question_slug
stringlengths 3
77
| title
stringlengths 1
183
| slug
stringlengths 12
45
| summary
stringlengths 1
160
⌀ | author
stringlengths 2
30
| certification
stringclasses 2
values | created_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| updated_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| hit_count
int64 0
10.6M
| has_video
bool 2
classes | content
stringlengths 4
576k
| upvotes
int64 0
11.5k
| downvotes
int64 0
358
| tags
stringlengths 2
193
| comments
int64 0
2.56k
|
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
find-target-indices-after-sorting-array | C++ | Two Methods with explanation | O(N) and O(N log N) approach | Easy to understand | c-two-methods-with-explanation-on-and-on-m69x | Two methods discussed with explanation.\n\nMethod 1:\n\t1. First sort the array.\n\t2. Find the index of the target\n\t3. Push it into the "result" vector.\n\n\ | joyetahpr | NORMAL | 2021-12-03T10:15:46.537735+00:00 | 2021-12-03T10:15:46.537762+00:00 | 2,669 | false | **Two methods discussed with explanation.**\n\n**Method 1:**\n\t**1.** First sort the array.\n\t**2.** Find the index of the target\n\t**3.** Push it into the **"result"** vector.\n\n```\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n vector<int> result;\n sort(nums.begin(),nums.end());\n for(int i=0;i<nums.size();i++){\n if(nums[i]==target) result.push_back(i);\n }\n return result;\n }\n};\n```\n\n**Time Complexity :** O(N log N)\n\n\n**Method 2:**\n\t**1.** Count the occurences of elements lesser then the target and freq of target elements.\n* \t\t\t"small" contains the number of element lesser than the target.\n* \t\t\t"count" contains the number of occurences of the target element.\n**2.** Now run a loop from **"small"** ( means **"small"** elements are lesser than our target value, so our target value will come after **"small"** elements in sorted array ), upto **"count"**.\n**3.** Push the index into the **"result"** vector.\n\t\t\t\t\t\t\n```\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n int small=0,count=0,k=0;\n for(auto n:nums) {\n if(n<target) small++; // calculates the frequency of elements lesser than the target\n if(n==target) count++; // calculate the number of occurences of the target element\n }\n vector<int> result(count);\n for(int i=small;i<small+count;i++) \n result[k++]=i;\n return result;\n }\n};\n```\n\n**Time Complexity :** O(N)\n\nIf you liked the solution, then please upvote : ) | 38 | 1 | ['C', 'Sorting', 'C++'] | 4 |
find-target-indices-after-sorting-array | Easy C++ solution | Binary Search | Explained | easy-c-solution-binary-search-explained-urzwl | \nclass Solution {\npublic:\n vector <int> ans; //to store answers\n vector<int> targetIndices(vector<int>& nums, int target) {\n sort(nums.begin | ahanavish | NORMAL | 2022-02-04T19:37:39.725671+00:00 | 2022-02-04T19:37:39.725701+00:00 | 3,708 | false | ```\nclass Solution {\npublic:\n vector <int> ans; //to store answers\n vector<int> targetIndices(vector<int>& nums, int target) {\n sort(nums.begin(), nums.end()); //sorting arrays initially\n int start=0, end=nums.size()-1;\n binary(nums, start, end, target); //calling binary search on whole array \n return ans;\n }\n \n void binary(vector <int> nums, int start, int end, int target){\n if(start>end) //if array can\'t be divided into subarrays anymore\n return;\n \n int mid = start + (end-start)/2; //declaring mid element\n if(nums[mid] == target){ //when target is found, push it into ans and call binary on each halved subarrays\n binary(nums, start, mid-1, target);\n ans.push_back(mid);\n binary(nums, mid+1, end, target); \n }\n else if(nums[mid]>target) //calling binary on lower half of array\n binary(nums, start, mid-1, target);\n else //calling binary on upper half of array\n binary(nums, mid+1, end, target);\n }\n \n};\n``` | 33 | 0 | ['C', 'Binary Tree', 'C++'] | 6 |
find-target-indices-after-sorting-array | NO SEARCHING | NO SORTING 👑 | BEATS 100% | no-searching-no-sorting-beats-100-by-tej-g2mq | Intuition\nwe dont need actual indices, we dont care about sequence or order so we don\'t need sort.\nbinary search \n# Approach\nJust count number of elements | tejtharun625 | NORMAL | 2023-08-01T17:40:55.356685+00:00 | 2023-08-01T17:40:55.356712+00:00 | 1,647 | false | # Intuition\nwe dont need actual indices, we dont care about sequence or order so we don\'t need sort.\nbinary search \n# Approach\nJust count number of elements which are less than or equal to given target. say lessThanOrEqualCount\nThen count number of elements which are strictly less than given target onlyLessThanCount\n\nif lessThanOrEqualCount = onlyLessThanCount: return empty\nor else all numbers between them\n\n# Complexity\n- Time complexity:\n$$O(n)$$\n- Space complexity: O(1)\n$$O(1)$$\n\n# Code\n```\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n lessThanEqual = 0\n onlyLess = 0\n for i in nums:\n if i <= target:\n lessThanEqual += 1\n if i < target:\n onlyLess += 1\n return list(range(onlyLess, lessThanEqual))\n```\n\nPlease upvote if you like the solution.\n | 26 | 0 | ['Python3'] | 4 |
find-target-indices-after-sorting-array | Python O(n) simple and fast solution || O(nlogn) sorting solution | python-on-simple-and-fast-solution-onlog-5mo1 | Python :\nTime complexity: O(n)\n\ndef targetIndices(self, nums: List[int], target: int) -> List[int]:\n\tindex = 0\n\tcount = 0\n\n\tfor num in nums:\n\t\tif n | TovAm | NORMAL | 2022-01-08T22:10:37.780176+00:00 | 2022-01-08T22:10:37.780230+00:00 | 2,296 | false | **Python :**\nTime complexity: *O(n)*\n```\ndef targetIndices(self, nums: List[int], target: int) -> List[int]:\n\tindex = 0\n\tcount = 0\n\n\tfor num in nums:\n\t\tif num < target:\n\t\t\tindex += 1\n\n\t\tif num == target:\n\t\t\tcount += 1\n\n\treturn list(range(index, index + count))\n```\n\nTime complexity: *O(nlogn)*\n\n```\ndef targetIndices(self, nums: List[int], target: int) -> List[int]:\n\tnums = sorted(nums)\n\treturn [i for i in range(len(nums)) if nums[i]== target]\n```\n\n**Like it ? please upvote !**\n\n | 24 | 0 | ['Sorting', 'Python'] | 1 |
find-target-indices-after-sorting-array | [Java] 0ms + explanations | java-0ms-explanations-by-stefanelstan-ilux | \nclass Solution {\n /** Algorithm:\n - Parse the array once and count how many are lesser than target and how many are equal\n - DO NOT sort t | StefanelStan | NORMAL | 2021-11-29T21:50:54.091860+00:00 | 2021-11-29T21:51:53.514697+00:00 | 1,725 | false | ```\nclass Solution {\n /** Algorithm:\n - Parse the array once and count how many are lesser than target and how many are equal\n - DO NOT sort the array as we don\'t need it sorted.\n Just to know how many are lesser and how many are equal. O(N) better than O(NlogN - sorting)\n - The response list will have a size = with the number of equal elements (as their positions)\n - Loop from smaller to smaller+equal and add the values into the list. Return the list\n */\n public List<Integer> targetIndices(int[] nums, int target) {\n int smaller = 0;\n int equal = 0;\n for (int num : nums) {\n if (num < target) {\n smaller++;\n } else if (num == target) {\n equal++;\n }\n }\n\t\tList<Integer> indices = new ArrayList<>(equal);\n for (int i = smaller; i < smaller + equal; i++) {\n indices.add(i);\n }\n return indices;\n }\n}\n``` | 19 | 0 | ['Java'] | 2 |
find-target-indices-after-sorting-array | C++ || Easy || Binary Search | c-easy-binary-search-by-rohit192ranjan-zd23 | \uD83D\uDE4FUpvote if u like the solution \uD83D\uDE4F\n\n\nclass Solution {\npublic:\n int firstOccurence(vector<int>& nums, int target){\n int start | rohit192ranjan | NORMAL | 2022-07-27T18:09:32.875784+00:00 | 2022-07-27T18:09:32.875831+00:00 | 1,654 | false | \uD83D\uDE4FUpvote if u like the solution \uD83D\uDE4F\n\n```\nclass Solution {\npublic:\n int firstOccurence(vector<int>& nums, int target){\n int start = 0;\n int res = -1;\n int end = nums.size()-1;\n while(start<=end){\n int mid = start + (end-start)/2;\n if(target==nums[mid]){\n res = mid;\n end = mid-1;\n }\n else if(target<nums[mid])\n end = mid-1;\n else \n start = mid+1;\n }\n return res;\n }\n int lastOccurence(vector<int>& nums, int target){\n int start = 0;\n int res = -1;\n int end = nums.size()-1;\n while(start<=end){\n int mid = start + (end-start)/2;\n if(target==nums[mid]){\n res = mid;\n start = mid+1;\n }\n else if(target<nums[mid])\n end = mid-1;\n else \n start = mid+1;\n }\n return res;\n }\n vector<int> targetIndices(vector<int>& nums, int target) {\n vector<int> result;\n sort(nums.begin(), nums.end());\n int first = firstOccurence(nums, target);\n int last = lastOccurence(nums, target);\n if(first!=-1 && last!=-1){\n for(int i=first; i<=last; i++)\n result.push_back(i);}\n return result;\n }\n};\n``` | 15 | 0 | ['Binary Search', 'C', 'Binary Tree'] | 1 |
find-target-indices-after-sorting-array | Python - Solution + One-Line! | python-solution-one-line-by-domthedevelo-jynd | Solution - Time Complexity: O(n log(n)):\n\nclass Solution:\n def targetIndices(self, nums, target):\n ans = []\n for i,num in enumerate(sorted | domthedeveloper | NORMAL | 2022-05-10T04:41:50.948969+00:00 | 2022-05-10T04:53:49.753791+00:00 | 1,936 | false | **Solution - Time Complexity: O(n log(n))**:\n```\nclass Solution:\n def targetIndices(self, nums, target):\n ans = []\n for i,num in enumerate(sorted(nums)):\n if num == target: ans.append(i)\n return ans\n```\n\n**One-Line - Time Complexity: O(n log(n))**:\n```\nclass Solution:\n def targetIndices(self, nums, target):\n return [i for i,num in enumerate(sorted(nums)) if num==target]\n```\n\n**Solution - Time Complexity: O(n)**:\n```\nclass Solution:\n def targetIndices(self, nums, target):\n idx = cnt = 0\n for num in nums:\n idx += num < target\n cnt += num == target\n return list(range(idx, idx+cnt))\n```\n\n**Solution - Time Complexity: O(n) - Concise**:\n```\nclass Solution:\n def targetIndices(self, nums, target):\n idx = sum(num < target for num in nums)\n cnt = sum(num == target for num in nums)\n return list(range(idx, idx+cnt))\n``` | 15 | 0 | ['Sorting', 'Enumeration', 'Python', 'Python3'] | 1 |
find-target-indices-after-sorting-array | Javascript Solution using binary search | javascript-solution-using-binary-search-z363f | \nfunction binarySearch(lists, sorted, low, high, target){\n if(low > high) return;\n \n const mid = low + Math.floor((high - low) / 2);\n \n if( | nileshsaini_99 | NORMAL | 2022-01-31T07:37:41.583668+00:00 | 2022-01-31T07:37:41.583714+00:00 | 1,916 | false | ```\nfunction binarySearch(lists, sorted, low, high, target){\n if(low > high) return;\n \n const mid = low + Math.floor((high - low) / 2);\n \n if(sorted[mid] === target){\n lists.push(mid);\n }\n \n binarySearch(lists, sorted, low, mid-1, target);\n binarySearch(lists, sorted, mid+1, high, target);\n}\n\nvar targetIndices = function(nums, target) {\n let result = [];\n nums.sort((a,b)=>a-b);\n if(!nums.includes(target)) return [];\n\n binarySearch(result, nums, 0 , nums.length-1, target);\n return result.sort((a,b) => a-b);\n}\n``` | 12 | 0 | ['Binary Search', 'JavaScript'] | 1 |
find-target-indices-after-sorting-array | Just count and get index | just-count-and-get-index-by-xxvvpp-95ob | We can do it using simple sort , but that makes us learn a new way of finding the index of an element without sorting.\n\nSteps:\nJust count the number of eleme | xxvvpp | NORMAL | 2021-11-28T04:24:11.645646+00:00 | 2021-12-28T06:48:33.671854+00:00 | 1,272 | false | # We can do it using simple sort , but that makes us learn a new way of finding the index of an element without sorting.\n\n**Steps:**\nJust `count the number of elements smaller` than the target` as target element will have `it\'s index after them.\nIf `count of smaller element=n`, then the `index of target element will start from nth index because of 0-based indexing of array.`\n\n vector<int> targetIndices(vector<int>& nums, int target) {\n int cnt=0 , small=0 , k=0;\n\t\t\n for(auto i:nums){\n if(i<target) small++;\n if(i==target) cnt++;\n }\n\t\t\n vector<int> ans;\n\t\t//to make push_back work in O(1) TIME -> RESERVE SPACE\n\t\tans.reserve(cnt);\n \n for(int i=small;i<cnt+small;i++) v.push_back(i); \n\t\t\n return v;\n } | 11 | 0 | ['C'] | 1 |
find-target-indices-after-sorting-array | Java || 1000% beats ❤️❤️ || 0(N) || No Sorting || Unique Solution ❤️❤️❤️ | java-1000-beats-0n-no-sorting-unique-sol-7ujs | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \nFirst count no of eleme | saurabh_kumar1 | NORMAL | 2023-10-05T16:35:02.566399+00:00 | 2023-10-05T16:35:02.566428+00:00 | 738 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nFirst count no of element less than target and also count no of target element in nums array. then add lessTarget + countTarget....\n\n# Complexity\n- Time complexity:0(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:0(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n int countTarget = 0 , lessTarget = 0;\n for(int i=0; i<nums.length; i++){\n if(nums[i]<target) lessTarget++;\n if(nums[i]==target) countTarget++;\n }\n List<Integer> ans = new ArrayList<>();\n for(int i=0; i<countTarget; i++){\n ans.add(lessTarget + i);\n }\n return ans;\n\n\n\n\n // Arrays.sort(nums);\n // List<Integer> ans = new ArrayList();\n // int first = 0, last = nums.length-1, val = -1;\n // while(first<=last){\n // int mid = (last+first) / 2;\n // if(nums[mid] == target){\n // val=mid; last = mid -1;\n // }\n // else if(nums[mid]>target){\n // last = mid-1;\n // }else{\n // first = mid+1;\n // }\n // }\n // first = 0; last = nums.length-1;\n // int val1 =-1;\n // while(first<=last){\n // int mid = (last+first) / 2;\n // if(nums[mid] == target){\n // val1=mid; first = mid + 1;\n // }\n // else if(nums[mid]>target){\n // last = mid-1;\n // }else{\n // first = mid+1;\n // }\n // }\n // if(val==-1) return ans;\n // for(int i=val; i<=val1; i++){\n // ans.add(i); \n // }\n // return ans;\n }\n}\n\n// ANOTHER SOLUTION\n// Arrays.sort(nums);\n// List<Integer> ans = new ArrayList();\n// for(int i=0; i<nums.length; i++){\n// if(nums[i] == target) ans.add(i);\n// }\n// return ans;\n``` | 10 | 0 | ['Java'] | 2 |
find-target-indices-after-sorting-array | Python || 98.02% Faster || Binary Search || Sorting | python-9802-faster-binary-search-sorting-ahz6 | \nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n nums.sort()\n l,h=0,len(nums)-1\n left=right=- | pulkit_uppal | NORMAL | 2022-12-04T21:56:32.854904+00:00 | 2022-12-04T21:56:32.854932+00:00 | 2,883 | false | ```\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n nums.sort()\n l,h=0,len(nums)-1\n left=right=-1\n while l<=h: #For finding the leftmost index of the target element\n m=(l+h)>>1\n if nums[m]==target:\n left=m\n h=m-1\n elif nums[m]>target:\n h=m-1\n else:\n l=m+1\n l,h=left,len(nums)-1\n while l<=h: #For finding the rightmost index of the target element\n m=(l+h)>>1\n if nums[m]==target:\n right=m\n l=m+1\n elif nums[m]>target:\n h=m-1\n else:\n l=m+1\n if left==-1:\n return []\n ans=range(left,right+1)\n return ans \n```\n\n**An upovte will be encouraging** | 10 | 0 | ['Binary Search', 'Sorting', 'Binary Tree', 'Python', 'Python3'] | 4 |
find-target-indices-after-sorting-array | Java O(N) Single loop | java-on-single-loop-by-devn007-8dev | \nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n int low = 0;\n int high = nums.length-1;\n for(int n | devn007 | NORMAL | 2022-01-18T14:30:25.451135+00:00 | 2022-01-18T14:30:25.451161+00:00 | 841 | false | ```\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n int low = 0;\n int high = nums.length-1;\n for(int num: nums){\n if(num<target){\n low++;\n }else if(num>target){\n high--;\n }\n }\n List<Integer> list = new ArrayList<>();\n while(low<=high){\n list.add(low);\n low++;\n }\n return list;\n }\n}\n``` | 9 | 0 | ['Java'] | 1 |
find-target-indices-after-sorting-array | Easy Java | fast | For beginners | EXPLAINED!!! 💡 | easy-java-fast-for-beginners-explained-b-nhxo | Approach\n- Quick sort the array\n- Iterate through the list and add all the indexes for which target == nums[i].\n- return the arraylist\n\n# Complexity\n- Tim | AbirDey | NORMAL | 2023-04-22T05:26:13.201715+00:00 | 2023-04-26T11:19:59.463721+00:00 | 880 | false | # Approach\n- Quick sort the array\n- Iterate through the list and add all the indexes for which target == nums[i].\n- return the arraylist\n\n# Complexity\n- Time complexity:O(n log n)\n\n- Space complexity:O(n)\n\n# Code\n```\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n Arrays.sort(nums);\n List<Integer> a = new ArrayList<>();\n for(int i = 0;i<nums.length;i++){\n if (nums[i] == target){\n a.add(i);\n }else if(nums[i] > target){\n break;\n }\n }\n return a;\n }\n}\n```\n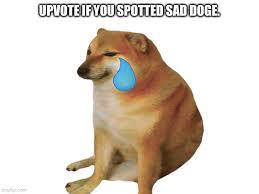\n | 8 | 0 | ['Array', 'Sorting', 'Java'] | 1 |
find-target-indices-after-sorting-array | Go O(n) one pass | go-on-one-pass-by-tuanbieber-al5o | \nfunc targetIndices(nums []int, target int) []int {\n var res []int\n \n count, left := 0, 0\n \n for i := 0; i < len(nums); i++ {\n if n | tuanbieber | NORMAL | 2022-06-30T01:57:10.292030+00:00 | 2022-06-30T01:57:10.292059+00:00 | 423 | false | ```\nfunc targetIndices(nums []int, target int) []int {\n var res []int\n \n count, left := 0, 0\n \n for i := 0; i < len(nums); i++ {\n if nums[i] == target {\n count++\n }\n \n if nums[i] < target {\n left++\n }\n }\n \n for i := 0; i < count; i++ {\n res = append(res, left)\n left++\n }\n \n return res\n}\n``` | 8 | 0 | ['C', 'Java', 'Go'] | 0 |
find-target-indices-after-sorting-array | Easy to Understand || C++ code | easy-to-understand-c-code-by-sunny_6289-3xg8 | Why Linear search better than Binary search in this case\n Describe your first thoughts on how to solve this problem. \nIn this case using binary search is not | sunny_6289 | NORMAL | 2023-05-04T17:05:06.930528+00:00 | 2023-08-06T18:19:14.137317+00:00 | 1,391 | false | # Why Linear search better than Binary search in this case\n<!-- Describe your first thoughts on how to solve this problem. -->\nIn this case using binary search is not an optimal solution because \n\n- The given array is not sorted so sorting it will take $$nlogn$$ time complexity itself.\n- if after sorting the array contains the target element in both halves of the given array then we will need to traverse again one of the half after using binary search on the array.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nBasic Linear Search\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(nlogn)$$\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(1)$$\n# Code\n```\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n sort(nums.begin(),nums.end());\n vector<int>ans;\n for(int i=0;i<nums.size();i++){\n if(nums[i]==target){\n ans.push_back(i);\n }\n }\n return ans;\n }\n};\n```\n# Please Upvote if it was helpful \u2B06\uFE0F | 7 | 0 | ['C++'] | 2 |
find-target-indices-after-sorting-array | Simple C++ Solution | Iteration :) | simple-c-solution-iteration-by-scaar-zlw6 | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach : \n- Simple Iteration Over sorted Array ! \n Describe your approach to so | Scaar | NORMAL | 2023-03-28T19:58:31.436861+00:00 | 2023-03-28T19:58:31.436895+00:00 | 616 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach : \n- Simple Iteration Over sorted Array ! \n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n sort(nums.begin(), nums.end());\n vector<int> result;\n for (int i=0;i<nums.size();i++){\n if(nums[i] == target){\n result.push_back(i);\n }\n }\n return result;\n \n }\n};\n``` | 7 | 1 | ['Iterator', 'C++'] | 0 |
find-target-indices-after-sorting-array | Java | Array | Sorting | Straightforward Solution | java-array-sorting-straightforward-solut-nq0j | \nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n Arrays.sort(nums);\n ArrayList<Integer> list = new ArrayLis | Divyansh__26 | NORMAL | 2022-09-17T08:01:19.191611+00:00 | 2022-09-17T08:01:19.191675+00:00 | 978 | false | ```\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n Arrays.sort(nums);\n ArrayList<Integer> list = new ArrayList<>();\n for(int i=0;i<nums.length;i++){\n if(nums[i]==target)\n list.add(i);\n if(nums[i]>target)\n break;\n }\n return list;\n }\n}\n```\nKindly upvote if you like the code. | 7 | 0 | ['Array', 'Sorting', 'Java'] | 2 |
find-target-indices-after-sorting-array | Python 3 (40ms) | O(nlogn) Sorting Simple Solution | python-3-40ms-onlogn-sorting-simple-solu-mhz1 | \nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n nums.sort()\n ans=[]\n for i in range(len(nums | MrShobhit | NORMAL | 2022-01-21T09:50:04.829343+00:00 | 2022-01-21T09:50:04.829371+00:00 | 731 | false | ```\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n nums.sort()\n ans=[]\n for i in range(len(nums)):\n if nums[i]==target:\n ans.append(i)\n return ans\n``` | 7 | 1 | ['Array', 'Sorting', 'Python'] | 4 |
find-target-indices-after-sorting-array | [C++] Naive and Linear Single-Pass Solutions Compared, 100% Time, ~99% Space | c-naive-and-linear-single-pass-solutions-e0lm | This problem is very trivial to be solved just following the requirement and, without even bothering to overthink, we might just do what we are told to do, give | Ajna2 | NORMAL | 2021-12-05T10:27:39.033110+00:00 | 2021-12-05T10:28:31.230794+00:00 | 508 | false | This problem is very trivial to be solved just following the requirement and, without even bothering to overthink, we might just do what we are told to do, given the very low constraints.\n\nWe will first of all declare a result variable `res`, then sort `nums`, proceed to iterate through them through an index `i` and record the position of any number `n` (`= nums[i]`) matching `target`, then finalle `return` `res`.\n\nThe naive code:\n\n```cpp\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n // support variables\n vector<int> res;\n // finding matches\n sort(begin(nums), end(nums));\n for (int i = 0, lmt = nums.size(), n; i < lmt; i++) {\n n = nums[i];\n if (n == target) res.push_back(i);\n else if (n > target) break;\n }\n return res;\n }\n};\n```\n\nDo we really need to sort the whole input vector? Probably not in a number of cases, so we might be instead first compute how many elements are before `target` and then `partial_sort` accordingly, bearing in mind that partial-sorting has an approssimate complexity which approximates to `(end - begin) * log(begin - mid)`, thus it might easily give us some improvements when the amount of elements to sort (`mid` in the above formula, `notOverTarget` in the code) is close to the start of the vector (`begin`), for the mere cost of an extra pass.\n\nThe code, which performs hardly any better with the short input we get, but still worth discussing in interviews - if you ask me:\n\n```cpp\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n // support variables\n vector<int> res;\n int notOverTarget = accumulate(begin(nums), end(nums), 0, [target](int a, int b){return a + (b <= target);});\n // finding matches\n partial_sort(begin(nums), begin(nums) + notOverTarget, end(nums));\n for (int i = 0, lmt = nums.size(), n; i < lmt; i++) {\n n = nums[i];\n if (n == target) res.push_back(i);\n }\n return res;\n }\n};\n```\n\nBut... Wait a moment! If we know how many elements are going to be not greater than `target`, we might not needt to sort at all!\n\nChanging our logic a bit, we might still iterate once through `nums`, figure out how many elements are strictly less than `target` (`lower`) and how many are equal to it (`matches`) and then just create `res` to be of the proper size (`matches`, again) and set its value to be starting from the amount of lesser elements onwards!\n\nIf that helps, think about implicitly having done a bucket-sorting of `nums`, but without actually rearranging the cell (and consuming space and time to do so), focusing only on the three buckets: lesser, equal and greater values, with no need to even bother counting the third ones.\n\nThe linear time code, which performs significantly better even on the small inputs we were provided:\n\n```cpp\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n // counting lesser and equal elements than target in nums\n int less = 0, matches = 0;\n for_each(begin(nums), end(nums), [&](int n){\n if (n < target) less++;\n else if (n == target) matches++;\n });\n // setting and filling res accordingly\n vector<int> res(matches);\n iota(begin(res), end(res), less);\n return res;\n }\n};\n``` | 7 | 1 | ['C', 'Bucket Sort', 'C++'] | 2 |
find-target-indices-after-sorting-array | Python || 98.02% Faster || O(n) Solution || Without Sorting | python-9802-faster-on-solution-without-s-wsnl | \nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n l=c=0\n for i in nums:\n if i==target:\n | pulkit_uppal | NORMAL | 2022-12-04T22:03:43.409866+00:00 | 2022-12-04T22:03:43.409899+00:00 | 758 | false | ```\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n l=c=0\n for i in nums:\n if i==target:\n c+=1\n elif i<target:\n l+=1\n ans=[]\n for i in range(c):\n ans.append(l)\n l+=1\n return ans\n```\n\n**An upvote will be encouraging** | 6 | 0 | ['Python', 'Python3'] | 0 |
find-target-indices-after-sorting-array | Python binSearch | python-binsearch-by-arkataev-usp1 | \nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n nums.sort()\n \n def bin_search(arr, t, lower= | arkataev | NORMAL | 2022-03-22T16:26:30.732885+00:00 | 2022-03-22T16:26:30.732930+00:00 | 702 | false | ```\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n nums.sort()\n \n def bin_search(arr, t, lower=True):\n left, right = 0, len(arr) -1 \n \n while left <= right:\n\n mid = (left + right) // 2\n\n if arr[mid] == t:\n if lower:\n if mid == left or nums[mid] != arr[mid - 1]:\n return mid\n else:\n right = mid - 1\n else:\n if mid == right or arr[mid] != arr[mid + 1]:\n return mid\n else:\n left = mid + 1\n \n elif arr[mid] > t:\n right = mid - 1 \n elif nums[mid] < t:\n left = mid + 1\n \n return -1\n \n start, end = bin_search(nums, target), bin_search(nums, target, False)\n \n if start < 0 and end < 0:\n return []\n \n return list(range(start, end + 1))\n \n``` | 6 | 0 | ['Binary Tree', 'Python'] | 1 |
find-target-indices-after-sorting-array | EASY SOLUTION IN JAVA BEATING SPACE AND TIME COMPLEXITY | easy-solution-in-java-beating-space-and-sq05p | Complexity
Time complexity: O(n*(logn))
Space complexity: O(n)
Code | arshi_bansal | NORMAL | 2025-01-28T07:51:21.589791+00:00 | 2025-01-28T07:51:21.589791+00:00 | 508 | false | # Complexity
- Time complexity: O(n*(logn))
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: O(n)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
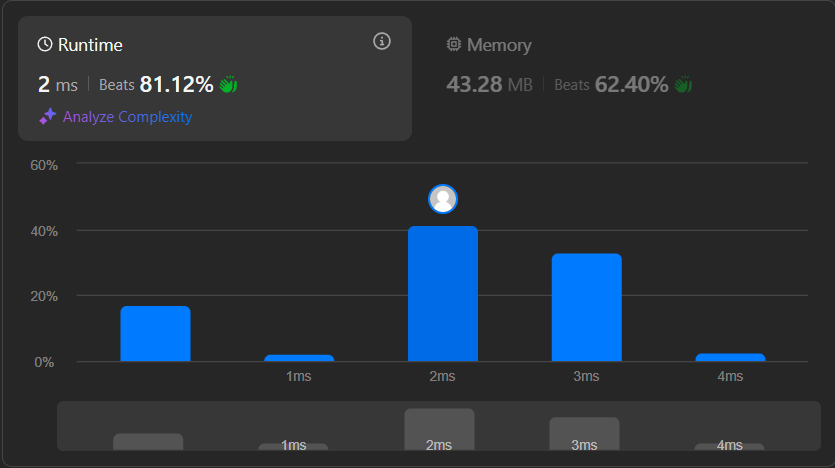
# Code
```java []
class Solution {
public List<Integer> targetIndices(int[] nums, int target) {
List<Integer> result=new ArrayList<>();
Arrays.sort(nums);
int n=nums.length;
for(int i=0;i<n;i++){
if(nums[i]==target){
result.add(i);
}
}
return result;
}
}
```
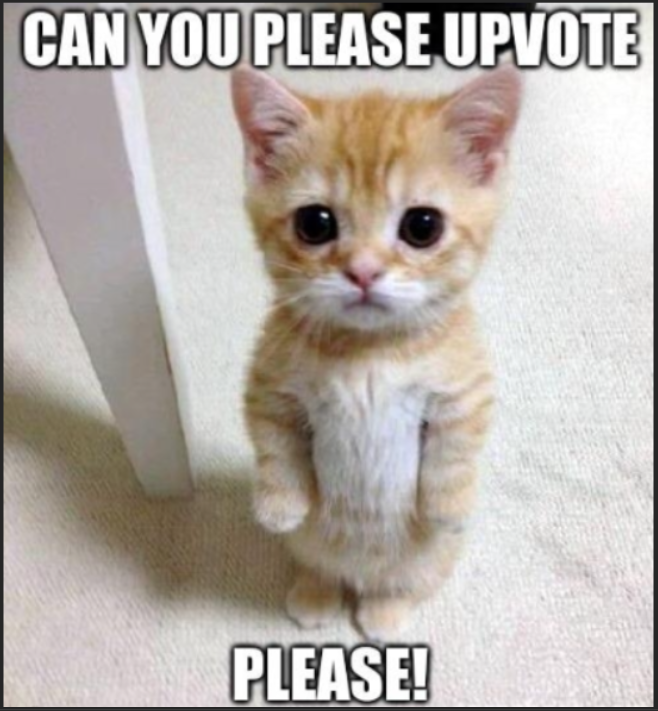
| 5 | 0 | ['Array', 'Binary Search', 'Sorting', 'Java'] | 0 |
find-target-indices-after-sorting-array | Count Sort with Linear Solution and Constant Space with 0 ms RunTime. | count-sort-with-linear-solution-and-cons-ovga | Intuition\n- Count the number of elements which are less than the target and get the count of total number of occurences of target.\n- Then we can calculate the | Guru_Prasath_K_S | NORMAL | 2024-05-31T03:48:53.508966+00:00 | 2024-06-01T16:59:58.259041+00:00 | 195 | false | # Intuition\n- Count the number of elements which are less than the target and get the count of total number of occurences of target.\n- Then we can calculate the indices of the target using the above.\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n---\n\n\n\n# Approach\n# Initialize Counters :\n- `countLessThanTarget` to keep track of the number of elements in the array that are less than the `target`.\n- `TargetTotalCount` to keep track of the total occurrences of the target element in the array.\n\n---\n\n\n# First Pass - Count Elements :\n\n- Iterate through the array and update `countLessThanTarget` and `TargetTotalCount` accordingly :\n - If the current element is less than the `target`, increment `countLessThanTarget`.\n - If the current element is equal to the `target`, increment `TargetTotalCount`.\n\n---\n\n\n# Determine Target Indices :\n\n- The starting index for the target elements in the sorted array is `countLessThanTarget`.\n- The target elements will span from `countLessThanTarget` to `countLessThanTarget + TargetTotalCount - 1`.\n\n---\n\n\n# Generate Result :\n\n- Create a vector `targetIndices` and populate it with the indices from `countLessThanTarget` to `countLessThanTarget + TargetTotalCount - 1`.\n\n\n---\n\n\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n---\n\n\n# Code\n```\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n\n int countLessThanTarget = 0 , TargetTotalCount = 0;\n\n for(int i = 0 ; i < nums.size() ; i++) {\n\n if(nums[i] < target) countLessThanTarget++;\n if(nums[i] == target) TargetTotalCount++;\n\n }\n\n vector<int> targetIndices;\n\n for(int i = countLessThanTarget ; i < countLessThanTarget + TargetTotalCount ; i++) {\n targetIndices.push_back(i);\n }\n\n return targetIndices;\n }\n};\n``` | 5 | 0 | ['Counting Sort', 'C++'] | 1 |
find-target-indices-after-sorting-array | Python3 || Easy beginner solution. | python3-easy-beginner-solution-by-kalyan-9pa0 | Please upvote if you find the solution helpful\n\n# Code\n\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n l= | Kalyan_2003 | NORMAL | 2023-02-28T13:25:05.643958+00:00 | 2023-02-28T13:25:05.643997+00:00 | 938 | false | # Please upvote if you find the solution helpful\n\n# Code\n```\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n l=[]\n nums.sort()\n for i in range(len(nums)):\n if nums[i]==target:\n l.append(i)\n return l\n \n``` | 5 | 0 | ['Array', 'Sorting', 'Python3'] | 1 |
find-target-indices-after-sorting-array | JAVA || Easily understandable || properly explained | java-easily-understandable-properly-expl-3bbt | \n# Approach\n1st: sorting the array;\n2nd: using a for loop, if any value matches with target then inserting that into the list.\n\n\n\n# Code\n\nclass Solutio | sharforaz_rahman | NORMAL | 2022-11-19T06:42:20.202051+00:00 | 2022-11-19T06:42:46.437872+00:00 | 541 | false | \n# Approach\n1st: sorting the array;\n2nd: using a for loop, if any value matches with target then inserting that into the list.\n\n\n\n# Code\n```\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n Arrays.sort(nums);\n ArrayList<Integer> list = new ArrayList<>();\n for (int i = 0; i < nums.length; i++) {\n if (nums[i] == target) {\n list.add(i);\n }\n }\n return list;\n }\n}\n``` | 5 | 0 | ['Java'] | 0 |
find-target-indices-after-sorting-array | ✔️Python, C++,Java|| Beginner level||Simple-Short-Solution✔️ | python-cjava-beginner-levelsimple-short-8eund | Please upvote to motivate me in my quest of documenting all leetcode solutions. HAPPY CODING:)\nAny suggestions and improvements are always welcome.\n___\n__\nQ | Anos | NORMAL | 2022-09-13T08:30:38.224175+00:00 | 2022-09-13T08:30:38.224218+00:00 | 670 | false | ***Please upvote to motivate me in my quest of documenting all leetcode solutions. HAPPY CODING:)\nAny suggestions and improvements are always welcome*.**\n___________________\n_________________\n***Q2089. Find Target Indices After Sorting Array***\n____________________________________________________________________________________________________________________\n____________________________________________________________________________________________________________________\n\u2705 **Python Code** :\n```\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n l,h=0,len(nums)\n for n in nums:\n if n>target:\n h-=1\n elif n<target:\n l+=1\n return (range(l,h))\n```\n**Runtime:** 55 ms\t\n**Memory Usage:** 13.6 MB\n____________________________________________________________________________________________________________________\n____________________________________________________________________________________________________________________\n\n\u2705 **Java Code** :\n```\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n List<Integer> ans=new ArrayList<>();\n Arrays.sort(nums);\n int l=0,h=nums.length-1;\n for(int num:nums)\n {\n if(num<target)\n l++;\n else if(num>target)\n h--;\n }\n while(l<=h)\n ans.add(l++);\n \n return ans;\n }\n}\n```\n**Runtime:** 3 ms\t\t\n**Memory Usage:** 43..8 MB\t\n____________________________________________________________________________________________________________________\n____________________________________________________________________________________________________________________\n\u2705 **C++ Code** :\n```\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n vector<int> ans;\n int l=0,h=nums.size()-1;\n sort(nums.begin(),nums.end());\n for(auto num:nums)\n {\n if (num>target)\n h--;\n else if(num<target)\n l++;\n }\n while(l<=h)\n {\n ans.push_back(l++);\n }\n return ans;\n }\n};\n```\n**Runtime:** 7 ms\t\n**Memory Usage:** 11.3 MB\t\t\n____________________________________________________________________________________________________________________\n____________________________________________________________________________________________________________________\nIf you like the solution, please upvote \uD83D\uDD3C\nFor any questions, or discussions, comment below. \uD83D\uDC47\uFE0F\n | 5 | 0 | ['C', 'Binary Tree', 'Python', 'Java'] | 1 |
find-target-indices-after-sorting-array | C++ | Two Methods with explanation | O(N) and O(N log N) approach | Easy to understand | c-two-methods-with-explanation-on-and-on-9gdx | O(N) Solution\n\n\nclass Solution {\npublic:\n\n vector<int> targetIndices(vector<int>& nums, int target) {\n vector<int> vec;\n int c1=0, c2=0 | AdityaBhate | NORMAL | 2022-06-26T07:18:04.108080+00:00 | 2022-11-08T05:47:46.469040+00:00 | 1,420 | false | ## **O(N) Solution**\n\n```\nclass Solution {\npublic:\n\n vector<int> targetIndices(vector<int>& nums, int target) {\n vector<int> vec;\n int c1=0, c2=0;\n for(auto i=0;i<nums.size();i++){\n if(nums[i]==target)\n c2++;\n else if(nums[i]<target)\n c1++;\n }\n for(auto i=c1;i<c2+c1;i++)\n vec.emplace_back(i);\n return vec;\n }\n};\n```\n\n## **O(N logN) Solution**\n```\nclass Solution {\npublic:\n\n vector<int> targetIndices(vector<int>& nums, int target) {\n vector<int> vec;\n sort(nums.begin(),nums.end());\n for(auto i=0;i<nums.size();i++){\n if(nums[i]==target)\n vec.emplace_back(i);\n }\n return vec;\n }\n};\n```\n\n# **Please Upvote if it helps \uD83D\uDE4F** | 5 | 0 | ['Array', 'Binary Search', 'C++', 'Java', 'Python3'] | 0 |
find-target-indices-after-sorting-array | C++ || Easy Fast || Explained || Binary Search | c-easy-fast-explained-binary-search-by-s-51pb | Approach\n Sort the nums vector\n Find the index where target is found using a binary search function\n in case no such index found return empty vector\n Otherw | shriya27 | NORMAL | 2022-02-08T19:46:43.474980+00:00 | 2022-02-08T19:47:27.812613+00:00 | 460 | false | **Approach**\n* Sort the nums vector\n* Find the index where target is found using a binary search function\n* in case no such index found return empty vector\n* Otherwise, take a temp variable with value of srch-1 and iterate till temp reaches 0 and push all such temp index in ans vector\n* Reverse the ans vector and tehn store srch index\n* iterate from srch+1 till right end and store all such index havng target stored\n````\nclass Solution {\npublic:\n int bs(vector<int>& nums, int target){\n int l=0,m;\n int r=nums.size()-1;\n while(l<=r)\n {\n m=l+(r-l)/2;\n if(nums[m]==target)return m;\n else if(nums[m]<target) l=m+1;\n else r=m-1;\n }\n return -1;\n }\n vector<int> targetIndices(vector<int>& nums, int target) {\n sort(nums.begin(),nums.end());\n vector<int> ans;\n int srch=bs(nums,target);\n if(srch==-1)return {};\n int l=0,r=nums.size()-1;\n int temp=srch-1;\n while(temp>=0 && nums[temp]==target)\n {\n ans.push_back(temp);\n temp--;\n }\n reverse(ans.begin(),ans.end());\n ans.push_back(srch);\n temp=srch+1;\n while(temp<=r && nums[temp]==target)\n {\n ans.push_back(temp);\n temp++;\n }\n return ans;\n }\n};\n````\n\nTC: O(NlogN)+O(logN) +O(N) +O(N)\nSC: O(N)\n**Please Upvote if you like the solution! :)** | 5 | 0 | ['Binary Search', 'C'] | 0 |
find-target-indices-after-sorting-array | binary search. Easy solution. | binary-search-easy-solution-by-error_adp-0n4h | Intuition\n Describe your first thoughts on how to solve this problem. \nfirst find the lower_bound then the upper_bound then handle some corner case and ultima | error_adp | NORMAL | 2024-05-25T02:09:07.159776+00:00 | 2024-05-25T02:09:07.159798+00:00 | 902 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nfirst find the lower_bound then the upper_bound then handle some corner case and ultimately i found the answer...\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int findLow(vector<int> &nums, int target, int low,int high){\n int ans=-1;\n while(low<=high){\n int mid = (low+high)/2;\n if(nums[mid] == target){\n ans = mid;\n high = mid-1;\n }\n else if(target > nums[mid]){\n low = mid+1;\n }else{\n high = mid-1;\n }\n }\n return ans;\n }\n int findHigh(vector<int> &nums, int target, int low,int high){\n int ans=-1;\n while(low<=high){\n int mid = (low+high)/2;\n if(nums[mid] == target){\n ans = mid;\n low=mid+1;\n }\n else if(target > nums[mid]){\n low = mid+1;\n }else{\n high = mid-1;\n }\n }\n return ans;\n }\n vector<int> targetIndices(vector<int>& nums, int target) {\n sort(nums.begin(), nums.end());\n int low = 0;\n int high = nums.size()-1;\n int x = findLow(nums,target,low,high);\n int y = findHigh(nums,target,low,high);\n if(x==y and x!=(-1) and y!=(-1)){\n return {x};\n }\n if(x==(-1) and y==(-1))return {};\n if(y-x == 1)return {x,y};\n vector<int> ans;\n if((y-x) > 1){\n for(int i=x; i<=y; i++){\n ans.push_back(i);\n }\n }\n return ans;\n }\n};\n``` | 4 | 0 | ['C++'] | 0 |
find-target-indices-after-sorting-array | Easy JAVA Solution [ 42% ][ 3ms ] | easy-java-solution-42-3ms-by-rajarshimit-6bey | Approach\n1. Initialize an empty ArrayList to store the indices of elements that match the target value.\n2. Loop through the array nums using a for loop.\n3. F | RajarshiMitra | NORMAL | 2024-04-15T15:06:05.075775+00:00 | 2024-06-16T08:32:46.935953+00:00 | 39 | false | # Approach\n1. Initialize an empty `ArrayList` to store the indices of elements that match the target value.\n2. Loop through the array `nums` using a for loop.\n3. For each element in the array, check if it matches the target value.\n4. If the element matches the target, add its index to the `ArrayList`.\n5. After iterating through the array, return the `ArrayList` containing the indices of elements that match the target value.\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(k)\nwhere k is the number of occurrences of the target in the array nums.\n\n# Code\n```\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n Arrays.sort(nums);\n ArrayList<Integer> res = new ArrayList<>();\n for(int i=0; i<nums.length; i++){\n if(nums[i] == target) res.add(i);\n }\n return res;\n }\n}\n```\n\n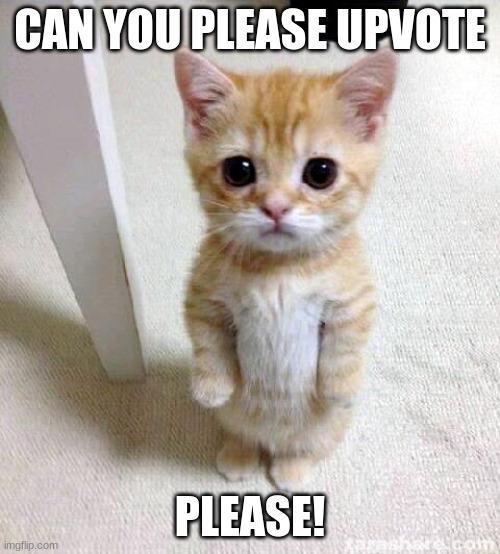\n | 4 | 0 | ['Java'] | 0 |
find-target-indices-after-sorting-array | JAVA Easy Solution 🔥 100% Faster Code 🔥 With and Without Using Sorting 🔥 | java-easy-solution-100-faster-code-with-xrd3c | Two solutions with and without using sorting.\n\n# Complexity\n- Time complexity: O(n)\n Add your time complexity here, e.g. O(n) \n\n- Space complexity: O(1)\n | diyordev | NORMAL | 2023-12-04T11:21:46.211611+00:00 | 2023-12-04T11:21:46.211652+00:00 | 288 | false | # Two solutions with and without using sorting.\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# First Solution [Without Sorting]\n```\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n int countTarget = 0, lessTarget = 0;\n for (int num : nums) {\n if (num < target) lessTarget++;\n if (num == target) countTarget++;\n }\n List<Integer> result = new ArrayList<>();\n for (int i = 0; i < countTarget; i++)\n result.add(lessTarget + i);\n return result;\n }\n} \n```\n\n# Complexity\n- Time complexity: $$O(n log(n))$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Second Solution [Using Sorting]\n```\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n List<Integer> result = new ArrayList<>();\n Arrays.sort(nums);\n for (int i = 0; i < nums.length; i++)\n if (nums[i] == target)\n result.add(i);\n return result;\n }\n} \n``` | 4 | 0 | ['Java'] | 0 |
find-target-indices-after-sorting-array | Easiest Solution(100%/87%) | easiest-solution10087-by-kubatabdrakhman-h77d | \n# Code\n```\n/*\n * @param {number[]} nums\n * @param {number} target\n * @return {number[]}\n /\nvar targetIndices = function(nums, target) {\n let result | kubatabdrakhmanov | NORMAL | 2023-08-31T06:21:05.495774+00:00 | 2023-08-31T06:21:05.495809+00:00 | 947 | false | \n# Code\n```\n/**\n * @param {number[]} nums\n * @param {number} target\n * @return {number[]}\n */\nvar targetIndices = function(nums, target) {\n let result = [];\n\n nums.sort((a,b) => a-b).forEach((item, idx) => {\n if(item === target){\n result.push(idx);\n }\n })\n return result\n}; | 4 | 0 | ['Array', 'JavaScript'] | 0 |
find-target-indices-after-sorting-array | NO SEARCHING | NO SORTING 👑 | BEATS 100% | no-searching-no-sorting-beats-100-by-tej-ud9z | Intuition\nwe dont need actual indices, we dont care about sequence or order so we don\'t need sort.\nbinary search \n# Approach\nJust count number of elements | tejtharun625 | NORMAL | 2023-08-01T17:41:58.803048+00:00 | 2023-08-01T17:41:58.803078+00:00 | 422 | false | # Intuition\nwe dont need actual indices, we dont care about sequence or order so we don\'t need sort.\nbinary search \n# Approach\nJust count number of elements which are less than or equal to given target. say lessThanOrEqualCount\nThen count number of elements which are strictly less than given target onlyLessThanCount\n\nif lessThanOrEqualCount = onlyLessThanCount: return empty\nor else all numbers between them\n\n# Complexity\n- Time complexity:\n$$O(n)$$\n- Space complexity: O(1)\n$$O(1)$$\n\n# Code\n```\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n lessThanEqual = 0\n onlyLess = 0\n for i in nums:\n if i <= target:\n lessThanEqual += 1\n if i < target:\n onlyLess += 1\n return list(range(onlyLess, lessThanEqual))\n```\n\nPlease upvote if you like the solution.\n | 4 | 0 | ['Binary Search', 'Sorting', 'Python3'] | 0 |
find-target-indices-after-sorting-array | Simple O(n) solution. No sorting. | simple-on-solution-no-sorting-by-vmittal-epo8 | Intuition\nYou don\'t need to sort the Array, you only need to find numbers bigger than target and smaller than target. Once you have that you can easily count | vmittal91 | NORMAL | 2023-04-25T03:32:33.020248+00:00 | 2023-04-25T03:32:33.020295+00:00 | 217 | false | # Intuition\nYou don\'t need to sort the Array, you only need to find numbers bigger than target and smaller than target. Once you have that you can easily count the indices.\n\n# Approach\nIterate over each number in the array and keep track of numbers lesser than target in the variable startSkip and numbers greater than target in the variable endSkip. \n\nRun a for look starting from the first index where the first occurance of target will occur in the sorted array and iteratively keep adding indices until you reach the last index of target number, which will be endSkip numbers away from the last index of the array.\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(n) to store the elements.\n\n# Code\n```\npublic class Solution {\n public IList<int> TargetIndices(int[] nums, int target) {\n List<int> nos = new List<int>();\n int startSkip = 0;\n int endSkip = 0;\n foreach(int num in nums){\n if (num > target){\n endSkip++;\n }\n if (num < target){\n startSkip++;\n }\n }\n \n for(int i = startSkip; i<nums.Length-endSkip; i++){\n nos.Add(i);\n }\n\n return nos;\n }\n}\n``` | 4 | 0 | ['C#'] | 3 |
find-target-indices-after-sorting-array | 2 Lines of Code--->Using BST Logic | 2-lines-of-code-using-bst-logic-by-ganji-dt5c | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | GANJINAVEEN | NORMAL | 2023-03-11T20:01:59.524544+00:00 | 2023-03-11T20:01:59.524570+00:00 | 735 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n n=sorted(nums)\n index1,index2=bisect.bisect_left(n,target),bisect.bisect_right(n,target)\n return list(range(index1,index2))\n #please upvote me it would encourage me alot\n\n\n\n``` | 4 | 0 | ['Python3'] | 0 |
find-target-indices-after-sorting-array | How **Binary Search** 🤔🤔 || Simple *time efficient* approach || Explained in detail || Code in C++ | how-binary-search-simple-time-efficient-e5l83 | Intuition\n Describe your first thoughts on how to solve this problem. \n1. The problem is labelled as \'Binary Search\' but since the array is not given in sor | user7863d | NORMAL | 2023-02-23T16:28:56.173888+00:00 | 2023-02-23T16:28:56.173930+00:00 | 1,402 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n1. The problem is labelled as \'Binary Search\' but since the array is not given in sorted form, we can\'t use binary search directly and sorting it to apply binary search will give time complexity of $$O(n logn)$$ (due to sorting).\n\n2. And even after sorting, it the target element lies in both the sub-divisions of the array, then also we will have to traverse the entire array in order to count its total occurances.\n\n3. Hence, using binary search would not be an ideal approach for this problem. \n\n--> The most efficient time complexity which can be achieved in this problem is $$O(n)$$. \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Traverse the entire array in one go in $$O(n)$$ time and store the **count of occurances of target element** (*let say in variable count*) and **elements which are lesser than it** (*let say in variable lesser*).\n\n2. Create a vector (array) and enter the target indices which will hold the target element as per the values obtained from lesser and count variables in non-decreasing order.\n\n3. Finally return the output vector. \n\n# Complexity\n- Time complexity = $$O(n)$$, due to linear traversal in array.\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$, due to the output vector used.\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n vector<int> ans ;\n\n int n = nums.size() ;\n\n int count = 0, lesser = 0 ;\n\n for(int i=0; i<n; i++){\n if(nums[i] < target) lesser++ ;\n else if(nums[i] == target) count++ ;\n }\n\n int start = lesser, end = start + count - 1 ;\n for(int i=start; i<=end; i++){\n ans.push_back(i) ;\n }\n\n return ans ;\n }\n};\n``` | 4 | 0 | ['C++'] | 3 |
find-target-indices-after-sorting-array | O(n) Solution Python | on-solution-python-by-ayon_ssp-o1wg | Count Operation: \n\n# Author: github.com/Ayon-SSP\n# Time: O(N)\n# Space: O(1)\nclass Solution(object):\n def targetIndices(self, nums, target):\n lo | ayon_ssp | NORMAL | 2022-12-08T15:59:28.468641+00:00 | 2022-12-08T15:59:28.468678+00:00 | 756 | false | ## Count Operation: \n```\n# Author: github.com/Ayon-SSP\n# Time: O(N)\n# Space: O(1)\nclass Solution(object):\n def targetIndices(self, nums, target):\n lowVals = 0; repOfTg = 0\n for val in nums:\n if val < target:\n lowVals += 1\n elif val == target:\n repOfTg += 1\n ans = []\n for _ in range(repOfTg):\n ans.append(lowVals)\n lowVals += 1\n return ans\n```\nCheck Out SDE Prep full Road Map[`GitHub`](https://github.com/Ayon-SSP/The-SDE-Prep)\n | 4 | 0 | ['Python'] | 0 |
find-target-indices-after-sorting-array | C++ Easy Solution using Binary Search || Well Explained using comments || Understandable | c-easy-solution-using-binary-search-well-kvsb | \nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n \n sort (nums.begin(), nums.end());\n \n | bipsig | NORMAL | 2022-07-12T21:30:29.070908+00:00 | 2022-07-12T21:30:29.070937+00:00 | 638 | false | ```\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n \n sort (nums.begin(), nums.end());\n \n int low = 0;\n int high = nums.size() - 1;\n int mid;\n vector <int> ans;\n \n /*So the logic that we are going to use is:\n Binary Search is successful in finding only 1 instance of an element if there are multiple duplicates. So how do we find the rest instances. \n What I thought is that binary search randomly finds one instance of an element from the sorted array.\n So the other instances of that element will either be on its right or on its left and no where else.\n What if we store the index of the element found and use that index to find if the same element lies on it sright and left.\n NOTE: The boundary conditions, index must not go below 0 or higher than size of array which can give runtime error*/\n \n \n while (low <= high)\n {\n mid = (high+low)/2;\n \n if (nums[mid] == target)\n {\n ans.push_back (mid);\n break;\n }\n else if (nums[mid] < target)\n {\n low = mid + 1;\n }\n else\n {\n high = mid - 1;\n }\n }\n \n /*If no instance is found then we return the empty array as there cannot be any other duplicate*/\n if (ans.size() == 0)\n return ans;\n \n int tmid = mid;\n \n /*Checking the left side of the found element*/\n mid--;\n while (mid >= 0 && nums[mid] == target)\n {\n ans.push_back (mid);\n mid--;\n }\n \n mid = tmid;\n \n \n /*Checking the right side of the found element*/\n mid++;\n while (mid < nums.size() && nums[mid] == target)\n {\n ans.push_back (mid);\n mid++;\n }\n \n \n //Sorting the found element in ascending order\n sort (ans.begin(), ans.end());\n \n return ans;\n \n }\n};\n\n``` | 4 | 0 | ['C', 'Sorting', 'Binary Tree', 'C++'] | 1 |
find-target-indices-after-sorting-array | C++ Explained Binary Search Approach | c-explained-binary-search-approach-by-ga-ss12 | Kindly upvote if you find it helpful : )\n\nclass Solution {\npublic:\n //separate function to calculate first occurence of target using binary search\n i | gargiii | NORMAL | 2022-02-26T09:37:39.956793+00:00 | 2022-02-26T09:37:39.956820+00:00 | 367 | false | Kindly **upvote** if you find it helpful **: )**\n```\nclass Solution {\npublic:\n //separate function to calculate first occurence of target using binary search\n int firstOccurence(vector<int>& nums, int k){\n int s = 0, e = nums.size()-1;\n int m = s + (e-s)/2;\n int ans = -1; // if target not found in the given array then function will return -1\n while(s<=e){\n if(nums[m]==k){\n ans = m;\n e = m-1;\n }\n else if(nums[m]<k){\n s = m+1;\n }\n else if(nums[m]>k){\n e = m-1;\n }\n m = s + (e-s)/2;\n }\n return ans;\n }\n //separate function to calculate last occurence of target using binary search\n int lastOccurence(vector<int>& nums, int k){\n int s = 0, e = nums.size()-1;\n int m = s + (e-s)/2;\n int ans = -1; // if target not found in the given array then function will return -1\n while(s<=e){\n if(nums[m]==k){\n ans = m;\n s = m+1;\n }\n else if(nums[m]<k){\n s = m+1;\n }\n else if(nums[m]>k){\n e = m-1;\n }\n m = s + (e-s)/2;\n }\n return ans;\n }\n vector<int> targetIndices(vector<int>& nums, int target) {\n vector<int> ans; // creating ans vector for storing target indices\n sort(nums.begin(),nums.end()); // sorting given vector array\n \n int first = firstOccurence(nums,target); \n int last = lastOccurence(nums,target);\n \n if(first != -1 && last != -1){ // check if target is found \n for(int i=first; i<=last; i++){ // iterate from first to last and push all the indices in ans vector\n ans.push_back(i);\n }\n }\n return ans;\n }\n};\n``` | 4 | 1 | ['C', 'Binary Tree'] | 1 |
find-target-indices-after-sorting-array | Simple python code with explanation | simple-python-code-with-explanation-by-p-b8ut | Simple O(nlogn) Solution:\nIf we sort the array, simply we can search through array and see at which index target exists and we just update it in our result. \n | punitvara | NORMAL | 2022-01-28T13:37:25.662110+00:00 | 2022-01-28T13:37:25.662136+00:00 | 342 | false | **Simple O(nlogn) Solution:**\nIf we sort the array, simply we can search through array and see at which index target exists and we just update it in our result. \n\n```\nclass Solution:\n \n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n res = []\n nums.sort()\n for i in range(len(nums)):\n if nums[i] == target:\n res.append(i)\n return res\n```\n\n**O(N) Solution**\nWe can traverse through array and see how many elements exist which are less than target and how many target elements are present. Then we dont need to serach the array \n\nLets take an example:\nnums = [1 3 4 2 2 5]\n\nIf we sort above array then output would look something like this\nnums = [1 2 2 3 4 5]\n\nWhat information is useful if we sort array is how many numbers are present less than target. Once we know it and number of targets. We can get it without sorting too. We can just iterate through array and see get it. Once we have that we can simply push target index after no of elements which are less than target. \n```\nclass Solution:\n \n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n res = []\n equal = 0\n less = 0\n for i in range(len(nums)):\n if nums[i] == target:\n equal += 1\n elif nums[i] < target:\n less += 1\n \n for i in range(equal):\n res.append(less)\n less += 1\n return res\n \n``` | 4 | 0 | ['Python'] | 0 |
find-target-indices-after-sorting-array | Two Method Soln in Python Without Sorting | two-method-soln-in-python-without-sortin-3rh2 | Method - 1 \nWithout Sorting Simple logic\nTC - O(N)\n\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n \n | gamitejpratapsingh998 | NORMAL | 2022-01-07T12:11:59.641506+00:00 | 2022-01-07T12:16:34.909040+00:00 | 610 | false | Method - 1 \nWithout Sorting Simple logic\nTC - O(N)\n```\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n \n m=c=0 \n for i in nums:\n if i<target:\n m+=1\n if i==target:\n c+= 1\n if target not in nums:\n return []\n \n ans=[]\n while c > 0:\n ans.append(m)\n m+=1\n c-=1\n return ans\n```\n\nMethod - 2\nUsing Min Heap :-\nTC - O(NlogN)\n\n```\nclass Solution:\n \n import heapq\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n \n heapq.heapify(nums)\n i=0\n ans=[]\n while nums!=[]:\n x = heapq.heappop(nums)\n if x==target:\n ans.append(i)\n if x > target:\n break\n i+=1\n return ans\n``` | 4 | 0 | ['Heap (Priority Queue)', 'Python', 'Python3'] | 0 |
find-target-indices-after-sorting-array | C++ O(N) time without sorting | c-on-time-without-sorting-by-ralphcoder-gj78 | \nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n vector<int> ans;\n int start=0,fre=0;\n for(a | ralphcoder | NORMAL | 2021-12-12T08:14:25.592654+00:00 | 2021-12-12T08:14:25.592691+00:00 | 274 | false | ```\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n vector<int> ans;\n int start=0,fre=0;\n for(auto i: nums)\n { if(i<target)start++;//counted elements less than the target\n if(i==target)fre++;}//frequency of the target\n while(fre--)\n ans.push_back(start++);\n return ans; \n }\n};\n``` | 4 | 0 | ['C'] | 0 |
find-target-indices-after-sorting-array | Java Code Both Approach | java-code-both-approach-by-rizon__kumar-6ech | Brute Force - \nT.C => nlog(n) + O(n)\n\n\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n \n //nlog(n)\n | rizon__kumar | NORMAL | 2021-12-05T04:24:04.925644+00:00 | 2021-12-07T12:35:16.977747+00:00 | 187 | false | **Brute Force - **\nT.C => nlog(n) + O(n)\n\n```\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n \n //nlog(n)\n Arrays.sort(nums);\n \n List<Integer> ans = new ArrayList<>();\n \n int n = nums.length;\n \n //O(n)\n for(int i=0; i<n; i++){\n if(nums[i] == target) ans.add(i);\n }\n \n return ans;\n }\n}\n```\n**Optimized Approach - Count Sort**\nCounting Sort\nOne iteration to count less than target, and equal target. Build output based on the first index at lessthan.\nT.C => O(N) \n\n```\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n int count = 0, lessthan = 0;\n for (int n : nums) {\n if (n == target) count++;\n if (n < target) lessthan++;\n }\n \n List<Integer> result = new ArrayList<>();\n for (int i = 0; i < count; i++) {\n result.add(lessthan++);\n }\n return result;\n }\n}\n```\n\n\n | 4 | 0 | [] | 2 |
find-target-indices-after-sorting-array | Python one pass simple NO SORTING | Beats 100% , 0ms 🔥 | python-one-pass-simple-no-sorting-beats-yz63n | IntuitionWithout sorting only single pass lookup with memory can solve this problem, no need to complicate the solution.ApproachKeep 2 variables -
First to note | nilesh0103 | NORMAL | 2025-02-26T04:18:53.016460+00:00 | 2025-02-26T04:18:53.016460+00:00 | 279 | false | # Intuition
Without sorting only single pass lookup with memory can solve this problem, no need to complicate the solution.
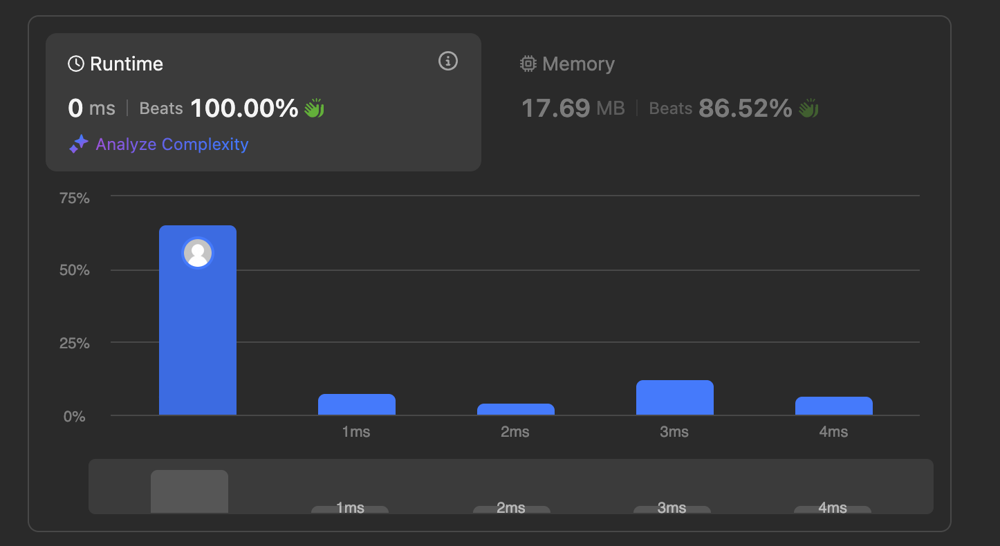
# Approach
Keep 2 variables -
First to note down the element count less than target.
Second to note the repetition of target.
Return array starting from startCount to startCount+repetition.
# Complexity
- Time complexity:
O(n)
- Space complexity:
O(1)
# Code
```python3 []
class Solution:
def targetIndices(self, nums: List[int], target: int) -> List[int]:
startIndex = 0
repetition = 0
for i in range(len(nums)):
if nums[i] < target:
startIndex+=1
elif nums[i] == target:
repetition +=1
return [i for i in range(startIndex,startIndex+repetition)]
``` | 3 | 0 | ['Python3'] | 2 |
find-target-indices-after-sorting-array | Simple Solution | Beginner Friendly | Easy Approach with Explanation✅ | simple-solution-beginner-friendly-easy-a-64au | Intuition\n Describe your first thoughts on how to solve this problem. \nTo find all the indices of a target number in an array, we can sort the array and then | Jithinp96 | NORMAL | 2024-11-27T13:52:06.803513+00:00 | 2024-11-27T13:52:06.803544+00:00 | 236 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nTo find all the indices of a target number in an array, we can sort the array and then iterate through it to collect indices where the value matches the target.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Sort the `nums` array in ascending order.\n2. Traverse the sorted array and check if each element is equal to the `target`.\n3. If it matches, add the index to the result array.\n4. Return the result array containing all indices.\n\n# Code\n```javascript []\n/**\n * @param {number[]} nums\n * @param {number} target\n * @return {number[]}\n */\nvar targetIndices = function(nums, target) {\n let number = [];\n nums.sort((a,b)=>a-b);\n for(let i=0; i<nums.length; i++){\n if(nums[i]=== target){\n number.push(i)\n }\n }\n return number;\n};\n```\n\n```typescript []\nfunction targetIndices(nums: number[], target: number): number[] {\n const result: number[] = [];\n nums.sort((a, b) => a - b);\n\n for (let i = 0; i < nums.length; i++) {\n if (nums[i] === target) {\n result.push(i);\n }\n }\n\n return result;\n}; | 3 | 0 | ['TypeScript', 'JavaScript'] | 0 |
find-target-indices-after-sorting-array | Efficient Index Calculation for Target in Unsorted Array Without Sorting | efficient-index-calculation-for-target-i-kp3o | Intuition\n Describe your first thoughts on how to solve this problem. \nThe problem asks us to find all the indices where the target value would appear in a so | prasannaprassadshenoy | NORMAL | 2024-10-02T09:50:10.497256+00:00 | 2024-10-02T09:50:10.497285+00:00 | 199 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe problem asks us to find all the indices where the target value would appear in a sorted array. However, instead of sorting the array, we can use a more efficient approach by simply counting how many numbers are less than the target (which helps us find the starting index) and how many times the target appears (which helps us find the rest of the indices). This way, we avoid the overhead of sorting the array.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1) Counting elements: First, iterate through the array and count two things:\n\n2) How many elements are strictly less than the target (lower).\nHow many times the target appears (count).\nConstructing the result: The indices where the target would appear are consecutive, starting from lower (i.e., the number of elements less than the target) and going up to lower + count - 1.\n\n3) Return result: After counting, if the target exists (count > 0), generate and return the list of indices from lower to lower + count - \n\n\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n1) The time complexity is O(n), where n is the size of the input array nums. We only need to iterate through the array once to count the necessary values.\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n1) The space complexity is O(1) if we ignore the space needed for the result, as we are only using a few integer variables (lower, count).\n2) The space complexity is O(k), where k is the number of target occurrences in the result, as we need to store the target indices.\n\n# Code\n```cpp []\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n int lower=0, count=0;\n for(int i: nums){\n if(i<target){\n lower++;\n }\n else if(i==target){\n count++;\n }\n } \n vector<int> result;\n for(int i=0; i<count; i++){\n result.push_back(lower+i);\n }\n return result;\n }\n};\n``` | 3 | 0 | ['C++'] | 0 |
find-target-indices-after-sorting-array | [JAVA] beats 100% solution without sorting | java-beats-100-solution-without-sorting-l4j62 | Intuition\nThe task is to find the indices of a target element if the array were sorted. Instead of sorting the array, which takes O(nlog\u2061n)O(nlogn), we ca | Shubhash_Singh | NORMAL | 2024-10-02T04:38:34.866965+00:00 | 2024-10-02T04:38:34.866996+00:00 | 241 | false | # Intuition\nThe task is to find the indices of a target element if the array were sorted. Instead of sorting the array, which takes O(nlog\u2061n)O(nlogn), we can count:\n1. The number of elements that are smaller than the target.\n2. How many times the target appears.\n\nWith these two pieces of information, we can directly calculate the target indices in the sorted array.\n# Approach\n1. Count Elements: Loop through the array and count:\n\n - `lowerElement`: The number of elements smaller than the target.\n - `elemCount`: The number of occurrences of the target element.\n\n2. Generate Result: The target element would start appearing at the index `lowerElement` in the sorted array, and its indices will be continuous for `elemCount` times. So, generate the indices starting from `lowerElement`.\n\n# Complexity\n- Time complexity:\n - The algorithm runs in a single pass through the array, so the time complexity is `O(n)`, where n is the number of elements in the array.\n- Space complexity:\n - We only use a few additional variables and a result list that stores indices. The space complexity is `O(1)` for the variables and O(k)O(k) for the result list, where k is the number of target occurrences (at most equal to n in the worst case).\n# Code\n```java []\nimport java.util.*;\n\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n List<Integer> res = new ArrayList<>();\n int lowerElement = 0;\n int elemCount = 0;\n\n for(int i = 0;i<nums.length;i++){\n if(nums[i] < target){\n lowerElement ++;\n }\n if(nums[i] == target){\n elemCount++;\n }\n }\n for(int i = 0;i<elemCount;i++){\n res.add(lowerElement++);\n }\n\n return res;\n\n }\n\n}\n``` | 3 | 0 | ['Java'] | 1 |
find-target-indices-after-sorting-array | 💢☠💫Easiest👾Faster✅💯 Lesser🧠 🎯 C++✅Python3🐍✅Java✅C✅Python🐍✅C#✅💥🔥💫Explained☠💥🔥 Beats 100 | easiestfaster-lesser-cpython3javacpython-pyyt | Intuition\n\n\n\n\n\n Describe your first thoughts on how to solve this problem. \nJavaScript []\n//JavaScript\n/**\n * @param {number[]} nums\n * @param {numbe | Edwards310 | NORMAL | 2024-08-26T04:00:14.983956+00:00 | 2024-08-26T04:00:14.983988+00:00 | 667 | false | # Intuition\n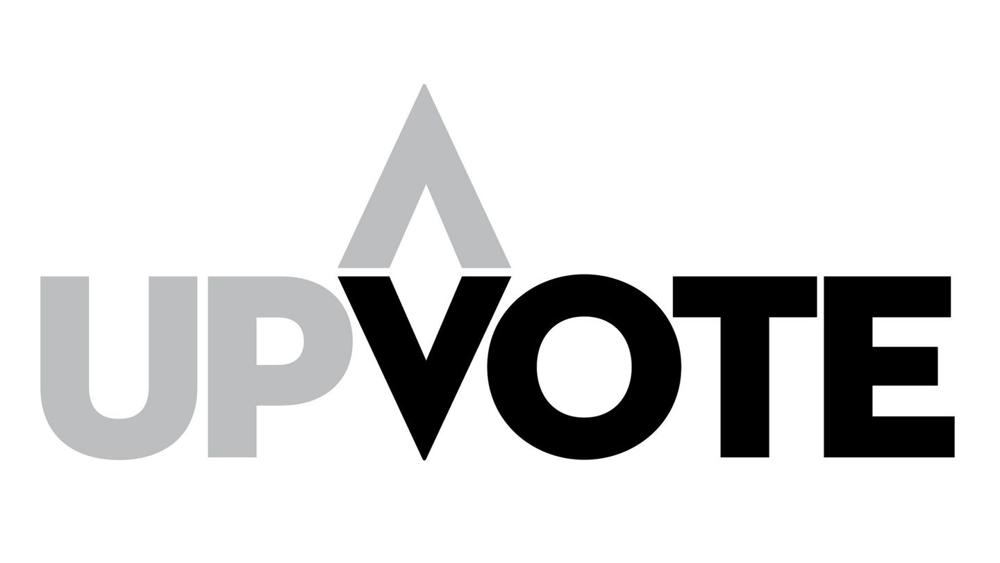\n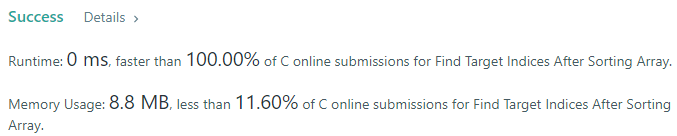\n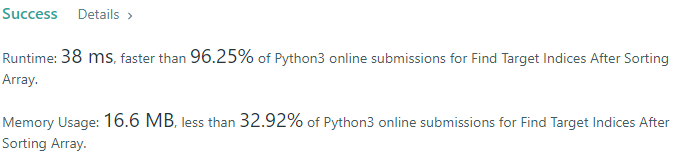\n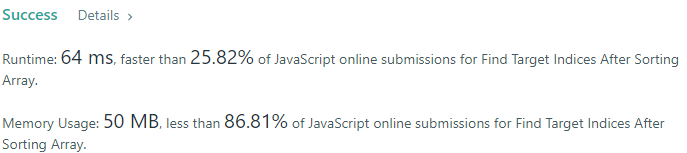\n\n<!-- Describe your first thoughts on how to solve this problem. -->\n```JavaScript []\n//JavaScript\n/**\n * @param {number[]} nums\n * @param {number} target\n * @return {number[]}\n */\nvar targetIndices = function(nums, target) {\n const arr = []\n let n = nums.length\n nums.sort((a, b) =>{\n return a - b;\n })\n for(let i = 0; i < n; i++)\n if(nums[i] == target)\n arr.push(i)\n return arr\n};\n```\n```C++ []\n//C++ Code\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n vector<int> res;\n int n = nums.size();\n sort(nums.begin(), nums.end());\n for (int i = 0; i < n; i++)\n if (nums[i] == target)\n res.push_back(i);\n\n return res;\n }\n};\n```\n```Python3 []\n#Python3 Code\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n nums.sort()\n n = len(nums)\n return [s for s in range(n) if nums[s] == target]\n```\n```Java []\n//Java Code\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n List<Integer> res = new ArrayList<>();\n Arrays.sort(nums);\n for (int i = 0; i < nums.length; i++) {\n if (nums[i] == target)\n res.add(i);\n }\n return res;\n }\n}\n```\n```C []\n//C Code\n/**\n * Note: The returned array must be malloced, assume caller calls free().\n */\nint cmp(const void* a, const void* b) { \n return *(int*)a - *(int*)b;\n}\n\nint* targetIndices(int* nums, int numsSize, int target, int* returnSize) {\n int* arr = (int*)calloc(sizeof(int), 101);\n *returnSize = 0;\n\n qsort(nums, numsSize, sizeof(int), cmp);\n \n for (int i = 0; i < numsSize; i++)\n if (nums[i] == target)\n arr[(*returnSize)++] = i;\n return arr;\n}\n```\n```Python []\n#Python Code\nclass Solution(object):\n def targetIndices(self, nums, target):\n """\n :type nums: List[int]\n :type target: int\n :rtype: List[int]\n """\n res = []\n nums = sorted(nums)\n for i in range(len(nums)):\n if nums[i] == target:\n res.append(i)\n return res\n```\n```C# []\n//C# Code\npublic class Solution {\n public IList<int> TargetIndices(int[] nums, int target) {\n List<int> res = new List<int>();\n int n = nums.Length;\n Array.Sort(nums);\n for (int i = 0; i < n; ++i)\n if (nums[i] == target)\n res.Add(i);\n return res;\n }\n}\n```\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n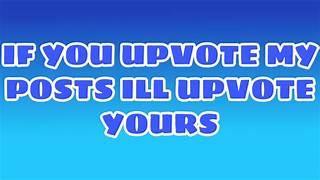\n | 3 | 1 | ['Array', 'Binary Search', 'C', 'Sorting', 'Python', 'C++', 'Java', 'Python3', 'JavaScript', 'C#'] | 2 |
find-target-indices-after-sorting-array | Math based, linear solution | math-based-linear-solution-by-2pp0ereztt-ps42 | Intuition\nWe do not need to sort the array, we only need to know how many smaller items are there. That way we can tell what is the index of the first target v | 2PP0erEZTT68w6N | NORMAL | 2024-07-16T12:57:21.142265+00:00 | 2024-07-16T12:57:21.142310+00:00 | 86 | false | # Intuition\nWe do not need to sort the array, we only need to know how many smaller items are there. That way we can tell what is the index of the first `target` value in the sorted array.\n\n# Approach\nGo over the list of numbers. Count how many smaller numbers are present, and have many times `target` is listed. We will return that many indices from the first index that is assigned after "all smaller items". \n\n# Complexity\n- Time complexity:\nWe go over the array once -> `linear`\n\n- Space complexity:\nWe do not use any other data structure, but the returned vector. If we do not count that, then the algorithm is `constant` space. If we count it, worst case scenario we need to return all items of the input array -> `linear`.\n# Code\n```\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n int targetCount = 0; // will match how many values we will need to return\n int smallerCount = 0; // count of smaller ints\n\n for (auto num : nums) {\n if (num == target) {\n targetCount++;\n } else if (num < target) {\n smallerCount++;\n }\n }\n\n // here we know how many indices we need to return, and what is the smallest of them. Let\'s create a vector based on this info\n vector<int> result;\n\n for (int idx = 0; idx < targetCount; idx++) {\n result.push_back(smallerCount);\n smallerCount++;\n }\n\n return result;\n }\n};\n``` | 3 | 0 | ['C++'] | 0 |
find-target-indices-after-sorting-array | Counting sort python3 easy solution | counting-sort-python3-easy-solution-by-a-0wvh | \n\n# Code\n\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n # implementing counting sort\n d = defaul | annulet | NORMAL | 2024-05-12T06:21:31.328845+00:00 | 2024-05-12T06:21:31.328867+00:00 | 332 | false | \n\n# Code\n```\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n # implementing counting sort\n d = defaultdict(int)\n\n for num in nums:\n d[num] += 1\n\n if target not in d:\n return []\n\n # bounding indices\n start = sum([v for k, v in d.items() if k < target])\n end = start + d[target]\n\n return list(range(start, end))\n\n``` | 3 | 0 | ['Sorting', 'Counting', 'Python3'] | 0 |
find-target-indices-after-sorting-array | 1 ms Beats 85.70% of users with Java | 1-ms-beats-8570-of-users-with-java-by-su-6qzk | Intuition\n Describe your first thoughts on how to solve this problem. \nSort the array and then traverse the array and if arr[i]==arr[i+1]\nthen i and i+1 are | suyalneeraj09 | NORMAL | 2024-03-31T11:20:17.115061+00:00 | 2024-03-31T11:20:17.115090+00:00 | 93 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nSort the array and then traverse the array and if arr[i]==arr[i+1]\nthen i and i+1 are the target indices\n# Approach\n<!-- Describe your approach to solving the problem. -->\nSort & then intutive \n# Complexity\n- Time complexity : O(nlogn).\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity : O(log n).\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n if (nums == null || nums.length == 0) {\n return null;\n }\n List<Integer> ans = new ArrayList<>();\n quicksort(nums,0,nums.length-1);\n for (int i = 0; i < nums.length; i++) {\n if (nums[i] == target)\n ans.add(i);\n }\n return ans;\n\n }\n\n private static void quicksort(int[] arr, int low, int hi) {\n if (low >= hi)\n return;\n\n int s = low;\n int e = hi;\n int m = s + (e - s) / 2;\n int pivot = arr[m];\n\n while (s <= e) {\n while (arr[s] < pivot) {\n s++;\n }\n while (arr[e] > pivot) {\n e--;\n }\n if (s <= e) {\n swap(arr, s, e);\n s++;\n e--;\n }\n }\n quicksort(arr, s, hi);\n quicksort(arr, low, e);\n }\n\n static void swap(int[] arr, int f, int s) {\n int t = arr[f];\n arr[f] = arr[s];\n arr[s] = t;\n }\n}\n``` | 3 | 0 | ['Sorting', 'Java'] | 1 |
find-target-indices-after-sorting-array | C++ best Approach For beginners ( 100% fast solution ) | c-best-approach-for-beginners-100-fast-s-xrob | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | abhirajpratapsingh | NORMAL | 2023-09-30T12:44:33.654975+00:00 | 2023-09-30T12:44:33.655001+00:00 | 10 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n int leftMostIndex(vector<int>& nums,int target)\n {\n int start=0;\n int end=nums.size()-1;\n int mid=start+(end-start)/2;\n int leftmostindex=-1;\n while(start<=end)\n {\n if(nums[mid]==target)\n {\n leftmostindex=mid;\n end=mid-1;\n }\n else if(nums[mid]>target)\n end=mid-1;\n else if(nums[mid]<target)\n start=mid+1;\n mid=start+(end-start)/2;\n }\n return leftmostindex;\n }\n int rightMostIndex(vector<int>& nums,int target)\n {\n int start=0;\n int end=nums.size()-1;\n int mid=start+(end-start)/2;\n int rightmostindex=-1;\n while(start<=end)\n {\n if(nums[mid]==target)\n {\n rightmostindex=mid;\n start=mid+1;\n }\n else if(nums[mid]<target)\n start=mid+1;\n else if(nums[mid]>target)\n end=mid-1;\n mid=start+(end-start)/2;\n }\n return rightmostindex;\n }\n vector<int> targetIndices(vector<int>& nums, int target) \n { \n int first,last;\n vector<int>v;\n sort(nums.begin(),nums.end());\n first=leftMostIndex(nums,target);\n last=rightMostIndex(nums,target);\n while(first<=last &&(first!=-1 && last!=-1))\n {\n v.push_back(first);\n first++;\n }\n return v;\n }\n};\n``` | 3 | 0 | ['C++'] | 0 |
find-target-indices-after-sorting-array | TypeScript/JavaScript O(NLogN) Solution | typescriptjavascript-onlogn-solution-by-qbhps | \n\n# Complexity\n- Time complexity: O(NLogN)\n Add your time complexity here, e.g. O(n) \n\n- Space complexity: O(N)\n Add your space complexity here, e.g. O(n | halshar | NORMAL | 2023-09-28T17:56:27.684595+00:00 | 2023-09-28T17:56:27.684620+00:00 | 211 | false | \n\n# Complexity\n- Time complexity: $$O(NLogN)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(N)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfunction targetIndices(nums: number[], target: number): number[] {\n // first we\'ll sort the array\n nums.sort((a, b) => a - b);\n\n // then we\'ll apply binary search and find the\n // first index of the target\n const n = nums.length;\n let low = 0;\n let high = n - 1;\n\n while (low <= high) {\n const mid = Math.floor((low + high) / 2);\n\n // we need to find the lowest index of\n // the target, so even if we find our\n // target there is still a possibility\n // that our target might be available at\n // the lower index, so search left\n if (nums[mid] >= target) high = mid - 1;\n // else the value at mid is lower than\n // the target so search right side\n else low = mid + 1;\n }\n\n // after the end of the iteration the `low`\n // pointer will point to the lowest index\n // so we\'ll iterate from the lowest index\n // and for every index we find our target\n // we push that into array and once we\n // find other element than target then\n // we break out of the iteration\n const ans: number[] = [];\n for (let i = low; i < n; i++) {\n if (nums[i] !== target) break;\n ans.push(i);\n }\n\n return ans;\n}\n\n``` | 3 | 0 | ['TypeScript', 'JavaScript'] | 2 |
find-target-indices-after-sorting-array | Simple Binary Search Solution(100%/91%) | simple-binary-search-solution10091-by-ku-oboj | bins\n# Code\n\n/**\n * @param {number[]} nums\n * @param {number} target\n * @return {number[]}\n */\nvar targetIndices = function(nums, target) {\n let res | kubatabdrakhmanov | NORMAL | 2023-08-31T07:25:59.118921+00:00 | 2023-08-31T07:25:59.118941+00:00 | 659 | false | bins\n# Code\n```\n/**\n * @param {number[]} nums\n * @param {number} target\n * @return {number[]}\n */\nvar targetIndices = function(nums, target) {\n let result = [];\n if(!nums.includes(target)) return result;\n \n let sortedArr = nums;\n sortedArr.sort((a,b) => a-b);\n let left = 0;\n let right = sortedArr.length-1;\n let start = -1;\n let end = -1;\n\n while(left <= right){\n let mid = Math.round((right + left) / 2);\n if(sortedArr[mid] === target){\n start = mid-1;\n end = mid+1;\n\n result.push(mid)\n while(sortedArr[start] === target){\n result.push(start)\n start -= 1\n }\n while(sortedArr[end] === target){\n result.push(end)\n end += 1\n }\n break;\n }else if(sortedArr[mid] < target){\n left = mid+1;\n }else if(sortedArr[mid] > target){\n right = mid-1;\n }\n }\n return result.sort((a,b) => a-b)\n};\n\n``` | 3 | 0 | ['Array', 'Binary Search', 'Sorting', 'JavaScript'] | 2 |
find-target-indices-after-sorting-array | Optimized Solution with explanation || C || C++ || Java | optimized-solution-with-explanation-c-c-xx3of | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n This approach is based | Ayush-Rawat | NORMAL | 2023-08-30T15:32:59.374527+00:00 | 2023-08-30T15:32:59.374550+00:00 | 829 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n This approach is based on the observation of a sorted array. In an array which is sorted in increasing order all the elements before val are less than val. So, to get the indices of val in a sorted array, instead of performing sort operation, we can simply count the elements less than val. If the count is x then val will start from x-th index (because of 0-based indexing). Follow the steps mentioned below: \n\nTraverse the array. Use variables to store the count of elements less than val (smallCount) and elements having a value same as val (sameCount).\nIf the value of an element is less than val increment smallCount by one.\nIf the value is same as val increment sameCount by one.\nAfter traversal is complete, the indices will start from smallCount and end at (smallCount+sameCount-1)\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(N)\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(1)\n# Code\n```\n/**\n * Note: The returned array must be malloced, assume caller calls free().\n */\nint* targetIndices(int* nums, int numsSize, int target, int* returnSize){\n int small=0,same=0;\n for(int i=0;i<numsSize;i++){\n if(nums[i] < target) small++;\n if(nums[i] == target) same++;\n }\n *returnSize=same;\n int*ans=(int*)malloc(sizeof(int)*same);\n int i=0;\n while(same--){\n ans[i++]=small++;\n }\n return ans;\n}\n``` | 3 | 0 | ['Array', 'Two Pointers', 'Binary Search', 'C', 'C++', 'Java'] | 0 |
find-target-indices-after-sorting-array | Binary Search Solution || First and Last position || C++ | binary-search-solution-first-and-last-po-g91o | \n\n\n class Solution {\n public:\n \n int left(vector&nums,int k,int n){\n int low=0,high=n-1,ans=-1;\n while(low<=high){\n | harsh_patell21 | NORMAL | 2023-07-26T04:41:33.333062+00:00 | 2023-07-26T04:41:33.333091+00:00 | 87 | false | \n\n\n class Solution {\n public:\n \n int left(vector<int>&nums,int k,int n){\n int low=0,high=n-1,ans=-1;\n while(low<=high){\n int mid=low+(high-low)/2;\n if(nums[mid]==k){\n ans=mid;\n high=mid-1;\n }\n else if(nums[mid]<k){\n low=mid+1;\n }\n else{\n high=mid-1;\n }\n }\n return ans;\n }\n \n int right(vector<int>&nums,int k,int n){\n int low=0,high=n-1,ans=-1;\n while(low<=high){\n int mid=low+(high-low)/2;\n if(nums[mid]==k){\n ans=mid;\n low=mid+1;\n }\n else if(nums[mid]<k){\n low=mid+1;\n }\n else{\n high=mid-1;\n }\n }\n return ans;\n }\n \n vector<int> targetIndices(vector<int>& nums, int target) {\n sort(nums.begin(),nums.end());\n int n=nums.size();\n int l=left(nums,target,n);\n int r=right(nums,target,n);\n if(l==-1)return {};\n vector<int>vect;\n for(int i=l;i<=r;i++){\n vect.push_back(i);\n }\n return vect;\n }\n }; | 3 | 0 | ['Binary Tree'] | 0 |
find-target-indices-after-sorting-array | Find Target Indices After Sorting Array 🧑💻🧑💻 || JAVA solution code 💁💁... | find-target-indices-after-sorting-array-gys5n | Code\n\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n ArrayList <Integer> arr = new ArrayList<>();\n Arrays | Jayakumar__S | NORMAL | 2023-07-03T10:42:19.489938+00:00 | 2023-07-03T10:42:19.489958+00:00 | 455 | false | # Code\n```\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n ArrayList <Integer> arr = new ArrayList<>();\n Arrays.sort(nums);\n for(int i=0; i<nums.length; i++){\n if(nums[i] == target){\n arr.add(i);\n }\n }\n return arr;\n }\n}\n``` | 3 | 0 | ['Java'] | 0 |
find-target-indices-after-sorting-array | Binary Search and Iteration-based Solution for Finding Target Indices in Sorted Array | binary-search-and-iteration-based-soluti-rzgi | \n\n\n# Approach\n Describe your approach to solving the problem. \nThis code uses binary search to find the first and last occurrences of the target value in t | ashutosh75 | NORMAL | 2023-04-20T20:57:48.869907+00:00 | 2023-08-15T20:43:57.716788+00:00 | 280 | false | \n\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nThis code uses binary search to find the first and last occurrences of the target value in the sorted input array. It then iterates over the indices between these two occurrences and adds the ones that contain the target value to a list, which is then returned.\n\nThe algorithm first sorts the input array in non-decreasing order using the Arrays.sort method. Then, it uses two helper functions findFirst and findLast to find the first and last occurrences of the target value in the sorted array, respectively. Both functions use binary search to find the index of the target value in the array. The findFirst function returns the index of the first occurrence of the target value, or -1 if it is not found. The findLast function returns the index of the last occurrence of the target value, or -1 if it is not found.\n\nIf either the findFirst or findLast function returns -1, indicating that the target value does not exist in the array, the algorithm returns an empty list.\n\nIf the target value exists in the array, the algorithm initializes an empty list called targetIndices to store the indices where the target value occurs. It then iterates from the index of the first occurrence of the target value to the index of the last occurrence of the target value and adds the indices where the target value occurs to the targetIndices list.\n\nFinally, the algorithm returns the targetIndices list, which contains the indices of the target value in the sorted array in increasing order.\n\n# Complexity\n- Time complexity: O((log n) * n) \n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n Arrays.sort(nums); // sort the array in non-decreasing order\n \n // find the first and last occurrences of the target value using binary search\n int left = findFirst(nums, target);\n int right = findLast(nums, target);\n \n // if target value does not exist in the array, return an empty list\n if (left == -1 || right == -1) {\n return new ArrayList<Integer>();\n }\n \n List<Integer> targetIndices = new ArrayList<Integer>(); // initialize an empty list\n \n // iterate from left to right and append indices where the target value occurs\n for (int i = left; i <= right; i++) {\n if (nums[i] == target) {\n targetIndices.add(i);\n }\n }\n \n return targetIndices; // return the list of target indices\n }\n \n // helper function to find the first occurrence of the target value using binary search\n private int findFirst(int[] nums, int target) {\n int left = 0, right = nums.length - 1;\n while (left <= right) {\n int mid = left + (right - left) / 2;\n if (nums[mid] < target) {\n left = mid + 1;\n } else {\n right = mid - 1;\n }\n }\n return (left < nums.length && nums[left] == target) ? left : -1;\n }\n \n // helper function to find the last occurrence of the target value using binary search\n private int findLast(int[] nums, int target) {\n int left = 0, right = nums.length - 1;\n while (left <= right) {\n int mid = left + (right - left) / 2;\n if (nums[mid] <= target) {\n left = mid + 1;\n } else {\n right = mid - 1;\n }\n }\n return (right >= 0 && nums[right] == target) ? right : -1;\n }\n}\n\n``` | 3 | 0 | ['Array', 'Binary Search', 'Sorting', 'Java'] | 1 |
find-target-indices-after-sorting-array | Binary Search | C++ | binary-search-c-by-_kitish-rejn | Code\n\nclass Solution {\nprivate:\n int bs(vector<int>&v,int k,bool first=true)\n {\n int l = 0, h = size(v)-1, md,ans = -1;\n while(h >= l | _kitish | NORMAL | 2023-04-01T22:45:27.338657+00:00 | 2023-04-01T22:45:27.338684+00:00 | 1,448 | false | # Code\n```\nclass Solution {\nprivate:\n int bs(vector<int>&v,int k,bool first=true)\n {\n int l = 0, h = size(v)-1, md,ans = -1;\n while(h >= l){\n md = l + (h-l)/2;\n if(v[md] == k){\n ans = md;\n first ? h = md-1 : l = md + 1;\n }\n else if(v[md] > k) h = md-1;\n else l = md+1;\n }\n return ans;\n }\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n sort(begin(nums),end(nums));\n int first = bs(nums,target);\n if(first == -1) return {};\n int second = bs(nums,target,false);\n vector<int> ans(second-first+1);\n iota(begin(ans),end(ans),first);\n return ans;\n }\n};\n``` | 3 | 0 | ['Array', 'Binary Search', 'Sorting', 'C++'] | 0 |
find-target-indices-after-sorting-array | C++ ✔|| Beats 94% 🚀 || Beginner Friendly 💥 | c-beats-94-beginner-friendly-by-mithiles-26yi | Brute Force Solution \n\nclass Solution \n{\n public:\n vector<int> targetIndices(vector<int>& nums, int target) \n {\n sort(nums.be | mithileshl_6 | NORMAL | 2023-02-01T12:54:51.497355+00:00 | 2023-02-01T12:54:51.497401+00:00 | 476 | false | # Brute Force Solution \n```\nclass Solution \n{\n public:\n vector<int> targetIndices(vector<int>& nums, int target) \n {\n sort(nums.begin(), nums.end()); // first Sort the vector\n \n int N = nums.size();\n vector<int> v;\n\n // find the target element by traversing the loop and push the index in the vector\n for(int i=0; i<N; i++)\n {\n if(nums[i] == target) \n {\n v.push_back(i);\n }\n }\n return v;\n }\n};\n```\n\n# Using Binary Search \n\n```\nclass Solution \n{\n public:\n vector<int> targetIndices(vector<int>& nums, int target) \n {\n sort(nums.begin(), nums.end());\n \n vector<int> v;\n \n int first = occurance(nums, target, true); // find the first Occurrence of the element\n int last = occurance(nums, target, false); // find the last Occurrence of the element\n \n if(first == -1 && last == -1)\n {\n return {};\n }\n \n for(int i=first; i<=last; i++)\n {\n v.push_back(i);\n }\n return v;\n }\n \n int occurance(vector<int>& nums, int target, bool isTrue)\n {\n int low = 0;\n int high = nums.size()-1;\n int ans = -1;\n \n while(low <= high)\n {\n int mid = low + ((high-low)/2);\n \n if(nums[mid] == target)\n {\n ans = mid;\n if(isTrue)\n {\n high = mid - 1;\n }\n else\n {\n low = mid + 1;\n } \n }\n else if(nums[mid] > target)\n {\n high = mid - 1;\n }\n else\n {\n low = mid + 1;\n }\n }\n return ans;\n }\n};\n``` | 3 | 0 | ['Array', 'Binary Search', 'Sorting', 'C++'] | 1 |
find-target-indices-after-sorting-array | Simple solution on Swift | simple-solution-on-swift-by-blackbirdng-c969 | Code\n\nclass Solution {\n func targetIndices(_ nums: [Int], _ target: Int) -> [Int] {\n var result = [Int]()\n for (index, item) in nums.sorte | blackbirdNG | NORMAL | 2023-01-08T10:02:46.675833+00:00 | 2023-01-08T10:02:46.675883+00:00 | 271 | false | # Code\n```\nclass Solution {\n func targetIndices(_ nums: [Int], _ target: Int) -> [Int] {\n var result = [Int]()\n for (index, item) in nums.sorted().enumerated() where item == target {\n result.append(index)\n }\n return result\n }\n}\n```\n### Please upvote if you found the solution useful! | 3 | 0 | ['Swift'] | 0 |
find-target-indices-after-sorting-array | Beats 98% - Easy Python Solution | beats-98-easy-python-solution-by-pranavb-fjhi | \nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n if len(nums) == 1:\n if target in nums:\n | PranavBhatt | NORMAL | 2022-12-01T00:26:20.387052+00:00 | 2022-12-01T00:26:20.387117+00:00 | 733 | false | ```\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n if len(nums) == 1:\n if target in nums:\n return [0]\n else:\n return []\n else:\n if target in nums:\n nums.sort()\n count = nums.count(target)\n reference = nums.index(target)\n values = [reference]\n for i in range(1, count):\n reference += 1\n values += [nums.index(target, reference)]\n return values\n else:\n return []\n``` | 3 | 0 | ['Python'] | 0 |
find-target-indices-after-sorting-array | BruetForce JAVA || Easy | bruetforce-java-easy-by-harshitrai_3768-bvpr | class Solution {\n public List targetIndices(int[] nums, int target) {\n List al=new ArrayList<>();\n Arrays.sort(nums);\n for(int i=0;i | HarshitRai_3768 | NORMAL | 2022-10-08T17:45:03.328805+00:00 | 2022-10-08T17:45:03.328846+00:00 | 233 | false | class Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n List<Integer> al=new ArrayList<>();\n Arrays.sort(nums);\n for(int i=0;i<nums.length;i++)\n {\n if(nums[i]==target)\n al.add(i);\n \n }\n return al;\n }\n} | 3 | 0 | [] | 0 |
find-target-indices-after-sorting-array | c++ binary search | c-binary-search-by-soni_ankita_1111-fabq | class Solution {\npublic:\n \n // first occurence\n int firstocc(vectorv,int k)\n { int n=v.size();\n int s=0,e=n-1;\n int ans=-1;\n | soni_ankita_1111 | NORMAL | 2022-10-04T12:28:35.940809+00:00 | 2022-10-04T12:28:35.940852+00:00 | 345 | false | class Solution {\npublic:\n \n // first occurence\n int firstocc(vector<int>v,int k)\n { int n=v.size();\n int s=0,e=n-1;\n int ans=-1;\n int mid=s+(e-s)/2;\n \n while(s<=e)\n {\n if(v[mid]==k)\n {\n ans=mid;\n e=mid-1;\n }\n else if(v[mid]>k) e=mid-1;\n else s=mid+1;\n mid=s+(e-s)/2;\n\n }\n return ans;\n }\n // last occurence \n \n int lastocc(vector<int>v,int k)\n { int n=v.size();\n int s=0,e=n-1;\n int ans=-1;\n int mid=s+(e-s)/2;\n \n while(s<=e)\n {\n if(v[mid]==k)\n {\n ans=mid;\n s=mid+1;\n }\n else if(v[mid]>k) e=mid-1;\n else s=mid+1;\n mid=s+(e-s)/2;\n\n }\n return ans;\n }\n \n \n vector<int> targetIndices(vector<int>& nums, int target) {\n sort(nums.begin(),nums.end());\n vector<int >Ans;\n int n1=firstocc(nums,target);\n int n2=lastocc(nums,target) ;\n if(n1!=-1 && n2!=-1)\n {\n for(int i=n1;i<=n2;i++)\n {\n Ans.push_back(i);\n }\n }\n return Ans;\n \n }\n}; | 3 | 0 | [] | 0 |
find-target-indices-after-sorting-array | Faster | No Sorting | Easy | C++ Solution | faster-no-sorting-easy-c-solution-by-ite-pfsp | If you find it helpful, Please Up-Vote it.\n\n\n### Intution :-\n We can get the answer without sorting the array\n We need to keep count the number of elements | iteshgavel | NORMAL | 2022-09-06T13:26:13.430221+00:00 | 2022-09-06T13:27:54.237306+00:00 | 135 | false | **If you find it helpful, Please Up-Vote it.**\n\n\n### **Intution :-**\n* We can get the answer **without sorting the array**\n* We need to **keep count the number of elements smaller than the target**, present in array, because the will definetitly come before the target when sorted.\n* We also need tho **count the number of occurence of target element** in array, so that we can return the array having position of all ocurrence.\n* We can get the position of target element by using simple formula :-\n**Position** **=** **Number of elements smaller than target element** \n* We can iterate a loop for all occurence of target, and just increase the position by 1;\n\n### **Approach :-**\n* Keep two variables, `small` and `count`.\n* `small` will keep count of all the elements which are lesser than the `target` element.\n* `count` will keep count of number of occurence of the `target` element.\n* Now, we can iterate a loop till we get the toal number of `target` element present in array (i.e., `count`).\n* In each iteration, we will store the the position of `target` element in sorted array, which will be `small`+`iterator`.\n\n### **C++ Code**\n\n```\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n int count=0;\n int small = 0;\n \n\t\t//------Iterating to get the count and small elements\n for(auto e : nums){\n if(e==target){\n count++;\n }\n else if(e<target){\n small++;\n }\n }\n \n vector<int> ans;\n\t\t\n\t\t//-------generating the answer vector\n for(int i=0; i<count; i++){\n ans.push_back(small+i);\n }\n \n return ans;\n }\n};\n```\n\n\n**If you find it helpful, Please Up-Vote it.** | 3 | 0 | ['C'] | 0 |
find-target-indices-after-sorting-array | 2089. Find Target Indices After Sorting Array||EASY JAVA SOLUTION | 2089-find-target-indices-after-sorting-a-5y9w | \nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n ArrayList<Integer> arr=new ArrayList<>();\n Arrays.sort(num | parulsahni621 | NORMAL | 2022-09-02T16:16:01.599989+00:00 | 2022-09-02T16:16:01.600032+00:00 | 344 | false | ```\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n ArrayList<Integer> arr=new ArrayList<>();\n Arrays.sort(nums);\n for(int i=0;i<nums.length;i++)\n {\n if(nums[i]==target)\n arr.add(i);\n }\n return arr;\n }\n}\n``` | 3 | 0 | ['Array', 'Java'] | 0 |
find-target-indices-after-sorting-array | Java, Using Binary Search - Basic | java-using-binary-search-basic-by-vlack_-9zpj | \npublic void helper(int[] nums, int start, int end, int target, List<Integer> list){\n if(start > end) return;\n int mid = (start + end)/2;\n | vlack_panther | NORMAL | 2022-05-30T16:43:59.765063+00:00 | 2022-05-30T16:43:59.765107+00:00 | 747 | false | ```\npublic void helper(int[] nums, int start, int end, int target, List<Integer> list){\n if(start > end) return;\n int mid = (start + end)/2;\n if(nums[mid] == target){\n list.add(mid);\n }\n helper(nums, start, mid-1, target, list);\n helper(nums, mid+1, end, target, list);\n }\n \npublic List<Integer> targetIndices(int[] nums, int target) {\n Arrays.sort(nums);\n List<Integer> list = new ArrayList<>();\n helper(nums, 0, nums.length-1, target, list);\n Collections.sort(list);\n return list;\n }\n``` | 3 | 0 | ['Binary Tree', 'Java'] | 2 |
find-target-indices-after-sorting-array | C++ O(N) without sorting | c-on-without-sorting-by-d911-4mb0 | We do not need to sort the array - we can just count elements smaller (ind) than the target.\ncnt is storing number of time target element is present.\n\n\nC++\ | d911 | NORMAL | 2022-03-06T18:39:05.802166+00:00 | 2022-03-06T18:39:05.802212+00:00 | 141 | false | We do not need to sort the array - we can just count elements smaller (`ind`) than the target.\n`cnt` is storing number of time target element is present.\n\n\n**C++**\n```\nvector<int> targetIndices(vector<int>& nums, int target) {\n vector<int> ans;\n int ind=0,cnt=0;\n int n=nums.size();\n for(int i=0;i<n;i++){\n if(nums[i]<target)ind++;\n else if(nums[i]==target)cnt++;\n }\n \n while(cnt--){\n ans.push_back(ind);\n ind++;\n }\n return ans; \n }\n``` | 3 | 0 | ['C', 'Counting Sort'] | 0 |
find-target-indices-after-sorting-array | 2 Approaches : O(NlogN) & O(N) || Binary Search || Counting || C++ | 2-approaches-onlogn-on-binary-search-cou-wwgo | Method 1:\n1. First sort the array.\n2. Find the index of the target\n3. Push it into the "result" vector.\n\n\nclass Solution {\npublic:\n vector<int> targe | deleted_user | NORMAL | 2022-02-26T04:58:21.531960+00:00 | 2022-02-26T04:58:48.918655+00:00 | 217 | false | **Method 1:**\n**1. First sort the array.\n2. Find the index of the target\n3. Push it into the "result" vector.**\n\n```\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n vector<int> result;\n sort(nums.begin(),nums.end());\n for(int i=0;i<nums.size();i++){\n if(nums[i]==target) result.push_back(i);\n }\n return result;\n }\n};\n```\n**Time Complexity : O(N log N)**\n\n**Method 2:**\n1. Count the occurences of elements lesser then the target and freq of target elements.\n \t"small" contains the number of element lesser than the target.\n \t"count" contains the number of occurences of the target element.\n2. Now run a loop from "small" ( means "small" elements are lesser than our target value, so our target value will come after "small" elements in sorted array ), upto "count".\n3. Push the index into the "result" vector.\n\n```\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n int small=0,count=0,k=0;\n for(auto n:nums) {\n if(n<target) small++; // calculates the frequency of elements lesser than the target\n if(n==target) count++; // calculate the number of occurences of the target element\n }\n vector<int> result(count);\n for(int i=small;i<small+count;i++) \n result[k++]=i;\n return result;\n }\n};\n```\n**Time Complexity : O(N)**\n\nPlease upvote \uD83D\uDC4D\uD83C\uDFFB : ) | 3 | 0 | ['Binary Search', 'C', 'Counting'] | 1 |
find-target-indices-after-sorting-array | Binary Search || Java || Fast-Efficient | binary-search-java-fast-efficient-by-fut-g4wv | First we are sorting the array then we find first and last occurence of target then we add from first to last all indexes in arrayList Hope this will help other | futureprogrammer28 | NORMAL | 2022-01-31T06:06:11.540337+00:00 | 2022-01-31T06:06:11.540394+00:00 | 384 | false | **First we are sorting the array then we find first and last occurence of target then we add from first to last all indexes in arrayList** Hope this will help other :)\n```\nclass Solution {\n public int first(int[] a,int t){\n int l=0;\n int r=a.length-1;\n int mid;\n while(l<=r){\n mid=l+(r-l)/2;\n if(a[mid]>t){\n r=mid-1;\n }else if(a[mid]<t){\n l=mid+1;\n }else{\n if(mid==0 || a[mid]!=a[mid-1])\n return mid;\n else\n r=mid-1;\n }\n }\n return -1;\n }\n public int last(int[] a,int t){\n int l=0;\n int r=a.length-1;\n int mid;\n while(l<=r){\n mid=l+(r-l)/2;\n if(a[mid]>t){\n r=mid-1;\n }else if(a[mid]<t){\n l=mid+1;\n }else{\n if(mid==a.length-1 || a[mid]!=a[mid+1])\n return mid;\n else\n l=mid+1;\n }\n }\n return -1;\n }\n public List<Integer> targetIndices(int[] nums, int target) {\n Arrays.sort(nums);\n List<Integer> l=new ArrayList<>();\n int f=first(nums,target);\n int la=last(nums,target);\n if(f==-1 || la==-1)\n return l;\n for(int i=f;i<=la;i++)\n l.add(i);\n return l;\n }\n}\n```\n\nif you like the logic pls upvote me :) | 3 | 2 | ['Sorting', 'Binary Tree', 'Java'] | 0 |
find-target-indices-after-sorting-array | python3 | easiest | one liner | | python3-easiest-one-liner-by-anilchouhan-zwc3 | \nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n nums.sort()\n return [i for i, v in enumerate(nums) i | Anilchouhan181 | NORMAL | 2022-01-31T05:15:10.551536+00:00 | 2022-01-31T05:15:10.551566+00:00 | 65 | false | ```\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n nums.sort()\n return [i for i, v in enumerate(nums) if v == target]\n``` | 3 | 1 | [] | 2 |
find-target-indices-after-sorting-array | simple python beats 100% spead, modified binary search | simple-python-beats-100-spead-modified-b-qudv | i used modified version of binary search to find both first and last occurence of the target\nstarting from low, high equals start and end of the list, but then | msaoudallah | NORMAL | 2022-01-10T16:28:52.616511+00:00 | 2022-01-10T16:28:52.616552+00:00 | 572 | false | i used modified version of binary search to find both first and last occurence of the target\nstarting from low, high equals start and end of the list, but then i noticed in the second trial we don\'t need to scan the whole array to get the last occurence, and made l = start\n\n```\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n l = 0\n r = len(nums) -1\n nums.sort()\n # print(nums)\n start,end=-1,-1\n\n # first \n while (l <=r):\n m = (l+r)//2\n \n if (target== nums[m]):\n start=m\n r = m-1\n \n if target > nums[m]:\n l = m+1\n \n elif target < nums[m]:\n r = m-1\n \n # last\n l,r=start,len(nums)-1\n while (l <=r):\n m = (l+r)//2\n \n if (target== nums[m]):\n end=m\n l = m+1\n \n if target > nums[m]:\n l = m+1\n \n elif target < nums[m]:\n r = m-1\n \n \n # print(start,end)\n if start==end==-1:\n return []\n else:\n res = list(range(start,end+1))\n return res\n```\n | 3 | 0 | ['Binary Search', 'Binary Tree', 'Python'] | 0 |
find-target-indices-after-sorting-array | javascript O(N) | javascript-on-by-martinjoseph225-ym6v | \nvar targetIndices = function(nums, target) {\n let lownums=repeat=0;\n for(i=0;i<nums.length;i++){\n if(nums[i]<target){\n lownums++;\ | martinjoseph225 | NORMAL | 2022-01-09T19:30:17.142682+00:00 | 2022-01-09T19:33:26.143285+00:00 | 855 | false | ```\nvar targetIndices = function(nums, target) {\n let lownums=repeat=0;\n for(i=0;i<nums.length;i++){\n if(nums[i]<target){\n lownums++;\n }\n if(nums[i]==target){\n repeat++}\n }\n let arr=[];\n\t\n if(repeat<1){\n return arr;\n }\n else{\n for(i=0;i<repeat;i++){\n arr.push(lownums+i)\n }\n return arr;\n }\n \n};\n``` | 3 | 0 | ['JavaScript'] | 1 |
find-target-indices-after-sorting-array | [Java] Two Approaches | java-two-approaches-by-pranjaldeshmukh-3t16 | Approach 1\n\nTime Complexity : O(nlogn)\nSpace Complexity : [> O(1) ] https://stackoverflow.com/questions/22571586/will-arrays-sort-increase-time-complexity-a | PranjalDeshmukh | NORMAL | 2022-01-05T20:20:33.131831+00:00 | 2022-01-05T20:20:33.131878+00:00 | 426 | false | *Approach 1*\n\n**Time Complexity** : O(nlogn)\n**Space Complexity** : [> O(1) ] [https://stackoverflow.com/questions/22571586/will-arrays-sort-increase-time-complexity-and-space-time-complexity](http://)\n ```\n class Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n Arrays.sort(nums);\n List<Integer> result = new ArrayList<>();\n for(int i = 0;i < nums.length;i++) {\n if(nums[i] == target) {\n result.add(i);\n } \n }\n return result;\n }\n}\n ```\n \n *Approach 2*\n \n **Time Complexity** : O(n)\n **Space Complexity**: O(1)\n \n ```\n class Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n List<Integer>result = new ArrayList<>();\n int number_of_targets = 0;\n int less_than_target_values = 0;\n for(int i : nums) {\n if(i < target) {\n less_than_target_values++;\n }\n else if(i == target) {\n number_of_targets++;\n }\n }\n while(number_of_targets > 0) {\n result.add(less_than_target_values);\n less_than_target_values++;\n number_of_targets--;\n }\n return result;\n }\n}\n ``` | 3 | 0 | ['Java'] | 0 |
find-target-indices-after-sorting-array | Java | 0 ms | Simple | java-0-ms-simple-by-prashant404-069t | \nT/S: O(n)/O(1)\n Ignoring space for output\n Count frequency of target and number of numbers smaller than target\n Create a list of indices based on the above | prashant404 | NORMAL | 2021-12-22T20:19:16.192347+00:00 | 2022-01-05T03:05:52.310190+00:00 | 267 | false | \n**T/S:** O(n)/O(1)\n* Ignoring space for output\n* Count frequency of target and number of numbers smaller than target\n* Create a list of indices based on the above calculation\n```\npublic List<Integer> targetIndices(int[] nums, int target) {\n\tvar targetCount = 0;\n\tvar smallerCount = 0;\n\n\tfor (var num : nums)\n\t\tif (num == target)\n\t\t\ttargetCount++;\n\t\telse if (num < target)\n\t\t\tsmallerCount++;\n\n\tvar targets = new ArrayList<Integer>();\n\tfor (var i = smallerCount; i < targetCount + smallerCount; i++)\n\t\ttargets.add(i);\n\treturn targets;\n}\n```\n**Variation 2:** Same idea using Streams\n```\npublic List<Integer> targetIndices(int[] nums, int target) {\n\tvar targetCount = 0;\n\tvar smallerCount = 0;\n\t\n\tfor (var num : nums)\n\t\tif (num == target)\n\t\t\ttargetCount++;\n\t\telse if (num < target)\n\t\t\tsmallerCount++;\n\n\treturn IntStream.range(smallerCount, targetCount + smallerCount)\n\t\t\t\t\t.boxed()\n\t\t\t\t\t.collect(Collectors.toCollection(ArrayList::new));\n}\n```\n***Please upvote if this helps*** | 3 | 0 | ['Java'] | 1 |
find-target-indices-after-sorting-array | o(n) simple python solution | on-simple-python-solution-by-sahana__63-azc6 | Some observations about the problem:\n\n1. We do not need to sort the array since we only have to find the index of target element if it were in a sorted array. | sahana__63 | NORMAL | 2021-12-10T15:10:06.418152+00:00 | 2021-12-10T15:10:06.418196+00:00 | 278 | false | Some observations about the problem:\n\n1. We do not need to sort the array since we only have to find the index of target element if it were in a sorted array. This is nothing but the number of elements that are less than the target. Let number of such elements be ` lt_ct`. Then `lt_ct+1` would be the next element and `lt_ct+1-1= lt_ct` since its zero indexed\n\n2. if there more than 1 elements equal to target (say `ct` times), the resultant array will have consecutive elements with length equal to `ct` which cab be generated using `range` function\n\n```\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n lt_ct, ct=0, 0\n\t\t\n for i in nums:\n if i < target: lt_ct+=1\n elif i == target: ct+=1\n \n res=list(range(lt_ct,lt_ct+ct))\n return res\n``` | 3 | 0 | ['Python'] | 0 |
find-target-indices-after-sorting-array | Python one liner | python-one-liner-by-kidchorus-9jlp | return list(filter(lambda x: (x != -1),[[-1,i][sorted(nums)[i] == target] for i in range(len(nums))])) | Kidchorus | NORMAL | 2021-12-06T09:47:21.099484+00:00 | 2021-12-06T09:47:21.099522+00:00 | 144 | false | ```return list(filter(lambda x: (x != -1),[[-1,i][sorted(nums)[i] == target] for i in range(len(nums))]))``` | 3 | 0 | [] | 1 |
find-target-indices-after-sorting-array | [Rust] Simple Solution without sort | rust-simple-solution-without-sort-by-koh-04xa | rust\nimpl Solution {\n pub fn target_indices(nums: Vec<i32>, target: i32) -> Vec<i32> {\n let mut target_count = 0;\n let mut smaller_count = | kohbis | NORMAL | 2021-12-02T10:31:51.897402+00:00 | 2021-12-03T01:40:07.944119+00:00 | 116 | false | ```rust\nimpl Solution {\n pub fn target_indices(nums: Vec<i32>, target: i32) -> Vec<i32> {\n let mut target_count = 0;\n let mut smaller_count = 0;\n\n for n in nums {\n if target == n {\n target_count += 1;\n } else if target > n {\n smaller_count += 1;\n }\n }\n\n (smaller_count..smaller_count + target_count).collect()\n }\n}\n``` | 3 | 0 | ['Rust'] | 1 |
find-target-indices-after-sorting-array | [Python3] Short O(N) Solution + 2 Liners + Numpy 1-Liner | python3-short-on-solution-2-liners-numpy-aien | Just simply count how many elements are less than the target and you will get the lowest index for target element. Then rest of it should be straightforward.\n\ | blackspinner | NORMAL | 2021-11-28T04:18:00.683857+00:00 | 2021-12-01T10:01:21.068375+00:00 | 256 | false | Just simply count how many elements are less than the target and you will get the lowest index for target element. Then rest of it should be straightforward.\n```\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n cnt_smaller = 0\n cnt_equal = 0\n for x in nums:\n if x < target:\n cnt_smaller += 1\n elif x == target:\n cnt_equal += 1\n return range(cnt_smaller, cnt_smaller + cnt_equal)\n```\n\n2-Liners version (by @stefan4trivia aka @StefanPochmann):\n```\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n smaller = sum(map(target.__gt__, nums))\n return islice(count(smaller), nums.count(target))\n```\n\nShorter 1-Liner O(N log N) version (also by @stefan4trivia aka @StefanPochmann):\n```\nimport numpy as np\n\nclass Solution(object):\n def targetIndices(self, nums, target):\n return np.where(np.sort(nums) == target)[0]\n``` | 3 | 0 | [] | 1 |
find-target-indices-after-sorting-array | c++ || eASY SOLUTION | c-easy-solution-by-vineet_raosahab-98yi | \nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n sort(nums.begin(),nums.end());\n vector<int> result; | VineetKumar2023 | NORMAL | 2021-11-28T04:02:45.315490+00:00 | 2021-11-28T04:02:45.315520+00:00 | 193 | false | ```\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n sort(nums.begin(),nums.end());\n vector<int> result;\n for(int i=0;i<nums.size();i++)\n {\n if(nums[i]==target)\n result.push_back(i);\n }\n return result;\n }\n};\n``` | 3 | 1 | [] | 0 |
find-target-indices-after-sorting-array | Beats 100%, simple solution | beats-100-simple-solution-by-adarshtec-rcwe | Intuitionsimple aprroach that would hit is to simply sort and find the indices of the targetApproachsort the array using inbuilt function and then find the targ | Adarshtec | NORMAL | 2025-02-12T08:50:08.220663+00:00 | 2025-02-12T08:50:08.220663+00:00 | 252 | false | # Intuition
simple aprroach that would hit is to simply sort and find the indices of the target
# Approach
sort the array using inbuilt function and then find the target element using for loop
# Complexity
- Time complexity:
o(nlogn)
- Space complexity:
o(n)
# Code
```cpp []
class Solution {
public:
vector<int> targetIndices(vector<int>& nums, int target) {
vector<int> c;
sort(nums.begin(),nums.end());
for(int i = 0 ; i < nums.size() ;i++){
if(nums[i]==target){
c.push_back(i);
}
}
return c;
}
};
``` | 2 | 0 | ['C++'] | 1 |
find-target-indices-after-sorting-array | last submission beat 100% of other submissions' runtime.|| O(N) || O(1)|| easy and simple solution| | last-submission-beat-100-of-other-submis-92u8 | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | Rohan07_6473 | NORMAL | 2025-02-05T01:59:47.893571+00:00 | 2025-02-05T01:59:47.893571+00:00 | 154 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
vector<int> targetIndices(vector<int>& nums, int target) {
int n = nums.size();
vector<int>p;
sort(nums.begin() , nums.end());
for(int i =0 ;i<n ;i++){
if(nums[i] == target){
p.push_back(i);
}
}
return p;
}
};
``` | 2 | 0 | ['C++'] | 1 |
find-target-indices-after-sorting-array | Very Easy | Beginner Friendly | 100% Beat | Cpp | Optimized | very-easy-beginner-friendly-100-beat-cpp-22eu | ApproachSort the array, iterate through it, and collect indices of elements equal to the target in a result vector. Stop iteration when elements exceed the targ | Ujjwal_Saini007 | NORMAL | 2025-01-27T08:42:21.967282+00:00 | 2025-01-27T08:42:21.967282+00:00 | 149 | false | # Approach
Sort the array, iterate through it, and collect indices of elements equal to the target in a result vector. Stop iteration when elements exceed the target value. At last return the result.
# Complexity
- Time complexity: **O(nlogn)**
- Space complexity: **O(n)**
# Code
```cpp []
class Solution {
public:
vector<int> targetIndices(vector<int>& nums, int target) {
int size = nums.size();
vector<int> res;
sort(nums.begin(), nums.end());
for(int i=0; i<size; i++){
if(nums[i] == target){
res.push_back(i);
}
else if(nums[i]>target)
break;
}
return res;
}
};
``` | 2 | 0 | ['C++'] | 0 |
find-target-indices-after-sorting-array | Easier Java Solution || Using Sorting | easier-java-solution-using-sorting-by-uc-qyp7 | IntuitionThe elements are in ascending order, which allows us to easily traverse and find all occurrences of the target.ApproachSorting the array ensures that a | ucontactpawan | NORMAL | 2025-01-14T20:15:06.119445+00:00 | 2025-01-14T20:15:06.119445+00:00 | 224 | false | # Intuition
The elements are in ascending order, which allows us to easily traverse and find all occurrences of the target.
# Approach
Sorting the array ensures that all occurrences of the target value are grouped together. This simplifies the process of finding and collecting the indices.
Use a for loop to iterate through the sorted array.
Check if the current element is equal to the target.
If true, add the current **index (i)** to the result list.
# Complexity
- Time complexity:
O(nlogn).
- Space complexity:
O(n)
# Code
```java []
class Solution {
public List<Integer> targetIndices(int[] nums, int target) {
ArrayList<Integer> list = new ArrayList<>();
Arrays.sort(nums);
for(int i=0; i<nums.length; i++){
if(nums[i]==target){
list.add(i);
}
}
return list;
}
}
``` | 2 | 0 | ['Array', 'Sorting', 'Java'] | 2 |
find-target-indices-after-sorting-array | easy c++ | easy-c-by-rajeev_22-cw3x | Complexity
Time complexity:
O(nlogn)
Code | Rajeev_22 | NORMAL | 2024-12-23T12:51:48.861720+00:00 | 2024-12-23T12:51:48.861720+00:00 | 151 | false |
# Complexity
- Time complexity:
O(nlogn)
# Code
```cpp []
class Solution {
public:
vector<int> targetIndices(vector<int>& nums, int target)
{
vector<int> ans;
sort(begin(nums),end(nums));
auto x = lower_bound(nums.begin(),nums.end(),target);
auto y = upper_bound(nums.begin(),nums.end(),target);
for(auto i =x;i!=y;i++)
{
ans.push_back(i-nums.begin());
}
return ans;
}
};
``` | 2 | 0 | ['C++'] | 0 |
find-target-indices-after-sorting-array | leetcodedaybyday - Beats 100% with C++ and Python3 | leetcodedaybyday-beats-100-with-c-and-py-hmj8 | IntuitionThe problem requires finding the indices of a specific target in a sorted version of the input array. Sorting the array ensures that all occurrences of | tuanlong1106 | NORMAL | 2024-12-15T08:18:38.948171+00:00 | 2024-12-15T08:18:38.948171+00:00 | 236 | false | # Intuition\nThe problem requires finding the indices of a specific `target` in a sorted version of the input array. Sorting the array ensures that all occurrences of the `target` are contiguous, allowing for a simple linear scan to collect the indices.\n\n# Approach\n1. **Sort the Array**: \n Sort the input array `nums` in non-decreasing order to make all occurrences of `target` contiguous.\n\n2. **Iterate Through the Array**: \n Perform a single pass through the sorted array and check if each element equals the `target`. If it does, add its index to the result.\n\n3. **Return the Result**: \n Once the loop is complete, return the list of collected indices.\n\n# Complexity\n- **Time Complexity**: \n - Sorting the array takes \\(O(n \\log n)\\), where \\(n\\) is the size of `nums`.\n - The subsequent iteration to collect indices takes \\(O(n)\\).\n - Total: \\(O(n \\log n)\\).\n\n- **Space Complexity**: \n - Sorting the array is done in-place, requiring \\(O(1)\\) additional space.\n - The output vector `idx` requires \\(O(k)\\), where \\(k\\) is the number of indices that match the `target`.\n - Total: \\(O(k)\\).\n\n# Code\n```cpp []\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n sort(nums.begin(), nums.end());\n vector<int> idx;\n for (int i = 0; i < nums.size(); i++){\n if (nums[i] == target)\n idx.push_back(i);\n }\n return idx;\n }\n};\n```\n```python3 []\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n nums.sort()\n idx = []\n for i in range(len(nums)):\n if nums[i] == target:\n idx.append(i)\n return idx\n```\n | 2 | 0 | ['C++', 'Python3'] | 0 |
find-target-indices-after-sorting-array | Solution | solution-by-vijay_sathappan-1f4p | Intuition\nYou are given a 0-indexed integer array nums and a target element target.\nA target index is an index i such that nums[i] == target.\nReturn a list o | vijay_sathappan | NORMAL | 2024-10-26T05:35:29.114323+00:00 | 2024-10-26T05:35:29.114351+00:00 | 117 | false | # Intuition\nYou are given a 0-indexed integer array nums and a target element target.\nA target index is an index i such that nums[i] == target.\nReturn a list of the target indices of nums after sorting nums in non-decreasing order. If there are no target indices, return an empty list. The returned list must be sorted in increasing order.\n\n# Approach\nSort the List and check if the element equals target, append the index of the element.\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(n)\n\n# Code\n```python []\nclass Solution(object):\n def targetIndices(self, nums, target):\n nums.sort()\n out=[]\n for i in range(len(nums)):\n if nums[i]==target:\n out.append(i)\n return out\n \n``` | 2 | 0 | ['Array', 'Sorting', 'Python', 'Python3'] | 0 |
find-target-indices-after-sorting-array | 🎯✨ "Find Target Indices in a Sorted Array: Sort ➡️ Search ➡️ Collect!" 📊 | find-target-indices-in-a-sorted-array-so-pih0 | Intuition \u2728:\n1. Sorting the Array \uD83E\uDDE9:\n First, we sort the array to easily find the target\u2019s indices. Sorting helps to bring all occurren | sajidmujawar | NORMAL | 2024-10-06T17:34:33.836036+00:00 | 2024-10-06T17:34:33.836069+00:00 | 57 | false | # Intuition \u2728:\n1. Sorting the Array \uD83E\uDDE9:\n First, we sort the array to easily find the target\u2019s indices. Sorting helps to bring all occurrences of the target together in one place.\n\n2. Find the First Occurrence of Target \uD83C\uDFAF:\n After sorting, we use lower_bound to locate the first position where the target might appear. Think of it as a radar \uD83D\uDEF0\uFE0F scanning the array for the first potential match.\n\n3. Collect Target Indices \uD83D\uDCCA:\n Starting from the first occurrence found, we move forward \u23E9 and check if each element is the target. If yes, we collect \uD83D\uDED2 its index in a result list. Once we find a different number, we stop \uD83D\uDEAB.\n\n4. Return the Indices \uD83C\uDFC1:\n Finally, we return the list of indices where the target appears.\n\n# Approach \uD83D\uDEE0\uFE0F:\n1. Sort the Array \uD83D\uDD04:\n```\nsort(nums.begin(), nums.end());\n```\nSorting ensures the target elements are together.\n\n2. Find the First Match \uD83C\uDFAF:\n\n```\nint a = lower_bound(nums.begin(), nums.end(), target) - nums.begin();\n```\nThis finds where the target could start appearing in the sorted array.\n\n3. Loop to Gather Indices \u27BF:\n\n```\nfor(int i = a; i < nums.size(); i++) {\n if (nums[i] == target) {\n ans.push_back(i); // Add index to result \uD83D\uDCE5\n } else {\n break; // Stop if we see a different number \uD83D\uDED1\n }\n}\n```\n4. Return the Result \uD83C\uDF89:\n\n```\nreturn ans;\n```\nThis approach ensures you find and store the correct indices after sorting, and it breaks out of the loop when the target stops appearing.\n\n# Complexity\u23F1\uFE0F\n- Time complexity:\u23F3\n$$O(nlogn)$$ \uD83C\uDF00\n\n- Space complexity:\uD83D\uDCBE\n$$O(n)$$\n\n# Code\n```cpp []\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n sort(nums.begin(),nums.end());\n \n int a=lower_bound(nums.begin(),nums.end(),target)-nums.begin();\n vector<int> ans;\n\n for(int i=a;i<nums.size();i++)\n { if(nums[i]==target)\n ans.push_back(i);\n else \n break;}\n \n return ans;\n }\n};\n``` | 2 | 0 | ['C++'] | 0 |
find-target-indices-after-sorting-array | easy c++ solution 🚀 | easy-c-solution-by-harshith173-jcfc | \n Add your space complexity here, e.g. O(n) \n\n# Code\ncpp []\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n | harshith173 | NORMAL | 2024-09-06T14:27:16.922826+00:00 | 2024-09-06T14:27:16.922844+00:00 | 188 | false | \n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```cpp []\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n sort(nums.begin(),nums.end()); \n int n=nums.size();\n vector<int> ans;\n for(int i=0;i<n;i++){\n if(nums[i]==target){\n ans.push_back(i);\n }\n }\n return ans;\n }\n};\n``` | 2 | 0 | ['C++'] | 0 |
find-target-indices-after-sorting-array | Find Target Indices After Sorting Array | find-target-indices-after-sorting-array-y3zxh | Intuition\nMy first thought on solving this problem is to leverage sorting and a linear scan. By sorting the array first, we can then iterate through it and col | tejdekiwadiya | NORMAL | 2024-07-21T05:53:43.327471+00:00 | 2024-07-21T05:53:43.327494+00:00 | 571 | false | # Intuition\nMy first thought on solving this problem is to leverage sorting and a linear scan. By sorting the array first, we can then iterate through it and collect all indices where the elements are equal to the target value. Sorting helps us ensure that all instances of the target are contiguous, making it easy to collect their indices in a single pass.\n\n# Approach\n1. **Sort the Array**: First, sort the array. This will arrange all elements in non-decreasing order.\n2. **Initialize Result List**: Create a list `ans` to store the indices of the target value.\n3. **Iterate and Collect Indices**: Iterate through the sorted array. For each element that matches the target, add its index to the `ans` list.\n4. **Return Result**: Finally, return the `ans` list containing all indices where the target value appears.\n\n# Complexity\n- **Time Complexity**: The time complexity is dominated by the sorting step, which is (O(n log n)), where (n) is the number of elements in the array. The subsequent linear scan through the array is (O(n)), but this does not change the overall complexity since (O(n log n)) is the more significant term.\n- **Space Complexity**: The space complexity is (O(1)) for the sorting operation if we do not consider the space required for the input and output. However, the space complexity for the output list `ans` is (O(k)), where (k) is the number of occurrences of the target in the array.\n\n# Code\n\n```java\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n List<Integer> ans = new ArrayList<>();\n Arrays.sort(nums); // Sort the array\n System.out.println(Arrays.toString(nums)); // Print the sorted array for debugging\n for (int i = 0; i < nums.length; i++) { // Iterate through the sorted array\n if (nums[i] == target) { // Check if the current element is equal to the target\n ans.add(i); // Add the index to the result list\n }\n }\n return ans; // Return the list of indices\n }\n}\n``` | 2 | 0 | ['Array', 'Binary Search', 'Sorting', 'Java'] | 0 |
find-target-indices-after-sorting-array | for beginner solution. | for-beginner-solution-by-anishg018-6eit | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Anishg018 | NORMAL | 2024-07-02T10:58:23.969953+00:00 | 2024-07-02T10:58:23.969985+00:00 | 182 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n vector<int> ans;\n sort(nums.begin(),nums.end());\n for(int i=0; i<nums.size(); i++){\n if(nums[i]==target){\n ans.push_back(i);\n }\n }\n \n return ans;\n }\n};\n``` | 2 | 0 | ['C++'] | 0 |
find-target-indices-after-sorting-array | Beginners solution | beginners-solution-by-shreya_kumari_24-i7hc | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | Shreya_Kumari_24 | NORMAL | 2024-04-03T13:01:47.126969+00:00 | 2024-04-03T13:01:47.127014+00:00 | 254 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n ArrayList<Integer>li = new ArrayList<>();\n Arrays.sort(nums);\n for(int i = 0;i<nums.length;i++){\n if(nums[i]==target)\n li.add(i);\n }\n return li;\n }\n}\n``` | 2 | 0 | ['Java'] | 0 |
find-target-indices-after-sorting-array | ✔️✔️✔️easy to understand 1 liner PYTHON code || C++ || JAVA || DART || KOTLIN⚡⚡⚡ | easy-to-understand-1-liner-python-code-c-xy6p | Code\nPython []\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n nums.sort()\n return [i for i in range | anish_sule | NORMAL | 2024-03-19T06:33:22.497830+00:00 | 2024-03-19T07:21:03.486422+00:00 | 112 | false | # Code\n```Python []\nclass Solution:\n def targetIndices(self, nums: List[int], target: int) -> List[int]:\n nums.sort()\n return [i for i in range(len(nums)) if nums[i] == target]\n```\n```C++ []\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n vector<int> fin;\n sort(nums.begin(), nums.end());\n for (int i = 0; i < nums.size(); i++) {\n if (nums[i] == target) {\n fin.push_back(i);\n }\n }\n return fin;\n }\n};\n```\n```Java []\nclass Solution {\n public List<Integer> targetIndices(int[] nums, int target) {\n Arrays.sort(nums);\n ArrayList<Integer> fin = new ArrayList<Integer>();\n for(int i=0;i<nums.length;i++){\n if(nums[i]==target)fin.add(i);\n }\n return fin;\n }\n}\n```\n```Dart []\nclass Solution {\n List<int> targetIndices(List<int> nums, int target) {\n nums.sort();\n List<int> fin=[];\n for(int i=0;i<nums.length; i++){\n if(nums[i]==target){\n fin.add(i);\n }\n }\n return fin ;\n }\n}\n```\n```Kotlin []\nclass Solution {\n fun targetIndices(nums: IntArray, target: Int): List<Int> {\n var fin=arrayListOf<Int>()\n nums.sort()\n for(i in 0 until nums.size){\n if(nums[i]==target){\n fin.add(i)\n }\n }\n return fin\n }\n}\n``` | 2 | 0 | ['Sorting', 'C++', 'Java', 'Python3', 'Dart'] | 1 |
find-target-indices-after-sorting-array | C++ || Linear Search || Simple Approach | c-linear-search-simple-approach-by-meuru-qbxb | \n# Complexity\n- Time complexity: O(nlogn)\n Add your time complexity here, e.g. O(n) \n\n- Space complexity: O(1)\n Add your space complexity here, e.g. O(n) | meurudesu | NORMAL | 2024-02-09T15:12:26.355602+00:00 | 2024-02-23T02:21:52.945144+00:00 | 590 | false | \n# Complexity\n- Time complexity: $$O(nlogn)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n vector<int> targetIndices(vector<int>& nums, int target) {\n sort(nums.begin(), nums.end());\n vector<int> ans;\n for(int i=0; i<nums.size(); i++) {\n if(nums[i] == target) {\n ans.push_back(i);\n }\n }\n return ans;\n }\n};\n``` | 2 | 0 | ['C++'] | 1 |
describe-the-painting | [Python] Easy solution in O(n*logn) with detailed explanation | python-easy-solution-in-onlogn-with-deta-5btx | Idea\nwe can iterate on coordinates of each [start_i, end_i) from small to large and maintain the current mixed color.\n\n### How can we maintain the mixed colo | fishballlin | NORMAL | 2021-07-24T16:01:35.389474+00:00 | 2022-03-18T00:07:27.373792+00:00 | 3,950 | false | ### Idea\nwe can iterate on coordinates of each `[start_i, end_i)` from small to large and maintain the current mixed color.\n\n### How can we maintain the mixed color?\nWe can do addition if the current coordinate is the beginning of a segment.\nIn contrast, We do subtraction if the current coordinate is the end of a segment.\n\n### Examples\nLet\'s give the example for understanding it easily.\n\n#### Example 1:\n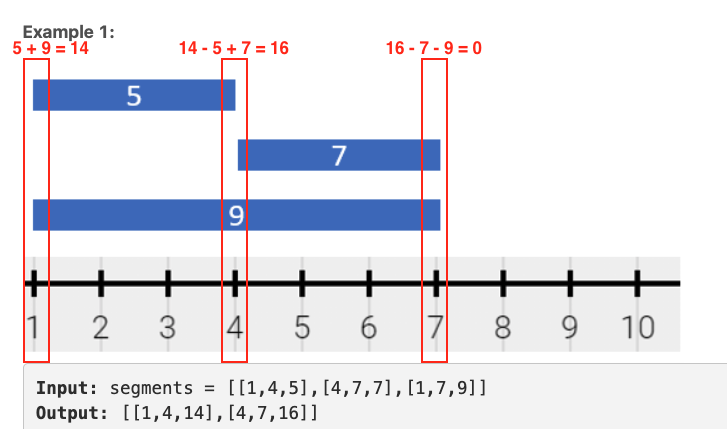\n\nThere are 3 coordinates get involved. 1, 4, 7 respetively.\nAt coordinate 1, there are two segment starting here. Thus, the current mixed color will be 5 + 9 = 14\nAt coordinate 4, the segment [1, 4, 5] is end here and the segment [4, 7, 7] also start here. Thus, the current mixed color will be 14 - 5 + 7 = 16\nAt coordinate 7, two segments are end here. Thus, the current mixed color will be 0\nSo we know the final answer should be [1, 4, 14] and [4, 7, 16].\n\n\n#### Example 2:\n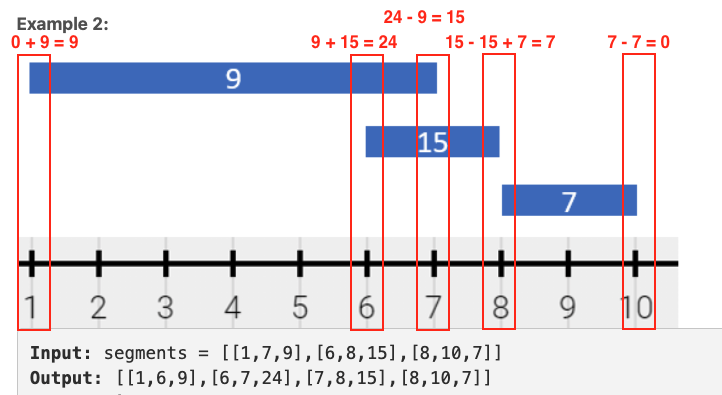\n\nThere are 5 coordinates get involved. 1, 6, 7, 8, 10 respetively.\nAt coordinate 1, only the segment [1, 7, 9] start here. Thus, the current mixed color will be 0 + 9 = 9\nAt coordinate 6, another segment [6, 8, 15] start here. Thus, the current mixed color will be 9 + 15 = 24\nAt coordinate 7, the segment [1, 7, 9] is end here. Thus, the current mixed color will be 24 - 9 = 15\nAt coordinate 8, the segment [6, 8, 15] is end here and the segment [8, 10, 7] also start here. Thus, the current mixed color will be 15 - 15 + 7 = 7\nAt coordinate 10, the segment [8, 10, 7] is end here. Thus, the current mixed color will be 0\nSo we know the final answer should be [1,6,9], [6,7,24], [7,8,15], [8,10,7].\n\n### Complexity\n- Time complexity: O(n * log(n)) # We can use a fixed size array with length 10^5 to replace the default dict and bound the time complexity to O(n).\n- Space complexity: O(n)\n\n### Code\n\n```python\nclass Solution:\n def splitPainting(self, segments: List[List[int]]) -> List[List[int]]:\n\t\t# via this mapping, we can easily know which coordinates should be took into consideration.\n mapping = defaultdict(int)\n for s, e, c in segments:\n mapping[s] += c\n mapping[e] -= c\n \n res = []\n prev, color = None, 0\n for now in sorted(mapping):\n if color: # if color == 0, it means this part isn\'t painted.\n res.append((prev, now, color))\n \n color += mapping[now]\n prev = now\n \n return res\n```\n\nPlease upvote if it\'s helpful or leave comments if I can do better. Thank you in advance.\n | 145 | 0 | ['Python3'] | 13 |
describe-the-painting | [Java/C++/Python] Sweep Line | javacpython-sweep-line-by-lee215-1kfj | Intuition\nSimilar to the problem of Meeting Rooms.\n\n\n# Explanation\nWe first record the delta change in the map d,\nthen we sweep the vertex i of segments f | lee215 | NORMAL | 2021-07-24T16:12:55.411522+00:00 | 2021-07-24T17:05:37.316082+00:00 | 5,257 | false | # **Intuition**\nSimilar to the problem of Meeting Rooms.\n<br>\n\n# **Explanation**\nWe first record the delta change in the map `d`,\nthen we sweep the vertex `i` of segments from small to big,\nand we accmulate the delta change `d[i]`.\nFor each segment, we push its values to result `res`.\n<br>\n\n# **Complexity**\nTime `O(nlogn)`\nSpace `O(n)`\n<br>\n\n**Java**\n```java\n public List<List<Long>> splitPainting(int[][] A) {\n Map<Integer, Long> d = new TreeMap<>();\n for (int[] a : A) {\n d.put(a[0], d.getOrDefault(a[0], 0L) + a[2]);\n d.put(a[1], d.getOrDefault(a[1], 0L) - a[2]);\n }\n List<List<Long>> res = new ArrayList<>();;\n long i = 0, sum = 0;\n for (int j : d.keySet()) {\n if (sum > 0)\n res.add(Arrays.asList(i, (long)j, sum));\n sum += d.get(j);\n i = j;\n }\n return res;\n }\n```\n**C++**\n```cpp\n vector<vector<long long>> splitPainting(vector<vector<int>>& A) {\n map<int, long long> d;\n for (auto& a : A) {\n d[a[0]] += a[2];\n d[a[1]] -= a[2];\n }\n vector<vector<long long> > res;\n int i = 0;\n for (auto& it : d) {\n if (d[i] > 0)\n res.push_back({i, it.first, d[i]});\n d[it.first] += d[i];\n i = it.first;\n }\n return res;\n }\n```\n\n**Python**\n```py\n def splitPainting(self, segs):\n d = collections.defaultdict(int)\n for i, j, c in segs:\n d[i] += c\n d[j] -= c\n res = []\n i = 0\n for j in sorted(d):\n if d[i]:\n res.append([i, j, d[i]])\n d[j] += d[i]\n i = j\n return res\n```\n | 88 | 4 | [] | 12 |
describe-the-painting | Line Sweep | line-sweep-by-votrubac-otky | We can use the line sweep approach to compute the sum (color mix) for each point. We use this line to check when a sum changes (a segment starts or finishes) an | votrubac | NORMAL | 2021-07-24T16:01:43.482145+00:00 | 2021-07-24T18:23:22.581106+00:00 | 5,761 | false | We can use the line sweep approach to compute the sum (color mix) for each point. We use this line to check when a sum changes (a segment starts or finishes) and describe each mix. But there is a catch: different colors could produce the same sum. \n\nI was puzzled for a moment, and then I noticed that the color for each segment is distinct. So, every time a segment starts or ends, the color mix is different (even if the sum is the same). So we also mark `ends` of color segments on our line.\n\n**Java**\n```java\npublic List<List<Long>> splitPainting(int[][] segments) {\n long mix[] = new long[100002], sum = 0, last_i = 0;\n boolean ends[] = new boolean[100002];\n List<List<Long>> res = new ArrayList<>();\n for (var s : segments) {\n mix[s[0]] += s[2];\n mix[s[1]] -= s[2];\n ends[s[0]] = ends[s[1]] = true;\n }\n for (int i = 1; i < 100002; ++i) {\n if (ends[i] && sum > 0)\n res.add(Arrays.asList(last_i, (long)i, sum));\n last_i = ends[i] ? i : last_i;\n sum += mix[i];\n } \n return res;\n}\n```\n**C++**\n```cpp\nvector<vector<long long>> splitPainting(vector<vector<int>>& segments) {\n long long mix[100002] = {}, sum = 0, last_i = 0;\n bool ends[100002] = {};\n vector<vector<long long>> res;\n for (auto &s : segments) {\n mix[s[0]] += s[2];\n mix[s[1]] -= s[2];\n ends[s[0]] = ends[s[1]] = true;\n }\n for (int i = 1; i < 100002; ++i) {\n if (ends[i] && sum)\n res.push_back({last_i, i, sum});\n last_i = ends[i] ? i : last_i;\n sum += mix[i];\n }\n return res;\n}\n```\n**Python 3**\n```python\nclass Solution:\n def splitPainting(self, segments: List[List[int]]) -> List[List[int]]:\n mix, res, last_i = DefaultDict(int), [], 0\n for start, end, color in segments:\n mix[start] += color\n mix[end] -= color\n for i in sorted(mix.keys()):\n if last_i in mix and mix[last_i]:\n res.append([last_i, i, mix[last_i]])\n mix[i] += mix[last_i]\n last_i = i\n return res\n``` | 81 | 1 | ['C', 'Java', 'Python3'] | 25 |
describe-the-painting | JAVA Easiest Solution. O(NlogN). Same as Car Pooling and Meeting Rooms II | java-easiest-solution-onlogn-same-as-car-znpy | Iterate on all the segments(start, end, color) of the painting and add color value at start and subtract it at end. Use TreeMap to store the points sorted. Keep | rohitjoins | NORMAL | 2021-07-24T16:13:29.775505+00:00 | 2021-07-25T19:40:05.558438+00:00 | 1,660 | false | Iterate on all the segments(start, end, color) of the painting and add color value at start and subtract it at end. Use TreeMap to store the points sorted. Keep adding the value of color at each point to know the current value at that point.\n\n```\npublic List<List<Long>> splitPainting(int[][] segments) {\n\tTreeMap<Integer, Long> map = new TreeMap<>();\n\n\tfor(int segment[]: segments) {\n\t\tmap.put(segment[0], map.getOrDefault(segment[0], 0L) + segment[2]);\n\t\tmap.put(segment[1], map.getOrDefault(segment[1], 0L) - segment[2]);\n\t}\n\n\tList<List<Long>> result = new ArrayList<>();\n\n\tint prev = 0;\n\tlong sum = 0;\n\n\tfor(int key: map.keySet()) {\n\t\tif(sum != 0) { // Ignore the unpainted interval\n\t\t\tresult.add(Arrays.asList((long)prev, (long)key, sum)); // Add the interval\n\t\t}\n\n\t\tsum += map.get(key);\n\t\tprev = key;\n\t}\n\n\treturn result;\n}\n```\n\nPlease upvote if you find it useful. | 28 | 1 | ['Java'] | 5 |
describe-the-painting | c++ using map with explanation in simple way | c-using-map-with-explanation-in-simple-w-xyhc | This explanation is for when we are given arrival and leave time of some persons in room. And we have to calculate the maximum number of persons in room at a ti | abaddu_21 | NORMAL | 2021-07-27T04:04:51.792456+00:00 | 2021-07-27T18:51:36.150596+00:00 | 1,096 | false | This explanation is for when we are given arrival and leave time of some persons in room. And we have to calculate the maximum number of persons in room at a time. Or calculate interval wise how many persons currently present in room. I think these two are similar.\nWe go through the events from left to right and maintain a counter. Always when\na person arrives, we increase the value of the counter by one, and when a person\nleaves, we decrease the value of the counter by one. The answer to the problem is\nthe maximum value of the counter during the algorithm.\nIn case of color when new color added/removed then previous interval end at that point and new interval starts.\nIf you don\'t understand add comment. Else if you like it? upvote.\n```\nclass Solution {\npublic:\n vector<vector<long long>> splitPainting(vector<vector<int>>& seg) {\n vector<vector<long long>> v;\n map<long long,long long> m;\n long long int i=0;\n while(i<seg.size())\n {\n m[seg[i][0]]+=seg[i][2];\n m[seg[i][1]]-=seg[i][2];\n i++;\n }\n long long int j=0,k=0;\n for(auto x:m)\n {\n long long int prev=j;\n j+=x.second;\n if(prev>0)\n v.push_back({k,x.first,prev});\n k=x.first;\n }\n return v;\n }\n};\n``` | 16 | 0 | ['C'] | 1 |
describe-the-painting | [Python3] sweeping again | python3-sweeping-again-by-ye15-0nl4 | \n\nclass Solution:\n def splitPainting(self, segments: List[List[int]]) -> List[List[int]]:\n vals = []\n for start, end, color in segments: \ | ye15 | NORMAL | 2021-07-24T16:02:15.245245+00:00 | 2021-07-24T16:02:15.245290+00:00 | 956 | false | \n```\nclass Solution:\n def splitPainting(self, segments: List[List[int]]) -> List[List[int]]:\n vals = []\n for start, end, color in segments: \n vals.append((start, +color))\n vals.append((end, -color))\n \n ans = []\n prefix = prev = 0 \n for x, c in sorted(vals): \n if prev < x and prefix: ans.append([prev, x, prefix])\n prev = x\n prefix += c \n return ans \n``` | 15 | 1 | ['Python3'] | 2 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.