question_slug
stringlengths 3
77
| title
stringlengths 1
183
| slug
stringlengths 12
45
| summary
stringlengths 1
160
⌀ | author
stringlengths 2
30
| certification
stringclasses 2
values | created_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| updated_at
stringdate 2013-10-25 17:32:12
2025-04-12 09:38:24
| hit_count
int64 0
10.6M
| has_video
bool 2
classes | content
stringlengths 4
576k
| upvotes
int64 0
11.5k
| downvotes
int64 0
358
| tags
stringlengths 2
193
| comments
int64 0
2.56k
|
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
largest-number-after-digit-swaps-by-parity | Simple Map Approach | simple-map-approach-by-msegal347-veli | Code\n\nclass Solution:\n def largestInteger(self, num: int) -> int:\n # Convert number to a list of digits\n digits = [int(d) for d in str(num | msegal347 | NORMAL | 2024-06-12T19:50:15.302357+00:00 | 2024-06-12T19:50:15.302380+00:00 | 29 | false | # Code\n```\nclass Solution:\n def largestInteger(self, num: int) -> int:\n # Convert number to a list of digits\n digits = [int(d) for d in str(num)]\n \n # Separate the digits by parity\n evens = sorted((d for d in digits if d % 2 == 0), reverse=True)\n odds = sorted((d for d in digits if d % 2 == 1), reverse=True)\n \n # Reconstruct the number\n result = []\n for d in digits:\n if d % 2 == 0:\n # Place the largest even digit available\n result.append(evens.pop(0))\n else:\n # Place the largest odd digit available\n result.append(odds.pop(0))\n \n # Join the digits to form the final number\n return int(\'\'.join(map(str, result)))\n``` | 1 | 0 | ['Python3'] | 0 |
largest-number-after-digit-swaps-by-parity | Python Max Heap Simple Solution | python-max-heap-simple-solution-by-asu2s-uqrl | Complexity\n- Time complexity: O(n*logn)\n\n- Space complexity: O(n)\n\n# Code\n\nclass Solution:\n def largestInteger(self, num: int) -> int:\n even, | asu2sh | NORMAL | 2024-05-08T14:03:08.559043+00:00 | 2024-05-08T14:52:16.089889+00:00 | 255 | false | # Complexity\n- Time complexity: $$O(n*logn)$$\n\n- Space complexity: $$O(n)$$\n\n# Code\n```\nclass Solution:\n def largestInteger(self, num: int) -> int:\n even, odd = [], []\n nums = [-int(n) for n in str(num)]\n\n for n in nums:\n if n % 2:\n heapq.heappush(even, n)\n else:\n heapq.heappush(odd, n)\n\n res = 0\n for n in nums:\n if n % 2:\n x = -heapq.heappop(even)\n else:\n x = -heapq.heappop(odd)\n res = res * 10 + x\n \n return res\n\n``` | 1 | 0 | ['Heap (Priority Queue)', 'Python3'] | 0 |
largest-number-after-digit-swaps-by-parity | Simple Java Code 0 ms beats 100 % | simple-java-code-0-ms-beats-100-by-arobh-14mn | Complexity\n\n# Code\n\nclass Solution {\n public int largestInteger(int num) {\n int count=0;\n int temp=num;\n while(temp>0){\n | Arobh | NORMAL | 2024-05-07T01:27:49.602270+00:00 | 2024-05-07T01:27:49.602290+00:00 | 251 | false | # Complexity\n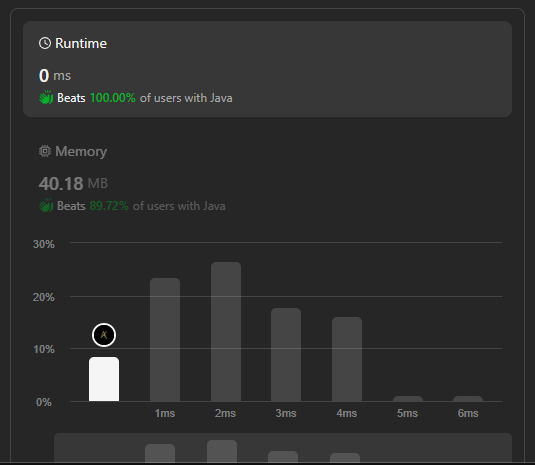\n# Code\n```\nclass Solution {\n public int largestInteger(int num) {\n int count=0;\n int temp=num;\n while(temp>0){\n count++;\n temp/=10;\n }\n int arr[]=new int[count];\n int k=count-1;\n temp=num;\n while(temp>0){\n arr[k--]=temp%10;\n temp/=10;\n }\n for(int i=0;i<count-1;i++){\n for(int j=i+1;j<count;j++){\n if(arr[i]%2==0&&arr[j]%2==0&&arr[j]>arr[i]){\n int z=arr[i];\n arr[i]=arr[j];\n arr[j]=z;\n }\n else if(arr[i]%2!=0&&arr[j]%2!=0&&arr[j]>arr[i]){\n int z=arr[i];\n arr[i]=arr[j];\n arr[j]=z;\n }\n }\n }\n int ans=0;\n for(int i: arr){\n ans=ans*10 + i;\n }\n return ans;\n }\n}\n``` | 1 | 0 | ['Java'] | 0 |
largest-number-after-digit-swaps-by-parity | C++ Solution || 100%beats || using priority_queue || easy to understand | c-solution-100beats-using-priority_queue-5dqr | Code\n\nclass Solution {\npublic:\n int largestInteger(int num) {\n string numStr = to_string(num);\n priority_queue<int> oddDigits, evenDigits | harshil_sutariya | NORMAL | 2024-04-11T09:20:53.695292+00:00 | 2024-04-11T09:22:34.414351+00:00 | 586 | false | # Code\n```\nclass Solution {\npublic:\n int largestInteger(int num) {\n string numStr = to_string(num);\n priority_queue<int> oddDigits, evenDigits;\n\n for (char digit : numStr) {\n if ((digit - \'0\') % 2 == 0) {\n evenDigits.push(digit - \'0\');\n } else {\n oddDigits.push(digit - \'0\');\n }\n }\n for (char& digit : numStr) {\n if ((digit - \'0\') % 2 == 0) {\n digit = evenDigits.top() + \'0\';\n evenDigits.pop();\n } else {\n digit = oddDigits.top() + \'0\';\n oddDigits.pop();\n }\n }\n return stoi(numStr);\n }\n};\n\n``` | 1 | 0 | ['Heap (Priority Queue)', 'C++'] | 0 |
largest-number-after-digit-swaps-by-parity | Easiest Understandable Soln | easiest-understandable-soln-by-sumo25-fz4o | \n\n# Code\n\nclass Solution {\n public int largestInteger(int num) {\n PriorityQueue<Integer> odd=new PriorityQueue<>(Collections.reverseOrder());\n | sumo25 | NORMAL | 2024-03-06T21:02:15.028723+00:00 | 2024-03-06T21:02:15.028775+00:00 | 922 | false | \n\n# Code\n```\nclass Solution {\n public int largestInteger(int num) {\n PriorityQueue<Integer> odd=new PriorityQueue<>(Collections.reverseOrder());\n PriorityQueue<Integer> even=new PriorityQueue<>(Collections.reverseOrder());\n String s=""+num;\n char[] ch=s.toCharArray();\n for(int i=0;i<ch.length;i++){\n if(Character.getNumericValue(ch[i])%2==0){\n even.add(Character.getNumericValue(ch[i]));\n }\n else{\n odd.add(Character.getNumericValue(ch[i]));\n }\n }\n\n String str="";\n for(int i=0;i<ch.length;i++){\n if(Character.getNumericValue(ch[i])%2==0){\n str+=""+even.poll();\n }\n else{\n str+=""+odd.poll();\n }\n }\n int ans=Integer.parseInt(str);\n return ans;\n\n }\n}\n``` | 1 | 0 | ['Java'] | 0 |
largest-number-after-digit-swaps-by-parity | Two Different Solutions -- Simple and Easy | two-different-solutions-simple-and-easy-idp93 | We first record the frequency of each digit in digitFreq list.\nNow we go through each digit of the number and replace it with the max number we have of the sam | mohit94596 | NORMAL | 2023-11-12T04:57:57.421970+00:00 | 2023-11-12T04:57:57.421994+00:00 | 175 | false | We first record the frequency of each digit in digitFreq list.\nNow we go through each digit of the number and replace it with the max number we have of the same parity in digitFreq. Don\'t forget to decrease the freq once you reaplce. \nTC: 5 * O(n), because we have to go through a list of 5(out of 10, 5 are same parity)\n\n```\nclass Solution:\n def largestInteger(self, num: int) -> int:\n digitFreq = [0] * 10 #Lets record the frequency of each digit. \n \n for digit in str(num): #We record here\n digit = int(digit)\n digitFreq[digit] += 1\n \n maxNum = \'\' #max number will be a string, we will later convert to int\n for digit in str(num):\n digit = int(digit)\n \n #If digit is even, we will put the max even number here\n if digit%2 == 0:\n for i in range(8, -1, -2):\n if digitFreq[i] > 0:\n maxNum += str(i)\n digitFreq[i] -= 1\n break\n \n #If digit is odd, we will put the max odd number here. \n else:\n for i in range(9, 0, -2):\n if digitFreq[i] > 0:\n maxNum += str(i)\n digitFreq[i] -= 1\n break\n return int(maxNum)\n```\n\n\nInstead of using a list and going to a list of 10 each time, we can build a max heap and go though that. We go though the nums and for every num we replace it with the max number of the same parity. If there is no digit with that parity then keep the current number. \nTC: O(n Logn), for building heap and popping from heap. \n\n```\nclass Solution:\n def largestInteger(self, num: int) -> int:\n oddNumberHeap = []\n evenNumberHeap = []\n \n for digit in str(num): #Push onto heaps\n digit = int(digit)\n if digit%2 == 0:\n heappush(evenNumberHeap, -digit)\n else:\n heappush(oddNumberHeap, -digit)\n \n maxNum = \'\' #max number will be a string, we will later convert to int\n for digit in str(num):\n digit = int(digit)\n \n #If digit is even, we will put the max even number here\n if digit%2 == 0 and evenNumberHeap:\n maxEvenNum = -heappop(evenNumberHeap)\n maxNum += str(maxEvenNum)\n \n #If digit is odd, we will put the max odd number here. \n elif digit%2 == 1 and oddNumberHeap:\n maxEvenNum = -heappop(oddNumberHeap)\n maxNum += str(maxEvenNum)\n \n #If parity not found, we keep the same number\n else:\n maxNum += str(digit)\n \n return int(maxNum)\n`` | 1 | 0 | ['Heap (Priority Queue)', 'Python'] | 0 |
largest-number-after-digit-swaps-by-parity | Largest Number After Digit Swaps by Parity | largest-number-after-digit-swaps-by-pari-iplf | \n\n# Code\n\nclass Solution {\n public int largestInteger(int num) {\n ArrayList<Integer> lstEven=new ArrayList<>();\n ArrayList<Integer> lstO | riya1202 | NORMAL | 2023-11-01T13:01:13.890160+00:00 | 2023-11-01T13:01:13.890181+00:00 | 666 | false | \n\n# Code\n```\nclass Solution {\n public int largestInteger(int num) {\n ArrayList<Integer> lstEven=new ArrayList<>();\n ArrayList<Integer> lstOdd=new ArrayList<>();\n ArrayList<Character> pos=new ArrayList<>();\n\n String s=""+num;\n\n for(int i=0;i<s.length();i++){\n if(Character.getNumericValue(s.charAt(i))%2==0){\n lstEven.add(Character.getNumericValue(s.charAt(i)));\n pos.add(\'e\');\n }\n else{\n lstOdd.add(Character.getNumericValue(s.charAt(i)));\n pos.add(\'o\');\n }\n }\n Collections.sort(lstEven,Collections.reverseOrder());\n Collections.sort(lstOdd,Collections.reverseOrder());\n\n int oi=0,ei=0;\n s="";\n for(int i=0;i<pos.size();i++){\n if(pos.get(i)==\'e\'){\n s+=lstEven.get(ei++);\n }\n else{\n s+=lstOdd.get(oi++);\n }\n }\n\n return Integer.valueOf(s);\n }\n}\n``` | 1 | 0 | ['Sorting', 'Heap (Priority Queue)', 'Java'] | 2 |
largest-number-after-digit-swaps-by-parity | Java int array (faster than 100%) | java-int-array-faster-than-100-by-coffee-q2ud | Code\n\nclass Solution {\n\n private int summarize(int[] digits, int length) {\n int result = 0;\n\n int multiplier = 1;\n for (int i = | coffeeminator | NORMAL | 2023-10-30T14:44:41.767545+00:00 | 2023-10-30T14:44:41.767566+00:00 | 1,217 | false | # Code\n```\nclass Solution {\n\n private int summarize(int[] digits, int length) {\n int result = 0;\n\n int multiplier = 1;\n for (int i = length - 1; i >= 0; i--) {\n result += digits[i] * multiplier;\n multiplier *= 10;\n }\n\n return result;\n }\n\n public int largestInteger(int num) {\n int result = num;\n\n // calc length\n int temp = num;\n int length = 0;\n while (temp > 0) {\n ++length;\n temp /= 10;\n }\n\n // fill the digits array \n final int[] digits = new int[length];\n int index = length;\n while (num > 0) {\n digits[--index] = num % 10;\n num /= 10;\n }\n\n for (index = 0; index < length - 1; index++) {\n // find the max digit with the same parity\n final int parity = digits[index] % 2;\n int max = digits[index];\n int swapIndex = 0;\n for (int i = index + 1; i <= length - 1; i++) {\n final int digit = digits[i];\n if ((digit % 2 == parity) && (digit > max)) {\n max = digit;\n swapIndex = i;\n }\n }\n\n // swap them\n if (max > digits[index]) {\n digits[index] += digits[swapIndex];\n digits[swapIndex] = digits[index] - digits[swapIndex];\n digits[index] -= digits[swapIndex];\n }\n\n final int candidate = summarize(digits, length);\n if (candidate > result) {\n result = candidate;\n }\n }\n\n return result;\n }\n}\n``` | 1 | 0 | ['Java'] | 1 |
largest-number-after-digit-swaps-by-parity | JS || Easy to understand | js-easy-to-understand-by-k4zhymukhan-ztun | Intuition\n Describe your first thoughts on how to solve this problem. \n\n# Approach\n Describe your approach to solving the problem. \n\n# Complexity\n- Time | K4zhymukhan | NORMAL | 2023-10-22T15:55:42.289739+00:00 | 2023-10-22T15:55:42.289764+00:00 | 200 | false | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n/**\n * @param {number} num\n * @return {number}\n */\nvar largestInteger = function(num) {\n\n let answer = \'\';\n const sorted = [...String(num)].sort((a, b) => a - b);\n\n const odd = sorted.filter((i) => i % 2 === 1);\n const even = sorted.filter((i) => i % 2 === 0);\n \n for (let i = 0; i < sorted.length; i++) {\n if (String(num)[i] % 2 === 0) {\n answer += even.pop();\n } else {\n answer += odd.pop();\n }\n }\n\n return answer;\n};\n``` | 1 | 0 | ['JavaScript'] | 0 |
largest-number-after-digit-swaps-by-parity | Very slow solution based on PQueue. Also version for odd and even digits positions. | very-slow-solution-based-on-pqueue-also-wnx05 | Code\n\npublic class Solution {\n public int LargestInteger(int n) {\n int num = n;\n var odd = new PriorityQueue<int, int>();\n var eve | thrillobit | NORMAL | 2023-07-24T19:06:31.120709+00:00 | 2023-07-24T19:06:31.120737+00:00 | 49 | false | # Code\n```\npublic class Solution {\n public int LargestInteger(int n) {\n int num = n;\n var odd = new PriorityQueue<int, int>();\n var even = new PriorityQueue<int, int>();\n\n while (num>0) {\n if ( (num%10)%2 == 0)\n odd.Enqueue(num%10, num%10);\n else\n even.Enqueue(num%10, num%10);\n \n num = num/10;\n }\n\n int ans = 0, multiple = 1;\n while (n>0) {\n if ( (n%10)%2 == 0)\n ans += multiple * odd.Dequeue();\n else\n ans += multiple * even.Dequeue();\n\n multiple = multiple*10;\n n = n/10;\n }\n \n return ans;\n }\n}\n\n\n/*\nSolution for odd and even digits POSITIONS\npublic int LargestInteger(int num) {\n var odd = new PriorityQueue<int, int>();\n var even = new PriorityQueue<int, int>();\n bool oddEven = true;\n\n while (num>0) {\n if (oddEven)\n odd.Enqueue(num%10, -num%10);\n else\n even.Enqueue(num%10, -num%10);\n \n oddEven = !oddEven;\n num = num/10;\n }\n\n int ans = 0;\n if (odd.Count>even.Count)\n ans = odd.Dequeue();\n while (odd.Count>0) {\n ans = ans*10;\n ans += even.Dequeue();\n ans = ans*10;\n ans += odd.Dequeue();\n }\n \n return ans;\n }\n*/\n``` | 1 | 0 | ['Heap (Priority Queue)', 'C#'] | 0 |
largest-number-after-digit-swaps-by-parity | JS | Priority Queue | Heap | js-priority-queue-heap-by-darcyrush-57ub | Code\n\n/**\n * @param {number} num\n * @return {number}\n */\nvar largestInteger = function(num) {\n let numArr = num.toString().split(\'\').map(d => Number(d | darcyrush | NORMAL | 2023-04-20T10:58:02.379936+00:00 | 2023-04-20T10:58:02.379974+00:00 | 262 | false | # Code\n```\n/**\n * @param {number} num\n * @return {number}\n */\nvar largestInteger = function(num) {\n let numArr = num.toString().split(\'\').map(d => Number(d))\n\n let oddQ = new MaxPriorityQueue({\n compare: (a, b) => (a < b)\n })\n \n let evenQ = new MaxPriorityQueue({\n compare: (a, b) => (a < b)\n })\n\n numArr.forEach(value => {\n if (value % 2 === 0) {\n evenQ.enqueue(value)\n } else {\n oddQ.enqueue(value)\n }\n })\n \n let resArr = []\n\n for (let n of numArr) {\n if (n % 2 === 0) {\n resArr.push(evenQ.dequeue())\n } else {\n resArr.push(oddQ.dequeue())\n }\n }\n\n return resArr.reduce((prev, curr) => \'\' + prev + curr)\n};\n\n``` | 1 | 0 | ['Heap (Priority Queue)', 'JavaScript'] | 0 |
largest-number-after-digit-swaps-by-parity | Python: Optimal and Clean with explanation: O(nlogn) time and O(n) space | python-optimal-and-clean-with-explanatio-yhx2 | Approach\n Describe your approach to solving the problem. \n1. get the set of indicies of odd parity elem. \n2. sort the values there descending\n3. same with r | topswe | NORMAL | 2022-12-20T00:34:30.267584+00:00 | 2023-02-02T04:07:07.389813+00:00 | 183 | false | # Approach\n<!-- Describe your approach to solving the problem. -->\n1. get the set of indicies of odd parity elem. \n2. sort the values there descending\n3. same with remaining set of indicies of even parity elem\n4. now rewire each index according to the sorted order of its odd/even list.\n# Complexity\n$$n = \\log(num)$$, the number of digits of $$num$$.\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(nlogn)$$\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$\n# Code\n```\nclass Solution:\n # 1. get the set of indicies of odd parity elem. \n # 2. sort the values there descending\n # 3. same with remaining set of indicies of even parity elem\n # 4. now rewire each index according to the sorted order of its odd/even list.\n\n def largestInteger(self, num: int) -> int:\n s = str(num)\n n = len(s)\n odd_indicies = dict((i,s[i]) for i in range(n) if int(s[i]) % 2 == 1)\n even_indicies = dict((i,s[i]) for i in range(n) if int(s[i]) % 2 == 0)\n res = [\'\'] * n\n sorted_odds = sorted(odd_indicies.values(), reverse=True)\n sorted_evens = sorted(even_indicies.values(),reverse=True)\n idx_odd, idx_even = 0,0\n\n for i in range(n):\n if i in odd_indicies:\n res[i] = sorted_odds[idx_odd]\n idx_odd+=1\n else:\n res[i] = sorted_evens[idx_even]\n idx_even+=1\n\n return int("".join(res))\n \n``` | 1 | 0 | ['Python3'] | 1 |
largest-number-after-digit-swaps-by-parity | Typescript | Javascript, 100% faster | typescript-javascript-100-faster-by-toha-nz30 | \nfunction largestInteger(num: number): number {\n const oddSet = new Set(\'13579\'.split(\'\'));\n const digits = num.toString().split(\'\');\n const | tohasan | NORMAL | 2022-09-28T19:35:26.478027+00:00 | 2022-09-28T19:35:26.478068+00:00 | 86 | false | ```\nfunction largestInteger(num: number): number {\n const oddSet = new Set(\'13579\'.split(\'\'));\n const digits = num.toString().split(\'\');\n const oddDigits = sort(digits.filter(d => oddSet.has(d)));\n const evenDigits = sort(digits.filter(d => !oddSet.has(d)));\n \n let numStr = \'\';\n for (let i = 0; i < digits.length; i++) {\n numStr += oddSet.has(digits[i]) ? oddDigits.pop() : evenDigits.pop();\n }\n return Number(numStr);\n}\n\nfunction sort(digits: string[]): string[] {\n return digits.sort((d1, d2) => d1.localeCompare(d2));\n}\n``` | 1 | 0 | ['TypeScript', 'JavaScript'] | 0 |
largest-number-after-digit-swaps-by-parity | ✅ [Rust] 0 ms, two solutions (with detailed comments) | rust-0-ms-two-solutions-with-detailed-co-08if | This solution employs quadtratic-time swaps that are cheap due to the small size of array of digits. It demonstrated 0 ms runtime (100%) and used 2.0 MB memory | stanislav-iablokov | NORMAL | 2022-09-10T15:02:46.600990+00:00 | 2022-10-23T12:58:26.700425+00:00 | 77 | false | This [solution](https://leetcode.com/submissions/detail/796326742/) employs quadtratic-time swaps that are cheap due to the small size of array of digits. It demonstrated **0 ms runtime (100%)** and used **2.0 MB memory (71.43%)**. Detailed comments are provided.\n\n**IF YOU LIKE THIS SOLUTION, PLEASE UPVOTE.**\n```\nimpl Solution \n{\n pub fn largest_integer(mut num: i32) -> i32 \n {\n // [1] first, break number into digits\n let mut digits: Vec<i32>= Vec::with_capacity(10);\n while num > 0\n {\n digits.push(num % 10);\n num /= 10;\n }\n \n // [2] provided that we do not expect more than 10 digits (num <= 10^9),\n // performing swaps using nested loops is cheap\n for i in 0..digits.len()\n {\n for j in i+1..digits.len()\n {\n // [3] swaps are performed separately for each parity\n if digits[i] % 2 == digits[j] % 2 && digits[i] > digits[j]\n {\n digits.swap(i, j);\n }\n }\n }\n \n // [4] finally, reconstruct the number\n digits.iter().rev().fold(0, |acc, d| d+acc*10)\n }\n}\n```\n\nFor perfectionists, I provide a more optimized [solution](https://leetcode.com/submissions/detail/796347785/) with binary heaps.\n```\nuse std::collections::BinaryHeap;\n\nimpl Solution \n{\n pub fn largest_integer(num: i32) -> i32 \n {\n let mut num = num as usize;\n // [1] store two groups of digits, even and odd, in binary heaps\n let mut digits = [BinaryHeap::new(), BinaryHeap::new()];\n \n // [2] store information on the positions of digits,\n // whether the corresponding digit belongs to evens or odds\n let mut positions = Vec::with_capacity(10);\n\n // [3] partition digits into two groups while keeping track\n // of each position\'s group \n while num > 0 \n {\n digits[num % 2].push(num % 10);\n positions.push(num % 2);\n num /= 10;\n }\n\n // [4] reconstruct the number\n positions.iter().rev()\n .fold(0, |acc, &d| 10 * acc + digits[d].pop().unwrap()) as i32\n }\n}\n``` | 1 | 0 | ['Rust'] | 0 |
largest-number-after-digit-swaps-by-parity | JAVA easy solution using List | java-easy-solution-using-list-by-21arka2-vlhq | \nclass Solution {\n public int largestInteger(int n) {\n List<Integer>e=new ArrayList<>();\n List<Integer>o=new ArrayList<>();\n List<I | 21Arka2002 | NORMAL | 2022-09-09T15:28:43.135972+00:00 | 2022-09-09T15:28:43.136008+00:00 | 603 | false | ```\nclass Solution {\n public int largestInteger(int n) {\n List<Integer>e=new ArrayList<>();\n List<Integer>o=new ArrayList<>();\n List<Integer>num=new ArrayList<>();\n while(n>0)\n {\n num.add(n%10);\n if((n%10)%2==0)e.add(n%10);\n else o.add(n%10);\n n/=10;\n }\n Collections.reverse(num);\n Collections.sort(e);\n Collections.reverse(e);\n Collections.sort(o);\n Collections.reverse(o);\n int eve=0,odd=0,s=0;\n for(int i=0;i<num.size();i++)\n {\n if(num.get(i)%2==0)\n {\n s=(s*10)+e.get(eve);\n eve+=1;\n }\n else \n {\n s=(s*10)+o.get(odd);\n odd+=1;\n }\n }\n return s;\n }\n}\n``` | 1 | 0 | ['Array', 'Java'] | 0 |
largest-number-after-digit-swaps-by-parity | Python MaxHeap Solution | python-maxheap-solution-by-sharmanihal96-8bp9 | \nclass Solution:\n def largestInteger(self, num: int) -> int:\n evenHeap=[]\n oddHeap=[]\n digits = [int(x) for x in str(num)]\n | sharmanihal96 | NORMAL | 2022-08-21T07:46:21.361380+00:00 | 2022-08-21T07:46:21.361412+00:00 | 25 | false | ```\nclass Solution:\n def largestInteger(self, num: int) -> int:\n evenHeap=[]\n oddHeap=[]\n digits = [int(x) for x in str(num)]\n for i in digits:\n if i %2==0:\n heappush(evenHeap,i*-1)\n else:\n heappush(oddHeap,i*-1)\n max=\'\'\n for i in digits:\n if i%2==0: \n temp=heappop(evenHeap)*-1\n max+=str(temp)\n else:\n temp=(heappop(oddHeap)*-1)\n max+=str(temp)\n return int(max)\n``` | 1 | 0 | [] | 0 |
largest-number-after-digit-swaps-by-parity | C++ easy solution for beginner using priority queue | c-easy-solution-for-beginner-using-prior-zz3z | Pls upvote if it\'s helpful\n\nint largestInteger(int num) \n {\n priority_queue<int>e;\n priority_queue<int>o ;\n int temp=0 ;\n | ayushpanchal0523 | NORMAL | 2022-07-29T19:22:02.882840+00:00 | 2022-07-29T19:22:02.882880+00:00 | 102 | false | **Pls upvote if it\'s helpful**\n```\nint largestInteger(int num) \n {\n priority_queue<int>e;\n priority_queue<int>o ;\n int temp=0 ;\n while(num>0)\n {\n int x=num%10 ;\n if(x%2==0)\n e.push(x);\n else \n o.push(x) ;\n temp=temp*10 ;\n temp+=x ;\n num=num/10 ;\n }\n \n while(!o.empty() && !e.empty())\n {\n num=num*10 ;\n int x=temp%10 ;\n if(x%2!=0)\n {\n num+=o.top() ;\n o.pop() ;\n }\n else \n {\n num+=e.top() ;\n e.pop() ;\n }\n temp=temp/10 ;\n }\n \n while(!e.empty())\n {\n num=num*10 ;\n num+=e.top() ;\n e.pop() ;\n }\n while(!o.empty())\n {\n num=num*10 ;\n num+=o.top() ;\n o.pop() ;\n }\n return num ;\n }\n``` | 1 | 0 | ['C', 'Heap (Priority Queue)'] | 0 |
largest-number-after-digit-swaps-by-parity | 3ms Java Solution (Priority Queue) | 3ms-java-solution-priority-queue-by-gite-n6hd | \tclass Solution {\n\t\tpublic int largestInteger(int num) {\n\t\t\tQueue q1 = new PriorityQueue<>(Collections.reverseOrder());\n\t\t\tQueue q2 = new PriorityQu | Gitesh054 | NORMAL | 2022-07-24T08:43:59.957118+00:00 | 2022-07-24T08:43:59.957161+00:00 | 115 | false | \tclass Solution {\n\t\tpublic int largestInteger(int num) {\n\t\t\tQueue<Integer> q1 = new PriorityQueue<>(Collections.reverseOrder());\n\t\t\tQueue<Integer> q2 = new PriorityQueue<>(Collections.reverseOrder());\n\t\t\tString x = num+"";\n\t\t\tint[] nums = new int[x.length()];\n\t\t\tfor(int i=0 ;i<nums.length; i++) {\n\t\t\t\tnums[i] = Integer.parseInt(x.charAt(i)+"");\n\t\t\t\tif((nums[i])%2==0) {\n\t\t\t\t\tq1.add(nums[i]);\n\t\t\t\t}else {\n\t\t\t\t\tq2.add(nums[i]);\n\t\t\t\t}\n\t\t\t}\n\t\t\tStringBuilder ans = new StringBuilder();\n\n\t\t\tfor(int i=0 ;i<nums.length; i++) {\n\t\t\t\tif(nums[i]%2==0) {\n\t\t\t\t\tans.append(q1.remove()+"");\n\t\t\t\t}else {\n\t\t\t\t\tans.append(q2.remove()+"");\n\t\t\t\t}\n\t\t\t}\n\t\t\treturn Integer.parseInt(ans.toString());\n\t\t}\n\t} | 1 | 0 | ['Heap (Priority Queue)'] | 1 |
largest-number-after-digit-swaps-by-parity | 100% FASTER AND EASY TO UNDERSTAND ( USNIG PRIORITY QUEUE) | 100-faster-and-easy-to-understand-usnig-1wlge | \tclass Solution {\n\tpublic:\n\t\tint largestInteger(int num) {\n\t\t\tvectorv;\n\t\t\tpriority_queuepqe;// EVEN PRIORITY QUEUE\n\t\t\tpriority_queuepqo;// ODD | mahan07122001 | NORMAL | 2022-07-24T08:41:35.226849+00:00 | 2022-07-24T08:51:02.455809+00:00 | 122 | false | \tclass Solution {\n\tpublic:\n\t\tint largestInteger(int num) {\n\t\t\tvector<int>v;\n\t\t\tpriority_queue<int>pqe;// EVEN PRIORITY QUEUE\n\t\t\tpriority_queue<int>pqo;// ODD PRIORITY QUEUE\n\t\t\tlong long sum=0;\n\t\t\twhile(num){\n\t\t\t\tint k=num%10;\n\t\t\t\tv.push_back(k); // PUSHING IN VECTOR\n\t\t\t\tif(k%2==0)pqe.push(k); // PUSHING IN EVEN PRIORITY QUEUE\n\t\t\t\telse pqo.push(k); // PUSHING IN ODD PRIORITY QUEUE\n\t\t\t\tnum/=10;\n\t\t\t}\n\t\t\t// NOW WE WILL REVERSE OUR VECTOR \n\t\t\tfor(int i=0;i<v.size()/2;i++){\n\t\t\t\tswap(v[i],v[v.size()-i-1]);\n\t\t\t}\n\t\t\tfor(int i=0;i<v.size();i++){\n\t\t\t// If v[i] is even we will swap v[i] with top of even priority queue\n\t\t\t\tif(v[i]%2==0){\n\t\t\t\t\tint m=pqe.top();\n\t\t\t\t\tswap(v[i],m);\n\t\t\t\t\tpqe.pop();\n\t\t\t\t}\n\t\t // If v[i] is odd we will swap v[i] with top of odd priority queue\n\t\t\t\telse{\n\t\t\t\t\tint m=pqo.top();\n\t\t\t\t\tswap(v[i],m);\n\t\t\t\t\tpqo.pop();\n\t\t\t\t}\n\n\t\t\t}\n\t\t\tfor(int i=0;i<v.size();i++){\n\t\t\t\tsum=sum*10+v[i];\n\t\t\t}\n\t\t\treturn sum;\n\t\t}\n\t}; | 1 | 0 | ['C', 'Heap (Priority Queue)'] | 0 |
largest-number-after-digit-swaps-by-parity | Java - PriorityQueue - 1 ms | java-priorityqueue-1-ms-by-abanoubcs-a74s | \nclass Solution {\n public int largestInteger(int num) {\n PriorityQueue < Integer > odd = new PriorityQueue < Integer > ();\n PriorityQueue < | abanoubcs | NORMAL | 2022-07-20T19:06:43.632839+00:00 | 2022-07-20T19:07:45.902568+00:00 | 66 | false | ```\nclass Solution {\n public int largestInteger(int num) {\n PriorityQueue < Integer > odd = new PriorityQueue < Integer > ();\n PriorityQueue < Integer > even = new PriorityQueue < Integer > ();\n int n = num;\n while (n > 0) {\n if (n % 2 == 0) {\n even.offer(n % 10);\n } else {\n odd.offer(n % 10);\n }\n n /= 10;\n }\n int newNum = 0;\n int factor = 1;\n while (num > 0) {\n newNum += factor * (num % 2 == 0 ? even.poll() : odd.poll());\n num /= 10;\n factor *= 10;\n }\n\n\n return newNum;\n }\n}\n```\n\n\nOR\n\n\n```\nclass Solution {\n public int largestInteger(int num) {\n char[] arr = Integer.toString(num).toCharArray();\n\n PriorityQueue < Character > odd = new PriorityQueue();\n PriorityQueue < Character > even = new PriorityQueue();\n\n for (char c: arr) {\n if (c % 2 == 0) {\n even.offer(c);\n } else {\n odd.offer(c);\n }\n }\n\n for (int i = arr.length - 1; i >= 0; i--) {\n arr[i] = arr[i] % 2 == 0 ? even.poll() : odd.poll();\n }\n\n return Integer.parseInt(new String(arr));\n }\n}\n``` | 1 | 0 | [] | 0 |
largest-number-after-digit-swaps-by-parity | C / 0ms / 100% t / 93% s | c-0ms-100-t-93-s-by-tonicaletti-usmm | \nint cmp(void* u, void* v)\n{\n return *(int*)u - *(int*)v;\n}\n\nint largestInteger(int num){\n\n int* u = malloc(10*sizeof(int));\n int* g = malloc( | tonicaletti | NORMAL | 2022-07-17T09:49:54.953879+00:00 | 2022-07-17T09:49:54.953921+00:00 | 86 | false | ```\nint cmp(void* u, void* v)\n{\n return *(int*)u - *(int*)v;\n}\n\nint largestInteger(int num){\n\n int* u = malloc(10*sizeof(int));\n int* g = malloc(10*sizeof(int));\n int* f = malloc(10*sizeof(int));\n \n int i_g = 0, i_u = 0, i_f = 0;\n while(num > 0)\n {\n int r = num % 10;\n f[i_f++] = r % 2;\n if(r % 2 == 0)\n g[i_g++] = r;\n else\n u[i_u++] = r;\n num = num -r;\n num = num/10;\n }\n qsort(u, i_u, sizeof(int), cmp);\n qsort(g, i_g, sizeof(int), cmp);\n \n int out = 0; int k_g = 0; int k_u = 0; long p = 1;\n for(int i = 0; i < i_f; i++)\n {\n if(f[i] == 0)\n out = out + g[k_g++]*p; \n else\n out = out + u[k_u++]*p;\n p *= 10;\n }\n return (int)out;\n}\n``` | 1 | 0 | [] | 0 |
largest-number-after-digit-swaps-by-parity | python easy selection sort | python-easy-selection-sort-by-sonusahu05-k0f2 | \tclass Solution:\n\t\tdef largestInteger(self, num: int) -> int:\n\t\t\tarr=[int(i) for i in str(num)]\n\t\t\tprint(arr)\n\t\t\t#selection sort\n\t\t\tfor i in | sonusahu050502 | NORMAL | 2022-07-14T09:28:04.565521+00:00 | 2022-07-14T09:28:04.565556+00:00 | 310 | false | \tclass Solution:\n\t\tdef largestInteger(self, num: int) -> int:\n\t\t\tarr=[int(i) for i in str(num)]\n\t\t\tprint(arr)\n\t\t\t#selection sort\n\t\t\tfor i in range(0,len(arr)):\n\t\t\t#selection sort for odd numbers\n\t\t\t\tif arr[i]%2!=0:\n\t\t\t\t\tmaxi=i\n\t\t\t\t\tfor j in range(i+1,len(arr)):\n\t\t\t\t\t\tif arr[j]%2!=0 and arr[j]>arr[maxi]:\n\t\t\t\t\t\t\tmaxi=j\n\t\t\t\t\tarr[i],arr[maxi]=arr[maxi],arr[i]\n\t\t\t\n\t\t\t#selection sort for even numbers\n\t\t\t\tif arr[i]%2==0:\n\t\t\t\t\tmaxi=i\n\t\t\t\t\tfor j in range(i+1,len(arr)):\n\t\t\t\t\t\tif arr[j]%2==0 and arr[j]>arr[maxi]:\n\t\t\t\t\t\t\tmaxi=j\n\t\t\t\t\tarr[i],arr[maxi]=arr[maxi],arr[i]\n\t\t\t\t\t\n\t\t\treturn int("".join([str(i) for i in arr])) | 1 | 0 | ['Python'] | 0 |
largest-number-after-digit-swaps-by-parity | Faster Than 100% || Easy || Priority Queue | faster-than-100-easy-priority-queue-by-a-pa29 | \nclass Solution {\npublic:\n int largestInteger(int num) {\n string snum = to_string(num), result;\n priority_queue<int> even, odd;\n i | Aakarsh_Inamdar | NORMAL | 2022-07-01T08:29:06.957054+00:00 | 2022-07-01T08:29:06.957091+00:00 | 140 | false | ```\nclass Solution {\npublic:\n int largestInteger(int num) {\n string snum = to_string(num), result;\n priority_queue<int> even, odd;\n int rtn_val;\n \n for(int i=0; i < snum.length(); i++){\n if(((int)snum[i]) % 2 == 0){\n even.push((int)snum[i]-48);\n }else{\n odd.push((int)snum[i]-48);\n }\n }\n for(int i=0; i < snum.length(); i++){\n if(((int)snum[i]) % 2 == 0){\n string s = to_string(even.top());\n even.pop();\n result += s;\n }else{\n string s = to_string(odd.top());\n odd.pop();\n result += s;\n }\n }\n rtn_val = stoi(result);\n return rtn_val;\n }\n};\n``` | 1 | 0 | ['String', 'C', 'Heap (Priority Queue)'] | 0 |
largest-number-after-digit-swaps-by-parity | C++||0ms || Easy to Understand | c0ms-easy-to-understand-by-adnor-cbkz | ```\nclass Solution {\npublic:\n int largestInteger(int num) {\n int n=num;\n vectorv;\n priority_queueheap1, heap2;\n while(n){\ | Adnor | NORMAL | 2022-06-25T18:15:41.619275+00:00 | 2022-06-25T18:15:41.619321+00:00 | 140 | false | ```\nclass Solution {\npublic:\n int largestInteger(int num) {\n int n=num;\n vector<int>v;\n priority_queue<int>heap1, heap2;\n while(n){\n int remain = n%10;\n v.push_back(remain);\n if(remain%2==0){\n heap1.push(remain);\n }\n else if(remain%2!=0){\n heap2.push(remain);\n }\n n = (n-remain)/10;\n }\n reverse(v.begin(), v.end());\n for(int i=0; i<v.size(); i++){\n if(v[i]%2==0){\n v[i]=heap1.top();\n heap1.pop();\n }\n else{\n v[i]=heap2.top();\n heap2.pop();\n }\n }\n reverse(v.begin(), v.end());\n long temp=1, ans=0;\n for(int i=0; i<v.size(); i++){\n ans+=temp*v[i];\n temp=temp*10;\n }\n return ans;\n }\n}; | 1 | 0 | ['C'] | 0 |
largest-number-after-digit-swaps-by-parity | C++ || priority queue | c-priority-queue-by-jatin837-ufbk | use two priority queue(one for odd and other for even) and replace each digit according to it\'s parity from queue.\n\nc++\n\nclass Solution {\npublic:\n int | jatin837 | NORMAL | 2022-06-21T11:33:50.733125+00:00 | 2022-06-24T11:36:36.634759+00:00 | 158 | false | use two priority queue(one for odd and other for even) and replace each digit according to it\'s parity from queue.\n\n```c++\n\nclass Solution {\npublic:\n int largestInteger(int num) {\n string n = to_string(num);\n priority_queue<char>opq;\n priority_queue<char>epq;\n for(char ch:n){\n if((ch - \'0\')%2)\n epq.push(ch);\n else\n opq.push(ch);\n }\n for(char &ch:n){\n if((ch - \'0\')%2){\n ch = epq.top();\n epq.pop();\n }\n else{\n ch = opq.top();\n opq.pop();\n }\n }\n return stoi(n);\n }\n};\n``` | 1 | 0 | ['C', 'Heap (Priority Queue)'] | 1 |
construct-k-palindrome-strings | [Java/C++/Python] Straight Forward | javacpython-straight-forward-by-lee215-9hfu | Intuition\nCondition 1. odd characters <= k\nCount the occurrences of all characters.\nIf one character has odd times occurrences,\nthere must be at least one p | lee215 | NORMAL | 2020-04-04T16:09:29.099269+00:00 | 2020-07-15T15:11:45.919619+00:00 | 20,426 | false | # **Intuition**\nCondition 1. `odd characters <= k`\nCount the occurrences of all characters.\nIf one character has odd times occurrences,\nthere must be at least one palindrome,\nwith odd length and this character in the middle.\nSo we count the characters that appear odd times,\nthe number of odd character should not be bigger than `k`.\n\nCondition 2. `k <= s.length()`\nAlso, if we have one character in each palindrome,\nwe will have at most `s.length()` palindromes,\nso we need `k <= s.length()`.\n\nThe above two condition are necessary and sufficient conditions for this problem.\nSo we return `odd <= k <= n`\n<br>\n\n# **Construction**\n@spjparmar immediately got a question like why this works always for all strings.\nHe gave the some following dry runs. :)\nFor any string with 0 odd character count , we can form k no. of palindrome strings for sure with k<=n\n(This is why k<=n)\n\n**eg**\naabb, k=1| abba\naabb, k=2 | aa, bb\naabb, k=3 | a, a, bb\naabb, k=4 | a, a, b, b\n\nFor any string with odd character count <=k , we can always form k palindrome string for sure with k<=n\n**eg2**\naabbc, k=1 | aacbb\naabbc, k=2 | aca, bb\naabbc, k=3 | a,a, bcb\naabbc, k=4 | a, a, c ,bb\naabbc, k=5 | a, a, c, b, b\n\n**eg3**\naabc, k=1 | N/A\naabc, k=2 | aba, c\naabc, k=3 | aa, b, c\naabc, k=4 | a, a, b, c\n\nHope this helps somebody.\n<br>\n\n# **Complexity**\nTime `O(N)`\nSpace `O(1)`\n<br>\n\n**Java:**\n```java\n public boolean canConstruct(String s, int k) {\n int odd = 0, n = s.length(), count[] = new int[26];\n for (int i = 0; i < n; ++i) {\n count[s.charAt(i) - \'a\'] ^= 1;\n odd += count[s.charAt(i) - \'a\'] > 0 ? 1 : -1;\n }\n return odd <= k && k <= n;\n }\n```\n\n**C++:**\n```cpp\n bool canConstruct(string s, int k) {\n bitset<26> odd;\n for (char& c : s)\n odd.flip(c - \'a\');\n return odd.count() <= k && k <= s.length();\n }\n```\n\n**Python:**\n```py\n def canConstruct(self, s, k):\n return sum(i & 1 for i in collections.Counter(s).values()) <= k <= len(s)\n``` | 253 | 5 | [] | 35 |
construct-k-palindrome-strings | ✅🔥BEATS 99%🔥 || 3 UNIQUE APPROACHES TO Construct K Palindrome Strings 💡|| CONCISE CODE ✅ | beats-99-3-unique-approaches-to-construc-yi9j | 💡IntuitionThe problem revolves around the frequency of characters in a string. To construct palindromes:
Characters with odd frequencies must be the centers of | RSPRIMES1234 | NORMAL | 2025-01-11T02:18:38.385546+00:00 | 2025-01-11T14:15:26.320764+00:00 | 18,663 | false | 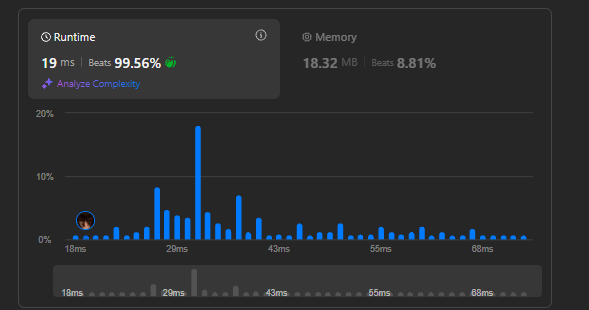
# 💡Intuition
The problem revolves around the frequency of characters in a string. To construct palindromes:
1. Characters with **odd frequencies** must be the centers of palindromes.
2. The number of odd frequencies determines the **minimum number of palindromes** required.
3. If the total odd frequencies ≤ `k`, the task is feasible.
---
# 🔍Approach 1: Sorting and Grouping
## Explanation
- **Sort the String**: Group identical characters together.
- **Count Odd Frequencies**: Traverse the sorted characters, counting the ones with odd frequencies.
- **Check Feasibility**: Ensure the number of odd frequencies is ≤ `k`.
---
# 💻Complexity
- **Time Complexity**:
$$O(n \log n)$$ — Sorting the string.
- **Space Complexity**:
$$O(n)$$ — For storing the sorted characters.
---
# Code
```python []
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
if len(s) < k:
return False
sorted_s = sorted(s)
odd_count = 0
i = 0
while i < len(sorted_s):
char = sorted_s[i]
count = 0
while i < len(sorted_s) and sorted_s[i] == char:
count += 1
i += 1
if count % 2 == 1:
odd_count += 1
return odd_count <= k
```
``` cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
if (s.length() < k) return false;
sort(s.begin(), s.end());
int oddCount = 0;
for (int i = 0; i < s.length(); ) {
char current = s[i];
int count = 0;
while (i < s.length() && s[i] == current) {
count++;
i++;
}
if (count % 2 != 0) oddCount++;
}
return oddCount <= k;
}
};
```
``` java []
import java.util.Arrays;
class Solution {
public boolean canConstruct(String s, int k) {
if (s.length() < k) return false;
char[] chars = s.toCharArray();
Arrays.sort(chars);
int oddCount = 0;
for (int i = 0; i < chars.length; ) {
char current = chars[i];
int count = 0;
while (i < chars.length && chars[i] == current) {
count++;
i++;
}
if (count % 2 != 0) oddCount++;
}
return oddCount <= k;
}
}
```
``` javascript []
var canConstruct = function(s, k) {
if (s.length < k) return false;
let chars = [...s].sort();
let oddCount = 0;
for (let i = 0; i < chars.length; ) {
let current = chars[i];
let count = 0;
while (i < chars.length && chars[i] === current) {
count++;
i++;
}
if (count % 2 !== 0) oddCount++;
}
return oddCount <= k;
};
```
``` ruby []
def can_construct(s, k)
return false if s.length < k
chars = s.chars.sort
odd_count = 0
i = 0
while i < chars.length
current = chars[i]
count = 0
while i < chars.length && chars[i] == current
count += 1
i += 1
end
odd_count += 1 if count.odd?
end
odd_count <= k
end
```
``` go []
func canConstruct(s string, k int) bool {
if len(s) < k {
return false
}
chars := []rune(s)
sort.Slice(chars, func(i, j int) bool { return chars[i] < chars[j] })
oddCount := 0
for i := 0; i < len(chars); {
current := chars[i]
count := 0
for i < len(chars) && chars[i] == current {
count++
i++
}
if count%2 != 0 {
oddCount++
}
}
return oddCount <= k
}
```
``` csharp []
using System;
public class Solution {
public bool CanConstruct(string s, int k) {
if (s.Length < k) return false;
char[] chars = s.ToCharArray();
Array.Sort(chars);
int oddCount = 0;
for (int i = 0; i < chars.Length; ) {
char current = chars[i];
int count = 0;
while (i < chars.Length && chars[i] == current) {
count++;
i++;
}
if (count % 2 != 0) oddCount++;
}
return oddCount <= k;
}
}
```
# 🔍Approach 2: Bitmasking
## Explanation
- Each character corresponds to a specific bit in a bitmask (e.g., `'a'` is the 0th bit, `'b'` is the 1st bit, etc.).
- Toggle the bits for each character in the string:
- If a bit is `1`, it indicates an odd frequency.
- If a bit is `0`, it indicates an even frequency.
- Count the number of `1`s in the bitmask to determine how many characters have odd frequencies.
- If the number of odd frequencies is ≤ `k`, constructing `k` palindromes is possible.
---
## 💻Complexity
- **Time Complexity**:
$$O(n)$$ — Iterate through the string and count set bits.
- **Space Complexity**:
$$O(1)$$ — Constant space used for the bitmask.
---
# Code
```python []
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
if len(s) < k:
return False
bitmask = 0
for char in s:
bitmask ^= (1 << (ord(char) - ord('a')))
odd_count = bin(bitmask).count('1')
return odd_count <= k
```
``` cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
if (s.length() < k) return false;
int bitmask = 0;
for (char c : s) {
bitmask ^= (1 << (c - 'a'));
}
int oddCount = __builtin_popcount(bitmask);
return oddCount <= k;
}
};
```
``` java []
class Solution {
public boolean canConstruct(String s, int k) {
if (s.length() < k) return false;
int bitmask = 0;
for (char c : s.toCharArray()) {
bitmask ^= (1 << (c - 'a'));
}
int oddCount = Integer.bitCount(bitmask);
return oddCount <= k;
}
}
```
``` javascript []
var canConstruct = function(s, k) {
if (s.length < k) return false;
let bitmask = 0;
for (let char of s) {
bitmask ^= (1 << (char.charCodeAt(0) - 'a'.charCodeAt(0)));
}
let oddCount = bitmask.toString(2).split('1').length - 1;
return oddCount <= k;
};
```
``` ruby []
def can_construct(s, k)
return false if s.length < k
bitmask = 0
s.each_char do |char|
bitmask ^= (1 << (char.ord - 'a'.ord))
end
odd_count = bitmask.to_s(2).count('1')
odd_count <= k
end
```
``` go []
func canConstruct(s string, k int) bool {
if len(s) < k {
return false
}
bitmask := 0
for _, char := range s {
bitmask ^= (1 << (char - 'a'))
}
oddCount := 0
for bitmask > 0 {
oddCount += bitmask & 1
bitmask >>= 1
}
return oddCount <= k
}
```
``` csharp []
using System;
public class Solution {
public bool CanConstruct(string s, int k) {
if (s.Length < k) return false;
int bitmask = 0;
foreach (char c in s) {
bitmask ^= (1 << (c - 'a'));
}
int oddCount = CountSetBits(bitmask);
return oddCount <= k;
}
private int CountSetBits(int n) {
int count = 0;
while (n > 0) {
count += n & 1;
n >>= 1;
}
return count;
}
}
```
# 🔍Approach 3: Using a Counter
## Explanation
- Count the frequency of each character in the string using a `Counter`.
- Determine how many characters have an odd frequency.
- If the number of odd frequencies is greater than `k`, constructing `k` palindromes is not possible.
- Otherwise, it is possible.
---
## 💻Complexity
- **Time Complexity**:
$$O(n)$$ — Counting characters and calculating the number of odd frequencies.
- **Space Complexity**:
$$O(1)$$ — Fixed space for the character count (max 26 letters for English alphabet).
---
# Code
```python []
from collections import Counter
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
if len(s) < k:
return False
char_count = Counter(s)
odd_count = sum(freq % 2 for freq in char_count.values())
return odd_count <= k
```
``` cpp []
#include <unordered_map>
using namespace std;
class Solution {
public:
bool canConstruct(string s, int k) {
if (s.length() < k) return false;
unordered_map<char, int> charCount;
for (char c : s) {
charCount[c]++;
}
int oddCount = 0;
for (auto& entry : charCount) {
if (entry.second % 2 != 0) oddCount++;
}
return oddCount <= k;
}
};
```
``` java []
import java.util.HashMap;
class Solution {
public boolean canConstruct(String s, int k) {
if (s.length() < k) return false;
HashMap<Character, Integer> charCount = new HashMap<>();
for (char c : s.toCharArray()) {
charCount.put(c, charCount.getOrDefault(c, 0) + 1);
}
int oddCount = 0;
for (int freq : charCount.values()) {
if (freq % 2 != 0) oddCount++;
}
return oddCount <= k;
}
}
```
``` javascript []
var canConstruct = function(s, k) {
if (s.length < k) return false;
let charCount = {};
for (let char of s) {
charCount[char] = (charCount[char] || 0) + 1;
}
let oddCount = 0;
for (let count of Object.values(charCount)) {
if (count % 2 !== 0) oddCount++;
}
return oddCount <= k;
};
```
``` ruby []
def can_construct(s, k)
return false if s.length < k
char_count = Hash.new(0)
s.each_char { |char| char_count[char] += 1 }
odd_count = char_count.values.count { |count| count.odd? }
odd_count <= k
end
```
``` go []
func canConstruct(s string, k int) bool {
if len(s) < k {
return false
}
charCount := make(map[rune]int)
for _, char := range s {
charCount[char]++
}
oddCount := 0
for _, count := range charCount {
if count%2 != 0 {
oddCount++
}
}
return oddCount <= k
}
```
``` csharp []
using System;
using System.Collections.Generic;
public class Solution {
public bool CanConstruct(string s, int k) {
if (s.Length < k) return false;
Dictionary<char, int> charCount = new Dictionary<char, int>();
foreach (char c in s) {
if (!charCount.ContainsKey(c)) {
charCount[c] = 0;
}
charCount[c]++;
}
int oddCount = 0;
foreach (int count in charCount.Values) {
if (count % 2 != 0) oddCount++;
}
return oddCount <= k;
}
}
```
# ✅Conclusion
---
We explored three distinct approaches to solving the "Construct K Palindrome Strings" problem. Each approach has its unique strengths and trade-offs:
1. **Approach 1: Sorting the String**
- Simple and intuitive, but has a time complexity of \(O(n \log n)\), making it less efficient for larger inputs.
2. **Approach 2: Bitmasking**
- Efficient in terms of space (\(O(1)\)) and time (\(O(n)\)) as we utilize bit manipulation to track character frequencies. Best for problems where we're working with small alphabets (like lowercase English letters).
3. **Approach 3: Using a Counter**
- Very clear and easy to implement using a counter to track character frequencies. Time complexity is linear \(O(n)\) and space complexity is constant \(O(1)\) for the alphabet size.
In practice, **Approach 3 (Using a Counter)** is often the most straightforward and efficient for typical use cases, especially when combined with the simplicity of built-in data structures. However, **Approach 2 (Bitmasking)** shines when optimization is a top priority, particularly with respect to space usage.
Each approach is valid and the best one depends on the problem's constraints and the developer's familiarity with the concepts.
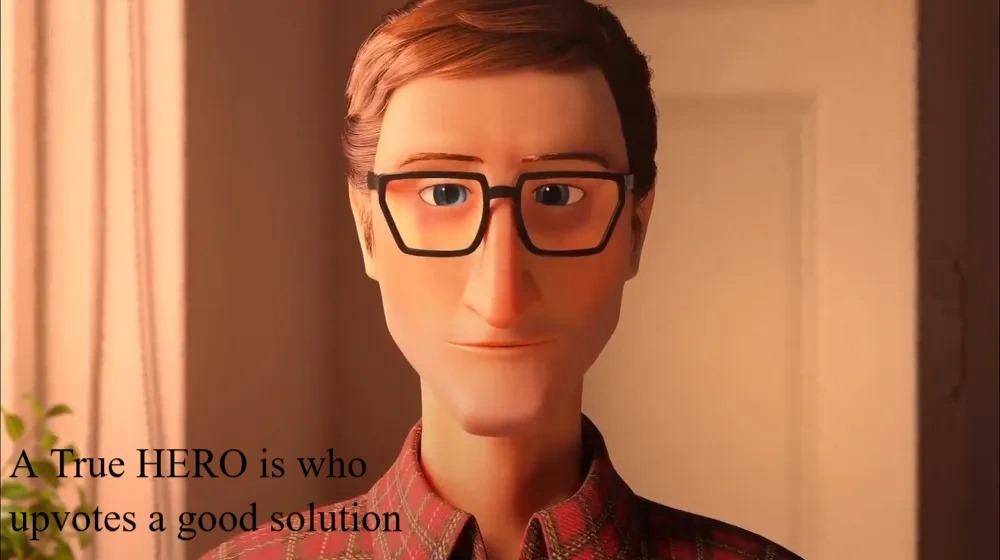
| 104 | 2 | ['C++', 'Java', 'Go', 'Python3', 'Ruby', 'C#'] | 9 |
construct-k-palindrome-strings | [java/Python 3] Count odd occurrences - 2 codes w/ brief explanation and analysis. | javapython-3-count-odd-occurrences-2-cod-63d5 | Explanation of the method - credit to @lionkingeatapple\nIf we need to construct k palindromes, and each palindrome at most has1 character with odd times of occ | rock | NORMAL | 2020-04-04T16:02:00.765058+00:00 | 2022-09-04T05:56:32.595619+00:00 | 4,454 | false | **Explanation of the method** - credit to **@lionkingeatapple**\nIf we need to construct `k` palindromes, and each palindrome at most has`1` character with odd times of occurrence. The oddest times of occurrence we can have is `1 * k = k`. Therefore, `oddNum <= k`. And it is impossible to construct `k` palindromes when the input string s\'s length is less than `k`. So we can conclude that in order to construct `k` palindromes it must satisfy the condition `oddNum <= k` && `k <= s.length()`.\n\n----\n\n1. Each palindrome at most has one character with odd times of occurrence;\n2. `Non-empty` means each palindrome at least has one character, hence `k <= s.length()`.\n\n**Method 1:**\n```java\n public boolean canConstruct(String s, int k) {\n int[] count = new int[26];\n for (int i = 0; i < s.length(); ++i) {\n ++count[s.charAt(i) - \'a\'];\n }\n int oddNum = 0;\n for (int i = 0; i < 26; ++i) {\n oddNum += count[i] % 2;\n }\n return oddNum <= k && k <= s.length();\n }\n```\nOr make the above 2 for loops as 1:\n```java\n public boolean canConstruct(String s, int k) {\n int[] count = new int[26];\n int oddNum = 0;\n for (int i = 0; i < s.length(); ++i) {\n oddNum += ++count[s.charAt(i) - \'a\'] % 2 == 0 ? -1 : 1;\n }\n return oddNum <= k && k <= s.length();\n }\n```\n```python\n def canConstruct(self, s: str, k: int) -> bool:\n return sum(v % 2 for v in collections.Counter(s).values()) <= k <= len(s)\n```\n\n----\n\n**Method 2:**\nOr use bit manipulation:\n```java\n public boolean canConstruct(String s, int k) {\n int odd = 0;\n for (int i = 0; i < s.length(); ++i) {\n odd ^= 1 << (s.charAt(i) - \'a\');\n }\n return Integer.bitCount(odd) <= k && k <= s.length();\n }\n```\n```python\n def canConstruct(self, s: str, k: int) -> bool:\n odd = 0\n for c in s:\n odd ^= 1 << (ord(c) - ord(\'a\'))\n return bin(odd).count(\'1\') <= k <= len(s)\n```\n\n**Analysis:**\nTime: O(n), space: O(1), where n = s.length(). | 44 | 2 | [] | 6 |
construct-k-palindrome-strings | Clear Python 3 solution faster than 91% with explanation | clear-python-3-solution-faster-than-91-w-4wxy | \nfrom collections import Counter\n\nclass Solution:\n def canConstruct(self, s: str, k: int) -> bool:\n if k > len(s):\n return False\n | swap24 | NORMAL | 2020-08-24T08:33:18.152121+00:00 | 2020-08-25T14:04:57.538767+00:00 | 3,084 | false | ```\nfrom collections import Counter\n\nclass Solution:\n def canConstruct(self, s: str, k: int) -> bool:\n if k > len(s):\n return False\n h = Counter(s)\n countOdd = 0\n for value in h.values():\n if value % 2:\n countOdd += 1\n if countOdd > k:\n return False\n return True\n```\nThe solution is based on the understanding that a string can be a palindrome only if it has **at most 1 character** whose frequency is odd. So if the number of characters having an odd frequency is greater than the number of palindromes we need to form, then naturally it\'s impossible to do so. | 38 | 0 | ['Python', 'Python3'] | 5 |
construct-k-palindrome-strings | ✅||Easy solutions||[with proof]||Beats 100% in most||🔥|🐍|C#️⃣|C➕➕|☕|💎|🔥🔥 | easy-solutionswith-proofbeats-100-in-mos-4cp0 | HELLOW PLEZ UPVOTE!!
Intuition💡
A palindrome is a string that reads the same forward and backward.
For constructing a palindrome:
Characters must appear in | AbyssReaper | NORMAL | 2025-01-11T07:53:54.394063+00:00 | 2025-01-11T07:53:54.394063+00:00 | 149 | false | - # ***********************HELLOW PLEZ UPVOTE!!***********************
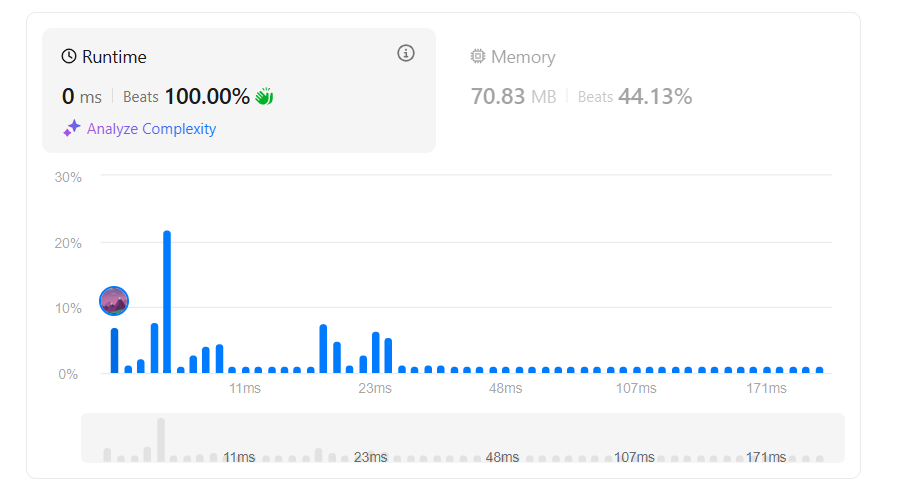
# Intuition💡
- A palindrome is a string that reads the same forward and backward.
- For constructing a palindrome:
1. Characters must appear in pairs (even frequency).
1. At most one character with an odd frequency can be placed at the center of the palindrome.
- The challenge is to determine if it's possible to create exactly `k` palindromes from the string `s`.
---
# Approach🚀
1. First check if k is larger than string length - if yes, return false
1. Count frequency of each character using an array of size 26
1. Count total number of characters with odd frequencies
1. Return true if odd count ≤ k and k ≤ string length
---
# Complexity
# 1. Time Complexity 🕒
O(n): Iterate through the string to update the bitmask.
# 2. Space Complexity 🗄️
O(1): Constant space for the bitmask.
---
---
---
*****THESE ARE VERY SMALL AND EASY CODES!*****
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
if (s.length() < k) return false;
sort(s.begin(), s.end());
int oddCount = 0;
for (int i = 0; i < s.length(); ) {
char current = s[i];
int count = 0;
while (i < s.length() && s[i] == current) {
count++;
i++;
}
if (count % 2 != 0) oddCount++;
}
return oddCount <= k;
}
};
```
```java []
class Solution {
public boolean canConstruct(String s, int k) {
if (s.length() < k)
return false;
if (s.length() == k)
return true;
int odd = 0;
for (char chr : s.toCharArray())
odd ^= 1 << (chr - 'a');
return Integer.bitCount(odd) <= k;
}
}
```
```python []
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
if len(s) < k:
return False
if len(s) == k:
return True
odd = 0
for chr in s:
odd ^= (1 << (ord(chr) - ord('a')))
return bin(odd).count('1') <= k
```
```javascript []
class Solution {
canConstruct(s, k) {
if (k > s.length) return false;
const charCount = new Array(26).fill(0);
for (let c of s) {
charCount[c.charCodeAt(0) - 'a'.charCodeAt(0)]++;
}
let oddCount = 0;
for (let i = 0; i < 26; i++) {
if (charCount[i] % 2 === 1) oddCount++;
}
return oddCount <= k && k <= s.length;
}
}
```
```ruby []
def can_construct(s, k)
return false if s.length < k
chars = s.chars.sort
odd_count = 0
i = 0
while i < chars.length
current = chars[i]
count = 0
while i < chars.length && chars[i] == current
count += 1
i += 1
end
odd_count += 1 if count.odd?
end
odd_count <= k
end
```
```C# []
using System;
public class Solution {
public bool CanConstruct(string s, int k) {
if (s.Length < k) return false;
char[] chars = s.ToCharArray();
Array.Sort(chars);
int oddCount = 0;
for (int i = 0; i < chars.Length; ) {
char current = chars[i];
int count = 0;
while (i < chars.Length && chars[i] == current) {
count++;
i++;
}
if (count % 2 != 0) oddCount++;
}
return oddCount <= k;
}
}
```
```c []
bool canConstruct(char* s, int k) {
int freq[256] = {0};
int single_count = 0;
for (int i = 0; s[i] != '\0'; i++) {
freq[s[i]]++;
}
for (int i = 0; i < 256; i++) {
if (freq[i] % 2 == 1) {
single_count++;
}
}
return single_count <= k && k <= strlen(s);
}
```
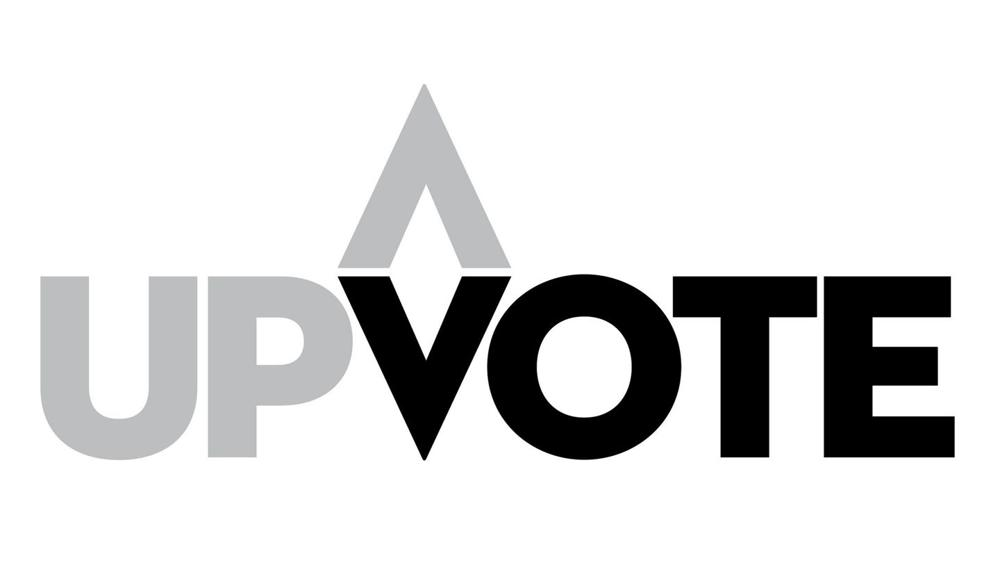
| 29 | 0 | ['C', 'Python', 'C++', 'Java', 'Ruby', 'JavaScript', 'C#'] | 2 |
construct-k-palindrome-strings | ✅ Simple Freq Count Detailed Solution | easy-by-sumeet_sharma-1-a5qb | Can Construct Palindromes 🏗️Problem Understanding 🤔Given a string s and a number k, we need to determine if we can construct exactly k palindromes using the cha | Sumeet_Sharma-1 | NORMAL | 2025-01-11T05:01:42.544772+00:00 | 2025-01-11T05:02:32.150407+00:00 | 4,106 | false | # **Can Construct Palindromes** 🏗️
### **Problem Understanding** 🤔
Given a string `s` and a number `k`, we need to determine if we can construct exactly `k` palindromes using the characters from `s`.
---
## **Intuition** 💡
- A **palindrome** is a string that reads the same forward and backward.
- For constructing a palindrome:
- Characters must appear in **pairs** (even frequency).
- At most **one** character with an **odd frequency** can be placed at the center of the palindrome.
- The challenge is to determine if it's possible to create exactly `k` palindromes from the string `s`.
---
## **Approach** 🚀
### **Step 1: Count Character Frequencies** 🧮
- Count how many times each character appears in the string `s`.
- Use an unordered map `M` to store the frequency of each character.
### **Step 2: Count Odd Frequencies (Singles)** 🔢
- A character with an **odd frequency** will need to be placed at the center of a palindrome.
- Count how many characters have odd frequencies (`Single` count).
### **Step 3: Determine Feasibility** ✅
- If `Single <= k` and `k <= s.size()`, it's possible to create exactly `k` palindromes.
- `Single <= k`: There must be enough "odd characters" to form the palindromes.
- `k <= s.size()`: You cannot form more palindromes than there are characters in the string.
- 'a': 2, 'b': 2, 'c': 2, 'd': 2, 'e': 2, 'f': 2, 'g': 1
2. **Count Singles (Odd Frequencies)**:
- `'g'` has an odd frequency (`1`), so `Single = 1`.
3. **Feasibility Check**:
- `Single = 1`
- `k = 4` (we can place the odd character `g` in one of the palindromes).
- `k = 4` is valid because it is less than or equal to the number of characters in `s` (13).
**Output:**
Yes, it is possible to form exactly 4 palindromes. 🎉
---
## **Time and Space Complexity** ⏳
### **Time Complexity** 🕒
- Counting character frequencies takes **O(n)** where `n` is the length of the string `s`.
- Iterating over the frequencies takes **O(m)** where `m` is the number of distinct characters in `s` (which is at most `26` for lowercase English letters).
- **Overall Time Complexity: O(n)**.
### **Space Complexity** 🧑💻
- We are using an unordered map to store the frequency of each character.
- The space complexity is **O(m)** where `m` is the number of distinct characters in `s` (maximum `26` for lowercase letters).
- **Overall Space Complexity: O(m)**.
# [Telegram](https://t.me/leetmath)
---
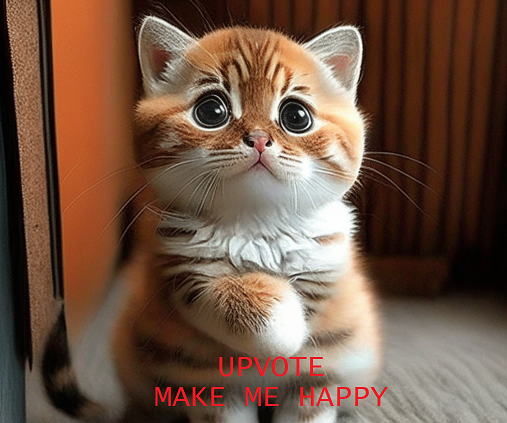
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
unordered_map<char, int> M;
int Single = 0;
// Count frequencies of each character
for (char c : s) {
M[c]++;
}
// Count pairs and singles
for (auto &[ch, freq] : M) {
if (freq % 2 == 1) {
Single++; // Count remaining single characters
}
}
return (Single <= k && k <= s.size());
}
};
```
```Java []
class Solution {
public boolean canConstruct(String s, int k) {
Map<Character, Integer> M = new HashMap<>();
int Single = 0;
for (char c : s.toCharArray()) {
M.put(c, M.getOrDefault(c, 0) + 1);
}
for (int freq : M.values()) {
if (freq % 2 == 1) {
Single++;
}
}
return Single <= k && k <= s.length();
}
}
```
```Python3 []
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
freq_map = {}
single_count = 0
for c in s:
freq_map[c] = freq_map.get(c, 0) + 1
for freq in freq_map.values():
if freq % 2 == 1:
single_count += 1
return single_count <= k and k <= len(s)
```
```C []
bool canConstruct(char* s, int k) {
int freq[256] = {0};
int single_count = 0;
for (int i = 0; s[i] != '\0'; i++) {
freq[s[i]]++;
}
for (int i = 0; i < 256; i++) {
if (freq[i] % 2 == 1) {
single_count++;
}
}
return single_count <= k && k <= strlen(s);
}
```
```C# []
public class Solution {
public bool CanConstruct(string s, int k) {
var freqMap = new Dictionary<char, int>();
int singleCount = 0;
foreach (char c in s) {
if (freqMap.ContainsKey(c)) {
freqMap[c]++;
} else {
freqMap[c] = 1;
}
}
foreach (var freq in freqMap.Values) {
if (freq % 2 == 1) {
singleCount++;
}
}
return singleCount <= k && k <= s.Length;
}
}
```
```JavaScript []
/**
* @param {string} s
* @param {number} k
* @return {boolean}
*/
var canConstruct = function(s, k) {
const freqMap = {};
let singleCount = 0;
// Count frequencies of each character
for (const c of s) {
freqMap[c] = (freqMap[c] || 0) + 1;
}
// Count how many characters have an odd frequency
for (const freq of Object.values(freqMap)) {
if (freq % 2 === 1) {
singleCount++;
}
}
// Check if we can construct exactly k palindromes
return singleCount <= k && k <= s.length;
};
```
| 25 | 1 | ['String', 'C', 'Counting', 'C++', 'Java', 'Python3', 'JavaScript', 'C#'] | 5 |
construct-k-palindrome-strings | only consider the parity of freq+bitset|Py3 1-liner|C++ beats 100% | only-consider-the-parity-of-freq-bitsetb-3tk3 | IntuitionFollow the hints.
This problem becomes fairly easy.The key observation: palindrome strings of even lengths have all letters even occurrences, palindrom | anwendeng | NORMAL | 2025-01-11T00:33:12.478355+00:00 | 2025-01-11T13:08:03.808279+00:00 | 1,955 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
Follow the hints.
This problem becomes fairly easy.
The key observation: palindrome strings of even lengths have all letters even occurrences, palindrome strings of odd lengths have all letters even occurrences but except one letter with odd occurrence.
If n is big enough, say n>=k, it's only to consider the number of odd occurrences for chars.
Python 1-liner is made.
# Approach
<!-- Describe your approach to solving the problem. -->
[Please Turn on English subtitles if necessary]
[https://youtu.be/zCV4ZiBNlKQ?si=4erkSpNzpDiUM4Xs](https://youtu.be/zCV4ZiBNlKQ?si=4erkSpNzpDiUM4Xs)
1. let `n=|s|`
2. If `n<k` return false
3. Initialize the `bitset<26> freq=0`
4. Set `freq.flip(c-'a')` same as `freq[c-'a']^=1` for each c in s
5. if `bitcount(freq)<=k` return `true` else `false`
6. Python code is made
7. 2nd version for C++ is made by using C++20 popcount
8. Python 1-liner is made in use of Counter which is a submodule of dict
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
$O(n+26)$
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
$O(26)$
# Code||C++ bitset 0ms beats 100%
```cpp []
class Solution {
public:
bool canConstruct(string& s, int k) {
const int n=s.size();
if (n<k) return 0;
bitset<26> freq=0;
for(char c: s)
freq.flip(c-'a');
return freq.count()<=k;
}
};
```
```cpp [C++ 2nd ver]
class Solution {
public:
bool canConstruct(string& s, int k) {
const int n=s.size();
if (n<k) return 0;
unsigned freq=0;
for(char c: s)
freq^=(1<<(c-'a'));
return popcount(freq)<=k;
}
};
```
# Python
```
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
n=len(s)
if n<k: return False
freq=[False]*26
for c in s:
freq[ord(c)-97]^=1
return freq.count(True)<=k
```
# Python 1-liner
```
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
return (freq:=Counter(s)) and sum(f&1 for f in freq.values())<=k<=len(s)
```
# Some other Palindrome Problems
If you think this medium question too easy, try the following!
[2108. Find First Palindromic String in the Array](https://leetcode.com/problems/find-first-palindromic-string-in-the-array/solutions/4717919/python-1-liner-vs-several-c-c-sols-19ms-beats-99-86/)<- easy
[1457. Pseudo-Palindromic Paths in a Binary Tree](https://leetcode.com/problems/pseudo-palindromic-paths-in-a-binary-tree/solutions/4616431/dfs-bitset-parity-check-beats-100/)<- in combination with binary tree
[3260. Find the Largest Palindrome Divisible by K](https://leetcode.com/problems/find-the-largest-palindrome-divisible-by-k/solutions/5654462/modular-arithmetic-only-k-7-is-hard-number-theoretical-sol/)<- really hard | 22 | 4 | ['Greedy', 'Bit Manipulation', 'C++', 'Python3'] | 5 |
construct-k-palindrome-strings | ✅🔥BEATS 100% PROOF🔥||🌟JAVA | C++ | C# | PYTHON💡|| CONCISE CODE✅|| | beats-100-proofconcise-codejava-beginner-k96r | Proof 💯Problem Analysis 🧠We are given a string s and an integer k.
The goal is to determine if we can use all the characters in s to construct exactly k palindr | mukund_rakholiya | NORMAL | 2025-01-11T00:10:57.258378+00:00 | 2025-01-11T00:27:11.533185+00:00 | 1,649 | false | # Proof 💯
---
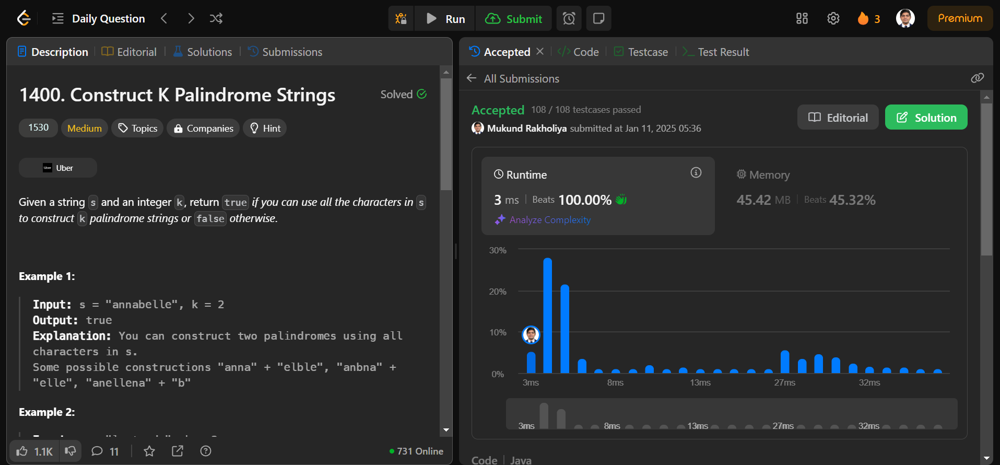
```
```
# Problem Analysis 🧠
---
We are given a string `s` and an integer `k`.
The goal is to determine if we can use all the characters in `s` to construct exactly `k` palindrome strings.
**Key Observations:**
1. A palindrome allows at most one character to have an odd frequency.
2. Therefore, the minimum number of palindromes required is equal to the number of characters with odd frequencies.
3. If the number of odd frequencies is greater than `k`, it is **impossible** to construct `k` palindromes.
```
```
# Intuition 💡
---
1. Count the frequency of each character in the string `s`.
2. Determine the number of characters with **odd frequencies** (let's call this `oddCount`).
3. Check the conditions:
- If `k > s.length()`, return `false` (not enough characters to form `k` palindromes).
- If `oddCount > k`, return `false` (cannot form enough palindromes due to odd characters).
- Otherwise, return `true`.
```
```
# Approach 🚀
---
### 1. Count Odd Frequencies
- Use a **bitmask** to track the parity (odd or even) of character frequencies efficiently.
- Toggle the bit corresponding to each character in the bitmask.
- Use `Integer.bitCount()` to count the number of odd frequencies.
### 2. Verify Conditions
- If the number of odd frequencies is less than or equal to `k`, return `true`.
- Otherwise, return `false`.
```
```
# Complexity 🔍
---
### 1. Time Complexity 🕒
- **O(n):** Iterate through the string to update the bitmask.
### 2. Space Complexity 🗄️
- **O(1):** Constant space for the bitmask.
# Concept of Tags Used 📌
---
- **Bit Manipulation:** Efficiently tracks the odd/even frequency of characters.
- **Counting and Validation:** Verifies constraints to decide the output.
```
```
# Code 📒
```java []
class Solution {
public boolean canConstruct(String s, int k) {
if (s.length() < k)
return false;
if (s.length() == k)
return true;
int odd = 0;
for (char chr : s.toCharArray())
odd ^= 1 << (chr - 'a');
return Integer.bitCount(odd) <= k;
}
}
```
```C++ []
class Solution {
public:
bool canConstruct(string s, int k) {
if (s.length() < k)
return false;
if (s.length() == k)
return true;
int odd = 0;
for (char chr : s)
odd ^= (1 << (chr - 'a'));
return __builtin_popcount(odd) <= k;
}
};
```
```python []
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
if len(s) < k:
return False
if len(s) == k:
return True
odd = 0
for chr in s:
odd ^= (1 << (ord(chr) - ord('a')))
return bin(odd).count('1') <= k
```
```Csharp []
public class Solution {
public bool CanConstruct(string s, int k) {
if (s.Length < k)
return false;
if (s.Length == k)
return true;
int odd = 0;
foreach (char chr in s)
odd ^= (1 << (chr - 'a'));
return CountSetBits(odd) <= k;
}
private int CountSetBits(int n) {
int count = 0;
while (n > 0) {
count += (n & 1);
n >>= 1;
}
return count;
}
}
```
```
```
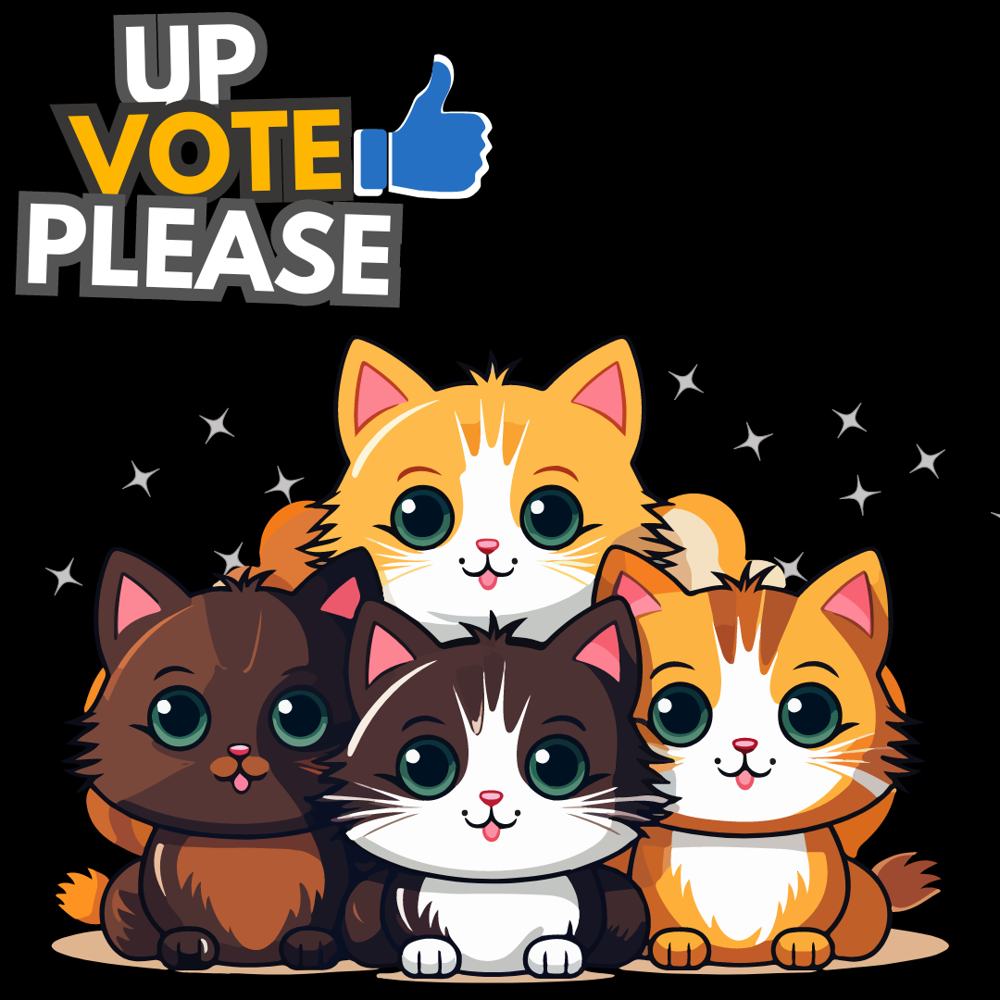
```
```
| 16 | 3 | ['String', 'Bit Manipulation', 'Counting', 'Python', 'C++', 'Java', 'C#'] | 8 |
construct-k-palindrome-strings | C++ Super Simple Solution, Only 6 Short Lines | c-super-simple-solution-only-6-short-lin-jr76 | \nclass Solution {\npublic:\n bool canConstruct(string s, int k) {\n if (s.size() < k) return false;\n \n vector<int> freq(26);\n | yehudisk | NORMAL | 2021-07-14T16:07:50.948189+00:00 | 2021-07-14T16:07:50.948219+00:00 | 1,538 | false | ```\nclass Solution {\npublic:\n bool canConstruct(string s, int k) {\n if (s.size() < k) return false;\n \n vector<int> freq(26);\n for (auto ch : s) freq[ch - \'a\']++;\n \n int odd = 0;\n for (auto f : freq) odd += (f % 2);\n \n return odd <= k;\n }\n};\n```\n**Like it? please upvote!** | 16 | 2 | ['C'] | 4 |
construct-k-palindrome-strings | Counting | String | Solution for LeetCode#1400 | counting-string-solution-for-leetcode140-w5qq | IntuitionThe problem asks if it's possible to construct k palindromic strings using all characters from the given string s. The key insight is that palindromes | samir023041 | NORMAL | 2025-01-11T00:27:15.273722+00:00 | 2025-01-11T00:53:31.248430+00:00 | 1,360 | false | 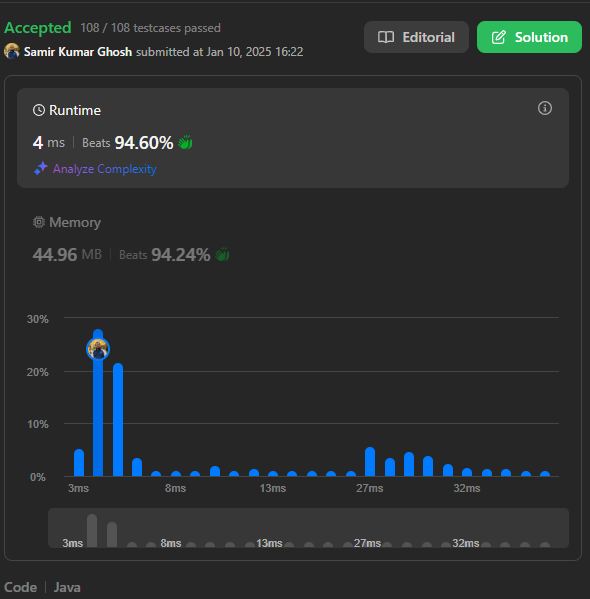
# Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
The problem asks if it's possible to construct k palindromic strings using all characters from the given string s. The key insight is that palindromes can have at most one character with an odd count.
# Approach
<!-- Describe your approach to solving the problem. -->
1. First, check if the length of s is less than k. If so, it's impossible to create k strings.
2. Count the frequency of each character in s.
3. Count the number of characters with odd frequencies.
4. If the number of odd-frequency characters is greater than k, it's impossible to create k palindromes.
5. Otherwise, it's always possible to construct k palindromes.
# Complexity
- Time complexity: O(n)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: O(1)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public boolean canConstruct(String s, int k) {
int n=s.length();
if(n<k){
return false;
}
int[] arr=new int[26];
for(char ch:s.toCharArray()){
arr[ch-'a']++;
}
int oddCharCnt=0;
for(int i=0; i<26; i++){
if(arr[i]==0) continue;
if(arr[i]%2==1){
oddCharCnt++;
}
}
if(oddCharCnt>k){
return false;
}
return true;
}
}
```
```python []
class Solution(object):
def canConstruct(self, s, k):
"""
:type s: str
:type k: int
:rtype: bool
"""
n = len(s)
if n < k:
return False
if n == k:
return True
arr = [0] * 26
for ch in s:
arr[ord(ch) - ord('a')] += 1
oddCharCnt = sum(1 for count in arr if count % 2 == 1)
return oddCharCnt <= k
``` | 15 | 6 | ['Python', 'Java', 'Python3'] | 3 |
construct-k-palindrome-strings | 25ms | 100% Beats | PHP | 25ms-100-beats-php-by-ma7med-m8xd | Code | Ma7med | NORMAL | 2025-01-11T09:07:03.365788+00:00 | 2025-01-15T08:39:33.742627+00:00 | 480 | false |
# Code
```php []
class Solution {
/**
* @param String $s
* @param Integer $k
* @return Boolean
*/
function canConstruct($s, $k) {
// If k is greater than the length of s, it's impossible to construct k palindromes
if ($k > strlen($s)) {
return false;
}
// Count the frequency of each character
$charCount = array_count_values(str_split($s));
$oddCount = 0;
// Count the number of characters with odd frequencies
foreach ($charCount as $count) {
if ($count % 2 !== 0) {
$oddCount++;
}
}
// The number of palindromes we can construct must be at least the number
// of odd frequency characters, and at most the length of the string.
return $oddCount <= $k;
}
}
``` | 14 | 0 | ['PHP'] | 0 |
construct-k-palindrome-strings | ✅🔥BEATS 100%🔥|| 🌟✅ Efficient Palindrome Building: A Beginner-Friendly Solution! 🧩💡|| Approved ✅ | beats-100-efficient-palindrome-building-54bpx | IntuitionThe problem is to check whether we can rearrange the characters of the string s to form exactly k palindrome strings.
Key observations:
A palindrome ca | venkat_pasapuleti | NORMAL | 2025-01-11T04:05:14.287232+00:00 | 2025-01-11T04:49:36.469749+00:00 | 502 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
The problem is to check whether we can rearrange the characters of the string `s` to form exactly `k` palindrome strings.
Key observations:
- A **palindrome** can have at most one character with an odd frequency.
- To form `k` palindromes, the string must have at most `k` characters with odd frequencies.
- If the length of `s` is less than `k`, it’s impossible to form `k` palindromes since there aren't enough characters.
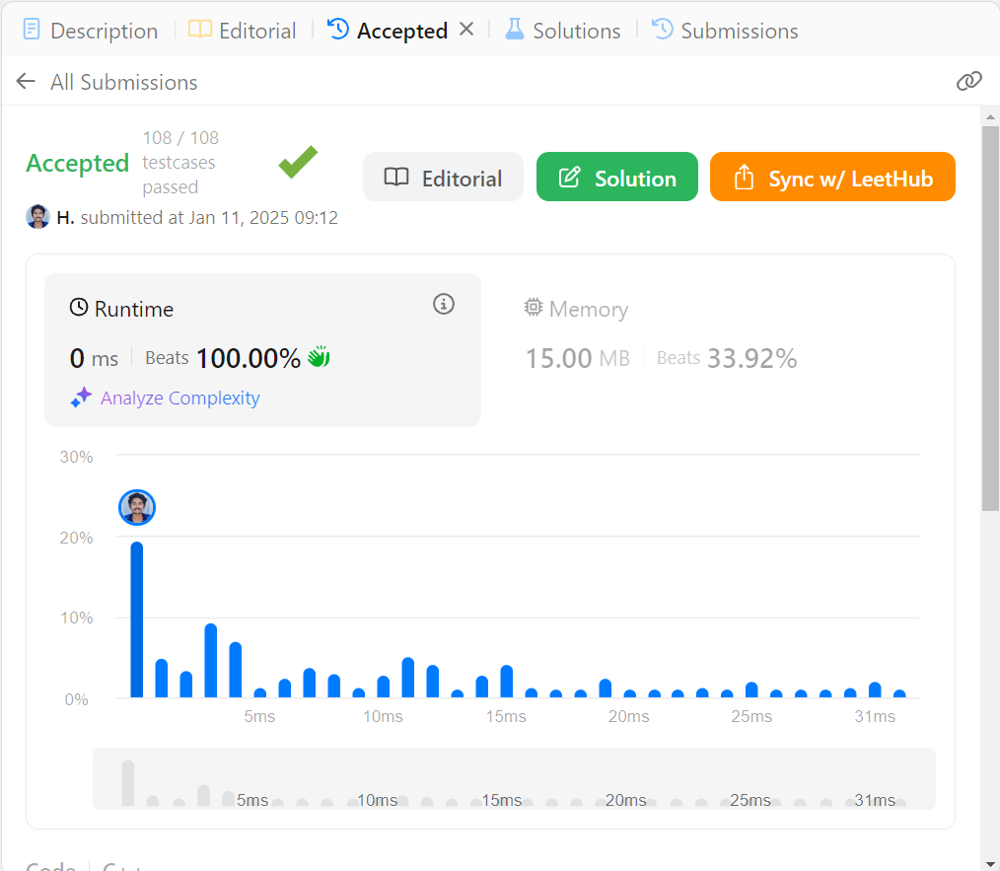
# Approach
<!-- Describe your approach to solving the problem. -->
1. **Initial Check:**
- If the size of the string `s` is less than `k`, return `false` because it's impossible to form more palindromes than the number of characters available.
2. **Frequency Count:**
- Use a frequency array of size 26 to count the occurrences of each character in `s` (since there are 26 lowercase English letters).
3. **Count Odd Frequencies:**
- Traverse the frequency array to count how many characters have an odd frequency. Each odd frequency requires its own palindrome.
4. **Decision:**
- If the number of odd frequencies (`odds`) is greater than `k`, return `false` because there aren't enough palindromes to accommodate the odd frequencies.
- Otherwise, return `true`, as it's feasible to form exactly `k` palindromes.
# Complexity
- **Time Complexity:**
- Calculating the frequency array takes \(O(n)\), where \(n\) is the size of the string.
- Traversing the frequency array (size 26) takes \(O(26)\), which is constant.
**Total:** O(n)
- **Space Complexity:**
- The frequency array requires \(O(26)\), which is constant and considered \(O(1)\).
**Total:** O(1)
# Code
``` c []
bool canConstruct(char* s, int k) {
int length = strlen(s);
if (length < k)
return false;
int freq[26] = {0};
for (int i = 0; s[i] != '\0'; i++) {
freq[s[i] - 'a']++;
}
int odds = 0;
for (int i = 0; i < 26; i++) {
if (freq[i] % 2 != 0)
odds++;
}
return odds <= k;
}
```
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
if (s.size() < k)
return false;
vector<int> freq(26, 0);
for (const char& c : s) {
freq[c - 'a']++;
}
int odds = 0;
for (int i = 0; i < 26; i++) {
if (freq[i] % 2 != 0)
odds++;
}
return odds <= k;
}
};
```
```python3 []
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
if len(s) < k:
return False
from collections import Counter
freq = Counter(s)
odds = sum(1 for count in freq.values() if count % 2 != 0)
return odds <= k
```
``` java []
class Solution {
public boolean canConstruct(String s, int k) {
if (s.length() < k)
return false;
int[] freq = new int[26];
for (char c : s.toCharArray()) {
freq[c - 'a']++;
}
int odds = 0;
for (int count : freq) {
if (count % 2 != 0)
odds++;
}
return odds <= k;
}
}
```
``` javascript []
var canConstruct = function(s, k) {
if (s.length < k)
return false;
const freq = new Array(26).fill(0);
for (const char of s) {
freq[char.charCodeAt(0) - 'a'.charCodeAt(0)]++;
}
let odds = 0;
for (const count of freq) {
if (count % 2 !== 0)
odds++;
}
return odds <= k;
};
```
### Example 1:
**Input:**
`s = "annabelle", k = 2`
**Steps:**
1. **Initial Check:**
- The length of `s` is 9, which is greater than `k` (2), so we proceed.
2. **Frequency Count:**
- `{'a': 2, 'n': 2, 'b': 1, 'e': 3, 'l': 2}`
3. **Count Odd Frequencies:**
- Characters `b` and `e` have odd frequencies (`odds = 2`).
4. **Decision:**
- Since `odds (2) <= k (2)`, it is possible to form 2 palindromes.
**Output:**
`true`
**Explanation:**
You can construct two palindromes using all characters in `s`.
Some possible constructions:
- `"anna" + "elble"`
- `"anbna" + "elle"`
- `"anellena" + "b"`
---
### Example 2:
**Input:**
`s = "leetcode", k = 3`
**Steps:**
1. **Initial Check:**
- The length of `s` is 8, which is greater than `k` (3), so we proceed.
2. **Frequency Count:**
- `{'l': 1, 'e': 3, 't': 1, 'c': 1, 'o': 1, 'd': 1}`
3. **Count Odd Frequencies:**
- Characters `l`, `t`, `c`, `o`, and `d` have odd frequencies (`odds = 5`).
4. **Decision:**
- Since `odds (5) > k (3)`, it is not possible to form 3 palindromes.
**Output:**
`false`
**Explanation:**
It is impossible to construct 3 palindromes using all the characters of `s`.
---
### Example 3:
**Input:**
`s = "true", k = 4`
**Steps:**
1. **Initial Check:**
- The length of `s` is 4, which is equal to `k` (4), so we proceed.
2. **Frequency Count:**
- `{'t': 1, 'r': 1, 'u': 1, 'e': 1}`
3. **Count Odd Frequencies:**
- All characters have odd frequencies (`odds = 4`).
4. **Decision:**
- Since `odds (4) = k (4)`, it is possible to form 4 palindromes.
**Output:**
`true`
**Explanation:**
The only possible solution is to put each character in a separate string.
Result: `"t"`, `"r"`, `"u"`, `"e"`.
# Key Points
- **Odd Frequency Check:** Counts characters with odd frequencies to determine palindrome feasibility.
- **Efficient Filtering:** Skips unnecessary checks by comparing string length with `k` upfront, ensuring early exits for invalid cases.
- **Handles Edge Cases:** Works seamlessly for strings shorter than `k` or with all odd frequencies.
- **Optimal for Large Inputs:** Uses a single pass for frequency calculation and a fixed-size array, ensuring efficiency even for long strings.
- **Clean & Readable Code:** Well-structured logic with comments, making it easy to understand and maintain.
🌟 **Why You'll Love This Solution!**
- **Clear & Simple 💡:** Designed for maximum readability.
- **100% Efficiency Approved 🏆:** Handles both edge cases and large datasets effectively.
- **Reusable 🎨:** Can be used for any string and `k` combinations.
💖 **Show Your Support!** 👍
If this explanation helped you understand the problem and solution, hit that like button and share your feedback below. Your encouragement fuels more optimized solutions! 🚀✨
# UpVote 🏆
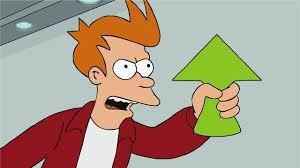
| 11 | 0 | ['C', 'C++', 'Java', 'Python3', 'JavaScript'] | 4 |
construct-k-palindrome-strings | 0ms | Beats 100%| Using Bitmask Based (13Line) | Efficient & Easy C++ Solu| Full Explain| With Proof | 0ms-beats-100-using-bitmask-based-13line-ux3m | IntuitionThe problem revolves around determining whether a given string can be split into k palindromic substrings. A palindrome is a string that reads the same | HarshitSinha | NORMAL | 2025-01-11T01:31:46.256875+00:00 | 2025-01-11T01:31:46.256875+00:00 | 422 | false | 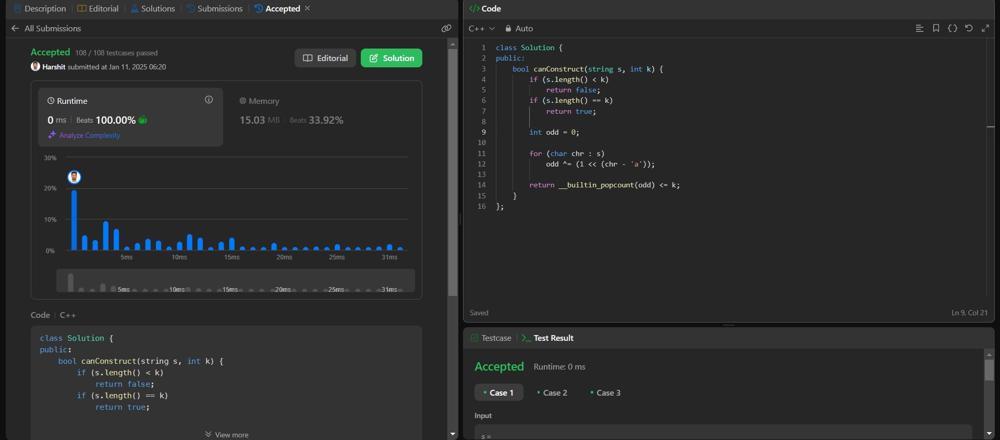
# Intuition
The problem revolves around determining whether a given string can be split into k palindromic substrings. A palindrome is a string that reads the same forward and backward. The key to solving this problem lies in understanding the frequency of characters in the string:
1. Palindrome Character Properties:
- In a palindrome, characters must pair up to form the string symmetrically. This means most characters should appear an even number of times.
- The only exception is that one character can appear an odd number of times and sit in the middle of the palindrome if the length of the palindrome is odd.
2. Odd Frequencies and Palindromes:
- To form palindromes from the string, the number of characters that appear an odd number of times is crucial.
- Specifically, we can have at most k characters with odd frequencies, because in the worst case, each odd frequency can form the middle of a distinct palindrome. If we have more than k characters with odd frequencies, it becomes impossible to split the string into k palindromes.
3. Tracking Odd Frequencies Efficiently:
- We need to count how many characters in the string have an odd frequency. Instead of explicitly counting frequencies, we can use a bitmask to efficiently track this information.
- Each bit in the bitmask corresponds to a character ('a' to 'z'). The bit is set to 1 if the character has an odd frequency and 0 if it has an even frequency.
- After processing the string, the number of set bits (1s) in the bitmask represents how many characters have an odd frequency.
4. Final Check:
- If the number of characters with odd frequencies (i.e., the number of set bits in the bitmask) is less than or equal to k, then it is possible to split the string into k or more palindromic substrings. Otherwise, it’s not possible.
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
1. Edge Case Handling:
- If the length of the string `s` is less than` k`, return false. You can’t have more palindromes than the number of characters.
- If the length of` s` equals `k`, return true. Each character can be its own palindrome.
2. Track Odd Frequencies Using Bitmasking:
- Initialize an integer `oddCount` to represent a bitmask. Each bit corresponds to a character ('a' to 'z'), and toggling the bits will track whether a character’s frequency is odd or even.
- For each character in the string, use XOR operation to toggle the corresponding bit in` oddCount`:
-- If a character’s frequency is odd, its corresponding bit will be set to 1.
-- If a character’s frequency is even, its corresponding bit will be set to 0.
3. Count Set Bits (Odd Frequencies):
- The number of set bits in the` oddCount` bitmask directly represents the number of characters that have odd frequencies.
- Use `__builtin_popcount() `to efficiently count the number of set bits in oddCount.
4. Final Check:
- If the number of odd frequencies (set bits in the bitmask) is less than or equal to` k`, return true. It is possible to split the string into k or more palindromic substrings.
- Otherwise, return false.
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity: **𝑂(𝑛)** , where 𝑛 is the length of the string.
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: **𝑂(1)** , where it is Constant because does not depend on the size of the input string
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
if (s.length() < k) return false;
if (s.length() == k) return true;
int oddCount = 0;
for (char chr : s) {
oddCount ^= 1 << (chr - 'a');
}
int setBits = __builtin_popcount(oddCount);
return setBits <= k;
}
};
```
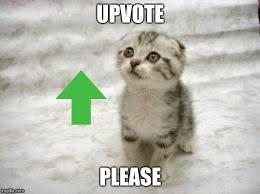
| 10 | 1 | ['Hash Table', 'String', 'Greedy', 'Bit Manipulation', 'Counting', 'Ordered Set', 'Counting Sort', 'Bitmask', 'C++'] | 2 |
construct-k-palindrome-strings | ⚡⚡⚡One-Liner Solution with Efficient Checks ✅⚡⚡⚡ | one-liner-solution-with-efficient-checks-oqap | IntuitionThe problem involves determining if a string s can be rearranged into k palindromes. Each palindrome can have at most one character with an odd frequen | suhas_sr7 | NORMAL | 2025-01-11T15:14:11.281991+00:00 | 2025-01-11T15:14:11.281991+00:00 | 194 | false |
# Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
The problem involves determining if a string `s` can be rearranged into `k` palindromes. Each palindrome can have at most one character with an odd frequency (in the middle), and the rest must appear in pairs. This means the number of characters with odd frequencies must not exceed `k`.
# Approach
<!-- Describe your approach to solving the problem. -->
1. First, check if the length of the string `s` is at least `k`. If not, it's impossible to form `k` non-empty palindromes.
2. Count the frequency of each character in `s`.
3. Calculate how many characters have an odd frequency.
4. If the number of odd frequencies is greater than `k`, return `False`. Otherwise, return `True`.
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
$$O(n)$$, where $$n$$ is the length of the string. Calculating character frequencies involves iterating through the string, and the `set` operation adds a negligible overhead for unique character extraction.
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
$$O(1)$$ if we consider the set of characters fixed (e.g., ASCII or Unicode range). Otherwise, $$O(u)$$, where $$u$$ is the number of unique characters in `s`.
# Code
```python
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
return len(s) >= k and sum(s.count(i) % 2 for i in set(s)) <= k | 9 | 0 | ['Python3'] | 0 |
construct-k-palindrome-strings | Java, counting, simple, explained | java-counting-simple-explained-by-gthor1-6guy | Idea is following: count of chars can be even or odd. Those that are even - they can be used in one of substrings to form the palindrome, so we don\'t care abou | gthor10 | NORMAL | 2020-04-05T03:50:06.888346+00:00 | 2020-04-05T03:50:06.888401+00:00 | 1,848 | false | Idea is following: count of chars can be even or odd. Those that are even - they can be used in one of substrings to form the palindrome, so we don\'t care about those.\nIf character has odd frequency - it can be inserted only 1 or 3 or 5... etc. to form the palindrome. However number of such characters cannot be more than number of strings, in other words - only one such char is allowed per substring. \nThis leads to solution - count all frequencies, count those that are odd. If number of such chars > then k - it\'s not possible to balance it to form all palindromes.\n\nO(n) time - iterate over every char in string to count freq.\nO(1) space - use fixed size array to count freq.\n\n```\n public boolean canConstruct(String s, int k) {\n if (s == null || s.isEmpty() || s.length() < k)\n return false;\n \n int[] count = new int[26];\n //count freq of every character\n for (char ch : s.toCharArray()) ++count[ch - \'a\'];\n //count chars that has odd freq\n int countOfOdd = 0;\n for (int i = 0; i < 26; i++) \n if (count[i] % 2 == 1)\n ++countOfOdd;\n return countOfOdd <= k;\n }\n``` | 9 | 0 | ['String', 'Java'] | 2 |
construct-k-palindrome-strings | Most Optimized Solution | ✅Beats 100% | C++| Java | Python | JavaScript | most-optimized-solution-beats-100-c-java-2p5w | IntuitionTo construct k palindromes, we need to understand that each palindrome can have at most one character with odd frequency (in the middle). Therefore, th | BijoySingh7 | NORMAL | 2025-01-11T04:28:36.629306+00:00 | 2025-01-11T04:28:36.629306+00:00 | 1,214 | false | ```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
if (k > s.length()) return false;
int charCount[26] = {0};
for (char c : s) {
charCount[c - 'a']++;
}
int oddCount = 0;
for (int i = 0; i < 26; i++) {
if (charCount[i] % 2 == 1) oddCount++;
}
return oddCount <= k;
}
};
```
```python []
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
if k > len(s): return False
charCount = [0] * 26
for c in s:
charCount[ord(c) - ord('a')] += 1
oddCount = 0
for i in range(26):
if charCount[i] % 2 == 1:
oddCount += 1
return oddCount <= k and k <= len(s)
```
```java []
class Solution {
public boolean canConstruct(String s, int k) {
if (k > s.length()) return false;
int[] charCount = new int[26];
for (char c : s.toCharArray()) {
charCount[c - 'a']++;
}
int oddCount = 0;
for (int i = 0; i < 26; i++) {
if (charCount[i] % 2 == 1) oddCount++;
}
return oddCount <= k && k <= s.length();
}
}
```
```javascript []
class Solution {
canConstruct(s, k) {
if (k > s.length) return false;
const charCount = new Array(26).fill(0);
for (let c of s) {
charCount[c.charCodeAt(0) - 'a'.charCodeAt(0)]++;
}
let oddCount = 0;
for (let i = 0; i < 26; i++) {
if (charCount[i] % 2 === 1) oddCount++;
}
return oddCount <= k && k <= s.length;
}
}
```
# Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
To construct k palindromes, we need to understand that each palindrome can have at most one character with odd frequency (in the middle). Therefore, the total number of characters with odd frequencies must not exceed k.
# Approach
<!-- Describe your approach to solving the problem. -->
1. First check if k is larger than string length - if yes, return false
2. Count frequency of each character using an array of size 26
3. Count total number of characters with odd frequencies
4. Return true if odd count ≤ k and k ≤ string length
# Complexity
- Time complexity: $$O(n)$$ where n is the length of string s
- Space complexity: $$O(1)$$ as we use fixed size array of 26 characters
### *If you have any questions or need further clarification, feel free to drop a comment! 😊* | 8 | 1 | ['String', 'Greedy', 'Counting', 'Python', 'C++', 'Java', 'Python3', 'JavaScript'] | 13 |
construct-k-palindrome-strings | Hash | Simple Logic | Beats 100% | hash-simple-logic-beats-100-by-sparker_7-v7dl | IntuitionOdd count characters will create problems for palindrome.All the odd frequency characters can be used as a single character string which is always a pa | Sparker_7242 | NORMAL | 2025-01-11T10:49:34.086834+00:00 | 2025-01-11T10:49:34.086834+00:00 | 46 | false | 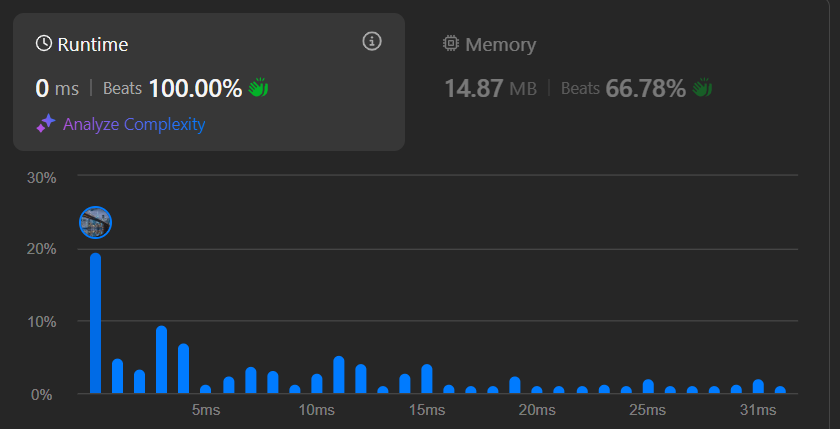
# Intuition
Odd count characters will create problems for palindrome.
**All the odd frequency characters can be used as a single character string which is always a palindrome. E.g. "a","g","l","v", etc. and one of them can be used with the remaining characters in between.**
# Approach
If string size is < k then it is not possible.
Count the frequency of each character.
Count which characters have an odd frequency and compare them with k.
E.g. s = "aabcccdd"
For k = 1 --> Not possible as b and c have odd frequencies.
For k = 2 --> b & c are the between characters and we can put remaining on both sides of them by our choice. E.g. "aca" & "dcbcd" or "adcccda" & "b" or any other combination.
For k = 3 --> "b","c" and "acddca" or "aa", "ccc" and "dbd" or any other combination.
and so on.
> So even frequency characters can be placed anywhere and only odd ones will have to be taken care of.
# Complexity
- Time complexity: O(n)
- Space complexity: O(1)
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
int n = s.size();
if (n < k) return false;
vector<int> v(26,0);
for (char &c: s) v[c-'a']++;
int ct = 0;
for (int i = 0; i < 26; i++){
if (v[i]&1) ct++;
}
if (ct > k) return false;
return true;
}
};
```
# Upvote 👍 if you found this useful | 7 | 0 | ['Hash Table', 'String', 'Counting', 'C++'] | 1 |
construct-k-palindrome-strings | 💻🧠BEST SOLUTION✅| BEATS 100%⌛⚡| (C++/Java/Python3/Javascript) | best-solution-beats-100-cjavapython3java-pa1i | IntuitionTo form a palindrome, all characters must have even counts, except for at most one character with an odd count (which can be placed in the middle of th | Fawz-Haaroon | NORMAL | 2025-01-11T08:45:50.630835+00:00 | 2025-01-11T10:04:38.610259+00:00 | 47 | false | # **Intuition**
To form a palindrome, all characters must have even counts, except for at most one character with an odd count (which can be placed in the middle of the palindrome).
To construct `k` palindromes:
1. The number of palindromes cannot exceed the number of characters in the string (`k <= s.length`).
2. The number of palindromes must be at least equal to the number of characters with odd frequencies (`oddCount <= k`).
# **Approach**
1. Count the frequency of each character in the string.
2. Calculate the number of characters with odd frequencies (`oddCount`).
3. Return `true` if the following conditions are met:
- `k <= s.length` (not enough characters to form `k` palindromes).
- `oddCount <= k` (each palindrome can accommodate one odd-count character).
4. Otherwise, return `false`.
# **Complexity**
- **Time Complexity:**
- `O(n)`, where `n` is the length of the string. Counting frequencies and odd counts requires a single traversal.
- **Space Complexity:**
- `O(1)` (for English letters, since the frequency array is fixed at size 26).
# **Code**
``` C++ []
class Solution {
public:
bool canConstruct(string s, int k) {
if (k > s.size()) return false;
int letterCount[26] = {0}, oddCount = 0;
for (char c : s) {
oddCount += (++letterCount[c - 'a'] & 1) ? 1 : -1;
}return oddCount <= k;
}
};
```
``` Python []
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
if len(s) == k:
return True
if len(s) < k:
return False
odd = 0
for char in set(s):
if s.count(char) % 2:
odd += 1
if odd > k:
return False
return True
```
```Java []
class Solution {
public boolean canConstruct(String s, int k) {
if (s.length() < k) return false;
if (s.length() == k) return true;
int oddCount = 0;
for (char chr : s.toCharArray()) {
oddCount ^= 1 << (chr - 'a');
}return Integer.bitCount(oddCount) <= k;
}
}
```
```Javascript []
/**
* @param {string} s
* @param {number} k
* @return {boolean}
*/
var canConstruct = function(s, k) {
if (s.length < k) return false;
const letters = new Array(26).fill(0);
for (const char of s) {
letters[char.charCodeAt(0) - 'a'.charCodeAt(0)]++;
}
const oddCount = letters.reduce((count, freq) => count + (freq % 2), 0);
return oddCount <= k;
};
};
```
```
✨ AN UPVOTE WILL BE APPRECIATED ^_~ ✨
```
``` | 7 | 1 | ['Python', 'C++', 'Java', 'Python3', 'JavaScript'] | 0 |
construct-k-palindrome-strings | [Java] O(n) Time and O(1) Space Beats 100% | java-on-time-and-o1-space-beats-100-by-b-a8kt | We can just simply use a 26 bit integer to store the set bits and then use XOR to flip the bits. \n\nclass Solution {\n public boolean canConstruct(String s, | blackspinner | NORMAL | 2020-04-04T18:20:33.007619+00:00 | 2022-08-20T01:02:07.700115+00:00 | 1,257 | false | We can just simply use a 26 bit integer to store the set bits and then use XOR to flip the bits. \n```\nclass Solution {\n public boolean canConstruct(String s, int k) {\n int odd = 0;\n \n for (int i = 0; i < s.length(); i++) {\n odd ^= 1 << (s.charAt(i) - \'a\');\n }\n \n return Integer.bitCount(odd) <= k && k <= s.length(); \n }\n}\n``` | 7 | 0 | [] | 1 |
construct-k-palindrome-strings | ✅100% || C++ || Python || 🎈Hash Table || ✨Bit-Set || 🚀Beginner-Friendly | 100-c-python-hash-table-bit-set-beginner-os2r | IntuitionThe main idea is to count the number of characters with odd frequencies in the string s. A palindrome can have at most one character with an odd freque | Rohithaaishu16 | NORMAL | 2025-01-11T04:56:34.154725+00:00 | 2025-01-11T04:56:34.154725+00:00 | 143 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
The main idea is to count the number of characters with odd frequencies in the string `s`. A palindrome can have at most one character with an odd frequency (this would be the middle character in case of odd-length palindromes). Therefore, if the number of characters with odd frequencies (`odd`) is less than or equal to `k`, we can construct `k` palindrome strings.
# Approach 1: Using unordered_map
- Count Character Frequencies: Using an unordered_map, count the frequency of each character in the string `s`.
- Odd Count Characters: Count how many of these characters have an odd frequency.
- Check Constructibility: Compare the count of odd frequency characters to `k`. If `odd` ≤ `k` and the length of `s` is at least `k`, return true. Otherwise, return false.
# Approach 2: Using bitset (Optimized)
- Use bitset for Frequencies: Using a bitset to keep track of odd frequencies of characters. Every time a character appears, flip its corresponding bit.
- Odd Count Characters: The number of set bits in the bitset will give the count of characters with odd frequencies.
- Check Constructibility: Compare the count of odd frequency characters to `k`. If `odd`≤ `𝑘` and the length of `𝑠` is at least `𝑘`, return true. Otherwise, return false.
<!-- Describe your approach to solving the problem. -->
# Complexity
**Approach 1: unordered_map**
- Time Complexity: $$O(n)$$, where `𝑛` is the length of the string `𝑠`.
- Space Complexity: $$O(n)$$.
**Approach 2: bitset**
- Time Complexity: $$O(n)$$.
- Space Complexity: $$O(1)$$ (more optimized).
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
if (s.length() < k) return false;
unordered_map<char, int> freq;
for (char c : s) { freq[c]++; }
int odd = 0;
for (auto& pair : freq)
if (pair.second % 2 != 0)
odd++;
return odd <= k;
}
};
```
```python []
from collections import Counter
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
if len(s) < k:
return False
freq = Counter(s)
odd = sum(1 for count in freq.values() if count % 2 != 0)
return odd <= k
```
#
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
bitset<26>odd;
for(char& c:s)
odd.flip(c-'a');
return odd.count()<=k && k<=s.length();
}
};
```
```python []
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
if len(s) < k:
return False
odd = set()
for c in s:
if c in odd:
odd.remove(c)
else:
odd.add(c)
return len(odd) <= k
``` | 6 | 0 | ['Hash Table', 'String', 'Greedy', 'Counting', 'C++', 'Python3'] | 0 |
construct-k-palindrome-strings | ✅✅ easiest approach ever 🔥🔥 | easiest-approach-ever-by-ishanbagra-nd7g | Intuition
The problem revolves around the properties of palindromes. A string can only form a palindrome if it has at most one character with an odd frequency. | ishanbagra | NORMAL | 2025-01-11T04:21:15.667218+00:00 | 2025-01-11T04:21:15.667218+00:00 | 328 | false | Intuition
The problem revolves around the properties of palindromes. A string can only form a palindrome if it has at most one character with an odd frequency. Hence, the number of palindromes we can form from a string depends on the frequency of its characters.
The key insight is:
If the length of the string is less than k, it is impossible to form k substrings.
The number of characters with an odd frequency determines the minimum number of palindromes required.
Approach
Base Cases:
If the length of s (n) is less than k, return false since we cannot split the string into more substrings than its total characters.
If n == k, return true because each character can be a palindrome substring.
Character Frequency Count:
Use an unordered map to count the frequency of each character in s.
Count Odd Frequencies:
Traverse the frequency map and count the characters with odd frequencies (count).
Check Feasibility:
If the number of odd frequencies (count) exceeds k, return false since we cannot reduce the odd frequencies below this number.
Otherwise, return true.
Complexity
Time Complexity:
Building the frequency map takes O(n), where 𝑛n is the length of the string.Traversing the map to count odd frequencies takes
O(26), as the map contains at most 26 entries (for lowercase English letters).
Overall: O(n).
Space Complexity:
The map stores at most 26 entries for the lowercase alphabet.
Overall: O(1) (constant space, independent of n).
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
int n=s.length();
if(n<k)
{
return false;
}
if(n==k)
{
return true;
}
unordered_map<char,int>map;
int count=0;
for(auto it:s)
{
map[it]++;
}
for(auto it:map)
{
if(it.second%2==1)
{
count++;
}
}
if(count>k)
{
return false;
}
return true;
}
};
```
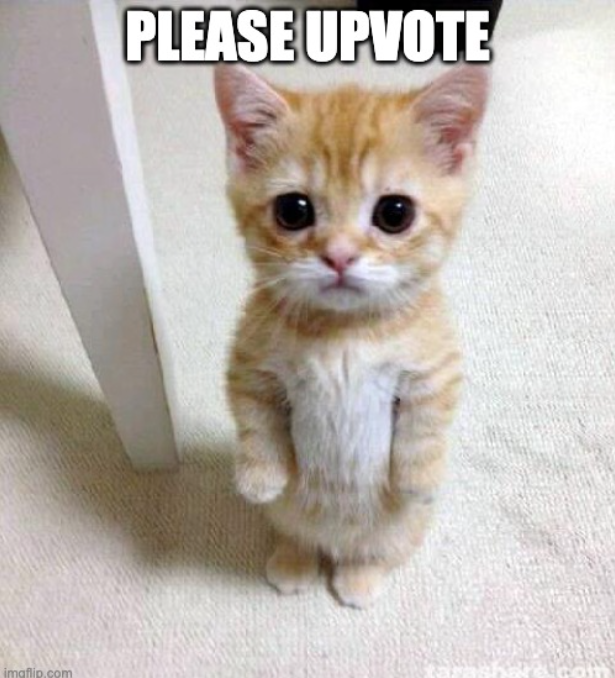
| 6 | 0 | ['C++'] | 3 |
construct-k-palindrome-strings | C++ Easy T.C.:O(n) and S.C:O(1), beats 98.4% in T.C. and 95% in S.C. | c-easy-tcon-and-sco1-beats-984-in-tc-and-dkes | Intuition: \nCount for the number of charachters having odd number of occurences\n Describe your first thoughts on how to solve this problem. \n\n# Approach:\nI | aryanshsingla | NORMAL | 2022-12-14T13:10:11.812747+00:00 | 2022-12-14T17:22:26.833179+00:00 | 1,317 | false | # Intuition: \nCount for the number of charachters having odd number of occurences\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach:\nIf the number of odd occurences of charachters are less than k, we can partition the string in k no.of palndromes.We are counting for odd occurences because eve occurences can be arranged in any order around the \'centre\' of the palindrome.And we need to check additionally, if the k is less than the length of the string to be partiotined, then we don\'t have any way do partition it.\n\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n bool canConstruct(string s, int k) {\n\n int a[26]; // to store the count of charachters\n memset(a,0,sizeof(a));\n int n=s.length();\n if(k>n)return 0;\n for(int i=0;i<n;i++)\n {\n a[s[i]-\'a\']++;\n }\n int res=0;\n for(int i=0;i<26;i++)\n {\n // if count is odd, then increment the resultant\n if(a[i]%2)res++;\n }\n return res<=k;\n }\n};\n``` | 6 | 0 | ['Greedy', 'C++'] | 0 |
construct-k-palindrome-strings | Easy C++ Explaination O(N) using Map | easy-c-explaination-on-using-map-by-avi1-mqux | class Solution {\npublic:\n\n// we can have only odd number of a particular char only one time in a pallindrome\n ```\n bool canConstruct(string s, in | avi1273619 | NORMAL | 2022-08-05T11:01:21.573137+00:00 | 2022-08-05T11:05:55.984465+00:00 | 616 | false | class Solution {\npublic:\n\n// we can have only odd number of a particular char only one time in a pallindrome\n ```\n bool canConstruct(string s, int k) \n{\n\t\t if(s.size()<k)\n\t\t\t\treturn false;\n unordered_map<char,int> frq;\n for(int i=0;i<s.size();i++)\n {\n frq[s[i]]++;\n }\n int cnt=0;\n for(auto x: frq)\n \n {\n if(x.second%2==1)\n cnt++;\n }\n if(cnt>k)\n return false;\n else\n return true;\n }\n}; | 6 | 1 | ['C'] | 1 |
construct-k-palindrome-strings | Simple JAVA Solution | simple-java-solution-by-ruchita56-jhgj | -Count all odd number of characters in string and check whether they are less or equal to k\n\npublic boolean canConstruct(String s, int k) {\n\t\tif(s.length() | ruchita56 | NORMAL | 2021-07-20T18:13:30.354024+00:00 | 2021-07-20T18:13:30.354068+00:00 | 686 | false | -Count all odd number of characters in string and check whether they are less or equal to k\n```\npublic boolean canConstruct(String s, int k) {\n\t\tif(s.length()<k) {\n\t\t\treturn false;\n\t\t}\n\t\tHashMap<Character,Integer> map=new HashMap<>();\n\t\tfor(char c:s.toCharArray()) {\n\t\t\tmap.put(c,map.getOrDefault(c, 0)+1);\n\t\t}\n\t\tint odd=0;\n\t\tfor(int count:map.values()) {\n\t\t\tif(count%2==1) {\n\t\t\t\todd++;\n\t\t\t}\n\t\t}\n\t\treturn odd<=k;\n \n }\n``` | 6 | 0 | ['Java'] | 0 |
construct-k-palindrome-strings | [Python] count odd | python-count-odd-by-zy07621-ggh8 | \nclass Solution:\n def canConstruct(self, s: str, k: int) -> bool:\n \n if len(s) < k:\n return False\n \n dic = {}\n | zy07621 | NORMAL | 2020-04-04T16:04:23.442196+00:00 | 2020-04-04T16:04:23.442249+00:00 | 708 | false | ```\nclass Solution:\n def canConstruct(self, s: str, k: int) -> bool:\n \n if len(s) < k:\n return False\n \n dic = {}\n for x in s:\n if x not in dic:\n dic[x] = 1\n else:\n dic[x] += 1\n \n odd = 0\n for key,val in dic.items(): \n odd += val % 2\n even = len(dic) - odd\n \n \n return True if odd <= k else False\n``` | 6 | 1 | [] | 2 |
construct-k-palindrome-strings | [C++] Number of Odd frequency character | c-number-of-odd-frequency-character-by-n-i1mb | If k is more than n then palindromes are never possible.\nOtherwise, if the odd frequency character count is less than k, you can always make k palindromes.\n\n | nicmit | NORMAL | 2020-04-04T16:02:44.348437+00:00 | 2020-04-04T16:07:53.691812+00:00 | 1,074 | false | If `k` is more than `n` then palindromes are never possible.\nOtherwise, if the odd frequency character count is less than `k`, you can always make `k` palindromes.\n\nFor example : `s = Leetcode` and `k = 5`\n\nWe have odd freq characters : `{L, e, t, c, o, d}` , we can create 5 palindromes, but then atleast one of the characters from these odd frequency ones, will be left, Hence false.\n\n\n```\nclass Solution {\npublic:\n bool canConstruct(string s, int k) {\n int n = s.size();\n if(k > n)\n return 0;\n if(k == n)\n return 1;\n vector<int> f(26);\n for(int i = 0; i < n; i++)\n f[s[i] - \'a\']++;\n \n int c = 0;\n for(int i = 0; i < 26; i++)\n {\n if(f[i] % 2 == 1)\n c++;\n }\n return c <= k;\n }\n};\n``` | 6 | 0 | [] | 2 |
construct-k-palindrome-strings | 3 Line | Best Easy Solution | Beginner Friendly | O(n) Time :) | 3-line-best-easy-solution-beginner-frien-jlu8 | IntuitionThe problem revolves around the concept of constructing palindromes, which is heavily influenced by the frequency of characters in the string. A palind | azhan-born-to-win | NORMAL | 2025-01-11T02:38:37.234512+00:00 | 2025-01-11T02:38:37.234512+00:00 | 227 | false | ---
# Intuition
The problem revolves around the concept of constructing palindromes, which is heavily influenced by the frequency of characters in the string. A palindrome can tolerate at most one character with an odd frequency, as this odd character can occupy the center of the palindrome. The rest of the characters must have even frequencies to ensure symmetry. Thus, the number of characters with odd frequencies dictates the minimum number of palindromes required.
---
# Approach
1. **Character Frequency Count**:
- Use a hash map (or `Counter` from Python's `collections`) to count the occurrences of each character in the string.
2. **Count Odd Frequencies**:
- Iterate through the frequency map and count how many characters have an odd frequency. This count determines the minimum number of palindromes needed.
3. **Validate Conditions**:
- Check if \( k \) is at least the number of odd frequencies (to satisfy the minimum palindrome requirement).
- Check if \( k \) is less than or equal to the length of the string (as you cannot form more palindromes than the available characters).
4. **Return the Result**:
- If both conditions are satisfied, return `True`; otherwise, return `False`.
---
# Complexity
- **Time complexity**:
- Computing the frequency of characters takes \( O(n) \), where \( n \) is the length of the string.
- Counting odd frequencies involves iterating through the hash map, which has a constant size of 26 (for lowercase English letters), making it \( O(1) \).
- Overall, the time complexity is **\( O(n) \)**.
- **Space complexity**:
- Storing the frequency count requires \( O(1) \) space since the hash map has a maximum of 26 entries (one for each lowercase English letter).
- Overall, the space complexity is **\( O(1) \)**.
---
# Code
```python3 []
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
freq = Counter(s)
odd_count = sum(1 for count in freq.values() if count % 2 != 0)
return odd_count <= k <= len(s)
``` | 5 | 0 | ['Python3'] | 1 |
construct-k-palindrome-strings | Swift 100% | swift-100-by-shafiq-bd-04-5mjo | IntuitionThe goal is to determine if it's possible to construct k palindromes using all characters of the string s. Palindromes have at most one character with | shafiq-bd-04 | NORMAL | 2025-01-11T00:27:46.158588+00:00 | 2025-01-11T00:27:46.158588+00:00 | 88 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
The goal is to determine if it's possible to construct k palindromes using all characters of the string s. Palindromes have at most one character with an odd frequency (for odd-length palindromes) or all even frequencies (for even-length palindromes). Thus, the key insight is to count the characters with odd frequencies and ensure that there are at most k of them.
# Approach
<!-- Describe your approach to solving the problem. -->
Guard Clause for Basic Check:
- If the length of the string s is less than k, it's impossible to form k palindromes because each palindrome requires at least one character.
Frequency Count:
- Use a hashmap to count the frequency of each character in s.
Count Odd Frequencies:
- Loop through the hashmap and count the number of characters that have an odd frequency. Each odd frequency corresponds to a character that would need to be placed in the middle of a palindrome.
Check Feasibility:
- If the number of odd-frequency characters (count) is less than or equal to k, it's possible to construct k palindromes. Otherwise, it’s not.
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
O(n+m)
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
O(n)
# Code
```swift []
class Solution {
func canConstruct(_ s: String, _ k: Int) -> Bool {
var hashMap : [Character : Int] = [:]
guard s.count >= k else{
return false
}
for ch in s {
hashMap[ch, default : 0] += 1
}
var count = 0
for (key, value) in hashMap {
count += (value % 2)
}
if count <= k{
return true
}else{
return false
}
}
}
``` | 5 | 0 | ['Swift'] | 1 |
construct-k-palindrome-strings | Best Solution for Arrays 🚀 in C++, Python and Java || 100% working | best-solution-for-arrays-in-c-python-and-ra0k | Intuition💡 First, we observe that a palindrome can only have at most one character with an odd frequency. If there are more odd-frequency characters than k, it' | BladeRunner150 | NORMAL | 2025-01-12T05:53:15.328990+00:00 | 2025-01-12T05:53:15.328990+00:00 | 27 | false | # Intuition
💡 First, we observe that a palindrome can only have at most **one character with an odd frequency**. If there are more odd-frequency characters than `k`, it's impossible to split the string into `k` palindromes.
# Approach
1. 🧮 Count the frequency of each character.
2. 🔢 Count how many characters have odd frequencies.
3. ❌ If the number of odd frequencies is greater than `k`, return `False`. Otherwise, return `True`.
# Complexity
- Time complexity: $$O(n)$$, where $$n$$ is the length of the string.
- Space complexity: $$O(1)$$ (using a fixed array for character frequency).
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
vector<int> arr(26, 0);
int n = s.length();
if (n < k) return false;
for (char &x : s) arr[x - 'a']++;
int odd = 0;
for (int b : arr) if (b % 2 == 1) odd++;
return (k >= odd);
}
};
```
```python []
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
from collections import Counter
n = len(s)
if n < k: return False
odd = sum(1 for count in Counter(s).values() if count % 2 == 1)
return k >= odd
```
```java []
class Solution {
public boolean canConstruct(String s, int k) {
int[] arr = new int[26];
int n = s.length();
if (n < k) return false;
for (char x : s.toCharArray()) arr[x - 'a']++;
int odd = 0;
for (int b : arr) if (b % 2 == 1) odd++;
return k >= odd;
}
}
```
<img src="https://assets.leetcode.com/users/images/7b864aef-f8a2-4d0b-a376-37cdcc64e38c_1735298989.3319144.jpeg" alt="upvote" width="150px">
# Connect with me on LinkedIn for more insights! 🌟 Link in bio | 4 | 0 | ['Hash Table', 'String', 'Greedy', 'Counting', 'Python', 'C++', 'Java', 'Python3'] | 0 |
construct-k-palindrome-strings | Chill Solution for a chill guy || 😶🌫 Chill efficienty BEATS 100%!!! 😶🌫 | chill-solution-for-a-chill-guy-chill-eff-7l9z | I'm just a chill guy that solved this problem...Chill Codeme:Please Upvote 🙏🏽🙏🏽🙏🏽!!!! | mattebiroo | NORMAL | 2025-01-11T23:15:48.043924+00:00 | 2025-01-11T23:15:48.043924+00:00 | 15 | false | I'm just a chill guy that solved this problem...
# Chill Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
unordered_map<char, int> hMap;
set<char> cKeys;
int cOdds = 0;
int cEven = 0;
int totCE = 0;
for(char ch : s){
cKeys.insert(ch);
if (hMap.find(ch) != hMap.end()) {
hMap[ch]++;
}else{
hMap[ch] = 1;
}
}
for(char ch : cKeys){
if (hMap[ch] % 2 != 0) {
cOdds++;
totCE += hMap[ch]-1;
}else{
cEven++;
totCE += hMap[ch];
}
}
if (cOdds > k) return false;
if (cOdds == k || cOdds + totCE >= k) return true;
return false;
}
};
```
### me:
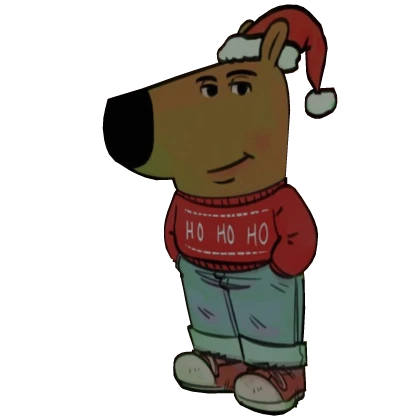
## Please Upvote 🙏🏽🙏🏽🙏🏽!!!!
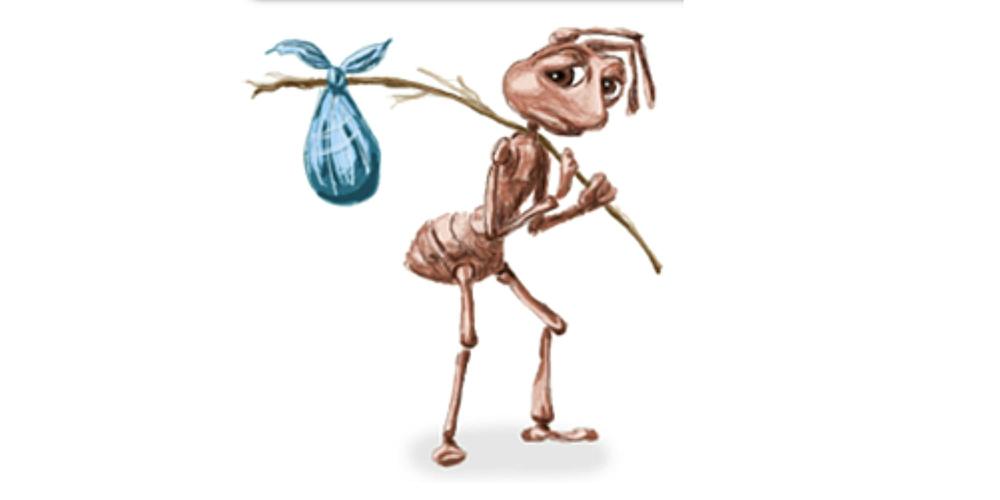
| 4 | 0 | ['C++'] | 1 |
construct-k-palindrome-strings | Simple py,JAVA code explained in detail! | simple-pyjava-code-explained-in-detail-b-e1vy | Problem understanding
Given a string s and integer k.
They are asking us to find whether it is possible to construct k palindromes.
Approach:Counting+GreedyIntu | arjunprabhakar1910 | NORMAL | 2025-01-11T14:51:31.087029+00:00 | 2025-01-11T14:51:31.087029+00:00 | 52 | false | # Problem understanding
- Given a string `s` and integer `k`.
- They are asking us to find whether it is possible to construct `k` palindromes.
---
# Approach:Counting+Greedy
<!-- Describe your approach to solving the problem. -->
# Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
- There are only *3 ways* to form a palindrome.
- All the characters frequency is ***even***.
- ***Single*** character.
- Single ***odd*** and any number of ***even*** characters.
- what we should be focused on here is that if there are more number of *odd frequency* of chars than `k` we can't distribute them within `k` palindromic sequences.
- The above logic is implemented using `hash` to find frequency and `count` to calculate the *odd freq.*
- If `count`>`k` then we can't form palindromic subsequence within `k`.
# Complexity
- Time complexity:$O(N)$
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:$O(N)$
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```Python3 []
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
if len(s)<k:
return False
hash=Counter(s)
print(hash)
count=0
for v in hash.values():
if v%2==1:
count+=1
return True if count<=k else False
```
```java []
class Solution {
public boolean canConstruct(String s, int k) {
if(s.length()<k){
return false;
}
Map<Character,Integer> hash=new HashMap<>();
for(char c:s.toCharArray()){
hash.put(c,hash.getOrDefault(c,0)+1);
}
ArrayList<Integer> val=new ArrayList<>(hash.values());
int count=0;
for(int i:val){
if(i%2==1){
count++;
}
}
if(count<=k){
return true;
}
else{
return false;
}
}
}
``` | 4 | 0 | ['Hash Table', 'String', 'Greedy', 'Counting', 'Python', 'Java'] | 0 |
construct-k-palindrome-strings | ✅ One Line Solution | one-line-solution-by-mikposp-wc4m | (Disclaimer: this is not an example to follow in a real project - it is written for fun and training mostly)Code #1Time complexity: O(n). Space complexity: O(1) | MikPosp | NORMAL | 2025-01-11T10:57:41.367499+00:00 | 2025-01-11T11:25:23.650429+00:00 | 205 | false | (Disclaimer: this is not an example to follow in a real project - it is written for fun and training mostly)
# Code #1
Time complexity: $$O(n)$$. Space complexity: $$O(1)$$.
```python3
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
return sum(f&1 for f in Counter(s).values())<=k<=len(s)
```
# Code #2
Time complexity: $$O(n)$$. Space complexity: $$O(1)$$.
```python3
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
return reduce(xor,(1<<ord(c)-97 for c in s)).bit_count()<=k<=len(s)
```
(Disclaimer 2: code above is just a product of fantasy packed into one line, it is not claimed to be 'true' oneliners - please, remind about drawbacks only if you know how to make it better) | 4 | 0 | ['Hash Table', 'String', 'Counting', 'Python', 'Python3'] | 1 |
construct-k-palindrome-strings | 🎯Easy explanation || O(n) || Greedy Approach 🚀 | easy-explanation-on-greedy-approach-by-c-jovw | Intuition
If the s.length < k we cannot construct k strings from s and answer is false.
If the number of characters that have odd counts is > k then the minimum | cs_balotiya | NORMAL | 2025-01-11T10:48:01.419589+00:00 | 2025-01-11T10:48:01.419589+00:00 | 5 | false | # Intuition
1. If the s.length < k we cannot construct k strings from s and answer is false.
2. If the number of characters that have odd counts is > k then the minimum number of palindrome strings we can construct is > k and answer is false.
3. Otherwise you can construct exactly k palindrome strings and answer is true.
# Complexity
- Time complexity:
$$O(n)$$
- Space complexity:
$$O(n)$$
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
if (s.size() < k) return false;
unordered_map<char, int> hash;
for (char ch : s) hash[ch]++;
int count = 0, optional = 0;
for (auto x : hash) {
if (x.second % 2 == 1) count++;
}
return count <= k;
}
};
```
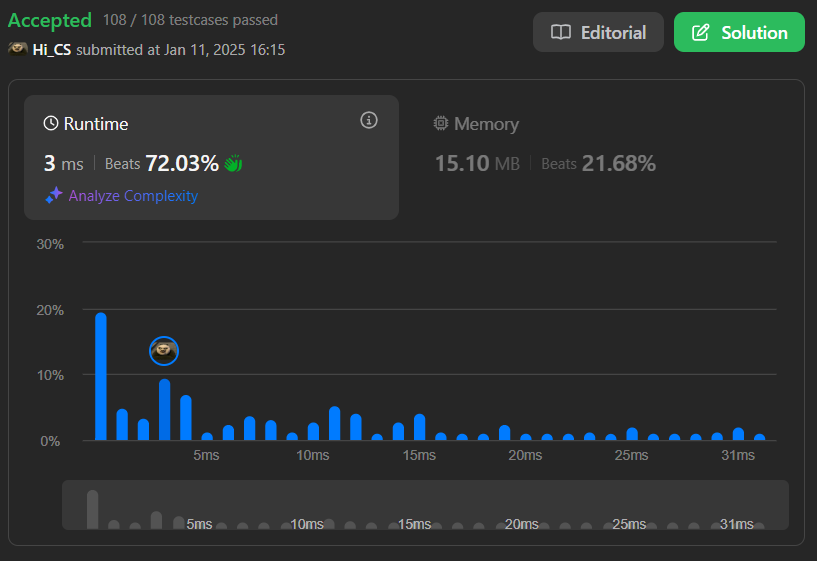
| 4 | 0 | ['C++'] | 0 |
construct-k-palindrome-strings | Eassyyy Pessyyy Solution ✅ | Runtime 91.82%🔥| TC = O(n) 🔥| SC = O(1) 🔥 | eassyyy-pessyyy-solution-runtime-9182-tc-3je3 | IntuitionThe problem involves determining whether it's possible to construct k palindrome strings from the given string s. Palindromes have specific properties: | jaitaneja333 | NORMAL | 2025-01-11T04:14:49.336665+00:00 | 2025-01-11T04:15:27.626152+00:00 | 178 | false | # Intuition
#### The problem involves determining whether it's possible to construct k palindrome strings from the given string s. Palindromes have specific properties:
* A string can be rearranged into a palindrome if at most one character has an odd frequency (for a single palindrome).
* For multiple palindromes, the total number of characters with odd frequencies must be less than or equal to the number of palindromes (k).
# Approach
1. Check the base cases:
* If the length of s equals k, return true.
* If the length of s is less than k, return false.
2. Count the frequency of each character in s using a hash map (unordered_map).
3. Count how many characters have an odd frequency.
4. If the number of characters with odd frequencies is greater than k, return false. Otherwise, return true.
# Complexity
### Time Complexity: `O(n)`
* Building the frequency map takes O(n), where n is the length of the string s.
* Iterating through the hash map to count odd frequencies takes O(m), where m is the number of unique characters in s.
* In the worst case, m=26 for lowercase English letters, making it O(1) effectively.
* Overall: O(n).
### Space Complexity: `O(1)`
* The hash map requires O(m) space, where
m is the number of unique characters in s.
* In the worst case, m=26 for lowercase English letters, making it O(1) effectively.
* Overall: O(1) additional space.
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
if(s.size() == k) return true;
if(s.size() < k) return false;
unordered_map<int,int> hmap;
int count = 0;
for(int i = 0; i < s.size(); i++){
hmap[s[i]]++;
}
for(auto it = hmap.begin(); it != hmap.end(); it++){
if(it->second % 2 == 1) count++;
}
if(count > k) return false;
return true;
}
};
```
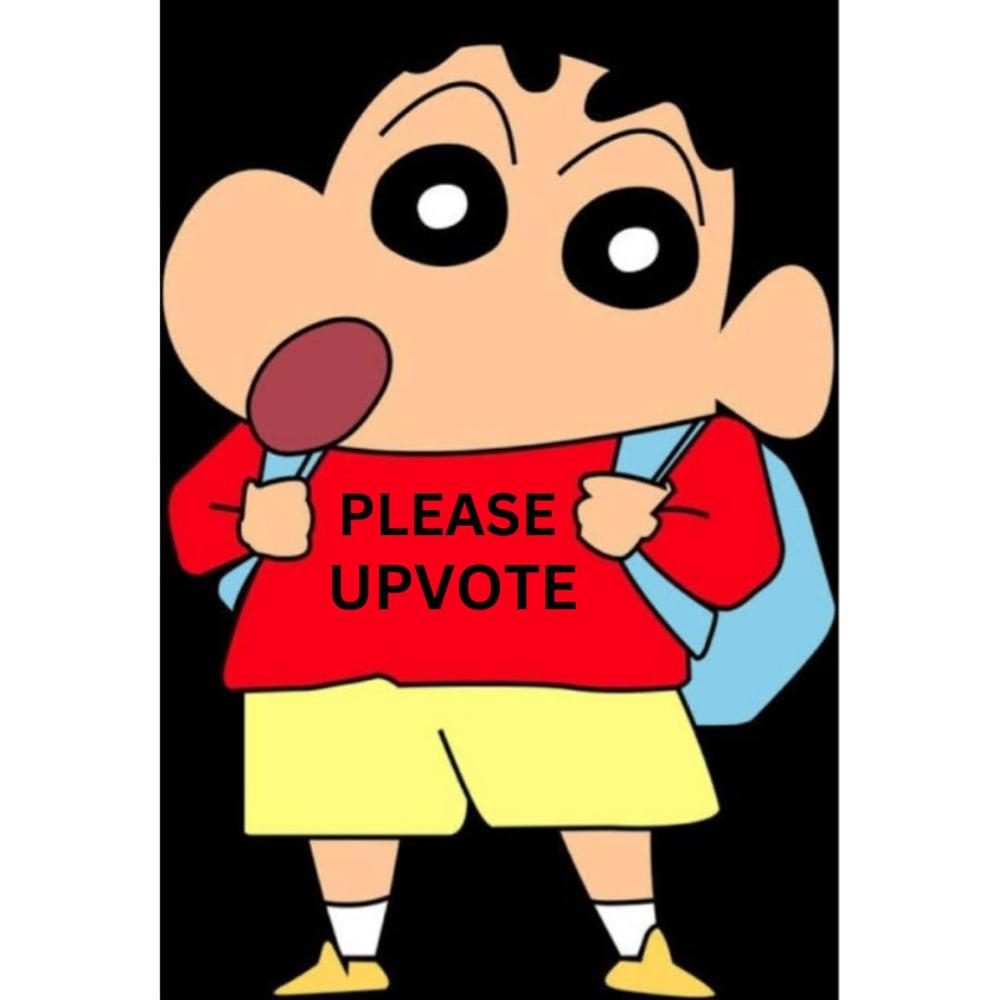
| 4 | 0 | ['Hash Table', 'String', 'Counting', 'C++'] | 3 |
construct-k-palindrome-strings | Easy C++ solution with explanation | easy-c-solution-with-explanation-by-itsm-yxv1 | IntuitionWe can make a palindrome if we have one character which occurs a single time and rest occur in pairs, or if all the characters occur in pairs.
example | itsme_S | NORMAL | 2025-01-11T03:02:11.651362+00:00 | 2025-01-11T03:02:11.651362+00:00 | 78 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
We can make a palindrome if we have one character which occurs a single time and rest occur in pairs, or if all the characters occur in pairs.
example - in aabaa: a occurs in pairs two times while b occurs a single time
# Approach
<!-- Describe your approach to solving the problem. -->
We will count the number of times single character occurs and since we have to form k palindrome strings, we want that number to be less than or equal to k.
Also we want the string's length to atleast be equal to k.
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
O(n) because we traverse the string once and we also travel the map but since we are storing lowercase english letters hence it will be O(n + 26) which simplifies to O(n).
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
We are using a map which can at most be O(26) which is constant hence space complexity comes down to O(1).
# Code
```cpp []
class Solution {
public:
//here we can first count the number of times characters in the string.
//then if the number of characters that occur 1 times is > k then it is not possible to construct the string
//we will consider the characters in pairs
bool canConstruct(string s, int k) {
if(s.length() < k){
return false;
}
unordered_map<char, int> mp;
for( int i = 0; i < s.length(); i++ ){
mp[s[i]]++;
}
int single = 0;
for( auto val: mp ){
single += (val.second % 2);
}
return single <= k ? true : false;
}
};
``` | 4 | 0 | ['C++'] | 0 |
construct-k-palindrome-strings | 🚀 Efficient Single-Pass Solution | Fully Explained & Well-Commented 🔍| Check this out once | efficient-single-pass-solution-fully-exp-widg | IntuitionIn a palindrome, all characters must appear an even number of times, except for at most one character that can appear an odd number of times (to occupy | Bulba_Bulbasar | NORMAL | 2025-01-11T00:54:16.897402+00:00 | 2025-01-11T00:54:16.897402+00:00 | 262 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
In a palindrome, all characters must appear an even number of times, except for at most one character that can appear an odd number of times (to occupy the middle position). Therefore, the number of characters with odd frequencies determines the minimum number of palindromic strings that can be formed. Each character with an odd frequency must be placed in the center of a separate palindrome.
---
# Approach
<!-- Describe your approach to solving the problem. -->
1. Edge Case Check: If the length of the string s is less than k, it's impossible to split it into k non-empty palindromic strings.
2. Frequency Count: Iterate through the string and maintain a frequency array to count occurrences of each character.
3. Odd Frequency Count: Iterate through the frequency array and count how many characters have an odd frequency.
4. Validation:
- If the number of odd-frequency characters is greater than k, forming k palindromic strings is impossible.
- If it's less than or equal to k, it's possible, so return true.
---
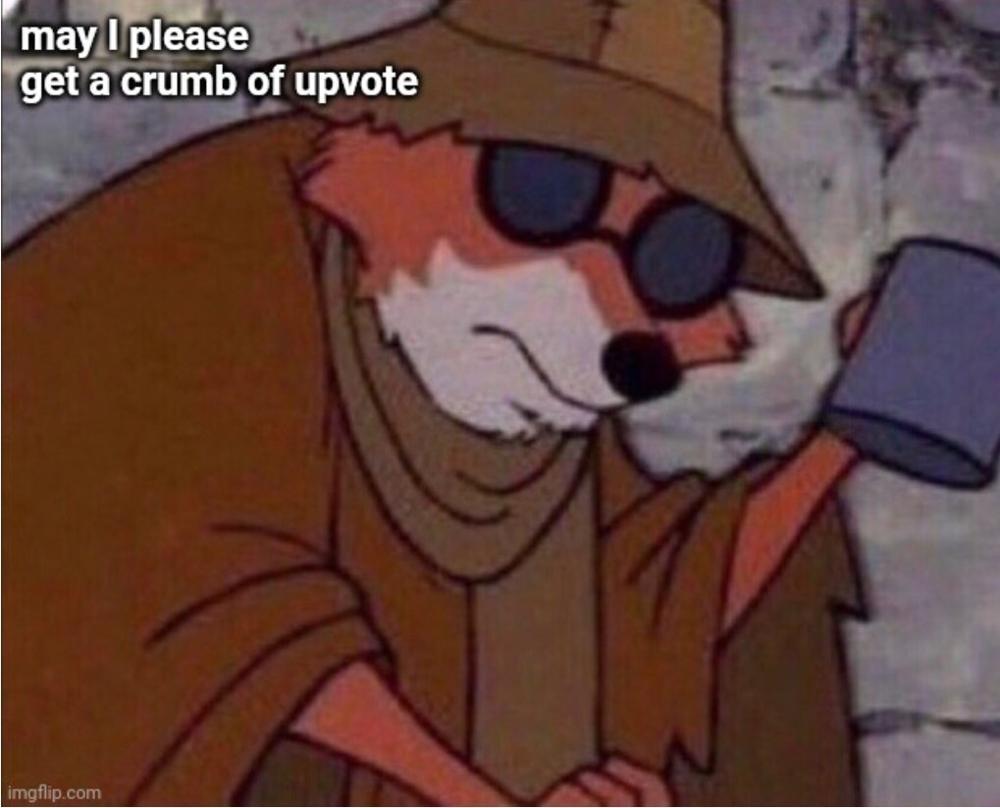
---
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
O(n)
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
O(1)
---
# Code
```java []
class Solution {
public boolean canConstruct(String s, int k) {
// If the length of the string is less than k, it's impossible to form k non-empty palindromic strings
if (s.length() < k) {
return false;
}
// Frequency array to count occurrences of each character (assuming lowercase English letters)
int[] freq = new int[26];
// Convert the string to a character array for easy iteration
char[] characters = s.toCharArray();
// Variable to count the number of characters with odd frequencies
int unique = 0;
// Count the frequency of each character in the string
for (char c : characters) {
freq[c - 'a']++;
}
// Count how many characters have an odd frequency
for (int i : freq) {
if (i % 2 != 0) {
unique++;
}
}
// The minimum number of palindromic strings needed is equal to the number of odd frequency characters
// If unique <= k, it's possible to form k palindrome strings
return unique <= k;
}
}
```
```C# []
public class Solution {
public bool CanConstruct(string s, int k) {
// If the string length is less than k, it's impossible to form k palindromic strings
if (s.Length < k) {
return false;
}
// Frequency array for lowercase English letters
int[] freq = new int[26];
// Count the frequency of each character
foreach (char c in s) {
freq[c - 'a']++;
}
// Count characters with odd frequencies
int oddCount = 0;
foreach (int count in freq) {
if (count % 2 != 0) {
oddCount++;
}
}
// If odd frequencies are <= k, it's possible to form k palindromic strings
return oddCount <= k;
}
}
```
```C++ []
class Solution {
public:
bool canConstruct(string s, int k) {
// If the string length is less than k, it's impossible to form k palindromic strings
if (s.length() < k) {
return false;
}
// Frequency array for lowercase English letters
int freq[26] = {0};
// Count the frequency of each character
for (char c : s) {
freq[c - 'a']++;
}
// Count characters with odd frequencies
int oddCount = 0;
for (int i = 0; i < 26; i++) {
if (freq[i] % 2 != 0) {
oddCount++;
}
}
// If odd frequencies are <= k, it's possible to form k palindromic strings
return oddCount <= k;
}
};
```
```C []
bool canConstruct(char* s, int k) {
int len = strlen(s);
// If the string length is less than k, it's impossible to form k palindromic strings
if (len < k) {
return false;
}
// Frequency array for lowercase English letters
int freq[26] = {0};
// Count the frequency of each character
for (int i = 0; i < len; i++) {
freq[s[i] - 'a']++;
}
// Count characters with odd frequencies
int oddCount = 0;
for (int i = 0; i < 26; i++) {
if (freq[i] % 2 != 0) {
oddCount++;
}
}
// If odd frequencies are <= k, it's possible to form k palindromic strings
return oddCount <= k;
}
```
```javascript []
/**
* @param {string} s
* @param {number} k
* @return {boolean}
*/
var canConstruct = function(s, k) {
// If the string length is less than k, it's impossible to form k palindromic strings
if (s.length < k) {
return false;
}
// Frequency map for character counts
const freq = new Array(26).fill(0);
// Count the frequency of each character
for (let char of s) {
freq[char.charCodeAt(0) - 'a'.charCodeAt(0)]++;
}
// Count characters with odd frequencies
let oddCount = 0;
for (let count of freq) {
if (count % 2 !== 0) {
oddCount++;
}
}
// If odd frequencies are <= k, it's possible to form k palindromic strings
return oddCount <= k;
};
```
---
| 4 | 0 | ['Hash Table', 'String', 'C', 'C++', 'Java', 'JavaScript', 'C#'] | 2 |
construct-k-palindrome-strings | simple bitmask | simple-bitmask-by-theyta-v8dp | IntuitionApproachComplexity
Time complexity:
O(n)
Space complexity:
O(1)Code | theyta | NORMAL | 2025-01-11T00:23:35.368907+00:00 | 2025-01-11T00:23:35.368907+00:00 | 80 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
$$O(n)$$
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
$$O(1)$$
# Code
```rust []
impl Solution {
pub fn can_construct(s: String, k: i32) -> bool {
// we can pair letters together to extend palendromes
// so we only need to care about if theres an odd or even number of each letter
// we will use a bitmask to store this information.
// for each palendrome we can use up one extra odd count char,
// so all we need to do is check if theres <= k odd letters.
//
// the question wants *exactly* k palendromes
// instead of *up to* k palendromes,
// so we need to check theres at least k characters in s first.
s.len() >= k as usize
&& s.bytes()
.fold(0u32, |acc, b| acc ^ (1 << b-b'a'))
.count_ones() <= k as u32
}
}
``` | 4 | 0 | ['Rust'] | 2 |
construct-k-palindrome-strings | [javascript] -One Line Solution | javascript-one-line-solution-by-charnavo-rfev | please upvote, you motivate me to solve problems in original ways | charnavoki | NORMAL | 2025-01-11T00:20:18.657737+00:00 | 2025-01-11T00:20:18.657737+00:00 | 191 | false |
```javascript []
const canConstruct = (s, k, frequencies = Object.values([...s].reduce((o, v) => (o[v] = (o[v]|0) + 1, o), {}))) =>
s.length >= k && frequencies.filter(n => n%2).length <= k;
```
#### please upvote, you motivate me to solve problems in original ways | 4 | 0 | ['JavaScript'] | 0 |
construct-k-palindrome-strings | JAVA SOLUTION | java-solution-by-tejasmesta-ok7c | class Solution {\n public boolean canConstruct(String s, int k) {\n\t\n int n = s.length();\n \n if(n<k)\n {\n return | tejasmesta | NORMAL | 2022-09-12T14:30:20.691785+00:00 | 2022-09-12T14:30:20.691846+00:00 | 745 | false | class Solution {\n public boolean canConstruct(String s, int k) {\n\t\n int n = s.length();\n \n if(n<k)\n {\n return false;\n }\n \n HashMap<Character,Integer> map = new HashMap<>();\n \n for(int i=0;i<n;i++)\n {\n char c = s.charAt(i);\n map.put(c,map.getOrDefault(c,0)+1);\n }\n \n int ans = 0; \n \n for(Map.Entry<Character,Integer> e:map.entrySet())\n {\n int v = e.getValue();\n \n if(v%2!=0)\n {\n ans++;\n }\n }\n \n return ans<=k;\n }\n} | 4 | 0 | ['Java'] | 1 |
construct-k-palindrome-strings | C++ solution based on pidgeonhole principle | c-solution-based-on-pidgeonhole-principl-z4rn | If we have more odd frequency characters than palindromes, then at least 2 odd frequency characters must be in the same palindrome. Since this is not possible, | nc_ | NORMAL | 2020-07-07T17:52:15.758997+00:00 | 2020-07-07T17:52:15.759029+00:00 | 347 | false | If we have more odd frequency characters than palindromes, then at least 2 odd frequency characters must be in the same palindrome. Since this is not possible, we return true if we have fewer or equal to k odd frequency characters.\n\n```\nclass Solution {\npublic:\n bool canConstruct(string s, int k) {\n if (s.size() < k) return false;\n \n vector<int> count(26, 0);\n for (char c: s)\n count[c-\'a\']++;\n \n int countOdd = count_if(count.begin(), count.end(), [](int i) {\n return i % 2;\n });\n \n // Pidgeonhole, 2 odd freq chars must be in a palindrome, which is impossible \n return countOdd <= k;\n }\n};\n``` | 4 | 0 | [] | 0 |
construct-k-palindrome-strings | python 1line | python-1line-by-shtarkon-lzic | \nreturn len(s) >= k and sum(i % 2 for i in Counter(s).values()) <= k\n | shtarkon | NORMAL | 2020-04-04T16:08:55.695758+00:00 | 2020-04-04T16:08:55.695812+00:00 | 192 | false | ```\nreturn len(s) >= k and sum(i % 2 for i in Counter(s).values()) <= k\n``` | 4 | 2 | [] | 1 |
construct-k-palindrome-strings | 💢Faster✅💯 Lesser C++✅Python3🐍✅Java✅C✅Python🐍✅C#✅💥🔥💫Explained☠💥🔥 Beats 💯 | faster-lesser-cpython3javacpythoncexplai-bnow | IntuitionApproach
JavaScript Code --> https://leetcode.com/problems/construct-k-palindrome-strings/submissions/1505165242
C++ Code --> https://leetcode.com/prob | Edwards310 | NORMAL | 2025-01-11T15:10:27.148430+00:00 | 2025-01-11T15:10:27.148430+00:00 | 57 | false | 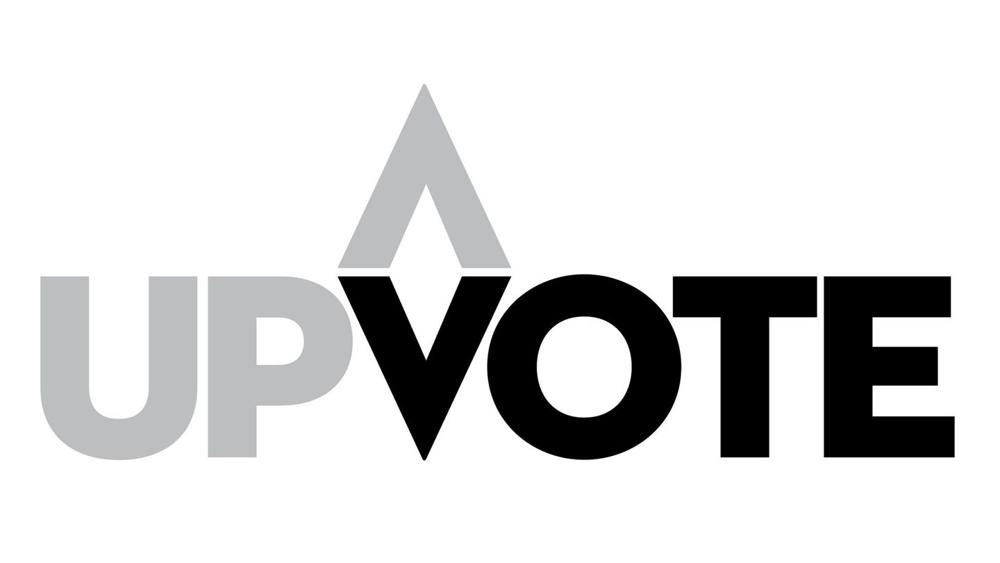
# Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your first thoughts on how to solve this problem. -->
- ***JavaScript Code -->*** https://leetcode.com/problems/construct-k-palindrome-strings/submissions/1505165242
- ***C++ Code -->*** https://leetcode.com/problems/construct-k-palindrome-strings/submissions/1505140603
- ***Python3 Code -->*** https://leetcode.com/problems/construct-k-palindrome-strings/submissions/1505140291
- ***Java Code -->*** https://leetcode.com/problems/construct-k-palindrome-strings/submissions/1505144477
- ***C Code -->*** https://leetcode.com/problems/construct-k-palindrome-strings/submissions/1505148100
- ***Python Code -->*** https://leetcode.com/problems/construct-k-palindrome-strings/submissions/1505139783
- ***C# Code -->*** https://leetcode.com/problems/construct-k-palindrome-strings/submissions/1505155248
- ***Go Code -->*** https://leetcode.com/problems/construct-k-palindrome-strings/submissions/1505168958
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
O(N)
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
O(1)
# Code
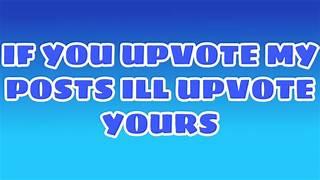 | 3 | 0 | ['Hash Table', 'C', 'Counting', 'Python', 'C++', 'Java', 'Go', 'Python3', 'JavaScript', 'C#'] | 0 |
construct-k-palindrome-strings | Easiest C++ Solution | Count Method | easiest-c-solution-count-method-by-vinee-id0a | IntuitionApproachComplexity
Time complexity:
Space complexity:
Code | vineetvermaa30 | NORMAL | 2025-01-11T11:45:38.454872+00:00 | 2025-01-11T11:45:38.454872+00:00 | 84 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
// If the s.length < k we cannot construct k strings from s, hence answer is false.
if(s.length()<k) return false;
if(s.length()==k) return true;
unordered_map<char,int> mp;
for(auto ch: s) mp[ch]++;
int oddCharactersCount = 0;
for(auto ch: mp) if(ch.second%2 == 1) oddCharactersCount++;
// If the number of characters that have odd counts is > k then, k palindrome
// string can't be form, hence return false
// Otherwise you can construct exactly k palindrome strings and answer is true
return oddCharactersCount<=k;
}
};
``` | 3 | 0 | ['C++'] | 0 |
construct-k-palindrome-strings | 🔥BEATS 💯 % 🎯 |✨SUPER EASY BEGINNERS 👏| With illustrative diagram | beats-super-easy-beginners-with-illustra-sfkp | Problem: Construct k Palindrome StringsGiven a string s and an integer k, determine if it is possible to construct exactly k palindrome strings using all the ch | CodeWithSparsh | NORMAL | 2025-01-11T11:39:19.583973+00:00 | 2025-01-11T11:42:53.384929+00:00 | 63 | false | 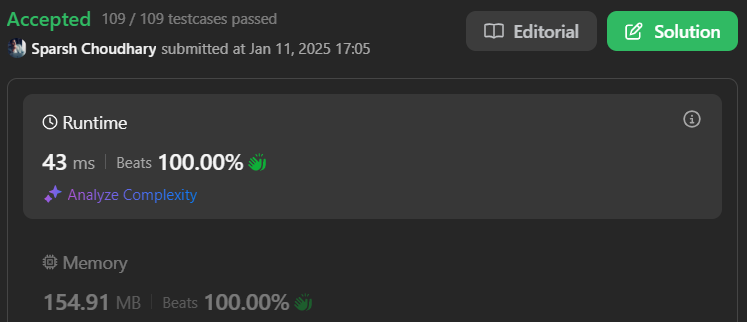
---
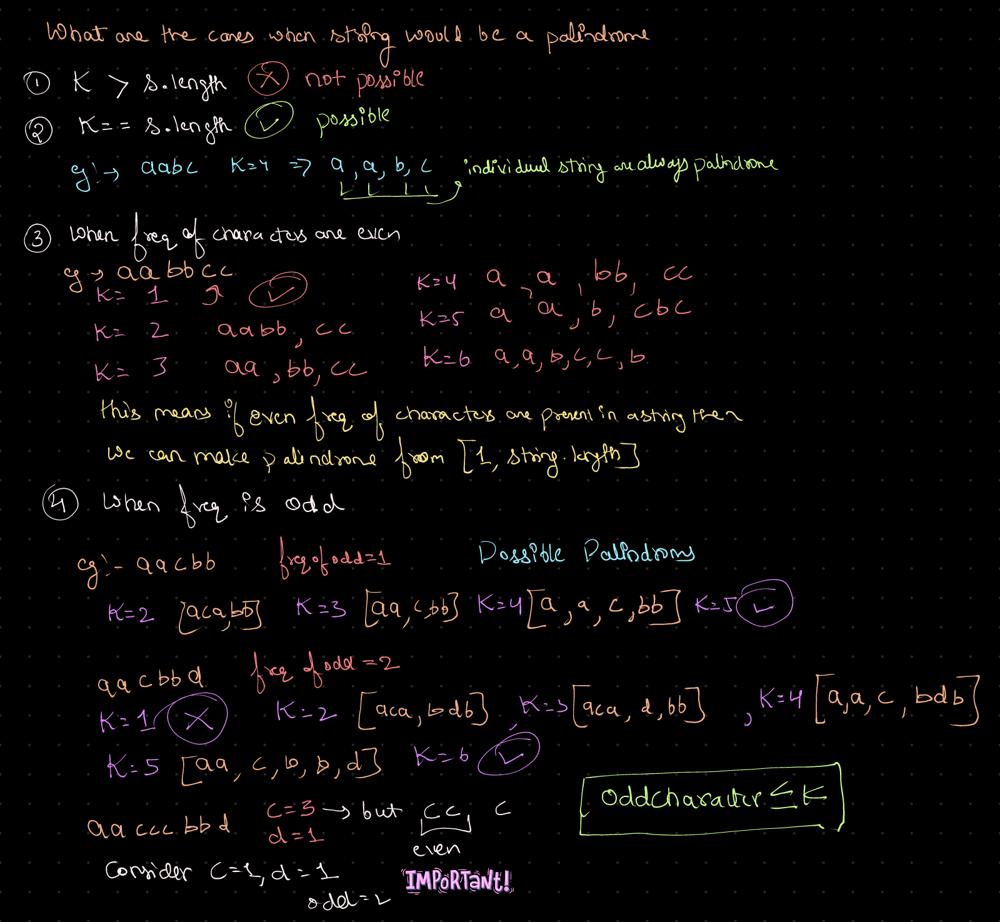
---
### Problem: Construct k Palindrome Strings
Given a string `s` and an integer `k`, determine if it is possible to construct exactly `k` palindrome strings using all the characters of `s`.
---
### **Intuition**
1. **Palindrome Basics**:
- A palindrome requires characters to appear in pairs, except for at most one character that can appear an odd number of times (for the middle of the palindrome).
2. **Core Idea**:
- If the number of characters with odd frequencies in `s` is greater than `k`, it's impossible to construct `k` palindromes, as each palindrome can only tolerate one odd frequency character.
- Additionally, if the total length of the string is less than `k`, it's impossible to construct `k` palindromes because each palindrome must have at least one character.
---
### **Approach**
1. **Initial Checks**:
- If the length of `s` is less than `k`, return `false` (not enough characters for `k` palindromes).
- If the length of `s` equals `k`, return `true` (each character can be its own palindrome).
2. **Count Character Frequencies**:
- Use a map to count the frequency of each character in `s`.
3. **Count Odd Frequencies**:
- Iterate over the frequency map to count characters with odd frequencies.
4. **Final Check**:
- If the number of odd-frequency characters is less than or equal to `k`, return `true` (it's possible to group them into palindromes).
- Otherwise, return `false`.
---
### **Complexity**
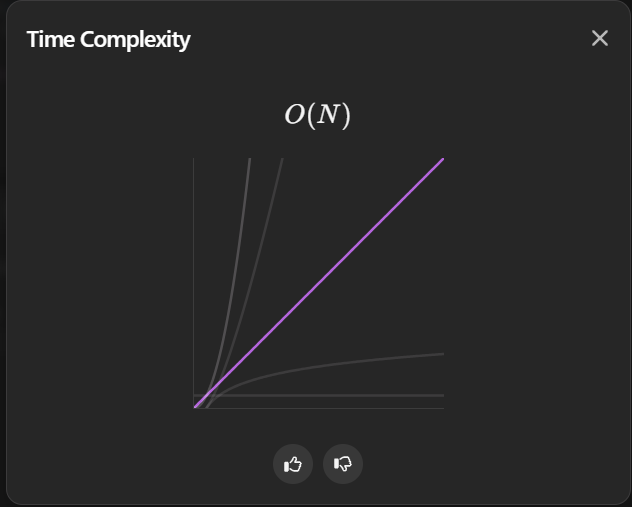
- Counting character frequencies: $$O(n)$$
- Iterating through the frequency map: $$O(26)$$ (constant for a fixed alphabet size, e.g., lowercase English letters).
**Overall**: $$O(n)$$.
- **Space Complexity**:
- Frequency map requires $$O(26)$$ space for a fixed alphabet.
**Overall**: $$O(1)$$ for constant space (fixed alphabet).
---
```dart []
class Solution {
bool canConstruct(String s, int k) {
// If length of string is less than k, it's not possible
if (s.length < k) return false;
// If length equals k, every character can form its own palindrome
if (s.length == k) return true;
// Count character frequencies
Map<String, int> freqMap = {};
for (String char in s.split('')) {
freqMap[char] = (freqMap[char] ?? 0) + 1;
}
// Count characters with odd frequencies
int oddCount = 0;
for (var freq in freqMap.values) {
if (freq.isOdd) oddCount++;
}
// If odd frequencies are less than or equal to k, return true
return oddCount <= k;
}
}
```
```python []
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
# If length of string is less than k, it's not possible
if len(s) < k:
return False
# If length equals k, each character can form its own palindrome
if len(s) == k:
return True
# Count character frequencies
from collections import Counter
freq = Counter(s)
# Count characters with odd frequencies
odd_count = sum(1 for count in freq.values() if count % 2 != 0)
# If odd frequencies are less than or equal to k, return true
return odd_count <= k
```
```java []
import java.util.*;
class Solution {
public boolean canConstruct(String s, int k) {
// If length of string is less than k, it's not possible
if (s.length() < k) return false;
// If length equals k, each character can form its own palindrome
if (s.length() == k) return true;
// Count character frequencies
Map<Character, Integer> freqMap = new HashMap<>();
for (char c : s.toCharArray()) {
freqMap.put(c, freqMap.getOrDefault(c, 0) + 1);
}
// Count characters with odd frequencies
int oddCount = 0;
for (int freq : freqMap.values()) {
if (freq % 2 != 0) oddCount++;
}
// If odd frequencies are less than or equal to k, return true
return oddCount <= k;
}
}
```
```cpp []
#include <unordered_map>
#include <string>
using namespace std;
class Solution {
public:
bool canConstruct(string s, int k) {
// If length of string is less than k, it's not possible
if (s.length() < k) return false;
// If length equals k, each character can form its own palindrome
if (s.length() == k) return true;
// Count character frequencies
unordered_map<char, int> freqMap;
for (char c : s) {
freqMap[c]++;
}
// Count characters with odd frequencies
int oddCount = 0;
for (auto& [char, freq] : freqMap) {
if (freq % 2 != 0) oddCount++;
}
// If odd frequencies are less than or equal to k, return true
return oddCount <= k;
}
};
```
```javascript []
var canConstruct = function(s, k) {
// If length of string is less than k, it's not possible
if (s.length < k) return false;
// If length equals k, each character can form its own palindrome
if (s.length === k) return true;
// Count character frequencies
const freqMap = {};
for (let char of s) {
freqMap[char] = (freqMap[char] || 0) + 1;
}
// Count characters with odd frequencies
let oddCount = 0;
for (let freq of Object.values(freqMap)) {
if (freq % 2 !== 0) oddCount++;
}
// If odd frequencies are less than or equal to k, return true
return oddCount <= k;
};
```
```go []
func canConstruct(s string, k int) bool {
// If length of string is less than k, it's not possible
if len(s) < k {
return false
}
// If length equals k, each character can form its own palindrome
if len(s) == k {
return true
}
// Count character frequencies
freq := make(map[rune]int)
for _, char := range s {
freq[char]++
}
// Count characters with odd frequencies
oddCount := 0
for _, count := range freq {
if count%2 != 0 {
oddCount++
}
}
// If odd frequencies are less than or equal to k, return true
return oddCount <= k
}
```
---
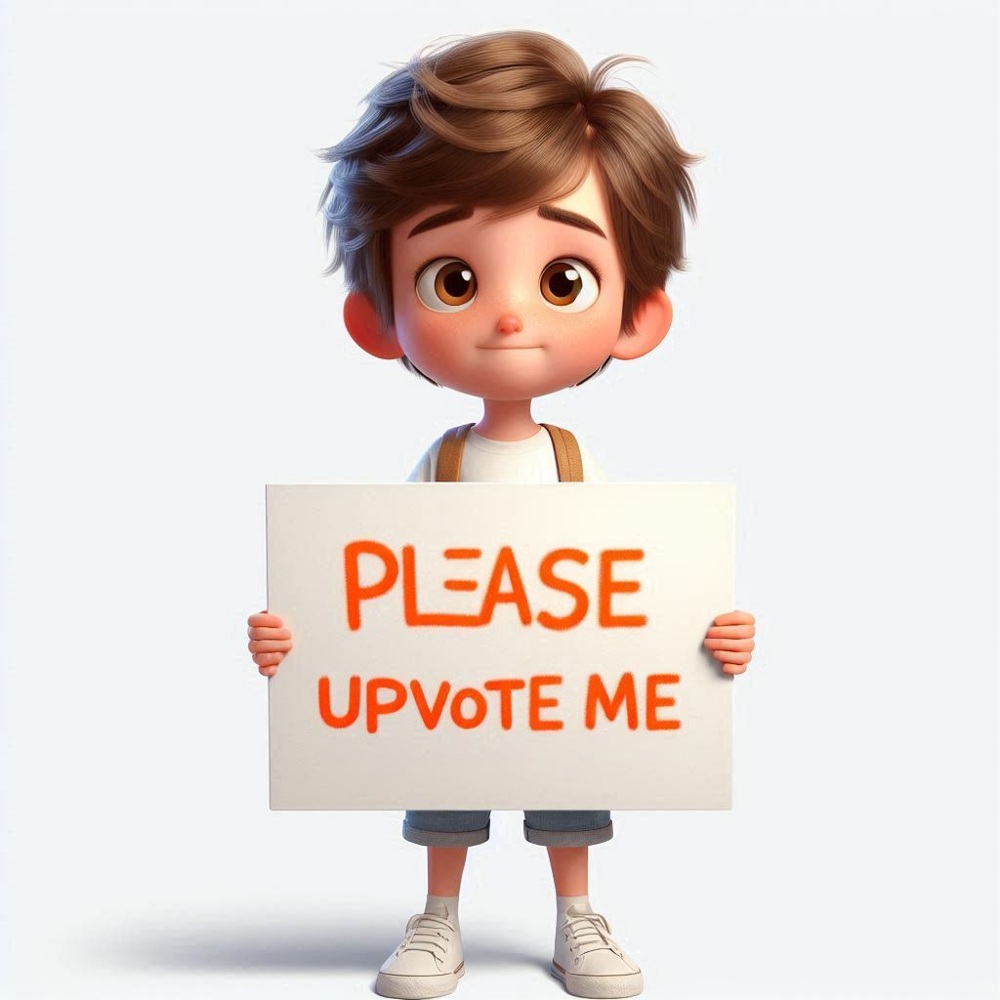 {:style='width:250px'} | 3 | 0 | ['Hash Table', 'Greedy', 'C', 'Counting', 'C++', 'Java', 'Go', 'Python3', 'JavaScript', 'Dart'] | 2 |
construct-k-palindrome-strings | ✅ Easiest & Efficient code | Beats 100% 💯 | easiest-efficient-code-beats-100-by-yoge-0k0b | IntuitionTo form a palindrome:
Characters with even frequencies can be fully used without issues.
Characters with odd frequencies require at least one separate | yogeshm01 | NORMAL | 2025-01-11T11:39:11.177841+00:00 | 2025-01-11T11:39:11.177841+00:00 | 14 | false | # Intuition
To form a palindrome:
- Characters with even frequencies can be fully used without issues.
- Characters with odd frequencies require at least one separate palindrome to fit their "extra" character.
So, the key idea is:
- Count how many characters have odd frequencies.
- If the number of these "odd characters" is less than or equal to k, it's possible to form k palindromes.
- If k is greater than the length of the string s, it's impossible because you can't create more palindromes than available characters.
# Approach
Initial Check:
1. If k is greater than the length of the string s, it's impossible to form k palindromes, as each palindrome requires at least one character.
2. Character Frequency Count:
Use a dictionary (or a collections.Counter) to count the frequency of each character in the string.
3. Count Odd Frequencies:
Iterate through the frequency counts and count how many characters have an odd frequency.
4. Decision:
If the number of odd-frequency characters is less than or equal to k, it's possible to form k palindromes. Otherwise, it's not.
# Complexity
- Time complexity:
$$O(n)$$, where 𝑛 is the length of the string s.
Counting the frequency of characters takes 𝑂(𝑛).
- Space complexity:
O(1) (constant)
# Code
```python []
class Solution(object):
def canConstruct(self, s, k):
if len(s)<k:
return False
char = {}
for i in s:
if i in char:
char[i]+=1
else:
char[i] = 1
odd_count = 0
for i in char.values():
if i % 2 != 0:
odd_count += 1
return odd_count <= k
``` | 3 | 0 | ['Python'] | 0 |
construct-k-palindrome-strings | construct-k-palindrome-strings | construct-k-palindrome-strings-by-yadav_-brkj | Code | Yadav_Akash_ | NORMAL | 2025-01-11T09:30:12.748723+00:00 | 2025-01-11T09:30:12.748723+00:00 | 14 | false |
# Code
```java []
class Solution {
public boolean canConstruct(String s, int k) {
if(k>s.length()){
return false;
}
int freq[]=new int[26];
for(int i=0;i<s.length();i++){
freq[s.charAt(i)-'a']++;
}
int count=0;
for(int i=0;i<freq.length;i++){
if(freq[i]%2!=0){
count++;
}
}
return count<=k;
}
}
``` | 3 | 0 | ['Java'] | 0 |
construct-k-palindrome-strings | Kotlin. Beats 100% (3 ms). Counting odd bits (bitwise solution). | kotlin-beats-100-3-ms-counting-odd-bits-83h02 | Code | mobdev778 | NORMAL | 2025-01-11T08:24:49.506667+00:00 | 2025-01-11T08:24:49.506667+00:00 | 39 | false | 
# Code
```kotlin []
class Solution {
fun canConstruct(s: String, k: Int): Boolean {
if (s.length < k) return false
var bits = 0
val s = s.toCharArray()
for (c in s) {
bits = bits xor (1 shl (c - 'a'))
}
return Integer.bitCount(bits) <= k
}
}
``` | 3 | 0 | ['Kotlin'] | 1 |
construct-k-palindrome-strings | 100% faster code in O(n) time and O(1) space | 100-faster-code-in-on-time-and-o1-space-11qmj | IntuitionWe need to find if we can make k palindromes by shuffling the alphabets of a string.ApproachSo we will count the number of alphabets those are present | madhavgarg2213 | NORMAL | 2025-01-11T08:09:49.347395+00:00 | 2025-01-11T08:09:49.347395+00:00 | 70 | false | # Intuition
We need to find if we can make k palindromes by shuffling the alphabets of a string.
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
So we will count the number of alphabets those are present odd number of times, as each palindrome can have at most 1 odd occurance character. hence we will output the answer.
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity: O(N)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: O(1)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
if(s.length()==k){
return true;
}
if(s.length()<k){
return false;
}
vector<int> v(26,0);
for(char c : s){
v[c-'a']++;
}
int count = 0;
for(int i : v){
if(i%2==1){
count++;
}
}
if(count<=k){
return true;
}
return false;
}
};
``` | 3 | 0 | ['String', 'Greedy', 'Counting', 'C++'] | 0 |
construct-k-palindrome-strings | 🔥 BEATS 100 %🔥✅ || Construct K Palindrome Strings in ONE PASS 💡|| Optimal Code Approach✅ | beats-100-construct-k-palindrome-strings-7bth | IntuitionA string can be rearranged into a palindrome if at most one character has an odd frequency. Given k, we need to determine if we can construct exactly k | EmPty063 | NORMAL | 2025-01-11T05:30:56.781207+00:00 | 2025-01-11T05:30:56.781207+00:00 | 45 | false | 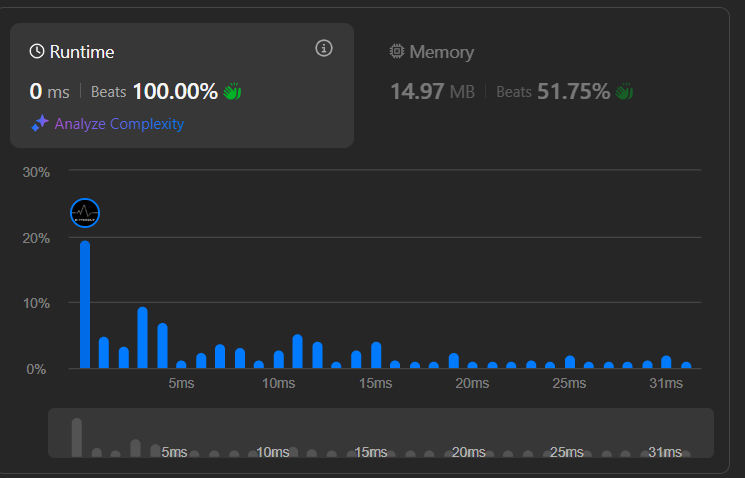
# Intuition
A string can be rearranged into a palindrome if at most one character has an odd frequency. Given `k`, we need to determine if we can construct exactly `k` palindromic strings.
1. If `s.length() < k`, it's impossible to form` k` non-empty strings, so return false.
2. We count the frequency of each character in `s`.
3. To form `k` palindromic strings, the minimum required is the number of characters with odd frequencies (since each must be placed in a separate palindrome).
4. If the number of characters with odd frequency `(oddCount) `is less than or equal to `k` , it's possible to construct k palindromes. Otherwise, return `false`.
# Approach
1. Edge Case: If `s.length() < k`, return `false`.
2. Character Frequency Count: Use an array `freq[26]` to store the count of each character.
3. Count Odd Frequencies: Traverse `freq` and count characters appearing an odd number of times.
4. Final Condition: If `oddCnt <= k`, return `true`, otherwise return `false`.
# Complexity
- Time complexity:
`𝑂(𝑛)`,where n is the length of `s` (we iterate through `s` and `freq` once).
- Space complexity:
`O(1)` (constant extra space for `freq`, since it's fixed at size `26`)
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
if(s.length()<k) return false;
vector<int> freq(26,0);
for(char c: s) freq[c-'a']++;
int oddcnt = 0 ;
for(int cnt:freq){
if(cnt%2 == 1) oddcnt++;
}
return oddcnt<=k;
}
};
```
### Upvote Plz
| 3 | 0 | ['String', 'C++'] | 0 |
construct-k-palindrome-strings | Construct k palindrome strings - Easy Explanation 🔜 | construct-k-palindrome-strings-easy-expl-0smr | Intuition
If the number of palindrome k is greater than the length of the string s, then no such k palindrome can be made from the string s.
If the number of | RAJESWARI_P | NORMAL | 2025-01-11T05:27:36.650336+00:00 | 2025-01-11T05:27:36.650336+00:00 | 25 | false | # Intuition
1. If the number of palindrome k is greater than the length of the string s, then no such k palindrome can be made from the string s.
1. If the number of palindrome k is equal to the length of the string s, then each single character in the string s is actually palindrome.
1. Atleast each palindrome constructed can have atmost one character with the odd Frequency.
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
**Initialization**:
Initialize n to be the number of characters in the string s.
int n=s.length();
**Precondition**:
Check if the number of palindrome k is greater than the length of the string s , then return false.
if(k>n)
return false;
Check if the number of palindrome k equals the length of the string s, then return true.
if(k==n)
return true;
**Calculate frequency of each character in string s**:
Initialize count to be the integer array with the size of 26.
int[] count=new int[26];
Iterate through all the characters in the string s,then update the count of each characters in the count array.
for(char ch:s.toCharArray())
{
count[ch-'a']++;
}
**Find number of characters with odd Frequency**:
Iterate through all the elements of the count array and update the OddCnt if the count of the frequency of each character is odd
(count[i]%2!=0).
int oddCnt=0;
for(int i=0;i<26;i++)
{
if(count[i]%2!=0)
oddCnt++;
}
**Finalizing result**:
If the characters with odd frequency is less than or equal to k and k should be less than or equal to n(this means that each palindrome must have atmost one odd Frequency), then return true.
if(oddCnt<=k && k<=n)
return true;
Otherwise return false, since no such k palindromic strings can be formed from the string of length n.
return false;
<!-- Describe your approach to solving the problem. -->
# Complexity
- **Time complexity**: **O(n)**.
n -> number of characters in the string s.
**Length of the string s**: **O(n)**.
Calculating the length of the string s iterates through all the elements of the string s.Hence it's time complexity is **O(n)**.
**Iterate through string to populate frequency**: **O(n)**.
To populate frequency of the characters in the count array, iterates through all the characters of the string s, Hence it takes
**O(n) time**.
**Iterate through count array**: **O(1)**.
Iterate through the count array 26 times and hence it takes constant time complexity of **O(26) which will be O(1)**.
**Overall Time Complexity**: **O(n)**.
Hence the overall time complexity of the above program can be
**O(n)+O(n)+O(1) = O(n) time**.
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- ***Space complexity***: **O(1)**.
The count array is used whose size is independent of the input size n, hence it takes **constant space**.
Hence it's space complexity is **O(1)**.
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public boolean canConstruct(String s, int k) {
int n=s.length();
if(k>n)
return false;
if(k==n)
return true;
int[] count=new int[26];
for(char ch:s.toCharArray())
{
count[ch-'a']++;
}
int oddCnt=0;
for(int i=0;i<26;i++)
{
if(count[i]%2!=0)
oddCnt++;
}
if(oddCnt<=k && k<=n)
return true;
return false;
}
}
``` | 3 | 0 | ['String', 'Counting', 'Java'] | 0 |
construct-k-palindrome-strings | Java 🍵 | Easy ✅ | Beats 95% 🔥 | TC: O(n) 📈 | SC: O(1) 🤯 - Optimal Solution | java-easy-beats-95-tc-on-sc-o1-optimal-s-17zu | ComplexityCode | mani-26 | NORMAL | 2025-01-11T03:06:31.191550+00:00 | 2025-01-11T03:06:31.191550+00:00 | 117 | false | # Complexity
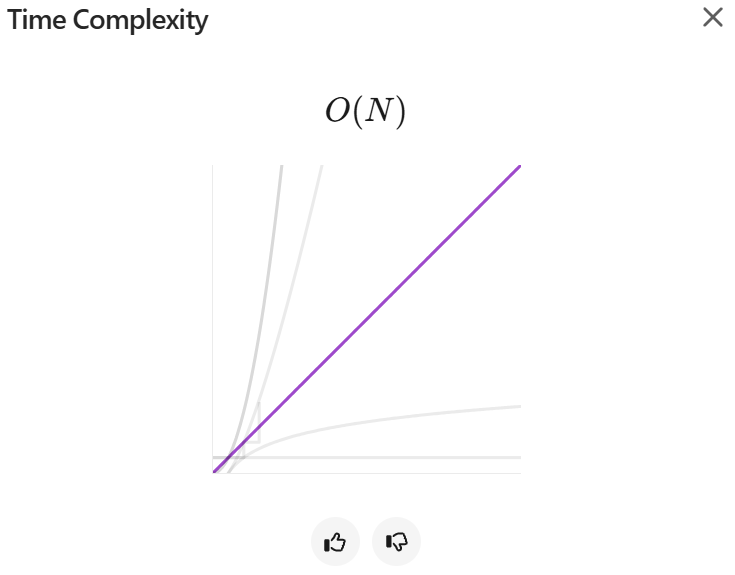
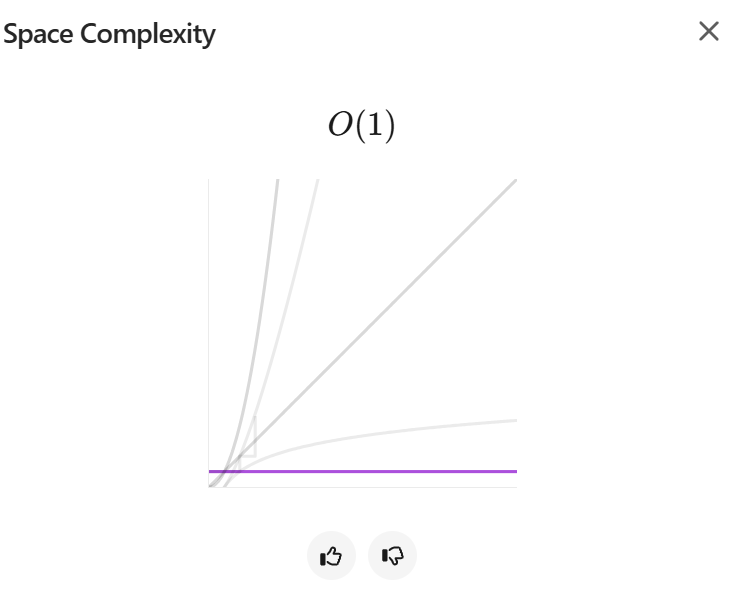
# Code
```java []
class Solution {
public boolean canConstruct(String s, int k) {
int n=s.length();
if(k>n) return false;
int freq[]=new int[26];
int oddCount=0;
for(int i=0;i<n;i++){
freq[s.charAt(i)-'a']++;
}
for(int i=0;i<26;i++){
if(freq[i]%2==1){
oddCount++;
}
}
if(oddCount>k) return false;
return true;
}
}
```
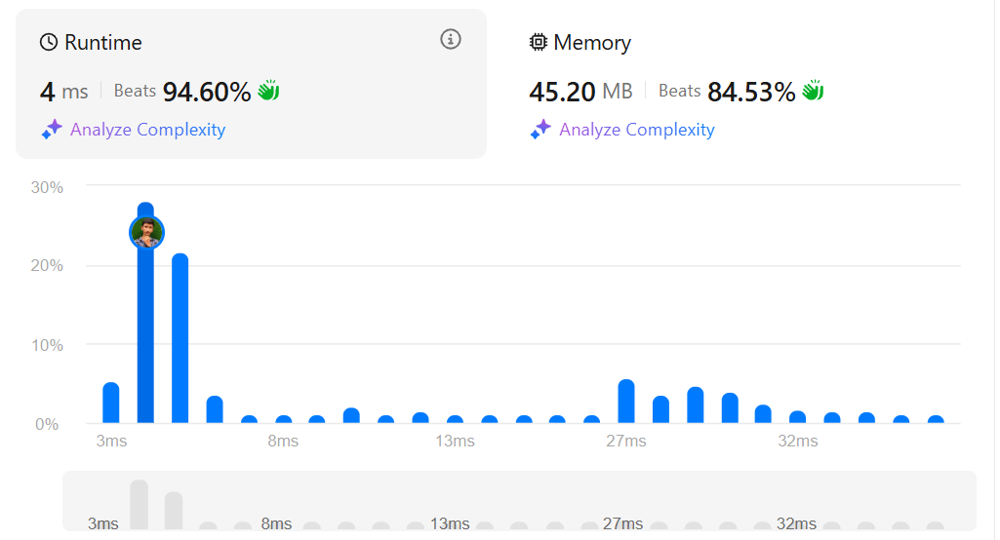
| 3 | 0 | ['Hash Table', 'String', 'Greedy', 'Counting', 'Java'] | 0 |
construct-k-palindrome-strings | Only Count Odd Frequencies - Explanation | C++, Python, Java | only-count-odd-frequencies-explanation-c-b0hy | ApproachWe can use a greedy approach to find our solution. In our greedy approach, we'll mostly only focus on palindromes consisting of one specific character u | not_yl3 | NORMAL | 2025-01-11T00:43:43.283561+00:00 | 2025-01-11T00:43:43.283561+00:00 | 281 | false | # Approach
<!-- Describe your approach to solving the problem. -->
We can use a greedy approach to find our solution. In our greedy approach, we'll mostly only focus on palindromes consisting of one specific character until the end. First we'll get the frequency of each character in `s` with a frequency array `freq[26]`.
Firstly, if `s.length()` we can return False immediately since it would be impossible to form $$k$$ palindromes with less than $$k$$ characters.
For this problem, we only need to check the number of characters with odd frequencies is less than `k`. In a valid palindrome, only one character can have an odd frequency with every other having an even frequency to help mirror the string around the center. Therefore, when we have characters with odd frequencies in our greedy approach, they must stand alone. This makes the minimum number of palindromes we can form with all the characters in `s` equal to the number of characters with odd frequencies.
But what about characters with even frequencies. These actually don't create any additional constraints on the problem as in the end they can be distributed in any way needed. For example, if we have 5 characters with odd frequencies (meaning 5 palindromes already), `k = 6`, and 20 other unique characters each with even frequencies, we can simply create 1 palindrome with the rest of the characters since they all have even frequencies.
The same is true for the odd frequency characters. If there's less tha k of them then we can create the other needed palindromes either with single characters or combining them with the other even-frequency characters.
# Complexity
- Time complexity: $$O(n)$$
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: $$O(1)$$
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```C++ []
class Solution {
public:
bool canConstruct(string s, int k) {
if (s.length() < k)
return false;
int freq[26] = {};
for (char c : s)
freq[c - 'a']++;
for (int i = 0; i < 26; i++)
if ((k -= (freq[i] & 1)) < 0)
return false;
return true;
}
};
```
```python []
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
return len(s) >= k and sum(c & 1 for c in Counter(s).values()) <= k
```
```Java []
class Solution {
public boolean canConstruct(String s, int k) {
if (s.length() < k)
return false;
int[] freq = new int[26];
for (int i = 0; i < s.length(); i++)
freq[s.charAt(i) - 'a']++;
for (int i = 0; i < 26; i++)
if ((k -= freq[i] % 2) < 0)
return false;
return true;
}
}
```
**Little more elegant and in one iteration:**
```cpp
bool canConstruct(string s, int k) {
if (s.length() < k)
return false;
int freq[26] = {};
for (char c : s)
k += (++freq[c - 'a'] & 1 ? -1 : 1);
return k >= 0;
}
```
| 3 | 0 | ['Hash Table', 'String', 'Greedy', 'C', 'Counting', 'Python', 'C++', 'Java', 'Python3'] | 0 |
construct-k-palindrome-strings | Beginner Friendly[c++] | beginner-friendlyc-by-gopalagks-h444 | \nclass Solution {\npublic:\n bool canConstruct(string s, int k) {\n unordered_map<char,int>mp;\n int counte=0,counto=0;\n for(auto ch:s | gopalagks | NORMAL | 2022-01-08T03:24:08.465488+00:00 | 2022-06-03T05:48:35.987156+00:00 | 174 | false | ```\nclass Solution {\npublic:\n bool canConstruct(string s, int k) {\n unordered_map<char,int>mp;\n int counte=0,counto=0;\n for(auto ch:s){\n mp[ch]++;\n }\n for(auto it:mp){\n if(it.second%2==0){\n counte++;\n }else{\n counto++;\n }\n }\n if(k>=counto&&s.length()>=k){\n return true;\n }else{\n return false;\n }\n \n }\n};\n``` | 3 | 0 | [] | 0 |
construct-k-palindrome-strings | [JS] O(n) solution with comments | js-on-solution-with-comments-by-wintryle-xse3 | \nvar canConstruct = function(s, k) {\n\t// if string length is less than k, we cannot form k palindromes from the string, so return false\n if(k > s.length) | wintryleo | NORMAL | 2021-08-22T18:28:48.057362+00:00 | 2021-08-22T21:41:21.969656+00:00 | 374 | false | ```\nvar canConstruct = function(s, k) {\n\t// if string length is less than k, we cannot form k palindromes from the string, so return false\n if(k > s.length) {\n return false;\n }\n\t// created a map to keep count of each letter in the string\n const map = new Map(); // O(26)\n for(let i = 0; i < s.length; ++i) { // O(n)\n map.set(s[i], map.has(s[i]) ? map.get(s[i]) + 1 : 1);\n }\n\t// check how many letters have odd count\n let oddCount = 0;\n map.forEach((value, key) => { // O(26)\n if(value % 2 !== 0) {\n ++oddCount;\n }\n })\n\t// if letters with odd count are greater than k, that means more than k palindromes need to be formed to\n\t// make palindromes from the string where all the letters are utilised, so return false\n\t// palindrome can be of 2 form: `aaaaa` and `aabaa`, one letter can have odd and even occurences, but \n\t// there will be at least x palindromes if x letters are there with odd number of frequency\n if(oddCount > k) {\n return false;\n }\n\t// since the previous conditions do not hold, we can make k palindromes from the string, so return true\n return true;\n};\n```\nTime Complexity = O(n)\nSpace Complexity = O(26) ~ O(1) | 3 | 0 | ['JavaScript'] | 0 |
construct-k-palindrome-strings | [Java] Short & Concise | java-short-concise-by-manrajsingh007-wzd8 | ```\nclass Solution {\n public boolean canConstruct(String s, int k) {\n int n = s.length();\n if(k > n) return false;\n if(k == n) retu | manrajsingh007 | NORMAL | 2020-04-04T16:02:36.282789+00:00 | 2020-04-04T16:02:36.282829+00:00 | 142 | false | ```\nclass Solution {\n public boolean canConstruct(String s, int k) {\n int n = s.length();\n if(k > n) return false;\n if(k == n) return true;\n int[] arr = new int[26];\n for(int i = 0; i < n; i++) arr[s.charAt(i) - \'a\']++;\n int singles = 0;\n for(int i = 0; i < 26; i++) {\n if(arr[i] % 2 != 0) singles++;\n }\n return singles <= k;\n }\n} | 3 | 3 | [] | 0 |
construct-k-palindrome-strings | C++ Check Odd Count | c-check-odd-count-by-votrubac-8784 | Intuition: a palindrome can have no more than one odd letter in the middle. If a string has n odd letters, we cannot construct less than n palindromes.\n\ncpp\n | votrubac | NORMAL | 2020-04-04T16:02:33.085130+00:00 | 2020-04-04T16:11:27.315742+00:00 | 182 | false | Intuition: a palindrome can have no more than one odd letter in the middle. If a string has `n` odd letters, we cannot construct less than `n` palindromes.\n\n```cpp\nbool canConstruct(string s, int k) {\n if (k >= s.size())\n return k == s.size();\n bool cnt[26] = {};\n for (auto ch : s)\n cnt[ch - \'a\'] = !cnt[ch - \'a\'];\n auto odd = count_if(begin(cnt), end(cnt), [](int n) { return n % 2; });\n return odd <= k;\n}\n``` | 3 | 3 | [] | 0 |
construct-k-palindrome-strings | PYTHON💡|| CONCISE CODE✅|| | python-concise-code-by-pbs_gopi-0imc | Complexity
Time complexity:
Space complexity:
Code | pbs_gopi | NORMAL | 2025-01-12T06:22:44.167767+00:00 | 2025-01-12T06:22:44.167767+00:00 | 5 | false | # Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
O(n)
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
O(1)
# Code
```python3 []
from collections import Counter
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
num=0
if len(s)==k:
return True
elif len(s)<k:
return False
count=Counter(s)
for i in range(len(set(s))):
if count[s[i]]%2==1:
num+=1
if num>k:
return False
else:
return True
``` | 2 | 0 | ['Python3'] | 0 |
construct-k-palindrome-strings | 2-Approaches : Better | Optimal. | 2-approaches-better-optimal-by-priyans_r-a979 | Approach 1:
Store the count of all characters in vector.
Run a loop till 26-time.
If any count of character occur odd time so add in the 'cnt' variable.
If 'cnt | priyans_raj | NORMAL | 2025-01-11T23:00:46.295633+00:00 | 2025-01-11T23:00:46.295633+00:00 | 3 | false | # Approach 1:
<!-- Describe your approach to solving the problem. -->
1. Store the count of all characters in vector.
2. Run a loop till 26-time.
3. If any count of character occur odd time so add in the 'cnt' variable.
4. If 'cnt' cross the 'k' so it cannot be palindrome.
# Complexity
- Time complexity: O(N + 26)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: O(26)
Taking constant space for storing the count of small characters.
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
if(k>s.size()) return 0;
vector<int> words(26,0);
for(auto it:s) words[it - 'a']++;
int cnt = 0;
for(int i=0;i<26;i++) if(words[i]%2==1) cnt++;
return cnt<=k;
}
};
```
# Approach 2:
# Complexity
- Time complexity: O(NlogN + N)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: O(1)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
if (k > s.size())
return 0;
sort(s.begin(), s.end());
int cnt = 1, check = 0;
char pre = s[0];
for (int i = 1; i < s.size() ; i++) {
if (pre != s[i]) {
check+=cnt%2;
pre = s[i];
cnt = 1;
}
else cnt++;
if(check>k) return false;
}
check+=cnt%2;
return check<=k;
}
};
``` | 2 | 0 | ['C++'] | 0 |
construct-k-palindrome-strings | Easy C++ Beats 100% | easy-c-beats-100-by-abhishek-verma01-zxx0 | IntuitionThe problem boils down to understanding the properties of palindromes and how character frequencies contribute to their formation:
A palindrome can hav | Abhishek-Verma01 | NORMAL | 2025-01-11T19:48:01.665832+00:00 | 2025-01-11T19:48:01.665832+00:00 | 18 | false | # Intuition
<!-- Describe your first thoughts on how to solve this problem. -->
The problem boils down to understanding the properties of palindromes and how character frequencies contribute to their formation:
1. A palindrome can have at most one character with an odd frequency.
2. To create multiple palindromes, each palindrome can "use" one character with an odd frequency as its center.
3. Therefore, the number of characters with odd frequencies `(oddCount) `must not exceed the required number of palindromes `(k).`
# Approach
<!-- Describe your approach to solving the problem. -->
1. Edge Case Checks:
* If the string length equals` k, `each character can act as its own palindrome.
* If the string length is less than` k,` it's impossible to create `k` palindromes.
2. Frequency Count:
* Count the frequency of each character in the string.
3. Odd Frequency Calculation:
* Iterate over the frequency array to count characters with odd frequencies.
4. Validation:
* If the number of odd-frequency characters is greater than `k,` return` false.`
* Otherwise, return `true. `
# Complexity
- Time complexity:
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
Overall -- O(N)
- Space complexity:
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
O(1)
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
// If the string length equals the number of palindromes required,
// it means each character can act as its own palindrome.
if(s.size() == k) {
return true;
}
// If the string length is less than the number of palindromes required,
// it's impossible to form `k` palindromes.
if(s.size() < k) {
return false;
}
// Create a frequency array for all 26 lowercase letters.
vector<int> freq(26, 0);
int oddCount = 0; // Counts characters with odd frequencies.
// Calculate the frequency of each character in the string.
for(char ch : s) {
freq[ch - 'a']++;
}
// Count how many characters have odd frequencies.
for(int count : freq) {
if(count % 2 != 0) {
oddCount++;
}
}
// If the number of odd-frequency characters exceeds `k`,
// it's impossible to form `k` palindromes.
if(oddCount > k) {
return false;
}
// Otherwise, it's possible to form `k` palindromes.
return true;
}
};
``` | 2 | 0 | ['String', 'Greedy', 'Counting', 'C++'] | 0 |
construct-k-palindrome-strings | Solutions - Count Odd Frequencies and Bit manipulation | solutions-count-odd-frequencies-and-bit-yzlp1 | IntuitionWe are given a string s composed of lowercase letters and an integer k. Our goal is to determine if it's possible to rearrange the characters of the st | rohity821 | NORMAL | 2025-01-11T15:12:31.901019+00:00 | 2025-01-11T15:12:31.901019+00:00 | 14 | false | # Intuition
We are given a string `s` composed of lowercase letters and an integer `k`. Our goal is to determine if it's possible to rearrange the characters of the string into exactly `k` palindromic substrings.
A palindrome is a string that reads the same forward and backward, showing symmetry with respect to its center.
# Approach
1. Handle initial edge cases, comparing the length of s to k.
- If the length of s is less than k, we return false, as we do not have enough characters to form k palindromes.
- If the length of s is equal to k, we return true, as we can simply use each character of s to form a palindrome.
2. Initialize:
- an array freq of size 26, representing the frequencies of each alphabetical character.
- an integer oddCount, representing the number of odd frequencies found in the string.
3. Iterate through s, incrementing the value of the index in freq corresponding to the character.
4. Iterate through the freq, incrementing oddCount when a frequency is odd.
5. Return true if oddCount is less than or equal to k; return false otherwise.
# Complexity
- Time complexity: O(N)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
We traverse the string of length n only once.
All other operations performed happen in constant time. This includes traversing freq, as the size of the array is a fixed size of 26.
- Space complexity: O(1)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
The space required does not depend on the size of the input string, so only constant space is used.
Since we are limited to only lowercase letters in s, we can store the frequencies in constant space with an array of size 26, freq
# Code
```swift []
class Solution {
func canConstruct(_ s: String, _ k: Int) -> Bool {
if s.count < k { return false }
if s.count == k { return true }
var array = Array(repeating:0, count: 26)
var oddCount = 0
var charA = Character("a")
for char in s {
let index = char.asciiValue! - charA.asciiValue!
let val = array[Int(index)]
array[Int(index)] = val + 1
}
for count in array {
if count % 2 == 1 {
oddCount += 1
}
}
return oddCount <= k
}
}
```
# Approach
<!-- Describe your approach to solving the problem. -->
1. Handle initial edge cases, comparing the length of s to k.
- If the length of s is less than k, we return false, as we do not have enough characters to form k palindromes.
- If the length of s is equal to k, we return true, as we can simply use each character of s to form a palindrome.
2. Initialize an integer oddCount, which is used as a bitmask to track characters with odd frequencies
3. Iterate through s. For each character, we flip the bit tracking that character, with a set bit of 1 representing an odd frequency and a cleared bit 0 representing an even frequency
4. Return true if the number of 1 bits is less than or equal to k; return false otherwise.
# Complexity
- Time complexity: O(n)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
The loop iterates over each character in the string s, which takes O(n) time. The built-in function to count bits operates in O(1) time since it works on a fixed-size integer (32 bits). Therefore, the overall time complexity is dominated by the loop, resulting in O(n).
- Space complexity: O(1)
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
The space required does not depend on the size of the input string, so only constant space is used.
# Code
```swift []
class Solution {
func canConstruct(_ s: String, _ k: Int) -> Bool {
if s.count < k { return false }
if s.count == k { return true }
var oddCount = 0
var charA = Character("a")
for char in s {
oddCount ^= 1 << (char.asciiValue! - charA.asciiValue!)
}
return oddCount.nonzeroBitCount <= k
}
}
``` | 2 | 0 | ['Hash Table', 'String', 'Greedy', 'Swift', 'Counting'] | 1 |
construct-k-palindrome-strings | "Checking Feasibility of Constructing 𝑘 k Palindromes from a String" | checking-feasibility-of-constructing-k-k-6o4h | IntuitionThe problem revolves around understanding the properties of palindromes. A palindrome can have at most one character with an odd frequency. Therefore, | ankita_molak21 | NORMAL | 2025-01-11T15:02:18.170424+00:00 | 2025-01-11T15:02:18.170424+00:00 | 6 | false | # Intuition
The problem revolves around understanding the properties of palindromes. A palindrome can have at most one character with an odd frequency. Therefore, to construct k palindrome strings, the minimum number of palindromes required is determined by the number of characters with odd frequencies. If k is less than this number, it’s impossible to form k palindromes. Similarly, if k exceeds the total number of characters in the string, forming k palindromes is also not feasible.
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
The solution begins by counting the frequency of each character in the string s. A hash map is used to store these frequencies. Next, the algorithm calculates how many characters have an odd frequency, as each odd-frequency character must be the center of its own palindrome. Finally, the function checks whether k falls between the number of odd frequencies and the total length of s. If k meets this condition, the function returns true, indicating it is possible to form k palindromes. Otherwise, it returns false.
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity:
- Counting character frequencies:
O(n), where
n is the length of 𝑠.
Counting odd frequencies:
O(26)=O(1), since there are at most 26 lowercase English letters.
Overall: O(n)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity:
- Hash map to store frequencies:
O(26)=O(1) because the character set is limited to lowercase English letters.
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
#include <unordered_map>
#include <string>
using namespace std;
class Solution {
public:
bool canConstruct(string s, int k) {
// If k is greater than the length of s, it's impossible to create k palindromes
if (k > s.size()) return false;
// Count the frequency of each character
unordered_map<char, int> freq;
for (char c : s) {
freq[c]++;
}
// Count characters with odd frequencies
int oddCount = 0;
for (auto [char_, count] : freq) {
if (count % 2 != 0) {
oddCount++;
}
}
// If k is less than the number of odd frequencies, we can't construct k palindromes
return k >= oddCount;
}
};
``` | 2 | 0 | ['Hash Table', 'String', 'Greedy', 'Counting', 'C++'] | 0 |
construct-k-palindrome-strings | 🔥Explanations No One Will Give You🎓🧠 Very Easy C++ Solution✅✨ 🚀Beats 💯 % on LeetCode🚀🎉!! | explanations-no-one-will-give-you-very-e-2x9i | IntuitionFirst of all on reading the question, I noticed a few conditions for getting a palindrome like the smallest palindrome string can be a letter itself an | codonaut_anant_05 | NORMAL | 2025-01-11T14:21:31.437284+00:00 | 2025-01-11T14:21:31.437284+00:00 | 10 | false | # Intuition
First of all on reading the question, I noticed a few conditions for getting a palindrome like the smallest palindrome string can be a letter itself and the letter at the middle of the palindrome string is having an odd frequency always. Now keeping these conditions in mind I tried to code the solution to the problem.
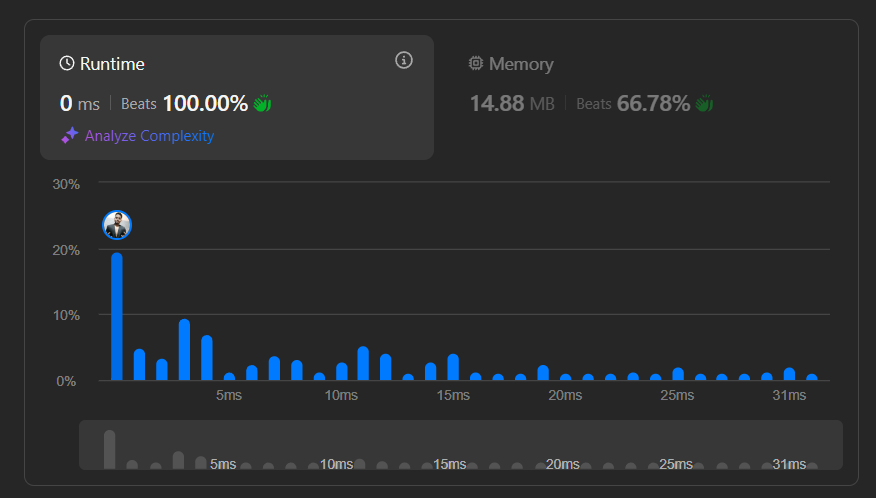
# Approach
- Create a variable named `n` where `n=s.size()`.
- Now, `if(n==k)` return `true` as at max we can make n palindrome strings of single character.
- `if(n<k)` then return false.
- Now, create a `freq` array to keep a track of the frequency of each character in the given string `s`.
- Then create a counter variable to count characters with odd frequency, i.e `cnt_odd=0`.
- Now, traverse through the `freq` array and in each iteration increment `cnt_odd` if the the frequency is odd and also check that `if (cnt_odd>k)` then return `false`.
- Finally, return `true`.
# Complexity
- **Time complexity:** O(N)⏳
- **Space complexity:** O(1)🚀
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
int n=s.size();
if(n==k) return true;
if(n<k) return false;
int freq[26]={0};
for(auto x: s) ++freq[x-'a'];
int cnt_odd=0;
for(int i=0; i<26; ++i){
cnt_odd+=(freq[i]&1);
if(cnt_odd>k) return false;
}
return true;
}
};
auto init=[](){
ios_base::sync_with_stdio(0);
cin.tie(0);
cout.tie(0);
return 0;
}();
``` | 2 | 0 | ['C++'] | 0 |
construct-k-palindrome-strings | Easy Approach | easy-approach-by-sriharshabhoomandla-u8f3 | IntuitionTo construct k palindromes from the string s, we need:
At least k characters in the string. If s.size() < k, it is impossible, so return false.
Each pa | sriharshabhoomandla | NORMAL | 2025-01-11T13:55:20.493806+00:00 | 2025-01-11T13:55:20.493806+00:00 | 10 | false | # Intuition
To construct k palindromes from the string s, we need:
1. At least k characters in the string. If s.size() < k, it is impossible, so return false.
2. Each palindrome can have at most one character with an odd frequency. Therefore, the total number of characters with odd frequencies in s must not exceed k.
# Approach
1. Check string size: If the length of s is less than k, return false since it's impossible to construct k palindromes.
2. Count character frequencies: Use an array freq to store the frequency of each character in s.
3. Count odd frequencies: Count how many characters have an odd frequency using the variable cnt.
4. Compare odd frequencies with k: If the number of odd frequencies (cnt) exceeds k, return false. Otherwise, return true.
# Complexity
- Time complexity: O(n), where 𝑛 is the length of the string s. We iterate through the string once to count frequencies and through the array of size 26 to count odd frequencies.
- Space complexity: O(1), since the array freq has a fixed size of 26.
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
if(s.size()<k) return false;
int freq[26]={0};
for(auto& i:s){
freq[i-'a']++;
}
int cnt=0;
for(int i=0;i<26;i++){
if(freq[i]%2) cnt++;
}
if(cnt>k) return false;
return true;
}
};
``` | 2 | 0 | ['C++'] | 0 |
construct-k-palindrome-strings | 🌟 HashMap & Counting Made Simple! 🔢 | Beginner-Friendly 🐣 | O(N) Solution 🚀 | hashmap-counting-made-simple-beginner-fr-4e3z | Intuition : 💡We aim to split the string s into exactly k palindromic substrings.
Palindromes need at most 1 odd-frequency character 🔑.
Count odd-frequency chara | mansimittal935 | NORMAL | 2025-01-11T13:52:44.878267+00:00 | 2025-01-11T13:52:44.878267+00:00 | 88 | false | # Intuition : 💡
We aim to split the `string` `s` into exactly `k` palindromic substrings.
- Palindromes need `at most 1 odd-frequency` character 🔑.
- Count odd-frequency characters . If there are more odd characters than `k`, it’s impossible to form `k` palindromes.
---
# Approach 🔍
1. ***Edge Check***: If s.size() < k ➡️ return false 🙅♂️ (not enough characters to form k substrings).
2. ***Frequency Count*** 📊:
- Use a vector<int> of size 26 to store the frequency of each letter 🅰️🅱️...
3. ***Count Odds*** 🔢:
Iterate over the frequency array to count characters with odd frequencies .
4. ***Decision***:
- If cnt > k ➡️ false 🚫 (too many odd characters).
- Otherwise, return true ✅.
---
# Complexity
- Time complexity:
Iteration -> O(n)
- Space complexity:
O(1)
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
if(s.size() < k) return false;
vector<int> count (26, 0);
for(char &c: s) {
count[c - 'a']++;
}
int cnt = 0;
for(int &val : count) {
if(val%2) cnt++;
}
if(cnt > k) return false;
return true;
}
};
```
```java []
public class Solution {
public boolean canConstruct(String s, int k) {
if (s.length() < k) return false;
int[] count = new int[26];
for (char c : s.toCharArray()) {
count[c - 'a']++;
}
int oddCount = 0;
for (int val : count) {
if (val % 2 != 0) oddCount++;
}
return oddCount <= k;
}
}
```
```python []
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
if len(s) < k:
return False
count = [0] * 26
for c in s:
count[ord(c) - ord('a')] += 1
odd_count = sum(1 for val in count if val % 2 != 0)
return odd_count <= k
```
```ruby []
def can_construct(s, k)
return false if s.length < k
count = Array.new(26, 0)
s.each_char { |c| count[c.ord - 'a'.ord] += 1 }
odd_count = count.count { |val| val.odd? }
odd_count <= k
end
```
```javascript []
class Solution {
canConstruct(s, k) {
if (s.length < k) return false;
const count = Array(26).fill(0);
for (const c of s) {
count[c.charCodeAt(0) - 'a'.charCodeAt(0)]++;
}
const oddCount = count.filter(val => val % 2 !== 0).length;
return oddCount <= k;
}
}
```
```typescript []
class Solution {
canConstruct(s: string, k: number): boolean {
if (s.length < k) return false;
const count = Array(26).fill(0);
for (const c of s) {
count[c.charCodeAt(0) - 'a'.charCodeAt(0)]++;
}
const oddCount = count.filter(val => val % 2 !== 0).length;
return oddCount <= k;
}
}
```
```rust []
impl Solution {
pub fn can_construct(s: String, k: i32) -> bool {
if s.len() < k as usize {
return false;
}
let mut count = [0; 26];
for c in s.chars() {
count[(c as usize) - ('a' as usize)] += 1;
}
let odd_count = count.iter().filter(|&&val| val % 2 != 0).count();
odd_count <= k as usize
}
}
```
```kotlin []
class Solution {
fun canConstruct(s: String, k: Int): Boolean {
if (s.length < k) return false
val count = IntArray(26)
for (c in s) {
count[c - 'a']++
}
val oddCount = count.count { it % 2 != 0 }
return oddCount <= k
}
}
```
```dart []
class Solution {
bool canConstruct(String s, int k) {
if (s.length < k) return false;
List<int> count = List.filled(26, 0);
for (var c in s.runes) {
count[c - 'a'.runes.first]++;
}
int oddCount = count.where((val) => val % 2 != 0).length;
return oddCount <= k;
}
}
```
```C# []
public class Solution {
public bool CanConstruct(string s, int k) {
if (s.Length < k) return false;
int[] count = new int[26];
foreach (char c in s) {
count[c - 'a']++;
}
int oddCount = count.Count(val => val % 2 != 0);
return oddCount <= k;
}
}
```
| 2 | 0 | ['Hash Table', 'String', 'Greedy', 'Counting', 'C++', 'Java', 'TypeScript', 'Rust', 'JavaScript', 'Dart'] | 0 |
construct-k-palindrome-strings | 🧩Form K Palindromes ✨with Minimal Effort 🧠✅ | form-k-palindromes-with-minimal-effort-b-r2zi | Intuition 🤔✨We need to check if the string s can be split into k palindromes.
Palindrome Fact: Only odd-frequency characters matter when forming palindromes (si | shubhamrajpoot_ | NORMAL | 2025-01-11T13:45:02.356580+00:00 | 2025-01-11T13:45:02.356580+00:00 | 49 | false | # Intuition 🤔✨
We need to check if the string s can be split into k palindromes.
- Palindrome Fact: Only odd-frequency characters matter when forming palindromes (since the middle character can stay unmatched).
- So, the question boils down to:
1. Count the characters with odd frequencies.
2. Check if we can manage them within the given k palindromes.
---
# Approach 🛠️📝
1. Base Case:
- If s.length < k, it's impossible to form k substrings. ❌
2. Frequency Count:
- Count how often each character appears using a frequency array (or hash map). 📊
3. Count Odds:
- Count the characters with odd frequencies. 🔢
4. Check Feasibility:
- If the number of odd-frequency characters is greater than k, return false. Otherwise, it's possible! ✅
---
# Complexity ⏱️⚡
## Time Complexity:
- Counting frequencies 🕒: O(n), where n is the length of the string.
- Checking odd frequencies 🕒: O(26) ≈ O(1) (since the alphabet size is constant).
- Total: O(n).
## Space Complexity:
- Frequency storage 📂: O(26) ≈ O(1).
---
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
if(s.size() < k) return false ;
vector<int> freq(26, 0);
int cnt = 0;
for (auto& ch : s) {
freq[ch - 'a']++;
}
for (auto& it : freq) {
if (it % 2 != 0) {
cnt++;
}
}
if (cnt <= k)
return true;
return false;
}
};
```
```java []
class Solution {
public boolean canConstruct(String s, int k) {
if (s.length() < k) return false;
int[] freq = new int[26];
int oddCount = 0;
for (char ch : s.toCharArray()) {
freq[ch - 'a']++;
}
for (int count : freq) {
if (count % 2 != 0) {
oddCount++;
}
}
return oddCount <= k;
}
}
```
```javascript []
var canConstruct = function(s, k) {
if (s.length < k) return false;
let freq = Array(26).fill(0);
let oddCount = 0;
for (let ch of s) {
freq[ch.charCodeAt(0) - 'a'.charCodeAt(0)]++;
}
for (let count of freq) {
if (count % 2 !== 0) {
oddCount++;
}
}
return oddCount <= k;
};
```
```python []
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
if len(s) < k:
return False
freq = [0] * 26
for ch in s:
freq[ord(ch) - ord('a')] += 1
odd_count = sum(1 for count in freq if count % 2 != 0)
return odd_count <= k
```
```ruby []
def can_construct(s, k)
return false if s.length < k
freq = Array.new(26, 0)
s.each_char { |ch| freq[ch.ord - 'a'.ord] += 1 }
odd_count = freq.count { |count| count % 2 != 0 }
odd_count <= k
end
```
```rust []
impl Solution {
pub fn can_construct(s: String, k: i32) -> bool {
if s.len() < k as usize {
return false;
}
let mut freq = vec![0; 26];
let mut odd_count = 0;
for ch in s.chars() {
freq[(ch as usize - 'a' as usize)] += 1;
}
for count in freq {
if count % 2 != 0 {
odd_count += 1;
}
}
odd_count <= k
}
}
```
```c# []
public class Solution {
public bool CanConstruct(string s, int k) {
if (s.Length < k) return false;
int[] freq = new int[26];
int oddCount = 0;
foreach (char ch in s) {
freq[ch - 'a']++;
}
foreach (int count in freq) {
if (count % 2 != 0) {
oddCount++;
}
}
return oddCount <= k;
}
}
```
```go []
func canConstruct(s string, k int) bool {
if len(s) < k {
return false
}
freq := make([]int, 26)
oddCount := 0
for _, ch := range s {
freq[ch-'a']++
}
for _, count := range freq {
if count%2 != 0 {
oddCount++
}
}
return oddCount <= k
}
```
```swift []
class Solution {
func canConstruct(_ s: String, _ k: Int) -> Bool {
if s.count < k {
return false
}
var freq = [Int](repeating: 0, count: 26)
var oddCount = 0
for ch in s {
freq[Int(ch.asciiValue! - Character("a").asciiValue!)] += 1
}
for count in freq {
if count % 2 != 0 {
oddCount += 1
}
}
return oddCount <= k
}
}
```
| 2 | 0 | ['Swift', 'Counting', 'Python', 'C++', 'Java', 'Go', 'Rust', 'Ruby', 'JavaScript', 'C#'] | 0 |
construct-k-palindrome-strings | C++ Solution + YouTube Tutorial 🎬💖 | c-solution-youtube-tutorial-by-ra_zan-hlch | IntuitionTo determine if a string can be split into exactly k palindromes, the key insight is understanding the properties of palindromes:
A palindrome can have | ra_zan | NORMAL | 2025-01-11T13:40:56.146505+00:00 | 2025-01-11T13:40:56.146505+00:00 | 7 | false | # Intuition
To determine if a string can be split into exactly `k` palindromes, the key insight is understanding the properties of palindromes:
1. A palindrome can have at most **one character** with an odd frequency.
2. The number of characters with odd frequencies determines the **minimum number of palindromes** needed.
Using these observations, we can infer that:
- If the number of odd-frequency characters (`single`) exceeds `k`, it’s impossible to create `k` palindromes.
- If `k` is greater than the length of the string, it’s also impossible because there aren't enough characters.
Thus, we need to:
1. Count the frequency of each character.
2. Count how many characters have an odd frequency.
3. Check if `k` lies within the valid range: `single <= k <= s.size()`.
---
# Approach
1. Use a hash map to count the frequency of each character in the string.
2. Count the number of characters with odd frequencies (`single`).
3. Validate the range:
- If `k` is greater than or equal to `single` (minimum required palindromes) and less than or equal to the string length (`s.size()`), return `true`.
- Otherwise, return `false`.
---
# Complexity
- **Time complexity**:
$$O(n)$$
- Iterating through the string to count frequencies takes \(O(n)\).
- Iterating through the hash map (maximum 26 entries for lowercase English letters) takes \(O(1)\).
- **Space complexity**:
$$O(1)$$
- The hash map stores at most 26 keys (fixed size for lowercase English letters).
---
# YouTube Tutorial
🎥 **Watch the full explanation on YouTube**:
<iframe width="560" height="315" src="https://www.youtube.com/embed/sqKDUZ3yLpY" title="YouTube video tutorial for Word Subsets - LeetCode 916" frameborder="0" allow="accelerometer; autoplay; clipboard-write; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe>
In this tutorial, we:
- Explain the intuition behind the solution.
- Walk through examples step-by-step.
- Write and analyze the C++ code.
---
# Code
```cpp
class Solution {
public:
bool canConstruct(string s, int k) {
unordered_map<char, int> freq;
int single = 0;
// Count character frequencies
for (auto c : s) {
freq[c]++;
}
// Count odd frequencies
for (auto &[c, count] : freq) {
if (count % 2 == 1) {
single++;
}
}
// Check if k is in the valid range
return k >= single && k <= s.size();
}
};
```
Don’t forget to **like**, **subscribe**, and **comment** with your thoughts or questions!
| 2 | 0 | ['Hash Table', 'String', 'Greedy', 'Counting', 'C++'] | 0 |
construct-k-palindrome-strings | beats 100% || frequency array | beats-100-frequency-array-by-akshatchawl-rcc3 | IntuitionEach palindrmic string can have only one non pair element. So there must be max k elements with odd frequency in the given string.ApproachComplexity
Ti | akshatchawla1307 | NORMAL | 2025-01-11T13:20:30.421683+00:00 | 2025-01-11T13:20:30.421683+00:00 | 4 | false | # Intuition
Each palindrmic string can have only one non pair element. So there must be max k elements with odd frequency in the given string.
<!-- Describe your first thoughts on how to solve this problem. -->
# Approach
<!-- Describe your approach to solving the problem. -->
# Complexity
- Time complexity: O(N)
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: O(1) // array of size 26 is required
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
if(s.size()<k)
return 0;
vector<int>freq(26,0);
for(char i:s)
freq[i-'a']++;
int count=0;
for(int i:freq)
{
if(i%2)
count++;
if(count>k)
return 0;
}
return 1;
}
};
``` | 2 | 0 | ['C++'] | 0 |
construct-k-palindrome-strings | ✅ ⟣ Java Solution ⟢ | java-solution-by-harsh__005-n8da | Code | Harsh__005 | NORMAL | 2025-01-11T10:35:27.584450+00:00 | 2025-01-11T10:35:27.584450+00:00 | 13 | false | # Code
```java []
class Solution {
public boolean canConstruct(String s, int k) {
int n = s.length();
if(n < k) return false;
else if(n == k) return true;
int[] ct = new int[26];
for(char ch : s.toCharArray()) {
ct[ch-'a']++;
}
int oddct = 0;
for(int i=0; i<26; i++) {
if(ct[i]%2 != 0) oddct++;
}
return oddct <= k;
}
}
``` | 2 | 0 | ['Java'] | 1 |
construct-k-palindrome-strings | ✅✅Beats 100%🔥Java🔥Python|| 🚀🚀Super Simple and Efficient Solution🚀🚀||🔥Python🔥Java✅✅ | beats-100javapython-super-simple-and-eff-rr9u | Complexity
Time complexity: O(n)
Space complexity: O(1)
Code | shobhit_yadav | NORMAL | 2025-01-11T09:46:43.231148+00:00 | 2025-01-11T09:46:43.231148+00:00 | 24 | false | # Complexity
- Time complexity: $$O(n)$$
<!-- Add your time complexity here, e.g. $$O(n)$$ -->
- Space complexity: $$O(1)$$
<!-- Add your space complexity here, e.g. $$O(n)$$ -->
# Code
```java []
class Solution {
public boolean canConstruct(String s, int k) {
if (s.length() < k)
return false;
int[] count = new int[26];
for (final char c : s.toCharArray())
count[c - 'a'] ^= 1;
return Arrays.stream(count).filter(c -> c % 2 == 1).count() <= k;
}
}
```
```python []
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
return sum(freq & 1
for freq in collections.Counter(s).values()) <= k <= len(s)
``` | 2 | 0 | ['Hash Table', 'String', 'Greedy', 'Counting', 'Java', 'Python3'] | 0 |
construct-k-palindrome-strings | Understand how palindromes work! Easiest possible way! | understand-how-palindromes-work-easiest-4uvuo | IntuitionAt first, this problem might seem a bit tricky if you haven’t worked with palindromes before. But the key idea here is to understand how the frequencie | pankajpatwal1224 | NORMAL | 2025-01-11T08:56:01.493916+00:00 | 2025-01-11T08:56:01.493916+00:00 | 12 | false | # Intuition
At first, this problem might seem a bit tricky if you haven’t worked with palindromes before. But the key idea here is to understand how the frequencies of characters in a string determine whether it can form a palindrome.
So, what’s special about a palindrome? It’s a string that reads the same forwards and backward, like "aba" or "abba". For that to happen, the characters need to follow a specific pattern:
Characters with even frequencies are easy to work with—they can always form symmetric pairs on both sides of the palindrome.
Characters with odd frequencies are trickier. Each one needs to sit in the middle of its own palindrome (because it can’t pair with anything else).
Let’s look at some examples:
The string abc isn’t a palindrome because all characters have odd frequencies.
The string aba is a palindrome—a appears twice (even), and b appears once (odd, so it sits in the middle).
The string abca isn’t a palindrome, but if we rearrange it to abba, it becomes one because all characters can pair symmetrically.
For this problem, we’re trying to figure out if we can split the string into exactly k palindromes. The trick is to think about how the odd and even frequencies will contribute to the palindromes.
# Approach
Count character frequencies:
First, I counted how many times each character appears in the string. This gives us a clear picture of how many odd and even frequencies we’re dealing with.
---
Categorize frequencies:
Characters with even frequencies can form pairs and are easy to fit into palindromes.
Characters with odd frequencies are the ones that define the minimum number of palindromes we need, because each odd frequency requires its own palindrome (or at least a central spot in one).
---
Find the range of possible palindromes:
The minimum number of palindromes is equal to the count of characters with odd frequencies.
The maximum number of palindromes is the total number of characters since, in the worst case, we could just make single-character palindromes.
---
Check if k is valid:
If k is at least the minimum and at most the maximum number of palindromes, we return true. Otherwise, it’s impossible to split the string into k palindromes.
# Complexity
- Time complexity:
O(n) because we only loop through the string once to count the frequencies and then process a fixed-size array of 26 letters.
- Space complexity:
O(1) since the character frequency array is always of size 26, no matter how long the string is.
# Code
```java []
class Solution {
public boolean canConstruct(String s, int k) {
int []arr=new int[26];
int ind=0;
for(char ch:s.toCharArray()){
arr[ch-'a']++;
}
int total_x=0;
int total_y=0;
int only_x=0;
int only_y=0;
for(int i=0;i<26;i++){
if(arr[i]%2==0){
total_x+=arr[i];
only_x++;
}else{
total_y+=arr[i];
only_y++;
}
}
int min=only_y;
int max=total_x+total_y;
if(k>=min && k<=max ){
return true;
}
return false;
}
}
```
```python []
class Solution:
def canConstruct(self, s: str, k: int) -> bool:
from collections import Counter
# Count character frequencies
freq = Counter(s)
# Count characters with odd frequencies
odd_count = sum(1 for count in freq.values() if count % 2 != 0)
# Minimum and maximum possible palindromes
min_palindromes = odd_count
max_palindromes = len(s)
# Check if k is within range
return min_palindromes <= k <= max_palindromes
```
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
vector<int> freq(26, 0);
for (char ch : s) {
freq[ch - 'a']++;
}
int oddCount = 0;
for (int count : freq) {
if (count % 2 != 0) {
oddCount++;
}
}
int minPalindromes = oddCount;
int maxPalindromes = s.length();
return k >= minPalindromes && k <= maxPalindromes;
}
};
```
| 2 | 0 | ['Hash Table', 'String', 'Greedy', 'Counting', 'C++', 'Java', 'Python3'] | 0 |
construct-k-palindrome-strings | Easy Approach Solution Optimized Complexity 🚀 | easy-approach-solution-optimized-complex-h272 | IntuitionThe problem is about determining if we can construct k palindromic strings from the given string s. The key property of palindromes is that they can ha | hamza_p2 | NORMAL | 2025-01-11T07:16:38.250022+00:00 | 2025-01-11T07:16:38.250022+00:00 | 43 | false | # Intuition
The problem is about determining if we can construct k palindromic strings from the given string s. The key property of palindromes is that they can have at most one character with an odd frequency (for the center of the palindrome), and the remaining characters must appear an even number of times.
# Approach
Check Base Case:
If the length of the string s is less than k, it's impossible to construct k palindromes because each palindrome needs at least one character.
Character Frequency Count:
Use an array of size 26 to count the frequency of each character in the string s. Each index in the array corresponds to a character ('a' to 'z').
Count Odd Frequencies:
Iterate over the frequency array and count how many characters have an odd frequency. These characters can only be used in the center of a palindrome.
If there are more than k characters with odd frequencies, it is impossible to construct k palindromes.
Decision:
If the number of odd frequencies is less than or equal to k, then constructing k palindromes is possible, so return true. Otherwise, return false.
# Complexity
- Time complexity:
𝑂(𝑛), where n is the length of the string s. We iterate through the string once to count frequencies and then iterate through the fixed-size array of size 26.
- Space complexity:
𝑂(1), since the space required is constant and only depends on the fixed-size frequency array for 26 characters.
# Code
```java []
class Solution {
public boolean canConstruct(String s, int k) {
int n=s.length();
if(s.length()<k){
return false;
}
int arr[]=new int[26];
for(int i=0;i<n;i++){
arr[s.charAt(i)-'a']+=1;
}
int count=0;
for(int i=0;i<arr.length;i++){
if(arr[i]%2!=0){
count ++;
}
}
if(count>k){
return false;
}else{
return true;
}
}
}
``` | 2 | 0 | ['Java'] | 0 |
construct-k-palindrome-strings | A Dance of Odd Frequencies | One Pass Solution | Beats 100% | a-dance-of-odd-frequencies-one-pass-solu-vzsz | IntuitionTo determine if it's possible to construct exactly k palindrome strings using all the characters in s, I focused on the properties of palindromes. A pa | mahfuz2411 | NORMAL | 2025-01-11T07:15:51.171617+00:00 | 2025-01-11T07:15:51.171617+00:00 | 7 | false | # Intuition
To determine if it's possible to construct exactly `k` palindrome strings using all the characters in `s`, I focused on the properties of palindromes. A palindrome can have at most one odd-frequency character. Thus, the number of odd-frequency characters in `s` dictates the minimum number of palindromes required.
# Approach
1. **Check if `k` exceeds the length of `s`**: If `k > s.size()`, it's impossible to construct `k` palindromes, as there aren't enough characters.
2. **Count odd-frequency characters**:
- Use a frequency array to track the character counts.
- For each character, toggle between odd and even frequency.
3. **Compare odd frequencies with `k`**:
- If `odd <= k`, we can form the required palindromes.
- Otherwise, it's not possible.
# Complexity
- Time complexity:
$$O(n)$$, where \(n\) is the length of `s`, as we traverse the string and process character frequencies.
- Space complexity:
$$O(1)$$, since the frequency array has a fixed size of 26 (for lowercase letters).
# Code
```cpp
class Solution {
public:
bool canConstruct(string s, int k) {
if(k > s.size()) return 0;
vector<int> v(26, 0);
int odd = 0;
for(auto d: s) {
v[d - 'a']++;
if(v[d - 'a'] % 2) odd++;
else odd--;
}
if(k >= odd) return 1;
return 0;
}
};
```
#### If you have any questions or concerns about my solution, feel free to leave a comment instead of downvoting—I'd love to help! 😊 And if you found my solution helpful, please consider giving it an upvote. Your support means a lot and lets me know I made a difference! 🙌 | 2 | 0 | ['Hash Table', 'String', 'Greedy', 'Counting', 'C++'] | 0 |
construct-k-palindrome-strings | Beats 100% || 2Approaches | beats-100-2approaches-by-sarvpreet_kaur-igg5 | Complexity
Time complexity:
Approach 1 - O(N)
Approach 2 - O(N)
Code | Sarvpreet_Kaur | NORMAL | 2025-01-11T07:12:16.167059+00:00 | 2025-01-11T07:12:16.167059+00:00 | 4 | false |
# Complexity
- Time complexity:
Approach 1 - O(N)
Approach 2 - O(N)
# Code
```cpp []
//Approach 1
class Solution {
public:
bool canConstruct(string s, int k) {
int n = s.size();
if(k>n)return false;
int odd = 0;
int even = 0;
vector<int>freq(26,0);
for(int i=0;i<n;i++){
freq[s[i]-'a']++;
}
for(int i=0;i<freq.size();i++){
if(freq[i]%2==0)even++;
else odd++;
}
if(odd>k)return false;
return true;
}
};
//Approach 2
class Solution {
public:
bool canConstruct(string s, int k) {
int n = s.size();
if(k>n)return false;
int oddchar = 0;
for(int i=0;i<s.size();i++){
oddchar ^= 1<<(s[i]-'a');
}
int odd = __builtin_popcount(oddchar);
if(odd>k)return false;
return true;
}
};
``` | 2 | 0 | ['Hash Table', 'String', 'Greedy', 'Counting', 'C++'] | 0 |
construct-k-palindrome-strings | Simple Java Solution - Using HashMap | simple-java-solution-using-hashmap-by-ru-w3kb | Complexity
Time complexity:
O(n), where n = s.length()
Space complexity:
O(n), where n = s.length()
Code | ruch21 | NORMAL | 2025-01-11T07:02:17.142310+00:00 | 2025-01-11T07:02:17.142310+00:00 | 12 | false | # Complexity
- Time complexity:
$$O(n)$$, where n = s.length()
- Space complexity:
$$O(n)$$, where n = s.length()
# Code
```java []
class Solution {
public boolean canConstruct(String s, int k) {
if(s.length() < k) {
return false;
}
HashMap<Character, Integer> map = new HashMap<>();
for(char ch: s.toCharArray()) {
map.put(ch, map.getOrDefault(ch,0)+1);
}
int oddCount = 0;
for(char ch: map.keySet()) {
if(map.get(ch) % 2 != 0) {
oddCount++;
}
if(oddCount > k) {
return false;
}
}
return true;
}
}
``` | 2 | 0 | ['Java'] | 1 |
construct-k-palindrome-strings | Odd Characters Assemble (But Not Too Many)! | odd-characters-assemble-but-not-too-many-6lck | IntuitionThe problem revolves around determining if a given string s can be rearranged into exactly k palindromes. A palindrome has certain properties, such as | ParthSaini1353 | NORMAL | 2025-01-11T06:22:37.078325+00:00 | 2025-01-11T06:22:37.078325+00:00 | 42 | false | # Intuition
The problem revolves around determining if a given string s can be rearranged into exactly k palindromes. A palindrome has certain properties, such as the number of odd-frequency characters. This observation drives the solution.
# Approach
Initial Constraints:
If the length of the string s is less than k, it's impossible to create k palindromes. Return false in this case.
Frequency Count:
Use a hash map (unordered_map) to count the frequency of each character in s.
Odd Frequency Analysis:
Palindromes allow at most one character with an odd frequency in the middle. For k palindromes, at most k characters can have odd frequencies. Count the number of odd-frequency characters.
Validation:
If the number of odd-frequency characters is less than or equal to k, the string can be rearranged into k palindromes. Otherwise, it cannot.
# Code
```cpp []
class Solution {
public:
bool canConstruct(string s, int k) {
unordered_map<char,int>mmp;
if(s.length()<k)
return 0;
for(auto it:s)
{
mmp[it]++;
}
if(mmp.size()==k)
return 1;
int cnt=0;
for(auto it:mmp)
if(it.second%2!=0)
{
cnt++;
}
if(cnt<=k)
return 1;
else return 0;
}
};
``` | 2 | 0 | ['C++'] | 0 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.