScore ->
( model, postScore model )
NewScore (Ok score) ->
let
message =
"Your score of "
++ (toString score.score)
++ " was successfully shared!"
in
( { model | alertMessage = Just message }, Cmd.none )
NewScore (Err error) ->
( { model | alertMessage = Just (httpErrorMessage error) }, Cmd.none )
NewGame ->
( { model | gameNumber = model.gameNumber + 1 }, getEntries )
NewEntries (Ok randomEntries) ->
( { model | entries = randomEntries }, Cmd.none)
NewEntries (Err error) ->
( {model | alertMessage = Just (httpErrorMessage error)}, Cmd.none)
CloseAlert ->
( {model | alertMessage = Nothing}, Cmd.none )
Mark id ->
({ model | entries = Entry.markEntryWithId model.entries id }, Cmd.none )
httpErrorMessage: Http.Error -> String
httpErrorMessage error =
case error of
Http.NetworkError ->
"Is the server running?"
Http.BadStatus response ->
(toString response.status)
Http.BadPayload message _ ->
"Decoding Failed: " ++ message
_ ->
(toString error)
-- DECODERS / ENCODERS
encodeScore : Model -> Encode.Value
encodeScore model =
Encode.object
[ ("name", Encode.string model.name)
, ("score", Encode.int ( Entry.sumMarkedPoints model.entries ) )
]
scoreDecoder : Decoder Score
scoreDecoder =
Decode.map3 Score
(field "id" Decode.int)
(field "name" Decode.string)
(field "score" Decode.int)
-- COMMANDS
generateRandomNumber : Cmd Msg
generateRandomNumber =
Random.generate NewRandom (Random.int 1 100)
getEntries : Cmd Msg
getEntries =
Entry.getEntries NewEntries "http://localhost:3000/random-entries"
postScore : Model -> Cmd Msg
postScore model =
let
url =
"http://localhost:3000/scores"
body =
encodeScore model
|> Http.jsonBody
request =
Http.post url body scoreDecoder
in
Http.send NewScore request
-- VIEW
view : Model -> Html Msg
view model =
div [ class "content" ]
[ viewHeader "Buzzword Bingo"
, viewPlayer model.name model.gameNumber
, alert CloseAlert model.alertMessage
, viewNameInput model
, Entry.viewEntryList Mark model.entries
, viewScore ( Entry.sumMarkedPoints model.entries )
, div [ class "button-group" ]
[ primaryButton NewGame "New Game"
, primaryButton ShareScore "Save Score"
]
, div [ class "debug" ] [ text (toString model) ]
, viewFooter
]
viewPlayer : PlayerName -> GameNumber -> Html Msg
viewPlayer name gameNumber =
h2 [ id "info", class "classy" ]
[ a [ href "#", onClick (ChangeGameState EnteringName) ]
[ text name ]
, text (" - Game #" ++ (toString gameNumber))
]
viewHeader : String -> Html Msg
viewHeader title =
header []
[ h1 [] [ text title ] ]
viewFooter : Html Msg
viewFooter =
footer []
[ a [ href "http://elm-lang.org" ]
[ text "Powered by Elm." ]
]
viewScore : Int -> Html Msg
viewScore score =
div
[ class "score" ]
[ span [ class "label" ] [ text "Score" ]
, span [ class "value" ] [ text (toString score) ]
]
viewNameInput : Model -> Html Msg
viewNameInput model =
case model.gameState of
EnteringName ->
div [ class "name-input" ]
[ input
[ type_ "text"
, placeholder "Who's playing?"
, autofocus True
, value model.nameInput
, onInput SetNameInput
]
[ ]
, primaryButton SaveName "Save"
, primaryButton CancelName "Cancel"
]
Playing ->
text ""
main : Program Never Model Msg
main =
Html.program
{ init = ( initialModel, getEntries )
, view = view
, update = update
, subscriptions = (\_ -> Sub.none )
}
| 59557 | module Bingo exposing (..)
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick, onInput)
import Random
import Http
import Json.Decode as Decode exposing (Decoder, field, succeed)
import Json.Encode as Encode
import ViewHelpers exposing (..)
import Entry
-- MODEL
type GameState = EnteringName | Playing
type alias Model =
{ name : PlayerName
, gameNumber : GameNumber
, entries : List Entry.Entry
, alertMessage : Maybe String
, nameInput : String
, gameState : GameState
}
type alias PlayerName = String
type alias GameNumber = Int
type alias Score =
{ id : Int
, name : String
, score : Int
}
initialModel : Model
initialModel =
{ name = "<NAME>"
, gameNumber = 1
, entries = []
, alertMessage = Nothing
, nameInput = ""
, gameState = EnteringName
}
-- UPDATE
type Msg
= NewGame
| Mark Int
| NewRandom Int
| NewEntries (Result Http.Error (List Entry.Entry))
| CloseAlert
| ShareScore
| NewScore (Result Http.Error Score)
| SetNameInput String
| SaveName
| CancelName
| ChangeGameState GameState
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
ChangeGameState state ->
( { model | gameState = state }, Cmd.none )
SaveName ->
if String.isEmpty model.nameInput then
( model, Cmd.none )
else
( { model | name = model.nameInput,
nameInput = "",
gameState = Playing }, Cmd.none )
CancelName ->
( { model | nameInput = "", gameState = Playing }, Cmd.none )
SetNameInput value ->
( { model | nameInput = value }, Cmd.none )
NewRandom randomNumber ->
( { model | gameNumber = randomNumber }, Cmd.none )
ShareScore ->
( model, postScore model )
NewScore (Ok score) ->
let
message =
"Your score of "
++ (toString score.score)
++ " was successfully shared!"
in
( { model | alertMessage = Just message }, Cmd.none )
NewScore (Err error) ->
( { model | alertMessage = Just (httpErrorMessage error) }, Cmd.none )
NewGame ->
( { model | gameNumber = model.gameNumber + 1 }, getEntries )
NewEntries (Ok randomEntries) ->
( { model | entries = randomEntries }, Cmd.none)
NewEntries (Err error) ->
( {model | alertMessage = Just (httpErrorMessage error)}, Cmd.none)
CloseAlert ->
( {model | alertMessage = Nothing}, Cmd.none )
Mark id ->
({ model | entries = Entry.markEntryWithId model.entries id }, Cmd.none )
httpErrorMessage: Http.Error -> String
httpErrorMessage error =
case error of
Http.NetworkError ->
"Is the server running?"
Http.BadStatus response ->
(toString response.status)
Http.BadPayload message _ ->
"Decoding Failed: " ++ message
_ ->
(toString error)
-- DECODERS / ENCODERS
encodeScore : Model -> Encode.Value
encodeScore model =
Encode.object
[ ("name", Encode.string model.name)
, ("score", Encode.int ( Entry.sumMarkedPoints model.entries ) )
]
scoreDecoder : Decoder Score
scoreDecoder =
Decode.map3 Score
(field "id" Decode.int)
(field "name" Decode.string)
(field "score" Decode.int)
-- COMMANDS
generateRandomNumber : Cmd Msg
generateRandomNumber =
Random.generate NewRandom (Random.int 1 100)
getEntries : Cmd Msg
getEntries =
Entry.getEntries NewEntries "http://localhost:3000/random-entries"
postScore : Model -> Cmd Msg
postScore model =
let
url =
"http://localhost:3000/scores"
body =
encodeScore model
|> Http.jsonBody
request =
Http.post url body scoreDecoder
in
Http.send NewScore request
-- VIEW
view : Model -> Html Msg
view model =
div [ class "content" ]
[ viewHeader "Buzzword Bingo"
, viewPlayer model.name model.gameNumber
, alert CloseAlert model.alertMessage
, viewNameInput model
, Entry.viewEntryList Mark model.entries
, viewScore ( Entry.sumMarkedPoints model.entries )
, div [ class "button-group" ]
[ primaryButton NewGame "New Game"
, primaryButton ShareScore "Save Score"
]
, div [ class "debug" ] [ text (toString model) ]
, viewFooter
]
viewPlayer : PlayerName -> GameNumber -> Html Msg
viewPlayer name gameNumber =
h2 [ id "info", class "classy" ]
[ a [ href "#", onClick (ChangeGameState EnteringName) ]
[ text name ]
, text (" - Game #" ++ (toString gameNumber))
]
viewHeader : String -> Html Msg
viewHeader title =
header []
[ h1 [] [ text title ] ]
viewFooter : Html Msg
viewFooter =
footer []
[ a [ href "http://elm-lang.org" ]
[ text "Powered by Elm." ]
]
viewScore : Int -> Html Msg
viewScore score =
div
[ class "score" ]
[ span [ class "label" ] [ text "Score" ]
, span [ class "value" ] [ text (toString score) ]
]
viewNameInput : Model -> Html Msg
viewNameInput model =
case model.gameState of
EnteringName ->
div [ class "name-input" ]
[ input
[ type_ "text"
, placeholder "Who's playing?"
, autofocus True
, value model.nameInput
, onInput SetNameInput
]
[ ]
, primaryButton SaveName "Save"
, primaryButton CancelName "Cancel"
]
Playing ->
text ""
main : Program Never Model Msg
main =
Html.program
{ init = ( initialModel, getEntries )
, view = view
, update = update
, subscriptions = (\_ -> Sub.none )
}
| true | module Bingo exposing (..)
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick, onInput)
import Random
import Http
import Json.Decode as Decode exposing (Decoder, field, succeed)
import Json.Encode as Encode
import ViewHelpers exposing (..)
import Entry
-- MODEL
type GameState = EnteringName | Playing
type alias Model =
{ name : PlayerName
, gameNumber : GameNumber
, entries : List Entry.Entry
, alertMessage : Maybe String
, nameInput : String
, gameState : GameState
}
type alias PlayerName = String
type alias GameNumber = Int
type alias Score =
{ id : Int
, name : String
, score : Int
}
initialModel : Model
initialModel =
{ name = "PI:NAME:<NAME>END_PI"
, gameNumber = 1
, entries = []
, alertMessage = Nothing
, nameInput = ""
, gameState = EnteringName
}
-- UPDATE
type Msg
= NewGame
| Mark Int
| NewRandom Int
| NewEntries (Result Http.Error (List Entry.Entry))
| CloseAlert
| ShareScore
| NewScore (Result Http.Error Score)
| SetNameInput String
| SaveName
| CancelName
| ChangeGameState GameState
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
ChangeGameState state ->
( { model | gameState = state }, Cmd.none )
SaveName ->
if String.isEmpty model.nameInput then
( model, Cmd.none )
else
( { model | name = model.nameInput,
nameInput = "",
gameState = Playing }, Cmd.none )
CancelName ->
( { model | nameInput = "", gameState = Playing }, Cmd.none )
SetNameInput value ->
( { model | nameInput = value }, Cmd.none )
NewRandom randomNumber ->
( { model | gameNumber = randomNumber }, Cmd.none )
ShareScore ->
( model, postScore model )
NewScore (Ok score) ->
let
message =
"Your score of "
++ (toString score.score)
++ " was successfully shared!"
in
( { model | alertMessage = Just message }, Cmd.none )
NewScore (Err error) ->
( { model | alertMessage = Just (httpErrorMessage error) }, Cmd.none )
NewGame ->
( { model | gameNumber = model.gameNumber + 1 }, getEntries )
NewEntries (Ok randomEntries) ->
( { model | entries = randomEntries }, Cmd.none)
NewEntries (Err error) ->
( {model | alertMessage = Just (httpErrorMessage error)}, Cmd.none)
CloseAlert ->
( {model | alertMessage = Nothing}, Cmd.none )
Mark id ->
({ model | entries = Entry.markEntryWithId model.entries id }, Cmd.none )
httpErrorMessage: Http.Error -> String
httpErrorMessage error =
case error of
Http.NetworkError ->
"Is the server running?"
Http.BadStatus response ->
(toString response.status)
Http.BadPayload message _ ->
"Decoding Failed: " ++ message
_ ->
(toString error)
-- DECODERS / ENCODERS
encodeScore : Model -> Encode.Value
encodeScore model =
Encode.object
[ ("name", Encode.string model.name)
, ("score", Encode.int ( Entry.sumMarkedPoints model.entries ) )
]
scoreDecoder : Decoder Score
scoreDecoder =
Decode.map3 Score
(field "id" Decode.int)
(field "name" Decode.string)
(field "score" Decode.int)
-- COMMANDS
generateRandomNumber : Cmd Msg
generateRandomNumber =
Random.generate NewRandom (Random.int 1 100)
getEntries : Cmd Msg
getEntries =
Entry.getEntries NewEntries "http://localhost:3000/random-entries"
postScore : Model -> Cmd Msg
postScore model =
let
url =
"http://localhost:3000/scores"
body =
encodeScore model
|> Http.jsonBody
request =
Http.post url body scoreDecoder
in
Http.send NewScore request
-- VIEW
view : Model -> Html Msg
view model =
div [ class "content" ]
[ viewHeader "Buzzword Bingo"
, viewPlayer model.name model.gameNumber
, alert CloseAlert model.alertMessage
, viewNameInput model
, Entry.viewEntryList Mark model.entries
, viewScore ( Entry.sumMarkedPoints model.entries )
, div [ class "button-group" ]
[ primaryButton NewGame "New Game"
, primaryButton ShareScore "Save Score"
]
, div [ class "debug" ] [ text (toString model) ]
, viewFooter
]
viewPlayer : PlayerName -> GameNumber -> Html Msg
viewPlayer name gameNumber =
h2 [ id "info", class "classy" ]
[ a [ href "#", onClick (ChangeGameState EnteringName) ]
[ text name ]
, text (" - Game #" ++ (toString gameNumber))
]
viewHeader : String -> Html Msg
viewHeader title =
header []
[ h1 [] [ text title ] ]
viewFooter : Html Msg
viewFooter =
footer []
[ a [ href "http://elm-lang.org" ]
[ text "Powered by Elm." ]
]
viewScore : Int -> Html Msg
viewScore score =
div
[ class "score" ]
[ span [ class "label" ] [ text "Score" ]
, span [ class "value" ] [ text (toString score) ]
]
viewNameInput : Model -> Html Msg
viewNameInput model =
case model.gameState of
EnteringName ->
div [ class "name-input" ]
[ input
[ type_ "text"
, placeholder "Who's playing?"
, autofocus True
, value model.nameInput
, onInput SetNameInput
]
[ ]
, primaryButton SaveName "Save"
, primaryButton CancelName "Cancel"
]
Playing ->
text ""
main : Program Never Model Msg
main =
Html.program
{ init = ( initialModel, getEntries )
, view = view
, update = update
, subscriptions = (\_ -> Sub.none )
}
| elm |
[
{
"context": "should go here instead of this sample token:\n \"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\"\n",
"end": 147,
"score": 0.9990240932,
"start": 107,
"tag": "KEY",
"value": "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"
}
] | Auth.elm | pepsm/practice-elm | 0 | module Auth exposing (token)
token =
-- Your token should go here instead of this sample token:
"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"
| 44295 | module Auth exposing (token)
token =
-- Your token should go here instead of this sample token:
"<KEY>"
| true | module Auth exposing (token)
token =
-- Your token should go here instead of this sample token:
"PI:KEY:<KEY>END_PI"
| elm |
[
{
"context": "-- Elm-chat\n-- Developed by Christian Visintin <christian.visintin1997@gmail.com>\n-- Copyright ",
"end": 48,
"score": 0.9998890758,
"start": 30,
"tag": "NAME",
"value": "Christian Visintin"
},
{
"context": "-- Elm-chat\n-- Developed by Christian Visintin <christian.visintin1997@gmail.com>\n-- Copyright (C) 2021 - Christian Visintin\n-- ",
"end": 82,
"score": 0.9999250174,
"start": 50,
"tag": "EMAIL",
"value": "christian.visintin1997@gmail.com"
},
{
"context": "n.visintin1997@gmail.com>\n-- Copyright (C) 2021 - Christian Visintin\n-- Distribuited under \"The Unlicense\" license\n--",
"end": 127,
"score": 0.9998824,
"start": 109,
"tag": "NAME",
"value": "Christian Visintin"
}
] | src/Ports.elm | veeso/elm-chat | 0 | -- Elm-chat
-- Developed by Christian Visintin <christian.visintin1997@gmail.com>
-- Copyright (C) 2021 - Christian Visintin
-- Distribuited under "The Unlicense" license
-- for more information, please refer to <https://unlicense.org>
port module Ports exposing (chatMessageReceiver, sessionChanged, setSession, startChat)
import Json.Decode exposing (Value)
-- Ports
{-| Tells to JsRuntime to connect to remote via websockets
-}
port startChat : () -> Cmd msg
{-| Receiver for message from websockets
-}
port chatMessageReceiver : (String -> msg) -> Sub msg
{-| This port is called when the session in the storage changes
-}
port sessionChanged : (Value -> msg) -> Sub msg
{-| This port is called from Elm to set the session in the local storage
-}
port setSession : Value -> Cmd msg
| 42925 | -- Elm-chat
-- Developed by <NAME> <<EMAIL>>
-- Copyright (C) 2021 - <NAME>
-- Distribuited under "The Unlicense" license
-- for more information, please refer to <https://unlicense.org>
port module Ports exposing (chatMessageReceiver, sessionChanged, setSession, startChat)
import Json.Decode exposing (Value)
-- Ports
{-| Tells to JsRuntime to connect to remote via websockets
-}
port startChat : () -> Cmd msg
{-| Receiver for message from websockets
-}
port chatMessageReceiver : (String -> msg) -> Sub msg
{-| This port is called when the session in the storage changes
-}
port sessionChanged : (Value -> msg) -> Sub msg
{-| This port is called from Elm to set the session in the local storage
-}
port setSession : Value -> Cmd msg
| true | -- Elm-chat
-- Developed by PI:NAME:<NAME>END_PI <PI:EMAIL:<EMAIL>END_PI>
-- Copyright (C) 2021 - PI:NAME:<NAME>END_PI
-- Distribuited under "The Unlicense" license
-- for more information, please refer to <https://unlicense.org>
port module Ports exposing (chatMessageReceiver, sessionChanged, setSession, startChat)
import Json.Decode exposing (Value)
-- Ports
{-| Tells to JsRuntime to connect to remote via websockets
-}
port startChat : () -> Cmd msg
{-| Receiver for message from websockets
-}
port chatMessageReceiver : (String -> msg) -> Sub msg
{-| This port is called when the session in the storage changes
-}
port sessionChanged : (Value -> msg) -> Sub msg
{-| This port is called from Elm to set the session in the local storage
-}
port setSession : Value -> Cmd msg
| elm |
[
{
"context": "g and evictions.\"\n , author = \"Greg Ziegan\"\n , programmingLanguage = Stru",
"end": 2049,
"score": 0.9998855591,
"start": 2038,
"tag": "NAME",
"value": "Greg Ziegan"
}
] | pages/src/Page/Glossary.elm | gregziegan/eviction-tracker | 5 | module Page.Glossary exposing (Data, Model, Msg, page)
import Cloudinary
import DataSource exposing (DataSource)
import Date exposing (Date)
import Element exposing (Element, alignLeft, alignRight, alignTop, centerX, column, fill, fillPortion, height, maximum, minimum, paddingXY, px, row, spacing, textColumn, width, wrappedRow)
import Element.Font as Font
import Head
import Head.Seo as Seo
import Logo
import Markdown.Html
import MarkdownCodec
import MarkdownRenderer
import OptimizedDecoder
import Page exposing (Page, StaticPayload)
import Pages.PageUrl exposing (PageUrl)
import Pages.Url
import Path
import Shared
import Site
import StructuredData
import View exposing (View)
type alias Model =
()
type alias Msg =
Never
type alias RouteParams =
{}
page : Page RouteParams Data
page =
Page.single
{ head = head
, data = data
}
|> Page.buildNoState { view = view }
data : DataSource Data
data =
MarkdownCodec.withFrontmatter Data
frontmatterDecoder
blogRenderer
"content/glossary.md"
head :
StaticPayload Data RouteParams
-> List Head.Tag
head static =
let
metadata =
static.data.metadata
in
Head.structuredData
(StructuredData.aboutPage
{ title = metadata.title
, description = metadata.description
, author = StructuredData.person { name = "Red Door Collective" }
, publisher = StructuredData.person { name = "Red Door Collective" }
, url = Site.config.canonicalUrl ++ Path.toAbsolute static.path
, imageUrl = metadata.image
, lastReviewed = Date.toIsoString metadata.lastReviewed
, mainEntityOfPage =
StructuredData.softwareSourceCode
{ codeRepositoryUrl = "https://github.com/red-door-collective/eviction-tracker"
, description = "A free website that keeps the people informed about housing and evictions."
, author = "Greg Ziegan"
, programmingLanguage = StructuredData.elmLang
}
}
)
:: (Seo.summary
{ canonicalUrlOverride = Nothing
, siteName = "Red Door Collective"
, image = Logo.smallImage
, description = metadata.description
, locale = Just "en-us"
, title = metadata.title
}
|> Seo.website
)
type alias Data =
{ metadata : Metadata
, body : List (Element Msg)
}
view :
Maybe PageUrl
-> Shared.Model
-> StaticPayload Data RouteParams
-> View Msg
view maybeUrl sharedModel static =
{ title = static.data.metadata.title
, body =
[ column
[ width (fill |> minimum 300 |> maximum 750)
, centerX
, spacing 10
, paddingXY 0 10
]
[ row
[ width fill ]
[ textColumn [ width fill ] static.data.body
]
]
]
}
elmUiRenderer =
MarkdownRenderer.renderer
viewAlignLeft : List (Element msg) -> Element msg
viewAlignLeft renderedChildren =
row [ alignLeft, width (fill |> maximum 375), height fill ]
renderedChildren
viewAlignRight : List (Element msg) -> Element msg
viewAlignRight renderedChildren =
row [ alignRight, width (fill |> maximum 375), height fill ]
renderedChildren
viewTextColumn : List (Element msg) -> Element msg
viewTextColumn renderedChildren =
textColumn
[ width fill
, Element.spacingXY 10 10
]
renderedChildren
viewRow : List (Element msg) -> Element msg
viewRow renderedChildren =
wrappedRow
[ width fill
, Element.spacingXY 0 10
, alignTop
]
renderedChildren
viewColumn : String -> List (Element msg) -> Element msg
viewColumn portion renderedChildren =
let
fillAttr =
width <|
case String.toInt portion of
Nothing ->
fill
Just number ->
fillPortion number
in
column [ alignTop, fillAttr ] renderedChildren
viewSizedImage : Maybe String -> Maybe String -> Maybe String -> String -> String -> Element msg
viewSizedImage title widthInPx heightInPx src alt =
let
( w, h ) =
( Maybe.andThen String.toInt widthInPx
, Maybe.andThen String.toInt heightInPx
)
widthAttr =
case w of
Just number ->
width (px number)
Nothing ->
width fill
heightAttr =
case h of
Just number ->
height (px number)
Nothing ->
height fill
in
case title of
Just _ ->
Element.image [ centerX, widthAttr, heightAttr ] { src = src, description = alt }
Nothing ->
row [ width fill ] [ Element.image [ centerX, widthAttr, heightAttr ] { src = src, description = alt } ]
viewLegend : String -> Element msg
viewLegend title =
column
[ Element.padding 20
, Element.spacing 30
, Element.centerX
]
[ Element.row [ Element.spacing 20 ]
[ Element.el
[ Font.bold
, Font.size 30
]
(Element.text title)
]
]
blogRenderer =
{ elmUiRenderer
| html =
Markdown.Html.oneOf
[ Markdown.Html.tag "legend"
(\title _ ->
viewLegend title
)
|> Markdown.Html.withAttribute "title"
, Markdown.Html.tag "sized-image"
(\title widthInPx heightInPx src alt _ ->
viewSizedImage title widthInPx heightInPx src alt
)
|> Markdown.Html.withOptionalAttribute "title"
|> Markdown.Html.withOptionalAttribute "width"
|> Markdown.Html.withOptionalAttribute "height"
|> Markdown.Html.withAttribute "src"
|> Markdown.Html.withAttribute "alt"
, Markdown.Html.tag "column"
(\portion renderedChildren ->
viewColumn portion renderedChildren
)
|> Markdown.Html.withAttribute "portion"
, Markdown.Html.tag "text-column"
(\renderedChildren ->
viewTextColumn renderedChildren
)
, Markdown.Html.tag "align-left"
(\renderedChildren ->
viewAlignLeft renderedChildren
)
, Markdown.Html.tag "align-right"
(\renderedChildren ->
viewAlignRight renderedChildren
)
, Markdown.Html.tag "row"
(\renderedChildren ->
viewRow renderedChildren
)
]
}
type alias Metadata =
{ title : String
, description : String
, lastReviewed : Date
, image : Pages.Url.Url
}
frontmatterDecoder : OptimizedDecoder.Decoder Metadata
frontmatterDecoder =
OptimizedDecoder.map4 Metadata
(OptimizedDecoder.field "title" OptimizedDecoder.string)
(OptimizedDecoder.field "description" OptimizedDecoder.string)
(OptimizedDecoder.field "lastReviewed"
(OptimizedDecoder.string
|> OptimizedDecoder.andThen
(\isoString ->
Date.fromIsoString isoString
|> OptimizedDecoder.fromResult
)
)
)
(OptimizedDecoder.field "image" imageDecoder)
imageDecoder : OptimizedDecoder.Decoder Pages.Url.Url
imageDecoder =
OptimizedDecoder.string
|> OptimizedDecoder.map (\asset -> Cloudinary.url asset Nothing 400 300)
| 3508 | module Page.Glossary exposing (Data, Model, Msg, page)
import Cloudinary
import DataSource exposing (DataSource)
import Date exposing (Date)
import Element exposing (Element, alignLeft, alignRight, alignTop, centerX, column, fill, fillPortion, height, maximum, minimum, paddingXY, px, row, spacing, textColumn, width, wrappedRow)
import Element.Font as Font
import Head
import Head.Seo as Seo
import Logo
import Markdown.Html
import MarkdownCodec
import MarkdownRenderer
import OptimizedDecoder
import Page exposing (Page, StaticPayload)
import Pages.PageUrl exposing (PageUrl)
import Pages.Url
import Path
import Shared
import Site
import StructuredData
import View exposing (View)
type alias Model =
()
type alias Msg =
Never
type alias RouteParams =
{}
page : Page RouteParams Data
page =
Page.single
{ head = head
, data = data
}
|> Page.buildNoState { view = view }
data : DataSource Data
data =
MarkdownCodec.withFrontmatter Data
frontmatterDecoder
blogRenderer
"content/glossary.md"
head :
StaticPayload Data RouteParams
-> List Head.Tag
head static =
let
metadata =
static.data.metadata
in
Head.structuredData
(StructuredData.aboutPage
{ title = metadata.title
, description = metadata.description
, author = StructuredData.person { name = "Red Door Collective" }
, publisher = StructuredData.person { name = "Red Door Collective" }
, url = Site.config.canonicalUrl ++ Path.toAbsolute static.path
, imageUrl = metadata.image
, lastReviewed = Date.toIsoString metadata.lastReviewed
, mainEntityOfPage =
StructuredData.softwareSourceCode
{ codeRepositoryUrl = "https://github.com/red-door-collective/eviction-tracker"
, description = "A free website that keeps the people informed about housing and evictions."
, author = "<NAME>"
, programmingLanguage = StructuredData.elmLang
}
}
)
:: (Seo.summary
{ canonicalUrlOverride = Nothing
, siteName = "Red Door Collective"
, image = Logo.smallImage
, description = metadata.description
, locale = Just "en-us"
, title = metadata.title
}
|> Seo.website
)
type alias Data =
{ metadata : Metadata
, body : List (Element Msg)
}
view :
Maybe PageUrl
-> Shared.Model
-> StaticPayload Data RouteParams
-> View Msg
view maybeUrl sharedModel static =
{ title = static.data.metadata.title
, body =
[ column
[ width (fill |> minimum 300 |> maximum 750)
, centerX
, spacing 10
, paddingXY 0 10
]
[ row
[ width fill ]
[ textColumn [ width fill ] static.data.body
]
]
]
}
elmUiRenderer =
MarkdownRenderer.renderer
viewAlignLeft : List (Element msg) -> Element msg
viewAlignLeft renderedChildren =
row [ alignLeft, width (fill |> maximum 375), height fill ]
renderedChildren
viewAlignRight : List (Element msg) -> Element msg
viewAlignRight renderedChildren =
row [ alignRight, width (fill |> maximum 375), height fill ]
renderedChildren
viewTextColumn : List (Element msg) -> Element msg
viewTextColumn renderedChildren =
textColumn
[ width fill
, Element.spacingXY 10 10
]
renderedChildren
viewRow : List (Element msg) -> Element msg
viewRow renderedChildren =
wrappedRow
[ width fill
, Element.spacingXY 0 10
, alignTop
]
renderedChildren
viewColumn : String -> List (Element msg) -> Element msg
viewColumn portion renderedChildren =
let
fillAttr =
width <|
case String.toInt portion of
Nothing ->
fill
Just number ->
fillPortion number
in
column [ alignTop, fillAttr ] renderedChildren
viewSizedImage : Maybe String -> Maybe String -> Maybe String -> String -> String -> Element msg
viewSizedImage title widthInPx heightInPx src alt =
let
( w, h ) =
( Maybe.andThen String.toInt widthInPx
, Maybe.andThen String.toInt heightInPx
)
widthAttr =
case w of
Just number ->
width (px number)
Nothing ->
width fill
heightAttr =
case h of
Just number ->
height (px number)
Nothing ->
height fill
in
case title of
Just _ ->
Element.image [ centerX, widthAttr, heightAttr ] { src = src, description = alt }
Nothing ->
row [ width fill ] [ Element.image [ centerX, widthAttr, heightAttr ] { src = src, description = alt } ]
viewLegend : String -> Element msg
viewLegend title =
column
[ Element.padding 20
, Element.spacing 30
, Element.centerX
]
[ Element.row [ Element.spacing 20 ]
[ Element.el
[ Font.bold
, Font.size 30
]
(Element.text title)
]
]
blogRenderer =
{ elmUiRenderer
| html =
Markdown.Html.oneOf
[ Markdown.Html.tag "legend"
(\title _ ->
viewLegend title
)
|> Markdown.Html.withAttribute "title"
, Markdown.Html.tag "sized-image"
(\title widthInPx heightInPx src alt _ ->
viewSizedImage title widthInPx heightInPx src alt
)
|> Markdown.Html.withOptionalAttribute "title"
|> Markdown.Html.withOptionalAttribute "width"
|> Markdown.Html.withOptionalAttribute "height"
|> Markdown.Html.withAttribute "src"
|> Markdown.Html.withAttribute "alt"
, Markdown.Html.tag "column"
(\portion renderedChildren ->
viewColumn portion renderedChildren
)
|> Markdown.Html.withAttribute "portion"
, Markdown.Html.tag "text-column"
(\renderedChildren ->
viewTextColumn renderedChildren
)
, Markdown.Html.tag "align-left"
(\renderedChildren ->
viewAlignLeft renderedChildren
)
, Markdown.Html.tag "align-right"
(\renderedChildren ->
viewAlignRight renderedChildren
)
, Markdown.Html.tag "row"
(\renderedChildren ->
viewRow renderedChildren
)
]
}
type alias Metadata =
{ title : String
, description : String
, lastReviewed : Date
, image : Pages.Url.Url
}
frontmatterDecoder : OptimizedDecoder.Decoder Metadata
frontmatterDecoder =
OptimizedDecoder.map4 Metadata
(OptimizedDecoder.field "title" OptimizedDecoder.string)
(OptimizedDecoder.field "description" OptimizedDecoder.string)
(OptimizedDecoder.field "lastReviewed"
(OptimizedDecoder.string
|> OptimizedDecoder.andThen
(\isoString ->
Date.fromIsoString isoString
|> OptimizedDecoder.fromResult
)
)
)
(OptimizedDecoder.field "image" imageDecoder)
imageDecoder : OptimizedDecoder.Decoder Pages.Url.Url
imageDecoder =
OptimizedDecoder.string
|> OptimizedDecoder.map (\asset -> Cloudinary.url asset Nothing 400 300)
| true | module Page.Glossary exposing (Data, Model, Msg, page)
import Cloudinary
import DataSource exposing (DataSource)
import Date exposing (Date)
import Element exposing (Element, alignLeft, alignRight, alignTop, centerX, column, fill, fillPortion, height, maximum, minimum, paddingXY, px, row, spacing, textColumn, width, wrappedRow)
import Element.Font as Font
import Head
import Head.Seo as Seo
import Logo
import Markdown.Html
import MarkdownCodec
import MarkdownRenderer
import OptimizedDecoder
import Page exposing (Page, StaticPayload)
import Pages.PageUrl exposing (PageUrl)
import Pages.Url
import Path
import Shared
import Site
import StructuredData
import View exposing (View)
type alias Model =
()
type alias Msg =
Never
type alias RouteParams =
{}
page : Page RouteParams Data
page =
Page.single
{ head = head
, data = data
}
|> Page.buildNoState { view = view }
data : DataSource Data
data =
MarkdownCodec.withFrontmatter Data
frontmatterDecoder
blogRenderer
"content/glossary.md"
head :
StaticPayload Data RouteParams
-> List Head.Tag
head static =
let
metadata =
static.data.metadata
in
Head.structuredData
(StructuredData.aboutPage
{ title = metadata.title
, description = metadata.description
, author = StructuredData.person { name = "Red Door Collective" }
, publisher = StructuredData.person { name = "Red Door Collective" }
, url = Site.config.canonicalUrl ++ Path.toAbsolute static.path
, imageUrl = metadata.image
, lastReviewed = Date.toIsoString metadata.lastReviewed
, mainEntityOfPage =
StructuredData.softwareSourceCode
{ codeRepositoryUrl = "https://github.com/red-door-collective/eviction-tracker"
, description = "A free website that keeps the people informed about housing and evictions."
, author = "PI:NAME:<NAME>END_PI"
, programmingLanguage = StructuredData.elmLang
}
}
)
:: (Seo.summary
{ canonicalUrlOverride = Nothing
, siteName = "Red Door Collective"
, image = Logo.smallImage
, description = metadata.description
, locale = Just "en-us"
, title = metadata.title
}
|> Seo.website
)
type alias Data =
{ metadata : Metadata
, body : List (Element Msg)
}
view :
Maybe PageUrl
-> Shared.Model
-> StaticPayload Data RouteParams
-> View Msg
view maybeUrl sharedModel static =
{ title = static.data.metadata.title
, body =
[ column
[ width (fill |> minimum 300 |> maximum 750)
, centerX
, spacing 10
, paddingXY 0 10
]
[ row
[ width fill ]
[ textColumn [ width fill ] static.data.body
]
]
]
}
elmUiRenderer =
MarkdownRenderer.renderer
viewAlignLeft : List (Element msg) -> Element msg
viewAlignLeft renderedChildren =
row [ alignLeft, width (fill |> maximum 375), height fill ]
renderedChildren
viewAlignRight : List (Element msg) -> Element msg
viewAlignRight renderedChildren =
row [ alignRight, width (fill |> maximum 375), height fill ]
renderedChildren
viewTextColumn : List (Element msg) -> Element msg
viewTextColumn renderedChildren =
textColumn
[ width fill
, Element.spacingXY 10 10
]
renderedChildren
viewRow : List (Element msg) -> Element msg
viewRow renderedChildren =
wrappedRow
[ width fill
, Element.spacingXY 0 10
, alignTop
]
renderedChildren
viewColumn : String -> List (Element msg) -> Element msg
viewColumn portion renderedChildren =
let
fillAttr =
width <|
case String.toInt portion of
Nothing ->
fill
Just number ->
fillPortion number
in
column [ alignTop, fillAttr ] renderedChildren
viewSizedImage : Maybe String -> Maybe String -> Maybe String -> String -> String -> Element msg
viewSizedImage title widthInPx heightInPx src alt =
let
( w, h ) =
( Maybe.andThen String.toInt widthInPx
, Maybe.andThen String.toInt heightInPx
)
widthAttr =
case w of
Just number ->
width (px number)
Nothing ->
width fill
heightAttr =
case h of
Just number ->
height (px number)
Nothing ->
height fill
in
case title of
Just _ ->
Element.image [ centerX, widthAttr, heightAttr ] { src = src, description = alt }
Nothing ->
row [ width fill ] [ Element.image [ centerX, widthAttr, heightAttr ] { src = src, description = alt } ]
viewLegend : String -> Element msg
viewLegend title =
column
[ Element.padding 20
, Element.spacing 30
, Element.centerX
]
[ Element.row [ Element.spacing 20 ]
[ Element.el
[ Font.bold
, Font.size 30
]
(Element.text title)
]
]
blogRenderer =
{ elmUiRenderer
| html =
Markdown.Html.oneOf
[ Markdown.Html.tag "legend"
(\title _ ->
viewLegend title
)
|> Markdown.Html.withAttribute "title"
, Markdown.Html.tag "sized-image"
(\title widthInPx heightInPx src alt _ ->
viewSizedImage title widthInPx heightInPx src alt
)
|> Markdown.Html.withOptionalAttribute "title"
|> Markdown.Html.withOptionalAttribute "width"
|> Markdown.Html.withOptionalAttribute "height"
|> Markdown.Html.withAttribute "src"
|> Markdown.Html.withAttribute "alt"
, Markdown.Html.tag "column"
(\portion renderedChildren ->
viewColumn portion renderedChildren
)
|> Markdown.Html.withAttribute "portion"
, Markdown.Html.tag "text-column"
(\renderedChildren ->
viewTextColumn renderedChildren
)
, Markdown.Html.tag "align-left"
(\renderedChildren ->
viewAlignLeft renderedChildren
)
, Markdown.Html.tag "align-right"
(\renderedChildren ->
viewAlignRight renderedChildren
)
, Markdown.Html.tag "row"
(\renderedChildren ->
viewRow renderedChildren
)
]
}
type alias Metadata =
{ title : String
, description : String
, lastReviewed : Date
, image : Pages.Url.Url
}
frontmatterDecoder : OptimizedDecoder.Decoder Metadata
frontmatterDecoder =
OptimizedDecoder.map4 Metadata
(OptimizedDecoder.field "title" OptimizedDecoder.string)
(OptimizedDecoder.field "description" OptimizedDecoder.string)
(OptimizedDecoder.field "lastReviewed"
(OptimizedDecoder.string
|> OptimizedDecoder.andThen
(\isoString ->
Date.fromIsoString isoString
|> OptimizedDecoder.fromResult
)
)
)
(OptimizedDecoder.field "image" imageDecoder)
imageDecoder : OptimizedDecoder.Decoder Pages.Url.Url
imageDecoder =
OptimizedDecoder.string
|> OptimizedDecoder.map (\asset -> Cloudinary.url asset Nothing 400 300)
| elm |
[
{
"context": " name : String\n name =\n \"Hermann\"\n\n increment : Int -> Int\n incremen",
"end": 11126,
"score": 0.9947600365,
"start": 11119,
"tag": "NAME",
"value": "Hermann"
}
] | snippets/elm-examples/TypeExamples.elm | lyddonb/dont_worry_about_monads | 4 | -- The last type is the return type.
-- A single type means it takes 0 arguments
thing : Int
thing = 0
-- You can alias a type
type alias Model = Int
-- This allows us to make more readable types.
model : Model
model = 0
-- This is a sum type (specifially a boolean type).
-- You may also see: tagged or disjoint unions or variant types
type Msg = Increment | Decrement
-- Why boolean and sum?
-- Because there are 2 possible options
type Bool = False | True
-- Sum Types can have as many options as you'd like
type Cars = Mustang | Camero | Taurus | Fit | Focus
-- Why called Sum Type?
-- You can find the total number of possible values by adding them
-- Bool has 2 possible options (False + True)
-- Cars has 5 possible options (Mustang + Camero + Taurus + Fit + Focus)
-- Note this is not the "space". To get that you need to use the max possible space of Int
-- FUNCTION TYPES AND ARROWS
-- PARTIALLY APPLIED FUNCTIONS AND CURRYING
-- This takes 2 integers and returns and Int
add1 : Int -> Int -> Int
add1 x y = x + y
-- But it doesn't have to. You can pass only one argument
add1 1
-- What happens. Well you can read that like
add1 : Int -> (Int -> Int)
-- This means when you don't pass all of the arguments in you will get back a function.
-- So:
add1 1
-- Returns a function
-- Let's set that to a variable
let add2 = add1
-- Now add2 is a function that takes a single argument. It looks like
add2 : Int -> Int
add2 x = add1 1
-- or
add2 x = 1 + x
-- So if we call it
add2 2 -- 3
-- Btw this can be done in Javascript, Python, etc
-- Javascript:
> var myCurriableFunction = function (x, y, z){
return function (y, z){
x + y + z;
}
}
> myCurriableFunction(2);
function (y, z){
x + y + z;
}
-- Back to our code
-- This takes 2 arguments, a Msg and a Model and returns a Model
update : Msg -> Model -> Model
update msg model =
case msg of
Increment ->
model + 1
Decrement ->
model - 1
-- This takes a single type of Model and returns a single type of Html Msg
view : Model -> Html Msg
view model =
div []
[ button [ onClick Decrement ] [ text "-" ]
, div [] [ text (toString model) ]
, button [ onClick Increment ] [ text "+" ]
]
-- TYPE ALIASES
-- Html is another type alias for:
type alias Html msg = VirtualDom.Node msg
-- This type as a "generic" type.
-- The msg could be anything:
type alias Html a = VirtualDom.Node a
-- RECORDS
-- What if we want to store multiple values?
-- Record Types!
type alias Record =
{ value : Int
, count : Int
}
-- Records are often called Product Types or Tuples
-- Why?
-- It's a compound type that is formed by a sequence of types and is commonly denoted:
-- (T1, T2, ..., Tn) or T1 x T2 x ... x Tn
-- They correspond to cartesian products thus products types
-- By allowing you to be named they become records
-- Or potentially in other languages ... structs, classes, etc
-- So you find the total number of options by multiplying the maximum value of each option.
-- https://www.stephanboyer.com/post/18/algebraic-data-types
record : Record
record =
{ value = 0
, count = 0
}
-- Accessing properties
getValue : Record -> Int
getValue record = record.value
-- Updating Properties
updateValue : Int -> Record -> Record
updateValue newValue existingRecord = { existingRecord | value = newValue }
-- Shorthand accessors
getValueShort : Record -> Int
getValueShort record = .value record
-- We can do neat stuff
type alias Record2 =
{ value : Int
, count : Int
, foo : String
}
type alias Record3 =
{ value : Int
, count : Int
, bar : String
}
type alias Record4 =
{ value : Int
, foobar : String
}
type alias ValueRecord a =
{ a | value : Int }
getValue2 : ValueRecord a -> Int
getValue2 valRec = valRec.value
-- COMPILES: getValue2 record2
-- COMPILES: getValue2 record3
-- COMPILES: getValue2 record4
updateRecord : Int -> { a | value : Int, count : Int } -> { a | value : Int, count : Int }
updateRecord rec newValue =
{ rec
| value = newValue
, count = count + 1
}
-- COMPILES: updateRecord 1 record2
-- COMPILES: updateRecord 1 record3
-- DOESN'T COMPILE: updateRecord 1 record4
-- NULLS
-- DON'T EXIST!!!!
-- If we can't have a null we need something to help us
-- What does a NULL mean?
-- It meas we either have something or we don'to
-- So what about something called either
type Either a b
= Left a
| Right b
-- But really it's not THAT generic. How about?
type Result error value
= Ok value
| Err error
-- Well this makes a ton of since for an error but this isn't an error.
-- It's expected and it means Nothing, so what about maybe ...
type Maybe a
= Just a
| Nothing
-- Now we have something. Maybe we Just have a value (of type a) or we have Nothing
giveMeIfGreatherThan0 : Int -> Maybe Int
giveMeIfGreatherThan0 val =
if val > 0 then
Just val
else
Nothing
-- But now there is this structure that w ehave to deal with.
-- Thankfully there are many functions in the maybe package to help us out
withDefault : a -> Maybe a -> a
withDefault default maybe =
case maybe of
Just value -> value
Nothing -> default
withDefault 10 (Just 15) -- 15
withDefault "foo" (Just "bar") -- "bar"
withDefault 10 (Nothing) -- Nothing
withDefault "foo" (Nothing) -- Nothing
-- Map
map : (a -> b) -> Maybe a -> Maybe b
map f maybe =
case maybe of
Just value -> Just (f value)
Nothing -> Nothing
-- Hmm so we take in a function a maybe and return a slightly modified maybe
-- Let's create a function from (a -> b)
-- This could be:
add1 : Int -> Int
add1 val = val + 1
-- In this case we use the same type. It doesn't have to be 2 different types
-- but we can do that
positiveMessage : Int -> String
positiveMessage val =
if val > 0 then
"I'm a positive message!"
else
"I'm not so postivie :("
-- Now we can "map" that function over our Maybe ... wut!
map add1 (Just 10) -- Just 11
map add1 Nothing -- Nothing
map positiveMessage (Just 10) -- Just "I'm a positive message!"
map positiveMessage (Just -23) -- Just "I'm not so positive :("
map positiveMessage Nothing -- Nothing
-- LISTS
simpleList : List Int
simpleList = [1, 2, 3, 4]
insertIntoSimpleList : Int -> List Int
insertIntoSimpleList num = num :: [1, 2, 3, 4]
-- LOOPS?
-- Elm doesn't have them
-- We use functions. It is functional programming after all
-- FOLDS
-- We start with a fold
-- This is a fold left of Ints
-- The left means we reduce (or traverse) from the left
foldlInt : (Int -> Int -> Int) -> Int -> List Int -> Int
foldlInt func aggVal list =
case list of
[] ->
aggVal
x :: xs ->
foldl func (func x aggVal) xs
-- It takes a function of two values that returns one
-- It then takes some value to accumulate into. Something that can accumulate like a list, a string, a number
-- It also takes a starting value
-- So this takes a function of prepend (insert into the 0 index of a list)
-- as well as an empty list to accumlate into
-- and of course our starting list
-- Thus starting from the left we prepend 1 we have [1]
-- And the inserting 2 into the 0 index we have [2, 1]
-- And then we finish by prepending the 3: [3,2,1]
foldlInt (::) [] [1,2,3] == [3,2,1]
-- We also can fold from the right
foldrInt : (Int -> Int -> Int) -> Int -> List Int -> Int
-- Using the same arugments as before we end up with the same list we started
-- Since we started from the right we prepend 3 we have [3]
-- And the inserting 2 into the 0 index we have [2, 3]
-- And then we finish by prepending the 1: [1,2,3]
foldrInt (::) [] [1,2,3] == [1,2,3]
-- We of course can pass in different types of functions
-- What if we use the max function
foldlInt max 0 [1,2,3] == 3
-- We give it the builtin max function that is a more generic version of:
max : Int -> Int
max x y = if x > y then x else y
-- We can then make this a nice function.
maximumInt : List Int -> Int
maximumInt list =
case list of
x :: xs ->
Just (foldl max x xs)
[x] ->
x
[] ->
0
-- But this kinda sucks. If we give an empty list we get back a 0.
-- Which might be ok but isn't obvious.
maximumInt [1,2] == 2
maximumInt [1] == 1
maximumInt [] == 0 -- Blech!
-- How about using a Maybe?
-- Why Maybe?
maximumInt : List Int -> Maybe Int
maximumInt list =
case list of
x :: xs ->
Just (foldl max x xs)
_ ->
Nothing
maximumInt [1,2] == Just 2
maximumInt [1] == Just 1
maximumInt [] == Nothing
-- Now if we want to get a 0 value when the list is empty we just use what we already have:
withDefault 0 (maximumInt [1,2]) == 2
withDefault 0 (maximumInt [1]) == 1
withDefault 0 (maximumInt []) == 0
-- While we're here, we do this function chaining quite a bit.
-- And we're not a lisp so we'd like to avoid all of the parentheses.
-- Thankfully elm gives us some nice symbols to use
withDefault 0 <| maximumInt [1,2] == 2
withDefault 0 <| maximumInt [1] == 1
withDefault 0 <| maximumInt [] == 0
-- This allows us to do nice chaining when we have many functions
withDefault 0
<| maximumInt
<| range 0 10
== 10
-- or
[1,2,3]
|> maximumInt
|> withDefault 0
== 3
-- Also while we're here let's make the fold more generic:
foldl : (a -> b -> b) -> b -> List a -> b
foldl func acc list =
case list of
[] ->
acc
x :: xs ->
foldl func (func x acc) xs
-- Now our fold function can work on many different data types.
-- With generic fold functions what can we do?
-- It turns out, quite a lot.
map : (a -> b) -> List a -> List b
map f xs =
foldr (\x acc -> f x :: acc) [] xs
map (\x -> x + 1) [1,2,3] == [2,3,4]
add1 : Int -> Int
add1 x = x + 1
map add1 [1,2,3] == [2,3,4]
-- There are all kinds of functions for working with lists in the List.elm module in the standard library
-- Just like Python, Javascript and Ruby
-- isEmpty, length, reverse, member, head, tail, filter, take, drop, sum, all, etc
-- Elm has documentation to help you transition
-- http://elm-lang.org/docs/from-javascript
-- IMMUTABILITY
-- One last thing before we get to the real fun
-- Purescript is functional and immutable ... so can we store state?
-- Yes
-- It's no different than const in js but you must do it within a let expression.
forty : Int
forty =
let
twentyFour =
3 * 8
sixteen =
4 ^ 2
in
twentyFour + sixteen
-- You just can't reassign the same variable
bad : Int
bad =
let
twentyFour =
3 * 8
twentyFour =
20 + 4
in
twentyFour
-- This will not compile.
good : Int
good =
let
twentyFour =
3 * 8
newTwentyFour =
20 + 4
in
newTwentyFour
-- This will compile.
-- 2 other notes
-- You can assign functions
-- And you can provide type annotations
letFunctions : Int
letFunctions =
let
hypotenuse a b =
sqrt (a^2 + b^2)
name : String
name =
"Hermann"
increment : Int -> Int
increment n =
n + 1
in
increment 10
| 23183 | -- The last type is the return type.
-- A single type means it takes 0 arguments
thing : Int
thing = 0
-- You can alias a type
type alias Model = Int
-- This allows us to make more readable types.
model : Model
model = 0
-- This is a sum type (specifially a boolean type).
-- You may also see: tagged or disjoint unions or variant types
type Msg = Increment | Decrement
-- Why boolean and sum?
-- Because there are 2 possible options
type Bool = False | True
-- Sum Types can have as many options as you'd like
type Cars = Mustang | Camero | Taurus | Fit | Focus
-- Why called Sum Type?
-- You can find the total number of possible values by adding them
-- Bool has 2 possible options (False + True)
-- Cars has 5 possible options (Mustang + Camero + Taurus + Fit + Focus)
-- Note this is not the "space". To get that you need to use the max possible space of Int
-- FUNCTION TYPES AND ARROWS
-- PARTIALLY APPLIED FUNCTIONS AND CURRYING
-- This takes 2 integers and returns and Int
add1 : Int -> Int -> Int
add1 x y = x + y
-- But it doesn't have to. You can pass only one argument
add1 1
-- What happens. Well you can read that like
add1 : Int -> (Int -> Int)
-- This means when you don't pass all of the arguments in you will get back a function.
-- So:
add1 1
-- Returns a function
-- Let's set that to a variable
let add2 = add1
-- Now add2 is a function that takes a single argument. It looks like
add2 : Int -> Int
add2 x = add1 1
-- or
add2 x = 1 + x
-- So if we call it
add2 2 -- 3
-- Btw this can be done in Javascript, Python, etc
-- Javascript:
> var myCurriableFunction = function (x, y, z){
return function (y, z){
x + y + z;
}
}
> myCurriableFunction(2);
function (y, z){
x + y + z;
}
-- Back to our code
-- This takes 2 arguments, a Msg and a Model and returns a Model
update : Msg -> Model -> Model
update msg model =
case msg of
Increment ->
model + 1
Decrement ->
model - 1
-- This takes a single type of Model and returns a single type of Html Msg
view : Model -> Html Msg
view model =
div []
[ button [ onClick Decrement ] [ text "-" ]
, div [] [ text (toString model) ]
, button [ onClick Increment ] [ text "+" ]
]
-- TYPE ALIASES
-- Html is another type alias for:
type alias Html msg = VirtualDom.Node msg
-- This type as a "generic" type.
-- The msg could be anything:
type alias Html a = VirtualDom.Node a
-- RECORDS
-- What if we want to store multiple values?
-- Record Types!
type alias Record =
{ value : Int
, count : Int
}
-- Records are often called Product Types or Tuples
-- Why?
-- It's a compound type that is formed by a sequence of types and is commonly denoted:
-- (T1, T2, ..., Tn) or T1 x T2 x ... x Tn
-- They correspond to cartesian products thus products types
-- By allowing you to be named they become records
-- Or potentially in other languages ... structs, classes, etc
-- So you find the total number of options by multiplying the maximum value of each option.
-- https://www.stephanboyer.com/post/18/algebraic-data-types
record : Record
record =
{ value = 0
, count = 0
}
-- Accessing properties
getValue : Record -> Int
getValue record = record.value
-- Updating Properties
updateValue : Int -> Record -> Record
updateValue newValue existingRecord = { existingRecord | value = newValue }
-- Shorthand accessors
getValueShort : Record -> Int
getValueShort record = .value record
-- We can do neat stuff
type alias Record2 =
{ value : Int
, count : Int
, foo : String
}
type alias Record3 =
{ value : Int
, count : Int
, bar : String
}
type alias Record4 =
{ value : Int
, foobar : String
}
type alias ValueRecord a =
{ a | value : Int }
getValue2 : ValueRecord a -> Int
getValue2 valRec = valRec.value
-- COMPILES: getValue2 record2
-- COMPILES: getValue2 record3
-- COMPILES: getValue2 record4
updateRecord : Int -> { a | value : Int, count : Int } -> { a | value : Int, count : Int }
updateRecord rec newValue =
{ rec
| value = newValue
, count = count + 1
}
-- COMPILES: updateRecord 1 record2
-- COMPILES: updateRecord 1 record3
-- DOESN'T COMPILE: updateRecord 1 record4
-- NULLS
-- DON'T EXIST!!!!
-- If we can't have a null we need something to help us
-- What does a NULL mean?
-- It meas we either have something or we don'to
-- So what about something called either
type Either a b
= Left a
| Right b
-- But really it's not THAT generic. How about?
type Result error value
= Ok value
| Err error
-- Well this makes a ton of since for an error but this isn't an error.
-- It's expected and it means Nothing, so what about maybe ...
type Maybe a
= Just a
| Nothing
-- Now we have something. Maybe we Just have a value (of type a) or we have Nothing
giveMeIfGreatherThan0 : Int -> Maybe Int
giveMeIfGreatherThan0 val =
if val > 0 then
Just val
else
Nothing
-- But now there is this structure that w ehave to deal with.
-- Thankfully there are many functions in the maybe package to help us out
withDefault : a -> Maybe a -> a
withDefault default maybe =
case maybe of
Just value -> value
Nothing -> default
withDefault 10 (Just 15) -- 15
withDefault "foo" (Just "bar") -- "bar"
withDefault 10 (Nothing) -- Nothing
withDefault "foo" (Nothing) -- Nothing
-- Map
map : (a -> b) -> Maybe a -> Maybe b
map f maybe =
case maybe of
Just value -> Just (f value)
Nothing -> Nothing
-- Hmm so we take in a function a maybe and return a slightly modified maybe
-- Let's create a function from (a -> b)
-- This could be:
add1 : Int -> Int
add1 val = val + 1
-- In this case we use the same type. It doesn't have to be 2 different types
-- but we can do that
positiveMessage : Int -> String
positiveMessage val =
if val > 0 then
"I'm a positive message!"
else
"I'm not so postivie :("
-- Now we can "map" that function over our Maybe ... wut!
map add1 (Just 10) -- Just 11
map add1 Nothing -- Nothing
map positiveMessage (Just 10) -- Just "I'm a positive message!"
map positiveMessage (Just -23) -- Just "I'm not so positive :("
map positiveMessage Nothing -- Nothing
-- LISTS
simpleList : List Int
simpleList = [1, 2, 3, 4]
insertIntoSimpleList : Int -> List Int
insertIntoSimpleList num = num :: [1, 2, 3, 4]
-- LOOPS?
-- Elm doesn't have them
-- We use functions. It is functional programming after all
-- FOLDS
-- We start with a fold
-- This is a fold left of Ints
-- The left means we reduce (or traverse) from the left
foldlInt : (Int -> Int -> Int) -> Int -> List Int -> Int
foldlInt func aggVal list =
case list of
[] ->
aggVal
x :: xs ->
foldl func (func x aggVal) xs
-- It takes a function of two values that returns one
-- It then takes some value to accumulate into. Something that can accumulate like a list, a string, a number
-- It also takes a starting value
-- So this takes a function of prepend (insert into the 0 index of a list)
-- as well as an empty list to accumlate into
-- and of course our starting list
-- Thus starting from the left we prepend 1 we have [1]
-- And the inserting 2 into the 0 index we have [2, 1]
-- And then we finish by prepending the 3: [3,2,1]
foldlInt (::) [] [1,2,3] == [3,2,1]
-- We also can fold from the right
foldrInt : (Int -> Int -> Int) -> Int -> List Int -> Int
-- Using the same arugments as before we end up with the same list we started
-- Since we started from the right we prepend 3 we have [3]
-- And the inserting 2 into the 0 index we have [2, 3]
-- And then we finish by prepending the 1: [1,2,3]
foldrInt (::) [] [1,2,3] == [1,2,3]
-- We of course can pass in different types of functions
-- What if we use the max function
foldlInt max 0 [1,2,3] == 3
-- We give it the builtin max function that is a more generic version of:
max : Int -> Int
max x y = if x > y then x else y
-- We can then make this a nice function.
maximumInt : List Int -> Int
maximumInt list =
case list of
x :: xs ->
Just (foldl max x xs)
[x] ->
x
[] ->
0
-- But this kinda sucks. If we give an empty list we get back a 0.
-- Which might be ok but isn't obvious.
maximumInt [1,2] == 2
maximumInt [1] == 1
maximumInt [] == 0 -- Blech!
-- How about using a Maybe?
-- Why Maybe?
maximumInt : List Int -> Maybe Int
maximumInt list =
case list of
x :: xs ->
Just (foldl max x xs)
_ ->
Nothing
maximumInt [1,2] == Just 2
maximumInt [1] == Just 1
maximumInt [] == Nothing
-- Now if we want to get a 0 value when the list is empty we just use what we already have:
withDefault 0 (maximumInt [1,2]) == 2
withDefault 0 (maximumInt [1]) == 1
withDefault 0 (maximumInt []) == 0
-- While we're here, we do this function chaining quite a bit.
-- And we're not a lisp so we'd like to avoid all of the parentheses.
-- Thankfully elm gives us some nice symbols to use
withDefault 0 <| maximumInt [1,2] == 2
withDefault 0 <| maximumInt [1] == 1
withDefault 0 <| maximumInt [] == 0
-- This allows us to do nice chaining when we have many functions
withDefault 0
<| maximumInt
<| range 0 10
== 10
-- or
[1,2,3]
|> maximumInt
|> withDefault 0
== 3
-- Also while we're here let's make the fold more generic:
foldl : (a -> b -> b) -> b -> List a -> b
foldl func acc list =
case list of
[] ->
acc
x :: xs ->
foldl func (func x acc) xs
-- Now our fold function can work on many different data types.
-- With generic fold functions what can we do?
-- It turns out, quite a lot.
map : (a -> b) -> List a -> List b
map f xs =
foldr (\x acc -> f x :: acc) [] xs
map (\x -> x + 1) [1,2,3] == [2,3,4]
add1 : Int -> Int
add1 x = x + 1
map add1 [1,2,3] == [2,3,4]
-- There are all kinds of functions for working with lists in the List.elm module in the standard library
-- Just like Python, Javascript and Ruby
-- isEmpty, length, reverse, member, head, tail, filter, take, drop, sum, all, etc
-- Elm has documentation to help you transition
-- http://elm-lang.org/docs/from-javascript
-- IMMUTABILITY
-- One last thing before we get to the real fun
-- Purescript is functional and immutable ... so can we store state?
-- Yes
-- It's no different than const in js but you must do it within a let expression.
forty : Int
forty =
let
twentyFour =
3 * 8
sixteen =
4 ^ 2
in
twentyFour + sixteen
-- You just can't reassign the same variable
bad : Int
bad =
let
twentyFour =
3 * 8
twentyFour =
20 + 4
in
twentyFour
-- This will not compile.
good : Int
good =
let
twentyFour =
3 * 8
newTwentyFour =
20 + 4
in
newTwentyFour
-- This will compile.
-- 2 other notes
-- You can assign functions
-- And you can provide type annotations
letFunctions : Int
letFunctions =
let
hypotenuse a b =
sqrt (a^2 + b^2)
name : String
name =
"<NAME>"
increment : Int -> Int
increment n =
n + 1
in
increment 10
| true | -- The last type is the return type.
-- A single type means it takes 0 arguments
thing : Int
thing = 0
-- You can alias a type
type alias Model = Int
-- This allows us to make more readable types.
model : Model
model = 0
-- This is a sum type (specifially a boolean type).
-- You may also see: tagged or disjoint unions or variant types
type Msg = Increment | Decrement
-- Why boolean and sum?
-- Because there are 2 possible options
type Bool = False | True
-- Sum Types can have as many options as you'd like
type Cars = Mustang | Camero | Taurus | Fit | Focus
-- Why called Sum Type?
-- You can find the total number of possible values by adding them
-- Bool has 2 possible options (False + True)
-- Cars has 5 possible options (Mustang + Camero + Taurus + Fit + Focus)
-- Note this is not the "space". To get that you need to use the max possible space of Int
-- FUNCTION TYPES AND ARROWS
-- PARTIALLY APPLIED FUNCTIONS AND CURRYING
-- This takes 2 integers and returns and Int
add1 : Int -> Int -> Int
add1 x y = x + y
-- But it doesn't have to. You can pass only one argument
add1 1
-- What happens. Well you can read that like
add1 : Int -> (Int -> Int)
-- This means when you don't pass all of the arguments in you will get back a function.
-- So:
add1 1
-- Returns a function
-- Let's set that to a variable
let add2 = add1
-- Now add2 is a function that takes a single argument. It looks like
add2 : Int -> Int
add2 x = add1 1
-- or
add2 x = 1 + x
-- So if we call it
add2 2 -- 3
-- Btw this can be done in Javascript, Python, etc
-- Javascript:
> var myCurriableFunction = function (x, y, z){
return function (y, z){
x + y + z;
}
}
> myCurriableFunction(2);
function (y, z){
x + y + z;
}
-- Back to our code
-- This takes 2 arguments, a Msg and a Model and returns a Model
update : Msg -> Model -> Model
update msg model =
case msg of
Increment ->
model + 1
Decrement ->
model - 1
-- This takes a single type of Model and returns a single type of Html Msg
view : Model -> Html Msg
view model =
div []
[ button [ onClick Decrement ] [ text "-" ]
, div [] [ text (toString model) ]
, button [ onClick Increment ] [ text "+" ]
]
-- TYPE ALIASES
-- Html is another type alias for:
type alias Html msg = VirtualDom.Node msg
-- This type as a "generic" type.
-- The msg could be anything:
type alias Html a = VirtualDom.Node a
-- RECORDS
-- What if we want to store multiple values?
-- Record Types!
type alias Record =
{ value : Int
, count : Int
}
-- Records are often called Product Types or Tuples
-- Why?
-- It's a compound type that is formed by a sequence of types and is commonly denoted:
-- (T1, T2, ..., Tn) or T1 x T2 x ... x Tn
-- They correspond to cartesian products thus products types
-- By allowing you to be named they become records
-- Or potentially in other languages ... structs, classes, etc
-- So you find the total number of options by multiplying the maximum value of each option.
-- https://www.stephanboyer.com/post/18/algebraic-data-types
record : Record
record =
{ value = 0
, count = 0
}
-- Accessing properties
getValue : Record -> Int
getValue record = record.value
-- Updating Properties
updateValue : Int -> Record -> Record
updateValue newValue existingRecord = { existingRecord | value = newValue }
-- Shorthand accessors
getValueShort : Record -> Int
getValueShort record = .value record
-- We can do neat stuff
type alias Record2 =
{ value : Int
, count : Int
, foo : String
}
type alias Record3 =
{ value : Int
, count : Int
, bar : String
}
type alias Record4 =
{ value : Int
, foobar : String
}
type alias ValueRecord a =
{ a | value : Int }
getValue2 : ValueRecord a -> Int
getValue2 valRec = valRec.value
-- COMPILES: getValue2 record2
-- COMPILES: getValue2 record3
-- COMPILES: getValue2 record4
updateRecord : Int -> { a | value : Int, count : Int } -> { a | value : Int, count : Int }
updateRecord rec newValue =
{ rec
| value = newValue
, count = count + 1
}
-- COMPILES: updateRecord 1 record2
-- COMPILES: updateRecord 1 record3
-- DOESN'T COMPILE: updateRecord 1 record4
-- NULLS
-- DON'T EXIST!!!!
-- If we can't have a null we need something to help us
-- What does a NULL mean?
-- It meas we either have something or we don'to
-- So what about something called either
type Either a b
= Left a
| Right b
-- But really it's not THAT generic. How about?
type Result error value
= Ok value
| Err error
-- Well this makes a ton of since for an error but this isn't an error.
-- It's expected and it means Nothing, so what about maybe ...
type Maybe a
= Just a
| Nothing
-- Now we have something. Maybe we Just have a value (of type a) or we have Nothing
giveMeIfGreatherThan0 : Int -> Maybe Int
giveMeIfGreatherThan0 val =
if val > 0 then
Just val
else
Nothing
-- But now there is this structure that w ehave to deal with.
-- Thankfully there are many functions in the maybe package to help us out
withDefault : a -> Maybe a -> a
withDefault default maybe =
case maybe of
Just value -> value
Nothing -> default
withDefault 10 (Just 15) -- 15
withDefault "foo" (Just "bar") -- "bar"
withDefault 10 (Nothing) -- Nothing
withDefault "foo" (Nothing) -- Nothing
-- Map
map : (a -> b) -> Maybe a -> Maybe b
map f maybe =
case maybe of
Just value -> Just (f value)
Nothing -> Nothing
-- Hmm so we take in a function a maybe and return a slightly modified maybe
-- Let's create a function from (a -> b)
-- This could be:
add1 : Int -> Int
add1 val = val + 1
-- In this case we use the same type. It doesn't have to be 2 different types
-- but we can do that
positiveMessage : Int -> String
positiveMessage val =
if val > 0 then
"I'm a positive message!"
else
"I'm not so postivie :("
-- Now we can "map" that function over our Maybe ... wut!
map add1 (Just 10) -- Just 11
map add1 Nothing -- Nothing
map positiveMessage (Just 10) -- Just "I'm a positive message!"
map positiveMessage (Just -23) -- Just "I'm not so positive :("
map positiveMessage Nothing -- Nothing
-- LISTS
simpleList : List Int
simpleList = [1, 2, 3, 4]
insertIntoSimpleList : Int -> List Int
insertIntoSimpleList num = num :: [1, 2, 3, 4]
-- LOOPS?
-- Elm doesn't have them
-- We use functions. It is functional programming after all
-- FOLDS
-- We start with a fold
-- This is a fold left of Ints
-- The left means we reduce (or traverse) from the left
foldlInt : (Int -> Int -> Int) -> Int -> List Int -> Int
foldlInt func aggVal list =
case list of
[] ->
aggVal
x :: xs ->
foldl func (func x aggVal) xs
-- It takes a function of two values that returns one
-- It then takes some value to accumulate into. Something that can accumulate like a list, a string, a number
-- It also takes a starting value
-- So this takes a function of prepend (insert into the 0 index of a list)
-- as well as an empty list to accumlate into
-- and of course our starting list
-- Thus starting from the left we prepend 1 we have [1]
-- And the inserting 2 into the 0 index we have [2, 1]
-- And then we finish by prepending the 3: [3,2,1]
foldlInt (::) [] [1,2,3] == [3,2,1]
-- We also can fold from the right
foldrInt : (Int -> Int -> Int) -> Int -> List Int -> Int
-- Using the same arugments as before we end up with the same list we started
-- Since we started from the right we prepend 3 we have [3]
-- And the inserting 2 into the 0 index we have [2, 3]
-- And then we finish by prepending the 1: [1,2,3]
foldrInt (::) [] [1,2,3] == [1,2,3]
-- We of course can pass in different types of functions
-- What if we use the max function
foldlInt max 0 [1,2,3] == 3
-- We give it the builtin max function that is a more generic version of:
max : Int -> Int
max x y = if x > y then x else y
-- We can then make this a nice function.
maximumInt : List Int -> Int
maximumInt list =
case list of
x :: xs ->
Just (foldl max x xs)
[x] ->
x
[] ->
0
-- But this kinda sucks. If we give an empty list we get back a 0.
-- Which might be ok but isn't obvious.
maximumInt [1,2] == 2
maximumInt [1] == 1
maximumInt [] == 0 -- Blech!
-- How about using a Maybe?
-- Why Maybe?
maximumInt : List Int -> Maybe Int
maximumInt list =
case list of
x :: xs ->
Just (foldl max x xs)
_ ->
Nothing
maximumInt [1,2] == Just 2
maximumInt [1] == Just 1
maximumInt [] == Nothing
-- Now if we want to get a 0 value when the list is empty we just use what we already have:
withDefault 0 (maximumInt [1,2]) == 2
withDefault 0 (maximumInt [1]) == 1
withDefault 0 (maximumInt []) == 0
-- While we're here, we do this function chaining quite a bit.
-- And we're not a lisp so we'd like to avoid all of the parentheses.
-- Thankfully elm gives us some nice symbols to use
withDefault 0 <| maximumInt [1,2] == 2
withDefault 0 <| maximumInt [1] == 1
withDefault 0 <| maximumInt [] == 0
-- This allows us to do nice chaining when we have many functions
withDefault 0
<| maximumInt
<| range 0 10
== 10
-- or
[1,2,3]
|> maximumInt
|> withDefault 0
== 3
-- Also while we're here let's make the fold more generic:
foldl : (a -> b -> b) -> b -> List a -> b
foldl func acc list =
case list of
[] ->
acc
x :: xs ->
foldl func (func x acc) xs
-- Now our fold function can work on many different data types.
-- With generic fold functions what can we do?
-- It turns out, quite a lot.
map : (a -> b) -> List a -> List b
map f xs =
foldr (\x acc -> f x :: acc) [] xs
map (\x -> x + 1) [1,2,3] == [2,3,4]
add1 : Int -> Int
add1 x = x + 1
map add1 [1,2,3] == [2,3,4]
-- There are all kinds of functions for working with lists in the List.elm module in the standard library
-- Just like Python, Javascript and Ruby
-- isEmpty, length, reverse, member, head, tail, filter, take, drop, sum, all, etc
-- Elm has documentation to help you transition
-- http://elm-lang.org/docs/from-javascript
-- IMMUTABILITY
-- One last thing before we get to the real fun
-- Purescript is functional and immutable ... so can we store state?
-- Yes
-- It's no different than const in js but you must do it within a let expression.
forty : Int
forty =
let
twentyFour =
3 * 8
sixteen =
4 ^ 2
in
twentyFour + sixteen
-- You just can't reassign the same variable
bad : Int
bad =
let
twentyFour =
3 * 8
twentyFour =
20 + 4
in
twentyFour
-- This will not compile.
good : Int
good =
let
twentyFour =
3 * 8
newTwentyFour =
20 + 4
in
newTwentyFour
-- This will compile.
-- 2 other notes
-- You can assign functions
-- And you can provide type annotations
letFunctions : Int
letFunctions =
let
hypotenuse a b =
sqrt (a^2 + b^2)
name : String
name =
"PI:NAME:<NAME>END_PI"
increment : Int -> Int
increment n =
n + 1
in
increment 10
| elm |
[
{
"context": "ute properties\n-}\npropKey : String\npropKey =\n \"a2\"\n\n\n{-| Internal key for style\n-}\nstyleKey : Strin",
"end": 395,
"score": 0.9084502459,
"start": 393,
"tag": "KEY",
"value": "a2"
},
{
"context": "ey for style\n-}\nstyleKey : String\nstyleKey =\n \"a1\"\n\n\n{-| Internal key for style\n-}\neventKey : Strin",
"end": 465,
"score": 0.9388908744,
"start": 463,
"tag": "KEY",
"value": "a1"
},
{
"context": "ey for style\n-}\neventKey : String\neventKey =\n \"a0\"\n\n\n{-| Internal key for style\n-}\nattributeKey : S",
"end": 535,
"score": 0.9569196701,
"start": 533,
"tag": "KEY",
"value": "a0"
},
{
"context": "tyle\n-}\nattributeKey : String\nattributeKey =\n \"a3\"\n\n\n{-| Internal key for style\n-}\nattributeNamespa",
"end": 613,
"score": 0.9615436792,
"start": 611,
"tag": "KEY",
"value": "a3"
},
{
"context": "mespaceKey : String\nattributeNamespaceKey =\n \"a4\"\n\n\n{-| Keys that we are aware of and should pay a",
"end": 709,
"score": 0.9607892632,
"start": 708,
"tag": "KEY",
"value": "4"
}
] | src/Test/Html/Internal/ElmHtml/Constants.elm | marc136/test | 494 | module Test.Html.Internal.ElmHtml.Constants exposing
( propKey, styleKey, eventKey, attributeKey, attributeNamespaceKey
, knownKeys
)
{-| Constants for representing internal keys for Elm's vdom implementation
@docs propKey, styleKey, eventKey, attributeKey, attributeNamespaceKey
@docs knownKeys
-}
{-| Internal key for attribute properties
-}
propKey : String
propKey =
"a2"
{-| Internal key for style
-}
styleKey : String
styleKey =
"a1"
{-| Internal key for style
-}
eventKey : String
eventKey =
"a0"
{-| Internal key for style
-}
attributeKey : String
attributeKey =
"a3"
{-| Internal key for style
-}
attributeNamespaceKey : String
attributeNamespaceKey =
"a4"
{-| Keys that we are aware of and should pay attention to
-}
knownKeys : List String
knownKeys =
[ styleKey, eventKey, attributeKey, attributeNamespaceKey ]
| 627 | module Test.Html.Internal.ElmHtml.Constants exposing
( propKey, styleKey, eventKey, attributeKey, attributeNamespaceKey
, knownKeys
)
{-| Constants for representing internal keys for Elm's vdom implementation
@docs propKey, styleKey, eventKey, attributeKey, attributeNamespaceKey
@docs knownKeys
-}
{-| Internal key for attribute properties
-}
propKey : String
propKey =
"<KEY>"
{-| Internal key for style
-}
styleKey : String
styleKey =
"<KEY>"
{-| Internal key for style
-}
eventKey : String
eventKey =
"<KEY>"
{-| Internal key for style
-}
attributeKey : String
attributeKey =
"<KEY>"
{-| Internal key for style
-}
attributeNamespaceKey : String
attributeNamespaceKey =
"a<KEY>"
{-| Keys that we are aware of and should pay attention to
-}
knownKeys : List String
knownKeys =
[ styleKey, eventKey, attributeKey, attributeNamespaceKey ]
| true | module Test.Html.Internal.ElmHtml.Constants exposing
( propKey, styleKey, eventKey, attributeKey, attributeNamespaceKey
, knownKeys
)
{-| Constants for representing internal keys for Elm's vdom implementation
@docs propKey, styleKey, eventKey, attributeKey, attributeNamespaceKey
@docs knownKeys
-}
{-| Internal key for attribute properties
-}
propKey : String
propKey =
"PI:KEY:<KEY>END_PI"
{-| Internal key for style
-}
styleKey : String
styleKey =
"PI:KEY:<KEY>END_PI"
{-| Internal key for style
-}
eventKey : String
eventKey =
"PI:KEY:<KEY>END_PI"
{-| Internal key for style
-}
attributeKey : String
attributeKey =
"PI:KEY:<KEY>END_PI"
{-| Internal key for style
-}
attributeNamespaceKey : String
attributeNamespaceKey =
"aPI:KEY:<KEY>END_PI"
{-| Keys that we are aware of and should pay attention to
-}
knownKeys : List String
knownKeys =
[ styleKey, eventKey, attributeKey, attributeNamespaceKey ]
| elm |
[
{
"context": "{- Copyright (C) 2018 Mark D. Blackwell.\n All rights reserved.\n This program is distr",
"end": 39,
"score": 0.9998607635,
"start": 22,
"tag": "NAME",
"value": "Mark D. Blackwell"
},
{
"context": "sg2Cmd msg =\n --See:\n -- http://github.com/billstclair/elm-dynamodb/blob/7ac30d60b98fbe7ea253be13f5f9df4",
"end": 1453,
"score": 0.9994635582,
"start": 1442,
"tag": "USERNAME",
"value": "billstclair"
}
] | src/front-end/utility/Utilities.elm | MarkDBlackwell/qplaylist-remember | 0 | {- Copyright (C) 2018 Mark D. Blackwell.
All rights reserved.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
-}
module Utilities exposing
( attributeIdFromMaybe
, attributesEmpty
, cmdMsg2Cmd
, field2String
, goldenRatio
, htmlNodeNull
, idMorphString
, idRefreshString
, innerHtmlEmpty
, matchingIndexes
, pred
, succ
)
import ElmCycle
import Html
import Html.Attributes
import Json.Decode
import Task
import ViewType
exposing
( Id
, IdMaybe
)
field2String : String -> Json.Decode.Decoder String
field2String text =
Json.Decode.string
|> Json.Decode.field
text
matchingIndexes : List a -> a -> List Int
matchingIndexes listOfThings thing =
let
matchWithIndexMaybe : Int -> a -> Maybe Int
matchWithIndexMaybe index variable =
if thing /= variable then
Nothing
else
Just index
in
listOfThings
|> List.indexedMap matchWithIndexMaybe
|> List.filterMap identity
pred : Int -> Int
pred x =
--Predecessor
x - 1
succ : Int -> Int
succ x =
--Successor
x + 1
-- UPDATE
cmdMsg2Cmd : ElmCycle.Msg -> Cmd ElmCycle.Msg
cmdMsg2Cmd msg =
--See:
-- http://github.com/billstclair/elm-dynamodb/blob/7ac30d60b98fbe7ea253be13f5f9df4d9c661b92/src/DynamoBackend.elm
--For wrapping a message as a Cmd:
msg
|> Task.succeed
|> Task.perform
identity
-- VIEW
attributeIdFromMaybe : IdMaybe -> List (Html.Attribute ElmCycle.Msg)
attributeIdFromMaybe attributeIdMaybe =
case attributeIdMaybe of
Nothing ->
attributesEmpty
Just x ->
[ Html.Attributes.id x ]
attributesEmpty : List (Html.Attribute ElmCycle.Msg)
attributesEmpty =
[]
goldenRatio : Float
goldenRatio =
--See:
-- http://en.wikipedia.org/w/index.php?title=Golden_ratio&oldid=790709344
0.6180339887498949
htmlNodeNull : Html.Html ElmCycle.Msg
htmlNodeNull =
Html.text ""
idMorphString : Id
idMorphString =
"morph"
idRefreshString : Id
idRefreshString =
"refresh"
innerHtmlEmpty : List (Html.Html ElmCycle.Msg)
innerHtmlEmpty =
[]
| 28 | {- Copyright (C) 2018 <NAME>.
All rights reserved.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
-}
module Utilities exposing
( attributeIdFromMaybe
, attributesEmpty
, cmdMsg2Cmd
, field2String
, goldenRatio
, htmlNodeNull
, idMorphString
, idRefreshString
, innerHtmlEmpty
, matchingIndexes
, pred
, succ
)
import ElmCycle
import Html
import Html.Attributes
import Json.Decode
import Task
import ViewType
exposing
( Id
, IdMaybe
)
field2String : String -> Json.Decode.Decoder String
field2String text =
Json.Decode.string
|> Json.Decode.field
text
matchingIndexes : List a -> a -> List Int
matchingIndexes listOfThings thing =
let
matchWithIndexMaybe : Int -> a -> Maybe Int
matchWithIndexMaybe index variable =
if thing /= variable then
Nothing
else
Just index
in
listOfThings
|> List.indexedMap matchWithIndexMaybe
|> List.filterMap identity
pred : Int -> Int
pred x =
--Predecessor
x - 1
succ : Int -> Int
succ x =
--Successor
x + 1
-- UPDATE
cmdMsg2Cmd : ElmCycle.Msg -> Cmd ElmCycle.Msg
cmdMsg2Cmd msg =
--See:
-- http://github.com/billstclair/elm-dynamodb/blob/7ac30d60b98fbe7ea253be13f5f9df4d9c661b92/src/DynamoBackend.elm
--For wrapping a message as a Cmd:
msg
|> Task.succeed
|> Task.perform
identity
-- VIEW
attributeIdFromMaybe : IdMaybe -> List (Html.Attribute ElmCycle.Msg)
attributeIdFromMaybe attributeIdMaybe =
case attributeIdMaybe of
Nothing ->
attributesEmpty
Just x ->
[ Html.Attributes.id x ]
attributesEmpty : List (Html.Attribute ElmCycle.Msg)
attributesEmpty =
[]
goldenRatio : Float
goldenRatio =
--See:
-- http://en.wikipedia.org/w/index.php?title=Golden_ratio&oldid=790709344
0.6180339887498949
htmlNodeNull : Html.Html ElmCycle.Msg
htmlNodeNull =
Html.text ""
idMorphString : Id
idMorphString =
"morph"
idRefreshString : Id
idRefreshString =
"refresh"
innerHtmlEmpty : List (Html.Html ElmCycle.Msg)
innerHtmlEmpty =
[]
| true | {- Copyright (C) 2018 PI:NAME:<NAME>END_PI.
All rights reserved.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
-}
module Utilities exposing
( attributeIdFromMaybe
, attributesEmpty
, cmdMsg2Cmd
, field2String
, goldenRatio
, htmlNodeNull
, idMorphString
, idRefreshString
, innerHtmlEmpty
, matchingIndexes
, pred
, succ
)
import ElmCycle
import Html
import Html.Attributes
import Json.Decode
import Task
import ViewType
exposing
( Id
, IdMaybe
)
field2String : String -> Json.Decode.Decoder String
field2String text =
Json.Decode.string
|> Json.Decode.field
text
matchingIndexes : List a -> a -> List Int
matchingIndexes listOfThings thing =
let
matchWithIndexMaybe : Int -> a -> Maybe Int
matchWithIndexMaybe index variable =
if thing /= variable then
Nothing
else
Just index
in
listOfThings
|> List.indexedMap matchWithIndexMaybe
|> List.filterMap identity
pred : Int -> Int
pred x =
--Predecessor
x - 1
succ : Int -> Int
succ x =
--Successor
x + 1
-- UPDATE
cmdMsg2Cmd : ElmCycle.Msg -> Cmd ElmCycle.Msg
cmdMsg2Cmd msg =
--See:
-- http://github.com/billstclair/elm-dynamodb/blob/7ac30d60b98fbe7ea253be13f5f9df4d9c661b92/src/DynamoBackend.elm
--For wrapping a message as a Cmd:
msg
|> Task.succeed
|> Task.perform
identity
-- VIEW
attributeIdFromMaybe : IdMaybe -> List (Html.Attribute ElmCycle.Msg)
attributeIdFromMaybe attributeIdMaybe =
case attributeIdMaybe of
Nothing ->
attributesEmpty
Just x ->
[ Html.Attributes.id x ]
attributesEmpty : List (Html.Attribute ElmCycle.Msg)
attributesEmpty =
[]
goldenRatio : Float
goldenRatio =
--See:
-- http://en.wikipedia.org/w/index.php?title=Golden_ratio&oldid=790709344
0.6180339887498949
htmlNodeNull : Html.Html ElmCycle.Msg
htmlNodeNull =
Html.text ""
idMorphString : Id
idMorphString =
"morph"
idRefreshString : Id
idRefreshString =
"refresh"
innerHtmlEmpty : List (Html.Html ElmCycle.Msg)
innerHtmlEmpty =
[]
| elm |
[
{
"context": "{-\n Copyright 2020 Morgan Stanley\n\n Licensed under the Apache License, Version 2.",
"end": 35,
"score": 0.9998221993,
"start": 21,
"tag": "NAME",
"value": "Morgan Stanley"
}
] | src/Morphir/IR/SDK/LocalDate.elm | bekand/morphir-elm | 6 | {-
Copyright 2020 Morgan Stanley
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-}
module Morphir.IR.SDK.LocalDate exposing (..)
import Dict
import Morphir.IR.Documented exposing (Documented)
import Morphir.IR.Module as Module exposing (ModuleName)
import Morphir.IR.Name as Name
import Morphir.IR.Path as Path exposing (Path)
import Morphir.IR.SDK.Basics exposing (intType)
import Morphir.IR.SDK.Common exposing (toFQName, vSpec)
import Morphir.IR.SDK.Maybe exposing (maybeType)
import Morphir.IR.SDK.String exposing (stringType)
import Morphir.IR.Type as Type exposing (Specification(..), Type(..))
import Morphir.IR.Value as Value
moduleName : ModuleName
moduleName =
Path.fromString "LocalDate"
moduleSpec : Module.Specification ()
moduleSpec =
{ types =
Dict.fromList
[ ( Name.fromString "LocalDate", OpaqueTypeSpecification [] |> Documented "Type that represents a date concept." )
]
, values =
Dict.fromList
[ vSpec "fromISO" [ ( "iso", stringType () ) ] (maybeType () (localDateType ()))
, vSpec "fromParts" [ ( "year", intType () ), ( "month", intType () ), ( "day", intType () ) ] (maybeType () (localDateType ()))
, vSpec "diffInDays" [ ( "date1", localDateType () ), ( "date2", localDateType () ) ] (intType ())
, vSpec "diffInWeeks" [ ( "date1", localDateType () ), ( "date2", localDateType () ) ] (intType ())
, vSpec "diffInMonths" [ ( "date1", localDateType () ), ( "date2", localDateType () ) ] (intType ())
, vSpec "diffInYears" [ ( "date1", localDateType () ), ( "date2", localDateType () ) ] (intType ())
, vSpec "addDays" [ ( "offset", intType () ), ( "startDate", localDateType () ) ] (localDateType ())
, vSpec "addWeeks" [ ( "offset", intType () ), ( "startDate", localDateType () ) ] (localDateType ())
, vSpec "addMonths" [ ( "offset", intType () ), ( "startDate", localDateType () ) ] (localDateType ())
, vSpec "addYears" [ ( "offset", intType () ), ( "startDate", localDateType () ) ] (localDateType ())
]
}
localDateType : a -> Type a
localDateType attributes =
Reference attributes (toFQName moduleName "LocalDate") []
| 529 | {-
Copyright 2020 <NAME>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-}
module Morphir.IR.SDK.LocalDate exposing (..)
import Dict
import Morphir.IR.Documented exposing (Documented)
import Morphir.IR.Module as Module exposing (ModuleName)
import Morphir.IR.Name as Name
import Morphir.IR.Path as Path exposing (Path)
import Morphir.IR.SDK.Basics exposing (intType)
import Morphir.IR.SDK.Common exposing (toFQName, vSpec)
import Morphir.IR.SDK.Maybe exposing (maybeType)
import Morphir.IR.SDK.String exposing (stringType)
import Morphir.IR.Type as Type exposing (Specification(..), Type(..))
import Morphir.IR.Value as Value
moduleName : ModuleName
moduleName =
Path.fromString "LocalDate"
moduleSpec : Module.Specification ()
moduleSpec =
{ types =
Dict.fromList
[ ( Name.fromString "LocalDate", OpaqueTypeSpecification [] |> Documented "Type that represents a date concept." )
]
, values =
Dict.fromList
[ vSpec "fromISO" [ ( "iso", stringType () ) ] (maybeType () (localDateType ()))
, vSpec "fromParts" [ ( "year", intType () ), ( "month", intType () ), ( "day", intType () ) ] (maybeType () (localDateType ()))
, vSpec "diffInDays" [ ( "date1", localDateType () ), ( "date2", localDateType () ) ] (intType ())
, vSpec "diffInWeeks" [ ( "date1", localDateType () ), ( "date2", localDateType () ) ] (intType ())
, vSpec "diffInMonths" [ ( "date1", localDateType () ), ( "date2", localDateType () ) ] (intType ())
, vSpec "diffInYears" [ ( "date1", localDateType () ), ( "date2", localDateType () ) ] (intType ())
, vSpec "addDays" [ ( "offset", intType () ), ( "startDate", localDateType () ) ] (localDateType ())
, vSpec "addWeeks" [ ( "offset", intType () ), ( "startDate", localDateType () ) ] (localDateType ())
, vSpec "addMonths" [ ( "offset", intType () ), ( "startDate", localDateType () ) ] (localDateType ())
, vSpec "addYears" [ ( "offset", intType () ), ( "startDate", localDateType () ) ] (localDateType ())
]
}
localDateType : a -> Type a
localDateType attributes =
Reference attributes (toFQName moduleName "LocalDate") []
| true | {-
Copyright 2020 PI:NAME:<NAME>END_PI
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-}
module Morphir.IR.SDK.LocalDate exposing (..)
import Dict
import Morphir.IR.Documented exposing (Documented)
import Morphir.IR.Module as Module exposing (ModuleName)
import Morphir.IR.Name as Name
import Morphir.IR.Path as Path exposing (Path)
import Morphir.IR.SDK.Basics exposing (intType)
import Morphir.IR.SDK.Common exposing (toFQName, vSpec)
import Morphir.IR.SDK.Maybe exposing (maybeType)
import Morphir.IR.SDK.String exposing (stringType)
import Morphir.IR.Type as Type exposing (Specification(..), Type(..))
import Morphir.IR.Value as Value
moduleName : ModuleName
moduleName =
Path.fromString "LocalDate"
moduleSpec : Module.Specification ()
moduleSpec =
{ types =
Dict.fromList
[ ( Name.fromString "LocalDate", OpaqueTypeSpecification [] |> Documented "Type that represents a date concept." )
]
, values =
Dict.fromList
[ vSpec "fromISO" [ ( "iso", stringType () ) ] (maybeType () (localDateType ()))
, vSpec "fromParts" [ ( "year", intType () ), ( "month", intType () ), ( "day", intType () ) ] (maybeType () (localDateType ()))
, vSpec "diffInDays" [ ( "date1", localDateType () ), ( "date2", localDateType () ) ] (intType ())
, vSpec "diffInWeeks" [ ( "date1", localDateType () ), ( "date2", localDateType () ) ] (intType ())
, vSpec "diffInMonths" [ ( "date1", localDateType () ), ( "date2", localDateType () ) ] (intType ())
, vSpec "diffInYears" [ ( "date1", localDateType () ), ( "date2", localDateType () ) ] (intType ())
, vSpec "addDays" [ ( "offset", intType () ), ( "startDate", localDateType () ) ] (localDateType ())
, vSpec "addWeeks" [ ( "offset", intType () ), ( "startDate", localDateType () ) ] (localDateType ())
, vSpec "addMonths" [ ( "offset", intType () ), ( "startDate", localDateType () ) ] (localDateType ())
, vSpec "addYears" [ ( "offset", intType () ), ( "startDate", localDateType () ) ] (localDateType ())
]
}
localDateType : a -> Type a
localDateType attributes =
Reference attributes (toFQName moduleName "LocalDate") []
| elm |
[
{
"context": "ord password ->\n ( { model | password = password }, Cmd.none )\n\n InputMessage message ->\n ",
"end": 2198,
"score": 0.998927772,
"start": 2190,
"tag": "PASSWORD",
"value": "password"
}
] | examples/Example1.elm | gege251/elm-result-validators | 4 | module Example1 exposing (main)
import Browser
import Dict
import Html exposing (Html, button, div, form, input, label, text)
import Html.Attributes exposing (checked, style, type_, value)
import Html.Events exposing (onCheck, onInput, onSubmit)
import Validator.Bool
import Validator.Named exposing (Validated, checkOnly, noCheck, validate, validateMany)
import Validator.String
type Msg
= InputName String
| InputEmail String
| InputAge String
| InputPassword String
| InputMessage String
| ToggleApproved Bool
| Submitted
type alias Model =
{ name : String
, email : String
, age : String
, password : String
, message : String
, approved : Bool
, validated : Validated String ValidForm
}
init : ( Model, Cmd Msg )
init =
( { name = ""
, email = ""
, age = ""
, password = ""
, message = ""
, approved = False
, validated = Err Dict.empty
}
, Cmd.none
)
type alias ValidForm =
{ name : String
, email : String
, age : Int
, password : String
, message : String
}
validateForm : Model -> Validated String ValidForm
validateForm model =
Ok ValidForm
|> validate "name" (Validator.String.notEmpty "name is required") model.name
|> validate "email" (Validator.String.isEmail "email is invalid") model.email
|> validate "age" (Validator.String.isInt "age is not a number") model.age
|> validateMany "password"
[ Validator.String.hasLetter "password needs to contain letters"
, Validator.String.hasNumber "password needs to contain numbers"
]
model.password
|> noCheck model.message
|> checkOnly "approved" (Validator.Bool.isTrue "please approve") model.approved
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
InputName name ->
( { model | name = name }, Cmd.none )
InputEmail email ->
( { model | email = email }, Cmd.none )
InputAge age ->
( { model | age = age }, Cmd.none )
InputPassword password ->
( { model | password = password }, Cmd.none )
InputMessage message ->
( { model | message = message }, Cmd.none )
ToggleApproved approved ->
( { model | approved = approved }, Cmd.none )
Submitted ->
let
validated =
validateForm model
in
-- We save the validation result, so we can display errors in the view function
( { model | validated = validated }
, case validated of
Err _ ->
Cmd.none
Ok validForm ->
postForm validForm
)
postForm : ValidForm -> Cmd Msg
postForm validForm =
-- postForm only accepts validated forms
Debug.log "form submitted" validForm
|> (\_ -> Cmd.none)
view : Model -> Html Msg
view model =
form [ onSubmit Submitted ]
[ div []
[ label []
[ div [] [ text "Name" ]
, input [ onInput InputName, value model.name ] []
, viewErrors "name" model.validated
]
]
, div []
[ label [ style "width" "200px" ]
[ div [] [ text "Email" ]
, input [ onInput InputEmail, value model.email ] []
, viewErrors "email" model.validated
]
]
, div []
[ label [ style "width" "200px" ]
[ div [] [ text "Age" ]
, input [ onInput InputAge, value model.age ] []
, viewErrors "age" model.validated
]
]
, div []
[ label [ style "width" "200px" ]
[ div [] [ text "Password" ]
, input [ onInput InputPassword, value model.password ] []
, viewErrors "password" model.validated
]
]
, div []
[ label [ style "width" "200px" ]
[ div [] [ text "Message" ]
, input [ onInput InputMessage, value model.message ] []
, viewErrors "message" model.validated
]
]
, div []
[ label [ style "width" "200px" ]
[ div [] [ text "Approve stuff" ]
, input [ type_ "checkbox", onCheck ToggleApproved, checked model.approved ] []
, viewErrors "approved" model.validated
]
]
, button [] [ text "Submit" ]
]
viewErrors : String -> Validated String ValidForm -> Html Msg
viewErrors fieldName validated =
case Validator.Named.getErrors fieldName validated of
Nothing ->
text ""
Just errors ->
div []
(List.map
(\error ->
div [ style "color" "red" ] [ text error ]
)
errors
)
main : Program () Model Msg
main =
Browser.element
{ init = \_ -> init
, view = view
, update = update
, subscriptions = \_ -> Sub.none
}
| 15944 | module Example1 exposing (main)
import Browser
import Dict
import Html exposing (Html, button, div, form, input, label, text)
import Html.Attributes exposing (checked, style, type_, value)
import Html.Events exposing (onCheck, onInput, onSubmit)
import Validator.Bool
import Validator.Named exposing (Validated, checkOnly, noCheck, validate, validateMany)
import Validator.String
type Msg
= InputName String
| InputEmail String
| InputAge String
| InputPassword String
| InputMessage String
| ToggleApproved Bool
| Submitted
type alias Model =
{ name : String
, email : String
, age : String
, password : String
, message : String
, approved : Bool
, validated : Validated String ValidForm
}
init : ( Model, Cmd Msg )
init =
( { name = ""
, email = ""
, age = ""
, password = ""
, message = ""
, approved = False
, validated = Err Dict.empty
}
, Cmd.none
)
type alias ValidForm =
{ name : String
, email : String
, age : Int
, password : String
, message : String
}
validateForm : Model -> Validated String ValidForm
validateForm model =
Ok ValidForm
|> validate "name" (Validator.String.notEmpty "name is required") model.name
|> validate "email" (Validator.String.isEmail "email is invalid") model.email
|> validate "age" (Validator.String.isInt "age is not a number") model.age
|> validateMany "password"
[ Validator.String.hasLetter "password needs to contain letters"
, Validator.String.hasNumber "password needs to contain numbers"
]
model.password
|> noCheck model.message
|> checkOnly "approved" (Validator.Bool.isTrue "please approve") model.approved
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
InputName name ->
( { model | name = name }, Cmd.none )
InputEmail email ->
( { model | email = email }, Cmd.none )
InputAge age ->
( { model | age = age }, Cmd.none )
InputPassword password ->
( { model | password = <PASSWORD> }, Cmd.none )
InputMessage message ->
( { model | message = message }, Cmd.none )
ToggleApproved approved ->
( { model | approved = approved }, Cmd.none )
Submitted ->
let
validated =
validateForm model
in
-- We save the validation result, so we can display errors in the view function
( { model | validated = validated }
, case validated of
Err _ ->
Cmd.none
Ok validForm ->
postForm validForm
)
postForm : ValidForm -> Cmd Msg
postForm validForm =
-- postForm only accepts validated forms
Debug.log "form submitted" validForm
|> (\_ -> Cmd.none)
view : Model -> Html Msg
view model =
form [ onSubmit Submitted ]
[ div []
[ label []
[ div [] [ text "Name" ]
, input [ onInput InputName, value model.name ] []
, viewErrors "name" model.validated
]
]
, div []
[ label [ style "width" "200px" ]
[ div [] [ text "Email" ]
, input [ onInput InputEmail, value model.email ] []
, viewErrors "email" model.validated
]
]
, div []
[ label [ style "width" "200px" ]
[ div [] [ text "Age" ]
, input [ onInput InputAge, value model.age ] []
, viewErrors "age" model.validated
]
]
, div []
[ label [ style "width" "200px" ]
[ div [] [ text "Password" ]
, input [ onInput InputPassword, value model.password ] []
, viewErrors "password" model.validated
]
]
, div []
[ label [ style "width" "200px" ]
[ div [] [ text "Message" ]
, input [ onInput InputMessage, value model.message ] []
, viewErrors "message" model.validated
]
]
, div []
[ label [ style "width" "200px" ]
[ div [] [ text "Approve stuff" ]
, input [ type_ "checkbox", onCheck ToggleApproved, checked model.approved ] []
, viewErrors "approved" model.validated
]
]
, button [] [ text "Submit" ]
]
viewErrors : String -> Validated String ValidForm -> Html Msg
viewErrors fieldName validated =
case Validator.Named.getErrors fieldName validated of
Nothing ->
text ""
Just errors ->
div []
(List.map
(\error ->
div [ style "color" "red" ] [ text error ]
)
errors
)
main : Program () Model Msg
main =
Browser.element
{ init = \_ -> init
, view = view
, update = update
, subscriptions = \_ -> Sub.none
}
| true | module Example1 exposing (main)
import Browser
import Dict
import Html exposing (Html, button, div, form, input, label, text)
import Html.Attributes exposing (checked, style, type_, value)
import Html.Events exposing (onCheck, onInput, onSubmit)
import Validator.Bool
import Validator.Named exposing (Validated, checkOnly, noCheck, validate, validateMany)
import Validator.String
type Msg
= InputName String
| InputEmail String
| InputAge String
| InputPassword String
| InputMessage String
| ToggleApproved Bool
| Submitted
type alias Model =
{ name : String
, email : String
, age : String
, password : String
, message : String
, approved : Bool
, validated : Validated String ValidForm
}
init : ( Model, Cmd Msg )
init =
( { name = ""
, email = ""
, age = ""
, password = ""
, message = ""
, approved = False
, validated = Err Dict.empty
}
, Cmd.none
)
type alias ValidForm =
{ name : String
, email : String
, age : Int
, password : String
, message : String
}
validateForm : Model -> Validated String ValidForm
validateForm model =
Ok ValidForm
|> validate "name" (Validator.String.notEmpty "name is required") model.name
|> validate "email" (Validator.String.isEmail "email is invalid") model.email
|> validate "age" (Validator.String.isInt "age is not a number") model.age
|> validateMany "password"
[ Validator.String.hasLetter "password needs to contain letters"
, Validator.String.hasNumber "password needs to contain numbers"
]
model.password
|> noCheck model.message
|> checkOnly "approved" (Validator.Bool.isTrue "please approve") model.approved
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
InputName name ->
( { model | name = name }, Cmd.none )
InputEmail email ->
( { model | email = email }, Cmd.none )
InputAge age ->
( { model | age = age }, Cmd.none )
InputPassword password ->
( { model | password = PI:PASSWORD:<PASSWORD>END_PI }, Cmd.none )
InputMessage message ->
( { model | message = message }, Cmd.none )
ToggleApproved approved ->
( { model | approved = approved }, Cmd.none )
Submitted ->
let
validated =
validateForm model
in
-- We save the validation result, so we can display errors in the view function
( { model | validated = validated }
, case validated of
Err _ ->
Cmd.none
Ok validForm ->
postForm validForm
)
postForm : ValidForm -> Cmd Msg
postForm validForm =
-- postForm only accepts validated forms
Debug.log "form submitted" validForm
|> (\_ -> Cmd.none)
view : Model -> Html Msg
view model =
form [ onSubmit Submitted ]
[ div []
[ label []
[ div [] [ text "Name" ]
, input [ onInput InputName, value model.name ] []
, viewErrors "name" model.validated
]
]
, div []
[ label [ style "width" "200px" ]
[ div [] [ text "Email" ]
, input [ onInput InputEmail, value model.email ] []
, viewErrors "email" model.validated
]
]
, div []
[ label [ style "width" "200px" ]
[ div [] [ text "Age" ]
, input [ onInput InputAge, value model.age ] []
, viewErrors "age" model.validated
]
]
, div []
[ label [ style "width" "200px" ]
[ div [] [ text "Password" ]
, input [ onInput InputPassword, value model.password ] []
, viewErrors "password" model.validated
]
]
, div []
[ label [ style "width" "200px" ]
[ div [] [ text "Message" ]
, input [ onInput InputMessage, value model.message ] []
, viewErrors "message" model.validated
]
]
, div []
[ label [ style "width" "200px" ]
[ div [] [ text "Approve stuff" ]
, input [ type_ "checkbox", onCheck ToggleApproved, checked model.approved ] []
, viewErrors "approved" model.validated
]
]
, button [] [ text "Submit" ]
]
viewErrors : String -> Validated String ValidForm -> Html Msg
viewErrors fieldName validated =
case Validator.Named.getErrors fieldName validated of
Nothing ->
text ""
Just errors ->
div []
(List.map
(\error ->
div [ style "color" "red" ] [ text error ]
)
errors
)
main : Program () Model Msg
main =
Browser.element
{ init = \_ -> init
, view = view
, update = update
, subscriptions = \_ -> Sub.none
}
| elm |
[
{
"context": "\ntodos : List Todo\ntodos =\n [ Todo 0 \"Dinner at John's\" False\n , Todo 1 \"Mom's birthday soon\" False\n ",
"end": 816,
"score": 0.9542362094,
"start": 810,
"tag": "NAME",
"value": "John's"
},
{
"context": " [ Todo 0 \"Dinner at John's\" False\n , Todo 1 \"Mom's birthday soon\" False\n , Todo 2 \"Call Jerry a",
"end": 841,
"score": 0.6994559169,
"start": 838,
"tag": "NAME",
"value": "Mom"
},
{
"context": "o 1 \"Mom's birthday soon\" False\n , Todo 2 \"Call Jerry asap!\" False\n , Todo 3 \"Meeting at Dublin on S",
"end": 889,
"score": 0.9698264003,
"start": 884,
"tag": "NAME",
"value": "Jerry"
},
{
"context": " \"Call Jerry asap!\" False\n , Todo 3 \"Meeting at Dublin on Saturday\" True\n , Todo 4 \"Feed the dog\" Fal",
"end": 934,
"score": 0.8080727458,
"start": 928,
"tag": "NAME",
"value": "Dublin"
}
] | src/Main.elm | Saanglao/todosmile_elm | 0 | -- Tutorial todo for blog you can find at
module Main exposing (..)
import Browser exposing (sandbox)
import Html exposing (Html, Attribute, header, div, ul, li, h1, h3, text, input)
import Html.Attributes exposing (class, id, value, placeholder)
import Html.Events exposing (onInput, onClick, onDoubleClick, on, keyCode)
import Json.Decode as Json
main : Program () Model Msg
main =
Browser.sandbox
{init = init
, update = update
, view = view
}
-- MODEL
-- Consider the Model as all the states of our app, any update be made to it.
type alias Model =
{todos: List Todo
, field: String
}
type alias Todo =
{id : Int
, text : String
, isDone: Bool
}
-- Prepopulate list with initial values
todos : List Todo
todos =
[ Todo 0 "Dinner at John's" False
, Todo 1 "Mom's birthday soon" False
, Todo 2 "Call Jerry asap!" False
, Todo 3 "Meeting at Dublin on Saturday" True
, Todo 4 "Feed the dog" False
, Todo 5 "" False
, Todo 6 "www.littlescrawl.com" False
, Todo 7 "Thank you" False
, Todo 8 "" False
]
init : Model
init =
{todos = todos
, field = ""
}
-- END MODEL
-- UPDATE
type Msg =
AddTodo
| AddText String
| RemoveTodo Int
| KeyDown Int
| ToggleTodo Int
onKeyDown : (Int -> msg) -> Attribute msg
onKeyDown tagger =
on "keydown" (Json.map tagger keyCode)
update : Msg -> Model -> Model
update msg model =
case msg of
AddText text ->
{model | field = text}
AddTodo ->
{model | todos = model.todos ++ [Todo (List.length model.todos) model.field False], field = "" }
KeyDown key ->
if key == 13 then
{model | todos = model.todos ++ [Todo (List.length model.todos) model.field False], field = "" }
else
model
RemoveTodo id ->
{model | todos = List.filter (\x->x.id /= id ) model.todos}
ToggleTodo id ->
{model | todos = List.map (\x -> if (x.id == id) then {x | isDone = not (x.isDone) } else x ) model.todos}
-- END UPDATE
-- VIEWS
appHeader : Html Msg
appHeader =
header []
[h1 [] [ text "TO:DO" ]
, h3 [] [ text "Do it with Elm!" ]
]
appInput : Model -> Html Msg
appInput model =
input [ placeholder "Enter todo", value model.field, onInput AddText, onKeyDown KeyDown] []
todoList : Model -> Html Msg
todoList model =
ul [ class "todos-container" ]
(List.map (\l ->
li [ class (if l.isDone then "is-done" else ""), onClick (ToggleTodo l.id), onDoubleClick (RemoveTodo l.id) ]
[ text l.text ])
model.todos)
view : Model -> Html Msg
view model =
div [ id "app" ]
[appHeader
, appInput model
, todoList model
]
-- END VIEW | 50668 | -- Tutorial todo for blog you can find at
module Main exposing (..)
import Browser exposing (sandbox)
import Html exposing (Html, Attribute, header, div, ul, li, h1, h3, text, input)
import Html.Attributes exposing (class, id, value, placeholder)
import Html.Events exposing (onInput, onClick, onDoubleClick, on, keyCode)
import Json.Decode as Json
main : Program () Model Msg
main =
Browser.sandbox
{init = init
, update = update
, view = view
}
-- MODEL
-- Consider the Model as all the states of our app, any update be made to it.
type alias Model =
{todos: List Todo
, field: String
}
type alias Todo =
{id : Int
, text : String
, isDone: Bool
}
-- Prepopulate list with initial values
todos : List Todo
todos =
[ Todo 0 "Dinner at <NAME>" False
, Todo 1 "<NAME>'s birthday soon" False
, Todo 2 "Call <NAME> asap!" False
, Todo 3 "Meeting at <NAME> on Saturday" True
, Todo 4 "Feed the dog" False
, Todo 5 "" False
, Todo 6 "www.littlescrawl.com" False
, Todo 7 "Thank you" False
, Todo 8 "" False
]
init : Model
init =
{todos = todos
, field = ""
}
-- END MODEL
-- UPDATE
type Msg =
AddTodo
| AddText String
| RemoveTodo Int
| KeyDown Int
| ToggleTodo Int
onKeyDown : (Int -> msg) -> Attribute msg
onKeyDown tagger =
on "keydown" (Json.map tagger keyCode)
update : Msg -> Model -> Model
update msg model =
case msg of
AddText text ->
{model | field = text}
AddTodo ->
{model | todos = model.todos ++ [Todo (List.length model.todos) model.field False], field = "" }
KeyDown key ->
if key == 13 then
{model | todos = model.todos ++ [Todo (List.length model.todos) model.field False], field = "" }
else
model
RemoveTodo id ->
{model | todos = List.filter (\x->x.id /= id ) model.todos}
ToggleTodo id ->
{model | todos = List.map (\x -> if (x.id == id) then {x | isDone = not (x.isDone) } else x ) model.todos}
-- END UPDATE
-- VIEWS
appHeader : Html Msg
appHeader =
header []
[h1 [] [ text "TO:DO" ]
, h3 [] [ text "Do it with Elm!" ]
]
appInput : Model -> Html Msg
appInput model =
input [ placeholder "Enter todo", value model.field, onInput AddText, onKeyDown KeyDown] []
todoList : Model -> Html Msg
todoList model =
ul [ class "todos-container" ]
(List.map (\l ->
li [ class (if l.isDone then "is-done" else ""), onClick (ToggleTodo l.id), onDoubleClick (RemoveTodo l.id) ]
[ text l.text ])
model.todos)
view : Model -> Html Msg
view model =
div [ id "app" ]
[appHeader
, appInput model
, todoList model
]
-- END VIEW | true | -- Tutorial todo for blog you can find at
module Main exposing (..)
import Browser exposing (sandbox)
import Html exposing (Html, Attribute, header, div, ul, li, h1, h3, text, input)
import Html.Attributes exposing (class, id, value, placeholder)
import Html.Events exposing (onInput, onClick, onDoubleClick, on, keyCode)
import Json.Decode as Json
main : Program () Model Msg
main =
Browser.sandbox
{init = init
, update = update
, view = view
}
-- MODEL
-- Consider the Model as all the states of our app, any update be made to it.
type alias Model =
{todos: List Todo
, field: String
}
type alias Todo =
{id : Int
, text : String
, isDone: Bool
}
-- Prepopulate list with initial values
todos : List Todo
todos =
[ Todo 0 "Dinner at PI:NAME:<NAME>END_PI" False
, Todo 1 "PI:NAME:<NAME>END_PI's birthday soon" False
, Todo 2 "Call PI:NAME:<NAME>END_PI asap!" False
, Todo 3 "Meeting at PI:NAME:<NAME>END_PI on Saturday" True
, Todo 4 "Feed the dog" False
, Todo 5 "" False
, Todo 6 "www.littlescrawl.com" False
, Todo 7 "Thank you" False
, Todo 8 "" False
]
init : Model
init =
{todos = todos
, field = ""
}
-- END MODEL
-- UPDATE
type Msg =
AddTodo
| AddText String
| RemoveTodo Int
| KeyDown Int
| ToggleTodo Int
onKeyDown : (Int -> msg) -> Attribute msg
onKeyDown tagger =
on "keydown" (Json.map tagger keyCode)
update : Msg -> Model -> Model
update msg model =
case msg of
AddText text ->
{model | field = text}
AddTodo ->
{model | todos = model.todos ++ [Todo (List.length model.todos) model.field False], field = "" }
KeyDown key ->
if key == 13 then
{model | todos = model.todos ++ [Todo (List.length model.todos) model.field False], field = "" }
else
model
RemoveTodo id ->
{model | todos = List.filter (\x->x.id /= id ) model.todos}
ToggleTodo id ->
{model | todos = List.map (\x -> if (x.id == id) then {x | isDone = not (x.isDone) } else x ) model.todos}
-- END UPDATE
-- VIEWS
appHeader : Html Msg
appHeader =
header []
[h1 [] [ text "TO:DO" ]
, h3 [] [ text "Do it with Elm!" ]
]
appInput : Model -> Html Msg
appInput model =
input [ placeholder "Enter todo", value model.field, onInput AddText, onKeyDown KeyDown] []
todoList : Model -> Html Msg
todoList model =
ul [ class "todos-container" ]
(List.map (\l ->
li [ class (if l.isDone then "is-done" else ""), onClick (ToggleTodo l.id), onDoubleClick (RemoveTodo l.id) ]
[ text l.text ])
model.todos)
view : Model -> Html Msg
view model =
div [ id "app" ]
[appHeader
, appInput model
, todoList model
]
-- END VIEW | elm |
[
{
"context": "nputEmail\n , viewInput \"password\" \"Password\" model.form.password InputPassword\n ",
"end": 4701,
"score": 0.9941838384,
"start": 4693,
"tag": "PASSWORD",
"value": "Password"
}
] | web/src/Main.elm | zweimach/niur | 0 | module Main exposing (main)
import Browser exposing (Document)
import Bytes exposing (Bytes)
import File.Download as DL
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (..)
import Http
import Key exposing (Key)
import VitePluginHelper as V
main : Program Flags Model Msg
main =
Browser.document
{ init = init
, view = view
, update = update
, subscriptions = subscriptions
}
type alias Flags =
{ apiUrl : Maybe String
}
type alias Model =
{ form : Key
, status : Status
, apiUrl : String
}
type Status
= Ready
| Failure Error
| Loading
| Success
type Error
= ServerError String
| EnvError
type Msg
= InputCompanyName String
| InputTaxId String
| InputEmail String
| InputPassword String
| SubmitForm
| ClearForm
| GotBytes Bytes
| ChangeStatus Status
init : Flags -> ( Model, Cmd Msg )
init flags =
let
( apiUrl, status ) =
case flags.apiUrl of
Nothing ->
( "", Failure EnvError )
Just "" ->
( "", Failure EnvError )
Just s ->
( s, Ready )
in
( { form = Key.empty
, status = status
, apiUrl = apiUrl
}
, Cmd.none
)
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
InputCompanyName companyName ->
updateForm (\form -> { form | companyName = companyName }) model
InputTaxId taxId ->
updateForm (\form -> { form | taxId = taxId |> String.replace "." "" |> String.replace "-" "" }) model
InputEmail email ->
updateForm (\form -> { form | email = email }) model
InputPassword password ->
updateForm (\form -> { form | password = password }) model
SubmitForm ->
submitForm model
ClearForm ->
clearForm model
GotBytes bytes ->
downloadFile bytes model
ChangeStatus status ->
( changeStatus status model, Cmd.none )
changeStatus : Status -> Model -> Model
changeStatus status model =
{ model | status = status }
downloadFile : Bytes -> Model -> ( Model, Cmd msg )
downloadFile bytes model =
( changeStatus Success model, DL.bytes (model.form.taxId ++ ".p12") "application/x-pkcs12" bytes )
updateForm : (Key -> Key) -> Model -> ( Model, Cmd msg )
updateForm transform model =
( { model | form = transform model.form }, Cmd.none )
submitForm : Model -> ( Model, Cmd Msg )
submitForm model =
let
toMsg result =
case result of
Ok b ->
GotBytes b
Err e ->
ChangeStatus <| Failure e
toResult response =
case response of
Http.BadUrl_ s ->
Err (ServerError <| "You did not provide a valid URL: (" ++ s ++ ").")
Http.Timeout_ ->
Err (ServerError "It took too long to get a response.")
Http.NetworkError_ ->
Err (ServerError "Cannot reach the server.")
Http.BadStatus_ data _ ->
Err (ServerError <| "Error " ++ String.fromInt data.statusCode ++ ": " ++ data.statusText ++ ".")
Http.GoodStatus_ _ body ->
Ok body
in
( changeStatus Loading model
, Http.riskyRequest
{ method = "POST"
, headers = []
, url = model.apiUrl ++ "/pkcs12"
, body = Http.jsonBody <| Key.encode model.form
, expect = Http.expectBytesResponse toMsg toResult
, timeout = Just 30000
, tracker = Nothing
}
)
clearForm : Model -> ( Model, Cmd msg )
clearForm model =
( { model | form = Key.empty }, Cmd.none )
subscriptions : Model -> Sub Msg
subscriptions _ =
Sub.none
view : Model -> Document Msg
view model =
Document "Genkey Impact"
[ div [ class "h-full flex flex-col justify-start items-center gap-4 p-4 text-sm md:text-lg" ]
[ h1 [ class "my-4 font-serif text-3xl font-bold text-sky-700" ] [ text "Genkey Impact" ]
, Html.form [ novalidate True, onSubmit SubmitForm, class "w-full sm:max-w-screen-sm flex flex-col justify-start items-stretch gap-4 p-2 md:p-8" ]
[ viewInput "text" "Company Name" model.form.companyName InputCompanyName
, viewInput "text" "NPWP" model.form.taxId InputTaxId
, viewInput "email" "Email" model.form.email InputEmail
, viewInput "password" "Password" model.form.password InputPassword
, button
[ type_ "submit"
, class "px-4 py-2 rounded border-2 border-sky-400 bg-sky-300 focus:outline-none hover:bg-sky-500 active:border-indigo-600 focus:bg-sky-500 font-semibold text-blue-800 disabled:bg-gray-300 disabled:border-gray-300 disabled:text-gray-700"
, disabled (model.status == Loading || model.status == Failure EnvError)
]
[ text "Submit" ]
, button
[ type_ "button"
, onClick ClearForm
, class "px-4 py-2 rounded border-2 border-sky-400 bg-sky-100 focus:outline-none hover:bg-sky-200 active:border-indigo-600 focus:bg-sky-200 font-semibold text-blue-800"
, disabled (model.status == Loading)
]
[ text "Clear" ]
]
, viewMessage model.status
]
]
viewInput : String -> String -> String -> (String -> msg) -> Html msg
viewInput t p v toMsg =
input
[ class "px-4 py-2 rounded bg-white border-2 border-sky-400 hover:border-indigo-500 focus:outline-none focus:border-blue-500 placeholder:text-sky-300"
, type_ t
, placeholder p
, value v
, onInput toMsg
]
[]
viewMessage : Status -> Html msg
viewMessage status =
let
( icon, primary, secondary ) =
case status of
Failure _ ->
( V.asset "/assets/error.svg", "border-red-500 bg-rose-50", "text-red-800" )
Success ->
( V.asset "/assets/success.svg", "border-emerald-600 bg-emerald-50", "text-green-800" )
Ready ->
( V.asset "/assets/info.svg", "border-blue-400 bg-sky-50", "text-blue-800" )
Loading ->
( V.asset "/assets/loading.svg", "border-cyan-300 bg-cyan-50", "text-cyan-700" )
message =
case status of
Ready ->
"Please input your digital certificate information."
Loading ->
"Loading ..."
Failure e ->
case e of
EnvError ->
"The API URL is empty. You can't send information to the server."
ServerError s ->
s
Success ->
"Your digital certificate has been created. Please check your downloads."
in
div [ class primary, class "max-w-xl flex justify-center items-center gap-4 p-4 rounded-lg border-4" ]
[ img [ src icon, width 48, height 48 ] []
, p [ class secondary, class "text-ellipsis overflow-hidden" ] [ text message ]
]
| 39546 | module Main exposing (main)
import Browser exposing (Document)
import Bytes exposing (Bytes)
import File.Download as DL
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (..)
import Http
import Key exposing (Key)
import VitePluginHelper as V
main : Program Flags Model Msg
main =
Browser.document
{ init = init
, view = view
, update = update
, subscriptions = subscriptions
}
type alias Flags =
{ apiUrl : Maybe String
}
type alias Model =
{ form : Key
, status : Status
, apiUrl : String
}
type Status
= Ready
| Failure Error
| Loading
| Success
type Error
= ServerError String
| EnvError
type Msg
= InputCompanyName String
| InputTaxId String
| InputEmail String
| InputPassword String
| SubmitForm
| ClearForm
| GotBytes Bytes
| ChangeStatus Status
init : Flags -> ( Model, Cmd Msg )
init flags =
let
( apiUrl, status ) =
case flags.apiUrl of
Nothing ->
( "", Failure EnvError )
Just "" ->
( "", Failure EnvError )
Just s ->
( s, Ready )
in
( { form = Key.empty
, status = status
, apiUrl = apiUrl
}
, Cmd.none
)
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
InputCompanyName companyName ->
updateForm (\form -> { form | companyName = companyName }) model
InputTaxId taxId ->
updateForm (\form -> { form | taxId = taxId |> String.replace "." "" |> String.replace "-" "" }) model
InputEmail email ->
updateForm (\form -> { form | email = email }) model
InputPassword password ->
updateForm (\form -> { form | password = password }) model
SubmitForm ->
submitForm model
ClearForm ->
clearForm model
GotBytes bytes ->
downloadFile bytes model
ChangeStatus status ->
( changeStatus status model, Cmd.none )
changeStatus : Status -> Model -> Model
changeStatus status model =
{ model | status = status }
downloadFile : Bytes -> Model -> ( Model, Cmd msg )
downloadFile bytes model =
( changeStatus Success model, DL.bytes (model.form.taxId ++ ".p12") "application/x-pkcs12" bytes )
updateForm : (Key -> Key) -> Model -> ( Model, Cmd msg )
updateForm transform model =
( { model | form = transform model.form }, Cmd.none )
submitForm : Model -> ( Model, Cmd Msg )
submitForm model =
let
toMsg result =
case result of
Ok b ->
GotBytes b
Err e ->
ChangeStatus <| Failure e
toResult response =
case response of
Http.BadUrl_ s ->
Err (ServerError <| "You did not provide a valid URL: (" ++ s ++ ").")
Http.Timeout_ ->
Err (ServerError "It took too long to get a response.")
Http.NetworkError_ ->
Err (ServerError "Cannot reach the server.")
Http.BadStatus_ data _ ->
Err (ServerError <| "Error " ++ String.fromInt data.statusCode ++ ": " ++ data.statusText ++ ".")
Http.GoodStatus_ _ body ->
Ok body
in
( changeStatus Loading model
, Http.riskyRequest
{ method = "POST"
, headers = []
, url = model.apiUrl ++ "/pkcs12"
, body = Http.jsonBody <| Key.encode model.form
, expect = Http.expectBytesResponse toMsg toResult
, timeout = Just 30000
, tracker = Nothing
}
)
clearForm : Model -> ( Model, Cmd msg )
clearForm model =
( { model | form = Key.empty }, Cmd.none )
subscriptions : Model -> Sub Msg
subscriptions _ =
Sub.none
view : Model -> Document Msg
view model =
Document "Genkey Impact"
[ div [ class "h-full flex flex-col justify-start items-center gap-4 p-4 text-sm md:text-lg" ]
[ h1 [ class "my-4 font-serif text-3xl font-bold text-sky-700" ] [ text "Genkey Impact" ]
, Html.form [ novalidate True, onSubmit SubmitForm, class "w-full sm:max-w-screen-sm flex flex-col justify-start items-stretch gap-4 p-2 md:p-8" ]
[ viewInput "text" "Company Name" model.form.companyName InputCompanyName
, viewInput "text" "NPWP" model.form.taxId InputTaxId
, viewInput "email" "Email" model.form.email InputEmail
, viewInput "password" "<PASSWORD>" model.form.password InputPassword
, button
[ type_ "submit"
, class "px-4 py-2 rounded border-2 border-sky-400 bg-sky-300 focus:outline-none hover:bg-sky-500 active:border-indigo-600 focus:bg-sky-500 font-semibold text-blue-800 disabled:bg-gray-300 disabled:border-gray-300 disabled:text-gray-700"
, disabled (model.status == Loading || model.status == Failure EnvError)
]
[ text "Submit" ]
, button
[ type_ "button"
, onClick ClearForm
, class "px-4 py-2 rounded border-2 border-sky-400 bg-sky-100 focus:outline-none hover:bg-sky-200 active:border-indigo-600 focus:bg-sky-200 font-semibold text-blue-800"
, disabled (model.status == Loading)
]
[ text "Clear" ]
]
, viewMessage model.status
]
]
viewInput : String -> String -> String -> (String -> msg) -> Html msg
viewInput t p v toMsg =
input
[ class "px-4 py-2 rounded bg-white border-2 border-sky-400 hover:border-indigo-500 focus:outline-none focus:border-blue-500 placeholder:text-sky-300"
, type_ t
, placeholder p
, value v
, onInput toMsg
]
[]
viewMessage : Status -> Html msg
viewMessage status =
let
( icon, primary, secondary ) =
case status of
Failure _ ->
( V.asset "/assets/error.svg", "border-red-500 bg-rose-50", "text-red-800" )
Success ->
( V.asset "/assets/success.svg", "border-emerald-600 bg-emerald-50", "text-green-800" )
Ready ->
( V.asset "/assets/info.svg", "border-blue-400 bg-sky-50", "text-blue-800" )
Loading ->
( V.asset "/assets/loading.svg", "border-cyan-300 bg-cyan-50", "text-cyan-700" )
message =
case status of
Ready ->
"Please input your digital certificate information."
Loading ->
"Loading ..."
Failure e ->
case e of
EnvError ->
"The API URL is empty. You can't send information to the server."
ServerError s ->
s
Success ->
"Your digital certificate has been created. Please check your downloads."
in
div [ class primary, class "max-w-xl flex justify-center items-center gap-4 p-4 rounded-lg border-4" ]
[ img [ src icon, width 48, height 48 ] []
, p [ class secondary, class "text-ellipsis overflow-hidden" ] [ text message ]
]
| true | module Main exposing (main)
import Browser exposing (Document)
import Bytes exposing (Bytes)
import File.Download as DL
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (..)
import Http
import Key exposing (Key)
import VitePluginHelper as V
main : Program Flags Model Msg
main =
Browser.document
{ init = init
, view = view
, update = update
, subscriptions = subscriptions
}
type alias Flags =
{ apiUrl : Maybe String
}
type alias Model =
{ form : Key
, status : Status
, apiUrl : String
}
type Status
= Ready
| Failure Error
| Loading
| Success
type Error
= ServerError String
| EnvError
type Msg
= InputCompanyName String
| InputTaxId String
| InputEmail String
| InputPassword String
| SubmitForm
| ClearForm
| GotBytes Bytes
| ChangeStatus Status
init : Flags -> ( Model, Cmd Msg )
init flags =
let
( apiUrl, status ) =
case flags.apiUrl of
Nothing ->
( "", Failure EnvError )
Just "" ->
( "", Failure EnvError )
Just s ->
( s, Ready )
in
( { form = Key.empty
, status = status
, apiUrl = apiUrl
}
, Cmd.none
)
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
InputCompanyName companyName ->
updateForm (\form -> { form | companyName = companyName }) model
InputTaxId taxId ->
updateForm (\form -> { form | taxId = taxId |> String.replace "." "" |> String.replace "-" "" }) model
InputEmail email ->
updateForm (\form -> { form | email = email }) model
InputPassword password ->
updateForm (\form -> { form | password = password }) model
SubmitForm ->
submitForm model
ClearForm ->
clearForm model
GotBytes bytes ->
downloadFile bytes model
ChangeStatus status ->
( changeStatus status model, Cmd.none )
changeStatus : Status -> Model -> Model
changeStatus status model =
{ model | status = status }
downloadFile : Bytes -> Model -> ( Model, Cmd msg )
downloadFile bytes model =
( changeStatus Success model, DL.bytes (model.form.taxId ++ ".p12") "application/x-pkcs12" bytes )
updateForm : (Key -> Key) -> Model -> ( Model, Cmd msg )
updateForm transform model =
( { model | form = transform model.form }, Cmd.none )
submitForm : Model -> ( Model, Cmd Msg )
submitForm model =
let
toMsg result =
case result of
Ok b ->
GotBytes b
Err e ->
ChangeStatus <| Failure e
toResult response =
case response of
Http.BadUrl_ s ->
Err (ServerError <| "You did not provide a valid URL: (" ++ s ++ ").")
Http.Timeout_ ->
Err (ServerError "It took too long to get a response.")
Http.NetworkError_ ->
Err (ServerError "Cannot reach the server.")
Http.BadStatus_ data _ ->
Err (ServerError <| "Error " ++ String.fromInt data.statusCode ++ ": " ++ data.statusText ++ ".")
Http.GoodStatus_ _ body ->
Ok body
in
( changeStatus Loading model
, Http.riskyRequest
{ method = "POST"
, headers = []
, url = model.apiUrl ++ "/pkcs12"
, body = Http.jsonBody <| Key.encode model.form
, expect = Http.expectBytesResponse toMsg toResult
, timeout = Just 30000
, tracker = Nothing
}
)
clearForm : Model -> ( Model, Cmd msg )
clearForm model =
( { model | form = Key.empty }, Cmd.none )
subscriptions : Model -> Sub Msg
subscriptions _ =
Sub.none
view : Model -> Document Msg
view model =
Document "Genkey Impact"
[ div [ class "h-full flex flex-col justify-start items-center gap-4 p-4 text-sm md:text-lg" ]
[ h1 [ class "my-4 font-serif text-3xl font-bold text-sky-700" ] [ text "Genkey Impact" ]
, Html.form [ novalidate True, onSubmit SubmitForm, class "w-full sm:max-w-screen-sm flex flex-col justify-start items-stretch gap-4 p-2 md:p-8" ]
[ viewInput "text" "Company Name" model.form.companyName InputCompanyName
, viewInput "text" "NPWP" model.form.taxId InputTaxId
, viewInput "email" "Email" model.form.email InputEmail
, viewInput "password" "PI:PASSWORD:<PASSWORD>END_PI" model.form.password InputPassword
, button
[ type_ "submit"
, class "px-4 py-2 rounded border-2 border-sky-400 bg-sky-300 focus:outline-none hover:bg-sky-500 active:border-indigo-600 focus:bg-sky-500 font-semibold text-blue-800 disabled:bg-gray-300 disabled:border-gray-300 disabled:text-gray-700"
, disabled (model.status == Loading || model.status == Failure EnvError)
]
[ text "Submit" ]
, button
[ type_ "button"
, onClick ClearForm
, class "px-4 py-2 rounded border-2 border-sky-400 bg-sky-100 focus:outline-none hover:bg-sky-200 active:border-indigo-600 focus:bg-sky-200 font-semibold text-blue-800"
, disabled (model.status == Loading)
]
[ text "Clear" ]
]
, viewMessage model.status
]
]
viewInput : String -> String -> String -> (String -> msg) -> Html msg
viewInput t p v toMsg =
input
[ class "px-4 py-2 rounded bg-white border-2 border-sky-400 hover:border-indigo-500 focus:outline-none focus:border-blue-500 placeholder:text-sky-300"
, type_ t
, placeholder p
, value v
, onInput toMsg
]
[]
viewMessage : Status -> Html msg
viewMessage status =
let
( icon, primary, secondary ) =
case status of
Failure _ ->
( V.asset "/assets/error.svg", "border-red-500 bg-rose-50", "text-red-800" )
Success ->
( V.asset "/assets/success.svg", "border-emerald-600 bg-emerald-50", "text-green-800" )
Ready ->
( V.asset "/assets/info.svg", "border-blue-400 bg-sky-50", "text-blue-800" )
Loading ->
( V.asset "/assets/loading.svg", "border-cyan-300 bg-cyan-50", "text-cyan-700" )
message =
case status of
Ready ->
"Please input your digital certificate information."
Loading ->
"Loading ..."
Failure e ->
case e of
EnvError ->
"The API URL is empty. You can't send information to the server."
ServerError s ->
s
Success ->
"Your digital certificate has been created. Please check your downloads."
in
div [ class primary, class "max-w-xl flex justify-center items-center gap-4 p-4 rounded-lg border-4" ]
[ img [ src icon, width 48, height 48 ] []
, p [ class secondary, class "text-ellipsis overflow-hidden" ] [ text message ]
]
| elm |
[
{
"context": "s : List Quiz.Question\nquestions =\n [ ( True, \"Aero\" )\n , ( False, \"Mars Bar\" )\n , ( True, \"All",
"end": 418,
"score": 0.9977430105,
"start": 414,
"tag": "NAME",
"value": "Aero"
},
{
"context": "uestions =\n [ ( True, \"Aero\" )\n , ( False, \"Mars Bar\" )\n , ( True, \"Allens\" )\n , ( False, \"Cadbu",
"end": 446,
"score": 0.9996714592,
"start": 438,
"tag": "NAME",
"value": "Mars Bar"
},
{
"context": "ero\" )\n , ( False, \"Mars Bar\" )\n , ( True, \"Allens\" )\n , ( False, \"Cadbury\" )\n , ( True, \"Uncl",
"end": 471,
"score": 0.9995825291,
"start": 465,
"tag": "NAME",
"value": "Allens"
},
{
"context": "s Bar\" )\n , ( True, \"Allens\" )\n , ( False, \"Cadbury\" )\n , ( True, \"Uncle Tobys\" )\n , ( False, \"",
"end": 498,
"score": 0.998931706,
"start": 491,
"tag": "NAME",
"value": "Cadbury"
},
{
"context": "lens\" )\n , ( False, \"Cadbury\" )\n , ( True, \"Uncle Tobys\" )\n , ( False, \"Weet-Bix\" )\n , ( True, \"Che",
"end": 528,
"score": 0.9994571805,
"start": 517,
"tag": "NAME",
"value": "Uncle Tobys"
},
{
"context": "\" )\n , ( True, \"Uncle Tobys\" )\n , ( False, \"Weet-Bix\" )\n , ( True, \"Cheerios\" )\n , ( False, \"Kel",
"end": 556,
"score": 0.991546154,
"start": 548,
"tag": "NAME",
"value": "Weet-Bix"
},
{
"context": "bys\" )\n , ( False, \"Weet-Bix\" )\n , ( True, \"Cheerios\" )\n , ( False, \"Kellogg's Corn Flakes\" )\n ,",
"end": 583,
"score": 0.9995430112,
"start": 575,
"tag": "NAME",
"value": "Cheerios"
},
{
"context": "Bix\" )\n , ( True, \"Cheerios\" )\n , ( False, \"Kellogg's Corn Flakes\" )\n , ( True, \"Quick-eze\" )\n , ( False, \"Re",
"end": 624,
"score": 0.9939541221,
"start": 603,
"tag": "NAME",
"value": "Kellogg's Corn Flakes"
},
{
"context": "( False, \"Kellogg's Corn Flakes\" )\n , ( True, \"Quick-eze\" )\n , ( False, \"Rennie\" )\n , ( True, \"Butte",
"end": 652,
"score": 0.8546785116,
"start": 643,
"tag": "NAME",
"value": "Quick-eze"
},
{
"context": "es\" )\n , ( True, \"Quick-eze\" )\n , ( False, \"Rennie\" )\n , ( True, \"Butter Menthols\" )\n , ( Fals",
"end": 678,
"score": 0.9996576905,
"start": 672,
"tag": "NAME",
"value": "Rennie"
},
{
"context": "k-eze\" )\n , ( False, \"Rennie\" )\n , ( True, \"Butter Menthols\" )\n , ( False, \"Fisherman's Friend\" )\n , ( ",
"end": 712,
"score": 0.9996768236,
"start": 697,
"tag": "NAME",
"value": "Butter Menthols"
},
{
"context": " , ( True, \"Butter Menthols\" )\n , ( False, \"Fisherman's Friend\" )\n , ( True, \"Crunch\" )\n , ( False, \"Krack",
"end": 750,
"score": 0.9814406633,
"start": 732,
"tag": "NAME",
"value": "Fisherman's Friend"
},
{
"context": " , ( False, \"Fisherman's Friend\" )\n , ( True, \"Crunch\" )\n , ( False, \"Krackel\" )\n , ( True, \"Fanc",
"end": 775,
"score": 0.9994556308,
"start": 769,
"tag": "NAME",
"value": "Crunch"
},
{
"context": "riend\" )\n , ( True, \"Crunch\" )\n , ( False, \"Krackel\" )\n , ( True, \"Fancy Feast\" )\n , ( True, \"F",
"end": 802,
"score": 0.9994707704,
"start": 795,
"tag": "NAME",
"value": "Krackel"
},
{
"context": "unch\" )\n , ( False, \"Krackel\" )\n , ( True, \"Fancy Feast\" )\n , ( True, \"Friskies\" )\n , ( False, \"Whi",
"end": 832,
"score": 0.9987371564,
"start": 821,
"tag": "NAME",
"value": "Fancy Feast"
},
{
"context": "l\" )\n , ( True, \"Fancy Feast\" )\n , ( True, \"Friskies\" )\n , ( False, \"Whiskas\" )\n , ( False, \"Pur",
"end": 859,
"score": 0.9992542863,
"start": 851,
"tag": "NAME",
"value": "Friskies"
},
{
"context": "ast\" )\n , ( True, \"Friskies\" )\n , ( False, \"Whiskas\" )\n , ( False, \"Purina\" )\n , ( True, \"Gerbe",
"end": 886,
"score": 0.9979540706,
"start": 879,
"tag": "NAME",
"value": "Whiskas"
},
{
"context": "ies\" )\n , ( False, \"Whiskas\" )\n , ( False, \"Purina\" )\n , ( True, \"Gerber (Baby Formula)\" )\n , ",
"end": 912,
"score": 0.9992548227,
"start": 906,
"tag": "NAME",
"value": "Purina"
},
{
"context": "iskas\" )\n , ( False, \"Purina\" )\n , ( True, \"Gerber (Baby Formula)\" )\n , ( False, \"Enfamil (Baby F",
"end": 937,
"score": 0.9965955019,
"start": 931,
"tag": "NAME",
"value": "Gerber"
},
{
"context": "( True, \"Gerber (Baby Formula)\" )\n , ( False, \"Enfamil (Baby Formula)\" )\n , ( True, \"Häagen-Dazs\" )\n ",
"end": 979,
"score": 0.9761007428,
"start": 972,
"tag": "NAME",
"value": "Enfamil"
},
{
"context": " False, \"Enfamil (Baby Formula)\" )\n , ( True, \"Häagen-Dazs\" )\n , ( False, \"Ben and Jerry\" )\n , ( True,",
"end": 1024,
"score": 0.9993828535,
"start": 1013,
"tag": "NAME",
"value": "Häagen-Dazs"
},
{
"context": "\" )\n , ( True, \"Häagen-Dazs\" )\n , ( False, \"Ben and Jerry\" )\n , ( True, \"Kit Kat\" )\n , ( False, \"Snic",
"end": 1057,
"score": 0.9989228249,
"start": 1044,
"tag": "NAME",
"value": "Ben and Jerry"
},
{
"context": ")\n , ( False, \"Ben and Jerry\" )\n , ( True, \"Kit Kat\" )\n , ( False, \"Snickers\" )\n , ( True, \"Lea",
"end": 1083,
"score": 0.9996514916,
"start": 1076,
"tag": "NAME",
"value": "Kit Kat"
},
{
"context": "erry\" )\n , ( True, \"Kit Kat\" )\n , ( False, \"Snickers\" )\n , ( True, \"Lean Cuisine\" )\n , ( False, ",
"end": 1111,
"score": 0.9994246364,
"start": 1103,
"tag": "NAME",
"value": "Snickers"
},
{
"context": "Kat\" )\n , ( False, \"Snickers\" )\n , ( True, \"Lean Cuisine\" )\n , ( False, \"Weight Watchers\" )\n , ( Tru",
"end": 1142,
"score": 0.9997301102,
"start": 1130,
"tag": "NAME",
"value": "Lean Cuisine"
},
{
"context": " )\n , ( True, \"Lean Cuisine\" )\n , ( False, \"Weight Watchers\" )\n , ( True, \"Maggi\" )\n , ( False, \"Top Ra",
"end": 1177,
"score": 0.8856537938,
"start": 1162,
"tag": "NAME",
"value": "Weight Watchers"
},
{
"context": " , ( False, \"Weight Watchers\" )\n , ( True, \"Maggi\" )\n , ( False, \"Top Ramen\" )\n , ( True, \"Mi",
"end": 1201,
"score": 0.9995113611,
"start": 1196,
"tag": "NAME",
"value": "Maggi"
},
{
"context": "tchers\" )\n , ( True, \"Maggi\" )\n , ( False, \"Top Ramen\" )\n , ( True, \"Milo\" )\n , ( False, \"Moove\" ",
"end": 1230,
"score": 0.9092059731,
"start": 1221,
"tag": "NAME",
"value": "Top Ramen"
},
{
"context": "gi\" )\n , ( False, \"Top Ramen\" )\n , ( True, \"Milo\" )\n , ( False, \"Moove\" )\n , ( True, \"Mövenp",
"end": 1253,
"score": 0.9993562698,
"start": 1249,
"tag": "NAME",
"value": "Milo"
},
{
"context": "p Ramen\" )\n , ( True, \"Milo\" )\n , ( False, \"Moove\" )\n , ( True, \"Mövenpick\" )\n , ( False, \"Ba",
"end": 1278,
"score": 0.9994788766,
"start": 1273,
"tag": "NAME",
"value": "Moove"
},
{
"context": " \"Milo\" )\n , ( False, \"Moove\" )\n , ( True, \"Mövenpick\" )\n , ( False, \"Baskin Robbins\" )\n , ( True",
"end": 1306,
"score": 0.9997440577,
"start": 1297,
"tag": "NAME",
"value": "Mövenpick"
},
{
"context": "ve\" )\n , ( True, \"Mövenpick\" )\n , ( False, \"Baskin Robbins\" )\n , ( True, \"Nescafé\" )\n , ( False, \"Star",
"end": 1340,
"score": 0.9997816086,
"start": 1326,
"tag": "NAME",
"value": "Baskin Robbins"
},
{
"context": "\n , ( False, \"Baskin Robbins\" )\n , ( True, \"Nescafé\" )\n , ( False, \"Starbucks\" )\n , ( True, \"Ne",
"end": 1366,
"score": 0.999729991,
"start": 1359,
"tag": "NAME",
"value": "Nescafé"
},
{
"context": "bins\" )\n , ( True, \"Nescafé\" )\n , ( False, \"Starbucks\" )\n , ( True, \"Nespresso\" )\n , ( False, \"Il",
"end": 1395,
"score": 0.7881798148,
"start": 1386,
"tag": "NAME",
"value": "Starbucks"
},
{
"context": "fé\" )\n , ( False, \"Starbucks\" )\n , ( True, \"Nespresso\" )\n , ( False, \"Illy\" )\n , ( True, ",
"end": 1415,
"score": 0.9696807265,
"start": 1414,
"tag": "NAME",
"value": "N"
},
{
"context": "é\" )\n , ( False, \"Starbucks\" )\n , ( True, \"Nespresso\" )\n , ( False, \"Illy\" )\n , ( True, \"Nesquic",
"end": 1423,
"score": 0.9849293828,
"start": 1415,
"tag": "NAME",
"value": "espresso"
},
{
"context": "ks\" )\n , ( True, \"Nespresso\" )\n , ( False, \"Illy\" )\n , ( True, \"Nesquick\" )\n , ( False, \"Ova",
"end": 1447,
"score": 0.9935471416,
"start": 1443,
"tag": "NAME",
"value": "Illy"
},
{
"context": "spresso\" )\n , ( False, \"Illy\" )\n , ( True, \"Nesquick\" )\n , ( False, \"Ovaltine\" )\n , ( True, \"Per",
"end": 1474,
"score": 0.9862937927,
"start": 1466,
"tag": "NAME",
"value": "Nesquick"
},
{
"context": "lly\" )\n , ( True, \"Nesquick\" )\n , ( False, \"Ovaltine\" )\n , ( True, \"Perrier\" )\n , ( False, \"Evia",
"end": 1502,
"score": 0.9900281429,
"start": 1494,
"tag": "NAME",
"value": "Ovaltine"
},
{
"context": "ick\" )\n , ( False, \"Ovaltine\" )\n , ( True, \"Perrier\" )\n , ( False, \"Evian\" )\n , ( True, \"San Pe",
"end": 1528,
"score": 0.9964630008,
"start": 1521,
"tag": "NAME",
"value": "Perrier"
},
{
"context": "tine\" )\n , ( True, \"Perrier\" )\n , ( False, \"Evian\" )\n , ( True, \"San Pellegrino\" )\n , ( False",
"end": 1553,
"score": 0.9954420328,
"start": 1548,
"tag": "NAME",
"value": "Evian"
},
{
"context": "errier\" )\n , ( False, \"Evian\" )\n , ( True, \"San Pellegrino\" )\n , ( False, \"Cool Ridge\" )\n , ( True, \"S",
"end": 1586,
"score": 0.9990539551,
"start": 1572,
"tag": "NAME",
"value": "San Pellegrino"
},
{
"context": "\n , ( True, \"San Pellegrino\" )\n , ( False, \"Cool Ridge\" )\n , ( True, \"Smarties\" )\n , ( False, \"M&M",
"end": 1616,
"score": 0.9641652703,
"start": 1606,
"tag": "NAME",
"value": "Cool Ridge"
},
{
"context": "o\" )\n , ( False, \"Cool Ridge\" )\n , ( True, \"Smarties\" )\n , ( False, \"M&Ms\" )\n , ( True, \"Nerds\" ",
"end": 1643,
"score": 0.9856632948,
"start": 1635,
"tag": "NAME",
"value": "Smarties"
},
{
"context": "dge\" )\n , ( True, \"Smarties\" )\n , ( False, \"M&Ms\" )\n , ( True, \"Nerds\" )\n , ( False, \"Skittl",
"end": 1667,
"score": 0.6429620385,
"start": 1663,
"tag": "NAME",
"value": "M&Ms"
},
{
"context": "marties\" )\n , ( False, \"M&Ms\" )\n , ( True, \"Nerds\" )\n , ( False, \"Skittles\" )\n , ( True, \"Won",
"end": 1691,
"score": 0.9711052775,
"start": 1686,
"tag": "NAME",
"value": "Nerds"
},
{
"context": " \"M&Ms\" )\n , ( True, \"Nerds\" )\n , ( False, \"Skittles\" )\n , ( True, \"Wonka\" )\n , ( False, \"Hershe",
"end": 1719,
"score": 0.9690733552,
"start": 1711,
"tag": "NAME",
"value": "Skittles"
},
{
"context": "rds\" )\n , ( False, \"Skittles\" )\n , ( True, \"Wonka\" )\n , ( False, \"Hersheys\" )\n ]\n\n\nshuffleLis",
"end": 1743,
"score": 0.9876864552,
"start": 1738,
"tag": "NAME",
"value": "Wonka"
},
{
"context": "ittles\" )\n , ( True, \"Wonka\" )\n , ( False, \"Hersheys\" )\n ]\n\n\nshuffleListToArray : List ( Bool, Stri",
"end": 1771,
"score": 0.9839018583,
"start": 1763,
"tag": "NAME",
"value": "Hersheys"
}
] | Main.elm | camjc/nestle-or-not | 1 | module Main exposing (main)
import Quiz exposing (update, view, Question, Model, Msg)
import Array
import Html exposing (Html, beginnerProgram)
import Markdown
import Random
import Random.Array
-- True is Nestle, False is Other.
-- I haven’t vetted the practices of all other examples yet,
-- but just list the main competitor to each Nestle product.
questions : List Quiz.Question
questions =
[ ( True, "Aero" )
, ( False, "Mars Bar" )
, ( True, "Allens" )
, ( False, "Cadbury" )
, ( True, "Uncle Tobys" )
, ( False, "Weet-Bix" )
, ( True, "Cheerios" )
, ( False, "Kellogg's Corn Flakes" )
, ( True, "Quick-eze" )
, ( False, "Rennie" )
, ( True, "Butter Menthols" )
, ( False, "Fisherman's Friend" )
, ( True, "Crunch" )
, ( False, "Krackel" )
, ( True, "Fancy Feast" )
, ( True, "Friskies" )
, ( False, "Whiskas" )
, ( False, "Purina" )
, ( True, "Gerber (Baby Formula)" )
, ( False, "Enfamil (Baby Formula)" )
, ( True, "Häagen-Dazs" )
, ( False, "Ben and Jerry" )
, ( True, "Kit Kat" )
, ( False, "Snickers" )
, ( True, "Lean Cuisine" )
, ( False, "Weight Watchers" )
, ( True, "Maggi" )
, ( False, "Top Ramen" )
, ( True, "Milo" )
, ( False, "Moove" )
, ( True, "Mövenpick" )
, ( False, "Baskin Robbins" )
, ( True, "Nescafé" )
, ( False, "Starbucks" )
, ( True, "Nespresso" )
, ( False, "Illy" )
, ( True, "Nesquick" )
, ( False, "Ovaltine" )
, ( True, "Perrier" )
, ( False, "Evian" )
, ( True, "San Pellegrino" )
, ( False, "Cool Ridge" )
, ( True, "Smarties" )
, ( False, "M&Ms" )
, ( True, "Nerds" )
, ( False, "Skittles" )
, ( True, "Wonka" )
, ( False, "Hersheys" )
]
shuffleListToArray : List ( Bool, String ) -> Array.Array Quiz.Question
shuffleListToArray list =
Tuple.first (Random.step (Random.Array.shuffle (Array.fromList list)) (Random.initialSeed 204862737))
instructionsComponent : Html a
instructionsComponent =
Markdown.toHtml [] """
# Nestle or Not
## Reasons I wanted to learn what products Nestle makes
- [Alleged child slavery](https://www.rt.com/usa/328682-child-slavery-scotus-nestle/)
- [Alleged water crisis profiteering](https://www.salon.com/2015/04/07/nestles_despicable_water_crisis_profiteering_how_its_making_a_killing_%E2%80%94%C2%A0while_california_is_dying_of_thirst/)
- [Discouraging breast feeding](http://www.theguardian.com/sustainable-business/nestle-baby-milk-scandal-food-industry-standards)
## How to Play
- A product name will appear.
- Click *Nestle* if you think they made it.
- Click *Other* if you think they didn't.
"""
model : Quiz.Model
model =
{ questionId =
-1
-- Show Instructions
, questions = shuffleListToArray questions
, correctAnswers = Array.empty
, wrongAnswers = Array.empty
, buttonTrue = ( "Nestle", "#005a97" )
, buttonFalse = ( "Other", "#00975a" )
, instructionsComponent = instructionsComponent
}
main : Program Never Model Msg
main =
Html.beginnerProgram { model = model, view = view, update = update }
| 55840 | module Main exposing (main)
import Quiz exposing (update, view, Question, Model, Msg)
import Array
import Html exposing (Html, beginnerProgram)
import Markdown
import Random
import Random.Array
-- True is Nestle, False is Other.
-- I haven’t vetted the practices of all other examples yet,
-- but just list the main competitor to each Nestle product.
questions : List Quiz.Question
questions =
[ ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME>" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME> (Baby Formula)" )
, ( False, "<NAME> (Baby Formula)" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME> <NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
, ( True, "<NAME>" )
, ( False, "<NAME>" )
]
shuffleListToArray : List ( Bool, String ) -> Array.Array Quiz.Question
shuffleListToArray list =
Tuple.first (Random.step (Random.Array.shuffle (Array.fromList list)) (Random.initialSeed 204862737))
instructionsComponent : Html a
instructionsComponent =
Markdown.toHtml [] """
# Nestle or Not
## Reasons I wanted to learn what products Nestle makes
- [Alleged child slavery](https://www.rt.com/usa/328682-child-slavery-scotus-nestle/)
- [Alleged water crisis profiteering](https://www.salon.com/2015/04/07/nestles_despicable_water_crisis_profiteering_how_its_making_a_killing_%E2%80%94%C2%A0while_california_is_dying_of_thirst/)
- [Discouraging breast feeding](http://www.theguardian.com/sustainable-business/nestle-baby-milk-scandal-food-industry-standards)
## How to Play
- A product name will appear.
- Click *Nestle* if you think they made it.
- Click *Other* if you think they didn't.
"""
model : Quiz.Model
model =
{ questionId =
-1
-- Show Instructions
, questions = shuffleListToArray questions
, correctAnswers = Array.empty
, wrongAnswers = Array.empty
, buttonTrue = ( "Nestle", "#005a97" )
, buttonFalse = ( "Other", "#00975a" )
, instructionsComponent = instructionsComponent
}
main : Program Never Model Msg
main =
Html.beginnerProgram { model = model, view = view, update = update }
| true | module Main exposing (main)
import Quiz exposing (update, view, Question, Model, Msg)
import Array
import Html exposing (Html, beginnerProgram)
import Markdown
import Random
import Random.Array
-- True is Nestle, False is Other.
-- I haven’t vetted the practices of all other examples yet,
-- but just list the main competitor to each Nestle product.
questions : List Quiz.Question
questions =
[ ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI (Baby Formula)" )
, ( False, "PI:NAME:<NAME>END_PI (Baby Formula)" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
, ( True, "PI:NAME:<NAME>END_PI" )
, ( False, "PI:NAME:<NAME>END_PI" )
]
shuffleListToArray : List ( Bool, String ) -> Array.Array Quiz.Question
shuffleListToArray list =
Tuple.first (Random.step (Random.Array.shuffle (Array.fromList list)) (Random.initialSeed 204862737))
instructionsComponent : Html a
instructionsComponent =
Markdown.toHtml [] """
# Nestle or Not
## Reasons I wanted to learn what products Nestle makes
- [Alleged child slavery](https://www.rt.com/usa/328682-child-slavery-scotus-nestle/)
- [Alleged water crisis profiteering](https://www.salon.com/2015/04/07/nestles_despicable_water_crisis_profiteering_how_its_making_a_killing_%E2%80%94%C2%A0while_california_is_dying_of_thirst/)
- [Discouraging breast feeding](http://www.theguardian.com/sustainable-business/nestle-baby-milk-scandal-food-industry-standards)
## How to Play
- A product name will appear.
- Click *Nestle* if you think they made it.
- Click *Other* if you think they didn't.
"""
model : Quiz.Model
model =
{ questionId =
-1
-- Show Instructions
, questions = shuffleListToArray questions
, correctAnswers = Array.empty
, wrongAnswers = Array.empty
, buttonTrue = ( "Nestle", "#005a97" )
, buttonFalse = ( "Other", "#00975a" )
, instructionsComponent = instructionsComponent
}
main : Program Never Model Msg
main =
Html.beginnerProgram { model = model, view = view, update = update }
| elm |
[
{
"context": "y javascript to work-around\n-- https://github.com/elm-explorations/markdown/issues/1\n-- See https://github.com/dmy/e",
"end": 1101,
"score": 0.9043254852,
"start": 1085,
"tag": "USERNAME",
"value": "elm-explorations"
},
{
"context": "tions/markdown/issues/1\n-- See https://github.com/dmy/elm-doc-preview/issues/1\n--\n-- Local documentatio",
"end": 1149,
"score": 0.9996647835,
"start": 1146,
"tag": "USERNAME",
"value": "dmy"
},
{
"context": "-link\"\n , href \"https://github.com/dmy/elm-doc-preview\"\n ]\n ",
"end": 18826,
"score": 0.9987286329,
"start": 18823,
"tag": "USERNAME",
"value": "dmy"
},
{
"context": "ext \" \"\n , span [] [ text \"Copyright © 2019 Rémi Lefèvre.\" ]\n ]\n\n\n\n-- Files handling\n\n\nselectFiles ",
"end": 18990,
"score": 0.9998754263,
"start": 18978,
"tag": "NAME",
"value": "Rémi Lefèvre"
}
] | src/Main.elm | avh4/elm-doc-preview | 0 | port module Main exposing (main)
import Block
import Browser exposing (UrlRequest(..))
import Browser.Dom as Dom
import Browser.Navigation as Nav
import Dict exposing (Dict)
import Elm.Docs as Docs
import Elm.Error as Error
import Elm.Type as Type
import Errors exposing (viewError)
import File exposing (File)
import File.Select as Select
import Html exposing (Html, a, div, h1, input, li, span, text, ul)
import Html.Attributes exposing (class, href, id, placeholder, style, title, value)
import Html.Events exposing (on, onClick, onInput, preventDefaultOn)
import Html.Extra as Html exposing (viewIf)
import Http
import Json.Decode as Decode exposing (Decoder)
import Markdown
import Maybe.Extra as Maybe
import Online
import Regex
import Svg exposing (svg)
import Svg.Attributes exposing (d, fill, height, viewBox, width)
import Task
import Url exposing (Url)
import Url.Builder
import Url.Parser exposing (Parser)
import Url.Parser.Query as Query
import Utils.Markdown as Markdown
-- PORTS
--
-- Urls changes are intercepted by javascript to work-around
-- https://github.com/elm-explorations/markdown/issues/1
-- See https://github.com/dmy/elm-doc-preview/issues/1
--
-- Local documentation is stored in local storage to improve
-- navigation (mainly when going back from external links).
port locationHrefRequested : (String -> msg) -> Sub msg
port nameUpdated : (String -> msg) -> Sub msg
port compilationUpdated : (String -> msg) -> Sub msg
port readmeUpdated : (String -> msg) -> Sub msg
port docsUpdated : (String -> msg) -> Sub msg
port depsUpdated : (Decode.Value -> msg) -> Sub msg
port storeReadme : String -> Cmd msg
port storeDocs : String -> Cmd msg
port clearStorage : () -> Cmd msg
-- TYPES
type Msg
= Ignored String
| NameUpdated String
| CompilationCompleted String
| DepsUpdated Decode.Value
| OpenFilesClicked
| GotFiles File (List File)
| ClosePreviewClicked
| DocsLoaded String
| DocsRequestCompleted (Result Http.Error String)
| ReadmeLoaded String
| ReadmeRequestCompleted (Result Http.Error String)
| FilterChanged String
| OwnerChanged Owner
| LocationHrefRequested String
| UrlRequested UrlRequest
| UrlChanged Url
type alias Model =
{ name : Maybe String
, readme : Maybe String
, modules : List Docs.Module
, deps : Dict String Dep
, navKey : Nav.Key
, url : Url
, page : Page
, source : Source
, online : Bool
, error : Maybe Error.Error
, filter : String
}
type alias Dep =
{ version : String
, readme : String
, modules : List Docs.Module
}
type Page
= Readme Owner
| Module Owner String
type Owner
= Main
| Package String
type Source
= Local
| Remote LoadingState Repo
type LoadingState
= Loading
| LoadingReadme
| LoadingDocs
| Loaded
type alias Repo =
{ name : String
, version : String
}
type alias Flags =
{ readme : Maybe String
, docs : Maybe String
, online : Bool
}
-- INIT
init : Flags -> Url -> Nav.Key -> ( Model, Cmd Msg )
init flags url navKey =
let
source =
urlToSource url
( readme, modules ) =
initDoc source flags
in
( { name = Nothing
, readme = readme
, modules = modules
, deps = Dict.empty
, navKey = navKey
, url = url
, page = urlToPage url Main
, source = source
, online = flags.online
, error = Nothing
, filter = ""
}
, Cmd.batch
[ focusOpenFilesLink
, getDoc source
, scrollToFragment url
]
)
initDoc : Source -> Flags -> ( Maybe String, List Docs.Module )
initDoc source flags =
case source of
Remote _ _ ->
( Nothing, [] )
Local ->
( flags.readme
, flags.docs
|> Maybe.map decodeDocs
|> Maybe.withDefault []
)
focusOpenFilesLink : Cmd Msg
focusOpenFilesLink =
Task.attempt (\_ -> Ignored "focus") (Dom.focus "open-link")
urlToSource : Url -> Source
urlToSource url =
Url.Parser.parse repoParser { url | path = "" }
|> Maybe.join
|> Maybe.map (Remote Loading)
|> Maybe.withDefault Local
repoParser : Parser (Maybe Repo -> Maybe Repo) (Maybe Repo)
repoParser =
Url.Parser.query <|
Query.map2 repoParserHelper
(Query.string "repo")
(Query.string "version")
repoParserHelper : Maybe String -> Maybe String -> Maybe Repo
repoParserHelper maybeRepo maybeVersion =
case ( maybeRepo, maybeVersion ) of
( Just repo, Just version ) ->
Just (Repo repo version)
( Just repo, Nothing ) ->
Just (Repo repo "master")
_ ->
Nothing
getDoc : Source -> Cmd Msg
getDoc source =
case source of
Remote _ repo ->
Cmd.batch
[ Http.get
{ url = githubFileUrl repo "docs.json"
, expect = Http.expectString DocsRequestCompleted
}
, Http.get
{ url = githubFileUrl repo "README.md"
, expect = Http.expectString ReadmeRequestCompleted
}
, clearStorage ()
]
Local ->
Cmd.none
githubFileUrl : Repo -> String -> String
githubFileUrl repo file =
Url.Builder.crossOrigin "https://raw.githubusercontent.com"
[ repo.name
, repo.version
, file
]
[]
sourceQuery : Source -> String
sourceQuery source =
case source of
Remote _ repo ->
Url.Builder.toQuery
[ Url.Builder.string "repo" repo.name
, Url.Builder.string "version" repo.version
]
Local ->
""
-- VIEW
view : Model -> Browser.Document Msg
view model =
{ title = "Elm Doc Preview"
, body =
[ div
[ style "min-width" "100%"
, style "min-height" "100%"
, style "display" "flex"
, style "flex-direction" "column"
, stopDragOver
, preventDefaultOn "drop"
(Decode.map alwaysPreventDefault dropDecoder)
]
[ viewMain model
, footer
]
]
}
viewMain : Model -> Html Msg
viewMain model =
div
[ class "center"
, style "flex" "1"
, style "flex-wrap" "wrap-reverse"
, style "display" "flex"
, style "align-items" "flex-end"
]
[ case ( model.error, isLoading model ) of
( Just error, _ ) ->
compilationError error
( Nothing, True ) ->
spinner
_ ->
page model
, navigation (pageOwner model.page) model
]
dropDecoder : Decoder Msg
dropDecoder =
Decode.at [ "dataTransfer", "files" ]
(Decode.oneOrMore GotFiles File.decoder)
stopDragOver : Html.Attribute Msg
stopDragOver =
preventDefaultOn "dragover"
(Decode.map alwaysPreventDefault (Decode.succeed <| Ignored "dragover"))
alwaysPreventDefault : msg -> ( msg, Bool )
alwaysPreventDefault msg =
( msg, True )
isLoading : Model -> Bool
isLoading model =
-- Offline, waiting for websocket update
if not model.online && model.readme == Nothing then
True
else
case model.source of
Remote Loading _ ->
True
Remote LoadingReadme _ ->
True
Remote LoadingDocs _ ->
True
_ ->
False
page : Model -> Html Msg
page model =
div [ class "block-list" ] <|
case model.page of
Readme owner ->
[ Markdown.block (pageReadme owner model) ]
Module owner name ->
filteredModule model.filter
(pageModules owner model)
model.source
name
pageReadme : Owner -> Model -> String
pageReadme owner model =
case ( owner, model.readme, model.online ) of
( Main, Nothing, True ) ->
case model.modules of
[] ->
Online.readme
_ ->
Online.readmeWithModules
( Main, Just readme, _ ) ->
readme
( Package pkg, _, _ ) ->
case Dict.get pkg model.deps of
Just dep ->
dep.readme
Nothing ->
""
_ ->
""
pageModules : Owner -> Model -> List Docs.Module
pageModules owner model =
case owner of
Main ->
model.modules
Package pkg ->
case Dict.get pkg model.deps of
Just dep ->
dep.modules
Nothing ->
[]
filteredModule : String -> List Docs.Module -> Source -> String -> List (Html Msg)
filteredModule filter modules source name =
case List.filter (\m -> m.name == name) modules of
currentModule :: _ ->
doc filter modules currentModule (sourceQuery source)
_ ->
[ h1 [] [ text "Error" ]
, text ("Module \"" ++ name ++ "\" not found.")
]
spinner : Html msg
spinner =
div [ class "block-list" ] <|
[ div [ class "spinner" ]
[ div [ class "bounce1" ] []
, div [ class "bounce2" ] []
, div [ class "bounce3" ] []
]
]
compilationError : Error.Error -> Html msg
compilationError error =
div
[ class "block-list"
, style "margin-top" "24px"
]
[ viewError error ]
doc : String -> List Docs.Module -> Docs.Module -> String -> List (Html msg)
doc filter modules currentModule query =
let
info =
Block.makeInfo currentModule.name modules query
in
h1 [ class "block-list-title" ] [ text currentModule.name ]
:: List.map (Block.view filter info) (Docs.toBlocks currentModule)
-- Side navigation bar
navigation : Owner -> Model -> Html Msg
navigation owner model =
div [ class "pkg-nav" ]
[ logo
, viewIf (model.online && not (isLoading model)) <|
openLink
, viewIf model.online <|
closeLink model
, filterBox model.filter model.modules
, viewIf (model.readme /= Nothing) <|
navLinks model.source model.page [ Readme owner ]
, browseSourceLink model.source
, if String.isEmpty model.filter then
pageModules owner model
|> List.map (\m -> Module owner m.name)
|> navLinks model.source model.page
else
search model.source model.page model.filter (pageModules owner model)
, packages owner model.name model.filter model.deps
]
logo : Html msg
logo =
div
[ style "display" "flex"
, style "transform" "translateX(-8px)"
]
[ svg
[ width "50px", height "70px", viewBox "0 0 210 297" ]
[ Svg.path [ d "M61.8 132v92.7l46.3-46.3-46.3-46.3z", fill "#60b5cc" ] []
, Svg.path [ d "M83 59.9L62.9 80.2 83 100.5z", fill "#f0ad00" ] []
, Svg.path [ d "M109 184.1l-65.4 65.5H109v-65.5z", fill "#5a6378" ] []
, Svg.path [ d "M145 217.6l-32.1 32H143l32-32z", fill "#7fd13b" ] []
, Svg.path [ d "M59.4 83.5l-22.7 22.7 22.7 22.7 22.7-22.7z", fill "#7fd13b" ] []
, Svg.path [ d "M35.8 59.9v40.6L56 80.2z", fill "#f0ad00" ] []
, Svg.path [ d "M57 132l-31 31.2 31 31v-62.1z", fill "#60b5cc" ] []
]
, div
[ style "color" "#5a6378ff"
, style "line-height" "16px"
, style "padding-top" "12px"
, style "transform" "translateX(-20px)"
]
[ div [] [ text "elm doc" ]
, div [] [ text "preview" ]
]
]
openLink : Html Msg
openLink =
div []
[ a
[ id "open-link"
, style "cursor" "pointer"
, href ""
, onEnterOrSpace OpenFilesClicked
, onClick OpenFilesClicked
]
[ text "Open Files" ]
]
closeLink : Model -> Html Msg
closeLink model =
if model.readme == Nothing && model.modules == [] then
Html.nothing
else
div [ style "margin-bottom" "10px" ]
[ a
[ style "cursor" "pointer"
, href "/"
, onClick ClosePreviewClicked
]
[ text "Close Preview" ]
]
browseSourceLink : Source -> Html Msg
browseSourceLink source =
case source of
Local ->
Html.nothing
Remote _ repo ->
div [ style "margin-bottom" "20px" ]
[ a
[ style "cursor" "pointer"
, href (githubSource repo)
]
[ text "Browse Source" ]
]
filterBox : String -> List Docs.Module -> Html Msg
filterBox filter modules =
input
[ placeholder "Filter with regex"
, value filter
, onInput FilterChanged
]
[]
search : Source -> Page -> String -> List Docs.Module -> Html Msg
search source currentPage filter modules =
ul []
(List.filterMap (searchModule source currentPage filter) modules)
searchModule : Source -> Page -> String -> Docs.Module -> Maybe (Html Msg)
searchModule source currentPage filter m =
let
results =
List.concat
[ searchFrom m.binops filter
, searchFrom m.unions filter
, searchFrom m.aliases filter
, searchFrom m.values filter
]
in
if List.isEmpty results then
Nothing
else
Just <|
li [ class "pkg-nav-search-chunk" ]
[ pageLink source currentPage (Module (pageOwner currentPage) m.name)
, ul [] (List.map (searchResult source m.name) results)
]
searchFrom : List { r | name : String } -> String -> List String
searchFrom records filter =
List.filterMap
(\r ->
if contains filter r.name then
Just r.name
else
Nothing
)
records
contains : String -> String -> Bool
contains pattern str =
pattern
|> Regex.fromStringWith { caseInsensitive = True, multiline = False }
|> Maybe.map (\regex -> Regex.contains regex str)
|> Maybe.withDefault False
searchResult : Source -> String -> String -> Html Msg
searchResult source module_ symbol =
resultLink source module_ symbol
githubSource : Repo -> String
githubSource repo =
Url.Builder.crossOrigin "https://github.com"
[ repo.name
, "tree"
, repo.version
]
[]
onEnterOrSpace : msg -> Html.Attribute msg
onEnterOrSpace msg =
on "keyup"
(Decode.field "key" Decode.string
|> Decode.andThen
(\key ->
if key == "Enter" || key == " " then
Decode.succeed msg
else
Decode.fail ""
)
)
navLinks : Source -> Page -> List Page -> Html msg
navLinks source currentPage pages =
ul []
(List.map (pageLink source currentPage) pages)
pageLink : Source -> Page -> Page -> Html msg
pageLink source currentPage targetPage =
li
[ case targetPage of
Readme _ ->
style "" ""
_ ->
style "margin-left" "10px"
]
[ a
[ class "pkg-nav-module"
, href (pagePath targetPage ++ sourceQuery source)
, styleIf (currentPage == targetPage) "font-weight" "bold"
, styleIf (currentPage == targetPage) "text-decoration" "underline"
]
[ case targetPage of
Readme _ ->
text "README"
Module _ name ->
text name
]
]
resultLink : Source -> String -> String -> Html Msg
resultLink source module_ symbol =
li []
[ a
[ class "pkg-nav-module"
, (href << String.concat) <|
[ modulePath module_
, sourceQuery source
, "#"
, symbol
]
]
[ text symbol
]
]
packages : Owner -> Maybe String -> String -> Dict String Dep -> Html Msg
packages owner maybeDefault filter deps =
let
default =
Maybe.map (mainPackage owner) maybeDefault
|> Maybe.withDefault Html.nothing
dependencies =
Dict.toList deps
|> List.filter (\( name, _ ) -> contains filter name)
|> List.sortBy (String.toLower << Tuple.first)
in
ul [ style "margin-top" "20px" ] <|
(default :: List.map (dependency owner) dependencies)
dependency : Owner -> ( String, Dep ) -> Html Msg
dependency owner ( name, { version, modules } ) =
package owner name version
mainPackage : Owner -> String -> Html Msg
mainPackage owner name =
li
[ styleIf (owner == Main) "font-weight" "bold"
, styleIf (owner == Main) "text-decoration" "underline"
]
[ a [ onClick (OwnerChanged Main) ]
[ text name ]
]
package : Owner -> String -> String -> Html Msg
package owner name version =
li
[ styleIf (Package name == owner) "font-weight" "bold"
, styleIf (Package name == owner) "text-decoration" "underline"
, style "margin-left" "10px"
]
[ a [ onClick (OwnerChanged <| Package name) ]
[ text (" " ++ name ++ " " ++ version) ]
]
pagePath : Page -> String
pagePath page_ =
case page_ of
Module _ name ->
"/" ++ modulePath name
Readme _ ->
"/"
modulePath : String -> String
modulePath name =
slugify name
styleIf : Bool -> String -> String -> Html.Attribute msg
styleIf cond property value =
if cond then
style property value
else
style "" ""
slugify : String -> String
slugify str =
String.replace "." "-" str
unslugify : String -> String
unslugify str =
String.replace "-" "." str
-- Footer
footer : Html msg
footer =
div [ class "footer" ]
[ span []
[ text "elm-doc-preview is "
, a
[ class "grey-link"
, href "https://github.com/dmy/elm-doc-preview"
]
[ text "open source." ]
]
, text " "
, span [] [ text "Copyright © 2019 Rémi Lefèvre." ]
]
-- Files handling
selectFiles : Cmd Msg
selectFiles =
Select.files
-- Browsers do not agree on mime types :/
[ "text/plain", "text/markdown", "application/json", ".md" ]
GotFiles
readFiles : List File -> Cmd Msg
readFiles files =
Cmd.batch (List.map readFile files)
readFile : File -> Cmd Msg
readFile file =
case ( File.name file, File.mime file ) of
( "README.md", _ ) ->
Task.perform ReadmeLoaded (File.toString file)
( "docs.json", "application/json" ) ->
Task.perform DocsLoaded (File.toString file)
_ ->
Cmd.none
decodeDocs : String -> List Docs.Module
decodeDocs docs =
case Decode.decodeString (Decode.list Docs.decoder) docs of
Ok modules ->
modules
Err _ ->
[]
decodeDeps : Decode.Value -> Dict String Dep
decodeDeps value =
case Decode.decodeValue (Decode.dict depDecoder) value of
Ok deps ->
deps
Err err ->
Dict.empty
depDecoder : Decoder Dep
depDecoder =
Decode.map3 Dep
(Decode.field "version" Decode.string)
(Decode.field "readme" Decode.string)
(Decode.field "docs" <| Decode.map decodeDocs Decode.string)
-- UPDATE
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
Ignored _ ->
( model, Cmd.none )
NameUpdated name ->
( { model | name = Just name }, Cmd.none )
CompilationCompleted error ->
( setError error model, Cmd.none )
DepsUpdated deps ->
( { model | deps = decodeDeps deps }, Cmd.none )
OpenFilesClicked ->
( model, selectFiles )
GotFiles file files ->
( { model | source = Local }, readFiles (file :: files) )
ClosePreviewClicked ->
( closePreview model, clearStorage () )
DocsLoaded docs ->
( setModules (decodeDocs docs) model
, Cmd.batch
[ scrollToFragment model.url
, storeDocs docs
]
)
DocsRequestCompleted (Ok docs) ->
( setModules (decodeDocs docs) model, scrollToFragment model.url )
DocsRequestCompleted (Err error) ->
( setModules [] model, Cmd.none )
ReadmeLoaded readme ->
( setReadme (Just readme) model
, Cmd.batch
[ scrollToFragment model.url
, storeReadme readme
]
)
ReadmeRequestCompleted (Ok readme) ->
( setReadme (Just readme) model, scrollToFragment model.url )
ReadmeRequestCompleted (Err error) ->
( setReadme Nothing model, Cmd.none )
FilterChanged filterValue ->
( { model | filter = filterValue }, Cmd.none )
OwnerChanged owner ->
( { model | page = Readme owner, filter = "" }, Cmd.none )
LocationHrefRequested href ->
( model, requestLocationHref model.navKey href )
UrlRequested request ->
-- Url requests are intercepted by javascript
-- and handled by LocationHrefRequested to work-around
-- unhandled links in markdown blocks.
( model, Cmd.none )
UrlChanged url ->
( { model
| page = urlToPage url (pageOwner model.page)
, url = url
}
, addUrlQuery model url
)
addUrlQuery : Model -> Url -> Cmd Msg
addUrlQuery model url =
case ( url /= model.url, sourceQuery model.source ) of
( False, _ ) ->
Cmd.none
( True, "" ) ->
scrollToFragment url
( True, query ) ->
Nav.replaceUrl model.navKey <|
Url.toString <|
{ url | query = Just (String.dropLeft 1 query) }
setError : String -> Model -> Model
setError errorJsonString model =
case Decode.decodeString Error.decoder errorJsonString of
Ok error ->
{ model | error = Just error }
Err _ ->
{ model | error = Nothing }
setReadme : Maybe String -> Model -> Model
setReadme readme model =
{ model
| readme = readme
, source =
case model.source of
Remote Loading repo ->
Remote LoadingDocs repo
Remote LoadingReadme repo ->
Remote Loaded repo
_ ->
model.source
}
setModules : List Docs.Module -> Model -> Model
setModules modules model =
{ model
| modules = modules
, source =
case model.source of
Remote Loading repo ->
Remote LoadingReadme repo
Remote LoadingDocs repo ->
Remote Loaded repo
_ ->
model.source
}
scrollToFragment : Url -> Cmd Msg
scrollToFragment url =
case url.fragment of
Just id ->
Dom.getElement id
|> Task.andThen (\e -> Dom.setViewport 0 e.element.y)
|> Task.attempt (\_ -> Ignored "scroll")
Nothing ->
Cmd.none
closePreview : Model -> Model
closePreview model =
{ model
| readme = Nothing
, modules = []
, page = Readme Main
, source = Local
, error = Nothing
, filter = ""
}
requestLocationHref : Nav.Key -> String -> Cmd Msg
requestLocationHref navKey href =
case Url.fromString href of
Just url ->
-- Complete URL are expected to be external
Nav.load href
Nothing ->
Nav.pushUrl navKey href
urlToPage : Url -> Owner -> Page
urlToPage url owner =
case url.path of
"/" ->
Readme owner
_ ->
Module owner (unslugify <| String.dropLeft 1 <| url.path)
pageOwner : Page -> Owner
pageOwner page_ =
case page_ of
Readme readmeOwner ->
readmeOwner
Module moduleOwner _ ->
moduleOwner
-- SUBSCRIPTIONS
subscriptions : Model -> Sub Msg
subscriptions model =
Sub.batch
[ locationHrefRequested LocationHrefRequested
, nameUpdated NameUpdated
, compilationUpdated CompilationCompleted
, readmeUpdated ReadmeLoaded
, docsUpdated DocsLoaded
, depsUpdated DepsUpdated
]
-- MAIN
main : Program Flags Model Msg
main =
Browser.application
{ init = init
, update = update
, view = view
, subscriptions = subscriptions
, onUrlRequest = UrlRequested
, onUrlChange = UrlChanged
}
| 17556 | port module Main exposing (main)
import Block
import Browser exposing (UrlRequest(..))
import Browser.Dom as Dom
import Browser.Navigation as Nav
import Dict exposing (Dict)
import Elm.Docs as Docs
import Elm.Error as Error
import Elm.Type as Type
import Errors exposing (viewError)
import File exposing (File)
import File.Select as Select
import Html exposing (Html, a, div, h1, input, li, span, text, ul)
import Html.Attributes exposing (class, href, id, placeholder, style, title, value)
import Html.Events exposing (on, onClick, onInput, preventDefaultOn)
import Html.Extra as Html exposing (viewIf)
import Http
import Json.Decode as Decode exposing (Decoder)
import Markdown
import Maybe.Extra as Maybe
import Online
import Regex
import Svg exposing (svg)
import Svg.Attributes exposing (d, fill, height, viewBox, width)
import Task
import Url exposing (Url)
import Url.Builder
import Url.Parser exposing (Parser)
import Url.Parser.Query as Query
import Utils.Markdown as Markdown
-- PORTS
--
-- Urls changes are intercepted by javascript to work-around
-- https://github.com/elm-explorations/markdown/issues/1
-- See https://github.com/dmy/elm-doc-preview/issues/1
--
-- Local documentation is stored in local storage to improve
-- navigation (mainly when going back from external links).
port locationHrefRequested : (String -> msg) -> Sub msg
port nameUpdated : (String -> msg) -> Sub msg
port compilationUpdated : (String -> msg) -> Sub msg
port readmeUpdated : (String -> msg) -> Sub msg
port docsUpdated : (String -> msg) -> Sub msg
port depsUpdated : (Decode.Value -> msg) -> Sub msg
port storeReadme : String -> Cmd msg
port storeDocs : String -> Cmd msg
port clearStorage : () -> Cmd msg
-- TYPES
type Msg
= Ignored String
| NameUpdated String
| CompilationCompleted String
| DepsUpdated Decode.Value
| OpenFilesClicked
| GotFiles File (List File)
| ClosePreviewClicked
| DocsLoaded String
| DocsRequestCompleted (Result Http.Error String)
| ReadmeLoaded String
| ReadmeRequestCompleted (Result Http.Error String)
| FilterChanged String
| OwnerChanged Owner
| LocationHrefRequested String
| UrlRequested UrlRequest
| UrlChanged Url
type alias Model =
{ name : Maybe String
, readme : Maybe String
, modules : List Docs.Module
, deps : Dict String Dep
, navKey : Nav.Key
, url : Url
, page : Page
, source : Source
, online : Bool
, error : Maybe Error.Error
, filter : String
}
type alias Dep =
{ version : String
, readme : String
, modules : List Docs.Module
}
type Page
= Readme Owner
| Module Owner String
type Owner
= Main
| Package String
type Source
= Local
| Remote LoadingState Repo
type LoadingState
= Loading
| LoadingReadme
| LoadingDocs
| Loaded
type alias Repo =
{ name : String
, version : String
}
type alias Flags =
{ readme : Maybe String
, docs : Maybe String
, online : Bool
}
-- INIT
init : Flags -> Url -> Nav.Key -> ( Model, Cmd Msg )
init flags url navKey =
let
source =
urlToSource url
( readme, modules ) =
initDoc source flags
in
( { name = Nothing
, readme = readme
, modules = modules
, deps = Dict.empty
, navKey = navKey
, url = url
, page = urlToPage url Main
, source = source
, online = flags.online
, error = Nothing
, filter = ""
}
, Cmd.batch
[ focusOpenFilesLink
, getDoc source
, scrollToFragment url
]
)
initDoc : Source -> Flags -> ( Maybe String, List Docs.Module )
initDoc source flags =
case source of
Remote _ _ ->
( Nothing, [] )
Local ->
( flags.readme
, flags.docs
|> Maybe.map decodeDocs
|> Maybe.withDefault []
)
focusOpenFilesLink : Cmd Msg
focusOpenFilesLink =
Task.attempt (\_ -> Ignored "focus") (Dom.focus "open-link")
urlToSource : Url -> Source
urlToSource url =
Url.Parser.parse repoParser { url | path = "" }
|> Maybe.join
|> Maybe.map (Remote Loading)
|> Maybe.withDefault Local
repoParser : Parser (Maybe Repo -> Maybe Repo) (Maybe Repo)
repoParser =
Url.Parser.query <|
Query.map2 repoParserHelper
(Query.string "repo")
(Query.string "version")
repoParserHelper : Maybe String -> Maybe String -> Maybe Repo
repoParserHelper maybeRepo maybeVersion =
case ( maybeRepo, maybeVersion ) of
( Just repo, Just version ) ->
Just (Repo repo version)
( Just repo, Nothing ) ->
Just (Repo repo "master")
_ ->
Nothing
getDoc : Source -> Cmd Msg
getDoc source =
case source of
Remote _ repo ->
Cmd.batch
[ Http.get
{ url = githubFileUrl repo "docs.json"
, expect = Http.expectString DocsRequestCompleted
}
, Http.get
{ url = githubFileUrl repo "README.md"
, expect = Http.expectString ReadmeRequestCompleted
}
, clearStorage ()
]
Local ->
Cmd.none
githubFileUrl : Repo -> String -> String
githubFileUrl repo file =
Url.Builder.crossOrigin "https://raw.githubusercontent.com"
[ repo.name
, repo.version
, file
]
[]
sourceQuery : Source -> String
sourceQuery source =
case source of
Remote _ repo ->
Url.Builder.toQuery
[ Url.Builder.string "repo" repo.name
, Url.Builder.string "version" repo.version
]
Local ->
""
-- VIEW
view : Model -> Browser.Document Msg
view model =
{ title = "Elm Doc Preview"
, body =
[ div
[ style "min-width" "100%"
, style "min-height" "100%"
, style "display" "flex"
, style "flex-direction" "column"
, stopDragOver
, preventDefaultOn "drop"
(Decode.map alwaysPreventDefault dropDecoder)
]
[ viewMain model
, footer
]
]
}
viewMain : Model -> Html Msg
viewMain model =
div
[ class "center"
, style "flex" "1"
, style "flex-wrap" "wrap-reverse"
, style "display" "flex"
, style "align-items" "flex-end"
]
[ case ( model.error, isLoading model ) of
( Just error, _ ) ->
compilationError error
( Nothing, True ) ->
spinner
_ ->
page model
, navigation (pageOwner model.page) model
]
dropDecoder : Decoder Msg
dropDecoder =
Decode.at [ "dataTransfer", "files" ]
(Decode.oneOrMore GotFiles File.decoder)
stopDragOver : Html.Attribute Msg
stopDragOver =
preventDefaultOn "dragover"
(Decode.map alwaysPreventDefault (Decode.succeed <| Ignored "dragover"))
alwaysPreventDefault : msg -> ( msg, Bool )
alwaysPreventDefault msg =
( msg, True )
isLoading : Model -> Bool
isLoading model =
-- Offline, waiting for websocket update
if not model.online && model.readme == Nothing then
True
else
case model.source of
Remote Loading _ ->
True
Remote LoadingReadme _ ->
True
Remote LoadingDocs _ ->
True
_ ->
False
page : Model -> Html Msg
page model =
div [ class "block-list" ] <|
case model.page of
Readme owner ->
[ Markdown.block (pageReadme owner model) ]
Module owner name ->
filteredModule model.filter
(pageModules owner model)
model.source
name
pageReadme : Owner -> Model -> String
pageReadme owner model =
case ( owner, model.readme, model.online ) of
( Main, Nothing, True ) ->
case model.modules of
[] ->
Online.readme
_ ->
Online.readmeWithModules
( Main, Just readme, _ ) ->
readme
( Package pkg, _, _ ) ->
case Dict.get pkg model.deps of
Just dep ->
dep.readme
Nothing ->
""
_ ->
""
pageModules : Owner -> Model -> List Docs.Module
pageModules owner model =
case owner of
Main ->
model.modules
Package pkg ->
case Dict.get pkg model.deps of
Just dep ->
dep.modules
Nothing ->
[]
filteredModule : String -> List Docs.Module -> Source -> String -> List (Html Msg)
filteredModule filter modules source name =
case List.filter (\m -> m.name == name) modules of
currentModule :: _ ->
doc filter modules currentModule (sourceQuery source)
_ ->
[ h1 [] [ text "Error" ]
, text ("Module \"" ++ name ++ "\" not found.")
]
spinner : Html msg
spinner =
div [ class "block-list" ] <|
[ div [ class "spinner" ]
[ div [ class "bounce1" ] []
, div [ class "bounce2" ] []
, div [ class "bounce3" ] []
]
]
compilationError : Error.Error -> Html msg
compilationError error =
div
[ class "block-list"
, style "margin-top" "24px"
]
[ viewError error ]
doc : String -> List Docs.Module -> Docs.Module -> String -> List (Html msg)
doc filter modules currentModule query =
let
info =
Block.makeInfo currentModule.name modules query
in
h1 [ class "block-list-title" ] [ text currentModule.name ]
:: List.map (Block.view filter info) (Docs.toBlocks currentModule)
-- Side navigation bar
navigation : Owner -> Model -> Html Msg
navigation owner model =
div [ class "pkg-nav" ]
[ logo
, viewIf (model.online && not (isLoading model)) <|
openLink
, viewIf model.online <|
closeLink model
, filterBox model.filter model.modules
, viewIf (model.readme /= Nothing) <|
navLinks model.source model.page [ Readme owner ]
, browseSourceLink model.source
, if String.isEmpty model.filter then
pageModules owner model
|> List.map (\m -> Module owner m.name)
|> navLinks model.source model.page
else
search model.source model.page model.filter (pageModules owner model)
, packages owner model.name model.filter model.deps
]
logo : Html msg
logo =
div
[ style "display" "flex"
, style "transform" "translateX(-8px)"
]
[ svg
[ width "50px", height "70px", viewBox "0 0 210 297" ]
[ Svg.path [ d "M61.8 132v92.7l46.3-46.3-46.3-46.3z", fill "#60b5cc" ] []
, Svg.path [ d "M83 59.9L62.9 80.2 83 100.5z", fill "#f0ad00" ] []
, Svg.path [ d "M109 184.1l-65.4 65.5H109v-65.5z", fill "#5a6378" ] []
, Svg.path [ d "M145 217.6l-32.1 32H143l32-32z", fill "#7fd13b" ] []
, Svg.path [ d "M59.4 83.5l-22.7 22.7 22.7 22.7 22.7-22.7z", fill "#7fd13b" ] []
, Svg.path [ d "M35.8 59.9v40.6L56 80.2z", fill "#f0ad00" ] []
, Svg.path [ d "M57 132l-31 31.2 31 31v-62.1z", fill "#60b5cc" ] []
]
, div
[ style "color" "#5a6378ff"
, style "line-height" "16px"
, style "padding-top" "12px"
, style "transform" "translateX(-20px)"
]
[ div [] [ text "elm doc" ]
, div [] [ text "preview" ]
]
]
openLink : Html Msg
openLink =
div []
[ a
[ id "open-link"
, style "cursor" "pointer"
, href ""
, onEnterOrSpace OpenFilesClicked
, onClick OpenFilesClicked
]
[ text "Open Files" ]
]
closeLink : Model -> Html Msg
closeLink model =
if model.readme == Nothing && model.modules == [] then
Html.nothing
else
div [ style "margin-bottom" "10px" ]
[ a
[ style "cursor" "pointer"
, href "/"
, onClick ClosePreviewClicked
]
[ text "Close Preview" ]
]
browseSourceLink : Source -> Html Msg
browseSourceLink source =
case source of
Local ->
Html.nothing
Remote _ repo ->
div [ style "margin-bottom" "20px" ]
[ a
[ style "cursor" "pointer"
, href (githubSource repo)
]
[ text "Browse Source" ]
]
filterBox : String -> List Docs.Module -> Html Msg
filterBox filter modules =
input
[ placeholder "Filter with regex"
, value filter
, onInput FilterChanged
]
[]
search : Source -> Page -> String -> List Docs.Module -> Html Msg
search source currentPage filter modules =
ul []
(List.filterMap (searchModule source currentPage filter) modules)
searchModule : Source -> Page -> String -> Docs.Module -> Maybe (Html Msg)
searchModule source currentPage filter m =
let
results =
List.concat
[ searchFrom m.binops filter
, searchFrom m.unions filter
, searchFrom m.aliases filter
, searchFrom m.values filter
]
in
if List.isEmpty results then
Nothing
else
Just <|
li [ class "pkg-nav-search-chunk" ]
[ pageLink source currentPage (Module (pageOwner currentPage) m.name)
, ul [] (List.map (searchResult source m.name) results)
]
searchFrom : List { r | name : String } -> String -> List String
searchFrom records filter =
List.filterMap
(\r ->
if contains filter r.name then
Just r.name
else
Nothing
)
records
contains : String -> String -> Bool
contains pattern str =
pattern
|> Regex.fromStringWith { caseInsensitive = True, multiline = False }
|> Maybe.map (\regex -> Regex.contains regex str)
|> Maybe.withDefault False
searchResult : Source -> String -> String -> Html Msg
searchResult source module_ symbol =
resultLink source module_ symbol
githubSource : Repo -> String
githubSource repo =
Url.Builder.crossOrigin "https://github.com"
[ repo.name
, "tree"
, repo.version
]
[]
onEnterOrSpace : msg -> Html.Attribute msg
onEnterOrSpace msg =
on "keyup"
(Decode.field "key" Decode.string
|> Decode.andThen
(\key ->
if key == "Enter" || key == " " then
Decode.succeed msg
else
Decode.fail ""
)
)
navLinks : Source -> Page -> List Page -> Html msg
navLinks source currentPage pages =
ul []
(List.map (pageLink source currentPage) pages)
pageLink : Source -> Page -> Page -> Html msg
pageLink source currentPage targetPage =
li
[ case targetPage of
Readme _ ->
style "" ""
_ ->
style "margin-left" "10px"
]
[ a
[ class "pkg-nav-module"
, href (pagePath targetPage ++ sourceQuery source)
, styleIf (currentPage == targetPage) "font-weight" "bold"
, styleIf (currentPage == targetPage) "text-decoration" "underline"
]
[ case targetPage of
Readme _ ->
text "README"
Module _ name ->
text name
]
]
resultLink : Source -> String -> String -> Html Msg
resultLink source module_ symbol =
li []
[ a
[ class "pkg-nav-module"
, (href << String.concat) <|
[ modulePath module_
, sourceQuery source
, "#"
, symbol
]
]
[ text symbol
]
]
packages : Owner -> Maybe String -> String -> Dict String Dep -> Html Msg
packages owner maybeDefault filter deps =
let
default =
Maybe.map (mainPackage owner) maybeDefault
|> Maybe.withDefault Html.nothing
dependencies =
Dict.toList deps
|> List.filter (\( name, _ ) -> contains filter name)
|> List.sortBy (String.toLower << Tuple.first)
in
ul [ style "margin-top" "20px" ] <|
(default :: List.map (dependency owner) dependencies)
dependency : Owner -> ( String, Dep ) -> Html Msg
dependency owner ( name, { version, modules } ) =
package owner name version
mainPackage : Owner -> String -> Html Msg
mainPackage owner name =
li
[ styleIf (owner == Main) "font-weight" "bold"
, styleIf (owner == Main) "text-decoration" "underline"
]
[ a [ onClick (OwnerChanged Main) ]
[ text name ]
]
package : Owner -> String -> String -> Html Msg
package owner name version =
li
[ styleIf (Package name == owner) "font-weight" "bold"
, styleIf (Package name == owner) "text-decoration" "underline"
, style "margin-left" "10px"
]
[ a [ onClick (OwnerChanged <| Package name) ]
[ text (" " ++ name ++ " " ++ version) ]
]
pagePath : Page -> String
pagePath page_ =
case page_ of
Module _ name ->
"/" ++ modulePath name
Readme _ ->
"/"
modulePath : String -> String
modulePath name =
slugify name
styleIf : Bool -> String -> String -> Html.Attribute msg
styleIf cond property value =
if cond then
style property value
else
style "" ""
slugify : String -> String
slugify str =
String.replace "." "-" str
unslugify : String -> String
unslugify str =
String.replace "-" "." str
-- Footer
footer : Html msg
footer =
div [ class "footer" ]
[ span []
[ text "elm-doc-preview is "
, a
[ class "grey-link"
, href "https://github.com/dmy/elm-doc-preview"
]
[ text "open source." ]
]
, text " "
, span [] [ text "Copyright © 2019 <NAME>." ]
]
-- Files handling
selectFiles : Cmd Msg
selectFiles =
Select.files
-- Browsers do not agree on mime types :/
[ "text/plain", "text/markdown", "application/json", ".md" ]
GotFiles
readFiles : List File -> Cmd Msg
readFiles files =
Cmd.batch (List.map readFile files)
readFile : File -> Cmd Msg
readFile file =
case ( File.name file, File.mime file ) of
( "README.md", _ ) ->
Task.perform ReadmeLoaded (File.toString file)
( "docs.json", "application/json" ) ->
Task.perform DocsLoaded (File.toString file)
_ ->
Cmd.none
decodeDocs : String -> List Docs.Module
decodeDocs docs =
case Decode.decodeString (Decode.list Docs.decoder) docs of
Ok modules ->
modules
Err _ ->
[]
decodeDeps : Decode.Value -> Dict String Dep
decodeDeps value =
case Decode.decodeValue (Decode.dict depDecoder) value of
Ok deps ->
deps
Err err ->
Dict.empty
depDecoder : Decoder Dep
depDecoder =
Decode.map3 Dep
(Decode.field "version" Decode.string)
(Decode.field "readme" Decode.string)
(Decode.field "docs" <| Decode.map decodeDocs Decode.string)
-- UPDATE
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
Ignored _ ->
( model, Cmd.none )
NameUpdated name ->
( { model | name = Just name }, Cmd.none )
CompilationCompleted error ->
( setError error model, Cmd.none )
DepsUpdated deps ->
( { model | deps = decodeDeps deps }, Cmd.none )
OpenFilesClicked ->
( model, selectFiles )
GotFiles file files ->
( { model | source = Local }, readFiles (file :: files) )
ClosePreviewClicked ->
( closePreview model, clearStorage () )
DocsLoaded docs ->
( setModules (decodeDocs docs) model
, Cmd.batch
[ scrollToFragment model.url
, storeDocs docs
]
)
DocsRequestCompleted (Ok docs) ->
( setModules (decodeDocs docs) model, scrollToFragment model.url )
DocsRequestCompleted (Err error) ->
( setModules [] model, Cmd.none )
ReadmeLoaded readme ->
( setReadme (Just readme) model
, Cmd.batch
[ scrollToFragment model.url
, storeReadme readme
]
)
ReadmeRequestCompleted (Ok readme) ->
( setReadme (Just readme) model, scrollToFragment model.url )
ReadmeRequestCompleted (Err error) ->
( setReadme Nothing model, Cmd.none )
FilterChanged filterValue ->
( { model | filter = filterValue }, Cmd.none )
OwnerChanged owner ->
( { model | page = Readme owner, filter = "" }, Cmd.none )
LocationHrefRequested href ->
( model, requestLocationHref model.navKey href )
UrlRequested request ->
-- Url requests are intercepted by javascript
-- and handled by LocationHrefRequested to work-around
-- unhandled links in markdown blocks.
( model, Cmd.none )
UrlChanged url ->
( { model
| page = urlToPage url (pageOwner model.page)
, url = url
}
, addUrlQuery model url
)
addUrlQuery : Model -> Url -> Cmd Msg
addUrlQuery model url =
case ( url /= model.url, sourceQuery model.source ) of
( False, _ ) ->
Cmd.none
( True, "" ) ->
scrollToFragment url
( True, query ) ->
Nav.replaceUrl model.navKey <|
Url.toString <|
{ url | query = Just (String.dropLeft 1 query) }
setError : String -> Model -> Model
setError errorJsonString model =
case Decode.decodeString Error.decoder errorJsonString of
Ok error ->
{ model | error = Just error }
Err _ ->
{ model | error = Nothing }
setReadme : Maybe String -> Model -> Model
setReadme readme model =
{ model
| readme = readme
, source =
case model.source of
Remote Loading repo ->
Remote LoadingDocs repo
Remote LoadingReadme repo ->
Remote Loaded repo
_ ->
model.source
}
setModules : List Docs.Module -> Model -> Model
setModules modules model =
{ model
| modules = modules
, source =
case model.source of
Remote Loading repo ->
Remote LoadingReadme repo
Remote LoadingDocs repo ->
Remote Loaded repo
_ ->
model.source
}
scrollToFragment : Url -> Cmd Msg
scrollToFragment url =
case url.fragment of
Just id ->
Dom.getElement id
|> Task.andThen (\e -> Dom.setViewport 0 e.element.y)
|> Task.attempt (\_ -> Ignored "scroll")
Nothing ->
Cmd.none
closePreview : Model -> Model
closePreview model =
{ model
| readme = Nothing
, modules = []
, page = Readme Main
, source = Local
, error = Nothing
, filter = ""
}
requestLocationHref : Nav.Key -> String -> Cmd Msg
requestLocationHref navKey href =
case Url.fromString href of
Just url ->
-- Complete URL are expected to be external
Nav.load href
Nothing ->
Nav.pushUrl navKey href
urlToPage : Url -> Owner -> Page
urlToPage url owner =
case url.path of
"/" ->
Readme owner
_ ->
Module owner (unslugify <| String.dropLeft 1 <| url.path)
pageOwner : Page -> Owner
pageOwner page_ =
case page_ of
Readme readmeOwner ->
readmeOwner
Module moduleOwner _ ->
moduleOwner
-- SUBSCRIPTIONS
subscriptions : Model -> Sub Msg
subscriptions model =
Sub.batch
[ locationHrefRequested LocationHrefRequested
, nameUpdated NameUpdated
, compilationUpdated CompilationCompleted
, readmeUpdated ReadmeLoaded
, docsUpdated DocsLoaded
, depsUpdated DepsUpdated
]
-- MAIN
main : Program Flags Model Msg
main =
Browser.application
{ init = init
, update = update
, view = view
, subscriptions = subscriptions
, onUrlRequest = UrlRequested
, onUrlChange = UrlChanged
}
| true | port module Main exposing (main)
import Block
import Browser exposing (UrlRequest(..))
import Browser.Dom as Dom
import Browser.Navigation as Nav
import Dict exposing (Dict)
import Elm.Docs as Docs
import Elm.Error as Error
import Elm.Type as Type
import Errors exposing (viewError)
import File exposing (File)
import File.Select as Select
import Html exposing (Html, a, div, h1, input, li, span, text, ul)
import Html.Attributes exposing (class, href, id, placeholder, style, title, value)
import Html.Events exposing (on, onClick, onInput, preventDefaultOn)
import Html.Extra as Html exposing (viewIf)
import Http
import Json.Decode as Decode exposing (Decoder)
import Markdown
import Maybe.Extra as Maybe
import Online
import Regex
import Svg exposing (svg)
import Svg.Attributes exposing (d, fill, height, viewBox, width)
import Task
import Url exposing (Url)
import Url.Builder
import Url.Parser exposing (Parser)
import Url.Parser.Query as Query
import Utils.Markdown as Markdown
-- PORTS
--
-- Urls changes are intercepted by javascript to work-around
-- https://github.com/elm-explorations/markdown/issues/1
-- See https://github.com/dmy/elm-doc-preview/issues/1
--
-- Local documentation is stored in local storage to improve
-- navigation (mainly when going back from external links).
port locationHrefRequested : (String -> msg) -> Sub msg
port nameUpdated : (String -> msg) -> Sub msg
port compilationUpdated : (String -> msg) -> Sub msg
port readmeUpdated : (String -> msg) -> Sub msg
port docsUpdated : (String -> msg) -> Sub msg
port depsUpdated : (Decode.Value -> msg) -> Sub msg
port storeReadme : String -> Cmd msg
port storeDocs : String -> Cmd msg
port clearStorage : () -> Cmd msg
-- TYPES
type Msg
= Ignored String
| NameUpdated String
| CompilationCompleted String
| DepsUpdated Decode.Value
| OpenFilesClicked
| GotFiles File (List File)
| ClosePreviewClicked
| DocsLoaded String
| DocsRequestCompleted (Result Http.Error String)
| ReadmeLoaded String
| ReadmeRequestCompleted (Result Http.Error String)
| FilterChanged String
| OwnerChanged Owner
| LocationHrefRequested String
| UrlRequested UrlRequest
| UrlChanged Url
type alias Model =
{ name : Maybe String
, readme : Maybe String
, modules : List Docs.Module
, deps : Dict String Dep
, navKey : Nav.Key
, url : Url
, page : Page
, source : Source
, online : Bool
, error : Maybe Error.Error
, filter : String
}
type alias Dep =
{ version : String
, readme : String
, modules : List Docs.Module
}
type Page
= Readme Owner
| Module Owner String
type Owner
= Main
| Package String
type Source
= Local
| Remote LoadingState Repo
type LoadingState
= Loading
| LoadingReadme
| LoadingDocs
| Loaded
type alias Repo =
{ name : String
, version : String
}
type alias Flags =
{ readme : Maybe String
, docs : Maybe String
, online : Bool
}
-- INIT
init : Flags -> Url -> Nav.Key -> ( Model, Cmd Msg )
init flags url navKey =
let
source =
urlToSource url
( readme, modules ) =
initDoc source flags
in
( { name = Nothing
, readme = readme
, modules = modules
, deps = Dict.empty
, navKey = navKey
, url = url
, page = urlToPage url Main
, source = source
, online = flags.online
, error = Nothing
, filter = ""
}
, Cmd.batch
[ focusOpenFilesLink
, getDoc source
, scrollToFragment url
]
)
initDoc : Source -> Flags -> ( Maybe String, List Docs.Module )
initDoc source flags =
case source of
Remote _ _ ->
( Nothing, [] )
Local ->
( flags.readme
, flags.docs
|> Maybe.map decodeDocs
|> Maybe.withDefault []
)
focusOpenFilesLink : Cmd Msg
focusOpenFilesLink =
Task.attempt (\_ -> Ignored "focus") (Dom.focus "open-link")
urlToSource : Url -> Source
urlToSource url =
Url.Parser.parse repoParser { url | path = "" }
|> Maybe.join
|> Maybe.map (Remote Loading)
|> Maybe.withDefault Local
repoParser : Parser (Maybe Repo -> Maybe Repo) (Maybe Repo)
repoParser =
Url.Parser.query <|
Query.map2 repoParserHelper
(Query.string "repo")
(Query.string "version")
repoParserHelper : Maybe String -> Maybe String -> Maybe Repo
repoParserHelper maybeRepo maybeVersion =
case ( maybeRepo, maybeVersion ) of
( Just repo, Just version ) ->
Just (Repo repo version)
( Just repo, Nothing ) ->
Just (Repo repo "master")
_ ->
Nothing
getDoc : Source -> Cmd Msg
getDoc source =
case source of
Remote _ repo ->
Cmd.batch
[ Http.get
{ url = githubFileUrl repo "docs.json"
, expect = Http.expectString DocsRequestCompleted
}
, Http.get
{ url = githubFileUrl repo "README.md"
, expect = Http.expectString ReadmeRequestCompleted
}
, clearStorage ()
]
Local ->
Cmd.none
githubFileUrl : Repo -> String -> String
githubFileUrl repo file =
Url.Builder.crossOrigin "https://raw.githubusercontent.com"
[ repo.name
, repo.version
, file
]
[]
sourceQuery : Source -> String
sourceQuery source =
case source of
Remote _ repo ->
Url.Builder.toQuery
[ Url.Builder.string "repo" repo.name
, Url.Builder.string "version" repo.version
]
Local ->
""
-- VIEW
view : Model -> Browser.Document Msg
view model =
{ title = "Elm Doc Preview"
, body =
[ div
[ style "min-width" "100%"
, style "min-height" "100%"
, style "display" "flex"
, style "flex-direction" "column"
, stopDragOver
, preventDefaultOn "drop"
(Decode.map alwaysPreventDefault dropDecoder)
]
[ viewMain model
, footer
]
]
}
viewMain : Model -> Html Msg
viewMain model =
div
[ class "center"
, style "flex" "1"
, style "flex-wrap" "wrap-reverse"
, style "display" "flex"
, style "align-items" "flex-end"
]
[ case ( model.error, isLoading model ) of
( Just error, _ ) ->
compilationError error
( Nothing, True ) ->
spinner
_ ->
page model
, navigation (pageOwner model.page) model
]
dropDecoder : Decoder Msg
dropDecoder =
Decode.at [ "dataTransfer", "files" ]
(Decode.oneOrMore GotFiles File.decoder)
stopDragOver : Html.Attribute Msg
stopDragOver =
preventDefaultOn "dragover"
(Decode.map alwaysPreventDefault (Decode.succeed <| Ignored "dragover"))
alwaysPreventDefault : msg -> ( msg, Bool )
alwaysPreventDefault msg =
( msg, True )
isLoading : Model -> Bool
isLoading model =
-- Offline, waiting for websocket update
if not model.online && model.readme == Nothing then
True
else
case model.source of
Remote Loading _ ->
True
Remote LoadingReadme _ ->
True
Remote LoadingDocs _ ->
True
_ ->
False
page : Model -> Html Msg
page model =
div [ class "block-list" ] <|
case model.page of
Readme owner ->
[ Markdown.block (pageReadme owner model) ]
Module owner name ->
filteredModule model.filter
(pageModules owner model)
model.source
name
pageReadme : Owner -> Model -> String
pageReadme owner model =
case ( owner, model.readme, model.online ) of
( Main, Nothing, True ) ->
case model.modules of
[] ->
Online.readme
_ ->
Online.readmeWithModules
( Main, Just readme, _ ) ->
readme
( Package pkg, _, _ ) ->
case Dict.get pkg model.deps of
Just dep ->
dep.readme
Nothing ->
""
_ ->
""
pageModules : Owner -> Model -> List Docs.Module
pageModules owner model =
case owner of
Main ->
model.modules
Package pkg ->
case Dict.get pkg model.deps of
Just dep ->
dep.modules
Nothing ->
[]
filteredModule : String -> List Docs.Module -> Source -> String -> List (Html Msg)
filteredModule filter modules source name =
case List.filter (\m -> m.name == name) modules of
currentModule :: _ ->
doc filter modules currentModule (sourceQuery source)
_ ->
[ h1 [] [ text "Error" ]
, text ("Module \"" ++ name ++ "\" not found.")
]
spinner : Html msg
spinner =
div [ class "block-list" ] <|
[ div [ class "spinner" ]
[ div [ class "bounce1" ] []
, div [ class "bounce2" ] []
, div [ class "bounce3" ] []
]
]
compilationError : Error.Error -> Html msg
compilationError error =
div
[ class "block-list"
, style "margin-top" "24px"
]
[ viewError error ]
doc : String -> List Docs.Module -> Docs.Module -> String -> List (Html msg)
doc filter modules currentModule query =
let
info =
Block.makeInfo currentModule.name modules query
in
h1 [ class "block-list-title" ] [ text currentModule.name ]
:: List.map (Block.view filter info) (Docs.toBlocks currentModule)
-- Side navigation bar
navigation : Owner -> Model -> Html Msg
navigation owner model =
div [ class "pkg-nav" ]
[ logo
, viewIf (model.online && not (isLoading model)) <|
openLink
, viewIf model.online <|
closeLink model
, filterBox model.filter model.modules
, viewIf (model.readme /= Nothing) <|
navLinks model.source model.page [ Readme owner ]
, browseSourceLink model.source
, if String.isEmpty model.filter then
pageModules owner model
|> List.map (\m -> Module owner m.name)
|> navLinks model.source model.page
else
search model.source model.page model.filter (pageModules owner model)
, packages owner model.name model.filter model.deps
]
logo : Html msg
logo =
div
[ style "display" "flex"
, style "transform" "translateX(-8px)"
]
[ svg
[ width "50px", height "70px", viewBox "0 0 210 297" ]
[ Svg.path [ d "M61.8 132v92.7l46.3-46.3-46.3-46.3z", fill "#60b5cc" ] []
, Svg.path [ d "M83 59.9L62.9 80.2 83 100.5z", fill "#f0ad00" ] []
, Svg.path [ d "M109 184.1l-65.4 65.5H109v-65.5z", fill "#5a6378" ] []
, Svg.path [ d "M145 217.6l-32.1 32H143l32-32z", fill "#7fd13b" ] []
, Svg.path [ d "M59.4 83.5l-22.7 22.7 22.7 22.7 22.7-22.7z", fill "#7fd13b" ] []
, Svg.path [ d "M35.8 59.9v40.6L56 80.2z", fill "#f0ad00" ] []
, Svg.path [ d "M57 132l-31 31.2 31 31v-62.1z", fill "#60b5cc" ] []
]
, div
[ style "color" "#5a6378ff"
, style "line-height" "16px"
, style "padding-top" "12px"
, style "transform" "translateX(-20px)"
]
[ div [] [ text "elm doc" ]
, div [] [ text "preview" ]
]
]
openLink : Html Msg
openLink =
div []
[ a
[ id "open-link"
, style "cursor" "pointer"
, href ""
, onEnterOrSpace OpenFilesClicked
, onClick OpenFilesClicked
]
[ text "Open Files" ]
]
closeLink : Model -> Html Msg
closeLink model =
if model.readme == Nothing && model.modules == [] then
Html.nothing
else
div [ style "margin-bottom" "10px" ]
[ a
[ style "cursor" "pointer"
, href "/"
, onClick ClosePreviewClicked
]
[ text "Close Preview" ]
]
browseSourceLink : Source -> Html Msg
browseSourceLink source =
case source of
Local ->
Html.nothing
Remote _ repo ->
div [ style "margin-bottom" "20px" ]
[ a
[ style "cursor" "pointer"
, href (githubSource repo)
]
[ text "Browse Source" ]
]
filterBox : String -> List Docs.Module -> Html Msg
filterBox filter modules =
input
[ placeholder "Filter with regex"
, value filter
, onInput FilterChanged
]
[]
search : Source -> Page -> String -> List Docs.Module -> Html Msg
search source currentPage filter modules =
ul []
(List.filterMap (searchModule source currentPage filter) modules)
searchModule : Source -> Page -> String -> Docs.Module -> Maybe (Html Msg)
searchModule source currentPage filter m =
let
results =
List.concat
[ searchFrom m.binops filter
, searchFrom m.unions filter
, searchFrom m.aliases filter
, searchFrom m.values filter
]
in
if List.isEmpty results then
Nothing
else
Just <|
li [ class "pkg-nav-search-chunk" ]
[ pageLink source currentPage (Module (pageOwner currentPage) m.name)
, ul [] (List.map (searchResult source m.name) results)
]
searchFrom : List { r | name : String } -> String -> List String
searchFrom records filter =
List.filterMap
(\r ->
if contains filter r.name then
Just r.name
else
Nothing
)
records
contains : String -> String -> Bool
contains pattern str =
pattern
|> Regex.fromStringWith { caseInsensitive = True, multiline = False }
|> Maybe.map (\regex -> Regex.contains regex str)
|> Maybe.withDefault False
searchResult : Source -> String -> String -> Html Msg
searchResult source module_ symbol =
resultLink source module_ symbol
githubSource : Repo -> String
githubSource repo =
Url.Builder.crossOrigin "https://github.com"
[ repo.name
, "tree"
, repo.version
]
[]
onEnterOrSpace : msg -> Html.Attribute msg
onEnterOrSpace msg =
on "keyup"
(Decode.field "key" Decode.string
|> Decode.andThen
(\key ->
if key == "Enter" || key == " " then
Decode.succeed msg
else
Decode.fail ""
)
)
navLinks : Source -> Page -> List Page -> Html msg
navLinks source currentPage pages =
ul []
(List.map (pageLink source currentPage) pages)
pageLink : Source -> Page -> Page -> Html msg
pageLink source currentPage targetPage =
li
[ case targetPage of
Readme _ ->
style "" ""
_ ->
style "margin-left" "10px"
]
[ a
[ class "pkg-nav-module"
, href (pagePath targetPage ++ sourceQuery source)
, styleIf (currentPage == targetPage) "font-weight" "bold"
, styleIf (currentPage == targetPage) "text-decoration" "underline"
]
[ case targetPage of
Readme _ ->
text "README"
Module _ name ->
text name
]
]
resultLink : Source -> String -> String -> Html Msg
resultLink source module_ symbol =
li []
[ a
[ class "pkg-nav-module"
, (href << String.concat) <|
[ modulePath module_
, sourceQuery source
, "#"
, symbol
]
]
[ text symbol
]
]
packages : Owner -> Maybe String -> String -> Dict String Dep -> Html Msg
packages owner maybeDefault filter deps =
let
default =
Maybe.map (mainPackage owner) maybeDefault
|> Maybe.withDefault Html.nothing
dependencies =
Dict.toList deps
|> List.filter (\( name, _ ) -> contains filter name)
|> List.sortBy (String.toLower << Tuple.first)
in
ul [ style "margin-top" "20px" ] <|
(default :: List.map (dependency owner) dependencies)
dependency : Owner -> ( String, Dep ) -> Html Msg
dependency owner ( name, { version, modules } ) =
package owner name version
mainPackage : Owner -> String -> Html Msg
mainPackage owner name =
li
[ styleIf (owner == Main) "font-weight" "bold"
, styleIf (owner == Main) "text-decoration" "underline"
]
[ a [ onClick (OwnerChanged Main) ]
[ text name ]
]
package : Owner -> String -> String -> Html Msg
package owner name version =
li
[ styleIf (Package name == owner) "font-weight" "bold"
, styleIf (Package name == owner) "text-decoration" "underline"
, style "margin-left" "10px"
]
[ a [ onClick (OwnerChanged <| Package name) ]
[ text (" " ++ name ++ " " ++ version) ]
]
pagePath : Page -> String
pagePath page_ =
case page_ of
Module _ name ->
"/" ++ modulePath name
Readme _ ->
"/"
modulePath : String -> String
modulePath name =
slugify name
styleIf : Bool -> String -> String -> Html.Attribute msg
styleIf cond property value =
if cond then
style property value
else
style "" ""
slugify : String -> String
slugify str =
String.replace "." "-" str
unslugify : String -> String
unslugify str =
String.replace "-" "." str
-- Footer
footer : Html msg
footer =
div [ class "footer" ]
[ span []
[ text "elm-doc-preview is "
, a
[ class "grey-link"
, href "https://github.com/dmy/elm-doc-preview"
]
[ text "open source." ]
]
, text " "
, span [] [ text "Copyright © 2019 PI:NAME:<NAME>END_PI." ]
]
-- Files handling
selectFiles : Cmd Msg
selectFiles =
Select.files
-- Browsers do not agree on mime types :/
[ "text/plain", "text/markdown", "application/json", ".md" ]
GotFiles
readFiles : List File -> Cmd Msg
readFiles files =
Cmd.batch (List.map readFile files)
readFile : File -> Cmd Msg
readFile file =
case ( File.name file, File.mime file ) of
( "README.md", _ ) ->
Task.perform ReadmeLoaded (File.toString file)
( "docs.json", "application/json" ) ->
Task.perform DocsLoaded (File.toString file)
_ ->
Cmd.none
decodeDocs : String -> List Docs.Module
decodeDocs docs =
case Decode.decodeString (Decode.list Docs.decoder) docs of
Ok modules ->
modules
Err _ ->
[]
decodeDeps : Decode.Value -> Dict String Dep
decodeDeps value =
case Decode.decodeValue (Decode.dict depDecoder) value of
Ok deps ->
deps
Err err ->
Dict.empty
depDecoder : Decoder Dep
depDecoder =
Decode.map3 Dep
(Decode.field "version" Decode.string)
(Decode.field "readme" Decode.string)
(Decode.field "docs" <| Decode.map decodeDocs Decode.string)
-- UPDATE
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
Ignored _ ->
( model, Cmd.none )
NameUpdated name ->
( { model | name = Just name }, Cmd.none )
CompilationCompleted error ->
( setError error model, Cmd.none )
DepsUpdated deps ->
( { model | deps = decodeDeps deps }, Cmd.none )
OpenFilesClicked ->
( model, selectFiles )
GotFiles file files ->
( { model | source = Local }, readFiles (file :: files) )
ClosePreviewClicked ->
( closePreview model, clearStorage () )
DocsLoaded docs ->
( setModules (decodeDocs docs) model
, Cmd.batch
[ scrollToFragment model.url
, storeDocs docs
]
)
DocsRequestCompleted (Ok docs) ->
( setModules (decodeDocs docs) model, scrollToFragment model.url )
DocsRequestCompleted (Err error) ->
( setModules [] model, Cmd.none )
ReadmeLoaded readme ->
( setReadme (Just readme) model
, Cmd.batch
[ scrollToFragment model.url
, storeReadme readme
]
)
ReadmeRequestCompleted (Ok readme) ->
( setReadme (Just readme) model, scrollToFragment model.url )
ReadmeRequestCompleted (Err error) ->
( setReadme Nothing model, Cmd.none )
FilterChanged filterValue ->
( { model | filter = filterValue }, Cmd.none )
OwnerChanged owner ->
( { model | page = Readme owner, filter = "" }, Cmd.none )
LocationHrefRequested href ->
( model, requestLocationHref model.navKey href )
UrlRequested request ->
-- Url requests are intercepted by javascript
-- and handled by LocationHrefRequested to work-around
-- unhandled links in markdown blocks.
( model, Cmd.none )
UrlChanged url ->
( { model
| page = urlToPage url (pageOwner model.page)
, url = url
}
, addUrlQuery model url
)
addUrlQuery : Model -> Url -> Cmd Msg
addUrlQuery model url =
case ( url /= model.url, sourceQuery model.source ) of
( False, _ ) ->
Cmd.none
( True, "" ) ->
scrollToFragment url
( True, query ) ->
Nav.replaceUrl model.navKey <|
Url.toString <|
{ url | query = Just (String.dropLeft 1 query) }
setError : String -> Model -> Model
setError errorJsonString model =
case Decode.decodeString Error.decoder errorJsonString of
Ok error ->
{ model | error = Just error }
Err _ ->
{ model | error = Nothing }
setReadme : Maybe String -> Model -> Model
setReadme readme model =
{ model
| readme = readme
, source =
case model.source of
Remote Loading repo ->
Remote LoadingDocs repo
Remote LoadingReadme repo ->
Remote Loaded repo
_ ->
model.source
}
setModules : List Docs.Module -> Model -> Model
setModules modules model =
{ model
| modules = modules
, source =
case model.source of
Remote Loading repo ->
Remote LoadingReadme repo
Remote LoadingDocs repo ->
Remote Loaded repo
_ ->
model.source
}
scrollToFragment : Url -> Cmd Msg
scrollToFragment url =
case url.fragment of
Just id ->
Dom.getElement id
|> Task.andThen (\e -> Dom.setViewport 0 e.element.y)
|> Task.attempt (\_ -> Ignored "scroll")
Nothing ->
Cmd.none
closePreview : Model -> Model
closePreview model =
{ model
| readme = Nothing
, modules = []
, page = Readme Main
, source = Local
, error = Nothing
, filter = ""
}
requestLocationHref : Nav.Key -> String -> Cmd Msg
requestLocationHref navKey href =
case Url.fromString href of
Just url ->
-- Complete URL are expected to be external
Nav.load href
Nothing ->
Nav.pushUrl navKey href
urlToPage : Url -> Owner -> Page
urlToPage url owner =
case url.path of
"/" ->
Readme owner
_ ->
Module owner (unslugify <| String.dropLeft 1 <| url.path)
pageOwner : Page -> Owner
pageOwner page_ =
case page_ of
Readme readmeOwner ->
readmeOwner
Module moduleOwner _ ->
moduleOwner
-- SUBSCRIPTIONS
subscriptions : Model -> Sub Msg
subscriptions model =
Sub.batch
[ locationHrefRequested LocationHrefRequested
, nameUpdated NameUpdated
, compilationUpdated CompilationCompleted
, readmeUpdated ReadmeLoaded
, docsUpdated DocsLoaded
, depsUpdated DepsUpdated
]
-- MAIN
main : Program Flags Model Msg
main =
Browser.application
{ init = init
, update = update
, view = view
, subscriptions = subscriptions
, onUrlRequest = UrlRequested
, onUrlChange = UrlChanged
}
| elm |
[
{
"context": " \\_ ->\n Scores.register \"Paul\"\n |> Expect.all\n ",
"end": 1323,
"score": 0.9986045957,
"start": 1319,
"tag": "NAME",
"value": "Paul"
},
{
"context": "\n |> Expect.equal \"Paul\"\n , \\score ->\n ",
"end": 1529,
"score": 0.9988070726,
"start": 1525,
"tag": "NAME",
"value": "Paul"
},
{
"context": " \\_ ->\n Scores.register \"Paul\"\n |> Scores.incrementScore 10\n",
"end": 1816,
"score": 0.9979066253,
"start": 1812,
"tag": "NAME",
"value": "Paul"
},
{
"context": " \\_ ->\n Scores.register \"Paul\"\n |> Scores.decrementScore 10\n",
"end": 2032,
"score": 0.9985734224,
"start": 2028,
"tag": "NAME",
"value": "Paul"
},
{
"context": " Scores.scoreChange\n (\"Paul\"\n |> Scores.regist",
"end": 2371,
"score": 0.9985517859,
"start": 2367,
"tag": "NAME",
"value": "Paul"
},
{
"context": " Scores.scoreChange\n (\"Paul\"\n |> Scores.regist",
"end": 2785,
"score": 0.9982489347,
"start": 2781,
"tag": "NAME",
"value": "Paul"
},
{
"context": " |> Scores.gameOver\n (\"Paul\"\n |> Scores.regist",
"end": 3273,
"score": 0.9985471368,
"start": 3269,
"tag": "NAME",
"value": "Paul"
},
{
"context": " |> Scores.gameOver\n (\"Paul\"\n |> Scores.regist",
"end": 3676,
"score": 0.9991022348,
"start": 3672,
"tag": "NAME",
"value": "Paul"
},
{
"context": " |> Scores.gameOver\n (\"Paul\"\n |> Scores.register\n ",
"end": 4102,
"score": 0.998703599,
"start": 4098,
"tag": "NAME",
"value": "Paul"
},
{
"context": " Expect.equal\n [ { name = \"Paul\", points = 10 }\n , { name ",
"end": 4354,
"score": 0.99720186,
"start": 4350,
"tag": "NAME",
"value": "Paul"
},
{
"context": " |> Scores.gameOver\n (\"Paul\"\n |> Scores.register\n ",
"end": 5028,
"score": 0.998508513,
"start": 5024,
"tag": "NAME",
"value": "Paul"
},
{
"context": " |> Scores.gameOver\n (\"Paul\"\n |> Scores.register\n ",
"end": 5408,
"score": 0.9987900257,
"start": 5404,
"tag": "NAME",
"value": "Paul"
},
{
"context": " |> Scores.gameOver\n (\"Paul\"\n |> Scores.register\n ",
"end": 5784,
"score": 0.9981335998,
"start": 5780,
"tag": "NAME",
"value": "Paul"
},
{
"context": " Expect.equal\n [ { name = \"Paul\", points = 10 }\n , { name ",
"end": 6405,
"score": 0.9980289936,
"start": 6401,
"tag": "NAME",
"value": "Paul"
},
{
"context": " |> Scores.scoreChange\n (\"Paul\"\n |> Scores.register\n ",
"end": 7090,
"score": 0.9993824959,
"start": 7086,
"tag": "NAME",
"value": "Paul"
},
{
"context": "test \"name\" <|\n \\_ ->\n \"Paul\"\n |> Scores.register\n ",
"end": 7372,
"score": 0.9992309809,
"start": 7368,
"tag": "NAME",
"value": "Paul"
},
{
"context": " Scores.name\n |> Expect.equal \"Paul\"\n , test \"points\" <|\n \\_ ->\n ",
"end": 7489,
"score": 0.9980287552,
"start": 7485,
"tag": "NAME",
"value": "Paul"
},
{
"context": "st \"points\" <|\n \\_ ->\n \"Paul\"\n |> Scores.register\n ",
"end": 7557,
"score": 0.999002099,
"start": 7553,
"tag": "NAME",
"value": "Paul"
},
{
"context": "updateName\" <|\n \\_ ->\n \"Paul\"\n |> Scores.register\n ",
"end": 7932,
"score": 0.9994374514,
"start": 7928,
"tag": "NAME",
"value": "Paul"
},
{
"context": "egister\n |> Scores.updateName \"Steve\"\n |> Scores.name\n ",
"end": 8020,
"score": 0.9993528128,
"start": 8015,
"tag": "NAME",
"value": "Steve"
},
{
"context": " Scores.name\n |> Expect.equal \"Steve\"\n ]\n",
"end": 8099,
"score": 0.9994241595,
"start": 8094,
"tag": "NAME",
"value": "Steve"
}
] | tests/Shared/ScoresTests.elm | phollyer/elm-space-invaders | 0 | module Shared.ScoresTests exposing (suite)
import Expect
import Fuzz exposing (intRange)
import Shared.Scores as Scores exposing (Score, Scores)
import Test exposing (Test, describe, fuzz, fuzz2, test)
init : Scores
init =
Scores.init
10
[]
suite : Test
suite =
describe "The Scores Module"
[ describe "init"
[ fuzz (intRange 0 100) "with no highscores" <|
\total ->
Scores.init
total
[]
|> Scores.total
|> Expect.equal total
, fuzz2 (intRange 1 10) (intRange 10 100) "with too many highscores" <|
\total range ->
Scores.init
total
(range
|> List.range 10
|> List.map
(\index ->
{ name = ""
, points = 0
}
)
)
|> Scores.total
|> Expect.equal total
]
, test "register" <|
\_ ->
Scores.register "Paul"
|> Expect.all
[ \score ->
score
|> Scores.name
|> Expect.equal "Paul"
, \score ->
score
|> Scores.points
|> Expect.equal 0
]
, test "incrementScore" <|
\_ ->
Scores.register "Paul"
|> Scores.incrementScore 10
|> Scores.points
|> Expect.equal 10
, test "decrementScore" <|
\_ ->
Scores.register "Paul"
|> Scores.decrementScore 10
|> Scores.points
|> Expect.equal -10
, describe "scoreChange"
[ test "updates the current highest" <|
\_ ->
init
|> Scores.scoreChange
("Paul"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.highestToString
|> Expect.equal "10"
, test "does not update the list" <|
\_ ->
init
|> Scores.scoreChange
("Paul"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.highest
|> Scores.points
|> Expect.equal 0
]
, describe "gameOver"
[ test "updates the current highest" <|
\_ ->
init
|> Scores.gameOver
("Paul"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.highestToString
|> Expect.equal "10"
, test "updates the list" <|
\_ ->
init
|> Scores.gameOver
("Paul"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.highest
|> Scores.points
|> Expect.equal 10
]
, test "prepareForSave" <|
\_ ->
init
|> Scores.gameOver
("Paul"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.prepareForSave
|> Expect.equal
[ { name = "Paul", points = 10 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
]
, test "highest" <|
\_ ->
init
|> Scores.gameOver
("Paul"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.highest
|> Scores.points
|> Expect.equal 10
, test "lowest" <|
\_ ->
init
|> Scores.gameOver
("Paul"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.lowest
|> Scores.points
|> Expect.equal 0
, test "list" <|
\_ ->
init
|> Scores.gameOver
("Paul"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.list
|> List.map
(\score ->
{ name =
score
|> Scores.name
, points =
score
|> Scores.points
}
)
|> Expect.equal
[ { name = "Paul", points = 10 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
]
, test "highestToString" <|
\_ ->
init
|> Scores.scoreChange
("Paul"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.highestToString
|> Expect.equal "10"
, test "name" <|
\_ ->
"Paul"
|> Scores.register
|> Scores.name
|> Expect.equal "Paul"
, test "points" <|
\_ ->
"Paul"
|> Scores.register
|> Scores.incrementScore 10
|> Scores.points
|> Expect.equal 10
, test "total" <|
\_ ->
init
|> Scores.total
|> Expect.equal 10
, test "updateName" <|
\_ ->
"Paul"
|> Scores.register
|> Scores.updateName "Steve"
|> Scores.name
|> Expect.equal "Steve"
]
| 36515 | module Shared.ScoresTests exposing (suite)
import Expect
import Fuzz exposing (intRange)
import Shared.Scores as Scores exposing (Score, Scores)
import Test exposing (Test, describe, fuzz, fuzz2, test)
init : Scores
init =
Scores.init
10
[]
suite : Test
suite =
describe "The Scores Module"
[ describe "init"
[ fuzz (intRange 0 100) "with no highscores" <|
\total ->
Scores.init
total
[]
|> Scores.total
|> Expect.equal total
, fuzz2 (intRange 1 10) (intRange 10 100) "with too many highscores" <|
\total range ->
Scores.init
total
(range
|> List.range 10
|> List.map
(\index ->
{ name = ""
, points = 0
}
)
)
|> Scores.total
|> Expect.equal total
]
, test "register" <|
\_ ->
Scores.register "<NAME>"
|> Expect.all
[ \score ->
score
|> Scores.name
|> Expect.equal "<NAME>"
, \score ->
score
|> Scores.points
|> Expect.equal 0
]
, test "incrementScore" <|
\_ ->
Scores.register "<NAME>"
|> Scores.incrementScore 10
|> Scores.points
|> Expect.equal 10
, test "decrementScore" <|
\_ ->
Scores.register "<NAME>"
|> Scores.decrementScore 10
|> Scores.points
|> Expect.equal -10
, describe "scoreChange"
[ test "updates the current highest" <|
\_ ->
init
|> Scores.scoreChange
("<NAME>"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.highestToString
|> Expect.equal "10"
, test "does not update the list" <|
\_ ->
init
|> Scores.scoreChange
("<NAME>"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.highest
|> Scores.points
|> Expect.equal 0
]
, describe "gameOver"
[ test "updates the current highest" <|
\_ ->
init
|> Scores.gameOver
("<NAME>"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.highestToString
|> Expect.equal "10"
, test "updates the list" <|
\_ ->
init
|> Scores.gameOver
("<NAME>"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.highest
|> Scores.points
|> Expect.equal 10
]
, test "prepareForSave" <|
\_ ->
init
|> Scores.gameOver
("<NAME>"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.prepareForSave
|> Expect.equal
[ { name = "<NAME>", points = 10 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
]
, test "highest" <|
\_ ->
init
|> Scores.gameOver
("<NAME>"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.highest
|> Scores.points
|> Expect.equal 10
, test "lowest" <|
\_ ->
init
|> Scores.gameOver
("<NAME>"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.lowest
|> Scores.points
|> Expect.equal 0
, test "list" <|
\_ ->
init
|> Scores.gameOver
("<NAME>"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.list
|> List.map
(\score ->
{ name =
score
|> Scores.name
, points =
score
|> Scores.points
}
)
|> Expect.equal
[ { name = "<NAME>", points = 10 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
]
, test "highestToString" <|
\_ ->
init
|> Scores.scoreChange
("<NAME>"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.highestToString
|> Expect.equal "10"
, test "name" <|
\_ ->
"<NAME>"
|> Scores.register
|> Scores.name
|> Expect.equal "<NAME>"
, test "points" <|
\_ ->
"<NAME>"
|> Scores.register
|> Scores.incrementScore 10
|> Scores.points
|> Expect.equal 10
, test "total" <|
\_ ->
init
|> Scores.total
|> Expect.equal 10
, test "updateName" <|
\_ ->
"<NAME>"
|> Scores.register
|> Scores.updateName "<NAME>"
|> Scores.name
|> Expect.equal "<NAME>"
]
| true | module Shared.ScoresTests exposing (suite)
import Expect
import Fuzz exposing (intRange)
import Shared.Scores as Scores exposing (Score, Scores)
import Test exposing (Test, describe, fuzz, fuzz2, test)
init : Scores
init =
Scores.init
10
[]
suite : Test
suite =
describe "The Scores Module"
[ describe "init"
[ fuzz (intRange 0 100) "with no highscores" <|
\total ->
Scores.init
total
[]
|> Scores.total
|> Expect.equal total
, fuzz2 (intRange 1 10) (intRange 10 100) "with too many highscores" <|
\total range ->
Scores.init
total
(range
|> List.range 10
|> List.map
(\index ->
{ name = ""
, points = 0
}
)
)
|> Scores.total
|> Expect.equal total
]
, test "register" <|
\_ ->
Scores.register "PI:NAME:<NAME>END_PI"
|> Expect.all
[ \score ->
score
|> Scores.name
|> Expect.equal "PI:NAME:<NAME>END_PI"
, \score ->
score
|> Scores.points
|> Expect.equal 0
]
, test "incrementScore" <|
\_ ->
Scores.register "PI:NAME:<NAME>END_PI"
|> Scores.incrementScore 10
|> Scores.points
|> Expect.equal 10
, test "decrementScore" <|
\_ ->
Scores.register "PI:NAME:<NAME>END_PI"
|> Scores.decrementScore 10
|> Scores.points
|> Expect.equal -10
, describe "scoreChange"
[ test "updates the current highest" <|
\_ ->
init
|> Scores.scoreChange
("PI:NAME:<NAME>END_PI"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.highestToString
|> Expect.equal "10"
, test "does not update the list" <|
\_ ->
init
|> Scores.scoreChange
("PI:NAME:<NAME>END_PI"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.highest
|> Scores.points
|> Expect.equal 0
]
, describe "gameOver"
[ test "updates the current highest" <|
\_ ->
init
|> Scores.gameOver
("PI:NAME:<NAME>END_PI"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.highestToString
|> Expect.equal "10"
, test "updates the list" <|
\_ ->
init
|> Scores.gameOver
("PI:NAME:<NAME>END_PI"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.highest
|> Scores.points
|> Expect.equal 10
]
, test "prepareForSave" <|
\_ ->
init
|> Scores.gameOver
("PI:NAME:<NAME>END_PI"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.prepareForSave
|> Expect.equal
[ { name = "PI:NAME:<NAME>END_PI", points = 10 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
]
, test "highest" <|
\_ ->
init
|> Scores.gameOver
("PI:NAME:<NAME>END_PI"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.highest
|> Scores.points
|> Expect.equal 10
, test "lowest" <|
\_ ->
init
|> Scores.gameOver
("PI:NAME:<NAME>END_PI"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.lowest
|> Scores.points
|> Expect.equal 0
, test "list" <|
\_ ->
init
|> Scores.gameOver
("PI:NAME:<NAME>END_PI"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.list
|> List.map
(\score ->
{ name =
score
|> Scores.name
, points =
score
|> Scores.points
}
)
|> Expect.equal
[ { name = "PI:NAME:<NAME>END_PI", points = 10 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
, { name = "---", points = 0 }
]
, test "highestToString" <|
\_ ->
init
|> Scores.scoreChange
("PI:NAME:<NAME>END_PI"
|> Scores.register
|> Scores.incrementScore 10
)
|> Scores.highestToString
|> Expect.equal "10"
, test "name" <|
\_ ->
"PI:NAME:<NAME>END_PI"
|> Scores.register
|> Scores.name
|> Expect.equal "PI:NAME:<NAME>END_PI"
, test "points" <|
\_ ->
"PI:NAME:<NAME>END_PI"
|> Scores.register
|> Scores.incrementScore 10
|> Scores.points
|> Expect.equal 10
, test "total" <|
\_ ->
init
|> Scores.total
|> Expect.equal 10
, test "updateName" <|
\_ ->
"PI:NAME:<NAME>END_PI"
|> Scores.register
|> Scores.updateName "PI:NAME:<NAME>END_PI"
|> Scores.name
|> Expect.equal "PI:NAME:<NAME>END_PI"
]
| elm |
[
{
"context": "opLevelDomains =\n [ \"cosmic\" ]\n\n\ninput1 =\n \"test@ohomail.co\"\n\n\nmailcheckResult1 =\n suggestWith domains sec",
"end": 365,
"score": 0.9999092817,
"start": 350,
"tag": "EMAIL",
"value": "test@ohomail.co"
},
{
"context": "ilcheckResult1 == Just ( \"test\", \"yohomail.com\", \"test@yohomail.com\" )\n\n\ninput2 =\n \"test@fakedomain.comic\"\n\n\nmailc",
"end": 541,
"score": 0.9999040961,
"start": 524,
"tag": "EMAIL",
"value": "test@yohomail.com"
},
{
"context": "omail.com\", \"test@yohomail.com\" )\n\n\ninput2 =\n \"test@fakedomain.comic\"\n\n\nmailcheckResult2 =\n suggestWith domains sec",
"end": 582,
"score": 0.9997785091,
"start": 561,
"tag": "EMAIL",
"value": "test@fakedomain.comic"
},
{
"context": "ckResult2 == Just ( \"test\", \"fakedomain.cosmic\", \"test@fakedomain.cosmic\" )\n\n\ninput3 =\n \"test@supermail.tld\"\n\n\nmailchec",
"end": 768,
"score": 0.999625802,
"start": 746,
"tag": "EMAIL",
"value": "test@fakedomain.cosmic"
},
{
"context": "smic\", \"test@fakedomain.cosmic\" )\n\n\ninput3 =\n \"test@supermail.tld\"\n\n\nmailcheckResult3 =\n suggestWith domains sec",
"end": 806,
"score": 0.9998890758,
"start": 788,
"tag": "EMAIL",
"value": "test@supermail.tld"
},
{
"context": "ilcheckResult3 == Just ( \"test\", \"supamail.tld\", \"test@supamail.tld\" )\n\n\nd1 =\n Debug.log \"mailcheckResult1\" ( inpu",
"end": 982,
"score": 0.9998857379,
"start": 965,
"tag": "EMAIL",
"value": "test@supamail.tld"
}
] | Example2.elm | rluiten/mailcheck | 28 | module Main exposing (domains, input1, input2, input3, mailcheckResult1, mailcheckResult2, mailcheckResult3, secondLevelDomains, test1Pass, test2Pass, test3Pass, topLevelDomains)
import Mailcheck exposing (suggestWith)
domains =
[ "yohomail.com" ]
secondLevelDomains =
[ "supamail" ]
topLevelDomains =
[ "cosmic" ]
input1 =
"test@ohomail.co"
mailcheckResult1 =
suggestWith domains secondLevelDomains topLevelDomains input1
test1Pass =
mailcheckResult1 == Just ( "test", "yohomail.com", "test@yohomail.com" )
input2 =
"test@fakedomain.comic"
mailcheckResult2 =
suggestWith domains secondLevelDomains topLevelDomains input2
test2Pass =
mailcheckResult2 == Just ( "test", "fakedomain.cosmic", "test@fakedomain.cosmic" )
input3 =
"test@supermail.tld"
mailcheckResult3 =
suggestWith domains secondLevelDomains topLevelDomains input3
test3Pass =
mailcheckResult3 == Just ( "test", "supamail.tld", "test@supamail.tld" )
d1 =
Debug.log "mailcheckResult1" ( input1, mailcheckResult1, test1Pass )
d2 =
Debug.log "mailcheckResult2" ( input2, mailcheckResult2, test2Pass )
d3 =
Debug.log "mailcheckResult3" ( input3, mailcheckResult3, test3Pass )
| 7100 | module Main exposing (domains, input1, input2, input3, mailcheckResult1, mailcheckResult2, mailcheckResult3, secondLevelDomains, test1Pass, test2Pass, test3Pass, topLevelDomains)
import Mailcheck exposing (suggestWith)
domains =
[ "yohomail.com" ]
secondLevelDomains =
[ "supamail" ]
topLevelDomains =
[ "cosmic" ]
input1 =
"<EMAIL>"
mailcheckResult1 =
suggestWith domains secondLevelDomains topLevelDomains input1
test1Pass =
mailcheckResult1 == Just ( "test", "yohomail.com", "<EMAIL>" )
input2 =
"<EMAIL>"
mailcheckResult2 =
suggestWith domains secondLevelDomains topLevelDomains input2
test2Pass =
mailcheckResult2 == Just ( "test", "fakedomain.cosmic", "<EMAIL>" )
input3 =
"<EMAIL>"
mailcheckResult3 =
suggestWith domains secondLevelDomains topLevelDomains input3
test3Pass =
mailcheckResult3 == Just ( "test", "supamail.tld", "<EMAIL>" )
d1 =
Debug.log "mailcheckResult1" ( input1, mailcheckResult1, test1Pass )
d2 =
Debug.log "mailcheckResult2" ( input2, mailcheckResult2, test2Pass )
d3 =
Debug.log "mailcheckResult3" ( input3, mailcheckResult3, test3Pass )
| true | module Main exposing (domains, input1, input2, input3, mailcheckResult1, mailcheckResult2, mailcheckResult3, secondLevelDomains, test1Pass, test2Pass, test3Pass, topLevelDomains)
import Mailcheck exposing (suggestWith)
domains =
[ "yohomail.com" ]
secondLevelDomains =
[ "supamail" ]
topLevelDomains =
[ "cosmic" ]
input1 =
"PI:EMAIL:<EMAIL>END_PI"
mailcheckResult1 =
suggestWith domains secondLevelDomains topLevelDomains input1
test1Pass =
mailcheckResult1 == Just ( "test", "yohomail.com", "PI:EMAIL:<EMAIL>END_PI" )
input2 =
"PI:EMAIL:<EMAIL>END_PI"
mailcheckResult2 =
suggestWith domains secondLevelDomains topLevelDomains input2
test2Pass =
mailcheckResult2 == Just ( "test", "fakedomain.cosmic", "PI:EMAIL:<EMAIL>END_PI" )
input3 =
"PI:EMAIL:<EMAIL>END_PI"
mailcheckResult3 =
suggestWith domains secondLevelDomains topLevelDomains input3
test3Pass =
mailcheckResult3 == Just ( "test", "supamail.tld", "PI:EMAIL:<EMAIL>END_PI" )
d1 =
Debug.log "mailcheckResult1" ( input1, mailcheckResult1, test1Pass )
d2 =
Debug.log "mailcheckResult2" ( input2, mailcheckResult2, test2Pass )
d3 =
Debug.log "mailcheckResult3" ( input3, mailcheckResult3, test3Pass )
| elm |
[
{
"context": "ed = True\n }\n in\n [ { name = \"Tenacity\"\n , id = 1\n , rarity = 1\n , ",
"end": 2883,
"score": 0.9981548786,
"start": 2875,
"tag": "NAME",
"value": "Tenacity"
},
{
"context": " , limited = False\n }\n , { name = \"Meditation\"\n , id = 2\n , rarity = 1\n , ",
"end": 3220,
"score": 0.9980675578,
"start": 3210,
"tag": "NAME",
"value": "Meditation"
},
{
"context": " , limited = False\n }\n , { name = \"Technique\"\n , id = 3\n , rarity = 1\n , ",
"end": 3558,
"score": 0.9687386155,
"start": 3549,
"tag": "NAME",
"value": "Technique"
},
{
"context": " , limited = False\n }\n , { name = \"Concentration\"\n , id = 9\n , rarity = 2\n , ",
"end": 5591,
"score": 0.9991087914,
"start": 5578,
"tag": "NAME",
"value": "Concentration"
},
{
"context": " , limited = False\n }\n , { name = \"Divine Oracle\"\n , id = 10\n , rarity = 2\n ,",
"end": 5931,
"score": 0.9993363023,
"start": 5918,
"tag": "NAME",
"value": "Divine Oracle"
},
{
"context": " , limited = False\n }\n , { name = \"Azoth Blade\"\n , id = 11\n , rarity = 3\n ,",
"end": 6264,
"score": 0.9995744228,
"start": 6253,
"tag": "NAME",
"value": "Azoth Blade"
},
{
"context": "False\n }\n , { name = \"False Attendant's Writings\"\n , id = 12\n , rarity = 3\n ,",
"end": 6620,
"score": 0.7626376152,
"start": 6612,
"tag": "NAME",
"value": "Writings"
},
{
"context": " , limited = False\n }\n , { name = \"Rin's Pendant\"\n , id = 16\n , rarity = 3",
"end": 8025,
"score": 0.8466709256,
"start": 8020,
"tag": "NAME",
"value": "Rin's"
},
{
"context": " , limited = False\n }\n , { name = \"Dragon's Meridian\"\n , id = 18\n , rarity = 3\n ,",
"end": 8727,
"score": 0.9981910586,
"start": 8710,
"tag": "NAME",
"value": "Dragon's Meridian"
},
{
"context": " , limited = False\n }\n , { name = \"Sorcery Ore\"\n , id = 19\n , rarity = 3\n ,",
"end": 9062,
"score": 0.9992984533,
"start": 9051,
"tag": "NAME",
"value": "Sorcery Ore"
},
{
"context": " , limited = False\n }\n , { name = \"Dragonkin\"\n , id = 20\n , rarity = 3\n ,",
"end": 9400,
"score": 0.9992050529,
"start": 9391,
"tag": "NAME",
"value": "Dragonkin"
},
{
"context": "ted = False\n }\n , { name = \"Iron-Willed Training\"\n , id = 21\n , rarity = 4\n ,",
"end": 9745,
"score": 0.6116060615,
"start": 9737,
"tag": "NAME",
"value": "Training"
},
{
"context": " , limited = False\n }\n , { name = \"Primeval Curse\"\n , id = 22\n , rarity = 4\n ,",
"end": 10090,
"score": 0.9998624325,
"start": 10076,
"tag": "NAME",
"value": "Primeval Curse"
},
{
"context": " , limited = False\n }\n , { name = \"Projection\"\n , id = 23\n , rarity = 4\n ,",
"end": 10432,
"score": 0.9997013211,
"start": 10422,
"tag": "NAME",
"value": "Projection"
},
{
"context": " , limited = False\n }\n , { name = \"Gandr\"\n , id = 24\n , rarity = 4\n ,",
"end": 10770,
"score": 0.9998520017,
"start": 10765,
"tag": "NAME",
"value": "Gandr"
},
{
"context": " , limited = False\n }\n , { name = \"Verdant Sound of Destruction\"\n , id = 25\n , rarity = 4\n ,",
"end": 11133,
"score": 0.9980063438,
"start": 11105,
"tag": "NAME",
"value": "Verdant Sound of Destruction"
},
{
"context": " , limited = False\n }\n , { name = \"Divine Banquet\"\n , id = 29\n , rarity = 4\n ,",
"end": 12532,
"score": 0.9805251956,
"start": 12518,
"tag": "NAME",
"value": "Divine Banquet"
},
{
"context": " , limited = False\n }\n , { name = \"Angel's Song\"\n , id = 30\n , rarity = 4\n ,",
"end": 12874,
"score": 0.9996570945,
"start": 12862,
"tag": "NAME",
"value": "Angel's Song"
},
{
"context": " , limited = True\n }\n , { name = \"Parted Sea\"\n , id = 37\n , rarity = 3\n ,",
"end": 15283,
"score": 0.8383612633,
"start": 15273,
"tag": "NAME",
"value": "Parted Sea"
},
{
"context": " , limited = False\n }\n , { name = \"Holy Shroud of Magdalene\"\n , id = 39\n , rarity = 4\n ,",
"end": 16068,
"score": 0.9937670231,
"start": 16044,
"tag": "NAME",
"value": "Holy Shroud of Magdalene"
},
{
"context": " , limited = False\n }\n , { name = \"Prisma Cosmos\"\n , id = 40\n , rarity = 5\n ,",
"end": 16438,
"score": 0.9990448952,
"start": 16425,
"tag": "NAME",
"value": "Prisma Cosmos"
},
{
"context": " , limited = False\n }\n , { name = \"Nightless Rose\"\n , id = 41\n , rarity = 5\n ,",
"end": 16792,
"score": 0.9936177135,
"start": 16778,
"tag": "NAME",
"value": "Nightless Rose"
},
{
"context": " , limited = True\n }\n , { name = \"Mooncell Automaton\"\n , id = 42\n , rarity = 3\n ,",
"end": 17146,
"score": 0.9254307747,
"start": 17128,
"tag": "NAME",
"value": "Mooncell Automaton"
},
{
"context": " , limited = False\n }\n , { name = \"Moony Jewel\"\n , id = 43\n , rarity = 4\n ,",
"end": 17629,
"score": 0.9998787642,
"start": 17618,
"tag": "NAME",
"value": "Moony Jewel"
},
{
"context": " , limited = True\n }\n , { name = \"Moonlight Fest\"\n , id = 45\n , rarity =",
"end": 18319,
"score": 0.7356476784,
"start": 18315,
"tag": "NAME",
"value": "Moon"
},
{
"context": " , limited = True\n }\n , { name = \"Runestone\"\n , id = 46\n , rarity = 3\n ",
"end": 18740,
"score": 0.6136439443,
"start": 18737,
"tag": "NAME",
"value": "Run"
},
{
"context": " , limited = False\n }\n , { name = \"The Black Grail\"\n , id = 48\n , rarity = 5\n ,",
"end": 19587,
"score": 0.8263773918,
"start": 19576,
"tag": "NAME",
"value": "Black Grail"
},
{
"context": " , limited = False\n }\n , { name = \"Jack-o'-lantern\"\n , id = 49\n , rarity = 3\n ,",
"end": 20002,
"score": 0.9996271729,
"start": 19987,
"tag": "NAME",
"value": "Jack-o'-lantern"
},
{
"context": " , limited = True\n }\n , { name = \"Halloween Arrangement\"\n , id = 51\n , rarity = 4\n ,",
"end": 20700,
"score": 0.9993712306,
"start": 20679,
"tag": "NAME",
"value": "Halloween Arrangement"
},
{
"context": " , limited = True\n }\n , { name = \"Halloween Princess\"\n , id = 52\n , rarity = 5\n ,",
"end": 21113,
"score": 0.9997875094,
"start": 21095,
"tag": "NAME",
"value": "Halloween Princess"
},
{
"context": " , limited = True\n }\n , { name = \"Little Halloween Devil\"\n , id = 53\n , rarity = 5\n ,",
"end": 21533,
"score": 0.9998462796,
"start": 21511,
"tag": "NAME",
"value": "Little Halloween Devil"
},
{
"context": " , limited = True\n }\n , { name = \"Maid in Halloween\"\n , id = 54\n , rarity = 5\n ,",
"end": 21945,
"score": 0.9981641173,
"start": 21928,
"tag": "NAME",
"value": "Maid in Halloween"
},
{
"context": " , limited = True\n }\n , { name = \"Anchors Aweigh\"\n , id = 55\n , rarity = 3\n ,",
"end": 22293,
"score": 0.9996185303,
"start": 22279,
"tag": "NAME",
"value": "Anchors Aweigh"
},
{
"context": " , limited = False\n }\n , { name = \"Code Cast\"\n , id = 56\n , rarity = 4\n ,",
"end": 22638,
"score": 0.9995228052,
"start": 22629,
"tag": "NAME",
"value": "Code Cast"
},
{
"context": " , limited = False\n }\n , { name = \"Victor of the Moon\"\n , id = 57\n , rarity = 5\n ,",
"end": 23071,
"score": 0.9998066425,
"start": 23053,
"tag": "NAME",
"value": "Victor of the Moon"
},
{
"context": " , limited = False\n }\n , { name = \"Another Ending\"\n , id = 58\n , rarity = 5\n ,",
"end": 23496,
"score": 0.9889781475,
"start": 23482,
"tag": "NAME",
"value": "Another Ending"
},
{
"context": " , limited = False\n }\n , { name = \"Fate GUDAGUDA Order\"\n , id = 59\n , rarity = 3\n ,",
"end": 23922,
"score": 0.9970706701,
"start": 23903,
"tag": "NAME",
"value": "Fate GUDAGUDA Order"
},
{
"context": " , limited = True\n }\n , { name = \"Guda-o\"\n , id = 61\n , rarity = 5\n ",
"end": 25165,
"score": 0.9654858112,
"start": 25161,
"tag": "NAME",
"value": "Guda"
},
{
"context": " , limited = True\n }\n , { name = \"GUDAGUDA Poster Girl\"\n , id = 62\n , rarity = 5\n ,",
"end": 25586,
"score": 0.9998414516,
"start": 25566,
"tag": "NAME",
"value": "GUDAGUDA Poster Girl"
},
{
"context": " , limited = True\n }\n , { name = \"Demon Boar\"\n , id = 65\n , rarity = 3\n ,",
"end": 25990,
"score": 0.9998509884,
"start": 25980,
"tag": "NAME",
"value": "Demon Boar"
},
{
"context": " , limited = False\n }\n , { name = \"Knight's Dignity\"\n , id = 66\n , rarity = 4\n ,",
"end": 26342,
"score": 0.9997807145,
"start": 26326,
"tag": "NAME",
"value": "Knight's Dignity"
},
{
"context": " , limited = False\n }\n , { name = \"A Fragment of 2030\"\n , id = 67\n , rarity = 5\n ",
"end": 26767,
"score": 0.8798420429,
"start": 26754,
"tag": "NAME",
"value": "A Fragment of"
},
{
"context": " False\n }\n , { name = \"A Fragment of 2030\"\n , id = 67\n , rarity = 5\n ",
"end": 26771,
"score": 0.5914471149,
"start": 26770,
"tag": "NAME",
"value": "3"
},
{
"context": " , limited = False\n }\n , { name = \"Lightning Reindeer\"\n , id = 68\n , rarity = 3\n ,",
"end": 27123,
"score": 0.9971784949,
"start": 27105,
"tag": "NAME",
"value": "Lightning Reindeer"
},
{
"context": " , limited = True\n }\n , { name = \"March of the Saint\"\n , id = 69\n , rarity = 4\n ,",
"end": 27478,
"score": 0.9948275685,
"start": 27460,
"tag": "NAME",
"value": "March of the Saint"
},
{
"context": " , limited = True\n }\n , { name = \"Present For My Master\"\n , id = 70\n , rarity = 5\n ,",
"end": 27910,
"score": 0.9412875772,
"start": 27889,
"tag": "NAME",
"value": "Present For My Master"
},
{
"context": " , limited = True\n }\n , { name = \"Holy Night Sign\"\n , id = 71\n , rarity = 5\n ,",
"end": 28336,
"score": 0.9993011951,
"start": 28321,
"tag": "NAME",
"value": "Holy Night Sign"
},
{
"context": " , limited = True\n }\n , { name = \"Clock Tower\"\n , id = 72\n , rarity = 3\n ,",
"end": 28759,
"score": 0.9994235635,
"start": 28748,
"tag": "NAME",
"value": "Clock Tower"
},
{
"context": " , limited = False\n }\n , { name = \"Necromancy\"\n , id = 73\n , rarity = 4\n ,",
"end": 29102,
"score": 0.9997932911,
"start": 29092,
"tag": "NAME",
"value": "Necromancy"
},
{
"context": " , limited = False\n }\n , { name = \"Awakened Will\"\n , id = 74\n , rarity = 4\n ,",
"end": 29465,
"score": 0.9997676015,
"start": 29452,
"tag": "NAME",
"value": "Awakened Will"
},
{
"context": " , limited = True\n }\n , { name = \"Grand New Year\"\n , id = 79\n , rarity = 5\n ,",
"end": 31610,
"score": 0.9996121526,
"start": 31596,
"tag": "NAME",
"value": "Grand New Year"
},
{
"context": " , limited = True\n }\n , { name = \"Mona Lisa\"\n , id = 80\n , rarity = 5\n ,",
"end": 32064,
"score": 0.9998823404,
"start": 32055,
"tag": "NAME",
"value": "Mona Lisa"
},
{
"context": " , limited = True\n }\n , { name = \"Happy x3 Order\"\n , id = 81\n , rarity = 4\n ,",
"end": 32410,
"score": 0.9988046885,
"start": 32396,
"tag": "NAME",
"value": "Happy x3 Order"
},
{
"context": " , limited = True\n }\n , { name = \"Purely Bloom\"\n , id = 82\n , rarity = 5\n ,",
"end": 32754,
"score": 0.9993209243,
"start": 32742,
"tag": "NAME",
"value": "Purely Bloom"
},
{
"context": " , limited = True\n }\n , { name = \"Star of Altria\"\n , id = 83\n , rarity = 5\n ,",
"end": 33110,
"score": 0.8565363288,
"start": 33096,
"tag": "NAME",
"value": "Star of Altria"
},
{
"context": " , limited = True\n }\n , { name = \"Trueshot\"\n , id = 84\n , rarity = 3\n ,",
"end": 33525,
"score": 0.9996668696,
"start": 33517,
"tag": "NAME",
"value": "Trueshot"
},
{
"context": " , limited = True\n }\n , { name = \"Mikotto! Bride Training\"\n , id = 85\n , rari",
"end": 33921,
"score": 0.9991592765,
"start": 33914,
"tag": "NAME",
"value": "Mikotto"
},
{
"context": "limited = True\n }\n , { name = \"Mikotto! Bride Training\"\n , id = 85\n , rarity = 4\n ,",
"end": 33937,
"score": 0.8807353973,
"start": 33923,
"tag": "NAME",
"value": "Bride Training"
},
{
"context": " , limited = True\n }\n , { name = \"Ryudoji Temple\"\n , id = 89\n , rarity = 3\n ,",
"end": 34685,
"score": 0.9996623397,
"start": 34671,
"tag": "NAME",
"value": "Ryudoji Temple"
},
{
"context": " , limited = False\n }\n , { name = \"Mana Gauge\"\n , id = 90\n , rarity = 3\n ,",
"end": 35091,
"score": 0.9997045994,
"start": 35081,
"tag": "NAME",
"value": "Mana Gauge"
},
{
"context": " , limited = False\n }\n , { name = \"Elixir of Love\"\n , id = 91\n , rarity = 3\n ,",
"end": 35464,
"score": 0.9834095836,
"start": 35450,
"tag": "NAME",
"value": "Elixir of Love"
},
{
"context": " , limited = False\n }\n , { name = \"Storch Ritter\"\n , id = 92\n , rarity = 3\n ,",
"end": 35814,
"score": 0.9958019853,
"start": 35801,
"tag": "NAME",
"value": "Storch Ritter"
},
{
"context": " , limited = False\n }\n , { name = \"Hermitage\"\n , id = 93\n , rarity = 3\n ,",
"end": 36170,
"score": 0.9998211265,
"start": 36161,
"tag": "NAME",
"value": "Hermitage"
},
{
"context": " , limited = False\n }\n , { name = \"Motored Cuirassier\"\n , id = 94\n , rarity = 3\n ,",
"end": 36522,
"score": 0.9944226146,
"start": 36504,
"tag": "NAME",
"value": "Motored Cuirassier"
},
{
"context": " , limited = False\n }\n , { name = \"Stuffed Lion\"\n , id = 95\n , rarity = 3\n ,",
"end": 36891,
"score": 0.9997389913,
"start": 36879,
"tag": "NAME",
"value": "Stuffed Lion"
},
{
"context": " , limited = False\n }\n , { name = \"Lugh's Halo\"\n , id = 96\n , rarity = 3\n ,",
"end": 37242,
"score": 0.9998493195,
"start": 37231,
"tag": "NAME",
"value": "Lugh's Halo"
},
{
"context": " , limited = False\n }\n , { name = \"Vessel of the Saint\"\n , id = 97\n , rarity = 5\n ,",
"end": 37594,
"score": 0.6391885877,
"start": 37575,
"tag": "NAME",
"value": "Vessel of the Saint"
},
{
"context": " , limited = False\n }\n , { name = \"Golden Millennium Tree\"\n , id = 98\n , rarity = 4\n ,",
"end": 38027,
"score": 0.9858411551,
"start": 38005,
"tag": "NAME",
"value": "Golden Millennium Tree"
},
{
"context": " False\n }\n , { name = \"Heroic Portrait: Mash Kyrielight\"\n , id = 99\n , rarity = 4\n ,",
"end": 38404,
"score": 0.9993975163,
"start": 38389,
"tag": "NAME",
"value": "Mash Kyrielight"
},
{
"context": "= True\n }\n , { name = \"Heroic Portrait: Altria Pendragon\"\n , id = 100\n , rarity = 4\n ",
"end": 38759,
"score": 0.9992992878,
"start": 38743,
"tag": "NAME",
"value": "Altria Pendragon"
},
{
"context": "= True\n }\n , { name = \"Heroic Portrait: Jeanne d'Arc\"\n , id = 101\n , rarity = 4\n ",
"end": 39111,
"score": 0.9993267059,
"start": 39099,
"tag": "NAME",
"value": "Jeanne d'Arc"
},
{
"context": "= True\n }\n , { name = \"Heroic Portrait: Altera\"\n , id = 102\n , rarity = 4\n ",
"end": 39457,
"score": 0.979998529,
"start": 39451,
"tag": "NAME",
"value": "Altera"
},
{
"context": "= True\n }\n , { name = \"Heroic Portrait: Arjuna\"\n , id = 103\n , rarity = 4\n ",
"end": 39803,
"score": 0.985853374,
"start": 39797,
"tag": "NAME",
"value": "Arjuna"
},
{
"context": "= True\n }\n , { name = \"Heroic Portrait: Scathach\"\n , id = 104\n , rarity = 4\n ",
"end": 40151,
"score": 0.9154908657,
"start": 40143,
"tag": "NAME",
"value": "Scathach"
},
{
"context": "= True\n }\n , { name = \"Heroic Portrait: Ushiwakamaru\"\n , id = 105\n , rarity = 4\n ",
"end": 40503,
"score": 0.9261247516,
"start": 40491,
"tag": "NAME",
"value": "Ushiwakamaru"
},
{
"context": "= True\n }\n , { name = \"Heroic Portrait: Henry Jekyll & Hyde\"\n , id = 106\n , rarity = 4",
"end": 40855,
"score": 0.9991150498,
"start": 40843,
"tag": "NAME",
"value": "Henry Jekyll"
},
{
"context": " , { name = \"Heroic Portrait: Henry Jekyll & Hyde\"\n , id = 106\n , rarity = 4\n ",
"end": 40862,
"score": 0.995654881,
"start": 40858,
"tag": "NAME",
"value": "Hyde"
},
{
"context": "= True\n }\n , { name = \"Heroic Portrait: Mephistopheles\"\n , id = 107\n , rarity = 4\n ",
"end": 41216,
"score": 0.9088717103,
"start": 41202,
"tag": "NAME",
"value": "Mephistopheles"
},
{
"context": "= True\n }\n , { name = \"Heroic Portrait: Darius III\"\n , id = 108\n , rarity = 4\n ",
"end": 41566,
"score": 0.9993805885,
"start": 41556,
"tag": "NAME",
"value": "Darius III"
},
{
"context": " , limited = True\n }\n , { name = \"Valentine Dojo of Tears\"\n , id = 109\n ",
"end": 41892,
"score": 0.5214415193,
"start": 41889,
"tag": "NAME",
"value": "Val"
},
{
"context": "rue\n }\n , { name = \"Valentine Dojo of Tears\"\n , id = 109\n , rarity = 3\n ",
"end": 41912,
"score": 0.6966547966,
"start": 41908,
"tag": "NAME",
"value": "ears"
},
{
"context": " , limited = True\n }\n , { name = \"Kitchen ☆ Patissiere\"\n , id = 110\n , rarity ",
"end": 42371,
"score": 0.8988472223,
"start": 42364,
"tag": "NAME",
"value": "Kitchen"
},
{
"context": "imited = True\n }\n , { name = \"Kitchen ☆ Patissiere\"\n , id = 110\n , rarity = 4\n ",
"end": 42384,
"score": 0.9513232112,
"start": 42374,
"tag": "NAME",
"value": "Patissiere"
},
{
"context": " , limited = True\n }\n , { name = \"Street Choco-Maid\"\n , id = 111\n , rarity = 5\n ",
"end": 42807,
"score": 0.9990718961,
"start": 42790,
"tag": "NAME",
"value": "Street Choco-Maid"
},
{
"context": " , limited = True\n }\n , { name = \"Melty Sweetheart\"\n , id = 112\n , rarity = 5\n ",
"end": 43311,
"score": 0.9998676181,
"start": 43295,
"tag": "NAME",
"value": "Melty Sweetheart"
},
{
"context": " , limited = True\n }\n , { name = \"Decapitating Bunny 2018\"\n , id = 154\n , rarity = 5\n ",
"end": 43772,
"score": 0.9987329841,
"start": 43749,
"tag": "NAME",
"value": "Decapitating Bunny 2018"
},
{
"context": " , limited = True\n }\n , { name = \"Mature Gentleman\"\n , id = 155\n , rarity = 5\n ",
"end": 44196,
"score": 0.9998283386,
"start": 44180,
"tag": "NAME",
"value": "Mature Gentleman"
},
{
"context": " , limited = True\n }\n , { name = \"Grand Puppeteer\"\n , id = 156\n , rarity = 5\n ",
"end": 44542,
"score": 0.9997799993,
"start": 44527,
"tag": "NAME",
"value": "Grand Puppeteer"
},
{
"context": " , limited = True\n }\n , { name = \"Threefold Barrier\"\n , id = 157\n , rarity = 5\n ",
"end": 44966,
"score": 0.9990473986,
"start": 44949,
"tag": "NAME",
"value": "Threefold Barrier"
},
{
"context": " , limited = True\n }\n , { name = \"Vivid Dance of Fists\"\n , id = 158\n , rarity = 4\n ",
"end": 45332,
"score": 0.993322134,
"start": 45312,
"tag": "NAME",
"value": "Vivid Dance of Fists"
},
{
"context": " , limited = True\n }\n , { name = \"Mystic Eyes of Distortion\"\n , id = 159\n , r",
"end": 45674,
"score": 0.7262436748,
"start": 45666,
"tag": "NAME",
"value": "Mystic E"
},
{
"context": " , limited = True\n }\n , { name = \"Summer's Precognition\"\n , id = 160\n , rarity = 4\n ",
"end": 46125,
"score": 0.994289875,
"start": 46104,
"tag": "NAME",
"value": "Summer's Precognition"
},
{
"context": " , limited = True\n }\n , { name = \"Chorus\"\n , id = 161\n , rarity = 4\n ",
"end": 46531,
"score": 0.9992661476,
"start": 46525,
"tag": "NAME",
"value": "Chorus"
},
{
"context": " , limited = True\n }\n , { name = \"Sprinter\"\n , id = 162\n , rarity = 3\n ",
"end": 46952,
"score": 0.9991834164,
"start": 46944,
"tag": "NAME",
"value": "Sprinter"
},
{
"context": " , limited = True\n }\n , { name = \"Kiss Your Hand\"\n , id = 165\n , rarit",
"end": 47771,
"score": 0.8618792892,
"start": 47770,
"tag": "NAME",
"value": "K"
},
{
"context": " , limited = True\n }\n , { name = \"Teacher and I\"\n , id = 166\n , rarity = 5\n ",
"end": 48275,
"score": 0.9893286824,
"start": 48262,
"tag": "NAME",
"value": "Teacher and I"
},
{
"context": " , limited = True\n }\n , { name = \"Versus\"\n , id = 167\n , rarity = 5\n ",
"end": 48678,
"score": 0.9963468313,
"start": 48672,
"tag": "NAME",
"value": "Versus"
},
{
"context": " , limited = True\n }\n , { name = \"Salon de Marie\"\n , id = 170\n , rarity = 3\n ",
"end": 50125,
"score": 0.9998939633,
"start": 50111,
"tag": "NAME",
"value": "Salon de Marie"
},
{
"context": " , limited = True\n }\n , { name = \"Prince of Slayer\"\n , id = 171\n , rarity = 3\n ",
"end": 50604,
"score": 0.9998608828,
"start": 50588,
"tag": "NAME",
"value": "Prince of Slayer"
},
{
"context": " , limited = True\n }\n , { name = \"Noisy Obsession\"\n , id = 172\n , rarity = 4\n ",
"end": 51056,
"score": 0.9992268682,
"start": 51041,
"tag": "NAME",
"value": "Noisy Obsession"
},
{
"context": "0, To Self GaugeUp <| Flat 40]\n , { name = \"Ideal Holy King\"\n , id = 175\n , rarity = 5\n ",
"end": 51645,
"score": 0.9927160144,
"start": 51630,
"tag": "NAME",
"value": "Ideal Holy King"
},
{
"context": " , limited = False\n }\n , { name = \"Record Holder\"\n , id = 176\n , rarity = 4\n ",
"end": 51992,
"score": 0.9963775277,
"start": 51979,
"tag": "NAME",
"value": "Record Holder"
},
{
"context": " , limited = False\n }\n , { name = \"Beast of Billows\"\n , id = 177\n , rarity = 3\n ",
"end": 52339,
"score": 0.9929609299,
"start": 52323,
"tag": "NAME",
"value": "Beast of Billows"
},
{
"context": " , limited = False\n }\n , { name = \"Personal Training\"\n , id = 178\n , rarity = 5\n ",
"end": 52701,
"score": 0.9822297096,
"start": 52684,
"tag": "NAME",
"value": "Personal Training"
},
{
"context": " , limited = True\n }\n , { name = \"The Scholars of Chaldea\"\n , id = 179\n , rarity = 5\n ",
"end": 53051,
"score": 0.9907740355,
"start": 53028,
"tag": "NAME",
"value": "The Scholars of Chaldea"
},
{
"context": " , limited = True\n }\n , { name = \"Maiden Leading Chaldea\"\n , id = 180\n , rarity = 5\n ",
"end": 53482,
"score": 0.9985332489,
"start": 53460,
"tag": "NAME",
"value": "Maiden Leading Chaldea"
},
{
"context": " , limited = True\n }\n , { name = \"The Merciless One\"\n , id = 181\n , rarity = 5\n ",
"end": 53914,
"score": 0.9503563046,
"start": 53897,
"tag": "NAME",
"value": "The Merciless One"
},
{
"context": " , limited = False\n }\n , { name = \"Art of Death\"\n , id = 183\n , rarity =",
"end": 54705,
"score": 0.5289886594,
"start": 54702,
"tag": "NAME",
"value": "Art"
},
{
"context": " , limited = False\n }\n , { name = \"Gentle Affection\"\n , id = 184\n , rarity = 4\n ",
"end": 55092,
"score": 0.9824624658,
"start": 55076,
"tag": "NAME",
"value": "Gentle Affection"
},
{
"context": " , limited = False\n }\n , { name = \"Volumen Hydrargyrum\"\n , id = 185\n , rarity = 5\n ",
"end": 55438,
"score": 0.9987229705,
"start": 55419,
"tag": "NAME",
"value": "Volumen Hydrargyrum"
},
{
"context": " , limited = False\n }\n , { name = \"Innocent Maiden\"\n , id = 186\n , rarity = 4\n ",
"end": 55865,
"score": 0.7487158179,
"start": 55850,
"tag": "NAME",
"value": "Innocent Maiden"
},
{
"context": " True\n }\n , bond 191 \"Crown of the Star\" \"Altria Pendragon\" Icon.DamageUp\n [party AttackUp 15]\n , bo",
"end": 57643,
"score": 0.9872121215,
"start": 57627,
"tag": "NAME",
"value": "Altria Pendragon"
},
{
"context": " AttackUp 15]\n , bond 192 \"Relic of the King\" \"Zhuge Liang (Lord El-Melloi II)\" Icon.BusterUp\n [party_ ",
"end": 57732,
"score": 0.9993001819,
"start": 57721,
"tag": "NAME",
"value": "Zhuge Liang"
},
{
"context": "15]\n , bond 193 \"Triumph of the Lord Impaler\" \"Vlad III\" Icon.BeamUp\n [self NPUp 30, atkChanc",
"end": 57840,
"score": 0.6064891815,
"start": 57839,
"tag": "NAME",
"value": "V"
},
{
"context": " Flat 5]\n , bond 194 \"Revelation from Heaven\" \"Jeanne d'Arc\" Icon.BusterUp\n [party_ Buster 15]\n , bon",
"end": 57978,
"score": 0.8808526397,
"start": 57966,
"tag": "NAME",
"value": "Jeanne d'Arc"
},
{
"context": "ster 15]\n , bond 195 \"Memories of the Dragon\" \"Altria Pendragon (Alter)\" Icon.BeamUp\n [self NPUp 30, atkChan",
"end": 58076,
"score": 0.9912939668,
"start": 58060,
"tag": "NAME",
"value": "Altria Pendragon"
},
{
"context": "rn <| Flat 500]\n , bond 200 \"Worthless Jewel\" \"Mata Hari\" Icon.NobleUp\n [party NPGen 15]\n , bond 2",
"end": 58692,
"score": 0.9883393645,
"start": 58683,
"tag": "NAME",
"value": "Mata Hari"
},
{
"context": "arty NPGen 15]\n , bond 201 \"Eternal Solitude\" \"Altera\" Icon.SwordUp\n [party AttackUp 15]\n , bon",
"end": 58771,
"score": 0.9960963726,
"start": 58765,
"tag": "NAME",
"value": "Altera"
},
{
"context": "ty AttackUp 15]\n , bond 202 \"Queen's Present\" \"Chevalier d'Eon\" Icon.ArtsUp\n [party_ Arts 15]\n , bond 20",
"end": 58861,
"score": 0.9994708896,
"start": 58846,
"tag": "NAME",
"value": "Chevalier d'Eon"
},
{
"context": "p\n [party_ Arts 15]\n , bond 203 \"Elixir\" \"Elisabeth Bathory\" Icon.HealTurn\n [party HealPerTurn 500]\n ",
"end": 58940,
"score": 0.9997963309,
"start": 58923,
"tag": "NAME",
"value": "Elisabeth Bathory"
},
{
"context": "ty HealPerTurn 500]\n , bond 204 \"My Necklace\" \"Marie Antoinette\" Icon.StarHaloUp\n [party StarUp 20]\n , bo",
"end": 59032,
"score": 0.9997594357,
"start": 59016,
"tag": "NAME",
"value": "Marie Antoinette"
},
{
"context": "rty StarUp 20]\n , bond 205 \"Staff He Gave Me\" \"Martha\" Icon.HealUp\n [party HealingReceived 30]\n ",
"end": 59115,
"score": 0.9995148182,
"start": 59109,
"tag": "NAME",
"value": "Martha"
},
{
"context": "\n [party HealingReceived 30]\n , bond 206 \"Iron Maiden\" \"Carmilla\" Icon.BeamUp\n [self NPUp 30, atkC",
"end": 59189,
"score": 0.999499917,
"start": 59178,
"tag": "NAME",
"value": "Iron Maiden"
},
{
"context": "HealingReceived 30]\n , bond 206 \"Iron Maiden\" \"Carmilla\" Icon.BeamUp\n [self NPUp 30, atkChance 10 <|",
"end": 59200,
"score": 0.9985564351,
"start": 59192,
"tag": "NAME",
"value": "Carmilla"
},
{
"context": "0 <| Debuff Target 1 SealNP Full]\n , bond 207 \"Cat Apron\" \"Tamamo Cat\" Icon.Heal\n [party HPUp 2000]\n ",
"end": 59305,
"score": 0.9893522859,
"start": 59296,
"tag": "NAME",
"value": "Cat Apron"
},
{
"context": "Target 1 SealNP Full]\n , bond 207 \"Cat Apron\" \"Tamamo Cat\" Icon.Heal\n [party HPUp 2000]\n , bond 208",
"end": 59318,
"score": 0.9681236744,
"start": 59308,
"tag": "NAME",
"value": "Tamamo Cat"
},
{
"context": "Icon.Heal\n [party HPUp 2000]\n , bond 208 \"Thirst for Victory\" \"Boudica\" Icon.StarHaloUp\n ",
"end": 59372,
"score": 0.8676201105,
"start": 59370,
"tag": "NAME",
"value": "Th"
},
{
"context": "y HPUp 2000]\n , bond 208 \"Thirst for Victory\" \"Boudica\" Icon.StarHaloUp\n [party StarUp 20]\n , bo",
"end": 59398,
"score": 0.9930369854,
"start": 59391,
"tag": "NAME",
"value": "Boudica"
},
{
"context": "ty StarUp 20]\n , bond 209 \"To My Dear Friend\" \"Hans Christian Andersen\" Icon.HoodUp\n [party DebuffResist 30]\n , ",
"end": 59499,
"score": 0.998916924,
"start": 59476,
"tag": "NAME",
"value": "Hans Christian Andersen"
},
{
"context": "dUp\n [party DebuffResist 30]\n , bond 210 \"Sacred Devotion\" \"Arash\" Icon.Heal\n [ When \"defeated\" <| To ",
"end": 59574,
"score": 0.9923032522,
"start": 59559,
"tag": "NAME",
"value": "Sacred Devotion"
},
{
"context": "ebuffResist 30]\n , bond 210 \"Sacred Devotion\" \"Arash\" Icon.Heal\n [ When \"defeated\" <| To Party Re",
"end": 59582,
"score": 0.993698597,
"start": 59577,
"tag": "NAME",
"value": "Arash"
},
{
"context": "arty Heal <| Flat 5000\n ]\n , { name = \"The Wandering Tales of Shana-oh\"\n , id = 211\n , rarity =",
"end": 59752,
"score": 0.8757660985,
"start": 59730,
"tag": "NAME",
"value": "The Wandering Tales of"
},
{
"context": "\n ]\n , { name = \"The Wandering Tales of Shana-oh\"\n , id = 211\n , rarity = 5\n ",
"end": 59761,
"score": 0.9006229639,
"start": 59753,
"tag": "NAME",
"value": "Shana-oh"
},
{
"context": " , limited = True\n }\n , { name = \"Golden Captures the Carp\"\n , id = 212\n ",
"end": 60227,
"score": 0.5219669342,
"start": 60223,
"tag": "NAME",
"value": "Gold"
},
{
"context": "e\n }\n , bond 216 \"Key of the King's Law\" \"Gilgamesh\" Icon.BeamUp\n [self NPUp 30, atkChance 30 <<",
"end": 61914,
"score": 0.9990389943,
"start": 61905,
"tag": "NAME",
"value": "Gilgamesh"
},
{
"context": "CritUp <| Flat 10]\n , bond 217 \"Golden Glass\" \"Sakata Kintoki\" Icon.BeamUp\n [self NPUp 30, atkChance 30 <<",
"end": 62042,
"score": 0.9995425344,
"start": 62028,
"tag": "NAME",
"value": "Sakata Kintoki"
},
{
"context": " <| Flat 5]\n , bond 218 \"Thunderous Applause\" \"Nero Claudius\" Icon.ArtsUp\n [party_ Arts 15]\n , bond 21",
"end": 62171,
"score": 0.9997882843,
"start": 62158,
"tag": "NAME",
"value": "Nero Claudius"
},
{
"context": "con.ArtsUp\n [party_ Arts 15]\n , bond 219 \"Das Rheingold\" \"Siegfried\" Icon.NobleUp\n [party NPGen 15]\n",
"end": 62237,
"score": 0.9992280006,
"start": 62224,
"tag": "NAME",
"value": "Das Rheingold"
},
{
"context": " [party_ Arts 15]\n , bond 219 \"Das Rheingold\" \"Siegfried\" Icon.NobleUp\n [party NPGen 15]\n , bond 2",
"end": 62249,
"score": 0.9995326996,
"start": 62240,
"tag": "NAME",
"value": "Siegfried"
},
{
"context": "Gen 15]\n , bond 220 \"Radiance of the Goddess\" \"Stheno\" Icon.QuickUp\n [party_ Quick 15]\n , bond ",
"end": 62335,
"score": 0.9981089234,
"start": 62329,
"tag": "NAME",
"value": "Stheno"
},
{
"context": "Quick 15]\n , bond 221 \"Voyage of the Flowers\" \"Altria Pendragon (Lily)\" Icon.SwordUp\n [party AttackUp 10, pa",
"end": 62430,
"score": 0.9997962713,
"start": 62414,
"tag": "NAME",
"value": "Altria Pendragon"
},
{
"context": "nd 221 \"Voyage of the Flowers\" \"Altria Pendragon (Lily)\" Icon.SwordUp\n [party AttackUp 10, party St",
"end": 62436,
"score": 0.9826453924,
"start": 62432,
"tag": "NAME",
"value": "Lily"
},
{
"context": " StarUp 10]\n , bond 222 \"Ark of the Covenant\" \"David\" Icon.BeamUp\n [self NPUp 30, When \"attacking",
"end": 62538,
"score": 0.9996057749,
"start": 62533,
"tag": "NAME",
"value": "David"
},
{
"context": "ath <| Flat 10]\n , bond 223 \"Door to Babylon\" \"Darius III\" Icon.BusterUp\n [party_ Buster 15]\n , bon",
"end": 62664,
"score": 0.9991309643,
"start": 62654,
"tag": "NAME",
"value": "Darius III"
},
{
"context": " Buster 15]\n , bond 224 \"Blood-Thirsting Axe\" \"Eric Bloodaxe\" Icon.ExclamationUp\n [party CritUp 25]\n ,",
"end": 62756,
"score": 0.9992921948,
"start": 62743,
"tag": "NAME",
"value": "Eric Bloodaxe"
},
{
"context": " [party CritUp 25]\n , bond 225 \"Insurrection\" \"Spartacus\" Icon.Kneel\n [gutsPercent 50]\n , { name ",
"end": 62841,
"score": 0.9874463677,
"start": 62832,
"tag": "NAME",
"value": "Spartacus"
},
{
"context": "n.Kneel\n [gutsPercent 50]\n , { name = \"Go West!!\"\n , id = 226\n , rarity = 5\n ",
"end": 62903,
"score": 0.8422684073,
"start": 62896,
"tag": "NAME",
"value": "Go West"
},
{
"context": " , limited = True\n }\n , { name = \"True Samadhi Fire\"\n , id = 228\n , rarity = 4\n ",
"end": 63813,
"score": 0.9990457296,
"start": 63796,
"tag": "NAME",
"value": "True Samadhi Fire"
},
{
"context": "ng\n , limited = True\n }\n , bond 230 \"Tristar Belt\" \"Orion\" Icon.ExclamationUp\n [party CritUp 2",
"end": 64649,
"score": 0.8081123829,
"start": 64637,
"tag": "NAME",
"value": "Tristar Belt"
},
{
"context": "ted = True\n }\n , bond 230 \"Tristar Belt\" \"Orion\" Icon.ExclamationUp\n [party CritUp 25]\n ,",
"end": 64657,
"score": 0.8960481882,
"start": 64652,
"tag": "NAME",
"value": "Orion"
},
{
"context": "amationUp\n [party CritUp 25]\n , bond 231 \"Golden Helm\" \"Francis Drake\" Icon.BeamUp\n [party NPUp 20",
"end": 64729,
"score": 0.7259371877,
"start": 64718,
"tag": "NAME",
"value": "Golden Helm"
},
{
"context": " [party CritUp 25]\n , bond 231 \"Golden Helm\" \"Francis Drake\" Icon.BeamUp\n [party NPUp 20]\n , bond 232",
"end": 64745,
"score": 0.9870765805,
"start": 64732,
"tag": "NAME",
"value": "Francis Drake"
},
{
"context": " NPUp 20]\n , bond 232 \"Black Knight's Helmet\" \"Lancelot\" Icon.BeamUp\n [self NPUp 30, atkChance 30 <<",
"end": 64829,
"score": 0.9044687152,
"start": 64821,
"tag": "NAME",
"value": "Lancelot"
},
{
"context": "f Target 3 CritChance <| Flat 30]\n , bond 233 \"Golden Apple\" \"Atalante\" Icon.QuickUp\n [party_ Quick 15]\n",
"end": 64947,
"score": 0.6544100046,
"start": 64935,
"tag": "NAME",
"value": "Golden Apple"
},
{
"context": "Chance <| Flat 30]\n , bond 233 \"Golden Apple\" \"Atalante\" Icon.QuickUp\n [party_ Quick 15]\n , bond ",
"end": 64958,
"score": 0.9517299533,
"start": 64950,
"tag": "NAME",
"value": "Atalante"
},
{
"context": "y_ Quick 15]\n , bond 234 \"Holy Pumpkin Grail\" \"Elisabeth Bathory (Halloween)\" Icon.HoodUp\n [party DebuffResis",
"end": 65051,
"score": 0.8703578711,
"start": 65034,
"tag": "NAME",
"value": "Elisabeth Bathory"
},
{
"context": "dUp\n [party DebuffResist 30]\n , bond 235 \"Rotary Matchlock\" \"Oda Nobunaga\" Icon.ExclamationUp\n [party C",
"end": 65139,
"score": 0.9844780564,
"start": 65123,
"tag": "NAME",
"value": "Rotary Matchlock"
},
{
"context": "buffResist 30]\n , bond 235 \"Rotary Matchlock\" \"Oda Nobunaga\" Icon.ExclamationUp\n [party CritUp 25]\n ,",
"end": 65154,
"score": 0.9982640743,
"start": 65142,
"tag": "NAME",
"value": "Oda Nobunaga"
},
{
"context": "amationUp\n [party CritUp 25]\n , bond 236 \"Llamrei Unit II\" \"Altria Pendragon (Santa Alter)\" Icon.StarHal",
"end": 65227,
"score": 0.6711776853,
"start": 65215,
"tag": "NAME",
"value": "Llamrei Unit"
},
{
"context": "arty CritUp 25]\n , bond 236 \"Llamrei Unit II\" \"Altria Pendragon (Santa Alter)\" Icon.StarHaloUp\n [party StarU",
"end": 65249,
"score": 0.9976771474,
"start": 65233,
"tag": "NAME",
"value": "Altria Pendragon"
},
{
"context": " 15]\n , bond 239 \"Heaven Among the Mountains\" \"Sasaki Kojirou\" Icon.QuickUp\n [party_ Quick 15]\n , { nam",
"end": 65561,
"score": 0.9992811084,
"start": 65547,
"tag": "NAME",
"value": "Sasaki Kojirou"
},
{
"context": "uickUp\n [party_ Quick 15]\n , { name = \"Divine Princess of the Storm\"\n , id = 240\n , rarity = 5\n ",
"end": 65647,
"score": 0.9987027049,
"start": 65619,
"tag": "NAME",
"value": "Divine Princess of the Storm"
},
{
"context": " , limited = True\n }\n , { name = \"Ox-Demon King\"\n , id = 241\n , rarity = 5\n ",
"end": 66011,
"score": 0.9980807304,
"start": 65998,
"tag": "NAME",
"value": "Ox-Demon King"
},
{
"context": " , limited = True\n }\n , { name = \"Personal Lesson\"\n , id = 242\n , rarity = 5\n ",
"end": 66365,
"score": 0.9920516014,
"start": 66350,
"tag": "NAME",
"value": "Personal Lesson"
},
{
"context": " , limited = True\n }\n , { name = \"Bronze-Link Manipulator\"\n , id = 243\n , rarity = 3\n ",
"end": 66713,
"score": 0.9993537068,
"start": 66690,
"tag": "NAME",
"value": "Bronze-Link Manipulator"
},
{
"context": " , limited = False\n }\n , { name = \"Ath nGabla\"\n , id = 244\n , rarity = 3\n ",
"end": 67054,
"score": 0.9998280406,
"start": 67044,
"tag": "NAME",
"value": "Ath nGabla"
},
{
"context": " , limited = False\n }\n , { name = \"Bygone Dream\"\n , id = 245\n , rarity = 3\n ",
"end": 67480,
"score": 0.9993706942,
"start": 67468,
"tag": "NAME",
"value": "Bygone Dream"
},
{
"context": " , limited = False\n }\n , { name = \"Extremely Spicy Mapo Tofu\"\n , id = 246\n , rarity = 3\n ",
"end": 67852,
"score": 0.9852466583,
"start": 67827,
"tag": "NAME",
"value": "Extremely Spicy Mapo Tofu"
},
{
"context": " , limited = False\n }\n , { name = \"Jeweled Sword Zelretch\"\n , id = 247\n , rarity = 3\n ",
"end": 68211,
"score": 0.9986079931,
"start": 68189,
"tag": "NAME",
"value": "Jeweled Sword Zelretch"
},
{
"context": "y_ Arts 15]\n , bond 249 \"Headband of Resolve\" \"Okita Souji\" Icon.ExclamationUp\n [party CritUp 25]\n ,",
"end": 68721,
"score": 0.9993324876,
"start": 68710,
"tag": "NAME",
"value": "Okita Souji"
},
{
"context": "amationUp\n [party CritUp 25]\n , bond 250 \"Calico Jack\" \"Anne Bonny & Mary Read\" Icon.ExclamationUp\n ",
"end": 68793,
"score": 0.9998480678,
"start": 68782,
"tag": "NAME",
"value": "Calico Jack"
},
{
"context": " [party CritUp 25]\n , bond 250 \"Calico Jack\" \"Anne Bonny & Mary Read\" Icon.ExclamationUp\n [party Crit",
"end": 68806,
"score": 0.9998570681,
"start": 68796,
"tag": "NAME",
"value": "Anne Bonny"
},
{
"context": "tUp 25]\n , bond 250 \"Calico Jack\" \"Anne Bonny & Mary Read\" Icon.ExclamationUp\n [party CritUp 25]\n ,",
"end": 68818,
"score": 0.9998366237,
"start": 68809,
"tag": "NAME",
"value": "Mary Read"
},
{
"context": " [party CritUp 25]\n , bond 251 \"Gazing Upon Dun Scaith\" \"Scathach\" Icon.QuickUp\n [party_ Quick 15]\n",
"end": 68901,
"score": 0.9956924915,
"start": 68891,
"tag": "NAME",
"value": "Dun Scaith"
},
{
"context": "itUp 25]\n , bond 251 \"Gazing Upon Dun Scaith\" \"Scathach\" Icon.QuickUp\n [party_ Quick 15]\n , bond ",
"end": 68912,
"score": 0.9985679984,
"start": 68904,
"tag": "NAME",
"value": "Scathach"
},
{
"context": "rty_ Quick 15]\n , bond 252 \"Star of Prophecy\" \"Cu Chulainn\" Icon.BeamUp\n [self NPUp 30, atkChance 30 <<",
"end": 68997,
"score": 0.9979464412,
"start": 68986,
"tag": "NAME",
"value": "Cu Chulainn"
},
{
"context": "< Grant Self 3 CritUp <| Flat 10]\n , bond 253 \"Hekate's Staff\" \"Medea\" Icon.ArtsUp\n [party_ Ar",
"end": 69098,
"score": 0.7740362883,
"start": 69096,
"tag": "NAME",
"value": "He"
},
{
"context": "itUp <| Flat 10]\n , bond 253 \"Hekate's Staff\" \"Medea\" Icon.ArtsUp\n [party_ Arts 15]\n , bond 25",
"end": 69118,
"score": 0.9990558624,
"start": 69113,
"tag": "NAME",
"value": "Medea"
},
{
"context": "party_ Arts 15]\n , bond 254 \"Formless Island\" \"Medusa\" Icon.NobleUp\n [party NPGen 15]\n , bond 2",
"end": 69195,
"score": 0.9975857139,
"start": 69189,
"tag": "NAME",
"value": "Medusa"
},
{
"context": "arty NPGen 15]\n , bond 255 \"Cask of the Wise\" \"Alexander\" Icon.QuickUp\n [party_ Quick 15]\n , bond ",
"end": 69277,
"score": 0.9992307425,
"start": 69268,
"tag": "NAME",
"value": "Alexander"
},
{
"context": "n.QuickUp\n [party_ Quick 15]\n , bond 256 \"Shaytan's Arm\" \"Hassan of the Cursed Arm\" Icon.ReaperUp\n ",
"end": 69339,
"score": 0.8521304727,
"start": 69332,
"tag": "NAME",
"value": "Shaytan"
},
{
"context": "ReaperUp\n [party DeathUp 20]\n , bond 257 \"Ariadne's Thread\" \"Asterios\" Icon.QuickUp\n [party_ Quick 15]\n",
"end": 69445,
"score": 0.9559099674,
"start": 69429,
"tag": "NAME",
"value": "Ariadne's Thread"
},
{
"context": "ty DeathUp 20]\n , bond 257 \"Ariadne's Thread\" \"Asterios\" Icon.QuickUp\n [party_ Quick 15]\n , { nam",
"end": 69456,
"score": 0.9988396764,
"start": 69448,
"tag": "NAME",
"value": "Asterios"
},
{
"context": "uickUp\n [party_ Quick 15]\n , { name = \"Dumplings Over Flowers\"\n , id = 258\n , rarity = 5\n ",
"end": 69536,
"score": 0.9778637886,
"start": 69514,
"tag": "NAME",
"value": "Dumplings Over Flowers"
},
{
"context": " , limited = True\n }\n , { name = \"Faithful Companions\"\n , id = 259\n , rarity = 4\n ",
"end": 69967,
"score": 0.9080836177,
"start": 69948,
"tag": "NAME",
"value": "Faithful Companions"
},
{
"context": " , limited = False\n }\n , { name = \"Covering Fire\"\n , id = 264\n , rarity = 4\n ",
"end": 72132,
"score": 0.7434981465,
"start": 72119,
"tag": "NAME",
"value": "Covering Fire"
},
{
"context": " , limited = False\n }\n , { name = \"Battle of Camlann\"\n , id = 265\n , rarity = 3\n ",
"end": 72559,
"score": 0.9982149005,
"start": 72542,
"tag": "NAME",
"value": "Battle of Camlann"
},
{
"context": "mited = False\n }\n , bond 266 \"Who Am I?\" \"Mordred\" Icon.BeamUp\n [party NPUp 20]\n , bond 267",
"end": 72917,
"score": 0.9998250008,
"start": 72910,
"tag": "NAME",
"value": "Mordred"
},
{
"context": "p 20]\n , bond 267 \"The Misty Night of London\" \"Jack the Ripper\" Icon.ExclamationUp\n [party CritUp 25]\n ,",
"end": 73012,
"score": 0.9998173714,
"start": 72997,
"tag": "NAME",
"value": "Jack the Ripper"
},
{
"context": " [party CritUp 25]\n , bond 268 \"Wonderland\" \"Nursery Rhyme\" Icon.ExclamationUp\n [party CritUp 15, party",
"end": 73099,
"score": 0.9992137551,
"start": 73086,
"tag": "NAME",
"value": "Nursery Rhyme"
},
{
"context": "tUp 15, party HealingReceived 10]\n , bond 269 \"Faceless King\" \"Robin Hood\" Icon.ArtsUp\n [party_ Arts",
"end": 73194,
"score": 0.7888357043,
"start": 73186,
"tag": "NAME",
"value": "Faceless"
},
{
"context": "alingReceived 10]\n , bond 269 \"Faceless King\" \"Robin Hood\" Icon.ArtsUp\n [party_ Arts 15]\n , bond 27",
"end": 73212,
"score": 0.9998134375,
"start": 73202,
"tag": "NAME",
"value": "Robin Hood"
},
{
"context": "con.ArtsUp\n [party_ Arts 15]\n , bond 270 \"Usumidori\" \"Ushiwakamaru\" Icon.QuickUp\n [party_ Qui",
"end": 73271,
"score": 0.8048710823,
"start": 73265,
"tag": "NAME",
"value": "Usumid"
},
{
"context": " [party_ Arts 15]\n , bond 270 \"Usumidori\" \"Ushiwakamaru\" Icon.QuickUp\n [party_ Quick 15]\n , bond ",
"end": 73289,
"score": 0.963988781,
"start": 73277,
"tag": "NAME",
"value": "Ushiwakamaru"
},
{
"context": "ck 15]\n , bond 271 \"Etiquette of Nine Guests\" \"Jing Ke\" Icon.BeamUp\n [self NPUp 30, atkChance 30 <<",
"end": 73378,
"score": 0.9997553229,
"start": 73371,
"tag": "NAME",
"value": "Jing Ke"
},
{
"context": " Grant Self 3 DeathUp <| Flat 30]\n , bond 272 \"Heaven Scorcher Halberd\" \"Lu Bu Fengxian\" Icon.BusterUp\n [party_ Bus",
"end": 73501,
"score": 0.9994863868,
"start": 73478,
"tag": "NAME",
"value": "Heaven Scorcher Halberd"
},
{
"context": "lat 30]\n , bond 272 \"Heaven Scorcher Halberd\" \"Lu Bu Fengxian\" Icon.BusterUp\n [party_ Buster 15]\n , bon",
"end": 73518,
"score": 0.9997702241,
"start": 73504,
"tag": "NAME",
"value": "Lu Bu Fengxian"
},
{
"context": "ter 15]\n , bond 273 \"What can be Left Behind\" \"Georgios\" Icon.Shield\n [ When \"defeated\" << Times 1 <",
"end": 73609,
"score": 0.9762658477,
"start": 73601,
"tag": "NAME",
"value": "Georgios"
},
{
"context": " 3 DamageCut <| Flat 1000\n ]\n , bond 274 \"Thermopylae\" \"Leonidas I\" Icon.BusterUp\n [party_ Buster ",
"end": 73793,
"score": 0.9996221662,
"start": 73782,
"tag": "NAME",
"value": "Thermopylae"
},
{
"context": "| Flat 1000\n ]\n , bond 274 \"Thermopylae\" \"Leonidas I\" Icon.BusterUp\n [party_ Buster 15]\n , bon",
"end": 73806,
"score": 0.9997572899,
"start": 73796,
"tag": "NAME",
"value": "Leonidas I"
},
{
"context": "BusterUp\n [party_ Buster 15]\n , bond 275 \"Haydn Quartets\" \"Wolfgang Amadeus Mozart\" Icon.BeamUp\n [par",
"end": 73877,
"score": 0.9998601079,
"start": 73863,
"tag": "NAME",
"value": "Haydn Quartets"
},
{
"context": "arty_ Buster 15]\n , bond 275 \"Haydn Quartets\" \"Wolfgang Amadeus Mozart\" Icon.BeamUp\n [party NPUp 20]\n , gift 276",
"end": 73903,
"score": 0.999892354,
"start": 73880,
"tag": "NAME",
"value": "Wolfgang Amadeus Mozart"
},
{
"context": "Icon.BeamUp\n [party NPUp 20]\n , gift 276 \"Anniversary Heroines\" Icon.SwordUp\n [self AttackUp 10, self Stars",
"end": 73975,
"score": 0.9994602203,
"start": 73955,
"tag": "NAME",
"value": "Anniversary Heroines"
},
{
"context": "ackUp 10, self StarsPerTurn 3]\n , { name = \"Leisure Stroll\"\n , id = 277\n , rarity = 5\n ",
"end": 74069,
"score": 0.9998733401,
"start": 74055,
"tag": "NAME",
"value": "Leisure Stroll"
},
{
"context": " , limited = True\n }\n , { name = \"Partake with the King\"\n , id = 278\n , rarity = 5\n ",
"end": 74506,
"score": 0.9990615845,
"start": 74485,
"tag": "NAME",
"value": "Partake with the King"
},
{
"context": " , limited = True\n }\n , { name = \"Goldfish Scooping\"\n , id = 279\n , rarity = 4\n ",
"end": 74930,
"score": 0.9996711612,
"start": 74913,
"tag": "NAME",
"value": "Goldfish Scooping"
},
{
"context": " , limited = True\n }\n , { name = \"Fire Flower\"\n , id = 280\n , rarity = 3\n ",
"end": 75345,
"score": 0.9990447164,
"start": 75334,
"tag": "NAME",
"value": "Fire Flower"
},
{
"context": "ed = True\n }\n , bond 281 \"Arm of Raiden\" \"Nikola Tesla\" Icon.BeamUp\n [party NPUp 20]\n , bond 282",
"end": 75771,
"score": 0.9996081591,
"start": 75759,
"tag": "NAME",
"value": "Nikola Tesla"
},
{
"context": " [party NPUp 20]\n , bond 282 \"Endowed Hero\" \"Arjuna\" Icon.BeamUp\n [self NPUp 30, self GatherUp 1",
"end": 75844,
"score": 0.9995192885,
"start": 75838,
"tag": "NAME",
"value": "Arjuna"
},
{
"context": "rUp 1000]\n , bond 283 \"Light of the Deprived\" \"Karna\" Icon.AllUp\n [party_ Quick 8, party_ Arts 8,",
"end": 75944,
"score": 0.9996685386,
"start": 75939,
"tag": "NAME",
"value": "Karna"
},
{
"context": "Buster 8]\n , bond 284 \"Procedure to Humanity\" \"Frankenstein\" Icon.QuickUp\n [party_ Quick 15]\n , bond ",
"end": 76064,
"score": 0.9994729757,
"start": 76052,
"tag": "NAME",
"value": "Frankenstein"
},
{
"context": " [party_ Quick 15]\n , bond 285 \"Black Helmet\" \"Altria Pendragon (Lancer Alter)\" Icon.NobleUp\n [party NPGen 1",
"end": 76150,
"score": 0.9900129437,
"start": 76134,
"tag": "NAME",
"value": "Altria Pendragon"
},
{
"context": "en 15]\n , bond 286 \"Legend of the Gallic War\" \"Gaius Julius Caesar\" Icon.QuickUp\n [party_ Quick 15]\n , bond ",
"end": 76265,
"score": 0.9659478664,
"start": 76246,
"tag": "NAME",
"value": "Gaius Julius Caesar"
},
{
"context": "n.QuickUp\n [party_ Quick 15]\n , bond 287 \"Rome\" \"Romulus\" Icon.BeamUp\n [party NPUp 20]\n ",
"end": 76324,
"score": 0.8526991606,
"start": 76320,
"tag": "NAME",
"value": "Rome"
},
{
"context": "Up\n [party_ Quick 15]\n , bond 287 \"Rome\" \"Romulus\" Icon.BeamUp\n [party NPUp 20]\n , bond 288",
"end": 76334,
"score": 0.9996934533,
"start": 76327,
"tag": "NAME",
"value": "Romulus"
},
{
"context": "p 20]\n , bond 288 \"Encounter at Gojou Bridge\" \"Musashibou Benkei\" Icon.NobleRedUp\n [party NPFromDamage 20]\n ",
"end": 76431,
"score": 0.9998735189,
"start": 76414,
"tag": "NAME",
"value": "Musashibou Benkei"
},
{
"context": "ty_ Quick 15]\n , bond 290 \"Really Convenient\" \"William Shakespeare\" Icon.NobleUp\n [party NPGen 15]\n , { name",
"end": 76629,
"score": 0.9998796582,
"start": 76610,
"tag": "NAME",
"value": "William Shakespeare"
},
{
"context": "NobleUp\n [party NPGen 15]\n , { name = \"Pirates Party!\"\n , id = 291\n , rarity = 5\n ,",
"end": 76699,
"score": 0.9419038892,
"start": 76686,
"tag": "NAME",
"value": "Pirates Party"
},
{
"context": " , limited = True\n }\n , { name = \"Summertime Mistress\"\n , id = 292\n , rarity = 5\n ",
"end": 77124,
"score": 0.9955790639,
"start": 77105,
"tag": "NAME",
"value": "Summertime Mistress"
},
{
"context": " , limited = True\n }\n , { name = \"Twilight Memory\"\n , id = 293\n , rarity = 4\n ",
"end": 77542,
"score": 0.9660633206,
"start": 77527,
"tag": "NAME",
"value": "Twilight Memory"
},
{
"context": " , limited = True\n }\n , { name = \"Shiny Goddess\"\n , id = 294\n , rarity = 3\n ",
"end": 77968,
"score": 0.9911950827,
"start": 77955,
"tag": "NAME",
"value": "Shiny Goddess"
},
{
"context": " , limited = True\n }\n , { name = \"Knights of Marines\"\n , id = 295\n , rarity = 5\n ",
"end": 78391,
"score": 0.9601723552,
"start": 78373,
"tag": "NAME",
"value": "Knights of Marines"
},
{
"context": " , limited = True\n }\n , { name = \"Chaldea Lifesavers\"\n , id = 296\n , rarity = 5\n ",
"end": 78814,
"score": 0.9997831583,
"start": 78796,
"tag": "NAME",
"value": "Chaldea Lifesavers"
},
{
"context": " , limited = True\n }\n , { name = \"Meat Wars\"\n , id = 297\n , rarity = 4\n ",
"end": 79219,
"score": 0.7821455598,
"start": 79215,
"tag": "NAME",
"value": "Meat"
},
{
"context": ", limited = True\n }\n , { name = \"Meat Wars\"\n , id = 297\n , rarity = 4\n ",
"end": 79224,
"score": 0.9022116661,
"start": 79221,
"tag": "NAME",
"value": "ars"
},
{
"context": " , limited = True\n }\n , { name = \"Shaved Ice (Void's Dust Flavor)\"\n , id = 298\n ",
"end": 79652,
"score": 0.9824430943,
"start": 79642,
"tag": "NAME",
"value": "Shaved Ice"
},
{
"context": "ty NPGen 10]\n , bond 301 \"Ring of Bay Laurel\" \"Nero Claudius (Bride)\" Icon.ArtsUp\n [party_ Arts 15]\n ,",
"end": 80343,
"score": 0.980753243,
"start": 80330,
"tag": "NAME",
"value": "Nero Claudius"
},
{
"context": "it Dragon) 20]\n , bond 303 \"Bratan of Wisdom\" \"Fionn mac Cumhaill\" Icon.ArtsUp\n [party_ Arts ",
"end": 80532,
"score": 0.5377396345,
"start": 80531,
"tag": "NAME",
"value": "F"
},
{
"context": "ty NPUp 10]\n , bond 304 \"Prelati's Spellbook\" \"Gilles de Rais\" Icon.BusterUp\n [party_ Buster 20, demeritAl",
"end": 80653,
"score": 0.710773468,
"start": 80639,
"tag": "NAME",
"value": "Gilles de Rais"
},
{
"context": "l DebuffVuln 20]\n , bond 305 \"Parasitic Bomb\" \"Mephistopheles\" Icon.BeamUp\n [party NPUp 20]\n ",
"end": 80755,
"score": 0.5061617494,
"start": 80753,
"tag": "NAME",
"value": "Me"
},
{
"context": "uln 20]\n , bond 305 \"Parasitic Bomb\" \"Mephistopheles\" Icon.BeamUp\n [party NPUp 20]\n , bond 306",
"end": 80767,
"score": 0.5556640625,
"start": 80762,
"tag": "NAME",
"value": "heles"
},
{
"context": "ty NPUp 20]\n , bond 306 \"Seethe of a Warrior\" \"Fergus mac Roich\" Icon.BusterUp\n [party_ Buster 10, party NPU",
"end": 80857,
"score": 0.9998517632,
"start": 80841,
"tag": "NAME",
"value": "Fergus mac Roich"
},
{
"context": "arty NPUp 10]\n , bond 307 \"My Loathsome Life\" \"Charles-Henri Sanson\" Icon.ReaperUp\n [party DeathUp 10, party NPG",
"end": 80969,
"score": 0.9998554587,
"start": 80949,
"tag": "NAME",
"value": "Charles-Henri Sanson"
},
{
"context": "NPGen 10]\n , bond 308 \"There is No Love Here\" \"Caligula\" Icon.BusterUp\n [party_ Buster 20, demeritAl",
"end": 81074,
"score": 0.9994537234,
"start": 81066,
"tag": "NAME",
"value": "Caligula"
},
{
"context": "20, demeritAll DefenseDown 10]\n , { name = \"Magical Girl of Sapphire\"\n , id = 309\n , rarity = 5\n ",
"end": 81185,
"score": 0.9997580051,
"start": 81161,
"tag": "NAME",
"value": "Magical Girl of Sapphire"
},
{
"context": " , limited = True\n }\n , { name = \"Kill on Sight\"\n , id = 310\n , rarity =",
"end": 81585,
"score": 0.6973616481,
"start": 81581,
"tag": "NAME",
"value": "Kill"
},
{
"context": " , limited = True\n }\n , { name = \"Zunga Zunga!\"\n , id = 311\n , rarity = 3\n ",
"end": 82012,
"score": 0.9981896281,
"start": 82001,
"tag": "NAME",
"value": "Zunga Zunga"
},
{
"context": " , limited = True\n }\n , { name = \"Kaleid Ruby\"\n , id = 312\n , rarity = 4\n ",
"end": 82438,
"score": 0.9998830557,
"start": 82427,
"tag": "NAME",
"value": "Kaleid Ruby"
},
{
"context": " , limited = True\n }\n , { name = \"Kaleid Sapphire\"\n , id = 313\n , rarity = 4\n ",
"end": 82861,
"score": 0.9998914003,
"start": 82846,
"tag": "NAME",
"value": "Kaleid Sapphire"
},
{
"context": "\n }\n , bond 315 \"Mugashiki—Shinkuu Myou\" \"Ryougi Shiki (Saber)\" Icon.ArtsUp\n [party_ Arts 15]\n ,",
"end": 83299,
"score": 0.9987327456,
"start": 83287,
"tag": "NAME",
"value": "Ryougi Shiki"
},
{
"context": "rty_ Arts 15]\n , bond 316 \"Frontliner's Flag\" \"Amakusa Shirou\" Icon.DamageUp\n [ party (Special AttackUp <|",
"end": 83394,
"score": 0.9989449978,
"start": 83380,
"tag": "NAME",
"value": "Amakusa Shirou"
},
{
"context": "emonic) 20\n ]\n , bond 317 \"Chateau d'If\" \"Edmond Dantes\" Icon.QuickUp\n [party_ Quick 15]\n , bond ",
"end": 83571,
"score": 0.9988850355,
"start": 83558,
"tag": "NAME",
"value": "Edmond Dantes"
},
{
"context": "Received 30]\n , bond 319 \"Red Leather Jacket\" \"Ryougi Shiki (Assassin)\" Icon.ReaperUp\n [party DeathUp 30",
"end": 83755,
"score": 0.996896565,
"start": 83743,
"tag": "NAME",
"value": "Ryougi Shiki"
},
{
"context": "30]\n , bond 320 \"Otherworldly Mystical Horse\" \"Astolfo\" Icon.Dodge\n [self NPUp 30, Times 1 <| Grant",
"end": 83860,
"score": 0.5851439834,
"start": 83853,
"tag": "NAME",
"value": "Astolfo"
},
{
"context": "sion Full]\n , bond 321 \"Letter From a Friend\" \"Gilles de Rais (Caster)\" Icon.BusterUp\n [party_ Buster 20, ",
"end": 83986,
"score": 0.9981479645,
"start": 83972,
"tag": "NAME",
"value": "Gilles de Rais"
},
{
"context": " StarDown 20]\n , bond 322 \"Hound of Culann\" \"Cu Chulainn (Prototype)\" Icon.DamageUp\n [party (Sp",
"end": 84099,
"score": 0.757653594,
"start": 84097,
"tag": "NAME",
"value": "Ch"
},
{
"context": "Down 20]\n , bond 322 \"Hound of Culann\" \"Cu Chulainn (Prototype)\" Icon.DamageUp\n [party (Special ",
"end": 84105,
"score": 0.6490334272,
"start": 84101,
"tag": "NAME",
"value": "ainn"
},
{
"context": "n.BeamUp\n [party NPUp 20]\n , { name = \"Glory Is With Me\"\n , id = 325\n , rarity = 5\n ",
"end": 84397,
"score": 0.9933802485,
"start": 84381,
"tag": "NAME",
"value": "Glory Is With Me"
},
{
"context": " , limited = True\n }\n , { name = \"Original Legion\"\n , id = 326\n , rarity = 4\n ",
"end": 84869,
"score": 0.9887023568,
"start": 84854,
"tag": "NAME",
"value": "Original Legion"
},
{
"context": " , limited = True\n }\n , { name = \"Howl at the Moon\"\n , id = 327\n , rarity = 3\n ",
"end": 85290,
"score": 0.9991411567,
"start": 85274,
"tag": "NAME",
"value": "Howl at the Moon"
},
{
"context": " , limited = True\n }\n , { name = \"Princess of the White Rose\"\n , id = 328\n , rarity = 5\n ",
"end": 85728,
"score": 0.999565959,
"start": 85702,
"tag": "NAME",
"value": "Princess of the White Rose"
},
{
"context": " , limited = True\n }\n , { name = \"Joint Recital\"\n , id = 329\n , rarity = 5\n ",
"end": 86145,
"score": 0.9997242093,
"start": 86132,
"tag": "NAME",
"value": "Joint Recital"
},
{
"context": " , limited = True\n }\n , { name = \"Chaldea Lunchtime\"\n , id = 330\n , rarity = 5\n ",
"end": 86573,
"score": 0.9997239709,
"start": 86556,
"tag": "NAME",
"value": "Chaldea Lunchtime"
},
{
"context": " , limited = True\n }\n , { name = \"Fragarach\"\n , id = 331\n , rarity = 3\n ",
"end": 86913,
"score": 0.9997019172,
"start": 86904,
"tag": "NAME",
"value": "Fragarach"
},
{
"context": " , limited = False\n }\n , { name = \"Inverted Moon of the Heavens\"\n , id = 332\n",
"end": 87247,
"score": 0.888931334,
"start": 87245,
"tag": "NAME",
"value": "In"
},
{
"context": "ted = False\n }\n , { name = \"Inverted Moon of the Heavens\"\n , id = 332\n , rarity",
"end": 87261,
"score": 0.5491181016,
"start": 87256,
"tag": "NAME",
"value": "on of"
},
{
"context": "se\n }\n , { name = \"Inverted Moon of the Heavens\"\n , id = 332\n , rarity = 3\n ",
"end": 87273,
"score": 0.8839473724,
"start": 87266,
"tag": "NAME",
"value": "Heavens"
},
{
"context": " , limited = False\n }\n , { name = \"Hydra Dagger\"\n , id = 333\n , rarity = 3\n ",
"end": 87696,
"score": 0.9997246265,
"start": 87684,
"tag": "NAME",
"value": "Hydra Dagger"
},
{
"context": " [self NPUp 30, gutsPercent 20]\n , bond 336 \"Sacred Spring\" \"Queen Medb\" Icon.NobleUp\n [party NPGen 15]",
"end": 88254,
"score": 0.9601261616,
"start": 88241,
"tag": "NAME",
"value": "Sacred Spring"
},
{
"context": ", gutsPercent 20]\n , bond 336 \"Sacred Spring\" \"Queen Medb\" Icon.NobleUp\n [party NPGen 15]\n , bond 3",
"end": 88267,
"score": 0.9929506779,
"start": 88257,
"tag": "NAME",
"value": "Queen Medb"
},
{
"context": " NPGen 15]\n , bond 337 \"Indestructible Blade\" \"Rama\" Icon.ExclamationUp\n [party CritUp 25]\n ,",
"end": 88348,
"score": 0.914639473,
"start": 88344,
"tag": "NAME",
"value": "Rama"
},
{
"context": "ty CritUp 25]\n , bond 338 \"Concealed Goddess\" \"Helena Blavatsky\" Icon.DamageUp\n [party (Special AttackUp <| ",
"end": 88445,
"score": 0.9996982217,
"start": 88429,
"tag": "NAME",
"value": "Helena Blavatsky"
},
{
"context": "sin) 20]\n , bond 339 \"Lights of Civilization\" \"Thomas Edison\" Icon.NobleUp\n [party NPGen 15]\n , bond 3",
"end": 88571,
"score": 0.9996609688,
"start": 88558,
"tag": "NAME",
"value": "Thomas Edison"
},
{
"context": " , bond 340 \"Reaching the Zenith of My Skill\" \"Li Shuwen\" Icon.ArtsUp\n [party_ Arts 15]\n , bond 34",
"end": 88668,
"score": 0.9996032715,
"start": 88659,
"tag": "NAME",
"value": "Li Shuwen"
},
{
"context": "on.ArtsUp\n [party_ Arts 15]\n , bond 341 \"Knight's Oath\" \"Diarmuid Ua Duibhne\" Icon.ArtsUp\n [",
"end": 88727,
"score": 0.6431926489,
"start": 88722,
"tag": "NAME",
"value": "night"
},
{
"context": " [party_ Arts 15]\n , bond 341 \"Knight's Oath\" \"Diarmuid Ua Duibhne\" Icon.ArtsUp\n [party_ Quick 10, party_ Arts ",
"end": 88756,
"score": 0.9990559816,
"start": 88737,
"tag": "NAME",
"value": "Diarmuid Ua Duibhne"
},
{
"context": "k 10, party_ Arts 10]\n , bond 342 \"Elemental\" \"Paracelsus von Hohenheim\" Icon.ArtsUp\n [party_ Arts 10, party NPUp 10",
"end": 88862,
"score": 0.9993531704,
"start": 88838,
"tag": "NAME",
"value": "Paracelsus von Hohenheim"
},
{
"context": " NPUp 10]\n , bond 343 \"NEO Difference Engine\" \"Charles Babbage\" Icon.BusterUp\n [party_ Buster 20, demeritAl",
"end": 88969,
"score": 0.9997040629,
"start": 88954,
"tag": "NAME",
"value": "Charles Babbage"
},
{
"context": "20, demeritAll DefenseDown 10]\n , { name = \"Dangerous Beast\"\n , id = 344\n , rarity = 5\n ",
"end": 89071,
"score": 0.9990655184,
"start": 89056,
"tag": "NAME",
"value": "Dangerous Beast"
},
{
"context": " , limited = True\n }\n , { name = \"Witch Under the Moonlight\"\n , id = 345\n , rarity = 4\n ",
"end": 89509,
"score": 0.8899387717,
"start": 89484,
"tag": "NAME",
"value": "Witch Under the Moonlight"
},
{
"context": " , limited = True\n }\n , { name = \"Count Romani Archaman's Hospitality\"\n , id = 346\n , rari",
"end": 89938,
"score": 0.8136156201,
"start": 89917,
"tag": "NAME",
"value": "Count Romani Archaman"
},
{
"context": " , limited = True\n }\n , { name = \"Hero Elly's Adventure\"\n , id = 347\n , rarity = 5\n ",
"end": 90372,
"score": 0.9806252122,
"start": 90351,
"tag": "NAME",
"value": "Hero Elly's Adventure"
},
{
"context": " , limited = True\n }\n , { name = \"Wizard & Priest\"\n , id = 348\n , rarity = 4\n ",
"end": 90796,
"score": 0.9806857109,
"start": 90781,
"tag": "NAME",
"value": "Wizard & Priest"
},
{
"context": " , limited = True\n }\n , { name = \"Mata Hari's Tavern\"\n , id = 349\n , rarity = 3\n ",
"end": 91224,
"score": 0.9998750091,
"start": 91206,
"tag": "NAME",
"value": "Mata Hari's Tavern"
},
{
"context": " }\n , bond 350 \"Hell of Blazing Punishment\" \"Jeanne d'Arc (Alter)\" Icon.BusterUp\n [party_ Buster 15]\n ",
"end": 91788,
"score": 0.8228011131,
"start": 91776,
"tag": "NAME",
"value": "Jeanne d'Arc"
},
{
"context": "BusterUp\n [party_ Buster 15]\n , bond 351 \"Gordian Knot\" \"Iskandar\" Icon.SwordUp\n [party AttackUp 15",
"end": 91865,
"score": 0.9915493727,
"start": 91853,
"tag": "NAME",
"value": "Gordian Knot"
},
{
"context": "[party_ Buster 15]\n , bond 351 \"Gordian Knot\" \"Iskandar\" Icon.SwordUp\n [party AttackUp 15]\n , bon",
"end": 91876,
"score": 0.9962066412,
"start": 91868,
"tag": "NAME",
"value": "Iskandar"
},
{
"context": "party AttackUp 15]\n , bond 352 \"White Dragon\" \"Xuanzang Sanzang\" Icon.BusterUp\n [party_ Buster 20, demeritAl",
"end": 91964,
"score": 0.9995402694,
"start": 91948,
"tag": "NAME",
"value": "Xuanzang Sanzang"
},
{
"context": "party_ Arts 10]\n , bond 354 \"Dress of Heaven\" \"Irisviel (Holy Grail)\" Icon.HealUp\n [party HealingRec",
"end": 92187,
"score": 0.9972005486,
"start": 92179,
"tag": "NAME",
"value": "Irisviel"
},
{
"context": "Gen 15]\n , bond 356 \"Spirit of the Vast Land\" \"Geronimo\" Icon.NobleUp\n [party NPGen 15]\n , bond 3",
"end": 92403,
"score": 0.9957367778,
"start": 92395,
"tag": "NAME",
"value": "Geronimo"
},
{
"context": ", party_ Arts 8]\n , bond 359 \"Final Fragment\" \"Angra Mainyu\" Icon.DamageUp\n [ gutsPercent 20\n , sel",
"end": 92724,
"score": 0.9989103079,
"start": 92712,
"tag": "NAME",
"value": "Angra Mainyu"
},
{
"context": "tarsPerTurn 3]\n , gift 361 \"Spiritron Portrait: Nero Claudius\" Icon.Road\n [Bonus EXP Units <| Flat 50]\n ",
"end": 93131,
"score": 0.8952264786,
"start": 93118,
"tag": "NAME",
"value": "Nero Claudius"
},
{
"context": "EXP Units <| Flat 50]\n , bond 367 \"Divine Wine—Shinpen Kidoku\" \"Shuten-Douji\" Icon.ArtsQuickUp\n [party_ Qu",
"end": 93676,
"score": 0.9995530248,
"start": 93662,
"tag": "NAME",
"value": "Shinpen Kidoku"
},
{
"context": " 50]\n , bond 367 \"Divine Wine—Shinpen Kidoku\" \"Shuten-Douji\" Icon.ArtsQuickUp\n [party_ Quick 10, p",
"end": 93685,
"score": 0.9345678091,
"start": 93679,
"tag": "NAME",
"value": "Shuten"
},
{
"context": " , bond 367 \"Divine Wine—Shinpen Kidoku\" \"Shuten-Douji\" Icon.ArtsQuickUp\n [party_ Quick 10, party_ ",
"end": 93691,
"score": 0.9030432701,
"start": 93686,
"tag": "NAME",
"value": "Douji"
},
{
"context": "[party_ Quick 10, party_ Arts 10]\n , bond 368 \"Doujigiri Yasutsuna\" \"Minamoto-no-Raikou\" Icon.BusterUp\n [party_",
"end": 93785,
"score": 0.9997159839,
"start": 93766,
"tag": "NAME",
"value": "Doujigiri Yasutsuna"
},
{
"context": "y_ Arts 10]\n , bond 368 \"Doujigiri Yasutsuna\" \"Minamoto-no-Raikou\" Icon.BusterUp\n [party_ Buster 10,",
"end": 93796,
"score": 0.7110593915,
"start": 93788,
"tag": "NAME",
"value": "Minamoto"
},
{
"context": "0]\n , bond 368 \"Doujigiri Yasutsuna\" \"Minamoto-no-Raikou\" Icon.BusterUp\n [party_ Buster 10, pa",
"end": 93799,
"score": 0.6065253019,
"start": 93797,
"tag": "NAME",
"value": "no"
},
{
"context": " , bond 368 \"Doujigiri Yasutsuna\" \"Minamoto-no-Raikou\" Icon.BusterUp\n [party_ Buster 10, party",
"end": 93802,
"score": 0.6057173014,
"start": 93800,
"tag": "NAME",
"value": "Ra"
},
{
"context": ", bond 368 \"Doujigiri Yasutsuna\" \"Minamoto-no-Raikou\" Icon.BusterUp\n [party_ Buster 10, party Cri",
"end": 93806,
"score": 0.5149791837,
"start": 93804,
"tag": "NAME",
"value": "ou"
},
{
"context": "arty_ Buster 10, party CritUp 15]\n , bond 369 \"Ramesseum\" \"Ozymandias\" Icon.BusterArtsUp\n [party_ Art",
"end": 93889,
"score": 0.8412767053,
"start": 93880,
"tag": "NAME",
"value": "Ramesseum"
},
{
"context": " 10, party CritUp 15]\n , bond 369 \"Ramesseum\" \"Ozymandias\" Icon.BusterArtsUp\n [party_ Arts 10, party_ ",
"end": 93902,
"score": 0.9901670814,
"start": 93892,
"tag": "NAME",
"value": "Ozymandias"
},
{
"context": "uster 10]\n , bond 370 \"Bone Sword (Nameless)\" \"Ibaraki-Douji\" Icon.BusterUp\n [party_ Buster 20, ",
"end": 94007,
"score": 0.6922149658,
"start": 94003,
"tag": "NAME",
"value": "Ibar"
},
{
"context": "\n , bond 370 \"Bone Sword (Nameless)\" \"Ibaraki-Douji\" Icon.BusterUp\n [party_ Buster 20, demeritAl",
"end": 94016,
"score": 0.7235819697,
"start": 94012,
"tag": "NAME",
"value": "ouji"
},
{
"context": " [party StarUp 20]\n , bond 372 \"Gringolet\" \"Gawain\" Icon.BusterUp\n [party_ Buster 15]\n , bon",
"end": 94217,
"score": 0.8877429366,
"start": 94211,
"tag": "NAME",
"value": "Gawain"
},
{
"context": "rty_ Buster 15]\n , bond 373 \"But I Lied Once\" \"Tristan\" Icon.ExclamationUp\n [party CritUp 25]\n ,",
"end": 94299,
"score": 0.9957527518,
"start": 94292,
"tag": "NAME",
"value": "Tristan"
},
{
"context": "\n , bond 374 \"Exercising the Royal Authority\" \"Nitocris\" Icon.NobleUp\n [party NPGen 10, party NPUp 1",
"end": 94401,
"score": 0.9989981651,
"start": 94393,
"tag": "NAME",
"value": "Nitocris"
},
{
"context": " 15]\n , bond 376 \"Cook Despite of Exhaustion\" \"Tawara Touta\" Icon.HealTurn\n [party HealPerTurn 500]\n ",
"end": 94611,
"score": 0.9485381246,
"start": 94599,
"tag": "NAME",
"value": "Tawara Touta"
},
{
"context": "y HealPerTurn 500]\n , bond 377 \"King's Horse\" \"Altria Pendragon (Lancer)\" Icon.SwordUp\n [party AttackUp 10, ",
"end": 94704,
"score": 0.9801806211,
"start": 94688,
"tag": "NAME",
"value": "Altria Pendragon"
},
{
"context": "PUp 10]\n , bond 378 \"All-Encompassing Wisdom\" \"Leonardo da Vinci\" Icon.BeamUp\n [party NPUp 20]\n , bond 379",
"end": 94828,
"score": 0.9996886849,
"start": 94811,
"tag": "NAME",
"value": "Leonardo da Vinci"
},
{
"context": "PGen 10, party CritUp 10]\n , bond 381 \"Reminiscence of the Summer\"\n \"Marie Antoinette (C",
"end": 95114,
"score": 0.5690693259,
"start": 95110,
"tag": "NAME",
"value": "ence"
},
{
"context": "d 381 \"Reminiscence of the Summer\"\n \"Marie Antoinette (Caster)\" Icon.ExclamationUp\n [party CritUp ",
"end": 95161,
"score": 0.9998515248,
"start": 95145,
"tag": "NAME",
"value": "Marie Antoinette"
},
{
"context": "rrently in the Middle of a Shower\"\n \"Anne Bonny & Mary Read (Archer)\" Icon.BusterArtsUp\n [pa",
"end": 95293,
"score": 0.999804616,
"start": 95283,
"tag": "NAME",
"value": "Anne Bonny"
},
{
"context": "he Middle of a Shower\"\n \"Anne Bonny & Mary Read (Archer)\" Icon.BusterArtsUp\n [party_ Buster ",
"end": 95305,
"score": 0.999777317,
"start": 95296,
"tag": "NAME",
"value": "Mary Read"
},
{
"context": "party_ Buster 10, party_ Arts 10]\n , bond 383 \"Prydwen\" \"Mordred (Rider)\" Icon.BeamUp\n [party NPUp ",
"end": 95398,
"score": 0.9984796643,
"start": 95391,
"tag": "NAME",
"value": "Prydwen"
},
{
"context": "ter 10, party_ Arts 10]\n , bond 383 \"Prydwen\" \"Mordred (Rider)\" Icon.BeamUp\n [party NPUp 20]\n , ",
"end": 95408,
"score": 0.9997189641,
"start": 95401,
"tag": "NAME",
"value": "Mordred"
},
{
"context": " 20]\n , bond 384 \"Beach Love Letter (Terror)\" \"Kiyohime (Lancer)\" Icon.BusterUp\n [party_ Buster 20, ",
"end": 95505,
"score": 0.9967421293,
"start": 95497,
"tag": "NAME",
"value": "Kiyohime"
},
{
"context": " DefenseDown 10]\n , bond 385 \"Lost Right Arm\" \"Bedivere\" Icon.BusterUp\n [party_ Buster 10, party NPG",
"end": 95623,
"score": 0.9721657634,
"start": 95615,
"tag": "NAME",
"value": "Bedivere"
},
{
"context": "uickUp\n [party_ Quick 15]\n , { name = \"A Moment of Tranquility\"\n , id = 387\n , rarity = 5\n ",
"end": 95815,
"score": 0.7772837877,
"start": 95797,
"tag": "NAME",
"value": "A Moment of Tranqu"
},
{
"context": " , limited = True\n }\n , { name = \"Saint's Invitation\"\n , id = 389\n , rarity = 3\n ",
"end": 96738,
"score": 0.9989830852,
"start": 96720,
"tag": "NAME",
"value": "Saint's Invitation"
},
{
"context": " , limited = True\n }\n , { name = \"Holy Night Supper\"\n , id = 390\n , rarity = 5\n ",
"end": 97162,
"score": 0.998852849,
"start": 97145,
"tag": "NAME",
"value": "Holy Night Supper"
},
{
"context": "ted = True\n }\n , bond 391 \"Champion Cup\" \"Altria Pendragon (Archer)\" Icon.SwordUp\n [party AttackUp 15]\n",
"end": 97638,
"score": 0.9997847676,
"start": 97622,
"tag": "NAME",
"value": "Altria Pendragon"
},
{
"context": "\n , bond 392 \"Phantasmal Summoning (Install)\" \"Illyasviel von Einzbern\" Icon.AllUp\n [party_ Buster 8, party_ Quick ",
"end": 97760,
"score": 0.9991226196,
"start": 97737,
"tag": "NAME",
"value": "Illyasviel von Einzbern"
},
{
"context": " party_ Arts 8]\n , bond 393 \"Serpent of Fate\" \"Cleopatra\" Icon.BeamUp\n [party NPUp 25, demeritAll Def",
"end": 97871,
"score": 0.958781898,
"start": 97862,
"tag": "NAME",
"value": "Cleopatra"
},
{
"context": "ll DefenseDown 10]\n , bond 394 \"Holy Knuckle\" \"Martha (Ruler)\" Icon.BusterUp\n [party_ Buster 15]\n ",
"end": 97971,
"score": 0.9991496801,
"start": 97965,
"tag": "NAME",
"value": "Martha"
},
{
"context": "ty_ Buster 15]\n , bond 395 \"Minimal Prudence\" \"Scathach (Assassin)\" Icon.QuickUp\n [party_ Quick 15]\n",
"end": 98063,
"score": 0.992882669,
"start": 98055,
"tag": "NAME",
"value": "Scathach"
},
{
"context": "arty_ Quick 15]\n , bond 396 \"Sharing of Pain\" \"Chloe von Einzbern\" Icon.ExclamationUp\n [party CritUp 30, demer",
"end": 98165,
"score": 0.9994164109,
"start": 98147,
"tag": "NAME",
"value": "Chloe von Einzbern"
},
{
"context": " , bond 397 \"Creed at the Bottom of the Earth\" \"Vlad III (EXTRA)\" Icon.QuickBusterUp\n [party_ Quick 1",
"end": 98296,
"score": 0.9827768803,
"start": 98288,
"tag": "NAME",
"value": "Vlad III"
},
{
"context": "ter 10]\n , bond 398 \"Invitation to Halloween\" \"Elisabeth Bathory (Brave)\" Icon.BusterUp\n [party_ Buster 20, d",
"end": 98426,
"score": 0.998026073,
"start": 98409,
"tag": "NAME",
"value": "Elisabeth Bathory"
},
{
"context": "s MysticCode Units <| Flat 50]\n , { name = \"Devilish Bodhisattva\"\n , id = 400\n , rarity = 5\n ",
"end": 98622,
"score": 0.9998806715,
"start": 98602,
"tag": "NAME",
"value": "Devilish Bodhisattva"
},
{
"context": " , limited = False\n }\n , { name = \"Room Guard\"\n , id = 401\n , rarity = 4\n ",
"end": 99043,
"score": 0.9492673874,
"start": 99033,
"tag": "NAME",
"value": "Room Guard"
},
{
"context": " , limited = True\n }\n , { name = \"Cheers to 2019\"\n , id = 415\n , rarity = 4\n ",
"end": 99888,
"score": 0.9980109334,
"start": 99874,
"tag": "NAME",
"value": "Cheers to 2019"
},
{
"context": " , limited = True\n }\n , { name = \"Reality Marble\"\n , id = 418\n , rarity = 3\n ",
"end": 100233,
"score": 0.9983270764,
"start": 100219,
"tag": "NAME",
"value": "Reality Marble"
},
{
"context": " , limited = False\n }\n , { name = \"Potion of Youth\"\n , id = 419\n , rarity = 3\n",
"end": 100589,
"score": 0.8457753062,
"start": 100580,
"tag": "NAME",
"value": "Potion of"
},
{
"context": "ted = False\n }\n , { name = \"Potion of Youth\"\n , id = 419\n , rarity = 3\n ",
"end": 100595,
"score": 0.591417253,
"start": 100591,
"tag": "NAME",
"value": "outh"
},
{
"context": " , limited = False\n }\n , { name = \"Cute Orangette\"\n , id = 421\n , rarity = 5\n ",
"end": 101385,
"score": 0.9998227954,
"start": 101371,
"tag": "NAME",
"value": "Cute Orangette"
},
{
"context": " , limited = True\n }\n , { name = \"Chocolatier\"\n , id = 422\n , rarity = 5\n ",
"end": 101794,
"score": 0.9998283386,
"start": 101783,
"tag": "NAME",
"value": "Chocolatier"
},
{
"context": " , limited = True\n }\n , { name = \"Bitter Black\"\n , id = 424\n , rarity = 4\n ",
"end": 102222,
"score": 0.9998070598,
"start": 102210,
"tag": "NAME",
"value": "Bitter Black"
},
{
"context": " , limited = True\n }\n , { name = \"Blissful Time\"\n , id = 425\n , rarity = 3\n ",
"end": 102646,
"score": 0.6034252644,
"start": 102633,
"tag": "NAME",
"value": "Blissful Time"
},
{
"context": " , limited = True\n }\n , { name = \"Arrogance of a Victor\"\n , id = 426\n , ra",
"end": 103058,
"score": 0.754483819,
"start": 103053,
"tag": "NAME",
"value": "Arrog"
},
{
"context": "= True\n }\n , { name = \"Arrogance of a Victor\"\n , id = 426\n , rarity = 3\n ",
"end": 103074,
"score": 0.5911276937,
"start": 103069,
"tag": "NAME",
"value": "ictor"
},
{
"context": " , limited = True\n }\n , { name = \"Sweet Crystal\"\n , id = 427\n , rarity = 5\n ",
"end": 103492,
"score": 0.9949580431,
"start": 103479,
"tag": "NAME",
"value": "Sweet Crystal"
},
{
"context": " , limited = True\n }\n , { name = \"Fondant au Chocolat\"\n , id = 428\n , rarity = 5\n ",
"end": 103908,
"score": 0.9981160164,
"start": 103889,
"tag": "NAME",
"value": "Fondant au Chocolat"
},
{
"context": " }\n , bond 403 \"Seven-Headed Warhammer Sita\" \"Ishtar\" Icon.BusterUp\n [party_ Buster 20, demeritAl",
"end": 104369,
"score": 0.981084168,
"start": 104363,
"tag": "NAME",
"value": "Ishtar"
},
{
"context": "\n [party HealingReceived 30]\n , bond 407 \"Dup Shimati\" \"Gilgamesh (Caster)\" Icon.BeamUp\n [party NP",
"end": 104769,
"score": 0.9997742176,
"start": 104758,
"tag": "NAME",
"value": "Dup Shimati"
},
{
"context": "HealingReceived 30]\n , bond 407 \"Dup Shimati\" \"Gilgamesh (Caster)\" Icon.BeamUp\n [party NPUp 20]\n ,",
"end": 104781,
"score": 0.9993363023,
"start": 104772,
"tag": "NAME",
"value": "Gilgamesh"
},
{
"context": "Icon.BeamUp\n [party NPUp 20]\n , bond 408 \"Chrysaor\" \"Gorgon\" Icon.BusterUp\n [party_ Buster 10, ",
"end": 104850,
"score": 0.9991889,
"start": 104842,
"tag": "NAME",
"value": "Chrysaor"
},
{
"context": "\n [party NPUp 20]\n , bond 408 \"Chrysaor\" \"Gorgon\" Icon.BusterUp\n [party_ Buster 10, party NPG",
"end": 104859,
"score": 0.9996700287,
"start": 104853,
"tag": "NAME",
"value": "Gorgon"
},
{
"context": "_ Buster 15]\n , bond 411 \"The Furthest Tower\" \"Merlin\" Icon.BusterUp\n [party_ Buster 10, party Cri",
"end": 105164,
"score": 0.9996461868,
"start": 105158,
"tag": "NAME",
"value": "Merlin"
},
{
"context": "ritUp 15]\n , bond 416 \"Buddhism of Emptiness\" \"Miyamoto Musashi\" Icon.HoodUp\n [self NPUp 30, Times 3 <| Gran",
"end": 105278,
"score": 0.9992213249,
"start": 105262,
"tag": "NAME",
"value": "Miyamoto Musashi"
},
{
"context": "20]\n , bond 546 \"The Dynamics of an Asteroid\" \"James Moriarty\" Icon.BeamUp\n [Grant (AlliesType Evil) 0 NPU",
"end": 105648,
"score": 0.9997736216,
"start": 105634,
"tag": "NAME",
"value": "James Moriarty"
},
{
"context": ", party_ Arts 8, party_ Buster 8]\n , bond 548 \"Jenseits der Wildnis\" \"Hessian Lobo\" Icon.QuickUp\n [self (CardUp ",
"end": 105876,
"score": 0.9949373007,
"start": 105856,
"tag": "NAME",
"value": "Jenseits der Wildnis"
},
{
"context": " Buster 8]\n , bond 548 \"Jenseits der Wildnis\" \"Hessian Lobo\" Icon.QuickUp\n [self (CardUp Quick) 10, part",
"end": 105891,
"score": 0.9997943044,
"start": 105879,
"tag": "NAME",
"value": "Hessian Lobo"
},
{
"context": " NPGen 10]\n , bond 549 \"108 Stars of Destiny\" \"Yan Qing\" Icon.QuickUp\n [party_ Quick 10, party CritU",
"end": 106000,
"score": 0.9993687272,
"start": 105992,
"tag": "NAME",
"value": "Yan Qing"
},
{
"context": " , limited = True\n }\n , { name = \"Cafe Camelot\"\n , id = 553\n , rarity = 4\n ",
"end": 106978,
"score": 0.9998583794,
"start": 106966,
"tag": "NAME",
"value": "Cafe Camelot"
},
{
"context": " , limited = True\n }\n , { name = \"Outrage\"\n , id = 554\n , rarity = 4\n ",
"end": 107454,
"score": 0.9998521209,
"start": 107447,
"tag": "NAME",
"value": "Outrage"
},
{
"context": " , limited = True\n }\n , { name = \"Operation Fianna\"\n , id = 555\n , rarity = 4\n ",
"end": 107863,
"score": 0.9995820522,
"start": 107847,
"tag": "NAME",
"value": "Operation Fianna"
},
{
"context": " , limited = True\n }\n , { name = \"Quatre Feuilles\"\n , id = 556\n , rarity = 3\n ",
"end": 108286,
"score": 0.999892652,
"start": 108271,
"tag": "NAME",
"value": "Quatre Feuilles"
},
{
"context": " , limited = True\n }\n , { name = \"Neverland\"\n , id = 557\n , rarity = 3\n ",
"end": 108828,
"score": 0.9217792153,
"start": 108819,
"tag": "NAME",
"value": "Neverland"
},
{
"context": ", limited = True\n }\n , bond 559 \"Garden\" \"Arthur Pendragon (Prototype)\" Icon.SwordUp\n [party AttackUp 1",
"end": 109301,
"score": 0.9660765529,
"start": 109285,
"tag": "NAME",
"value": "Arthur Pendragon"
},
{
"context": "rdUp\n [party AttackUp 15]\n , { name = \"Demon King of the Sixth Heaven\"\n , id = 560\n , r",
"end": 109386,
"score": 0.8783855438,
"start": 109373,
"tag": "NAME",
"value": "Demon King of"
},
{
"context": "ackUp 15]\n , { name = \"Demon King of the Sixth Heaven\"\n , id = 560\n , rarity = 5",
"end": 109396,
"score": 0.5379197598,
"start": 109394,
"tag": "NAME",
"value": "th"
},
{
"context": " , limited = True\n }\n , { name = \"Fortress of the Sun\"\n , id = 561\n , rar",
"end": 109816,
"score": 0.6564613581,
"start": 109812,
"tag": "NAME",
"value": "Fort"
},
{
"context": "ted = True\n }\n , { name = \"Wolves of Mibu\"\n , id = 562\n , rarity = 5\n ",
"end": 110308,
"score": 0.5983684063,
"start": 110306,
"tag": "NAME",
"value": "bu"
},
{
"context": " }\n , bond 567 \"Haori of the Oath\" \"Hijikata Toshizo\" Icon.Kneel\n [Times 1 << Grant Self 0 Guts <",
"end": 111699,
"score": 0.9587182999,
"start": 111693,
"tag": "NAME",
"value": "oshizo"
},
{
"context": "0 Guts <| Flat 1, self CritUp 30]\n , bond 568 \"Adzuki Beanbag\" \"Chacha\" Icon.BeamUp\n [party NPUp 25, demer",
"end": 111805,
"score": 0.9991133809,
"start": 111791,
"tag": "NAME",
"value": "Adzuki Beanbag"
},
{
"context": " self CritUp 30]\n , bond 568 \"Adzuki Beanbag\" \"Chacha\" Icon.BeamUp\n [party NPUp 25, demeritAll Def",
"end": 111814,
"score": 0.9977909327,
"start": 111808,
"tag": "NAME",
"value": "Chacha"
},
{
"context": "25, demeritAll DefenseDown 10]\n , { name = \"Conquering the Great Sea of Stars\"\n , id = ",
"end": 111899,
"score": 0.8338614106,
"start": 111896,
"tag": "NAME",
"value": "Con"
},
{
"context": " , limited = True\n }\n , { name = \"One Summer\"\n , id = 570\n , rarity = 5\n ",
"end": 112349,
"score": 0.8978086114,
"start": 112339,
"tag": "NAME",
"value": "One Summer"
},
{
"context": " , limited = True\n }\n , { name = \"Premonition of Beginnings\"\n , id = 572\n ",
"end": 112807,
"score": 0.8947919011,
"start": 112804,
"tag": "NAME",
"value": "Pre"
},
{
"context": "ster 8]\n , bond 578 \"Tenma's Spring Training\" \"Suzuka Gozen\" Icon.BusterUp\n [self (CardUp Buster) 10, pa",
"end": 113647,
"score": 0.9958645105,
"start": 113635,
"tag": "NAME",
"value": "Suzuka Gozen"
},
{
"context": " NPUp 10]\n , bond 579 \"Dream from the Cradle\" \"Sessyoin Kiara\" Icon.ArtsUp\n [self (CardUp Arts) 20, self H",
"end": 113764,
"score": 0.9994599223,
"start": 113750,
"tag": "NAME",
"value": "Sessyoin Kiara"
},
{
"context": "00, demeritOthers HPDown 1000]\n , { name = \"Marugoshi Shinji\"\n , id = 581\n , rarity = 3\n ",
"end": 113886,
"score": 0.9998201728,
"start": 113870,
"tag": "NAME",
"value": "Marugoshi Shinji"
},
{
"context": " 50]\n , bond 586 \"King Shahryay's Bedchamber\" \"Scheherazade\" Icon.HoodUp\n [self DebuffResist 100]\n , ",
"end": 114723,
"score": 0.8425836563,
"start": 114711,
"tag": "NAME",
"value": "Scheherazade"
},
{
"context": "sist 100]\n , bond 587 \"The Palace of Luoyang\" \"Wu Zetian\" Icon.QuickUp\n [party_ Quick 20, demeritAll ",
"end": 114816,
"score": 0.9795457721,
"start": 114807,
"tag": "NAME",
"value": "Wu Zetian"
},
{
"context": "0]\n , bond 588 \"Military Sash of the War God\" \"Penthesilea\" Icon.BusterUp\n [party_ Buster 20, demeritAl",
"end": 114940,
"score": 0.7742638588,
"start": 114929,
"tag": "NAME",
"value": "Penthesilea"
},
{
"context": "er 20, demeritAll DefenseDown 10]\n , bond 589 \"Nina\" \"Christopher Columbus\" Icon.BusterUp\n [part",
"end": 115028,
"score": 0.9996268749,
"start": 115024,
"tag": "NAME",
"value": "Nina"
},
{
"context": "demeritAll DefenseDown 10]\n , bond 589 \"Nina\" \"Christopher Columbus\" Icon.BusterUp\n [party_ Buster 10, party NPG",
"end": 115051,
"score": 0.9998434782,
"start": 115031,
"tag": "NAME",
"value": "Christopher Columbus"
},
{
"context": "ty_ Buster 10, party NPGen 10]\n , { name = \"Chaldea Anniversary\"\n , id = 590\n , rarity = 5\n ",
"end": 115146,
"score": 0.9997019172,
"start": 115127,
"tag": "NAME",
"value": "Chaldea Anniversary"
},
{
"context": " , limited = True\n }\n , { name = \"Afternoon Party\"\n , id = 591\n , rarity = 4\n ",
"end": 115635,
"score": 0.9970060587,
"start": 115620,
"tag": "NAME",
"value": "Afternoon Party"
},
{
"context": " , limited = True\n }\n , { name = \"Starlight Fest\"\n , id = 592\n , rarity = 3\n ",
"end": 116066,
"score": 0.9998746514,
"start": 116052,
"tag": "NAME",
"value": "Starlight Fest"
},
{
"context": "Code Units <| Flat 50]\n , bond 641 \"Frontier\" \"Paul Bunyan\" Icon.BusterUp\n [party_ Buster 15]\n , bon",
"end": 116679,
"score": 0.9998931885,
"start": 116668,
"tag": "NAME",
"value": "Paul Bunyan"
},
{
"context": "rty_ Buster 15]\n , bond 642 \"The Vaunted One\" \"Sherlock Holmes\" Icon.QuickUp\n [party_ Quick 10, party CritU",
"end": 116769,
"score": 0.9998885989,
"start": 116754,
"tag": "NAME",
"value": "Sherlock Holmes"
},
{
"context": "ty_ Quick 10, party CritUp 15]\n , { name = \"Summer Little\"\n , id = 643\n , rarity = 5\n ",
"end": 116857,
"score": 0.9998881817,
"start": 116844,
"tag": "NAME",
"value": "Summer Little"
},
{
"context": " , limited = True\n }\n , { name = \"White Cruising\"\n , id = 644\n , rarity = 4\n ",
"end": 117329,
"score": 0.9998333454,
"start": 117315,
"tag": "NAME",
"value": "White Cruising"
},
{
"context": " , limited = True\n }\n , { name = \"Sugar Vacation\"\n , id = 645\n , rarity = 3\n ",
"end": 117819,
"score": 0.9985767603,
"start": 117805,
"tag": "NAME",
"value": "Sugar Vacation"
},
{
"context": " , limited = True\n }\n , { name = \"Seaside Luxury\"\n , id = 646\n , rarity = 5\n ",
"end": 118241,
"score": 0.9995967746,
"start": 118227,
"tag": "NAME",
"value": "Seaside Luxury"
},
{
"context": "ng\n , limited = True\n }\n , bond 647 \"Domus Aurea de Curcubeu Mare\" \"Nero Claudius (Caster)\" Icon.BusterUp\n [pa",
"end": 118674,
"score": 0.9998776913,
"start": 118646,
"tag": "NAME",
"value": "Domus Aurea de Curcubeu Mare"
},
{
"context": " }\n , bond 647 \"Domus Aurea de Curcubeu Mare\" \"Nero Claudius (Caster)\" Icon.BusterUp\n [party_ Buster 10, ",
"end": 118690,
"score": 0.9998558164,
"start": 118677,
"tag": "NAME",
"value": "Nero Claudius"
},
{
"context": " party NPGen 10]\n , bond 648 \"Handy-Bandages\" \"Frankenstein (Saber)\" Icon.QuickUp\n [self (CardUp Quick) ",
"end": 118801,
"score": 0.9579603076,
"start": 118789,
"tag": "NAME",
"value": "Frankenstein"
},
{
"context": " party NPGen 10]\n , bond 649 \"A Gift From Ra\" \"Nitocris (Assassin)\" Icon.ArtsUp\n [party_ Arts",
"end": 118905,
"score": 0.8890740871,
"start": 118904,
"tag": "NAME",
"value": "N"
},
{
"context": " [party_ Arts 10, party NPGen 10]\n , bond 650 \"Tenka Fubu -2017 Summer.ver-\" \"Oda Nobunaga (Berserker)",
"end": 118997,
"score": 0.9886097908,
"start": 118992,
"tag": "NAME",
"value": "Tenka"
},
{
"context": "0]\n , bond 650 \"Tenka Fubu -2017 Summer.ver-\" \"Oda Nobunaga (Berserker)\" Icon.BusterUp\n [party_ Buster 1",
"end": 119035,
"score": 0.9945600033,
"start": 119023,
"tag": "NAME",
"value": "Oda Nobunaga"
},
{
"context": "]\n , bond 651 \"Champion's Cup of the Goddess\" \"Ishtar (Rider)\" Icon.QuickUp\n [party_ Quick 10, par",
"end": 119186,
"score": 0.8934326172,
"start": 119180,
"tag": "NAME",
"value": "Ishtar"
},
{
"context": "party NPUp 10]\n , bond 656 \"Mop of Selection\" \"Altria Pendragon (Rider Alter)\" Icon.SwordUp\n [party AttackUp",
"end": 119299,
"score": 0.9911071062,
"start": 119283,
"tag": "NAME",
"value": "Altria Pendragon"
},
{
"context": "meritAll DefenseDown 15]\n , bond 657 \"NYARF!\" \"Helena Blavatsky (Archer)\" Icon.ArtsQuickUp\n [party_ Quick 10",
"end": 119422,
"score": 0.99915272,
"start": 119406,
"tag": "NAME",
"value": "Helena Blavatsky"
},
{
"context": "[party_ Quick 10, party_ Arts 10]\n , bond 658 \"Kyougoku\" \"Minamoto-no-Raikou (Lancer)\" Icon.QuickBusterUp",
"end": 119514,
"score": 0.9297592044,
"start": 119506,
"tag": "NAME",
"value": "Kyougoku"
},
{
"context": "ck 10, party_ Arts 10]\n , bond 658 \"Kyougoku\" \"Minamoto-no-Raikou (Lancer)\" Icon.QuickBusterUp\n [party_ Quick ",
"end": 119535,
"score": 0.8007105589,
"start": 119517,
"tag": "NAME",
"value": "Minamoto-no-Raikou"
},
{
"context": "y_ Quick 10, party_ Buster 10]\n , { name = \"Battle Olympia\"\n , id = 660\n , rarity = 5\n ",
"end": 119640,
"score": 0.9686875343,
"start": 119626,
"tag": "NAME",
"value": "Battle Olympia"
},
{
"context": " , limited = True\n }\n , { name = \"At Wish's End\"\n , id = 666\n , rarity = 5\n ",
"end": 120545,
"score": 0.7305570841,
"start": 120536,
"tag": "NAME",
"value": "At Wish's"
},
{
"context": " , limited = True\n }\n , { name = \"Comrades\"\n , id = 667\n , rarity = 4\n ",
"end": 120975,
"score": 0.9996747375,
"start": 120967,
"tag": "NAME",
"value": "Comrades"
},
{
"context": "ng\n , limited = True\n }\n , bond 669 \"Urvara Nandi\" \"Parvati\" Icon.QuickUp\n [party_ Quick 10, p",
"end": 121456,
"score": 0.9998043776,
"start": 121444,
"tag": "NAME",
"value": "Urvara Nandi"
},
{
"context": "ted = True\n }\n , bond 669 \"Urvara Nandi\" \"Parvati\" Icon.QuickUp\n [party_ Quick 10, party NPGen",
"end": 121466,
"score": 0.9996368289,
"start": 121459,
"tag": "NAME",
"value": "Parvati"
},
{
"context": " Units <| Flat 50]\n , gift 671 \"Heroic Costume: Leonardo da Vinci\" Icon.Road\n [Bonus MysticCode Units <| Flat ",
"end": 121662,
"score": 0.9997797608,
"start": 121645,
"tag": "NAME",
"value": "Leonardo da Vinci"
},
{
"context": " Units <| Flat 50]\n , gift 672 \"Heroic Costume: Jeanne d'Arc\" Icon.Road\n [Bonus MysticCode Units <| Flat ",
"end": 121760,
"score": 0.9918051958,
"start": 121748,
"tag": "NAME",
"value": "Jeanne d'Arc"
},
{
"context": " Units <| Flat 50]\n , gift 673 \"Heroic Costume: Nero Claudius\" Icon.Road\n [Bonus MysticCode Units <| Flat ",
"end": 121859,
"score": 0.9995845556,
"start": 121846,
"tag": "NAME",
"value": "Nero Claudius"
},
{
"context": "]\n , bond 675 \"A Wish Spanning 3 Generations\" \"Tomoe Gozen\" Icon.BusterUp\n [party_ Buster 10, party NPU",
"end": 121972,
"score": 0.998192668,
"start": 121961,
"tag": "NAME",
"value": "Tomoe Gozen"
},
{
"context": "[party_ Buster 10, party NPUp 10]\n , bond 676 \"Getsurin Kuyou\" \"Mochizuki Chiyome\" Icon.ArtsQuickUp\n [part",
"end": 122058,
"score": 0.9998025298,
"start": 122044,
"tag": "NAME",
"value": "Getsurin Kuyou"
},
{
"context": ", party NPUp 10]\n , bond 676 \"Getsurin Kuyou\" \"Mochizuki Chiyome\" Icon.ArtsQuickUp\n [party_ Quick 10, party_ ",
"end": 122078,
"score": 0.9998044372,
"start": 122061,
"tag": "NAME",
"value": "Mochizuki Chiyome"
},
{
"context": "[party_ Quick 10, party_ Arts 10]\n , bond 677 \"Jumonji Yari\" \"Houzouin Inshun\" Icon.QuickUp\n [party_ Qui",
"end": 122165,
"score": 0.9997265935,
"start": 122153,
"tag": "NAME",
"value": "Jumonji Yari"
},
{
"context": "0, party_ Arts 10]\n , bond 677 \"Jumonji Yari\" \"Houzouin Inshun\" Icon.QuickUp\n [party_ Quick 10, party CritU",
"end": 122183,
"score": 0.9997092485,
"start": 122168,
"tag": "NAME",
"value": "Houzouin Inshun"
},
{
"context": "10, party CritUp 15]\n , bond 678 \"Double-Tiered Kasa\" \"Yagyu Munenori\" Icon.ArtsUp\n [party_ Ar",
"end": 122270,
"score": 0.950686872,
"start": 122269,
"tag": "NAME",
"value": "K"
},
{
"context": "y CritUp 15]\n , bond 678 \"Double-Tiered Kasa\" \"Yagyu Munenori\" Icon.ArtsUp\n [party_ Arts 10, party NPUp 10",
"end": 122290,
"score": 0.9996801019,
"start": 122276,
"tag": "NAME",
"value": "Yagyu Munenori"
},
{
"context": " [party_ Arts 10, party NPUp 10]\n , bond 679 \"Danzou's Ox\" \"Katou Danzo\" Icon.QuickBusterUp\n [party_ Q",
"end": 122369,
"score": 0.9869157672,
"start": 122358,
"tag": "NAME",
"value": "Danzou's Ox"
},
{
"context": " 10, party NPUp 10]\n , bond 679 \"Danzou's Ox\" \"Katou Danzo\" Icon.QuickBusterUp\n [party_ Quick 10, party",
"end": 122383,
"score": 0.9996002913,
"start": 122372,
"tag": "NAME",
"value": "Katou Danzo"
},
{
"context": "arty_ Quick 10, party_ Buster 10]\n , bond 686 \"Princess' Origami\" \"Osakabehime\" Icon.NobleUp\n [party NPGen 15",
"end": 122479,
"score": 0.9986360073,
"start": 122462,
"tag": "NAME",
"value": "Princess' Origami"
},
{
"context": "y_ Buster 10]\n , bond 686 \"Princess' Origami\" \"Osakabehime\" Icon.NobleUp\n [party NPGen 15]\n , bond 6",
"end": 122493,
"score": 0.998919487,
"start": 122482,
"tag": "NAME",
"value": "Osakabehime"
},
{
"context": "ter 20, demeritAll DebuffVuln 20]\n , bond 688 \"Guardian Gigantic\" \"Mecha Eli-chan MkII\" Icon.BusterUp\n [party",
"end": 122685,
"score": 0.954140842,
"start": 122668,
"tag": "NAME",
"value": "Guardian Gigantic"
},
{
"context": " [Bonus EXP Units <| Flat 50]\n , { name = \"Soul Eater\"\n , id = 691\n , rarity = 3\n ",
"end": 122901,
"score": 0.9997155666,
"start": 122891,
"tag": "NAME",
"value": "Soul Eater"
},
{
"context": " , limited = False\n }\n , { name = \"Divine Construct\"\n , id = 674\n , rarity = 3\n ",
"end": 123262,
"score": 0.9988815188,
"start": 123246,
"tag": "NAME",
"value": "Divine Construct"
},
{
"context": " [Bonus EXP Units <| Flat 50 ]\n , bond 692 \"Falcon Witch's Banquet\" \"Circe\" Icon.NobleUp\n [party NPGen 10, part",
"end": 123711,
"score": 0.9880262613,
"start": 123689,
"tag": "NAME",
"value": "Falcon Witch's Banquet"
},
{
"context": "lat 50 ]\n , bond 692 \"Falcon Witch's Banquet\" \"Circe\" Icon.NobleUp\n [party NPGen 10, party NPUp 1",
"end": 123719,
"score": 0.972001493,
"start": 123714,
"tag": "NAME",
"value": "Circe"
},
{
"context": "0, party NPUp 10]\n , bond 693 \"Universe Ring\" \"Nezha\" Icon.QuickUp\n [party_ Quick 10, party NPUp ",
"end": 123809,
"score": 0.9834394455,
"start": 123804,
"tag": "NAME",
"value": "Nezha"
},
{
"context": "0, party NPUp 10]\n , bond 694 \"Tributes to King Solomon\" \"Queen of Sheba\" Icon.BusterArtsUp\n [party_",
"end": 123903,
"score": 0.9362462163,
"start": 123896,
"tag": "NAME",
"value": "Solomon"
},
{
"context": "Up 10]\n , bond 694 \"Tributes to King Solomon\" \"Queen of Sheba\" Icon.BusterArtsUp\n [party_ Arts 10, party_ ",
"end": 123920,
"score": 0.6603270173,
"start": 123906,
"tag": "NAME",
"value": "Queen of Sheba"
},
{
"context": "party_ Arts 10, party_ Buster 10]\n , bond 695 \"Rosarium de Clavis Argenteus\" \"Abigail Williams\" Icon.BeamUp\n [Times 3 <|",
"end": 124025,
"score": 0.9977783561,
"start": 123997,
"tag": "NAME",
"value": "Rosarium de Clavis Argenteus"
},
{
"context": "0]\n , bond 695 \"Rosarium de Clavis Argenteus\" \"Abigail Williams\" Icon.BeamUp\n [Times 3 <| Grant Self 0 Death",
"end": 124044,
"score": 0.999551475,
"start": 124028,
"tag": "NAME",
"value": "Abigail Williams"
},
{
"context": "t Full]\n , bond 700 \"Blooming Flowers in Kur\" \"Ereshkigal\" Icon.BusterUp\n [party_ Buster 10, party NPU",
"end": 124159,
"score": 0.9433829188,
"start": 124149,
"tag": "NAME",
"value": "Ereshkigal"
},
{
"context": "ty_ Quick 10, party CritUp 15]\n , { name = \"New Beginning\"\n , id = 704\n , rarity = 5\n ",
"end": 124379,
"score": 0.9986560941,
"start": 124366,
"tag": "NAME",
"value": "New Beginning"
},
{
"context": "9 \"Painting of a Dragon Passing Over Mount Fuji\" \"Katsushika Hokusai\" Icon.Kneel\n [Times 1 << Grant Self 0 GutsPe",
"end": 124904,
"score": 0.9969841242,
"start": 124886,
"tag": "NAME",
"value": "Katsushika Hokusai"
},
{
"context": "nt <| Flat 20]\n , bond 717 \"Mysterious Glass\" \"Semiramis\" Icon.BusterUp\n [party_ Buster 10, party NPU",
"end": 125016,
"score": 0.780795157,
"start": 125007,
"tag": "NAME",
"value": "Semiramis"
},
{
"context": " 10, party NPUp 10]\n , bond 762 \"Summer Rain\" \"Asagami Fujino\" Icon.BusterArtsUp\n [party_ Arts 10, party_ ",
"end": 125116,
"score": 0.9991397858,
"start": 125102,
"tag": "NAME",
"value": "Asagami Fujino"
},
{
"context": " , limited = True\n }\n , { name = \"The Dantes Files: Undercover in a Foreign Land\"\n ",
"end": 126123,
"score": 0.5687887669,
"start": 126120,
"tag": "NAME",
"value": "The"
},
{
"context": " , limited = True\n }\n , bond 787 \"OTMA\" \"Anastasia Nikolaevna Romanova\" Icon.ArtsUp\n [party_ Arts 10, party NPUp 10",
"end": 126680,
"score": 0.9998788238,
"start": 126651,
"tag": "NAME",
"value": "Anastasia Nikolaevna Romanova"
},
{
"context": "arty_ Buster 10]\n , bond 790 \"Wildfire Blade\" \"Antonio Salieri\" Icon.ArtsUp\n [party_ Arts 10, party NPGen 1",
"end": 127016,
"score": 0.9998520613,
"start": 127001,
"tag": "NAME",
"value": "Antonio Salieri"
},
{
"context": "rty_ Buster 10, party NPUp 10]\n , { name = \"Distant Pilgrimage\"\n , id = 792\n , rarity = 5\n ",
"end": 127226,
"score": 0.9998056293,
"start": 127208,
"tag": "NAME",
"value": "Distant Pilgrimage"
},
{
"context": " , limited = True\n }\n , { name = \"At Trifas\"\n , id = 796\n , rarity = 5\n ",
"end": 129099,
"score": 0.9995108247,
"start": 129090,
"tag": "NAME",
"value": "At Trifas"
},
{
"context": " bond 799 \"The Purpose of Learning and Teaching\" \"Chiron\" Icon.ArtsQuickUp\n [party_ Arts 10, party_ Q",
"end": 129742,
"score": 0.6862896681,
"start": 129736,
"tag": "NAME",
"value": "Chiron"
},
{
"context": "party_ Quick 10]\n , bond 800 \"Nameless Death\" \"Sieg\" Icon.NobleUp\n [party NPGen 10, party NPUp 1",
"end": 129838,
"score": 0.8016216755,
"start": 129834,
"tag": "NAME",
"value": "Sieg"
}
] | src/Database/CraftEssences.elm | jnbooth/chaldeas | 18 | module Database.CraftEssences exposing
( db
, getBond
, getAll
)
{-| All Craft Essences. -}
-- Unlike Servants, which are divided into multiple subfiles,
-- Craft Essences are all included in this one file
-- along with their data model definition.
import Dict exposing (Dict)
import Maybe.Extra as Maybe
import Class.Has exposing (Has)
import Model.Icon as Icon
import Model.Card exposing (Card(..))
import Model.Class as Class exposing (Class(..))
import Model.CraftEssence exposing (CraftEssence)
import Model.Trait exposing (Trait(..))
import Model.Servant exposing (Servant)
import Model.Skill.Amount exposing (Amount(..))
import Model.Skill.BonusEffect exposing (BonusEffect(..), BonusType(..))
import Model.Skill.BuffEffect exposing (BuffEffect(..))
import Model.Skill.DebuffEffect exposing (DebuffEffect(..))
import Model.Skill.InstantEffect exposing (InstantEffect(..))
import Model.Skill.SkillEffect exposing (SkillEffect(..))
import Model.Skill.Special exposing (Special(..))
import Model.Skill.Target exposing (Target(..))
import Database
getAll : Has CraftEssence a -> List a
getAll =
Database.genericGetAll db
{-| All Craft Essences available in EN. -}
-- Note: Names _must_ be true to their EN localization.
-- GrandOrder.Wiki is only trustworthy for CEs that have been in the game
-- for a while. Craft Essences introduced during events and the like should be
-- checked against the official announcement.
db : List CraftEssence
db =
let
gutsPercent =
Times 1 <<
Grant Self 0 GutsPercent <<
Flat
self buff =
Grant Self 0 buff <<
Flat
party buff =
Grant Party 0 buff <<
Flat
party_ card =
party (CardUp card)
demeritAll debuff =
Debuff Party 0 debuff <<
Flat
demeritOthers debuff =
Debuff Others 0 debuff <<
Flat
atkChance chance =
When "attacking" <<
Chance chance
bond id name servant icon effect =
{ name = name
, id = id
, rarity = 4
, icon = icon
, stats = { base = { atk = 100, hp = 100 }
, max = { atk = 100, hp = 100 }
}
, effect = effect
, bond = Just servant
, limited = False
}
gift id name icon effect =
{ name = name
, id = id
, rarity = 4
, icon = icon
, stats = { base = { atk = 100, hp = 100 }
, max = { atk = 100, hp = 100 }
}
, effect = effect
, bond = Nothing
, limited = True
}
in
[ { name = "Tenacity"
, id = 1
, rarity = 1
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 100 }
, max = { atk = 0, hp = 300 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 3 5 ]
, bond = Nothing
, limited = False
}
, { name = "Meditation"
, id = 2
, rarity = 1
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 150 }
, max = { atk = 0, hp = 450 }
}
, effect = [ Grant Self 0 DebuffResist <| Range 5 10 ]
, bond = Nothing
, limited = False
}
, { name = "Technique"
, id = 3
, rarity = 1
, icon = Icon.ArtsUp
, stats = { base = { atk = 100, hp = 0 }
, max = { atk = 300, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 3 5 ]
, bond = Nothing
, limited = False
}
, { name = "Preemption"
, id = 4
, rarity = 1
, icon = Icon.QuickUp
, stats = { base = { atk = 100, hp = 0 }
, max = { atk = 300, hp = 0 }
}
, effect = [Grant Self 0 (CardUp Quick) <| Range 3 5]
, bond = Nothing
, limited = False
}
, { name = "Destruction"
, id = 5
, rarity = 1
, icon = Icon.BusterUp
, stats = { base = { atk = 100, hp = 0 }
, max = { atk = 300, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 3 5 ]
, bond = Nothing
, limited = False
}
, { name = "Flash"
, id = 6
, rarity = 2
, icon = Icon.ExclamationUp
, stats = { base = { atk = 150, hp = 0 }
, max = { atk = 500, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 5 10 ]
, bond = Nothing
, limited = False
}
, { name = "Opportunity"
, id = 7
, rarity = 2
, icon = Icon.StarHaloUp
, stats = { base = { atk = 75, hp = 112 }
, max = { atk = 250, hp = 375 }
}
, effect = [ Grant Self 0 StarUp <| Range 5 10 ]
, bond = Nothing
, limited = False
}
, { name = "Fruitful"
, id = 8
, rarity = 2
, icon = Icon.Noble
, stats = { base = { atk = 75, hp = 112 }
, max = { atk = 250, hp = 375 }
}
, effect = [ To Self GaugeUp <| Range 10 20 ]
, bond = Nothing
, limited = False
}
, { name = "Concentration"
, id = 9
, rarity = 2
, icon = Icon.NobleUp
, stats = { base = { atk = 75, hp = 112 }
, max = { atk = 250, hp = 375 }
}
, effect = [ Grant Self 0 NPGen <| Range 5 10 ]
, bond = Nothing
, limited = False
}
, { name = "Divine Oracle"
, id = 10
, rarity = 2
, icon = Icon.BeamUp
, stats = { base = { atk = 150, hp = 0 }
, max = { atk = 500, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 5 10 ]
, bond = Nothing
, limited = False
}
, { name = "Azoth Blade"
, id = 11
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 200 }
, max = { atk = 0, hp = 1000 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "False Attendant's Writings"
, id = 12
, rarity = 3
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DebuffResist <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "The Azure Black Keys"
, id = 13
, rarity = 3
, icon = Icon.ArtsUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "The Verdant Black Keys"
, id = 14
, rarity = 3
, icon = Icon.QuickUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "The Crimson Black Keys"
, id = 15
, rarity = 3
, icon = Icon.BusterUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "Rin's Pendant"
, id = 16
, rarity = 3
, icon = Icon.ExclamationUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "Spell Tome"
, id = 17
, rarity = 3
, icon = Icon.StarHaloUp
, stats = { base = { atk = 100, hp = 150 }
, max = { atk = 500, hp = 750 }
}
, effect = [ Grant Self 0 StarUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "Dragon's Meridian"
, id = 18
, rarity = 3
, icon = Icon.Noble
, stats = { base = { atk = 100, hp = 150 }
, max = { atk = 500, hp = 750 }
}
, effect = [ To Self GaugeUp <| Range 30 50 ]
, bond = Nothing
, limited = False
}
, { name = "Sorcery Ore"
, id = 19
, rarity = 3
, icon = Icon.NobleUp
, stats = { base = { atk = 100, hp = 150 }
, max = { atk = 500, hp = 750 }
}
, effect = [ Grant Self 0 NPGen <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "Dragonkin"
, id = 20
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "Iron-Willed Training"
, id = 21
, rarity = 4
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 400 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "Primeval Curse"
, id = 22
, rarity = 4
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 DebuffResist <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Projection"
, id = 23
, rarity = 4
, icon = Icon.ArtsUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "Gandr"
, id = 24
, rarity = 4
, icon = Icon.QuickUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "Verdant Sound of Destruction"
, id = 25
, rarity = 4
, icon = Icon.BusterUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "Gem Magecraft: Antumbra"
, id = 26
, rarity = 4
, icon = Icon.ExclamationUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Be Elegant"
, id = 27
, rarity = 4
, icon = Icon.StarHaloUp
, stats = { base = { atk = 200, hp = 300 }
, max = { atk = 750, hp = 1125 }
}
, effect = [ Grant Self 0 StarUp <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "The Imaginary Element"
, id = 28
, rarity = 4
, icon = Icon.Noble
, stats = { base = { atk = 200, hp = 300 }
, max = { atk = 750, hp = 1125 }
}
, effect = [ To Self GaugeUp <| Range 60 75 ]
, bond = Nothing
, limited = False
}
, { name = "Divine Banquet"
, id = 29
, rarity = 4
, icon = Icon.NobleUp
, stats = { base = { atk = 200, hp = 300 }
, max = { atk = 750, hp = 1125 }
}
, effect = [ Grant Self 0 NPGen <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Angel's Song"
, id = 30
, rarity = 4
, icon = Icon.BeamUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Formal Craft"
, id = 31
, rarity = 5
, icon = Icon.ArtsUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Imaginary Around"
, id = 32
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Limited/Zero Over"
, id = 33
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Kaleidoscope"
, id = 34
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ To Self GaugeUp <| Range 80 100 ]
, bond = Nothing
, limited = False
}
, { name = "Heaven's Feel"
, id = 35
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 40 50 ]
, bond = Nothing
, limited = False
}
, { name = "Beginning of the Journey"
, id = 36
, rarity = 4
, icon = Icon.Road
, stats = { base = { atk = 50, hp = 50 }
, max = { atk = 50, hp = 50 }
}
, effect = [ Bonus FriendPoints Units <| Flat 75 ]
, bond = Nothing
, limited = True
}
, { name = "Parted Sea"
, id = 37
, rarity = 3
, icon = Icon.Dodge
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Times 1 <| Grant Self 0 Evasion Full
, Grant Self 0 DebuffResist <| Range 5 10
]
, bond = Nothing
, limited = False
}
, { name = "Seal Designation Enforcer"
, id = 38
, rarity = 4
, icon = Icon.StarUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 GatherUp <| Range 600 800 ]
, bond = Nothing
, limited = False
}
, { name = "Holy Shroud of Magdalene"
, id = 39
, rarity = 4
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 400 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 (Special DefenseUp <| VsTrait Male) <| Range 25 35 ]
, bond = Nothing
, limited = False
}
, { name = "Prisma Cosmos"
, id = 40
, rarity = 5
, icon = Icon.NobleTurn
, stats = { base = { atk = 250, hp = 375 }
, max = { atk = 1000, hp = 1500 }
}
, effect = [ Grant Self 0 GaugePerTurn <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "Nightless Rose"
, id = 41
, rarity = 5
, icon = Icon.Kneel
, stats = { base = { atk = 0, hp = 500 }
, max = { atk = 0, hp = 2000 }
}
, effect = [ Times 1 << Grant Self 0 Guts <| Range 500 1000 ]
, bond = Nothing
, limited = True
}
, { name = "Mooncell Automaton"
, id = 42
, rarity = 3
, icon = Icon.AllUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 3 5
, Grant Self 0 (CardUp Buster) <| Range 3 5
, Grant Self 0 (CardUp Quick) <| Range 3 5
]
, bond = Nothing
, limited = False
}
, { name = "Moony Jewel"
, id = 43
, rarity = 4
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 (Resist Charm) <| Range 80 100 ]
, bond = Nothing
, limited = True
}
, { name = "Moon Goddess' Bath"
, id = 44
, rarity = 5
, icon = Icon.HealTurn
, stats = { base = { atk = 0, hp = 500 }
, max = { atk = 0, hp = 2000 }
}
, effect = [ Grant Self 0 HealPerTurn <| Range 500 750 ]
, bond = Nothing
, limited = True
}
, { name = "Moonlight Fest"
, id = 45
, rarity = 5
, icon = Icon.StarHaloUp
, stats = { base = { atk = 250, hp = 375 }
, max = { atk = 1000, hp = 1500 }
}
, effect = [ Grant Self 0 StarUp <| Range 15 20
, Grant Self 0 CritUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "Runestone"
, id = 46
, rarity = 3
, icon = Icon.HoodUp
, stats = { base = { atk = 100, hp = 150 }
, max = { atk = 500, hp = 750 }
}
, effect = [ Grant Self 0 DebuffResist <| Range 5 10
, Grant Self 0 GatherUp <| Range 100 200
]
, bond = Nothing
, limited = False
}
, { name = "With One Strike"
, id = 47
, rarity = 4
, icon = Icon.Bullseye
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 SureHit Full
, Grant Self 0 (CardUp Quick) <| Range 8 10
]
, bond = Nothing
, limited = False
}
, { name = "The Black Grail"
, id = 48
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 600, hp = 0 }
, max = { atk = 2400, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 60 80
, Debuff Self 0 HealthLoss <| Flat 500
]
, bond = Nothing
, limited = False
}
, { name = "Jack-o'-lantern"
, id = 49
, rarity = 3
, icon = Icon.DamageUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 DamageUp <| Range 100 200 ]
, bond = Nothing
, limited = True
}
, { name = "Trick or Treat"
, id = 50
, rarity = 3
, icon = Icon.StaffUp
, stats = { base = { atk = 100, hp = 150 }
, max = { atk = 500, hp = 750 }
}
, effect = [ Grant Self 0 DebuffUp <| Range 10 12 ]
, bond = Nothing
, limited = True
}
, { name = "Halloween Arrangement"
, id = 51
, rarity = 4
, icon = Icon.CrosshairUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 1 Taunt Full
, Grant Self 1 DefenseUp <| Range 60 80
]
, bond = Nothing
, limited = True
}
, { name = "Halloween Princess"
, id = 52
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 250, hp = 375 }
, max = { atk = 1000, hp = 1500 }
}
, effect = [ Grant Self 0 NPUp <| Range 15 20
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, { name = "Little Halloween Devil"
, id = 53
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 NPGen <| Range 20 25
, To Self GaugeUp <| Range 50 60
]
, bond = Nothing
, limited = True
}
, { name = "Maid in Halloween"
, id = 54
, rarity = 5
, icon = Icon.HealUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 0 HealingReceived <| Range 60 75 ]
, bond = Nothing
, limited = True
}
, { name = "Anchors Aweigh"
, id = 55
, rarity = 3
, icon = Icon.HealTurn
, stats = { base = { atk = 300, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 HealPerTurn <| Range 100 200 ]
, bond = Nothing
, limited = False
}
, { name = "Code Cast"
, id = 56
, rarity = 4
, icon = Icon.SwordShieldUp
, stats = { base = { atk = 200, hp = 300 }
, max = { atk = 750, hp = 1125 }
}
, effect = [ Grant Self 3 AttackUp <| Range 25 30
, Grant Self 3 DefenseUp <| Range 25 30
]
, bond = Nothing
, limited = False
}
, { name = "Victor of the Moon"
, id = 57
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 600, hp = 0 }
, max = { atk = 2400, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 10 15
, Grant Self 0 CritUp <| Range 20 25
]
, bond = Nothing
, limited = False
}
, { name = "Another Ending"
, id = 58
, rarity = 5
, icon = Icon.ArtsUp
, stats = { base = { atk = 600, hp = 0 }
, max = { atk = 2400, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 CritUp <| Range 20 25
]
, bond = Nothing
, limited = False
}
, { name = "Fate GUDAGUDA Order"
, id = 59
, rarity = 3
, icon = Icon.AllUp
, stats = { base = { atk = 100, hp = 150 }
, max = { atk = 500, hp = 750 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 1 2
, Grant Self 0 (CardUp Arts) <| Range 1 2
, Grant Self 0 (CardUp Buster) <| Range 1 2
, Grant Self 0 StarUp <| Range 1 2
, Grant Self 0 GatherUp <| Range 1 2
, Grant Self 0 NPGen <| Range 1 2
, Grant Self 0 NPUp <| Range 1 2
, Grant Self 0 DebuffUp <| Range 1 2
, Grant Self 0 DebuffResist <| Range 1 2
]
, bond = Nothing
, limited = True
}
, { name = "After-Party Order!"
, id = 60
, rarity = 4
, icon = Icon.QuickBusterUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 10 15
, Grant Self 0 (CardUp Buster) <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "Guda-o"
, id = 61
, rarity = 5
, icon = Icon.DamageUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 15 20
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "GUDAGUDA Poster Girl"
, id = 62
, rarity = 5
, icon = Icon.CrosshairUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 3 Taunt Full
, Grant Self 3 AttackUp <| Range 60 80
]
, bond = Nothing
, limited = True
}
, { name = "Demon Boar"
, id = 65
, rarity = 3
, icon = Icon.QuickUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "Knight's Dignity"
, id = 66
, rarity = 4
, icon = Icon.ExclamationUp
, stats = { base = { atk = 200, hp = 300 }
, max = { atk = 750, hp = 1125 }
}
, effect = [ Grant Self 0 CritUp <| Range 40 50
, Debuff Self 0 DefenseDown <| Flat 20
]
, bond = Nothing
, limited = False
}
, { name = "A Fragment of 2030"
, id = 67
, rarity = 5
, icon = Icon.StarTurn
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "Lightning Reindeer"
, id = 68
, rarity = 3
, icon = Icon.BusterUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 3 (CardUp Buster) <| Range 15 20 ]
, bond = Nothing
, limited = True
}
, { name = "March of the Saint"
, id = 69
, rarity = 4
, icon = Icon.HealTurn
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 HealPerTurn <| Range 200 300
, Grant Self 0 GaugePerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "Present For My Master"
, id = 70
, rarity = 5
, icon = Icon.StarUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 0 GatherUp <| Range 100 200
, Grant Self 0 HealingReceived <| Range 40 50
]
, bond = Nothing
, limited = True
}
, { name = "Holy Night Sign"
, id = 71
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 250, hp = 375 }
, max = { atk = 1000, hp = 1500 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 8 10
, Grant Self 0 CritUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "Clock Tower"
, id = 72
, rarity = 3
, icon = Icon.NobleTurn
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 GaugePerTurn <| Range 2 3 ]
, bond = Nothing
, limited = False
}
, { name = "Necromancy"
, id = 73
, rarity = 4
, icon = Icon.Kneel
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2400 }
}
, effect = [ Chance 50 << Times 1 << Grant Self 0 Guts <| Range 500 1000 ]
, bond = Nothing
, limited = False
}
, { name = "Awakened Will"
, id = 74
, rarity = 4
, icon = Icon.NobleTurn
, stats = { base = { atk = 200, hp = 300 }
, max = { atk = 750, hp = 1125 }
}
, effect = [ Chance 60 << Grant Self 0 GaugePerTurn <| Range 12 15
, Debuff Self 0 HealthLoss <| Flat 500
]
, bond = Nothing
, limited = False
}
, { name = "500-Year Obsession"
, id = 75
, rarity = 5
, icon = Icon.Circuits
, stats = { base = { atk = 600, hp = 0 }
, max = { atk = 2400, hp = 0 }
}
, effect = [ When "defeated by an enemy" <| Debuff Target 2 SealNP Full
, When "defeated by an enemy" <<
Debuff Target 10 Curse <| Range 1000 2000
]
, bond = Nothing
, limited = False
}
, { name = "Peacefulness of 2018"
, id = 76
, rarity = 3
, icon = Icon.HealTurn
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 HealPerTurn <| Range 200 300
, Debuff Self 0 AttackDown <| Flat 10
]
, bond = Nothing
, limited = True
}
, { name = "Heroic New Year"
, id = 77
, rarity = 4
, icon = Icon.HoodUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Times 1 <| Grant Self 0 DebuffResist Full
, Grant Self 0 DefenseUp <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "Law of the Jungle"
, id = 78
, rarity = 3
, icon = Icon.Crystal
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Bonus QPQuest Units <| Range 2017 2018 ]
, bond = Nothing
, limited = True
}
, { name = "Grand New Year"
, id = 79
, rarity = 5
, icon = Icon.Shield
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 1 Taunt Full
, Grant Self 1 Invincibility Full
, Grant Self 0 DebuffResist <| Range 10 20
]
, bond = Nothing
, limited = True
}
, { name = "Mona Lisa"
, id = 80
, rarity = 5
, icon = Icon.Crystal
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Bonus QPDrop Percent <| Range 2 10 ]
, bond = Nothing
, limited = True
}
, { name = "Happy x3 Order"
, id = 81
, rarity = 4
, icon = Icon.StarTurn
, stats = { base = { atk = 0, hp = 2018 }
, max = { atk = 0, hp = 2018 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 0 1 ]
, bond = Nothing
, limited = True
}
, { name = "Purely Bloom"
, id = 82
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ ToMax (Range 40 50) << Grant Self 0 NPUp <| Flat 5 ]
, bond = Nothing
, limited = True
}
, { name = "Star of Altria"
, id = 83
, rarity = 5
, icon = Icon.Kneel
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Times 1 << Grant Self 0 Guts <| Flat 1
, Grant Self 0 DebuffResist <| Range 5 10
]
, bond = Nothing
, limited = True
}
, { name = "Trueshot"
, id = 84
, rarity = 3
, icon = Icon.Bullseye
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 SureHit Full
, Grant Self 0 CritUp <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "Mikotto! Bride Training"
, id = 85
, rarity = 4
, icon = Icon.HealTurn
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Chance 65 << Grant Self 0 HealPerTurn <| Range 750 1000 ]
, bond = Nothing
, limited = True
}
, { name = "The Crimson Land of Shadows"
, id = 86
, rarity = 5
, icon = Icon.DamageUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ ToMax (Range 1000 1200) << Grant Self 0 DamageUp <| Flat 100 ]
, bond = Nothing
, limited = True
}
, { name = "Ryudoji Temple"
, id = 89
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 NPUp <| Range 10 15
, To Self GaugeUp <| Range 20 30
]
, bond = Nothing
, limited = False
}
, { name = "Mana Gauge"
, id = 90
, rarity = 3
, icon = Icon.DamageUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (Special AttackUp <| VsClass Caster) <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "Elixir of Love"
, id = 91
, rarity = 3
, icon = Icon.Heart
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 (Success Charm) <| Range 12 15 ]
, bond = Nothing
, limited = False
}
, { name = "Storch Ritter"
, id = 92
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ equipped Berserker << Grant Self 0 NPUp <| Range 15 25 ]
, bond = Nothing
, limited = False
}
, { name = "Hermitage"
, id = 93
, rarity = 3
, icon = Icon.ArtsUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 3 (CardUp Arts) <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "Motored Cuirassier"
, id = 94
, rarity = 3
, icon = Icon.DamageUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (Special AttackUp <| VsClass Rider) <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "Stuffed Lion"
, id = 95
, rarity = 3
, icon = Icon.Heal
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ When "defeated" << To Party Heal <| Range 800 1000 ]
, bond = Nothing
, limited = False
}
, { name = "Lugh's Halo"
, id = 96
, rarity = 3
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 (Resist Stun) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Vessel of the Saint"
, id = 97
, rarity = 5
, icon = Icon.HoodUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Times 3 <| Grant Self 0 DebuffResist Full
, Grant Self 0 NPGen <| Range 15 20
]
, bond = Nothing
, limited = False
}
, { name = "Golden Millennium Tree"
, id = 98
, rarity = 4
, icon = Icon.HPUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ ToMax (Flat 3000) << Grant Self 0 HPUp <| Range 200 300 ]
, bond = Nothing
, limited = False
}
, { name = "Heroic Portrait: Mash Kyrielight"
, id = 99
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: Altria Pendragon"
, id = 100
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: Jeanne d'Arc"
, id = 101
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: Altera"
, id = 102
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: Arjuna"
, id = 103
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: Scathach"
, id = 104
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: Ushiwakamaru"
, id = 105
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: Henry Jekyll & Hyde"
, id = 106
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: Mephistopheles"
, id = 107
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: Darius III"
, id = 108
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Valentine Dojo of Tears"
, id = 109
, rarity = 3
, icon = Icon.Bullseye
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 SureHit Full
, Grant Self 0 GaugePerTurn <| Range 3 5
, Debuff Self 0 CharmVuln <| Flat 10
]
, bond = Nothing
, limited = True
}
, { name = "Kitchen ☆ Patissiere"
, id = 110
, rarity = 4
, icon = Icon.StarHaloUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 StarUp <| Range 15 20
, Grant Self 0 NPGen <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "Street Choco-Maid"
, id = 111
, rarity = 5
, icon = Icon.ArtsQuickUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 (CardUp Quick) <| Range 10 15
, Grant Self 0 HealingReceived <| Range 20 30
]
, bond = Nothing
, limited = True
}
, { name = "Melty Sweetheart"
, id = 112
, rarity = 5
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Times 3 << Grant Self 0 (Special DefenseUp <| VsTrait Male) <| Flat 100
, Grant Self 0 StarUp <| Range 10 20
]
, bond = Nothing
, limited = True
}
, { name = "Decapitating Bunny 2018"
, id = 154
, rarity = 5
, icon = Icon.ShieldBreak
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 IgnoreInvinc Full
, Grant Self 0 (CardUp Quick) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "Mature Gentleman"
, id = 155
, rarity = 5
, icon = Icon.FireUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 0 DeathResist <| Range 60 80 ]
, bond = Nothing
, limited = True
}
, { name = "Grand Puppeteer"
, id = 156
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ To Self GaugeUp <| Range 50 60
, Grant Self 3 (CardUp Arts) <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "Threefold Barrier"
, id = 157
, rarity = 5
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Times 3 << Grant Self 0 DamageCut <| Range 1000 1200 ]
, bond = Nothing
, limited = True
}
, { name = "Vivid Dance of Fists"
, id = 158
, rarity = 4
, icon = Icon.DamageUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 DamageUp <| Range 800 1000 ]
, bond = Nothing
, limited = True
}
, { name = "Mystic Eyes of Distortion"
, id = 159
, rarity = 4
, icon = Icon.BusterUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 20 25
, Debuff Self 0 DefenseDown <| Flat 15
]
, bond = Nothing
, limited = True
}
, { name = "Summer's Precognition"
, id = 160
, rarity = 4
, icon = Icon.Dodge
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Times 1 <| Grant Self 0 Evasion Full
, Grant Self 0 StarUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "Chorus"
, id = 161
, rarity = 4
, icon = Icon.StarUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 GatherUp <| Range 300 400
, Grant Self 3 DebuffResist <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "Sprinter"
, id = 162
, rarity = 3
, icon = Icon.QuickUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 5 8
, Grant Self 0 DebuffResist <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "Repeat Magic"
, id = 163
, rarity = 3
, icon = Icon.Noble
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ To Self GaugeUp <| Range 20 30
, Grant Self 0 NPGen <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "Kiss Your Hand"
, id = 165
, rarity = 5
, icon = Icon.AllUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 12
, Grant Self 0 (CardUp Buster) <| Range 10 12
, Grant Self 0 (CardUp Quick) <| Range 10 12
]
, bond = Nothing
, limited = True
}
, { name = "Teacher and I"
, id = 166
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ To Self GaugeUp <| Range 5 60
, Grant Self 0 GatherUp <| Range 300 400
]
, bond = Nothing
, limited = True
}
, { name = "Versus"
, id = 167
, rarity = 5
, icon = Icon.DamageUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 3 (Special AttackUp <| VsTrait Divine) <| Range 80 100
, Grant Self 3 (Special DefenseUp <| VsTrait Divine) <| Range 40 50
]
, bond = Nothing
, limited = True
}
, { name = "Beasts Under the Moon"
, id = 168
, rarity = 4
, icon = Icon.NobleUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 NPGen <| Range 12 15
, Grant Self 0 StarUp <| Range 12 15
, Grant Self 0 HealPerTurn <| Range 200 300
]
, bond = Nothing
, limited = True
}
, { name = "Glass Full Sweet Time"
, id = 169
, rarity = 4
, icon = Icon.Bullseye
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 SureHit Full
, Grant Self 0 DamageUp <| Range 400 600
, Grant Self 0 DamageCut <| Range 200 300
]
, bond = Nothing
, limited = True
}
, { name = "Salon de Marie"
, id = 170
, rarity = 3
, icon = Icon.Dodge
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Times 1 <| Grant Self 0 Evasion Full
, Grant Self 0 HealingReceived <| Range 5 10
, Grant Self 0 DebuffUp <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "Prince of Slayer"
, id = 171
, rarity = 3
, icon = Icon.StarTurn
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 1 2
, Grant Self 0 (Special AttackUp <| VsTrait Dragon) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "Noisy Obsession"
, id = 172
, rarity = 4
, icon = Icon.ExclamationUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 15 20
, Grant Self 0 NPUp <| Range 15 20
, Grant Self 0 (Success Charm) <| Range 12 15
]
, bond = Nothing
, limited = True
}
, gift 174 "[Heaven's Feel]" Icon.QuickUp
[self (CardUp Quick) 10, To Self GaugeUp <| Flat 40]
, { name = "Ideal Holy King"
, id = 175
, rarity = 5
, icon = Icon.HPUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Party 0 HPUp <| Range 1000 1200 ]
, bond = Nothing
, limited = False
}
, { name = "Record Holder"
, id = 176
, rarity = 4
, icon = Icon.StaffUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 DebuffUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "Beast of Billows"
, id = 177
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ equipped Lancer << Grant Self 0 NPUp <| Range 15 25 ]
, bond = Nothing
, limited = False
}
, { name = "Personal Training"
, id = 178
, rarity = 5
, icon = Icon.Road
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Bonus EXP Percent <| Range 2 10 ]
, bond = Nothing
, limited = True
}
, { name = "The Scholars of Chaldea"
, id = 179
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ To Self GaugeUp <| Range 30 50
, Grant Self 0 HealingReceived <| Range 20 30
]
, bond = Nothing
, limited = True
}
, { name = "Maiden Leading Chaldea"
, id = 180
, rarity = 5
, icon = Icon.StarTurn
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 3 4
, Grant Self 0 (CardUp Buster) <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "The Merciless One"
, id = 181
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ When "defeated" << To Party GaugeUp <| Range 15 20
, Grant Self 0 (CardUp Buster) <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "Art of the Poisonous Snake"
, id = 182
, rarity = 4
, icon = Icon.ArtsUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 3 (CardUp Arts) <| Range 30 40 ]
, bond = Nothing
, limited = False
}
, { name = "Art of Death"
, id = 183
, rarity = 4
, icon = Icon.DamageUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (Special AttackUp <| VsTrait Humanoid) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Gentle Affection"
, id = 184
, rarity = 4
, icon = Icon.HealUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 HealUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "Volumen Hydrargyrum"
, id = 185
, rarity = 5
, icon = Icon.Shield
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Times 3 <| Grant Self 0 Invincibility Full
, Grant Self 0 DamageUp <| Range 200 500
]
, bond = Nothing
, limited = False
}
, { name = "Innocent Maiden"
, id = 186
, rarity = 4
, icon = Icon.NobleTurn
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 GaugePerTurn <| Range 4 5
, Grant Self 0 (CardUp Quick) <| Range 10 12
]
, bond = Nothing
, limited = False
}
, { name = "Self Geas Scroll"
, id = 187
, rarity = 3
, icon = Icon.StaffUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 (Success Stun) <| Range 12 15 ]
, bond = Nothing
, limited = False
}
, { name = "Before Awakening"
, id = 188
, rarity = 5
, icon = Icon.AllUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 (CardUp Buster) <| Range 8 10
, Grant Self 0 (CardUp Quick) <| Range 8 10
, Grant Self 0 DefenseUp <| Range 8 10
]
, bond = Nothing
, limited = False
}
, { name = "His Rightful Place"
, id = 189
, rarity = 5
, icon = Icon.StarTurn
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 3 4
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, bond 191 "Crown of the Star" "Altria Pendragon" Icon.DamageUp
[party AttackUp 15]
, bond 192 "Relic of the King" "Zhuge Liang (Lord El-Melloi II)" Icon.BusterUp
[party_ Buster 15]
, bond 193 "Triumph of the Lord Impaler" "Vlad III" Icon.BeamUp
[self NPUp 30, atkChance 30 << To Self GaugeUp <| Flat 5]
, bond 194 "Revelation from Heaven" "Jeanne d'Arc" Icon.BusterUp
[party_ Buster 15]
, bond 195 "Memories of the Dragon" "Altria Pendragon (Alter)" Icon.BeamUp
[self NPUp 30, atkChance 30 << Debuff Target 3 DefenseDown <| Flat 5]
, bond 196 "Hunter of the Red Plains" "EMIYA" Icon.BeamUp
[self NPUp 30, atkChance 30 << To Party GainStars <| Flat 5]
, bond 197 "Castle of Snow" "Heracles" Icon.Kneel
[Times 3 <| self Guts 500]
, bond 198 "Yggdrasil Tree" "Cu Chulainn (Caster)" Icon.BeamUp
[self NPUp 30, atkChance 30 << To Self Heal <| Flat 500]
, bond 199 "Scorching Embrace" "Kiyohime" Icon.BeamUp
[self NPUp 30, atkChance 30 << Debuff Target 5 Burn <| Flat 500]
, bond 200 "Worthless Jewel" "Mata Hari" Icon.NobleUp
[party NPGen 15]
, bond 201 "Eternal Solitude" "Altera" Icon.SwordUp
[party AttackUp 15]
, bond 202 "Queen's Present" "Chevalier d'Eon" Icon.ArtsUp
[party_ Arts 15]
, bond 203 "Elixir" "Elisabeth Bathory" Icon.HealTurn
[party HealPerTurn 500]
, bond 204 "My Necklace" "Marie Antoinette" Icon.StarHaloUp
[party StarUp 20]
, bond 205 "Staff He Gave Me" "Martha" Icon.HealUp
[party HealingReceived 30]
, bond 206 "Iron Maiden" "Carmilla" Icon.BeamUp
[self NPUp 30, atkChance 10 <| Debuff Target 1 SealNP Full]
, bond 207 "Cat Apron" "Tamamo Cat" Icon.Heal
[party HPUp 2000]
, bond 208 "Thirst for Victory" "Boudica" Icon.StarHaloUp
[party StarUp 20]
, bond 209 "To My Dear Friend" "Hans Christian Andersen" Icon.HoodUp
[party DebuffResist 30]
, bond 210 "Sacred Devotion" "Arash" Icon.Heal
[ When "defeated" <| To Party RemoveDebuffs Full
, When "defeated" <| To Party Heal <| Flat 5000
]
, { name = "The Wandering Tales of Shana-oh"
, id = 211
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 10 15
, When "defeated" <<
Grant Party 1 (CardUp Quick) <| Range 20 30
]
, bond = Nothing
, limited = True
}
, { name = "Golden Captures the Carp"
, id = 212
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ To Self GaugeUp <| Range 30 50
, To Party GainStars <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "A Fox Night's Dream"
, id = 213
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 NPGen <| Range 20 25
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "Burning Tale of Love"
, id = 214
, rarity = 4
, icon = Icon.DamageUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (Special AttackUp <| VsTrait Male) <| Range 25 30
, Grant Self 0 DebuffUp <| Range 12 15
]
, bond = Nothing
, limited = True
}
, { name = "Reciting the Subscription List"
, id = 215
, rarity = 3
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Times 1 <| Grant Self 0 DebuffResist Full ]
, bond = Nothing
, limited = True
}
, bond 216 "Key of the King's Law" "Gilgamesh" Icon.BeamUp
[self NPUp 30, atkChance 30 << Grant Self 3 CritUp <| Flat 10]
, bond 217 "Golden Glass" "Sakata Kintoki" Icon.BeamUp
[self NPUp 30, atkChance 30 << To Self GaugeUp <| Flat 5]
, bond 218 "Thunderous Applause" "Nero Claudius" Icon.ArtsUp
[party_ Arts 15]
, bond 219 "Das Rheingold" "Siegfried" Icon.NobleUp
[party NPGen 15]
, bond 220 "Radiance of the Goddess" "Stheno" Icon.QuickUp
[party_ Quick 15]
, bond 221 "Voyage of the Flowers" "Altria Pendragon (Lily)" Icon.SwordUp
[party AttackUp 10, party StarUp 10]
, bond 222 "Ark of the Covenant" "David" Icon.BeamUp
[self NPUp 30, When "attacking" << To Enemy Death <| Flat 10]
, bond 223 "Door to Babylon" "Darius III" Icon.BusterUp
[party_ Buster 15]
, bond 224 "Blood-Thirsting Axe" "Eric Bloodaxe" Icon.ExclamationUp
[party CritUp 25]
, bond 225 "Insurrection" "Spartacus" Icon.Kneel
[gutsPercent 50]
, { name = "Go West!!"
, id = 226
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 NPUp <| Range 20 25
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "The Classic Three Great Heroes"
, id = 227
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 NPUp <| Range 15 20
, Grant Self 0 StarUp <| Range 15 20
, To Self GaugeUp <| Range 25 40
]
, bond = Nothing
, limited = True
}
, { name = "True Samadhi Fire"
, id = 228
, rarity = 4
, icon = Icon.BeamUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 15 20
, Grant Self 0 (CardUp Buster) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "All Three Together"
, id = 229
, rarity = 3
, icon = Icon.StarUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 GatherUp <| Range 100 200
, Grant Self 0 CritUp <| Range 5 10
]
, bond = Nothing
, limited = True
}
, bond 230 "Tristar Belt" "Orion" Icon.ExclamationUp
[party CritUp 25]
, bond 231 "Golden Helm" "Francis Drake" Icon.BeamUp
[party NPUp 20]
, bond 232 "Black Knight's Helmet" "Lancelot" Icon.BeamUp
[self NPUp 30, atkChance 30 << Debuff Target 3 CritChance <| Flat 30]
, bond 233 "Golden Apple" "Atalante" Icon.QuickUp
[party_ Quick 15]
, bond 234 "Holy Pumpkin Grail" "Elisabeth Bathory (Halloween)" Icon.HoodUp
[party DebuffResist 30]
, bond 235 "Rotary Matchlock" "Oda Nobunaga" Icon.ExclamationUp
[party CritUp 25]
, bond 236 "Llamrei Unit II" "Altria Pendragon (Santa Alter)" Icon.StarHaloUp
[party StarUp 20]
, bond 237 "Things to Calm the Heart" "Henry Jekyll & Hyde" Icon.BusterUp
[party_ Buster 15]
, bond 238 "Glory of the Past Days" "Edward Teach" Icon.BusterUp
[party_ Buster 15]
, bond 239 "Heaven Among the Mountains" "Sasaki Kojirou" Icon.QuickUp
[party_ Quick 15]
, { name = "Divine Princess of the Storm"
, id = 240
, rarity = 5
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ When "defeated" << Grant Party 3 DefenseUp <| Range 20 25 ]
, bond = Nothing
, limited = True
}
, { name = "Ox-Demon King"
, id = 241
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Party 3 (CardUp Buster) <| Range 10 15 ]
, bond = Nothing
, limited = True
}
, { name = "Personal Lesson"
, id = 242
, rarity = 5
, icon = Icon.Road
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Bonus MysticCode Percent <| Flat 2 ]
, bond = Nothing
, limited = True
}
, { name = "Bronze-Link Manipulator"
, id = 243
, rarity = 3
, icon = Icon.SwordUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 3 AttackUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "Ath nGabla"
, id = 244
, rarity = 3
, icon = Icon.QuickUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 10 15
, Debuff Self 0 DefenseDown <| Flat 10
]
, bond = Nothing
, limited = False
}
, { name = "Bygone Dream"
, id = 245
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ equipped Assassin << Grant Self 0 NPUp <| Range 15 25 ]
, bond = Nothing
, limited = False
}
, { name = "Extremely Spicy Mapo Tofu"
, id = 246
, rarity = 3
, icon = Icon.HealUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 HealingReceived <| Range 10 20 ]
, bond = Nothing
, limited = False
}
, { name = "Jeweled Sword Zelretch"
, id = 247
, rarity = 3
, icon = Icon.NobleUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 NPGen <| Range 5 10
, To Self GaugeUp <| Range 25 40
]
, bond = Nothing
, limited = False
}
, bond 248 "Tamamo's Club" "Tamamo-no-Mae" Icon.ArtsUp
[party_ Arts 15]
, bond 249 "Headband of Resolve" "Okita Souji" Icon.ExclamationUp
[party CritUp 25]
, bond 250 "Calico Jack" "Anne Bonny & Mary Read" Icon.ExclamationUp
[party CritUp 25]
, bond 251 "Gazing Upon Dun Scaith" "Scathach" Icon.QuickUp
[party_ Quick 15]
, bond 252 "Star of Prophecy" "Cu Chulainn" Icon.BeamUp
[self NPUp 30, atkChance 30 << Grant Self 3 CritUp <| Flat 10]
, bond 253 "Hekate's Staff" "Medea" Icon.ArtsUp
[party_ Arts 15]
, bond 254 "Formless Island" "Medusa" Icon.NobleUp
[party NPGen 15]
, bond 255 "Cask of the Wise" "Alexander" Icon.QuickUp
[party_ Quick 15]
, bond 256 "Shaytan's Arm" "Hassan of the Cursed Arm" Icon.ReaperUp
[party DeathUp 20]
, bond 257 "Ariadne's Thread" "Asterios" Icon.QuickUp
[party_ Quick 15]
, { name = "Dumplings Over Flowers"
, id = 258
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 15 20
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "Faithful Companions"
, id = 259
, rarity = 4
, icon = Icon.ArtsUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 NPGen <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "Hidden Sword: Pheasant Reversal"
, id = 260
, rarity = 3
, icon = Icon.QuickUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 3 5
, Grant Self 0 CritUp <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "Golden Sumo: Boulder Tournament"
, id = 261
, rarity = 5
, icon = Icon.SwordUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 AttackUp <| Range 10 15
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, { name = "Hot Spring Under the Moon"
, id = 262
, rarity = 5
, icon = Icon.ExclamationUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 20 25
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "Origin Bullet"
, id = 263
, rarity = 5
, icon = Icon.ShieldBreak
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 IgnoreInvinc Full
, Grant Self 0 (Special AttackUp <| VsClass Caster) <| Range 35 40
]
, bond = Nothing
, limited = False
}
, { name = "Covering Fire"
, id = 264
, rarity = 4
, icon = Icon.DamageUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 DamageUp <| Range 400 600
, Grant Self 0 CritUp <| Range 15 20
]
, bond = Nothing
, limited = False
}
, { name = "Battle of Camlann"
, id = 265
, rarity = 3
, icon = Icon.Noble
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ When "defeated" << To Party GaugeUp <| Range 10 15 ]
, bond = Nothing
, limited = False
}
, bond 266 "Who Am I?" "Mordred" Icon.BeamUp
[party NPUp 20]
, bond 267 "The Misty Night of London" "Jack the Ripper" Icon.ExclamationUp
[party CritUp 25]
, bond 268 "Wonderland" "Nursery Rhyme" Icon.ExclamationUp
[party CritUp 15, party HealingReceived 10]
, bond 269 "Faceless King" "Robin Hood" Icon.ArtsUp
[party_ Arts 15]
, bond 270 "Usumidori" "Ushiwakamaru" Icon.QuickUp
[party_ Quick 15]
, bond 271 "Etiquette of Nine Guests" "Jing Ke" Icon.BeamUp
[self NPUp 30, atkChance 30 << Grant Self 3 DeathUp <| Flat 30]
, bond 272 "Heaven Scorcher Halberd" "Lu Bu Fengxian" Icon.BusterUp
[party_ Buster 15]
, bond 273 "What can be Left Behind" "Georgios" Icon.Shield
[ When "defeated" << Times 1 <| Grant Party 0 Invincibility Full
, When "defeated" << Grant Party 3 DamageCut <| Flat 1000
]
, bond 274 "Thermopylae" "Leonidas I" Icon.BusterUp
[party_ Buster 15]
, bond 275 "Haydn Quartets" "Wolfgang Amadeus Mozart" Icon.BeamUp
[party NPUp 20]
, gift 276 "Anniversary Heroines" Icon.SwordUp
[self AttackUp 10, self StarsPerTurn 3]
, { name = "Leisure Stroll"
, id = 277
, rarity = 5
, icon = Icon.StarUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 GatherUp <| Range 400 600
, Grant Self 0 (CardUp Arts) <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "Partake with the King"
, id = 278
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 10 15
, To Self GaugeUp <| Range 50 60
]
, bond = Nothing
, limited = True
}
, { name = "Goldfish Scooping"
, id = 279
, rarity = 4
, icon = Icon.Bullseye
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 SureHit Full
, Grant Self 0 (CardUp Buster) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "Fire Flower"
, id = 280
, rarity = 3
, icon = Icon.StarHaloUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 StarUp <| Range 5 10
, Grant Self 0 CritUp <| Range 5 10
]
, bond = Nothing
, limited = True
}
, bond 281 "Arm of Raiden" "Nikola Tesla" Icon.BeamUp
[party NPUp 20]
, bond 282 "Endowed Hero" "Arjuna" Icon.BeamUp
[self NPUp 30, self GatherUp 1000]
, bond 283 "Light of the Deprived" "Karna" Icon.AllUp
[party_ Quick 8, party_ Arts 8, party_ Buster 8]
, bond 284 "Procedure to Humanity" "Frankenstein" Icon.QuickUp
[party_ Quick 15]
, bond 285 "Black Helmet" "Altria Pendragon (Lancer Alter)" Icon.NobleUp
[party NPGen 15]
, bond 286 "Legend of the Gallic War" "Gaius Julius Caesar" Icon.QuickUp
[party_ Quick 15]
, bond 287 "Rome" "Romulus" Icon.BeamUp
[party NPUp 20]
, bond 288 "Encounter at Gojou Bridge" "Musashibou Benkei" Icon.NobleRedUp
[party NPFromDamage 20]
, bond 289 "Impure Death Mask" "Phantom of the Opera" Icon.QuickUp
[party_ Quick 15]
, bond 290 "Really Convenient" "William Shakespeare" Icon.NobleUp
[party NPGen 15]
, { name = "Pirates Party!"
, id = 291
, rarity = 5
, icon = Icon.ShieldBreak
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 IgnoreInvinc Full
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "Summertime Mistress"
, id = 292
, rarity = 5
, icon = Icon.ExclamationUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 15 20
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, { name = "Twilight Memory"
, id = 293
, rarity = 4
, icon = Icon.QuickUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Times 1 <| Grant Self 0 Evasion Full
, Grant Self 0 (CardUp Quick) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "Shiny Goddess"
, id = 294
, rarity = 3
, icon = Icon.ArtsUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 3 5
, Grant Self 0 (CardUp Arts) <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "Knights of Marines"
, id = 295
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 10 15
, To Self GaugeUp <| Range 50 60
]
, bond = Nothing
, limited = True
}
, { name = "Chaldea Lifesavers"
, id = 296
, rarity = 5
, icon = Icon.Kneel
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Times 1 << Grant Self 0 Guts <| Flat 1
, Grant Self 0 NPGen <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "Meat Wars"
, id = 297
, rarity = 4
, icon = Icon.HealTurn
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 HealPerTurn <| Range 200 300
, Grant Self 0 (CardUp Arts) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "Shaved Ice (Void's Dust Flavor)"
, id = 298
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DamageCut <| Range 100 200
, Grant Self 0 DebuffResist <| Range 5 10
]
, bond = Nothing
, limited = True
}
, bond 299 "Annihilation List" "Mysterious Heroine X" Icon.DamageUp
[party (Special AttackUp <| VsClass Saber) 20]
, bond 300 "Imperishable Flames" "Brynhild" Icon.BusterUp
[party_ Buster 10, party NPGen 10]
, bond 301 "Ring of Bay Laurel" "Nero Claudius (Bride)" Icon.ArtsUp
[party_ Arts 15]
, bond 302 "Final Battle" "Beowulf" Icon.DamageUp
[party (Special AttackUp <| VsTrait Dragon) 20]
, bond 303 "Bratan of Wisdom" "Fionn mac Cumhaill" Icon.ArtsUp
[party_ Arts 10, party NPUp 10]
, bond 304 "Prelati's Spellbook" "Gilles de Rais" Icon.BusterUp
[party_ Buster 20, demeritAll DebuffVuln 20]
, bond 305 "Parasitic Bomb" "Mephistopheles" Icon.BeamUp
[party NPUp 20]
, bond 306 "Seethe of a Warrior" "Fergus mac Roich" Icon.BusterUp
[party_ Buster 10, party NPUp 10]
, bond 307 "My Loathsome Life" "Charles-Henri Sanson" Icon.ReaperUp
[party DeathUp 10, party NPGen 10]
, bond 308 "There is No Love Here" "Caligula" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, { name = "Magical Girl of Sapphire"
, id = 309
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 NPGen <| Range 25 30
, To Self GaugeUp <| Range 40 50
]
, bond = Nothing
, limited = True
}
, { name = "Kill on Sight"
, id = 310
, rarity = 4
, icon = Icon.ArtsUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "Zunga Zunga!"
, id = 311
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DamageCut <| Range 100 200
, Grant Self 0 HealingReceived <| Range 5 10
]
, bond = Nothing
, limited = True
}
, { name = "Kaleid Ruby"
, id = 312
, rarity = 4
, icon = Icon.BusterUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 10 15
, Grant Self 0 NPUp <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "Kaleid Sapphire"
, id = 313
, rarity = 4
, icon = Icon.ArtsUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 NPUp <| Range 8 10
]
, bond = Nothing
, limited = True
}
, bond 315 "Mugashiki—Shinkuu Myou" "Ryougi Shiki (Saber)" Icon.ArtsUp
[party_ Arts 15]
, bond 316 "Frontliner's Flag" "Amakusa Shirou" Icon.DamageUp
[ party (Special AttackUp <| VsTrait Undead) 20
, party (Special AttackUp <| VsTrait Demonic) 20
]
, bond 317 "Chateau d'If" "Edmond Dantes" Icon.QuickUp
[party_ Quick 15]
, bond 318 "Unlimited Pancakes" "Medea (Lily)" Icon.HealUp
[party HealingReceived 30]
, bond 319 "Red Leather Jacket" "Ryougi Shiki (Assassin)" Icon.ReaperUp
[party DeathUp 30]
, bond 320 "Otherworldly Mystical Horse" "Astolfo" Icon.Dodge
[self NPUp 30, Times 1 <| Grant Party 0 Evasion Full]
, bond 321 "Letter From a Friend" "Gilles de Rais (Caster)" Icon.BusterUp
[party_ Buster 20, demeritAll StarDown 20]
, bond 322 "Hound of Culann" "Cu Chulainn (Prototype)" Icon.DamageUp
[party (Special AttackUp <| VsTrait WildBeast) 20]
, bond 323 "Radiance of the Goddess (Euryale)" "Euryale" Icon.ArtsUp
[party_ Arts 15]
, bond 324 "Hero's Armament" "Hektor" Icon.BeamUp
[party NPUp 20]
, { name = "Glory Is With Me"
, id = 325
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 15 20
, Grant Self 0 CritUp <| Range 15 20
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "Original Legion"
, id = 326
, rarity = 4
, icon = Icon.ShieldUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 8 20
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "Howl at the Moon"
, id = 327
, rarity = 3
, icon = Icon.BusterUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 10 15
, Debuff Self 0 DebuffVuln <| Flat 20
]
, bond = Nothing
, limited = True
}
, { name = "Princess of the White Rose"
, id = 328
, rarity = 5
, icon = Icon.Kneel
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Times 1 << Grant Self 0 Guts <| Flat 1
, To Self GaugeUp <| Range 10 20
]
, bond = Nothing
, limited = True
}
, { name = "Joint Recital"
, id = 329
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 15 20
, Grant Self 0 CritUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "Chaldea Lunchtime"
, id = 330
, rarity = 5
, icon = Icon.Rainbow
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Bonus Bond Percent <| Range 2 10 ]
, bond = Nothing
, limited = True
}
, { name = "Fragarach"
, id = 331
, rarity = 3
, icon = Icon.StarUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 GatherUp <| Range 200 300 ]
, bond = Nothing
, limited = False
}
, { name = "Inverted Moon of the Heavens"
, id = 332
, rarity = 3
, icon = Icon.StarTurn
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 1 2
, Grant Self 0 DebuffResist <| Range 5 10
]
, bond = Nothing
, limited = False
}
, { name = "Hydra Dagger"
, id = 333
, rarity = 3
, icon = Icon.ReaperUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 DeathUp <| Range 5 10 ]
, bond = Nothing
, limited = False
}
, bond 334 "Indefatigable" "Florence Nightingale" Icon.BusterUp
[party_ Buster 10, party HealingReceived 20]
, bond 335 "One-Man War" "Cu Chulainn (Alter)" Icon.Kneel
[self NPUp 30, gutsPercent 20]
, bond 336 "Sacred Spring" "Queen Medb" Icon.NobleUp
[party NPGen 15]
, bond 337 "Indestructible Blade" "Rama" Icon.ExclamationUp
[party CritUp 25]
, bond 338 "Concealed Goddess" "Helena Blavatsky" Icon.DamageUp
[party (Special AttackUp <| VsClass Assassin) 20]
, bond 339 "Lights of Civilization" "Thomas Edison" Icon.NobleUp
[party NPGen 15]
, bond 340 "Reaching the Zenith of My Skill" "Li Shuwen" Icon.ArtsUp
[party_ Arts 15]
, bond 341 "Knight's Oath" "Diarmuid Ua Duibhne" Icon.ArtsUp
[party_ Quick 10, party_ Arts 10]
, bond 342 "Elemental" "Paracelsus von Hohenheim" Icon.ArtsUp
[party_ Arts 10, party NPUp 10]
, bond 343 "NEO Difference Engine" "Charles Babbage" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, { name = "Dangerous Beast"
, id = 344
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 15 20
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "Witch Under the Moonlight"
, id = 345
, rarity = 4
, icon = Icon.ArtsUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 NPGen <| Range 5 10
]
, bond = Nothing
, limited = True
}
, { name = "Count Romani Archaman's Hospitality"
, id = 346
, rarity = 3
, icon = Icon.NobleUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 NPGen <| Range 5 10
, Grant Self 0 DefenseUp <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "Hero Elly's Adventure"
, id = 347
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 10 15
, Grant Self 0 NPUp <| Range 20 25
]
, bond = Nothing
, limited = True
}
, { name = "Wizard & Priest"
, id = 348
, rarity = 4
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 NPUp <| Range 15 20
, Grant Self 0 HealingReceived <| Range 10 20
]
, bond = Nothing
, limited = True
}
, { name = "Mata Hari's Tavern"
, id = 349
, rarity = 3
, icon = Icon.AllUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 2 3
, Grant Self 0 (CardUp Buster) <| Range 2 3
, Grant Self 0 (CardUp Quick) <| Range 2 3
, Grant Self 0 CritUp <| Range 5 10
]
, bond = Nothing
, limited = True
}
, bond 350 "Hell of Blazing Punishment" "Jeanne d'Arc (Alter)" Icon.BusterUp
[party_ Buster 15]
, bond 351 "Gordian Knot" "Iskandar" Icon.SwordUp
[party AttackUp 15]
, bond 352 "White Dragon" "Xuanzang Sanzang" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, bond 353 "The Sun Shines Here" "Emiya (Assassin)" Icon.ArtsQuickUp
[party_ Quick 10, party_ Arts 10]
, bond 354 "Dress of Heaven" "Irisviel (Holy Grail)" Icon.HealUp
[party HealingReceived 30]
, bond 355 "Manifestation of the Golden Rule" "Gilgamesh (Child)" Icon.NobleUp
[party NPGen 15]
, bond 356 "Spirit of the Vast Land" "Geronimo" Icon.NobleUp
[party NPGen 15]
, bond 357 "Extolling the Revolver" "Billy the Kid" Icon.ExclamationUp
[party CritUp 25]
, bond 358 "Library of Hundred Men" "Hassan of the Hundred Personas" Icon.AllUp
[party_ Buster 8, party_ Quick 8, party_ Arts 8]
, bond 359 "Final Fragment" "Angra Mainyu" Icon.DamageUp
[ gutsPercent 20
, self (Special AttackUp <| VsClass Beast1) 200
, self (Special AttackUp <| VsClass Beast2) 200
, self (Special AttackUp <| VsClass Beast3L) 200
, self (Special AttackUp <| VsClass Beast3R) 200
]
, gift 360 "Fate/EXTELLA" Icon.ExclamationUp
[self CritUp 15, self StarsPerTurn 3]
, gift 361 "Spiritron Portrait: Nero Claudius" Icon.Road
[Bonus EXP Units <| Flat 50]
, gift 362 "Spiritron Portrait: Nameless" Icon.Road
[Bonus EXP Units <| Flat 50]
, gift 363 "Spiritron Portrait: Tamamo-no-Mae" Icon.Road
[Bonus EXP Units <| Flat 50]
, gift 364 "Spiritron Portrait: Karna" Icon.Road
[Bonus EXP Units <| Flat 50]
, gift 365 "Spiritron Portrait: Altera" Icon.Road
[Bonus EXP Units <| Flat 50]
, gift 366 "Spiritron Portrait: Gilgamesh" Icon.Road
[Bonus EXP Units <| Flat 50]
, bond 367 "Divine Wine—Shinpen Kidoku" "Shuten-Douji" Icon.ArtsQuickUp
[party_ Quick 10, party_ Arts 10]
, bond 368 "Doujigiri Yasutsuna" "Minamoto-no-Raikou" Icon.BusterUp
[party_ Buster 10, party CritUp 15]
, bond 369 "Ramesseum" "Ozymandias" Icon.BusterArtsUp
[party_ Arts 10, party_ Buster 10]
, bond 370 "Bone Sword (Nameless)" "Ibaraki-Douji" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, bond 371 "Unit Golden Bear" "Sakata Kintoki (Rider)" Icon.StarHaloUp
[party StarUp 20]
, bond 372 "Gringolet" "Gawain" Icon.BusterUp
[party_ Buster 15]
, bond 373 "But I Lied Once" "Tristan" Icon.ExclamationUp
[party CritUp 25]
, bond 374 "Exercising the Royal Authority" "Nitocris" Icon.NobleUp
[party NPGen 10, party NPUp 10]
, bond 375 "Mask of a Demon" "Fuuma \"Evil-wind\" Kotarou" Icon.QuickUp
[party_ Quick 15]
, bond 376 "Cook Despite of Exhaustion" "Tawara Touta" Icon.HealTurn
[party HealPerTurn 500]
, bond 377 "King's Horse" "Altria Pendragon (Lancer)" Icon.SwordUp
[party AttackUp 10, party NPUp 10]
, bond 378 "All-Encompassing Wisdom" "Leonardo da Vinci" Icon.BeamUp
[party NPUp 20]
, bond 379 "Sunset Beach" "Tamamo-no-Mae (Lancer)" Icon.QuickBusterUp
[party_ Quick 10, party_ Buster 10]
, bond 380 "Lady of the Lake" "Lancelot (Saber)" Icon.NobleUp
[party NPGen 10, party CritUp 10]
, bond 381 "Reminiscence of the Summer"
"Marie Antoinette (Caster)" Icon.ExclamationUp
[party CritUp 25]
, bond 382 "Currently in the Middle of a Shower"
"Anne Bonny & Mary Read (Archer)" Icon.BusterArtsUp
[party_ Buster 10, party_ Arts 10]
, bond 383 "Prydwen" "Mordred (Rider)" Icon.BeamUp
[party NPUp 20]
, bond 384 "Beach Love Letter (Terror)" "Kiyohime (Lancer)" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, bond 385 "Lost Right Arm" "Bedivere" Icon.BusterUp
[party_ Buster 10, party NPGen 10]
, bond 386 "Proof of Existence" "Hassan of the Serenity" Icon.QuickUp
[party_ Quick 15]
, { name = "A Moment of Tranquility"
, id = 387
, rarity = 5
, icon = Icon.ArtsQuickUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 10 15
, Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 NPGen <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "Reading on the Holy Night"
, id = 388
, rarity = 4
, icon = Icon.NobleUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 NPGen <| Range 15 20
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "Saint's Invitation"
, id = 389
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 3 5
, Grant Self 0 DamageCut <| Range 100 200
]
, bond = Nothing
, limited = True
}
, { name = "Holy Night Supper"
, id = 390
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ To Self GaugeUp <| Range 30 50
, Grant Self 0 CritUp <| Range 10 15
, Grant Self 0 NPUp <| Range 10 15
]
, bond = Nothing
, limited = True
}
, bond 391 "Champion Cup" "Altria Pendragon (Archer)" Icon.SwordUp
[party AttackUp 15]
, bond 392 "Phantasmal Summoning (Install)" "Illyasviel von Einzbern" Icon.AllUp
[party_ Buster 8, party_ Quick 8, party_ Arts 8]
, bond 393 "Serpent of Fate" "Cleopatra" Icon.BeamUp
[party NPUp 25, demeritAll DefenseDown 10]
, bond 394 "Holy Knuckle" "Martha (Ruler)" Icon.BusterUp
[party_ Buster 15]
, bond 395 "Minimal Prudence" "Scathach (Assassin)" Icon.QuickUp
[party_ Quick 15]
, bond 396 "Sharing of Pain" "Chloe von Einzbern" Icon.ExclamationUp
[party CritUp 30, demeritAll HealthLoss 200]
, bond 397 "Creed at the Bottom of the Earth" "Vlad III (EXTRA)" Icon.QuickBusterUp
[party_ Quick 10, party_ Buster 10]
, bond 398 "Invitation to Halloween" "Elisabeth Bathory (Brave)" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, gift 399 "First Order" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, { name = "Devilish Bodhisattva"
, id = 400
, rarity = 5
, icon = Icon.SunUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ To Self GaugeUp <| Range 50 60
, Times 1 << Grant Self 0 Overcharge <| Flat 2
]
, bond = Nothing
, limited = False
}
, { name = "Room Guard"
, id = 401
, rarity = 4
, icon = Icon.StarUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 GatherUp <| Range 300 400
, Grant Self 0 DamageCut <| Range 300 400
]
, bond = Nothing
, limited = False
}
, { name = "Over the Cuckoo's Nest"
, id = 414
, rarity = 3
, icon = Icon.Crystal
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Bonus QPQuest Units <| Flat 2019
, Grant Self 0 DamageUp <| Range 0 19
]
, bond = Nothing
, limited = True
}
, { name = "Cheers to 2019"
, id = 415
, rarity = 4
, icon = Icon.HealTurn
, stats = { base = { atk = 0, hp = 2019 }
, max = { atk = 0, hp = 2019 }
}
, effect = [ Grant Self 0 HealPerTurn <| Flat 100 ]
, bond = Nothing
, limited = True
}
, { name = "Reality Marble"
, id = 418
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ equipped Archer << Grant Self 0 NPUp <| Range 15 25 ]
, bond = Nothing
, limited = False
}
, { name = "Potion of Youth"
, id = 419
, rarity = 3
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 (Resist AttackDown) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Collection of Mysterious Masks"
, id = 420
, rarity = 3
, icon = Icon.StarUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 GatherUp <| Range 100 200
, Grant Self 0 StarUp <| Range 5 10
]
, bond = Nothing
, limited = False
}
, { name = "Cute Orangette"
, id = 421
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 NPGen <| Range 25 30
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "Chocolatier"
, id = 422
, rarity = 5
, icon = Icon.ArtsUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 15 20
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "Bitter Black"
, id = 424
, rarity = 4
, icon = Icon.HealUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 HealingReceived <| Range 15 20
, Grant Self 0 NPGen <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "Blissful Time"
, id = 425
, rarity = 3
, icon = Icon.HealUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 HealingReceived <| Range 5 10
, Grant Self 0 StarUp <| Range 5 10
]
, bond = Nothing
, limited = True
}
, { name = "Arrogance of a Victor"
, id = 426
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DamageCut <| Range 100 200
, Grant Self 0 StarUp <| Range 5 10
]
, bond = Nothing
, limited = True
}
, { name = "Sweet Crystal"
, id = 427
, rarity = 5
, icon = Icon.ShieldBreak
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 IgnoreInvinc Full
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, { name = "Fondant au Chocolat"
, id = 428
, rarity = 5
, icon = Icon.DamageUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (Special AttackUp <| VsTrait Divine) <| Range 25 30
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, bond 403 "Seven-Headed Warhammer Sita" "Ishtar" Icon.BusterUp
[party_ Buster 20, demeritAll DebuffVuln 20]
, bond 404 "Flower of Humbaba" "Enkidu" Icon.HealTurn
[party HealPerTurn 500]
, bond 405 "Piedra del Sol" "Quetzalcoatl" Icon.BusterArtsUp
[party_ Arts 10, party_ Buster 10]
, bond 406 "Door to the Ocean" "Jeanne d'Arc Alter Santa Lily" Icon.HealUp
[party HealingReceived 30]
, bond 407 "Dup Shimati" "Gilgamesh (Caster)" Icon.BeamUp
[party NPUp 20]
, bond 408 "Chrysaor" "Gorgon" Icon.BusterUp
[party_ Buster 10, party NPGen 10]
, bond 409 "Uncertainty About the Future" "Medusa (Lancer)" Icon.QuickUp
[party_ Quick 10, party NPGen 10]
, bond 410 "Primeval Flame" "Jaguar Warrior" Icon.BusterUp
[party_ Buster 15]
, bond 411 "The Furthest Tower" "Merlin" Icon.BusterUp
[party_ Buster 10, party CritUp 15]
, bond 416 "Buddhism of Emptiness" "Miyamoto Musashi" Icon.HoodUp
[self NPUp 30, Times 3 <| Grant Self 0 DebuffResist Full]
, bond 417 "The Rift of the Valley" "\"First Hassan\"" Icon.HoodUp
[self DebuffResist 100]
, bond 429 "Dark Knight-Kun" "Mysterious Heroine X (Alter)" Icon.DamageUp
[party (Special AttackUp <| VsClass Saber) 20]
, bond 546 "The Dynamics of an Asteroid" "James Moriarty" Icon.BeamUp
[Grant (AlliesType Evil) 0 NPUp <| Flat 25]
, bond 547 "Kanshou & Bakuya (Revolvers)" "EMIYA (Alter)" Icon.AllUp
[party_ Quick 8, party_ Arts 8, party_ Buster 8]
, bond 548 "Jenseits der Wildnis" "Hessian Lobo" Icon.QuickUp
[self (CardUp Quick) 10, party NPGen 10]
, bond 549 "108 Stars of Destiny" "Yan Qing" Icon.QuickUp
[party_ Quick 10, party CritUp 15]
, { name = "Conversation on the Hot Sands"
, id = 550
, rarity = 5
, icon = Icon.StarUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 GatherUp <| Range 400 600
, Grant Self 0 CritUp <| Range 20 25
]
, bond = Nothing
, limited = True
}
, { name = "The Dantes Files: The Case of the Spring Jaunt"
, id = 551
, rarity = 5
, icon = Icon.ShieldBreak
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 IgnoreInvinc Full
, Grant Self 0 (CardUp Arts) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "Cafe Camelot"
, id = 553
, rarity = 4
, icon = Icon.HealUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 HealingReceived <| Range 8 10
, Grant Self 0 DebuffResist <| Range 8 10
, Grant Self 0 DefenseUp <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "Outrage"
, id = 554
, rarity = 4
, icon = Icon.CrosshairUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 1 Taunt Full
, Grant Self 1 NPGen <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "Operation Fianna"
, id = 555
, rarity = 4
, icon = Icon.Star
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ To Party GainStars <| Range 10 20
, Grant Self 0 (CardUp Buster) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "Quatre Feuilles"
, id = 556
, rarity = 3
, icon = Icon.AllUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 2 3
, Grant Self 0 (CardUp Buster) <| Range 2 3
, Grant Self 0 (CardUp Quick) <| Range 2 3
, Grant Self 0 MentalResist <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "Neverland"
, id = 557
, rarity = 3
, icon = Icon.StarHaloUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 4 8
, Grant Self 0 StarUp <| Range 4 8
, Grant Self 0 NPUp <| Range 4 8
]
, bond = Nothing
, limited = True
}
, bond 559 "Garden" "Arthur Pendragon (Prototype)" Icon.SwordUp
[party AttackUp 15]
, { name = "Demon King of the Sixth Heaven"
, id = 560
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 15 20
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "Fortress of the Sun"
, id = 561
, rarity = 5
, icon = Icon.ExclamationUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 CritUp <| Range 15 20
, Grant Self 0 StarUp <| Range 15 20
, To Self GaugeUp <| Range 25 40
]
, bond = Nothing
, limited = True
}
, { name = "Wolves of Mibu"
, id = 562
, rarity = 5
, icon = Icon.QuickBusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 8 10
, Grant Self 0 (CardUp Quick) <| Range 8 10
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "A Stroll in the Spring Breeze"
, id = 563
, rarity = 4
, icon = Icon.NobleUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 NPGen <| Range 15 20
, Grant Self 0 DamageCut <| Range 300 400
]
, bond = Nothing
, limited = True
}
, { name = "The Flower of High Society"
, id = 564
, rarity = 3
, icon = Icon.ArtsQuickUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 4 6
, Grant Self 0 (CardUp Quick) <| Range 4 6
]
, bond = Nothing
, limited = True
}
, bond 567 "Haori of the Oath" "Hijikata Toshizo" Icon.Kneel
[Times 1 << Grant Self 0 Guts <| Flat 1, self CritUp 30]
, bond 568 "Adzuki Beanbag" "Chacha" Icon.BeamUp
[party NPUp 25, demeritAll DefenseDown 10]
, { name = "Conquering the Great Sea of Stars"
, id = 569
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 15 20
, Grant Self 0 NPGen <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "One Summer"
, id = 570
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 8 10
, Grant Self 0 NPUp <| Range 8 10
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, { name = "Premonition of Beginnings"
, id = 572
, rarity = 4
, icon = Icon.StarTurn
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 2 3
, Grant Self 0 GaugePerTurn <| Range 2 3
]
, bond = Nothing
, limited = True
}
, bond 575 "The Radiant One" "Meltryllis" Icon.QuickUp
[self (CardUp Quick) 20, self CritUp 30, demeritOthers CritDown 10]
, bond 576 "Don't Avert Your Eyes Away From Me" "Passionlip" Icon.BusterUp
[party_ Buster 20, demeritAll DebuffVuln 20]
, bond 577 "A Passerby's Dream" "BB" Icon.AllUp
[party_ Quick 8, party_ Arts 8, party_ Buster 8]
, bond 578 "Tenma's Spring Training" "Suzuka Gozen" Icon.BusterUp
[self (CardUp Buster) 10, party NPUp 10]
, bond 579 "Dream from the Cradle" "Sessyoin Kiara" Icon.ArtsUp
[self (CardUp Arts) 20, self HPUp 3000, demeritOthers HPDown 1000]
, { name = "Marugoshi Shinji"
, id = 581
, rarity = 3
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 (Resist DefenseDown) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Ruined Church"
, id = 580
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 (Special DefenseUp <| VsTrait Male) <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, gift 583 "Fate/Apocrypha" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, bond 586 "King Shahryay's Bedchamber" "Scheherazade" Icon.HoodUp
[self DebuffResist 100]
, bond 587 "The Palace of Luoyang" "Wu Zetian" Icon.QuickUp
[party_ Quick 20, demeritAll DefenseDown 10]
, bond 588 "Military Sash of the War God" "Penthesilea" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, bond 589 "Nina" "Christopher Columbus" Icon.BusterUp
[party_ Buster 10, party NPGen 10]
, { name = "Chaldea Anniversary"
, id = 590
, rarity = 5
, icon = Icon.ArtsQuickUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 (CardUp Quick) <| Range 8 10
, To Self GaugeUp <| Range 50 60
]
, bond = Nothing
, limited = True
}
, { name = "Afternoon Party"
, id = 591
, rarity = 4
, icon = Icon.NobleTurn
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 GaugePerTurn <| Range 4 5
, Grant Self 0 (CardUp Arts) <| Range 10 12
]
, bond = Nothing
, limited = True
}
, { name = "Starlight Fest"
, id = 592
, rarity = 3
, icon = Icon.StarUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 GatherUp <| Range 100 200
, Grant Self 0 (CardUp Quick) <| Range 2 3
]
, bond = Nothing
, limited = True
}
, gift 593 "Learning With Manga! FGO" Icon.Road
[Bonus EXP Units <| Flat 50]
, gift 607 "Formal Portrait: Atalante" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, bond 641 "Frontier" "Paul Bunyan" Icon.BusterUp
[party_ Buster 15]
, bond 642 "The Vaunted One" "Sherlock Holmes" Icon.QuickUp
[party_ Quick 10, party CritUp 15]
, { name = "Summer Little"
, id = 643
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 NPGen <| Range 25 30
, Grant Self 0 NPUp <| Range 10 15
, Grant Self 0 CritUp <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "White Cruising"
, id = 644
, rarity = 4
, icon = Icon.BusterArtsUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 (CardUp Buster) <| Range 8 10
, Grant Self 0 NPGen <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "Sugar Vacation"
, id = 645
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 3 5
, Grant Self 0 (CardUp Quick) <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "Seaside Luxury"
, id = 646
, rarity = 5
, icon = Icon.StarHaloUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 StarUp <| Range 20 25
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, bond 647 "Domus Aurea de Curcubeu Mare" "Nero Claudius (Caster)" Icon.BusterUp
[party_ Buster 10, party NPGen 10]
, bond 648 "Handy-Bandages" "Frankenstein (Saber)" Icon.QuickUp
[self (CardUp Quick) 10, party NPGen 10]
, bond 649 "A Gift From Ra" "Nitocris (Assassin)" Icon.ArtsUp
[party_ Arts 10, party NPGen 10]
, bond 650 "Tenka Fubu -2017 Summer.ver-" "Oda Nobunaga (Berserker)" Icon.BusterUp
[party_ Buster 15, party CritUp 15, demeritAll DefenseDown 10]
, bond 651 "Champion's Cup of the Goddess" "Ishtar (Rider)" Icon.QuickUp
[party_ Quick 10, party NPUp 10]
, bond 656 "Mop of Selection" "Altria Pendragon (Rider Alter)" Icon.SwordUp
[party AttackUp 20, demeritAll DefenseDown 15]
, bond 657 "NYARF!" "Helena Blavatsky (Archer)" Icon.ArtsQuickUp
[party_ Quick 10, party_ Arts 10]
, bond 658 "Kyougoku" "Minamoto-no-Raikou (Lancer)" Icon.QuickBusterUp
[party_ Quick 10, party_ Buster 10]
, { name = "Battle Olympia"
, id = 660
, rarity = 5
, icon = Icon.BusterArtsUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 (CardUp Buster) <| Range 8 10
, To Self GaugeUp <| Range 50 60
]
, bond = Nothing
, limited = True
}
, { name = "Divine Three-Legged Race"
, id = 661
, rarity = 5
, icon = Icon.Kneel
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Times 1 << Grant Self 0 Guts <| Flat 1
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "At Wish's End"
, id = 666
, rarity = 5
, icon = Icon.StarUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 GatherUp <| Range 400 600
, Grant Self 0 (CardUp Buster) <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "Comrades"
, id = 667
, rarity = 4
, icon = Icon.BusterArtsUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 (CardUp Buster) <| Range 8 10
, Grant Self 0 NPUp <| Range 5 10
]
, bond = Nothing
, limited = True
}
, bond 669 "Urvara Nandi" "Parvati" Icon.QuickUp
[party_ Quick 10, party NPGen 10]
, gift 670 "Heroic Costume: Medusa" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, gift 671 "Heroic Costume: Leonardo da Vinci" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, gift 672 "Heroic Costume: Jeanne d'Arc" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, gift 673 "Heroic Costume: Nero Claudius" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, bond 675 "A Wish Spanning 3 Generations" "Tomoe Gozen" Icon.BusterUp
[party_ Buster 10, party NPUp 10]
, bond 676 "Getsurin Kuyou" "Mochizuki Chiyome" Icon.ArtsQuickUp
[party_ Quick 10, party_ Arts 10]
, bond 677 "Jumonji Yari" "Houzouin Inshun" Icon.QuickUp
[party_ Quick 10, party CritUp 15]
, bond 678 "Double-Tiered Kasa" "Yagyu Munenori" Icon.ArtsUp
[party_ Arts 10, party NPUp 10]
, bond 679 "Danzou's Ox" "Katou Danzo" Icon.QuickBusterUp
[party_ Quick 10, party_ Buster 10]
, bond 686 "Princess' Origami" "Osakabehime" Icon.NobleUp
[party NPGen 15]
, bond 687 "Electrologica Diagram" "Mecha Eli-chan" Icon.BusterUp
[party_ Buster 20, demeritAll DebuffVuln 20]
, bond 688 "Guardian Gigantic" "Mecha Eli-chan MkII" Icon.BusterUp
[party_ Buster 20, demeritAll DebuffVuln 20]
, gift 689 "Pray Upon the Sword, Wish Upon Life" Icon.Road
[Bonus EXP Units <| Flat 50]
, { name = "Soul Eater"
, id = 691
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ equipped Caster << Grant Self 0 NPUp <| Range 15 25 ]
, bond = Nothing
, limited = False
}
, { name = "Divine Construct"
, id = 674
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ equipped Saber << Grant Self 0 NPUp <| Range 15 25 ]
, bond = Nothing
, limited = False
}
, gift 690 "FGO VR Mash Kyrielight" Icon.Road
[Bonus EXP Units <| Flat 50 ]
, bond 692 "Falcon Witch's Banquet" "Circe" Icon.NobleUp
[party NPGen 10, party NPUp 10]
, bond 693 "Universe Ring" "Nezha" Icon.QuickUp
[party_ Quick 10, party NPUp 10]
, bond 694 "Tributes to King Solomon" "Queen of Sheba" Icon.BusterArtsUp
[party_ Arts 10, party_ Buster 10]
, bond 695 "Rosarium de Clavis Argenteus" "Abigail Williams" Icon.BeamUp
[Times 3 <| Grant Self 0 DeathResist Full]
, bond 700 "Blooming Flowers in Kur" "Ereshkigal" Icon.BusterUp
[party_ Buster 10, party NPUp 10]
, bond 701 "The Rainbow Soaring Under the Night Sky" "Attila the San(ta)" Icon.QuickUp
[party_ Quick 10, party CritUp 15]
, { name = "New Beginning"
, id = 704
, rarity = 5
, icon = Icon.ArtsUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 CritUp <| Range 10 15
, To Self GaugeUp <| Range 40 50
]
, bond = Nothing
, limited = True
}
, bond 709 "Painting of a Dragon Passing Over Mount Fuji" "Katsushika Hokusai" Icon.Kneel
[Times 1 << Grant Self 0 GutsPercent <| Flat 20]
, bond 717 "Mysterious Glass" "Semiramis" Icon.BusterUp
[party_ Buster 10, party NPUp 10]
, bond 762 "Summer Rain" "Asagami Fujino" Icon.BusterArtsUp
[party_ Arts 10, party_ Buster 10]
, { name = "Chaldea Special Investigation Unit"
, id = 768
, rarity = 4
, icon = Icon.Bullseye
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 SureHit Full
, Grant Self 0 (CardUp Arts) <| Range 5 8
, Grant Self 0 (CardUp Buster) <| Range 5 8
]
, bond = Nothing
, limited = True
}
, { name = "The Star of Camelot"
, id = 764
, rarity = 5
, icon = Icon.StarUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 GatherUp <| Range 400 600
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "The Dantes Files: Undercover in a Foreign Land"
, id = 765
, rarity = 5
, icon = Icon.BusterArtsUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 (CardUp Buster) <| Range 10 15
, Grant Self 0 NPGen <| Range 10 15
]
, bond = Nothing
, limited = True
}
, bond 787 "OTMA" "Anastasia Nikolaevna Romanova" Icon.ArtsUp
[party_ Arts 10, party NPUp 10]
, bond 788 "Calydon's Hide" "Atalante (Alter)" Icon.QuickUp
[party_ Quick 15, party CritUp 25, demeritAll DefenseDown 10]
, bond 789 "Truth and Death" "Avicebron" Icon.BusterArtsUp
[party_ Arts 10, party_ Buster 10]
, bond 790 "Wildfire Blade" "Antonio Salieri" Icon.ArtsUp
[party_ Arts 10, party NPGen 10]
, bond 791 "Library of Ivan the Terrible" "Ivan the Terrible" Icon.BusterUp
[party_ Buster 10, party NPUp 10]
, { name = "Distant Pilgrimage"
, id = 792
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 NPGen <| Range 10 15
, Grant Self 0 NPUp <| Range 10 15
, To Self GaugeUp <| Range 40 50
]
, bond = Nothing
, limited = True
}
, { name = "Moment of Bliss"
, id = 793
, rarity = 4
, icon = Icon.BusterArtsUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 8 10
, Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 DefenseUp <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "Away We Go!"
, id = 794
, rarity = 3
, icon = Icon.QuickUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 3 5
, Grant Self 0 NPGen <| Range 5 10
]
, bond = Nothing
, limited = True
}
, { name = "An Afternoon at the Fortress"
, id = 795
, rarity = 5
, icon = Icon.BusterArtsUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 10 15
, Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 NPUp <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "At Trifas"
, id = 796
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 NPGen <| Range 10 15
, Grant Self 0 CritUp <| Range 10 15
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, bond 798 "The Object That Can Hold a Universe" "Achilles" Icon.Shield
[self NPUp 30, Times 1 <| Grant Party 3 Invincibility Full]
, bond 799 "The Purpose of Learning and Teaching" "Chiron" Icon.ArtsQuickUp
[party_ Arts 10, party_ Quick 10]
, bond 800 "Nameless Death" "Sieg" Icon.NobleUp
[party NPGen 10, party NPUp 10]
]
equipped : Class -> SkillEffect -> SkillEffect
equipped =
When << (++) "equipped by a " << Class.show
{-| Retrieves the corresponding Bond CE. Memoized for performance. -}
getBond : Servant -> Maybe CraftEssence
getBond s =
Dict.get s.name bondMap
{-| Memoization table for `getBond`. -}
bondMap : Dict String CraftEssence
bondMap =
let
go ce =
Maybe.map (\bond -> (bond, ce)) ce.bond
in
db
|> List.map go
>> Maybe.values
>> Dict.fromList
| 27911 | module Database.CraftEssences exposing
( db
, getBond
, getAll
)
{-| All Craft Essences. -}
-- Unlike Servants, which are divided into multiple subfiles,
-- Craft Essences are all included in this one file
-- along with their data model definition.
import Dict exposing (Dict)
import Maybe.Extra as Maybe
import Class.Has exposing (Has)
import Model.Icon as Icon
import Model.Card exposing (Card(..))
import Model.Class as Class exposing (Class(..))
import Model.CraftEssence exposing (CraftEssence)
import Model.Trait exposing (Trait(..))
import Model.Servant exposing (Servant)
import Model.Skill.Amount exposing (Amount(..))
import Model.Skill.BonusEffect exposing (BonusEffect(..), BonusType(..))
import Model.Skill.BuffEffect exposing (BuffEffect(..))
import Model.Skill.DebuffEffect exposing (DebuffEffect(..))
import Model.Skill.InstantEffect exposing (InstantEffect(..))
import Model.Skill.SkillEffect exposing (SkillEffect(..))
import Model.Skill.Special exposing (Special(..))
import Model.Skill.Target exposing (Target(..))
import Database
getAll : Has CraftEssence a -> List a
getAll =
Database.genericGetAll db
{-| All Craft Essences available in EN. -}
-- Note: Names _must_ be true to their EN localization.
-- GrandOrder.Wiki is only trustworthy for CEs that have been in the game
-- for a while. Craft Essences introduced during events and the like should be
-- checked against the official announcement.
db : List CraftEssence
db =
let
gutsPercent =
Times 1 <<
Grant Self 0 GutsPercent <<
Flat
self buff =
Grant Self 0 buff <<
Flat
party buff =
Grant Party 0 buff <<
Flat
party_ card =
party (CardUp card)
demeritAll debuff =
Debuff Party 0 debuff <<
Flat
demeritOthers debuff =
Debuff Others 0 debuff <<
Flat
atkChance chance =
When "attacking" <<
Chance chance
bond id name servant icon effect =
{ name = name
, id = id
, rarity = 4
, icon = icon
, stats = { base = { atk = 100, hp = 100 }
, max = { atk = 100, hp = 100 }
}
, effect = effect
, bond = Just servant
, limited = False
}
gift id name icon effect =
{ name = name
, id = id
, rarity = 4
, icon = icon
, stats = { base = { atk = 100, hp = 100 }
, max = { atk = 100, hp = 100 }
}
, effect = effect
, bond = Nothing
, limited = True
}
in
[ { name = "<NAME>"
, id = 1
, rarity = 1
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 100 }
, max = { atk = 0, hp = 300 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 3 5 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 2
, rarity = 1
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 150 }
, max = { atk = 0, hp = 450 }
}
, effect = [ Grant Self 0 DebuffResist <| Range 5 10 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 3
, rarity = 1
, icon = Icon.ArtsUp
, stats = { base = { atk = 100, hp = 0 }
, max = { atk = 300, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 3 5 ]
, bond = Nothing
, limited = False
}
, { name = "Preemption"
, id = 4
, rarity = 1
, icon = Icon.QuickUp
, stats = { base = { atk = 100, hp = 0 }
, max = { atk = 300, hp = 0 }
}
, effect = [Grant Self 0 (CardUp Quick) <| Range 3 5]
, bond = Nothing
, limited = False
}
, { name = "Destruction"
, id = 5
, rarity = 1
, icon = Icon.BusterUp
, stats = { base = { atk = 100, hp = 0 }
, max = { atk = 300, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 3 5 ]
, bond = Nothing
, limited = False
}
, { name = "Flash"
, id = 6
, rarity = 2
, icon = Icon.ExclamationUp
, stats = { base = { atk = 150, hp = 0 }
, max = { atk = 500, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 5 10 ]
, bond = Nothing
, limited = False
}
, { name = "Opportunity"
, id = 7
, rarity = 2
, icon = Icon.StarHaloUp
, stats = { base = { atk = 75, hp = 112 }
, max = { atk = 250, hp = 375 }
}
, effect = [ Grant Self 0 StarUp <| Range 5 10 ]
, bond = Nothing
, limited = False
}
, { name = "Fruitful"
, id = 8
, rarity = 2
, icon = Icon.Noble
, stats = { base = { atk = 75, hp = 112 }
, max = { atk = 250, hp = 375 }
}
, effect = [ To Self GaugeUp <| Range 10 20 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 9
, rarity = 2
, icon = Icon.NobleUp
, stats = { base = { atk = 75, hp = 112 }
, max = { atk = 250, hp = 375 }
}
, effect = [ Grant Self 0 NPGen <| Range 5 10 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 10
, rarity = 2
, icon = Icon.BeamUp
, stats = { base = { atk = 150, hp = 0 }
, max = { atk = 500, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 5 10 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 11
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 200 }
, max = { atk = 0, hp = 1000 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "False Attendant's <NAME>"
, id = 12
, rarity = 3
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DebuffResist <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "The Azure Black Keys"
, id = 13
, rarity = 3
, icon = Icon.ArtsUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "The Verdant Black Keys"
, id = 14
, rarity = 3
, icon = Icon.QuickUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "The Crimson Black Keys"
, id = 15
, rarity = 3
, icon = Icon.BusterUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME> Pendant"
, id = 16
, rarity = 3
, icon = Icon.ExclamationUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "Spell Tome"
, id = 17
, rarity = 3
, icon = Icon.StarHaloUp
, stats = { base = { atk = 100, hp = 150 }
, max = { atk = 500, hp = 750 }
}
, effect = [ Grant Self 0 StarUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 18
, rarity = 3
, icon = Icon.Noble
, stats = { base = { atk = 100, hp = 150 }
, max = { atk = 500, hp = 750 }
}
, effect = [ To Self GaugeUp <| Range 30 50 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 19
, rarity = 3
, icon = Icon.NobleUp
, stats = { base = { atk = 100, hp = 150 }
, max = { atk = 500, hp = 750 }
}
, effect = [ Grant Self 0 NPGen <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 20
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "Iron-Willed <NAME>"
, id = 21
, rarity = 4
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 400 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 22
, rarity = 4
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 DebuffResist <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 23
, rarity = 4
, icon = Icon.ArtsUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 24
, rarity = 4
, icon = Icon.QuickUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 25
, rarity = 4
, icon = Icon.BusterUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "Gem Magecraft: Antumbra"
, id = 26
, rarity = 4
, icon = Icon.ExclamationUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Be Elegant"
, id = 27
, rarity = 4
, icon = Icon.StarHaloUp
, stats = { base = { atk = 200, hp = 300 }
, max = { atk = 750, hp = 1125 }
}
, effect = [ Grant Self 0 StarUp <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "The Imaginary Element"
, id = 28
, rarity = 4
, icon = Icon.Noble
, stats = { base = { atk = 200, hp = 300 }
, max = { atk = 750, hp = 1125 }
}
, effect = [ To Self GaugeUp <| Range 60 75 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 29
, rarity = 4
, icon = Icon.NobleUp
, stats = { base = { atk = 200, hp = 300 }
, max = { atk = 750, hp = 1125 }
}
, effect = [ Grant Self 0 NPGen <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 30
, rarity = 4
, icon = Icon.BeamUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Formal Craft"
, id = 31
, rarity = 5
, icon = Icon.ArtsUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Imaginary Around"
, id = 32
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Limited/Zero Over"
, id = 33
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Kaleidoscope"
, id = 34
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ To Self GaugeUp <| Range 80 100 ]
, bond = Nothing
, limited = False
}
, { name = "Heaven's Feel"
, id = 35
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 40 50 ]
, bond = Nothing
, limited = False
}
, { name = "Beginning of the Journey"
, id = 36
, rarity = 4
, icon = Icon.Road
, stats = { base = { atk = 50, hp = 50 }
, max = { atk = 50, hp = 50 }
}
, effect = [ Bonus FriendPoints Units <| Flat 75 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 37
, rarity = 3
, icon = Icon.Dodge
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Times 1 <| Grant Self 0 Evasion Full
, Grant Self 0 DebuffResist <| Range 5 10
]
, bond = Nothing
, limited = False
}
, { name = "Seal Designation Enforcer"
, id = 38
, rarity = 4
, icon = Icon.StarUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 GatherUp <| Range 600 800 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 39
, rarity = 4
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 400 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 (Special DefenseUp <| VsTrait Male) <| Range 25 35 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 40
, rarity = 5
, icon = Icon.NobleTurn
, stats = { base = { atk = 250, hp = 375 }
, max = { atk = 1000, hp = 1500 }
}
, effect = [ Grant Self 0 GaugePerTurn <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 41
, rarity = 5
, icon = Icon.Kneel
, stats = { base = { atk = 0, hp = 500 }
, max = { atk = 0, hp = 2000 }
}
, effect = [ Times 1 << Grant Self 0 Guts <| Range 500 1000 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 42
, rarity = 3
, icon = Icon.AllUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 3 5
, Grant Self 0 (CardUp Buster) <| Range 3 5
, Grant Self 0 (CardUp Quick) <| Range 3 5
]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 43
, rarity = 4
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 (Resist Charm) <| Range 80 100 ]
, bond = Nothing
, limited = True
}
, { name = "Moon Goddess' Bath"
, id = 44
, rarity = 5
, icon = Icon.HealTurn
, stats = { base = { atk = 0, hp = 500 }
, max = { atk = 0, hp = 2000 }
}
, effect = [ Grant Self 0 HealPerTurn <| Range 500 750 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>light Fest"
, id = 45
, rarity = 5
, icon = Icon.StarHaloUp
, stats = { base = { atk = 250, hp = 375 }
, max = { atk = 1000, hp = 1500 }
}
, effect = [ Grant Self 0 StarUp <| Range 15 20
, Grant Self 0 CritUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>estone"
, id = 46
, rarity = 3
, icon = Icon.HoodUp
, stats = { base = { atk = 100, hp = 150 }
, max = { atk = 500, hp = 750 }
}
, effect = [ Grant Self 0 DebuffResist <| Range 5 10
, Grant Self 0 GatherUp <| Range 100 200
]
, bond = Nothing
, limited = False
}
, { name = "With One Strike"
, id = 47
, rarity = 4
, icon = Icon.Bullseye
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 SureHit Full
, Grant Self 0 (CardUp Quick) <| Range 8 10
]
, bond = Nothing
, limited = False
}
, { name = "The <NAME>"
, id = 48
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 600, hp = 0 }
, max = { atk = 2400, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 60 80
, Debuff Self 0 HealthLoss <| Flat 500
]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 49
, rarity = 3
, icon = Icon.DamageUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 DamageUp <| Range 100 200 ]
, bond = Nothing
, limited = True
}
, { name = "Trick or Treat"
, id = 50
, rarity = 3
, icon = Icon.StaffUp
, stats = { base = { atk = 100, hp = 150 }
, max = { atk = 500, hp = 750 }
}
, effect = [ Grant Self 0 DebuffUp <| Range 10 12 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 51
, rarity = 4
, icon = Icon.CrosshairUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 1 Taunt Full
, Grant Self 1 DefenseUp <| Range 60 80
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 52
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 250, hp = 375 }
, max = { atk = 1000, hp = 1500 }
}
, effect = [ Grant Self 0 NPUp <| Range 15 20
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 53
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 NPGen <| Range 20 25
, To Self GaugeUp <| Range 50 60
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 54
, rarity = 5
, icon = Icon.HealUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 0 HealingReceived <| Range 60 75 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 55
, rarity = 3
, icon = Icon.HealTurn
, stats = { base = { atk = 300, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 HealPerTurn <| Range 100 200 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 56
, rarity = 4
, icon = Icon.SwordShieldUp
, stats = { base = { atk = 200, hp = 300 }
, max = { atk = 750, hp = 1125 }
}
, effect = [ Grant Self 3 AttackUp <| Range 25 30
, Grant Self 3 DefenseUp <| Range 25 30
]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 57
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 600, hp = 0 }
, max = { atk = 2400, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 10 15
, Grant Self 0 CritUp <| Range 20 25
]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 58
, rarity = 5
, icon = Icon.ArtsUp
, stats = { base = { atk = 600, hp = 0 }
, max = { atk = 2400, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 CritUp <| Range 20 25
]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 59
, rarity = 3
, icon = Icon.AllUp
, stats = { base = { atk = 100, hp = 150 }
, max = { atk = 500, hp = 750 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 1 2
, Grant Self 0 (CardUp Arts) <| Range 1 2
, Grant Self 0 (CardUp Buster) <| Range 1 2
, Grant Self 0 StarUp <| Range 1 2
, Grant Self 0 GatherUp <| Range 1 2
, Grant Self 0 NPGen <| Range 1 2
, Grant Self 0 NPUp <| Range 1 2
, Grant Self 0 DebuffUp <| Range 1 2
, Grant Self 0 DebuffResist <| Range 1 2
]
, bond = Nothing
, limited = True
}
, { name = "After-Party Order!"
, id = 60
, rarity = 4
, icon = Icon.QuickBusterUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 10 15
, Grant Self 0 (CardUp Buster) <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>-o"
, id = 61
, rarity = 5
, icon = Icon.DamageUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 15 20
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 62
, rarity = 5
, icon = Icon.CrosshairUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 3 Taunt Full
, Grant Self 3 AttackUp <| Range 60 80
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 65
, rarity = 3
, icon = Icon.QuickUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 66
, rarity = 4
, icon = Icon.ExclamationUp
, stats = { base = { atk = 200, hp = 300 }
, max = { atk = 750, hp = 1125 }
}
, effect = [ Grant Self 0 CritUp <| Range 40 50
, Debuff Self 0 DefenseDown <| Flat 20
]
, bond = Nothing
, limited = False
}
, { name = "<NAME> 20<NAME>0"
, id = 67
, rarity = 5
, icon = Icon.StarTurn
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 68
, rarity = 3
, icon = Icon.BusterUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 3 (CardUp Buster) <| Range 15 20 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 69
, rarity = 4
, icon = Icon.HealTurn
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 HealPerTurn <| Range 200 300
, Grant Self 0 GaugePerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 70
, rarity = 5
, icon = Icon.StarUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 0 GatherUp <| Range 100 200
, Grant Self 0 HealingReceived <| Range 40 50
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 71
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 250, hp = 375 }
, max = { atk = 1000, hp = 1500 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 8 10
, Grant Self 0 CritUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 72
, rarity = 3
, icon = Icon.NobleTurn
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 GaugePerTurn <| Range 2 3 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 73
, rarity = 4
, icon = Icon.Kneel
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2400 }
}
, effect = [ Chance 50 << Times 1 << Grant Self 0 Guts <| Range 500 1000 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 74
, rarity = 4
, icon = Icon.NobleTurn
, stats = { base = { atk = 200, hp = 300 }
, max = { atk = 750, hp = 1125 }
}
, effect = [ Chance 60 << Grant Self 0 GaugePerTurn <| Range 12 15
, Debuff Self 0 HealthLoss <| Flat 500
]
, bond = Nothing
, limited = False
}
, { name = "500-Year Obsession"
, id = 75
, rarity = 5
, icon = Icon.Circuits
, stats = { base = { atk = 600, hp = 0 }
, max = { atk = 2400, hp = 0 }
}
, effect = [ When "defeated by an enemy" <| Debuff Target 2 SealNP Full
, When "defeated by an enemy" <<
Debuff Target 10 Curse <| Range 1000 2000
]
, bond = Nothing
, limited = False
}
, { name = "Peacefulness of 2018"
, id = 76
, rarity = 3
, icon = Icon.HealTurn
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 HealPerTurn <| Range 200 300
, Debuff Self 0 AttackDown <| Flat 10
]
, bond = Nothing
, limited = True
}
, { name = "Heroic New Year"
, id = 77
, rarity = 4
, icon = Icon.HoodUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Times 1 <| Grant Self 0 DebuffResist Full
, Grant Self 0 DefenseUp <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "Law of the Jungle"
, id = 78
, rarity = 3
, icon = Icon.Crystal
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Bonus QPQuest Units <| Range 2017 2018 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 79
, rarity = 5
, icon = Icon.Shield
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 1 Taunt Full
, Grant Self 1 Invincibility Full
, Grant Self 0 DebuffResist <| Range 10 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 80
, rarity = 5
, icon = Icon.Crystal
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Bonus QPDrop Percent <| Range 2 10 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 81
, rarity = 4
, icon = Icon.StarTurn
, stats = { base = { atk = 0, hp = 2018 }
, max = { atk = 0, hp = 2018 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 0 1 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 82
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ ToMax (Range 40 50) << Grant Self 0 NPUp <| Flat 5 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 83
, rarity = 5
, icon = Icon.Kneel
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Times 1 << Grant Self 0 Guts <| Flat 1
, Grant Self 0 DebuffResist <| Range 5 10
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 84
, rarity = 3
, icon = Icon.Bullseye
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 SureHit Full
, Grant Self 0 CritUp <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>! <NAME>"
, id = 85
, rarity = 4
, icon = Icon.HealTurn
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Chance 65 << Grant Self 0 HealPerTurn <| Range 750 1000 ]
, bond = Nothing
, limited = True
}
, { name = "The Crimson Land of Shadows"
, id = 86
, rarity = 5
, icon = Icon.DamageUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ ToMax (Range 1000 1200) << Grant Self 0 DamageUp <| Flat 100 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 89
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 NPUp <| Range 10 15
, To Self GaugeUp <| Range 20 30
]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 90
, rarity = 3
, icon = Icon.DamageUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (Special AttackUp <| VsClass Caster) <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 91
, rarity = 3
, icon = Icon.Heart
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 (Success Charm) <| Range 12 15 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 92
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ equipped Berserker << Grant Self 0 NPUp <| Range 15 25 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 93
, rarity = 3
, icon = Icon.ArtsUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 3 (CardUp Arts) <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 94
, rarity = 3
, icon = Icon.DamageUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (Special AttackUp <| VsClass Rider) <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 95
, rarity = 3
, icon = Icon.Heal
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ When "defeated" << To Party Heal <| Range 800 1000 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 96
, rarity = 3
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 (Resist Stun) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 97
, rarity = 5
, icon = Icon.HoodUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Times 3 <| Grant Self 0 DebuffResist Full
, Grant Self 0 NPGen <| Range 15 20
]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 98
, rarity = 4
, icon = Icon.HPUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ ToMax (Flat 3000) << Grant Self 0 HPUp <| Range 200 300 ]
, bond = Nothing
, limited = False
}
, { name = "Heroic Portrait: <NAME>"
, id = 99
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: <NAME>"
, id = 100
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: <NAME>"
, id = 101
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: <NAME>"
, id = 102
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: <NAME>"
, id = 103
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: <NAME>"
, id = 104
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: <NAME>"
, id = 105
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: <NAME> & <NAME>"
, id = 106
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: <NAME>"
, id = 107
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: <NAME>"
, id = 108
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>entine Dojo of T<NAME>"
, id = 109
, rarity = 3
, icon = Icon.Bullseye
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 SureHit Full
, Grant Self 0 GaugePerTurn <| Range 3 5
, Debuff Self 0 CharmVuln <| Flat 10
]
, bond = Nothing
, limited = True
}
, { name = "<NAME> ☆ <NAME>"
, id = 110
, rarity = 4
, icon = Icon.StarHaloUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 StarUp <| Range 15 20
, Grant Self 0 NPGen <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 111
, rarity = 5
, icon = Icon.ArtsQuickUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 (CardUp Quick) <| Range 10 15
, Grant Self 0 HealingReceived <| Range 20 30
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 112
, rarity = 5
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Times 3 << Grant Self 0 (Special DefenseUp <| VsTrait Male) <| Flat 100
, Grant Self 0 StarUp <| Range 10 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 154
, rarity = 5
, icon = Icon.ShieldBreak
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 IgnoreInvinc Full
, Grant Self 0 (CardUp Quick) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 155
, rarity = 5
, icon = Icon.FireUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 0 DeathResist <| Range 60 80 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 156
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ To Self GaugeUp <| Range 50 60
, Grant Self 3 (CardUp Arts) <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 157
, rarity = 5
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Times 3 << Grant Self 0 DamageCut <| Range 1000 1200 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 158
, rarity = 4
, icon = Icon.DamageUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 DamageUp <| Range 800 1000 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>yes of Distortion"
, id = 159
, rarity = 4
, icon = Icon.BusterUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 20 25
, Debuff Self 0 DefenseDown <| Flat 15
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 160
, rarity = 4
, icon = Icon.Dodge
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Times 1 <| Grant Self 0 Evasion Full
, Grant Self 0 StarUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 161
, rarity = 4
, icon = Icon.StarUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 GatherUp <| Range 300 400
, Grant Self 3 DebuffResist <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 162
, rarity = 3
, icon = Icon.QuickUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 5 8
, Grant Self 0 DebuffResist <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "Repeat Magic"
, id = 163
, rarity = 3
, icon = Icon.Noble
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ To Self GaugeUp <| Range 20 30
, Grant Self 0 NPGen <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>iss Your Hand"
, id = 165
, rarity = 5
, icon = Icon.AllUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 12
, Grant Self 0 (CardUp Buster) <| Range 10 12
, Grant Self 0 (CardUp Quick) <| Range 10 12
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 166
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ To Self GaugeUp <| Range 5 60
, Grant Self 0 GatherUp <| Range 300 400
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 167
, rarity = 5
, icon = Icon.DamageUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 3 (Special AttackUp <| VsTrait Divine) <| Range 80 100
, Grant Self 3 (Special DefenseUp <| VsTrait Divine) <| Range 40 50
]
, bond = Nothing
, limited = True
}
, { name = "Beasts Under the Moon"
, id = 168
, rarity = 4
, icon = Icon.NobleUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 NPGen <| Range 12 15
, Grant Self 0 StarUp <| Range 12 15
, Grant Self 0 HealPerTurn <| Range 200 300
]
, bond = Nothing
, limited = True
}
, { name = "Glass Full Sweet Time"
, id = 169
, rarity = 4
, icon = Icon.Bullseye
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 SureHit Full
, Grant Self 0 DamageUp <| Range 400 600
, Grant Self 0 DamageCut <| Range 200 300
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 170
, rarity = 3
, icon = Icon.Dodge
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Times 1 <| Grant Self 0 Evasion Full
, Grant Self 0 HealingReceived <| Range 5 10
, Grant Self 0 DebuffUp <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 171
, rarity = 3
, icon = Icon.StarTurn
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 1 2
, Grant Self 0 (Special AttackUp <| VsTrait Dragon) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 172
, rarity = 4
, icon = Icon.ExclamationUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 15 20
, Grant Self 0 NPUp <| Range 15 20
, Grant Self 0 (Success Charm) <| Range 12 15
]
, bond = Nothing
, limited = True
}
, gift 174 "[Heaven's Feel]" Icon.QuickUp
[self (CardUp Quick) 10, To Self GaugeUp <| Flat 40]
, { name = "<NAME>"
, id = 175
, rarity = 5
, icon = Icon.HPUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Party 0 HPUp <| Range 1000 1200 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 176
, rarity = 4
, icon = Icon.StaffUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 DebuffUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 177
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ equipped Lancer << Grant Self 0 NPUp <| Range 15 25 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 178
, rarity = 5
, icon = Icon.Road
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Bonus EXP Percent <| Range 2 10 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 179
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ To Self GaugeUp <| Range 30 50
, Grant Self 0 HealingReceived <| Range 20 30
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 180
, rarity = 5
, icon = Icon.StarTurn
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 3 4
, Grant Self 0 (CardUp Buster) <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 181
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ When "defeated" << To Party GaugeUp <| Range 15 20
, Grant Self 0 (CardUp Buster) <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "Art of the Poisonous Snake"
, id = 182
, rarity = 4
, icon = Icon.ArtsUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 3 (CardUp Arts) <| Range 30 40 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME> of Death"
, id = 183
, rarity = 4
, icon = Icon.DamageUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (Special AttackUp <| VsTrait Humanoid) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 184
, rarity = 4
, icon = Icon.HealUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 HealUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 185
, rarity = 5
, icon = Icon.Shield
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Times 3 <| Grant Self 0 Invincibility Full
, Grant Self 0 DamageUp <| Range 200 500
]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 186
, rarity = 4
, icon = Icon.NobleTurn
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 GaugePerTurn <| Range 4 5
, Grant Self 0 (CardUp Quick) <| Range 10 12
]
, bond = Nothing
, limited = False
}
, { name = "Self Geas Scroll"
, id = 187
, rarity = 3
, icon = Icon.StaffUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 (Success Stun) <| Range 12 15 ]
, bond = Nothing
, limited = False
}
, { name = "Before Awakening"
, id = 188
, rarity = 5
, icon = Icon.AllUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 (CardUp Buster) <| Range 8 10
, Grant Self 0 (CardUp Quick) <| Range 8 10
, Grant Self 0 DefenseUp <| Range 8 10
]
, bond = Nothing
, limited = False
}
, { name = "His Rightful Place"
, id = 189
, rarity = 5
, icon = Icon.StarTurn
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 3 4
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, bond 191 "Crown of the Star" "<NAME>" Icon.DamageUp
[party AttackUp 15]
, bond 192 "Relic of the King" "<NAME> (Lord El-Melloi II)" Icon.BusterUp
[party_ Buster 15]
, bond 193 "Triumph of the Lord Impaler" "<NAME>lad III" Icon.BeamUp
[self NPUp 30, atkChance 30 << To Self GaugeUp <| Flat 5]
, bond 194 "Revelation from Heaven" "<NAME>" Icon.BusterUp
[party_ Buster 15]
, bond 195 "Memories of the Dragon" "<NAME> (Alter)" Icon.BeamUp
[self NPUp 30, atkChance 30 << Debuff Target 3 DefenseDown <| Flat 5]
, bond 196 "Hunter of the Red Plains" "EMIYA" Icon.BeamUp
[self NPUp 30, atkChance 30 << To Party GainStars <| Flat 5]
, bond 197 "Castle of Snow" "Heracles" Icon.Kneel
[Times 3 <| self Guts 500]
, bond 198 "Yggdrasil Tree" "Cu Chulainn (Caster)" Icon.BeamUp
[self NPUp 30, atkChance 30 << To Self Heal <| Flat 500]
, bond 199 "Scorching Embrace" "Kiyohime" Icon.BeamUp
[self NPUp 30, atkChance 30 << Debuff Target 5 Burn <| Flat 500]
, bond 200 "Worthless Jewel" "<NAME>" Icon.NobleUp
[party NPGen 15]
, bond 201 "Eternal Solitude" "<NAME>" Icon.SwordUp
[party AttackUp 15]
, bond 202 "Queen's Present" "<NAME>" Icon.ArtsUp
[party_ Arts 15]
, bond 203 "Elixir" "<NAME>" Icon.HealTurn
[party HealPerTurn 500]
, bond 204 "My Necklace" "<NAME>" Icon.StarHaloUp
[party StarUp 20]
, bond 205 "Staff He Gave Me" "<NAME>" Icon.HealUp
[party HealingReceived 30]
, bond 206 "<NAME>" "<NAME>" Icon.BeamUp
[self NPUp 30, atkChance 10 <| Debuff Target 1 SealNP Full]
, bond 207 "<NAME>" "<NAME>" Icon.Heal
[party HPUp 2000]
, bond 208 "<NAME>irst for Victory" "<NAME>" Icon.StarHaloUp
[party StarUp 20]
, bond 209 "To My Dear Friend" "<NAME>" Icon.HoodUp
[party DebuffResist 30]
, bond 210 "<NAME>" "<NAME>" Icon.Heal
[ When "defeated" <| To Party RemoveDebuffs Full
, When "defeated" <| To Party Heal <| Flat 5000
]
, { name = "<NAME> <NAME>"
, id = 211
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 10 15
, When "defeated" <<
Grant Party 1 (CardUp Quick) <| Range 20 30
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>en Captures the Carp"
, id = 212
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ To Self GaugeUp <| Range 30 50
, To Party GainStars <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "A Fox Night's Dream"
, id = 213
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 NPGen <| Range 20 25
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "Burning Tale of Love"
, id = 214
, rarity = 4
, icon = Icon.DamageUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (Special AttackUp <| VsTrait Male) <| Range 25 30
, Grant Self 0 DebuffUp <| Range 12 15
]
, bond = Nothing
, limited = True
}
, { name = "Reciting the Subscription List"
, id = 215
, rarity = 3
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Times 1 <| Grant Self 0 DebuffResist Full ]
, bond = Nothing
, limited = True
}
, bond 216 "Key of the King's Law" "<NAME>" Icon.BeamUp
[self NPUp 30, atkChance 30 << Grant Self 3 CritUp <| Flat 10]
, bond 217 "Golden Glass" "<NAME>" Icon.BeamUp
[self NPUp 30, atkChance 30 << To Self GaugeUp <| Flat 5]
, bond 218 "Thunderous Applause" "<NAME>" Icon.ArtsUp
[party_ Arts 15]
, bond 219 "<NAME>" "<NAME>" Icon.NobleUp
[party NPGen 15]
, bond 220 "Radiance of the Goddess" "<NAME>" Icon.QuickUp
[party_ Quick 15]
, bond 221 "Voyage of the Flowers" "<NAME> (<NAME>)" Icon.SwordUp
[party AttackUp 10, party StarUp 10]
, bond 222 "Ark of the Covenant" "<NAME>" Icon.BeamUp
[self NPUp 30, When "attacking" << To Enemy Death <| Flat 10]
, bond 223 "Door to Babylon" "<NAME>" Icon.BusterUp
[party_ Buster 15]
, bond 224 "Blood-Thirsting Axe" "<NAME>" Icon.ExclamationUp
[party CritUp 25]
, bond 225 "Insurrection" "<NAME>" Icon.Kneel
[gutsPercent 50]
, { name = "<NAME>!!"
, id = 226
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 NPUp <| Range 20 25
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "The Classic Three Great Heroes"
, id = 227
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 NPUp <| Range 15 20
, Grant Self 0 StarUp <| Range 15 20
, To Self GaugeUp <| Range 25 40
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 228
, rarity = 4
, icon = Icon.BeamUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 15 20
, Grant Self 0 (CardUp Buster) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "All Three Together"
, id = 229
, rarity = 3
, icon = Icon.StarUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 GatherUp <| Range 100 200
, Grant Self 0 CritUp <| Range 5 10
]
, bond = Nothing
, limited = True
}
, bond 230 "<NAME>" "<NAME>" Icon.ExclamationUp
[party CritUp 25]
, bond 231 "<NAME>" "<NAME>" Icon.BeamUp
[party NPUp 20]
, bond 232 "Black Knight's Helmet" "<NAME>" Icon.BeamUp
[self NPUp 30, atkChance 30 << Debuff Target 3 CritChance <| Flat 30]
, bond 233 "<NAME>" "<NAME>" Icon.QuickUp
[party_ Quick 15]
, bond 234 "Holy Pumpkin Grail" "<NAME> (Halloween)" Icon.HoodUp
[party DebuffResist 30]
, bond 235 "<NAME>" "<NAME>" Icon.ExclamationUp
[party CritUp 25]
, bond 236 "<NAME> II" "<NAME> (Santa Alter)" Icon.StarHaloUp
[party StarUp 20]
, bond 237 "Things to Calm the Heart" "Henry Jekyll & Hyde" Icon.BusterUp
[party_ Buster 15]
, bond 238 "Glory of the Past Days" "Edward Teach" Icon.BusterUp
[party_ Buster 15]
, bond 239 "Heaven Among the Mountains" "<NAME>" Icon.QuickUp
[party_ Quick 15]
, { name = "<NAME>"
, id = 240
, rarity = 5
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ When "defeated" << Grant Party 3 DefenseUp <| Range 20 25 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 241
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Party 3 (CardUp Buster) <| Range 10 15 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 242
, rarity = 5
, icon = Icon.Road
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Bonus MysticCode Percent <| Flat 2 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 243
, rarity = 3
, icon = Icon.SwordUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 3 AttackUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 244
, rarity = 3
, icon = Icon.QuickUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 10 15
, Debuff Self 0 DefenseDown <| Flat 10
]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 245
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ equipped Assassin << Grant Self 0 NPUp <| Range 15 25 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 246
, rarity = 3
, icon = Icon.HealUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 HealingReceived <| Range 10 20 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 247
, rarity = 3
, icon = Icon.NobleUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 NPGen <| Range 5 10
, To Self GaugeUp <| Range 25 40
]
, bond = Nothing
, limited = False
}
, bond 248 "Tamamo's Club" "Tamamo-no-Mae" Icon.ArtsUp
[party_ Arts 15]
, bond 249 "Headband of Resolve" "<NAME>" Icon.ExclamationUp
[party CritUp 25]
, bond 250 "<NAME>" "<NAME> & <NAME>" Icon.ExclamationUp
[party CritUp 25]
, bond 251 "Gazing Upon <NAME>" "<NAME>" Icon.QuickUp
[party_ Quick 15]
, bond 252 "Star of Prophecy" "<NAME>" Icon.BeamUp
[self NPUp 30, atkChance 30 << Grant Self 3 CritUp <| Flat 10]
, bond 253 "<NAME>kate's Staff" "<NAME>" Icon.ArtsUp
[party_ Arts 15]
, bond 254 "Formless Island" "<NAME>" Icon.NobleUp
[party NPGen 15]
, bond 255 "Cask of the Wise" "<NAME>" Icon.QuickUp
[party_ Quick 15]
, bond 256 "<NAME>'s Arm" "Hassan of the Cursed Arm" Icon.ReaperUp
[party DeathUp 20]
, bond 257 "<NAME>" "<NAME>" Icon.QuickUp
[party_ Quick 15]
, { name = "<NAME>"
, id = 258
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 15 20
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 259
, rarity = 4
, icon = Icon.ArtsUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 NPGen <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "Hidden Sword: Pheasant Reversal"
, id = 260
, rarity = 3
, icon = Icon.QuickUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 3 5
, Grant Self 0 CritUp <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "Golden Sumo: Boulder Tournament"
, id = 261
, rarity = 5
, icon = Icon.SwordUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 AttackUp <| Range 10 15
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, { name = "Hot Spring Under the Moon"
, id = 262
, rarity = 5
, icon = Icon.ExclamationUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 20 25
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "Origin Bullet"
, id = 263
, rarity = 5
, icon = Icon.ShieldBreak
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 IgnoreInvinc Full
, Grant Self 0 (Special AttackUp <| VsClass Caster) <| Range 35 40
]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 264
, rarity = 4
, icon = Icon.DamageUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 DamageUp <| Range 400 600
, Grant Self 0 CritUp <| Range 15 20
]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 265
, rarity = 3
, icon = Icon.Noble
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ When "defeated" << To Party GaugeUp <| Range 10 15 ]
, bond = Nothing
, limited = False
}
, bond 266 "Who Am I?" "<NAME>" Icon.BeamUp
[party NPUp 20]
, bond 267 "The Misty Night of London" "<NAME>" Icon.ExclamationUp
[party CritUp 25]
, bond 268 "Wonderland" "<NAME>" Icon.ExclamationUp
[party CritUp 15, party HealingReceived 10]
, bond 269 "<NAME> King" "<NAME>" Icon.ArtsUp
[party_ Arts 15]
, bond 270 "<NAME>ori" "<NAME>" Icon.QuickUp
[party_ Quick 15]
, bond 271 "Etiquette of Nine Guests" "<NAME>" Icon.BeamUp
[self NPUp 30, atkChance 30 << Grant Self 3 DeathUp <| Flat 30]
, bond 272 "<NAME>" "<NAME>" Icon.BusterUp
[party_ Buster 15]
, bond 273 "What can be Left Behind" "<NAME>" Icon.Shield
[ When "defeated" << Times 1 <| Grant Party 0 Invincibility Full
, When "defeated" << Grant Party 3 DamageCut <| Flat 1000
]
, bond 274 "<NAME>" "<NAME>" Icon.BusterUp
[party_ Buster 15]
, bond 275 "<NAME>" "<NAME>" Icon.BeamUp
[party NPUp 20]
, gift 276 "<NAME>" Icon.SwordUp
[self AttackUp 10, self StarsPerTurn 3]
, { name = "<NAME>"
, id = 277
, rarity = 5
, icon = Icon.StarUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 GatherUp <| Range 400 600
, Grant Self 0 (CardUp Arts) <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 278
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 10 15
, To Self GaugeUp <| Range 50 60
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 279
, rarity = 4
, icon = Icon.Bullseye
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 SureHit Full
, Grant Self 0 (CardUp Buster) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 280
, rarity = 3
, icon = Icon.StarHaloUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 StarUp <| Range 5 10
, Grant Self 0 CritUp <| Range 5 10
]
, bond = Nothing
, limited = True
}
, bond 281 "Arm of Raiden" "<NAME>" Icon.BeamUp
[party NPUp 20]
, bond 282 "Endowed Hero" "<NAME>" Icon.BeamUp
[self NPUp 30, self GatherUp 1000]
, bond 283 "Light of the Deprived" "<NAME>" Icon.AllUp
[party_ Quick 8, party_ Arts 8, party_ Buster 8]
, bond 284 "Procedure to Humanity" "<NAME>" Icon.QuickUp
[party_ Quick 15]
, bond 285 "Black Helmet" "<NAME> (Lancer Alter)" Icon.NobleUp
[party NPGen 15]
, bond 286 "Legend of the Gallic War" "<NAME>" Icon.QuickUp
[party_ Quick 15]
, bond 287 "<NAME>" "<NAME>" Icon.BeamUp
[party NPUp 20]
, bond 288 "Encounter at Gojou Bridge" "<NAME>" Icon.NobleRedUp
[party NPFromDamage 20]
, bond 289 "Impure Death Mask" "Phantom of the Opera" Icon.QuickUp
[party_ Quick 15]
, bond 290 "Really Convenient" "<NAME>" Icon.NobleUp
[party NPGen 15]
, { name = "<NAME>!"
, id = 291
, rarity = 5
, icon = Icon.ShieldBreak
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 IgnoreInvinc Full
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 292
, rarity = 5
, icon = Icon.ExclamationUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 15 20
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 293
, rarity = 4
, icon = Icon.QuickUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Times 1 <| Grant Self 0 Evasion Full
, Grant Self 0 (CardUp Quick) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 294
, rarity = 3
, icon = Icon.ArtsUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 3 5
, Grant Self 0 (CardUp Arts) <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 295
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 10 15
, To Self GaugeUp <| Range 50 60
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 296
, rarity = 5
, icon = Icon.Kneel
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Times 1 << Grant Self 0 Guts <| Flat 1
, Grant Self 0 NPGen <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME> W<NAME>"
, id = 297
, rarity = 4
, icon = Icon.HealTurn
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 HealPerTurn <| Range 200 300
, Grant Self 0 (CardUp Arts) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "<NAME> (Void's Dust Flavor)"
, id = 298
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DamageCut <| Range 100 200
, Grant Self 0 DebuffResist <| Range 5 10
]
, bond = Nothing
, limited = True
}
, bond 299 "Annihilation List" "Mysterious Heroine X" Icon.DamageUp
[party (Special AttackUp <| VsClass Saber) 20]
, bond 300 "Imperishable Flames" "Brynhild" Icon.BusterUp
[party_ Buster 10, party NPGen 10]
, bond 301 "Ring of Bay Laurel" "<NAME> (Bride)" Icon.ArtsUp
[party_ Arts 15]
, bond 302 "Final Battle" "Beowulf" Icon.DamageUp
[party (Special AttackUp <| VsTrait Dragon) 20]
, bond 303 "Bratan of Wisdom" "<NAME>ionn mac Cumhaill" Icon.ArtsUp
[party_ Arts 10, party NPUp 10]
, bond 304 "Prelati's Spellbook" "<NAME>" Icon.BusterUp
[party_ Buster 20, demeritAll DebuffVuln 20]
, bond 305 "Parasitic Bomb" "<NAME>phistop<NAME>" Icon.BeamUp
[party NPUp 20]
, bond 306 "Seethe of a Warrior" "<NAME>" Icon.BusterUp
[party_ Buster 10, party NPUp 10]
, bond 307 "My Loathsome Life" "<NAME>" Icon.ReaperUp
[party DeathUp 10, party NPGen 10]
, bond 308 "There is No Love Here" "<NAME>" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, { name = "<NAME>"
, id = 309
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 NPGen <| Range 25 30
, To Self GaugeUp <| Range 40 50
]
, bond = Nothing
, limited = True
}
, { name = "<NAME> on Sight"
, id = 310
, rarity = 4
, icon = Icon.ArtsUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>!"
, id = 311
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DamageCut <| Range 100 200
, Grant Self 0 HealingReceived <| Range 5 10
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 312
, rarity = 4
, icon = Icon.BusterUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 10 15
, Grant Self 0 NPUp <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 313
, rarity = 4
, icon = Icon.ArtsUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 NPUp <| Range 8 10
]
, bond = Nothing
, limited = True
}
, bond 315 "Mugashiki—Shinkuu Myou" "<NAME> (Saber)" Icon.ArtsUp
[party_ Arts 15]
, bond 316 "Frontliner's Flag" "<NAME>" Icon.DamageUp
[ party (Special AttackUp <| VsTrait Undead) 20
, party (Special AttackUp <| VsTrait Demonic) 20
]
, bond 317 "Chateau d'If" "<NAME>" Icon.QuickUp
[party_ Quick 15]
, bond 318 "Unlimited Pancakes" "Medea (Lily)" Icon.HealUp
[party HealingReceived 30]
, bond 319 "Red Leather Jacket" "<NAME> (Assassin)" Icon.ReaperUp
[party DeathUp 30]
, bond 320 "Otherworldly Mystical Horse" "<NAME>" Icon.Dodge
[self NPUp 30, Times 1 <| Grant Party 0 Evasion Full]
, bond 321 "Letter From a Friend" "<NAME> (Caster)" Icon.BusterUp
[party_ Buster 20, demeritAll StarDown 20]
, bond 322 "Hound of Culann" "Cu <NAME>ul<NAME> (Prototype)" Icon.DamageUp
[party (Special AttackUp <| VsTrait WildBeast) 20]
, bond 323 "Radiance of the Goddess (Euryale)" "Euryale" Icon.ArtsUp
[party_ Arts 15]
, bond 324 "Hero's Armament" "Hektor" Icon.BeamUp
[party NPUp 20]
, { name = "<NAME>"
, id = 325
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 15 20
, Grant Self 0 CritUp <| Range 15 20
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 326
, rarity = 4
, icon = Icon.ShieldUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 8 20
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 327
, rarity = 3
, icon = Icon.BusterUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 10 15
, Debuff Self 0 DebuffVuln <| Flat 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 328
, rarity = 5
, icon = Icon.Kneel
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Times 1 << Grant Self 0 Guts <| Flat 1
, To Self GaugeUp <| Range 10 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 329
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 15 20
, Grant Self 0 CritUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 330
, rarity = 5
, icon = Icon.Rainbow
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Bonus Bond Percent <| Range 2 10 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 331
, rarity = 3
, icon = Icon.StarUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 GatherUp <| Range 200 300 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>verted Mo<NAME> the <NAME>"
, id = 332
, rarity = 3
, icon = Icon.StarTurn
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 1 2
, Grant Self 0 DebuffResist <| Range 5 10
]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 333
, rarity = 3
, icon = Icon.ReaperUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 DeathUp <| Range 5 10 ]
, bond = Nothing
, limited = False
}
, bond 334 "Indefatigable" "Florence Nightingale" Icon.BusterUp
[party_ Buster 10, party HealingReceived 20]
, bond 335 "One-Man War" "Cu Chulainn (Alter)" Icon.Kneel
[self NPUp 30, gutsPercent 20]
, bond 336 "<NAME>" "<NAME>" Icon.NobleUp
[party NPGen 15]
, bond 337 "Indestructible Blade" "<NAME>" Icon.ExclamationUp
[party CritUp 25]
, bond 338 "Concealed Goddess" "<NAME>" Icon.DamageUp
[party (Special AttackUp <| VsClass Assassin) 20]
, bond 339 "Lights of Civilization" "<NAME>" Icon.NobleUp
[party NPGen 15]
, bond 340 "Reaching the Zenith of My Skill" "<NAME>" Icon.ArtsUp
[party_ Arts 15]
, bond 341 "K<NAME>'s Oath" "<NAME>" Icon.ArtsUp
[party_ Quick 10, party_ Arts 10]
, bond 342 "Elemental" "<NAME>" Icon.ArtsUp
[party_ Arts 10, party NPUp 10]
, bond 343 "NEO Difference Engine" "<NAME>" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, { name = "<NAME>"
, id = 344
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 15 20
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 345
, rarity = 4
, icon = Icon.ArtsUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 NPGen <| Range 5 10
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>'s Hospitality"
, id = 346
, rarity = 3
, icon = Icon.NobleUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 NPGen <| Range 5 10
, Grant Self 0 DefenseUp <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 347
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 10 15
, Grant Self 0 NPUp <| Range 20 25
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 348
, rarity = 4
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 NPUp <| Range 15 20
, Grant Self 0 HealingReceived <| Range 10 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 349
, rarity = 3
, icon = Icon.AllUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 2 3
, Grant Self 0 (CardUp Buster) <| Range 2 3
, Grant Self 0 (CardUp Quick) <| Range 2 3
, Grant Self 0 CritUp <| Range 5 10
]
, bond = Nothing
, limited = True
}
, bond 350 "Hell of Blazing Punishment" "<NAME> (Alter)" Icon.BusterUp
[party_ Buster 15]
, bond 351 "<NAME>" "<NAME>" Icon.SwordUp
[party AttackUp 15]
, bond 352 "White Dragon" "<NAME>" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, bond 353 "The Sun Shines Here" "Emiya (Assassin)" Icon.ArtsQuickUp
[party_ Quick 10, party_ Arts 10]
, bond 354 "Dress of Heaven" "<NAME> (Holy Grail)" Icon.HealUp
[party HealingReceived 30]
, bond 355 "Manifestation of the Golden Rule" "Gilgamesh (Child)" Icon.NobleUp
[party NPGen 15]
, bond 356 "Spirit of the Vast Land" "<NAME>" Icon.NobleUp
[party NPGen 15]
, bond 357 "Extolling the Revolver" "Billy the Kid" Icon.ExclamationUp
[party CritUp 25]
, bond 358 "Library of Hundred Men" "Hassan of the Hundred Personas" Icon.AllUp
[party_ Buster 8, party_ Quick 8, party_ Arts 8]
, bond 359 "Final Fragment" "<NAME>" Icon.DamageUp
[ gutsPercent 20
, self (Special AttackUp <| VsClass Beast1) 200
, self (Special AttackUp <| VsClass Beast2) 200
, self (Special AttackUp <| VsClass Beast3L) 200
, self (Special AttackUp <| VsClass Beast3R) 200
]
, gift 360 "Fate/EXTELLA" Icon.ExclamationUp
[self CritUp 15, self StarsPerTurn 3]
, gift 361 "Spiritron Portrait: <NAME>" Icon.Road
[Bonus EXP Units <| Flat 50]
, gift 362 "Spiritron Portrait: Nameless" Icon.Road
[Bonus EXP Units <| Flat 50]
, gift 363 "Spiritron Portrait: Tamamo-no-Mae" Icon.Road
[Bonus EXP Units <| Flat 50]
, gift 364 "Spiritron Portrait: Karna" Icon.Road
[Bonus EXP Units <| Flat 50]
, gift 365 "Spiritron Portrait: Altera" Icon.Road
[Bonus EXP Units <| Flat 50]
, gift 366 "Spiritron Portrait: Gilgamesh" Icon.Road
[Bonus EXP Units <| Flat 50]
, bond 367 "Divine Wine—<NAME>" "<NAME>-<NAME>" Icon.ArtsQuickUp
[party_ Quick 10, party_ Arts 10]
, bond 368 "<NAME>" "<NAME>-<NAME>-<NAME>ik<NAME>" Icon.BusterUp
[party_ Buster 10, party CritUp 15]
, bond 369 "<NAME>" "<NAME>" Icon.BusterArtsUp
[party_ Arts 10, party_ Buster 10]
, bond 370 "Bone Sword (Nameless)" "<NAME>aki-D<NAME>" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, bond 371 "Unit Golden Bear" "Sakata Kintoki (Rider)" Icon.StarHaloUp
[party StarUp 20]
, bond 372 "Gringolet" "<NAME>" Icon.BusterUp
[party_ Buster 15]
, bond 373 "But I Lied Once" "<NAME>" Icon.ExclamationUp
[party CritUp 25]
, bond 374 "Exercising the Royal Authority" "<NAME>" Icon.NobleUp
[party NPGen 10, party NPUp 10]
, bond 375 "Mask of a Demon" "Fuuma \"Evil-wind\" Kotarou" Icon.QuickUp
[party_ Quick 15]
, bond 376 "Cook Despite of Exhaustion" "<NAME>" Icon.HealTurn
[party HealPerTurn 500]
, bond 377 "King's Horse" "<NAME> (Lancer)" Icon.SwordUp
[party AttackUp 10, party NPUp 10]
, bond 378 "All-Encompassing Wisdom" "<NAME>" Icon.BeamUp
[party NPUp 20]
, bond 379 "Sunset Beach" "Tamamo-no-Mae (Lancer)" Icon.QuickBusterUp
[party_ Quick 10, party_ Buster 10]
, bond 380 "Lady of the Lake" "Lancelot (Saber)" Icon.NobleUp
[party NPGen 10, party CritUp 10]
, bond 381 "Reminisc<NAME> of the Summer"
"<NAME> (Caster)" Icon.ExclamationUp
[party CritUp 25]
, bond 382 "Currently in the Middle of a Shower"
"<NAME> & <NAME> (Archer)" Icon.BusterArtsUp
[party_ Buster 10, party_ Arts 10]
, bond 383 "<NAME>" "<NAME> (Rider)" Icon.BeamUp
[party NPUp 20]
, bond 384 "Beach Love Letter (Terror)" "<NAME> (Lancer)" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, bond 385 "Lost Right Arm" "<NAME>" Icon.BusterUp
[party_ Buster 10, party NPGen 10]
, bond 386 "Proof of Existence" "Hassan of the Serenity" Icon.QuickUp
[party_ Quick 15]
, { name = "<NAME>ility"
, id = 387
, rarity = 5
, icon = Icon.ArtsQuickUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 10 15
, Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 NPGen <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "Reading on the Holy Night"
, id = 388
, rarity = 4
, icon = Icon.NobleUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 NPGen <| Range 15 20
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 389
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 3 5
, Grant Self 0 DamageCut <| Range 100 200
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 390
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ To Self GaugeUp <| Range 30 50
, Grant Self 0 CritUp <| Range 10 15
, Grant Self 0 NPUp <| Range 10 15
]
, bond = Nothing
, limited = True
}
, bond 391 "Champion Cup" "<NAME> (Archer)" Icon.SwordUp
[party AttackUp 15]
, bond 392 "Phantasmal Summoning (Install)" "<NAME>" Icon.AllUp
[party_ Buster 8, party_ Quick 8, party_ Arts 8]
, bond 393 "Serpent of Fate" "<NAME>" Icon.BeamUp
[party NPUp 25, demeritAll DefenseDown 10]
, bond 394 "Holy Knuckle" "<NAME> (Ruler)" Icon.BusterUp
[party_ Buster 15]
, bond 395 "Minimal Prudence" "<NAME> (Assassin)" Icon.QuickUp
[party_ Quick 15]
, bond 396 "Sharing of Pain" "<NAME>" Icon.ExclamationUp
[party CritUp 30, demeritAll HealthLoss 200]
, bond 397 "Creed at the Bottom of the Earth" "<NAME> (EXTRA)" Icon.QuickBusterUp
[party_ Quick 10, party_ Buster 10]
, bond 398 "Invitation to Halloween" "<NAME> (Brave)" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, gift 399 "First Order" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, { name = "<NAME>"
, id = 400
, rarity = 5
, icon = Icon.SunUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ To Self GaugeUp <| Range 50 60
, Times 1 << Grant Self 0 Overcharge <| Flat 2
]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 401
, rarity = 4
, icon = Icon.StarUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 GatherUp <| Range 300 400
, Grant Self 0 DamageCut <| Range 300 400
]
, bond = Nothing
, limited = False
}
, { name = "Over the Cuckoo's Nest"
, id = 414
, rarity = 3
, icon = Icon.Crystal
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Bonus QPQuest Units <| Flat 2019
, Grant Self 0 DamageUp <| Range 0 19
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 415
, rarity = 4
, icon = Icon.HealTurn
, stats = { base = { atk = 0, hp = 2019 }
, max = { atk = 0, hp = 2019 }
}
, effect = [ Grant Self 0 HealPerTurn <| Flat 100 ]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 418
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ equipped Archer << Grant Self 0 NPUp <| Range 15 25 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME> Y<NAME>"
, id = 419
, rarity = 3
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 (Resist AttackDown) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Collection of Mysterious Masks"
, id = 420
, rarity = 3
, icon = Icon.StarUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 GatherUp <| Range 100 200
, Grant Self 0 StarUp <| Range 5 10
]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 421
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 NPGen <| Range 25 30
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 422
, rarity = 5
, icon = Icon.ArtsUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 15 20
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 424
, rarity = 4
, icon = Icon.HealUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 HealingReceived <| Range 15 20
, Grant Self 0 NPGen <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 425
, rarity = 3
, icon = Icon.HealUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 HealingReceived <| Range 5 10
, Grant Self 0 StarUp <| Range 5 10
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>ance of a V<NAME>"
, id = 426
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DamageCut <| Range 100 200
, Grant Self 0 StarUp <| Range 5 10
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 427
, rarity = 5
, icon = Icon.ShieldBreak
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 IgnoreInvinc Full
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 428
, rarity = 5
, icon = Icon.DamageUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (Special AttackUp <| VsTrait Divine) <| Range 25 30
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, bond 403 "Seven-Headed Warhammer Sita" "<NAME>" Icon.BusterUp
[party_ Buster 20, demeritAll DebuffVuln 20]
, bond 404 "Flower of Humbaba" "Enkidu" Icon.HealTurn
[party HealPerTurn 500]
, bond 405 "Piedra del Sol" "Quetzalcoatl" Icon.BusterArtsUp
[party_ Arts 10, party_ Buster 10]
, bond 406 "Door to the Ocean" "Jeanne d'Arc Alter Santa Lily" Icon.HealUp
[party HealingReceived 30]
, bond 407 "<NAME>" "<NAME> (Caster)" Icon.BeamUp
[party NPUp 20]
, bond 408 "<NAME>" "<NAME>" Icon.BusterUp
[party_ Buster 10, party NPGen 10]
, bond 409 "Uncertainty About the Future" "Medusa (Lancer)" Icon.QuickUp
[party_ Quick 10, party NPGen 10]
, bond 410 "Primeval Flame" "Jaguar Warrior" Icon.BusterUp
[party_ Buster 15]
, bond 411 "The Furthest Tower" "<NAME>" Icon.BusterUp
[party_ Buster 10, party CritUp 15]
, bond 416 "Buddhism of Emptiness" "<NAME>" Icon.HoodUp
[self NPUp 30, Times 3 <| Grant Self 0 DebuffResist Full]
, bond 417 "The Rift of the Valley" "\"First Hassan\"" Icon.HoodUp
[self DebuffResist 100]
, bond 429 "Dark Knight-Kun" "Mysterious Heroine X (Alter)" Icon.DamageUp
[party (Special AttackUp <| VsClass Saber) 20]
, bond 546 "The Dynamics of an Asteroid" "<NAME>" Icon.BeamUp
[Grant (AlliesType Evil) 0 NPUp <| Flat 25]
, bond 547 "Kanshou & Bakuya (Revolvers)" "EMIYA (Alter)" Icon.AllUp
[party_ Quick 8, party_ Arts 8, party_ Buster 8]
, bond 548 "<NAME>" "<NAME>" Icon.QuickUp
[self (CardUp Quick) 10, party NPGen 10]
, bond 549 "108 Stars of Destiny" "<NAME>" Icon.QuickUp
[party_ Quick 10, party CritUp 15]
, { name = "Conversation on the Hot Sands"
, id = 550
, rarity = 5
, icon = Icon.StarUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 GatherUp <| Range 400 600
, Grant Self 0 CritUp <| Range 20 25
]
, bond = Nothing
, limited = True
}
, { name = "The Dantes Files: The Case of the Spring Jaunt"
, id = 551
, rarity = 5
, icon = Icon.ShieldBreak
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 IgnoreInvinc Full
, Grant Self 0 (CardUp Arts) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 553
, rarity = 4
, icon = Icon.HealUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 HealingReceived <| Range 8 10
, Grant Self 0 DebuffResist <| Range 8 10
, Grant Self 0 DefenseUp <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 554
, rarity = 4
, icon = Icon.CrosshairUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 1 Taunt Full
, Grant Self 1 NPGen <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 555
, rarity = 4
, icon = Icon.Star
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ To Party GainStars <| Range 10 20
, Grant Self 0 (CardUp Buster) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 556
, rarity = 3
, icon = Icon.AllUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 2 3
, Grant Self 0 (CardUp Buster) <| Range 2 3
, Grant Self 0 (CardUp Quick) <| Range 2 3
, Grant Self 0 MentalResist <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 557
, rarity = 3
, icon = Icon.StarHaloUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 4 8
, Grant Self 0 StarUp <| Range 4 8
, Grant Self 0 NPUp <| Range 4 8
]
, bond = Nothing
, limited = True
}
, bond 559 "Garden" "<NAME> (Prototype)" Icon.SwordUp
[party AttackUp 15]
, { name = "<NAME> the Six<NAME> Heaven"
, id = 560
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 15 20
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>ress of the Sun"
, id = 561
, rarity = 5
, icon = Icon.ExclamationUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 CritUp <| Range 15 20
, Grant Self 0 StarUp <| Range 15 20
, To Self GaugeUp <| Range 25 40
]
, bond = Nothing
, limited = True
}
, { name = "Wolves of Mi<NAME>"
, id = 562
, rarity = 5
, icon = Icon.QuickBusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 8 10
, Grant Self 0 (CardUp Quick) <| Range 8 10
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "A Stroll in the Spring Breeze"
, id = 563
, rarity = 4
, icon = Icon.NobleUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 NPGen <| Range 15 20
, Grant Self 0 DamageCut <| Range 300 400
]
, bond = Nothing
, limited = True
}
, { name = "The Flower of High Society"
, id = 564
, rarity = 3
, icon = Icon.ArtsQuickUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 4 6
, Grant Self 0 (CardUp Quick) <| Range 4 6
]
, bond = Nothing
, limited = True
}
, bond 567 "Haori of the Oath" "Hijikata T<NAME>" Icon.Kneel
[Times 1 << Grant Self 0 Guts <| Flat 1, self CritUp 30]
, bond 568 "<NAME>" "<NAME>" Icon.BeamUp
[party NPUp 25, demeritAll DefenseDown 10]
, { name = "<NAME>quering the Great Sea of Stars"
, id = 569
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 15 20
, Grant Self 0 NPGen <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 570
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 8 10
, Grant Self 0 NPUp <| Range 8 10
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>monition of Beginnings"
, id = 572
, rarity = 4
, icon = Icon.StarTurn
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 2 3
, Grant Self 0 GaugePerTurn <| Range 2 3
]
, bond = Nothing
, limited = True
}
, bond 575 "The Radiant One" "Meltryllis" Icon.QuickUp
[self (CardUp Quick) 20, self CritUp 30, demeritOthers CritDown 10]
, bond 576 "Don't Avert Your Eyes Away From Me" "Passionlip" Icon.BusterUp
[party_ Buster 20, demeritAll DebuffVuln 20]
, bond 577 "A Passerby's Dream" "BB" Icon.AllUp
[party_ Quick 8, party_ Arts 8, party_ Buster 8]
, bond 578 "Tenma's Spring Training" "<NAME>" Icon.BusterUp
[self (CardUp Buster) 10, party NPUp 10]
, bond 579 "Dream from the Cradle" "<NAME>" Icon.ArtsUp
[self (CardUp Arts) 20, self HPUp 3000, demeritOthers HPDown 1000]
, { name = "<NAME>"
, id = 581
, rarity = 3
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 (Resist DefenseDown) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Ruined Church"
, id = 580
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 (Special DefenseUp <| VsTrait Male) <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, gift 583 "Fate/Apocrypha" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, bond 586 "King Shahryay's Bedchamber" "<NAME>" Icon.HoodUp
[self DebuffResist 100]
, bond 587 "The Palace of Luoyang" "<NAME>" Icon.QuickUp
[party_ Quick 20, demeritAll DefenseDown 10]
, bond 588 "Military Sash of the War God" "<NAME>" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, bond 589 "<NAME>" "<NAME>" Icon.BusterUp
[party_ Buster 10, party NPGen 10]
, { name = "<NAME>"
, id = 590
, rarity = 5
, icon = Icon.ArtsQuickUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 (CardUp Quick) <| Range 8 10
, To Self GaugeUp <| Range 50 60
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 591
, rarity = 4
, icon = Icon.NobleTurn
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 GaugePerTurn <| Range 4 5
, Grant Self 0 (CardUp Arts) <| Range 10 12
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 592
, rarity = 3
, icon = Icon.StarUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 GatherUp <| Range 100 200
, Grant Self 0 (CardUp Quick) <| Range 2 3
]
, bond = Nothing
, limited = True
}
, gift 593 "Learning With Manga! FGO" Icon.Road
[Bonus EXP Units <| Flat 50]
, gift 607 "Formal Portrait: Atalante" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, bond 641 "Frontier" "<NAME>" Icon.BusterUp
[party_ Buster 15]
, bond 642 "The Vaunted One" "<NAME>" Icon.QuickUp
[party_ Quick 10, party CritUp 15]
, { name = "<NAME>"
, id = 643
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 NPGen <| Range 25 30
, Grant Self 0 NPUp <| Range 10 15
, Grant Self 0 CritUp <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 644
, rarity = 4
, icon = Icon.BusterArtsUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 (CardUp Buster) <| Range 8 10
, Grant Self 0 NPGen <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 645
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 3 5
, Grant Self 0 (CardUp Quick) <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 646
, rarity = 5
, icon = Icon.StarHaloUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 StarUp <| Range 20 25
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, bond 647 "<NAME>" "<NAME> (Caster)" Icon.BusterUp
[party_ Buster 10, party NPGen 10]
, bond 648 "Handy-Bandages" "<NAME> (Saber)" Icon.QuickUp
[self (CardUp Quick) 10, party NPGen 10]
, bond 649 "A Gift From Ra" "<NAME>itocris (Assassin)" Icon.ArtsUp
[party_ Arts 10, party NPGen 10]
, bond 650 "<NAME> Fubu -2017 Summer.ver-" "<NAME> (Berserker)" Icon.BusterUp
[party_ Buster 15, party CritUp 15, demeritAll DefenseDown 10]
, bond 651 "Champion's Cup of the Goddess" "<NAME> (Rider)" Icon.QuickUp
[party_ Quick 10, party NPUp 10]
, bond 656 "Mop of Selection" "<NAME> (Rider Alter)" Icon.SwordUp
[party AttackUp 20, demeritAll DefenseDown 15]
, bond 657 "NYARF!" "<NAME> (Archer)" Icon.ArtsQuickUp
[party_ Quick 10, party_ Arts 10]
, bond 658 "<NAME>" "<NAME> (Lancer)" Icon.QuickBusterUp
[party_ Quick 10, party_ Buster 10]
, { name = "<NAME>"
, id = 660
, rarity = 5
, icon = Icon.BusterArtsUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 (CardUp Buster) <| Range 8 10
, To Self GaugeUp <| Range 50 60
]
, bond = Nothing
, limited = True
}
, { name = "Divine Three-Legged Race"
, id = 661
, rarity = 5
, icon = Icon.Kneel
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Times 1 << Grant Self 0 Guts <| Flat 1
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "<NAME> End"
, id = 666
, rarity = 5
, icon = Icon.StarUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 GatherUp <| Range 400 600
, Grant Self 0 (CardUp Buster) <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 667
, rarity = 4
, icon = Icon.BusterArtsUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 (CardUp Buster) <| Range 8 10
, Grant Self 0 NPUp <| Range 5 10
]
, bond = Nothing
, limited = True
}
, bond 669 "<NAME>" "<NAME>" Icon.QuickUp
[party_ Quick 10, party NPGen 10]
, gift 670 "Heroic Costume: Medusa" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, gift 671 "Heroic Costume: <NAME>" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, gift 672 "Heroic Costume: <NAME>" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, gift 673 "Heroic Costume: <NAME>" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, bond 675 "A Wish Spanning 3 Generations" "<NAME>" Icon.BusterUp
[party_ Buster 10, party NPUp 10]
, bond 676 "<NAME>" "<NAME>" Icon.ArtsQuickUp
[party_ Quick 10, party_ Arts 10]
, bond 677 "<NAME>" "<NAME>" Icon.QuickUp
[party_ Quick 10, party CritUp 15]
, bond 678 "Double-Tiered <NAME>asa" "<NAME>" Icon.ArtsUp
[party_ Arts 10, party NPUp 10]
, bond 679 "<NAME>" "<NAME>" Icon.QuickBusterUp
[party_ Quick 10, party_ Buster 10]
, bond 686 "<NAME>" "<NAME>" Icon.NobleUp
[party NPGen 15]
, bond 687 "Electrologica Diagram" "Mecha Eli-chan" Icon.BusterUp
[party_ Buster 20, demeritAll DebuffVuln 20]
, bond 688 "<NAME>" "Mecha Eli-chan MkII" Icon.BusterUp
[party_ Buster 20, demeritAll DebuffVuln 20]
, gift 689 "Pray Upon the Sword, Wish Upon Life" Icon.Road
[Bonus EXP Units <| Flat 50]
, { name = "<NAME>"
, id = 691
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ equipped Caster << Grant Self 0 NPUp <| Range 15 25 ]
, bond = Nothing
, limited = False
}
, { name = "<NAME>"
, id = 674
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ equipped Saber << Grant Self 0 NPUp <| Range 15 25 ]
, bond = Nothing
, limited = False
}
, gift 690 "FGO VR Mash Kyrielight" Icon.Road
[Bonus EXP Units <| Flat 50 ]
, bond 692 "<NAME>" "<NAME>" Icon.NobleUp
[party NPGen 10, party NPUp 10]
, bond 693 "Universe Ring" "<NAME>" Icon.QuickUp
[party_ Quick 10, party NPUp 10]
, bond 694 "Tributes to King <NAME>" "<NAME>" Icon.BusterArtsUp
[party_ Arts 10, party_ Buster 10]
, bond 695 "<NAME>" "<NAME>" Icon.BeamUp
[Times 3 <| Grant Self 0 DeathResist Full]
, bond 700 "Blooming Flowers in Kur" "<NAME>" Icon.BusterUp
[party_ Buster 10, party NPUp 10]
, bond 701 "The Rainbow Soaring Under the Night Sky" "Attila the San(ta)" Icon.QuickUp
[party_ Quick 10, party CritUp 15]
, { name = "<NAME>"
, id = 704
, rarity = 5
, icon = Icon.ArtsUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 CritUp <| Range 10 15
, To Self GaugeUp <| Range 40 50
]
, bond = Nothing
, limited = True
}
, bond 709 "Painting of a Dragon Passing Over Mount Fuji" "<NAME>" Icon.Kneel
[Times 1 << Grant Self 0 GutsPercent <| Flat 20]
, bond 717 "Mysterious Glass" "<NAME>" Icon.BusterUp
[party_ Buster 10, party NPUp 10]
, bond 762 "Summer Rain" "<NAME>" Icon.BusterArtsUp
[party_ Arts 10, party_ Buster 10]
, { name = "Chaldea Special Investigation Unit"
, id = 768
, rarity = 4
, icon = Icon.Bullseye
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 SureHit Full
, Grant Self 0 (CardUp Arts) <| Range 5 8
, Grant Self 0 (CardUp Buster) <| Range 5 8
]
, bond = Nothing
, limited = True
}
, { name = "The Star of Camelot"
, id = 764
, rarity = 5
, icon = Icon.StarUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 GatherUp <| Range 400 600
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "<NAME> Dantes Files: Undercover in a Foreign Land"
, id = 765
, rarity = 5
, icon = Icon.BusterArtsUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 (CardUp Buster) <| Range 10 15
, Grant Self 0 NPGen <| Range 10 15
]
, bond = Nothing
, limited = True
}
, bond 787 "OTMA" "<NAME>" Icon.ArtsUp
[party_ Arts 10, party NPUp 10]
, bond 788 "Calydon's Hide" "Atalante (Alter)" Icon.QuickUp
[party_ Quick 15, party CritUp 25, demeritAll DefenseDown 10]
, bond 789 "Truth and Death" "Avicebron" Icon.BusterArtsUp
[party_ Arts 10, party_ Buster 10]
, bond 790 "Wildfire Blade" "<NAME>" Icon.ArtsUp
[party_ Arts 10, party NPGen 10]
, bond 791 "Library of Ivan the Terrible" "Ivan the Terrible" Icon.BusterUp
[party_ Buster 10, party NPUp 10]
, { name = "<NAME>"
, id = 792
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 NPGen <| Range 10 15
, Grant Self 0 NPUp <| Range 10 15
, To Self GaugeUp <| Range 40 50
]
, bond = Nothing
, limited = True
}
, { name = "Moment of Bliss"
, id = 793
, rarity = 4
, icon = Icon.BusterArtsUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 8 10
, Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 DefenseUp <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "Away We Go!"
, id = 794
, rarity = 3
, icon = Icon.QuickUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 3 5
, Grant Self 0 NPGen <| Range 5 10
]
, bond = Nothing
, limited = True
}
, { name = "An Afternoon at the Fortress"
, id = 795
, rarity = 5
, icon = Icon.BusterArtsUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 10 15
, Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 NPUp <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "<NAME>"
, id = 796
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 NPGen <| Range 10 15
, Grant Self 0 CritUp <| Range 10 15
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, bond 798 "The Object That Can Hold a Universe" "Achilles" Icon.Shield
[self NPUp 30, Times 1 <| Grant Party 3 Invincibility Full]
, bond 799 "The Purpose of Learning and Teaching" "<NAME>" Icon.ArtsQuickUp
[party_ Arts 10, party_ Quick 10]
, bond 800 "Nameless Death" "<NAME>" Icon.NobleUp
[party NPGen 10, party NPUp 10]
]
equipped : Class -> SkillEffect -> SkillEffect
equipped =
When << (++) "equipped by a " << Class.show
{-| Retrieves the corresponding Bond CE. Memoized for performance. -}
getBond : Servant -> Maybe CraftEssence
getBond s =
Dict.get s.name bondMap
{-| Memoization table for `getBond`. -}
bondMap : Dict String CraftEssence
bondMap =
let
go ce =
Maybe.map (\bond -> (bond, ce)) ce.bond
in
db
|> List.map go
>> Maybe.values
>> Dict.fromList
| true | module Database.CraftEssences exposing
( db
, getBond
, getAll
)
{-| All Craft Essences. -}
-- Unlike Servants, which are divided into multiple subfiles,
-- Craft Essences are all included in this one file
-- along with their data model definition.
import Dict exposing (Dict)
import Maybe.Extra as Maybe
import Class.Has exposing (Has)
import Model.Icon as Icon
import Model.Card exposing (Card(..))
import Model.Class as Class exposing (Class(..))
import Model.CraftEssence exposing (CraftEssence)
import Model.Trait exposing (Trait(..))
import Model.Servant exposing (Servant)
import Model.Skill.Amount exposing (Amount(..))
import Model.Skill.BonusEffect exposing (BonusEffect(..), BonusType(..))
import Model.Skill.BuffEffect exposing (BuffEffect(..))
import Model.Skill.DebuffEffect exposing (DebuffEffect(..))
import Model.Skill.InstantEffect exposing (InstantEffect(..))
import Model.Skill.SkillEffect exposing (SkillEffect(..))
import Model.Skill.Special exposing (Special(..))
import Model.Skill.Target exposing (Target(..))
import Database
getAll : Has CraftEssence a -> List a
getAll =
Database.genericGetAll db
{-| All Craft Essences available in EN. -}
-- Note: Names _must_ be true to their EN localization.
-- GrandOrder.Wiki is only trustworthy for CEs that have been in the game
-- for a while. Craft Essences introduced during events and the like should be
-- checked against the official announcement.
db : List CraftEssence
db =
let
gutsPercent =
Times 1 <<
Grant Self 0 GutsPercent <<
Flat
self buff =
Grant Self 0 buff <<
Flat
party buff =
Grant Party 0 buff <<
Flat
party_ card =
party (CardUp card)
demeritAll debuff =
Debuff Party 0 debuff <<
Flat
demeritOthers debuff =
Debuff Others 0 debuff <<
Flat
atkChance chance =
When "attacking" <<
Chance chance
bond id name servant icon effect =
{ name = name
, id = id
, rarity = 4
, icon = icon
, stats = { base = { atk = 100, hp = 100 }
, max = { atk = 100, hp = 100 }
}
, effect = effect
, bond = Just servant
, limited = False
}
gift id name icon effect =
{ name = name
, id = id
, rarity = 4
, icon = icon
, stats = { base = { atk = 100, hp = 100 }
, max = { atk = 100, hp = 100 }
}
, effect = effect
, bond = Nothing
, limited = True
}
in
[ { name = "PI:NAME:<NAME>END_PI"
, id = 1
, rarity = 1
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 100 }
, max = { atk = 0, hp = 300 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 3 5 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 2
, rarity = 1
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 150 }
, max = { atk = 0, hp = 450 }
}
, effect = [ Grant Self 0 DebuffResist <| Range 5 10 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 3
, rarity = 1
, icon = Icon.ArtsUp
, stats = { base = { atk = 100, hp = 0 }
, max = { atk = 300, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 3 5 ]
, bond = Nothing
, limited = False
}
, { name = "Preemption"
, id = 4
, rarity = 1
, icon = Icon.QuickUp
, stats = { base = { atk = 100, hp = 0 }
, max = { atk = 300, hp = 0 }
}
, effect = [Grant Self 0 (CardUp Quick) <| Range 3 5]
, bond = Nothing
, limited = False
}
, { name = "Destruction"
, id = 5
, rarity = 1
, icon = Icon.BusterUp
, stats = { base = { atk = 100, hp = 0 }
, max = { atk = 300, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 3 5 ]
, bond = Nothing
, limited = False
}
, { name = "Flash"
, id = 6
, rarity = 2
, icon = Icon.ExclamationUp
, stats = { base = { atk = 150, hp = 0 }
, max = { atk = 500, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 5 10 ]
, bond = Nothing
, limited = False
}
, { name = "Opportunity"
, id = 7
, rarity = 2
, icon = Icon.StarHaloUp
, stats = { base = { atk = 75, hp = 112 }
, max = { atk = 250, hp = 375 }
}
, effect = [ Grant Self 0 StarUp <| Range 5 10 ]
, bond = Nothing
, limited = False
}
, { name = "Fruitful"
, id = 8
, rarity = 2
, icon = Icon.Noble
, stats = { base = { atk = 75, hp = 112 }
, max = { atk = 250, hp = 375 }
}
, effect = [ To Self GaugeUp <| Range 10 20 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 9
, rarity = 2
, icon = Icon.NobleUp
, stats = { base = { atk = 75, hp = 112 }
, max = { atk = 250, hp = 375 }
}
, effect = [ Grant Self 0 NPGen <| Range 5 10 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 10
, rarity = 2
, icon = Icon.BeamUp
, stats = { base = { atk = 150, hp = 0 }
, max = { atk = 500, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 5 10 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 11
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 200 }
, max = { atk = 0, hp = 1000 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "False Attendant's PI:NAME:<NAME>END_PI"
, id = 12
, rarity = 3
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DebuffResist <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "The Azure Black Keys"
, id = 13
, rarity = 3
, icon = Icon.ArtsUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "The Verdant Black Keys"
, id = 14
, rarity = 3
, icon = Icon.QuickUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "The Crimson Black Keys"
, id = 15
, rarity = 3
, icon = Icon.BusterUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI Pendant"
, id = 16
, rarity = 3
, icon = Icon.ExclamationUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "Spell Tome"
, id = 17
, rarity = 3
, icon = Icon.StarHaloUp
, stats = { base = { atk = 100, hp = 150 }
, max = { atk = 500, hp = 750 }
}
, effect = [ Grant Self 0 StarUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 18
, rarity = 3
, icon = Icon.Noble
, stats = { base = { atk = 100, hp = 150 }
, max = { atk = 500, hp = 750 }
}
, effect = [ To Self GaugeUp <| Range 30 50 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 19
, rarity = 3
, icon = Icon.NobleUp
, stats = { base = { atk = 100, hp = 150 }
, max = { atk = 500, hp = 750 }
}
, effect = [ Grant Self 0 NPGen <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 20
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "Iron-Willed PI:NAME:<NAME>END_PI"
, id = 21
, rarity = 4
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 400 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 22
, rarity = 4
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 DebuffResist <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 23
, rarity = 4
, icon = Icon.ArtsUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 24
, rarity = 4
, icon = Icon.QuickUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 25
, rarity = 4
, icon = Icon.BusterUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "Gem Magecraft: Antumbra"
, id = 26
, rarity = 4
, icon = Icon.ExclamationUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Be Elegant"
, id = 27
, rarity = 4
, icon = Icon.StarHaloUp
, stats = { base = { atk = 200, hp = 300 }
, max = { atk = 750, hp = 1125 }
}
, effect = [ Grant Self 0 StarUp <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "The Imaginary Element"
, id = 28
, rarity = 4
, icon = Icon.Noble
, stats = { base = { atk = 200, hp = 300 }
, max = { atk = 750, hp = 1125 }
}
, effect = [ To Self GaugeUp <| Range 60 75 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 29
, rarity = 4
, icon = Icon.NobleUp
, stats = { base = { atk = 200, hp = 300 }
, max = { atk = 750, hp = 1125 }
}
, effect = [ Grant Self 0 NPGen <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 30
, rarity = 4
, icon = Icon.BeamUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Formal Craft"
, id = 31
, rarity = 5
, icon = Icon.ArtsUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Imaginary Around"
, id = 32
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Limited/Zero Over"
, id = 33
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Kaleidoscope"
, id = 34
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ To Self GaugeUp <| Range 80 100 ]
, bond = Nothing
, limited = False
}
, { name = "Heaven's Feel"
, id = 35
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 40 50 ]
, bond = Nothing
, limited = False
}
, { name = "Beginning of the Journey"
, id = 36
, rarity = 4
, icon = Icon.Road
, stats = { base = { atk = 50, hp = 50 }
, max = { atk = 50, hp = 50 }
}
, effect = [ Bonus FriendPoints Units <| Flat 75 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 37
, rarity = 3
, icon = Icon.Dodge
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Times 1 <| Grant Self 0 Evasion Full
, Grant Self 0 DebuffResist <| Range 5 10
]
, bond = Nothing
, limited = False
}
, { name = "Seal Designation Enforcer"
, id = 38
, rarity = 4
, icon = Icon.StarUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 GatherUp <| Range 600 800 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 39
, rarity = 4
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 400 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 (Special DefenseUp <| VsTrait Male) <| Range 25 35 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 40
, rarity = 5
, icon = Icon.NobleTurn
, stats = { base = { atk = 250, hp = 375 }
, max = { atk = 1000, hp = 1500 }
}
, effect = [ Grant Self 0 GaugePerTurn <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 41
, rarity = 5
, icon = Icon.Kneel
, stats = { base = { atk = 0, hp = 500 }
, max = { atk = 0, hp = 2000 }
}
, effect = [ Times 1 << Grant Self 0 Guts <| Range 500 1000 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 42
, rarity = 3
, icon = Icon.AllUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 3 5
, Grant Self 0 (CardUp Buster) <| Range 3 5
, Grant Self 0 (CardUp Quick) <| Range 3 5
]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 43
, rarity = 4
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 (Resist Charm) <| Range 80 100 ]
, bond = Nothing
, limited = True
}
, { name = "Moon Goddess' Bath"
, id = 44
, rarity = 5
, icon = Icon.HealTurn
, stats = { base = { atk = 0, hp = 500 }
, max = { atk = 0, hp = 2000 }
}
, effect = [ Grant Self 0 HealPerTurn <| Range 500 750 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PIlight Fest"
, id = 45
, rarity = 5
, icon = Icon.StarHaloUp
, stats = { base = { atk = 250, hp = 375 }
, max = { atk = 1000, hp = 1500 }
}
, effect = [ Grant Self 0 StarUp <| Range 15 20
, Grant Self 0 CritUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PIestone"
, id = 46
, rarity = 3
, icon = Icon.HoodUp
, stats = { base = { atk = 100, hp = 150 }
, max = { atk = 500, hp = 750 }
}
, effect = [ Grant Self 0 DebuffResist <| Range 5 10
, Grant Self 0 GatherUp <| Range 100 200
]
, bond = Nothing
, limited = False
}
, { name = "With One Strike"
, id = 47
, rarity = 4
, icon = Icon.Bullseye
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 SureHit Full
, Grant Self 0 (CardUp Quick) <| Range 8 10
]
, bond = Nothing
, limited = False
}
, { name = "The PI:NAME:<NAME>END_PI"
, id = 48
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 600, hp = 0 }
, max = { atk = 2400, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 60 80
, Debuff Self 0 HealthLoss <| Flat 500
]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 49
, rarity = 3
, icon = Icon.DamageUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 DamageUp <| Range 100 200 ]
, bond = Nothing
, limited = True
}
, { name = "Trick or Treat"
, id = 50
, rarity = 3
, icon = Icon.StaffUp
, stats = { base = { atk = 100, hp = 150 }
, max = { atk = 500, hp = 750 }
}
, effect = [ Grant Self 0 DebuffUp <| Range 10 12 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 51
, rarity = 4
, icon = Icon.CrosshairUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 1 Taunt Full
, Grant Self 1 DefenseUp <| Range 60 80
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 52
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 250, hp = 375 }
, max = { atk = 1000, hp = 1500 }
}
, effect = [ Grant Self 0 NPUp <| Range 15 20
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 53
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 NPGen <| Range 20 25
, To Self GaugeUp <| Range 50 60
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 54
, rarity = 5
, icon = Icon.HealUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 0 HealingReceived <| Range 60 75 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 55
, rarity = 3
, icon = Icon.HealTurn
, stats = { base = { atk = 300, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 HealPerTurn <| Range 100 200 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 56
, rarity = 4
, icon = Icon.SwordShieldUp
, stats = { base = { atk = 200, hp = 300 }
, max = { atk = 750, hp = 1125 }
}
, effect = [ Grant Self 3 AttackUp <| Range 25 30
, Grant Self 3 DefenseUp <| Range 25 30
]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 57
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 600, hp = 0 }
, max = { atk = 2400, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 10 15
, Grant Self 0 CritUp <| Range 20 25
]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 58
, rarity = 5
, icon = Icon.ArtsUp
, stats = { base = { atk = 600, hp = 0 }
, max = { atk = 2400, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 CritUp <| Range 20 25
]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 59
, rarity = 3
, icon = Icon.AllUp
, stats = { base = { atk = 100, hp = 150 }
, max = { atk = 500, hp = 750 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 1 2
, Grant Self 0 (CardUp Arts) <| Range 1 2
, Grant Self 0 (CardUp Buster) <| Range 1 2
, Grant Self 0 StarUp <| Range 1 2
, Grant Self 0 GatherUp <| Range 1 2
, Grant Self 0 NPGen <| Range 1 2
, Grant Self 0 NPUp <| Range 1 2
, Grant Self 0 DebuffUp <| Range 1 2
, Grant Self 0 DebuffResist <| Range 1 2
]
, bond = Nothing
, limited = True
}
, { name = "After-Party Order!"
, id = 60
, rarity = 4
, icon = Icon.QuickBusterUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 10 15
, Grant Self 0 (CardUp Buster) <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI-o"
, id = 61
, rarity = 5
, icon = Icon.DamageUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 15 20
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 62
, rarity = 5
, icon = Icon.CrosshairUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 3 Taunt Full
, Grant Self 3 AttackUp <| Range 60 80
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 65
, rarity = 3
, icon = Icon.QuickUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 66
, rarity = 4
, icon = Icon.ExclamationUp
, stats = { base = { atk = 200, hp = 300 }
, max = { atk = 750, hp = 1125 }
}
, effect = [ Grant Self 0 CritUp <| Range 40 50
, Debuff Self 0 DefenseDown <| Flat 20
]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI 20PI:NAME:<NAME>END_PI0"
, id = 67
, rarity = 5
, icon = Icon.StarTurn
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 68
, rarity = 3
, icon = Icon.BusterUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 3 (CardUp Buster) <| Range 15 20 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 69
, rarity = 4
, icon = Icon.HealTurn
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 HealPerTurn <| Range 200 300
, Grant Self 0 GaugePerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 70
, rarity = 5
, icon = Icon.StarUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 0 GatherUp <| Range 100 200
, Grant Self 0 HealingReceived <| Range 40 50
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 71
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 250, hp = 375 }
, max = { atk = 1000, hp = 1500 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 8 10
, Grant Self 0 CritUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 72
, rarity = 3
, icon = Icon.NobleTurn
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 GaugePerTurn <| Range 2 3 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 73
, rarity = 4
, icon = Icon.Kneel
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2400 }
}
, effect = [ Chance 50 << Times 1 << Grant Self 0 Guts <| Range 500 1000 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 74
, rarity = 4
, icon = Icon.NobleTurn
, stats = { base = { atk = 200, hp = 300 }
, max = { atk = 750, hp = 1125 }
}
, effect = [ Chance 60 << Grant Self 0 GaugePerTurn <| Range 12 15
, Debuff Self 0 HealthLoss <| Flat 500
]
, bond = Nothing
, limited = False
}
, { name = "500-Year Obsession"
, id = 75
, rarity = 5
, icon = Icon.Circuits
, stats = { base = { atk = 600, hp = 0 }
, max = { atk = 2400, hp = 0 }
}
, effect = [ When "defeated by an enemy" <| Debuff Target 2 SealNP Full
, When "defeated by an enemy" <<
Debuff Target 10 Curse <| Range 1000 2000
]
, bond = Nothing
, limited = False
}
, { name = "Peacefulness of 2018"
, id = 76
, rarity = 3
, icon = Icon.HealTurn
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 HealPerTurn <| Range 200 300
, Debuff Self 0 AttackDown <| Flat 10
]
, bond = Nothing
, limited = True
}
, { name = "Heroic New Year"
, id = 77
, rarity = 4
, icon = Icon.HoodUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Times 1 <| Grant Self 0 DebuffResist Full
, Grant Self 0 DefenseUp <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "Law of the Jungle"
, id = 78
, rarity = 3
, icon = Icon.Crystal
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Bonus QPQuest Units <| Range 2017 2018 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 79
, rarity = 5
, icon = Icon.Shield
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 1 Taunt Full
, Grant Self 1 Invincibility Full
, Grant Self 0 DebuffResist <| Range 10 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 80
, rarity = 5
, icon = Icon.Crystal
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Bonus QPDrop Percent <| Range 2 10 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 81
, rarity = 4
, icon = Icon.StarTurn
, stats = { base = { atk = 0, hp = 2018 }
, max = { atk = 0, hp = 2018 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 0 1 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 82
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ ToMax (Range 40 50) << Grant Self 0 NPUp <| Flat 5 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 83
, rarity = 5
, icon = Icon.Kneel
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Times 1 << Grant Self 0 Guts <| Flat 1
, Grant Self 0 DebuffResist <| Range 5 10
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 84
, rarity = 3
, icon = Icon.Bullseye
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 SureHit Full
, Grant Self 0 CritUp <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI! PI:NAME:<NAME>END_PI"
, id = 85
, rarity = 4
, icon = Icon.HealTurn
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Chance 65 << Grant Self 0 HealPerTurn <| Range 750 1000 ]
, bond = Nothing
, limited = True
}
, { name = "The Crimson Land of Shadows"
, id = 86
, rarity = 5
, icon = Icon.DamageUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ ToMax (Range 1000 1200) << Grant Self 0 DamageUp <| Flat 100 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 89
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 NPUp <| Range 10 15
, To Self GaugeUp <| Range 20 30
]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 90
, rarity = 3
, icon = Icon.DamageUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (Special AttackUp <| VsClass Caster) <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 91
, rarity = 3
, icon = Icon.Heart
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 (Success Charm) <| Range 12 15 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 92
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ equipped Berserker << Grant Self 0 NPUp <| Range 15 25 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 93
, rarity = 3
, icon = Icon.ArtsUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 3 (CardUp Arts) <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 94
, rarity = 3
, icon = Icon.DamageUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (Special AttackUp <| VsClass Rider) <| Range 8 10 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 95
, rarity = 3
, icon = Icon.Heal
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ When "defeated" << To Party Heal <| Range 800 1000 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 96
, rarity = 3
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 (Resist Stun) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 97
, rarity = 5
, icon = Icon.HoodUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Times 3 <| Grant Self 0 DebuffResist Full
, Grant Self 0 NPGen <| Range 15 20
]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 98
, rarity = 4
, icon = Icon.HPUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ ToMax (Flat 3000) << Grant Self 0 HPUp <| Range 200 300 ]
, bond = Nothing
, limited = False
}
, { name = "Heroic Portrait: PI:NAME:<NAME>END_PI"
, id = 99
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: PI:NAME:<NAME>END_PI"
, id = 100
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: PI:NAME:<NAME>END_PI"
, id = 101
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: PI:NAME:<NAME>END_PI"
, id = 102
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: PI:NAME:<NAME>END_PI"
, id = 103
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: PI:NAME:<NAME>END_PI"
, id = 104
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: PI:NAME:<NAME>END_PI"
, id = 105
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: PI:NAME:<NAME>END_PI & PI:NAME:<NAME>END_PI"
, id = 106
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: PI:NAME:<NAME>END_PI"
, id = 107
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "Heroic Portrait: PI:NAME:<NAME>END_PI"
, id = 108
, rarity = 4
, icon = Icon.Rainbow
, stats = { base = { atk = 500, hp = 500 }
, max = { atk = 500, hp = 500 }
}
, effect = [ Bonus Bond Units <| Flat 50 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PIentine Dojo of TPI:NAME:<NAME>END_PI"
, id = 109
, rarity = 3
, icon = Icon.Bullseye
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 SureHit Full
, Grant Self 0 GaugePerTurn <| Range 3 5
, Debuff Self 0 CharmVuln <| Flat 10
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI ☆ PI:NAME:<NAME>END_PI"
, id = 110
, rarity = 4
, icon = Icon.StarHaloUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 StarUp <| Range 15 20
, Grant Self 0 NPGen <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 111
, rarity = 5
, icon = Icon.ArtsQuickUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 (CardUp Quick) <| Range 10 15
, Grant Self 0 HealingReceived <| Range 20 30
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 112
, rarity = 5
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Times 3 << Grant Self 0 (Special DefenseUp <| VsTrait Male) <| Flat 100
, Grant Self 0 StarUp <| Range 10 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 154
, rarity = 5
, icon = Icon.ShieldBreak
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 IgnoreInvinc Full
, Grant Self 0 (CardUp Quick) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 155
, rarity = 5
, icon = Icon.FireUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 0 DeathResist <| Range 60 80 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 156
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ To Self GaugeUp <| Range 50 60
, Grant Self 3 (CardUp Arts) <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 157
, rarity = 5
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Times 3 << Grant Self 0 DamageCut <| Range 1000 1200 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 158
, rarity = 4
, icon = Icon.DamageUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 DamageUp <| Range 800 1000 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PIyes of Distortion"
, id = 159
, rarity = 4
, icon = Icon.BusterUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 20 25
, Debuff Self 0 DefenseDown <| Flat 15
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 160
, rarity = 4
, icon = Icon.Dodge
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Times 1 <| Grant Self 0 Evasion Full
, Grant Self 0 StarUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 161
, rarity = 4
, icon = Icon.StarUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 GatherUp <| Range 300 400
, Grant Self 3 DebuffResist <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 162
, rarity = 3
, icon = Icon.QuickUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 5 8
, Grant Self 0 DebuffResist <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "Repeat Magic"
, id = 163
, rarity = 3
, icon = Icon.Noble
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ To Self GaugeUp <| Range 20 30
, Grant Self 0 NPGen <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PIiss Your Hand"
, id = 165
, rarity = 5
, icon = Icon.AllUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 12
, Grant Self 0 (CardUp Buster) <| Range 10 12
, Grant Self 0 (CardUp Quick) <| Range 10 12
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 166
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ To Self GaugeUp <| Range 5 60
, Grant Self 0 GatherUp <| Range 300 400
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 167
, rarity = 5
, icon = Icon.DamageUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 3 (Special AttackUp <| VsTrait Divine) <| Range 80 100
, Grant Self 3 (Special DefenseUp <| VsTrait Divine) <| Range 40 50
]
, bond = Nothing
, limited = True
}
, { name = "Beasts Under the Moon"
, id = 168
, rarity = 4
, icon = Icon.NobleUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 NPGen <| Range 12 15
, Grant Self 0 StarUp <| Range 12 15
, Grant Self 0 HealPerTurn <| Range 200 300
]
, bond = Nothing
, limited = True
}
, { name = "Glass Full Sweet Time"
, id = 169
, rarity = 4
, icon = Icon.Bullseye
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 SureHit Full
, Grant Self 0 DamageUp <| Range 400 600
, Grant Self 0 DamageCut <| Range 200 300
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 170
, rarity = 3
, icon = Icon.Dodge
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Times 1 <| Grant Self 0 Evasion Full
, Grant Self 0 HealingReceived <| Range 5 10
, Grant Self 0 DebuffUp <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 171
, rarity = 3
, icon = Icon.StarTurn
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 1 2
, Grant Self 0 (Special AttackUp <| VsTrait Dragon) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 172
, rarity = 4
, icon = Icon.ExclamationUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 15 20
, Grant Self 0 NPUp <| Range 15 20
, Grant Self 0 (Success Charm) <| Range 12 15
]
, bond = Nothing
, limited = True
}
, gift 174 "[Heaven's Feel]" Icon.QuickUp
[self (CardUp Quick) 10, To Self GaugeUp <| Flat 40]
, { name = "PI:NAME:<NAME>END_PI"
, id = 175
, rarity = 5
, icon = Icon.HPUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Party 0 HPUp <| Range 1000 1200 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 176
, rarity = 4
, icon = Icon.StaffUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 DebuffUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 177
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ equipped Lancer << Grant Self 0 NPUp <| Range 15 25 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 178
, rarity = 5
, icon = Icon.Road
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Bonus EXP Percent <| Range 2 10 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 179
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ To Self GaugeUp <| Range 30 50
, Grant Self 0 HealingReceived <| Range 20 30
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 180
, rarity = 5
, icon = Icon.StarTurn
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 3 4
, Grant Self 0 (CardUp Buster) <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 181
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ When "defeated" << To Party GaugeUp <| Range 15 20
, Grant Self 0 (CardUp Buster) <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "Art of the Poisonous Snake"
, id = 182
, rarity = 4
, icon = Icon.ArtsUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 3 (CardUp Arts) <| Range 30 40 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI of Death"
, id = 183
, rarity = 4
, icon = Icon.DamageUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (Special AttackUp <| VsTrait Humanoid) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 184
, rarity = 4
, icon = Icon.HealUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 HealUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 185
, rarity = 5
, icon = Icon.Shield
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Times 3 <| Grant Self 0 Invincibility Full
, Grant Self 0 DamageUp <| Range 200 500
]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 186
, rarity = 4
, icon = Icon.NobleTurn
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 GaugePerTurn <| Range 4 5
, Grant Self 0 (CardUp Quick) <| Range 10 12
]
, bond = Nothing
, limited = False
}
, { name = "Self Geas Scroll"
, id = 187
, rarity = 3
, icon = Icon.StaffUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 (Success Stun) <| Range 12 15 ]
, bond = Nothing
, limited = False
}
, { name = "Before Awakening"
, id = 188
, rarity = 5
, icon = Icon.AllUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 (CardUp Buster) <| Range 8 10
, Grant Self 0 (CardUp Quick) <| Range 8 10
, Grant Self 0 DefenseUp <| Range 8 10
]
, bond = Nothing
, limited = False
}
, { name = "His Rightful Place"
, id = 189
, rarity = 5
, icon = Icon.StarTurn
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 3 4
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, bond 191 "Crown of the Star" "PI:NAME:<NAME>END_PI" Icon.DamageUp
[party AttackUp 15]
, bond 192 "Relic of the King" "PI:NAME:<NAME>END_PI (Lord El-Melloi II)" Icon.BusterUp
[party_ Buster 15]
, bond 193 "Triumph of the Lord Impaler" "PI:NAME:<NAME>END_PIlad III" Icon.BeamUp
[self NPUp 30, atkChance 30 << To Self GaugeUp <| Flat 5]
, bond 194 "Revelation from Heaven" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 15]
, bond 195 "Memories of the Dragon" "PI:NAME:<NAME>END_PI (Alter)" Icon.BeamUp
[self NPUp 30, atkChance 30 << Debuff Target 3 DefenseDown <| Flat 5]
, bond 196 "Hunter of the Red Plains" "EMIYA" Icon.BeamUp
[self NPUp 30, atkChance 30 << To Party GainStars <| Flat 5]
, bond 197 "Castle of Snow" "Heracles" Icon.Kneel
[Times 3 <| self Guts 500]
, bond 198 "Yggdrasil Tree" "Cu Chulainn (Caster)" Icon.BeamUp
[self NPUp 30, atkChance 30 << To Self Heal <| Flat 500]
, bond 199 "Scorching Embrace" "Kiyohime" Icon.BeamUp
[self NPUp 30, atkChance 30 << Debuff Target 5 Burn <| Flat 500]
, bond 200 "Worthless Jewel" "PI:NAME:<NAME>END_PI" Icon.NobleUp
[party NPGen 15]
, bond 201 "Eternal Solitude" "PI:NAME:<NAME>END_PI" Icon.SwordUp
[party AttackUp 15]
, bond 202 "Queen's Present" "PI:NAME:<NAME>END_PI" Icon.ArtsUp
[party_ Arts 15]
, bond 203 "Elixir" "PI:NAME:<NAME>END_PI" Icon.HealTurn
[party HealPerTurn 500]
, bond 204 "My Necklace" "PI:NAME:<NAME>END_PI" Icon.StarHaloUp
[party StarUp 20]
, bond 205 "Staff He Gave Me" "PI:NAME:<NAME>END_PI" Icon.HealUp
[party HealingReceived 30]
, bond 206 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.BeamUp
[self NPUp 30, atkChance 10 <| Debuff Target 1 SealNP Full]
, bond 207 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.Heal
[party HPUp 2000]
, bond 208 "PI:NAME:<NAME>END_PIirst for Victory" "PI:NAME:<NAME>END_PI" Icon.StarHaloUp
[party StarUp 20]
, bond 209 "To My Dear Friend" "PI:NAME:<NAME>END_PI" Icon.HoodUp
[party DebuffResist 30]
, bond 210 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.Heal
[ When "defeated" <| To Party RemoveDebuffs Full
, When "defeated" <| To Party Heal <| Flat 5000
]
, { name = "PI:NAME:<NAME>END_PI PI:NAME:<NAME>END_PI"
, id = 211
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 10 15
, When "defeated" <<
Grant Party 1 (CardUp Quick) <| Range 20 30
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PIen Captures the Carp"
, id = 212
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ To Self GaugeUp <| Range 30 50
, To Party GainStars <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "A Fox Night's Dream"
, id = 213
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 NPGen <| Range 20 25
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "Burning Tale of Love"
, id = 214
, rarity = 4
, icon = Icon.DamageUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (Special AttackUp <| VsTrait Male) <| Range 25 30
, Grant Self 0 DebuffUp <| Range 12 15
]
, bond = Nothing
, limited = True
}
, { name = "Reciting the Subscription List"
, id = 215
, rarity = 3
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Times 1 <| Grant Self 0 DebuffResist Full ]
, bond = Nothing
, limited = True
}
, bond 216 "Key of the King's Law" "PI:NAME:<NAME>END_PI" Icon.BeamUp
[self NPUp 30, atkChance 30 << Grant Self 3 CritUp <| Flat 10]
, bond 217 "Golden Glass" "PI:NAME:<NAME>END_PI" Icon.BeamUp
[self NPUp 30, atkChance 30 << To Self GaugeUp <| Flat 5]
, bond 218 "Thunderous Applause" "PI:NAME:<NAME>END_PI" Icon.ArtsUp
[party_ Arts 15]
, bond 219 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.NobleUp
[party NPGen 15]
, bond 220 "Radiance of the Goddess" "PI:NAME:<NAME>END_PI" Icon.QuickUp
[party_ Quick 15]
, bond 221 "Voyage of the Flowers" "PI:NAME:<NAME>END_PI (PI:NAME:<NAME>END_PI)" Icon.SwordUp
[party AttackUp 10, party StarUp 10]
, bond 222 "Ark of the Covenant" "PI:NAME:<NAME>END_PI" Icon.BeamUp
[self NPUp 30, When "attacking" << To Enemy Death <| Flat 10]
, bond 223 "Door to Babylon" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 15]
, bond 224 "Blood-Thirsting Axe" "PI:NAME:<NAME>END_PI" Icon.ExclamationUp
[party CritUp 25]
, bond 225 "Insurrection" "PI:NAME:<NAME>END_PI" Icon.Kneel
[gutsPercent 50]
, { name = "PI:NAME:<NAME>END_PI!!"
, id = 226
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 NPUp <| Range 20 25
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "The Classic Three Great Heroes"
, id = 227
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 NPUp <| Range 15 20
, Grant Self 0 StarUp <| Range 15 20
, To Self GaugeUp <| Range 25 40
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 228
, rarity = 4
, icon = Icon.BeamUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 15 20
, Grant Self 0 (CardUp Buster) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "All Three Together"
, id = 229
, rarity = 3
, icon = Icon.StarUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 GatherUp <| Range 100 200
, Grant Self 0 CritUp <| Range 5 10
]
, bond = Nothing
, limited = True
}
, bond 230 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.ExclamationUp
[party CritUp 25]
, bond 231 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.BeamUp
[party NPUp 20]
, bond 232 "Black Knight's Helmet" "PI:NAME:<NAME>END_PI" Icon.BeamUp
[self NPUp 30, atkChance 30 << Debuff Target 3 CritChance <| Flat 30]
, bond 233 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.QuickUp
[party_ Quick 15]
, bond 234 "Holy Pumpkin Grail" "PI:NAME:<NAME>END_PI (Halloween)" Icon.HoodUp
[party DebuffResist 30]
, bond 235 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.ExclamationUp
[party CritUp 25]
, bond 236 "PI:NAME:<NAME>END_PI II" "PI:NAME:<NAME>END_PI (Santa Alter)" Icon.StarHaloUp
[party StarUp 20]
, bond 237 "Things to Calm the Heart" "Henry Jekyll & Hyde" Icon.BusterUp
[party_ Buster 15]
, bond 238 "Glory of the Past Days" "Edward Teach" Icon.BusterUp
[party_ Buster 15]
, bond 239 "Heaven Among the Mountains" "PI:NAME:<NAME>END_PI" Icon.QuickUp
[party_ Quick 15]
, { name = "PI:NAME:<NAME>END_PI"
, id = 240
, rarity = 5
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ When "defeated" << Grant Party 3 DefenseUp <| Range 20 25 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 241
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Party 3 (CardUp Buster) <| Range 10 15 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 242
, rarity = 5
, icon = Icon.Road
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Bonus MysticCode Percent <| Flat 2 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 243
, rarity = 3
, icon = Icon.SwordUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 3 AttackUp <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 244
, rarity = 3
, icon = Icon.QuickUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 10 15
, Debuff Self 0 DefenseDown <| Flat 10
]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 245
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ equipped Assassin << Grant Self 0 NPUp <| Range 15 25 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 246
, rarity = 3
, icon = Icon.HealUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 HealingReceived <| Range 10 20 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 247
, rarity = 3
, icon = Icon.NobleUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 NPGen <| Range 5 10
, To Self GaugeUp <| Range 25 40
]
, bond = Nothing
, limited = False
}
, bond 248 "Tamamo's Club" "Tamamo-no-Mae" Icon.ArtsUp
[party_ Arts 15]
, bond 249 "Headband of Resolve" "PI:NAME:<NAME>END_PI" Icon.ExclamationUp
[party CritUp 25]
, bond 250 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI & PI:NAME:<NAME>END_PI" Icon.ExclamationUp
[party CritUp 25]
, bond 251 "Gazing Upon PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.QuickUp
[party_ Quick 15]
, bond 252 "Star of Prophecy" "PI:NAME:<NAME>END_PI" Icon.BeamUp
[self NPUp 30, atkChance 30 << Grant Self 3 CritUp <| Flat 10]
, bond 253 "PI:NAME:<NAME>END_PIkate's Staff" "PI:NAME:<NAME>END_PI" Icon.ArtsUp
[party_ Arts 15]
, bond 254 "Formless Island" "PI:NAME:<NAME>END_PI" Icon.NobleUp
[party NPGen 15]
, bond 255 "Cask of the Wise" "PI:NAME:<NAME>END_PI" Icon.QuickUp
[party_ Quick 15]
, bond 256 "PI:NAME:<NAME>END_PI's Arm" "Hassan of the Cursed Arm" Icon.ReaperUp
[party DeathUp 20]
, bond 257 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.QuickUp
[party_ Quick 15]
, { name = "PI:NAME:<NAME>END_PI"
, id = 258
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 15 20
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 259
, rarity = 4
, icon = Icon.ArtsUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 NPGen <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "Hidden Sword: Pheasant Reversal"
, id = 260
, rarity = 3
, icon = Icon.QuickUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 3 5
, Grant Self 0 CritUp <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "Golden Sumo: Boulder Tournament"
, id = 261
, rarity = 5
, icon = Icon.SwordUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 AttackUp <| Range 10 15
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, { name = "Hot Spring Under the Moon"
, id = 262
, rarity = 5
, icon = Icon.ExclamationUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 20 25
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "Origin Bullet"
, id = 263
, rarity = 5
, icon = Icon.ShieldBreak
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 IgnoreInvinc Full
, Grant Self 0 (Special AttackUp <| VsClass Caster) <| Range 35 40
]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 264
, rarity = 4
, icon = Icon.DamageUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 DamageUp <| Range 400 600
, Grant Self 0 CritUp <| Range 15 20
]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 265
, rarity = 3
, icon = Icon.Noble
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ When "defeated" << To Party GaugeUp <| Range 10 15 ]
, bond = Nothing
, limited = False
}
, bond 266 "Who Am I?" "PI:NAME:<NAME>END_PI" Icon.BeamUp
[party NPUp 20]
, bond 267 "The Misty Night of London" "PI:NAME:<NAME>END_PI" Icon.ExclamationUp
[party CritUp 25]
, bond 268 "Wonderland" "PI:NAME:<NAME>END_PI" Icon.ExclamationUp
[party CritUp 15, party HealingReceived 10]
, bond 269 "PI:NAME:<NAME>END_PI King" "PI:NAME:<NAME>END_PI" Icon.ArtsUp
[party_ Arts 15]
, bond 270 "PI:NAME:<NAME>END_PIori" "PI:NAME:<NAME>END_PI" Icon.QuickUp
[party_ Quick 15]
, bond 271 "Etiquette of Nine Guests" "PI:NAME:<NAME>END_PI" Icon.BeamUp
[self NPUp 30, atkChance 30 << Grant Self 3 DeathUp <| Flat 30]
, bond 272 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 15]
, bond 273 "What can be Left Behind" "PI:NAME:<NAME>END_PI" Icon.Shield
[ When "defeated" << Times 1 <| Grant Party 0 Invincibility Full
, When "defeated" << Grant Party 3 DamageCut <| Flat 1000
]
, bond 274 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 15]
, bond 275 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.BeamUp
[party NPUp 20]
, gift 276 "PI:NAME:<NAME>END_PI" Icon.SwordUp
[self AttackUp 10, self StarsPerTurn 3]
, { name = "PI:NAME:<NAME>END_PI"
, id = 277
, rarity = 5
, icon = Icon.StarUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 GatherUp <| Range 400 600
, Grant Self 0 (CardUp Arts) <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 278
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 10 15
, To Self GaugeUp <| Range 50 60
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 279
, rarity = 4
, icon = Icon.Bullseye
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 SureHit Full
, Grant Self 0 (CardUp Buster) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 280
, rarity = 3
, icon = Icon.StarHaloUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 StarUp <| Range 5 10
, Grant Self 0 CritUp <| Range 5 10
]
, bond = Nothing
, limited = True
}
, bond 281 "Arm of Raiden" "PI:NAME:<NAME>END_PI" Icon.BeamUp
[party NPUp 20]
, bond 282 "Endowed Hero" "PI:NAME:<NAME>END_PI" Icon.BeamUp
[self NPUp 30, self GatherUp 1000]
, bond 283 "Light of the Deprived" "PI:NAME:<NAME>END_PI" Icon.AllUp
[party_ Quick 8, party_ Arts 8, party_ Buster 8]
, bond 284 "Procedure to Humanity" "PI:NAME:<NAME>END_PI" Icon.QuickUp
[party_ Quick 15]
, bond 285 "Black Helmet" "PI:NAME:<NAME>END_PI (Lancer Alter)" Icon.NobleUp
[party NPGen 15]
, bond 286 "Legend of the Gallic War" "PI:NAME:<NAME>END_PI" Icon.QuickUp
[party_ Quick 15]
, bond 287 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.BeamUp
[party NPUp 20]
, bond 288 "Encounter at Gojou Bridge" "PI:NAME:<NAME>END_PI" Icon.NobleRedUp
[party NPFromDamage 20]
, bond 289 "Impure Death Mask" "Phantom of the Opera" Icon.QuickUp
[party_ Quick 15]
, bond 290 "Really Convenient" "PI:NAME:<NAME>END_PI" Icon.NobleUp
[party NPGen 15]
, { name = "PI:NAME:<NAME>END_PI!"
, id = 291
, rarity = 5
, icon = Icon.ShieldBreak
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 IgnoreInvinc Full
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 292
, rarity = 5
, icon = Icon.ExclamationUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 15 20
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 293
, rarity = 4
, icon = Icon.QuickUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Times 1 <| Grant Self 0 Evasion Full
, Grant Self 0 (CardUp Quick) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 294
, rarity = 3
, icon = Icon.ArtsUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 3 5
, Grant Self 0 (CardUp Arts) <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 295
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 10 15
, To Self GaugeUp <| Range 50 60
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 296
, rarity = 5
, icon = Icon.Kneel
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Times 1 << Grant Self 0 Guts <| Flat 1
, Grant Self 0 NPGen <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI WPI:NAME:<NAME>END_PI"
, id = 297
, rarity = 4
, icon = Icon.HealTurn
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 HealPerTurn <| Range 200 300
, Grant Self 0 (CardUp Arts) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI (Void's Dust Flavor)"
, id = 298
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DamageCut <| Range 100 200
, Grant Self 0 DebuffResist <| Range 5 10
]
, bond = Nothing
, limited = True
}
, bond 299 "Annihilation List" "Mysterious Heroine X" Icon.DamageUp
[party (Special AttackUp <| VsClass Saber) 20]
, bond 300 "Imperishable Flames" "Brynhild" Icon.BusterUp
[party_ Buster 10, party NPGen 10]
, bond 301 "Ring of Bay Laurel" "PI:NAME:<NAME>END_PI (Bride)" Icon.ArtsUp
[party_ Arts 15]
, bond 302 "Final Battle" "Beowulf" Icon.DamageUp
[party (Special AttackUp <| VsTrait Dragon) 20]
, bond 303 "Bratan of Wisdom" "PI:NAME:<NAME>END_PIionn mac Cumhaill" Icon.ArtsUp
[party_ Arts 10, party NPUp 10]
, bond 304 "Prelati's Spellbook" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 20, demeritAll DebuffVuln 20]
, bond 305 "Parasitic Bomb" "PI:NAME:<NAME>END_PIphistopPI:NAME:<NAME>END_PI" Icon.BeamUp
[party NPUp 20]
, bond 306 "Seethe of a Warrior" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 10, party NPUp 10]
, bond 307 "My Loathsome Life" "PI:NAME:<NAME>END_PI" Icon.ReaperUp
[party DeathUp 10, party NPGen 10]
, bond 308 "There is No Love Here" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, { name = "PI:NAME:<NAME>END_PI"
, id = 309
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 NPGen <| Range 25 30
, To Self GaugeUp <| Range 40 50
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI on Sight"
, id = 310
, rarity = 4
, icon = Icon.ArtsUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI!"
, id = 311
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DamageCut <| Range 100 200
, Grant Self 0 HealingReceived <| Range 5 10
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 312
, rarity = 4
, icon = Icon.BusterUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 10 15
, Grant Self 0 NPUp <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 313
, rarity = 4
, icon = Icon.ArtsUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 NPUp <| Range 8 10
]
, bond = Nothing
, limited = True
}
, bond 315 "Mugashiki—Shinkuu Myou" "PI:NAME:<NAME>END_PI (Saber)" Icon.ArtsUp
[party_ Arts 15]
, bond 316 "Frontliner's Flag" "PI:NAME:<NAME>END_PI" Icon.DamageUp
[ party (Special AttackUp <| VsTrait Undead) 20
, party (Special AttackUp <| VsTrait Demonic) 20
]
, bond 317 "Chateau d'If" "PI:NAME:<NAME>END_PI" Icon.QuickUp
[party_ Quick 15]
, bond 318 "Unlimited Pancakes" "Medea (Lily)" Icon.HealUp
[party HealingReceived 30]
, bond 319 "Red Leather Jacket" "PI:NAME:<NAME>END_PI (Assassin)" Icon.ReaperUp
[party DeathUp 30]
, bond 320 "Otherworldly Mystical Horse" "PI:NAME:<NAME>END_PI" Icon.Dodge
[self NPUp 30, Times 1 <| Grant Party 0 Evasion Full]
, bond 321 "Letter From a Friend" "PI:NAME:<NAME>END_PI (Caster)" Icon.BusterUp
[party_ Buster 20, demeritAll StarDown 20]
, bond 322 "Hound of Culann" "Cu PI:NAME:<NAME>END_PIulPI:NAME:<NAME>END_PI (Prototype)" Icon.DamageUp
[party (Special AttackUp <| VsTrait WildBeast) 20]
, bond 323 "Radiance of the Goddess (Euryale)" "Euryale" Icon.ArtsUp
[party_ Arts 15]
, bond 324 "Hero's Armament" "Hektor" Icon.BeamUp
[party NPUp 20]
, { name = "PI:NAME:<NAME>END_PI"
, id = 325
, rarity = 5
, icon = Icon.BeamUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 NPUp <| Range 15 20
, Grant Self 0 CritUp <| Range 15 20
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 326
, rarity = 4
, icon = Icon.ShieldUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 8 20
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 327
, rarity = 3
, icon = Icon.BusterUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 10 15
, Debuff Self 0 DebuffVuln <| Flat 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 328
, rarity = 5
, icon = Icon.Kneel
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Times 1 << Grant Self 0 Guts <| Flat 1
, To Self GaugeUp <| Range 10 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 329
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 15 20
, Grant Self 0 CritUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 330
, rarity = 5
, icon = Icon.Rainbow
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Bonus Bond Percent <| Range 2 10 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 331
, rarity = 3
, icon = Icon.StarUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 GatherUp <| Range 200 300 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PIverted MoPI:NAME:<NAME>END_PI the PI:NAME:<NAME>END_PI"
, id = 332
, rarity = 3
, icon = Icon.StarTurn
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 1 2
, Grant Self 0 DebuffResist <| Range 5 10
]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 333
, rarity = 3
, icon = Icon.ReaperUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 DeathUp <| Range 5 10 ]
, bond = Nothing
, limited = False
}
, bond 334 "Indefatigable" "Florence Nightingale" Icon.BusterUp
[party_ Buster 10, party HealingReceived 20]
, bond 335 "One-Man War" "Cu Chulainn (Alter)" Icon.Kneel
[self NPUp 30, gutsPercent 20]
, bond 336 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.NobleUp
[party NPGen 15]
, bond 337 "Indestructible Blade" "PI:NAME:<NAME>END_PI" Icon.ExclamationUp
[party CritUp 25]
, bond 338 "Concealed Goddess" "PI:NAME:<NAME>END_PI" Icon.DamageUp
[party (Special AttackUp <| VsClass Assassin) 20]
, bond 339 "Lights of Civilization" "PI:NAME:<NAME>END_PI" Icon.NobleUp
[party NPGen 15]
, bond 340 "Reaching the Zenith of My Skill" "PI:NAME:<NAME>END_PI" Icon.ArtsUp
[party_ Arts 15]
, bond 341 "KPI:NAME:<NAME>END_PI's Oath" "PI:NAME:<NAME>END_PI" Icon.ArtsUp
[party_ Quick 10, party_ Arts 10]
, bond 342 "Elemental" "PI:NAME:<NAME>END_PI" Icon.ArtsUp
[party_ Arts 10, party NPUp 10]
, bond 343 "NEO Difference Engine" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, { name = "PI:NAME:<NAME>END_PI"
, id = 344
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 15 20
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 345
, rarity = 4
, icon = Icon.ArtsUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 NPGen <| Range 5 10
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI's Hospitality"
, id = 346
, rarity = 3
, icon = Icon.NobleUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 NPGen <| Range 5 10
, Grant Self 0 DefenseUp <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 347
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 10 15
, Grant Self 0 NPUp <| Range 20 25
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 348
, rarity = 4
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 NPUp <| Range 15 20
, Grant Self 0 HealingReceived <| Range 10 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 349
, rarity = 3
, icon = Icon.AllUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 2 3
, Grant Self 0 (CardUp Buster) <| Range 2 3
, Grant Self 0 (CardUp Quick) <| Range 2 3
, Grant Self 0 CritUp <| Range 5 10
]
, bond = Nothing
, limited = True
}
, bond 350 "Hell of Blazing Punishment" "PI:NAME:<NAME>END_PI (Alter)" Icon.BusterUp
[party_ Buster 15]
, bond 351 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.SwordUp
[party AttackUp 15]
, bond 352 "White Dragon" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, bond 353 "The Sun Shines Here" "Emiya (Assassin)" Icon.ArtsQuickUp
[party_ Quick 10, party_ Arts 10]
, bond 354 "Dress of Heaven" "PI:NAME:<NAME>END_PI (Holy Grail)" Icon.HealUp
[party HealingReceived 30]
, bond 355 "Manifestation of the Golden Rule" "Gilgamesh (Child)" Icon.NobleUp
[party NPGen 15]
, bond 356 "Spirit of the Vast Land" "PI:NAME:<NAME>END_PI" Icon.NobleUp
[party NPGen 15]
, bond 357 "Extolling the Revolver" "Billy the Kid" Icon.ExclamationUp
[party CritUp 25]
, bond 358 "Library of Hundred Men" "Hassan of the Hundred Personas" Icon.AllUp
[party_ Buster 8, party_ Quick 8, party_ Arts 8]
, bond 359 "Final Fragment" "PI:NAME:<NAME>END_PI" Icon.DamageUp
[ gutsPercent 20
, self (Special AttackUp <| VsClass Beast1) 200
, self (Special AttackUp <| VsClass Beast2) 200
, self (Special AttackUp <| VsClass Beast3L) 200
, self (Special AttackUp <| VsClass Beast3R) 200
]
, gift 360 "Fate/EXTELLA" Icon.ExclamationUp
[self CritUp 15, self StarsPerTurn 3]
, gift 361 "Spiritron Portrait: PI:NAME:<NAME>END_PI" Icon.Road
[Bonus EXP Units <| Flat 50]
, gift 362 "Spiritron Portrait: Nameless" Icon.Road
[Bonus EXP Units <| Flat 50]
, gift 363 "Spiritron Portrait: Tamamo-no-Mae" Icon.Road
[Bonus EXP Units <| Flat 50]
, gift 364 "Spiritron Portrait: Karna" Icon.Road
[Bonus EXP Units <| Flat 50]
, gift 365 "Spiritron Portrait: Altera" Icon.Road
[Bonus EXP Units <| Flat 50]
, gift 366 "Spiritron Portrait: Gilgamesh" Icon.Road
[Bonus EXP Units <| Flat 50]
, bond 367 "Divine Wine—PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI-PI:NAME:<NAME>END_PI" Icon.ArtsQuickUp
[party_ Quick 10, party_ Arts 10]
, bond 368 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI-PI:NAME:<NAME>END_PI-PI:NAME:<NAME>END_PIikPI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 10, party CritUp 15]
, bond 369 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.BusterArtsUp
[party_ Arts 10, party_ Buster 10]
, bond 370 "Bone Sword (Nameless)" "PI:NAME:<NAME>END_PIaki-DPI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, bond 371 "Unit Golden Bear" "Sakata Kintoki (Rider)" Icon.StarHaloUp
[party StarUp 20]
, bond 372 "Gringolet" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 15]
, bond 373 "But I Lied Once" "PI:NAME:<NAME>END_PI" Icon.ExclamationUp
[party CritUp 25]
, bond 374 "Exercising the Royal Authority" "PI:NAME:<NAME>END_PI" Icon.NobleUp
[party NPGen 10, party NPUp 10]
, bond 375 "Mask of a Demon" "Fuuma \"Evil-wind\" Kotarou" Icon.QuickUp
[party_ Quick 15]
, bond 376 "Cook Despite of Exhaustion" "PI:NAME:<NAME>END_PI" Icon.HealTurn
[party HealPerTurn 500]
, bond 377 "King's Horse" "PI:NAME:<NAME>END_PI (Lancer)" Icon.SwordUp
[party AttackUp 10, party NPUp 10]
, bond 378 "All-Encompassing Wisdom" "PI:NAME:<NAME>END_PI" Icon.BeamUp
[party NPUp 20]
, bond 379 "Sunset Beach" "Tamamo-no-Mae (Lancer)" Icon.QuickBusterUp
[party_ Quick 10, party_ Buster 10]
, bond 380 "Lady of the Lake" "Lancelot (Saber)" Icon.NobleUp
[party NPGen 10, party CritUp 10]
, bond 381 "ReminiscPI:NAME:<NAME>END_PI of the Summer"
"PI:NAME:<NAME>END_PI (Caster)" Icon.ExclamationUp
[party CritUp 25]
, bond 382 "Currently in the Middle of a Shower"
"PI:NAME:<NAME>END_PI & PI:NAME:<NAME>END_PI (Archer)" Icon.BusterArtsUp
[party_ Buster 10, party_ Arts 10]
, bond 383 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI (Rider)" Icon.BeamUp
[party NPUp 20]
, bond 384 "Beach Love Letter (Terror)" "PI:NAME:<NAME>END_PI (Lancer)" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, bond 385 "Lost Right Arm" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 10, party NPGen 10]
, bond 386 "Proof of Existence" "Hassan of the Serenity" Icon.QuickUp
[party_ Quick 15]
, { name = "PI:NAME:<NAME>END_PIility"
, id = 387
, rarity = 5
, icon = Icon.ArtsQuickUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 10 15
, Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 NPGen <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "Reading on the Holy Night"
, id = 388
, rarity = 4
, icon = Icon.NobleUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 NPGen <| Range 15 20
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 389
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 3 5
, Grant Self 0 DamageCut <| Range 100 200
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 390
, rarity = 5
, icon = Icon.Noble
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ To Self GaugeUp <| Range 30 50
, Grant Self 0 CritUp <| Range 10 15
, Grant Self 0 NPUp <| Range 10 15
]
, bond = Nothing
, limited = True
}
, bond 391 "Champion Cup" "PI:NAME:<NAME>END_PI (Archer)" Icon.SwordUp
[party AttackUp 15]
, bond 392 "Phantasmal Summoning (Install)" "PI:NAME:<NAME>END_PI" Icon.AllUp
[party_ Buster 8, party_ Quick 8, party_ Arts 8]
, bond 393 "Serpent of Fate" "PI:NAME:<NAME>END_PI" Icon.BeamUp
[party NPUp 25, demeritAll DefenseDown 10]
, bond 394 "Holy Knuckle" "PI:NAME:<NAME>END_PI (Ruler)" Icon.BusterUp
[party_ Buster 15]
, bond 395 "Minimal Prudence" "PI:NAME:<NAME>END_PI (Assassin)" Icon.QuickUp
[party_ Quick 15]
, bond 396 "Sharing of Pain" "PI:NAME:<NAME>END_PI" Icon.ExclamationUp
[party CritUp 30, demeritAll HealthLoss 200]
, bond 397 "Creed at the Bottom of the Earth" "PI:NAME:<NAME>END_PI (EXTRA)" Icon.QuickBusterUp
[party_ Quick 10, party_ Buster 10]
, bond 398 "Invitation to Halloween" "PI:NAME:<NAME>END_PI (Brave)" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, gift 399 "First Order" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, { name = "PI:NAME:<NAME>END_PI"
, id = 400
, rarity = 5
, icon = Icon.SunUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ To Self GaugeUp <| Range 50 60
, Times 1 << Grant Self 0 Overcharge <| Flat 2
]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 401
, rarity = 4
, icon = Icon.StarUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 GatherUp <| Range 300 400
, Grant Self 0 DamageCut <| Range 300 400
]
, bond = Nothing
, limited = False
}
, { name = "Over the Cuckoo's Nest"
, id = 414
, rarity = 3
, icon = Icon.Crystal
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Bonus QPQuest Units <| Flat 2019
, Grant Self 0 DamageUp <| Range 0 19
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 415
, rarity = 4
, icon = Icon.HealTurn
, stats = { base = { atk = 0, hp = 2019 }
, max = { atk = 0, hp = 2019 }
}
, effect = [ Grant Self 0 HealPerTurn <| Flat 100 ]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 418
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ equipped Archer << Grant Self 0 NPUp <| Range 15 25 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI YPI:NAME:<NAME>END_PI"
, id = 419
, rarity = 3
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 (Resist AttackDown) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Collection of Mysterious Masks"
, id = 420
, rarity = 3
, icon = Icon.StarUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 GatherUp <| Range 100 200
, Grant Self 0 StarUp <| Range 5 10
]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 421
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 NPGen <| Range 25 30
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 422
, rarity = 5
, icon = Icon.ArtsUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 15 20
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 424
, rarity = 4
, icon = Icon.HealUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 HealingReceived <| Range 15 20
, Grant Self 0 NPGen <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 425
, rarity = 3
, icon = Icon.HealUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 HealingReceived <| Range 5 10
, Grant Self 0 StarUp <| Range 5 10
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PIance of a VPI:NAME:<NAME>END_PI"
, id = 426
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DamageCut <| Range 100 200
, Grant Self 0 StarUp <| Range 5 10
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 427
, rarity = 5
, icon = Icon.ShieldBreak
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 IgnoreInvinc Full
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 428
, rarity = 5
, icon = Icon.DamageUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (Special AttackUp <| VsTrait Divine) <| Range 25 30
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, bond 403 "Seven-Headed Warhammer Sita" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 20, demeritAll DebuffVuln 20]
, bond 404 "Flower of Humbaba" "Enkidu" Icon.HealTurn
[party HealPerTurn 500]
, bond 405 "Piedra del Sol" "Quetzalcoatl" Icon.BusterArtsUp
[party_ Arts 10, party_ Buster 10]
, bond 406 "Door to the Ocean" "Jeanne d'Arc Alter Santa Lily" Icon.HealUp
[party HealingReceived 30]
, bond 407 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI (Caster)" Icon.BeamUp
[party NPUp 20]
, bond 408 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 10, party NPGen 10]
, bond 409 "Uncertainty About the Future" "Medusa (Lancer)" Icon.QuickUp
[party_ Quick 10, party NPGen 10]
, bond 410 "Primeval Flame" "Jaguar Warrior" Icon.BusterUp
[party_ Buster 15]
, bond 411 "The Furthest Tower" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 10, party CritUp 15]
, bond 416 "Buddhism of Emptiness" "PI:NAME:<NAME>END_PI" Icon.HoodUp
[self NPUp 30, Times 3 <| Grant Self 0 DebuffResist Full]
, bond 417 "The Rift of the Valley" "\"First Hassan\"" Icon.HoodUp
[self DebuffResist 100]
, bond 429 "Dark Knight-Kun" "Mysterious Heroine X (Alter)" Icon.DamageUp
[party (Special AttackUp <| VsClass Saber) 20]
, bond 546 "The Dynamics of an Asteroid" "PI:NAME:<NAME>END_PI" Icon.BeamUp
[Grant (AlliesType Evil) 0 NPUp <| Flat 25]
, bond 547 "Kanshou & Bakuya (Revolvers)" "EMIYA (Alter)" Icon.AllUp
[party_ Quick 8, party_ Arts 8, party_ Buster 8]
, bond 548 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.QuickUp
[self (CardUp Quick) 10, party NPGen 10]
, bond 549 "108 Stars of Destiny" "PI:NAME:<NAME>END_PI" Icon.QuickUp
[party_ Quick 10, party CritUp 15]
, { name = "Conversation on the Hot Sands"
, id = 550
, rarity = 5
, icon = Icon.StarUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 GatherUp <| Range 400 600
, Grant Self 0 CritUp <| Range 20 25
]
, bond = Nothing
, limited = True
}
, { name = "The Dantes Files: The Case of the Spring Jaunt"
, id = 551
, rarity = 5
, icon = Icon.ShieldBreak
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 IgnoreInvinc Full
, Grant Self 0 (CardUp Arts) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 553
, rarity = 4
, icon = Icon.HealUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 HealingReceived <| Range 8 10
, Grant Self 0 DebuffResist <| Range 8 10
, Grant Self 0 DefenseUp <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 554
, rarity = 4
, icon = Icon.CrosshairUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 1 Taunt Full
, Grant Self 1 NPGen <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 555
, rarity = 4
, icon = Icon.Star
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ To Party GainStars <| Range 10 20
, Grant Self 0 (CardUp Buster) <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 556
, rarity = 3
, icon = Icon.AllUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 2 3
, Grant Self 0 (CardUp Buster) <| Range 2 3
, Grant Self 0 (CardUp Quick) <| Range 2 3
, Grant Self 0 MentalResist <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 557
, rarity = 3
, icon = Icon.StarHaloUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ Grant Self 0 CritUp <| Range 4 8
, Grant Self 0 StarUp <| Range 4 8
, Grant Self 0 NPUp <| Range 4 8
]
, bond = Nothing
, limited = True
}
, bond 559 "Garden" "PI:NAME:<NAME>END_PI (Prototype)" Icon.SwordUp
[party AttackUp 15]
, { name = "PI:NAME:<NAME>END_PI the SixPI:NAME:<NAME>END_PI Heaven"
, id = 560
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 15 20
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PIress of the Sun"
, id = 561
, rarity = 5
, icon = Icon.ExclamationUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 CritUp <| Range 15 20
, Grant Self 0 StarUp <| Range 15 20
, To Self GaugeUp <| Range 25 40
]
, bond = Nothing
, limited = True
}
, { name = "Wolves of MiPI:NAME:<NAME>END_PI"
, id = 562
, rarity = 5
, icon = Icon.QuickBusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 8 10
, Grant Self 0 (CardUp Quick) <| Range 8 10
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "A Stroll in the Spring Breeze"
, id = 563
, rarity = 4
, icon = Icon.NobleUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 NPGen <| Range 15 20
, Grant Self 0 DamageCut <| Range 300 400
]
, bond = Nothing
, limited = True
}
, { name = "The Flower of High Society"
, id = 564
, rarity = 3
, icon = Icon.ArtsQuickUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 4 6
, Grant Self 0 (CardUp Quick) <| Range 4 6
]
, bond = Nothing
, limited = True
}
, bond 567 "Haori of the Oath" "Hijikata TPI:NAME:<NAME>END_PI" Icon.Kneel
[Times 1 << Grant Self 0 Guts <| Flat 1, self CritUp 30]
, bond 568 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.BeamUp
[party NPUp 25, demeritAll DefenseDown 10]
, { name = "PI:NAME:<NAME>END_PIquering the Great Sea of Stars"
, id = 569
, rarity = 5
, icon = Icon.BusterUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 15 20
, Grant Self 0 NPGen <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 570
, rarity = 5
, icon = Icon.QuickUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 8 10
, Grant Self 0 NPUp <| Range 8 10
, To Self GaugeUp <| Range 30 50
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PImonition of Beginnings"
, id = 572
, rarity = 4
, icon = Icon.StarTurn
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 StarsPerTurn <| Range 2 3
, Grant Self 0 GaugePerTurn <| Range 2 3
]
, bond = Nothing
, limited = True
}
, bond 575 "The Radiant One" "Meltryllis" Icon.QuickUp
[self (CardUp Quick) 20, self CritUp 30, demeritOthers CritDown 10]
, bond 576 "Don't Avert Your Eyes Away From Me" "Passionlip" Icon.BusterUp
[party_ Buster 20, demeritAll DebuffVuln 20]
, bond 577 "A Passerby's Dream" "BB" Icon.AllUp
[party_ Quick 8, party_ Arts 8, party_ Buster 8]
, bond 578 "Tenma's Spring Training" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[self (CardUp Buster) 10, party NPUp 10]
, bond 579 "Dream from the Cradle" "PI:NAME:<NAME>END_PI" Icon.ArtsUp
[self (CardUp Arts) 20, self HPUp 3000, demeritOthers HPDown 1000]
, { name = "PI:NAME:<NAME>END_PI"
, id = 581
, rarity = 3
, icon = Icon.HoodUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 (Resist DefenseDown) <| Range 25 30 ]
, bond = Nothing
, limited = False
}
, { name = "Ruined Church"
, id = 580
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 (Special DefenseUp <| VsTrait Male) <| Range 15 20 ]
, bond = Nothing
, limited = False
}
, gift 583 "Fate/Apocrypha" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, bond 586 "King Shahryay's Bedchamber" "PI:NAME:<NAME>END_PI" Icon.HoodUp
[self DebuffResist 100]
, bond 587 "The Palace of Luoyang" "PI:NAME:<NAME>END_PI" Icon.QuickUp
[party_ Quick 20, demeritAll DefenseDown 10]
, bond 588 "Military Sash of the War God" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 20, demeritAll DefenseDown 10]
, bond 589 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 10, party NPGen 10]
, { name = "PI:NAME:<NAME>END_PI"
, id = 590
, rarity = 5
, icon = Icon.ArtsQuickUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 (CardUp Quick) <| Range 8 10
, To Self GaugeUp <| Range 50 60
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 591
, rarity = 4
, icon = Icon.NobleTurn
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 GaugePerTurn <| Range 4 5
, Grant Self 0 (CardUp Arts) <| Range 10 12
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 592
, rarity = 3
, icon = Icon.StarUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 GatherUp <| Range 100 200
, Grant Self 0 (CardUp Quick) <| Range 2 3
]
, bond = Nothing
, limited = True
}
, gift 593 "Learning With Manga! FGO" Icon.Road
[Bonus EXP Units <| Flat 50]
, gift 607 "Formal Portrait: Atalante" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, bond 641 "Frontier" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 15]
, bond 642 "The Vaunted One" "PI:NAME:<NAME>END_PI" Icon.QuickUp
[party_ Quick 10, party CritUp 15]
, { name = "PI:NAME:<NAME>END_PI"
, id = 643
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 NPGen <| Range 25 30
, Grant Self 0 NPUp <| Range 10 15
, Grant Self 0 CritUp <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 644
, rarity = 4
, icon = Icon.BusterArtsUp
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 (CardUp Buster) <| Range 8 10
, Grant Self 0 NPGen <| Range 8 10
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 645
, rarity = 3
, icon = Icon.ShieldUp
, stats = { base = { atk = 0, hp = 300 }
, max = { atk = 0, hp = 1500 }
}
, effect = [ Grant Self 0 DefenseUp <| Range 3 5
, Grant Self 0 (CardUp Quick) <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 646
, rarity = 5
, icon = Icon.StarHaloUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 StarUp <| Range 20 25
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, bond 647 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI (Caster)" Icon.BusterUp
[party_ Buster 10, party NPGen 10]
, bond 648 "Handy-Bandages" "PI:NAME:<NAME>END_PI (Saber)" Icon.QuickUp
[self (CardUp Quick) 10, party NPGen 10]
, bond 649 "A Gift From Ra" "PI:NAME:<NAME>END_PIitocris (Assassin)" Icon.ArtsUp
[party_ Arts 10, party NPGen 10]
, bond 650 "PI:NAME:<NAME>END_PI Fubu -2017 Summer.ver-" "PI:NAME:<NAME>END_PI (Berserker)" Icon.BusterUp
[party_ Buster 15, party CritUp 15, demeritAll DefenseDown 10]
, bond 651 "Champion's Cup of the Goddess" "PI:NAME:<NAME>END_PI (Rider)" Icon.QuickUp
[party_ Quick 10, party NPUp 10]
, bond 656 "Mop of Selection" "PI:NAME:<NAME>END_PI (Rider Alter)" Icon.SwordUp
[party AttackUp 20, demeritAll DefenseDown 15]
, bond 657 "NYARF!" "PI:NAME:<NAME>END_PI (Archer)" Icon.ArtsQuickUp
[party_ Quick 10, party_ Arts 10]
, bond 658 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI (Lancer)" Icon.QuickBusterUp
[party_ Quick 10, party_ Buster 10]
, { name = "PI:NAME:<NAME>END_PI"
, id = 660
, rarity = 5
, icon = Icon.BusterArtsUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 (CardUp Buster) <| Range 8 10
, To Self GaugeUp <| Range 50 60
]
, bond = Nothing
, limited = True
}
, { name = "Divine Three-Legged Race"
, id = 661
, rarity = 5
, icon = Icon.Kneel
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Times 1 << Grant Self 0 Guts <| Flat 1
, Grant Self 0 NPUp <| Range 15 20
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI End"
, id = 666
, rarity = 5
, icon = Icon.StarUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 GatherUp <| Range 400 600
, Grant Self 0 (CardUp Buster) <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 667
, rarity = 4
, icon = Icon.BusterArtsUp
, stats = { base = { atk = 400, hp = 0 }
, max = { atk = 1500, hp = 0 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 (CardUp Buster) <| Range 8 10
, Grant Self 0 NPUp <| Range 5 10
]
, bond = Nothing
, limited = True
}
, bond 669 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.QuickUp
[party_ Quick 10, party NPGen 10]
, gift 670 "Heroic Costume: Medusa" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, gift 671 "Heroic Costume: PI:NAME:<NAME>END_PI" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, gift 672 "Heroic Costume: PI:NAME:<NAME>END_PI" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, gift 673 "Heroic Costume: PI:NAME:<NAME>END_PI" Icon.Road
[Bonus MysticCode Units <| Flat 50]
, bond 675 "A Wish Spanning 3 Generations" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 10, party NPUp 10]
, bond 676 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.ArtsQuickUp
[party_ Quick 10, party_ Arts 10]
, bond 677 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.QuickUp
[party_ Quick 10, party CritUp 15]
, bond 678 "Double-Tiered PI:NAME:<NAME>END_PIasa" "PI:NAME:<NAME>END_PI" Icon.ArtsUp
[party_ Arts 10, party NPUp 10]
, bond 679 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.QuickBusterUp
[party_ Quick 10, party_ Buster 10]
, bond 686 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.NobleUp
[party NPGen 15]
, bond 687 "Electrologica Diagram" "Mecha Eli-chan" Icon.BusterUp
[party_ Buster 20, demeritAll DebuffVuln 20]
, bond 688 "PI:NAME:<NAME>END_PI" "Mecha Eli-chan MkII" Icon.BusterUp
[party_ Buster 20, demeritAll DebuffVuln 20]
, gift 689 "Pray Upon the Sword, Wish Upon Life" Icon.Road
[Bonus EXP Units <| Flat 50]
, { name = "PI:NAME:<NAME>END_PI"
, id = 691
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ equipped Caster << Grant Self 0 NPUp <| Range 15 25 ]
, bond = Nothing
, limited = False
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 674
, rarity = 3
, icon = Icon.BeamUp
, stats = { base = { atk = 200, hp = 0 }
, max = { atk = 1000, hp = 0 }
}
, effect = [ equipped Saber << Grant Self 0 NPUp <| Range 15 25 ]
, bond = Nothing
, limited = False
}
, gift 690 "FGO VR Mash Kyrielight" Icon.Road
[Bonus EXP Units <| Flat 50 ]
, bond 692 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.NobleUp
[party NPGen 10, party NPUp 10]
, bond 693 "Universe Ring" "PI:NAME:<NAME>END_PI" Icon.QuickUp
[party_ Quick 10, party NPUp 10]
, bond 694 "Tributes to King PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.BusterArtsUp
[party_ Arts 10, party_ Buster 10]
, bond 695 "PI:NAME:<NAME>END_PI" "PI:NAME:<NAME>END_PI" Icon.BeamUp
[Times 3 <| Grant Self 0 DeathResist Full]
, bond 700 "Blooming Flowers in Kur" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 10, party NPUp 10]
, bond 701 "The Rainbow Soaring Under the Night Sky" "Attila the San(ta)" Icon.QuickUp
[party_ Quick 10, party CritUp 15]
, { name = "PI:NAME:<NAME>END_PI"
, id = 704
, rarity = 5
, icon = Icon.ArtsUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 CritUp <| Range 10 15
, To Self GaugeUp <| Range 40 50
]
, bond = Nothing
, limited = True
}
, bond 709 "Painting of a Dragon Passing Over Mount Fuji" "PI:NAME:<NAME>END_PI" Icon.Kneel
[Times 1 << Grant Self 0 GutsPercent <| Flat 20]
, bond 717 "Mysterious Glass" "PI:NAME:<NAME>END_PI" Icon.BusterUp
[party_ Buster 10, party NPUp 10]
, bond 762 "Summer Rain" "PI:NAME:<NAME>END_PI" Icon.BusterArtsUp
[party_ Arts 10, party_ Buster 10]
, { name = "Chaldea Special Investigation Unit"
, id = 768
, rarity = 4
, icon = Icon.Bullseye
, stats = { base = { atk = 200, hp = 320 }
, max = { atk = 750, hp = 1200 }
}
, effect = [ Grant Self 0 SureHit Full
, Grant Self 0 (CardUp Arts) <| Range 5 8
, Grant Self 0 (CardUp Buster) <| Range 5 8
]
, bond = Nothing
, limited = True
}
, { name = "The Star of Camelot"
, id = 764
, rarity = 5
, icon = Icon.StarUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 GatherUp <| Range 400 600
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI Dantes Files: Undercover in a Foreign Land"
, id = 765
, rarity = 5
, icon = Icon.BusterArtsUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 (CardUp Buster) <| Range 10 15
, Grant Self 0 NPGen <| Range 10 15
]
, bond = Nothing
, limited = True
}
, bond 787 "OTMA" "PI:NAME:<NAME>END_PI" Icon.ArtsUp
[party_ Arts 10, party NPUp 10]
, bond 788 "Calydon's Hide" "Atalante (Alter)" Icon.QuickUp
[party_ Quick 15, party CritUp 25, demeritAll DefenseDown 10]
, bond 789 "Truth and Death" "Avicebron" Icon.BusterArtsUp
[party_ Arts 10, party_ Buster 10]
, bond 790 "Wildfire Blade" "PI:NAME:<NAME>END_PI" Icon.ArtsUp
[party_ Arts 10, party NPGen 10]
, bond 791 "Library of Ivan the Terrible" "Ivan the Terrible" Icon.BusterUp
[party_ Buster 10, party NPUp 10]
, { name = "PI:NAME:<NAME>END_PI"
, id = 792
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 250, hp = 400 }
, max = { atk = 1000, hp = 1600 }
}
, effect = [ Grant Self 0 NPGen <| Range 10 15
, Grant Self 0 NPUp <| Range 10 15
, To Self GaugeUp <| Range 40 50
]
, bond = Nothing
, limited = True
}
, { name = "Moment of Bliss"
, id = 793
, rarity = 4
, icon = Icon.BusterArtsUp
, stats = { base = { atk = 0, hp = 600 }
, max = { atk = 0, hp = 2250 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 8 10
, Grant Self 0 (CardUp Arts) <| Range 8 10
, Grant Self 0 DefenseUp <| Range 3 5
]
, bond = Nothing
, limited = True
}
, { name = "Away We Go!"
, id = 794
, rarity = 3
, icon = Icon.QuickUp
, stats = { base = { atk = 100, hp = 160 }
, max = { atk = 500, hp = 800 }
}
, effect = [ Grant Self 0 (CardUp Quick) <| Range 3 5
, Grant Self 0 NPGen <| Range 5 10
]
, bond = Nothing
, limited = True
}
, { name = "An Afternoon at the Fortress"
, id = 795
, rarity = 5
, icon = Icon.BusterArtsUp
, stats = { base = { atk = 0, hp = 750 }
, max = { atk = 0, hp = 3000 }
}
, effect = [ Grant Self 0 (CardUp Buster) <| Range 10 15
, Grant Self 0 (CardUp Arts) <| Range 10 15
, Grant Self 0 NPUp <| Range 10 15
]
, bond = Nothing
, limited = True
}
, { name = "PI:NAME:<NAME>END_PI"
, id = 796
, rarity = 5
, icon = Icon.NobleUp
, stats = { base = { atk = 500, hp = 0 }
, max = { atk = 2000, hp = 0 }
}
, effect = [ Grant Self 0 NPGen <| Range 10 15
, Grant Self 0 CritUp <| Range 10 15
, Grant Self 0 StarsPerTurn <| Range 3 4
]
, bond = Nothing
, limited = True
}
, bond 798 "The Object That Can Hold a Universe" "Achilles" Icon.Shield
[self NPUp 30, Times 1 <| Grant Party 3 Invincibility Full]
, bond 799 "The Purpose of Learning and Teaching" "PI:NAME:<NAME>END_PI" Icon.ArtsQuickUp
[party_ Arts 10, party_ Quick 10]
, bond 800 "Nameless Death" "PI:NAME:<NAME>END_PI" Icon.NobleUp
[party NPGen 10, party NPUp 10]
]
equipped : Class -> SkillEffect -> SkillEffect
equipped =
When << (++) "equipped by a " << Class.show
{-| Retrieves the corresponding Bond CE. Memoized for performance. -}
getBond : Servant -> Maybe CraftEssence
getBond s =
Dict.get s.name bondMap
{-| Memoization table for `getBond`. -}
bondMap : Dict String CraftEssence
bondMap =
let
go ce =
Maybe.map (\bond -> (bond, ce)) ce.bond
in
db
|> List.map go
>> Maybe.values
>> Dict.fromList
| elm |
[
{
"context": "{-\nCopyright 2020 Morgan Stanley\n\nLicensed under the Apache License, Version 2.0 (",
"end": 32,
"score": 0.9998161793,
"start": 18,
"tag": "NAME",
"value": "Morgan Stanley"
}
] | src/Morphir/Sample/Apps/Upstream/Market/App.elm | maoo/morphir-examples | 0 | {-
Copyright 2020 Morgan Stanley
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-}
module Morphir.Sample.Apps.Upstream.Market.App exposing (..)
{-| This is a stub for an external Market app. Normally this would
live in an external library but it's included for simplicity. The
application's API is exposed here and in the external library the
implementation would be included as well.
-}
import Dict exposing (Dict)
import Morphir.SDK.App exposing (StatefulApp, statefulApp, cmdNone)
import Morphir.Sample.Apps.Shared.Market as Market
import Morphir.Sample.Apps.Shared.Product as Product
import Morphir.Sample.Apps.Shared.Price exposing(Price)
type alias Market =
{ benchmarkRate : Float
, gcRate : Float
}
type alias State =
Dict Market.ID Market
-- Types
type alias App =
StatefulApp API RemoteState LocalState Event
type alias API =
{}
type alias RemoteState =
()
type alias LocalState =
Dict Market.ID Market
type Event =
Event
| 49407 | {-
Copyright 2020 <NAME>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-}
module Morphir.Sample.Apps.Upstream.Market.App exposing (..)
{-| This is a stub for an external Market app. Normally this would
live in an external library but it's included for simplicity. The
application's API is exposed here and in the external library the
implementation would be included as well.
-}
import Dict exposing (Dict)
import Morphir.SDK.App exposing (StatefulApp, statefulApp, cmdNone)
import Morphir.Sample.Apps.Shared.Market as Market
import Morphir.Sample.Apps.Shared.Product as Product
import Morphir.Sample.Apps.Shared.Price exposing(Price)
type alias Market =
{ benchmarkRate : Float
, gcRate : Float
}
type alias State =
Dict Market.ID Market
-- Types
type alias App =
StatefulApp API RemoteState LocalState Event
type alias API =
{}
type alias RemoteState =
()
type alias LocalState =
Dict Market.ID Market
type Event =
Event
| true | {-
Copyright 2020 PI:NAME:<NAME>END_PI
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-}
module Morphir.Sample.Apps.Upstream.Market.App exposing (..)
{-| This is a stub for an external Market app. Normally this would
live in an external library but it's included for simplicity. The
application's API is exposed here and in the external library the
implementation would be included as well.
-}
import Dict exposing (Dict)
import Morphir.SDK.App exposing (StatefulApp, statefulApp, cmdNone)
import Morphir.Sample.Apps.Shared.Market as Market
import Morphir.Sample.Apps.Shared.Product as Product
import Morphir.Sample.Apps.Shared.Price exposing(Price)
type alias Market =
{ benchmarkRate : Float
, gcRate : Float
}
type alias State =
Dict Market.ID Market
-- Types
type alias App =
StatefulApp API RemoteState LocalState Event
type alias API =
{}
type alias RemoteState =
()
type alias LocalState =
Dict Market.ID Market
type Event =
Event
| elm |
[
{
"context": "then\n [ commitButton\n { name = \"Mark as new\"\n , class = \"mark-as-new\"\n ",
"end": 4699,
"score": 0.6573029757,
"start": 4695,
"tag": "NAME",
"value": "Mark"
}
] | web/elm/CommitList/View.elm | joakimk/exremit | 27 | module CommitList.View exposing (view)
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick)
import String
import VirtualDom exposing (Node, Property)
import Shared.Formatting exposing (formattedTime, authorName)
import Shared.Types exposing (..)
import Settings.Types exposing (..)
import Shared.Change exposing (changeMsg)
import Shared.Avatar exposing (avatarUrl)
import Shared.Helpers exposing (onClickWithPreventDefault)
import CommitList.Header.View
view : Model -> Node Msg
view model =
let
commits =
commitsToShow model
toggleButtonText =
(if model.settings.showAllReviewedCommits then
"Hide most reviewed commits"
else
"Show more reviewed commits"
)
in
div []
[ CommitList.Header.View.view model
, ul [ class "commits-list" ] (List.map (renderCommit model) commits)
, ul [ class "commits-list" ]
[ li [ class "centered-button-commit-row" ]
[ button [ onClick (ChangeSettings ToggleShowAllReviewedCommits) ] [ text toggleButtonText ]
]
]
]
commitsToShow : Model -> List Commit
commitsToShow model =
let
list =
model.commits |> List.take (model.commitsToShowCount)
in
if model.settings.showAllReviewedCommits then
list
else
limitShownReviewedCommits list
limitShownReviewedCommits : List Commit -> List Commit
limitShownReviewedCommits list =
let
oldestUnreviewedCommit =
list |> List.filter (\commit -> not commit.isReviewed) |> List.sortBy .timestamp |> List.head
minReviewedCommitsToShowCount =
5
in
case oldestUnreviewedCommit of
Just commit ->
Tuple.first (list |> List.partition (\c -> c.id + minReviewedCommitsToShowCount >= commit.id))
Nothing ->
list |> List.take (minReviewedCommitsToShowCount)
renderCommit : Model -> Commit -> Node Msg
renderCommit model commit =
li [ id (commitId commit), (commitClassList model commit) ]
[ a [ class "block-link", href (commitUrl model commit) ]
[ div [ class "commit-wrapper", onClick (ShowCommit commit.id) ]
[ div [ class "commit-controls" ] (renderButtons model commit)
, img [ class "commit-avatar", src (avatarUrl commit.authorGravatarHash) ] []
, div [ class "commit-summary-and-details" ]
[ div [ class "commit-summary test-summary" ] [ text commit.summary ]
, renderCommitDetails commit
]
]
]
]
renderCommitDetails : Commit -> Node a
renderCommitDetails commit =
div [ class "commit-details" ]
[ text " in "
, strong [] [ text commit.repository ]
, span [ class "by-author" ]
[ text " by "
, strong [] [ text (authorName commit.authorName) ]
, text " on "
, span [ class "test-timestamp" ] [ text (formattedTime commit.timestamp) ]
]
]
-- don't link to github in tests since that makes testing difficult
commitUrl : Model -> Commit -> String
commitUrl model commit =
if model.environment == "test" || model.environment == "dev" then
"#"
else
commit.url
renderButtons : Model -> Commit -> List (Node Msg)
renderButtons model commit =
if commit.isNew then
[ commitButton
{ name = "Start review"
, class = "start-review"
, iconClass = "fa-eye"
, msg = (changeMsg StartReview model commit)
, openCommitOnGithub = (model.lastClickedCommitId /= commit.id)
}
]
else if commit.isBeingReviewed then
[ commitButton
{ name = "Abandon review"
, class = "abandon-review"
, iconClass = "fa-eye-slash"
, msg = (changeMsg AbandonReview model commit)
, openCommitOnGithub = False
}
, commitButton
{ name = "Mark as reviewed"
, class = "mark-as-reviewed"
, iconClass = "fa-eye-slash"
, msg = (changeMsg MarkAsReviewed model commit)
, openCommitOnGithub = False
}
, img [ class "commit-reviewer-avatar test-reviewer", src (avatarUrl commit.pendingReviewerGravatarHash), reviewerDataAttribute (commit.pendingReviewerEmail) ] []
]
else if commit.isReviewed then
[ commitButton
{ name = "Mark as new"
, class = "mark-as-new"
, iconClass = "fa-eye-slash"
, msg = (changeMsg MarkAsNew model commit)
, openCommitOnGithub = False
}
, img [ class "commit-reviewer-avatar test-reviewer", src (avatarUrl commit.reviewerGravatarHash), reviewerDataAttribute (commit.reviewerEmail) ] []
]
else
-- This should never happen
[]
reviewerDataAttribute : Maybe String -> Attribute a
reviewerDataAttribute email =
attribute "data-test-reviewer-email" (Maybe.withDefault "" email)
commitButton : CommitButton -> Node Msg
commitButton commitButton =
button
[ class ("small test-button" ++ " " ++ commitButton.class)
, onClickWithPreventDefault (not commitButton.openCommitOnGithub) commitButton.msg
]
[ i [ class ("fa" ++ " " ++ commitButton.iconClass) ] []
, text " "
, text commitButton.name
]
commitClassList : Model -> Commit -> Property a
commitClassList model commit =
classList
[ ( "commit", True )
, ( "your-last-clicked", model.lastClickedCommitId == commit.id )
, ( "authored-by-you", authoredByYou model commit )
, ( "is-being-reviewed", commit.isBeingReviewed )
, ( "is-reviewed", commit.isReviewed )
, ( "test-is-new", commit.isNew )
, ( "test-is-being-reviewed", commit.isBeingReviewed )
, ( "test-is-reviewed", commit.isReviewed )
, ( "test-commit", True )
, ( "test-authored-by-you", authoredByYou model commit )
]
authoredByYou : Model -> Commit -> Bool
authoredByYou model commit =
String.contains model.settings.name (authorName commit.authorName)
commitId : Commit -> String
commitId commit =
"commit-" ++ toString commit.id
| 39263 | module CommitList.View exposing (view)
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick)
import String
import VirtualDom exposing (Node, Property)
import Shared.Formatting exposing (formattedTime, authorName)
import Shared.Types exposing (..)
import Settings.Types exposing (..)
import Shared.Change exposing (changeMsg)
import Shared.Avatar exposing (avatarUrl)
import Shared.Helpers exposing (onClickWithPreventDefault)
import CommitList.Header.View
view : Model -> Node Msg
view model =
let
commits =
commitsToShow model
toggleButtonText =
(if model.settings.showAllReviewedCommits then
"Hide most reviewed commits"
else
"Show more reviewed commits"
)
in
div []
[ CommitList.Header.View.view model
, ul [ class "commits-list" ] (List.map (renderCommit model) commits)
, ul [ class "commits-list" ]
[ li [ class "centered-button-commit-row" ]
[ button [ onClick (ChangeSettings ToggleShowAllReviewedCommits) ] [ text toggleButtonText ]
]
]
]
commitsToShow : Model -> List Commit
commitsToShow model =
let
list =
model.commits |> List.take (model.commitsToShowCount)
in
if model.settings.showAllReviewedCommits then
list
else
limitShownReviewedCommits list
limitShownReviewedCommits : List Commit -> List Commit
limitShownReviewedCommits list =
let
oldestUnreviewedCommit =
list |> List.filter (\commit -> not commit.isReviewed) |> List.sortBy .timestamp |> List.head
minReviewedCommitsToShowCount =
5
in
case oldestUnreviewedCommit of
Just commit ->
Tuple.first (list |> List.partition (\c -> c.id + minReviewedCommitsToShowCount >= commit.id))
Nothing ->
list |> List.take (minReviewedCommitsToShowCount)
renderCommit : Model -> Commit -> Node Msg
renderCommit model commit =
li [ id (commitId commit), (commitClassList model commit) ]
[ a [ class "block-link", href (commitUrl model commit) ]
[ div [ class "commit-wrapper", onClick (ShowCommit commit.id) ]
[ div [ class "commit-controls" ] (renderButtons model commit)
, img [ class "commit-avatar", src (avatarUrl commit.authorGravatarHash) ] []
, div [ class "commit-summary-and-details" ]
[ div [ class "commit-summary test-summary" ] [ text commit.summary ]
, renderCommitDetails commit
]
]
]
]
renderCommitDetails : Commit -> Node a
renderCommitDetails commit =
div [ class "commit-details" ]
[ text " in "
, strong [] [ text commit.repository ]
, span [ class "by-author" ]
[ text " by "
, strong [] [ text (authorName commit.authorName) ]
, text " on "
, span [ class "test-timestamp" ] [ text (formattedTime commit.timestamp) ]
]
]
-- don't link to github in tests since that makes testing difficult
commitUrl : Model -> Commit -> String
commitUrl model commit =
if model.environment == "test" || model.environment == "dev" then
"#"
else
commit.url
renderButtons : Model -> Commit -> List (Node Msg)
renderButtons model commit =
if commit.isNew then
[ commitButton
{ name = "Start review"
, class = "start-review"
, iconClass = "fa-eye"
, msg = (changeMsg StartReview model commit)
, openCommitOnGithub = (model.lastClickedCommitId /= commit.id)
}
]
else if commit.isBeingReviewed then
[ commitButton
{ name = "Abandon review"
, class = "abandon-review"
, iconClass = "fa-eye-slash"
, msg = (changeMsg AbandonReview model commit)
, openCommitOnGithub = False
}
, commitButton
{ name = "Mark as reviewed"
, class = "mark-as-reviewed"
, iconClass = "fa-eye-slash"
, msg = (changeMsg MarkAsReviewed model commit)
, openCommitOnGithub = False
}
, img [ class "commit-reviewer-avatar test-reviewer", src (avatarUrl commit.pendingReviewerGravatarHash), reviewerDataAttribute (commit.pendingReviewerEmail) ] []
]
else if commit.isReviewed then
[ commitButton
{ name = "<NAME> as new"
, class = "mark-as-new"
, iconClass = "fa-eye-slash"
, msg = (changeMsg MarkAsNew model commit)
, openCommitOnGithub = False
}
, img [ class "commit-reviewer-avatar test-reviewer", src (avatarUrl commit.reviewerGravatarHash), reviewerDataAttribute (commit.reviewerEmail) ] []
]
else
-- This should never happen
[]
reviewerDataAttribute : Maybe String -> Attribute a
reviewerDataAttribute email =
attribute "data-test-reviewer-email" (Maybe.withDefault "" email)
commitButton : CommitButton -> Node Msg
commitButton commitButton =
button
[ class ("small test-button" ++ " " ++ commitButton.class)
, onClickWithPreventDefault (not commitButton.openCommitOnGithub) commitButton.msg
]
[ i [ class ("fa" ++ " " ++ commitButton.iconClass) ] []
, text " "
, text commitButton.name
]
commitClassList : Model -> Commit -> Property a
commitClassList model commit =
classList
[ ( "commit", True )
, ( "your-last-clicked", model.lastClickedCommitId == commit.id )
, ( "authored-by-you", authoredByYou model commit )
, ( "is-being-reviewed", commit.isBeingReviewed )
, ( "is-reviewed", commit.isReviewed )
, ( "test-is-new", commit.isNew )
, ( "test-is-being-reviewed", commit.isBeingReviewed )
, ( "test-is-reviewed", commit.isReviewed )
, ( "test-commit", True )
, ( "test-authored-by-you", authoredByYou model commit )
]
authoredByYou : Model -> Commit -> Bool
authoredByYou model commit =
String.contains model.settings.name (authorName commit.authorName)
commitId : Commit -> String
commitId commit =
"commit-" ++ toString commit.id
| true | module CommitList.View exposing (view)
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick)
import String
import VirtualDom exposing (Node, Property)
import Shared.Formatting exposing (formattedTime, authorName)
import Shared.Types exposing (..)
import Settings.Types exposing (..)
import Shared.Change exposing (changeMsg)
import Shared.Avatar exposing (avatarUrl)
import Shared.Helpers exposing (onClickWithPreventDefault)
import CommitList.Header.View
view : Model -> Node Msg
view model =
let
commits =
commitsToShow model
toggleButtonText =
(if model.settings.showAllReviewedCommits then
"Hide most reviewed commits"
else
"Show more reviewed commits"
)
in
div []
[ CommitList.Header.View.view model
, ul [ class "commits-list" ] (List.map (renderCommit model) commits)
, ul [ class "commits-list" ]
[ li [ class "centered-button-commit-row" ]
[ button [ onClick (ChangeSettings ToggleShowAllReviewedCommits) ] [ text toggleButtonText ]
]
]
]
commitsToShow : Model -> List Commit
commitsToShow model =
let
list =
model.commits |> List.take (model.commitsToShowCount)
in
if model.settings.showAllReviewedCommits then
list
else
limitShownReviewedCommits list
limitShownReviewedCommits : List Commit -> List Commit
limitShownReviewedCommits list =
let
oldestUnreviewedCommit =
list |> List.filter (\commit -> not commit.isReviewed) |> List.sortBy .timestamp |> List.head
minReviewedCommitsToShowCount =
5
in
case oldestUnreviewedCommit of
Just commit ->
Tuple.first (list |> List.partition (\c -> c.id + minReviewedCommitsToShowCount >= commit.id))
Nothing ->
list |> List.take (minReviewedCommitsToShowCount)
renderCommit : Model -> Commit -> Node Msg
renderCommit model commit =
li [ id (commitId commit), (commitClassList model commit) ]
[ a [ class "block-link", href (commitUrl model commit) ]
[ div [ class "commit-wrapper", onClick (ShowCommit commit.id) ]
[ div [ class "commit-controls" ] (renderButtons model commit)
, img [ class "commit-avatar", src (avatarUrl commit.authorGravatarHash) ] []
, div [ class "commit-summary-and-details" ]
[ div [ class "commit-summary test-summary" ] [ text commit.summary ]
, renderCommitDetails commit
]
]
]
]
renderCommitDetails : Commit -> Node a
renderCommitDetails commit =
div [ class "commit-details" ]
[ text " in "
, strong [] [ text commit.repository ]
, span [ class "by-author" ]
[ text " by "
, strong [] [ text (authorName commit.authorName) ]
, text " on "
, span [ class "test-timestamp" ] [ text (formattedTime commit.timestamp) ]
]
]
-- don't link to github in tests since that makes testing difficult
commitUrl : Model -> Commit -> String
commitUrl model commit =
if model.environment == "test" || model.environment == "dev" then
"#"
else
commit.url
renderButtons : Model -> Commit -> List (Node Msg)
renderButtons model commit =
if commit.isNew then
[ commitButton
{ name = "Start review"
, class = "start-review"
, iconClass = "fa-eye"
, msg = (changeMsg StartReview model commit)
, openCommitOnGithub = (model.lastClickedCommitId /= commit.id)
}
]
else if commit.isBeingReviewed then
[ commitButton
{ name = "Abandon review"
, class = "abandon-review"
, iconClass = "fa-eye-slash"
, msg = (changeMsg AbandonReview model commit)
, openCommitOnGithub = False
}
, commitButton
{ name = "Mark as reviewed"
, class = "mark-as-reviewed"
, iconClass = "fa-eye-slash"
, msg = (changeMsg MarkAsReviewed model commit)
, openCommitOnGithub = False
}
, img [ class "commit-reviewer-avatar test-reviewer", src (avatarUrl commit.pendingReviewerGravatarHash), reviewerDataAttribute (commit.pendingReviewerEmail) ] []
]
else if commit.isReviewed then
[ commitButton
{ name = "PI:NAME:<NAME>END_PI as new"
, class = "mark-as-new"
, iconClass = "fa-eye-slash"
, msg = (changeMsg MarkAsNew model commit)
, openCommitOnGithub = False
}
, img [ class "commit-reviewer-avatar test-reviewer", src (avatarUrl commit.reviewerGravatarHash), reviewerDataAttribute (commit.reviewerEmail) ] []
]
else
-- This should never happen
[]
reviewerDataAttribute : Maybe String -> Attribute a
reviewerDataAttribute email =
attribute "data-test-reviewer-email" (Maybe.withDefault "" email)
commitButton : CommitButton -> Node Msg
commitButton commitButton =
button
[ class ("small test-button" ++ " " ++ commitButton.class)
, onClickWithPreventDefault (not commitButton.openCommitOnGithub) commitButton.msg
]
[ i [ class ("fa" ++ " " ++ commitButton.iconClass) ] []
, text " "
, text commitButton.name
]
commitClassList : Model -> Commit -> Property a
commitClassList model commit =
classList
[ ( "commit", True )
, ( "your-last-clicked", model.lastClickedCommitId == commit.id )
, ( "authored-by-you", authoredByYou model commit )
, ( "is-being-reviewed", commit.isBeingReviewed )
, ( "is-reviewed", commit.isReviewed )
, ( "test-is-new", commit.isNew )
, ( "test-is-being-reviewed", commit.isBeingReviewed )
, ( "test-is-reviewed", commit.isReviewed )
, ( "test-commit", True )
, ( "test-authored-by-you", authoredByYou model commit )
]
authoredByYou : Model -> Commit -> Bool
authoredByYou model commit =
String.contains model.settings.name (authorName commit.authorName)
commitId : Commit -> String
commitId commit =
"commit-" ++ toString commit.id
| elm |
[
{
"context": "ncoder for Trie\n\n@docs encoder\n\nCopyright (c) 2015 Robin Luiten\n\n-}\n\nimport Dict exposing (Dict)\nimport Json.Enco",
"end": 118,
"score": 0.9998416901,
"start": 106,
"tag": "NAME",
"value": "Robin Luiten"
}
] | src/Trie/Json/Encoder.elm | harrysarson/trie | 5 | module Trie.Json.Encoder exposing (encoder)
{-| Json Encoder for Trie
@docs encoder
Copyright (c) 2015 Robin Luiten
-}
import Dict exposing (Dict)
import Json.Encode as Encode
import Set
import String
import TrieModel exposing (Trie(..))
{-| Encoder for Trie.
-}
encoder : (f -> Encode.Value) -> Trie f -> Encode.Value
encoder valEnc trie =
case trie of
EmptyTrie ->
Encode.null
ValNode refValues ->
encoderValDict valEnc refValues
TrieNode trieDict ->
encoderTrieDict valEnc trieDict
ValTrieNode ( refValues, trieDict ) ->
let
encodedValues =
encoderValDict valEnc refValues
encodedDict =
encoderTrieDict valEnc trieDict
in
Encode.list identity [ encodedValues, encodedDict ]
{-| Encode the Dict of Value references.
-}
encoderValDict : (f -> Encode.Value) -> Dict String f -> Encode.Value
encoderValDict valEnc refValues =
Dict.toList refValues
|> List.map (\( key, val ) -> ( key, valEnc val ))
|> Encode.object
{-| Encode the core Trie structure Dict.
-}
encoderTrieDict : (f -> Encode.Value) -> Dict String (Trie f) -> Encode.Value
encoderTrieDict valEnc trieDict =
Dict.toList trieDict
|> List.map (\( key, val ) -> ( key, encoder valEnc val ))
|> Encode.object
| 45082 | module Trie.Json.Encoder exposing (encoder)
{-| Json Encoder for Trie
@docs encoder
Copyright (c) 2015 <NAME>
-}
import Dict exposing (Dict)
import Json.Encode as Encode
import Set
import String
import TrieModel exposing (Trie(..))
{-| Encoder for Trie.
-}
encoder : (f -> Encode.Value) -> Trie f -> Encode.Value
encoder valEnc trie =
case trie of
EmptyTrie ->
Encode.null
ValNode refValues ->
encoderValDict valEnc refValues
TrieNode trieDict ->
encoderTrieDict valEnc trieDict
ValTrieNode ( refValues, trieDict ) ->
let
encodedValues =
encoderValDict valEnc refValues
encodedDict =
encoderTrieDict valEnc trieDict
in
Encode.list identity [ encodedValues, encodedDict ]
{-| Encode the Dict of Value references.
-}
encoderValDict : (f -> Encode.Value) -> Dict String f -> Encode.Value
encoderValDict valEnc refValues =
Dict.toList refValues
|> List.map (\( key, val ) -> ( key, valEnc val ))
|> Encode.object
{-| Encode the core Trie structure Dict.
-}
encoderTrieDict : (f -> Encode.Value) -> Dict String (Trie f) -> Encode.Value
encoderTrieDict valEnc trieDict =
Dict.toList trieDict
|> List.map (\( key, val ) -> ( key, encoder valEnc val ))
|> Encode.object
| true | module Trie.Json.Encoder exposing (encoder)
{-| Json Encoder for Trie
@docs encoder
Copyright (c) 2015 PI:NAME:<NAME>END_PI
-}
import Dict exposing (Dict)
import Json.Encode as Encode
import Set
import String
import TrieModel exposing (Trie(..))
{-| Encoder for Trie.
-}
encoder : (f -> Encode.Value) -> Trie f -> Encode.Value
encoder valEnc trie =
case trie of
EmptyTrie ->
Encode.null
ValNode refValues ->
encoderValDict valEnc refValues
TrieNode trieDict ->
encoderTrieDict valEnc trieDict
ValTrieNode ( refValues, trieDict ) ->
let
encodedValues =
encoderValDict valEnc refValues
encodedDict =
encoderTrieDict valEnc trieDict
in
Encode.list identity [ encodedValues, encodedDict ]
{-| Encode the Dict of Value references.
-}
encoderValDict : (f -> Encode.Value) -> Dict String f -> Encode.Value
encoderValDict valEnc refValues =
Dict.toList refValues
|> List.map (\( key, val ) -> ( key, valEnc val ))
|> Encode.object
{-| Encode the core Trie structure Dict.
-}
encoderTrieDict : (f -> Encode.Value) -> Dict String (Trie f) -> Encode.Value
encoderTrieDict valEnc trieDict =
Dict.toList trieDict
|> List.map (\( key, val ) -> ( key, encoder valEnc val ))
|> Encode.object
| elm |
[
{
"context": "\n { check = scan\n , info =\n { key = \"DuplicateRecordFieldUpdate\"\n , name = \"Duplicate Record Field Update\"",
"end": 631,
"score": 0.9731521606,
"start": 605,
"tag": "KEY",
"value": "DuplicateRecordFieldUpdate"
}
] | src/Analyser/Checks/DuplicateRecordFieldUpdate.elm | arowM/elm-analyse | 0 | module Analyser.Checks.DuplicateRecordFieldUpdate exposing (checker)
import ASTUtil.Inspector as Inspector exposing (Order(Post), defaultConfig)
import Analyser.Checks.Base exposing (Checker)
import Analyser.Configuration exposing (Configuration)
import Analyser.FileContext exposing (FileContext)
import Analyser.Messages.Data as Data exposing (MessageData)
import Analyser.Messages.Schema as Schema
import Dict
import Dict.Extra as Dict
import Elm.Syntax.Expression exposing (RecordUpdate)
import Elm.Syntax.Range as Range
checker : Checker
checker =
{ check = scan
, info =
{ key = "DuplicateRecordFieldUpdate"
, name = "Duplicate Record Field Update"
, description = "You only want to update a field once in the record update syntax. Doing twice may only cause bugs."
, schema =
Schema.schema
|> Schema.rangeListProp "ranges"
|> Schema.varProp "fieldName"
}
}
type alias Context =
List MessageData
scan : FileContext -> Configuration -> List MessageData
scan fileContext _ =
Inspector.inspect
{ defaultConfig
| onRecordUpdate = Post onRecordUpdate
}
fileContext.ast
[]
onRecordUpdate : RecordUpdate -> Context -> Context
onRecordUpdate { updates } context =
updates
|> Dict.groupBy Tuple.first
|> Dict.filter (\_ v -> List.length v > 1)
|> Dict.map (\_ v -> List.map (Tuple.second >> Tuple.first) v)
|> Dict.toList
|> List.map buildMessageData
|> (++) context
buildMessageData : ( String, List Range.Range ) -> MessageData
buildMessageData ( fieldName, ranges ) =
Data.init
(String.concat
[ "The '"
, fieldName
, "' field for a record is updated multiple times in one expression."
]
)
|> Data.addVarName "fieldName" fieldName
|> Data.addRanges "ranges" ranges
| 26026 | module Analyser.Checks.DuplicateRecordFieldUpdate exposing (checker)
import ASTUtil.Inspector as Inspector exposing (Order(Post), defaultConfig)
import Analyser.Checks.Base exposing (Checker)
import Analyser.Configuration exposing (Configuration)
import Analyser.FileContext exposing (FileContext)
import Analyser.Messages.Data as Data exposing (MessageData)
import Analyser.Messages.Schema as Schema
import Dict
import Dict.Extra as Dict
import Elm.Syntax.Expression exposing (RecordUpdate)
import Elm.Syntax.Range as Range
checker : Checker
checker =
{ check = scan
, info =
{ key = "<KEY>"
, name = "Duplicate Record Field Update"
, description = "You only want to update a field once in the record update syntax. Doing twice may only cause bugs."
, schema =
Schema.schema
|> Schema.rangeListProp "ranges"
|> Schema.varProp "fieldName"
}
}
type alias Context =
List MessageData
scan : FileContext -> Configuration -> List MessageData
scan fileContext _ =
Inspector.inspect
{ defaultConfig
| onRecordUpdate = Post onRecordUpdate
}
fileContext.ast
[]
onRecordUpdate : RecordUpdate -> Context -> Context
onRecordUpdate { updates } context =
updates
|> Dict.groupBy Tuple.first
|> Dict.filter (\_ v -> List.length v > 1)
|> Dict.map (\_ v -> List.map (Tuple.second >> Tuple.first) v)
|> Dict.toList
|> List.map buildMessageData
|> (++) context
buildMessageData : ( String, List Range.Range ) -> MessageData
buildMessageData ( fieldName, ranges ) =
Data.init
(String.concat
[ "The '"
, fieldName
, "' field for a record is updated multiple times in one expression."
]
)
|> Data.addVarName "fieldName" fieldName
|> Data.addRanges "ranges" ranges
| true | module Analyser.Checks.DuplicateRecordFieldUpdate exposing (checker)
import ASTUtil.Inspector as Inspector exposing (Order(Post), defaultConfig)
import Analyser.Checks.Base exposing (Checker)
import Analyser.Configuration exposing (Configuration)
import Analyser.FileContext exposing (FileContext)
import Analyser.Messages.Data as Data exposing (MessageData)
import Analyser.Messages.Schema as Schema
import Dict
import Dict.Extra as Dict
import Elm.Syntax.Expression exposing (RecordUpdate)
import Elm.Syntax.Range as Range
checker : Checker
checker =
{ check = scan
, info =
{ key = "PI:KEY:<KEY>END_PI"
, name = "Duplicate Record Field Update"
, description = "You only want to update a field once in the record update syntax. Doing twice may only cause bugs."
, schema =
Schema.schema
|> Schema.rangeListProp "ranges"
|> Schema.varProp "fieldName"
}
}
type alias Context =
List MessageData
scan : FileContext -> Configuration -> List MessageData
scan fileContext _ =
Inspector.inspect
{ defaultConfig
| onRecordUpdate = Post onRecordUpdate
}
fileContext.ast
[]
onRecordUpdate : RecordUpdate -> Context -> Context
onRecordUpdate { updates } context =
updates
|> Dict.groupBy Tuple.first
|> Dict.filter (\_ v -> List.length v > 1)
|> Dict.map (\_ v -> List.map (Tuple.second >> Tuple.first) v)
|> Dict.toList
|> List.map buildMessageData
|> (++) context
buildMessageData : ( String, List Range.Range ) -> MessageData
buildMessageData ( fieldName, ranges ) =
Data.init
(String.concat
[ "The '"
, fieldName
, "' field for a record is updated multiple times in one expression."
]
)
|> Data.addVarName "fieldName" fieldName
|> Data.addRanges "ranges" ranges
| elm |
[
{
"context": "Username\"\n , input ChangeUsername \"username\" \"text\" model.username\n , username",
"end": 1805,
"score": 0.9941349626,
"start": 1797,
"tag": "USERNAME",
"value": "username"
},
{
"context": "ing }\nvalidate model =\n { username = required \"Username\" model.username\n , password = required \"Passwo",
"end": 2771,
"score": 0.9958642721,
"start": 2763,
"tag": "USERNAME",
"value": "Username"
},
{
"context": "odel =\n { username = required \"Username\" model.username\n , password = required \"Password\" model.passwo",
"end": 2787,
"score": 0.9789571166,
"start": 2779,
"tag": "USERNAME",
"value": "username"
},
{
"context": "ername\" model.username\n , password = required \"Password\" model.password\n }\n\n\nrequired : String -> Stri",
"end": 2823,
"score": 0.9549464583,
"start": 2815,
"tag": "PASSWORD",
"value": "Password"
}
] | elm/Components/LoginForm.elm | JamesHageman/rangle-elm-starter | 1 | module Components.LoginForm exposing (..)
import Html exposing (Html, form, text, button)
import Html.Attributes exposing (class, type')
import Html.Events exposing (onSubmit, onClick)
import Components.UI.Form exposing (input, label, group, error)
import Components.UI.Alert as Alert
import String
-- Model
type alias Model =
{ username : String
, password : String
}
type alias SubmitModel =
Model
type Msg
= ChangeUsername String
| ChangePassword String
| Clear
| Submit
init : Model
init =
Model "" ""
-- Update
update : Msg -> Model -> ( Model, Maybe SubmitModel )
update msg model =
case msg of
ChangeUsername name ->
( { model | username = name }, Nothing )
ChangePassword pass ->
( { model | password = pass }, Nothing )
Clear ->
( init, Nothing )
Submit ->
let
{ username, password } =
validate model
in
case ( username, password ) of
( Nothing, Nothing ) ->
( model, Just model )
_ ->
( model, Nothing )
-- View
view : Maybe String -> Model -> Html Msg
view errorMessage model =
let
validations =
validate model
usernameMsg =
validationMessage validations.username
passwordMsg =
validationMessage validations.password
in
form [ onSubmit Submit ]
[ case errorMessage of
Nothing ->
text ""
Just msg ->
Alert.view "error" [ text msg ]
, group
[ label "Username"
, input ChangeUsername "username" "text" model.username
, usernameMsg
]
, group
[ label "Password"
, input ChangePassword "password" "password" model.password
, passwordMsg
]
, group
[ button
[ class "btn btn-primary mr1"
, type' "submit"
]
[ text "Login" ]
, button
[ class "btn btn-primary bg-red"
, onClick Clear
]
[ text "Clear" ]
]
]
validationMessage : Maybe String -> Html msg
validationMessage validation =
case validation of
Nothing ->
text ""
Just msg ->
error [ text msg ]
validate : Model -> { username : Maybe String, password : Maybe String }
validate model =
{ username = required "Username" model.username
, password = required "Password" model.password
}
required : String -> String -> Maybe String
required label str =
if String.length str > 0 then
Nothing
else
Just (label ++ " is required.")
| 24803 | module Components.LoginForm exposing (..)
import Html exposing (Html, form, text, button)
import Html.Attributes exposing (class, type')
import Html.Events exposing (onSubmit, onClick)
import Components.UI.Form exposing (input, label, group, error)
import Components.UI.Alert as Alert
import String
-- Model
type alias Model =
{ username : String
, password : String
}
type alias SubmitModel =
Model
type Msg
= ChangeUsername String
| ChangePassword String
| Clear
| Submit
init : Model
init =
Model "" ""
-- Update
update : Msg -> Model -> ( Model, Maybe SubmitModel )
update msg model =
case msg of
ChangeUsername name ->
( { model | username = name }, Nothing )
ChangePassword pass ->
( { model | password = pass }, Nothing )
Clear ->
( init, Nothing )
Submit ->
let
{ username, password } =
validate model
in
case ( username, password ) of
( Nothing, Nothing ) ->
( model, Just model )
_ ->
( model, Nothing )
-- View
view : Maybe String -> Model -> Html Msg
view errorMessage model =
let
validations =
validate model
usernameMsg =
validationMessage validations.username
passwordMsg =
validationMessage validations.password
in
form [ onSubmit Submit ]
[ case errorMessage of
Nothing ->
text ""
Just msg ->
Alert.view "error" [ text msg ]
, group
[ label "Username"
, input ChangeUsername "username" "text" model.username
, usernameMsg
]
, group
[ label "Password"
, input ChangePassword "password" "password" model.password
, passwordMsg
]
, group
[ button
[ class "btn btn-primary mr1"
, type' "submit"
]
[ text "Login" ]
, button
[ class "btn btn-primary bg-red"
, onClick Clear
]
[ text "Clear" ]
]
]
validationMessage : Maybe String -> Html msg
validationMessage validation =
case validation of
Nothing ->
text ""
Just msg ->
error [ text msg ]
validate : Model -> { username : Maybe String, password : Maybe String }
validate model =
{ username = required "Username" model.username
, password = required "<PASSWORD>" model.password
}
required : String -> String -> Maybe String
required label str =
if String.length str > 0 then
Nothing
else
Just (label ++ " is required.")
| true | module Components.LoginForm exposing (..)
import Html exposing (Html, form, text, button)
import Html.Attributes exposing (class, type')
import Html.Events exposing (onSubmit, onClick)
import Components.UI.Form exposing (input, label, group, error)
import Components.UI.Alert as Alert
import String
-- Model
type alias Model =
{ username : String
, password : String
}
type alias SubmitModel =
Model
type Msg
= ChangeUsername String
| ChangePassword String
| Clear
| Submit
init : Model
init =
Model "" ""
-- Update
update : Msg -> Model -> ( Model, Maybe SubmitModel )
update msg model =
case msg of
ChangeUsername name ->
( { model | username = name }, Nothing )
ChangePassword pass ->
( { model | password = pass }, Nothing )
Clear ->
( init, Nothing )
Submit ->
let
{ username, password } =
validate model
in
case ( username, password ) of
( Nothing, Nothing ) ->
( model, Just model )
_ ->
( model, Nothing )
-- View
view : Maybe String -> Model -> Html Msg
view errorMessage model =
let
validations =
validate model
usernameMsg =
validationMessage validations.username
passwordMsg =
validationMessage validations.password
in
form [ onSubmit Submit ]
[ case errorMessage of
Nothing ->
text ""
Just msg ->
Alert.view "error" [ text msg ]
, group
[ label "Username"
, input ChangeUsername "username" "text" model.username
, usernameMsg
]
, group
[ label "Password"
, input ChangePassword "password" "password" model.password
, passwordMsg
]
, group
[ button
[ class "btn btn-primary mr1"
, type' "submit"
]
[ text "Login" ]
, button
[ class "btn btn-primary bg-red"
, onClick Clear
]
[ text "Clear" ]
]
]
validationMessage : Maybe String -> Html msg
validationMessage validation =
case validation of
Nothing ->
text ""
Just msg ->
error [ text msg ]
validate : Model -> { username : Maybe String, password : Maybe String }
validate model =
{ username = required "Username" model.username
, password = required "PI:PASSWORD:<PASSWORD>END_PI" model.password
}
required : String -> String -> Maybe String
required label str =
if String.length str > 0 then
Nothing
else
Just (label ++ " is required.")
| elm |
[
{
"context": "\"Authors\" ]\n , p [] [ text \"Proudly made by Neil Ashford, Simon Gordon, Callum Hays and Damian Van Kranend",
"end": 256,
"score": 0.9998730421,
"start": 244,
"tag": "NAME",
"value": "Neil Ashford"
},
{
"context": " , p [] [ text \"Proudly made by Neil Ashford, Simon Gordon, Callum Hays and Damian Van Kranendonk.\" ]\n ",
"end": 270,
"score": 0.9998776913,
"start": 258,
"tag": "NAME",
"value": "Simon Gordon"
},
{
"context": " text \"Proudly made by Neil Ashford, Simon Gordon, Callum Hays and Damian Van Kranendonk.\" ]\n , h3 [] [ t",
"end": 283,
"score": 0.9998666048,
"start": 272,
"tag": "NAME",
"value": "Callum Hays"
},
{
"context": "ade by Neil Ashford, Simon Gordon, Callum Hays and Damian Van Kranendonk.\" ]\n , h3 [] [ text \"About this applicatio",
"end": 309,
"score": 0.9998692274,
"start": 288,
"tag": "NAME",
"value": "Damian Van Kranendonk"
},
{
"context": " a\n [ href \"https://github.com/ashfordneil/state-machina/\"\n , target \"_bl",
"end": 1630,
"score": 0.8316724896,
"start": 1619,
"tag": "USERNAME",
"value": "ashfordneil"
}
] | frontend/src/Errata.elm | ashfordneil/state-machina | 2 | module Errata exposing (..)
import Html exposing (..)
import Html.Attributes exposing (href, target)
stateMachines =
div []
[ h2 [] [ text "Information" ]
, h3 [] [ text "Authors" ]
, p [] [ text "Proudly made by Neil Ashford, Simon Gordon, Callum Hays and Damian Van Kranendonk." ]
, h3 [] [ text "About this application" ]
, div []
[ p [] [ text "State Machina is an automated tool to assist in the design of finite state machines." ]
, p []
[ text
("The green state is the starting state of your machine. It cannot be deleted. "
++ "Red states are the valid accepting / final states of your machine. "
++ "The blue states are the other (non-accepting) states. "
)
]
, p []
[ text
("Transitions between states are represented by arrows. The label \"input 1/input 2\" on "
++ "a transition means that that transition can take place on input 1 or input 2."
)
]
]
, h3 [] [ text "Resources" ]
, ul []
[ li []
[ a
[ href "http://blog.markshead.com/869/state-machines-computer-science/"
, target "_blank"
]
[ text "State Machines - Basics of Computer Science"
]
]
, li []
[ a
[ href "https://github.com/ashfordneil/state-machina/"
, target "_blank"
]
[ text "Source code of this application"
]
]
]
]
| 23422 | module Errata exposing (..)
import Html exposing (..)
import Html.Attributes exposing (href, target)
stateMachines =
div []
[ h2 [] [ text "Information" ]
, h3 [] [ text "Authors" ]
, p [] [ text "Proudly made by <NAME>, <NAME>, <NAME> and <NAME>." ]
, h3 [] [ text "About this application" ]
, div []
[ p [] [ text "State Machina is an automated tool to assist in the design of finite state machines." ]
, p []
[ text
("The green state is the starting state of your machine. It cannot be deleted. "
++ "Red states are the valid accepting / final states of your machine. "
++ "The blue states are the other (non-accepting) states. "
)
]
, p []
[ text
("Transitions between states are represented by arrows. The label \"input 1/input 2\" on "
++ "a transition means that that transition can take place on input 1 or input 2."
)
]
]
, h3 [] [ text "Resources" ]
, ul []
[ li []
[ a
[ href "http://blog.markshead.com/869/state-machines-computer-science/"
, target "_blank"
]
[ text "State Machines - Basics of Computer Science"
]
]
, li []
[ a
[ href "https://github.com/ashfordneil/state-machina/"
, target "_blank"
]
[ text "Source code of this application"
]
]
]
]
| true | module Errata exposing (..)
import Html exposing (..)
import Html.Attributes exposing (href, target)
stateMachines =
div []
[ h2 [] [ text "Information" ]
, h3 [] [ text "Authors" ]
, p [] [ text "Proudly made by PI:NAME:<NAME>END_PI, PI:NAME:<NAME>END_PI, PI:NAME:<NAME>END_PI and PI:NAME:<NAME>END_PI." ]
, h3 [] [ text "About this application" ]
, div []
[ p [] [ text "State Machina is an automated tool to assist in the design of finite state machines." ]
, p []
[ text
("The green state is the starting state of your machine. It cannot be deleted. "
++ "Red states are the valid accepting / final states of your machine. "
++ "The blue states are the other (non-accepting) states. "
)
]
, p []
[ text
("Transitions between states are represented by arrows. The label \"input 1/input 2\" on "
++ "a transition means that that transition can take place on input 1 or input 2."
)
]
]
, h3 [] [ text "Resources" ]
, ul []
[ li []
[ a
[ href "http://blog.markshead.com/869/state-machines-computer-science/"
, target "_blank"
]
[ text "State Machines - Basics of Computer Science"
]
]
, li []
[ a
[ href "https://github.com/ashfordneil/state-machina/"
, target "_blank"
]
[ text "Source code of this application"
]
]
]
]
| elm |
[
{
"context": "38891\"\n , placeholder \"测试2\"\n , style \"display\" \"b",
"end": 30893,
"score": 0.6773758531,
"start": 30890,
"tag": "NAME",
"value": "测试2"
},
{
"context": "38891\"\n , placeholder \"验证2\"\n , style \"display\" \"b",
"end": 31975,
"score": 0.692085743,
"start": 31972,
"tag": "NAME",
"value": "验证2"
},
{
"context": "38891\"\n , placeholder \"测试3\"\n , style \"display\" \"b",
"end": 33308,
"score": 0.6846853495,
"start": 33305,
"tag": "NAME",
"value": "测试3"
}
] | static/elm/clash_of_code.elm | haskell4brina/CodeGame | 7 | module Main exposing (main)
import Array exposing (..)
import Browser
import Debug exposing (..)
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick, onInput)
import Http exposing (..)
import Json.Encode as Encode
main =
Browser.element
{ init = init
, update = update
, view = view
, subscriptions = subscriptions
}
type alias Title =
String
type alias Statement =
String
type alias InputDescription =
String
type alias OutputDescription =
String
type alias Constraints =
String
type alias TestCases_Test =
{ input : String, output : String }
type alias TestCases_Validater =
{ input : String, output : String }
type alias TestCases =
{ testName : String
, validator : String
, test : TestCases_Test
, validater : TestCases_Validater
}
type alias Model =
{ title : Title --标题
, statement : Statement --声明
, inputDescription : InputDescription --输入说明
, outputDescription : OutputDescription --输出说明
, constraints : Constraints --限制条件
, testCases1 : TestCases --测试用例
, testCases2 : TestCases
, testCases3 : TestCases
, testCases4 : TestCases
, testCases5 : TestCases
, radioValue : String --用于构建单选框
, dataJave : String --用于更改选框中的语言的时候储存文本框中的String
, dataPython : String
, dataHaskell : String
, dataJave_ : String --用于更改选框中的语言的时候储存文本框中的String
, dataPython_ : String
, dataHaskell_ : String
, selectJava : String
, selectPython : String
, selectHaskell : String
}
init : () -> ( Model, Cmd Msg )
init _ =
( { title = ""
, statement = ""
, inputDescription = ""
, outputDescription = ""
, constraints = ""
, testCases1 =
{ testName = ""
, validator = ""
, test =
{ input = ""
, output = ""
}
, validater =
{ input = ""
, output = ""
}
}
, testCases2 =
{ testName = ""
, validator = ""
, test =
{ input = ""
, output = ""
}
, validater =
{ input = ""
, output = ""
}
}
, testCases3 =
{ testName = ""
, validator = ""
, test =
{ input = ""
, output = ""
}
, validater =
{ input = ""
, output = ""
}
}
, testCases4 =
{ testName = ""
, validator = ""
, test =
{ input = ""
, output = ""
}
, validater =
{ input = ""
, output = ""
}
}
, testCases5 =
{ testName = ""
, validator = ""
, test =
{ input = ""
, output = ""
}
, validater =
{ input = ""
, output = ""
}
}
, radioValue = "Easy"
, dataJave = ""
, dataPython = ""
, dataHaskell = ""
, dataJave_ = ""
, dataPython_ = ""
, dataHaskell_ = ""
, selectJava = "Java"
, selectPython = "Python"
, selectHaskell = "Haskell"
}
, Cmd.none
)
type Msg
= Display (Result Http.Error String)
| PushDate
| ChangeTitle String
| ChangeStatemen String
| ChangeInputDescription String
| ChangeOutputDescription String
| ChangeConstraints String
| ChangeTestCasesTestName1 String
| ChangeTestCasesValidator1 String
| ChangeTestCasesTestName2 String
| ChangeTestCasesValidator2 String
| ChangeTestCasesTestName3 String
| ChangeTestCasesValidator3 String
| ChangeTestCasesTestName4 String
| ChangeTestCasesValidator4 String
| ChangeTestCasesTestName5 String
| ChangeTestCasesValidator5 String
| ChangeTestCasesTestInput1 String
| ChangeTestCasesTestOutput1 String
| ChangeTestCasesValidaterInput1 String
| ChangeTestCasesValidaterOutput1 String
| ChangeTestCasesTestInput2 String
| ChangeTestCasesTestOutput2 String
| ChangeTestCasesValidaterInput2 String
| ChangeTestCasesValidaterOutput2 String
| ChangeTestCasesTestInput3 String
| ChangeTestCasesTestOutput3 String
| ChangeTestCasesValidaterInput3 String
| ChangeTestCasesValidaterOutput3 String
| ChangeTestCasesTestInput4 String
| ChangeTestCasesTestOutput4 String
| ChangeTestCasesValidaterInput4 String
| ChangeTestCasesValidaterOutput4 String
| ChangeTestCasesTestInput5 String
| ChangeTestCasesTestOutput5 String
| ChangeTestCasesValidaterInput5 String
| ChangeTestCasesValidaterOutput5 String
| ChangeTestCases TestCases
| TranRadio String
| StorageInputJ String --储存解决方案
| StorageInputJ_ String
| StorageInputP String
| StorageInputP_ String
| StorageInputH String
| StorageInputH_ String
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
Display result ->
case result of
Ok fullt ->
( model, Cmd.none )
Err _ ->
( model, Cmd.none )
PushDate ->
Debug.log "ceshi"
( model
, Http.post
{ url = "/code"
, body =
Http.multipartBody
[ stringPart "test"
(Encode.encode 1
(Encode.object
[ ( "title", Encode.string model.title )
, ( "statement", Encode.string model.statement )
, ( "inputDescription", Encode.string model.inputDescription )
, ( "outputDescription", Encode.string model.outputDescription )
, ( "constraints", Encode.string model.constraints )
, ( "game_difficulty", Encode.string model.radioValue)
, ( "testCases1"
, Encode.object
[ ( "testName1", Encode.string model.testCases1.testName )
, ( "validator1", Encode.string model.testCases1.validator )
, ( "test1"
, Encode.object
[ ( "input", Encode.string model.testCases1.test.input )
, ( "output", Encode.string model.testCases1.test.output )
]
)
, ( "validater1"
, Encode.object
[ ( "input", Encode.string model.testCases1.validater.input )
, ( "output", Encode.string model.testCases1.validater.output )
]
)
]
)
, ( "testCases2"
, Encode.object
[ ( "testName2", Encode.string model.testCases2.testName )
, ( "validator2", Encode.string model.testCases2.validator )
, ( "test2"
, Encode.object
[ ( "input", Encode.string model.testCases2.test.input )
, ( "output", Encode.string model.testCases2.test.output )
]
)
, ( "validater2"
, Encode.object
[ ( "input", Encode.string model.testCases2.validater.input )
, ( "output", Encode.string model.testCases2.validater.output )
]
)
]
)
, ( "testCases3"
, Encode.object
[ ( "testName3", Encode.string model.testCases3.testName )
, ( "validator3", Encode.string model.testCases3.validator )
, ( "test3"
, Encode.object
[ ( "input", Encode.string model.testCases3.test.input )
, ( "output", Encode.string model.testCases3.test.output )
]
)
, ( "validater3"
, Encode.object
[ ( "input", Encode.string model.testCases3.validater.input )
, ( "output", Encode.string model.testCases3.validater.output )
]
)
]
)
, ( "testCases4"
, Encode.object
[ ( "testName4", Encode.string model.testCases4.testName )
, ( "validator4", Encode.string model.testCases4.validator )
, ( "test4"
, Encode.object
[ ( "input", Encode.string model.testCases4.test.input )
, ( "output", Encode.string model.testCases4.test.output )
]
)
, ( "validater4"
, Encode.object
[ ( "input", Encode.string model.testCases4.validater.input )
, ( "output", Encode.string model.testCases4.validater.output )
]
)
]
)
, ( "testCases5"
, Encode.object
[ ( "testName5", Encode.string model.testCases5.testName )
, ( "validator5", Encode.string model.testCases5.validator )
, ( "test5"
, Encode.object
[ ( "input", Encode.string model.testCases5.test.input )
, ( "output", Encode.string model.testCases5.test.output )
]
)
, ( "validater5"
, Encode.object
[ ( "input", Encode.string model.testCases5.validater.input )
, ( "output", Encode.string model.testCases5.validater.output )
]
)
]
)
, ( "Solution_language_Java",Encode.string model.selectJava)
, ( "Solution_Java",Encode.string model.dataJave)
, ( "Unresolved_Java",Encode.string model.dataJave_)
, ( "Solution_language_Python",Encode.string model.selectPython)
, ( "Solution_Python",Encode.string model.dataPython)
, ( "Unresolved_Python",Encode.string model.dataPython_)
, ( "Solution_language_Haskell",Encode.string model.selectHaskell)
, ( "Solution_Haskell",Encode.string model.dataHaskell)
, ( "Unresolved_Haskell",Encode.string model.dataHaskell_)
]
)
)
]
, expect = Http.expectString Display
}
)
ChangeTitle newTitle ->
( { model | title = newTitle }, Cmd.none )
ChangeStatemen newStatement ->
( { model | statement = newStatement }, Cmd.none )
ChangeInputDescription newInputDescription ->
( { model | inputDescription = newInputDescription }, Cmd.none )
ChangeOutputDescription newOutputDescription ->
( { model | outputDescription = newOutputDescription }, Cmd.none )
ChangeConstraints newConstraints ->
( { model | constraints = newConstraints }, Cmd.none )
ChangeTestCasesTestName1 newTestCasesTestName ->
updateTestName1 (\testCases -> { testCases | testName = newTestCasesTestName }) model
ChangeTestCasesValidator1 newTestCasesValidator ->
updateTestName1 (\testCases -> { testCases | validator = newTestCasesValidator }) model
ChangeTestCasesTestName2 newTestCasesTestName ->
updateTestName2 (\testCases -> { testCases | testName = newTestCasesTestName }) model
ChangeTestCasesValidator2 newTestCasesValidator ->
updateTestName2 (\testCases -> { testCases | validator = newTestCasesValidator }) model
ChangeTestCasesTestName3 newTestCasesTestName ->
updateTestName3 (\testCases -> { testCases | testName = newTestCasesTestName }) model
ChangeTestCasesValidator3 newTestCasesValidator ->
updateTestName3 (\testCases -> { testCases | validator = newTestCasesValidator }) model
ChangeTestCasesTestName4 newTestCasesTestName ->
updateTestName4 (\testCases -> { testCases | testName = newTestCasesTestName }) model
ChangeTestCasesValidator4 newTestCasesValidator ->
updateTestName4 (\testCases -> { testCases | validator = newTestCasesValidator }) model
ChangeTestCasesTestName5 newTestCasesTestName ->
updateTestName5 (\testCases -> { testCases | testName = newTestCasesTestName }) model
ChangeTestCasesValidator5 newTestCasesValidator ->
updateTestName5 (\testCases -> { testCases | validator = newTestCasesValidator }) model
ChangeTestCasesTestInput1 newTestCasesTestInput ->
updateTestCasesTestInput1 (\test -> { test | input = newTestCasesTestInput }) model.testCases1 model
ChangeTestCasesTestOutput1 newTestCasesTestOutput ->
updateTestCasesTestInput1 (\test -> { test | output = newTestCasesTestOutput }) model.testCases1 model
ChangeTestCasesValidaterInput1 newTestCasesValidaterInput ->
updateTestCasesValidater1 (\validater -> { validater | input = newTestCasesValidaterInput }) model.testCases1 model
ChangeTestCasesValidaterOutput1 newTestCasesValidaterOutput ->
updateTestCasesValidater1 (\validater -> { validater | output = newTestCasesValidaterOutput }) model.testCases1 model
ChangeTestCasesTestInput2 newTestCasesTestInput ->
updateTestCasesTestInput2 (\test -> { test | input = newTestCasesTestInput }) model.testCases2 model
ChangeTestCasesTestOutput2 newTestCasesTestOutput ->
updateTestCasesTestInput2 (\test -> { test | output = newTestCasesTestOutput }) model.testCases2 model
ChangeTestCasesValidaterInput2 newTestCasesValidaterInput ->
updateTestCasesValidater2 (\validater -> { validater | input = newTestCasesValidaterInput }) model.testCases2 model
ChangeTestCasesValidaterOutput2 newTestCasesValidaterOutput ->
updateTestCasesValidater2 (\validater -> { validater | output = newTestCasesValidaterOutput }) model.testCases2 model
ChangeTestCasesTestInput3 newTestCasesTestInput ->
updateTestCasesTestInput3 (\test -> { test | input = newTestCasesTestInput }) model.testCases3 model
ChangeTestCasesTestOutput3 newTestCasesTestOutput ->
updateTestCasesTestInput3 (\test -> { test | output = newTestCasesTestOutput }) model.testCases3 model
ChangeTestCasesValidaterInput3 newTestCasesValidaterInput ->
updateTestCasesValidater3 (\validater -> { validater | input = newTestCasesValidaterInput }) model.testCases3 model
ChangeTestCasesValidaterOutput3 newTestCasesValidaterOutput ->
updateTestCasesValidater3 (\validater -> { validater | output = newTestCasesValidaterOutput }) model.testCases3 model
ChangeTestCasesTestInput4 newTestCasesTestInput ->
updateTestCasesTestInput4 (\test -> { test | input = newTestCasesTestInput }) model.testCases4 model
ChangeTestCasesTestOutput4 newTestCasesTestOutput ->
updateTestCasesTestInput4 (\test -> { test | output = newTestCasesTestOutput }) model.testCases4 model
ChangeTestCasesValidaterInput4 newTestCasesValidaterInput ->
updateTestCasesValidater4 (\validater -> { validater | input = newTestCasesValidaterInput }) model.testCases4 model
ChangeTestCasesValidaterOutput4 newTestCasesValidaterOutput ->
updateTestCasesValidater4 (\validater -> { validater | output = newTestCasesValidaterOutput }) model.testCases4 model
ChangeTestCasesTestInput5 newTestCasesTestInput ->
updateTestCasesTestInput5 (\test -> { test | input = newTestCasesTestInput }) model.testCases5 model
ChangeTestCasesTestOutput5 newTestCasesTestOutput ->
updateTestCasesTestInput5 (\test -> { test | output = newTestCasesTestOutput }) model.testCases5 model
ChangeTestCasesValidaterInput5 newTestCasesValidaterInput ->
updateTestCasesValidater5 (\validater -> { validater | input = newTestCasesValidaterInput }) model.testCases5 model
ChangeTestCasesValidaterOutput5 newTestCasesValidaterOutput ->
updateTestCasesValidater5 (\validater -> { validater | output = newTestCasesValidaterOutput }) model.testCases5 model
ChangeTestCases newTestCases ->
( model, Cmd.none )
TranRadio value ->
( { model | radioValue = value }, Cmd.none )
StorageInputJ inputData ->
({model | dataJave = inputData},Cmd.none)
StorageInputJ_ inputData ->
({model | dataJave_ = inputData},Cmd.none)
StorageInputP inputData ->
({model | dataPython = inputData},Cmd.none)
StorageInputP_ inputData ->
({model | dataPython_ = inputData},Cmd.none)
StorageInputH inputData ->
({model | dataHaskell = inputData},Cmd.none)
StorageInputH_ inputData ->
({model | dataHaskell_ = inputData},Cmd.none)
updateTestName1 : (TestCases -> TestCases) -> Model -> ( Model, Cmd Msg )
updateTestName1 transform model =
( { model | testCases1 = transform model.testCases1 }, Cmd.none )
updateTestName2 : (TestCases -> TestCases) -> Model -> ( Model, Cmd Msg )
updateTestName2 transform model =
( { model | testCases2 = transform model.testCases2 }, Cmd.none )
updateTestName3 : (TestCases -> TestCases) -> Model -> ( Model, Cmd Msg )
updateTestName3 transform model =
( { model | testCases3 = transform model.testCases3 }, Cmd.none )
updateTestName4 : (TestCases -> TestCases) -> Model -> ( Model, Cmd Msg )
updateTestName4 transform model =
( { model | testCases4 = transform model.testCases4 }, Cmd.none )
updateTestName5 : (TestCases -> TestCases) -> Model -> ( Model, Cmd Msg )
updateTestName5 transform model =
( { model | testCases5 = transform model.testCases5 }, Cmd.none )
--像json中低层级的key中添加值的一组方法
updateTestCasesTestInput1 : (TestCases_Test -> TestCases_Test) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesTestInput1 transdate testCases1 model =
( { model | testCases1 = { testCases1 | test = transdate model.testCases1.test } }, Cmd.none )
updateTestCasesTestInput2 : (TestCases_Test -> TestCases_Test) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesTestInput2 transdate testCases2 model =
( { model | testCases2 = { testCases2 | test = transdate model.testCases2.test } }, Cmd.none )
updateTestCasesTestInput3 : (TestCases_Test -> TestCases_Test) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesTestInput3 transdate testCases3 model =
( { model | testCases3 = { testCases3 | test = transdate model.testCases3.test } }, Cmd.none )
updateTestCasesTestInput4 : (TestCases_Test -> TestCases_Test) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesTestInput4 transdate testCases4 model =
( { model | testCases4 = { testCases4 | test = transdate model.testCases4.test } }, Cmd.none )
updateTestCasesTestInput5 : (TestCases_Test -> TestCases_Test) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesTestInput5 transdate testCases5 model =
( { model | testCases5 = { testCases5 | test = transdate model.testCases5.test } }, Cmd.none )
updateTestCasesValidater1 : (TestCases_Validater -> TestCases_Validater) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesValidater1 transdate testCases1 model =
( { model | testCases1 = { testCases1 | validater = transdate model.testCases1.validater } }, Cmd.none )
updateTestCasesValidater2 : (TestCases_Validater -> TestCases_Validater) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesValidater2 transdate testCases2 model =
( { model | testCases2 = { testCases2 | validater = transdate model.testCases2.validater } }, Cmd.none )
updateTestCasesValidater3 : (TestCases_Validater -> TestCases_Validater) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesValidater3 transdate testCases3 model =
( { model | testCases3 = { testCases3 | validater = transdate model.testCases3.validater } }, Cmd.none )
updateTestCasesValidater4 : (TestCases_Validater -> TestCases_Validater) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesValidater4 transdate testCases4 model =
( { model | testCases4 = { testCases4 | validater = transdate model.testCases4.validater } }, Cmd.none )
updateTestCasesValidater5 : (TestCases_Validater -> TestCases_Validater) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesValidater5 transdate testCases5 model =
( { model | testCases5 = { testCases5 | validater = transdate model.testCases5.validater } }, Cmd.none )
view : Model -> Html Msg
view model =
div
[ style "background-color" "#454c55"
, style "width" "100%"
, style "height" "4500px"
]
[ div
[ style "position" "absolute"
, style "top" "50%"
, style "left" "50%"
, style "height" "4300px"
, style "width" "50%"
, style "margin" "-15% 0 0 -25%"
, style "border" "1px solid #aaaaaa"
, style "background-color" "#ffffff"
]
[ Html.form
[ style "margin" "50px"
, style "display" "block"
, style "width" "90%"
, style "height" "90%"
]
[ p
[]
[ text "在这个页面上你可以设计自己的拼图!点击"
, a
[ href "#" ]
[ text "这里" ]
, text
"获取更多有关信息"
]
, p
[]
[ text "标题" ]
, input
[ style "border" "1px solid #bbbbbb"
, style "width" "100%"
, style "height" "40px"
, value model.title
, onInput ChangeTitle
]
[]
, p [] [ text "声明" ]
, input
[ style "border" "1px solid #bbbbbb"
, style "width" "100%"
, style "height" "80px"
, value model.statement
, onInput ChangeStatemen
]
[]
, p [] [ text "输入说明" ]
, input
[ style "border" "1px solid #bbbbbb"
, style "width" "100%"
, style "height" "80px"
, value model.inputDescription
, onInput ChangeInputDescription
]
[]
, p [] [ text "输出说明" ]
, input
[ style "border" "1px solid #bbbbbb"
, style "width" "100%"
, style "height" "80px"
, value model.outputDescription
, onInput ChangeOutputDescription
]
[]
, p [] [ text "限制条件" ]
, input
[ style "border" "1px solid #bbbbbb"
, style "width" "100%"
, style "height" "80px"
, value model.constraints
, onInput ChangeConstraints
]
[]
, div
--Game modes 已转变成游戏难度
[ style "width" "100%"
, style "height" "120px"
]
[ p [] [ text "游戏难度" ]
, label [] [ input [ type_ "radio", value "Easy", checked (model.radioValue == "Easy"), onClick (TranRadio "Easy") ] [], text "简单" ]
, label [] [ input [ type_ "radio", value "Medium", checked (model.radioValue == "Medium"), onClick (TranRadio "Medium") ] [], text "中等" ]
, label [] [ input [ type_ "radio", value "Difficult", checked (model.radioValue == "Difficult"), onClick (TranRadio "Difficult") ] [], text "困难" ]
, label [] [ input [ type_ "radio", value "Expert", checked (model.radioValue == "Expert"), onClick (TranRadio "Expert") ] [], text "专家" ]
]
, div
--Test cases
[ style "width" "100%"
]
[ text "测试用例"
, input [ style "color" "#838891"
, placeholder "测试1"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases1.testName
, onInput ChangeTestCasesTestName1 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases1.test.input
, onInput ChangeTestCasesTestInput1
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases1.test.output
, onInput ChangeTestCasesTestOutput1
]
[]
, input [ style "color" "#838891"
, placeholder "验证1"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases1.validator
, onInput ChangeTestCasesValidator1 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases1.validater.input
, onInput ChangeTestCasesValidaterInput1
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases1.validater.output
, onInput ChangeTestCasesValidaterOutput1
]
[]
, div
--分割线 测试和验证2
[ style "border" "1px solid #bbbbbb"
, style "margin-top" "20px"
]
[]
, input [ style "color" "#838891"
, placeholder "测试2"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases2.testName
, onInput ChangeTestCasesTestName2 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases2.test.input
, onInput ChangeTestCasesTestInput2
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases2.test.output
, onInput ChangeTestCasesTestOutput2
]
[]
, input [ style "color" "#838891"
, placeholder "验证2"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases2.validator
, onInput ChangeTestCasesValidator2 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases2.validater.input
, onInput ChangeTestCasesValidaterInput2
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases2.validater.output
, onInput ChangeTestCasesValidaterOutput2
]
[]
, div
--分割线 测试和验证3
[ style "border" "1px solid #bbbbbb"
, style "margin-top" "20px"
]
[]
, input [ style "color" "#838891"
, placeholder "测试3"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases3.testName
, onInput ChangeTestCasesTestName3 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases3.test.input
, onInput ChangeTestCasesTestInput3
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases3.test.output
, onInput ChangeTestCasesTestOutput3
]
[]
, input [ style "color" "#838891"
, placeholder "验证3"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases3.validator
, onInput ChangeTestCasesValidator3 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases3.validater.input
, onInput ChangeTestCasesValidaterInput3
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases3.validater.output
, onInput ChangeTestCasesValidaterOutput3
]
[]
, div
--分割线 测试和验证4
[ style "border" "1px solid #bbbbbb"
, style "margin-top" "20px"
]
[]
, input [ style "color" "#838891"
, placeholder "测试4"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases4.testName
, onInput ChangeTestCasesTestName4 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases4.test.input
, onInput ChangeTestCasesTestInput4
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases4.test.output
, onInput ChangeTestCasesTestOutput4
]
[]
, input [ style "color" "#838891"
, placeholder "验证4"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases4.validator
, onInput ChangeTestCasesValidator4 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases4.validater.input
, onInput ChangeTestCasesValidaterInput4
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases4.validater.output
, onInput ChangeTestCasesValidaterOutput4
]
[]
, div
--分割线 测试和验证5
[ style "border" "1px solid #bbbbbb"
, style "margin-top" "20px"
]
[]
, input [ style "color" "#838891"
, placeholder "测试5"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases5.testName
, onInput ChangeTestCasesTestName5 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases5.validater.input
, onInput ChangeTestCasesTestInput5
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases5.validater.output
, onInput ChangeTestCasesTestOutput5
]
[]
, input [ style "color" "#838891"
, placeholder "验证5"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases5.validator
, onInput ChangeTestCasesValidator5 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases5.validater.input
, onInput ChangeTestCasesValidaterInput5
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases5.validater.output
, onInput ChangeTestCasesValidaterOutput5
]
[]
, div
[ style "width" "150px"
, style "height" "30px"
, style "background-color" "rgba(69,76,85,0.15)"
]
[ text "添加一个测试用例" ]
]
, div
[ style "width" "100%"
, style "margin-top" "20px"
]
[ p [] [ text "解决语言" ]
, p [] [text "Java"]
]
, div []
--
[ p [] [ text "解决方案" ]
, textarea
[ style "width" "100%"
, style "height" "200px"
, value model.dataJave
, onInput StorageInputJ
]
[]
]
, div []
--Stub generator input
[ p [] [ text "未解决方案" ]
, textarea
[ style "width" "100%"
, style "height" "200px"
, value model.dataJave_
, onInput StorageInputJ_
]
[]
]
, div
[ style "width" "100%"
, style "margin-top" "20px"
]
[ p [] [ text "解决语言" ]
, p [] [text "Python"]
]
, div []
--
[ p [] [ text "解决方案" ]
, textarea
[ style "width" "100%"
, style "height" "200px"
, value model.dataPython
, onInput StorageInputP
]
[]
]
, div []
--Stub generator input
[ p [] [ text "未解决方案" ]
, textarea
[ style "width" "100%"
, style "height" "200px"
, value model.dataPython_
, onInput StorageInputP_
]
[]
]
, div
[ style "width" "100%"
, style "margin-top" "20px"
]
[ p [] [ text "解决语言" ]
, p [] [text "Haskell"]
]
, div []
--
[ p [] [ text "解决方案" ]
, textarea
[ style "width" "100%"
, style "height" "200px"
, value model.dataHaskell
, onInput StorageInputH
]
[]
]
, div []
--Stub generator input
[ p [] [ text "未解决方案" ]
, textarea
[ style "width" "100%"
, style "height" "200px"
, value model.dataHaskell_
, onInput StorageInputH_
]
[]
]
, div [
style "display" "inline-block"
, style "width" "100%"
, style "height" "50px"
, style "background-color" "rgba(242,187,19,0.7)"
, style "margin-bottom" "20px"
] [text "提交方案"]
, div []
[
div
[ style "display" "inline-block"
, style "width" "50%"
, style "height" "50px"
, style "background-color" "rgba(242,187,19,0.7)"
, onClick PushDate
]
[ text "保存" ]
, div
[ style "display" "inline-block"
, style "width" "50%"
, style "height" "50px"
, style "background-color" "rgba(69,76,85,0.1)"
]
[ text "测试在IDE" ]
]
]
]
]
subscriptions : Model -> Sub Msg
subscriptions model =
Sub.none
| 59708 | module Main exposing (main)
import Array exposing (..)
import Browser
import Debug exposing (..)
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick, onInput)
import Http exposing (..)
import Json.Encode as Encode
main =
Browser.element
{ init = init
, update = update
, view = view
, subscriptions = subscriptions
}
type alias Title =
String
type alias Statement =
String
type alias InputDescription =
String
type alias OutputDescription =
String
type alias Constraints =
String
type alias TestCases_Test =
{ input : String, output : String }
type alias TestCases_Validater =
{ input : String, output : String }
type alias TestCases =
{ testName : String
, validator : String
, test : TestCases_Test
, validater : TestCases_Validater
}
type alias Model =
{ title : Title --标题
, statement : Statement --声明
, inputDescription : InputDescription --输入说明
, outputDescription : OutputDescription --输出说明
, constraints : Constraints --限制条件
, testCases1 : TestCases --测试用例
, testCases2 : TestCases
, testCases3 : TestCases
, testCases4 : TestCases
, testCases5 : TestCases
, radioValue : String --用于构建单选框
, dataJave : String --用于更改选框中的语言的时候储存文本框中的String
, dataPython : String
, dataHaskell : String
, dataJave_ : String --用于更改选框中的语言的时候储存文本框中的String
, dataPython_ : String
, dataHaskell_ : String
, selectJava : String
, selectPython : String
, selectHaskell : String
}
init : () -> ( Model, Cmd Msg )
init _ =
( { title = ""
, statement = ""
, inputDescription = ""
, outputDescription = ""
, constraints = ""
, testCases1 =
{ testName = ""
, validator = ""
, test =
{ input = ""
, output = ""
}
, validater =
{ input = ""
, output = ""
}
}
, testCases2 =
{ testName = ""
, validator = ""
, test =
{ input = ""
, output = ""
}
, validater =
{ input = ""
, output = ""
}
}
, testCases3 =
{ testName = ""
, validator = ""
, test =
{ input = ""
, output = ""
}
, validater =
{ input = ""
, output = ""
}
}
, testCases4 =
{ testName = ""
, validator = ""
, test =
{ input = ""
, output = ""
}
, validater =
{ input = ""
, output = ""
}
}
, testCases5 =
{ testName = ""
, validator = ""
, test =
{ input = ""
, output = ""
}
, validater =
{ input = ""
, output = ""
}
}
, radioValue = "Easy"
, dataJave = ""
, dataPython = ""
, dataHaskell = ""
, dataJave_ = ""
, dataPython_ = ""
, dataHaskell_ = ""
, selectJava = "Java"
, selectPython = "Python"
, selectHaskell = "Haskell"
}
, Cmd.none
)
type Msg
= Display (Result Http.Error String)
| PushDate
| ChangeTitle String
| ChangeStatemen String
| ChangeInputDescription String
| ChangeOutputDescription String
| ChangeConstraints String
| ChangeTestCasesTestName1 String
| ChangeTestCasesValidator1 String
| ChangeTestCasesTestName2 String
| ChangeTestCasesValidator2 String
| ChangeTestCasesTestName3 String
| ChangeTestCasesValidator3 String
| ChangeTestCasesTestName4 String
| ChangeTestCasesValidator4 String
| ChangeTestCasesTestName5 String
| ChangeTestCasesValidator5 String
| ChangeTestCasesTestInput1 String
| ChangeTestCasesTestOutput1 String
| ChangeTestCasesValidaterInput1 String
| ChangeTestCasesValidaterOutput1 String
| ChangeTestCasesTestInput2 String
| ChangeTestCasesTestOutput2 String
| ChangeTestCasesValidaterInput2 String
| ChangeTestCasesValidaterOutput2 String
| ChangeTestCasesTestInput3 String
| ChangeTestCasesTestOutput3 String
| ChangeTestCasesValidaterInput3 String
| ChangeTestCasesValidaterOutput3 String
| ChangeTestCasesTestInput4 String
| ChangeTestCasesTestOutput4 String
| ChangeTestCasesValidaterInput4 String
| ChangeTestCasesValidaterOutput4 String
| ChangeTestCasesTestInput5 String
| ChangeTestCasesTestOutput5 String
| ChangeTestCasesValidaterInput5 String
| ChangeTestCasesValidaterOutput5 String
| ChangeTestCases TestCases
| TranRadio String
| StorageInputJ String --储存解决方案
| StorageInputJ_ String
| StorageInputP String
| StorageInputP_ String
| StorageInputH String
| StorageInputH_ String
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
Display result ->
case result of
Ok fullt ->
( model, Cmd.none )
Err _ ->
( model, Cmd.none )
PushDate ->
Debug.log "ceshi"
( model
, Http.post
{ url = "/code"
, body =
Http.multipartBody
[ stringPart "test"
(Encode.encode 1
(Encode.object
[ ( "title", Encode.string model.title )
, ( "statement", Encode.string model.statement )
, ( "inputDescription", Encode.string model.inputDescription )
, ( "outputDescription", Encode.string model.outputDescription )
, ( "constraints", Encode.string model.constraints )
, ( "game_difficulty", Encode.string model.radioValue)
, ( "testCases1"
, Encode.object
[ ( "testName1", Encode.string model.testCases1.testName )
, ( "validator1", Encode.string model.testCases1.validator )
, ( "test1"
, Encode.object
[ ( "input", Encode.string model.testCases1.test.input )
, ( "output", Encode.string model.testCases1.test.output )
]
)
, ( "validater1"
, Encode.object
[ ( "input", Encode.string model.testCases1.validater.input )
, ( "output", Encode.string model.testCases1.validater.output )
]
)
]
)
, ( "testCases2"
, Encode.object
[ ( "testName2", Encode.string model.testCases2.testName )
, ( "validator2", Encode.string model.testCases2.validator )
, ( "test2"
, Encode.object
[ ( "input", Encode.string model.testCases2.test.input )
, ( "output", Encode.string model.testCases2.test.output )
]
)
, ( "validater2"
, Encode.object
[ ( "input", Encode.string model.testCases2.validater.input )
, ( "output", Encode.string model.testCases2.validater.output )
]
)
]
)
, ( "testCases3"
, Encode.object
[ ( "testName3", Encode.string model.testCases3.testName )
, ( "validator3", Encode.string model.testCases3.validator )
, ( "test3"
, Encode.object
[ ( "input", Encode.string model.testCases3.test.input )
, ( "output", Encode.string model.testCases3.test.output )
]
)
, ( "validater3"
, Encode.object
[ ( "input", Encode.string model.testCases3.validater.input )
, ( "output", Encode.string model.testCases3.validater.output )
]
)
]
)
, ( "testCases4"
, Encode.object
[ ( "testName4", Encode.string model.testCases4.testName )
, ( "validator4", Encode.string model.testCases4.validator )
, ( "test4"
, Encode.object
[ ( "input", Encode.string model.testCases4.test.input )
, ( "output", Encode.string model.testCases4.test.output )
]
)
, ( "validater4"
, Encode.object
[ ( "input", Encode.string model.testCases4.validater.input )
, ( "output", Encode.string model.testCases4.validater.output )
]
)
]
)
, ( "testCases5"
, Encode.object
[ ( "testName5", Encode.string model.testCases5.testName )
, ( "validator5", Encode.string model.testCases5.validator )
, ( "test5"
, Encode.object
[ ( "input", Encode.string model.testCases5.test.input )
, ( "output", Encode.string model.testCases5.test.output )
]
)
, ( "validater5"
, Encode.object
[ ( "input", Encode.string model.testCases5.validater.input )
, ( "output", Encode.string model.testCases5.validater.output )
]
)
]
)
, ( "Solution_language_Java",Encode.string model.selectJava)
, ( "Solution_Java",Encode.string model.dataJave)
, ( "Unresolved_Java",Encode.string model.dataJave_)
, ( "Solution_language_Python",Encode.string model.selectPython)
, ( "Solution_Python",Encode.string model.dataPython)
, ( "Unresolved_Python",Encode.string model.dataPython_)
, ( "Solution_language_Haskell",Encode.string model.selectHaskell)
, ( "Solution_Haskell",Encode.string model.dataHaskell)
, ( "Unresolved_Haskell",Encode.string model.dataHaskell_)
]
)
)
]
, expect = Http.expectString Display
}
)
ChangeTitle newTitle ->
( { model | title = newTitle }, Cmd.none )
ChangeStatemen newStatement ->
( { model | statement = newStatement }, Cmd.none )
ChangeInputDescription newInputDescription ->
( { model | inputDescription = newInputDescription }, Cmd.none )
ChangeOutputDescription newOutputDescription ->
( { model | outputDescription = newOutputDescription }, Cmd.none )
ChangeConstraints newConstraints ->
( { model | constraints = newConstraints }, Cmd.none )
ChangeTestCasesTestName1 newTestCasesTestName ->
updateTestName1 (\testCases -> { testCases | testName = newTestCasesTestName }) model
ChangeTestCasesValidator1 newTestCasesValidator ->
updateTestName1 (\testCases -> { testCases | validator = newTestCasesValidator }) model
ChangeTestCasesTestName2 newTestCasesTestName ->
updateTestName2 (\testCases -> { testCases | testName = newTestCasesTestName }) model
ChangeTestCasesValidator2 newTestCasesValidator ->
updateTestName2 (\testCases -> { testCases | validator = newTestCasesValidator }) model
ChangeTestCasesTestName3 newTestCasesTestName ->
updateTestName3 (\testCases -> { testCases | testName = newTestCasesTestName }) model
ChangeTestCasesValidator3 newTestCasesValidator ->
updateTestName3 (\testCases -> { testCases | validator = newTestCasesValidator }) model
ChangeTestCasesTestName4 newTestCasesTestName ->
updateTestName4 (\testCases -> { testCases | testName = newTestCasesTestName }) model
ChangeTestCasesValidator4 newTestCasesValidator ->
updateTestName4 (\testCases -> { testCases | validator = newTestCasesValidator }) model
ChangeTestCasesTestName5 newTestCasesTestName ->
updateTestName5 (\testCases -> { testCases | testName = newTestCasesTestName }) model
ChangeTestCasesValidator5 newTestCasesValidator ->
updateTestName5 (\testCases -> { testCases | validator = newTestCasesValidator }) model
ChangeTestCasesTestInput1 newTestCasesTestInput ->
updateTestCasesTestInput1 (\test -> { test | input = newTestCasesTestInput }) model.testCases1 model
ChangeTestCasesTestOutput1 newTestCasesTestOutput ->
updateTestCasesTestInput1 (\test -> { test | output = newTestCasesTestOutput }) model.testCases1 model
ChangeTestCasesValidaterInput1 newTestCasesValidaterInput ->
updateTestCasesValidater1 (\validater -> { validater | input = newTestCasesValidaterInput }) model.testCases1 model
ChangeTestCasesValidaterOutput1 newTestCasesValidaterOutput ->
updateTestCasesValidater1 (\validater -> { validater | output = newTestCasesValidaterOutput }) model.testCases1 model
ChangeTestCasesTestInput2 newTestCasesTestInput ->
updateTestCasesTestInput2 (\test -> { test | input = newTestCasesTestInput }) model.testCases2 model
ChangeTestCasesTestOutput2 newTestCasesTestOutput ->
updateTestCasesTestInput2 (\test -> { test | output = newTestCasesTestOutput }) model.testCases2 model
ChangeTestCasesValidaterInput2 newTestCasesValidaterInput ->
updateTestCasesValidater2 (\validater -> { validater | input = newTestCasesValidaterInput }) model.testCases2 model
ChangeTestCasesValidaterOutput2 newTestCasesValidaterOutput ->
updateTestCasesValidater2 (\validater -> { validater | output = newTestCasesValidaterOutput }) model.testCases2 model
ChangeTestCasesTestInput3 newTestCasesTestInput ->
updateTestCasesTestInput3 (\test -> { test | input = newTestCasesTestInput }) model.testCases3 model
ChangeTestCasesTestOutput3 newTestCasesTestOutput ->
updateTestCasesTestInput3 (\test -> { test | output = newTestCasesTestOutput }) model.testCases3 model
ChangeTestCasesValidaterInput3 newTestCasesValidaterInput ->
updateTestCasesValidater3 (\validater -> { validater | input = newTestCasesValidaterInput }) model.testCases3 model
ChangeTestCasesValidaterOutput3 newTestCasesValidaterOutput ->
updateTestCasesValidater3 (\validater -> { validater | output = newTestCasesValidaterOutput }) model.testCases3 model
ChangeTestCasesTestInput4 newTestCasesTestInput ->
updateTestCasesTestInput4 (\test -> { test | input = newTestCasesTestInput }) model.testCases4 model
ChangeTestCasesTestOutput4 newTestCasesTestOutput ->
updateTestCasesTestInput4 (\test -> { test | output = newTestCasesTestOutput }) model.testCases4 model
ChangeTestCasesValidaterInput4 newTestCasesValidaterInput ->
updateTestCasesValidater4 (\validater -> { validater | input = newTestCasesValidaterInput }) model.testCases4 model
ChangeTestCasesValidaterOutput4 newTestCasesValidaterOutput ->
updateTestCasesValidater4 (\validater -> { validater | output = newTestCasesValidaterOutput }) model.testCases4 model
ChangeTestCasesTestInput5 newTestCasesTestInput ->
updateTestCasesTestInput5 (\test -> { test | input = newTestCasesTestInput }) model.testCases5 model
ChangeTestCasesTestOutput5 newTestCasesTestOutput ->
updateTestCasesTestInput5 (\test -> { test | output = newTestCasesTestOutput }) model.testCases5 model
ChangeTestCasesValidaterInput5 newTestCasesValidaterInput ->
updateTestCasesValidater5 (\validater -> { validater | input = newTestCasesValidaterInput }) model.testCases5 model
ChangeTestCasesValidaterOutput5 newTestCasesValidaterOutput ->
updateTestCasesValidater5 (\validater -> { validater | output = newTestCasesValidaterOutput }) model.testCases5 model
ChangeTestCases newTestCases ->
( model, Cmd.none )
TranRadio value ->
( { model | radioValue = value }, Cmd.none )
StorageInputJ inputData ->
({model | dataJave = inputData},Cmd.none)
StorageInputJ_ inputData ->
({model | dataJave_ = inputData},Cmd.none)
StorageInputP inputData ->
({model | dataPython = inputData},Cmd.none)
StorageInputP_ inputData ->
({model | dataPython_ = inputData},Cmd.none)
StorageInputH inputData ->
({model | dataHaskell = inputData},Cmd.none)
StorageInputH_ inputData ->
({model | dataHaskell_ = inputData},Cmd.none)
updateTestName1 : (TestCases -> TestCases) -> Model -> ( Model, Cmd Msg )
updateTestName1 transform model =
( { model | testCases1 = transform model.testCases1 }, Cmd.none )
updateTestName2 : (TestCases -> TestCases) -> Model -> ( Model, Cmd Msg )
updateTestName2 transform model =
( { model | testCases2 = transform model.testCases2 }, Cmd.none )
updateTestName3 : (TestCases -> TestCases) -> Model -> ( Model, Cmd Msg )
updateTestName3 transform model =
( { model | testCases3 = transform model.testCases3 }, Cmd.none )
updateTestName4 : (TestCases -> TestCases) -> Model -> ( Model, Cmd Msg )
updateTestName4 transform model =
( { model | testCases4 = transform model.testCases4 }, Cmd.none )
updateTestName5 : (TestCases -> TestCases) -> Model -> ( Model, Cmd Msg )
updateTestName5 transform model =
( { model | testCases5 = transform model.testCases5 }, Cmd.none )
--像json中低层级的key中添加值的一组方法
updateTestCasesTestInput1 : (TestCases_Test -> TestCases_Test) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesTestInput1 transdate testCases1 model =
( { model | testCases1 = { testCases1 | test = transdate model.testCases1.test } }, Cmd.none )
updateTestCasesTestInput2 : (TestCases_Test -> TestCases_Test) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesTestInput2 transdate testCases2 model =
( { model | testCases2 = { testCases2 | test = transdate model.testCases2.test } }, Cmd.none )
updateTestCasesTestInput3 : (TestCases_Test -> TestCases_Test) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesTestInput3 transdate testCases3 model =
( { model | testCases3 = { testCases3 | test = transdate model.testCases3.test } }, Cmd.none )
updateTestCasesTestInput4 : (TestCases_Test -> TestCases_Test) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesTestInput4 transdate testCases4 model =
( { model | testCases4 = { testCases4 | test = transdate model.testCases4.test } }, Cmd.none )
updateTestCasesTestInput5 : (TestCases_Test -> TestCases_Test) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesTestInput5 transdate testCases5 model =
( { model | testCases5 = { testCases5 | test = transdate model.testCases5.test } }, Cmd.none )
updateTestCasesValidater1 : (TestCases_Validater -> TestCases_Validater) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesValidater1 transdate testCases1 model =
( { model | testCases1 = { testCases1 | validater = transdate model.testCases1.validater } }, Cmd.none )
updateTestCasesValidater2 : (TestCases_Validater -> TestCases_Validater) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesValidater2 transdate testCases2 model =
( { model | testCases2 = { testCases2 | validater = transdate model.testCases2.validater } }, Cmd.none )
updateTestCasesValidater3 : (TestCases_Validater -> TestCases_Validater) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesValidater3 transdate testCases3 model =
( { model | testCases3 = { testCases3 | validater = transdate model.testCases3.validater } }, Cmd.none )
updateTestCasesValidater4 : (TestCases_Validater -> TestCases_Validater) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesValidater4 transdate testCases4 model =
( { model | testCases4 = { testCases4 | validater = transdate model.testCases4.validater } }, Cmd.none )
updateTestCasesValidater5 : (TestCases_Validater -> TestCases_Validater) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesValidater5 transdate testCases5 model =
( { model | testCases5 = { testCases5 | validater = transdate model.testCases5.validater } }, Cmd.none )
view : Model -> Html Msg
view model =
div
[ style "background-color" "#454c55"
, style "width" "100%"
, style "height" "4500px"
]
[ div
[ style "position" "absolute"
, style "top" "50%"
, style "left" "50%"
, style "height" "4300px"
, style "width" "50%"
, style "margin" "-15% 0 0 -25%"
, style "border" "1px solid #aaaaaa"
, style "background-color" "#ffffff"
]
[ Html.form
[ style "margin" "50px"
, style "display" "block"
, style "width" "90%"
, style "height" "90%"
]
[ p
[]
[ text "在这个页面上你可以设计自己的拼图!点击"
, a
[ href "#" ]
[ text "这里" ]
, text
"获取更多有关信息"
]
, p
[]
[ text "标题" ]
, input
[ style "border" "1px solid #bbbbbb"
, style "width" "100%"
, style "height" "40px"
, value model.title
, onInput ChangeTitle
]
[]
, p [] [ text "声明" ]
, input
[ style "border" "1px solid #bbbbbb"
, style "width" "100%"
, style "height" "80px"
, value model.statement
, onInput ChangeStatemen
]
[]
, p [] [ text "输入说明" ]
, input
[ style "border" "1px solid #bbbbbb"
, style "width" "100%"
, style "height" "80px"
, value model.inputDescription
, onInput ChangeInputDescription
]
[]
, p [] [ text "输出说明" ]
, input
[ style "border" "1px solid #bbbbbb"
, style "width" "100%"
, style "height" "80px"
, value model.outputDescription
, onInput ChangeOutputDescription
]
[]
, p [] [ text "限制条件" ]
, input
[ style "border" "1px solid #bbbbbb"
, style "width" "100%"
, style "height" "80px"
, value model.constraints
, onInput ChangeConstraints
]
[]
, div
--Game modes 已转变成游戏难度
[ style "width" "100%"
, style "height" "120px"
]
[ p [] [ text "游戏难度" ]
, label [] [ input [ type_ "radio", value "Easy", checked (model.radioValue == "Easy"), onClick (TranRadio "Easy") ] [], text "简单" ]
, label [] [ input [ type_ "radio", value "Medium", checked (model.radioValue == "Medium"), onClick (TranRadio "Medium") ] [], text "中等" ]
, label [] [ input [ type_ "radio", value "Difficult", checked (model.radioValue == "Difficult"), onClick (TranRadio "Difficult") ] [], text "困难" ]
, label [] [ input [ type_ "radio", value "Expert", checked (model.radioValue == "Expert"), onClick (TranRadio "Expert") ] [], text "专家" ]
]
, div
--Test cases
[ style "width" "100%"
]
[ text "测试用例"
, input [ style "color" "#838891"
, placeholder "测试1"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases1.testName
, onInput ChangeTestCasesTestName1 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases1.test.input
, onInput ChangeTestCasesTestInput1
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases1.test.output
, onInput ChangeTestCasesTestOutput1
]
[]
, input [ style "color" "#838891"
, placeholder "验证1"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases1.validator
, onInput ChangeTestCasesValidator1 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases1.validater.input
, onInput ChangeTestCasesValidaterInput1
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases1.validater.output
, onInput ChangeTestCasesValidaterOutput1
]
[]
, div
--分割线 测试和验证2
[ style "border" "1px solid #bbbbbb"
, style "margin-top" "20px"
]
[]
, input [ style "color" "#838891"
, placeholder "<NAME>"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases2.testName
, onInput ChangeTestCasesTestName2 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases2.test.input
, onInput ChangeTestCasesTestInput2
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases2.test.output
, onInput ChangeTestCasesTestOutput2
]
[]
, input [ style "color" "#838891"
, placeholder "<NAME>"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases2.validator
, onInput ChangeTestCasesValidator2 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases2.validater.input
, onInput ChangeTestCasesValidaterInput2
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases2.validater.output
, onInput ChangeTestCasesValidaterOutput2
]
[]
, div
--分割线 测试和验证3
[ style "border" "1px solid #bbbbbb"
, style "margin-top" "20px"
]
[]
, input [ style "color" "#838891"
, placeholder "<NAME>"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases3.testName
, onInput ChangeTestCasesTestName3 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases3.test.input
, onInput ChangeTestCasesTestInput3
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases3.test.output
, onInput ChangeTestCasesTestOutput3
]
[]
, input [ style "color" "#838891"
, placeholder "验证3"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases3.validator
, onInput ChangeTestCasesValidator3 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases3.validater.input
, onInput ChangeTestCasesValidaterInput3
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases3.validater.output
, onInput ChangeTestCasesValidaterOutput3
]
[]
, div
--分割线 测试和验证4
[ style "border" "1px solid #bbbbbb"
, style "margin-top" "20px"
]
[]
, input [ style "color" "#838891"
, placeholder "测试4"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases4.testName
, onInput ChangeTestCasesTestName4 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases4.test.input
, onInput ChangeTestCasesTestInput4
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases4.test.output
, onInput ChangeTestCasesTestOutput4
]
[]
, input [ style "color" "#838891"
, placeholder "验证4"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases4.validator
, onInput ChangeTestCasesValidator4 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases4.validater.input
, onInput ChangeTestCasesValidaterInput4
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases4.validater.output
, onInput ChangeTestCasesValidaterOutput4
]
[]
, div
--分割线 测试和验证5
[ style "border" "1px solid #bbbbbb"
, style "margin-top" "20px"
]
[]
, input [ style "color" "#838891"
, placeholder "测试5"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases5.testName
, onInput ChangeTestCasesTestName5 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases5.validater.input
, onInput ChangeTestCasesTestInput5
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases5.validater.output
, onInput ChangeTestCasesTestOutput5
]
[]
, input [ style "color" "#838891"
, placeholder "验证5"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases5.validator
, onInput ChangeTestCasesValidator5 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases5.validater.input
, onInput ChangeTestCasesValidaterInput5
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases5.validater.output
, onInput ChangeTestCasesValidaterOutput5
]
[]
, div
[ style "width" "150px"
, style "height" "30px"
, style "background-color" "rgba(69,76,85,0.15)"
]
[ text "添加一个测试用例" ]
]
, div
[ style "width" "100%"
, style "margin-top" "20px"
]
[ p [] [ text "解决语言" ]
, p [] [text "Java"]
]
, div []
--
[ p [] [ text "解决方案" ]
, textarea
[ style "width" "100%"
, style "height" "200px"
, value model.dataJave
, onInput StorageInputJ
]
[]
]
, div []
--Stub generator input
[ p [] [ text "未解决方案" ]
, textarea
[ style "width" "100%"
, style "height" "200px"
, value model.dataJave_
, onInput StorageInputJ_
]
[]
]
, div
[ style "width" "100%"
, style "margin-top" "20px"
]
[ p [] [ text "解决语言" ]
, p [] [text "Python"]
]
, div []
--
[ p [] [ text "解决方案" ]
, textarea
[ style "width" "100%"
, style "height" "200px"
, value model.dataPython
, onInput StorageInputP
]
[]
]
, div []
--Stub generator input
[ p [] [ text "未解决方案" ]
, textarea
[ style "width" "100%"
, style "height" "200px"
, value model.dataPython_
, onInput StorageInputP_
]
[]
]
, div
[ style "width" "100%"
, style "margin-top" "20px"
]
[ p [] [ text "解决语言" ]
, p [] [text "Haskell"]
]
, div []
--
[ p [] [ text "解决方案" ]
, textarea
[ style "width" "100%"
, style "height" "200px"
, value model.dataHaskell
, onInput StorageInputH
]
[]
]
, div []
--Stub generator input
[ p [] [ text "未解决方案" ]
, textarea
[ style "width" "100%"
, style "height" "200px"
, value model.dataHaskell_
, onInput StorageInputH_
]
[]
]
, div [
style "display" "inline-block"
, style "width" "100%"
, style "height" "50px"
, style "background-color" "rgba(242,187,19,0.7)"
, style "margin-bottom" "20px"
] [text "提交方案"]
, div []
[
div
[ style "display" "inline-block"
, style "width" "50%"
, style "height" "50px"
, style "background-color" "rgba(242,187,19,0.7)"
, onClick PushDate
]
[ text "保存" ]
, div
[ style "display" "inline-block"
, style "width" "50%"
, style "height" "50px"
, style "background-color" "rgba(69,76,85,0.1)"
]
[ text "测试在IDE" ]
]
]
]
]
subscriptions : Model -> Sub Msg
subscriptions model =
Sub.none
| true | module Main exposing (main)
import Array exposing (..)
import Browser
import Debug exposing (..)
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick, onInput)
import Http exposing (..)
import Json.Encode as Encode
main =
Browser.element
{ init = init
, update = update
, view = view
, subscriptions = subscriptions
}
type alias Title =
String
type alias Statement =
String
type alias InputDescription =
String
type alias OutputDescription =
String
type alias Constraints =
String
type alias TestCases_Test =
{ input : String, output : String }
type alias TestCases_Validater =
{ input : String, output : String }
type alias TestCases =
{ testName : String
, validator : String
, test : TestCases_Test
, validater : TestCases_Validater
}
type alias Model =
{ title : Title --标题
, statement : Statement --声明
, inputDescription : InputDescription --输入说明
, outputDescription : OutputDescription --输出说明
, constraints : Constraints --限制条件
, testCases1 : TestCases --测试用例
, testCases2 : TestCases
, testCases3 : TestCases
, testCases4 : TestCases
, testCases5 : TestCases
, radioValue : String --用于构建单选框
, dataJave : String --用于更改选框中的语言的时候储存文本框中的String
, dataPython : String
, dataHaskell : String
, dataJave_ : String --用于更改选框中的语言的时候储存文本框中的String
, dataPython_ : String
, dataHaskell_ : String
, selectJava : String
, selectPython : String
, selectHaskell : String
}
init : () -> ( Model, Cmd Msg )
init _ =
( { title = ""
, statement = ""
, inputDescription = ""
, outputDescription = ""
, constraints = ""
, testCases1 =
{ testName = ""
, validator = ""
, test =
{ input = ""
, output = ""
}
, validater =
{ input = ""
, output = ""
}
}
, testCases2 =
{ testName = ""
, validator = ""
, test =
{ input = ""
, output = ""
}
, validater =
{ input = ""
, output = ""
}
}
, testCases3 =
{ testName = ""
, validator = ""
, test =
{ input = ""
, output = ""
}
, validater =
{ input = ""
, output = ""
}
}
, testCases4 =
{ testName = ""
, validator = ""
, test =
{ input = ""
, output = ""
}
, validater =
{ input = ""
, output = ""
}
}
, testCases5 =
{ testName = ""
, validator = ""
, test =
{ input = ""
, output = ""
}
, validater =
{ input = ""
, output = ""
}
}
, radioValue = "Easy"
, dataJave = ""
, dataPython = ""
, dataHaskell = ""
, dataJave_ = ""
, dataPython_ = ""
, dataHaskell_ = ""
, selectJava = "Java"
, selectPython = "Python"
, selectHaskell = "Haskell"
}
, Cmd.none
)
type Msg
= Display (Result Http.Error String)
| PushDate
| ChangeTitle String
| ChangeStatemen String
| ChangeInputDescription String
| ChangeOutputDescription String
| ChangeConstraints String
| ChangeTestCasesTestName1 String
| ChangeTestCasesValidator1 String
| ChangeTestCasesTestName2 String
| ChangeTestCasesValidator2 String
| ChangeTestCasesTestName3 String
| ChangeTestCasesValidator3 String
| ChangeTestCasesTestName4 String
| ChangeTestCasesValidator4 String
| ChangeTestCasesTestName5 String
| ChangeTestCasesValidator5 String
| ChangeTestCasesTestInput1 String
| ChangeTestCasesTestOutput1 String
| ChangeTestCasesValidaterInput1 String
| ChangeTestCasesValidaterOutput1 String
| ChangeTestCasesTestInput2 String
| ChangeTestCasesTestOutput2 String
| ChangeTestCasesValidaterInput2 String
| ChangeTestCasesValidaterOutput2 String
| ChangeTestCasesTestInput3 String
| ChangeTestCasesTestOutput3 String
| ChangeTestCasesValidaterInput3 String
| ChangeTestCasesValidaterOutput3 String
| ChangeTestCasesTestInput4 String
| ChangeTestCasesTestOutput4 String
| ChangeTestCasesValidaterInput4 String
| ChangeTestCasesValidaterOutput4 String
| ChangeTestCasesTestInput5 String
| ChangeTestCasesTestOutput5 String
| ChangeTestCasesValidaterInput5 String
| ChangeTestCasesValidaterOutput5 String
| ChangeTestCases TestCases
| TranRadio String
| StorageInputJ String --储存解决方案
| StorageInputJ_ String
| StorageInputP String
| StorageInputP_ String
| StorageInputH String
| StorageInputH_ String
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
Display result ->
case result of
Ok fullt ->
( model, Cmd.none )
Err _ ->
( model, Cmd.none )
PushDate ->
Debug.log "ceshi"
( model
, Http.post
{ url = "/code"
, body =
Http.multipartBody
[ stringPart "test"
(Encode.encode 1
(Encode.object
[ ( "title", Encode.string model.title )
, ( "statement", Encode.string model.statement )
, ( "inputDescription", Encode.string model.inputDescription )
, ( "outputDescription", Encode.string model.outputDescription )
, ( "constraints", Encode.string model.constraints )
, ( "game_difficulty", Encode.string model.radioValue)
, ( "testCases1"
, Encode.object
[ ( "testName1", Encode.string model.testCases1.testName )
, ( "validator1", Encode.string model.testCases1.validator )
, ( "test1"
, Encode.object
[ ( "input", Encode.string model.testCases1.test.input )
, ( "output", Encode.string model.testCases1.test.output )
]
)
, ( "validater1"
, Encode.object
[ ( "input", Encode.string model.testCases1.validater.input )
, ( "output", Encode.string model.testCases1.validater.output )
]
)
]
)
, ( "testCases2"
, Encode.object
[ ( "testName2", Encode.string model.testCases2.testName )
, ( "validator2", Encode.string model.testCases2.validator )
, ( "test2"
, Encode.object
[ ( "input", Encode.string model.testCases2.test.input )
, ( "output", Encode.string model.testCases2.test.output )
]
)
, ( "validater2"
, Encode.object
[ ( "input", Encode.string model.testCases2.validater.input )
, ( "output", Encode.string model.testCases2.validater.output )
]
)
]
)
, ( "testCases3"
, Encode.object
[ ( "testName3", Encode.string model.testCases3.testName )
, ( "validator3", Encode.string model.testCases3.validator )
, ( "test3"
, Encode.object
[ ( "input", Encode.string model.testCases3.test.input )
, ( "output", Encode.string model.testCases3.test.output )
]
)
, ( "validater3"
, Encode.object
[ ( "input", Encode.string model.testCases3.validater.input )
, ( "output", Encode.string model.testCases3.validater.output )
]
)
]
)
, ( "testCases4"
, Encode.object
[ ( "testName4", Encode.string model.testCases4.testName )
, ( "validator4", Encode.string model.testCases4.validator )
, ( "test4"
, Encode.object
[ ( "input", Encode.string model.testCases4.test.input )
, ( "output", Encode.string model.testCases4.test.output )
]
)
, ( "validater4"
, Encode.object
[ ( "input", Encode.string model.testCases4.validater.input )
, ( "output", Encode.string model.testCases4.validater.output )
]
)
]
)
, ( "testCases5"
, Encode.object
[ ( "testName5", Encode.string model.testCases5.testName )
, ( "validator5", Encode.string model.testCases5.validator )
, ( "test5"
, Encode.object
[ ( "input", Encode.string model.testCases5.test.input )
, ( "output", Encode.string model.testCases5.test.output )
]
)
, ( "validater5"
, Encode.object
[ ( "input", Encode.string model.testCases5.validater.input )
, ( "output", Encode.string model.testCases5.validater.output )
]
)
]
)
, ( "Solution_language_Java",Encode.string model.selectJava)
, ( "Solution_Java",Encode.string model.dataJave)
, ( "Unresolved_Java",Encode.string model.dataJave_)
, ( "Solution_language_Python",Encode.string model.selectPython)
, ( "Solution_Python",Encode.string model.dataPython)
, ( "Unresolved_Python",Encode.string model.dataPython_)
, ( "Solution_language_Haskell",Encode.string model.selectHaskell)
, ( "Solution_Haskell",Encode.string model.dataHaskell)
, ( "Unresolved_Haskell",Encode.string model.dataHaskell_)
]
)
)
]
, expect = Http.expectString Display
}
)
ChangeTitle newTitle ->
( { model | title = newTitle }, Cmd.none )
ChangeStatemen newStatement ->
( { model | statement = newStatement }, Cmd.none )
ChangeInputDescription newInputDescription ->
( { model | inputDescription = newInputDescription }, Cmd.none )
ChangeOutputDescription newOutputDescription ->
( { model | outputDescription = newOutputDescription }, Cmd.none )
ChangeConstraints newConstraints ->
( { model | constraints = newConstraints }, Cmd.none )
ChangeTestCasesTestName1 newTestCasesTestName ->
updateTestName1 (\testCases -> { testCases | testName = newTestCasesTestName }) model
ChangeTestCasesValidator1 newTestCasesValidator ->
updateTestName1 (\testCases -> { testCases | validator = newTestCasesValidator }) model
ChangeTestCasesTestName2 newTestCasesTestName ->
updateTestName2 (\testCases -> { testCases | testName = newTestCasesTestName }) model
ChangeTestCasesValidator2 newTestCasesValidator ->
updateTestName2 (\testCases -> { testCases | validator = newTestCasesValidator }) model
ChangeTestCasesTestName3 newTestCasesTestName ->
updateTestName3 (\testCases -> { testCases | testName = newTestCasesTestName }) model
ChangeTestCasesValidator3 newTestCasesValidator ->
updateTestName3 (\testCases -> { testCases | validator = newTestCasesValidator }) model
ChangeTestCasesTestName4 newTestCasesTestName ->
updateTestName4 (\testCases -> { testCases | testName = newTestCasesTestName }) model
ChangeTestCasesValidator4 newTestCasesValidator ->
updateTestName4 (\testCases -> { testCases | validator = newTestCasesValidator }) model
ChangeTestCasesTestName5 newTestCasesTestName ->
updateTestName5 (\testCases -> { testCases | testName = newTestCasesTestName }) model
ChangeTestCasesValidator5 newTestCasesValidator ->
updateTestName5 (\testCases -> { testCases | validator = newTestCasesValidator }) model
ChangeTestCasesTestInput1 newTestCasesTestInput ->
updateTestCasesTestInput1 (\test -> { test | input = newTestCasesTestInput }) model.testCases1 model
ChangeTestCasesTestOutput1 newTestCasesTestOutput ->
updateTestCasesTestInput1 (\test -> { test | output = newTestCasesTestOutput }) model.testCases1 model
ChangeTestCasesValidaterInput1 newTestCasesValidaterInput ->
updateTestCasesValidater1 (\validater -> { validater | input = newTestCasesValidaterInput }) model.testCases1 model
ChangeTestCasesValidaterOutput1 newTestCasesValidaterOutput ->
updateTestCasesValidater1 (\validater -> { validater | output = newTestCasesValidaterOutput }) model.testCases1 model
ChangeTestCasesTestInput2 newTestCasesTestInput ->
updateTestCasesTestInput2 (\test -> { test | input = newTestCasesTestInput }) model.testCases2 model
ChangeTestCasesTestOutput2 newTestCasesTestOutput ->
updateTestCasesTestInput2 (\test -> { test | output = newTestCasesTestOutput }) model.testCases2 model
ChangeTestCasesValidaterInput2 newTestCasesValidaterInput ->
updateTestCasesValidater2 (\validater -> { validater | input = newTestCasesValidaterInput }) model.testCases2 model
ChangeTestCasesValidaterOutput2 newTestCasesValidaterOutput ->
updateTestCasesValidater2 (\validater -> { validater | output = newTestCasesValidaterOutput }) model.testCases2 model
ChangeTestCasesTestInput3 newTestCasesTestInput ->
updateTestCasesTestInput3 (\test -> { test | input = newTestCasesTestInput }) model.testCases3 model
ChangeTestCasesTestOutput3 newTestCasesTestOutput ->
updateTestCasesTestInput3 (\test -> { test | output = newTestCasesTestOutput }) model.testCases3 model
ChangeTestCasesValidaterInput3 newTestCasesValidaterInput ->
updateTestCasesValidater3 (\validater -> { validater | input = newTestCasesValidaterInput }) model.testCases3 model
ChangeTestCasesValidaterOutput3 newTestCasesValidaterOutput ->
updateTestCasesValidater3 (\validater -> { validater | output = newTestCasesValidaterOutput }) model.testCases3 model
ChangeTestCasesTestInput4 newTestCasesTestInput ->
updateTestCasesTestInput4 (\test -> { test | input = newTestCasesTestInput }) model.testCases4 model
ChangeTestCasesTestOutput4 newTestCasesTestOutput ->
updateTestCasesTestInput4 (\test -> { test | output = newTestCasesTestOutput }) model.testCases4 model
ChangeTestCasesValidaterInput4 newTestCasesValidaterInput ->
updateTestCasesValidater4 (\validater -> { validater | input = newTestCasesValidaterInput }) model.testCases4 model
ChangeTestCasesValidaterOutput4 newTestCasesValidaterOutput ->
updateTestCasesValidater4 (\validater -> { validater | output = newTestCasesValidaterOutput }) model.testCases4 model
ChangeTestCasesTestInput5 newTestCasesTestInput ->
updateTestCasesTestInput5 (\test -> { test | input = newTestCasesTestInput }) model.testCases5 model
ChangeTestCasesTestOutput5 newTestCasesTestOutput ->
updateTestCasesTestInput5 (\test -> { test | output = newTestCasesTestOutput }) model.testCases5 model
ChangeTestCasesValidaterInput5 newTestCasesValidaterInput ->
updateTestCasesValidater5 (\validater -> { validater | input = newTestCasesValidaterInput }) model.testCases5 model
ChangeTestCasesValidaterOutput5 newTestCasesValidaterOutput ->
updateTestCasesValidater5 (\validater -> { validater | output = newTestCasesValidaterOutput }) model.testCases5 model
ChangeTestCases newTestCases ->
( model, Cmd.none )
TranRadio value ->
( { model | radioValue = value }, Cmd.none )
StorageInputJ inputData ->
({model | dataJave = inputData},Cmd.none)
StorageInputJ_ inputData ->
({model | dataJave_ = inputData},Cmd.none)
StorageInputP inputData ->
({model | dataPython = inputData},Cmd.none)
StorageInputP_ inputData ->
({model | dataPython_ = inputData},Cmd.none)
StorageInputH inputData ->
({model | dataHaskell = inputData},Cmd.none)
StorageInputH_ inputData ->
({model | dataHaskell_ = inputData},Cmd.none)
updateTestName1 : (TestCases -> TestCases) -> Model -> ( Model, Cmd Msg )
updateTestName1 transform model =
( { model | testCases1 = transform model.testCases1 }, Cmd.none )
updateTestName2 : (TestCases -> TestCases) -> Model -> ( Model, Cmd Msg )
updateTestName2 transform model =
( { model | testCases2 = transform model.testCases2 }, Cmd.none )
updateTestName3 : (TestCases -> TestCases) -> Model -> ( Model, Cmd Msg )
updateTestName3 transform model =
( { model | testCases3 = transform model.testCases3 }, Cmd.none )
updateTestName4 : (TestCases -> TestCases) -> Model -> ( Model, Cmd Msg )
updateTestName4 transform model =
( { model | testCases4 = transform model.testCases4 }, Cmd.none )
updateTestName5 : (TestCases -> TestCases) -> Model -> ( Model, Cmd Msg )
updateTestName5 transform model =
( { model | testCases5 = transform model.testCases5 }, Cmd.none )
--像json中低层级的key中添加值的一组方法
updateTestCasesTestInput1 : (TestCases_Test -> TestCases_Test) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesTestInput1 transdate testCases1 model =
( { model | testCases1 = { testCases1 | test = transdate model.testCases1.test } }, Cmd.none )
updateTestCasesTestInput2 : (TestCases_Test -> TestCases_Test) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesTestInput2 transdate testCases2 model =
( { model | testCases2 = { testCases2 | test = transdate model.testCases2.test } }, Cmd.none )
updateTestCasesTestInput3 : (TestCases_Test -> TestCases_Test) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesTestInput3 transdate testCases3 model =
( { model | testCases3 = { testCases3 | test = transdate model.testCases3.test } }, Cmd.none )
updateTestCasesTestInput4 : (TestCases_Test -> TestCases_Test) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesTestInput4 transdate testCases4 model =
( { model | testCases4 = { testCases4 | test = transdate model.testCases4.test } }, Cmd.none )
updateTestCasesTestInput5 : (TestCases_Test -> TestCases_Test) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesTestInput5 transdate testCases5 model =
( { model | testCases5 = { testCases5 | test = transdate model.testCases5.test } }, Cmd.none )
updateTestCasesValidater1 : (TestCases_Validater -> TestCases_Validater) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesValidater1 transdate testCases1 model =
( { model | testCases1 = { testCases1 | validater = transdate model.testCases1.validater } }, Cmd.none )
updateTestCasesValidater2 : (TestCases_Validater -> TestCases_Validater) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesValidater2 transdate testCases2 model =
( { model | testCases2 = { testCases2 | validater = transdate model.testCases2.validater } }, Cmd.none )
updateTestCasesValidater3 : (TestCases_Validater -> TestCases_Validater) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesValidater3 transdate testCases3 model =
( { model | testCases3 = { testCases3 | validater = transdate model.testCases3.validater } }, Cmd.none )
updateTestCasesValidater4 : (TestCases_Validater -> TestCases_Validater) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesValidater4 transdate testCases4 model =
( { model | testCases4 = { testCases4 | validater = transdate model.testCases4.validater } }, Cmd.none )
updateTestCasesValidater5 : (TestCases_Validater -> TestCases_Validater) -> TestCases -> Model -> ( Model, Cmd Msg )
updateTestCasesValidater5 transdate testCases5 model =
( { model | testCases5 = { testCases5 | validater = transdate model.testCases5.validater } }, Cmd.none )
view : Model -> Html Msg
view model =
div
[ style "background-color" "#454c55"
, style "width" "100%"
, style "height" "4500px"
]
[ div
[ style "position" "absolute"
, style "top" "50%"
, style "left" "50%"
, style "height" "4300px"
, style "width" "50%"
, style "margin" "-15% 0 0 -25%"
, style "border" "1px solid #aaaaaa"
, style "background-color" "#ffffff"
]
[ Html.form
[ style "margin" "50px"
, style "display" "block"
, style "width" "90%"
, style "height" "90%"
]
[ p
[]
[ text "在这个页面上你可以设计自己的拼图!点击"
, a
[ href "#" ]
[ text "这里" ]
, text
"获取更多有关信息"
]
, p
[]
[ text "标题" ]
, input
[ style "border" "1px solid #bbbbbb"
, style "width" "100%"
, style "height" "40px"
, value model.title
, onInput ChangeTitle
]
[]
, p [] [ text "声明" ]
, input
[ style "border" "1px solid #bbbbbb"
, style "width" "100%"
, style "height" "80px"
, value model.statement
, onInput ChangeStatemen
]
[]
, p [] [ text "输入说明" ]
, input
[ style "border" "1px solid #bbbbbb"
, style "width" "100%"
, style "height" "80px"
, value model.inputDescription
, onInput ChangeInputDescription
]
[]
, p [] [ text "输出说明" ]
, input
[ style "border" "1px solid #bbbbbb"
, style "width" "100%"
, style "height" "80px"
, value model.outputDescription
, onInput ChangeOutputDescription
]
[]
, p [] [ text "限制条件" ]
, input
[ style "border" "1px solid #bbbbbb"
, style "width" "100%"
, style "height" "80px"
, value model.constraints
, onInput ChangeConstraints
]
[]
, div
--Game modes 已转变成游戏难度
[ style "width" "100%"
, style "height" "120px"
]
[ p [] [ text "游戏难度" ]
, label [] [ input [ type_ "radio", value "Easy", checked (model.radioValue == "Easy"), onClick (TranRadio "Easy") ] [], text "简单" ]
, label [] [ input [ type_ "radio", value "Medium", checked (model.radioValue == "Medium"), onClick (TranRadio "Medium") ] [], text "中等" ]
, label [] [ input [ type_ "radio", value "Difficult", checked (model.radioValue == "Difficult"), onClick (TranRadio "Difficult") ] [], text "困难" ]
, label [] [ input [ type_ "radio", value "Expert", checked (model.radioValue == "Expert"), onClick (TranRadio "Expert") ] [], text "专家" ]
]
, div
--Test cases
[ style "width" "100%"
]
[ text "测试用例"
, input [ style "color" "#838891"
, placeholder "测试1"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases1.testName
, onInput ChangeTestCasesTestName1 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases1.test.input
, onInput ChangeTestCasesTestInput1
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases1.test.output
, onInput ChangeTestCasesTestOutput1
]
[]
, input [ style "color" "#838891"
, placeholder "验证1"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases1.validator
, onInput ChangeTestCasesValidator1 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases1.validater.input
, onInput ChangeTestCasesValidaterInput1
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases1.validater.output
, onInput ChangeTestCasesValidaterOutput1
]
[]
, div
--分割线 测试和验证2
[ style "border" "1px solid #bbbbbb"
, style "margin-top" "20px"
]
[]
, input [ style "color" "#838891"
, placeholder "PI:NAME:<NAME>END_PI"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases2.testName
, onInput ChangeTestCasesTestName2 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases2.test.input
, onInput ChangeTestCasesTestInput2
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases2.test.output
, onInput ChangeTestCasesTestOutput2
]
[]
, input [ style "color" "#838891"
, placeholder "PI:NAME:<NAME>END_PI"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases2.validator
, onInput ChangeTestCasesValidator2 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases2.validater.input
, onInput ChangeTestCasesValidaterInput2
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases2.validater.output
, onInput ChangeTestCasesValidaterOutput2
]
[]
, div
--分割线 测试和验证3
[ style "border" "1px solid #bbbbbb"
, style "margin-top" "20px"
]
[]
, input [ style "color" "#838891"
, placeholder "PI:NAME:<NAME>END_PI"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases3.testName
, onInput ChangeTestCasesTestName3 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases3.test.input
, onInput ChangeTestCasesTestInput3
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases3.test.output
, onInput ChangeTestCasesTestOutput3
]
[]
, input [ style "color" "#838891"
, placeholder "验证3"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases3.validator
, onInput ChangeTestCasesValidator3 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases3.validater.input
, onInput ChangeTestCasesValidaterInput3
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases3.validater.output
, onInput ChangeTestCasesValidaterOutput3
]
[]
, div
--分割线 测试和验证4
[ style "border" "1px solid #bbbbbb"
, style "margin-top" "20px"
]
[]
, input [ style "color" "#838891"
, placeholder "测试4"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases4.testName
, onInput ChangeTestCasesTestName4 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases4.test.input
, onInput ChangeTestCasesTestInput4
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases4.test.output
, onInput ChangeTestCasesTestOutput4
]
[]
, input [ style "color" "#838891"
, placeholder "验证4"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases4.validator
, onInput ChangeTestCasesValidator4 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases4.validater.input
, onInput ChangeTestCasesValidaterInput4
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases4.validater.output
, onInput ChangeTestCasesValidaterOutput4
]
[]
, div
--分割线 测试和验证5
[ style "border" "1px solid #bbbbbb"
, style "margin-top" "20px"
]
[]
, input [ style "color" "#838891"
, placeholder "测试5"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases5.testName
, onInput ChangeTestCasesTestName5 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases5.validater.input
, onInput ChangeTestCasesTestInput5
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases5.validater.output
, onInput ChangeTestCasesTestOutput5
]
[]
, input [ style "color" "#838891"
, placeholder "验证5"
, style "display" "block"
, style "margin-bottom" "10px"
, value model.testCases5.validator
, onInput ChangeTestCasesValidator5 ] []
, textarea
[ placeholder "输入"
, style "width" "45%"
, style "height" "80px"
, value model.testCases5.validater.input
, onInput ChangeTestCasesValidaterInput5
]
[]
, textarea
[ placeholder "输出"
, style "width" "45%"
, style "height" "80px"
, style "margin-left" "20px"
, value model.testCases5.validater.output
, onInput ChangeTestCasesValidaterOutput5
]
[]
, div
[ style "width" "150px"
, style "height" "30px"
, style "background-color" "rgba(69,76,85,0.15)"
]
[ text "添加一个测试用例" ]
]
, div
[ style "width" "100%"
, style "margin-top" "20px"
]
[ p [] [ text "解决语言" ]
, p [] [text "Java"]
]
, div []
--
[ p [] [ text "解决方案" ]
, textarea
[ style "width" "100%"
, style "height" "200px"
, value model.dataJave
, onInput StorageInputJ
]
[]
]
, div []
--Stub generator input
[ p [] [ text "未解决方案" ]
, textarea
[ style "width" "100%"
, style "height" "200px"
, value model.dataJave_
, onInput StorageInputJ_
]
[]
]
, div
[ style "width" "100%"
, style "margin-top" "20px"
]
[ p [] [ text "解决语言" ]
, p [] [text "Python"]
]
, div []
--
[ p [] [ text "解决方案" ]
, textarea
[ style "width" "100%"
, style "height" "200px"
, value model.dataPython
, onInput StorageInputP
]
[]
]
, div []
--Stub generator input
[ p [] [ text "未解决方案" ]
, textarea
[ style "width" "100%"
, style "height" "200px"
, value model.dataPython_
, onInput StorageInputP_
]
[]
]
, div
[ style "width" "100%"
, style "margin-top" "20px"
]
[ p [] [ text "解决语言" ]
, p [] [text "Haskell"]
]
, div []
--
[ p [] [ text "解决方案" ]
, textarea
[ style "width" "100%"
, style "height" "200px"
, value model.dataHaskell
, onInput StorageInputH
]
[]
]
, div []
--Stub generator input
[ p [] [ text "未解决方案" ]
, textarea
[ style "width" "100%"
, style "height" "200px"
, value model.dataHaskell_
, onInput StorageInputH_
]
[]
]
, div [
style "display" "inline-block"
, style "width" "100%"
, style "height" "50px"
, style "background-color" "rgba(242,187,19,0.7)"
, style "margin-bottom" "20px"
] [text "提交方案"]
, div []
[
div
[ style "display" "inline-block"
, style "width" "50%"
, style "height" "50px"
, style "background-color" "rgba(242,187,19,0.7)"
, onClick PushDate
]
[ text "保存" ]
, div
[ style "display" "inline-block"
, style "width" "50%"
, style "height" "50px"
, style "background-color" "rgba(69,76,85,0.1)"
]
[ text "测试在IDE" ]
]
]
]
]
subscriptions : Model -> Sub Msg
subscriptions model =
Sub.none
| elm |
[
{
"context": "splayNameInput\n , placeholder \"My Cool Nickname\"\n , value <| Maybe.withDefault",
"end": 2680,
"score": 0.9937714338,
"start": 2664,
"tag": "NAME",
"value": "My Cool Nickname"
},
{
"context": "splayNameInput\n , placeholder \"My Cool Nickname\"\n , value data.newDisplayName\n",
"end": 3000,
"score": 0.991112411,
"start": 2984,
"tag": "NAME",
"value": "My Cool Nickname"
}
] | client/src/Pages/Profile.elm | lsocrate/rivalsdb | 0 | module Pages.Profile exposing (Model, Msg, page)
import API.User
import Auth
import Effect exposing (Effect)
import Gen.Params.Profile exposing (Params)
import Html exposing (Html, button, form, h1, input, label, text)
import Html.Attributes exposing (disabled, placeholder, type_, value)
import Html.Events exposing (onInput, onSubmit)
import Page
import Request
import Shared
import UI.Layout.Template
import View exposing (View)
page : Shared.Model -> Request.With Params -> Page.With Model Msg
page shared _ =
Page.protected.advanced
(\user ->
{ init = init user
, update = update user
, view = view shared
, subscriptions = always Sub.none
}
)
-- INIT
type Model
= Loading
| Loaded { displayName : Maybe String }
| Editing { previousDisplayName : Maybe String, newDisplayName : String }
init : Auth.User -> ( Model, Effect Msg )
init user =
( Loading, Effect.fromCmd <| API.User.read FetchedUser user.id )
-- UPDATE
type Msg
= FromShared Shared.Msg
| DisplayNameInput String
| DisplayNameSave
| FetchedUser API.User.ResultRead
| SavedDisplayName API.User.ResultSaveDisplayName
update : Auth.User -> Msg -> Model -> ( Model, Effect Msg )
update user msg model =
case ( model, msg ) of
( _, FromShared subMsg ) ->
( model, Effect.fromShared subMsg )
( _, FetchedUser (Ok { displayName }) ) ->
( Loaded { displayName = displayName }, Effect.none )
( _, FetchedUser (Err _) ) ->
( Loading, Effect.none )
( Loading, _ ) ->
( model, Effect.none )
( Loaded data, DisplayNameInput text ) ->
( Editing { previousDisplayName = data.displayName, newDisplayName = String.trim text }, Effect.none )
( Editing data, DisplayNameInput text ) ->
( Editing { data | newDisplayName = String.trim text }, Effect.none )
( Editing { newDisplayName }, DisplayNameSave ) ->
( model, Effect.fromCmd <| API.User.saveDisplayName SavedDisplayName user.token user.id newDisplayName )
( _, DisplayNameSave ) ->
( model, Effect.none )
( _, SavedDisplayName _ ) ->
( model, Effect.none )
-- VIEW
view : Shared.Model -> Model -> View Msg
view shared model =
UI.Layout.Template.view FromShared
shared
(case model of
Loading ->
[ text "Loading..." ]
Loaded data ->
[ viewHeader
, viewForm
[ onInput DisplayNameInput
, placeholder "My Cool Nickname"
, value <| Maybe.withDefault "" data.displayName
]
False
]
Editing data ->
[ viewHeader
, viewForm
[ onInput DisplayNameInput
, placeholder "My Cool Nickname"
, value data.newDisplayName
]
True
]
)
viewHeader : Html msg
viewHeader =
h1 [] [ text "My Profile" ]
viewForm : List (Html.Attribute Msg) -> Bool -> Html Msg
viewForm inputAttrs canSave =
form [ onSubmit DisplayNameSave ]
[ label [] [ text "Display Name" ]
, input inputAttrs []
, button [ type_ "submit", disabled (not canSave) ] [ text "save" ]
]
| 446 | module Pages.Profile exposing (Model, Msg, page)
import API.User
import Auth
import Effect exposing (Effect)
import Gen.Params.Profile exposing (Params)
import Html exposing (Html, button, form, h1, input, label, text)
import Html.Attributes exposing (disabled, placeholder, type_, value)
import Html.Events exposing (onInput, onSubmit)
import Page
import Request
import Shared
import UI.Layout.Template
import View exposing (View)
page : Shared.Model -> Request.With Params -> Page.With Model Msg
page shared _ =
Page.protected.advanced
(\user ->
{ init = init user
, update = update user
, view = view shared
, subscriptions = always Sub.none
}
)
-- INIT
type Model
= Loading
| Loaded { displayName : Maybe String }
| Editing { previousDisplayName : Maybe String, newDisplayName : String }
init : Auth.User -> ( Model, Effect Msg )
init user =
( Loading, Effect.fromCmd <| API.User.read FetchedUser user.id )
-- UPDATE
type Msg
= FromShared Shared.Msg
| DisplayNameInput String
| DisplayNameSave
| FetchedUser API.User.ResultRead
| SavedDisplayName API.User.ResultSaveDisplayName
update : Auth.User -> Msg -> Model -> ( Model, Effect Msg )
update user msg model =
case ( model, msg ) of
( _, FromShared subMsg ) ->
( model, Effect.fromShared subMsg )
( _, FetchedUser (Ok { displayName }) ) ->
( Loaded { displayName = displayName }, Effect.none )
( _, FetchedUser (Err _) ) ->
( Loading, Effect.none )
( Loading, _ ) ->
( model, Effect.none )
( Loaded data, DisplayNameInput text ) ->
( Editing { previousDisplayName = data.displayName, newDisplayName = String.trim text }, Effect.none )
( Editing data, DisplayNameInput text ) ->
( Editing { data | newDisplayName = String.trim text }, Effect.none )
( Editing { newDisplayName }, DisplayNameSave ) ->
( model, Effect.fromCmd <| API.User.saveDisplayName SavedDisplayName user.token user.id newDisplayName )
( _, DisplayNameSave ) ->
( model, Effect.none )
( _, SavedDisplayName _ ) ->
( model, Effect.none )
-- VIEW
view : Shared.Model -> Model -> View Msg
view shared model =
UI.Layout.Template.view FromShared
shared
(case model of
Loading ->
[ text "Loading..." ]
Loaded data ->
[ viewHeader
, viewForm
[ onInput DisplayNameInput
, placeholder "<NAME>"
, value <| Maybe.withDefault "" data.displayName
]
False
]
Editing data ->
[ viewHeader
, viewForm
[ onInput DisplayNameInput
, placeholder "<NAME>"
, value data.newDisplayName
]
True
]
)
viewHeader : Html msg
viewHeader =
h1 [] [ text "My Profile" ]
viewForm : List (Html.Attribute Msg) -> Bool -> Html Msg
viewForm inputAttrs canSave =
form [ onSubmit DisplayNameSave ]
[ label [] [ text "Display Name" ]
, input inputAttrs []
, button [ type_ "submit", disabled (not canSave) ] [ text "save" ]
]
| true | module Pages.Profile exposing (Model, Msg, page)
import API.User
import Auth
import Effect exposing (Effect)
import Gen.Params.Profile exposing (Params)
import Html exposing (Html, button, form, h1, input, label, text)
import Html.Attributes exposing (disabled, placeholder, type_, value)
import Html.Events exposing (onInput, onSubmit)
import Page
import Request
import Shared
import UI.Layout.Template
import View exposing (View)
page : Shared.Model -> Request.With Params -> Page.With Model Msg
page shared _ =
Page.protected.advanced
(\user ->
{ init = init user
, update = update user
, view = view shared
, subscriptions = always Sub.none
}
)
-- INIT
type Model
= Loading
| Loaded { displayName : Maybe String }
| Editing { previousDisplayName : Maybe String, newDisplayName : String }
init : Auth.User -> ( Model, Effect Msg )
init user =
( Loading, Effect.fromCmd <| API.User.read FetchedUser user.id )
-- UPDATE
type Msg
= FromShared Shared.Msg
| DisplayNameInput String
| DisplayNameSave
| FetchedUser API.User.ResultRead
| SavedDisplayName API.User.ResultSaveDisplayName
update : Auth.User -> Msg -> Model -> ( Model, Effect Msg )
update user msg model =
case ( model, msg ) of
( _, FromShared subMsg ) ->
( model, Effect.fromShared subMsg )
( _, FetchedUser (Ok { displayName }) ) ->
( Loaded { displayName = displayName }, Effect.none )
( _, FetchedUser (Err _) ) ->
( Loading, Effect.none )
( Loading, _ ) ->
( model, Effect.none )
( Loaded data, DisplayNameInput text ) ->
( Editing { previousDisplayName = data.displayName, newDisplayName = String.trim text }, Effect.none )
( Editing data, DisplayNameInput text ) ->
( Editing { data | newDisplayName = String.trim text }, Effect.none )
( Editing { newDisplayName }, DisplayNameSave ) ->
( model, Effect.fromCmd <| API.User.saveDisplayName SavedDisplayName user.token user.id newDisplayName )
( _, DisplayNameSave ) ->
( model, Effect.none )
( _, SavedDisplayName _ ) ->
( model, Effect.none )
-- VIEW
view : Shared.Model -> Model -> View Msg
view shared model =
UI.Layout.Template.view FromShared
shared
(case model of
Loading ->
[ text "Loading..." ]
Loaded data ->
[ viewHeader
, viewForm
[ onInput DisplayNameInput
, placeholder "PI:NAME:<NAME>END_PI"
, value <| Maybe.withDefault "" data.displayName
]
False
]
Editing data ->
[ viewHeader
, viewForm
[ onInput DisplayNameInput
, placeholder "PI:NAME:<NAME>END_PI"
, value data.newDisplayName
]
True
]
)
viewHeader : Html msg
viewHeader =
h1 [] [ text "My Profile" ]
viewForm : List (Html.Attribute Msg) -> Bool -> Html Msg
viewForm inputAttrs canSave =
form [ onSubmit DisplayNameSave ]
[ label [] [ text "Display Name" ]
, input inputAttrs []
, button [ type_ "submit", disabled (not canSave) ] [ text "save" ]
]
| elm |
[
{
"context": "akeExample \"Triangles\" equiTri\n , makeExample \"Frank Lloyd Wright\" flw1\n , makeExample \"Frank Lloyd Wright B\" fl",
"end": 13229,
"score": 0.9993174672,
"start": 13211,
"tag": "NAME",
"value": "Frank Lloyd Wright"
},
{
"context": "mple \"Frank Lloyd Wright\" flw1\n , makeExample \"Frank Lloyd Wright B\" flw2\n , makeExample \"Bezier Curves\" bezier\n ",
"end": 13275,
"score": 0.994125247,
"start": 13255,
"tag": "NAME",
"value": "Frank Lloyd Wright B"
}
] | src/ExamplesTemplate.elm | digitalsatori/sketch-n-sketch | 565 | module ExamplesGenerated exposing
( list, templateCategories
, blankSvgTemplate, blankHtmlTemplate, initTemplate
, badPreludeTemplate
, fromleo_markdown
, fromleo_markdown_optimized
, fromleo_markdown_optimized_lensless
, fromleo_recipe2
, fromleo_conference_budgetting
, fromleo_modelviewcontroller
, fromleo_latexeditor
, repl
, tableOfStatesA
, tableOfStatesB
, tableOfStatesC
, fromleo_linkedtexteditor
, fromleo_translatabledoc
, fromleo_dixit
, christmas_song_3_after_translation
, mapMaybeLens
, listAppendLens
, Example
)
import Lang exposing (Exp, Val, Widget, Env)
import FastParser
import LeoParser
import Eval
import Utils
import PreludeGenerated as Prelude
import DefaultIconTheme
import Syntax
import EvalUpdate
import Parser
import ParserUtils
type alias Example = {
e: Exp,
v: Val,
ws: List Widget,
env: Env}
--------------------------------------------------------------------------------
initTemplate = blankHtmlTemplate
getStartedTemplate = "Get Started"
blankSvgTemplate = "Blank Svg Document"
blankHtmlTemplate = "Blank Html Document"
badPreludeTemplate = "Bad Prelude"
--------------------------------------------------------------------------------
makeExample = makeExample_ FastParser.parseE Syntax.Little
makeLeoExample = makeExample_ LeoParser.parse Syntax.Leo
makeExample_: (String -> Result Parser.Error Exp) -> Syntax.Syntax -> String -> String -> (String, (String, () -> Result String Example))
makeExample_ parser syntax name s =
let thunk () =
-- TODO tolerate parse errors, change Select Example
parser s |> Result.mapError (\pmsg -> "Error parsing example " ++ name ++"\n" ++ ParserUtils.showError pmsg) |> Result.map (\e ->
-----------------------------------------------------
-- if name == "*Prelude*" then
-- {e=e, v=LangSvg.dummySvgVal, ws=[], ati=ati}
-- else
-----------------------------------------------------
let ((v,ws), env) = Utils.fromOk ("Error executing example " ++ name) <| EvalUpdate.runWithEnv syntax e in
{e=e, v=v, ws=ws, env=env}
)
in
(name, (s, thunk))
LITTLE_TO_ELM threeBoxes
LITTLE_TO_ELM nBoxesH2
LITTLE_TO_ELM sineWaveOfBoxes
LITTLE_TO_ELM sineWaveGrid
-- LITTLE_TO_ELM waveOfBoxes
-- LITTLE_TO_ELM waveOfBoxesTokens
-- LITTLE_TO_ELM waveOfBoxes3
LITTLE_TO_ELM nBoxes
LITTLE_TO_ELM groupOfBoxes
-- LITTLE_TO_ELM sixBoxesA
-- LITTLE_TO_ELM sixBoxesB
LITTLE_TO_ELM basicSlides
LITTLE_TO_ELM logo
LITTLE_TO_ELM logo2
-- TODO stack overflow for some reason?
-- LITTLE_TO_ELM logoSizes
LITTLE_TO_ELM elmLogo
-- LITTLE_TO_ELM activeTrans
LITTLE_TO_ELM activeTrans2
LITTLE_TO_ELM botanic
LITTLE_TO_ELM rings
LITTLE_TO_ELM polygons
LITTLE_TO_ELM stars
LITTLE_TO_ELM sliders
LITTLE_TO_ELM buttons
LITTLE_TO_ELM widgets
LITTLE_TO_ELM xySlider
LITTLE_TO_ELM offsets
LITTLE_TO_ELM rgba
-- LITTLE_TO_ELM boxGrid
LITTLE_TO_ELM boxGridTokenFilter
LITTLE_TO_ELM usFlag13
LITTLE_TO_ELM usFlag50
LITTLE_TO_ELM chicago
-- LITTLE_TO_ELM chicagoColors
LITTLE_TO_ELM frenchSudan
LITTLE_TO_ELM flw1
LITTLE_TO_ELM flw2
LITTLE_TO_ELM ferris
LITTLE_TO_ELM ferris2
LITTLE_TO_ELM ferris2target
LITTLE_TO_ELM ferrisWheelSlideshow
LITTLE_TO_ELM pieChart1
LITTLE_TO_ELM solarSystem
LITTLE_TO_ELM fractalTree
LITTLE_TO_ELM hilbertCurveAnimation
LITTLE_TO_ELM stickFigures
-- TODO stack overflow for some reason?
--LITTLE_TO_ELM cultOfLambda
LITTLE_TO_ELM clique
LITTLE_TO_ELM miscShapes
LITTLE_TO_ELM paths1
LITTLE_TO_ELM paths2
LITTLE_TO_ELM paths3
LITTLE_TO_ELM paths4
LITTLE_TO_ELM paths5
LITTLE_TO_ELM sailBoat
LITTLE_TO_ELM eyeIcon
LITTLE_TO_ELM wikimedia
LITTLE_TO_ELM haskell
LITTLE_TO_ELM matrices
LITTLE_TO_ELM rotTest
LITTLE_TO_ELM interfaceButtons
LITTLE_TO_ELM barGraph
LITTLE_TO_ELM thawFreeze
LITTLE_TO_ELM dictionaries
-- LITTLE_TO_ELM deleteBoxes
LITTLE_TO_ELM cover
LITTLE_TO_ELM poppl
LITTLE_TO_ELM bezier
-- LITTLE_TO_ELM surveyResultsTriBubbles
-- LITTLE_TO_ELM surveyResultsTriHist
LITTLE_TO_ELM surveyResultsTriHist2
LITTLE_TO_ELM equiTri
LITTLE_TO_ELM gridTile
LITTLE_TO_ELM lilliconP
LITTLE_TO_ELM lilliconP2
LITTLE_TO_ELM keyboard
LITTLE_TO_ELM keyboard2
LITTLE_TO_ELM keyboard2target
LITTLE_TO_ELM tessellation
LITTLE_TO_ELM tessellationTarget
LITTLE_TO_ELM tessellation2
LITTLE_TO_ELM floralLogo
LITTLE_TO_ELM floralLogo2
LITTLE_TO_ELM zones
LITTLE_TO_ELM roundedRect
LITTLE_TO_ELM spiralSpiralGraph
-- LITTLE_TO_ELM relateRects0
-- LITTLE_TO_ELM relateRects1
-- LITTLE_TO_ELM relateCircles0
-- LITTLE_TO_ELM relateLines0
-- LITTLE_TO_ELM relatePoints0
-- LITTLE_TO_ELM relatePoints1
-- LITTLE_TO_ELM relatePoints2
-- LITTLE_TO_ELM relatePoints3
-- LITTLE_TO_ELM relatePoints4
LITTLE_TO_ELM blank
LITTLE_TO_ELM horrorFilms0
LITTLE_TO_ELM cyclingAssociation0
LITTLE_TO_ELM snsLogoWheel
LITTLE_TO_ELM sns_UIST
LITTLE_TO_ELM sns_revisited_UIST
LITTLE_TO_ELM botanic_UIST
LITTLE_TO_ELM coffee_UIST
LITTLE_TO_ELM rectangleTrisection
LITTLE_TO_ELM battery
LITTLE_TO_ELM batteryDynamic
LITTLE_TO_ELM mondrianArch
LITTLE_TO_ELM ladder
LITTLE_TO_ELM rails
LITTLE_TO_ELM target
LITTLE_TO_ELM xs
LITTLE_TO_ELM conifer
LITTLE_TO_ELM ferris3
LITTLE_TO_ELM gear
LITTLE_TO_ELM kochSnowflake
LITTLE_TO_ELM replaceTerminalsWithWorkstations
LITTLE_TO_ELM balanceScale
LITTLE_TO_ELM pencilTip
LITTLE_TO_ELM calendarIcon
LITTLE_TO_ELM sns_deuce
LITTLE_TO_ELM target_deuce
LITTLE_TO_ELM battery_deuce
LITTLE_TO_ELM coffee_deuce
LITTLE_TO_ELM mondrian_arch_deuce
LEO_TO_ELM cat
--------------------------------------------------------------------------------
-- Deuce User Study Files
-- these .little files are generated from somewhere else;
-- don't change them manually
LITTLE_TO_ELM study_start
LITTLE_TO_ELM study_transition_1
LITTLE_TO_ELM study_transition_2
LITTLE_TO_ELM study_end
LITTLE_TO_ELM tutorial_step_01
LITTLE_TO_ELM tutorial_step_02
LITTLE_TO_ELM tutorial_step_03
LITTLE_TO_ELM tutorial_step_04
LITTLE_TO_ELM tutorial_step_05
LITTLE_TO_ELM tutorial_step_06
LITTLE_TO_ELM tutorial_step_07
LITTLE_TO_ELM tutorial_step_08
LITTLE_TO_ELM tutorial_step_09
LITTLE_TO_ELM tutorial_step_10
LITTLE_TO_ELM tutorial_step_11
LITTLE_TO_ELM tutorial_step_12
LITTLE_TO_ELM tutorial_step_13
LITTLE_TO_ELM tutorial_step_14
LITTLE_TO_ELM tutorial_step_15
LITTLE_TO_ELM tutorial_step_16
LITTLE_TO_ELM tutorial_step_17
LITTLE_TO_ELM tutorial_step_18
LITTLE_TO_ELM tutorial_step_19
LITTLE_TO_ELM tutorial_step_20
LITTLE_TO_ELM tutorial_step_21
LITTLE_TO_ELM tutorial_step_22
LITTLE_TO_ELM tutorial_step_23
LITTLE_TO_ELM task_one_rectangle
LITTLE_TO_ELM task_two_circles
LITTLE_TO_ELM task_three_rectangles
LITTLE_TO_ELM task_target
LITTLE_TO_ELM task_four_squares
LITTLE_TO_ELM task_lambda
--------------------------------------------------------------------------------
LEO_TO_ELM badPrelude
LEO_TO_ELM blankSvg
LEO_TO_ELM blankDoc
LEO_TO_ELM welcome1
LEO_TO_ELM tableOfStatesA
LEO_TO_ELM tableOfStatesB
LEO_TO_ELM tableOfStatesC
LEO_TO_ELM tableOfStatesE
LEO_TO_ELM simpleBudget
LEO_TO_ELM mapMaybeLens
LEO_TO_ELM mapListLens_1
LEO_TO_ELM mapListLens_2
LEO_TO_ELM listAppendLens
LEO_TO_ELM fromleo/markdown
LEO_TO_ELM fromleo/markdown_optimized
LEO_TO_ELM fromleo/markdown_optimized_lensless
LEO_TO_ELM fromleo/conference_budgetting
LEO_TO_ELM fromleo/recipe
LEO_TO_ELM fromleo/recipe2
LEO_TO_ELM fromleo/modelviewcontroller
LEO_TO_ELM fromleo/linkedtexteditor
LEO_TO_ELM fromleo/translatabledoc
LEO_TO_ELM fromleo/latexeditor
LEO_TO_ELM fromleo/dixit
LEO_TO_ELM christmas_song_3_after_translation
LEO_TO_ELM fromleo/pizzas_doodle
LEO_TO_ELM fromleo/universal_number
LEO_TO_ELM repl
LEO_TO_ELM slides
LEO_TO_ELM docs
LEO_TO_ELM sync
LEO_TO_ELM foldl_reversible_join
LEO_TO_ELM references_in_text
LEO_TO_ELM tutorialStudentGrades
--------------------------------------------------------------------------------
welcomeCategory =
( "Welcome"
, [ makeLeoExample blankSvgTemplate blankSvg
, makeLeoExample blankHtmlTemplate blankDoc
, makeLeoExample getStartedTemplate welcome1
-- , makeLeoExample "Tutorial" blankDoc
]
)
docsCategory =
( "Examples (OOPSLA 2018 Submission)"
, [ makeLeoExample "1a: Table of States" tableOfStatesA
, makeLeoExample "1b: Table of States" tableOfStatesC
, makeLeoExample "1c: Table of States" tableOfStatesE
] ++
(
List.indexedMap
(\i (caption, program) ->
makeLeoExample (toString (2+i) ++ ": " ++ caption) program
)
[ ("ICFP tutorial", tutorialStudentGrades)
, ("Conference Budget", fromleo_conference_budgetting)
, ("Model View Controller", fromleo_modelviewcontroller)
, ("Scalable Recipe Editor", fromleo_recipe2)
, ("Cloning Editor", fromleo_linkedtexteditor)
, ("Translation Editor", fromleo_translatabledoc)
, ("Markdown Editor", fromleo_markdown)
, ("Dixit Scoresheet", fromleo_dixit)
, ("Doodle", fromleo_pizzas_doodle)
, ("Universal numbers", fromleo_universal_number)
, ("LaTeX Editor", fromleo_latexeditor)
, ("REPL with SVG", repl)
, ("Slides", slides)
, ("Docs", docs)
-- TODO maybe Conference?
, ("Html regex replace: references", references_in_text)
, ("String join, concatMap", foldl_reversible_join)
, ("Lens: Maybe Map", mapMaybeLens)
, ("Lens: List Map 1", mapListLens_1)
, ("Lens: List Map 2", mapListLens_2)
, ("Lens: List Append", listAppendLens)
]
)
)
defaultIconCategory =
( "Default Icons"
, [ makeExample "Icon: Cursor" DefaultIconTheme.cursor
, makeExample "Icon: Point Or Offset" DefaultIconTheme.pointOrOffset
, makeExample "Icon: Text" DefaultIconTheme.text
, makeExample "Icon: Line" DefaultIconTheme.line
, makeExample "Icon: Rect" DefaultIconTheme.rect
, makeExample "Icon: Ellipse" DefaultIconTheme.ellipse
, makeExample "Icon: Polygon" DefaultIconTheme.polygon
, makeExample "Icon: Path" DefaultIconTheme.path
]
)
deuceCategory =
( "Deuce Examples"
, [ makeExample "Sketch-n-Sketch Logo" sns_deuce
, makeExample "Target" target_deuce
, makeExample "Battery" battery_deuce
, makeExample "Coffee Mug" coffee_deuce
, makeExample "Mondrian Arch" mondrian_arch_deuce
]
)
logoCategory =
( "Logos"
, List.sortBy Tuple.first
[ makeExample "SnS Logo (UIST)" sns_UIST
, makeExample "SnS Logo Revisited (UIST)" sns_revisited_UIST
, makeExample "Botanic Garden Logo (UIST)" botanic_UIST
, makeExample "Logo" logo
, makeExample "Botanic Garden Logo" botanic
, makeExample "Active Trans Logo" activeTrans2
, makeExample "SnS Logo Wheel" snsLogoWheel
, makeExample "Haskell.org Logo" haskell
, makeExample "Cover Logo" cover
, makeExample "POP-PL Logo" poppl
, makeExample "Floral Logo 1" floralLogo
, makeExample "Floral Logo 2" floralLogo2
, makeExample "Elm Logo" elmLogo
, makeExample "Logo 2" logo2
-- , makeExample "Logo Sizes" logoSizes
]
)
flagCategory =
( "Flags"
, List.sortBy Tuple.first
[ makeExample "Chicago Flag" chicago
, makeExample "US-13 Flag" usFlag13
, makeExample "US-50 Flag" usFlag50
, makeExample "French Sudan Flag" frenchSudan
]
)
otherCategory =
( "Other"
, [ makeExample "Coffee Mugs (UIST)" coffee_UIST
, makeExample "Wave Boxes" sineWaveOfBoxes
, makeExample "Wave Boxes Grid" sineWaveGrid
, makeExample "Basic Slides" basicSlides
, makeExample "Sailboat" sailBoat
, makeExample "Sliders" sliders
, makeExample "Buttons" buttons
, makeExample "Widgets" widgets
, makeExample "xySlider" xySlider
, makeExample "Offsets" offsets
, makeExample "Tile Pattern" boxGridTokenFilter
, makeExample "Color Picker" rgba
, makeExample "Ferris Wheel" ferris
, makeExample "Ferris Task Before" ferris2
, makeExample "Ferris Task After" ferris2target
, makeExample "Ferris Wheel Slideshow" ferrisWheelSlideshow
, makeExample "Survey Results" surveyResultsTriHist2
, makeExample "Hilbert Curve Animation" hilbertCurveAnimation
, makeExample "Bar Graph" barGraph
, makeExample "Pie Chart" pieChart1
, makeExample "Solar System" solarSystem
, makeExample "Clique" clique
, makeExample "Eye Icon" eyeIcon
, makeExample "Horror Films" horrorFilms0
, makeExample "Cycling Association" cyclingAssociation0
, makeExample "Lillicon P" lilliconP
, makeExample "Lillicon P, v2" lilliconP2
, makeExample "Keyboard" keyboard
, makeExample "Keyboard Task Before" keyboard2
, makeExample "Keyboard Task After" keyboard2target
, makeExample "Tessellation Task Before" tessellation
, makeExample "Tessellation Task After" tessellationTarget
, makeExample "Tessellation 2" tessellation2
, makeExample "Spiral Spiral-Graph" spiralSpiralGraph
, makeExample "Rounded Rect" roundedRect
, makeExample "Thaw/Freeze" thawFreeze
, makeExample "Dictionaries" dictionaries
, makeExample "3 Boxes" threeBoxes
, makeExample "N Boxes Sli" nBoxes
, makeExample "N Boxes" groupOfBoxes
, makeExample "Rings" rings
, makeExample "Polygons" polygons
, makeExample "Stars" stars
, makeExample "Triangles" equiTri
, makeExample "Frank Lloyd Wright" flw1
, makeExample "Frank Lloyd Wright B" flw2
, makeExample "Bezier Curves" bezier
, makeExample "Fractal Tree" fractalTree
, makeExample "Stick Figures" stickFigures
-- , makeExample "Cult of Lambda" cultOfLambda
, makeExample "Matrix Transformations" matrices
, makeExample "Misc Shapes" miscShapes
, makeExample "Interface Buttons" interfaceButtons
, makeExample "Paths 1" paths1
, makeExample "Paths 2" paths2
, makeExample "Paths 3" paths3
, makeExample "Paths 4" paths4
, makeExample "Paths 5" paths5
, makeExample "Sample Rotations" rotTest
, makeExample "Grid Tile" gridTile
, makeExample "Zones" zones
, makeExample "Rectangle Trisection" rectangleTrisection
, makeExample "Battery" battery
, makeExample "Battery (Dynamic)" batteryDynamic
, makeExample "Mondrian Arch" mondrianArch
, makeExample "Ladder" ladder
, makeExample "Rails" rails
, makeExample "Target" target
, makeExample "Xs" xs
, makeExample "Conifer" conifer
, makeExample "Ferris Wheel 3" ferris3
, makeExample "Gear" gear
, makeExample "Koch Snowflake" kochSnowflake
, makeExample "Replace Terminals With Workstations" replaceTerminalsWithWorkstations
, makeExample "Balance Scale" balanceScale
, makeExample "Pencil Tip" pencilTip
, makeExample "Calendar Icon" calendarIcon
, makeLeoExample "SVG Cat" cat
]
)
deuceUserStudyCategory =
( "Deuce User Study"
, [ makeExample "Deuce Study Start" study_start
, makeExample "Step 01" tutorial_step_01
, makeExample "Step 02" tutorial_step_02
, makeExample "Step 03" tutorial_step_03
, makeExample "Step 04" tutorial_step_04
, makeExample "Step 05" tutorial_step_05
, makeExample "Step 06" tutorial_step_06
, makeExample "Step 07" tutorial_step_07
, makeExample "Step 08" tutorial_step_08
, makeExample "Step 09" tutorial_step_09
, makeExample "Step 10" tutorial_step_10
, makeExample "Step 11" tutorial_step_11
, makeExample "Step 12" tutorial_step_12
, makeExample "Step 13" tutorial_step_13
, makeExample "Step 14" tutorial_step_14
, makeExample "Step 15" tutorial_step_15
, makeExample "Step 16" tutorial_step_16
, makeExample "Step 17" tutorial_step_17
, makeExample "Step 18" tutorial_step_18
, makeExample "Step 19" tutorial_step_19
, makeExample "Step 20" tutorial_step_20
, makeExample "Step 21" tutorial_step_21
, makeExample "Step 22" tutorial_step_22
, makeExample "Step 23" tutorial_step_23
, makeExample "Deuce Study Transition 1" study_transition_1
, makeExample "One Rectangle" task_one_rectangle
, makeExample "Two Circles" task_two_circles
, makeExample "Three Rectangles" task_three_rectangles
, makeExample "Target Icon" task_target
, makeExample "Deuce Study Transition 2" study_transition_2
, makeExample "Four Squares" task_four_squares
, makeExample "Lambda Icon" task_lambda
, makeExample "Deuce Study End" study_end
]
)
internalCategory =
( "(Internal Things...)"
, [ makeLeoExample "Standard Prelude" Prelude.preludeLeo
, makeLeoExample badPreludeTemplate badPrelude
]
)
templateCategories =
[ welcomeCategory
, docsCategory
, deuceCategory
, defaultIconCategory
, logoCategory
, flagCategory
, otherCategory
, deuceUserStudyCategory
, internalCategory
]
list =
templateCategories
|> List.map Tuple.second
|> List.concat
| 47385 | module ExamplesGenerated exposing
( list, templateCategories
, blankSvgTemplate, blankHtmlTemplate, initTemplate
, badPreludeTemplate
, fromleo_markdown
, fromleo_markdown_optimized
, fromleo_markdown_optimized_lensless
, fromleo_recipe2
, fromleo_conference_budgetting
, fromleo_modelviewcontroller
, fromleo_latexeditor
, repl
, tableOfStatesA
, tableOfStatesB
, tableOfStatesC
, fromleo_linkedtexteditor
, fromleo_translatabledoc
, fromleo_dixit
, christmas_song_3_after_translation
, mapMaybeLens
, listAppendLens
, Example
)
import Lang exposing (Exp, Val, Widget, Env)
import FastParser
import LeoParser
import Eval
import Utils
import PreludeGenerated as Prelude
import DefaultIconTheme
import Syntax
import EvalUpdate
import Parser
import ParserUtils
type alias Example = {
e: Exp,
v: Val,
ws: List Widget,
env: Env}
--------------------------------------------------------------------------------
initTemplate = blankHtmlTemplate
getStartedTemplate = "Get Started"
blankSvgTemplate = "Blank Svg Document"
blankHtmlTemplate = "Blank Html Document"
badPreludeTemplate = "Bad Prelude"
--------------------------------------------------------------------------------
makeExample = makeExample_ FastParser.parseE Syntax.Little
makeLeoExample = makeExample_ LeoParser.parse Syntax.Leo
makeExample_: (String -> Result Parser.Error Exp) -> Syntax.Syntax -> String -> String -> (String, (String, () -> Result String Example))
makeExample_ parser syntax name s =
let thunk () =
-- TODO tolerate parse errors, change Select Example
parser s |> Result.mapError (\pmsg -> "Error parsing example " ++ name ++"\n" ++ ParserUtils.showError pmsg) |> Result.map (\e ->
-----------------------------------------------------
-- if name == "*Prelude*" then
-- {e=e, v=LangSvg.dummySvgVal, ws=[], ati=ati}
-- else
-----------------------------------------------------
let ((v,ws), env) = Utils.fromOk ("Error executing example " ++ name) <| EvalUpdate.runWithEnv syntax e in
{e=e, v=v, ws=ws, env=env}
)
in
(name, (s, thunk))
LITTLE_TO_ELM threeBoxes
LITTLE_TO_ELM nBoxesH2
LITTLE_TO_ELM sineWaveOfBoxes
LITTLE_TO_ELM sineWaveGrid
-- LITTLE_TO_ELM waveOfBoxes
-- LITTLE_TO_ELM waveOfBoxesTokens
-- LITTLE_TO_ELM waveOfBoxes3
LITTLE_TO_ELM nBoxes
LITTLE_TO_ELM groupOfBoxes
-- LITTLE_TO_ELM sixBoxesA
-- LITTLE_TO_ELM sixBoxesB
LITTLE_TO_ELM basicSlides
LITTLE_TO_ELM logo
LITTLE_TO_ELM logo2
-- TODO stack overflow for some reason?
-- LITTLE_TO_ELM logoSizes
LITTLE_TO_ELM elmLogo
-- LITTLE_TO_ELM activeTrans
LITTLE_TO_ELM activeTrans2
LITTLE_TO_ELM botanic
LITTLE_TO_ELM rings
LITTLE_TO_ELM polygons
LITTLE_TO_ELM stars
LITTLE_TO_ELM sliders
LITTLE_TO_ELM buttons
LITTLE_TO_ELM widgets
LITTLE_TO_ELM xySlider
LITTLE_TO_ELM offsets
LITTLE_TO_ELM rgba
-- LITTLE_TO_ELM boxGrid
LITTLE_TO_ELM boxGridTokenFilter
LITTLE_TO_ELM usFlag13
LITTLE_TO_ELM usFlag50
LITTLE_TO_ELM chicago
-- LITTLE_TO_ELM chicagoColors
LITTLE_TO_ELM frenchSudan
LITTLE_TO_ELM flw1
LITTLE_TO_ELM flw2
LITTLE_TO_ELM ferris
LITTLE_TO_ELM ferris2
LITTLE_TO_ELM ferris2target
LITTLE_TO_ELM ferrisWheelSlideshow
LITTLE_TO_ELM pieChart1
LITTLE_TO_ELM solarSystem
LITTLE_TO_ELM fractalTree
LITTLE_TO_ELM hilbertCurveAnimation
LITTLE_TO_ELM stickFigures
-- TODO stack overflow for some reason?
--LITTLE_TO_ELM cultOfLambda
LITTLE_TO_ELM clique
LITTLE_TO_ELM miscShapes
LITTLE_TO_ELM paths1
LITTLE_TO_ELM paths2
LITTLE_TO_ELM paths3
LITTLE_TO_ELM paths4
LITTLE_TO_ELM paths5
LITTLE_TO_ELM sailBoat
LITTLE_TO_ELM eyeIcon
LITTLE_TO_ELM wikimedia
LITTLE_TO_ELM haskell
LITTLE_TO_ELM matrices
LITTLE_TO_ELM rotTest
LITTLE_TO_ELM interfaceButtons
LITTLE_TO_ELM barGraph
LITTLE_TO_ELM thawFreeze
LITTLE_TO_ELM dictionaries
-- LITTLE_TO_ELM deleteBoxes
LITTLE_TO_ELM cover
LITTLE_TO_ELM poppl
LITTLE_TO_ELM bezier
-- LITTLE_TO_ELM surveyResultsTriBubbles
-- LITTLE_TO_ELM surveyResultsTriHist
LITTLE_TO_ELM surveyResultsTriHist2
LITTLE_TO_ELM equiTri
LITTLE_TO_ELM gridTile
LITTLE_TO_ELM lilliconP
LITTLE_TO_ELM lilliconP2
LITTLE_TO_ELM keyboard
LITTLE_TO_ELM keyboard2
LITTLE_TO_ELM keyboard2target
LITTLE_TO_ELM tessellation
LITTLE_TO_ELM tessellationTarget
LITTLE_TO_ELM tessellation2
LITTLE_TO_ELM floralLogo
LITTLE_TO_ELM floralLogo2
LITTLE_TO_ELM zones
LITTLE_TO_ELM roundedRect
LITTLE_TO_ELM spiralSpiralGraph
-- LITTLE_TO_ELM relateRects0
-- LITTLE_TO_ELM relateRects1
-- LITTLE_TO_ELM relateCircles0
-- LITTLE_TO_ELM relateLines0
-- LITTLE_TO_ELM relatePoints0
-- LITTLE_TO_ELM relatePoints1
-- LITTLE_TO_ELM relatePoints2
-- LITTLE_TO_ELM relatePoints3
-- LITTLE_TO_ELM relatePoints4
LITTLE_TO_ELM blank
LITTLE_TO_ELM horrorFilms0
LITTLE_TO_ELM cyclingAssociation0
LITTLE_TO_ELM snsLogoWheel
LITTLE_TO_ELM sns_UIST
LITTLE_TO_ELM sns_revisited_UIST
LITTLE_TO_ELM botanic_UIST
LITTLE_TO_ELM coffee_UIST
LITTLE_TO_ELM rectangleTrisection
LITTLE_TO_ELM battery
LITTLE_TO_ELM batteryDynamic
LITTLE_TO_ELM mondrianArch
LITTLE_TO_ELM ladder
LITTLE_TO_ELM rails
LITTLE_TO_ELM target
LITTLE_TO_ELM xs
LITTLE_TO_ELM conifer
LITTLE_TO_ELM ferris3
LITTLE_TO_ELM gear
LITTLE_TO_ELM kochSnowflake
LITTLE_TO_ELM replaceTerminalsWithWorkstations
LITTLE_TO_ELM balanceScale
LITTLE_TO_ELM pencilTip
LITTLE_TO_ELM calendarIcon
LITTLE_TO_ELM sns_deuce
LITTLE_TO_ELM target_deuce
LITTLE_TO_ELM battery_deuce
LITTLE_TO_ELM coffee_deuce
LITTLE_TO_ELM mondrian_arch_deuce
LEO_TO_ELM cat
--------------------------------------------------------------------------------
-- Deuce User Study Files
-- these .little files are generated from somewhere else;
-- don't change them manually
LITTLE_TO_ELM study_start
LITTLE_TO_ELM study_transition_1
LITTLE_TO_ELM study_transition_2
LITTLE_TO_ELM study_end
LITTLE_TO_ELM tutorial_step_01
LITTLE_TO_ELM tutorial_step_02
LITTLE_TO_ELM tutorial_step_03
LITTLE_TO_ELM tutorial_step_04
LITTLE_TO_ELM tutorial_step_05
LITTLE_TO_ELM tutorial_step_06
LITTLE_TO_ELM tutorial_step_07
LITTLE_TO_ELM tutorial_step_08
LITTLE_TO_ELM tutorial_step_09
LITTLE_TO_ELM tutorial_step_10
LITTLE_TO_ELM tutorial_step_11
LITTLE_TO_ELM tutorial_step_12
LITTLE_TO_ELM tutorial_step_13
LITTLE_TO_ELM tutorial_step_14
LITTLE_TO_ELM tutorial_step_15
LITTLE_TO_ELM tutorial_step_16
LITTLE_TO_ELM tutorial_step_17
LITTLE_TO_ELM tutorial_step_18
LITTLE_TO_ELM tutorial_step_19
LITTLE_TO_ELM tutorial_step_20
LITTLE_TO_ELM tutorial_step_21
LITTLE_TO_ELM tutorial_step_22
LITTLE_TO_ELM tutorial_step_23
LITTLE_TO_ELM task_one_rectangle
LITTLE_TO_ELM task_two_circles
LITTLE_TO_ELM task_three_rectangles
LITTLE_TO_ELM task_target
LITTLE_TO_ELM task_four_squares
LITTLE_TO_ELM task_lambda
--------------------------------------------------------------------------------
LEO_TO_ELM badPrelude
LEO_TO_ELM blankSvg
LEO_TO_ELM blankDoc
LEO_TO_ELM welcome1
LEO_TO_ELM tableOfStatesA
LEO_TO_ELM tableOfStatesB
LEO_TO_ELM tableOfStatesC
LEO_TO_ELM tableOfStatesE
LEO_TO_ELM simpleBudget
LEO_TO_ELM mapMaybeLens
LEO_TO_ELM mapListLens_1
LEO_TO_ELM mapListLens_2
LEO_TO_ELM listAppendLens
LEO_TO_ELM fromleo/markdown
LEO_TO_ELM fromleo/markdown_optimized
LEO_TO_ELM fromleo/markdown_optimized_lensless
LEO_TO_ELM fromleo/conference_budgetting
LEO_TO_ELM fromleo/recipe
LEO_TO_ELM fromleo/recipe2
LEO_TO_ELM fromleo/modelviewcontroller
LEO_TO_ELM fromleo/linkedtexteditor
LEO_TO_ELM fromleo/translatabledoc
LEO_TO_ELM fromleo/latexeditor
LEO_TO_ELM fromleo/dixit
LEO_TO_ELM christmas_song_3_after_translation
LEO_TO_ELM fromleo/pizzas_doodle
LEO_TO_ELM fromleo/universal_number
LEO_TO_ELM repl
LEO_TO_ELM slides
LEO_TO_ELM docs
LEO_TO_ELM sync
LEO_TO_ELM foldl_reversible_join
LEO_TO_ELM references_in_text
LEO_TO_ELM tutorialStudentGrades
--------------------------------------------------------------------------------
welcomeCategory =
( "Welcome"
, [ makeLeoExample blankSvgTemplate blankSvg
, makeLeoExample blankHtmlTemplate blankDoc
, makeLeoExample getStartedTemplate welcome1
-- , makeLeoExample "Tutorial" blankDoc
]
)
docsCategory =
( "Examples (OOPSLA 2018 Submission)"
, [ makeLeoExample "1a: Table of States" tableOfStatesA
, makeLeoExample "1b: Table of States" tableOfStatesC
, makeLeoExample "1c: Table of States" tableOfStatesE
] ++
(
List.indexedMap
(\i (caption, program) ->
makeLeoExample (toString (2+i) ++ ": " ++ caption) program
)
[ ("ICFP tutorial", tutorialStudentGrades)
, ("Conference Budget", fromleo_conference_budgetting)
, ("Model View Controller", fromleo_modelviewcontroller)
, ("Scalable Recipe Editor", fromleo_recipe2)
, ("Cloning Editor", fromleo_linkedtexteditor)
, ("Translation Editor", fromleo_translatabledoc)
, ("Markdown Editor", fromleo_markdown)
, ("Dixit Scoresheet", fromleo_dixit)
, ("Doodle", fromleo_pizzas_doodle)
, ("Universal numbers", fromleo_universal_number)
, ("LaTeX Editor", fromleo_latexeditor)
, ("REPL with SVG", repl)
, ("Slides", slides)
, ("Docs", docs)
-- TODO maybe Conference?
, ("Html regex replace: references", references_in_text)
, ("String join, concatMap", foldl_reversible_join)
, ("Lens: Maybe Map", mapMaybeLens)
, ("Lens: List Map 1", mapListLens_1)
, ("Lens: List Map 2", mapListLens_2)
, ("Lens: List Append", listAppendLens)
]
)
)
defaultIconCategory =
( "Default Icons"
, [ makeExample "Icon: Cursor" DefaultIconTheme.cursor
, makeExample "Icon: Point Or Offset" DefaultIconTheme.pointOrOffset
, makeExample "Icon: Text" DefaultIconTheme.text
, makeExample "Icon: Line" DefaultIconTheme.line
, makeExample "Icon: Rect" DefaultIconTheme.rect
, makeExample "Icon: Ellipse" DefaultIconTheme.ellipse
, makeExample "Icon: Polygon" DefaultIconTheme.polygon
, makeExample "Icon: Path" DefaultIconTheme.path
]
)
deuceCategory =
( "Deuce Examples"
, [ makeExample "Sketch-n-Sketch Logo" sns_deuce
, makeExample "Target" target_deuce
, makeExample "Battery" battery_deuce
, makeExample "Coffee Mug" coffee_deuce
, makeExample "Mondrian Arch" mondrian_arch_deuce
]
)
logoCategory =
( "Logos"
, List.sortBy Tuple.first
[ makeExample "SnS Logo (UIST)" sns_UIST
, makeExample "SnS Logo Revisited (UIST)" sns_revisited_UIST
, makeExample "Botanic Garden Logo (UIST)" botanic_UIST
, makeExample "Logo" logo
, makeExample "Botanic Garden Logo" botanic
, makeExample "Active Trans Logo" activeTrans2
, makeExample "SnS Logo Wheel" snsLogoWheel
, makeExample "Haskell.org Logo" haskell
, makeExample "Cover Logo" cover
, makeExample "POP-PL Logo" poppl
, makeExample "Floral Logo 1" floralLogo
, makeExample "Floral Logo 2" floralLogo2
, makeExample "Elm Logo" elmLogo
, makeExample "Logo 2" logo2
-- , makeExample "Logo Sizes" logoSizes
]
)
flagCategory =
( "Flags"
, List.sortBy Tuple.first
[ makeExample "Chicago Flag" chicago
, makeExample "US-13 Flag" usFlag13
, makeExample "US-50 Flag" usFlag50
, makeExample "French Sudan Flag" frenchSudan
]
)
otherCategory =
( "Other"
, [ makeExample "Coffee Mugs (UIST)" coffee_UIST
, makeExample "Wave Boxes" sineWaveOfBoxes
, makeExample "Wave Boxes Grid" sineWaveGrid
, makeExample "Basic Slides" basicSlides
, makeExample "Sailboat" sailBoat
, makeExample "Sliders" sliders
, makeExample "Buttons" buttons
, makeExample "Widgets" widgets
, makeExample "xySlider" xySlider
, makeExample "Offsets" offsets
, makeExample "Tile Pattern" boxGridTokenFilter
, makeExample "Color Picker" rgba
, makeExample "Ferris Wheel" ferris
, makeExample "Ferris Task Before" ferris2
, makeExample "Ferris Task After" ferris2target
, makeExample "Ferris Wheel Slideshow" ferrisWheelSlideshow
, makeExample "Survey Results" surveyResultsTriHist2
, makeExample "Hilbert Curve Animation" hilbertCurveAnimation
, makeExample "Bar Graph" barGraph
, makeExample "Pie Chart" pieChart1
, makeExample "Solar System" solarSystem
, makeExample "Clique" clique
, makeExample "Eye Icon" eyeIcon
, makeExample "Horror Films" horrorFilms0
, makeExample "Cycling Association" cyclingAssociation0
, makeExample "Lillicon P" lilliconP
, makeExample "Lillicon P, v2" lilliconP2
, makeExample "Keyboard" keyboard
, makeExample "Keyboard Task Before" keyboard2
, makeExample "Keyboard Task After" keyboard2target
, makeExample "Tessellation Task Before" tessellation
, makeExample "Tessellation Task After" tessellationTarget
, makeExample "Tessellation 2" tessellation2
, makeExample "Spiral Spiral-Graph" spiralSpiralGraph
, makeExample "Rounded Rect" roundedRect
, makeExample "Thaw/Freeze" thawFreeze
, makeExample "Dictionaries" dictionaries
, makeExample "3 Boxes" threeBoxes
, makeExample "N Boxes Sli" nBoxes
, makeExample "N Boxes" groupOfBoxes
, makeExample "Rings" rings
, makeExample "Polygons" polygons
, makeExample "Stars" stars
, makeExample "Triangles" equiTri
, makeExample "<NAME>" flw1
, makeExample "<NAME>" flw2
, makeExample "Bezier Curves" bezier
, makeExample "Fractal Tree" fractalTree
, makeExample "Stick Figures" stickFigures
-- , makeExample "Cult of Lambda" cultOfLambda
, makeExample "Matrix Transformations" matrices
, makeExample "Misc Shapes" miscShapes
, makeExample "Interface Buttons" interfaceButtons
, makeExample "Paths 1" paths1
, makeExample "Paths 2" paths2
, makeExample "Paths 3" paths3
, makeExample "Paths 4" paths4
, makeExample "Paths 5" paths5
, makeExample "Sample Rotations" rotTest
, makeExample "Grid Tile" gridTile
, makeExample "Zones" zones
, makeExample "Rectangle Trisection" rectangleTrisection
, makeExample "Battery" battery
, makeExample "Battery (Dynamic)" batteryDynamic
, makeExample "Mondrian Arch" mondrianArch
, makeExample "Ladder" ladder
, makeExample "Rails" rails
, makeExample "Target" target
, makeExample "Xs" xs
, makeExample "Conifer" conifer
, makeExample "Ferris Wheel 3" ferris3
, makeExample "Gear" gear
, makeExample "Koch Snowflake" kochSnowflake
, makeExample "Replace Terminals With Workstations" replaceTerminalsWithWorkstations
, makeExample "Balance Scale" balanceScale
, makeExample "Pencil Tip" pencilTip
, makeExample "Calendar Icon" calendarIcon
, makeLeoExample "SVG Cat" cat
]
)
deuceUserStudyCategory =
( "Deuce User Study"
, [ makeExample "Deuce Study Start" study_start
, makeExample "Step 01" tutorial_step_01
, makeExample "Step 02" tutorial_step_02
, makeExample "Step 03" tutorial_step_03
, makeExample "Step 04" tutorial_step_04
, makeExample "Step 05" tutorial_step_05
, makeExample "Step 06" tutorial_step_06
, makeExample "Step 07" tutorial_step_07
, makeExample "Step 08" tutorial_step_08
, makeExample "Step 09" tutorial_step_09
, makeExample "Step 10" tutorial_step_10
, makeExample "Step 11" tutorial_step_11
, makeExample "Step 12" tutorial_step_12
, makeExample "Step 13" tutorial_step_13
, makeExample "Step 14" tutorial_step_14
, makeExample "Step 15" tutorial_step_15
, makeExample "Step 16" tutorial_step_16
, makeExample "Step 17" tutorial_step_17
, makeExample "Step 18" tutorial_step_18
, makeExample "Step 19" tutorial_step_19
, makeExample "Step 20" tutorial_step_20
, makeExample "Step 21" tutorial_step_21
, makeExample "Step 22" tutorial_step_22
, makeExample "Step 23" tutorial_step_23
, makeExample "Deuce Study Transition 1" study_transition_1
, makeExample "One Rectangle" task_one_rectangle
, makeExample "Two Circles" task_two_circles
, makeExample "Three Rectangles" task_three_rectangles
, makeExample "Target Icon" task_target
, makeExample "Deuce Study Transition 2" study_transition_2
, makeExample "Four Squares" task_four_squares
, makeExample "Lambda Icon" task_lambda
, makeExample "Deuce Study End" study_end
]
)
internalCategory =
( "(Internal Things...)"
, [ makeLeoExample "Standard Prelude" Prelude.preludeLeo
, makeLeoExample badPreludeTemplate badPrelude
]
)
templateCategories =
[ welcomeCategory
, docsCategory
, deuceCategory
, defaultIconCategory
, logoCategory
, flagCategory
, otherCategory
, deuceUserStudyCategory
, internalCategory
]
list =
templateCategories
|> List.map Tuple.second
|> List.concat
| true | module ExamplesGenerated exposing
( list, templateCategories
, blankSvgTemplate, blankHtmlTemplate, initTemplate
, badPreludeTemplate
, fromleo_markdown
, fromleo_markdown_optimized
, fromleo_markdown_optimized_lensless
, fromleo_recipe2
, fromleo_conference_budgetting
, fromleo_modelviewcontroller
, fromleo_latexeditor
, repl
, tableOfStatesA
, tableOfStatesB
, tableOfStatesC
, fromleo_linkedtexteditor
, fromleo_translatabledoc
, fromleo_dixit
, christmas_song_3_after_translation
, mapMaybeLens
, listAppendLens
, Example
)
import Lang exposing (Exp, Val, Widget, Env)
import FastParser
import LeoParser
import Eval
import Utils
import PreludeGenerated as Prelude
import DefaultIconTheme
import Syntax
import EvalUpdate
import Parser
import ParserUtils
type alias Example = {
e: Exp,
v: Val,
ws: List Widget,
env: Env}
--------------------------------------------------------------------------------
initTemplate = blankHtmlTemplate
getStartedTemplate = "Get Started"
blankSvgTemplate = "Blank Svg Document"
blankHtmlTemplate = "Blank Html Document"
badPreludeTemplate = "Bad Prelude"
--------------------------------------------------------------------------------
makeExample = makeExample_ FastParser.parseE Syntax.Little
makeLeoExample = makeExample_ LeoParser.parse Syntax.Leo
makeExample_: (String -> Result Parser.Error Exp) -> Syntax.Syntax -> String -> String -> (String, (String, () -> Result String Example))
makeExample_ parser syntax name s =
let thunk () =
-- TODO tolerate parse errors, change Select Example
parser s |> Result.mapError (\pmsg -> "Error parsing example " ++ name ++"\n" ++ ParserUtils.showError pmsg) |> Result.map (\e ->
-----------------------------------------------------
-- if name == "*Prelude*" then
-- {e=e, v=LangSvg.dummySvgVal, ws=[], ati=ati}
-- else
-----------------------------------------------------
let ((v,ws), env) = Utils.fromOk ("Error executing example " ++ name) <| EvalUpdate.runWithEnv syntax e in
{e=e, v=v, ws=ws, env=env}
)
in
(name, (s, thunk))
LITTLE_TO_ELM threeBoxes
LITTLE_TO_ELM nBoxesH2
LITTLE_TO_ELM sineWaveOfBoxes
LITTLE_TO_ELM sineWaveGrid
-- LITTLE_TO_ELM waveOfBoxes
-- LITTLE_TO_ELM waveOfBoxesTokens
-- LITTLE_TO_ELM waveOfBoxes3
LITTLE_TO_ELM nBoxes
LITTLE_TO_ELM groupOfBoxes
-- LITTLE_TO_ELM sixBoxesA
-- LITTLE_TO_ELM sixBoxesB
LITTLE_TO_ELM basicSlides
LITTLE_TO_ELM logo
LITTLE_TO_ELM logo2
-- TODO stack overflow for some reason?
-- LITTLE_TO_ELM logoSizes
LITTLE_TO_ELM elmLogo
-- LITTLE_TO_ELM activeTrans
LITTLE_TO_ELM activeTrans2
LITTLE_TO_ELM botanic
LITTLE_TO_ELM rings
LITTLE_TO_ELM polygons
LITTLE_TO_ELM stars
LITTLE_TO_ELM sliders
LITTLE_TO_ELM buttons
LITTLE_TO_ELM widgets
LITTLE_TO_ELM xySlider
LITTLE_TO_ELM offsets
LITTLE_TO_ELM rgba
-- LITTLE_TO_ELM boxGrid
LITTLE_TO_ELM boxGridTokenFilter
LITTLE_TO_ELM usFlag13
LITTLE_TO_ELM usFlag50
LITTLE_TO_ELM chicago
-- LITTLE_TO_ELM chicagoColors
LITTLE_TO_ELM frenchSudan
LITTLE_TO_ELM flw1
LITTLE_TO_ELM flw2
LITTLE_TO_ELM ferris
LITTLE_TO_ELM ferris2
LITTLE_TO_ELM ferris2target
LITTLE_TO_ELM ferrisWheelSlideshow
LITTLE_TO_ELM pieChart1
LITTLE_TO_ELM solarSystem
LITTLE_TO_ELM fractalTree
LITTLE_TO_ELM hilbertCurveAnimation
LITTLE_TO_ELM stickFigures
-- TODO stack overflow for some reason?
--LITTLE_TO_ELM cultOfLambda
LITTLE_TO_ELM clique
LITTLE_TO_ELM miscShapes
LITTLE_TO_ELM paths1
LITTLE_TO_ELM paths2
LITTLE_TO_ELM paths3
LITTLE_TO_ELM paths4
LITTLE_TO_ELM paths5
LITTLE_TO_ELM sailBoat
LITTLE_TO_ELM eyeIcon
LITTLE_TO_ELM wikimedia
LITTLE_TO_ELM haskell
LITTLE_TO_ELM matrices
LITTLE_TO_ELM rotTest
LITTLE_TO_ELM interfaceButtons
LITTLE_TO_ELM barGraph
LITTLE_TO_ELM thawFreeze
LITTLE_TO_ELM dictionaries
-- LITTLE_TO_ELM deleteBoxes
LITTLE_TO_ELM cover
LITTLE_TO_ELM poppl
LITTLE_TO_ELM bezier
-- LITTLE_TO_ELM surveyResultsTriBubbles
-- LITTLE_TO_ELM surveyResultsTriHist
LITTLE_TO_ELM surveyResultsTriHist2
LITTLE_TO_ELM equiTri
LITTLE_TO_ELM gridTile
LITTLE_TO_ELM lilliconP
LITTLE_TO_ELM lilliconP2
LITTLE_TO_ELM keyboard
LITTLE_TO_ELM keyboard2
LITTLE_TO_ELM keyboard2target
LITTLE_TO_ELM tessellation
LITTLE_TO_ELM tessellationTarget
LITTLE_TO_ELM tessellation2
LITTLE_TO_ELM floralLogo
LITTLE_TO_ELM floralLogo2
LITTLE_TO_ELM zones
LITTLE_TO_ELM roundedRect
LITTLE_TO_ELM spiralSpiralGraph
-- LITTLE_TO_ELM relateRects0
-- LITTLE_TO_ELM relateRects1
-- LITTLE_TO_ELM relateCircles0
-- LITTLE_TO_ELM relateLines0
-- LITTLE_TO_ELM relatePoints0
-- LITTLE_TO_ELM relatePoints1
-- LITTLE_TO_ELM relatePoints2
-- LITTLE_TO_ELM relatePoints3
-- LITTLE_TO_ELM relatePoints4
LITTLE_TO_ELM blank
LITTLE_TO_ELM horrorFilms0
LITTLE_TO_ELM cyclingAssociation0
LITTLE_TO_ELM snsLogoWheel
LITTLE_TO_ELM sns_UIST
LITTLE_TO_ELM sns_revisited_UIST
LITTLE_TO_ELM botanic_UIST
LITTLE_TO_ELM coffee_UIST
LITTLE_TO_ELM rectangleTrisection
LITTLE_TO_ELM battery
LITTLE_TO_ELM batteryDynamic
LITTLE_TO_ELM mondrianArch
LITTLE_TO_ELM ladder
LITTLE_TO_ELM rails
LITTLE_TO_ELM target
LITTLE_TO_ELM xs
LITTLE_TO_ELM conifer
LITTLE_TO_ELM ferris3
LITTLE_TO_ELM gear
LITTLE_TO_ELM kochSnowflake
LITTLE_TO_ELM replaceTerminalsWithWorkstations
LITTLE_TO_ELM balanceScale
LITTLE_TO_ELM pencilTip
LITTLE_TO_ELM calendarIcon
LITTLE_TO_ELM sns_deuce
LITTLE_TO_ELM target_deuce
LITTLE_TO_ELM battery_deuce
LITTLE_TO_ELM coffee_deuce
LITTLE_TO_ELM mondrian_arch_deuce
LEO_TO_ELM cat
--------------------------------------------------------------------------------
-- Deuce User Study Files
-- these .little files are generated from somewhere else;
-- don't change them manually
LITTLE_TO_ELM study_start
LITTLE_TO_ELM study_transition_1
LITTLE_TO_ELM study_transition_2
LITTLE_TO_ELM study_end
LITTLE_TO_ELM tutorial_step_01
LITTLE_TO_ELM tutorial_step_02
LITTLE_TO_ELM tutorial_step_03
LITTLE_TO_ELM tutorial_step_04
LITTLE_TO_ELM tutorial_step_05
LITTLE_TO_ELM tutorial_step_06
LITTLE_TO_ELM tutorial_step_07
LITTLE_TO_ELM tutorial_step_08
LITTLE_TO_ELM tutorial_step_09
LITTLE_TO_ELM tutorial_step_10
LITTLE_TO_ELM tutorial_step_11
LITTLE_TO_ELM tutorial_step_12
LITTLE_TO_ELM tutorial_step_13
LITTLE_TO_ELM tutorial_step_14
LITTLE_TO_ELM tutorial_step_15
LITTLE_TO_ELM tutorial_step_16
LITTLE_TO_ELM tutorial_step_17
LITTLE_TO_ELM tutorial_step_18
LITTLE_TO_ELM tutorial_step_19
LITTLE_TO_ELM tutorial_step_20
LITTLE_TO_ELM tutorial_step_21
LITTLE_TO_ELM tutorial_step_22
LITTLE_TO_ELM tutorial_step_23
LITTLE_TO_ELM task_one_rectangle
LITTLE_TO_ELM task_two_circles
LITTLE_TO_ELM task_three_rectangles
LITTLE_TO_ELM task_target
LITTLE_TO_ELM task_four_squares
LITTLE_TO_ELM task_lambda
--------------------------------------------------------------------------------
LEO_TO_ELM badPrelude
LEO_TO_ELM blankSvg
LEO_TO_ELM blankDoc
LEO_TO_ELM welcome1
LEO_TO_ELM tableOfStatesA
LEO_TO_ELM tableOfStatesB
LEO_TO_ELM tableOfStatesC
LEO_TO_ELM tableOfStatesE
LEO_TO_ELM simpleBudget
LEO_TO_ELM mapMaybeLens
LEO_TO_ELM mapListLens_1
LEO_TO_ELM mapListLens_2
LEO_TO_ELM listAppendLens
LEO_TO_ELM fromleo/markdown
LEO_TO_ELM fromleo/markdown_optimized
LEO_TO_ELM fromleo/markdown_optimized_lensless
LEO_TO_ELM fromleo/conference_budgetting
LEO_TO_ELM fromleo/recipe
LEO_TO_ELM fromleo/recipe2
LEO_TO_ELM fromleo/modelviewcontroller
LEO_TO_ELM fromleo/linkedtexteditor
LEO_TO_ELM fromleo/translatabledoc
LEO_TO_ELM fromleo/latexeditor
LEO_TO_ELM fromleo/dixit
LEO_TO_ELM christmas_song_3_after_translation
LEO_TO_ELM fromleo/pizzas_doodle
LEO_TO_ELM fromleo/universal_number
LEO_TO_ELM repl
LEO_TO_ELM slides
LEO_TO_ELM docs
LEO_TO_ELM sync
LEO_TO_ELM foldl_reversible_join
LEO_TO_ELM references_in_text
LEO_TO_ELM tutorialStudentGrades
--------------------------------------------------------------------------------
welcomeCategory =
( "Welcome"
, [ makeLeoExample blankSvgTemplate blankSvg
, makeLeoExample blankHtmlTemplate blankDoc
, makeLeoExample getStartedTemplate welcome1
-- , makeLeoExample "Tutorial" blankDoc
]
)
docsCategory =
( "Examples (OOPSLA 2018 Submission)"
, [ makeLeoExample "1a: Table of States" tableOfStatesA
, makeLeoExample "1b: Table of States" tableOfStatesC
, makeLeoExample "1c: Table of States" tableOfStatesE
] ++
(
List.indexedMap
(\i (caption, program) ->
makeLeoExample (toString (2+i) ++ ": " ++ caption) program
)
[ ("ICFP tutorial", tutorialStudentGrades)
, ("Conference Budget", fromleo_conference_budgetting)
, ("Model View Controller", fromleo_modelviewcontroller)
, ("Scalable Recipe Editor", fromleo_recipe2)
, ("Cloning Editor", fromleo_linkedtexteditor)
, ("Translation Editor", fromleo_translatabledoc)
, ("Markdown Editor", fromleo_markdown)
, ("Dixit Scoresheet", fromleo_dixit)
, ("Doodle", fromleo_pizzas_doodle)
, ("Universal numbers", fromleo_universal_number)
, ("LaTeX Editor", fromleo_latexeditor)
, ("REPL with SVG", repl)
, ("Slides", slides)
, ("Docs", docs)
-- TODO maybe Conference?
, ("Html regex replace: references", references_in_text)
, ("String join, concatMap", foldl_reversible_join)
, ("Lens: Maybe Map", mapMaybeLens)
, ("Lens: List Map 1", mapListLens_1)
, ("Lens: List Map 2", mapListLens_2)
, ("Lens: List Append", listAppendLens)
]
)
)
defaultIconCategory =
( "Default Icons"
, [ makeExample "Icon: Cursor" DefaultIconTheme.cursor
, makeExample "Icon: Point Or Offset" DefaultIconTheme.pointOrOffset
, makeExample "Icon: Text" DefaultIconTheme.text
, makeExample "Icon: Line" DefaultIconTheme.line
, makeExample "Icon: Rect" DefaultIconTheme.rect
, makeExample "Icon: Ellipse" DefaultIconTheme.ellipse
, makeExample "Icon: Polygon" DefaultIconTheme.polygon
, makeExample "Icon: Path" DefaultIconTheme.path
]
)
deuceCategory =
( "Deuce Examples"
, [ makeExample "Sketch-n-Sketch Logo" sns_deuce
, makeExample "Target" target_deuce
, makeExample "Battery" battery_deuce
, makeExample "Coffee Mug" coffee_deuce
, makeExample "Mondrian Arch" mondrian_arch_deuce
]
)
logoCategory =
( "Logos"
, List.sortBy Tuple.first
[ makeExample "SnS Logo (UIST)" sns_UIST
, makeExample "SnS Logo Revisited (UIST)" sns_revisited_UIST
, makeExample "Botanic Garden Logo (UIST)" botanic_UIST
, makeExample "Logo" logo
, makeExample "Botanic Garden Logo" botanic
, makeExample "Active Trans Logo" activeTrans2
, makeExample "SnS Logo Wheel" snsLogoWheel
, makeExample "Haskell.org Logo" haskell
, makeExample "Cover Logo" cover
, makeExample "POP-PL Logo" poppl
, makeExample "Floral Logo 1" floralLogo
, makeExample "Floral Logo 2" floralLogo2
, makeExample "Elm Logo" elmLogo
, makeExample "Logo 2" logo2
-- , makeExample "Logo Sizes" logoSizes
]
)
flagCategory =
( "Flags"
, List.sortBy Tuple.first
[ makeExample "Chicago Flag" chicago
, makeExample "US-13 Flag" usFlag13
, makeExample "US-50 Flag" usFlag50
, makeExample "French Sudan Flag" frenchSudan
]
)
otherCategory =
( "Other"
, [ makeExample "Coffee Mugs (UIST)" coffee_UIST
, makeExample "Wave Boxes" sineWaveOfBoxes
, makeExample "Wave Boxes Grid" sineWaveGrid
, makeExample "Basic Slides" basicSlides
, makeExample "Sailboat" sailBoat
, makeExample "Sliders" sliders
, makeExample "Buttons" buttons
, makeExample "Widgets" widgets
, makeExample "xySlider" xySlider
, makeExample "Offsets" offsets
, makeExample "Tile Pattern" boxGridTokenFilter
, makeExample "Color Picker" rgba
, makeExample "Ferris Wheel" ferris
, makeExample "Ferris Task Before" ferris2
, makeExample "Ferris Task After" ferris2target
, makeExample "Ferris Wheel Slideshow" ferrisWheelSlideshow
, makeExample "Survey Results" surveyResultsTriHist2
, makeExample "Hilbert Curve Animation" hilbertCurveAnimation
, makeExample "Bar Graph" barGraph
, makeExample "Pie Chart" pieChart1
, makeExample "Solar System" solarSystem
, makeExample "Clique" clique
, makeExample "Eye Icon" eyeIcon
, makeExample "Horror Films" horrorFilms0
, makeExample "Cycling Association" cyclingAssociation0
, makeExample "Lillicon P" lilliconP
, makeExample "Lillicon P, v2" lilliconP2
, makeExample "Keyboard" keyboard
, makeExample "Keyboard Task Before" keyboard2
, makeExample "Keyboard Task After" keyboard2target
, makeExample "Tessellation Task Before" tessellation
, makeExample "Tessellation Task After" tessellationTarget
, makeExample "Tessellation 2" tessellation2
, makeExample "Spiral Spiral-Graph" spiralSpiralGraph
, makeExample "Rounded Rect" roundedRect
, makeExample "Thaw/Freeze" thawFreeze
, makeExample "Dictionaries" dictionaries
, makeExample "3 Boxes" threeBoxes
, makeExample "N Boxes Sli" nBoxes
, makeExample "N Boxes" groupOfBoxes
, makeExample "Rings" rings
, makeExample "Polygons" polygons
, makeExample "Stars" stars
, makeExample "Triangles" equiTri
, makeExample "PI:NAME:<NAME>END_PI" flw1
, makeExample "PI:NAME:<NAME>END_PI" flw2
, makeExample "Bezier Curves" bezier
, makeExample "Fractal Tree" fractalTree
, makeExample "Stick Figures" stickFigures
-- , makeExample "Cult of Lambda" cultOfLambda
, makeExample "Matrix Transformations" matrices
, makeExample "Misc Shapes" miscShapes
, makeExample "Interface Buttons" interfaceButtons
, makeExample "Paths 1" paths1
, makeExample "Paths 2" paths2
, makeExample "Paths 3" paths3
, makeExample "Paths 4" paths4
, makeExample "Paths 5" paths5
, makeExample "Sample Rotations" rotTest
, makeExample "Grid Tile" gridTile
, makeExample "Zones" zones
, makeExample "Rectangle Trisection" rectangleTrisection
, makeExample "Battery" battery
, makeExample "Battery (Dynamic)" batteryDynamic
, makeExample "Mondrian Arch" mondrianArch
, makeExample "Ladder" ladder
, makeExample "Rails" rails
, makeExample "Target" target
, makeExample "Xs" xs
, makeExample "Conifer" conifer
, makeExample "Ferris Wheel 3" ferris3
, makeExample "Gear" gear
, makeExample "Koch Snowflake" kochSnowflake
, makeExample "Replace Terminals With Workstations" replaceTerminalsWithWorkstations
, makeExample "Balance Scale" balanceScale
, makeExample "Pencil Tip" pencilTip
, makeExample "Calendar Icon" calendarIcon
, makeLeoExample "SVG Cat" cat
]
)
deuceUserStudyCategory =
( "Deuce User Study"
, [ makeExample "Deuce Study Start" study_start
, makeExample "Step 01" tutorial_step_01
, makeExample "Step 02" tutorial_step_02
, makeExample "Step 03" tutorial_step_03
, makeExample "Step 04" tutorial_step_04
, makeExample "Step 05" tutorial_step_05
, makeExample "Step 06" tutorial_step_06
, makeExample "Step 07" tutorial_step_07
, makeExample "Step 08" tutorial_step_08
, makeExample "Step 09" tutorial_step_09
, makeExample "Step 10" tutorial_step_10
, makeExample "Step 11" tutorial_step_11
, makeExample "Step 12" tutorial_step_12
, makeExample "Step 13" tutorial_step_13
, makeExample "Step 14" tutorial_step_14
, makeExample "Step 15" tutorial_step_15
, makeExample "Step 16" tutorial_step_16
, makeExample "Step 17" tutorial_step_17
, makeExample "Step 18" tutorial_step_18
, makeExample "Step 19" tutorial_step_19
, makeExample "Step 20" tutorial_step_20
, makeExample "Step 21" tutorial_step_21
, makeExample "Step 22" tutorial_step_22
, makeExample "Step 23" tutorial_step_23
, makeExample "Deuce Study Transition 1" study_transition_1
, makeExample "One Rectangle" task_one_rectangle
, makeExample "Two Circles" task_two_circles
, makeExample "Three Rectangles" task_three_rectangles
, makeExample "Target Icon" task_target
, makeExample "Deuce Study Transition 2" study_transition_2
, makeExample "Four Squares" task_four_squares
, makeExample "Lambda Icon" task_lambda
, makeExample "Deuce Study End" study_end
]
)
internalCategory =
( "(Internal Things...)"
, [ makeLeoExample "Standard Prelude" Prelude.preludeLeo
, makeLeoExample badPreludeTemplate badPrelude
]
)
templateCategories =
[ welcomeCategory
, docsCategory
, deuceCategory
, defaultIconCategory
, logoCategory
, flagCategory
, otherCategory
, deuceUserStudyCategory
, internalCategory
]
list =
templateCategories
|> List.map Tuple.second
|> List.concat
| elm |
[
{
"context": " about images? 
import Expect exposing (Expectation)
import Fuzz exposing (Fuzzer, int, list, string)
import Markdown.Block as Markdown
import Markdown.Html
import Markdown.Parser as Markdown
import Markdown.PrettyTables as Tables
import Markdown.Renderer as Markdown
import Markdown.Scaffolded as Scaffolded
import Test exposing (..)
{-
Unfortunately, there are few tests, right now. You're welcome to contribute tests!
I personally test this project using the /examples and another semi-production app.
If you want to contribute: There are good opportunities for creating property-based
tests for this application. For that it would be neccessary to write a fuzzer:
`Fuzzer children -> Fuzzer (Scaffolded.Block children)` and then identify the
mathematical properties of functions like `map`, `foldFunction`, etc. and test them.
-}
suite : Test
suite =
describe "Markdown"
[ describe "Formatting"
[ test "round-trip" (testRoundTrip prettyprint exampleMarkdown)
, test "idempotency" (testIdempotency prettyprint exampleMarkdown)
]
, describe "PrettyTables"
[ -- Examples
test "basic example"
(testConcrete (prettyprintTables Tables.defaultStyle)
{ input =
[ "Person | Age"
, "--|-:"
, "Someone I used to know | No idea"
, "Me | 23"
]
, expect =
[ "| Person | Age |"
, "|------------------------|--------:|"
, "| Someone I used to know | No idea |"
, "| Me | 23 |"
]
}
)
, test "no additional newlines (issue #4)"
(testConcrete (prettyprintTables Tables.compactStyle)
{ input =
[ "one | two"
, "--- | ---"
, ""
, ""
, "para after table"
]
, expect =
[ "one | two"
, "--- | ---"
, ""
, "para after table"
]
}
)
-- Round-Trips
, test "round-trip (small, compact)"
(testRoundTrip
(prettyprintTables Tables.compactStyle)
exampleTables
)
, test "round-trip (big, compact)"
(testRoundTrip
(prettyprintTables Tables.compactStyle)
exampleTablesMarkdown
)
, test "round-trip (small, default)"
(testRoundTrip
(prettyprintTables Tables.defaultStyle)
exampleTables
)
, test "round-trip (big, default)"
(testRoundTrip
(prettyprintTables Tables.defaultStyle)
exampleTablesMarkdown
)
-- Idempotency
, test "idempotency (small, compact)"
(testRoundTrip
(prettyprintTables Tables.compactStyle)
exampleTables
)
, test "idempotency (big, compact)"
(testRoundTrip
(prettyprintTables Tables.compactStyle)
exampleTablesMarkdown
)
, test "idempotency (small, default)"
(testRoundTrip
(prettyprintTables Tables.defaultStyle)
exampleTables
)
, test "idempotency (big, default)"
(testRoundTrip
(prettyprintTables Tables.defaultStyle)
exampleTablesMarkdown
)
]
, describe "Scaffolded"
[ test "foldIndexed" <|
\_ ->
let
renderPath : List Int -> String
renderPath path =
"(" ++ String.join "," (List.map String.fromInt path) ++ ") "
reduceWithIndices : Scaffolded.Block (List Int -> String) -> List Int -> String
reduceWithIndices block path =
case Scaffolded.foldIndexed block path of
Scaffolded.Heading { level, rawText, children } ->
Scaffolded.Heading
{ level = level
, rawText = rawText
, children = renderPath path :: children
}
|> Scaffolded.reducePretty
other ->
Scaffolded.reducePretty other
input =
String.join "\n"
[ "# Everything has indices."
, ""
, "If you write some text in between, you'll see that following headings' indices change."
, ""
, "## Another heading."
]
in
parseMarkdown input
|> Result.andThen
(Markdown.render
(Scaffolded.toRenderer
{ renderHtml = Markdown.Html.oneOf []
, renderMarkdown = reduceWithIndices
}
)
)
|> Result.map (List.indexedMap (\index expectsPath -> expectsPath [ index ]))
|> Result.map
(Expect.equalLists
[ "# (0) Everything has indices."
, "If you write some text in between, you'll see that following headings' indices change."
, "## (2) Another heading."
]
)
|> expectOk
]
]
testConcrete : (String -> Result String String) -> { input : List String, expect : List String } -> () -> Expectation
testConcrete prettyprinter { input, expect } () =
input
|> String.join "\n"
|> prettyprinter
|> Result.map (Expect.equal (String.join "\n" expect))
|> expectOk
testRoundTrip : (String -> Result String String) -> String -> () -> Expectation
testRoundTrip prettyprinter markdown () =
Result.map2
(\original prettyprinted ->
Expect.equalLists original prettyprinted
)
(markdown
|> parseMarkdown
|> Result.mapError ((++) "Error in normal Markdown: ")
)
(markdown
|> prettyprinter
|> Result.andThen parseMarkdown
|> Result.mapError ((++) "Error in pretty-printed Markdown: ")
)
|> expectOk
testIdempotency : (String -> Result String String) -> String -> () -> Expectation
testIdempotency prettyprinter markdown () =
Result.map2
(\prettyOnce prettyTwice ->
Expect.equal prettyOnce prettyTwice
)
(markdown
|> prettyprinter
|> Result.mapError ((++) "Error after prettyprinting: ")
)
(markdown
|> prettyprinter
|> Result.andThen prettyprinter
|> Result.mapError ((++) "Error after prettyprinting twice: ")
)
|> expectOk
expectOk : Result String Expectation -> Expectation
expectOk result =
case result of
Ok e ->
e
Err error ->
Expect.fail error
parseMarkdown : String -> Result String (List Markdown.Block)
parseMarkdown markdown =
markdown
|> Markdown.parse
|> Result.mapError (List.map Markdown.deadEndToString >> String.join "\n")
prettyprint : String -> Result String String
prettyprint markdown =
markdown
|> parseMarkdown
|> Result.andThen
(Markdown.render
(Scaffolded.toRenderer
{ renderHtml = Markdown.Html.oneOf []
, renderMarkdown = Scaffolded.reducePretty
}
)
)
|> Result.map (String.join "\n\n")
prettyprintTables : Tables.TableStyle -> String -> Result String String
prettyprintTables style markdown =
markdown
|> parseMarkdown
|> Result.andThen
(Markdown.render
(Scaffolded.toRenderer
{ renderHtml = Markdown.Html.oneOf []
, renderMarkdown = Tables.reducePrettyTable style
}
)
)
|> Result.map Tables.finishReduction
-- Test data
exampleMarkdown : String
exampleMarkdown =
"""# Format _beautiful_ Markdown
> Markdown is only fine
> When *pretty printed*
> It has to be **BEAUTIFUL** I SAID.
## Links
Let's try [a `link`](https://example.com)
What about images? 
## Lists
Markdown formatting is:
* [ ] Stupid
* [X] Okay-ish
* [ ] Works
1. Don't use this project
1. What are you doing?
1. Ok. Please go now.
- Let's not do checkboxes.
---------
0. Write library
1. Publish
2. ???
3. Profit!
## Code blocks
```elm
viewMarkdown : String -> List (Html Msg)
viewMarkdown markdown =
[ Html.h2 [] [ Html.text "Prettyprinted:" ]
, Html.hr [] []
, Html.pre [ Attr.style "word-wrap" "pre-wrap" ] [ Html.text markdown ]
]
```
```
Please use more beautiful
Code blocks!
```
"""
exampleTables : String
exampleTables =
"""
Name | Year of birth
--|-:
Tom Hanks | 1956
Philipp | 1997
Jesus | -4
Caesar | -100
Appliance | Cost | Own
:-:|-:|:-
Dishwasher | 500€ | Yes
Toaster | 40€ | No
Refrigerator | 600€ | Yes
Washing Machine | 1200€ | Yes
This is some text to ensure the right amount of newlines
Table | Without Rows
--|--
More text to ensure we generate the right spacing between markdown blocks
"""
exampleTablesMarkdown : String
exampleTablesMarkdown =
"""People
| :bowtie: `:bowtie:` | :smile: `:smile:` | :laughing: `:laughing:` |
|---|---|---|
| :blush: `:blush:` | :smiley: `:smiley:` | :relaxed: `:relaxed:` |
| :smirk: `:smirk:` | :heart_eyes: `:heart_eyes:` | :kissing_heart: `:kissing_heart:` |
| :kissing_closed_eyes: `:kissing_closed_eyes:` | :flushed: `:flushed:` | :relieved: `:relieved:` |
| :satisfied: `:satisfied:` | :grin: `:grin:` | :wink: `:wink:` |
| :stuck_out_tongue_winking_eye: `:stuck_out_tongue_winking_eye:` | :stuck_out_tongue_closed_eyes: `:stuck_out_tongue_closed_eyes:` | :grinning: `:grinning:` |
| :kissing: `:kissing:` | :kissing_smiling_eyes: `:kissing_smiling_eyes:` | :stuck_out_tongue: `:stuck_out_tongue:` |
| :sleeping: `:sleeping:` | :worried: `:worried:` | :frowning: `:frowning:` |
| :anguished: `:anguished:` | :open_mouth: `:open_mouth:` | :grimacing: `:grimacing:` |
| :confused: `:confused:` | :hushed: `:hushed:` | :expressionless: `:expressionless:` |
| :unamused: `:unamused:` | :sweat_smile: `:sweat_smile:` | :sweat: `:sweat:` |
| :disappointed_relieved: `:disappointed_relieved:` | :weary: `:weary:` | :pensive: `:pensive:` |
| :disappointed: `:disappointed:` | :confounded: `:confounded:` | :fearful: `:fearful:` |
| :cold_sweat: `:cold_sweat:` | :persevere: `:persevere:` | :cry: `:cry:` |
| :sob: `:sob:` | :joy: `:joy:` | :astonished: `:astonished:` |
| :scream: `:scream:` | :neckbeard: `:neckbeard:` | :tired_face: `:tired_face:` |
| :angry: `:angry:` | :rage: `:rage:` | :triumph: `:triumph:` |
| :sleepy: `:sleepy:` | :yum: `:yum:` | :mask: `:mask:` |
| :sunglasses: `:sunglasses:` | :dizzy_face: `:dizzy_face:` | :imp: `:imp:` |
| :smiling_imp: `:smiling_imp:` | :neutral_face: `:neutral_face:` | :no_mouth: `:no_mouth:` |
| :innocent: `:innocent:` | :alien: `:alien:` | :yellow_heart: `:yellow_heart:` |
| :blue_heart: `:blue_heart:` | :purple_heart: `:purple_heart:` | :heart: `:heart:` |
| :green_heart: `:green_heart:` | :broken_heart: `:broken_heart:` | :heartbeat: `:heartbeat:` |
| :heartpulse: `:heartpulse:` | :two_hearts: `:two_hearts:` | :revolving_hearts: `:revolving_hearts:` |
| :cupid: `:cupid:` | :sparkling_heart: `:sparkling_heart:` | :sparkles: `:sparkles:` |
| :star: `:star:` | :star2: `:star2:` | :dizzy: `:dizzy:` |
| :boom: `:boom:` | :collision: `:collision:` | :anger: `:anger:` |
| :exclamation: `:exclamation:` | :question: `:question:` | :grey_exclamation: `:grey_exclamation:` |
| :grey_question: `:grey_question:` | :zzz: `:zzz:` | :dash: `:dash:` |
| :sweat_drops: `:sweat_drops:` | :notes: `:notes:` | :musical_note: `:musical_note:` |
| :fire: `:fire:` | :hankey: `:hankey:` | :poop: `:poop:` |
| :shit: `:shit:` | :+1: `:+1:` | :thumbsup: `:thumbsup:` |
| :-1: `:-1:` | :thumbsdown: `:thumbsdown:` | :ok_hand: `:ok_hand:` |
| :punch: `:punch:` | :facepunch: `:facepunch:` | :fist: `:fist:` |
| :v: `:v:` | :wave: `:wave:` | :hand: `:hand:` |
| :raised_hand: `:raised_hand:` | :open_hands: `:open_hands:` | :point_up: `:point_up:` |
| :point_down: `:point_down:` | :point_left: `:point_left:` | :point_right: `:point_right:` |
| :raised_hands: `:raised_hands:` | :pray: `:pray:` | :point_up_2: `:point_up_2:` |
| :clap: `:clap:` | :muscle: `:muscle:` | :metal: `:metal:` |
| :fu: `:fu:` | :walking: `:walking:` | :runner: `:runner:` |
| :running: `:running:` | :couple: `:couple:` | :family: `:family:` |
| :two_men_holding_hands: `:two_men_holding_hands:` | :two_women_holding_hands: `:two_women_holding_hands:` | :dancer: `:dancer:` |
| :dancers: `:dancers:` | :ok_woman: `:ok_woman:` | :no_good: `:no_good:` |
| :information_desk_person: `:information_desk_person:` | :raising_hand: `:raising_hand:` | :bride_with_veil: `:bride_with_veil:` |
| :person_with_pouting_face: `:person_with_pouting_face:` | :person_frowning: `:person_frowning:` | :bow: `:bow:` |
| :couplekiss: `:couplekiss:` | :couple_with_heart: `:couple_with_heart:` | :massage: `:massage:` |
| :haircut: `:haircut:` | :nail_care: `:nail_care:` | :boy: `:boy:` |
| :girl: `:girl:` | :woman: `:woman:` | :man: `:man:` |
| :baby: `:baby:` | :older_woman: `:older_woman:` | :older_man: `:older_man:` |
| :person_with_blond_hair: `:person_with_blond_hair:` | :man_with_gua_pi_mao: `:man_with_gua_pi_mao:` | :man_with_turban: `:man_with_turban:` |
| :construction_worker: `:construction_worker:` | :cop: `:cop:` | :angel: `:angel:` |
| :princess: `:princess:` | :smiley_cat: `:smiley_cat:` | :smile_cat: `:smile_cat:` |
| :heart_eyes_cat: `:heart_eyes_cat:` | :kissing_cat: `:kissing_cat:` | :smirk_cat: `:smirk_cat:` |
| :scream_cat: `:scream_cat:` | :crying_cat_face: `:crying_cat_face:` | :joy_cat: `:joy_cat:` |
| :pouting_cat: `:pouting_cat:` | :japanese_ogre: `:japanese_ogre:` | :japanese_goblin: `:japanese_goblin:` |
| :see_no_evil: `:see_no_evil:` | :hear_no_evil: `:hear_no_evil:` | :speak_no_evil: `:speak_no_evil:` |
| :guardsman: `:guardsman:` | :skull: `:skull:` | :feet: `:feet:` |
| :lips: `:lips:` | :kiss: `:kiss:` | :droplet: `:droplet:` |
| :ear: `:ear:` | :eyes: `:eyes:` | :nose: `:nose:` |
| :tongue: `:tongue:` | :love_letter: `:love_letter:` | :bust_in_silhouette: `:bust_in_silhouette:` |
| :busts_in_silhouette: `:busts_in_silhouette:` | :speech_balloon: `:speech_balloon:` | :thought_balloon: `:thought_balloon:` |
| :feelsgood: `:feelsgood:` | :finnadie: `:finnadie:` | :goberserk: `:goberserk:` |
| :godmode: `:godmode:` | :hurtrealbad: `:hurtrealbad:` | :rage1: `:rage1:` |
| :rage2: `:rage2:` | :rage3: `:rage3:` | :rage4: `:rage4:` |
| :suspect: `:suspect:` | :trollface: `:trollface:` |
Nature
| :sunny: `:sunny:` | :umbrella: `:umbrella:` | :cloud: `:cloud:` |
|---|---|---|
| :snowflake: `:snowflake:` | :snowman: `:snowman:` | :zap: `:zap:` |
| :cyclone: `:cyclone:` | :foggy: `:foggy:` | :ocean: `:ocean:` |
| :cat: `:cat:` | :dog: `:dog:` | :mouse: `:mouse:` |
| :hamster: `:hamster:` | :rabbit: `:rabbit:` | :wolf: `:wolf:` |
| :frog: `:frog:` | :tiger: `:tiger:` | :koala: `:koala:` |
| :bear: `:bear:` | :pig: `:pig:` | :pig_nose: `:pig_nose:` |
| :cow: `:cow:` | :boar: `:boar:` | :monkey_face: `:monkey_face:` |
| :monkey: `:monkey:` | :horse: `:horse:` | :racehorse: `:racehorse:` |
| :camel: `:camel:` | :sheep: `:sheep:` | :elephant: `:elephant:` |
| :panda_face: `:panda_face:` | :snake: `:snake:` | :bird: `:bird:` |
| :baby_chick: `:baby_chick:` | :hatched_chick: `:hatched_chick:` | :hatching_chick: `:hatching_chick:` |
| :chicken: `:chicken:` | :penguin: `:penguin:` | :turtle: `:turtle:` |
| :bug: `:bug:` | :honeybee: `:honeybee:` | :ant: `:ant:` |
| :beetle: `:beetle:` | :snail: `:snail:` | :octopus: `:octopus:` |
| :tropical_fish: `:tropical_fish:` | :fish: `:fish:` | :whale: `:whale:` |
| :whale2: `:whale2:` | :dolphin: `:dolphin:` | :cow2: `:cow2:` |
| :ram: `:ram:` | :rat: `:rat:` | :water_buffalo: `:water_buffalo:` |
| :tiger2: `:tiger2:` | :rabbit2: `:rabbit2:` | :dragon: `:dragon:` |
| :goat: `:goat:` | :rooster: `:rooster:` | :dog2: `:dog2:` |
| :pig2: `:pig2:` | :mouse2: `:mouse2:` | :ox: `:ox:` |
| :dragon_face: `:dragon_face:` | :blowfish: `:blowfish:` | :crocodile: `:crocodile:` |
| :dromedary_camel: `:dromedary_camel:` | :leopard: `:leopard:` | :cat2: `:cat2:` |
| :poodle: `:poodle:` | :paw_prints: `:paw_prints:` | :bouquet: `:bouquet:` |
| :cherry_blossom: `:cherry_blossom:` | :tulip: `:tulip:` | :four_leaf_clover: `:four_leaf_clover:` |
| :rose: `:rose:` | :sunflower: `:sunflower:` | :hibiscus: `:hibiscus:` |
| :maple_leaf: `:maple_leaf:` | :leaves: `:leaves:` | :fallen_leaf: `:fallen_leaf:` |
| :herb: `:herb:` | :mushroom: `:mushroom:` | :cactus: `:cactus:` |
| :palm_tree: `:palm_tree:` | :evergreen_tree: `:evergreen_tree:` | :deciduous_tree: `:deciduous_tree:` |
| :chestnut: `:chestnut:` | :seedling: `:seedling:` | :blossom: `:blossom:` |
| :ear_of_rice: `:ear_of_rice:` | :shell: `:shell:` | :globe_with_meridians: `:globe_with_meridians:` |
| :sun_with_face: `:sun_with_face:` | :full_moon_with_face: `:full_moon_with_face:` | :new_moon_with_face: `:new_moon_with_face:` |
| :new_moon: `:new_moon:` | :waxing_crescent_moon: `:waxing_crescent_moon:` | :first_quarter_moon: `:first_quarter_moon:` |
| :waxing_gibbous_moon: `:waxing_gibbous_moon:` | :full_moon: `:full_moon:` | :waning_gibbous_moon: `:waning_gibbous_moon:` |
| :last_quarter_moon: `:last_quarter_moon:` | :waning_crescent_moon: `:waning_crescent_moon:` | :last_quarter_moon_with_face: `:last_quarter_moon_with_face:` |
| :first_quarter_moon_with_face: `:first_quarter_moon_with_face:` | :moon: `:moon:` | :earth_africa: `:earth_africa:` |
| :earth_americas: `:earth_americas:` | :earth_asia: `:earth_asia:` | :volcano: `:volcano:` |
| :milky_way: `:milky_way:` | :partly_sunny: `:partly_sunny:` | :octocat: `:octocat:` |
| :squirrel: `:squirrel:` |
Objects
| :bamboo: `:bamboo:` | :gift_heart: `:gift_heart:` | :dolls: `:dolls:` |
|---|---|---|
| :school_satchel: `:school_satchel:` | :mortar_board: `:mortar_board:` | :flags: `:flags:` |
| :fireworks: `:fireworks:` | :sparkler: `:sparkler:` | :wind_chime: `:wind_chime:` |
| :rice_scene: `:rice_scene:` | :jack_o_lantern: `:jack_o_lantern:` | :ghost: `:ghost:` |
| :santa: `:santa:` | :christmas_tree: `:christmas_tree:` | :gift: `:gift:` |
| :bell: `:bell:` | :no_bell: `:no_bell:` | :tanabata_tree: `:tanabata_tree:` |
| :tada: `:tada:` | :confetti_ball: `:confetti_ball:` | :balloon: `:balloon:` |
| :crystal_ball: `:crystal_ball:` | :cd: `:cd:` | :dvd: `:dvd:` |
| :floppy_disk: `:floppy_disk:` | :camera: `:camera:` | :video_camera: `:video_camera:` |
| :movie_camera: `:movie_camera:` | :computer: `:computer:` | :tv: `:tv:` |
| :iphone: `:iphone:` | :phone: `:phone:` | :telephone: `:telephone:` |
| :telephone_receiver: `:telephone_receiver:` | :pager: `:pager:` | :fax: `:fax:` |
| :minidisc: `:minidisc:` | :vhs: `:vhs:` | :sound: `:sound:` |
| :speaker: `:speaker:` | :mute: `:mute:` | :loudspeaker: `:loudspeaker:` |
| :mega: `:mega:` | :hourglass: `:hourglass:` | :hourglass_flowing_sand: `:hourglass_flowing_sand:` |
| :alarm_clock: `:alarm_clock:` | :watch: `:watch:` | :radio: `:radio:` |
| :satellite: `:satellite:` | :loop: `:loop:` | :mag: `:mag:` |
| :mag_right: `:mag_right:` | :unlock: `:unlock:` | :lock: `:lock:` |
| :lock_with_ink_pen: `:lock_with_ink_pen:` | :closed_lock_with_key: `:closed_lock_with_key:` | :key: `:key:` |
| :bulb: `:bulb:` | :flashlight: `:flashlight:` | :high_brightness: `:high_brightness:` |
| :low_brightness: `:low_brightness:` | :electric_plug: `:electric_plug:` | :battery: `:battery:` |
| :calling: `:calling:` | :email: `:email:` | :mailbox: `:mailbox:` |
| :postbox: `:postbox:` | :bath: `:bath:` | :bathtub: `:bathtub:` |
| :shower: `:shower:` | :toilet: `:toilet:` | :wrench: `:wrench:` |
| :nut_and_bolt: `:nut_and_bolt:` | :hammer: `:hammer:` | :seat: `:seat:` |
| :moneybag: `:moneybag:` | :yen: `:yen:` | :dollar: `:dollar:` |
| :pound: `:pound:` | :euro: `:euro:` | :credit_card: `:credit_card:` |
| :money_with_wings: `:money_with_wings:` | :e-mail: `:e-mail:` | :inbox_tray: `:inbox_tray:` |
| :outbox_tray: `:outbox_tray:` | :envelope: `:envelope:` | :incoming_envelope: `:incoming_envelope:` |
| :postal_horn: `:postal_horn:` | :mailbox_closed: `:mailbox_closed:` | :mailbox_with_mail: `:mailbox_with_mail:` |
| :mailbox_with_no_mail: `:mailbox_with_no_mail:` | :door: `:door:` | :smoking: `:smoking:` |
| :bomb: `:bomb:` | :gun: `:gun:` | :hocho: `:hocho:` |
| :pill: `:pill:` | :syringe: `:syringe:` | :page_facing_up: `:page_facing_up:` |
| :page_with_curl: `:page_with_curl:` | :bookmark_tabs: `:bookmark_tabs:` | :bar_chart: `:bar_chart:` |
| :chart_with_upwards_trend: `:chart_with_upwards_trend:` | :chart_with_downwards_trend: `:chart_with_downwards_trend:` | :scroll: `:scroll:` |
| :clipboard: `:clipboard:` | :calendar: `:calendar:` | :date: `:date:` |
| :card_index: `:card_index:` | :file_folder: `:file_folder:` | :open_file_folder: `:open_file_folder:` |
| :scissors: `:scissors:` | :pushpin: `:pushpin:` | :paperclip: `:paperclip:` |
| :black_nib: `:black_nib:` | :pencil2: `:pencil2:` | :straight_ruler: `:straight_ruler:` |
| :triangular_ruler: `:triangular_ruler:` | :closed_book: `:closed_book:` | :green_book: `:green_book:` |
| :blue_book: `:blue_book:` | :orange_book: `:orange_book:` | :notebook: `:notebook:` |
| :notebook_with_decorative_cover: `:notebook_with_decorative_cover:` | :ledger: `:ledger:` | :books: `:books:` |
| :bookmark: `:bookmark:` | :name_badge: `:name_badge:` | :microscope: `:microscope:` |
| :telescope: `:telescope:` | :newspaper: `:newspaper:` | :football: `:football:` |
| :basketball: `:basketball:` | :soccer: `:soccer:` | :baseball: `:baseball:` |
| :tennis: `:tennis:` | :8ball: `:8ball:` | :rugby_football: `:rugby_football:` |
| :bowling: `:bowling:` | :golf: `:golf:` | :mountain_bicyclist: `:mountain_bicyclist:` |
| :bicyclist: `:bicyclist:` | :horse_racing: `:horse_racing:` | :snowboarder: `:snowboarder:` |
| :swimmer: `:swimmer:` | :surfer: `:surfer:` | :ski: `:ski:` |
| :spades: `:spades:` | :hearts: `:hearts:` | :clubs: `:clubs:` |
| :diamonds: `:diamonds:` | :gem: `:gem:` | :ring: `:ring:` |
| :trophy: `:trophy:` | :musical_score: `:musical_score:` | :musical_keyboard: `:musical_keyboard:` |
| :violin: `:violin:` | :space_invader: `:space_invader:` | :video_game: `:video_game:` |
| :black_joker: `:black_joker:` | :flower_playing_cards: `:flower_playing_cards:` | :game_die: `:game_die:` |
| :dart: `:dart:` | :mahjong: `:mahjong:` | :clapper: `:clapper:` |
| :memo: `:memo:` | :pencil: `:pencil:` | :book: `:book:` |
| :art: `:art:` | :microphone: `:microphone:` | :headphones: `:headphones:` |
| :trumpet: `:trumpet:` | :saxophone: `:saxophone:` | :guitar: `:guitar:` |
| :shoe: `:shoe:` | :sandal: `:sandal:` | :high_heel: `:high_heel:` |
| :lipstick: `:lipstick:` | :boot: `:boot:` | :shirt: `:shirt:` |
| :tshirt: `:tshirt:` | :necktie: `:necktie:` | :womans_clothes: `:womans_clothes:` |
| :dress: `:dress:` | :running_shirt_with_sash: `:running_shirt_with_sash:` | :jeans: `:jeans:` |
| :kimono: `:kimono:` | :bikini: `:bikini:` | :ribbon: `:ribbon:` |
| :tophat: `:tophat:` | :crown: `:crown:` | :womans_hat: `:womans_hat:` |
| :mans_shoe: `:mans_shoe:` | :closed_umbrella: `:closed_umbrella:` | :briefcase: `:briefcase:` |
| :handbag: `:handbag:` | :pouch: `:pouch:` | :purse: `:purse:` |
| :eyeglasses: `:eyeglasses:` | :fishing_pole_and_fish: `:fishing_pole_and_fish:` | :coffee: `:coffee:` |
| :tea: `:tea:` | :sake: `:sake:` | :baby_bottle: `:baby_bottle:` |
| :beer: `:beer:` | :beers: `:beers:` | :cocktail: `:cocktail:` |
| :tropical_drink: `:tropical_drink:` | :wine_glass: `:wine_glass:` | :fork_and_knife: `:fork_and_knife:` |
| :pizza: `:pizza:` | :hamburger: `:hamburger:` | :fries: `:fries:` |
| :poultry_leg: `:poultry_leg:` | :meat_on_bone: `:meat_on_bone:` | :spaghetti: `:spaghetti:` |
| :curry: `:curry:` | :fried_shrimp: `:fried_shrimp:` | :bento: `:bento:` |
| :sushi: `:sushi:` | :fish_cake: `:fish_cake:` | :rice_ball: `:rice_ball:` |
| :rice_cracker: `:rice_cracker:` | :rice: `:rice:` | :ramen: `:ramen:` |
| :stew: `:stew:` | :oden: `:oden:` | :dango: `:dango:` |
| :egg: `:egg:` | :bread: `:bread:` | :doughnut: `:doughnut:` |
| :custard: `:custard:` | :icecream: `:icecream:` | :ice_cream: `:ice_cream:` |
| :shaved_ice: `:shaved_ice:` | :birthday: `:birthday:` | :cake: `:cake:` |
| :cookie: `:cookie:` | :chocolate_bar: `:chocolate_bar:` | :candy: `:candy:` |
| :lollipop: `:lollipop:` | :honey_pot: `:honey_pot:` | :apple: `:apple:` |
| :green_apple: `:green_apple:` | :tangerine: `:tangerine:` | :lemon: `:lemon:` |
| :cherries: `:cherries:` | :grapes: `:grapes:` | :watermelon: `:watermelon:` |
| :strawberry: `:strawberry:` | :peach: `:peach:` | :melon: `:melon:` |
| :banana: `:banana:` | :pear: `:pear:` | :pineapple: `:pineapple:` |
| :sweet_potato: `:sweet_potato:` | :eggplant: `:eggplant:` | :tomato: `:tomato:` |
| :corn: `:corn:` |
Places
| :house: `:house:` | :house_with_garden: `:house_with_garden:` | :school: `:school:` |
|---|---|---|
| :office: `:office:` | :post_office: `:post_office:` | :hospital: `:hospital:` |
| :bank: `:bank:` | :convenience_store: `:convenience_store:` | :love_hotel: `:love_hotel:` |
| :hotel: `:hotel:` | :wedding: `:wedding:` | :church: `:church:` |
| :department_store: `:department_store:` | :european_post_office: `:european_post_office:` | :city_sunrise: `:city_sunrise:` |
| :city_sunset: `:city_sunset:` | :japanese_castle: `:japanese_castle:` | :european_castle: `:european_castle:` |
| :tent: `:tent:` | :factory: `:factory:` | :tokyo_tower: `:tokyo_tower:` |
| :japan: `:japan:` | :mount_fuji: `:mount_fuji:` | :sunrise_over_mountains: `:sunrise_over_mountains:` |
| :sunrise: `:sunrise:` | :stars: `:stars:` | :statue_of_liberty: `:statue_of_liberty:` |
| :bridge_at_night: `:bridge_at_night:` | :carousel_horse: `:carousel_horse:` | :rainbow: `:rainbow:` |
| :ferris_wheel: `:ferris_wheel:` | :fountain: `:fountain:` | :roller_coaster: `:roller_coaster:` |
| :ship: `:ship:` | :speedboat: `:speedboat:` | :boat: `:boat:` |
| :sailboat: `:sailboat:` | :rowboat: `:rowboat:` | :anchor: `:anchor:` |
| :rocket: `:rocket:` | :airplane: `:airplane:` | :helicopter: `:helicopter:` |
| :steam_locomotive: `:steam_locomotive:` | :tram: `:tram:` | :mountain_railway: `:mountain_railway:` |
| :bike: `:bike:` | :aerial_tramway: `:aerial_tramway:` | :suspension_railway: `:suspension_railway:` |
| :mountain_cableway: `:mountain_cableway:` | :tractor: `:tractor:` | :blue_car: `:blue_car:` |
| :oncoming_automobile: `:oncoming_automobile:` | :car: `:car:` | :red_car: `:red_car:` |
| :taxi: `:taxi:` | :oncoming_taxi: `:oncoming_taxi:` | :articulated_lorry: `:articulated_lorry:` |
| :bus: `:bus:` | :oncoming_bus: `:oncoming_bus:` | :rotating_light: `:rotating_light:` |
| :police_car: `:police_car:` | :oncoming_police_car: `:oncoming_police_car:` | :fire_engine: `:fire_engine:` |
| :ambulance: `:ambulance:` | :minibus: `:minibus:` | :truck: `:truck:` |
| :train: `:train:` | :station: `:station:` | :train2: `:train2:` |
| :bullettrain_front: `:bullettrain_front:` | :bullettrain_side: `:bullettrain_side:` | :light_rail: `:light_rail:` |
| :monorail: `:monorail:` | :railway_car: `:railway_car:` | :trolleybus: `:trolleybus:` |
| :ticket: `:ticket:` | :fuelpump: `:fuelpump:` | :vertical_traffic_light: `:vertical_traffic_light:` |
| :traffic_light: `:traffic_light:` | :warning: `:warning:` | :construction: `:construction:` |
| :beginner: `:beginner:` | :atm: `:atm:` | :slot_machine: `:slot_machine:` |
| :busstop: `:busstop:` | :barber: `:barber:` | :hotsprings: `:hotsprings:` |
| :checkered_flag: `:checkered_flag:` | :crossed_flags: `:crossed_flags:` | :izakaya_lantern: `:izakaya_lantern:` |
| :moyai: `:moyai:` | :circus_tent: `:circus_tent:` | :performing_arts: `:performing_arts:` |
| :round_pushpin: `:round_pushpin:` | :triangular_flag_on_post: `:triangular_flag_on_post:` | :jp: `:jp:` |
| :kr: `:kr:` | :cn: `:cn:` | :us: `:us:` |
| :fr: `:fr:` | :es: `:es:` | :it: `:it:` |
| :ru: `:ru:` | :gb: `:gb:` | :uk: `:uk:` |
| :de: `:de:` |
Symbols
| :one: `:one:` | :two: `:two:` | :three: `:three:` |
|---|---|---|
| :four: `:four:` | :five: `:five:` | :six: `:six:` |
| :seven: `:seven:` | :eight: `:eight:` | :nine: `:nine:` |
| :keycap_ten: `:keycap_ten:` | :1234: `:1234:` | :zero: `:zero:` |
| :hash: `:hash:` | :symbols: `:symbols:` | :arrow_backward: `:arrow_backward:` |
| :arrow_down: `:arrow_down:` | :arrow_forward: `:arrow_forward:` | :arrow_left: `:arrow_left:` |
| :capital_abcd: `:capital_abcd:` | :abcd: `:abcd:` | :abc: `:abc:` |
| :arrow_lower_left: `:arrow_lower_left:` | :arrow_lower_right: `:arrow_lower_right:` | :arrow_right: `:arrow_right:` |
| :arrow_up: `:arrow_up:` | :arrow_upper_left: `:arrow_upper_left:` | :arrow_upper_right: `:arrow_upper_right:` |
| :arrow_double_down: `:arrow_double_down:` | :arrow_double_up: `:arrow_double_up:` | :arrow_down_small: `:arrow_down_small:` |
| :arrow_heading_down: `:arrow_heading_down:` | :arrow_heading_up: `:arrow_heading_up:` | :leftwards_arrow_with_hook: `:leftwards_arrow_with_hook:` |
| :arrow_right_hook: `:arrow_right_hook:` | :left_right_arrow: `:left_right_arrow:` | :arrow_up_down: `:arrow_up_down:` |
| :arrow_up_small: `:arrow_up_small:` | :arrows_clockwise: `:arrows_clockwise:` | :arrows_counterclockwise: `:arrows_counterclockwise:` |
| :rewind: `:rewind:` | :fast_forward: `:fast_forward:` | :information_source: `:information_source:` |
| :ok: `:ok:` | :twisted_rightwards_arrows: `:twisted_rightwards_arrows:` | :repeat: `:repeat:` |
| :repeat_one: `:repeat_one:` | :new: `:new:` | :top: `:top:` |
| :up: `:up:` | :cool: `:cool:` | :free: `:free:` |
| :ng: `:ng:` | :cinema: `:cinema:` | :koko: `:koko:` |
| :signal_strength: `:signal_strength:` | :u5272: `:u5272:` | :u5408: `:u5408:` |
| :u55b6: `:u55b6:` | :u6307: `:u6307:` | :u6708: `:u6708:` |
| :u6709: `:u6709:` | :u6e80: `:u6e80:` | :u7121: `:u7121:` |
| :u7533: `:u7533:` | :u7a7a: `:u7a7a:` | :u7981: `:u7981:` |
| :sa: `:sa:` | :restroom: `:restroom:` | :mens: `:mens:` |
| :womens: `:womens:` | :baby_symbol: `:baby_symbol:` | :no_smoking: `:no_smoking:` |
| :parking: `:parking:` | :wheelchair: `:wheelchair:` | :metro: `:metro:` |
| :baggage_claim: `:baggage_claim:` | :accept: `:accept:` | :wc: `:wc:` |
| :potable_water: `:potable_water:` | :put_litter_in_its_place: `:put_litter_in_its_place:` | :secret: `:secret:` |
| :congratulations: `:congratulations:` | :m: `:m:` | :passport_control: `:passport_control:` |
| :left_luggage: `:left_luggage:` | :customs: `:customs:` | :ideograph_advantage: `:ideograph_advantage:` |
| :cl: `:cl:` | :sos: `:sos:` | :id: `:id:` |
| :no_entry_sign: `:no_entry_sign:` | :underage: `:underage:` | :no_mobile_phones: `:no_mobile_phones:` |
| :do_not_litter: `:do_not_litter:` | :non-potable_water: `:non-potable_water:` | :no_bicycles: `:no_bicycles:` |
| :no_pedestrians: `:no_pedestrians:` | :children_crossing: `:children_crossing:` | :no_entry: `:no_entry:` |
| :eight_spoked_asterisk: `:eight_spoked_asterisk:` | :eight_pointed_black_star: `:eight_pointed_black_star:` | :heart_decoration: `:heart_decoration:` |
| :vs: `:vs:` | :vibration_mode: `:vibration_mode:` | :mobile_phone_off: `:mobile_phone_off:` |
| :chart: `:chart:` | :currency_exchange: `:currency_exchange:` | :aries: `:aries:` |
| :taurus: `:taurus:` | :gemini: `:gemini:` | :cancer: `:cancer:` |
| :leo: `:leo:` | :virgo: `:virgo:` | :libra: `:libra:` |
| :scorpius: `:scorpius:` | :sagittarius: `:sagittarius:` | :capricorn: `:capricorn:` |
| :aquarius: `:aquarius:` | :pisces: `:pisces:` | :ophiuchus: `:ophiuchus:` |
| :six_pointed_star: `:six_pointed_star:` | :negative_squared_cross_mark: `:negative_squared_cross_mark:` | :a: `:a:` |
| :b: `:b:` | :ab: `:ab:` | :o2: `:o2:` |
| :diamond_shape_with_a_dot_inside: `:diamond_shape_with_a_dot_inside:` | :recycle: `:recycle:` | :end: `:end:` |
| :on: `:on:` | :soon: `:soon:` | :clock1: `:clock1:` |
| :clock130: `:clock130:` | :clock10: `:clock10:` | :clock1030: `:clock1030:` |
| :clock11: `:clock11:` | :clock1130: `:clock1130:` | :clock12: `:clock12:` |
| :clock1230: `:clock1230:` | :clock2: `:clock2:` | :clock230: `:clock230:` |
| :clock3: `:clock3:` | :clock330: `:clock330:` | :clock4: `:clock4:` |
| :clock430: `:clock430:` | :clock5: `:clock5:` | :clock530: `:clock530:` |
| :clock6: `:clock6:` | :clock630: `:clock630:` | :clock7: `:clock7:` |
| :clock730: `:clock730:` | :clock8: `:clock8:` | :clock830: `:clock830:` |
| :clock9: `:clock9:` | :clock930: `:clock930:` | :heavy_dollar_sign: `:heavy_dollar_sign:` |
| :copyright: `:copyright:` | :registered: `:registered:` | :tm: `:tm:` |
| :x: `:x:` | :heavy_exclamation_mark: `:heavy_exclamation_mark:` | :bangbang: `:bangbang:` |
| :interrobang: `:interrobang:` | :o: `:o:` | :heavy_multiplication_x: `:heavy_multiplication_x:` |
| :heavy_plus_sign: `:heavy_plus_sign:` | :heavy_minus_sign: `:heavy_minus_sign:` | :heavy_division_sign: `:heavy_division_sign:` |
| :white_flower: `:white_flower:` | :100: `:100:` | :heavy_check_mark: `:heavy_check_mark:` |
| :ballot_box_with_check: `:ballot_box_with_check:` | :radio_button: `:radio_button:` | :link: `:link:` |
| :curly_loop: `:curly_loop:` | :wavy_dash: `:wavy_dash:` | :part_alternation_mark: `:part_alternation_mark:` |
| :trident: `:trident:` | :black_square: `:black_square:` | :white_square: `:white_square:` |
| :white_check_mark: `:white_check_mark:` | :black_square_button: `:black_square_button:` | :white_square_button: `:white_square_button:` |
| :black_circle: `:black_circle:` | :white_circle: `:white_circle:` | :red_circle: `:red_circle:` |
| :large_blue_circle: `:large_blue_circle:` | :large_blue_diamond: `:large_blue_diamond:` | :large_orange_diamond: `:large_orange_diamond:` |
| :small_blue_diamond: `:small_blue_diamond:` | :small_orange_diamond: `:small_orange_diamond:` | :small_red_triangle: `:small_red_triangle:` |
| :small_red_triangle_down: `:small_red_triangle_down:` | :shipit: `:shipit:` |
"""
| 25141 | module Tests exposing (..)
import Expect exposing (Expectation)
import Fuzz exposing (Fuzzer, int, list, string)
import Markdown.Block as Markdown
import Markdown.Html
import Markdown.Parser as Markdown
import Markdown.PrettyTables as Tables
import Markdown.Renderer as Markdown
import Markdown.Scaffolded as Scaffolded
import Test exposing (..)
{-
Unfortunately, there are few tests, right now. You're welcome to contribute tests!
I personally test this project using the /examples and another semi-production app.
If you want to contribute: There are good opportunities for creating property-based
tests for this application. For that it would be neccessary to write a fuzzer:
`Fuzzer children -> Fuzzer (Scaffolded.Block children)` and then identify the
mathematical properties of functions like `map`, `foldFunction`, etc. and test them.
-}
suite : Test
suite =
describe "Markdown"
[ describe "Formatting"
[ test "round-trip" (testRoundTrip prettyprint exampleMarkdown)
, test "idempotency" (testIdempotency prettyprint exampleMarkdown)
]
, describe "PrettyTables"
[ -- Examples
test "basic example"
(testConcrete (prettyprintTables Tables.defaultStyle)
{ input =
[ "Person | Age"
, "--|-:"
, "Someone I used to know | No idea"
, "Me | 23"
]
, expect =
[ "| Person | Age |"
, "|------------------------|--------:|"
, "| Someone I used to know | No idea |"
, "| Me | 23 |"
]
}
)
, test "no additional newlines (issue #4)"
(testConcrete (prettyprintTables Tables.compactStyle)
{ input =
[ "one | two"
, "--- | ---"
, ""
, ""
, "para after table"
]
, expect =
[ "one | two"
, "--- | ---"
, ""
, "para after table"
]
}
)
-- Round-Trips
, test "round-trip (small, compact)"
(testRoundTrip
(prettyprintTables Tables.compactStyle)
exampleTables
)
, test "round-trip (big, compact)"
(testRoundTrip
(prettyprintTables Tables.compactStyle)
exampleTablesMarkdown
)
, test "round-trip (small, default)"
(testRoundTrip
(prettyprintTables Tables.defaultStyle)
exampleTables
)
, test "round-trip (big, default)"
(testRoundTrip
(prettyprintTables Tables.defaultStyle)
exampleTablesMarkdown
)
-- Idempotency
, test "idempotency (small, compact)"
(testRoundTrip
(prettyprintTables Tables.compactStyle)
exampleTables
)
, test "idempotency (big, compact)"
(testRoundTrip
(prettyprintTables Tables.compactStyle)
exampleTablesMarkdown
)
, test "idempotency (small, default)"
(testRoundTrip
(prettyprintTables Tables.defaultStyle)
exampleTables
)
, test "idempotency (big, default)"
(testRoundTrip
(prettyprintTables Tables.defaultStyle)
exampleTablesMarkdown
)
]
, describe "Scaffolded"
[ test "foldIndexed" <|
\_ ->
let
renderPath : List Int -> String
renderPath path =
"(" ++ String.join "," (List.map String.fromInt path) ++ ") "
reduceWithIndices : Scaffolded.Block (List Int -> String) -> List Int -> String
reduceWithIndices block path =
case Scaffolded.foldIndexed block path of
Scaffolded.Heading { level, rawText, children } ->
Scaffolded.Heading
{ level = level
, rawText = rawText
, children = renderPath path :: children
}
|> Scaffolded.reducePretty
other ->
Scaffolded.reducePretty other
input =
String.join "\n"
[ "# Everything has indices."
, ""
, "If you write some text in between, you'll see that following headings' indices change."
, ""
, "## Another heading."
]
in
parseMarkdown input
|> Result.andThen
(Markdown.render
(Scaffolded.toRenderer
{ renderHtml = Markdown.Html.oneOf []
, renderMarkdown = reduceWithIndices
}
)
)
|> Result.map (List.indexedMap (\index expectsPath -> expectsPath [ index ]))
|> Result.map
(Expect.equalLists
[ "# (0) Everything has indices."
, "If you write some text in between, you'll see that following headings' indices change."
, "## (2) Another heading."
]
)
|> expectOk
]
]
testConcrete : (String -> Result String String) -> { input : List String, expect : List String } -> () -> Expectation
testConcrete prettyprinter { input, expect } () =
input
|> String.join "\n"
|> prettyprinter
|> Result.map (Expect.equal (String.join "\n" expect))
|> expectOk
testRoundTrip : (String -> Result String String) -> String -> () -> Expectation
testRoundTrip prettyprinter markdown () =
Result.map2
(\original prettyprinted ->
Expect.equalLists original prettyprinted
)
(markdown
|> parseMarkdown
|> Result.mapError ((++) "Error in normal Markdown: ")
)
(markdown
|> prettyprinter
|> Result.andThen parseMarkdown
|> Result.mapError ((++) "Error in pretty-printed Markdown: ")
)
|> expectOk
testIdempotency : (String -> Result String String) -> String -> () -> Expectation
testIdempotency prettyprinter markdown () =
Result.map2
(\prettyOnce prettyTwice ->
Expect.equal prettyOnce prettyTwice
)
(markdown
|> prettyprinter
|> Result.mapError ((++) "Error after prettyprinting: ")
)
(markdown
|> prettyprinter
|> Result.andThen prettyprinter
|> Result.mapError ((++) "Error after prettyprinting twice: ")
)
|> expectOk
expectOk : Result String Expectation -> Expectation
expectOk result =
case result of
Ok e ->
e
Err error ->
Expect.fail error
parseMarkdown : String -> Result String (List Markdown.Block)
parseMarkdown markdown =
markdown
|> Markdown.parse
|> Result.mapError (List.map Markdown.deadEndToString >> String.join "\n")
prettyprint : String -> Result String String
prettyprint markdown =
markdown
|> parseMarkdown
|> Result.andThen
(Markdown.render
(Scaffolded.toRenderer
{ renderHtml = Markdown.Html.oneOf []
, renderMarkdown = Scaffolded.reducePretty
}
)
)
|> Result.map (String.join "\n\n")
prettyprintTables : Tables.TableStyle -> String -> Result String String
prettyprintTables style markdown =
markdown
|> parseMarkdown
|> Result.andThen
(Markdown.render
(Scaffolded.toRenderer
{ renderHtml = Markdown.Html.oneOf []
, renderMarkdown = Tables.reducePrettyTable style
}
)
)
|> Result.map Tables.finishReduction
-- Test data
exampleMarkdown : String
exampleMarkdown =
"""# Format _beautiful_ Markdown
> Markdown is only fine
> When *pretty printed*
> It has to be **BEAUTIFUL** I SAID.
## Links
Let's try [a `link`](https://example.com)
What about images? 
## Lists
Markdown formatting is:
* [ ] Stupid
* [X] Okay-ish
* [ ] Works
1. Don't use this project
1. What are you doing?
1. Ok. Please go now.
- Let's not do checkboxes.
---------
0. Write library
1. Publish
2. ???
3. Profit!
## Code blocks
```elm
viewMarkdown : String -> List (Html Msg)
viewMarkdown markdown =
[ Html.h2 [] [ Html.text "Prettyprinted:" ]
, Html.hr [] []
, Html.pre [ Attr.style "word-wrap" "pre-wrap" ] [ Html.text markdown ]
]
```
```
Please use more beautiful
Code blocks!
```
"""
exampleTables : String
exampleTables =
"""
Name | Year of birth
--|-:
<NAME> | 1956
<NAME> | 1997
<NAME>esus | -4
Caesar | -100
Appliance | Cost | Own
:-:|-:|:-
Dishwasher | 500€ | Yes
Toaster | 40€ | No
Refrigerator | 600€ | Yes
Washing Machine | 1200€ | Yes
This is some text to ensure the right amount of newlines
Table | Without Rows
--|--
More text to ensure we generate the right spacing between markdown blocks
"""
exampleTablesMarkdown : String
exampleTablesMarkdown =
"""People
| :bowtie: `:bowtie:` | :smile: `:smile:` | :laughing: `:laughing:` |
|---|---|---|
| :blush: `:blush:` | :smiley: `:smiley:` | :relaxed: `:relaxed:` |
| :smirk: `:smirk:` | :heart_eyes: `:heart_eyes:` | :kissing_heart: `:kissing_heart:` |
| :kissing_closed_eyes: `:kissing_closed_eyes:` | :flushed: `:flushed:` | :relieved: `:relieved:` |
| :satisfied: `:satisfied:` | :grin: `:grin:` | :wink: `:wink:` |
| :stuck_out_tongue_winking_eye: `:stuck_out_tongue_winking_eye:` | :stuck_out_tongue_closed_eyes: `:stuck_out_tongue_closed_eyes:` | :grinning: `:grinning:` |
| :kissing: `:kissing:` | :kissing_smiling_eyes: `:kissing_smiling_eyes:` | :stuck_out_tongue: `:stuck_out_tongue:` |
| :sleeping: `:sleeping:` | :worried: `:worried:` | :frowning: `:frowning:` |
| :anguished: `:anguished:` | :open_mouth: `:open_mouth:` | :grimacing: `:grimacing:` |
| :confused: `:confused:` | :hushed: `:hushed:` | :expressionless: `:expressionless:` |
| :unamused: `:unamused:` | :sweat_smile: `:sweat_smile:` | :sweat: `:sweat:` |
| :disappointed_relieved: `:disappointed_relieved:` | :weary: `:weary:` | :pensive: `:pensive:` |
| :disappointed: `:disappointed:` | :confounded: `:confounded:` | :fearful: `:fearful:` |
| :cold_sweat: `:cold_sweat:` | :persevere: `:persevere:` | :cry: `:cry:` |
| :sob: `:sob:` | :joy: `:joy:` | :astonished: `:astonished:` |
| :scream: `:scream:` | :neckbeard: `:neckbeard:` | :tired_face: `:tired_face:` |
| :angry: `:angry:` | :rage: `:rage:` | :triumph: `:triumph:` |
| :sleepy: `:sleepy:` | :yum: `:yum:` | :mask: `:mask:` |
| :sunglasses: `:sunglasses:` | :dizzy_face: `:dizzy_face:` | :imp: `:imp:` |
| :smiling_imp: `:smiling_imp:` | :neutral_face: `:neutral_face:` | :no_mouth: `:no_mouth:` |
| :innocent: `:innocent:` | :alien: `:alien:` | :yellow_heart: `:yellow_heart:` |
| :blue_heart: `:blue_heart:` | :purple_heart: `:purple_heart:` | :heart: `:heart:` |
| :green_heart: `:green_heart:` | :broken_heart: `:broken_heart:` | :heartbeat: `:heartbeat:` |
| :heartpulse: `:heartpulse:` | :two_hearts: `:two_hearts:` | :revolving_hearts: `:revolving_hearts:` |
| :cupid: `:cupid:` | :sparkling_heart: `:sparkling_heart:` | :sparkles: `:sparkles:` |
| :star: `:star:` | :star2: `:star2:` | :dizzy: `:dizzy:` |
| :boom: `:boom:` | :collision: `:collision:` | :anger: `:anger:` |
| :exclamation: `:exclamation:` | :question: `:question:` | :grey_exclamation: `:grey_exclamation:` |
| :grey_question: `:grey_question:` | :zzz: `:zzz:` | :dash: `:dash:` |
| :sweat_drops: `:sweat_drops:` | :notes: `:notes:` | :musical_note: `:musical_note:` |
| :fire: `:fire:` | :hankey: `:hankey:` | :poop: `:poop:` |
| :shit: `:shit:` | :+1: `:+1:` | :thumbsup: `:thumbsup:` |
| :-1: `:-1:` | :thumbsdown: `:thumbsdown:` | :ok_hand: `:ok_hand:` |
| :punch: `:punch:` | :facepunch: `:facepunch:` | :fist: `:fist:` |
| :v: `:v:` | :wave: `:wave:` | :hand: `:hand:` |
| :raised_hand: `:raised_hand:` | :open_hands: `:open_hands:` | :point_up: `:point_up:` |
| :point_down: `:point_down:` | :point_left: `:point_left:` | :point_right: `:point_right:` |
| :raised_hands: `:raised_hands:` | :pray: `:pray:` | :point_up_2: `:point_up_2:` |
| :clap: `:clap:` | :muscle: `:muscle:` | :metal: `:metal:` |
| :fu: `:fu:` | :walking: `:walking:` | :runner: `:runner:` |
| :running: `:running:` | :couple: `:couple:` | :family: `:family:` |
| :two_men_holding_hands: `:two_men_holding_hands:` | :two_women_holding_hands: `:two_women_holding_hands:` | :dancer: `:dancer:` |
| :dancers: `:dancers:` | :ok_woman: `:ok_woman:` | :no_good: `:no_good:` |
| :information_desk_person: `:information_desk_person:` | :raising_hand: `:raising_hand:` | :bride_with_veil: `:bride_with_veil:` |
| :person_with_pouting_face: `:person_with_pouting_face:` | :person_frowning: `:person_frowning:` | :bow: `:bow:` |
| :couplekiss: `:couplekiss:` | :couple_with_heart: `:couple_with_heart:` | :massage: `:massage:` |
| :haircut: `:haircut:` | :nail_care: `:nail_care:` | :boy: `:boy:` |
| :girl: `:girl:` | :woman: `:woman:` | :man: `:man:` |
| :baby: `:baby:` | :older_woman: `:older_woman:` | :older_man: `:older_man:` |
| :person_with_blond_hair: `:person_with_blond_hair:` | :man_with_gua_pi_mao: `:man_with_gua_pi_mao:` | :man_with_turban: `:man_with_turban:` |
| :construction_worker: `:construction_worker:` | :cop: `:cop:` | :angel: `:angel:` |
| :princess: `:princess:` | :smiley_cat: `:smiley_cat:` | :smile_cat: `:smile_cat:` |
| :heart_eyes_cat: `:heart_eyes_cat:` | :kissing_cat: `:kissing_cat:` | :smirk_cat: `:smirk_cat:` |
| :scream_cat: `:scream_cat:` | :crying_cat_face: `:crying_cat_face:` | :joy_cat: `:joy_cat:` |
| :pouting_cat: `:pouting_cat:` | :japanese_ogre: `:japanese_ogre:` | :japanese_goblin: `:japanese_goblin:` |
| :see_no_evil: `:see_no_evil:` | :hear_no_evil: `:hear_no_evil:` | :speak_no_evil: `:speak_no_evil:` |
| :guardsman: `:guardsman:` | :skull: `:skull:` | :feet: `:feet:` |
| :lips: `:lips:` | :kiss: `:kiss:` | :droplet: `:droplet:` |
| :ear: `:ear:` | :eyes: `:eyes:` | :nose: `:nose:` |
| :tongue: `:tongue:` | :love_letter: `:love_letter:` | :bust_in_silhouette: `:bust_in_silhouette:` |
| :busts_in_silhouette: `:busts_in_silhouette:` | :speech_balloon: `:speech_balloon:` | :thought_balloon: `:thought_balloon:` |
| :feelsgood: `:feelsgood:` | :finnadie: `:finnadie:` | :goberserk: `:goberserk:` |
| :godmode: `:godmode:` | :hurtrealbad: `:hurtrealbad:` | :rage1: `:rage1:` |
| :rage2: `:rage2:` | :rage3: `:rage3:` | :rage4: `:rage4:` |
| :suspect: `:suspect:` | :trollface: `:trollface:` |
Nature
| :sunny: `:sunny:` | :umbrella: `:umbrella:` | :cloud: `:cloud:` |
|---|---|---|
| :snowflake: `:snowflake:` | :snowman: `:snowman:` | :zap: `:zap:` |
| :cyclone: `:cyclone:` | :foggy: `:foggy:` | :ocean: `:ocean:` |
| :cat: `:cat:` | :dog: `:dog:` | :mouse: `:mouse:` |
| :hamster: `:hamster:` | :rabbit: `:rabbit:` | :wolf: `:wolf:` |
| :frog: `:frog:` | :tiger: `:tiger:` | :koala: `:koala:` |
| :bear: `:bear:` | :pig: `:pig:` | :pig_nose: `:pig_nose:` |
| :cow: `:cow:` | :boar: `:boar:` | :monkey_face: `:monkey_face:` |
| :monkey: `:monkey:` | :horse: `:horse:` | :racehorse: `:racehorse:` |
| :camel: `:camel:` | :sheep: `:sheep:` | :elephant: `:elephant:` |
| :panda_face: `:panda_face:` | :snake: `:snake:` | :bird: `:bird:` |
| :baby_chick: `:baby_chick:` | :hatched_chick: `:hatched_chick:` | :hatching_chick: `:hatching_chick:` |
| :chicken: `:chicken:` | :penguin: `:penguin:` | :turtle: `:turtle:` |
| :bug: `:bug:` | :honeybee: `:honeybee:` | :ant: `:ant:` |
| :beetle: `:beetle:` | :snail: `:snail:` | :octopus: `:octopus:` |
| :tropical_fish: `:tropical_fish:` | :fish: `:fish:` | :whale: `:whale:` |
| :whale2: `:whale2:` | :dolphin: `:dolphin:` | :cow2: `:cow2:` |
| :ram: `:ram:` | :rat: `:rat:` | :water_buffalo: `:water_buffalo:` |
| :tiger2: `:tiger2:` | :rabbit2: `:rabbit2:` | :dragon: `:dragon:` |
| :goat: `:goat:` | :rooster: `:rooster:` | :dog2: `:dog2:` |
| :pig2: `:pig2:` | :mouse2: `:mouse2:` | :ox: `:ox:` |
| :dragon_face: `:dragon_face:` | :blowfish: `:blowfish:` | :crocodile: `:crocodile:` |
| :dromedary_camel: `:dromedary_camel:` | :leopard: `:leopard:` | :cat2: `:cat2:` |
| :poodle: `:poodle:` | :paw_prints: `:paw_prints:` | :bouquet: `:bouquet:` |
| :cherry_blossom: `:cherry_blossom:` | :tulip: `:tulip:` | :four_leaf_clover: `:four_leaf_clover:` |
| :rose: `:rose:` | :sunflower: `:sunflower:` | :hibiscus: `:hibiscus:` |
| :maple_leaf: `:maple_leaf:` | :leaves: `:leaves:` | :fallen_leaf: `:fallen_leaf:` |
| :herb: `:herb:` | :mushroom: `:mushroom:` | :cactus: `:cactus:` |
| :palm_tree: `:palm_tree:` | :evergreen_tree: `:evergreen_tree:` | :deciduous_tree: `:deciduous_tree:` |
| :chestnut: `:chestnut:` | :seedling: `:seedling:` | :blossom: `:blossom:` |
| :ear_of_rice: `:ear_of_rice:` | :shell: `:shell:` | :globe_with_meridians: `:globe_with_meridians:` |
| :sun_with_face: `:sun_with_face:` | :full_moon_with_face: `:full_moon_with_face:` | :new_moon_with_face: `:new_moon_with_face:` |
| :new_moon: `:new_moon:` | :waxing_crescent_moon: `:waxing_crescent_moon:` | :first_quarter_moon: `:first_quarter_moon:` |
| :waxing_gibbous_moon: `:waxing_gibbous_moon:` | :full_moon: `:full_moon:` | :waning_gibbous_moon: `:waning_gibbous_moon:` |
| :last_quarter_moon: `:last_quarter_moon:` | :waning_crescent_moon: `:waning_crescent_moon:` | :last_quarter_moon_with_face: `:last_quarter_moon_with_face:` |
| :first_quarter_moon_with_face: `:first_quarter_moon_with_face:` | :moon: `:moon:` | :earth_africa: `:earth_africa:` |
| :earth_americas: `:earth_americas:` | :earth_asia: `:earth_asia:` | :volcano: `:volcano:` |
| :milky_way: `:milky_way:` | :partly_sunny: `:partly_sunny:` | :octocat: `:octocat:` |
| :squirrel: `:squirrel:` |
Objects
| :bamboo: `:bamboo:` | :gift_heart: `:gift_heart:` | :dolls: `:dolls:` |
|---|---|---|
| :school_satchel: `:school_satchel:` | :mortar_board: `:mortar_board:` | :flags: `:flags:` |
| :fireworks: `:fireworks:` | :sparkler: `:sparkler:` | :wind_chime: `:wind_chime:` |
| :rice_scene: `:rice_scene:` | :jack_o_lantern: `:jack_o_lantern:` | :ghost: `:ghost:` |
| :santa: `:santa:` | :christmas_tree: `:christmas_tree:` | :gift: `:gift:` |
| :bell: `:bell:` | :no_bell: `:no_bell:` | :tanabata_tree: `:tanabata_tree:` |
| :tada: `:tada:` | :confetti_ball: `:confetti_ball:` | :balloon: `:balloon:` |
| :crystal_ball: `:crystal_ball:` | :cd: `:cd:` | :dvd: `:dvd:` |
| :floppy_disk: `:floppy_disk:` | :camera: `:camera:` | :video_camera: `:video_camera:` |
| :movie_camera: `:movie_camera:` | :computer: `:computer:` | :tv: `:tv:` |
| :iphone: `:iphone:` | :phone: `:phone:` | :telephone: `:telephone:` |
| :telephone_receiver: `:telephone_receiver:` | :pager: `:pager:` | :fax: `:fax:` |
| :minidisc: `:minidisc:` | :vhs: `:vhs:` | :sound: `:sound:` |
| :speaker: `:speaker:` | :mute: `:mute:` | :loudspeaker: `:loudspeaker:` |
| :mega: `:mega:` | :hourglass: `:hourglass:` | :hourglass_flowing_sand: `:hourglass_flowing_sand:` |
| :alarm_clock: `:alarm_clock:` | :watch: `:watch:` | :radio: `:radio:` |
| :satellite: `:satellite:` | :loop: `:loop:` | :mag: `:mag:` |
| :mag_right: `:mag_right:` | :unlock: `:unlock:` | :lock: `:lock:` |
| :lock_with_ink_pen: `:lock_with_ink_pen:` | :closed_lock_with_key: `:closed_lock_with_key:` | :key: `:key:` |
| :bulb: `:bulb:` | :flashlight: `:flashlight:` | :high_brightness: `:high_brightness:` |
| :low_brightness: `:low_brightness:` | :electric_plug: `:electric_plug:` | :battery: `:battery:` |
| :calling: `:calling:` | :email: `:email:` | :mailbox: `:mailbox:` |
| :postbox: `:postbox:` | :bath: `:bath:` | :bathtub: `:bathtub:` |
| :shower: `:shower:` | :toilet: `:toilet:` | :wrench: `:wrench:` |
| :nut_and_bolt: `:nut_and_bolt:` | :hammer: `:hammer:` | :seat: `:seat:` |
| :moneybag: `:moneybag:` | :yen: `:yen:` | :dollar: `:dollar:` |
| :pound: `:pound:` | :euro: `:euro:` | :credit_card: `:credit_card:` |
| :money_with_wings: `:money_with_wings:` | :e-mail: `:e-mail:` | :inbox_tray: `:inbox_tray:` |
| :outbox_tray: `:outbox_tray:` | :envelope: `:envelope:` | :incoming_envelope: `:incoming_envelope:` |
| :postal_horn: `:postal_horn:` | :mailbox_closed: `:mailbox_closed:` | :mailbox_with_mail: `:mailbox_with_mail:` |
| :mailbox_with_no_mail: `:mailbox_with_no_mail:` | :door: `:door:` | :smoking: `:smoking:` |
| :bomb: `:bomb:` | :gun: `:gun:` | :hocho: `:hocho:` |
| :pill: `:pill:` | :syringe: `:syringe:` | :page_facing_up: `:page_facing_up:` |
| :page_with_curl: `:page_with_curl:` | :bookmark_tabs: `:bookmark_tabs:` | :bar_chart: `:bar_chart:` |
| :chart_with_upwards_trend: `:chart_with_upwards_trend:` | :chart_with_downwards_trend: `:chart_with_downwards_trend:` | :scroll: `:scroll:` |
| :clipboard: `:clipboard:` | :calendar: `:calendar:` | :date: `:date:` |
| :card_index: `:card_index:` | :file_folder: `:file_folder:` | :open_file_folder: `:open_file_folder:` |
| :scissors: `:scissors:` | :pushpin: `:pushpin:` | :paperclip: `:paperclip:` |
| :black_nib: `:black_nib:` | :pencil2: `:pencil2:` | :straight_ruler: `:straight_ruler:` |
| :triangular_ruler: `:triangular_ruler:` | :closed_book: `:closed_book:` | :green_book: `:green_book:` |
| :blue_book: `:blue_book:` | :orange_book: `:orange_book:` | :notebook: `:notebook:` |
| :notebook_with_decorative_cover: `:notebook_with_decorative_cover:` | :ledger: `:ledger:` | :books: `:books:` |
| :bookmark: `:bookmark:` | :name_badge: `:name_badge:` | :microscope: `:microscope:` |
| :telescope: `:telescope:` | :newspaper: `:newspaper:` | :football: `:football:` |
| :basketball: `:basketball:` | :soccer: `:soccer:` | :baseball: `:baseball:` |
| :tennis: `:tennis:` | :8ball: `:8ball:` | :rugby_football: `:rugby_football:` |
| :bowling: `:bowling:` | :golf: `:golf:` | :mountain_bicyclist: `:mountain_bicyclist:` |
| :bicyclist: `:bicyclist:` | :horse_racing: `:horse_racing:` | :snowboarder: `:snowboarder:` |
| :swimmer: `:swimmer:` | :surfer: `:surfer:` | :ski: `:ski:` |
| :spades: `:spades:` | :hearts: `:hearts:` | :clubs: `:clubs:` |
| :diamonds: `:diamonds:` | :gem: `:gem:` | :ring: `:ring:` |
| :trophy: `:trophy:` | :musical_score: `:musical_score:` | :musical_keyboard: `:musical_keyboard:` |
| :violin: `:violin:` | :space_invader: `:space_invader:` | :video_game: `:video_game:` |
| :black_joker: `:black_joker:` | :flower_playing_cards: `:flower_playing_cards:` | :game_die: `:game_die:` |
| :dart: `:dart:` | :mahjong: `:mahjong:` | :clapper: `:clapper:` |
| :memo: `:memo:` | :pencil: `:pencil:` | :book: `:book:` |
| :art: `:art:` | :microphone: `:microphone:` | :headphones: `:headphones:` |
| :trumpet: `:trumpet:` | :saxophone: `:saxophone:` | :guitar: `:guitar:` |
| :shoe: `:shoe:` | :sandal: `:sandal:` | :high_heel: `:high_heel:` |
| :lipstick: `:lipstick:` | :boot: `:boot:` | :shirt: `:shirt:` |
| :tshirt: `:tshirt:` | :necktie: `:necktie:` | :womans_clothes: `:womans_clothes:` |
| :dress: `:dress:` | :running_shirt_with_sash: `:running_shirt_with_sash:` | :jeans: `:jeans:` |
| :kimono: `:kimono:` | :bikini: `:bikini:` | :ribbon: `:ribbon:` |
| :tophat: `:tophat:` | :crown: `:crown:` | :womans_hat: `:womans_hat:` |
| :mans_shoe: `:mans_shoe:` | :closed_umbrella: `:closed_umbrella:` | :briefcase: `:briefcase:` |
| :handbag: `:handbag:` | :pouch: `:pouch:` | :purse: `:purse:` |
| :eyeglasses: `:eyeglasses:` | :fishing_pole_and_fish: `:fishing_pole_and_fish:` | :coffee: `:coffee:` |
| :tea: `:tea:` | :sake: `:sake:` | :baby_bottle: `:baby_bottle:` |
| :beer: `:beer:` | :beers: `:beers:` | :cocktail: `:cocktail:` |
| :tropical_drink: `:tropical_drink:` | :wine_glass: `:wine_glass:` | :fork_and_knife: `:fork_and_knife:` |
| :pizza: `:pizza:` | :hamburger: `:hamburger:` | :fries: `:fries:` |
| :poultry_leg: `:poultry_leg:` | :meat_on_bone: `:meat_on_bone:` | :spaghetti: `:spaghetti:` |
| :curry: `:curry:` | :fried_shrimp: `:fried_shrimp:` | :bento: `:bento:` |
| :sushi: `:sushi:` | :fish_cake: `:fish_cake:` | :rice_ball: `:rice_ball:` |
| :rice_cracker: `:rice_cracker:` | :rice: `:rice:` | :ramen: `:ramen:` |
| :stew: `:stew:` | :oden: `:oden:` | :dango: `:dango:` |
| :egg: `:egg:` | :bread: `:bread:` | :doughnut: `:doughnut:` |
| :custard: `:custard:` | :icecream: `:icecream:` | :ice_cream: `:ice_cream:` |
| :shaved_ice: `:shaved_ice:` | :birthday: `:birthday:` | :cake: `:cake:` |
| :cookie: `:cookie:` | :chocolate_bar: `:chocolate_bar:` | :candy: `:candy:` |
| :lollipop: `:lollipop:` | :honey_pot: `:honey_pot:` | :apple: `:apple:` |
| :green_apple: `:green_apple:` | :tangerine: `:tangerine:` | :lemon: `:lemon:` |
| :cherries: `:cherries:` | :grapes: `:grapes:` | :watermelon: `:watermelon:` |
| :strawberry: `:strawberry:` | :peach: `:peach:` | :melon: `:melon:` |
| :banana: `:banana:` | :pear: `:pear:` | :pineapple: `:pineapple:` |
| :sweet_potato: `:sweet_potato:` | :eggplant: `:eggplant:` | :tomato: `:tomato:` |
| :corn: `:corn:` |
Places
| :house: `:house:` | :house_with_garden: `:house_with_garden:` | :school: `:school:` |
|---|---|---|
| :office: `:office:` | :post_office: `:post_office:` | :hospital: `:hospital:` |
| :bank: `:bank:` | :convenience_store: `:convenience_store:` | :love_hotel: `:love_hotel:` |
| :hotel: `:hotel:` | :wedding: `:wedding:` | :church: `:church:` |
| :department_store: `:department_store:` | :european_post_office: `:european_post_office:` | :city_sunrise: `:city_sunrise:` |
| :city_sunset: `:city_sunset:` | :japanese_castle: `:japanese_castle:` | :european_castle: `:european_castle:` |
| :tent: `:tent:` | :factory: `:factory:` | :tokyo_tower: `:tokyo_tower:` |
| :japan: `:japan:` | :mount_fuji: `:mount_fuji:` | :sunrise_over_mountains: `:sunrise_over_mountains:` |
| :sunrise: `:sunrise:` | :stars: `:stars:` | :statue_of_liberty: `:statue_of_liberty:` |
| :bridge_at_night: `:bridge_at_night:` | :carousel_horse: `:carousel_horse:` | :rainbow: `:rainbow:` |
| :ferris_wheel: `:ferris_wheel:` | :fountain: `:fountain:` | :roller_coaster: `:roller_coaster:` |
| :ship: `:ship:` | :speedboat: `:speedboat:` | :boat: `:boat:` |
| :sailboat: `:sailboat:` | :rowboat: `:rowboat:` | :anchor: `:anchor:` |
| :rocket: `:rocket:` | :airplane: `:airplane:` | :helicopter: `:helicopter:` |
| :steam_locomotive: `:steam_locomotive:` | :tram: `:tram:` | :mountain_railway: `:mountain_railway:` |
| :bike: `:bike:` | :aerial_tramway: `:aerial_tramway:` | :suspension_railway: `:suspension_railway:` |
| :mountain_cableway: `:mountain_cableway:` | :tractor: `:tractor:` | :blue_car: `:blue_car:` |
| :oncoming_automobile: `:oncoming_automobile:` | :car: `:car:` | :red_car: `:red_car:` |
| :taxi: `:taxi:` | :oncoming_taxi: `:oncoming_taxi:` | :articulated_lorry: `:articulated_lorry:` |
| :bus: `:bus:` | :oncoming_bus: `:oncoming_bus:` | :rotating_light: `:rotating_light:` |
| :police_car: `:police_car:` | :oncoming_police_car: `:oncoming_police_car:` | :fire_engine: `:fire_engine:` |
| :ambulance: `:ambulance:` | :minibus: `:minibus:` | :truck: `:truck:` |
| :train: `:train:` | :station: `:station:` | :train2: `:train2:` |
| :bullettrain_front: `:bullettrain_front:` | :bullettrain_side: `:bullettrain_side:` | :light_rail: `:light_rail:` |
| :monorail: `:monorail:` | :railway_car: `:railway_car:` | :trolleybus: `:trolleybus:` |
| :ticket: `:ticket:` | :fuelpump: `:fuelpump:` | :vertical_traffic_light: `:vertical_traffic_light:` |
| :traffic_light: `:traffic_light:` | :warning: `:warning:` | :construction: `:construction:` |
| :beginner: `:beginner:` | :atm: `:atm:` | :slot_machine: `:slot_machine:` |
| :busstop: `:busstop:` | :barber: `:barber:` | :hotsprings: `:hotsprings:` |
| :checkered_flag: `:checkered_flag:` | :crossed_flags: `:crossed_flags:` | :izakaya_lantern: `:izakaya_lantern:` |
| :moyai: `:moyai:` | :circus_tent: `:circus_tent:` | :performing_arts: `:performing_arts:` |
| :round_pushpin: `:round_pushpin:` | :triangular_flag_on_post: `:triangular_flag_on_post:` | :jp: `:jp:` |
| :kr: `:kr:` | :cn: `:cn:` | :us: `:us:` |
| :fr: `:fr:` | :es: `:es:` | :it: `:it:` |
| :ru: `:ru:` | :gb: `:gb:` | :uk: `:uk:` |
| :de: `:de:` |
Symbols
| :one: `:one:` | :two: `:two:` | :three: `:three:` |
|---|---|---|
| :four: `:four:` | :five: `:five:` | :six: `:six:` |
| :seven: `:seven:` | :eight: `:eight:` | :nine: `:nine:` |
| :keycap_ten: `:keycap_ten:` | :1234: `:1234:` | :zero: `:zero:` |
| :hash: `:hash:` | :symbols: `:symbols:` | :arrow_backward: `:arrow_backward:` |
| :arrow_down: `:arrow_down:` | :arrow_forward: `:arrow_forward:` | :arrow_left: `:arrow_left:` |
| :capital_abcd: `:capital_abcd:` | :abcd: `:abcd:` | :abc: `:abc:` |
| :arrow_lower_left: `:arrow_lower_left:` | :arrow_lower_right: `:arrow_lower_right:` | :arrow_right: `:arrow_right:` |
| :arrow_up: `:arrow_up:` | :arrow_upper_left: `:arrow_upper_left:` | :arrow_upper_right: `:arrow_upper_right:` |
| :arrow_double_down: `:arrow_double_down:` | :arrow_double_up: `:arrow_double_up:` | :arrow_down_small: `:arrow_down_small:` |
| :arrow_heading_down: `:arrow_heading_down:` | :arrow_heading_up: `:arrow_heading_up:` | :leftwards_arrow_with_hook: `:leftwards_arrow_with_hook:` |
| :arrow_right_hook: `:arrow_right_hook:` | :left_right_arrow: `:left_right_arrow:` | :arrow_up_down: `:arrow_up_down:` |
| :arrow_up_small: `:arrow_up_small:` | :arrows_clockwise: `:arrows_clockwise:` | :arrows_counterclockwise: `:arrows_counterclockwise:` |
| :rewind: `:rewind:` | :fast_forward: `:fast_forward:` | :information_source: `:information_source:` |
| :ok: `:ok:` | :twisted_rightwards_arrows: `:twisted_rightwards_arrows:` | :repeat: `:repeat:` |
| :repeat_one: `:repeat_one:` | :new: `:new:` | :top: `:top:` |
| :up: `:up:` | :cool: `:cool:` | :free: `:free:` |
| :ng: `:ng:` | :cinema: `:cinema:` | :koko: `:koko:` |
| :signal_strength: `:signal_strength:` | :u5272: `:u5272:` | :u5408: `:u5408:` |
| :u55b6: `:u55b6:` | :u6307: `:u6307:` | :u6708: `:u6708:` |
| :u6709: `:u6709:` | :u6e80: `:u6e80:` | :u7121: `:u7121:` |
| :u7533: `:u7533:` | :u7a7a: `:u7a7a:` | :u7981: `:u7981:` |
| :sa: `:sa:` | :restroom: `:restroom:` | :mens: `:mens:` |
| :womens: `:womens:` | :baby_symbol: `:baby_symbol:` | :no_smoking: `:no_smoking:` |
| :parking: `:parking:` | :wheelchair: `:wheelchair:` | :metro: `:metro:` |
| :baggage_claim: `:baggage_claim:` | :accept: `:accept:` | :wc: `:wc:` |
| :potable_water: `:potable_water:` | :put_litter_in_its_place: `:put_litter_in_its_place:` | :secret: `:secret:` |
| :congratulations: `:congratulations:` | :m: `:m:` | :passport_control: `:passport_control:` |
| :left_luggage: `:left_luggage:` | :customs: `:customs:` | :ideograph_advantage: `:ideograph_advantage:` |
| :cl: `:cl:` | :sos: `:sos:` | :id: `:id:` |
| :no_entry_sign: `:no_entry_sign:` | :underage: `:underage:` | :no_mobile_phones: `:no_mobile_phones:` |
| :do_not_litter: `:do_not_litter:` | :non-potable_water: `:non-potable_water:` | :no_bicycles: `:no_bicycles:` |
| :no_pedestrians: `:no_pedestrians:` | :children_crossing: `:children_crossing:` | :no_entry: `:no_entry:` |
| :eight_spoked_asterisk: `:eight_spoked_asterisk:` | :eight_pointed_black_star: `:eight_pointed_black_star:` | :heart_decoration: `:heart_decoration:` |
| :vs: `:vs:` | :vibration_mode: `:vibration_mode:` | :mobile_phone_off: `:mobile_phone_off:` |
| :chart: `:chart:` | :currency_exchange: `:currency_exchange:` | :aries: `:aries:` |
| :taurus: `:taurus:` | :gemini: `:gemini:` | :cancer: `:cancer:` |
| :leo: `:leo:` | :virgo: `:virgo:` | :libra: `:libra:` |
| :scorpius: `:scorpius:` | :sagittarius: `:sagittarius:` | :capricorn: `:capricorn:` |
| :aquarius: `:aquarius:` | :pisces: `:pisces:` | :ophiuchus: `:ophiuchus:` |
| :six_pointed_star: `:six_pointed_star:` | :negative_squared_cross_mark: `:negative_squared_cross_mark:` | :a: `:a:` |
| :b: `:b:` | :ab: `:ab:` | :o2: `:o2:` |
| :diamond_shape_with_a_dot_inside: `:diamond_shape_with_a_dot_inside:` | :recycle: `:recycle:` | :end: `:end:` |
| :on: `:on:` | :soon: `:soon:` | :clock1: `:clock1:` |
| :clock130: `:clock130:` | :clock10: `:clock10:` | :clock1030: `:clock1030:` |
| :clock11: `:clock11:` | :clock1130: `:clock1130:` | :clock12: `:clock12:` |
| :clock1230: `:clock1230:` | :clock2: `:clock2:` | :clock230: `:clock230:` |
| :clock3: `:clock3:` | :clock330: `:clock330:` | :clock4: `:clock4:` |
| :clock430: `:clock430:` | :clock5: `:clock5:` | :clock530: `:clock530:` |
| :clock6: `:clock6:` | :clock630: `:clock630:` | :clock7: `:clock7:` |
| :clock730: `:clock730:` | :clock8: `:clock8:` | :clock830: `:clock830:` |
| :clock9: `:clock9:` | :clock930: `:clock930:` | :heavy_dollar_sign: `:heavy_dollar_sign:` |
| :copyright: `:copyright:` | :registered: `:registered:` | :tm: `:tm:` |
| :x: `:x:` | :heavy_exclamation_mark: `:heavy_exclamation_mark:` | :bangbang: `:bangbang:` |
| :interrobang: `:interrobang:` | :o: `:o:` | :heavy_multiplication_x: `:heavy_multiplication_x:` |
| :heavy_plus_sign: `:heavy_plus_sign:` | :heavy_minus_sign: `:heavy_minus_sign:` | :heavy_division_sign: `:heavy_division_sign:` |
| :white_flower: `:white_flower:` | :100: `:100:` | :heavy_check_mark: `:heavy_check_mark:` |
| :ballot_box_with_check: `:ballot_box_with_check:` | :radio_button: `:radio_button:` | :link: `:link:` |
| :curly_loop: `:curly_loop:` | :wavy_dash: `:wavy_dash:` | :part_alternation_mark: `:part_alternation_mark:` |
| :trident: `:trident:` | :black_square: `:black_square:` | :white_square: `:white_square:` |
| :white_check_mark: `:white_check_mark:` | :black_square_button: `:black_square_button:` | :white_square_button: `:white_square_button:` |
| :black_circle: `:black_circle:` | :white_circle: `:white_circle:` | :red_circle: `:red_circle:` |
| :large_blue_circle: `:large_blue_circle:` | :large_blue_diamond: `:large_blue_diamond:` | :large_orange_diamond: `:large_orange_diamond:` |
| :small_blue_diamond: `:small_blue_diamond:` | :small_orange_diamond: `:small_orange_diamond:` | :small_red_triangle: `:small_red_triangle:` |
| :small_red_triangle_down: `:small_red_triangle_down:` | :shipit: `:shipit:` |
"""
| true | module Tests exposing (..)
import Expect exposing (Expectation)
import Fuzz exposing (Fuzzer, int, list, string)
import Markdown.Block as Markdown
import Markdown.Html
import Markdown.Parser as Markdown
import Markdown.PrettyTables as Tables
import Markdown.Renderer as Markdown
import Markdown.Scaffolded as Scaffolded
import Test exposing (..)
{-
Unfortunately, there are few tests, right now. You're welcome to contribute tests!
I personally test this project using the /examples and another semi-production app.
If you want to contribute: There are good opportunities for creating property-based
tests for this application. For that it would be neccessary to write a fuzzer:
`Fuzzer children -> Fuzzer (Scaffolded.Block children)` and then identify the
mathematical properties of functions like `map`, `foldFunction`, etc. and test them.
-}
suite : Test
suite =
describe "Markdown"
[ describe "Formatting"
[ test "round-trip" (testRoundTrip prettyprint exampleMarkdown)
, test "idempotency" (testIdempotency prettyprint exampleMarkdown)
]
, describe "PrettyTables"
[ -- Examples
test "basic example"
(testConcrete (prettyprintTables Tables.defaultStyle)
{ input =
[ "Person | Age"
, "--|-:"
, "Someone I used to know | No idea"
, "Me | 23"
]
, expect =
[ "| Person | Age |"
, "|------------------------|--------:|"
, "| Someone I used to know | No idea |"
, "| Me | 23 |"
]
}
)
, test "no additional newlines (issue #4)"
(testConcrete (prettyprintTables Tables.compactStyle)
{ input =
[ "one | two"
, "--- | ---"
, ""
, ""
, "para after table"
]
, expect =
[ "one | two"
, "--- | ---"
, ""
, "para after table"
]
}
)
-- Round-Trips
, test "round-trip (small, compact)"
(testRoundTrip
(prettyprintTables Tables.compactStyle)
exampleTables
)
, test "round-trip (big, compact)"
(testRoundTrip
(prettyprintTables Tables.compactStyle)
exampleTablesMarkdown
)
, test "round-trip (small, default)"
(testRoundTrip
(prettyprintTables Tables.defaultStyle)
exampleTables
)
, test "round-trip (big, default)"
(testRoundTrip
(prettyprintTables Tables.defaultStyle)
exampleTablesMarkdown
)
-- Idempotency
, test "idempotency (small, compact)"
(testRoundTrip
(prettyprintTables Tables.compactStyle)
exampleTables
)
, test "idempotency (big, compact)"
(testRoundTrip
(prettyprintTables Tables.compactStyle)
exampleTablesMarkdown
)
, test "idempotency (small, default)"
(testRoundTrip
(prettyprintTables Tables.defaultStyle)
exampleTables
)
, test "idempotency (big, default)"
(testRoundTrip
(prettyprintTables Tables.defaultStyle)
exampleTablesMarkdown
)
]
, describe "Scaffolded"
[ test "foldIndexed" <|
\_ ->
let
renderPath : List Int -> String
renderPath path =
"(" ++ String.join "," (List.map String.fromInt path) ++ ") "
reduceWithIndices : Scaffolded.Block (List Int -> String) -> List Int -> String
reduceWithIndices block path =
case Scaffolded.foldIndexed block path of
Scaffolded.Heading { level, rawText, children } ->
Scaffolded.Heading
{ level = level
, rawText = rawText
, children = renderPath path :: children
}
|> Scaffolded.reducePretty
other ->
Scaffolded.reducePretty other
input =
String.join "\n"
[ "# Everything has indices."
, ""
, "If you write some text in between, you'll see that following headings' indices change."
, ""
, "## Another heading."
]
in
parseMarkdown input
|> Result.andThen
(Markdown.render
(Scaffolded.toRenderer
{ renderHtml = Markdown.Html.oneOf []
, renderMarkdown = reduceWithIndices
}
)
)
|> Result.map (List.indexedMap (\index expectsPath -> expectsPath [ index ]))
|> Result.map
(Expect.equalLists
[ "# (0) Everything has indices."
, "If you write some text in between, you'll see that following headings' indices change."
, "## (2) Another heading."
]
)
|> expectOk
]
]
testConcrete : (String -> Result String String) -> { input : List String, expect : List String } -> () -> Expectation
testConcrete prettyprinter { input, expect } () =
input
|> String.join "\n"
|> prettyprinter
|> Result.map (Expect.equal (String.join "\n" expect))
|> expectOk
testRoundTrip : (String -> Result String String) -> String -> () -> Expectation
testRoundTrip prettyprinter markdown () =
Result.map2
(\original prettyprinted ->
Expect.equalLists original prettyprinted
)
(markdown
|> parseMarkdown
|> Result.mapError ((++) "Error in normal Markdown: ")
)
(markdown
|> prettyprinter
|> Result.andThen parseMarkdown
|> Result.mapError ((++) "Error in pretty-printed Markdown: ")
)
|> expectOk
testIdempotency : (String -> Result String String) -> String -> () -> Expectation
testIdempotency prettyprinter markdown () =
Result.map2
(\prettyOnce prettyTwice ->
Expect.equal prettyOnce prettyTwice
)
(markdown
|> prettyprinter
|> Result.mapError ((++) "Error after prettyprinting: ")
)
(markdown
|> prettyprinter
|> Result.andThen prettyprinter
|> Result.mapError ((++) "Error after prettyprinting twice: ")
)
|> expectOk
expectOk : Result String Expectation -> Expectation
expectOk result =
case result of
Ok e ->
e
Err error ->
Expect.fail error
parseMarkdown : String -> Result String (List Markdown.Block)
parseMarkdown markdown =
markdown
|> Markdown.parse
|> Result.mapError (List.map Markdown.deadEndToString >> String.join "\n")
prettyprint : String -> Result String String
prettyprint markdown =
markdown
|> parseMarkdown
|> Result.andThen
(Markdown.render
(Scaffolded.toRenderer
{ renderHtml = Markdown.Html.oneOf []
, renderMarkdown = Scaffolded.reducePretty
}
)
)
|> Result.map (String.join "\n\n")
prettyprintTables : Tables.TableStyle -> String -> Result String String
prettyprintTables style markdown =
markdown
|> parseMarkdown
|> Result.andThen
(Markdown.render
(Scaffolded.toRenderer
{ renderHtml = Markdown.Html.oneOf []
, renderMarkdown = Tables.reducePrettyTable style
}
)
)
|> Result.map Tables.finishReduction
-- Test data
exampleMarkdown : String
exampleMarkdown =
"""# Format _beautiful_ Markdown
> Markdown is only fine
> When *pretty printed*
> It has to be **BEAUTIFUL** I SAID.
## Links
Let's try [a `link`](https://example.com)
What about images? 
## Lists
Markdown formatting is:
* [ ] Stupid
* [X] Okay-ish
* [ ] Works
1. Don't use this project
1. What are you doing?
1. Ok. Please go now.
- Let's not do checkboxes.
---------
0. Write library
1. Publish
2. ???
3. Profit!
## Code blocks
```elm
viewMarkdown : String -> List (Html Msg)
viewMarkdown markdown =
[ Html.h2 [] [ Html.text "Prettyprinted:" ]
, Html.hr [] []
, Html.pre [ Attr.style "word-wrap" "pre-wrap" ] [ Html.text markdown ]
]
```
```
Please use more beautiful
Code blocks!
```
"""
exampleTables : String
exampleTables =
"""
Name | Year of birth
--|-:
PI:NAME:<NAME>END_PI | 1956
PI:NAME:<NAME>END_PI | 1997
PI:NAME:<NAME>END_PIesus | -4
Caesar | -100
Appliance | Cost | Own
:-:|-:|:-
Dishwasher | 500€ | Yes
Toaster | 40€ | No
Refrigerator | 600€ | Yes
Washing Machine | 1200€ | Yes
This is some text to ensure the right amount of newlines
Table | Without Rows
--|--
More text to ensure we generate the right spacing between markdown blocks
"""
exampleTablesMarkdown : String
exampleTablesMarkdown =
"""People
| :bowtie: `:bowtie:` | :smile: `:smile:` | :laughing: `:laughing:` |
|---|---|---|
| :blush: `:blush:` | :smiley: `:smiley:` | :relaxed: `:relaxed:` |
| :smirk: `:smirk:` | :heart_eyes: `:heart_eyes:` | :kissing_heart: `:kissing_heart:` |
| :kissing_closed_eyes: `:kissing_closed_eyes:` | :flushed: `:flushed:` | :relieved: `:relieved:` |
| :satisfied: `:satisfied:` | :grin: `:grin:` | :wink: `:wink:` |
| :stuck_out_tongue_winking_eye: `:stuck_out_tongue_winking_eye:` | :stuck_out_tongue_closed_eyes: `:stuck_out_tongue_closed_eyes:` | :grinning: `:grinning:` |
| :kissing: `:kissing:` | :kissing_smiling_eyes: `:kissing_smiling_eyes:` | :stuck_out_tongue: `:stuck_out_tongue:` |
| :sleeping: `:sleeping:` | :worried: `:worried:` | :frowning: `:frowning:` |
| :anguished: `:anguished:` | :open_mouth: `:open_mouth:` | :grimacing: `:grimacing:` |
| :confused: `:confused:` | :hushed: `:hushed:` | :expressionless: `:expressionless:` |
| :unamused: `:unamused:` | :sweat_smile: `:sweat_smile:` | :sweat: `:sweat:` |
| :disappointed_relieved: `:disappointed_relieved:` | :weary: `:weary:` | :pensive: `:pensive:` |
| :disappointed: `:disappointed:` | :confounded: `:confounded:` | :fearful: `:fearful:` |
| :cold_sweat: `:cold_sweat:` | :persevere: `:persevere:` | :cry: `:cry:` |
| :sob: `:sob:` | :joy: `:joy:` | :astonished: `:astonished:` |
| :scream: `:scream:` | :neckbeard: `:neckbeard:` | :tired_face: `:tired_face:` |
| :angry: `:angry:` | :rage: `:rage:` | :triumph: `:triumph:` |
| :sleepy: `:sleepy:` | :yum: `:yum:` | :mask: `:mask:` |
| :sunglasses: `:sunglasses:` | :dizzy_face: `:dizzy_face:` | :imp: `:imp:` |
| :smiling_imp: `:smiling_imp:` | :neutral_face: `:neutral_face:` | :no_mouth: `:no_mouth:` |
| :innocent: `:innocent:` | :alien: `:alien:` | :yellow_heart: `:yellow_heart:` |
| :blue_heart: `:blue_heart:` | :purple_heart: `:purple_heart:` | :heart: `:heart:` |
| :green_heart: `:green_heart:` | :broken_heart: `:broken_heart:` | :heartbeat: `:heartbeat:` |
| :heartpulse: `:heartpulse:` | :two_hearts: `:two_hearts:` | :revolving_hearts: `:revolving_hearts:` |
| :cupid: `:cupid:` | :sparkling_heart: `:sparkling_heart:` | :sparkles: `:sparkles:` |
| :star: `:star:` | :star2: `:star2:` | :dizzy: `:dizzy:` |
| :boom: `:boom:` | :collision: `:collision:` | :anger: `:anger:` |
| :exclamation: `:exclamation:` | :question: `:question:` | :grey_exclamation: `:grey_exclamation:` |
| :grey_question: `:grey_question:` | :zzz: `:zzz:` | :dash: `:dash:` |
| :sweat_drops: `:sweat_drops:` | :notes: `:notes:` | :musical_note: `:musical_note:` |
| :fire: `:fire:` | :hankey: `:hankey:` | :poop: `:poop:` |
| :shit: `:shit:` | :+1: `:+1:` | :thumbsup: `:thumbsup:` |
| :-1: `:-1:` | :thumbsdown: `:thumbsdown:` | :ok_hand: `:ok_hand:` |
| :punch: `:punch:` | :facepunch: `:facepunch:` | :fist: `:fist:` |
| :v: `:v:` | :wave: `:wave:` | :hand: `:hand:` |
| :raised_hand: `:raised_hand:` | :open_hands: `:open_hands:` | :point_up: `:point_up:` |
| :point_down: `:point_down:` | :point_left: `:point_left:` | :point_right: `:point_right:` |
| :raised_hands: `:raised_hands:` | :pray: `:pray:` | :point_up_2: `:point_up_2:` |
| :clap: `:clap:` | :muscle: `:muscle:` | :metal: `:metal:` |
| :fu: `:fu:` | :walking: `:walking:` | :runner: `:runner:` |
| :running: `:running:` | :couple: `:couple:` | :family: `:family:` |
| :two_men_holding_hands: `:two_men_holding_hands:` | :two_women_holding_hands: `:two_women_holding_hands:` | :dancer: `:dancer:` |
| :dancers: `:dancers:` | :ok_woman: `:ok_woman:` | :no_good: `:no_good:` |
| :information_desk_person: `:information_desk_person:` | :raising_hand: `:raising_hand:` | :bride_with_veil: `:bride_with_veil:` |
| :person_with_pouting_face: `:person_with_pouting_face:` | :person_frowning: `:person_frowning:` | :bow: `:bow:` |
| :couplekiss: `:couplekiss:` | :couple_with_heart: `:couple_with_heart:` | :massage: `:massage:` |
| :haircut: `:haircut:` | :nail_care: `:nail_care:` | :boy: `:boy:` |
| :girl: `:girl:` | :woman: `:woman:` | :man: `:man:` |
| :baby: `:baby:` | :older_woman: `:older_woman:` | :older_man: `:older_man:` |
| :person_with_blond_hair: `:person_with_blond_hair:` | :man_with_gua_pi_mao: `:man_with_gua_pi_mao:` | :man_with_turban: `:man_with_turban:` |
| :construction_worker: `:construction_worker:` | :cop: `:cop:` | :angel: `:angel:` |
| :princess: `:princess:` | :smiley_cat: `:smiley_cat:` | :smile_cat: `:smile_cat:` |
| :heart_eyes_cat: `:heart_eyes_cat:` | :kissing_cat: `:kissing_cat:` | :smirk_cat: `:smirk_cat:` |
| :scream_cat: `:scream_cat:` | :crying_cat_face: `:crying_cat_face:` | :joy_cat: `:joy_cat:` |
| :pouting_cat: `:pouting_cat:` | :japanese_ogre: `:japanese_ogre:` | :japanese_goblin: `:japanese_goblin:` |
| :see_no_evil: `:see_no_evil:` | :hear_no_evil: `:hear_no_evil:` | :speak_no_evil: `:speak_no_evil:` |
| :guardsman: `:guardsman:` | :skull: `:skull:` | :feet: `:feet:` |
| :lips: `:lips:` | :kiss: `:kiss:` | :droplet: `:droplet:` |
| :ear: `:ear:` | :eyes: `:eyes:` | :nose: `:nose:` |
| :tongue: `:tongue:` | :love_letter: `:love_letter:` | :bust_in_silhouette: `:bust_in_silhouette:` |
| :busts_in_silhouette: `:busts_in_silhouette:` | :speech_balloon: `:speech_balloon:` | :thought_balloon: `:thought_balloon:` |
| :feelsgood: `:feelsgood:` | :finnadie: `:finnadie:` | :goberserk: `:goberserk:` |
| :godmode: `:godmode:` | :hurtrealbad: `:hurtrealbad:` | :rage1: `:rage1:` |
| :rage2: `:rage2:` | :rage3: `:rage3:` | :rage4: `:rage4:` |
| :suspect: `:suspect:` | :trollface: `:trollface:` |
Nature
| :sunny: `:sunny:` | :umbrella: `:umbrella:` | :cloud: `:cloud:` |
|---|---|---|
| :snowflake: `:snowflake:` | :snowman: `:snowman:` | :zap: `:zap:` |
| :cyclone: `:cyclone:` | :foggy: `:foggy:` | :ocean: `:ocean:` |
| :cat: `:cat:` | :dog: `:dog:` | :mouse: `:mouse:` |
| :hamster: `:hamster:` | :rabbit: `:rabbit:` | :wolf: `:wolf:` |
| :frog: `:frog:` | :tiger: `:tiger:` | :koala: `:koala:` |
| :bear: `:bear:` | :pig: `:pig:` | :pig_nose: `:pig_nose:` |
| :cow: `:cow:` | :boar: `:boar:` | :monkey_face: `:monkey_face:` |
| :monkey: `:monkey:` | :horse: `:horse:` | :racehorse: `:racehorse:` |
| :camel: `:camel:` | :sheep: `:sheep:` | :elephant: `:elephant:` |
| :panda_face: `:panda_face:` | :snake: `:snake:` | :bird: `:bird:` |
| :baby_chick: `:baby_chick:` | :hatched_chick: `:hatched_chick:` | :hatching_chick: `:hatching_chick:` |
| :chicken: `:chicken:` | :penguin: `:penguin:` | :turtle: `:turtle:` |
| :bug: `:bug:` | :honeybee: `:honeybee:` | :ant: `:ant:` |
| :beetle: `:beetle:` | :snail: `:snail:` | :octopus: `:octopus:` |
| :tropical_fish: `:tropical_fish:` | :fish: `:fish:` | :whale: `:whale:` |
| :whale2: `:whale2:` | :dolphin: `:dolphin:` | :cow2: `:cow2:` |
| :ram: `:ram:` | :rat: `:rat:` | :water_buffalo: `:water_buffalo:` |
| :tiger2: `:tiger2:` | :rabbit2: `:rabbit2:` | :dragon: `:dragon:` |
| :goat: `:goat:` | :rooster: `:rooster:` | :dog2: `:dog2:` |
| :pig2: `:pig2:` | :mouse2: `:mouse2:` | :ox: `:ox:` |
| :dragon_face: `:dragon_face:` | :blowfish: `:blowfish:` | :crocodile: `:crocodile:` |
| :dromedary_camel: `:dromedary_camel:` | :leopard: `:leopard:` | :cat2: `:cat2:` |
| :poodle: `:poodle:` | :paw_prints: `:paw_prints:` | :bouquet: `:bouquet:` |
| :cherry_blossom: `:cherry_blossom:` | :tulip: `:tulip:` | :four_leaf_clover: `:four_leaf_clover:` |
| :rose: `:rose:` | :sunflower: `:sunflower:` | :hibiscus: `:hibiscus:` |
| :maple_leaf: `:maple_leaf:` | :leaves: `:leaves:` | :fallen_leaf: `:fallen_leaf:` |
| :herb: `:herb:` | :mushroom: `:mushroom:` | :cactus: `:cactus:` |
| :palm_tree: `:palm_tree:` | :evergreen_tree: `:evergreen_tree:` | :deciduous_tree: `:deciduous_tree:` |
| :chestnut: `:chestnut:` | :seedling: `:seedling:` | :blossom: `:blossom:` |
| :ear_of_rice: `:ear_of_rice:` | :shell: `:shell:` | :globe_with_meridians: `:globe_with_meridians:` |
| :sun_with_face: `:sun_with_face:` | :full_moon_with_face: `:full_moon_with_face:` | :new_moon_with_face: `:new_moon_with_face:` |
| :new_moon: `:new_moon:` | :waxing_crescent_moon: `:waxing_crescent_moon:` | :first_quarter_moon: `:first_quarter_moon:` |
| :waxing_gibbous_moon: `:waxing_gibbous_moon:` | :full_moon: `:full_moon:` | :waning_gibbous_moon: `:waning_gibbous_moon:` |
| :last_quarter_moon: `:last_quarter_moon:` | :waning_crescent_moon: `:waning_crescent_moon:` | :last_quarter_moon_with_face: `:last_quarter_moon_with_face:` |
| :first_quarter_moon_with_face: `:first_quarter_moon_with_face:` | :moon: `:moon:` | :earth_africa: `:earth_africa:` |
| :earth_americas: `:earth_americas:` | :earth_asia: `:earth_asia:` | :volcano: `:volcano:` |
| :milky_way: `:milky_way:` | :partly_sunny: `:partly_sunny:` | :octocat: `:octocat:` |
| :squirrel: `:squirrel:` |
Objects
| :bamboo: `:bamboo:` | :gift_heart: `:gift_heart:` | :dolls: `:dolls:` |
|---|---|---|
| :school_satchel: `:school_satchel:` | :mortar_board: `:mortar_board:` | :flags: `:flags:` |
| :fireworks: `:fireworks:` | :sparkler: `:sparkler:` | :wind_chime: `:wind_chime:` |
| :rice_scene: `:rice_scene:` | :jack_o_lantern: `:jack_o_lantern:` | :ghost: `:ghost:` |
| :santa: `:santa:` | :christmas_tree: `:christmas_tree:` | :gift: `:gift:` |
| :bell: `:bell:` | :no_bell: `:no_bell:` | :tanabata_tree: `:tanabata_tree:` |
| :tada: `:tada:` | :confetti_ball: `:confetti_ball:` | :balloon: `:balloon:` |
| :crystal_ball: `:crystal_ball:` | :cd: `:cd:` | :dvd: `:dvd:` |
| :floppy_disk: `:floppy_disk:` | :camera: `:camera:` | :video_camera: `:video_camera:` |
| :movie_camera: `:movie_camera:` | :computer: `:computer:` | :tv: `:tv:` |
| :iphone: `:iphone:` | :phone: `:phone:` | :telephone: `:telephone:` |
| :telephone_receiver: `:telephone_receiver:` | :pager: `:pager:` | :fax: `:fax:` |
| :minidisc: `:minidisc:` | :vhs: `:vhs:` | :sound: `:sound:` |
| :speaker: `:speaker:` | :mute: `:mute:` | :loudspeaker: `:loudspeaker:` |
| :mega: `:mega:` | :hourglass: `:hourglass:` | :hourglass_flowing_sand: `:hourglass_flowing_sand:` |
| :alarm_clock: `:alarm_clock:` | :watch: `:watch:` | :radio: `:radio:` |
| :satellite: `:satellite:` | :loop: `:loop:` | :mag: `:mag:` |
| :mag_right: `:mag_right:` | :unlock: `:unlock:` | :lock: `:lock:` |
| :lock_with_ink_pen: `:lock_with_ink_pen:` | :closed_lock_with_key: `:closed_lock_with_key:` | :key: `:key:` |
| :bulb: `:bulb:` | :flashlight: `:flashlight:` | :high_brightness: `:high_brightness:` |
| :low_brightness: `:low_brightness:` | :electric_plug: `:electric_plug:` | :battery: `:battery:` |
| :calling: `:calling:` | :email: `:email:` | :mailbox: `:mailbox:` |
| :postbox: `:postbox:` | :bath: `:bath:` | :bathtub: `:bathtub:` |
| :shower: `:shower:` | :toilet: `:toilet:` | :wrench: `:wrench:` |
| :nut_and_bolt: `:nut_and_bolt:` | :hammer: `:hammer:` | :seat: `:seat:` |
| :moneybag: `:moneybag:` | :yen: `:yen:` | :dollar: `:dollar:` |
| :pound: `:pound:` | :euro: `:euro:` | :credit_card: `:credit_card:` |
| :money_with_wings: `:money_with_wings:` | :e-mail: `:e-mail:` | :inbox_tray: `:inbox_tray:` |
| :outbox_tray: `:outbox_tray:` | :envelope: `:envelope:` | :incoming_envelope: `:incoming_envelope:` |
| :postal_horn: `:postal_horn:` | :mailbox_closed: `:mailbox_closed:` | :mailbox_with_mail: `:mailbox_with_mail:` |
| :mailbox_with_no_mail: `:mailbox_with_no_mail:` | :door: `:door:` | :smoking: `:smoking:` |
| :bomb: `:bomb:` | :gun: `:gun:` | :hocho: `:hocho:` |
| :pill: `:pill:` | :syringe: `:syringe:` | :page_facing_up: `:page_facing_up:` |
| :page_with_curl: `:page_with_curl:` | :bookmark_tabs: `:bookmark_tabs:` | :bar_chart: `:bar_chart:` |
| :chart_with_upwards_trend: `:chart_with_upwards_trend:` | :chart_with_downwards_trend: `:chart_with_downwards_trend:` | :scroll: `:scroll:` |
| :clipboard: `:clipboard:` | :calendar: `:calendar:` | :date: `:date:` |
| :card_index: `:card_index:` | :file_folder: `:file_folder:` | :open_file_folder: `:open_file_folder:` |
| :scissors: `:scissors:` | :pushpin: `:pushpin:` | :paperclip: `:paperclip:` |
| :black_nib: `:black_nib:` | :pencil2: `:pencil2:` | :straight_ruler: `:straight_ruler:` |
| :triangular_ruler: `:triangular_ruler:` | :closed_book: `:closed_book:` | :green_book: `:green_book:` |
| :blue_book: `:blue_book:` | :orange_book: `:orange_book:` | :notebook: `:notebook:` |
| :notebook_with_decorative_cover: `:notebook_with_decorative_cover:` | :ledger: `:ledger:` | :books: `:books:` |
| :bookmark: `:bookmark:` | :name_badge: `:name_badge:` | :microscope: `:microscope:` |
| :telescope: `:telescope:` | :newspaper: `:newspaper:` | :football: `:football:` |
| :basketball: `:basketball:` | :soccer: `:soccer:` | :baseball: `:baseball:` |
| :tennis: `:tennis:` | :8ball: `:8ball:` | :rugby_football: `:rugby_football:` |
| :bowling: `:bowling:` | :golf: `:golf:` | :mountain_bicyclist: `:mountain_bicyclist:` |
| :bicyclist: `:bicyclist:` | :horse_racing: `:horse_racing:` | :snowboarder: `:snowboarder:` |
| :swimmer: `:swimmer:` | :surfer: `:surfer:` | :ski: `:ski:` |
| :spades: `:spades:` | :hearts: `:hearts:` | :clubs: `:clubs:` |
| :diamonds: `:diamonds:` | :gem: `:gem:` | :ring: `:ring:` |
| :trophy: `:trophy:` | :musical_score: `:musical_score:` | :musical_keyboard: `:musical_keyboard:` |
| :violin: `:violin:` | :space_invader: `:space_invader:` | :video_game: `:video_game:` |
| :black_joker: `:black_joker:` | :flower_playing_cards: `:flower_playing_cards:` | :game_die: `:game_die:` |
| :dart: `:dart:` | :mahjong: `:mahjong:` | :clapper: `:clapper:` |
| :memo: `:memo:` | :pencil: `:pencil:` | :book: `:book:` |
| :art: `:art:` | :microphone: `:microphone:` | :headphones: `:headphones:` |
| :trumpet: `:trumpet:` | :saxophone: `:saxophone:` | :guitar: `:guitar:` |
| :shoe: `:shoe:` | :sandal: `:sandal:` | :high_heel: `:high_heel:` |
| :lipstick: `:lipstick:` | :boot: `:boot:` | :shirt: `:shirt:` |
| :tshirt: `:tshirt:` | :necktie: `:necktie:` | :womans_clothes: `:womans_clothes:` |
| :dress: `:dress:` | :running_shirt_with_sash: `:running_shirt_with_sash:` | :jeans: `:jeans:` |
| :kimono: `:kimono:` | :bikini: `:bikini:` | :ribbon: `:ribbon:` |
| :tophat: `:tophat:` | :crown: `:crown:` | :womans_hat: `:womans_hat:` |
| :mans_shoe: `:mans_shoe:` | :closed_umbrella: `:closed_umbrella:` | :briefcase: `:briefcase:` |
| :handbag: `:handbag:` | :pouch: `:pouch:` | :purse: `:purse:` |
| :eyeglasses: `:eyeglasses:` | :fishing_pole_and_fish: `:fishing_pole_and_fish:` | :coffee: `:coffee:` |
| :tea: `:tea:` | :sake: `:sake:` | :baby_bottle: `:baby_bottle:` |
| :beer: `:beer:` | :beers: `:beers:` | :cocktail: `:cocktail:` |
| :tropical_drink: `:tropical_drink:` | :wine_glass: `:wine_glass:` | :fork_and_knife: `:fork_and_knife:` |
| :pizza: `:pizza:` | :hamburger: `:hamburger:` | :fries: `:fries:` |
| :poultry_leg: `:poultry_leg:` | :meat_on_bone: `:meat_on_bone:` | :spaghetti: `:spaghetti:` |
| :curry: `:curry:` | :fried_shrimp: `:fried_shrimp:` | :bento: `:bento:` |
| :sushi: `:sushi:` | :fish_cake: `:fish_cake:` | :rice_ball: `:rice_ball:` |
| :rice_cracker: `:rice_cracker:` | :rice: `:rice:` | :ramen: `:ramen:` |
| :stew: `:stew:` | :oden: `:oden:` | :dango: `:dango:` |
| :egg: `:egg:` | :bread: `:bread:` | :doughnut: `:doughnut:` |
| :custard: `:custard:` | :icecream: `:icecream:` | :ice_cream: `:ice_cream:` |
| :shaved_ice: `:shaved_ice:` | :birthday: `:birthday:` | :cake: `:cake:` |
| :cookie: `:cookie:` | :chocolate_bar: `:chocolate_bar:` | :candy: `:candy:` |
| :lollipop: `:lollipop:` | :honey_pot: `:honey_pot:` | :apple: `:apple:` |
| :green_apple: `:green_apple:` | :tangerine: `:tangerine:` | :lemon: `:lemon:` |
| :cherries: `:cherries:` | :grapes: `:grapes:` | :watermelon: `:watermelon:` |
| :strawberry: `:strawberry:` | :peach: `:peach:` | :melon: `:melon:` |
| :banana: `:banana:` | :pear: `:pear:` | :pineapple: `:pineapple:` |
| :sweet_potato: `:sweet_potato:` | :eggplant: `:eggplant:` | :tomato: `:tomato:` |
| :corn: `:corn:` |
Places
| :house: `:house:` | :house_with_garden: `:house_with_garden:` | :school: `:school:` |
|---|---|---|
| :office: `:office:` | :post_office: `:post_office:` | :hospital: `:hospital:` |
| :bank: `:bank:` | :convenience_store: `:convenience_store:` | :love_hotel: `:love_hotel:` |
| :hotel: `:hotel:` | :wedding: `:wedding:` | :church: `:church:` |
| :department_store: `:department_store:` | :european_post_office: `:european_post_office:` | :city_sunrise: `:city_sunrise:` |
| :city_sunset: `:city_sunset:` | :japanese_castle: `:japanese_castle:` | :european_castle: `:european_castle:` |
| :tent: `:tent:` | :factory: `:factory:` | :tokyo_tower: `:tokyo_tower:` |
| :japan: `:japan:` | :mount_fuji: `:mount_fuji:` | :sunrise_over_mountains: `:sunrise_over_mountains:` |
| :sunrise: `:sunrise:` | :stars: `:stars:` | :statue_of_liberty: `:statue_of_liberty:` |
| :bridge_at_night: `:bridge_at_night:` | :carousel_horse: `:carousel_horse:` | :rainbow: `:rainbow:` |
| :ferris_wheel: `:ferris_wheel:` | :fountain: `:fountain:` | :roller_coaster: `:roller_coaster:` |
| :ship: `:ship:` | :speedboat: `:speedboat:` | :boat: `:boat:` |
| :sailboat: `:sailboat:` | :rowboat: `:rowboat:` | :anchor: `:anchor:` |
| :rocket: `:rocket:` | :airplane: `:airplane:` | :helicopter: `:helicopter:` |
| :steam_locomotive: `:steam_locomotive:` | :tram: `:tram:` | :mountain_railway: `:mountain_railway:` |
| :bike: `:bike:` | :aerial_tramway: `:aerial_tramway:` | :suspension_railway: `:suspension_railway:` |
| :mountain_cableway: `:mountain_cableway:` | :tractor: `:tractor:` | :blue_car: `:blue_car:` |
| :oncoming_automobile: `:oncoming_automobile:` | :car: `:car:` | :red_car: `:red_car:` |
| :taxi: `:taxi:` | :oncoming_taxi: `:oncoming_taxi:` | :articulated_lorry: `:articulated_lorry:` |
| :bus: `:bus:` | :oncoming_bus: `:oncoming_bus:` | :rotating_light: `:rotating_light:` |
| :police_car: `:police_car:` | :oncoming_police_car: `:oncoming_police_car:` | :fire_engine: `:fire_engine:` |
| :ambulance: `:ambulance:` | :minibus: `:minibus:` | :truck: `:truck:` |
| :train: `:train:` | :station: `:station:` | :train2: `:train2:` |
| :bullettrain_front: `:bullettrain_front:` | :bullettrain_side: `:bullettrain_side:` | :light_rail: `:light_rail:` |
| :monorail: `:monorail:` | :railway_car: `:railway_car:` | :trolleybus: `:trolleybus:` |
| :ticket: `:ticket:` | :fuelpump: `:fuelpump:` | :vertical_traffic_light: `:vertical_traffic_light:` |
| :traffic_light: `:traffic_light:` | :warning: `:warning:` | :construction: `:construction:` |
| :beginner: `:beginner:` | :atm: `:atm:` | :slot_machine: `:slot_machine:` |
| :busstop: `:busstop:` | :barber: `:barber:` | :hotsprings: `:hotsprings:` |
| :checkered_flag: `:checkered_flag:` | :crossed_flags: `:crossed_flags:` | :izakaya_lantern: `:izakaya_lantern:` |
| :moyai: `:moyai:` | :circus_tent: `:circus_tent:` | :performing_arts: `:performing_arts:` |
| :round_pushpin: `:round_pushpin:` | :triangular_flag_on_post: `:triangular_flag_on_post:` | :jp: `:jp:` |
| :kr: `:kr:` | :cn: `:cn:` | :us: `:us:` |
| :fr: `:fr:` | :es: `:es:` | :it: `:it:` |
| :ru: `:ru:` | :gb: `:gb:` | :uk: `:uk:` |
| :de: `:de:` |
Symbols
| :one: `:one:` | :two: `:two:` | :three: `:three:` |
|---|---|---|
| :four: `:four:` | :five: `:five:` | :six: `:six:` |
| :seven: `:seven:` | :eight: `:eight:` | :nine: `:nine:` |
| :keycap_ten: `:keycap_ten:` | :1234: `:1234:` | :zero: `:zero:` |
| :hash: `:hash:` | :symbols: `:symbols:` | :arrow_backward: `:arrow_backward:` |
| :arrow_down: `:arrow_down:` | :arrow_forward: `:arrow_forward:` | :arrow_left: `:arrow_left:` |
| :capital_abcd: `:capital_abcd:` | :abcd: `:abcd:` | :abc: `:abc:` |
| :arrow_lower_left: `:arrow_lower_left:` | :arrow_lower_right: `:arrow_lower_right:` | :arrow_right: `:arrow_right:` |
| :arrow_up: `:arrow_up:` | :arrow_upper_left: `:arrow_upper_left:` | :arrow_upper_right: `:arrow_upper_right:` |
| :arrow_double_down: `:arrow_double_down:` | :arrow_double_up: `:arrow_double_up:` | :arrow_down_small: `:arrow_down_small:` |
| :arrow_heading_down: `:arrow_heading_down:` | :arrow_heading_up: `:arrow_heading_up:` | :leftwards_arrow_with_hook: `:leftwards_arrow_with_hook:` |
| :arrow_right_hook: `:arrow_right_hook:` | :left_right_arrow: `:left_right_arrow:` | :arrow_up_down: `:arrow_up_down:` |
| :arrow_up_small: `:arrow_up_small:` | :arrows_clockwise: `:arrows_clockwise:` | :arrows_counterclockwise: `:arrows_counterclockwise:` |
| :rewind: `:rewind:` | :fast_forward: `:fast_forward:` | :information_source: `:information_source:` |
| :ok: `:ok:` | :twisted_rightwards_arrows: `:twisted_rightwards_arrows:` | :repeat: `:repeat:` |
| :repeat_one: `:repeat_one:` | :new: `:new:` | :top: `:top:` |
| :up: `:up:` | :cool: `:cool:` | :free: `:free:` |
| :ng: `:ng:` | :cinema: `:cinema:` | :koko: `:koko:` |
| :signal_strength: `:signal_strength:` | :u5272: `:u5272:` | :u5408: `:u5408:` |
| :u55b6: `:u55b6:` | :u6307: `:u6307:` | :u6708: `:u6708:` |
| :u6709: `:u6709:` | :u6e80: `:u6e80:` | :u7121: `:u7121:` |
| :u7533: `:u7533:` | :u7a7a: `:u7a7a:` | :u7981: `:u7981:` |
| :sa: `:sa:` | :restroom: `:restroom:` | :mens: `:mens:` |
| :womens: `:womens:` | :baby_symbol: `:baby_symbol:` | :no_smoking: `:no_smoking:` |
| :parking: `:parking:` | :wheelchair: `:wheelchair:` | :metro: `:metro:` |
| :baggage_claim: `:baggage_claim:` | :accept: `:accept:` | :wc: `:wc:` |
| :potable_water: `:potable_water:` | :put_litter_in_its_place: `:put_litter_in_its_place:` | :secret: `:secret:` |
| :congratulations: `:congratulations:` | :m: `:m:` | :passport_control: `:passport_control:` |
| :left_luggage: `:left_luggage:` | :customs: `:customs:` | :ideograph_advantage: `:ideograph_advantage:` |
| :cl: `:cl:` | :sos: `:sos:` | :id: `:id:` |
| :no_entry_sign: `:no_entry_sign:` | :underage: `:underage:` | :no_mobile_phones: `:no_mobile_phones:` |
| :do_not_litter: `:do_not_litter:` | :non-potable_water: `:non-potable_water:` | :no_bicycles: `:no_bicycles:` |
| :no_pedestrians: `:no_pedestrians:` | :children_crossing: `:children_crossing:` | :no_entry: `:no_entry:` |
| :eight_spoked_asterisk: `:eight_spoked_asterisk:` | :eight_pointed_black_star: `:eight_pointed_black_star:` | :heart_decoration: `:heart_decoration:` |
| :vs: `:vs:` | :vibration_mode: `:vibration_mode:` | :mobile_phone_off: `:mobile_phone_off:` |
| :chart: `:chart:` | :currency_exchange: `:currency_exchange:` | :aries: `:aries:` |
| :taurus: `:taurus:` | :gemini: `:gemini:` | :cancer: `:cancer:` |
| :leo: `:leo:` | :virgo: `:virgo:` | :libra: `:libra:` |
| :scorpius: `:scorpius:` | :sagittarius: `:sagittarius:` | :capricorn: `:capricorn:` |
| :aquarius: `:aquarius:` | :pisces: `:pisces:` | :ophiuchus: `:ophiuchus:` |
| :six_pointed_star: `:six_pointed_star:` | :negative_squared_cross_mark: `:negative_squared_cross_mark:` | :a: `:a:` |
| :b: `:b:` | :ab: `:ab:` | :o2: `:o2:` |
| :diamond_shape_with_a_dot_inside: `:diamond_shape_with_a_dot_inside:` | :recycle: `:recycle:` | :end: `:end:` |
| :on: `:on:` | :soon: `:soon:` | :clock1: `:clock1:` |
| :clock130: `:clock130:` | :clock10: `:clock10:` | :clock1030: `:clock1030:` |
| :clock11: `:clock11:` | :clock1130: `:clock1130:` | :clock12: `:clock12:` |
| :clock1230: `:clock1230:` | :clock2: `:clock2:` | :clock230: `:clock230:` |
| :clock3: `:clock3:` | :clock330: `:clock330:` | :clock4: `:clock4:` |
| :clock430: `:clock430:` | :clock5: `:clock5:` | :clock530: `:clock530:` |
| :clock6: `:clock6:` | :clock630: `:clock630:` | :clock7: `:clock7:` |
| :clock730: `:clock730:` | :clock8: `:clock8:` | :clock830: `:clock830:` |
| :clock9: `:clock9:` | :clock930: `:clock930:` | :heavy_dollar_sign: `:heavy_dollar_sign:` |
| :copyright: `:copyright:` | :registered: `:registered:` | :tm: `:tm:` |
| :x: `:x:` | :heavy_exclamation_mark: `:heavy_exclamation_mark:` | :bangbang: `:bangbang:` |
| :interrobang: `:interrobang:` | :o: `:o:` | :heavy_multiplication_x: `:heavy_multiplication_x:` |
| :heavy_plus_sign: `:heavy_plus_sign:` | :heavy_minus_sign: `:heavy_minus_sign:` | :heavy_division_sign: `:heavy_division_sign:` |
| :white_flower: `:white_flower:` | :100: `:100:` | :heavy_check_mark: `:heavy_check_mark:` |
| :ballot_box_with_check: `:ballot_box_with_check:` | :radio_button: `:radio_button:` | :link: `:link:` |
| :curly_loop: `:curly_loop:` | :wavy_dash: `:wavy_dash:` | :part_alternation_mark: `:part_alternation_mark:` |
| :trident: `:trident:` | :black_square: `:black_square:` | :white_square: `:white_square:` |
| :white_check_mark: `:white_check_mark:` | :black_square_button: `:black_square_button:` | :white_square_button: `:white_square_button:` |
| :black_circle: `:black_circle:` | :white_circle: `:white_circle:` | :red_circle: `:red_circle:` |
| :large_blue_circle: `:large_blue_circle:` | :large_blue_diamond: `:large_blue_diamond:` | :large_orange_diamond: `:large_orange_diamond:` |
| :small_blue_diamond: `:small_blue_diamond:` | :small_orange_diamond: `:small_orange_diamond:` | :small_red_triangle: `:small_red_triangle:` |
| :small_red_triangle_down: `:small_red_triangle_down:` | :shipit: `:shipit:` |
"""
| elm |
[
{
"context": "{-\nCopyright 2020 Morgan Stanley\n\nLicensed under the Apache License, Version 2.0 (",
"end": 32,
"score": 0.9998053312,
"start": 18,
"tag": "NAME",
"value": "Morgan Stanley"
}
] | draft/DevBot/Source.elm | aszenz/morphir-elm | 6 | {-
Copyright 2020 Morgan Stanley
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-}
module SlateX.DevBot.Source exposing (..)
{-| Utilities related to generating source code.
-}
empty : String
empty =
""
space : String
space =
" "
newLine : String
newLine =
"\n"
semi : String
semi =
";"
dot : String
dot =
"."
{-| Indent the specified string. If the string contains multiple lines they will all be indented.
-}
indent : Int -> String -> String
indent depth string =
string
|> String.lines
|> indentLines depth
{-| Indent the specified list of string. If the string contains multiple lines they will all be indented.
-}
indentLines : Int -> List String -> String
indentLines depth lines =
lines
|> List.concatMap String.lines
|> List.map
(\line ->
(String.repeat depth space) ++ line
)
|> String.join newLine
dotSep : List String -> String
dotSep parts =
parts |> String.join dot
parens : String -> String
parens string =
"(" ++ string ++ ")" | 43414 | {-
Copyright 2020 <NAME>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-}
module SlateX.DevBot.Source exposing (..)
{-| Utilities related to generating source code.
-}
empty : String
empty =
""
space : String
space =
" "
newLine : String
newLine =
"\n"
semi : String
semi =
";"
dot : String
dot =
"."
{-| Indent the specified string. If the string contains multiple lines they will all be indented.
-}
indent : Int -> String -> String
indent depth string =
string
|> String.lines
|> indentLines depth
{-| Indent the specified list of string. If the string contains multiple lines they will all be indented.
-}
indentLines : Int -> List String -> String
indentLines depth lines =
lines
|> List.concatMap String.lines
|> List.map
(\line ->
(String.repeat depth space) ++ line
)
|> String.join newLine
dotSep : List String -> String
dotSep parts =
parts |> String.join dot
parens : String -> String
parens string =
"(" ++ string ++ ")" | true | {-
Copyright 2020 PI:NAME:<NAME>END_PI
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-}
module SlateX.DevBot.Source exposing (..)
{-| Utilities related to generating source code.
-}
empty : String
empty =
""
space : String
space =
" "
newLine : String
newLine =
"\n"
semi : String
semi =
";"
dot : String
dot =
"."
{-| Indent the specified string. If the string contains multiple lines they will all be indented.
-}
indent : Int -> String -> String
indent depth string =
string
|> String.lines
|> indentLines depth
{-| Indent the specified list of string. If the string contains multiple lines they will all be indented.
-}
indentLines : Int -> List String -> String
indentLines depth lines =
lines
|> List.concatMap String.lines
|> List.map
(\line ->
(String.repeat depth space) ++ line
)
|> String.join newLine
dotSep : List String -> String
dotSep parts =
parts |> String.join dot
parens : String -> String
parens string =
"(" ++ string ++ ")" | elm |
[
{
"context": "\npopular entries:\n\n[elm-html]: https://github.com/evancz/elm-html\n[todo]: http://evancz.github.io/elm-todo",
"end": 1254,
"score": 0.9994258285,
"start": 1248,
"tag": "USERNAME",
"value": "evancz"
},
{
"context": "github.io/elm-todomvc/\n[code]: https://github.com/evancz/elm-todomvc/blob/master/Todo.elm\n[bench]: https:/",
"end": 1342,
"score": 0.999466002,
"start": 1336,
"tag": "USERNAME",
"value": "evancz"
},
{
"context": "great numbers.\n\n[virtual-dom]: https://github.com/Matt-Esch/virtual-dom\n\nPerformance is a good hook, but the ",
"end": 2163,
"score": 0.9657669067,
"start": 2154,
"tag": "USERNAME",
"value": "Matt-Esch"
},
{
"context": " new ways to make views more modular and pleasant. Paul Chiusano\nexplains this aspiration very nicely in his [prov",
"end": 9812,
"score": 0.9997596741,
"start": 9799,
"tag": "NAME",
"value": "Paul Chiusano"
},
{
"context": "izing these techniques.\nThank you in particular to Sebastian Markbage, David Nolen, Matt Esch, and Jake\nVerbaten who he",
"end": 10758,
"score": 0.9999054074,
"start": 10740,
"tag": "NAME",
"value": "Sebastian Markbage"
},
{
"context": "es.\nThank you in particular to Sebastian Markbage, David Nolen, Matt Esch, and Jake\nVerbaten who helped me *unde",
"end": 10771,
"score": 0.9998955131,
"start": 10760,
"tag": "NAME",
"value": "David Nolen"
},
{
"context": " in particular to Sebastian Markbage, David Nolen, Matt Esch, and Jake\nVerbaten who helped me *understand* the",
"end": 10782,
"score": 0.9998925924,
"start": 10773,
"tag": "NAME",
"value": "Matt Esch"
},
{
"context": "to Sebastian Markbage, David Nolen, Matt Esch, and Jake\nVerbaten who helped me *understand* them.\n\nHuge thanks to ",
"end": 10801,
"score": 0.9837720394,
"start": 10788,
"tag": "NAME",
"value": "Jake\nVerbaten"
},
{
"context": "n who helped me *understand* them.\n\nHuge thanks to Matt Esch and Jake Verbaten who created [virtual-dom][] and",
"end": 10860,
"score": 0.9998706579,
"start": 10851,
"tag": "NAME",
"value": "Matt Esch"
},
{
"context": "e *understand* them.\n\nHuge thanks to Matt Esch and Jake Verbaten who created [virtual-dom][] and\n[mercury][], whic",
"end": 10878,
"score": 0.999153018,
"start": 10865,
"tag": "NAME",
"value": "Jake Verbaten"
}
] | frontend/public/blog/Blazing-Fast-Html.elm | arsatiki/elm-lang.org | 0 | import Graphics.Element (..)
import Markdown
import Signal (Signal, (<~))
import Website.Skeleton (skeleton)
import Window
port title : String
port title = "Blazing Fast HTML"
main : Signal Element
main =
skeleton "Blog" everything <~ Window.dimensions
everything wid =
let w = truncate (toFloat wid * 0.8)
w' = min 600 w
section txt =
let words = width w' txt in
container w (heightOf words) middle words
in
flow down
[ width w pageTitle
, section intro
]
pageTitle = Markdown.toElement """
<br/>
<div style="font-family: futura, 'century gothic', 'twentieth century', calibri, verdana, helvetica, arial; text-align: center;">
<div style="font-size: 4em;">Blazing Fast HTML</div>
<div style="font-size: 1.5em;">Virtual DOM in Elm</div>
</div>
"""
intro = Markdown.toElement """
<br/>
The new [elm-html][] library lets you use
HTML and CSS directly in Elm. Want to use flexbox? Want to keep using existing
style sheets? Elm now makes all of this pleasant and *fast*. For example, when
recreating the [TodoMVC][todo] app, the [code][code] is quite simple and our
[preliminary benchmarks][bench] show that it is extremely fast compared to other
popular entries:
[elm-html]: https://github.com/evancz/elm-html
[todo]: http://evancz.github.io/elm-todomvc/
[code]: https://github.com/evancz/elm-todomvc/blob/master/Todo.elm
[bench]: https://evancz.github.io/todomvc-perf-comparison
<a href="https://evancz.github.io/todomvc-perf-comparison">
<img src="/diagrams/sampleResults.png"
alt="sample results with Firefox 30 on a Macbook Air with OSX 10.9.4"
title="sample results with Firefox 30 on a Macbook Air with OSX 10.9.4"
style="width:500px; height:380px; margin-left: auto; margin-right: auto; display:block;"></a>
Both [elm-html][] and Mercury are based on the [virtual-dom][] project, which is
responsible for the great performance. The first half of this post will explore
what “virtual DOM” means and how **purity** and **immutability**
make it extremely fast. This will explain why Om, Mercury, and Elm all get such
great numbers.
[virtual-dom]: https://github.com/Matt-Esch/virtual-dom
Performance is a good hook, but the real benefit is that this approach leads to
code that is easier to understand and maintain. In short, it becomes very simple
to create reusable HTML widgets and abstract out common patterns. *This* is why
people with larger code bases should be interested in virtual DOM approaches.
This library is also great news for people who have been thinking about using
Elm. It means you can use Elm *and* keep using the same CSS and
designer/developer workflow that you are comfortable with. It is simpler than
ever to get the benefits of Elm in your project. Let’s see how it works.
[FRP]: /learn/What-is-FRP.elm
[std]: package.elm-lang.org/packages/elm-lang/core/latest/
## Virtual DOM
This library is based on the idea of a “virtual DOM”. Rather than
touching the DOM directly, we build an abstract version of it on each frame. We
use the `node` function to create a cheap representation of what we want:
```haskell
node : String -> List Attribute -> List Html -> Html
```
This lets us specify a tag, a list of HTML attributes, and a list of children.
For HTML5 tags, there are helper functions such as `(div = node "div")` to make
things look a lot cleaner. For example, here is how you can build a simple
`profile` widget that shows a user’s picture and name:
```haskell
profile : User -> Html
profile user =
div [ class "profile" ] [
img [ src user.picture ] [],
span [] [ text user.name ]
]
```
Notice that we set a class so the whole thing can be styled from CSS. Paired
with Elm’s module system, this makes it easy to abstract out common
patterns and reuse code. You can check out the full API and documentation
[here](http://package.elm-lang.org/packages/evancz/elm-html/latest/) and we will
explore more example uses soon in the section on [reusable
widgets](#reusable-widgets).
## Making Virtual DOM Fast
Virtual DOM sounds pretty slow, right? Create a whole new scene on every frame?
This technique is actually [widely used in the game industry][scene] and
performs shockingly well for DOM updates when you use two relatively simple
techniques: diffing and laziness.
[scene]: http://en.wikipedia.org/wiki/Scene_graph
React popularized the idea of “diffing” to figure out how the DOM
needs to be modified. **Diffing means taking the *current* virtual DOM and the
*new* virtual DOM and looking for changes.** It sounds kind of fancy at first,
but it is a very simple process. We first make a big list of all the
differences, like if someone has changed the color of a particular `<div>` or
added an entirely new one. After all of the differences are found, we use them
as instructions for modifying the DOM in one big batch using
[`requestAnimationFrame`][raf]. This means we do the dirty work of modifying
the DOM and making sure everything is fast. You can focus on writing code
that is easy to understand and maintain.
[raf]: https://developer.mozilla.org/en/docs/Web/API/window.requestAnimationFrame
This approach created a clear path to fully supporting HTML and CSS in a way
that is perfect for Elm! Even better, Elm already has great facilities for
purity and immutability, which are vital for optimizations that make diffing
*way* faster.
As pioneered by React and Om, being *lazy* about diffing can lead to great
performance improvements, especially if you have immutable data. For example,
let’s say we are rendering a list of tasks:
```haskell
todoList : List Task -> Html
todoList tasks =
div [] (map todoItem tasks)
```
But we may know that on many updates, none of the tasks are changing. And if no
task changes, the view must not be changing either. This is a perfect time to be
`lazy`:
```haskell
lazy : (a -> Html) -> a -> Html
todoWidget : State -> Html
todoWidget state =
lazy todoList state.tasks
```
Instead of calling the `todoList` function on every frame, we check to see if
`state.tasks` has changed since last frame. If not, we can skip everything.
No need to call the function, do any diffing, or touch the DOM at all!
This optimization is safe in Elm because functions are [pure][] and data is
[immutable][].
* **Purity** means that the `todoList` function will *always* have
the same output given the same input. So if we know `state.tasks` is the same,
we can skip `todoList` entirely.
* **Immutability** makes it cheap to figure out when things are “the
same”. Immutability guarantees that if two things are referentially
equal, they *must* be structurally equal.
So we just check to see if `todoList` and `state.tasks` are the same as last
frame by comparing the old and new values by *reference*. This is super cheap,
and if they are the same, the `lazy` function can often avoid a ton of work.
This is a pretty simple trick that can speed things up significantly.
[pure]: http://en.wikipedia.org/wiki/Pure_function
[immutable]: http://en.wikipedia.org/wiki/Immutable_object
If you have been following Elm, you may begin to see a pattern:
purity and immutability are kind of a big deal. Read about [hot-swapping in
Elm](/blog/Interactive-Programming.elm) and the [time traveling
debugger](http://debug.elm-lang.org/) to learn more about this.
<h2 id="reusable-widgets">Reusable Widgets</h2>
This approach makes it incredibly simple to create reusable widgets. For
example, a list of user profiles can be nicely abstracted with something like
this:
```haskell
import Html (..)
profiles : List User -> Html
profiles users =
div [] (map profile users)
profile : User -> Html
profile user =
div [] [
img [ src user.picture ] [],
text user.name
]
```
We now have a `profiles` widget that takes a list of users and gives us back
some HTML. It is easy to reuse anywhere, and unlike templating languages, we can
use any part of Elm to help create widgets like this. We can even begin to
create community libraries for common widgets or patterns.
If you want to create complex styles, those can be abstracted out and reused
too! In the following example, we define a `font` and `background` that
can be mixed and matched on any node.
```haskell
-- small reusable CSS properties
font : List CssProperty
font = [
prop "font-family" "futura, sans-serif",
prop "color" "rgb(42, 42, 42)",
prop "font-size" "2em"
]
background : List CssProperty
background = [
prop "background-color" "rgb(245, 245, 245)"
]
-- combine them to make individual nodes
profiles : List User -> Html
profiles users =
div [ style (font ++ background) ] (map profile users)
```
So creating reusable widgets and abstracting out common patterns is extremely
simple now, but we can do much more than this!
## Freedom of Abstraction
When I started working on the project that would become Elm, HTML was about 20
years old and people still had to read three blog posts and five Stack Overflow
questions to figure out how to vertically center things. My initial goal with
Elm was to rethink GUIs from scratch. **What would web programming look like if
we could restart?**
[elm-html][] has two very important strengths in pursuing that goal. First, it
gives you access to HTML and CSS, so you can always take full advantage of the
latest features. Second, it makes it possible to create *new* abstractions.
This means **HTML and CSS become the basic building blocks for *nicer*
abstractions.** For example, it may be possible to recreate Elm’s
`Element` abstraction using this library. But most importantly, *anyone* can
experiment with new ways to make views more modular and pleasant. Paul Chiusano
explains this aspiration very nicely in his [provocative post on CSS][css].
[css]: http://pchiusano.github.io/2014-07-02/css-is-unnecessary.html
My goal with Elm is still to rethink web programming, and in a weird and twisted
way, fully supporting HTML and CSS is a big step in that direction. I am excited
to see what we can do with [elm-html][]!
## Notes on Architecture
As with any new approach, one of the first questions people ask is “what
does it look like for a large project?” The general approach is in the
same ballpark as architecting large applications with Om or Facebook’s Flux. I
have informally outlined [how this works in Elm][architecture] and plan to
create more formal documentation and examples soon.
[architecture]: https://gist.github.com/evancz/2b2ba366cae1887fe621
## Thank you
Thank you to React and Om for discovering and popularizing these techniques.
Thank you in particular to Sebastian Markbage, David Nolen, Matt Esch, and Jake
Verbaten who helped me *understand* them.
Huge thanks to Matt Esch and Jake Verbaten who created [virtual-dom][] and
[mercury][], which this library is based on. They are fully responsible for
the great performance!
[mercury]: https://github.com/Raynos/mercury
"""
| 1697 | import Graphics.Element (..)
import Markdown
import Signal (Signal, (<~))
import Website.Skeleton (skeleton)
import Window
port title : String
port title = "Blazing Fast HTML"
main : Signal Element
main =
skeleton "Blog" everything <~ Window.dimensions
everything wid =
let w = truncate (toFloat wid * 0.8)
w' = min 600 w
section txt =
let words = width w' txt in
container w (heightOf words) middle words
in
flow down
[ width w pageTitle
, section intro
]
pageTitle = Markdown.toElement """
<br/>
<div style="font-family: futura, 'century gothic', 'twentieth century', calibri, verdana, helvetica, arial; text-align: center;">
<div style="font-size: 4em;">Blazing Fast HTML</div>
<div style="font-size: 1.5em;">Virtual DOM in Elm</div>
</div>
"""
intro = Markdown.toElement """
<br/>
The new [elm-html][] library lets you use
HTML and CSS directly in Elm. Want to use flexbox? Want to keep using existing
style sheets? Elm now makes all of this pleasant and *fast*. For example, when
recreating the [TodoMVC][todo] app, the [code][code] is quite simple and our
[preliminary benchmarks][bench] show that it is extremely fast compared to other
popular entries:
[elm-html]: https://github.com/evancz/elm-html
[todo]: http://evancz.github.io/elm-todomvc/
[code]: https://github.com/evancz/elm-todomvc/blob/master/Todo.elm
[bench]: https://evancz.github.io/todomvc-perf-comparison
<a href="https://evancz.github.io/todomvc-perf-comparison">
<img src="/diagrams/sampleResults.png"
alt="sample results with Firefox 30 on a Macbook Air with OSX 10.9.4"
title="sample results with Firefox 30 on a Macbook Air with OSX 10.9.4"
style="width:500px; height:380px; margin-left: auto; margin-right: auto; display:block;"></a>
Both [elm-html][] and Mercury are based on the [virtual-dom][] project, which is
responsible for the great performance. The first half of this post will explore
what “virtual DOM” means and how **purity** and **immutability**
make it extremely fast. This will explain why Om, Mercury, and Elm all get such
great numbers.
[virtual-dom]: https://github.com/Matt-Esch/virtual-dom
Performance is a good hook, but the real benefit is that this approach leads to
code that is easier to understand and maintain. In short, it becomes very simple
to create reusable HTML widgets and abstract out common patterns. *This* is why
people with larger code bases should be interested in virtual DOM approaches.
This library is also great news for people who have been thinking about using
Elm. It means you can use Elm *and* keep using the same CSS and
designer/developer workflow that you are comfortable with. It is simpler than
ever to get the benefits of Elm in your project. Let’s see how it works.
[FRP]: /learn/What-is-FRP.elm
[std]: package.elm-lang.org/packages/elm-lang/core/latest/
## Virtual DOM
This library is based on the idea of a “virtual DOM”. Rather than
touching the DOM directly, we build an abstract version of it on each frame. We
use the `node` function to create a cheap representation of what we want:
```haskell
node : String -> List Attribute -> List Html -> Html
```
This lets us specify a tag, a list of HTML attributes, and a list of children.
For HTML5 tags, there are helper functions such as `(div = node "div")` to make
things look a lot cleaner. For example, here is how you can build a simple
`profile` widget that shows a user’s picture and name:
```haskell
profile : User -> Html
profile user =
div [ class "profile" ] [
img [ src user.picture ] [],
span [] [ text user.name ]
]
```
Notice that we set a class so the whole thing can be styled from CSS. Paired
with Elm’s module system, this makes it easy to abstract out common
patterns and reuse code. You can check out the full API and documentation
[here](http://package.elm-lang.org/packages/evancz/elm-html/latest/) and we will
explore more example uses soon in the section on [reusable
widgets](#reusable-widgets).
## Making Virtual DOM Fast
Virtual DOM sounds pretty slow, right? Create a whole new scene on every frame?
This technique is actually [widely used in the game industry][scene] and
performs shockingly well for DOM updates when you use two relatively simple
techniques: diffing and laziness.
[scene]: http://en.wikipedia.org/wiki/Scene_graph
React popularized the idea of “diffing” to figure out how the DOM
needs to be modified. **Diffing means taking the *current* virtual DOM and the
*new* virtual DOM and looking for changes.** It sounds kind of fancy at first,
but it is a very simple process. We first make a big list of all the
differences, like if someone has changed the color of a particular `<div>` or
added an entirely new one. After all of the differences are found, we use them
as instructions for modifying the DOM in one big batch using
[`requestAnimationFrame`][raf]. This means we do the dirty work of modifying
the DOM and making sure everything is fast. You can focus on writing code
that is easy to understand and maintain.
[raf]: https://developer.mozilla.org/en/docs/Web/API/window.requestAnimationFrame
This approach created a clear path to fully supporting HTML and CSS in a way
that is perfect for Elm! Even better, Elm already has great facilities for
purity and immutability, which are vital for optimizations that make diffing
*way* faster.
As pioneered by React and Om, being *lazy* about diffing can lead to great
performance improvements, especially if you have immutable data. For example,
let’s say we are rendering a list of tasks:
```haskell
todoList : List Task -> Html
todoList tasks =
div [] (map todoItem tasks)
```
But we may know that on many updates, none of the tasks are changing. And if no
task changes, the view must not be changing either. This is a perfect time to be
`lazy`:
```haskell
lazy : (a -> Html) -> a -> Html
todoWidget : State -> Html
todoWidget state =
lazy todoList state.tasks
```
Instead of calling the `todoList` function on every frame, we check to see if
`state.tasks` has changed since last frame. If not, we can skip everything.
No need to call the function, do any diffing, or touch the DOM at all!
This optimization is safe in Elm because functions are [pure][] and data is
[immutable][].
* **Purity** means that the `todoList` function will *always* have
the same output given the same input. So if we know `state.tasks` is the same,
we can skip `todoList` entirely.
* **Immutability** makes it cheap to figure out when things are “the
same”. Immutability guarantees that if two things are referentially
equal, they *must* be structurally equal.
So we just check to see if `todoList` and `state.tasks` are the same as last
frame by comparing the old and new values by *reference*. This is super cheap,
and if they are the same, the `lazy` function can often avoid a ton of work.
This is a pretty simple trick that can speed things up significantly.
[pure]: http://en.wikipedia.org/wiki/Pure_function
[immutable]: http://en.wikipedia.org/wiki/Immutable_object
If you have been following Elm, you may begin to see a pattern:
purity and immutability are kind of a big deal. Read about [hot-swapping in
Elm](/blog/Interactive-Programming.elm) and the [time traveling
debugger](http://debug.elm-lang.org/) to learn more about this.
<h2 id="reusable-widgets">Reusable Widgets</h2>
This approach makes it incredibly simple to create reusable widgets. For
example, a list of user profiles can be nicely abstracted with something like
this:
```haskell
import Html (..)
profiles : List User -> Html
profiles users =
div [] (map profile users)
profile : User -> Html
profile user =
div [] [
img [ src user.picture ] [],
text user.name
]
```
We now have a `profiles` widget that takes a list of users and gives us back
some HTML. It is easy to reuse anywhere, and unlike templating languages, we can
use any part of Elm to help create widgets like this. We can even begin to
create community libraries for common widgets or patterns.
If you want to create complex styles, those can be abstracted out and reused
too! In the following example, we define a `font` and `background` that
can be mixed and matched on any node.
```haskell
-- small reusable CSS properties
font : List CssProperty
font = [
prop "font-family" "futura, sans-serif",
prop "color" "rgb(42, 42, 42)",
prop "font-size" "2em"
]
background : List CssProperty
background = [
prop "background-color" "rgb(245, 245, 245)"
]
-- combine them to make individual nodes
profiles : List User -> Html
profiles users =
div [ style (font ++ background) ] (map profile users)
```
So creating reusable widgets and abstracting out common patterns is extremely
simple now, but we can do much more than this!
## Freedom of Abstraction
When I started working on the project that would become Elm, HTML was about 20
years old and people still had to read three blog posts and five Stack Overflow
questions to figure out how to vertically center things. My initial goal with
Elm was to rethink GUIs from scratch. **What would web programming look like if
we could restart?**
[elm-html][] has two very important strengths in pursuing that goal. First, it
gives you access to HTML and CSS, so you can always take full advantage of the
latest features. Second, it makes it possible to create *new* abstractions.
This means **HTML and CSS become the basic building blocks for *nicer*
abstractions.** For example, it may be possible to recreate Elm’s
`Element` abstraction using this library. But most importantly, *anyone* can
experiment with new ways to make views more modular and pleasant. <NAME>
explains this aspiration very nicely in his [provocative post on CSS][css].
[css]: http://pchiusano.github.io/2014-07-02/css-is-unnecessary.html
My goal with Elm is still to rethink web programming, and in a weird and twisted
way, fully supporting HTML and CSS is a big step in that direction. I am excited
to see what we can do with [elm-html][]!
## Notes on Architecture
As with any new approach, one of the first questions people ask is “what
does it look like for a large project?” The general approach is in the
same ballpark as architecting large applications with Om or Facebook’s Flux. I
have informally outlined [how this works in Elm][architecture] and plan to
create more formal documentation and examples soon.
[architecture]: https://gist.github.com/evancz/2b2ba366cae1887fe621
## Thank you
Thank you to React and Om for discovering and popularizing these techniques.
Thank you in particular to <NAME>, <NAME>, <NAME>, and <NAME> who helped me *understand* them.
Huge thanks to <NAME> and <NAME> who created [virtual-dom][] and
[mercury][], which this library is based on. They are fully responsible for
the great performance!
[mercury]: https://github.com/Raynos/mercury
"""
| true | import Graphics.Element (..)
import Markdown
import Signal (Signal, (<~))
import Website.Skeleton (skeleton)
import Window
port title : String
port title = "Blazing Fast HTML"
main : Signal Element
main =
skeleton "Blog" everything <~ Window.dimensions
everything wid =
let w = truncate (toFloat wid * 0.8)
w' = min 600 w
section txt =
let words = width w' txt in
container w (heightOf words) middle words
in
flow down
[ width w pageTitle
, section intro
]
pageTitle = Markdown.toElement """
<br/>
<div style="font-family: futura, 'century gothic', 'twentieth century', calibri, verdana, helvetica, arial; text-align: center;">
<div style="font-size: 4em;">Blazing Fast HTML</div>
<div style="font-size: 1.5em;">Virtual DOM in Elm</div>
</div>
"""
intro = Markdown.toElement """
<br/>
The new [elm-html][] library lets you use
HTML and CSS directly in Elm. Want to use flexbox? Want to keep using existing
style sheets? Elm now makes all of this pleasant and *fast*. For example, when
recreating the [TodoMVC][todo] app, the [code][code] is quite simple and our
[preliminary benchmarks][bench] show that it is extremely fast compared to other
popular entries:
[elm-html]: https://github.com/evancz/elm-html
[todo]: http://evancz.github.io/elm-todomvc/
[code]: https://github.com/evancz/elm-todomvc/blob/master/Todo.elm
[bench]: https://evancz.github.io/todomvc-perf-comparison
<a href="https://evancz.github.io/todomvc-perf-comparison">
<img src="/diagrams/sampleResults.png"
alt="sample results with Firefox 30 on a Macbook Air with OSX 10.9.4"
title="sample results with Firefox 30 on a Macbook Air with OSX 10.9.4"
style="width:500px; height:380px; margin-left: auto; margin-right: auto; display:block;"></a>
Both [elm-html][] and Mercury are based on the [virtual-dom][] project, which is
responsible for the great performance. The first half of this post will explore
what “virtual DOM” means and how **purity** and **immutability**
make it extremely fast. This will explain why Om, Mercury, and Elm all get such
great numbers.
[virtual-dom]: https://github.com/Matt-Esch/virtual-dom
Performance is a good hook, but the real benefit is that this approach leads to
code that is easier to understand and maintain. In short, it becomes very simple
to create reusable HTML widgets and abstract out common patterns. *This* is why
people with larger code bases should be interested in virtual DOM approaches.
This library is also great news for people who have been thinking about using
Elm. It means you can use Elm *and* keep using the same CSS and
designer/developer workflow that you are comfortable with. It is simpler than
ever to get the benefits of Elm in your project. Let’s see how it works.
[FRP]: /learn/What-is-FRP.elm
[std]: package.elm-lang.org/packages/elm-lang/core/latest/
## Virtual DOM
This library is based on the idea of a “virtual DOM”. Rather than
touching the DOM directly, we build an abstract version of it on each frame. We
use the `node` function to create a cheap representation of what we want:
```haskell
node : String -> List Attribute -> List Html -> Html
```
This lets us specify a tag, a list of HTML attributes, and a list of children.
For HTML5 tags, there are helper functions such as `(div = node "div")` to make
things look a lot cleaner. For example, here is how you can build a simple
`profile` widget that shows a user’s picture and name:
```haskell
profile : User -> Html
profile user =
div [ class "profile" ] [
img [ src user.picture ] [],
span [] [ text user.name ]
]
```
Notice that we set a class so the whole thing can be styled from CSS. Paired
with Elm’s module system, this makes it easy to abstract out common
patterns and reuse code. You can check out the full API and documentation
[here](http://package.elm-lang.org/packages/evancz/elm-html/latest/) and we will
explore more example uses soon in the section on [reusable
widgets](#reusable-widgets).
## Making Virtual DOM Fast
Virtual DOM sounds pretty slow, right? Create a whole new scene on every frame?
This technique is actually [widely used in the game industry][scene] and
performs shockingly well for DOM updates when you use two relatively simple
techniques: diffing and laziness.
[scene]: http://en.wikipedia.org/wiki/Scene_graph
React popularized the idea of “diffing” to figure out how the DOM
needs to be modified. **Diffing means taking the *current* virtual DOM and the
*new* virtual DOM and looking for changes.** It sounds kind of fancy at first,
but it is a very simple process. We first make a big list of all the
differences, like if someone has changed the color of a particular `<div>` or
added an entirely new one. After all of the differences are found, we use them
as instructions for modifying the DOM in one big batch using
[`requestAnimationFrame`][raf]. This means we do the dirty work of modifying
the DOM and making sure everything is fast. You can focus on writing code
that is easy to understand and maintain.
[raf]: https://developer.mozilla.org/en/docs/Web/API/window.requestAnimationFrame
This approach created a clear path to fully supporting HTML and CSS in a way
that is perfect for Elm! Even better, Elm already has great facilities for
purity and immutability, which are vital for optimizations that make diffing
*way* faster.
As pioneered by React and Om, being *lazy* about diffing can lead to great
performance improvements, especially if you have immutable data. For example,
let’s say we are rendering a list of tasks:
```haskell
todoList : List Task -> Html
todoList tasks =
div [] (map todoItem tasks)
```
But we may know that on many updates, none of the tasks are changing. And if no
task changes, the view must not be changing either. This is a perfect time to be
`lazy`:
```haskell
lazy : (a -> Html) -> a -> Html
todoWidget : State -> Html
todoWidget state =
lazy todoList state.tasks
```
Instead of calling the `todoList` function on every frame, we check to see if
`state.tasks` has changed since last frame. If not, we can skip everything.
No need to call the function, do any diffing, or touch the DOM at all!
This optimization is safe in Elm because functions are [pure][] and data is
[immutable][].
* **Purity** means that the `todoList` function will *always* have
the same output given the same input. So if we know `state.tasks` is the same,
we can skip `todoList` entirely.
* **Immutability** makes it cheap to figure out when things are “the
same”. Immutability guarantees that if two things are referentially
equal, they *must* be structurally equal.
So we just check to see if `todoList` and `state.tasks` are the same as last
frame by comparing the old and new values by *reference*. This is super cheap,
and if they are the same, the `lazy` function can often avoid a ton of work.
This is a pretty simple trick that can speed things up significantly.
[pure]: http://en.wikipedia.org/wiki/Pure_function
[immutable]: http://en.wikipedia.org/wiki/Immutable_object
If you have been following Elm, you may begin to see a pattern:
purity and immutability are kind of a big deal. Read about [hot-swapping in
Elm](/blog/Interactive-Programming.elm) and the [time traveling
debugger](http://debug.elm-lang.org/) to learn more about this.
<h2 id="reusable-widgets">Reusable Widgets</h2>
This approach makes it incredibly simple to create reusable widgets. For
example, a list of user profiles can be nicely abstracted with something like
this:
```haskell
import Html (..)
profiles : List User -> Html
profiles users =
div [] (map profile users)
profile : User -> Html
profile user =
div [] [
img [ src user.picture ] [],
text user.name
]
```
We now have a `profiles` widget that takes a list of users and gives us back
some HTML. It is easy to reuse anywhere, and unlike templating languages, we can
use any part of Elm to help create widgets like this. We can even begin to
create community libraries for common widgets or patterns.
If you want to create complex styles, those can be abstracted out and reused
too! In the following example, we define a `font` and `background` that
can be mixed and matched on any node.
```haskell
-- small reusable CSS properties
font : List CssProperty
font = [
prop "font-family" "futura, sans-serif",
prop "color" "rgb(42, 42, 42)",
prop "font-size" "2em"
]
background : List CssProperty
background = [
prop "background-color" "rgb(245, 245, 245)"
]
-- combine them to make individual nodes
profiles : List User -> Html
profiles users =
div [ style (font ++ background) ] (map profile users)
```
So creating reusable widgets and abstracting out common patterns is extremely
simple now, but we can do much more than this!
## Freedom of Abstraction
When I started working on the project that would become Elm, HTML was about 20
years old and people still had to read three blog posts and five Stack Overflow
questions to figure out how to vertically center things. My initial goal with
Elm was to rethink GUIs from scratch. **What would web programming look like if
we could restart?**
[elm-html][] has two very important strengths in pursuing that goal. First, it
gives you access to HTML and CSS, so you can always take full advantage of the
latest features. Second, it makes it possible to create *new* abstractions.
This means **HTML and CSS become the basic building blocks for *nicer*
abstractions.** For example, it may be possible to recreate Elm’s
`Element` abstraction using this library. But most importantly, *anyone* can
experiment with new ways to make views more modular and pleasant. PI:NAME:<NAME>END_PI
explains this aspiration very nicely in his [provocative post on CSS][css].
[css]: http://pchiusano.github.io/2014-07-02/css-is-unnecessary.html
My goal with Elm is still to rethink web programming, and in a weird and twisted
way, fully supporting HTML and CSS is a big step in that direction. I am excited
to see what we can do with [elm-html][]!
## Notes on Architecture
As with any new approach, one of the first questions people ask is “what
does it look like for a large project?” The general approach is in the
same ballpark as architecting large applications with Om or Facebook’s Flux. I
have informally outlined [how this works in Elm][architecture] and plan to
create more formal documentation and examples soon.
[architecture]: https://gist.github.com/evancz/2b2ba366cae1887fe621
## Thank you
Thank you to React and Om for discovering and popularizing these techniques.
Thank you in particular to PI:NAME:<NAME>END_PI, PI:NAME:<NAME>END_PI, PI:NAME:<NAME>END_PI, and PI:NAME:<NAME>END_PI who helped me *understand* them.
Huge thanks to PI:NAME:<NAME>END_PI and PI:NAME:<NAME>END_PI who created [virtual-dom][] and
[mercury][], which this library is based on. They are fully responsible for
the great performance!
[mercury]: https://github.com/Raynos/mercury
"""
| elm |
[
{
"context": " [ ( \"firstName\", GraphQL.Argument.string \"Tom\" )\n ]\n (GraphQL.Selector.su",
"end": 2252,
"score": 0.9996955395,
"start": 2249,
"tag": "NAME",
"value": "Tom"
},
{
"context": "ation RenameUser {\n updateMe(\"firstName\": \"Tom\") {\n id\n firstName\n ",
"end": 2706,
"score": 0.9995902181,
"start": 2703,
"tag": "NAME",
"value": "Tom"
},
{
"context": "/example.com/graphql\"\n |> withBearerToken \"eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJhIjoiYSJ9.MvhYYpYBuN1rUaV0GGnQGvr889zY0xSc20Lnt8nMTfE\"\n\n-}\nwithBearerToken : String -> Request a -> Req",
"end": 8193,
"score": 0.9997226596,
"start": 8100,
"tag": "KEY",
"value": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJhIjoiYSJ9.MvhYYpYBuN1rUaV0GGnQGvr889zY0xSc20Lnt8nMTfE"
}
] | src/GraphQL.elm | owanturist/elm-graphql | 1 | module GraphQL exposing
( GraphQL, query, mutation, subscription, render, toDecoder
, Request, get, post
, withHeader, withHeaders, withBearerToken
, withQueryParam, withQueryParams, withTimeout, withCredentials, withDataPath
, Error(..), send, sendCancelable, cancel, toTask, toTaskWithCacheBuster
)
{-| Building and sending GraphQL.
Building of HTTP request has been based on [`elm-http-builder`](http://package.elm-lang.org/packages/lukewestby/elm-http-builder/latest).
# Build a GraphQL
@docs GraphQL, query, mutation, subscription, render, toDecoder
# Build a Request
@docs Request, get, post
@docs withHeader, withHeaders, withBearerToken
@docs withQueryParam, withQueryParams, withTimeout, withCredentials, withDataPath
# Make a Request
@docs Error, send, sendCancelable, cancel, toTask, toTaskWithCacheBuster
-}
import GraphQL.Selector as Selector exposing (Selector)
import Http
import Internal
import Json.Decode as Decode exposing (Decoder, decodeString, errorToString)
import Json.Encode as Encode
import Task exposing (Task)
import Time
import Url
{-| -}
type GraphQL a
= GraphQL String String (Selector a)
{-| Build a GraphQL named query.
GraphQL.Selector.succeed identity
|> GraphQL.Selector.field "me"
[]
(GraphQL.Selector.succeed User
|> GraphQL.Selector.field "id" [] GraphQL.Selector.string
|> GraphQL.Selector.field "firstName" [] GraphQL.Selector.string
|> GraphQL.Selector.field "lastName" [] GraphQL.Selector.string
)
|> query "Me"
It collects a GraphQL which is equal to:
"""
query Me {
me {
id
firstName
lastName
}
}
"""
and decoder is equal to:
Decode.field "me"
(Decode.map3
(Decode.field "id" Decode.string)
(Decode.field "firstName" Decode.string)
(Decode.field "lastName" Decode.string)
)
-}
query : String -> Selector a -> GraphQL a
query =
GraphQL "query"
{-| Build a GraphQL named mutation.
GraphQL.Selector.succeed identity
|> GraphQL.Selector.field "updateMe"
[ ( "firstName", GraphQL.Argument.string "Tom" )
]
(GraphQL.Selector.succeed User
|> GraphQL.Selector.field "id" [] GraphQL.Selector.string
|> GraphQL.Selector.field "firstName" [] GraphQL.Selector.string
|> GraphQL.Selector.field "lastName" [] GraphQL.Selector.string
)
|> mutation "RenameUser"
It collects a GraphQL which is equal to:
"""
mutation RenameUser {
updateMe("firstName": "Tom") {
id
firstName
lastName
}
}
"""
and decoder is equal to:
Decode.field "updateMe"
(Decode.map3
(Decode.field "id" Decode.string)
(Decode.field "firstName" Decode.string)
(Decode.field "lastName" Decode.string)
)
-}
mutation : String -> Selector a -> GraphQL a
mutation =
GraphQL "mutation"
{-| Build a GraphQL named subscription.
GraphQL.Selector.succeed identity
|> GraphQL.Selector.field "onUpdateMe"
[]
(GraphQL.Selector.succeed User
|> GraphQL.Selector.field "id" [] GraphQL.Selector.string
|> GraphQL.Selector.field "firstName" [] GraphQL.Selector.string
|> GraphQL.Selector.field "lastName" [] GraphQL.Selector.string
)
|> subscription "OnUpdateUser"
It collects a GraphQL which is equal to:
"""
subscription OnUpdateUser {
onUpdateMe {
id
firstName
lastName
}
}
"""
and decoder is equal to:
Decode.field "onUpdateMe"
(Decode.map3
(Decode.field "id" Decode.string)
(Decode.field "firstName" Decode.string)
(Decode.field "lastName" Decode.string)
)
-}
subscription : String -> Selector a -> GraphQL a
subscription =
GraphQL "subscription"
{-| Render GraphQL string representation from a GraphQL.
-}
render : GraphQL a -> String
render (GraphQL operation name selector) =
(operation ++ " " ++ name) ++ Internal.wrap "{" "}" (Selector.render selector)
{-| Build a Decoder from a GraphQL.
-}
toDecoder : GraphQL a -> Decoder a
toDecoder (GraphQL _ _ selector) =
Selector.toDecoder selector
{-| A type for chaining request configuration.
-}
type Request a
= Request
{ method : String
, url : String
, headers : List Http.Header
, body : Http.Body
, decoder : Decoder a
, dataPath : List String
, timeout : Maybe Float
, withCredentials : Bool
, queryParams : List ( String, String )
}
initRequest : Bool -> String -> GraphQL a -> Request a
initRequest isGetMethod url graphql =
let
( method, queryParams, body ) =
if isGetMethod then
( "GET"
, [ ( "query", render graphql ) ]
, Http.emptyBody
)
else
( "POST"
, []
, [ ( "query", Encode.string (render graphql) ) ]
|> Encode.object
|> Http.jsonBody
)
in
Request
{ method = method
, url = url
, headers = []
, body = body
, decoder = toDecoder graphql
, dataPath = [ "data" ]
, timeout = Nothing
, withCredentials = False
, queryParams = queryParams
}
{-| Start building a GET request with a given URL.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
-}
get : String -> GraphQL a -> Request a
get =
initRequest True
{-| Start building a POST request with a given URL.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> post "https://example.com/graphql"
-}
post : String -> GraphQL a -> Request a
post =
initRequest False
{-| Add a single header to a request.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withHeader "Content-Type" "application/json"
|> withHeader "Accept" "application/json"
-}
withHeader : String -> String -> Request a -> Request a
withHeader key value (Request builder) =
Request { builder | headers = Http.header key value :: builder.headers }
{-| Add many headers to a request.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withHeaders
[ ( "Content-Type", "application/json" )
, ( "Accept", "application/json" )
]
-}
withHeaders : List ( String, String ) -> Request a -> Request a
withHeaders headerPairs (Request builder) =
case List.map (\( key, value ) -> Http.header key value) headerPairs of
[] ->
Request builder
newHeaders ->
Request { builder | headers = builder.headers ++ newHeaders }
{-| Add a bearer token to a request.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withBearerToken "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJhIjoiYSJ9.MvhYYpYBuN1rUaV0GGnQGvr889zY0xSc20Lnt8nMTfE"
-}
withBearerToken : String -> Request a -> Request a
withBearerToken value request =
withHeader "Authorization" ("Bearer " ++ value) request
{-| Add a query param to the url for the request.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withQueryParams "hello" "world"
|> withQueryParams "baz" "qux"
-- sends a request to https://example.com/graphql?hello=world&baz=qux
-}
withQueryParam : String -> String -> Request a -> Request a
withQueryParam key value (Request builder) =
Request { builder | queryParams = builder.queryParams ++ [ ( key, value ) ] }
{-| Add some query params to the url for the request.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withQueryParams [ ( "hello", "world" ), ( "foo", "bar" ) ]
|> withQueryParams [ ( "baz", "qux" ) ]
-- sends a request to https://example.com/graphql?hello=world&foo=bar&baz=qux
-}
withQueryParams : List ( String, String ) -> Request a -> Request a
withQueryParams queryParams (Request builder) =
Request { builder | queryParams = builder.queryParams ++ queryParams }
{-| Set the `timeout` setting on the request.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withTimeout (10 * Time.second)
-}
withTimeout : Float -> Request a -> Request a
withTimeout timeout (Request builder) =
Request { builder | timeout = Just timeout }
{-| Set the `withCredentials` flag on the request to True. Works via
[`XMLHttpRequest#withCredentials`](https://developer.mozilla.org/en-US/docs/Web/API/XMLHttpRequest/withCredentials).
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withCredentials True
-}
withCredentials : Bool -> Request a -> Request a
withCredentials with (Request builder) =
Request { builder | withCredentials = with }
{-| Set a path of graphql data. Default value is `[ "data" ]`.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withDataPath [ "my", "custom", "data", "path" ]
-}
withDataPath : List String -> Request a -> Request a
withDataPath dataPath (Request builder) =
Request { builder | dataPath = dataPath }
{-| A Request can fail in a couple ways:
- BadUrl means you did not provide a valid URL.
- Timeout means it took too long to get a response.
- NetworkError means the user turned off their wifi, went in a cave, etc.
- BadStatus means you got a response back, but the status code indicates failure.
- BadPayload means you got a response back with a nice status code,
but the body of the response was something unexpected.
The String in this case is a debugging message that
explains what went wrong with your JSON decoder or whatever.
-}
type Error
= BadUrl String
| Timeout
| NetworkError
| BadStatus Int
| BadBody String
responseHandler : Decoder a -> Http.Response String -> Result Error a
responseHandler decoder response =
case response of
Http.BadUrl_ url ->
Err (BadUrl url)
Http.Timeout_ ->
Err Timeout
Http.NetworkError_ ->
Err NetworkError
Http.BadStatus_ meta _ ->
Err (BadStatus meta.statusCode)
Http.GoodStatus_ _ body ->
case decodeString decoder body of
Ok value ->
Ok value
Err err ->
Err (BadBody (errorToString err))
sendHttpRequest : Maybe String -> (Result Error a -> msg) -> Request a -> Cmd msg
sendHttpRequest tracker tagger (Request builder) =
let
config =
{ method = builder.method
, headers = builder.headers
, url = buildFullUrl builder.url builder.queryParams
, body = builder.body
, expect = Http.expectStringResponse tagger (responseHandler (Decode.at builder.dataPath builder.decoder))
, timeout = builder.timeout
, tracker = tracker
}
in
if builder.withCredentials then
Http.riskyRequest config
else
Http.request config
{-| Send the Http request.
-}
send : (Result Error a -> msg) -> Request a -> Cmd msg
send =
sendHttpRequest Nothing
{-| Send the cancelable Http request.
-}
sendCancelable : String -> (Result Error a -> msg) -> Request a -> Cmd msg
sendCancelable =
sendHttpRequest << Just
{-| Try to cancel an ongoing cancelable Http request.
-}
cancel : String -> Cmd msg
cancel =
Http.cancel
toHttpTask : Maybe String -> Request a -> Task Error a
toHttpTask cacheBuster request =
Task.andThen
(\(Request builder) ->
let
config =
{ method = builder.method
, headers = builder.headers
, url = buildFullUrl builder.url builder.queryParams
, body = builder.body
, resolver = Http.stringResolver (responseHandler (Decode.at builder.dataPath builder.decoder))
, timeout = builder.timeout
}
in
if builder.withCredentials then
Http.riskyTask config
else
Http.task config
)
(case cacheBuster of
Nothing ->
Task.succeed request
Just buster ->
Task.map
((|>) request << withQueryParam buster << String.fromInt << Time.posixToMillis)
Time.now
)
{-| Convert the `Request` to a `Task` with all options applied.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> toTask
-- makes a task of request to https://example.com/graphql
-}
toTask : Request a -> Task Error a
toTask =
toHttpTask Nothing
{-| Convert the `Request` to a `Task` with a Time based cache buster added to the URL.
You provide a key for an extra query param, and when the request is sent that
query param will be given a value with the current timestamp.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> toTaskWithCacheBuster "cache_buster"
-- makes a task of request to https://example.com/graphql?cache_buster=1481633217383
-}
toTaskWithCacheBuster : String -> Request a -> Task Error a
toTaskWithCacheBuster =
toHttpTask << Just
buildFullUrl : String -> List ( String, String ) -> String
buildFullUrl url queryParams =
if List.isEmpty queryParams then
url
else
url ++ "?" ++ joinUrlEncoded queryParams
joinUrlEncoded : List ( String, String ) -> String
joinUrlEncoded args =
String.join "&" (List.map queryPair args)
queryPair : ( String, String ) -> String
queryPair ( key, value ) =
queryEscape key ++ "=" ++ queryEscape value
queryEscape : String -> String
queryEscape =
Url.percentEncode >> replace "%20" "+"
replace : String -> String -> String -> String
replace old new =
String.split old >> String.join new
| 7730 | module GraphQL exposing
( GraphQL, query, mutation, subscription, render, toDecoder
, Request, get, post
, withHeader, withHeaders, withBearerToken
, withQueryParam, withQueryParams, withTimeout, withCredentials, withDataPath
, Error(..), send, sendCancelable, cancel, toTask, toTaskWithCacheBuster
)
{-| Building and sending GraphQL.
Building of HTTP request has been based on [`elm-http-builder`](http://package.elm-lang.org/packages/lukewestby/elm-http-builder/latest).
# Build a GraphQL
@docs GraphQL, query, mutation, subscription, render, toDecoder
# Build a Request
@docs Request, get, post
@docs withHeader, withHeaders, withBearerToken
@docs withQueryParam, withQueryParams, withTimeout, withCredentials, withDataPath
# Make a Request
@docs Error, send, sendCancelable, cancel, toTask, toTaskWithCacheBuster
-}
import GraphQL.Selector as Selector exposing (Selector)
import Http
import Internal
import Json.Decode as Decode exposing (Decoder, decodeString, errorToString)
import Json.Encode as Encode
import Task exposing (Task)
import Time
import Url
{-| -}
type GraphQL a
= GraphQL String String (Selector a)
{-| Build a GraphQL named query.
GraphQL.Selector.succeed identity
|> GraphQL.Selector.field "me"
[]
(GraphQL.Selector.succeed User
|> GraphQL.Selector.field "id" [] GraphQL.Selector.string
|> GraphQL.Selector.field "firstName" [] GraphQL.Selector.string
|> GraphQL.Selector.field "lastName" [] GraphQL.Selector.string
)
|> query "Me"
It collects a GraphQL which is equal to:
"""
query Me {
me {
id
firstName
lastName
}
}
"""
and decoder is equal to:
Decode.field "me"
(Decode.map3
(Decode.field "id" Decode.string)
(Decode.field "firstName" Decode.string)
(Decode.field "lastName" Decode.string)
)
-}
query : String -> Selector a -> GraphQL a
query =
GraphQL "query"
{-| Build a GraphQL named mutation.
GraphQL.Selector.succeed identity
|> GraphQL.Selector.field "updateMe"
[ ( "firstName", GraphQL.Argument.string "<NAME>" )
]
(GraphQL.Selector.succeed User
|> GraphQL.Selector.field "id" [] GraphQL.Selector.string
|> GraphQL.Selector.field "firstName" [] GraphQL.Selector.string
|> GraphQL.Selector.field "lastName" [] GraphQL.Selector.string
)
|> mutation "RenameUser"
It collects a GraphQL which is equal to:
"""
mutation RenameUser {
updateMe("firstName": "<NAME>") {
id
firstName
lastName
}
}
"""
and decoder is equal to:
Decode.field "updateMe"
(Decode.map3
(Decode.field "id" Decode.string)
(Decode.field "firstName" Decode.string)
(Decode.field "lastName" Decode.string)
)
-}
mutation : String -> Selector a -> GraphQL a
mutation =
GraphQL "mutation"
{-| Build a GraphQL named subscription.
GraphQL.Selector.succeed identity
|> GraphQL.Selector.field "onUpdateMe"
[]
(GraphQL.Selector.succeed User
|> GraphQL.Selector.field "id" [] GraphQL.Selector.string
|> GraphQL.Selector.field "firstName" [] GraphQL.Selector.string
|> GraphQL.Selector.field "lastName" [] GraphQL.Selector.string
)
|> subscription "OnUpdateUser"
It collects a GraphQL which is equal to:
"""
subscription OnUpdateUser {
onUpdateMe {
id
firstName
lastName
}
}
"""
and decoder is equal to:
Decode.field "onUpdateMe"
(Decode.map3
(Decode.field "id" Decode.string)
(Decode.field "firstName" Decode.string)
(Decode.field "lastName" Decode.string)
)
-}
subscription : String -> Selector a -> GraphQL a
subscription =
GraphQL "subscription"
{-| Render GraphQL string representation from a GraphQL.
-}
render : GraphQL a -> String
render (GraphQL operation name selector) =
(operation ++ " " ++ name) ++ Internal.wrap "{" "}" (Selector.render selector)
{-| Build a Decoder from a GraphQL.
-}
toDecoder : GraphQL a -> Decoder a
toDecoder (GraphQL _ _ selector) =
Selector.toDecoder selector
{-| A type for chaining request configuration.
-}
type Request a
= Request
{ method : String
, url : String
, headers : List Http.Header
, body : Http.Body
, decoder : Decoder a
, dataPath : List String
, timeout : Maybe Float
, withCredentials : Bool
, queryParams : List ( String, String )
}
initRequest : Bool -> String -> GraphQL a -> Request a
initRequest isGetMethod url graphql =
let
( method, queryParams, body ) =
if isGetMethod then
( "GET"
, [ ( "query", render graphql ) ]
, Http.emptyBody
)
else
( "POST"
, []
, [ ( "query", Encode.string (render graphql) ) ]
|> Encode.object
|> Http.jsonBody
)
in
Request
{ method = method
, url = url
, headers = []
, body = body
, decoder = toDecoder graphql
, dataPath = [ "data" ]
, timeout = Nothing
, withCredentials = False
, queryParams = queryParams
}
{-| Start building a GET request with a given URL.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
-}
get : String -> GraphQL a -> Request a
get =
initRequest True
{-| Start building a POST request with a given URL.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> post "https://example.com/graphql"
-}
post : String -> GraphQL a -> Request a
post =
initRequest False
{-| Add a single header to a request.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withHeader "Content-Type" "application/json"
|> withHeader "Accept" "application/json"
-}
withHeader : String -> String -> Request a -> Request a
withHeader key value (Request builder) =
Request { builder | headers = Http.header key value :: builder.headers }
{-| Add many headers to a request.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withHeaders
[ ( "Content-Type", "application/json" )
, ( "Accept", "application/json" )
]
-}
withHeaders : List ( String, String ) -> Request a -> Request a
withHeaders headerPairs (Request builder) =
case List.map (\( key, value ) -> Http.header key value) headerPairs of
[] ->
Request builder
newHeaders ->
Request { builder | headers = builder.headers ++ newHeaders }
{-| Add a bearer token to a request.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withBearerToken "<KEY>"
-}
withBearerToken : String -> Request a -> Request a
withBearerToken value request =
withHeader "Authorization" ("Bearer " ++ value) request
{-| Add a query param to the url for the request.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withQueryParams "hello" "world"
|> withQueryParams "baz" "qux"
-- sends a request to https://example.com/graphql?hello=world&baz=qux
-}
withQueryParam : String -> String -> Request a -> Request a
withQueryParam key value (Request builder) =
Request { builder | queryParams = builder.queryParams ++ [ ( key, value ) ] }
{-| Add some query params to the url for the request.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withQueryParams [ ( "hello", "world" ), ( "foo", "bar" ) ]
|> withQueryParams [ ( "baz", "qux" ) ]
-- sends a request to https://example.com/graphql?hello=world&foo=bar&baz=qux
-}
withQueryParams : List ( String, String ) -> Request a -> Request a
withQueryParams queryParams (Request builder) =
Request { builder | queryParams = builder.queryParams ++ queryParams }
{-| Set the `timeout` setting on the request.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withTimeout (10 * Time.second)
-}
withTimeout : Float -> Request a -> Request a
withTimeout timeout (Request builder) =
Request { builder | timeout = Just timeout }
{-| Set the `withCredentials` flag on the request to True. Works via
[`XMLHttpRequest#withCredentials`](https://developer.mozilla.org/en-US/docs/Web/API/XMLHttpRequest/withCredentials).
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withCredentials True
-}
withCredentials : Bool -> Request a -> Request a
withCredentials with (Request builder) =
Request { builder | withCredentials = with }
{-| Set a path of graphql data. Default value is `[ "data" ]`.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withDataPath [ "my", "custom", "data", "path" ]
-}
withDataPath : List String -> Request a -> Request a
withDataPath dataPath (Request builder) =
Request { builder | dataPath = dataPath }
{-| A Request can fail in a couple ways:
- BadUrl means you did not provide a valid URL.
- Timeout means it took too long to get a response.
- NetworkError means the user turned off their wifi, went in a cave, etc.
- BadStatus means you got a response back, but the status code indicates failure.
- BadPayload means you got a response back with a nice status code,
but the body of the response was something unexpected.
The String in this case is a debugging message that
explains what went wrong with your JSON decoder or whatever.
-}
type Error
= BadUrl String
| Timeout
| NetworkError
| BadStatus Int
| BadBody String
responseHandler : Decoder a -> Http.Response String -> Result Error a
responseHandler decoder response =
case response of
Http.BadUrl_ url ->
Err (BadUrl url)
Http.Timeout_ ->
Err Timeout
Http.NetworkError_ ->
Err NetworkError
Http.BadStatus_ meta _ ->
Err (BadStatus meta.statusCode)
Http.GoodStatus_ _ body ->
case decodeString decoder body of
Ok value ->
Ok value
Err err ->
Err (BadBody (errorToString err))
sendHttpRequest : Maybe String -> (Result Error a -> msg) -> Request a -> Cmd msg
sendHttpRequest tracker tagger (Request builder) =
let
config =
{ method = builder.method
, headers = builder.headers
, url = buildFullUrl builder.url builder.queryParams
, body = builder.body
, expect = Http.expectStringResponse tagger (responseHandler (Decode.at builder.dataPath builder.decoder))
, timeout = builder.timeout
, tracker = tracker
}
in
if builder.withCredentials then
Http.riskyRequest config
else
Http.request config
{-| Send the Http request.
-}
send : (Result Error a -> msg) -> Request a -> Cmd msg
send =
sendHttpRequest Nothing
{-| Send the cancelable Http request.
-}
sendCancelable : String -> (Result Error a -> msg) -> Request a -> Cmd msg
sendCancelable =
sendHttpRequest << Just
{-| Try to cancel an ongoing cancelable Http request.
-}
cancel : String -> Cmd msg
cancel =
Http.cancel
toHttpTask : Maybe String -> Request a -> Task Error a
toHttpTask cacheBuster request =
Task.andThen
(\(Request builder) ->
let
config =
{ method = builder.method
, headers = builder.headers
, url = buildFullUrl builder.url builder.queryParams
, body = builder.body
, resolver = Http.stringResolver (responseHandler (Decode.at builder.dataPath builder.decoder))
, timeout = builder.timeout
}
in
if builder.withCredentials then
Http.riskyTask config
else
Http.task config
)
(case cacheBuster of
Nothing ->
Task.succeed request
Just buster ->
Task.map
((|>) request << withQueryParam buster << String.fromInt << Time.posixToMillis)
Time.now
)
{-| Convert the `Request` to a `Task` with all options applied.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> toTask
-- makes a task of request to https://example.com/graphql
-}
toTask : Request a -> Task Error a
toTask =
toHttpTask Nothing
{-| Convert the `Request` to a `Task` with a Time based cache buster added to the URL.
You provide a key for an extra query param, and when the request is sent that
query param will be given a value with the current timestamp.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> toTaskWithCacheBuster "cache_buster"
-- makes a task of request to https://example.com/graphql?cache_buster=1481633217383
-}
toTaskWithCacheBuster : String -> Request a -> Task Error a
toTaskWithCacheBuster =
toHttpTask << Just
buildFullUrl : String -> List ( String, String ) -> String
buildFullUrl url queryParams =
if List.isEmpty queryParams then
url
else
url ++ "?" ++ joinUrlEncoded queryParams
joinUrlEncoded : List ( String, String ) -> String
joinUrlEncoded args =
String.join "&" (List.map queryPair args)
queryPair : ( String, String ) -> String
queryPair ( key, value ) =
queryEscape key ++ "=" ++ queryEscape value
queryEscape : String -> String
queryEscape =
Url.percentEncode >> replace "%20" "+"
replace : String -> String -> String -> String
replace old new =
String.split old >> String.join new
| true | module GraphQL exposing
( GraphQL, query, mutation, subscription, render, toDecoder
, Request, get, post
, withHeader, withHeaders, withBearerToken
, withQueryParam, withQueryParams, withTimeout, withCredentials, withDataPath
, Error(..), send, sendCancelable, cancel, toTask, toTaskWithCacheBuster
)
{-| Building and sending GraphQL.
Building of HTTP request has been based on [`elm-http-builder`](http://package.elm-lang.org/packages/lukewestby/elm-http-builder/latest).
# Build a GraphQL
@docs GraphQL, query, mutation, subscription, render, toDecoder
# Build a Request
@docs Request, get, post
@docs withHeader, withHeaders, withBearerToken
@docs withQueryParam, withQueryParams, withTimeout, withCredentials, withDataPath
# Make a Request
@docs Error, send, sendCancelable, cancel, toTask, toTaskWithCacheBuster
-}
import GraphQL.Selector as Selector exposing (Selector)
import Http
import Internal
import Json.Decode as Decode exposing (Decoder, decodeString, errorToString)
import Json.Encode as Encode
import Task exposing (Task)
import Time
import Url
{-| -}
type GraphQL a
= GraphQL String String (Selector a)
{-| Build a GraphQL named query.
GraphQL.Selector.succeed identity
|> GraphQL.Selector.field "me"
[]
(GraphQL.Selector.succeed User
|> GraphQL.Selector.field "id" [] GraphQL.Selector.string
|> GraphQL.Selector.field "firstName" [] GraphQL.Selector.string
|> GraphQL.Selector.field "lastName" [] GraphQL.Selector.string
)
|> query "Me"
It collects a GraphQL which is equal to:
"""
query Me {
me {
id
firstName
lastName
}
}
"""
and decoder is equal to:
Decode.field "me"
(Decode.map3
(Decode.field "id" Decode.string)
(Decode.field "firstName" Decode.string)
(Decode.field "lastName" Decode.string)
)
-}
query : String -> Selector a -> GraphQL a
query =
GraphQL "query"
{-| Build a GraphQL named mutation.
GraphQL.Selector.succeed identity
|> GraphQL.Selector.field "updateMe"
[ ( "firstName", GraphQL.Argument.string "PI:NAME:<NAME>END_PI" )
]
(GraphQL.Selector.succeed User
|> GraphQL.Selector.field "id" [] GraphQL.Selector.string
|> GraphQL.Selector.field "firstName" [] GraphQL.Selector.string
|> GraphQL.Selector.field "lastName" [] GraphQL.Selector.string
)
|> mutation "RenameUser"
It collects a GraphQL which is equal to:
"""
mutation RenameUser {
updateMe("firstName": "PI:NAME:<NAME>END_PI") {
id
firstName
lastName
}
}
"""
and decoder is equal to:
Decode.field "updateMe"
(Decode.map3
(Decode.field "id" Decode.string)
(Decode.field "firstName" Decode.string)
(Decode.field "lastName" Decode.string)
)
-}
mutation : String -> Selector a -> GraphQL a
mutation =
GraphQL "mutation"
{-| Build a GraphQL named subscription.
GraphQL.Selector.succeed identity
|> GraphQL.Selector.field "onUpdateMe"
[]
(GraphQL.Selector.succeed User
|> GraphQL.Selector.field "id" [] GraphQL.Selector.string
|> GraphQL.Selector.field "firstName" [] GraphQL.Selector.string
|> GraphQL.Selector.field "lastName" [] GraphQL.Selector.string
)
|> subscription "OnUpdateUser"
It collects a GraphQL which is equal to:
"""
subscription OnUpdateUser {
onUpdateMe {
id
firstName
lastName
}
}
"""
and decoder is equal to:
Decode.field "onUpdateMe"
(Decode.map3
(Decode.field "id" Decode.string)
(Decode.field "firstName" Decode.string)
(Decode.field "lastName" Decode.string)
)
-}
subscription : String -> Selector a -> GraphQL a
subscription =
GraphQL "subscription"
{-| Render GraphQL string representation from a GraphQL.
-}
render : GraphQL a -> String
render (GraphQL operation name selector) =
(operation ++ " " ++ name) ++ Internal.wrap "{" "}" (Selector.render selector)
{-| Build a Decoder from a GraphQL.
-}
toDecoder : GraphQL a -> Decoder a
toDecoder (GraphQL _ _ selector) =
Selector.toDecoder selector
{-| A type for chaining request configuration.
-}
type Request a
= Request
{ method : String
, url : String
, headers : List Http.Header
, body : Http.Body
, decoder : Decoder a
, dataPath : List String
, timeout : Maybe Float
, withCredentials : Bool
, queryParams : List ( String, String )
}
initRequest : Bool -> String -> GraphQL a -> Request a
initRequest isGetMethod url graphql =
let
( method, queryParams, body ) =
if isGetMethod then
( "GET"
, [ ( "query", render graphql ) ]
, Http.emptyBody
)
else
( "POST"
, []
, [ ( "query", Encode.string (render graphql) ) ]
|> Encode.object
|> Http.jsonBody
)
in
Request
{ method = method
, url = url
, headers = []
, body = body
, decoder = toDecoder graphql
, dataPath = [ "data" ]
, timeout = Nothing
, withCredentials = False
, queryParams = queryParams
}
{-| Start building a GET request with a given URL.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
-}
get : String -> GraphQL a -> Request a
get =
initRequest True
{-| Start building a POST request with a given URL.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> post "https://example.com/graphql"
-}
post : String -> GraphQL a -> Request a
post =
initRequest False
{-| Add a single header to a request.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withHeader "Content-Type" "application/json"
|> withHeader "Accept" "application/json"
-}
withHeader : String -> String -> Request a -> Request a
withHeader key value (Request builder) =
Request { builder | headers = Http.header key value :: builder.headers }
{-| Add many headers to a request.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withHeaders
[ ( "Content-Type", "application/json" )
, ( "Accept", "application/json" )
]
-}
withHeaders : List ( String, String ) -> Request a -> Request a
withHeaders headerPairs (Request builder) =
case List.map (\( key, value ) -> Http.header key value) headerPairs of
[] ->
Request builder
newHeaders ->
Request { builder | headers = builder.headers ++ newHeaders }
{-| Add a bearer token to a request.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withBearerToken "PI:KEY:<KEY>END_PI"
-}
withBearerToken : String -> Request a -> Request a
withBearerToken value request =
withHeader "Authorization" ("Bearer " ++ value) request
{-| Add a query param to the url for the request.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withQueryParams "hello" "world"
|> withQueryParams "baz" "qux"
-- sends a request to https://example.com/graphql?hello=world&baz=qux
-}
withQueryParam : String -> String -> Request a -> Request a
withQueryParam key value (Request builder) =
Request { builder | queryParams = builder.queryParams ++ [ ( key, value ) ] }
{-| Add some query params to the url for the request.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withQueryParams [ ( "hello", "world" ), ( "foo", "bar" ) ]
|> withQueryParams [ ( "baz", "qux" ) ]
-- sends a request to https://example.com/graphql?hello=world&foo=bar&baz=qux
-}
withQueryParams : List ( String, String ) -> Request a -> Request a
withQueryParams queryParams (Request builder) =
Request { builder | queryParams = builder.queryParams ++ queryParams }
{-| Set the `timeout` setting on the request.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withTimeout (10 * Time.second)
-}
withTimeout : Float -> Request a -> Request a
withTimeout timeout (Request builder) =
Request { builder | timeout = Just timeout }
{-| Set the `withCredentials` flag on the request to True. Works via
[`XMLHttpRequest#withCredentials`](https://developer.mozilla.org/en-US/docs/Web/API/XMLHttpRequest/withCredentials).
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withCredentials True
-}
withCredentials : Bool -> Request a -> Request a
withCredentials with (Request builder) =
Request { builder | withCredentials = with }
{-| Set a path of graphql data. Default value is `[ "data" ]`.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> withDataPath [ "my", "custom", "data", "path" ]
-}
withDataPath : List String -> Request a -> Request a
withDataPath dataPath (Request builder) =
Request { builder | dataPath = dataPath }
{-| A Request can fail in a couple ways:
- BadUrl means you did not provide a valid URL.
- Timeout means it took too long to get a response.
- NetworkError means the user turned off their wifi, went in a cave, etc.
- BadStatus means you got a response back, but the status code indicates failure.
- BadPayload means you got a response back with a nice status code,
but the body of the response was something unexpected.
The String in this case is a debugging message that
explains what went wrong with your JSON decoder or whatever.
-}
type Error
= BadUrl String
| Timeout
| NetworkError
| BadStatus Int
| BadBody String
responseHandler : Decoder a -> Http.Response String -> Result Error a
responseHandler decoder response =
case response of
Http.BadUrl_ url ->
Err (BadUrl url)
Http.Timeout_ ->
Err Timeout
Http.NetworkError_ ->
Err NetworkError
Http.BadStatus_ meta _ ->
Err (BadStatus meta.statusCode)
Http.GoodStatus_ _ body ->
case decodeString decoder body of
Ok value ->
Ok value
Err err ->
Err (BadBody (errorToString err))
sendHttpRequest : Maybe String -> (Result Error a -> msg) -> Request a -> Cmd msg
sendHttpRequest tracker tagger (Request builder) =
let
config =
{ method = builder.method
, headers = builder.headers
, url = buildFullUrl builder.url builder.queryParams
, body = builder.body
, expect = Http.expectStringResponse tagger (responseHandler (Decode.at builder.dataPath builder.decoder))
, timeout = builder.timeout
, tracker = tracker
}
in
if builder.withCredentials then
Http.riskyRequest config
else
Http.request config
{-| Send the Http request.
-}
send : (Result Error a -> msg) -> Request a -> Cmd msg
send =
sendHttpRequest Nothing
{-| Send the cancelable Http request.
-}
sendCancelable : String -> (Result Error a -> msg) -> Request a -> Cmd msg
sendCancelable =
sendHttpRequest << Just
{-| Try to cancel an ongoing cancelable Http request.
-}
cancel : String -> Cmd msg
cancel =
Http.cancel
toHttpTask : Maybe String -> Request a -> Task Error a
toHttpTask cacheBuster request =
Task.andThen
(\(Request builder) ->
let
config =
{ method = builder.method
, headers = builder.headers
, url = buildFullUrl builder.url builder.queryParams
, body = builder.body
, resolver = Http.stringResolver (responseHandler (Decode.at builder.dataPath builder.decoder))
, timeout = builder.timeout
}
in
if builder.withCredentials then
Http.riskyTask config
else
Http.task config
)
(case cacheBuster of
Nothing ->
Task.succeed request
Just buster ->
Task.map
((|>) request << withQueryParam buster << String.fromInt << Time.posixToMillis)
Time.now
)
{-| Convert the `Request` to a `Task` with all options applied.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> toTask
-- makes a task of request to https://example.com/graphql
-}
toTask : Request a -> Task Error a
toTask =
toHttpTask Nothing
{-| Convert the `Request` to a `Task` with a Time based cache buster added to the URL.
You provide a key for an extra query param, and when the request is sent that
query param will be given a value with the current timestamp.
GraphQL.Selector.succeed Tuple.pair
|> GraphQL.Selector.field "me" [] userSelector
|> GraphQL.Selector.field "articles" [] (GraphQL.Selector.list articleSelector)
|> query "InitialData"
|> get "https://example.com/graphql"
|> toTaskWithCacheBuster "cache_buster"
-- makes a task of request to https://example.com/graphql?cache_buster=1481633217383
-}
toTaskWithCacheBuster : String -> Request a -> Task Error a
toTaskWithCacheBuster =
toHttpTask << Just
buildFullUrl : String -> List ( String, String ) -> String
buildFullUrl url queryParams =
if List.isEmpty queryParams then
url
else
url ++ "?" ++ joinUrlEncoded queryParams
joinUrlEncoded : List ( String, String ) -> String
joinUrlEncoded args =
String.join "&" (List.map queryPair args)
queryPair : ( String, String ) -> String
queryPair ( key, value ) =
queryEscape key ++ "=" ++ queryEscape value
queryEscape : String -> String
queryEscape =
Url.percentEncode >> replace "%20" "+"
replace : String -> String -> String -> String
replace old new =
String.split old >> String.join new
| elm |
[
{
"context": " \"\"\"This is *italicized inline HTML <bio name=\"Dillon Kearns\" photo=\"https://avatars2.githubusercontent.com/u/",
"end": 21291,
"score": 0.9998727441,
"start": 21278,
"tag": "NAME",
"value": "Dillon Kearns"
},
{
"context": " , { name = \"name\", value = \"Dillon Kearns\" }\n ]\n",
"end": 22109,
"score": 0.9998202324,
"start": 22096,
"tag": "NAME",
"value": "Dillon Kearns"
},
{
"context": "\" <|\n \\() ->\n \"<foo@bar.example.com>\\n\"\n |> expectOk\n ",
"end": 30299,
"score": 0.9999085665,
"start": 30280,
"tag": "EMAIL",
"value": "foo@bar.example.com"
},
{
"context": "ph\n [ Link \"mailto:foo@bar.example.com\" Nothing [ Text \"foo@bar.example.com\" ]\n ",
"end": 30446,
"score": 0.9999068379,
"start": 30427,
"tag": "EMAIL",
"value": "foo@bar.example.com"
},
{
"context": "Link \"mailto:foo@bar.example.com\" Nothing [ Text \"foo@bar.example.com\" ]\n ]\n ",
"end": 30483,
"score": 0.9998999834,
"start": 30464,
"tag": "EMAIL",
"value": "foo@bar.example.com"
}
] | tests/Tests.elm | klaftertief/elm-markdown | 0 | module Tests exposing (suite)
import Expect exposing (Expectation)
import Markdown.Block as Block exposing (..)
import Markdown.Parser as Markdown exposing (..)
import Parser
import Parser.Advanced as Advanced exposing ((|.), (|=))
import Test exposing (..)
type alias Parser a =
Advanced.Parser String Parser.Problem a
parse : String -> Result (List (Advanced.DeadEnd String Parser.Problem)) (List Block)
parse markdown =
markdown
|> Markdown.parse
suite : Test
suite =
describe "parsing"
[ describe "headings"
[ test "Heading 1" <|
\() ->
"# Hello!"
|> parse
|> Expect.equal (Ok [ Block.Heading Block.H1 (unstyledText "Hello!") ])
, test "heading can end with trailing #'s'" <|
\() ->
"# Hello! ###"
|> parse
|> Expect.equal (Ok [ Block.Heading Block.H1 (unstyledText "Hello!") ])
, test "Heading 2" <|
\() ->
"## Hello!"
|> parse
|> Expect.equal (Ok [ Block.Heading Block.H2 (unstyledText "Hello!") ])
, test "Emphasis line is not interpreted as a list" <|
\() ->
"*This is not a list, it's a paragraph with emphasis*\n"
|> parse
|> Expect.equal (Ok [ Block.Paragraph (emphasisText "This is not a list, it's a paragraph with emphasis") ])
, test "Line starting with a decimal is not interpreted as a list" <|
\() ->
"3.5 is a number - is not a list\n"
|> parse
|> Expect.equal (Ok [ Block.Paragraph (unstyledText "3.5 is a number - is not a list") ])
, test "Heading 7 is parsed using fallback parsing" <|
\() ->
"####### Hello!"
|> expectOk [ Block.Paragraph [ Text "####### Hello!" ] ]
]
, test "plain text" <|
\() ->
"This is just some text"
|> parse
|> Expect.equal (Ok [ Block.Paragraph (unstyledText "This is just some text") ])
, test "parse heading then plain text" <|
\() ->
"""# Heading
This is just some text
"""
|> parse
|> Expect.equal
(Ok
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.Paragraph (unstyledText "This is just some text")
]
)
, test "doesn't need to end in newline" <|
\() ->
"""# Heading
This is just some text"""
|> parse
|> Expect.equal
(Ok
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.Paragraph (unstyledText "This is just some text")
]
)
, test "long example" <|
\() ->
"""# Heading
This is just some text.
## Subheading
Body of the subheading.
"""
|> parse
|> Expect.equal
(Ok
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.Paragraph (unstyledText "This is just some text.")
, Block.Heading Block.H2 (unstyledText "Subheading")
, Block.Paragraph (unstyledText "Body of the subheading.")
]
)
, test "embedded HTML" <|
\() ->
"""# Heading
<div>
Hello!
</div>
"""
|> parse
|> Expect.equal
(Ok
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.HtmlBlock
(Block.HtmlElement "div"
[]
[ Block.Paragraph (unstyledText "Hello!")
]
)
]
)
, test "heading within HTML" <|
\() ->
"""# Heading
<div>
# Heading in a div!
</div>
"""
|> parse
|> Expect.equal
(Ok
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.HtmlBlock
(Block.HtmlElement "div"
[]
[ Block.Heading Block.H1 (unstyledText "Heading in a div!")
]
)
]
)
, test "simple list" <|
\() ->
"""- One
- Two
- Three
"""
|> parse
|> Expect.equal
(Ok
[ Block.UnorderedList
[ plainListItem "One"
, plainListItem "Two"
, plainListItem "Three"
]
-- TODO why is this extra block here? Fix
-- , ListBlock []
]
)
, test "sibling unordered lists with different markers" <|
\() ->
"""- Item 1
- Item 2
- Item 3
+ Item 4
+ Item 5
+ Item 6
* Item 7
* Item 8
* Item 9
"""
|> parse
|> Expect.equal
(Ok
[ Block.UnorderedList
[ plainListItem "Item 1"
, plainListItem "Item 2"
, plainListItem "Item 3"
]
, Block.UnorderedList
[ plainListItem "Item 4"
, plainListItem "Item 5"
, plainListItem "Item 6"
]
, Block.UnorderedList
[ plainListItem "Item 7"
, plainListItem "Item 8"
, plainListItem "Item 9"
]
]
)
, test "sibling ordered lists with different markers" <|
\() ->
"""1. foo
2. bar
3) baz
"""
|> parse
|> Expect.equal
(Ok
[ Block.OrderedList 1
[ unstyledText "foo"
, unstyledText "bar"
]
, Block.OrderedList 3
[ unstyledText "baz"
]
]
)
, test "A paragraph with a numeral that is NOT 1 in the text before a blank line" <|
\() ->
"""The number of windows in my house is
14. The number of doors is 6."""
|> parse
|> Expect.equal
(Ok
[ Block.Paragraph (unstyledText "The number of windows in my house is\n14. The number of doors is 6.")
]
)
, test "A paragraph with a numeral that IS 1 in the text" <|
\() ->
"""The number of windows in my house is
1. The number of doors is 6.
"""
|> parse
|> Expect.equal
(Ok
[ Block.Paragraph (unstyledText "The number of windows in my house is")
, Block.OrderedList 1
[ unstyledText "The number of doors is 6."
]
]
)
, test "thematic break" <|
\() ->
"""---"""
|> parse
|> Expect.equal
(Ok
[ Block.ThematicBreak
]
)
, test "simple table" <|
\() ->
"""| abc | def |
|---|---|
"""
|> parse
|> Expect.equal
(Ok
[ Block.Table
[ { label = [ Text "abc" ], alignment = Nothing }
, { label = [ Text "def" ], alignment = Nothing }
]
[]
]
)
, test "simple table with data" <|
\() ->
"""| abc | def |
|---|---|
| foo | bar |
| bar | baz |
"""
|> parse
|> Expect.equal
(Ok
[ Block.Table
[ { label = [ Text "abc" ], alignment = Nothing }
, { label = [ Text "def" ], alignment = Nothing }
]
[ [ [ Text "foo" ], [ Text "bar" ] ]
, [ [ Text "bar" ], [ Text "baz" ] ]
]
]
)
, test "table with alignment" <|
\() ->
"""| abc | def | ghi | jkl
|:---|:------:|--:|---|
| foo | bar | baz | boo |
| bar | baz | boo | foo |
"""
|> parse
|> Expect.equal
(Ok
[ Block.Table
[ { label = [ Text "abc" ], alignment = Just AlignLeft }
, { label = [ Text "def" ], alignment = Just AlignCenter }
, { label = [ Text "ghi" ], alignment = Just AlignRight }
, { label = [ Text "jkl" ], alignment = Nothing }
]
[ [ [ Text "foo" ], [ Text "bar" ], [ Text "baz" ], [ Text "boo" ] ]
, [ [ Text "bar" ], [ Text "baz" ], [ Text "boo" ], [ Text "foo" ] ]
]
]
)
, test "table with a cell that looks like a heading but isn't" <|
\() ->
"""| abc | def |
| --- | --- |
| bar | baz |
####### asdf
"""
|> parse
|> Expect.equal
(Ok
[ Block.Table
[ { label = [ Text "abc" ], alignment = Nothing }
, { label = [ Text "def" ], alignment = Nothing }
]
[ [ [ Text "bar" ], [ Text "baz" ] ]
, [ [ Text "####### asdf" ], [] ]
]
]
)
, test "table ended by a heading" <|
\() ->
"""| abc | def |
| --- | --- |
| bar | baz |
###### asdf
"""
|> parse
|> Expect.equal
(Ok
[ Block.Table
[ { label = [ Text "abc" ], alignment = Nothing }
, { label = [ Text "def" ], alignment = Nothing }
]
[ [ [ Text "bar" ], [ Text "baz" ] ]
]
, Block.Heading Block.H6 [ Text "asdf" ]
]
)
, test "tables separated by a blank line should be separate" <|
\() ->
"""| abc | def |
| --- | --- |
| bar | baz |
| abc | def |
| --- | --- |
| bar | baz |
"""
|> parse
|> Expect.equal
(Ok
[ Block.Table
[ { label = [ Text "abc" ], alignment = Nothing }
, { label = [ Text "def" ], alignment = Nothing }
]
[ [ [ Text "bar" ], [ Text "baz" ] ]
]
, Block.Table
[ { label = [ Text "abc" ], alignment = Nothing }
, { label = [ Text "def" ], alignment = Nothing }
]
[ [ [ Text "bar" ], [ Text "baz" ] ]
]
]
)
, test "multiple thematic breaks" <|
\() ->
"""***
---
___"""
|> parse
|> Expect.equal
(Ok
[ Block.ThematicBreak
, Block.ThematicBreak
, Block.ThematicBreak
]
)
, test "thematic break followed by newline" <|
\() ->
"""---
"""
|> parse
|> Expect.equal
(Ok
[ Block.ThematicBreak
]
)
, test "blank lines are ignored" <|
\() ->
" \n \n \n\t\n"
|> parse
|> Expect.equal (Ok [])
, test "mixed content with list" <|
\() ->
"""# Title
- This is an item
- And so is this
Text after
"""
|> parse
|> Expect.equal
(Ok
[ Block.Heading Block.H1 (unstyledText "Title")
, Block.UnorderedList
[ plainListItem "This is an item"
, plainListItem "And so is this"
]
, Block.Paragraph (unstyledText "Text after")
-- TODO why is this extra block here? Fix
-- , ListBlock []
]
)
, test "code fence with paragraph and heading below" <|
\() ->
"""```shell
.
├── content/
├── elm.json
├── images/
├── static/
├── index.js
├── package.json
└── src/
└── Main.elm
```
This is more stuff
## h2
qwer
"""
|> parse
|> Expect.equal
(Ok
[ Block.CodeBlock
{ body = ".\n├── content/\n├── elm.json\n├── images/\n├── static/\n├── index.js\n├── package.json\n└── src/\n └── Main.elm"
, language = Just "shell"
}
, Block.Paragraph (unstyledText "This is more stuff")
, Block.Heading Block.H2 (unstyledText "h2")
, Block.Paragraph (unstyledText "qwer")
]
)
, test "indented code block" <|
\() ->
""" foo = 123"""
|> parse
|> Expect.equal (Ok [ Block.CodeBlock { body = "foo = 123", language = Nothing } ])
, test "indented code block with tab" <|
\() ->
"""\tfoo = 123"""
|> parse
|> Expect.equal (Ok [ Block.CodeBlock { body = "foo = 123", language = Nothing } ])
, test "image" <|
\() ->
""""""
|> parse
|> Expect.equal
(Ok
[ Block.Paragraph
[ Block.Image "/my/image.jpg" Nothing [ Block.Text "This is an image" ]
--{ string = "This is an image"
-- , style =
-- { isBold = False
-- , isCode = False
-- , isItalic = False
-- , link = Just { destination = Block.Image "/my/image.jpg", title = Nothing }
-- }
--
-- }
]
]
)
--, skip <|
-- test "autolink" <|
-- \() ->
-- "<http://foo.bar.baz>\n"
-- |> parse
-- |> Expect.equal
-- (Ok
-- [ Block.Paragraph
-- [ Block.Link "http://foo.bar.baz" Nothing [ Block.Text "http://foo.bar.baz" ] ]
-- ]
-- )
, describe "blank line"
[ test "even though paragraphs can start with blank lines, it is not a paragraph if there are only blanks" <|
\() ->
" \n"
|> parse
|> Expect.equal (Ok [])
]
, describe "block quotes"
[ test "Simple block quote" <|
\() ->
">This is a quote\n"
|> parse
|> Expect.equal (Ok [ Block.BlockQuote [ Block.Paragraph (unstyledText "This is a quote") ] ])
, test "block quote with a space after" <|
\() ->
"> This is a quote\n"
|> parse
|> Expect.equal (Ok [ Block.BlockQuote [ Block.Paragraph (unstyledText "This is a quote") ] ])
, test "consecutive block quote lines are combined" <|
\() ->
"""> # Heading
> Body
"""
|> parse
|> Expect.equal
(Ok
[ Block.BlockQuote
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.Paragraph (unstyledText "Body")
]
]
)
, test "plain lines immediately after block quote lines are combined" <|
\() ->
"""> # Heading
I'm part of the block quote
"""
|> parse
|> Expect.equal
(Ok
[ Block.BlockQuote
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.Paragraph (unstyledText "I'm part of the block quote")
]
]
)
]
, test "indented code" <|
\() ->
""" sum a b =
a + b
"""
|> parse
|> Expect.equal
(Ok
[ Block.CodeBlock
{ body = "sum a b =\n a + b"
, language = Nothing
}
]
)
, test "block quotes eat the first space and allow paragraphs to start with 3 spaces" <|
\() ->
"""> code
> not code
"""
|> parse
|> Expect.equal
(Ok
[ Block.BlockQuote
[ Block.CodeBlock
{ body = "code"
, language = Nothing
}
]
, Block.BlockQuote
[ Block.Paragraph (unstyledText "not code")
]
]
)
, test "inline HTML" <|
\() ->
"""This is *italicized inline HTML <bio name="Dillon Kearns" photo="https://avatars2.githubusercontent.com/u/1384166" />*"""
|> parse
|> Expect.equal
(Ok
[ Block.Paragraph
[ Block.Text "This is "
, Block.Emphasis
[ Block.Text "italicized inline HTML "
, Block.HtmlInline
(Block.HtmlElement "bio"
-- NOTE: attribute names are in reverse alphabetical order
[ { name = "photo", value = "https://avatars2.githubusercontent.com/u/1384166" }
, { name = "name", value = "Dillon Kearns" }
]
[]
)
]
]
]
)
, test "blank lines separate paragraphs within block quote" <|
\() ->
"""> foo
>
> bar
"""
|> parse
|> Expect.equal
(Ok
[ Block.BlockQuote
[ Block.Paragraph (unstyledText "foo")
, Block.Paragraph (unstyledText "bar")
]
]
)
, test "hard line break with two spaces" <|
\() ->
"foo \nbaz"
|> parse
|> Expect.equal
(Ok
[ Paragraph
[ Text "foo"
, HardLineBreak
, Text "baz"
]
]
)
, test "indented code blocks cannot interrupt paragraphs" <|
\() ->
"""aaa
bbb
ccc"""
|> parse
|> Expect.equal
(Ok
[ Paragraph
[ Text
"""aaa
bbb
ccc"""
]
]
)
, test "keeps items grouped in a paragraph within block quotes when there are no blank lines separating them" <|
\() ->
"""> # Foo
> bar
> baz
"""
|> parse
|> Expect.equal
(Ok
[ Block.BlockQuote
[ Block.Heading Block.H1 (unstyledText "Foo")
, Block.Paragraph (unstyledText "bar\nbaz")
]
]
)
, test "backslash line break" <|
\() ->
"Before\\\nAfter"
|> parse
|> Expect.equal
(Ok
[ Block.Paragraph
[ Block.Text "Before"
, Block.HardLineBreak
, Block.Text "After"
]
]
)
, describe "html"
[ test "html comment" <|
\() ->
"<!-- hello! -->"
|> parse
|> Expect.equal
(Ok
[ Block.HtmlBlock (Block.HtmlComment " hello! ") ]
)
, test "nested html comment" <|
\() ->
"""<Resources>
<Book title="Crime and Punishment">
<!-- this is the book review -->
This is my review...
</Book>
</Resources>
"""
|> parse
|> Expect.equal
(Ok
[ HtmlBlock
(HtmlElement "resources"
[]
[ HtmlBlock
(HtmlElement "book"
[ { name = "title", value = "Crime and Punishment" } ]
[ HtmlBlock (HtmlComment " this is the book review ")
, Paragraph [ Text "This is my review..." ]
]
)
]
)
]
)
]
, test "HTML declaration" <|
\() ->
"""<!DOCTYPE html>"""
|> parse
|> Expect.equal
(Ok
[ HtmlBlock (HtmlDeclaration "DOCTYPE" "html") ]
)
, describe "inline html"
[ test "cdata sections" <|
\() ->
"foo <![CDATA[>&<]]>"
|> parse
|> Expect.equal
(Ok
[ Paragraph
[ Text "foo "
, HtmlInline (Cdata ">&<")
]
]
)
, test "nested HTML" <|
\() ->
"""foo <Resources><Resource type="book" title="Notes From Underground" /></Resources>"""
|> parse
|> Expect.equal
(Ok
[ Paragraph
[ Text "foo "
, HtmlInline
(HtmlElement "resources"
[]
[ HtmlBlock
(HtmlElement "resource"
[ { name = "type", value = "book" }
, { name = "title", value = "Notes From Underground" }
]
[]
)
]
)
]
]
)
, test "nested markdown within nested HTML" <|
\() ->
"""foo <Resources><Resource type="book" title="Notes From Underground" />9/10 interesting read!</Resources>"""
|> parse
|> Expect.equal
(Ok
[ Paragraph
[ Text "foo "
, HtmlInline
(HtmlElement "resources"
[]
[ HtmlBlock
(HtmlElement "resource"
[ { name = "type", value = "book" }
, { name = "title", value = "Notes From Underground" }
]
[]
)
, Paragraph [ Text "9/10 interesting read!" ]
]
)
]
]
)
]
, describe "beginning with autolink"
[ test "simple autolink" <|
\() ->
"<https://elm-lang.org>\n"
|> expectOk
[ Paragraph
[ Link "https://elm-lang.org" Nothing [ Text "https://elm-lang.org" ]
]
]
, test "email autolink" <|
\() ->
"<foo@bar.example.com>\n"
|> expectOk
[ Paragraph
[ Link "mailto:foo@bar.example.com" Nothing [ Text "foo@bar.example.com" ]
]
]
]
, describe "link reference definitions"
[ test "basic example" <|
\() ->
"""[foo]: /url "title"
[foo]
"""
|> parse
|> Expect.equal
(Ok [ Paragraph [ Link "/url" (Just "title") [ Text "foo" ] ] ])
, test "invalid reference uses fallback paragraph parsing" <|
\() ->
"""[foo]:
[foo]
"""
|> parse
|> Expect.equal
(Ok
[ Paragraph [ Text "[foo]:" ]
, Paragraph [ Text "[foo]" ]
]
)
]
]
expectOk : List Block -> String -> Expectation
expectOk expected input =
case input |> parse of
Ok actual ->
actual
|> Expect.equal expected
Err error ->
Expect.fail (Debug.toString error)
plainListItem : String -> Block.ListItem Block.Inline
plainListItem body =
Block.ListItem Block.NoTask [ Block.Text body ]
unstyledText : String -> List Inline
unstyledText body =
[ Block.Text body ]
emphasisText : String -> List Inline
emphasisText body =
[ Block.Emphasis <|
[ Block.Text body ]
]
parserError : String -> Expect.Expectation
parserError markdown =
case parse markdown of
Ok _ ->
Expect.fail "Expected a parser failure!"
Err _ ->
Expect.pass
| 55685 | module Tests exposing (suite)
import Expect exposing (Expectation)
import Markdown.Block as Block exposing (..)
import Markdown.Parser as Markdown exposing (..)
import Parser
import Parser.Advanced as Advanced exposing ((|.), (|=))
import Test exposing (..)
type alias Parser a =
Advanced.Parser String Parser.Problem a
parse : String -> Result (List (Advanced.DeadEnd String Parser.Problem)) (List Block)
parse markdown =
markdown
|> Markdown.parse
suite : Test
suite =
describe "parsing"
[ describe "headings"
[ test "Heading 1" <|
\() ->
"# Hello!"
|> parse
|> Expect.equal (Ok [ Block.Heading Block.H1 (unstyledText "Hello!") ])
, test "heading can end with trailing #'s'" <|
\() ->
"# Hello! ###"
|> parse
|> Expect.equal (Ok [ Block.Heading Block.H1 (unstyledText "Hello!") ])
, test "Heading 2" <|
\() ->
"## Hello!"
|> parse
|> Expect.equal (Ok [ Block.Heading Block.H2 (unstyledText "Hello!") ])
, test "Emphasis line is not interpreted as a list" <|
\() ->
"*This is not a list, it's a paragraph with emphasis*\n"
|> parse
|> Expect.equal (Ok [ Block.Paragraph (emphasisText "This is not a list, it's a paragraph with emphasis") ])
, test "Line starting with a decimal is not interpreted as a list" <|
\() ->
"3.5 is a number - is not a list\n"
|> parse
|> Expect.equal (Ok [ Block.Paragraph (unstyledText "3.5 is a number - is not a list") ])
, test "Heading 7 is parsed using fallback parsing" <|
\() ->
"####### Hello!"
|> expectOk [ Block.Paragraph [ Text "####### Hello!" ] ]
]
, test "plain text" <|
\() ->
"This is just some text"
|> parse
|> Expect.equal (Ok [ Block.Paragraph (unstyledText "This is just some text") ])
, test "parse heading then plain text" <|
\() ->
"""# Heading
This is just some text
"""
|> parse
|> Expect.equal
(Ok
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.Paragraph (unstyledText "This is just some text")
]
)
, test "doesn't need to end in newline" <|
\() ->
"""# Heading
This is just some text"""
|> parse
|> Expect.equal
(Ok
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.Paragraph (unstyledText "This is just some text")
]
)
, test "long example" <|
\() ->
"""# Heading
This is just some text.
## Subheading
Body of the subheading.
"""
|> parse
|> Expect.equal
(Ok
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.Paragraph (unstyledText "This is just some text.")
, Block.Heading Block.H2 (unstyledText "Subheading")
, Block.Paragraph (unstyledText "Body of the subheading.")
]
)
, test "embedded HTML" <|
\() ->
"""# Heading
<div>
Hello!
</div>
"""
|> parse
|> Expect.equal
(Ok
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.HtmlBlock
(Block.HtmlElement "div"
[]
[ Block.Paragraph (unstyledText "Hello!")
]
)
]
)
, test "heading within HTML" <|
\() ->
"""# Heading
<div>
# Heading in a div!
</div>
"""
|> parse
|> Expect.equal
(Ok
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.HtmlBlock
(Block.HtmlElement "div"
[]
[ Block.Heading Block.H1 (unstyledText "Heading in a div!")
]
)
]
)
, test "simple list" <|
\() ->
"""- One
- Two
- Three
"""
|> parse
|> Expect.equal
(Ok
[ Block.UnorderedList
[ plainListItem "One"
, plainListItem "Two"
, plainListItem "Three"
]
-- TODO why is this extra block here? Fix
-- , ListBlock []
]
)
, test "sibling unordered lists with different markers" <|
\() ->
"""- Item 1
- Item 2
- Item 3
+ Item 4
+ Item 5
+ Item 6
* Item 7
* Item 8
* Item 9
"""
|> parse
|> Expect.equal
(Ok
[ Block.UnorderedList
[ plainListItem "Item 1"
, plainListItem "Item 2"
, plainListItem "Item 3"
]
, Block.UnorderedList
[ plainListItem "Item 4"
, plainListItem "Item 5"
, plainListItem "Item 6"
]
, Block.UnorderedList
[ plainListItem "Item 7"
, plainListItem "Item 8"
, plainListItem "Item 9"
]
]
)
, test "sibling ordered lists with different markers" <|
\() ->
"""1. foo
2. bar
3) baz
"""
|> parse
|> Expect.equal
(Ok
[ Block.OrderedList 1
[ unstyledText "foo"
, unstyledText "bar"
]
, Block.OrderedList 3
[ unstyledText "baz"
]
]
)
, test "A paragraph with a numeral that is NOT 1 in the text before a blank line" <|
\() ->
"""The number of windows in my house is
14. The number of doors is 6."""
|> parse
|> Expect.equal
(Ok
[ Block.Paragraph (unstyledText "The number of windows in my house is\n14. The number of doors is 6.")
]
)
, test "A paragraph with a numeral that IS 1 in the text" <|
\() ->
"""The number of windows in my house is
1. The number of doors is 6.
"""
|> parse
|> Expect.equal
(Ok
[ Block.Paragraph (unstyledText "The number of windows in my house is")
, Block.OrderedList 1
[ unstyledText "The number of doors is 6."
]
]
)
, test "thematic break" <|
\() ->
"""---"""
|> parse
|> Expect.equal
(Ok
[ Block.ThematicBreak
]
)
, test "simple table" <|
\() ->
"""| abc | def |
|---|---|
"""
|> parse
|> Expect.equal
(Ok
[ Block.Table
[ { label = [ Text "abc" ], alignment = Nothing }
, { label = [ Text "def" ], alignment = Nothing }
]
[]
]
)
, test "simple table with data" <|
\() ->
"""| abc | def |
|---|---|
| foo | bar |
| bar | baz |
"""
|> parse
|> Expect.equal
(Ok
[ Block.Table
[ { label = [ Text "abc" ], alignment = Nothing }
, { label = [ Text "def" ], alignment = Nothing }
]
[ [ [ Text "foo" ], [ Text "bar" ] ]
, [ [ Text "bar" ], [ Text "baz" ] ]
]
]
)
, test "table with alignment" <|
\() ->
"""| abc | def | ghi | jkl
|:---|:------:|--:|---|
| foo | bar | baz | boo |
| bar | baz | boo | foo |
"""
|> parse
|> Expect.equal
(Ok
[ Block.Table
[ { label = [ Text "abc" ], alignment = Just AlignLeft }
, { label = [ Text "def" ], alignment = Just AlignCenter }
, { label = [ Text "ghi" ], alignment = Just AlignRight }
, { label = [ Text "jkl" ], alignment = Nothing }
]
[ [ [ Text "foo" ], [ Text "bar" ], [ Text "baz" ], [ Text "boo" ] ]
, [ [ Text "bar" ], [ Text "baz" ], [ Text "boo" ], [ Text "foo" ] ]
]
]
)
, test "table with a cell that looks like a heading but isn't" <|
\() ->
"""| abc | def |
| --- | --- |
| bar | baz |
####### asdf
"""
|> parse
|> Expect.equal
(Ok
[ Block.Table
[ { label = [ Text "abc" ], alignment = Nothing }
, { label = [ Text "def" ], alignment = Nothing }
]
[ [ [ Text "bar" ], [ Text "baz" ] ]
, [ [ Text "####### asdf" ], [] ]
]
]
)
, test "table ended by a heading" <|
\() ->
"""| abc | def |
| --- | --- |
| bar | baz |
###### asdf
"""
|> parse
|> Expect.equal
(Ok
[ Block.Table
[ { label = [ Text "abc" ], alignment = Nothing }
, { label = [ Text "def" ], alignment = Nothing }
]
[ [ [ Text "bar" ], [ Text "baz" ] ]
]
, Block.Heading Block.H6 [ Text "asdf" ]
]
)
, test "tables separated by a blank line should be separate" <|
\() ->
"""| abc | def |
| --- | --- |
| bar | baz |
| abc | def |
| --- | --- |
| bar | baz |
"""
|> parse
|> Expect.equal
(Ok
[ Block.Table
[ { label = [ Text "abc" ], alignment = Nothing }
, { label = [ Text "def" ], alignment = Nothing }
]
[ [ [ Text "bar" ], [ Text "baz" ] ]
]
, Block.Table
[ { label = [ Text "abc" ], alignment = Nothing }
, { label = [ Text "def" ], alignment = Nothing }
]
[ [ [ Text "bar" ], [ Text "baz" ] ]
]
]
)
, test "multiple thematic breaks" <|
\() ->
"""***
---
___"""
|> parse
|> Expect.equal
(Ok
[ Block.ThematicBreak
, Block.ThematicBreak
, Block.ThematicBreak
]
)
, test "thematic break followed by newline" <|
\() ->
"""---
"""
|> parse
|> Expect.equal
(Ok
[ Block.ThematicBreak
]
)
, test "blank lines are ignored" <|
\() ->
" \n \n \n\t\n"
|> parse
|> Expect.equal (Ok [])
, test "mixed content with list" <|
\() ->
"""# Title
- This is an item
- And so is this
Text after
"""
|> parse
|> Expect.equal
(Ok
[ Block.Heading Block.H1 (unstyledText "Title")
, Block.UnorderedList
[ plainListItem "This is an item"
, plainListItem "And so is this"
]
, Block.Paragraph (unstyledText "Text after")
-- TODO why is this extra block here? Fix
-- , ListBlock []
]
)
, test "code fence with paragraph and heading below" <|
\() ->
"""```shell
.
├── content/
├── elm.json
├── images/
├── static/
├── index.js
├── package.json
└── src/
└── Main.elm
```
This is more stuff
## h2
qwer
"""
|> parse
|> Expect.equal
(Ok
[ Block.CodeBlock
{ body = ".\n├── content/\n├── elm.json\n├── images/\n├── static/\n├── index.js\n├── package.json\n└── src/\n └── Main.elm"
, language = Just "shell"
}
, Block.Paragraph (unstyledText "This is more stuff")
, Block.Heading Block.H2 (unstyledText "h2")
, Block.Paragraph (unstyledText "qwer")
]
)
, test "indented code block" <|
\() ->
""" foo = 123"""
|> parse
|> Expect.equal (Ok [ Block.CodeBlock { body = "foo = 123", language = Nothing } ])
, test "indented code block with tab" <|
\() ->
"""\tfoo = 123"""
|> parse
|> Expect.equal (Ok [ Block.CodeBlock { body = "foo = 123", language = Nothing } ])
, test "image" <|
\() ->
""""""
|> parse
|> Expect.equal
(Ok
[ Block.Paragraph
[ Block.Image "/my/image.jpg" Nothing [ Block.Text "This is an image" ]
--{ string = "This is an image"
-- , style =
-- { isBold = False
-- , isCode = False
-- , isItalic = False
-- , link = Just { destination = Block.Image "/my/image.jpg", title = Nothing }
-- }
--
-- }
]
]
)
--, skip <|
-- test "autolink" <|
-- \() ->
-- "<http://foo.bar.baz>\n"
-- |> parse
-- |> Expect.equal
-- (Ok
-- [ Block.Paragraph
-- [ Block.Link "http://foo.bar.baz" Nothing [ Block.Text "http://foo.bar.baz" ] ]
-- ]
-- )
, describe "blank line"
[ test "even though paragraphs can start with blank lines, it is not a paragraph if there are only blanks" <|
\() ->
" \n"
|> parse
|> Expect.equal (Ok [])
]
, describe "block quotes"
[ test "Simple block quote" <|
\() ->
">This is a quote\n"
|> parse
|> Expect.equal (Ok [ Block.BlockQuote [ Block.Paragraph (unstyledText "This is a quote") ] ])
, test "block quote with a space after" <|
\() ->
"> This is a quote\n"
|> parse
|> Expect.equal (Ok [ Block.BlockQuote [ Block.Paragraph (unstyledText "This is a quote") ] ])
, test "consecutive block quote lines are combined" <|
\() ->
"""> # Heading
> Body
"""
|> parse
|> Expect.equal
(Ok
[ Block.BlockQuote
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.Paragraph (unstyledText "Body")
]
]
)
, test "plain lines immediately after block quote lines are combined" <|
\() ->
"""> # Heading
I'm part of the block quote
"""
|> parse
|> Expect.equal
(Ok
[ Block.BlockQuote
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.Paragraph (unstyledText "I'm part of the block quote")
]
]
)
]
, test "indented code" <|
\() ->
""" sum a b =
a + b
"""
|> parse
|> Expect.equal
(Ok
[ Block.CodeBlock
{ body = "sum a b =\n a + b"
, language = Nothing
}
]
)
, test "block quotes eat the first space and allow paragraphs to start with 3 spaces" <|
\() ->
"""> code
> not code
"""
|> parse
|> Expect.equal
(Ok
[ Block.BlockQuote
[ Block.CodeBlock
{ body = "code"
, language = Nothing
}
]
, Block.BlockQuote
[ Block.Paragraph (unstyledText "not code")
]
]
)
, test "inline HTML" <|
\() ->
"""This is *italicized inline HTML <bio name="<NAME>" photo="https://avatars2.githubusercontent.com/u/1384166" />*"""
|> parse
|> Expect.equal
(Ok
[ Block.Paragraph
[ Block.Text "This is "
, Block.Emphasis
[ Block.Text "italicized inline HTML "
, Block.HtmlInline
(Block.HtmlElement "bio"
-- NOTE: attribute names are in reverse alphabetical order
[ { name = "photo", value = "https://avatars2.githubusercontent.com/u/1384166" }
, { name = "name", value = "<NAME>" }
]
[]
)
]
]
]
)
, test "blank lines separate paragraphs within block quote" <|
\() ->
"""> foo
>
> bar
"""
|> parse
|> Expect.equal
(Ok
[ Block.BlockQuote
[ Block.Paragraph (unstyledText "foo")
, Block.Paragraph (unstyledText "bar")
]
]
)
, test "hard line break with two spaces" <|
\() ->
"foo \nbaz"
|> parse
|> Expect.equal
(Ok
[ Paragraph
[ Text "foo"
, HardLineBreak
, Text "baz"
]
]
)
, test "indented code blocks cannot interrupt paragraphs" <|
\() ->
"""aaa
bbb
ccc"""
|> parse
|> Expect.equal
(Ok
[ Paragraph
[ Text
"""aaa
bbb
ccc"""
]
]
)
, test "keeps items grouped in a paragraph within block quotes when there are no blank lines separating them" <|
\() ->
"""> # Foo
> bar
> baz
"""
|> parse
|> Expect.equal
(Ok
[ Block.BlockQuote
[ Block.Heading Block.H1 (unstyledText "Foo")
, Block.Paragraph (unstyledText "bar\nbaz")
]
]
)
, test "backslash line break" <|
\() ->
"Before\\\nAfter"
|> parse
|> Expect.equal
(Ok
[ Block.Paragraph
[ Block.Text "Before"
, Block.HardLineBreak
, Block.Text "After"
]
]
)
, describe "html"
[ test "html comment" <|
\() ->
"<!-- hello! -->"
|> parse
|> Expect.equal
(Ok
[ Block.HtmlBlock (Block.HtmlComment " hello! ") ]
)
, test "nested html comment" <|
\() ->
"""<Resources>
<Book title="Crime and Punishment">
<!-- this is the book review -->
This is my review...
</Book>
</Resources>
"""
|> parse
|> Expect.equal
(Ok
[ HtmlBlock
(HtmlElement "resources"
[]
[ HtmlBlock
(HtmlElement "book"
[ { name = "title", value = "Crime and Punishment" } ]
[ HtmlBlock (HtmlComment " this is the book review ")
, Paragraph [ Text "This is my review..." ]
]
)
]
)
]
)
]
, test "HTML declaration" <|
\() ->
"""<!DOCTYPE html>"""
|> parse
|> Expect.equal
(Ok
[ HtmlBlock (HtmlDeclaration "DOCTYPE" "html") ]
)
, describe "inline html"
[ test "cdata sections" <|
\() ->
"foo <![CDATA[>&<]]>"
|> parse
|> Expect.equal
(Ok
[ Paragraph
[ Text "foo "
, HtmlInline (Cdata ">&<")
]
]
)
, test "nested HTML" <|
\() ->
"""foo <Resources><Resource type="book" title="Notes From Underground" /></Resources>"""
|> parse
|> Expect.equal
(Ok
[ Paragraph
[ Text "foo "
, HtmlInline
(HtmlElement "resources"
[]
[ HtmlBlock
(HtmlElement "resource"
[ { name = "type", value = "book" }
, { name = "title", value = "Notes From Underground" }
]
[]
)
]
)
]
]
)
, test "nested markdown within nested HTML" <|
\() ->
"""foo <Resources><Resource type="book" title="Notes From Underground" />9/10 interesting read!</Resources>"""
|> parse
|> Expect.equal
(Ok
[ Paragraph
[ Text "foo "
, HtmlInline
(HtmlElement "resources"
[]
[ HtmlBlock
(HtmlElement "resource"
[ { name = "type", value = "book" }
, { name = "title", value = "Notes From Underground" }
]
[]
)
, Paragraph [ Text "9/10 interesting read!" ]
]
)
]
]
)
]
, describe "beginning with autolink"
[ test "simple autolink" <|
\() ->
"<https://elm-lang.org>\n"
|> expectOk
[ Paragraph
[ Link "https://elm-lang.org" Nothing [ Text "https://elm-lang.org" ]
]
]
, test "email autolink" <|
\() ->
"<<EMAIL>>\n"
|> expectOk
[ Paragraph
[ Link "mailto:<EMAIL>" Nothing [ Text "<EMAIL>" ]
]
]
]
, describe "link reference definitions"
[ test "basic example" <|
\() ->
"""[foo]: /url "title"
[foo]
"""
|> parse
|> Expect.equal
(Ok [ Paragraph [ Link "/url" (Just "title") [ Text "foo" ] ] ])
, test "invalid reference uses fallback paragraph parsing" <|
\() ->
"""[foo]:
[foo]
"""
|> parse
|> Expect.equal
(Ok
[ Paragraph [ Text "[foo]:" ]
, Paragraph [ Text "[foo]" ]
]
)
]
]
expectOk : List Block -> String -> Expectation
expectOk expected input =
case input |> parse of
Ok actual ->
actual
|> Expect.equal expected
Err error ->
Expect.fail (Debug.toString error)
plainListItem : String -> Block.ListItem Block.Inline
plainListItem body =
Block.ListItem Block.NoTask [ Block.Text body ]
unstyledText : String -> List Inline
unstyledText body =
[ Block.Text body ]
emphasisText : String -> List Inline
emphasisText body =
[ Block.Emphasis <|
[ Block.Text body ]
]
parserError : String -> Expect.Expectation
parserError markdown =
case parse markdown of
Ok _ ->
Expect.fail "Expected a parser failure!"
Err _ ->
Expect.pass
| true | module Tests exposing (suite)
import Expect exposing (Expectation)
import Markdown.Block as Block exposing (..)
import Markdown.Parser as Markdown exposing (..)
import Parser
import Parser.Advanced as Advanced exposing ((|.), (|=))
import Test exposing (..)
type alias Parser a =
Advanced.Parser String Parser.Problem a
parse : String -> Result (List (Advanced.DeadEnd String Parser.Problem)) (List Block)
parse markdown =
markdown
|> Markdown.parse
suite : Test
suite =
describe "parsing"
[ describe "headings"
[ test "Heading 1" <|
\() ->
"# Hello!"
|> parse
|> Expect.equal (Ok [ Block.Heading Block.H1 (unstyledText "Hello!") ])
, test "heading can end with trailing #'s'" <|
\() ->
"# Hello! ###"
|> parse
|> Expect.equal (Ok [ Block.Heading Block.H1 (unstyledText "Hello!") ])
, test "Heading 2" <|
\() ->
"## Hello!"
|> parse
|> Expect.equal (Ok [ Block.Heading Block.H2 (unstyledText "Hello!") ])
, test "Emphasis line is not interpreted as a list" <|
\() ->
"*This is not a list, it's a paragraph with emphasis*\n"
|> parse
|> Expect.equal (Ok [ Block.Paragraph (emphasisText "This is not a list, it's a paragraph with emphasis") ])
, test "Line starting with a decimal is not interpreted as a list" <|
\() ->
"3.5 is a number - is not a list\n"
|> parse
|> Expect.equal (Ok [ Block.Paragraph (unstyledText "3.5 is a number - is not a list") ])
, test "Heading 7 is parsed using fallback parsing" <|
\() ->
"####### Hello!"
|> expectOk [ Block.Paragraph [ Text "####### Hello!" ] ]
]
, test "plain text" <|
\() ->
"This is just some text"
|> parse
|> Expect.equal (Ok [ Block.Paragraph (unstyledText "This is just some text") ])
, test "parse heading then plain text" <|
\() ->
"""# Heading
This is just some text
"""
|> parse
|> Expect.equal
(Ok
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.Paragraph (unstyledText "This is just some text")
]
)
, test "doesn't need to end in newline" <|
\() ->
"""# Heading
This is just some text"""
|> parse
|> Expect.equal
(Ok
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.Paragraph (unstyledText "This is just some text")
]
)
, test "long example" <|
\() ->
"""# Heading
This is just some text.
## Subheading
Body of the subheading.
"""
|> parse
|> Expect.equal
(Ok
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.Paragraph (unstyledText "This is just some text.")
, Block.Heading Block.H2 (unstyledText "Subheading")
, Block.Paragraph (unstyledText "Body of the subheading.")
]
)
, test "embedded HTML" <|
\() ->
"""# Heading
<div>
Hello!
</div>
"""
|> parse
|> Expect.equal
(Ok
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.HtmlBlock
(Block.HtmlElement "div"
[]
[ Block.Paragraph (unstyledText "Hello!")
]
)
]
)
, test "heading within HTML" <|
\() ->
"""# Heading
<div>
# Heading in a div!
</div>
"""
|> parse
|> Expect.equal
(Ok
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.HtmlBlock
(Block.HtmlElement "div"
[]
[ Block.Heading Block.H1 (unstyledText "Heading in a div!")
]
)
]
)
, test "simple list" <|
\() ->
"""- One
- Two
- Three
"""
|> parse
|> Expect.equal
(Ok
[ Block.UnorderedList
[ plainListItem "One"
, plainListItem "Two"
, plainListItem "Three"
]
-- TODO why is this extra block here? Fix
-- , ListBlock []
]
)
, test "sibling unordered lists with different markers" <|
\() ->
"""- Item 1
- Item 2
- Item 3
+ Item 4
+ Item 5
+ Item 6
* Item 7
* Item 8
* Item 9
"""
|> parse
|> Expect.equal
(Ok
[ Block.UnorderedList
[ plainListItem "Item 1"
, plainListItem "Item 2"
, plainListItem "Item 3"
]
, Block.UnorderedList
[ plainListItem "Item 4"
, plainListItem "Item 5"
, plainListItem "Item 6"
]
, Block.UnorderedList
[ plainListItem "Item 7"
, plainListItem "Item 8"
, plainListItem "Item 9"
]
]
)
, test "sibling ordered lists with different markers" <|
\() ->
"""1. foo
2. bar
3) baz
"""
|> parse
|> Expect.equal
(Ok
[ Block.OrderedList 1
[ unstyledText "foo"
, unstyledText "bar"
]
, Block.OrderedList 3
[ unstyledText "baz"
]
]
)
, test "A paragraph with a numeral that is NOT 1 in the text before a blank line" <|
\() ->
"""The number of windows in my house is
14. The number of doors is 6."""
|> parse
|> Expect.equal
(Ok
[ Block.Paragraph (unstyledText "The number of windows in my house is\n14. The number of doors is 6.")
]
)
, test "A paragraph with a numeral that IS 1 in the text" <|
\() ->
"""The number of windows in my house is
1. The number of doors is 6.
"""
|> parse
|> Expect.equal
(Ok
[ Block.Paragraph (unstyledText "The number of windows in my house is")
, Block.OrderedList 1
[ unstyledText "The number of doors is 6."
]
]
)
, test "thematic break" <|
\() ->
"""---"""
|> parse
|> Expect.equal
(Ok
[ Block.ThematicBreak
]
)
, test "simple table" <|
\() ->
"""| abc | def |
|---|---|
"""
|> parse
|> Expect.equal
(Ok
[ Block.Table
[ { label = [ Text "abc" ], alignment = Nothing }
, { label = [ Text "def" ], alignment = Nothing }
]
[]
]
)
, test "simple table with data" <|
\() ->
"""| abc | def |
|---|---|
| foo | bar |
| bar | baz |
"""
|> parse
|> Expect.equal
(Ok
[ Block.Table
[ { label = [ Text "abc" ], alignment = Nothing }
, { label = [ Text "def" ], alignment = Nothing }
]
[ [ [ Text "foo" ], [ Text "bar" ] ]
, [ [ Text "bar" ], [ Text "baz" ] ]
]
]
)
, test "table with alignment" <|
\() ->
"""| abc | def | ghi | jkl
|:---|:------:|--:|---|
| foo | bar | baz | boo |
| bar | baz | boo | foo |
"""
|> parse
|> Expect.equal
(Ok
[ Block.Table
[ { label = [ Text "abc" ], alignment = Just AlignLeft }
, { label = [ Text "def" ], alignment = Just AlignCenter }
, { label = [ Text "ghi" ], alignment = Just AlignRight }
, { label = [ Text "jkl" ], alignment = Nothing }
]
[ [ [ Text "foo" ], [ Text "bar" ], [ Text "baz" ], [ Text "boo" ] ]
, [ [ Text "bar" ], [ Text "baz" ], [ Text "boo" ], [ Text "foo" ] ]
]
]
)
, test "table with a cell that looks like a heading but isn't" <|
\() ->
"""| abc | def |
| --- | --- |
| bar | baz |
####### asdf
"""
|> parse
|> Expect.equal
(Ok
[ Block.Table
[ { label = [ Text "abc" ], alignment = Nothing }
, { label = [ Text "def" ], alignment = Nothing }
]
[ [ [ Text "bar" ], [ Text "baz" ] ]
, [ [ Text "####### asdf" ], [] ]
]
]
)
, test "table ended by a heading" <|
\() ->
"""| abc | def |
| --- | --- |
| bar | baz |
###### asdf
"""
|> parse
|> Expect.equal
(Ok
[ Block.Table
[ { label = [ Text "abc" ], alignment = Nothing }
, { label = [ Text "def" ], alignment = Nothing }
]
[ [ [ Text "bar" ], [ Text "baz" ] ]
]
, Block.Heading Block.H6 [ Text "asdf" ]
]
)
, test "tables separated by a blank line should be separate" <|
\() ->
"""| abc | def |
| --- | --- |
| bar | baz |
| abc | def |
| --- | --- |
| bar | baz |
"""
|> parse
|> Expect.equal
(Ok
[ Block.Table
[ { label = [ Text "abc" ], alignment = Nothing }
, { label = [ Text "def" ], alignment = Nothing }
]
[ [ [ Text "bar" ], [ Text "baz" ] ]
]
, Block.Table
[ { label = [ Text "abc" ], alignment = Nothing }
, { label = [ Text "def" ], alignment = Nothing }
]
[ [ [ Text "bar" ], [ Text "baz" ] ]
]
]
)
, test "multiple thematic breaks" <|
\() ->
"""***
---
___"""
|> parse
|> Expect.equal
(Ok
[ Block.ThematicBreak
, Block.ThematicBreak
, Block.ThematicBreak
]
)
, test "thematic break followed by newline" <|
\() ->
"""---
"""
|> parse
|> Expect.equal
(Ok
[ Block.ThematicBreak
]
)
, test "blank lines are ignored" <|
\() ->
" \n \n \n\t\n"
|> parse
|> Expect.equal (Ok [])
, test "mixed content with list" <|
\() ->
"""# Title
- This is an item
- And so is this
Text after
"""
|> parse
|> Expect.equal
(Ok
[ Block.Heading Block.H1 (unstyledText "Title")
, Block.UnorderedList
[ plainListItem "This is an item"
, plainListItem "And so is this"
]
, Block.Paragraph (unstyledText "Text after")
-- TODO why is this extra block here? Fix
-- , ListBlock []
]
)
, test "code fence with paragraph and heading below" <|
\() ->
"""```shell
.
├── content/
├── elm.json
├── images/
├── static/
├── index.js
├── package.json
└── src/
└── Main.elm
```
This is more stuff
## h2
qwer
"""
|> parse
|> Expect.equal
(Ok
[ Block.CodeBlock
{ body = ".\n├── content/\n├── elm.json\n├── images/\n├── static/\n├── index.js\n├── package.json\n└── src/\n └── Main.elm"
, language = Just "shell"
}
, Block.Paragraph (unstyledText "This is more stuff")
, Block.Heading Block.H2 (unstyledText "h2")
, Block.Paragraph (unstyledText "qwer")
]
)
, test "indented code block" <|
\() ->
""" foo = 123"""
|> parse
|> Expect.equal (Ok [ Block.CodeBlock { body = "foo = 123", language = Nothing } ])
, test "indented code block with tab" <|
\() ->
"""\tfoo = 123"""
|> parse
|> Expect.equal (Ok [ Block.CodeBlock { body = "foo = 123", language = Nothing } ])
, test "image" <|
\() ->
""""""
|> parse
|> Expect.equal
(Ok
[ Block.Paragraph
[ Block.Image "/my/image.jpg" Nothing [ Block.Text "This is an image" ]
--{ string = "This is an image"
-- , style =
-- { isBold = False
-- , isCode = False
-- , isItalic = False
-- , link = Just { destination = Block.Image "/my/image.jpg", title = Nothing }
-- }
--
-- }
]
]
)
--, skip <|
-- test "autolink" <|
-- \() ->
-- "<http://foo.bar.baz>\n"
-- |> parse
-- |> Expect.equal
-- (Ok
-- [ Block.Paragraph
-- [ Block.Link "http://foo.bar.baz" Nothing [ Block.Text "http://foo.bar.baz" ] ]
-- ]
-- )
, describe "blank line"
[ test "even though paragraphs can start with blank lines, it is not a paragraph if there are only blanks" <|
\() ->
" \n"
|> parse
|> Expect.equal (Ok [])
]
, describe "block quotes"
[ test "Simple block quote" <|
\() ->
">This is a quote\n"
|> parse
|> Expect.equal (Ok [ Block.BlockQuote [ Block.Paragraph (unstyledText "This is a quote") ] ])
, test "block quote with a space after" <|
\() ->
"> This is a quote\n"
|> parse
|> Expect.equal (Ok [ Block.BlockQuote [ Block.Paragraph (unstyledText "This is a quote") ] ])
, test "consecutive block quote lines are combined" <|
\() ->
"""> # Heading
> Body
"""
|> parse
|> Expect.equal
(Ok
[ Block.BlockQuote
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.Paragraph (unstyledText "Body")
]
]
)
, test "plain lines immediately after block quote lines are combined" <|
\() ->
"""> # Heading
I'm part of the block quote
"""
|> parse
|> Expect.equal
(Ok
[ Block.BlockQuote
[ Block.Heading Block.H1 (unstyledText "Heading")
, Block.Paragraph (unstyledText "I'm part of the block quote")
]
]
)
]
, test "indented code" <|
\() ->
""" sum a b =
a + b
"""
|> parse
|> Expect.equal
(Ok
[ Block.CodeBlock
{ body = "sum a b =\n a + b"
, language = Nothing
}
]
)
, test "block quotes eat the first space and allow paragraphs to start with 3 spaces" <|
\() ->
"""> code
> not code
"""
|> parse
|> Expect.equal
(Ok
[ Block.BlockQuote
[ Block.CodeBlock
{ body = "code"
, language = Nothing
}
]
, Block.BlockQuote
[ Block.Paragraph (unstyledText "not code")
]
]
)
, test "inline HTML" <|
\() ->
"""This is *italicized inline HTML <bio name="PI:NAME:<NAME>END_PI" photo="https://avatars2.githubusercontent.com/u/1384166" />*"""
|> parse
|> Expect.equal
(Ok
[ Block.Paragraph
[ Block.Text "This is "
, Block.Emphasis
[ Block.Text "italicized inline HTML "
, Block.HtmlInline
(Block.HtmlElement "bio"
-- NOTE: attribute names are in reverse alphabetical order
[ { name = "photo", value = "https://avatars2.githubusercontent.com/u/1384166" }
, { name = "name", value = "PI:NAME:<NAME>END_PI" }
]
[]
)
]
]
]
)
, test "blank lines separate paragraphs within block quote" <|
\() ->
"""> foo
>
> bar
"""
|> parse
|> Expect.equal
(Ok
[ Block.BlockQuote
[ Block.Paragraph (unstyledText "foo")
, Block.Paragraph (unstyledText "bar")
]
]
)
, test "hard line break with two spaces" <|
\() ->
"foo \nbaz"
|> parse
|> Expect.equal
(Ok
[ Paragraph
[ Text "foo"
, HardLineBreak
, Text "baz"
]
]
)
, test "indented code blocks cannot interrupt paragraphs" <|
\() ->
"""aaa
bbb
ccc"""
|> parse
|> Expect.equal
(Ok
[ Paragraph
[ Text
"""aaa
bbb
ccc"""
]
]
)
, test "keeps items grouped in a paragraph within block quotes when there are no blank lines separating them" <|
\() ->
"""> # Foo
> bar
> baz
"""
|> parse
|> Expect.equal
(Ok
[ Block.BlockQuote
[ Block.Heading Block.H1 (unstyledText "Foo")
, Block.Paragraph (unstyledText "bar\nbaz")
]
]
)
, test "backslash line break" <|
\() ->
"Before\\\nAfter"
|> parse
|> Expect.equal
(Ok
[ Block.Paragraph
[ Block.Text "Before"
, Block.HardLineBreak
, Block.Text "After"
]
]
)
, describe "html"
[ test "html comment" <|
\() ->
"<!-- hello! -->"
|> parse
|> Expect.equal
(Ok
[ Block.HtmlBlock (Block.HtmlComment " hello! ") ]
)
, test "nested html comment" <|
\() ->
"""<Resources>
<Book title="Crime and Punishment">
<!-- this is the book review -->
This is my review...
</Book>
</Resources>
"""
|> parse
|> Expect.equal
(Ok
[ HtmlBlock
(HtmlElement "resources"
[]
[ HtmlBlock
(HtmlElement "book"
[ { name = "title", value = "Crime and Punishment" } ]
[ HtmlBlock (HtmlComment " this is the book review ")
, Paragraph [ Text "This is my review..." ]
]
)
]
)
]
)
]
, test "HTML declaration" <|
\() ->
"""<!DOCTYPE html>"""
|> parse
|> Expect.equal
(Ok
[ HtmlBlock (HtmlDeclaration "DOCTYPE" "html") ]
)
, describe "inline html"
[ test "cdata sections" <|
\() ->
"foo <![CDATA[>&<]]>"
|> parse
|> Expect.equal
(Ok
[ Paragraph
[ Text "foo "
, HtmlInline (Cdata ">&<")
]
]
)
, test "nested HTML" <|
\() ->
"""foo <Resources><Resource type="book" title="Notes From Underground" /></Resources>"""
|> parse
|> Expect.equal
(Ok
[ Paragraph
[ Text "foo "
, HtmlInline
(HtmlElement "resources"
[]
[ HtmlBlock
(HtmlElement "resource"
[ { name = "type", value = "book" }
, { name = "title", value = "Notes From Underground" }
]
[]
)
]
)
]
]
)
, test "nested markdown within nested HTML" <|
\() ->
"""foo <Resources><Resource type="book" title="Notes From Underground" />9/10 interesting read!</Resources>"""
|> parse
|> Expect.equal
(Ok
[ Paragraph
[ Text "foo "
, HtmlInline
(HtmlElement "resources"
[]
[ HtmlBlock
(HtmlElement "resource"
[ { name = "type", value = "book" }
, { name = "title", value = "Notes From Underground" }
]
[]
)
, Paragraph [ Text "9/10 interesting read!" ]
]
)
]
]
)
]
, describe "beginning with autolink"
[ test "simple autolink" <|
\() ->
"<https://elm-lang.org>\n"
|> expectOk
[ Paragraph
[ Link "https://elm-lang.org" Nothing [ Text "https://elm-lang.org" ]
]
]
, test "email autolink" <|
\() ->
"<PI:EMAIL:<EMAIL>END_PI>\n"
|> expectOk
[ Paragraph
[ Link "mailto:PI:EMAIL:<EMAIL>END_PI" Nothing [ Text "PI:EMAIL:<EMAIL>END_PI" ]
]
]
]
, describe "link reference definitions"
[ test "basic example" <|
\() ->
"""[foo]: /url "title"
[foo]
"""
|> parse
|> Expect.equal
(Ok [ Paragraph [ Link "/url" (Just "title") [ Text "foo" ] ] ])
, test "invalid reference uses fallback paragraph parsing" <|
\() ->
"""[foo]:
[foo]
"""
|> parse
|> Expect.equal
(Ok
[ Paragraph [ Text "[foo]:" ]
, Paragraph [ Text "[foo]" ]
]
)
]
]
expectOk : List Block -> String -> Expectation
expectOk expected input =
case input |> parse of
Ok actual ->
actual
|> Expect.equal expected
Err error ->
Expect.fail (Debug.toString error)
plainListItem : String -> Block.ListItem Block.Inline
plainListItem body =
Block.ListItem Block.NoTask [ Block.Text body ]
unstyledText : String -> List Inline
unstyledText body =
[ Block.Text body ]
emphasisText : String -> List Inline
emphasisText body =
[ Block.Emphasis <|
[ Block.Text body ]
]
parserError : String -> Expect.Expectation
parserError markdown =
case parse markdown of
Ok _ ->
Expect.fail "Expected a parser failure!"
Err _ ->
Expect.pass
| elm |
[
{
"context": "ers at the start\" <|\n \\() -> root \"Aaron\" |> equal \"aaron\"\n , test \"with some p",
"end": 2942,
"score": 0.9819784164,
"start": 2937,
"tag": "NAME",
"value": "Aaron"
},
{
"context": " <|\n \\() -> root \"Aaron\" |> equal \"aaron\"\n , test \"with some punctuation and sp",
"end": 2959,
"score": 0.9474295378,
"start": 2954,
"tag": "NAME",
"value": "aaron"
}
] | tests/Tests.elm | NoRedInk/elm-simple-fuzzy | 20 | module Tests exposing (..)
import Test exposing (..)
import Expect exposing (Expectation, equal)
import Simple.Fuzzy exposing (match, root, filter)
matchTests : Test
matchTests =
Test.describe "Simple.Fuzzy.match"
[ Test.describe "should match"
[ test "when no needle" <|
\() -> match "" "word" |> equal True
, test "when single letter starts haystack" <|
\() -> match "w" "word" |> equal True
, test "when single letter is in haystack" <|
\() -> match "o" "word" |> equal True
, test "when multiple letters start haystack" <|
\() -> match "wo" "word" |> equal True
, test "when multiple letters are in haystack" <|
\() -> match "or" "word" |> equal True
, test "when multiple letters spread throughout word" <|
\() -> match "od" "word" |> equal True
, test "when needle exactly matches haystack" <|
\() -> match "word" "word" |> equal True
, test "even when cases do not" <|
\() -> match "wOrD" "woRd" |> equal True
, test "even when punctuation does not" <|
\() -> match "wOr,D" "w,ord" |> equal True
, test "even when spaces do not" <|
\() -> match "lst" "its a list" |> equal True
, test "numbers are matched" <|
\() -> match "1" "number 1" |> equal True
]
, Test.describe "should not match"
[ test "when needle letters are not in right order" <|
\() -> match "ro" "word" |> equal False
, test "when needle letters are duplicated" <|
\() -> match "rr" "word" |> equal False
]
]
filterTests : Test
filterTests =
Test.describe "Simple.Fuzzy.filter"
[ test "filters for all the matching strings" <|
\() -> Simple.Fuzzy.filter .name "el" dummyList |> equal expectedList
]
type alias Language =
{ name : String }
dummyList : List Language
dummyList =
[ Language "Elm"
, Language "Javascript"
, Language "Ruby"
, Language "Elixir"
, Language "Ruby"
]
expectedList : List Language
expectedList =
[ Language "Elm"
, Language "Elixir"
]
rootTests : Test
rootTests =
Test.describe "Simple.Fuzzy.root"
[ Test.describe "should break a word down to its canonical self"
[ test "with an empty string" <|
\() -> root "" |> equal ""
, test "with a single letter" <|
\() -> root "a" |> equal "a"
, test "with many letters" <|
\() -> root "abc" |> equal "abc"
, test "with capital letters in the middle" <|
\() -> root "aBc" |> equal "abc"
, test "with capital letters at the start" <|
\() -> root "Aaron" |> equal "aaron"
, test "with some punctuation and spaces letters" <|
\() -> root "abc!! f" |> equal "abcf"
, test "with some punctuation and spaces letters and numbers" <|
\() -> root "abc!! 123" |> equal "abc123"
]
]
| 60125 | module Tests exposing (..)
import Test exposing (..)
import Expect exposing (Expectation, equal)
import Simple.Fuzzy exposing (match, root, filter)
matchTests : Test
matchTests =
Test.describe "Simple.Fuzzy.match"
[ Test.describe "should match"
[ test "when no needle" <|
\() -> match "" "word" |> equal True
, test "when single letter starts haystack" <|
\() -> match "w" "word" |> equal True
, test "when single letter is in haystack" <|
\() -> match "o" "word" |> equal True
, test "when multiple letters start haystack" <|
\() -> match "wo" "word" |> equal True
, test "when multiple letters are in haystack" <|
\() -> match "or" "word" |> equal True
, test "when multiple letters spread throughout word" <|
\() -> match "od" "word" |> equal True
, test "when needle exactly matches haystack" <|
\() -> match "word" "word" |> equal True
, test "even when cases do not" <|
\() -> match "wOrD" "woRd" |> equal True
, test "even when punctuation does not" <|
\() -> match "wOr,D" "w,ord" |> equal True
, test "even when spaces do not" <|
\() -> match "lst" "its a list" |> equal True
, test "numbers are matched" <|
\() -> match "1" "number 1" |> equal True
]
, Test.describe "should not match"
[ test "when needle letters are not in right order" <|
\() -> match "ro" "word" |> equal False
, test "when needle letters are duplicated" <|
\() -> match "rr" "word" |> equal False
]
]
filterTests : Test
filterTests =
Test.describe "Simple.Fuzzy.filter"
[ test "filters for all the matching strings" <|
\() -> Simple.Fuzzy.filter .name "el" dummyList |> equal expectedList
]
type alias Language =
{ name : String }
dummyList : List Language
dummyList =
[ Language "Elm"
, Language "Javascript"
, Language "Ruby"
, Language "Elixir"
, Language "Ruby"
]
expectedList : List Language
expectedList =
[ Language "Elm"
, Language "Elixir"
]
rootTests : Test
rootTests =
Test.describe "Simple.Fuzzy.root"
[ Test.describe "should break a word down to its canonical self"
[ test "with an empty string" <|
\() -> root "" |> equal ""
, test "with a single letter" <|
\() -> root "a" |> equal "a"
, test "with many letters" <|
\() -> root "abc" |> equal "abc"
, test "with capital letters in the middle" <|
\() -> root "aBc" |> equal "abc"
, test "with capital letters at the start" <|
\() -> root "<NAME>" |> equal "<NAME>"
, test "with some punctuation and spaces letters" <|
\() -> root "abc!! f" |> equal "abcf"
, test "with some punctuation and spaces letters and numbers" <|
\() -> root "abc!! 123" |> equal "abc123"
]
]
| true | module Tests exposing (..)
import Test exposing (..)
import Expect exposing (Expectation, equal)
import Simple.Fuzzy exposing (match, root, filter)
matchTests : Test
matchTests =
Test.describe "Simple.Fuzzy.match"
[ Test.describe "should match"
[ test "when no needle" <|
\() -> match "" "word" |> equal True
, test "when single letter starts haystack" <|
\() -> match "w" "word" |> equal True
, test "when single letter is in haystack" <|
\() -> match "o" "word" |> equal True
, test "when multiple letters start haystack" <|
\() -> match "wo" "word" |> equal True
, test "when multiple letters are in haystack" <|
\() -> match "or" "word" |> equal True
, test "when multiple letters spread throughout word" <|
\() -> match "od" "word" |> equal True
, test "when needle exactly matches haystack" <|
\() -> match "word" "word" |> equal True
, test "even when cases do not" <|
\() -> match "wOrD" "woRd" |> equal True
, test "even when punctuation does not" <|
\() -> match "wOr,D" "w,ord" |> equal True
, test "even when spaces do not" <|
\() -> match "lst" "its a list" |> equal True
, test "numbers are matched" <|
\() -> match "1" "number 1" |> equal True
]
, Test.describe "should not match"
[ test "when needle letters are not in right order" <|
\() -> match "ro" "word" |> equal False
, test "when needle letters are duplicated" <|
\() -> match "rr" "word" |> equal False
]
]
filterTests : Test
filterTests =
Test.describe "Simple.Fuzzy.filter"
[ test "filters for all the matching strings" <|
\() -> Simple.Fuzzy.filter .name "el" dummyList |> equal expectedList
]
type alias Language =
{ name : String }
dummyList : List Language
dummyList =
[ Language "Elm"
, Language "Javascript"
, Language "Ruby"
, Language "Elixir"
, Language "Ruby"
]
expectedList : List Language
expectedList =
[ Language "Elm"
, Language "Elixir"
]
rootTests : Test
rootTests =
Test.describe "Simple.Fuzzy.root"
[ Test.describe "should break a word down to its canonical self"
[ test "with an empty string" <|
\() -> root "" |> equal ""
, test "with a single letter" <|
\() -> root "a" |> equal "a"
, test "with many letters" <|
\() -> root "abc" |> equal "abc"
, test "with capital letters in the middle" <|
\() -> root "aBc" |> equal "abc"
, test "with capital letters at the start" <|
\() -> root "PI:NAME:<NAME>END_PI" |> equal "PI:NAME:<NAME>END_PI"
, test "with some punctuation and spaces letters" <|
\() -> root "abc!! f" |> equal "abcf"
, test "with some punctuation and spaces letters and numbers" <|
\() -> root "abc!! 123" |> equal "abc123"
]
]
| elm |
[
{
"context": "généraux: \"\n , a [href (\"mailto:\"++\"mairie.murol@wanadoo.fr\")]\n [text \"mairie.murol@wanadoo",
"end": 2102,
"score": 0.9999265671,
"start": 2079,
"tag": "EMAIL",
"value": "mairie.murol@wanadoo.fr"
},
{
"context": "rie.murol@wanadoo.fr\")]\n [text \"mairie.murol@wanadoo.fr\"]\n ]\n , br [] []\n , s",
"end": 2155,
"score": 0.9999284744,
"start": 2132,
"tag": "EMAIL",
"value": "mairie.murol@wanadoo.fr"
},
{
"context": "nimation: \"\n , a [href (\"mailto:\"++\"murolanimation@orange.fr\")]\n [text \"murolanimation@orang",
"end": 2331,
"score": 0.9999219179,
"start": 2307,
"tag": "EMAIL",
"value": "murolanimation@orange.fr"
},
{
"context": "lanimation@orange.fr\")]\n [text \"murolanimation@orange.fr\"]\n ]\n , h5 [] [text \"Horaire",
"end": 2385,
"score": 0.9999235868,
"start": 2361,
"tag": "EMAIL",
"value": "murolanimation@orange.fr"
}
] | src/HorairesContact.elm | eniac314/mairieMurol | 0 | module HorairesContact where
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (..)
import StartApp.Simple as StartApp
import List exposing (..)
import String exposing (words, join, cons, uncons)
import Char
import Dict exposing (..)
import Utils exposing (mainMenu,
renderMainMenu,
pageFooter,
capitalize,
renderListImg,
logos,
Action (..),
renderSubMenu,
mail,
site,
link)
-- Model
subMenu = []
initialModel =
{ mainMenu = mainMenu
, subMenu = subMenu
, mainContent = initialContent
}
-- View
view address model =
div [ id "container"]
[ renderMainMenu
["Mairie", "Horaires et contact"]
(.mainMenu model)
, div [ id "subContainer"]
[ .mainContent model
]
, pageFooter
]
-- Update
contentMap =
fromList []
update action model =
case action of
NoOp -> model
Entry s -> changeMain model s
changeMain model s =
let newContent = get s contentMap
in case newContent of
Nothing -> model
Just c -> { model | mainContent = c }
--Main
main =
StartApp.start
{ model = initialModel
, view = view
, update = update
}
initialContent =
div [ class "subContainerData noSubmenu", id "horairesEtContact"]
[ h2 [] [text "Contacter la mairie"]
, div []
[ figure []
[img [src "/images/Mairie.jpg"] []]
, h5 [] [text "Par courrier:"]
, p [] [text "Mairie de Murol - Place de l'hôtel de ville - 63790 Murol"]
, h5 [] [text "Par téléphone :"]
, p [] [text "04 73 88 60 67 / Fax : 04 73 88 65 03 "]
, h5 [] [text "Par mail:"]
, span [class "email"]
[ text "Services généraux: "
, a [href ("mailto:"++"mairie.murol@wanadoo.fr")]
[text "mairie.murol@wanadoo.fr"]
]
, br [] []
, span [class "email"]
[ text "Service animation: "
, a [href ("mailto:"++"murolanimation@orange.fr")]
[text "murolanimation@orange.fr"]
]
, h5 [] [text "Horaires d'ouverture:"]
, p [] [text "du lundi au vendredi : 9h à 12h30 / 13h30 à 17h"]
--, p [] [text "Permanence maire/adjoints samedi matin"]
, p [] [text "Rendez-vous possibles avec le maire ou les adjoints le samedi matin"]
--, h5 [] [text "Location de salles des fêtes municipales"]
--, p [] [text "La commune dispose de 3 salles polyvalentes.
-- 2 situés sur le bourg de Murol et la 3ème sur le bourg de Beaune le froid."]
--, p [] [text "Ces salles sont mises à la location au tarif de 150€ la journée pour vos évènements"]
--, p [] [text "Elles restent à disposition à titre gracieux pour les murolais."]
]
]
-- Data
| 27970 | module HorairesContact where
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (..)
import StartApp.Simple as StartApp
import List exposing (..)
import String exposing (words, join, cons, uncons)
import Char
import Dict exposing (..)
import Utils exposing (mainMenu,
renderMainMenu,
pageFooter,
capitalize,
renderListImg,
logos,
Action (..),
renderSubMenu,
mail,
site,
link)
-- Model
subMenu = []
initialModel =
{ mainMenu = mainMenu
, subMenu = subMenu
, mainContent = initialContent
}
-- View
view address model =
div [ id "container"]
[ renderMainMenu
["Mairie", "Horaires et contact"]
(.mainMenu model)
, div [ id "subContainer"]
[ .mainContent model
]
, pageFooter
]
-- Update
contentMap =
fromList []
update action model =
case action of
NoOp -> model
Entry s -> changeMain model s
changeMain model s =
let newContent = get s contentMap
in case newContent of
Nothing -> model
Just c -> { model | mainContent = c }
--Main
main =
StartApp.start
{ model = initialModel
, view = view
, update = update
}
initialContent =
div [ class "subContainerData noSubmenu", id "horairesEtContact"]
[ h2 [] [text "Contacter la mairie"]
, div []
[ figure []
[img [src "/images/Mairie.jpg"] []]
, h5 [] [text "Par courrier:"]
, p [] [text "Mairie de Murol - Place de l'hôtel de ville - 63790 Murol"]
, h5 [] [text "Par téléphone :"]
, p [] [text "04 73 88 60 67 / Fax : 04 73 88 65 03 "]
, h5 [] [text "Par mail:"]
, span [class "email"]
[ text "Services généraux: "
, a [href ("mailto:"++"<EMAIL>")]
[text "<EMAIL>"]
]
, br [] []
, span [class "email"]
[ text "Service animation: "
, a [href ("mailto:"++"<EMAIL>")]
[text "<EMAIL>"]
]
, h5 [] [text "Horaires d'ouverture:"]
, p [] [text "du lundi au vendredi : 9h à 12h30 / 13h30 à 17h"]
--, p [] [text "Permanence maire/adjoints samedi matin"]
, p [] [text "Rendez-vous possibles avec le maire ou les adjoints le samedi matin"]
--, h5 [] [text "Location de salles des fêtes municipales"]
--, p [] [text "La commune dispose de 3 salles polyvalentes.
-- 2 situés sur le bourg de Murol et la 3ème sur le bourg de Beaune le froid."]
--, p [] [text "Ces salles sont mises à la location au tarif de 150€ la journée pour vos évènements"]
--, p [] [text "Elles restent à disposition à titre gracieux pour les murolais."]
]
]
-- Data
| true | module HorairesContact where
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (..)
import StartApp.Simple as StartApp
import List exposing (..)
import String exposing (words, join, cons, uncons)
import Char
import Dict exposing (..)
import Utils exposing (mainMenu,
renderMainMenu,
pageFooter,
capitalize,
renderListImg,
logos,
Action (..),
renderSubMenu,
mail,
site,
link)
-- Model
subMenu = []
initialModel =
{ mainMenu = mainMenu
, subMenu = subMenu
, mainContent = initialContent
}
-- View
view address model =
div [ id "container"]
[ renderMainMenu
["Mairie", "Horaires et contact"]
(.mainMenu model)
, div [ id "subContainer"]
[ .mainContent model
]
, pageFooter
]
-- Update
contentMap =
fromList []
update action model =
case action of
NoOp -> model
Entry s -> changeMain model s
changeMain model s =
let newContent = get s contentMap
in case newContent of
Nothing -> model
Just c -> { model | mainContent = c }
--Main
main =
StartApp.start
{ model = initialModel
, view = view
, update = update
}
initialContent =
div [ class "subContainerData noSubmenu", id "horairesEtContact"]
[ h2 [] [text "Contacter la mairie"]
, div []
[ figure []
[img [src "/images/Mairie.jpg"] []]
, h5 [] [text "Par courrier:"]
, p [] [text "Mairie de Murol - Place de l'hôtel de ville - 63790 Murol"]
, h5 [] [text "Par téléphone :"]
, p [] [text "04 73 88 60 67 / Fax : 04 73 88 65 03 "]
, h5 [] [text "Par mail:"]
, span [class "email"]
[ text "Services généraux: "
, a [href ("mailto:"++"PI:EMAIL:<EMAIL>END_PI")]
[text "PI:EMAIL:<EMAIL>END_PI"]
]
, br [] []
, span [class "email"]
[ text "Service animation: "
, a [href ("mailto:"++"PI:EMAIL:<EMAIL>END_PI")]
[text "PI:EMAIL:<EMAIL>END_PI"]
]
, h5 [] [text "Horaires d'ouverture:"]
, p [] [text "du lundi au vendredi : 9h à 12h30 / 13h30 à 17h"]
--, p [] [text "Permanence maire/adjoints samedi matin"]
, p [] [text "Rendez-vous possibles avec le maire ou les adjoints le samedi matin"]
--, h5 [] [text "Location de salles des fêtes municipales"]
--, p [] [text "La commune dispose de 3 salles polyvalentes.
-- 2 situés sur le bourg de Murol et la 3ème sur le bourg de Beaune le froid."]
--, p [] [text "Ces salles sont mises à la location au tarif de 150€ la journée pour vos évènements"]
--, p [] [text "Elles restent à disposition à titre gracieux pour les murolais."]
]
]
-- Data
| elm |
[
{
"context": "{-\nCopyright 2020 Morgan Stanley\n\nLicensed under the Apache License, Version 2.0 (",
"end": 32,
"score": 0.9998083115,
"start": 18,
"tag": "NAME",
"value": "Morgan Stanley"
}
] | src/Morphir/IR/Type/Codec.elm | aszenz/morphir-elm | 0 | {-
Copyright 2020 Morgan Stanley
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-}
module Morphir.IR.Type.Codec exposing (..)
import Json.Decode as Decode
import Json.Encode as Encode
import Morphir.IR.AccessControlled.Codec exposing (decodeAccessControlled, encodeAccessControlled)
import Morphir.IR.FQName.Codec exposing (decodeFQName, encodeFQName)
import Morphir.IR.Name.Codec exposing (decodeName, encodeName)
import Morphir.IR.Type exposing (Constructor(..), Constructors, Definition(..), Field, Specification(..), Type(..))
{-| Encode a type into JSON.
-}
encodeType : (a -> Encode.Value) -> Type a -> Encode.Value
encodeType encodeAttributes tpe =
case tpe of
Variable a name ->
Encode.list identity
[ Encode.string "variable"
, encodeAttributes a
, encodeName name
]
Reference a typeName typeParameters ->
Encode.list identity
[ Encode.string "reference"
, encodeAttributes a
, encodeFQName typeName
, Encode.list (encodeType encodeAttributes) typeParameters
]
Tuple a elementTypes ->
Encode.list identity
[ Encode.string "tuple"
, encodeAttributes a
, Encode.list (encodeType encodeAttributes) elementTypes
]
Record a fieldTypes ->
Encode.list identity
[ Encode.string "record"
, encodeAttributes a
, Encode.list (encodeField encodeAttributes) fieldTypes
]
ExtensibleRecord a variableName fieldTypes ->
Encode.list identity
[ Encode.string "extensible_record"
, encodeAttributes a
, encodeName variableName
, Encode.list (encodeField encodeAttributes) fieldTypes
]
Function a argumentType returnType ->
Encode.list identity
[ Encode.string "function"
, encodeAttributes a
, encodeType encodeAttributes argumentType
, encodeType encodeAttributes returnType
]
Unit a ->
Encode.list identity
[ Encode.string "unit"
, encodeAttributes a
]
{-| Decode a type from JSON.
-}
decodeType : Decode.Decoder a -> Decode.Decoder (Type a)
decodeType decodeAttributes =
let
lazyDecodeType =
Decode.lazy
(\_ ->
decodeType decodeAttributes
)
lazyDecodeField =
Decode.lazy
(\_ ->
decodeField decodeAttributes
)
in
Decode.index 0 Decode.string
|> Decode.andThen
(\kind ->
case kind of
"variable" ->
Decode.map2 Variable
(Decode.index 1 decodeAttributes)
(Decode.index 2 decodeName)
"reference" ->
Decode.map3 Reference
(Decode.index 1 decodeAttributes)
(Decode.index 2 decodeFQName)
(Decode.index 3 (Decode.list (Decode.lazy (\_ -> decodeType decodeAttributes))))
"tuple" ->
Decode.map2 Tuple
(Decode.index 1 decodeAttributes)
(Decode.index 2 (Decode.list lazyDecodeType))
"record" ->
Decode.map2 Record
(Decode.index 1 decodeAttributes)
(Decode.index 2 (Decode.list lazyDecodeField))
"extensible_record" ->
Decode.map3 ExtensibleRecord
(Decode.index 1 decodeAttributes)
(Decode.index 2 decodeName)
(Decode.index 3 (Decode.list lazyDecodeField))
"function" ->
Decode.map3 Function
(Decode.index 1 decodeAttributes)
(Decode.index 2 lazyDecodeType)
(Decode.index 3 lazyDecodeType)
"unit" ->
Decode.map Unit
(Decode.index 1 decodeAttributes)
_ ->
Decode.fail ("Unknown kind: " ++ kind)
)
encodeField : (a -> Encode.Value) -> Field a -> Encode.Value
encodeField encodeAttributes field =
Encode.list identity
[ encodeName field.name
, encodeType encodeAttributes field.tpe
]
decodeField : Decode.Decoder a -> Decode.Decoder (Field a)
decodeField decodeAttributes =
Decode.map2 Field
(Decode.index 0 decodeName)
(Decode.index 1 (decodeType decodeAttributes))
{-| -}
encodeSpecification : (a -> Encode.Value) -> Specification a -> Encode.Value
encodeSpecification encodeAttributes spec =
case spec of
TypeAliasSpecification params exp ->
Encode.list identity
[ Encode.string "type_alias_specification"
, Encode.list encodeName params
, encodeType encodeAttributes exp
]
OpaqueTypeSpecification params ->
Encode.list identity
[ Encode.string "opaque_type_specification"
, Encode.list encodeName params
]
CustomTypeSpecification params ctors ->
Encode.list identity
[ Encode.string "custom_type_specification"
, Encode.list encodeName params
, encodeConstructors encodeAttributes ctors
]
{-| -}
encodeDefinition : (a -> Encode.Value) -> Definition a -> Encode.Value
encodeDefinition encodeAttributes def =
case def of
TypeAliasDefinition params exp ->
Encode.list identity
[ Encode.string "type_alias_definition"
, Encode.list encodeName params
, encodeType encodeAttributes exp
]
CustomTypeDefinition params ctors ->
Encode.list identity
[ Encode.string "custom_type_definition"
, Encode.list encodeName params
, encodeAccessControlled (encodeConstructors encodeAttributes) ctors
]
decodeDefinition : Decode.Decoder a -> Decode.Decoder (Definition a)
decodeDefinition decodeAttributes =
Decode.index 0 Decode.string
|> Decode.andThen
(\kind ->
case kind of
"type_alias_definition" ->
Decode.map2 TypeAliasDefinition
(Decode.index 1 (Decode.list decodeName))
(Decode.index 2 (decodeType decodeAttributes))
"custom_type_definition" ->
Decode.map2 CustomTypeDefinition
(Decode.index 1 (Decode.list decodeName))
(Decode.index 2 (decodeAccessControlled (decodeConstructors decodeAttributes)))
_ ->
Decode.fail ("Unknown kind: " ++ kind)
)
encodeConstructors : (a -> Encode.Value) -> Constructors a -> Encode.Value
encodeConstructors encodeAttributes ctors =
ctors
|> Encode.list
(\(Constructor ctorName ctorArgs) ->
Encode.list identity
[ Encode.string "constructor"
, encodeName ctorName
, ctorArgs
|> Encode.list
(\( argName, argType ) ->
Encode.list identity
[ encodeName argName
, encodeType encodeAttributes argType
]
)
]
)
decodeConstructors : Decode.Decoder a -> Decode.Decoder (Constructors a)
decodeConstructors decodeAttributes =
Decode.list
(Decode.index 0 Decode.string
|> Decode.andThen
(\kind ->
case kind of
"constructor" ->
Decode.map2 Constructor
(Decode.index 1 decodeName)
(Decode.index 2
(Decode.list
(Decode.map2 Tuple.pair
(Decode.index 0 decodeName)
(Decode.index 1 (decodeType decodeAttributes))
)
)
)
_ ->
Decode.fail ("Unknown kind: " ++ kind)
)
)
| 22017 | {-
Copyright 2020 <NAME>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-}
module Morphir.IR.Type.Codec exposing (..)
import Json.Decode as Decode
import Json.Encode as Encode
import Morphir.IR.AccessControlled.Codec exposing (decodeAccessControlled, encodeAccessControlled)
import Morphir.IR.FQName.Codec exposing (decodeFQName, encodeFQName)
import Morphir.IR.Name.Codec exposing (decodeName, encodeName)
import Morphir.IR.Type exposing (Constructor(..), Constructors, Definition(..), Field, Specification(..), Type(..))
{-| Encode a type into JSON.
-}
encodeType : (a -> Encode.Value) -> Type a -> Encode.Value
encodeType encodeAttributes tpe =
case tpe of
Variable a name ->
Encode.list identity
[ Encode.string "variable"
, encodeAttributes a
, encodeName name
]
Reference a typeName typeParameters ->
Encode.list identity
[ Encode.string "reference"
, encodeAttributes a
, encodeFQName typeName
, Encode.list (encodeType encodeAttributes) typeParameters
]
Tuple a elementTypes ->
Encode.list identity
[ Encode.string "tuple"
, encodeAttributes a
, Encode.list (encodeType encodeAttributes) elementTypes
]
Record a fieldTypes ->
Encode.list identity
[ Encode.string "record"
, encodeAttributes a
, Encode.list (encodeField encodeAttributes) fieldTypes
]
ExtensibleRecord a variableName fieldTypes ->
Encode.list identity
[ Encode.string "extensible_record"
, encodeAttributes a
, encodeName variableName
, Encode.list (encodeField encodeAttributes) fieldTypes
]
Function a argumentType returnType ->
Encode.list identity
[ Encode.string "function"
, encodeAttributes a
, encodeType encodeAttributes argumentType
, encodeType encodeAttributes returnType
]
Unit a ->
Encode.list identity
[ Encode.string "unit"
, encodeAttributes a
]
{-| Decode a type from JSON.
-}
decodeType : Decode.Decoder a -> Decode.Decoder (Type a)
decodeType decodeAttributes =
let
lazyDecodeType =
Decode.lazy
(\_ ->
decodeType decodeAttributes
)
lazyDecodeField =
Decode.lazy
(\_ ->
decodeField decodeAttributes
)
in
Decode.index 0 Decode.string
|> Decode.andThen
(\kind ->
case kind of
"variable" ->
Decode.map2 Variable
(Decode.index 1 decodeAttributes)
(Decode.index 2 decodeName)
"reference" ->
Decode.map3 Reference
(Decode.index 1 decodeAttributes)
(Decode.index 2 decodeFQName)
(Decode.index 3 (Decode.list (Decode.lazy (\_ -> decodeType decodeAttributes))))
"tuple" ->
Decode.map2 Tuple
(Decode.index 1 decodeAttributes)
(Decode.index 2 (Decode.list lazyDecodeType))
"record" ->
Decode.map2 Record
(Decode.index 1 decodeAttributes)
(Decode.index 2 (Decode.list lazyDecodeField))
"extensible_record" ->
Decode.map3 ExtensibleRecord
(Decode.index 1 decodeAttributes)
(Decode.index 2 decodeName)
(Decode.index 3 (Decode.list lazyDecodeField))
"function" ->
Decode.map3 Function
(Decode.index 1 decodeAttributes)
(Decode.index 2 lazyDecodeType)
(Decode.index 3 lazyDecodeType)
"unit" ->
Decode.map Unit
(Decode.index 1 decodeAttributes)
_ ->
Decode.fail ("Unknown kind: " ++ kind)
)
encodeField : (a -> Encode.Value) -> Field a -> Encode.Value
encodeField encodeAttributes field =
Encode.list identity
[ encodeName field.name
, encodeType encodeAttributes field.tpe
]
decodeField : Decode.Decoder a -> Decode.Decoder (Field a)
decodeField decodeAttributes =
Decode.map2 Field
(Decode.index 0 decodeName)
(Decode.index 1 (decodeType decodeAttributes))
{-| -}
encodeSpecification : (a -> Encode.Value) -> Specification a -> Encode.Value
encodeSpecification encodeAttributes spec =
case spec of
TypeAliasSpecification params exp ->
Encode.list identity
[ Encode.string "type_alias_specification"
, Encode.list encodeName params
, encodeType encodeAttributes exp
]
OpaqueTypeSpecification params ->
Encode.list identity
[ Encode.string "opaque_type_specification"
, Encode.list encodeName params
]
CustomTypeSpecification params ctors ->
Encode.list identity
[ Encode.string "custom_type_specification"
, Encode.list encodeName params
, encodeConstructors encodeAttributes ctors
]
{-| -}
encodeDefinition : (a -> Encode.Value) -> Definition a -> Encode.Value
encodeDefinition encodeAttributes def =
case def of
TypeAliasDefinition params exp ->
Encode.list identity
[ Encode.string "type_alias_definition"
, Encode.list encodeName params
, encodeType encodeAttributes exp
]
CustomTypeDefinition params ctors ->
Encode.list identity
[ Encode.string "custom_type_definition"
, Encode.list encodeName params
, encodeAccessControlled (encodeConstructors encodeAttributes) ctors
]
decodeDefinition : Decode.Decoder a -> Decode.Decoder (Definition a)
decodeDefinition decodeAttributes =
Decode.index 0 Decode.string
|> Decode.andThen
(\kind ->
case kind of
"type_alias_definition" ->
Decode.map2 TypeAliasDefinition
(Decode.index 1 (Decode.list decodeName))
(Decode.index 2 (decodeType decodeAttributes))
"custom_type_definition" ->
Decode.map2 CustomTypeDefinition
(Decode.index 1 (Decode.list decodeName))
(Decode.index 2 (decodeAccessControlled (decodeConstructors decodeAttributes)))
_ ->
Decode.fail ("Unknown kind: " ++ kind)
)
encodeConstructors : (a -> Encode.Value) -> Constructors a -> Encode.Value
encodeConstructors encodeAttributes ctors =
ctors
|> Encode.list
(\(Constructor ctorName ctorArgs) ->
Encode.list identity
[ Encode.string "constructor"
, encodeName ctorName
, ctorArgs
|> Encode.list
(\( argName, argType ) ->
Encode.list identity
[ encodeName argName
, encodeType encodeAttributes argType
]
)
]
)
decodeConstructors : Decode.Decoder a -> Decode.Decoder (Constructors a)
decodeConstructors decodeAttributes =
Decode.list
(Decode.index 0 Decode.string
|> Decode.andThen
(\kind ->
case kind of
"constructor" ->
Decode.map2 Constructor
(Decode.index 1 decodeName)
(Decode.index 2
(Decode.list
(Decode.map2 Tuple.pair
(Decode.index 0 decodeName)
(Decode.index 1 (decodeType decodeAttributes))
)
)
)
_ ->
Decode.fail ("Unknown kind: " ++ kind)
)
)
| true | {-
Copyright 2020 PI:NAME:<NAME>END_PI
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-}
module Morphir.IR.Type.Codec exposing (..)
import Json.Decode as Decode
import Json.Encode as Encode
import Morphir.IR.AccessControlled.Codec exposing (decodeAccessControlled, encodeAccessControlled)
import Morphir.IR.FQName.Codec exposing (decodeFQName, encodeFQName)
import Morphir.IR.Name.Codec exposing (decodeName, encodeName)
import Morphir.IR.Type exposing (Constructor(..), Constructors, Definition(..), Field, Specification(..), Type(..))
{-| Encode a type into JSON.
-}
encodeType : (a -> Encode.Value) -> Type a -> Encode.Value
encodeType encodeAttributes tpe =
case tpe of
Variable a name ->
Encode.list identity
[ Encode.string "variable"
, encodeAttributes a
, encodeName name
]
Reference a typeName typeParameters ->
Encode.list identity
[ Encode.string "reference"
, encodeAttributes a
, encodeFQName typeName
, Encode.list (encodeType encodeAttributes) typeParameters
]
Tuple a elementTypes ->
Encode.list identity
[ Encode.string "tuple"
, encodeAttributes a
, Encode.list (encodeType encodeAttributes) elementTypes
]
Record a fieldTypes ->
Encode.list identity
[ Encode.string "record"
, encodeAttributes a
, Encode.list (encodeField encodeAttributes) fieldTypes
]
ExtensibleRecord a variableName fieldTypes ->
Encode.list identity
[ Encode.string "extensible_record"
, encodeAttributes a
, encodeName variableName
, Encode.list (encodeField encodeAttributes) fieldTypes
]
Function a argumentType returnType ->
Encode.list identity
[ Encode.string "function"
, encodeAttributes a
, encodeType encodeAttributes argumentType
, encodeType encodeAttributes returnType
]
Unit a ->
Encode.list identity
[ Encode.string "unit"
, encodeAttributes a
]
{-| Decode a type from JSON.
-}
decodeType : Decode.Decoder a -> Decode.Decoder (Type a)
decodeType decodeAttributes =
let
lazyDecodeType =
Decode.lazy
(\_ ->
decodeType decodeAttributes
)
lazyDecodeField =
Decode.lazy
(\_ ->
decodeField decodeAttributes
)
in
Decode.index 0 Decode.string
|> Decode.andThen
(\kind ->
case kind of
"variable" ->
Decode.map2 Variable
(Decode.index 1 decodeAttributes)
(Decode.index 2 decodeName)
"reference" ->
Decode.map3 Reference
(Decode.index 1 decodeAttributes)
(Decode.index 2 decodeFQName)
(Decode.index 3 (Decode.list (Decode.lazy (\_ -> decodeType decodeAttributes))))
"tuple" ->
Decode.map2 Tuple
(Decode.index 1 decodeAttributes)
(Decode.index 2 (Decode.list lazyDecodeType))
"record" ->
Decode.map2 Record
(Decode.index 1 decodeAttributes)
(Decode.index 2 (Decode.list lazyDecodeField))
"extensible_record" ->
Decode.map3 ExtensibleRecord
(Decode.index 1 decodeAttributes)
(Decode.index 2 decodeName)
(Decode.index 3 (Decode.list lazyDecodeField))
"function" ->
Decode.map3 Function
(Decode.index 1 decodeAttributes)
(Decode.index 2 lazyDecodeType)
(Decode.index 3 lazyDecodeType)
"unit" ->
Decode.map Unit
(Decode.index 1 decodeAttributes)
_ ->
Decode.fail ("Unknown kind: " ++ kind)
)
encodeField : (a -> Encode.Value) -> Field a -> Encode.Value
encodeField encodeAttributes field =
Encode.list identity
[ encodeName field.name
, encodeType encodeAttributes field.tpe
]
decodeField : Decode.Decoder a -> Decode.Decoder (Field a)
decodeField decodeAttributes =
Decode.map2 Field
(Decode.index 0 decodeName)
(Decode.index 1 (decodeType decodeAttributes))
{-| -}
encodeSpecification : (a -> Encode.Value) -> Specification a -> Encode.Value
encodeSpecification encodeAttributes spec =
case spec of
TypeAliasSpecification params exp ->
Encode.list identity
[ Encode.string "type_alias_specification"
, Encode.list encodeName params
, encodeType encodeAttributes exp
]
OpaqueTypeSpecification params ->
Encode.list identity
[ Encode.string "opaque_type_specification"
, Encode.list encodeName params
]
CustomTypeSpecification params ctors ->
Encode.list identity
[ Encode.string "custom_type_specification"
, Encode.list encodeName params
, encodeConstructors encodeAttributes ctors
]
{-| -}
encodeDefinition : (a -> Encode.Value) -> Definition a -> Encode.Value
encodeDefinition encodeAttributes def =
case def of
TypeAliasDefinition params exp ->
Encode.list identity
[ Encode.string "type_alias_definition"
, Encode.list encodeName params
, encodeType encodeAttributes exp
]
CustomTypeDefinition params ctors ->
Encode.list identity
[ Encode.string "custom_type_definition"
, Encode.list encodeName params
, encodeAccessControlled (encodeConstructors encodeAttributes) ctors
]
decodeDefinition : Decode.Decoder a -> Decode.Decoder (Definition a)
decodeDefinition decodeAttributes =
Decode.index 0 Decode.string
|> Decode.andThen
(\kind ->
case kind of
"type_alias_definition" ->
Decode.map2 TypeAliasDefinition
(Decode.index 1 (Decode.list decodeName))
(Decode.index 2 (decodeType decodeAttributes))
"custom_type_definition" ->
Decode.map2 CustomTypeDefinition
(Decode.index 1 (Decode.list decodeName))
(Decode.index 2 (decodeAccessControlled (decodeConstructors decodeAttributes)))
_ ->
Decode.fail ("Unknown kind: " ++ kind)
)
encodeConstructors : (a -> Encode.Value) -> Constructors a -> Encode.Value
encodeConstructors encodeAttributes ctors =
ctors
|> Encode.list
(\(Constructor ctorName ctorArgs) ->
Encode.list identity
[ Encode.string "constructor"
, encodeName ctorName
, ctorArgs
|> Encode.list
(\( argName, argType ) ->
Encode.list identity
[ encodeName argName
, encodeType encodeAttributes argType
]
)
]
)
decodeConstructors : Decode.Decoder a -> Decode.Decoder (Constructors a)
decodeConstructors decodeAttributes =
Decode.list
(Decode.index 0 Decode.string
|> Decode.andThen
(\kind ->
case kind of
"constructor" ->
Decode.map2 Constructor
(Decode.index 1 decodeName)
(Decode.index 2
(Decode.list
(Decode.map2 Tuple.pair
(Decode.index 0 decodeName)
(Decode.index 1 (decodeType decodeAttributes))
)
)
)
_ ->
Decode.fail ("Unknown kind: " ++ kind)
)
)
| elm |
[
{
"context": "odel : Model\ninitialModel =\n { characters = [ \"Luke\", \"Leia\", \"Han\" ]\n , selectedCharacter = \"\"\n ",
"end": 294,
"score": 0.9996899366,
"start": 290,
"tag": "NAME",
"value": "Luke"
},
{
"context": "odel\ninitialModel =\n { characters = [ \"Luke\", \"Leia\", \"Han\" ]\n , selectedCharacter = \"\"\n }\n\n\nup",
"end": 302,
"score": 0.999628067,
"start": 298,
"tag": "NAME",
"value": "Leia"
},
{
"context": "tialModel =\n { characters = [ \"Luke\", \"Leia\", \"Han\" ]\n , selectedCharacter = \"\"\n }\n\n\nupdate : ",
"end": 309,
"score": 0.9995620847,
"start": 306,
"tag": "NAME",
"value": "Han"
}
] | main.elm | PascalLeMerrer/elm-goodbye-js-talk | 0 | module Main exposing (main)
import Html exposing (..)
import Html.Events exposing (onClick)
type alias Model =
{ characters : List String
, selectedCharacter : String
}
type Msg
= Select String
| Unselect
initialModel : Model
initialModel =
{ characters = [ "Luke", "Leia", "Han" ]
, selectedCharacter = ""
}
update : Msg -> Model -> Model
update msg model =
case msg of
Select name ->
{ model | selectedCharacter = name }
Unselect ->
{ model | selectedCharacter = "" }
view : Model -> Html Msg
view model =
let
unselectButton =
if model.selectedCharacter == "" then
p [] [ text "Select a character" ]
else
button [ onClick Unselect ] [ text "unselect character" ]
in
div []
[ ul [] <|
List.map viewItem model.characters
, p [] [ text model.selectedCharacter ]
, unselectButton
]
viewItem : String -> Html Msg
viewItem name =
li [ onClick <| Select name ] [ text name ]
main =
Html.beginnerProgram
{ model = initialModel
, view = view
, update = update
}
| 33205 | module Main exposing (main)
import Html exposing (..)
import Html.Events exposing (onClick)
type alias Model =
{ characters : List String
, selectedCharacter : String
}
type Msg
= Select String
| Unselect
initialModel : Model
initialModel =
{ characters = [ "<NAME>", "<NAME>", "<NAME>" ]
, selectedCharacter = ""
}
update : Msg -> Model -> Model
update msg model =
case msg of
Select name ->
{ model | selectedCharacter = name }
Unselect ->
{ model | selectedCharacter = "" }
view : Model -> Html Msg
view model =
let
unselectButton =
if model.selectedCharacter == "" then
p [] [ text "Select a character" ]
else
button [ onClick Unselect ] [ text "unselect character" ]
in
div []
[ ul [] <|
List.map viewItem model.characters
, p [] [ text model.selectedCharacter ]
, unselectButton
]
viewItem : String -> Html Msg
viewItem name =
li [ onClick <| Select name ] [ text name ]
main =
Html.beginnerProgram
{ model = initialModel
, view = view
, update = update
}
| true | module Main exposing (main)
import Html exposing (..)
import Html.Events exposing (onClick)
type alias Model =
{ characters : List String
, selectedCharacter : String
}
type Msg
= Select String
| Unselect
initialModel : Model
initialModel =
{ characters = [ "PI:NAME:<NAME>END_PI", "PI:NAME:<NAME>END_PI", "PI:NAME:<NAME>END_PI" ]
, selectedCharacter = ""
}
update : Msg -> Model -> Model
update msg model =
case msg of
Select name ->
{ model | selectedCharacter = name }
Unselect ->
{ model | selectedCharacter = "" }
view : Model -> Html Msg
view model =
let
unselectButton =
if model.selectedCharacter == "" then
p [] [ text "Select a character" ]
else
button [ onClick Unselect ] [ text "unselect character" ]
in
div []
[ ul [] <|
List.map viewItem model.characters
, p [] [ text model.selectedCharacter ]
, unselectButton
]
viewItem : String -> Html Msg
viewItem name =
li [ onClick <| Select name ] [ text name ]
main =
Html.beginnerProgram
{ model = initialModel
, view = view
, update = update
}
| elm |
[
{
"context": "g was inspired by ruby-i18n\n-- https://github.com/ruby-i18n/i18n\n\n\ntrimComments : String -> String\ntrimCommen",
"end": 11521,
"score": 0.999397099,
"start": 11512,
"tag": "USERNAME",
"value": "ruby-i18n"
},
{
"context": "uage.Translations\n lastName =\n { key = \"fieldLastName\"\n , en_us = \"Last name[[R]]\"\n , zh_",
"end": 12098,
"score": 0.9231692553,
"start": 12085,
"tag": "KEY",
"value": "fieldLastName"
}
] | src/R10/I18n.elm | rakutentech/rakuten-ui | 0 | module R10.I18n exposing (t, replace, text, paragraph, RenderingMode(..), paragraphFromString)
{-| Internationalization stuff
@docs t, replace, text, paragraph, RenderingMode, paragraphFromString
-}
import Dict
import Element.WithContext exposing (..)
import Element.WithContext.Border as Border
import Element.WithContext.Font as Font
import Html.Attributes
import Html.Events
import Json.Decode
import List.Extra
import R10.Color.AttrsBackground
import R10.Color.AttrsFont
import R10.Color.Svg
import R10.Context exposing (..)
import R10.Language
import R10.Paragraph
import R10.SimpleMarkdown
import R10.Svg.Others
import R10.Transition
import Regex
import Url
-- There are two types of substitutions:
--
-- * Tag subsitituton, that use culry brackets: {tag}
-- * Link substitution, similar to markdown: [label](tag)
--
-- Rulses
--
-- * "tag" can be a pre-defined value, such as "notifyText",
-- "company_name", "cookie", etc. or a numeric value, such as "1", "2", etc.
-- Pre-defined value are replaced with fix values coming from flags,
-- numeric values are replaced by elements contained in a list.
--
-- * Links are created composing the "label", that contain the text of the
-- link already translated in the proper language, and the tag, that
-- is the url of the link.
--
-- * If the url start with "http" then is considered an external link and
-- and icon is attached.
--
-- * There are special cases where link cannot be clicked, in this case
-- we show in clear what the content of the link is
--
{-| -}
paragraph :
List (Attribute (R10.Context.ContextInternal z) msg)
->
{ renderingMode : RenderingMode
, tagReplacer : R10.Context.ContextInternal z -> String -> String
, translation : R10.Language.Translations
}
-> Element (R10.Context.ContextInternal z) msg
paragraph attrs { renderingMode, tagReplacer, translation } =
withContext <|
\c ->
paragraphFromString
attrs
{ renderingMode = renderingMode
, tagReplacer = tagReplacer
, string = t c.contextR10.language translation
, msgNoOp = Nothing
}
{-| -}
paragraphFromString :
List (Attribute (R10.Context.ContextInternal z) msg)
->
{ renderingMode : RenderingMode
, tagReplacer : R10.Context.ContextInternal z -> String -> String
, string : String
, msgNoOp : Maybe msg
}
-> Element (R10.Context.ContextInternal z) msg
paragraphFromString attrs { renderingMode, tagReplacer, string, msgNoOp } =
withContext <|
\c ->
case renderingMode of
Normal ->
R10.Paragraph.normal
attrs
(string
|> applySubstitutions
{ tagReplacer = tagReplacer
, context = c
, renderingMode = renderingMode
, msgNoOp = msgNoOp
}
)
Error ->
R10.Paragraph.small
([ htmlAttribute <| Html.Attributes.id "ie-flex-fix-320"
, R10.Color.AttrsFont.error
]
++ attrs
)
(string
|> applySubstitutions
{ tagReplacer = tagReplacer
, context = c
, renderingMode = renderingMode
, msgNoOp = msgNoOp
}
)
applySubstitutions :
{ a
| context : R10.Context.ContextInternal z
, renderingMode : RenderingMode
, tagReplacer : R10.Context.ContextInternal z -> String -> String
, msgNoOp : Maybe msg
}
-> String
-> List (Element (R10.Context.ContextInternal z) msg)
applySubstitutions { tagReplacer, context, renderingMode, msgNoOp } translationAsString =
translationAsString
|> tagReplacer context
|> specialMarkdown
{ tagReplacer = tagReplacer
, renderingMode = renderingMode
, msgNoOp = msgNoOp
}
{-| -}
type RenderingMode
= Normal
| Error
replaceStartOver : (a -> String) -> a -> String -> String
replaceStartOver tagReplacer c string =
string
|> String.replace "{start_over}" (tagReplacer c)
specialMarkdown :
{ a
| renderingMode : RenderingMode
, tagReplacer : R10.Context.ContextInternal z -> String -> String
, msgNoOp : Maybe msg
}
-> String
-> List (Element (R10.Context.ContextInternal z) msg)
specialMarkdown { tagReplacer, renderingMode, msgNoOp } translationAsString =
let
boldGenerator : String -> Element (R10.Context.ContextInternal z) msg
boldGenerator string_ =
el [ Font.bold ] <| Element.WithContext.text string_
textGenerator : String -> Element (R10.Context.ContextInternal z) msg
textGenerator string_ =
Element.WithContext.text string_
elementLabelGenerator : String -> Element (R10.Context.ContextInternal z) msg
elementLabelGenerator string_ =
Element.WithContext.text string_
linkGenerator : String -> String -> Element (R10.Context.ContextInternal z) msg
linkGenerator label tag =
withContext <|
\c ->
let
newTag : String
newTag =
("{" ++ tag ++ "}")
|> tagReplacer c
|> (\tag_ ->
-- If the tag has not been replace, we put back the original tag
if tag_ == "{" ++ tag ++ "}" then
tag
else
tag_
)
focusedOrOver : List (Decoration (R10.Context.ContextInternal z))
focusedOrOver =
case renderingMode of
Normal ->
[ R10.Color.AttrsFont.linkOver ]
Error ->
[]
isInternal =
isInternalLink c.contextR10.currentUrl newTag
in
if tag == "fake_link" then
case renderingMode of
Normal ->
el [ R10.Color.AttrsFont.link ] <| Element.WithContext.text label
Error ->
el [ Font.underline ] <| Element.WithContext.text label
else
row
([ spacing 5 ]
++ (case msgNoOp of
Just msg ->
[ htmlAttribute <| Html.Events.stopPropagationOn "click" <| Json.Decode.map (\a -> ( a, True )) (Json.Decode.succeed msg) ]
Nothing ->
[]
)
)
([ (if isInternal then
link
else
newTabLink
)
--
-- There was a tabindex 0 before, not sure why this
-- was needed. Removing it now.
--
-- [ htmlAttribute <| Html.Attributes.tabindex 0 ]
--
([]
++ (case renderingMode of
Normal ->
[ R10.Color.AttrsFont.link ]
Error ->
[ Font.underline ]
)
++ [ focused focusedOrOver
, mouseOver focusedOrOver
, R10.Transition.transition "all 0.15s"
]
)
{ url = newTag, label = elementLabelGenerator label }
-- , el [ Font.color <| rgb 0 0.6 0 ] <| Element.WithContext.text <| "<TEMP : " ++ newTag ++ ">"
]
++ (if isInternal then
[]
else
[ R10.Svg.Others.externalLink
[ htmlAttribute <| Html.Attributes.style "vertical-align" "middle"
, paddingEach { top = 0, right = 3, bottom = 0, left = 0 }
]
((case renderingMode of
Normal ->
R10.Color.Svg.link
Error ->
R10.Color.Svg.error
)
c.contextR10.theme
)
16
]
)
)
in
translationAsString
|> R10.SimpleMarkdown.markdown boldGenerator textGenerator linkGenerator
isInternalLink : Url.Url -> String -> Bool
isInternalLink current target =
if String.startsWith "#" target then
-- Only fragment, so it is internal link
True
else
target
|> Url.fromString
|> Maybe.map
(\target_ ->
-- Check if the url is all the same, except the fragment
target_.host
== current.host
&& target_.port_
== current.port_
&& target_.path
== current.path
&& target_.protocol
== current.protocol
&& target_.query
== current.query
)
-- In case we cannot compare, we assume it is internal
|> Maybe.withDefault False
dictUrls : Dict.Dict String String
dictUrls =
Dict.fromList [ ( "cookie", "https://example.com/cookies" ) ]
--
--
--
--
--
--
--
--
--
--
--
--
--
--
--
--
--
--
-- OLD STUFF
--
-- Naming was inspired by ruby-i18n
-- https://github.com/ruby-i18n/i18n
trimComments : String -> String
trimComments =
-- Removing all pairs of square brackets that just contain comments
Regex.replace
(Maybe.withDefault Regex.never <|
Regex.fromStringWith
{ caseInsensitive = True
, multiline = True
}
"\\[\\[[^]]*\\]\\]"
)
(\_ -> "")
{- Util to find translation based on given locale and trim unnecessary comments on translation.
@example
lastName : R10.Language.Translations
lastName =
{ key = "fieldLastName"
, en_us = "Last name[[R]]"
, zh_tw = "姓氏"
, ja_jp = "姓"
, fr_fr = ""
, de_de = ""
...
, da_dk = ""
, sv_se = ""
}
R10.I18n.t R10.Language.EN_US lastName
#=> "Last name"
-}
{-| Translate some text
-}
t : R10.Language.Language -> R10.Language.Translations -> String
t language translation =
R10.Language.select language translation
|> trimComments
{-| Shorthand to transform a translation into an `Element.text`
-}
text : R10.Language.Translations -> Element (R10.Context.ContextInternal z) msg
text translation =
withContext <| \c -> Element.WithContext.text <| t c.contextR10.language translation
{-| Utility for variable replacement in translation.
raw : String
raw =
"Hello my name is '{firstName}, {lastName}'"
result =
raw
|> R10.I18n.replace [ ( "{firstName}", "foo" ), ( "{lastName}", "bar" ) ]
-- result == "Hello my name is 'foo, bar'"
-}
replace : List ( String, String ) -> String -> String
replace variableList translation =
let
replacement =
\( pattern, value ) acc ->
String.join value <| String.split pattern acc
in
List.foldl replacement translation variableList
| 8189 | module R10.I18n exposing (t, replace, text, paragraph, RenderingMode(..), paragraphFromString)
{-| Internationalization stuff
@docs t, replace, text, paragraph, RenderingMode, paragraphFromString
-}
import Dict
import Element.WithContext exposing (..)
import Element.WithContext.Border as Border
import Element.WithContext.Font as Font
import Html.Attributes
import Html.Events
import Json.Decode
import List.Extra
import R10.Color.AttrsBackground
import R10.Color.AttrsFont
import R10.Color.Svg
import R10.Context exposing (..)
import R10.Language
import R10.Paragraph
import R10.SimpleMarkdown
import R10.Svg.Others
import R10.Transition
import Regex
import Url
-- There are two types of substitutions:
--
-- * Tag subsitituton, that use culry brackets: {tag}
-- * Link substitution, similar to markdown: [label](tag)
--
-- Rulses
--
-- * "tag" can be a pre-defined value, such as "notifyText",
-- "company_name", "cookie", etc. or a numeric value, such as "1", "2", etc.
-- Pre-defined value are replaced with fix values coming from flags,
-- numeric values are replaced by elements contained in a list.
--
-- * Links are created composing the "label", that contain the text of the
-- link already translated in the proper language, and the tag, that
-- is the url of the link.
--
-- * If the url start with "http" then is considered an external link and
-- and icon is attached.
--
-- * There are special cases where link cannot be clicked, in this case
-- we show in clear what the content of the link is
--
{-| -}
paragraph :
List (Attribute (R10.Context.ContextInternal z) msg)
->
{ renderingMode : RenderingMode
, tagReplacer : R10.Context.ContextInternal z -> String -> String
, translation : R10.Language.Translations
}
-> Element (R10.Context.ContextInternal z) msg
paragraph attrs { renderingMode, tagReplacer, translation } =
withContext <|
\c ->
paragraphFromString
attrs
{ renderingMode = renderingMode
, tagReplacer = tagReplacer
, string = t c.contextR10.language translation
, msgNoOp = Nothing
}
{-| -}
paragraphFromString :
List (Attribute (R10.Context.ContextInternal z) msg)
->
{ renderingMode : RenderingMode
, tagReplacer : R10.Context.ContextInternal z -> String -> String
, string : String
, msgNoOp : Maybe msg
}
-> Element (R10.Context.ContextInternal z) msg
paragraphFromString attrs { renderingMode, tagReplacer, string, msgNoOp } =
withContext <|
\c ->
case renderingMode of
Normal ->
R10.Paragraph.normal
attrs
(string
|> applySubstitutions
{ tagReplacer = tagReplacer
, context = c
, renderingMode = renderingMode
, msgNoOp = msgNoOp
}
)
Error ->
R10.Paragraph.small
([ htmlAttribute <| Html.Attributes.id "ie-flex-fix-320"
, R10.Color.AttrsFont.error
]
++ attrs
)
(string
|> applySubstitutions
{ tagReplacer = tagReplacer
, context = c
, renderingMode = renderingMode
, msgNoOp = msgNoOp
}
)
applySubstitutions :
{ a
| context : R10.Context.ContextInternal z
, renderingMode : RenderingMode
, tagReplacer : R10.Context.ContextInternal z -> String -> String
, msgNoOp : Maybe msg
}
-> String
-> List (Element (R10.Context.ContextInternal z) msg)
applySubstitutions { tagReplacer, context, renderingMode, msgNoOp } translationAsString =
translationAsString
|> tagReplacer context
|> specialMarkdown
{ tagReplacer = tagReplacer
, renderingMode = renderingMode
, msgNoOp = msgNoOp
}
{-| -}
type RenderingMode
= Normal
| Error
replaceStartOver : (a -> String) -> a -> String -> String
replaceStartOver tagReplacer c string =
string
|> String.replace "{start_over}" (tagReplacer c)
specialMarkdown :
{ a
| renderingMode : RenderingMode
, tagReplacer : R10.Context.ContextInternal z -> String -> String
, msgNoOp : Maybe msg
}
-> String
-> List (Element (R10.Context.ContextInternal z) msg)
specialMarkdown { tagReplacer, renderingMode, msgNoOp } translationAsString =
let
boldGenerator : String -> Element (R10.Context.ContextInternal z) msg
boldGenerator string_ =
el [ Font.bold ] <| Element.WithContext.text string_
textGenerator : String -> Element (R10.Context.ContextInternal z) msg
textGenerator string_ =
Element.WithContext.text string_
elementLabelGenerator : String -> Element (R10.Context.ContextInternal z) msg
elementLabelGenerator string_ =
Element.WithContext.text string_
linkGenerator : String -> String -> Element (R10.Context.ContextInternal z) msg
linkGenerator label tag =
withContext <|
\c ->
let
newTag : String
newTag =
("{" ++ tag ++ "}")
|> tagReplacer c
|> (\tag_ ->
-- If the tag has not been replace, we put back the original tag
if tag_ == "{" ++ tag ++ "}" then
tag
else
tag_
)
focusedOrOver : List (Decoration (R10.Context.ContextInternal z))
focusedOrOver =
case renderingMode of
Normal ->
[ R10.Color.AttrsFont.linkOver ]
Error ->
[]
isInternal =
isInternalLink c.contextR10.currentUrl newTag
in
if tag == "fake_link" then
case renderingMode of
Normal ->
el [ R10.Color.AttrsFont.link ] <| Element.WithContext.text label
Error ->
el [ Font.underline ] <| Element.WithContext.text label
else
row
([ spacing 5 ]
++ (case msgNoOp of
Just msg ->
[ htmlAttribute <| Html.Events.stopPropagationOn "click" <| Json.Decode.map (\a -> ( a, True )) (Json.Decode.succeed msg) ]
Nothing ->
[]
)
)
([ (if isInternal then
link
else
newTabLink
)
--
-- There was a tabindex 0 before, not sure why this
-- was needed. Removing it now.
--
-- [ htmlAttribute <| Html.Attributes.tabindex 0 ]
--
([]
++ (case renderingMode of
Normal ->
[ R10.Color.AttrsFont.link ]
Error ->
[ Font.underline ]
)
++ [ focused focusedOrOver
, mouseOver focusedOrOver
, R10.Transition.transition "all 0.15s"
]
)
{ url = newTag, label = elementLabelGenerator label }
-- , el [ Font.color <| rgb 0 0.6 0 ] <| Element.WithContext.text <| "<TEMP : " ++ newTag ++ ">"
]
++ (if isInternal then
[]
else
[ R10.Svg.Others.externalLink
[ htmlAttribute <| Html.Attributes.style "vertical-align" "middle"
, paddingEach { top = 0, right = 3, bottom = 0, left = 0 }
]
((case renderingMode of
Normal ->
R10.Color.Svg.link
Error ->
R10.Color.Svg.error
)
c.contextR10.theme
)
16
]
)
)
in
translationAsString
|> R10.SimpleMarkdown.markdown boldGenerator textGenerator linkGenerator
isInternalLink : Url.Url -> String -> Bool
isInternalLink current target =
if String.startsWith "#" target then
-- Only fragment, so it is internal link
True
else
target
|> Url.fromString
|> Maybe.map
(\target_ ->
-- Check if the url is all the same, except the fragment
target_.host
== current.host
&& target_.port_
== current.port_
&& target_.path
== current.path
&& target_.protocol
== current.protocol
&& target_.query
== current.query
)
-- In case we cannot compare, we assume it is internal
|> Maybe.withDefault False
dictUrls : Dict.Dict String String
dictUrls =
Dict.fromList [ ( "cookie", "https://example.com/cookies" ) ]
--
--
--
--
--
--
--
--
--
--
--
--
--
--
--
--
--
--
-- OLD STUFF
--
-- Naming was inspired by ruby-i18n
-- https://github.com/ruby-i18n/i18n
trimComments : String -> String
trimComments =
-- Removing all pairs of square brackets that just contain comments
Regex.replace
(Maybe.withDefault Regex.never <|
Regex.fromStringWith
{ caseInsensitive = True
, multiline = True
}
"\\[\\[[^]]*\\]\\]"
)
(\_ -> "")
{- Util to find translation based on given locale and trim unnecessary comments on translation.
@example
lastName : R10.Language.Translations
lastName =
{ key = "<KEY>"
, en_us = "Last name[[R]]"
, zh_tw = "姓氏"
, ja_jp = "姓"
, fr_fr = ""
, de_de = ""
...
, da_dk = ""
, sv_se = ""
}
R10.I18n.t R10.Language.EN_US lastName
#=> "Last name"
-}
{-| Translate some text
-}
t : R10.Language.Language -> R10.Language.Translations -> String
t language translation =
R10.Language.select language translation
|> trimComments
{-| Shorthand to transform a translation into an `Element.text`
-}
text : R10.Language.Translations -> Element (R10.Context.ContextInternal z) msg
text translation =
withContext <| \c -> Element.WithContext.text <| t c.contextR10.language translation
{-| Utility for variable replacement in translation.
raw : String
raw =
"Hello my name is '{firstName}, {lastName}'"
result =
raw
|> R10.I18n.replace [ ( "{firstName}", "foo" ), ( "{lastName}", "bar" ) ]
-- result == "Hello my name is 'foo, bar'"
-}
replace : List ( String, String ) -> String -> String
replace variableList translation =
let
replacement =
\( pattern, value ) acc ->
String.join value <| String.split pattern acc
in
List.foldl replacement translation variableList
| true | module R10.I18n exposing (t, replace, text, paragraph, RenderingMode(..), paragraphFromString)
{-| Internationalization stuff
@docs t, replace, text, paragraph, RenderingMode, paragraphFromString
-}
import Dict
import Element.WithContext exposing (..)
import Element.WithContext.Border as Border
import Element.WithContext.Font as Font
import Html.Attributes
import Html.Events
import Json.Decode
import List.Extra
import R10.Color.AttrsBackground
import R10.Color.AttrsFont
import R10.Color.Svg
import R10.Context exposing (..)
import R10.Language
import R10.Paragraph
import R10.SimpleMarkdown
import R10.Svg.Others
import R10.Transition
import Regex
import Url
-- There are two types of substitutions:
--
-- * Tag subsitituton, that use culry brackets: {tag}
-- * Link substitution, similar to markdown: [label](tag)
--
-- Rulses
--
-- * "tag" can be a pre-defined value, such as "notifyText",
-- "company_name", "cookie", etc. or a numeric value, such as "1", "2", etc.
-- Pre-defined value are replaced with fix values coming from flags,
-- numeric values are replaced by elements contained in a list.
--
-- * Links are created composing the "label", that contain the text of the
-- link already translated in the proper language, and the tag, that
-- is the url of the link.
--
-- * If the url start with "http" then is considered an external link and
-- and icon is attached.
--
-- * There are special cases where link cannot be clicked, in this case
-- we show in clear what the content of the link is
--
{-| -}
paragraph :
List (Attribute (R10.Context.ContextInternal z) msg)
->
{ renderingMode : RenderingMode
, tagReplacer : R10.Context.ContextInternal z -> String -> String
, translation : R10.Language.Translations
}
-> Element (R10.Context.ContextInternal z) msg
paragraph attrs { renderingMode, tagReplacer, translation } =
withContext <|
\c ->
paragraphFromString
attrs
{ renderingMode = renderingMode
, tagReplacer = tagReplacer
, string = t c.contextR10.language translation
, msgNoOp = Nothing
}
{-| -}
paragraphFromString :
List (Attribute (R10.Context.ContextInternal z) msg)
->
{ renderingMode : RenderingMode
, tagReplacer : R10.Context.ContextInternal z -> String -> String
, string : String
, msgNoOp : Maybe msg
}
-> Element (R10.Context.ContextInternal z) msg
paragraphFromString attrs { renderingMode, tagReplacer, string, msgNoOp } =
withContext <|
\c ->
case renderingMode of
Normal ->
R10.Paragraph.normal
attrs
(string
|> applySubstitutions
{ tagReplacer = tagReplacer
, context = c
, renderingMode = renderingMode
, msgNoOp = msgNoOp
}
)
Error ->
R10.Paragraph.small
([ htmlAttribute <| Html.Attributes.id "ie-flex-fix-320"
, R10.Color.AttrsFont.error
]
++ attrs
)
(string
|> applySubstitutions
{ tagReplacer = tagReplacer
, context = c
, renderingMode = renderingMode
, msgNoOp = msgNoOp
}
)
applySubstitutions :
{ a
| context : R10.Context.ContextInternal z
, renderingMode : RenderingMode
, tagReplacer : R10.Context.ContextInternal z -> String -> String
, msgNoOp : Maybe msg
}
-> String
-> List (Element (R10.Context.ContextInternal z) msg)
applySubstitutions { tagReplacer, context, renderingMode, msgNoOp } translationAsString =
translationAsString
|> tagReplacer context
|> specialMarkdown
{ tagReplacer = tagReplacer
, renderingMode = renderingMode
, msgNoOp = msgNoOp
}
{-| -}
type RenderingMode
= Normal
| Error
replaceStartOver : (a -> String) -> a -> String -> String
replaceStartOver tagReplacer c string =
string
|> String.replace "{start_over}" (tagReplacer c)
specialMarkdown :
{ a
| renderingMode : RenderingMode
, tagReplacer : R10.Context.ContextInternal z -> String -> String
, msgNoOp : Maybe msg
}
-> String
-> List (Element (R10.Context.ContextInternal z) msg)
specialMarkdown { tagReplacer, renderingMode, msgNoOp } translationAsString =
let
boldGenerator : String -> Element (R10.Context.ContextInternal z) msg
boldGenerator string_ =
el [ Font.bold ] <| Element.WithContext.text string_
textGenerator : String -> Element (R10.Context.ContextInternal z) msg
textGenerator string_ =
Element.WithContext.text string_
elementLabelGenerator : String -> Element (R10.Context.ContextInternal z) msg
elementLabelGenerator string_ =
Element.WithContext.text string_
linkGenerator : String -> String -> Element (R10.Context.ContextInternal z) msg
linkGenerator label tag =
withContext <|
\c ->
let
newTag : String
newTag =
("{" ++ tag ++ "}")
|> tagReplacer c
|> (\tag_ ->
-- If the tag has not been replace, we put back the original tag
if tag_ == "{" ++ tag ++ "}" then
tag
else
tag_
)
focusedOrOver : List (Decoration (R10.Context.ContextInternal z))
focusedOrOver =
case renderingMode of
Normal ->
[ R10.Color.AttrsFont.linkOver ]
Error ->
[]
isInternal =
isInternalLink c.contextR10.currentUrl newTag
in
if tag == "fake_link" then
case renderingMode of
Normal ->
el [ R10.Color.AttrsFont.link ] <| Element.WithContext.text label
Error ->
el [ Font.underline ] <| Element.WithContext.text label
else
row
([ spacing 5 ]
++ (case msgNoOp of
Just msg ->
[ htmlAttribute <| Html.Events.stopPropagationOn "click" <| Json.Decode.map (\a -> ( a, True )) (Json.Decode.succeed msg) ]
Nothing ->
[]
)
)
([ (if isInternal then
link
else
newTabLink
)
--
-- There was a tabindex 0 before, not sure why this
-- was needed. Removing it now.
--
-- [ htmlAttribute <| Html.Attributes.tabindex 0 ]
--
([]
++ (case renderingMode of
Normal ->
[ R10.Color.AttrsFont.link ]
Error ->
[ Font.underline ]
)
++ [ focused focusedOrOver
, mouseOver focusedOrOver
, R10.Transition.transition "all 0.15s"
]
)
{ url = newTag, label = elementLabelGenerator label }
-- , el [ Font.color <| rgb 0 0.6 0 ] <| Element.WithContext.text <| "<TEMP : " ++ newTag ++ ">"
]
++ (if isInternal then
[]
else
[ R10.Svg.Others.externalLink
[ htmlAttribute <| Html.Attributes.style "vertical-align" "middle"
, paddingEach { top = 0, right = 3, bottom = 0, left = 0 }
]
((case renderingMode of
Normal ->
R10.Color.Svg.link
Error ->
R10.Color.Svg.error
)
c.contextR10.theme
)
16
]
)
)
in
translationAsString
|> R10.SimpleMarkdown.markdown boldGenerator textGenerator linkGenerator
isInternalLink : Url.Url -> String -> Bool
isInternalLink current target =
if String.startsWith "#" target then
-- Only fragment, so it is internal link
True
else
target
|> Url.fromString
|> Maybe.map
(\target_ ->
-- Check if the url is all the same, except the fragment
target_.host
== current.host
&& target_.port_
== current.port_
&& target_.path
== current.path
&& target_.protocol
== current.protocol
&& target_.query
== current.query
)
-- In case we cannot compare, we assume it is internal
|> Maybe.withDefault False
dictUrls : Dict.Dict String String
dictUrls =
Dict.fromList [ ( "cookie", "https://example.com/cookies" ) ]
--
--
--
--
--
--
--
--
--
--
--
--
--
--
--
--
--
--
-- OLD STUFF
--
-- Naming was inspired by ruby-i18n
-- https://github.com/ruby-i18n/i18n
trimComments : String -> String
trimComments =
-- Removing all pairs of square brackets that just contain comments
Regex.replace
(Maybe.withDefault Regex.never <|
Regex.fromStringWith
{ caseInsensitive = True
, multiline = True
}
"\\[\\[[^]]*\\]\\]"
)
(\_ -> "")
{- Util to find translation based on given locale and trim unnecessary comments on translation.
@example
lastName : R10.Language.Translations
lastName =
{ key = "PI:KEY:<KEY>END_PI"
, en_us = "Last name[[R]]"
, zh_tw = "姓氏"
, ja_jp = "姓"
, fr_fr = ""
, de_de = ""
...
, da_dk = ""
, sv_se = ""
}
R10.I18n.t R10.Language.EN_US lastName
#=> "Last name"
-}
{-| Translate some text
-}
t : R10.Language.Language -> R10.Language.Translations -> String
t language translation =
R10.Language.select language translation
|> trimComments
{-| Shorthand to transform a translation into an `Element.text`
-}
text : R10.Language.Translations -> Element (R10.Context.ContextInternal z) msg
text translation =
withContext <| \c -> Element.WithContext.text <| t c.contextR10.language translation
{-| Utility for variable replacement in translation.
raw : String
raw =
"Hello my name is '{firstName}, {lastName}'"
result =
raw
|> R10.I18n.replace [ ( "{firstName}", "foo" ), ( "{lastName}", "bar" ) ]
-- result == "Hello my name is 'foo, bar'"
-}
replace : List ( String, String ) -> String -> String
replace variableList translation =
let
replacement =
\( pattern, value ) acc ->
String.join value <| String.split pattern acc
in
List.foldl replacement translation variableList
| elm |
[
{
"context": "on\"\n\n\ntypeFirstName : String\ntypeFirstName =\n \"firstName\"\n\n\ntypeLastName : String\ntypeLastName =\n \"last",
"end": 4234,
"score": 0.9376443624,
"start": 4225,
"tag": "NAME",
"value": "firstName"
},
{
"context": "Name\"\n\n\ntypeLastName : String\ntypeLastName =\n \"lastName\"\n\n\ntypeEmail : String\ntypeEmail =\n \"email\"\n\n\nt",
"end": 4288,
"score": 0.9719708562,
"start": 4280,
"tag": "NAME",
"value": "lastName"
}
] | frontend/src/Api/Point.elm | nathan-415/simpleiot | 0 | module Api.Point exposing
( Point
, blankMajicValue
, clearText
, decode
, empty
, encode
, encodeList
, filterSpecialPoints
, get
, getBestDesc
, getBool
, getLatest
, getText
, getValue
, newText
, newValue
, renderPoint
, typeActionType
, typeActive
, typeAddress
, typeAppVersion
, typeAuthToken
, typeBaud
, typeBucket
, typeChannel
, typeClientServer
, typeCmdPending
, typeConditionType
, typeDataFormat
, typeDebug
, typeDescription
, typeDevice
, typeEmail
, typeEnd
, typeErrorCount
, typeErrorCountCRC
, typeErrorCountCRCReset
, typeErrorCountEOF
, typeErrorCountEOFReset
, typeErrorCountReset
, typeFilePath
, typeFirstName
, typeFrom
, typeHwVersion
, typeID
, typeLastName
, typeMinActive
, typeModbusIOType
, typeNodeType
, typeOSVersion
, typeOffset
, typeOperator
, typeOrg
, typePass
, typePhone
, typePointID
, typePointIndex
, typePointType
, typePollPeriod
, typePort
, typeProtocol
, typeReadOnly
, typeSID
, typeScale
, typeService
, typeStart
, typeStartApp
, typeStartSystem
, typeSwUpdateError
, typeSwUpdatePercComplete
, typeSwUpdateRunning
, typeSwUpdateState
, typeSysState
, typeTombstone
, typeURI
, typeUnits
, typeUpdateApp
, typeUpdateOS
, typeValue
, typeValueSet
, typeValueType
, typeVariableType
, typeWeekday
, updatePoint
, updatePoints
, valueActionNotify
, valueActionPlayAudio
, valueActionSetValue
, valueActionSetValueBool
, valueActionSetValueText
, valueClient
, valueContains
, valueEqual
, valueFLOAT32
, valueGreaterThan
, valueINT16
, valueINT32
, valueLessThan
, valueModbusCoil
, valueModbusDiscreteInput
, valueModbusHoldingRegister
, valueModbusInputRegister
, valueNotEqual
, valueNumber
, valueOff
, valueOn
, valueOnOff
, valuePointValue
, valueRTU
, valueSMTP
, valueSchedule
, valueServer
, valueSysStateOffline
, valueSysStateOnline
, valueSysStatePowerOff
, valueSysStateUnknown
, valueTCP
, valueText
, valueTwilio
, valueUINT16
, valueUINT32
)
import Iso8601
import Json.Decode as Decode
import Json.Decode.Extra
import Json.Decode.Pipeline exposing (optional)
import Json.Encode
import List.Extra
import Round
import Time
typeChannel : String
typeChannel =
"channel"
typeDevice : String
typeDevice =
"device"
typeDescription : String
typeDescription =
"description"
typeFilePath : String
typeFilePath =
"filePath"
typeScale : String
typeScale =
"scale"
typeOffset : String
typeOffset =
"offset"
typeUnits : String
typeUnits =
"units"
typeValue : String
typeValue =
"value"
typeValueSet : String
typeValueSet =
"valueSet"
typeReadOnly : String
typeReadOnly =
"readOnly"
typeCmdPending : String
typeCmdPending =
"cmdPending"
typeSwUpdateState : String
typeSwUpdateState =
"swUpdateState"
typeStartApp : String
typeStartApp =
"startApp"
typeStartSystem : String
typeStartSystem =
"startSystem"
typeUpdateOS : String
typeUpdateOS =
"updateOS"
typeUpdateApp : String
typeUpdateApp =
"updateApp"
typeSysState : String
typeSysState =
"sysState"
valueSysStateUnknown : String
valueSysStateUnknown =
"unknown"
valueSysStatePowerOff : String
valueSysStatePowerOff =
"powerOff"
valueSysStateOffline : String
valueSysStateOffline =
"offline"
valueSysStateOnline : String
valueSysStateOnline =
"online"
typeSwUpdateRunning : String
typeSwUpdateRunning =
"swUpdateRunning"
typeSwUpdateError : String
typeSwUpdateError =
"swUpdateError"
typeSwUpdatePercComplete : String
typeSwUpdatePercComplete =
"swUpdatePercComplete"
typeOSVersion : String
typeOSVersion =
"osVersion"
typeAppVersion : String
typeAppVersion =
"appVersion"
typeHwVersion : String
typeHwVersion =
"hwVersion"
typeFirstName : String
typeFirstName =
"firstName"
typeLastName : String
typeLastName =
"lastName"
typeEmail : String
typeEmail =
"email"
typePhone : String
typePhone =
"phone"
typePass : String
typePass =
"pass"
typePort : String
typePort =
"port"
typeBaud : String
typeBaud =
"baud"
typeID : String
typeID =
"id"
typeAddress : String
typeAddress =
"address"
typeErrorCount : String
typeErrorCount =
"errorCount"
typeErrorCountEOF : String
typeErrorCountEOF =
"errorCountEOF"
typeErrorCountCRC : String
typeErrorCountCRC =
"errorCountCRC"
typeErrorCountReset : String
typeErrorCountReset =
"errorCountReset"
typeErrorCountEOFReset : String
typeErrorCountEOFReset =
"errorCountEOFReset"
typeErrorCountCRCReset : String
typeErrorCountCRCReset =
"errorCountCRCReset"
typeProtocol : String
typeProtocol =
"protocol"
valueRTU : String
valueRTU =
"RTU"
valueTCP : String
valueTCP =
"TCP"
typeModbusIOType : String
typeModbusIOType =
"modbusIoType"
valueModbusDiscreteInput : String
valueModbusDiscreteInput =
"modbusDiscreteInput"
valueModbusCoil : String
valueModbusCoil =
"modbusCoil"
valueModbusInputRegister : String
valueModbusInputRegister =
"modbusInputRegister"
valueModbusHoldingRegister : String
valueModbusHoldingRegister =
"modbusHoldingRegister"
typeDataFormat : String
typeDataFormat =
"dataFormat"
typeDebug : String
typeDebug =
"debug"
typePollPeriod : String
typePollPeriod =
"pollPeriod"
valueUINT16 : String
valueUINT16 =
"uint16"
valueINT16 : String
valueINT16 =
"int16"
valueUINT32 : String
valueUINT32 =
"uint32"
valueINT32 : String
valueINT32 =
"int32"
valueFLOAT32 : String
valueFLOAT32 =
"float32"
typeClientServer : String
typeClientServer =
"clientServer"
valueClient : String
valueClient =
"client"
valueServer : String
valueServer =
"server"
typeURI : String
typeURI =
"uri"
typeConditionType : String
typeConditionType =
"conditionType"
valuePointValue : String
valuePointValue =
"pointValue"
valueSchedule : String
valueSchedule =
"schedule"
typeValueType : String
typeValueType =
"valueType"
typeStart : String
typeStart =
"start"
typeEnd : String
typeEnd =
"end"
typeWeekday : String
typeWeekday =
"weekday"
typePointID : String
typePointID =
"pointID"
typePointType : String
typePointType =
"pointType"
typePointIndex : String
typePointIndex =
"pointIndex"
typeOperator : String
typeOperator =
"operator"
valueGreaterThan : String
valueGreaterThan =
">"
valueLessThan : String
valueLessThan =
"<"
valueEqual : String
valueEqual =
"="
valueNotEqual : String
valueNotEqual =
"!="
valueOn : String
valueOn =
"on"
valueOff : String
valueOff =
"off"
valueContains : String
valueContains =
"contains"
typeMinActive : String
typeMinActive =
"minActive"
typeActionType : String
typeActionType =
"actionType"
valueActionNotify : String
valueActionNotify =
"notify"
valueActionSetValue : String
valueActionSetValue =
"setValue"
valueActionPlayAudio : String
valueActionPlayAudio =
"playAudio"
valueActionSetValueBool : String
valueActionSetValueBool =
"setValueBool"
valueActionSetValueText : String
valueActionSetValueText =
"setValueText"
typeService : String
typeService =
"service"
valueTwilio : String
valueTwilio =
"twilio"
valueSMTP : String
valueSMTP =
"smtp"
typeSID : String
typeSID =
"sid"
typeAuthToken : String
typeAuthToken =
"authToken"
typeFrom : String
typeFrom =
"from"
typeVariableType : String
typeVariableType =
"variableType"
typeNodeType : String
typeNodeType =
"nodeType"
typeTombstone : String
typeTombstone =
"tombstone"
valueOnOff : String
valueOnOff =
"onOff"
valueNumber : String
valueNumber =
"number"
valueText : String
valueText =
"text"
typeActive : String
typeActive =
"active"
typeBucket : String
typeBucket =
"bucket"
typeOrg : String
typeOrg =
"org"
-- Point should match data/Point.go
type alias Point =
{ id : String
, index : Int
, typ : String
, time : Time.Posix
, value : Float
, text : String
, min : Float
, max : Float
}
empty : Point
empty =
Point
""
0
""
(Time.millisToPosix 0)
0
""
0
0
newValue : String -> String -> Float -> Point
newValue id typ value =
{ id = id
, typ = typ
, index = 0
, time = Time.millisToPosix 0
, value = value
, text = ""
, min = 0
, max = 0
}
newText : String -> String -> String -> Point
newText id typ text =
{ id = id
, typ = typ
, index = 0
, time = Time.millisToPosix 0
, value = 0
, text = text
, min = 0
, max = 0
}
specialPoints : List String
specialPoints =
[ typeDescription
, typeHwVersion
, typeOSVersion
, typeAppVersion
]
filterSpecialPoints : List Point -> List Point
filterSpecialPoints points =
List.filter (\p -> not <| List.member p.typ specialPoints) points
encode : Point -> Json.Encode.Value
encode s =
Json.Encode.object
[ ( "id", Json.Encode.string <| s.id )
, ( "index", Json.Encode.int <| s.index )
, ( "type", Json.Encode.string <| s.typ )
, ( "time", Iso8601.encode <| s.time )
, ( "value", Json.Encode.float <| s.value )
, ( "text", Json.Encode.string <| s.text )
, ( "min", Json.Encode.float <| s.min )
, ( "max", Json.Encode.float <| s.max )
]
encodeList : List Point -> Json.Encode.Value
encodeList p =
Json.Encode.list encode p
decode : Decode.Decoder Point
decode =
Decode.succeed Point
|> optional "id" Decode.string ""
|> optional "index" Decode.int 0
|> optional "type" Decode.string ""
|> optional "time" Json.Decode.Extra.datetime (Time.millisToPosix 0)
|> optional "value" Decode.float 0
|> optional "text" Decode.string ""
|> optional "min" Decode.float 0
|> optional "max" Decode.float 0
renderPoint : Point -> String
renderPoint s =
let
id =
if s.id == "" then
""
else
s.id ++ ": "
value =
if s.text /= "" then
s.text
else
Round.round 2 s.value
in
id ++ value ++ " (" ++ s.typ ++ ")"
updatePoint : List Point -> Point -> List Point
updatePoint points point =
case
List.Extra.findIndex
(\p ->
point.id == p.id && point.typ == p.typ && point.index == p.index
)
points
of
Just index ->
List.Extra.setAt index point points
Nothing ->
point :: points
updatePoints : List Point -> List Point -> List Point
updatePoints points newPoints =
List.foldr
(\newPoint updatedPoints -> updatePoint updatedPoints newPoint)
points
newPoints
get : List Point -> String -> Int -> String -> Maybe Point
get points id index typ =
List.Extra.find
(\p ->
id == p.id && typ == p.typ && index == p.index
)
points
getText : List Point -> String -> Int -> String -> String
getText points id index typ =
case
get points id index typ
of
Just found ->
found.text
Nothing ->
""
getBestDesc : List Point -> String
getBestDesc points =
let
firstName =
getText points "" 0 typeFirstName
desc =
getText points "" 0 typeDescription
in
if firstName /= "" then
firstName ++ " " ++ getText points "" 0 typeLastName
else if desc /= "" then
desc
else
"no description"
getValue : List Point -> String -> Int -> String -> Float
getValue points id index typ =
case
get points id index typ
of
Just found ->
found.value
Nothing ->
0
getBool : List Point -> String -> Int -> String -> Bool
getBool points id index typ =
getValue points id index typ == 1
getLatest : List Point -> Maybe Point
getLatest points =
List.foldl
(\p result ->
case result of
Just point ->
if Time.posixToMillis p.time > Time.posixToMillis point.time then
Just p
else
Just point
Nothing ->
Just p
)
Nothing
points
-- clearText is used to sanitize points that have number values before saving.
-- the text value is used by the form when editting things like decimal points
blankMajicValue : String
blankMajicValue =
"123BLANK123"
clearText : List Point -> List Point
clearText points =
List.map
(\p ->
if p.value /= 0 || p.text == blankMajicValue then
{ p | text = "" }
else
p
)
points
| 4692 | module Api.Point exposing
( Point
, blankMajicValue
, clearText
, decode
, empty
, encode
, encodeList
, filterSpecialPoints
, get
, getBestDesc
, getBool
, getLatest
, getText
, getValue
, newText
, newValue
, renderPoint
, typeActionType
, typeActive
, typeAddress
, typeAppVersion
, typeAuthToken
, typeBaud
, typeBucket
, typeChannel
, typeClientServer
, typeCmdPending
, typeConditionType
, typeDataFormat
, typeDebug
, typeDescription
, typeDevice
, typeEmail
, typeEnd
, typeErrorCount
, typeErrorCountCRC
, typeErrorCountCRCReset
, typeErrorCountEOF
, typeErrorCountEOFReset
, typeErrorCountReset
, typeFilePath
, typeFirstName
, typeFrom
, typeHwVersion
, typeID
, typeLastName
, typeMinActive
, typeModbusIOType
, typeNodeType
, typeOSVersion
, typeOffset
, typeOperator
, typeOrg
, typePass
, typePhone
, typePointID
, typePointIndex
, typePointType
, typePollPeriod
, typePort
, typeProtocol
, typeReadOnly
, typeSID
, typeScale
, typeService
, typeStart
, typeStartApp
, typeStartSystem
, typeSwUpdateError
, typeSwUpdatePercComplete
, typeSwUpdateRunning
, typeSwUpdateState
, typeSysState
, typeTombstone
, typeURI
, typeUnits
, typeUpdateApp
, typeUpdateOS
, typeValue
, typeValueSet
, typeValueType
, typeVariableType
, typeWeekday
, updatePoint
, updatePoints
, valueActionNotify
, valueActionPlayAudio
, valueActionSetValue
, valueActionSetValueBool
, valueActionSetValueText
, valueClient
, valueContains
, valueEqual
, valueFLOAT32
, valueGreaterThan
, valueINT16
, valueINT32
, valueLessThan
, valueModbusCoil
, valueModbusDiscreteInput
, valueModbusHoldingRegister
, valueModbusInputRegister
, valueNotEqual
, valueNumber
, valueOff
, valueOn
, valueOnOff
, valuePointValue
, valueRTU
, valueSMTP
, valueSchedule
, valueServer
, valueSysStateOffline
, valueSysStateOnline
, valueSysStatePowerOff
, valueSysStateUnknown
, valueTCP
, valueText
, valueTwilio
, valueUINT16
, valueUINT32
)
import Iso8601
import Json.Decode as Decode
import Json.Decode.Extra
import Json.Decode.Pipeline exposing (optional)
import Json.Encode
import List.Extra
import Round
import Time
typeChannel : String
typeChannel =
"channel"
typeDevice : String
typeDevice =
"device"
typeDescription : String
typeDescription =
"description"
typeFilePath : String
typeFilePath =
"filePath"
typeScale : String
typeScale =
"scale"
typeOffset : String
typeOffset =
"offset"
typeUnits : String
typeUnits =
"units"
typeValue : String
typeValue =
"value"
typeValueSet : String
typeValueSet =
"valueSet"
typeReadOnly : String
typeReadOnly =
"readOnly"
typeCmdPending : String
typeCmdPending =
"cmdPending"
typeSwUpdateState : String
typeSwUpdateState =
"swUpdateState"
typeStartApp : String
typeStartApp =
"startApp"
typeStartSystem : String
typeStartSystem =
"startSystem"
typeUpdateOS : String
typeUpdateOS =
"updateOS"
typeUpdateApp : String
typeUpdateApp =
"updateApp"
typeSysState : String
typeSysState =
"sysState"
valueSysStateUnknown : String
valueSysStateUnknown =
"unknown"
valueSysStatePowerOff : String
valueSysStatePowerOff =
"powerOff"
valueSysStateOffline : String
valueSysStateOffline =
"offline"
valueSysStateOnline : String
valueSysStateOnline =
"online"
typeSwUpdateRunning : String
typeSwUpdateRunning =
"swUpdateRunning"
typeSwUpdateError : String
typeSwUpdateError =
"swUpdateError"
typeSwUpdatePercComplete : String
typeSwUpdatePercComplete =
"swUpdatePercComplete"
typeOSVersion : String
typeOSVersion =
"osVersion"
typeAppVersion : String
typeAppVersion =
"appVersion"
typeHwVersion : String
typeHwVersion =
"hwVersion"
typeFirstName : String
typeFirstName =
"<NAME>"
typeLastName : String
typeLastName =
"<NAME>"
typeEmail : String
typeEmail =
"email"
typePhone : String
typePhone =
"phone"
typePass : String
typePass =
"pass"
typePort : String
typePort =
"port"
typeBaud : String
typeBaud =
"baud"
typeID : String
typeID =
"id"
typeAddress : String
typeAddress =
"address"
typeErrorCount : String
typeErrorCount =
"errorCount"
typeErrorCountEOF : String
typeErrorCountEOF =
"errorCountEOF"
typeErrorCountCRC : String
typeErrorCountCRC =
"errorCountCRC"
typeErrorCountReset : String
typeErrorCountReset =
"errorCountReset"
typeErrorCountEOFReset : String
typeErrorCountEOFReset =
"errorCountEOFReset"
typeErrorCountCRCReset : String
typeErrorCountCRCReset =
"errorCountCRCReset"
typeProtocol : String
typeProtocol =
"protocol"
valueRTU : String
valueRTU =
"RTU"
valueTCP : String
valueTCP =
"TCP"
typeModbusIOType : String
typeModbusIOType =
"modbusIoType"
valueModbusDiscreteInput : String
valueModbusDiscreteInput =
"modbusDiscreteInput"
valueModbusCoil : String
valueModbusCoil =
"modbusCoil"
valueModbusInputRegister : String
valueModbusInputRegister =
"modbusInputRegister"
valueModbusHoldingRegister : String
valueModbusHoldingRegister =
"modbusHoldingRegister"
typeDataFormat : String
typeDataFormat =
"dataFormat"
typeDebug : String
typeDebug =
"debug"
typePollPeriod : String
typePollPeriod =
"pollPeriod"
valueUINT16 : String
valueUINT16 =
"uint16"
valueINT16 : String
valueINT16 =
"int16"
valueUINT32 : String
valueUINT32 =
"uint32"
valueINT32 : String
valueINT32 =
"int32"
valueFLOAT32 : String
valueFLOAT32 =
"float32"
typeClientServer : String
typeClientServer =
"clientServer"
valueClient : String
valueClient =
"client"
valueServer : String
valueServer =
"server"
typeURI : String
typeURI =
"uri"
typeConditionType : String
typeConditionType =
"conditionType"
valuePointValue : String
valuePointValue =
"pointValue"
valueSchedule : String
valueSchedule =
"schedule"
typeValueType : String
typeValueType =
"valueType"
typeStart : String
typeStart =
"start"
typeEnd : String
typeEnd =
"end"
typeWeekday : String
typeWeekday =
"weekday"
typePointID : String
typePointID =
"pointID"
typePointType : String
typePointType =
"pointType"
typePointIndex : String
typePointIndex =
"pointIndex"
typeOperator : String
typeOperator =
"operator"
valueGreaterThan : String
valueGreaterThan =
">"
valueLessThan : String
valueLessThan =
"<"
valueEqual : String
valueEqual =
"="
valueNotEqual : String
valueNotEqual =
"!="
valueOn : String
valueOn =
"on"
valueOff : String
valueOff =
"off"
valueContains : String
valueContains =
"contains"
typeMinActive : String
typeMinActive =
"minActive"
typeActionType : String
typeActionType =
"actionType"
valueActionNotify : String
valueActionNotify =
"notify"
valueActionSetValue : String
valueActionSetValue =
"setValue"
valueActionPlayAudio : String
valueActionPlayAudio =
"playAudio"
valueActionSetValueBool : String
valueActionSetValueBool =
"setValueBool"
valueActionSetValueText : String
valueActionSetValueText =
"setValueText"
typeService : String
typeService =
"service"
valueTwilio : String
valueTwilio =
"twilio"
valueSMTP : String
valueSMTP =
"smtp"
typeSID : String
typeSID =
"sid"
typeAuthToken : String
typeAuthToken =
"authToken"
typeFrom : String
typeFrom =
"from"
typeVariableType : String
typeVariableType =
"variableType"
typeNodeType : String
typeNodeType =
"nodeType"
typeTombstone : String
typeTombstone =
"tombstone"
valueOnOff : String
valueOnOff =
"onOff"
valueNumber : String
valueNumber =
"number"
valueText : String
valueText =
"text"
typeActive : String
typeActive =
"active"
typeBucket : String
typeBucket =
"bucket"
typeOrg : String
typeOrg =
"org"
-- Point should match data/Point.go
type alias Point =
{ id : String
, index : Int
, typ : String
, time : Time.Posix
, value : Float
, text : String
, min : Float
, max : Float
}
empty : Point
empty =
Point
""
0
""
(Time.millisToPosix 0)
0
""
0
0
newValue : String -> String -> Float -> Point
newValue id typ value =
{ id = id
, typ = typ
, index = 0
, time = Time.millisToPosix 0
, value = value
, text = ""
, min = 0
, max = 0
}
newText : String -> String -> String -> Point
newText id typ text =
{ id = id
, typ = typ
, index = 0
, time = Time.millisToPosix 0
, value = 0
, text = text
, min = 0
, max = 0
}
specialPoints : List String
specialPoints =
[ typeDescription
, typeHwVersion
, typeOSVersion
, typeAppVersion
]
filterSpecialPoints : List Point -> List Point
filterSpecialPoints points =
List.filter (\p -> not <| List.member p.typ specialPoints) points
encode : Point -> Json.Encode.Value
encode s =
Json.Encode.object
[ ( "id", Json.Encode.string <| s.id )
, ( "index", Json.Encode.int <| s.index )
, ( "type", Json.Encode.string <| s.typ )
, ( "time", Iso8601.encode <| s.time )
, ( "value", Json.Encode.float <| s.value )
, ( "text", Json.Encode.string <| s.text )
, ( "min", Json.Encode.float <| s.min )
, ( "max", Json.Encode.float <| s.max )
]
encodeList : List Point -> Json.Encode.Value
encodeList p =
Json.Encode.list encode p
decode : Decode.Decoder Point
decode =
Decode.succeed Point
|> optional "id" Decode.string ""
|> optional "index" Decode.int 0
|> optional "type" Decode.string ""
|> optional "time" Json.Decode.Extra.datetime (Time.millisToPosix 0)
|> optional "value" Decode.float 0
|> optional "text" Decode.string ""
|> optional "min" Decode.float 0
|> optional "max" Decode.float 0
renderPoint : Point -> String
renderPoint s =
let
id =
if s.id == "" then
""
else
s.id ++ ": "
value =
if s.text /= "" then
s.text
else
Round.round 2 s.value
in
id ++ value ++ " (" ++ s.typ ++ ")"
updatePoint : List Point -> Point -> List Point
updatePoint points point =
case
List.Extra.findIndex
(\p ->
point.id == p.id && point.typ == p.typ && point.index == p.index
)
points
of
Just index ->
List.Extra.setAt index point points
Nothing ->
point :: points
updatePoints : List Point -> List Point -> List Point
updatePoints points newPoints =
List.foldr
(\newPoint updatedPoints -> updatePoint updatedPoints newPoint)
points
newPoints
get : List Point -> String -> Int -> String -> Maybe Point
get points id index typ =
List.Extra.find
(\p ->
id == p.id && typ == p.typ && index == p.index
)
points
getText : List Point -> String -> Int -> String -> String
getText points id index typ =
case
get points id index typ
of
Just found ->
found.text
Nothing ->
""
getBestDesc : List Point -> String
getBestDesc points =
let
firstName =
getText points "" 0 typeFirstName
desc =
getText points "" 0 typeDescription
in
if firstName /= "" then
firstName ++ " " ++ getText points "" 0 typeLastName
else if desc /= "" then
desc
else
"no description"
getValue : List Point -> String -> Int -> String -> Float
getValue points id index typ =
case
get points id index typ
of
Just found ->
found.value
Nothing ->
0
getBool : List Point -> String -> Int -> String -> Bool
getBool points id index typ =
getValue points id index typ == 1
getLatest : List Point -> Maybe Point
getLatest points =
List.foldl
(\p result ->
case result of
Just point ->
if Time.posixToMillis p.time > Time.posixToMillis point.time then
Just p
else
Just point
Nothing ->
Just p
)
Nothing
points
-- clearText is used to sanitize points that have number values before saving.
-- the text value is used by the form when editting things like decimal points
blankMajicValue : String
blankMajicValue =
"123BLANK123"
clearText : List Point -> List Point
clearText points =
List.map
(\p ->
if p.value /= 0 || p.text == blankMajicValue then
{ p | text = "" }
else
p
)
points
| true | module Api.Point exposing
( Point
, blankMajicValue
, clearText
, decode
, empty
, encode
, encodeList
, filterSpecialPoints
, get
, getBestDesc
, getBool
, getLatest
, getText
, getValue
, newText
, newValue
, renderPoint
, typeActionType
, typeActive
, typeAddress
, typeAppVersion
, typeAuthToken
, typeBaud
, typeBucket
, typeChannel
, typeClientServer
, typeCmdPending
, typeConditionType
, typeDataFormat
, typeDebug
, typeDescription
, typeDevice
, typeEmail
, typeEnd
, typeErrorCount
, typeErrorCountCRC
, typeErrorCountCRCReset
, typeErrorCountEOF
, typeErrorCountEOFReset
, typeErrorCountReset
, typeFilePath
, typeFirstName
, typeFrom
, typeHwVersion
, typeID
, typeLastName
, typeMinActive
, typeModbusIOType
, typeNodeType
, typeOSVersion
, typeOffset
, typeOperator
, typeOrg
, typePass
, typePhone
, typePointID
, typePointIndex
, typePointType
, typePollPeriod
, typePort
, typeProtocol
, typeReadOnly
, typeSID
, typeScale
, typeService
, typeStart
, typeStartApp
, typeStartSystem
, typeSwUpdateError
, typeSwUpdatePercComplete
, typeSwUpdateRunning
, typeSwUpdateState
, typeSysState
, typeTombstone
, typeURI
, typeUnits
, typeUpdateApp
, typeUpdateOS
, typeValue
, typeValueSet
, typeValueType
, typeVariableType
, typeWeekday
, updatePoint
, updatePoints
, valueActionNotify
, valueActionPlayAudio
, valueActionSetValue
, valueActionSetValueBool
, valueActionSetValueText
, valueClient
, valueContains
, valueEqual
, valueFLOAT32
, valueGreaterThan
, valueINT16
, valueINT32
, valueLessThan
, valueModbusCoil
, valueModbusDiscreteInput
, valueModbusHoldingRegister
, valueModbusInputRegister
, valueNotEqual
, valueNumber
, valueOff
, valueOn
, valueOnOff
, valuePointValue
, valueRTU
, valueSMTP
, valueSchedule
, valueServer
, valueSysStateOffline
, valueSysStateOnline
, valueSysStatePowerOff
, valueSysStateUnknown
, valueTCP
, valueText
, valueTwilio
, valueUINT16
, valueUINT32
)
import Iso8601
import Json.Decode as Decode
import Json.Decode.Extra
import Json.Decode.Pipeline exposing (optional)
import Json.Encode
import List.Extra
import Round
import Time
typeChannel : String
typeChannel =
"channel"
typeDevice : String
typeDevice =
"device"
typeDescription : String
typeDescription =
"description"
typeFilePath : String
typeFilePath =
"filePath"
typeScale : String
typeScale =
"scale"
typeOffset : String
typeOffset =
"offset"
typeUnits : String
typeUnits =
"units"
typeValue : String
typeValue =
"value"
typeValueSet : String
typeValueSet =
"valueSet"
typeReadOnly : String
typeReadOnly =
"readOnly"
typeCmdPending : String
typeCmdPending =
"cmdPending"
typeSwUpdateState : String
typeSwUpdateState =
"swUpdateState"
typeStartApp : String
typeStartApp =
"startApp"
typeStartSystem : String
typeStartSystem =
"startSystem"
typeUpdateOS : String
typeUpdateOS =
"updateOS"
typeUpdateApp : String
typeUpdateApp =
"updateApp"
typeSysState : String
typeSysState =
"sysState"
valueSysStateUnknown : String
valueSysStateUnknown =
"unknown"
valueSysStatePowerOff : String
valueSysStatePowerOff =
"powerOff"
valueSysStateOffline : String
valueSysStateOffline =
"offline"
valueSysStateOnline : String
valueSysStateOnline =
"online"
typeSwUpdateRunning : String
typeSwUpdateRunning =
"swUpdateRunning"
typeSwUpdateError : String
typeSwUpdateError =
"swUpdateError"
typeSwUpdatePercComplete : String
typeSwUpdatePercComplete =
"swUpdatePercComplete"
typeOSVersion : String
typeOSVersion =
"osVersion"
typeAppVersion : String
typeAppVersion =
"appVersion"
typeHwVersion : String
typeHwVersion =
"hwVersion"
typeFirstName : String
typeFirstName =
"PI:NAME:<NAME>END_PI"
typeLastName : String
typeLastName =
"PI:NAME:<NAME>END_PI"
typeEmail : String
typeEmail =
"email"
typePhone : String
typePhone =
"phone"
typePass : String
typePass =
"pass"
typePort : String
typePort =
"port"
typeBaud : String
typeBaud =
"baud"
typeID : String
typeID =
"id"
typeAddress : String
typeAddress =
"address"
typeErrorCount : String
typeErrorCount =
"errorCount"
typeErrorCountEOF : String
typeErrorCountEOF =
"errorCountEOF"
typeErrorCountCRC : String
typeErrorCountCRC =
"errorCountCRC"
typeErrorCountReset : String
typeErrorCountReset =
"errorCountReset"
typeErrorCountEOFReset : String
typeErrorCountEOFReset =
"errorCountEOFReset"
typeErrorCountCRCReset : String
typeErrorCountCRCReset =
"errorCountCRCReset"
typeProtocol : String
typeProtocol =
"protocol"
valueRTU : String
valueRTU =
"RTU"
valueTCP : String
valueTCP =
"TCP"
typeModbusIOType : String
typeModbusIOType =
"modbusIoType"
valueModbusDiscreteInput : String
valueModbusDiscreteInput =
"modbusDiscreteInput"
valueModbusCoil : String
valueModbusCoil =
"modbusCoil"
valueModbusInputRegister : String
valueModbusInputRegister =
"modbusInputRegister"
valueModbusHoldingRegister : String
valueModbusHoldingRegister =
"modbusHoldingRegister"
typeDataFormat : String
typeDataFormat =
"dataFormat"
typeDebug : String
typeDebug =
"debug"
typePollPeriod : String
typePollPeriod =
"pollPeriod"
valueUINT16 : String
valueUINT16 =
"uint16"
valueINT16 : String
valueINT16 =
"int16"
valueUINT32 : String
valueUINT32 =
"uint32"
valueINT32 : String
valueINT32 =
"int32"
valueFLOAT32 : String
valueFLOAT32 =
"float32"
typeClientServer : String
typeClientServer =
"clientServer"
valueClient : String
valueClient =
"client"
valueServer : String
valueServer =
"server"
typeURI : String
typeURI =
"uri"
typeConditionType : String
typeConditionType =
"conditionType"
valuePointValue : String
valuePointValue =
"pointValue"
valueSchedule : String
valueSchedule =
"schedule"
typeValueType : String
typeValueType =
"valueType"
typeStart : String
typeStart =
"start"
typeEnd : String
typeEnd =
"end"
typeWeekday : String
typeWeekday =
"weekday"
typePointID : String
typePointID =
"pointID"
typePointType : String
typePointType =
"pointType"
typePointIndex : String
typePointIndex =
"pointIndex"
typeOperator : String
typeOperator =
"operator"
valueGreaterThan : String
valueGreaterThan =
">"
valueLessThan : String
valueLessThan =
"<"
valueEqual : String
valueEqual =
"="
valueNotEqual : String
valueNotEqual =
"!="
valueOn : String
valueOn =
"on"
valueOff : String
valueOff =
"off"
valueContains : String
valueContains =
"contains"
typeMinActive : String
typeMinActive =
"minActive"
typeActionType : String
typeActionType =
"actionType"
valueActionNotify : String
valueActionNotify =
"notify"
valueActionSetValue : String
valueActionSetValue =
"setValue"
valueActionPlayAudio : String
valueActionPlayAudio =
"playAudio"
valueActionSetValueBool : String
valueActionSetValueBool =
"setValueBool"
valueActionSetValueText : String
valueActionSetValueText =
"setValueText"
typeService : String
typeService =
"service"
valueTwilio : String
valueTwilio =
"twilio"
valueSMTP : String
valueSMTP =
"smtp"
typeSID : String
typeSID =
"sid"
typeAuthToken : String
typeAuthToken =
"authToken"
typeFrom : String
typeFrom =
"from"
typeVariableType : String
typeVariableType =
"variableType"
typeNodeType : String
typeNodeType =
"nodeType"
typeTombstone : String
typeTombstone =
"tombstone"
valueOnOff : String
valueOnOff =
"onOff"
valueNumber : String
valueNumber =
"number"
valueText : String
valueText =
"text"
typeActive : String
typeActive =
"active"
typeBucket : String
typeBucket =
"bucket"
typeOrg : String
typeOrg =
"org"
-- Point should match data/Point.go
type alias Point =
{ id : String
, index : Int
, typ : String
, time : Time.Posix
, value : Float
, text : String
, min : Float
, max : Float
}
empty : Point
empty =
Point
""
0
""
(Time.millisToPosix 0)
0
""
0
0
newValue : String -> String -> Float -> Point
newValue id typ value =
{ id = id
, typ = typ
, index = 0
, time = Time.millisToPosix 0
, value = value
, text = ""
, min = 0
, max = 0
}
newText : String -> String -> String -> Point
newText id typ text =
{ id = id
, typ = typ
, index = 0
, time = Time.millisToPosix 0
, value = 0
, text = text
, min = 0
, max = 0
}
specialPoints : List String
specialPoints =
[ typeDescription
, typeHwVersion
, typeOSVersion
, typeAppVersion
]
filterSpecialPoints : List Point -> List Point
filterSpecialPoints points =
List.filter (\p -> not <| List.member p.typ specialPoints) points
encode : Point -> Json.Encode.Value
encode s =
Json.Encode.object
[ ( "id", Json.Encode.string <| s.id )
, ( "index", Json.Encode.int <| s.index )
, ( "type", Json.Encode.string <| s.typ )
, ( "time", Iso8601.encode <| s.time )
, ( "value", Json.Encode.float <| s.value )
, ( "text", Json.Encode.string <| s.text )
, ( "min", Json.Encode.float <| s.min )
, ( "max", Json.Encode.float <| s.max )
]
encodeList : List Point -> Json.Encode.Value
encodeList p =
Json.Encode.list encode p
decode : Decode.Decoder Point
decode =
Decode.succeed Point
|> optional "id" Decode.string ""
|> optional "index" Decode.int 0
|> optional "type" Decode.string ""
|> optional "time" Json.Decode.Extra.datetime (Time.millisToPosix 0)
|> optional "value" Decode.float 0
|> optional "text" Decode.string ""
|> optional "min" Decode.float 0
|> optional "max" Decode.float 0
renderPoint : Point -> String
renderPoint s =
let
id =
if s.id == "" then
""
else
s.id ++ ": "
value =
if s.text /= "" then
s.text
else
Round.round 2 s.value
in
id ++ value ++ " (" ++ s.typ ++ ")"
updatePoint : List Point -> Point -> List Point
updatePoint points point =
case
List.Extra.findIndex
(\p ->
point.id == p.id && point.typ == p.typ && point.index == p.index
)
points
of
Just index ->
List.Extra.setAt index point points
Nothing ->
point :: points
updatePoints : List Point -> List Point -> List Point
updatePoints points newPoints =
List.foldr
(\newPoint updatedPoints -> updatePoint updatedPoints newPoint)
points
newPoints
get : List Point -> String -> Int -> String -> Maybe Point
get points id index typ =
List.Extra.find
(\p ->
id == p.id && typ == p.typ && index == p.index
)
points
getText : List Point -> String -> Int -> String -> String
getText points id index typ =
case
get points id index typ
of
Just found ->
found.text
Nothing ->
""
getBestDesc : List Point -> String
getBestDesc points =
let
firstName =
getText points "" 0 typeFirstName
desc =
getText points "" 0 typeDescription
in
if firstName /= "" then
firstName ++ " " ++ getText points "" 0 typeLastName
else if desc /= "" then
desc
else
"no description"
getValue : List Point -> String -> Int -> String -> Float
getValue points id index typ =
case
get points id index typ
of
Just found ->
found.value
Nothing ->
0
getBool : List Point -> String -> Int -> String -> Bool
getBool points id index typ =
getValue points id index typ == 1
getLatest : List Point -> Maybe Point
getLatest points =
List.foldl
(\p result ->
case result of
Just point ->
if Time.posixToMillis p.time > Time.posixToMillis point.time then
Just p
else
Just point
Nothing ->
Just p
)
Nothing
points
-- clearText is used to sanitize points that have number values before saving.
-- the text value is used by the form when editting things like decimal points
blankMajicValue : String
blankMajicValue =
"123BLANK123"
clearText : List Point -> List Point
clearText points =
List.map
(\p ->
if p.value /= 0 || p.text == blankMajicValue then
{ p | text = "" }
else
p
)
points
| elm |
[
{
"context": " String, String, Int )\ntuplaMultiple = ( \"Name\", \"Last name\", 1 )\n\n{-\nRecords\n-}\n\n-- Record sin tipo\ncuentaSi",
"end": 455,
"score": 0.9624155164,
"start": 446,
"tag": "NAME",
"value": "Last name"
},
{
"context": "-- Record sin tipo\ncuentaSinTipo = \n { nombre = \"Name\",\n apellido = \"lastName\",\n id = 1\n }\n\n-- Reco",
"end": 532,
"score": 0.999715507,
"start": 528,
"tag": "NAME",
"value": "Name"
},
{
"context": "ntaSinTipo = \n { nombre = \"Name\",\n apellido = \"lastName\",\n id = 1\n }\n\n-- Record con tipo\ntype alias Nom",
"end": 558,
"score": 0.999104023,
"start": 550,
"tag": "NAME",
"value": "lastName"
}
] | ejemplos/06-tipos/colecciones.elm | flandrade/introduccion-elm | 0 | module Colecciones exposing (..)
{-
Listas
-}
-- Números
listaNumero : List number
listaNumero = [1, 2, 3]
-- Lista deben tener el mismo tipo.
listaNumeroError : List number
listaNumeroError = [1, "2", 3]
-- Texto
listaString : List String
listaString = ["Hola", "Elm", "lista"]
{-
Tuplas
-}
-- Mismo tipo
tuplaTipo : ( Int, Int )
tuplaTipo = ( 2, 5 )
-- Diferentes tipos
tuplaMultiple : ( String, String, Int )
tuplaMultiple = ( "Name", "Last name", 1 )
{-
Records
-}
-- Record sin tipo
cuentaSinTipo =
{ nombre = "Name",
apellido = "lastName",
id = 1
}
-- Record con tipo
type alias Nombre = String
type alias Cuenta =
{ nombre : Nombre
, apellido : String
, id : Int
}
cuentaConTipo : Cuenta
cuentaConTipo = Cuenta "Nombre" "Apellido" 2
-- Obtener valor
nombreActual : Cuenta -> Nombre
nombreActual cuenta = cuenta.nombre
-- Sólo se tiene acceso a campos existentes.
cedulaActual : Cuenta -> Int
cedulaActual cuenta = cuenta.cedula
-- Actualizar valor
actualizarNombre : Cuenta -> Nombre -> Cuenta
actualizarNombre cuenta nuevoNombre =
{ cuenta | nombre = nuevoNombre }
| 20182 | module Colecciones exposing (..)
{-
Listas
-}
-- Números
listaNumero : List number
listaNumero = [1, 2, 3]
-- Lista deben tener el mismo tipo.
listaNumeroError : List number
listaNumeroError = [1, "2", 3]
-- Texto
listaString : List String
listaString = ["Hola", "Elm", "lista"]
{-
Tuplas
-}
-- Mismo tipo
tuplaTipo : ( Int, Int )
tuplaTipo = ( 2, 5 )
-- Diferentes tipos
tuplaMultiple : ( String, String, Int )
tuplaMultiple = ( "Name", "<NAME>", 1 )
{-
Records
-}
-- Record sin tipo
cuentaSinTipo =
{ nombre = "<NAME>",
apellido = "<NAME>",
id = 1
}
-- Record con tipo
type alias Nombre = String
type alias Cuenta =
{ nombre : Nombre
, apellido : String
, id : Int
}
cuentaConTipo : Cuenta
cuentaConTipo = Cuenta "Nombre" "Apellido" 2
-- Obtener valor
nombreActual : Cuenta -> Nombre
nombreActual cuenta = cuenta.nombre
-- Sólo se tiene acceso a campos existentes.
cedulaActual : Cuenta -> Int
cedulaActual cuenta = cuenta.cedula
-- Actualizar valor
actualizarNombre : Cuenta -> Nombre -> Cuenta
actualizarNombre cuenta nuevoNombre =
{ cuenta | nombre = nuevoNombre }
| true | module Colecciones exposing (..)
{-
Listas
-}
-- Números
listaNumero : List number
listaNumero = [1, 2, 3]
-- Lista deben tener el mismo tipo.
listaNumeroError : List number
listaNumeroError = [1, "2", 3]
-- Texto
listaString : List String
listaString = ["Hola", "Elm", "lista"]
{-
Tuplas
-}
-- Mismo tipo
tuplaTipo : ( Int, Int )
tuplaTipo = ( 2, 5 )
-- Diferentes tipos
tuplaMultiple : ( String, String, Int )
tuplaMultiple = ( "Name", "PI:NAME:<NAME>END_PI", 1 )
{-
Records
-}
-- Record sin tipo
cuentaSinTipo =
{ nombre = "PI:NAME:<NAME>END_PI",
apellido = "PI:NAME:<NAME>END_PI",
id = 1
}
-- Record con tipo
type alias Nombre = String
type alias Cuenta =
{ nombre : Nombre
, apellido : String
, id : Int
}
cuentaConTipo : Cuenta
cuentaConTipo = Cuenta "Nombre" "Apellido" 2
-- Obtener valor
nombreActual : Cuenta -> Nombre
nombreActual cuenta = cuenta.nombre
-- Sólo se tiene acceso a campos existentes.
cedulaActual : Cuenta -> Int
cedulaActual cuenta = cuenta.cedula
-- Actualizar valor
actualizarNombre : Cuenta -> Nombre -> Cuenta
actualizarNombre cuenta nuevoNombre =
{ cuenta | nombre = nuevoNombre }
| elm |
[
{
"context": "ia JUG\"]\n[Date \"1992.11.04\"]\n[Round \"29\"]\n[White \"Fischer, Robert J.\"]\n[Black \"Spassky, Boris V.\"]\n[Result \"1/2-1/2\"]",
"end": 12930,
"score": 0.9992333651,
"start": 12913,
"tag": "NAME",
"value": "Fischer, Robert J"
},
{
"context": "[Round \"29\"]\n[White \"Fischer, Robert J.\"]\n[Black \"Spassky, Boris V.\"]\n[Result \"1/2-1/2\"]\n\n1. e4 e5 (1... c5 2. Nf3 d",
"end": 12958,
"score": 0.9920225143,
"start": 12942,
"tag": "NAME",
"value": "Spassky, Boris V"
}
] | src/Internal/AnnotatedGame.elm | romstad/elm-chess | 6 | module Internal.AnnotatedGame exposing
( Game
, addMove
, addSanMove
, addSanMoveSequence
, back
, buildMoveText
, children
, continuations
, empty
, examplePgn
, forward
, fromPgn
, fromPgnString
, goToMove
, isAtBeginning
, isAtBeginningOfVariation
, isAtEnd
, moves
, nextMove
, position
, previousMove
, tagValue
, toBeginning
, toBeginningOfVariation
, toEnd
, toEndOfVariation
, toPgn
)
import Array exposing (Array)
import Internal.AnnotatedPgn as Pgn
exposing
( GameResult(..)
, MoveText
, MoveTextItem(..)
, PgnGame
, TagPair
)
import Internal.Move as Move exposing (Move, Variation)
import Internal.Notation exposing (fromSan, toSan)
import Internal.PieceColor as PieceColor exposing (PieceColor)
import Internal.Position as Position exposing (Position)
import Internal.Util exposing (failableFoldl)
import Parser
import Tree exposing (Tree)
import Tree.Zipper as Zipper exposing (Zipper)
type alias GameNode =
{ position : Position
, comment : Maybe String
, precomment : Maybe String
, nag : Maybe Int
, id : Int
, ply : Int
}
type alias Game =
{ result : GameResult
, tags : List TagPair
, tree : Tree GameNode
, focus : Zipper GameNode
, nodeCount : Int
}
empty : Game
empty =
let
tree =
Tree.singleton
{ position = Position.initial
, comment = Nothing
, precomment = Nothing
, nag = Nothing
, id = 0
, ply = 0
}
in
{ result = UnknownResult
, tags = []
, tree = tree
, focus = Zipper.fromTree tree
, nodeCount = 1
}
{-| Get the value of a game tag.
-}
tagValue : String -> Game -> Maybe String
tagValue tagName game =
game.tags
|> List.filter (\t -> Tuple.first t == tagName)
|> List.head
|> Maybe.map Tuple.second
lastMove : GameNode -> Move
lastMove node =
Maybe.withDefault (Move.Move 0) (Position.lastMove node.position)
{-| The current node in the game tree
-}
currentNode : Game -> GameNode
currentNode game =
Zipper.label game.focus
{-| Children of the current node in the game tree
-}
children : Game -> List GameNode
children game =
List.map Tree.label (Zipper.children game.focus)
{-| Continuations from the current node in the game tree
-}
continuations : Game -> List Move
continuations game =
List.map
(\n ->
Maybe.withDefault
(Move.Move 0)
(Position.lastMove n.position)
)
(children game)
{-| The position at the current move of the game.
-}
position : Game -> Position
position game =
(currentNode game).position
{-| List of all moves in the game.
-}
moves : Game -> List Move
moves game =
let
zipper =
Zipper.fromTree game.tree
movesInternal z =
case Zipper.firstChild z of
Nothing ->
[]
Just zz ->
case Position.lastMove (Zipper.label zz).position of
Nothing ->
[]
Just m ->
m :: movesInternal zz
in
movesInternal (Zipper.fromTree game.tree)
{-| The previous move in the game, i.e. the move that resulted in the
current game position. Returns `Nothing` if we're at the beginning of the
game.
-}
previousMove : Game -> Maybe Move
previousMove game =
Position.lastMove (position game)
{-| The next move in the game from the current position. Returns `Nothing` if
we're at the end of the game.
-}
nextMove : Game -> Maybe Move
nextMove game =
Zipper.firstChild game.focus
|> Maybe.andThen (\z -> Position.lastMove (Zipper.label z).position)
{-| Jump to the given game ply number.
-}
goToMove : Int -> Game -> Game
goToMove moveIndex game =
if moveIndex <= 0 then
{ game | focus = Zipper.fromTree game.tree }
else
let
descendant zipper generations =
if generations == 0 then
zipper
else
case Zipper.firstChild zipper of
Nothing ->
zipper
Just z ->
descendant z (generations - 1)
in
{ game | focus = descendant (Zipper.fromTree game.tree) moveIndex }
{-| Jump to the node with the given node ID, if it exists. If not, return the
game unchanged. Perhaps this one should return a `Maybe Game` instead?
-}
goToNode : Int -> Game -> Game
goToNode nodeId game =
{ game
| focus =
Maybe.withDefault game.focus <|
Zipper.findFromRoot (\n -> n.id == nodeId) game.focus
}
{-| Are we at the beginning of the game?
-}
isAtBeginning : Game -> Bool
isAtBeginning game =
Zipper.label game.focus == Tree.label game.tree
{-| Are we at the end of the game?
-}
isAtEnd : Game -> Bool
isAtEnd game =
Zipper.firstChild game.focus == Nothing
{-| Step one move forward, unless we are already at the end of the game.
If we are already at the end, do nothing.
-}
forward : Game -> Game
forward game =
{ game
| focus = Maybe.withDefault game.focus (Zipper.firstChild game.focus)
}
{-| Step one move backward, unless we are already at the beginning of the game.
If we are already at the beginning, do nothing.
-}
back : Game -> Game
back game =
{ game
| focus = Maybe.withDefault game.focus (Zipper.parent game.focus)
}
{-| Go to the beginning of the game.
-}
toBeginning : Game -> Game
toBeginning game =
{ game | focus = Zipper.root game.focus }
{-| Go to the end of the game.
-}
toEnd : Game -> Game
toEnd game =
toEndOfVariation <| toBeginning game
isAtBeginningOfVariation : Game -> Bool
isAtBeginningOfVariation game =
case Zipper.parent game.focus of
Nothing ->
True
Just parent ->
Zipper.firstChild parent /= Just game.focus
{-| Go to the beginning of the current variation.
-}
toBeginningOfVariation : Game -> Game
toBeginningOfVariation game =
if isAtBeginningOfVariation game then
game
else
toBeginningOfVariation (back game)
{-| Go to the end of the current variation.
-}
toEndOfVariation : Game -> Game
toEndOfVariation game =
{ game | focus = Zipper.lastDescendant game.focus }
{-| Add a move to the game at the current location. If we're not at a leaf
node, the move is added as an alternative variation.
-}
addMove : Move -> Game -> Game
addMove move game =
let
pos =
Position.doMove move (position game)
node =
{ position = pos
, comment = Nothing
, precomment = Nothing
, nag = Nothing
, id = game.nodeCount
, ply = (Zipper.label game.focus).ply + 1
}
z =
let
forest =
Zipper.children game.focus
in
Zipper.replaceTree
(Tree.tree
(Zipper.label game.focus)
(forest ++ [ Tree.singleton node ])
)
game.focus
in
{ game
| tree = Zipper.toTree z
, focus = Maybe.withDefault z <| Zipper.lastChild z
, nodeCount = game.nodeCount + 1
}
{-| Tries to add a move in short algebraic notation at the current move index.
If the input string is not a legal, unambiguous move in short algebraic notation
from the current game position, returns Nothing.
-}
addSanMove : String -> Game -> Maybe Game
addSanMove sanMove game =
Maybe.map (\m -> addMove m game) <|
fromSan sanMove (position game)
{-| Tries to add a sequence of moves in short algebraic notation at the current
move index. If one of the strings in the input list is not a legal, unambiguous
move in short algebraic notation, returns `Nothing`.
-}
addSanMoveSequence : List String -> Game -> Maybe Game
addSanMoveSequence sanMoves game =
failableFoldl addSanMove game sanMoves
fromPgn : PgnGame -> Game
fromPgn pgnGame =
Tuple.first <|
List.foldl
addMoveTextItem
( { empty | tags = pgnGame.headers }, Nothing )
pgnGame.moveText
fromPgnString : String -> Maybe Game
fromPgnString pgnString =
Maybe.map fromPgn (Pgn.fromString pgnString)
toPgn : Game -> PgnGame
toPgn game =
{ headers = game.tags
, moveText =
(if Position.sideToMove (position <| toBeginning game) == PieceColor.black then
[ MoveNumber "1." ]
else
[]
)
++ (buildMoveText <|
Zipper.fromTree <|
game.tree
)
++ [ Termination game.result ]
}
addMoveTextItem : MoveTextItem -> ( Game, Maybe String ) -> ( Game, Maybe String )
addMoveTextItem mti ( game, preCmt ) =
case mti of
Move move ->
case preCmt of
Nothing ->
( Maybe.withDefault game (addSanMove move game), Nothing )
Just pc ->
( addPreComment pc
(Maybe.withDefault
game
(addSanMove move game)
)
, Nothing
)
MoveNumber _ ->
( game, preCmt )
Variation var ->
( addVariation var game, Nothing )
Comment cmt ->
if isAtBeginning game || not (isAtEnd game) then
( game, Just cmt )
else
( addComment cmt game, Nothing )
Nag nag ->
( addNag nag game, Nothing )
Termination t ->
( { game | result = t }, Nothing )
addPreComment : String -> Game -> Game
addPreComment cmt game =
{ game
| focus =
Zipper.mapLabel
(\n -> { n | precomment = Just cmt })
game.focus
}
addComment : String -> Game -> Game
addComment cmt game =
{ game
| focus =
Zipper.mapLabel
(\n -> { n | comment = Just cmt })
game.focus
}
addNag : Int -> Game -> Game
addNag nag game =
{ game
| focus =
Zipper.mapLabel
(\n -> { n | nag = Just nag })
game.focus
}
addVariation : MoveText -> Game -> Game
addVariation variation game =
game
|> back
|> (\g -> Tuple.first <| List.foldl addMoveTextItem ( g, Nothing ) variation)
|> toBeginningOfVariation
|> back
|> forward
buildMoveText : Zipper GameNode -> MoveText
buildMoveText zip =
case Zipper.firstChild zip of
Nothing ->
[]
Just ch ->
let
pos =
(Zipper.label zip).position
in
moveToMoveText
pos
(Zipper.label ch)
(Position.sideToMove pos == PieceColor.white)
++ -- Alternative variations
List.map
(\t ->
Variation <|
moveToMoveText pos (Tree.label t) True
++ buildMoveText (Zipper.fromTree t)
)
(Zipper.siblingsAfterFocus ch)
++ -- Game continuation
buildMoveText ch
moveToMoveText : Position -> GameNode -> Bool -> MoveText
moveToMoveText pos node moveNum =
let
move =
lastMove node
in
-- Pre-comment
(case node.precomment of
Nothing ->
[]
Just cmt ->
[ Comment cmt ]
)
-- Move number
++ (if moveNum then
[ MoveNumber
(if Position.sideToMove pos == PieceColor.white then
String.fromInt (node.ply // 2 + 1) ++ "."
else
String.fromInt (node.ply // 2 + 1) ++ "..."
)
]
else
[]
)
-- Move
++ [ Move <| toSan move pos ]
-- Numeric Annotation Glyph
++ (case node.nag of
Nothing ->
[]
Just nag ->
[ Nag nag ]
)
-- Comment
++ (case node.comment of
Nothing ->
[]
Just cmt ->
[ Comment cmt ]
)
examplePgn =
"""[Event "F/S Return Match"]
[Site "Belgrade, Serbia JUG"]
[Date "1992.11.04"]
[Round "29"]
[White "Fischer, Robert J."]
[Black "Spassky, Boris V."]
[Result "1/2-1/2"]
1. e4 e5 (1... c5 2. Nf3 d6) 2. Nf3 Nc6 3. Bb5 a6 {This opening is called the Ruy Lopez.}
4. Ba4 ({If } 4. Bxc6 dxc6 { visible? } 5. O-O (5. Nxe5 Qd4) f6 {End of var}) Nf6 {After var} 5. O-O Be7 6. Re1 b5 7. Bb3 d6 8. c3 O-O 9. h3 Nb8 10. d4 Nbd7
11. c4 c6 12. cxb5 axb5 13. Nc3 Bb7 14. Bg5 b4 { boring } 15. Nb1 h6 16. Bh4 c5 17. dxe5
Nxe4 18. Bxe7 Qxe7 19. exd6 Qf6 20. Nbd2 Nxd6 21. Nc4 Nxc4 22. Bxc4 Nb6
23. Ne5 Rae8 24. Bxf7+ Rxf7 25. Nxf7 Rxe1+ 26. Qxe1 Kxf7 27. Qe3 Qg5 28. Qxg5
hxg5 29. b3 Ke6 30. a3 Kd6 31. axb4 cxb4 32. Ra5 Nd5 33. f3 Bc8 34. Kf2 Bf5
35. Ra7 g6 36. Ra6+ Kc5 37. Ke1 Nf4 38. g3 Nxh3 39. Kd2 Kb5 40. Rd6 Kc5 41. Ra6
Nf2 42. g4 Bd3 43. Re6 1/2-1/2"""
| 40676 | module Internal.AnnotatedGame exposing
( Game
, addMove
, addSanMove
, addSanMoveSequence
, back
, buildMoveText
, children
, continuations
, empty
, examplePgn
, forward
, fromPgn
, fromPgnString
, goToMove
, isAtBeginning
, isAtBeginningOfVariation
, isAtEnd
, moves
, nextMove
, position
, previousMove
, tagValue
, toBeginning
, toBeginningOfVariation
, toEnd
, toEndOfVariation
, toPgn
)
import Array exposing (Array)
import Internal.AnnotatedPgn as Pgn
exposing
( GameResult(..)
, MoveText
, MoveTextItem(..)
, PgnGame
, TagPair
)
import Internal.Move as Move exposing (Move, Variation)
import Internal.Notation exposing (fromSan, toSan)
import Internal.PieceColor as PieceColor exposing (PieceColor)
import Internal.Position as Position exposing (Position)
import Internal.Util exposing (failableFoldl)
import Parser
import Tree exposing (Tree)
import Tree.Zipper as Zipper exposing (Zipper)
type alias GameNode =
{ position : Position
, comment : Maybe String
, precomment : Maybe String
, nag : Maybe Int
, id : Int
, ply : Int
}
type alias Game =
{ result : GameResult
, tags : List TagPair
, tree : Tree GameNode
, focus : Zipper GameNode
, nodeCount : Int
}
empty : Game
empty =
let
tree =
Tree.singleton
{ position = Position.initial
, comment = Nothing
, precomment = Nothing
, nag = Nothing
, id = 0
, ply = 0
}
in
{ result = UnknownResult
, tags = []
, tree = tree
, focus = Zipper.fromTree tree
, nodeCount = 1
}
{-| Get the value of a game tag.
-}
tagValue : String -> Game -> Maybe String
tagValue tagName game =
game.tags
|> List.filter (\t -> Tuple.first t == tagName)
|> List.head
|> Maybe.map Tuple.second
lastMove : GameNode -> Move
lastMove node =
Maybe.withDefault (Move.Move 0) (Position.lastMove node.position)
{-| The current node in the game tree
-}
currentNode : Game -> GameNode
currentNode game =
Zipper.label game.focus
{-| Children of the current node in the game tree
-}
children : Game -> List GameNode
children game =
List.map Tree.label (Zipper.children game.focus)
{-| Continuations from the current node in the game tree
-}
continuations : Game -> List Move
continuations game =
List.map
(\n ->
Maybe.withDefault
(Move.Move 0)
(Position.lastMove n.position)
)
(children game)
{-| The position at the current move of the game.
-}
position : Game -> Position
position game =
(currentNode game).position
{-| List of all moves in the game.
-}
moves : Game -> List Move
moves game =
let
zipper =
Zipper.fromTree game.tree
movesInternal z =
case Zipper.firstChild z of
Nothing ->
[]
Just zz ->
case Position.lastMove (Zipper.label zz).position of
Nothing ->
[]
Just m ->
m :: movesInternal zz
in
movesInternal (Zipper.fromTree game.tree)
{-| The previous move in the game, i.e. the move that resulted in the
current game position. Returns `Nothing` if we're at the beginning of the
game.
-}
previousMove : Game -> Maybe Move
previousMove game =
Position.lastMove (position game)
{-| The next move in the game from the current position. Returns `Nothing` if
we're at the end of the game.
-}
nextMove : Game -> Maybe Move
nextMove game =
Zipper.firstChild game.focus
|> Maybe.andThen (\z -> Position.lastMove (Zipper.label z).position)
{-| Jump to the given game ply number.
-}
goToMove : Int -> Game -> Game
goToMove moveIndex game =
if moveIndex <= 0 then
{ game | focus = Zipper.fromTree game.tree }
else
let
descendant zipper generations =
if generations == 0 then
zipper
else
case Zipper.firstChild zipper of
Nothing ->
zipper
Just z ->
descendant z (generations - 1)
in
{ game | focus = descendant (Zipper.fromTree game.tree) moveIndex }
{-| Jump to the node with the given node ID, if it exists. If not, return the
game unchanged. Perhaps this one should return a `Maybe Game` instead?
-}
goToNode : Int -> Game -> Game
goToNode nodeId game =
{ game
| focus =
Maybe.withDefault game.focus <|
Zipper.findFromRoot (\n -> n.id == nodeId) game.focus
}
{-| Are we at the beginning of the game?
-}
isAtBeginning : Game -> Bool
isAtBeginning game =
Zipper.label game.focus == Tree.label game.tree
{-| Are we at the end of the game?
-}
isAtEnd : Game -> Bool
isAtEnd game =
Zipper.firstChild game.focus == Nothing
{-| Step one move forward, unless we are already at the end of the game.
If we are already at the end, do nothing.
-}
forward : Game -> Game
forward game =
{ game
| focus = Maybe.withDefault game.focus (Zipper.firstChild game.focus)
}
{-| Step one move backward, unless we are already at the beginning of the game.
If we are already at the beginning, do nothing.
-}
back : Game -> Game
back game =
{ game
| focus = Maybe.withDefault game.focus (Zipper.parent game.focus)
}
{-| Go to the beginning of the game.
-}
toBeginning : Game -> Game
toBeginning game =
{ game | focus = Zipper.root game.focus }
{-| Go to the end of the game.
-}
toEnd : Game -> Game
toEnd game =
toEndOfVariation <| toBeginning game
isAtBeginningOfVariation : Game -> Bool
isAtBeginningOfVariation game =
case Zipper.parent game.focus of
Nothing ->
True
Just parent ->
Zipper.firstChild parent /= Just game.focus
{-| Go to the beginning of the current variation.
-}
toBeginningOfVariation : Game -> Game
toBeginningOfVariation game =
if isAtBeginningOfVariation game then
game
else
toBeginningOfVariation (back game)
{-| Go to the end of the current variation.
-}
toEndOfVariation : Game -> Game
toEndOfVariation game =
{ game | focus = Zipper.lastDescendant game.focus }
{-| Add a move to the game at the current location. If we're not at a leaf
node, the move is added as an alternative variation.
-}
addMove : Move -> Game -> Game
addMove move game =
let
pos =
Position.doMove move (position game)
node =
{ position = pos
, comment = Nothing
, precomment = Nothing
, nag = Nothing
, id = game.nodeCount
, ply = (Zipper.label game.focus).ply + 1
}
z =
let
forest =
Zipper.children game.focus
in
Zipper.replaceTree
(Tree.tree
(Zipper.label game.focus)
(forest ++ [ Tree.singleton node ])
)
game.focus
in
{ game
| tree = Zipper.toTree z
, focus = Maybe.withDefault z <| Zipper.lastChild z
, nodeCount = game.nodeCount + 1
}
{-| Tries to add a move in short algebraic notation at the current move index.
If the input string is not a legal, unambiguous move in short algebraic notation
from the current game position, returns Nothing.
-}
addSanMove : String -> Game -> Maybe Game
addSanMove sanMove game =
Maybe.map (\m -> addMove m game) <|
fromSan sanMove (position game)
{-| Tries to add a sequence of moves in short algebraic notation at the current
move index. If one of the strings in the input list is not a legal, unambiguous
move in short algebraic notation, returns `Nothing`.
-}
addSanMoveSequence : List String -> Game -> Maybe Game
addSanMoveSequence sanMoves game =
failableFoldl addSanMove game sanMoves
fromPgn : PgnGame -> Game
fromPgn pgnGame =
Tuple.first <|
List.foldl
addMoveTextItem
( { empty | tags = pgnGame.headers }, Nothing )
pgnGame.moveText
fromPgnString : String -> Maybe Game
fromPgnString pgnString =
Maybe.map fromPgn (Pgn.fromString pgnString)
toPgn : Game -> PgnGame
toPgn game =
{ headers = game.tags
, moveText =
(if Position.sideToMove (position <| toBeginning game) == PieceColor.black then
[ MoveNumber "1." ]
else
[]
)
++ (buildMoveText <|
Zipper.fromTree <|
game.tree
)
++ [ Termination game.result ]
}
addMoveTextItem : MoveTextItem -> ( Game, Maybe String ) -> ( Game, Maybe String )
addMoveTextItem mti ( game, preCmt ) =
case mti of
Move move ->
case preCmt of
Nothing ->
( Maybe.withDefault game (addSanMove move game), Nothing )
Just pc ->
( addPreComment pc
(Maybe.withDefault
game
(addSanMove move game)
)
, Nothing
)
MoveNumber _ ->
( game, preCmt )
Variation var ->
( addVariation var game, Nothing )
Comment cmt ->
if isAtBeginning game || not (isAtEnd game) then
( game, Just cmt )
else
( addComment cmt game, Nothing )
Nag nag ->
( addNag nag game, Nothing )
Termination t ->
( { game | result = t }, Nothing )
addPreComment : String -> Game -> Game
addPreComment cmt game =
{ game
| focus =
Zipper.mapLabel
(\n -> { n | precomment = Just cmt })
game.focus
}
addComment : String -> Game -> Game
addComment cmt game =
{ game
| focus =
Zipper.mapLabel
(\n -> { n | comment = Just cmt })
game.focus
}
addNag : Int -> Game -> Game
addNag nag game =
{ game
| focus =
Zipper.mapLabel
(\n -> { n | nag = Just nag })
game.focus
}
addVariation : MoveText -> Game -> Game
addVariation variation game =
game
|> back
|> (\g -> Tuple.first <| List.foldl addMoveTextItem ( g, Nothing ) variation)
|> toBeginningOfVariation
|> back
|> forward
buildMoveText : Zipper GameNode -> MoveText
buildMoveText zip =
case Zipper.firstChild zip of
Nothing ->
[]
Just ch ->
let
pos =
(Zipper.label zip).position
in
moveToMoveText
pos
(Zipper.label ch)
(Position.sideToMove pos == PieceColor.white)
++ -- Alternative variations
List.map
(\t ->
Variation <|
moveToMoveText pos (Tree.label t) True
++ buildMoveText (Zipper.fromTree t)
)
(Zipper.siblingsAfterFocus ch)
++ -- Game continuation
buildMoveText ch
moveToMoveText : Position -> GameNode -> Bool -> MoveText
moveToMoveText pos node moveNum =
let
move =
lastMove node
in
-- Pre-comment
(case node.precomment of
Nothing ->
[]
Just cmt ->
[ Comment cmt ]
)
-- Move number
++ (if moveNum then
[ MoveNumber
(if Position.sideToMove pos == PieceColor.white then
String.fromInt (node.ply // 2 + 1) ++ "."
else
String.fromInt (node.ply // 2 + 1) ++ "..."
)
]
else
[]
)
-- Move
++ [ Move <| toSan move pos ]
-- Numeric Annotation Glyph
++ (case node.nag of
Nothing ->
[]
Just nag ->
[ Nag nag ]
)
-- Comment
++ (case node.comment of
Nothing ->
[]
Just cmt ->
[ Comment cmt ]
)
examplePgn =
"""[Event "F/S Return Match"]
[Site "Belgrade, Serbia JUG"]
[Date "1992.11.04"]
[Round "29"]
[White "<NAME>."]
[Black "<NAME>."]
[Result "1/2-1/2"]
1. e4 e5 (1... c5 2. Nf3 d6) 2. Nf3 Nc6 3. Bb5 a6 {This opening is called the Ruy Lopez.}
4. Ba4 ({If } 4. Bxc6 dxc6 { visible? } 5. O-O (5. Nxe5 Qd4) f6 {End of var}) Nf6 {After var} 5. O-O Be7 6. Re1 b5 7. Bb3 d6 8. c3 O-O 9. h3 Nb8 10. d4 Nbd7
11. c4 c6 12. cxb5 axb5 13. Nc3 Bb7 14. Bg5 b4 { boring } 15. Nb1 h6 16. Bh4 c5 17. dxe5
Nxe4 18. Bxe7 Qxe7 19. exd6 Qf6 20. Nbd2 Nxd6 21. Nc4 Nxc4 22. Bxc4 Nb6
23. Ne5 Rae8 24. Bxf7+ Rxf7 25. Nxf7 Rxe1+ 26. Qxe1 Kxf7 27. Qe3 Qg5 28. Qxg5
hxg5 29. b3 Ke6 30. a3 Kd6 31. axb4 cxb4 32. Ra5 Nd5 33. f3 Bc8 34. Kf2 Bf5
35. Ra7 g6 36. Ra6+ Kc5 37. Ke1 Nf4 38. g3 Nxh3 39. Kd2 Kb5 40. Rd6 Kc5 41. Ra6
Nf2 42. g4 Bd3 43. Re6 1/2-1/2"""
| true | module Internal.AnnotatedGame exposing
( Game
, addMove
, addSanMove
, addSanMoveSequence
, back
, buildMoveText
, children
, continuations
, empty
, examplePgn
, forward
, fromPgn
, fromPgnString
, goToMove
, isAtBeginning
, isAtBeginningOfVariation
, isAtEnd
, moves
, nextMove
, position
, previousMove
, tagValue
, toBeginning
, toBeginningOfVariation
, toEnd
, toEndOfVariation
, toPgn
)
import Array exposing (Array)
import Internal.AnnotatedPgn as Pgn
exposing
( GameResult(..)
, MoveText
, MoveTextItem(..)
, PgnGame
, TagPair
)
import Internal.Move as Move exposing (Move, Variation)
import Internal.Notation exposing (fromSan, toSan)
import Internal.PieceColor as PieceColor exposing (PieceColor)
import Internal.Position as Position exposing (Position)
import Internal.Util exposing (failableFoldl)
import Parser
import Tree exposing (Tree)
import Tree.Zipper as Zipper exposing (Zipper)
type alias GameNode =
{ position : Position
, comment : Maybe String
, precomment : Maybe String
, nag : Maybe Int
, id : Int
, ply : Int
}
type alias Game =
{ result : GameResult
, tags : List TagPair
, tree : Tree GameNode
, focus : Zipper GameNode
, nodeCount : Int
}
empty : Game
empty =
let
tree =
Tree.singleton
{ position = Position.initial
, comment = Nothing
, precomment = Nothing
, nag = Nothing
, id = 0
, ply = 0
}
in
{ result = UnknownResult
, tags = []
, tree = tree
, focus = Zipper.fromTree tree
, nodeCount = 1
}
{-| Get the value of a game tag.
-}
tagValue : String -> Game -> Maybe String
tagValue tagName game =
game.tags
|> List.filter (\t -> Tuple.first t == tagName)
|> List.head
|> Maybe.map Tuple.second
lastMove : GameNode -> Move
lastMove node =
Maybe.withDefault (Move.Move 0) (Position.lastMove node.position)
{-| The current node in the game tree
-}
currentNode : Game -> GameNode
currentNode game =
Zipper.label game.focus
{-| Children of the current node in the game tree
-}
children : Game -> List GameNode
children game =
List.map Tree.label (Zipper.children game.focus)
{-| Continuations from the current node in the game tree
-}
continuations : Game -> List Move
continuations game =
List.map
(\n ->
Maybe.withDefault
(Move.Move 0)
(Position.lastMove n.position)
)
(children game)
{-| The position at the current move of the game.
-}
position : Game -> Position
position game =
(currentNode game).position
{-| List of all moves in the game.
-}
moves : Game -> List Move
moves game =
let
zipper =
Zipper.fromTree game.tree
movesInternal z =
case Zipper.firstChild z of
Nothing ->
[]
Just zz ->
case Position.lastMove (Zipper.label zz).position of
Nothing ->
[]
Just m ->
m :: movesInternal zz
in
movesInternal (Zipper.fromTree game.tree)
{-| The previous move in the game, i.e. the move that resulted in the
current game position. Returns `Nothing` if we're at the beginning of the
game.
-}
previousMove : Game -> Maybe Move
previousMove game =
Position.lastMove (position game)
{-| The next move in the game from the current position. Returns `Nothing` if
we're at the end of the game.
-}
nextMove : Game -> Maybe Move
nextMove game =
Zipper.firstChild game.focus
|> Maybe.andThen (\z -> Position.lastMove (Zipper.label z).position)
{-| Jump to the given game ply number.
-}
goToMove : Int -> Game -> Game
goToMove moveIndex game =
if moveIndex <= 0 then
{ game | focus = Zipper.fromTree game.tree }
else
let
descendant zipper generations =
if generations == 0 then
zipper
else
case Zipper.firstChild zipper of
Nothing ->
zipper
Just z ->
descendant z (generations - 1)
in
{ game | focus = descendant (Zipper.fromTree game.tree) moveIndex }
{-| Jump to the node with the given node ID, if it exists. If not, return the
game unchanged. Perhaps this one should return a `Maybe Game` instead?
-}
goToNode : Int -> Game -> Game
goToNode nodeId game =
{ game
| focus =
Maybe.withDefault game.focus <|
Zipper.findFromRoot (\n -> n.id == nodeId) game.focus
}
{-| Are we at the beginning of the game?
-}
isAtBeginning : Game -> Bool
isAtBeginning game =
Zipper.label game.focus == Tree.label game.tree
{-| Are we at the end of the game?
-}
isAtEnd : Game -> Bool
isAtEnd game =
Zipper.firstChild game.focus == Nothing
{-| Step one move forward, unless we are already at the end of the game.
If we are already at the end, do nothing.
-}
forward : Game -> Game
forward game =
{ game
| focus = Maybe.withDefault game.focus (Zipper.firstChild game.focus)
}
{-| Step one move backward, unless we are already at the beginning of the game.
If we are already at the beginning, do nothing.
-}
back : Game -> Game
back game =
{ game
| focus = Maybe.withDefault game.focus (Zipper.parent game.focus)
}
{-| Go to the beginning of the game.
-}
toBeginning : Game -> Game
toBeginning game =
{ game | focus = Zipper.root game.focus }
{-| Go to the end of the game.
-}
toEnd : Game -> Game
toEnd game =
toEndOfVariation <| toBeginning game
isAtBeginningOfVariation : Game -> Bool
isAtBeginningOfVariation game =
case Zipper.parent game.focus of
Nothing ->
True
Just parent ->
Zipper.firstChild parent /= Just game.focus
{-| Go to the beginning of the current variation.
-}
toBeginningOfVariation : Game -> Game
toBeginningOfVariation game =
if isAtBeginningOfVariation game then
game
else
toBeginningOfVariation (back game)
{-| Go to the end of the current variation.
-}
toEndOfVariation : Game -> Game
toEndOfVariation game =
{ game | focus = Zipper.lastDescendant game.focus }
{-| Add a move to the game at the current location. If we're not at a leaf
node, the move is added as an alternative variation.
-}
addMove : Move -> Game -> Game
addMove move game =
let
pos =
Position.doMove move (position game)
node =
{ position = pos
, comment = Nothing
, precomment = Nothing
, nag = Nothing
, id = game.nodeCount
, ply = (Zipper.label game.focus).ply + 1
}
z =
let
forest =
Zipper.children game.focus
in
Zipper.replaceTree
(Tree.tree
(Zipper.label game.focus)
(forest ++ [ Tree.singleton node ])
)
game.focus
in
{ game
| tree = Zipper.toTree z
, focus = Maybe.withDefault z <| Zipper.lastChild z
, nodeCount = game.nodeCount + 1
}
{-| Tries to add a move in short algebraic notation at the current move index.
If the input string is not a legal, unambiguous move in short algebraic notation
from the current game position, returns Nothing.
-}
addSanMove : String -> Game -> Maybe Game
addSanMove sanMove game =
Maybe.map (\m -> addMove m game) <|
fromSan sanMove (position game)
{-| Tries to add a sequence of moves in short algebraic notation at the current
move index. If one of the strings in the input list is not a legal, unambiguous
move in short algebraic notation, returns `Nothing`.
-}
addSanMoveSequence : List String -> Game -> Maybe Game
addSanMoveSequence sanMoves game =
failableFoldl addSanMove game sanMoves
fromPgn : PgnGame -> Game
fromPgn pgnGame =
Tuple.first <|
List.foldl
addMoveTextItem
( { empty | tags = pgnGame.headers }, Nothing )
pgnGame.moveText
fromPgnString : String -> Maybe Game
fromPgnString pgnString =
Maybe.map fromPgn (Pgn.fromString pgnString)
toPgn : Game -> PgnGame
toPgn game =
{ headers = game.tags
, moveText =
(if Position.sideToMove (position <| toBeginning game) == PieceColor.black then
[ MoveNumber "1." ]
else
[]
)
++ (buildMoveText <|
Zipper.fromTree <|
game.tree
)
++ [ Termination game.result ]
}
addMoveTextItem : MoveTextItem -> ( Game, Maybe String ) -> ( Game, Maybe String )
addMoveTextItem mti ( game, preCmt ) =
case mti of
Move move ->
case preCmt of
Nothing ->
( Maybe.withDefault game (addSanMove move game), Nothing )
Just pc ->
( addPreComment pc
(Maybe.withDefault
game
(addSanMove move game)
)
, Nothing
)
MoveNumber _ ->
( game, preCmt )
Variation var ->
( addVariation var game, Nothing )
Comment cmt ->
if isAtBeginning game || not (isAtEnd game) then
( game, Just cmt )
else
( addComment cmt game, Nothing )
Nag nag ->
( addNag nag game, Nothing )
Termination t ->
( { game | result = t }, Nothing )
addPreComment : String -> Game -> Game
addPreComment cmt game =
{ game
| focus =
Zipper.mapLabel
(\n -> { n | precomment = Just cmt })
game.focus
}
addComment : String -> Game -> Game
addComment cmt game =
{ game
| focus =
Zipper.mapLabel
(\n -> { n | comment = Just cmt })
game.focus
}
addNag : Int -> Game -> Game
addNag nag game =
{ game
| focus =
Zipper.mapLabel
(\n -> { n | nag = Just nag })
game.focus
}
addVariation : MoveText -> Game -> Game
addVariation variation game =
game
|> back
|> (\g -> Tuple.first <| List.foldl addMoveTextItem ( g, Nothing ) variation)
|> toBeginningOfVariation
|> back
|> forward
buildMoveText : Zipper GameNode -> MoveText
buildMoveText zip =
case Zipper.firstChild zip of
Nothing ->
[]
Just ch ->
let
pos =
(Zipper.label zip).position
in
moveToMoveText
pos
(Zipper.label ch)
(Position.sideToMove pos == PieceColor.white)
++ -- Alternative variations
List.map
(\t ->
Variation <|
moveToMoveText pos (Tree.label t) True
++ buildMoveText (Zipper.fromTree t)
)
(Zipper.siblingsAfterFocus ch)
++ -- Game continuation
buildMoveText ch
moveToMoveText : Position -> GameNode -> Bool -> MoveText
moveToMoveText pos node moveNum =
let
move =
lastMove node
in
-- Pre-comment
(case node.precomment of
Nothing ->
[]
Just cmt ->
[ Comment cmt ]
)
-- Move number
++ (if moveNum then
[ MoveNumber
(if Position.sideToMove pos == PieceColor.white then
String.fromInt (node.ply // 2 + 1) ++ "."
else
String.fromInt (node.ply // 2 + 1) ++ "..."
)
]
else
[]
)
-- Move
++ [ Move <| toSan move pos ]
-- Numeric Annotation Glyph
++ (case node.nag of
Nothing ->
[]
Just nag ->
[ Nag nag ]
)
-- Comment
++ (case node.comment of
Nothing ->
[]
Just cmt ->
[ Comment cmt ]
)
examplePgn =
"""[Event "F/S Return Match"]
[Site "Belgrade, Serbia JUG"]
[Date "1992.11.04"]
[Round "29"]
[White "PI:NAME:<NAME>END_PI."]
[Black "PI:NAME:<NAME>END_PI."]
[Result "1/2-1/2"]
1. e4 e5 (1... c5 2. Nf3 d6) 2. Nf3 Nc6 3. Bb5 a6 {This opening is called the Ruy Lopez.}
4. Ba4 ({If } 4. Bxc6 dxc6 { visible? } 5. O-O (5. Nxe5 Qd4) f6 {End of var}) Nf6 {After var} 5. O-O Be7 6. Re1 b5 7. Bb3 d6 8. c3 O-O 9. h3 Nb8 10. d4 Nbd7
11. c4 c6 12. cxb5 axb5 13. Nc3 Bb7 14. Bg5 b4 { boring } 15. Nb1 h6 16. Bh4 c5 17. dxe5
Nxe4 18. Bxe7 Qxe7 19. exd6 Qf6 20. Nbd2 Nxd6 21. Nc4 Nxc4 22. Bxc4 Nb6
23. Ne5 Rae8 24. Bxf7+ Rxf7 25. Nxf7 Rxe1+ 26. Qxe1 Kxf7 27. Qe3 Qg5 28. Qxg5
hxg5 29. b3 Ke6 30. a3 Kd6 31. axb4 cxb4 32. Ra5 Nd5 33. f3 Bc8 34. Kf2 Bf5
35. Ra7 g6 36. Ra6+ Kc5 37. Ke1 Nf4 38. g3 Nxh3 39. Kd2 Kb5 40. Rd6 Kc5 41. Ra6
Nf2 42. g4 Bd3 43. Re6 1/2-1/2"""
| elm |
[
{
"context": ", LatLng )\n | NoResults String\n\n\napiKey =\n \"AIzaSyCkOFxL5NF1feuebbB6PW8fP3SDg1aa6tM\"\n\n\nmain =\n program\n { init = init\n ",
"end": 556,
"score": 0.9997757077,
"start": 517,
"tag": "KEY",
"value": "AIzaSyCkOFxL5NF1feuebbB6PW8fP3SDg1aa6tM"
}
] | examples/SearchMarkers.elm | elm-review-bot/elm-mapbox | 1 | module Markers exposing (Msg(..), apiKey, geocode, getFirstLocation, init, main, subscriptions, update, view)
import Geocoding
import Html.Styled as Html exposing (program)
import Html.Styled.Events as E exposing (onInput)
import Http
import Maps exposing (Msg)
import Maps.Geo exposing (Bounds, LatLng)
import Maps.Map as Map
import Maps.Marker as Marker exposing (Marker)
type Msg
= MapMsg (Maps.Msg ())
| GeoCode String
| GoToGeoCode String ( Bounds, LatLng )
| NoResults String
apiKey =
"AIzaSyCkOFxL5NF1feuebbB6PW8fP3SDg1aa6tM"
main =
program
{ init = init
, update = update
, subscriptions = subscriptions
, view = view
}
init =
( { map =
Maps.defaultModel
|> Maps.updateMap (Map.setHeight 600)
|> Maps.updateMap (Map.setWidth 1000)
, place = ""
}
, Cmd.none
)
update msg model =
case msg of
MapMsg mapMsg ->
Maps.update mapMsg model.map
|> Tuple.mapFirst (\map -> { model | map = map })
|> Tuple.mapSecond (Cmd.map MapMsg)
GeoCode place ->
( { model | place = place }, geocode place )
GoToGeoCode place ( bounds, latLng ) ->
if place == model.place then
model.map
|> Maps.updateMap (Map.viewBounds bounds)
|> Maps.updateMarkers (\_ -> [ Marker.create latLng ])
|> (\map -> ( { model | map = map }, Cmd.none ))
else
( model, Cmd.none )
NoResults place ->
( model, Cmd.none )
geocode : String -> Cmd Msg
geocode place =
Geocoding.requestForAddress apiKey place
|> Geocoding.send (Maybe.withDefault (NoResults place) << Maybe.map (GoToGeoCode place) << getFirstLocation)
getFirstLocation : Result Http.Error Geocoding.Response -> Maybe ( Bounds, LatLng )
getFirstLocation result =
result
|> Result.toMaybe
|> Maybe.map .results
|> Maybe.andThen List.head
|> Maybe.map .geometry
|> Maybe.map
(\geometry ->
let
bounds =
Maps.Geo.bounds
{ northEast = { lat = geometry.viewport.northeast.latitude, lng = geometry.viewport.northeast.longitude }
, southWest = { lat = geometry.viewport.southwest.latitude, lng = geometry.viewport.southwest.longitude }
}
latLng =
{ lat = geometry.location.latitude
, lng = geometry.location.longitude
}
in
( bounds, latLng )
)
subscriptions model =
Sub.map MapMsg <| Maps.subscriptions model.map
view model =
Html.div
[]
[ Html.map MapMsg <| Maps.view model.map
, Html.input
[ onInput GeoCode
]
[]
]
| 55384 | module Markers exposing (Msg(..), apiKey, geocode, getFirstLocation, init, main, subscriptions, update, view)
import Geocoding
import Html.Styled as Html exposing (program)
import Html.Styled.Events as E exposing (onInput)
import Http
import Maps exposing (Msg)
import Maps.Geo exposing (Bounds, LatLng)
import Maps.Map as Map
import Maps.Marker as Marker exposing (Marker)
type Msg
= MapMsg (Maps.Msg ())
| GeoCode String
| GoToGeoCode String ( Bounds, LatLng )
| NoResults String
apiKey =
"<KEY>"
main =
program
{ init = init
, update = update
, subscriptions = subscriptions
, view = view
}
init =
( { map =
Maps.defaultModel
|> Maps.updateMap (Map.setHeight 600)
|> Maps.updateMap (Map.setWidth 1000)
, place = ""
}
, Cmd.none
)
update msg model =
case msg of
MapMsg mapMsg ->
Maps.update mapMsg model.map
|> Tuple.mapFirst (\map -> { model | map = map })
|> Tuple.mapSecond (Cmd.map MapMsg)
GeoCode place ->
( { model | place = place }, geocode place )
GoToGeoCode place ( bounds, latLng ) ->
if place == model.place then
model.map
|> Maps.updateMap (Map.viewBounds bounds)
|> Maps.updateMarkers (\_ -> [ Marker.create latLng ])
|> (\map -> ( { model | map = map }, Cmd.none ))
else
( model, Cmd.none )
NoResults place ->
( model, Cmd.none )
geocode : String -> Cmd Msg
geocode place =
Geocoding.requestForAddress apiKey place
|> Geocoding.send (Maybe.withDefault (NoResults place) << Maybe.map (GoToGeoCode place) << getFirstLocation)
getFirstLocation : Result Http.Error Geocoding.Response -> Maybe ( Bounds, LatLng )
getFirstLocation result =
result
|> Result.toMaybe
|> Maybe.map .results
|> Maybe.andThen List.head
|> Maybe.map .geometry
|> Maybe.map
(\geometry ->
let
bounds =
Maps.Geo.bounds
{ northEast = { lat = geometry.viewport.northeast.latitude, lng = geometry.viewport.northeast.longitude }
, southWest = { lat = geometry.viewport.southwest.latitude, lng = geometry.viewport.southwest.longitude }
}
latLng =
{ lat = geometry.location.latitude
, lng = geometry.location.longitude
}
in
( bounds, latLng )
)
subscriptions model =
Sub.map MapMsg <| Maps.subscriptions model.map
view model =
Html.div
[]
[ Html.map MapMsg <| Maps.view model.map
, Html.input
[ onInput GeoCode
]
[]
]
| true | module Markers exposing (Msg(..), apiKey, geocode, getFirstLocation, init, main, subscriptions, update, view)
import Geocoding
import Html.Styled as Html exposing (program)
import Html.Styled.Events as E exposing (onInput)
import Http
import Maps exposing (Msg)
import Maps.Geo exposing (Bounds, LatLng)
import Maps.Map as Map
import Maps.Marker as Marker exposing (Marker)
type Msg
= MapMsg (Maps.Msg ())
| GeoCode String
| GoToGeoCode String ( Bounds, LatLng )
| NoResults String
apiKey =
"PI:KEY:<KEY>END_PI"
main =
program
{ init = init
, update = update
, subscriptions = subscriptions
, view = view
}
init =
( { map =
Maps.defaultModel
|> Maps.updateMap (Map.setHeight 600)
|> Maps.updateMap (Map.setWidth 1000)
, place = ""
}
, Cmd.none
)
update msg model =
case msg of
MapMsg mapMsg ->
Maps.update mapMsg model.map
|> Tuple.mapFirst (\map -> { model | map = map })
|> Tuple.mapSecond (Cmd.map MapMsg)
GeoCode place ->
( { model | place = place }, geocode place )
GoToGeoCode place ( bounds, latLng ) ->
if place == model.place then
model.map
|> Maps.updateMap (Map.viewBounds bounds)
|> Maps.updateMarkers (\_ -> [ Marker.create latLng ])
|> (\map -> ( { model | map = map }, Cmd.none ))
else
( model, Cmd.none )
NoResults place ->
( model, Cmd.none )
geocode : String -> Cmd Msg
geocode place =
Geocoding.requestForAddress apiKey place
|> Geocoding.send (Maybe.withDefault (NoResults place) << Maybe.map (GoToGeoCode place) << getFirstLocation)
getFirstLocation : Result Http.Error Geocoding.Response -> Maybe ( Bounds, LatLng )
getFirstLocation result =
result
|> Result.toMaybe
|> Maybe.map .results
|> Maybe.andThen List.head
|> Maybe.map .geometry
|> Maybe.map
(\geometry ->
let
bounds =
Maps.Geo.bounds
{ northEast = { lat = geometry.viewport.northeast.latitude, lng = geometry.viewport.northeast.longitude }
, southWest = { lat = geometry.viewport.southwest.latitude, lng = geometry.viewport.southwest.longitude }
}
latLng =
{ lat = geometry.location.latitude
, lng = geometry.location.longitude
}
in
( bounds, latLng )
)
subscriptions model =
Sub.map MapMsg <| Maps.subscriptions model.map
view model =
Html.div
[]
[ Html.map MapMsg <| Maps.view model.map
, Html.input
[ onInput GeoCode
]
[]
]
| elm |
[
{
"context": " Just { userUi | displayName = newDisplayName }\n\n Nothing ->\n",
"end": 3234,
"score": 0.9037141204,
"start": 3231,
"tag": "NAME",
"value": "new"
},
{
"context": " Just { userUi | password = newPassword }\n\n Nothing ->\n ",
"end": 3743,
"score": 0.9972233772,
"start": 3732,
"tag": "PASSWORD",
"value": "newPassword"
}
] | app/UserManagementApp/Update.elm | digitalsatori/narrows | 116 | module UserManagementApp.Update exposing (..)
import Http
import Core.Routes exposing (Route(..))
import Common.Models exposing (errorBanner, bannerForHttpError)
import UserManagementApp.Api
import UserManagementApp.Messages exposing (..)
import UserManagementApp.Models exposing (..)
urlUpdate : Route -> Model -> ( Model, Cmd Msg )
urlUpdate route model =
case route of
UserManagementPage ->
( { model | banner = Nothing
, users = Nothing
}
, UserManagementApp.Api.fetchUsers
)
_ ->
( model, Cmd.none )
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
NoOp ->
( model, Cmd.none )
UsersFetchResult (Err error) ->
case error of
Http.BadBody parserError ->
( { model | banner = errorBanner <| "Error parsing fetched users: " ++ parserError }
, Cmd.none
)
Http.BadStatus status ->
( { model | banner = errorBanner <| "Error fetching users: " ++ (String.fromInt status) }
, Cmd.none
)
_ ->
( { model | banner = errorBanner <| "Error fetching users, network error" }
, Cmd.none
)
UsersFetchResult (Ok resp) ->
( { model | users = Just resp.users }
, Cmd.none
)
SelectUser userId ->
case model.users of
Just users ->
let
selectedUser =
List.head <| List.filter (\u -> u.id == userId) users
displayName =
case selectedUser of
Just user ->
user.displayName
Nothing ->
""
isUserAdmin =
case selectedUser of
Just user ->
user.role == "admin"
Nothing ->
False
in
( { model
| userUi =
Just
{ userId = userId
, displayName = displayName
, password = ""
, isAdmin = isUserAdmin
}
, banner = Nothing
}
, Cmd.none
)
Nothing ->
( model, Cmd.none )
UnselectUser ->
( { model
| userUi = Nothing
, banner = Nothing
}
, Cmd.none
)
UpdateDisplayName newDisplayName ->
let
updatedUserUi =
case model.userUi of
Just userUi ->
Just { userUi | displayName = newDisplayName }
Nothing ->
Nothing
in
( { model
| userUi = updatedUserUi
, banner = Nothing
}
, Cmd.none
)
UpdatePassword newPassword ->
let
updatedUserUi =
case model.userUi of
Just userUi ->
Just { userUi | password = newPassword }
Nothing ->
Nothing
in
( { model
| userUi = updatedUserUi
, banner = Nothing
}
, Cmd.none
)
UpdateIsAdmin isAdmin ->
let
updatedUserUi =
case model.userUi of
Just userUi ->
Just { userUi | isAdmin = isAdmin }
Nothing ->
Nothing
in
( { model
| userUi = updatedUserUi
, banner = Nothing
}
, Cmd.none
)
SaveUser ->
case model.userUi of
Just userUi ->
( model, UserManagementApp.Api.saveUser userUi )
Nothing ->
( model, Cmd.none )
SaveUserResult (Err err) ->
let
errorString =
case err of
Http.BadBody parserError ->
"Bad payload: " ++ parserError
Http.BadStatus status ->
"Got status " ++ (String.fromInt status)
_ ->
"Cannot connect to server"
in
( { model | banner = errorBanner errorString }, Cmd.none )
SaveUserResult (Ok resp) ->
case resp of
Http.GoodStatus_ _ _ ->
( { model | userUi = Nothing }
, UserManagementApp.Api.fetchUsers
)
Http.BadStatus_ metadata _ ->
( { model | banner = errorBanner <| "Error updating user, status code " ++ (String.fromInt metadata.statusCode) }
, Cmd.none
)
_ ->
( { model | banner = errorBanner "Error updating user, network error" }
, Cmd.none
)
DeleteUserDialog ->
( { model | showDeleteUserDialog = True }
, Cmd.none
)
CancelDeleteUser ->
( { model | showDeleteUserDialog = False }
, Cmd.none
)
DeleteUser ->
case model.userUi of
Just userUi ->
( { model | showDeleteUserDialog = False }
, UserManagementApp.Api.deleteUser userUi.userId
)
Nothing ->
( model, Cmd.none )
DeleteUserResult (Err err) ->
let
errorString =
case err of
Http.BadBody parserError ->
"Bad payload: " ++ parserError
Http.BadStatus status ->
"Got status " ++ (String.fromInt status)
_ ->
"Cannot connect to server"
in
( { model | banner = errorBanner errorString }, Cmd.none )
DeleteUserResult (Ok resp) ->
case resp of
Http.GoodStatus_ _ _ ->
( { model | userUi = Nothing }
, UserManagementApp.Api.fetchUsers
)
Http.BadStatus_ metadata _ ->
( { model | banner = errorBanner <| "Error deleting user, status code " ++ (String.fromInt metadata.statusCode) }
, Cmd.none
)
_ ->
( { model | banner = errorBanner "Error deleting user, network error" }
, Cmd.none
)
UpdateNewUserEmail newEmail ->
( { model | newUserEmail = newEmail, banner = Nothing }
, Cmd.none
)
UpdateNewUserDisplayName newDisplayName ->
( { model | newUserDisplayName = newDisplayName, banner = Nothing }
, Cmd.none
)
UpdateNewUserIsAdmin newIsAdmin ->
( { model | newUserIsAdmin = newIsAdmin, banner = Nothing }
, Cmd.none
)
SaveNewUser ->
( model
, UserManagementApp.Api.saveNewUser model.newUserEmail model.newUserDisplayName model.newUserIsAdmin
)
SaveNewUserResult (Err err) ->
( { model | banner = bannerForHttpError err }
, Cmd.none
)
SaveNewUserResult (Ok user) ->
let
updatedUsers = case model.users of
Just users -> Just (List.append users [ user ])
Nothing -> Just [ user ]
in
( { model | users = updatedUsers
, newUserEmail = ""
, newUserDisplayName = ""
, newUserIsAdmin = False
}
, Cmd.none
)
| 10247 | module UserManagementApp.Update exposing (..)
import Http
import Core.Routes exposing (Route(..))
import Common.Models exposing (errorBanner, bannerForHttpError)
import UserManagementApp.Api
import UserManagementApp.Messages exposing (..)
import UserManagementApp.Models exposing (..)
urlUpdate : Route -> Model -> ( Model, Cmd Msg )
urlUpdate route model =
case route of
UserManagementPage ->
( { model | banner = Nothing
, users = Nothing
}
, UserManagementApp.Api.fetchUsers
)
_ ->
( model, Cmd.none )
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
NoOp ->
( model, Cmd.none )
UsersFetchResult (Err error) ->
case error of
Http.BadBody parserError ->
( { model | banner = errorBanner <| "Error parsing fetched users: " ++ parserError }
, Cmd.none
)
Http.BadStatus status ->
( { model | banner = errorBanner <| "Error fetching users: " ++ (String.fromInt status) }
, Cmd.none
)
_ ->
( { model | banner = errorBanner <| "Error fetching users, network error" }
, Cmd.none
)
UsersFetchResult (Ok resp) ->
( { model | users = Just resp.users }
, Cmd.none
)
SelectUser userId ->
case model.users of
Just users ->
let
selectedUser =
List.head <| List.filter (\u -> u.id == userId) users
displayName =
case selectedUser of
Just user ->
user.displayName
Nothing ->
""
isUserAdmin =
case selectedUser of
Just user ->
user.role == "admin"
Nothing ->
False
in
( { model
| userUi =
Just
{ userId = userId
, displayName = displayName
, password = ""
, isAdmin = isUserAdmin
}
, banner = Nothing
}
, Cmd.none
)
Nothing ->
( model, Cmd.none )
UnselectUser ->
( { model
| userUi = Nothing
, banner = Nothing
}
, Cmd.none
)
UpdateDisplayName newDisplayName ->
let
updatedUserUi =
case model.userUi of
Just userUi ->
Just { userUi | displayName = <NAME>DisplayName }
Nothing ->
Nothing
in
( { model
| userUi = updatedUserUi
, banner = Nothing
}
, Cmd.none
)
UpdatePassword newPassword ->
let
updatedUserUi =
case model.userUi of
Just userUi ->
Just { userUi | password = <PASSWORD> }
Nothing ->
Nothing
in
( { model
| userUi = updatedUserUi
, banner = Nothing
}
, Cmd.none
)
UpdateIsAdmin isAdmin ->
let
updatedUserUi =
case model.userUi of
Just userUi ->
Just { userUi | isAdmin = isAdmin }
Nothing ->
Nothing
in
( { model
| userUi = updatedUserUi
, banner = Nothing
}
, Cmd.none
)
SaveUser ->
case model.userUi of
Just userUi ->
( model, UserManagementApp.Api.saveUser userUi )
Nothing ->
( model, Cmd.none )
SaveUserResult (Err err) ->
let
errorString =
case err of
Http.BadBody parserError ->
"Bad payload: " ++ parserError
Http.BadStatus status ->
"Got status " ++ (String.fromInt status)
_ ->
"Cannot connect to server"
in
( { model | banner = errorBanner errorString }, Cmd.none )
SaveUserResult (Ok resp) ->
case resp of
Http.GoodStatus_ _ _ ->
( { model | userUi = Nothing }
, UserManagementApp.Api.fetchUsers
)
Http.BadStatus_ metadata _ ->
( { model | banner = errorBanner <| "Error updating user, status code " ++ (String.fromInt metadata.statusCode) }
, Cmd.none
)
_ ->
( { model | banner = errorBanner "Error updating user, network error" }
, Cmd.none
)
DeleteUserDialog ->
( { model | showDeleteUserDialog = True }
, Cmd.none
)
CancelDeleteUser ->
( { model | showDeleteUserDialog = False }
, Cmd.none
)
DeleteUser ->
case model.userUi of
Just userUi ->
( { model | showDeleteUserDialog = False }
, UserManagementApp.Api.deleteUser userUi.userId
)
Nothing ->
( model, Cmd.none )
DeleteUserResult (Err err) ->
let
errorString =
case err of
Http.BadBody parserError ->
"Bad payload: " ++ parserError
Http.BadStatus status ->
"Got status " ++ (String.fromInt status)
_ ->
"Cannot connect to server"
in
( { model | banner = errorBanner errorString }, Cmd.none )
DeleteUserResult (Ok resp) ->
case resp of
Http.GoodStatus_ _ _ ->
( { model | userUi = Nothing }
, UserManagementApp.Api.fetchUsers
)
Http.BadStatus_ metadata _ ->
( { model | banner = errorBanner <| "Error deleting user, status code " ++ (String.fromInt metadata.statusCode) }
, Cmd.none
)
_ ->
( { model | banner = errorBanner "Error deleting user, network error" }
, Cmd.none
)
UpdateNewUserEmail newEmail ->
( { model | newUserEmail = newEmail, banner = Nothing }
, Cmd.none
)
UpdateNewUserDisplayName newDisplayName ->
( { model | newUserDisplayName = newDisplayName, banner = Nothing }
, Cmd.none
)
UpdateNewUserIsAdmin newIsAdmin ->
( { model | newUserIsAdmin = newIsAdmin, banner = Nothing }
, Cmd.none
)
SaveNewUser ->
( model
, UserManagementApp.Api.saveNewUser model.newUserEmail model.newUserDisplayName model.newUserIsAdmin
)
SaveNewUserResult (Err err) ->
( { model | banner = bannerForHttpError err }
, Cmd.none
)
SaveNewUserResult (Ok user) ->
let
updatedUsers = case model.users of
Just users -> Just (List.append users [ user ])
Nothing -> Just [ user ]
in
( { model | users = updatedUsers
, newUserEmail = ""
, newUserDisplayName = ""
, newUserIsAdmin = False
}
, Cmd.none
)
| true | module UserManagementApp.Update exposing (..)
import Http
import Core.Routes exposing (Route(..))
import Common.Models exposing (errorBanner, bannerForHttpError)
import UserManagementApp.Api
import UserManagementApp.Messages exposing (..)
import UserManagementApp.Models exposing (..)
urlUpdate : Route -> Model -> ( Model, Cmd Msg )
urlUpdate route model =
case route of
UserManagementPage ->
( { model | banner = Nothing
, users = Nothing
}
, UserManagementApp.Api.fetchUsers
)
_ ->
( model, Cmd.none )
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
NoOp ->
( model, Cmd.none )
UsersFetchResult (Err error) ->
case error of
Http.BadBody parserError ->
( { model | banner = errorBanner <| "Error parsing fetched users: " ++ parserError }
, Cmd.none
)
Http.BadStatus status ->
( { model | banner = errorBanner <| "Error fetching users: " ++ (String.fromInt status) }
, Cmd.none
)
_ ->
( { model | banner = errorBanner <| "Error fetching users, network error" }
, Cmd.none
)
UsersFetchResult (Ok resp) ->
( { model | users = Just resp.users }
, Cmd.none
)
SelectUser userId ->
case model.users of
Just users ->
let
selectedUser =
List.head <| List.filter (\u -> u.id == userId) users
displayName =
case selectedUser of
Just user ->
user.displayName
Nothing ->
""
isUserAdmin =
case selectedUser of
Just user ->
user.role == "admin"
Nothing ->
False
in
( { model
| userUi =
Just
{ userId = userId
, displayName = displayName
, password = ""
, isAdmin = isUserAdmin
}
, banner = Nothing
}
, Cmd.none
)
Nothing ->
( model, Cmd.none )
UnselectUser ->
( { model
| userUi = Nothing
, banner = Nothing
}
, Cmd.none
)
UpdateDisplayName newDisplayName ->
let
updatedUserUi =
case model.userUi of
Just userUi ->
Just { userUi | displayName = PI:NAME:<NAME>END_PIDisplayName }
Nothing ->
Nothing
in
( { model
| userUi = updatedUserUi
, banner = Nothing
}
, Cmd.none
)
UpdatePassword newPassword ->
let
updatedUserUi =
case model.userUi of
Just userUi ->
Just { userUi | password = PI:PASSWORD:<PASSWORD>END_PI }
Nothing ->
Nothing
in
( { model
| userUi = updatedUserUi
, banner = Nothing
}
, Cmd.none
)
UpdateIsAdmin isAdmin ->
let
updatedUserUi =
case model.userUi of
Just userUi ->
Just { userUi | isAdmin = isAdmin }
Nothing ->
Nothing
in
( { model
| userUi = updatedUserUi
, banner = Nothing
}
, Cmd.none
)
SaveUser ->
case model.userUi of
Just userUi ->
( model, UserManagementApp.Api.saveUser userUi )
Nothing ->
( model, Cmd.none )
SaveUserResult (Err err) ->
let
errorString =
case err of
Http.BadBody parserError ->
"Bad payload: " ++ parserError
Http.BadStatus status ->
"Got status " ++ (String.fromInt status)
_ ->
"Cannot connect to server"
in
( { model | banner = errorBanner errorString }, Cmd.none )
SaveUserResult (Ok resp) ->
case resp of
Http.GoodStatus_ _ _ ->
( { model | userUi = Nothing }
, UserManagementApp.Api.fetchUsers
)
Http.BadStatus_ metadata _ ->
( { model | banner = errorBanner <| "Error updating user, status code " ++ (String.fromInt metadata.statusCode) }
, Cmd.none
)
_ ->
( { model | banner = errorBanner "Error updating user, network error" }
, Cmd.none
)
DeleteUserDialog ->
( { model | showDeleteUserDialog = True }
, Cmd.none
)
CancelDeleteUser ->
( { model | showDeleteUserDialog = False }
, Cmd.none
)
DeleteUser ->
case model.userUi of
Just userUi ->
( { model | showDeleteUserDialog = False }
, UserManagementApp.Api.deleteUser userUi.userId
)
Nothing ->
( model, Cmd.none )
DeleteUserResult (Err err) ->
let
errorString =
case err of
Http.BadBody parserError ->
"Bad payload: " ++ parserError
Http.BadStatus status ->
"Got status " ++ (String.fromInt status)
_ ->
"Cannot connect to server"
in
( { model | banner = errorBanner errorString }, Cmd.none )
DeleteUserResult (Ok resp) ->
case resp of
Http.GoodStatus_ _ _ ->
( { model | userUi = Nothing }
, UserManagementApp.Api.fetchUsers
)
Http.BadStatus_ metadata _ ->
( { model | banner = errorBanner <| "Error deleting user, status code " ++ (String.fromInt metadata.statusCode) }
, Cmd.none
)
_ ->
( { model | banner = errorBanner "Error deleting user, network error" }
, Cmd.none
)
UpdateNewUserEmail newEmail ->
( { model | newUserEmail = newEmail, banner = Nothing }
, Cmd.none
)
UpdateNewUserDisplayName newDisplayName ->
( { model | newUserDisplayName = newDisplayName, banner = Nothing }
, Cmd.none
)
UpdateNewUserIsAdmin newIsAdmin ->
( { model | newUserIsAdmin = newIsAdmin, banner = Nothing }
, Cmd.none
)
SaveNewUser ->
( model
, UserManagementApp.Api.saveNewUser model.newUserEmail model.newUserDisplayName model.newUserIsAdmin
)
SaveNewUserResult (Err err) ->
( { model | banner = bannerForHttpError err }
, Cmd.none
)
SaveNewUserResult (Ok user) ->
let
updatedUsers = case model.users of
Just users -> Just (List.append users [ user ])
Nothing -> Just [ user ]
in
( { model | users = updatedUsers
, newUserEmail = ""
, newUserDisplayName = ""
, newUserIsAdmin = False
}
, Cmd.none
)
| elm |
[
{
"context": "giphy.com/v1/gifs/random\"\n [ \"api_key\" => \"dc6zaTOxFJmzC\"\n , \"tag\" => topic\n ]\n\n\ndecodeUrl :",
"end": 1878,
"score": 0.9995625019,
"start": 1865,
"tag": "KEY",
"value": "dc6zaTOxFJmzC"
}
] | examples/6/RandomGif.elm | richard-gebbia/start-app-alt | 3 | module RandomGif where
import Html exposing (..)
import Html.Attributes exposing (style)
import Html.Events exposing (onClick)
import Http
import Json.Decode as Json
import Task exposing (Task)
-- Model
type alias Model =
{ topic : String
, gifUrl : String
, shouldRequest : Bool
}
init : String -> Model
init topic =
{ topic = topic
, gifUrl = "assets/waiting.gif"
, shouldRequest = True
}
-- Action
type Action
= RequestMore
| NewGif (Maybe String)
-- Update
update : Action -> Model -> Model
update action model =
case action of
RequestMore ->
{ model | shouldRequest = True }
NewGif maybeGifUrl ->
Model model.topic (Maybe.withDefault model.gifUrl maybeGifUrl) False
-- View
(=>) = (,)
view : Signal.Address Action -> Model -> Html
view address model =
div [ style [ "width" => "200px" ] ]
[ h2 [headerStyle] [text model.topic]
, div [imgStyle model.gifUrl] []
, button [ onClick address RequestMore ] [ text "More Please!" ]
]
headerStyle : Attribute
headerStyle =
style
[ "width" => "200px"
, "text-align" => "center"
]
imgStyle : String -> Attribute
imgStyle url =
style
[ "display" => "inline-block"
, "width" => "200px"
, "height" => "200px"
, "background-position" => "center center"
, "background-size" => "cover"
, "background-image" => ("url('" ++ url ++ "')")
]
-- Effects
getRandomGif : Signal.Address Action -> String -> Task () ()
getRandomGif address topic =
Http.get decodeUrl (randomUrl topic)
|> Task.toMaybe
|> Task.map NewGif
|> flip Task.andThen (Signal.send address)
randomUrl : String -> String
randomUrl topic =
Http.url "http://api.giphy.com/v1/gifs/random"
[ "api_key" => "dc6zaTOxFJmzC"
, "tag" => topic
]
decodeUrl : Json.Decoder String
decodeUrl =
Json.at ["data", "image_url"] Json.string
request : Signal.Address Action -> Model -> Task () ()
request address model =
if model.shouldRequest then
getRandomGif address model.topic
else
Task.succeed ()
-- Step
type alias Output =
{ html: Html
, http: Task () ()
}
stepOutput : Signal.Address Action -> Model -> Output
stepOutput address model =
{ html = view address model
, http = request address model
}
| 53029 | module RandomGif where
import Html exposing (..)
import Html.Attributes exposing (style)
import Html.Events exposing (onClick)
import Http
import Json.Decode as Json
import Task exposing (Task)
-- Model
type alias Model =
{ topic : String
, gifUrl : String
, shouldRequest : Bool
}
init : String -> Model
init topic =
{ topic = topic
, gifUrl = "assets/waiting.gif"
, shouldRequest = True
}
-- Action
type Action
= RequestMore
| NewGif (Maybe String)
-- Update
update : Action -> Model -> Model
update action model =
case action of
RequestMore ->
{ model | shouldRequest = True }
NewGif maybeGifUrl ->
Model model.topic (Maybe.withDefault model.gifUrl maybeGifUrl) False
-- View
(=>) = (,)
view : Signal.Address Action -> Model -> Html
view address model =
div [ style [ "width" => "200px" ] ]
[ h2 [headerStyle] [text model.topic]
, div [imgStyle model.gifUrl] []
, button [ onClick address RequestMore ] [ text "More Please!" ]
]
headerStyle : Attribute
headerStyle =
style
[ "width" => "200px"
, "text-align" => "center"
]
imgStyle : String -> Attribute
imgStyle url =
style
[ "display" => "inline-block"
, "width" => "200px"
, "height" => "200px"
, "background-position" => "center center"
, "background-size" => "cover"
, "background-image" => ("url('" ++ url ++ "')")
]
-- Effects
getRandomGif : Signal.Address Action -> String -> Task () ()
getRandomGif address topic =
Http.get decodeUrl (randomUrl topic)
|> Task.toMaybe
|> Task.map NewGif
|> flip Task.andThen (Signal.send address)
randomUrl : String -> String
randomUrl topic =
Http.url "http://api.giphy.com/v1/gifs/random"
[ "api_key" => "<KEY>"
, "tag" => topic
]
decodeUrl : Json.Decoder String
decodeUrl =
Json.at ["data", "image_url"] Json.string
request : Signal.Address Action -> Model -> Task () ()
request address model =
if model.shouldRequest then
getRandomGif address model.topic
else
Task.succeed ()
-- Step
type alias Output =
{ html: Html
, http: Task () ()
}
stepOutput : Signal.Address Action -> Model -> Output
stepOutput address model =
{ html = view address model
, http = request address model
}
| true | module RandomGif where
import Html exposing (..)
import Html.Attributes exposing (style)
import Html.Events exposing (onClick)
import Http
import Json.Decode as Json
import Task exposing (Task)
-- Model
type alias Model =
{ topic : String
, gifUrl : String
, shouldRequest : Bool
}
init : String -> Model
init topic =
{ topic = topic
, gifUrl = "assets/waiting.gif"
, shouldRequest = True
}
-- Action
type Action
= RequestMore
| NewGif (Maybe String)
-- Update
update : Action -> Model -> Model
update action model =
case action of
RequestMore ->
{ model | shouldRequest = True }
NewGif maybeGifUrl ->
Model model.topic (Maybe.withDefault model.gifUrl maybeGifUrl) False
-- View
(=>) = (,)
view : Signal.Address Action -> Model -> Html
view address model =
div [ style [ "width" => "200px" ] ]
[ h2 [headerStyle] [text model.topic]
, div [imgStyle model.gifUrl] []
, button [ onClick address RequestMore ] [ text "More Please!" ]
]
headerStyle : Attribute
headerStyle =
style
[ "width" => "200px"
, "text-align" => "center"
]
imgStyle : String -> Attribute
imgStyle url =
style
[ "display" => "inline-block"
, "width" => "200px"
, "height" => "200px"
, "background-position" => "center center"
, "background-size" => "cover"
, "background-image" => ("url('" ++ url ++ "')")
]
-- Effects
getRandomGif : Signal.Address Action -> String -> Task () ()
getRandomGif address topic =
Http.get decodeUrl (randomUrl topic)
|> Task.toMaybe
|> Task.map NewGif
|> flip Task.andThen (Signal.send address)
randomUrl : String -> String
randomUrl topic =
Http.url "http://api.giphy.com/v1/gifs/random"
[ "api_key" => "PI:KEY:<KEY>END_PI"
, "tag" => topic
]
decodeUrl : Json.Decoder String
decodeUrl =
Json.at ["data", "image_url"] Json.string
request : Signal.Address Action -> Model -> Task () ()
request address model =
if model.shouldRequest then
getRandomGif address model.topic
else
Task.succeed ()
-- Step
type alias Output =
{ html: Html
, http: Task () ()
}
stepOutput : Signal.Address Action -> Model -> Output
stepOutput address model =
{ html = view address model
, http = request address model
}
| elm |
[
{
"context": " ]\n\n\nshowDebtor : Debtor -> Html msg\nshowDebtor { firstName, lastName, birthDate, birthPlace, cnp, debts } =\n",
"end": 3837,
"score": 0.998971343,
"start": 3828,
"tag": "NAME",
"value": "firstName"
},
{
"context": "ebtor : Debtor -> Html msg\nshowDebtor { firstName, lastName, birthDate, birthPlace, cnp, debts } =\n let\n ",
"end": 3847,
"score": 0.9993692636,
"start": 3839,
"tag": "NAME",
"value": "lastName"
},
{
"context": " in\n H.tr []\n [ H.td [] [ H.text firstName ]\n , H.td [] [ H.text lastName ]\n ",
"end": 4040,
"score": 0.9993641376,
"start": 4031,
"tag": "NAME",
"value": "firstName"
},
{
"context": " H.text firstName ]\n , H.td [] [ H.text lastName ]\n , H.td [] [ H.text birthDate ]\n ",
"end": 4082,
"score": 0.9994674325,
"start": 4074,
"tag": "NAME",
"value": "lastName"
}
] | web/src/Views/Watcher.elm | jshacks/challenge-show-me-the-money | 2 | module Views.Watcher exposing (..)
import Html as H exposing (Html)
import Html.Attributes as A
import Watcher.Model as Watcher exposing (..)
view : Watcher.State -> Html msg
view state =
H.div []
[ addDebtor
, showDebtors state
]
addDebtor : Html msg
addDebtor =
H.section [ A.class "add-debtor form-s" ]
[ H.h3 [] [ H.text "Add New Debtor" ]
, H.input [ A.type' "text", A.placeholder "First Name" ] []
, H.input [ A.type' "text", A.placeholder "Last Name" ] []
, H.input [ A.type' "date", A.placeholder "Birth Date" ] []
, H.input [ A.type' "text", A.placeholder "CNP" ] []
, H.button [ A.class "submit" ] [ H.text "Add Debtor" ]
]
showDebtors : Watcher.State -> Html msg
showDebtors { debtors } =
H.section [ A.class "debtors-table" ]
[ H.h2 [] [ H.text "Debtors" ]
, H.table []
[ H.thead []
[ H.tr []
[ H.th [] [ H.text "First Name" ]
, H.th [] [ H.text "Last Name" ]
, H.th [] [ H.text "Birth Date" ]
, H.th [] [ H.text "CNP" ]
, H.th [] [ H.text "Total Debt" ]
, H.th [] [ H.text "" ]
]
]
, H.tbody [] (debtors |> List.map showDebtor)
]
]
debtorDetails =
H.section
[ A.class "debtor-view" ]
[ H.h2 [] [ H.text "Debtor: Sile" ]
, H.article [ A.class "debtor-info" ]
[ H.h3 [] [ H.text "Personal Details" ]
, H.p [] [ H.label [] [ H.text "First Name: " ], H.span [] [ H.text "<<fname>>" ] ]
, H.p [] [ H.label [] [ H.text "Last Name: " ], H.span [] [ H.text "<<L name>>" ] ]
, H.p [] [ H.label [] [ H.text "Birth Date: " ], H.span [] [ H.text "<<birth date>>" ] ]
, H.p [] [ H.label [] [ H.text "CNP: " ], H.span [] [ H.text "<<cnp>>" ] ]
, H.p [] [ H.label [] [ H.text "Total Debt: " ], H.span [] [ H.text "<<total debt>>" ] ]
, H.button [] [ H.text "Edit Personal Details" ]
]
, H.article [ A.class "add-debt form-s" ]
[ H.h3 [] [ H.text "Add New Debt for Sile" ]
, H.input [ A.type' "text", A.placeholder "Amount" ] []
, H.textarea [ A.placeholder "Reason" ] []
, H.button [ A.class "submit" ] [ H.text "Add Debt" ]
]
]
debtsList =
H.section
[ A.class "debts-list" ]
[ H.h3 [] [ H.text "Incurred Debts" ]
, H.article [ A.class "debts" ]
[ H.p [] [ H.label [] [ H.text "Amount: " ], H.span [] [ H.text "514851 lei" ] ]
, H.p [] [ H.label [] [ H.text "Reason " ], H.span [] [ H.text "Lorem ipsum dummy text" ] ]
, H.button [] [ H.text "Edit Debt" ]
]
, H.article [ A.class "debts" ]
[ H.p [] [ H.label [] [ H.text "Amount: " ], H.span [] [ H.text "514851 lei" ] ]
, H.p [] [ H.label [] [ H.text "Reason " ], H.span [] [ H.text "Lorem ipsum dummy text" ] ]
, H.button [] [ H.text "Edit Debt" ]
]
, H.article [ A.class "debts" ]
[ H.p [] [ H.label [] [ H.text "Amount: " ], H.span [] [ H.text "514851 lei" ] ]
, H.p [] [ H.label [] [ H.text "Reason " ], H.span [] [ H.text "Lorem ipsum dummy text" ] ]
, H.button [] [ H.text "Edit Debt" ]
]
, H.article [ A.class "debts" ]
[ H.p [] [ H.label [] [ H.text "Amount: " ], H.span [] [ H.text "514851 lei" ] ]
, H.p [] [ H.label [] [ H.text "Reason " ], H.span [] [ H.text "Lorem ipsum dummy text" ] ]
, H.button [] [ H.text "Edit / Update" ]
]
]
showDebtor : Debtor -> Html msg
showDebtor { firstName, lastName, birthDate, birthPlace, cnp, debts } =
let
totalDebt =
debts |> List.map .amount |> List.sum |> toString
in
H.tr []
[ H.td [] [ H.text firstName ]
, H.td [] [ H.text lastName ]
, H.td [] [ H.text birthDate ]
, H.td [] [ H.text cnp ]
, H.td [] [ H.text <| totalDebt ++ " RON" ]
, H.td [] [ H.button [] [ H.text "Edit" ] ]
]
| 2038 | module Views.Watcher exposing (..)
import Html as H exposing (Html)
import Html.Attributes as A
import Watcher.Model as Watcher exposing (..)
view : Watcher.State -> Html msg
view state =
H.div []
[ addDebtor
, showDebtors state
]
addDebtor : Html msg
addDebtor =
H.section [ A.class "add-debtor form-s" ]
[ H.h3 [] [ H.text "Add New Debtor" ]
, H.input [ A.type' "text", A.placeholder "First Name" ] []
, H.input [ A.type' "text", A.placeholder "Last Name" ] []
, H.input [ A.type' "date", A.placeholder "Birth Date" ] []
, H.input [ A.type' "text", A.placeholder "CNP" ] []
, H.button [ A.class "submit" ] [ H.text "Add Debtor" ]
]
showDebtors : Watcher.State -> Html msg
showDebtors { debtors } =
H.section [ A.class "debtors-table" ]
[ H.h2 [] [ H.text "Debtors" ]
, H.table []
[ H.thead []
[ H.tr []
[ H.th [] [ H.text "First Name" ]
, H.th [] [ H.text "Last Name" ]
, H.th [] [ H.text "Birth Date" ]
, H.th [] [ H.text "CNP" ]
, H.th [] [ H.text "Total Debt" ]
, H.th [] [ H.text "" ]
]
]
, H.tbody [] (debtors |> List.map showDebtor)
]
]
debtorDetails =
H.section
[ A.class "debtor-view" ]
[ H.h2 [] [ H.text "Debtor: Sile" ]
, H.article [ A.class "debtor-info" ]
[ H.h3 [] [ H.text "Personal Details" ]
, H.p [] [ H.label [] [ H.text "First Name: " ], H.span [] [ H.text "<<fname>>" ] ]
, H.p [] [ H.label [] [ H.text "Last Name: " ], H.span [] [ H.text "<<L name>>" ] ]
, H.p [] [ H.label [] [ H.text "Birth Date: " ], H.span [] [ H.text "<<birth date>>" ] ]
, H.p [] [ H.label [] [ H.text "CNP: " ], H.span [] [ H.text "<<cnp>>" ] ]
, H.p [] [ H.label [] [ H.text "Total Debt: " ], H.span [] [ H.text "<<total debt>>" ] ]
, H.button [] [ H.text "Edit Personal Details" ]
]
, H.article [ A.class "add-debt form-s" ]
[ H.h3 [] [ H.text "Add New Debt for Sile" ]
, H.input [ A.type' "text", A.placeholder "Amount" ] []
, H.textarea [ A.placeholder "Reason" ] []
, H.button [ A.class "submit" ] [ H.text "Add Debt" ]
]
]
debtsList =
H.section
[ A.class "debts-list" ]
[ H.h3 [] [ H.text "Incurred Debts" ]
, H.article [ A.class "debts" ]
[ H.p [] [ H.label [] [ H.text "Amount: " ], H.span [] [ H.text "514851 lei" ] ]
, H.p [] [ H.label [] [ H.text "Reason " ], H.span [] [ H.text "Lorem ipsum dummy text" ] ]
, H.button [] [ H.text "Edit Debt" ]
]
, H.article [ A.class "debts" ]
[ H.p [] [ H.label [] [ H.text "Amount: " ], H.span [] [ H.text "514851 lei" ] ]
, H.p [] [ H.label [] [ H.text "Reason " ], H.span [] [ H.text "Lorem ipsum dummy text" ] ]
, H.button [] [ H.text "Edit Debt" ]
]
, H.article [ A.class "debts" ]
[ H.p [] [ H.label [] [ H.text "Amount: " ], H.span [] [ H.text "514851 lei" ] ]
, H.p [] [ H.label [] [ H.text "Reason " ], H.span [] [ H.text "Lorem ipsum dummy text" ] ]
, H.button [] [ H.text "Edit Debt" ]
]
, H.article [ A.class "debts" ]
[ H.p [] [ H.label [] [ H.text "Amount: " ], H.span [] [ H.text "514851 lei" ] ]
, H.p [] [ H.label [] [ H.text "Reason " ], H.span [] [ H.text "Lorem ipsum dummy text" ] ]
, H.button [] [ H.text "Edit / Update" ]
]
]
showDebtor : Debtor -> Html msg
showDebtor { <NAME>, <NAME>, birthDate, birthPlace, cnp, debts } =
let
totalDebt =
debts |> List.map .amount |> List.sum |> toString
in
H.tr []
[ H.td [] [ H.text <NAME> ]
, H.td [] [ H.text <NAME> ]
, H.td [] [ H.text birthDate ]
, H.td [] [ H.text cnp ]
, H.td [] [ H.text <| totalDebt ++ " RON" ]
, H.td [] [ H.button [] [ H.text "Edit" ] ]
]
| true | module Views.Watcher exposing (..)
import Html as H exposing (Html)
import Html.Attributes as A
import Watcher.Model as Watcher exposing (..)
view : Watcher.State -> Html msg
view state =
H.div []
[ addDebtor
, showDebtors state
]
addDebtor : Html msg
addDebtor =
H.section [ A.class "add-debtor form-s" ]
[ H.h3 [] [ H.text "Add New Debtor" ]
, H.input [ A.type' "text", A.placeholder "First Name" ] []
, H.input [ A.type' "text", A.placeholder "Last Name" ] []
, H.input [ A.type' "date", A.placeholder "Birth Date" ] []
, H.input [ A.type' "text", A.placeholder "CNP" ] []
, H.button [ A.class "submit" ] [ H.text "Add Debtor" ]
]
showDebtors : Watcher.State -> Html msg
showDebtors { debtors } =
H.section [ A.class "debtors-table" ]
[ H.h2 [] [ H.text "Debtors" ]
, H.table []
[ H.thead []
[ H.tr []
[ H.th [] [ H.text "First Name" ]
, H.th [] [ H.text "Last Name" ]
, H.th [] [ H.text "Birth Date" ]
, H.th [] [ H.text "CNP" ]
, H.th [] [ H.text "Total Debt" ]
, H.th [] [ H.text "" ]
]
]
, H.tbody [] (debtors |> List.map showDebtor)
]
]
debtorDetails =
H.section
[ A.class "debtor-view" ]
[ H.h2 [] [ H.text "Debtor: Sile" ]
, H.article [ A.class "debtor-info" ]
[ H.h3 [] [ H.text "Personal Details" ]
, H.p [] [ H.label [] [ H.text "First Name: " ], H.span [] [ H.text "<<fname>>" ] ]
, H.p [] [ H.label [] [ H.text "Last Name: " ], H.span [] [ H.text "<<L name>>" ] ]
, H.p [] [ H.label [] [ H.text "Birth Date: " ], H.span [] [ H.text "<<birth date>>" ] ]
, H.p [] [ H.label [] [ H.text "CNP: " ], H.span [] [ H.text "<<cnp>>" ] ]
, H.p [] [ H.label [] [ H.text "Total Debt: " ], H.span [] [ H.text "<<total debt>>" ] ]
, H.button [] [ H.text "Edit Personal Details" ]
]
, H.article [ A.class "add-debt form-s" ]
[ H.h3 [] [ H.text "Add New Debt for Sile" ]
, H.input [ A.type' "text", A.placeholder "Amount" ] []
, H.textarea [ A.placeholder "Reason" ] []
, H.button [ A.class "submit" ] [ H.text "Add Debt" ]
]
]
debtsList =
H.section
[ A.class "debts-list" ]
[ H.h3 [] [ H.text "Incurred Debts" ]
, H.article [ A.class "debts" ]
[ H.p [] [ H.label [] [ H.text "Amount: " ], H.span [] [ H.text "514851 lei" ] ]
, H.p [] [ H.label [] [ H.text "Reason " ], H.span [] [ H.text "Lorem ipsum dummy text" ] ]
, H.button [] [ H.text "Edit Debt" ]
]
, H.article [ A.class "debts" ]
[ H.p [] [ H.label [] [ H.text "Amount: " ], H.span [] [ H.text "514851 lei" ] ]
, H.p [] [ H.label [] [ H.text "Reason " ], H.span [] [ H.text "Lorem ipsum dummy text" ] ]
, H.button [] [ H.text "Edit Debt" ]
]
, H.article [ A.class "debts" ]
[ H.p [] [ H.label [] [ H.text "Amount: " ], H.span [] [ H.text "514851 lei" ] ]
, H.p [] [ H.label [] [ H.text "Reason " ], H.span [] [ H.text "Lorem ipsum dummy text" ] ]
, H.button [] [ H.text "Edit Debt" ]
]
, H.article [ A.class "debts" ]
[ H.p [] [ H.label [] [ H.text "Amount: " ], H.span [] [ H.text "514851 lei" ] ]
, H.p [] [ H.label [] [ H.text "Reason " ], H.span [] [ H.text "Lorem ipsum dummy text" ] ]
, H.button [] [ H.text "Edit / Update" ]
]
]
showDebtor : Debtor -> Html msg
showDebtor { PI:NAME:<NAME>END_PI, PI:NAME:<NAME>END_PI, birthDate, birthPlace, cnp, debts } =
let
totalDebt =
debts |> List.map .amount |> List.sum |> toString
in
H.tr []
[ H.td [] [ H.text PI:NAME:<NAME>END_PI ]
, H.td [] [ H.text PI:NAME:<NAME>END_PI ]
, H.td [] [ H.text birthDate ]
, H.td [] [ H.text cnp ]
, H.td [] [ H.text <| totalDebt ++ " RON" ]
, H.td [] [ H.button [] [ H.text "Edit" ] ]
]
| elm |
[
{
"context": "le = \"Le corbeau et le renard\"\n , content = \"Maître Corbeau, sur un arbre perché,\\nTenait en son bec un froma",
"end": 8106,
"score": 0.998688519,
"start": 8092,
"tag": "NAME",
"value": "Maître Corbeau"
},
{
"context": " un arbre perché,\\nTenait en son bec un fromage.\\nMaître Renard, par l'odeur alléché,\\nLui tint à peu près ce lan",
"end": 8174,
"score": 0.9366439581,
"start": 8161,
"tag": "NAME",
"value": "Maître Renard"
}
] | boiler_plate.elm | goldenson/graphql-rails-api | 26 | module Main exposing (main)
import BodyBuilder exposing (..)
import BodyBuilder.Attributes as Attributes exposing (..)
import BodyBuilder.Events as Events
import BodyBuilder.Router as Router
exposing
( History
, Page
, StandardHistoryMsg(..)
, Transition
, handleStandardHistory
, historyView
, initHistoryAndData
, maybeTransitionSubscription
, pageWithDefaultTransition
, push
)
import BodyBuilder.Style as Style
import Browser
import Browser.Navigation as Nav
import Color
import Elegant exposing (SizeUnit, percent, pt, px, vh)
import Elegant.Border as Border
import Elegant.Box as Box
import Elegant.Constants as Constants
import Elegant.Corner as Corner
import Elegant.Cursor as Cursor
import Elegant.Dimensions as Dimensions
import Elegant.Display as Display
import Elegant.Outline as Outline
import Elegant.Padding as Padding
import Elegant.Typography as Typography
import Modifiers exposing (..)
import Time
import Url
find_by : (a -> b) -> b -> List a -> Maybe a
find_by insideDataFun data =
List.filter (\e -> insideDataFun e == data)
>> List.head
type Route
= BlogpostsIndex
| BlogpostsShow Int
type alias Data =
{ blogposts : List Blogpost
, key : Nav.Key
, url : Url.Url
}
type alias MyHistory =
History Route Msg
type alias Model =
{ history : MyHistory
, data : Data
}
type HistoryMsg
= BlogpostShow Int
type Msg
= HistoryMsgWrapper HistoryMsg
| StandardHistoryWrapper StandardHistoryMsg
| UrlChanged Url.Url
| LinkClicked Browser.UrlRequest
type alias MarkdownString =
String
type alias Blogpost =
{ id : Int
, title : String
, content : MarkdownString
, publishedAt : Maybe Time.Posix
, image : String
}
handleHistory : HistoryMsg -> MyHistory -> MyHistory
handleHistory route history =
case route of
BlogpostShow id ->
history |> push (Router.pageWithDefaultTransition (BlogpostsShow id))
gray : Color.Color
gray =
Color.grayscale 0.9
titleView : Blogpost -> NodeWithStyle Msg
titleView blogpost =
button
[ Events.onClick <| HistoryMsgWrapper <| BlogpostShow blogpost.id
, standardCellStyle
]
[ text blogpost.title ]
showView : { b | maybeBlogpost : Maybe Blogpost } -> NodeWithStyle Msg
showView data =
case data.maybeBlogpost of
Nothing ->
node [] [ text "Error" ]
Just blogPost ->
Router.pageWithHeader
(Router.headerElement
{ left = Router.headerButton (StandardHistoryWrapper Router.Back) "← BACK"
, center = Router.headerButton (StandardHistoryWrapper Router.Back) "Blog"
, right = node [] []
}
)
(blogpostView blogPost)
blogpostView : Blogpost -> NodeWithStyle msg
blogpostView blogpost =
node []
[ img "" blogpost.image [ style [ Style.block [ Display.fullWidth ] ] ]
, node
[ style
[ Style.block []
, Style.box [ Box.padding [ Padding.horizontal Constants.medium ] ]
]
]
(textToHtml blogpost.content)
]
textToHtml : String -> List (NodeWithStyle msg)
textToHtml =
(>>)
(String.split "\n")
(List.foldr (\e accu -> [ text e, br ] ++ accu) [])
standardCellStyle : Modifiers.Modifier (Attributes.BoxContainer (Attributes.MaybeBlockContainer a))
standardCellStyle =
style
[ Style.block
[ Display.alignment Display.left
, Display.fullWidth
]
, Style.box
[ Box.cursor Cursor.pointer
, Box.border
[ Border.all [ Border.none ]
, Border.bottom [ Border.solid, Elegant.color gray ]
]
, Box.outline [ Outline.none ]
, Box.typography
[ Typography.fontFamilyInherit
, Typography.size Constants.zeta
]
, Box.corner [ Corner.circular Corner.all (px 0) ]
, Box.padding [ Padding.all Constants.large ]
, Box.background [ Elegant.color Color.white ]
]
]
blogpostsIndex : List Blogpost -> NodeWithStyle Msg
blogpostsIndex blogposts =
node
[ style
[ Style.block [ Display.dimensions [ Dimensions.height (vh 100) ] ]
, Style.box [ Box.background [ Elegant.color gray ] ]
]
]
(blogposts |> List.map titleView)
blogpostsShow : Int -> List Blogpost -> NodeWithStyle Msg
blogpostsShow id blogposts =
node [] [ showView { maybeBlogpost = blogposts |> find_by .id id } ]
pageView : Data -> Page Route Msg -> Maybe (Transition Route Msg) -> NodeWithStyle Msg
pageView { blogposts } { route } transition =
case route of
BlogpostsIndex ->
blogpostsIndex blogposts
BlogpostsShow id ->
blogpostsShow id blogposts
chooseView : Model -> Document Msg
chooseView model =
case model.data.url.path of
"/admin" ->
adminView model
_ ->
homeView model
homeView : Model -> Document Msg
homeView ({ history, data } as model) =
{ title = "ProjectTest"
, body =
div
[ style
[ Style.box
[ Box.typography
[ Typography.fontFamilySansSerif
, Typography.size Constants.zeta
]
]
]
]
[ historyView (pageView data) history ]
}
adminView : Model -> Document Msg
adminView ({ history, data } as model) =
{ title = "Admin ProjectTest"
, body =
div
[ style
[ Style.box
[ Box.typography
[ Typography.fontFamilySansSerif
, Typography.size Constants.zeta
]
]
]
]
[ text "ADMIN is going to be here :)" ]
}
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
let
data =
model.data
in
case msg of
HistoryMsgWrapper historyMsg ->
( { model | history = handleHistory historyMsg model.history }, Cmd.none )
StandardHistoryWrapper historyMsg ->
model |> handleStandardHistory historyMsg
LinkClicked urlRequest ->
case urlRequest of
Browser.Internal url ->
( model, Nav.pushUrl data.key (Url.toString url) )
Browser.External href ->
( model, Nav.load href )
UrlChanged url ->
( { model | data = { data | url = url } }
, Cmd.none
)
subscriptions : Model -> Sub Msg
subscriptions model =
maybeTransitionSubscription model.history
initBlogposts : List Blogpost
initBlogposts =
[ { id = 1
, title = "La cigale et la fourmi"
, publishedAt = Just <| Time.millisToPosix 1502323200
, content = "La Cigale, ayant chanté\nTout l'Été,\nSe trouva fort dépourvue\nQuand la bise fut venue.\nPas un seul petit morceau\nDe mouche ou de vermisseau.\nElle alla crier famine\nChez la Fourmi sa voisine,\nLa priant de lui prêter\nQuelque grain pour subsister\nJusqu'à la saison nouvelle.\nJe vous paierai, lui dit-elle,\nAvant l'Oût, foi d'animal,\nIntérêt et principal.\nLa Fourmi n'est pas prêteuse ;\nC'est là son moindre défaut.\n« Que faisiez-vous au temps chaud ?\nDit-elle à cette emprunteuse.\n— Nuit et jour à tout venant\nJe chantais, ne vous déplaise.\n— Vous chantiez ? j'en suis fort aise.\nEh bien !dansez maintenant. »\n"
, image = "https://upload.wikimedia.org/wikipedia/commons/thumb/c/c1/Snodgrass_Magicicada_septendecim.jpg/1024px-Snodgrass_Magicicada_septendecim.jpg"
}
, { id = 2
, title = "Le corbeau et le renard"
, content = "Maître Corbeau, sur un arbre perché,\nTenait en son bec un fromage.\nMaître Renard, par l'odeur alléché,\nLui tint à peu près ce langage :\nEt bonjour, Monsieur du Corbeau.\nQue vous êtes joli ! que vous me semblez beau !\nSans mentir, si votre ramage\nSe rapporte à votre plumage,\nVous êtes le Phénix des hôtes de ces bois.\nÀ ces mots, le Corbeau ne se sent pas de joie ;\nEt pour montrer sa belle voix,\nIl ouvre un large bec, laisse tomber sa proie.\nLe Renard s'en saisit, et dit : Mon bon Monsieur,\nApprenez que tout flatteur\nVit aux dépens de celui qui l'écoute.\nCette leçon vaut bien un fromage, sans doute.\nLe Corbeau honteux et confus\nJura, mais un peu tard, qu'on ne l'y prendrait plus."
, publishedAt = Just <| Time.millisToPosix 1502323200
, image = "https://upload.wikimedia.org/wikipedia/commons/4/47/Karga_9107.svg"
}
]
initData : () -> Url.Url -> Nav.Key -> Data
initData flags url key =
{ blogposts = initBlogposts
, key = key
, url = url
}
init : () -> Url.Url -> Nav.Key -> { data : Data, history : MyHistory }
init flags url key =
initHistoryAndData BlogpostsIndex (initData flags url key) StandardHistoryWrapper
main : Program () Model Msg
main =
application
{ init = \flags -> \url -> \key -> ( init flags url key, Cmd.none )
, onUrlChange = UrlChanged
, onUrlRequest = LinkClicked
, update = update
, subscriptions = subscriptions
, view = chooseView
}
| 2600 | module Main exposing (main)
import BodyBuilder exposing (..)
import BodyBuilder.Attributes as Attributes exposing (..)
import BodyBuilder.Events as Events
import BodyBuilder.Router as Router
exposing
( History
, Page
, StandardHistoryMsg(..)
, Transition
, handleStandardHistory
, historyView
, initHistoryAndData
, maybeTransitionSubscription
, pageWithDefaultTransition
, push
)
import BodyBuilder.Style as Style
import Browser
import Browser.Navigation as Nav
import Color
import Elegant exposing (SizeUnit, percent, pt, px, vh)
import Elegant.Border as Border
import Elegant.Box as Box
import Elegant.Constants as Constants
import Elegant.Corner as Corner
import Elegant.Cursor as Cursor
import Elegant.Dimensions as Dimensions
import Elegant.Display as Display
import Elegant.Outline as Outline
import Elegant.Padding as Padding
import Elegant.Typography as Typography
import Modifiers exposing (..)
import Time
import Url
find_by : (a -> b) -> b -> List a -> Maybe a
find_by insideDataFun data =
List.filter (\e -> insideDataFun e == data)
>> List.head
type Route
= BlogpostsIndex
| BlogpostsShow Int
type alias Data =
{ blogposts : List Blogpost
, key : Nav.Key
, url : Url.Url
}
type alias MyHistory =
History Route Msg
type alias Model =
{ history : MyHistory
, data : Data
}
type HistoryMsg
= BlogpostShow Int
type Msg
= HistoryMsgWrapper HistoryMsg
| StandardHistoryWrapper StandardHistoryMsg
| UrlChanged Url.Url
| LinkClicked Browser.UrlRequest
type alias MarkdownString =
String
type alias Blogpost =
{ id : Int
, title : String
, content : MarkdownString
, publishedAt : Maybe Time.Posix
, image : String
}
handleHistory : HistoryMsg -> MyHistory -> MyHistory
handleHistory route history =
case route of
BlogpostShow id ->
history |> push (Router.pageWithDefaultTransition (BlogpostsShow id))
gray : Color.Color
gray =
Color.grayscale 0.9
titleView : Blogpost -> NodeWithStyle Msg
titleView blogpost =
button
[ Events.onClick <| HistoryMsgWrapper <| BlogpostShow blogpost.id
, standardCellStyle
]
[ text blogpost.title ]
showView : { b | maybeBlogpost : Maybe Blogpost } -> NodeWithStyle Msg
showView data =
case data.maybeBlogpost of
Nothing ->
node [] [ text "Error" ]
Just blogPost ->
Router.pageWithHeader
(Router.headerElement
{ left = Router.headerButton (StandardHistoryWrapper Router.Back) "← BACK"
, center = Router.headerButton (StandardHistoryWrapper Router.Back) "Blog"
, right = node [] []
}
)
(blogpostView blogPost)
blogpostView : Blogpost -> NodeWithStyle msg
blogpostView blogpost =
node []
[ img "" blogpost.image [ style [ Style.block [ Display.fullWidth ] ] ]
, node
[ style
[ Style.block []
, Style.box [ Box.padding [ Padding.horizontal Constants.medium ] ]
]
]
(textToHtml blogpost.content)
]
textToHtml : String -> List (NodeWithStyle msg)
textToHtml =
(>>)
(String.split "\n")
(List.foldr (\e accu -> [ text e, br ] ++ accu) [])
standardCellStyle : Modifiers.Modifier (Attributes.BoxContainer (Attributes.MaybeBlockContainer a))
standardCellStyle =
style
[ Style.block
[ Display.alignment Display.left
, Display.fullWidth
]
, Style.box
[ Box.cursor Cursor.pointer
, Box.border
[ Border.all [ Border.none ]
, Border.bottom [ Border.solid, Elegant.color gray ]
]
, Box.outline [ Outline.none ]
, Box.typography
[ Typography.fontFamilyInherit
, Typography.size Constants.zeta
]
, Box.corner [ Corner.circular Corner.all (px 0) ]
, Box.padding [ Padding.all Constants.large ]
, Box.background [ Elegant.color Color.white ]
]
]
blogpostsIndex : List Blogpost -> NodeWithStyle Msg
blogpostsIndex blogposts =
node
[ style
[ Style.block [ Display.dimensions [ Dimensions.height (vh 100) ] ]
, Style.box [ Box.background [ Elegant.color gray ] ]
]
]
(blogposts |> List.map titleView)
blogpostsShow : Int -> List Blogpost -> NodeWithStyle Msg
blogpostsShow id blogposts =
node [] [ showView { maybeBlogpost = blogposts |> find_by .id id } ]
pageView : Data -> Page Route Msg -> Maybe (Transition Route Msg) -> NodeWithStyle Msg
pageView { blogposts } { route } transition =
case route of
BlogpostsIndex ->
blogpostsIndex blogposts
BlogpostsShow id ->
blogpostsShow id blogposts
chooseView : Model -> Document Msg
chooseView model =
case model.data.url.path of
"/admin" ->
adminView model
_ ->
homeView model
homeView : Model -> Document Msg
homeView ({ history, data } as model) =
{ title = "ProjectTest"
, body =
div
[ style
[ Style.box
[ Box.typography
[ Typography.fontFamilySansSerif
, Typography.size Constants.zeta
]
]
]
]
[ historyView (pageView data) history ]
}
adminView : Model -> Document Msg
adminView ({ history, data } as model) =
{ title = "Admin ProjectTest"
, body =
div
[ style
[ Style.box
[ Box.typography
[ Typography.fontFamilySansSerif
, Typography.size Constants.zeta
]
]
]
]
[ text "ADMIN is going to be here :)" ]
}
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
let
data =
model.data
in
case msg of
HistoryMsgWrapper historyMsg ->
( { model | history = handleHistory historyMsg model.history }, Cmd.none )
StandardHistoryWrapper historyMsg ->
model |> handleStandardHistory historyMsg
LinkClicked urlRequest ->
case urlRequest of
Browser.Internal url ->
( model, Nav.pushUrl data.key (Url.toString url) )
Browser.External href ->
( model, Nav.load href )
UrlChanged url ->
( { model | data = { data | url = url } }
, Cmd.none
)
subscriptions : Model -> Sub Msg
subscriptions model =
maybeTransitionSubscription model.history
initBlogposts : List Blogpost
initBlogposts =
[ { id = 1
, title = "La cigale et la fourmi"
, publishedAt = Just <| Time.millisToPosix 1502323200
, content = "La Cigale, ayant chanté\nTout l'Été,\nSe trouva fort dépourvue\nQuand la bise fut venue.\nPas un seul petit morceau\nDe mouche ou de vermisseau.\nElle alla crier famine\nChez la Fourmi sa voisine,\nLa priant de lui prêter\nQuelque grain pour subsister\nJusqu'à la saison nouvelle.\nJe vous paierai, lui dit-elle,\nAvant l'Oût, foi d'animal,\nIntérêt et principal.\nLa Fourmi n'est pas prêteuse ;\nC'est là son moindre défaut.\n« Que faisiez-vous au temps chaud ?\nDit-elle à cette emprunteuse.\n— Nuit et jour à tout venant\nJe chantais, ne vous déplaise.\n— Vous chantiez ? j'en suis fort aise.\nEh bien !dansez maintenant. »\n"
, image = "https://upload.wikimedia.org/wikipedia/commons/thumb/c/c1/Snodgrass_Magicicada_septendecim.jpg/1024px-Snodgrass_Magicicada_septendecim.jpg"
}
, { id = 2
, title = "Le corbeau et le renard"
, content = "<NAME>, sur un arbre perché,\nTenait en son bec un fromage.\n<NAME>, par l'odeur alléché,\nLui tint à peu près ce langage :\nEt bonjour, Monsieur du Corbeau.\nQue vous êtes joli ! que vous me semblez beau !\nSans mentir, si votre ramage\nSe rapporte à votre plumage,\nVous êtes le Phénix des hôtes de ces bois.\nÀ ces mots, le Corbeau ne se sent pas de joie ;\nEt pour montrer sa belle voix,\nIl ouvre un large bec, laisse tomber sa proie.\nLe Renard s'en saisit, et dit : Mon bon Monsieur,\nApprenez que tout flatteur\nVit aux dépens de celui qui l'écoute.\nCette leçon vaut bien un fromage, sans doute.\nLe Corbeau honteux et confus\nJura, mais un peu tard, qu'on ne l'y prendrait plus."
, publishedAt = Just <| Time.millisToPosix 1502323200
, image = "https://upload.wikimedia.org/wikipedia/commons/4/47/Karga_9107.svg"
}
]
initData : () -> Url.Url -> Nav.Key -> Data
initData flags url key =
{ blogposts = initBlogposts
, key = key
, url = url
}
init : () -> Url.Url -> Nav.Key -> { data : Data, history : MyHistory }
init flags url key =
initHistoryAndData BlogpostsIndex (initData flags url key) StandardHistoryWrapper
main : Program () Model Msg
main =
application
{ init = \flags -> \url -> \key -> ( init flags url key, Cmd.none )
, onUrlChange = UrlChanged
, onUrlRequest = LinkClicked
, update = update
, subscriptions = subscriptions
, view = chooseView
}
| true | module Main exposing (main)
import BodyBuilder exposing (..)
import BodyBuilder.Attributes as Attributes exposing (..)
import BodyBuilder.Events as Events
import BodyBuilder.Router as Router
exposing
( History
, Page
, StandardHistoryMsg(..)
, Transition
, handleStandardHistory
, historyView
, initHistoryAndData
, maybeTransitionSubscription
, pageWithDefaultTransition
, push
)
import BodyBuilder.Style as Style
import Browser
import Browser.Navigation as Nav
import Color
import Elegant exposing (SizeUnit, percent, pt, px, vh)
import Elegant.Border as Border
import Elegant.Box as Box
import Elegant.Constants as Constants
import Elegant.Corner as Corner
import Elegant.Cursor as Cursor
import Elegant.Dimensions as Dimensions
import Elegant.Display as Display
import Elegant.Outline as Outline
import Elegant.Padding as Padding
import Elegant.Typography as Typography
import Modifiers exposing (..)
import Time
import Url
find_by : (a -> b) -> b -> List a -> Maybe a
find_by insideDataFun data =
List.filter (\e -> insideDataFun e == data)
>> List.head
type Route
= BlogpostsIndex
| BlogpostsShow Int
type alias Data =
{ blogposts : List Blogpost
, key : Nav.Key
, url : Url.Url
}
type alias MyHistory =
History Route Msg
type alias Model =
{ history : MyHistory
, data : Data
}
type HistoryMsg
= BlogpostShow Int
type Msg
= HistoryMsgWrapper HistoryMsg
| StandardHistoryWrapper StandardHistoryMsg
| UrlChanged Url.Url
| LinkClicked Browser.UrlRequest
type alias MarkdownString =
String
type alias Blogpost =
{ id : Int
, title : String
, content : MarkdownString
, publishedAt : Maybe Time.Posix
, image : String
}
handleHistory : HistoryMsg -> MyHistory -> MyHistory
handleHistory route history =
case route of
BlogpostShow id ->
history |> push (Router.pageWithDefaultTransition (BlogpostsShow id))
gray : Color.Color
gray =
Color.grayscale 0.9
titleView : Blogpost -> NodeWithStyle Msg
titleView blogpost =
button
[ Events.onClick <| HistoryMsgWrapper <| BlogpostShow blogpost.id
, standardCellStyle
]
[ text blogpost.title ]
showView : { b | maybeBlogpost : Maybe Blogpost } -> NodeWithStyle Msg
showView data =
case data.maybeBlogpost of
Nothing ->
node [] [ text "Error" ]
Just blogPost ->
Router.pageWithHeader
(Router.headerElement
{ left = Router.headerButton (StandardHistoryWrapper Router.Back) "← BACK"
, center = Router.headerButton (StandardHistoryWrapper Router.Back) "Blog"
, right = node [] []
}
)
(blogpostView blogPost)
blogpostView : Blogpost -> NodeWithStyle msg
blogpostView blogpost =
node []
[ img "" blogpost.image [ style [ Style.block [ Display.fullWidth ] ] ]
, node
[ style
[ Style.block []
, Style.box [ Box.padding [ Padding.horizontal Constants.medium ] ]
]
]
(textToHtml blogpost.content)
]
textToHtml : String -> List (NodeWithStyle msg)
textToHtml =
(>>)
(String.split "\n")
(List.foldr (\e accu -> [ text e, br ] ++ accu) [])
standardCellStyle : Modifiers.Modifier (Attributes.BoxContainer (Attributes.MaybeBlockContainer a))
standardCellStyle =
style
[ Style.block
[ Display.alignment Display.left
, Display.fullWidth
]
, Style.box
[ Box.cursor Cursor.pointer
, Box.border
[ Border.all [ Border.none ]
, Border.bottom [ Border.solid, Elegant.color gray ]
]
, Box.outline [ Outline.none ]
, Box.typography
[ Typography.fontFamilyInherit
, Typography.size Constants.zeta
]
, Box.corner [ Corner.circular Corner.all (px 0) ]
, Box.padding [ Padding.all Constants.large ]
, Box.background [ Elegant.color Color.white ]
]
]
blogpostsIndex : List Blogpost -> NodeWithStyle Msg
blogpostsIndex blogposts =
node
[ style
[ Style.block [ Display.dimensions [ Dimensions.height (vh 100) ] ]
, Style.box [ Box.background [ Elegant.color gray ] ]
]
]
(blogposts |> List.map titleView)
blogpostsShow : Int -> List Blogpost -> NodeWithStyle Msg
blogpostsShow id blogposts =
node [] [ showView { maybeBlogpost = blogposts |> find_by .id id } ]
pageView : Data -> Page Route Msg -> Maybe (Transition Route Msg) -> NodeWithStyle Msg
pageView { blogposts } { route } transition =
case route of
BlogpostsIndex ->
blogpostsIndex blogposts
BlogpostsShow id ->
blogpostsShow id blogposts
chooseView : Model -> Document Msg
chooseView model =
case model.data.url.path of
"/admin" ->
adminView model
_ ->
homeView model
homeView : Model -> Document Msg
homeView ({ history, data } as model) =
{ title = "ProjectTest"
, body =
div
[ style
[ Style.box
[ Box.typography
[ Typography.fontFamilySansSerif
, Typography.size Constants.zeta
]
]
]
]
[ historyView (pageView data) history ]
}
adminView : Model -> Document Msg
adminView ({ history, data } as model) =
{ title = "Admin ProjectTest"
, body =
div
[ style
[ Style.box
[ Box.typography
[ Typography.fontFamilySansSerif
, Typography.size Constants.zeta
]
]
]
]
[ text "ADMIN is going to be here :)" ]
}
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
let
data =
model.data
in
case msg of
HistoryMsgWrapper historyMsg ->
( { model | history = handleHistory historyMsg model.history }, Cmd.none )
StandardHistoryWrapper historyMsg ->
model |> handleStandardHistory historyMsg
LinkClicked urlRequest ->
case urlRequest of
Browser.Internal url ->
( model, Nav.pushUrl data.key (Url.toString url) )
Browser.External href ->
( model, Nav.load href )
UrlChanged url ->
( { model | data = { data | url = url } }
, Cmd.none
)
subscriptions : Model -> Sub Msg
subscriptions model =
maybeTransitionSubscription model.history
initBlogposts : List Blogpost
initBlogposts =
[ { id = 1
, title = "La cigale et la fourmi"
, publishedAt = Just <| Time.millisToPosix 1502323200
, content = "La Cigale, ayant chanté\nTout l'Été,\nSe trouva fort dépourvue\nQuand la bise fut venue.\nPas un seul petit morceau\nDe mouche ou de vermisseau.\nElle alla crier famine\nChez la Fourmi sa voisine,\nLa priant de lui prêter\nQuelque grain pour subsister\nJusqu'à la saison nouvelle.\nJe vous paierai, lui dit-elle,\nAvant l'Oût, foi d'animal,\nIntérêt et principal.\nLa Fourmi n'est pas prêteuse ;\nC'est là son moindre défaut.\n« Que faisiez-vous au temps chaud ?\nDit-elle à cette emprunteuse.\n— Nuit et jour à tout venant\nJe chantais, ne vous déplaise.\n— Vous chantiez ? j'en suis fort aise.\nEh bien !dansez maintenant. »\n"
, image = "https://upload.wikimedia.org/wikipedia/commons/thumb/c/c1/Snodgrass_Magicicada_septendecim.jpg/1024px-Snodgrass_Magicicada_septendecim.jpg"
}
, { id = 2
, title = "Le corbeau et le renard"
, content = "PI:NAME:<NAME>END_PI, sur un arbre perché,\nTenait en son bec un fromage.\nPI:NAME:<NAME>END_PI, par l'odeur alléché,\nLui tint à peu près ce langage :\nEt bonjour, Monsieur du Corbeau.\nQue vous êtes joli ! que vous me semblez beau !\nSans mentir, si votre ramage\nSe rapporte à votre plumage,\nVous êtes le Phénix des hôtes de ces bois.\nÀ ces mots, le Corbeau ne se sent pas de joie ;\nEt pour montrer sa belle voix,\nIl ouvre un large bec, laisse tomber sa proie.\nLe Renard s'en saisit, et dit : Mon bon Monsieur,\nApprenez que tout flatteur\nVit aux dépens de celui qui l'écoute.\nCette leçon vaut bien un fromage, sans doute.\nLe Corbeau honteux et confus\nJura, mais un peu tard, qu'on ne l'y prendrait plus."
, publishedAt = Just <| Time.millisToPosix 1502323200
, image = "https://upload.wikimedia.org/wikipedia/commons/4/47/Karga_9107.svg"
}
]
initData : () -> Url.Url -> Nav.Key -> Data
initData flags url key =
{ blogposts = initBlogposts
, key = key
, url = url
}
init : () -> Url.Url -> Nav.Key -> { data : Data, history : MyHistory }
init flags url key =
initHistoryAndData BlogpostsIndex (initData flags url key) StandardHistoryWrapper
main : Program () Model Msg
main =
application
{ init = \flags -> \url -> \key -> ( init flags url key, Cmd.none )
, onUrlChange = UrlChanged
, onUrlRequest = LinkClicked
, update = update
, subscriptions = subscriptions
, view = chooseView
}
| elm |
[
{
"context": "ck\n (Callback.UserFetched <| Ok sampleUser)\n >> Tuple.first\n ",
"end": 6767,
"score": 0.9945634604,
"start": 6757,
"tag": "USERNAME",
"value": "sampleUser"
},
{
"context": "ck\n (Callback.UserFetched <| Ok sampleUser)\n >> Tuple.first\n ",
"end": 21184,
"score": 0.6443737745,
"start": 21174,
"tag": "USERNAME",
"value": "sampleUser"
},
{
"context": "ck\n (Callback.UserFetched <| Ok sampleUser)\n >> Tuple.first\n ",
"end": 21663,
"score": 0.5007032156,
"start": 21653,
"tag": "USERNAME",
"value": "sampleUser"
},
{
"context": "ck\n (Callback.UserFetched <| Ok sampleUser)\n >> Tuple.first\n ",
"end": 23136,
"score": 0.9962351918,
"start": 23126,
"tag": "USERNAME",
"value": "sampleUser"
},
{
"context": "st\"\n , userName = \"test\"\n , name = \"test\"\n",
"end": 36589,
"score": 0.9991148114,
"start": 36585,
"tag": "USERNAME",
"value": "test"
},
{
"context": " \"test\"\n , name = \"test\"\n , email = \"test\"",
"end": 36637,
"score": 0.9457865953,
"start": 36633,
"tag": "NAME",
"value": "test"
},
{
"context": " , displayUserId = \"displayUserIdTest\"\n }\n ",
"end": 36964,
"score": 0.994348228,
"start": 36947,
"tag": "USERNAME",
"value": "displayUserIdTest"
},
{
"context": "st\"\n , userName = \"test\"\n , name = \"test\"\n",
"end": 37329,
"score": 0.9986261129,
"start": 37325,
"tag": "USERNAME",
"value": "test"
},
{
"context": " \"test\"\n , name = \"test\"\n , email = \"test\"",
"end": 37377,
"score": 0.9990324974,
"start": 37373,
"tag": "NAME",
"value": "test"
},
{
"context": " , displayUserId = \"displayUserIdTest\"\n }\n ",
"end": 37704,
"score": 0.998174727,
"start": 37687,
"tag": "USERNAME",
"value": "displayUserIdTest"
},
{
"context": "rse.User\nsampleUser =\n { id = \"1\", userName = \"test\", name = \"Bob\", isAdmin = False, email = \"bob@bob",
"end": 47144,
"score": 0.9991191626,
"start": 47140,
"tag": "USERNAME",
"value": "test"
},
{
"context": "User =\n { id = \"1\", userName = \"test\", name = \"Bob\", isAdmin = False, email = \"bob@bob.com\", teams =",
"end": 47158,
"score": 0.9993768334,
"start": 47155,
"tag": "NAME",
"value": "Bob"
},
{
"context": "= \"test\", name = \"Bob\", isAdmin = False, email = \"bob@bob.com\", teams = Dict.empty , displayUserId = \"displayUs",
"end": 47198,
"score": 0.9999130368,
"start": 47187,
"tag": "EMAIL",
"value": "bob@bob.com"
},
{
"context": "b@bob.com\", teams = Dict.empty , displayUserId = \"displayUserIdTest\" }\n\n\npipelineBreadcrumbSelector : List Selector.S",
"end": 47256,
"score": 0.9986240864,
"start": 47239,
"tag": "USERNAME",
"value": "displayUserIdTest"
}
] | web/elm/tests/TopBarTests.elm | YodaLyfe/concourse | 1 | module TopBarTests exposing (all)
import Application.Application as Application
import Assets
import Char
import ColorValues
import Common exposing (defineHoverBehaviour, hoverOver, queryView)
import Concourse exposing (JsonValue(..))
import Dashboard.SearchBar as SearchBar
import DashboardTests exposing (iconSelector)
import Data
import Dict
import Expect exposing (..)
import Html.Attributes as Attr
import Http
import Keyboard
import Login.Login as Login
import Message.Callback as Callback exposing (Callback(..))
import Message.Effects as Effects
import Message.Message as Msgs
import Message.Subscription exposing (Delivery(..))
import Message.TopLevelMessage as ApplicationMsgs
import Routes
import Set
import Test exposing (..)
import Test.Html.Event as Event
import Test.Html.Query as Query
import Test.Html.Selector as Selector
exposing
( attribute
, class
, containing
, id
, style
, tag
, text
)
import Time
import Url
import Views.Styles
rspecStyleDescribe : String -> subject -> List (subject -> Test) -> Test
rspecStyleDescribe description beforeEach subTests =
Test.describe description
(subTests |> List.map ((|>) beforeEach))
context : String -> (setup -> subject) -> List (subject -> Test) -> (setup -> Test)
context description beforeEach subTests setup =
Test.describe description
(subTests |> List.map ((|>) (beforeEach setup)))
it : String -> (subject -> Expectation) -> subject -> Test
it desc expectationFunc subject =
Test.test desc <|
\_ -> expectationFunc subject
update : Msgs.Message -> Login.Model {} -> ( Login.Model {}, List Effects.Effect )
update msg =
(\a -> ( a, [] )) >> Login.update msg
lineHeight : String
lineHeight =
"54px"
borderGrey : String
borderGrey =
"#3d3c3c"
backgroundGrey : String
backgroundGrey =
"#1e1d1d"
pausedBlue : String
pausedBlue =
"#3498db"
topBarHeight : String
topBarHeight =
"54px"
searchBarBorder : String -> String
searchBarBorder color =
"1px solid " ++ color
searchBarHeight : String
searchBarHeight =
"30px"
searchBarWidth : String
searchBarWidth =
"251px"
searchBarPadding : String
searchBarPadding =
"0 42px"
flags : Application.Flags
flags =
{ turbulenceImgSrc = ""
, notFoundImgSrc = ""
, csrfToken = ""
, authToken = ""
, pipelineRunningKeyframes = ""
}
instanceVars : Concourse.InstanceVars
instanceVars =
Dict.fromList [ ( "var1", JsonString "v1" ), ( "var2", JsonString "v2" ) ]
pipelineInstance : Concourse.Pipeline
pipelineInstance =
Data.pipeline "team" 1
|> Data.withName "pipeline"
|> Data.withInstanceVars instanceVars
archivedPipelineInstance : Concourse.Pipeline
archivedPipelineInstance =
Data.pipeline "team" 1
|> Data.withName "pipeline"
|> Data.withInstanceVars (Dict.fromList [ ( "foo", JsonString "bar" ) ])
|> Data.withArchived True
all : Test
all =
describe "TopBar"
[ rspecStyleDescribe "when on pipeline page"
(Common.init "/teams/team/pipelines/pipeline"
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ Data.pipeline "team" 1 |> Data.withName "pipeline" ]
)
|> Tuple.first
)
[ context "when login state unknown"
queryView
[ it "shows concourse logo" <|
Query.has
[ style "background-image" <|
Assets.backgroundImage <|
Just Assets.ConcourseLogoWhite
, style "background-position" "50% 50%"
, style "background-repeat" "no-repeat"
, style "background-size" "42px 42px"
, style "width" topBarHeight
, style "height" topBarHeight
]
, it "does not shows instance group breadcrumb" <|
Query.hasNot [ id "breadcrumb-instance-group" ]
, it "shows pipeline breadcrumb" <|
Query.has [ id "breadcrumb-pipeline" ]
, context "pipeline breadcrumb"
(Query.find [ id "breadcrumb-pipeline" ])
[ it "renders icon first" <|
Query.children []
>> Query.first
>> Query.has pipelineBreadcrumbSelector
, it "renders pipeline name second" <|
Query.children []
>> Query.index 1
>> Query.has
[ text "pipeline" ]
, it "has pointer cursor" <|
Query.has [ style "cursor" "pointer" ]
, it "is a link to the relevant pipeline page" <|
Query.has
[ tag "a"
, attribute <|
Attr.href
"/teams/team/pipelines/pipeline"
]
]
, it "has dark grey background" <|
Query.has [ style "background-color" ColorValues.grey100 ]
, it "lays out contents horizontally" <|
Query.has [ style "display" "flex" ]
, it "maximizes spacing between the left and right navs" <|
Query.has [ style "justify-content" "space-between" ]
, it "renders the login component last" <|
Query.children []
>> Query.index -1
>> Query.has [ id "login-component" ]
]
, context "when logged out"
(Application.handleCallback
(Callback.UserFetched <| Data.httpUnauthorized)
>> Tuple.first
>> queryView
)
[ it "renders the login component last" <|
Query.children []
>> Query.index -1
>> Query.has [ id "login-component" ]
, it "has a link to login" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-item" ]
>> Query.has [ tag "a", attribute <| Attr.href "/sky/login" ]
]
, context "when logged in"
(Application.handleCallback
(Callback.UserFetched <| Ok sampleUser)
>> Tuple.first
>> queryView
)
[ it "renders the login component last" <|
Query.children []
>> Query.index -1
>> Query.has [ id "login-component" ]
, it "renders login component with a maximum width" <|
Query.find [ id "login-component" ]
>> Query.has [ style "max-width" "20%" ]
, it "renders login container with relative position" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-container" ]
>> Query.has
[ style "position" "relative" ]
, it "lays out login container contents vertically" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-container" ]
>> Query.has
[ style "display" "flex"
, style "flex-direction" "column"
]
, it "draws lighter grey line to the left of login container" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-container" ]
>> Query.has
[ style "border-left" <| "1px solid " ++ borderGrey ]
, it "renders login container tall enough" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-container" ]
>> Query.has
[ style "line-height" lineHeight ]
, it "has the login username styles" <|
Query.children []
>> Query.index -1
>> Query.find [ id "user-id" ]
>> Expect.all
[ Query.has
[ style "padding" "0 30px"
, style "cursor" "pointer"
, style "display" "flex"
, style "align-items" "center"
, style "justify-content" "center"
, style "flex-grow" "1"
]
, Query.children []
>> Query.index 0
>> Query.has
[ style "overflow" "hidden"
, style "text-overflow" "ellipsis"
]
]
, it "shows the logged in displayUserId when the user is logged in" <|
Query.children []
>> Query.index -1
>> Query.find [ id "user-id" ]
>> Query.has [ text "displayUserIdTest" ]
, it "Click UserMenu message is received when login menu is clicked" <|
Query.find [ id "login-container" ]
>> Event.simulate Event.click
>> Event.expect
(ApplicationMsgs.Update <| Msgs.Click Msgs.UserMenu)
, it "does not render the logout button" <|
Query.children []
>> Query.index -1
>> Query.find [ id "user-id" ]
>> Query.hasNot [ id "logout-button" ]
, it "renders pause pipeline button" <|
Query.find [ id "top-bar-pause-toggle" ]
>> Query.has
[ style "background-image" <|
Assets.backgroundImage <|
Just Assets.PauseIcon
]
]
, it "clicking a pinned resource navigates to the pinned resource page" <|
Application.update
(ApplicationMsgs.Update <|
Msgs.GoToRoute
(Routes.Resource
{ id = Data.shortResourceId
, page = Nothing
, version = Nothing
}
)
)
>> Tuple.second
>> Expect.equal
[ Effects.NavigateTo <|
Routes.toString <|
Routes.Resource
{ id = Data.shortResourceId
, page = Nothing
, version = Nothing
}
]
, context "when pipeline is paused"
(Application.handleCallback
(Callback.PipelineFetched <|
Ok <|
(Data.pipeline "t" 0
|> Data.withName "p"
|> Data.withPaused True
)
)
>> Tuple.first
>> Application.handleCallback
(Callback.UserFetched <| Ok sampleUser)
>> Tuple.first
>> queryView
)
[ it "has blue background" <|
Query.has [ style "background-color" pausedBlue ]
]
, context "when pipeline is archived"
(Application.handleCallback
(Callback.PipelineFetched <|
Ok <|
(Data.pipeline "t" 0
|> Data.withName "p"
|> Data.withPaused True
|> Data.withArchived True
)
)
>> Tuple.first
>> Application.handleCallback
(Callback.UserFetched <| Ok sampleUser)
>> Tuple.first
>> queryView
)
[ it "does not render pause toggle" <|
Query.hasNot [ id "top-bar-pause-toggle" ]
, it "draws uses the normal border colour for the login container" <|
Query.find [ id "login-container" ]
>> Query.has
[ style "border-left" <| "1px solid " ++ borderGrey ]
]
, context "when hovering over the pinned icon"
(hoverOver Msgs.TopBarPinIcon >> Tuple.first)
<|
let
testWithPinnedVersion version tooltipText =
Application.handleCallback
(Callback.ResourcesFetched <|
Ok
[ Data.resource version ]
)
>> Tuple.first
>> queryView
>> Query.find [ id "tooltips" ]
>> Query.has [ text tooltipText ]
in
[ it "shows correct text when there's resources pinned" <|
testWithPinnedVersion (Just "v1")
"view pinned resources"
, it "shows correct text when there's no resources pinned" <|
testWithPinnedVersion Nothing
"no pinned resources"
]
, context "when hovering over the favorited icon"
(hoverOver (Msgs.TopBarFavoritedIcon 0) >> Tuple.first)
<|
let
testWithFavoritedPipelines ids tooltipText =
Application.handleDelivery
(FavoritedPipelinesReceived <|
Ok ids
)
>> Tuple.first
>> Application.handleCallback
(Callback.PipelineFetched <|
Ok <|
(Data.pipeline "t" 0 |> Data.withName "p")
)
>> Tuple.first
>> queryView
>> Query.find [ id "tooltips" ]
>> Query.has [ text tooltipText ]
in
[ it "shows correct text when the pipeline is not favorited" <|
testWithFavoritedPipelines Set.empty
"favorite pipeline"
, it "shows correct text when the pipeline is favorited" <|
testWithFavoritedPipelines (Set.singleton 0)
"unfavorite pipeline"
]
, context "when hovering over the pause toggle icon"
(hoverOver (Msgs.TopBarPauseToggle Data.shortPipelineId) >> Tuple.first)
<|
let
testWithPaused paused tooltipText =
Application.handleCallback
(PipelineFetched <|
Ok
(Data.pipeline "t" 0
|> Data.withName "p"
|> Data.withPaused paused
)
)
>> Tuple.first
>> queryView
>> Query.find [ id "tooltips" ]
>> Query.has [ text tooltipText ]
in
[ it "shows correct text when the pipeline is not paused" <|
testWithPaused False
"pause pipeline"
, it "shows correct text when the pipeline is paused" <|
testWithPaused True
"unpause pipeline"
]
]
, rspecStyleDescribe
"when on pipeline page for an instanced pipeline"
(Common.initRoute (Routes.Pipeline { id = Concourse.toPipelineId pipelineInstance, groups = [] })
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok
[ pipelineInstance
, archivedPipelineInstance
, Data.pipeline "team" 2 |> Data.withName "pipeline"
]
)
|> Tuple.first
|> queryView
)
[ it "shows instance group breadcrumb" <|
Query.has [ id "breadcrumb-instance-group" ]
, context "instance group breadcrumb"
(Query.find [ id "breadcrumb-instance-group" ])
[ it "displays badge containing number of pipelines in group" <|
Query.children []
>> Query.first
>> Query.has [ text "2" ]
, it "contains the name of the instance group" <|
Query.has [ text "pipeline" ]
, it "is a link to the instance group view" <|
Query.has
[ tag "a"
, Common.routeHref <|
Routes.Dashboard
{ searchType = Routes.Normal "team:\"team\" group:\"pipeline\""
, dashboardView = Routes.ViewNonArchivedPipelines
}
]
]
, it "has a pipeline breadcrumb" <|
Query.has [ id "breadcrumb-pipeline" ]
, context "pipeline breadcrumb"
(Query.find [ id "breadcrumb-pipeline" ])
[ it "renders icon first" <|
Query.children []
>> Query.first
>> Query.has pipelineBreadcrumbSelector
, it "renders instance vars in hyphen notation second" <|
Query.children []
>> Query.index 1
>> Query.has [ text "v1-v2" ]
, it "has pointer cursor" <|
Query.has [ style "cursor" "pointer" ]
, it "is a link to the relevant pipeline page" <|
Query.has
[ tag "a"
, Common.routeHref (Routes.Pipeline { id = Concourse.toPipelineId pipelineInstance, groups = [] })
]
]
]
, rspecStyleDescribe
"when on pipeline page for an instanced pipeline with no instance vars"
(Common.init "/teams/team/pipelines/pipeline"
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok
[ pipelineInstance
, Data.pipeline "team" 2 |> Data.withName "pipeline"
]
)
|> Tuple.first
|> queryView
)
[ it "shows instance group breadcrumb" <|
Query.has [ id "breadcrumb-instance-group" ]
, context "pipeline breadcrumb"
(Query.find [ id "breadcrumb-pipeline" ])
[ it "renders the empty set" <|
Query.children []
>> Query.index 1
>> Query.has [ text "{}" ]
]
]
, rspecStyleDescribe "rendering user menus on clicks"
(Common.init "/teams/team/pipelines/pipeline")
[ it "shows user menu when ToggleUserMenu msg is received" <|
Application.handleCallback
(Callback.UserFetched <| Ok sampleUser)
>> Tuple.first
>> Application.update
(ApplicationMsgs.Update <| Msgs.Click Msgs.UserMenu)
>> Tuple.first
>> queryView
>> Query.has [ id "logout-button" ]
, it "renders user menu content when click UserMenu msg is received and logged in" <|
Application.handleCallback
(Callback.UserFetched <| Ok sampleUser)
>> Tuple.first
>> Application.update
(ApplicationMsgs.Update <| Msgs.Click Msgs.UserMenu)
>> Tuple.first
>> queryView
>> Expect.all
[ Query.has [ id "logout-button" ]
, Query.find [ id "logout-button" ]
>> Query.has [ text "logout" ]
, Query.find [ id "logout-button" ]
>> Query.has
[ style "position" "absolute"
, style "top" "55px"
, style "background-color" ColorValues.grey100
, style "height" topBarHeight
, style "width" "100%"
, style "border-top" <| "1px solid " ++ borderGrey
, style "cursor" "pointer"
, style "display" "flex"
, style "align-items" "center"
, style "justify-content" "center"
, style "flex-grow" "1"
]
]
, it "when logout is clicked, a Click LogoutButton msg is sent" <|
Application.handleCallback
(Callback.UserFetched <| Ok sampleUser)
>> Tuple.first
>> Application.update
(ApplicationMsgs.Update <| Msgs.Click Msgs.UserMenu)
>> Tuple.first
>> queryView
>> Query.find [ id "logout-button" ]
>> Event.simulate Event.click
>> Event.expect
(ApplicationMsgs.Update <| Msgs.Click Msgs.LogoutButton)
, it "shows 'login' when LoggedOut TopLevelMessage is successful" <|
Application.handleCallback
(Callback.LoggedOut <| Ok ())
>> Tuple.first
>> queryView
>> Query.find [ id "login-item" ]
>> Query.has [ text "login" ]
]
, rspecStyleDescribe "login component when user is logged out"
(Common.init "/teams/team/pipelines/pipeline"
|> Application.handleCallback
(Callback.LoggedOut (Ok ()))
|> Tuple.first
|> queryView
)
[ it "has a link to login" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-item" ]
>> Query.has [ tag "a", attribute <| Attr.href "/sky/login" ]
, it "has the login container styles" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-container" ]
>> Query.has
[ style "position" "relative"
, style "display" "flex"
, style "flex-direction" "column"
, style "border-left" <| "1px solid " ++ borderGrey
, style "line-height" lineHeight
]
, it "has the login username styles" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-item" ]
>> Query.has
[ style "padding" "0 30px"
, style "cursor" "pointer"
, style "display" "flex"
, style "align-items" "center"
, style "justify-content" "center"
, style "flex-grow" "1"
]
]
, rspecStyleDescribe "when triggering a log in message"
(Common.init "/"
|> Application.handleCallback
(Callback.LoggedOut (Ok ()))
)
[ it "redirects to login page when you click login" <|
Tuple.first
>> Application.update
(ApplicationMsgs.Update <| Msgs.Click Msgs.LoginButton)
>> Tuple.second
>> Expect.equal [ Effects.RedirectToLogin ]
]
, rspecStyleDescribe "rendering top bar on build page"
(Common.init "/teams/team/pipelines/pipeline/jobs/job/builds/1"
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ Data.pipeline "team" 1 |> Data.withName "pipeline" ]
)
|> Tuple.first
|> queryView
)
[ it "should pad the breadcrumbs to max size so they can be left-aligned" <|
Query.find
[ id "breadcrumbs" ]
>> Query.has [ style "flex-grow" "1" ]
, it "pipeline breadcrumb should have a link to the pipeline page when viewing build details" <|
Query.find [ id "breadcrumb-pipeline" ]
>> Query.has
[ tag "a"
, attribute <|
Attr.href
"/teams/team/pipelines/pipeline"
]
, context "job breadcrumb"
(Query.find [ id "breadcrumb-job" ])
[ it "is laid out horizontally with appropriate spacing" <|
Query.has
[ style "display" "inline-block"
, style "padding" "0 10px"
]
, it "has job icon rendered first" <|
Query.has jobBreadcrumbSelector
, it "has build name after job icon" <|
Query.has [ text "job" ]
, it "does not appear clickable" <|
Query.hasNot [ style "cursor" "pointer" ]
]
]
, rspecStyleDescribe
"when on build page for an instanced pipeline"
(Common.initRoute
(Routes.Build
{ id =
{ teamName = "team"
, pipelineName = "pipeline"
, pipelineInstanceVars = instanceVars
, jobName = "job"
, buildName = "1"
}
, highlight = Routes.HighlightNothing
}
)
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ pipelineInstance ]
)
|> Tuple.first
|> queryView
)
[ it "shows instance group breadcrumb" <|
Query.has [ id "breadcrumb-instance-group" ]
, it "has a pipeline breadcrumb" <|
Query.has [ id "breadcrumb-pipeline" ]
, context "pipeline breadcrumb"
(Query.find [ id "breadcrumb-pipeline" ])
[ it "renders instance vars in hyphen notation second" <|
Query.children []
>> Query.index 1
>> Query.has [ text "v1-v2" ]
]
]
, rspecStyleDescribe "rendering top bar on resource page"
(Common.init "/teams/team/pipelines/pipeline/resources/resource"
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ Data.pipeline "team" 1 |> Data.withName "pipeline" ]
)
|> Tuple.first
|> queryView
)
[ it "should pad the breadcrumbs to max size so they can be left-aligned" <|
Query.find
[ id "breadcrumbs" ]
>> Query.has [ style "flex-grow" "1" ]
, it "pipeline breadcrumb should have a link to the pipeline page when viewing resource details" <|
Query.find [ id "breadcrumb-pipeline" ]
>> Query.has
[ tag "a"
, attribute <|
Attr.href
"/teams/team/pipelines/pipeline"
]
, it "there is a / between pipeline and resource in breadcrumb" <|
Query.find [ id "breadcrumbs" ]
>> Query.children []
>> Expect.all
[ Query.index 1
>> Query.has [ class "breadcrumb-separator" ]
, Query.index 1 >> Query.has [ text "/" ]
, Query.index 2 >> Query.has [ id "breadcrumb-resource" ]
]
, it "resource breadcrumb is laid out horizontally with appropriate spacing" <|
Query.find [ id "breadcrumb-resource" ]
>> Query.has
[ style "display" "inline-block"
, style "padding" "0 10px"
]
, it "top bar has resource breadcrumb with resource icon rendered first" <|
Query.find [ id "breadcrumb-resource" ]
>> Query.children []
>> Query.index 0
>> Query.has resourceBreadcrumbSelector
, it "top bar has resource name after resource icon" <|
Query.find [ id "breadcrumb-resource" ]
>> Query.children []
>> Query.index 1
>> Query.has
[ text "resource" ]
]
, rspecStyleDescribe
"when on resource page for an instanced pipeline"
(Common.initRoute
(Routes.Resource
{ id =
{ teamName = "team"
, pipelineName = "pipeline"
, pipelineInstanceVars = instanceVars
, resourceName = "resource"
}
, page = Nothing
, version = Nothing
}
)
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ pipelineInstance ]
)
|> Tuple.first
|> queryView
)
[ it "shows instance group breadcrumb" <|
Query.has [ id "breadcrumb-instance-group" ]
, it "has a pipeline breadcrumb" <|
Query.has [ id "breadcrumb-pipeline" ]
, context "pipeline breadcrumb"
(Query.find [ id "breadcrumb-pipeline" ])
[ it "renders instance vars in hyphen notation second" <|
Query.children []
>> Query.index 1
>> Query.has [ text "v1-v2" ]
]
]
, rspecStyleDescribe "rendering top bar on job page"
(Common.init "/teams/team/pipelines/pipeline/jobs/job"
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ Data.pipeline "team" 1 |> Data.withName "pipeline" ]
)
|> Tuple.first
|> queryView
)
[ it "should pad the breadcrumbs to max size so they can be left-aligned" <|
Query.find
[ id "breadcrumbs" ]
>> Query.has [ style "flex-grow" "1" ]
, it "pipeline breadcrumb should have a link to the pipeline page when viewing job details" <|
Query.find [ id "breadcrumb-pipeline" ]
>> Query.has
[ tag "a"
, attribute <|
Attr.href
"/teams/team/pipelines/pipeline"
]
, it "there is a / between pipeline and job in breadcrumb" <|
Query.find [ id "breadcrumbs" ]
>> Query.children []
>> Expect.all
[ Query.index 1
>> Query.has [ class "breadcrumb-separator" ]
, Query.index 0 >> Query.has [ id "breadcrumb-pipeline" ]
, Query.index 2 >> Query.has [ id "breadcrumb-job" ]
]
]
, rspecStyleDescribe
"when on job page for an instanced pipeline"
(Common.initRoute
(Routes.Job
{ id =
{ teamName = "team"
, pipelineName = "pipeline"
, pipelineInstanceVars = instanceVars
, jobName = "job"
}
, page = Nothing
}
)
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ pipelineInstance ]
)
|> Tuple.first
|> queryView
)
[ it "shows instance group breadcrumb" <|
Query.has [ id "breadcrumb-instance-group" ]
, it "has a pipeline breadcrumb" <|
Query.has [ id "breadcrumb-pipeline" ]
, context "pipeline breadcrumb"
(Query.find [ id "breadcrumb-pipeline" ])
[ it "renders instance vars in hyphen notation second" <|
Query.children []
>> Query.index 1
>> Query.has [ text "v1-v2" ]
]
]
, describe "pause toggle" <|
let
givenPipelinePaused =
Common.init "/teams/t/pipelines/p"
|> Application.handleCallback
(Callback.PipelineFetched <|
Ok
(Data.pipeline "t" 0
|> Data.withName "p"
|> Data.withPaused True
)
)
|> Tuple.first
givenUserAuthorized =
Application.handleCallback
(Callback.UserFetched <|
Ok
{ id = "test"
, userName = "test"
, name = "test"
, email = "test"
, isAdmin = False
, teams =
Dict.fromList
[ ( "t", [ "member" ] ) ]
, displayUserId = "displayUserIdTest"
}
)
>> Tuple.first
givenUserUnauthorized =
Application.handleCallback
(Callback.UserFetched <|
Ok
{ id = "test"
, userName = "test"
, name = "test"
, email = "test"
, isAdmin = False
, teams =
Dict.fromList
[ ( "s", [ "member" ] ) ]
, displayUserId = "displayUserIdTest"
}
)
>> Tuple.first
pipelineIdentifier =
Data.shortPipelineId
toggleMsg =
ApplicationMsgs.Update <|
Msgs.Click <|
Msgs.TopBarPauseToggle
pipelineIdentifier
in
[ defineHoverBehaviour
{ name = "play pipeline icon when authorized"
, setup = givenPipelinePaused |> givenUserAuthorized
, query = queryView >> Query.find [ id "top-bar-pause-toggle" ]
, unhoveredSelector =
{ description = "faded play button with light border"
, selector =
[ style "opacity" "0.5"
, style "margin" "17px"
, style "cursor" "pointer"
]
++ iconSelector
{ size = "20px"
, image = Assets.PlayIcon
}
}
, hoveredSelector =
{ description = "white play button with light border"
, selector =
[ style "opacity" "1"
, style "margin" "17px"
, style "cursor" "pointer"
]
++ iconSelector
{ size = "20px"
, image = Assets.PlayIcon
}
}
, hoverable =
Msgs.TopBarPauseToggle pipelineIdentifier
}
, defineHoverBehaviour
{ name = "play pipeline icon when unauthenticated"
, setup = givenPipelinePaused
, query = queryView >> Query.find [ id "top-bar-pause-toggle" ]
, unhoveredSelector =
{ description = "faded play button with light border"
, selector =
[ style "opacity" "0.5"
, style "margin" "17px"
, style "cursor" "pointer"
]
++ iconSelector
{ size = "20px"
, image = Assets.PlayIcon
}
}
, hoveredSelector =
{ description = "white play button with light border"
, selector =
[ style "opacity" "1"
, style "margin" "17px"
, style "cursor" "pointer"
]
++ iconSelector
{ size = "20px"
, image = Assets.PlayIcon
}
}
, hoverable =
Msgs.TopBarPauseToggle pipelineIdentifier
}
, defineHoverBehaviour
{ name = "play pipeline icon when unauthorized"
, setup = givenPipelinePaused |> givenUserUnauthorized
, query = queryView >> Query.find [ id "top-bar-pause-toggle" ]
, unhoveredSelector =
{ description = "faded play button with light border"
, selector =
[ style "opacity" "0.2"
, style "margin" "17px"
, style "cursor" "default"
]
++ iconSelector
{ size = "20px"
, image = Assets.PlayIcon
}
}
, hoveredSelector =
{ description = "faded play button with tooltip below"
, selector =
[ containing
([ style "cursor" "default"
, style "opacity" "0.2"
]
++ iconSelector
{ size = "20px"
, image = Assets.PlayIcon
}
)
, containing
[ style "position" "absolute"
, style "top" "100%"
]
, style "position" "relative"
, style "margin" "17px"
]
}
, hoverable =
Msgs.TopBarPauseToggle pipelineIdentifier
}
, test "clicking play button sends TogglePipelinePaused msg" <|
\_ ->
givenPipelinePaused
|> queryView
|> Query.find [ id "top-bar-pause-toggle" ]
|> Event.simulate Event.click
|> Event.expect toggleMsg
, test "play button unclickable for non-members" <|
\_ ->
givenPipelinePaused
|> givenUserUnauthorized
|> queryView
|> Query.find [ id "top-bar-pause-toggle" ]
|> Event.simulate Event.click
|> Event.toResult
|> Expect.err
, test "play button click msg sends api call" <|
\_ ->
givenPipelinePaused
|> Application.update toggleMsg
|> Tuple.second
|> Expect.equal
[ Effects.SendTogglePipelineRequest
pipelineIdentifier
True
]
, test "play button click msg turns icon into spinner" <|
\_ ->
givenPipelinePaused
|> Application.update toggleMsg
|> Tuple.first
|> queryView
|> Query.has
[ style "animation"
"container-rotate 1568ms linear infinite"
, style "height" "20px"
, style "width" "20px"
]
, test "successful PipelineToggled callback turns topbar dark" <|
\_ ->
givenPipelinePaused
|> Application.update toggleMsg
|> Tuple.first
|> Application.handleCallback
(Callback.PipelineToggled pipelineIdentifier <| Ok ())
|> Tuple.first
|> queryView
|> Query.find [ id "top-bar-app" ]
|> Query.has
[ style "background-color" ColorValues.grey100 ]
, test "successful callback turns spinner into pause button" <|
\_ ->
givenPipelinePaused
|> Application.update toggleMsg
|> Tuple.first
|> Application.handleCallback
(Callback.PipelineToggled pipelineIdentifier <| Ok ())
|> Tuple.first
|> queryView
|> Query.find [ id "top-bar-pause-toggle" ]
|> Query.has
(iconSelector
{ size = "20px"
, image = Assets.PauseIcon
}
)
, test "Unauthorized PipelineToggled callback redirects to login" <|
\_ ->
givenPipelinePaused
|> Application.handleCallback
(Callback.PipelineToggled pipelineIdentifier <|
Data.httpUnauthorized
)
|> Tuple.second
|> Expect.equal
[ Effects.RedirectToLogin ]
, test "erroring PipelineToggled callback leaves topbar blue" <|
\_ ->
givenPipelinePaused
|> Application.handleCallback
(Callback.PipelineToggled pipelineIdentifier <|
Data.httpInternalServerError
)
|> Tuple.first
|> queryView
|> Query.find [ id "top-bar-app" ]
|> Query.has
[ style "background-color" pausedBlue ]
]
]
eachHasStyle : String -> String -> Query.Multiple msg -> Expectation
eachHasStyle property value =
Query.each <| Query.has [ style property value ]
sampleUser : Concourse.User
sampleUser =
{ id = "1", userName = "test", name = "Bob", isAdmin = False, email = "bob@bob.com", teams = Dict.empty , displayUserId = "displayUserIdTest" }
pipelineBreadcrumbSelector : List Selector.Selector
pipelineBreadcrumbSelector =
[ style "background-image" <|
Assets.backgroundImage <|
Just (Assets.BreadcrumbIcon Assets.PipelineComponent)
, style "background-repeat" "no-repeat"
]
jobBreadcrumbSelector : List Selector.Selector
jobBreadcrumbSelector =
[ style "background-image" <|
Assets.backgroundImage <|
Just (Assets.BreadcrumbIcon Assets.JobComponent)
, style "background-repeat" "no-repeat"
]
resourceBreadcrumbSelector : List Selector.Selector
resourceBreadcrumbSelector =
[ style "background-image" <|
Assets.backgroundImage <|
Just (Assets.BreadcrumbIcon Assets.ResourceComponent)
, style "background-repeat" "no-repeat"
]
| 57183 | module TopBarTests exposing (all)
import Application.Application as Application
import Assets
import Char
import ColorValues
import Common exposing (defineHoverBehaviour, hoverOver, queryView)
import Concourse exposing (JsonValue(..))
import Dashboard.SearchBar as SearchBar
import DashboardTests exposing (iconSelector)
import Data
import Dict
import Expect exposing (..)
import Html.Attributes as Attr
import Http
import Keyboard
import Login.Login as Login
import Message.Callback as Callback exposing (Callback(..))
import Message.Effects as Effects
import Message.Message as Msgs
import Message.Subscription exposing (Delivery(..))
import Message.TopLevelMessage as ApplicationMsgs
import Routes
import Set
import Test exposing (..)
import Test.Html.Event as Event
import Test.Html.Query as Query
import Test.Html.Selector as Selector
exposing
( attribute
, class
, containing
, id
, style
, tag
, text
)
import Time
import Url
import Views.Styles
rspecStyleDescribe : String -> subject -> List (subject -> Test) -> Test
rspecStyleDescribe description beforeEach subTests =
Test.describe description
(subTests |> List.map ((|>) beforeEach))
context : String -> (setup -> subject) -> List (subject -> Test) -> (setup -> Test)
context description beforeEach subTests setup =
Test.describe description
(subTests |> List.map ((|>) (beforeEach setup)))
it : String -> (subject -> Expectation) -> subject -> Test
it desc expectationFunc subject =
Test.test desc <|
\_ -> expectationFunc subject
update : Msgs.Message -> Login.Model {} -> ( Login.Model {}, List Effects.Effect )
update msg =
(\a -> ( a, [] )) >> Login.update msg
lineHeight : String
lineHeight =
"54px"
borderGrey : String
borderGrey =
"#3d3c3c"
backgroundGrey : String
backgroundGrey =
"#1e1d1d"
pausedBlue : String
pausedBlue =
"#3498db"
topBarHeight : String
topBarHeight =
"54px"
searchBarBorder : String -> String
searchBarBorder color =
"1px solid " ++ color
searchBarHeight : String
searchBarHeight =
"30px"
searchBarWidth : String
searchBarWidth =
"251px"
searchBarPadding : String
searchBarPadding =
"0 42px"
flags : Application.Flags
flags =
{ turbulenceImgSrc = ""
, notFoundImgSrc = ""
, csrfToken = ""
, authToken = ""
, pipelineRunningKeyframes = ""
}
instanceVars : Concourse.InstanceVars
instanceVars =
Dict.fromList [ ( "var1", JsonString "v1" ), ( "var2", JsonString "v2" ) ]
pipelineInstance : Concourse.Pipeline
pipelineInstance =
Data.pipeline "team" 1
|> Data.withName "pipeline"
|> Data.withInstanceVars instanceVars
archivedPipelineInstance : Concourse.Pipeline
archivedPipelineInstance =
Data.pipeline "team" 1
|> Data.withName "pipeline"
|> Data.withInstanceVars (Dict.fromList [ ( "foo", JsonString "bar" ) ])
|> Data.withArchived True
all : Test
all =
describe "TopBar"
[ rspecStyleDescribe "when on pipeline page"
(Common.init "/teams/team/pipelines/pipeline"
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ Data.pipeline "team" 1 |> Data.withName "pipeline" ]
)
|> Tuple.first
)
[ context "when login state unknown"
queryView
[ it "shows concourse logo" <|
Query.has
[ style "background-image" <|
Assets.backgroundImage <|
Just Assets.ConcourseLogoWhite
, style "background-position" "50% 50%"
, style "background-repeat" "no-repeat"
, style "background-size" "42px 42px"
, style "width" topBarHeight
, style "height" topBarHeight
]
, it "does not shows instance group breadcrumb" <|
Query.hasNot [ id "breadcrumb-instance-group" ]
, it "shows pipeline breadcrumb" <|
Query.has [ id "breadcrumb-pipeline" ]
, context "pipeline breadcrumb"
(Query.find [ id "breadcrumb-pipeline" ])
[ it "renders icon first" <|
Query.children []
>> Query.first
>> Query.has pipelineBreadcrumbSelector
, it "renders pipeline name second" <|
Query.children []
>> Query.index 1
>> Query.has
[ text "pipeline" ]
, it "has pointer cursor" <|
Query.has [ style "cursor" "pointer" ]
, it "is a link to the relevant pipeline page" <|
Query.has
[ tag "a"
, attribute <|
Attr.href
"/teams/team/pipelines/pipeline"
]
]
, it "has dark grey background" <|
Query.has [ style "background-color" ColorValues.grey100 ]
, it "lays out contents horizontally" <|
Query.has [ style "display" "flex" ]
, it "maximizes spacing between the left and right navs" <|
Query.has [ style "justify-content" "space-between" ]
, it "renders the login component last" <|
Query.children []
>> Query.index -1
>> Query.has [ id "login-component" ]
]
, context "when logged out"
(Application.handleCallback
(Callback.UserFetched <| Data.httpUnauthorized)
>> Tuple.first
>> queryView
)
[ it "renders the login component last" <|
Query.children []
>> Query.index -1
>> Query.has [ id "login-component" ]
, it "has a link to login" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-item" ]
>> Query.has [ tag "a", attribute <| Attr.href "/sky/login" ]
]
, context "when logged in"
(Application.handleCallback
(Callback.UserFetched <| Ok sampleUser)
>> Tuple.first
>> queryView
)
[ it "renders the login component last" <|
Query.children []
>> Query.index -1
>> Query.has [ id "login-component" ]
, it "renders login component with a maximum width" <|
Query.find [ id "login-component" ]
>> Query.has [ style "max-width" "20%" ]
, it "renders login container with relative position" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-container" ]
>> Query.has
[ style "position" "relative" ]
, it "lays out login container contents vertically" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-container" ]
>> Query.has
[ style "display" "flex"
, style "flex-direction" "column"
]
, it "draws lighter grey line to the left of login container" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-container" ]
>> Query.has
[ style "border-left" <| "1px solid " ++ borderGrey ]
, it "renders login container tall enough" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-container" ]
>> Query.has
[ style "line-height" lineHeight ]
, it "has the login username styles" <|
Query.children []
>> Query.index -1
>> Query.find [ id "user-id" ]
>> Expect.all
[ Query.has
[ style "padding" "0 30px"
, style "cursor" "pointer"
, style "display" "flex"
, style "align-items" "center"
, style "justify-content" "center"
, style "flex-grow" "1"
]
, Query.children []
>> Query.index 0
>> Query.has
[ style "overflow" "hidden"
, style "text-overflow" "ellipsis"
]
]
, it "shows the logged in displayUserId when the user is logged in" <|
Query.children []
>> Query.index -1
>> Query.find [ id "user-id" ]
>> Query.has [ text "displayUserIdTest" ]
, it "Click UserMenu message is received when login menu is clicked" <|
Query.find [ id "login-container" ]
>> Event.simulate Event.click
>> Event.expect
(ApplicationMsgs.Update <| Msgs.Click Msgs.UserMenu)
, it "does not render the logout button" <|
Query.children []
>> Query.index -1
>> Query.find [ id "user-id" ]
>> Query.hasNot [ id "logout-button" ]
, it "renders pause pipeline button" <|
Query.find [ id "top-bar-pause-toggle" ]
>> Query.has
[ style "background-image" <|
Assets.backgroundImage <|
Just Assets.PauseIcon
]
]
, it "clicking a pinned resource navigates to the pinned resource page" <|
Application.update
(ApplicationMsgs.Update <|
Msgs.GoToRoute
(Routes.Resource
{ id = Data.shortResourceId
, page = Nothing
, version = Nothing
}
)
)
>> Tuple.second
>> Expect.equal
[ Effects.NavigateTo <|
Routes.toString <|
Routes.Resource
{ id = Data.shortResourceId
, page = Nothing
, version = Nothing
}
]
, context "when pipeline is paused"
(Application.handleCallback
(Callback.PipelineFetched <|
Ok <|
(Data.pipeline "t" 0
|> Data.withName "p"
|> Data.withPaused True
)
)
>> Tuple.first
>> Application.handleCallback
(Callback.UserFetched <| Ok sampleUser)
>> Tuple.first
>> queryView
)
[ it "has blue background" <|
Query.has [ style "background-color" pausedBlue ]
]
, context "when pipeline is archived"
(Application.handleCallback
(Callback.PipelineFetched <|
Ok <|
(Data.pipeline "t" 0
|> Data.withName "p"
|> Data.withPaused True
|> Data.withArchived True
)
)
>> Tuple.first
>> Application.handleCallback
(Callback.UserFetched <| Ok sampleUser)
>> Tuple.first
>> queryView
)
[ it "does not render pause toggle" <|
Query.hasNot [ id "top-bar-pause-toggle" ]
, it "draws uses the normal border colour for the login container" <|
Query.find [ id "login-container" ]
>> Query.has
[ style "border-left" <| "1px solid " ++ borderGrey ]
]
, context "when hovering over the pinned icon"
(hoverOver Msgs.TopBarPinIcon >> Tuple.first)
<|
let
testWithPinnedVersion version tooltipText =
Application.handleCallback
(Callback.ResourcesFetched <|
Ok
[ Data.resource version ]
)
>> Tuple.first
>> queryView
>> Query.find [ id "tooltips" ]
>> Query.has [ text tooltipText ]
in
[ it "shows correct text when there's resources pinned" <|
testWithPinnedVersion (Just "v1")
"view pinned resources"
, it "shows correct text when there's no resources pinned" <|
testWithPinnedVersion Nothing
"no pinned resources"
]
, context "when hovering over the favorited icon"
(hoverOver (Msgs.TopBarFavoritedIcon 0) >> Tuple.first)
<|
let
testWithFavoritedPipelines ids tooltipText =
Application.handleDelivery
(FavoritedPipelinesReceived <|
Ok ids
)
>> Tuple.first
>> Application.handleCallback
(Callback.PipelineFetched <|
Ok <|
(Data.pipeline "t" 0 |> Data.withName "p")
)
>> Tuple.first
>> queryView
>> Query.find [ id "tooltips" ]
>> Query.has [ text tooltipText ]
in
[ it "shows correct text when the pipeline is not favorited" <|
testWithFavoritedPipelines Set.empty
"favorite pipeline"
, it "shows correct text when the pipeline is favorited" <|
testWithFavoritedPipelines (Set.singleton 0)
"unfavorite pipeline"
]
, context "when hovering over the pause toggle icon"
(hoverOver (Msgs.TopBarPauseToggle Data.shortPipelineId) >> Tuple.first)
<|
let
testWithPaused paused tooltipText =
Application.handleCallback
(PipelineFetched <|
Ok
(Data.pipeline "t" 0
|> Data.withName "p"
|> Data.withPaused paused
)
)
>> Tuple.first
>> queryView
>> Query.find [ id "tooltips" ]
>> Query.has [ text tooltipText ]
in
[ it "shows correct text when the pipeline is not paused" <|
testWithPaused False
"pause pipeline"
, it "shows correct text when the pipeline is paused" <|
testWithPaused True
"unpause pipeline"
]
]
, rspecStyleDescribe
"when on pipeline page for an instanced pipeline"
(Common.initRoute (Routes.Pipeline { id = Concourse.toPipelineId pipelineInstance, groups = [] })
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok
[ pipelineInstance
, archivedPipelineInstance
, Data.pipeline "team" 2 |> Data.withName "pipeline"
]
)
|> Tuple.first
|> queryView
)
[ it "shows instance group breadcrumb" <|
Query.has [ id "breadcrumb-instance-group" ]
, context "instance group breadcrumb"
(Query.find [ id "breadcrumb-instance-group" ])
[ it "displays badge containing number of pipelines in group" <|
Query.children []
>> Query.first
>> Query.has [ text "2" ]
, it "contains the name of the instance group" <|
Query.has [ text "pipeline" ]
, it "is a link to the instance group view" <|
Query.has
[ tag "a"
, Common.routeHref <|
Routes.Dashboard
{ searchType = Routes.Normal "team:\"team\" group:\"pipeline\""
, dashboardView = Routes.ViewNonArchivedPipelines
}
]
]
, it "has a pipeline breadcrumb" <|
Query.has [ id "breadcrumb-pipeline" ]
, context "pipeline breadcrumb"
(Query.find [ id "breadcrumb-pipeline" ])
[ it "renders icon first" <|
Query.children []
>> Query.first
>> Query.has pipelineBreadcrumbSelector
, it "renders instance vars in hyphen notation second" <|
Query.children []
>> Query.index 1
>> Query.has [ text "v1-v2" ]
, it "has pointer cursor" <|
Query.has [ style "cursor" "pointer" ]
, it "is a link to the relevant pipeline page" <|
Query.has
[ tag "a"
, Common.routeHref (Routes.Pipeline { id = Concourse.toPipelineId pipelineInstance, groups = [] })
]
]
]
, rspecStyleDescribe
"when on pipeline page for an instanced pipeline with no instance vars"
(Common.init "/teams/team/pipelines/pipeline"
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok
[ pipelineInstance
, Data.pipeline "team" 2 |> Data.withName "pipeline"
]
)
|> Tuple.first
|> queryView
)
[ it "shows instance group breadcrumb" <|
Query.has [ id "breadcrumb-instance-group" ]
, context "pipeline breadcrumb"
(Query.find [ id "breadcrumb-pipeline" ])
[ it "renders the empty set" <|
Query.children []
>> Query.index 1
>> Query.has [ text "{}" ]
]
]
, rspecStyleDescribe "rendering user menus on clicks"
(Common.init "/teams/team/pipelines/pipeline")
[ it "shows user menu when ToggleUserMenu msg is received" <|
Application.handleCallback
(Callback.UserFetched <| Ok sampleUser)
>> Tuple.first
>> Application.update
(ApplicationMsgs.Update <| Msgs.Click Msgs.UserMenu)
>> Tuple.first
>> queryView
>> Query.has [ id "logout-button" ]
, it "renders user menu content when click UserMenu msg is received and logged in" <|
Application.handleCallback
(Callback.UserFetched <| Ok sampleUser)
>> Tuple.first
>> Application.update
(ApplicationMsgs.Update <| Msgs.Click Msgs.UserMenu)
>> Tuple.first
>> queryView
>> Expect.all
[ Query.has [ id "logout-button" ]
, Query.find [ id "logout-button" ]
>> Query.has [ text "logout" ]
, Query.find [ id "logout-button" ]
>> Query.has
[ style "position" "absolute"
, style "top" "55px"
, style "background-color" ColorValues.grey100
, style "height" topBarHeight
, style "width" "100%"
, style "border-top" <| "1px solid " ++ borderGrey
, style "cursor" "pointer"
, style "display" "flex"
, style "align-items" "center"
, style "justify-content" "center"
, style "flex-grow" "1"
]
]
, it "when logout is clicked, a Click LogoutButton msg is sent" <|
Application.handleCallback
(Callback.UserFetched <| Ok sampleUser)
>> Tuple.first
>> Application.update
(ApplicationMsgs.Update <| Msgs.Click Msgs.UserMenu)
>> Tuple.first
>> queryView
>> Query.find [ id "logout-button" ]
>> Event.simulate Event.click
>> Event.expect
(ApplicationMsgs.Update <| Msgs.Click Msgs.LogoutButton)
, it "shows 'login' when LoggedOut TopLevelMessage is successful" <|
Application.handleCallback
(Callback.LoggedOut <| Ok ())
>> Tuple.first
>> queryView
>> Query.find [ id "login-item" ]
>> Query.has [ text "login" ]
]
, rspecStyleDescribe "login component when user is logged out"
(Common.init "/teams/team/pipelines/pipeline"
|> Application.handleCallback
(Callback.LoggedOut (Ok ()))
|> Tuple.first
|> queryView
)
[ it "has a link to login" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-item" ]
>> Query.has [ tag "a", attribute <| Attr.href "/sky/login" ]
, it "has the login container styles" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-container" ]
>> Query.has
[ style "position" "relative"
, style "display" "flex"
, style "flex-direction" "column"
, style "border-left" <| "1px solid " ++ borderGrey
, style "line-height" lineHeight
]
, it "has the login username styles" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-item" ]
>> Query.has
[ style "padding" "0 30px"
, style "cursor" "pointer"
, style "display" "flex"
, style "align-items" "center"
, style "justify-content" "center"
, style "flex-grow" "1"
]
]
, rspecStyleDescribe "when triggering a log in message"
(Common.init "/"
|> Application.handleCallback
(Callback.LoggedOut (Ok ()))
)
[ it "redirects to login page when you click login" <|
Tuple.first
>> Application.update
(ApplicationMsgs.Update <| Msgs.Click Msgs.LoginButton)
>> Tuple.second
>> Expect.equal [ Effects.RedirectToLogin ]
]
, rspecStyleDescribe "rendering top bar on build page"
(Common.init "/teams/team/pipelines/pipeline/jobs/job/builds/1"
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ Data.pipeline "team" 1 |> Data.withName "pipeline" ]
)
|> Tuple.first
|> queryView
)
[ it "should pad the breadcrumbs to max size so they can be left-aligned" <|
Query.find
[ id "breadcrumbs" ]
>> Query.has [ style "flex-grow" "1" ]
, it "pipeline breadcrumb should have a link to the pipeline page when viewing build details" <|
Query.find [ id "breadcrumb-pipeline" ]
>> Query.has
[ tag "a"
, attribute <|
Attr.href
"/teams/team/pipelines/pipeline"
]
, context "job breadcrumb"
(Query.find [ id "breadcrumb-job" ])
[ it "is laid out horizontally with appropriate spacing" <|
Query.has
[ style "display" "inline-block"
, style "padding" "0 10px"
]
, it "has job icon rendered first" <|
Query.has jobBreadcrumbSelector
, it "has build name after job icon" <|
Query.has [ text "job" ]
, it "does not appear clickable" <|
Query.hasNot [ style "cursor" "pointer" ]
]
]
, rspecStyleDescribe
"when on build page for an instanced pipeline"
(Common.initRoute
(Routes.Build
{ id =
{ teamName = "team"
, pipelineName = "pipeline"
, pipelineInstanceVars = instanceVars
, jobName = "job"
, buildName = "1"
}
, highlight = Routes.HighlightNothing
}
)
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ pipelineInstance ]
)
|> Tuple.first
|> queryView
)
[ it "shows instance group breadcrumb" <|
Query.has [ id "breadcrumb-instance-group" ]
, it "has a pipeline breadcrumb" <|
Query.has [ id "breadcrumb-pipeline" ]
, context "pipeline breadcrumb"
(Query.find [ id "breadcrumb-pipeline" ])
[ it "renders instance vars in hyphen notation second" <|
Query.children []
>> Query.index 1
>> Query.has [ text "v1-v2" ]
]
]
, rspecStyleDescribe "rendering top bar on resource page"
(Common.init "/teams/team/pipelines/pipeline/resources/resource"
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ Data.pipeline "team" 1 |> Data.withName "pipeline" ]
)
|> Tuple.first
|> queryView
)
[ it "should pad the breadcrumbs to max size so they can be left-aligned" <|
Query.find
[ id "breadcrumbs" ]
>> Query.has [ style "flex-grow" "1" ]
, it "pipeline breadcrumb should have a link to the pipeline page when viewing resource details" <|
Query.find [ id "breadcrumb-pipeline" ]
>> Query.has
[ tag "a"
, attribute <|
Attr.href
"/teams/team/pipelines/pipeline"
]
, it "there is a / between pipeline and resource in breadcrumb" <|
Query.find [ id "breadcrumbs" ]
>> Query.children []
>> Expect.all
[ Query.index 1
>> Query.has [ class "breadcrumb-separator" ]
, Query.index 1 >> Query.has [ text "/" ]
, Query.index 2 >> Query.has [ id "breadcrumb-resource" ]
]
, it "resource breadcrumb is laid out horizontally with appropriate spacing" <|
Query.find [ id "breadcrumb-resource" ]
>> Query.has
[ style "display" "inline-block"
, style "padding" "0 10px"
]
, it "top bar has resource breadcrumb with resource icon rendered first" <|
Query.find [ id "breadcrumb-resource" ]
>> Query.children []
>> Query.index 0
>> Query.has resourceBreadcrumbSelector
, it "top bar has resource name after resource icon" <|
Query.find [ id "breadcrumb-resource" ]
>> Query.children []
>> Query.index 1
>> Query.has
[ text "resource" ]
]
, rspecStyleDescribe
"when on resource page for an instanced pipeline"
(Common.initRoute
(Routes.Resource
{ id =
{ teamName = "team"
, pipelineName = "pipeline"
, pipelineInstanceVars = instanceVars
, resourceName = "resource"
}
, page = Nothing
, version = Nothing
}
)
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ pipelineInstance ]
)
|> Tuple.first
|> queryView
)
[ it "shows instance group breadcrumb" <|
Query.has [ id "breadcrumb-instance-group" ]
, it "has a pipeline breadcrumb" <|
Query.has [ id "breadcrumb-pipeline" ]
, context "pipeline breadcrumb"
(Query.find [ id "breadcrumb-pipeline" ])
[ it "renders instance vars in hyphen notation second" <|
Query.children []
>> Query.index 1
>> Query.has [ text "v1-v2" ]
]
]
, rspecStyleDescribe "rendering top bar on job page"
(Common.init "/teams/team/pipelines/pipeline/jobs/job"
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ Data.pipeline "team" 1 |> Data.withName "pipeline" ]
)
|> Tuple.first
|> queryView
)
[ it "should pad the breadcrumbs to max size so they can be left-aligned" <|
Query.find
[ id "breadcrumbs" ]
>> Query.has [ style "flex-grow" "1" ]
, it "pipeline breadcrumb should have a link to the pipeline page when viewing job details" <|
Query.find [ id "breadcrumb-pipeline" ]
>> Query.has
[ tag "a"
, attribute <|
Attr.href
"/teams/team/pipelines/pipeline"
]
, it "there is a / between pipeline and job in breadcrumb" <|
Query.find [ id "breadcrumbs" ]
>> Query.children []
>> Expect.all
[ Query.index 1
>> Query.has [ class "breadcrumb-separator" ]
, Query.index 0 >> Query.has [ id "breadcrumb-pipeline" ]
, Query.index 2 >> Query.has [ id "breadcrumb-job" ]
]
]
, rspecStyleDescribe
"when on job page for an instanced pipeline"
(Common.initRoute
(Routes.Job
{ id =
{ teamName = "team"
, pipelineName = "pipeline"
, pipelineInstanceVars = instanceVars
, jobName = "job"
}
, page = Nothing
}
)
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ pipelineInstance ]
)
|> Tuple.first
|> queryView
)
[ it "shows instance group breadcrumb" <|
Query.has [ id "breadcrumb-instance-group" ]
, it "has a pipeline breadcrumb" <|
Query.has [ id "breadcrumb-pipeline" ]
, context "pipeline breadcrumb"
(Query.find [ id "breadcrumb-pipeline" ])
[ it "renders instance vars in hyphen notation second" <|
Query.children []
>> Query.index 1
>> Query.has [ text "v1-v2" ]
]
]
, describe "pause toggle" <|
let
givenPipelinePaused =
Common.init "/teams/t/pipelines/p"
|> Application.handleCallback
(Callback.PipelineFetched <|
Ok
(Data.pipeline "t" 0
|> Data.withName "p"
|> Data.withPaused True
)
)
|> Tuple.first
givenUserAuthorized =
Application.handleCallback
(Callback.UserFetched <|
Ok
{ id = "test"
, userName = "test"
, name = "<NAME>"
, email = "test"
, isAdmin = False
, teams =
Dict.fromList
[ ( "t", [ "member" ] ) ]
, displayUserId = "displayUserIdTest"
}
)
>> Tuple.first
givenUserUnauthorized =
Application.handleCallback
(Callback.UserFetched <|
Ok
{ id = "test"
, userName = "test"
, name = "<NAME>"
, email = "test"
, isAdmin = False
, teams =
Dict.fromList
[ ( "s", [ "member" ] ) ]
, displayUserId = "displayUserIdTest"
}
)
>> Tuple.first
pipelineIdentifier =
Data.shortPipelineId
toggleMsg =
ApplicationMsgs.Update <|
Msgs.Click <|
Msgs.TopBarPauseToggle
pipelineIdentifier
in
[ defineHoverBehaviour
{ name = "play pipeline icon when authorized"
, setup = givenPipelinePaused |> givenUserAuthorized
, query = queryView >> Query.find [ id "top-bar-pause-toggle" ]
, unhoveredSelector =
{ description = "faded play button with light border"
, selector =
[ style "opacity" "0.5"
, style "margin" "17px"
, style "cursor" "pointer"
]
++ iconSelector
{ size = "20px"
, image = Assets.PlayIcon
}
}
, hoveredSelector =
{ description = "white play button with light border"
, selector =
[ style "opacity" "1"
, style "margin" "17px"
, style "cursor" "pointer"
]
++ iconSelector
{ size = "20px"
, image = Assets.PlayIcon
}
}
, hoverable =
Msgs.TopBarPauseToggle pipelineIdentifier
}
, defineHoverBehaviour
{ name = "play pipeline icon when unauthenticated"
, setup = givenPipelinePaused
, query = queryView >> Query.find [ id "top-bar-pause-toggle" ]
, unhoveredSelector =
{ description = "faded play button with light border"
, selector =
[ style "opacity" "0.5"
, style "margin" "17px"
, style "cursor" "pointer"
]
++ iconSelector
{ size = "20px"
, image = Assets.PlayIcon
}
}
, hoveredSelector =
{ description = "white play button with light border"
, selector =
[ style "opacity" "1"
, style "margin" "17px"
, style "cursor" "pointer"
]
++ iconSelector
{ size = "20px"
, image = Assets.PlayIcon
}
}
, hoverable =
Msgs.TopBarPauseToggle pipelineIdentifier
}
, defineHoverBehaviour
{ name = "play pipeline icon when unauthorized"
, setup = givenPipelinePaused |> givenUserUnauthorized
, query = queryView >> Query.find [ id "top-bar-pause-toggle" ]
, unhoveredSelector =
{ description = "faded play button with light border"
, selector =
[ style "opacity" "0.2"
, style "margin" "17px"
, style "cursor" "default"
]
++ iconSelector
{ size = "20px"
, image = Assets.PlayIcon
}
}
, hoveredSelector =
{ description = "faded play button with tooltip below"
, selector =
[ containing
([ style "cursor" "default"
, style "opacity" "0.2"
]
++ iconSelector
{ size = "20px"
, image = Assets.PlayIcon
}
)
, containing
[ style "position" "absolute"
, style "top" "100%"
]
, style "position" "relative"
, style "margin" "17px"
]
}
, hoverable =
Msgs.TopBarPauseToggle pipelineIdentifier
}
, test "clicking play button sends TogglePipelinePaused msg" <|
\_ ->
givenPipelinePaused
|> queryView
|> Query.find [ id "top-bar-pause-toggle" ]
|> Event.simulate Event.click
|> Event.expect toggleMsg
, test "play button unclickable for non-members" <|
\_ ->
givenPipelinePaused
|> givenUserUnauthorized
|> queryView
|> Query.find [ id "top-bar-pause-toggle" ]
|> Event.simulate Event.click
|> Event.toResult
|> Expect.err
, test "play button click msg sends api call" <|
\_ ->
givenPipelinePaused
|> Application.update toggleMsg
|> Tuple.second
|> Expect.equal
[ Effects.SendTogglePipelineRequest
pipelineIdentifier
True
]
, test "play button click msg turns icon into spinner" <|
\_ ->
givenPipelinePaused
|> Application.update toggleMsg
|> Tuple.first
|> queryView
|> Query.has
[ style "animation"
"container-rotate 1568ms linear infinite"
, style "height" "20px"
, style "width" "20px"
]
, test "successful PipelineToggled callback turns topbar dark" <|
\_ ->
givenPipelinePaused
|> Application.update toggleMsg
|> Tuple.first
|> Application.handleCallback
(Callback.PipelineToggled pipelineIdentifier <| Ok ())
|> Tuple.first
|> queryView
|> Query.find [ id "top-bar-app" ]
|> Query.has
[ style "background-color" ColorValues.grey100 ]
, test "successful callback turns spinner into pause button" <|
\_ ->
givenPipelinePaused
|> Application.update toggleMsg
|> Tuple.first
|> Application.handleCallback
(Callback.PipelineToggled pipelineIdentifier <| Ok ())
|> Tuple.first
|> queryView
|> Query.find [ id "top-bar-pause-toggle" ]
|> Query.has
(iconSelector
{ size = "20px"
, image = Assets.PauseIcon
}
)
, test "Unauthorized PipelineToggled callback redirects to login" <|
\_ ->
givenPipelinePaused
|> Application.handleCallback
(Callback.PipelineToggled pipelineIdentifier <|
Data.httpUnauthorized
)
|> Tuple.second
|> Expect.equal
[ Effects.RedirectToLogin ]
, test "erroring PipelineToggled callback leaves topbar blue" <|
\_ ->
givenPipelinePaused
|> Application.handleCallback
(Callback.PipelineToggled pipelineIdentifier <|
Data.httpInternalServerError
)
|> Tuple.first
|> queryView
|> Query.find [ id "top-bar-app" ]
|> Query.has
[ style "background-color" pausedBlue ]
]
]
eachHasStyle : String -> String -> Query.Multiple msg -> Expectation
eachHasStyle property value =
Query.each <| Query.has [ style property value ]
sampleUser : Concourse.User
sampleUser =
{ id = "1", userName = "test", name = "<NAME>", isAdmin = False, email = "<EMAIL>", teams = Dict.empty , displayUserId = "displayUserIdTest" }
pipelineBreadcrumbSelector : List Selector.Selector
pipelineBreadcrumbSelector =
[ style "background-image" <|
Assets.backgroundImage <|
Just (Assets.BreadcrumbIcon Assets.PipelineComponent)
, style "background-repeat" "no-repeat"
]
jobBreadcrumbSelector : List Selector.Selector
jobBreadcrumbSelector =
[ style "background-image" <|
Assets.backgroundImage <|
Just (Assets.BreadcrumbIcon Assets.JobComponent)
, style "background-repeat" "no-repeat"
]
resourceBreadcrumbSelector : List Selector.Selector
resourceBreadcrumbSelector =
[ style "background-image" <|
Assets.backgroundImage <|
Just (Assets.BreadcrumbIcon Assets.ResourceComponent)
, style "background-repeat" "no-repeat"
]
| true | module TopBarTests exposing (all)
import Application.Application as Application
import Assets
import Char
import ColorValues
import Common exposing (defineHoverBehaviour, hoverOver, queryView)
import Concourse exposing (JsonValue(..))
import Dashboard.SearchBar as SearchBar
import DashboardTests exposing (iconSelector)
import Data
import Dict
import Expect exposing (..)
import Html.Attributes as Attr
import Http
import Keyboard
import Login.Login as Login
import Message.Callback as Callback exposing (Callback(..))
import Message.Effects as Effects
import Message.Message as Msgs
import Message.Subscription exposing (Delivery(..))
import Message.TopLevelMessage as ApplicationMsgs
import Routes
import Set
import Test exposing (..)
import Test.Html.Event as Event
import Test.Html.Query as Query
import Test.Html.Selector as Selector
exposing
( attribute
, class
, containing
, id
, style
, tag
, text
)
import Time
import Url
import Views.Styles
rspecStyleDescribe : String -> subject -> List (subject -> Test) -> Test
rspecStyleDescribe description beforeEach subTests =
Test.describe description
(subTests |> List.map ((|>) beforeEach))
context : String -> (setup -> subject) -> List (subject -> Test) -> (setup -> Test)
context description beforeEach subTests setup =
Test.describe description
(subTests |> List.map ((|>) (beforeEach setup)))
it : String -> (subject -> Expectation) -> subject -> Test
it desc expectationFunc subject =
Test.test desc <|
\_ -> expectationFunc subject
update : Msgs.Message -> Login.Model {} -> ( Login.Model {}, List Effects.Effect )
update msg =
(\a -> ( a, [] )) >> Login.update msg
lineHeight : String
lineHeight =
"54px"
borderGrey : String
borderGrey =
"#3d3c3c"
backgroundGrey : String
backgroundGrey =
"#1e1d1d"
pausedBlue : String
pausedBlue =
"#3498db"
topBarHeight : String
topBarHeight =
"54px"
searchBarBorder : String -> String
searchBarBorder color =
"1px solid " ++ color
searchBarHeight : String
searchBarHeight =
"30px"
searchBarWidth : String
searchBarWidth =
"251px"
searchBarPadding : String
searchBarPadding =
"0 42px"
flags : Application.Flags
flags =
{ turbulenceImgSrc = ""
, notFoundImgSrc = ""
, csrfToken = ""
, authToken = ""
, pipelineRunningKeyframes = ""
}
instanceVars : Concourse.InstanceVars
instanceVars =
Dict.fromList [ ( "var1", JsonString "v1" ), ( "var2", JsonString "v2" ) ]
pipelineInstance : Concourse.Pipeline
pipelineInstance =
Data.pipeline "team" 1
|> Data.withName "pipeline"
|> Data.withInstanceVars instanceVars
archivedPipelineInstance : Concourse.Pipeline
archivedPipelineInstance =
Data.pipeline "team" 1
|> Data.withName "pipeline"
|> Data.withInstanceVars (Dict.fromList [ ( "foo", JsonString "bar" ) ])
|> Data.withArchived True
all : Test
all =
describe "TopBar"
[ rspecStyleDescribe "when on pipeline page"
(Common.init "/teams/team/pipelines/pipeline"
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ Data.pipeline "team" 1 |> Data.withName "pipeline" ]
)
|> Tuple.first
)
[ context "when login state unknown"
queryView
[ it "shows concourse logo" <|
Query.has
[ style "background-image" <|
Assets.backgroundImage <|
Just Assets.ConcourseLogoWhite
, style "background-position" "50% 50%"
, style "background-repeat" "no-repeat"
, style "background-size" "42px 42px"
, style "width" topBarHeight
, style "height" topBarHeight
]
, it "does not shows instance group breadcrumb" <|
Query.hasNot [ id "breadcrumb-instance-group" ]
, it "shows pipeline breadcrumb" <|
Query.has [ id "breadcrumb-pipeline" ]
, context "pipeline breadcrumb"
(Query.find [ id "breadcrumb-pipeline" ])
[ it "renders icon first" <|
Query.children []
>> Query.first
>> Query.has pipelineBreadcrumbSelector
, it "renders pipeline name second" <|
Query.children []
>> Query.index 1
>> Query.has
[ text "pipeline" ]
, it "has pointer cursor" <|
Query.has [ style "cursor" "pointer" ]
, it "is a link to the relevant pipeline page" <|
Query.has
[ tag "a"
, attribute <|
Attr.href
"/teams/team/pipelines/pipeline"
]
]
, it "has dark grey background" <|
Query.has [ style "background-color" ColorValues.grey100 ]
, it "lays out contents horizontally" <|
Query.has [ style "display" "flex" ]
, it "maximizes spacing between the left and right navs" <|
Query.has [ style "justify-content" "space-between" ]
, it "renders the login component last" <|
Query.children []
>> Query.index -1
>> Query.has [ id "login-component" ]
]
, context "when logged out"
(Application.handleCallback
(Callback.UserFetched <| Data.httpUnauthorized)
>> Tuple.first
>> queryView
)
[ it "renders the login component last" <|
Query.children []
>> Query.index -1
>> Query.has [ id "login-component" ]
, it "has a link to login" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-item" ]
>> Query.has [ tag "a", attribute <| Attr.href "/sky/login" ]
]
, context "when logged in"
(Application.handleCallback
(Callback.UserFetched <| Ok sampleUser)
>> Tuple.first
>> queryView
)
[ it "renders the login component last" <|
Query.children []
>> Query.index -1
>> Query.has [ id "login-component" ]
, it "renders login component with a maximum width" <|
Query.find [ id "login-component" ]
>> Query.has [ style "max-width" "20%" ]
, it "renders login container with relative position" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-container" ]
>> Query.has
[ style "position" "relative" ]
, it "lays out login container contents vertically" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-container" ]
>> Query.has
[ style "display" "flex"
, style "flex-direction" "column"
]
, it "draws lighter grey line to the left of login container" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-container" ]
>> Query.has
[ style "border-left" <| "1px solid " ++ borderGrey ]
, it "renders login container tall enough" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-container" ]
>> Query.has
[ style "line-height" lineHeight ]
, it "has the login username styles" <|
Query.children []
>> Query.index -1
>> Query.find [ id "user-id" ]
>> Expect.all
[ Query.has
[ style "padding" "0 30px"
, style "cursor" "pointer"
, style "display" "flex"
, style "align-items" "center"
, style "justify-content" "center"
, style "flex-grow" "1"
]
, Query.children []
>> Query.index 0
>> Query.has
[ style "overflow" "hidden"
, style "text-overflow" "ellipsis"
]
]
, it "shows the logged in displayUserId when the user is logged in" <|
Query.children []
>> Query.index -1
>> Query.find [ id "user-id" ]
>> Query.has [ text "displayUserIdTest" ]
, it "Click UserMenu message is received when login menu is clicked" <|
Query.find [ id "login-container" ]
>> Event.simulate Event.click
>> Event.expect
(ApplicationMsgs.Update <| Msgs.Click Msgs.UserMenu)
, it "does not render the logout button" <|
Query.children []
>> Query.index -1
>> Query.find [ id "user-id" ]
>> Query.hasNot [ id "logout-button" ]
, it "renders pause pipeline button" <|
Query.find [ id "top-bar-pause-toggle" ]
>> Query.has
[ style "background-image" <|
Assets.backgroundImage <|
Just Assets.PauseIcon
]
]
, it "clicking a pinned resource navigates to the pinned resource page" <|
Application.update
(ApplicationMsgs.Update <|
Msgs.GoToRoute
(Routes.Resource
{ id = Data.shortResourceId
, page = Nothing
, version = Nothing
}
)
)
>> Tuple.second
>> Expect.equal
[ Effects.NavigateTo <|
Routes.toString <|
Routes.Resource
{ id = Data.shortResourceId
, page = Nothing
, version = Nothing
}
]
, context "when pipeline is paused"
(Application.handleCallback
(Callback.PipelineFetched <|
Ok <|
(Data.pipeline "t" 0
|> Data.withName "p"
|> Data.withPaused True
)
)
>> Tuple.first
>> Application.handleCallback
(Callback.UserFetched <| Ok sampleUser)
>> Tuple.first
>> queryView
)
[ it "has blue background" <|
Query.has [ style "background-color" pausedBlue ]
]
, context "when pipeline is archived"
(Application.handleCallback
(Callback.PipelineFetched <|
Ok <|
(Data.pipeline "t" 0
|> Data.withName "p"
|> Data.withPaused True
|> Data.withArchived True
)
)
>> Tuple.first
>> Application.handleCallback
(Callback.UserFetched <| Ok sampleUser)
>> Tuple.first
>> queryView
)
[ it "does not render pause toggle" <|
Query.hasNot [ id "top-bar-pause-toggle" ]
, it "draws uses the normal border colour for the login container" <|
Query.find [ id "login-container" ]
>> Query.has
[ style "border-left" <| "1px solid " ++ borderGrey ]
]
, context "when hovering over the pinned icon"
(hoverOver Msgs.TopBarPinIcon >> Tuple.first)
<|
let
testWithPinnedVersion version tooltipText =
Application.handleCallback
(Callback.ResourcesFetched <|
Ok
[ Data.resource version ]
)
>> Tuple.first
>> queryView
>> Query.find [ id "tooltips" ]
>> Query.has [ text tooltipText ]
in
[ it "shows correct text when there's resources pinned" <|
testWithPinnedVersion (Just "v1")
"view pinned resources"
, it "shows correct text when there's no resources pinned" <|
testWithPinnedVersion Nothing
"no pinned resources"
]
, context "when hovering over the favorited icon"
(hoverOver (Msgs.TopBarFavoritedIcon 0) >> Tuple.first)
<|
let
testWithFavoritedPipelines ids tooltipText =
Application.handleDelivery
(FavoritedPipelinesReceived <|
Ok ids
)
>> Tuple.first
>> Application.handleCallback
(Callback.PipelineFetched <|
Ok <|
(Data.pipeline "t" 0 |> Data.withName "p")
)
>> Tuple.first
>> queryView
>> Query.find [ id "tooltips" ]
>> Query.has [ text tooltipText ]
in
[ it "shows correct text when the pipeline is not favorited" <|
testWithFavoritedPipelines Set.empty
"favorite pipeline"
, it "shows correct text when the pipeline is favorited" <|
testWithFavoritedPipelines (Set.singleton 0)
"unfavorite pipeline"
]
, context "when hovering over the pause toggle icon"
(hoverOver (Msgs.TopBarPauseToggle Data.shortPipelineId) >> Tuple.first)
<|
let
testWithPaused paused tooltipText =
Application.handleCallback
(PipelineFetched <|
Ok
(Data.pipeline "t" 0
|> Data.withName "p"
|> Data.withPaused paused
)
)
>> Tuple.first
>> queryView
>> Query.find [ id "tooltips" ]
>> Query.has [ text tooltipText ]
in
[ it "shows correct text when the pipeline is not paused" <|
testWithPaused False
"pause pipeline"
, it "shows correct text when the pipeline is paused" <|
testWithPaused True
"unpause pipeline"
]
]
, rspecStyleDescribe
"when on pipeline page for an instanced pipeline"
(Common.initRoute (Routes.Pipeline { id = Concourse.toPipelineId pipelineInstance, groups = [] })
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok
[ pipelineInstance
, archivedPipelineInstance
, Data.pipeline "team" 2 |> Data.withName "pipeline"
]
)
|> Tuple.first
|> queryView
)
[ it "shows instance group breadcrumb" <|
Query.has [ id "breadcrumb-instance-group" ]
, context "instance group breadcrumb"
(Query.find [ id "breadcrumb-instance-group" ])
[ it "displays badge containing number of pipelines in group" <|
Query.children []
>> Query.first
>> Query.has [ text "2" ]
, it "contains the name of the instance group" <|
Query.has [ text "pipeline" ]
, it "is a link to the instance group view" <|
Query.has
[ tag "a"
, Common.routeHref <|
Routes.Dashboard
{ searchType = Routes.Normal "team:\"team\" group:\"pipeline\""
, dashboardView = Routes.ViewNonArchivedPipelines
}
]
]
, it "has a pipeline breadcrumb" <|
Query.has [ id "breadcrumb-pipeline" ]
, context "pipeline breadcrumb"
(Query.find [ id "breadcrumb-pipeline" ])
[ it "renders icon first" <|
Query.children []
>> Query.first
>> Query.has pipelineBreadcrumbSelector
, it "renders instance vars in hyphen notation second" <|
Query.children []
>> Query.index 1
>> Query.has [ text "v1-v2" ]
, it "has pointer cursor" <|
Query.has [ style "cursor" "pointer" ]
, it "is a link to the relevant pipeline page" <|
Query.has
[ tag "a"
, Common.routeHref (Routes.Pipeline { id = Concourse.toPipelineId pipelineInstance, groups = [] })
]
]
]
, rspecStyleDescribe
"when on pipeline page for an instanced pipeline with no instance vars"
(Common.init "/teams/team/pipelines/pipeline"
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok
[ pipelineInstance
, Data.pipeline "team" 2 |> Data.withName "pipeline"
]
)
|> Tuple.first
|> queryView
)
[ it "shows instance group breadcrumb" <|
Query.has [ id "breadcrumb-instance-group" ]
, context "pipeline breadcrumb"
(Query.find [ id "breadcrumb-pipeline" ])
[ it "renders the empty set" <|
Query.children []
>> Query.index 1
>> Query.has [ text "{}" ]
]
]
, rspecStyleDescribe "rendering user menus on clicks"
(Common.init "/teams/team/pipelines/pipeline")
[ it "shows user menu when ToggleUserMenu msg is received" <|
Application.handleCallback
(Callback.UserFetched <| Ok sampleUser)
>> Tuple.first
>> Application.update
(ApplicationMsgs.Update <| Msgs.Click Msgs.UserMenu)
>> Tuple.first
>> queryView
>> Query.has [ id "logout-button" ]
, it "renders user menu content when click UserMenu msg is received and logged in" <|
Application.handleCallback
(Callback.UserFetched <| Ok sampleUser)
>> Tuple.first
>> Application.update
(ApplicationMsgs.Update <| Msgs.Click Msgs.UserMenu)
>> Tuple.first
>> queryView
>> Expect.all
[ Query.has [ id "logout-button" ]
, Query.find [ id "logout-button" ]
>> Query.has [ text "logout" ]
, Query.find [ id "logout-button" ]
>> Query.has
[ style "position" "absolute"
, style "top" "55px"
, style "background-color" ColorValues.grey100
, style "height" topBarHeight
, style "width" "100%"
, style "border-top" <| "1px solid " ++ borderGrey
, style "cursor" "pointer"
, style "display" "flex"
, style "align-items" "center"
, style "justify-content" "center"
, style "flex-grow" "1"
]
]
, it "when logout is clicked, a Click LogoutButton msg is sent" <|
Application.handleCallback
(Callback.UserFetched <| Ok sampleUser)
>> Tuple.first
>> Application.update
(ApplicationMsgs.Update <| Msgs.Click Msgs.UserMenu)
>> Tuple.first
>> queryView
>> Query.find [ id "logout-button" ]
>> Event.simulate Event.click
>> Event.expect
(ApplicationMsgs.Update <| Msgs.Click Msgs.LogoutButton)
, it "shows 'login' when LoggedOut TopLevelMessage is successful" <|
Application.handleCallback
(Callback.LoggedOut <| Ok ())
>> Tuple.first
>> queryView
>> Query.find [ id "login-item" ]
>> Query.has [ text "login" ]
]
, rspecStyleDescribe "login component when user is logged out"
(Common.init "/teams/team/pipelines/pipeline"
|> Application.handleCallback
(Callback.LoggedOut (Ok ()))
|> Tuple.first
|> queryView
)
[ it "has a link to login" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-item" ]
>> Query.has [ tag "a", attribute <| Attr.href "/sky/login" ]
, it "has the login container styles" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-container" ]
>> Query.has
[ style "position" "relative"
, style "display" "flex"
, style "flex-direction" "column"
, style "border-left" <| "1px solid " ++ borderGrey
, style "line-height" lineHeight
]
, it "has the login username styles" <|
Query.children []
>> Query.index -1
>> Query.find [ id "login-item" ]
>> Query.has
[ style "padding" "0 30px"
, style "cursor" "pointer"
, style "display" "flex"
, style "align-items" "center"
, style "justify-content" "center"
, style "flex-grow" "1"
]
]
, rspecStyleDescribe "when triggering a log in message"
(Common.init "/"
|> Application.handleCallback
(Callback.LoggedOut (Ok ()))
)
[ it "redirects to login page when you click login" <|
Tuple.first
>> Application.update
(ApplicationMsgs.Update <| Msgs.Click Msgs.LoginButton)
>> Tuple.second
>> Expect.equal [ Effects.RedirectToLogin ]
]
, rspecStyleDescribe "rendering top bar on build page"
(Common.init "/teams/team/pipelines/pipeline/jobs/job/builds/1"
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ Data.pipeline "team" 1 |> Data.withName "pipeline" ]
)
|> Tuple.first
|> queryView
)
[ it "should pad the breadcrumbs to max size so they can be left-aligned" <|
Query.find
[ id "breadcrumbs" ]
>> Query.has [ style "flex-grow" "1" ]
, it "pipeline breadcrumb should have a link to the pipeline page when viewing build details" <|
Query.find [ id "breadcrumb-pipeline" ]
>> Query.has
[ tag "a"
, attribute <|
Attr.href
"/teams/team/pipelines/pipeline"
]
, context "job breadcrumb"
(Query.find [ id "breadcrumb-job" ])
[ it "is laid out horizontally with appropriate spacing" <|
Query.has
[ style "display" "inline-block"
, style "padding" "0 10px"
]
, it "has job icon rendered first" <|
Query.has jobBreadcrumbSelector
, it "has build name after job icon" <|
Query.has [ text "job" ]
, it "does not appear clickable" <|
Query.hasNot [ style "cursor" "pointer" ]
]
]
, rspecStyleDescribe
"when on build page for an instanced pipeline"
(Common.initRoute
(Routes.Build
{ id =
{ teamName = "team"
, pipelineName = "pipeline"
, pipelineInstanceVars = instanceVars
, jobName = "job"
, buildName = "1"
}
, highlight = Routes.HighlightNothing
}
)
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ pipelineInstance ]
)
|> Tuple.first
|> queryView
)
[ it "shows instance group breadcrumb" <|
Query.has [ id "breadcrumb-instance-group" ]
, it "has a pipeline breadcrumb" <|
Query.has [ id "breadcrumb-pipeline" ]
, context "pipeline breadcrumb"
(Query.find [ id "breadcrumb-pipeline" ])
[ it "renders instance vars in hyphen notation second" <|
Query.children []
>> Query.index 1
>> Query.has [ text "v1-v2" ]
]
]
, rspecStyleDescribe "rendering top bar on resource page"
(Common.init "/teams/team/pipelines/pipeline/resources/resource"
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ Data.pipeline "team" 1 |> Data.withName "pipeline" ]
)
|> Tuple.first
|> queryView
)
[ it "should pad the breadcrumbs to max size so they can be left-aligned" <|
Query.find
[ id "breadcrumbs" ]
>> Query.has [ style "flex-grow" "1" ]
, it "pipeline breadcrumb should have a link to the pipeline page when viewing resource details" <|
Query.find [ id "breadcrumb-pipeline" ]
>> Query.has
[ tag "a"
, attribute <|
Attr.href
"/teams/team/pipelines/pipeline"
]
, it "there is a / between pipeline and resource in breadcrumb" <|
Query.find [ id "breadcrumbs" ]
>> Query.children []
>> Expect.all
[ Query.index 1
>> Query.has [ class "breadcrumb-separator" ]
, Query.index 1 >> Query.has [ text "/" ]
, Query.index 2 >> Query.has [ id "breadcrumb-resource" ]
]
, it "resource breadcrumb is laid out horizontally with appropriate spacing" <|
Query.find [ id "breadcrumb-resource" ]
>> Query.has
[ style "display" "inline-block"
, style "padding" "0 10px"
]
, it "top bar has resource breadcrumb with resource icon rendered first" <|
Query.find [ id "breadcrumb-resource" ]
>> Query.children []
>> Query.index 0
>> Query.has resourceBreadcrumbSelector
, it "top bar has resource name after resource icon" <|
Query.find [ id "breadcrumb-resource" ]
>> Query.children []
>> Query.index 1
>> Query.has
[ text "resource" ]
]
, rspecStyleDescribe
"when on resource page for an instanced pipeline"
(Common.initRoute
(Routes.Resource
{ id =
{ teamName = "team"
, pipelineName = "pipeline"
, pipelineInstanceVars = instanceVars
, resourceName = "resource"
}
, page = Nothing
, version = Nothing
}
)
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ pipelineInstance ]
)
|> Tuple.first
|> queryView
)
[ it "shows instance group breadcrumb" <|
Query.has [ id "breadcrumb-instance-group" ]
, it "has a pipeline breadcrumb" <|
Query.has [ id "breadcrumb-pipeline" ]
, context "pipeline breadcrumb"
(Query.find [ id "breadcrumb-pipeline" ])
[ it "renders instance vars in hyphen notation second" <|
Query.children []
>> Query.index 1
>> Query.has [ text "v1-v2" ]
]
]
, rspecStyleDescribe "rendering top bar on job page"
(Common.init "/teams/team/pipelines/pipeline/jobs/job"
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ Data.pipeline "team" 1 |> Data.withName "pipeline" ]
)
|> Tuple.first
|> queryView
)
[ it "should pad the breadcrumbs to max size so they can be left-aligned" <|
Query.find
[ id "breadcrumbs" ]
>> Query.has [ style "flex-grow" "1" ]
, it "pipeline breadcrumb should have a link to the pipeline page when viewing job details" <|
Query.find [ id "breadcrumb-pipeline" ]
>> Query.has
[ tag "a"
, attribute <|
Attr.href
"/teams/team/pipelines/pipeline"
]
, it "there is a / between pipeline and job in breadcrumb" <|
Query.find [ id "breadcrumbs" ]
>> Query.children []
>> Expect.all
[ Query.index 1
>> Query.has [ class "breadcrumb-separator" ]
, Query.index 0 >> Query.has [ id "breadcrumb-pipeline" ]
, Query.index 2 >> Query.has [ id "breadcrumb-job" ]
]
]
, rspecStyleDescribe
"when on job page for an instanced pipeline"
(Common.initRoute
(Routes.Job
{ id =
{ teamName = "team"
, pipelineName = "pipeline"
, pipelineInstanceVars = instanceVars
, jobName = "job"
}
, page = Nothing
}
)
|> Application.handleCallback
(Callback.AllPipelinesFetched <|
Ok [ pipelineInstance ]
)
|> Tuple.first
|> queryView
)
[ it "shows instance group breadcrumb" <|
Query.has [ id "breadcrumb-instance-group" ]
, it "has a pipeline breadcrumb" <|
Query.has [ id "breadcrumb-pipeline" ]
, context "pipeline breadcrumb"
(Query.find [ id "breadcrumb-pipeline" ])
[ it "renders instance vars in hyphen notation second" <|
Query.children []
>> Query.index 1
>> Query.has [ text "v1-v2" ]
]
]
, describe "pause toggle" <|
let
givenPipelinePaused =
Common.init "/teams/t/pipelines/p"
|> Application.handleCallback
(Callback.PipelineFetched <|
Ok
(Data.pipeline "t" 0
|> Data.withName "p"
|> Data.withPaused True
)
)
|> Tuple.first
givenUserAuthorized =
Application.handleCallback
(Callback.UserFetched <|
Ok
{ id = "test"
, userName = "test"
, name = "PI:NAME:<NAME>END_PI"
, email = "test"
, isAdmin = False
, teams =
Dict.fromList
[ ( "t", [ "member" ] ) ]
, displayUserId = "displayUserIdTest"
}
)
>> Tuple.first
givenUserUnauthorized =
Application.handleCallback
(Callback.UserFetched <|
Ok
{ id = "test"
, userName = "test"
, name = "PI:NAME:<NAME>END_PI"
, email = "test"
, isAdmin = False
, teams =
Dict.fromList
[ ( "s", [ "member" ] ) ]
, displayUserId = "displayUserIdTest"
}
)
>> Tuple.first
pipelineIdentifier =
Data.shortPipelineId
toggleMsg =
ApplicationMsgs.Update <|
Msgs.Click <|
Msgs.TopBarPauseToggle
pipelineIdentifier
in
[ defineHoverBehaviour
{ name = "play pipeline icon when authorized"
, setup = givenPipelinePaused |> givenUserAuthorized
, query = queryView >> Query.find [ id "top-bar-pause-toggle" ]
, unhoveredSelector =
{ description = "faded play button with light border"
, selector =
[ style "opacity" "0.5"
, style "margin" "17px"
, style "cursor" "pointer"
]
++ iconSelector
{ size = "20px"
, image = Assets.PlayIcon
}
}
, hoveredSelector =
{ description = "white play button with light border"
, selector =
[ style "opacity" "1"
, style "margin" "17px"
, style "cursor" "pointer"
]
++ iconSelector
{ size = "20px"
, image = Assets.PlayIcon
}
}
, hoverable =
Msgs.TopBarPauseToggle pipelineIdentifier
}
, defineHoverBehaviour
{ name = "play pipeline icon when unauthenticated"
, setup = givenPipelinePaused
, query = queryView >> Query.find [ id "top-bar-pause-toggle" ]
, unhoveredSelector =
{ description = "faded play button with light border"
, selector =
[ style "opacity" "0.5"
, style "margin" "17px"
, style "cursor" "pointer"
]
++ iconSelector
{ size = "20px"
, image = Assets.PlayIcon
}
}
, hoveredSelector =
{ description = "white play button with light border"
, selector =
[ style "opacity" "1"
, style "margin" "17px"
, style "cursor" "pointer"
]
++ iconSelector
{ size = "20px"
, image = Assets.PlayIcon
}
}
, hoverable =
Msgs.TopBarPauseToggle pipelineIdentifier
}
, defineHoverBehaviour
{ name = "play pipeline icon when unauthorized"
, setup = givenPipelinePaused |> givenUserUnauthorized
, query = queryView >> Query.find [ id "top-bar-pause-toggle" ]
, unhoveredSelector =
{ description = "faded play button with light border"
, selector =
[ style "opacity" "0.2"
, style "margin" "17px"
, style "cursor" "default"
]
++ iconSelector
{ size = "20px"
, image = Assets.PlayIcon
}
}
, hoveredSelector =
{ description = "faded play button with tooltip below"
, selector =
[ containing
([ style "cursor" "default"
, style "opacity" "0.2"
]
++ iconSelector
{ size = "20px"
, image = Assets.PlayIcon
}
)
, containing
[ style "position" "absolute"
, style "top" "100%"
]
, style "position" "relative"
, style "margin" "17px"
]
}
, hoverable =
Msgs.TopBarPauseToggle pipelineIdentifier
}
, test "clicking play button sends TogglePipelinePaused msg" <|
\_ ->
givenPipelinePaused
|> queryView
|> Query.find [ id "top-bar-pause-toggle" ]
|> Event.simulate Event.click
|> Event.expect toggleMsg
, test "play button unclickable for non-members" <|
\_ ->
givenPipelinePaused
|> givenUserUnauthorized
|> queryView
|> Query.find [ id "top-bar-pause-toggle" ]
|> Event.simulate Event.click
|> Event.toResult
|> Expect.err
, test "play button click msg sends api call" <|
\_ ->
givenPipelinePaused
|> Application.update toggleMsg
|> Tuple.second
|> Expect.equal
[ Effects.SendTogglePipelineRequest
pipelineIdentifier
True
]
, test "play button click msg turns icon into spinner" <|
\_ ->
givenPipelinePaused
|> Application.update toggleMsg
|> Tuple.first
|> queryView
|> Query.has
[ style "animation"
"container-rotate 1568ms linear infinite"
, style "height" "20px"
, style "width" "20px"
]
, test "successful PipelineToggled callback turns topbar dark" <|
\_ ->
givenPipelinePaused
|> Application.update toggleMsg
|> Tuple.first
|> Application.handleCallback
(Callback.PipelineToggled pipelineIdentifier <| Ok ())
|> Tuple.first
|> queryView
|> Query.find [ id "top-bar-app" ]
|> Query.has
[ style "background-color" ColorValues.grey100 ]
, test "successful callback turns spinner into pause button" <|
\_ ->
givenPipelinePaused
|> Application.update toggleMsg
|> Tuple.first
|> Application.handleCallback
(Callback.PipelineToggled pipelineIdentifier <| Ok ())
|> Tuple.first
|> queryView
|> Query.find [ id "top-bar-pause-toggle" ]
|> Query.has
(iconSelector
{ size = "20px"
, image = Assets.PauseIcon
}
)
, test "Unauthorized PipelineToggled callback redirects to login" <|
\_ ->
givenPipelinePaused
|> Application.handleCallback
(Callback.PipelineToggled pipelineIdentifier <|
Data.httpUnauthorized
)
|> Tuple.second
|> Expect.equal
[ Effects.RedirectToLogin ]
, test "erroring PipelineToggled callback leaves topbar blue" <|
\_ ->
givenPipelinePaused
|> Application.handleCallback
(Callback.PipelineToggled pipelineIdentifier <|
Data.httpInternalServerError
)
|> Tuple.first
|> queryView
|> Query.find [ id "top-bar-app" ]
|> Query.has
[ style "background-color" pausedBlue ]
]
]
eachHasStyle : String -> String -> Query.Multiple msg -> Expectation
eachHasStyle property value =
Query.each <| Query.has [ style property value ]
sampleUser : Concourse.User
sampleUser =
{ id = "1", userName = "test", name = "PI:NAME:<NAME>END_PI", isAdmin = False, email = "PI:EMAIL:<EMAIL>END_PI", teams = Dict.empty , displayUserId = "displayUserIdTest" }
pipelineBreadcrumbSelector : List Selector.Selector
pipelineBreadcrumbSelector =
[ style "background-image" <|
Assets.backgroundImage <|
Just (Assets.BreadcrumbIcon Assets.PipelineComponent)
, style "background-repeat" "no-repeat"
]
jobBreadcrumbSelector : List Selector.Selector
jobBreadcrumbSelector =
[ style "background-image" <|
Assets.backgroundImage <|
Just (Assets.BreadcrumbIcon Assets.JobComponent)
, style "background-repeat" "no-repeat"
]
resourceBreadcrumbSelector : List Selector.Selector
resourceBreadcrumbSelector =
[ style "background-image" <|
Assets.backgroundImage <|
Just (Assets.BreadcrumbIcon Assets.ResourceComponent)
, style "background-repeat" "no-repeat"
]
| elm |
[
{
"context": "oken = \"\" })\n { placeholder = \"example@5apps.com\"\n , question = \"\"\"\n ",
"end": 9061,
"score": 0.9999098182,
"start": 9044,
"tag": "EMAIL",
"value": "example@5apps.com"
},
{
"context": "false\"\n\n --\n , placeholder \"anQLS9Usw24gxUi11IgVBg76z8SCWZgLKkoWIeJ1ClVmBHLRlaiA0CtvONVA",
"end": 13301,
"score": 0.643327117,
"start": 13291,
"tag": "KEY",
"value": "anQLS9Usw2"
},
{
"context": " --\n , placeholder \"anQLS9Usw24gxUi11IgVBg76z8SCWZgLKkoWIeJ1ClVmBHLRlaiA0CtvONVAMGritbgd3U45cPTxrhFU0WXaOAa8pVt1",
"end": 13333,
"score": 0.6152857542,
"start": 13306,
"tag": "KEY",
"value": "11IgVBg76z8SCWZgLKkoWIeJ1Cl"
},
{
"context": "lder \"anQLS9Usw24gxUi11IgVBg76z8SCWZgLKkoWIeJ1ClVmBHLRlaiA0CtvONVAMGritbgd3U45cPTxrhFU0WXaOAa8pVt186KyEccfUNyA",
"end": 13345,
"score": 0.6482318044,
"start": 13335,
"tag": "KEY",
"value": "BHLRlaiA0C"
},
{
"context": "Usw24gxUi11IgVBg76z8SCWZgLKkoWIeJ1ClVmBHLRlaiA0CtvONVAMGritbgd3U45cPTxrhFU0WXaOAa8pVt186KyEccfUNyAq97\"\n\n ",
"end": 13353,
"score": 0.6446177363,
"start": 13347,
"tag": "KEY",
"value": "ONVAMG"
},
{
"context": "11IgVBg76z8SCWZgLKkoWIeJ1ClVmBHLRlaiA0CtvONVAMGritbgd3U45cPTxrhFU0WXaOAa8pVt186KyEccfUNyAq97\"\n\n --\n , style \"-webkit-tex",
"end": 13398,
"score": 0.7062501907,
"start": 13356,
"tag": "KEY",
"value": "bgd3U45cPTxrhFU0WXaOAa8pVt186KyEccfUNyAq97"
}
] | src/Applications/UI/Authentication/View.elm | icidasset/isotach | 8 | module UI.Authentication.View exposing (view)
import Chunky exposing (..)
import Conditional exposing (..)
import Html exposing (Html, a, button, text)
import Html.Attributes exposing (attribute, href, placeholder, src, style, target, title, value, width)
import Html.Events exposing (onClick, onSubmit)
import Html.Events.Extra exposing (onClickStopPropagation)
import Html.Events.Extra.Mouse as Mouse
import Html.Extra as Html
import Html.Lazy as Lazy
import Markdown
import Material.Icons as Icons
import Material.Icons.Types exposing (Coloring(..))
import Svg exposing (Svg)
import UI.Authentication.Types as Authentication exposing (..)
import UI.Kit
import UI.Svg.Elements
import UI.Types as UI exposing (..)
import User.Layer exposing (..)
-- 🗺
view : Model -> Html UI.Msg
view =
Html.map AuthenticationMsg << Lazy.lazy view_ << .authentication
view_ : State -> Html Authentication.Msg
view_ state =
chunk
[ "flex"
, "flex-col"
, "h-full"
, "items-center"
]
[ brick
[ style "height" "42%" ]
[ "flex"
, "items-center"
, "pb-8"
--
, "md:pb-0"
]
[ -- Logo
-------
chunk
[ "py-5", "relative" ]
[ slab
Html.img
[ onClick CancelFlow
, src "images/diffuse-light.svg"
, width 190
--
, case state of
Welcome ->
title "Diffuse"
_ ->
title "Go back"
]
[ case state of
Welcome ->
"cursor-default"
_ ->
"cursor-pointer"
]
[]
-- Speech bubble
----------------
, case state of
Authenticating ->
speechBubble negotiating
InputScreen _ { question } ->
question
|> String.lines
|> List.map String.trimLeft
|> String.join "\n"
|> Markdown.toHtmlWith
{ githubFlavored = Nothing
, defaultHighlighting = Nothing
, sanitize = False
, smartypants = True
}
[]
|> speechBubble
NewEncryptionKeyScreen _ _ ->
[ text "I need a passphrase to encrypt your personal data."
, lineBreak
, inline
[ "font-normal", "text-white-60" ]
[ text "This'll prevent other people from reading your data." ]
]
|> chunk []
|> speechBubble
UpdateEncryptionKeyScreen _ _ ->
[ text "I need a new passphrase to encrypt your personal data."
, lineBreak
, inline
[ "font-normal", "text-white-60" ]
[ text "This'll prevent other people from reading your data." ]
]
|> chunk []
|> speechBubble
Welcome ->
[ text "Diffuse plays music"
, inline [ "not-italic", "font-normal", "mr-px" ] [ text " ♫ " ]
, inline [ "font-normal", "text-white-60" ]
[ text "from your Dropbox,"
, lineBreak
, text "IPFS node, Amazon S3 bucket, or any other"
, lineBreak
, text "cloud/distributed storage service you use."
]
]
|> chunk []
|> speechBubble
_ ->
[ text "Where would you like to keep your personal data?"
, lineBreak
, inline
[ "font-normal", "text-white-60" ]
[ text "That's things like your favourites, your playlists, etc."
, lineBreak
, text "After this you'll be able add some music ♫"
]
]
|> chunk []
|> speechBubble
]
]
-----------------------------------------
-- Content
-----------------------------------------
, case state of
InputScreen _ opts ->
inputScreen opts
NewEncryptionKeyScreen method pass ->
encryptionKeyScreen
{ withEncryption = SignInWithPassphrase method (Maybe.withDefault "" pass)
, withoutEncryption = SignIn method
}
UpdateEncryptionKeyScreen method pass ->
encryptionKeyScreen
{ withEncryption = UpdateEncryptionKey method (Maybe.withDefault "" pass)
, withoutEncryption = RemoveEncryptionKey method
}
Unauthenticated ->
choicesScreen
Authenticated _ ->
choicesScreen
Authenticating ->
Html.nothing
Welcome ->
welcomeScreen
-----------------------------------------
-- Link to about page
-----------------------------------------
, chunk
[ "antialiased"
, "font-semibold"
, "flex"
, "flex-grow"
, "items-end"
, "leading-snug"
, "pb-8"
, "pt-3"
, "text-sm"
]
[ slab
a
[ href "about/" ]
[ "border-b"
, "border-white-60"
, "italic"
, "no-underline"
, "text-white-60"
]
[ text "More info" ]
]
]
-- WELCOME
welcomeScreen : Html Authentication.Msg
welcomeScreen =
chunk
[ "mt-3"
, "relative"
, "z-10"
]
[ UI.Kit.buttonWithColor
UI.Kit.Blank
UI.Kit.Filled
GetStarted
(slab
Html.span
[ style "letter-spacing" "0.25em"
]
[ "align-middle"
, "inline-block"
, "text-nearly-sm"
]
[ text "SIGN IN" ]
)
]
-- LOADING
negotiating : Html Authentication.Msg
negotiating =
chunk
[ "flex"
, "items-center"
]
[ chunk
[ "transform", "-translate-y-px" ]
[ Html.map never (UI.Svg.Elements.loadingWithSize 14) ]
, chunk
[ "italic"
, "ml-2"
, "text-opacity-80"
, "text-sm"
, "text-white"
]
[ Html.text "Negotiating with service" ]
]
-- CHOICES
choicesScreen : Html Authentication.Msg
choicesScreen =
chunk
[ "bg-white"
, "rounded"
, "px-4"
, "py-2"
, "relative"
, "z-10"
-- Dark mode
------------
, "dark:bg-darkest-hour"
]
[ choiceButton
{ action = ShowNewEncryptionKeyScreen Local
, icon = Icons.web
, infoLink = Nothing
, label = "My Browser"
, outOfOrder = False
}
, choiceButton
{ action = TriggerExternalAuth (Fission { initialised = False }) ""
, icon = \_ _ -> Svg.map never UI.Svg.Elements.fissionLogo
, infoLink = Just "https://fission.codes/"
, label = "Fission"
, outOfOrder = False
}
, choiceButton
{ action = TriggerExternalAuth (Dropbox { accessToken = "", expiresAt = 0, refreshToken = "" }) ""
, icon = \_ _ -> Svg.map never UI.Svg.Elements.dropboxLogo
, infoLink = Just "https://dropbox.com/"
, label = "Dropbox"
, outOfOrder = False
}
, choiceButton
{ action =
AskForInput
(RemoteStorage { userAddress = "", token = "" })
{ placeholder = "example@5apps.com"
, question = """
What's your user address?
<span class="font-normal text-white-60">
<br />The format's
<span class="font-semibold">username@server.domain</span>
</span>
"""
, value = ""
}
, icon = \_ _ -> Svg.map never UI.Svg.Elements.remoteStorageLogo
, infoLink = Just "https://remotestorage.io/"
, label = "RemoteStorage"
, outOfOrder = False
}
-- More options
---------------
, chunk
[ "pb-px", "pt-4", "text-center" ]
[ slab
Html.span
[ title "More options"
, Mouse.onClick ShowMoreOptions
]
[ "inline-block", "px-1", "cursor-pointer", "leading-none" ]
[ chunk
[ "pointer-events-none" ]
[ Icons.more_horiz 22 Inherit ]
]
]
]
choiceButton :
{ action : msg
, icon : Int -> Coloring -> Svg msg
, infoLink : Maybe String
, label : String
, outOfOrder : Bool
}
-> Html msg
choiceButton { action, icon, infoLink, label, outOfOrder } =
chunk
[ "border-b"
, "border-gray-300"
, "relative"
--
, "last:border-b-0"
-- Dark mode
------------
, "dark:border-base01"
]
[ -----------------------------------------
-- Button
-----------------------------------------
slab
button
[ onClick action ]
[ "bg-transparent"
, "cursor-pointer"
, "flex"
, "items-center"
, "leading-none"
, "min-w-tiny"
, "outline-none"
, "px-2"
, "py-4"
, "text-left"
, "text-sm"
]
[ chunk
[ "flex"
, "items-center"
--
, ifThenElse outOfOrder "opacity-20" "opacity-100"
]
[ inline
[ "inline-flex", "mr-4" ]
[ icon 16 Inherit ]
, text label
]
]
-----------------------------------------
-- Info icon
-----------------------------------------
, case infoLink of
Just link ->
slab
Html.a
[ style "left" "100%"
, style "top" "50%"
, style "transform" "translateY(-50%)"
--
, href link
, target "_blank"
, title ("Learn more about " ++ label)
]
[ "absolute"
, "cursor-pointer"
, "duration-100"
, "leading-none"
, "ml-4"
, "opacity-40"
, "pl-4"
, "text-white"
, "transition-opacity"
, "transform"
, "-translate-y-1/2"
--
, "hocus:opacity-100"
]
[ Icons.help 17 Inherit ]
Nothing ->
nothing
]
-- ENCRYPTION KEY
encryptionKeyScreen : { withEncryption : Authentication.Msg, withoutEncryption : Authentication.Msg } -> Html Authentication.Msg
encryptionKeyScreen { withEncryption, withoutEncryption } =
slab
Html.form
[ onSubmit withEncryption ]
[ "flex"
, "flex-col"
, "max-w-xs"
, "px-3"
, "w-screen"
--
, "sm:px-0"
]
[ UI.Kit.textArea
[ attribute "autocapitalize" "none"
, attribute "autocomplete" "off"
, attribute "autocorrect" "off"
, attribute "rows" "4"
, attribute "spellcheck" "false"
--
, placeholder "anQLS9Usw24gxUi11IgVBg76z8SCWZgLKkoWIeJ1ClVmBHLRlaiA0CtvONVAMGritbgd3U45cPTxrhFU0WXaOAa8pVt186KyEccfUNyAq97"
--
, style "-webkit-text-security" "disc"
--
, Html.Events.onInput KeepPassphraseInMemory
]
, UI.Kit.button
UI.Kit.Filled
Authentication.Bypass
(text "Continue")
, brick
[ onClickStopPropagation withoutEncryption ]
[ "cursor-pointer"
, "flex"
, "items-center"
, "justify-center"
, "leading-snug"
, "mt-3"
, "opacity-50"
, "text-white"
, "text-xs"
]
[ inline [ "inline-block", "leading-none", "mr-2" ] [ Icons.warning 13 Inherit ]
, text "Continue without encryption"
]
]
-- INPUT SCREEN
inputScreen : Question -> Html Authentication.Msg
inputScreen question =
slab
Html.form
[ onSubmit ConfirmInput ]
[ "flex"
, "flex-col"
, "max-w-xs"
, "px-3"
, "w-screen"
--
, "sm:px-0"
]
[ UI.Kit.textFieldAlt
[ attribute "autocapitalize" "off"
, placeholder question.placeholder
, Html.Events.onInput Input
, value question.value
]
, UI.Kit.button
UI.Kit.Filled
Authentication.Bypass
(text "Continue")
]
-- SPEECH BUBBLE
speechBubble : Html msg -> Html msg
speechBubble contents =
chunk
[ "absolute"
, "antialiased"
, "bg-background"
, "border-b"
, "border-transparent"
, "font-semibold"
, "italic"
, "leading-snug"
, "left-1/2"
, "max-w-screen"
, "-translate-x-1/2"
, "px-4"
, "py-1"
, "rounded"
, "text-center"
, "text-sm"
, "text-white"
, "top-full"
, "transform"
, "whitespace-nowrap"
-- Dark mode
------------
, "dark:bg-darkest-hour"
, "dark:text-gray-600"
]
[ chunk
[ "mb-px", "pb-px", "pt-1" ]
[ contents ]
--
, brick
speechBubbleArrowStyles
[ "absolute"
, "border-background"
, "h-0"
, "left-1/2"
, "-translate-x-1/2"
, "-translate-y-full"
, "top-0"
, "transform"
, "w-0"
-- Dark mode
------------
, "dark:border-darkest-hour"
]
[]
]
-- 🖼
speechBubbleArrowStyles : List (Html.Attribute msg)
speechBubbleArrowStyles =
[ style "border-top-color" "transparent"
, style "border-left-color" "transparent"
, style "border-right-color" "transparent"
, style "border-width" "0 6px 5px 6px"
]
| 55565 | module UI.Authentication.View exposing (view)
import Chunky exposing (..)
import Conditional exposing (..)
import Html exposing (Html, a, button, text)
import Html.Attributes exposing (attribute, href, placeholder, src, style, target, title, value, width)
import Html.Events exposing (onClick, onSubmit)
import Html.Events.Extra exposing (onClickStopPropagation)
import Html.Events.Extra.Mouse as Mouse
import Html.Extra as Html
import Html.Lazy as Lazy
import Markdown
import Material.Icons as Icons
import Material.Icons.Types exposing (Coloring(..))
import Svg exposing (Svg)
import UI.Authentication.Types as Authentication exposing (..)
import UI.Kit
import UI.Svg.Elements
import UI.Types as UI exposing (..)
import User.Layer exposing (..)
-- 🗺
view : Model -> Html UI.Msg
view =
Html.map AuthenticationMsg << Lazy.lazy view_ << .authentication
view_ : State -> Html Authentication.Msg
view_ state =
chunk
[ "flex"
, "flex-col"
, "h-full"
, "items-center"
]
[ brick
[ style "height" "42%" ]
[ "flex"
, "items-center"
, "pb-8"
--
, "md:pb-0"
]
[ -- Logo
-------
chunk
[ "py-5", "relative" ]
[ slab
Html.img
[ onClick CancelFlow
, src "images/diffuse-light.svg"
, width 190
--
, case state of
Welcome ->
title "Diffuse"
_ ->
title "Go back"
]
[ case state of
Welcome ->
"cursor-default"
_ ->
"cursor-pointer"
]
[]
-- Speech bubble
----------------
, case state of
Authenticating ->
speechBubble negotiating
InputScreen _ { question } ->
question
|> String.lines
|> List.map String.trimLeft
|> String.join "\n"
|> Markdown.toHtmlWith
{ githubFlavored = Nothing
, defaultHighlighting = Nothing
, sanitize = False
, smartypants = True
}
[]
|> speechBubble
NewEncryptionKeyScreen _ _ ->
[ text "I need a passphrase to encrypt your personal data."
, lineBreak
, inline
[ "font-normal", "text-white-60" ]
[ text "This'll prevent other people from reading your data." ]
]
|> chunk []
|> speechBubble
UpdateEncryptionKeyScreen _ _ ->
[ text "I need a new passphrase to encrypt your personal data."
, lineBreak
, inline
[ "font-normal", "text-white-60" ]
[ text "This'll prevent other people from reading your data." ]
]
|> chunk []
|> speechBubble
Welcome ->
[ text "Diffuse plays music"
, inline [ "not-italic", "font-normal", "mr-px" ] [ text " ♫ " ]
, inline [ "font-normal", "text-white-60" ]
[ text "from your Dropbox,"
, lineBreak
, text "IPFS node, Amazon S3 bucket, or any other"
, lineBreak
, text "cloud/distributed storage service you use."
]
]
|> chunk []
|> speechBubble
_ ->
[ text "Where would you like to keep your personal data?"
, lineBreak
, inline
[ "font-normal", "text-white-60" ]
[ text "That's things like your favourites, your playlists, etc."
, lineBreak
, text "After this you'll be able add some music ♫"
]
]
|> chunk []
|> speechBubble
]
]
-----------------------------------------
-- Content
-----------------------------------------
, case state of
InputScreen _ opts ->
inputScreen opts
NewEncryptionKeyScreen method pass ->
encryptionKeyScreen
{ withEncryption = SignInWithPassphrase method (Maybe.withDefault "" pass)
, withoutEncryption = SignIn method
}
UpdateEncryptionKeyScreen method pass ->
encryptionKeyScreen
{ withEncryption = UpdateEncryptionKey method (Maybe.withDefault "" pass)
, withoutEncryption = RemoveEncryptionKey method
}
Unauthenticated ->
choicesScreen
Authenticated _ ->
choicesScreen
Authenticating ->
Html.nothing
Welcome ->
welcomeScreen
-----------------------------------------
-- Link to about page
-----------------------------------------
, chunk
[ "antialiased"
, "font-semibold"
, "flex"
, "flex-grow"
, "items-end"
, "leading-snug"
, "pb-8"
, "pt-3"
, "text-sm"
]
[ slab
a
[ href "about/" ]
[ "border-b"
, "border-white-60"
, "italic"
, "no-underline"
, "text-white-60"
]
[ text "More info" ]
]
]
-- WELCOME
welcomeScreen : Html Authentication.Msg
welcomeScreen =
chunk
[ "mt-3"
, "relative"
, "z-10"
]
[ UI.Kit.buttonWithColor
UI.Kit.Blank
UI.Kit.Filled
GetStarted
(slab
Html.span
[ style "letter-spacing" "0.25em"
]
[ "align-middle"
, "inline-block"
, "text-nearly-sm"
]
[ text "SIGN IN" ]
)
]
-- LOADING
negotiating : Html Authentication.Msg
negotiating =
chunk
[ "flex"
, "items-center"
]
[ chunk
[ "transform", "-translate-y-px" ]
[ Html.map never (UI.Svg.Elements.loadingWithSize 14) ]
, chunk
[ "italic"
, "ml-2"
, "text-opacity-80"
, "text-sm"
, "text-white"
]
[ Html.text "Negotiating with service" ]
]
-- CHOICES
choicesScreen : Html Authentication.Msg
choicesScreen =
chunk
[ "bg-white"
, "rounded"
, "px-4"
, "py-2"
, "relative"
, "z-10"
-- Dark mode
------------
, "dark:bg-darkest-hour"
]
[ choiceButton
{ action = ShowNewEncryptionKeyScreen Local
, icon = Icons.web
, infoLink = Nothing
, label = "My Browser"
, outOfOrder = False
}
, choiceButton
{ action = TriggerExternalAuth (Fission { initialised = False }) ""
, icon = \_ _ -> Svg.map never UI.Svg.Elements.fissionLogo
, infoLink = Just "https://fission.codes/"
, label = "Fission"
, outOfOrder = False
}
, choiceButton
{ action = TriggerExternalAuth (Dropbox { accessToken = "", expiresAt = 0, refreshToken = "" }) ""
, icon = \_ _ -> Svg.map never UI.Svg.Elements.dropboxLogo
, infoLink = Just "https://dropbox.com/"
, label = "Dropbox"
, outOfOrder = False
}
, choiceButton
{ action =
AskForInput
(RemoteStorage { userAddress = "", token = "" })
{ placeholder = "<EMAIL>"
, question = """
What's your user address?
<span class="font-normal text-white-60">
<br />The format's
<span class="font-semibold">username@server.domain</span>
</span>
"""
, value = ""
}
, icon = \_ _ -> Svg.map never UI.Svg.Elements.remoteStorageLogo
, infoLink = Just "https://remotestorage.io/"
, label = "RemoteStorage"
, outOfOrder = False
}
-- More options
---------------
, chunk
[ "pb-px", "pt-4", "text-center" ]
[ slab
Html.span
[ title "More options"
, Mouse.onClick ShowMoreOptions
]
[ "inline-block", "px-1", "cursor-pointer", "leading-none" ]
[ chunk
[ "pointer-events-none" ]
[ Icons.more_horiz 22 Inherit ]
]
]
]
choiceButton :
{ action : msg
, icon : Int -> Coloring -> Svg msg
, infoLink : Maybe String
, label : String
, outOfOrder : Bool
}
-> Html msg
choiceButton { action, icon, infoLink, label, outOfOrder } =
chunk
[ "border-b"
, "border-gray-300"
, "relative"
--
, "last:border-b-0"
-- Dark mode
------------
, "dark:border-base01"
]
[ -----------------------------------------
-- Button
-----------------------------------------
slab
button
[ onClick action ]
[ "bg-transparent"
, "cursor-pointer"
, "flex"
, "items-center"
, "leading-none"
, "min-w-tiny"
, "outline-none"
, "px-2"
, "py-4"
, "text-left"
, "text-sm"
]
[ chunk
[ "flex"
, "items-center"
--
, ifThenElse outOfOrder "opacity-20" "opacity-100"
]
[ inline
[ "inline-flex", "mr-4" ]
[ icon 16 Inherit ]
, text label
]
]
-----------------------------------------
-- Info icon
-----------------------------------------
, case infoLink of
Just link ->
slab
Html.a
[ style "left" "100%"
, style "top" "50%"
, style "transform" "translateY(-50%)"
--
, href link
, target "_blank"
, title ("Learn more about " ++ label)
]
[ "absolute"
, "cursor-pointer"
, "duration-100"
, "leading-none"
, "ml-4"
, "opacity-40"
, "pl-4"
, "text-white"
, "transition-opacity"
, "transform"
, "-translate-y-1/2"
--
, "hocus:opacity-100"
]
[ Icons.help 17 Inherit ]
Nothing ->
nothing
]
-- ENCRYPTION KEY
encryptionKeyScreen : { withEncryption : Authentication.Msg, withoutEncryption : Authentication.Msg } -> Html Authentication.Msg
encryptionKeyScreen { withEncryption, withoutEncryption } =
slab
Html.form
[ onSubmit withEncryption ]
[ "flex"
, "flex-col"
, "max-w-xs"
, "px-3"
, "w-screen"
--
, "sm:px-0"
]
[ UI.Kit.textArea
[ attribute "autocapitalize" "none"
, attribute "autocomplete" "off"
, attribute "autocorrect" "off"
, attribute "rows" "4"
, attribute "spellcheck" "false"
--
, placeholder "<KEY>4gxUi<KEY>Vm<KEY>tv<KEY>rit<KEY>"
--
, style "-webkit-text-security" "disc"
--
, Html.Events.onInput KeepPassphraseInMemory
]
, UI.Kit.button
UI.Kit.Filled
Authentication.Bypass
(text "Continue")
, brick
[ onClickStopPropagation withoutEncryption ]
[ "cursor-pointer"
, "flex"
, "items-center"
, "justify-center"
, "leading-snug"
, "mt-3"
, "opacity-50"
, "text-white"
, "text-xs"
]
[ inline [ "inline-block", "leading-none", "mr-2" ] [ Icons.warning 13 Inherit ]
, text "Continue without encryption"
]
]
-- INPUT SCREEN
inputScreen : Question -> Html Authentication.Msg
inputScreen question =
slab
Html.form
[ onSubmit ConfirmInput ]
[ "flex"
, "flex-col"
, "max-w-xs"
, "px-3"
, "w-screen"
--
, "sm:px-0"
]
[ UI.Kit.textFieldAlt
[ attribute "autocapitalize" "off"
, placeholder question.placeholder
, Html.Events.onInput Input
, value question.value
]
, UI.Kit.button
UI.Kit.Filled
Authentication.Bypass
(text "Continue")
]
-- SPEECH BUBBLE
speechBubble : Html msg -> Html msg
speechBubble contents =
chunk
[ "absolute"
, "antialiased"
, "bg-background"
, "border-b"
, "border-transparent"
, "font-semibold"
, "italic"
, "leading-snug"
, "left-1/2"
, "max-w-screen"
, "-translate-x-1/2"
, "px-4"
, "py-1"
, "rounded"
, "text-center"
, "text-sm"
, "text-white"
, "top-full"
, "transform"
, "whitespace-nowrap"
-- Dark mode
------------
, "dark:bg-darkest-hour"
, "dark:text-gray-600"
]
[ chunk
[ "mb-px", "pb-px", "pt-1" ]
[ contents ]
--
, brick
speechBubbleArrowStyles
[ "absolute"
, "border-background"
, "h-0"
, "left-1/2"
, "-translate-x-1/2"
, "-translate-y-full"
, "top-0"
, "transform"
, "w-0"
-- Dark mode
------------
, "dark:border-darkest-hour"
]
[]
]
-- 🖼
speechBubbleArrowStyles : List (Html.Attribute msg)
speechBubbleArrowStyles =
[ style "border-top-color" "transparent"
, style "border-left-color" "transparent"
, style "border-right-color" "transparent"
, style "border-width" "0 6px 5px 6px"
]
| true | module UI.Authentication.View exposing (view)
import Chunky exposing (..)
import Conditional exposing (..)
import Html exposing (Html, a, button, text)
import Html.Attributes exposing (attribute, href, placeholder, src, style, target, title, value, width)
import Html.Events exposing (onClick, onSubmit)
import Html.Events.Extra exposing (onClickStopPropagation)
import Html.Events.Extra.Mouse as Mouse
import Html.Extra as Html
import Html.Lazy as Lazy
import Markdown
import Material.Icons as Icons
import Material.Icons.Types exposing (Coloring(..))
import Svg exposing (Svg)
import UI.Authentication.Types as Authentication exposing (..)
import UI.Kit
import UI.Svg.Elements
import UI.Types as UI exposing (..)
import User.Layer exposing (..)
-- 🗺
view : Model -> Html UI.Msg
view =
Html.map AuthenticationMsg << Lazy.lazy view_ << .authentication
view_ : State -> Html Authentication.Msg
view_ state =
chunk
[ "flex"
, "flex-col"
, "h-full"
, "items-center"
]
[ brick
[ style "height" "42%" ]
[ "flex"
, "items-center"
, "pb-8"
--
, "md:pb-0"
]
[ -- Logo
-------
chunk
[ "py-5", "relative" ]
[ slab
Html.img
[ onClick CancelFlow
, src "images/diffuse-light.svg"
, width 190
--
, case state of
Welcome ->
title "Diffuse"
_ ->
title "Go back"
]
[ case state of
Welcome ->
"cursor-default"
_ ->
"cursor-pointer"
]
[]
-- Speech bubble
----------------
, case state of
Authenticating ->
speechBubble negotiating
InputScreen _ { question } ->
question
|> String.lines
|> List.map String.trimLeft
|> String.join "\n"
|> Markdown.toHtmlWith
{ githubFlavored = Nothing
, defaultHighlighting = Nothing
, sanitize = False
, smartypants = True
}
[]
|> speechBubble
NewEncryptionKeyScreen _ _ ->
[ text "I need a passphrase to encrypt your personal data."
, lineBreak
, inline
[ "font-normal", "text-white-60" ]
[ text "This'll prevent other people from reading your data." ]
]
|> chunk []
|> speechBubble
UpdateEncryptionKeyScreen _ _ ->
[ text "I need a new passphrase to encrypt your personal data."
, lineBreak
, inline
[ "font-normal", "text-white-60" ]
[ text "This'll prevent other people from reading your data." ]
]
|> chunk []
|> speechBubble
Welcome ->
[ text "Diffuse plays music"
, inline [ "not-italic", "font-normal", "mr-px" ] [ text " ♫ " ]
, inline [ "font-normal", "text-white-60" ]
[ text "from your Dropbox,"
, lineBreak
, text "IPFS node, Amazon S3 bucket, or any other"
, lineBreak
, text "cloud/distributed storage service you use."
]
]
|> chunk []
|> speechBubble
_ ->
[ text "Where would you like to keep your personal data?"
, lineBreak
, inline
[ "font-normal", "text-white-60" ]
[ text "That's things like your favourites, your playlists, etc."
, lineBreak
, text "After this you'll be able add some music ♫"
]
]
|> chunk []
|> speechBubble
]
]
-----------------------------------------
-- Content
-----------------------------------------
, case state of
InputScreen _ opts ->
inputScreen opts
NewEncryptionKeyScreen method pass ->
encryptionKeyScreen
{ withEncryption = SignInWithPassphrase method (Maybe.withDefault "" pass)
, withoutEncryption = SignIn method
}
UpdateEncryptionKeyScreen method pass ->
encryptionKeyScreen
{ withEncryption = UpdateEncryptionKey method (Maybe.withDefault "" pass)
, withoutEncryption = RemoveEncryptionKey method
}
Unauthenticated ->
choicesScreen
Authenticated _ ->
choicesScreen
Authenticating ->
Html.nothing
Welcome ->
welcomeScreen
-----------------------------------------
-- Link to about page
-----------------------------------------
, chunk
[ "antialiased"
, "font-semibold"
, "flex"
, "flex-grow"
, "items-end"
, "leading-snug"
, "pb-8"
, "pt-3"
, "text-sm"
]
[ slab
a
[ href "about/" ]
[ "border-b"
, "border-white-60"
, "italic"
, "no-underline"
, "text-white-60"
]
[ text "More info" ]
]
]
-- WELCOME
welcomeScreen : Html Authentication.Msg
welcomeScreen =
chunk
[ "mt-3"
, "relative"
, "z-10"
]
[ UI.Kit.buttonWithColor
UI.Kit.Blank
UI.Kit.Filled
GetStarted
(slab
Html.span
[ style "letter-spacing" "0.25em"
]
[ "align-middle"
, "inline-block"
, "text-nearly-sm"
]
[ text "SIGN IN" ]
)
]
-- LOADING
negotiating : Html Authentication.Msg
negotiating =
chunk
[ "flex"
, "items-center"
]
[ chunk
[ "transform", "-translate-y-px" ]
[ Html.map never (UI.Svg.Elements.loadingWithSize 14) ]
, chunk
[ "italic"
, "ml-2"
, "text-opacity-80"
, "text-sm"
, "text-white"
]
[ Html.text "Negotiating with service" ]
]
-- CHOICES
choicesScreen : Html Authentication.Msg
choicesScreen =
chunk
[ "bg-white"
, "rounded"
, "px-4"
, "py-2"
, "relative"
, "z-10"
-- Dark mode
------------
, "dark:bg-darkest-hour"
]
[ choiceButton
{ action = ShowNewEncryptionKeyScreen Local
, icon = Icons.web
, infoLink = Nothing
, label = "My Browser"
, outOfOrder = False
}
, choiceButton
{ action = TriggerExternalAuth (Fission { initialised = False }) ""
, icon = \_ _ -> Svg.map never UI.Svg.Elements.fissionLogo
, infoLink = Just "https://fission.codes/"
, label = "Fission"
, outOfOrder = False
}
, choiceButton
{ action = TriggerExternalAuth (Dropbox { accessToken = "", expiresAt = 0, refreshToken = "" }) ""
, icon = \_ _ -> Svg.map never UI.Svg.Elements.dropboxLogo
, infoLink = Just "https://dropbox.com/"
, label = "Dropbox"
, outOfOrder = False
}
, choiceButton
{ action =
AskForInput
(RemoteStorage { userAddress = "", token = "" })
{ placeholder = "PI:EMAIL:<EMAIL>END_PI"
, question = """
What's your user address?
<span class="font-normal text-white-60">
<br />The format's
<span class="font-semibold">username@server.domain</span>
</span>
"""
, value = ""
}
, icon = \_ _ -> Svg.map never UI.Svg.Elements.remoteStorageLogo
, infoLink = Just "https://remotestorage.io/"
, label = "RemoteStorage"
, outOfOrder = False
}
-- More options
---------------
, chunk
[ "pb-px", "pt-4", "text-center" ]
[ slab
Html.span
[ title "More options"
, Mouse.onClick ShowMoreOptions
]
[ "inline-block", "px-1", "cursor-pointer", "leading-none" ]
[ chunk
[ "pointer-events-none" ]
[ Icons.more_horiz 22 Inherit ]
]
]
]
choiceButton :
{ action : msg
, icon : Int -> Coloring -> Svg msg
, infoLink : Maybe String
, label : String
, outOfOrder : Bool
}
-> Html msg
choiceButton { action, icon, infoLink, label, outOfOrder } =
chunk
[ "border-b"
, "border-gray-300"
, "relative"
--
, "last:border-b-0"
-- Dark mode
------------
, "dark:border-base01"
]
[ -----------------------------------------
-- Button
-----------------------------------------
slab
button
[ onClick action ]
[ "bg-transparent"
, "cursor-pointer"
, "flex"
, "items-center"
, "leading-none"
, "min-w-tiny"
, "outline-none"
, "px-2"
, "py-4"
, "text-left"
, "text-sm"
]
[ chunk
[ "flex"
, "items-center"
--
, ifThenElse outOfOrder "opacity-20" "opacity-100"
]
[ inline
[ "inline-flex", "mr-4" ]
[ icon 16 Inherit ]
, text label
]
]
-----------------------------------------
-- Info icon
-----------------------------------------
, case infoLink of
Just link ->
slab
Html.a
[ style "left" "100%"
, style "top" "50%"
, style "transform" "translateY(-50%)"
--
, href link
, target "_blank"
, title ("Learn more about " ++ label)
]
[ "absolute"
, "cursor-pointer"
, "duration-100"
, "leading-none"
, "ml-4"
, "opacity-40"
, "pl-4"
, "text-white"
, "transition-opacity"
, "transform"
, "-translate-y-1/2"
--
, "hocus:opacity-100"
]
[ Icons.help 17 Inherit ]
Nothing ->
nothing
]
-- ENCRYPTION KEY
encryptionKeyScreen : { withEncryption : Authentication.Msg, withoutEncryption : Authentication.Msg } -> Html Authentication.Msg
encryptionKeyScreen { withEncryption, withoutEncryption } =
slab
Html.form
[ onSubmit withEncryption ]
[ "flex"
, "flex-col"
, "max-w-xs"
, "px-3"
, "w-screen"
--
, "sm:px-0"
]
[ UI.Kit.textArea
[ attribute "autocapitalize" "none"
, attribute "autocomplete" "off"
, attribute "autocorrect" "off"
, attribute "rows" "4"
, attribute "spellcheck" "false"
--
, placeholder "PI:KEY:<KEY>END_PI4gxUiPI:KEY:<KEY>END_PIVmPI:KEY:<KEY>END_PItvPI:KEY:<KEY>END_PIritPI:KEY:<KEY>END_PI"
--
, style "-webkit-text-security" "disc"
--
, Html.Events.onInput KeepPassphraseInMemory
]
, UI.Kit.button
UI.Kit.Filled
Authentication.Bypass
(text "Continue")
, brick
[ onClickStopPropagation withoutEncryption ]
[ "cursor-pointer"
, "flex"
, "items-center"
, "justify-center"
, "leading-snug"
, "mt-3"
, "opacity-50"
, "text-white"
, "text-xs"
]
[ inline [ "inline-block", "leading-none", "mr-2" ] [ Icons.warning 13 Inherit ]
, text "Continue without encryption"
]
]
-- INPUT SCREEN
inputScreen : Question -> Html Authentication.Msg
inputScreen question =
slab
Html.form
[ onSubmit ConfirmInput ]
[ "flex"
, "flex-col"
, "max-w-xs"
, "px-3"
, "w-screen"
--
, "sm:px-0"
]
[ UI.Kit.textFieldAlt
[ attribute "autocapitalize" "off"
, placeholder question.placeholder
, Html.Events.onInput Input
, value question.value
]
, UI.Kit.button
UI.Kit.Filled
Authentication.Bypass
(text "Continue")
]
-- SPEECH BUBBLE
speechBubble : Html msg -> Html msg
speechBubble contents =
chunk
[ "absolute"
, "antialiased"
, "bg-background"
, "border-b"
, "border-transparent"
, "font-semibold"
, "italic"
, "leading-snug"
, "left-1/2"
, "max-w-screen"
, "-translate-x-1/2"
, "px-4"
, "py-1"
, "rounded"
, "text-center"
, "text-sm"
, "text-white"
, "top-full"
, "transform"
, "whitespace-nowrap"
-- Dark mode
------------
, "dark:bg-darkest-hour"
, "dark:text-gray-600"
]
[ chunk
[ "mb-px", "pb-px", "pt-1" ]
[ contents ]
--
, brick
speechBubbleArrowStyles
[ "absolute"
, "border-background"
, "h-0"
, "left-1/2"
, "-translate-x-1/2"
, "-translate-y-full"
, "top-0"
, "transform"
, "w-0"
-- Dark mode
------------
, "dark:border-darkest-hour"
]
[]
]
-- 🖼
speechBubbleArrowStyles : List (Html.Attribute msg)
speechBubbleArrowStyles =
[ style "border-top-color" "transparent"
, style "border-left-color" "transparent"
, style "border-right-color" "transparent"
, style "border-width" "0 6px 5px 6px"
]
| elm |
[
{
"context": "onEmailKey : String\r\nconfirmationEmailKey =\r\n \"abc\"\r\n\r\n\r\nstartPointX : String\r\nstartPointX =\r\n \"1",
"end": 3269,
"score": 0.853167057,
"start": 3266,
"tag": "KEY",
"value": "abc"
}
] | src/Env.elm | MartinSStewart/ascii-art | 28 | module Env exposing (Mode(..), adminEmail, adminEmail_, adminUserId, adminUserId_, confirmationEmailKey, domain, hyperlinkWhitelist, isProduction, isProduction_, mapDrawAt, mapDrawAtX, mapDrawAtY, mode, notifyAdminWaitInHours, sendGridKey, sendGridKey_, startPointAt, startPointX, startPointY, statisticsBounds, statisticsDrawAt, statisticsDrawAtX, statisticsDrawAtY, statisticsX0, statisticsX1, statisticsY0, statisticsY1)
-- The Env.elm file is for per-environment configuration.
-- See https://dashboard.lamdera.app/docs/environment for more info.
import Bounds exposing (Bounds)
import EmailAddress exposing (EmailAddress)
import Helper exposing (Coord)
import SendGrid
import Units exposing (AsciiUnit)
import User exposing (UserId)
adminUserId_ : String
adminUserId_ =
"0"
adminUserId : Maybe UserId
adminUserId =
String.toInt adminUserId_ |> Maybe.map User.userId
isProduction_ : String
isProduction_ =
"False"
isProduction : Bool
isProduction =
case String.toLower isProduction_ |> String.trim of
"true" ->
True
"false" ->
False
_ ->
False
adminEmail_ : String
adminEmail_ =
""
adminEmail : Maybe EmailAddress
adminEmail =
EmailAddress.fromString adminEmail_
sendGridKey_ : String
sendGridKey_ =
""
sendGridKey : SendGrid.ApiKey
sendGridKey =
SendGrid.apiKey sendGridKey_
domain : String
domain =
"localhost:8000"
statisticsX0 : String
statisticsX0 =
"-258"
statisticsY0 : String
statisticsY0 =
"-78"
statisticsX1 : String
statisticsX1 =
"1136"
statisticsY1 : String
statisticsY1 =
"209"
statisticsBounds : Bounds AsciiUnit
statisticsBounds =
Maybe.map4
(\x0 y0 x1 y1 -> Bounds.bounds (Helper.fromRawCoord ( x0, y0 )) (Helper.fromRawCoord ( x1, y1 )))
(String.toInt statisticsX0)
(String.toInt statisticsY0)
(String.toInt statisticsX1)
(String.toInt statisticsY1)
|> Maybe.withDefault (Bounds.bounds (Helper.fromRawCoord ( 0, 0 )) (Helper.fromRawCoord ( 0, 0 )))
statisticsDrawAtX : String
statisticsDrawAtX =
"32"
statisticsDrawAtY : String
statisticsDrawAtY =
"0"
statisticsDrawAt : Coord AsciiUnit
statisticsDrawAt =
Maybe.map2
(\x y -> Helper.fromRawCoord ( x, y ))
(String.toInt statisticsDrawAtX)
(String.toInt statisticsDrawAtY)
|> Maybe.withDefault (Helper.fromRawCoord ( 32, 0 ))
mapDrawAtX : String
mapDrawAtX =
"759"
mapDrawAtY : String
mapDrawAtY =
"-71"
mapDrawAt : Coord AsciiUnit
mapDrawAt =
Maybe.map2
(\x y -> Helper.fromRawCoord ( x, y ))
(String.toInt mapDrawAtX)
(String.toInt mapDrawAtY)
|> Maybe.withDefault (Helper.fromRawCoord ( 32, 100 ))
notifyAdminWaitInHours : String
notifyAdminWaitInHours =
"0.05"
hyperlinkWhitelist : String
hyperlinkWhitelist =
"www.patorjk.com/software/taag, ro-box.netlify.app, the-best-color.lamdera.app, agirg.com, yourworldoftext.com, www.yourworldoftext.com, meetdown.app, ellie-app.com"
confirmationEmailKey : String
confirmationEmailKey =
"abc"
startPointX : String
startPointX =
"123"
startPointY : String
startPointY =
"44"
startPointAt : Coord AsciiUnit
startPointAt =
Maybe.map2
(\x y -> Helper.fromRawCoord ( x, y ))
(String.toInt startPointX)
(String.toInt startPointY)
|> Maybe.withDefault (Helper.fromRawCoord ( 0, 0 ))
| 21893 | module Env exposing (Mode(..), adminEmail, adminEmail_, adminUserId, adminUserId_, confirmationEmailKey, domain, hyperlinkWhitelist, isProduction, isProduction_, mapDrawAt, mapDrawAtX, mapDrawAtY, mode, notifyAdminWaitInHours, sendGridKey, sendGridKey_, startPointAt, startPointX, startPointY, statisticsBounds, statisticsDrawAt, statisticsDrawAtX, statisticsDrawAtY, statisticsX0, statisticsX1, statisticsY0, statisticsY1)
-- The Env.elm file is for per-environment configuration.
-- See https://dashboard.lamdera.app/docs/environment for more info.
import Bounds exposing (Bounds)
import EmailAddress exposing (EmailAddress)
import Helper exposing (Coord)
import SendGrid
import Units exposing (AsciiUnit)
import User exposing (UserId)
adminUserId_ : String
adminUserId_ =
"0"
adminUserId : Maybe UserId
adminUserId =
String.toInt adminUserId_ |> Maybe.map User.userId
isProduction_ : String
isProduction_ =
"False"
isProduction : Bool
isProduction =
case String.toLower isProduction_ |> String.trim of
"true" ->
True
"false" ->
False
_ ->
False
adminEmail_ : String
adminEmail_ =
""
adminEmail : Maybe EmailAddress
adminEmail =
EmailAddress.fromString adminEmail_
sendGridKey_ : String
sendGridKey_ =
""
sendGridKey : SendGrid.ApiKey
sendGridKey =
SendGrid.apiKey sendGridKey_
domain : String
domain =
"localhost:8000"
statisticsX0 : String
statisticsX0 =
"-258"
statisticsY0 : String
statisticsY0 =
"-78"
statisticsX1 : String
statisticsX1 =
"1136"
statisticsY1 : String
statisticsY1 =
"209"
statisticsBounds : Bounds AsciiUnit
statisticsBounds =
Maybe.map4
(\x0 y0 x1 y1 -> Bounds.bounds (Helper.fromRawCoord ( x0, y0 )) (Helper.fromRawCoord ( x1, y1 )))
(String.toInt statisticsX0)
(String.toInt statisticsY0)
(String.toInt statisticsX1)
(String.toInt statisticsY1)
|> Maybe.withDefault (Bounds.bounds (Helper.fromRawCoord ( 0, 0 )) (Helper.fromRawCoord ( 0, 0 )))
statisticsDrawAtX : String
statisticsDrawAtX =
"32"
statisticsDrawAtY : String
statisticsDrawAtY =
"0"
statisticsDrawAt : Coord AsciiUnit
statisticsDrawAt =
Maybe.map2
(\x y -> Helper.fromRawCoord ( x, y ))
(String.toInt statisticsDrawAtX)
(String.toInt statisticsDrawAtY)
|> Maybe.withDefault (Helper.fromRawCoord ( 32, 0 ))
mapDrawAtX : String
mapDrawAtX =
"759"
mapDrawAtY : String
mapDrawAtY =
"-71"
mapDrawAt : Coord AsciiUnit
mapDrawAt =
Maybe.map2
(\x y -> Helper.fromRawCoord ( x, y ))
(String.toInt mapDrawAtX)
(String.toInt mapDrawAtY)
|> Maybe.withDefault (Helper.fromRawCoord ( 32, 100 ))
notifyAdminWaitInHours : String
notifyAdminWaitInHours =
"0.05"
hyperlinkWhitelist : String
hyperlinkWhitelist =
"www.patorjk.com/software/taag, ro-box.netlify.app, the-best-color.lamdera.app, agirg.com, yourworldoftext.com, www.yourworldoftext.com, meetdown.app, ellie-app.com"
confirmationEmailKey : String
confirmationEmailKey =
"<KEY>"
startPointX : String
startPointX =
"123"
startPointY : String
startPointY =
"44"
startPointAt : Coord AsciiUnit
startPointAt =
Maybe.map2
(\x y -> Helper.fromRawCoord ( x, y ))
(String.toInt startPointX)
(String.toInt startPointY)
|> Maybe.withDefault (Helper.fromRawCoord ( 0, 0 ))
| true | module Env exposing (Mode(..), adminEmail, adminEmail_, adminUserId, adminUserId_, confirmationEmailKey, domain, hyperlinkWhitelist, isProduction, isProduction_, mapDrawAt, mapDrawAtX, mapDrawAtY, mode, notifyAdminWaitInHours, sendGridKey, sendGridKey_, startPointAt, startPointX, startPointY, statisticsBounds, statisticsDrawAt, statisticsDrawAtX, statisticsDrawAtY, statisticsX0, statisticsX1, statisticsY0, statisticsY1)
-- The Env.elm file is for per-environment configuration.
-- See https://dashboard.lamdera.app/docs/environment for more info.
import Bounds exposing (Bounds)
import EmailAddress exposing (EmailAddress)
import Helper exposing (Coord)
import SendGrid
import Units exposing (AsciiUnit)
import User exposing (UserId)
adminUserId_ : String
adminUserId_ =
"0"
adminUserId : Maybe UserId
adminUserId =
String.toInt adminUserId_ |> Maybe.map User.userId
isProduction_ : String
isProduction_ =
"False"
isProduction : Bool
isProduction =
case String.toLower isProduction_ |> String.trim of
"true" ->
True
"false" ->
False
_ ->
False
adminEmail_ : String
adminEmail_ =
""
adminEmail : Maybe EmailAddress
adminEmail =
EmailAddress.fromString adminEmail_
sendGridKey_ : String
sendGridKey_ =
""
sendGridKey : SendGrid.ApiKey
sendGridKey =
SendGrid.apiKey sendGridKey_
domain : String
domain =
"localhost:8000"
statisticsX0 : String
statisticsX0 =
"-258"
statisticsY0 : String
statisticsY0 =
"-78"
statisticsX1 : String
statisticsX1 =
"1136"
statisticsY1 : String
statisticsY1 =
"209"
statisticsBounds : Bounds AsciiUnit
statisticsBounds =
Maybe.map4
(\x0 y0 x1 y1 -> Bounds.bounds (Helper.fromRawCoord ( x0, y0 )) (Helper.fromRawCoord ( x1, y1 )))
(String.toInt statisticsX0)
(String.toInt statisticsY0)
(String.toInt statisticsX1)
(String.toInt statisticsY1)
|> Maybe.withDefault (Bounds.bounds (Helper.fromRawCoord ( 0, 0 )) (Helper.fromRawCoord ( 0, 0 )))
statisticsDrawAtX : String
statisticsDrawAtX =
"32"
statisticsDrawAtY : String
statisticsDrawAtY =
"0"
statisticsDrawAt : Coord AsciiUnit
statisticsDrawAt =
Maybe.map2
(\x y -> Helper.fromRawCoord ( x, y ))
(String.toInt statisticsDrawAtX)
(String.toInt statisticsDrawAtY)
|> Maybe.withDefault (Helper.fromRawCoord ( 32, 0 ))
mapDrawAtX : String
mapDrawAtX =
"759"
mapDrawAtY : String
mapDrawAtY =
"-71"
mapDrawAt : Coord AsciiUnit
mapDrawAt =
Maybe.map2
(\x y -> Helper.fromRawCoord ( x, y ))
(String.toInt mapDrawAtX)
(String.toInt mapDrawAtY)
|> Maybe.withDefault (Helper.fromRawCoord ( 32, 100 ))
notifyAdminWaitInHours : String
notifyAdminWaitInHours =
"0.05"
hyperlinkWhitelist : String
hyperlinkWhitelist =
"www.patorjk.com/software/taag, ro-box.netlify.app, the-best-color.lamdera.app, agirg.com, yourworldoftext.com, www.yourworldoftext.com, meetdown.app, ellie-app.com"
confirmationEmailKey : String
confirmationEmailKey =
"PI:KEY:<KEY>END_PI"
startPointX : String
startPointX =
"123"
startPointY : String
startPointY =
"44"
startPointAt : Coord AsciiUnit
startPointAt =
Maybe.map2
(\x y -> Helper.fromRawCoord ( x, y ))
(String.toInt startPointX)
(String.toInt startPointY)
|> Maybe.withDefault (Helper.fromRawCoord ( 0, 0 ))
| elm |
[
{
"context": "報\" ]\n , div [ class \"label-kana\" ] [ text \"フリガナ\" ]\n , div [ class \"kana\" ] [ text char.kan",
"end": 5083,
"score": 0.9899145365,
"start": 5079,
"tag": "NAME",
"value": "フリガナ"
}
] | src/assets/js/Page/MyPages/CharacterCreate.elm | hibohiboo/garden | 0 | port module Page.MyPages.CharacterCreate exposing (Model, Msg, init, subscriptions, update, view)
import Browser.Navigation as Navigation
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick, onInput)
import Http
import Models.Card as Card
import Models.Character exposing (..)
import Models.CharacterEditor as CharacterEditor exposing (..)
import Page.MyPages.CharacterEditor as CharacterEditor exposing (editArea)
import Page.MyPages.CharacterView exposing (cardsView)
import Session
import Skeleton exposing (viewLink, viewMain)
import Url
import Url.Builder
import Utils.ModalWindow as Modal
import Utils.NavigationMenu exposing (NaviState(..), NavigationMenu, closeNavigationButton, getNavigationPageClass, openNavigationButton, toggleNavigationState, viewNav)
port saveNewCharacter : String -> Cmd msg
port createdCharacter : (Bool -> msg) -> Sub msg
subscriptions : Sub Msg
subscriptions =
Sub.batch
[ createdCharacter CreatedCharacter
]
type alias Model =
{ session : Session.Data
, naviState : NaviState
, character : Character
, editorModel : EditorModel CharacterEditor.Msg
}
init : Session.Data -> String -> String -> ( Model, Cmd Msg )
init session apiKey storeUserId =
let
cards =
case Session.getCards session of
Just sheet ->
case Card.cardDataListDecodeFromJson sheet of
Ok list ->
list
Err _ ->
[]
Nothing ->
[]
cardsCmd =
if cards == [] then
Session.fetchCards GotCards apiKey
else
Cmd.none
initChar =
initCharacter storeUserId
char =
if cards == [] then
initChar
else
Models.Character.initBaseCards initChar cards
editor =
initEditor
in
( Model session Close char { editor | cards = cards }
, Cmd.batch [ cardsCmd ]
)
type Msg
= ToggleNavigation
| EditorMsg CharacterEditor.Msg
| Save
| CreatedCharacter Bool
| GotCards (Result Http.Error String)
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
ToggleNavigation ->
( { model | naviState = toggleNavigationState model.naviState }, Cmd.none )
-- キャラクターデータの更新
EditorMsg emsg ->
let
( ( char, editor ), s ) =
CharacterEditor.update emsg model.character model.editorModel
in
( { model | character = char, editorModel = editor }, Cmd.map EditorMsg s )
Save ->
( model, model.character |> encodeCharacter |> saveNewCharacter )
CreatedCharacter _ ->
( model, Navigation.load (Url.Builder.absolute [ "mypage" ] []) )
GotCards (Ok json) ->
case Card.cardDataListDecodeFromJson json of
Ok cards ->
let
oldEditorModel =
model.editorModel
newEditorModel =
{ oldEditorModel | cards = cards }
newCharacter =
Models.Character.initBaseCards model.character cards
in
( { model
| editorModel = newEditorModel
, character = newCharacter
, session = Session.addCards model.session json
}
, Cmd.none
)
Err _ ->
( model, Cmd.none )
GotCards (Err _) ->
( model, Cmd.none )
view : Model -> Skeleton.Details Msg
view model =
let
-- ナビゲーションの状態によってページに持たせるクラスを変える
naviClass =
getNavigationPageClass
model.naviState
in
{ title = "新規作成"
, attrs = [ class naviClass, class "character-sheet" ]
, kids =
[ viewMain <| viewHelper model
]
}
viewHelper : Model -> Html Msg
viewHelper model =
div [ class "" ]
[ h1 []
[ text "新規作成" ]
, div
[ class "edit-karte" ]
[ edit model
, cardsView model.character
-- , karte model
]
]
edit : Model -> Html Msg
edit model =
div [ class "edit-area" ]
[ Html.map EditorMsg (editArea model.character model.editorModel)
, button [ onClick Save, class "btn waves-effect waves-light", type_ "button", name "save" ]
[ text "保存"
, i [ class "material-icons right" ] [ text "send" ]
]
]
karte : Model -> Html Msg
karte model =
let
char =
model.character
in
div [ class "karte" ]
[ div [ class "label-personal" ] [ text "個体識別用情報" ]
, div [ class "label-kana" ] [ text "フリガナ" ]
, div [ class "kana" ] [ text char.kana ]
, div [ class "label-name" ] [ text "名前" ]
, div [ class "name" ] [ text char.name ]
, div [ class "outer-line" ] []
]
| 936 | port module Page.MyPages.CharacterCreate exposing (Model, Msg, init, subscriptions, update, view)
import Browser.Navigation as Navigation
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick, onInput)
import Http
import Models.Card as Card
import Models.Character exposing (..)
import Models.CharacterEditor as CharacterEditor exposing (..)
import Page.MyPages.CharacterEditor as CharacterEditor exposing (editArea)
import Page.MyPages.CharacterView exposing (cardsView)
import Session
import Skeleton exposing (viewLink, viewMain)
import Url
import Url.Builder
import Utils.ModalWindow as Modal
import Utils.NavigationMenu exposing (NaviState(..), NavigationMenu, closeNavigationButton, getNavigationPageClass, openNavigationButton, toggleNavigationState, viewNav)
port saveNewCharacter : String -> Cmd msg
port createdCharacter : (Bool -> msg) -> Sub msg
subscriptions : Sub Msg
subscriptions =
Sub.batch
[ createdCharacter CreatedCharacter
]
type alias Model =
{ session : Session.Data
, naviState : NaviState
, character : Character
, editorModel : EditorModel CharacterEditor.Msg
}
init : Session.Data -> String -> String -> ( Model, Cmd Msg )
init session apiKey storeUserId =
let
cards =
case Session.getCards session of
Just sheet ->
case Card.cardDataListDecodeFromJson sheet of
Ok list ->
list
Err _ ->
[]
Nothing ->
[]
cardsCmd =
if cards == [] then
Session.fetchCards GotCards apiKey
else
Cmd.none
initChar =
initCharacter storeUserId
char =
if cards == [] then
initChar
else
Models.Character.initBaseCards initChar cards
editor =
initEditor
in
( Model session Close char { editor | cards = cards }
, Cmd.batch [ cardsCmd ]
)
type Msg
= ToggleNavigation
| EditorMsg CharacterEditor.Msg
| Save
| CreatedCharacter Bool
| GotCards (Result Http.Error String)
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
ToggleNavigation ->
( { model | naviState = toggleNavigationState model.naviState }, Cmd.none )
-- キャラクターデータの更新
EditorMsg emsg ->
let
( ( char, editor ), s ) =
CharacterEditor.update emsg model.character model.editorModel
in
( { model | character = char, editorModel = editor }, Cmd.map EditorMsg s )
Save ->
( model, model.character |> encodeCharacter |> saveNewCharacter )
CreatedCharacter _ ->
( model, Navigation.load (Url.Builder.absolute [ "mypage" ] []) )
GotCards (Ok json) ->
case Card.cardDataListDecodeFromJson json of
Ok cards ->
let
oldEditorModel =
model.editorModel
newEditorModel =
{ oldEditorModel | cards = cards }
newCharacter =
Models.Character.initBaseCards model.character cards
in
( { model
| editorModel = newEditorModel
, character = newCharacter
, session = Session.addCards model.session json
}
, Cmd.none
)
Err _ ->
( model, Cmd.none )
GotCards (Err _) ->
( model, Cmd.none )
view : Model -> Skeleton.Details Msg
view model =
let
-- ナビゲーションの状態によってページに持たせるクラスを変える
naviClass =
getNavigationPageClass
model.naviState
in
{ title = "新規作成"
, attrs = [ class naviClass, class "character-sheet" ]
, kids =
[ viewMain <| viewHelper model
]
}
viewHelper : Model -> Html Msg
viewHelper model =
div [ class "" ]
[ h1 []
[ text "新規作成" ]
, div
[ class "edit-karte" ]
[ edit model
, cardsView model.character
-- , karte model
]
]
edit : Model -> Html Msg
edit model =
div [ class "edit-area" ]
[ Html.map EditorMsg (editArea model.character model.editorModel)
, button [ onClick Save, class "btn waves-effect waves-light", type_ "button", name "save" ]
[ text "保存"
, i [ class "material-icons right" ] [ text "send" ]
]
]
karte : Model -> Html Msg
karte model =
let
char =
model.character
in
div [ class "karte" ]
[ div [ class "label-personal" ] [ text "個体識別用情報" ]
, div [ class "label-kana" ] [ text "<NAME>" ]
, div [ class "kana" ] [ text char.kana ]
, div [ class "label-name" ] [ text "名前" ]
, div [ class "name" ] [ text char.name ]
, div [ class "outer-line" ] []
]
| true | port module Page.MyPages.CharacterCreate exposing (Model, Msg, init, subscriptions, update, view)
import Browser.Navigation as Navigation
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick, onInput)
import Http
import Models.Card as Card
import Models.Character exposing (..)
import Models.CharacterEditor as CharacterEditor exposing (..)
import Page.MyPages.CharacterEditor as CharacterEditor exposing (editArea)
import Page.MyPages.CharacterView exposing (cardsView)
import Session
import Skeleton exposing (viewLink, viewMain)
import Url
import Url.Builder
import Utils.ModalWindow as Modal
import Utils.NavigationMenu exposing (NaviState(..), NavigationMenu, closeNavigationButton, getNavigationPageClass, openNavigationButton, toggleNavigationState, viewNav)
port saveNewCharacter : String -> Cmd msg
port createdCharacter : (Bool -> msg) -> Sub msg
subscriptions : Sub Msg
subscriptions =
Sub.batch
[ createdCharacter CreatedCharacter
]
type alias Model =
{ session : Session.Data
, naviState : NaviState
, character : Character
, editorModel : EditorModel CharacterEditor.Msg
}
init : Session.Data -> String -> String -> ( Model, Cmd Msg )
init session apiKey storeUserId =
let
cards =
case Session.getCards session of
Just sheet ->
case Card.cardDataListDecodeFromJson sheet of
Ok list ->
list
Err _ ->
[]
Nothing ->
[]
cardsCmd =
if cards == [] then
Session.fetchCards GotCards apiKey
else
Cmd.none
initChar =
initCharacter storeUserId
char =
if cards == [] then
initChar
else
Models.Character.initBaseCards initChar cards
editor =
initEditor
in
( Model session Close char { editor | cards = cards }
, Cmd.batch [ cardsCmd ]
)
type Msg
= ToggleNavigation
| EditorMsg CharacterEditor.Msg
| Save
| CreatedCharacter Bool
| GotCards (Result Http.Error String)
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
ToggleNavigation ->
( { model | naviState = toggleNavigationState model.naviState }, Cmd.none )
-- キャラクターデータの更新
EditorMsg emsg ->
let
( ( char, editor ), s ) =
CharacterEditor.update emsg model.character model.editorModel
in
( { model | character = char, editorModel = editor }, Cmd.map EditorMsg s )
Save ->
( model, model.character |> encodeCharacter |> saveNewCharacter )
CreatedCharacter _ ->
( model, Navigation.load (Url.Builder.absolute [ "mypage" ] []) )
GotCards (Ok json) ->
case Card.cardDataListDecodeFromJson json of
Ok cards ->
let
oldEditorModel =
model.editorModel
newEditorModel =
{ oldEditorModel | cards = cards }
newCharacter =
Models.Character.initBaseCards model.character cards
in
( { model
| editorModel = newEditorModel
, character = newCharacter
, session = Session.addCards model.session json
}
, Cmd.none
)
Err _ ->
( model, Cmd.none )
GotCards (Err _) ->
( model, Cmd.none )
view : Model -> Skeleton.Details Msg
view model =
let
-- ナビゲーションの状態によってページに持たせるクラスを変える
naviClass =
getNavigationPageClass
model.naviState
in
{ title = "新規作成"
, attrs = [ class naviClass, class "character-sheet" ]
, kids =
[ viewMain <| viewHelper model
]
}
viewHelper : Model -> Html Msg
viewHelper model =
div [ class "" ]
[ h1 []
[ text "新規作成" ]
, div
[ class "edit-karte" ]
[ edit model
, cardsView model.character
-- , karte model
]
]
edit : Model -> Html Msg
edit model =
div [ class "edit-area" ]
[ Html.map EditorMsg (editArea model.character model.editorModel)
, button [ onClick Save, class "btn waves-effect waves-light", type_ "button", name "save" ]
[ text "保存"
, i [ class "material-icons right" ] [ text "send" ]
]
]
karte : Model -> Html Msg
karte model =
let
char =
model.character
in
div [ class "karte" ]
[ div [ class "label-personal" ] [ text "個体識別用情報" ]
, div [ class "label-kana" ] [ text "PI:NAME:<NAME>END_PI" ]
, div [ class "kana" ] [ text char.kana ]
, div [ class "label-name" ] [ text "名前" ]
, div [ class "name" ] [ text char.name ]
, div [ class "outer-line" ] []
]
| elm |
[
{
"context": "ak h)\n , \"Bessie says moooo\" == (let c = cow(\"Bessie\") in speak c)\n , \"Little Lamb says baaaa\" ==",
"end": 587,
"score": 0.6193509698,
"start": 584,
"tag": "NAME",
"value": "ess"
}
] | elm/animals.elm | idrougge/ple | 59 | import SimpleAssert exposing (assertAll)
type Kind = Cow | Horse | Sheep
type alias Animal = { name : String, kind : Kind }
speak : Animal -> String
speak a = a.name ++ " says " ++ sound a
sound : Animal -> String
sound a =
case a.kind of
Cow ->
"moooo"
Horse ->
"neigh"
Sheep ->
"baaaa"
horse n = { name = n, kind = Horse }
cow n = { name = n, kind = Cow }
sheep n = { name = n, kind = Sheep }
main = assertAll
[ "CJ says neigh" == (let h = horse("CJ") in speak h)
, "Bessie says moooo" == (let c = cow("Bessie") in speak c)
, "Little Lamb says baaaa" == speak (sheep "Little Lamb")
]
| 35480 | import SimpleAssert exposing (assertAll)
type Kind = Cow | Horse | Sheep
type alias Animal = { name : String, kind : Kind }
speak : Animal -> String
speak a = a.name ++ " says " ++ sound a
sound : Animal -> String
sound a =
case a.kind of
Cow ->
"moooo"
Horse ->
"neigh"
Sheep ->
"baaaa"
horse n = { name = n, kind = Horse }
cow n = { name = n, kind = Cow }
sheep n = { name = n, kind = Sheep }
main = assertAll
[ "CJ says neigh" == (let h = horse("CJ") in speak h)
, "Bessie says moooo" == (let c = cow("B<NAME>ie") in speak c)
, "Little Lamb says baaaa" == speak (sheep "Little Lamb")
]
| true | import SimpleAssert exposing (assertAll)
type Kind = Cow | Horse | Sheep
type alias Animal = { name : String, kind : Kind }
speak : Animal -> String
speak a = a.name ++ " says " ++ sound a
sound : Animal -> String
sound a =
case a.kind of
Cow ->
"moooo"
Horse ->
"neigh"
Sheep ->
"baaaa"
horse n = { name = n, kind = Horse }
cow n = { name = n, kind = Cow }
sheep n = { name = n, kind = Sheep }
main = assertAll
[ "CJ says neigh" == (let h = horse("CJ") in speak h)
, "Bessie says moooo" == (let c = cow("BPI:NAME:<NAME>END_PIie") in speak c)
, "Little Lamb says baaaa" == speak (sheep "Little Lamb")
]
| elm |
[
{
"context": "d\" <|\n \\_ ->\n let\n calculated = [ \"Dawn\"\n , \"Brian\"\n ",
"end": 874,
"score": 0.9981392622,
"start": 870,
"tag": "NAME",
"value": "Dawn"
},
{
"context": " calculated = [ \"Dawn\"\n , \"Brian\"\n , \"Paul\"\n ",
"end": 905,
"score": 0.9995783567,
"start": 900,
"tag": "NAME",
"value": "Brian"
},
{
"context": " , \"Brian\"\n , \"Paul\"\n , \"Sophie\"\n ",
"end": 935,
"score": 0.999612689,
"start": 931,
"tag": "NAME",
"value": "Paul"
},
{
"context": " , \"Paul\"\n , \"Sophie\"\n ]\n in\n calculat",
"end": 967,
"score": 0.9996678233,
"start": 961,
"tag": "NAME",
"value": "Sophie"
},
{
"context": " |> Expect.all\n [ List.member \"Dawn\" >> Expect.true \"has Dawn\"\n , List.me",
"end": 1076,
"score": 0.5524948835,
"start": 1073,
"tag": "NAME",
"value": "awn"
},
{
"context": "xpect.true \"has Dawn\"\n , List.member \"Brian\" >> Expect.true \"has Brian\"\n ]\n ",
"end": 1137,
"score": 0.9995447397,
"start": 1132,
"tag": "NAME",
"value": "Brian"
},
{
"context": " , List.member \"Brian\" >> Expect.true \"has Brian\"\n ]\n \n",
"end": 1164,
"score": 0.9990590811,
"start": 1159,
"tag": "NAME",
"value": "Brian"
}
] | elm/tests/ToInt/TestExamples.elm | marick/static-fp | 29 | module ToInt.TestExamples exposing (..)
import Test exposing (..)
import Expect exposing (Expectation)
import Result.Extra as Result
suite : Test
suite =
describe "arithmetic"
[ test "+" <|
\_ ->
1 + 1 |> Expect.equal 2
, test "-" <|
\_ ->
1 - 1 |> Expect.equal 0
]
errTest : Test
errTest =
test "an empty string is rejected" <|
\_ ->
String.toInt ""
|> Result.isErr
|> Expect.true ""
isErr : Result a b -> Expectation
isErr result =
case result of
Ok _ ->
Expect.fail ("Actual: " ++ toString result)
Err _ ->
Expect.pass
anotherErrTest : Test
anotherErrTest =
test "another empty string is rejected" <|
\_ ->
String.toInt "" |> isErr
doubleCheck : Test
doubleCheck =
test "parents are included" <|
\_ ->
let
calculated = [ "Dawn"
, "Brian"
, "Paul"
, "Sophie"
]
in
calculated
|> Expect.all
[ List.member "Dawn" >> Expect.true "has Dawn"
, List.member "Brian" >> Expect.true "has Brian"
]
| 36150 | module ToInt.TestExamples exposing (..)
import Test exposing (..)
import Expect exposing (Expectation)
import Result.Extra as Result
suite : Test
suite =
describe "arithmetic"
[ test "+" <|
\_ ->
1 + 1 |> Expect.equal 2
, test "-" <|
\_ ->
1 - 1 |> Expect.equal 0
]
errTest : Test
errTest =
test "an empty string is rejected" <|
\_ ->
String.toInt ""
|> Result.isErr
|> Expect.true ""
isErr : Result a b -> Expectation
isErr result =
case result of
Ok _ ->
Expect.fail ("Actual: " ++ toString result)
Err _ ->
Expect.pass
anotherErrTest : Test
anotherErrTest =
test "another empty string is rejected" <|
\_ ->
String.toInt "" |> isErr
doubleCheck : Test
doubleCheck =
test "parents are included" <|
\_ ->
let
calculated = [ "<NAME>"
, "<NAME>"
, "<NAME>"
, "<NAME>"
]
in
calculated
|> Expect.all
[ List.member "D<NAME>" >> Expect.true "has Dawn"
, List.member "<NAME>" >> Expect.true "has <NAME>"
]
| true | module ToInt.TestExamples exposing (..)
import Test exposing (..)
import Expect exposing (Expectation)
import Result.Extra as Result
suite : Test
suite =
describe "arithmetic"
[ test "+" <|
\_ ->
1 + 1 |> Expect.equal 2
, test "-" <|
\_ ->
1 - 1 |> Expect.equal 0
]
errTest : Test
errTest =
test "an empty string is rejected" <|
\_ ->
String.toInt ""
|> Result.isErr
|> Expect.true ""
isErr : Result a b -> Expectation
isErr result =
case result of
Ok _ ->
Expect.fail ("Actual: " ++ toString result)
Err _ ->
Expect.pass
anotherErrTest : Test
anotherErrTest =
test "another empty string is rejected" <|
\_ ->
String.toInt "" |> isErr
doubleCheck : Test
doubleCheck =
test "parents are included" <|
\_ ->
let
calculated = [ "PI:NAME:<NAME>END_PI"
, "PI:NAME:<NAME>END_PI"
, "PI:NAME:<NAME>END_PI"
, "PI:NAME:<NAME>END_PI"
]
in
calculated
|> Expect.all
[ List.member "DPI:NAME:<NAME>END_PI" >> Expect.true "has Dawn"
, List.member "PI:NAME:<NAME>END_PI" >> Expect.true "has PI:NAME:<NAME>END_PI"
]
| elm |
[
{
"context": " [ id \"txtPwd\", placeholder \"Password\" ]\n []\n ",
"end": 5436,
"score": 0.9917362928,
"start": 5428,
"tag": "PASSWORD",
"value": "Password"
},
{
"context": " [ id \"txtPwd\", placeholder \"Password\" ,onInput (CheckPwd pwd)]\n ",
"end": 7087,
"score": 0.9823015928,
"start": 7079,
"tag": "PASSWORD",
"value": "Password"
}
] | static/elm/login.elm | AccountYi/game | 0 | module Main exposing (main, view)
import Browser
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick)
import Http
main =
Browser.sandbox { init = init, update = update, view = view }
type alias Model =
{ loadState : StateModel
, login : Login
, singup : Singup
, emailState : Int
, pwdState : Int
}
-- 0未填写 默认
-- 1 填写正确
-- 2 填写错误
type alias Login =
{ email : String
, password : String
}
type alias Singup =
{ email : String
, password : String
}
init : () -> (Model, Cmd Msg)
init _ =
({loadState = StateModel
, login =
{ email = ""
, password = ""
}
, singup =
{ email = ""
, password = ""
}
,emailState=0
,pwdState=0
}
,
Cmd.none
-- Http.post
-- { url = "/login"
-- , expect = Http.expectString GotText
-- }
)
type StateModel
= Fail
| Loading
| Success
type Msg
= GotText (Result Http.Error String)
| LoginSubmit --登录提交
| SingupSubmit Singup --注册提交
| CheckEmail String --验证邮箱
| CheckPwd String --验证密码
loginUser : String -> String -> Cmd Msg
loginUser email pwd =
Http.post
{
url="/loginUser",
body=
multipleBody
[
stringPart "" email,
stringPart "" pwd
]
, expect = Http.f
}
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
GotText result ->
case result of
Ok fullText ->
Err _ ->
(Fail,Cmd.none)
LoginSubmit ->
if Bool.not (String.isEmpty email) && Bool.not (String.isEmpty pwd) then
-- email && pwd both not empty
--check correct
if (checkEmail model.email) && (checkPwd model.pwd) then
-- can post req
({model|emailState=1,pwdState=1},)
else
if checkEmail email then
({model|emailState=1},Cmd.none)
else
({model|emailState=2},Cmd.none)
if checkPwd pwd then
({model|pwdState=1},Cmd.none)
else
({model|pwdState=2},Cmd.none)
else
-- check one is empty
if String.isEmpty email then
({model|emailState=0},Cmd.none)
else
(model,Cmd.none)
if String.isEmpty pwd then
({model|pwdState=0},Cmd.none)
else
(model,Cmd.none)
CheckEmail email->
if String.isEmpty email then
({model|emailState=0},Cmd.none)
else
if checkEmail email then
({model|emailState=1},Cmd.none)
else
({model|emailState=2},Cmd.none)
CheckPwd pwd->
if String.isEmpty pwd then
({model|pwdState=0},Cmd.none)
else
if checkPwd pwd then
({model|pwdState=1},Cmd.none)
else
({model|pwdState=2},Cmd.none)
checkEmail : String -> Bool
checkEmail email=email==/^([a-zA-Z0-9_-])+@([a-zA-Z0-9_-])+(.[a-zA-Z0-9_-])+/
checkPwd : String -> Bool
checkPwd pwd=pwd==/(a-zA-Z0-9){7,}/
view : Model -> Html Msg
view model =
case model.loadState of
Loading ->
div
[ class "all" ]
[ div
[ class "logo" ]
[ button
[ id "btn2" ]
[ text "SING UP" ]
, button
[ id "btn1" ]
[ text "LOG IN" ]
, div
[ class "word1" ]
[ a
[ href "#" ]
[ text "COMPNIES" ]
]
, div
[ class "icon" ]
[ img
[ src "/static/images/icon.png" ]
[]
]
]
, div
[ id "LoginBox" ]
[ div
[ class "row1" ]
[ span
[]
[ text "Sign in to an existing CodinGame account" ]
, a
[ href "#", title "关闭窗口", class "close_btn", id "closeBtn" ]
[ text "×" ]
]
, div
[ class "row" ]
[ span
[]
[ text "Email:" ]
, span
[ class "inputBox" ]
[ input
[ id "txtName", placeholder "Email" ]
[]
]
, a
[ href "#", title "提示", class "warning", id "warn" ]
[ text "*" ]
]
, div
[ class "row" ]
[ span
[]
[ text "Password:" ]
, span
[ class "inputBox" ]
[ input
[ id "txtPwd", placeholder "Password" ]
[]
]
, a
[ href "#", title "提示", class "warning", id "warn2" ]
[ text "*" ]
]
, div
[ class "row" ]
[ a
[ href "#", id "loginbtn", onClick LoginSubmit ]
[ text "LOG IN" ]
]
]
, div
[ id "SingupBox" ]
[ div
[ class "row1" ]
[ span
[]
[ text "Sign up and start playing, for free" ]
, a
[ href "#", title "关闭窗口", class "close_btn", id "closeBtn1" ]
[ text "×" ]
]
, div
[ class "row" ]
[ span
[]
[ text "Email:" ]
, span
[ class "inputBox" ]
[ input
-- TODO check email
[ id "txtName1", placeholder "Email",onInput (CheckEmail email) ]
[]
]
, a
[ href "#", title "提示", class "warning", id "warn3" ]
[ text "*" ]
]
, div
[ class "row" ]
[ span
[]
[ text "Password:" ]
, span
[ class "inputBox" ]
[ input
-- TODO check password
[ id "txtPwd", placeholder "Password" ,onInput (CheckPwd pwd)]
[]
]
, a
[ href "#", title "提示", class "warning", id "warn4" ]
[ text "*" ]
]
, div
[ class "row" ]
[ a
-- TODO check both email and pwd then post
[ href "#", id "singupbtn", onClick SingupSubmit ]
[ text "SING UP" ]
]
]
, div
[ class "banner" ]
[]
, div
[ class "bottom" ]
[]
]
| 6002 | module Main exposing (main, view)
import Browser
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick)
import Http
main =
Browser.sandbox { init = init, update = update, view = view }
type alias Model =
{ loadState : StateModel
, login : Login
, singup : Singup
, emailState : Int
, pwdState : Int
}
-- 0未填写 默认
-- 1 填写正确
-- 2 填写错误
type alias Login =
{ email : String
, password : String
}
type alias Singup =
{ email : String
, password : String
}
init : () -> (Model, Cmd Msg)
init _ =
({loadState = StateModel
, login =
{ email = ""
, password = ""
}
, singup =
{ email = ""
, password = ""
}
,emailState=0
,pwdState=0
}
,
Cmd.none
-- Http.post
-- { url = "/login"
-- , expect = Http.expectString GotText
-- }
)
type StateModel
= Fail
| Loading
| Success
type Msg
= GotText (Result Http.Error String)
| LoginSubmit --登录提交
| SingupSubmit Singup --注册提交
| CheckEmail String --验证邮箱
| CheckPwd String --验证密码
loginUser : String -> String -> Cmd Msg
loginUser email pwd =
Http.post
{
url="/loginUser",
body=
multipleBody
[
stringPart "" email,
stringPart "" pwd
]
, expect = Http.f
}
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
GotText result ->
case result of
Ok fullText ->
Err _ ->
(Fail,Cmd.none)
LoginSubmit ->
if Bool.not (String.isEmpty email) && Bool.not (String.isEmpty pwd) then
-- email && pwd both not empty
--check correct
if (checkEmail model.email) && (checkPwd model.pwd) then
-- can post req
({model|emailState=1,pwdState=1},)
else
if checkEmail email then
({model|emailState=1},Cmd.none)
else
({model|emailState=2},Cmd.none)
if checkPwd pwd then
({model|pwdState=1},Cmd.none)
else
({model|pwdState=2},Cmd.none)
else
-- check one is empty
if String.isEmpty email then
({model|emailState=0},Cmd.none)
else
(model,Cmd.none)
if String.isEmpty pwd then
({model|pwdState=0},Cmd.none)
else
(model,Cmd.none)
CheckEmail email->
if String.isEmpty email then
({model|emailState=0},Cmd.none)
else
if checkEmail email then
({model|emailState=1},Cmd.none)
else
({model|emailState=2},Cmd.none)
CheckPwd pwd->
if String.isEmpty pwd then
({model|pwdState=0},Cmd.none)
else
if checkPwd pwd then
({model|pwdState=1},Cmd.none)
else
({model|pwdState=2},Cmd.none)
checkEmail : String -> Bool
checkEmail email=email==/^([a-zA-Z0-9_-])+@([a-zA-Z0-9_-])+(.[a-zA-Z0-9_-])+/
checkPwd : String -> Bool
checkPwd pwd=pwd==/(a-zA-Z0-9){7,}/
view : Model -> Html Msg
view model =
case model.loadState of
Loading ->
div
[ class "all" ]
[ div
[ class "logo" ]
[ button
[ id "btn2" ]
[ text "SING UP" ]
, button
[ id "btn1" ]
[ text "LOG IN" ]
, div
[ class "word1" ]
[ a
[ href "#" ]
[ text "COMPNIES" ]
]
, div
[ class "icon" ]
[ img
[ src "/static/images/icon.png" ]
[]
]
]
, div
[ id "LoginBox" ]
[ div
[ class "row1" ]
[ span
[]
[ text "Sign in to an existing CodinGame account" ]
, a
[ href "#", title "关闭窗口", class "close_btn", id "closeBtn" ]
[ text "×" ]
]
, div
[ class "row" ]
[ span
[]
[ text "Email:" ]
, span
[ class "inputBox" ]
[ input
[ id "txtName", placeholder "Email" ]
[]
]
, a
[ href "#", title "提示", class "warning", id "warn" ]
[ text "*" ]
]
, div
[ class "row" ]
[ span
[]
[ text "Password:" ]
, span
[ class "inputBox" ]
[ input
[ id "txtPwd", placeholder "<PASSWORD>" ]
[]
]
, a
[ href "#", title "提示", class "warning", id "warn2" ]
[ text "*" ]
]
, div
[ class "row" ]
[ a
[ href "#", id "loginbtn", onClick LoginSubmit ]
[ text "LOG IN" ]
]
]
, div
[ id "SingupBox" ]
[ div
[ class "row1" ]
[ span
[]
[ text "Sign up and start playing, for free" ]
, a
[ href "#", title "关闭窗口", class "close_btn", id "closeBtn1" ]
[ text "×" ]
]
, div
[ class "row" ]
[ span
[]
[ text "Email:" ]
, span
[ class "inputBox" ]
[ input
-- TODO check email
[ id "txtName1", placeholder "Email",onInput (CheckEmail email) ]
[]
]
, a
[ href "#", title "提示", class "warning", id "warn3" ]
[ text "*" ]
]
, div
[ class "row" ]
[ span
[]
[ text "Password:" ]
, span
[ class "inputBox" ]
[ input
-- TODO check password
[ id "txtPwd", placeholder "<PASSWORD>" ,onInput (CheckPwd pwd)]
[]
]
, a
[ href "#", title "提示", class "warning", id "warn4" ]
[ text "*" ]
]
, div
[ class "row" ]
[ a
-- TODO check both email and pwd then post
[ href "#", id "singupbtn", onClick SingupSubmit ]
[ text "SING UP" ]
]
]
, div
[ class "banner" ]
[]
, div
[ class "bottom" ]
[]
]
| true | module Main exposing (main, view)
import Browser
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onClick)
import Http
main =
Browser.sandbox { init = init, update = update, view = view }
type alias Model =
{ loadState : StateModel
, login : Login
, singup : Singup
, emailState : Int
, pwdState : Int
}
-- 0未填写 默认
-- 1 填写正确
-- 2 填写错误
type alias Login =
{ email : String
, password : String
}
type alias Singup =
{ email : String
, password : String
}
init : () -> (Model, Cmd Msg)
init _ =
({loadState = StateModel
, login =
{ email = ""
, password = ""
}
, singup =
{ email = ""
, password = ""
}
,emailState=0
,pwdState=0
}
,
Cmd.none
-- Http.post
-- { url = "/login"
-- , expect = Http.expectString GotText
-- }
)
type StateModel
= Fail
| Loading
| Success
type Msg
= GotText (Result Http.Error String)
| LoginSubmit --登录提交
| SingupSubmit Singup --注册提交
| CheckEmail String --验证邮箱
| CheckPwd String --验证密码
loginUser : String -> String -> Cmd Msg
loginUser email pwd =
Http.post
{
url="/loginUser",
body=
multipleBody
[
stringPart "" email,
stringPart "" pwd
]
, expect = Http.f
}
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
GotText result ->
case result of
Ok fullText ->
Err _ ->
(Fail,Cmd.none)
LoginSubmit ->
if Bool.not (String.isEmpty email) && Bool.not (String.isEmpty pwd) then
-- email && pwd both not empty
--check correct
if (checkEmail model.email) && (checkPwd model.pwd) then
-- can post req
({model|emailState=1,pwdState=1},)
else
if checkEmail email then
({model|emailState=1},Cmd.none)
else
({model|emailState=2},Cmd.none)
if checkPwd pwd then
({model|pwdState=1},Cmd.none)
else
({model|pwdState=2},Cmd.none)
else
-- check one is empty
if String.isEmpty email then
({model|emailState=0},Cmd.none)
else
(model,Cmd.none)
if String.isEmpty pwd then
({model|pwdState=0},Cmd.none)
else
(model,Cmd.none)
CheckEmail email->
if String.isEmpty email then
({model|emailState=0},Cmd.none)
else
if checkEmail email then
({model|emailState=1},Cmd.none)
else
({model|emailState=2},Cmd.none)
CheckPwd pwd->
if String.isEmpty pwd then
({model|pwdState=0},Cmd.none)
else
if checkPwd pwd then
({model|pwdState=1},Cmd.none)
else
({model|pwdState=2},Cmd.none)
checkEmail : String -> Bool
checkEmail email=email==/^([a-zA-Z0-9_-])+@([a-zA-Z0-9_-])+(.[a-zA-Z0-9_-])+/
checkPwd : String -> Bool
checkPwd pwd=pwd==/(a-zA-Z0-9){7,}/
view : Model -> Html Msg
view model =
case model.loadState of
Loading ->
div
[ class "all" ]
[ div
[ class "logo" ]
[ button
[ id "btn2" ]
[ text "SING UP" ]
, button
[ id "btn1" ]
[ text "LOG IN" ]
, div
[ class "word1" ]
[ a
[ href "#" ]
[ text "COMPNIES" ]
]
, div
[ class "icon" ]
[ img
[ src "/static/images/icon.png" ]
[]
]
]
, div
[ id "LoginBox" ]
[ div
[ class "row1" ]
[ span
[]
[ text "Sign in to an existing CodinGame account" ]
, a
[ href "#", title "关闭窗口", class "close_btn", id "closeBtn" ]
[ text "×" ]
]
, div
[ class "row" ]
[ span
[]
[ text "Email:" ]
, span
[ class "inputBox" ]
[ input
[ id "txtName", placeholder "Email" ]
[]
]
, a
[ href "#", title "提示", class "warning", id "warn" ]
[ text "*" ]
]
, div
[ class "row" ]
[ span
[]
[ text "Password:" ]
, span
[ class "inputBox" ]
[ input
[ id "txtPwd", placeholder "PI:PASSWORD:<PASSWORD>END_PI" ]
[]
]
, a
[ href "#", title "提示", class "warning", id "warn2" ]
[ text "*" ]
]
, div
[ class "row" ]
[ a
[ href "#", id "loginbtn", onClick LoginSubmit ]
[ text "LOG IN" ]
]
]
, div
[ id "SingupBox" ]
[ div
[ class "row1" ]
[ span
[]
[ text "Sign up and start playing, for free" ]
, a
[ href "#", title "关闭窗口", class "close_btn", id "closeBtn1" ]
[ text "×" ]
]
, div
[ class "row" ]
[ span
[]
[ text "Email:" ]
, span
[ class "inputBox" ]
[ input
-- TODO check email
[ id "txtName1", placeholder "Email",onInput (CheckEmail email) ]
[]
]
, a
[ href "#", title "提示", class "warning", id "warn3" ]
[ text "*" ]
]
, div
[ class "row" ]
[ span
[]
[ text "Password:" ]
, span
[ class "inputBox" ]
[ input
-- TODO check password
[ id "txtPwd", placeholder "PI:PASSWORD:<PASSWORD>END_PI" ,onInput (CheckPwd pwd)]
[]
]
, a
[ href "#", title "提示", class "warning", id "warn4" ]
[ text "*" ]
]
, div
[ class "row" ]
[ a
-- TODO check both email and pwd then post
[ href "#", id "singupbtn", onClick SingupSubmit ]
[ text "SING UP" ]
]
]
, div
[ class "banner" ]
[]
, div
[ class "bottom" ]
[]
]
| elm |
[
{
"context": "value\n , name = name\n , id = parent",
"end": 24435,
"score": 0.9891989827,
"start": 24431,
"tag": "NAME",
"value": "name"
},
{
"context": "Block\n { name = name\n , id = parent",
"end": 24665,
"score": 0.9847564101,
"start": 24661,
"tag": "NAME",
"value": "name"
}
] | src/Mark.elm | digitalsatori/elm-markup | 166 | module Mark exposing
( Document, document, documentWith
, Block, block
, string, int, float, bool
, Styles, text, textWith
, Replacement, commonReplacements, replacement, balanced
, annotation, verbatim
, Record, record, field, toBlock
, oneOf, manyOf
, tree, Enumerated(..), Item(..), Icon(..)
, Outcome(..), Partial
, metadata, compile, parse, Parsed, toString, render
, map, verify, onError
, withId, withAttr, documentId, idToString, stringToId
, lookup
)
{-|
# Building Documents
@docs Document, document, documentWith
@docs Block, block
# Primitives
@docs string, int, float, bool
# Text
@docs Styles, text, textWith
# Text Replacements
@docs Replacement, commonReplacements, replacement, balanced
# Text Annotations
Along with basic [`styling`](#text) and [`replacements`](#replacement), we also have a few ways to annotate text.
@docs annotation, verbatim
# Records
@docs Record, record, field, toBlock
# Higher Level
@docs oneOf, manyOf
# Trees
@docs tree, Enumerated, Item, Icon
# Rendering
@docs Outcome, Partial
@docs metadata, compile, parse, Parsed, toString, render
# Constraining and Recovering Blocks
@docs map, verify, onError
@docs withId, withAttr, documentId, idToString, stringToId
@docs lookup
-}
import Mark.Edit
import Mark.Error
import Mark.Internal.Description as Desc exposing (..)
import Mark.Internal.Error as Error exposing (AstError(..), Context(..), Problem(..))
import Mark.Internal.Id as Id exposing (..)
import Mark.Internal.Index as Index
import Mark.Internal.Outcome as Outcome
import Mark.Internal.Parser as Parse
import Mark.New exposing (block)
import Parser.Advanced as Parser exposing ((|.), (|=), Parser)
{-| -}
type alias Parsed =
Desc.Parsed
{- INTERFACE -}
{-| -}
toString : Parsed -> String
toString =
Desc.toString
{-| -}
parse : Document metadata data -> String -> Outcome (List Mark.Error.Error) (Partial Parsed) Parsed
parse doc source =
Desc.compile doc source
|> moveParsedToResult
{-| -}
metadata : Document metadata document -> String -> Result Mark.Error.Error metadata
metadata (Desc.Document doc) source =
case Parser.run doc.metadata source of
Ok parsed ->
parsed
|> Result.mapError
(Error.render source)
Err irrecoverableParsingErrors ->
Err (Error.renderParsingErrors source irrecoverableParsingErrors)
moveParsedToResult :
Result
(Outcome.Outcome (List Error.Rendered)
{ errors : List Error.Rendered
, result : data
}
data
)
( Parsed
, Outcome.Outcome (List Error.Rendered)
{ errors : List Error.Rendered
, result : data
}
data
)
-> Outcome (List Mark.Error.Error) (Partial Parsed) Parsed
moveParsedToResult result =
case result of
Ok ( parsed, Outcome.Success success ) ->
Success parsed
Ok ( parsed, Outcome.Almost almost ) ->
Almost
{ errors = almost.errors
, result = parsed
}
Ok ( parsed, Outcome.Failure errors ) ->
Failure errors
Err (Outcome.Success success) ->
Failure []
Err (Outcome.Almost almost) ->
Failure almost.errors
Err (Outcome.Failure fail) ->
Failure fail
{-| -}
render : Document meta data -> Parsed -> Outcome (List Mark.Error.Error) (Partial ( meta, List data )) ( meta, List data )
render doc ((Parsed parsedDetails) as parsed) =
Desc.render doc parsed
|> rewrapOutcome
{-| -}
compile : Document meta data -> String -> Outcome (List Mark.Error.Error) (Partial ( meta, List data )) ( meta, List data )
compile doc source =
Desc.compile doc source
|> flattenErrors
|> rewrapOutcome
flattenErrors result =
case result of
Ok ( parsed, outcome ) ->
outcome
Err outcome ->
outcome
rewrapOutcome : Outcome.Outcome x y z -> Outcome x y z
rewrapOutcome outcome =
case outcome of
Outcome.Success s ->
Success s
Outcome.Almost x ->
Almost x
Outcome.Failure f ->
Failure f
{-| -}
type alias Partial data =
{ errors : List Mark.Error.Error
, result : data
}
{-| -}
startDocRange : Range
startDocRange =
{ start =
{ offset = 0
, line = 1
, column = 1
}
, end =
{ offset = 0
, line = 1
, column = 1
}
}
{-| -}
type alias Document meta data =
Desc.Document meta data
{-| -}
type Outcome failure almost success
= Success success
| Almost almost
| Failure failure
{-| -}
type alias Block data =
Desc.Block data
getUnexpecteds : Description -> List Error.UnexpectedDetails
getUnexpecteds description =
case description of
DescribeBlock details ->
getUnexpecteds details.found
Record details ->
List.concatMap
(Tuple.second >> getUnexpecteds)
details.found
Group many ->
List.concatMap getUnexpecteds many.children
StartsWith details ->
getUnexpecteds details.first
++ getUnexpecteds details.second
DescribeItem details ->
List.concatMap getUnexpecteds details.content
++ List.concatMap getUnexpecteds details.children
-- Primitives
DescribeBoolean details ->
[]
DescribeInteger details ->
[]
DescribeFloat details ->
[]
DescribeText details ->
[]
DescribeString _ str ->
[]
DescribeUnexpected _ details ->
[ details ]
{-| -}
type alias Position =
{ offset : Int
, line : Int
, column : Int
}
{-| -}
type alias Range =
{ start : Position
, end : Position
}
{- BLOCKS -}
{-| Create a markup `Document`. You're first goal is to describe a document in terms of the blocks you're expecting.
Here's an overly simple document that captures one block, a Title, and wraps it in some `Html`
document : Mark.Document (Html msg)
document =
Mark.document
(\title -> Html.main [] [ title ])
(Mark.block "Title"
(Html.h1 [])
Mark.string
)
will parse the following markup:
```markup
|> Title
Here is my title!
```
and ultimately render it as
```html
<main>
<h1>Here is my title!</h1>
</main>
```
-}
document :
List (Block block)
-> Document () block
document blocks =
createDocument (\_ -> "none")
(Parser.succeed (Ok ()))
(map (Tuple.pair ()) (manyOf blocks))
createDocument :
(meta -> String)
-> Parser Error.Context Error.Problem (Result Error.UnexpectedDetails meta)
-> Block ( meta, List data )
-> Document meta data
createDocument toDocumentId meta child =
Document
{ expect =
getBlockExpectation child
, metadata =
meta
, converter =
\(Parsed parsed) ->
Desc.renderBlock child parsed.found
, parser =
Parser.getSource
|> Parser.andThen
(\src ->
let
docId : String
docId =
case Parser.run meta src of
Ok (Ok m) ->
toDocumentId m
_ ->
-- This should return an error!
-- it means the metadata is invalid
""
seed : Seed
seed =
Id.initialSeed docId
( currentSeed, blockParser ) =
Parse.getFailableBlock Desc.ParseBlock seed child
in
Parser.succeed
(\source ( range, value ) ->
Parsed
{ errors =
List.map (Error.render source) (getUnexpecteds value)
, found = value
, expected = getBlockExpectation child
, initialSeed = seed
, currentSeed = currentSeed
, attributes = []
}
)
|. Parser.chompWhile (\c -> c == '\n')
|= Parser.getSource
|= Parse.withRange (Parser.withIndent 0 blockParser)
|. Parser.chompWhile (\c -> c == ' ' || c == '\n')
|. Parser.end End
)
}
{-| Capture some metadata at the start of your document, followed by the body.
import Mark
Mark.documentWith
{ id = \metadata -> metadata.id
, metadata =
Mark.record
(\id author publishedAt ->
{ author = author
, publishedAt = publishedAt
}
)
|> Mark.field "id" Mark.string
|> Mark.field "author" Mark.string
|> Mark.field "publishedAt" Mark.string
, blocks =
[
]
}
**Note** - You can also specify an `id`, which is a document identifier and is included in `Mark.Edit.Id`. This is really only necessary if you're building an editor that can edit multiple documents at once.
Otherwise, feel free to simple put `id = \_ -> "doc"`
-}
documentWith :
{ id : metadata -> String
, metadata : Record metadata
, blocks : List (Block block)
}
-> Document metadata block
documentWith config =
let
metadataBlock =
toBlock config.metadata
in
createDocument config.id
(getMetadataParser metadataBlock)
(startWith
Tuple.pair
metadataBlock
(manyOf config.blocks)
)
getMetadataParser metadataBlock =
let
( _, metadataParser ) =
Parse.getFailableBlock Desc.ParseBlock (Id.initialSeed "") metadataBlock
in
Parser.andThen
(\description ->
case renderBlock metadataBlock description of
Outcome.Success meta ->
Parser.succeed (Ok meta.data)
Outcome.Failure astError ->
Parser.succeed
(Err
{ problem = Error.DocumentMismatch
, range = Desc.emptyRange
}
)
Outcome.Almost (Uncertain ( unexpected, otherUnexpecteds )) ->
Parser.succeed (Err unexpected)
Outcome.Almost (Recovered ( unexpected, otherUnexpecteds ) renderedChild) ->
Parser.succeed (Err unexpected)
)
(Parser.withIndent 0 metadataParser)
{-| Change the result of a block by applying a function to it.
-}
map : (a -> b) -> Block a -> Block b
map fn (Block details) =
Block
{ kind = details.kind
, converter = mapSuccessAndRecovered fn << details.converter
, parser = details.parser
, expect = details.expect
}
{-| -}
type alias CustomError =
{ title : String
, message : List String
}
{-| `Mark.verify` lets you put constraints on a block.
Let's say you don't just want a `Mark.string`, you actually want a date.
So, you install the [`ISO8601`](https://package.elm-lang.org/packages/rtfeldman/elm-iso8601-date-strings/latest/) and you write something that looks like:
import Iso8601
import Mark
import Mark.Error
import Time
date : Mark.Block Time.Posix
date =
Mark.verify
(\str ->
str
|> Iso8601.toTime
|> Result.mapError
(\_ -> illformatedDateMessage)
)
Mark.string
illformatedDateMessage =
Mark.Error.custom
{ title = "Bad Date"
, message =
[ "I was trying to parse a date, but this format looks off.\n\n"
, "Dates should be in ISO 8601 format:\n\n"
, "YYYY-MM-DDTHH:mm:ss.SSSZ"
]
}
Now you can use `date` whever you actually want dates and the error message will be shown if something goes wrong.
More importantly, you now know if a document parses successfully, that all your dates are correctly formatted.
`Mark.verify` is a very nice way to extend your markup however you'd like.
You could use it to
- add units to numbers
- parse a custom format, like [Latex mathematical equations](https://en.wikibooks.org/wiki/LaTeX/Mathematics#Operators)
- ensure that numbers are between a range or are always positive.
How exciting! Seriously, I think this is pretty cool.
-}
verify : (a -> Result Mark.Error.Custom b) -> Block a -> Block b
verify fn (Block details) =
Block
{ kind = details.kind
, expect = details.expect
, parser =
\ctxt seed ->
details.parser ctxt seed
|> Tuple.mapSecond
(\parser ->
parser
|> Parse.withRange
|> Parser.andThen
(\( range, desc ) ->
-- we only care about reporting if applying `fn` was a problem
-- not other errors, which will shake out normally
case details.converter desc of
Outcome.Success a ->
case fn a.data of
Ok new ->
Parser.succeed desc
Err newErr ->
Parser.succeed
(DescribeUnexpected (getId desc)
{ problem = Error.Custom newErr
, range = range
}
)
Outcome.Almost (Recovered err a) ->
case fn a.data of
Ok new ->
Parser.succeed desc
Err newErr ->
Parser.succeed
(DescribeUnexpected (getId desc)
{ problem = Error.Custom newErr
, range = range
}
)
Outcome.Almost (Uncertain x) ->
Parser.succeed desc
Outcome.Failure f ->
Parser.succeed desc
)
)
, converter =
\desc ->
case details.converter desc of
Outcome.Success a ->
case fn a.data of
Ok new ->
Outcome.Success { data = new, attrs = a.attrs }
Err newErr ->
uncertain
{ problem = Error.Custom newErr
-- TODO: Does this mean we need to thread source snippets everywhere to get them here?
, range = startDocRange
}
Outcome.Almost (Recovered err a) ->
case fn a.data of
Ok new ->
Outcome.Almost (Recovered err { data = new, attrs = a.attrs })
Err newErr ->
uncertain
{ problem = Error.Custom newErr
, range = startDocRange
}
Outcome.Almost (Uncertain x) ->
Outcome.Almost (Uncertain x)
Outcome.Failure f ->
Outcome.Failure f
}
{-| -}
documentId : Mark.Edit.Id -> String
documentId (Id.Id str _) =
str
{-| Look up a specific block in your document by id.
-}
lookup : Mark.Edit.Id -> Document meta block -> Parsed -> Outcome (List Mark.Error.Error) (Partial block) block
lookup id doc parsed =
Desc.lookup id doc parsed
|> rewrapOutcome
{-| Get an `Id` associated with a `Block`, which can be used to make updates through `Mark.Edit`.
Mark.withId
(\id str ->
Html.span
[ onClick (Mark.Edit.delete id) ]
[ Html.text str ]
)
Mark.string
-}
withId : (Mark.Edit.Id -> a -> b) -> Block a -> Block b
withId fn (Block details) =
Block
{ kind = details.kind
, converter =
\desc ->
let
id : Id
id =
Desc.getId desc
in
Desc.mapSuccessAndRecovered
(fn id)
(details.converter desc)
, parser = details.parser
, expect = details.expect
}
{-| Get an `Id` associated with a `Block`, which can be used to make updates through `Mark.Edit`.
Mark.string
|> Mark.withAttr
(\str -> ("link", str))
-}
withAttr : (a -> ( String, String )) -> Block a -> Block a
withAttr fn (Block details) =
Block
{ kind = details.kind
, converter =
\desc ->
let
id : Id
id =
Desc.getId desc
in
case details.converter desc of
Outcome.Success deets ->
let
new =
case fn deets.data of
( key, value ) ->
{ name = key
, value = value
, block = id
}
in
Outcome.Success
{ data = deets.data
, attrs = new :: deets.attrs
}
Outcome.Almost (Uncertain x) ->
Outcome.Almost (Uncertain x)
Outcome.Almost (Recovered errs deets) ->
let
new =
case fn deets.data of
( key, value ) ->
{ name = key
, value = value
, block = id
}
in
Outcome.Almost
(Recovered errs
{ data = deets.data
, attrs = new :: deets.attrs
}
)
Outcome.Failure errs ->
Outcome.Failure errs
, parser = details.parser
, expect = details.expect
}
{-| It may be necessary to convert an `Id` to a `String` and back in order attach it as an `Html.Attributes.id` and read it back.
See the editor example for more details.
**Note** be aware that the actual string format of an `Id` is an implementation detail and may change even on patch releases of a library.
-}
idToString : Mark.Edit.Id -> String
idToString =
Id.toString
{-| -}
stringToId : String -> Maybe Mark.Edit.Id
stringToId =
Id.fromString
{-| Parsing any given `Block` can fail.
However sometimes we don't want the _whole document_ to be unable to render just because there was a small error somewhere.
So, we need some way to say "Hey, if you run into an issue, here's a placeholder value to use."
And that's what `Mark.onError` does.
Mark.int
|> Mark.onError 5
This means if we fail to parse an integer (let's say we added a decimal), that this block would still be renderable with a default value of `5`.
**Note** If there _is_ an error that is fixed using `onError`, we'll get a [`Partial`](#Partial) when we render the document. This will let us see the _full rendered document_, but also see the _error_ that actually occurred.
-}
onError : a -> Block a -> Block a
onError newValue (Block details) =
Block
{ kind = details.kind
, expect = details.expect
, parser = details.parser
, converter =
\desc ->
case details.converter desc of
Outcome.Success a ->
Outcome.Success a
Outcome.Almost (Recovered err a) ->
Outcome.Almost (Recovered err a)
Outcome.Almost (Uncertain x) ->
Outcome.Almost
(Recovered x { data = newValue, attrs = [] })
Outcome.Failure f ->
Outcome.Failure f
}
{-| A named block.
Mark.block "MyBlock"
Html.text
Mark.string
Will parse the following and render it using `Html.text`
|> MyBlock
Here is an unformatted string!
**Note** block names should be capitalized. In the future this may be enforced.
-}
block : String -> (child -> result) -> Block child -> Block result
block name view child =
Block
{ kind = Named name
, expect = ExpectBlock name (getBlockExpectation child)
, converter =
\desc ->
case desc of
DescribeBlock details ->
if details.name == name then
renderBlock child details.found
|> mapSuccessAndRecovered view
else
-- This is not the block that was expected.
Outcome.Failure NoMatch
_ ->
Outcome.Failure NoMatch
, parser =
\context seed ->
let
( newSeed, childParser ) =
getParser context (Id.indent seed) child
( parentId, parentSeed ) =
Id.step seed
( errorId, finalSeed ) =
Id.step parentSeed
in
( finalSeed
, Parser.map
(\result ->
case result of
Ok details ->
DescribeBlock
{ found = details.value
, name = name
, id = parentId
}
Err details ->
DescribeBlock
{ name = name
, id = parentId
, found =
DescribeUnexpected errorId
{ range = details.range
, problem = details.error
}
}
)
<|
Parse.withRangeResult
(Parse.withIndent
(\indentation ->
Parser.succeed identity
|. Parser.keyword
(Parser.Token name
(ExpectingBlockName name)
)
|. Parser.chompWhile (\c -> c == ' ')
|. Parse.skipBlankLineWith ()
|= Parser.oneOf
[ (Parser.succeed identity
|= Parse.getPosition
|. Parser.token
(Parser.Token
(String.repeat (indentation + 4) " ")
(ExpectingIndentation (indentation + 4))
)
)
|> Parser.andThen
(\start ->
Parser.oneOf
-- ALERT: This first parser will fail because `Parse.raggedIndentedStringAbove`
-- expects indentation for the first line.
[ Parser.succeed
(\end ->
Err (Error.ExpectingIndent (indentation + 4))
)
|. Parser.chompIf (\c -> c == ' ') Space
|. Parser.chompWhile (\c -> c == ' ')
|= Parse.getPosition
|. Parser.loop "" (Parse.raggedIndentedStringAbove indentation)
, Parser.map Ok <|
Parser.withIndent
(indentation + 4)
(Parser.inContext (InBlock name) childParser)
]
)
-- If we're here, it's because the indentation failed.
-- If the child parser failed in some way, it would
-- take care of that itself by returning Unexpected
, Parser.succeed
(Err (Error.ExpectingIndent (indentation + 4)))
|. Parser.loop "" (Parse.raggedIndentedStringAbove indentation)
]
)
)
)
}
{-| -}
startWith :
(start -> rest -> result)
-> Block start
-> Block rest
-> Block result
startWith fn startBlock endBlock =
Block
{ kind = Value
, expect = ExpectStartsWith (getBlockExpectation startBlock) (getBlockExpectation endBlock)
, converter =
\desc ->
case desc of
StartsWith details ->
Desc.mergeWithAttrs fn
(Desc.renderBlock startBlock details.first)
(Desc.renderBlock endBlock details.second)
_ ->
Outcome.Failure NoMatch
, parser =
\context seed ->
let
( parentId, newSeed ) =
Id.step seed
( startSeed, startParser ) =
getParser ParseBlock newSeed startBlock
( remainSeed, endParser ) =
getParser ParseBlock startSeed endBlock
in
( remainSeed
, Parser.succeed
(\( range, ( begin, end ) ) ->
StartsWith
{ id = parentId
, first = begin
, second = end
}
)
|= Parse.withRange
(Parser.succeed Tuple.pair
|= startParser
|. Parser.loop 0 manyBlankLines
|= endParser
)
)
}
{-| Skip all blank lines.
-}
manyBlankLines lineCount =
Parser.oneOf
[ Parse.skipBlankLineWith (Parser.Loop (lineCount + 1))
, Parser.succeed (Parser.Done ())
]
{-| -}
oneOf : List (Block a) -> Block a
oneOf blocks =
let
expectations =
List.map getBlockExpectation blocks
in
Block
{ kind = Value
, expect = ExpectOneOf expectations
, converter =
\desc ->
Desc.findMatch desc blocks
, parser =
Parse.oneOf blocks expectations
}
{-| Many blocks that are all at the same indentation level.
-}
manyOf : List (Block a) -> Block (List a)
manyOf blocks =
let
expectations =
List.map getBlockExpectation blocks
in
Block
{ kind = Value
, expect = ExpectManyOf expectations
, converter =
\desc ->
let
getRendered id existingResult children =
case children of
[] ->
mapSuccessAndRecovered List.reverse existingResult
top :: remain ->
getRendered id
(Desc.mergeWithAttrs (::)
(Desc.findMatch top blocks)
existingResult
)
remain
in
case desc of
Group many ->
getRendered many.id
(Outcome.Success { data = [], attrs = [] })
many.children
_ ->
Outcome.Failure NoMatch
, parser =
\context seed ->
let
( parentId, newSeed ) =
Id.step seed
indentedSeed : Seed
indentedSeed =
Id.indent seed
in
( newSeed
, Parser.succeed
(\children ->
Group
{ id = parentId
, children = children
}
)
|= Parse.withIndent
(\indentation ->
Parser.loop
{ parsedSomething = False
, found = []
, seed =
indentedSeed
}
(Parse.manyOf indentation blocks)
)
)
}
{-| -}
type Icon
= Bullet
| Number
{-| -}
type Enumerated item
= Enumerated
{ icon : Icon
, items : List (Item item)
}
{-| **Note** `index` is our position within the nested list.
The first `Int` in the tuple is our current position in the current sub list.
The `List Int` that follows are the indices for the parent list.
For example, given this list
```markup
|> List
1. First element
-- Second Element
1. Element #2.1
-- Element #2.1.1
-- Element #2.2
-- Third Element
```
here are the indices:
```markup
1. (1, [])
-- (2, [])
1. (1, [2])
-- (1, [1,2])
-- (2, [2])
-- (3, [])
```
-}
type Item item
= Item
{ index : ( Int, List Int )
, content : List item
, children : Enumerated item
}
{-| Would you believe that a markdown list is actually a tree?
Here's an example of a nested list in `elm-markup`:
```markup
|> List
1. This is definitely the first thing.
With some additional content.
--- Another thing.
And some more content
1. A sublist
With it's content
And some other content
--- Second item
```
**Note** As before, the indentation is always a multiple of 4.
In `elm-markup` you can make a nested section either `Bulleted` or `Numbered` by having the first element of the section start with `-` or `1.`.
The rest of the icons at that level are ignored. So this:
|> List
1. First
-- Second
-- Third
Is a numbered list. And this:
|> List
-- First
1. sublist one
-- sublist two
-- sublist three
-- Second
-- Third
is a bulleted list with a numbered list inside of it.
**Note** You can use as many dashes(`-`) as you want to start an item. This can be useful to make the indentation match up. Similarly, you can also use spaces after the dash or number.
Here's how to render the above list:
import Mark
myTree =
Mark.tree "List" renderList text
-- Note: we have to define this as a separate function because
-- `Items` and `Node` are a pair of mutually recursive data structures.
-- It's easiest to render them using two separate functions:
-- renderList and renderItem
renderList (Mark.Enumerated list) =
let
group =
case list.icon of
Mark.Bullet ->
Html.ul
Mark.Number ->
Html.ol
in
group []
(List.map renderItem list.items)
renderItem (Mark.Item item) =
Html.li []
[ Html.div [] item.content
, renderList item.children
]
-}
tree :
(Enumerated item -> result)
-> Block item
-> Block result
tree view contentBlock =
let
blockExpectation =
getBlockExpectation contentBlock
expectation : Expectation
expectation =
ExpectTree blockExpectation
in
Block
{ kind = Value
, expect = expectation
, converter =
\description ->
case description of
Group details ->
details.children
|> reduceRender Index.zero
getItemIcon
(renderTreeNodeSmall contentBlock)
|> (\( _, icon, outcome ) ->
case outcome of
Outcome.Success nodes ->
Outcome.Success
{ data =
view
(Enumerated
{ icon =
case icon of
Desc.Bullet ->
Bullet
Desc.AutoNumber _ ->
Number
, items = List.map .data nodes
}
)
, attrs = List.concatMap .attrs nodes
}
Outcome.Almost (Uncertain u) ->
Outcome.Almost (Uncertain u)
Outcome.Almost (Recovered errs nodes) ->
Outcome.Almost
(Recovered errs
{ data =
view
(Enumerated
{ icon =
case icon of
Desc.Bullet ->
Bullet
Desc.AutoNumber _ ->
Number
, items = List.map .data nodes
}
)
, attrs = List.concatMap .attrs nodes
}
)
Outcome.Failure f ->
Outcome.Failure f
)
_ ->
Outcome.Failure Error.NoMatch
, parser =
\context seed ->
let
( newId, newSeed ) =
Id.step seed
indentedSeed : Seed
indentedSeed =
Id.indent seed
in
( newSeed
, Parse.withIndent
(\baseIndent ->
let
( secondSeed, itemParser ) =
getParser context indentedSeed contentBlock
in
Parser.map
(\( pos, builtTree ) ->
Group
{ id = newId
, children = builtTree
}
)
(Parse.withRange
(parseTree baseIndent secondSeed contentBlock itemParser)
)
)
)
}
parseTree baseIndent seed contentBlock itemParser =
(Parser.succeed
(\start icon item end ->
{ start = start
, end = end
, item = item
, icon = icon
}
)
|= Parse.getPosition
|= Parse.iconParser
|= itemParser
|= Parse.getPosition
)
|> Parser.andThen
(\details ->
let
( startId, startSeed ) =
Id.step seed
in
Parser.loop
( { base = baseIndent
, prev = baseIndent
, seed = startSeed
}
, { previouslyAdded = Parse.AddedItem
, captured = []
, stack =
[ { start = details.start
, description =
DescribeItem
{ id = startId
, icon = details.icon
, content =
[ details.item
]
, children = []
}
}
]
}
)
(Parse.fullTree
ParseInTree
contentBlock
)
)
getItemIcon desc =
case desc of
DescribeItem item ->
item.icon
_ ->
Desc.Bullet
{-| -}
renderTreeNodeSmall :
Block item
-> Desc.Icon
-> Index.Index
-> Description
-> Desc.BlockOutcome (Item item)
renderTreeNodeSmall contentBlock icon index found =
case found of
DescribeItem item ->
let
( newIndex, childrenIcon, renderedChildren ) =
reduceRender (Index.indent index)
getItemIcon
(renderTreeNodeSmall contentBlock)
item.children
( contentIndex, _, renderedContent ) =
reduceRender (Index.dedent newIndex)
(always Desc.Bullet)
(\icon_ i foundItem ->
renderBlock contentBlock foundItem
)
item.content
in
Desc.mergeListWithAttrs
(\content children ->
Item
{ index = Index.toList index
, content = content
, children =
Enumerated
{ icon =
case childrenIcon of
Desc.Bullet ->
Bullet
Desc.AutoNumber _ ->
Number
, items =
children
}
}
)
renderedContent
renderedChildren
_ ->
Outcome.Failure Error.NoMatch
reduceRender :
Index.Index
-> (thing -> Desc.Icon)
-> (Desc.Icon -> Index.Index -> thing -> Outcome.Outcome Error.AstError (Uncertain other) other)
-> List thing
-> ( Index.Index, Desc.Icon, Outcome.Outcome Error.AstError (Uncertain (List other)) (List other) )
reduceRender index getIcon fn list =
list
|> List.foldl
(\item ( i, existingIcon, gathered ) ->
let
icon =
if Index.top i == 0 then
getIcon item
else
existingIcon
newItem =
case gathered of
Outcome.Success remain ->
case fn icon i item of
Outcome.Success newThing ->
Outcome.Success (newThing :: remain)
Outcome.Almost (Uncertain err) ->
Outcome.Almost (Uncertain err)
Outcome.Almost (Recovered err data) ->
Outcome.Almost
(Recovered err
(data :: remain)
)
Outcome.Failure f ->
Outcome.Failure f
almostOrfailure ->
almostOrfailure
in
( Index.increment i
, icon
, newItem
)
)
( index, Desc.Bullet, Outcome.Success [] )
|> (\( i, ic, outcome ) ->
( i, ic, Outcome.mapSuccess List.reverse outcome )
)
{-| -}
type alias Index =
List Int
{- TEXT BLOCKS -}
{-| -}
type alias Styles =
{ bold : Bool
, italic : Bool
, strike : Bool
}
{-| One of the first things that's interesting about a markup language is how to handle _styled text_.
In `elm-markup` there are only a limited number of special characters for formatting text.
- `/italic/` results in _italics_
- `*bold*` results in **bold**
- and `~strike~` results in ~~strike~~
Here's an example of how to convert markup text into `Html` using `Mark.text`:
Mark.text
(\styles string ->
Html.span
[ Html.Attributes.classList
[ ( "bold", styles.bold )
, ( "italic", styles.italic )
, ( "strike", styles.strike )
]
]
[ Html.text string ]
)
Though you might be thinking that `bold`, `italic`, and `strike` are not nearly enough!
And you're right, this is just to get you started. Your next stop is [`Mark.textWith`](#textWith), which is more involved to use but can represent everything you're used to having in a markup language.
**Note:** Text blocks stop when two consecutive newline characters are encountered.
-}
text :
(Styles -> String -> text)
-> Block (List text)
text view =
textWith
{ view = view
, inlines = []
, replacements = commonReplacements
}
{-| -}
type alias Selection =
{ anchor : Offset
, focus : Offset
}
{-| -}
type alias Offset =
Int
{-| Handling formatted text is a little more involved than may be initially apparent, but have no fear!
`textWith` is where a lot of things come together. Let's check out what these fields actually mean.
- `view` is the function to render an individual fragment of text.
- This is mostly what [`Mark.text`](#text) does, so it should seem familiar.
- `replacements` will replace characters before rendering.
- For example, we can replace `...` with the real ellipses unicode character, `…`.
- `inlines` are custom inline blocks. You can use these to render things like links or emojis :D.
-}
textWith :
{ view :
Styles
-> String
-> rendered
, replacements : List Replacement
, inlines : List (Record rendered)
}
-> Block (List rendered)
textWith options =
let
inlineRecords =
List.map recordToInlineBlock options.inlines
inlineExpectations =
List.map
(\(ProtoRecord rec) ->
ExpectInlineBlock
{ name = rec.name
, kind =
blockKindToSelection rec.blockKind
, fields = rec.expectations
}
)
options.inlines
in
Block
{ kind = Value
, expect = ExpectTextBlock inlineExpectations
, converter =
renderText
{ view = always options.view
, inlines = inlineRecords
}
, parser =
\context seed ->
let
-- Note #1 - seed for styled text is advanced here
-- instead of within the styledText parser
( _, newSeed ) =
Id.step seed
in
( newSeed
, Parse.getPosition
|> Parser.andThen
(\pos ->
Parse.styledText
{ inlines = List.map (\x -> x Desc.EmptyAnnotation) inlineRecords
, replacements = options.replacements
}
context
seed
pos
emptyStyles
)
)
}
recordToInlineBlock (Desc.ProtoRecord details) annotationType =
let
expectations : Expectation
expectations =
Desc.ExpectRecord details.name
details.expectations
in
Desc.Block
{ kind = details.blockKind
, expect = expectations
, converter =
\desc ->
case details.fieldConverter desc annotationType of
Outcome.Success fields ->
case fields.data of
( fieldDescriptions, rendered ) ->
Outcome.Success
{ data = rendered
, attrs = fields.attrs
}
Outcome.Failure fail ->
Outcome.Failure fail
Outcome.Almost (Desc.Uncertain e) ->
Outcome.Almost (Desc.Uncertain e)
Outcome.Almost (Desc.Recovered e fields) ->
case fields.data of
( fieldDescriptions, rendered ) ->
Outcome.Almost
(Desc.Recovered e
{ data = rendered
, attrs = fields.attrs
}
)
, parser =
\context seed ->
let
( parentId, parentSeed ) =
Id.step seed
( newSeed, fields ) =
Id.thread (Id.indent seed) (List.foldl (\f ls -> f ParseInline :: ls) [] details.fields)
( failureId, finalSeed ) =
Id.step newSeed
in
( finalSeed
, Parse.record Parse.InlineRecord
parentId
failureId
details.name
expectations
fields
)
}
type alias Cursor data =
{ outcome : BlockOutcome data
, lastOffset : Int
}
type alias TextOutcome data =
Outcome.Outcome Error.AstError (Uncertain data) data
renderText :
{ view :
{ id : Id
, selection : Selection
}
-> Styles
-> String
-> rendered
, inlines : List (InlineSelection -> Block rendered)
}
-> Description
-> BlockOutcome (List rendered)
renderText options description =
case description of
DescribeText details ->
let
outcome =
details.text
|> List.foldl (convertTextDescription details.id options)
{ outcome = Outcome.Success { data = [], attrs = [] }
, lastOffset = 0
}
|> .outcome
in
outcome
|> mapSuccessAndRecovered List.reverse
_ ->
Outcome.Failure Error.NoMatch
convertTextDescription :
Id
->
{ view :
{ id : Id
, selection : Selection
}
-> Styles
-> String
-> rendered
, inlines : List (InlineSelection -> Block rendered)
}
-> TextDescription
-> Cursor (List rendered)
-> Cursor (List rendered)
convertTextDescription id options comp cursor =
let
blockLength : Int
blockLength =
length comp
in
case comp of
Styled (Desc.Text styling str) ->
{ outcome =
Desc.mergeWithAttrs (::)
(Outcome.Success
{ data =
options.view
{ id = id
, selection =
{ anchor = cursor.lastOffset
, focus = cursor.lastOffset + blockLength
}
}
styling
str
, attrs = []
}
)
cursor.outcome
, lastOffset =
cursor.lastOffset + blockLength
}
InlineBlock details ->
case details.record of
DescribeUnexpected unexpId unexpDetails ->
{ outcome =
Desc.uncertain unexpDetails
, lastOffset = cursor.lastOffset + blockLength
}
_ ->
let
recordName =
Desc.recordName details.record
|> Maybe.withDefault ""
matchInlineName name almostInlineBlock maybeFound =
case maybeFound of
Nothing ->
let
(Block inlineDetails) =
almostInlineBlock details.kind
in
if matchKinds details inlineDetails.kind then
Just inlineDetails
else
Nothing
_ ->
maybeFound
maybeMatched =
List.foldl
(matchInlineName recordName)
Nothing
options.inlines
in
case maybeMatched of
Nothing ->
{ outcome =
Outcome.Failure NoMatch
, lastOffset = cursor.lastOffset + blockLength
}
Just matched ->
{ outcome =
Desc.mergeWithAttrs (::)
(matched.converter details.record)
cursor.outcome
, lastOffset = cursor.lastOffset + blockLength
}
matchKinds inline blockKind =
let
recordName =
case inline.record of
Record rec ->
Just rec.name
_ ->
Nothing
in
case ( recordName, inline.kind, blockKind ) of
( Just inlineName, SelectString str, VerbatimNamed vertName ) ->
inlineName == vertName
( Just inlineName, SelectText _, AnnotationNamed annName ) ->
inlineName == annName
( Just inlineName, EmptyAnnotation, Named name ) ->
inlineName == name
_ ->
False
{-| -}
type alias Replacement =
Parse.Replacement
{-| An annotation is some _styled text_, a _name_, and zero or more _attributes_.
So, we can make a `link` that looks like this in markup:
```markup
Here is my [*cool* sentence]{link| url = website.com }.
```
and rendered in elm-land via:
link =
Mark.annotation "link"
(\id styles url ->
Html.a
[ Html.Attributes.href url ]
(List.map renderStyles styles)
)
|> Mark.field "url" Mark.string
-}
annotation : String -> (Id -> List ( Styles, String ) -> result) -> Record result
annotation name view =
Desc.ProtoRecord
{ name = name
, blockKind = Desc.AnnotationNamed name
, expectations = []
, fieldConverter =
\desc selected ->
case desc of
Desc.Record details ->
if details.name == name then
Outcome.Success
{ data =
( details.found
, view (getId desc) (selectedText selected)
)
, attrs = []
}
else
Outcome.Failure NoMatch
_ ->
Outcome.Failure NoMatch
, fields = []
}
selectedText sel =
case sel of
EmptyAnnotation ->
[]
SelectText txts ->
List.map textToTuple txts
SelectString _ ->
[]
textToTuple (Desc.Text style str) =
( style, str )
selectedString sel =
case sel of
EmptyAnnotation ->
""
SelectText txts ->
""
SelectString str ->
str
{-| A `verbatim` annotation is denoted by backticks(\`) and allows you to capture a literal string.
Just like `annotation`, a `verbatim` can have a name and attributes attached to it.
Let's say we wanted to embed an inline piece of elm code. We could write
inlineElm =
Mark.verbatim "elm"
(\id str ->
Html.span
[ Html.Attributes.class "elm-code" ]
[ Html.text str ]
)
Which would capture the following
Here's an inline function: `\you -> Awesome`{elm}.
**Note** A verbatim can be written without a name or attributes and will capture the contents as a literal string, ignoring any special characters.
Let's take a look at `http://elm-lang.com`.
-}
verbatim : String -> (Id -> String -> result) -> Record result
verbatim name view =
Desc.ProtoRecord
{ name = name
, blockKind = Desc.VerbatimNamed name
, expectations = []
, fieldConverter =
\desc selected ->
case desc of
Desc.Record details ->
if details.name == name then
Outcome.Success
{ attrs = []
, data = ( details.found, view (getId desc) (selectedString selected) )
}
else
Outcome.Failure NoMatch
_ ->
Outcome.Failure NoMatch
, fields = []
}
{- PRIMITIVE BLOCKS -}
{-| This will capture a multiline string.
For example:
Mark.block "Poem"
(\str -> str)
Mark.string
will capture
```markup
|> Poem
Whose woods these are I think I know.
His house is in the village though;
He will not see me stopping here
To watch his woods fill up with snow.
```
Where `str` in the above function will be
"""Whose woods these are I think I know.
His house is in the village though;
He will not see me stopping here
To watch his woods fill up with snow."""
**Note** If you're looking for styled text, you probably want [`Mark.text`](#text) or [`Mark.textWith`](#textWith).
-}
string : Block String
string =
Block
{ kind = Value
, expect = ExpectString "REPLACE"
, converter =
\desc ->
case desc of
DescribeString id str ->
Outcome.Success { data = String.trim str, attrs = [] }
_ ->
Outcome.Failure NoMatch
, parser =
\context seed ->
let
( id, newSeed ) =
Id.step seed
in
( newSeed
, case context of
ParseInline ->
Parser.succeed
(\start str end ->
DescribeString id
(String.trim str)
)
|= Parse.getPosition
|= Parser.getChompedString
(Parser.chompWhile
(\c -> c /= '\n' && c /= ',' && c /= '}')
)
|= Parse.getPosition
ParseBlock ->
Parser.map
(\( pos, str ) ->
DescribeString id str
)
(Parse.withRange
(Parse.withIndent
(\indentation ->
Parser.loop "" (Parse.indentedString indentation)
)
)
)
ParseInTree ->
Parser.map
(DescribeString id)
(Parse.withIndent
(\indentation ->
Parser.loop "" (Parse.indentedString indentation)
)
)
)
}
{-| Capture either `True` or `False`.
`elm-markup` doesn't infer truthiness in other values, so it needs to be exactly `True` or `False`.
-}
bool : Block Bool
bool =
Block
{ kind = Value
, expect = ExpectBoolean False
, converter =
\desc ->
case desc of
DescribeBoolean details ->
Outcome.Success
{ data = details.found
, attrs = []
}
_ ->
Outcome.Failure NoMatch
, parser =
\context seed ->
let
( id, newSeed ) =
Id.step seed
in
( newSeed
, Parser.map
(\boolResult ->
case boolResult of
Err err ->
DescribeUnexpected id
{ range = err.range
, problem = Error.BadBool
}
Ok details ->
DescribeBoolean
{ id = id
, found = details.value
}
)
(Parse.withRangeResult
(Parser.oneOf
[ Parser.token (Parser.Token "True" (Expecting "True"))
|> Parser.map (always (Ok True))
, Parser.token (Parser.Token "False" (Expecting "False"))
|> Parser.map (always (Ok False))
, Parser.map Err Parse.word
]
)
)
)
}
{-| -}
int : Block Int
int =
Block
{ kind = Value
, converter =
\desc ->
case desc of
DescribeInteger details ->
Outcome.Success
{ data = details.found
, attrs = []
}
_ ->
Outcome.Failure NoMatch
, expect = ExpectInteger 0
, parser =
\context seed ->
let
( id, newSeed ) =
Id.step seed
in
( newSeed
, Parse.int id
)
}
{-| -}
float : Block Float
float =
Block
{ kind = Value
, converter =
\desc ->
case desc of
DescribeFloat details ->
Outcome.Success
{ data = Tuple.second details.found
, attrs = []
}
_ ->
Outcome.Failure NoMatch
, expect = ExpectFloat 0
, parser =
\context seed ->
let
( id, newSeed ) =
Id.step seed
in
( newSeed
, Parse.float id
)
}
{- Parser Heleprs -}
{-| This is a set of common character replacements with some typographical niceties.
- `...` is converted to the ellipses unicode character(`…`).
- `"` Straight double quotes are [replaced with curly quotes](https://practicaltypography.com/straight-and-curly-quotes.html) (`“`, `”`)
- `'` Single Quotes are replaced with apostrophes(`’`).
- `--` is replaced with an [en-dash(`–`)](https://practicaltypography.com/hyphens-and-dashes.html).
- `---` is replaced with an [em-dash(`—`)](https://practicaltypography.com/hyphens-and-dashes.html).
- `<>` also known as "glue", will create a non-breaking space (` `). This is not for manually increasing space (sequential `<>` tokens will only render as one ` `), but to signify that the space between two words shouldn't break when wrapping. Like glueing two words together!
**Note** this is included by default in `Mark.text`
-}
commonReplacements : List Replacement
commonReplacements =
[ Parse.Replacement "..." "…"
, Parse.Replacement "<>" "\u{00A0}"
, Parse.Replacement "---" "—"
, Parse.Replacement "--" "–"
, Parse.Replacement "'" "’"
, Parse.Balanced
{ start = ( "\"", "“" )
, end = ( "\"", "”" )
}
]
{-| Replace a string with another string. This can be useful to have shortcuts to unicode characters.
For example, we could use this to replace `...` with the unicode ellipses character: `…`.
-}
replacement : String -> String -> Replacement
replacement =
Parse.Replacement
{-| A balanced replacement. This is used for replacing parentheses or to do auto-curly quotes.
Mark.balanced
{ start = ( "\"", "“" )
, end = ( "\"", "”" )
}
-}
balanced :
{ start : ( String, String )
, end : ( String, String )
}
-> Replacement
balanced =
Parse.Balanced
{- RECORDS -}
{-| -}
type alias Record a =
Desc.Record a
{-| Parse a record with any number of fields.
Mark.record "Image"
(\src description ->
Html.img
[ Html.Attributes.src src
, Html.Attributes.alt description
]
[]
)
|> Mark.field "src" Mark.string
|> Mark.field "description" Mark.string
|> Mark.toBlock
would parse the following markup:
```markup
|> Image
src = http://placekitten/200/500
description = What a cutie.
```
Fields can be in any order in the markup. Also, by convention field names should be `camelCase`. This might be enforced in the future.
-}
record : String -> data -> Record data
record name view =
Desc.ProtoRecord
{ name = name
, blockKind = Desc.Named name
, expectations = []
, fieldConverter =
\desc ann ->
case desc of
Desc.Record details ->
if details.name == name && ann == Desc.EmptyAnnotation then
Outcome.Success { data = ( details.found, view ), attrs = [] }
else
Outcome.Failure NoMatch
_ ->
Outcome.Failure NoMatch
, fields = []
}
{-| -}
field : String -> Block value -> Record (value -> result) -> Record result
field name value (Desc.ProtoRecord details) =
let
newField =
Field name value
in
Desc.ProtoRecord
{ name = details.name
, blockKind = details.blockKind
, expectations = fieldExpectation newField :: details.expectations
, fieldConverter =
\desc ann ->
case details.fieldConverter desc ann of
Outcome.Success fields ->
case fields.data of
( fieldDescriptions, rendered ) ->
case getField newField fieldDescriptions of
Just outcome ->
mapSuccessAndRecovered
(\myField ->
( fieldDescriptions
, rendered myField
)
)
outcome
Nothing ->
Desc.uncertain
{ problem = Error.MissingFields [ fieldName newField ]
, range = Desc.emptyRange
}
Outcome.Almost (Desc.Recovered e fields) ->
case fields.data of
( fieldDescriptions, rendered ) ->
case getField newField fieldDescriptions of
Just outcome ->
mapSuccessAndRecovered
(\myField ->
( fieldDescriptions
, rendered myField
)
)
outcome
Nothing ->
Desc.uncertain
{ problem = Error.MissingFields [ fieldName newField ]
, range = Desc.emptyRange
}
Outcome.Failure fail ->
Outcome.Failure fail
Outcome.Almost (Desc.Uncertain e) ->
Outcome.Almost (Desc.Uncertain e)
, fields =
fieldParser newField :: details.fields
}
fieldName (Field name _) =
name
{-| TODO: convert to recursion
-}
getField :
Field value
-> List ( String, Desc.Description )
-> Maybe (Desc.BlockOutcome value)
getField (Field name fieldBlock) fields =
List.foldl (matchField name fieldBlock) Nothing fields
matchField :
String
-> Block value
-> ( String, Desc.Description )
-> Maybe (Desc.BlockOutcome value)
-> Maybe (Desc.BlockOutcome value)
matchField targetName targetBlock ( name, description ) existing =
case existing of
Just _ ->
existing
Nothing ->
if name == targetName then
Just (Desc.renderBlock targetBlock description)
else
existing
{-| Convert a `Record` to a `Block`.
-}
toBlock : Record a -> Block a
toBlock (Desc.ProtoRecord details) =
let
expectations : Expectation
expectations =
Desc.ExpectRecord details.name
details.expectations
in
Desc.Block
{ kind = details.blockKind
, expect = expectations
, converter =
\desc ->
case details.fieldConverter desc Desc.EmptyAnnotation of
Outcome.Success fields ->
case fields.data of
( fieldDescriptions, rendered ) ->
Outcome.Success { data = rendered, attrs = fields.attrs }
Outcome.Failure fail ->
Outcome.Failure fail
Outcome.Almost (Desc.Uncertain e) ->
Outcome.Almost (Desc.Uncertain e)
Outcome.Almost (Desc.Recovered e fields) ->
case fields.data of
( fieldDescriptions, rendered ) ->
Outcome.Almost
(Desc.Recovered e
{ data = rendered
, attrs = fields.attrs
}
)
, parser =
\context seed ->
let
( parentId, parentSeed ) =
Id.step seed
( newSeed, fields ) =
Id.thread (Id.indent seed)
(List.foldl (\f ls -> f ParseBlock :: ls) [] details.fields)
( failureId, finalSeed ) =
Id.step newSeed
in
( parentSeed
, Parse.record Parse.BlockRecord
parentId
failureId
details.name
expectations
fields
)
}
{-| -}
type Field value
= Field String (Block value)
fieldParser :
Field value
-> Desc.ParseContext
-> Id.Seed
-> ( Id.Seed, ( String, Parser Context Problem ( String, Desc.Description ) ) )
fieldParser (Field name myBlock) context seed =
let
( newSeed, blockParser ) =
Desc.getParser context seed myBlock
in
( newSeed
, ( name
, fieldContentParser
name
blockParser
)
)
fieldExpectation (Field name fieldBlock) =
( name, Desc.getBlockExpectation fieldBlock )
fieldContentParser : String -> Parser Error.Context Error.Problem Desc.Description -> Parser Error.Context Error.Problem ( String, Desc.Description )
fieldContentParser name parser =
Parse.withIndent
(\indentation ->
Parser.map
(\( pos, description ) ->
( name, description )
)
(Parse.withRange
(Parser.oneOf
[ Parser.withIndent (indentation + 4) (Parser.inContext (InRecordField name) parser)
, Parser.succeed identity
|. Parser.chompWhile (\c -> c == '\n')
|. Parser.token (Parser.Token (String.repeat (indentation + 4) " ") (ExpectingIndentation indentation))
|= Parser.withIndent (indentation + 4) (Parser.inContext (InRecordField name) parser)
]
)
)
)
| 4069 | module Mark exposing
( Document, document, documentWith
, Block, block
, string, int, float, bool
, Styles, text, textWith
, Replacement, commonReplacements, replacement, balanced
, annotation, verbatim
, Record, record, field, toBlock
, oneOf, manyOf
, tree, Enumerated(..), Item(..), Icon(..)
, Outcome(..), Partial
, metadata, compile, parse, Parsed, toString, render
, map, verify, onError
, withId, withAttr, documentId, idToString, stringToId
, lookup
)
{-|
# Building Documents
@docs Document, document, documentWith
@docs Block, block
# Primitives
@docs string, int, float, bool
# Text
@docs Styles, text, textWith
# Text Replacements
@docs Replacement, commonReplacements, replacement, balanced
# Text Annotations
Along with basic [`styling`](#text) and [`replacements`](#replacement), we also have a few ways to annotate text.
@docs annotation, verbatim
# Records
@docs Record, record, field, toBlock
# Higher Level
@docs oneOf, manyOf
# Trees
@docs tree, Enumerated, Item, Icon
# Rendering
@docs Outcome, Partial
@docs metadata, compile, parse, Parsed, toString, render
# Constraining and Recovering Blocks
@docs map, verify, onError
@docs withId, withAttr, documentId, idToString, stringToId
@docs lookup
-}
import Mark.Edit
import Mark.Error
import Mark.Internal.Description as Desc exposing (..)
import Mark.Internal.Error as Error exposing (AstError(..), Context(..), Problem(..))
import Mark.Internal.Id as Id exposing (..)
import Mark.Internal.Index as Index
import Mark.Internal.Outcome as Outcome
import Mark.Internal.Parser as Parse
import Mark.New exposing (block)
import Parser.Advanced as Parser exposing ((|.), (|=), Parser)
{-| -}
type alias Parsed =
Desc.Parsed
{- INTERFACE -}
{-| -}
toString : Parsed -> String
toString =
Desc.toString
{-| -}
parse : Document metadata data -> String -> Outcome (List Mark.Error.Error) (Partial Parsed) Parsed
parse doc source =
Desc.compile doc source
|> moveParsedToResult
{-| -}
metadata : Document metadata document -> String -> Result Mark.Error.Error metadata
metadata (Desc.Document doc) source =
case Parser.run doc.metadata source of
Ok parsed ->
parsed
|> Result.mapError
(Error.render source)
Err irrecoverableParsingErrors ->
Err (Error.renderParsingErrors source irrecoverableParsingErrors)
moveParsedToResult :
Result
(Outcome.Outcome (List Error.Rendered)
{ errors : List Error.Rendered
, result : data
}
data
)
( Parsed
, Outcome.Outcome (List Error.Rendered)
{ errors : List Error.Rendered
, result : data
}
data
)
-> Outcome (List Mark.Error.Error) (Partial Parsed) Parsed
moveParsedToResult result =
case result of
Ok ( parsed, Outcome.Success success ) ->
Success parsed
Ok ( parsed, Outcome.Almost almost ) ->
Almost
{ errors = almost.errors
, result = parsed
}
Ok ( parsed, Outcome.Failure errors ) ->
Failure errors
Err (Outcome.Success success) ->
Failure []
Err (Outcome.Almost almost) ->
Failure almost.errors
Err (Outcome.Failure fail) ->
Failure fail
{-| -}
render : Document meta data -> Parsed -> Outcome (List Mark.Error.Error) (Partial ( meta, List data )) ( meta, List data )
render doc ((Parsed parsedDetails) as parsed) =
Desc.render doc parsed
|> rewrapOutcome
{-| -}
compile : Document meta data -> String -> Outcome (List Mark.Error.Error) (Partial ( meta, List data )) ( meta, List data )
compile doc source =
Desc.compile doc source
|> flattenErrors
|> rewrapOutcome
flattenErrors result =
case result of
Ok ( parsed, outcome ) ->
outcome
Err outcome ->
outcome
rewrapOutcome : Outcome.Outcome x y z -> Outcome x y z
rewrapOutcome outcome =
case outcome of
Outcome.Success s ->
Success s
Outcome.Almost x ->
Almost x
Outcome.Failure f ->
Failure f
{-| -}
type alias Partial data =
{ errors : List Mark.Error.Error
, result : data
}
{-| -}
startDocRange : Range
startDocRange =
{ start =
{ offset = 0
, line = 1
, column = 1
}
, end =
{ offset = 0
, line = 1
, column = 1
}
}
{-| -}
type alias Document meta data =
Desc.Document meta data
{-| -}
type Outcome failure almost success
= Success success
| Almost almost
| Failure failure
{-| -}
type alias Block data =
Desc.Block data
getUnexpecteds : Description -> List Error.UnexpectedDetails
getUnexpecteds description =
case description of
DescribeBlock details ->
getUnexpecteds details.found
Record details ->
List.concatMap
(Tuple.second >> getUnexpecteds)
details.found
Group many ->
List.concatMap getUnexpecteds many.children
StartsWith details ->
getUnexpecteds details.first
++ getUnexpecteds details.second
DescribeItem details ->
List.concatMap getUnexpecteds details.content
++ List.concatMap getUnexpecteds details.children
-- Primitives
DescribeBoolean details ->
[]
DescribeInteger details ->
[]
DescribeFloat details ->
[]
DescribeText details ->
[]
DescribeString _ str ->
[]
DescribeUnexpected _ details ->
[ details ]
{-| -}
type alias Position =
{ offset : Int
, line : Int
, column : Int
}
{-| -}
type alias Range =
{ start : Position
, end : Position
}
{- BLOCKS -}
{-| Create a markup `Document`. You're first goal is to describe a document in terms of the blocks you're expecting.
Here's an overly simple document that captures one block, a Title, and wraps it in some `Html`
document : Mark.Document (Html msg)
document =
Mark.document
(\title -> Html.main [] [ title ])
(Mark.block "Title"
(Html.h1 [])
Mark.string
)
will parse the following markup:
```markup
|> Title
Here is my title!
```
and ultimately render it as
```html
<main>
<h1>Here is my title!</h1>
</main>
```
-}
document :
List (Block block)
-> Document () block
document blocks =
createDocument (\_ -> "none")
(Parser.succeed (Ok ()))
(map (Tuple.pair ()) (manyOf blocks))
createDocument :
(meta -> String)
-> Parser Error.Context Error.Problem (Result Error.UnexpectedDetails meta)
-> Block ( meta, List data )
-> Document meta data
createDocument toDocumentId meta child =
Document
{ expect =
getBlockExpectation child
, metadata =
meta
, converter =
\(Parsed parsed) ->
Desc.renderBlock child parsed.found
, parser =
Parser.getSource
|> Parser.andThen
(\src ->
let
docId : String
docId =
case Parser.run meta src of
Ok (Ok m) ->
toDocumentId m
_ ->
-- This should return an error!
-- it means the metadata is invalid
""
seed : Seed
seed =
Id.initialSeed docId
( currentSeed, blockParser ) =
Parse.getFailableBlock Desc.ParseBlock seed child
in
Parser.succeed
(\source ( range, value ) ->
Parsed
{ errors =
List.map (Error.render source) (getUnexpecteds value)
, found = value
, expected = getBlockExpectation child
, initialSeed = seed
, currentSeed = currentSeed
, attributes = []
}
)
|. Parser.chompWhile (\c -> c == '\n')
|= Parser.getSource
|= Parse.withRange (Parser.withIndent 0 blockParser)
|. Parser.chompWhile (\c -> c == ' ' || c == '\n')
|. Parser.end End
)
}
{-| Capture some metadata at the start of your document, followed by the body.
import Mark
Mark.documentWith
{ id = \metadata -> metadata.id
, metadata =
Mark.record
(\id author publishedAt ->
{ author = author
, publishedAt = publishedAt
}
)
|> Mark.field "id" Mark.string
|> Mark.field "author" Mark.string
|> Mark.field "publishedAt" Mark.string
, blocks =
[
]
}
**Note** - You can also specify an `id`, which is a document identifier and is included in `Mark.Edit.Id`. This is really only necessary if you're building an editor that can edit multiple documents at once.
Otherwise, feel free to simple put `id = \_ -> "doc"`
-}
documentWith :
{ id : metadata -> String
, metadata : Record metadata
, blocks : List (Block block)
}
-> Document metadata block
documentWith config =
let
metadataBlock =
toBlock config.metadata
in
createDocument config.id
(getMetadataParser metadataBlock)
(startWith
Tuple.pair
metadataBlock
(manyOf config.blocks)
)
getMetadataParser metadataBlock =
let
( _, metadataParser ) =
Parse.getFailableBlock Desc.ParseBlock (Id.initialSeed "") metadataBlock
in
Parser.andThen
(\description ->
case renderBlock metadataBlock description of
Outcome.Success meta ->
Parser.succeed (Ok meta.data)
Outcome.Failure astError ->
Parser.succeed
(Err
{ problem = Error.DocumentMismatch
, range = Desc.emptyRange
}
)
Outcome.Almost (Uncertain ( unexpected, otherUnexpecteds )) ->
Parser.succeed (Err unexpected)
Outcome.Almost (Recovered ( unexpected, otherUnexpecteds ) renderedChild) ->
Parser.succeed (Err unexpected)
)
(Parser.withIndent 0 metadataParser)
{-| Change the result of a block by applying a function to it.
-}
map : (a -> b) -> Block a -> Block b
map fn (Block details) =
Block
{ kind = details.kind
, converter = mapSuccessAndRecovered fn << details.converter
, parser = details.parser
, expect = details.expect
}
{-| -}
type alias CustomError =
{ title : String
, message : List String
}
{-| `Mark.verify` lets you put constraints on a block.
Let's say you don't just want a `Mark.string`, you actually want a date.
So, you install the [`ISO8601`](https://package.elm-lang.org/packages/rtfeldman/elm-iso8601-date-strings/latest/) and you write something that looks like:
import Iso8601
import Mark
import Mark.Error
import Time
date : Mark.Block Time.Posix
date =
Mark.verify
(\str ->
str
|> Iso8601.toTime
|> Result.mapError
(\_ -> illformatedDateMessage)
)
Mark.string
illformatedDateMessage =
Mark.Error.custom
{ title = "Bad Date"
, message =
[ "I was trying to parse a date, but this format looks off.\n\n"
, "Dates should be in ISO 8601 format:\n\n"
, "YYYY-MM-DDTHH:mm:ss.SSSZ"
]
}
Now you can use `date` whever you actually want dates and the error message will be shown if something goes wrong.
More importantly, you now know if a document parses successfully, that all your dates are correctly formatted.
`Mark.verify` is a very nice way to extend your markup however you'd like.
You could use it to
- add units to numbers
- parse a custom format, like [Latex mathematical equations](https://en.wikibooks.org/wiki/LaTeX/Mathematics#Operators)
- ensure that numbers are between a range or are always positive.
How exciting! Seriously, I think this is pretty cool.
-}
verify : (a -> Result Mark.Error.Custom b) -> Block a -> Block b
verify fn (Block details) =
Block
{ kind = details.kind
, expect = details.expect
, parser =
\ctxt seed ->
details.parser ctxt seed
|> Tuple.mapSecond
(\parser ->
parser
|> Parse.withRange
|> Parser.andThen
(\( range, desc ) ->
-- we only care about reporting if applying `fn` was a problem
-- not other errors, which will shake out normally
case details.converter desc of
Outcome.Success a ->
case fn a.data of
Ok new ->
Parser.succeed desc
Err newErr ->
Parser.succeed
(DescribeUnexpected (getId desc)
{ problem = Error.Custom newErr
, range = range
}
)
Outcome.Almost (Recovered err a) ->
case fn a.data of
Ok new ->
Parser.succeed desc
Err newErr ->
Parser.succeed
(DescribeUnexpected (getId desc)
{ problem = Error.Custom newErr
, range = range
}
)
Outcome.Almost (Uncertain x) ->
Parser.succeed desc
Outcome.Failure f ->
Parser.succeed desc
)
)
, converter =
\desc ->
case details.converter desc of
Outcome.Success a ->
case fn a.data of
Ok new ->
Outcome.Success { data = new, attrs = a.attrs }
Err newErr ->
uncertain
{ problem = Error.Custom newErr
-- TODO: Does this mean we need to thread source snippets everywhere to get them here?
, range = startDocRange
}
Outcome.Almost (Recovered err a) ->
case fn a.data of
Ok new ->
Outcome.Almost (Recovered err { data = new, attrs = a.attrs })
Err newErr ->
uncertain
{ problem = Error.Custom newErr
, range = startDocRange
}
Outcome.Almost (Uncertain x) ->
Outcome.Almost (Uncertain x)
Outcome.Failure f ->
Outcome.Failure f
}
{-| -}
documentId : Mark.Edit.Id -> String
documentId (Id.Id str _) =
str
{-| Look up a specific block in your document by id.
-}
lookup : Mark.Edit.Id -> Document meta block -> Parsed -> Outcome (List Mark.Error.Error) (Partial block) block
lookup id doc parsed =
Desc.lookup id doc parsed
|> rewrapOutcome
{-| Get an `Id` associated with a `Block`, which can be used to make updates through `Mark.Edit`.
Mark.withId
(\id str ->
Html.span
[ onClick (Mark.Edit.delete id) ]
[ Html.text str ]
)
Mark.string
-}
withId : (Mark.Edit.Id -> a -> b) -> Block a -> Block b
withId fn (Block details) =
Block
{ kind = details.kind
, converter =
\desc ->
let
id : Id
id =
Desc.getId desc
in
Desc.mapSuccessAndRecovered
(fn id)
(details.converter desc)
, parser = details.parser
, expect = details.expect
}
{-| Get an `Id` associated with a `Block`, which can be used to make updates through `Mark.Edit`.
Mark.string
|> Mark.withAttr
(\str -> ("link", str))
-}
withAttr : (a -> ( String, String )) -> Block a -> Block a
withAttr fn (Block details) =
Block
{ kind = details.kind
, converter =
\desc ->
let
id : Id
id =
Desc.getId desc
in
case details.converter desc of
Outcome.Success deets ->
let
new =
case fn deets.data of
( key, value ) ->
{ name = key
, value = value
, block = id
}
in
Outcome.Success
{ data = deets.data
, attrs = new :: deets.attrs
}
Outcome.Almost (Uncertain x) ->
Outcome.Almost (Uncertain x)
Outcome.Almost (Recovered errs deets) ->
let
new =
case fn deets.data of
( key, value ) ->
{ name = key
, value = value
, block = id
}
in
Outcome.Almost
(Recovered errs
{ data = deets.data
, attrs = new :: deets.attrs
}
)
Outcome.Failure errs ->
Outcome.Failure errs
, parser = details.parser
, expect = details.expect
}
{-| It may be necessary to convert an `Id` to a `String` and back in order attach it as an `Html.Attributes.id` and read it back.
See the editor example for more details.
**Note** be aware that the actual string format of an `Id` is an implementation detail and may change even on patch releases of a library.
-}
idToString : Mark.Edit.Id -> String
idToString =
Id.toString
{-| -}
stringToId : String -> Maybe Mark.Edit.Id
stringToId =
Id.fromString
{-| Parsing any given `Block` can fail.
However sometimes we don't want the _whole document_ to be unable to render just because there was a small error somewhere.
So, we need some way to say "Hey, if you run into an issue, here's a placeholder value to use."
And that's what `Mark.onError` does.
Mark.int
|> Mark.onError 5
This means if we fail to parse an integer (let's say we added a decimal), that this block would still be renderable with a default value of `5`.
**Note** If there _is_ an error that is fixed using `onError`, we'll get a [`Partial`](#Partial) when we render the document. This will let us see the _full rendered document_, but also see the _error_ that actually occurred.
-}
onError : a -> Block a -> Block a
onError newValue (Block details) =
Block
{ kind = details.kind
, expect = details.expect
, parser = details.parser
, converter =
\desc ->
case details.converter desc of
Outcome.Success a ->
Outcome.Success a
Outcome.Almost (Recovered err a) ->
Outcome.Almost (Recovered err a)
Outcome.Almost (Uncertain x) ->
Outcome.Almost
(Recovered x { data = newValue, attrs = [] })
Outcome.Failure f ->
Outcome.Failure f
}
{-| A named block.
Mark.block "MyBlock"
Html.text
Mark.string
Will parse the following and render it using `Html.text`
|> MyBlock
Here is an unformatted string!
**Note** block names should be capitalized. In the future this may be enforced.
-}
block : String -> (child -> result) -> Block child -> Block result
block name view child =
Block
{ kind = Named name
, expect = ExpectBlock name (getBlockExpectation child)
, converter =
\desc ->
case desc of
DescribeBlock details ->
if details.name == name then
renderBlock child details.found
|> mapSuccessAndRecovered view
else
-- This is not the block that was expected.
Outcome.Failure NoMatch
_ ->
Outcome.Failure NoMatch
, parser =
\context seed ->
let
( newSeed, childParser ) =
getParser context (Id.indent seed) child
( parentId, parentSeed ) =
Id.step seed
( errorId, finalSeed ) =
Id.step parentSeed
in
( finalSeed
, Parser.map
(\result ->
case result of
Ok details ->
DescribeBlock
{ found = details.value
, name = <NAME>
, id = parentId
}
Err details ->
DescribeBlock
{ name = <NAME>
, id = parentId
, found =
DescribeUnexpected errorId
{ range = details.range
, problem = details.error
}
}
)
<|
Parse.withRangeResult
(Parse.withIndent
(\indentation ->
Parser.succeed identity
|. Parser.keyword
(Parser.Token name
(ExpectingBlockName name)
)
|. Parser.chompWhile (\c -> c == ' ')
|. Parse.skipBlankLineWith ()
|= Parser.oneOf
[ (Parser.succeed identity
|= Parse.getPosition
|. Parser.token
(Parser.Token
(String.repeat (indentation + 4) " ")
(ExpectingIndentation (indentation + 4))
)
)
|> Parser.andThen
(\start ->
Parser.oneOf
-- ALERT: This first parser will fail because `Parse.raggedIndentedStringAbove`
-- expects indentation for the first line.
[ Parser.succeed
(\end ->
Err (Error.ExpectingIndent (indentation + 4))
)
|. Parser.chompIf (\c -> c == ' ') Space
|. Parser.chompWhile (\c -> c == ' ')
|= Parse.getPosition
|. Parser.loop "" (Parse.raggedIndentedStringAbove indentation)
, Parser.map Ok <|
Parser.withIndent
(indentation + 4)
(Parser.inContext (InBlock name) childParser)
]
)
-- If we're here, it's because the indentation failed.
-- If the child parser failed in some way, it would
-- take care of that itself by returning Unexpected
, Parser.succeed
(Err (Error.ExpectingIndent (indentation + 4)))
|. Parser.loop "" (Parse.raggedIndentedStringAbove indentation)
]
)
)
)
}
{-| -}
startWith :
(start -> rest -> result)
-> Block start
-> Block rest
-> Block result
startWith fn startBlock endBlock =
Block
{ kind = Value
, expect = ExpectStartsWith (getBlockExpectation startBlock) (getBlockExpectation endBlock)
, converter =
\desc ->
case desc of
StartsWith details ->
Desc.mergeWithAttrs fn
(Desc.renderBlock startBlock details.first)
(Desc.renderBlock endBlock details.second)
_ ->
Outcome.Failure NoMatch
, parser =
\context seed ->
let
( parentId, newSeed ) =
Id.step seed
( startSeed, startParser ) =
getParser ParseBlock newSeed startBlock
( remainSeed, endParser ) =
getParser ParseBlock startSeed endBlock
in
( remainSeed
, Parser.succeed
(\( range, ( begin, end ) ) ->
StartsWith
{ id = parentId
, first = begin
, second = end
}
)
|= Parse.withRange
(Parser.succeed Tuple.pair
|= startParser
|. Parser.loop 0 manyBlankLines
|= endParser
)
)
}
{-| Skip all blank lines.
-}
manyBlankLines lineCount =
Parser.oneOf
[ Parse.skipBlankLineWith (Parser.Loop (lineCount + 1))
, Parser.succeed (Parser.Done ())
]
{-| -}
oneOf : List (Block a) -> Block a
oneOf blocks =
let
expectations =
List.map getBlockExpectation blocks
in
Block
{ kind = Value
, expect = ExpectOneOf expectations
, converter =
\desc ->
Desc.findMatch desc blocks
, parser =
Parse.oneOf blocks expectations
}
{-| Many blocks that are all at the same indentation level.
-}
manyOf : List (Block a) -> Block (List a)
manyOf blocks =
let
expectations =
List.map getBlockExpectation blocks
in
Block
{ kind = Value
, expect = ExpectManyOf expectations
, converter =
\desc ->
let
getRendered id existingResult children =
case children of
[] ->
mapSuccessAndRecovered List.reverse existingResult
top :: remain ->
getRendered id
(Desc.mergeWithAttrs (::)
(Desc.findMatch top blocks)
existingResult
)
remain
in
case desc of
Group many ->
getRendered many.id
(Outcome.Success { data = [], attrs = [] })
many.children
_ ->
Outcome.Failure NoMatch
, parser =
\context seed ->
let
( parentId, newSeed ) =
Id.step seed
indentedSeed : Seed
indentedSeed =
Id.indent seed
in
( newSeed
, Parser.succeed
(\children ->
Group
{ id = parentId
, children = children
}
)
|= Parse.withIndent
(\indentation ->
Parser.loop
{ parsedSomething = False
, found = []
, seed =
indentedSeed
}
(Parse.manyOf indentation blocks)
)
)
}
{-| -}
type Icon
= Bullet
| Number
{-| -}
type Enumerated item
= Enumerated
{ icon : Icon
, items : List (Item item)
}
{-| **Note** `index` is our position within the nested list.
The first `Int` in the tuple is our current position in the current sub list.
The `List Int` that follows are the indices for the parent list.
For example, given this list
```markup
|> List
1. First element
-- Second Element
1. Element #2.1
-- Element #2.1.1
-- Element #2.2
-- Third Element
```
here are the indices:
```markup
1. (1, [])
-- (2, [])
1. (1, [2])
-- (1, [1,2])
-- (2, [2])
-- (3, [])
```
-}
type Item item
= Item
{ index : ( Int, List Int )
, content : List item
, children : Enumerated item
}
{-| Would you believe that a markdown list is actually a tree?
Here's an example of a nested list in `elm-markup`:
```markup
|> List
1. This is definitely the first thing.
With some additional content.
--- Another thing.
And some more content
1. A sublist
With it's content
And some other content
--- Second item
```
**Note** As before, the indentation is always a multiple of 4.
In `elm-markup` you can make a nested section either `Bulleted` or `Numbered` by having the first element of the section start with `-` or `1.`.
The rest of the icons at that level are ignored. So this:
|> List
1. First
-- Second
-- Third
Is a numbered list. And this:
|> List
-- First
1. sublist one
-- sublist two
-- sublist three
-- Second
-- Third
is a bulleted list with a numbered list inside of it.
**Note** You can use as many dashes(`-`) as you want to start an item. This can be useful to make the indentation match up. Similarly, you can also use spaces after the dash or number.
Here's how to render the above list:
import Mark
myTree =
Mark.tree "List" renderList text
-- Note: we have to define this as a separate function because
-- `Items` and `Node` are a pair of mutually recursive data structures.
-- It's easiest to render them using two separate functions:
-- renderList and renderItem
renderList (Mark.Enumerated list) =
let
group =
case list.icon of
Mark.Bullet ->
Html.ul
Mark.Number ->
Html.ol
in
group []
(List.map renderItem list.items)
renderItem (Mark.Item item) =
Html.li []
[ Html.div [] item.content
, renderList item.children
]
-}
tree :
(Enumerated item -> result)
-> Block item
-> Block result
tree view contentBlock =
let
blockExpectation =
getBlockExpectation contentBlock
expectation : Expectation
expectation =
ExpectTree blockExpectation
in
Block
{ kind = Value
, expect = expectation
, converter =
\description ->
case description of
Group details ->
details.children
|> reduceRender Index.zero
getItemIcon
(renderTreeNodeSmall contentBlock)
|> (\( _, icon, outcome ) ->
case outcome of
Outcome.Success nodes ->
Outcome.Success
{ data =
view
(Enumerated
{ icon =
case icon of
Desc.Bullet ->
Bullet
Desc.AutoNumber _ ->
Number
, items = List.map .data nodes
}
)
, attrs = List.concatMap .attrs nodes
}
Outcome.Almost (Uncertain u) ->
Outcome.Almost (Uncertain u)
Outcome.Almost (Recovered errs nodes) ->
Outcome.Almost
(Recovered errs
{ data =
view
(Enumerated
{ icon =
case icon of
Desc.Bullet ->
Bullet
Desc.AutoNumber _ ->
Number
, items = List.map .data nodes
}
)
, attrs = List.concatMap .attrs nodes
}
)
Outcome.Failure f ->
Outcome.Failure f
)
_ ->
Outcome.Failure Error.NoMatch
, parser =
\context seed ->
let
( newId, newSeed ) =
Id.step seed
indentedSeed : Seed
indentedSeed =
Id.indent seed
in
( newSeed
, Parse.withIndent
(\baseIndent ->
let
( secondSeed, itemParser ) =
getParser context indentedSeed contentBlock
in
Parser.map
(\( pos, builtTree ) ->
Group
{ id = newId
, children = builtTree
}
)
(Parse.withRange
(parseTree baseIndent secondSeed contentBlock itemParser)
)
)
)
}
parseTree baseIndent seed contentBlock itemParser =
(Parser.succeed
(\start icon item end ->
{ start = start
, end = end
, item = item
, icon = icon
}
)
|= Parse.getPosition
|= Parse.iconParser
|= itemParser
|= Parse.getPosition
)
|> Parser.andThen
(\details ->
let
( startId, startSeed ) =
Id.step seed
in
Parser.loop
( { base = baseIndent
, prev = baseIndent
, seed = startSeed
}
, { previouslyAdded = Parse.AddedItem
, captured = []
, stack =
[ { start = details.start
, description =
DescribeItem
{ id = startId
, icon = details.icon
, content =
[ details.item
]
, children = []
}
}
]
}
)
(Parse.fullTree
ParseInTree
contentBlock
)
)
getItemIcon desc =
case desc of
DescribeItem item ->
item.icon
_ ->
Desc.Bullet
{-| -}
renderTreeNodeSmall :
Block item
-> Desc.Icon
-> Index.Index
-> Description
-> Desc.BlockOutcome (Item item)
renderTreeNodeSmall contentBlock icon index found =
case found of
DescribeItem item ->
let
( newIndex, childrenIcon, renderedChildren ) =
reduceRender (Index.indent index)
getItemIcon
(renderTreeNodeSmall contentBlock)
item.children
( contentIndex, _, renderedContent ) =
reduceRender (Index.dedent newIndex)
(always Desc.Bullet)
(\icon_ i foundItem ->
renderBlock contentBlock foundItem
)
item.content
in
Desc.mergeListWithAttrs
(\content children ->
Item
{ index = Index.toList index
, content = content
, children =
Enumerated
{ icon =
case childrenIcon of
Desc.Bullet ->
Bullet
Desc.AutoNumber _ ->
Number
, items =
children
}
}
)
renderedContent
renderedChildren
_ ->
Outcome.Failure Error.NoMatch
reduceRender :
Index.Index
-> (thing -> Desc.Icon)
-> (Desc.Icon -> Index.Index -> thing -> Outcome.Outcome Error.AstError (Uncertain other) other)
-> List thing
-> ( Index.Index, Desc.Icon, Outcome.Outcome Error.AstError (Uncertain (List other)) (List other) )
reduceRender index getIcon fn list =
list
|> List.foldl
(\item ( i, existingIcon, gathered ) ->
let
icon =
if Index.top i == 0 then
getIcon item
else
existingIcon
newItem =
case gathered of
Outcome.Success remain ->
case fn icon i item of
Outcome.Success newThing ->
Outcome.Success (newThing :: remain)
Outcome.Almost (Uncertain err) ->
Outcome.Almost (Uncertain err)
Outcome.Almost (Recovered err data) ->
Outcome.Almost
(Recovered err
(data :: remain)
)
Outcome.Failure f ->
Outcome.Failure f
almostOrfailure ->
almostOrfailure
in
( Index.increment i
, icon
, newItem
)
)
( index, Desc.Bullet, Outcome.Success [] )
|> (\( i, ic, outcome ) ->
( i, ic, Outcome.mapSuccess List.reverse outcome )
)
{-| -}
type alias Index =
List Int
{- TEXT BLOCKS -}
{-| -}
type alias Styles =
{ bold : Bool
, italic : Bool
, strike : Bool
}
{-| One of the first things that's interesting about a markup language is how to handle _styled text_.
In `elm-markup` there are only a limited number of special characters for formatting text.
- `/italic/` results in _italics_
- `*bold*` results in **bold**
- and `~strike~` results in ~~strike~~
Here's an example of how to convert markup text into `Html` using `Mark.text`:
Mark.text
(\styles string ->
Html.span
[ Html.Attributes.classList
[ ( "bold", styles.bold )
, ( "italic", styles.italic )
, ( "strike", styles.strike )
]
]
[ Html.text string ]
)
Though you might be thinking that `bold`, `italic`, and `strike` are not nearly enough!
And you're right, this is just to get you started. Your next stop is [`Mark.textWith`](#textWith), which is more involved to use but can represent everything you're used to having in a markup language.
**Note:** Text blocks stop when two consecutive newline characters are encountered.
-}
text :
(Styles -> String -> text)
-> Block (List text)
text view =
textWith
{ view = view
, inlines = []
, replacements = commonReplacements
}
{-| -}
type alias Selection =
{ anchor : Offset
, focus : Offset
}
{-| -}
type alias Offset =
Int
{-| Handling formatted text is a little more involved than may be initially apparent, but have no fear!
`textWith` is where a lot of things come together. Let's check out what these fields actually mean.
- `view` is the function to render an individual fragment of text.
- This is mostly what [`Mark.text`](#text) does, so it should seem familiar.
- `replacements` will replace characters before rendering.
- For example, we can replace `...` with the real ellipses unicode character, `…`.
- `inlines` are custom inline blocks. You can use these to render things like links or emojis :D.
-}
textWith :
{ view :
Styles
-> String
-> rendered
, replacements : List Replacement
, inlines : List (Record rendered)
}
-> Block (List rendered)
textWith options =
let
inlineRecords =
List.map recordToInlineBlock options.inlines
inlineExpectations =
List.map
(\(ProtoRecord rec) ->
ExpectInlineBlock
{ name = rec.name
, kind =
blockKindToSelection rec.blockKind
, fields = rec.expectations
}
)
options.inlines
in
Block
{ kind = Value
, expect = ExpectTextBlock inlineExpectations
, converter =
renderText
{ view = always options.view
, inlines = inlineRecords
}
, parser =
\context seed ->
let
-- Note #1 - seed for styled text is advanced here
-- instead of within the styledText parser
( _, newSeed ) =
Id.step seed
in
( newSeed
, Parse.getPosition
|> Parser.andThen
(\pos ->
Parse.styledText
{ inlines = List.map (\x -> x Desc.EmptyAnnotation) inlineRecords
, replacements = options.replacements
}
context
seed
pos
emptyStyles
)
)
}
recordToInlineBlock (Desc.ProtoRecord details) annotationType =
let
expectations : Expectation
expectations =
Desc.ExpectRecord details.name
details.expectations
in
Desc.Block
{ kind = details.blockKind
, expect = expectations
, converter =
\desc ->
case details.fieldConverter desc annotationType of
Outcome.Success fields ->
case fields.data of
( fieldDescriptions, rendered ) ->
Outcome.Success
{ data = rendered
, attrs = fields.attrs
}
Outcome.Failure fail ->
Outcome.Failure fail
Outcome.Almost (Desc.Uncertain e) ->
Outcome.Almost (Desc.Uncertain e)
Outcome.Almost (Desc.Recovered e fields) ->
case fields.data of
( fieldDescriptions, rendered ) ->
Outcome.Almost
(Desc.Recovered e
{ data = rendered
, attrs = fields.attrs
}
)
, parser =
\context seed ->
let
( parentId, parentSeed ) =
Id.step seed
( newSeed, fields ) =
Id.thread (Id.indent seed) (List.foldl (\f ls -> f ParseInline :: ls) [] details.fields)
( failureId, finalSeed ) =
Id.step newSeed
in
( finalSeed
, Parse.record Parse.InlineRecord
parentId
failureId
details.name
expectations
fields
)
}
type alias Cursor data =
{ outcome : BlockOutcome data
, lastOffset : Int
}
type alias TextOutcome data =
Outcome.Outcome Error.AstError (Uncertain data) data
renderText :
{ view :
{ id : Id
, selection : Selection
}
-> Styles
-> String
-> rendered
, inlines : List (InlineSelection -> Block rendered)
}
-> Description
-> BlockOutcome (List rendered)
renderText options description =
case description of
DescribeText details ->
let
outcome =
details.text
|> List.foldl (convertTextDescription details.id options)
{ outcome = Outcome.Success { data = [], attrs = [] }
, lastOffset = 0
}
|> .outcome
in
outcome
|> mapSuccessAndRecovered List.reverse
_ ->
Outcome.Failure Error.NoMatch
convertTextDescription :
Id
->
{ view :
{ id : Id
, selection : Selection
}
-> Styles
-> String
-> rendered
, inlines : List (InlineSelection -> Block rendered)
}
-> TextDescription
-> Cursor (List rendered)
-> Cursor (List rendered)
convertTextDescription id options comp cursor =
let
blockLength : Int
blockLength =
length comp
in
case comp of
Styled (Desc.Text styling str) ->
{ outcome =
Desc.mergeWithAttrs (::)
(Outcome.Success
{ data =
options.view
{ id = id
, selection =
{ anchor = cursor.lastOffset
, focus = cursor.lastOffset + blockLength
}
}
styling
str
, attrs = []
}
)
cursor.outcome
, lastOffset =
cursor.lastOffset + blockLength
}
InlineBlock details ->
case details.record of
DescribeUnexpected unexpId unexpDetails ->
{ outcome =
Desc.uncertain unexpDetails
, lastOffset = cursor.lastOffset + blockLength
}
_ ->
let
recordName =
Desc.recordName details.record
|> Maybe.withDefault ""
matchInlineName name almostInlineBlock maybeFound =
case maybeFound of
Nothing ->
let
(Block inlineDetails) =
almostInlineBlock details.kind
in
if matchKinds details inlineDetails.kind then
Just inlineDetails
else
Nothing
_ ->
maybeFound
maybeMatched =
List.foldl
(matchInlineName recordName)
Nothing
options.inlines
in
case maybeMatched of
Nothing ->
{ outcome =
Outcome.Failure NoMatch
, lastOffset = cursor.lastOffset + blockLength
}
Just matched ->
{ outcome =
Desc.mergeWithAttrs (::)
(matched.converter details.record)
cursor.outcome
, lastOffset = cursor.lastOffset + blockLength
}
matchKinds inline blockKind =
let
recordName =
case inline.record of
Record rec ->
Just rec.name
_ ->
Nothing
in
case ( recordName, inline.kind, blockKind ) of
( Just inlineName, SelectString str, VerbatimNamed vertName ) ->
inlineName == vertName
( Just inlineName, SelectText _, AnnotationNamed annName ) ->
inlineName == annName
( Just inlineName, EmptyAnnotation, Named name ) ->
inlineName == name
_ ->
False
{-| -}
type alias Replacement =
Parse.Replacement
{-| An annotation is some _styled text_, a _name_, and zero or more _attributes_.
So, we can make a `link` that looks like this in markup:
```markup
Here is my [*cool* sentence]{link| url = website.com }.
```
and rendered in elm-land via:
link =
Mark.annotation "link"
(\id styles url ->
Html.a
[ Html.Attributes.href url ]
(List.map renderStyles styles)
)
|> Mark.field "url" Mark.string
-}
annotation : String -> (Id -> List ( Styles, String ) -> result) -> Record result
annotation name view =
Desc.ProtoRecord
{ name = name
, blockKind = Desc.AnnotationNamed name
, expectations = []
, fieldConverter =
\desc selected ->
case desc of
Desc.Record details ->
if details.name == name then
Outcome.Success
{ data =
( details.found
, view (getId desc) (selectedText selected)
)
, attrs = []
}
else
Outcome.Failure NoMatch
_ ->
Outcome.Failure NoMatch
, fields = []
}
selectedText sel =
case sel of
EmptyAnnotation ->
[]
SelectText txts ->
List.map textToTuple txts
SelectString _ ->
[]
textToTuple (Desc.Text style str) =
( style, str )
selectedString sel =
case sel of
EmptyAnnotation ->
""
SelectText txts ->
""
SelectString str ->
str
{-| A `verbatim` annotation is denoted by backticks(\`) and allows you to capture a literal string.
Just like `annotation`, a `verbatim` can have a name and attributes attached to it.
Let's say we wanted to embed an inline piece of elm code. We could write
inlineElm =
Mark.verbatim "elm"
(\id str ->
Html.span
[ Html.Attributes.class "elm-code" ]
[ Html.text str ]
)
Which would capture the following
Here's an inline function: `\you -> Awesome`{elm}.
**Note** A verbatim can be written without a name or attributes and will capture the contents as a literal string, ignoring any special characters.
Let's take a look at `http://elm-lang.com`.
-}
verbatim : String -> (Id -> String -> result) -> Record result
verbatim name view =
Desc.ProtoRecord
{ name = name
, blockKind = Desc.VerbatimNamed name
, expectations = []
, fieldConverter =
\desc selected ->
case desc of
Desc.Record details ->
if details.name == name then
Outcome.Success
{ attrs = []
, data = ( details.found, view (getId desc) (selectedString selected) )
}
else
Outcome.Failure NoMatch
_ ->
Outcome.Failure NoMatch
, fields = []
}
{- PRIMITIVE BLOCKS -}
{-| This will capture a multiline string.
For example:
Mark.block "Poem"
(\str -> str)
Mark.string
will capture
```markup
|> Poem
Whose woods these are I think I know.
His house is in the village though;
He will not see me stopping here
To watch his woods fill up with snow.
```
Where `str` in the above function will be
"""Whose woods these are I think I know.
His house is in the village though;
He will not see me stopping here
To watch his woods fill up with snow."""
**Note** If you're looking for styled text, you probably want [`Mark.text`](#text) or [`Mark.textWith`](#textWith).
-}
string : Block String
string =
Block
{ kind = Value
, expect = ExpectString "REPLACE"
, converter =
\desc ->
case desc of
DescribeString id str ->
Outcome.Success { data = String.trim str, attrs = [] }
_ ->
Outcome.Failure NoMatch
, parser =
\context seed ->
let
( id, newSeed ) =
Id.step seed
in
( newSeed
, case context of
ParseInline ->
Parser.succeed
(\start str end ->
DescribeString id
(String.trim str)
)
|= Parse.getPosition
|= Parser.getChompedString
(Parser.chompWhile
(\c -> c /= '\n' && c /= ',' && c /= '}')
)
|= Parse.getPosition
ParseBlock ->
Parser.map
(\( pos, str ) ->
DescribeString id str
)
(Parse.withRange
(Parse.withIndent
(\indentation ->
Parser.loop "" (Parse.indentedString indentation)
)
)
)
ParseInTree ->
Parser.map
(DescribeString id)
(Parse.withIndent
(\indentation ->
Parser.loop "" (Parse.indentedString indentation)
)
)
)
}
{-| Capture either `True` or `False`.
`elm-markup` doesn't infer truthiness in other values, so it needs to be exactly `True` or `False`.
-}
bool : Block Bool
bool =
Block
{ kind = Value
, expect = ExpectBoolean False
, converter =
\desc ->
case desc of
DescribeBoolean details ->
Outcome.Success
{ data = details.found
, attrs = []
}
_ ->
Outcome.Failure NoMatch
, parser =
\context seed ->
let
( id, newSeed ) =
Id.step seed
in
( newSeed
, Parser.map
(\boolResult ->
case boolResult of
Err err ->
DescribeUnexpected id
{ range = err.range
, problem = Error.BadBool
}
Ok details ->
DescribeBoolean
{ id = id
, found = details.value
}
)
(Parse.withRangeResult
(Parser.oneOf
[ Parser.token (Parser.Token "True" (Expecting "True"))
|> Parser.map (always (Ok True))
, Parser.token (Parser.Token "False" (Expecting "False"))
|> Parser.map (always (Ok False))
, Parser.map Err Parse.word
]
)
)
)
}
{-| -}
int : Block Int
int =
Block
{ kind = Value
, converter =
\desc ->
case desc of
DescribeInteger details ->
Outcome.Success
{ data = details.found
, attrs = []
}
_ ->
Outcome.Failure NoMatch
, expect = ExpectInteger 0
, parser =
\context seed ->
let
( id, newSeed ) =
Id.step seed
in
( newSeed
, Parse.int id
)
}
{-| -}
float : Block Float
float =
Block
{ kind = Value
, converter =
\desc ->
case desc of
DescribeFloat details ->
Outcome.Success
{ data = Tuple.second details.found
, attrs = []
}
_ ->
Outcome.Failure NoMatch
, expect = ExpectFloat 0
, parser =
\context seed ->
let
( id, newSeed ) =
Id.step seed
in
( newSeed
, Parse.float id
)
}
{- Parser Heleprs -}
{-| This is a set of common character replacements with some typographical niceties.
- `...` is converted to the ellipses unicode character(`…`).
- `"` Straight double quotes are [replaced with curly quotes](https://practicaltypography.com/straight-and-curly-quotes.html) (`“`, `”`)
- `'` Single Quotes are replaced with apostrophes(`’`).
- `--` is replaced with an [en-dash(`–`)](https://practicaltypography.com/hyphens-and-dashes.html).
- `---` is replaced with an [em-dash(`—`)](https://practicaltypography.com/hyphens-and-dashes.html).
- `<>` also known as "glue", will create a non-breaking space (` `). This is not for manually increasing space (sequential `<>` tokens will only render as one ` `), but to signify that the space between two words shouldn't break when wrapping. Like glueing two words together!
**Note** this is included by default in `Mark.text`
-}
commonReplacements : List Replacement
commonReplacements =
[ Parse.Replacement "..." "…"
, Parse.Replacement "<>" "\u{00A0}"
, Parse.Replacement "---" "—"
, Parse.Replacement "--" "–"
, Parse.Replacement "'" "’"
, Parse.Balanced
{ start = ( "\"", "“" )
, end = ( "\"", "”" )
}
]
{-| Replace a string with another string. This can be useful to have shortcuts to unicode characters.
For example, we could use this to replace `...` with the unicode ellipses character: `…`.
-}
replacement : String -> String -> Replacement
replacement =
Parse.Replacement
{-| A balanced replacement. This is used for replacing parentheses or to do auto-curly quotes.
Mark.balanced
{ start = ( "\"", "“" )
, end = ( "\"", "”" )
}
-}
balanced :
{ start : ( String, String )
, end : ( String, String )
}
-> Replacement
balanced =
Parse.Balanced
{- RECORDS -}
{-| -}
type alias Record a =
Desc.Record a
{-| Parse a record with any number of fields.
Mark.record "Image"
(\src description ->
Html.img
[ Html.Attributes.src src
, Html.Attributes.alt description
]
[]
)
|> Mark.field "src" Mark.string
|> Mark.field "description" Mark.string
|> Mark.toBlock
would parse the following markup:
```markup
|> Image
src = http://placekitten/200/500
description = What a cutie.
```
Fields can be in any order in the markup. Also, by convention field names should be `camelCase`. This might be enforced in the future.
-}
record : String -> data -> Record data
record name view =
Desc.ProtoRecord
{ name = name
, blockKind = Desc.Named name
, expectations = []
, fieldConverter =
\desc ann ->
case desc of
Desc.Record details ->
if details.name == name && ann == Desc.EmptyAnnotation then
Outcome.Success { data = ( details.found, view ), attrs = [] }
else
Outcome.Failure NoMatch
_ ->
Outcome.Failure NoMatch
, fields = []
}
{-| -}
field : String -> Block value -> Record (value -> result) -> Record result
field name value (Desc.ProtoRecord details) =
let
newField =
Field name value
in
Desc.ProtoRecord
{ name = details.name
, blockKind = details.blockKind
, expectations = fieldExpectation newField :: details.expectations
, fieldConverter =
\desc ann ->
case details.fieldConverter desc ann of
Outcome.Success fields ->
case fields.data of
( fieldDescriptions, rendered ) ->
case getField newField fieldDescriptions of
Just outcome ->
mapSuccessAndRecovered
(\myField ->
( fieldDescriptions
, rendered myField
)
)
outcome
Nothing ->
Desc.uncertain
{ problem = Error.MissingFields [ fieldName newField ]
, range = Desc.emptyRange
}
Outcome.Almost (Desc.Recovered e fields) ->
case fields.data of
( fieldDescriptions, rendered ) ->
case getField newField fieldDescriptions of
Just outcome ->
mapSuccessAndRecovered
(\myField ->
( fieldDescriptions
, rendered myField
)
)
outcome
Nothing ->
Desc.uncertain
{ problem = Error.MissingFields [ fieldName newField ]
, range = Desc.emptyRange
}
Outcome.Failure fail ->
Outcome.Failure fail
Outcome.Almost (Desc.Uncertain e) ->
Outcome.Almost (Desc.Uncertain e)
, fields =
fieldParser newField :: details.fields
}
fieldName (Field name _) =
name
{-| TODO: convert to recursion
-}
getField :
Field value
-> List ( String, Desc.Description )
-> Maybe (Desc.BlockOutcome value)
getField (Field name fieldBlock) fields =
List.foldl (matchField name fieldBlock) Nothing fields
matchField :
String
-> Block value
-> ( String, Desc.Description )
-> Maybe (Desc.BlockOutcome value)
-> Maybe (Desc.BlockOutcome value)
matchField targetName targetBlock ( name, description ) existing =
case existing of
Just _ ->
existing
Nothing ->
if name == targetName then
Just (Desc.renderBlock targetBlock description)
else
existing
{-| Convert a `Record` to a `Block`.
-}
toBlock : Record a -> Block a
toBlock (Desc.ProtoRecord details) =
let
expectations : Expectation
expectations =
Desc.ExpectRecord details.name
details.expectations
in
Desc.Block
{ kind = details.blockKind
, expect = expectations
, converter =
\desc ->
case details.fieldConverter desc Desc.EmptyAnnotation of
Outcome.Success fields ->
case fields.data of
( fieldDescriptions, rendered ) ->
Outcome.Success { data = rendered, attrs = fields.attrs }
Outcome.Failure fail ->
Outcome.Failure fail
Outcome.Almost (Desc.Uncertain e) ->
Outcome.Almost (Desc.Uncertain e)
Outcome.Almost (Desc.Recovered e fields) ->
case fields.data of
( fieldDescriptions, rendered ) ->
Outcome.Almost
(Desc.Recovered e
{ data = rendered
, attrs = fields.attrs
}
)
, parser =
\context seed ->
let
( parentId, parentSeed ) =
Id.step seed
( newSeed, fields ) =
Id.thread (Id.indent seed)
(List.foldl (\f ls -> f ParseBlock :: ls) [] details.fields)
( failureId, finalSeed ) =
Id.step newSeed
in
( parentSeed
, Parse.record Parse.BlockRecord
parentId
failureId
details.name
expectations
fields
)
}
{-| -}
type Field value
= Field String (Block value)
fieldParser :
Field value
-> Desc.ParseContext
-> Id.Seed
-> ( Id.Seed, ( String, Parser Context Problem ( String, Desc.Description ) ) )
fieldParser (Field name myBlock) context seed =
let
( newSeed, blockParser ) =
Desc.getParser context seed myBlock
in
( newSeed
, ( name
, fieldContentParser
name
blockParser
)
)
fieldExpectation (Field name fieldBlock) =
( name, Desc.getBlockExpectation fieldBlock )
fieldContentParser : String -> Parser Error.Context Error.Problem Desc.Description -> Parser Error.Context Error.Problem ( String, Desc.Description )
fieldContentParser name parser =
Parse.withIndent
(\indentation ->
Parser.map
(\( pos, description ) ->
( name, description )
)
(Parse.withRange
(Parser.oneOf
[ Parser.withIndent (indentation + 4) (Parser.inContext (InRecordField name) parser)
, Parser.succeed identity
|. Parser.chompWhile (\c -> c == '\n')
|. Parser.token (Parser.Token (String.repeat (indentation + 4) " ") (ExpectingIndentation indentation))
|= Parser.withIndent (indentation + 4) (Parser.inContext (InRecordField name) parser)
]
)
)
)
| true | module Mark exposing
( Document, document, documentWith
, Block, block
, string, int, float, bool
, Styles, text, textWith
, Replacement, commonReplacements, replacement, balanced
, annotation, verbatim
, Record, record, field, toBlock
, oneOf, manyOf
, tree, Enumerated(..), Item(..), Icon(..)
, Outcome(..), Partial
, metadata, compile, parse, Parsed, toString, render
, map, verify, onError
, withId, withAttr, documentId, idToString, stringToId
, lookup
)
{-|
# Building Documents
@docs Document, document, documentWith
@docs Block, block
# Primitives
@docs string, int, float, bool
# Text
@docs Styles, text, textWith
# Text Replacements
@docs Replacement, commonReplacements, replacement, balanced
# Text Annotations
Along with basic [`styling`](#text) and [`replacements`](#replacement), we also have a few ways to annotate text.
@docs annotation, verbatim
# Records
@docs Record, record, field, toBlock
# Higher Level
@docs oneOf, manyOf
# Trees
@docs tree, Enumerated, Item, Icon
# Rendering
@docs Outcome, Partial
@docs metadata, compile, parse, Parsed, toString, render
# Constraining and Recovering Blocks
@docs map, verify, onError
@docs withId, withAttr, documentId, idToString, stringToId
@docs lookup
-}
import Mark.Edit
import Mark.Error
import Mark.Internal.Description as Desc exposing (..)
import Mark.Internal.Error as Error exposing (AstError(..), Context(..), Problem(..))
import Mark.Internal.Id as Id exposing (..)
import Mark.Internal.Index as Index
import Mark.Internal.Outcome as Outcome
import Mark.Internal.Parser as Parse
import Mark.New exposing (block)
import Parser.Advanced as Parser exposing ((|.), (|=), Parser)
{-| -}
type alias Parsed =
Desc.Parsed
{- INTERFACE -}
{-| -}
toString : Parsed -> String
toString =
Desc.toString
{-| -}
parse : Document metadata data -> String -> Outcome (List Mark.Error.Error) (Partial Parsed) Parsed
parse doc source =
Desc.compile doc source
|> moveParsedToResult
{-| -}
metadata : Document metadata document -> String -> Result Mark.Error.Error metadata
metadata (Desc.Document doc) source =
case Parser.run doc.metadata source of
Ok parsed ->
parsed
|> Result.mapError
(Error.render source)
Err irrecoverableParsingErrors ->
Err (Error.renderParsingErrors source irrecoverableParsingErrors)
moveParsedToResult :
Result
(Outcome.Outcome (List Error.Rendered)
{ errors : List Error.Rendered
, result : data
}
data
)
( Parsed
, Outcome.Outcome (List Error.Rendered)
{ errors : List Error.Rendered
, result : data
}
data
)
-> Outcome (List Mark.Error.Error) (Partial Parsed) Parsed
moveParsedToResult result =
case result of
Ok ( parsed, Outcome.Success success ) ->
Success parsed
Ok ( parsed, Outcome.Almost almost ) ->
Almost
{ errors = almost.errors
, result = parsed
}
Ok ( parsed, Outcome.Failure errors ) ->
Failure errors
Err (Outcome.Success success) ->
Failure []
Err (Outcome.Almost almost) ->
Failure almost.errors
Err (Outcome.Failure fail) ->
Failure fail
{-| -}
render : Document meta data -> Parsed -> Outcome (List Mark.Error.Error) (Partial ( meta, List data )) ( meta, List data )
render doc ((Parsed parsedDetails) as parsed) =
Desc.render doc parsed
|> rewrapOutcome
{-| -}
compile : Document meta data -> String -> Outcome (List Mark.Error.Error) (Partial ( meta, List data )) ( meta, List data )
compile doc source =
Desc.compile doc source
|> flattenErrors
|> rewrapOutcome
flattenErrors result =
case result of
Ok ( parsed, outcome ) ->
outcome
Err outcome ->
outcome
rewrapOutcome : Outcome.Outcome x y z -> Outcome x y z
rewrapOutcome outcome =
case outcome of
Outcome.Success s ->
Success s
Outcome.Almost x ->
Almost x
Outcome.Failure f ->
Failure f
{-| -}
type alias Partial data =
{ errors : List Mark.Error.Error
, result : data
}
{-| -}
startDocRange : Range
startDocRange =
{ start =
{ offset = 0
, line = 1
, column = 1
}
, end =
{ offset = 0
, line = 1
, column = 1
}
}
{-| -}
type alias Document meta data =
Desc.Document meta data
{-| -}
type Outcome failure almost success
= Success success
| Almost almost
| Failure failure
{-| -}
type alias Block data =
Desc.Block data
getUnexpecteds : Description -> List Error.UnexpectedDetails
getUnexpecteds description =
case description of
DescribeBlock details ->
getUnexpecteds details.found
Record details ->
List.concatMap
(Tuple.second >> getUnexpecteds)
details.found
Group many ->
List.concatMap getUnexpecteds many.children
StartsWith details ->
getUnexpecteds details.first
++ getUnexpecteds details.second
DescribeItem details ->
List.concatMap getUnexpecteds details.content
++ List.concatMap getUnexpecteds details.children
-- Primitives
DescribeBoolean details ->
[]
DescribeInteger details ->
[]
DescribeFloat details ->
[]
DescribeText details ->
[]
DescribeString _ str ->
[]
DescribeUnexpected _ details ->
[ details ]
{-| -}
type alias Position =
{ offset : Int
, line : Int
, column : Int
}
{-| -}
type alias Range =
{ start : Position
, end : Position
}
{- BLOCKS -}
{-| Create a markup `Document`. You're first goal is to describe a document in terms of the blocks you're expecting.
Here's an overly simple document that captures one block, a Title, and wraps it in some `Html`
document : Mark.Document (Html msg)
document =
Mark.document
(\title -> Html.main [] [ title ])
(Mark.block "Title"
(Html.h1 [])
Mark.string
)
will parse the following markup:
```markup
|> Title
Here is my title!
```
and ultimately render it as
```html
<main>
<h1>Here is my title!</h1>
</main>
```
-}
document :
List (Block block)
-> Document () block
document blocks =
createDocument (\_ -> "none")
(Parser.succeed (Ok ()))
(map (Tuple.pair ()) (manyOf blocks))
createDocument :
(meta -> String)
-> Parser Error.Context Error.Problem (Result Error.UnexpectedDetails meta)
-> Block ( meta, List data )
-> Document meta data
createDocument toDocumentId meta child =
Document
{ expect =
getBlockExpectation child
, metadata =
meta
, converter =
\(Parsed parsed) ->
Desc.renderBlock child parsed.found
, parser =
Parser.getSource
|> Parser.andThen
(\src ->
let
docId : String
docId =
case Parser.run meta src of
Ok (Ok m) ->
toDocumentId m
_ ->
-- This should return an error!
-- it means the metadata is invalid
""
seed : Seed
seed =
Id.initialSeed docId
( currentSeed, blockParser ) =
Parse.getFailableBlock Desc.ParseBlock seed child
in
Parser.succeed
(\source ( range, value ) ->
Parsed
{ errors =
List.map (Error.render source) (getUnexpecteds value)
, found = value
, expected = getBlockExpectation child
, initialSeed = seed
, currentSeed = currentSeed
, attributes = []
}
)
|. Parser.chompWhile (\c -> c == '\n')
|= Parser.getSource
|= Parse.withRange (Parser.withIndent 0 blockParser)
|. Parser.chompWhile (\c -> c == ' ' || c == '\n')
|. Parser.end End
)
}
{-| Capture some metadata at the start of your document, followed by the body.
import Mark
Mark.documentWith
{ id = \metadata -> metadata.id
, metadata =
Mark.record
(\id author publishedAt ->
{ author = author
, publishedAt = publishedAt
}
)
|> Mark.field "id" Mark.string
|> Mark.field "author" Mark.string
|> Mark.field "publishedAt" Mark.string
, blocks =
[
]
}
**Note** - You can also specify an `id`, which is a document identifier and is included in `Mark.Edit.Id`. This is really only necessary if you're building an editor that can edit multiple documents at once.
Otherwise, feel free to simple put `id = \_ -> "doc"`
-}
documentWith :
{ id : metadata -> String
, metadata : Record metadata
, blocks : List (Block block)
}
-> Document metadata block
documentWith config =
let
metadataBlock =
toBlock config.metadata
in
createDocument config.id
(getMetadataParser metadataBlock)
(startWith
Tuple.pair
metadataBlock
(manyOf config.blocks)
)
getMetadataParser metadataBlock =
let
( _, metadataParser ) =
Parse.getFailableBlock Desc.ParseBlock (Id.initialSeed "") metadataBlock
in
Parser.andThen
(\description ->
case renderBlock metadataBlock description of
Outcome.Success meta ->
Parser.succeed (Ok meta.data)
Outcome.Failure astError ->
Parser.succeed
(Err
{ problem = Error.DocumentMismatch
, range = Desc.emptyRange
}
)
Outcome.Almost (Uncertain ( unexpected, otherUnexpecteds )) ->
Parser.succeed (Err unexpected)
Outcome.Almost (Recovered ( unexpected, otherUnexpecteds ) renderedChild) ->
Parser.succeed (Err unexpected)
)
(Parser.withIndent 0 metadataParser)
{-| Change the result of a block by applying a function to it.
-}
map : (a -> b) -> Block a -> Block b
map fn (Block details) =
Block
{ kind = details.kind
, converter = mapSuccessAndRecovered fn << details.converter
, parser = details.parser
, expect = details.expect
}
{-| -}
type alias CustomError =
{ title : String
, message : List String
}
{-| `Mark.verify` lets you put constraints on a block.
Let's say you don't just want a `Mark.string`, you actually want a date.
So, you install the [`ISO8601`](https://package.elm-lang.org/packages/rtfeldman/elm-iso8601-date-strings/latest/) and you write something that looks like:
import Iso8601
import Mark
import Mark.Error
import Time
date : Mark.Block Time.Posix
date =
Mark.verify
(\str ->
str
|> Iso8601.toTime
|> Result.mapError
(\_ -> illformatedDateMessage)
)
Mark.string
illformatedDateMessage =
Mark.Error.custom
{ title = "Bad Date"
, message =
[ "I was trying to parse a date, but this format looks off.\n\n"
, "Dates should be in ISO 8601 format:\n\n"
, "YYYY-MM-DDTHH:mm:ss.SSSZ"
]
}
Now you can use `date` whever you actually want dates and the error message will be shown if something goes wrong.
More importantly, you now know if a document parses successfully, that all your dates are correctly formatted.
`Mark.verify` is a very nice way to extend your markup however you'd like.
You could use it to
- add units to numbers
- parse a custom format, like [Latex mathematical equations](https://en.wikibooks.org/wiki/LaTeX/Mathematics#Operators)
- ensure that numbers are between a range or are always positive.
How exciting! Seriously, I think this is pretty cool.
-}
verify : (a -> Result Mark.Error.Custom b) -> Block a -> Block b
verify fn (Block details) =
Block
{ kind = details.kind
, expect = details.expect
, parser =
\ctxt seed ->
details.parser ctxt seed
|> Tuple.mapSecond
(\parser ->
parser
|> Parse.withRange
|> Parser.andThen
(\( range, desc ) ->
-- we only care about reporting if applying `fn` was a problem
-- not other errors, which will shake out normally
case details.converter desc of
Outcome.Success a ->
case fn a.data of
Ok new ->
Parser.succeed desc
Err newErr ->
Parser.succeed
(DescribeUnexpected (getId desc)
{ problem = Error.Custom newErr
, range = range
}
)
Outcome.Almost (Recovered err a) ->
case fn a.data of
Ok new ->
Parser.succeed desc
Err newErr ->
Parser.succeed
(DescribeUnexpected (getId desc)
{ problem = Error.Custom newErr
, range = range
}
)
Outcome.Almost (Uncertain x) ->
Parser.succeed desc
Outcome.Failure f ->
Parser.succeed desc
)
)
, converter =
\desc ->
case details.converter desc of
Outcome.Success a ->
case fn a.data of
Ok new ->
Outcome.Success { data = new, attrs = a.attrs }
Err newErr ->
uncertain
{ problem = Error.Custom newErr
-- TODO: Does this mean we need to thread source snippets everywhere to get them here?
, range = startDocRange
}
Outcome.Almost (Recovered err a) ->
case fn a.data of
Ok new ->
Outcome.Almost (Recovered err { data = new, attrs = a.attrs })
Err newErr ->
uncertain
{ problem = Error.Custom newErr
, range = startDocRange
}
Outcome.Almost (Uncertain x) ->
Outcome.Almost (Uncertain x)
Outcome.Failure f ->
Outcome.Failure f
}
{-| -}
documentId : Mark.Edit.Id -> String
documentId (Id.Id str _) =
str
{-| Look up a specific block in your document by id.
-}
lookup : Mark.Edit.Id -> Document meta block -> Parsed -> Outcome (List Mark.Error.Error) (Partial block) block
lookup id doc parsed =
Desc.lookup id doc parsed
|> rewrapOutcome
{-| Get an `Id` associated with a `Block`, which can be used to make updates through `Mark.Edit`.
Mark.withId
(\id str ->
Html.span
[ onClick (Mark.Edit.delete id) ]
[ Html.text str ]
)
Mark.string
-}
withId : (Mark.Edit.Id -> a -> b) -> Block a -> Block b
withId fn (Block details) =
Block
{ kind = details.kind
, converter =
\desc ->
let
id : Id
id =
Desc.getId desc
in
Desc.mapSuccessAndRecovered
(fn id)
(details.converter desc)
, parser = details.parser
, expect = details.expect
}
{-| Get an `Id` associated with a `Block`, which can be used to make updates through `Mark.Edit`.
Mark.string
|> Mark.withAttr
(\str -> ("link", str))
-}
withAttr : (a -> ( String, String )) -> Block a -> Block a
withAttr fn (Block details) =
Block
{ kind = details.kind
, converter =
\desc ->
let
id : Id
id =
Desc.getId desc
in
case details.converter desc of
Outcome.Success deets ->
let
new =
case fn deets.data of
( key, value ) ->
{ name = key
, value = value
, block = id
}
in
Outcome.Success
{ data = deets.data
, attrs = new :: deets.attrs
}
Outcome.Almost (Uncertain x) ->
Outcome.Almost (Uncertain x)
Outcome.Almost (Recovered errs deets) ->
let
new =
case fn deets.data of
( key, value ) ->
{ name = key
, value = value
, block = id
}
in
Outcome.Almost
(Recovered errs
{ data = deets.data
, attrs = new :: deets.attrs
}
)
Outcome.Failure errs ->
Outcome.Failure errs
, parser = details.parser
, expect = details.expect
}
{-| It may be necessary to convert an `Id` to a `String` and back in order attach it as an `Html.Attributes.id` and read it back.
See the editor example for more details.
**Note** be aware that the actual string format of an `Id` is an implementation detail and may change even on patch releases of a library.
-}
idToString : Mark.Edit.Id -> String
idToString =
Id.toString
{-| -}
stringToId : String -> Maybe Mark.Edit.Id
stringToId =
Id.fromString
{-| Parsing any given `Block` can fail.
However sometimes we don't want the _whole document_ to be unable to render just because there was a small error somewhere.
So, we need some way to say "Hey, if you run into an issue, here's a placeholder value to use."
And that's what `Mark.onError` does.
Mark.int
|> Mark.onError 5
This means if we fail to parse an integer (let's say we added a decimal), that this block would still be renderable with a default value of `5`.
**Note** If there _is_ an error that is fixed using `onError`, we'll get a [`Partial`](#Partial) when we render the document. This will let us see the _full rendered document_, but also see the _error_ that actually occurred.
-}
onError : a -> Block a -> Block a
onError newValue (Block details) =
Block
{ kind = details.kind
, expect = details.expect
, parser = details.parser
, converter =
\desc ->
case details.converter desc of
Outcome.Success a ->
Outcome.Success a
Outcome.Almost (Recovered err a) ->
Outcome.Almost (Recovered err a)
Outcome.Almost (Uncertain x) ->
Outcome.Almost
(Recovered x { data = newValue, attrs = [] })
Outcome.Failure f ->
Outcome.Failure f
}
{-| A named block.
Mark.block "MyBlock"
Html.text
Mark.string
Will parse the following and render it using `Html.text`
|> MyBlock
Here is an unformatted string!
**Note** block names should be capitalized. In the future this may be enforced.
-}
block : String -> (child -> result) -> Block child -> Block result
block name view child =
Block
{ kind = Named name
, expect = ExpectBlock name (getBlockExpectation child)
, converter =
\desc ->
case desc of
DescribeBlock details ->
if details.name == name then
renderBlock child details.found
|> mapSuccessAndRecovered view
else
-- This is not the block that was expected.
Outcome.Failure NoMatch
_ ->
Outcome.Failure NoMatch
, parser =
\context seed ->
let
( newSeed, childParser ) =
getParser context (Id.indent seed) child
( parentId, parentSeed ) =
Id.step seed
( errorId, finalSeed ) =
Id.step parentSeed
in
( finalSeed
, Parser.map
(\result ->
case result of
Ok details ->
DescribeBlock
{ found = details.value
, name = PI:NAME:<NAME>END_PI
, id = parentId
}
Err details ->
DescribeBlock
{ name = PI:NAME:<NAME>END_PI
, id = parentId
, found =
DescribeUnexpected errorId
{ range = details.range
, problem = details.error
}
}
)
<|
Parse.withRangeResult
(Parse.withIndent
(\indentation ->
Parser.succeed identity
|. Parser.keyword
(Parser.Token name
(ExpectingBlockName name)
)
|. Parser.chompWhile (\c -> c == ' ')
|. Parse.skipBlankLineWith ()
|= Parser.oneOf
[ (Parser.succeed identity
|= Parse.getPosition
|. Parser.token
(Parser.Token
(String.repeat (indentation + 4) " ")
(ExpectingIndentation (indentation + 4))
)
)
|> Parser.andThen
(\start ->
Parser.oneOf
-- ALERT: This first parser will fail because `Parse.raggedIndentedStringAbove`
-- expects indentation for the first line.
[ Parser.succeed
(\end ->
Err (Error.ExpectingIndent (indentation + 4))
)
|. Parser.chompIf (\c -> c == ' ') Space
|. Parser.chompWhile (\c -> c == ' ')
|= Parse.getPosition
|. Parser.loop "" (Parse.raggedIndentedStringAbove indentation)
, Parser.map Ok <|
Parser.withIndent
(indentation + 4)
(Parser.inContext (InBlock name) childParser)
]
)
-- If we're here, it's because the indentation failed.
-- If the child parser failed in some way, it would
-- take care of that itself by returning Unexpected
, Parser.succeed
(Err (Error.ExpectingIndent (indentation + 4)))
|. Parser.loop "" (Parse.raggedIndentedStringAbove indentation)
]
)
)
)
}
{-| -}
startWith :
(start -> rest -> result)
-> Block start
-> Block rest
-> Block result
startWith fn startBlock endBlock =
Block
{ kind = Value
, expect = ExpectStartsWith (getBlockExpectation startBlock) (getBlockExpectation endBlock)
, converter =
\desc ->
case desc of
StartsWith details ->
Desc.mergeWithAttrs fn
(Desc.renderBlock startBlock details.first)
(Desc.renderBlock endBlock details.second)
_ ->
Outcome.Failure NoMatch
, parser =
\context seed ->
let
( parentId, newSeed ) =
Id.step seed
( startSeed, startParser ) =
getParser ParseBlock newSeed startBlock
( remainSeed, endParser ) =
getParser ParseBlock startSeed endBlock
in
( remainSeed
, Parser.succeed
(\( range, ( begin, end ) ) ->
StartsWith
{ id = parentId
, first = begin
, second = end
}
)
|= Parse.withRange
(Parser.succeed Tuple.pair
|= startParser
|. Parser.loop 0 manyBlankLines
|= endParser
)
)
}
{-| Skip all blank lines.
-}
manyBlankLines lineCount =
Parser.oneOf
[ Parse.skipBlankLineWith (Parser.Loop (lineCount + 1))
, Parser.succeed (Parser.Done ())
]
{-| -}
oneOf : List (Block a) -> Block a
oneOf blocks =
let
expectations =
List.map getBlockExpectation blocks
in
Block
{ kind = Value
, expect = ExpectOneOf expectations
, converter =
\desc ->
Desc.findMatch desc blocks
, parser =
Parse.oneOf blocks expectations
}
{-| Many blocks that are all at the same indentation level.
-}
manyOf : List (Block a) -> Block (List a)
manyOf blocks =
let
expectations =
List.map getBlockExpectation blocks
in
Block
{ kind = Value
, expect = ExpectManyOf expectations
, converter =
\desc ->
let
getRendered id existingResult children =
case children of
[] ->
mapSuccessAndRecovered List.reverse existingResult
top :: remain ->
getRendered id
(Desc.mergeWithAttrs (::)
(Desc.findMatch top blocks)
existingResult
)
remain
in
case desc of
Group many ->
getRendered many.id
(Outcome.Success { data = [], attrs = [] })
many.children
_ ->
Outcome.Failure NoMatch
, parser =
\context seed ->
let
( parentId, newSeed ) =
Id.step seed
indentedSeed : Seed
indentedSeed =
Id.indent seed
in
( newSeed
, Parser.succeed
(\children ->
Group
{ id = parentId
, children = children
}
)
|= Parse.withIndent
(\indentation ->
Parser.loop
{ parsedSomething = False
, found = []
, seed =
indentedSeed
}
(Parse.manyOf indentation blocks)
)
)
}
{-| -}
type Icon
= Bullet
| Number
{-| -}
type Enumerated item
= Enumerated
{ icon : Icon
, items : List (Item item)
}
{-| **Note** `index` is our position within the nested list.
The first `Int` in the tuple is our current position in the current sub list.
The `List Int` that follows are the indices for the parent list.
For example, given this list
```markup
|> List
1. First element
-- Second Element
1. Element #2.1
-- Element #2.1.1
-- Element #2.2
-- Third Element
```
here are the indices:
```markup
1. (1, [])
-- (2, [])
1. (1, [2])
-- (1, [1,2])
-- (2, [2])
-- (3, [])
```
-}
type Item item
= Item
{ index : ( Int, List Int )
, content : List item
, children : Enumerated item
}
{-| Would you believe that a markdown list is actually a tree?
Here's an example of a nested list in `elm-markup`:
```markup
|> List
1. This is definitely the first thing.
With some additional content.
--- Another thing.
And some more content
1. A sublist
With it's content
And some other content
--- Second item
```
**Note** As before, the indentation is always a multiple of 4.
In `elm-markup` you can make a nested section either `Bulleted` or `Numbered` by having the first element of the section start with `-` or `1.`.
The rest of the icons at that level are ignored. So this:
|> List
1. First
-- Second
-- Third
Is a numbered list. And this:
|> List
-- First
1. sublist one
-- sublist two
-- sublist three
-- Second
-- Third
is a bulleted list with a numbered list inside of it.
**Note** You can use as many dashes(`-`) as you want to start an item. This can be useful to make the indentation match up. Similarly, you can also use spaces after the dash or number.
Here's how to render the above list:
import Mark
myTree =
Mark.tree "List" renderList text
-- Note: we have to define this as a separate function because
-- `Items` and `Node` are a pair of mutually recursive data structures.
-- It's easiest to render them using two separate functions:
-- renderList and renderItem
renderList (Mark.Enumerated list) =
let
group =
case list.icon of
Mark.Bullet ->
Html.ul
Mark.Number ->
Html.ol
in
group []
(List.map renderItem list.items)
renderItem (Mark.Item item) =
Html.li []
[ Html.div [] item.content
, renderList item.children
]
-}
tree :
(Enumerated item -> result)
-> Block item
-> Block result
tree view contentBlock =
let
blockExpectation =
getBlockExpectation contentBlock
expectation : Expectation
expectation =
ExpectTree blockExpectation
in
Block
{ kind = Value
, expect = expectation
, converter =
\description ->
case description of
Group details ->
details.children
|> reduceRender Index.zero
getItemIcon
(renderTreeNodeSmall contentBlock)
|> (\( _, icon, outcome ) ->
case outcome of
Outcome.Success nodes ->
Outcome.Success
{ data =
view
(Enumerated
{ icon =
case icon of
Desc.Bullet ->
Bullet
Desc.AutoNumber _ ->
Number
, items = List.map .data nodes
}
)
, attrs = List.concatMap .attrs nodes
}
Outcome.Almost (Uncertain u) ->
Outcome.Almost (Uncertain u)
Outcome.Almost (Recovered errs nodes) ->
Outcome.Almost
(Recovered errs
{ data =
view
(Enumerated
{ icon =
case icon of
Desc.Bullet ->
Bullet
Desc.AutoNumber _ ->
Number
, items = List.map .data nodes
}
)
, attrs = List.concatMap .attrs nodes
}
)
Outcome.Failure f ->
Outcome.Failure f
)
_ ->
Outcome.Failure Error.NoMatch
, parser =
\context seed ->
let
( newId, newSeed ) =
Id.step seed
indentedSeed : Seed
indentedSeed =
Id.indent seed
in
( newSeed
, Parse.withIndent
(\baseIndent ->
let
( secondSeed, itemParser ) =
getParser context indentedSeed contentBlock
in
Parser.map
(\( pos, builtTree ) ->
Group
{ id = newId
, children = builtTree
}
)
(Parse.withRange
(parseTree baseIndent secondSeed contentBlock itemParser)
)
)
)
}
parseTree baseIndent seed contentBlock itemParser =
(Parser.succeed
(\start icon item end ->
{ start = start
, end = end
, item = item
, icon = icon
}
)
|= Parse.getPosition
|= Parse.iconParser
|= itemParser
|= Parse.getPosition
)
|> Parser.andThen
(\details ->
let
( startId, startSeed ) =
Id.step seed
in
Parser.loop
( { base = baseIndent
, prev = baseIndent
, seed = startSeed
}
, { previouslyAdded = Parse.AddedItem
, captured = []
, stack =
[ { start = details.start
, description =
DescribeItem
{ id = startId
, icon = details.icon
, content =
[ details.item
]
, children = []
}
}
]
}
)
(Parse.fullTree
ParseInTree
contentBlock
)
)
getItemIcon desc =
case desc of
DescribeItem item ->
item.icon
_ ->
Desc.Bullet
{-| -}
renderTreeNodeSmall :
Block item
-> Desc.Icon
-> Index.Index
-> Description
-> Desc.BlockOutcome (Item item)
renderTreeNodeSmall contentBlock icon index found =
case found of
DescribeItem item ->
let
( newIndex, childrenIcon, renderedChildren ) =
reduceRender (Index.indent index)
getItemIcon
(renderTreeNodeSmall contentBlock)
item.children
( contentIndex, _, renderedContent ) =
reduceRender (Index.dedent newIndex)
(always Desc.Bullet)
(\icon_ i foundItem ->
renderBlock contentBlock foundItem
)
item.content
in
Desc.mergeListWithAttrs
(\content children ->
Item
{ index = Index.toList index
, content = content
, children =
Enumerated
{ icon =
case childrenIcon of
Desc.Bullet ->
Bullet
Desc.AutoNumber _ ->
Number
, items =
children
}
}
)
renderedContent
renderedChildren
_ ->
Outcome.Failure Error.NoMatch
reduceRender :
Index.Index
-> (thing -> Desc.Icon)
-> (Desc.Icon -> Index.Index -> thing -> Outcome.Outcome Error.AstError (Uncertain other) other)
-> List thing
-> ( Index.Index, Desc.Icon, Outcome.Outcome Error.AstError (Uncertain (List other)) (List other) )
reduceRender index getIcon fn list =
list
|> List.foldl
(\item ( i, existingIcon, gathered ) ->
let
icon =
if Index.top i == 0 then
getIcon item
else
existingIcon
newItem =
case gathered of
Outcome.Success remain ->
case fn icon i item of
Outcome.Success newThing ->
Outcome.Success (newThing :: remain)
Outcome.Almost (Uncertain err) ->
Outcome.Almost (Uncertain err)
Outcome.Almost (Recovered err data) ->
Outcome.Almost
(Recovered err
(data :: remain)
)
Outcome.Failure f ->
Outcome.Failure f
almostOrfailure ->
almostOrfailure
in
( Index.increment i
, icon
, newItem
)
)
( index, Desc.Bullet, Outcome.Success [] )
|> (\( i, ic, outcome ) ->
( i, ic, Outcome.mapSuccess List.reverse outcome )
)
{-| -}
type alias Index =
List Int
{- TEXT BLOCKS -}
{-| -}
type alias Styles =
{ bold : Bool
, italic : Bool
, strike : Bool
}
{-| One of the first things that's interesting about a markup language is how to handle _styled text_.
In `elm-markup` there are only a limited number of special characters for formatting text.
- `/italic/` results in _italics_
- `*bold*` results in **bold**
- and `~strike~` results in ~~strike~~
Here's an example of how to convert markup text into `Html` using `Mark.text`:
Mark.text
(\styles string ->
Html.span
[ Html.Attributes.classList
[ ( "bold", styles.bold )
, ( "italic", styles.italic )
, ( "strike", styles.strike )
]
]
[ Html.text string ]
)
Though you might be thinking that `bold`, `italic`, and `strike` are not nearly enough!
And you're right, this is just to get you started. Your next stop is [`Mark.textWith`](#textWith), which is more involved to use but can represent everything you're used to having in a markup language.
**Note:** Text blocks stop when two consecutive newline characters are encountered.
-}
text :
(Styles -> String -> text)
-> Block (List text)
text view =
textWith
{ view = view
, inlines = []
, replacements = commonReplacements
}
{-| -}
type alias Selection =
{ anchor : Offset
, focus : Offset
}
{-| -}
type alias Offset =
Int
{-| Handling formatted text is a little more involved than may be initially apparent, but have no fear!
`textWith` is where a lot of things come together. Let's check out what these fields actually mean.
- `view` is the function to render an individual fragment of text.
- This is mostly what [`Mark.text`](#text) does, so it should seem familiar.
- `replacements` will replace characters before rendering.
- For example, we can replace `...` with the real ellipses unicode character, `…`.
- `inlines` are custom inline blocks. You can use these to render things like links or emojis :D.
-}
textWith :
{ view :
Styles
-> String
-> rendered
, replacements : List Replacement
, inlines : List (Record rendered)
}
-> Block (List rendered)
textWith options =
let
inlineRecords =
List.map recordToInlineBlock options.inlines
inlineExpectations =
List.map
(\(ProtoRecord rec) ->
ExpectInlineBlock
{ name = rec.name
, kind =
blockKindToSelection rec.blockKind
, fields = rec.expectations
}
)
options.inlines
in
Block
{ kind = Value
, expect = ExpectTextBlock inlineExpectations
, converter =
renderText
{ view = always options.view
, inlines = inlineRecords
}
, parser =
\context seed ->
let
-- Note #1 - seed for styled text is advanced here
-- instead of within the styledText parser
( _, newSeed ) =
Id.step seed
in
( newSeed
, Parse.getPosition
|> Parser.andThen
(\pos ->
Parse.styledText
{ inlines = List.map (\x -> x Desc.EmptyAnnotation) inlineRecords
, replacements = options.replacements
}
context
seed
pos
emptyStyles
)
)
}
recordToInlineBlock (Desc.ProtoRecord details) annotationType =
let
expectations : Expectation
expectations =
Desc.ExpectRecord details.name
details.expectations
in
Desc.Block
{ kind = details.blockKind
, expect = expectations
, converter =
\desc ->
case details.fieldConverter desc annotationType of
Outcome.Success fields ->
case fields.data of
( fieldDescriptions, rendered ) ->
Outcome.Success
{ data = rendered
, attrs = fields.attrs
}
Outcome.Failure fail ->
Outcome.Failure fail
Outcome.Almost (Desc.Uncertain e) ->
Outcome.Almost (Desc.Uncertain e)
Outcome.Almost (Desc.Recovered e fields) ->
case fields.data of
( fieldDescriptions, rendered ) ->
Outcome.Almost
(Desc.Recovered e
{ data = rendered
, attrs = fields.attrs
}
)
, parser =
\context seed ->
let
( parentId, parentSeed ) =
Id.step seed
( newSeed, fields ) =
Id.thread (Id.indent seed) (List.foldl (\f ls -> f ParseInline :: ls) [] details.fields)
( failureId, finalSeed ) =
Id.step newSeed
in
( finalSeed
, Parse.record Parse.InlineRecord
parentId
failureId
details.name
expectations
fields
)
}
type alias Cursor data =
{ outcome : BlockOutcome data
, lastOffset : Int
}
type alias TextOutcome data =
Outcome.Outcome Error.AstError (Uncertain data) data
renderText :
{ view :
{ id : Id
, selection : Selection
}
-> Styles
-> String
-> rendered
, inlines : List (InlineSelection -> Block rendered)
}
-> Description
-> BlockOutcome (List rendered)
renderText options description =
case description of
DescribeText details ->
let
outcome =
details.text
|> List.foldl (convertTextDescription details.id options)
{ outcome = Outcome.Success { data = [], attrs = [] }
, lastOffset = 0
}
|> .outcome
in
outcome
|> mapSuccessAndRecovered List.reverse
_ ->
Outcome.Failure Error.NoMatch
convertTextDescription :
Id
->
{ view :
{ id : Id
, selection : Selection
}
-> Styles
-> String
-> rendered
, inlines : List (InlineSelection -> Block rendered)
}
-> TextDescription
-> Cursor (List rendered)
-> Cursor (List rendered)
convertTextDescription id options comp cursor =
let
blockLength : Int
blockLength =
length comp
in
case comp of
Styled (Desc.Text styling str) ->
{ outcome =
Desc.mergeWithAttrs (::)
(Outcome.Success
{ data =
options.view
{ id = id
, selection =
{ anchor = cursor.lastOffset
, focus = cursor.lastOffset + blockLength
}
}
styling
str
, attrs = []
}
)
cursor.outcome
, lastOffset =
cursor.lastOffset + blockLength
}
InlineBlock details ->
case details.record of
DescribeUnexpected unexpId unexpDetails ->
{ outcome =
Desc.uncertain unexpDetails
, lastOffset = cursor.lastOffset + blockLength
}
_ ->
let
recordName =
Desc.recordName details.record
|> Maybe.withDefault ""
matchInlineName name almostInlineBlock maybeFound =
case maybeFound of
Nothing ->
let
(Block inlineDetails) =
almostInlineBlock details.kind
in
if matchKinds details inlineDetails.kind then
Just inlineDetails
else
Nothing
_ ->
maybeFound
maybeMatched =
List.foldl
(matchInlineName recordName)
Nothing
options.inlines
in
case maybeMatched of
Nothing ->
{ outcome =
Outcome.Failure NoMatch
, lastOffset = cursor.lastOffset + blockLength
}
Just matched ->
{ outcome =
Desc.mergeWithAttrs (::)
(matched.converter details.record)
cursor.outcome
, lastOffset = cursor.lastOffset + blockLength
}
matchKinds inline blockKind =
let
recordName =
case inline.record of
Record rec ->
Just rec.name
_ ->
Nothing
in
case ( recordName, inline.kind, blockKind ) of
( Just inlineName, SelectString str, VerbatimNamed vertName ) ->
inlineName == vertName
( Just inlineName, SelectText _, AnnotationNamed annName ) ->
inlineName == annName
( Just inlineName, EmptyAnnotation, Named name ) ->
inlineName == name
_ ->
False
{-| -}
type alias Replacement =
Parse.Replacement
{-| An annotation is some _styled text_, a _name_, and zero or more _attributes_.
So, we can make a `link` that looks like this in markup:
```markup
Here is my [*cool* sentence]{link| url = website.com }.
```
and rendered in elm-land via:
link =
Mark.annotation "link"
(\id styles url ->
Html.a
[ Html.Attributes.href url ]
(List.map renderStyles styles)
)
|> Mark.field "url" Mark.string
-}
annotation : String -> (Id -> List ( Styles, String ) -> result) -> Record result
annotation name view =
Desc.ProtoRecord
{ name = name
, blockKind = Desc.AnnotationNamed name
, expectations = []
, fieldConverter =
\desc selected ->
case desc of
Desc.Record details ->
if details.name == name then
Outcome.Success
{ data =
( details.found
, view (getId desc) (selectedText selected)
)
, attrs = []
}
else
Outcome.Failure NoMatch
_ ->
Outcome.Failure NoMatch
, fields = []
}
selectedText sel =
case sel of
EmptyAnnotation ->
[]
SelectText txts ->
List.map textToTuple txts
SelectString _ ->
[]
textToTuple (Desc.Text style str) =
( style, str )
selectedString sel =
case sel of
EmptyAnnotation ->
""
SelectText txts ->
""
SelectString str ->
str
{-| A `verbatim` annotation is denoted by backticks(\`) and allows you to capture a literal string.
Just like `annotation`, a `verbatim` can have a name and attributes attached to it.
Let's say we wanted to embed an inline piece of elm code. We could write
inlineElm =
Mark.verbatim "elm"
(\id str ->
Html.span
[ Html.Attributes.class "elm-code" ]
[ Html.text str ]
)
Which would capture the following
Here's an inline function: `\you -> Awesome`{elm}.
**Note** A verbatim can be written without a name or attributes and will capture the contents as a literal string, ignoring any special characters.
Let's take a look at `http://elm-lang.com`.
-}
verbatim : String -> (Id -> String -> result) -> Record result
verbatim name view =
Desc.ProtoRecord
{ name = name
, blockKind = Desc.VerbatimNamed name
, expectations = []
, fieldConverter =
\desc selected ->
case desc of
Desc.Record details ->
if details.name == name then
Outcome.Success
{ attrs = []
, data = ( details.found, view (getId desc) (selectedString selected) )
}
else
Outcome.Failure NoMatch
_ ->
Outcome.Failure NoMatch
, fields = []
}
{- PRIMITIVE BLOCKS -}
{-| This will capture a multiline string.
For example:
Mark.block "Poem"
(\str -> str)
Mark.string
will capture
```markup
|> Poem
Whose woods these are I think I know.
His house is in the village though;
He will not see me stopping here
To watch his woods fill up with snow.
```
Where `str` in the above function will be
"""Whose woods these are I think I know.
His house is in the village though;
He will not see me stopping here
To watch his woods fill up with snow."""
**Note** If you're looking for styled text, you probably want [`Mark.text`](#text) or [`Mark.textWith`](#textWith).
-}
string : Block String
string =
Block
{ kind = Value
, expect = ExpectString "REPLACE"
, converter =
\desc ->
case desc of
DescribeString id str ->
Outcome.Success { data = String.trim str, attrs = [] }
_ ->
Outcome.Failure NoMatch
, parser =
\context seed ->
let
( id, newSeed ) =
Id.step seed
in
( newSeed
, case context of
ParseInline ->
Parser.succeed
(\start str end ->
DescribeString id
(String.trim str)
)
|= Parse.getPosition
|= Parser.getChompedString
(Parser.chompWhile
(\c -> c /= '\n' && c /= ',' && c /= '}')
)
|= Parse.getPosition
ParseBlock ->
Parser.map
(\( pos, str ) ->
DescribeString id str
)
(Parse.withRange
(Parse.withIndent
(\indentation ->
Parser.loop "" (Parse.indentedString indentation)
)
)
)
ParseInTree ->
Parser.map
(DescribeString id)
(Parse.withIndent
(\indentation ->
Parser.loop "" (Parse.indentedString indentation)
)
)
)
}
{-| Capture either `True` or `False`.
`elm-markup` doesn't infer truthiness in other values, so it needs to be exactly `True` or `False`.
-}
bool : Block Bool
bool =
Block
{ kind = Value
, expect = ExpectBoolean False
, converter =
\desc ->
case desc of
DescribeBoolean details ->
Outcome.Success
{ data = details.found
, attrs = []
}
_ ->
Outcome.Failure NoMatch
, parser =
\context seed ->
let
( id, newSeed ) =
Id.step seed
in
( newSeed
, Parser.map
(\boolResult ->
case boolResult of
Err err ->
DescribeUnexpected id
{ range = err.range
, problem = Error.BadBool
}
Ok details ->
DescribeBoolean
{ id = id
, found = details.value
}
)
(Parse.withRangeResult
(Parser.oneOf
[ Parser.token (Parser.Token "True" (Expecting "True"))
|> Parser.map (always (Ok True))
, Parser.token (Parser.Token "False" (Expecting "False"))
|> Parser.map (always (Ok False))
, Parser.map Err Parse.word
]
)
)
)
}
{-| -}
int : Block Int
int =
Block
{ kind = Value
, converter =
\desc ->
case desc of
DescribeInteger details ->
Outcome.Success
{ data = details.found
, attrs = []
}
_ ->
Outcome.Failure NoMatch
, expect = ExpectInteger 0
, parser =
\context seed ->
let
( id, newSeed ) =
Id.step seed
in
( newSeed
, Parse.int id
)
}
{-| -}
float : Block Float
float =
Block
{ kind = Value
, converter =
\desc ->
case desc of
DescribeFloat details ->
Outcome.Success
{ data = Tuple.second details.found
, attrs = []
}
_ ->
Outcome.Failure NoMatch
, expect = ExpectFloat 0
, parser =
\context seed ->
let
( id, newSeed ) =
Id.step seed
in
( newSeed
, Parse.float id
)
}
{- Parser Heleprs -}
{-| This is a set of common character replacements with some typographical niceties.
- `...` is converted to the ellipses unicode character(`…`).
- `"` Straight double quotes are [replaced with curly quotes](https://practicaltypography.com/straight-and-curly-quotes.html) (`“`, `”`)
- `'` Single Quotes are replaced with apostrophes(`’`).
- `--` is replaced with an [en-dash(`–`)](https://practicaltypography.com/hyphens-and-dashes.html).
- `---` is replaced with an [em-dash(`—`)](https://practicaltypography.com/hyphens-and-dashes.html).
- `<>` also known as "glue", will create a non-breaking space (` `). This is not for manually increasing space (sequential `<>` tokens will only render as one ` `), but to signify that the space between two words shouldn't break when wrapping. Like glueing two words together!
**Note** this is included by default in `Mark.text`
-}
commonReplacements : List Replacement
commonReplacements =
[ Parse.Replacement "..." "…"
, Parse.Replacement "<>" "\u{00A0}"
, Parse.Replacement "---" "—"
, Parse.Replacement "--" "–"
, Parse.Replacement "'" "’"
, Parse.Balanced
{ start = ( "\"", "“" )
, end = ( "\"", "”" )
}
]
{-| Replace a string with another string. This can be useful to have shortcuts to unicode characters.
For example, we could use this to replace `...` with the unicode ellipses character: `…`.
-}
replacement : String -> String -> Replacement
replacement =
Parse.Replacement
{-| A balanced replacement. This is used for replacing parentheses or to do auto-curly quotes.
Mark.balanced
{ start = ( "\"", "“" )
, end = ( "\"", "”" )
}
-}
balanced :
{ start : ( String, String )
, end : ( String, String )
}
-> Replacement
balanced =
Parse.Balanced
{- RECORDS -}
{-| -}
type alias Record a =
Desc.Record a
{-| Parse a record with any number of fields.
Mark.record "Image"
(\src description ->
Html.img
[ Html.Attributes.src src
, Html.Attributes.alt description
]
[]
)
|> Mark.field "src" Mark.string
|> Mark.field "description" Mark.string
|> Mark.toBlock
would parse the following markup:
```markup
|> Image
src = http://placekitten/200/500
description = What a cutie.
```
Fields can be in any order in the markup. Also, by convention field names should be `camelCase`. This might be enforced in the future.
-}
record : String -> data -> Record data
record name view =
Desc.ProtoRecord
{ name = name
, blockKind = Desc.Named name
, expectations = []
, fieldConverter =
\desc ann ->
case desc of
Desc.Record details ->
if details.name == name && ann == Desc.EmptyAnnotation then
Outcome.Success { data = ( details.found, view ), attrs = [] }
else
Outcome.Failure NoMatch
_ ->
Outcome.Failure NoMatch
, fields = []
}
{-| -}
field : String -> Block value -> Record (value -> result) -> Record result
field name value (Desc.ProtoRecord details) =
let
newField =
Field name value
in
Desc.ProtoRecord
{ name = details.name
, blockKind = details.blockKind
, expectations = fieldExpectation newField :: details.expectations
, fieldConverter =
\desc ann ->
case details.fieldConverter desc ann of
Outcome.Success fields ->
case fields.data of
( fieldDescriptions, rendered ) ->
case getField newField fieldDescriptions of
Just outcome ->
mapSuccessAndRecovered
(\myField ->
( fieldDescriptions
, rendered myField
)
)
outcome
Nothing ->
Desc.uncertain
{ problem = Error.MissingFields [ fieldName newField ]
, range = Desc.emptyRange
}
Outcome.Almost (Desc.Recovered e fields) ->
case fields.data of
( fieldDescriptions, rendered ) ->
case getField newField fieldDescriptions of
Just outcome ->
mapSuccessAndRecovered
(\myField ->
( fieldDescriptions
, rendered myField
)
)
outcome
Nothing ->
Desc.uncertain
{ problem = Error.MissingFields [ fieldName newField ]
, range = Desc.emptyRange
}
Outcome.Failure fail ->
Outcome.Failure fail
Outcome.Almost (Desc.Uncertain e) ->
Outcome.Almost (Desc.Uncertain e)
, fields =
fieldParser newField :: details.fields
}
fieldName (Field name _) =
name
{-| TODO: convert to recursion
-}
getField :
Field value
-> List ( String, Desc.Description )
-> Maybe (Desc.BlockOutcome value)
getField (Field name fieldBlock) fields =
List.foldl (matchField name fieldBlock) Nothing fields
matchField :
String
-> Block value
-> ( String, Desc.Description )
-> Maybe (Desc.BlockOutcome value)
-> Maybe (Desc.BlockOutcome value)
matchField targetName targetBlock ( name, description ) existing =
case existing of
Just _ ->
existing
Nothing ->
if name == targetName then
Just (Desc.renderBlock targetBlock description)
else
existing
{-| Convert a `Record` to a `Block`.
-}
toBlock : Record a -> Block a
toBlock (Desc.ProtoRecord details) =
let
expectations : Expectation
expectations =
Desc.ExpectRecord details.name
details.expectations
in
Desc.Block
{ kind = details.blockKind
, expect = expectations
, converter =
\desc ->
case details.fieldConverter desc Desc.EmptyAnnotation of
Outcome.Success fields ->
case fields.data of
( fieldDescriptions, rendered ) ->
Outcome.Success { data = rendered, attrs = fields.attrs }
Outcome.Failure fail ->
Outcome.Failure fail
Outcome.Almost (Desc.Uncertain e) ->
Outcome.Almost (Desc.Uncertain e)
Outcome.Almost (Desc.Recovered e fields) ->
case fields.data of
( fieldDescriptions, rendered ) ->
Outcome.Almost
(Desc.Recovered e
{ data = rendered
, attrs = fields.attrs
}
)
, parser =
\context seed ->
let
( parentId, parentSeed ) =
Id.step seed
( newSeed, fields ) =
Id.thread (Id.indent seed)
(List.foldl (\f ls -> f ParseBlock :: ls) [] details.fields)
( failureId, finalSeed ) =
Id.step newSeed
in
( parentSeed
, Parse.record Parse.BlockRecord
parentId
failureId
details.name
expectations
fields
)
}
{-| -}
type Field value
= Field String (Block value)
fieldParser :
Field value
-> Desc.ParseContext
-> Id.Seed
-> ( Id.Seed, ( String, Parser Context Problem ( String, Desc.Description ) ) )
fieldParser (Field name myBlock) context seed =
let
( newSeed, blockParser ) =
Desc.getParser context seed myBlock
in
( newSeed
, ( name
, fieldContentParser
name
blockParser
)
)
fieldExpectation (Field name fieldBlock) =
( name, Desc.getBlockExpectation fieldBlock )
fieldContentParser : String -> Parser Error.Context Error.Problem Desc.Description -> Parser Error.Context Error.Problem ( String, Desc.Description )
fieldContentParser name parser =
Parse.withIndent
(\indentation ->
Parser.map
(\( pos, description ) ->
( name, description )
)
(Parse.withRange
(Parser.oneOf
[ Parser.withIndent (indentation + 4) (Parser.inContext (InRecordField name) parser)
, Parser.succeed identity
|. Parser.chompWhile (\c -> c == '\n')
|. Parser.token (Parser.Token (String.repeat (indentation + 4) " ") (ExpectingIndentation indentation))
|= Parser.withIndent (indentation + 4) (Parser.inContext (InRecordField name) parser)
]
)
)
)
| elm |
[
{
"context": " decoding implementation in pure Elm.\n\nThanks to [Ning Sun](https://github.com/sunng87) for the [JavaScript ",
"end": 170,
"score": 0.9882705808,
"start": 162,
"tag": "NAME",
"value": "Ning Sun"
},
{
"context": "ure Elm.\n\nThanks to [Ning Sun](https://github.com/sunng87) for the [JavaScript implementation](https://gith",
"end": 198,
"score": 0.9996545315,
"start": 191,
"tag": "USERNAME",
"value": "sunng87"
},
{
"context": "he [JavaScript implementation](https://github.com/sunng87/node-geohash).\n\n\n# Functions\n\n@docs encode, decod",
"end": 262,
"score": 0.9995199442,
"start": 255,
"tag": "USERNAME",
"value": "sunng87"
}
] | src/Geohash.elm | elm-review-bot/elm-geohash | 1 | module Geohash exposing (encode, decodeCoordinate, decodeBoundingBox)
{-| This module is a Geohash encoding and decoding implementation in pure Elm.
Thanks to [Ning Sun](https://github.com/sunng87) for the [JavaScript implementation](https://github.com/sunng87/node-geohash).
# Functions
@docs encode, decodeCoordinate, decodeBoundingBox
-}
import GeohashDecode
import GeohashEncode
{-| Encodes coordinate (latitude, longitude, precision) to geohash.
encode 57.648 10.41 6 == "u4pruy"
-}
encode : Float -> Float -> Int -> String
encode =
GeohashEncode.encode
{-| Decodes geohash and returns center coordinate.
decodeCoordinate "u281zk"
== { lat = 48.14483642578125
, lon = 11.5740966796875
, latError = 0.00274658203125
, lonError = 0.0054931640625
}
-}
decodeCoordinate : String -> { lat : Float, lon : Float, latError : Float, lonError : Float }
decodeCoordinate =
GeohashDecode.decode
{-| Decodes a geohash and returns the bounding box.
decodeBoundingBox "u281zk" =
{ minLat = 48.14208984375
, minLon = 11.568603515625
, maxLat = 48.1475830078125
, maxLon = 11.57958984375
}
-}
decodeBoundingBox : String -> { minLat : Float, minLon : Float, maxLat : Float, maxLon : Float }
decodeBoundingBox =
GeohashDecode.decodeBoundingBox
| 8726 | module Geohash exposing (encode, decodeCoordinate, decodeBoundingBox)
{-| This module is a Geohash encoding and decoding implementation in pure Elm.
Thanks to [<NAME>](https://github.com/sunng87) for the [JavaScript implementation](https://github.com/sunng87/node-geohash).
# Functions
@docs encode, decodeCoordinate, decodeBoundingBox
-}
import GeohashDecode
import GeohashEncode
{-| Encodes coordinate (latitude, longitude, precision) to geohash.
encode 57.648 10.41 6 == "u4pruy"
-}
encode : Float -> Float -> Int -> String
encode =
GeohashEncode.encode
{-| Decodes geohash and returns center coordinate.
decodeCoordinate "u281zk"
== { lat = 48.14483642578125
, lon = 11.5740966796875
, latError = 0.00274658203125
, lonError = 0.0054931640625
}
-}
decodeCoordinate : String -> { lat : Float, lon : Float, latError : Float, lonError : Float }
decodeCoordinate =
GeohashDecode.decode
{-| Decodes a geohash and returns the bounding box.
decodeBoundingBox "u281zk" =
{ minLat = 48.14208984375
, minLon = 11.568603515625
, maxLat = 48.1475830078125
, maxLon = 11.57958984375
}
-}
decodeBoundingBox : String -> { minLat : Float, minLon : Float, maxLat : Float, maxLon : Float }
decodeBoundingBox =
GeohashDecode.decodeBoundingBox
| true | module Geohash exposing (encode, decodeCoordinate, decodeBoundingBox)
{-| This module is a Geohash encoding and decoding implementation in pure Elm.
Thanks to [PI:NAME:<NAME>END_PI](https://github.com/sunng87) for the [JavaScript implementation](https://github.com/sunng87/node-geohash).
# Functions
@docs encode, decodeCoordinate, decodeBoundingBox
-}
import GeohashDecode
import GeohashEncode
{-| Encodes coordinate (latitude, longitude, precision) to geohash.
encode 57.648 10.41 6 == "u4pruy"
-}
encode : Float -> Float -> Int -> String
encode =
GeohashEncode.encode
{-| Decodes geohash and returns center coordinate.
decodeCoordinate "u281zk"
== { lat = 48.14483642578125
, lon = 11.5740966796875
, latError = 0.00274658203125
, lonError = 0.0054931640625
}
-}
decodeCoordinate : String -> { lat : Float, lon : Float, latError : Float, lonError : Float }
decodeCoordinate =
GeohashDecode.decode
{-| Decodes a geohash and returns the bounding box.
decodeBoundingBox "u281zk" =
{ minLat = 48.14208984375
, minLon = 11.568603515625
, maxLat = 48.1475830078125
, maxLon = 11.57958984375
}
-}
decodeBoundingBox : String -> { minLat : Float, minLon : Float, maxLat : Float, maxLon : Float }
decodeBoundingBox =
GeohashDecode.decodeBoundingBox
| elm |
[
{
"context": " , name = \"0\"\n , teamName = \"team\"\n , job = Nothing\n ",
"end": 22798,
"score": 0.7292987108,
"start": 22794,
"tag": "USERNAME",
"value": "team"
},
{
"context": "ge = OneOffBuildPage 0\n , id = 0\n , name = \"0\"\n , now = Nothing\n , job = Nothing\n , di",
"end": 24020,
"score": 0.8631783128,
"start": 24019,
"tag": "NAME",
"value": "0"
}
] | web/elm/benchmarks/Benchmarks.elm | pd/concourse | 0 | module Benchmarks exposing (main)
import Ansi.Log
import Application.Models exposing (Session)
import Array
import Assets
import Benchmark
import Benchmark.Runner exposing (BenchmarkProgram, program)
import Build.Build as Build
import Build.Header.Models exposing (BuildPageType(..), CommentBarVisibility(..), CurrentOutput(..))
import Build.Models
import Build.Output.Models
import Build.Output.Output
import Build.StepTree.Models as STModels
import Build.Styles
import Concourse
import Concourse.BuildStatus
import Concourse.Pagination exposing (Page)
import Dashboard.DashboardPreview as DP
import DateFormat
import Dict exposing (Dict)
import HoverState
import Html exposing (Html)
import Html.Attributes
exposing
( attribute
, class
, classList
, href
, id
, style
, tabindex
, title
)
import Html.Events exposing (onBlur, onFocus, onMouseEnter, onMouseLeave)
import Html.Lazy
import Keyboard
import Login.Login as Login
import Maybe.Extra
import Message.Message exposing (DomID(..), Message(..), PipelinesSection(..))
import RemoteData exposing (WebData)
import Routes exposing (Highlight)
import ScreenSize
import Set
import SideBar.SideBar as SideBar
import StrictEvents exposing (onLeftClick, onScroll, onWheel)
import Time
import UserState
import Views.BuildDuration as BuildDuration
import Views.Icon as Icon
import Views.LoadingIndicator as LoadingIndicator
import Views.NotAuthorized as NotAuthorized
import Views.Spinner as Spinner
import Views.Styles
import Views.TopBar as TopBar
type alias Model =
Login.Model
{ page : BuildPageType
, now : Maybe Time.Posix
, disableManualTrigger : Bool
, history : List Concourse.Build
, nextPage : Maybe Page
, currentBuild : WebData CurrentBuild
, autoScroll : Bool
, previousKeyPress : Maybe Keyboard.KeyEvent
, shiftDown : Bool
, isTriggerBuildKeyDown : Bool
, showHelp : Bool
, highlight : Highlight
, hoveredCounter : Int
, fetchingHistory : Bool
, scrolledToCurrentBuild : Bool
, authorized : Bool
}
type alias CurrentBuild =
{ build : Concourse.Build
, prep : Maybe Concourse.BuildPrep
, output : CurrentOutput
}
main : BenchmarkProgram
main =
program <|
Benchmark.describe "benchmark suite"
[ Benchmark.compare "DashboardPreview.view"
"current"
(\_ -> DP.view AllPipelinesSection HoverState.NoHover (DP.groupByRank sampleJobs))
"old"
(\_ -> dashboardPreviewView sampleJobs)
, Benchmark.compare "Build.view"
"current"
(\_ -> Build.view sampleSession sampleModel)
"old"
(\_ -> buildView sampleSession sampleOldModel)
]
bodyId : String
bodyId =
"build-body"
historyId : String
historyId =
"builds"
buildView : Session -> Model -> Html Message
buildView session model =
let
route =
case model.page of
OneOffBuildPage buildId ->
Routes.OneOffBuild
{ id = buildId
, highlight = model.highlight
}
JobBuildPage buildId ->
Routes.Build
{ id = buildId
, highlight = model.highlight
}
in
Html.div
(id "page-including-top-bar" :: Views.Styles.pageIncludingTopBar)
[ Html.div
(id "top-bar-app" :: Views.Styles.topBar False)
[ SideBar.sideBarIcon session
, TopBar.concourseLogo
, breadcrumbs session model
, Login.view session.userState model
]
, Html.div
(id "page-below-top-bar" :: Views.Styles.pageBelowTopBar route)
[ SideBar.view session
(currentJob model
|> Maybe.map
(\j ->
{ pipelineName = j.pipelineName
, pipelineInstanceVars = j.pipelineInstanceVars
, teamName = j.teamName
}
)
)
, viewBuildPage session model
]
]
viewBuildPage : Session -> Model -> Html Message
viewBuildPage session model =
case model.currentBuild |> RemoteData.toMaybe of
Just currentBuild ->
Html.div
[ class "with-fixed-header"
, attribute "data-build-name" currentBuild.build.name
, style "flex-grow" "1"
, style "display" "flex"
, style "flex-direction" "column"
, style "overflow" "hidden"
]
[ viewBuildHeader session model currentBuild.build
, body
session
{ currentBuild = currentBuild
, authorized = model.authorized
, showHelp = model.showHelp
}
]
_ ->
LoadingIndicator.view
currentJob : Model -> Maybe Concourse.JobIdentifier
currentJob =
.currentBuild
>> RemoteData.toMaybe
>> Maybe.map .build
>> Maybe.andThen .job
breadcrumbs : Session -> Model -> Html Message
breadcrumbs session model =
case ( currentJob model, model.page ) of
( Just jobId, _ ) ->
TopBar.breadcrumbs session <|
Routes.Job
{ id = jobId
, page = Nothing
}
( _, JobBuildPage buildId ) ->
TopBar.breadcrumbs session <|
Routes.Build
{ id = buildId
, highlight = model.highlight
}
_ ->
Html.text ""
body :
Session
->
{ currentBuild : CurrentBuild
, authorized : Bool
, showHelp : Bool
}
-> Html Message
body session { currentBuild, authorized, showHelp } =
Html.div
([ class "scrollable-body build-body"
, id bodyId
, tabindex 0
, onScroll Scrolled
]
++ Build.Styles.body
)
<|
if authorized then
[ viewBuildPrep currentBuild.prep
, Html.Lazy.lazy2 viewBuildOutput session currentBuild.output
, keyboardHelp showHelp
]
++ tombstone session.timeZone currentBuild
else
[ NotAuthorized.view ]
viewBuildHeader :
Session
-> Model
-> Concourse.Build
-> Html Message
viewBuildHeader session model build =
let
triggerButton =
case currentJob model of
Just _ ->
let
buttonDisabled =
model.disableManualTrigger
buttonHovered =
HoverState.isHovered
TriggerBuildButton
session.hovered
in
Html.button
([ attribute "role" "button"
, attribute "tabindex" "0"
, attribute "aria-label" "Trigger Build"
, attribute "title" "Trigger Build"
, onLeftClick <| Click TriggerBuildButton
, onMouseEnter <| Hover <| Just TriggerBuildButton
, onFocus <| Hover <| Just TriggerBuildButton
, onMouseLeave <| Hover Nothing
, onBlur <| Hover Nothing
]
++ Build.Styles.triggerButton
buttonDisabled
buttonHovered
build.status
)
<|
Icon.icon
{ sizePx = 40
, image = Assets.AddCircleIcon |> Assets.CircleOutlineIcon
}
[]
:: (if buttonDisabled && buttonHovered then
[ Html.div
[]
[ Html.text <|
"manual triggering disabled "
++ "in job config"
]
]
else
[]
)
Nothing ->
Html.text ""
abortHovered =
HoverState.isHovered AbortBuildButton session.hovered
abortButton =
if Concourse.BuildStatus.isRunning build.status then
Html.button
([ onLeftClick (Click <| AbortBuildButton)
, attribute "role" "button"
, attribute "tabindex" "0"
, attribute "aria-label" "Abort Build"
, attribute "title" "Abort Build"
, onMouseEnter <| Hover <| Just AbortBuildButton
, onFocus <| Hover <| Just AbortBuildButton
, onMouseLeave <| Hover Nothing
, onBlur <| Hover Nothing
]
++ Build.Styles.abortButton abortHovered
)
[ Icon.icon
{ sizePx = 40
, image = Assets.AbortCircleIcon |> Assets.CircleOutlineIcon
}
[]
]
else
Html.text ""
buildTitle =
case build.job of
Just jobId ->
let
jobRoute =
Routes.Job { id = jobId, page = Nothing }
in
Html.a
[ href <| Routes.toString jobRoute ]
[ Html.span [ class "build-name" ] [ Html.text jobId.jobName ]
, Html.text (" #" ++ build.name)
]
_ ->
Html.text ("build #" ++ String.fromInt build.id)
in
Html.div [ class "fixed-header" ]
[ Html.div
([ id "build-header"
, class "build-header"
]
++ Build.Styles.header build.status
)
[ Html.div []
[ Html.h1 [] [ buildTitle ]
, case model.now of
Just n ->
BuildDuration.view session.timeZone build.duration n
Nothing ->
Html.text ""
]
, Html.div
[ style "display" "flex" ]
[ abortButton, triggerButton ]
]
, Html.div
[ onWheel ScrollBuilds ]
[ lazyViewHistory build model.history ]
]
tombstone : Time.Zone -> CurrentBuild -> List (Html Message)
tombstone timeZone currentBuild =
let
build =
currentBuild.build
maybeBirthDate =
Maybe.Extra.or build.duration.startedAt build.duration.finishedAt
in
case ( maybeBirthDate, build.reapTime ) of
( Just birthDate, Just reapTime ) ->
[ Html.div
[ class "tombstone" ]
[ Html.div [ class "heading" ] [ Html.text "RIP" ]
, Html.div
[ class "job-name" ]
[ Html.text <|
Maybe.withDefault
"one-off build"
<|
Maybe.map .jobName build.job
]
, Html.div
[ class "build-name" ]
[ Html.text <|
"build #"
++ (case build.job of
Nothing ->
String.fromInt build.id
Just _ ->
build.name
)
]
, Html.div
[ class "date" ]
[ Html.text <|
mmDDYY timeZone birthDate
++ "-"
++ mmDDYY timeZone reapTime
]
, Html.div
[ class "epitaph" ]
[ Html.text <|
case build.status of
Concourse.BuildStatus.BuildStatusSucceeded ->
"It passed, and now it has passed on."
Concourse.BuildStatus.BuildStatusFailed ->
"It failed, and now has been forgotten."
Concourse.BuildStatus.BuildStatusErrored ->
"It errored, but has found forgiveness."
Concourse.BuildStatus.BuildStatusAborted ->
"It was never given a chance."
_ ->
"I'm not dead yet."
]
]
, Html.div
[ class "explanation" ]
[ Html.text "This log has been "
, Html.a
[ Html.Attributes.href "https://concourse-ci.org/jobs.html#job-build-log-retention" ]
[ Html.text "reaped." ]
]
]
_ ->
[]
keyboardHelp : Bool -> Html Message
keyboardHelp showHelp =
Html.div
[ classList
[ ( "keyboard-help", True )
, ( "hidden", not showHelp )
]
]
[ Html.div
[ class "help-title" ]
[ Html.text "keyboard shortcuts" ]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "h" ]
, Html.span [ class "key" ] [ Html.text "l" ]
]
, Html.text "previous/next build"
]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "j" ]
, Html.span [ class "key" ] [ Html.text "k" ]
]
, Html.text "scroll down/up"
]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "T" ] ]
, Html.text "trigger a new build"
]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "A" ] ]
, Html.text "abort build"
]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "gg" ] ]
, Html.text "scroll to the top"
]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "G" ] ]
, Html.text "scroll to the bottom"
]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "?" ] ]
, Html.text "hide/show help"
]
]
viewBuildOutput : Session -> CurrentOutput -> Html Message
viewBuildOutput session output =
case output of
Output o ->
Build.Output.Output.view
{ timeZone = session.timeZone, hovered = session.hovered }
o
Cancelled ->
Html.div
Build.Styles.errorLog
[ Html.text "build cancelled" ]
Empty ->
Html.div [] []
viewBuildPrep : Maybe Concourse.BuildPrep -> Html Message
viewBuildPrep buildPrep =
case buildPrep of
Just prep ->
Html.div [ class "build-step" ]
[ Html.div
[ class "header"
, style "display" "flex"
, style "align-items" "center"
]
[ Icon.icon
{ sizePx = 15, image = Assets.CogsIcon }
[ style "margin" "6.5px"
, style "margin-right" "0.5px"
, style "background-size" "contain"
]
, Html.h3 [] [ Html.text "preparing build" ]
]
, Html.div []
[ Html.ul [ class "prep-status-list" ]
([ viewBuildPrepLi "checking pipeline is not paused" prep.pausedPipeline Dict.empty
, viewBuildPrepLi "checking job is not paused" prep.pausedJob Dict.empty
]
++ viewBuildPrepInputs prep.inputs
++ [ viewBuildPrepLi "waiting for a suitable set of input versions" prep.inputsSatisfied prep.missingInputReasons
, viewBuildPrepLi "checking max-in-flight is not reached" prep.maxRunningBuilds Dict.empty
]
)
]
]
Nothing ->
Html.div [] []
lazyViewHistory : Concourse.Build -> List Concourse.Build -> Html Message
lazyViewHistory currentBuild builds =
Html.Lazy.lazy2 viewHistory currentBuild builds
viewHistory : Concourse.Build -> List Concourse.Build -> Html Message
viewHistory currentBuild builds =
Html.ul [ id historyId ]
(List.map (viewHistoryItem currentBuild) builds)
viewHistoryItem : Concourse.Build -> Concourse.Build -> Html Message
viewHistoryItem currentBuild build =
Html.li
([ classList [ ( "current", build.id == currentBuild.id ) ]
, id <| String.fromInt build.id
]
++ Build.Styles.historyItem
currentBuild.status
(build.id == currentBuild.id)
build.status
)
[ Html.a
[ onLeftClick <| Click <| BuildTab build.id build.name
, href <| Routes.toString <| Routes.buildRoute build.id build.name build.job
]
[ Html.text build.name ]
]
mmDDYY : Time.Zone -> Time.Posix -> String
mmDDYY =
DateFormat.format
[ DateFormat.monthFixed
, DateFormat.text "/"
, DateFormat.dayOfMonthFixed
, DateFormat.text "/"
, DateFormat.yearNumberLastTwo
]
viewBuildPrepLi :
String
-> Concourse.BuildPrepStatus
-> Dict String String
-> Html Message
viewBuildPrepLi text status details =
Html.li
[ classList
[ ( "prep-status", True )
, ( "inactive", status == Concourse.BuildPrepStatusUnknown )
]
]
[ Html.div
[ style "align-items" "center"
, style "display" "flex"
]
[ viewBuildPrepStatus status
, Html.span []
[ Html.text text ]
]
, viewBuildPrepDetails details
]
viewBuildPrepInputs : Dict String Concourse.BuildPrepStatus -> List (Html Message)
viewBuildPrepInputs inputs =
List.map viewBuildPrepInput (Dict.toList inputs)
viewBuildPrepInput : ( String, Concourse.BuildPrepStatus ) -> Html Message
viewBuildPrepInput ( name, status ) =
viewBuildPrepLi ("discovering any new versions of " ++ name) status Dict.empty
viewBuildPrepDetails : Dict String String -> Html Message
viewBuildPrepDetails details =
Html.ul [ class "details" ]
(List.map viewDetailItem (Dict.toList details))
viewBuildPrepStatus : Concourse.BuildPrepStatus -> Html Message
viewBuildPrepStatus status =
case status of
Concourse.BuildPrepStatusUnknown ->
Html.div
[ title "thinking..." ]
[ Spinner.spinner
{ sizePx = 12
, margin = "0 5px 0 0"
}
]
Concourse.BuildPrepStatusBlocking ->
Html.div
[ title "blocking" ]
[ Spinner.spinner
{ sizePx = 12
, margin = "0 5px 0 0"
}
]
Concourse.BuildPrepStatusNotBlocking ->
Icon.icon
{ sizePx = 12
, image = Assets.NotBlockingCheckIcon
}
[ style "margin-right" "5px"
, style "background-size" "contain"
, title "not blocking"
]
viewDetailItem : ( String, String ) -> Html Message
viewDetailItem ( name, status ) =
Html.li []
[ Html.text (name ++ " - " ++ status) ]
sampleSession : Session
sampleSession =
{ authToken = ""
, csrfToken = ""
, expandedTeamsInAllPipelines = Set.empty
, collapsedTeamsInFavorites = Set.empty
, favoritedPipelines = Set.empty
, favoritedInstanceGroups = Set.empty
, hovered = HoverState.NoHover
, sideBarState =
{ isOpen = False
, width = 275
}
, draggingSideBar = False
, notFoundImgSrc = ""
, pipelineRunningKeyframes = ""
, pipelines = RemoteData.NotAsked
, screenSize = ScreenSize.Desktop
, timeZone = Time.utc
, turbulenceImgSrc = ""
, userState = UserState.UserStateLoggedOut
, clusterName = ""
, version = ""
, featureFlags = Concourse.defaultFeatureFlags
, route =
Routes.OneOffBuild
{ id = 1
, highlight = Routes.HighlightNothing
}
}
sampleOldModel : Model
sampleOldModel =
{ page = OneOffBuildPage 0
, now = Nothing
, disableManualTrigger = False
, history = []
, nextPage = Nothing
, currentBuild =
RemoteData.Success
{ build =
{ id = 0
, name = "0"
, teamName = "team"
, job = Nothing
, status = Concourse.BuildStatus.BuildStatusStarted
, duration =
{ startedAt = Nothing
, finishedAt = Nothing
}
, reapTime = Nothing
, createdBy = Nothing
, comment = ""
}
, prep = Nothing
, output =
Output
{ steps = stepsModel
, state = Build.Output.Models.StepsLiveUpdating
, eventSourceOpened = True
, eventStreamUrlPath = Nothing
, highlight = Routes.HighlightNothing
, buildId = Nothing
}
}
, autoScroll = True
, previousKeyPress = Nothing
, shiftDown = False
, isTriggerBuildKeyDown = False
, showHelp = False
, highlight = Routes.HighlightNothing
, hoveredCounter = 0
, fetchingHistory = False
, scrolledToCurrentBuild = True
, authorized = True
, isUserMenuExpanded = False
}
sampleModel : Build.Models.Model
sampleModel =
{ page = OneOffBuildPage 0
, id = 0
, name = "0"
, now = Nothing
, job = Nothing
, disableManualTrigger = False
, history = []
, nextPage = Nothing
, prep = Nothing
, duration = { startedAt = Nothing, finishedAt = Nothing }
, status = Concourse.BuildStatus.BuildStatusStarted
, output =
Output
{ steps = stepsModel
, state = Build.Output.Models.StepsLiveUpdating
, eventSourceOpened = True
, eventStreamUrlPath = Nothing
, highlight = Routes.HighlightNothing
, buildId = Nothing
}
, autoScroll = True
, isScrollToIdInProgress = False
, previousKeyPress = Nothing
, isTriggerBuildKeyDown = False
, showHelp = False
, highlight = Routes.HighlightNothing
, authorized = True
, fetchingHistory = False
, scrolledToCurrentBuild = False
, shiftDown = False
, isUserMenuExpanded = False
, hasLoadedYet = True
, notFound = False
, reapTime = Nothing
, createdBy = Nothing
, comment = Hidden ""
}
ansiLogStyle : Ansi.Log.Style
ansiLogStyle =
{ foreground = Nothing
, background = Nothing
, bold = False
, faint = False
, italic = False
, underline = False
, blink = False
, inverted = False
, fraktur = False
, framed = False
}
position : Ansi.Log.CursorPosition
position =
{ row = 0
, column = 0
}
log : Ansi.Log.Model
log =
{ lineDiscipline = Ansi.Log.Cooked
, lines = Array.empty
, position = position
, savedPosition = Nothing
, style = ansiLogStyle
, remainder = ""
}
tree : STModels.StepTree
tree =
STModels.Task "stepid"
steps : Dict Routes.StepID STModels.Step
steps =
Dict.singleton "stepid"
{ id = "stepid"
, buildStep = Concourse.BuildStepTask "task_step"
, state = STModels.StepStateRunning
, log = log
, error = Nothing
, expanded = True
, version = Nothing
, metadata = []
, changed = False
, timestamps = Dict.empty
, initialize = Nothing
, start = Nothing
, finish = Nothing
, tabFocus = STModels.Auto
, expandedHeaders = Dict.empty
, initializationExpanded = False
, imageCheck = Nothing
, imageGet = Nothing
}
stepsModel : Maybe STModels.StepTreeModel
stepsModel =
Just
{ tree = tree
, steps = steps
, highlight = Routes.HighlightNothing
, resources = { inputs = [], outputs = [] }
, buildId = Nothing
}
sampleJob : String -> List String -> Concourse.Job
sampleJob name passed =
{ name = name
, pipelineId = 1
, pipelineName = "pipeline"
, pipelineInstanceVars = Dict.empty
, teamName = "team"
, nextBuild = Nothing
, finishedBuild = Nothing
, transitionBuild = Nothing
, paused = False
, disableManualTrigger = False
, inputs =
[ { name = "input"
, resource = "resource"
, passed = passed
, trigger = True
}
]
, outputs = []
, groups = []
}
sampleJobs : List Concourse.Job
sampleJobs =
[ sampleJob "job1" []
, sampleJob "job2a" [ "job1" ]
, sampleJob "job2b" [ "job1" ]
, sampleJob "job3" [ "job2a" ]
, sampleJob "job4" [ "job3" ]
]
dashboardPreviewView : List Concourse.Job -> Html msg
dashboardPreviewView jobs =
let
groups =
jobGroups jobs
width =
Dict.size groups
height =
Maybe.withDefault 0 <| List.maximum (List.map List.length (Dict.values groups))
in
Html.div
[ classList
[ ( "pipeline-grid", True )
, ( "pipeline-grid-wide", width > 12 )
, ( "pipeline-grid-tall", height > 12 )
, ( "pipeline-grid-super-wide", width > 24 )
, ( "pipeline-grid-super-tall", height > 24 )
]
]
<|
List.map
(\js ->
List.map viewJob js
|> Html.div [ class "parallel-grid" ]
)
(Dict.values groups)
viewJob : Concourse.Job -> Html msg
viewJob job =
let
jobStatus =
case job.finishedBuild of
Just fb ->
Concourse.BuildStatus.show fb.status
Nothing ->
"no-builds"
isJobRunning =
job.nextBuild /= Nothing
latestBuild =
if job.nextBuild == Nothing then
job.finishedBuild
else
job.nextBuild
in
Html.div
[ classList
[ ( "node " ++ jobStatus, True )
, ( "running", isJobRunning )
, ( "paused", job.paused )
]
, attribute "data-tooltip" job.name
]
<|
case latestBuild of
Nothing ->
[ Html.a [ href <| Routes.toString <| Routes.jobRoute job ] [ Html.text "" ] ]
Just build ->
[ Html.a [ href <| Routes.toString <| Routes.buildRoute build.id build.name build.job ] [ Html.text "" ] ]
jobGroups : List Concourse.Job -> Dict Int (List Concourse.Job)
jobGroups jobs =
let
jobLookup =
jobByName <| List.foldl (\job byName -> Dict.insert job.name job byName) Dict.empty jobs
in
Dict.foldl
(\jobName depth byDepth ->
Dict.update depth
(\jobsA ->
Just (jobLookup jobName :: Maybe.withDefault [] jobsA)
)
byDepth
)
Dict.empty
(jobDepths jobs Dict.empty)
jobByName : Dict String Concourse.Job -> String -> Concourse.Job
jobByName jobs job =
case Dict.get job jobs of
Just a ->
a
Nothing ->
{ name = ""
, pipelineId = 0
, pipelineName = ""
, pipelineInstanceVars = Dict.empty
, teamName = ""
, nextBuild = Nothing
, finishedBuild = Nothing
, transitionBuild = Nothing
, paused = False
, disableManualTrigger = False
, inputs = []
, outputs = []
, groups = []
}
jobDepths : List Concourse.Job -> Dict String Int -> Dict String Int
jobDepths jobs dict =
case jobs of
[] ->
dict
job :: otherJobs ->
let
passedJobs =
List.concatMap .passed job.inputs
in
case List.length passedJobs of
0 ->
jobDepths otherJobs <| Dict.insert job.name 0 dict
_ ->
let
passedJobDepths =
List.map (\passedJob -> Dict.get passedJob dict) passedJobs
in
if List.member Nothing passedJobDepths then
jobDepths (List.append otherJobs [ job ]) dict
else
let
depths =
List.map (\depth -> Maybe.withDefault 0 depth) passedJobDepths
maxPassedJobDepth =
Maybe.withDefault 0 <| List.maximum depths
in
jobDepths otherJobs <| Dict.insert job.name (maxPassedJobDepth + 1) dict
| 6262 | module Benchmarks exposing (main)
import Ansi.Log
import Application.Models exposing (Session)
import Array
import Assets
import Benchmark
import Benchmark.Runner exposing (BenchmarkProgram, program)
import Build.Build as Build
import Build.Header.Models exposing (BuildPageType(..), CommentBarVisibility(..), CurrentOutput(..))
import Build.Models
import Build.Output.Models
import Build.Output.Output
import Build.StepTree.Models as STModels
import Build.Styles
import Concourse
import Concourse.BuildStatus
import Concourse.Pagination exposing (Page)
import Dashboard.DashboardPreview as DP
import DateFormat
import Dict exposing (Dict)
import HoverState
import Html exposing (Html)
import Html.Attributes
exposing
( attribute
, class
, classList
, href
, id
, style
, tabindex
, title
)
import Html.Events exposing (onBlur, onFocus, onMouseEnter, onMouseLeave)
import Html.Lazy
import Keyboard
import Login.Login as Login
import Maybe.Extra
import Message.Message exposing (DomID(..), Message(..), PipelinesSection(..))
import RemoteData exposing (WebData)
import Routes exposing (Highlight)
import ScreenSize
import Set
import SideBar.SideBar as SideBar
import StrictEvents exposing (onLeftClick, onScroll, onWheel)
import Time
import UserState
import Views.BuildDuration as BuildDuration
import Views.Icon as Icon
import Views.LoadingIndicator as LoadingIndicator
import Views.NotAuthorized as NotAuthorized
import Views.Spinner as Spinner
import Views.Styles
import Views.TopBar as TopBar
type alias Model =
Login.Model
{ page : BuildPageType
, now : Maybe Time.Posix
, disableManualTrigger : Bool
, history : List Concourse.Build
, nextPage : Maybe Page
, currentBuild : WebData CurrentBuild
, autoScroll : Bool
, previousKeyPress : Maybe Keyboard.KeyEvent
, shiftDown : Bool
, isTriggerBuildKeyDown : Bool
, showHelp : Bool
, highlight : Highlight
, hoveredCounter : Int
, fetchingHistory : Bool
, scrolledToCurrentBuild : Bool
, authorized : Bool
}
type alias CurrentBuild =
{ build : Concourse.Build
, prep : Maybe Concourse.BuildPrep
, output : CurrentOutput
}
main : BenchmarkProgram
main =
program <|
Benchmark.describe "benchmark suite"
[ Benchmark.compare "DashboardPreview.view"
"current"
(\_ -> DP.view AllPipelinesSection HoverState.NoHover (DP.groupByRank sampleJobs))
"old"
(\_ -> dashboardPreviewView sampleJobs)
, Benchmark.compare "Build.view"
"current"
(\_ -> Build.view sampleSession sampleModel)
"old"
(\_ -> buildView sampleSession sampleOldModel)
]
bodyId : String
bodyId =
"build-body"
historyId : String
historyId =
"builds"
buildView : Session -> Model -> Html Message
buildView session model =
let
route =
case model.page of
OneOffBuildPage buildId ->
Routes.OneOffBuild
{ id = buildId
, highlight = model.highlight
}
JobBuildPage buildId ->
Routes.Build
{ id = buildId
, highlight = model.highlight
}
in
Html.div
(id "page-including-top-bar" :: Views.Styles.pageIncludingTopBar)
[ Html.div
(id "top-bar-app" :: Views.Styles.topBar False)
[ SideBar.sideBarIcon session
, TopBar.concourseLogo
, breadcrumbs session model
, Login.view session.userState model
]
, Html.div
(id "page-below-top-bar" :: Views.Styles.pageBelowTopBar route)
[ SideBar.view session
(currentJob model
|> Maybe.map
(\j ->
{ pipelineName = j.pipelineName
, pipelineInstanceVars = j.pipelineInstanceVars
, teamName = j.teamName
}
)
)
, viewBuildPage session model
]
]
viewBuildPage : Session -> Model -> Html Message
viewBuildPage session model =
case model.currentBuild |> RemoteData.toMaybe of
Just currentBuild ->
Html.div
[ class "with-fixed-header"
, attribute "data-build-name" currentBuild.build.name
, style "flex-grow" "1"
, style "display" "flex"
, style "flex-direction" "column"
, style "overflow" "hidden"
]
[ viewBuildHeader session model currentBuild.build
, body
session
{ currentBuild = currentBuild
, authorized = model.authorized
, showHelp = model.showHelp
}
]
_ ->
LoadingIndicator.view
currentJob : Model -> Maybe Concourse.JobIdentifier
currentJob =
.currentBuild
>> RemoteData.toMaybe
>> Maybe.map .build
>> Maybe.andThen .job
breadcrumbs : Session -> Model -> Html Message
breadcrumbs session model =
case ( currentJob model, model.page ) of
( Just jobId, _ ) ->
TopBar.breadcrumbs session <|
Routes.Job
{ id = jobId
, page = Nothing
}
( _, JobBuildPage buildId ) ->
TopBar.breadcrumbs session <|
Routes.Build
{ id = buildId
, highlight = model.highlight
}
_ ->
Html.text ""
body :
Session
->
{ currentBuild : CurrentBuild
, authorized : Bool
, showHelp : Bool
}
-> Html Message
body session { currentBuild, authorized, showHelp } =
Html.div
([ class "scrollable-body build-body"
, id bodyId
, tabindex 0
, onScroll Scrolled
]
++ Build.Styles.body
)
<|
if authorized then
[ viewBuildPrep currentBuild.prep
, Html.Lazy.lazy2 viewBuildOutput session currentBuild.output
, keyboardHelp showHelp
]
++ tombstone session.timeZone currentBuild
else
[ NotAuthorized.view ]
viewBuildHeader :
Session
-> Model
-> Concourse.Build
-> Html Message
viewBuildHeader session model build =
let
triggerButton =
case currentJob model of
Just _ ->
let
buttonDisabled =
model.disableManualTrigger
buttonHovered =
HoverState.isHovered
TriggerBuildButton
session.hovered
in
Html.button
([ attribute "role" "button"
, attribute "tabindex" "0"
, attribute "aria-label" "Trigger Build"
, attribute "title" "Trigger Build"
, onLeftClick <| Click TriggerBuildButton
, onMouseEnter <| Hover <| Just TriggerBuildButton
, onFocus <| Hover <| Just TriggerBuildButton
, onMouseLeave <| Hover Nothing
, onBlur <| Hover Nothing
]
++ Build.Styles.triggerButton
buttonDisabled
buttonHovered
build.status
)
<|
Icon.icon
{ sizePx = 40
, image = Assets.AddCircleIcon |> Assets.CircleOutlineIcon
}
[]
:: (if buttonDisabled && buttonHovered then
[ Html.div
[]
[ Html.text <|
"manual triggering disabled "
++ "in job config"
]
]
else
[]
)
Nothing ->
Html.text ""
abortHovered =
HoverState.isHovered AbortBuildButton session.hovered
abortButton =
if Concourse.BuildStatus.isRunning build.status then
Html.button
([ onLeftClick (Click <| AbortBuildButton)
, attribute "role" "button"
, attribute "tabindex" "0"
, attribute "aria-label" "Abort Build"
, attribute "title" "Abort Build"
, onMouseEnter <| Hover <| Just AbortBuildButton
, onFocus <| Hover <| Just AbortBuildButton
, onMouseLeave <| Hover Nothing
, onBlur <| Hover Nothing
]
++ Build.Styles.abortButton abortHovered
)
[ Icon.icon
{ sizePx = 40
, image = Assets.AbortCircleIcon |> Assets.CircleOutlineIcon
}
[]
]
else
Html.text ""
buildTitle =
case build.job of
Just jobId ->
let
jobRoute =
Routes.Job { id = jobId, page = Nothing }
in
Html.a
[ href <| Routes.toString jobRoute ]
[ Html.span [ class "build-name" ] [ Html.text jobId.jobName ]
, Html.text (" #" ++ build.name)
]
_ ->
Html.text ("build #" ++ String.fromInt build.id)
in
Html.div [ class "fixed-header" ]
[ Html.div
([ id "build-header"
, class "build-header"
]
++ Build.Styles.header build.status
)
[ Html.div []
[ Html.h1 [] [ buildTitle ]
, case model.now of
Just n ->
BuildDuration.view session.timeZone build.duration n
Nothing ->
Html.text ""
]
, Html.div
[ style "display" "flex" ]
[ abortButton, triggerButton ]
]
, Html.div
[ onWheel ScrollBuilds ]
[ lazyViewHistory build model.history ]
]
tombstone : Time.Zone -> CurrentBuild -> List (Html Message)
tombstone timeZone currentBuild =
let
build =
currentBuild.build
maybeBirthDate =
Maybe.Extra.or build.duration.startedAt build.duration.finishedAt
in
case ( maybeBirthDate, build.reapTime ) of
( Just birthDate, Just reapTime ) ->
[ Html.div
[ class "tombstone" ]
[ Html.div [ class "heading" ] [ Html.text "RIP" ]
, Html.div
[ class "job-name" ]
[ Html.text <|
Maybe.withDefault
"one-off build"
<|
Maybe.map .jobName build.job
]
, Html.div
[ class "build-name" ]
[ Html.text <|
"build #"
++ (case build.job of
Nothing ->
String.fromInt build.id
Just _ ->
build.name
)
]
, Html.div
[ class "date" ]
[ Html.text <|
mmDDYY timeZone birthDate
++ "-"
++ mmDDYY timeZone reapTime
]
, Html.div
[ class "epitaph" ]
[ Html.text <|
case build.status of
Concourse.BuildStatus.BuildStatusSucceeded ->
"It passed, and now it has passed on."
Concourse.BuildStatus.BuildStatusFailed ->
"It failed, and now has been forgotten."
Concourse.BuildStatus.BuildStatusErrored ->
"It errored, but has found forgiveness."
Concourse.BuildStatus.BuildStatusAborted ->
"It was never given a chance."
_ ->
"I'm not dead yet."
]
]
, Html.div
[ class "explanation" ]
[ Html.text "This log has been "
, Html.a
[ Html.Attributes.href "https://concourse-ci.org/jobs.html#job-build-log-retention" ]
[ Html.text "reaped." ]
]
]
_ ->
[]
keyboardHelp : Bool -> Html Message
keyboardHelp showHelp =
Html.div
[ classList
[ ( "keyboard-help", True )
, ( "hidden", not showHelp )
]
]
[ Html.div
[ class "help-title" ]
[ Html.text "keyboard shortcuts" ]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "h" ]
, Html.span [ class "key" ] [ Html.text "l" ]
]
, Html.text "previous/next build"
]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "j" ]
, Html.span [ class "key" ] [ Html.text "k" ]
]
, Html.text "scroll down/up"
]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "T" ] ]
, Html.text "trigger a new build"
]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "A" ] ]
, Html.text "abort build"
]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "gg" ] ]
, Html.text "scroll to the top"
]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "G" ] ]
, Html.text "scroll to the bottom"
]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "?" ] ]
, Html.text "hide/show help"
]
]
viewBuildOutput : Session -> CurrentOutput -> Html Message
viewBuildOutput session output =
case output of
Output o ->
Build.Output.Output.view
{ timeZone = session.timeZone, hovered = session.hovered }
o
Cancelled ->
Html.div
Build.Styles.errorLog
[ Html.text "build cancelled" ]
Empty ->
Html.div [] []
viewBuildPrep : Maybe Concourse.BuildPrep -> Html Message
viewBuildPrep buildPrep =
case buildPrep of
Just prep ->
Html.div [ class "build-step" ]
[ Html.div
[ class "header"
, style "display" "flex"
, style "align-items" "center"
]
[ Icon.icon
{ sizePx = 15, image = Assets.CogsIcon }
[ style "margin" "6.5px"
, style "margin-right" "0.5px"
, style "background-size" "contain"
]
, Html.h3 [] [ Html.text "preparing build" ]
]
, Html.div []
[ Html.ul [ class "prep-status-list" ]
([ viewBuildPrepLi "checking pipeline is not paused" prep.pausedPipeline Dict.empty
, viewBuildPrepLi "checking job is not paused" prep.pausedJob Dict.empty
]
++ viewBuildPrepInputs prep.inputs
++ [ viewBuildPrepLi "waiting for a suitable set of input versions" prep.inputsSatisfied prep.missingInputReasons
, viewBuildPrepLi "checking max-in-flight is not reached" prep.maxRunningBuilds Dict.empty
]
)
]
]
Nothing ->
Html.div [] []
lazyViewHistory : Concourse.Build -> List Concourse.Build -> Html Message
lazyViewHistory currentBuild builds =
Html.Lazy.lazy2 viewHistory currentBuild builds
viewHistory : Concourse.Build -> List Concourse.Build -> Html Message
viewHistory currentBuild builds =
Html.ul [ id historyId ]
(List.map (viewHistoryItem currentBuild) builds)
viewHistoryItem : Concourse.Build -> Concourse.Build -> Html Message
viewHistoryItem currentBuild build =
Html.li
([ classList [ ( "current", build.id == currentBuild.id ) ]
, id <| String.fromInt build.id
]
++ Build.Styles.historyItem
currentBuild.status
(build.id == currentBuild.id)
build.status
)
[ Html.a
[ onLeftClick <| Click <| BuildTab build.id build.name
, href <| Routes.toString <| Routes.buildRoute build.id build.name build.job
]
[ Html.text build.name ]
]
mmDDYY : Time.Zone -> Time.Posix -> String
mmDDYY =
DateFormat.format
[ DateFormat.monthFixed
, DateFormat.text "/"
, DateFormat.dayOfMonthFixed
, DateFormat.text "/"
, DateFormat.yearNumberLastTwo
]
viewBuildPrepLi :
String
-> Concourse.BuildPrepStatus
-> Dict String String
-> Html Message
viewBuildPrepLi text status details =
Html.li
[ classList
[ ( "prep-status", True )
, ( "inactive", status == Concourse.BuildPrepStatusUnknown )
]
]
[ Html.div
[ style "align-items" "center"
, style "display" "flex"
]
[ viewBuildPrepStatus status
, Html.span []
[ Html.text text ]
]
, viewBuildPrepDetails details
]
viewBuildPrepInputs : Dict String Concourse.BuildPrepStatus -> List (Html Message)
viewBuildPrepInputs inputs =
List.map viewBuildPrepInput (Dict.toList inputs)
viewBuildPrepInput : ( String, Concourse.BuildPrepStatus ) -> Html Message
viewBuildPrepInput ( name, status ) =
viewBuildPrepLi ("discovering any new versions of " ++ name) status Dict.empty
viewBuildPrepDetails : Dict String String -> Html Message
viewBuildPrepDetails details =
Html.ul [ class "details" ]
(List.map viewDetailItem (Dict.toList details))
viewBuildPrepStatus : Concourse.BuildPrepStatus -> Html Message
viewBuildPrepStatus status =
case status of
Concourse.BuildPrepStatusUnknown ->
Html.div
[ title "thinking..." ]
[ Spinner.spinner
{ sizePx = 12
, margin = "0 5px 0 0"
}
]
Concourse.BuildPrepStatusBlocking ->
Html.div
[ title "blocking" ]
[ Spinner.spinner
{ sizePx = 12
, margin = "0 5px 0 0"
}
]
Concourse.BuildPrepStatusNotBlocking ->
Icon.icon
{ sizePx = 12
, image = Assets.NotBlockingCheckIcon
}
[ style "margin-right" "5px"
, style "background-size" "contain"
, title "not blocking"
]
viewDetailItem : ( String, String ) -> Html Message
viewDetailItem ( name, status ) =
Html.li []
[ Html.text (name ++ " - " ++ status) ]
sampleSession : Session
sampleSession =
{ authToken = ""
, csrfToken = ""
, expandedTeamsInAllPipelines = Set.empty
, collapsedTeamsInFavorites = Set.empty
, favoritedPipelines = Set.empty
, favoritedInstanceGroups = Set.empty
, hovered = HoverState.NoHover
, sideBarState =
{ isOpen = False
, width = 275
}
, draggingSideBar = False
, notFoundImgSrc = ""
, pipelineRunningKeyframes = ""
, pipelines = RemoteData.NotAsked
, screenSize = ScreenSize.Desktop
, timeZone = Time.utc
, turbulenceImgSrc = ""
, userState = UserState.UserStateLoggedOut
, clusterName = ""
, version = ""
, featureFlags = Concourse.defaultFeatureFlags
, route =
Routes.OneOffBuild
{ id = 1
, highlight = Routes.HighlightNothing
}
}
sampleOldModel : Model
sampleOldModel =
{ page = OneOffBuildPage 0
, now = Nothing
, disableManualTrigger = False
, history = []
, nextPage = Nothing
, currentBuild =
RemoteData.Success
{ build =
{ id = 0
, name = "0"
, teamName = "team"
, job = Nothing
, status = Concourse.BuildStatus.BuildStatusStarted
, duration =
{ startedAt = Nothing
, finishedAt = Nothing
}
, reapTime = Nothing
, createdBy = Nothing
, comment = ""
}
, prep = Nothing
, output =
Output
{ steps = stepsModel
, state = Build.Output.Models.StepsLiveUpdating
, eventSourceOpened = True
, eventStreamUrlPath = Nothing
, highlight = Routes.HighlightNothing
, buildId = Nothing
}
}
, autoScroll = True
, previousKeyPress = Nothing
, shiftDown = False
, isTriggerBuildKeyDown = False
, showHelp = False
, highlight = Routes.HighlightNothing
, hoveredCounter = 0
, fetchingHistory = False
, scrolledToCurrentBuild = True
, authorized = True
, isUserMenuExpanded = False
}
sampleModel : Build.Models.Model
sampleModel =
{ page = OneOffBuildPage 0
, id = 0
, name = "<NAME>"
, now = Nothing
, job = Nothing
, disableManualTrigger = False
, history = []
, nextPage = Nothing
, prep = Nothing
, duration = { startedAt = Nothing, finishedAt = Nothing }
, status = Concourse.BuildStatus.BuildStatusStarted
, output =
Output
{ steps = stepsModel
, state = Build.Output.Models.StepsLiveUpdating
, eventSourceOpened = True
, eventStreamUrlPath = Nothing
, highlight = Routes.HighlightNothing
, buildId = Nothing
}
, autoScroll = True
, isScrollToIdInProgress = False
, previousKeyPress = Nothing
, isTriggerBuildKeyDown = False
, showHelp = False
, highlight = Routes.HighlightNothing
, authorized = True
, fetchingHistory = False
, scrolledToCurrentBuild = False
, shiftDown = False
, isUserMenuExpanded = False
, hasLoadedYet = True
, notFound = False
, reapTime = Nothing
, createdBy = Nothing
, comment = Hidden ""
}
ansiLogStyle : Ansi.Log.Style
ansiLogStyle =
{ foreground = Nothing
, background = Nothing
, bold = False
, faint = False
, italic = False
, underline = False
, blink = False
, inverted = False
, fraktur = False
, framed = False
}
position : Ansi.Log.CursorPosition
position =
{ row = 0
, column = 0
}
log : Ansi.Log.Model
log =
{ lineDiscipline = Ansi.Log.Cooked
, lines = Array.empty
, position = position
, savedPosition = Nothing
, style = ansiLogStyle
, remainder = ""
}
tree : STModels.StepTree
tree =
STModels.Task "stepid"
steps : Dict Routes.StepID STModels.Step
steps =
Dict.singleton "stepid"
{ id = "stepid"
, buildStep = Concourse.BuildStepTask "task_step"
, state = STModels.StepStateRunning
, log = log
, error = Nothing
, expanded = True
, version = Nothing
, metadata = []
, changed = False
, timestamps = Dict.empty
, initialize = Nothing
, start = Nothing
, finish = Nothing
, tabFocus = STModels.Auto
, expandedHeaders = Dict.empty
, initializationExpanded = False
, imageCheck = Nothing
, imageGet = Nothing
}
stepsModel : Maybe STModels.StepTreeModel
stepsModel =
Just
{ tree = tree
, steps = steps
, highlight = Routes.HighlightNothing
, resources = { inputs = [], outputs = [] }
, buildId = Nothing
}
sampleJob : String -> List String -> Concourse.Job
sampleJob name passed =
{ name = name
, pipelineId = 1
, pipelineName = "pipeline"
, pipelineInstanceVars = Dict.empty
, teamName = "team"
, nextBuild = Nothing
, finishedBuild = Nothing
, transitionBuild = Nothing
, paused = False
, disableManualTrigger = False
, inputs =
[ { name = "input"
, resource = "resource"
, passed = passed
, trigger = True
}
]
, outputs = []
, groups = []
}
sampleJobs : List Concourse.Job
sampleJobs =
[ sampleJob "job1" []
, sampleJob "job2a" [ "job1" ]
, sampleJob "job2b" [ "job1" ]
, sampleJob "job3" [ "job2a" ]
, sampleJob "job4" [ "job3" ]
]
dashboardPreviewView : List Concourse.Job -> Html msg
dashboardPreviewView jobs =
let
groups =
jobGroups jobs
width =
Dict.size groups
height =
Maybe.withDefault 0 <| List.maximum (List.map List.length (Dict.values groups))
in
Html.div
[ classList
[ ( "pipeline-grid", True )
, ( "pipeline-grid-wide", width > 12 )
, ( "pipeline-grid-tall", height > 12 )
, ( "pipeline-grid-super-wide", width > 24 )
, ( "pipeline-grid-super-tall", height > 24 )
]
]
<|
List.map
(\js ->
List.map viewJob js
|> Html.div [ class "parallel-grid" ]
)
(Dict.values groups)
viewJob : Concourse.Job -> Html msg
viewJob job =
let
jobStatus =
case job.finishedBuild of
Just fb ->
Concourse.BuildStatus.show fb.status
Nothing ->
"no-builds"
isJobRunning =
job.nextBuild /= Nothing
latestBuild =
if job.nextBuild == Nothing then
job.finishedBuild
else
job.nextBuild
in
Html.div
[ classList
[ ( "node " ++ jobStatus, True )
, ( "running", isJobRunning )
, ( "paused", job.paused )
]
, attribute "data-tooltip" job.name
]
<|
case latestBuild of
Nothing ->
[ Html.a [ href <| Routes.toString <| Routes.jobRoute job ] [ Html.text "" ] ]
Just build ->
[ Html.a [ href <| Routes.toString <| Routes.buildRoute build.id build.name build.job ] [ Html.text "" ] ]
jobGroups : List Concourse.Job -> Dict Int (List Concourse.Job)
jobGroups jobs =
let
jobLookup =
jobByName <| List.foldl (\job byName -> Dict.insert job.name job byName) Dict.empty jobs
in
Dict.foldl
(\jobName depth byDepth ->
Dict.update depth
(\jobsA ->
Just (jobLookup jobName :: Maybe.withDefault [] jobsA)
)
byDepth
)
Dict.empty
(jobDepths jobs Dict.empty)
jobByName : Dict String Concourse.Job -> String -> Concourse.Job
jobByName jobs job =
case Dict.get job jobs of
Just a ->
a
Nothing ->
{ name = ""
, pipelineId = 0
, pipelineName = ""
, pipelineInstanceVars = Dict.empty
, teamName = ""
, nextBuild = Nothing
, finishedBuild = Nothing
, transitionBuild = Nothing
, paused = False
, disableManualTrigger = False
, inputs = []
, outputs = []
, groups = []
}
jobDepths : List Concourse.Job -> Dict String Int -> Dict String Int
jobDepths jobs dict =
case jobs of
[] ->
dict
job :: otherJobs ->
let
passedJobs =
List.concatMap .passed job.inputs
in
case List.length passedJobs of
0 ->
jobDepths otherJobs <| Dict.insert job.name 0 dict
_ ->
let
passedJobDepths =
List.map (\passedJob -> Dict.get passedJob dict) passedJobs
in
if List.member Nothing passedJobDepths then
jobDepths (List.append otherJobs [ job ]) dict
else
let
depths =
List.map (\depth -> Maybe.withDefault 0 depth) passedJobDepths
maxPassedJobDepth =
Maybe.withDefault 0 <| List.maximum depths
in
jobDepths otherJobs <| Dict.insert job.name (maxPassedJobDepth + 1) dict
| true | module Benchmarks exposing (main)
import Ansi.Log
import Application.Models exposing (Session)
import Array
import Assets
import Benchmark
import Benchmark.Runner exposing (BenchmarkProgram, program)
import Build.Build as Build
import Build.Header.Models exposing (BuildPageType(..), CommentBarVisibility(..), CurrentOutput(..))
import Build.Models
import Build.Output.Models
import Build.Output.Output
import Build.StepTree.Models as STModels
import Build.Styles
import Concourse
import Concourse.BuildStatus
import Concourse.Pagination exposing (Page)
import Dashboard.DashboardPreview as DP
import DateFormat
import Dict exposing (Dict)
import HoverState
import Html exposing (Html)
import Html.Attributes
exposing
( attribute
, class
, classList
, href
, id
, style
, tabindex
, title
)
import Html.Events exposing (onBlur, onFocus, onMouseEnter, onMouseLeave)
import Html.Lazy
import Keyboard
import Login.Login as Login
import Maybe.Extra
import Message.Message exposing (DomID(..), Message(..), PipelinesSection(..))
import RemoteData exposing (WebData)
import Routes exposing (Highlight)
import ScreenSize
import Set
import SideBar.SideBar as SideBar
import StrictEvents exposing (onLeftClick, onScroll, onWheel)
import Time
import UserState
import Views.BuildDuration as BuildDuration
import Views.Icon as Icon
import Views.LoadingIndicator as LoadingIndicator
import Views.NotAuthorized as NotAuthorized
import Views.Spinner as Spinner
import Views.Styles
import Views.TopBar as TopBar
type alias Model =
Login.Model
{ page : BuildPageType
, now : Maybe Time.Posix
, disableManualTrigger : Bool
, history : List Concourse.Build
, nextPage : Maybe Page
, currentBuild : WebData CurrentBuild
, autoScroll : Bool
, previousKeyPress : Maybe Keyboard.KeyEvent
, shiftDown : Bool
, isTriggerBuildKeyDown : Bool
, showHelp : Bool
, highlight : Highlight
, hoveredCounter : Int
, fetchingHistory : Bool
, scrolledToCurrentBuild : Bool
, authorized : Bool
}
type alias CurrentBuild =
{ build : Concourse.Build
, prep : Maybe Concourse.BuildPrep
, output : CurrentOutput
}
main : BenchmarkProgram
main =
program <|
Benchmark.describe "benchmark suite"
[ Benchmark.compare "DashboardPreview.view"
"current"
(\_ -> DP.view AllPipelinesSection HoverState.NoHover (DP.groupByRank sampleJobs))
"old"
(\_ -> dashboardPreviewView sampleJobs)
, Benchmark.compare "Build.view"
"current"
(\_ -> Build.view sampleSession sampleModel)
"old"
(\_ -> buildView sampleSession sampleOldModel)
]
bodyId : String
bodyId =
"build-body"
historyId : String
historyId =
"builds"
buildView : Session -> Model -> Html Message
buildView session model =
let
route =
case model.page of
OneOffBuildPage buildId ->
Routes.OneOffBuild
{ id = buildId
, highlight = model.highlight
}
JobBuildPage buildId ->
Routes.Build
{ id = buildId
, highlight = model.highlight
}
in
Html.div
(id "page-including-top-bar" :: Views.Styles.pageIncludingTopBar)
[ Html.div
(id "top-bar-app" :: Views.Styles.topBar False)
[ SideBar.sideBarIcon session
, TopBar.concourseLogo
, breadcrumbs session model
, Login.view session.userState model
]
, Html.div
(id "page-below-top-bar" :: Views.Styles.pageBelowTopBar route)
[ SideBar.view session
(currentJob model
|> Maybe.map
(\j ->
{ pipelineName = j.pipelineName
, pipelineInstanceVars = j.pipelineInstanceVars
, teamName = j.teamName
}
)
)
, viewBuildPage session model
]
]
viewBuildPage : Session -> Model -> Html Message
viewBuildPage session model =
case model.currentBuild |> RemoteData.toMaybe of
Just currentBuild ->
Html.div
[ class "with-fixed-header"
, attribute "data-build-name" currentBuild.build.name
, style "flex-grow" "1"
, style "display" "flex"
, style "flex-direction" "column"
, style "overflow" "hidden"
]
[ viewBuildHeader session model currentBuild.build
, body
session
{ currentBuild = currentBuild
, authorized = model.authorized
, showHelp = model.showHelp
}
]
_ ->
LoadingIndicator.view
currentJob : Model -> Maybe Concourse.JobIdentifier
currentJob =
.currentBuild
>> RemoteData.toMaybe
>> Maybe.map .build
>> Maybe.andThen .job
breadcrumbs : Session -> Model -> Html Message
breadcrumbs session model =
case ( currentJob model, model.page ) of
( Just jobId, _ ) ->
TopBar.breadcrumbs session <|
Routes.Job
{ id = jobId
, page = Nothing
}
( _, JobBuildPage buildId ) ->
TopBar.breadcrumbs session <|
Routes.Build
{ id = buildId
, highlight = model.highlight
}
_ ->
Html.text ""
body :
Session
->
{ currentBuild : CurrentBuild
, authorized : Bool
, showHelp : Bool
}
-> Html Message
body session { currentBuild, authorized, showHelp } =
Html.div
([ class "scrollable-body build-body"
, id bodyId
, tabindex 0
, onScroll Scrolled
]
++ Build.Styles.body
)
<|
if authorized then
[ viewBuildPrep currentBuild.prep
, Html.Lazy.lazy2 viewBuildOutput session currentBuild.output
, keyboardHelp showHelp
]
++ tombstone session.timeZone currentBuild
else
[ NotAuthorized.view ]
viewBuildHeader :
Session
-> Model
-> Concourse.Build
-> Html Message
viewBuildHeader session model build =
let
triggerButton =
case currentJob model of
Just _ ->
let
buttonDisabled =
model.disableManualTrigger
buttonHovered =
HoverState.isHovered
TriggerBuildButton
session.hovered
in
Html.button
([ attribute "role" "button"
, attribute "tabindex" "0"
, attribute "aria-label" "Trigger Build"
, attribute "title" "Trigger Build"
, onLeftClick <| Click TriggerBuildButton
, onMouseEnter <| Hover <| Just TriggerBuildButton
, onFocus <| Hover <| Just TriggerBuildButton
, onMouseLeave <| Hover Nothing
, onBlur <| Hover Nothing
]
++ Build.Styles.triggerButton
buttonDisabled
buttonHovered
build.status
)
<|
Icon.icon
{ sizePx = 40
, image = Assets.AddCircleIcon |> Assets.CircleOutlineIcon
}
[]
:: (if buttonDisabled && buttonHovered then
[ Html.div
[]
[ Html.text <|
"manual triggering disabled "
++ "in job config"
]
]
else
[]
)
Nothing ->
Html.text ""
abortHovered =
HoverState.isHovered AbortBuildButton session.hovered
abortButton =
if Concourse.BuildStatus.isRunning build.status then
Html.button
([ onLeftClick (Click <| AbortBuildButton)
, attribute "role" "button"
, attribute "tabindex" "0"
, attribute "aria-label" "Abort Build"
, attribute "title" "Abort Build"
, onMouseEnter <| Hover <| Just AbortBuildButton
, onFocus <| Hover <| Just AbortBuildButton
, onMouseLeave <| Hover Nothing
, onBlur <| Hover Nothing
]
++ Build.Styles.abortButton abortHovered
)
[ Icon.icon
{ sizePx = 40
, image = Assets.AbortCircleIcon |> Assets.CircleOutlineIcon
}
[]
]
else
Html.text ""
buildTitle =
case build.job of
Just jobId ->
let
jobRoute =
Routes.Job { id = jobId, page = Nothing }
in
Html.a
[ href <| Routes.toString jobRoute ]
[ Html.span [ class "build-name" ] [ Html.text jobId.jobName ]
, Html.text (" #" ++ build.name)
]
_ ->
Html.text ("build #" ++ String.fromInt build.id)
in
Html.div [ class "fixed-header" ]
[ Html.div
([ id "build-header"
, class "build-header"
]
++ Build.Styles.header build.status
)
[ Html.div []
[ Html.h1 [] [ buildTitle ]
, case model.now of
Just n ->
BuildDuration.view session.timeZone build.duration n
Nothing ->
Html.text ""
]
, Html.div
[ style "display" "flex" ]
[ abortButton, triggerButton ]
]
, Html.div
[ onWheel ScrollBuilds ]
[ lazyViewHistory build model.history ]
]
tombstone : Time.Zone -> CurrentBuild -> List (Html Message)
tombstone timeZone currentBuild =
let
build =
currentBuild.build
maybeBirthDate =
Maybe.Extra.or build.duration.startedAt build.duration.finishedAt
in
case ( maybeBirthDate, build.reapTime ) of
( Just birthDate, Just reapTime ) ->
[ Html.div
[ class "tombstone" ]
[ Html.div [ class "heading" ] [ Html.text "RIP" ]
, Html.div
[ class "job-name" ]
[ Html.text <|
Maybe.withDefault
"one-off build"
<|
Maybe.map .jobName build.job
]
, Html.div
[ class "build-name" ]
[ Html.text <|
"build #"
++ (case build.job of
Nothing ->
String.fromInt build.id
Just _ ->
build.name
)
]
, Html.div
[ class "date" ]
[ Html.text <|
mmDDYY timeZone birthDate
++ "-"
++ mmDDYY timeZone reapTime
]
, Html.div
[ class "epitaph" ]
[ Html.text <|
case build.status of
Concourse.BuildStatus.BuildStatusSucceeded ->
"It passed, and now it has passed on."
Concourse.BuildStatus.BuildStatusFailed ->
"It failed, and now has been forgotten."
Concourse.BuildStatus.BuildStatusErrored ->
"It errored, but has found forgiveness."
Concourse.BuildStatus.BuildStatusAborted ->
"It was never given a chance."
_ ->
"I'm not dead yet."
]
]
, Html.div
[ class "explanation" ]
[ Html.text "This log has been "
, Html.a
[ Html.Attributes.href "https://concourse-ci.org/jobs.html#job-build-log-retention" ]
[ Html.text "reaped." ]
]
]
_ ->
[]
keyboardHelp : Bool -> Html Message
keyboardHelp showHelp =
Html.div
[ classList
[ ( "keyboard-help", True )
, ( "hidden", not showHelp )
]
]
[ Html.div
[ class "help-title" ]
[ Html.text "keyboard shortcuts" ]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "h" ]
, Html.span [ class "key" ] [ Html.text "l" ]
]
, Html.text "previous/next build"
]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "j" ]
, Html.span [ class "key" ] [ Html.text "k" ]
]
, Html.text "scroll down/up"
]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "T" ] ]
, Html.text "trigger a new build"
]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "A" ] ]
, Html.text "abort build"
]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "gg" ] ]
, Html.text "scroll to the top"
]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "G" ] ]
, Html.text "scroll to the bottom"
]
, Html.div
[ class "help-line" ]
[ Html.div
[ class "keys" ]
[ Html.span [ class "key" ] [ Html.text "?" ] ]
, Html.text "hide/show help"
]
]
viewBuildOutput : Session -> CurrentOutput -> Html Message
viewBuildOutput session output =
case output of
Output o ->
Build.Output.Output.view
{ timeZone = session.timeZone, hovered = session.hovered }
o
Cancelled ->
Html.div
Build.Styles.errorLog
[ Html.text "build cancelled" ]
Empty ->
Html.div [] []
viewBuildPrep : Maybe Concourse.BuildPrep -> Html Message
viewBuildPrep buildPrep =
case buildPrep of
Just prep ->
Html.div [ class "build-step" ]
[ Html.div
[ class "header"
, style "display" "flex"
, style "align-items" "center"
]
[ Icon.icon
{ sizePx = 15, image = Assets.CogsIcon }
[ style "margin" "6.5px"
, style "margin-right" "0.5px"
, style "background-size" "contain"
]
, Html.h3 [] [ Html.text "preparing build" ]
]
, Html.div []
[ Html.ul [ class "prep-status-list" ]
([ viewBuildPrepLi "checking pipeline is not paused" prep.pausedPipeline Dict.empty
, viewBuildPrepLi "checking job is not paused" prep.pausedJob Dict.empty
]
++ viewBuildPrepInputs prep.inputs
++ [ viewBuildPrepLi "waiting for a suitable set of input versions" prep.inputsSatisfied prep.missingInputReasons
, viewBuildPrepLi "checking max-in-flight is not reached" prep.maxRunningBuilds Dict.empty
]
)
]
]
Nothing ->
Html.div [] []
lazyViewHistory : Concourse.Build -> List Concourse.Build -> Html Message
lazyViewHistory currentBuild builds =
Html.Lazy.lazy2 viewHistory currentBuild builds
viewHistory : Concourse.Build -> List Concourse.Build -> Html Message
viewHistory currentBuild builds =
Html.ul [ id historyId ]
(List.map (viewHistoryItem currentBuild) builds)
viewHistoryItem : Concourse.Build -> Concourse.Build -> Html Message
viewHistoryItem currentBuild build =
Html.li
([ classList [ ( "current", build.id == currentBuild.id ) ]
, id <| String.fromInt build.id
]
++ Build.Styles.historyItem
currentBuild.status
(build.id == currentBuild.id)
build.status
)
[ Html.a
[ onLeftClick <| Click <| BuildTab build.id build.name
, href <| Routes.toString <| Routes.buildRoute build.id build.name build.job
]
[ Html.text build.name ]
]
mmDDYY : Time.Zone -> Time.Posix -> String
mmDDYY =
DateFormat.format
[ DateFormat.monthFixed
, DateFormat.text "/"
, DateFormat.dayOfMonthFixed
, DateFormat.text "/"
, DateFormat.yearNumberLastTwo
]
viewBuildPrepLi :
String
-> Concourse.BuildPrepStatus
-> Dict String String
-> Html Message
viewBuildPrepLi text status details =
Html.li
[ classList
[ ( "prep-status", True )
, ( "inactive", status == Concourse.BuildPrepStatusUnknown )
]
]
[ Html.div
[ style "align-items" "center"
, style "display" "flex"
]
[ viewBuildPrepStatus status
, Html.span []
[ Html.text text ]
]
, viewBuildPrepDetails details
]
viewBuildPrepInputs : Dict String Concourse.BuildPrepStatus -> List (Html Message)
viewBuildPrepInputs inputs =
List.map viewBuildPrepInput (Dict.toList inputs)
viewBuildPrepInput : ( String, Concourse.BuildPrepStatus ) -> Html Message
viewBuildPrepInput ( name, status ) =
viewBuildPrepLi ("discovering any new versions of " ++ name) status Dict.empty
viewBuildPrepDetails : Dict String String -> Html Message
viewBuildPrepDetails details =
Html.ul [ class "details" ]
(List.map viewDetailItem (Dict.toList details))
viewBuildPrepStatus : Concourse.BuildPrepStatus -> Html Message
viewBuildPrepStatus status =
case status of
Concourse.BuildPrepStatusUnknown ->
Html.div
[ title "thinking..." ]
[ Spinner.spinner
{ sizePx = 12
, margin = "0 5px 0 0"
}
]
Concourse.BuildPrepStatusBlocking ->
Html.div
[ title "blocking" ]
[ Spinner.spinner
{ sizePx = 12
, margin = "0 5px 0 0"
}
]
Concourse.BuildPrepStatusNotBlocking ->
Icon.icon
{ sizePx = 12
, image = Assets.NotBlockingCheckIcon
}
[ style "margin-right" "5px"
, style "background-size" "contain"
, title "not blocking"
]
viewDetailItem : ( String, String ) -> Html Message
viewDetailItem ( name, status ) =
Html.li []
[ Html.text (name ++ " - " ++ status) ]
sampleSession : Session
sampleSession =
{ authToken = ""
, csrfToken = ""
, expandedTeamsInAllPipelines = Set.empty
, collapsedTeamsInFavorites = Set.empty
, favoritedPipelines = Set.empty
, favoritedInstanceGroups = Set.empty
, hovered = HoverState.NoHover
, sideBarState =
{ isOpen = False
, width = 275
}
, draggingSideBar = False
, notFoundImgSrc = ""
, pipelineRunningKeyframes = ""
, pipelines = RemoteData.NotAsked
, screenSize = ScreenSize.Desktop
, timeZone = Time.utc
, turbulenceImgSrc = ""
, userState = UserState.UserStateLoggedOut
, clusterName = ""
, version = ""
, featureFlags = Concourse.defaultFeatureFlags
, route =
Routes.OneOffBuild
{ id = 1
, highlight = Routes.HighlightNothing
}
}
sampleOldModel : Model
sampleOldModel =
{ page = OneOffBuildPage 0
, now = Nothing
, disableManualTrigger = False
, history = []
, nextPage = Nothing
, currentBuild =
RemoteData.Success
{ build =
{ id = 0
, name = "0"
, teamName = "team"
, job = Nothing
, status = Concourse.BuildStatus.BuildStatusStarted
, duration =
{ startedAt = Nothing
, finishedAt = Nothing
}
, reapTime = Nothing
, createdBy = Nothing
, comment = ""
}
, prep = Nothing
, output =
Output
{ steps = stepsModel
, state = Build.Output.Models.StepsLiveUpdating
, eventSourceOpened = True
, eventStreamUrlPath = Nothing
, highlight = Routes.HighlightNothing
, buildId = Nothing
}
}
, autoScroll = True
, previousKeyPress = Nothing
, shiftDown = False
, isTriggerBuildKeyDown = False
, showHelp = False
, highlight = Routes.HighlightNothing
, hoveredCounter = 0
, fetchingHistory = False
, scrolledToCurrentBuild = True
, authorized = True
, isUserMenuExpanded = False
}
sampleModel : Build.Models.Model
sampleModel =
{ page = OneOffBuildPage 0
, id = 0
, name = "PI:NAME:<NAME>END_PI"
, now = Nothing
, job = Nothing
, disableManualTrigger = False
, history = []
, nextPage = Nothing
, prep = Nothing
, duration = { startedAt = Nothing, finishedAt = Nothing }
, status = Concourse.BuildStatus.BuildStatusStarted
, output =
Output
{ steps = stepsModel
, state = Build.Output.Models.StepsLiveUpdating
, eventSourceOpened = True
, eventStreamUrlPath = Nothing
, highlight = Routes.HighlightNothing
, buildId = Nothing
}
, autoScroll = True
, isScrollToIdInProgress = False
, previousKeyPress = Nothing
, isTriggerBuildKeyDown = False
, showHelp = False
, highlight = Routes.HighlightNothing
, authorized = True
, fetchingHistory = False
, scrolledToCurrentBuild = False
, shiftDown = False
, isUserMenuExpanded = False
, hasLoadedYet = True
, notFound = False
, reapTime = Nothing
, createdBy = Nothing
, comment = Hidden ""
}
ansiLogStyle : Ansi.Log.Style
ansiLogStyle =
{ foreground = Nothing
, background = Nothing
, bold = False
, faint = False
, italic = False
, underline = False
, blink = False
, inverted = False
, fraktur = False
, framed = False
}
position : Ansi.Log.CursorPosition
position =
{ row = 0
, column = 0
}
log : Ansi.Log.Model
log =
{ lineDiscipline = Ansi.Log.Cooked
, lines = Array.empty
, position = position
, savedPosition = Nothing
, style = ansiLogStyle
, remainder = ""
}
tree : STModels.StepTree
tree =
STModels.Task "stepid"
steps : Dict Routes.StepID STModels.Step
steps =
Dict.singleton "stepid"
{ id = "stepid"
, buildStep = Concourse.BuildStepTask "task_step"
, state = STModels.StepStateRunning
, log = log
, error = Nothing
, expanded = True
, version = Nothing
, metadata = []
, changed = False
, timestamps = Dict.empty
, initialize = Nothing
, start = Nothing
, finish = Nothing
, tabFocus = STModels.Auto
, expandedHeaders = Dict.empty
, initializationExpanded = False
, imageCheck = Nothing
, imageGet = Nothing
}
stepsModel : Maybe STModels.StepTreeModel
stepsModel =
Just
{ tree = tree
, steps = steps
, highlight = Routes.HighlightNothing
, resources = { inputs = [], outputs = [] }
, buildId = Nothing
}
sampleJob : String -> List String -> Concourse.Job
sampleJob name passed =
{ name = name
, pipelineId = 1
, pipelineName = "pipeline"
, pipelineInstanceVars = Dict.empty
, teamName = "team"
, nextBuild = Nothing
, finishedBuild = Nothing
, transitionBuild = Nothing
, paused = False
, disableManualTrigger = False
, inputs =
[ { name = "input"
, resource = "resource"
, passed = passed
, trigger = True
}
]
, outputs = []
, groups = []
}
sampleJobs : List Concourse.Job
sampleJobs =
[ sampleJob "job1" []
, sampleJob "job2a" [ "job1" ]
, sampleJob "job2b" [ "job1" ]
, sampleJob "job3" [ "job2a" ]
, sampleJob "job4" [ "job3" ]
]
dashboardPreviewView : List Concourse.Job -> Html msg
dashboardPreviewView jobs =
let
groups =
jobGroups jobs
width =
Dict.size groups
height =
Maybe.withDefault 0 <| List.maximum (List.map List.length (Dict.values groups))
in
Html.div
[ classList
[ ( "pipeline-grid", True )
, ( "pipeline-grid-wide", width > 12 )
, ( "pipeline-grid-tall", height > 12 )
, ( "pipeline-grid-super-wide", width > 24 )
, ( "pipeline-grid-super-tall", height > 24 )
]
]
<|
List.map
(\js ->
List.map viewJob js
|> Html.div [ class "parallel-grid" ]
)
(Dict.values groups)
viewJob : Concourse.Job -> Html msg
viewJob job =
let
jobStatus =
case job.finishedBuild of
Just fb ->
Concourse.BuildStatus.show fb.status
Nothing ->
"no-builds"
isJobRunning =
job.nextBuild /= Nothing
latestBuild =
if job.nextBuild == Nothing then
job.finishedBuild
else
job.nextBuild
in
Html.div
[ classList
[ ( "node " ++ jobStatus, True )
, ( "running", isJobRunning )
, ( "paused", job.paused )
]
, attribute "data-tooltip" job.name
]
<|
case latestBuild of
Nothing ->
[ Html.a [ href <| Routes.toString <| Routes.jobRoute job ] [ Html.text "" ] ]
Just build ->
[ Html.a [ href <| Routes.toString <| Routes.buildRoute build.id build.name build.job ] [ Html.text "" ] ]
jobGroups : List Concourse.Job -> Dict Int (List Concourse.Job)
jobGroups jobs =
let
jobLookup =
jobByName <| List.foldl (\job byName -> Dict.insert job.name job byName) Dict.empty jobs
in
Dict.foldl
(\jobName depth byDepth ->
Dict.update depth
(\jobsA ->
Just (jobLookup jobName :: Maybe.withDefault [] jobsA)
)
byDepth
)
Dict.empty
(jobDepths jobs Dict.empty)
jobByName : Dict String Concourse.Job -> String -> Concourse.Job
jobByName jobs job =
case Dict.get job jobs of
Just a ->
a
Nothing ->
{ name = ""
, pipelineId = 0
, pipelineName = ""
, pipelineInstanceVars = Dict.empty
, teamName = ""
, nextBuild = Nothing
, finishedBuild = Nothing
, transitionBuild = Nothing
, paused = False
, disableManualTrigger = False
, inputs = []
, outputs = []
, groups = []
}
jobDepths : List Concourse.Job -> Dict String Int -> Dict String Int
jobDepths jobs dict =
case jobs of
[] ->
dict
job :: otherJobs ->
let
passedJobs =
List.concatMap .passed job.inputs
in
case List.length passedJobs of
0 ->
jobDepths otherJobs <| Dict.insert job.name 0 dict
_ ->
let
passedJobDepths =
List.map (\passedJob -> Dict.get passedJob dict) passedJobs
in
if List.member Nothing passedJobDepths then
jobDepths (List.append otherJobs [ job ]) dict
else
let
depths =
List.map (\depth -> Maybe.withDefault 0 depth) passedJobDepths
maxPassedJobDepth =
Maybe.withDefault 0 <| List.maximum depths
in
jobDepths otherJobs <| Dict.insert job.name (maxPassedJobDepth + 1) dict
| elm |
[
{
"context": "created.\n\n init\n 10\n [ { name = \"Paul\"\n , points = 1000\n }\n , ",
"end": 1789,
"score": 0.9965245724,
"start": 1785,
"tag": "NAME",
"value": "Paul"
},
{
"context": " , points = 1000\n }\n , { name = \"Steve\"\n , points = 900\n }\n ]\n ",
"end": 1854,
"score": 0.9995518327,
"start": 1849,
"tag": "NAME",
"value": "Steve"
},
{
"context": " dropped.\n\n init\n 2\n [ { name = \"Paul\"\n , points = 1000\n }\n , ",
"end": 2129,
"score": 0.9994157553,
"start": 2125,
"tag": "NAME",
"value": "Paul"
},
{
"context": " , points = 1000\n }\n , { name = \"Steve\"\n , points = 900\n }\n , {",
"end": 2194,
"score": 0.9995453954,
"start": 2189,
"tag": "NAME",
"value": "Steve"
},
{
"context": " , points = 900\n }\n , { name = \"Jeremy\"\n , points = 800\n }\n ]\n ",
"end": 2259,
"score": 0.9996340275,
"start": 2253,
"tag": "NAME",
"value": "Jeremy"
},
{
"context": " --> 2\n\n init\n 2\n [ { name = \"Paul\"\n , points = 1000\n }\n , ",
"end": 2405,
"score": 0.9995566607,
"start": 2401,
"tag": "NAME",
"value": "Paul"
},
{
"context": " , points = 1000\n }\n , { name = \"Steve\"\n , points = 900\n }\n , {",
"end": 2470,
"score": 0.9995512962,
"start": 2465,
"tag": "NAME",
"value": "Steve"
},
{
"context": " , points = 900\n }\n , { name = \"Jeremy\"\n , points = 800\n }\n ]\n ",
"end": 2535,
"score": 0.9996703267,
"start": 2529,
"tag": "NAME",
"value": "Jeremy"
},
{
"context": " ]\n |> lowest\n |> name\n --> \"Steve\"\n\n-}\ninit : Max -> List { name : String, points :",
"end": 2636,
"score": 0.9995753765,
"start": 2631,
"tag": "NAME",
"value": "Steve"
},
{
"context": "ule this is taken care of\nfor you.\n\n register \"Paul\"\n |> name\n --> \"Paul\"\n\n register",
"end": 3987,
"score": 0.9994122386,
"start": 3983,
"tag": "NAME",
"value": "Paul"
},
{
"context": "\n register \"Paul\"\n |> name\n --> \"Paul\"\n\n register \"Paul\"\n |> points\n -",
"end": 4022,
"score": 0.9996564388,
"start": 4018,
"tag": "NAME",
"value": "Paul"
},
{
"context": " |> name\n --> \"Paul\"\n\n register \"Paul\"\n |> points\n --> 0\n\n-}\nregister : S",
"end": 4043,
"score": 0.9996355772,
"start": 4039,
"tag": "NAME",
"value": "Paul"
},
{
"context": "ule this is taken care of\nfor you.\n\n register \"Paul\"\n |> incrementScore 10\n |> incremen",
"end": 4367,
"score": 0.999632597,
"start": 4363,
"tag": "NAME",
"value": "Paul"
},
{
"context": "ule this is taken care of\nfor you.\n\n register \"Paul\"\n |> incrementScore 10\n |> decremen",
"end": 4831,
"score": 0.9996106625,
"start": 4827,
"tag": "NAME",
"value": "Paul"
},
{
"context": "st score.\n\n init\n 2\n [ { name = \"Paul\"\n , points = 1000\n }\n , ",
"end": 5876,
"score": 0.9994157553,
"start": 5872,
"tag": "NAME",
"value": "Paul"
},
{
"context": " , points = 1000\n }\n , { name = \"Steve\"\n , points = 900\n }\n ]\n ",
"end": 5941,
"score": 0.998801887,
"start": 5936,
"tag": "NAME",
"value": "Steve"
},
{
"context": "(#Score).\n\n init\n 2\n [ { name = \"Paul\"\n , points = 1000\n }\n , ",
"end": 6239,
"score": 0.9990084171,
"start": 6235,
"tag": "NAME",
"value": "Paul"
},
{
"context": " , points = 1000\n }\n , { name = \"Steve\"\n , points = 900\n }\n ]\n ",
"end": 6304,
"score": 0.9983931184,
"start": 6299,
"tag": "NAME",
"value": "Steve"
},
{
"context": "database.\n\n init\n 3\n [ { name = \"Paul\"\n , points = 1000\n }\n , ",
"end": 8627,
"score": 0.9991402626,
"start": 8623,
"tag": "NAME",
"value": "Paul"
},
{
"context": " , points = 1000\n }\n , { name = \"Steve\"\n , points = 900\n }\n ]\n ",
"end": 8692,
"score": 0.9990785122,
"start": 8687,
"tag": "NAME",
"value": "Steve"
},
{
"context": "\n |> prepareForSave\n --> [ {name = \"Paul\", points = 1000}, {name = \"Steve\", points = 900},",
"end": 8794,
"score": 0.9994046688,
"start": 8790,
"tag": "NAME",
"value": "Paul"
},
{
"context": " --> [ {name = \"Paul\", points = 1000}, {name = \"Steve\", points = 900}, {name = \"---\", points = 0}]\n\n-}\n",
"end": 8827,
"score": 0.9994678497,
"start": 8822,
"tag": "NAME",
"value": "Steve"
},
{
"context": "a `view`.\n\n init\n 3\n [ { name = \"Paul\"\n , points = 1000\n }\n , ",
"end": 9185,
"score": 0.9992021918,
"start": 9181,
"tag": "NAME",
"value": "Paul"
},
{
"context": " , points = 1000\n }\n , { name = \"Steve\"\n , points = 900\n }\n ]\n ",
"end": 9250,
"score": 0.9990916848,
"start": 9245,
"tag": "NAME",
"value": "Steve"
},
{
"context": "(#Score).\n\n init\n 3\n [ { name = \"Paul\"\n , points = 1000\n }\n , ",
"end": 9799,
"score": 0.9987932444,
"start": 9795,
"tag": "NAME",
"value": "Paul"
},
{
"context": " , points = 1000\n }\n , { name = \"Steve\"\n , points = 900\n }\n ]\n ",
"end": 9864,
"score": 0.9991629124,
"start": 9859,
"tag": "NAME",
"value": "Steve"
},
{
"context": "]\n |> highest\n |> name\n --> \"Paul\"\n\n-}\nname : Score -> String\nname (Score score) =\n",
"end": 9965,
"score": 0.9991266727,
"start": 9961,
"tag": "NAME",
"value": "Paul"
},
{
"context": "(#Score).\n\n init\n 3\n [ { name = \"Paul\"\n , points = 1000\n }\n , ",
"end": 10126,
"score": 0.9989617467,
"start": 10122,
"tag": "NAME",
"value": "Paul"
},
{
"context": " , points = 1000\n }\n , { name = \"Steve\"\n , points = 900\n }\n ]\n ",
"end": 10191,
"score": 0.9991098642,
"start": 10186,
"tag": "NAME",
"value": "Steve"
},
{
"context": "#Score)s.\n\n init\n 3\n [ { name = \"Paul\"\n , points = 1000\n }\n , ",
"end": 10448,
"score": 0.9990347624,
"start": 10444,
"tag": "NAME",
"value": "Paul"
},
{
"context": " , points = 1000\n }\n , { name = \"Steve\"\n , points = 900\n }\n ]\n ",
"end": 10513,
"score": 0.9986655116,
"start": 10508,
"tag": "NAME",
"value": "Steve"
}
] | src/Shared/Scores.elm | phollyer/elm-space-invaders | 0 | module Shared.Scores exposing
( Scores, Score
, Max, init
, register
, incrementScore, decrementScore, scoreChange, highest, lowest, list
, gameOver
, prepareForSave
, highestToString
, name, points, total
, updateName
)
{-| A module for managing game [Score](#Score)s and highscores.
This module is used by the [Players](Shared.Players) module to manage players
scores, so if you're using that module, you probably won't need many of the
functions in this module.
@docs Scores, Score
# CREATING
@docs Max, init
# REGISTERING
@docs register
# SCORING
@docs incrementScore, decrementScore, scoreChange, highest, lowest, list
# GAME OVER
@docs gameOver
# SAVING
@docs prepareForSave
# CONVERTING
@docs highestToString
# QUERYING
@docs name, points, total
# UPDATING
@docs updateName
-}
{-| A type representing a list of [Scores](#Scores).
This is an opaque type, use the exposed API to interact with it.
-}
type Scores
= Scores
{ currentHighest : Score
, list : List Score
, total : Int
}
{-| A type representing a [Player](Shared.Players#Player)'s [Score](#Score).
This is an opaque type, use the exposed API to interact with it.
-}
type Score
= Score
{ points : Int
, name : String
}
-- CREATING
{-| A type alias representing the maximum number of [Score](#Score)s to keep
track of.
-}
type alias Max =
Int
{-| Initialize a list of [Scores](#Scores).
**Note**, if you are using the [Players](Players#Players) module this is taken
care of for you.
If you want to record a total of 10 [Score](#Score)s, but the list contains
only 2, then an additional 8 zero [Score](#score)s will be created.
init
10
[ { name = "Paul"
, points = 1000
}
, { name = "Steve"
, points = 900
}
]
|> list
|> List.length
--> 10
If you want to record a total of 2 [Score](#Score)s, but the list contains 3,
then the lowest [Score](#Score) will be dropped.
init
2
[ { name = "Paul"
, points = 1000
}
, { name = "Steve"
, points = 900
}
, { name = "Jeremy"
, points = 800
}
]
|> list
|> List.length
--> 2
init
2
[ { name = "Paul"
, points = 1000
}
, { name = "Steve"
, points = 900
}
, { name = "Jeremy"
, points = 800
}
]
|> lowest
|> name
--> "Steve"
-}
init : Max -> List { name : String, points : Int } -> Scores
init max list_ =
let
newList =
list_
|> initScores
max
in
Scores
{ currentHighest =
newList
|> List.head
|> Maybe.withDefault
initScore
, list = newList
, total = max
}
initScores : Max -> List { name : String, points : Int } -> List Score
initScores max scores_ =
let
list_ =
scores_
|> List.map
Score
extras =
max - (list_ |> List.length)
in
case extras < 0 of
True ->
list_
|> List.sortWith
highToLow
|> List.take
max
False ->
list_
|> List.append
(initScore
|> List.repeat
extras
)
|> List.sortWith
highToLow
initScore : Score
initScore =
Score
{ name = "---"
, points = 0
}
-- REGISTERING
{-| Register a new player.
**Note**, if you are using the [Players](Players#Players) module this is taken care of
for you.
register "Paul"
|> name
--> "Paul"
register "Paul"
|> points
--> 0
-}
register : String -> Score
register name_ =
initScore
|> updateName
name_
-- SCORING
{-| Increase a [Score](#Score) by the specified amount.
**Note**, if you are using the [Players](Players#Players) module this is taken care of
for you.
register "Paul"
|> incrementScore 10
|> incrementScore 5
|> points
--> 15
-}
incrementScore : Int -> Score -> Score
incrementScore points_ score =
score
|> updatePoints
(score
|> points
|> (+) points_
)
{-| Decrease a [Score](#Score) by the specified amount.
**Note**, if you are using the [Players](Players#Players) module this is taken care of
for you.
register "Paul"
|> incrementScore 10
|> decrementScore 3
|> points
--> 7
-}
decrementScore : Int -> Score -> Score
decrementScore points_ score =
score
|> updatePoints
((score |> points) - points_)
{-| A players score has changed, so check to see if it replaces the current
`highest`.
**Note**, if you are using the [Players](Players#Players) module this is taken care of
for you.
This does not affect the whole list, only the `highest`
[Score](#Score). This is really intended for in-game use where you
just want to compare the current score to the highest, and display accordingly.
The whole list will be updated when [gameOver](#gameOver) is called.
-}
scoreChange : Score -> Scores -> Scores
scoreChange score scores =
case (score |> points) > (scores |> currentHighest |> points) of
True ->
scores
|> updateHighest
score
False ->
scores
{-| Get the highest score.
init
2
[ { name = "Paul"
, points = 1000
}
, { name = "Steve"
, points = 900
}
]
|> highest
|> points
--> 1000
-}
highest : Scores -> Score
highest scores =
scores
|> list
|> first
highToLow
{-| Get the lowest [Score](#Score).
init
2
[ { name = "Paul"
, points = 1000
}
, { name = "Steve"
, points = 900
}
]
|> lowest
|> points
--> 900
-}
lowest : Scores -> Score
lowest scores =
scores
|> list
|> first
lowToHigh
-- SORTING
first : (Score -> Score -> Order) -> List Score -> Score
first sorter list_ =
list_
|> List.sortWith
sorter
|> List.head
|> Maybe.withDefault
initScore
highToLow : Score -> Score -> Order
highToLow a b =
case compare (a |> points) (b |> points) of
GT ->
LT
EQ ->
EQ
LT ->
GT
lowToHigh : Score -> Score -> Order
lowToHigh a b =
case compare (a |> points) (b |> points) of
GT ->
GT
EQ ->
EQ
LT ->
LT
-- GAME OVER
{-| A player has finished their game so let's see if they make the highscores
list.
**Note**, if you are using the [Players](Players#Players) module this is taken care of
for you.
-}
gameOver : Score -> Scores -> Scores
gameOver score scores =
case scores |> list |> qualifies score of
True ->
let
newList =
scores
|> list
|> List.append
[ score ]
|> List.sortWith
highToLow
|> List.take
(scores
|> total
)
in
scores
|> updateList
newList
|> updateHighest
(newList
|> first
highToLow
)
False ->
scores
qualifies : Score -> List Score -> Bool
qualifies potential list_ =
let
filteredList =
list_
|> List.filter
(\score ->
(score |> points) > (potential |> points)
)
in
(filteredList |> List.length) /= (list_ |> List.length)
-- SAVING
{-| Prepare the list of [Scores](#Scores) for saving, either to
`localStorage` or a back end database.
init
3
[ { name = "Paul"
, points = 1000
}
, { name = "Steve"
, points = 900
}
]
|> prepareForSave
--> [ {name = "Paul", points = 1000}, {name = "Steve", points = 900}, {name = "---", points = 0}]
-}
prepareForSave : Scores -> List { name : String, points : Int }
prepareForSave scores =
scores
|> list
|> List.map
(\(Score score) -> score)
-- CONVERTING
{-| Convert the highest score to a `String` for displaying in a `view`.
init
3
[ { name = "Paul"
, points = 1000
}
, { name = "Steve"
, points = 900
}
]
|> highestToString
--> "1000"
-}
highestToString : Scores -> String
highestToString scores =
scores
|> currentHighest
|> points
|> String.fromInt
-- QUERYING
currentHighest : Scores -> Score
currentHighest (Scores scores) =
scores.currentHighest
{-| The list of [Score](#Score)s.
-}
list : Scores -> List Score
list (Scores scores) =
scores.list
{-| The name associated with a [Score](#Score).
init
3
[ { name = "Paul"
, points = 1000
}
, { name = "Steve"
, points = 900
}
]
|> highest
|> name
--> "Paul"
-}
name : Score -> String
name (Score score) =
score.name
{-| The points associated with a [Score](#Score).
init
3
[ { name = "Paul"
, points = 1000
}
, { name = "Steve"
, points = 900
}
]
|> highest
|> points
--> 1000
-}
points : Score -> Int
points (Score score) =
score.points
{-| The total number of [Score](#Score)s.
init
3
[ { name = "Paul"
, points = 1000
}
, { name = "Steve"
, points = 900
}
]
|> total
--> 3
-}
total : Scores -> Int
total (Scores scores) =
scores.total
-- UPDATING
updateHighest : Score -> Scores -> Scores
updateHighest score (Scores scores) =
Scores
{ scores
| currentHighest = score
}
updateList : List Score -> Scores -> Scores
updateList list_ (Scores scores) =
Scores
{ scores
| list = list_
}
{-| Update the name associated with a [Score](#Score).
-}
updateName : String -> Score -> Score
updateName name_ (Score score) =
Score
{ score
| name = name_
}
updatePoints : Int -> Score -> Score
updatePoints points_ (Score score) =
Score
{ score
| points = points_
}
| 1755 | module Shared.Scores exposing
( Scores, Score
, Max, init
, register
, incrementScore, decrementScore, scoreChange, highest, lowest, list
, gameOver
, prepareForSave
, highestToString
, name, points, total
, updateName
)
{-| A module for managing game [Score](#Score)s and highscores.
This module is used by the [Players](Shared.Players) module to manage players
scores, so if you're using that module, you probably won't need many of the
functions in this module.
@docs Scores, Score
# CREATING
@docs Max, init
# REGISTERING
@docs register
# SCORING
@docs incrementScore, decrementScore, scoreChange, highest, lowest, list
# GAME OVER
@docs gameOver
# SAVING
@docs prepareForSave
# CONVERTING
@docs highestToString
# QUERYING
@docs name, points, total
# UPDATING
@docs updateName
-}
{-| A type representing a list of [Scores](#Scores).
This is an opaque type, use the exposed API to interact with it.
-}
type Scores
= Scores
{ currentHighest : Score
, list : List Score
, total : Int
}
{-| A type representing a [Player](Shared.Players#Player)'s [Score](#Score).
This is an opaque type, use the exposed API to interact with it.
-}
type Score
= Score
{ points : Int
, name : String
}
-- CREATING
{-| A type alias representing the maximum number of [Score](#Score)s to keep
track of.
-}
type alias Max =
Int
{-| Initialize a list of [Scores](#Scores).
**Note**, if you are using the [Players](Players#Players) module this is taken
care of for you.
If you want to record a total of 10 [Score](#Score)s, but the list contains
only 2, then an additional 8 zero [Score](#score)s will be created.
init
10
[ { name = "<NAME>"
, points = 1000
}
, { name = "<NAME>"
, points = 900
}
]
|> list
|> List.length
--> 10
If you want to record a total of 2 [Score](#Score)s, but the list contains 3,
then the lowest [Score](#Score) will be dropped.
init
2
[ { name = "<NAME>"
, points = 1000
}
, { name = "<NAME>"
, points = 900
}
, { name = "<NAME>"
, points = 800
}
]
|> list
|> List.length
--> 2
init
2
[ { name = "<NAME>"
, points = 1000
}
, { name = "<NAME>"
, points = 900
}
, { name = "<NAME>"
, points = 800
}
]
|> lowest
|> name
--> "<NAME>"
-}
init : Max -> List { name : String, points : Int } -> Scores
init max list_ =
let
newList =
list_
|> initScores
max
in
Scores
{ currentHighest =
newList
|> List.head
|> Maybe.withDefault
initScore
, list = newList
, total = max
}
initScores : Max -> List { name : String, points : Int } -> List Score
initScores max scores_ =
let
list_ =
scores_
|> List.map
Score
extras =
max - (list_ |> List.length)
in
case extras < 0 of
True ->
list_
|> List.sortWith
highToLow
|> List.take
max
False ->
list_
|> List.append
(initScore
|> List.repeat
extras
)
|> List.sortWith
highToLow
initScore : Score
initScore =
Score
{ name = "---"
, points = 0
}
-- REGISTERING
{-| Register a new player.
**Note**, if you are using the [Players](Players#Players) module this is taken care of
for you.
register "<NAME>"
|> name
--> "<NAME>"
register "<NAME>"
|> points
--> 0
-}
register : String -> Score
register name_ =
initScore
|> updateName
name_
-- SCORING
{-| Increase a [Score](#Score) by the specified amount.
**Note**, if you are using the [Players](Players#Players) module this is taken care of
for you.
register "<NAME>"
|> incrementScore 10
|> incrementScore 5
|> points
--> 15
-}
incrementScore : Int -> Score -> Score
incrementScore points_ score =
score
|> updatePoints
(score
|> points
|> (+) points_
)
{-| Decrease a [Score](#Score) by the specified amount.
**Note**, if you are using the [Players](Players#Players) module this is taken care of
for you.
register "<NAME>"
|> incrementScore 10
|> decrementScore 3
|> points
--> 7
-}
decrementScore : Int -> Score -> Score
decrementScore points_ score =
score
|> updatePoints
((score |> points) - points_)
{-| A players score has changed, so check to see if it replaces the current
`highest`.
**Note**, if you are using the [Players](Players#Players) module this is taken care of
for you.
This does not affect the whole list, only the `highest`
[Score](#Score). This is really intended for in-game use where you
just want to compare the current score to the highest, and display accordingly.
The whole list will be updated when [gameOver](#gameOver) is called.
-}
scoreChange : Score -> Scores -> Scores
scoreChange score scores =
case (score |> points) > (scores |> currentHighest |> points) of
True ->
scores
|> updateHighest
score
False ->
scores
{-| Get the highest score.
init
2
[ { name = "<NAME>"
, points = 1000
}
, { name = "<NAME>"
, points = 900
}
]
|> highest
|> points
--> 1000
-}
highest : Scores -> Score
highest scores =
scores
|> list
|> first
highToLow
{-| Get the lowest [Score](#Score).
init
2
[ { name = "<NAME>"
, points = 1000
}
, { name = "<NAME>"
, points = 900
}
]
|> lowest
|> points
--> 900
-}
lowest : Scores -> Score
lowest scores =
scores
|> list
|> first
lowToHigh
-- SORTING
first : (Score -> Score -> Order) -> List Score -> Score
first sorter list_ =
list_
|> List.sortWith
sorter
|> List.head
|> Maybe.withDefault
initScore
highToLow : Score -> Score -> Order
highToLow a b =
case compare (a |> points) (b |> points) of
GT ->
LT
EQ ->
EQ
LT ->
GT
lowToHigh : Score -> Score -> Order
lowToHigh a b =
case compare (a |> points) (b |> points) of
GT ->
GT
EQ ->
EQ
LT ->
LT
-- GAME OVER
{-| A player has finished their game so let's see if they make the highscores
list.
**Note**, if you are using the [Players](Players#Players) module this is taken care of
for you.
-}
gameOver : Score -> Scores -> Scores
gameOver score scores =
case scores |> list |> qualifies score of
True ->
let
newList =
scores
|> list
|> List.append
[ score ]
|> List.sortWith
highToLow
|> List.take
(scores
|> total
)
in
scores
|> updateList
newList
|> updateHighest
(newList
|> first
highToLow
)
False ->
scores
qualifies : Score -> List Score -> Bool
qualifies potential list_ =
let
filteredList =
list_
|> List.filter
(\score ->
(score |> points) > (potential |> points)
)
in
(filteredList |> List.length) /= (list_ |> List.length)
-- SAVING
{-| Prepare the list of [Scores](#Scores) for saving, either to
`localStorage` or a back end database.
init
3
[ { name = "<NAME>"
, points = 1000
}
, { name = "<NAME>"
, points = 900
}
]
|> prepareForSave
--> [ {name = "<NAME>", points = 1000}, {name = "<NAME>", points = 900}, {name = "---", points = 0}]
-}
prepareForSave : Scores -> List { name : String, points : Int }
prepareForSave scores =
scores
|> list
|> List.map
(\(Score score) -> score)
-- CONVERTING
{-| Convert the highest score to a `String` for displaying in a `view`.
init
3
[ { name = "<NAME>"
, points = 1000
}
, { name = "<NAME>"
, points = 900
}
]
|> highestToString
--> "1000"
-}
highestToString : Scores -> String
highestToString scores =
scores
|> currentHighest
|> points
|> String.fromInt
-- QUERYING
currentHighest : Scores -> Score
currentHighest (Scores scores) =
scores.currentHighest
{-| The list of [Score](#Score)s.
-}
list : Scores -> List Score
list (Scores scores) =
scores.list
{-| The name associated with a [Score](#Score).
init
3
[ { name = "<NAME>"
, points = 1000
}
, { name = "<NAME>"
, points = 900
}
]
|> highest
|> name
--> "<NAME>"
-}
name : Score -> String
name (Score score) =
score.name
{-| The points associated with a [Score](#Score).
init
3
[ { name = "<NAME>"
, points = 1000
}
, { name = "<NAME>"
, points = 900
}
]
|> highest
|> points
--> 1000
-}
points : Score -> Int
points (Score score) =
score.points
{-| The total number of [Score](#Score)s.
init
3
[ { name = "<NAME>"
, points = 1000
}
, { name = "<NAME>"
, points = 900
}
]
|> total
--> 3
-}
total : Scores -> Int
total (Scores scores) =
scores.total
-- UPDATING
updateHighest : Score -> Scores -> Scores
updateHighest score (Scores scores) =
Scores
{ scores
| currentHighest = score
}
updateList : List Score -> Scores -> Scores
updateList list_ (Scores scores) =
Scores
{ scores
| list = list_
}
{-| Update the name associated with a [Score](#Score).
-}
updateName : String -> Score -> Score
updateName name_ (Score score) =
Score
{ score
| name = name_
}
updatePoints : Int -> Score -> Score
updatePoints points_ (Score score) =
Score
{ score
| points = points_
}
| true | module Shared.Scores exposing
( Scores, Score
, Max, init
, register
, incrementScore, decrementScore, scoreChange, highest, lowest, list
, gameOver
, prepareForSave
, highestToString
, name, points, total
, updateName
)
{-| A module for managing game [Score](#Score)s and highscores.
This module is used by the [Players](Shared.Players) module to manage players
scores, so if you're using that module, you probably won't need many of the
functions in this module.
@docs Scores, Score
# CREATING
@docs Max, init
# REGISTERING
@docs register
# SCORING
@docs incrementScore, decrementScore, scoreChange, highest, lowest, list
# GAME OVER
@docs gameOver
# SAVING
@docs prepareForSave
# CONVERTING
@docs highestToString
# QUERYING
@docs name, points, total
# UPDATING
@docs updateName
-}
{-| A type representing a list of [Scores](#Scores).
This is an opaque type, use the exposed API to interact with it.
-}
type Scores
= Scores
{ currentHighest : Score
, list : List Score
, total : Int
}
{-| A type representing a [Player](Shared.Players#Player)'s [Score](#Score).
This is an opaque type, use the exposed API to interact with it.
-}
type Score
= Score
{ points : Int
, name : String
}
-- CREATING
{-| A type alias representing the maximum number of [Score](#Score)s to keep
track of.
-}
type alias Max =
Int
{-| Initialize a list of [Scores](#Scores).
**Note**, if you are using the [Players](Players#Players) module this is taken
care of for you.
If you want to record a total of 10 [Score](#Score)s, but the list contains
only 2, then an additional 8 zero [Score](#score)s will be created.
init
10
[ { name = "PI:NAME:<NAME>END_PI"
, points = 1000
}
, { name = "PI:NAME:<NAME>END_PI"
, points = 900
}
]
|> list
|> List.length
--> 10
If you want to record a total of 2 [Score](#Score)s, but the list contains 3,
then the lowest [Score](#Score) will be dropped.
init
2
[ { name = "PI:NAME:<NAME>END_PI"
, points = 1000
}
, { name = "PI:NAME:<NAME>END_PI"
, points = 900
}
, { name = "PI:NAME:<NAME>END_PI"
, points = 800
}
]
|> list
|> List.length
--> 2
init
2
[ { name = "PI:NAME:<NAME>END_PI"
, points = 1000
}
, { name = "PI:NAME:<NAME>END_PI"
, points = 900
}
, { name = "PI:NAME:<NAME>END_PI"
, points = 800
}
]
|> lowest
|> name
--> "PI:NAME:<NAME>END_PI"
-}
init : Max -> List { name : String, points : Int } -> Scores
init max list_ =
let
newList =
list_
|> initScores
max
in
Scores
{ currentHighest =
newList
|> List.head
|> Maybe.withDefault
initScore
, list = newList
, total = max
}
initScores : Max -> List { name : String, points : Int } -> List Score
initScores max scores_ =
let
list_ =
scores_
|> List.map
Score
extras =
max - (list_ |> List.length)
in
case extras < 0 of
True ->
list_
|> List.sortWith
highToLow
|> List.take
max
False ->
list_
|> List.append
(initScore
|> List.repeat
extras
)
|> List.sortWith
highToLow
initScore : Score
initScore =
Score
{ name = "---"
, points = 0
}
-- REGISTERING
{-| Register a new player.
**Note**, if you are using the [Players](Players#Players) module this is taken care of
for you.
register "PI:NAME:<NAME>END_PI"
|> name
--> "PI:NAME:<NAME>END_PI"
register "PI:NAME:<NAME>END_PI"
|> points
--> 0
-}
register : String -> Score
register name_ =
initScore
|> updateName
name_
-- SCORING
{-| Increase a [Score](#Score) by the specified amount.
**Note**, if you are using the [Players](Players#Players) module this is taken care of
for you.
register "PI:NAME:<NAME>END_PI"
|> incrementScore 10
|> incrementScore 5
|> points
--> 15
-}
incrementScore : Int -> Score -> Score
incrementScore points_ score =
score
|> updatePoints
(score
|> points
|> (+) points_
)
{-| Decrease a [Score](#Score) by the specified amount.
**Note**, if you are using the [Players](Players#Players) module this is taken care of
for you.
register "PI:NAME:<NAME>END_PI"
|> incrementScore 10
|> decrementScore 3
|> points
--> 7
-}
decrementScore : Int -> Score -> Score
decrementScore points_ score =
score
|> updatePoints
((score |> points) - points_)
{-| A players score has changed, so check to see if it replaces the current
`highest`.
**Note**, if you are using the [Players](Players#Players) module this is taken care of
for you.
This does not affect the whole list, only the `highest`
[Score](#Score). This is really intended for in-game use where you
just want to compare the current score to the highest, and display accordingly.
The whole list will be updated when [gameOver](#gameOver) is called.
-}
scoreChange : Score -> Scores -> Scores
scoreChange score scores =
case (score |> points) > (scores |> currentHighest |> points) of
True ->
scores
|> updateHighest
score
False ->
scores
{-| Get the highest score.
init
2
[ { name = "PI:NAME:<NAME>END_PI"
, points = 1000
}
, { name = "PI:NAME:<NAME>END_PI"
, points = 900
}
]
|> highest
|> points
--> 1000
-}
highest : Scores -> Score
highest scores =
scores
|> list
|> first
highToLow
{-| Get the lowest [Score](#Score).
init
2
[ { name = "PI:NAME:<NAME>END_PI"
, points = 1000
}
, { name = "PI:NAME:<NAME>END_PI"
, points = 900
}
]
|> lowest
|> points
--> 900
-}
lowest : Scores -> Score
lowest scores =
scores
|> list
|> first
lowToHigh
-- SORTING
first : (Score -> Score -> Order) -> List Score -> Score
first sorter list_ =
list_
|> List.sortWith
sorter
|> List.head
|> Maybe.withDefault
initScore
highToLow : Score -> Score -> Order
highToLow a b =
case compare (a |> points) (b |> points) of
GT ->
LT
EQ ->
EQ
LT ->
GT
lowToHigh : Score -> Score -> Order
lowToHigh a b =
case compare (a |> points) (b |> points) of
GT ->
GT
EQ ->
EQ
LT ->
LT
-- GAME OVER
{-| A player has finished their game so let's see if they make the highscores
list.
**Note**, if you are using the [Players](Players#Players) module this is taken care of
for you.
-}
gameOver : Score -> Scores -> Scores
gameOver score scores =
case scores |> list |> qualifies score of
True ->
let
newList =
scores
|> list
|> List.append
[ score ]
|> List.sortWith
highToLow
|> List.take
(scores
|> total
)
in
scores
|> updateList
newList
|> updateHighest
(newList
|> first
highToLow
)
False ->
scores
qualifies : Score -> List Score -> Bool
qualifies potential list_ =
let
filteredList =
list_
|> List.filter
(\score ->
(score |> points) > (potential |> points)
)
in
(filteredList |> List.length) /= (list_ |> List.length)
-- SAVING
{-| Prepare the list of [Scores](#Scores) for saving, either to
`localStorage` or a back end database.
init
3
[ { name = "PI:NAME:<NAME>END_PI"
, points = 1000
}
, { name = "PI:NAME:<NAME>END_PI"
, points = 900
}
]
|> prepareForSave
--> [ {name = "PI:NAME:<NAME>END_PI", points = 1000}, {name = "PI:NAME:<NAME>END_PI", points = 900}, {name = "---", points = 0}]
-}
prepareForSave : Scores -> List { name : String, points : Int }
prepareForSave scores =
scores
|> list
|> List.map
(\(Score score) -> score)
-- CONVERTING
{-| Convert the highest score to a `String` for displaying in a `view`.
init
3
[ { name = "PI:NAME:<NAME>END_PI"
, points = 1000
}
, { name = "PI:NAME:<NAME>END_PI"
, points = 900
}
]
|> highestToString
--> "1000"
-}
highestToString : Scores -> String
highestToString scores =
scores
|> currentHighest
|> points
|> String.fromInt
-- QUERYING
currentHighest : Scores -> Score
currentHighest (Scores scores) =
scores.currentHighest
{-| The list of [Score](#Score)s.
-}
list : Scores -> List Score
list (Scores scores) =
scores.list
{-| The name associated with a [Score](#Score).
init
3
[ { name = "PI:NAME:<NAME>END_PI"
, points = 1000
}
, { name = "PI:NAME:<NAME>END_PI"
, points = 900
}
]
|> highest
|> name
--> "PI:NAME:<NAME>END_PI"
-}
name : Score -> String
name (Score score) =
score.name
{-| The points associated with a [Score](#Score).
init
3
[ { name = "PI:NAME:<NAME>END_PI"
, points = 1000
}
, { name = "PI:NAME:<NAME>END_PI"
, points = 900
}
]
|> highest
|> points
--> 1000
-}
points : Score -> Int
points (Score score) =
score.points
{-| The total number of [Score](#Score)s.
init
3
[ { name = "PI:NAME:<NAME>END_PI"
, points = 1000
}
, { name = "PI:NAME:<NAME>END_PI"
, points = 900
}
]
|> total
--> 3
-}
total : Scores -> Int
total (Scores scores) =
scores.total
-- UPDATING
updateHighest : Score -> Scores -> Scores
updateHighest score (Scores scores) =
Scores
{ scores
| currentHighest = score
}
updateList : List Score -> Scores -> Scores
updateList list_ (Scores scores) =
Scores
{ scores
| list = list_
}
{-| Update the name associated with a [Score](#Score).
-}
updateName : String -> Score -> Score
updateName name_ (Score score) =
Score
{ score
| name = name_
}
updatePoints : Int -> Score -> Score
updatePoints points_ (Score score) =
Score
{ score
| points = points_
}
| elm |
[
{
"context": "\n [ LineChart.line Color.orange Dots.triangle \"Chuck\" chuck\n , LineChart.line Color.yellow Dots.cir",
"end": 2136,
"score": 0.9987140298,
"start": 2131,
"tag": "NAME",
"value": "Chuck"
},
{
"context": "ck\n , LineChart.line Color.yellow Dots.circle \"Bobby\" bobby\n , LineChart.line Color.purple Dots.dia",
"end": 2196,
"score": 0.9995911121,
"start": 2191,
"tag": "NAME",
"value": "Bobby"
},
{
"context": " , LineChart.line Color.yellow Dots.circle \"Bobby\" bobby\n , LineChart.line Color.purple Dots.diamond \"A",
"end": 2203,
"score": 0.8584318161,
"start": 2198,
"tag": "NAME",
"value": "bobby"
},
{
"context": "y\n , LineChart.line Color.purple Dots.diamond \"Alice\" alice\n ]\n\n\ncustomLineConfig : Maybe Info -> L",
"end": 2257,
"score": 0.9997670054,
"start": 2252,
"tag": "NAME",
"value": "Alice"
},
{
"context": ", LineChart.line Color.purple Dots.diamond \"Alice\" alice\n ]\n\n\ncustomLineConfig : Maybe Info -> Line.Con",
"end": 2264,
"score": 0.9674978852,
"start": 2259,
"tag": "NAME",
"value": "alice"
}
] | examples/LineChart/Events1.elm | terezka/elm-charts-alpha | 7 | module Events1 exposing (main)
import Browser
import Html exposing (Html, div, h1, node, p, text)
import Html.Attributes exposing (class)
import Svg exposing (Attribute, Svg, g, text_, tspan)
import LineChart as LineChart
import Chart.Junk as Junk exposing (..)
import Chart.Dot as Dots
import Chart.Container as Container
import Chart.Interpolation as Interpolation
import Chart.Axis.Intersection as Intersection
import Chart.Axis as Axis
import Chart.Legends as Legends
import Chart.Line as Line
import Chart.Events as Events
import Chart.Axis.Unit as Unit
import Chart.Grid as Grid
import Chart.Legends as Legends
import Chart.Area as Area
import Chart.Element as Element
import Color
import Color.Manipulate
main : Program () Model Msg
main =
Browser.sandbox
{ init = init
, update = update
, view = view
}
-- MODEL
type alias Model =
{ hovering : Maybe (Events.Found Element.LineDot Info) }
init : Model
init =
{ hovering = Nothing }
-- UPDATE
type Msg
= Hover (Maybe (Events.Found Element.LineDot Info))
update : Msg -> Model -> Model
update msg model =
case msg of
Hover hovering ->
{ model | hovering = hovering }
-- VIEW
view : Model -> Svg Msg
view model =
Html.div
[ class "container" ]
[ chart model ]
chart : Model -> Html.Html Msg
chart model =
LineChart.viewCustom
{ y = Axis.default "Weight" Unit.kilograms (Just << .weight)
, x = Axis.default "Age" Unit.none .age
, container = Container.styled "line-chart-1" 700 450 [ ( "font-family", "monospace" ) ]
, interpolation = Interpolation.default
, intersection = Intersection.default
, legends = Legends.default
, events = Events.hoverDot Hover
, junk = Junk.default
, grid = Grid.default
, area = Area.default
, line =
-- Line.default
Line.hoverOne (Maybe.map Events.data model.hovering)
-- customLineConfig model.hovering
, dots =
-- Dots.default
Dots.hoverOne (Maybe.map Events.data model.hovering)
-- customDotsConfig model.hovering
}
[ LineChart.line Color.orange Dots.triangle "Chuck" chuck
, LineChart.line Color.yellow Dots.circle "Bobby" bobby
, LineChart.line Color.purple Dots.diamond "Alice" alice
]
customLineConfig : Maybe Info -> Line.Config Info
customLineConfig maybeHovered =
let
styleDefault =
Line.style 1 identity
styleHovered =
Line.style 2 identity
styleNotHovered =
Line.style 1 (Color.Manipulate.lighten 0.3)
lineConfig data = -- `data` being all the data for a line
case maybeHovered of
Just hovered ->
if List.any (dotIsHovered maybeHovered) data
-- This line is hovered
then styleHovered
-- Some line is hovered, but not this one
else styleNotHovered
Nothing ->
-- No line is hovered
styleDefault
in
Line.custom lineConfig
customDotsConfig : Maybe Info -> Dots.Config Info
customDotsConfig maybeHovered =
let
styleDefault =
Dots.empty 5 2
styleHover =
Dots.full 8
styleLegend data = -- `data` being all the data for a line
if List.any (dotIsHovered maybeHovered) data
then styleHover
else styleDefault
styleIndividual datum = -- `datum` being a single data point on a line
if dotIsHovered maybeHovered datum
then styleHover
else styleDefault
in
Dots.customAny
{ legend = styleLegend
, individual = styleIndividual
}
-- HELPERS
dotIsHovered : Maybe Info -> Info -> Bool
dotIsHovered maybeHovered datum =
Just datum == maybeHovered
-- DATA
type alias Info =
{ age : Float
, weight : Float
, height : Float
, income : Float
}
alice : List Info
alice =
[ Info 10 34 1.34 0
, Info 16 42 1.62 3000
, Info 25 75 1.73 25000
, Info 43 83 1.75 40000
]
bobby : List Info
bobby =
[ Info 10 38 1.32 0
, Info 17 69 1.75 2000
, Info 25 75 1.87 32000
, Info 43 77 1.87 52000
]
chuck : List Info
chuck =
[ Info 10 42 1.35 0
, Info 15 72 1.72 1800
, Info 25 89 1.83 85000
, Info 43 95 1.84 120000
]
| 14525 | module Events1 exposing (main)
import Browser
import Html exposing (Html, div, h1, node, p, text)
import Html.Attributes exposing (class)
import Svg exposing (Attribute, Svg, g, text_, tspan)
import LineChart as LineChart
import Chart.Junk as Junk exposing (..)
import Chart.Dot as Dots
import Chart.Container as Container
import Chart.Interpolation as Interpolation
import Chart.Axis.Intersection as Intersection
import Chart.Axis as Axis
import Chart.Legends as Legends
import Chart.Line as Line
import Chart.Events as Events
import Chart.Axis.Unit as Unit
import Chart.Grid as Grid
import Chart.Legends as Legends
import Chart.Area as Area
import Chart.Element as Element
import Color
import Color.Manipulate
main : Program () Model Msg
main =
Browser.sandbox
{ init = init
, update = update
, view = view
}
-- MODEL
type alias Model =
{ hovering : Maybe (Events.Found Element.LineDot Info) }
init : Model
init =
{ hovering = Nothing }
-- UPDATE
type Msg
= Hover (Maybe (Events.Found Element.LineDot Info))
update : Msg -> Model -> Model
update msg model =
case msg of
Hover hovering ->
{ model | hovering = hovering }
-- VIEW
view : Model -> Svg Msg
view model =
Html.div
[ class "container" ]
[ chart model ]
chart : Model -> Html.Html Msg
chart model =
LineChart.viewCustom
{ y = Axis.default "Weight" Unit.kilograms (Just << .weight)
, x = Axis.default "Age" Unit.none .age
, container = Container.styled "line-chart-1" 700 450 [ ( "font-family", "monospace" ) ]
, interpolation = Interpolation.default
, intersection = Intersection.default
, legends = Legends.default
, events = Events.hoverDot Hover
, junk = Junk.default
, grid = Grid.default
, area = Area.default
, line =
-- Line.default
Line.hoverOne (Maybe.map Events.data model.hovering)
-- customLineConfig model.hovering
, dots =
-- Dots.default
Dots.hoverOne (Maybe.map Events.data model.hovering)
-- customDotsConfig model.hovering
}
[ LineChart.line Color.orange Dots.triangle "<NAME>" chuck
, LineChart.line Color.yellow Dots.circle "<NAME>" <NAME>
, LineChart.line Color.purple Dots.diamond "<NAME>" <NAME>
]
customLineConfig : Maybe Info -> Line.Config Info
customLineConfig maybeHovered =
let
styleDefault =
Line.style 1 identity
styleHovered =
Line.style 2 identity
styleNotHovered =
Line.style 1 (Color.Manipulate.lighten 0.3)
lineConfig data = -- `data` being all the data for a line
case maybeHovered of
Just hovered ->
if List.any (dotIsHovered maybeHovered) data
-- This line is hovered
then styleHovered
-- Some line is hovered, but not this one
else styleNotHovered
Nothing ->
-- No line is hovered
styleDefault
in
Line.custom lineConfig
customDotsConfig : Maybe Info -> Dots.Config Info
customDotsConfig maybeHovered =
let
styleDefault =
Dots.empty 5 2
styleHover =
Dots.full 8
styleLegend data = -- `data` being all the data for a line
if List.any (dotIsHovered maybeHovered) data
then styleHover
else styleDefault
styleIndividual datum = -- `datum` being a single data point on a line
if dotIsHovered maybeHovered datum
then styleHover
else styleDefault
in
Dots.customAny
{ legend = styleLegend
, individual = styleIndividual
}
-- HELPERS
dotIsHovered : Maybe Info -> Info -> Bool
dotIsHovered maybeHovered datum =
Just datum == maybeHovered
-- DATA
type alias Info =
{ age : Float
, weight : Float
, height : Float
, income : Float
}
alice : List Info
alice =
[ Info 10 34 1.34 0
, Info 16 42 1.62 3000
, Info 25 75 1.73 25000
, Info 43 83 1.75 40000
]
bobby : List Info
bobby =
[ Info 10 38 1.32 0
, Info 17 69 1.75 2000
, Info 25 75 1.87 32000
, Info 43 77 1.87 52000
]
chuck : List Info
chuck =
[ Info 10 42 1.35 0
, Info 15 72 1.72 1800
, Info 25 89 1.83 85000
, Info 43 95 1.84 120000
]
| true | module Events1 exposing (main)
import Browser
import Html exposing (Html, div, h1, node, p, text)
import Html.Attributes exposing (class)
import Svg exposing (Attribute, Svg, g, text_, tspan)
import LineChart as LineChart
import Chart.Junk as Junk exposing (..)
import Chart.Dot as Dots
import Chart.Container as Container
import Chart.Interpolation as Interpolation
import Chart.Axis.Intersection as Intersection
import Chart.Axis as Axis
import Chart.Legends as Legends
import Chart.Line as Line
import Chart.Events as Events
import Chart.Axis.Unit as Unit
import Chart.Grid as Grid
import Chart.Legends as Legends
import Chart.Area as Area
import Chart.Element as Element
import Color
import Color.Manipulate
main : Program () Model Msg
main =
Browser.sandbox
{ init = init
, update = update
, view = view
}
-- MODEL
type alias Model =
{ hovering : Maybe (Events.Found Element.LineDot Info) }
init : Model
init =
{ hovering = Nothing }
-- UPDATE
type Msg
= Hover (Maybe (Events.Found Element.LineDot Info))
update : Msg -> Model -> Model
update msg model =
case msg of
Hover hovering ->
{ model | hovering = hovering }
-- VIEW
view : Model -> Svg Msg
view model =
Html.div
[ class "container" ]
[ chart model ]
chart : Model -> Html.Html Msg
chart model =
LineChart.viewCustom
{ y = Axis.default "Weight" Unit.kilograms (Just << .weight)
, x = Axis.default "Age" Unit.none .age
, container = Container.styled "line-chart-1" 700 450 [ ( "font-family", "monospace" ) ]
, interpolation = Interpolation.default
, intersection = Intersection.default
, legends = Legends.default
, events = Events.hoverDot Hover
, junk = Junk.default
, grid = Grid.default
, area = Area.default
, line =
-- Line.default
Line.hoverOne (Maybe.map Events.data model.hovering)
-- customLineConfig model.hovering
, dots =
-- Dots.default
Dots.hoverOne (Maybe.map Events.data model.hovering)
-- customDotsConfig model.hovering
}
[ LineChart.line Color.orange Dots.triangle "PI:NAME:<NAME>END_PI" chuck
, LineChart.line Color.yellow Dots.circle "PI:NAME:<NAME>END_PI" PI:NAME:<NAME>END_PI
, LineChart.line Color.purple Dots.diamond "PI:NAME:<NAME>END_PI" PI:NAME:<NAME>END_PI
]
customLineConfig : Maybe Info -> Line.Config Info
customLineConfig maybeHovered =
let
styleDefault =
Line.style 1 identity
styleHovered =
Line.style 2 identity
styleNotHovered =
Line.style 1 (Color.Manipulate.lighten 0.3)
lineConfig data = -- `data` being all the data for a line
case maybeHovered of
Just hovered ->
if List.any (dotIsHovered maybeHovered) data
-- This line is hovered
then styleHovered
-- Some line is hovered, but not this one
else styleNotHovered
Nothing ->
-- No line is hovered
styleDefault
in
Line.custom lineConfig
customDotsConfig : Maybe Info -> Dots.Config Info
customDotsConfig maybeHovered =
let
styleDefault =
Dots.empty 5 2
styleHover =
Dots.full 8
styleLegend data = -- `data` being all the data for a line
if List.any (dotIsHovered maybeHovered) data
then styleHover
else styleDefault
styleIndividual datum = -- `datum` being a single data point on a line
if dotIsHovered maybeHovered datum
then styleHover
else styleDefault
in
Dots.customAny
{ legend = styleLegend
, individual = styleIndividual
}
-- HELPERS
dotIsHovered : Maybe Info -> Info -> Bool
dotIsHovered maybeHovered datum =
Just datum == maybeHovered
-- DATA
type alias Info =
{ age : Float
, weight : Float
, height : Float
, income : Float
}
alice : List Info
alice =
[ Info 10 34 1.34 0
, Info 16 42 1.62 3000
, Info 25 75 1.73 25000
, Info 43 83 1.75 40000
]
bobby : List Info
bobby =
[ Info 10 38 1.32 0
, Info 17 69 1.75 2000
, Info 25 75 1.87 32000
, Info 43 77 1.87 52000
]
chuck : List Info
chuck =
[ Info 10 42 1.35 0
, Info 15 72 1.72 1800
, Info 25 89 1.83 85000
, Info 43 95 1.84 120000
]
| elm |
[
{
"context": "\nview model =\n div []\n [ h1 [] [ text \"Sinal\" ]\n , luz model green Verde\n , luz ",
"end": 2070,
"score": 0.5429047346,
"start": 2066,
"tag": "NAME",
"value": "inal"
}
] | 01-elm/Main.elm | soapdog/exemplinhos | 2 | module Exemplo exposing (main)
import Css exposing (..)
import Css.Colors exposing (..)
import Html
import Html.Styled exposing (..)
import Html.Styled.Attributes exposing (css, href, src)
import Html.Styled.Events exposing (onClick)
{-
O tipo apresentado abaixo simboliza os possiveis estados
do sinal. Note que é impossível mais que uma luz estar acesa
ao mesmo tempo pois o tipo algebraico abaixo não permite
esse tipo de representação.
Ao construir bons tipos, você elimina toda uma série de
bugs possíveis
-}
type Sinal
= TudoApagado
| Verde
| Amarelo
| Vermelho
type alias Model =
Sinal
{-
Quem tá vindo de React/Vue/Angular, essas são
as actions possíveis. No caso só tem uma.
-}
type Msg
= Acender Sinal
initialModel : Model
initialModel =
TudoApagado
{-
É quem processa as ações de ligar ou desligar uma luz.
Em React ou Vue seria quem lida com as actions.
O update é a única função que pode alterar o model.
-}
update : Msg -> Model -> Model
update msg model =
case msg of
Acender luz ->
luz
{-
Constroi uma luzinha. Ele gera HTML no formado:
tag [atributos] [filhos]
No nosso caso, cada luz é só uma div com um CSS, ou seja:
div [css] []
Além disso, como pode ser visto na descrição do tipo, nossas
luzinhas geram Msg, ou seja, actions para o update.
-}
luz : Model -> Color -> Sinal -> Html Msg
luz model cor tipo =
let
corzinha =
if model == tipo then
cor
else
silver
in
div
[ css
[ borderRadius (pct 50)
, width (px 40)
, height (px 40)
, margin (px 10)
, backgroundColor corzinha
]
, onClick (Acender tipo)
]
[]
{-
Essa é a view, ela gera HTML e Msg pro update.
Note que ela não tem como alterar o model, somente o
update pode alterar o model.
-}
view : Model -> Html Msg
view model =
div []
[ h1 [] [ text "Sinal" ]
, luz model green Verde
, luz model yellow Amarelo
, luz model red Vermelho
]
main : Program Never Model Msg
main =
Html.beginnerProgram
{ view = view >> toUnstyled
, update = update
, model = initialModel
}
| 44437 | module Exemplo exposing (main)
import Css exposing (..)
import Css.Colors exposing (..)
import Html
import Html.Styled exposing (..)
import Html.Styled.Attributes exposing (css, href, src)
import Html.Styled.Events exposing (onClick)
{-
O tipo apresentado abaixo simboliza os possiveis estados
do sinal. Note que é impossível mais que uma luz estar acesa
ao mesmo tempo pois o tipo algebraico abaixo não permite
esse tipo de representação.
Ao construir bons tipos, você elimina toda uma série de
bugs possíveis
-}
type Sinal
= TudoApagado
| Verde
| Amarelo
| Vermelho
type alias Model =
Sinal
{-
Quem tá vindo de React/Vue/Angular, essas são
as actions possíveis. No caso só tem uma.
-}
type Msg
= Acender Sinal
initialModel : Model
initialModel =
TudoApagado
{-
É quem processa as ações de ligar ou desligar uma luz.
Em React ou Vue seria quem lida com as actions.
O update é a única função que pode alterar o model.
-}
update : Msg -> Model -> Model
update msg model =
case msg of
Acender luz ->
luz
{-
Constroi uma luzinha. Ele gera HTML no formado:
tag [atributos] [filhos]
No nosso caso, cada luz é só uma div com um CSS, ou seja:
div [css] []
Além disso, como pode ser visto na descrição do tipo, nossas
luzinhas geram Msg, ou seja, actions para o update.
-}
luz : Model -> Color -> Sinal -> Html Msg
luz model cor tipo =
let
corzinha =
if model == tipo then
cor
else
silver
in
div
[ css
[ borderRadius (pct 50)
, width (px 40)
, height (px 40)
, margin (px 10)
, backgroundColor corzinha
]
, onClick (Acender tipo)
]
[]
{-
Essa é a view, ela gera HTML e Msg pro update.
Note que ela não tem como alterar o model, somente o
update pode alterar o model.
-}
view : Model -> Html Msg
view model =
div []
[ h1 [] [ text "S<NAME>" ]
, luz model green Verde
, luz model yellow Amarelo
, luz model red Vermelho
]
main : Program Never Model Msg
main =
Html.beginnerProgram
{ view = view >> toUnstyled
, update = update
, model = initialModel
}
| true | module Exemplo exposing (main)
import Css exposing (..)
import Css.Colors exposing (..)
import Html
import Html.Styled exposing (..)
import Html.Styled.Attributes exposing (css, href, src)
import Html.Styled.Events exposing (onClick)
{-
O tipo apresentado abaixo simboliza os possiveis estados
do sinal. Note que é impossível mais que uma luz estar acesa
ao mesmo tempo pois o tipo algebraico abaixo não permite
esse tipo de representação.
Ao construir bons tipos, você elimina toda uma série de
bugs possíveis
-}
type Sinal
= TudoApagado
| Verde
| Amarelo
| Vermelho
type alias Model =
Sinal
{-
Quem tá vindo de React/Vue/Angular, essas são
as actions possíveis. No caso só tem uma.
-}
type Msg
= Acender Sinal
initialModel : Model
initialModel =
TudoApagado
{-
É quem processa as ações de ligar ou desligar uma luz.
Em React ou Vue seria quem lida com as actions.
O update é a única função que pode alterar o model.
-}
update : Msg -> Model -> Model
update msg model =
case msg of
Acender luz ->
luz
{-
Constroi uma luzinha. Ele gera HTML no formado:
tag [atributos] [filhos]
No nosso caso, cada luz é só uma div com um CSS, ou seja:
div [css] []
Além disso, como pode ser visto na descrição do tipo, nossas
luzinhas geram Msg, ou seja, actions para o update.
-}
luz : Model -> Color -> Sinal -> Html Msg
luz model cor tipo =
let
corzinha =
if model == tipo then
cor
else
silver
in
div
[ css
[ borderRadius (pct 50)
, width (px 40)
, height (px 40)
, margin (px 10)
, backgroundColor corzinha
]
, onClick (Acender tipo)
]
[]
{-
Essa é a view, ela gera HTML e Msg pro update.
Note que ela não tem como alterar o model, somente o
update pode alterar o model.
-}
view : Model -> Html Msg
view model =
div []
[ h1 [] [ text "SPI:NAME:<NAME>END_PI" ]
, luz model green Verde
, luz model yellow Amarelo
, luz model red Vermelho
]
main : Program Never Model Msg
main =
Html.beginnerProgram
{ view = view >> toUnstyled
, update = update
, model = initialModel
}
| elm |
[
{
"context": "keShortName char =\n case char.name of\n \"Killer B\" -> \"B\"\n \"Shukaku Gaara\" -> \"Ga",
"end": 3971,
"score": 0.7954170704,
"start": 3963,
"tag": "NAME",
"value": "Killer B"
},
{
"context": "ame of\n \"Killer B\" -> \"B\"\n \"Shukaku Gaara\" -> \"Gaara\"\n \"Sage Mode Kabuto\" -> \"Kab",
"end": 4010,
"score": 0.9219371676,
"start": 3997,
"tag": "NAME",
"value": "Shukaku Gaara"
}
] | elm/src/Import/Flags.elm | robertvlasiu/naruto-unison | 4 | module Import.Flags exposing
( Characters
, Flags
, characterName
, decode
, failure
, printFailure
)
import Dict as Dict exposing (Dict)
import Json.Decode as D
import Json.Helpers as D
import List.Extra as List
import List.Nonempty as Nonempty exposing (Nonempty(..))
import Set exposing (Set)
import String.Extra as String
import Game.Chakra as Chakra
import Import.Model as Model exposing (Category(..), Chakras, Character, Failure(..), Skill, User)
import Util exposing (groupBy, unaccent)
type alias Flags =
{ url : String
, bg : String
, userTeam : List Character
, userPractice : List Character
, unlocked : Set String
, user : Maybe User
, avatars : List String
, characters : Characters
, visibles : Set String
, red : Set String
, blue : Set String
, csrf : String
, csrfParam : String
}
failure : Flags
failure =
{ url = ""
, bg = ""
, userTeam = []
, userPractice = []
, unlocked = Set.empty
, user = Nothing
, avatars = []
, characters = makeCharacters []
, visibles = Set.empty
, red = Set.empty
, blue = Set.empty
, csrf = ""
, csrfParam = ""
}
decode : D.Decoder Flags
decode =
D.succeed Flags
|> D.required "url" D.string
>> D.required "bg" D.string
>> D.required "userTeam" (D.list Model.jsonDecCharacter)
>> D.required "userPractice" (D.list Model.jsonDecCharacter)
>> D.required "unlocked" (D.list D.string |> D.map Set.fromList)
>> D.required "user" (D.maybe Model.jsonDecUser)
>> D.required "avatars" (D.list D.string)
>> D.required "characters" (D.list Model.jsonDecCharacter |> D.map makeCharacters)
>> D.required "visibles" (D.list D.string |> D.map Set.fromList)
>> D.required "red" (D.list D.string |> D.map Set.fromList)
>> D.required "blue" (D.list D.string |> D.map Set.fromList)
>> D.required "csrf" D.string
>> D.required "csrfParam" D.string
type alias Characters =
{ list : List Character
, dict : Dict String Character
, groupList : List (Nonempty Character)
, groupDict : Dict String (Nonempty Character)
, costs : Dict String Chakras
, shortName : Character -> String
}
makeCharacters : List Character -> Characters
makeCharacters chars =
let
shortNames =
chars
|> List.map (\x -> ( characterName x, makeShortName x ))
>> Dict.fromList
shortName char =
case Dict.get (characterName char) shortNames of
Just name -> name
Nothing -> makeShortName char
groupList =
groupBy (\x y -> shortName x == shortName y) chars
in
{ list =
chars
, dict =
Dict.fromList
<| withKey characterName chars
, groupList =
groupList
, groupDict =
Dict.fromList
<| withKey (Nonempty.head >> shortName) groupList
, costs =
Dict.fromList
<| List.map (\char -> (shortName char, characterCosts char)) chars
, shortName =
shortName
}
withKey : (a -> b) -> List a -> List ( b, a )
withKey f =
List.map <| \x -> ( f x, x )
clean : Char -> Char
clean x =
case x of
' ' -> '-'
_ -> unaccent x
characterName : Character -> String
characterName char =
String.map clean << String.toLower <| case char.category of
Original ->
char.name
Shippuden ->
char.name ++ " (S)"
Reanimated ->
char.name ++ " (R)"
makeShortName : Character -> String
makeShortName char =
case char.name of
"Killer B" -> "B"
"Shukaku Gaara" -> "Gaara"
"Sage Mode Kabuto" -> "Kabuto"
"Tobi" -> "Obito"
"Masked Man" -> "Obito"
"Nagato" -> "Pain"
_ ->
char.skills
|> List.getAt 3
>> Maybe.andThen List.head
>> Maybe.andThen shortFromInvuln
>> Maybe.withDefault char.name
shortFromInvuln : Skill -> Maybe String
shortFromInvuln x =
case String.words x.desc of
"The" :: name :: _ -> Just name
name :: _ -> Just name
[] -> Nothing
characterCosts : Character -> Chakras
characterCosts char =
char.skills
|> List.filterMap List.head
>> List.map .cost
>> Chakra.sum
printFailure : Failure -> String
printFailure x =
case x of
AlreadyQueued ->
"Your account is already queued"
Canceled ->
"Queue canceled"
Locked ->
"Character not unlocked"
InvalidTeam ->
"Invalid team"
NotFound ->
"User not found"
| 43540 | module Import.Flags exposing
( Characters
, Flags
, characterName
, decode
, failure
, printFailure
)
import Dict as Dict exposing (Dict)
import Json.Decode as D
import Json.Helpers as D
import List.Extra as List
import List.Nonempty as Nonempty exposing (Nonempty(..))
import Set exposing (Set)
import String.Extra as String
import Game.Chakra as Chakra
import Import.Model as Model exposing (Category(..), Chakras, Character, Failure(..), Skill, User)
import Util exposing (groupBy, unaccent)
type alias Flags =
{ url : String
, bg : String
, userTeam : List Character
, userPractice : List Character
, unlocked : Set String
, user : Maybe User
, avatars : List String
, characters : Characters
, visibles : Set String
, red : Set String
, blue : Set String
, csrf : String
, csrfParam : String
}
failure : Flags
failure =
{ url = ""
, bg = ""
, userTeam = []
, userPractice = []
, unlocked = Set.empty
, user = Nothing
, avatars = []
, characters = makeCharacters []
, visibles = Set.empty
, red = Set.empty
, blue = Set.empty
, csrf = ""
, csrfParam = ""
}
decode : D.Decoder Flags
decode =
D.succeed Flags
|> D.required "url" D.string
>> D.required "bg" D.string
>> D.required "userTeam" (D.list Model.jsonDecCharacter)
>> D.required "userPractice" (D.list Model.jsonDecCharacter)
>> D.required "unlocked" (D.list D.string |> D.map Set.fromList)
>> D.required "user" (D.maybe Model.jsonDecUser)
>> D.required "avatars" (D.list D.string)
>> D.required "characters" (D.list Model.jsonDecCharacter |> D.map makeCharacters)
>> D.required "visibles" (D.list D.string |> D.map Set.fromList)
>> D.required "red" (D.list D.string |> D.map Set.fromList)
>> D.required "blue" (D.list D.string |> D.map Set.fromList)
>> D.required "csrf" D.string
>> D.required "csrfParam" D.string
type alias Characters =
{ list : List Character
, dict : Dict String Character
, groupList : List (Nonempty Character)
, groupDict : Dict String (Nonempty Character)
, costs : Dict String Chakras
, shortName : Character -> String
}
makeCharacters : List Character -> Characters
makeCharacters chars =
let
shortNames =
chars
|> List.map (\x -> ( characterName x, makeShortName x ))
>> Dict.fromList
shortName char =
case Dict.get (characterName char) shortNames of
Just name -> name
Nothing -> makeShortName char
groupList =
groupBy (\x y -> shortName x == shortName y) chars
in
{ list =
chars
, dict =
Dict.fromList
<| withKey characterName chars
, groupList =
groupList
, groupDict =
Dict.fromList
<| withKey (Nonempty.head >> shortName) groupList
, costs =
Dict.fromList
<| List.map (\char -> (shortName char, characterCosts char)) chars
, shortName =
shortName
}
withKey : (a -> b) -> List a -> List ( b, a )
withKey f =
List.map <| \x -> ( f x, x )
clean : Char -> Char
clean x =
case x of
' ' -> '-'
_ -> unaccent x
characterName : Character -> String
characterName char =
String.map clean << String.toLower <| case char.category of
Original ->
char.name
Shippuden ->
char.name ++ " (S)"
Reanimated ->
char.name ++ " (R)"
makeShortName : Character -> String
makeShortName char =
case char.name of
"<NAME>" -> "B"
"<NAME>" -> "Gaara"
"Sage Mode Kabuto" -> "Kabuto"
"Tobi" -> "Obito"
"Masked Man" -> "Obito"
"Nagato" -> "Pain"
_ ->
char.skills
|> List.getAt 3
>> Maybe.andThen List.head
>> Maybe.andThen shortFromInvuln
>> Maybe.withDefault char.name
shortFromInvuln : Skill -> Maybe String
shortFromInvuln x =
case String.words x.desc of
"The" :: name :: _ -> Just name
name :: _ -> Just name
[] -> Nothing
characterCosts : Character -> Chakras
characterCosts char =
char.skills
|> List.filterMap List.head
>> List.map .cost
>> Chakra.sum
printFailure : Failure -> String
printFailure x =
case x of
AlreadyQueued ->
"Your account is already queued"
Canceled ->
"Queue canceled"
Locked ->
"Character not unlocked"
InvalidTeam ->
"Invalid team"
NotFound ->
"User not found"
| true | module Import.Flags exposing
( Characters
, Flags
, characterName
, decode
, failure
, printFailure
)
import Dict as Dict exposing (Dict)
import Json.Decode as D
import Json.Helpers as D
import List.Extra as List
import List.Nonempty as Nonempty exposing (Nonempty(..))
import Set exposing (Set)
import String.Extra as String
import Game.Chakra as Chakra
import Import.Model as Model exposing (Category(..), Chakras, Character, Failure(..), Skill, User)
import Util exposing (groupBy, unaccent)
type alias Flags =
{ url : String
, bg : String
, userTeam : List Character
, userPractice : List Character
, unlocked : Set String
, user : Maybe User
, avatars : List String
, characters : Characters
, visibles : Set String
, red : Set String
, blue : Set String
, csrf : String
, csrfParam : String
}
failure : Flags
failure =
{ url = ""
, bg = ""
, userTeam = []
, userPractice = []
, unlocked = Set.empty
, user = Nothing
, avatars = []
, characters = makeCharacters []
, visibles = Set.empty
, red = Set.empty
, blue = Set.empty
, csrf = ""
, csrfParam = ""
}
decode : D.Decoder Flags
decode =
D.succeed Flags
|> D.required "url" D.string
>> D.required "bg" D.string
>> D.required "userTeam" (D.list Model.jsonDecCharacter)
>> D.required "userPractice" (D.list Model.jsonDecCharacter)
>> D.required "unlocked" (D.list D.string |> D.map Set.fromList)
>> D.required "user" (D.maybe Model.jsonDecUser)
>> D.required "avatars" (D.list D.string)
>> D.required "characters" (D.list Model.jsonDecCharacter |> D.map makeCharacters)
>> D.required "visibles" (D.list D.string |> D.map Set.fromList)
>> D.required "red" (D.list D.string |> D.map Set.fromList)
>> D.required "blue" (D.list D.string |> D.map Set.fromList)
>> D.required "csrf" D.string
>> D.required "csrfParam" D.string
type alias Characters =
{ list : List Character
, dict : Dict String Character
, groupList : List (Nonempty Character)
, groupDict : Dict String (Nonempty Character)
, costs : Dict String Chakras
, shortName : Character -> String
}
makeCharacters : List Character -> Characters
makeCharacters chars =
let
shortNames =
chars
|> List.map (\x -> ( characterName x, makeShortName x ))
>> Dict.fromList
shortName char =
case Dict.get (characterName char) shortNames of
Just name -> name
Nothing -> makeShortName char
groupList =
groupBy (\x y -> shortName x == shortName y) chars
in
{ list =
chars
, dict =
Dict.fromList
<| withKey characterName chars
, groupList =
groupList
, groupDict =
Dict.fromList
<| withKey (Nonempty.head >> shortName) groupList
, costs =
Dict.fromList
<| List.map (\char -> (shortName char, characterCosts char)) chars
, shortName =
shortName
}
withKey : (a -> b) -> List a -> List ( b, a )
withKey f =
List.map <| \x -> ( f x, x )
clean : Char -> Char
clean x =
case x of
' ' -> '-'
_ -> unaccent x
characterName : Character -> String
characterName char =
String.map clean << String.toLower <| case char.category of
Original ->
char.name
Shippuden ->
char.name ++ " (S)"
Reanimated ->
char.name ++ " (R)"
makeShortName : Character -> String
makeShortName char =
case char.name of
"PI:NAME:<NAME>END_PI" -> "B"
"PI:NAME:<NAME>END_PI" -> "Gaara"
"Sage Mode Kabuto" -> "Kabuto"
"Tobi" -> "Obito"
"Masked Man" -> "Obito"
"Nagato" -> "Pain"
_ ->
char.skills
|> List.getAt 3
>> Maybe.andThen List.head
>> Maybe.andThen shortFromInvuln
>> Maybe.withDefault char.name
shortFromInvuln : Skill -> Maybe String
shortFromInvuln x =
case String.words x.desc of
"The" :: name :: _ -> Just name
name :: _ -> Just name
[] -> Nothing
characterCosts : Character -> Chakras
characterCosts char =
char.skills
|> List.filterMap List.head
>> List.map .cost
>> Chakra.sum
printFailure : Failure -> String
printFailure x =
case x of
AlreadyQueued ->
"Your account is already queued"
Canceled ->
"Queue canceled"
Locked ->
"Character not unlocked"
InvalidTeam ->
"Invalid team"
NotFound ->
"User not found"
| elm |
[
{
"context": "init : ( Model, Cmd Msg )\ninit =\n ( { title = \"Name\", asc = True }\n , Cmd.none\n )\n\n\nupdate : Ms",
"end": 571,
"score": 0.9931279421,
"start": 567,
"tag": "NAME",
"value": "Name"
},
{
"context": " { content =\n [ { id = 1, name = \"Antonio\", rating = 2.456, hash = Nothing }\n , ",
"end": 1340,
"score": 0.9648668766,
"start": 1333,
"tag": "NAME",
"value": "Antonio"
},
{
"context": " hash = Nothing }\n , { id = 2, name = \"Ana\", rating = 1.34, hash = Just \"45jf\" }\n ",
"end": 1411,
"score": 0.9952363968,
"start": 1408,
"tag": "NAME",
"value": "Ana"
},
{
"context": "h = Just \"45jf\" }\n , { id = 3, name = \"Alfred\", rating = 4.22, hash = Just \"6fs1\" }\n ",
"end": 1488,
"score": 0.9990862012,
"start": 1482,
"tag": "NAME",
"value": "Alfred"
},
{
"context": "h = Just \"6fs1\" }\n , { id = 4, name = \"Thomas\", rating = 3, hash = Just \"k52f\" }\n ]\n",
"end": 1565,
"score": 0.9400947094,
"start": 1559,
"tag": "NAME",
"value": "Thomas"
}
] | example/src/Example/SortTable.elm | elm-review-bot/elm-ui-widgets | 0 | module Example.SortTable exposing (Model, Msg, init, subscriptions, update, view)
import Browser
import Element exposing (Element)
import Widget exposing (SortTableStyle)
import Widget.Material as Material
type alias Style style msg =
{ style
| sortTable : SortTableStyle msg
}
materialStyle : Style {} msg
materialStyle =
{ sortTable = Material.sortTable Material.defaultPalette
}
type alias Model =
{ title : String
, asc : Bool
}
type Msg
= ChangedSorting String
init : ( Model, Cmd Msg )
init =
( { title = "Name", asc = True }
, Cmd.none
)
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
ChangedSorting string ->
( { title = string
, asc =
if model.title == string then
not model.asc
else
True
}
, Cmd.none
)
subscriptions : Model -> Sub Msg
subscriptions _ =
Sub.none
{-| You can remove the msgMapper. But by doing so, make sure to also change `msg` to `Msg` in the line below.
-}
view : (Msg -> msg) -> Style style msg -> Model -> Element msg
view msgMapper style model =
Widget.sortTable style.sortTable
{ content =
[ { id = 1, name = "Antonio", rating = 2.456, hash = Nothing }
, { id = 2, name = "Ana", rating = 1.34, hash = Just "45jf" }
, { id = 3, name = "Alfred", rating = 4.22, hash = Just "6fs1" }
, { id = 4, name = "Thomas", rating = 3, hash = Just "k52f" }
]
, columns =
[ Widget.intColumn
{ title = "Id"
, value = .id
, toString = \int -> "#" ++ String.fromInt int
, width = Element.fill
}
, Widget.stringColumn
{ title = "Name"
, value = .name
, toString = identity
, width = Element.fill
}
, Widget.floatColumn
{ title = "Rating"
, value = .rating
, toString = String.fromFloat
, width = Element.fill
}
, Widget.unsortableColumn
{ title = "Hash"
, toString = .hash >> Maybe.withDefault "None"
, width = Element.fill
}
]
, asc = model.asc
, sortBy = model.title
, onChange = ChangedSorting >> msgMapper
}
main : Program () Model Msg
main =
Browser.element
{ init = always init
, view = \model -> model |> view identity materialStyle |> Element.layout []
, update = update
, subscriptions = subscriptions
}
| 42822 | module Example.SortTable exposing (Model, Msg, init, subscriptions, update, view)
import Browser
import Element exposing (Element)
import Widget exposing (SortTableStyle)
import Widget.Material as Material
type alias Style style msg =
{ style
| sortTable : SortTableStyle msg
}
materialStyle : Style {} msg
materialStyle =
{ sortTable = Material.sortTable Material.defaultPalette
}
type alias Model =
{ title : String
, asc : Bool
}
type Msg
= ChangedSorting String
init : ( Model, Cmd Msg )
init =
( { title = "<NAME>", asc = True }
, Cmd.none
)
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
ChangedSorting string ->
( { title = string
, asc =
if model.title == string then
not model.asc
else
True
}
, Cmd.none
)
subscriptions : Model -> Sub Msg
subscriptions _ =
Sub.none
{-| You can remove the msgMapper. But by doing so, make sure to also change `msg` to `Msg` in the line below.
-}
view : (Msg -> msg) -> Style style msg -> Model -> Element msg
view msgMapper style model =
Widget.sortTable style.sortTable
{ content =
[ { id = 1, name = "<NAME>", rating = 2.456, hash = Nothing }
, { id = 2, name = "<NAME>", rating = 1.34, hash = Just "45jf" }
, { id = 3, name = "<NAME>", rating = 4.22, hash = Just "6fs1" }
, { id = 4, name = "<NAME>", rating = 3, hash = Just "k52f" }
]
, columns =
[ Widget.intColumn
{ title = "Id"
, value = .id
, toString = \int -> "#" ++ String.fromInt int
, width = Element.fill
}
, Widget.stringColumn
{ title = "Name"
, value = .name
, toString = identity
, width = Element.fill
}
, Widget.floatColumn
{ title = "Rating"
, value = .rating
, toString = String.fromFloat
, width = Element.fill
}
, Widget.unsortableColumn
{ title = "Hash"
, toString = .hash >> Maybe.withDefault "None"
, width = Element.fill
}
]
, asc = model.asc
, sortBy = model.title
, onChange = ChangedSorting >> msgMapper
}
main : Program () Model Msg
main =
Browser.element
{ init = always init
, view = \model -> model |> view identity materialStyle |> Element.layout []
, update = update
, subscriptions = subscriptions
}
| true | module Example.SortTable exposing (Model, Msg, init, subscriptions, update, view)
import Browser
import Element exposing (Element)
import Widget exposing (SortTableStyle)
import Widget.Material as Material
type alias Style style msg =
{ style
| sortTable : SortTableStyle msg
}
materialStyle : Style {} msg
materialStyle =
{ sortTable = Material.sortTable Material.defaultPalette
}
type alias Model =
{ title : String
, asc : Bool
}
type Msg
= ChangedSorting String
init : ( Model, Cmd Msg )
init =
( { title = "PI:NAME:<NAME>END_PI", asc = True }
, Cmd.none
)
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
ChangedSorting string ->
( { title = string
, asc =
if model.title == string then
not model.asc
else
True
}
, Cmd.none
)
subscriptions : Model -> Sub Msg
subscriptions _ =
Sub.none
{-| You can remove the msgMapper. But by doing so, make sure to also change `msg` to `Msg` in the line below.
-}
view : (Msg -> msg) -> Style style msg -> Model -> Element msg
view msgMapper style model =
Widget.sortTable style.sortTable
{ content =
[ { id = 1, name = "PI:NAME:<NAME>END_PI", rating = 2.456, hash = Nothing }
, { id = 2, name = "PI:NAME:<NAME>END_PI", rating = 1.34, hash = Just "45jf" }
, { id = 3, name = "PI:NAME:<NAME>END_PI", rating = 4.22, hash = Just "6fs1" }
, { id = 4, name = "PI:NAME:<NAME>END_PI", rating = 3, hash = Just "k52f" }
]
, columns =
[ Widget.intColumn
{ title = "Id"
, value = .id
, toString = \int -> "#" ++ String.fromInt int
, width = Element.fill
}
, Widget.stringColumn
{ title = "Name"
, value = .name
, toString = identity
, width = Element.fill
}
, Widget.floatColumn
{ title = "Rating"
, value = .rating
, toString = String.fromFloat
, width = Element.fill
}
, Widget.unsortableColumn
{ title = "Hash"
, toString = .hash >> Maybe.withDefault "None"
, width = Element.fill
}
]
, asc = model.asc
, sortBy = model.title
, onChange = ChangedSorting >> msgMapper
}
main : Program () Model Msg
main =
Browser.element
{ init = always init
, view = \model -> model |> view identity materialStyle |> Element.layout []
, update = update
, subscriptions = subscriptions
}
| elm |
[
{
"context": " very quickly.\\\"\" ]\n , div [] [ text \"- Sarah, Aldridge\" ]\n , img [ src model.session.images.t",
"end": 958,
"score": 0.9998698235,
"start": 943,
"tag": "NAME",
"value": "Sarah, Aldridge"
},
{
"context": "stimonials_01 ] []\n , div [] [ text \"\\\"Jane is very approachable and goes out of her way to m",
"end": 1063,
"score": 0.9983790517,
"start": 1059,
"tag": "NAME",
"value": "Jane"
},
{
"context": "and enjoyable.\\\"\" ]\n , div [] [ text \"- Dorothy, Brownhills\" ]\n , img [ src model.session.images.t",
"end": 1214,
"score": 0.9998639226,
"start": 1195,
"tag": "NAME",
"value": "Dorothy, Brownhills"
},
{
"context": "o our lessons.\\\"\" ]\n , div [] [ text \"- George, Cannock\" ]\n , br [] []\n , div [] [ ",
"end": 1565,
"score": 0.999848187,
"start": 1550,
"tag": "NAME",
"value": "George, Cannock"
},
{
"context": "iving it a go!\\\"\" ]\n , div [] [ text \"- Linzi, Cannock\" ]\n , br [] []\n , div [] [ ",
"end": 1847,
"score": 0.9998373985,
"start": 1833,
"tag": "NAME",
"value": "Linzi, Cannock"
},
{
"context": "ties on offer.\\\"\" ]\n , div [] [ text \"- Brendan, Great Wyrley\" ]\n , br [] []\n , div [] [ ",
"end": 2008,
"score": 0.9828292727,
"start": 1987,
"tag": "NAME",
"value": "Brendan, Great Wyrley"
},
{
"context": "ed each other.\\\"\" ]\n , div [] [ text \"- Christine, Great Wyrley\" ]\n , img [ src model.session.images.t",
"end": 2245,
"score": 0.9584312439,
"start": 2222,
"tag": "NAME",
"value": "Christine, Great Wyrley"
},
{
"context": "nterest to us.\\\"\" ]\n , div [] [ text \"- Neil, Chelsyn Hay\" ]\n , img [ src model.sess",
"end": 2591,
"score": 0.9947311878,
"start": 2587,
"tag": "NAME",
"value": "Neil"
},
{
"context": "t to us.\\\"\" ]\n , div [] [ text \"- Neil, Chelsyn Hay\" ]\n , img [ src model.session.images.t",
"end": 2604,
"score": 0.9935538173,
"start": 2593,
"tag": "NAME",
"value": "Chelsyn Hay"
}
] | src/Page/TestimonialsPage.elm | craig-handley/jlh-languages-elm | 0 | module Page.TestimonialsPage exposing (Model, Msg(..), init, update, view)
import Browser
import Html exposing (..)
import Html.Attributes exposing (..)
import Session
import Viewer
-- MODEL
type alias Model =
{ session : Session.Session
}
-- INIT
init : Session.Session -> ( Model, Cmd Msg )
init session =
( Model session, Cmd.none )
-- UPDATE
type Msg
= NoOp
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
NoOp ->
( model, Cmd.none )
-- VIEW
view : Model -> Viewer.Details Msg
view model =
{ title = toTitle
, body =
[ div [ class "content" ]
[ div [ class "heading" ] [ text "Testimonials" ]
, div [] [ text "\"It’s lively and I like the way we learn in complete sentences. The variety keeps your attention. It was fun. You learn quickly and the time passes very quickly.\"" ]
, div [] [ text "- Sarah, Aldridge" ]
, img [ src model.session.images.testimonials_01 ] []
, div [] [ text "\"Jane is very approachable and goes out of her way to make our sessions lively\u{00A0}and enjoyable.\"" ]
, div [] [ text "- Dorothy, Brownhills" ]
, img [ src model.session.images.testimonials_02 ] []
, div [] [ text "\"I have wanted to learn French for years but never managed to find the right class. Jane's method of teaching is one of mixing the learning with fun and empathy. I really look forward to our lessons.\"" ]
, div [] [ text "- George, Cannock" ]
, br [] []
, div [] [ text "\"Never any good with foreign languages at school or since but have found a new desire to learn French and Jane's lessons are encouraging me to be confident in giving it a go!\"" ]
, div [] [ text "- Linzi, Cannock" ]
, br [] []
, div [] [ text "\"I do love the variety of activities on offer.\"" ]
, div [] [ text "- Brendan, Great Wyrley" ]
, br [] []
, div [] [ text "\"It is relaxed and at times there are funny moments when we got it wrong. All participants gelled and helped each other.\"" ]
, div [] [ text "- Christine, Great Wyrley" ]
, img [ src model.session.images.testimonials_03 ] []
, div [] [ text "\"What I like about the lessons is that we are given information about how to learn and we are encouraged to look at things outside the lesson (a website or a radio programme) if they are of interest to us.\"" ]
, div [] [ text "- Neil, Chelsyn Hay" ]
, img [ src model.session.images.testimonials_04 ] []
]
]
}
-- HELPERS
toTitle : String
toTitle =
"Testimonials Page"
| 40634 | module Page.TestimonialsPage exposing (Model, Msg(..), init, update, view)
import Browser
import Html exposing (..)
import Html.Attributes exposing (..)
import Session
import Viewer
-- MODEL
type alias Model =
{ session : Session.Session
}
-- INIT
init : Session.Session -> ( Model, Cmd Msg )
init session =
( Model session, Cmd.none )
-- UPDATE
type Msg
= NoOp
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
NoOp ->
( model, Cmd.none )
-- VIEW
view : Model -> Viewer.Details Msg
view model =
{ title = toTitle
, body =
[ div [ class "content" ]
[ div [ class "heading" ] [ text "Testimonials" ]
, div [] [ text "\"It’s lively and I like the way we learn in complete sentences. The variety keeps your attention. It was fun. You learn quickly and the time passes very quickly.\"" ]
, div [] [ text "- <NAME>" ]
, img [ src model.session.images.testimonials_01 ] []
, div [] [ text "\"<NAME> is very approachable and goes out of her way to make our sessions lively\u{00A0}and enjoyable.\"" ]
, div [] [ text "- <NAME>" ]
, img [ src model.session.images.testimonials_02 ] []
, div [] [ text "\"I have wanted to learn French for years but never managed to find the right class. Jane's method of teaching is one of mixing the learning with fun and empathy. I really look forward to our lessons.\"" ]
, div [] [ text "- <NAME>" ]
, br [] []
, div [] [ text "\"Never any good with foreign languages at school or since but have found a new desire to learn French and Jane's lessons are encouraging me to be confident in giving it a go!\"" ]
, div [] [ text "- <NAME>" ]
, br [] []
, div [] [ text "\"I do love the variety of activities on offer.\"" ]
, div [] [ text "- <NAME>" ]
, br [] []
, div [] [ text "\"It is relaxed and at times there are funny moments when we got it wrong. All participants gelled and helped each other.\"" ]
, div [] [ text "- <NAME>" ]
, img [ src model.session.images.testimonials_03 ] []
, div [] [ text "\"What I like about the lessons is that we are given information about how to learn and we are encouraged to look at things outside the lesson (a website or a radio programme) if they are of interest to us.\"" ]
, div [] [ text "- <NAME>, <NAME>" ]
, img [ src model.session.images.testimonials_04 ] []
]
]
}
-- HELPERS
toTitle : String
toTitle =
"Testimonials Page"
| true | module Page.TestimonialsPage exposing (Model, Msg(..), init, update, view)
import Browser
import Html exposing (..)
import Html.Attributes exposing (..)
import Session
import Viewer
-- MODEL
type alias Model =
{ session : Session.Session
}
-- INIT
init : Session.Session -> ( Model, Cmd Msg )
init session =
( Model session, Cmd.none )
-- UPDATE
type Msg
= NoOp
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
NoOp ->
( model, Cmd.none )
-- VIEW
view : Model -> Viewer.Details Msg
view model =
{ title = toTitle
, body =
[ div [ class "content" ]
[ div [ class "heading" ] [ text "Testimonials" ]
, div [] [ text "\"It’s lively and I like the way we learn in complete sentences. The variety keeps your attention. It was fun. You learn quickly and the time passes very quickly.\"" ]
, div [] [ text "- PI:NAME:<NAME>END_PI" ]
, img [ src model.session.images.testimonials_01 ] []
, div [] [ text "\"PI:NAME:<NAME>END_PI is very approachable and goes out of her way to make our sessions lively\u{00A0}and enjoyable.\"" ]
, div [] [ text "- PI:NAME:<NAME>END_PI" ]
, img [ src model.session.images.testimonials_02 ] []
, div [] [ text "\"I have wanted to learn French for years but never managed to find the right class. Jane's method of teaching is one of mixing the learning with fun and empathy. I really look forward to our lessons.\"" ]
, div [] [ text "- PI:NAME:<NAME>END_PI" ]
, br [] []
, div [] [ text "\"Never any good with foreign languages at school or since but have found a new desire to learn French and Jane's lessons are encouraging me to be confident in giving it a go!\"" ]
, div [] [ text "- PI:NAME:<NAME>END_PI" ]
, br [] []
, div [] [ text "\"I do love the variety of activities on offer.\"" ]
, div [] [ text "- PI:NAME:<NAME>END_PI" ]
, br [] []
, div [] [ text "\"It is relaxed and at times there are funny moments when we got it wrong. All participants gelled and helped each other.\"" ]
, div [] [ text "- PI:NAME:<NAME>END_PI" ]
, img [ src model.session.images.testimonials_03 ] []
, div [] [ text "\"What I like about the lessons is that we are given information about how to learn and we are encouraged to look at things outside the lesson (a website or a radio programme) if they are of interest to us.\"" ]
, div [] [ text "- PI:NAME:<NAME>END_PI, PI:NAME:<NAME>END_PI" ]
, img [ src model.session.images.testimonials_04 ] []
]
]
}
-- HELPERS
toTitle : String
toTitle =
"Testimonials Page"
| elm |
[
{
"context": " \"\\\"Hella world\\\"\"\n [] \"a = \\\"Hella \\\"\\na = a + \\\"world\\\"\\na\"\n --|> onlyBefore\n |> ",
"end": 43859,
"score": 0.7102831602,
"start": 43854,
"tag": "NAME",
"value": "Hella"
}
] | tests/UpdateTests.elm | digitalsatori/sketch-n-sketch | 565 | module UpdateTests exposing (..)
import Helpers.Matchers exposing (..)
import Update exposing (..)
import UpdateRegex exposing (..)
import UpdateStack exposing (..)
import UpdateUtils exposing (..)
import Lang exposing (..)
import GroupStartMap exposing (..)
import Regex
import Utils
import Eval
import Syntax
import Lazy
import Results exposing (Results)
import LazyList
import LangUtils exposing (..)
import ParserUtils
import HTMLValParser
import Set
import ImpureGoodies
import EvalUpdate exposing (builtinEnv)
import ExamplesGenerated
import ElmParser
type StateChanger = StateChanger (State -> State)
type alias State = { numTests: Int, nthAssertion: Int, numSuccess: Int, numFailed: Int, currentName: String, errors: String, ignore: Bool, toLaunch: List StateChanger, onlyOnly: Bool }
init_state = State 0 0 0 0 "" "" False [] False
-- Metacommands to gather states without executing them
delay: (() -> State -> State) -> State -> State
delay thetest state =
if state.ignore then state else
thetest () state
only: (State -> State) -> State -> State
only stateChanger state =
let newState = stateChanger { state | toLaunch = [], onlyOnly = False} in
let newState2 =flush newState in
{newState2 | onlyOnly = True, toLaunch = []} -- wipe out other tests not launched
onlyLast: State -> State
onlyLast state =
case Utils.maybeLast state.toLaunch of
Just (StateChanger stateChanger) ->
only stateChanger state
_ -> Debug.crash "No tests to only test before the use of 'onlyLast'. Add '|> onlyLast' after a test you want to run alone"
onlyAfter = skipBefore
skipBefore: State -> State
skipBefore state = { state | toLaunch = [] }
onlyBefore: State -> State
onlyBefore =
flush >> ignore True
gather: (State -> State) -> State -> State
gather stateChanger state =
{ state | toLaunch = state.toLaunch ++ [StateChanger stateChanger] }
flush: State -> State
flush state =
let defaultState = {state | toLaunch = [] } in
if state.onlyOnly then defaultState else
List.foldl (\(StateChanger sc) s -> sc s) defaultState state.toLaunch
summary: State -> String
summary state_ =
let state = flush state_ in
Debug.log (state.errors ++ "\n-------------------\n "++toString state.numSuccess ++"/" ++ toString state.numTests++ " tests passed\n-------------------") "ok"
test_: String -> State -> State
test_ name state =
let _ = Debug.log name " [Start]" in
{state | nthAssertion = 1, currentName = name} -- Debug.log name "all tests passed"
test s = gather <| test_ s
ignore: Bool -> State -> State
ignore b state =
{state | ignore = b}
success state = { state |
numTests = state.numTests + 1,
numSuccess = state.numSuccess + 1,
nthAssertion = state.nthAssertion + 1
}
fail state newError = { state |
numTests = state.numTests + 1,
numFailed = state.numFailed + 1,
nthAssertion = state.nthAssertion + 1,
errors = state.errors ++ "\n" ++ newError
}
log state msg =
"[" ++ state.currentName ++ ", assertion #" ++ toString state.nthAssertion ++ "] " ++ msg
nthEnv = [("nth", "letrec nth list index = case list of head::tail -> if index == 0 then head else nth tail (index - 1); [] -> null in nth")]
genericAssertEqual: (a -> String) -> (a -> a -> Bool) -> a -> a -> State -> State
genericAssertEqual eToString isEqual obtained expected state =
if state.ignore then state else
if isEqual obtained expected then success state else fail state <| "[" ++ state.currentName ++ ", assertion #" ++ toString state.nthAssertion ++ "] Expected \n" ++
eToString expected ++ ", got\n" ++ eToString obtained
assertEqual_: a -> a -> State -> State
assertEqual_ = genericAssertEqual (toString) (==)
assertEqual a b = gather <| assertEqual_ a b
assertEqualVal_: Val -> Val -> State -> State
assertEqualVal_ = genericAssertEqual valToString (\x y -> valToString x == valToString y)
assertEqualVal v1 v2 = gather <| assertEqualVal_ v1 v2
updateAssert_: Bool -> Env -> Exp -> Val -> Val -> Env -> String -> State -> State
updateAssert_ checkEnv env exp origOut newOut expectedEnv expectedExpStr state =
if state.ignore then state else
let expected = (if checkEnv then envToString expectedEnv else "") ++ " |- " ++ expectedExpStr in
let problemdesc = ("\nFor problem:" ++
(if checkEnv then envToString env else "") ++ " |- " ++ unparse exp ++ " <-- " ++ valToString newOut ++
" (was " ++ valToString origOut ++ ")") in
case UpdateUtils.defaultVDiffs origOut newOut of
Err msg -> fail state <| log state <| "This diff is not allowed:" ++ msg
Ok (LazyList.Nil) -> fail state <| "Internal error: no diffs"
Ok (LazyList.Cons Nothing _) -> fail state <| log state <| "There was no diff between the previous output and the new output"
Ok ll->
--let _ = ImpureGoodies.log <| "Diffs observed: " ++ toString (LazyList.toList ll) in
case Ok (LazyList.filterMap identity ll) |> Results.andThen (\diffs ->
update LazyList.Nil LazyList.Nil (updateContext "initial" env exp [] origOut newOut diffs)) of
Ok (LazyList.Cons (envX, expX) lazyTail as ll) ->
let _ = LazyList.toList ll in
--let _ = ImpureGoodies.log <| toString expX.changes in
--let _ = ImpureGoodies.log <| eDiffsToString "" exp expX.val (expX.changes |> Maybe.withDefault (EConstDiffs EAnyDiffs)) in
let obtained = (if checkEnv then envToString envX.val else if envX.changes == [] then "" else toString envX.changes) ++ " |- " ++ unparse expX.val in
if obtained == expected then success state else
case Lazy.force lazyTail of
LazyList.Cons (envX2, expX2) lazyTail2 ->
let obtained2 = (
if checkEnv then envToString envX2.val else
if envX2.changes == [] then "" else toString envX2.changes) ++ " |- " ++ unparse expX2.val in
if obtained2 == expected then success state else
fail state <|
log state <| "Expected \n'" ++ expected ++ "'\n, got\n'" ++ obtained ++ "'\n and \n'" ++ obtained2 ++ problemdesc
LazyList.Nil ->
fail state <|
log state <| "Expected \n'" ++ expected ++ "'\n, got\n'" ++ obtained ++ "'\n" ++ problemdesc
Ok LazyList.Nil ->
fail state <| log state <| "Expected \n'" ++ expected ++ "', got no solutions without error" ++ problemdesc
Err msg ->
fail state <| log state <| "Expected \n'" ++ expected ++ "', got '" ++ msg ++ problemdesc
updateAssert env exp origOut newOut expectedEnv expectedExpStr =
gather <| updateAssert_ True env exp origOut newOut expectedEnv expectedExpStr
evalElmAssert_: List (String, String) -> String -> String -> State -> State
evalElmAssert_ envStr expStr expectedResStr state =
if state.ignore then state else
case Utils.projOk [parseEnv envStr] of
Err error -> fail state <| log state <| "Error while parsing environments: " ++ error
Ok [env] ->
case Utils.projOk [parse expStr, parse expectedResStr] of
Err error -> fail state <| log state <| "Error while parsing expressions or outputs: " ++ error
Ok [exp, res] ->
--let _ = Debug.log (log state <| toString exp) () in
case Utils.projOk [evalEnv env exp, eval res] of
Err error -> fail state <| log state <| "Error while evaluating the expression or the expected result: " ++ unparse exp ++ "," ++ unparse res ++ ": " ++ error
Ok [out, expectedOut] -> assertEqualVal_ out expectedOut state
Ok _ -> fail state "???"
Ok _ -> fail state "???"
Ok _ -> fail state "???"
evalElmAssert envStr expStr expectedResStr = gather <| evalElmAssert_ envStr expStr expectedResStr
evalElmAssert2_: Env -> String -> String -> State -> State
evalElmAssert2_ env expStr expectedResStr state =
if state.ignore then state else
case Utils.projOk [parse expStr, parse expectedResStr] of
Err error -> fail state <| log state <| "Error while parsing expressions or outputs: " ++ error
Ok [exp, res] ->
--let _ = Debug.log (log state <| toString exp) () in
case Utils.projOk [evalEnv env exp, eval res] of
Err error -> fail state <| log state <| "Error while evaluating the expression or the expected result: " ++ unparse exp ++ "," ++ unparse res ++ ": " ++ error
Ok [out, expectedOut] -> assertEqualVal_ out expectedOut state
Ok _ -> fail state "???"
Ok _ -> fail state "???"
evalElmAssert2 envStr expStr expectedResStr = gather <| evalElmAssert2_ envStr expStr expectedResStr
updateElmAssert_: List (String, String) -> String -> String -> List (String, String) -> String -> State -> State
updateElmAssert_ envStr expStr newOutStr expectedEnvStr expectedExpStr state =
if state.ignore then state else
case Utils.projOk [parseEnv envStr, parseEnv expectedEnvStr] of
Err error -> fail state <| log state <| "Error while parsing environments: " ++ error
Ok [env, expectedEnv] ->
case Utils.projOk [parse expStr, parse newOutStr] of
Err error -> fail state <| log state <| "Error while parsing expressions or outputs: " ++ error
Ok [exp, newOut] ->
--let _ = Debug.log (log state <| toString exp) () in
case Utils.projOk [evalEnv env exp, eval newOut] of
Err error ->
let _ = Debug.log (toString exp) () in
fail state <| log state <| "Error while evaluating the expression or the output: " ++ Syntax.unparser Syntax.Elm exp ++ " <- " ++ Syntax.unparser Syntax.Elm newOut ++ ": " ++ error
Ok [out, newOut] -> updateAssert_ True env exp out newOut expectedEnv expectedExpStr state
Ok _ -> fail state "???"
Ok _ -> fail state "???"
Ok _ -> fail state "???"
updateElmAssert envStr expStr newOutStr expectedEnvStr expectedExpStr = gather <| updateElmAssert_ envStr expStr newOutStr expectedEnvStr expectedExpStr
updateElmAssert2_: Env -> String -> String -> String -> State -> State
updateElmAssert2_ env expStr newOutStr expectedExpStr state =
if state.ignore then state else
case Utils.projOk [parse expStr, parse newOutStr] of
Err error -> fail state <| log state <| "Error while parsing expressions or outputs: " ++ error
Ok [exp, newOut] ->
--let _ = Debug.log (log state <| toString exp) () in
case Utils.projOk [evalEnv env exp, eval newOut] of
Err error -> fail state <| log state <| "Error while evaluating the expression or the output: " ++ Syntax.unparser Syntax.Elm exp ++ "," ++ Syntax.unparser Syntax.Elm newOut ++ ": " ++ error
Ok [out, newOut] -> updateAssert_ True env exp out newOut env expectedExpStr state
Ok _ -> fail state "???"
Ok _ -> fail state "???"
updateElmAssert2 env expStr newOutStr expectedExpStr = gather <| updateElmAssert2_ env expStr newOutStr expectedExpStr
updateElmPrelude_: String -> (String -> String) -> (String -> String) -> State -> State
updateElmPrelude_ expStr outReplacer expectedExpReplacer state =
if state.ignore then state else
case parse expStr of
Err error -> fail state <| log state <| "Error while parsing expressions or outputs: " ++ error
Ok exp ->
case evalEnv EvalUpdate.preludeEnv exp of
Err error -> fail state <| log state <| "Error while evaluating the expression: " ++ Syntax.unparser Syntax.Elm exp ++ ": " ++ error
Ok oldOut ->
case oldOut |> valToString |> outReplacer |> parse |> Result.andThen eval of
Err error -> fail state <| log state <| "Error while parsing expressions or outputs: " ++ error
Ok newOut ->
let expectedExpStr = expectedExpReplacer expStr in
updateAssert_ False EvalUpdate.preludeEnv exp oldOut newOut EvalUpdate.preludeEnv expectedExpStr state
updateElmPrelude expStr outReplacer expectedExpReplacer = gather <| updateElmPrelude_ expStr outReplacer expectedExpReplacer
replaceStr before after = Regex.replace Regex.All (Regex.regex before) (\_ -> after)
parse = Syntax.parser Syntax.Elm >> Result.mapError (\p -> ParserUtils.showError p)
unparse = Syntax.unparser Syntax.Elm
evalEnv env exp = Eval.doEval Syntax.Elm env exp |> Result.map (Tuple.first >> Tuple.first)
eval exp = Eval.doEval Syntax.Elm [] exp |> Result.map (Tuple.first >> Tuple.first)
parseEnv: List (String, String) -> Result String Env
parseEnv envStr =
envStr
|> List.map (\(name, exp) ->
parse exp
|> Result.mapError (\pError -> toString pError)
|> Result.andThen (\x ->
evalEnv [] x
|> Result.map (\x -> (name, x))))
|> Utils.projOk
|> Result.mapError (\pError -> toString pError)
dws = ws " "
dws2 = ws " "
dws1 = ws " "
dws3 = ws " "
dws4 = ws " "
dws5 = ws " "
dws6 = ws " "
val x = Val x (Provenance [] (tConst (ws "") 0) []) (Parents [])
tVal n = val <| (VConst Nothing (n, dummyTrace))
tVClosure maybeIndent pats body env = val <| VClosure maybeIndent pats body env
tVList vals = val <| VList vals
tVBool truth = val <| VBase (VBool truth)
tVStr chars = val <| VBase (VString chars)
tConst ws num = withDummyExpInfo <| EConst ws num dummyLoc noWidgetDecl
tBool space truth = withDummyExpInfo <| EBase space (EBool truth)
tString space chars = withDummyExpInfo <| EBase space (EString defaultQuoteChar chars)
tVar space name =withDummyExpInfo <| EVar space name
tFun sp0 pats body sp1 = (withDummyExpInfo <| EFun sp0 pats body sp1)
tApp sp0 fun args sp1 = withDummyExpInfo <| EApp sp0 fun args SpaceApp sp1
tList sp0 exps sp1 = withDummyExpInfo <| EList sp0 (List.map (\e -> (ws "", e)) exps) (ws "") Nothing sp1
--tListCons sp0 exps sp1 tail sp2 = EList sp0 exps sp1 (Just tail) sp2
tPVar space name = withDummyPatInfo <| PVar space name noWidgetDecl
tPAs sp0 name sp1 pat= withDummyPatInfo <| PAs sp0 name sp1 pat
tPList sp0 listPat sp1= withDummyPatInfo <| PList sp0 listPat (ws "") Nothing sp1
tPListCons sp0 listPat sp1 tailPat sp2 = withDummyPatInfo <| PList sp0 listPat sp1 (Just tailPat) sp1
onlySpecific = False
display_prelude_message = case ElmParser.preludeNotParsed of
Nothing -> ()
Just msg -> Debug.log msg ()
all_tests = init_state
|> ignore onlySpecific
|> test "triCombineTest"
|> delay (\() -> assertEqual
(mergeEnv
[("y", (tVal 2)), ("x", (tVal 1))]
[("y", (tVal 2)), ("x", (tVal 1))] []
[("y", (tVal 2)), ("x", (tVal 3))] [(1, VConstDiffs)]
) ([("y", (tVal 2)), ("x", (tVal 3))], [(1, VConstDiffs)]))
|> delay (\() -> assertEqual
(mergeEnv
[("x", (tVal 1)), ("y", (tVal 1)), ("z", (tVal 1))]
[("x", (tVal 1)), ("y", (tVal 2)), ("z", (tVal 2))] [(1, VConstDiffs), (2, VConstDiffs)]
[("x", (tVal 3)), ("y", (tVal 1)), ("z", (tVal 3))] [(0, VConstDiffs), (2, VConstDiffs)]
) ([("x", (tVal 3)), ("y", (tVal 2)), ("z", (tVal 3))], [(0, VConstDiffs), (1, VConstDiffs), (2, VConstDiffs)]))
|> test "Minus sign as binary or unary operator"
|> evalElmAssert [] "1-1" "0"
|> evalElmAssert [] "(\\x->x) -1" "-1"
|> test "update mutual recursion"
|> updateElmAssert [] "x =y\ny= 1\nx" "2"
[] "x =y\ny= 2\nx"
|> updateElmAssert [] "a = g 85\nf x = g (x - 17)\ng y = if y < 17 then y else f y\na" "2"
[] "a = g 87\nf x = g (x - 17)\ng y = if y < 17 then y else f y\na"
|> updateElmAssert [] "a = g 85\nf x = g (x - 17)\ng y = if y < 17 then y else f y\na" "2"
[] "a = g 85\nf x = g (x - 15)\ng y = if y < 17 then y else f y\na"
|> test "update const"
|> updateElmAssert [] " 1" "2"
[] " 2"
|> test "update boolean and strings"
|> updateElmAssert [] " True" "False"
[] " False"
|> updateElmAssert [] " 'Hello'" "'World'"
[] " 'World'"
|> test "update var"
|> updateElmAssert [("x", "1")] "x" "2"
[("x", "2")] "x"
|> updateElmAssert [("x", "1"), ("y", "3")] " x" "2"
[("x", "2"), ("y", "3")] " x"
|> updateElmAssert [("y", "3"), ("x", "1")] " x" "2"
[("y", "3"), ("x", "2")] " x"
|> test "update fun"
|> updateElmAssert [] "\\ x -> 1" "\\ x -> 2"
[] "\\ x -> 2"
|> test "update fun with 2 arguments"
|> updateElmAssert [] "\\x y -> 1" "\\y x -> 2"
[] "\\y x -> 2"
|> test "update nested fun with 2 and 1 arguments"
|> updateElmAssert [] "\\x y -> \\z -> 1" "\\y z -> \\x -> 3"
[] "\\y z -> \\x -> 3"
|> test "update app identity"
|> updateElmAssert [] "(\\x -> x) 1" "2"
[] "(\\x -> x) 2"
|> test "update app constant"
|> updateElmAssert [] "(\\x -> 1) 3" "2"
[] "(\\x -> 2) 3"
|> test "update pattern 'as' (\\(x as y) -> x [or y]) 1"
|> updateElmAssert [] "(\\x as y -> x) 1" "2"
[] "(\\x as y -> x) 2"
|> updateElmAssert [] "(\\(x as y) -> y) 1" "2"
[] "(\\(x as y) -> y) 2"
|> test "update pattern list (\\[x, y] -> x or y) [1, 2]"
|> updateElmAssert [] "(\\[x, y] -> x) [1, 2]" "3"
[] "(\\[x, y] -> x) [3, 2]"
|> updateElmAssert [] "(\\[x, y] -> y) [1, 2]" "3"
[] "(\\[x, y] -> y) [1, 3]"
|> test "update pattern list with tail (\\[x | [y]] -> x or y) [1, 2]"
|> updateElmAssert [] "(\\x :: y -> x) [1, 2]" "3"
[] "(\\x :: y -> x) [3, 2]"
|> updateElmAssert [] "(\\x :: [y] -> y) [1, 2]" "3"
[] "(\\x :: [y] -> y) [1, 3]"
|> test "update app (\\x y -> x) 1 2"
|> updateElmAssert [] "(\\x y -> x) 1 2" "3"
[] "(\\x y -> x) 3 2"
|> test "update app (\\x y -> y) 1 2"
|> updateElmAssert
[] "(\\x y -> y) 1 2" "3"
[] "(\\x y -> y) 1 3"
|> test "update if-then-else"
|> updateElmAssert
[] "if True then 1 else 2" "3"
[] "if True then 3 else 2"
|> updateElmAssert
[] "if False then 1 else 2" "3"
[] "if False then 1 else 3"
|> test "String concatenation"
|> updateElmAssert [] "'Hello' + 'world'" "'Hello world'"
[] "'Hello ' + 'world'"
|> test "update arithmetic operations"
|> updateElmAssert
[("x", "1"), ("y", "2")] " x+ y" "4"
[("x", "2"), ("y", "2")] " x+ y"
|> updateElmAssert
[("x", "1"), ("y", "2")] " x+ y" "4"
[("x", "1"), ("y", "3")] " x+ y"
|> updateElmAssert
[("x", "5"), ("y", "2")] " x- y" "1"
[("x", "3"), ("y", "2")] " x- y"
|> updateElmAssert
[("x", "2"), ("y", "3")] " x* y" "8"
[("x", "2"), ("y", "4")] " x* y"
|> updateElmAssert
[("x", "18"), ("y", "3")] " x/ y" "7"
[("x", "21"), ("y", "3")] " x/ y"
|> updateElmAssert
[("x", "3"), ("y", "4")] " x ^ y" "16"
[("x", "2"), ("y", "4")] " x ^ y"
|> updateElmAssert
[("x", "-1"), ("y", "3")] " x ^ y" "1"
[("x", "1"), ("y", "3")] " x ^ y"
|> updateElmAssert
[("x", "-2"), ("y", "3")] " x ^ y" "27"
[("x", "3"), ("y", "3")] " x ^ y"
|> updateElmAssert
[("x", "-1"), ("y", "0")] "x ^ y" "-1"
[("x", "-1"), ("y", "1")] "x ^ y"
|> updateElmAssert
[("x", "-1"), ("y", "3")] " x ^ y" "-8"
[("x", "-2"), ("y", "3")] " x ^ y"
|> updateElmAssert
[("x", "17"), ("y", "8")] " mod x y" "3"
[("x", "19"), ("y", "8")] " mod x y"
|> updateElmAssert
[("x", "1"), ("y", "0")] "arctan2 y x" "1.5707963267948966"
[("x", "6.123233995736766e-17"), ("y", "1")] "arctan2 y x"
|> updateElmAssert
[("x", "1"), ("y", "0")] "arctan2 y x" "3.141592653589793"
[("x", "-1"), ("y", "1.2246467991473532e-16")] "arctan2 y x"
|> updateElmAssert
[("x", "1"), ("y", "0")] "arctan2 y x" "-1.5707963267948966"
[("x", "6.123233995736766e-17"), ("y", "-1")] "arctan2 y x"
|> updateElmAssert
[("x", "0.1")] "cos x" "0"
[("x", "1.570796326794897")] "cos x"
|> updateElmAssert
[("x", "-0.1")] "cos x" "0"
[("x", "-1.570796326794897")] "cos x"
|> updateElmAssert
[("x", "0.1")] "cos x" "0"
[("x", "1.570796326794897")] "cos x"
|> updateElmAssert
[("x", "0.1")] "sin x" "1"
[("x", "1.570796326794897")] "sin x"
|> updateElmAssert
[("x", "-0.1")] "sin x" "-1"
[("x", "-1.570796326794897")] "sin x"
|> updateElmAssert
[("x", "0")] "arccos x" "0"
[("x", "1")] "arccos x"
|> updateElmAssert
[("x", "0")] "arcsin x" "1.5707963267948966"
[("x", "1")] "arcsin x"
|> updateElmAssert
[("x", "17.5")] "floor x" "15"
[("x", "15.5")] "floor x"
|> updateElmAssert
[("x", "17.5")] "ceiling x" "15"
[("x", "14.5")] "ceiling x"
|> updateElmAssert
[("x", "17.75")] "round x" "15"
[("x", "14.75")] "round x"
|> updateElmAssert
[("x", "17.25")] "round x" "15"
[("x", "15.25")] "round x"
|> updateElmAssert
[("x", "16")] "sqrt x" "3"
[("x", "9")] "sqrt x"
|> test "case of calls"
|> updateElmAssert
[("x", "[7, 1]")] "case x of\n [a, b] -> a + b\n u -> 0" "5"
[("x", "[4, 1]")] "case x of\n [a, b] -> a + b\n u -> 0"
|> updateElmAssert
[("x", "[7]")] "case x of\n [a, b] -> a + b\n u -> 0" "5"
[("x", "[7]")] "case x of\n [a, b] -> a + b\n u -> 5"
|> test "non-rec let"
|> updateElmAssert
[] "let x= 5 in\nlet y =2 in [x, y]" "[6, 3]"
[] "let x= 6 in\nlet y =3 in [x, y]"
|> test "list constructor"
|> updateElmAssert
[] "let x= 1 in\nlet y =[2] in x :: x :: y" "[3, 1, 2]"
[] "let x= 3 in\nlet y =[2] in x :: x :: y"
|> updateElmAssert
[] "let x= 1 in\nlet y =2 in [x, x, y]" "[3, 1, 2]"
[] "let x= 3 in\nlet y =2 in [x, x, y]"
|> updateElmAssert
[] "let x= 1 in\nlet y =2 in [x, x, y]" "[1, 3, 2]"
[] "let x= 3 in\nlet y =2 in [x, x, y]"
|> updateElmAssert
[] "let x= 1 in\nlet y =2 in [x, x, y]" "[1, 1, 3]"
[] "let x= 1 in\nlet y =3 in [x, x, y]"
|> updateElmAssert
[] "let x= 1 in\nlet y =[2] in x :: x :: y" "[1, 3, 2]"
[] "let x= 3 in\nlet y =[2] in x :: x :: y"
|> updateElmAssert
[] "let x= 1 in\nlet y =[2] in x :: x :: y" "[1, 1, 3]"
[] "let x= 1 in\nlet y =[3] in x :: x :: y"
|> test "rec let"
|> updateElmAssert
[] "let f x = if x == 0 then x else (f (x - 1)) in\n f 2" "3"
[] "let f x = if x == 0 then x else (f (x - 1)) in\n f 5"
|> test "Comments"
|> updateElmAssert
[] "--This is a comment\n 1" "2"
[] "--This is a comment\n 2"
|> test "Strings"
|> updateElmAssert
[] " \"This is a string\"" "\"Hello world\""
[] " \"Hello world\""
|> updateElmAssert
[] " \"This is a string\"" "\"Hello' \\\" wo\\\\rld\""
[] " \"Hello' \\\" wo\\\\rld\""
|> updateElmAssert
[] " 'This is a string'" "\"Hello world\""
[] " 'Hello world'"
|> updateElmAssert
[] " 'This is a string'" "\"Hello' \\\" wo\\\\rld\""
[] " 'Hello\\' \" wo\\\\rld'"
|> updateElmAssert
[] " 'Welcome to this world'" "\"Welcome again to this world\""
[] " 'Welcome again to this world'"
|> test "Strings concatenation"
|> updateElmAssert [] "(\"Hello\" + \" \") + \"world\"" "\"Good morning world\""
[] "(\"Good morning\" + \" \") + \"world\""
|> test "Many solutions"
|> updateElmAssert
[("h3", "\\text -> ['h3', [], [['TEXT', text]]]")] "x = 0 + 0\n\nh3 (toString x)" "['h3', [], [['TEXT', '1']]]"
[("h3", "\\text -> ['h3', [], [['TEXT', text]]]")] "x = 0 + 1\n\nh3 (toString x)"
|> updateElmAssert
[("h3", "\\text -> ['h3', [], [['TEXT', text]]]")] "x = 0 + 0\n\nh3 (toString x)" "['h3', [], [['TEXT', '1']]]"
[("h3", "\\text -> ['h3', [], [['TEXT', text]]]")] "x = 1 + 0\n\nh3 (toString x)"
|> test "Multiline string literals"
|> updateElmAssert
[] "\"\"\"Hello @(if 1 == 2 then \"big\" else \"\"\"very @(\"small\")\"\"\") world\"\"\"" "\"Hello very tiny world\""
[] "\"\"\"Hello @(if 1 == 2 then \"big\" else \"\"\"very @(\"tiny\")\"\"\") world\"\"\""
|> updateElmAssert
[] "let x = \"Hello\" in \"\"\"@x world\"\"\"" "\"Hello big world\""
[] "let x = \"Hello\" in \"\"\"@x big world\"\"\""
|> updateElmAssert
[] "let x = \"Hello\" in \"\"\"@x world\"\"\"" "\"Hello big world\""
[] "let x = \"Hello big\" in \"\"\"@x world\"\"\""
|> updateElmAssert
[] "let x = \"Hello\" in \"\"\"@x world\"\"\"" "\"Helloworld\""
[] "let x = \"Hello\" in \"\"\"@(x)world\"\"\""
|> updateElmAssert
[] "\"\"\"@let x = \"Hello\" in\n@x world\"\"\"" "\"Hello big world\""
[] "\"\"\"@let x = \"Hello big\" in\n@x world\"\"\""
|> updateElmAssert
[] "\"\"\"@let x = \"Hello\" in\n@x world\"\"\"" "\"Hello big world\""
[] "\"\"\"@let x = \"Hello\" in\n@x big world\"\"\""
|> updateElmAssert
[] "\"\"\"@let x = (\"Hello\" + \n \" big\") in\n@x world\"\"\"" "\"Hello tall world\""
[] "\"\"\"@let x = (\"Hello\" + \n \" tall\") in\n@x world\"\"\""
|> test "Finding all regex matches"
|> assertEqual (LazyList.toList <| allInterleavingsIn ["A", "BB", "C"] "xAyBBBzAoBBpCC")
[ ["x","y","BzAoBBp","C"]
, ["x","y","BzAoBBpC",""]
, ["x", "yB", "zAoBBp","C"]
, ["x", "yB", "zAoBBpC",""]
, ["x", "yBBBzAo", "p","C"]
, ["x", "yBBBzAo", "pC",""]
, ["xAyBBBz", "o", "p","C"]
, ["xAyBBBz", "o", "pC",""]
]
|> test "GroupStartMap"
|> assertEqual (List.map (\{submatches} -> submatches) (GroupStartMap.find Regex.All "a((bc)|cd)d" "aabcdacdd"))
[[{match = Just "bc", start = 2}, {match = Just "bc", start = 2}], [{match = Just "cd", start = 6}, {match = Nothing, start = -1}]]
|> assertEqual (GroupStartMap.replace Regex.All "a((bc)|cd)d" (
\match -> String.concat <| List.map (\subm -> toString subm.start ++ Maybe.withDefault "null" subm.match) match.submatches
) "aabcdacdd")
"a2bc2bc6cd-1null"
|> test "replaceAllIn"
|> evalElmAssert2 builtinEnv "replaceAllIn \"l\" \"L\" \"Hello world\"" "\"HeLLo worLd\""
|> evalElmAssert2 builtinEnv "replaceAllIn \"a(b|c)\" \"o$1\" \"This is acknowledgeable\"" "\"This is ocknowledgeoble\""
|> evalElmAssert2 builtinEnv "replaceAllIn \"a(b|c)\" (\\{group = [t, c]} -> \"oa\" + (if c == \"b\" then \"c\" else \"b\")) \"This is acknowledgeable\"" "\"This is oabknowledgeoacle\""
|> updateElmAssert2 builtinEnv "replaceAllIn \"e\" \"ee\" \"\"\"See some examples from File...\"\"\"" "\"Seeee somee emexamplees from Filee...\""
"replaceAllIn \"e\" \"eme\" \"\"\"See some examples from File...\"\"\""
|> updateElmAssert2 builtinEnv "[ 'div'\n , []\n , [ ['h2', [], [['TEXT', 'Welcome to Sketch-n-Sketch Docs!']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', 'Type something here...']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', replaceAllIn \"e\" \"ee\" \"\"\"\n See some examples from File -> New From Template in\n the menu bar, or by pressing the Previous and Next\n buttons in the top-right corner.\n \"\"\"]]]\n ]\n ]"
"[ 'div'\n , []\n , [ ['h2', [], [['TEXT', 'Welcome to Sketch-n-Sketch Docs!']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', 'Type something here...']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', \"\"\"\n Seeee somee eecxamplees from Filee -> Neew From Teemplatee in\n thee meenu bar, or by preessing thee Preevious and Neext\n buttons in thee top-right corneer.\n \"\"\"]]]\n ]\n ]"
"[ 'div'\n , []\n , [ ['h2', [], [['TEXT', 'Welcome to Sketch-n-Sketch Docs!']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', 'Type something here...']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', replaceAllIn \"e\" \"eec\" \"\"\"\n See some examples from File -> New From Template in\n the menu bar, or by pressing the Previous and Next\n buttons in the top-right corner.\n \"\"\"]]]\n ]\n ]"
|> updateElmAssert2 builtinEnv "[ 'div'\n , []\n , [ ['h2', [], [['TEXT', 'Welcome to Sketch-n-Sketch Docs!']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', 'Type something here...']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', replaceAllIn \"e\" \"ee\" \"\"\"\n See some examples from File -> New From Template in\n the menu bar, or by pressing the Previous and Next\n buttons in the top-right corner.\n \"\"\"]]]\n ]\n ]"
"[ 'div'\n , []\n , [ ['h2', [], [['TEXT', 'Welcome to Sketch-n-Sketch Docs!']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', 'Type something here...']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', \"\"\"\n Seeee somee eecxamplees from Filee -> Neew From Teemplatee in\n thee meenu bar, or by preessing thee Preevious and Neext\n buttons in thee top-right corneer.\n \"\"\"]]]\n ]\n ]"
"[ 'div'\n , []\n , [ ['h2', [], [['TEXT', 'Welcome to Sketch-n-Sketch Docs!']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', 'Type something here...']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', replaceAllIn \"e\" \"ee\" \"\"\"\n See some ecxamples from File -> New From Template in\n the menu bar, or by pressing the Previous and Next\n buttons in the top-right corner.\n \"\"\"]]]\n ]\n ]"
|>
updateElmAssert [] "extractFirstIn \"^\\\\s*(S(\\\\w)+ (\\\\w))\" \"\"\" See some examples\"\"\" |> case of Just [big, s1, s2] -> big + s1 + s2; e -> \"not the right shape\"" "\"Sea ses\""
[] "extractFirstIn \"^\\\\s*(S(\\\\w)+ (\\\\w))\" \"\"\" Sea some examples\"\"\" |> case of Just [big, s1, s2] -> big + s1 + s2; e -> \"not the right shape\""
|> evalElmAssert [] "extractFirstIn \"\"\"([\\w:_-]*)\"\"\" \"data-array=\\\"17\\\"\" |> case of Just [id] -> id; _ -> \"Nothing\" " "\"data-array\""
|> evalElmAssert2 builtinEnv "replaceAllIn \"\\\\[([^\\\\[]+)\\\\]\\\\(([^\\\\)]+)\\\\)\" \"<a href='$2'>$1</a>\" \"[Markdown](http://test)\"" "\"<a href='http://test'>Markdown</a>\""
|> evalElmAssert2 builtinEnv "replaceAllIn \"\\\\[([^\\\\[]+)\\\\]\\\\(([^\\\\)]+)\\\\)\" \"<a href='$2'>$1</a>\" \"[Markdown](https://fr.wikipedia.org/wiki/Markdown)\"" "\"<a href='https://fr.wikipedia.org/wiki/Markdown'>Markdown</a>\""
|> updateElmAssert2 builtinEnv "replaceAllIn \"\\\\[([^\\\\[]+)\\\\]\\\\(([^\\\\)]+)\\\\)\" \"<a href='$2'>$1</a>\" \"#[Markdown](https://fr.wikipedia.org/wiki/Markdown)\"" "\"#<a href='https://fr.wikipedia.org/wiki/Markdown'>Markdoooown</a>\""
"replaceAllIn \"\\\\[([^\\\\[]+)\\\\]\\\\(([^\\\\)]+)\\\\)\" \"<a href='$2'>$1</a>\" \"#[Markdoooown](https://fr.wikipedia.org/wiki/Markdown)\""
|> updateElmAssert2 builtinEnv "replaceAllIn \"x|y\" (\\{match} -> if match == \"x\" then \"5\" else \"7\") \"x,y\"" "\"6,8\""
"replaceAllIn \"x|y\" (\\{match} -> if match == \"x\" then \"6\" else \"8\") \"x,y\""
--|> test "Record construction, extraction and pattern "
-- |>
|> test "Partial application"
|> updateElmAssert
[] "let map f l = case l of head::tail -> f head::map f tail; [] -> [] in let td color txt = [ 'td', [['style', ['border', 'padding', ['background-color', color]]]], [['TEXT', txt]]] in map (td 'green') ['hello', 'world']"
"[[ 'td', [['style', ['border', 'padding', ['background-color', 'red']]]], [['TEXT', 'hello']]], [ 'td', [['style', ['border', 'padding', ['background-color', 'green']]]], [['TEXT', 'world']]]]"
[] "let map f l = case l of head::tail -> f head::map f tail; [] -> [] in let td color txt = [ 'td', [['style', ['border', 'padding', ['background-color', color]]]], [['TEXT', txt]]] in map (td 'red') ['hello', 'world']"
--|> ignore False
|> test "Multiple solutions"
|> updateElmAssert
[] "['A' + 'B', 'C' + 'D']" "['AGB', 'CGD']"
[] "['AG' + 'B', 'CG' + 'D']"
|> test "Records"
|> updateElmAssert
[] "{ a= 1, b= 2}.b" "3"
[] "{ a= 1, b= 3}.b"
|> updateElmAssert
[] "{ a= 1, b= 2}.a" "3"
[] "{ a= 3, b= 2}.a"
|> updateElmAssert
[] "let x = { a= 1, b= 2} in { x | a = 2 }.a" "3"
[] "let x = { a= 1, b= 2} in { x | a = 3 }.a"
|> updateElmAssert
[] "let x = { a= 1, b= 2} in { x | a = 2 }.b" "3"
[] "let x = { a= 1, b= 3} in { x | a = 2 }.b"
|> test "Indented lists, second position of list > 1 elements"
|> updateElmAssert
[] "[1, 2, 3, 4]" "[1, 5, 2, 3, 4]"
[] "[1, 5, 2, 3, 4]"
|> updateElmAssert
[] "[ 1,\n 2,\n 3,\n 4]" "[1, 5, 2, 3, 4]"
[] "[ 1,\n 5,\n 2,\n 3,\n 4]"
|> updateElmAssert
[] "[ 1\n, 2\n, 3\n, 4]" "[1, 5, 2, 3, 4]"
[] "[ 1\n, 5\n, 2\n, 3\n, 4]"
|> updateElmAssert
[] " [ 1\n, 2\n, 3\n, 4]" "[1, 5, 2, 3, 4]"
[] " [ 1\n, 5\n, 2\n, 3\n, 4]"
|> test "Indented lists, second position of list with 1 element"
|> updateElmAssert
[] "[1]" "[1, 2]"
[] "[1, 2]"
|> updateElmAssert
[] "[ 1]" "[ 1, 2]"
[] "[ 1, 2]"
|> updateElmAssert
[] "[ 1\n]" "[ 1, 2]"
[] "[ 1\n, 2\n]"
|> updateElmAssert
[] "[\n 1]" "[ 1, 2]"
[] "[\n 1,\n 2]"
|> test "Indented lists, first position of list with 1 element"
|> updateElmAssert
[] "[1]" "[2, 1]"
[] "[2, 1]"
|> updateElmAssert
[] "[ 1]" "[2, 1]"
[] "[ 2, 1]"
|> updateElmAssert
[] "[ 1\n]" "[2, 1]"
[] "[ 2\n, 1\n]"
|> updateElmAssert
[] "[\n 1]" "[2, 1]"
[] "[\n 2,\n 1]"
|> test "Indented lists, first position of list > 1 element"
|> updateElmAssert
[] "[1, 2]" "[0, 1, 2]"
[] "[0, 1, 2]"
|> updateElmAssert
[] "[ 1, 2]" "[0, 1, 2]"
[] "[ 0, 1, 2]"
|> updateElmAssert
[] "[ 1\n, 2\n, 3\n, 4]" "[0, 1, 2, 3, 4]"
[] "[ 0\n, 1\n, 2\n, 3\n, 4]"
|> test "Indented lists, first position of empty list"
|> updateElmAssert
[] "[]" "[1]"
[] "[1]"
|> updateElmAssert
[] "[ ]" "[1]"
[] "[1 ]"
|> updateElmAssert
[] " []" "[[1, 2]]"
[] " [[1, 2]]"
|> test "parsing HTML"
|> updateElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)]
"parseHTML \"hello\"" "[HTMLInner \"hello world\"]"
"parseHTML \"hello world\""
|> updateElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)]
"parseHTML \"hello<br>world\"" "[HTMLInner \"hello\", HTMLElement \"br\" [] \" \" RegularEndOpening [] VoidClosing, HTMLInner \"sworld\"]"
"parseHTML \"hello<br >sworld\""
|> updateElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)]
"parseHTML \"<?help>\"" "[HTMLComment (Less_Greater \"Injection: adding more chars like >, <, and -->\")]"
"parseHTML \"<!--Injection: adding more chars like >, <, and ~~>-->\""
|> evalElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)]
"parseHTML \"<h3>Hello world</h3>\"" "[HTMLElement \"h3\" [] \"\" RegularEndOpening [HTMLInner \"Hello world\"] (RegularClosing \"\")]"
|> evalElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)]
"parseHTML \"<a href='https://fr.wikipedia.org/wiki/Markdown'>Markdown</a>\"" "[HTMLElement \"a\" [HTMLAttribute \" \" \"href\" (HTMLAttributeString \"\" \"\" \"'\" \"https://fr.wikipedia.org/wiki/Markdown\")] \"\" RegularEndOpening [HTMLInner \"Markdown\"] (RegularClosing \"\")]"
|> updateElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)]
"parseHTML \"<a href='https://fr.wikipedia.org/wiki/Markdown'>Markdown</a> demo\""
"[HTMLElement \"a\" [HTMLAttribute \" \" \"href\" (HTMLAttributeString \"\" \"\" \"'\" \"https://fr.wikipedia.org/wiki/Markdown\")] \"\" RegularEndOpening [HTMLInner \"Markdown\"] (RegularClosing \"\"), HTMLInner \" demonstration\"]"
"parseHTML \"<a href='https://fr.wikipedia.org/wiki/Markdown'>Markdown</a> demonstration\""
|> evalElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)] "parseHTML \"Hello world\"" "[HTMLInner \"Hello world\"]"
|> evalElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)] "parseHTML \"<i><b>Hello</i></b>World\"" "[ HTMLElement \"i\" [] \"\" RegularEndOpening [ HTMLElement \"b\" [] \"\" RegularEndOpening [ HTMLInner \"Hello\"] ForgotClosing] (RegularClosing \"\"), HTMLInner \"</b>World\"]"
|> updateElmAssert [("color", "\"white\""), ("padding", "[\"padding\", \"3px\"]")] "[padding, [\"background-color\", color]]"
"[[\"padding\", \"3px\"], [ \"background-color\", \"lightgray\"]]"
[("color", "\"lightgray\""), ("padding", "[\"padding\", \"3px\"]")] "[padding, [\"background-color\", color]]"
|> test "freeze"
|> updateElmAssert [("freeze", "\\x -> x")] "freeze 0 + 1" "2"
[("freeze", "\\x -> x")] "freeze 0 + 2"
|> updateElmAssert [("freezeExpression", "\\x -> x")] "let x = 1 in freezeExpression (0 + x)" "2"
[("freezeExpression", "\\x -> x")] "let x = 2 in freezeExpression (0 + x)"
|> test "dictionary"
|> updateElmAssert [("x", "1")] "__DictGet__ \"a\" (__DictFromList__ [(\"a\", x)])" "Just 2"
[("x", "2")] "__DictGet__ \"a\" (__DictFromList__ [(\"a\", x)])"
|> updateElmAssert [("x", "1")] "__DictRemove__ \"b\" (__DictFromList__ [(\"a\", x), (\"b\", 2)])" "__DictFromList__ [(\"a\", 2)]"
[("x", "2")] "__DictRemove__ \"b\" (__DictFromList__ [(\"a\", x), (\"b\", 2)])"
|> updateElmAssert [("x", "1")] "__DictInsert__ \"b\" x (__DictFromList__ [(\"a\", x)])" "__DictFromList__ [(\"a\", 1), (\"b\", 2)]"
[("x", "2")] "__DictInsert__ \"b\" x (__DictFromList__ [(\"a\", x)])"
|> updateElmAssert [] "__DictInsert__ \"b\" 1 (__DictFromList__ [(\"a\", 2), (\"b\", 3)])" "__DictFromList__ [(\"a\", 1), (\"b\", 2)]"
[] "__DictInsert__ \"b\" 2 (__DictFromList__ [(\"a\", 1), (\"b\", 3)])"
|> test "recursive delayed"
|> updateElmAssert [] "f =\n let x = 1 in\n \\y -> x + y\n\nf 2" "4"
[] "f =\n let x = 2 in\n \\y -> x + y\n\nf 2"
|> updateElmAssert [] "f =\n let x = 1 in\n \\y -> x + y\n\nf 2" "4"
[] "f =\n let x = 1 in\n \\y -> x + y\n\nf 3"
|> test "All diffs"
|> assertEqual (allStringDiffs "abcabcabc" "abxabcabc" |> Results.toList)
[[DiffEqual "ab", DiffRemoved "c", DiffAdded "x", DiffEqual "abcabc"]]
|> assertEqual (allStringDiffs "ab3ab" "abab" |> Results.toList)
[[DiffEqual "ab", DiffRemoved "3", DiffEqual "ab"]]
|> assertEqual (allStringDiffs "aa3aa" "aa" |> Results.toList)
[[DiffEqual "aa", DiffRemoved "3aa"],
[DiffRemoved "aa3", DiffEqual "aa"]]
|> assertEqual (alldiffs identity ["a", "b", "3", "a", "b"] ["a", "b", "a", "b"] |> Results.toList)
[[DiffEqual ["a", "b"], DiffRemoved ["3"], DiffEqual ["a", "b"]]]
|> assertEqual (alldiffs identity ["a", "a", "3", "a", "a"] ["a", "a"] |> Results.toList)
[[DiffEqual ["a", "a"], DiffRemoved ["3", "a", "a"]],
[DiffRemoved ["a", "a", "3"], DiffEqual ["a", "a"]]]
|> test "Type parsing"
|> evalElmAssert [] "type List a = Nil | Cons a\n2" "2"
|> evalElmAssert [] "let type List a = Nil | Cons a in 2" "2"
|> evalElmAssert [] "let type List a = Nil | Cons a\nin 2" "2"
|> evalElmAssert [] "let\n type List a = Nil | Cons a\nin 2" "2"
--|> skipBefore
|> test "updateReplace"
-- newStart newEnd ... repStart repEnd
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"ab\" \"abc\" \"12ab567ab90\" (VStringDiffs [StringUpdate 0 1 2])"
"(\"12abc567abc90\", VStringDiffs [StringUpdate 0 1 2, StringUpdate 1 3 3, StringUpdate 6 8 3])"
-- repStart repEnd ... newStart newEnd
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"ab\" \"abc\" \"12ab567ab90\" (VStringDiffs [StringUpdate 5 5 1])"
"(\"12abc567abc90\", VStringDiffs [StringUpdate 2 4 3, StringUpdate 5 5 1, StringUpdate 6 8 3])"
-- newStart repStart repEnd ... newEnd
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"ab\" \"abc\" \"12ab567ab90\" (VStringDiffs [StringUpdate 1 2 4])"
"(\"12abc567abc90\", VStringDiffs [StringUpdate 1 2 5, StringUpdate 4 6 3])"
-- newStart repStart newEnd ... repEnd
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"ab\" \"abc\" \"12ab567ab90\" (VStringDiffs [StringUpdate 1 7 2])"
"(\"12abc567abc90\", VStringDiffs [StringUpdate 1 8 4, StringUpdate 11 13 3])"
-- repStart newStart newEnd ... repEnd
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"abc\" \"abcd\" \"12abc567abc90\" (VStringDiffs [StringUpdate 3 9 1])"
"(\"12abcd567abcd90\", VStringDiffs [StringUpdate 2 10 4, StringUpdate 13 16 4])"
-- repStart newStart repEnd ... newEnd
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"ab\" \"abc\" \"12ab567ab90\" (VStringDiffs [StringUpdate 3 7 2])"
"(\"12abc567abc90\", VStringDiffs [StringUpdate 2 7 4, StringUpdate 9 11 3])"
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"\"\"(<(ul|ol)>(?:(?!</\\2>)[\\s\\S])*)</li>\\s*<li>\"\"\" \"$1</li>\\n</$2>\\n<$2>\\n\\t<li>\" \"<ul><li>a</li><li>b</li></ul>\" (VStringDiffs [StringUpdate 14 14 10])"
"(\"<ul><li>a</li>\\n</ul>\\n<ul>\\n\\t<li>b</li></ul>\", VStringDiffs [StringUpdate 0 14 37])"
-- Add the test <i>Hello <b>world</span></i> --> <i>Hello <b>world</b></i> (make sure to capture all closing tags)
|> updateElmAssert2 builtinEnv "replaceAllIn \"\\\\$(\\\\w+|\\\\$)\" (\\m -> m.match) \"printer\"" "\"$translation1\""
"replaceAllIn \"\\\\$(\\\\w+|\\\\$)\" (\\m -> m.match) \"$translation1\""
|> test "imperative-like assignments"
|> updateElmAssert [] "a = \"Hello \"\na = a + \"world\"\na" "\"Hella world\""
[] "a = \"Hella \"\na = a + \"world\"\na"
--|> onlyBefore
|> updateElmPrelude (
ExamplesGenerated.mapMaybeLens
|> replaceStr "(\r?\n)+$" "" -- Remove newlines at the end
|> replaceStr "showValues \\[maybeRowA, maybeRowB, maybeRow1, maybeRow2\\]" "showValues [maybeRow1, maybeRow2]"
|> replaceStr "\r?\nmaybeRow(A|B).*" ""
) (replaceStr "New Jersey" "New Jersay")
(replaceStr "New Jersey" "New Jersay")
|> summary
| 36938 | module UpdateTests exposing (..)
import Helpers.Matchers exposing (..)
import Update exposing (..)
import UpdateRegex exposing (..)
import UpdateStack exposing (..)
import UpdateUtils exposing (..)
import Lang exposing (..)
import GroupStartMap exposing (..)
import Regex
import Utils
import Eval
import Syntax
import Lazy
import Results exposing (Results)
import LazyList
import LangUtils exposing (..)
import ParserUtils
import HTMLValParser
import Set
import ImpureGoodies
import EvalUpdate exposing (builtinEnv)
import ExamplesGenerated
import ElmParser
type StateChanger = StateChanger (State -> State)
type alias State = { numTests: Int, nthAssertion: Int, numSuccess: Int, numFailed: Int, currentName: String, errors: String, ignore: Bool, toLaunch: List StateChanger, onlyOnly: Bool }
init_state = State 0 0 0 0 "" "" False [] False
-- Metacommands to gather states without executing them
delay: (() -> State -> State) -> State -> State
delay thetest state =
if state.ignore then state else
thetest () state
only: (State -> State) -> State -> State
only stateChanger state =
let newState = stateChanger { state | toLaunch = [], onlyOnly = False} in
let newState2 =flush newState in
{newState2 | onlyOnly = True, toLaunch = []} -- wipe out other tests not launched
onlyLast: State -> State
onlyLast state =
case Utils.maybeLast state.toLaunch of
Just (StateChanger stateChanger) ->
only stateChanger state
_ -> Debug.crash "No tests to only test before the use of 'onlyLast'. Add '|> onlyLast' after a test you want to run alone"
onlyAfter = skipBefore
skipBefore: State -> State
skipBefore state = { state | toLaunch = [] }
onlyBefore: State -> State
onlyBefore =
flush >> ignore True
gather: (State -> State) -> State -> State
gather stateChanger state =
{ state | toLaunch = state.toLaunch ++ [StateChanger stateChanger] }
flush: State -> State
flush state =
let defaultState = {state | toLaunch = [] } in
if state.onlyOnly then defaultState else
List.foldl (\(StateChanger sc) s -> sc s) defaultState state.toLaunch
summary: State -> String
summary state_ =
let state = flush state_ in
Debug.log (state.errors ++ "\n-------------------\n "++toString state.numSuccess ++"/" ++ toString state.numTests++ " tests passed\n-------------------") "ok"
test_: String -> State -> State
test_ name state =
let _ = Debug.log name " [Start]" in
{state | nthAssertion = 1, currentName = name} -- Debug.log name "all tests passed"
test s = gather <| test_ s
ignore: Bool -> State -> State
ignore b state =
{state | ignore = b}
success state = { state |
numTests = state.numTests + 1,
numSuccess = state.numSuccess + 1,
nthAssertion = state.nthAssertion + 1
}
fail state newError = { state |
numTests = state.numTests + 1,
numFailed = state.numFailed + 1,
nthAssertion = state.nthAssertion + 1,
errors = state.errors ++ "\n" ++ newError
}
log state msg =
"[" ++ state.currentName ++ ", assertion #" ++ toString state.nthAssertion ++ "] " ++ msg
nthEnv = [("nth", "letrec nth list index = case list of head::tail -> if index == 0 then head else nth tail (index - 1); [] -> null in nth")]
genericAssertEqual: (a -> String) -> (a -> a -> Bool) -> a -> a -> State -> State
genericAssertEqual eToString isEqual obtained expected state =
if state.ignore then state else
if isEqual obtained expected then success state else fail state <| "[" ++ state.currentName ++ ", assertion #" ++ toString state.nthAssertion ++ "] Expected \n" ++
eToString expected ++ ", got\n" ++ eToString obtained
assertEqual_: a -> a -> State -> State
assertEqual_ = genericAssertEqual (toString) (==)
assertEqual a b = gather <| assertEqual_ a b
assertEqualVal_: Val -> Val -> State -> State
assertEqualVal_ = genericAssertEqual valToString (\x y -> valToString x == valToString y)
assertEqualVal v1 v2 = gather <| assertEqualVal_ v1 v2
updateAssert_: Bool -> Env -> Exp -> Val -> Val -> Env -> String -> State -> State
updateAssert_ checkEnv env exp origOut newOut expectedEnv expectedExpStr state =
if state.ignore then state else
let expected = (if checkEnv then envToString expectedEnv else "") ++ " |- " ++ expectedExpStr in
let problemdesc = ("\nFor problem:" ++
(if checkEnv then envToString env else "") ++ " |- " ++ unparse exp ++ " <-- " ++ valToString newOut ++
" (was " ++ valToString origOut ++ ")") in
case UpdateUtils.defaultVDiffs origOut newOut of
Err msg -> fail state <| log state <| "This diff is not allowed:" ++ msg
Ok (LazyList.Nil) -> fail state <| "Internal error: no diffs"
Ok (LazyList.Cons Nothing _) -> fail state <| log state <| "There was no diff between the previous output and the new output"
Ok ll->
--let _ = ImpureGoodies.log <| "Diffs observed: " ++ toString (LazyList.toList ll) in
case Ok (LazyList.filterMap identity ll) |> Results.andThen (\diffs ->
update LazyList.Nil LazyList.Nil (updateContext "initial" env exp [] origOut newOut diffs)) of
Ok (LazyList.Cons (envX, expX) lazyTail as ll) ->
let _ = LazyList.toList ll in
--let _ = ImpureGoodies.log <| toString expX.changes in
--let _ = ImpureGoodies.log <| eDiffsToString "" exp expX.val (expX.changes |> Maybe.withDefault (EConstDiffs EAnyDiffs)) in
let obtained = (if checkEnv then envToString envX.val else if envX.changes == [] then "" else toString envX.changes) ++ " |- " ++ unparse expX.val in
if obtained == expected then success state else
case Lazy.force lazyTail of
LazyList.Cons (envX2, expX2) lazyTail2 ->
let obtained2 = (
if checkEnv then envToString envX2.val else
if envX2.changes == [] then "" else toString envX2.changes) ++ " |- " ++ unparse expX2.val in
if obtained2 == expected then success state else
fail state <|
log state <| "Expected \n'" ++ expected ++ "'\n, got\n'" ++ obtained ++ "'\n and \n'" ++ obtained2 ++ problemdesc
LazyList.Nil ->
fail state <|
log state <| "Expected \n'" ++ expected ++ "'\n, got\n'" ++ obtained ++ "'\n" ++ problemdesc
Ok LazyList.Nil ->
fail state <| log state <| "Expected \n'" ++ expected ++ "', got no solutions without error" ++ problemdesc
Err msg ->
fail state <| log state <| "Expected \n'" ++ expected ++ "', got '" ++ msg ++ problemdesc
updateAssert env exp origOut newOut expectedEnv expectedExpStr =
gather <| updateAssert_ True env exp origOut newOut expectedEnv expectedExpStr
evalElmAssert_: List (String, String) -> String -> String -> State -> State
evalElmAssert_ envStr expStr expectedResStr state =
if state.ignore then state else
case Utils.projOk [parseEnv envStr] of
Err error -> fail state <| log state <| "Error while parsing environments: " ++ error
Ok [env] ->
case Utils.projOk [parse expStr, parse expectedResStr] of
Err error -> fail state <| log state <| "Error while parsing expressions or outputs: " ++ error
Ok [exp, res] ->
--let _ = Debug.log (log state <| toString exp) () in
case Utils.projOk [evalEnv env exp, eval res] of
Err error -> fail state <| log state <| "Error while evaluating the expression or the expected result: " ++ unparse exp ++ "," ++ unparse res ++ ": " ++ error
Ok [out, expectedOut] -> assertEqualVal_ out expectedOut state
Ok _ -> fail state "???"
Ok _ -> fail state "???"
Ok _ -> fail state "???"
evalElmAssert envStr expStr expectedResStr = gather <| evalElmAssert_ envStr expStr expectedResStr
evalElmAssert2_: Env -> String -> String -> State -> State
evalElmAssert2_ env expStr expectedResStr state =
if state.ignore then state else
case Utils.projOk [parse expStr, parse expectedResStr] of
Err error -> fail state <| log state <| "Error while parsing expressions or outputs: " ++ error
Ok [exp, res] ->
--let _ = Debug.log (log state <| toString exp) () in
case Utils.projOk [evalEnv env exp, eval res] of
Err error -> fail state <| log state <| "Error while evaluating the expression or the expected result: " ++ unparse exp ++ "," ++ unparse res ++ ": " ++ error
Ok [out, expectedOut] -> assertEqualVal_ out expectedOut state
Ok _ -> fail state "???"
Ok _ -> fail state "???"
evalElmAssert2 envStr expStr expectedResStr = gather <| evalElmAssert2_ envStr expStr expectedResStr
updateElmAssert_: List (String, String) -> String -> String -> List (String, String) -> String -> State -> State
updateElmAssert_ envStr expStr newOutStr expectedEnvStr expectedExpStr state =
if state.ignore then state else
case Utils.projOk [parseEnv envStr, parseEnv expectedEnvStr] of
Err error -> fail state <| log state <| "Error while parsing environments: " ++ error
Ok [env, expectedEnv] ->
case Utils.projOk [parse expStr, parse newOutStr] of
Err error -> fail state <| log state <| "Error while parsing expressions or outputs: " ++ error
Ok [exp, newOut] ->
--let _ = Debug.log (log state <| toString exp) () in
case Utils.projOk [evalEnv env exp, eval newOut] of
Err error ->
let _ = Debug.log (toString exp) () in
fail state <| log state <| "Error while evaluating the expression or the output: " ++ Syntax.unparser Syntax.Elm exp ++ " <- " ++ Syntax.unparser Syntax.Elm newOut ++ ": " ++ error
Ok [out, newOut] -> updateAssert_ True env exp out newOut expectedEnv expectedExpStr state
Ok _ -> fail state "???"
Ok _ -> fail state "???"
Ok _ -> fail state "???"
updateElmAssert envStr expStr newOutStr expectedEnvStr expectedExpStr = gather <| updateElmAssert_ envStr expStr newOutStr expectedEnvStr expectedExpStr
updateElmAssert2_: Env -> String -> String -> String -> State -> State
updateElmAssert2_ env expStr newOutStr expectedExpStr state =
if state.ignore then state else
case Utils.projOk [parse expStr, parse newOutStr] of
Err error -> fail state <| log state <| "Error while parsing expressions or outputs: " ++ error
Ok [exp, newOut] ->
--let _ = Debug.log (log state <| toString exp) () in
case Utils.projOk [evalEnv env exp, eval newOut] of
Err error -> fail state <| log state <| "Error while evaluating the expression or the output: " ++ Syntax.unparser Syntax.Elm exp ++ "," ++ Syntax.unparser Syntax.Elm newOut ++ ": " ++ error
Ok [out, newOut] -> updateAssert_ True env exp out newOut env expectedExpStr state
Ok _ -> fail state "???"
Ok _ -> fail state "???"
updateElmAssert2 env expStr newOutStr expectedExpStr = gather <| updateElmAssert2_ env expStr newOutStr expectedExpStr
updateElmPrelude_: String -> (String -> String) -> (String -> String) -> State -> State
updateElmPrelude_ expStr outReplacer expectedExpReplacer state =
if state.ignore then state else
case parse expStr of
Err error -> fail state <| log state <| "Error while parsing expressions or outputs: " ++ error
Ok exp ->
case evalEnv EvalUpdate.preludeEnv exp of
Err error -> fail state <| log state <| "Error while evaluating the expression: " ++ Syntax.unparser Syntax.Elm exp ++ ": " ++ error
Ok oldOut ->
case oldOut |> valToString |> outReplacer |> parse |> Result.andThen eval of
Err error -> fail state <| log state <| "Error while parsing expressions or outputs: " ++ error
Ok newOut ->
let expectedExpStr = expectedExpReplacer expStr in
updateAssert_ False EvalUpdate.preludeEnv exp oldOut newOut EvalUpdate.preludeEnv expectedExpStr state
updateElmPrelude expStr outReplacer expectedExpReplacer = gather <| updateElmPrelude_ expStr outReplacer expectedExpReplacer
replaceStr before after = Regex.replace Regex.All (Regex.regex before) (\_ -> after)
parse = Syntax.parser Syntax.Elm >> Result.mapError (\p -> ParserUtils.showError p)
unparse = Syntax.unparser Syntax.Elm
evalEnv env exp = Eval.doEval Syntax.Elm env exp |> Result.map (Tuple.first >> Tuple.first)
eval exp = Eval.doEval Syntax.Elm [] exp |> Result.map (Tuple.first >> Tuple.first)
parseEnv: List (String, String) -> Result String Env
parseEnv envStr =
envStr
|> List.map (\(name, exp) ->
parse exp
|> Result.mapError (\pError -> toString pError)
|> Result.andThen (\x ->
evalEnv [] x
|> Result.map (\x -> (name, x))))
|> Utils.projOk
|> Result.mapError (\pError -> toString pError)
dws = ws " "
dws2 = ws " "
dws1 = ws " "
dws3 = ws " "
dws4 = ws " "
dws5 = ws " "
dws6 = ws " "
val x = Val x (Provenance [] (tConst (ws "") 0) []) (Parents [])
tVal n = val <| (VConst Nothing (n, dummyTrace))
tVClosure maybeIndent pats body env = val <| VClosure maybeIndent pats body env
tVList vals = val <| VList vals
tVBool truth = val <| VBase (VBool truth)
tVStr chars = val <| VBase (VString chars)
tConst ws num = withDummyExpInfo <| EConst ws num dummyLoc noWidgetDecl
tBool space truth = withDummyExpInfo <| EBase space (EBool truth)
tString space chars = withDummyExpInfo <| EBase space (EString defaultQuoteChar chars)
tVar space name =withDummyExpInfo <| EVar space name
tFun sp0 pats body sp1 = (withDummyExpInfo <| EFun sp0 pats body sp1)
tApp sp0 fun args sp1 = withDummyExpInfo <| EApp sp0 fun args SpaceApp sp1
tList sp0 exps sp1 = withDummyExpInfo <| EList sp0 (List.map (\e -> (ws "", e)) exps) (ws "") Nothing sp1
--tListCons sp0 exps sp1 tail sp2 = EList sp0 exps sp1 (Just tail) sp2
tPVar space name = withDummyPatInfo <| PVar space name noWidgetDecl
tPAs sp0 name sp1 pat= withDummyPatInfo <| PAs sp0 name sp1 pat
tPList sp0 listPat sp1= withDummyPatInfo <| PList sp0 listPat (ws "") Nothing sp1
tPListCons sp0 listPat sp1 tailPat sp2 = withDummyPatInfo <| PList sp0 listPat sp1 (Just tailPat) sp1
onlySpecific = False
display_prelude_message = case ElmParser.preludeNotParsed of
Nothing -> ()
Just msg -> Debug.log msg ()
all_tests = init_state
|> ignore onlySpecific
|> test "triCombineTest"
|> delay (\() -> assertEqual
(mergeEnv
[("y", (tVal 2)), ("x", (tVal 1))]
[("y", (tVal 2)), ("x", (tVal 1))] []
[("y", (tVal 2)), ("x", (tVal 3))] [(1, VConstDiffs)]
) ([("y", (tVal 2)), ("x", (tVal 3))], [(1, VConstDiffs)]))
|> delay (\() -> assertEqual
(mergeEnv
[("x", (tVal 1)), ("y", (tVal 1)), ("z", (tVal 1))]
[("x", (tVal 1)), ("y", (tVal 2)), ("z", (tVal 2))] [(1, VConstDiffs), (2, VConstDiffs)]
[("x", (tVal 3)), ("y", (tVal 1)), ("z", (tVal 3))] [(0, VConstDiffs), (2, VConstDiffs)]
) ([("x", (tVal 3)), ("y", (tVal 2)), ("z", (tVal 3))], [(0, VConstDiffs), (1, VConstDiffs), (2, VConstDiffs)]))
|> test "Minus sign as binary or unary operator"
|> evalElmAssert [] "1-1" "0"
|> evalElmAssert [] "(\\x->x) -1" "-1"
|> test "update mutual recursion"
|> updateElmAssert [] "x =y\ny= 1\nx" "2"
[] "x =y\ny= 2\nx"
|> updateElmAssert [] "a = g 85\nf x = g (x - 17)\ng y = if y < 17 then y else f y\na" "2"
[] "a = g 87\nf x = g (x - 17)\ng y = if y < 17 then y else f y\na"
|> updateElmAssert [] "a = g 85\nf x = g (x - 17)\ng y = if y < 17 then y else f y\na" "2"
[] "a = g 85\nf x = g (x - 15)\ng y = if y < 17 then y else f y\na"
|> test "update const"
|> updateElmAssert [] " 1" "2"
[] " 2"
|> test "update boolean and strings"
|> updateElmAssert [] " True" "False"
[] " False"
|> updateElmAssert [] " 'Hello'" "'World'"
[] " 'World'"
|> test "update var"
|> updateElmAssert [("x", "1")] "x" "2"
[("x", "2")] "x"
|> updateElmAssert [("x", "1"), ("y", "3")] " x" "2"
[("x", "2"), ("y", "3")] " x"
|> updateElmAssert [("y", "3"), ("x", "1")] " x" "2"
[("y", "3"), ("x", "2")] " x"
|> test "update fun"
|> updateElmAssert [] "\\ x -> 1" "\\ x -> 2"
[] "\\ x -> 2"
|> test "update fun with 2 arguments"
|> updateElmAssert [] "\\x y -> 1" "\\y x -> 2"
[] "\\y x -> 2"
|> test "update nested fun with 2 and 1 arguments"
|> updateElmAssert [] "\\x y -> \\z -> 1" "\\y z -> \\x -> 3"
[] "\\y z -> \\x -> 3"
|> test "update app identity"
|> updateElmAssert [] "(\\x -> x) 1" "2"
[] "(\\x -> x) 2"
|> test "update app constant"
|> updateElmAssert [] "(\\x -> 1) 3" "2"
[] "(\\x -> 2) 3"
|> test "update pattern 'as' (\\(x as y) -> x [or y]) 1"
|> updateElmAssert [] "(\\x as y -> x) 1" "2"
[] "(\\x as y -> x) 2"
|> updateElmAssert [] "(\\(x as y) -> y) 1" "2"
[] "(\\(x as y) -> y) 2"
|> test "update pattern list (\\[x, y] -> x or y) [1, 2]"
|> updateElmAssert [] "(\\[x, y] -> x) [1, 2]" "3"
[] "(\\[x, y] -> x) [3, 2]"
|> updateElmAssert [] "(\\[x, y] -> y) [1, 2]" "3"
[] "(\\[x, y] -> y) [1, 3]"
|> test "update pattern list with tail (\\[x | [y]] -> x or y) [1, 2]"
|> updateElmAssert [] "(\\x :: y -> x) [1, 2]" "3"
[] "(\\x :: y -> x) [3, 2]"
|> updateElmAssert [] "(\\x :: [y] -> y) [1, 2]" "3"
[] "(\\x :: [y] -> y) [1, 3]"
|> test "update app (\\x y -> x) 1 2"
|> updateElmAssert [] "(\\x y -> x) 1 2" "3"
[] "(\\x y -> x) 3 2"
|> test "update app (\\x y -> y) 1 2"
|> updateElmAssert
[] "(\\x y -> y) 1 2" "3"
[] "(\\x y -> y) 1 3"
|> test "update if-then-else"
|> updateElmAssert
[] "if True then 1 else 2" "3"
[] "if True then 3 else 2"
|> updateElmAssert
[] "if False then 1 else 2" "3"
[] "if False then 1 else 3"
|> test "String concatenation"
|> updateElmAssert [] "'Hello' + 'world'" "'Hello world'"
[] "'Hello ' + 'world'"
|> test "update arithmetic operations"
|> updateElmAssert
[("x", "1"), ("y", "2")] " x+ y" "4"
[("x", "2"), ("y", "2")] " x+ y"
|> updateElmAssert
[("x", "1"), ("y", "2")] " x+ y" "4"
[("x", "1"), ("y", "3")] " x+ y"
|> updateElmAssert
[("x", "5"), ("y", "2")] " x- y" "1"
[("x", "3"), ("y", "2")] " x- y"
|> updateElmAssert
[("x", "2"), ("y", "3")] " x* y" "8"
[("x", "2"), ("y", "4")] " x* y"
|> updateElmAssert
[("x", "18"), ("y", "3")] " x/ y" "7"
[("x", "21"), ("y", "3")] " x/ y"
|> updateElmAssert
[("x", "3"), ("y", "4")] " x ^ y" "16"
[("x", "2"), ("y", "4")] " x ^ y"
|> updateElmAssert
[("x", "-1"), ("y", "3")] " x ^ y" "1"
[("x", "1"), ("y", "3")] " x ^ y"
|> updateElmAssert
[("x", "-2"), ("y", "3")] " x ^ y" "27"
[("x", "3"), ("y", "3")] " x ^ y"
|> updateElmAssert
[("x", "-1"), ("y", "0")] "x ^ y" "-1"
[("x", "-1"), ("y", "1")] "x ^ y"
|> updateElmAssert
[("x", "-1"), ("y", "3")] " x ^ y" "-8"
[("x", "-2"), ("y", "3")] " x ^ y"
|> updateElmAssert
[("x", "17"), ("y", "8")] " mod x y" "3"
[("x", "19"), ("y", "8")] " mod x y"
|> updateElmAssert
[("x", "1"), ("y", "0")] "arctan2 y x" "1.5707963267948966"
[("x", "6.123233995736766e-17"), ("y", "1")] "arctan2 y x"
|> updateElmAssert
[("x", "1"), ("y", "0")] "arctan2 y x" "3.141592653589793"
[("x", "-1"), ("y", "1.2246467991473532e-16")] "arctan2 y x"
|> updateElmAssert
[("x", "1"), ("y", "0")] "arctan2 y x" "-1.5707963267948966"
[("x", "6.123233995736766e-17"), ("y", "-1")] "arctan2 y x"
|> updateElmAssert
[("x", "0.1")] "cos x" "0"
[("x", "1.570796326794897")] "cos x"
|> updateElmAssert
[("x", "-0.1")] "cos x" "0"
[("x", "-1.570796326794897")] "cos x"
|> updateElmAssert
[("x", "0.1")] "cos x" "0"
[("x", "1.570796326794897")] "cos x"
|> updateElmAssert
[("x", "0.1")] "sin x" "1"
[("x", "1.570796326794897")] "sin x"
|> updateElmAssert
[("x", "-0.1")] "sin x" "-1"
[("x", "-1.570796326794897")] "sin x"
|> updateElmAssert
[("x", "0")] "arccos x" "0"
[("x", "1")] "arccos x"
|> updateElmAssert
[("x", "0")] "arcsin x" "1.5707963267948966"
[("x", "1")] "arcsin x"
|> updateElmAssert
[("x", "17.5")] "floor x" "15"
[("x", "15.5")] "floor x"
|> updateElmAssert
[("x", "17.5")] "ceiling x" "15"
[("x", "14.5")] "ceiling x"
|> updateElmAssert
[("x", "17.75")] "round x" "15"
[("x", "14.75")] "round x"
|> updateElmAssert
[("x", "17.25")] "round x" "15"
[("x", "15.25")] "round x"
|> updateElmAssert
[("x", "16")] "sqrt x" "3"
[("x", "9")] "sqrt x"
|> test "case of calls"
|> updateElmAssert
[("x", "[7, 1]")] "case x of\n [a, b] -> a + b\n u -> 0" "5"
[("x", "[4, 1]")] "case x of\n [a, b] -> a + b\n u -> 0"
|> updateElmAssert
[("x", "[7]")] "case x of\n [a, b] -> a + b\n u -> 0" "5"
[("x", "[7]")] "case x of\n [a, b] -> a + b\n u -> 5"
|> test "non-rec let"
|> updateElmAssert
[] "let x= 5 in\nlet y =2 in [x, y]" "[6, 3]"
[] "let x= 6 in\nlet y =3 in [x, y]"
|> test "list constructor"
|> updateElmAssert
[] "let x= 1 in\nlet y =[2] in x :: x :: y" "[3, 1, 2]"
[] "let x= 3 in\nlet y =[2] in x :: x :: y"
|> updateElmAssert
[] "let x= 1 in\nlet y =2 in [x, x, y]" "[3, 1, 2]"
[] "let x= 3 in\nlet y =2 in [x, x, y]"
|> updateElmAssert
[] "let x= 1 in\nlet y =2 in [x, x, y]" "[1, 3, 2]"
[] "let x= 3 in\nlet y =2 in [x, x, y]"
|> updateElmAssert
[] "let x= 1 in\nlet y =2 in [x, x, y]" "[1, 1, 3]"
[] "let x= 1 in\nlet y =3 in [x, x, y]"
|> updateElmAssert
[] "let x= 1 in\nlet y =[2] in x :: x :: y" "[1, 3, 2]"
[] "let x= 3 in\nlet y =[2] in x :: x :: y"
|> updateElmAssert
[] "let x= 1 in\nlet y =[2] in x :: x :: y" "[1, 1, 3]"
[] "let x= 1 in\nlet y =[3] in x :: x :: y"
|> test "rec let"
|> updateElmAssert
[] "let f x = if x == 0 then x else (f (x - 1)) in\n f 2" "3"
[] "let f x = if x == 0 then x else (f (x - 1)) in\n f 5"
|> test "Comments"
|> updateElmAssert
[] "--This is a comment\n 1" "2"
[] "--This is a comment\n 2"
|> test "Strings"
|> updateElmAssert
[] " \"This is a string\"" "\"Hello world\""
[] " \"Hello world\""
|> updateElmAssert
[] " \"This is a string\"" "\"Hello' \\\" wo\\\\rld\""
[] " \"Hello' \\\" wo\\\\rld\""
|> updateElmAssert
[] " 'This is a string'" "\"Hello world\""
[] " 'Hello world'"
|> updateElmAssert
[] " 'This is a string'" "\"Hello' \\\" wo\\\\rld\""
[] " 'Hello\\' \" wo\\\\rld'"
|> updateElmAssert
[] " 'Welcome to this world'" "\"Welcome again to this world\""
[] " 'Welcome again to this world'"
|> test "Strings concatenation"
|> updateElmAssert [] "(\"Hello\" + \" \") + \"world\"" "\"Good morning world\""
[] "(\"Good morning\" + \" \") + \"world\""
|> test "Many solutions"
|> updateElmAssert
[("h3", "\\text -> ['h3', [], [['TEXT', text]]]")] "x = 0 + 0\n\nh3 (toString x)" "['h3', [], [['TEXT', '1']]]"
[("h3", "\\text -> ['h3', [], [['TEXT', text]]]")] "x = 0 + 1\n\nh3 (toString x)"
|> updateElmAssert
[("h3", "\\text -> ['h3', [], [['TEXT', text]]]")] "x = 0 + 0\n\nh3 (toString x)" "['h3', [], [['TEXT', '1']]]"
[("h3", "\\text -> ['h3', [], [['TEXT', text]]]")] "x = 1 + 0\n\nh3 (toString x)"
|> test "Multiline string literals"
|> updateElmAssert
[] "\"\"\"Hello @(if 1 == 2 then \"big\" else \"\"\"very @(\"small\")\"\"\") world\"\"\"" "\"Hello very tiny world\""
[] "\"\"\"Hello @(if 1 == 2 then \"big\" else \"\"\"very @(\"tiny\")\"\"\") world\"\"\""
|> updateElmAssert
[] "let x = \"Hello\" in \"\"\"@x world\"\"\"" "\"Hello big world\""
[] "let x = \"Hello\" in \"\"\"@x big world\"\"\""
|> updateElmAssert
[] "let x = \"Hello\" in \"\"\"@x world\"\"\"" "\"Hello big world\""
[] "let x = \"Hello big\" in \"\"\"@x world\"\"\""
|> updateElmAssert
[] "let x = \"Hello\" in \"\"\"@x world\"\"\"" "\"Helloworld\""
[] "let x = \"Hello\" in \"\"\"@(x)world\"\"\""
|> updateElmAssert
[] "\"\"\"@let x = \"Hello\" in\n@x world\"\"\"" "\"Hello big world\""
[] "\"\"\"@let x = \"Hello big\" in\n@x world\"\"\""
|> updateElmAssert
[] "\"\"\"@let x = \"Hello\" in\n@x world\"\"\"" "\"Hello big world\""
[] "\"\"\"@let x = \"Hello\" in\n@x big world\"\"\""
|> updateElmAssert
[] "\"\"\"@let x = (\"Hello\" + \n \" big\") in\n@x world\"\"\"" "\"Hello tall world\""
[] "\"\"\"@let x = (\"Hello\" + \n \" tall\") in\n@x world\"\"\""
|> test "Finding all regex matches"
|> assertEqual (LazyList.toList <| allInterleavingsIn ["A", "BB", "C"] "xAyBBBzAoBBpCC")
[ ["x","y","BzAoBBp","C"]
, ["x","y","BzAoBBpC",""]
, ["x", "yB", "zAoBBp","C"]
, ["x", "yB", "zAoBBpC",""]
, ["x", "yBBBzAo", "p","C"]
, ["x", "yBBBzAo", "pC",""]
, ["xAyBBBz", "o", "p","C"]
, ["xAyBBBz", "o", "pC",""]
]
|> test "GroupStartMap"
|> assertEqual (List.map (\{submatches} -> submatches) (GroupStartMap.find Regex.All "a((bc)|cd)d" "aabcdacdd"))
[[{match = Just "bc", start = 2}, {match = Just "bc", start = 2}], [{match = Just "cd", start = 6}, {match = Nothing, start = -1}]]
|> assertEqual (GroupStartMap.replace Regex.All "a((bc)|cd)d" (
\match -> String.concat <| List.map (\subm -> toString subm.start ++ Maybe.withDefault "null" subm.match) match.submatches
) "aabcdacdd")
"a2bc2bc6cd-1null"
|> test "replaceAllIn"
|> evalElmAssert2 builtinEnv "replaceAllIn \"l\" \"L\" \"Hello world\"" "\"HeLLo worLd\""
|> evalElmAssert2 builtinEnv "replaceAllIn \"a(b|c)\" \"o$1\" \"This is acknowledgeable\"" "\"This is ocknowledgeoble\""
|> evalElmAssert2 builtinEnv "replaceAllIn \"a(b|c)\" (\\{group = [t, c]} -> \"oa\" + (if c == \"b\" then \"c\" else \"b\")) \"This is acknowledgeable\"" "\"This is oabknowledgeoacle\""
|> updateElmAssert2 builtinEnv "replaceAllIn \"e\" \"ee\" \"\"\"See some examples from File...\"\"\"" "\"Seeee somee emexamplees from Filee...\""
"replaceAllIn \"e\" \"eme\" \"\"\"See some examples from File...\"\"\""
|> updateElmAssert2 builtinEnv "[ 'div'\n , []\n , [ ['h2', [], [['TEXT', 'Welcome to Sketch-n-Sketch Docs!']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', 'Type something here...']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', replaceAllIn \"e\" \"ee\" \"\"\"\n See some examples from File -> New From Template in\n the menu bar, or by pressing the Previous and Next\n buttons in the top-right corner.\n \"\"\"]]]\n ]\n ]"
"[ 'div'\n , []\n , [ ['h2', [], [['TEXT', 'Welcome to Sketch-n-Sketch Docs!']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', 'Type something here...']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', \"\"\"\n Seeee somee eecxamplees from Filee -> Neew From Teemplatee in\n thee meenu bar, or by preessing thee Preevious and Neext\n buttons in thee top-right corneer.\n \"\"\"]]]\n ]\n ]"
"[ 'div'\n , []\n , [ ['h2', [], [['TEXT', 'Welcome to Sketch-n-Sketch Docs!']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', 'Type something here...']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', replaceAllIn \"e\" \"eec\" \"\"\"\n See some examples from File -> New From Template in\n the menu bar, or by pressing the Previous and Next\n buttons in the top-right corner.\n \"\"\"]]]\n ]\n ]"
|> updateElmAssert2 builtinEnv "[ 'div'\n , []\n , [ ['h2', [], [['TEXT', 'Welcome to Sketch-n-Sketch Docs!']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', 'Type something here...']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', replaceAllIn \"e\" \"ee\" \"\"\"\n See some examples from File -> New From Template in\n the menu bar, or by pressing the Previous and Next\n buttons in the top-right corner.\n \"\"\"]]]\n ]\n ]"
"[ 'div'\n , []\n , [ ['h2', [], [['TEXT', 'Welcome to Sketch-n-Sketch Docs!']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', 'Type something here...']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', \"\"\"\n Seeee somee eecxamplees from Filee -> Neew From Teemplatee in\n thee meenu bar, or by preessing thee Preevious and Neext\n buttons in thee top-right corneer.\n \"\"\"]]]\n ]\n ]"
"[ 'div'\n , []\n , [ ['h2', [], [['TEXT', 'Welcome to Sketch-n-Sketch Docs!']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', 'Type something here...']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', replaceAllIn \"e\" \"ee\" \"\"\"\n See some ecxamples from File -> New From Template in\n the menu bar, or by pressing the Previous and Next\n buttons in the top-right corner.\n \"\"\"]]]\n ]\n ]"
|>
updateElmAssert [] "extractFirstIn \"^\\\\s*(S(\\\\w)+ (\\\\w))\" \"\"\" See some examples\"\"\" |> case of Just [big, s1, s2] -> big + s1 + s2; e -> \"not the right shape\"" "\"Sea ses\""
[] "extractFirstIn \"^\\\\s*(S(\\\\w)+ (\\\\w))\" \"\"\" Sea some examples\"\"\" |> case of Just [big, s1, s2] -> big + s1 + s2; e -> \"not the right shape\""
|> evalElmAssert [] "extractFirstIn \"\"\"([\\w:_-]*)\"\"\" \"data-array=\\\"17\\\"\" |> case of Just [id] -> id; _ -> \"Nothing\" " "\"data-array\""
|> evalElmAssert2 builtinEnv "replaceAllIn \"\\\\[([^\\\\[]+)\\\\]\\\\(([^\\\\)]+)\\\\)\" \"<a href='$2'>$1</a>\" \"[Markdown](http://test)\"" "\"<a href='http://test'>Markdown</a>\""
|> evalElmAssert2 builtinEnv "replaceAllIn \"\\\\[([^\\\\[]+)\\\\]\\\\(([^\\\\)]+)\\\\)\" \"<a href='$2'>$1</a>\" \"[Markdown](https://fr.wikipedia.org/wiki/Markdown)\"" "\"<a href='https://fr.wikipedia.org/wiki/Markdown'>Markdown</a>\""
|> updateElmAssert2 builtinEnv "replaceAllIn \"\\\\[([^\\\\[]+)\\\\]\\\\(([^\\\\)]+)\\\\)\" \"<a href='$2'>$1</a>\" \"#[Markdown](https://fr.wikipedia.org/wiki/Markdown)\"" "\"#<a href='https://fr.wikipedia.org/wiki/Markdown'>Markdoooown</a>\""
"replaceAllIn \"\\\\[([^\\\\[]+)\\\\]\\\\(([^\\\\)]+)\\\\)\" \"<a href='$2'>$1</a>\" \"#[Markdoooown](https://fr.wikipedia.org/wiki/Markdown)\""
|> updateElmAssert2 builtinEnv "replaceAllIn \"x|y\" (\\{match} -> if match == \"x\" then \"5\" else \"7\") \"x,y\"" "\"6,8\""
"replaceAllIn \"x|y\" (\\{match} -> if match == \"x\" then \"6\" else \"8\") \"x,y\""
--|> test "Record construction, extraction and pattern "
-- |>
|> test "Partial application"
|> updateElmAssert
[] "let map f l = case l of head::tail -> f head::map f tail; [] -> [] in let td color txt = [ 'td', [['style', ['border', 'padding', ['background-color', color]]]], [['TEXT', txt]]] in map (td 'green') ['hello', 'world']"
"[[ 'td', [['style', ['border', 'padding', ['background-color', 'red']]]], [['TEXT', 'hello']]], [ 'td', [['style', ['border', 'padding', ['background-color', 'green']]]], [['TEXT', 'world']]]]"
[] "let map f l = case l of head::tail -> f head::map f tail; [] -> [] in let td color txt = [ 'td', [['style', ['border', 'padding', ['background-color', color]]]], [['TEXT', txt]]] in map (td 'red') ['hello', 'world']"
--|> ignore False
|> test "Multiple solutions"
|> updateElmAssert
[] "['A' + 'B', 'C' + 'D']" "['AGB', 'CGD']"
[] "['AG' + 'B', 'CG' + 'D']"
|> test "Records"
|> updateElmAssert
[] "{ a= 1, b= 2}.b" "3"
[] "{ a= 1, b= 3}.b"
|> updateElmAssert
[] "{ a= 1, b= 2}.a" "3"
[] "{ a= 3, b= 2}.a"
|> updateElmAssert
[] "let x = { a= 1, b= 2} in { x | a = 2 }.a" "3"
[] "let x = { a= 1, b= 2} in { x | a = 3 }.a"
|> updateElmAssert
[] "let x = { a= 1, b= 2} in { x | a = 2 }.b" "3"
[] "let x = { a= 1, b= 3} in { x | a = 2 }.b"
|> test "Indented lists, second position of list > 1 elements"
|> updateElmAssert
[] "[1, 2, 3, 4]" "[1, 5, 2, 3, 4]"
[] "[1, 5, 2, 3, 4]"
|> updateElmAssert
[] "[ 1,\n 2,\n 3,\n 4]" "[1, 5, 2, 3, 4]"
[] "[ 1,\n 5,\n 2,\n 3,\n 4]"
|> updateElmAssert
[] "[ 1\n, 2\n, 3\n, 4]" "[1, 5, 2, 3, 4]"
[] "[ 1\n, 5\n, 2\n, 3\n, 4]"
|> updateElmAssert
[] " [ 1\n, 2\n, 3\n, 4]" "[1, 5, 2, 3, 4]"
[] " [ 1\n, 5\n, 2\n, 3\n, 4]"
|> test "Indented lists, second position of list with 1 element"
|> updateElmAssert
[] "[1]" "[1, 2]"
[] "[1, 2]"
|> updateElmAssert
[] "[ 1]" "[ 1, 2]"
[] "[ 1, 2]"
|> updateElmAssert
[] "[ 1\n]" "[ 1, 2]"
[] "[ 1\n, 2\n]"
|> updateElmAssert
[] "[\n 1]" "[ 1, 2]"
[] "[\n 1,\n 2]"
|> test "Indented lists, first position of list with 1 element"
|> updateElmAssert
[] "[1]" "[2, 1]"
[] "[2, 1]"
|> updateElmAssert
[] "[ 1]" "[2, 1]"
[] "[ 2, 1]"
|> updateElmAssert
[] "[ 1\n]" "[2, 1]"
[] "[ 2\n, 1\n]"
|> updateElmAssert
[] "[\n 1]" "[2, 1]"
[] "[\n 2,\n 1]"
|> test "Indented lists, first position of list > 1 element"
|> updateElmAssert
[] "[1, 2]" "[0, 1, 2]"
[] "[0, 1, 2]"
|> updateElmAssert
[] "[ 1, 2]" "[0, 1, 2]"
[] "[ 0, 1, 2]"
|> updateElmAssert
[] "[ 1\n, 2\n, 3\n, 4]" "[0, 1, 2, 3, 4]"
[] "[ 0\n, 1\n, 2\n, 3\n, 4]"
|> test "Indented lists, first position of empty list"
|> updateElmAssert
[] "[]" "[1]"
[] "[1]"
|> updateElmAssert
[] "[ ]" "[1]"
[] "[1 ]"
|> updateElmAssert
[] " []" "[[1, 2]]"
[] " [[1, 2]]"
|> test "parsing HTML"
|> updateElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)]
"parseHTML \"hello\"" "[HTMLInner \"hello world\"]"
"parseHTML \"hello world\""
|> updateElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)]
"parseHTML \"hello<br>world\"" "[HTMLInner \"hello\", HTMLElement \"br\" [] \" \" RegularEndOpening [] VoidClosing, HTMLInner \"sworld\"]"
"parseHTML \"hello<br >sworld\""
|> updateElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)]
"parseHTML \"<?help>\"" "[HTMLComment (Less_Greater \"Injection: adding more chars like >, <, and -->\")]"
"parseHTML \"<!--Injection: adding more chars like >, <, and ~~>-->\""
|> evalElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)]
"parseHTML \"<h3>Hello world</h3>\"" "[HTMLElement \"h3\" [] \"\" RegularEndOpening [HTMLInner \"Hello world\"] (RegularClosing \"\")]"
|> evalElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)]
"parseHTML \"<a href='https://fr.wikipedia.org/wiki/Markdown'>Markdown</a>\"" "[HTMLElement \"a\" [HTMLAttribute \" \" \"href\" (HTMLAttributeString \"\" \"\" \"'\" \"https://fr.wikipedia.org/wiki/Markdown\")] \"\" RegularEndOpening [HTMLInner \"Markdown\"] (RegularClosing \"\")]"
|> updateElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)]
"parseHTML \"<a href='https://fr.wikipedia.org/wiki/Markdown'>Markdown</a> demo\""
"[HTMLElement \"a\" [HTMLAttribute \" \" \"href\" (HTMLAttributeString \"\" \"\" \"'\" \"https://fr.wikipedia.org/wiki/Markdown\")] \"\" RegularEndOpening [HTMLInner \"Markdown\"] (RegularClosing \"\"), HTMLInner \" demonstration\"]"
"parseHTML \"<a href='https://fr.wikipedia.org/wiki/Markdown'>Markdown</a> demonstration\""
|> evalElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)] "parseHTML \"Hello world\"" "[HTMLInner \"Hello world\"]"
|> evalElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)] "parseHTML \"<i><b>Hello</i></b>World\"" "[ HTMLElement \"i\" [] \"\" RegularEndOpening [ HTMLElement \"b\" [] \"\" RegularEndOpening [ HTMLInner \"Hello\"] ForgotClosing] (RegularClosing \"\"), HTMLInner \"</b>World\"]"
|> updateElmAssert [("color", "\"white\""), ("padding", "[\"padding\", \"3px\"]")] "[padding, [\"background-color\", color]]"
"[[\"padding\", \"3px\"], [ \"background-color\", \"lightgray\"]]"
[("color", "\"lightgray\""), ("padding", "[\"padding\", \"3px\"]")] "[padding, [\"background-color\", color]]"
|> test "freeze"
|> updateElmAssert [("freeze", "\\x -> x")] "freeze 0 + 1" "2"
[("freeze", "\\x -> x")] "freeze 0 + 2"
|> updateElmAssert [("freezeExpression", "\\x -> x")] "let x = 1 in freezeExpression (0 + x)" "2"
[("freezeExpression", "\\x -> x")] "let x = 2 in freezeExpression (0 + x)"
|> test "dictionary"
|> updateElmAssert [("x", "1")] "__DictGet__ \"a\" (__DictFromList__ [(\"a\", x)])" "Just 2"
[("x", "2")] "__DictGet__ \"a\" (__DictFromList__ [(\"a\", x)])"
|> updateElmAssert [("x", "1")] "__DictRemove__ \"b\" (__DictFromList__ [(\"a\", x), (\"b\", 2)])" "__DictFromList__ [(\"a\", 2)]"
[("x", "2")] "__DictRemove__ \"b\" (__DictFromList__ [(\"a\", x), (\"b\", 2)])"
|> updateElmAssert [("x", "1")] "__DictInsert__ \"b\" x (__DictFromList__ [(\"a\", x)])" "__DictFromList__ [(\"a\", 1), (\"b\", 2)]"
[("x", "2")] "__DictInsert__ \"b\" x (__DictFromList__ [(\"a\", x)])"
|> updateElmAssert [] "__DictInsert__ \"b\" 1 (__DictFromList__ [(\"a\", 2), (\"b\", 3)])" "__DictFromList__ [(\"a\", 1), (\"b\", 2)]"
[] "__DictInsert__ \"b\" 2 (__DictFromList__ [(\"a\", 1), (\"b\", 3)])"
|> test "recursive delayed"
|> updateElmAssert [] "f =\n let x = 1 in\n \\y -> x + y\n\nf 2" "4"
[] "f =\n let x = 2 in\n \\y -> x + y\n\nf 2"
|> updateElmAssert [] "f =\n let x = 1 in\n \\y -> x + y\n\nf 2" "4"
[] "f =\n let x = 1 in\n \\y -> x + y\n\nf 3"
|> test "All diffs"
|> assertEqual (allStringDiffs "abcabcabc" "abxabcabc" |> Results.toList)
[[DiffEqual "ab", DiffRemoved "c", DiffAdded "x", DiffEqual "abcabc"]]
|> assertEqual (allStringDiffs "ab3ab" "abab" |> Results.toList)
[[DiffEqual "ab", DiffRemoved "3", DiffEqual "ab"]]
|> assertEqual (allStringDiffs "aa3aa" "aa" |> Results.toList)
[[DiffEqual "aa", DiffRemoved "3aa"],
[DiffRemoved "aa3", DiffEqual "aa"]]
|> assertEqual (alldiffs identity ["a", "b", "3", "a", "b"] ["a", "b", "a", "b"] |> Results.toList)
[[DiffEqual ["a", "b"], DiffRemoved ["3"], DiffEqual ["a", "b"]]]
|> assertEqual (alldiffs identity ["a", "a", "3", "a", "a"] ["a", "a"] |> Results.toList)
[[DiffEqual ["a", "a"], DiffRemoved ["3", "a", "a"]],
[DiffRemoved ["a", "a", "3"], DiffEqual ["a", "a"]]]
|> test "Type parsing"
|> evalElmAssert [] "type List a = Nil | Cons a\n2" "2"
|> evalElmAssert [] "let type List a = Nil | Cons a in 2" "2"
|> evalElmAssert [] "let type List a = Nil | Cons a\nin 2" "2"
|> evalElmAssert [] "let\n type List a = Nil | Cons a\nin 2" "2"
--|> skipBefore
|> test "updateReplace"
-- newStart newEnd ... repStart repEnd
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"ab\" \"abc\" \"12ab567ab90\" (VStringDiffs [StringUpdate 0 1 2])"
"(\"12abc567abc90\", VStringDiffs [StringUpdate 0 1 2, StringUpdate 1 3 3, StringUpdate 6 8 3])"
-- repStart repEnd ... newStart newEnd
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"ab\" \"abc\" \"12ab567ab90\" (VStringDiffs [StringUpdate 5 5 1])"
"(\"12abc567abc90\", VStringDiffs [StringUpdate 2 4 3, StringUpdate 5 5 1, StringUpdate 6 8 3])"
-- newStart repStart repEnd ... newEnd
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"ab\" \"abc\" \"12ab567ab90\" (VStringDiffs [StringUpdate 1 2 4])"
"(\"12abc567abc90\", VStringDiffs [StringUpdate 1 2 5, StringUpdate 4 6 3])"
-- newStart repStart newEnd ... repEnd
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"ab\" \"abc\" \"12ab567ab90\" (VStringDiffs [StringUpdate 1 7 2])"
"(\"12abc567abc90\", VStringDiffs [StringUpdate 1 8 4, StringUpdate 11 13 3])"
-- repStart newStart newEnd ... repEnd
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"abc\" \"abcd\" \"12abc567abc90\" (VStringDiffs [StringUpdate 3 9 1])"
"(\"12abcd567abcd90\", VStringDiffs [StringUpdate 2 10 4, StringUpdate 13 16 4])"
-- repStart newStart repEnd ... newEnd
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"ab\" \"abc\" \"12ab567ab90\" (VStringDiffs [StringUpdate 3 7 2])"
"(\"12abc567abc90\", VStringDiffs [StringUpdate 2 7 4, StringUpdate 9 11 3])"
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"\"\"(<(ul|ol)>(?:(?!</\\2>)[\\s\\S])*)</li>\\s*<li>\"\"\" \"$1</li>\\n</$2>\\n<$2>\\n\\t<li>\" \"<ul><li>a</li><li>b</li></ul>\" (VStringDiffs [StringUpdate 14 14 10])"
"(\"<ul><li>a</li>\\n</ul>\\n<ul>\\n\\t<li>b</li></ul>\", VStringDiffs [StringUpdate 0 14 37])"
-- Add the test <i>Hello <b>world</span></i> --> <i>Hello <b>world</b></i> (make sure to capture all closing tags)
|> updateElmAssert2 builtinEnv "replaceAllIn \"\\\\$(\\\\w+|\\\\$)\" (\\m -> m.match) \"printer\"" "\"$translation1\""
"replaceAllIn \"\\\\$(\\\\w+|\\\\$)\" (\\m -> m.match) \"$translation1\""
|> test "imperative-like assignments"
|> updateElmAssert [] "a = \"Hello \"\na = a + \"world\"\na" "\"Hella world\""
[] "a = \"<NAME> \"\na = a + \"world\"\na"
--|> onlyBefore
|> updateElmPrelude (
ExamplesGenerated.mapMaybeLens
|> replaceStr "(\r?\n)+$" "" -- Remove newlines at the end
|> replaceStr "showValues \\[maybeRowA, maybeRowB, maybeRow1, maybeRow2\\]" "showValues [maybeRow1, maybeRow2]"
|> replaceStr "\r?\nmaybeRow(A|B).*" ""
) (replaceStr "New Jersey" "New Jersay")
(replaceStr "New Jersey" "New Jersay")
|> summary
| true | module UpdateTests exposing (..)
import Helpers.Matchers exposing (..)
import Update exposing (..)
import UpdateRegex exposing (..)
import UpdateStack exposing (..)
import UpdateUtils exposing (..)
import Lang exposing (..)
import GroupStartMap exposing (..)
import Regex
import Utils
import Eval
import Syntax
import Lazy
import Results exposing (Results)
import LazyList
import LangUtils exposing (..)
import ParserUtils
import HTMLValParser
import Set
import ImpureGoodies
import EvalUpdate exposing (builtinEnv)
import ExamplesGenerated
import ElmParser
type StateChanger = StateChanger (State -> State)
type alias State = { numTests: Int, nthAssertion: Int, numSuccess: Int, numFailed: Int, currentName: String, errors: String, ignore: Bool, toLaunch: List StateChanger, onlyOnly: Bool }
init_state = State 0 0 0 0 "" "" False [] False
-- Metacommands to gather states without executing them
delay: (() -> State -> State) -> State -> State
delay thetest state =
if state.ignore then state else
thetest () state
only: (State -> State) -> State -> State
only stateChanger state =
let newState = stateChanger { state | toLaunch = [], onlyOnly = False} in
let newState2 =flush newState in
{newState2 | onlyOnly = True, toLaunch = []} -- wipe out other tests not launched
onlyLast: State -> State
onlyLast state =
case Utils.maybeLast state.toLaunch of
Just (StateChanger stateChanger) ->
only stateChanger state
_ -> Debug.crash "No tests to only test before the use of 'onlyLast'. Add '|> onlyLast' after a test you want to run alone"
onlyAfter = skipBefore
skipBefore: State -> State
skipBefore state = { state | toLaunch = [] }
onlyBefore: State -> State
onlyBefore =
flush >> ignore True
gather: (State -> State) -> State -> State
gather stateChanger state =
{ state | toLaunch = state.toLaunch ++ [StateChanger stateChanger] }
flush: State -> State
flush state =
let defaultState = {state | toLaunch = [] } in
if state.onlyOnly then defaultState else
List.foldl (\(StateChanger sc) s -> sc s) defaultState state.toLaunch
summary: State -> String
summary state_ =
let state = flush state_ in
Debug.log (state.errors ++ "\n-------------------\n "++toString state.numSuccess ++"/" ++ toString state.numTests++ " tests passed\n-------------------") "ok"
test_: String -> State -> State
test_ name state =
let _ = Debug.log name " [Start]" in
{state | nthAssertion = 1, currentName = name} -- Debug.log name "all tests passed"
test s = gather <| test_ s
ignore: Bool -> State -> State
ignore b state =
{state | ignore = b}
success state = { state |
numTests = state.numTests + 1,
numSuccess = state.numSuccess + 1,
nthAssertion = state.nthAssertion + 1
}
fail state newError = { state |
numTests = state.numTests + 1,
numFailed = state.numFailed + 1,
nthAssertion = state.nthAssertion + 1,
errors = state.errors ++ "\n" ++ newError
}
log state msg =
"[" ++ state.currentName ++ ", assertion #" ++ toString state.nthAssertion ++ "] " ++ msg
nthEnv = [("nth", "letrec nth list index = case list of head::tail -> if index == 0 then head else nth tail (index - 1); [] -> null in nth")]
genericAssertEqual: (a -> String) -> (a -> a -> Bool) -> a -> a -> State -> State
genericAssertEqual eToString isEqual obtained expected state =
if state.ignore then state else
if isEqual obtained expected then success state else fail state <| "[" ++ state.currentName ++ ", assertion #" ++ toString state.nthAssertion ++ "] Expected \n" ++
eToString expected ++ ", got\n" ++ eToString obtained
assertEqual_: a -> a -> State -> State
assertEqual_ = genericAssertEqual (toString) (==)
assertEqual a b = gather <| assertEqual_ a b
assertEqualVal_: Val -> Val -> State -> State
assertEqualVal_ = genericAssertEqual valToString (\x y -> valToString x == valToString y)
assertEqualVal v1 v2 = gather <| assertEqualVal_ v1 v2
updateAssert_: Bool -> Env -> Exp -> Val -> Val -> Env -> String -> State -> State
updateAssert_ checkEnv env exp origOut newOut expectedEnv expectedExpStr state =
if state.ignore then state else
let expected = (if checkEnv then envToString expectedEnv else "") ++ " |- " ++ expectedExpStr in
let problemdesc = ("\nFor problem:" ++
(if checkEnv then envToString env else "") ++ " |- " ++ unparse exp ++ " <-- " ++ valToString newOut ++
" (was " ++ valToString origOut ++ ")") in
case UpdateUtils.defaultVDiffs origOut newOut of
Err msg -> fail state <| log state <| "This diff is not allowed:" ++ msg
Ok (LazyList.Nil) -> fail state <| "Internal error: no diffs"
Ok (LazyList.Cons Nothing _) -> fail state <| log state <| "There was no diff between the previous output and the new output"
Ok ll->
--let _ = ImpureGoodies.log <| "Diffs observed: " ++ toString (LazyList.toList ll) in
case Ok (LazyList.filterMap identity ll) |> Results.andThen (\diffs ->
update LazyList.Nil LazyList.Nil (updateContext "initial" env exp [] origOut newOut diffs)) of
Ok (LazyList.Cons (envX, expX) lazyTail as ll) ->
let _ = LazyList.toList ll in
--let _ = ImpureGoodies.log <| toString expX.changes in
--let _ = ImpureGoodies.log <| eDiffsToString "" exp expX.val (expX.changes |> Maybe.withDefault (EConstDiffs EAnyDiffs)) in
let obtained = (if checkEnv then envToString envX.val else if envX.changes == [] then "" else toString envX.changes) ++ " |- " ++ unparse expX.val in
if obtained == expected then success state else
case Lazy.force lazyTail of
LazyList.Cons (envX2, expX2) lazyTail2 ->
let obtained2 = (
if checkEnv then envToString envX2.val else
if envX2.changes == [] then "" else toString envX2.changes) ++ " |- " ++ unparse expX2.val in
if obtained2 == expected then success state else
fail state <|
log state <| "Expected \n'" ++ expected ++ "'\n, got\n'" ++ obtained ++ "'\n and \n'" ++ obtained2 ++ problemdesc
LazyList.Nil ->
fail state <|
log state <| "Expected \n'" ++ expected ++ "'\n, got\n'" ++ obtained ++ "'\n" ++ problemdesc
Ok LazyList.Nil ->
fail state <| log state <| "Expected \n'" ++ expected ++ "', got no solutions without error" ++ problemdesc
Err msg ->
fail state <| log state <| "Expected \n'" ++ expected ++ "', got '" ++ msg ++ problemdesc
updateAssert env exp origOut newOut expectedEnv expectedExpStr =
gather <| updateAssert_ True env exp origOut newOut expectedEnv expectedExpStr
evalElmAssert_: List (String, String) -> String -> String -> State -> State
evalElmAssert_ envStr expStr expectedResStr state =
if state.ignore then state else
case Utils.projOk [parseEnv envStr] of
Err error -> fail state <| log state <| "Error while parsing environments: " ++ error
Ok [env] ->
case Utils.projOk [parse expStr, parse expectedResStr] of
Err error -> fail state <| log state <| "Error while parsing expressions or outputs: " ++ error
Ok [exp, res] ->
--let _ = Debug.log (log state <| toString exp) () in
case Utils.projOk [evalEnv env exp, eval res] of
Err error -> fail state <| log state <| "Error while evaluating the expression or the expected result: " ++ unparse exp ++ "," ++ unparse res ++ ": " ++ error
Ok [out, expectedOut] -> assertEqualVal_ out expectedOut state
Ok _ -> fail state "???"
Ok _ -> fail state "???"
Ok _ -> fail state "???"
evalElmAssert envStr expStr expectedResStr = gather <| evalElmAssert_ envStr expStr expectedResStr
evalElmAssert2_: Env -> String -> String -> State -> State
evalElmAssert2_ env expStr expectedResStr state =
if state.ignore then state else
case Utils.projOk [parse expStr, parse expectedResStr] of
Err error -> fail state <| log state <| "Error while parsing expressions or outputs: " ++ error
Ok [exp, res] ->
--let _ = Debug.log (log state <| toString exp) () in
case Utils.projOk [evalEnv env exp, eval res] of
Err error -> fail state <| log state <| "Error while evaluating the expression or the expected result: " ++ unparse exp ++ "," ++ unparse res ++ ": " ++ error
Ok [out, expectedOut] -> assertEqualVal_ out expectedOut state
Ok _ -> fail state "???"
Ok _ -> fail state "???"
evalElmAssert2 envStr expStr expectedResStr = gather <| evalElmAssert2_ envStr expStr expectedResStr
updateElmAssert_: List (String, String) -> String -> String -> List (String, String) -> String -> State -> State
updateElmAssert_ envStr expStr newOutStr expectedEnvStr expectedExpStr state =
if state.ignore then state else
case Utils.projOk [parseEnv envStr, parseEnv expectedEnvStr] of
Err error -> fail state <| log state <| "Error while parsing environments: " ++ error
Ok [env, expectedEnv] ->
case Utils.projOk [parse expStr, parse newOutStr] of
Err error -> fail state <| log state <| "Error while parsing expressions or outputs: " ++ error
Ok [exp, newOut] ->
--let _ = Debug.log (log state <| toString exp) () in
case Utils.projOk [evalEnv env exp, eval newOut] of
Err error ->
let _ = Debug.log (toString exp) () in
fail state <| log state <| "Error while evaluating the expression or the output: " ++ Syntax.unparser Syntax.Elm exp ++ " <- " ++ Syntax.unparser Syntax.Elm newOut ++ ": " ++ error
Ok [out, newOut] -> updateAssert_ True env exp out newOut expectedEnv expectedExpStr state
Ok _ -> fail state "???"
Ok _ -> fail state "???"
Ok _ -> fail state "???"
updateElmAssert envStr expStr newOutStr expectedEnvStr expectedExpStr = gather <| updateElmAssert_ envStr expStr newOutStr expectedEnvStr expectedExpStr
updateElmAssert2_: Env -> String -> String -> String -> State -> State
updateElmAssert2_ env expStr newOutStr expectedExpStr state =
if state.ignore then state else
case Utils.projOk [parse expStr, parse newOutStr] of
Err error -> fail state <| log state <| "Error while parsing expressions or outputs: " ++ error
Ok [exp, newOut] ->
--let _ = Debug.log (log state <| toString exp) () in
case Utils.projOk [evalEnv env exp, eval newOut] of
Err error -> fail state <| log state <| "Error while evaluating the expression or the output: " ++ Syntax.unparser Syntax.Elm exp ++ "," ++ Syntax.unparser Syntax.Elm newOut ++ ": " ++ error
Ok [out, newOut] -> updateAssert_ True env exp out newOut env expectedExpStr state
Ok _ -> fail state "???"
Ok _ -> fail state "???"
updateElmAssert2 env expStr newOutStr expectedExpStr = gather <| updateElmAssert2_ env expStr newOutStr expectedExpStr
updateElmPrelude_: String -> (String -> String) -> (String -> String) -> State -> State
updateElmPrelude_ expStr outReplacer expectedExpReplacer state =
if state.ignore then state else
case parse expStr of
Err error -> fail state <| log state <| "Error while parsing expressions or outputs: " ++ error
Ok exp ->
case evalEnv EvalUpdate.preludeEnv exp of
Err error -> fail state <| log state <| "Error while evaluating the expression: " ++ Syntax.unparser Syntax.Elm exp ++ ": " ++ error
Ok oldOut ->
case oldOut |> valToString |> outReplacer |> parse |> Result.andThen eval of
Err error -> fail state <| log state <| "Error while parsing expressions or outputs: " ++ error
Ok newOut ->
let expectedExpStr = expectedExpReplacer expStr in
updateAssert_ False EvalUpdate.preludeEnv exp oldOut newOut EvalUpdate.preludeEnv expectedExpStr state
updateElmPrelude expStr outReplacer expectedExpReplacer = gather <| updateElmPrelude_ expStr outReplacer expectedExpReplacer
replaceStr before after = Regex.replace Regex.All (Regex.regex before) (\_ -> after)
parse = Syntax.parser Syntax.Elm >> Result.mapError (\p -> ParserUtils.showError p)
unparse = Syntax.unparser Syntax.Elm
evalEnv env exp = Eval.doEval Syntax.Elm env exp |> Result.map (Tuple.first >> Tuple.first)
eval exp = Eval.doEval Syntax.Elm [] exp |> Result.map (Tuple.first >> Tuple.first)
parseEnv: List (String, String) -> Result String Env
parseEnv envStr =
envStr
|> List.map (\(name, exp) ->
parse exp
|> Result.mapError (\pError -> toString pError)
|> Result.andThen (\x ->
evalEnv [] x
|> Result.map (\x -> (name, x))))
|> Utils.projOk
|> Result.mapError (\pError -> toString pError)
dws = ws " "
dws2 = ws " "
dws1 = ws " "
dws3 = ws " "
dws4 = ws " "
dws5 = ws " "
dws6 = ws " "
val x = Val x (Provenance [] (tConst (ws "") 0) []) (Parents [])
tVal n = val <| (VConst Nothing (n, dummyTrace))
tVClosure maybeIndent pats body env = val <| VClosure maybeIndent pats body env
tVList vals = val <| VList vals
tVBool truth = val <| VBase (VBool truth)
tVStr chars = val <| VBase (VString chars)
tConst ws num = withDummyExpInfo <| EConst ws num dummyLoc noWidgetDecl
tBool space truth = withDummyExpInfo <| EBase space (EBool truth)
tString space chars = withDummyExpInfo <| EBase space (EString defaultQuoteChar chars)
tVar space name =withDummyExpInfo <| EVar space name
tFun sp0 pats body sp1 = (withDummyExpInfo <| EFun sp0 pats body sp1)
tApp sp0 fun args sp1 = withDummyExpInfo <| EApp sp0 fun args SpaceApp sp1
tList sp0 exps sp1 = withDummyExpInfo <| EList sp0 (List.map (\e -> (ws "", e)) exps) (ws "") Nothing sp1
--tListCons sp0 exps sp1 tail sp2 = EList sp0 exps sp1 (Just tail) sp2
tPVar space name = withDummyPatInfo <| PVar space name noWidgetDecl
tPAs sp0 name sp1 pat= withDummyPatInfo <| PAs sp0 name sp1 pat
tPList sp0 listPat sp1= withDummyPatInfo <| PList sp0 listPat (ws "") Nothing sp1
tPListCons sp0 listPat sp1 tailPat sp2 = withDummyPatInfo <| PList sp0 listPat sp1 (Just tailPat) sp1
onlySpecific = False
display_prelude_message = case ElmParser.preludeNotParsed of
Nothing -> ()
Just msg -> Debug.log msg ()
all_tests = init_state
|> ignore onlySpecific
|> test "triCombineTest"
|> delay (\() -> assertEqual
(mergeEnv
[("y", (tVal 2)), ("x", (tVal 1))]
[("y", (tVal 2)), ("x", (tVal 1))] []
[("y", (tVal 2)), ("x", (tVal 3))] [(1, VConstDiffs)]
) ([("y", (tVal 2)), ("x", (tVal 3))], [(1, VConstDiffs)]))
|> delay (\() -> assertEqual
(mergeEnv
[("x", (tVal 1)), ("y", (tVal 1)), ("z", (tVal 1))]
[("x", (tVal 1)), ("y", (tVal 2)), ("z", (tVal 2))] [(1, VConstDiffs), (2, VConstDiffs)]
[("x", (tVal 3)), ("y", (tVal 1)), ("z", (tVal 3))] [(0, VConstDiffs), (2, VConstDiffs)]
) ([("x", (tVal 3)), ("y", (tVal 2)), ("z", (tVal 3))], [(0, VConstDiffs), (1, VConstDiffs), (2, VConstDiffs)]))
|> test "Minus sign as binary or unary operator"
|> evalElmAssert [] "1-1" "0"
|> evalElmAssert [] "(\\x->x) -1" "-1"
|> test "update mutual recursion"
|> updateElmAssert [] "x =y\ny= 1\nx" "2"
[] "x =y\ny= 2\nx"
|> updateElmAssert [] "a = g 85\nf x = g (x - 17)\ng y = if y < 17 then y else f y\na" "2"
[] "a = g 87\nf x = g (x - 17)\ng y = if y < 17 then y else f y\na"
|> updateElmAssert [] "a = g 85\nf x = g (x - 17)\ng y = if y < 17 then y else f y\na" "2"
[] "a = g 85\nf x = g (x - 15)\ng y = if y < 17 then y else f y\na"
|> test "update const"
|> updateElmAssert [] " 1" "2"
[] " 2"
|> test "update boolean and strings"
|> updateElmAssert [] " True" "False"
[] " False"
|> updateElmAssert [] " 'Hello'" "'World'"
[] " 'World'"
|> test "update var"
|> updateElmAssert [("x", "1")] "x" "2"
[("x", "2")] "x"
|> updateElmAssert [("x", "1"), ("y", "3")] " x" "2"
[("x", "2"), ("y", "3")] " x"
|> updateElmAssert [("y", "3"), ("x", "1")] " x" "2"
[("y", "3"), ("x", "2")] " x"
|> test "update fun"
|> updateElmAssert [] "\\ x -> 1" "\\ x -> 2"
[] "\\ x -> 2"
|> test "update fun with 2 arguments"
|> updateElmAssert [] "\\x y -> 1" "\\y x -> 2"
[] "\\y x -> 2"
|> test "update nested fun with 2 and 1 arguments"
|> updateElmAssert [] "\\x y -> \\z -> 1" "\\y z -> \\x -> 3"
[] "\\y z -> \\x -> 3"
|> test "update app identity"
|> updateElmAssert [] "(\\x -> x) 1" "2"
[] "(\\x -> x) 2"
|> test "update app constant"
|> updateElmAssert [] "(\\x -> 1) 3" "2"
[] "(\\x -> 2) 3"
|> test "update pattern 'as' (\\(x as y) -> x [or y]) 1"
|> updateElmAssert [] "(\\x as y -> x) 1" "2"
[] "(\\x as y -> x) 2"
|> updateElmAssert [] "(\\(x as y) -> y) 1" "2"
[] "(\\(x as y) -> y) 2"
|> test "update pattern list (\\[x, y] -> x or y) [1, 2]"
|> updateElmAssert [] "(\\[x, y] -> x) [1, 2]" "3"
[] "(\\[x, y] -> x) [3, 2]"
|> updateElmAssert [] "(\\[x, y] -> y) [1, 2]" "3"
[] "(\\[x, y] -> y) [1, 3]"
|> test "update pattern list with tail (\\[x | [y]] -> x or y) [1, 2]"
|> updateElmAssert [] "(\\x :: y -> x) [1, 2]" "3"
[] "(\\x :: y -> x) [3, 2]"
|> updateElmAssert [] "(\\x :: [y] -> y) [1, 2]" "3"
[] "(\\x :: [y] -> y) [1, 3]"
|> test "update app (\\x y -> x) 1 2"
|> updateElmAssert [] "(\\x y -> x) 1 2" "3"
[] "(\\x y -> x) 3 2"
|> test "update app (\\x y -> y) 1 2"
|> updateElmAssert
[] "(\\x y -> y) 1 2" "3"
[] "(\\x y -> y) 1 3"
|> test "update if-then-else"
|> updateElmAssert
[] "if True then 1 else 2" "3"
[] "if True then 3 else 2"
|> updateElmAssert
[] "if False then 1 else 2" "3"
[] "if False then 1 else 3"
|> test "String concatenation"
|> updateElmAssert [] "'Hello' + 'world'" "'Hello world'"
[] "'Hello ' + 'world'"
|> test "update arithmetic operations"
|> updateElmAssert
[("x", "1"), ("y", "2")] " x+ y" "4"
[("x", "2"), ("y", "2")] " x+ y"
|> updateElmAssert
[("x", "1"), ("y", "2")] " x+ y" "4"
[("x", "1"), ("y", "3")] " x+ y"
|> updateElmAssert
[("x", "5"), ("y", "2")] " x- y" "1"
[("x", "3"), ("y", "2")] " x- y"
|> updateElmAssert
[("x", "2"), ("y", "3")] " x* y" "8"
[("x", "2"), ("y", "4")] " x* y"
|> updateElmAssert
[("x", "18"), ("y", "3")] " x/ y" "7"
[("x", "21"), ("y", "3")] " x/ y"
|> updateElmAssert
[("x", "3"), ("y", "4")] " x ^ y" "16"
[("x", "2"), ("y", "4")] " x ^ y"
|> updateElmAssert
[("x", "-1"), ("y", "3")] " x ^ y" "1"
[("x", "1"), ("y", "3")] " x ^ y"
|> updateElmAssert
[("x", "-2"), ("y", "3")] " x ^ y" "27"
[("x", "3"), ("y", "3")] " x ^ y"
|> updateElmAssert
[("x", "-1"), ("y", "0")] "x ^ y" "-1"
[("x", "-1"), ("y", "1")] "x ^ y"
|> updateElmAssert
[("x", "-1"), ("y", "3")] " x ^ y" "-8"
[("x", "-2"), ("y", "3")] " x ^ y"
|> updateElmAssert
[("x", "17"), ("y", "8")] " mod x y" "3"
[("x", "19"), ("y", "8")] " mod x y"
|> updateElmAssert
[("x", "1"), ("y", "0")] "arctan2 y x" "1.5707963267948966"
[("x", "6.123233995736766e-17"), ("y", "1")] "arctan2 y x"
|> updateElmAssert
[("x", "1"), ("y", "0")] "arctan2 y x" "3.141592653589793"
[("x", "-1"), ("y", "1.2246467991473532e-16")] "arctan2 y x"
|> updateElmAssert
[("x", "1"), ("y", "0")] "arctan2 y x" "-1.5707963267948966"
[("x", "6.123233995736766e-17"), ("y", "-1")] "arctan2 y x"
|> updateElmAssert
[("x", "0.1")] "cos x" "0"
[("x", "1.570796326794897")] "cos x"
|> updateElmAssert
[("x", "-0.1")] "cos x" "0"
[("x", "-1.570796326794897")] "cos x"
|> updateElmAssert
[("x", "0.1")] "cos x" "0"
[("x", "1.570796326794897")] "cos x"
|> updateElmAssert
[("x", "0.1")] "sin x" "1"
[("x", "1.570796326794897")] "sin x"
|> updateElmAssert
[("x", "-0.1")] "sin x" "-1"
[("x", "-1.570796326794897")] "sin x"
|> updateElmAssert
[("x", "0")] "arccos x" "0"
[("x", "1")] "arccos x"
|> updateElmAssert
[("x", "0")] "arcsin x" "1.5707963267948966"
[("x", "1")] "arcsin x"
|> updateElmAssert
[("x", "17.5")] "floor x" "15"
[("x", "15.5")] "floor x"
|> updateElmAssert
[("x", "17.5")] "ceiling x" "15"
[("x", "14.5")] "ceiling x"
|> updateElmAssert
[("x", "17.75")] "round x" "15"
[("x", "14.75")] "round x"
|> updateElmAssert
[("x", "17.25")] "round x" "15"
[("x", "15.25")] "round x"
|> updateElmAssert
[("x", "16")] "sqrt x" "3"
[("x", "9")] "sqrt x"
|> test "case of calls"
|> updateElmAssert
[("x", "[7, 1]")] "case x of\n [a, b] -> a + b\n u -> 0" "5"
[("x", "[4, 1]")] "case x of\n [a, b] -> a + b\n u -> 0"
|> updateElmAssert
[("x", "[7]")] "case x of\n [a, b] -> a + b\n u -> 0" "5"
[("x", "[7]")] "case x of\n [a, b] -> a + b\n u -> 5"
|> test "non-rec let"
|> updateElmAssert
[] "let x= 5 in\nlet y =2 in [x, y]" "[6, 3]"
[] "let x= 6 in\nlet y =3 in [x, y]"
|> test "list constructor"
|> updateElmAssert
[] "let x= 1 in\nlet y =[2] in x :: x :: y" "[3, 1, 2]"
[] "let x= 3 in\nlet y =[2] in x :: x :: y"
|> updateElmAssert
[] "let x= 1 in\nlet y =2 in [x, x, y]" "[3, 1, 2]"
[] "let x= 3 in\nlet y =2 in [x, x, y]"
|> updateElmAssert
[] "let x= 1 in\nlet y =2 in [x, x, y]" "[1, 3, 2]"
[] "let x= 3 in\nlet y =2 in [x, x, y]"
|> updateElmAssert
[] "let x= 1 in\nlet y =2 in [x, x, y]" "[1, 1, 3]"
[] "let x= 1 in\nlet y =3 in [x, x, y]"
|> updateElmAssert
[] "let x= 1 in\nlet y =[2] in x :: x :: y" "[1, 3, 2]"
[] "let x= 3 in\nlet y =[2] in x :: x :: y"
|> updateElmAssert
[] "let x= 1 in\nlet y =[2] in x :: x :: y" "[1, 1, 3]"
[] "let x= 1 in\nlet y =[3] in x :: x :: y"
|> test "rec let"
|> updateElmAssert
[] "let f x = if x == 0 then x else (f (x - 1)) in\n f 2" "3"
[] "let f x = if x == 0 then x else (f (x - 1)) in\n f 5"
|> test "Comments"
|> updateElmAssert
[] "--This is a comment\n 1" "2"
[] "--This is a comment\n 2"
|> test "Strings"
|> updateElmAssert
[] " \"This is a string\"" "\"Hello world\""
[] " \"Hello world\""
|> updateElmAssert
[] " \"This is a string\"" "\"Hello' \\\" wo\\\\rld\""
[] " \"Hello' \\\" wo\\\\rld\""
|> updateElmAssert
[] " 'This is a string'" "\"Hello world\""
[] " 'Hello world'"
|> updateElmAssert
[] " 'This is a string'" "\"Hello' \\\" wo\\\\rld\""
[] " 'Hello\\' \" wo\\\\rld'"
|> updateElmAssert
[] " 'Welcome to this world'" "\"Welcome again to this world\""
[] " 'Welcome again to this world'"
|> test "Strings concatenation"
|> updateElmAssert [] "(\"Hello\" + \" \") + \"world\"" "\"Good morning world\""
[] "(\"Good morning\" + \" \") + \"world\""
|> test "Many solutions"
|> updateElmAssert
[("h3", "\\text -> ['h3', [], [['TEXT', text]]]")] "x = 0 + 0\n\nh3 (toString x)" "['h3', [], [['TEXT', '1']]]"
[("h3", "\\text -> ['h3', [], [['TEXT', text]]]")] "x = 0 + 1\n\nh3 (toString x)"
|> updateElmAssert
[("h3", "\\text -> ['h3', [], [['TEXT', text]]]")] "x = 0 + 0\n\nh3 (toString x)" "['h3', [], [['TEXT', '1']]]"
[("h3", "\\text -> ['h3', [], [['TEXT', text]]]")] "x = 1 + 0\n\nh3 (toString x)"
|> test "Multiline string literals"
|> updateElmAssert
[] "\"\"\"Hello @(if 1 == 2 then \"big\" else \"\"\"very @(\"small\")\"\"\") world\"\"\"" "\"Hello very tiny world\""
[] "\"\"\"Hello @(if 1 == 2 then \"big\" else \"\"\"very @(\"tiny\")\"\"\") world\"\"\""
|> updateElmAssert
[] "let x = \"Hello\" in \"\"\"@x world\"\"\"" "\"Hello big world\""
[] "let x = \"Hello\" in \"\"\"@x big world\"\"\""
|> updateElmAssert
[] "let x = \"Hello\" in \"\"\"@x world\"\"\"" "\"Hello big world\""
[] "let x = \"Hello big\" in \"\"\"@x world\"\"\""
|> updateElmAssert
[] "let x = \"Hello\" in \"\"\"@x world\"\"\"" "\"Helloworld\""
[] "let x = \"Hello\" in \"\"\"@(x)world\"\"\""
|> updateElmAssert
[] "\"\"\"@let x = \"Hello\" in\n@x world\"\"\"" "\"Hello big world\""
[] "\"\"\"@let x = \"Hello big\" in\n@x world\"\"\""
|> updateElmAssert
[] "\"\"\"@let x = \"Hello\" in\n@x world\"\"\"" "\"Hello big world\""
[] "\"\"\"@let x = \"Hello\" in\n@x big world\"\"\""
|> updateElmAssert
[] "\"\"\"@let x = (\"Hello\" + \n \" big\") in\n@x world\"\"\"" "\"Hello tall world\""
[] "\"\"\"@let x = (\"Hello\" + \n \" tall\") in\n@x world\"\"\""
|> test "Finding all regex matches"
|> assertEqual (LazyList.toList <| allInterleavingsIn ["A", "BB", "C"] "xAyBBBzAoBBpCC")
[ ["x","y","BzAoBBp","C"]
, ["x","y","BzAoBBpC",""]
, ["x", "yB", "zAoBBp","C"]
, ["x", "yB", "zAoBBpC",""]
, ["x", "yBBBzAo", "p","C"]
, ["x", "yBBBzAo", "pC",""]
, ["xAyBBBz", "o", "p","C"]
, ["xAyBBBz", "o", "pC",""]
]
|> test "GroupStartMap"
|> assertEqual (List.map (\{submatches} -> submatches) (GroupStartMap.find Regex.All "a((bc)|cd)d" "aabcdacdd"))
[[{match = Just "bc", start = 2}, {match = Just "bc", start = 2}], [{match = Just "cd", start = 6}, {match = Nothing, start = -1}]]
|> assertEqual (GroupStartMap.replace Regex.All "a((bc)|cd)d" (
\match -> String.concat <| List.map (\subm -> toString subm.start ++ Maybe.withDefault "null" subm.match) match.submatches
) "aabcdacdd")
"a2bc2bc6cd-1null"
|> test "replaceAllIn"
|> evalElmAssert2 builtinEnv "replaceAllIn \"l\" \"L\" \"Hello world\"" "\"HeLLo worLd\""
|> evalElmAssert2 builtinEnv "replaceAllIn \"a(b|c)\" \"o$1\" \"This is acknowledgeable\"" "\"This is ocknowledgeoble\""
|> evalElmAssert2 builtinEnv "replaceAllIn \"a(b|c)\" (\\{group = [t, c]} -> \"oa\" + (if c == \"b\" then \"c\" else \"b\")) \"This is acknowledgeable\"" "\"This is oabknowledgeoacle\""
|> updateElmAssert2 builtinEnv "replaceAllIn \"e\" \"ee\" \"\"\"See some examples from File...\"\"\"" "\"Seeee somee emexamplees from Filee...\""
"replaceAllIn \"e\" \"eme\" \"\"\"See some examples from File...\"\"\""
|> updateElmAssert2 builtinEnv "[ 'div'\n , []\n , [ ['h2', [], [['TEXT', 'Welcome to Sketch-n-Sketch Docs!']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', 'Type something here...']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', replaceAllIn \"e\" \"ee\" \"\"\"\n See some examples from File -> New From Template in\n the menu bar, or by pressing the Previous and Next\n buttons in the top-right corner.\n \"\"\"]]]\n ]\n ]"
"[ 'div'\n , []\n , [ ['h2', [], [['TEXT', 'Welcome to Sketch-n-Sketch Docs!']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', 'Type something here...']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', \"\"\"\n Seeee somee eecxamplees from Filee -> Neew From Teemplatee in\n thee meenu bar, or by preessing thee Preevious and Neext\n buttons in thee top-right corneer.\n \"\"\"]]]\n ]\n ]"
"[ 'div'\n , []\n , [ ['h2', [], [['TEXT', 'Welcome to Sketch-n-Sketch Docs!']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', 'Type something here...']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', replaceAllIn \"e\" \"eec\" \"\"\"\n See some examples from File -> New From Template in\n the menu bar, or by pressing the Previous and Next\n buttons in the top-right corner.\n \"\"\"]]]\n ]\n ]"
|> updateElmAssert2 builtinEnv "[ 'div'\n , []\n , [ ['h2', [], [['TEXT', 'Welcome to Sketch-n-Sketch Docs!']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', 'Type something here...']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', replaceAllIn \"e\" \"ee\" \"\"\"\n See some examples from File -> New From Template in\n the menu bar, or by pressing the Previous and Next\n buttons in the top-right corner.\n \"\"\"]]]\n ]\n ]"
"[ 'div'\n , []\n , [ ['h2', [], [['TEXT', 'Welcome to Sketch-n-Sketch Docs!']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', 'Type something here...']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', \"\"\"\n Seeee somee eecxamplees from Filee -> Neew From Teemplatee in\n thee meenu bar, or by preessing thee Preevious and Neext\n buttons in thee top-right corneer.\n \"\"\"]]]\n ]\n ]"
"[ 'div'\n , []\n , [ ['h2', [], [['TEXT', 'Welcome to Sketch-n-Sketch Docs!']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', 'Type something here...']]]\n , ['br', [], []]\n , ['p', [], [['TEXT', replaceAllIn \"e\" \"ee\" \"\"\"\n See some ecxamples from File -> New From Template in\n the menu bar, or by pressing the Previous and Next\n buttons in the top-right corner.\n \"\"\"]]]\n ]\n ]"
|>
updateElmAssert [] "extractFirstIn \"^\\\\s*(S(\\\\w)+ (\\\\w))\" \"\"\" See some examples\"\"\" |> case of Just [big, s1, s2] -> big + s1 + s2; e -> \"not the right shape\"" "\"Sea ses\""
[] "extractFirstIn \"^\\\\s*(S(\\\\w)+ (\\\\w))\" \"\"\" Sea some examples\"\"\" |> case of Just [big, s1, s2] -> big + s1 + s2; e -> \"not the right shape\""
|> evalElmAssert [] "extractFirstIn \"\"\"([\\w:_-]*)\"\"\" \"data-array=\\\"17\\\"\" |> case of Just [id] -> id; _ -> \"Nothing\" " "\"data-array\""
|> evalElmAssert2 builtinEnv "replaceAllIn \"\\\\[([^\\\\[]+)\\\\]\\\\(([^\\\\)]+)\\\\)\" \"<a href='$2'>$1</a>\" \"[Markdown](http://test)\"" "\"<a href='http://test'>Markdown</a>\""
|> evalElmAssert2 builtinEnv "replaceAllIn \"\\\\[([^\\\\[]+)\\\\]\\\\(([^\\\\)]+)\\\\)\" \"<a href='$2'>$1</a>\" \"[Markdown](https://fr.wikipedia.org/wiki/Markdown)\"" "\"<a href='https://fr.wikipedia.org/wiki/Markdown'>Markdown</a>\""
|> updateElmAssert2 builtinEnv "replaceAllIn \"\\\\[([^\\\\[]+)\\\\]\\\\(([^\\\\)]+)\\\\)\" \"<a href='$2'>$1</a>\" \"#[Markdown](https://fr.wikipedia.org/wiki/Markdown)\"" "\"#<a href='https://fr.wikipedia.org/wiki/Markdown'>Markdoooown</a>\""
"replaceAllIn \"\\\\[([^\\\\[]+)\\\\]\\\\(([^\\\\)]+)\\\\)\" \"<a href='$2'>$1</a>\" \"#[Markdoooown](https://fr.wikipedia.org/wiki/Markdown)\""
|> updateElmAssert2 builtinEnv "replaceAllIn \"x|y\" (\\{match} -> if match == \"x\" then \"5\" else \"7\") \"x,y\"" "\"6,8\""
"replaceAllIn \"x|y\" (\\{match} -> if match == \"x\" then \"6\" else \"8\") \"x,y\""
--|> test "Record construction, extraction and pattern "
-- |>
|> test "Partial application"
|> updateElmAssert
[] "let map f l = case l of head::tail -> f head::map f tail; [] -> [] in let td color txt = [ 'td', [['style', ['border', 'padding', ['background-color', color]]]], [['TEXT', txt]]] in map (td 'green') ['hello', 'world']"
"[[ 'td', [['style', ['border', 'padding', ['background-color', 'red']]]], [['TEXT', 'hello']]], [ 'td', [['style', ['border', 'padding', ['background-color', 'green']]]], [['TEXT', 'world']]]]"
[] "let map f l = case l of head::tail -> f head::map f tail; [] -> [] in let td color txt = [ 'td', [['style', ['border', 'padding', ['background-color', color]]]], [['TEXT', txt]]] in map (td 'red') ['hello', 'world']"
--|> ignore False
|> test "Multiple solutions"
|> updateElmAssert
[] "['A' + 'B', 'C' + 'D']" "['AGB', 'CGD']"
[] "['AG' + 'B', 'CG' + 'D']"
|> test "Records"
|> updateElmAssert
[] "{ a= 1, b= 2}.b" "3"
[] "{ a= 1, b= 3}.b"
|> updateElmAssert
[] "{ a= 1, b= 2}.a" "3"
[] "{ a= 3, b= 2}.a"
|> updateElmAssert
[] "let x = { a= 1, b= 2} in { x | a = 2 }.a" "3"
[] "let x = { a= 1, b= 2} in { x | a = 3 }.a"
|> updateElmAssert
[] "let x = { a= 1, b= 2} in { x | a = 2 }.b" "3"
[] "let x = { a= 1, b= 3} in { x | a = 2 }.b"
|> test "Indented lists, second position of list > 1 elements"
|> updateElmAssert
[] "[1, 2, 3, 4]" "[1, 5, 2, 3, 4]"
[] "[1, 5, 2, 3, 4]"
|> updateElmAssert
[] "[ 1,\n 2,\n 3,\n 4]" "[1, 5, 2, 3, 4]"
[] "[ 1,\n 5,\n 2,\n 3,\n 4]"
|> updateElmAssert
[] "[ 1\n, 2\n, 3\n, 4]" "[1, 5, 2, 3, 4]"
[] "[ 1\n, 5\n, 2\n, 3\n, 4]"
|> updateElmAssert
[] " [ 1\n, 2\n, 3\n, 4]" "[1, 5, 2, 3, 4]"
[] " [ 1\n, 5\n, 2\n, 3\n, 4]"
|> test "Indented lists, second position of list with 1 element"
|> updateElmAssert
[] "[1]" "[1, 2]"
[] "[1, 2]"
|> updateElmAssert
[] "[ 1]" "[ 1, 2]"
[] "[ 1, 2]"
|> updateElmAssert
[] "[ 1\n]" "[ 1, 2]"
[] "[ 1\n, 2\n]"
|> updateElmAssert
[] "[\n 1]" "[ 1, 2]"
[] "[\n 1,\n 2]"
|> test "Indented lists, first position of list with 1 element"
|> updateElmAssert
[] "[1]" "[2, 1]"
[] "[2, 1]"
|> updateElmAssert
[] "[ 1]" "[2, 1]"
[] "[ 2, 1]"
|> updateElmAssert
[] "[ 1\n]" "[2, 1]"
[] "[ 2\n, 1\n]"
|> updateElmAssert
[] "[\n 1]" "[2, 1]"
[] "[\n 2,\n 1]"
|> test "Indented lists, first position of list > 1 element"
|> updateElmAssert
[] "[1, 2]" "[0, 1, 2]"
[] "[0, 1, 2]"
|> updateElmAssert
[] "[ 1, 2]" "[0, 1, 2]"
[] "[ 0, 1, 2]"
|> updateElmAssert
[] "[ 1\n, 2\n, 3\n, 4]" "[0, 1, 2, 3, 4]"
[] "[ 0\n, 1\n, 2\n, 3\n, 4]"
|> test "Indented lists, first position of empty list"
|> updateElmAssert
[] "[]" "[1]"
[] "[1]"
|> updateElmAssert
[] "[ ]" "[1]"
[] "[1 ]"
|> updateElmAssert
[] " []" "[[1, 2]]"
[] " [[1, 2]]"
|> test "parsing HTML"
|> updateElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)]
"parseHTML \"hello\"" "[HTMLInner \"hello world\"]"
"parseHTML \"hello world\""
|> updateElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)]
"parseHTML \"hello<br>world\"" "[HTMLInner \"hello\", HTMLElement \"br\" [] \" \" RegularEndOpening [] VoidClosing, HTMLInner \"sworld\"]"
"parseHTML \"hello<br >sworld\""
|> updateElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)]
"parseHTML \"<?help>\"" "[HTMLComment (Less_Greater \"Injection: adding more chars like >, <, and -->\")]"
"parseHTML \"<!--Injection: adding more chars like >, <, and ~~>-->\""
|> evalElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)]
"parseHTML \"<h3>Hello world</h3>\"" "[HTMLElement \"h3\" [] \"\" RegularEndOpening [HTMLInner \"Hello world\"] (RegularClosing \"\")]"
|> evalElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)]
"parseHTML \"<a href='https://fr.wikipedia.org/wiki/Markdown'>Markdown</a>\"" "[HTMLElement \"a\" [HTMLAttribute \" \" \"href\" (HTMLAttributeString \"\" \"\" \"'\" \"https://fr.wikipedia.org/wiki/Markdown\")] \"\" RegularEndOpening [HTMLInner \"Markdown\"] (RegularClosing \"\")]"
|> updateElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)]
"parseHTML \"<a href='https://fr.wikipedia.org/wiki/Markdown'>Markdown</a> demo\""
"[HTMLElement \"a\" [HTMLAttribute \" \" \"href\" (HTMLAttributeString \"\" \"\" \"'\" \"https://fr.wikipedia.org/wiki/Markdown\")] \"\" RegularEndOpening [HTMLInner \"Markdown\"] (RegularClosing \"\"), HTMLInner \" demonstration\"]"
"parseHTML \"<a href='https://fr.wikipedia.org/wiki/Markdown'>Markdown</a> demonstration\""
|> evalElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)] "parseHTML \"Hello world\"" "[HTMLInner \"Hello world\"]"
|> evalElmAssert2 [("parseHTML", HTMLValParser.htmlValParser)] "parseHTML \"<i><b>Hello</i></b>World\"" "[ HTMLElement \"i\" [] \"\" RegularEndOpening [ HTMLElement \"b\" [] \"\" RegularEndOpening [ HTMLInner \"Hello\"] ForgotClosing] (RegularClosing \"\"), HTMLInner \"</b>World\"]"
|> updateElmAssert [("color", "\"white\""), ("padding", "[\"padding\", \"3px\"]")] "[padding, [\"background-color\", color]]"
"[[\"padding\", \"3px\"], [ \"background-color\", \"lightgray\"]]"
[("color", "\"lightgray\""), ("padding", "[\"padding\", \"3px\"]")] "[padding, [\"background-color\", color]]"
|> test "freeze"
|> updateElmAssert [("freeze", "\\x -> x")] "freeze 0 + 1" "2"
[("freeze", "\\x -> x")] "freeze 0 + 2"
|> updateElmAssert [("freezeExpression", "\\x -> x")] "let x = 1 in freezeExpression (0 + x)" "2"
[("freezeExpression", "\\x -> x")] "let x = 2 in freezeExpression (0 + x)"
|> test "dictionary"
|> updateElmAssert [("x", "1")] "__DictGet__ \"a\" (__DictFromList__ [(\"a\", x)])" "Just 2"
[("x", "2")] "__DictGet__ \"a\" (__DictFromList__ [(\"a\", x)])"
|> updateElmAssert [("x", "1")] "__DictRemove__ \"b\" (__DictFromList__ [(\"a\", x), (\"b\", 2)])" "__DictFromList__ [(\"a\", 2)]"
[("x", "2")] "__DictRemove__ \"b\" (__DictFromList__ [(\"a\", x), (\"b\", 2)])"
|> updateElmAssert [("x", "1")] "__DictInsert__ \"b\" x (__DictFromList__ [(\"a\", x)])" "__DictFromList__ [(\"a\", 1), (\"b\", 2)]"
[("x", "2")] "__DictInsert__ \"b\" x (__DictFromList__ [(\"a\", x)])"
|> updateElmAssert [] "__DictInsert__ \"b\" 1 (__DictFromList__ [(\"a\", 2), (\"b\", 3)])" "__DictFromList__ [(\"a\", 1), (\"b\", 2)]"
[] "__DictInsert__ \"b\" 2 (__DictFromList__ [(\"a\", 1), (\"b\", 3)])"
|> test "recursive delayed"
|> updateElmAssert [] "f =\n let x = 1 in\n \\y -> x + y\n\nf 2" "4"
[] "f =\n let x = 2 in\n \\y -> x + y\n\nf 2"
|> updateElmAssert [] "f =\n let x = 1 in\n \\y -> x + y\n\nf 2" "4"
[] "f =\n let x = 1 in\n \\y -> x + y\n\nf 3"
|> test "All diffs"
|> assertEqual (allStringDiffs "abcabcabc" "abxabcabc" |> Results.toList)
[[DiffEqual "ab", DiffRemoved "c", DiffAdded "x", DiffEqual "abcabc"]]
|> assertEqual (allStringDiffs "ab3ab" "abab" |> Results.toList)
[[DiffEqual "ab", DiffRemoved "3", DiffEqual "ab"]]
|> assertEqual (allStringDiffs "aa3aa" "aa" |> Results.toList)
[[DiffEqual "aa", DiffRemoved "3aa"],
[DiffRemoved "aa3", DiffEqual "aa"]]
|> assertEqual (alldiffs identity ["a", "b", "3", "a", "b"] ["a", "b", "a", "b"] |> Results.toList)
[[DiffEqual ["a", "b"], DiffRemoved ["3"], DiffEqual ["a", "b"]]]
|> assertEqual (alldiffs identity ["a", "a", "3", "a", "a"] ["a", "a"] |> Results.toList)
[[DiffEqual ["a", "a"], DiffRemoved ["3", "a", "a"]],
[DiffRemoved ["a", "a", "3"], DiffEqual ["a", "a"]]]
|> test "Type parsing"
|> evalElmAssert [] "type List a = Nil | Cons a\n2" "2"
|> evalElmAssert [] "let type List a = Nil | Cons a in 2" "2"
|> evalElmAssert [] "let type List a = Nil | Cons a\nin 2" "2"
|> evalElmAssert [] "let\n type List a = Nil | Cons a\nin 2" "2"
--|> skipBefore
|> test "updateReplace"
-- newStart newEnd ... repStart repEnd
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"ab\" \"abc\" \"12ab567ab90\" (VStringDiffs [StringUpdate 0 1 2])"
"(\"12abc567abc90\", VStringDiffs [StringUpdate 0 1 2, StringUpdate 1 3 3, StringUpdate 6 8 3])"
-- repStart repEnd ... newStart newEnd
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"ab\" \"abc\" \"12ab567ab90\" (VStringDiffs [StringUpdate 5 5 1])"
"(\"12abc567abc90\", VStringDiffs [StringUpdate 2 4 3, StringUpdate 5 5 1, StringUpdate 6 8 3])"
-- newStart repStart repEnd ... newEnd
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"ab\" \"abc\" \"12ab567ab90\" (VStringDiffs [StringUpdate 1 2 4])"
"(\"12abc567abc90\", VStringDiffs [StringUpdate 1 2 5, StringUpdate 4 6 3])"
-- newStart repStart newEnd ... repEnd
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"ab\" \"abc\" \"12ab567ab90\" (VStringDiffs [StringUpdate 1 7 2])"
"(\"12abc567abc90\", VStringDiffs [StringUpdate 1 8 4, StringUpdate 11 13 3])"
-- repStart newStart newEnd ... repEnd
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"abc\" \"abcd\" \"12abc567abc90\" (VStringDiffs [StringUpdate 3 9 1])"
"(\"12abcd567abcd90\", VStringDiffs [StringUpdate 2 10 4, StringUpdate 13 16 4])"
-- repStart newStart repEnd ... newEnd
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"ab\" \"abc\" \"12ab567ab90\" (VStringDiffs [StringUpdate 3 7 2])"
"(\"12abc567abc90\", VStringDiffs [StringUpdate 2 7 4, StringUpdate 9 11 3])"
|> evalElmAssert2 [("updateReplace", UpdateRegex.updateReplace EvalUpdate.eval EvalUpdate.update)]
"updateReplace \"\"\"(<(ul|ol)>(?:(?!</\\2>)[\\s\\S])*)</li>\\s*<li>\"\"\" \"$1</li>\\n</$2>\\n<$2>\\n\\t<li>\" \"<ul><li>a</li><li>b</li></ul>\" (VStringDiffs [StringUpdate 14 14 10])"
"(\"<ul><li>a</li>\\n</ul>\\n<ul>\\n\\t<li>b</li></ul>\", VStringDiffs [StringUpdate 0 14 37])"
-- Add the test <i>Hello <b>world</span></i> --> <i>Hello <b>world</b></i> (make sure to capture all closing tags)
|> updateElmAssert2 builtinEnv "replaceAllIn \"\\\\$(\\\\w+|\\\\$)\" (\\m -> m.match) \"printer\"" "\"$translation1\""
"replaceAllIn \"\\\\$(\\\\w+|\\\\$)\" (\\m -> m.match) \"$translation1\""
|> test "imperative-like assignments"
|> updateElmAssert [] "a = \"Hello \"\na = a + \"world\"\na" "\"Hella world\""
[] "a = \"PI:NAME:<NAME>END_PI \"\na = a + \"world\"\na"
--|> onlyBefore
|> updateElmPrelude (
ExamplesGenerated.mapMaybeLens
|> replaceStr "(\r?\n)+$" "" -- Remove newlines at the end
|> replaceStr "showValues \\[maybeRowA, maybeRowB, maybeRow1, maybeRow2\\]" "showValues [maybeRow1, maybeRow2]"
|> replaceStr "\r?\nmaybeRow(A|B).*" ""
) (replaceStr "New Jersey" "New Jersay")
(replaceStr "New Jersey" "New Jersay")
|> summary
| elm |
[
{
"context": " exposing (Html, text)\r\n\r\nmain =\r\n text \"Hello, Elm!\"",
"end": 3269,
"score": 0.8838254213,
"start": 3266,
"tag": "NAME",
"value": "Elm"
}
] | Chapter01/code-snippets-ch1.elm | PacktPublishing/Elm-Web-Development | 11 | -- the starting example
import Html exposing (text)
multiplyNumber x y =
x * y
main =
text ( toString ( multiplyNumber 8 10 ) )
-- another way to write the above
text ( toString ( multiplyNumber 2 ( multiplyNumber 2 ( multiplyNumber 2 10 ) ) ) )
-- piped syntax
import Html exposing (text)
multiplyNumber x y =
x * y
main =
multiplyNumber 2 10
|> multiplyNumber 2
|> multiplyNumber 2
|> toString
|> text
-- the starting example, re-written
import Html exposing (text)
multiplyNumber x y =
x * y
main =
multiplyNumber 8 10
|> toString
|> text
-- helpful type system
import Html exposing (text)
multiplyNumber: Int -> Int -> Int
multiplyNumber x y =
x * y
main =
multiplyNumber 8 10
|> toString
|> text
-- Get started writing Elm code
import Html exposing (text)
main =
text "Hello, World!"
-- With h1 function
import Html exposing (..)
main =
h1 [] [ text "Hello, Elm!" ]
-- With h2 function
h2 [] [ text "Hello, Elm!" ]
-- Making a link
main =
a [] [ text "Hello, Elm!" ]
-- Making an li
main =
li [] [ text "Hello, Elm!" ]
-- Making a paragraph
main =
p [] [ text "Hello, Elm!" ]
-- Making a paragraph
main =
p [] [ text "Hello, Elm!" ]
-- Nesting Html functions
import Html exposing (..)
main =
div []
[ p [] [text "1st paragraph" ]
, p [] [text "2nd paragraph" ]
]
-- Adding attributes
import Html exposing (..)
import Html.Attributes exposing (class)
main =
div [ class "danger" ]
[ p [] [text "1st paragraph" ]
, p [] [text "2nd paragraph" ]
]
-- Making a paragraph
main =
p [] [ text "Hello, Elm!" ]
-- Updating the style HTML tag in Ellie app
.danger {
background: red;
}
-- Adding type annotations
import Html exposing (..)
import Html.Attributes exposing (class)
main : Html msg
main =
div [ class "danger" ]
[ p [] [text "1st paragraph" ]
, p [] [text "2nd paragraph" ]
]
-- Get started fast with create-elm-app
npm install create-elm-app -g
create-elm-app elm-fun
elm-app start
-- Main.elm
import Html exposing (..)
import Html.Attributes exposing (class)
import Html exposing (..)
main : Html msg
main =
div [ ]
[ h1 [] [text "Elm is fun!" ]
, p [] [text "Let's learn some more!" ]
]
-- Get started with Elm on Windows 10
npm install -g elm
npm install -g elm-oracle
where.exe elm-oracle
C:\User\PC\AppData\Local\atom\app-1.19.7
C:\User\PC\AppData\Local\atom\app-1.19.7\resources\app\apm\bin
apm install atom-beautify
apm install elm-format
C:\Program Files (x86)\Elm Platform\0.18\bin
where.exe elm
C:\Program Files (x86)\Elm Platform\0.18\bin\elm-format.exe
-- testing elm-format with a poorly formatted elm file
-- command to run:
-- elm-format .\poorly-formatted-file.elm
module Main exposing (..)
import Html exposing (Html, text)
main =
text "Hello, Elm!"
apm install linter
C:\Users\PC\.atom\packages
elm-package install -y
-- Add some code to Main.elm to make sure it works:
module Main exposing (..)
import Html exposing (Html, text)
main =
text "Hello, Elm!" | 54399 | -- the starting example
import Html exposing (text)
multiplyNumber x y =
x * y
main =
text ( toString ( multiplyNumber 8 10 ) )
-- another way to write the above
text ( toString ( multiplyNumber 2 ( multiplyNumber 2 ( multiplyNumber 2 10 ) ) ) )
-- piped syntax
import Html exposing (text)
multiplyNumber x y =
x * y
main =
multiplyNumber 2 10
|> multiplyNumber 2
|> multiplyNumber 2
|> toString
|> text
-- the starting example, re-written
import Html exposing (text)
multiplyNumber x y =
x * y
main =
multiplyNumber 8 10
|> toString
|> text
-- helpful type system
import Html exposing (text)
multiplyNumber: Int -> Int -> Int
multiplyNumber x y =
x * y
main =
multiplyNumber 8 10
|> toString
|> text
-- Get started writing Elm code
import Html exposing (text)
main =
text "Hello, World!"
-- With h1 function
import Html exposing (..)
main =
h1 [] [ text "Hello, Elm!" ]
-- With h2 function
h2 [] [ text "Hello, Elm!" ]
-- Making a link
main =
a [] [ text "Hello, Elm!" ]
-- Making an li
main =
li [] [ text "Hello, Elm!" ]
-- Making a paragraph
main =
p [] [ text "Hello, Elm!" ]
-- Making a paragraph
main =
p [] [ text "Hello, Elm!" ]
-- Nesting Html functions
import Html exposing (..)
main =
div []
[ p [] [text "1st paragraph" ]
, p [] [text "2nd paragraph" ]
]
-- Adding attributes
import Html exposing (..)
import Html.Attributes exposing (class)
main =
div [ class "danger" ]
[ p [] [text "1st paragraph" ]
, p [] [text "2nd paragraph" ]
]
-- Making a paragraph
main =
p [] [ text "Hello, Elm!" ]
-- Updating the style HTML tag in Ellie app
.danger {
background: red;
}
-- Adding type annotations
import Html exposing (..)
import Html.Attributes exposing (class)
main : Html msg
main =
div [ class "danger" ]
[ p [] [text "1st paragraph" ]
, p [] [text "2nd paragraph" ]
]
-- Get started fast with create-elm-app
npm install create-elm-app -g
create-elm-app elm-fun
elm-app start
-- Main.elm
import Html exposing (..)
import Html.Attributes exposing (class)
import Html exposing (..)
main : Html msg
main =
div [ ]
[ h1 [] [text "Elm is fun!" ]
, p [] [text "Let's learn some more!" ]
]
-- Get started with Elm on Windows 10
npm install -g elm
npm install -g elm-oracle
where.exe elm-oracle
C:\User\PC\AppData\Local\atom\app-1.19.7
C:\User\PC\AppData\Local\atom\app-1.19.7\resources\app\apm\bin
apm install atom-beautify
apm install elm-format
C:\Program Files (x86)\Elm Platform\0.18\bin
where.exe elm
C:\Program Files (x86)\Elm Platform\0.18\bin\elm-format.exe
-- testing elm-format with a poorly formatted elm file
-- command to run:
-- elm-format .\poorly-formatted-file.elm
module Main exposing (..)
import Html exposing (Html, text)
main =
text "Hello, Elm!"
apm install linter
C:\Users\PC\.atom\packages
elm-package install -y
-- Add some code to Main.elm to make sure it works:
module Main exposing (..)
import Html exposing (Html, text)
main =
text "Hello, <NAME>!" | true | -- the starting example
import Html exposing (text)
multiplyNumber x y =
x * y
main =
text ( toString ( multiplyNumber 8 10 ) )
-- another way to write the above
text ( toString ( multiplyNumber 2 ( multiplyNumber 2 ( multiplyNumber 2 10 ) ) ) )
-- piped syntax
import Html exposing (text)
multiplyNumber x y =
x * y
main =
multiplyNumber 2 10
|> multiplyNumber 2
|> multiplyNumber 2
|> toString
|> text
-- the starting example, re-written
import Html exposing (text)
multiplyNumber x y =
x * y
main =
multiplyNumber 8 10
|> toString
|> text
-- helpful type system
import Html exposing (text)
multiplyNumber: Int -> Int -> Int
multiplyNumber x y =
x * y
main =
multiplyNumber 8 10
|> toString
|> text
-- Get started writing Elm code
import Html exposing (text)
main =
text "Hello, World!"
-- With h1 function
import Html exposing (..)
main =
h1 [] [ text "Hello, Elm!" ]
-- With h2 function
h2 [] [ text "Hello, Elm!" ]
-- Making a link
main =
a [] [ text "Hello, Elm!" ]
-- Making an li
main =
li [] [ text "Hello, Elm!" ]
-- Making a paragraph
main =
p [] [ text "Hello, Elm!" ]
-- Making a paragraph
main =
p [] [ text "Hello, Elm!" ]
-- Nesting Html functions
import Html exposing (..)
main =
div []
[ p [] [text "1st paragraph" ]
, p [] [text "2nd paragraph" ]
]
-- Adding attributes
import Html exposing (..)
import Html.Attributes exposing (class)
main =
div [ class "danger" ]
[ p [] [text "1st paragraph" ]
, p [] [text "2nd paragraph" ]
]
-- Making a paragraph
main =
p [] [ text "Hello, Elm!" ]
-- Updating the style HTML tag in Ellie app
.danger {
background: red;
}
-- Adding type annotations
import Html exposing (..)
import Html.Attributes exposing (class)
main : Html msg
main =
div [ class "danger" ]
[ p [] [text "1st paragraph" ]
, p [] [text "2nd paragraph" ]
]
-- Get started fast with create-elm-app
npm install create-elm-app -g
create-elm-app elm-fun
elm-app start
-- Main.elm
import Html exposing (..)
import Html.Attributes exposing (class)
import Html exposing (..)
main : Html msg
main =
div [ ]
[ h1 [] [text "Elm is fun!" ]
, p [] [text "Let's learn some more!" ]
]
-- Get started with Elm on Windows 10
npm install -g elm
npm install -g elm-oracle
where.exe elm-oracle
C:\User\PC\AppData\Local\atom\app-1.19.7
C:\User\PC\AppData\Local\atom\app-1.19.7\resources\app\apm\bin
apm install atom-beautify
apm install elm-format
C:\Program Files (x86)\Elm Platform\0.18\bin
where.exe elm
C:\Program Files (x86)\Elm Platform\0.18\bin\elm-format.exe
-- testing elm-format with a poorly formatted elm file
-- command to run:
-- elm-format .\poorly-formatted-file.elm
module Main exposing (..)
import Html exposing (Html, text)
main =
text "Hello, Elm!"
apm install linter
C:\Users\PC\.atom\packages
elm-package install -y
-- Add some code to Main.elm to make sure it works:
module Main exposing (..)
import Html exposing (Html, text)
main =
text "Hello, PI:NAME:<NAME>END_PI!" | elm |
[
{
"context": ")\n\n\ndevs : Array.Array Dev\ndevs =\n [ { name = \"Tomke\", color = \"Green\", fontColor = \"Black\" }\n , { ",
"end": 669,
"score": 0.9995081425,
"start": 664,
"tag": "NAME",
"value": "Tomke"
},
{
"context": " = \"Green\", fontColor = \"Black\" }\n , { name = \"Gregor\", color = \"Purple\", fontColor = \"White\" }\n , {",
"end": 733,
"score": 0.9995567799,
"start": 727,
"tag": "NAME",
"value": "Gregor"
},
{
"context": "= \"Purple\", fontColor = \"White\" }\n , { name = \"Jonas\", color = \"Red\", fontColor = \"Black\" }\n , { na",
"end": 797,
"score": 0.9995867014,
"start": 792,
"tag": "NAME",
"value": "Jonas"
},
{
"context": "or = \"Red\", fontColor = \"Black\" }\n , { name = \"Jens\", color = \"Black\", fontColor = \"White\" }\n , { ",
"end": 857,
"score": 0.999597013,
"start": 853,
"tag": "NAME",
"value": "Jens"
},
{
"context": " = \"Black\", fontColor = \"White\" }\n , { name = \"Tim\", color = \"Yellow\", fontColor = \"Black\" }\n , {",
"end": 918,
"score": 0.9998419881,
"start": 915,
"tag": "NAME",
"value": "Tim"
},
{
"context": "= \"Yellow\", fontColor = \"Black\" }\n , { name = \"Daniel\", color = \"Orange\", fontColor = \"Black\" }\n ]\n ",
"end": 983,
"score": 0.999245286,
"start": 977,
"tag": "NAME",
"value": "Daniel"
}
] | App.elm | MrMovl/dailyCodeReview | 0 | module App exposing (..)
import Html exposing (..)
import CSS
import Time exposing (Time)
import Date
import Task
import Array exposing (Array)
msPerDay : Time
msPerDay =
86400000
dayRange : Int
dayRange =
15
main : Program Never Model Msg
main =
Html.program
{ init = init
, view = view
, update = update
, subscriptions = subscriptions
}
-- MODEL
type alias Dev =
{ name : String, color : String, fontColor : String }
type alias Model =
{ today : Time }
init : ( Model, Cmd Msg )
init =
( { today = 0 }, Task.perform Today Time.now )
devs : Array.Array Dev
devs =
[ { name = "Tomke", color = "Green", fontColor = "Black" }
, { name = "Gregor", color = "Purple", fontColor = "White" }
, { name = "Jonas", color = "Red", fontColor = "Black" }
, { name = "Jens", color = "Black", fontColor = "White" }
, { name = "Tim", color = "Yellow", fontColor = "Black" }
, { name = "Daniel", color = "Orange", fontColor = "Black" }
]
|> Array.fromList
-- UPDATE
type Msg
= Today Time
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
Today today ->
( { model | today = today }, Cmd.none )
-- SUBSCRIPTIONS
subscriptions : Model -> Sub Msg
subscriptions model =
Sub.none
-- VIEW
view : Model -> Html Msg
view model =
div [ CSS.body ]
[ h1 [ CSS.header ] [ text "Daily Code Review" ]
, div [ CSS.column ] [ model.today |> todayToPrintedDevs ]
, div [ CSS.column ] [ model.today |> todayToPrintedDays ]
]
todayToPrintedDays : Time -> Html Msg
todayToPrintedDays today =
today |> nextTwoWeeks |> withoutWeekends |> printDays
todayToPrintedDevs : Time -> Html Msg
todayToPrintedDevs today =
today
|> nextTwoWeeks
|> accountForWeekends
|> timeToDays
|> daysToDevs
|> printDevs
accountForWeekends : List Time -> List Time
accountForWeekends days =
List.foldr skipWeekends days days
skipWeekends : Time -> List Time -> List Time
skipWeekends day days =
if isWorkday day then
days
else
days |> List.reverse |> List.drop 1 |> List.reverse
withoutWeekends : List Time -> List Time
withoutWeekends days =
List.filter (\x -> x |> isWorkday) days
isWorkday : Time -> Bool
isWorkday time =
let
day =
time |> Date.fromTime |> Date.dayOfWeek
in
day /= Date.Sun && day /= Date.Sat
printDays : List Time -> Html Msg
printDays days =
div [] (List.map printDay days)
printDay : Time -> Html Msg
printDay time =
div [] [ time |> Date.fromTime |> prettyDate |> text ]
nextTwoWeeks : Time -> List Time
nextTwoWeeks today =
let
fourteenDays =
List.range 0 dayRange
indexToDayFromToday =
indexToDay today
in
List.map indexToDayFromToday fourteenDays
indexToDay : Time -> Int -> Time
indexToDay today i =
today + ((toFloat i) * msPerDay)
timeToDays : List Time -> List Int
timeToDays =
List.map (\x -> Time.inHours x |> flippedDevide 24 |> floor)
daysToDevs : List Int -> List (Maybe Dev)
daysToDevs =
let
devCount =
Array.length devs
indexToDev day =
day |> flippedModulo devCount |> flippedGet devs
in
List.map indexToDev
printDevs : List (Maybe Dev) -> Html Msg
printDevs devs =
div [] (List.map printDev devs)
printDev : Maybe Dev -> Html Msg
printDev dev =
case dev of
Just dev ->
div
[ CSS.backgroundColor dev.color dev.fontColor ]
[ text dev.name, br [] [] ]
Nothing ->
div [] [ text "I don't know that guy" ]
--Helper
flippedModulo : Int -> Int -> Int
flippedModulo =
flip (%)
flippedDevide : Float -> Float -> Float
flippedDevide =
flip (/)
flippedGet : Array a -> Int -> Maybe a
flippedGet =
flip Array.get
prettyDate : Date.Date -> String
prettyDate date =
if (date |> Date.day |> toString) == "NaN" then
"That's no date!"
else
(toString (Date.day date)) ++ ". " ++ (toString (Date.month date)) ++ " " ++ (toString (Date.year date))
| 30529 | module App exposing (..)
import Html exposing (..)
import CSS
import Time exposing (Time)
import Date
import Task
import Array exposing (Array)
msPerDay : Time
msPerDay =
86400000
dayRange : Int
dayRange =
15
main : Program Never Model Msg
main =
Html.program
{ init = init
, view = view
, update = update
, subscriptions = subscriptions
}
-- MODEL
type alias Dev =
{ name : String, color : String, fontColor : String }
type alias Model =
{ today : Time }
init : ( Model, Cmd Msg )
init =
( { today = 0 }, Task.perform Today Time.now )
devs : Array.Array Dev
devs =
[ { name = "<NAME>", color = "Green", fontColor = "Black" }
, { name = "<NAME>", color = "Purple", fontColor = "White" }
, { name = "<NAME>", color = "Red", fontColor = "Black" }
, { name = "<NAME>", color = "Black", fontColor = "White" }
, { name = "<NAME>", color = "Yellow", fontColor = "Black" }
, { name = "<NAME>", color = "Orange", fontColor = "Black" }
]
|> Array.fromList
-- UPDATE
type Msg
= Today Time
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
Today today ->
( { model | today = today }, Cmd.none )
-- SUBSCRIPTIONS
subscriptions : Model -> Sub Msg
subscriptions model =
Sub.none
-- VIEW
view : Model -> Html Msg
view model =
div [ CSS.body ]
[ h1 [ CSS.header ] [ text "Daily Code Review" ]
, div [ CSS.column ] [ model.today |> todayToPrintedDevs ]
, div [ CSS.column ] [ model.today |> todayToPrintedDays ]
]
todayToPrintedDays : Time -> Html Msg
todayToPrintedDays today =
today |> nextTwoWeeks |> withoutWeekends |> printDays
todayToPrintedDevs : Time -> Html Msg
todayToPrintedDevs today =
today
|> nextTwoWeeks
|> accountForWeekends
|> timeToDays
|> daysToDevs
|> printDevs
accountForWeekends : List Time -> List Time
accountForWeekends days =
List.foldr skipWeekends days days
skipWeekends : Time -> List Time -> List Time
skipWeekends day days =
if isWorkday day then
days
else
days |> List.reverse |> List.drop 1 |> List.reverse
withoutWeekends : List Time -> List Time
withoutWeekends days =
List.filter (\x -> x |> isWorkday) days
isWorkday : Time -> Bool
isWorkday time =
let
day =
time |> Date.fromTime |> Date.dayOfWeek
in
day /= Date.Sun && day /= Date.Sat
printDays : List Time -> Html Msg
printDays days =
div [] (List.map printDay days)
printDay : Time -> Html Msg
printDay time =
div [] [ time |> Date.fromTime |> prettyDate |> text ]
nextTwoWeeks : Time -> List Time
nextTwoWeeks today =
let
fourteenDays =
List.range 0 dayRange
indexToDayFromToday =
indexToDay today
in
List.map indexToDayFromToday fourteenDays
indexToDay : Time -> Int -> Time
indexToDay today i =
today + ((toFloat i) * msPerDay)
timeToDays : List Time -> List Int
timeToDays =
List.map (\x -> Time.inHours x |> flippedDevide 24 |> floor)
daysToDevs : List Int -> List (Maybe Dev)
daysToDevs =
let
devCount =
Array.length devs
indexToDev day =
day |> flippedModulo devCount |> flippedGet devs
in
List.map indexToDev
printDevs : List (Maybe Dev) -> Html Msg
printDevs devs =
div [] (List.map printDev devs)
printDev : Maybe Dev -> Html Msg
printDev dev =
case dev of
Just dev ->
div
[ CSS.backgroundColor dev.color dev.fontColor ]
[ text dev.name, br [] [] ]
Nothing ->
div [] [ text "I don't know that guy" ]
--Helper
flippedModulo : Int -> Int -> Int
flippedModulo =
flip (%)
flippedDevide : Float -> Float -> Float
flippedDevide =
flip (/)
flippedGet : Array a -> Int -> Maybe a
flippedGet =
flip Array.get
prettyDate : Date.Date -> String
prettyDate date =
if (date |> Date.day |> toString) == "NaN" then
"That's no date!"
else
(toString (Date.day date)) ++ ". " ++ (toString (Date.month date)) ++ " " ++ (toString (Date.year date))
| true | module App exposing (..)
import Html exposing (..)
import CSS
import Time exposing (Time)
import Date
import Task
import Array exposing (Array)
msPerDay : Time
msPerDay =
86400000
dayRange : Int
dayRange =
15
main : Program Never Model Msg
main =
Html.program
{ init = init
, view = view
, update = update
, subscriptions = subscriptions
}
-- MODEL
type alias Dev =
{ name : String, color : String, fontColor : String }
type alias Model =
{ today : Time }
init : ( Model, Cmd Msg )
init =
( { today = 0 }, Task.perform Today Time.now )
devs : Array.Array Dev
devs =
[ { name = "PI:NAME:<NAME>END_PI", color = "Green", fontColor = "Black" }
, { name = "PI:NAME:<NAME>END_PI", color = "Purple", fontColor = "White" }
, { name = "PI:NAME:<NAME>END_PI", color = "Red", fontColor = "Black" }
, { name = "PI:NAME:<NAME>END_PI", color = "Black", fontColor = "White" }
, { name = "PI:NAME:<NAME>END_PI", color = "Yellow", fontColor = "Black" }
, { name = "PI:NAME:<NAME>END_PI", color = "Orange", fontColor = "Black" }
]
|> Array.fromList
-- UPDATE
type Msg
= Today Time
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
Today today ->
( { model | today = today }, Cmd.none )
-- SUBSCRIPTIONS
subscriptions : Model -> Sub Msg
subscriptions model =
Sub.none
-- VIEW
view : Model -> Html Msg
view model =
div [ CSS.body ]
[ h1 [ CSS.header ] [ text "Daily Code Review" ]
, div [ CSS.column ] [ model.today |> todayToPrintedDevs ]
, div [ CSS.column ] [ model.today |> todayToPrintedDays ]
]
todayToPrintedDays : Time -> Html Msg
todayToPrintedDays today =
today |> nextTwoWeeks |> withoutWeekends |> printDays
todayToPrintedDevs : Time -> Html Msg
todayToPrintedDevs today =
today
|> nextTwoWeeks
|> accountForWeekends
|> timeToDays
|> daysToDevs
|> printDevs
accountForWeekends : List Time -> List Time
accountForWeekends days =
List.foldr skipWeekends days days
skipWeekends : Time -> List Time -> List Time
skipWeekends day days =
if isWorkday day then
days
else
days |> List.reverse |> List.drop 1 |> List.reverse
withoutWeekends : List Time -> List Time
withoutWeekends days =
List.filter (\x -> x |> isWorkday) days
isWorkday : Time -> Bool
isWorkday time =
let
day =
time |> Date.fromTime |> Date.dayOfWeek
in
day /= Date.Sun && day /= Date.Sat
printDays : List Time -> Html Msg
printDays days =
div [] (List.map printDay days)
printDay : Time -> Html Msg
printDay time =
div [] [ time |> Date.fromTime |> prettyDate |> text ]
nextTwoWeeks : Time -> List Time
nextTwoWeeks today =
let
fourteenDays =
List.range 0 dayRange
indexToDayFromToday =
indexToDay today
in
List.map indexToDayFromToday fourteenDays
indexToDay : Time -> Int -> Time
indexToDay today i =
today + ((toFloat i) * msPerDay)
timeToDays : List Time -> List Int
timeToDays =
List.map (\x -> Time.inHours x |> flippedDevide 24 |> floor)
daysToDevs : List Int -> List (Maybe Dev)
daysToDevs =
let
devCount =
Array.length devs
indexToDev day =
day |> flippedModulo devCount |> flippedGet devs
in
List.map indexToDev
printDevs : List (Maybe Dev) -> Html Msg
printDevs devs =
div [] (List.map printDev devs)
printDev : Maybe Dev -> Html Msg
printDev dev =
case dev of
Just dev ->
div
[ CSS.backgroundColor dev.color dev.fontColor ]
[ text dev.name, br [] [] ]
Nothing ->
div [] [ text "I don't know that guy" ]
--Helper
flippedModulo : Int -> Int -> Int
flippedModulo =
flip (%)
flippedDevide : Float -> Float -> Float
flippedDevide =
flip (/)
flippedGet : Array a -> Int -> Maybe a
flippedGet =
flip Array.get
prettyDate : Date.Date -> String
prettyDate date =
if (date |> Date.day |> toString) == "NaN" then
"That's no date!"
else
(toString (Date.day date)) ++ ". " ++ (toString (Date.month date)) ++ " " ++ (toString (Date.year date))
| elm |
[
{
"context": "sword password ->\n { model | password = password }\n PasswordAgain password ->\n {",
"end": 623,
"score": 0.5893293619,
"start": 615,
"tag": "PASSWORD",
"value": "password"
}
] | elm/arc-form/src/Main.elm | Bigsby/js-lib-test | 0 | module Main exposing (..)
import Browser
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onInput)
main = Browser.sandbox { init = init, update = update, view = view }
type alias Model =
{ name : String
, password : String
, passwordAgain : String
}
init : Model
init =
Model "" "" ""
type Msg
= Name String
| Password String
| PasswordAgain String
update : Msg -> Model -> Model
update msg model =
case msg of
Name name ->
{ model | name = name }
Password password ->
{ model | password = password }
PasswordAgain password ->
{ model | passwordAgain = password }
view : Model -> Html Msg
view model =
div []
[ viewInput "text" "Name" model.name Name
, viewInput "password" "Password" model.password Password
, viewInput "password" "Re-enter Password" model.passwordAgain PasswordAgain
, viewValidation model
]
viewInput : String -> String -> String -> (String -> msg) -> Html msg
viewInput t p v toMsg =
input [ type_ t, placeholder p, value v, onInput toMsg ] []
viewValidation : Model -> Html msg
viewValidation model =
if String.length model.password < 8 then
viewErrorMessage "Passwords must be, at least 8 characters long."
else if not (isPasswordValid model.password) then
viewErrorMessage "Password must contain uppper, lower and digit characters"
else if model.password == model.passwordAgain then
div [ style "color" "green" ] [ text "OK" ]
else
viewErrorMessage "Passwords do not match!"
passwordValidationFuncs =
[ Char.isDigit
, Char.isUpper
, Char.isLower
]
isPasswordValid : String -> Bool
isPasswordValid password =
List.foldl (\test prev -> prev && (String.any test password)) True passwordValidationFuncs
-- String.any Char.isDigit password && String.any Char.isUpper password && String.any Char.isLower password
viewErrorMessage : String -> Html msg
viewErrorMessage error =
div [ style "color" "red" ] [ text error ]
| 51365 | module Main exposing (..)
import Browser
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onInput)
main = Browser.sandbox { init = init, update = update, view = view }
type alias Model =
{ name : String
, password : String
, passwordAgain : String
}
init : Model
init =
Model "" "" ""
type Msg
= Name String
| Password String
| PasswordAgain String
update : Msg -> Model -> Model
update msg model =
case msg of
Name name ->
{ model | name = name }
Password password ->
{ model | password = <PASSWORD> }
PasswordAgain password ->
{ model | passwordAgain = password }
view : Model -> Html Msg
view model =
div []
[ viewInput "text" "Name" model.name Name
, viewInput "password" "Password" model.password Password
, viewInput "password" "Re-enter Password" model.passwordAgain PasswordAgain
, viewValidation model
]
viewInput : String -> String -> String -> (String -> msg) -> Html msg
viewInput t p v toMsg =
input [ type_ t, placeholder p, value v, onInput toMsg ] []
viewValidation : Model -> Html msg
viewValidation model =
if String.length model.password < 8 then
viewErrorMessage "Passwords must be, at least 8 characters long."
else if not (isPasswordValid model.password) then
viewErrorMessage "Password must contain uppper, lower and digit characters"
else if model.password == model.passwordAgain then
div [ style "color" "green" ] [ text "OK" ]
else
viewErrorMessage "Passwords do not match!"
passwordValidationFuncs =
[ Char.isDigit
, Char.isUpper
, Char.isLower
]
isPasswordValid : String -> Bool
isPasswordValid password =
List.foldl (\test prev -> prev && (String.any test password)) True passwordValidationFuncs
-- String.any Char.isDigit password && String.any Char.isUpper password && String.any Char.isLower password
viewErrorMessage : String -> Html msg
viewErrorMessage error =
div [ style "color" "red" ] [ text error ]
| true | module Main exposing (..)
import Browser
import Html exposing (..)
import Html.Attributes exposing (..)
import Html.Events exposing (onInput)
main = Browser.sandbox { init = init, update = update, view = view }
type alias Model =
{ name : String
, password : String
, passwordAgain : String
}
init : Model
init =
Model "" "" ""
type Msg
= Name String
| Password String
| PasswordAgain String
update : Msg -> Model -> Model
update msg model =
case msg of
Name name ->
{ model | name = name }
Password password ->
{ model | password = PI:PASSWORD:<PASSWORD>END_PI }
PasswordAgain password ->
{ model | passwordAgain = password }
view : Model -> Html Msg
view model =
div []
[ viewInput "text" "Name" model.name Name
, viewInput "password" "Password" model.password Password
, viewInput "password" "Re-enter Password" model.passwordAgain PasswordAgain
, viewValidation model
]
viewInput : String -> String -> String -> (String -> msg) -> Html msg
viewInput t p v toMsg =
input [ type_ t, placeholder p, value v, onInput toMsg ] []
viewValidation : Model -> Html msg
viewValidation model =
if String.length model.password < 8 then
viewErrorMessage "Passwords must be, at least 8 characters long."
else if not (isPasswordValid model.password) then
viewErrorMessage "Password must contain uppper, lower and digit characters"
else if model.password == model.passwordAgain then
div [ style "color" "green" ] [ text "OK" ]
else
viewErrorMessage "Passwords do not match!"
passwordValidationFuncs =
[ Char.isDigit
, Char.isUpper
, Char.isLower
]
isPasswordValid : String -> Bool
isPasswordValid password =
List.foldl (\test prev -> prev && (String.any test password)) True passwordValidationFuncs
-- String.any Char.isDigit password && String.any Char.isUpper password && String.any Char.isLower password
viewErrorMessage : String -> Html msg
viewErrorMessage error =
div [ style "color" "red" ] [ text error ]
| elm |
[
{
"context": "n that is hiring this role.\" \n \"Ex. SciRate\"\n m.job.organisationName\n ",
"end": 9532,
"score": 0.6376373768,
"start": 9529,
"tag": "NAME",
"value": "Sci"
},
{
"context": "at is hiring this role.\" \n \"Ex. SciRate\"\n m.job.organisationName\n ",
"end": 9536,
"score": 0.5626891255,
"start": 9532,
"tag": "USERNAME",
"value": "Rate"
}
] | src/SciRate/Views/SubmitOrEdit.elm | scirate/scirate-jobs | 2 | port module SciRate.Views.SubmitOrEdit exposing (..)
import Browser
import Date exposing (Date, day, month, weekday, year)
import DatePicker exposing (DatePicker, DateEvent(..), defaultSettings)
import Html exposing ( Html, a, button, div, h2, h3
, input, label , li, p, span, text, ul
, node, small, hr
)
import Html.Attributes exposing ( checked, class, disabled, for
, href, id, placeholder, title, type_, value
, name
)
import Html.Events exposing (onInput, onClick)
import Json.Encode as Encode
import Url exposing (Url)
import Dict exposing (Dict)
import Http
import Json.Decode as Decode exposing (Decoder, bool, string, list)
import Json.Decode.Pipeline exposing (required)
import Json.Encode as Encode exposing (object)
import SciRate.Data exposing (Job, decodeOneJob, decodeAffectedRows
, decodeJobId, InitData, emptyJob
)
import SciRate.Misc exposing (queryStringItems)
import SciRate.Querying exposing (runQuery)
import SciRate.Views.Sidebar exposing (renderJob, fromJob)
port openPaymentForm : (String, Int) -> Cmd msg
port academicComplete : (String, Int) -> Cmd msg
mapDefault : b -> (a -> b) -> Maybe a -> b
mapDefault a f m
= Maybe.map f m
|> Maybe.withDefault a
main : Program InitData Model Msg
main =
Browser.element
{ view = view
, init = init
, update = update
, subscriptions = \_ -> Sub.none
}
-- | Returns a job.
getQuery : String -> String
getQuery token = """
query {
jobs(where: {token: {_eq: \"""" ++ token ++ """\"}}) {
academic
closingDate
contactEmail
contactName
created
jobTitle
jobUrl
location
organisationName
organisationUrl
remote
tags
withdrawn
approved
}
}
"""
-- | Adds a job.
insertQuery : Job -> String
insertQuery j = """
mutation {
insert_jobs(
objects:
{ organisationName: \"""" ++ j.organisationName ++ """\"
, organisationUrl: \"""" ++ j.organisationUrl ++ """\"
, jobTitle: \"""" ++ j.jobTitle ++ """\"
, jobUrl: \"""" ++ j.jobUrl ++ """\"
, remote: """ ++ (fromBool j.remote) ++ """
, academic: """ ++ (fromBool j.academic) ++ """
, contactName: \"""" ++ j.contactName ++ """\"
, contactEmail: \"""" ++ j.contactEmail ++ """\"
, location: \"""" ++ j.location ++ """\"
, tags: \"""" ++ j.tags ++ """\"
, closingDate: \"""" ++ j.closingDate ++ """\"
, withdrawn: false
, approved: false
}
) {
returning { id }
}
}
"""
-- | Updates an existing job.
updateQuery : String -> Job -> String
updateQuery token j = """
mutation {
update_jobs(
where: {token: {_eq: \"""" ++ token ++ """\"}},
_set:
{ organisationName: \"""" ++ j.organisationName ++ """\"
, organisationUrl: \"""" ++ j.organisationUrl ++ """\"
, jobTitle: \"""" ++ j.jobTitle ++ """\"
, jobUrl: \"""" ++ j.jobUrl ++ """\"
, remote: """ ++ (fromBool j.remote) ++ """
, contactName: \"""" ++ j.contactName ++ """\"
, location: \"""" ++ j.location ++ """\"
, tags: \"""" ++ j.tags ++ """\"
, closingDate: \"""" ++ j.closingDate ++ """\"
, withdrawn: """ ++ (fromBool j.withdrawn) ++ """
, approved: """ ++ (fromBool j.approved) ++ """
}) {
affected_rows
}
}
"""
fromBool : Bool -> String
fromBool b = case b of
True -> "true"
False -> "false"
type alias Model =
{ job : Job
, note : Maybe String
, dirty : Bool
, closingDate : Maybe Date
, datePicker : DatePicker
, editMode : Bool
, jobUuid : Maybe String
, initData : InitData
, error : Maybe String
}
emptyModel : InitData -> DatePicker -> Model
emptyModel id d
= { job = emptyJob
, note = Nothing
, dirty = False
, closingDate = Nothing
, datePicker = d
, editMode = False
, jobUuid = Nothing
, initData = id
, error = Nothing
}
init : InitData -> ( Model, Cmd Msg )
init d =
let
model = emptyModel d datePicker
( datePicker, datePickerFx ) = DatePicker.init
jobUuid = Dict.get "i" (queryStringItems d.queryString)
editMode = case jobUuid of
Nothing -> False
Just _ -> True
baseCmds = [ Cmd.map ToDatePicker datePickerFx ]
query = getQuery (Maybe.withDefault "" jobUuid)
getJobByUuid = runQuery d.graphqlUrl d.jwtToken query SelectJobResponse decodeOneJob
cmds =
if editMode
then getJobByUuid :: baseCmds
else baseCmds
in
( { model
| jobUuid = jobUuid
, editMode = editMode
, initData = d
}
, Cmd.batch cmds
)
requiredFieldsSatisfied : Model -> Bool
requiredFieldsSatisfied m =
List.all (not << String.isEmpty) <| List.map String.trim
[ m.job.jobTitle
, m.job.jobUrl
, m.job.organisationName
, m.job.organisationUrl
, m.job.contactEmail
, m.job.contactName
, m.job.location
, m.job.closingDate
]
view : Model -> Html Msg
view m =
let
elt = if m.job.withdrawn
then div [] [ text "This job has been withdrawn." ]
else div [ class "job-form" ]
[ jobEntryFields m
, jobPreview m
]
in
elt
jobPreview : Model -> Html Msg
jobPreview m
= div [ class "preview-content"]
[ div [ class "preview" ]
[ h3 [] [ text "Preview" ]
, renderJob (fromJob m.job)
]
]
jobEntryFields : Model -> Html Msg
jobEntryFields m =
let
maybeNote = case m.note of
Just n ->
if not m.dirty
then [ p [ class "submit-note" ] [ text n ] ]
else []
Nothing -> []
maybeError = case m.error of
Just n -> [ p [ class "submit-error" ] [ text n ] ]
Nothing -> []
paySection
= [ h3 [] [ text "Payment" ]
, paymentSection m
]
editSection
= [ h3 [] [ text "Update" ]
, div [ class "payment-section" ]
[ div []
[ button [ class "update"
, onClick UpdateJob
]
[ text "Update job details" ]
, button [ class "withdraw"
, onClick WithdrawJob
]
[ text "Withdraw Posting" ]
]
, p [ class "note" ] [ text """
Note: All job updates will go through a moderation queue. Unless
there is an issue (in which case you will be contacted), it will
appear on the site promptly!
""" ]
]
]
finalSection
= case m.editMode of
True -> editSection
_ -> paySection
topSection
= case m.editMode of
True -> h2 [] [ text "Update job posting" ]
_ -> h2 [] [ text "Post a new job" ]
in
div []
[ topSection
, div [ class "job-content" ]
(
[ h2 [] [ text "Details" ]
, small [ class "req-note" ] [ text "Fields marked with a '*' are required." ]
, h3 [] [ text "Position details" ]
, positionDetails m
, h3 [] [ text "Contact details" ]
, contactDetails m
] ++ finalSection ++ maybeNote ++ maybeError
)
]
contactDetails : Model -> Html Msg
contactDetails m =
let
maybeEmail =
if not m.editMode
then
[ textField
"Contact email"
"Your contact email. You will receive a link to edit to this address. This will not be shown to anyone."
""
m.job.contactEmail
UpdateContactEmail
]
else []
in
div [ class "job-form-fields" ]
(maybeEmail ++
[ textField
"Contact Name"
"Your contact name. Used only if we need to get in touch we you."
""
m.job.contactName
UpdateContactName
])
positionDetails : Model -> Html Msg
positionDetails m =
let
maybeAcademic =
if not m.editMode
then
[ boolField
"Academic or Industry role?"
"Is this an academic position?"
"Academic"
"Industry"
m.job.academic
UpdateAcademic
]
else []
in
div []
[ div [ class "job-form-fields" ] <|
[ textField
"Organsation name"
"The organisation that is hiring this role."
"Ex. SciRate"
m.job.organisationName
UpdateOrganisationName
, textField
"Link to organisation website"
"A link where people can learn more about your company."
"Ex. https://scirate.com"
m.job.organisationUrl
UpdateOrganisationUrl
, sep
, textField
"Job title"
"The job title."
"Ex. Senior Quantum Researcher"
m.job.jobTitle
UpdateJobTitle
, textField
"Link to job"
"A link to a website with a job description and details on how to apply."
"Ex. https://yourwebsite.com/jobs/?jobId=..."
m.job.jobUrl
UpdateJobUrl
, closingDateField m
, sep
, boolField
"Remote?"
"Can this job be undertaken remotely?"
"Yes/Maybe"
"No"
m.job.remote
UpdateRemote
, textField
"Location"
"""
Primary location/time-zone where the job is based or
'Multiple' if there are options.
"""
"Ex. Melbourne, AU or Delft, NL or Multiple"
m.job.location
UpdateLocation
] ++
maybeAcademic ++
[ sep
, textFieldR
False
"Tags"
"""
Tags seperated by commas. Useful to help people filter for
jobs using these skills/technologies.
"""
"Ex. cirq, python, surface-code"
m.job.tags
UpdateTags
]
]
paymentSection : Model -> Html Msg
paymentSection m =
let sat = requiredFieldsSatisfied m
r = case sat of
True -> div [ class "payment" ] [ payButton m ]
False -> div [ class "payment" ] [ text "Please complete all required fields." ]
in
div [ class "payment-section" ]
[ r
, p [ class "note" ] [ text """
Note: All jobs will go through a moderation queue. Unless there is
an issue (in which case you will be contacted), it will appear on
the site promptly!
""" ]
]
payButton : Model -> Html Msg
payButton m =
case m.job.academic of
True ->
div [] [ p [] [ text "Academic jobs are free, presently!" ]
, button [ class "pay"
, onClick SubmitAcademicJob
]
[ text "Submit an academic job" ]
]
False ->
div [] [ button [ class "pay"
, onClick StartStripePayment
]
[ text "Pay with Stripe" ]
]
sep : Html Msg
sep = span [] []
-- | Potential actions.
type Msg
-- >> Model updates
= UpdateContactEmail String
| UpdateContactName String
| UpdateOrganisationName String
| UpdateOrganisationUrl String
| UpdateJobTitle String
| UpdateJobUrl String
| UpdateLocation String
| UpdateTags String
| UpdateAcademic Bool
| UpdateRemote Bool
| StartStripePayment
| SubmitAcademicJob
--
| UpdateJob
| InsertJobResponse String (Result Http.Error (Maybe Int))
| UpdateJobResponse String (Result Http.Error Int)
| SelectJobResponse (Result Http.Error (Maybe Job))
| WithdrawJob
--
| ToDatePicker DatePicker.Msg
dateSettings : DatePicker.Settings
dateSettings =
{ defaultSettings
| inputId = Just "date-field"
, inputName = Just "date"
, inputClassList = [ ("form-control", True) ]
}
-- | Main model update.
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model
= let
noop = ( model, Cmd.none )
in case msg of
ToDatePicker m ->
let
( newDatePicker, event )
= DatePicker.update dateSettings m model.datePicker
newModel
= { model
| closingDate = case event of
Picked date -> Just date
_ -> model.closingDate
, dirty = True
, datePicker = newDatePicker
}
oldJob = model.job
-- We want an error later on.
mStrDate = newModel.closingDate |> Maybe.map (Date.format "yyyy-MM-dd")
strDate = Maybe.withDefault "" mStrDate
newJob = { oldJob | closingDate = strDate }
in
( { newModel | job = newJob }, Cmd.none )
-- State update busywork
UpdateContactEmail s ->
let oldJob = model.job
newJob = { oldJob | contactEmail = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateContactName s ->
let oldJob = model.job
newJob = { oldJob | contactName = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateOrganisationName s ->
let oldJob = model.job
newJob = { oldJob | organisationName = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateOrganisationUrl s ->
let oldJob = model.job
newJob = { oldJob | organisationUrl = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateJobTitle s ->
let oldJob = model.job
newJob = { oldJob | jobTitle = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateJobUrl s ->
let oldJob = model.job
newJob = { oldJob | jobUrl = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateLocation s ->
let oldJob = model.job
newJob = { oldJob | location = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateTags s ->
let oldJob = model.job
newJob = { oldJob | tags = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateAcademic b ->
let oldJob = model.job
newJob = { oldJob | academic = b }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateRemote b ->
let oldJob = model.job
newJob = { oldJob | remote = b }
in ( { model | job = newJob, dirty = True }, Cmd.none )
-- Job things
StartStripePayment ->
( model , runQuery model.initData.graphqlUrl model.initData.jwtToken (insertQuery model.job) (InsertJobResponse "Opening stripe ...") decodeJobId )
SubmitAcademicJob ->
( model , runQuery model.initData.graphqlUrl model.initData.jwtToken (insertQuery model.job) (InsertJobResponse "Job submitted!") decodeJobId )
WithdrawJob ->
let
oldJob = model.job
newJob = { oldJob | withdrawn = True }
query t = updateQuery t newJob
f token = runQuery model.initData.graphqlUrl model.initData.jwtToken (query token) (UpdateJobResponse "Job withdrawn.") decodeAffectedRows
cmd = mapDefault Cmd.none f model.jobUuid
in
( model, cmd )
UpdateJob ->
let
oldJob = model.job
newJob = { oldJob | approved = False }
query t = updateQuery t newJob
f token = runQuery model.initData.graphqlUrl model.initData.jwtToken (query token) (UpdateJobResponse "Job updated!") decodeAffectedRows
cmd = mapDefault Cmd.none f model.jobUuid
in
( model, cmd )
UpdateJobResponse s response ->
let
newModel
= case response of
Ok _ -> { model | note = Just s, dirty = False }
_ -> { model | error = Just "Unable to update job." }
in
( newModel, Cmd.none )
InsertJobResponse s response ->
let
newCmd =
case response of
-- We only open payment if it's an industry job.
Ok (Just jobId) -> if model.job.academic
then academicComplete (model.job.contactEmail, jobId)
else openPaymentForm (model.job.contactEmail, jobId)
_ -> Cmd.none
newModel =
case response of
Ok (Just _) -> { model | note = Just s, dirty = False }
_ -> { model | error = Just "Unable to submit job." }
in
( newModel, newCmd )
SelectJobResponse response ->
let
newModel =
case response of
Ok (Just j) -> { model | job = j
, closingDate = stringToDate j.closingDate
}
_ -> model
in
( newModel, Cmd.none )
stringToDate : String -> Maybe Date
stringToDate s =
String.split "T" s
|> List.head
|> Maybe.andThen (Result.toMaybe << Date.fromIsoString)
closingDateField : Model -> Html Msg
closingDateField model
= let
name_ = "closing-date"
txt = "Job closing date*"
in
div [ class "field" ]
[ label [ for name_ ] [ text txt ]
, div [ class "field-input" ]
[ DatePicker.view model.closingDate dateSettings model.datePicker
|> Html.map ToDatePicker
, div [ class "help" ]
[
p [] [ text "Closing date in yyyy-MM-dd "
, a [ href "https://time.is/Anywhere_on_Earth" ] [ text "anywhere on earth" ]
, text ". The furthest this can be is 3 months into the future."
]
]
]
]
textField = textFieldR True
-- | Make a text input field.
textFieldR : Bool -- ^ Is it required?
-> String -- ^ Name of the html field
-> String -- ^ Help text
-> String -- ^ Placeholder value
-> String -- ^ Current field value
-> (String -> Msg) -- ^ Command to run
-> Html Msg
textFieldR req label_ help placeholder_ val msg
= let
txt = if req then
label_ ++ "*"
else
label_
name_ = label_
in
div [ class "field" ]
[ label [ for name_ ] [ text txt ]
, div [ class "field-input" ]
[ input [ type_ "text"
, id name_
, placeholder placeholder_
, onInput msg
, value val
] []
, div [ class "help" ] [ p [] [ text help ] ]
]
]
-- | Make a boolean field with radio buttons.
boolField : String -- ^ Name of the html field
-> String -- ^ Help text
-> String -- ^ Label for 'true' radio button
-> String -- ^ Label for 'false' radio button
-> Bool -- ^ Present value
-> (Bool -> Msg) -- ^ Command to run
-> Html Msg
boolField label_ help labelTrue labelFalse val msg
= let
mkRadio txt v =
[ input [ type_ "radio"
, name label_
, id <| label_ ++ v
, value v
, onInput (msg << ((==) "yes"))
, checked ((v == "yes" && val) || (v == "no" && not val))
] []
, label [ for (label_ ++ v), class "radio" ] [ text txt ]
]
in
div [ class "field" ]
[ label [] [ text label_ ]
, div [ class "field-input" ]
<| mkRadio labelTrue "yes"
++ mkRadio labelFalse "no"
++ [ div [ class "help" ] [ p [] [ text help ] ] ]
]
| 28055 | port module SciRate.Views.SubmitOrEdit exposing (..)
import Browser
import Date exposing (Date, day, month, weekday, year)
import DatePicker exposing (DatePicker, DateEvent(..), defaultSettings)
import Html exposing ( Html, a, button, div, h2, h3
, input, label , li, p, span, text, ul
, node, small, hr
)
import Html.Attributes exposing ( checked, class, disabled, for
, href, id, placeholder, title, type_, value
, name
)
import Html.Events exposing (onInput, onClick)
import Json.Encode as Encode
import Url exposing (Url)
import Dict exposing (Dict)
import Http
import Json.Decode as Decode exposing (Decoder, bool, string, list)
import Json.Decode.Pipeline exposing (required)
import Json.Encode as Encode exposing (object)
import SciRate.Data exposing (Job, decodeOneJob, decodeAffectedRows
, decodeJobId, InitData, emptyJob
)
import SciRate.Misc exposing (queryStringItems)
import SciRate.Querying exposing (runQuery)
import SciRate.Views.Sidebar exposing (renderJob, fromJob)
port openPaymentForm : (String, Int) -> Cmd msg
port academicComplete : (String, Int) -> Cmd msg
mapDefault : b -> (a -> b) -> Maybe a -> b
mapDefault a f m
= Maybe.map f m
|> Maybe.withDefault a
main : Program InitData Model Msg
main =
Browser.element
{ view = view
, init = init
, update = update
, subscriptions = \_ -> Sub.none
}
-- | Returns a job.
getQuery : String -> String
getQuery token = """
query {
jobs(where: {token: {_eq: \"""" ++ token ++ """\"}}) {
academic
closingDate
contactEmail
contactName
created
jobTitle
jobUrl
location
organisationName
organisationUrl
remote
tags
withdrawn
approved
}
}
"""
-- | Adds a job.
insertQuery : Job -> String
insertQuery j = """
mutation {
insert_jobs(
objects:
{ organisationName: \"""" ++ j.organisationName ++ """\"
, organisationUrl: \"""" ++ j.organisationUrl ++ """\"
, jobTitle: \"""" ++ j.jobTitle ++ """\"
, jobUrl: \"""" ++ j.jobUrl ++ """\"
, remote: """ ++ (fromBool j.remote) ++ """
, academic: """ ++ (fromBool j.academic) ++ """
, contactName: \"""" ++ j.contactName ++ """\"
, contactEmail: \"""" ++ j.contactEmail ++ """\"
, location: \"""" ++ j.location ++ """\"
, tags: \"""" ++ j.tags ++ """\"
, closingDate: \"""" ++ j.closingDate ++ """\"
, withdrawn: false
, approved: false
}
) {
returning { id }
}
}
"""
-- | Updates an existing job.
updateQuery : String -> Job -> String
updateQuery token j = """
mutation {
update_jobs(
where: {token: {_eq: \"""" ++ token ++ """\"}},
_set:
{ organisationName: \"""" ++ j.organisationName ++ """\"
, organisationUrl: \"""" ++ j.organisationUrl ++ """\"
, jobTitle: \"""" ++ j.jobTitle ++ """\"
, jobUrl: \"""" ++ j.jobUrl ++ """\"
, remote: """ ++ (fromBool j.remote) ++ """
, contactName: \"""" ++ j.contactName ++ """\"
, location: \"""" ++ j.location ++ """\"
, tags: \"""" ++ j.tags ++ """\"
, closingDate: \"""" ++ j.closingDate ++ """\"
, withdrawn: """ ++ (fromBool j.withdrawn) ++ """
, approved: """ ++ (fromBool j.approved) ++ """
}) {
affected_rows
}
}
"""
fromBool : Bool -> String
fromBool b = case b of
True -> "true"
False -> "false"
type alias Model =
{ job : Job
, note : Maybe String
, dirty : Bool
, closingDate : Maybe Date
, datePicker : DatePicker
, editMode : Bool
, jobUuid : Maybe String
, initData : InitData
, error : Maybe String
}
emptyModel : InitData -> DatePicker -> Model
emptyModel id d
= { job = emptyJob
, note = Nothing
, dirty = False
, closingDate = Nothing
, datePicker = d
, editMode = False
, jobUuid = Nothing
, initData = id
, error = Nothing
}
init : InitData -> ( Model, Cmd Msg )
init d =
let
model = emptyModel d datePicker
( datePicker, datePickerFx ) = DatePicker.init
jobUuid = Dict.get "i" (queryStringItems d.queryString)
editMode = case jobUuid of
Nothing -> False
Just _ -> True
baseCmds = [ Cmd.map ToDatePicker datePickerFx ]
query = getQuery (Maybe.withDefault "" jobUuid)
getJobByUuid = runQuery d.graphqlUrl d.jwtToken query SelectJobResponse decodeOneJob
cmds =
if editMode
then getJobByUuid :: baseCmds
else baseCmds
in
( { model
| jobUuid = jobUuid
, editMode = editMode
, initData = d
}
, Cmd.batch cmds
)
requiredFieldsSatisfied : Model -> Bool
requiredFieldsSatisfied m =
List.all (not << String.isEmpty) <| List.map String.trim
[ m.job.jobTitle
, m.job.jobUrl
, m.job.organisationName
, m.job.organisationUrl
, m.job.contactEmail
, m.job.contactName
, m.job.location
, m.job.closingDate
]
view : Model -> Html Msg
view m =
let
elt = if m.job.withdrawn
then div [] [ text "This job has been withdrawn." ]
else div [ class "job-form" ]
[ jobEntryFields m
, jobPreview m
]
in
elt
jobPreview : Model -> Html Msg
jobPreview m
= div [ class "preview-content"]
[ div [ class "preview" ]
[ h3 [] [ text "Preview" ]
, renderJob (fromJob m.job)
]
]
jobEntryFields : Model -> Html Msg
jobEntryFields m =
let
maybeNote = case m.note of
Just n ->
if not m.dirty
then [ p [ class "submit-note" ] [ text n ] ]
else []
Nothing -> []
maybeError = case m.error of
Just n -> [ p [ class "submit-error" ] [ text n ] ]
Nothing -> []
paySection
= [ h3 [] [ text "Payment" ]
, paymentSection m
]
editSection
= [ h3 [] [ text "Update" ]
, div [ class "payment-section" ]
[ div []
[ button [ class "update"
, onClick UpdateJob
]
[ text "Update job details" ]
, button [ class "withdraw"
, onClick WithdrawJob
]
[ text "Withdraw Posting" ]
]
, p [ class "note" ] [ text """
Note: All job updates will go through a moderation queue. Unless
there is an issue (in which case you will be contacted), it will
appear on the site promptly!
""" ]
]
]
finalSection
= case m.editMode of
True -> editSection
_ -> paySection
topSection
= case m.editMode of
True -> h2 [] [ text "Update job posting" ]
_ -> h2 [] [ text "Post a new job" ]
in
div []
[ topSection
, div [ class "job-content" ]
(
[ h2 [] [ text "Details" ]
, small [ class "req-note" ] [ text "Fields marked with a '*' are required." ]
, h3 [] [ text "Position details" ]
, positionDetails m
, h3 [] [ text "Contact details" ]
, contactDetails m
] ++ finalSection ++ maybeNote ++ maybeError
)
]
contactDetails : Model -> Html Msg
contactDetails m =
let
maybeEmail =
if not m.editMode
then
[ textField
"Contact email"
"Your contact email. You will receive a link to edit to this address. This will not be shown to anyone."
""
m.job.contactEmail
UpdateContactEmail
]
else []
in
div [ class "job-form-fields" ]
(maybeEmail ++
[ textField
"Contact Name"
"Your contact name. Used only if we need to get in touch we you."
""
m.job.contactName
UpdateContactName
])
positionDetails : Model -> Html Msg
positionDetails m =
let
maybeAcademic =
if not m.editMode
then
[ boolField
"Academic or Industry role?"
"Is this an academic position?"
"Academic"
"Industry"
m.job.academic
UpdateAcademic
]
else []
in
div []
[ div [ class "job-form-fields" ] <|
[ textField
"Organsation name"
"The organisation that is hiring this role."
"Ex. <NAME>Rate"
m.job.organisationName
UpdateOrganisationName
, textField
"Link to organisation website"
"A link where people can learn more about your company."
"Ex. https://scirate.com"
m.job.organisationUrl
UpdateOrganisationUrl
, sep
, textField
"Job title"
"The job title."
"Ex. Senior Quantum Researcher"
m.job.jobTitle
UpdateJobTitle
, textField
"Link to job"
"A link to a website with a job description and details on how to apply."
"Ex. https://yourwebsite.com/jobs/?jobId=..."
m.job.jobUrl
UpdateJobUrl
, closingDateField m
, sep
, boolField
"Remote?"
"Can this job be undertaken remotely?"
"Yes/Maybe"
"No"
m.job.remote
UpdateRemote
, textField
"Location"
"""
Primary location/time-zone where the job is based or
'Multiple' if there are options.
"""
"Ex. Melbourne, AU or Delft, NL or Multiple"
m.job.location
UpdateLocation
] ++
maybeAcademic ++
[ sep
, textFieldR
False
"Tags"
"""
Tags seperated by commas. Useful to help people filter for
jobs using these skills/technologies.
"""
"Ex. cirq, python, surface-code"
m.job.tags
UpdateTags
]
]
paymentSection : Model -> Html Msg
paymentSection m =
let sat = requiredFieldsSatisfied m
r = case sat of
True -> div [ class "payment" ] [ payButton m ]
False -> div [ class "payment" ] [ text "Please complete all required fields." ]
in
div [ class "payment-section" ]
[ r
, p [ class "note" ] [ text """
Note: All jobs will go through a moderation queue. Unless there is
an issue (in which case you will be contacted), it will appear on
the site promptly!
""" ]
]
payButton : Model -> Html Msg
payButton m =
case m.job.academic of
True ->
div [] [ p [] [ text "Academic jobs are free, presently!" ]
, button [ class "pay"
, onClick SubmitAcademicJob
]
[ text "Submit an academic job" ]
]
False ->
div [] [ button [ class "pay"
, onClick StartStripePayment
]
[ text "Pay with Stripe" ]
]
sep : Html Msg
sep = span [] []
-- | Potential actions.
type Msg
-- >> Model updates
= UpdateContactEmail String
| UpdateContactName String
| UpdateOrganisationName String
| UpdateOrganisationUrl String
| UpdateJobTitle String
| UpdateJobUrl String
| UpdateLocation String
| UpdateTags String
| UpdateAcademic Bool
| UpdateRemote Bool
| StartStripePayment
| SubmitAcademicJob
--
| UpdateJob
| InsertJobResponse String (Result Http.Error (Maybe Int))
| UpdateJobResponse String (Result Http.Error Int)
| SelectJobResponse (Result Http.Error (Maybe Job))
| WithdrawJob
--
| ToDatePicker DatePicker.Msg
dateSettings : DatePicker.Settings
dateSettings =
{ defaultSettings
| inputId = Just "date-field"
, inputName = Just "date"
, inputClassList = [ ("form-control", True) ]
}
-- | Main model update.
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model
= let
noop = ( model, Cmd.none )
in case msg of
ToDatePicker m ->
let
( newDatePicker, event )
= DatePicker.update dateSettings m model.datePicker
newModel
= { model
| closingDate = case event of
Picked date -> Just date
_ -> model.closingDate
, dirty = True
, datePicker = newDatePicker
}
oldJob = model.job
-- We want an error later on.
mStrDate = newModel.closingDate |> Maybe.map (Date.format "yyyy-MM-dd")
strDate = Maybe.withDefault "" mStrDate
newJob = { oldJob | closingDate = strDate }
in
( { newModel | job = newJob }, Cmd.none )
-- State update busywork
UpdateContactEmail s ->
let oldJob = model.job
newJob = { oldJob | contactEmail = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateContactName s ->
let oldJob = model.job
newJob = { oldJob | contactName = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateOrganisationName s ->
let oldJob = model.job
newJob = { oldJob | organisationName = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateOrganisationUrl s ->
let oldJob = model.job
newJob = { oldJob | organisationUrl = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateJobTitle s ->
let oldJob = model.job
newJob = { oldJob | jobTitle = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateJobUrl s ->
let oldJob = model.job
newJob = { oldJob | jobUrl = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateLocation s ->
let oldJob = model.job
newJob = { oldJob | location = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateTags s ->
let oldJob = model.job
newJob = { oldJob | tags = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateAcademic b ->
let oldJob = model.job
newJob = { oldJob | academic = b }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateRemote b ->
let oldJob = model.job
newJob = { oldJob | remote = b }
in ( { model | job = newJob, dirty = True }, Cmd.none )
-- Job things
StartStripePayment ->
( model , runQuery model.initData.graphqlUrl model.initData.jwtToken (insertQuery model.job) (InsertJobResponse "Opening stripe ...") decodeJobId )
SubmitAcademicJob ->
( model , runQuery model.initData.graphqlUrl model.initData.jwtToken (insertQuery model.job) (InsertJobResponse "Job submitted!") decodeJobId )
WithdrawJob ->
let
oldJob = model.job
newJob = { oldJob | withdrawn = True }
query t = updateQuery t newJob
f token = runQuery model.initData.graphqlUrl model.initData.jwtToken (query token) (UpdateJobResponse "Job withdrawn.") decodeAffectedRows
cmd = mapDefault Cmd.none f model.jobUuid
in
( model, cmd )
UpdateJob ->
let
oldJob = model.job
newJob = { oldJob | approved = False }
query t = updateQuery t newJob
f token = runQuery model.initData.graphqlUrl model.initData.jwtToken (query token) (UpdateJobResponse "Job updated!") decodeAffectedRows
cmd = mapDefault Cmd.none f model.jobUuid
in
( model, cmd )
UpdateJobResponse s response ->
let
newModel
= case response of
Ok _ -> { model | note = Just s, dirty = False }
_ -> { model | error = Just "Unable to update job." }
in
( newModel, Cmd.none )
InsertJobResponse s response ->
let
newCmd =
case response of
-- We only open payment if it's an industry job.
Ok (Just jobId) -> if model.job.academic
then academicComplete (model.job.contactEmail, jobId)
else openPaymentForm (model.job.contactEmail, jobId)
_ -> Cmd.none
newModel =
case response of
Ok (Just _) -> { model | note = Just s, dirty = False }
_ -> { model | error = Just "Unable to submit job." }
in
( newModel, newCmd )
SelectJobResponse response ->
let
newModel =
case response of
Ok (Just j) -> { model | job = j
, closingDate = stringToDate j.closingDate
}
_ -> model
in
( newModel, Cmd.none )
stringToDate : String -> Maybe Date
stringToDate s =
String.split "T" s
|> List.head
|> Maybe.andThen (Result.toMaybe << Date.fromIsoString)
closingDateField : Model -> Html Msg
closingDateField model
= let
name_ = "closing-date"
txt = "Job closing date*"
in
div [ class "field" ]
[ label [ for name_ ] [ text txt ]
, div [ class "field-input" ]
[ DatePicker.view model.closingDate dateSettings model.datePicker
|> Html.map ToDatePicker
, div [ class "help" ]
[
p [] [ text "Closing date in yyyy-MM-dd "
, a [ href "https://time.is/Anywhere_on_Earth" ] [ text "anywhere on earth" ]
, text ". The furthest this can be is 3 months into the future."
]
]
]
]
textField = textFieldR True
-- | Make a text input field.
textFieldR : Bool -- ^ Is it required?
-> String -- ^ Name of the html field
-> String -- ^ Help text
-> String -- ^ Placeholder value
-> String -- ^ Current field value
-> (String -> Msg) -- ^ Command to run
-> Html Msg
textFieldR req label_ help placeholder_ val msg
= let
txt = if req then
label_ ++ "*"
else
label_
name_ = label_
in
div [ class "field" ]
[ label [ for name_ ] [ text txt ]
, div [ class "field-input" ]
[ input [ type_ "text"
, id name_
, placeholder placeholder_
, onInput msg
, value val
] []
, div [ class "help" ] [ p [] [ text help ] ]
]
]
-- | Make a boolean field with radio buttons.
boolField : String -- ^ Name of the html field
-> String -- ^ Help text
-> String -- ^ Label for 'true' radio button
-> String -- ^ Label for 'false' radio button
-> Bool -- ^ Present value
-> (Bool -> Msg) -- ^ Command to run
-> Html Msg
boolField label_ help labelTrue labelFalse val msg
= let
mkRadio txt v =
[ input [ type_ "radio"
, name label_
, id <| label_ ++ v
, value v
, onInput (msg << ((==) "yes"))
, checked ((v == "yes" && val) || (v == "no" && not val))
] []
, label [ for (label_ ++ v), class "radio" ] [ text txt ]
]
in
div [ class "field" ]
[ label [] [ text label_ ]
, div [ class "field-input" ]
<| mkRadio labelTrue "yes"
++ mkRadio labelFalse "no"
++ [ div [ class "help" ] [ p [] [ text help ] ] ]
]
| true | port module SciRate.Views.SubmitOrEdit exposing (..)
import Browser
import Date exposing (Date, day, month, weekday, year)
import DatePicker exposing (DatePicker, DateEvent(..), defaultSettings)
import Html exposing ( Html, a, button, div, h2, h3
, input, label , li, p, span, text, ul
, node, small, hr
)
import Html.Attributes exposing ( checked, class, disabled, for
, href, id, placeholder, title, type_, value
, name
)
import Html.Events exposing (onInput, onClick)
import Json.Encode as Encode
import Url exposing (Url)
import Dict exposing (Dict)
import Http
import Json.Decode as Decode exposing (Decoder, bool, string, list)
import Json.Decode.Pipeline exposing (required)
import Json.Encode as Encode exposing (object)
import SciRate.Data exposing (Job, decodeOneJob, decodeAffectedRows
, decodeJobId, InitData, emptyJob
)
import SciRate.Misc exposing (queryStringItems)
import SciRate.Querying exposing (runQuery)
import SciRate.Views.Sidebar exposing (renderJob, fromJob)
port openPaymentForm : (String, Int) -> Cmd msg
port academicComplete : (String, Int) -> Cmd msg
mapDefault : b -> (a -> b) -> Maybe a -> b
mapDefault a f m
= Maybe.map f m
|> Maybe.withDefault a
main : Program InitData Model Msg
main =
Browser.element
{ view = view
, init = init
, update = update
, subscriptions = \_ -> Sub.none
}
-- | Returns a job.
getQuery : String -> String
getQuery token = """
query {
jobs(where: {token: {_eq: \"""" ++ token ++ """\"}}) {
academic
closingDate
contactEmail
contactName
created
jobTitle
jobUrl
location
organisationName
organisationUrl
remote
tags
withdrawn
approved
}
}
"""
-- | Adds a job.
insertQuery : Job -> String
insertQuery j = """
mutation {
insert_jobs(
objects:
{ organisationName: \"""" ++ j.organisationName ++ """\"
, organisationUrl: \"""" ++ j.organisationUrl ++ """\"
, jobTitle: \"""" ++ j.jobTitle ++ """\"
, jobUrl: \"""" ++ j.jobUrl ++ """\"
, remote: """ ++ (fromBool j.remote) ++ """
, academic: """ ++ (fromBool j.academic) ++ """
, contactName: \"""" ++ j.contactName ++ """\"
, contactEmail: \"""" ++ j.contactEmail ++ """\"
, location: \"""" ++ j.location ++ """\"
, tags: \"""" ++ j.tags ++ """\"
, closingDate: \"""" ++ j.closingDate ++ """\"
, withdrawn: false
, approved: false
}
) {
returning { id }
}
}
"""
-- | Updates an existing job.
updateQuery : String -> Job -> String
updateQuery token j = """
mutation {
update_jobs(
where: {token: {_eq: \"""" ++ token ++ """\"}},
_set:
{ organisationName: \"""" ++ j.organisationName ++ """\"
, organisationUrl: \"""" ++ j.organisationUrl ++ """\"
, jobTitle: \"""" ++ j.jobTitle ++ """\"
, jobUrl: \"""" ++ j.jobUrl ++ """\"
, remote: """ ++ (fromBool j.remote) ++ """
, contactName: \"""" ++ j.contactName ++ """\"
, location: \"""" ++ j.location ++ """\"
, tags: \"""" ++ j.tags ++ """\"
, closingDate: \"""" ++ j.closingDate ++ """\"
, withdrawn: """ ++ (fromBool j.withdrawn) ++ """
, approved: """ ++ (fromBool j.approved) ++ """
}) {
affected_rows
}
}
"""
fromBool : Bool -> String
fromBool b = case b of
True -> "true"
False -> "false"
type alias Model =
{ job : Job
, note : Maybe String
, dirty : Bool
, closingDate : Maybe Date
, datePicker : DatePicker
, editMode : Bool
, jobUuid : Maybe String
, initData : InitData
, error : Maybe String
}
emptyModel : InitData -> DatePicker -> Model
emptyModel id d
= { job = emptyJob
, note = Nothing
, dirty = False
, closingDate = Nothing
, datePicker = d
, editMode = False
, jobUuid = Nothing
, initData = id
, error = Nothing
}
init : InitData -> ( Model, Cmd Msg )
init d =
let
model = emptyModel d datePicker
( datePicker, datePickerFx ) = DatePicker.init
jobUuid = Dict.get "i" (queryStringItems d.queryString)
editMode = case jobUuid of
Nothing -> False
Just _ -> True
baseCmds = [ Cmd.map ToDatePicker datePickerFx ]
query = getQuery (Maybe.withDefault "" jobUuid)
getJobByUuid = runQuery d.graphqlUrl d.jwtToken query SelectJobResponse decodeOneJob
cmds =
if editMode
then getJobByUuid :: baseCmds
else baseCmds
in
( { model
| jobUuid = jobUuid
, editMode = editMode
, initData = d
}
, Cmd.batch cmds
)
requiredFieldsSatisfied : Model -> Bool
requiredFieldsSatisfied m =
List.all (not << String.isEmpty) <| List.map String.trim
[ m.job.jobTitle
, m.job.jobUrl
, m.job.organisationName
, m.job.organisationUrl
, m.job.contactEmail
, m.job.contactName
, m.job.location
, m.job.closingDate
]
view : Model -> Html Msg
view m =
let
elt = if m.job.withdrawn
then div [] [ text "This job has been withdrawn." ]
else div [ class "job-form" ]
[ jobEntryFields m
, jobPreview m
]
in
elt
jobPreview : Model -> Html Msg
jobPreview m
= div [ class "preview-content"]
[ div [ class "preview" ]
[ h3 [] [ text "Preview" ]
, renderJob (fromJob m.job)
]
]
jobEntryFields : Model -> Html Msg
jobEntryFields m =
let
maybeNote = case m.note of
Just n ->
if not m.dirty
then [ p [ class "submit-note" ] [ text n ] ]
else []
Nothing -> []
maybeError = case m.error of
Just n -> [ p [ class "submit-error" ] [ text n ] ]
Nothing -> []
paySection
= [ h3 [] [ text "Payment" ]
, paymentSection m
]
editSection
= [ h3 [] [ text "Update" ]
, div [ class "payment-section" ]
[ div []
[ button [ class "update"
, onClick UpdateJob
]
[ text "Update job details" ]
, button [ class "withdraw"
, onClick WithdrawJob
]
[ text "Withdraw Posting" ]
]
, p [ class "note" ] [ text """
Note: All job updates will go through a moderation queue. Unless
there is an issue (in which case you will be contacted), it will
appear on the site promptly!
""" ]
]
]
finalSection
= case m.editMode of
True -> editSection
_ -> paySection
topSection
= case m.editMode of
True -> h2 [] [ text "Update job posting" ]
_ -> h2 [] [ text "Post a new job" ]
in
div []
[ topSection
, div [ class "job-content" ]
(
[ h2 [] [ text "Details" ]
, small [ class "req-note" ] [ text "Fields marked with a '*' are required." ]
, h3 [] [ text "Position details" ]
, positionDetails m
, h3 [] [ text "Contact details" ]
, contactDetails m
] ++ finalSection ++ maybeNote ++ maybeError
)
]
contactDetails : Model -> Html Msg
contactDetails m =
let
maybeEmail =
if not m.editMode
then
[ textField
"Contact email"
"Your contact email. You will receive a link to edit to this address. This will not be shown to anyone."
""
m.job.contactEmail
UpdateContactEmail
]
else []
in
div [ class "job-form-fields" ]
(maybeEmail ++
[ textField
"Contact Name"
"Your contact name. Used only if we need to get in touch we you."
""
m.job.contactName
UpdateContactName
])
positionDetails : Model -> Html Msg
positionDetails m =
let
maybeAcademic =
if not m.editMode
then
[ boolField
"Academic or Industry role?"
"Is this an academic position?"
"Academic"
"Industry"
m.job.academic
UpdateAcademic
]
else []
in
div []
[ div [ class "job-form-fields" ] <|
[ textField
"Organsation name"
"The organisation that is hiring this role."
"Ex. PI:NAME:<NAME>END_PIRate"
m.job.organisationName
UpdateOrganisationName
, textField
"Link to organisation website"
"A link where people can learn more about your company."
"Ex. https://scirate.com"
m.job.organisationUrl
UpdateOrganisationUrl
, sep
, textField
"Job title"
"The job title."
"Ex. Senior Quantum Researcher"
m.job.jobTitle
UpdateJobTitle
, textField
"Link to job"
"A link to a website with a job description and details on how to apply."
"Ex. https://yourwebsite.com/jobs/?jobId=..."
m.job.jobUrl
UpdateJobUrl
, closingDateField m
, sep
, boolField
"Remote?"
"Can this job be undertaken remotely?"
"Yes/Maybe"
"No"
m.job.remote
UpdateRemote
, textField
"Location"
"""
Primary location/time-zone where the job is based or
'Multiple' if there are options.
"""
"Ex. Melbourne, AU or Delft, NL or Multiple"
m.job.location
UpdateLocation
] ++
maybeAcademic ++
[ sep
, textFieldR
False
"Tags"
"""
Tags seperated by commas. Useful to help people filter for
jobs using these skills/technologies.
"""
"Ex. cirq, python, surface-code"
m.job.tags
UpdateTags
]
]
paymentSection : Model -> Html Msg
paymentSection m =
let sat = requiredFieldsSatisfied m
r = case sat of
True -> div [ class "payment" ] [ payButton m ]
False -> div [ class "payment" ] [ text "Please complete all required fields." ]
in
div [ class "payment-section" ]
[ r
, p [ class "note" ] [ text """
Note: All jobs will go through a moderation queue. Unless there is
an issue (in which case you will be contacted), it will appear on
the site promptly!
""" ]
]
payButton : Model -> Html Msg
payButton m =
case m.job.academic of
True ->
div [] [ p [] [ text "Academic jobs are free, presently!" ]
, button [ class "pay"
, onClick SubmitAcademicJob
]
[ text "Submit an academic job" ]
]
False ->
div [] [ button [ class "pay"
, onClick StartStripePayment
]
[ text "Pay with Stripe" ]
]
sep : Html Msg
sep = span [] []
-- | Potential actions.
type Msg
-- >> Model updates
= UpdateContactEmail String
| UpdateContactName String
| UpdateOrganisationName String
| UpdateOrganisationUrl String
| UpdateJobTitle String
| UpdateJobUrl String
| UpdateLocation String
| UpdateTags String
| UpdateAcademic Bool
| UpdateRemote Bool
| StartStripePayment
| SubmitAcademicJob
--
| UpdateJob
| InsertJobResponse String (Result Http.Error (Maybe Int))
| UpdateJobResponse String (Result Http.Error Int)
| SelectJobResponse (Result Http.Error (Maybe Job))
| WithdrawJob
--
| ToDatePicker DatePicker.Msg
dateSettings : DatePicker.Settings
dateSettings =
{ defaultSettings
| inputId = Just "date-field"
, inputName = Just "date"
, inputClassList = [ ("form-control", True) ]
}
-- | Main model update.
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model
= let
noop = ( model, Cmd.none )
in case msg of
ToDatePicker m ->
let
( newDatePicker, event )
= DatePicker.update dateSettings m model.datePicker
newModel
= { model
| closingDate = case event of
Picked date -> Just date
_ -> model.closingDate
, dirty = True
, datePicker = newDatePicker
}
oldJob = model.job
-- We want an error later on.
mStrDate = newModel.closingDate |> Maybe.map (Date.format "yyyy-MM-dd")
strDate = Maybe.withDefault "" mStrDate
newJob = { oldJob | closingDate = strDate }
in
( { newModel | job = newJob }, Cmd.none )
-- State update busywork
UpdateContactEmail s ->
let oldJob = model.job
newJob = { oldJob | contactEmail = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateContactName s ->
let oldJob = model.job
newJob = { oldJob | contactName = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateOrganisationName s ->
let oldJob = model.job
newJob = { oldJob | organisationName = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateOrganisationUrl s ->
let oldJob = model.job
newJob = { oldJob | organisationUrl = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateJobTitle s ->
let oldJob = model.job
newJob = { oldJob | jobTitle = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateJobUrl s ->
let oldJob = model.job
newJob = { oldJob | jobUrl = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateLocation s ->
let oldJob = model.job
newJob = { oldJob | location = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateTags s ->
let oldJob = model.job
newJob = { oldJob | tags = s }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateAcademic b ->
let oldJob = model.job
newJob = { oldJob | academic = b }
in ( { model | job = newJob, dirty = True }, Cmd.none )
UpdateRemote b ->
let oldJob = model.job
newJob = { oldJob | remote = b }
in ( { model | job = newJob, dirty = True }, Cmd.none )
-- Job things
StartStripePayment ->
( model , runQuery model.initData.graphqlUrl model.initData.jwtToken (insertQuery model.job) (InsertJobResponse "Opening stripe ...") decodeJobId )
SubmitAcademicJob ->
( model , runQuery model.initData.graphqlUrl model.initData.jwtToken (insertQuery model.job) (InsertJobResponse "Job submitted!") decodeJobId )
WithdrawJob ->
let
oldJob = model.job
newJob = { oldJob | withdrawn = True }
query t = updateQuery t newJob
f token = runQuery model.initData.graphqlUrl model.initData.jwtToken (query token) (UpdateJobResponse "Job withdrawn.") decodeAffectedRows
cmd = mapDefault Cmd.none f model.jobUuid
in
( model, cmd )
UpdateJob ->
let
oldJob = model.job
newJob = { oldJob | approved = False }
query t = updateQuery t newJob
f token = runQuery model.initData.graphqlUrl model.initData.jwtToken (query token) (UpdateJobResponse "Job updated!") decodeAffectedRows
cmd = mapDefault Cmd.none f model.jobUuid
in
( model, cmd )
UpdateJobResponse s response ->
let
newModel
= case response of
Ok _ -> { model | note = Just s, dirty = False }
_ -> { model | error = Just "Unable to update job." }
in
( newModel, Cmd.none )
InsertJobResponse s response ->
let
newCmd =
case response of
-- We only open payment if it's an industry job.
Ok (Just jobId) -> if model.job.academic
then academicComplete (model.job.contactEmail, jobId)
else openPaymentForm (model.job.contactEmail, jobId)
_ -> Cmd.none
newModel =
case response of
Ok (Just _) -> { model | note = Just s, dirty = False }
_ -> { model | error = Just "Unable to submit job." }
in
( newModel, newCmd )
SelectJobResponse response ->
let
newModel =
case response of
Ok (Just j) -> { model | job = j
, closingDate = stringToDate j.closingDate
}
_ -> model
in
( newModel, Cmd.none )
stringToDate : String -> Maybe Date
stringToDate s =
String.split "T" s
|> List.head
|> Maybe.andThen (Result.toMaybe << Date.fromIsoString)
closingDateField : Model -> Html Msg
closingDateField model
= let
name_ = "closing-date"
txt = "Job closing date*"
in
div [ class "field" ]
[ label [ for name_ ] [ text txt ]
, div [ class "field-input" ]
[ DatePicker.view model.closingDate dateSettings model.datePicker
|> Html.map ToDatePicker
, div [ class "help" ]
[
p [] [ text "Closing date in yyyy-MM-dd "
, a [ href "https://time.is/Anywhere_on_Earth" ] [ text "anywhere on earth" ]
, text ". The furthest this can be is 3 months into the future."
]
]
]
]
textField = textFieldR True
-- | Make a text input field.
textFieldR : Bool -- ^ Is it required?
-> String -- ^ Name of the html field
-> String -- ^ Help text
-> String -- ^ Placeholder value
-> String -- ^ Current field value
-> (String -> Msg) -- ^ Command to run
-> Html Msg
textFieldR req label_ help placeholder_ val msg
= let
txt = if req then
label_ ++ "*"
else
label_
name_ = label_
in
div [ class "field" ]
[ label [ for name_ ] [ text txt ]
, div [ class "field-input" ]
[ input [ type_ "text"
, id name_
, placeholder placeholder_
, onInput msg
, value val
] []
, div [ class "help" ] [ p [] [ text help ] ]
]
]
-- | Make a boolean field with radio buttons.
boolField : String -- ^ Name of the html field
-> String -- ^ Help text
-> String -- ^ Label for 'true' radio button
-> String -- ^ Label for 'false' radio button
-> Bool -- ^ Present value
-> (Bool -> Msg) -- ^ Command to run
-> Html Msg
boolField label_ help labelTrue labelFalse val msg
= let
mkRadio txt v =
[ input [ type_ "radio"
, name label_
, id <| label_ ++ v
, value v
, onInput (msg << ((==) "yes"))
, checked ((v == "yes" && val) || (v == "no" && not val))
] []
, label [ for (label_ ++ v), class "radio" ] [ text txt ]
]
in
div [ class "field" ]
[ label [] [ text label_ ]
, div [ class "field-input" ]
<| mkRadio labelTrue "yes"
++ mkRadio labelFalse "no"
++ [ div [ class "help" ] [ p [] [ text help ] ] ]
]
| elm |
[
{
"context": " |> Stateful.withItems\n [ Book \"Dan Brown\" \"Angels & Demons\" \"2000\"\n , Book \"Dan",
"end": 1535,
"score": 0.9997617602,
"start": 1526,
"tag": "NAME",
"value": "Dan Brown"
},
{
"context": "own\" \"Angels & Demons\" \"2000\"\n , Book \"Dan Brown\" \"The Da Vinci Code\" \"2003\"\n , Book \"D",
"end": 1591,
"score": 0.9997771978,
"start": 1582,
"tag": "NAME",
"value": "Dan Brown"
},
{
"context": "n\" \"The Da Vinci Code\" \"2003\"\n , Book \"Dan Brown\" \"The Lost Symbol\" \"2009\"\n , Book \"Dan",
"end": 1649,
"score": 0.9997388124,
"start": 1640,
"tag": "NAME",
"value": "Dan Brown"
},
{
"context": "own\" \"The Lost Symbol\" \"2009\"\n , Book \"Dan Brown\" \"Inferno\" \"2013\"\n , Book \"Dan Brown\" ",
"end": 1705,
"score": 0.9997490048,
"start": 1696,
"tag": "NAME",
"value": "Dan Brown"
},
{
"context": " \"Dan Brown\" \"Inferno\" \"2013\"\n , Book \"Dan Brown\" \"Origin\" \"2017\"\n ]\n |> Statefu",
"end": 1753,
"score": 0.9997905493,
"start": 1744,
"tag": "NAME",
"value": "Dan Brown"
},
{
"context": "title\n |> localSingleTextFilter (Just \"Dan\") .author\n |> localSingleTextFilter No",
"end": 2830,
"score": 0.9996385574,
"start": 2827,
"tag": "NAME",
"value": "Dan"
},
{
"context": "row in this table.\n\n withItems\n [ Book \"Dan Brown\" \"Angels & Demons\" \"2000\"\n , Book \"Dan Bro",
"end": 7094,
"score": 0.9996591806,
"start": 7085,
"tag": "NAME",
"value": "Dan Brown"
},
{
"context": "n Brown\" \"Angels & Demons\" \"2000\"\n , Book \"Dan Brown\" \"The Da Vinci Code\" \"2003\"\n , Book \"Dan B",
"end": 7146,
"score": 0.9996746778,
"start": 7137,
"tag": "NAME",
"value": "Dan Brown"
},
{
"context": "Brown\" \"The Da Vinci Code\" \"2003\"\n , Book \"Dan Brown\" \"The Lost Symbol\" \"2009\"\n , Book \"Dan Bro",
"end": 7200,
"score": 0.9996232986,
"start": 7191,
"tag": "NAME",
"value": "Dan Brown"
},
{
"context": "n Brown\" \"The Lost Symbol\" \"2009\"\n , Book \"Dan Brown\" \"Inferno\" \"2013\"\n , Book \"Dan Brown\" \"Ori",
"end": 7252,
"score": 0.9996017218,
"start": 7243,
"tag": "NAME",
"value": "Dan Brown"
},
{
"context": "Book \"Dan Brown\" \"Inferno\" \"2013\"\n , Book \"Dan Brown\" \"Origin\" \"2017\"\n ]\n someTable\n\n-}\n",
"end": 7296,
"score": 0.9995765686,
"start": 7287,
"tag": "NAME",
"value": "Dan Brown"
},
{
"context": "n this table.\n\n stateWithItems\n [ Book \"Dan Brown\" \"Angels & Demons\" \"2000\"\n , Book \"Dan Bro",
"end": 8942,
"score": 0.9997377396,
"start": 8933,
"tag": "NAME",
"value": "Dan Brown"
},
{
"context": "n Brown\" \"Angels & Demons\" \"2000\"\n , Book \"Dan Brown\" \"The Da Vinci Code\" \"2003\"\n , Book \"Dan B",
"end": 8994,
"score": 0.9996903539,
"start": 8985,
"tag": "NAME",
"value": "Dan Brown"
},
{
"context": "Brown\" \"The Da Vinci Code\" \"2003\"\n , Book \"Dan Brown\" \"The Lost Symbol\" \"2009\"\n , Book \"Dan Bro",
"end": 9048,
"score": 0.9996641874,
"start": 9039,
"tag": "NAME",
"value": "Dan Brown"
},
{
"context": "n Brown\" \"The Lost Symbol\" \"2009\"\n , Book \"Dan Brown\" \"Inferno\" \"2013\"\n , Book \"Dan Brown\" \"Ori",
"end": 9100,
"score": 0.9997011423,
"start": 9091,
"tag": "NAME",
"value": "Dan Brown"
},
{
"context": "Book \"Dan Brown\" \"Inferno\" \"2013\"\n , Book \"Dan Brown\" \"Origin\" \"2017\"\n ]\n someTableState",
"end": 9144,
"score": 0.9996927977,
"start": 9135,
"tag": "NAME",
"value": "Dan Brown"
},
{
"context": "title\n |> localSingleTextFilter (Just \"Dan\") .author\n |> localSingleTextFilter No",
"end": 19211,
"score": 0.9964719415,
"start": 19208,
"tag": "NAME",
"value": "Dan"
}
] | src/UI/Tables/Stateful.elm | gregziegan/paack-ui | 47 | module UI.Tables.Stateful exposing
( StatefulTable, StatefulConfig, table, withItems
, Responsive, Cover, Details, Detail, withResponsive, detailsEmpty, detailShown, detailHidden
, State, Msg, init, update, stateWithItems
, Filters, filtersEmpty, stateWithFilters
, localSingleTextFilter, remoteSingleTextFilter
, localMultiTextFilter, remoteMultiTextFilter
, localSingleDateFilter, remoteSingleDateFilter
, localRangeDateFilter, remoteRangeDateFilter
, periodSingle, pariodAfter, periodBefore, localPeriodDateFilter, remotePeriodDateFilter
, localSelectFilter, remoteSelectFilter
, Sorters, stateWithSorters
, sortersEmpty, sortBy, sortByFloat, sortByInt, sortByChar, sortWith, unsortable
, sortDecreasing, sortIncreasing
, withWidth
, stateWithSelection, stateIsSelected
, renderElement
)
{-| Tables are a matrixial data disposition with rows, columns, headers, and cells.
`UI.Tables` are type-safe, which means that every row needs to have the same number of columns (including the headers). Otherwise, compilation fails.
Stateful.table
{ toExternalMsg = Msg.ForTable
, columns = Book.tableColumns
, toRow = Book.toTableRow
, state = model.tableState
}
|> Stateful.withResponsive
{ toDetails = Book.toTableDetails
, toCover = Book.toTableCover
}
|> Stateful.withWidth (Element.fill |> Element.maximum 640)
|> Stateful.withItems
[ Book "Dan Brown" "Angels & Demons" "2000"
, Book "Dan Brown" "The Da Vinci Code" "2003"
, Book "Dan Brown" "The Lost Symbol" "2009"
, Book "Dan Brown" "Inferno" "2013"
, Book "Dan Brown" "Origin" "2017"
]
|> Stateful.renderElement renderConfig
Where `Book` is:
type alias Book =
{ author : String, title : String, year : String }
tableColumns =
columnsEmpty
|> column "Title" (columnWidthPixels 320)
|> column "Author" (columnWidthPixels 240)
|> column "Year" (columnWidthPixels 120)
toTableRow =
{ toKey = .title, toTableRowView}
toTableRowView { author, title, year } =
rowEmpty
|> rowCellText (Text.body1 title)
|> rowCellText (Text.body2 author)
|> rowCellText (Text.caption year)
toTableDetails { author, title } =
detailsEmpty
|> detailHidden
|> detailShown { label = "Author", content = cellFromText <| Text.body2 author }
|> detailHidden
toTableCover { title, year } =
{ title = title, caption = Just year }
someFilters =
filtersEmpty
|> localSingleTextFilter Nothing .title
|> localSingleTextFilter (Just "Dan") .author
|> localSingleTextFilter Nothing .year
And on model:
{ -...
, tableState : Stateful.Table Msg.Msg TypeNumbers.Three
}
{ -...
, tableState = Stateful.stateWithFilters Book.someFilters Stateful.init
}
# Stateful
@docs StatefulTable, StatefulConfig, table, withItems
## Mobile
@docs Responsive, Cover, Details, Detail, withResponsive, detailsEmpty, detailShown, detailHidden
## State
@docs State, Msg, init, update, stateWithItems
# Filters
@docs Filters, filtersEmpty, stateWithFilters
## Single Text
@docs localSingleTextFilter, remoteSingleTextFilter
## Multi Text
@docs localMultiTextFilter, remoteMultiTextFilter
## Single DateInput
@docs localSingleDateFilter, remoteSingleDateFilter
## Range Dates
@docs localRangeDateFilter, remoteRangeDateFilter
## Period Dates
@docs periodSingle, pariodAfter, periodBefore, localPeriodDateFilter, remotePeriodDateFilter
## Select (Radio Buttons)
@docs localSelectFilter, remoteSelectFilter
# Sorting
@docs Sorters, stateWithSorters
@docs sortersEmpty, sortBy, sortByFloat, sortByInt, sortByChar, sortWith, unsortable
@docs sortDecreasing, sortIncreasing
# Width
@docs withWidth
# Selection
## Local
@docs stateWithSelection, stateIsSelected
## Remote
TODO: withRemoteSelection
# Rendering
@docs renderElement
-}
import Element exposing (Element, fill, px, shrink)
import Element.Keyed as Keyed
import Set exposing (Set)
import Time
import UI.Checkbox as Checkbox exposing (checkbox)
import UI.Effects as Effects exposing (Effects)
import UI.Internal.Basics exposing (ifThenElse, maybeThen, prependMaybe)
import UI.Internal.DateInput as DateInput exposing (DateInput, PeriodDate, RangeDate)
import UI.Internal.Filter.Model as Filter
import UI.Internal.Filter.Sorter exposing (Sorter(..))
import UI.Internal.NArray as NArray exposing (NArray)
import UI.Internal.RenderConfig exposing (localeTerms)
import UI.Internal.Tables.Common exposing (..)
import UI.Internal.Tables.Filters as Filters
import UI.Internal.Tables.FiltersView as FiltersView
import UI.Internal.Tables.ListView as ListView
import UI.Internal.Tables.Sorters as Sorters exposing (Sorters)
import UI.Internal.Tables.View exposing (..)
import UI.RenderConfig as RenderConfig exposing (RenderConfig)
import UI.Tables.Common as Common exposing (..)
import UI.Utils.TypeNumbers as T
{-| The `StatefulTable msg item columns` type is used for describing the component for later rendering.
This is type that constrains type-safe sized-arrays.
See [`TypeNumbers`](UI-Utils-TypeNumbers) for how to compose its phantom type.
-}
type StatefulTable msg item columns
= Table (StatefulConfig msg item columns) (Options msg item columns)
{-| Record with parameters for the creation of a [`StatefulTable`](#table).
This is record that constrains type-safe sized-arrays.
See [`TypeNumbers`](UI-Utils-TypeNumbers) for how to compose its phantom type.
{ toExternalMsg = Msg.ForTable
, columns = Book.tableColumns
, toRow = Book.toTableRow
, state = model.tableState
}
-}
type alias StatefulConfig msg item columns =
{ columns : Columns columns
, toRow : ToRow msg item columns
, toExternalMsg : Msg item -> msg
, state : State msg item columns
}
type alias Options msg item columns =
{ overwriteItems : Maybe (List item)
, width : Element.Length
, responsive : Maybe (Responsive msg item columns)
}
{-| Constructs a stateful table from its columns and rows.
Also defines the handling function for messages, and the current table's state.
table
{ columns = Book.tableColumns
, toRow = Book.toTableRow
, toExternalMsg = Msg.ForTable
, state = model.tableState
}
-}
table : StatefulConfig msg item columns -> StatefulTable msg item columns
table config =
Table config defaultOptions
defaultOptions : Options msg item columns
defaultOptions =
{ overwriteItems = Nothing
, width = shrink
, responsive = Nothing
}
{-| **DEPRECATED**: Use [stateWithItems](#stateWithItems) instead.
Otherwise, by using this you'll be discarding sorting and fitlering.
Each of these items will become a row in this table.
withItems
[ Book "Dan Brown" "Angels & Demons" "2000"
, Book "Dan Brown" "The Da Vinci Code" "2003"
, Book "Dan Brown" "The Lost Symbol" "2009"
, Book "Dan Brown" "Inferno" "2013"
, Book "Dan Brown" "Origin" "2017"
]
someTable
-}
withItems : List item -> StatefulTable msg item columns -> StatefulTable msg item columns
withItems items (Table prop opt) =
Table prop { opt | overwriteItems = Just items }
-- State
{-| The `Stateful.Msg` handles stateful table's related messages.
-}
type Msg item
= MobileToggle Int
| ForFilters Filters.Msg
| ForSorters Sorters.Msg
| FilterDialogOpen Int
| FilterDialogClose
| SelectionToggleAll
| SelectionSet item Bool
{-| Keep this one in your Model, it holds the table's current state.
-}
type State msg item columns
= State (StateModel msg item columns)
type alias StateModel msg item columns =
{ filters : Maybe (Filters msg item columns)
, mobileSelected : Maybe Int
, filterDialog : Maybe Int
, localSelection : Maybe (Selection item)
, items : List item
, visibleItems : List item
, sorters : Maybe (Sorters item columns)
}
type Selections
= Individual (Set String)
| All
| Except (Set String)
type alias Selection item =
{ identifier : item -> String
, checks : Selections
}
{-| The correct way of instantiating a [`Table.State`](#State).
{ -- ...
, tableState = Stateful.init
-- ...
}
-}
init : State msg item columns
init =
State
{ filters = Nothing
, mobileSelected = Nothing
, filterDialog = Nothing
, localSelection = Nothing
, items = []
, visibleItems = []
, sorters = Nothing
}
{-| Each of these items will become a row in this table.
stateWithItems
[ Book "Dan Brown" "Angels & Demons" "2000"
, Book "Dan Brown" "The Da Vinci Code" "2003"
, Book "Dan Brown" "The Lost Symbol" "2009"
, Book "Dan Brown" "Inferno" "2013"
, Book "Dan Brown" "Origin" "2017"
]
someTableState
-}
stateWithItems : List item -> State msg item columns -> State msg item columns
stateWithItems items (State state) =
State
{ state
| items = items
, visibleItems =
items
|> maybeThen Filters.itemsApplyFilters state.filters
|> maybeThen Sorters.itemsApplySorting state.sorters
}
{-| Given a message, apply an update to the [`Table.State`](#State).
Do not ignore the returned `Cmd`, it may include remote filter's messages.
( newModel, newCmd ) =
Table.update subMsg oldModel.tableState
-}
update : Msg item -> State msg item columns -> ( State msg item columns, Effects msg )
update msg (State state) =
case msg of
MobileToggle index ->
updateMobileToggle state index
ForFilters subMsg ->
updateFilters state subMsg
ForSorters subMsg ->
updateSorters state subMsg
FilterDialogOpen index ->
( State { state | filterDialog = Just index }, Effects.none )
FilterDialogClose ->
( State { state | filterDialog = Nothing }, Effects.none )
SelectionToggleAll ->
updateSelectionToggleAll state
SelectionSet item value ->
updateSelectionSet state item value
updateMobileToggle : StateModel msg item columns -> Int -> ( State msg item columns, Effects msg )
updateMobileToggle state index =
( State
{ state
| mobileSelected =
if state.mobileSelected == Just index then
Nothing
else
Just index
}
, Effects.none
)
updateFilters : StateModel msg item columns -> Filters.Msg -> ( State msg item columns, Effects msg )
updateFilters state subMsg =
let
map ( newFilters, { effects, closeDialog } ) =
( applyFilters newFilters <|
if closeDialog then
{ state | filterDialog = Nothing }
else
state
, effects
)
in
case state.filters of
Just filters ->
filters
|> Filters.update subMsg
|> map
Nothing ->
( State state, Effects.none )
applyFilters : Filters msg item columns -> StateModel msg item columns -> State msg item columns
applyFilters newFilters state =
State
{ state
| visibleItems =
state.items
|> Filters.itemsApplyFilters newFilters
|> maybeThen Sorters.itemsApplySorting state.sorters
, filters = Just newFilters
}
updateSorters : StateModel msg item columns -> Sorters.Msg -> ( State msg item columns, Effects msg )
updateSorters state subMsg =
case state.sorters of
Just sorters ->
sorters
|> Sorters.update subMsg
|> (\( newSorters, subCmd ) ->
( state
|> applySorters newSorters
, subCmd
)
)
Nothing ->
( State state, Effects.none )
applySorters : Sorters item columns -> StateModel msg item columns -> State msg item columns
applySorters newSorters state =
State
{ state
| visibleItems =
state.items
|> maybeThen Filters.itemsApplyFilters state.filters
|> Sorters.itemsApplySorting newSorters
, sorters = Just newSorters
, filterDialog = Nothing
}
updateSelectionToggleAll : StateModel msg item columns -> ( State msg item columns, Effects msg )
updateSelectionToggleAll state =
let
invertAll old =
{ old
| checks =
case old.checks of
Individual _ ->
All
All ->
Individual Set.empty
Except set ->
if Set.isEmpty set then
Individual Set.empty
else
All
}
in
( State { state | localSelection = Maybe.map invertAll state.localSelection }
, Effects.none
)
updateSelectionSet : StateModel msg item columns -> item -> Bool -> ( State msg item columns, Effects msg )
updateSelectionSet state item value =
let
setItem old =
{ old
| checks =
case old.checks of
Individual set ->
selectIndividual old item value set
All ->
selectExcept old item value Set.empty
Except set ->
selectExcept old item value set
}
in
( State { state | localSelection = Maybe.map setItem state.localSelection }
, Effects.none
)
selectIndividual : Selection item -> item -> Bool -> Set String -> Selections
selectIndividual { identifier } item value set =
set
|> ifThenElse value
Set.insert
Set.remove
(identifier item)
|> Individual
selectExcept : Selection item -> item -> Bool -> Set String -> Selections
selectExcept { identifier } item value set =
set
|> ifThenElse value
Set.remove
Set.insert
(identifier item)
|> Except
-- Responsive
{-| Required information for displaying the mobile's layout.
This is record that constrains type-safe sized-arrays.
See [`TypeNumbers`](UI-Utils-TypeNumbers) for how to compose its phantom type.
{ toDetails = Book.toTableDetails
, toCover = Book.toTableCover
}
-}
type alias Responsive msg item columns =
{ toDetails : item -> Details msg columns
, toCover : item -> Cover
}
{-| What is displayed in a collapsed mobile's row.
{ title = "Foo Fighters - Everlong"
, caption = Just "Morumbi - São Paulo 2015-01-23"
}
-}
type alias Cover =
{ title : String, caption : Maybe String }
{-| Used to render a cell in the mobile's layout.
-}
type alias Detail msg =
{ label : String, content : Common.Cell msg }
{-| A set of [`Detail msg`](#Detail).
Must have the same amount of elements as cells do in the table's row.
-}
type alias Details msg columns =
NArray (Maybe (Detail msg)) columns
{-| Allows a table to have a responsive layout when on mobile.
withResponsive
{ toDetails = Book.toTableDetails
, toCover = Book.toTableCover
}
someTable
-}
withResponsive : Responsive msg item columns -> StatefulTable msg item columns -> StatefulTable msg item columns
withResponsive responsive (Table prop opt) =
Table prop { opt | responsive = Just responsive }
{-| An empty [`Details`](#Details) set.
toTableDetails { author, title } =
detailsEmpty
|> detailHidden
|> detailShown
{ label = "Author"
, content = cellFromText (Text.body2 author)
}
|> detailHidden
-}
detailsEmpty : Details msg T.Zero
detailsEmpty =
NArray.empty
{-| Defines that a cell will be shown in the mobile's layout.
detailShown
{ label = "Edit"
, content = cellFromButton editButton
}
detailsSet
-}
detailShown : Detail msg -> Details msg columns -> Details msg (T.Increase columns)
detailShown detail accu =
NArray.push (Just detail) accu
{-| Defines that a cell will be hidden in the mobile's layout.
detailsEmpty
|> detailHidden
|> detailHidden
|> detailHidden
-}
detailHidden : Details msg columns -> Details msg (T.Increase columns)
detailHidden accu =
NArray.push Nothing accu
-- Selection
{-| Apply selection defintion to a table's [`State`](#State).
model =
stateWithSelection Book.getISBN init
-}
stateWithSelection : (item -> String) -> Bool -> State msg item columns -> State msg item columns
stateWithSelection identifier default (State state) =
State
{ state
| localSelection =
Just
{ checks =
if default then
All
else
Individual Set.empty
, identifier = identifier
}
}
{-| Resolves if one item's row is or not selected.
isHungerGamesSelected =
Table.stateIsSelected hungerGamesBook tableState
-}
stateIsSelected : item -> State msg item columns -> Bool
stateIsSelected item (State state) =
case state.localSelection of
Just selection ->
internalIsSelected selection item
Nothing ->
False
-- Filters
type alias PeriodComparison =
DateInput.PeriodComparison
{-| Array with all the columns' filters and their initial state.
This is a type-safe sized-array.
See [`TypeNumbers`](UI-Utils-TypeNumbers) for how to compose its phantom type.
-}
type alias Filters msg item columns =
Filters.Filters msg item columns
{-| Apply filters defintion to a table's [`State`](#State).
model =
stateWithFilters Book.filtersInit init
-}
stateWithFilters : Filters msg item columns -> State msg item columns -> State msg item columns
stateWithFilters filters (State state) =
State
{ state
| filters = Just filters
, visibleItems =
state.items
|> Filters.itemsApplyFilters filters
|> maybeThen Sorters.itemsApplySorting state.sorters
}
{-| An empty [`Filters`](#Filters) set.
toTableDetails { author, title } =
filtersEmpty
|> localSingleTextFilter Nothing .title
|> localSingleTextFilter (Just "Dan") .author
|> localSingleTextFilter Nothing .year
-}
filtersEmpty : Filters msg item T.Zero
filtersEmpty =
Filters.empty
{-| A filter with one single text field.
Only part of the content must match the filter's input.
Filtering logic is applied internally by the component.
localSingleTextFilter
maybeInitialValue
mapItemToString
-}
localSingleTextFilter :
Maybe String
-> (item -> String)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
localSingleTextFilter initValue getData accu =
Filters.push
(Filter.singleTextLocal initValue getData)
accu
{-| A filter with one single text field.
Only part of the content must match the filter's input.
Filtering logic is applied through an external message.
When `Nothing` is applied to the message, it means to clear the current filter.
remoteSingleTextFilter
maybeInitialValue
Msg.ApplyFilter
-}
remoteSingleTextFilter :
Maybe String
-> (Maybe String -> msg)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
remoteSingleTextFilter initValue applyMsg accu =
Filters.push
(Filter.singleTextRemote initValue applyMsg)
accu
{-| A filter with multiple text field.
The content must match at least one of those fields otherwise it's filtered out.
Only part of the content must match the filter's input.
Filtering logic is applied internally by the component.
localMultiTextFilter
[]
mapItemToString
For having an initial filter applied:
localMultiTextFilter
[ "initial", "fields" ]
mapItemToString
-}
localMultiTextFilter :
List String
-> (item -> String)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
localMultiTextFilter initValue getData accu =
Filters.push
(Filter.multiTextLocal initValue getData)
accu
{-| A filter with multiple text field.
The content must match at least one of those fields otherwise it's filtered out.
Only part of the content must match the filter's input.
Filtering logic is applied through an external message.
When an empty list is applied to the message, it means to clear the current filter.
remoteMultiTextFilter
[ "initial", "fields" ]
Msg.ApplyFilter
-}
remoteMultiTextFilter :
List String
-> (List String -> msg)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
remoteMultiTextFilter initValue applyMsg accu =
Filters.push
(Filter.multiTextRemote initValue applyMsg)
accu
{-| A filter for dates with one single field.
Filtering logic is applied internally by the component.
localSingleDateFilter timeZone
(Just somePosixEpoch)
mapItemToPosixEpoch
-}
localSingleDateFilter :
Time.Zone
-> Maybe Time.Posix
-> (item -> Time.Posix)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
localSingleDateFilter timeZone initValue getData accu =
Filters.push
(Filter.singleDateLocal timeZone initValue getData)
accu
{-| A filter for dates with one single field.
Filtering logic is applied through an external message.
When `Nothing` is applied to the message, it means to clear the current filter.
remoteSingleDateFilter
maybeInitialPosix
Msg.ApplyFilter
-}
remoteSingleDateFilter :
Time.Zone
-> Maybe Time.Posix
-> (Maybe DateInput -> msg)
-> Filters.Filters msg item columns
-> Filters.Filters msg item (T.Increase columns)
remoteSingleDateFilter timeZone initValue applyMsg accu =
Filters.push
(Filter.singleDateRemote timeZone initValue applyMsg)
accu
{-| A filter for dates in an expected range.
The range is defined using two date fields.
Filtering logic is applied internally by the component.
localRangeDateFilter timeZone
(Just datesAfterThis)
(Just datesBeforeThis)
mapItemToPosixEpoch
**NOTE**: Hours, minutes and seconds are discarded from the range limits.
-}
localRangeDateFilter :
Time.Zone
-> Maybe Time.Posix
-> Maybe Time.Posix
-> (item -> Time.Posix)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
localRangeDateFilter timeZone fromTime toTime getData accu =
Filters.push
(Filter.rangeDateLocal timeZone fromTime toTime getData)
accu
{-| A filter for dates in an expected range.
The range is defined using two date fields.
Filtering logic is applied through an external message.
When `Nothing` is applied to the message, it means to clear the current filter.
remoteRangeDateFilter timeZone
(Just datesAfterThis)
(Just datesBeforeThis)
Msg.ApplyFilter
**NOTE**: Hours, minutes and seconds are discarded from the range limits.
-}
remoteRangeDateFilter :
Time.Zone
-> Maybe Time.Posix
-> Maybe Time.Posix
-> (Maybe RangeDate -> msg)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
remoteRangeDateFilter timeZone fromTime toTime applyMsg accu =
Filters.push
(Filter.rangeDateRemote timeZone fromTime toTime applyMsg)
accu
{-| A filter for a single date, dates before specified date, or dates after specified date.
The filter-case is defined using radio buttons.
Filtering logic is applied internally by the component.
localPeriodDateFilter timeZone
(Just somePosixEpoch)
(Just periodAfter)
mapItemToPosixEpoch
**NOTE**: Hours, minutes and seconds are discarded from the range limits.
-}
localPeriodDateFilter :
Time.Zone
-> Maybe Time.Posix
-> Maybe PeriodComparison
-> (item -> Time.Posix)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
localPeriodDateFilter timeZone initValue initComparison getData accu =
Filters.push
(Filter.periodDateLocal "table-date-period-filter" timeZone initValue initComparison getData)
accu
{-| A filter for a single date, dates before specified date, or dates after specified date.
The filter-case is defined using radio buttons.
Filtering logic is applied through an external message.
When `Nothing` is applied to the message, it means to clear the current filter.
remotePeriodDateFilter timeZone
(Just somePosixEpoch)
(Just periodAfter)
Msg.ApplyFilter
**NOTE**: Hours, minutes and seconds are discarded from the range limits.
-}
remotePeriodDateFilter :
Time.Zone
-> Maybe Time.Posix
-> Maybe PeriodComparison
-> (Maybe PeriodDate -> msg)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
remotePeriodDateFilter timeZone initValue initComparison applyMsg accu =
Filters.push
(Filter.periodDateRemote "table-date-period-filter" timeZone initValue initComparison applyMsg)
accu
{-| A filter for custom radio buttons.
Filtering logic is applied internally by the component.
localSelectFilter
[ "Option 1"
, "Option 2"
]
(Just 1)
mapItemEachOptionToBool
-}
localSelectFilter :
List String
-> Maybe Int
-> (item -> Int -> Bool)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
localSelectFilter initList initSelection getData accu =
Filters.push
(Filter.selectLocal "table-select-filter" initList initSelection getData)
accu
{-| A filter for custom radio buttons.
Filtering logic is applied through an external message.
When `Nothing` is applied to the message, it means to clear the current filter.
remoteSelectFilter
[ "Option 1"
, "Option 2"
]
(Just 1)
Msg.ApplyFilter
-}
remoteSelectFilter :
List String
-> Maybe Int
-> (Maybe Int -> msg)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
remoteSelectFilter initList initSelection applyMsg accu =
Filters.push
(Filter.selectRemote "table-select-filter" initList initSelection applyMsg)
accu
-- Periods
{-| When comparing if dates are the same.
-}
periodSingle : PeriodComparison
periodSingle =
DateInput.On
{-| When comparing if some date is after another.
-}
pariodAfter : PeriodComparison
pariodAfter =
DateInput.After
{-| When comparing if some date is before another.
-}
periodBefore : PeriodComparison
periodBefore =
DateInput.Before
-- Width
{-| Applies [`Element.width`](/packages/mdgriffith/elm-ui/latest/Element#width) to the component.
Table.withWidth
(Element.fill |> Element.minimum 220)
someTable
-}
withWidth : Element.Length -> StatefulTable msg item columns -> StatefulTable msg item columns
withWidth width (Table prop opt_) =
Table prop { opt_ | width = width }
-- Sorting
{-| Array with all the columns' sorting definitions.
This is a type-safe sized-array.
See [`TypeNumbers`](UI-Utils-TypeNumbers) for how to compose its phantom type.
-}
type alias Sorters item columns =
Sorters.Sorters item columns
{-| Apply sortings defintion to a table's [`State`](#State).
model =
stateWithSorters Book.sortersInit init
-}
stateWithSorters : Sorters item columns -> State msg item columns -> State msg item columns
stateWithSorters sorters (State state) =
State
{ state
| sorters = Just sorters
, visibleItems =
state.items
|> maybeThen Filters.itemsApplyFilters state.filters
|> Sorters.itemsApplySorting sorters
}
{-| Allow sorting a column alphabetically.
sortersInit =
sortersEmpty
|> sortBy .title
|> sortBy .author
|> unsortable
-}
sortBy :
(item -> String)
-> Sorters item columns
-> Sorters item (T.Increase columns)
sortBy fn =
Sorters.sortWith (AlphabeticalSortable fn)
{-| Allow sorting a column using a Float value.
sortersInit =
sortersEmpty
|> unsortable
|> sortByFloat .value
|> sortByFloat .timestamp
|> sortByFloat .average
-}
sortByFloat :
(item -> Float)
-> Sorters item columns
-> Sorters item (T.Increase columns)
sortByFloat fn =
Sorters.sortWith (FloatSortable fn)
{-| Allow sorting a column using an Integer value.
sortersInit =
sortersEmpty
|> unsortable
|> sortByInt .count
|> sortByInt .areaCode
|> sortByInt .hour
-}
sortByInt :
(item -> Int)
-> Sorters item columns
-> Sorters item (T.Increase columns)
sortByInt fn =
Sorters.sortWith (IntegerSortable fn)
{-| Allow sorting a column using a Char value.
sortersInit =
sortersEmpty
|> unsortable
|> sortByChar .firstLetter
-}
sortByChar :
(item -> Char)
-> Sorters item columns
-> Sorters item (T.Increase columns)
sortByChar fn =
Sorters.sortWith (CharSortable fn)
{-| Allow sorting a column with a custom function.
Check [`List.sortWith`](https://package.elm-lang.org/packages/elm/core/latest/List#sortWith)
sortersInit =
sortersEmpty
|> unsortable
|> sortWith flippedComparison
-}
sortWith :
(item -> item -> Order)
-> Sorters item columns
-> Sorters item (T.Increase columns)
sortWith fn =
Sorters.sortWith (CustomSortable fn)
{-| Changes the initial sorting to some columns as descreasing.
model =
stateWithSorters
(Book.sortersInit |> sortDecreasing 1)
init
-}
sortDecreasing : Int -> Sorters item columns -> Sorters item columns
sortDecreasing =
Sorters.sortDescending
{-| Changes the initial sorting to some columns as increasing.
model =
stateWithSorters
(Book.sortersInit |> sortIncreasing 1)
init
-}
sortIncreasing : Int -> Sorters item columns -> Sorters item columns
sortIncreasing =
Sorters.sortAscending
{-| An empty [`Sorters`](#Sorters) set.
sortersInit =
sortersEmpty
|> sortBy .title
|> sortBy .author
|> unsortable
-}
sortersEmpty : Sorters item T.Zero
sortersEmpty =
Sorters.sortersEmpty
{-| Describes that some column is not sortable.
sortersInit =
sortersEmpty
|> sortBy .title
|> sortBy .author
|> unsortable
-}
unsortable : Sorters item columns -> Sorters item (T.Increase columns)
unsortable =
Sorters.unsortable
-- Rendering
{-| End of the builder's life.
The result of this function is a ready-to-insert Elm UI's Element.
-}
renderElement : RenderConfig -> StatefulTable msg item columns -> Element msg
renderElement renderConfig (Table prop opt) =
case opt.responsive of
Just responsive ->
if RenderConfig.isMobile renderConfig then
mobileView renderConfig prop opt responsive
else
desktopView renderConfig prop opt
Nothing ->
desktopView renderConfig prop opt
-- Mobile rendering
mobileView :
RenderConfig
-> StatefulConfig msg item columns
-> Options msg item columns
-> Responsive msg item columns
-> Element msg
mobileView renderConfig prop opt responsive =
let
detailsTerms =
renderConfig |> localeTerms >> .tables >> .details
items =
viewGetItems (unwrapState prop.state) opt
|> List.indexedMap Tuple.pair
in
ListView.toggleableList
{ detailsShowLabel = detailsTerms.show
, detailsCollapseLabel = detailsTerms.collapse
, toKey = Tuple.second >> prop.toRow.toKey
, toCover = Tuple.second >> responsive.toCover
, toDetails =
Tuple.second
>> responsive.toDetails
>> NArray.toList
>> List.filterMap (Maybe.map (detailView renderConfig))
, selectMsg = Tuple.first >> MobileToggle >> prop.toExternalMsg
}
|> ListView.withItems items
|> ListView.withSelected (Tuple.first >> isSelected prop.state)
|> ListView.renderElement renderConfig
isSelected : State msg item columns -> Int -> Bool
isSelected (State { mobileSelected }) position =
Just position == mobileSelected
detailView : RenderConfig -> Detail msg -> ( String, Element msg )
detailView renderConfig { label, content } =
( label, cellContentRender renderConfig content )
-- Dekstop rendering
desktopView :
RenderConfig
-> StatefulConfig msg item columns
-> Options msg item columns
-> Element msg
desktopView renderConfig prop opt =
let
columns =
NArray.toList prop.columns
state =
unwrapState prop.state
items =
viewGetItems state opt
rows =
List.map (rowWithSelection renderConfig prop.toExternalMsg state prop.toRow columns) items
padding =
{ top = 20, left = 20, right = 20, bottom = 0 }
selectionHeader =
viewSelectionHeader renderConfig state prop.toExternalMsg
headers =
headersRender renderConfig
prop.toExternalMsg
state.filterDialog
state.filters
state.sorters
columns
selectionHeader
in
Keyed.column
[ Element.spacing 2
, Element.width opt.width
, Element.paddingEach padding
]
(headers :: rows)
viewSelectionHeader : RenderConfig -> StateModel msg item columns -> (Msg item -> msg) -> Maybe (Element msg)
viewSelectionHeader renderConfig state toExternalMsg =
Maybe.map
(SelectionToggleAll
|> toExternalMsg
|> FiltersView.headerSelectToggle renderConfig
|> always
)
state.localSelection
viewGetItems : StateModel msg item columns -> Options msg item columns -> List item
viewGetItems { visibleItems } opt =
Maybe.withDefault visibleItems opt.overwriteItems
unwrapState : State msg item columns -> StateModel msg item columns
unwrapState (State model) =
model
rowWithSelection :
RenderConfig
-> (Msg item -> msg)
-> StateModel msg item columns
-> ToRow msg item columns
-> List Column
-> item
-> ( String, Element msg )
rowWithSelection renderConfig msgMap state toRow columns item =
rowBox <|
case state.localSelection of
Just selection ->
item
|> rowRender renderConfig toRow columns
|> Tuple.mapSecond
((::)
(item
|> selectionCell renderConfig selection
|> Tuple.mapSecond (Element.map msgMap)
)
)
Nothing ->
rowRender renderConfig toRow columns item
headersRender :
RenderConfig
-> (Msg item -> msg)
-> Maybe Int
-> Maybe (Filters.Filters msg item columns)
-> Maybe (Sorters.Sorters item columns)
-> List Column
-> Maybe (Element msg)
-> ( String, Element msg )
headersRender renderConfig toExternalMsg selected filters sorters columns selectionHeader =
( "@headers"
, Element.row headersAttr <|
case filters of
Just filterArr ->
filterArr
|> NArray.toList
|> List.map2 (filterHeader renderConfig toExternalMsg selected) columns
|> List.indexedMap
(\index val ->
val (Maybe.andThen (Sorters.get index) sorters) index
)
|> prependMaybe selectionHeader
Nothing ->
List.map
(\(Column header { width }) ->
header
|> simpleHeaderRender renderConfig
|> cellSpace width
)
columns
)
filterHeader :
RenderConfig
-> (Msg item -> msg)
-> Maybe Int
-> Column
-> Filter.Filter msg item
-> Sorters.ColumnStatus item
-> Int
-> Element msg
filterHeader renderConfig toExternalMsg selected (Column header { width }) filter sorter index =
FiltersView.header renderConfig
filter
sorter
{ openMsg = toExternalMsg <| FilterDialogOpen index
, discardMsg = toExternalMsg <| FilterDialogClose
, fromFiltersMsg = ForFilters >> toExternalMsg
, fromSortersMsg = ForSorters >> toExternalMsg
, index = index
, label = header
, isOpen = selected == Just index
}
|> topCellSpace width
-- Selection
internalIsSelected : Selection item -> item -> Bool
internalIsSelected { identifier, checks } item =
case checks of
Individual set ->
Set.member (identifier item) set
All ->
True
Except set ->
set
|> Set.member (identifier item)
|> not
selectionCell : RenderConfig -> Selection item -> item -> ( String, Element (Msg item) )
selectionCell renderConfig selection item =
item
|> internalIsSelected selection
|> checkbox (localeTerms renderConfig |> .tables |> .selectRow) (SelectionSet item)
|> Checkbox.withHiddenLabel
|> Checkbox.renderElement renderConfig
|> Element.el
[ Element.centerX, Element.centerY ]
|> Element.el
[ Element.width (px 32), Element.height fill ]
|> Tuple.pair "@select"
| 36627 | module UI.Tables.Stateful exposing
( StatefulTable, StatefulConfig, table, withItems
, Responsive, Cover, Details, Detail, withResponsive, detailsEmpty, detailShown, detailHidden
, State, Msg, init, update, stateWithItems
, Filters, filtersEmpty, stateWithFilters
, localSingleTextFilter, remoteSingleTextFilter
, localMultiTextFilter, remoteMultiTextFilter
, localSingleDateFilter, remoteSingleDateFilter
, localRangeDateFilter, remoteRangeDateFilter
, periodSingle, pariodAfter, periodBefore, localPeriodDateFilter, remotePeriodDateFilter
, localSelectFilter, remoteSelectFilter
, Sorters, stateWithSorters
, sortersEmpty, sortBy, sortByFloat, sortByInt, sortByChar, sortWith, unsortable
, sortDecreasing, sortIncreasing
, withWidth
, stateWithSelection, stateIsSelected
, renderElement
)
{-| Tables are a matrixial data disposition with rows, columns, headers, and cells.
`UI.Tables` are type-safe, which means that every row needs to have the same number of columns (including the headers). Otherwise, compilation fails.
Stateful.table
{ toExternalMsg = Msg.ForTable
, columns = Book.tableColumns
, toRow = Book.toTableRow
, state = model.tableState
}
|> Stateful.withResponsive
{ toDetails = Book.toTableDetails
, toCover = Book.toTableCover
}
|> Stateful.withWidth (Element.fill |> Element.maximum 640)
|> Stateful.withItems
[ Book "<NAME>" "Angels & Demons" "2000"
, Book "<NAME>" "The Da Vinci Code" "2003"
, Book "<NAME>" "The Lost Symbol" "2009"
, Book "<NAME>" "Inferno" "2013"
, Book "<NAME>" "Origin" "2017"
]
|> Stateful.renderElement renderConfig
Where `Book` is:
type alias Book =
{ author : String, title : String, year : String }
tableColumns =
columnsEmpty
|> column "Title" (columnWidthPixels 320)
|> column "Author" (columnWidthPixels 240)
|> column "Year" (columnWidthPixels 120)
toTableRow =
{ toKey = .title, toTableRowView}
toTableRowView { author, title, year } =
rowEmpty
|> rowCellText (Text.body1 title)
|> rowCellText (Text.body2 author)
|> rowCellText (Text.caption year)
toTableDetails { author, title } =
detailsEmpty
|> detailHidden
|> detailShown { label = "Author", content = cellFromText <| Text.body2 author }
|> detailHidden
toTableCover { title, year } =
{ title = title, caption = Just year }
someFilters =
filtersEmpty
|> localSingleTextFilter Nothing .title
|> localSingleTextFilter (Just "<NAME>") .author
|> localSingleTextFilter Nothing .year
And on model:
{ -...
, tableState : Stateful.Table Msg.Msg TypeNumbers.Three
}
{ -...
, tableState = Stateful.stateWithFilters Book.someFilters Stateful.init
}
# Stateful
@docs StatefulTable, StatefulConfig, table, withItems
## Mobile
@docs Responsive, Cover, Details, Detail, withResponsive, detailsEmpty, detailShown, detailHidden
## State
@docs State, Msg, init, update, stateWithItems
# Filters
@docs Filters, filtersEmpty, stateWithFilters
## Single Text
@docs localSingleTextFilter, remoteSingleTextFilter
## Multi Text
@docs localMultiTextFilter, remoteMultiTextFilter
## Single DateInput
@docs localSingleDateFilter, remoteSingleDateFilter
## Range Dates
@docs localRangeDateFilter, remoteRangeDateFilter
## Period Dates
@docs periodSingle, pariodAfter, periodBefore, localPeriodDateFilter, remotePeriodDateFilter
## Select (Radio Buttons)
@docs localSelectFilter, remoteSelectFilter
# Sorting
@docs Sorters, stateWithSorters
@docs sortersEmpty, sortBy, sortByFloat, sortByInt, sortByChar, sortWith, unsortable
@docs sortDecreasing, sortIncreasing
# Width
@docs withWidth
# Selection
## Local
@docs stateWithSelection, stateIsSelected
## Remote
TODO: withRemoteSelection
# Rendering
@docs renderElement
-}
import Element exposing (Element, fill, px, shrink)
import Element.Keyed as Keyed
import Set exposing (Set)
import Time
import UI.Checkbox as Checkbox exposing (checkbox)
import UI.Effects as Effects exposing (Effects)
import UI.Internal.Basics exposing (ifThenElse, maybeThen, prependMaybe)
import UI.Internal.DateInput as DateInput exposing (DateInput, PeriodDate, RangeDate)
import UI.Internal.Filter.Model as Filter
import UI.Internal.Filter.Sorter exposing (Sorter(..))
import UI.Internal.NArray as NArray exposing (NArray)
import UI.Internal.RenderConfig exposing (localeTerms)
import UI.Internal.Tables.Common exposing (..)
import UI.Internal.Tables.Filters as Filters
import UI.Internal.Tables.FiltersView as FiltersView
import UI.Internal.Tables.ListView as ListView
import UI.Internal.Tables.Sorters as Sorters exposing (Sorters)
import UI.Internal.Tables.View exposing (..)
import UI.RenderConfig as RenderConfig exposing (RenderConfig)
import UI.Tables.Common as Common exposing (..)
import UI.Utils.TypeNumbers as T
{-| The `StatefulTable msg item columns` type is used for describing the component for later rendering.
This is type that constrains type-safe sized-arrays.
See [`TypeNumbers`](UI-Utils-TypeNumbers) for how to compose its phantom type.
-}
type StatefulTable msg item columns
= Table (StatefulConfig msg item columns) (Options msg item columns)
{-| Record with parameters for the creation of a [`StatefulTable`](#table).
This is record that constrains type-safe sized-arrays.
See [`TypeNumbers`](UI-Utils-TypeNumbers) for how to compose its phantom type.
{ toExternalMsg = Msg.ForTable
, columns = Book.tableColumns
, toRow = Book.toTableRow
, state = model.tableState
}
-}
type alias StatefulConfig msg item columns =
{ columns : Columns columns
, toRow : ToRow msg item columns
, toExternalMsg : Msg item -> msg
, state : State msg item columns
}
type alias Options msg item columns =
{ overwriteItems : Maybe (List item)
, width : Element.Length
, responsive : Maybe (Responsive msg item columns)
}
{-| Constructs a stateful table from its columns and rows.
Also defines the handling function for messages, and the current table's state.
table
{ columns = Book.tableColumns
, toRow = Book.toTableRow
, toExternalMsg = Msg.ForTable
, state = model.tableState
}
-}
table : StatefulConfig msg item columns -> StatefulTable msg item columns
table config =
Table config defaultOptions
defaultOptions : Options msg item columns
defaultOptions =
{ overwriteItems = Nothing
, width = shrink
, responsive = Nothing
}
{-| **DEPRECATED**: Use [stateWithItems](#stateWithItems) instead.
Otherwise, by using this you'll be discarding sorting and fitlering.
Each of these items will become a row in this table.
withItems
[ Book "<NAME>" "Angels & Demons" "2000"
, Book "<NAME>" "The Da Vinci Code" "2003"
, Book "<NAME>" "The Lost Symbol" "2009"
, Book "<NAME>" "Inferno" "2013"
, Book "<NAME>" "Origin" "2017"
]
someTable
-}
withItems : List item -> StatefulTable msg item columns -> StatefulTable msg item columns
withItems items (Table prop opt) =
Table prop { opt | overwriteItems = Just items }
-- State
{-| The `Stateful.Msg` handles stateful table's related messages.
-}
type Msg item
= MobileToggle Int
| ForFilters Filters.Msg
| ForSorters Sorters.Msg
| FilterDialogOpen Int
| FilterDialogClose
| SelectionToggleAll
| SelectionSet item Bool
{-| Keep this one in your Model, it holds the table's current state.
-}
type State msg item columns
= State (StateModel msg item columns)
type alias StateModel msg item columns =
{ filters : Maybe (Filters msg item columns)
, mobileSelected : Maybe Int
, filterDialog : Maybe Int
, localSelection : Maybe (Selection item)
, items : List item
, visibleItems : List item
, sorters : Maybe (Sorters item columns)
}
type Selections
= Individual (Set String)
| All
| Except (Set String)
type alias Selection item =
{ identifier : item -> String
, checks : Selections
}
{-| The correct way of instantiating a [`Table.State`](#State).
{ -- ...
, tableState = Stateful.init
-- ...
}
-}
init : State msg item columns
init =
State
{ filters = Nothing
, mobileSelected = Nothing
, filterDialog = Nothing
, localSelection = Nothing
, items = []
, visibleItems = []
, sorters = Nothing
}
{-| Each of these items will become a row in this table.
stateWithItems
[ Book "<NAME>" "Angels & Demons" "2000"
, Book "<NAME>" "The Da Vinci Code" "2003"
, Book "<NAME>" "The Lost Symbol" "2009"
, Book "<NAME>" "Inferno" "2013"
, Book "<NAME>" "Origin" "2017"
]
someTableState
-}
stateWithItems : List item -> State msg item columns -> State msg item columns
stateWithItems items (State state) =
State
{ state
| items = items
, visibleItems =
items
|> maybeThen Filters.itemsApplyFilters state.filters
|> maybeThen Sorters.itemsApplySorting state.sorters
}
{-| Given a message, apply an update to the [`Table.State`](#State).
Do not ignore the returned `Cmd`, it may include remote filter's messages.
( newModel, newCmd ) =
Table.update subMsg oldModel.tableState
-}
update : Msg item -> State msg item columns -> ( State msg item columns, Effects msg )
update msg (State state) =
case msg of
MobileToggle index ->
updateMobileToggle state index
ForFilters subMsg ->
updateFilters state subMsg
ForSorters subMsg ->
updateSorters state subMsg
FilterDialogOpen index ->
( State { state | filterDialog = Just index }, Effects.none )
FilterDialogClose ->
( State { state | filterDialog = Nothing }, Effects.none )
SelectionToggleAll ->
updateSelectionToggleAll state
SelectionSet item value ->
updateSelectionSet state item value
updateMobileToggle : StateModel msg item columns -> Int -> ( State msg item columns, Effects msg )
updateMobileToggle state index =
( State
{ state
| mobileSelected =
if state.mobileSelected == Just index then
Nothing
else
Just index
}
, Effects.none
)
updateFilters : StateModel msg item columns -> Filters.Msg -> ( State msg item columns, Effects msg )
updateFilters state subMsg =
let
map ( newFilters, { effects, closeDialog } ) =
( applyFilters newFilters <|
if closeDialog then
{ state | filterDialog = Nothing }
else
state
, effects
)
in
case state.filters of
Just filters ->
filters
|> Filters.update subMsg
|> map
Nothing ->
( State state, Effects.none )
applyFilters : Filters msg item columns -> StateModel msg item columns -> State msg item columns
applyFilters newFilters state =
State
{ state
| visibleItems =
state.items
|> Filters.itemsApplyFilters newFilters
|> maybeThen Sorters.itemsApplySorting state.sorters
, filters = Just newFilters
}
updateSorters : StateModel msg item columns -> Sorters.Msg -> ( State msg item columns, Effects msg )
updateSorters state subMsg =
case state.sorters of
Just sorters ->
sorters
|> Sorters.update subMsg
|> (\( newSorters, subCmd ) ->
( state
|> applySorters newSorters
, subCmd
)
)
Nothing ->
( State state, Effects.none )
applySorters : Sorters item columns -> StateModel msg item columns -> State msg item columns
applySorters newSorters state =
State
{ state
| visibleItems =
state.items
|> maybeThen Filters.itemsApplyFilters state.filters
|> Sorters.itemsApplySorting newSorters
, sorters = Just newSorters
, filterDialog = Nothing
}
updateSelectionToggleAll : StateModel msg item columns -> ( State msg item columns, Effects msg )
updateSelectionToggleAll state =
let
invertAll old =
{ old
| checks =
case old.checks of
Individual _ ->
All
All ->
Individual Set.empty
Except set ->
if Set.isEmpty set then
Individual Set.empty
else
All
}
in
( State { state | localSelection = Maybe.map invertAll state.localSelection }
, Effects.none
)
updateSelectionSet : StateModel msg item columns -> item -> Bool -> ( State msg item columns, Effects msg )
updateSelectionSet state item value =
let
setItem old =
{ old
| checks =
case old.checks of
Individual set ->
selectIndividual old item value set
All ->
selectExcept old item value Set.empty
Except set ->
selectExcept old item value set
}
in
( State { state | localSelection = Maybe.map setItem state.localSelection }
, Effects.none
)
selectIndividual : Selection item -> item -> Bool -> Set String -> Selections
selectIndividual { identifier } item value set =
set
|> ifThenElse value
Set.insert
Set.remove
(identifier item)
|> Individual
selectExcept : Selection item -> item -> Bool -> Set String -> Selections
selectExcept { identifier } item value set =
set
|> ifThenElse value
Set.remove
Set.insert
(identifier item)
|> Except
-- Responsive
{-| Required information for displaying the mobile's layout.
This is record that constrains type-safe sized-arrays.
See [`TypeNumbers`](UI-Utils-TypeNumbers) for how to compose its phantom type.
{ toDetails = Book.toTableDetails
, toCover = Book.toTableCover
}
-}
type alias Responsive msg item columns =
{ toDetails : item -> Details msg columns
, toCover : item -> Cover
}
{-| What is displayed in a collapsed mobile's row.
{ title = "Foo Fighters - Everlong"
, caption = Just "Morumbi - São Paulo 2015-01-23"
}
-}
type alias Cover =
{ title : String, caption : Maybe String }
{-| Used to render a cell in the mobile's layout.
-}
type alias Detail msg =
{ label : String, content : Common.Cell msg }
{-| A set of [`Detail msg`](#Detail).
Must have the same amount of elements as cells do in the table's row.
-}
type alias Details msg columns =
NArray (Maybe (Detail msg)) columns
{-| Allows a table to have a responsive layout when on mobile.
withResponsive
{ toDetails = Book.toTableDetails
, toCover = Book.toTableCover
}
someTable
-}
withResponsive : Responsive msg item columns -> StatefulTable msg item columns -> StatefulTable msg item columns
withResponsive responsive (Table prop opt) =
Table prop { opt | responsive = Just responsive }
{-| An empty [`Details`](#Details) set.
toTableDetails { author, title } =
detailsEmpty
|> detailHidden
|> detailShown
{ label = "Author"
, content = cellFromText (Text.body2 author)
}
|> detailHidden
-}
detailsEmpty : Details msg T.Zero
detailsEmpty =
NArray.empty
{-| Defines that a cell will be shown in the mobile's layout.
detailShown
{ label = "Edit"
, content = cellFromButton editButton
}
detailsSet
-}
detailShown : Detail msg -> Details msg columns -> Details msg (T.Increase columns)
detailShown detail accu =
NArray.push (Just detail) accu
{-| Defines that a cell will be hidden in the mobile's layout.
detailsEmpty
|> detailHidden
|> detailHidden
|> detailHidden
-}
detailHidden : Details msg columns -> Details msg (T.Increase columns)
detailHidden accu =
NArray.push Nothing accu
-- Selection
{-| Apply selection defintion to a table's [`State`](#State).
model =
stateWithSelection Book.getISBN init
-}
stateWithSelection : (item -> String) -> Bool -> State msg item columns -> State msg item columns
stateWithSelection identifier default (State state) =
State
{ state
| localSelection =
Just
{ checks =
if default then
All
else
Individual Set.empty
, identifier = identifier
}
}
{-| Resolves if one item's row is or not selected.
isHungerGamesSelected =
Table.stateIsSelected hungerGamesBook tableState
-}
stateIsSelected : item -> State msg item columns -> Bool
stateIsSelected item (State state) =
case state.localSelection of
Just selection ->
internalIsSelected selection item
Nothing ->
False
-- Filters
type alias PeriodComparison =
DateInput.PeriodComparison
{-| Array with all the columns' filters and their initial state.
This is a type-safe sized-array.
See [`TypeNumbers`](UI-Utils-TypeNumbers) for how to compose its phantom type.
-}
type alias Filters msg item columns =
Filters.Filters msg item columns
{-| Apply filters defintion to a table's [`State`](#State).
model =
stateWithFilters Book.filtersInit init
-}
stateWithFilters : Filters msg item columns -> State msg item columns -> State msg item columns
stateWithFilters filters (State state) =
State
{ state
| filters = Just filters
, visibleItems =
state.items
|> Filters.itemsApplyFilters filters
|> maybeThen Sorters.itemsApplySorting state.sorters
}
{-| An empty [`Filters`](#Filters) set.
toTableDetails { author, title } =
filtersEmpty
|> localSingleTextFilter Nothing .title
|> localSingleTextFilter (Just "<NAME>") .author
|> localSingleTextFilter Nothing .year
-}
filtersEmpty : Filters msg item T.Zero
filtersEmpty =
Filters.empty
{-| A filter with one single text field.
Only part of the content must match the filter's input.
Filtering logic is applied internally by the component.
localSingleTextFilter
maybeInitialValue
mapItemToString
-}
localSingleTextFilter :
Maybe String
-> (item -> String)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
localSingleTextFilter initValue getData accu =
Filters.push
(Filter.singleTextLocal initValue getData)
accu
{-| A filter with one single text field.
Only part of the content must match the filter's input.
Filtering logic is applied through an external message.
When `Nothing` is applied to the message, it means to clear the current filter.
remoteSingleTextFilter
maybeInitialValue
Msg.ApplyFilter
-}
remoteSingleTextFilter :
Maybe String
-> (Maybe String -> msg)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
remoteSingleTextFilter initValue applyMsg accu =
Filters.push
(Filter.singleTextRemote initValue applyMsg)
accu
{-| A filter with multiple text field.
The content must match at least one of those fields otherwise it's filtered out.
Only part of the content must match the filter's input.
Filtering logic is applied internally by the component.
localMultiTextFilter
[]
mapItemToString
For having an initial filter applied:
localMultiTextFilter
[ "initial", "fields" ]
mapItemToString
-}
localMultiTextFilter :
List String
-> (item -> String)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
localMultiTextFilter initValue getData accu =
Filters.push
(Filter.multiTextLocal initValue getData)
accu
{-| A filter with multiple text field.
The content must match at least one of those fields otherwise it's filtered out.
Only part of the content must match the filter's input.
Filtering logic is applied through an external message.
When an empty list is applied to the message, it means to clear the current filter.
remoteMultiTextFilter
[ "initial", "fields" ]
Msg.ApplyFilter
-}
remoteMultiTextFilter :
List String
-> (List String -> msg)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
remoteMultiTextFilter initValue applyMsg accu =
Filters.push
(Filter.multiTextRemote initValue applyMsg)
accu
{-| A filter for dates with one single field.
Filtering logic is applied internally by the component.
localSingleDateFilter timeZone
(Just somePosixEpoch)
mapItemToPosixEpoch
-}
localSingleDateFilter :
Time.Zone
-> Maybe Time.Posix
-> (item -> Time.Posix)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
localSingleDateFilter timeZone initValue getData accu =
Filters.push
(Filter.singleDateLocal timeZone initValue getData)
accu
{-| A filter for dates with one single field.
Filtering logic is applied through an external message.
When `Nothing` is applied to the message, it means to clear the current filter.
remoteSingleDateFilter
maybeInitialPosix
Msg.ApplyFilter
-}
remoteSingleDateFilter :
Time.Zone
-> Maybe Time.Posix
-> (Maybe DateInput -> msg)
-> Filters.Filters msg item columns
-> Filters.Filters msg item (T.Increase columns)
remoteSingleDateFilter timeZone initValue applyMsg accu =
Filters.push
(Filter.singleDateRemote timeZone initValue applyMsg)
accu
{-| A filter for dates in an expected range.
The range is defined using two date fields.
Filtering logic is applied internally by the component.
localRangeDateFilter timeZone
(Just datesAfterThis)
(Just datesBeforeThis)
mapItemToPosixEpoch
**NOTE**: Hours, minutes and seconds are discarded from the range limits.
-}
localRangeDateFilter :
Time.Zone
-> Maybe Time.Posix
-> Maybe Time.Posix
-> (item -> Time.Posix)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
localRangeDateFilter timeZone fromTime toTime getData accu =
Filters.push
(Filter.rangeDateLocal timeZone fromTime toTime getData)
accu
{-| A filter for dates in an expected range.
The range is defined using two date fields.
Filtering logic is applied through an external message.
When `Nothing` is applied to the message, it means to clear the current filter.
remoteRangeDateFilter timeZone
(Just datesAfterThis)
(Just datesBeforeThis)
Msg.ApplyFilter
**NOTE**: Hours, minutes and seconds are discarded from the range limits.
-}
remoteRangeDateFilter :
Time.Zone
-> Maybe Time.Posix
-> Maybe Time.Posix
-> (Maybe RangeDate -> msg)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
remoteRangeDateFilter timeZone fromTime toTime applyMsg accu =
Filters.push
(Filter.rangeDateRemote timeZone fromTime toTime applyMsg)
accu
{-| A filter for a single date, dates before specified date, or dates after specified date.
The filter-case is defined using radio buttons.
Filtering logic is applied internally by the component.
localPeriodDateFilter timeZone
(Just somePosixEpoch)
(Just periodAfter)
mapItemToPosixEpoch
**NOTE**: Hours, minutes and seconds are discarded from the range limits.
-}
localPeriodDateFilter :
Time.Zone
-> Maybe Time.Posix
-> Maybe PeriodComparison
-> (item -> Time.Posix)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
localPeriodDateFilter timeZone initValue initComparison getData accu =
Filters.push
(Filter.periodDateLocal "table-date-period-filter" timeZone initValue initComparison getData)
accu
{-| A filter for a single date, dates before specified date, or dates after specified date.
The filter-case is defined using radio buttons.
Filtering logic is applied through an external message.
When `Nothing` is applied to the message, it means to clear the current filter.
remotePeriodDateFilter timeZone
(Just somePosixEpoch)
(Just periodAfter)
Msg.ApplyFilter
**NOTE**: Hours, minutes and seconds are discarded from the range limits.
-}
remotePeriodDateFilter :
Time.Zone
-> Maybe Time.Posix
-> Maybe PeriodComparison
-> (Maybe PeriodDate -> msg)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
remotePeriodDateFilter timeZone initValue initComparison applyMsg accu =
Filters.push
(Filter.periodDateRemote "table-date-period-filter" timeZone initValue initComparison applyMsg)
accu
{-| A filter for custom radio buttons.
Filtering logic is applied internally by the component.
localSelectFilter
[ "Option 1"
, "Option 2"
]
(Just 1)
mapItemEachOptionToBool
-}
localSelectFilter :
List String
-> Maybe Int
-> (item -> Int -> Bool)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
localSelectFilter initList initSelection getData accu =
Filters.push
(Filter.selectLocal "table-select-filter" initList initSelection getData)
accu
{-| A filter for custom radio buttons.
Filtering logic is applied through an external message.
When `Nothing` is applied to the message, it means to clear the current filter.
remoteSelectFilter
[ "Option 1"
, "Option 2"
]
(Just 1)
Msg.ApplyFilter
-}
remoteSelectFilter :
List String
-> Maybe Int
-> (Maybe Int -> msg)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
remoteSelectFilter initList initSelection applyMsg accu =
Filters.push
(Filter.selectRemote "table-select-filter" initList initSelection applyMsg)
accu
-- Periods
{-| When comparing if dates are the same.
-}
periodSingle : PeriodComparison
periodSingle =
DateInput.On
{-| When comparing if some date is after another.
-}
pariodAfter : PeriodComparison
pariodAfter =
DateInput.After
{-| When comparing if some date is before another.
-}
periodBefore : PeriodComparison
periodBefore =
DateInput.Before
-- Width
{-| Applies [`Element.width`](/packages/mdgriffith/elm-ui/latest/Element#width) to the component.
Table.withWidth
(Element.fill |> Element.minimum 220)
someTable
-}
withWidth : Element.Length -> StatefulTable msg item columns -> StatefulTable msg item columns
withWidth width (Table prop opt_) =
Table prop { opt_ | width = width }
-- Sorting
{-| Array with all the columns' sorting definitions.
This is a type-safe sized-array.
See [`TypeNumbers`](UI-Utils-TypeNumbers) for how to compose its phantom type.
-}
type alias Sorters item columns =
Sorters.Sorters item columns
{-| Apply sortings defintion to a table's [`State`](#State).
model =
stateWithSorters Book.sortersInit init
-}
stateWithSorters : Sorters item columns -> State msg item columns -> State msg item columns
stateWithSorters sorters (State state) =
State
{ state
| sorters = Just sorters
, visibleItems =
state.items
|> maybeThen Filters.itemsApplyFilters state.filters
|> Sorters.itemsApplySorting sorters
}
{-| Allow sorting a column alphabetically.
sortersInit =
sortersEmpty
|> sortBy .title
|> sortBy .author
|> unsortable
-}
sortBy :
(item -> String)
-> Sorters item columns
-> Sorters item (T.Increase columns)
sortBy fn =
Sorters.sortWith (AlphabeticalSortable fn)
{-| Allow sorting a column using a Float value.
sortersInit =
sortersEmpty
|> unsortable
|> sortByFloat .value
|> sortByFloat .timestamp
|> sortByFloat .average
-}
sortByFloat :
(item -> Float)
-> Sorters item columns
-> Sorters item (T.Increase columns)
sortByFloat fn =
Sorters.sortWith (FloatSortable fn)
{-| Allow sorting a column using an Integer value.
sortersInit =
sortersEmpty
|> unsortable
|> sortByInt .count
|> sortByInt .areaCode
|> sortByInt .hour
-}
sortByInt :
(item -> Int)
-> Sorters item columns
-> Sorters item (T.Increase columns)
sortByInt fn =
Sorters.sortWith (IntegerSortable fn)
{-| Allow sorting a column using a Char value.
sortersInit =
sortersEmpty
|> unsortable
|> sortByChar .firstLetter
-}
sortByChar :
(item -> Char)
-> Sorters item columns
-> Sorters item (T.Increase columns)
sortByChar fn =
Sorters.sortWith (CharSortable fn)
{-| Allow sorting a column with a custom function.
Check [`List.sortWith`](https://package.elm-lang.org/packages/elm/core/latest/List#sortWith)
sortersInit =
sortersEmpty
|> unsortable
|> sortWith flippedComparison
-}
sortWith :
(item -> item -> Order)
-> Sorters item columns
-> Sorters item (T.Increase columns)
sortWith fn =
Sorters.sortWith (CustomSortable fn)
{-| Changes the initial sorting to some columns as descreasing.
model =
stateWithSorters
(Book.sortersInit |> sortDecreasing 1)
init
-}
sortDecreasing : Int -> Sorters item columns -> Sorters item columns
sortDecreasing =
Sorters.sortDescending
{-| Changes the initial sorting to some columns as increasing.
model =
stateWithSorters
(Book.sortersInit |> sortIncreasing 1)
init
-}
sortIncreasing : Int -> Sorters item columns -> Sorters item columns
sortIncreasing =
Sorters.sortAscending
{-| An empty [`Sorters`](#Sorters) set.
sortersInit =
sortersEmpty
|> sortBy .title
|> sortBy .author
|> unsortable
-}
sortersEmpty : Sorters item T.Zero
sortersEmpty =
Sorters.sortersEmpty
{-| Describes that some column is not sortable.
sortersInit =
sortersEmpty
|> sortBy .title
|> sortBy .author
|> unsortable
-}
unsortable : Sorters item columns -> Sorters item (T.Increase columns)
unsortable =
Sorters.unsortable
-- Rendering
{-| End of the builder's life.
The result of this function is a ready-to-insert Elm UI's Element.
-}
renderElement : RenderConfig -> StatefulTable msg item columns -> Element msg
renderElement renderConfig (Table prop opt) =
case opt.responsive of
Just responsive ->
if RenderConfig.isMobile renderConfig then
mobileView renderConfig prop opt responsive
else
desktopView renderConfig prop opt
Nothing ->
desktopView renderConfig prop opt
-- Mobile rendering
mobileView :
RenderConfig
-> StatefulConfig msg item columns
-> Options msg item columns
-> Responsive msg item columns
-> Element msg
mobileView renderConfig prop opt responsive =
let
detailsTerms =
renderConfig |> localeTerms >> .tables >> .details
items =
viewGetItems (unwrapState prop.state) opt
|> List.indexedMap Tuple.pair
in
ListView.toggleableList
{ detailsShowLabel = detailsTerms.show
, detailsCollapseLabel = detailsTerms.collapse
, toKey = Tuple.second >> prop.toRow.toKey
, toCover = Tuple.second >> responsive.toCover
, toDetails =
Tuple.second
>> responsive.toDetails
>> NArray.toList
>> List.filterMap (Maybe.map (detailView renderConfig))
, selectMsg = Tuple.first >> MobileToggle >> prop.toExternalMsg
}
|> ListView.withItems items
|> ListView.withSelected (Tuple.first >> isSelected prop.state)
|> ListView.renderElement renderConfig
isSelected : State msg item columns -> Int -> Bool
isSelected (State { mobileSelected }) position =
Just position == mobileSelected
detailView : RenderConfig -> Detail msg -> ( String, Element msg )
detailView renderConfig { label, content } =
( label, cellContentRender renderConfig content )
-- Dekstop rendering
desktopView :
RenderConfig
-> StatefulConfig msg item columns
-> Options msg item columns
-> Element msg
desktopView renderConfig prop opt =
let
columns =
NArray.toList prop.columns
state =
unwrapState prop.state
items =
viewGetItems state opt
rows =
List.map (rowWithSelection renderConfig prop.toExternalMsg state prop.toRow columns) items
padding =
{ top = 20, left = 20, right = 20, bottom = 0 }
selectionHeader =
viewSelectionHeader renderConfig state prop.toExternalMsg
headers =
headersRender renderConfig
prop.toExternalMsg
state.filterDialog
state.filters
state.sorters
columns
selectionHeader
in
Keyed.column
[ Element.spacing 2
, Element.width opt.width
, Element.paddingEach padding
]
(headers :: rows)
viewSelectionHeader : RenderConfig -> StateModel msg item columns -> (Msg item -> msg) -> Maybe (Element msg)
viewSelectionHeader renderConfig state toExternalMsg =
Maybe.map
(SelectionToggleAll
|> toExternalMsg
|> FiltersView.headerSelectToggle renderConfig
|> always
)
state.localSelection
viewGetItems : StateModel msg item columns -> Options msg item columns -> List item
viewGetItems { visibleItems } opt =
Maybe.withDefault visibleItems opt.overwriteItems
unwrapState : State msg item columns -> StateModel msg item columns
unwrapState (State model) =
model
rowWithSelection :
RenderConfig
-> (Msg item -> msg)
-> StateModel msg item columns
-> ToRow msg item columns
-> List Column
-> item
-> ( String, Element msg )
rowWithSelection renderConfig msgMap state toRow columns item =
rowBox <|
case state.localSelection of
Just selection ->
item
|> rowRender renderConfig toRow columns
|> Tuple.mapSecond
((::)
(item
|> selectionCell renderConfig selection
|> Tuple.mapSecond (Element.map msgMap)
)
)
Nothing ->
rowRender renderConfig toRow columns item
headersRender :
RenderConfig
-> (Msg item -> msg)
-> Maybe Int
-> Maybe (Filters.Filters msg item columns)
-> Maybe (Sorters.Sorters item columns)
-> List Column
-> Maybe (Element msg)
-> ( String, Element msg )
headersRender renderConfig toExternalMsg selected filters sorters columns selectionHeader =
( "@headers"
, Element.row headersAttr <|
case filters of
Just filterArr ->
filterArr
|> NArray.toList
|> List.map2 (filterHeader renderConfig toExternalMsg selected) columns
|> List.indexedMap
(\index val ->
val (Maybe.andThen (Sorters.get index) sorters) index
)
|> prependMaybe selectionHeader
Nothing ->
List.map
(\(Column header { width }) ->
header
|> simpleHeaderRender renderConfig
|> cellSpace width
)
columns
)
filterHeader :
RenderConfig
-> (Msg item -> msg)
-> Maybe Int
-> Column
-> Filter.Filter msg item
-> Sorters.ColumnStatus item
-> Int
-> Element msg
filterHeader renderConfig toExternalMsg selected (Column header { width }) filter sorter index =
FiltersView.header renderConfig
filter
sorter
{ openMsg = toExternalMsg <| FilterDialogOpen index
, discardMsg = toExternalMsg <| FilterDialogClose
, fromFiltersMsg = ForFilters >> toExternalMsg
, fromSortersMsg = ForSorters >> toExternalMsg
, index = index
, label = header
, isOpen = selected == Just index
}
|> topCellSpace width
-- Selection
internalIsSelected : Selection item -> item -> Bool
internalIsSelected { identifier, checks } item =
case checks of
Individual set ->
Set.member (identifier item) set
All ->
True
Except set ->
set
|> Set.member (identifier item)
|> not
selectionCell : RenderConfig -> Selection item -> item -> ( String, Element (Msg item) )
selectionCell renderConfig selection item =
item
|> internalIsSelected selection
|> checkbox (localeTerms renderConfig |> .tables |> .selectRow) (SelectionSet item)
|> Checkbox.withHiddenLabel
|> Checkbox.renderElement renderConfig
|> Element.el
[ Element.centerX, Element.centerY ]
|> Element.el
[ Element.width (px 32), Element.height fill ]
|> Tuple.pair "@select"
| true | module UI.Tables.Stateful exposing
( StatefulTable, StatefulConfig, table, withItems
, Responsive, Cover, Details, Detail, withResponsive, detailsEmpty, detailShown, detailHidden
, State, Msg, init, update, stateWithItems
, Filters, filtersEmpty, stateWithFilters
, localSingleTextFilter, remoteSingleTextFilter
, localMultiTextFilter, remoteMultiTextFilter
, localSingleDateFilter, remoteSingleDateFilter
, localRangeDateFilter, remoteRangeDateFilter
, periodSingle, pariodAfter, periodBefore, localPeriodDateFilter, remotePeriodDateFilter
, localSelectFilter, remoteSelectFilter
, Sorters, stateWithSorters
, sortersEmpty, sortBy, sortByFloat, sortByInt, sortByChar, sortWith, unsortable
, sortDecreasing, sortIncreasing
, withWidth
, stateWithSelection, stateIsSelected
, renderElement
)
{-| Tables are a matrixial data disposition with rows, columns, headers, and cells.
`UI.Tables` are type-safe, which means that every row needs to have the same number of columns (including the headers). Otherwise, compilation fails.
Stateful.table
{ toExternalMsg = Msg.ForTable
, columns = Book.tableColumns
, toRow = Book.toTableRow
, state = model.tableState
}
|> Stateful.withResponsive
{ toDetails = Book.toTableDetails
, toCover = Book.toTableCover
}
|> Stateful.withWidth (Element.fill |> Element.maximum 640)
|> Stateful.withItems
[ Book "PI:NAME:<NAME>END_PI" "Angels & Demons" "2000"
, Book "PI:NAME:<NAME>END_PI" "The Da Vinci Code" "2003"
, Book "PI:NAME:<NAME>END_PI" "The Lost Symbol" "2009"
, Book "PI:NAME:<NAME>END_PI" "Inferno" "2013"
, Book "PI:NAME:<NAME>END_PI" "Origin" "2017"
]
|> Stateful.renderElement renderConfig
Where `Book` is:
type alias Book =
{ author : String, title : String, year : String }
tableColumns =
columnsEmpty
|> column "Title" (columnWidthPixels 320)
|> column "Author" (columnWidthPixels 240)
|> column "Year" (columnWidthPixels 120)
toTableRow =
{ toKey = .title, toTableRowView}
toTableRowView { author, title, year } =
rowEmpty
|> rowCellText (Text.body1 title)
|> rowCellText (Text.body2 author)
|> rowCellText (Text.caption year)
toTableDetails { author, title } =
detailsEmpty
|> detailHidden
|> detailShown { label = "Author", content = cellFromText <| Text.body2 author }
|> detailHidden
toTableCover { title, year } =
{ title = title, caption = Just year }
someFilters =
filtersEmpty
|> localSingleTextFilter Nothing .title
|> localSingleTextFilter (Just "PI:NAME:<NAME>END_PI") .author
|> localSingleTextFilter Nothing .year
And on model:
{ -...
, tableState : Stateful.Table Msg.Msg TypeNumbers.Three
}
{ -...
, tableState = Stateful.stateWithFilters Book.someFilters Stateful.init
}
# Stateful
@docs StatefulTable, StatefulConfig, table, withItems
## Mobile
@docs Responsive, Cover, Details, Detail, withResponsive, detailsEmpty, detailShown, detailHidden
## State
@docs State, Msg, init, update, stateWithItems
# Filters
@docs Filters, filtersEmpty, stateWithFilters
## Single Text
@docs localSingleTextFilter, remoteSingleTextFilter
## Multi Text
@docs localMultiTextFilter, remoteMultiTextFilter
## Single DateInput
@docs localSingleDateFilter, remoteSingleDateFilter
## Range Dates
@docs localRangeDateFilter, remoteRangeDateFilter
## Period Dates
@docs periodSingle, pariodAfter, periodBefore, localPeriodDateFilter, remotePeriodDateFilter
## Select (Radio Buttons)
@docs localSelectFilter, remoteSelectFilter
# Sorting
@docs Sorters, stateWithSorters
@docs sortersEmpty, sortBy, sortByFloat, sortByInt, sortByChar, sortWith, unsortable
@docs sortDecreasing, sortIncreasing
# Width
@docs withWidth
# Selection
## Local
@docs stateWithSelection, stateIsSelected
## Remote
TODO: withRemoteSelection
# Rendering
@docs renderElement
-}
import Element exposing (Element, fill, px, shrink)
import Element.Keyed as Keyed
import Set exposing (Set)
import Time
import UI.Checkbox as Checkbox exposing (checkbox)
import UI.Effects as Effects exposing (Effects)
import UI.Internal.Basics exposing (ifThenElse, maybeThen, prependMaybe)
import UI.Internal.DateInput as DateInput exposing (DateInput, PeriodDate, RangeDate)
import UI.Internal.Filter.Model as Filter
import UI.Internal.Filter.Sorter exposing (Sorter(..))
import UI.Internal.NArray as NArray exposing (NArray)
import UI.Internal.RenderConfig exposing (localeTerms)
import UI.Internal.Tables.Common exposing (..)
import UI.Internal.Tables.Filters as Filters
import UI.Internal.Tables.FiltersView as FiltersView
import UI.Internal.Tables.ListView as ListView
import UI.Internal.Tables.Sorters as Sorters exposing (Sorters)
import UI.Internal.Tables.View exposing (..)
import UI.RenderConfig as RenderConfig exposing (RenderConfig)
import UI.Tables.Common as Common exposing (..)
import UI.Utils.TypeNumbers as T
{-| The `StatefulTable msg item columns` type is used for describing the component for later rendering.
This is type that constrains type-safe sized-arrays.
See [`TypeNumbers`](UI-Utils-TypeNumbers) for how to compose its phantom type.
-}
type StatefulTable msg item columns
= Table (StatefulConfig msg item columns) (Options msg item columns)
{-| Record with parameters for the creation of a [`StatefulTable`](#table).
This is record that constrains type-safe sized-arrays.
See [`TypeNumbers`](UI-Utils-TypeNumbers) for how to compose its phantom type.
{ toExternalMsg = Msg.ForTable
, columns = Book.tableColumns
, toRow = Book.toTableRow
, state = model.tableState
}
-}
type alias StatefulConfig msg item columns =
{ columns : Columns columns
, toRow : ToRow msg item columns
, toExternalMsg : Msg item -> msg
, state : State msg item columns
}
type alias Options msg item columns =
{ overwriteItems : Maybe (List item)
, width : Element.Length
, responsive : Maybe (Responsive msg item columns)
}
{-| Constructs a stateful table from its columns and rows.
Also defines the handling function for messages, and the current table's state.
table
{ columns = Book.tableColumns
, toRow = Book.toTableRow
, toExternalMsg = Msg.ForTable
, state = model.tableState
}
-}
table : StatefulConfig msg item columns -> StatefulTable msg item columns
table config =
Table config defaultOptions
defaultOptions : Options msg item columns
defaultOptions =
{ overwriteItems = Nothing
, width = shrink
, responsive = Nothing
}
{-| **DEPRECATED**: Use [stateWithItems](#stateWithItems) instead.
Otherwise, by using this you'll be discarding sorting and fitlering.
Each of these items will become a row in this table.
withItems
[ Book "PI:NAME:<NAME>END_PI" "Angels & Demons" "2000"
, Book "PI:NAME:<NAME>END_PI" "The Da Vinci Code" "2003"
, Book "PI:NAME:<NAME>END_PI" "The Lost Symbol" "2009"
, Book "PI:NAME:<NAME>END_PI" "Inferno" "2013"
, Book "PI:NAME:<NAME>END_PI" "Origin" "2017"
]
someTable
-}
withItems : List item -> StatefulTable msg item columns -> StatefulTable msg item columns
withItems items (Table prop opt) =
Table prop { opt | overwriteItems = Just items }
-- State
{-| The `Stateful.Msg` handles stateful table's related messages.
-}
type Msg item
= MobileToggle Int
| ForFilters Filters.Msg
| ForSorters Sorters.Msg
| FilterDialogOpen Int
| FilterDialogClose
| SelectionToggleAll
| SelectionSet item Bool
{-| Keep this one in your Model, it holds the table's current state.
-}
type State msg item columns
= State (StateModel msg item columns)
type alias StateModel msg item columns =
{ filters : Maybe (Filters msg item columns)
, mobileSelected : Maybe Int
, filterDialog : Maybe Int
, localSelection : Maybe (Selection item)
, items : List item
, visibleItems : List item
, sorters : Maybe (Sorters item columns)
}
type Selections
= Individual (Set String)
| All
| Except (Set String)
type alias Selection item =
{ identifier : item -> String
, checks : Selections
}
{-| The correct way of instantiating a [`Table.State`](#State).
{ -- ...
, tableState = Stateful.init
-- ...
}
-}
init : State msg item columns
init =
State
{ filters = Nothing
, mobileSelected = Nothing
, filterDialog = Nothing
, localSelection = Nothing
, items = []
, visibleItems = []
, sorters = Nothing
}
{-| Each of these items will become a row in this table.
stateWithItems
[ Book "PI:NAME:<NAME>END_PI" "Angels & Demons" "2000"
, Book "PI:NAME:<NAME>END_PI" "The Da Vinci Code" "2003"
, Book "PI:NAME:<NAME>END_PI" "The Lost Symbol" "2009"
, Book "PI:NAME:<NAME>END_PI" "Inferno" "2013"
, Book "PI:NAME:<NAME>END_PI" "Origin" "2017"
]
someTableState
-}
stateWithItems : List item -> State msg item columns -> State msg item columns
stateWithItems items (State state) =
State
{ state
| items = items
, visibleItems =
items
|> maybeThen Filters.itemsApplyFilters state.filters
|> maybeThen Sorters.itemsApplySorting state.sorters
}
{-| Given a message, apply an update to the [`Table.State`](#State).
Do not ignore the returned `Cmd`, it may include remote filter's messages.
( newModel, newCmd ) =
Table.update subMsg oldModel.tableState
-}
update : Msg item -> State msg item columns -> ( State msg item columns, Effects msg )
update msg (State state) =
case msg of
MobileToggle index ->
updateMobileToggle state index
ForFilters subMsg ->
updateFilters state subMsg
ForSorters subMsg ->
updateSorters state subMsg
FilterDialogOpen index ->
( State { state | filterDialog = Just index }, Effects.none )
FilterDialogClose ->
( State { state | filterDialog = Nothing }, Effects.none )
SelectionToggleAll ->
updateSelectionToggleAll state
SelectionSet item value ->
updateSelectionSet state item value
updateMobileToggle : StateModel msg item columns -> Int -> ( State msg item columns, Effects msg )
updateMobileToggle state index =
( State
{ state
| mobileSelected =
if state.mobileSelected == Just index then
Nothing
else
Just index
}
, Effects.none
)
updateFilters : StateModel msg item columns -> Filters.Msg -> ( State msg item columns, Effects msg )
updateFilters state subMsg =
let
map ( newFilters, { effects, closeDialog } ) =
( applyFilters newFilters <|
if closeDialog then
{ state | filterDialog = Nothing }
else
state
, effects
)
in
case state.filters of
Just filters ->
filters
|> Filters.update subMsg
|> map
Nothing ->
( State state, Effects.none )
applyFilters : Filters msg item columns -> StateModel msg item columns -> State msg item columns
applyFilters newFilters state =
State
{ state
| visibleItems =
state.items
|> Filters.itemsApplyFilters newFilters
|> maybeThen Sorters.itemsApplySorting state.sorters
, filters = Just newFilters
}
updateSorters : StateModel msg item columns -> Sorters.Msg -> ( State msg item columns, Effects msg )
updateSorters state subMsg =
case state.sorters of
Just sorters ->
sorters
|> Sorters.update subMsg
|> (\( newSorters, subCmd ) ->
( state
|> applySorters newSorters
, subCmd
)
)
Nothing ->
( State state, Effects.none )
applySorters : Sorters item columns -> StateModel msg item columns -> State msg item columns
applySorters newSorters state =
State
{ state
| visibleItems =
state.items
|> maybeThen Filters.itemsApplyFilters state.filters
|> Sorters.itemsApplySorting newSorters
, sorters = Just newSorters
, filterDialog = Nothing
}
updateSelectionToggleAll : StateModel msg item columns -> ( State msg item columns, Effects msg )
updateSelectionToggleAll state =
let
invertAll old =
{ old
| checks =
case old.checks of
Individual _ ->
All
All ->
Individual Set.empty
Except set ->
if Set.isEmpty set then
Individual Set.empty
else
All
}
in
( State { state | localSelection = Maybe.map invertAll state.localSelection }
, Effects.none
)
updateSelectionSet : StateModel msg item columns -> item -> Bool -> ( State msg item columns, Effects msg )
updateSelectionSet state item value =
let
setItem old =
{ old
| checks =
case old.checks of
Individual set ->
selectIndividual old item value set
All ->
selectExcept old item value Set.empty
Except set ->
selectExcept old item value set
}
in
( State { state | localSelection = Maybe.map setItem state.localSelection }
, Effects.none
)
selectIndividual : Selection item -> item -> Bool -> Set String -> Selections
selectIndividual { identifier } item value set =
set
|> ifThenElse value
Set.insert
Set.remove
(identifier item)
|> Individual
selectExcept : Selection item -> item -> Bool -> Set String -> Selections
selectExcept { identifier } item value set =
set
|> ifThenElse value
Set.remove
Set.insert
(identifier item)
|> Except
-- Responsive
{-| Required information for displaying the mobile's layout.
This is record that constrains type-safe sized-arrays.
See [`TypeNumbers`](UI-Utils-TypeNumbers) for how to compose its phantom type.
{ toDetails = Book.toTableDetails
, toCover = Book.toTableCover
}
-}
type alias Responsive msg item columns =
{ toDetails : item -> Details msg columns
, toCover : item -> Cover
}
{-| What is displayed in a collapsed mobile's row.
{ title = "Foo Fighters - Everlong"
, caption = Just "Morumbi - São Paulo 2015-01-23"
}
-}
type alias Cover =
{ title : String, caption : Maybe String }
{-| Used to render a cell in the mobile's layout.
-}
type alias Detail msg =
{ label : String, content : Common.Cell msg }
{-| A set of [`Detail msg`](#Detail).
Must have the same amount of elements as cells do in the table's row.
-}
type alias Details msg columns =
NArray (Maybe (Detail msg)) columns
{-| Allows a table to have a responsive layout when on mobile.
withResponsive
{ toDetails = Book.toTableDetails
, toCover = Book.toTableCover
}
someTable
-}
withResponsive : Responsive msg item columns -> StatefulTable msg item columns -> StatefulTable msg item columns
withResponsive responsive (Table prop opt) =
Table prop { opt | responsive = Just responsive }
{-| An empty [`Details`](#Details) set.
toTableDetails { author, title } =
detailsEmpty
|> detailHidden
|> detailShown
{ label = "Author"
, content = cellFromText (Text.body2 author)
}
|> detailHidden
-}
detailsEmpty : Details msg T.Zero
detailsEmpty =
NArray.empty
{-| Defines that a cell will be shown in the mobile's layout.
detailShown
{ label = "Edit"
, content = cellFromButton editButton
}
detailsSet
-}
detailShown : Detail msg -> Details msg columns -> Details msg (T.Increase columns)
detailShown detail accu =
NArray.push (Just detail) accu
{-| Defines that a cell will be hidden in the mobile's layout.
detailsEmpty
|> detailHidden
|> detailHidden
|> detailHidden
-}
detailHidden : Details msg columns -> Details msg (T.Increase columns)
detailHidden accu =
NArray.push Nothing accu
-- Selection
{-| Apply selection defintion to a table's [`State`](#State).
model =
stateWithSelection Book.getISBN init
-}
stateWithSelection : (item -> String) -> Bool -> State msg item columns -> State msg item columns
stateWithSelection identifier default (State state) =
State
{ state
| localSelection =
Just
{ checks =
if default then
All
else
Individual Set.empty
, identifier = identifier
}
}
{-| Resolves if one item's row is or not selected.
isHungerGamesSelected =
Table.stateIsSelected hungerGamesBook tableState
-}
stateIsSelected : item -> State msg item columns -> Bool
stateIsSelected item (State state) =
case state.localSelection of
Just selection ->
internalIsSelected selection item
Nothing ->
False
-- Filters
type alias PeriodComparison =
DateInput.PeriodComparison
{-| Array with all the columns' filters and their initial state.
This is a type-safe sized-array.
See [`TypeNumbers`](UI-Utils-TypeNumbers) for how to compose its phantom type.
-}
type alias Filters msg item columns =
Filters.Filters msg item columns
{-| Apply filters defintion to a table's [`State`](#State).
model =
stateWithFilters Book.filtersInit init
-}
stateWithFilters : Filters msg item columns -> State msg item columns -> State msg item columns
stateWithFilters filters (State state) =
State
{ state
| filters = Just filters
, visibleItems =
state.items
|> Filters.itemsApplyFilters filters
|> maybeThen Sorters.itemsApplySorting state.sorters
}
{-| An empty [`Filters`](#Filters) set.
toTableDetails { author, title } =
filtersEmpty
|> localSingleTextFilter Nothing .title
|> localSingleTextFilter (Just "PI:NAME:<NAME>END_PI") .author
|> localSingleTextFilter Nothing .year
-}
filtersEmpty : Filters msg item T.Zero
filtersEmpty =
Filters.empty
{-| A filter with one single text field.
Only part of the content must match the filter's input.
Filtering logic is applied internally by the component.
localSingleTextFilter
maybeInitialValue
mapItemToString
-}
localSingleTextFilter :
Maybe String
-> (item -> String)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
localSingleTextFilter initValue getData accu =
Filters.push
(Filter.singleTextLocal initValue getData)
accu
{-| A filter with one single text field.
Only part of the content must match the filter's input.
Filtering logic is applied through an external message.
When `Nothing` is applied to the message, it means to clear the current filter.
remoteSingleTextFilter
maybeInitialValue
Msg.ApplyFilter
-}
remoteSingleTextFilter :
Maybe String
-> (Maybe String -> msg)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
remoteSingleTextFilter initValue applyMsg accu =
Filters.push
(Filter.singleTextRemote initValue applyMsg)
accu
{-| A filter with multiple text field.
The content must match at least one of those fields otherwise it's filtered out.
Only part of the content must match the filter's input.
Filtering logic is applied internally by the component.
localMultiTextFilter
[]
mapItemToString
For having an initial filter applied:
localMultiTextFilter
[ "initial", "fields" ]
mapItemToString
-}
localMultiTextFilter :
List String
-> (item -> String)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
localMultiTextFilter initValue getData accu =
Filters.push
(Filter.multiTextLocal initValue getData)
accu
{-| A filter with multiple text field.
The content must match at least one of those fields otherwise it's filtered out.
Only part of the content must match the filter's input.
Filtering logic is applied through an external message.
When an empty list is applied to the message, it means to clear the current filter.
remoteMultiTextFilter
[ "initial", "fields" ]
Msg.ApplyFilter
-}
remoteMultiTextFilter :
List String
-> (List String -> msg)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
remoteMultiTextFilter initValue applyMsg accu =
Filters.push
(Filter.multiTextRemote initValue applyMsg)
accu
{-| A filter for dates with one single field.
Filtering logic is applied internally by the component.
localSingleDateFilter timeZone
(Just somePosixEpoch)
mapItemToPosixEpoch
-}
localSingleDateFilter :
Time.Zone
-> Maybe Time.Posix
-> (item -> Time.Posix)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
localSingleDateFilter timeZone initValue getData accu =
Filters.push
(Filter.singleDateLocal timeZone initValue getData)
accu
{-| A filter for dates with one single field.
Filtering logic is applied through an external message.
When `Nothing` is applied to the message, it means to clear the current filter.
remoteSingleDateFilter
maybeInitialPosix
Msg.ApplyFilter
-}
remoteSingleDateFilter :
Time.Zone
-> Maybe Time.Posix
-> (Maybe DateInput -> msg)
-> Filters.Filters msg item columns
-> Filters.Filters msg item (T.Increase columns)
remoteSingleDateFilter timeZone initValue applyMsg accu =
Filters.push
(Filter.singleDateRemote timeZone initValue applyMsg)
accu
{-| A filter for dates in an expected range.
The range is defined using two date fields.
Filtering logic is applied internally by the component.
localRangeDateFilter timeZone
(Just datesAfterThis)
(Just datesBeforeThis)
mapItemToPosixEpoch
**NOTE**: Hours, minutes and seconds are discarded from the range limits.
-}
localRangeDateFilter :
Time.Zone
-> Maybe Time.Posix
-> Maybe Time.Posix
-> (item -> Time.Posix)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
localRangeDateFilter timeZone fromTime toTime getData accu =
Filters.push
(Filter.rangeDateLocal timeZone fromTime toTime getData)
accu
{-| A filter for dates in an expected range.
The range is defined using two date fields.
Filtering logic is applied through an external message.
When `Nothing` is applied to the message, it means to clear the current filter.
remoteRangeDateFilter timeZone
(Just datesAfterThis)
(Just datesBeforeThis)
Msg.ApplyFilter
**NOTE**: Hours, minutes and seconds are discarded from the range limits.
-}
remoteRangeDateFilter :
Time.Zone
-> Maybe Time.Posix
-> Maybe Time.Posix
-> (Maybe RangeDate -> msg)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
remoteRangeDateFilter timeZone fromTime toTime applyMsg accu =
Filters.push
(Filter.rangeDateRemote timeZone fromTime toTime applyMsg)
accu
{-| A filter for a single date, dates before specified date, or dates after specified date.
The filter-case is defined using radio buttons.
Filtering logic is applied internally by the component.
localPeriodDateFilter timeZone
(Just somePosixEpoch)
(Just periodAfter)
mapItemToPosixEpoch
**NOTE**: Hours, minutes and seconds are discarded from the range limits.
-}
localPeriodDateFilter :
Time.Zone
-> Maybe Time.Posix
-> Maybe PeriodComparison
-> (item -> Time.Posix)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
localPeriodDateFilter timeZone initValue initComparison getData accu =
Filters.push
(Filter.periodDateLocal "table-date-period-filter" timeZone initValue initComparison getData)
accu
{-| A filter for a single date, dates before specified date, or dates after specified date.
The filter-case is defined using radio buttons.
Filtering logic is applied through an external message.
When `Nothing` is applied to the message, it means to clear the current filter.
remotePeriodDateFilter timeZone
(Just somePosixEpoch)
(Just periodAfter)
Msg.ApplyFilter
**NOTE**: Hours, minutes and seconds are discarded from the range limits.
-}
remotePeriodDateFilter :
Time.Zone
-> Maybe Time.Posix
-> Maybe PeriodComparison
-> (Maybe PeriodDate -> msg)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
remotePeriodDateFilter timeZone initValue initComparison applyMsg accu =
Filters.push
(Filter.periodDateRemote "table-date-period-filter" timeZone initValue initComparison applyMsg)
accu
{-| A filter for custom radio buttons.
Filtering logic is applied internally by the component.
localSelectFilter
[ "Option 1"
, "Option 2"
]
(Just 1)
mapItemEachOptionToBool
-}
localSelectFilter :
List String
-> Maybe Int
-> (item -> Int -> Bool)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
localSelectFilter initList initSelection getData accu =
Filters.push
(Filter.selectLocal "table-select-filter" initList initSelection getData)
accu
{-| A filter for custom radio buttons.
Filtering logic is applied through an external message.
When `Nothing` is applied to the message, it means to clear the current filter.
remoteSelectFilter
[ "Option 1"
, "Option 2"
]
(Just 1)
Msg.ApplyFilter
-}
remoteSelectFilter :
List String
-> Maybe Int
-> (Maybe Int -> msg)
-> Filters msg item columns
-> Filters msg item (T.Increase columns)
remoteSelectFilter initList initSelection applyMsg accu =
Filters.push
(Filter.selectRemote "table-select-filter" initList initSelection applyMsg)
accu
-- Periods
{-| When comparing if dates are the same.
-}
periodSingle : PeriodComparison
periodSingle =
DateInput.On
{-| When comparing if some date is after another.
-}
pariodAfter : PeriodComparison
pariodAfter =
DateInput.After
{-| When comparing if some date is before another.
-}
periodBefore : PeriodComparison
periodBefore =
DateInput.Before
-- Width
{-| Applies [`Element.width`](/packages/mdgriffith/elm-ui/latest/Element#width) to the component.
Table.withWidth
(Element.fill |> Element.minimum 220)
someTable
-}
withWidth : Element.Length -> StatefulTable msg item columns -> StatefulTable msg item columns
withWidth width (Table prop opt_) =
Table prop { opt_ | width = width }
-- Sorting
{-| Array with all the columns' sorting definitions.
This is a type-safe sized-array.
See [`TypeNumbers`](UI-Utils-TypeNumbers) for how to compose its phantom type.
-}
type alias Sorters item columns =
Sorters.Sorters item columns
{-| Apply sortings defintion to a table's [`State`](#State).
model =
stateWithSorters Book.sortersInit init
-}
stateWithSorters : Sorters item columns -> State msg item columns -> State msg item columns
stateWithSorters sorters (State state) =
State
{ state
| sorters = Just sorters
, visibleItems =
state.items
|> maybeThen Filters.itemsApplyFilters state.filters
|> Sorters.itemsApplySorting sorters
}
{-| Allow sorting a column alphabetically.
sortersInit =
sortersEmpty
|> sortBy .title
|> sortBy .author
|> unsortable
-}
sortBy :
(item -> String)
-> Sorters item columns
-> Sorters item (T.Increase columns)
sortBy fn =
Sorters.sortWith (AlphabeticalSortable fn)
{-| Allow sorting a column using a Float value.
sortersInit =
sortersEmpty
|> unsortable
|> sortByFloat .value
|> sortByFloat .timestamp
|> sortByFloat .average
-}
sortByFloat :
(item -> Float)
-> Sorters item columns
-> Sorters item (T.Increase columns)
sortByFloat fn =
Sorters.sortWith (FloatSortable fn)
{-| Allow sorting a column using an Integer value.
sortersInit =
sortersEmpty
|> unsortable
|> sortByInt .count
|> sortByInt .areaCode
|> sortByInt .hour
-}
sortByInt :
(item -> Int)
-> Sorters item columns
-> Sorters item (T.Increase columns)
sortByInt fn =
Sorters.sortWith (IntegerSortable fn)
{-| Allow sorting a column using a Char value.
sortersInit =
sortersEmpty
|> unsortable
|> sortByChar .firstLetter
-}
sortByChar :
(item -> Char)
-> Sorters item columns
-> Sorters item (T.Increase columns)
sortByChar fn =
Sorters.sortWith (CharSortable fn)
{-| Allow sorting a column with a custom function.
Check [`List.sortWith`](https://package.elm-lang.org/packages/elm/core/latest/List#sortWith)
sortersInit =
sortersEmpty
|> unsortable
|> sortWith flippedComparison
-}
sortWith :
(item -> item -> Order)
-> Sorters item columns
-> Sorters item (T.Increase columns)
sortWith fn =
Sorters.sortWith (CustomSortable fn)
{-| Changes the initial sorting to some columns as descreasing.
model =
stateWithSorters
(Book.sortersInit |> sortDecreasing 1)
init
-}
sortDecreasing : Int -> Sorters item columns -> Sorters item columns
sortDecreasing =
Sorters.sortDescending
{-| Changes the initial sorting to some columns as increasing.
model =
stateWithSorters
(Book.sortersInit |> sortIncreasing 1)
init
-}
sortIncreasing : Int -> Sorters item columns -> Sorters item columns
sortIncreasing =
Sorters.sortAscending
{-| An empty [`Sorters`](#Sorters) set.
sortersInit =
sortersEmpty
|> sortBy .title
|> sortBy .author
|> unsortable
-}
sortersEmpty : Sorters item T.Zero
sortersEmpty =
Sorters.sortersEmpty
{-| Describes that some column is not sortable.
sortersInit =
sortersEmpty
|> sortBy .title
|> sortBy .author
|> unsortable
-}
unsortable : Sorters item columns -> Sorters item (T.Increase columns)
unsortable =
Sorters.unsortable
-- Rendering
{-| End of the builder's life.
The result of this function is a ready-to-insert Elm UI's Element.
-}
renderElement : RenderConfig -> StatefulTable msg item columns -> Element msg
renderElement renderConfig (Table prop opt) =
case opt.responsive of
Just responsive ->
if RenderConfig.isMobile renderConfig then
mobileView renderConfig prop opt responsive
else
desktopView renderConfig prop opt
Nothing ->
desktopView renderConfig prop opt
-- Mobile rendering
mobileView :
RenderConfig
-> StatefulConfig msg item columns
-> Options msg item columns
-> Responsive msg item columns
-> Element msg
mobileView renderConfig prop opt responsive =
let
detailsTerms =
renderConfig |> localeTerms >> .tables >> .details
items =
viewGetItems (unwrapState prop.state) opt
|> List.indexedMap Tuple.pair
in
ListView.toggleableList
{ detailsShowLabel = detailsTerms.show
, detailsCollapseLabel = detailsTerms.collapse
, toKey = Tuple.second >> prop.toRow.toKey
, toCover = Tuple.second >> responsive.toCover
, toDetails =
Tuple.second
>> responsive.toDetails
>> NArray.toList
>> List.filterMap (Maybe.map (detailView renderConfig))
, selectMsg = Tuple.first >> MobileToggle >> prop.toExternalMsg
}
|> ListView.withItems items
|> ListView.withSelected (Tuple.first >> isSelected prop.state)
|> ListView.renderElement renderConfig
isSelected : State msg item columns -> Int -> Bool
isSelected (State { mobileSelected }) position =
Just position == mobileSelected
detailView : RenderConfig -> Detail msg -> ( String, Element msg )
detailView renderConfig { label, content } =
( label, cellContentRender renderConfig content )
-- Dekstop rendering
desktopView :
RenderConfig
-> StatefulConfig msg item columns
-> Options msg item columns
-> Element msg
desktopView renderConfig prop opt =
let
columns =
NArray.toList prop.columns
state =
unwrapState prop.state
items =
viewGetItems state opt
rows =
List.map (rowWithSelection renderConfig prop.toExternalMsg state prop.toRow columns) items
padding =
{ top = 20, left = 20, right = 20, bottom = 0 }
selectionHeader =
viewSelectionHeader renderConfig state prop.toExternalMsg
headers =
headersRender renderConfig
prop.toExternalMsg
state.filterDialog
state.filters
state.sorters
columns
selectionHeader
in
Keyed.column
[ Element.spacing 2
, Element.width opt.width
, Element.paddingEach padding
]
(headers :: rows)
viewSelectionHeader : RenderConfig -> StateModel msg item columns -> (Msg item -> msg) -> Maybe (Element msg)
viewSelectionHeader renderConfig state toExternalMsg =
Maybe.map
(SelectionToggleAll
|> toExternalMsg
|> FiltersView.headerSelectToggle renderConfig
|> always
)
state.localSelection
viewGetItems : StateModel msg item columns -> Options msg item columns -> List item
viewGetItems { visibleItems } opt =
Maybe.withDefault visibleItems opt.overwriteItems
unwrapState : State msg item columns -> StateModel msg item columns
unwrapState (State model) =
model
rowWithSelection :
RenderConfig
-> (Msg item -> msg)
-> StateModel msg item columns
-> ToRow msg item columns
-> List Column
-> item
-> ( String, Element msg )
rowWithSelection renderConfig msgMap state toRow columns item =
rowBox <|
case state.localSelection of
Just selection ->
item
|> rowRender renderConfig toRow columns
|> Tuple.mapSecond
((::)
(item
|> selectionCell renderConfig selection
|> Tuple.mapSecond (Element.map msgMap)
)
)
Nothing ->
rowRender renderConfig toRow columns item
headersRender :
RenderConfig
-> (Msg item -> msg)
-> Maybe Int
-> Maybe (Filters.Filters msg item columns)
-> Maybe (Sorters.Sorters item columns)
-> List Column
-> Maybe (Element msg)
-> ( String, Element msg )
headersRender renderConfig toExternalMsg selected filters sorters columns selectionHeader =
( "@headers"
, Element.row headersAttr <|
case filters of
Just filterArr ->
filterArr
|> NArray.toList
|> List.map2 (filterHeader renderConfig toExternalMsg selected) columns
|> List.indexedMap
(\index val ->
val (Maybe.andThen (Sorters.get index) sorters) index
)
|> prependMaybe selectionHeader
Nothing ->
List.map
(\(Column header { width }) ->
header
|> simpleHeaderRender renderConfig
|> cellSpace width
)
columns
)
filterHeader :
RenderConfig
-> (Msg item -> msg)
-> Maybe Int
-> Column
-> Filter.Filter msg item
-> Sorters.ColumnStatus item
-> Int
-> Element msg
filterHeader renderConfig toExternalMsg selected (Column header { width }) filter sorter index =
FiltersView.header renderConfig
filter
sorter
{ openMsg = toExternalMsg <| FilterDialogOpen index
, discardMsg = toExternalMsg <| FilterDialogClose
, fromFiltersMsg = ForFilters >> toExternalMsg
, fromSortersMsg = ForSorters >> toExternalMsg
, index = index
, label = header
, isOpen = selected == Just index
}
|> topCellSpace width
-- Selection
internalIsSelected : Selection item -> item -> Bool
internalIsSelected { identifier, checks } item =
case checks of
Individual set ->
Set.member (identifier item) set
All ->
True
Except set ->
set
|> Set.member (identifier item)
|> not
selectionCell : RenderConfig -> Selection item -> item -> ( String, Element (Msg item) )
selectionCell renderConfig selection item =
item
|> internalIsSelected selection
|> checkbox (localeTerms renderConfig |> .tables |> .selectRow) (SelectionSet item)
|> Checkbox.withHiddenLabel
|> Checkbox.renderElement renderConfig
|> Element.el
[ Element.centerX, Element.centerY ]
|> Element.el
[ Element.width (px 32), Element.height fill ]
|> Tuple.pair "@select"
| elm |