repo
stringlengths 8
123
| branch
stringclasses 178
values | readme
stringlengths 1
441k
โ | description
stringlengths 1
350
โ | topics
stringlengths 10
237
| createdAt
stringlengths 20
20
| lastCommitDate
stringlengths 20
20
| lastReleaseDate
stringlengths 20
20
โ | contributors
int64 0
10k
| pulls
int64 0
3.84k
| commits
int64 1
58.7k
| issues
int64 0
826
| forks
int64 0
13.1k
| stars
int64 2
49.2k
| diskUsage
float64 | license
stringclasses 24
values | language
stringclasses 80
values |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
riveraaj/Text-Encryptor | main | Link : https://riveraaj.github.io/Text-Encryptor/
[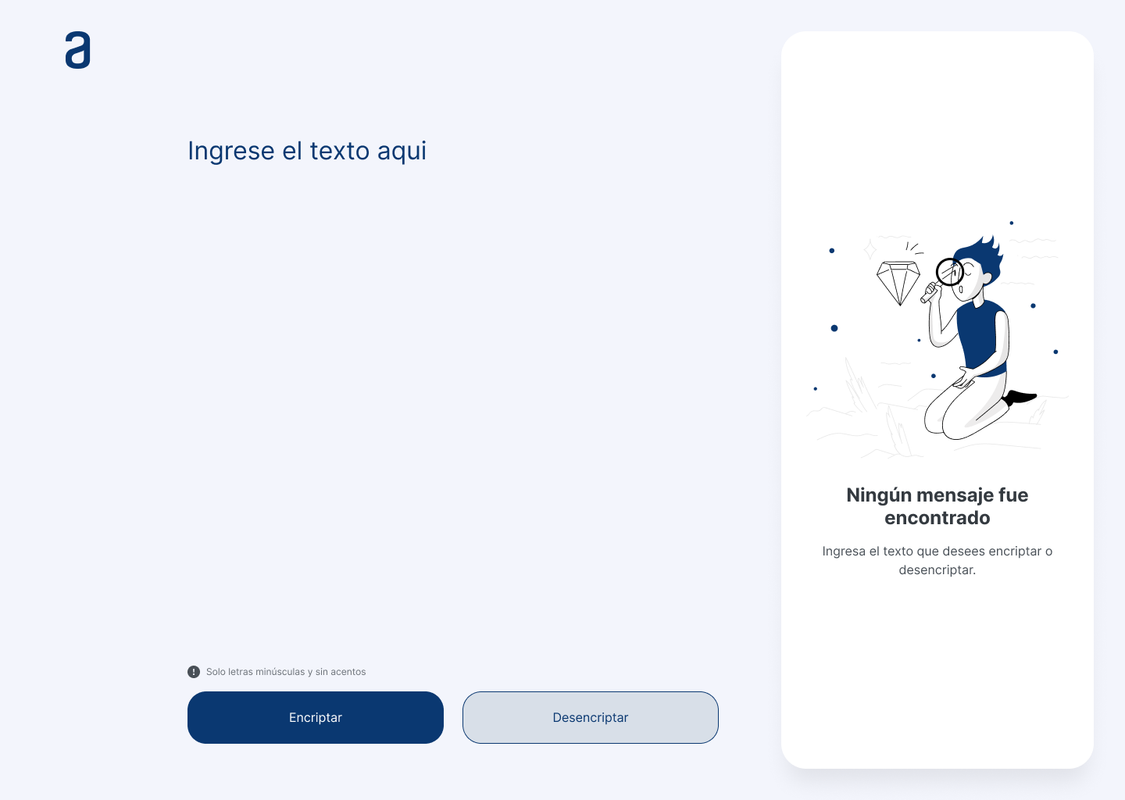](https://postimg.cc/Lqwfk6t0)
* Resume
This is the first Challenge of the ONE - Oracle Next Education program. This application has the function of encrypting and decoding text messages in a simple way. With the aim of exchanging information with other people in a safe and fun way.
* The encryption "keys" that we will use are the following:
La letra "e" es convertida para "enter"
La letra "i" es convertida para "imes"
La letra "a" es convertida para "ai"
La letra "o" es convertida para "ober"
La letra "u" es convertida para "ufat"
* Requirements:
- It should work only with lowercase letters
- Do not use letters with accents or special characters
- It must be possible to convert a word to the encrypted version also to return an encrypted word to its original version.
For example:
"cat" => "gaitober"
gaitober" => "cat"
- The page must have fields for insertion of the text that will be encrypted or decrypted, the user having to choose between the two options.
- The result should be displayed on the screen.
* Additional characteristics:
- A button that copies the encrypted/decrypted text for the transfer section, that is, it has the same functionality as ctrl+C or the "copy" option in the applications menu.
| Challenge ONE Sprint 01: Construye un encriptador de texto con Javascript | challengeonecodificador4,css3,html5,javascript | 2023-01-06T20:19:29Z | 2023-12-11T04:11:59Z | null | 1 | 0 | 18 | 0 | 0 | 5 | null | null | CSS |
leticialmbarros/ExpandOnePiece | main | # ExpandOnePiece
primeiro dia do projeto "50 Projects In 50 Days", este รฉ o primeiro dia e o 1 projeto.
| primeiro dia do projeto "50 Projects In 50 Days", este รฉ o primeiro dia e o 1 projeto. | css,html5,javascript | 2023-01-06T21:07:36Z | 2023-01-06T21:11:59Z | null | 1 | 0 | 3 | 0 | 1 | 5 | null | null | HTML |
otiai10/jstorm | main | # jstorm
[](https://www.npmjs.com/package/jstorm)
[](https://npm.runkit.com/jstorm)
[](https://www.npmjs.com/package/jstorm)
[](https://github.com/otiai10/jstorm/actions/workflows/node.yml)
[](https://github.com/otiai10/jstorm/actions/workflows/chrome-test.yml)
[](https://codecov.io/github/otiai10/jstorm)
[](https://codeclimate.com/github/otiai10/jstorm/maintainability)
ORM-like API provider for `window.localStorage` and `chrome.storage`, or any other data store.
```typescript
// For window.localStorage:
import { Model } from "jstorm/browser/local";
// For chrome.storage.sync:
import { Model } from "jstorm/chrome/sync";
```
```typescript
// For your custom storage:
import { Model } from "jstorm";
Model._area_ = yourCoolStorageAccessor;
// NOTE: It should implement `chrome.storage.StorageArea` interface.
```
# Getting started
```sh
npm install jstorm
# or yarn add, pnpm add, whatever
```
# Example Usage
```typescript
// In your JS/TS
import { Model } from "jstorm/chrome/local";
// Define your model,
class Player extends Model {
public name: string;
public age: number;
greet(): string {
return `Hello, my name is ${this.name}!`;
}
}
// and use it.
(async () => {
// Save records to chrome.storage.
const x = await Player.create({ name: "otiai10", age: 17 });
const y = await Player.create({ name: "hiromu", age: 32 });
// Retrieve records from chrome.storage.
console.log(await Player.list()); // [Player, Player] length 2
console.log(await Player.find(x._id)); // Player {name:"otiai10", age: 17}
})();
```
# Basic APIs
## Defining your model class
```typescript
import { Model } from "jstorm";
class Player extends Model {
// You can define your own members of your model.
public name: string;
public age: number;
// Optional: If you'd like to minify/mangle your JS,
// you'd better set the namespace of this mode explicitly.
static override _namespace_ = "Player";
}
```
That's all to get started. Let's enjoy.
## `new`
To construct new model object:
```typescript
const john = Player.new({name: "John", age: 17});
console.log(john._id); // null
```
NOTE: `new` does NOT save constructed object yet. Please use `save` to make it persistent.
## `save`
To save unsaved obejct to the storage:
```typescript
await john.save();
console.log(john._id); // 1672363730924
```
Now `_id` is generated because it's saved on the storage.
## `create`
Just a short-hand of `new` and `save`:
```typescript
const paul = await Player.create({name: "Paul", age: 16});
console.log(paul._id); // 1672968746499
```
## `list`
To list up all entities saved on this namespace:
```typescript
const all = await Player.list();
console.log(all.length); // 2
console.log(all[0].name); // John
```
## `dict`
To get all entities saved on this namespace as a dict:
```typescript
const dict = await Player.dict();
console.log(Object.entries(dict));
// [[1672363730924, Player], [1672968746499, Player]]
```
## `find`
To find specific object saved on this namespace:
```typescript
const found = await Player.find("1672968746499");
console.log(found?.name); // Paul
```
## `filter`
To find objects which should match a specific criteria:
```typescript
const criteria = (p: Player): bool => (Player.age > 16);
const filtered = await Player.filter(criteria);
console.log(filtered.length); // 1
```
## `update`
```typescript
await john.update({ age: 21 });
const found = await Player.find(john._id);
console.log(found.age); // 21
```
## `delete`
To delete a specific object:
```typescript
await john.delete();
const found = await Player.find(john._id);
console.log(found); // null
```
## `drop`
To delete all objects saved on this namespace:
```typescript
await Player.drop();
const list = await Player.list();
console.log(list.length); // 0
```
# Advanced properties
## `schema`
Use `static schema` to define relation between your `Model`s.
```typescript
import { Model, Types } from "jstorm/chrome/local";
class Team extends Model {
public name: string;
public captain: Player;
public admins: Player[];
static override schema = {
name: Types.string.isRequired,
captain: Types.model(Player, { eager: true }).isRequired,
admins: Types.arrayOf(Types.model(Player)),
}
}
```
> NOTE: When `{eager: true}` is provided in schema definition of `Types.model`, this model always look up your storage to populate specified fields, eagerly. Otherwise, this model just instantiate this field from what's saved under this model.
# Issues
- https://github.com/otiai10/jstorm/issues
| JavaScript Storage ORM (Object-Relational Mapper) for LocalStorage and Chrome Storage API | chrome-extension,chrome-storage,javascript,localstorage,orm,typescript | 2023-01-07T05:03:17Z | 2024-04-02T09:26:55Z | null | 1 | 19 | 62 | 0 | 0 | 5 | null | null | TypeScript |
ADITYAGABA1322/Daily-LeetCode-Challenge-Solution | main | # Daily-LeetCode-Challenge-Solution ๐

Hi Everyone. It's me Aditya and Here I'm providing you Daily LeetCode Challenge Solution with a full Explanation(Line to Line)
In Nine Languages:
C++๐ซถ && JAVA && Python && Python3 && C && C# && JavaScript && Swift && Dart ๐ฅ
<img align="right" src="https://media.giphy.com/media/2xE3cntFthsw6IR9OV/giphy.gif" width="400" />
<img align="bottom" src="https://appstickers-cdn.appadvice.com/1485748162/833420271/21c8eb2676cd8083729b7f85c616f97a-11.gif" width="40%" />
<img src="https://user-images.githubusercontent.com/73097560/115834477-dbab4500-a447-11eb-908a-139a6edaec5c.gif" width = "100%" >
<img align="bottom" src="https://media-exp1.licdn.com/dms/image/C4E12AQGhFbRMPvf0tg/article-cover_image-shrink_600_2000/0/1636293868291?e=2147483647&v=beta&t=Cmx0QZ0okPduLbfWorB2IIaBXhGelna6dtW0z9E8L34" width="100%" />
<img src="https://user-images.githubusercontent.com/73097560/115834477-dbab4500-a447-11eb-908a-139a6edaec5c.gif" width = "100%" >
<img align="bottom" src="https://wallpapercave.com/wp/wp7250277.jpg" width="100%" />
<img src="https://user-images.githubusercontent.com/73097560/115834477-dbab4500-a447-11eb-908a-139a6edaec5c.gif" width = "100%" >
| Let's Make Streak ๐ฅ | leetcode,streak,java,cpp,daily-coding-problem,csharp,javascript,python,python3,swift | 2023-01-09T16:42:22Z | 2024-05-22T18:16:30Z | null | 1 | 0 | 5,891 | 0 | 2 | 5 | null | MIT | C++ |
NicolasAndrehh/Nicolas-Olaya-Portfolio | main | <a name="readme-top"></a>
<!--
HOW TO USE:
This is an example of how you may give instructions on setting up your project locally.
Modify this file to match your project and remove sections that don't apply.
REQUIRED SECTIONS:
- Table of Contents
- About the Project
- Built With
- Live Demo
- Getting Started
- Authors
- Future Features
- Contributing
- Show your support
- Acknowledgements
- License
OPTIONAL SECTIONS:
- FAQ
After you're finished please remove all the comments and instructions!
-->
<div align="center">
<!-- You are encouraged to replace this logo with your own! Otherwise you can also remove it. -->
<img src="/images/shikimori.jpg" alt="logo" width="140" height="auto" />
<br/>
<h3><b>Nicolas Olaya - Portfolio Project</b></h3>
</div>
<!-- TABLE OF CONTENTS -->
# ๐ Table of Contents
- [๐ About the Project](#about-project)
- [๐ Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [๐ Live Demo](#live-demo)
- [๐ป Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#triangular_flag_on_post-deployment)
- [๐ฅ Authors](#authors)
- [๐ญ Future Features](#future-features)
- [๐ค Contributing](#contributing)
- [โญ๏ธ Show your support](#support)
- [๐ Acknowledgements](#acknowledgements)
- [๐ License](#license)
<!-- PROJECT DESCRIPTION -->
# ๐ [Nicolas-Olaya-Portfolio] <a name="about-project"></a>
**[Nicolas-Olaya-Portfolio]** is a project to present my knowledge and abilities in different programming languajes.
## ๐ Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
<details>
<summary>Client</summary>
<ul>
<li>HTML</li>
<li>CSS</li>
</ul>
</details>
<details>
<summary>Server</summary>
<ul>
<li>No server was required for this project</li>
</ul>
</details>
<details>
<summary>Database</summary>
<ul>
<li>No database was required for this project</li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
- **[This project use Linters for HTML and CSS]**
- **[This project does a correct use of GitHub Flows]**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## ๐ Live Demo <a name="live-demo"></a>
[Nicolas-Olaya-Portfolio - Live Demo](https://nicolasandrehh.github.io/Nicolas-Olaya-Portfolio/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## ๐ป Getting Started <a name="getting-started"></a>
To get a local copy up and running, follow these steps.
### Prerequisites
- You don't have to previously install nothing for this project
<!--
Example command:
```sh
gem install rails
```
-->
### Setup
Clone this repository to your desired folder:
```sh
cd my-folder
git clone git@github.com:NicolasAndrehh/Nicolas-Olaya-Portfolio.git
```
### Install
Install this project with:
```sh
// To install Webhint
npm install --save-dev hint@7.x
npx hint .
// To install Stylelint
npm install --save-dev stylelint@13.x stylelint-scss@3.x stylelint-config-standard@21.x stylelint-csstree-validator@1.x
```
### Usage
To run the project, execute the following command:
- This project doesn't need any command to run
### Run tests
To run tests, run the following command:
- This project doesn't have tests
### Deployment
You can deploy this project using:
- This project isn't deployable
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## ๐ฅ Authors <a name="authors"></a>
๐ค **Author1**
- GitHub: [@NicolasAndrehh](https://github.com/NicolasAndrehh)
- Twitter: [@nicolasolaya22](https://twitter.com/nicolasolaya22)
- LinkedIn: [LinkedIn](https://www.linkedin.com/in/nicolas-andres-olaya-gamba-3b032b248/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## ๐ญ Future Features <a name="future-features"></a>
- [ ] **[Put more projects in the projects section]**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## ๐ค Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## โญ๏ธ Show your support <a name="support"></a>
If you like this project you can share it to other people!
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## ๐ Acknowledgments <a name="acknowledgements"></a>
I would like to thank Microverse community.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FAQ (optional) -->
## ๐ License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| This is my portfolio, the place where you have to go if you want to know more about me and my skills. | css,html,javascript,linters | 2023-01-11T19:32:41Z | 2023-02-28T23:59:24Z | null | 3 | 11 | 85 | 3 | 0 | 5 | null | MIT | CSS |
raj03kumar/New-York | main | # New-York
This is New York Website with I created with parallax scrolling effect with JavaScript. The basic logic is to first cut the images from photoshop and use different z-index so that when we scroll images come one over other which will create that effect
| This is New York Website with I created with parallax scrolling effect with JavaScript | html-css-javascript,javascript,parallax-effect,parallax-scrolling,photoshop | 2023-01-10T13:50:06Z | 2023-01-10T14:11:28Z | null | 1 | 0 | 6 | 0 | 0 | 5 | null | GPL-3.0 | HTML |
ramNgithub/amazon.in-Clone | main |
# amazon.com
Amazon was founded by Jeff Bezos from his garage in Bellevue, Washington,[7] on July 5, 1994. Initially an online marketplace for books, it has expanded into a multitude of product categories, a strategy that has earned it the moniker The Everything Store.
## Authors
- [@ramNgithub](https://github.com/ramNgithub)
- [@abhayfaldu](https://github.com/abhayfaldu)
- [@AsifShaikh01](https://github.com/AsifShaikh01)
- [@owaisezarger](https://github.com/owaisezarger)
- [@atheist31](https://github.com/atheist31)
## Tech Stack used
- `REACT`
- `JAVA SCRIPT`
- `HTML`
- `CSS`
- `JSON SERVER`
## Deployed Link
https://endearing-tarsier-5faa41.netlify.app/
## Pages & Features
- Home Page(Stays)
- Add to cart functionality
- Sliding functionality
- Cart page
- Products pages
- Single Product page
# Snap shots
<b> Home Page </b>

<b> Best seller Page </b>

<b> Cart Page </b>

| An E-commerce website project developed by 5 developers in 6 days where user can singup, login with an email. User can see different pages and different products which are categorised well. Going forward user can add product to cart and can handle cart easily and finally can checkout and signout. | css,html,javascript,npm,reactjs | 2023-01-16T15:48:35Z | 2023-05-09T15:51:12Z | null | 6 | 25 | 96 | 0 | 2 | 5 | null | null | JavaScript |
AshDaGrat/Election-software | main | # Election-software
This system is a comprehensive solution for conducting secure and efficient voting over a LAN. Built using a combination of Python, JavaScript, HTML, CSS, and the Eel library. It includes a user-friendly interface, voter ID verification, and results calculation.
<img src="https://cdn.discordapp.com/attachments/1073895599910436915/1073897377997541426/image.png" >
<br>
# Installation
```
git clone https://github.com/AshDaGrat/Election-software
```
```
pip install -r requirements.txt
```
<br>
# Running
## On Server Side:
Edit the data\usn.json file with the voter IDs
<img src="https://cdn.discordapp.com/attachments/1073895599910436915/1073900366011322428/image.png">
Run Server.py and copy the IP address:
<img src="https://cdn.discordapp.com/attachments/1073895599910436915/1073898963746439258/image.png">
## On Client Side:
NOTE : Client and server should be connected to the same LAN
Edit the main.py file with the above IP address
<img src="https://cdn.discordapp.com/attachments/1073895599910436915/1073899663989673984/image.png">
Run main.py
<br>
# Vote Calculation
data\forfeit.json shows the list of voters who forfeit the election
data\done.json shows the list of voters who voted
data\recd.json shows the complete data of how every voter voted
Run data\counting.py to calculate votes
| Comprehensive solution for conducting secure and efficient voting over a LAN. | css,eel,election,html,javascript,json,python3,voting-application | 2023-01-12T02:58:48Z | 2024-04-06T17:42:06Z | null | 4 | 3 | 79 | 0 | 1 | 5 | null | CC0-1.0 | HTML |
tristan-greffe/portfolio | master |
<div align="center">

<p>My awesome personal portfolio created with React.js</p>
<p>
<a href="https://github.com/tristan-greffe/portfolio">
<img src="https://awesome.re/badge.svg" alt="awesome" />
</a>
<a href="https://github.com/tristan-greffe/portfolio/stargazers">
<img src="https://img.shields.io/github/stars/tristan-greffe/portfolio" alt="stars" />
</a>
<a href="https://github.com/tristan-greffe/portfolio/blob/master/LICENSE">
<img src="https://img.shields.io/github/license/tristan-greffe/portfolio.svg" alt="license" />
</a>
</p>
<h4>
<a href="https://portfolio.tristan-greffe.xyz/">View Demo</a>
<span> ยท </span>
<a href="https://github.com/tristan-greffe/portfolio/issues/">Report Bug</a>
<span> ยท </span>
<a href="https://github.com/tristan-greffe/portfolio/issues/">Request Feature</a>
</h4>
</div>
## About the Project
<div align="center">
<img src="./readme_assets/portfolio-project.png" height="auto" width="90%"/>
</div>
### Folder Structure
Here is the folder structure of Portfolio.
```bash
|- public/
|- src/
|-- assets/
|-- components/
|-- constants/
|-- scss/
|-- App.jsx
|-- index.js
|- package.json
```
### Tech Stack
[](https://skillicons.dev)
## Getting Started
#### Step 1:
At the main folder execute the following command in console to get the required dependencies:
```bash
npm install
```
or
```bash
yarn install
```
#### Step 2:
At the main folder execute the following command in console to run the development server:
```bash
npm run dev
```
or
```bash
yarn dev
```
## Contributing
Contributions are always welcome!
Contributions are what make the open source community such an amazing place to learn, inspire, and create. Any contributions you make are **greatly appreciated**.
If you have a suggestion that would make this better, please fork the repo and create a pull request. You can also simply open an issue with the tag "enhancement".
Don't forget to give the project a star! Thanks again!
1. Fork it! ๐ค
2. Create your feature branch: `git checkout -b my-new-feature`
3. Commit your changes: `git commit -m "Add some feature"`
4. Push to the branch: `git push origin my-new-feature`
5. Submit a pull request ๐
## License
This project is licensed under the MIT License - see the [license file](./LICENSE) for details
| My personal portfolio created with React.js | portfolio,portfolio-website,react,javascript,portfolio-project,developer-portfolio | 2023-01-11T18:04:44Z | 2024-04-10T00:14:40Z | null | 2 | 4 | 75 | 0 | 0 | 5 | null | MIT | JavaScript |
BahirHakimy/3D-Clock | main | # 3D-Clock
### Simple flipping clock made with HTML/CSS/JS
### [Live Demo](https://bahirhakimy.github.io/3D-Clock/)
| Simple flipping clock made with HTML/CSS/JS, in this project I have used CSS 3D features to build a beautiful flipping clock | css,html,javascript | 2023-01-03T08:48:44Z | 2023-01-10T19:05:49Z | null | 1 | 3 | 17 | 1 | 0 | 5 | null | null | HTML |
Tartiine/projet-web | main | # [projet-web] College project ENSISA 1A IR.
Made by [Isaac Dalberto](https://github.com/IDalb), [Corentin Leandre](https://github.com/corentinleandre), [Sofia Saadi](https://github.com/Tartiine)
Using `Python(Django)`, `Javascript`, `HTML` and `CSS`
January 2023
# Introduction
Website template based on `django` and `javascript` frameworks implementing an user authentification, administration and a chat system allowing emoticons :smile:.
# Prerequisite
# Quick start
```
python manage.py runserver
```
| null | django,ensisa,javascript | 2023-01-03T21:26:49Z | 2023-02-13T10:51:22Z | null | 3 | 0 | 119 | 0 | 0 | 5 | null | null | CSS |
AbeeraTahir/Crypto-Metrics | dev | <a name="readme-top"></a>
<!-- TABLE OF CONTENTS -->
# ๐ Table of Contents
- [๐ About the Project](#about-project)
- [๐ Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [๐ Live Demo](#live-demo)
- [๐ป Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [๐ฅ Authors](#authors)
- [๐ญ Future Features](#future-features)
- [๐ค Contributing](#contributing)
- [โญ๏ธ Show your support](#support)
- [๐ Acknowledgements](#acknowledgements)
- [๐ License](#license)
<!-- PROJECT DESCRIPTION -->
# ๐ Crypto Metrics <a name="about-project"></a>
**Crypto Metrics** is a mobile web application that uses [CoinStats API](https://cryptocointracker.com/api/coinstats) to display the list of cryptocurrencies and their market capitalization. Built with React and Redux.
## ๐ Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
<details>
<summary>Client</summary>
<ul>
<li><a href="https://reactjs.org/">React.js</a></li>
<li><a href="https://redux.js.org/">Redux</a></li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
- **Home Page displays a list of all cryptcurrencies**
- **User can search any cryptocurrency from the list**
- **Detail of each currency is displayed by clicking on the card on home page**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## ๐ Live Demo <a name="live-demo"></a>
- [Live Demo](https://incandescent-crepe-9d449b.netlify.app)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## ๐ป Getting Started <a name="getting-started"></a>
To get a local copy up and running, follow these steps.
### Prerequisites
In order to run this project you need:
- node.js
- git
### Setup
Clone this repository to your desired folder:
- Open your terminal
- Navigate to the directory where you want to clone the project.
- Clone the project using by running the following command:
`git clone https://github.com/AbeeraTahir/react-capstone.git`
### Install
Install this project using the following command:
`npm install`
### Usage
To run the project, execute the following command:
`npm start`
### Run tests
To run tests, run the following command:
`npm run test`
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## ๐ฅ Author <a name="authors"></a>
๐ค **Abeera Tahir**
- GitHub: [@AbeeraTahir](https://github.com/AbeeraTahir)
- Twitter: [@AbeeraTahir8]( https://twitter.com/AbeeraTahir8?t=z5CjMpmHMZmS98i09gUpYA&s=08)
- LinkedIn: [Abeera Tahir](https://www.linkedin.com/in/abeera-tahir/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## ๐ญ Future Features <a name="future-features"></a>
- **Cryptocurrency search can be done by price, market cap, or ranking etc.**
- **"Show more" button feature for the cryptocurrency list can be added in the Home Page**
- **Error page can be displayed on API failure**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## ๐ค Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## โญ๏ธ Show your support <a name="support"></a>
Give a โญ๏ธ if you like this project!
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## ๐ Acknowledgments <a name="acknowledgements"></a>
Original design idea by [Nelson Sakwa on Behance](https://www.behance.net/sakwadesignstudio)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## ๐ License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
_NOTE: we recommend using the [MIT license](https://choosealicense.com/licenses/mit/) - you can set it up quickly by [using templates available on GitHub](https://docs.github.com/en/communities/setting-up-your-project-for-healthy-contributions/adding-a-license-to-a-repository). You can also use [any other license](https://choosealicense.com/licenses/) if you wish._
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| Crypto Metrics is a mobile web application that uses CoinStats API to display the list of cryptocurrencies and their market capitalization. Built with React and Redux. | javascript,jest-tests,react,redux,webpack | 2023-01-02T10:22:29Z | 2023-06-25T08:40:59Z | null | 1 | 1 | 27 | 0 | 0 | 5 | null | MIT | JavaScript |
Amanmandal-M/SecondBackendProject | main | # SecondBackendProject
Products Related Backend
| Products Related Backend (Backend Deployed Link) | express,nodejs,bcrypt,dotenv,javascript,jwt-authentication,mongodb,mongoose | 2023-01-15T12:11:10Z | 2023-01-18T08:50:46Z | null | 1 | 0 | 15 | 0 | 1 | 5 | null | null | JavaScript |
Ghostfacefave/Best-working-blooket-hacks-for-IOS-and-PC-2023 | main | I love to code! Its been my favorite thing since ive been 5. And now i get to start coding big projects and all that stuff that I have to sadly say that i am quitting coding.
due to this, i hope you all like the project and live a very well but if this gets patched, i sadly wont be there to fix it, so enjoy it well you can.
| Working hacks for IOS and PC | blooket,blooket-hacks,hacks,ios,javascript,pc | 2023-01-07T06:13:29Z | 2023-01-07T07:26:56Z | null | 1 | 1 | 77 | 0 | 4 | 5 | null | NOASSERTION | JavaScript |
manq2010/beer-lovers | develop | <a name="readme-top"></a>
<!--
HOW TO USE:
This is an example of how you may give instructions on setting up your project locally.
Modify this file to match your project and remove sections that don't apply.
REQUIRED SECTIONS:
- Table of Contents
- About the Project
- Built With
- Live Demo
- Getting Started
- Authors
- Future Features
- Contributing
- Show your support
- Acknowledgements
- License
After you're finished please remove all the comments and instructions!
-->
<!-- TABLE OF CONTENTS -->
# ๐ Table of Contents
- [๐ About the Project](#about-project)
- [๐ Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [๐ Live Demo](#live-demo)
- [๐ป Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#deployment)
- [๐ฅ Authors](#authors)
- [๐ญ Future Features](#future-features)
- [๐ค Contributing](#contributing)
- [โญ๏ธ Show your support](#support)
- [๐ Acknowledgements](#acknowledgements)
- [โ FAQ](#faq)
- [๐ License](#license)
<!-- PROJECT DESCRIPTION -->
# ๐ Project <a name="about-project"></a>
> "Beers-Lovers" is a website that displays details of Brewdog beers. It allows users to search for their favorite beers or filter based on categories.
## ๐ Built With <a name="built-with"></a>
- HTML
- CSS
- JS
- React
### Tech Stack <a name="tech-stack"></a>
<details>
<summary>Client</summary>
<ul>
<li><a href="https://www.w3schools.com/js/">JavaScript</a></li>
<li><a href="https://www.w3schools.com/html/">HTML</a></li>
<li><a href="https://www.w3schools.com/css/">CSS</a></li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
> Using the project will help you to :
- **Filter beers by name and get their details**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## ๐ Live Demo <a name="live-demo"></a>
- [Beer Lovers Live Demo Link](https://beer-lovers.onrender.com/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## ๐ป Getting Started <a name="getting-started"></a>
To get a local copy up and running follow these simple example steps.
### Prerequisites
In order to run this project you need:
- Node js installed on your computer
- Npm Node package manager
- Terminal
### Setup
Clone this repository by using the command line :
- `git clone https://github.com/manq2010/beer-lovers.git`
### Install
Install this project with:
`npm install`
### Usage
To run the project, execute the following command:
`npm start`
### Run tests
To run tests, run the following command:
`npm test `
### Deployment
You can deploy this project using:
`npm run deploy`
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## ๐ฅ Authors <a name="authors"></a>
๐ค **Mancoba Sihlongonyane**
- GitHub: [@manq2010](https://github.com/manq2010/)
- Twitter: [@mancoba_c](https://twitter.com/mancoba_c/)
- LinkedIn: [mancobasihlongonyane](https://linkedin.com/in/mancobasihlongonyane/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## ๐ญ Future Features <a name="future-features"></a>
- **Add filter options for other catergories โณโ๏ธ**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## ๐ค Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## โญ๏ธ Show your support <a name="support"></a>
Give a โญ๏ธ if you like this project!
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## ๐ Acknowledgments <a name="acknowledgements"></a>
**Thank you**
- [Microverse](https://www.microverse.org/) for the project
- [Punk Api](https://punkapi.com/documentation/v2) for the API and documentation
- [Behance](https://www.behance.net/sakwadesignstudio) for the design
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FAQ (optional) -->
## โ FAQ <a name="faq"></a>
- **How to use the project**
- ```git clone https://github.com/manq2010/beer-lovers.git```
- **How to contribute to the project**
- Fork it by using ``fork`` button, or you can contact me.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## ๐ License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| "Beer-Lovers" is a website that displays details of Brewdog beers. It allows users to search for their favourite beers or filter based on categories. | javascript,jest-mocking,jest-snapshots,jest-tests,react,redux,slice | 2023-01-03T08:37:26Z | 2023-01-08T11:08:09Z | null | 1 | 3 | 47 | 0 | 0 | 5 | null | null | JavaScript |
Htetaungkyaw71/Gamery | master | <a name="readme-top"></a>
<!--
HOW TO USE:
This is an example of how you may give instructions on setting up your project locally.
Modify this file to match your project and remove sections that don't apply.
REQUIRED SECTIONS:
- Table of Contents
- About the Project
- Built With
- Live Demo
- Getting Started
- Authors
- Future Features
- Contributing
- Show your support
- Acknowledgements
- License
OPTIONAL SECTIONS:
- FAQ
After you're finished please remove all the comments and instructions!
-->
<div align="center">
<!-- You are encouraged to replace this logo with your own! Otherwise you can also remove it. -->
<img src="murple_logo.png" alt="logo" width="140" height="auto" />
<br/>
<h3><b>Gamery</b></h3>
</div>
<!-- TABLE OF CONTENTS -->
# ๐ Table of Contents
- [๐ About the Project](#about-project)
- [๐ Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [๐ Live Demo](#live-demo)
- [๐ป Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#triangular_flag_on_post-deployment)
- [๐ฅ Authors](#authors)
- [๐ญ Future Features](#future-features)
- [๐ค Contributing](#contributing)
- [โญ๏ธ Show your support](#support)
- [๐ Acknowledgements](#acknowledgements)
- [โ FAQ (OPTIONAL)](#faq)
- [๐ License](#license)
<!-- PROJECT DESCRIPTION -->
# ๐ [Gamery] <a name="about-project"></a>
> This is free to play games web application. Users can download free games in this app.
**[Gamery]** is a free to play game website
## ๐ Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
> HTML, CSS, React, Redux, React-testing library, API
<details>
<summary>Client</summary>
<ul>
<li><a href="https://reactjs.org/">React.js</a></li>
<li><a href="https://reactjs.org/">Redux.js</a></li>
</ul>
</details>
<details>
<summary>Server</summary>
<ul>
<li><a href="https://www.freetogame.com/api-doc">Free to game api</a></li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
> Describe between 1-3 key features of the application.
- **[Display games]**
- **[Dispay detail game]**
- **[direct link to game website]**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## ๐ Live Demo <a name="live-demo"></a>
> Add a link to your deployed project.
- [Live Demo Link](https://gamery.onrender.com/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## ๐ป Getting Started <a name="getting-started"></a>
> Describe how a new developer could make use of your project.
To get a local copy up and running, follow these steps.
## Screenshot

### Setup
Clone this repository to your desired folder:
```sh
cd my-folder
git clone git@github.com:Htetaungkyaw71/Gamery.git
```
-
### Install
Install this project with:
```sh
cd gamery
npm install
```
-
### Usage
To run the project, execute the following command:
```sh
npm start
```
-
### Run tests
To run tests, run the following command:
```sh
npm run test
```
-
### Deployment
You can deploy this project using:
```sh
npm run build
```
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## ๐ฅ Authors <a name="authors"></a>
> Mention all of the collaborators of this project.
๐ค **Htetaungkyaw**
- GitHub: [@githubhandle](https://github.com/Htetaungkyaw71)
- Twitter: [@twitterhandle](https://twitter.com/htetaun91907337)
- LinkedIn: [LinkedIn](https://www.linkedin.com/in/htet-aung-kyaw-9a77271a7/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## ๐ญ Future Features <a name="future-features"></a>
> Describe 1 - 3 features you will add to the project.
- [ ] **[Filter]**
- [ ] **[Browse games]**
- [ ] **[Search]**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## ๐ค Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](https://github.com/Htetaungkyaw71/Gamery/issues).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## โญ๏ธ Show your support <a name="support"></a>
> Write a message to encourage readers to support your project
If you like this project...
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## ๐ Acknowledgments <a name="acknowledgements"></a>
> Give credit to everyone who inspired your codebase.
I would like to thank...
- Inspiration api - [Freetogame api](https://freetogame.com/)
- Design is inspired from a design made by [Nelson Sakwa](https://www.behance.net/sakwadesignstudio).
- Linters are made by [Microverse Inc](https://github.com/microverseinc).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FAQ (optional) -->
## โ FAQ (OPTIONAL) <a name="faq"></a>
> Add at least 2 questions new developers would ask when they decide to use your project.
- **[Question_1]**
- [Answer_1]
- **[Question_2]**
- [Answer_2]
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## ๐ License <a name="license"></a>
This project is [MIT](./MIT.md) licensed.
_NOTE: we recommend using the [MIT license](https://choosealicense.com/licenses/mit/) - you can set it up quickly by [using templates available on GitHub](https://docs.github.com/en/communities/setting-up-your-project-for-healthy-contributions/adding-a-license-to-a-repository). You can also use [any other license](https://choosealicense.com/licenses/) if you wish._
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| A free-game app that pulls from the FreeToGame API to allow users to search for and view the free all games and view with categories. Built with React and Redux. | css,html,react,redux,redux-toolkit,javascript | 2023-01-01T07:55:38Z | 2023-01-03T15:58:21Z | null | 1 | 4 | 23 | 0 | 1 | 5 | null | null | JavaScript |
keanekwa/MathUwU | main | # MathUwU
Link to project: [MathUwU.com](https://mathuwu.com)
MathUwU aims on being both the Duolingo and Hackerrank of the quantitative finance world. Here's how:
Just like how [Duolingo](https://www.duolingo.com/) has revolutionalized learning languages and [MonkeyType](https://monkeytype.com/) has revolutionalized typing test, MathUwU aims on creating a fun platform for aspiring quant traders and developers to practice for their quantitative interviews.
One of the most popular practice tools in the market is the [Arithmetic Zetamac](https://arithmetic.zetamac.com/) game. While popular, Zetamac has a few limitations:
1. Lack of gamification
2. Lack of statistics to help players improve
3. Inability to save scores
4. Lack of more advanced game modes such as decimal and fraction arithmetic, or [Optiver's](https://optiver.com/) 80 in 8 game.
MathUwU introduces a fun, new way to practice for these mental arithmetic tests, which is an integral part of the quantitative finance world.
## How MathUwU Works
1. Choose a game mode
<img src="https://user-images.githubusercontent.com/8297863/235381623-f8a9e844-e9f9-40bf-b9ce-324fa4440438.png" width="800">
2. Customize game settings
<img src="https://user-images.githubusercontent.com/8297863/235381627-6c3a2556-684f-4a4b-8e89-412c0d13e50a.png" width="800">
3. Play the game
<img src="https://user-images.githubusercontent.com/8297863/235381634-d8863b76-0c0c-4806-afe8-2d0226663b13.png" width="800">
4. Review statistics and improve!
<img src="https://user-images.githubusercontent.com/8297863/235381616-97306cc2-a925-4722-8697-7ad96b76d9a4.png" width="800">
## Future Product Roadmap
[HackerRank](https://www.hackerrank.com/) and [CodeSignal](https://codesignal.com/) have become de-facto platforms when companies hire for programming focused roles. The first round of the interview process usually consists of sending an invitation to an applicant to complete an online test on one of these platforms.
Similarly, we hope that by incorporating invitation & testing administration features into MathUwU, we would be able to allow companies to incorporate MathUwU into the preliminary round of the hiring process.
| Gamified training platform for quantitative finance interviews. Full stack application developed with JavaScript (TypeScript & Next.js) and SQL (PostgreSQL). Deployed on Vercel and Railway. | javascript,nodejs,postgresql,quantitative-finance,reactjs,tailwindcss,typescript,railway,vercel | 2023-01-11T05:09:31Z | 2024-02-21T08:19:16Z | null | 1 | 25 | 169 | 1 | 0 | 5 | null | MIT | TypeScript |
Engelshell/armori | main | # armori - Alpha v1.0.9
## Unofficial mod to OMORI for Apple Silicon Macs
armori modifies OMORI so it can run on M1/2 Apple Silicon macs.
This mod will likely become unnecessary in the future when the game is officially fixed.
Alpha Quality software, there are likely bugs.

## Download
By using this software you agree to the MIT-0 License
Latest release: [armori-1.0.9-arm64.dmg](https://github.com/Engelshell/armori/releases/download/v1.0.9-alpha/armori-1.0.9-arm64.dmg)
## Install
Double click does not work! You must follow this:
<img src="open.png" alt="open image" width="400px" style="max-width:100%">
Right or two-finger click on armori.app, select 'open'.
## Support
Do not contact OMORI for support if you have this mod installed.
Uninstall and Reinstall OMORI through steam to remove this mod.
Post an issue here on Github if you run into issues.
[Discord Link](https://discord.gg/bJYqHRAg7A)
## Mods
Does not currently support oneloader. Oneloader does its own modifications and expects nwjs.
## License
[MIT No Attribution](LICENSE.md)
By using this software you agree to the license.
## Signing
Armori is self signed with key identity `armori - engelshell/armori`.
## Technical Details
- Full port of all components to apple silicon
- Ports from nwjs to Electron V26
- Greenworks compiled to apple silicon
- Steam SDK with apple silicon used
- Polyfill for nw.gui to electron
- Various fixes/wrappers to fix deprecated functionality
Looking to eventually port to other arm platforms
## TODO:
- Update nw.gui wrapper to include more functionality and correctness
- Rewrite installer as standalone app
- profile and discover potential performance issues caused by synchronous IPC.
## License
MIT No Attribution
Copyright 2022 Shelby Engels
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
| NWJS to Electron port for OMORI video game for M* Macs | arm64,electron,game,javascript,macos,nodejs,nwjs,omori | 2023-01-11T02:22:43Z | 2023-09-15T20:59:05Z | null | 1 | 0 | 17 | 1 | 0 | 5 | null | MIT-0 | JavaScript |
PowerLevel9000/Awesome-Books | dev | <a name="readme-top"></a>
<!-- the change -->
<div align="center">
<img src="./media/dilsher-logo.png" alt="logo" width="140" height="auto" />
<img src="./media/my-logo.png" alt="logo" width="140" height="auto" />
<br/>
<h1><b>Awesome Books</b><br><br></h1>
</div>
<div align="center">
<img src="./all-devices-black.png" alt="logo" width="100%" height="auto" />
</div>
<!-- TABLE OF CONTENTS -->
# ๐ Table of Contents
- [๐ About the Project](#about-project)
- [๐งช Linters And Deployment](#linters)
- [๐ Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [๐ Live Demo](#live-demo)
- [๐ป Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#triangular_flag_on_post-deployment)
- [๐ฅ Authors](#authors)
- [๐ญ Future Features](#future-features)
- [๐ค Contributing](#contributing)
- [โญ๏ธ Show your support](#support)
- [๐ Acknowledgements](#acknowledgements)
- [๐ License](#license)
<!-- PROJECT DESCRIPTION -->
# ๐ Awesome Books <a name="about-project"></a>
This Project for individual purpose and it organize your fav books
**Awesome Books** is just a javascript based simple project
## ๐งช Linters And Deployment <a name="linters"></a>
[](https://github.com/PowerLevel9000/Awesome-Books/actions/workflows/linters.yml)[](https://github.com/PowerLevel9000/Awesome-Books/actions/workflows/pages/pages-build-deployment)
## ๐ Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
In this project we used many skills, tech and libraries
<details>
<summary>Documentation</summary>
<ul>
<li><a href="https://html.com">HTML</a></li>
</ul>
</details>
<details>
<summary>Styling</summary>
<ul>
<li><a href="https://www.w3.org">CSS</a></li>
</ul>
</details>
<details>
<summary>Dynamics And Logics</summary>
<ul>
<li><a href="https://www.javascript.com/">JavaScript</a></li>
</ul>
</details>
### Key Features <a name="key-features"></a>
> Following features you should observe
- **You can add your fav book**
- **Yoc can remove it if you loose interest or mood swings**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## ๐ Live Demo <a name="live-demo"></a>
> This link will guide you to my project
- [Live Demo Link](https://powerlevel9000.github.io/Awesome-Books/dist/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## ๐ป Getting Started <a name="getting-started"></a>
For having local file and project you can fork this repo or clone it using `git clone https://github.com/PowerLevel9000/Awesome-Books.git`
And for making changes you you should follow prerequisites
### Prerequisites
In order to edit this project you need:
- Any text editor such as note pad and word pad
- A web browser
- Node js installed
- An IDE
#### Suggested IDE
- Visual studio code `I prefer this one ๐๐`
- Atom
- Sublime
- IntelliJ IDEA
- Visual code
### Setup
Clone this repository to your desired folder:
- Open your terminal there (bash cmd powershell run etc...) and run `npm i` to install node modules
- `npm run dev` or `npm run start` this will open project in browser
- If you to edit something edit in src dir and then run in terminal `npm run build` to build it for production
- Before deployment have some linter check
- [ ] `npx stylelint "**/*.{css,scss}"` for css and saas file
- [ ] `npx eslint .` for js files
- [ ] `npx hint .` for html files
- After fixing error build deploy for production
### Install
```
npm i
```
### Usage
- Navigation bar using SPA
- Book added to shelf
### Run tests
- Check whether your fav book added
- Check your mood swings works ๐งโ๐ซ๐ according to my project
### Deployment
You can deploy this project using:
- for this repo and use git hub pages to deploy it
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## ๐ฅ Authors <a name="authors"></a>
๐ค **Adarsh Pathak**
- GitHub: [@PowerLevel9000](https://github.com/githubhandle)
- Twitter: [@PowerLevel9002](https://twitter.com/PowerLevel9002?t=AIuSN7mTxk5a_MWpLolEjA&s=09)
- LinkedIn: [@Adarsh Pathak](https://www.linkedin.com/in/adarsh-pathak-56a831256/)
๐ค **Dilsher Balouch**
- GitHub: [@DilsherB](https://github.com/DilsherB)
- Twitter: [@_brilliantMindz](https://twitter.com/_brilliantMindz)
- LinkedIn: [@_brilliantMindz](https://www.linkedin.com/in/brilliantmindz/)
<!-- FUTURE FEATURES -->
## ๐ญ Future Features <a name="future-features"></a>
- [ ] **Add navigation bar**
- [ ] **improve functionality**
- [ ] **improve styling**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## ๐ค Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](https://github.com/PowerLevel9000/Awesome-Books/issues)).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## โญ๏ธ Show your support <a name="support"></a>
If you like my Project give it a Star โจ๐
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## ๐ Acknowledgments <a name="acknowledgements"></a>
I want to thank Microverse for giving me opportunity to show my skills
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## ๐ License <a name="license"></a>
This project is [MIT](https://github.com/PowerLevel9000/Awesome-Books/blob/master/MIT.md) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| It's for collection of your fav books with author | deployment,html-css-javascript,javascript,localstorage | 2023-01-16T07:57:07Z | 2023-05-27T10:33:09Z | null | 2 | 5 | 50 | 0 | 0 | 5 | null | null | JavaScript |
MrOwenovo/Verge3D-KarGoBang | master | ## ้กน็ฎไป็ป:
- ๅบไบverge3d-webGLๅผๅ็web3dๅบ็จ็จๅบ
- ๅฐblenderๅผๅ็ไบๅญๆฃๅๅจ็ปไธweb3dๆดๅ
- ๅบไบ็ฉบ้ดAI็ไธ็ปดๅๅญๆฃ
- ๅซๆๅ็ซฏๅ ่ฝฝๅๅๆฅไธๅฑ็ญ็้ข
- phๆฏๆๆบๆไฝ็ๆฌ๏ผไธๆฃๆไฝ้่ฆๅๅป้ฒ่ฏฏ่งฆใ
### ่ฏ็ฉๅฐๅ:
[ไธ็ปดๅๅญๆฃAI-ๅฐ้พ](https://f01-1309918226.file.myqcloud.com/42/2022/09/12/%E5%9B%B0%E9%9A%BE4/loading.html?x-cos-traffic-limit=819200) <br>
[ไธ็ปดๅๅญๆฃAI-ไธญ็บง](https://f01-1309918226.file.myqcloud.com/42/2022/09/12/%E5%9B%B0%E9%9A%BE4/loading.html?x-cos-traffic-limit=819200) <br>
[ไธ็ปดๅๅญๆฃAI-็ฎๅ](https://f01-1309918226.file.myqcloud.com/42/2022/09/12/%E5%9B%B0%E9%9A%BE4/loading.html?x-cos-traffic-limit=819200) <br>
[ไธ็ปดๅๅญๆฃAI-ๆฌๅฐ่ๆบ](https://f01-1309918226.file.myqcloud.com/42/2022/09/11/%E6%9C%AC%E5%9C%B01/loading.html?x-cos-traffic-limit=819200) <br>
## ้จ็ฝฒๆกไปถ
### ไธ่ฝฝverge3D
- verge3D-webGLๆกๆถ๏ผๆๅบไบblender็verge3d-blender,ๅบไบ3dMax็verge3d-3dMax
- verge3Dๅฎ็ฝ:[https://verge3d.funjoy.tech/verge3d](https://verge3d.funjoy.tech/verge3d)
### ็งปๅจ้กน็ฎๆไปถ
- ๆฏไธไธชๆไปถๅคน้ฝๆฏไธไธช้กน็ฎ๏ผๅฐๆๆ้กน็ฎ็งปๅจๅฐverge3D็applicationsๆไปถๅคนไธ
## ้กน็ฎๅ
ๅฎน๏ผ
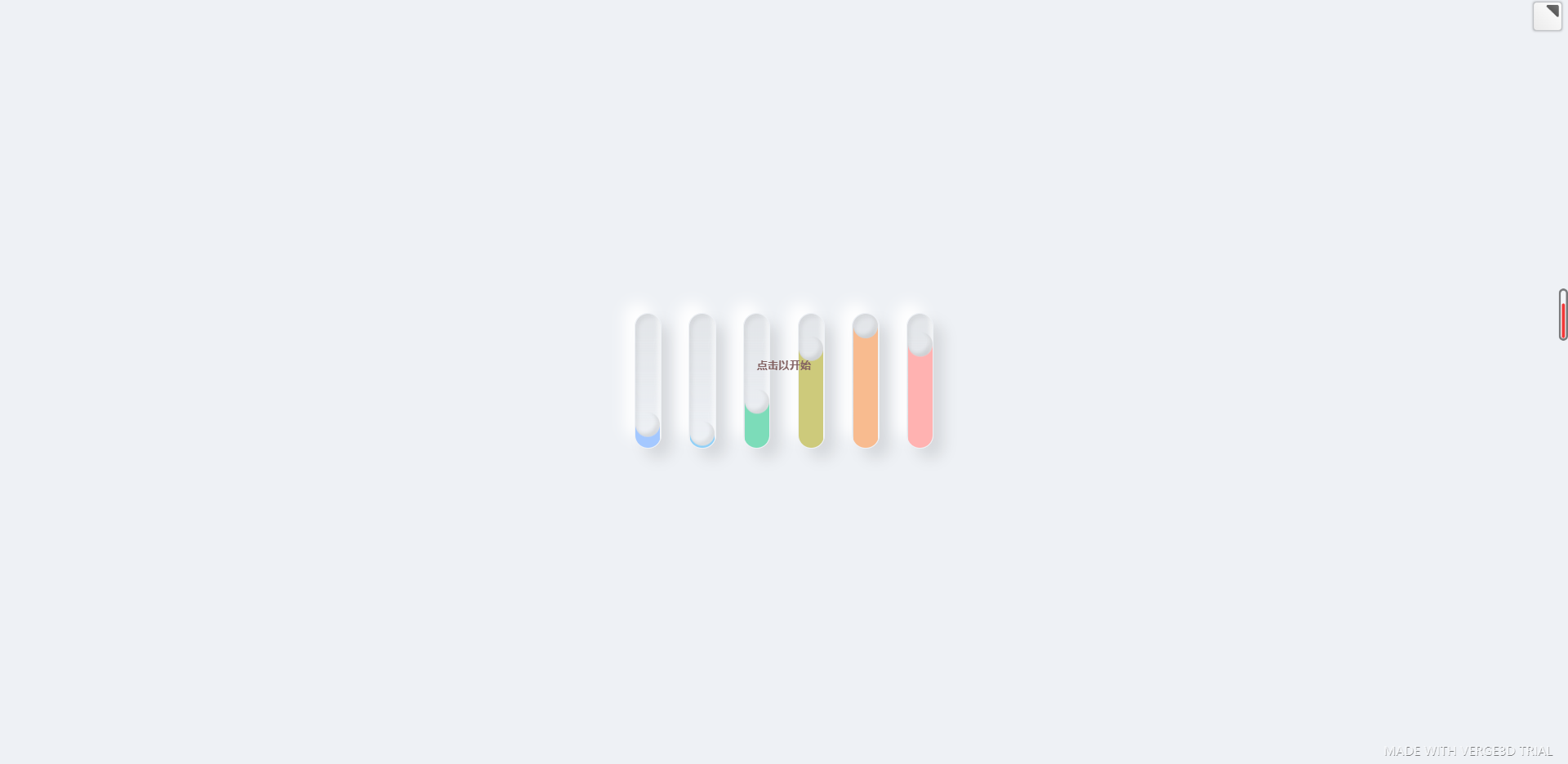
<p align="center">ไธ็ปดๅๅญๆฃๅ ่ฝฝ็้ข</p>
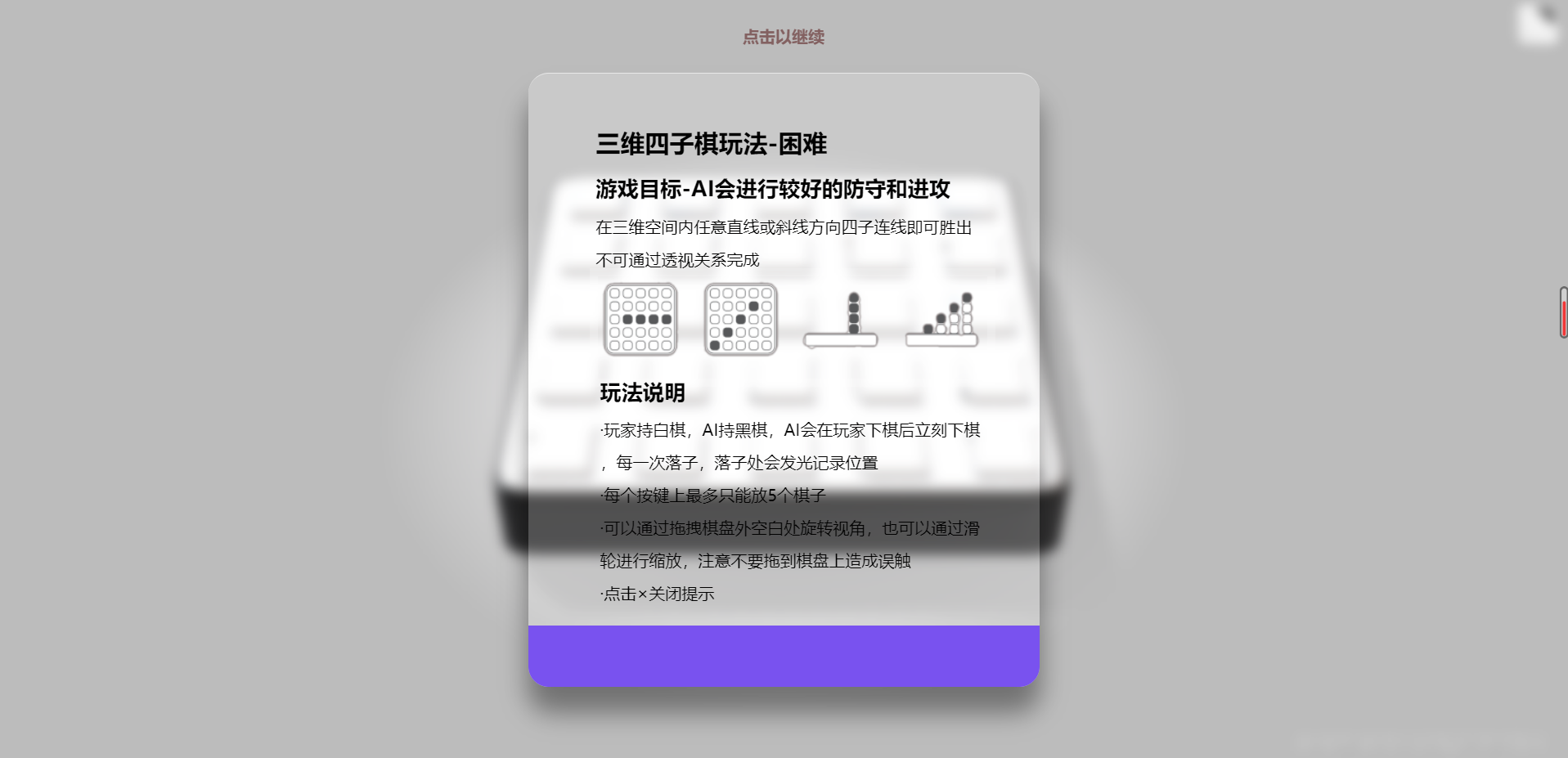
<p align="center">ไธ็ปดๅๅญๆฃๆไฝๆ็คบ</p>
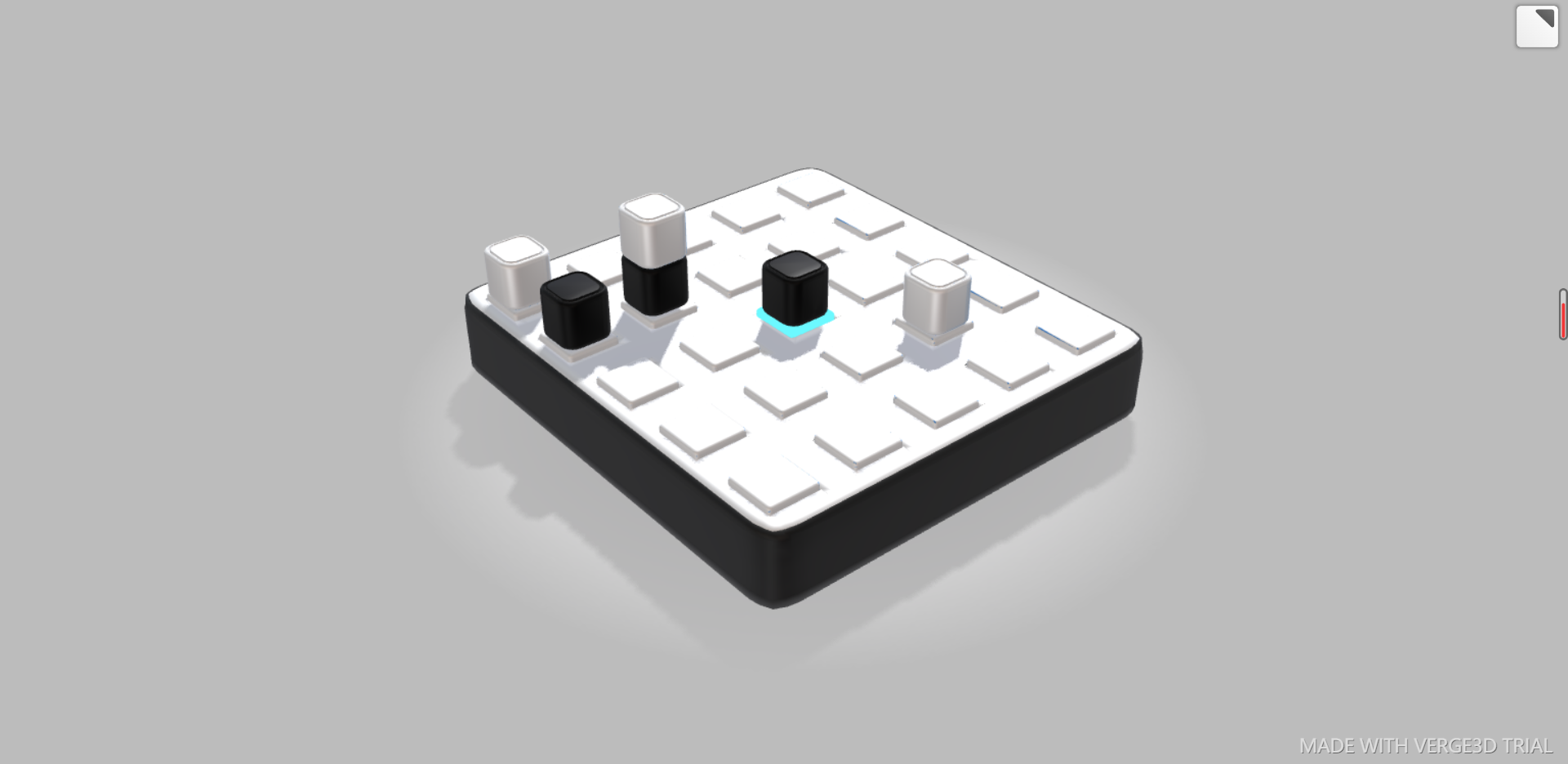
<p align="center">AIๆธธๆ่ฟ็จ็ป้ข</p>
| ไธ็ปดๅๅญๆฃ็verge3D ่ตๆบ,ๅซAI,่ๆบ็ญๅ
จ้จๆบ็ ๅๆจกๅ | javascript,blender,verge3d,web3d,webgl | 2023-01-12T03:49:16Z | 2023-12-01T14:02:26Z | null | 1 | 0 | 20 | 0 | 0 | 5 | null | null | JavaScript |
Learn-React-With-Harshi/chapter-01-inception-namaste-react | main | # `Learn React With Harshi ๐ฉ๐ปโ๐ป Series`
Documenting my learning journey of [Namaste React Live Course](https://learn.namastedev.com/) conducted by Akshay Saini
## Repository Structure in this series
All the repositories of this `Learn React With Harshi` series contains the class notes, coding and theory assignments of Namaste React Live course conducted by *Akshay Saini*. The project structure is
```
.
+-- class-notes.md
+-- theory-assignment.md
+-- README.md
+-- index.html
+-- app.js
+-- other files like react component(in upcoming repositories)
+-- .gitignore
+-- package.json
+-- package-lock.json
```
## Class Summary `Chapter-01 Inception` (24/12/2022)
Chapter-01 (Inception) was the first session of the Namaste React Live course. The whole class was excited about the beginning of a great course. We started learning react from scratch. Environment setup was clearly explained. Started with creating a simple Hello world program . First, using only html, then we tried to implement the same through javascript. Finally, we created a simple hello world program using React. Everything was done without installing any package. Unlike other courses which starts with create-react-app as first session, we tried writing the react code from zero. It was a true inception session for tranforming from ZERO to HERO in React.
One line about this chapter : `React is very simple, NO PANIC please.`
Check out [Chapter-01 Live](https://learn-react-with-harshi-chapter-01.netlify.app/) for the live demo of this chapter's coding assignment
If you are interested in learning other chapters of this series, please browse through the [my repositories](https://github.com/orgs/Learn-React-With-Harshi/repositories)
If you have any queries/suggestions about my version of this course, please feel free to raise PR. Happy coding !!!
| This was an introduction session to Namaste React Live Course. Wrote the first react program without any installation or create-react-app. | akshay-saini,create-react-app,css3,html5,javascript,namaste-react,react,react-dom,reactjs,react-coding-assignment | 2023-01-01T14:57:26Z | 2023-01-05T10:15:15Z | null | 1 | 0 | 21 | 0 | 5 | 5 | null | null | HTML |
shreshthkr/Craftsvilla-clone | main | null | It is a clone of Craftsvilla website which is an e-commerce platform for products related to Home-Decor. | chakra-ui,css,javascript,react | 2023-01-16T15:16:22Z | 2023-04-22T21:36:31Z | null | 2 | 7 | 21 | 0 | 0 | 5 | null | null | JavaScript |
Juanpa8830/Leaderboard | dev | <a name="readme-top"></a>
<div align="center">
<h3><b>Full Stack Developer</b></h3>
</div>
<!-- TABLE OF CONTENTS -->
# ๐ Table of Contents
- [๐ Table of Contents](#-table-of-contents)
- [๐ \[Leaderboard\] ](#-leaderboard-)
- [๐ Built With ](#-built-with-)
- [Tech Stack ](#tech-stack-)
- [Key Features ](#key-features-)
- [๐ Live Demo ](#-live-demo-)
- [๐ป Getting Started ](#-getting-started-)
- [Prerequisites](#prerequisites)
- [Setup](#setup)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Start the project](#start-the-project)
- [๐ฅ Author ](#-author-)
- [๐ญ Future Features ](#-future-features-)
- [๐ค Contributing ](#-contributing-)
- [โญ๏ธ Show your support ](#๏ธ-show-your-support-)
- [๐ Acknowledgments ](#-acknowledgments-)
- [๐ License ](#-license-)
<!-- PROJECT DESCRIPTION -->
# ๐ Leaderboard <a name="about-project"></a>
"Leaderboard" is an application that allows user to register user names and their repective scores.
## ๐ Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
- Webpack
- html
- JavaScript
- CSS
<!-- Features -->
### Key Features <a name="key-features"></a>
- **Javascript DOM manipulation**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## ๐ Live Demo <a name="live-demo"></a>
[click here for live-demo](https://juanpa8830.github.io/Leaderboard/dist/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## ๐ป Getting Started <a name="getting-started"></a>
### Prerequisites
* Have git installed. (For version control)
* Have text editor installed. (VSCode, sublime, atom)
* Have CSS,HTML & JS Linters installed and configured. For further information, visit [this link](https://github.com/microverseinc/linters-config/blob/master/README.md) to get better understanding on each linters used on this project.
### Setup
Clone this repository to your desired folder:
git clone git@github.com:Juanpa8830/Leaderboard.git
### Install
Install this project with:
- npm install (to install all the packages needed)
- npm run build (to generate the dist folder with files)
- npm run dev (to start the Webpack dev server)
### Usage
This page registers names and scores. To add a new line:
1. Type name and scores at the input fields.
2. click submit button.
3. The name and score will be added to the table and will be displayed.
<!-- AUTHORS -->
## ๐ฅ Author <a name="authors"></a>
๐ค **Juan Pablo Guerrero Rios**
- GitHub: [@Juanpa8830](https://github.com/Juanpa8830)
- Twitter: [@juanPa8830](https://https://twitter.com/JuanPa8830)
- LinkedIn: [Juan Pablo Guerrero](https://www.linkedin.com/in/juanguerrerorios)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## ๐ญ Future Features <a name="future-features"></a>
- [ ] **Database connection**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## ๐ค Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](https://github.com/Juank628/to_do_list/issues).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## โญ๏ธ Show your support <a name="support"></a>
Give a โญ๏ธ if you like this project!
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| "Leaderboard" is an application that allows user to register user names and their respective scores. These scores are displayed after getting the data from an API. Built with CSS, HTML, JavaScript | css3,html5,javascript | 2023-01-03T20:13:19Z | 2023-01-24T18:55:44Z | null | 1 | 3 | 26 | 0 | 0 | 5 | null | MIT | JavaScript |
KeremTAN/TodoAPP | master | null | This is an back-end application with is designed NodeJS | javascript,nodejs | 2023-01-10T10:49:01Z | 2023-01-12T07:32:51Z | null | 1 | 0 | 9 | 0 | 0 | 5 | null | null | JavaScript |
NikhilSharma1234/Mezzo | master |
# Mezzo 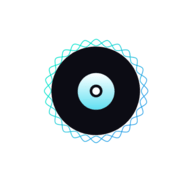
**Mezzo** is a twist on a typical musical application that helps users connect with both their friends and favorite artists. With Mezzo, you can discover new music and connect with like-minded people who share your musical interests. This application was created from a collaborative team of three within a 3-month timespan.
### Features
* **Personalized profiles**: Create a personalized profile that showcases your favorite genres, artists, and songs.
* **Music sharing**: Share your favorite songs or playlists with others on the platform and listen to other users' music selections.
* **Playlists**: Collaborate on playlists with friends and discover new music.
* **Like/Unlike**: Add music to playlists via liking or unliking your hand-picked songs.
* **Discover Tab**: Explore new music with our Discover Tab feature. Our app provides you with a curated selection of songs, artists, and playlists based on your listening history and preferences.
* **Login/Logout** Our app provides easy-to-use login/logout functionality. Simply log out of your account and log back in whenever you want to continue listening to your music.
* **Forgot Password** Forgot your password? No worries! Our app makes it easy to reset your password so you can get back to enjoying your music in no time.
### Future Developments
* **Lyrics**: Get the lyrics to your favorite songs right at your fingertips! Our app is currently working on a feature that will provide lyrics to millions of songs, so you can sing along and learn the words to your favorite tunes.
* **Social Tab**: Connect with other music lovers on our app's Social Tab. Soon you'll be able to share your favorite songs, playlists, and discover new music with friends.
* **Music Queue**: Our app is currently working on a Music Queue feature that will allow you to queue up your favorite songs and listen to them in the order you choose. Say goodbye to interruptions and enjoy your music uninterrupted.
* **Live Recommendations**: Looking for something new to listen to? Our app is working on a live recommendations feature that will provide you with personalized recommendations based on your listening history and preferences.
* **More Settings**: We're constantly improving our app and adding new settings to give you more control over your listening experience. Keep an eye out for our More Settings feature, where you'll be able to customize your app settings to your liking.
* **2FA**: Security is important to us, which is why we're currently working on adding 2FA (two-factor authentication) to our app. Soon you'll be able to enjoy your music with the peace of mind that comes from knowing your account is secure.
### Demo
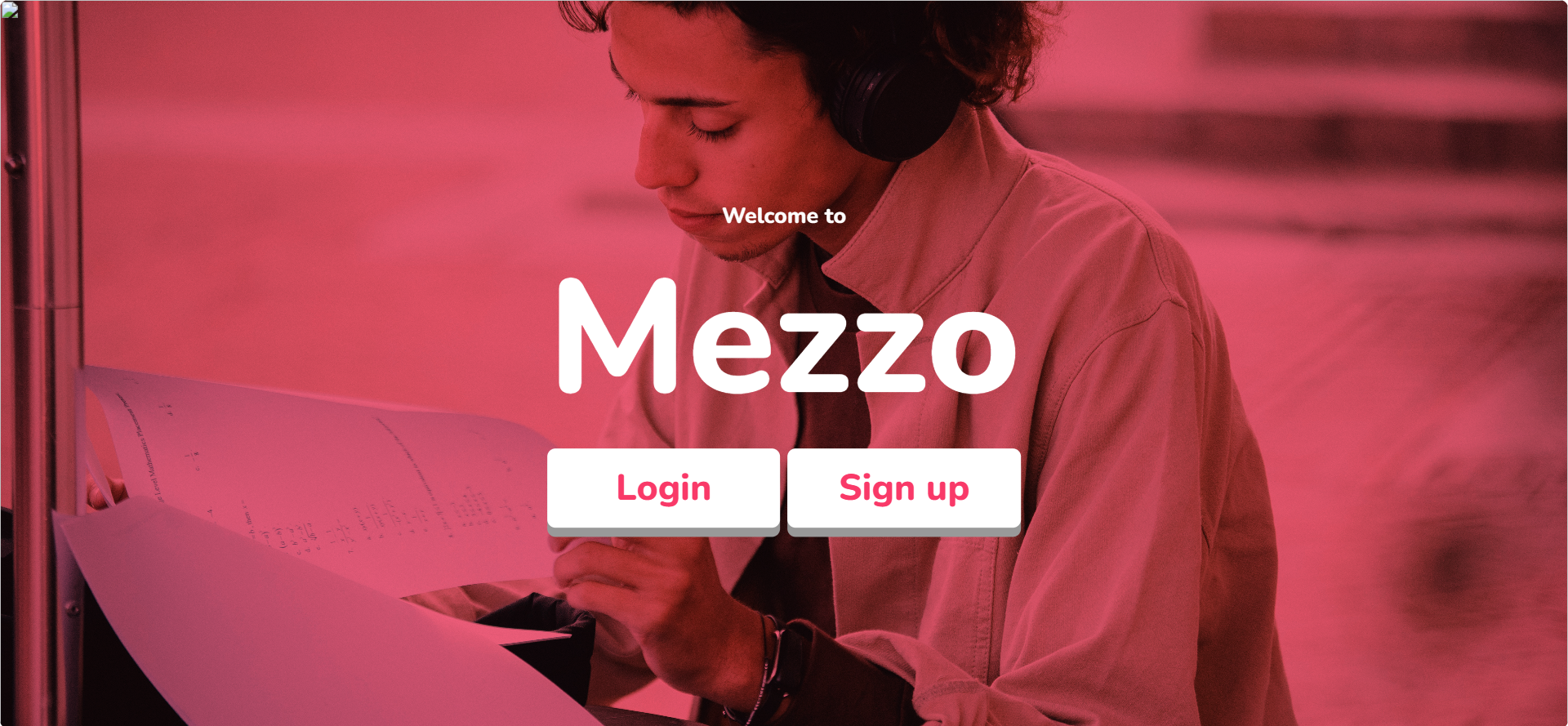
- Frontpage of Mezzo
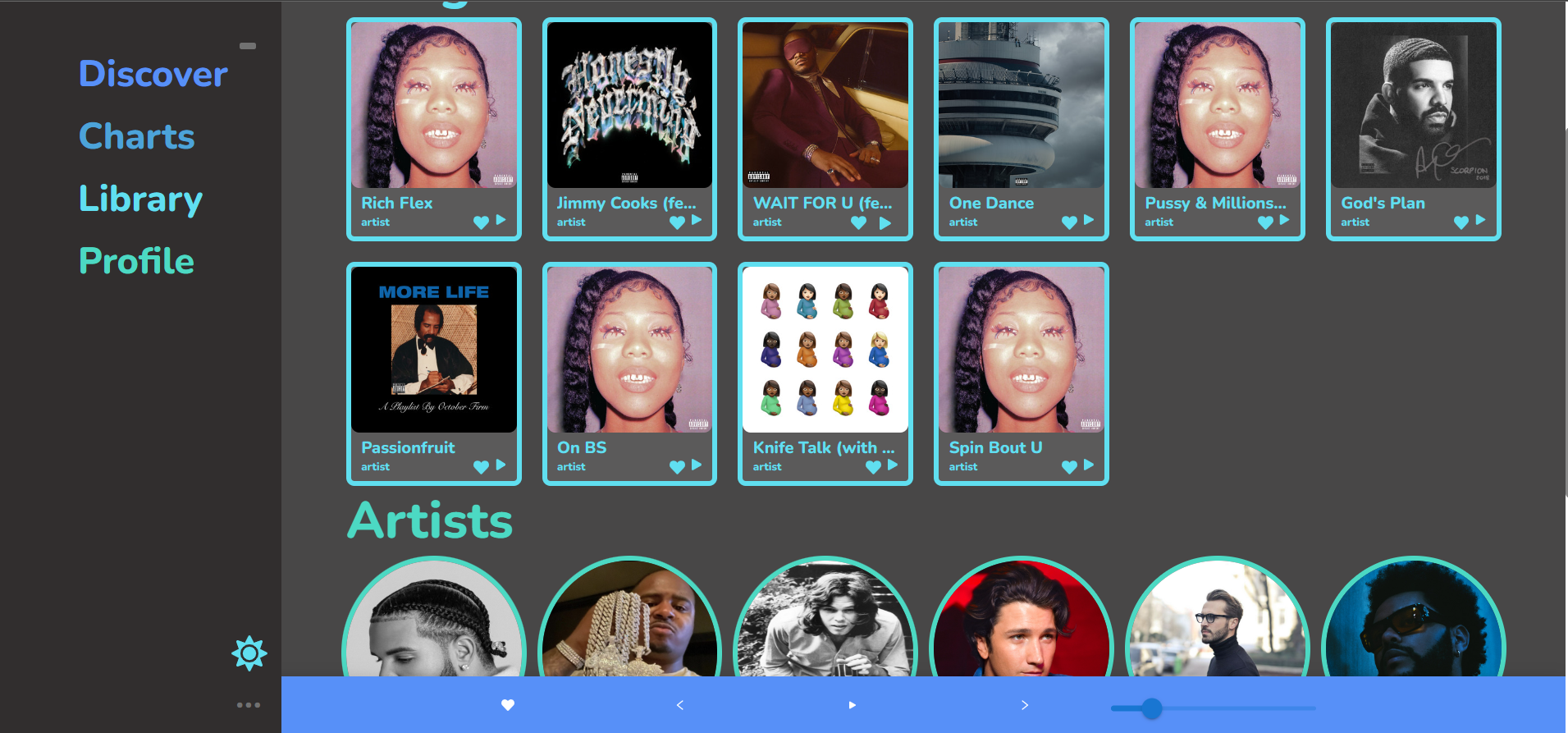
- Discover tab with a *'Drake'* query
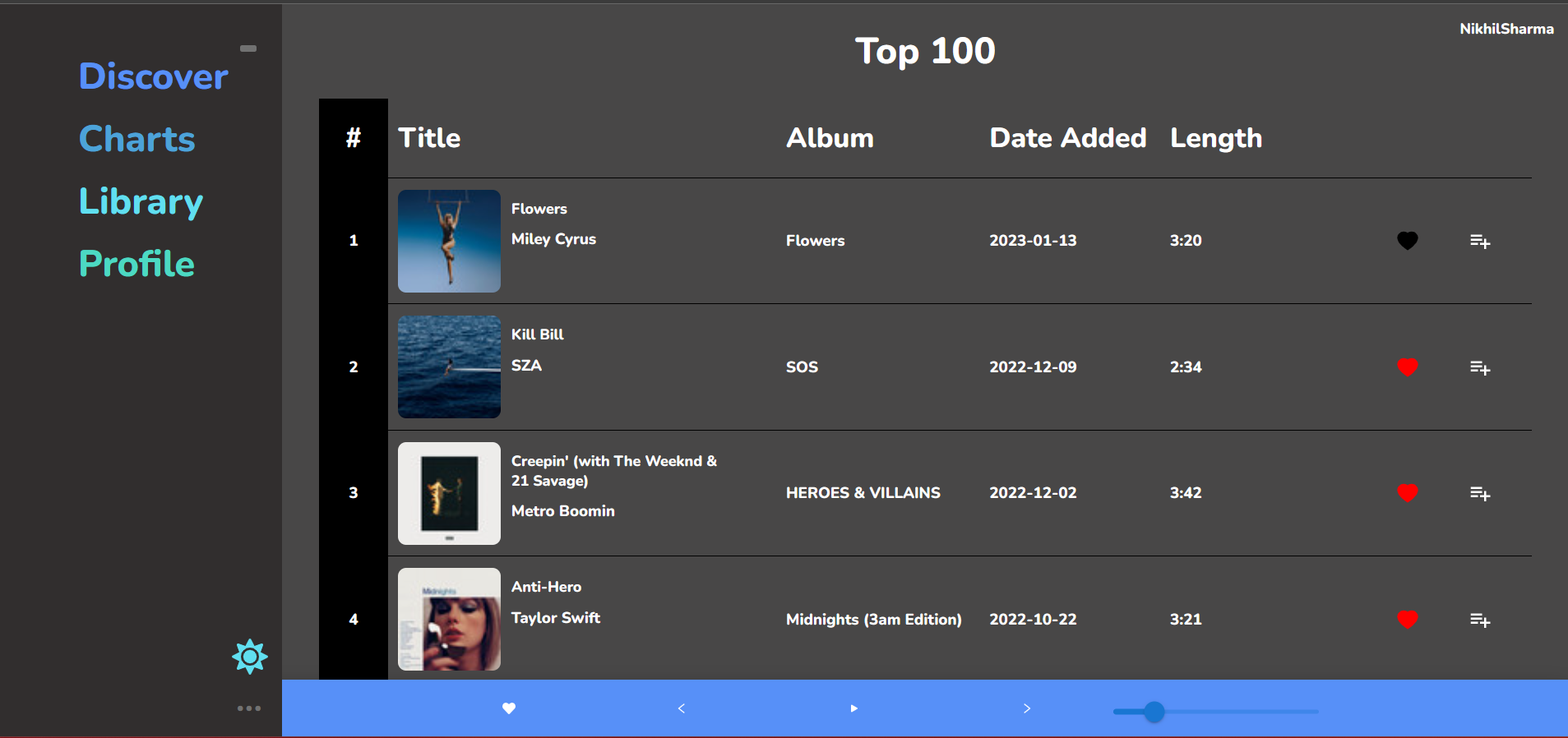
- Charts tab showcasing top 100 songs
## Deployment
Mezzo is currently deployed at *deployment_link*
## Technologies Used
**MERN Stack**:
* **MongoDB**: A popular NoSQL database that provides scalability, flexibility, and high availability.
* **Express**: A lightweight Node.js framework that simplifies the process of building APIs and web applications.
* **React**: A JavaScript library for building user interfaces.
* **Node.js**: A server-side JavaScript runtime built on the V8 engine that enables developers to build fast, scalable network applications.
## Additional Technologies
* **Socket.IO**: Work in progress, for 'Social Tab.' Socket.IO is an event-based communication library that allows us to eventually create a real-time chat platform.
* **JWT**: JSON web tokens allowed us to provide users with secure user authentication and authorization.
* **Spotify API**: Integrating the Spotify API into our web app allowed us to access the vast music library of Spotify and provide our users with a wide range of songs, artists, and playlists to choose from.
* **Postman / Insomnia**: Used to ensure all our API routes were functioning properly. This tool helped verify that our app was performing as expected.
* **Honorable Mentions**: Mongoose, cookies, user-sessions, etc.
## Contributing
We welcome contributions from anyone who is interested in improving Mezzo. To contribute, follow these steps:
1. Fork the repository.
2. Create a new branch for your feature or bug fix.
3. Make your changes and commit them with descriptive commit messages.
4. Push your changes to your fork.
5. Create a pull request and describe your changes.
## Authors
- [Nikhil Sharma](https://github.com/NikhilSharma1234)
- [Aidan Vancil](https://github.com/aidanvancil)
- [Fiorina Chau](https://github.com/fi0rina1)
| Project Two of the TechWise program by Google - Full Stack Application | express,javascript,mongodb,mongoose,nodejs | 2023-01-10T03:25:37Z | 2024-04-24T16:53:55Z | null | 4 | 36 | 387 | 0 | 0 | 5 | null | Apache-2.0 | JavaScript |
kaykeeb3/roquet.q | main | <p align="center">
<img src="https://github.com/rocketseat-education/nlw-06-discover/blob/main/.github/capa.png" width="100%" alt="Roquet.Q" />
</p>
<h1 align="center">Roquet.Q</h1>
<p align="center">
<a href="#-tecnologias">Tecnologias</a> |
<a href="#-projeto">Projeto</a> |
<a href="#-layout">Layout</a> |
<a href="#memo-licenรงa">Licenรงa</a>
</p>
<p align="center">
<img src="https://img.shields.io/static/v1?label=PRs&message=welcome&color=49AA26&labelColor=000000" alt="PRs welcome!" />
<img src="https://img.shields.io/static/v1?label=license&message=MIT&color=49AA26&labelColor=000000" alt="License" />
</p>
## ๐ Tecnologias
Este projeto foi desenvolvido utilizando as seguintes tecnologias:
- HTML
- CSS
- JavaScript
- NodeJS
- EJS
- Express
- SQLite
## ๐ป Projeto
O Roquet.Q รฉ uma aplicaรงรฃo de interaรงรฃo atravรฉs de perguntas, permitindo criar salas para que os usuรกrios anรดnimos faรงam perguntas. O criador da sala, usando uma senha, pode gerenciar essas perguntas e marcรก-las como lidas.
## ๐ Layout
Vocรช pode visualizar o layout do projeto [aqui](https://www.figma.com/community/file/1009821158959690135/Roquet.q). Uma conta no [Figma](https://figma.com) รฉ necessรกria para acessar o layout.
## :memo: Licenรงa
Este projeto estรก sob a licenรงa MIT. Veja o arquivo [LICENSE](.github/LICENSE.md) para mais detalhes.
| Roquet.Q รฉ uma aplicaรงรฃo de interaรงรฃo atravรฉs de perguntas, sendo possรญvel criar uma sala para internautas anรดnimos fazerem perguntas e o criador da sala em posse de uma senha gerenciar essas perguntas e marcar como lidas. | css,express,html,javascript,nodejs,rocketseat | 2023-01-08T12:52:24Z | 2023-10-20T00:19:43Z | null | 1 | 0 | 10 | 0 | 0 | 5 | null | null | EJS |
Abdullah-moiz/Pain-Gain-Fitness-App | main |
# Pain & Gain
Pain & Gain is a Fitness Web App based on MERN STACK TECHNOLOGIES ,
It consist of Three Main Modules
1 : Fitness E-commerce App that works with Rest API's
2 : Personal Trainee module you can hire Professional Trainer
3 : Chat Module is Added so You can chat with your Trainer
## Authors
- [@Abdullah Moiz](https://www.github.com/Abdullah-moiz)
# ScreenShots
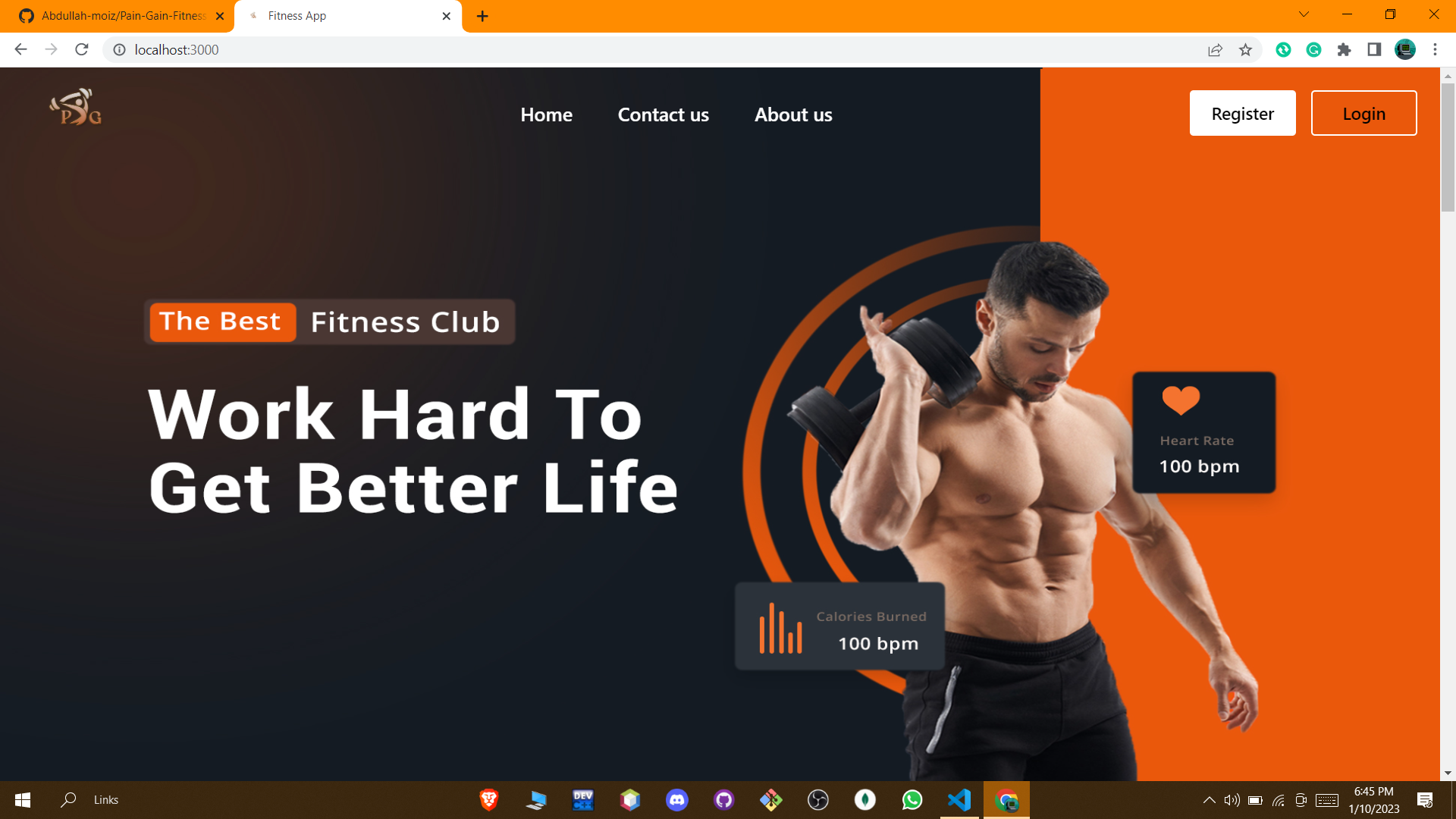
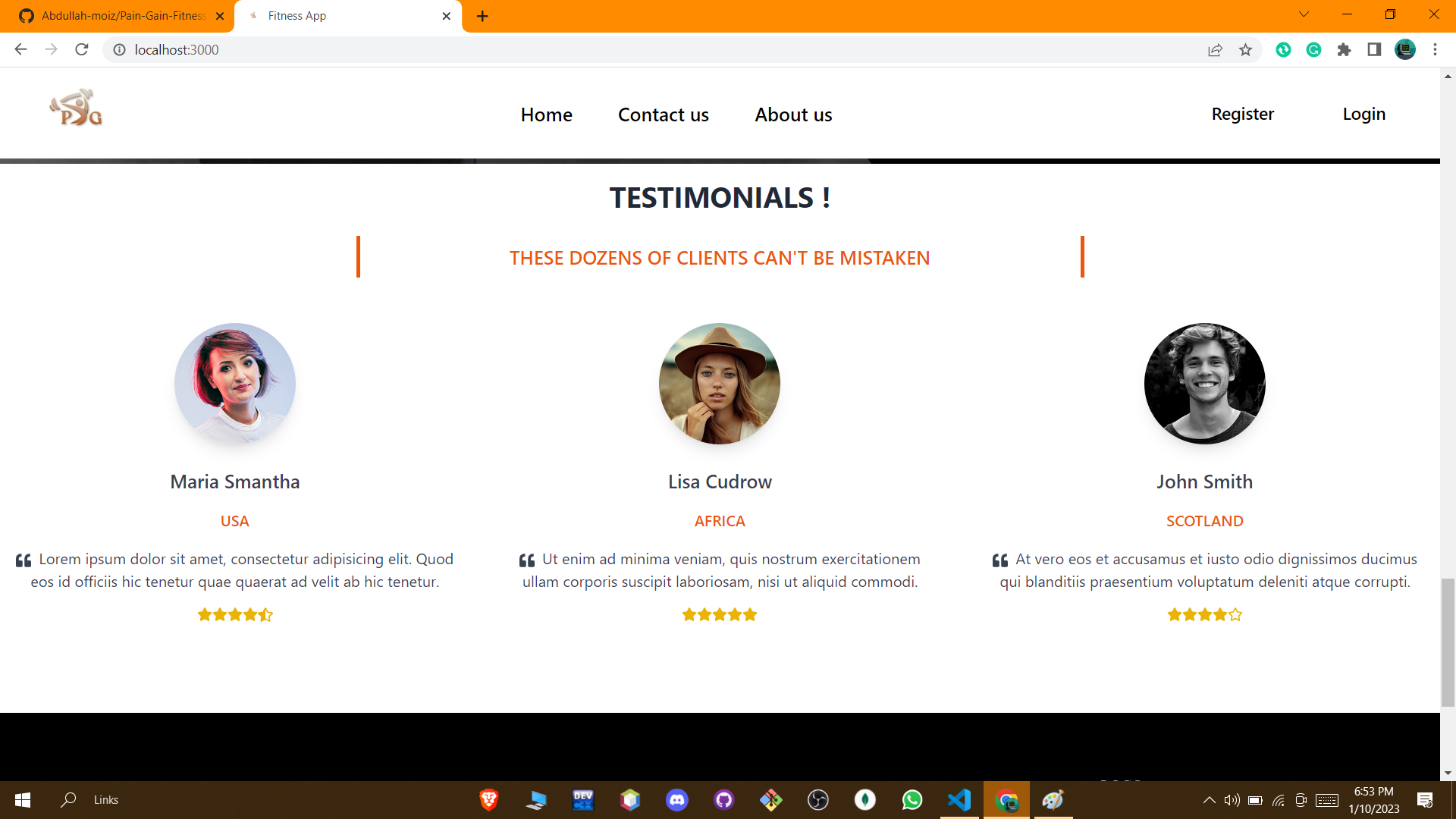
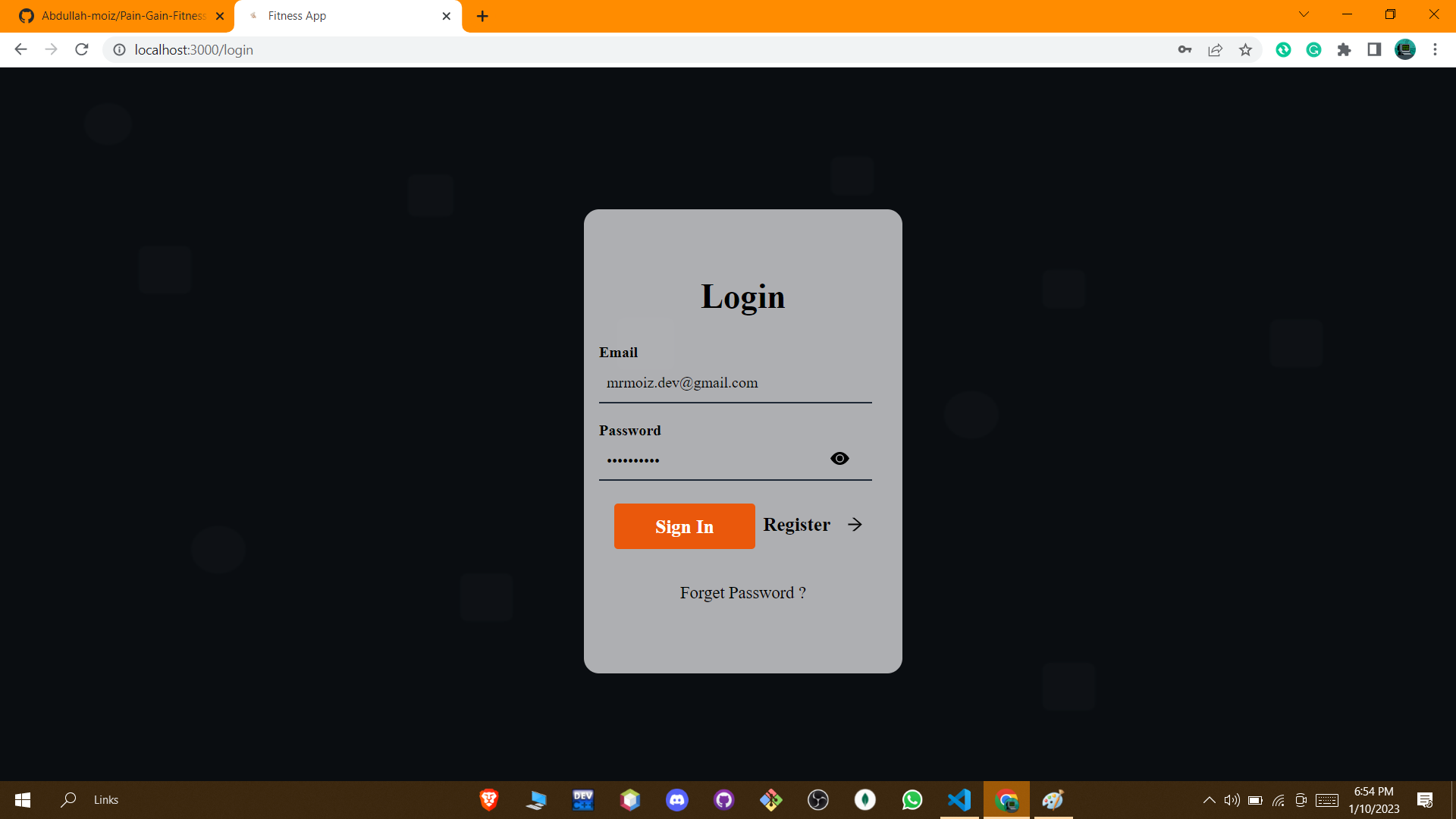
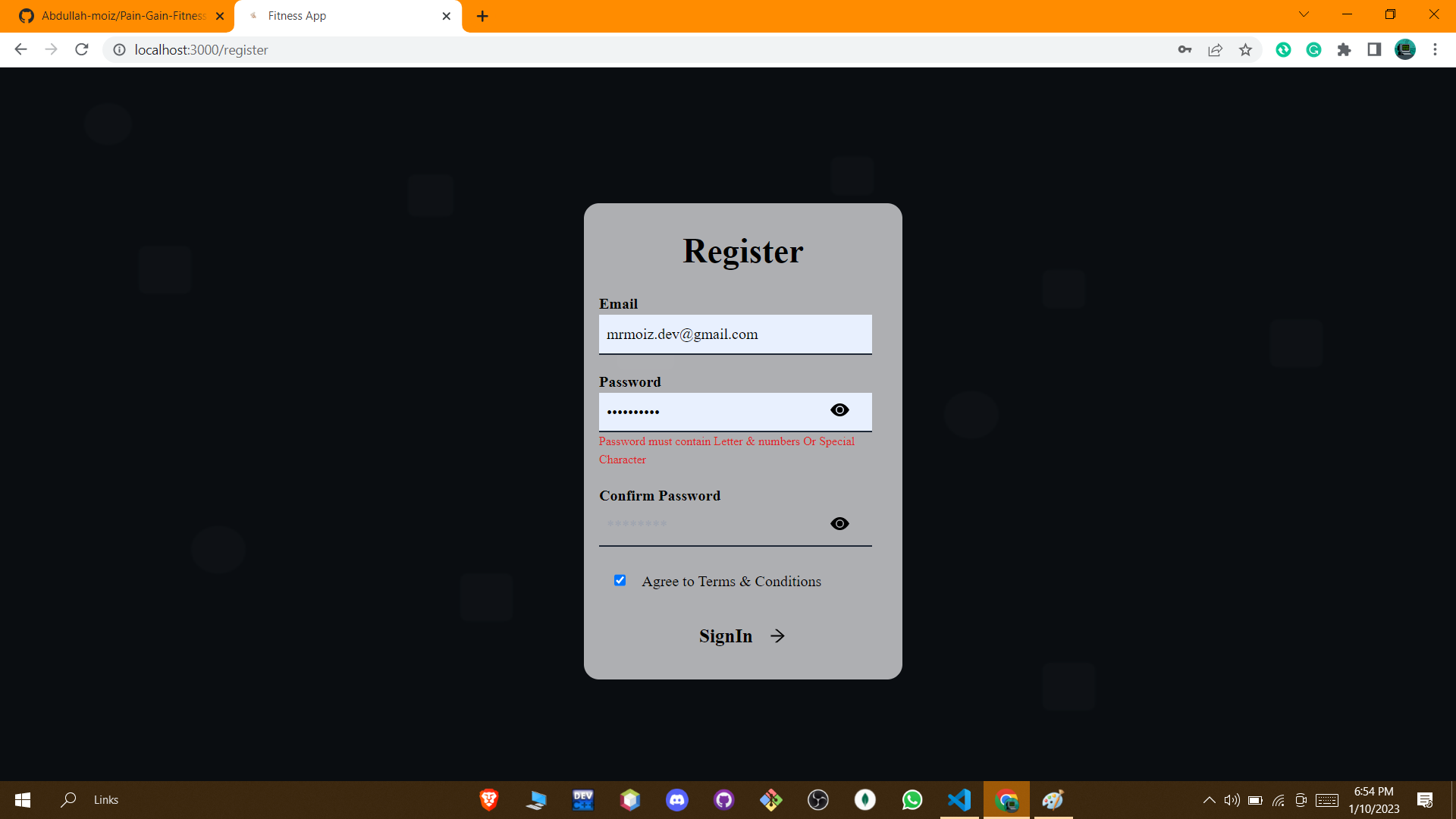
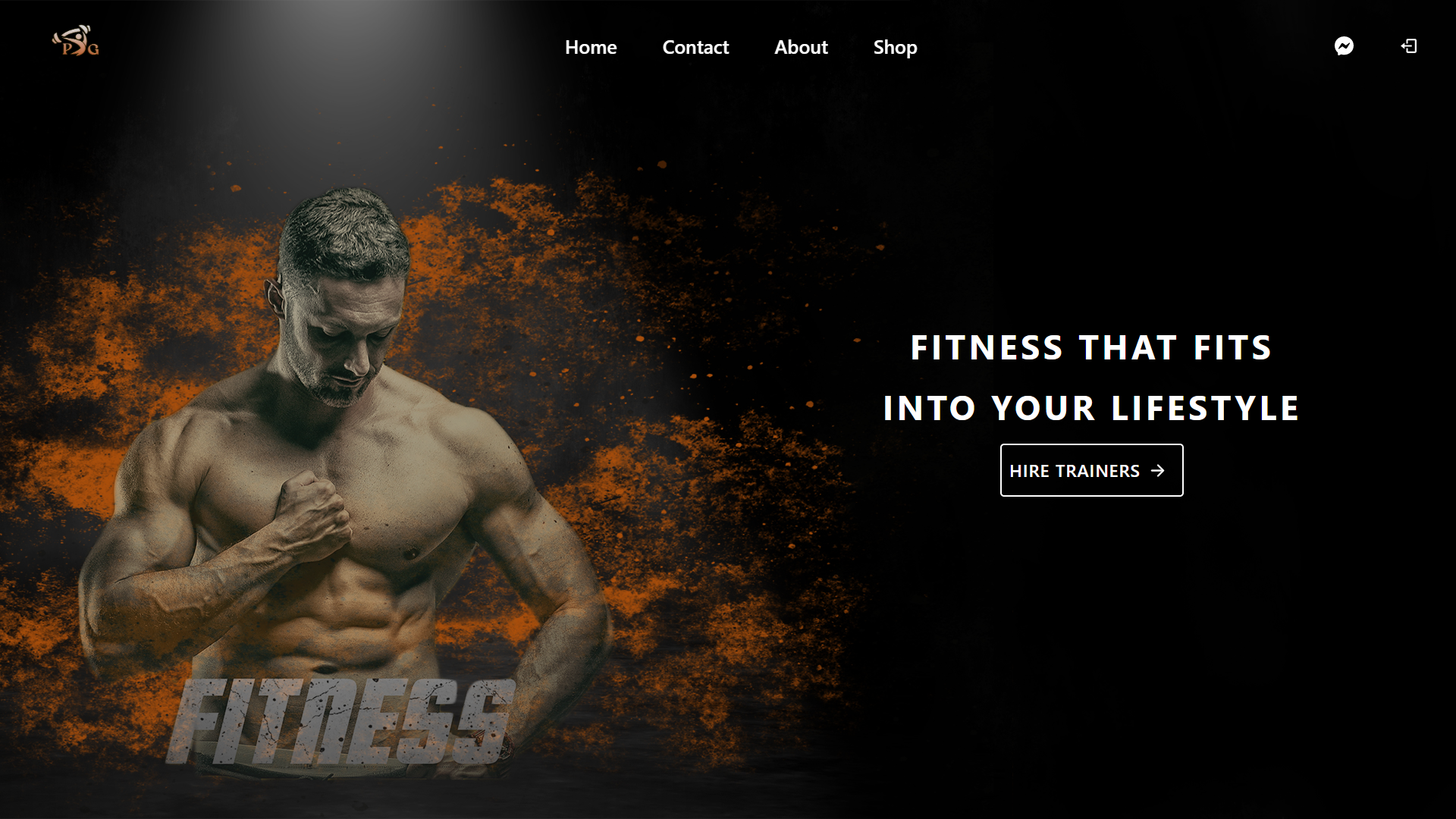
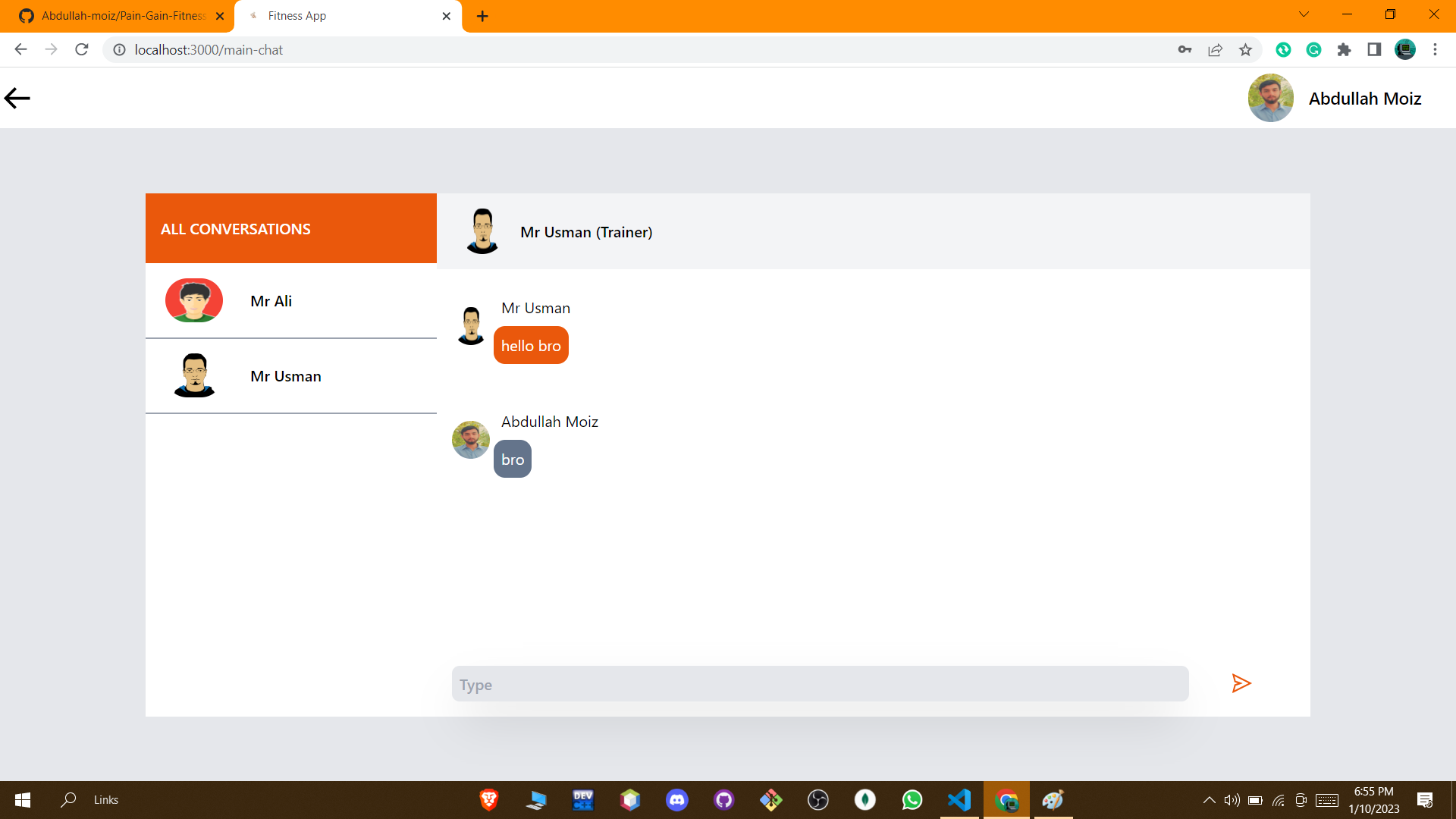
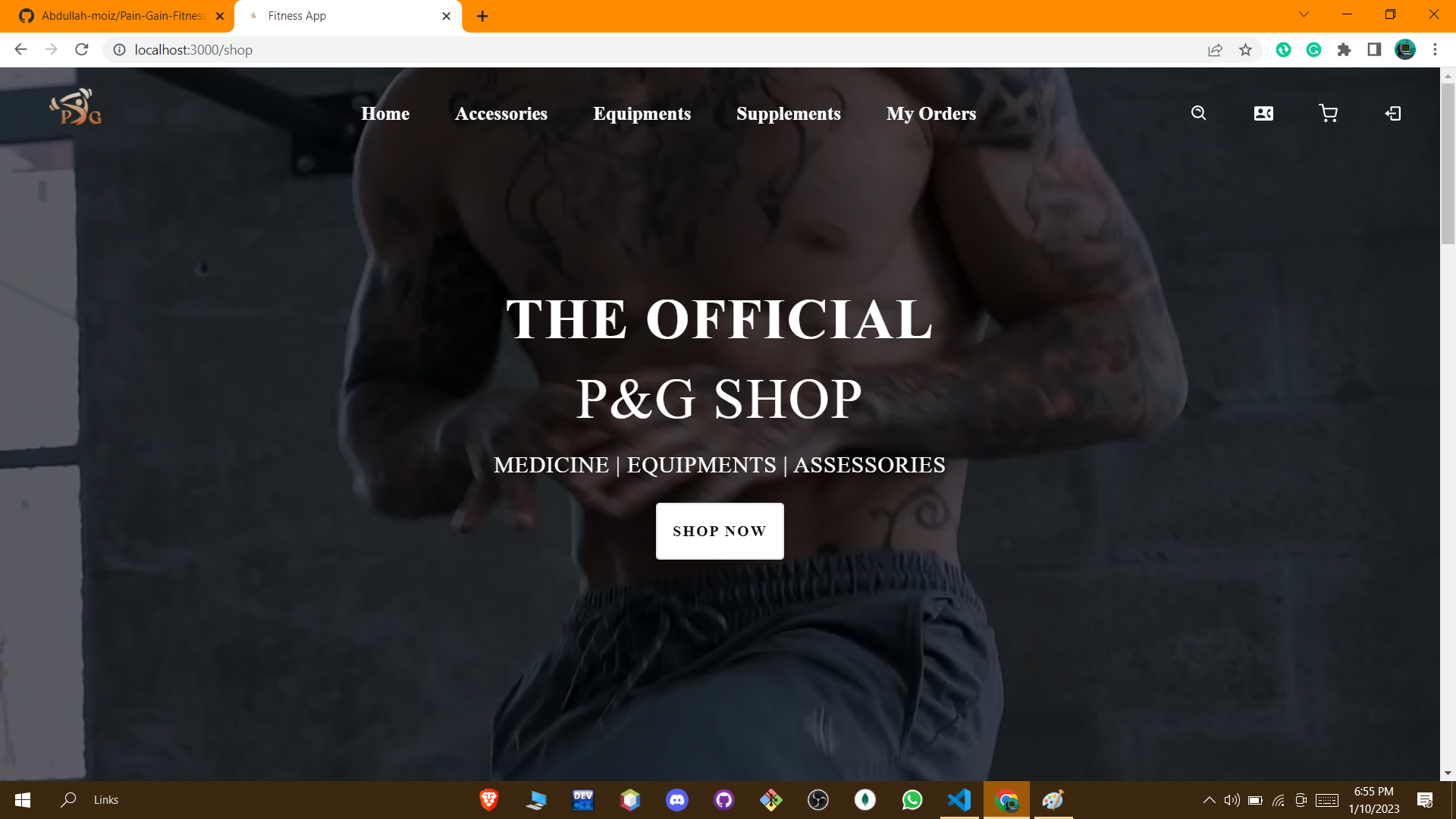
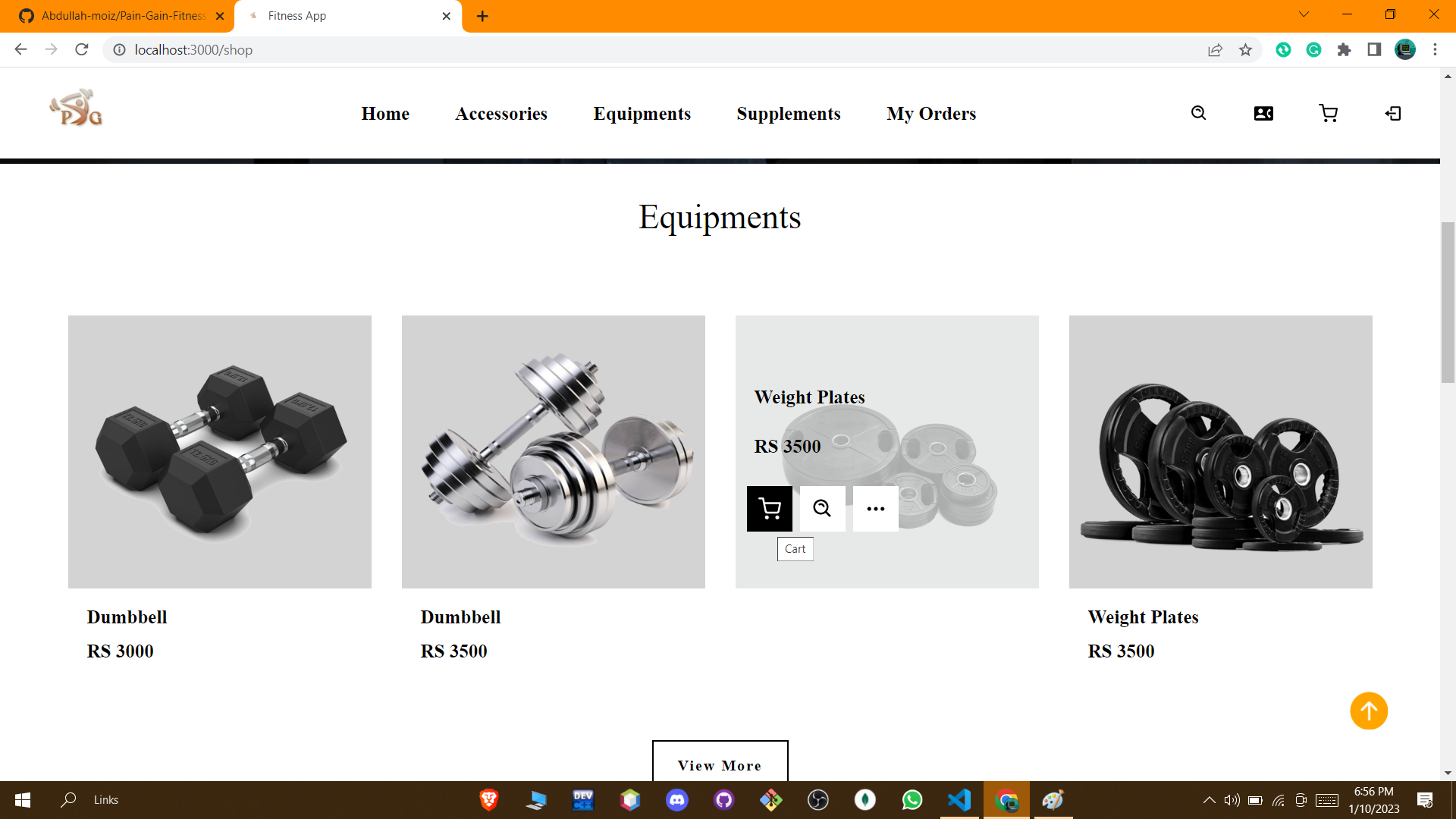
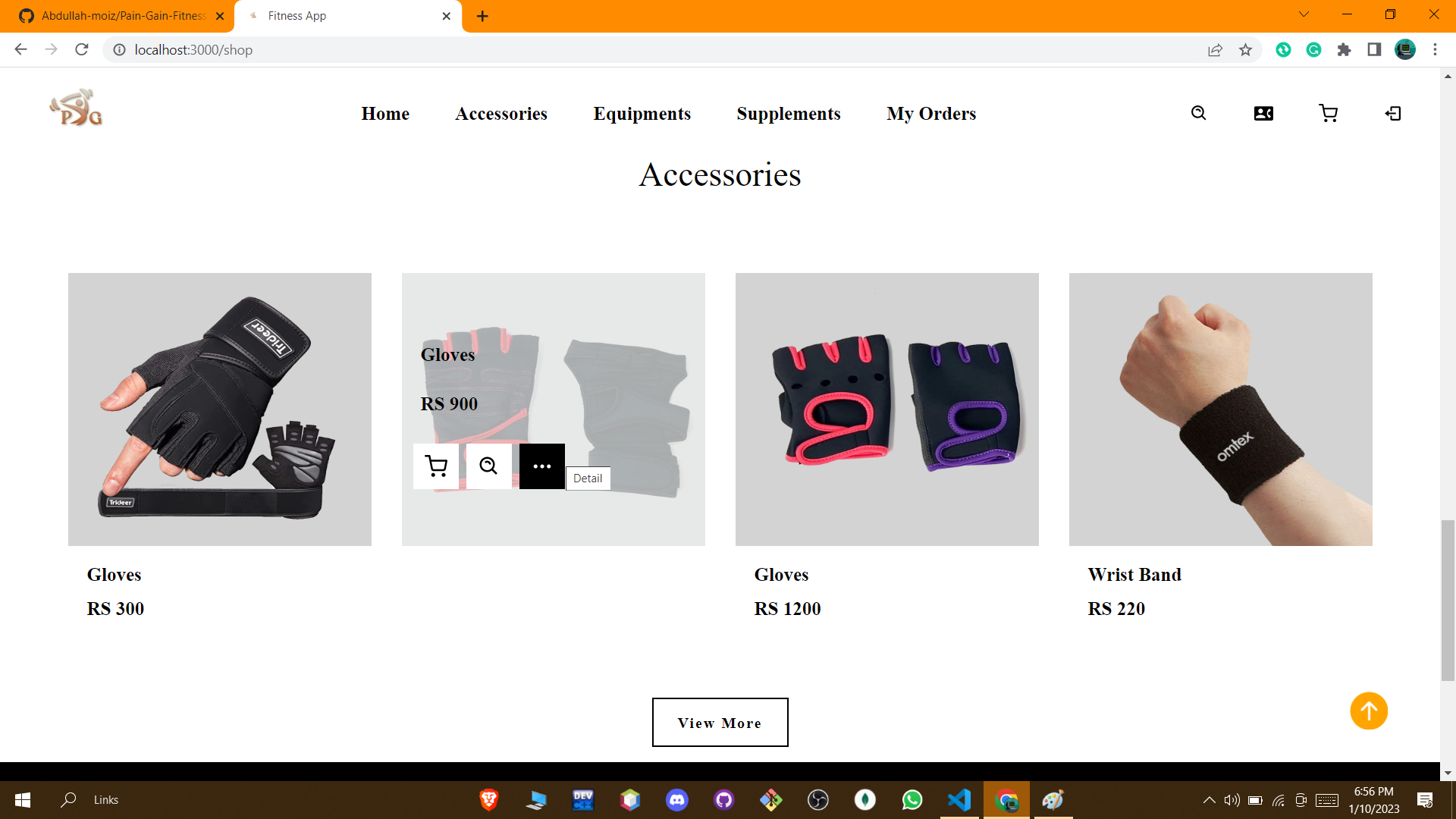
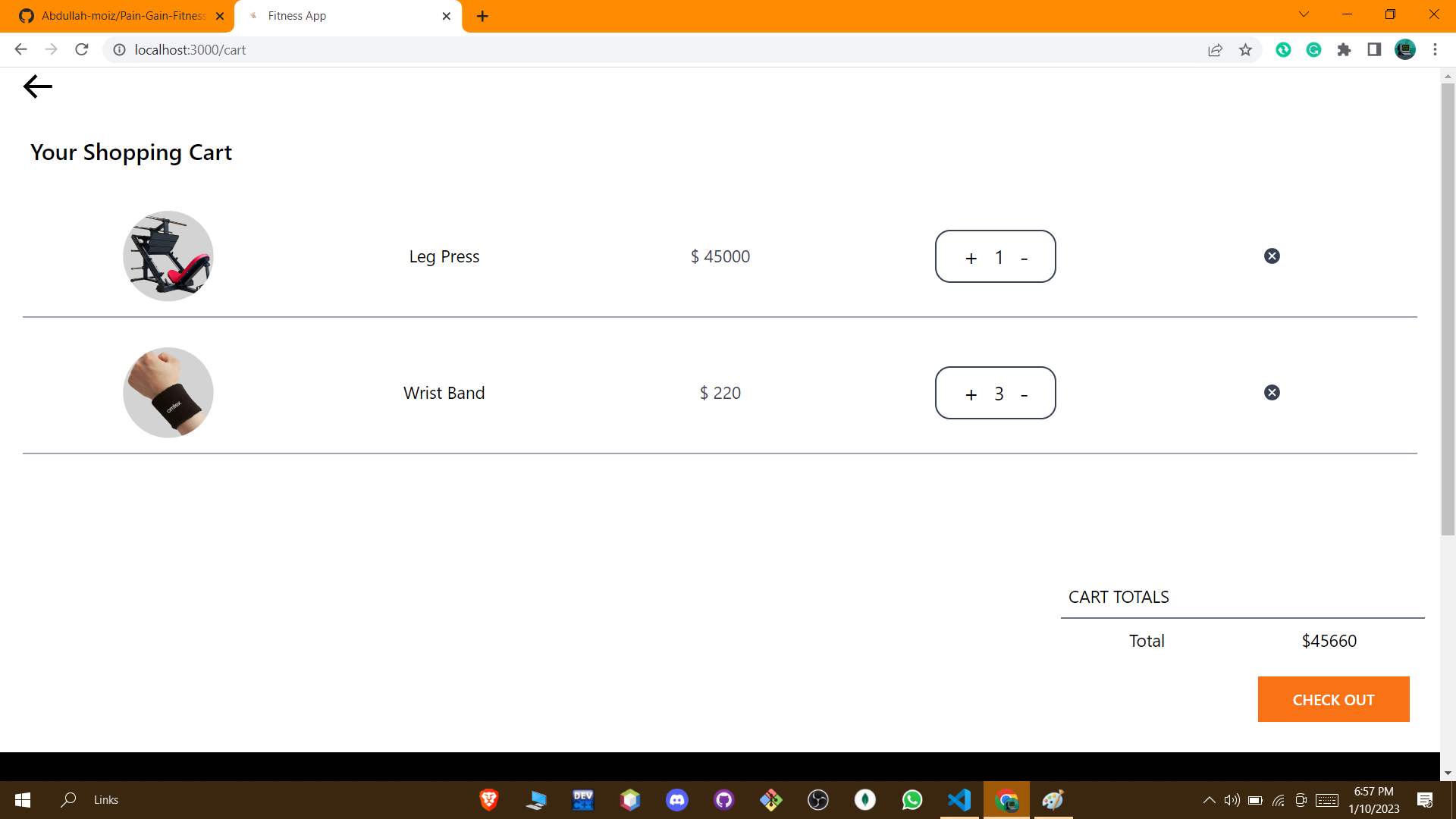
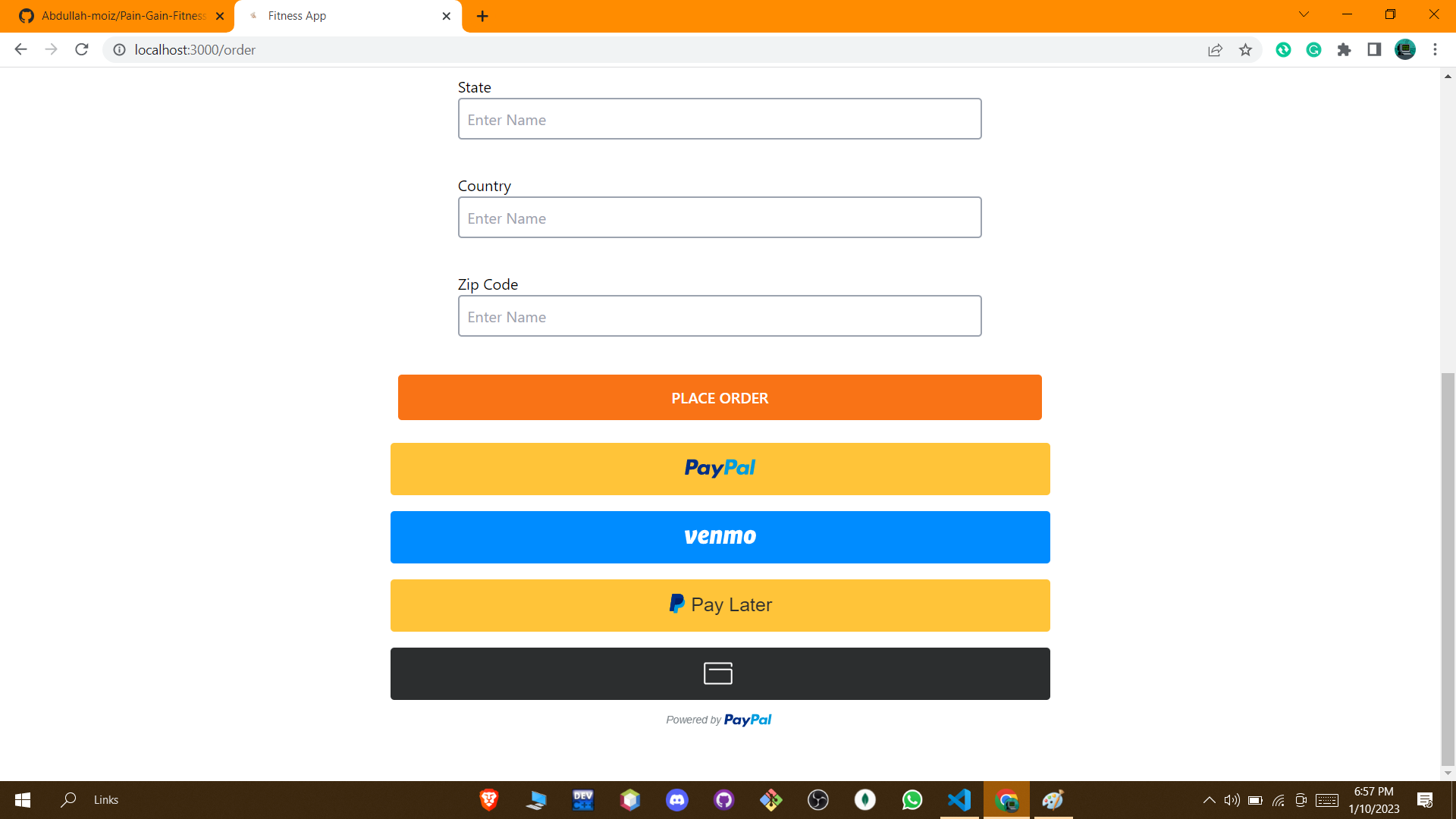
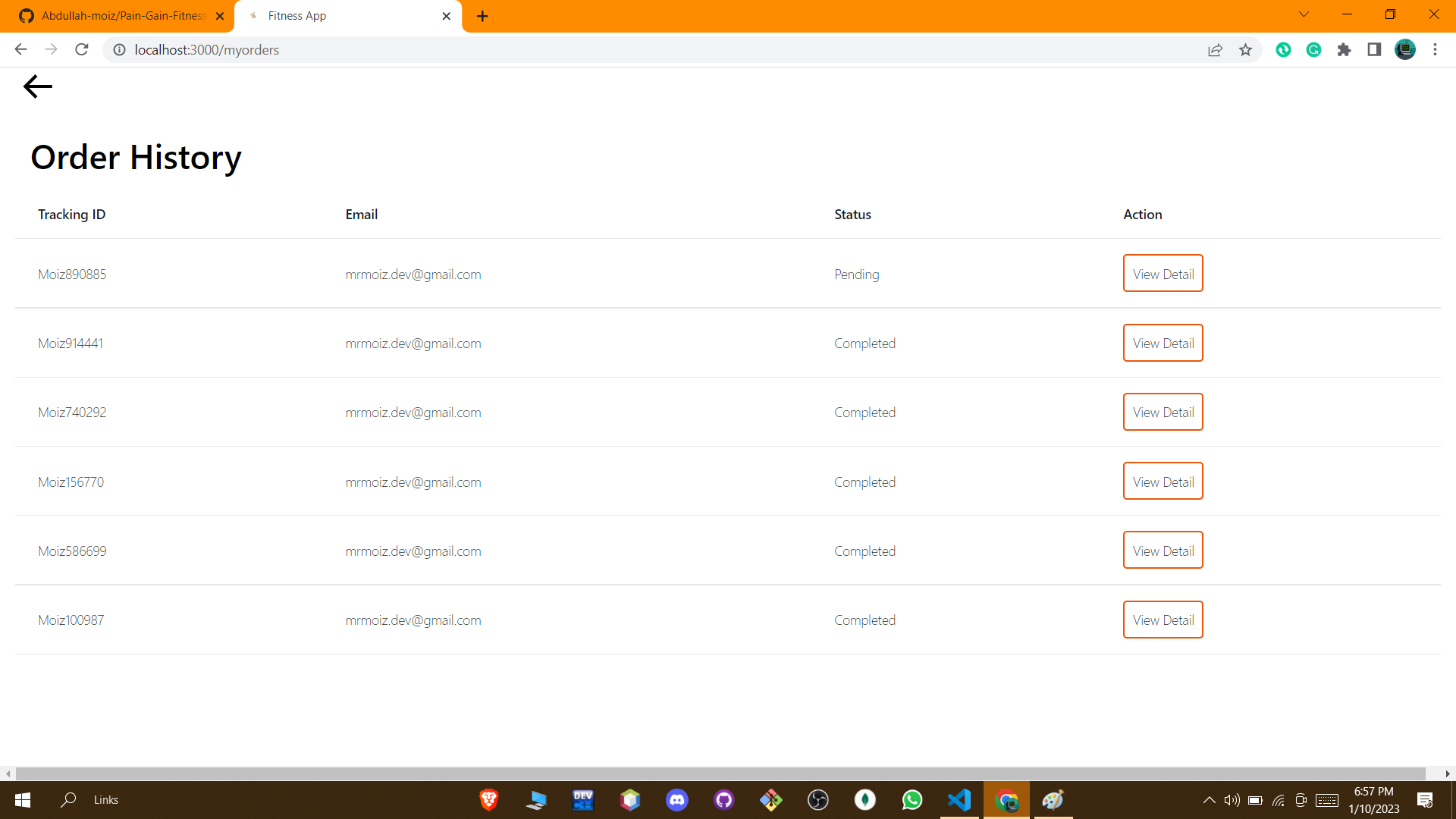
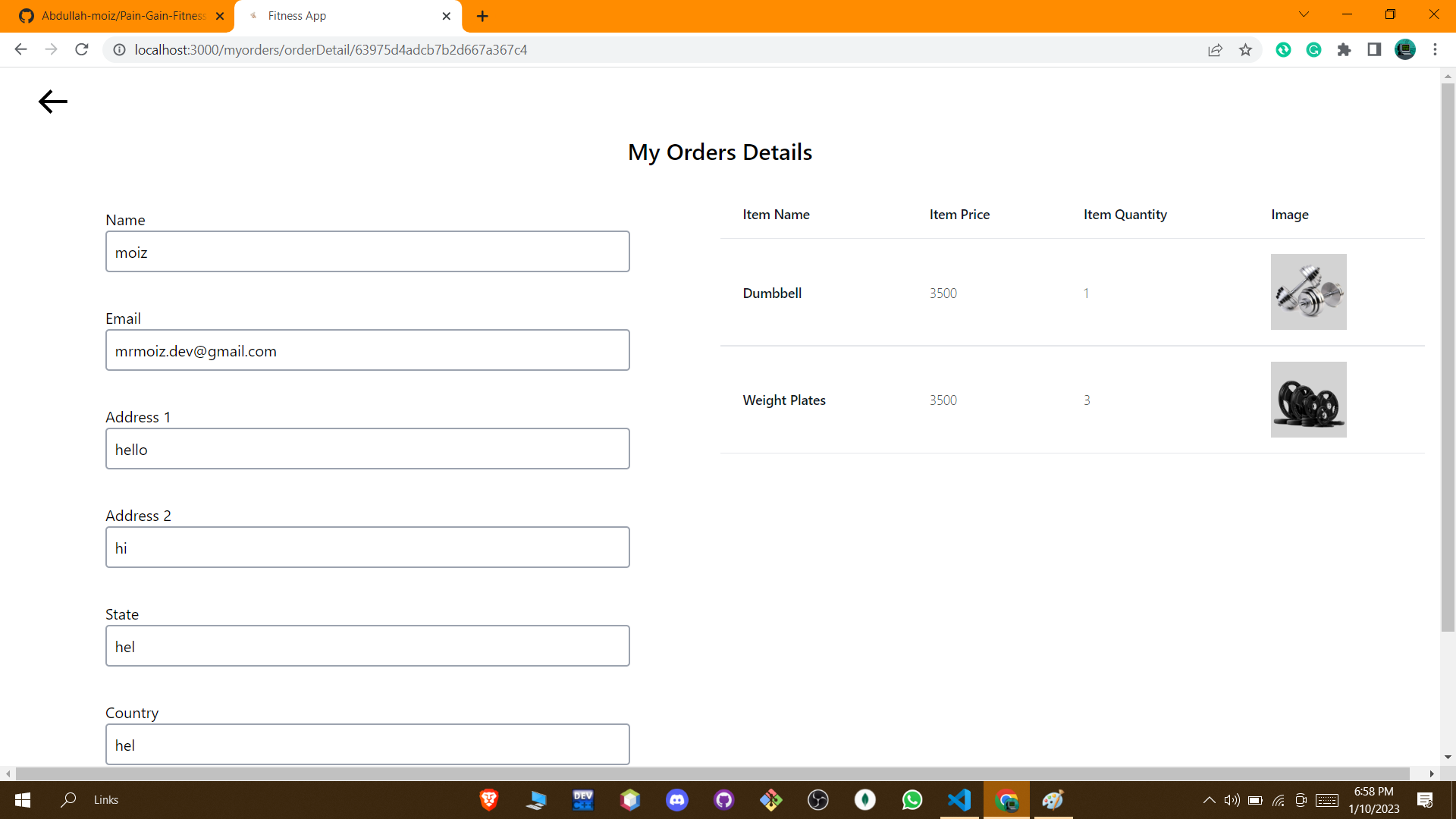
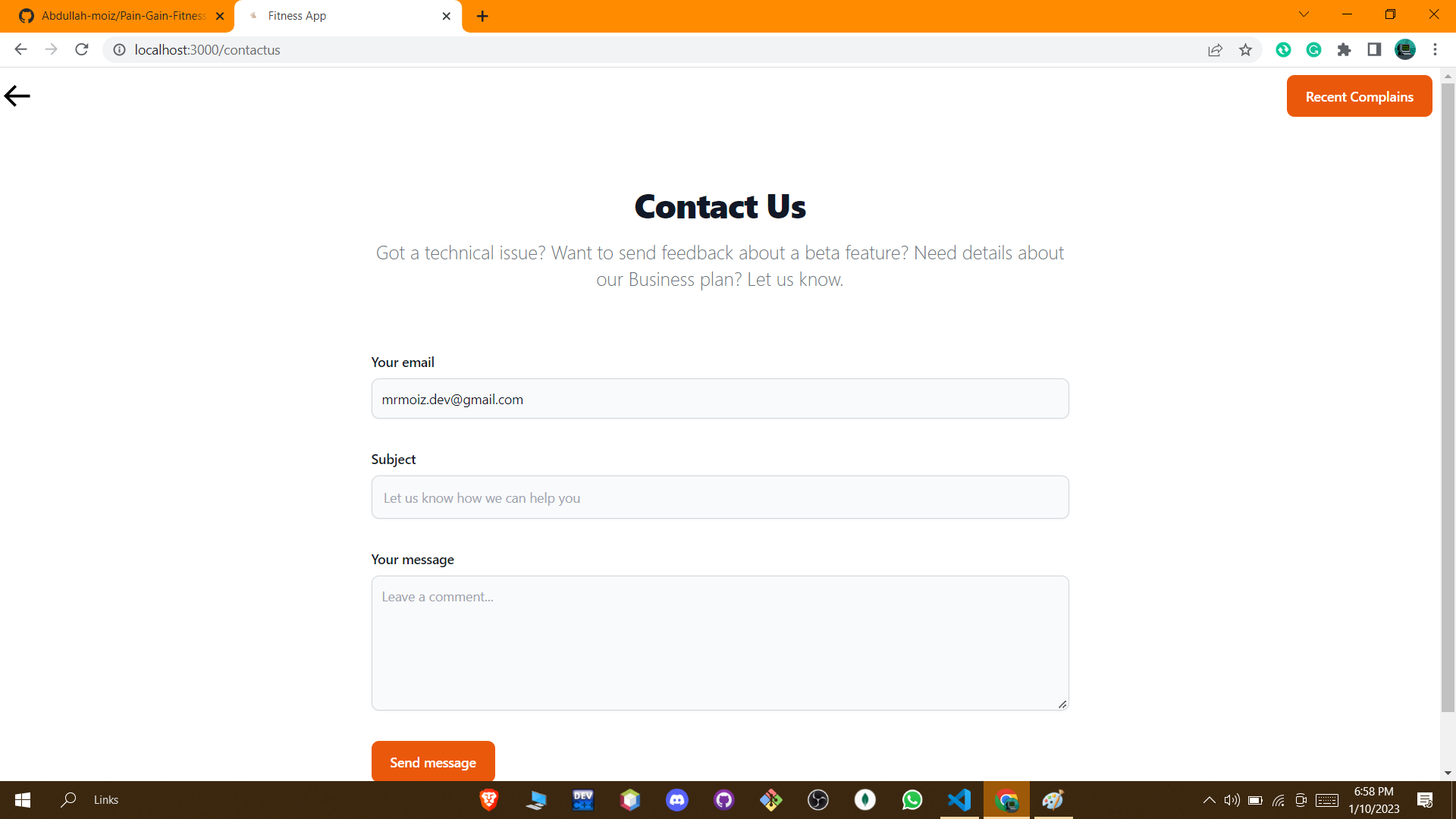
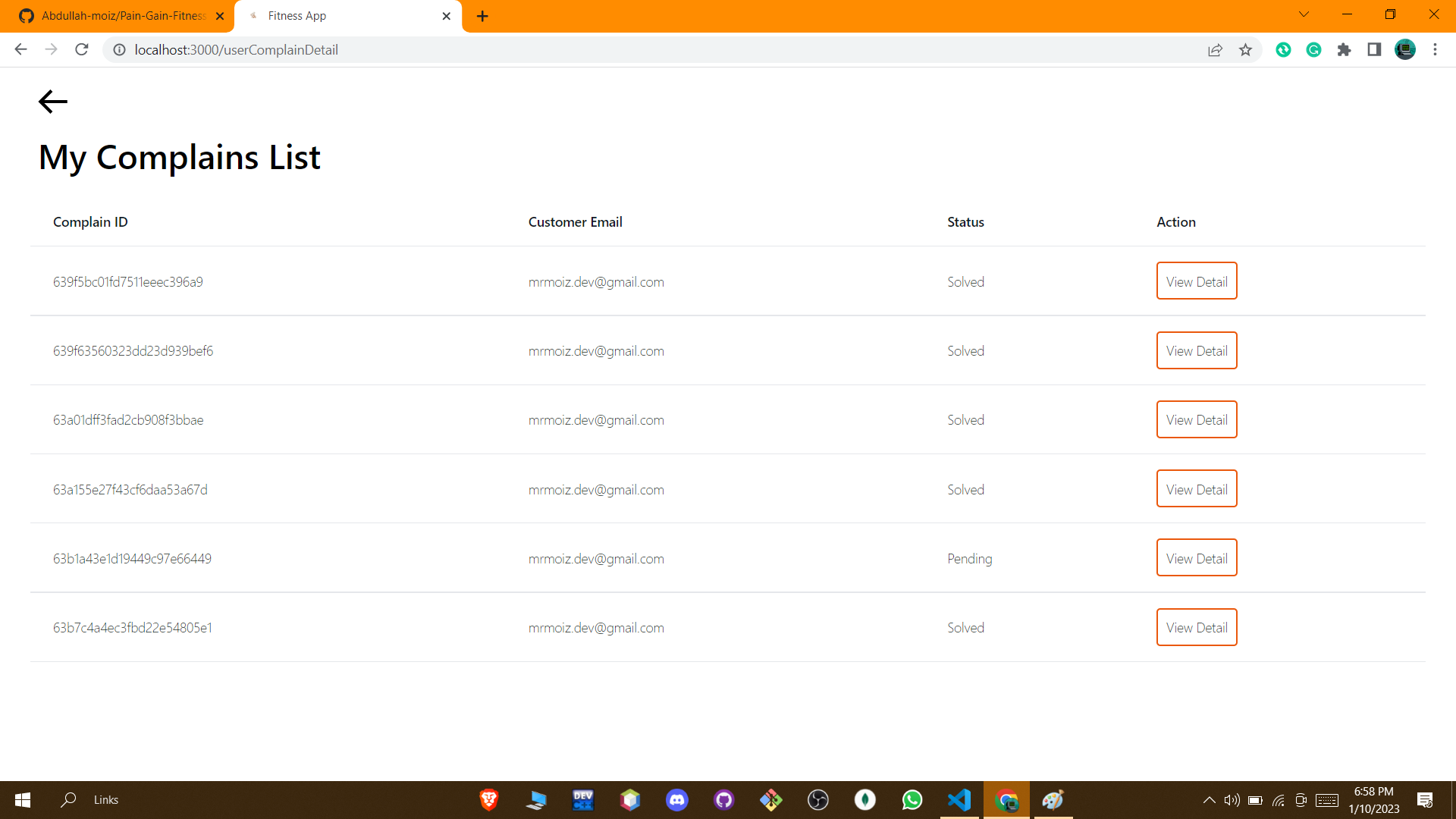
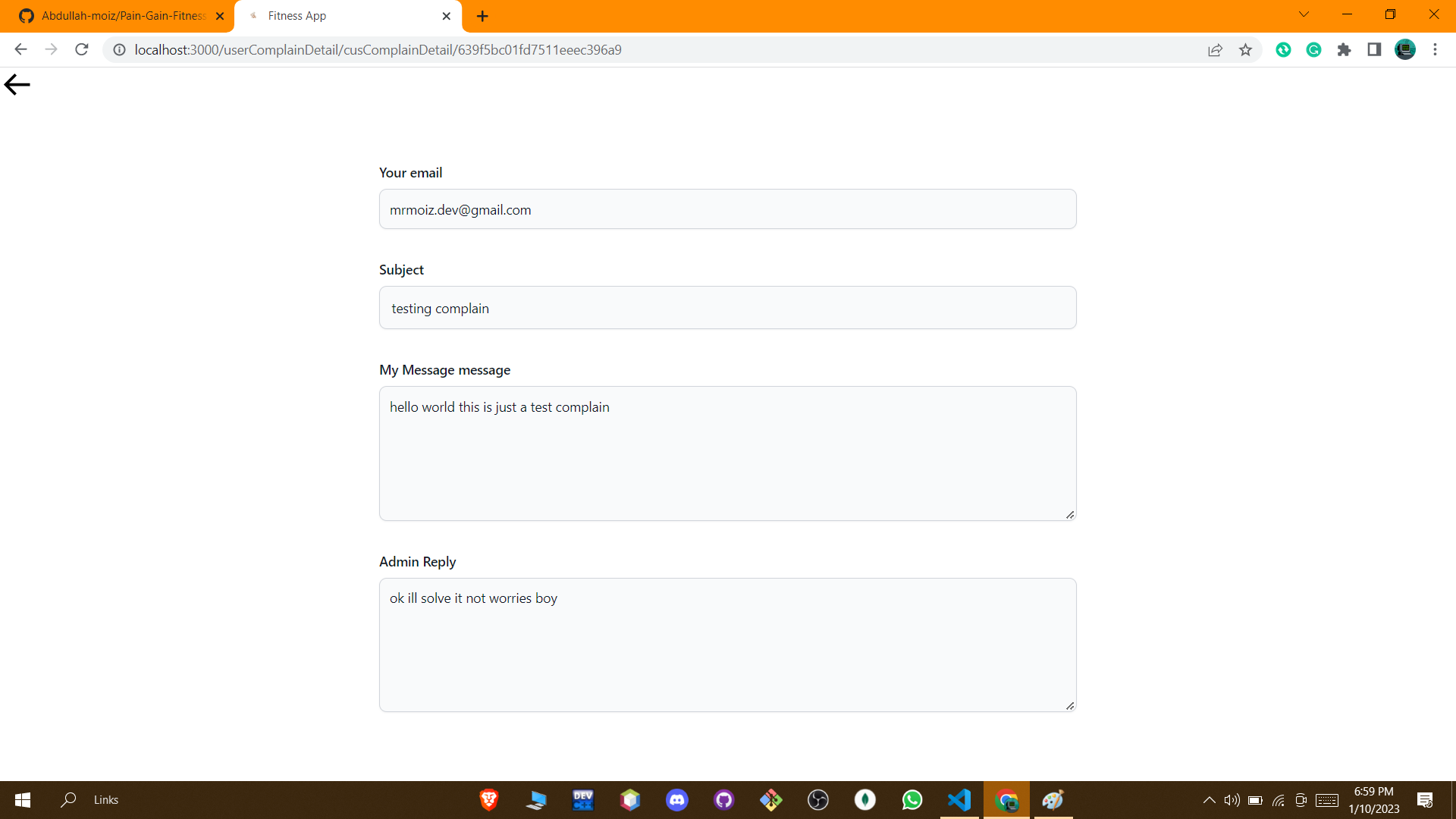
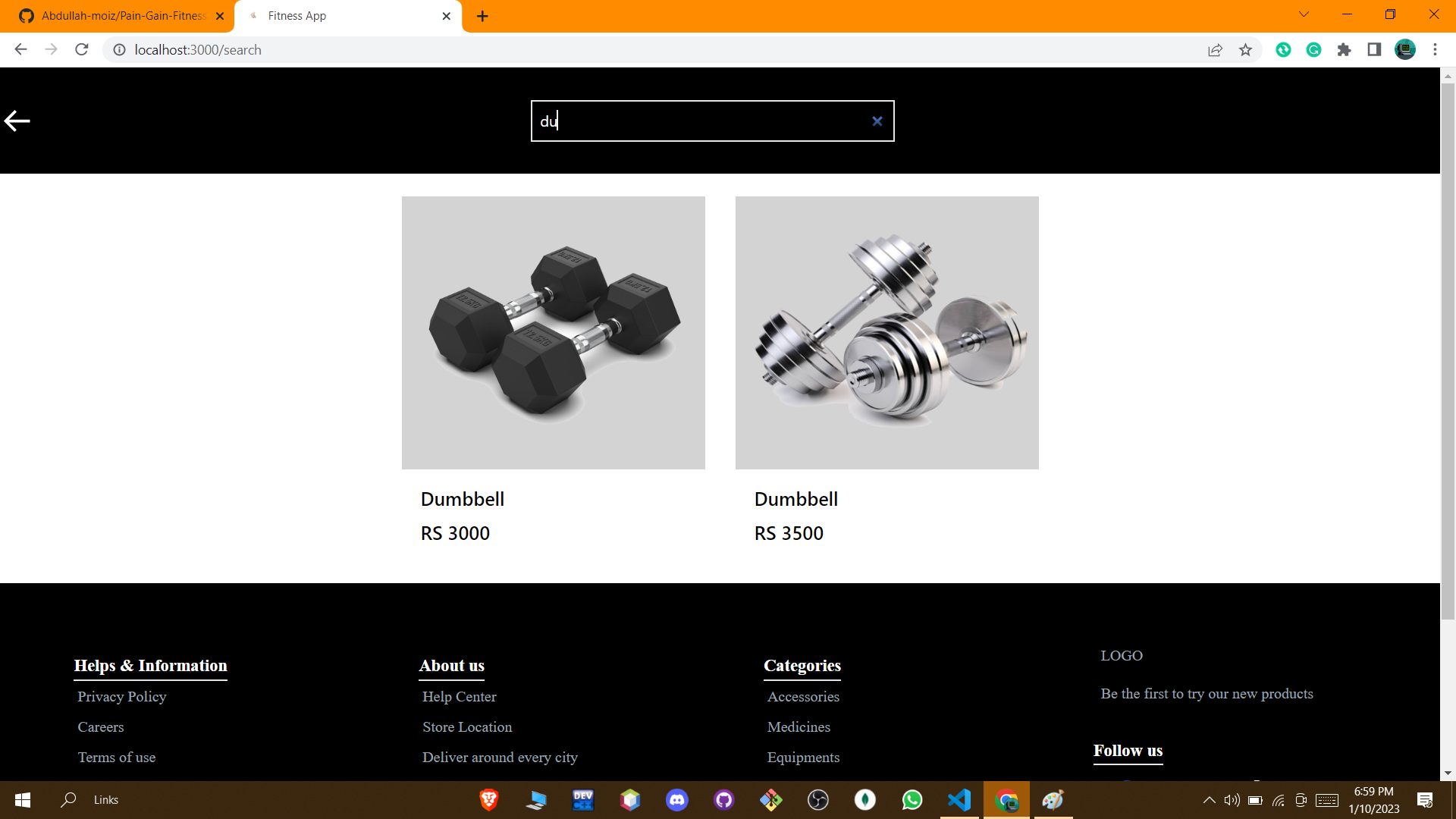
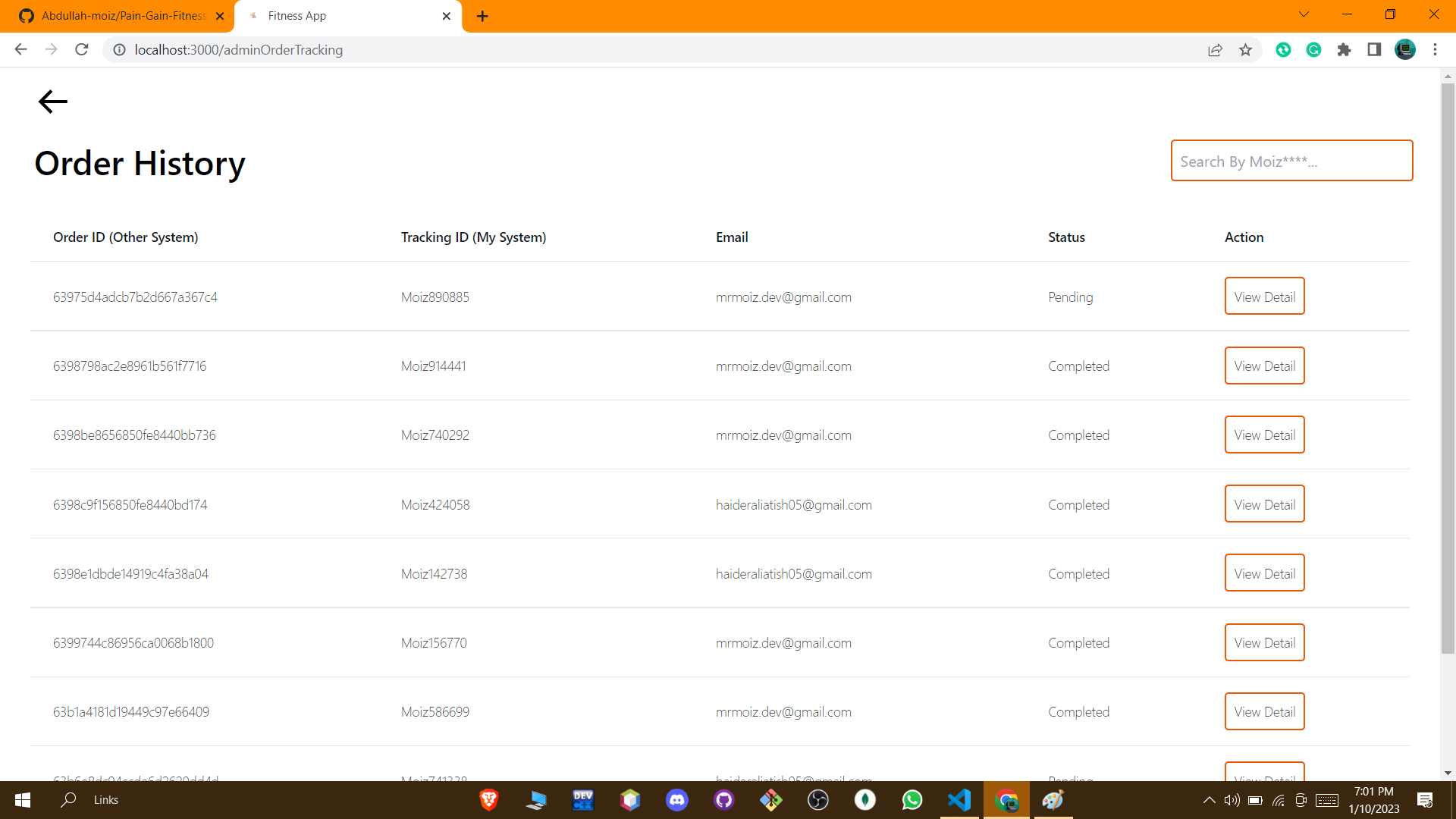
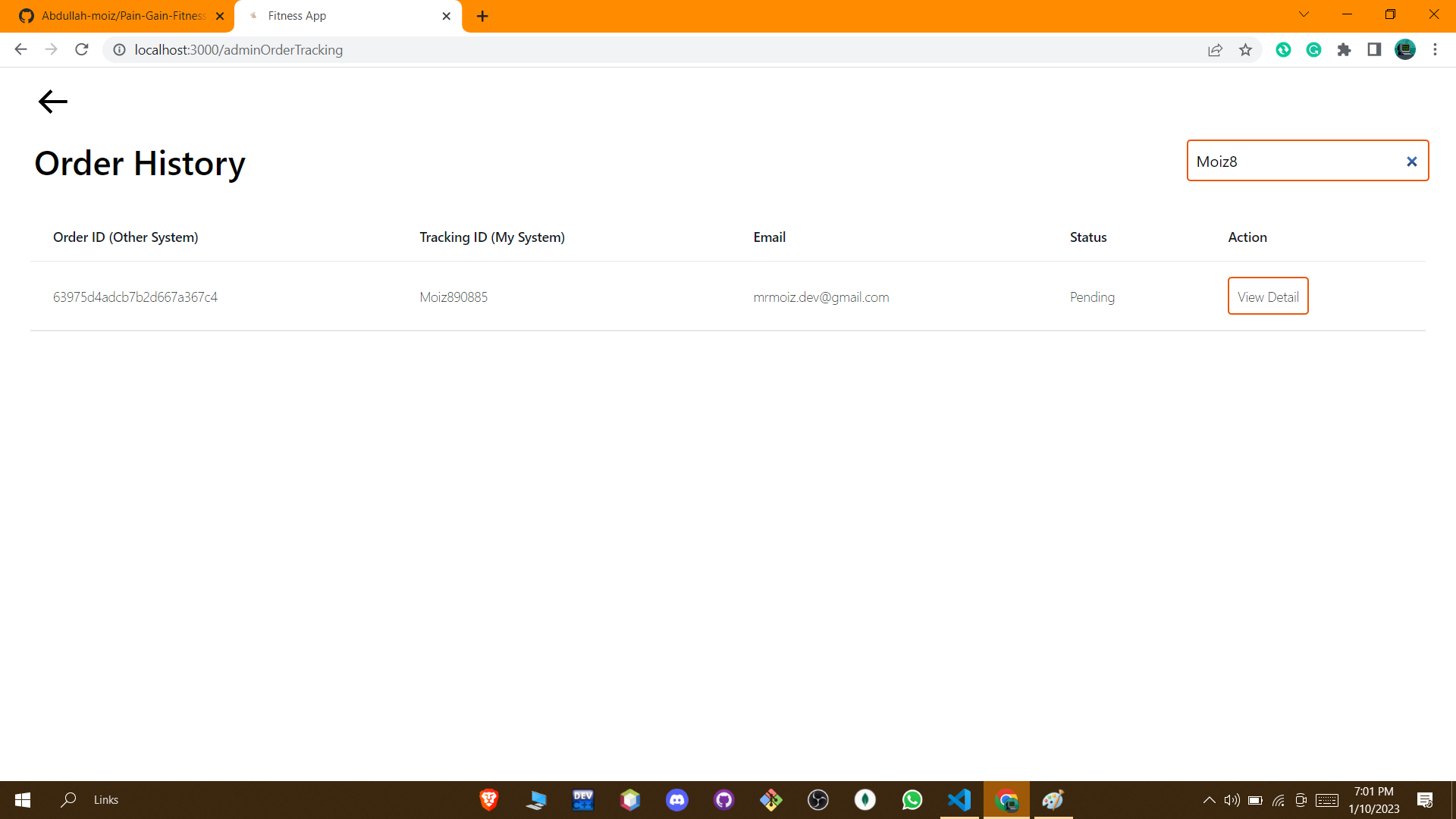
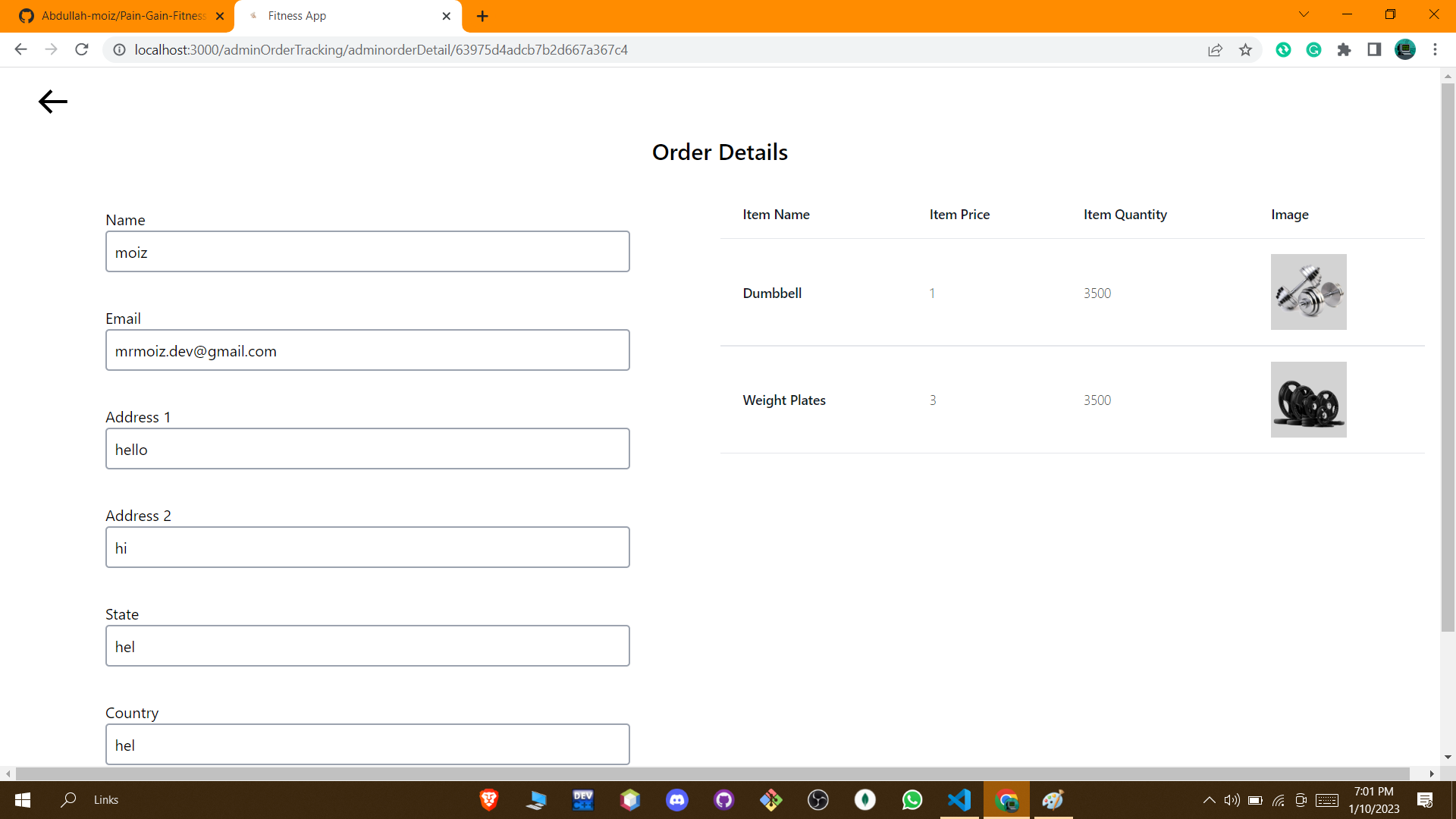
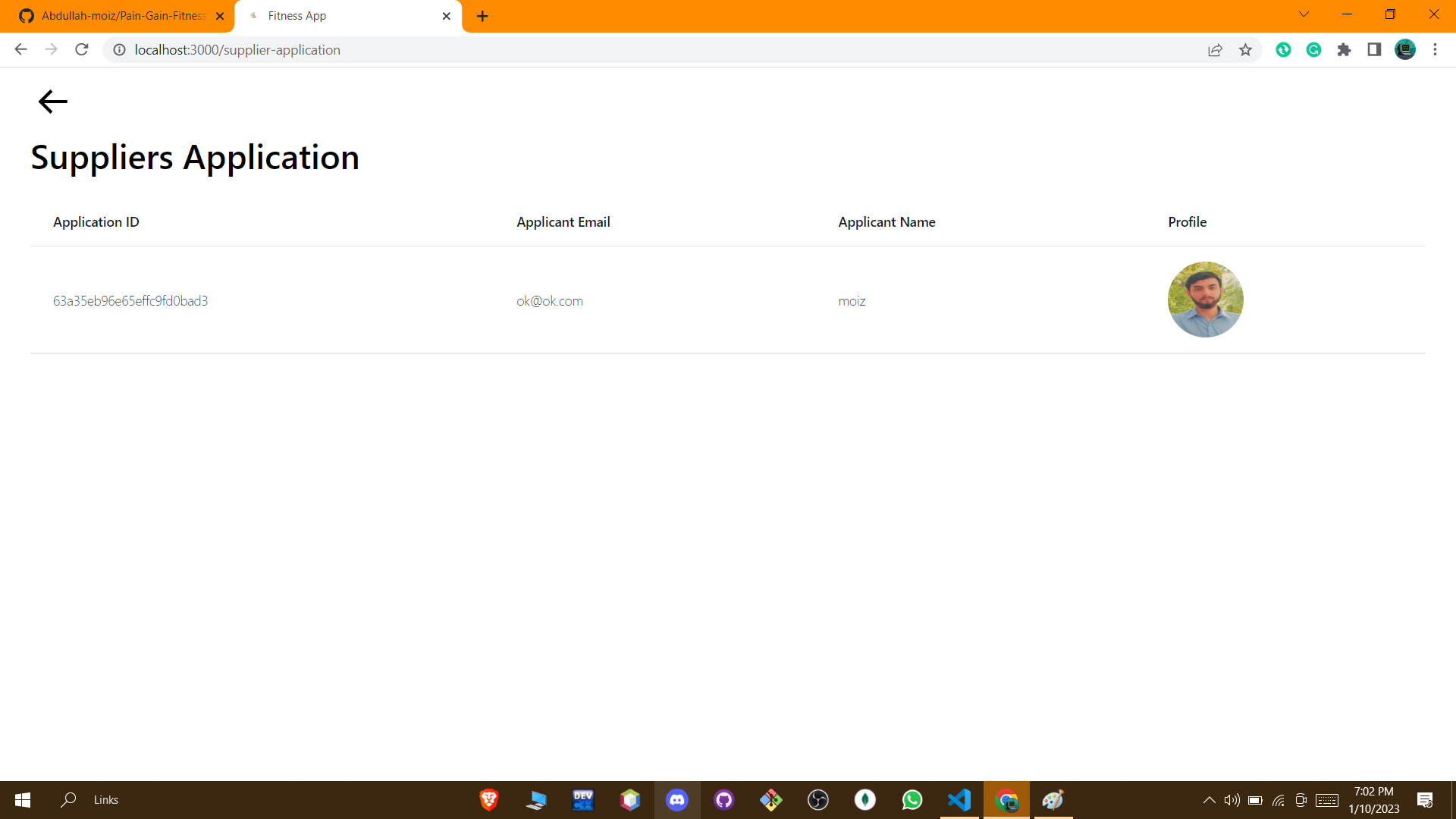
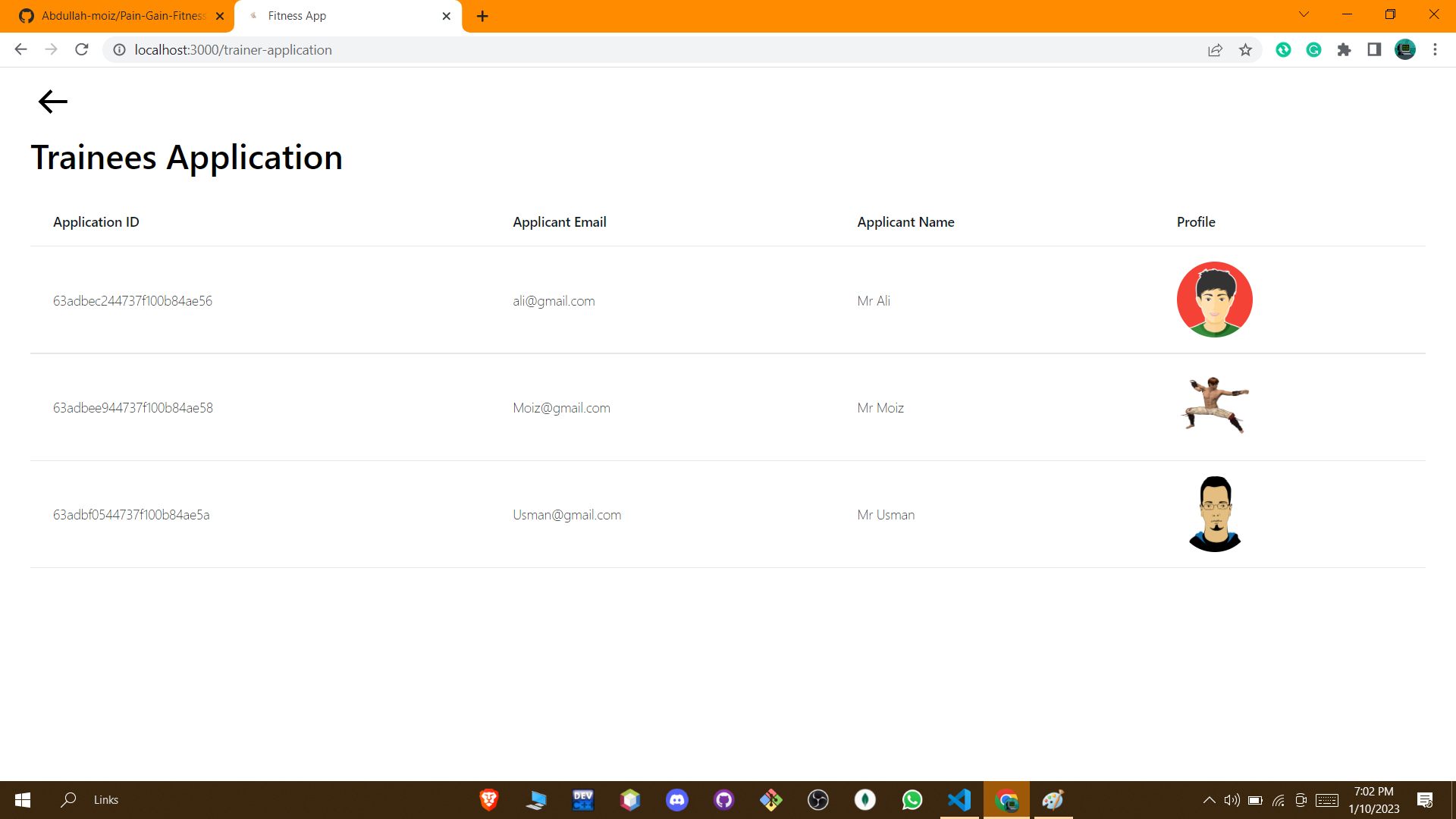
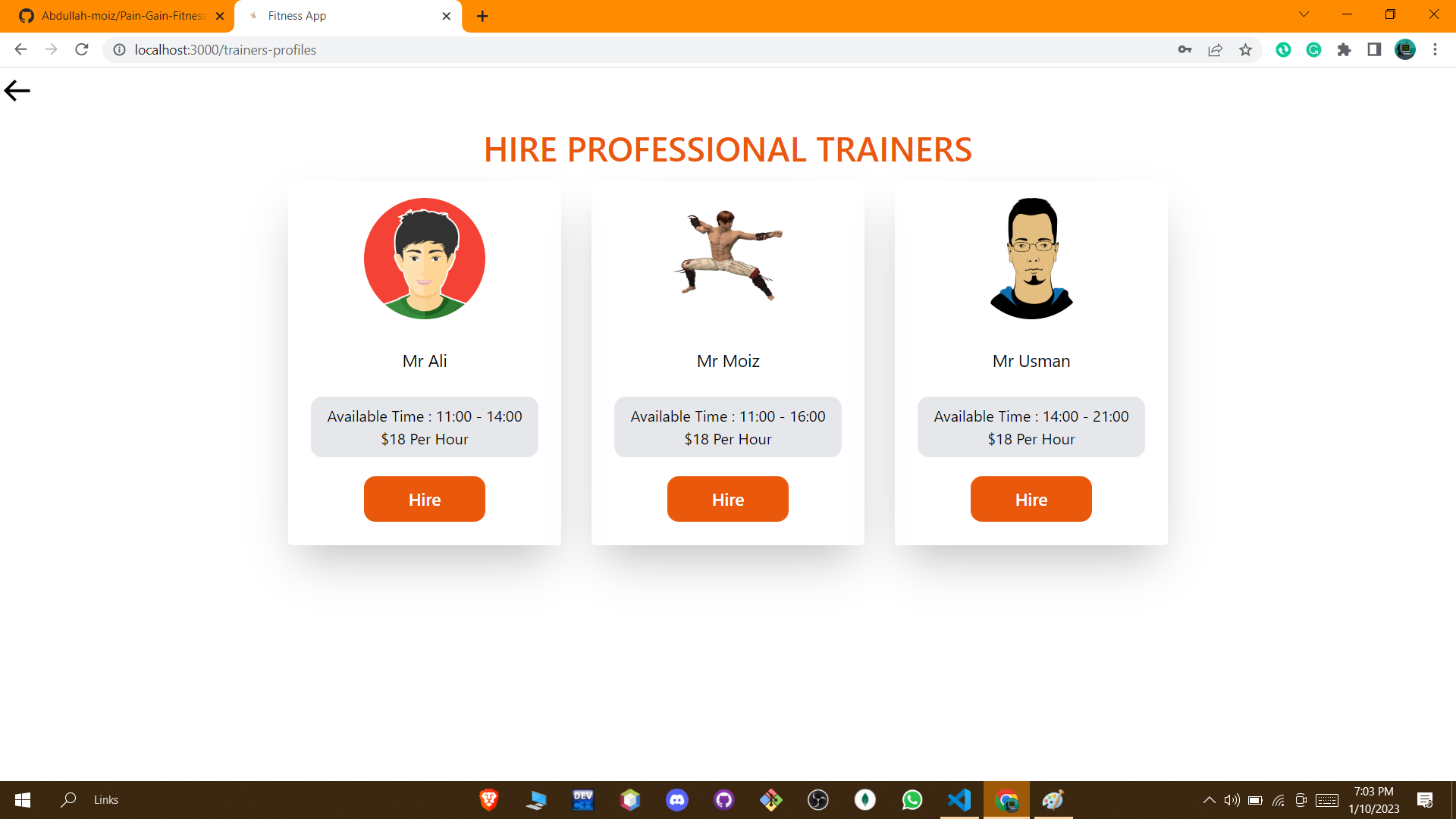
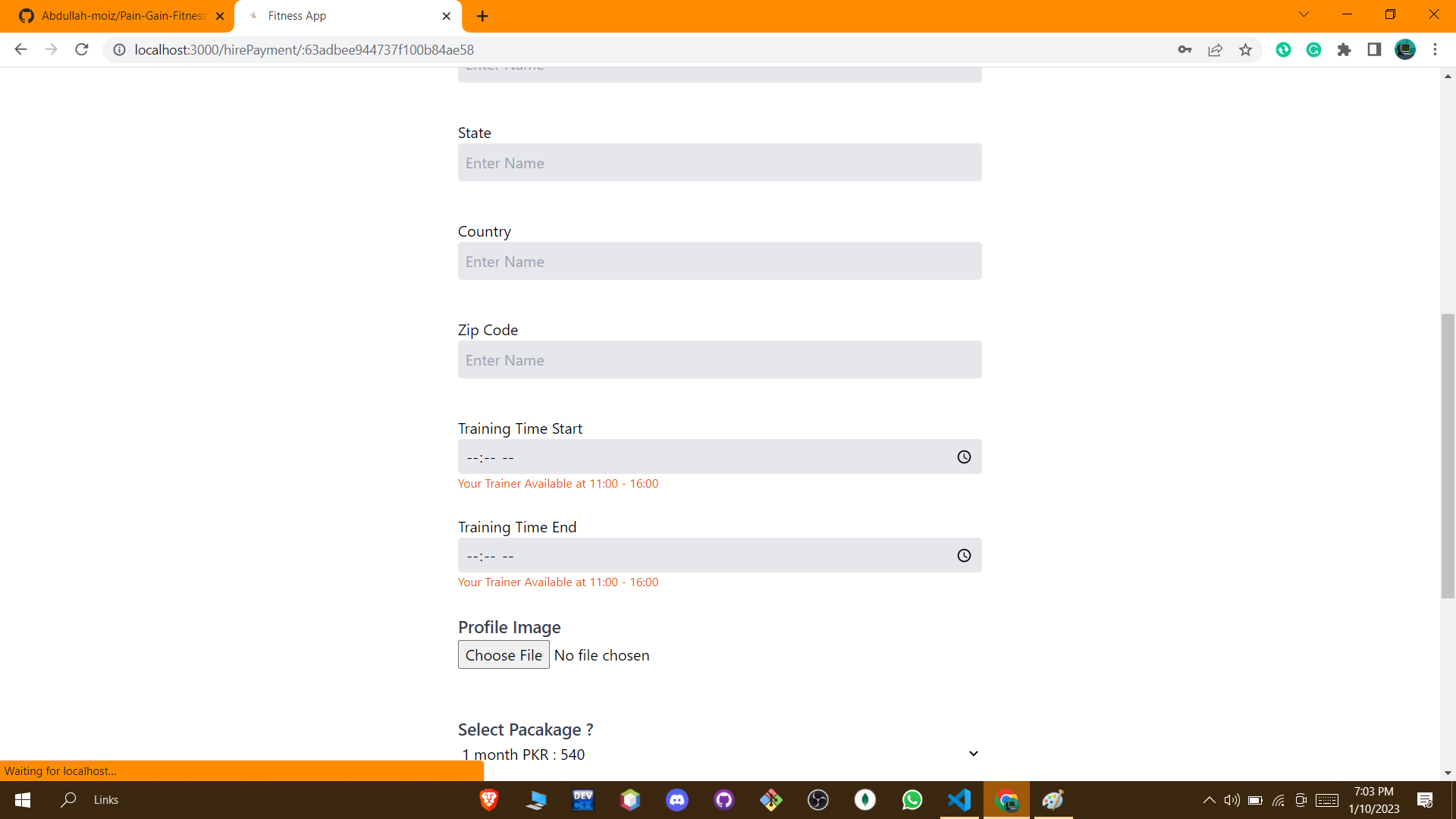
## Badges
Add badges from somewhere like: [shields.io](https://shields.io/)
[](https://choosealicense.com/licenses/mit/)
[](https://opensource.org/licenses/)
[](http://www.gnu.org/licenses/agpl-3.0)
# Getting Started with Create React App
- clone this repo
- cd client & cd server
- npm install in both folders
- npm start in both folders to start development server
| Builded E-commerce Module , Personal Trainee Module , Chat Module using Node JS , MongoDB , React JS , Firebase , Tailwindcss | api-rest,ecommerce,fitness-app,fitness-website-for-gym-freakers,javascript,json,mern-stack,mern-stack-app,node,tailwindcss | 2023-01-05T17:22:42Z | 2024-04-11T07:07:19Z | null | 4 | 1 | 24 | 0 | 2 | 5 | null | null | JavaScript |
Choaib-ELMADI/link-tree | main | <h1><a href='https://choaib-linktree.netlify.app' target='_blank'>Link Tree</a></h1>
<br>
<h3>Features of the website</h3>
<ul>
<li>Responsive</li>
<li>Simple</li>
</ul>
<h3>Reasons to develop this website</h3>
<ul>
<li>Make it easier to contact me</li>
<li>Seen paid versions, and decided to develop my own</li>
</ul>
| Simple LinkTree containing my socials and some of my projects. | javascript,links,tree,website | 2023-01-10T12:20:05Z | 2023-06-14T16:45:07Z | null | 1 | 0 | 10 | 0 | 0 | 5 | null | null | CSS |
SarahBass/VirtualPetShiba | main | # VirtualPetShiba
Clockface
Clockface for Fitbit Versa 3 Version 6.0
Versa3 Sense SDK 6.0 - Complete and Tested on Real Device
Stats heavy watch, filled with cute interactions, daily changing pictures, and fun surprises.

Contents: | Description:
--------- | ------------
index.js | Main Javascript Code for Watch
index.view | initializes JS library of resources
widgets.defs | imports to SDK library
utils.js | adds 0 to digit
styles.css | adds formatting
Images | Image Files and Folders not included on github
Developer: Sarah Bass
Link: [https://gallery.fitbit.com/details/6e255398-2919-4268-9ee5-a7674c3e4637]
Price : FREE
Fitbit clock filled with cute interactions, daily changing pictures, and fun surprises.
## DESCRIPTION:
Virtual Pet Shiba is a cute , animated dog on your watch that you can power up with your steps. Modeled after a real Shiba, this virtual dog has all the sass and attitude of the famous Japanese Breed known as a "doge." Mako, a real Shiba puppy from California, was used as the inspiration and model for the design.
Have fun exercising as this little dog gains hearts while you reach your goals. The virtual dog has three modes: sitting, walking, and sleeping. Based on your activity levels the dog will change what it does throughout the day and will have different moods.
Every month there is a new surprise for your virtual pet!
## Stats:
>Walk: Distance in Miles or KM
>Target : % of your goal reached
>Fire : Total Daily Calories Burned
>Stairs: Total Daily Floors
>Bolt: Total Daily Active Minutes
>Heart : Heart Rate (Click Star to Start)
>Footprints: Total Daily Steps
## Seasons
### Spring

### Summer

### Fall

### Winter

| Clockface Fitbit Versa 3 and Sense | clockface,fitbit,fitbit-clockface,fitbit-sdk,fitbit-sense,fitbit-versa3,javascript,shiba,shiba-inu,watchface | 2023-01-04T22:55:40Z | 2023-02-25T10:38:42Z | null | 1 | 0 | 34 | 0 | 0 | 5 | null | null | JavaScript |
Cinthia-Silva/jogo-mata-toupeiras | main | # Sobre o jogo Mata Toupeiras :otter:
> Desenvolvido por :woman_technologist: ```@Cinthia-Silva``` e :man_technologist: ```@Ruan-Narici```.
| :sparkles: Nome | Mata Toupeiras |
| - | - |
| :checkered_flag: INICIADO EM | 04/01/2023 |
| :white_check_mark: FINALIZADO EM | 05/01/2023 |
| :gear: TECNOLOGIAS | Adobe Photoshop, Figma, HTML5, CSS3, Javascript |
| :globe_with_meridians: URL | https://cinthia-silva.github.io/jogo-mata-toupeiras/ |
## Detalhes
O projeto foi criado com base no Mole Game. Porรฉm no projeto รฉ notรกvel um aperfeiรงoamento na qualidade das imagens e tambรฉm no layout.
## Objetivo
O objetivo do jogo รฉ acertar a toupeira com a marreta antes que ela volte ao buraco.
Sempre que o jogador acerta a toupeira, a pontuaรงรฃo dele aumenta.
No final do jogo รฉ exibido a pontuaรงรฃo final e o jogo รฉ reiniciado apรณs o jogador interagir.
## Atribuiรงรตes dos criadores
> ```~ Cinthia Silva```
>> Responsรกvel pelo layout e design do jogo.
>>> ADOBE PHOTOSHOP, FIGMA, HTML, CSS.
> ```~ Ruan Narici```
>> Responsรกvel pela programaรงรฃo e dinรขmica do jogo.
>>> JAVASCRIPT
Vale ressaltar que houve colaboraรงรฃo mรบtua em todo o processo de desenvolvimento. Finalizando assim um jogo bem divertido e bonito.
## Prรฉ-visualizaรงรฃo

| Jogo bem desafiador e bastante divertido! | css3,figma,game,html5,javascript | 2023-01-06T00:16:15Z | 2023-01-06T00:42:43Z | null | 2 | 0 | 8 | 0 | 1 | 5 | null | null | JavaScript |
JAILBREAK-101/Todo-App | main | # Todo-App
Welcome to my first Project built with HTML, CSS and JavaScript
Check out and store your Todo. https://jailbreak-101.github.io/Todo-App
### Features to be added
- Backend to be authenticated with Firebase.
- Focus mode to enable you to the Todo and complete it without getting distracted.
- Authentication with email account to save Todo on calendar to be notified of deadline and due date.
| A flexible Todo Application that uses the CRUD technique in viewing Todos created, designed and developed with HTML, CSS and JavaScript | css,html,javascript,todo | 2023-01-09T02:00:32Z | 2023-03-19T16:29:24Z | null | 1 | 0 | 20 | 0 | 0 | 5 | null | null | JavaScript |
kazaneza/African-Movies-Festival-Continental-Festival | main |
<!-- TABLE OF CONTENTS -->
# ๐ Table of Contents
- [๐ About the Project](#about-project)
- [๐ Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [๐ Live Demo](#live-demo)
- [๐ป Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#triangular_flag_on_post-deployment)
- [๐ฅ Authors](#authors)
- [๐ญ Future Features](#future-features)
- [๐ค Contributing](#contributing)
- [โญ๏ธ Show your support](#support)
- [๐ Acknowledgements](#acknowledgements)
- [โ FAQ (OPTIONAL)](#faq)
- [๐ License](#license)
<!-- PROJECT DESCRIPTION -->
# ๐ [African Movies Festival 2023] <a name="about-project"></a>
> African Movie festival is aimed to showcase African ability to produce their own movies, and recognizing Africans film makers
## ๐ Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
> I have used HTML and CSS in this project and also Javascript in implementing Js Dynamic function to take data and display the speakers info.
CSS
JAVASCRIPT
<details>
<summary>HTML</summary>
<ul>
<li><a href="#">HTML</a></li>
</ul>
</details>
<details>
<summary>Styles</summary>
<ul>
<li><a href="#">CSS</a></li>
</ul>
</details>
<details>
<summary>jAVASCRIPT</summary>
<ul>
<li><a href="#">JAVASCRIPT</a></li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
> African movies festival key features are.
- **[Register-project]**
- **[speakers]**
- **[sponsors]**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## ๐ Live Demo <a name="live-demo"></a>
> Add a link to your deployed project.
- [Live Demo Link](https://kazaneza.github.io/African-Movies-Festival-Continental-Festival/)
- [Recorded Loom Link](https://www.loom.com/share/6520d4922380490c81b4b85ab2fec03f)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## ๐ป Getting Started <a name="getting-started"></a>
>To get a local copy up and running, follow these steps.
### Prerequisites
In order to run this project you need:
```sh
npm install
Vs code or any code editor
```
### Setup
Clone this repository to your desired folder:
```sh
cd my-folder
git clone https://github.com/kazaneza/African-Movies-Festival-Continental-Festival
```
### Install
Install this project with:
```sh
cd African-Movies-Festival-Continental-Festival
```
### Usage
To run the project
```sh
Open in any browser
```
### Run tests
### Deployment
You can deploy this project using:
```sh
github pages
```
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## ๐ฅ Authors <a name="authors"></a>
๐ค **Author**
- GitHub: [@kazaneza](https://github.com/kazaneza)
- Twitter: [@kazaneza](https://twitter.com/kazaneza)
- LinkedIn: [@kazaneza](https://linkedin.com/in/kazaneza)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## ๐ญ Future Features <a name="future-features"></a>
> Describe 1 - 3 features you will add to the project.
- [ ] **[news_page]**
- [ ] **[Program_page]**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## ๐ค Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## โญ๏ธ Show your support <a name="support"></a>
> You are allowed to contribute on this project
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## ๐ Acknowledgments <a name="acknowledgements"></a>
> I would like to thank Cindy Shin in Behance for the amazing UX
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FAQ (optional) -->
## โ FAQ (OPTIONAL) <a name="faq"></a>
- **[how to clone the project?]**
- [git_clone_https://github.com/kazaneza/African-Movies-Festival-Continental-Festival]
- **[How to run project]**
- [use chrome]
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## ๐ License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
_NOTE: we recommend using the [MIT license](https://choosealicense.com/licenses/mit/) - you can set it up quickly by [using templates available on GitHub](https://docs.github.com/en/communities/setting-up-your-project-for-healthy-contributions/adding-a-license-to-a-repository). You can also use [any other license](https://choosealicense.com/licenses/) if you wish._
<p align="right">(<a href="#readme-top">back to top</a>)</p> | African Movie festival is aimed to showcase African ability to produce their own movies, and recognizing Africans film makers The project features a dynamic and interactive website that demonstrates my abilities in creating responsive design and implementing animations with CSS and JavaScript. The project features a dynamic and interactive website | css,html,javascript | 2023-01-09T07:24:32Z | 2023-01-31T13:25:57Z | null | 1 | 1 | 18 | 1 | 0 | 5 | null | MIT | CSS |
PrashantSathawane/fearful-doll-6867 | main | <!-- # fearful-doll-6867 -->
Zoomcar-clone-website
This was a collaborative project made by 5 members Executed in 5 days.
We did this project within 5 days in our unit-3 construct week.Flexi Wheels is a car rental company based in India that allows customers to rent cars on an hourly, daily, or weekly basis. It has expanded to several cities & states in India, as well as a few international locations. Flexi Wheels operates on a self-drive model, which means that customers can rent a car and drive it themselves, rather than hiring a driver. The company offers a variety of car models to choose from, and customers can book cars through the Flexi Wheels websiteย orย mobileย app.
Dharanesh <br>
Salman <br>
Akshay <br>
Upendra <br>
Prashant <br>
Deploy Link -https://644dfad2252c9713f067d005--lovely-pasca-14e6b1.netlify.app/
Tech Stack Used : -
Languages
HTML
CSS
JavaScript
Following are the Screenshots for the reference
Landing Page Landing Page
** Landing Page **

** Login Page **
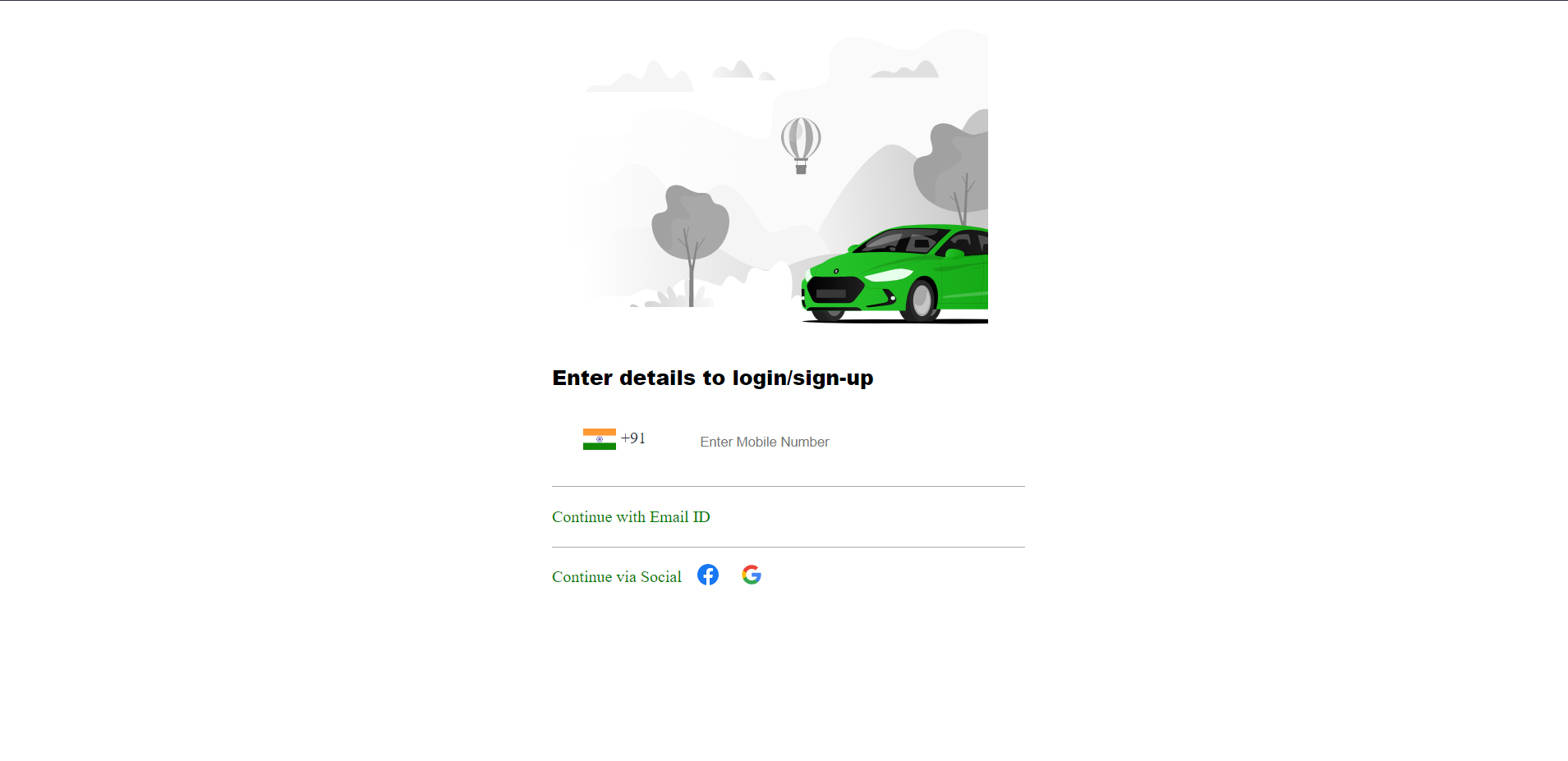
** All Product Page **
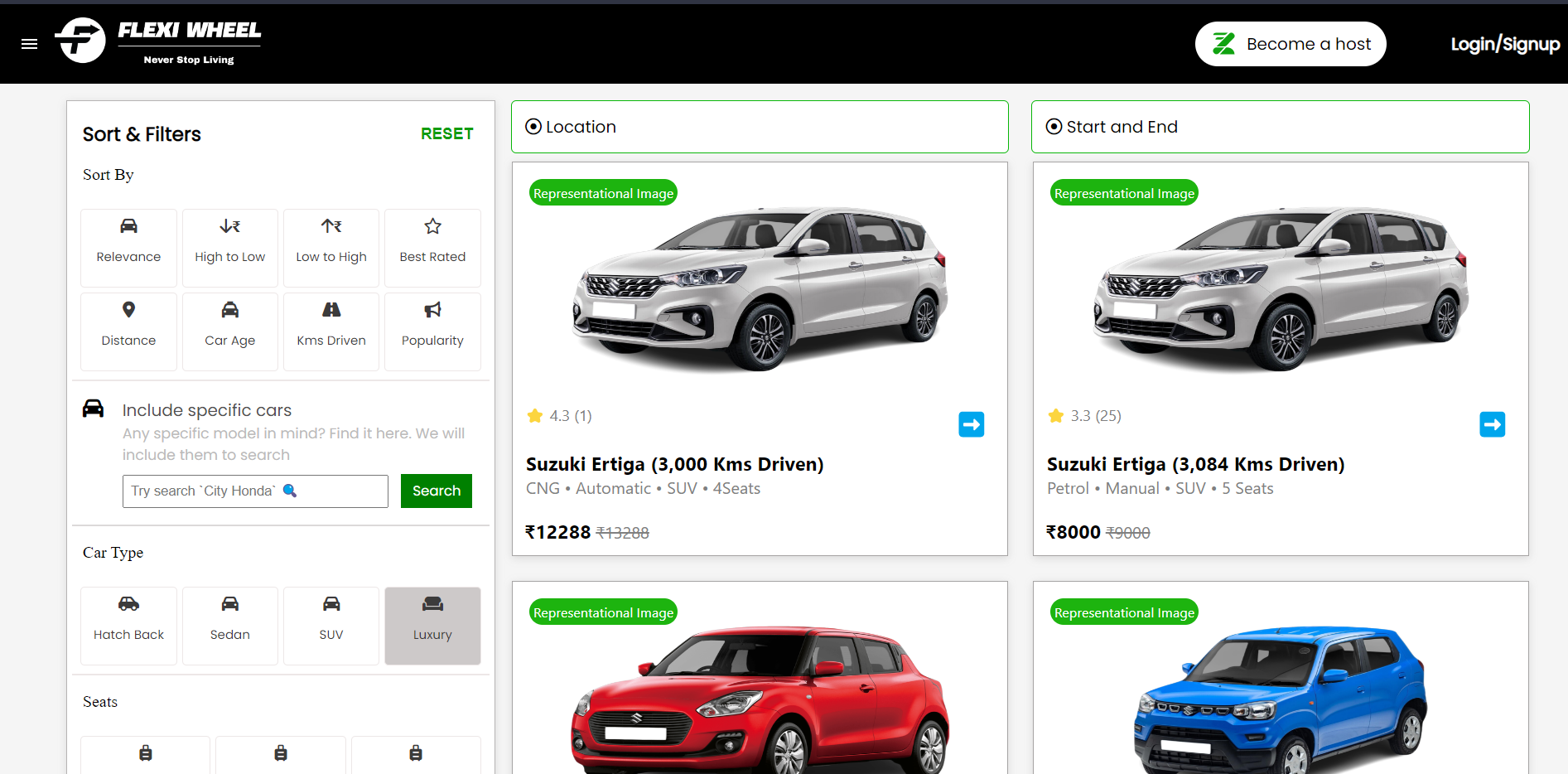
** Payment Page**
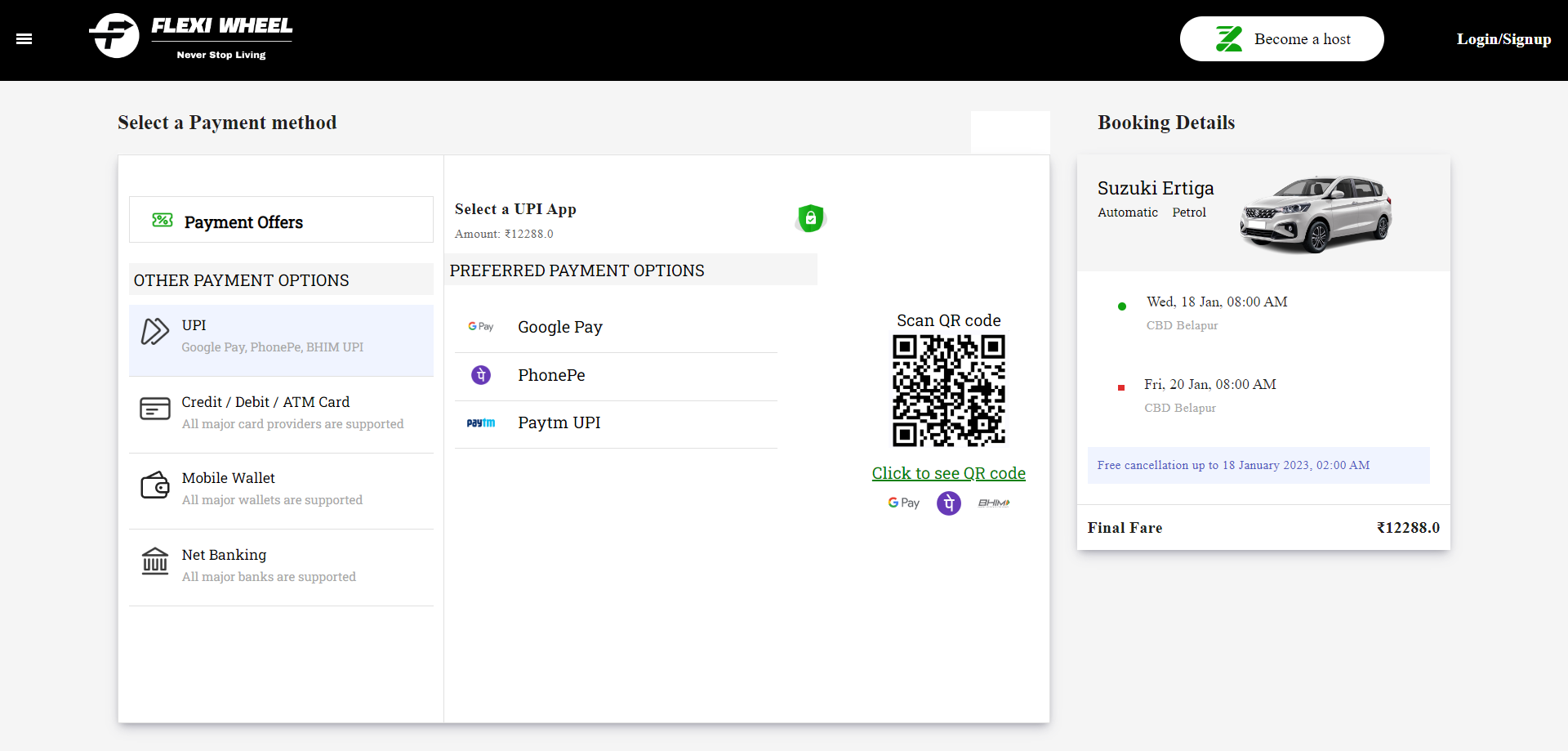
# About Project
We did this project within 5 days in our unit-3 construct week.Flexi Wheels is a car rental company based in India that allows customers to rent cars on an hourly, daily, or weekly basis. The company was founded in 2012 and has since expanded to several cities in India, as well as a few international locations. Flexi Wheels operates on a self-drive model, which means that customers can rent a car and drive it themselves, rather than hiring a driver. The company offers a variety of car models to choose from, and customers can book cars through the Flexi Wheels websiteย orย mobileย app.
| Flexi Wheels allows individuals to rent from a diverse pool of cars by the hourย orย byย theย day. | css3,html,javascript | 2023-01-16T15:56:59Z | 2023-05-24T20:50:30Z | null | 5 | 27 | 95 | 0 | 1 | 5 | null | null | HTML |
bhaveshkumar96/Snapdeal.com-Clone | main | # Snapdeal.com
Snapdeal is India's leading pure-play value Ecommerce platform.
It was a Collaborative project built in 5 days by 5 team members.
Team of 5 Members
1. Bhavesh Kumar
2. Vaishnavi Dilip Borkar
3. Surya prakash pokhriyal
4. Bhairavnath Gotam
5. Manish kumar Gupta cd
Area of Responsiblity
1. Bhavesh - Landing page / Admin page
2. Vaishnavi - Authentication ( Login / Sign-up / Checkout-page )
3. Surya Prakash - Products page ( men's)/ Product detail page / Cart page
4. Bhairavnath - Products page / Admin page (Beauty)
5. Manish - Add to Cart Page
<h4 align="center">Tech Stack used:</h4>
<p align="center">
<img src="https://img.shields.io/badge/React (18.2.0)-20232A?style=for-the-badge&logo=react&logoColor=61DAFB" alt="reactjs" />
<img src="https://img.shields.io/badge/Redux (4.2.0)-593D88?style=for-the-badge&logo=redux&logoColor=white" alt="redux" />
<img src="https://img.shields.io/badge/Chakra%20UI (2.2.8)-3bc7bd?style=for-the-badge&logo=chakraui&logoColor=white" alt="chakra-ui" />
<img src="https://img.shields.io/badge/JavaScript-323330?style=for-the-badge&logo=javascript&logoColor=F7DF1E" alt="javascript" />
<img src="https://img.shields.io/badge/CSS3-1572B6?style=for-the-badge&logo=css3&logoColor=white" alt="css3" />
<img src="https://img.shields.io/badge/HTML5-E34F26?style=for-the-badge&logo=html5&logoColor=white" alt="html5" />
</p>
HomePage:
<img src="https://cdn-images-1.medium.com/max/1000/1*k92cn9d1jhDWvuVEz1vwuQ.jpeg" alt="home"/>
Product Page
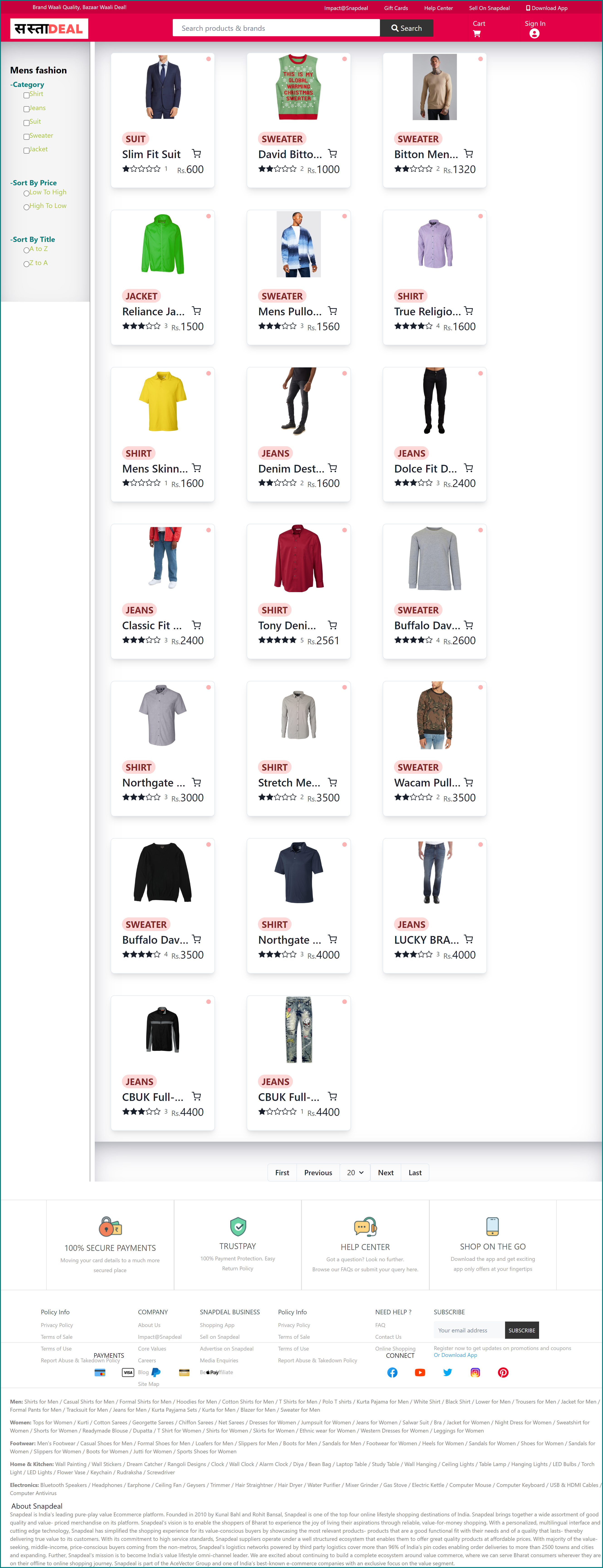
#login signup
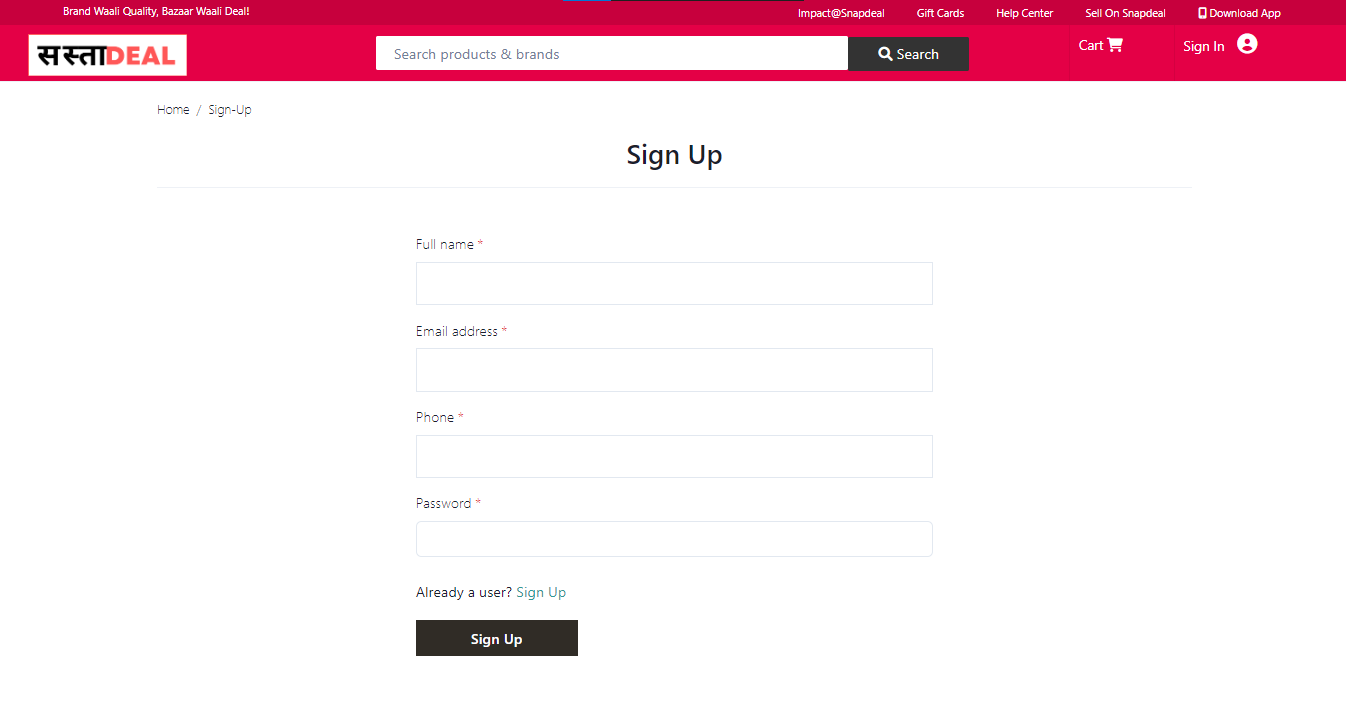
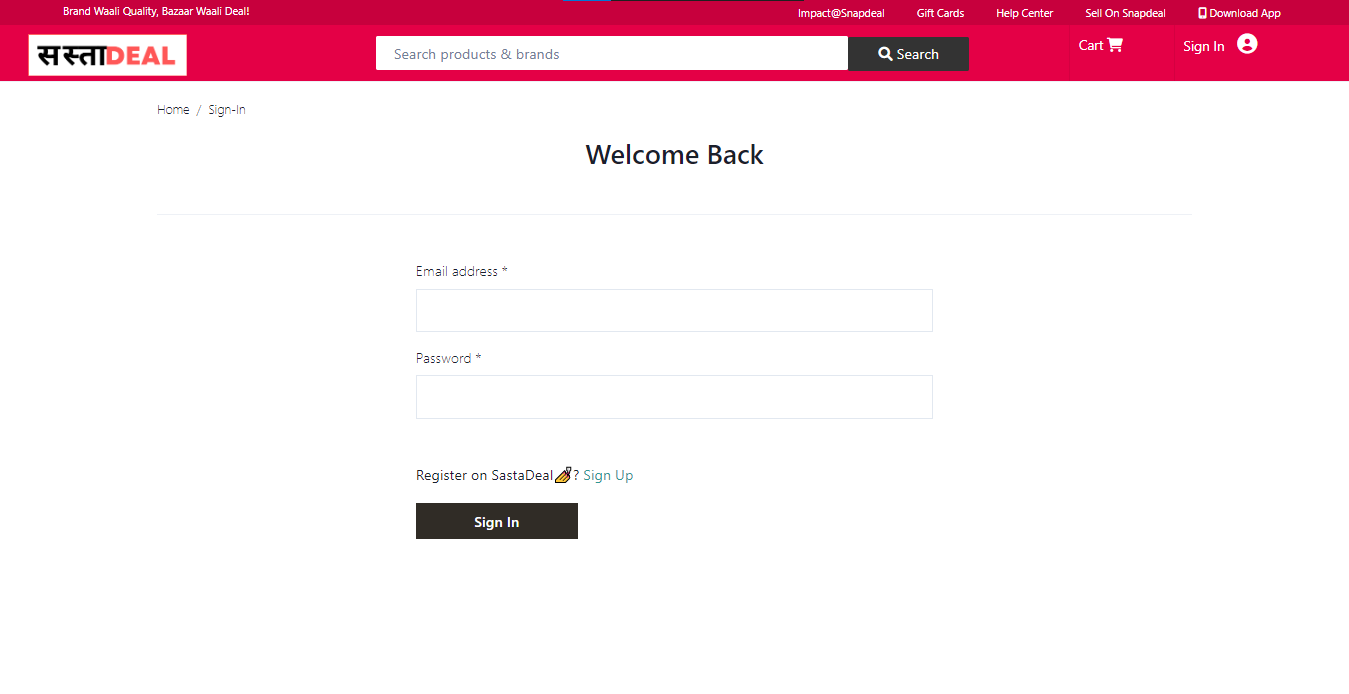
#Admin page
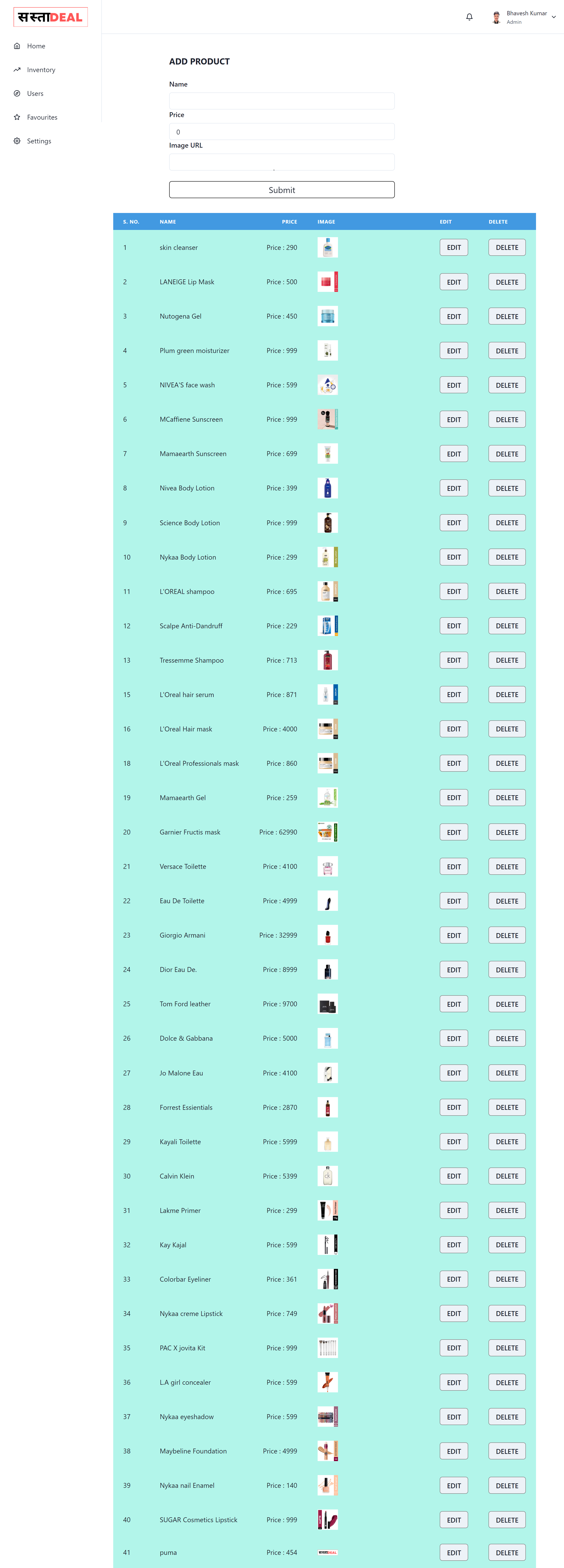
| Snapdeal is India's leading pure-play value Ecommerce platform. It was a Collaborative project built in 5 days by 5 team members using HTML,CSS, JavaScript, react ,react-redux | html,css,css3,react,react-redux,chakra-ui,javascript,reactjs,redux | 2023-01-14T08:51:32Z | 2023-11-17T07:23:15Z | null | 6 | 26 | 124 | 1 | 3 | 5 | null | null | JavaScript |
janishar/nodejs-bot-app | main | # Create Bot using JavaScript in NodeJs
Use this program to create bots for automating the daily and repetitive tasks
# Instructions
1. Clone this repo
2. Copy `.env.example` file and rename it `.env`
3. `npm install`
4. `npm start`
# Project Structure
```
.
โโโ .vscode
โ โโโ launch.json
โ โโโ settings.json
โโโ LICENSE
โโโ README.md
โโโ addons
โ โโโ config.test.js
โโโ package-lock.json
โโโ package.json
โโโ .env.example
โโโ .env
โโโ .gitignore
โโโ .prettierignore
โโโ .prettierrc
โโโ src
โโโ app.js
โโโ botbackup
โ โโโ index.js
โโโ botnotify
โ โโโ index.js
โโโ config.js
โโโ utils
โโโ debug.js
โโโ filelog.js
โโโ sleep.js
โโโ timetable.js
```
# About The Author
You can connect with me here:
* [Janishar Ali](https://janisharali.com)
* [Twitter](https://twitter.com/janisharali)
* [YouTube Channel](https://www.youtube.com/@unusualcode)
### Find this project useful ? :heart:
* Support it by clicking the :star: button on the upper right of this page. :v:
### License
```
Copyright (C) 2023 JANISHAR ALI ANWAR
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
```
| Create Bot using JavaScript in NodeJs. Use this program to create bots for automating the daily and repetitive tasks | automation,backup,backups,bot,bots,javascript,node,nodejs,process,repetitive-tasks | 2023-01-05T06:24:37Z | 2023-10-09T09:06:09Z | null | 1 | 0 | 9 | 0 | 0 | 5 | null | Apache-2.0 | JavaScript |
rainydevzz/Barri | master | null | A moderation bot in Typescript using the Oceanic Library. | discord-bot,moderation-bot,nodejs,oceanic,typescript,discord,postgresql,javascript | 2023-01-08T20:12:15Z | 2023-08-16T07:00:11Z | null | 1 | 0 | 112 | 0 | 0 | 5 | null | AGPL-3.0 | TypeScript |
Yosefgeda/My-Portfolio | new-branch-two | # My-Portfolio
<a name="readme-top"></a>
<div align="center">
<h3><b>Portfolio</b></h3>
</div>
<!-- TABLE OF CONTENTS -->
# ๐ Table of Contents
- [๐ About the Project](#about-project)
- [๐ Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [๐ Live Demo](#live-demo)
- [๐ป Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#triangular_flag_on_post-deployment)
- [๐ฅ Authors](#authors)
- [๐ญ Future Features](#future-features)
- [๐ค Contributing](#contributing)
- [โญ๏ธ Show your support](#support)
- [๐ Acknowledgements](#acknowledgements)
- [โ FAQ (OPTIONAL)](#faq)
- [๐ License](#license)
<!-- PROJECT DESCRIPTION -->
# ๐ [My-Portfolio] <a name="about-project"></a>
**[# ๐ [My-Portfolio] Project mainly focusing on building personal responsive portfolio page to showcase my skills and abiities and what I can offer.
## ๐ Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
> Describe the tech stack and include only the relevant sections that apply to your project.
<details>
<summary>Client</summary>
<ul>
<li>HTML5</li>
</ul>
</details>
<details>
<summary>Client</summary>
<ul>
<li>CSS3</li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
- Displays_the_first_two_sections_of_the_overall_portfolio_project
- Designed_to_be_responsive_and_mobile_friendly
- Have_a_navigation_bar_section_and_hero_section
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## ๐ Live Demo <a name="live-demo"></a>
>You can view the final page
<a href="https://gleaming-bombolone-0e6852.netlify.app/">HERE</a>
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## ๐ป Getting Started <a name="getting-started"></a>
> Click <a href="https://gleaming-bombolone-0e6852.netlify.app/">HERE</a> to view the page.
### Prerequisites
In order to run this project you need:
- Install Git in your computer [Git](https://git-scm.com/downloads)
- Any text editor [VSCode](https://code.visualstudio.com/download)
- Install linters on your local env't
### Setup
Clone this repository to your desired folder, access the directory and run the html file:
To clone: git clone git@github.com:Yosefgeda/My-portfolio.git
to change to directory: cd My-portfolio
Inside you will find the html file.
### Install
Linters have been installed for this project(npm install).
Added a gitignore file and included the node_modules and text.md files.
Linter actions are integreated witg github.
### Usage
execute the following steps:
To run the project on the html file run it through liveserver.
### Run tests
run npx hint .
and npx stylelint "**/*.{css,scss}"
and npx eslint .
### Deployment
You can view the Loom video of the page
<a href="https://www.loom.com/share/dfb599ec605b4dd285c94845543bd873">HERE</a>
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## ๐ฅ Authors <a name="authors"></a>
> Mention all of the collaborators of this project.
๐ค **Author1**
- GitHub: [@yosefgeda](https://github.com/yosefgeda)
- Twitter: [@yosefgeda](https://twitter.com/yosegeda)
- LinkedIn: [yosefgeda](https://linkedin.com/in/yosefgeda)
**Author2**
- GitHub: [github](https://github.com/alexiscyber14)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## ๐ญ Future Features <a name="future-features"></a>
- [ ] **[More_section_will_be_included]**
- [ ] **[The_coming_features_will_include_previous_works_done]**
<!-- CONTRIBUTING -->
## ๐ค Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issue page](https://github.com/Yosefgeda/My-portfolio/issues)
<!-- SUPPORT -->
If you like this project follow and give thumbs up on likedin
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## ๐ Acknowledgments <a name="acknowledgements"></a>
I would like to thank to my coding partener Precious Betine.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FAQ (optional) -->
## โ FAQ (OPTIONAL) <a name="faq"></a>
- **[What_version_of_chrome_do_I_need_to_view_the_page]**
- [All_versions_are_capable]
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ๐ License <a name="license"></a>
This project is [MIT](https://choosealicense.com/licenses/mit/) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| My personal portfolio, showcases JavaScript, HTML, and CSS projects with an engaging user experience. It includes detailed project descriptions, my skills, and contact information for easy communication. The design features a clean, minimal aesthetic for a professional appearance. | css,html,javascript | 2023-01-11T14:14:26Z | 2023-12-11T17:08:27Z | null | 3 | 9 | 65 | 0 | 0 | 5 | null | null | JavaScript |
amansingh456/Fraudy | main | # Fraudy
*About Website..*
*Fraudy is Ecommerce site for the newest & trending fashion accessories and online shop that offered high quality products at an affordable price. You can shop cloths,accessories.*
## Home-Page
.png)
.png)
## Login-Page && Signup-page
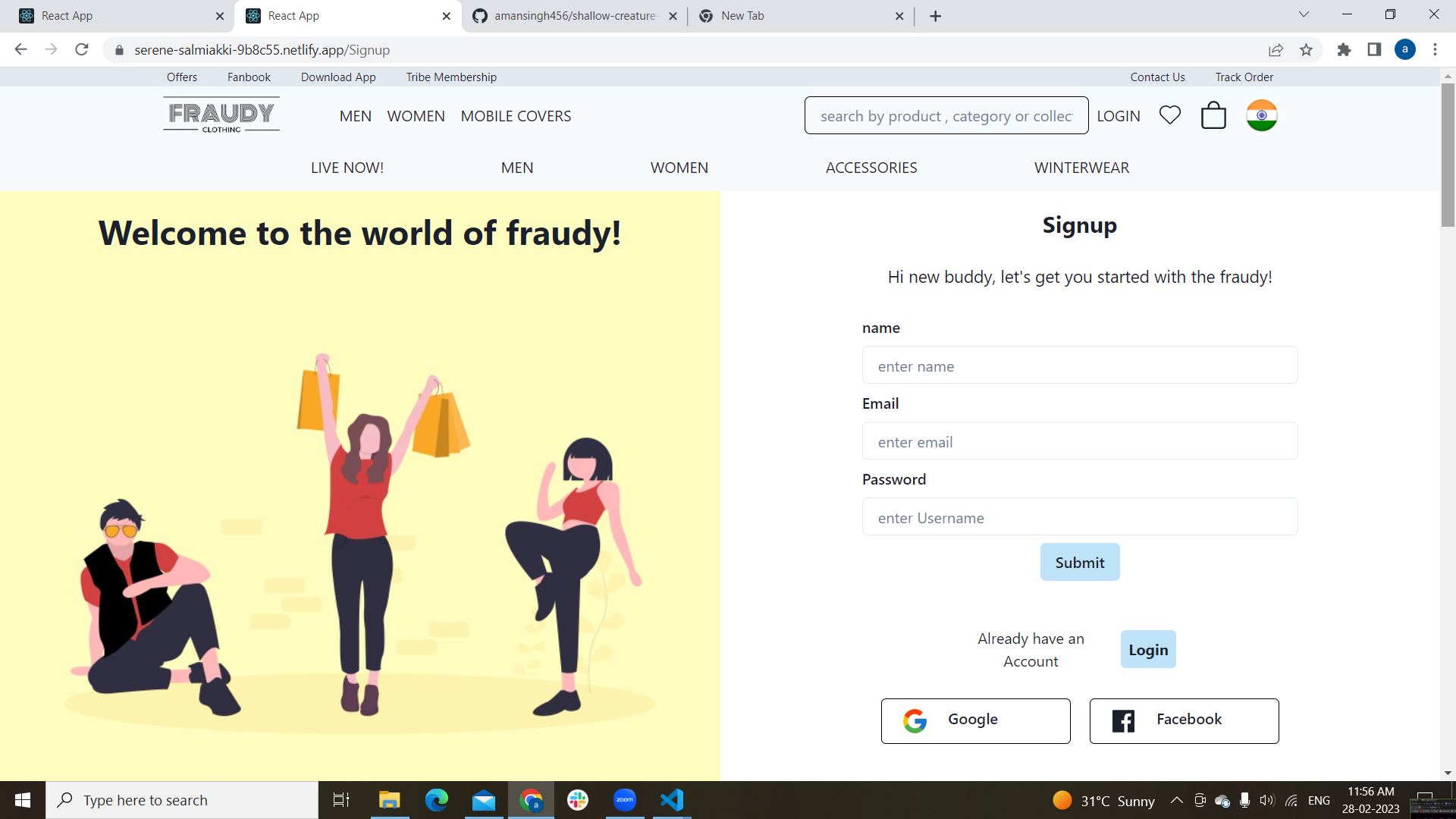
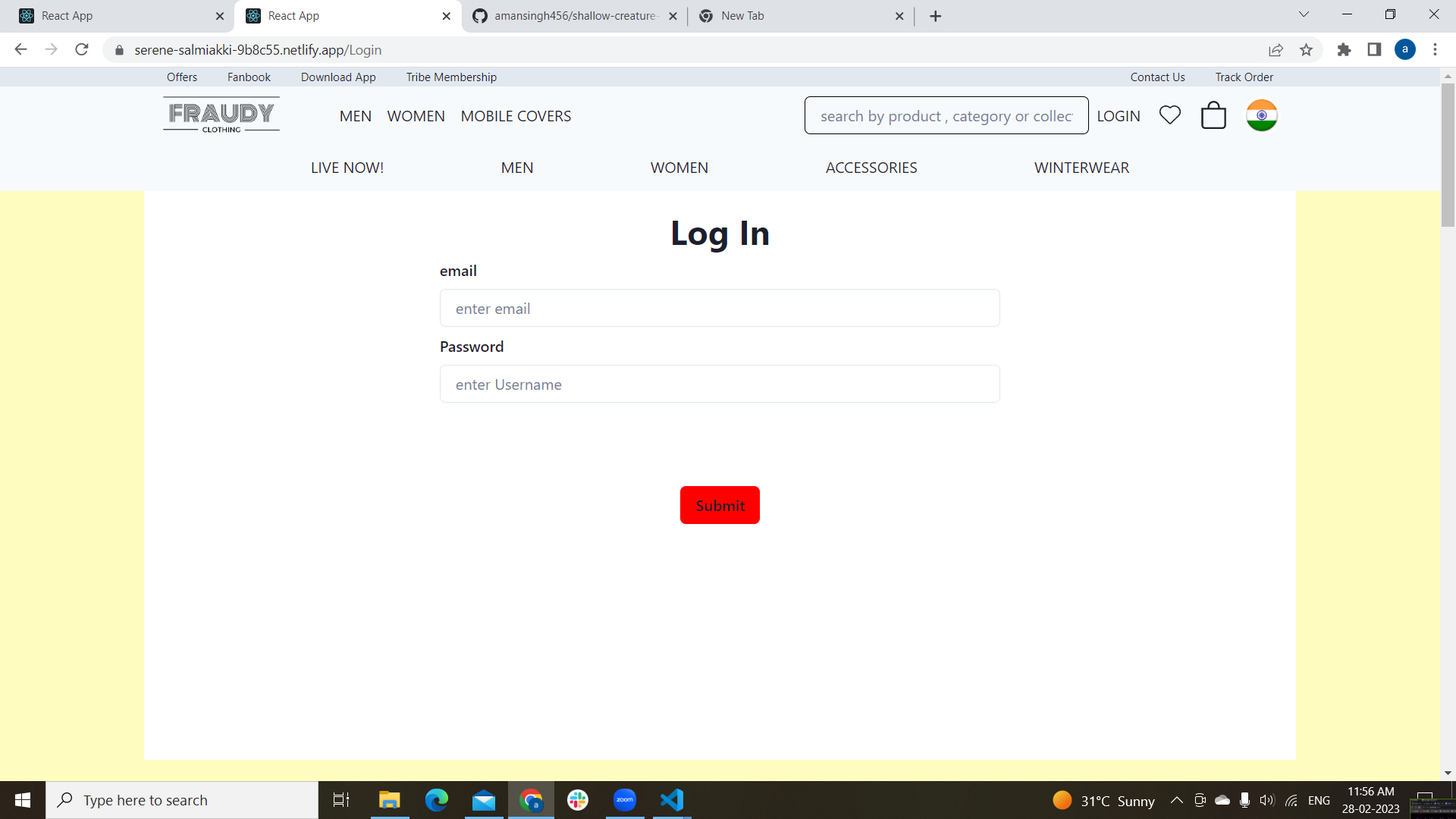
## Products-Page
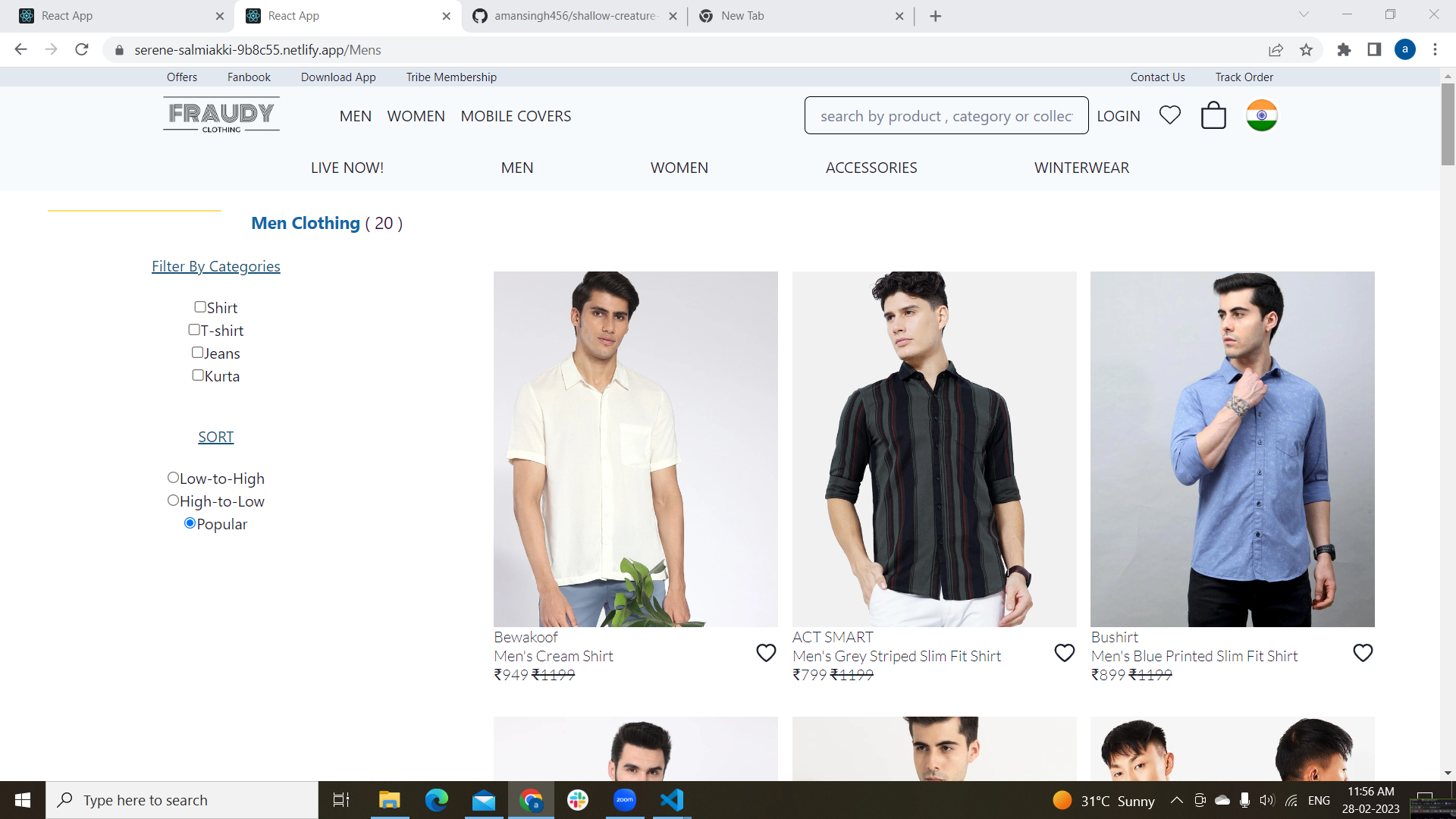
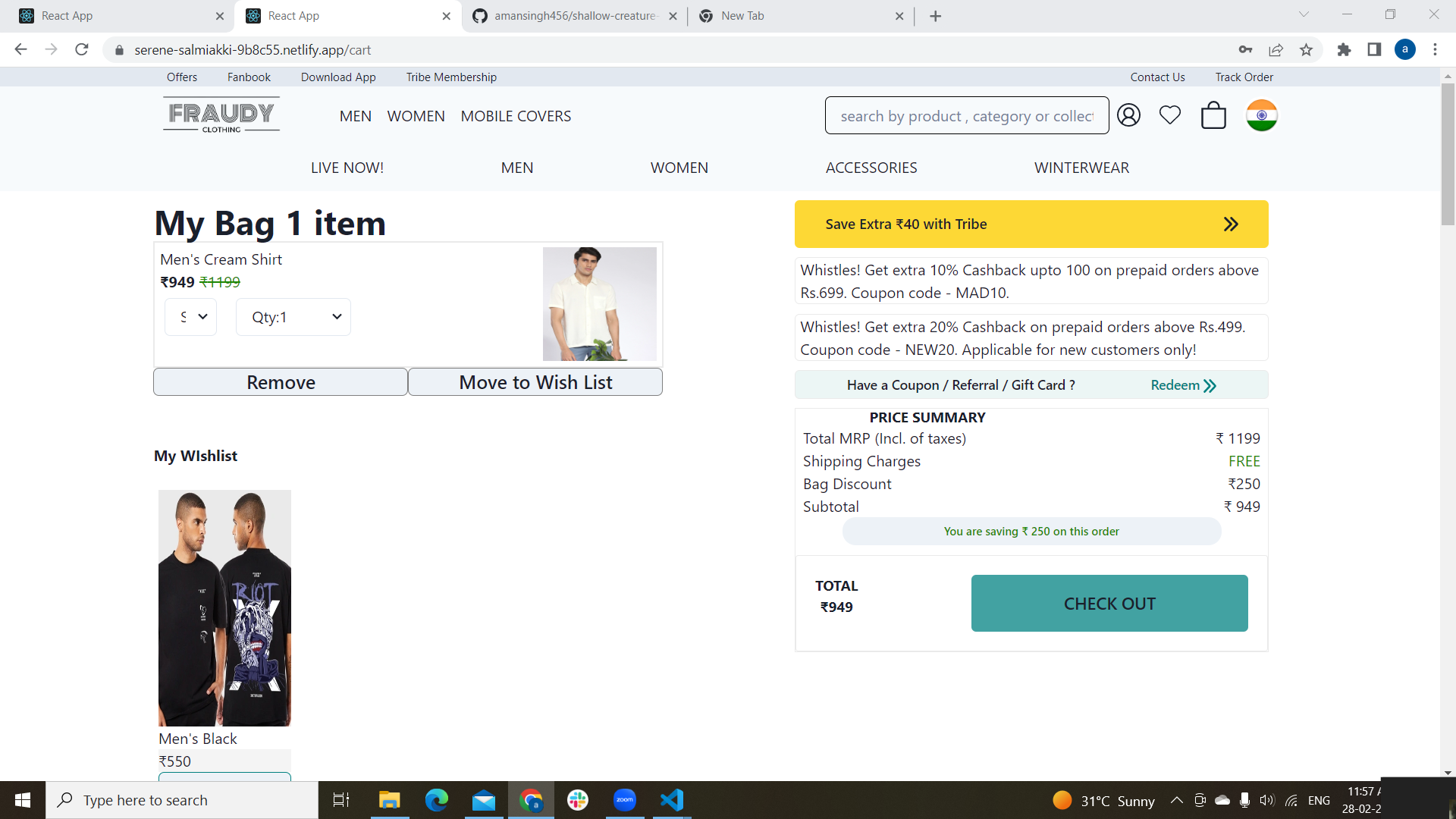
## ๐ค **_Collaborators_**
# ๐ง๐ป **Vivek Goswami** `IA Manager`
- Github:(https://github.com/vivekgoswami934)
- Linkedin:(https://www.linkedin.com/in/vivek-goswami-680a91203/)
# ๐ง๐ป **Aman Singh Rajawat** `Team Lead`
- Github:(https://github.com/amansingh456)
- Linkedin:(https://www.linkedin.com/in/aman690/)
- Email: (amansinghrajawat690@gmail.com)
# ๐ง๐ป **Abhijeet Kale**
- Github:(https://github.com/abhi5733)
- Linkedin:(https://www.linkedin.com/in/abhijeet-kale-588b77238/)
- Email: (abhijeetkale5733@gmail.com)
# ๐ฑ๐ปโโ๏ธ **Ravichandra Koli**
- Github:(https://github.co/Ravi515K)
- Linkedin:(https://www.linkedin.com/in/pratik-mate-a6a62919b/)
- Email: (koliravi95@gmail.com)
# ๐ง๐ปโ๐ฆฐ **Lipan Padhan**
- Github:(https://github.com/lipan1437)
- Linkedin:(https://www.linkedin.com/in/lipan-padhan-a0bb381a5/)
- Email: (lipanpadhan1432@gmail.com)
- api for backend >> https://mock-api-7p1z.onrender.com <<
| Fraudy is Ecommerce site for the newest & trending fashion accessories and online shop that offered high quality products at an affordable price. You can shop cloths,accessories. | css3,html5,javascript,reactjs,redux,redux-thunk | 2023-01-15T16:46:14Z | 2023-03-03T15:00:24Z | null | 6 | 18 | 89 | 0 | 3 | 5 | null | null | JavaScript |
hansemannn/titanium-giphy | main | # Giphy in Titanium
<img src="./.github/giphy-sdk.gif" height="300" />
Use the native Giphy iOS- and Android SDK's to select GIFs and display them in your app.
## Requirements
- [x] Titanium SDK 11.0.0+
## APIs
- `initialize({ apiKey })`: Initialize the SDK and provide your API key
- `showGIFDialog({ callback })`: Select a GIF and return it to the provided callback
## Example
See the example/app.js for details!
## Note
The default Android ImageView won't show the GIF animation. Check e.g. [av.imageview](https://github.com/AndreaVitale/av.imageview) for GIF support.
## Author
Hans Knรถchel
## License
MIT
| Use the native Giphy iOS- and Android SDK's to select GIFs and display them in your app. | giphy,titanium,android,ios,javascript,native,sdk | 2023-01-11T11:38:11Z | 2023-01-11T13:24:56Z | 2023-01-11T11:38:09Z | 2 | 1 | 7 | 0 | 1 | 5 | null | NOASSERTION | Objective-C |
piyush-agrawal6/Social-Media-Frontend | main | # Diverse - Lets get deversify.
Diverse is a social media chatting app where users can interact with different people, post images, comment, like and message each other. Users can add, edit and delete posts, comments and their details. Users can also follow and unfollow each other.
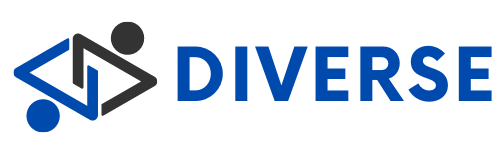
## Tech Stack
**Client:**
- **React**
- **Redux Thunk**
- **Axios**
- **CSS**
- **Ant-Designs**
**Server:**
- **Node Js**
- **Mongo DB**
- **Express Js**
- **Bcrypt**
- **JWT**
## Deployment
Client - Netlify
Server - Cyclic
## ๐ Links
Client - https://diversely.netlify.app
Server - https://busy-jade-sawfish-cape.cyclic.app
## Features
-Post, delete, and edit images
-Like, comment on the posts
-update profile and cover picture
-Delete the account and change the password
-Follow and unfollow users
-Proper Responsiveness
-Login/signup authentication
-Form validation
## Screenshots
1. Home page
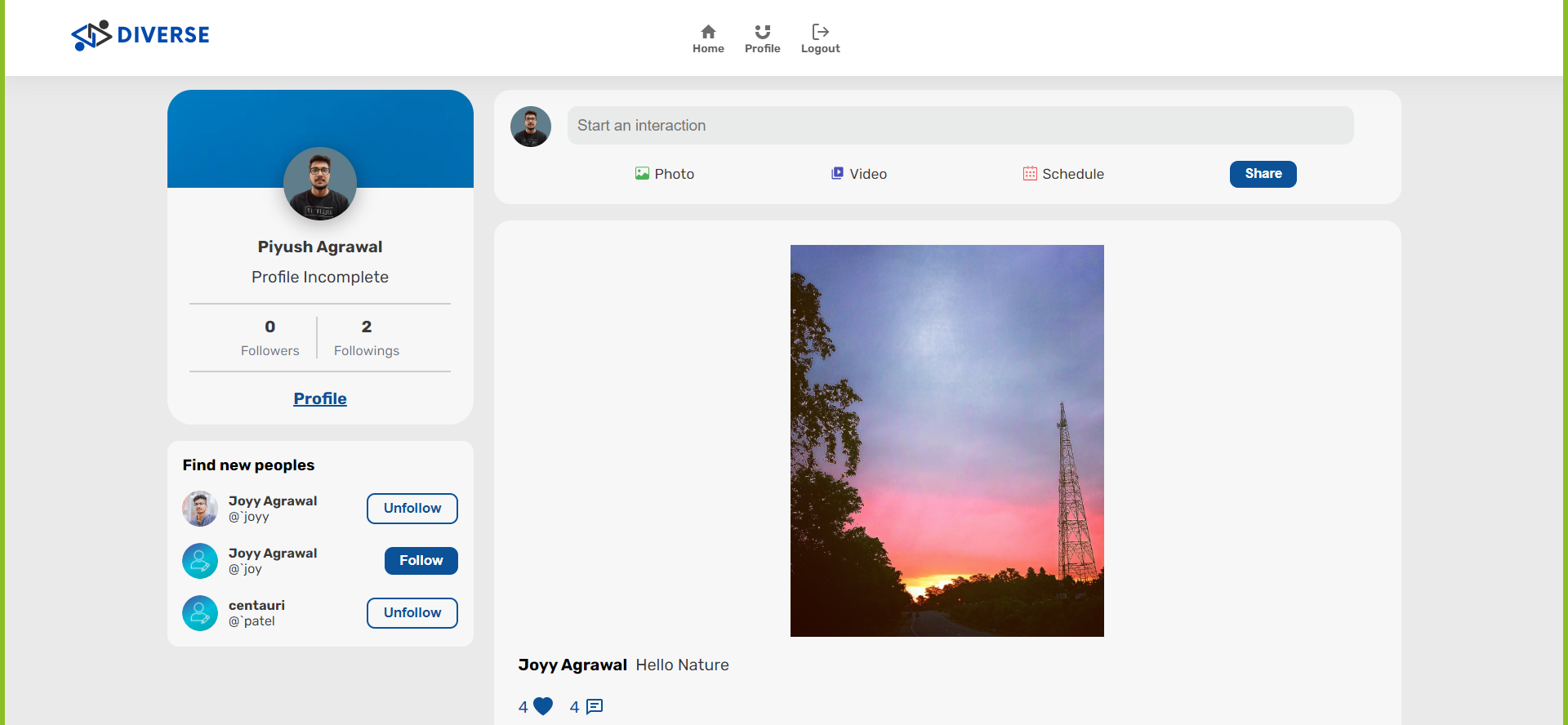
2. Profile page
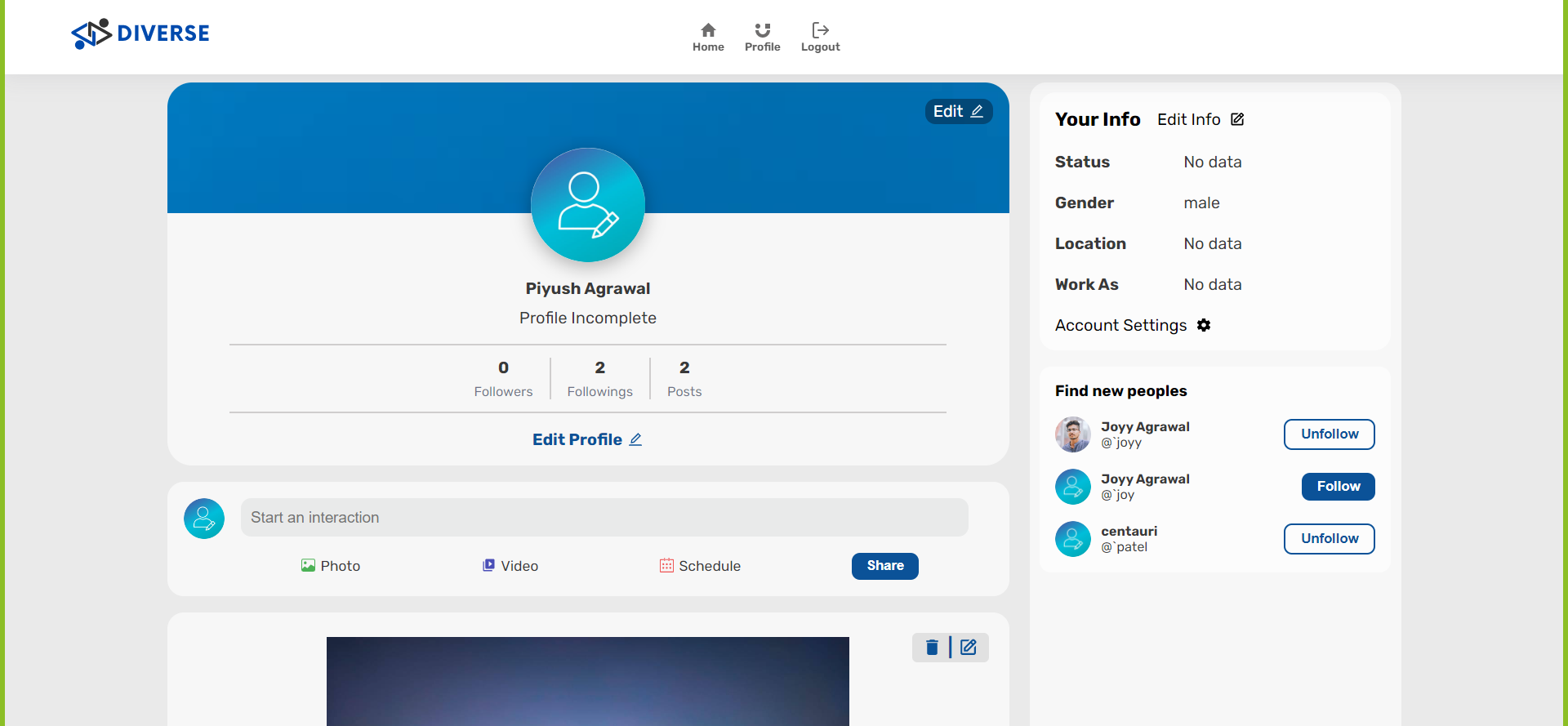
3. login page
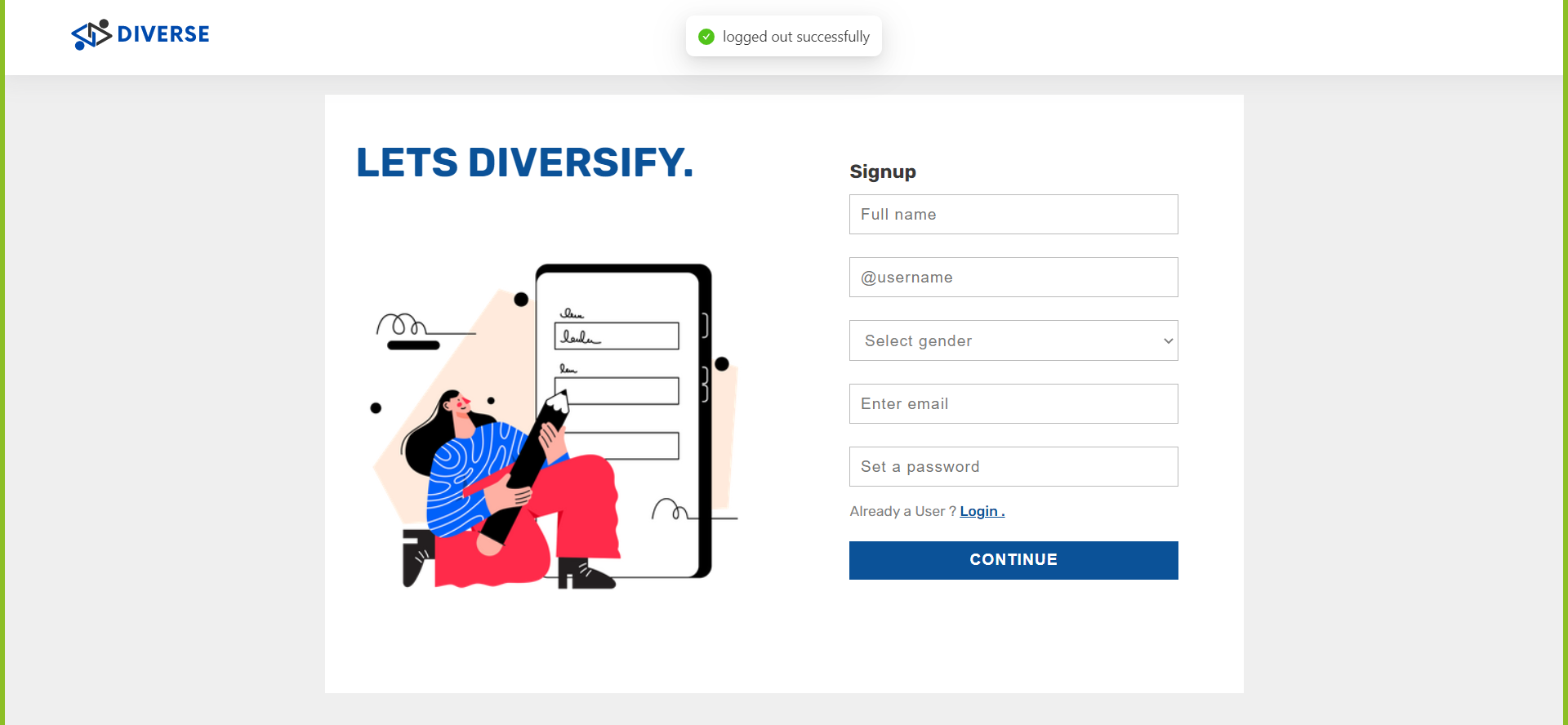
## Blog / Presentation
Presentation - https://drive.google.com/file/d/1qEAkelnlGGlWfVS7Gaa9-vaGtNlImhDg/view?usp=share_link
## Contributors
- [@Piyush Agrawal](https://github.com/piyush-agrawal6)
- [@Rajendra patel](https://github.com/centauricoder01)
| Diverse is a social media chatting app where users can interact with different people, post images, comment, like and message each other. Users can add, edit and delete posts, comments and their details. Users can also follow and unfollow each other. | antdesign,chat-application,chatting-app,javascript,react,reactjs,redux,redux-thunk,social-media | 2023-01-12T03:29:02Z | 2023-02-12T08:11:16Z | null | 2 | 0 | 55 | 0 | 0 | 5 | null | null | JavaScript |
alwaz-shahid/chrome-extension-to-copy-save-text | master | # Copy Highlighted Text
A simple chrome extension that allows you to copy highlighted text and save it to the browser's storage. The extension also allows you to export the saved text to a text file.
</hr>
### Installation
To install the extension, you need to download the source code and load it into your chrome browser as an "unpacked extension".
> - Download the source code from GitHub
> - Open the chrome browser and navigate to chrome://extensions/
> - Enable the "Developer mode" toggle in the top right corner
> - Click on the "Load unpacked" button and select the folder where you have the source code
> - The extension should now be installed and you should see it in the extension list
> - You should now see the extension's icon on the top right corner of your chrome browser
</hr>
### Usage
> - Click on the extension's icon after highlighting text on any website.
> - The text will be saved in the browser storage and an alert will be shown to confirm it.
> - To export the saved text to a text file, right-click on the extension's icon, and select "Export Highlighted Text" from the context menu.
</hr>
### Notes
> - The extension uses the browser's local storage to save the highlighted text.
> - The exported text file will be named "highlighted_text.txt" and it will be downloaded to the default download folder.
> - In case of any conflict, chrome will prompt to save the file with a different name or overwrite the existing one.
</hr>
### Functionalities
<p>
The provided code creates a context menu item "Export Highlighted Text" on browser_action and sets an onClicked event listener to it. Once the menu item is clicked, the function "exportData" is called which retrieves the highlightedText from chrome.storage.local and passes it to "downloadTextFile" function. The "downloadTextFile" function creates a text file with the passed text and downloads it.
Additionally, the code also sets an onClicked event listener for browser action which retrieves the currently selected text from the active tab and if there is any text selected, it concatenates it with previously saved highlightedText and saves it to chrome.storage.local.
</p>
</hr>
### Contribution
You are welcome to contribute to the project by submitting pull requests or by reporting issues.
</hr>
## License
This code is open-sourced under the MIT license
| This chrome extension allows you to easily save highlighted text from any website to the browser's storage. With a single click on the extension's icon, the highlighted text is saved, an alert is shown to confirm the action and the text is ready to be exported to a text file. | chrome-extension,copy-paste,extension,javascript,text | 2023-01-11T13:02:13Z | 2024-05-08T12:02:00Z | null | 1 | 0 | 8 | 0 | 0 | 5 | null | null | JavaScript |
mrprotocoll/html-capstone-microverse | main | <a name="readme-top"></a>
<!-- TABLE OF CONTENTS -->
# ๐ Table of Contents
- [๐ About the Project](#about-project)
- [๐ Built With](#built-with)
- [Tech Stack](#tech-stack)
- [๐ Live Demo](#live-demo)
- [๐ Project Live Presentation](#loom-demo)
- [๐ป Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [๐ฅ Authors](#authors)
- [๐ญ Future Features](#future-features)
- [๐ค Contributing](#contributing)
- [โญ๏ธ Show your support](#support)
- [๐ Acknowledgements](#acknowledgements)
- [๐ License](#license)
<!-- PROJECT DESCRIPTION -->
# ๐ MV Fullstack Graduation Website Project <a name="about-project"></a>
> Describe of project.
**MV Fullstack Graduation Website Project** is the capstone project in week 5 for day 1,2 and 3 of microverse fullstack development.
## ๐ Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
> Description of the tech stacks used
<details>
<summary>Client</summary>
<ul>
<li><a href="https://html.com/">Html</a></li>
<li><a href="https://www.w3.org/Style/CSS/Overview.en.html">CSS</a></li>
<li><a href="https://www.javascript.com/">JavaScript</a></li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
> Features of the application.
- **Home page**
- **About page**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## ๐ Live Demo <a name="live-demo"></a>
> Link to deployed project.
- [Live Demo Link](https://mrprotocoll.github.io/first-capstone-microverse/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- Project Presentation -->
## Project Presentatio <a name="loom-demo"></a>
> Link to Project Presentation.
- [Loom Walkthrough Link](https://www.loom.com/share/1656210cf90147c7965e1e62a5e00273)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## ๐ป Getting Started <a name="getting-started"></a>
> Describe how a new developer could make use of your project.
To get a local copy up and running, follow these steps.
### Prerequisites
In order to run this project you need:
1. npm
use the following link to setup npm if you dont have it already installed on your computer
<p align="left">(<a href="https://docs.npmjs.com/downloading-and-installing-node-js-and-npm">install npm</a>)</p>
### Setup
Clone this repository to your desired folder using the above command:
```sh
cd my-folder
git clone https://github.com/mrprotocoll/first-capstone-microverse.git
```
### Install
Install this project with:
```sh
cd my-project
npm install -y
```
### Usage
To run the project:
Open the index.html file using a web browser
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## ๐ฅ Authors <a name="authors"></a>
> Mention all of the collaborators of this project.
๐ค **mrprotocoll**
- GitHub: [@mrprotocoll](https://github.com/mrprotocoll)
- Twitter: [@dprotocoll](https://twitter.com/dprotocoll)
- LinkedIn: [@mrprotocoll](https://www.linkedin.com/in/mrprotocoll)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## ๐ค Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## โญ๏ธ Show your support <a name="support"></a>
If you like this project, please don't forget to follow me and give it a star.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## ๐ Acknowledgments <a name="acknowledgements"></a>
I would like to thank [Microverse](https://www.microverse.org/) , and [Cindy Shin](https://www.behance.net/adagio07) who is the owner of my project original template design.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## ๐ License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| A software trainee graduation event website that includes a landing page and the about page. | javascript | 2023-01-02T08:27:09Z | 2023-01-06T12:33:01Z | null | 1 | 1 | 23 | 1 | 0 | 5 | null | MIT | HTML |
Trast00/Capston-javascript | dev | <a name="readme-top"></a>
# ๐ Table of Contents
- [๐ Table of Contents](#-table-of-contents)
- [๐ ShowTime ](#-showtime-)
- [๐ Built With ](#-built-with-)
- [Tech Stack ](#tech-stack-)
- [Key Features ](#key-features-)
- [๐ Live Demo ](#-live-demo-)
- [๏ฟฝ Presentation Video ](#-presentation-video-)
- [๐ป Getting Started ](#-getting-started-)
- [Setup](#setup)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [๐ฅ Authors ](#-authors-)
- [๐ญ Future Features ](#-future-features-)
- [โญ๏ธ Show your support ](#๏ธ-show-your-support-)
- [๐ License ](#-license-)
# ๐ ShowTime <a name="about-project"></a>
**ShowTime** is Movie Streaming Website that use TV maze API to display a list of movies with detail. Users can leave comments and likes about their prefered movies.
[video of presentation](https://www.loom.com/share/99aaffee950643ba895293ae357802c2)
## ๐ Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
- HTML
- CSS
- JavaScript
### Key Features <a name="key-features"></a>
- **Use ES6**
- **Show Popup detail**
- **Use Api [tvmaze](https://www.tvmaze.com/api)**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## Preview
<details>
<summary>Preview</summary>

</details>
## ๐ Live Demo <a name="live-demo"></a>
[Live Demo Link](https://trast00.github.io/Capston-javascript/dist/index.html)
## ๐บ Presentation Video <a name="live-demo"></a>
[Presentation video](https://www.loom.com/share/99aaffee950643ba895293ae357802c2)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ๐ป Getting Started <a name="getting-started"></a>
To get a local copy up and running, follow these steps.
### Setup
Clone this repository to your desired folder:
```sh
cd my-folder
git clone git@github.com:myaccount/my-project.git
```
### Install
Install this project with:
Example command:
```sh
cd my-project
gem install
```
### Usage
To run the project, execute the following command:
```sh
npm start
```
### Run tests
To run tests, run the following command:
```sh
npm test
```
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ๐ฅ Authors <a name="authors"></a>
๐ค **Dicko Allasane**
- GitHub: [@githubhandle](https://github.com/Trast00)
- Twitter: [@twitterhandle](https://twitter.com/AllassaneDicko0/)
- LinkedIn: [LinkedIn](https://www.linkedin.com/in/allassane-dicko-744aaa224)
๐ค **Donald Akite**
- GitHub: [@githubhandle](https://github.com/quavo19)
- Twitter: [@twitterhandle](https://twitter.com/DonaldAkite)
- LinkedIn: [LinkedIn](https://www.linkedin.com/in/donald-akite-299a31222/)
## ๐ญ Future Features <a name="future-features"></a>
- [x] **Comment section**
- [ ] **Search bar**
- [ ] **List of episodes**
- [x] **More Personnalized UI**
## โญ๏ธ Show your support <a name="support"></a>
Give a โญ๏ธ if you like this project!
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ๐ License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| ShowTime is Movie Streaming Website that use TV maze API to display a list of movies with detail. Users can leave comments and likes about their prefered movies | html5,api,css,es6-javascript,javascript | 2023-01-02T12:27:30Z | 2023-06-09T13:09:30Z | null | 2 | 14 | 104 | 4 | 0 | 5 | null | MIT | JavaScript |
ziss11/notes-app-hapi | master | # notes-app-backend
Back-End dummy project using NodeJS with Hapi Framework
| Back-End dummy project using NodeJS with Hapi Framework | hapijs,javascript,nodejs | 2023-01-08T07:49:10Z | 2023-08-04T06:43:33Z | null | 1 | 0 | 27 | 0 | 0 | 5 | null | MIT | JavaScript |
MMGGYY66/awesome-books-solo | main | # my-awesome-books-solo
<p id="readme-top">My Microverse my-awesome-books-solo project (Module two)</p>
<!-- TABLE OF CONTENTS -->
# ๐ Table of Contents
- [my-awesome-books](#my-awesome-books)
- [๐ Table of Contents](#-table-of-contents)
- [๐ \[๐ฏ my-awesome-books ](#--my-awesome-books-)
- [๐ Built With ](#-built-with-)
- [Tech Stack ](#tech-stack-)
- [๐ Live Demo ](#-live-demo-)
- [๐ Presentation ](#-presentation-)
- [creating my-awesome-books:](#creating-my-awesome-books)
- [๐ Built With ](#-built-with--1)
- [Deploy my website with github pages":](#deploy-my-website-with-github-pages)
- [- Loom video link:](#--loom-video-link)
- [๐ป Getting Started ](#-getting-started-)
- [Prerequisites](#prerequisites)
- [Prerequisites](#prerequisites-1)
- [Install](#install)
- [Requirements](#requirements)
- [Run tests](#run-tests)
- [Deployment](#deployment)
- [๐ฅ Authors ](#-authors-)
- [๐ค Contributing ](#-contributing-)
- [๐ Show your support ](#-show-your-support-)
- [๐ญAcknowledgments ](#acknowledgments-)
- [๐ License ](#-license-)
<!-- PROJECT DESCRIPTION -->
# ๐ [๐ฏ my-awesome-books <a name="about-project"></a>
> "**Awesome books**" is a simple website that displays a list of books and allows you to add and remove books from that list. By building this application, you will learn how to manage data using JavaScript. Thanks to that your website will be more interactive. You will also use a medium-fidelity wireframe to build the UI.is a simple website that displays a list of books and allows you to add and remove books from that list. By building this application, you will learn how to manage data using JavaScript. Thanks to that your website will be more interactive. You will also use a medium-fidelity wireframe to build the UI.
## ๐ Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
## ๐ Live Demo <a name="live-demo"></a>
[link to my-awesome-books-solo: ](https://mmggyy66.github.io/awesome-books-solo/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ๐ Presentation <a name="presentation"></a>
- [Loom presentation Link]()
# creating my-awesome-books:
Re-create my Awesome books app following the rules:
Use ES6 syntax.
Divide my code into modules. Save each module in a separate file in the modules dir. Import modules in the index.js file. For this exercise
Keep all funcionalities of the app without errors.
Refactor my methods and functions to arrow functions.
Use let and const in a correct way.
## ๐ Built With <a name="built-with"></a>
<details>
<summary>Technology</summary>
<ul>
<li>HTML</li>
<li>CSS (**medium-fidelity** wireframe)</li>
<li>Javascript</li>
<li>Linters (Lighthouse, Webhint, Stylelint, Eslint)</li>
<li>Git/GitHub work-flow </li>
</ul>
</details>
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## Deploy my website with github pages":
# [link to my-awesome-books-solo: ](https://mmggyy66.github.io/awesome-books-solo/)
## - Loom video link:
[Loom walking through for my-awesome-books: ]()
## ๐ป Getting Started <a name="getting-started"></a>
To get a local copy up and running follow these simple example steps.
### Prerequisites
- IDE to edit and run the code (We use Visual Studio Code ๐ฅ).
- Git to versionning your work.
### Install
- first install package.json and node_modules run:
npm init -y
- npm install --save-dev hint
- npm install --save-dev stylelint@13.x stylelint-scss@3.x stylelint-config-standard@21.x stylelint-csstree-validator@1.x
<!-- or latest version -->
- npm install --save-dev stylelint stylelint-scss stylelint-config-standard stylelint-csstree-validator
- npm install --save-dev eslint@7.x eslint-config-airbnb-base@14.x eslint-plugin-import@2.x babel-eslint@10.x
<!-- or latest version -->
- npm install --save-dev eslint eslint-config-airbnb-base eslint-plugin-import babel-eslint
## Requirements
- Linters configuration.
- Part 1: Manage books collection (plain JS with objects).
- Part 2: Manage books collection (plain JS with classes) and add basic CSS.
- Part 3: Create a complete website with navigation.
Clone the repository to get start with project, then make sure to install dependencies in the linters file located in the [linter](https://github.com/Bateyjosue/linters-html-css/blob/main/.github/workflows/linters.yml) file
<p align="right">(<a href="#readme-top">back to top</a>)</p>
### Run tests
- to test and check the html file/s is error-free run:
npx hint .
- to fix errors run:
npx hint . -f
- to test and check the css file/s is error-free run:
npx stylelint "**/*.{css,scss}"
- to fix errors run:
npx stylelint "**/*.{css,scss}" --fix
- to test and check the js file/s is error-free run:
npx eslint .
- to fix errors run:
npx eslint . --fix
### Deployment
Check for the tests when you generate a pull request and fix the errors if any.
For stylelint error run:<code>sudo npx stylelint "\*_/_.{css,scss}" --fix</code> and it will the fix style issues automatically.
<!-- AUTHORS -->
## ๐ฅ Authors <a name="authors"></a>
๐ค **Mohamed Gamil Eldimardash**
- GitHub: [@github](https://github.com/MMGGYY66)
- LinkedIn: [LinkedIn](https://www.linkedin.com/in/mohamed-eldimardash-0023a3b5/)
- Twitter: [twitter](https://twitter.com/MOHAMEDELDIMARd)
- Facebook: [facebook](https://www.facebook.com/MOHAMED.ELDIMARDASH/)
<!-- CONTRIBUTING -->
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ๐ค Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the issues page
<!-- SUPPORT -->
## ๐ Show your support <a name="support"></a>
Give a โญ๏ธ if you like this project!
<p align="right"><a href="#readme-top">(back to top)</a></p>
<!-- ACKNOWLEDGEMENTS -->
## ๐ญAcknowledgments <a name="acknowledgements"></a>
- [Microverse Team](https://www.microverse.org/).
## ๐ License <a name="license"></a>
This project is [MIT](https://github.com/microverseinc/readme-template/blob/master/MIT.md) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| "Awesome books" is a simple website that displays a list of books and allows you to add remove books from that list. | css,eslint,javascript | 2023-01-15T15:04:02Z | 2023-02-02T20:31:00Z | null | 1 | 1 | 14 | 0 | 0 | 5 | null | null | JavaScript |
gaks1/Isaac-portfolio | main | <a name="readme-top"></a>
<div align="center">
<h1><b>Portfolio Page</b></h1>
</div>
# ๐ Table of Contents
- [๐ About the Project](#about-project)
- [๐ Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [๐ป Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#triangular_flag_on_post-deployment)
- [๐ฅ Authors](#authors)
- [๐ญ Future Features](#future-features)
- [๐ค Contributing](#contributing)
- [โญ๏ธ Show your support](#support)
- [๐ Acknowledgements](#acknowledgements)
- [๐ License](#license)
# ๐ [Isaac-portfolio] <a name="about-project"></a>
**[Portfolio website]** is a website that shows my skills in web development. It has links to my projects, resume and social media.<br>
A [loom video](https://www.loom.com/share/818e4b9451e240b29157082df20925e8) description of my project.
## ๐ Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
<details>
<summary>Client</summary>
<ul>
<li><a href="https://www.quackit.com/html/codes/html_code_library.cfm">Html</a></li>
<li><a href="https://www.w3docs.com/course/css-the-complete-guide-2020-incl-flexbox-grid-sass">css</a></li>
</ul>
</details>
### Key Features <a name="key-features"></a>
- **[Navbar]**-> a navigation bar.
- **[header]**-> general introduction to the programmer.
- **[Body]**-> general introduction to the projects done by programmer.
- **[About]**-> about myself.
- **[Contacts]**-> A form to contact me.
- **[Desktopversion]**-> Made a desktop version of the website.
- **[Mobile menu]**-> Added a mobile menu to the hamburger icon.
- **[Portfolio popup menu]**-> Added a pop up to the portfolio section.
- **[Form validation]**-> made a validation function for the form.
- **[Local Storage]**-> allow for local storage in the browser.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ๐ป Getting Started <a name="getting-started"></a>
To get a local copy up and running, follow these steps.
### Prerequisites
In order to run this project you need:
Git and Code editor.
### Setup
Clone this repository to your desired folder:
git clone git@github.com:gaks1/Isaac-portfolio.git
### Install
Install this project with:
http server:
npm install http-server -g
http-server
To run the project, execute the following command:
http-server public
### Run tests
To run tests, go to:
1. htmltester--> https://wave.webaim.org/
2. csstester-->https://jigsaw.w3.org/css-validator/
### Deployment
You can deploy this project using:
github pages.
Deployed [project](https://gaks1.github.io/Isaac-portfolio/) for view.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ๐ฅ Authors <a name="authors"></a>
๐ค Isaac Gakure Wanyoike
- GitHub: [@githubhandle](https://github.com/gaks1)
- Twitter: [@twitterhandle](https://twitter.com/bopplov)
- LinkedIn: [LinkedIn](https://www.linkedin.com/in/isaac-wanyoike-1841a8172/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ๐ญ Future Features <a name="future-features"></a>
- **[Bug fix]**-> Fix bugs.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ๐ค Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](https://github.com/gaks1/Isaac-portfolio/issues).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## โญ๏ธ Show your support <a name="support"></a>
If you like this project star my work
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ๐ Acknowledgments <a name="acknowledgements"></a>
I would like to thank Microverse.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ๐ License <a name="license"></a>
This project is [MIT](MIT.md) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p> | A portfolio website used to show all projects I have made. Built with JavaScript and is live through Github pages | css,html,javascript | 2023-01-11T15:48:48Z | 2023-02-28T15:20:46Z | null | 3 | 13 | 119 | 2 | 0 | 5 | null | null | CSS |
Channa47/AtoZ-Amazon.in--Clone | main |
<h1 align="center">AtoZ.in -Amazon Website Clone</h1>
<h4 align="center">AtoZ is an E-Commerce Web Application where users can able buy all kind of products through online
it is basically a clone of Amazon.in, Built with a team of Five members using MongoDB, Express, React, and NodeJS (MERN Stack) in Five Days</h4>
<br/>
<br/>
<h4 align="center">Deployed On:</h4>
<p align="center">
<img src="https://img.shields.io/badge/Netlify-00C7B7?style=for-the-badge&logo=netlify&logoColor=white" align="center" alt="netlify"/>
<img src="https://img.shields.io/badge/vercel-%23000000.svg?style=for-the-badge&logo=vercel&logoColor=whit" align="center" alt="vercel"/>
</p>
<br />
<h2 align="center">๐ฅ๏ธ Tech Stack</h2>
<h4 align="center">Frontend:</h4>
<p align="center">
<img src="https://img.shields.io/badge/React-20232A?style=for-the-badge&logo=react&logoColor=61DAFB" alt="reactjs" />
<img src="https://img.shields.io/badge/Redux-593D88?style=for-the-badge&logo=redux&logoColor=white" alt="redux" />
<img src="https://img.shields.io/badge/Chakra%20UI-3bc7bd?style=for-the-badge&logo=chakraui&logoColor=white" alt="chakra-ui" />
<img src="https://img.shields.io/badge/JavaScript-323330?style=for-the-badge&logo=javascript&logoColor=F7DF1E" alt="javascript" />
<img src="https://img.shields.io/badge/Rest_API-02303A?style=for-the-badge&logo=react-router&logoColor=white" alt="restAPI" />
<img src="https://img.shields.io/badge/CSS3-1572B6?style=for-the-badge&logo=css3&logoColor=white" alt="css3" />
<img src="https://img.shields.io/badge/HTML5-E34F26?style=for-the-badge&logo=html5&logoColor=white" alt="html5" />
</p>
<h4 align="center">Backend:</h4>
<p align="center">
<img src="https://img.shields.io/badge/Node.js-339933?style=for-the-badge&logo=nodedotjs&logoColor=white" alt="nodejs" />
<img src="https://img.shields.io/badge/Express.js-000000?style=for-the-badge&logo=express&logoColor=white" alt="expressjs" />
<img src="https://img.shields.io/badge/MongoDB-4EA94B?style=for-the-badge&logo=mongodb&logoColor=white" alt="mongodb" />
<img src="https://img.shields.io/badge/JWT-000000?style=for-the-badge&logo=JSON%20web%20tokens&logoColor=white" alt="JsonWebToken" />
</p>
<h3 align="center">Deployment</h3>
<h3 align="center"><a href="https://atozamazon.netlify.app/"><strong>Want to see live preview ยป</strong></a></h3>
<br />
## Screens/Pages
- Homepage / Landing Page
- Product Listing Page with Filters
- Product Description Page/Single Product Page
- Cart Management Page
- Checkout with Address Management Page
- Login Page
- Signup Page
- Admin Dashboard
<br />
## ๐ Features
- Login and Signup User Account
- JWT (Json Web Token) Authentication and BcryptJS Password Hashing
- Product Filters Based on Category
- Cart Management
- Address Management
- Payment/Checkout
- Admin Dashboard
- Add,Delete,Update Products
- Responsive Design
<br />
<h2>Screenshots</h2>
<h4>Homepage</h4>
<img src="https://i.imgur.com/w3NzfPt.png"/>
<br/>
<h4>Login and Signup Page</h4>
<img src="https://i.imgur.com/pRXNzU2.jpg"/>
<br/>
<h4>Products Page</h4>
<img src="https://i.imgur.com/1bQYyBA.jpg"/>
<br/>
<h4>Single Product Page</h4>
<img src="https://i.imgur.com/b2ArG88.jpg"/>
<br/>
<h4>Cart Page</h4>
<img src="https://i.imgur.com/UaDLiBD.jpg"/>
<br/>
<h4>Checkout Page</h4>
<img src="https://i.imgur.com/VwVqACn.jpg"/>
<br/>
<h4>Payment Page</h4>
<img src="https://i.imgur.com/1zBcEtU.jpg"/>
<br/>
<hr/>
<h1 align="center">Backend</h1>
### Products
## Get all products --Admin
localhost:8080/api/v1/products
for pagination limit is default 20
for sort you can just make price price[gt]=100 price[lt]=200 you get products
for filter you can apply filter at params ex: url?name=laptop
## Create a product(post)
localhost:8080/api/v1/products/new
req.body
{
"name":"product1",
"price":1200,
"description":"this is a sample product",
"category":"Laptop",
"images":{
"public_id":"sample Image",
"url":"sample url"
}
}
## Update product(put)
localhost:8080/api/v1/product/product_id
{
"name":"airbag",
"price":2500,
"description":"this is a sample product",
"category":"long lasting and safe",
"images":{
"public_id":"sample Image",
"url":"sample url"
}
}
## Delete product(delete)
localhost:8080/api/v1/product/product_id
## Get product details(get)
localhost:8080/api/v1/product/63b86748a213654da6108a61
## Authentication
## Regeister User(post)
localhost:8080/api/v1/register
req.body
{
"name":"shri",
"email":"geniusfactsinsta@gmail.com",
"password":"Shrinidhi@9999"
}
## Login User(post)
localhost:8080/api/v1/login
req.body
{
"email":"geniusfactsinsta@gmail.com",
"password":"shri12345678"
}
## Logout User(get)
localhost:8080/api/v1/logout
## forget password
localhost:8080/api/v1/password/forgot
req.body
{
"email":"geniusfactsinsta@gmail.com"
}
### User routes
## Update Profile(put)
localhost:8080/api/v1/me/update
{
"email":"shrideshpande9177@gmail.com",
"name":"shripad deshpande"
}
## get all reviews(get)
localhost:8080/api/v1/me/update
## delete reviews(delete)
localhost:8080/api/v1/reviews?id=63b86748a213654da6108a61&productId=63b86748a213654da6108a61
## update User role, name ,email --(admin) (put)
localhost:8080/api/v1/admin/users/63be5c31fe833b5cc5c0b825
req.body
{
"name":"shrivalli",
"email":"shrivalli@gmail.com",
"role":"admin"
}
## Update or Change user password (put)
localhost:8080/api/v1/logout
req.body
{
"oldPassword":"Shrinidhi@9999",
"newPassword":"shri12345678",
"confirmPassword":"shri12345678"
}
## Get Single User --(admin) (Get)
localhost:8080/api/v1/admin/users/63be5c31fe833b5cc5c0b825
## update or create reviews (put)
localhost:8080/api/v1/review
req.body
{
"productId":"63b86748a213654da6108a61",
"comment":"just ok product",
"rating":4
}
## Get all users (get)
localhost:8080/api/v1/admin/users
## Delete User --(admin) (delete)
localhost:8080/api/v1/admin/users/_id
## Get user Details(who is logged in)(get)
localhost:8080/api/v1/me
### Order
## Create new order (post)
localhost:8080/api/v1/order/new
{
"itemsPrice":200,
"taxPrice":120,
"shippingPrice":20,
"orderItems": [
{
"name": "laptop",
"price":20000,
"quantity":1,
"image":"sample Image",
"product": "63b86748a213654da6108a61"
}
],
"shippingInfo":{
"address":"deshpande galli tanaji chowk latur",
"city": "latur",
"state": "maharastra",
"country":"India",
"pinCode": 413512,
"phoneNo": 9284149182
},
"paymentInfo":{
"id":"sample payment Info",
"status":"succeede"
}
}
## get logged in user Orders/My Orders (get)
localhost:8080/api/v1/orders/me
## Get Single Order (get)
localhost:8080/api/v1/order/_orders_id
## Get all orders --admin (get)
localhost:8080/api/v1/admin/orders
## Delete order (delete)
localhost:8080/api/v1/admin/order/_orders_id
## Update order/process order (put)
localhost:8080/api/v1/admin/order/_orders_id
req.body
{
"status":"Delivered"
}
## Back End Deployement
[Back End] https://long-plum-ray-ring.cyclic.app/api/v1
admins Gmail : ```channa@gmail.com``` password : ```channa121```
NewDeployed Link : https://ato-z-amazon-in-clone.vercel.app/
| AtoZ is E-commercer web site were users can able buy different kind of products through online it is basically clone of Amazon.in | chakra-ui,css,expressjs,html,javascript,mongodb,nodejs,reactjs,redux,redux-thunk | 2023-01-16T14:06:39Z | 2023-07-15T07:16:32Z | null | 6 | 51 | 173 | 0 | 1 | 5 | null | null | JavaScript |
lorenzoworx/awesomeBooksEs6 | main | <a name="readme-top"></a>
<!-- TABLE OF CONTENTS -->
# ๐ Table of Contents
- [๐ About the Project](#about-project)
- [๐ Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [๐ Live Demo](#live-demo)
- [๐ป Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#triangular_flag_on_post-deployment)
- [๐ฅ Authors](#authors)
- [๐ญ Future Features](#future-features)
- [๐ค Contributing](#contributing)
- [โญ๏ธ Show your support](#support)
- [๐ Acknowledgements](#acknowledgements)
- [โ FAQ (OPTIONAL)](#faq)
- [๐ License](#license)
<!-- PROJECT DESCRIPTION -->
# ๐ [AWESOME LIBRARY] <a name="about-project"></a>
**[Awesome Library]** is a simple website that displays a list of books and allows you to add and remove books from that list.
## ๐ Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
<a href="https://www.w3.org/html/" target="_blank"><img align="center" src="https://raw.githubusercontent.com/devicons/devicon/master/icons/html5/html5-original-wordmark.svg" alt="html5" width="55" height="55"/></a><a href="https://www.w3schools.com/css/" target="_blank"><img align="center" src="https://raw.githubusercontent.com/devicons/devicon/master/icons/css3/css3-original-wordmark.svg" alt="css3" width="55" height="55"/></a><a href="https://developer.mozilla.org/en-US/docs/Web/JavaScript" target="_blank" rel="noreferrer"><img align="center" src="https://raw.githubusercontent.com/devicons/devicon/master/icons/javascript/javascript-original.svg" alt="javascript" width="55" height="55"/></a>
<!-- Features -->
### Key Features <a name="key-features"></a>
<!-- > Describe between 1-3 key features of the application.-->
- **Add book to the list**
- **Remove book from the list**
- **Wireframe UI design**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## ๐ Live Demo <a name="live-demo"></a>
For a live demo to our project, follow the link below
- [Live Demo Link](https://lorenzoworx.github.io/awesomeBooksEs6/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## ๐ป Getting Started <a name="getting-started"></a>
> Describe how a new developer could make use of your project.
To get a local copy up and running, follow these steps.
### Prerequisites
In order to run this project you need:
<!--
Example command:
```sh
gem install rails
```
-->
### Setup
- Clone this repository or download the Zip folder:
```
git clone https://github.com/lorenzoworx/awesomeBooksEs6
```
- Navigate to the location of the folder in your machine:
**``you@your-Pc-name:~$ cd <folder>``**
### Usage
- After Cloning this repo to your local machine
- Open the `index.html` in your browser
### Run tests
To track linter errors locally follow these steps:
Download all the dependencies run:
```
npm install
```
Track HTML linter errors run:
```
npx hint .
```
Track CSS linter errors run:
```
npx stylelint "**/*.{css,scss}"
```
Track JavaScript linter errors run:
```
npx eslint .
```
### Deployment
- GitHub Pages was used to deploy this website
- For more information about publishing sources, see "[About github page](https://docs.github.com/en/pages/getting-started-with-github-pages/about-github-pages#publishing-sources-for-github-pages-sites)"
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## ๐ฅ Authors <a name="authors"></a>
๐ค **Okolo Oshoke**
- GitHub: [@lorenzoworx](https://github.com/lorenzoworx)
- Twitter: [@lorenzoworx](https://twitter.com/lorenzoworx)
- LinkedIn: [Oshoke Okolo]https://www.linkedin.com/in/oshoke-okolo-665208108/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## ๐ญ Future Features <a name="future-features"></a>
- [ ] **[Navigation bar]**
- [ ] **[Use of classess]**
- [ ] **[Better style and design]**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## ๐ค Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## ๐ Acknowledgments <a name="acknowledgements"></a>
I would like to thank - [ Microverse]
****
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## โญ๏ธ Show your support <a name="support"></a>
<!-- > Write a message to encourage readers to support your project -->
Give a โญ๏ธ if you like this project!
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## ๐ License <a name="license"></a>
This project is [MIT](https://github.com/rivasbolinga/AwesomeBooks-library/blob/master/MIT.md) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| This is a books library app built using ES6, where users can browse and add books to their library.. It is a great tool for book lovers who want to keep track of their reading lists. | css,html,javascript | 2023-01-16T10:23:37Z | 2023-02-20T20:39:36Z | null | 1 | 2 | 14 | 0 | 4 | 5 | null | null | JavaScript |
mira713/medicine-hub | main | <h1 textAlign="center">Medical Hub</h1>
## Deployment
This section has moved here: vercel link [https://medical-hub-zeta.vercel.app] <br>
<hr><br>
<h3>Tech Stack<h3/>
<li>React.js</li>
<li>Redux</li>
<li>HTML</li>
<li>CSS</li>
<li>JavaScript</li>
<li>Rest API</li>
<li>Chakra UI</li>
<li>BootStarp</li>
<li>Styled Component</li>
<hr><br>
<h2>Features :</h2><br>
<li>Homepage - The landing Page</li>
<li>Login/Signup Page </li>
<li>Admin Panel</li>
<li>Product Page to browse all products based on categories</li>
<li>Sort products by prices and ratings</li>
<li>Filter products by brands and discounts</li>
<li>Pagination</li>
<li>Payment Page<li>
<li>Checkout Page</li>
<hr><br>
<h4>Homepage</h4>
<img src="medical-hub/screenshots/Screenshot (145).png" alt="homepage"/>
<P>Login form / sign-up form</p>
<img src="medical-hub/screenshots/Screenshot (147).png" alt="homepage"/>
<p>We have an admin panel in our website</P>
<img src="medical-hub/screenshots/Screenshot (148).png" alt="homepage"/>
<p>Some sort functions based on price and rating</P>
<img src="medical-hub/screenshots/s7sortFunction.png" alt="homepage"/>
<p>The main category/product page of our website</p>
<img src="medical-hub/screenshots/s6categorypage.png" alt="homepage"/>
<p>Search for the product here</p>
<img src="medical-hub/screenshots/s5search.png" alt="homepage"/>
<p>The main payment page</p>
<img src="medical-hub/screenshots/s2paymentPage.png" alt="homepage"/>
<hr>
<h4>Service Page</h4>
<h2>Team Members :</h2>
<li><a href="https://github.com/mira713">
Mitali Sinha</a>(project-lead)</li>
<li><a href="">Pallavi Jain</a></li>
<li><a href="">
Samruddhi Chavan</a></li>
<li><a href="">Priyanshu Pawar</a></li>
<li><a href="">Shreyash Birajdar</a></li>
<hr><hr>
| medicine-hub is an online medicine purchasing application that was built within a span of 5 days using react, JS, css, and npm packages redux, react router dom etc like | css,javascript,react,redux,chakra-ui | 2023-01-14T08:54:47Z | 2023-03-06T11:12:38Z | null | 6 | 12 | 82 | 0 | 0 | 5 | null | null | JavaScript |
HanaSabih/awesome-books | main | <a name="readme-top"></a>
<div align="center">
<h1><b>Awesome Books</b></h1>
</div>
<!-- TABLE OF CONTENTS -->
# ๐ Table of Contents
- [๐ About the Project](#about-project)
- [๐ Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [๐ Live Demo](#live-demo)
- [๐ป Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#triangular_flag_on_post-deployment)
- [๐ฅ Authors](#authors)
- [๐ญ Future Features](#future-features)
- [๐ค Contributing](#contributing)
- [โญ๏ธ Show your support](#support)
<!-- PROJECT DESCRIPTION -->
# ๐ Awesome Books <a name="about-project"></a>
This is a Awesome books: plain JavaScript with objects project in the Microverse program to test my understanding in different ways to create objects in JavaScript, and Create and access properties and methods of JavaScript objects.
## ๐ Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
<details>
<summary>Client</summary>
<ul>
<li>HTML</li>
<li>CSS</li>
<li>JAVASCRIPT</li>
<li>BOOTSTRAPL</li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
- **Responsive design**
- **Used only Bootstrap, CSS, html and Javascript**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## ๐ Live Demo <a name="live-demo"></a>
- [Live Demo Link](https://hanasabih.github.io/awesome-books/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## ๐ป Getting Started <a name="getting-started"></a>
To get a local copy up and running, follow these steps.
### Prerequisites
In order to run this project you need:
- A personal computer connected to the internet
- A Modern/Updated web browser
- Have a local version control like git installed on your computer and a Github account
- Code editor installed on your computer
### Setup
Clone this repository to your desired folder:
```sh
cd your-desired-folder
git clone https://github.com/HanaSabih/awesome-books.git
```
### Install
Install this project with:
```sh
cd your-desired-folder
git clone https://github.com/HanaSabih/awesome-books.git
```
### Usage
To run the project, open index.html with your preferred browser.
### Run tests
To run tests, run the following command in your `git bash` or CLI:
- `npx hint .` : for the html codebase and use of best practices.
- `npx stylelint "**/*.{css,scss}" `: for the css codebase and use of best practices. you can add.
- `npx eslint . `: for the JavaScript codebase and use of best practices.
<!-- AUTHORS -->
## ๐ฅ Authors <a name="authors"></a>
๐ค **Hana Sabih**
- GitHub: [@githubhandle](https://github.com/HanaSabih)
- LinkedIn: [LinkedIn](https://www.linkedin.com/in/hana-sabih/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## ๐ญ Future Features <a name="future-features"></a>
- [ ] **Program Page**
- [ ] **Register Page**
- [ ] **News Page**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## ๐ค Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## โญ๏ธ Show your support <a name="support"></a>
If you like this project, kindly give a โญ๏ธ.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| This website was an excellent learning experience for me because I used JavaScript to produce and manage the website, as well as OOP, modules, and local storage. The website allows visitors to see, add, and delete books from a list, as well as provide contact information. | bootstrap5,javascript,localstorage,oop | 2023-01-09T07:55:05Z | 2023-01-13T07:08:57Z | null | 2 | 3 | 20 | 1 | 0 | 5 | null | null | HTML |
sojinsamuel/InstantCopyPro | master | # InstantCopyPro
A simple web application that generates content based on a user-specified query.
## Features
- Accepts a user-specified query and generates content based on that query
- Allows the user to specify the number of words they want in the generated content
- Renders the generated content as Markdown
- Provides a slider to adjust the number of words in the generated content
- Allows the user to copy the generated content to their clipboard with a single click
- Displays a loading spinner while the content is being generated
## Technologies
This project was built with the following technologies:
* [React](https://reactjs.org/) for the frontend.
* [Marked](https://marked.js.org/) for rendering Markdown
* [RC Slider](https://www.npmjs.com/package/rc-slider) for the slider component
## Setup
1. Clone this repository to your local machine:
```
git clone https://github.com/sojinsamuel/InstantCopyPro.git
```
2. Navigate to the root directory of the project and install the dependencies:
```
npm install
```
3. Start the development server:
```
npm run dev
```
4. Open a web browser and go to http://localhost:5173 to view the application.
**NOTE:** The OpenAI API key used in this project has been removed for the purpose of preserving the privacy of the user who originally created the API key. If you wish to try out this project, you can simply replace the text `OPEN_AI_API_KEY` in the `FetchContent.js` file under `src` directory with your own API key. This will allow you to use the project as intended. Please note that an API key is necessary for the proper functioning of the project.
You can sign up for an API key at the [OpenAI Developer Portal](https://beta.openai.com/signup/)
| This web application allows you to generate content based on a user-specified query. Simply enter your desired query and choose the number of words you want in the generated content using the slider. The content will be generated and displayed as Markdown, and you can easily copy it to your clipboard with a single click. | marked,openai,react,campus-experts,javascript,openai-api,reactjs | 2023-01-06T06:38:43Z | 2023-01-06T07:10:55Z | null | 1 | 2 | 5 | 0 | 0 | 5 | null | null | JavaScript |
IndieCoderMM/math-magicians | develop | <a name="readme-top"></a>
## ๐ Table of Contents
- [๐งโโ๏ธ Math Magicians ](#๏ธ-math-magicians-)
- [๐ Built With ](#-built-with-)
- [Tech Stack ](#tech-stack-)
- [Key Features ](#key-features-)
- [๐ Live Demo ](#-live-demo-)
- [๐ป Getting Started ](#-getting-started-)
- [Prerequisites](#prerequisites)
- [Setup](#setup)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#deployment)
- [๐ง Contact ](#-contact-)
- [๐ญ Future Features ](#-future-features-)
- [๐ค Contributing ](#-contributing-)
- [๐ Show your support ](#-show-your-support-)
- [๐ Acknowledgments ](#-acknowledgments-)
- [๐ License ](#-license-)
<!-- PROJECT DESCRIPTION -->
# ๐งโโ๏ธ Math Magicians <a name="about-project"></a>
**Math Magicians** is a website that provides users with access to a calculator and a collection of math quotes.

## ๐ Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>









<!-- Features -->
### Key Features <a name="key-features"></a>
- **Make calculations**
- **Read random Math quotes**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## ๐ Live Demo <a name="live-demo"></a>
You can visit the live version of this website on [MathMagicians Webpage](https://math-magicians-oart.onrender.com/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## ๐ป Getting Started <a name="getting-started"></a>
To get a local copy up and running, follow these steps.
### Prerequisites
In order to run this project you need [Node.js](https://nodejs.org/en/) installed on your machine.
### Setup
Clone this repository to your desired folder:
```sh
cd my-folder
git clone git@github.com:IndieCoderMM/math-magicians.git
```
### Install
Install the dependencies with:
```sh
cd math-magicians
npm install
```
### Usage
To run the project, execute the following command:
```sh
npm start
```
### Run tests
To run tests, run the following command:
```sh
npm run test
```
### Deployment
You can deploy this project using:
```sh
npm run build
```
This will create a production-ready build of your website in `build/` folder, which you can use to deploy on a static site server.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## ๐ง Contact <a name="authors"></a>
I am always looking for ways to improve my project. If you have any suggestions or ideas, I would love to hear from you.
[](https://github.com/IndieCoderMM)
[](https://twitter.com/hthant_oo)
[](https://linkedin.com/in/hthantoo)
[](mailto:hthant00chk@gmail.com)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## ๐ญ Future Features <a name="future-features"></a>
- [x] **Mobile UI**
- [ ] **Add math quotes**
- [ ] **Add calculators**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## ๐ค Contributing <a name="contributing"></a>
I welcome any and all contributions to my website! If you have an idea for a new feature or have found a bug, please open an issue or submit a pull request.
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## ๐ Show your support <a name="support"></a>
If you like this project, please consider giving it a โญ.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## ๐ Acknowledgments <a name="acknowledgements"></a>
I would like to thank all code reviewers for making this project better.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## ๐ License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| A website for all math enthusiasts which provides an easy-to-use calculator and famous math quotes. Built with React. | javascript,reactjs,single-page-app,create-react-app,react-router | 2023-01-09T09:56:50Z | 2023-01-19T05:49:12Z | null | 1 | 5 | 33 | 0 | 1 | 4 | null | MIT | JavaScript |
AmolShelke2/e-commerce-website | master | # E-commerce website
An E-commerce website created with HTML CSS and JavaScript. It's Mobile friendly and
I use media quries for making it look good on every screen size
Star โญ The Repository if you love this project โค๏ธ.
# Tech stack used
As I wanted to practice my HTML CSS and basic JavaScript knowledge I used only those tools.
- HTML
- CSS
- JavaScript.
- For responsiveness I used the CSS @media queries.
- For layouts I used the CSS flexbox.
# Live Preview
[E-commerce website](https://e-commerce-website-rose-nu.vercel.app/)
# Preview
## Desktop
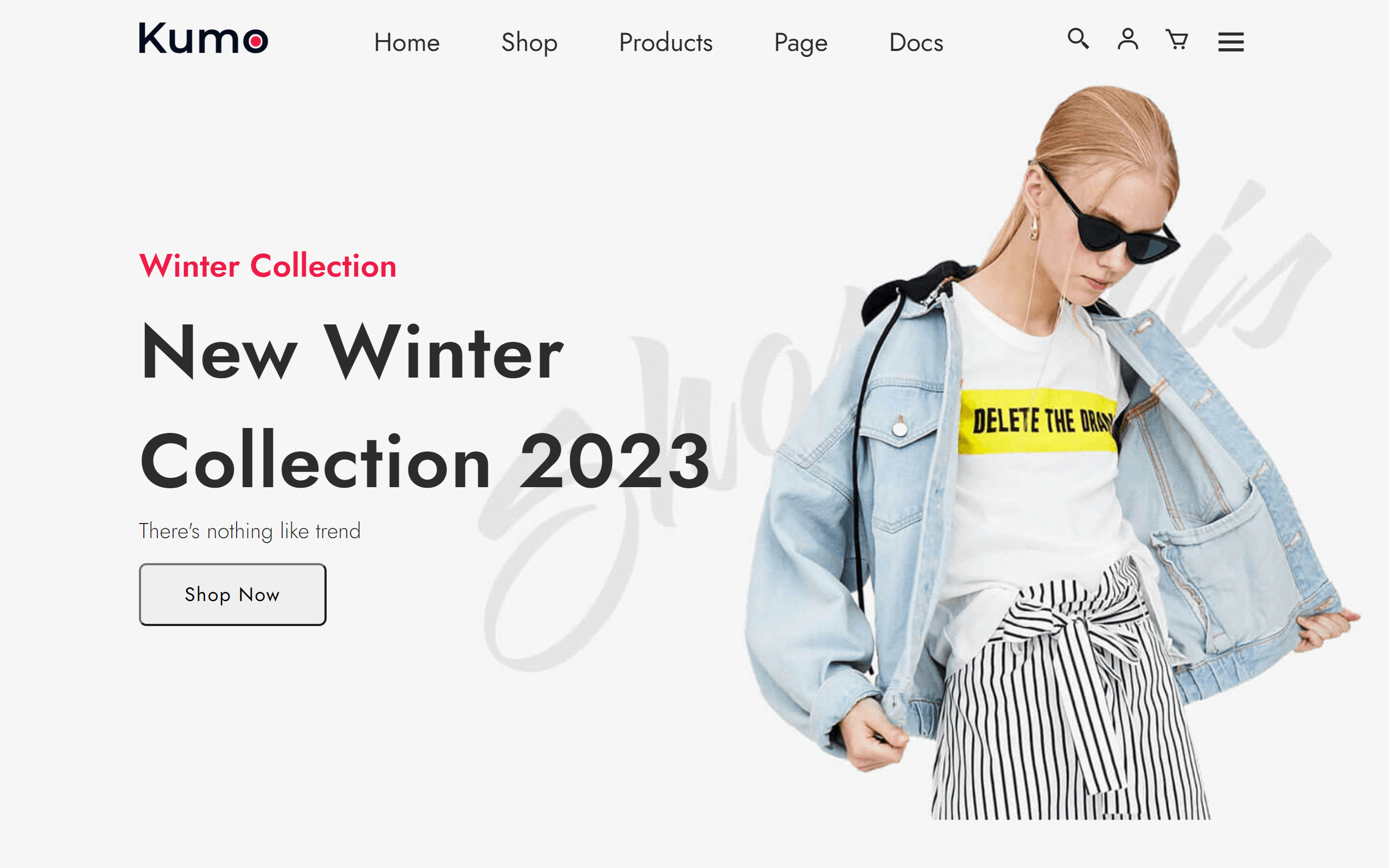
# More about Me.
I'm A self-tought Front End Developer having a good knowledge in HTML CSS and JavaScript and a basic understanding or REACT.jS
| An e-commerce website created with HTML, CSS and JavaScript. | css,css3,html,javascript | 2023-01-09T18:06:21Z | 2023-02-04T15:59:26Z | null | 1 | 0 | 63 | 0 | 0 | 4 | null | null | HTML |
AmanWorku/JS-Capstone | main | <a name="readme-top"></a>
<!-- TABLE OF CONTENTS -->
# ๐ Table of Contents
- [๐ Table of Contents](#-table-of-contents)
- [๐ป PixelBros ](#-pixelbros-)
- [๐ Built With ](#-built-with-)
- [Tech Stack ](#tech-stack-)
- [Key Features ](#key-features-)
- [๐ Live Demo ](#-live-demo-)
- [๐ป Getting Started ](#-getting-started-)
- [Prerequisites](#prerequisites)
- [Setup](#setup)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#deployment)
- [๐ฅ Authors ](#-authors-)
- [๐ค Contributing ](#-contributing-)
- [๐ Show your support ](#-show-your-support-)
- [๐ Acknowledgments ](#-acknowledgments-)
- [๐ License ](#-license-)
<!-- PROJECT DESCRIPTION -->
# ๐ป PixelBros <a name="about-project"></a>
**PixelBros** is a capstone project made using HTML, CSS and JavaScript at the end of Module 2 during our study in Microverse.
In this website, users can view all the retro game characters,and add likes/comments on their favourite ones.
## ๐ Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
- HTML, CSS, JavaScript
- [Amiibo API](https://www.amiiboapi.com/): API for characters data
- [Involvement API](https://www.notion.so/Involvement-API-869e60b5ad104603aa6db59e08150270): API for user likes and comments
- Webpack: Module bundler
- Jest: Unit testing
- GitHub Project: KanBan Board for tracking the progress
<!-- Features -->
### Key Features <a name="key-features"></a>
- **Browse through all of the different retro game characters**
- **Like and comment on your favourite characters**
- **View other users' likes and comments**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## ๐ Live Demo <a name="live-demo"></a>
You can visit the [Live Demo Website](https://indiecodermm.github.io/js-capstone/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## ๐ป Getting Started <a name="getting-started"></a>
To get a local copy up and running, follow these steps.
### Prerequisites
In order to run this project you need:
- [Node.js](https://nodejs.org/en/)
### Setup
Clone this repository to your desired folder:
```sh
cd my-project
git clone https://github.com/IndieCoderMM/js-capstone.git .
```
### Install
Install the dependencies with:
```sh
npm install
```
### Usage
To run the project, execute the following command:
```sh
npm start
```
### Run tests
To run tests, run the following command:
```sh
npm run test
```
### Deployment
You can deploy this project using:
```sh
npm run build
```
This will create a production ready build for website in the `dist` directory. You can then deploy this directory on the GitHub Pages.
<!-- AUTHORS -->
## ๐ฅ Authors <a name="authors"></a>
๐ค **Hein Thant**
- GitHub: [@IndieCoderMM](https://github.com/IndieCoderMM)
- Twitter: [@hThantO](https://twitter.com/hThantO)
- LinkedIn: [hthantoo](https://www.linkedin.com/in/hthantoo)
๐ค **Amanuel Worku**
- GitHub: [@AmanWorku](https://github.com/AmanWorku)
- Twitter: [@Amexworku](https://twitter.com/Amexworku)
- LinkedIn: [LinkedIn](https://www.linkedin.com/in/amanuel-worku-844903213/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## ๐ค Contributing <a name="contributing"></a>
We welcome any contributions to our website! If you have an idea for a new feature or have found a bug, please open an issue or submit a pull request.
Feel free to check the [issues page](https://github.com/IndieCoderMM/js-capstone/issues).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## ๐ Show your support <a name="support"></a>
If you like this project, please consider giving it a โญ. We really appreciate your support. Thank you!
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## ๐ Acknowledgments <a name="acknowledgements"></a>
I would like to thank Microverse for giving us this opportunity to work together and work on a project to understand what we have learned.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## ๐ License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| PixelBros is a capstone project made using HTML, CSS and JavaScript at the end of Module 2 during our study in Microverse. In this website, users can view all the retro game characters, and add likes/comments on their favorite ones. | javascript | 2023-01-09T09:14:12Z | 2023-01-05T14:26:42Z | null | 2 | 0 | 55 | 0 | 0 | 4 | null | MIT | JavaScript |
Chrystian-Calderon/Challenge_Encriptador | master | # Challenge-Encriptador
Es un encriptador y desencriptado para el challenge de One, esta hecho con html, css y javascript
Por otra parte tambien implemente un modo oscuro para la pagina.
Este proyecto no sรฉ si estoy usando bien los estilos o funciones pero voy aprendiendo.
| Es un encriptador y desencriptado para el challenge de One, esta hecho con html, css y javascript | challengeonecodificador4,css,html,javascript | 2023-01-09T15:22:21Z | 2023-01-13T20:00:40Z | null | 1 | 0 | 9 | 0 | 0 | 4 | null | null | JavaScript |
Ejajkhan613/Yummy-Pizza | main | # Yummy Pizza ๐
- This project is an online pizza ordering platform where users can register, log in, and order pizza online. The application allows users to browse different types of pizza, add them to their cart, and place an order.
## Developer Details ##
Name => Ejajul Ansari
Linkedin => www.linkedin.com/in/ejajul-ansari
## Project Details ##
Project Code = star-theory-7179
Project Name = Yummy Pizza
Project Backend Link = https://nice-outfit-tuna.cyclic.app/
Project Frontend Link = https://yummypizza-delivering-smile.netlify.app/
# Tech Stacks
- HTML
- CSS
- JavaScript
- MongoDB
- ExpressJS
- NodeJS
- MongooseJS
## Demo
# Signup Page
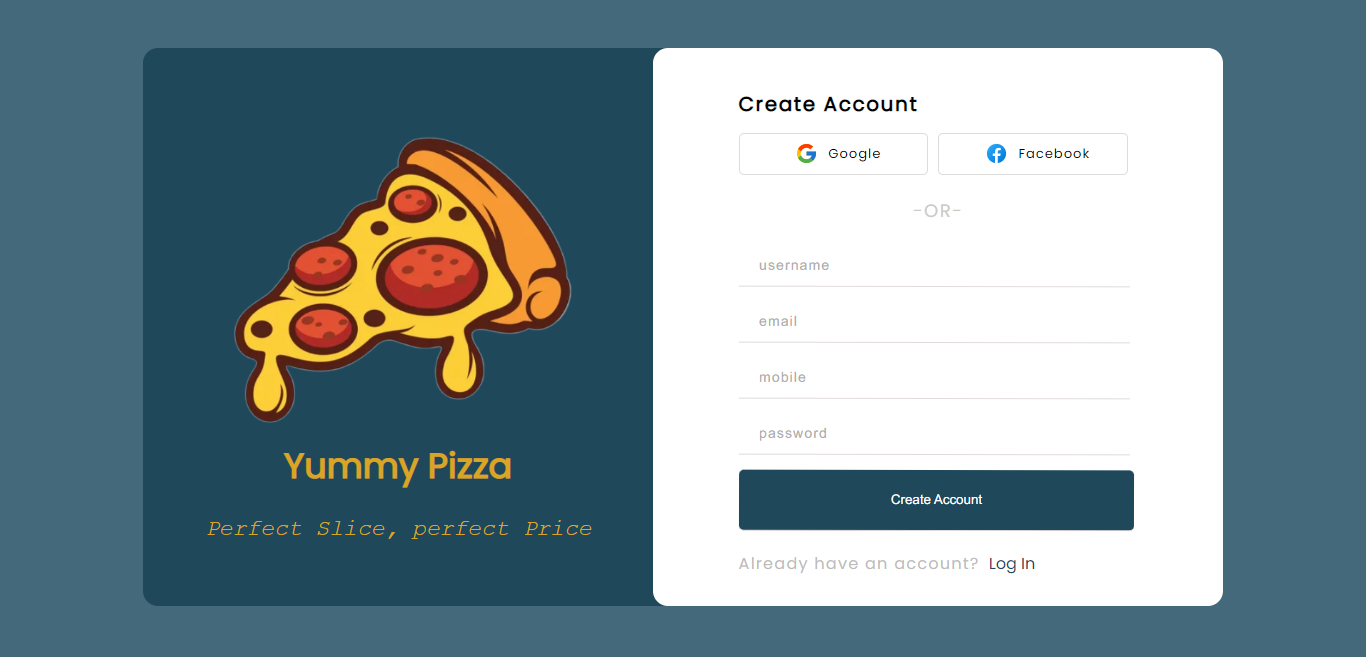
# Homepage
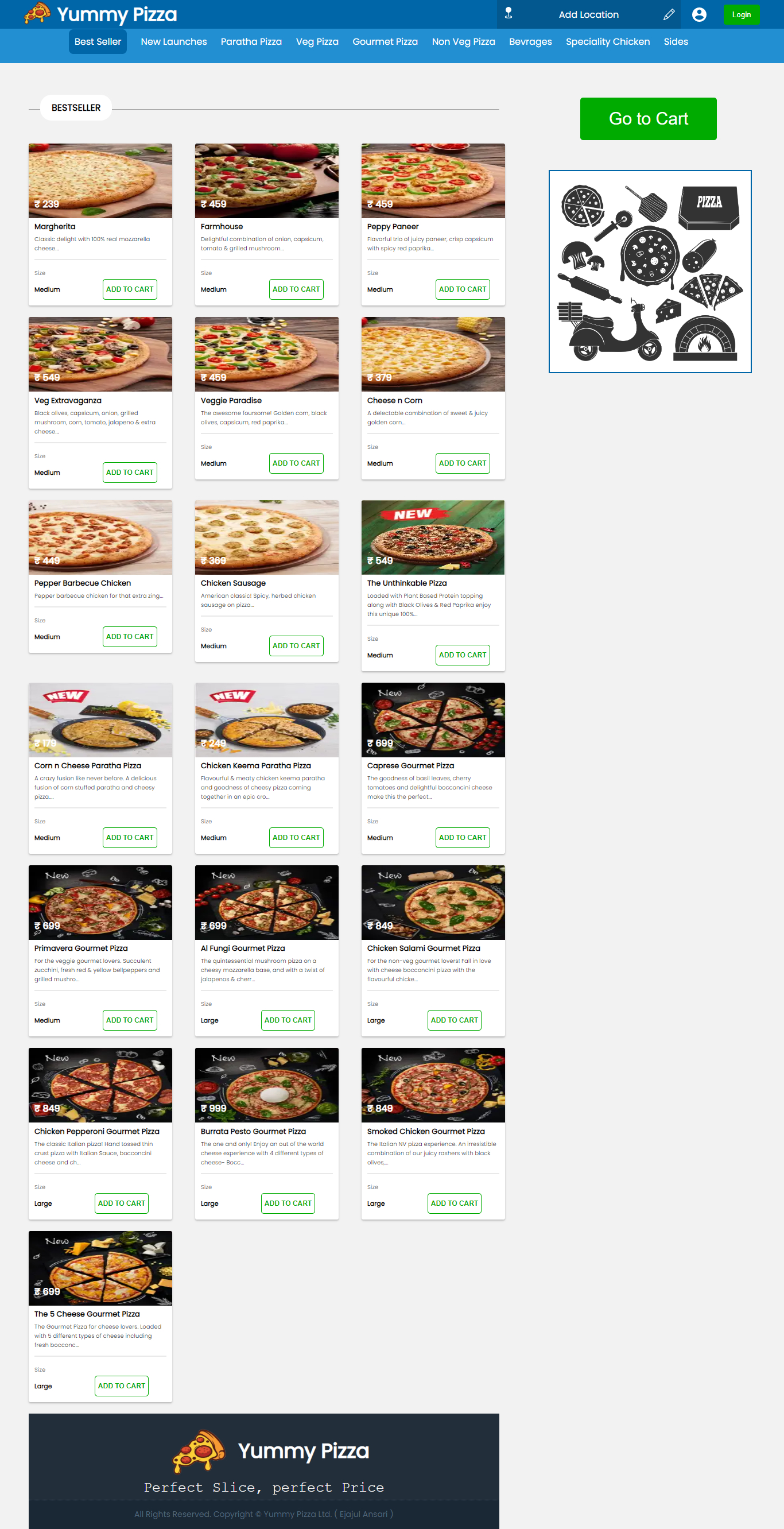
# Cart Page
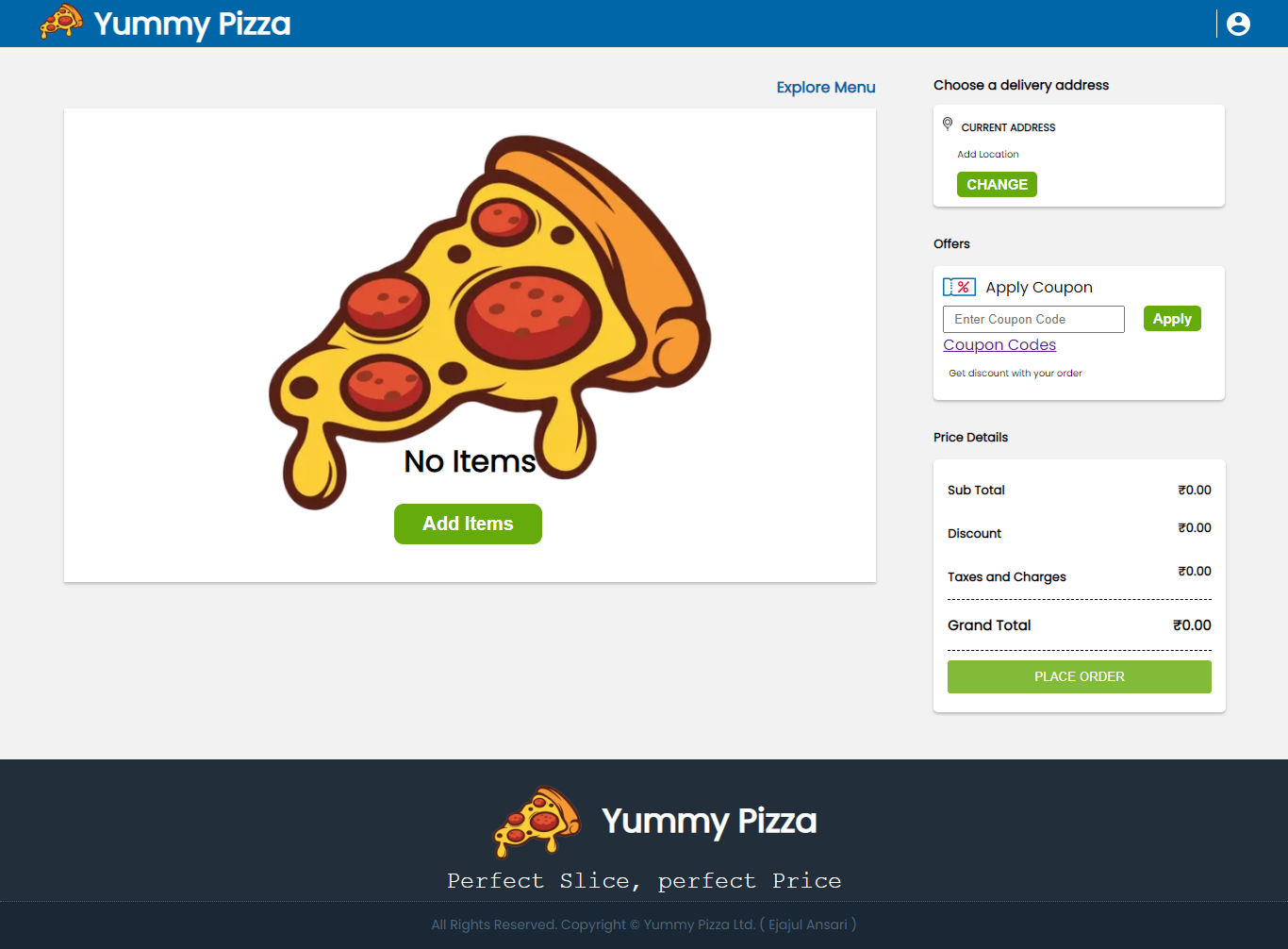
- These images are for Project
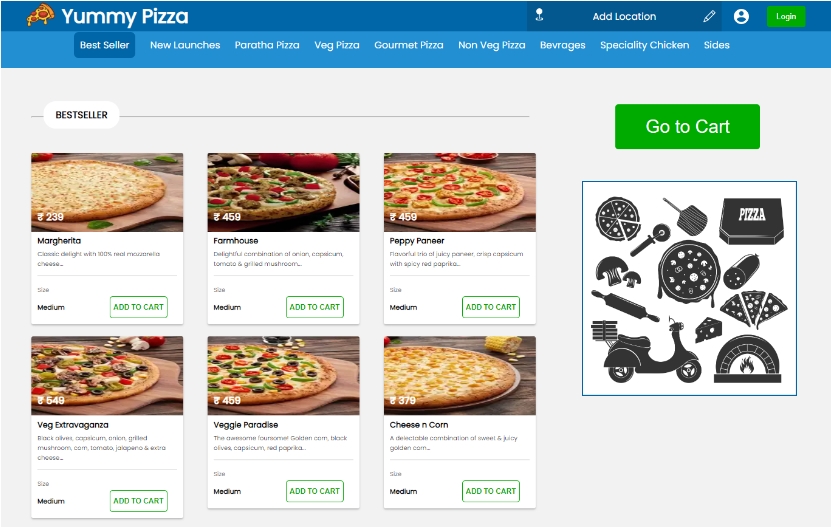
- Short Video
https://user-images.githubusercontent.com/112761880/216759468-65007752-10cf-4dbe-8cf3-89969df903da.mp4
| This is an online pizza ordering platform where users can register, log in, and order pizza online, users can also view their order history. this platform provides a convenient way for customers to keep track of their past purchases | nodejs,expressjs,mongodb-atlas,mongoosejs,css,html,javascript,nodemailer | 2023-01-14T07:14:03Z | 2023-05-25T07:10:28Z | null | 1 | 4 | 27 | 0 | 0 | 4 | null | null | JavaScript |
hayaabduljabbar/Responsive-Portfolio-Website | master | null | Reponsive Portfolio Website Using HTML, CSS, Bootstrap & JavaScript. | html,css,bootstrap,javascript,responsive-website | 2023-01-14T10:43:38Z | 2023-01-14T11:12:57Z | null | 1 | 0 | 1 | 0 | 0 | 4 | null | null | HTML |
imukeshkaushal/relianceDigital_clone | main | ## Project Name - adventurous-sweater-7809 (Digital Xpress)
Digital Xpress is ECommerce Website & is a clone of Reliance Digital. It is used for buying the electrical products, Home Appliances & other kind of products. Digital Express Digital Stores are bigger in size than the other format Digital Xpress Mini Stores. It is one of the largest electronics retailers in India with over 5,000 products in its inventory.
## Deployed Link
Visit :https://reliance-digital-beryl.vercel.app/
## Responsive for all screen sizes
## Tech Stack Used
Digital Express webiste looks very amazing and introduced with various type of languages like JavaScript, HTML, CSS etc. There are the some important details regarding with this project which is mentioned below:
<ul dir="auto">
<ol dir="auto">โ JavaScript : 94.6%</ol>
<ol dir="auto">โ HTML : 0.5%</ol>
<ol dir="auto">โ CSS : 4.9%</ol>
</ul>
## Functionalities
<ul dir="auto">
<ol dir="auto">1. Cart Functionality (like adding an item and removing) </ol>
<ol dir="auto">2. Admin Page</ol>
<ol dir="auto">3. Admin can add a product Will be shown on the latest page </ol>
<ol dir="auto">4. Login and Sign Up </ol>
</ul>
## Admin Account Details(Admin Email and Password)
<p>you can add products by admin account and all the users can see the product on latest page(which contain all the latest items)</p>
<ul dir="auto">
<ol dir="auto">Admin Email - eve.holt@reqres.in </ol>
<ol dir="auto">Password - Citysilicka</ol>
</ul>
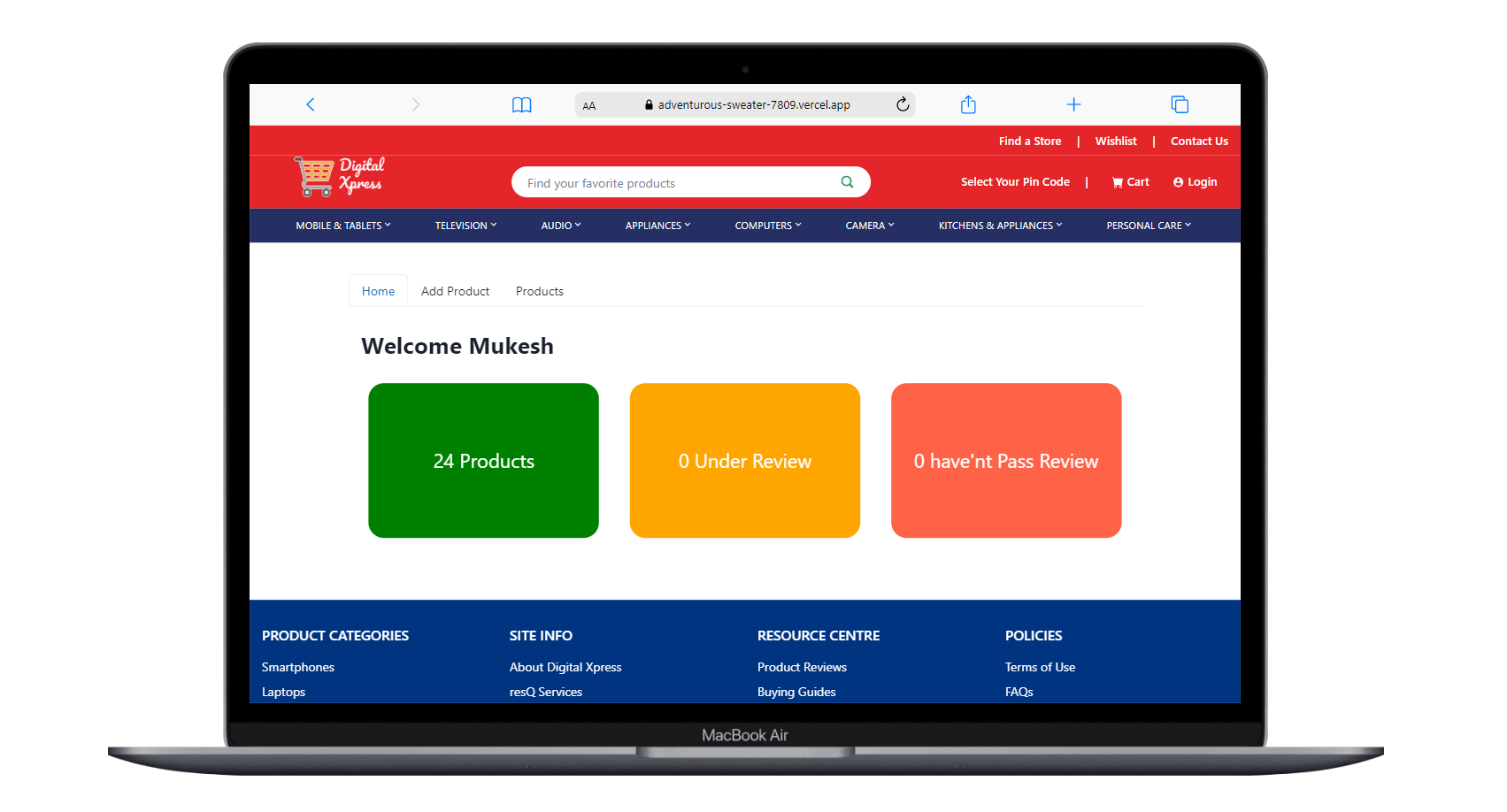
This report is provided by the Github language used stats. So, this is the total percentage of the coding languages. Now we explain our project in details.
## Features & Specifications
### Homepage
Homepage is one of the most important factor which also known by the name of Landing Page. There are different kinds of the Products and populer services are mentioned here. Take a look on it.
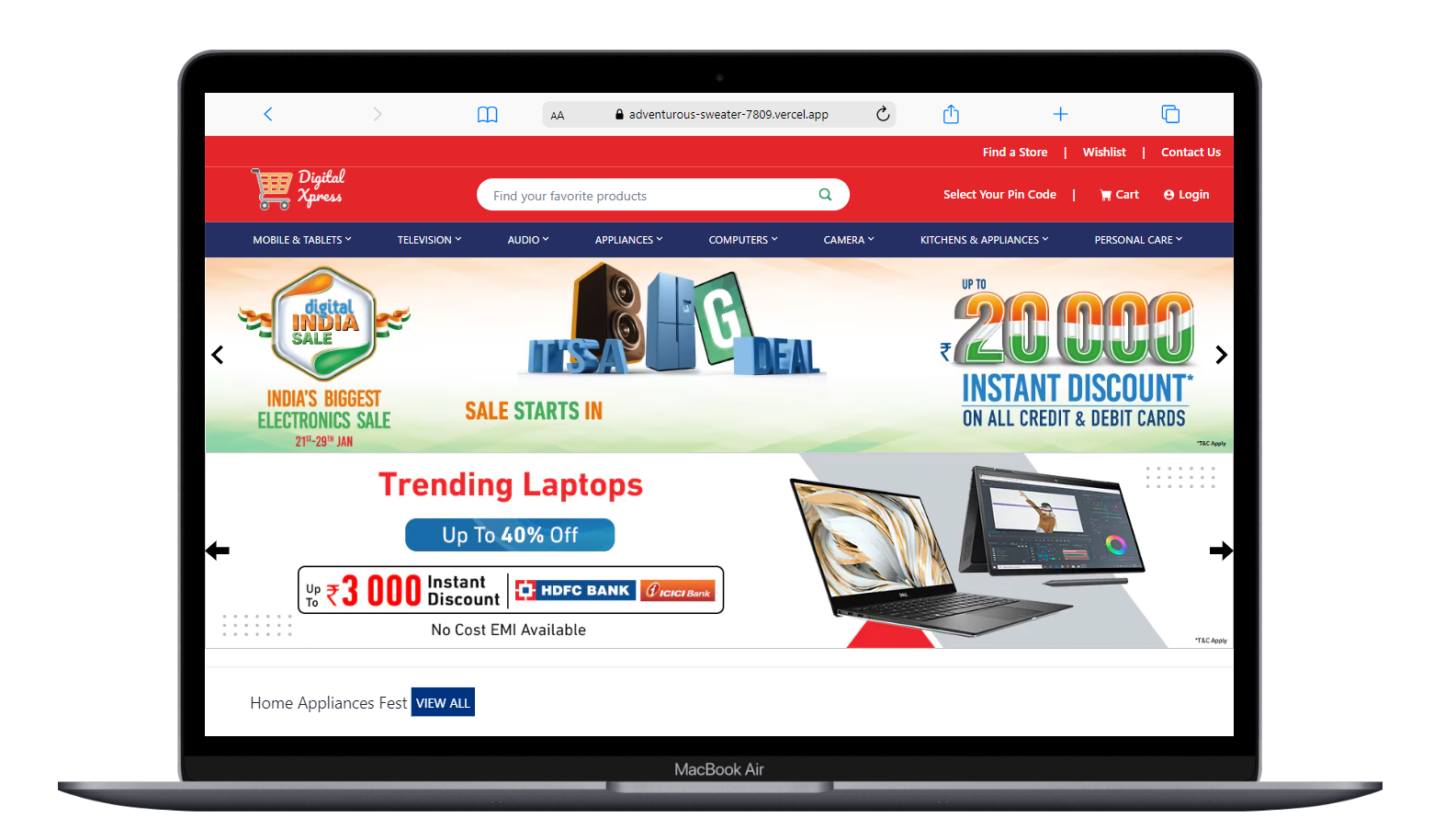
### Small Screen :
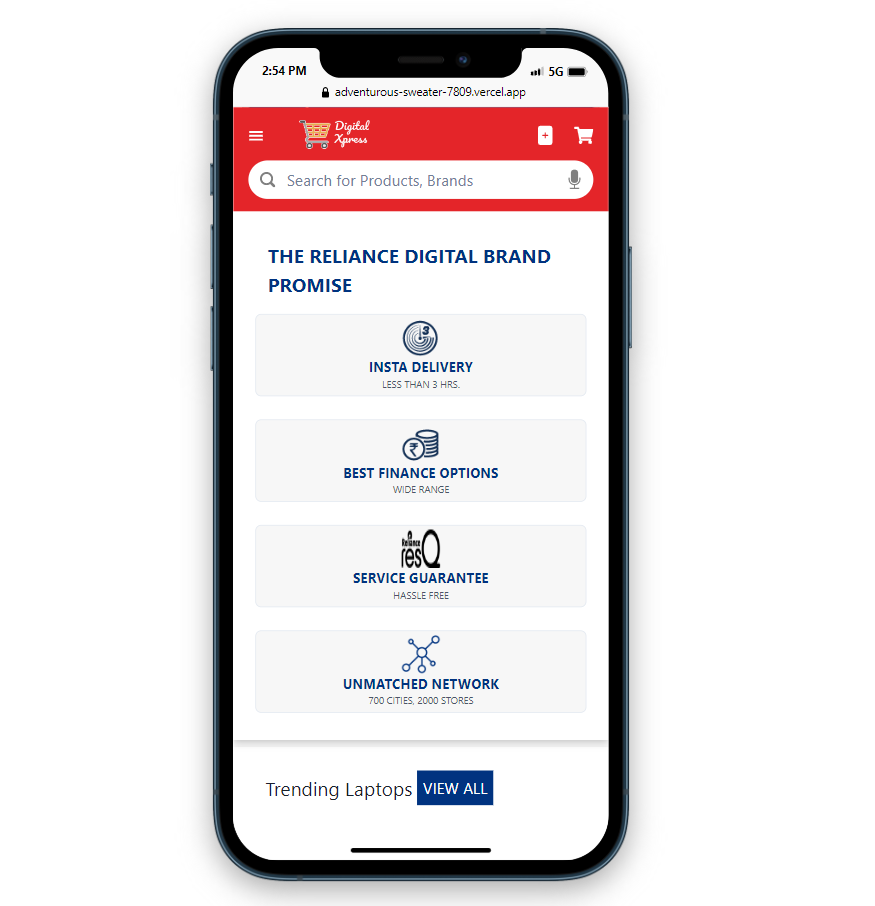
### Navbar
Navigation Bar is the topmost layer or bar of our website. In this bar, you find the various types of the menus like Mobile & Tablets , Television, Audio, Camera, Home Appliances, Accessories, Personal Care etc.
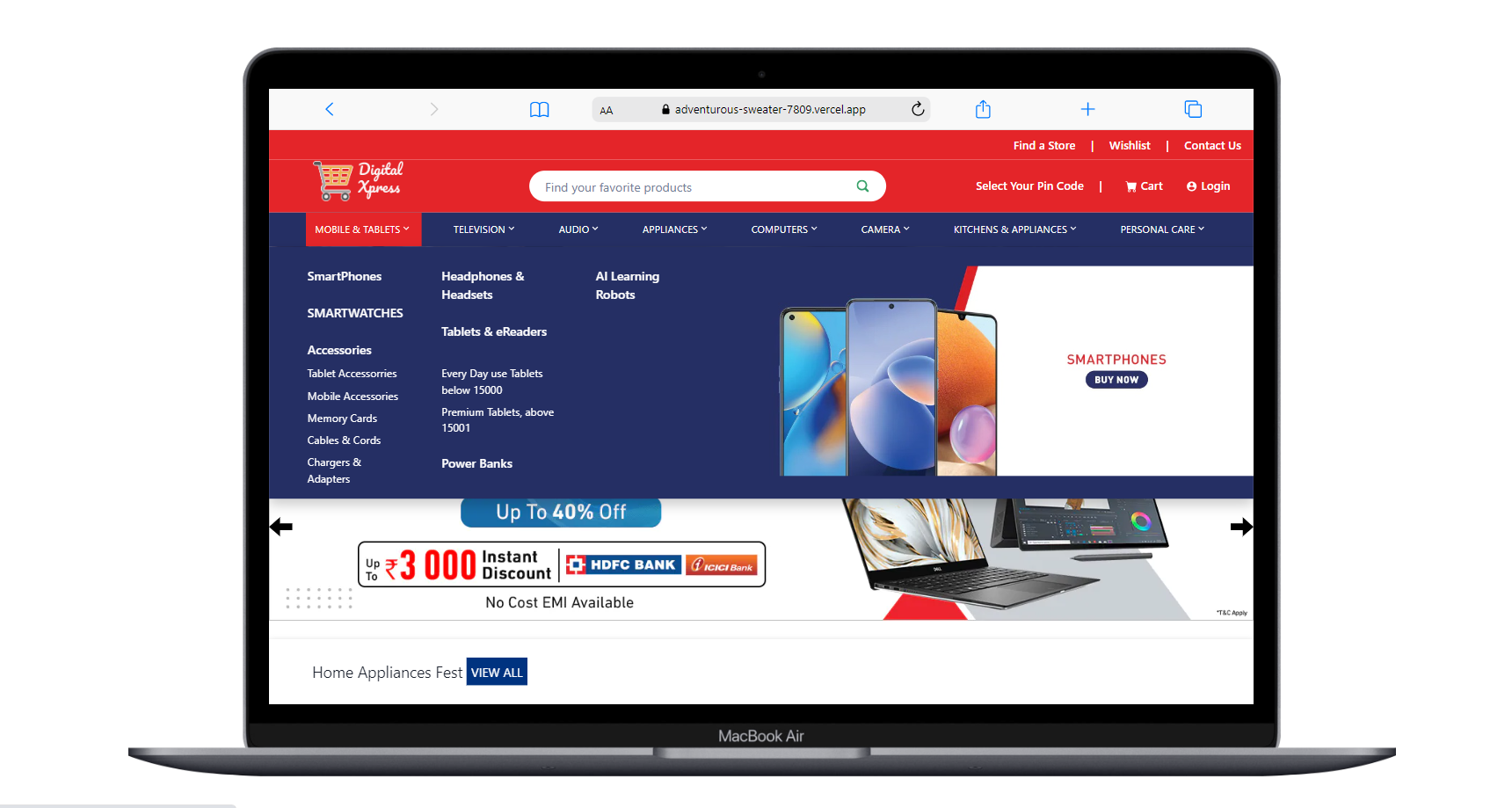
### Small Screen :
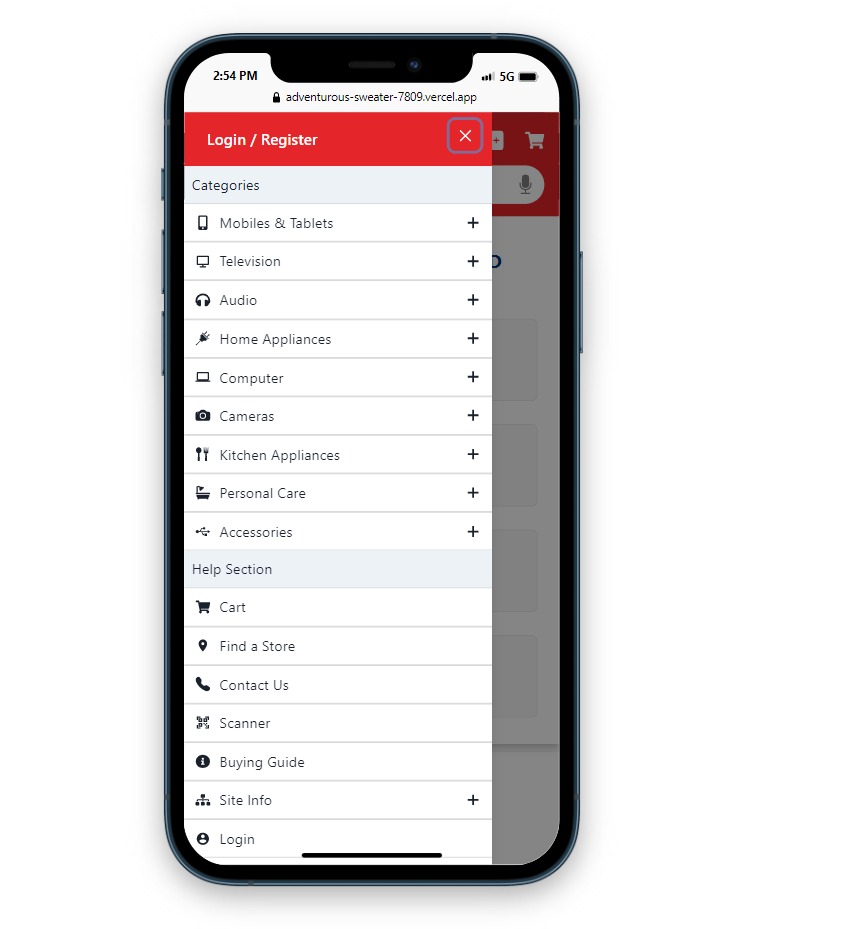
It is a cart Page where you find the different kinds of the products which products you added in your cart. Take a look on that.
## Product Page
In this section have different kinds of the products & various categories products etc. You easily find the product which you have to need.
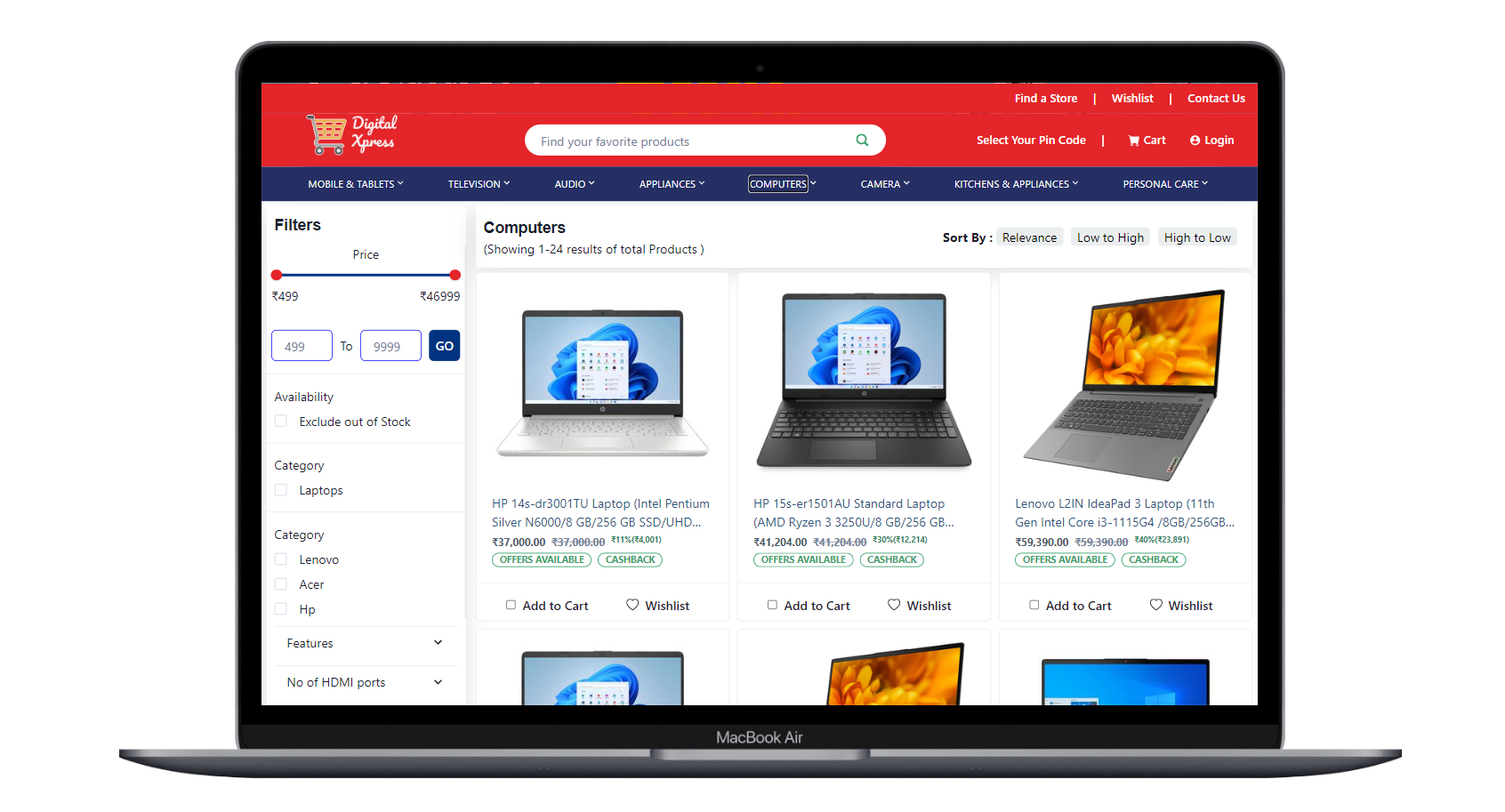
## Cart Page
Cart Page have all that product which you want to buy. In Product Page you have to click on add to cart. Product will added in cart Page. A cart page is an essential part of an e-commerce website. It is the page where users can pile up what they want to buy from the website and then simply checkout by paying online. To comprehend what a cart page does, think of it as a normal shopping cart in a store.
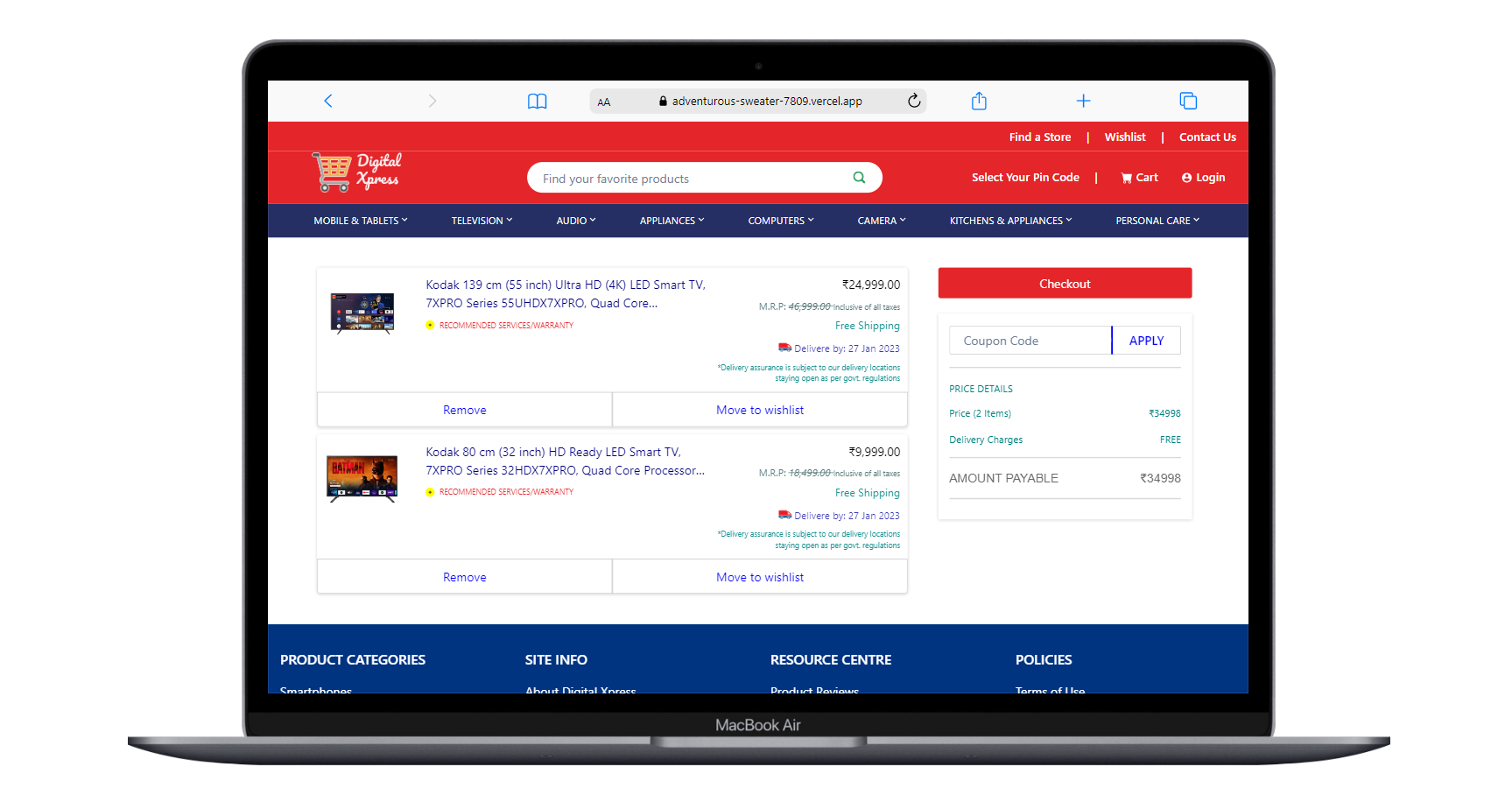
## Footer
As you know that every website have the header and footer. Also in this this website, we have a pritty footer which helps you to move the different kinds of the important pages.
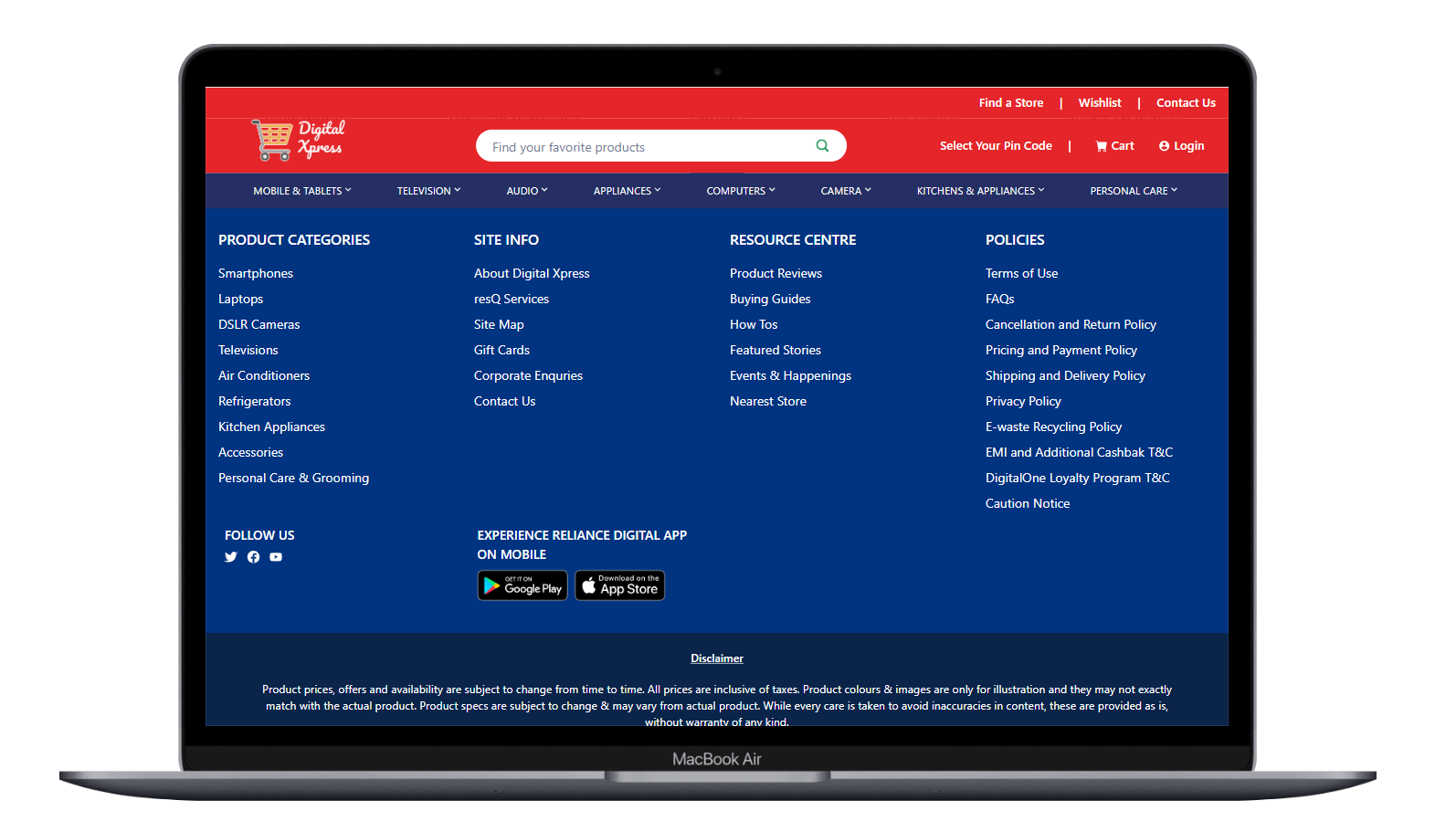
So, this is the footer of our website clone. All the things in quite easy to use as well as mobile, teblet and desktop. We hope you like these things.
## Note
This website is fully mobile friendly. You can use this website in mobile, tablet either desktop as well as. You feels very nice when you are using this website.
Things Used
We using different kinds of the technologies to make this website. These things are as follows:
- Javascript, React, Readux, Chakra UI, CSS, HTML etc.
## Contributers
Contributions are what make the open source community such an amazing place to learn, inspire, and create. Any contributions you make are greatly appreciated.
- [x] Abha Meshram - [GitHub Profile](https://github.com/abha2510)
- [x] Mukesh Kaushal - [GitHub Profile](https://github.com/imukeshkaushal)
- [x] Rajat Agrawal - [GitHub Profile](https://github.com/agrawalrajat310)
- [x] Tushit Saxena - [GitHub Profile](https://github.com/tushit99)
- [x] Brajmohan Verma - [GitHub Profile](https://github.com/vermabraj)
| Reliance Digital is one of the leading online shopping websites in India for electronic products, home & kitchen appliances etc. | chakra-ui,javascript,react,react-router,redux,redux-thunk | 2023-01-15T06:52:51Z | 2023-05-11T11:15:28Z | null | 7 | 40 | 140 | 0 | 2 | 4 | null | null | JavaScript |
gherrada22/PokeDex | main | <h3 align="center">
<img src="https://veekun.com/dex/media/pokemon/global-link/1.png" width="100" alt="Logo"/><br/>
<img src="https://veekun.com/dex/media/pokemon/global-link/1.png" height="30" width="0px"/>
PokeDex
<img src="https://veekun.com/dex/media/pokemon/global-link/1.png" height="30" width="0px"/>
</h3>
<p align="center">
<img src="https://user-images.githubusercontent.com/104341274/210186277-0d434bb0-80c0-43a9-b6b0-2e42e18c31a9.png" width="400" />
</p>
<p align="Center">
Welcome to Pokedex, your perfect companion to discover everything about pokemon!
Our project offers you a complete search for pokemon and their abilities, all developed with <a href="https://es.wikipedia.org/wiki/JavaScript"><b>JavaScript</b></a>, <a href="https://es.wikipedia.org/wiki/HTML5"><b>HTML5</b></a> and <a href="https://es.wikipedia.org/wiki/CSS"><b>CSS3</b></a> programming languages.
Don't get left behind in the pokemon world and start your adventure with Pokedex today!
<p>
## ๐ฝ๏ธ Demo
https://user-images.githubusercontent.com/104341274/210186251-9156a767-9169-49d4-80f8-943f0083959f.mp4
## ๐ง Installation instructions
1. Download the source code of the project to your local machine.
2. Open the index.html file in your browser to start the application.
## ๐พ Use
- Type the name of a pokemon in the search box.
- Click the "Search" button to perform the search.
- The pokemon's information will appear on the screen.
## ๐ Contributions
If you wish to contribute to the project, follow these steps:
1. Make a fork of the repository.
2. Create a branch for your contribution.
3. Commit your changes and push the branch to your fork repository.
4. Create a pull request from your repository to this main repository.
## โค๏ธCredits
- [PokeAPI](https://pokeapi.co/)
<p align="center">
<img src="https://user-images.githubusercontent.com/104341274/210186277-0d434bb0-80c0-43a9-b6b0-2e42e18c31a9.png" width="400" />
</p>
</p>
<p align="center">Copyright © 2023-present <a href="https://github.com/gherrada22" target="_blank">George Herrada Farfรกn</a>
| A web application that allows you to search for pokemon and view their corresponding information through a request to the pokemon API. | api,css3,html5,javascript | 2023-01-01T22:16:26Z | 2023-02-11T07:27:11Z | null | 1 | 0 | 5 | 0 | 2 | 4 | null | null | JavaScript |
Amanmandal-M/India-Mart-Clone | main | # haloed-ground-8588
Cloning <> India Mart <> Website
Project Name : HindBazaar
Project Logo : <a href="https://wonderful-tulumba-b2af93.netlify.app/Resources/Logo.png" target="_blank" >Project Logo</a>
**************************
<br>
**Backend Flow**
Deployed Link : `https://hindbazaar-mandal.up.railway.app`
<br>
<br>
*Tech Stacks*
<ul>
<li> <b>Nodejs</b></li>
<li> <b>Expressjs</b></li>
<li> <b>MongoDB</b></li>
</ul>
**Deployment Tools** <br>
<li><i>Railway</i></li>
-------------------------
**Api End Points**
<b>Default Endpoint For Products</b> : `/products`
Bricks Products
<b>Data of all Bricks</b> = `/BricksData`
<b>Post Data of all Excavator</b> = `/BricksPost`
<b>Get Data by Id</b> = `/Bricks/:id`
<b>Get Data by limits</b> = `/Brlimit?limit=`
<b>Get Data by Title</b>
= `/Brtitle?q=`
<b>Get Data of Price by Asc to Desc</b> = `/Brasctodesc`
<b>Get Data of Price by Desc to Asc</b> = `/Brdesctoasc`
Excavator Products
<b>Data of all Excavator</b> = `/ExcavatorData`
<b>Post Data of all Bricks</b> = `/ExcavatorPost`
<b>Get Data by Id</b> = `/Excavator/:id`
<b>Get Data by limit</b> = `/Exlimit?limit=`
<b>Get Data by Title</b> = `/Extitle?q=`
<b>Get Data of Price by Asc to Desc</b> = `/Exasctodesc`
<b>Get Data of Price by Desc to Asc</b> = `/Exdesctoasc`
--------------------------
**Frontend Flow**
Deployed Link : `https://wonderful-tulumba-b2af93.netlify.app`
<br>
<br>
*Tech Stacks*
<ul>
<li> <b>HTML</b></li>
<li> <b>CSS</b></li>
<li> <b>JAVASCRIPT</b></li>
</ul>
<br>
<i>Pages of Frontend</i>
<br>
<ul>
<li> Homepage</li>
<li> Product Page</li>
<li> Cart Page</li>
<li> Admin Page</li>
</ul>
<br>
** Logo **
<br>
<img width="40%" style="border-radius:100px" src="https://hind-bazaar.netlify.app/Resources/Logo.png" alt="Error">
<br>
<br>
** Images of Website **
<br>
** Homepage **
<br>
<img src="Frontend/Resources/first.png" alt="Error">
<br>
** Sign Up Page **
<br>
<img src="Frontend/Resources/second.png" alt="Error">
<br>
** Login Page **
<br>
<img src="Frontend/Resources/third.png" alt="Error">
<br>
** Product Page **
<br>
<img src="Frontend/Resources/fourth.png" alt="Error">
<br>
** Admin Page **
<br>
<img src="Frontend/Resources/fifth.png" alt="Error">
<br>
** Admin Crud Page **
<br>
<img src="Frontend/Resources/sixth.png" alt="Error">
| Hindmart is a clone of Indiamart. It is an Indian e-commerce website that provides B2C, B2B and customer to customer sales services via its web portal. | bcrypt,css,expressjs,html,javascript,jsonwebtoken,mongodb,mongoose,nodejs | 2023-01-16T19:42:23Z | 2023-07-19T20:27:16Z | null | 2 | 15 | 158 | 0 | 2 | 4 | null | null | HTML |
rachnarajaka040/PharmEasy_clone | main | # Pharmeasy Clone ๐๐ฉบ๐ฉน
https://myfitnesspal-clone.netlify.app/
This project is a clone of the popular online pharmacy website and mobile app, Pharmeasy. The goal of this project was to create a similar tool for ordering medicines and healthcare products online, as well as offering features for users to connect with doctors and book appointments.
## Technologies Used ๐งโ๐ป
* JavaScript
* HTML
* CSS
* Reactjs
* Redux
* REST API
## Features โจ
* User registration and login
* Ordering of medicines and healthcare products
* Order tracking feature
## Installation ๐ป
To run this project on your local machine, follow these steps:
1. Clone the repository using git clone https://github.com/your-username/fitnesspal-clone.git
2. Install dependencies using npm install
3. Start the development server using npm start
## Usage ๐ป
This project has been deployed on Netlify and can be accessed using this link: (https://pharmeasy-pt-web-08b.netlify.app/). From there, you can register as a new user or log in to an existing account. Once logged in, you can start ordering medicines and healthcare products, as well as connecting with doctors and booking appointments. You can also track the status of your orders.
## Team
- [Rachna Rajak](https://github.com/rachnarajaka040)
- [Ramanpreet Singh](https://github.com/Ramanpreet4718)
- [Suhail-K](https://github.com/SUHAIL-K)
- [Rajnesh Kumar](https://github.com/rajyadav0001)
- [Ankit Kumar](https://github.com/anandankitkumar1)
## Please watch the demo
https://user-images.githubusercontent.com/113717402/231686157-3ce3e23a-46c2-4205-9989-82620b603891.mp4
| PharmEasy is an Indian e-pharmacy company that operates as an online platform for purchasing medicines, diagnostics, and healthcare products. It provides a convenient and reliable solution for customers to order medicines from the comfort of their homes, saving them time and effort. | pharmacy,css3,html5,javascript | 2023-01-04T14:09:24Z | 2023-04-13T07:30:52Z | null | 6 | 15 | 45 | 0 | 3 | 4 | null | null | HTML |
RajlaxmiMeshram/Github-Bot | main | # github-bot | null | gitbot,javascript | 2023-01-11T05:05:40Z | 2023-12-24T19:55:26Z | null | 1 | 0 | 296 | 0 | 0 | 4 | null | null | JavaScript |
jacobhumston/sxcu.api | main | <img src="https://raw.githubusercontent.com/jacobhumston/sxcu.api/banner/new-banner.png" alt="sxcu.api">
# sxcu.api
Node.js library to interact with the sxcu.net API. _([A cli is also included!](https://github.com/jacobhumston/sxcu.api/blob/main/src/cli/README.md))_
sxcu.api was created to allow any developer of any skill set to easily create images, links, etc on sxcu.net without the hassle of learning the sxcu.net documentation directly.
> This module has **0** dependencies. _(excluding dev)_ [Check for yourself!](https://github.com/jacobhumston/sxcu.api/blob/main/package.json)
## Links
**sxcu.api:** [Docs](https://sxcu.api.lovelyjacob.com) / [Github](https://github.com/Lovely-Experiences/sxcu.api) / [npm](https://www.npmjs.com/package/sxcu.api)
**sxcu.net:** [Website](https://sxcu.net/) / [API Docs](https://sxcu.net/api/docs/) / [Discord](https://discord.gg/ZBcYQwMWTG) / [Donate](https://paypal.me/MisterFix)
## Updating to v2.0.0 from v1.x.x
The guide for doing so can be found [here](https://sxcu.api.lovelyjacob.com/guides/updating-to-v2.html).
## Installation
You can install sxcu.api using npm.
```bash
npm install sxcu.api
```
## Example Usage
Here is an example of uploading a file.
```js
// Import the package.
import * as sxcu from 'sxcu.api';
// Set the request user agent to the default.
// This will be done for you on the first request if you don't do it yourself.
sxcu.UserAgent.useDefault();
// Upload the file and log the response.
sxcu.uploadFile('image.png')
.then((response) => console.log(response))
.catch((err) => console.log(err));
```
In `v2.0.0` we made the switch to ESM and TypeScript. **However, we still support CommonJS.**
```js
// Use CommonJS's require method.
const { uploadFile, UserAgent, categorizeImports } = require('sxcu.api');
UserAgent.useDefault();
uploadFile('your-img')
.then((response) => console.log(response))
.catch((err) => console.log(err));
```
If you preferred categorized imports, then you can use `categorizeImports`. This works with ESM and CommonJS.
```js
// Import the package.
import { categorizeImports } from 'sxcu.api';
// Categorize imports.
const sxcu = categorizeImports();
// Set the request user agent to the default.
sxcu.userAgent.useDefault();
// Upload the file and log the response.
sxcu.files
.uploadFile('image.png')
.then((response) => console.log(response))
.catch((err) => console.log(err));
```
Respecting rate limits has been made extremely easy, all you need to do is enable the request queue.
```js
// Import methods.
import { toggleRequestQueue } from 'sxcu.api';
// Enable the request queue.
toggleRequestQueue(true, true);
```
Need to make a custom request? It's pretty simple as well. The request method allows you to make requests that respect rate limits as well (as long as `toggleRequestQueue` is used beforehand).
```js
// Import methods.
import { request, toggleRequestQueue } from 'sxcu.api';
// Enable the request queue.
toggleRequestQueue(true, true);
// Create your own request.
const response = await request({
type: 'GET',
statusErrors: [400, 429],
baseUrl: 'https://sxcu.net/api/',
path: `files/abc123`,
}).catch((error) => {
throw resolveError(error);
});
```
## Contributors
<!-- readme: collaborators,contributors,jacobhumston-school/- -start -->
<table>
<tr>
<td align="center">
<a href="https://github.com/jacobhumston">
<img src="https://avatars.githubusercontent.com/u/57332486?v=4" width="100;" alt="jacobhumston"/>
<br />
<sub><b>Jacob Humston</b></sub>
</a>
</td></tr>
</table>
<!-- readme: collaborators,contributors,jacobhumston-school/- -end -->
| Node.js library to interact with the sxcu.net API. | javascript,library,node,nodejs,npm,sxcu | 2023-01-14T05:46:33Z | 2024-04-19T22:43:13Z | 2024-04-19T22:43:13Z | 1 | 12 | 327 | 0 | 0 | 4 | null | MIT | TypeScript |
MNisarAli/Leaderboard | development | <a name="readme-top"></a>
<div align="center">
<img src="./src/favicon.ico" alt="logo" width="140" height="auto" />
<br/>
<h1><b>Leaderboard</b></h1>
</div>
# ๐ Table of Contents
- [๐ Table of Contents](#-table-of-contents)
- [๐ Leaderboard ](#-leaderboard-)
- [๐ Built With ](#-built-with-)
- [Tech Stack ](#tech-stack-)
- [Key Features ](#key-features-)
- [๐ Live Demo ](#-live-demo-)
- [๐ป Getting Started ](#-getting-started-)
- [Prerequisites](#prerequisites)
- [Setup](#setup)
- [Usage](#usage)
- [๐ฅ Authors ](#-authors-)
- [๐ค Contributing ](#-contributing-)
- [๐ Show your support ](#-show-your-support-)
- [๐ Acknowledgments ](#-acknowledgments-)
- [๐ License ](#-license-)
# ๐ Leaderboard <a name="about-project"></a>
"Leaderboard" is a website that displays scores submitted by various players and allows users to submit their own scores. The data is securely preserved using an external Leaderboard API service.
## ๐ Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
- HTML5
- CSS3
- JavaScript(ES6)
- Webpack
- Leaderboard API
### Key Features <a name="key-features"></a>
The key features of this website include.
- **Webpack**
- **ES6 Syntax**
- **Gitflow**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ๐ Live Demo <a name="live-demo"></a>
- [Live Demo Link](https://mnisarali.github.io/Leaderboard/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ๐ป Getting Started <a name="getting-started"></a>
To get a local copy up and running, follow these steps.
### Prerequisites
In order to run this project you need:
- [x] A code editor (like VSCode, Sublime, Atom, etc.)
- [x] Git and Node.js installed.
### Setup
To setup this project locally:
1. Open terminal in VScode.
2. Navigate to the directory where you want clone the copy of this repository.
3. Create new directory [optional].
4. Clone the repo using the below command.
```
git clone git@github.com:MNisarAli/Leaderboard.git
cd Leaderboard
```
### Usage
This project can be used to practice Webpack and Leaderboard API.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ๐ฅ Authors <a name="authors"></a>
The collaborators of this project.
๐จโ๐ **M Nisar Ali**
- GitHub: [@MNisarAli](https://github.com/MNisarAli)
- Gmail: [@dr.nisaralig@gmail.com](mailto:dr.nisaralig@gmail.com)
- LinkedIn: [@Muhammad Nisar Ali](https://www.linkedin.com/in/muhammad-nisar-ali)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ๐ค Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ๐ Show your support <a name="support"></a>
Give a โญ๏ธ, if you like this project!, also follow me on [GitHub](https://github.com/MNisarAli) & [LinkedIn](https://www.linkedin.com/in/muhammad-nisar-ali).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ๐ Acknowledgments <a name="acknowledgements"></a>
I would like to thank:
- [Microverseinc](https://github.com/microverseinc) for [Readme-template](https://github.com/microverseinc/readme-template).
- [Andrey Sitnik](https://github.com/ai), author of [PostCSS](https://github.com/postcss/postcss), [Autoprefixer](https://github.com/postcss/autoprefixer), and other awesome tools.
- [Leaderboard API](https://www.notion.so/Leaderboard-API-service-24c0c3c116974ac49488d4eb0267ade3)
- [Webpack](https://webpack.js.org/guides/getting-started/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ๐ License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| The Leaderboard website displays scores submitted by various players and allows users to submit their own scores. The data is securely preserved using an external Leaderboard API service. | css,html,javascript,rest-api,webpack | 2023-01-02T21:37:24Z | 2023-04-27T00:45:36Z | null | 1 | 5 | 44 | 0 | 1 | 4 | null | MIT | CSS |
bonaventureogeto/Learn-JavaScript | main | # Learn-JavaScript
Welcome to the "Learn JavaScript" repository! This repository is a collection of JavaScript concepts, projects, and their solutions, designed to help you learn and practice JavaScript programming. Whether you're a beginner looking to get started or an experienced developer seeking to sharpen your skills, you'll find a variety of projects here to challenge and inspire you.

## Table of Contents
1. [Introduction](#introduction)
2. [Projects](#projects)
3. [Getting Started](#getting-started)
4. [Contributing](#contributing)
5. [License](#license)
## Introduction
JavaScript is one of the most widely used programming languages for web development. It's essential for front-end web development, and with the rise of technologies like Node.js, it's become a prominent language for server-side development as well.
This repository is organized to guide you through your JavaScript learning journey progressively. You'll find projects of varying complexity, starting from simple exercises and gradually advancing to more challenging applications. Each project comes with a solution to help you understand how to approach and solve different problems using JavaScript.
## Projects
Here's a list of some of the projects available in this repository:
1. **Project 1: Basic JavaScript Exercises**
- Description: A collection of simple JavaScript exercises covering fundamental concepts such as variables, functions, conditionals, loops, and arrays.
- Solution: [Link to Project 1 Solutions](project1/README.md)
2. **Project 2: To-Do List Web App**
- Description: Build a basic to-do list web application using HTML, CSS, and JavaScript.
- Solution: [Link to Project 2 Solutions](project2/README.md)
3. **Project 3: Interactive Quiz Game**
- Description: Create an interactive quiz game where users can answer questions and see their scores.
- Solution: [Link to Project 3 Solutions](project3/README.md)
4. **Project 4: Weather App**
- Description: Develop a weather application that fetches weather data using an API and displays it to the user.
- Solution: [Link to Project 4 Solutions](project4/README.md)
<!-- Add more projects as needed -->
## Getting Started
To get started with these projects, follow these steps:
1. Clone this repository to your local machine:
```
git clone https://github.com/bonaventureogeto/learn-javascript.git
```
3. Navigate to the project folder you want to work on:
```
cd learn-javascript/<foldername>
```
4. Read the project's `README.md` file for instructions and guidance on the project.
5. Explore the solution provided in the `solutions` folder to understand the implementation.
6. Code, experiment, and have fun learning JavaScript!
## Contributing
Contributions to this repository are welcome! If you have additional JavaScript projects or improvements to existing ones, please consider contributing. Here's how you can contribute:
1. Fork this repository.
2. Create a new branch for your feature or bug fix:
```
git checkout -b feature/my-new-feature
```
3. Make your changes and commit them:
```
git commit -m "Add my new feature"
```
4. Push your changes to your forked repository:
```
git push origin feature/my-new-feature
```
5. Open a pull request to this repository with a detailed description of your changes.
6. Your contribution will be reviewed, and once accepted, it will be merged into the main repository.
## License
This repository is licensed under the MIT License - see the [LICENSE](LICENSE) file for details.
---
Happy coding and learning JavaScript!
| This repository is a collection of JavaScript concepts, projects, and their solutions, designed to help you learn and practice JavaScript programming. | es6,html-css-javascript,javascript,projects,vanilla-javascript | 2023-01-12T08:20:58Z | 2023-10-21T09:05:47Z | null | 1 | 0 | 173 | 0 | 9 | 4 | null | null | JavaScript |
Yuuuics/Coursera-Chinese-Subtitle | master | # Coursera-Chinese-Subtitle
ไธๆฌพ็ฎๅๆ็จ็ Coursera ไธญๆๅญๅน็ฟป่ฏๅจใ
## Docs
ๆๅผ Chrome ๆฉๅฑๅผๅ่
ๆจกๅผ๏ผๅฐๆๆฐ็ Release ไธ่ฝฝๅ็น้ โๅ ่ฝฝๅทฒ่งฃๅ็ๆฉๅฑ็จๅบโ๏ผๅฐ่งฃๅๅ็ๆไปถๅคน้ไธญ๏ผๅฎ่ฃ
ๅฎๆๅ๏ผๅจ Coursera ่ฏพ็จไธญ็นๅปๆๅฑๅพๆ ๏ผ**็จไฝ็ญๅพ
** ๅไพฟๅฏๆพ็คบไธญๆๅญๅนใ
็ฑไบๆฌๆฉๅฑๅบไบ manifest 2.0 ๆๅปบ๏ผๅจๅฎ่ฃ
ๆถๅฏ่ฝๅบ้๏ผๅฟฝ็ฅๅณๅฏใ
> Tips: ๆฌๆฉๅฑไฝฟ็จ Google Translate Api๏ผ็ฑไบ Google ็ฟป่ฏๅทฒ้ๅบไธญๅฝ๏ผๆไปฅๅฏ่ฝ้่ฆๆดๆน hosts ๆ่
็งๅญฆไธ็ฝใๅฝ็ถ๏ผๅฆๆๆๅฅฝ็ Google ้ๅไนๅฏไปฅๅจ Issue ไธญๆๅบๆ่
ๆไบค PRใ
| Coursera ๅญๅนไธญๆ็ฟป่ฏ Chrome ๆฉๅฑใ | chrome,chrome-extension,chrome-extensions,coursera,javascript,translater | 2023-01-10T08:50:03Z | 2023-01-12T12:48:49Z | 2023-01-10T08:49:20Z | 1 | 1 | 3 | 1 | 0 | 4 | null | GPL-3.0 | JavaScript |
sambeck87/group-capstone-project | develop | <a name="readme-top"></a>
<div align="center">
<img src="murple_logo.png" alt="logo" width="140" height="auto" />
<br/>
</div>
<!-- TABLE OF CONTENTS -->
# ๐ Table of Contents
- [๐ About the Project](#about-project)
- [๐ Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [๐ Live Demo](#live-demo)
- [๐ป Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#triangular_flag_on_post-deployment)
- [๐ฅ Authors](#authors)
- [๐ญ Future Features](#future-features)
- [๐ค Contributing](#contributing)
- [โญ๏ธ Show your support](#support)
- [๐ Acknowledgements](#acknowledgements)
- [โ FAQ](#faq)
- [๐ License](#license)
<!-- PROJECT DESCRIPTION -->
# ๐ Pokedex project <a name="about-project"></a>
**This first group capstone** is a project where we use the pokemon API to take 6 specific elements, showing in the main page the name of the pokemon and its image.
We implemented a likes function that stores the information in "Involvement API" which is called later to get the information and display it with the amount of likes of each element.
In the same way a button was implemented that opens a popup which shows in greater detail the characteristics of the selected pokemon.
In this popup you can view comments from other people and also create your own comment, all this thanks to the use of Involvement API.
Also two tests were created to verify the operation of the function that displays the number of comments and pokemon displayed in the DOM.
## ๐ Built With <a name="built-with"></a>
- HTML5
- CSS
- JavaScript
- Webpack
- Jest
### Tech Stack <a name="tech-stack"></a>
- Visual code
<!-- Features -->
### Key Features <a name="key-features"></a>
- **This project use API's to get and save information**
- **This project was created using asyncronous functions**
- **This project was implemented with calls and promises**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## ๐ Live Demo <a name="live-demo"></a>
- [Live Demo Link](https://sambeck87.github.io/group-capstone-project/)
- [Description video](https://drive.google.com/file/d/1ITx9sOrwqMaxeFMMvToJBXfK-PMGkRia/view?usp=share_link)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## ๐ป Getting Started <a name="getting-started"></a>
> Describe how a new developer could make use of your project.
To get a local copy up and running, follow these steps.
### Prerequisites
In order to run this project you need:
- A browser that supports html5
- Git
- A GitHub account
### Setup
Clone this repository to your desired folder:
- Go to this repo and copy the project link
https://github.com/sambeck87/group-capstone-project
- Open your terminal and clone the repo with this command "git clone https://github.com/sambeck87/group-capstone-project.git"
- Now, you have a copy on your pc.
### Install
Install this project with:
- You need to install npm to use the commands
- In your console type, npm install to install all the dependencies
### Usage
To run the project, execute the following command:
npm start
### Run tests
To run tests, run the following command:
npm test
### Deployment
You can deploy this project using:
Github Pages
This project use Github Pages to deploy the page, just type the command: "npm deploy"
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## ๐ฅ Authors <a name="authors"></a>
๐ค **Sandro Hernandez**
- GitHub: [@sambeck87](https://github.com/sambeck87)
- Twitter: [@sambeck4488](https://twitter.com/sambeck4488)
- LinkedIn: [LinkedIn](https://www.linkedin.com/in/sandro-israel-hern%C3%A1ndez-zamora-899386a4/)
๐ค **Glenda Diaz**
- GitHub: [@Gdiazdiaz](https://github.com/Gdiazdiaz)
- LinkedIn: [LinkedIn](www.linkedin.com/in/glendadiazz)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## ๐ญ Future Features <a name="future-features"></a>
- [ ] **It will allow just one like per persone**
- [ ] **It will contain more pokemons and features**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## ๐ค Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](https://github.com/sambeck87/Leaderboard-project/issues).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## โญ๏ธ Show your support <a name="support"></a>
> Write a message to encourage readers to support your project
If you like this project give a โญ๏ธ or send us a message. We will apreciate
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## ๐ Acknowledgments <a name="acknowledgements"></a>
I would like to thank to Microverse for the inspiration to be better every day.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FAQ (optional) -->
## โ FAQ <a name="faq"></a>
- **[Can this project be used in any browser?]**
- [This project was created and proved in firefox v107. You can try run it in older version but this project couldn't work well]
- **[Can I use the code of this project]**
- [Sure. We will be happy to colaborate with this project. This project has MIT license]
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## ๐ License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| This first group capstone is a project where we use the pokemon API to take 6 specific elements, showing in the main page the name of the pokemon and its image. We implemented a likes function that stores the information in "Involvement API" which is called later to get the information and display it with the amount of likes of each element | css,javascript,jest-tests,webpack | 2023-01-02T18:59:34Z | 2023-06-18T21:08:53Z | null | 2 | 15 | 57 | 0 | 1 | 4 | null | MIT | JavaScript |
kalialor/Programming-Notes | main | # Programming Notes
This repository contains my personal programming notes and projects. It serves as a reference for various technologies and concepts that I have learned during my programming journey.
## Technologies Used
- Javascript
- Java
- React
- React-Native
- Node.js
- Firebase
- Express.js
- MongoDB
- Mongoose
- PostgreSQL
- Git & GitHub
- Redux
- Blender
- Three.js
- WebGL
- Mapbox
## Lessons Learned
Throughout my experience working with these technologies, I have gained valuable insights and lessons. Some of the key lessons I've learned include:
- The importance of proper version control using Git and GitHub, enabling collaboration and tracking changes effectively.
- Understanding the fundamentals of JavaScript and how it powers dynamic web applications.
- Building responsive and interactive user interfaces with React and React Native.
- Developing server-side applications with Node.js, Express.js, and various databases like MongoDB and PostgreSQL.
- Leveraging Firebase to create scalable and real-time web and mobile applications.
- Utilizing Redux for efficient state management in complex JavaScript applications.
- Exploring 3D graphics and animation using Blender, Three.js, and WebGL.
- Incorporating Mapbox for integrating maps and location-based services into applications.
These are just a few of the lessons I've learned along the way, and this repository serves as a collection of my notes and projects to help others in their programming journey.
Feel free to explore the repository and use any code snippets or references that may be helpful to you. If you have any questions or suggestions, feel free to reach out! | My Notes From Learning How To Program | html,javascript,notes,css | 2023-01-05T19:42:15Z | 2023-07-12T21:38:09Z | null | 1 | 0 | 71 | 0 | 1 | 4 | null | null | Jupyter Notebook |
Pa1mekala37/ReactJs-Emoji-Game | main | In this project, let's build an **Emoji Game** by applying the concepts we have learned till now.
### Refer to the image below:
<br/>
<div style="text-align: center;">
<img src="https://assets.ccbp.in/frontend/content/react-js/emoji-game-output-v2.gif" alt="emoji-game-output" style="max-width:70%;box-shadow:0 2.8px 2.2px rgba(0, 0, 0, 0.12)">
</div>
<br/>
### Design Files
<details>
<summary>Click to view</summary>
- [Extra Small (Size < 576px), Small (Size >= 576px)](https://assets.ccbp.in/frontend/content/react-js/emoji-game-sm-outputs.png)
- [Medium (Size >= 768px), Large (Size >= 992px) and Extra Large (Size >= 1200px) - Game View](https://assets.ccbp.in/frontend/content/react-js/emoji-game-lg-output-v2.png)
- [Medium (Size >= 768px), Large (Size >= 992px) and Extra Large (Size >= 1200px) - Won Game](https://assets.ccbp.in/frontend/content/react-js/emoji-game-won-game-lg-output.png)
- [Medium (Size >= 768px), Large (Size >= 992px) and Extra Large (Size >= 1200px) - Lose Game](https://assets.ccbp.in/frontend/content/react-js/emoji-game-lose-game-lg-output.png)
</details>
### Set Up Instructions
<details>
<summary>Click to view</summary>
- Download dependencies by running `npm install`
- Start up the app using `npm start`
</details>
### Completion Instructions
<details>
<summary>Functionality to be added</summary>
<br/>
The app must have the following functionalities
- Initially, the _Score_ and _Total Score_ for the current game should be **0**
- When an **Emoji** is clicked,
- If it is not the same as any of the previously clicked emojis, then the _Score_ should be incremented by one
- If all the emojis are clicked exactly once
- [Won Game](https://assets.ccbp.in/frontend/content/react-js/emoji-game-won-game-lg-output.png) view should be displayed
- If it is the same as any of the previously clicked emojis
- [Lose Game](https://assets.ccbp.in/frontend/content/react-js/emoji-game-lose-game-lg-output.png) view should be displayed
- If the score achieved in the current game is higher than the previous scores then the _Top Score_ should be updated accordingly
- When the _Play Again_ button is clicked, then we should be able to play the game again
- The _Score_ value should be reset but not the _Top Score_ value
- The `EmojiGame` component receives the `emojisList` as a prop. It consists of a list of emoji objects with the following properties in each emoji object
| Key | Data Type |
| :-------: | :-------: |
| id | Number |
| emojiName | String |
| emojiUrl | String |
</details>
<details>
<summary>Components Structure</summary>
<br/>
<div style="text-align: center;">
<img src="https://assets.ccbp.in/frontend/content/react-js/emoji-game-game-view-component-breakdown-structure.png" alt="emoji game view component breakdown structure" style="max-width:100%;box-shadow:0 2.8px 2.2px rgba(0, 0, 0, 0.12)">
</div>
<br/>
<div style="text-align: center;">
<img src="https://assets.ccbp.in/frontend/content/react-js/emoji-game-win-lose-component-breakdown-structure.png" alt="emoji game win or lose component breakdown structure" style="max-width:100%;box-shadow:0 2.8px 2.2px rgba(0, 0, 0, 0.12)">
</div>
<br/>
</details>
<details>
<summary>Implementation Files</summary>
<br/>
Use these files to complete the implementation:
- `src/components/EmojiGame/index.js`
- `src/components/EmojiGame/index.css`
- `src/components/NavBar/index.js`
- `src/components/NavBar/index.css`
- `src/components/EmojiCard/index.js`
- `src/components/EmojiCard/index.css`
- `src/components/WinOrLoseCard/index.js`
- `src/components/WinOrLoseCard/index.css`
</details>
### Quick Tips
<details>
<summary>Click to view</summary>
<br>
- You can use the `cursor` CSS property to specify the mouse cursor to be displayed when pointing over an element
```
cursor: pointer;
```
<br/>
<img src="https://assets.ccbp.in/frontend/content/react-js/cursor-pointer-img.png" alt="cursor pointer" style="width:100px" />
- You can use the below `outline` CSS property for buttons and input elements to remove the highlighting when the elements are clicked
```
outline: none;
```
</details>
### Important Note
<details>
<summary>Click to view</summary>
<br/>
**The following instructions are required for the tests to pass**
- The emojis should have the alt as the value of the key `emojiName` from each emoji object
</details>
### Resources
<details>
<summary>Image URLs</summary>
- [https://assets.ccbp.in/frontend/react-js/game-logo-img.png](https://assets.ccbp.in/frontend/react-js/game-logo-img.png) alt should be **emoji logo**
- [https://assets.ccbp.in/frontend/react-js/won-game-img.png](https://assets.ccbp.in/frontend/react-js/won-game-img.png)
- [https://assets.ccbp.in/frontend/react-js/lose-game-img.png](https://assets.ccbp.in/frontend/react-js/lose-game-img.png)
</details>
<details>
<summary>Colors</summary>
<br/>
<div style="background-color: #6a59ff ; width: 150px; padding: 10px; color: white">Hex: #6a59ff</div>
<div style="background-color: #ffffff ; width: 150px; padding: 10px; color: black">Hex: #ffffff</div>
<div style="background-color: #3d3d3d ; width: 150px; padding: 10px; color: white">Hex: #3d3d3d</div>
#### Background Colors
<div style="background-color: #9796f0 ; width: 150px; padding: 10px; color: white">Hex: #9796f0</div>
<div style="background-color: #fbc7d4 ; width: 150px; padding: 10px; color: black">Hex: #fbc7d4</div>
<div style="background-color: #ffffff33 ; width: 150px; padding: 10px; color: black">Hex: #ffffff33</div>
<div style="background-color: #ffce27 ; width: 150px; padding: 10px; color: black">Hex: #ffce27</div>
#### Border Colors
<div style="background-color: #ffffff30 ; width: 150px; padding: 10px; color: black">Hex: #ffffff30</div>
</details>
<details>
<summary>Font-families</summary>
- Roboto
</details>
> ### _Things to Keep in Mind_
>
> - All components you implement should go in the `src/components` directory.
> - Don't change the component folder names as those are the files being imported into the tests.
> - **Do not remove the pre-filled code**
> - Want to quickly review some of the concepts youโve been learning? Take a look at the Cheat Sheets.
| Emoji Game using React.js | babel,css,front-end-development,html,javascript,node,npm,reactjs,uuidv4 | 2023-01-01T14:07:32Z | 2023-01-01T14:08:23Z | null | 1 | 0 | 1 | 0 | 0 | 4 | null | null | JavaScript |
ayame113/edia | main | # edia
[](https://github.com/ayame113/edia/actions/workflows/test.yml)
[](https://codecov.io/github/ayame113/edia)
ๆๅป่กจใChart.jsใซใใใใคใคใฐใฉใ ใซๅคๆใใพใใ
- ใฉใคใใฉใช๏ผhttps://deno.land/x/edia
- ใใญใฅใกใณใ๏ผhttps://deno.land/x/edia/lib/mod.ts
- Chromeๆกๅผตๆฉ่ฝ:
https://chrome.google.com/webstore/detail/edia/nggncccbpkmeojkfmhjjjimbfppfbgbe/related?hl=ja

```ts
import { generateDiagram } from "https://deno.land/x/edia@$MODULE_VERSION/lib/mod.ts";
const table = document.querySelector(".paper_table");
const el = await generateDiagram(table as HTMLTableElement);
document.body.insertAdjacentElement("afterbegin", el);
```
### ใใฃใฌใฏใใชๆงๆ
- `.vscode/`: vscode่จญๅฎใใกใคใซใ
- `build/`: TypeScriptใใซใ้ข้ฃใ
- `etension/`: Chromeๆกๅผต็จใใกใคใซใ
- `lib/`: ใฉใคใใฉใชใ
- `deno.json`: Deno่จญๅฎใใกใคใซใ
- `manifest.json`: Chromeๆกๅผต่จญๅฎใใกใคใซใ
### ้็บ
[Deno](https://deno.land/)ใใคใณในใใผใซใใๅฟ
่ฆใใใใพใใ
```shell
# TypeScriptใใใซใใใฆjsใใกใคใซใ็ๆ
> deno task build
# TypeScriptใใใซใใใฆjsใใกใคใซใ็ๆ๏ผใใกใคใซๅคๆดใฎๅบฆใซ่ชๅ็ๆ๏ผ
> deno task build:watch
# TypeScriptใใใซใ็ตๆใๆๆฐใใฉใใใใงใใฏ
> deno task build:check
# ใในใใๅฎ่ก
> deno task test
```
| ใใคใคใฐใฉใ ็ๆใฉใคใใฉใช | diagram,javascript | 2023-01-07T09:14:01Z | 2023-01-24T14:08:08Z | 2023-01-24T14:08:08Z | 1 | 9 | 28 | 1 | 0 | 4 | null | MIT | JavaScript |
8309h/homeDecor.com | main | # eminent-trucks-727
"welcome..."
# Getting Started
๐ Welcome to Notion!
landing page .
serch bar
adding logo wih help canva
welcomming login user
wishlist icon
cart icon
responsive images
responsive footer
// furniture page
show all product
adding stick property
filting with category
sorting with price
add to cart button
add to wishlit buttton
fotter parts
show admn adding prodeuct
add to them in cart
add to wishlist this
//kitchen page
show all product
adding stick property
filting with category
sorting with price
add to cart button
add to wishlit buttton
fotter parts
//wishlist page
show all prodect in wishlist
add them to cart page
able to remove from wishlist
// cart page
showing all product
stick static div in which we can see total of cart and offer part also
adding product quantity
decrese product quantity
remove product from cart
applying coupen code
for 30%
for 50%
buy now button
after clicking buy now redirecting to login page
// signup page
one email use at a time noe reuse of same email
after signup suceesful data store in localstore
adding mobile number
crete password
// login page
need to fill email
need to fill password
// payment page
after adding cart details on clicking submit
we receive otp
// otp page
after conform otp you can able to see recipte of your paymet with
your payment amount your name
// admin page
if you are the admin then you get unique otp
after conform otp
you get pruduct adding form
in the form after filling the product details
on clicking on sumbmit you get a pop up as product added succesfully
and product is shown in the furniture page
able to add to wishlist
able to add to cart page
| Description : Home Decor , that sells modern furniture, housewares, and decor. they offer high-quality products, exclusive designs, and seamless shopping solutions through digital design and visualization tools. | bootstrap,css,html,javascript,localstorage | 2023-01-16T14:56:27Z | 2023-01-24T05:33:59Z | null | 6 | 36 | 94 | 1 | 1 | 4 | null | null | CSS |
mateushsx/full-stack-course | main | # Curso Full Stack
## 
Anotaรงรตes do Curso de TypeScript e JavaScript do Luรญz Otavio Miranda
| Anotaรงรตes do Curso de TypeScript e JavaScript do Luรญz Otavio Miranda ๐ง๐ฟโ๐ป ๐ | annotations,book,course,css,full-stack,html,javascript,markdown,typescript,mateushsx | 2023-01-13T22:29:06Z | 2023-02-13T11:11:59Z | null | 1 | 0 | 21 | 0 | 0 | 4 | null | MIT | null |
tsparticles/editor | main | # tsParticles options GUI editor
tsParticles options GUI editor made with [Object GUI](https://github.com/matteobruni/object-gui)
## Usage
```javascript
tsParticles
.load("tsparticles", {
/* your options here */
})
.then((container) => {
showEditor(container).top().right().theme("dark");
});
```
or
```javascript
tsParticles.loadJSON("tsparticles", "particles.json").then((container) => {
showEditor(container).top().right().theme("dark");
});
```
| tsParticles official editor | hacktoberfest,javascript,tsparticles,typescript,object-gui | 2023-01-12T23:12:39Z | 2023-11-18T14:44:50Z | null | 1 | 37 | 66 | 1 | 0 | 4 | null | MIT | TypeScript |
MatheusPrudente/bora-codar | main | # bora-codar
<p>
<img src="https://img.shields.io/github/languages/count/MatheusPrudente/bora-codar"/>
<img src="https://img.shields.io/github/repo-size/MatheusPrudente/bora-codar"/>
<img src="https://img.shields.io/github/last-commit/MatheusPrudente/bora-codar"/>
<img src="https://img.shields.io/github/issues/MatheusPrudente/bora-codar"/>
</p>
>O #boraCodar รฉ um projeto da Rocketseat com o objetivo de estimular a prรกtica de programaรงรฃo semanal e ajudar na criaรงรฃo de projetos para portifรณlio.
Como participar do desafio:
- Toda quarta-feira, ร s 11h, serรก liberado um novo desafio no site boracodar.dev;
- Code a sua versรฃo do desafio, compartilhe o resultado e marque a Rocketseat nas redes sociais com a hashtag #boraCodar;
- Na semana seguinte (ou seja, na prรณxima quarta-feira, ร s 11h), no canal do YouTube da Rocketseat, vocรช encontra um vรญdeo da equipe da Rocket (um dos Boosters e educadores) com a resoluรงรฃo completa do desafio.
Resultados dos desafios:
| Semana | Desafio | Objetivo | Cรณdigo |
|---|---|---|---|
| Semana 01 | Music Player | #boraCodar um player de mรบsica | [Music Player](https://github.com/MatheusPrudente/bora-codar/tree/main/01-music-player) |
| Semana 02 | Product Card | #boraCodar um card de produto | [Product Card](https://github.com/MatheusPrudente/bora-codar/tree/main/02-product-card) |
| Semana 03 | Buttons and Cursors | #boraCodar botรตes e cursores | [Buttons and Cursors](https://github.com/MatheusPrudente/bora-codar/tree/main/03-buttons) |
| Semana 04 | Chat | #boraCodar um chat |[Chat](https://github.com/MatheusPrudente/bora-codar/tree/main/04-chat) |
| Semana 05 | Calculator | #boraCodar uma calculadora | [Calculator](https://github.com/MatheusPrudente/bora-codar/tree/main/05-calculator) |
| Semana 06 | Boarding Pass | #boraCodar um cartรฃo de embarque | [Boarding Pass](https://github.com/MatheusPrudente/bora-codar/tree/main/06-boarding-pass) |
| Semana 07 | Carnival Block | #boraCodar um site de blocos de carnaval | [Carnival Block](https://github.com/MatheusPrudente/bora-codar/tree/main/07-carnival-block) |
| Semana 08 | Dashboard | #boraCodar um Dashboard | [Dashboard](https://github.com/MatheusPrudente/bora-codar/tree/main/08-dashboard) |
| Semana 09 | Currency Converter | #boraCodar um conversor de moeda | [Currency Converter](https://github.com/MatheusPrudente/bora-codar/tree/main/09-currency-converter) |
| Semana 10 | Weather Forecast | #boraCodar uma pรกgina de previsรฃo de tempo | [Weather Forecast](https://github.com/MatheusPrudente/bora-codar/tree/main/10-weather-forecast)|
| Semana 11 | Login | #boraCodar uma pรกgina de Login | [Login](https://github.com/MatheusPrudente/bora-codar/tree/main/11-login)|
| Semana 12 | Kanban | #boraCodar um Kanban | [Kanban](https://github.com/MatheusPrudente/bora-codar/tree/main/12-kanban) |
| Semana 13 | Card Form | #boraCodar um formulรกrio de cartรฃo | [Card Form](https://github.com/MatheusPrudente/bora-codar/tree/main/12-card-form) |
| Semana 14 | Upload component| #boraCodar um componente de upload | [Upload component](https://github.com/MatheusPrudente/bora-codar/tree/main/14-upload-component) |
| Semana 15 | Pricing Table| #boraCodar uma Pricing Table | [Pricing Table](https://github.com/MatheusPrudente/bora-codar/tree/main/15-pricing-table) |
| Semana 16 | Contact page | #boraCodar uma pรกgina de contato | [Contact page](https://github.com/MatheusPrudente/bora-codar/tree/main/16-contact-page) |
| Semana 17 | Date Picker | #boraCodar um date picker | [Date Picker](https://github.com/MatheusPrudente/bora-codar/tree/main/17-date-picker) |
| Semana 18 | Character Card | #boraCodar um card de personagem | [Character Card](https://github.com/MatheusPrudente/bora-codar/tree/main/18-character-card) |
| Semana 19 | Transport Widget | #boraCodar um widget de transporte | [Transport Widget](https://github.com/MatheusPrudente/bora-codar/tree/main/19-transport-widget) |
| Semana 20 | Gallery | #boraCodar uma galeria com hover | [Gallery](https://github.com/MatheusPrudente/bora-codar/tree/main/20-gallery) |
| Semana 21 | Shopping Cart | #boraCodar um carrinho de compras | [Shopping Cart](https://github.com/MatheusPrudente/bora-codar/tree/main/21-shopping-cart) |
| Semana 22 | Profile Settings | #boraCodar um profile settings| [Profile Settings](https://github.com/MatheusPrudente/bora-codar/tree/main/22-profile-settings) |
| Semana 23 | Multi Step Form | #boraCodar um formulรกrio multi step | [Multi Step Form](https://github.com/MatheusPrudente/bora-codar/tree/main/23-multi-step-form) |
| Semana 24 | Lighting Adjustments | #boraCodar um ajuste de iluminaรงรฃo | [Lighting Adjustments](https://github.com/MatheusPrudente/bora-codar/tree/main/24-lighting-adjustments) |
| Semana 25 | VR Player | #boraCodar um ajuste de iluminaรงรฃo | [VR Player](https://github.com/MatheusPrudente/bora-codar/tree/main/25-vr-player) |
| Semana 26 | June Recipe | #boraCodar uma receita junina | [June Recipe](https://github.com/MatheusPrudente/bora-codar/tree/main/26-june-recipe) |
| Semana 27 | Page Not Found | #boraCodar uma pรกgina de erro 404 | [Page Not Found](https://github.com/MatheusPrudente/bora-codar/tree/main/27-page-not-found) |
| Semana 28 | IA Home Screen | #boraCodar uma plataforma de IA | [IA Home Screen](https://github.com/MatheusPrudente/bora-codar/tree/main/28-ia-home-screen) |
| Semana 29 | Before After IA | #boraCodar um antes e depois com IA | [Before After IA](https://github.com/MatheusPrudente/bora-codar/tree/main/29-before-after-ia) |
| Semana 30 | Movie Suggestion | #boraCodar Gerador de sugestรฃo de filmes com IA | [Movie Suggestion](https://github.com/MatheusPrudente/bora-codar/tree/main/30-movie-suggestion) |
| Semana 31 | Youtube Transcription | #boraCodar Transcriรงรฃo de vรญdeo com IA | [Youtube Transcription](https://github.com/MatheusPrudente/bora-codar/tree/main/31-youtube-transcription) |
| Semana 32 | SQL Translation App | #boraCodar um app de traduรงรฃo de SQL com IA | [SQL Translation App](https://github.com/MatheusPrudente/bora-codar/tree/main/32-sql-translation-app) |
| Semana 33 | Ticket Generator | #boraCodar Gerador de Ticket | [Ticket Generator](https://github.com/MatheusPrudente/bora-codar/tree/main/33-ticket-generator) |
| Semana 34 | Shopping List | #boraCodar uma lista de compras | [Shopping List](https://github.com/MatheusPrudente/bora-codar/tree/main/34-shopping-list) |
| Repositรณrio dos projetos do desafio Bora Codar da Rocketseat | boracodar,frontend,rocketseat,challenges,bora-codar,dom,js,html,html-css-javascript,html5 | 2023-01-15T14:52:40Z | 2023-09-04T01:13:37Z | null | 1 | 0 | 142 | 0 | 0 | 4 | null | null | HTML |
dev-aniketj/JSONToMarkdown-ReactJS | master | # JSON to Markdown
**It will automatically converts your JSON data into markdown code, but your have to make json file in the proper format**.
**[DOCUMENTATION](DOCUMENTATION.md)**
## How it is working ??
If you want to convert your JSON file then add the file into the `src/data/your_file.json`
<br/>
Go to **`App.js`** and add new item in it _OR_ just change the name of the file with is passed inside the **`createMarkdownFile()`** function.
<br/>
```
<div className="item" onClick={() => createMarkdownFile("your_file_name")}>
<h3>Title</h3>
<h5>File Name</h5>
</div>
```
## Installation
```sh
# Using npm
npm install --save json2md
# Using yarn
yarn add json2md
```
## Run
```sh
# Using npm
npm start
# Using yarn
yarn start
```
## Examples
### File 1
- [JSON](./src/data/file1.json)
- [Markdown](./src/markdown/file1.md)
<br/>
<br/>
<br/>
<br/>
<br/>
## NPM Packages
### I am using React Js and this NPM Package [json2md](https://www.npmjs.com/package/json2md)
| Convert JSON file to Markdown file | js,json,json-markdown,json-to-markdown,markdown,markdown-generator,react,reactjs,javascript,jsx | 2023-01-16T18:39:10Z | 2023-01-17T19:35:37Z | null | 1 | 1 | 12 | 0 | 1 | 4 | null | Apache-2.0 | JavaScript |
jugosack/HTML-CSS-JavaScript-capstone-project---Conference-page | main | <a name="readme-top"></a>
<div align="center">
<h3><b>First Microverse Capstone Project</b></h3>
</div>
# ๐ Table of Contents
- [๐ About the Project](#about-project)
- [๐ Video Description](#vid-desc)
- [๐ Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [๐ Live Demo](#live-demo)
- [๐ป Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Deployment](#deployment)
- [๐ฅ Authors](#authors)
- [๐ญ Future Features](#future-features)
- [๐ค Contributing](#contributing)
- [โญ๏ธ Show your support](#support)
- [๐ Acknowledgements](#acknowledgements)
- [๐ License](#license)
<!-- PROJECT DESCRIPTION -->
# ๐ Conference Page <a name="about-project"></a>
**Conference page** is the first Microverse Capstone at the end of Module 1
## Video Description <a name="vid-desc">
[Loom video describing project](https://www.loom.com/share/69349eb9e8de4197988d23b173c3f82b)
## ๐ Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
HTML, CSS and JavaScript
<details>
<summary>Client</summary>
<ul>
<li><a href="https://nodejs.org/">Node.js</a></li>
</ul>
</details>
<details>
<summary>Server</summary>
<ul>
<li><a href="#">Live Server</a></li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
- **Navigation Bar**
- **Mobile Menu**
- **Dynamic HTML for speakers section**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## ๐ Live Demo <a name="live-demo"></a>
- [Live Demo Link](https://jugosack.github.io/HTML-CSS-JavaScript-capstone-project---Conference-page/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## ๐ป Getting Started <a name="getting-started"></a>
To get a local copy up and running, follow these steps.
- Download and unzip the source files
- Open index.html with a browser to view
### Prerequisites
In order to run this project you need:
```sh
git clone https://github.com/jugosack/HTML-CSS-JavaScript-capstone-project---Conference-page
npm install
```
or
### Setup
Clone this repository to your desired folder:
```sh
cd my-folder
git clone https://github.com/jugosack/HTML-CSS-JavaScript-capstone-project---Conference-page
npm install
```
### Install
Install this project with:
```sh
cd my-project
npm install
```
### Usage
To run the project, kindly open index.html with your browser or run live server on VS Code:
### Deployment <a name="deployment"></a>
You can deploy this project with [GitHub Pages](https://pages.github.com/):
<p align="right"><a href="#readme-top">back to top</a>)</p>
<!-- AUTHOR -->
## ๐ฅ Author <a name="authors"></a>
- GitHub: [jugosack](https://github.com/jugosack)
- Twitter: [Jugoslav Achkoski](https://twitter.com/Jugosla22401325)
- LinkedIn: [Jugoslav Achkoski](https://www.linkedin.com/in/jugoslav-achkoski-3a074021/?originalSubdomain=mk)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## ๐ญ Future Features <a name="future-features"></a>
- [ ] **Contact Page**
- [ ] **Developers Page**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## ๐ค Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## โญ๏ธ Show your support <a name="support"></a>
If you like this project and would like to support, contact me.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## ๐ Acknowledgments <a name="acknowledgements"></a>
I would like to thank Microverse, [Cindy Shin](https://www.behance.net/adagio07) for this perfect design, my coding partners and the whole developer body in Microverse for helping me get this far.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## ๐ License <a name="license"></a>
This project is [Creative Commons](https://creativecommons.org/licenses/by-nc/4.0/legalcode) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| The "MILCON 2023" Capstone project is a website redesign for a conference of the same name. The website was built using HTML, CSS, and JavaScript, and it features a sleek and modern design that reflects the brand's aesthetic. The website includes information about the conference, including location, dates and featured speakers. | css,html,javascript | 2023-01-02T12:24:03Z | 2023-01-07T01:10:06Z | null | 1 | 1 | 24 | 1 | 0 | 4 | null | null | CSS |
hashmat-wani/tic-tac-toe | main | Tic-Tac-Toe is a game in which two players seek alternate turns to complete a row, a column, or a diagonal with either three Oโs or three Xโs drawn in the spaces of a grid of nine squares.
In our program or design [Tic Tac Toe Game], at first, on the webpage,
- There are three buttons which are labeled as **Play vs bot**, **Play vs friend**, and **Play online**. Users can select one option to continue the game, default is **Play vs bot**.
- If user selects the **play vs bot**, then user will be having three options of level _easy, medium, hard_ default is easy. And user can also select then the Player **X or O**,
- If the user selects the **X** then the bot will be **O** - _and first move will be played by user_. But if the user selects the **O** then the bot will be **X** - _and first move will be played by bot_.
- If user selects the **Play vs friend**, then both the **moves** will be played by User.
- User can also select board size between **3x3 and 5x5**.
- In **3x3** user will be having an option of 3 levels **easy, medium, and hard**. But in **5x5**, user can only select between **easy and medium**.
After selecting all the options, User can start the game by clicking on a button **Start Game**. Once the user starts, the selection box will disappear and the playboard is visible. There are the player names at the top in the playboard section and it indicates or shows whose turn is now. At the center of the webpage, there is a tic tac toe play area with **9** or **25** square boxes (depends on user's selection). Once you click on the particular box then there is visible a sign or icon which you have chosen on the selection box.
Once you click on any box then after a couple of seconds the bot will automatically select the box which is not selected by you or the bot before, and the opposite icon is visible there means if your icon is **X** then the bot will have **O**. Once a match is won by someone, the result box appears with.
* the winner sign and text.
* Dropdown box to change the first turn.
* A Replay and Quit button.
Once you click on the **Replay** button, the playboard will be restored and you can play again with the same settings. But, Once you click on the **Quit** button, the current page reloads and you've to select the options again.
If no one wins the match, the result box appears with **Tie Game!** and a replay and quit button. Once you click on the replay button, the current page reloads and you can play again.
Users can also **On/Off** Game audio by toggling the sound icon.
This game has 3 levels, **Easy, Medium, hard**.
- With the easy version, the **bot** chooses its move randomly.
- With the medium version, the bot chooses its move following some steps in order:
1. If a **bot** is at one move of winning, it chooses that move.
2. If the **player** is at one move of winning, it chooses that move.
3. Same as step 1, but if the bot is at two moves of winning.
4. Same as step 3, but with **player**.
5. Same as step 1, but if the bot is at three moves of winning.
6. Same as step 5, but with **player**.
7. **bot** goes for random move.
- With the hard version, I've implemented minimax algorithm
- Like a professional chess player, this algorithm sees a few steps ahead and puts itself in the shoes of its opponent. It keeps playing ahead until it reaches a terminal arrangement of the board (terminal state) resulting in a tie, a win, or a loss. Once in a terminal state, the AI will assign an arbitrary positive score (+10) for a win, a negative score (-10) for a loss, or a neutral score (0) for a tie. At the same time, the algorithm evaluates the moves that lead to a terminal state based on the playersโ turn. It will choose the move with maximum score when it is the AIโs turn and choose the move with the minimum score when it is the human playerโs turn. Using this strategy, Minimax avoids losing to the human player.
# Home Page

# 3x3 Board

# 5x5 Board

# Result box - winning

# Result box - lose

| Tic-Tac-Toe is a game in which two players seek alternate turns to complete a row, a column, or a diagonal with either three Oโs or three Xโs drawn in the spaces of a grid of nine squares. For more details, please go through readme. | css3,html5,javascript,minimax-algorithm | 2023-01-04T11:03:42Z | 2023-01-07T06:58:19Z | null | 1 | 0 | 9 | 0 | 0 | 4 | null | null | JavaScript |
pdelporte/inline-edit | main | # inline-edit
> Inline field editor for Django apps
Who has never dreamed about a simple inline edit mechanism? I know that they are many libraries or scripts available, but I found them not too easy to understand or with too many parameters to be able to quickly and easily use them. So, I decided to write my own library and let me here share the result with you.
The implementation that will be here describe is based on Javascript and use some Jquery feature. The back end to update effectively the database is based on the Django framework, but it can easily but translated to another framework, like Flask, or any other language like PHP.
The javascript file (*inline_edit.js*) and the css stylesheet file (*inline_edit.css*) can be found into the directory *static/inline_edit*.
## How does it work?
First of all, you have to add a reference to the stylesheet *inline_edit.css* and to the javascript file *inline_edit.js* into you HTML file.
<link href="/static/inline_edit/inline_edit.css" rel="stylesheet" type="text/css">
and
<script src="/static/inline_edit/inline_edit.js"></script>
Copy the directory *static/inline_edit* and its content into your Django *static* directory
Add the directory *lib* in the root of your Django application
Add an entry into your *urls.py* file in order to call the function to update your database
path("update/data/", upd_data, name="update_data" ),
Then modify you html code according to the description here after (add `<span>` attribute around the item you want to edit).
<span class="inline-edit"
data-id="{{ object.id }}"
data-name="bills.Product.name"
data-type="text"
data-value="{{ object.name }}">
{{ object.name }}
</span>
Restart your application, double-click on item... and that's it! Enjoy
## Step by step modification
### Modification in your html file
To implement the inline edit you have to add a few attributes around the element that you want to edit. For example, you want to edit the name of a product. Then around the name of the product you will add some html tags
<table class="table table-hover table-bordered">
<tr>
<td>{% trans "Product name" %}</td>
<td>{{ object.name }}</td>
</tr>
<tr>
<td>{% trans "Category" %}</td>
<td>{{ object.category }}</td>
</tr>
</table>
In django template's, `{{ object.name }}` will be effectively replace by the name of the product.
The same for `{{ object.category }}` which will display the name of the category of the product.
The above code will product something like this :

To enable inline edit we must change the html code to:
<td>
<span class="inline-edit"
data-id="{{ object.id }}"
data-name="bills.Product.name"
data-type="text"
data-value="{{ object.name }}">
{{ object.name }}
</span>
</td>
If we refresh the page, it will now look to something like this :

You do not see many changes, except the dotted line under the name of the product. This shows you that you can edit this information by double-clicking on it.
Of course, you can easily change this layout formatting by modifying the css file *inline_edit.css*.
Now if we double-click on the product name, an input field will replace the text, allowing to update the information

Change the information to, i.e., itโs English translation

To validate the change just click outside the field and thatโs it!

The **inline edit** now works with *text*, *number*, *checkbox*, *select* and *date* field.
Now that you have seen how easy it is easy to implement the inline edit, letโs come back to the parameter
<td>
<span class="inline-edit"
data-id="{{ object.id }}"
data-name="bills.Product.name"
data-type="text"
data-value="{{ object.name }}">
{{ object.name }}
</span>
</td>
The class name must be `class="inline-edit" of course you can add any other class definition to it.
`data-id` is the id of the record to which belong the field โobject.nameโ
`data-name="bills.Product.name" describe which field of the database must be updated.
The name is composed of the *app_name*, the *model_name* and the *field_name*.
So in the exemple `data-name="bills.Product.name"` the django *app_name* is **bills**.
In this Django app, in the file **models.py** there is a model called *โProductโ* (`class Product(models.Model):`) with a field called *name* (`name = models.CharField(max_length=255)`)
class Product(models.Model):
# Relationships
category = models.ForeignKey(ProductCategory, on_delete=models.CASCADE, related_name='products', default=1)
# Fields
name = models.CharField(max_length=255)
`data-type="text"` define that the input field is of type *text*.
The allowed values are *text*, *number*, *select*, *date* or *checkbox*.
`data-value="{{ object.name }}"` is a raw copy the value before it is edited.
I added this, because sometimes you add other information like a currency symbol or format the value to be more user-friendly.
## What about editing a foreign key?
It works pretty much the same way, excepted that the type of input field will be *โselectโ* and that we need to get the list of value for this *Select* field. This will be done by adding an extra attribute `data-url`. The result is :
<tr>
<td>{% trans "Category" %}</td>
<td>
<span class="inline-edit"
data-id="{{ object.id }}"
data-name="bills.Product.category_id"
data-type="select"
data-value="{{ object.category_id }}"
data-url="{% url 'bills:api-product-category-list' %}">
{{ object.category }}
</span>
</td>
</tr>
The attribute `data-url` will contain the url to retrieve the list of categories in json format.
The information returned must contain and *id* and *name* field.
Example or return values :
[{"id":7,"name":"Web"},{"id":8,"name":"Licences"},{"id":9,"name":"Devolo"},{"id":10,"name":"SSD"},{"id":11,"name":"Hardware"},{"id":1,"name":"Gรฉnรฉral"}]
You can easily use the Django *rest_framework* to carry on this.
Here is an example of the API view to return the list of ProductCategory (To have a very good documentation on Django Rest Framework [click here](https://simpleisbetterthancomplex.com/tutorial/2018/11/22/how-to-implement-token-authentication-using-django-rest-framework.html))
(file is : *app_name/views_api.py*)
from rest_framework import generics, permissions
from rest_framework.decorators import api_view
from rest_framework.response import Response
from rest_framework.reverse import reverse
from .serializers import *
from .permissions import IsOwnerOrReadOnly
class ProductCategoryList(generics.ListCreateAPIView):
queryset = ProductCategory.objects.all()
serializer_class = ProductCategorySerializer
permission_classes = (permissions.IsAuthenticated,)
The code for the serializer is (file : *app_name/serializers.py*):
from rest_framework import serializers
from .models import *
class ProductCategorySerializer(serializers.ModelSerializer):
class Meta:
model = ProductCategory
fields = ('id', 'name') :
Add in your *urls.py* the following line
path('product/unit/api', views_api.ProductUnitList.as_view(), name='api-product-unit-list'),
Of course, you have to create as many API function as you need to retrieve list of values for any *select* input item
When you double-click on the information to change, an input field with the actual value is shown

Click again into the field, the list of options is shown.

Select the wanted value

And click outside the field.
The value is changed

Letโs have a look for a **Boolean** value, like if the product is available in the webshop. In the HTML we had:
<tr>
<td>{% trans "Available in the webshop" %}</td>
<td>
{{ object.webshop|YesNo }}
</td>
</tr>
Letโs add the attributes to enable the inline edit. The code is now:
<tr>
<td>{% trans "Available in the webshop" %}</td>
<td>
<span class="inline-edit"
data-id="{{ object.id }}"
data-name="bills.Product.webshop"
data-type="checkbox"
data-value="{% if object.webshop %}1{% else %}0{% endif %}">
{{ object.webshop|YesNo }}
</span>
</td>
</tr>
The output is:

For a date the HTML attributes will be:
<tr>
<td>{% trans "Available from" %}</td>
<td>
<span class="inline-edit"
data-id="{{ object.id }}"
data-name="bills.Product.available_from"
data-type="date"
data-value="{{ object.available_from|default_if_none:""|date:"Y-m-d" }}">
{{ object.available_from|default_if_none:""|date:"d/m/Y" }}
</span>
</td>
</tr>

You can of course select the date with the drop-down calendar

To close and save the modification, click outside the input field.
For a **number**:
<tr>
<td>{% trans "Default quantity" %}</td>
<td>
<span class="text-end inline-edit"
data-id="{{ object.id }}"
data-name="bills.ProductPackaging.default_quantity"
data-type="number"
data-value="{{ object.default_quantity }}">
{{ object.default_quantity }}
</span>
</td>
</tr>

Double-click on the value to open the inline edit

Update the value and click outside to validate the change

## Callback function ##
Sometimes when you edit a field, there is a dependency on another one. For exemple, modifying a price excluding vat, you would like that the price with vat will be updated. It is now possible.
Add *data-post_func* to the definition with the name of the javascript function to be call.
*data-post_func="calc_price_vat_incl"*
<span class="text-end inline-edit"
id="product-price-vat-excl_{{ object.id }}"
data-id="{{ object.id }}"
data-name="bills.product.price_vat_excl"
data-type="number"
data-value="{{ object.price_vat_excl }}"
data-post_func="calc_price_vat_incl">
{{ object.price_vat_excl }}
</span>
The javascript function could be something like :
function calc_price_vat_incl(id) {
var price_vat_excl = parseFloat($("#product-price-vat-excl_" + id).data("value"));
var vat = parseFloat($("#vat_" + id).data("value"));
var price_vat_incl = price_vat_excl * (1 + (vat / 100));
$("#product-price-vat-incl_" + id).html(price_vat_incl);
$("#product-price-vat-incl_" + id).data('value', price_vat_incl);
}
Note : the function always receive as argument the id of the object, so the function muse avec a parameter, i.e. *id*.
## The back end script
The back end script is written in python, based on the Django framework.
You can find the source in the file *utils.py* into the directory *lib/*.
The function `def upd_data(request):` is the code responsible to update the value of the specified field into your database.
You can find the code in the file *lib/utils.py*
Do not forget to add in the *urls.py* a reference to the function :
path("update/data/", upd_data, name="update_data" ),
## Contributing
Inline Edit is an open source program. Feel free to fork and contribute.
In order to keep the match between this documentation and the last release, please contribute and pull requests on the dedicated `develop` branch.
## License
**Inline Edit - inline Editor**
**Copyright (c) 2023 Pierre Delporte**
[pierre.delporte@alf-solution.be](mailto:pierre.delporte@alf-solution.be)
[https://www.alf-solution.be](https://www.alf-solution.br)
MIT License
Copyright (c) [year] [fullname]
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
| Inline field editor for Django apps | django,inline-editing,javascript,bootstrap5 | 2023-01-07T14:48:58Z | 2023-06-08T06:36:52Z | null | 1 | 0 | 14 | 0 | 0 | 4 | null | MIT | JavaScript |
kkmk11/My-Portfolio | master | null | This is my Personal Portfolio attached with Resume in it that includes all my details along with my Education, Programming Skills, Work Experience ,Projects and Contact details etc. | bootstrap5,css,html5,javascript,portfolio-website | 2023-01-11T20:37:25Z | 2023-08-12T12:21:32Z | null | 1 | 0 | 22 | 0 | 0 | 4 | null | null | HTML |
Ashu0Singh/Sonic-Library-System-Frontend | master | # QuietZone: Sonic Library Sensor System
#### The Ultimate Library Discipline Solution
### Overview
We have developed a sound sensing device that produces three distinct outputs based on the decibel levels around each table in a library. This device is integrated with software, allowing the library manager to analyze and control the library in real-time. The device is connected to a network, which sends information to the backend and displays it dynamically on the front end. The result is an efficient and accurate integration of hardware and software.
### Problem with the Current System
As students, we often go to the library to have peaceful and productive study or work sessions. However, there is often still a problem with disturbance, and the librarian must manually go from table to table to maintain discipline, which is inefficient and time-consuming. To address this problem, we have developed a simple, one-stop solution for managing the library with our device and software.
### Solution
Our device consists of a decibel sensor encoded in an Arduino. It will be installed at each table and controlled unisystematically. If the decibel level at a table increases, our device will alert the people at the table visually, without disturbing them, and also notify the librarian through our developed software. This will help solve the issue of discipline in libraries, save time for the librarian, and enable the efficient use of the library for all users.
### Objectives
- Maintain discipline in the library
- Automate the issues faced in the library
- Track each table in the library
- Reduce the workload of the librarian
- Enable the efficient use of the library for all users
- Enhance a motivating study-work environment for everyone
- Make simple yet efficient use of hardware with low cost requirements
### Website
#### Front End
- JavaScript framework: React.js
- Style sheet language: CSS (Cascading Style Sheets)
#### Back End
- JavaScript library: Express.js
- Cloud service: Google Cloud Platform's App Engine
The front end of the website was built using React.js and CSS, while the back end was developed using Express.js. The back end handles authentication and processes raw data from the Arduino, which is then sent to the front end to update the view. The project also uses Google Cloud Platform's App Engine as a cloud service.
Deployed using Gcloud: http://sonic-library.el.r.appspot.com/SonicLibrarySystem/
## Screenshots
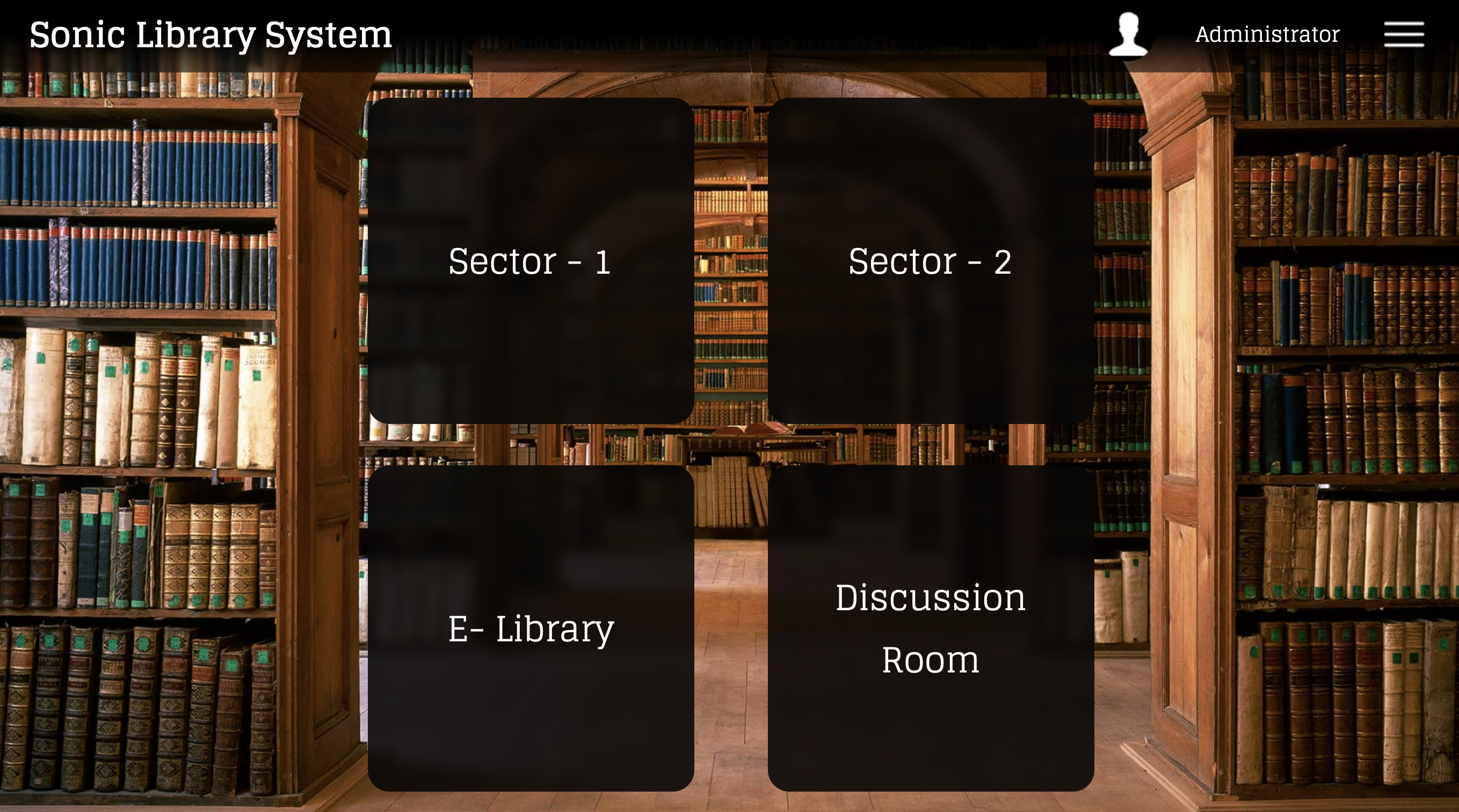
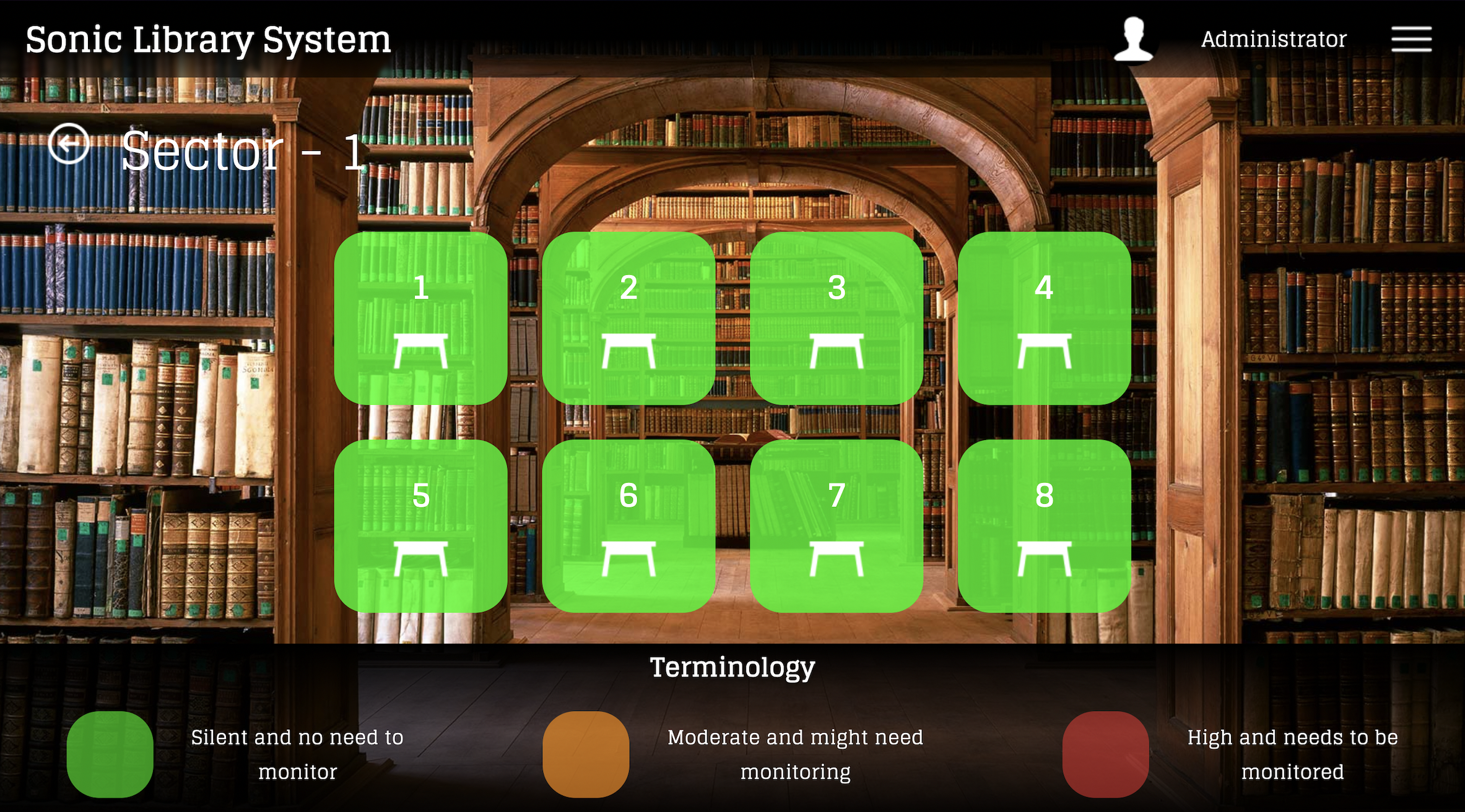
### Features
- Real-time monitoring: The website allows the library manager to view decibel levels at each table in real-time, allowing them to quickly identify and address any disruptions.
- Historical data analysis: The website provides a range of tools for analyzing decibel data over time, including graphs, charts, and other visualizations. This allows the library manager to identify trends and patterns, and make informed decisions on how to optimize the library environment.
- Alert notifications: The website can send notifications to the library manager when the decibel level at a table exceeds a certain threshold, alerting them to any disruptions that need to be addressed.
### One-Pager
[Document](https://docs.google.com/document/d/1v4ROqQ7UeUuHVVo-I8CKSAvSDu6iLb_uDSKcC_l0j2U/edit?usp=sharing)
### How to Deploy On Google Cloud?
First step, you have to enable App Engine Admin API in your Project. After enabling the API go to Cloud Shell and write the Command:
```
gcloud app create
```
Second step,open the Text Editor and create a new directory www and add the project folders and files in it.In the root directory create a file deploy.yaml add the following code in it:
```
runtime: python27
api_version: 1
threadsafe: true
handlers:
\- url: /
static_files: www/index.html
upload: www/index.html
\- url: /(.*)
static_files: www/\1
upload: www/(.*)
```
Third step,run the following commands:
```
gcloud app deploy deploy.yaml
gcloud app browse
```
(Remember to run this commands in the root directory)
<div align="center">
<h3>Connect With us on</h3>
<a href="https://twitter.com/gdscvitap" target="_blank"><img alt="Twitter" src="https://img.shields.io/badge/twitter-%231DA1F2.svg?&style=for-the-badge&logo=twitter&logoColor=white" /></a>
<a href="https://www.linkedin.com/company/dscvitap/" target="_blank"><img alt="LinkedIn" src="https://img.shields.io/badge/linkedin-%230077B5.svg?&style=for-the-badge&logo=linkedin&logoColor=white" /></a>
<a href="https://instagram.com/gdscvitap" target="_blank"><img alt="Instagram" src="https://img.shields.io/badge/instagram-%FF69B4.svg?&style=for-the-badge&logo=instagram&logoColor=white&color=cd486b" /></a>
</div>
----
GDSC VIT-AP projects adopt the [Contributor Covenant Code of Conduct](https://www.contributor-covenant.org/version/2/1/code_of_conduct.html). For more information see the [Code of Conduct FAQ](https://www.contributor-covenant.org/faq).
```javascript
if (youEnjoyed) {
starThisRepository();
}
```
-----------
[](https://forthebadge.com) [](https://forthebadge.com)
| We have created a sound sensing device integrated with software that monitors decibel levels at library tables. The device provides real-time analysis and control, alerting users visually and notifying the librarian when noise levels exceed a threshold. This efficient solution addresses the problem of maintaining discipline in libraries. | css,javascript,reactjs,rest-api | 2023-01-09T13:19:29Z | 2023-01-09T17:02:18Z | null | 2 | 1 | 3 | 0 | 1 | 4 | null | null | JavaScript |
Hasandev08/FYP-Individual-Part | master | # Getting Started with Create React App
This project was bootstrapped with [Create React App](https://github.com/facebook/create-react-app).
## Available Scripts
In the project directory, you can run:
### `npm start`
Runs the app in the development mode.\
Open [http://localhost:3000](http://localhost:3000) to view it in your browser.
The page will reload when you make changes.\
You may also see any lint errors in the console.
### `npm test`
Launches the test runner in the interactive watch mode.\
See the section about [running tests](https://facebook.github.io/create-react-app/docs/running-tests) for more information.
### `npm run build`
Builds the app for production to the `build` folder.\
It correctly bundles React in production mode and optimizes the build for the best performance.
The build is minified and the filenames include the hashes.\
Your app is ready to be deployed!
See the section about [deployment](https://facebook.github.io/create-react-app/docs/deployment) for more information.
### `npm run eject`
**Note: this is a one-way operation. Once you `eject`, you can't go back!**
If you aren't satisfied with the build tool and configuration choices, you can `eject` at any time. This command will remove the single build dependency from your project.
Instead, it will copy all the configuration files and the transitive dependencies (webpack, Babel, ESLint, etc) right into your project so you have full control over them. All of the commands except `eject` will still work, but they will point to the copied scripts so you can tweak them. At this point you're on your own.
You don't have to ever use `eject`. The curated feature set is suitable for small and middle deployments, and you shouldn't feel obligated to use this feature. However we understand that this tool wouldn't be useful if you couldn't customize it when you are ready for it.
## Learn More
You can learn more in the [Create React App documentation](https://facebook.github.io/create-react-app/docs/getting-started).
To learn React, check out the [React documentation](https://reactjs.org/).
### Code Splitting
This section has moved here: [https://facebook.github.io/create-react-app/docs/code-splitting](https://facebook.github.io/create-react-app/docs/code-splitting)
### Analyzing the Bundle Size
This section has moved here: [https://facebook.github.io/create-react-app/docs/analyzing-the-bundle-size](https://facebook.github.io/create-react-app/docs/analyzing-the-bundle-size)
### Making a Progressive Web App
This section has moved here: [https://facebook.github.io/create-react-app/docs/making-a-progressive-web-app](https://facebook.github.io/create-react-app/docs/making-a-progressive-web-app)
### Advanced Configuration
This section has moved here: [https://facebook.github.io/create-react-app/docs/advanced-configuration](https://facebook.github.io/create-react-app/docs/advanced-configuration)
### Deployment
This section has moved here: [https://facebook.github.io/create-react-app/docs/deployment](https://facebook.github.io/create-react-app/docs/deployment)
### `npm run build` fails to minify
This section has moved here: [https://facebook.github.io/create-react-app/docs/troubleshooting#npm-run-build-fails-to-minify](https://facebook.github.io/create-react-app/docs/troubleshooting#npm-run-build-fails-to-minify)
| It is the part of fyp that contains all of my work seperately. | bootstrap,css,front-end-development,html,javascript,material-ui,react,reactjs | 2023-01-09T21:02:45Z | 2023-05-22T19:49:53Z | null | 1 | 0 | 22 | 0 | 0 | 4 | null | null | JavaScript |
kalebzaki4/jogo-do-numero-secreto | main | # Jogo de Adivinhaรงรฃo por Voz
**Bem-vindo ao repositรณrio do Jogo de Adivinhaรงรฃo por Voz!** Este รฉ um jogo interativo em que o jogador precisa adivinhar um nรบmero gerado aleatoriamente atravรฉs de comandos de voz.
## Funcionalidades
- O jogo รฉ implementado em **JavaScript** usando tecnologias de reconhecimento de voz.
- Os jogadores podem interagir com o jogo usando comandos de voz para fazer suas tentativas de adivinhar o nรบmero.
- O jogo fornece feedback em tempo real sobre as tentativas dos jogadores, indicando se o nรบmero รฉ maior ou menor do que a tentativa atual.
- Ao acertar o nรบmero correto, o jogador recebe uma mensagem de parabรฉns e a pontuaรงรฃo รฉ registrada.
## Como Jogar
1. Clone este repositรณrio em sua mรกquina local.
2. Certifique-se de ter um navegador atualizado com suporte a reconhecimento de voz.
3. Abra o arquivo `index.html` em seu navegador.
4. Dรช permissรฃo para o navegador acessar o microfone.
5. Siga as instruรงรตes apresentadas na tela para comeรงar a jogar.
6. Use comandos de voz para fazer suas tentativas de adivinhaรงรฃo.
7. Receba feedback em tempo real sobre suas tentativas e continue a fazer novas tentativas atรฉ acertar o nรบmero correto.
8. Ao acertar o nรบmero, vocรช serรก parabenizado e sua pontuaรงรฃo serรก registrada.
## Dependรชncias
O jogo utiliza as seguintes dependรชncias:
- **JavaScript** - Linguagem de programaรงรฃo principal do jogo.
- **HTML** - Estrutura da pรกgina web do jogo.
- **CSS** - Estilizaรงรฃo e aparรชncia visual do jogo.
- **Web Speech API** - API de reconhecimento de voz do navegador.
## Contribuiรงรตes
Contribuiรงรตes sรฃo bem-vindas! Se vocรช deseja contribuir para o aprimoramento deste jogo, sinta-se ร vontade para enviar suas sugestรตes, correรงรตes de bugs ou novos recursos atravรฉs de pull requests.
Esperamos que vocรช se divirta jogando o **Jogo de Adivinhaรงรฃo por Voz!** Divirta-se e teste suas habilidades de adivinhaรงรฃo utilizando apenas sua voz.
| Bem-vindo ao repositรณrio do Jogo de Adivinhaรงรฃo por Voz! Este รฉ um jogo interativo em que o jogador precisa adivinhar um nรบmero gerado aleatoriamente atravรฉs de comandos de voz. | codigo,game,javascript,jogo | 2023-01-15T22:09:24Z | 2023-06-17T12:31:24Z | null | 1 | 0 | 1,420 | 0 | 2 | 4 | null | MIT | JavaScript |
Negar-La/Find-your-Food | main | <h1 align="center">๐๐ฒ Welcome to Find your Food! ๐ฒ๐</h1>
<p>๐ <strong> A Full-stack MERN App </strong></p>
<p>๐ Makes uses of <strong>HTML </strong>, <strong>CSS </strong>, <strong> ES6 JavaScript</strong>, and <strong> React</strong> on the FE, <strong> NodeJS</strong>, <strong> ExpressJS </strong>, and <strong> MongoDB </strong> on the BE. </p>
<p>๐ The APIs: <a href="https://developers.google.com/maps"> <strong>Google Map API </strong> </a>, <a href="https://auth0.com/docs/quickstart/spa/react/interactive"> <strong>Auth0</strong> </a>, <a href="https://cloudinary.com/documentation"> <strong>Cloudinary</strong></a>, and <a href="https://socket.io/"> <strong>Socket.io</strong> </a> </p>
<p>๐ In this platform, you can surf meals and get connected with the cook. </p>
<p>๐ User can add a post to favorite list or make a new post and edit/delete it or send a message to cook. </p>
<p>๐ <a href="https://socket.io/"> Socket.io </a> provides a live chat system. </p>
<p>๐ <a href="https://developers.google.com/maps"> Google Map API </a> provides location and based on origin address, the duration and distance is calculated. </p>
<p>๐ <a href="https://cloudinary.com/documentation"> Cloudinary </a> stores the pictures used accross the website as well as a widget to upload picture of the food. </p>
<p>๐ <a href="https://auth0.com/docs/quickstart/spa/react/interactive"> Auth0 </a> provides a secure and fast log in. </p>
<p align="center"> Click <a href="https://find-your-food.onrender.com"><strong> Here</strong> </a> to view the deployed website at render.com </p>
#### On Mobile device

#### Screenshot of Home page

#### Screenshot of Post a new Add

#### Screenshot of View Post Details

#### Screenshot of Showing on Google Map

#### Screenshot of My Favorite Posts

#### Screenshot of Live Chat

#### Screenshot of Menu

| A Full-stack MERN App where you can surf meals and get connected with the cook. | expressjs,javascript,mongodb,nodejs,reactjs | 2023-01-04T21:53:58Z | 2023-11-11T07:24:48Z | null | 1 | 16 | 95 | 0 | 0 | 4 | null | null | JavaScript |
CharlesCarr/react-data-fetch | main | ## Data Fetching in React Examples...
This GitHub repo is to serve as examples to the approaches in my article on data fetching in React on Dev.to.
[Dev.to Article](https://dev.to/char_carr_dev/6-approaches-for-data-fetching-in-react-1ec3)
### How to Navigate Repo
Each 'Approach' number in the article has a coordinating component in the src/components directory that is prefixed with 'Example'.
**Note:** for the last three examples there are additional files to review within this application (wrappers, redux store, hooks, etc.)
Hope this helps! | Data Fetch Example Repository (along with Dev.to article '6 Approaches to Data Fetching in React'). The app simply displays a random image of a dog using the random.dog external API | article,demo,dogs,fetch,fetch-api,fetching,javascript,react,reactjs | 2023-01-16T15:09:12Z | 2023-01-31T00:45:31Z | null | 1 | 0 | 9 | 0 | 1 | 4 | null | null | JavaScript |
Divya-Konala/Business-Landing-Page | master | null | A responsive business webpage which includes three section which are banner, about company, services and a form to contact | bootstrap5,css-animations,css3,html5,javascript | 2023-01-14T11:40:40Z | 2023-01-14T11:42:22Z | null | 1 | 0 | 1 | 0 | 0 | 4 | null | null | CSS |
runtfint/react-app-posts | master | # SIMPLY REACT APP
Fetching posts from REST API (axios)
Custom UI components with module.css
Using hooks and creating custom hooks
Routing by React Router
Simply login form / private & public routes
Sorting & Filtering posts
Dynamically navigation
Dynamically pagitation and endless feed (useObserver)
| Simply React App with posts from rest api | axios-react,javascript,react-components,react-hooks,reactrouter,reactrouterdom | 2023-01-11T14:47:49Z | 2023-05-19T06:02:38Z | null | 2 | 1 | 13 | 0 | 0 | 4 | null | null | JavaScript |
RileyXX/MeijerAutoCouponClipper | main | # MeijerAutoCouponClipper
This script allows you to automatically clip coupons on Meijer.com for user-desired categories.
## How to Use:
1. Copy the following [JavaScript code](https://github.com/RileyXX/MeijerAutoCouponClipper#javascript-code-preset-food-only-categories).
2. Login to Meijer.com and navigate to the [coupons page](https://www.meijer.com/shopping/coupons.html).
3. In your browser, press F12 or right-click and select "Inspect Element".
4. Click the console tab in the popup window.
5. Paste the JavaScript code in the box at the bottom and press Enter.
*Note: These instructions are for the Chrome browser. The hotkeys might vary depending on your browser/operating system.*
## Javascript Code (preset food only categories)
```javascript
const maxCoupons = 160;
const categories = ['Other', 'Bakery', 'Dairy', 'Deli', 'Dry Grocery', 'Fresh Meat', 'Fresh Seafood', 'Frozen Foods', 'Packaged Meat', 'Produce', 'Drinks'];
fetch("https://raw.githubusercontent.com/RileyXX/MeijerAutoCouponClipper/main/MeijerAutoCouponClipper.js")
.then((e => e.text()))
.then((e => {
let t = document.createElement("script");
t.innerHTML = e;
document.body.appendChild(t);
}))
.catch((e => console.error(e)));
```
Copy the code and go to [step 2](https://github.com/RileyXX/MeijerAutoCouponClipper#how-to-use) of the "How to use" section. Alternatively, to download this code as a text file for later use right click [this link](https://raw.githubusercontent.com/RileyXX/MeijerAutoCouponClipper/main/MeijerAutoCouponClipper.txt) > save link as > save. Or open link and ctrl+s to download.
<br><br>Source code can be found [here](https://github.com/RileyXX/MeijerAutoCouponClipper/blob/main/MeijerAutoCouponClipper.js).
## Alternative Javascript Code (manually select coupon categories from the meijer website before running the code)
```javascript
const maxCoupons = 160;
fetch("https://raw.githubusercontent.com/RileyXX/MeijerAutoCouponClipper/main/MeijerAutoCouponClipperNoCategories.js")
.then((e => e.text()))
.then((e => {
let t = document.createElement("script");
t.innerHTML = e;
document.body.appendChild(t);
}))
.catch((e => console.error(e)));
```
Copy the code and go to [step 2](https://github.com/RileyXX/MeijerAutoCouponClipper#how-to-use) of the "How to use" section. Alternatively, to download this code as a text file for later use right click [this link](https://raw.githubusercontent.com/RileyXX/MeijerAutoCouponClipper/main/MeijerAutoCouponClipperNoCategories.txt) > save link as > save. Or open link and ctrl+s to download.
<br><br>Source code can be found [here](https://github.com/RileyXX/MeijerAutoCouponClipper/blob/main/MeijerAutoCouponClipperNoCategories.js).
## How to Edit:
1. Open the text file you created with the code.
2. Replace the `maxCoupons` value `160` in line 1 with your desired amount of coupons to clip (e.g., 150).
3. Replace or add categories manually in line 2. Make sure to use the same format, with quotes around each category and a comma separator.
4. Save the .txt file.
## Notes/Limitations:
- Sending too many requests at once can result in your IP being temporarily banned from accessing the Meijer website. Try not to paste the code twice or run it too many times within a short time frame. It's recommended to use a VPN, but not necessary. If your IP gets banned, simply click the little shield button in the navigation bar of your browser and delete all the cookies for Meijer.com. Then change VPN server or wait (the duration of the ban is unknown, could range anywhere from a few minutes to 24 hours).
- Meijer.com allows clipping up to 160 coupons. By default, only the food categories are selected, but you can manually edit the categories. Select only the categories you know you will use the most.
## Demo:
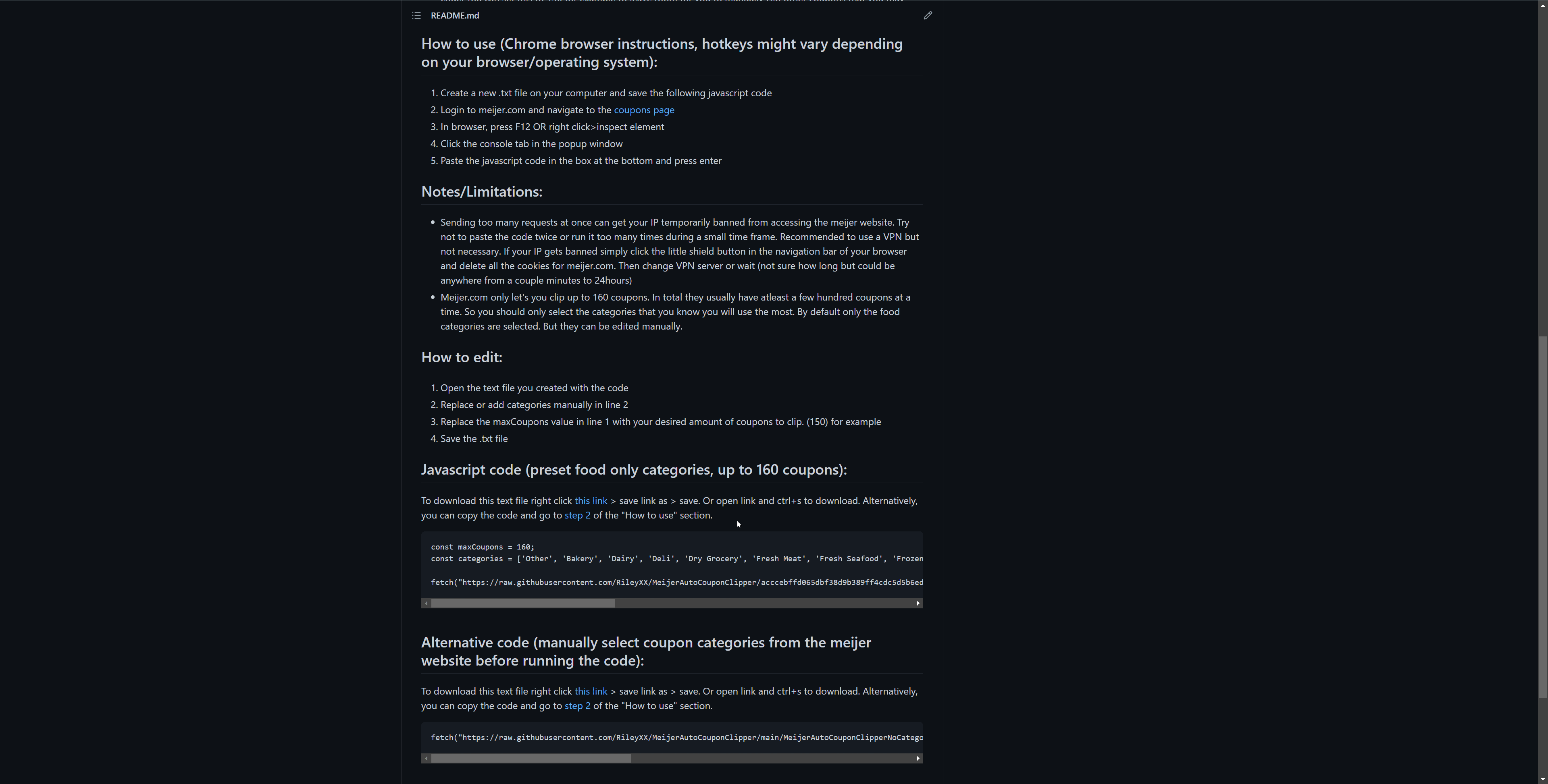
## Features:
- Automatically selects coupons on Meijer.com by category, with a maximum of 160 coupons. This is a limitation set by Meijer.
- Works on any browser (hotkeys might vary).
- Alternative categories can be selected by editing and saving the coupon categories in the code.
- The maximum number of coupons to clip can be modified by editing the `maxCoupons` value in the code's first line. The default is set to 160 (the maximum limit). Do not set this value higher than 160.
## Description:
I created this script to easily clip all the coupons on Meijer.com because they have hundreds of them, and manually going through them all to find what I wanted was time-consuming. With this script, it only takes a couple of seconds to clip all the coupons at once, allowing me to get the discounts automatically in-store or by placing an order on the website. I noticed that other users on Reddit were looking for similar scripts for different stores, but I couldn't find anything specifically for Meijer. That's why I created this script. I may improve this script and turn it into a Chrome extension at some point in the future.
## This Tool Was Also Posted On:
- [Reddit](https://www.reddit.com/r/meijer/comments/17xcxeh/auto_clip_all_coupons_script_for_meijercom_with/)
## Sponsorships, Donations, and Custom Projects:
If you find my scripts helpful, you can become a [sponsor](https://github.com/sponsors/RileyXX) and support my projects! If you need help with a project, open an issue, and I'll do my best to assist you. For other inquiries and custom projects, you can contact me on [Twitter](https://twitter.com/RileyxBell).
#### More Donation Options:
- Cashapp: `$rileyxx`
- Venmo: `@rileyxx`
- Bitcoin: `bc1qrjevwqv49z8y77len3azqfghxrjmrjvhy5zqau`
- Amazon Wishlist: [Link โ](https://www.amazon.com/hz/wishlist/ls/WURF5NWZ843U)
| This script will auto clip coupons on Meijer.com for user desired categories. | javascript,meijer | 2023-01-11T13:49:01Z | 2024-04-08T01:07:59Z | null | 1 | 0 | 51 | 0 | 0 | 4 | null | null | JavaScript |
Mujeeb4582/Air-Pollution-App | dev | <a name="readme-top"></a>
<!--
HOW TO USE:
This is an example of how you may give instructions on setting up your project locally.
Modify this file to match your project and remove sections that don't apply.
REQUIRED SECTIONS:
- Table of Contents
- About the Project
- Built With
- Live Demo
- Getting Started
- Authors
- Future Features
- Contributing
- Show your support
- Acknowledgements
- License
After you're finished please remove all the comments and instructions!
-->
<div align="center">
     
</div>
<!-- TABLE OF CONTENTS -->
# ๐ Table of Contents
- [๐ About the Project](#about-project)
- [Screen Shot](#app-screen-shots)
- [๐ Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [๐ Video Demo](#video-demo)
- [๐ Live Demo](#live-demo)
- [๐ป Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#triangular_flag_on_post-deployment)
- [๐ฅ Authors](#authors)
- [๐ญ Future Features](#future-features)
- [๐ค Contributing](#contributing)
- [โญ๏ธ Show your support](#support)
- [๐ Acknowledgements](#acknowledgements)
- [โ FAQ](#faq)
- [๐ License](#license)
<!-- PROJECT DESCRIPTION -->
# ๐ Air Pollution App <a name="about-project"></a>
This mobile-web application retrieves data on air pollution from an API and displays it in a web browser. It was designed using React and Redux.
## App Screen-Shots <a name="app-screen-shots"></a>
<div align="center">
<img src="./src/images/HomePage.jpeg" width="300px"</img>
<img src="./src/images/DetailsPage.jpeg" width="300px"</img>
</div>
## ๐ Built With <a name="built-with"></a>
<a name="built-with"> <br>  <br>  <br>  <br> </a>
### Tech Stack <a name="tech-stack"></a>
<details>
<summary>Client</summary>
<ul>
<li><a href="https://reactjs.org/">React.js</a></li>
</ul>
</details>
<details>
<summary>Database</summary>
<ul>
<li><a href="https://openweathermap.org/api/air-pollution#:~:text=Air%20Pollution%20API%20concept,-Air%20Pollution%20API&text=Besides%20basic%20Air%20Quality%20Index,PM2.5%20and%20PM10).">Air Pollution API</a></li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
- SPA
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## ๐ Video Demo <a name="video-demo"></a>
- 5 mint short [Video Demo Link](https://www.loom.com/share/88fd29d7c7fa4231a05c2790b3a14b6a)
## ๐ Live Demo <a name="live-demo"></a>
- [Live Demo Link](https://air-pollution.onrender.com/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## ๐ป Getting Started <a name="getting-started"></a>
To get a local copy up and running, follow these steps.
### Prerequisites
In order to run this project you need:
- [Node](https://nodejs.org/en/) installed in your computer
- [Git](https://git-scm.com/) installed in your computer
<!--
Example command:
```sh
gem install rails
```
-->
### Setup
Clone this repository to your desired folder:
```sh
cd my-folder
git clone https://github.com/Mujeeb4582/Air-Pollution-App.git
```
### Install
Install this project with:
```sh
cd my-project
npm install
```
### Usage
To run the project, execute the following command:
```sh
npm start
```
### Run tests
To run tests, run the following command:
```sh
npm test
```
### Deployment
You can deploy this project using:
```sh
npm build
```
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## ๐ฅ Authors <a name="authors"></a>
๐ค **Mujeeb ur Rahman**
- GitHub: [@Mujeeb4582](https://github.com/Mujeeb4582)
- Twitter: [@Mujeebu93992980](https://twitter.com/Mujeebu93992980)
- LinkedIn: [@Mujeeb](https://www.linkedin.com/in/rahman-mujeeb/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## ๐ญ Future Features <a name="future-features"></a>
- Add search country by voice
- Allow user to add new data to API
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## ๐ค Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](https://github.com/Mujeeb4582/Air-Pollution-App/issues).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## โญ๏ธ Show your support <a name="support"></a>
If you like this project give a Starโญ๏ธ.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## ๐ Acknowledgments <a name="acknowledgements"></a>
- Thanks to the Microverse team for the great curriculum.
- Thanks to the Code Reviewer(s) for the insightful feedback.
- Thanks to Nelson Sakwa on [Behance](https://www.behance.net/gallery/31579789/Ballhead-App-(Free-PSDs)) for providing us this template.
- Hat tip to anyone whose code was used.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FAQ (optional) -->
## โ FAQ <a name="faq"></a>
- **How to open the Air pollution details?**
- *You can click/tap on the country name or card it will navigate to details report page.*
- **How to return to the home page?**
- *You can click the left arrow located in the top-left corner of the page.*
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## ๐ License <a name="license"></a>
This project is [MIT](https://github.com/Mujeeb4582/Air-Pollution-App/blob/react-redux-capstone/Licence) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| This mobile-web application retrieves data on air pollution from an API and displays it in a web browser. It was designed using React and Redux. | bootstrap,css,eslint,html,javascript,reactjs,testing | 2023-01-02T12:17:16Z | 2023-01-14T07:56:06Z | null | 1 | 1 | 51 | 0 | 0 | 4 | null | MIT | JavaScript |
arpitmiahra4/Blue-Apple | main | # Blue Apple
Full-Stack Apple with Admin Dashboard & Paytm Payment Gateway.
Apple Inc. (formerly Apple Computer Inc.) is an American computer and consumer electronics company famous for creating the iPhone, iPad and Macintosh computers. Apple is one of the largest companies globally with a market cap of over 2 trillion dollars.
[Visit Now](https://blueapple.vercel.app/) ๐
## ๐ฅ๏ธ Tech Stack
**Frontend:**






**Backend:**



**Payment Gateway:**

**Deployed On:**
## Vercel
**Image Management:** [Cloudinary](https://cloudinary.com/)
## ๐ Features
- Login/Signup User Account
- Update Profile/Password User Account
- Cart Add/Remove Items
- My Orders (With All Filters)
- Order Details of All Ordered Item
- Review Products User Account
- Admin: Dashboard access to only admin roles
- Admin: Update Order Status | Delete Order
- Admin: Add/Update Products
- Admin: Update User Data | Delete User
- Admin: List Review of Product | Delete Review
- Stock Management: Decrease stock of product when shipped
## Wanna Look of Admin Dashboard And UI ๐ :

<table>
<tr>
<td><img src="https://i.ibb.co/0fYPt1v/Screenshot-20230122-164245.png" alt="mockup" /></td>
<td><img src="https://i.ibb.co/2hJvDVd/Screenshot-20230122-164254.png" alt="mockups" /></td>
</tr>
<tr>
<td><img src="https://i.ibb.co/7bfBYSg/Screenshot-20230122-164109.png" alt="mockup" /></td>
<td><img src="https://i.ibb.co/M1BKw7c/Screenshot-20230122-164131.png" alt="mockups" /></td>
</tr>
</table>
<h2>๐ฌ Contact</h2>
If you want to contact Our Team, you can reach us through below handles.
## Arpit Mishra
[](https://www.linkedin.com/in/arpit-mishra-662199222/)
## Vicky Kumar
[](https://www.linkedin.com/in/vicky-paswan/)
## Shiv Kant Dubey
[](https://www.linkedin.com/in/shiv-kant-dubey-94334b245/)
## Alok Verma
[](https://www.linkedin.com/in/alok-kumar-verma-2b4b43158/)
## Noor Mohammed M
[](https://www.linkedin.com/in/m-noor-mohammed-6130a4165/)
ยฉ 2023 arpit-mishra
[](https://forthebadge.com)
| Blue Apple. a online store to sale (macbook, iphone, ipad, watches) and many more | chakra-ui,javascript,mongodb-atlas,nodejs,reactjs | 2023-01-16T07:33:14Z | 2023-02-15T08:11:39Z | null | 6 | 12 | 81 | 0 | 0 | 4 | null | MIT | JavaScript |
raj03kumar/siren-jibaro | main | # siren-jibaro
This is a website related to a Netflix episode. In this repo i practiced how to embed YouTube video to our website
| This is a website related to a Netflix episode. In this repo i practiced how to embed YouTube video to our website | embedded,html-css-javascript,javascript,youtube-api,youtube-embed-plugin | 2023-01-15T18:40:46Z | 2023-01-15T18:43:41Z | null | 1 | 0 | 3 | 0 | 0 | 4 | null | GPL-3.0 | HTML |
Anchovia/HamstoryWebProject | master | # ๊ฒจ์ธ ์นํ์ด์ง ๊ฐ๋ฐ ์ต์ ํ๋ก์ ํธ
## 0. ํํ์ด์ง ์ฃผ์
* ### https://anchovia.github.io/HamstoryWebProject/
## 1. ํํ์ด์ง ์ด๋ฆ
* ### Hamstory
## 2. ํํ์ด์ง ์ฃผ์
* ### ํ์คํฐ ๊ด๋ จ ์ปค๋ฎค๋ํฐ ๋ฐ ์ํค
## 3. ์ ์ ์ธ์
| ์ญํ | ์ด๋ฆ(github) |
| ---- | ------------ |
| ํ์ฅ | Anchovia |
| UX/UI & ํ๋ก ํธ์๋ | Anchovia |
| ๋ฐฑ์๋ & QA | Jiyun82 |
| ๋ฐฑ์๋ & QA | tmdgus0228 |
## 4. ์คํ
์ดํฐ์ค
| Status | ์ค๋ช
|
| ------ | ---- |
| ๐ <span style="color:blue">New</span> | ํ์ธํ์ง ์์ ์๋ก์ด ์ด์ |
| ๐ <span style="color:ffd33d">Backlog</span> | ํด๋น ์ด์๋ฅผ ํ์ธํ๊ณ , ๋ฌธ์ ์ ์ ํ์์ค์ |
| ๐ <span style="color:orange">Ready</span> | ํด๋น ์ด์๋ฅผ ์์
ํ ์ค๋น๊ฐ ๋จ |
| ๐ <span style="color:red">In progress</span> | ํด๋น ์ด์์ ๋ํด ์์
์ค์ |
| ๐ <span style="color:violet">In review</span> | ํด๋น ์ด์๋ฅผ ํผ๋๋ฐฑ ํ๋ ๋ฑ ๋ง๋ฌด๋ฆฌ ์์
์ ํจ |
| โ
<span style="color:green">Done</span> | ์๋ฃ๋ ์ด์ |
## 5. ๋ ์ด๋ธ
| Label | ์ค๋ช
|
| ----- | ---- |
| ๐ <span style="color:blue">Feature</span> | ์๋ก์ด ๊ธฐ๋ฅ์ ์ถ๊ฐ |
| โป <span style="color:green">Refectoring</span> | ์ฝ๋ ๋ฆฌํฉํ ๋ง |
| ๐ <span style="color:green">CleanUp</span> | ํ์ผ ํน์ ํด๋๋ช
์ ์์ /์ญ์ ํ๊ฑฐ๋ ์ฎ๊ธฐ๋ ์์
๋ง์ธ ๊ฒฝ์ฐ |
| ๐จ <span style="color:ffd33d">Design</span> | CSS ๋ฑ ์ฌ์ฉ์ UI ๋์์ธ ๋ณ๊ฒฝ |
| ๐จ <span style="color:orange">Comment</span> | ์ฃผ์ ์ถ๊ฐ ๋ฐ ๋ณ๊ฒฝ |
| ๐ฐ <span style="color:orange">Document</span> | README ๋ฑ ๋ฌธ์ ์์ |
| ๐ <span style="color:red">BugFix</span> | ๋ฒ๊ทธ ์์ |
| โ <span style="color:red">HOTFIX</span> | ๊ธํ๊ฒ ์น๋ช
์ ์ธ ๋ฒ๊ทธ๋ฅผ ๊ณ ์ณ์ผํ๋ ๊ฒฝ์ฐ |
| ๐งช <span style="color:violet">Test</span> | ํ
์คํธ ์ฝ๋, ๋ฆฌํํ ๋ง ํ
์คํธ ์ฝ๋ ์ถ๊ฐ |
| โ <span style="color:violet">Chore</span> | ๋น๋ ์
๋ฌด ์์ , ํจํค์ง ๋งค๋์ ์์ , ํจํค์ง ๊ด๋ฆฌ์ ๊ตฌ์ฑ ๋ฑ ์
๋ฐ์ดํธ |
| โ <span style="color:blueviolet">Question</span> | ์ง๋ฌธ์ด ํ์ํ ์ด์์ธ ๊ฒฝ์ฐ |
| โ <span style="color:blueviolet">HelpWanted</span> | ๊ฐ์ธ์ ๋
ธ๋ ฅ์ผ๋ก๋ ํด๊ฒฐ์ด ์ด๋ ค์ด ๊ณผ์ ์ธ ๊ฒฝ์ฐ |
## 6. API ๋ช
์ธ์
* ### https://www.notion.so/fde421774beb41a1b05efdc1a0df83a7?v=8852182db97f4284a984258c88dd8c40 | ์น์ฌ์ดํธ ์ต์ ๊ฐ๋ฐ ํ๋ก์ ํธ | react,spring-boot,css,html,javascript,java | 2023-01-04T11:53:48Z | 2023-07-27T15:22:14Z | null | 3 | 92 | 272 | 8 | 2 | 4 | null | null | JavaScript |
Rafucho25/numeric-keyboard-amazfit-devices | master | # Numeric keyboard for amazfit devices
A simple numeric keyboard for amazfit band 7, GTS 4, GTS 4 mini and GTS 3.
## How to install
* Download the utils folder at the same level as the page folder.
## How it works
* The constructor accept one parameter: the coordinate "y" of the keyboard this is for example if you want to display something before the keyboard you can.
* You can use the file example.js(put in the page folder) to see the keyboard in action
## How to use(example for band 7)
* Add this line to the page you want to display the keyboard:
```javascript
import { keyboard_band7 } from '../utils/keyboard' //add or remove ../ depends of your page folder location
```
* Create an instance:
```javascript
const keyboard = new keyboard_band7()
```
* Get text:
```javascript
keyboard.text_value
```
* For GTS 4 and gts 3:
```javascript
import { keyboard_gts3_4 } from '../utils/keyboard'
```
* For GTS 4 mini:
```javascript
import { keyboard_gts4_mini } from '../utils/keyboard'
```
## Screenshot
<h3><img align="center" src="https://user-images.githubusercontent.com/16562078/211363831-de69a143-ae50-448a-95e7-9d684fc634e5.png"> Default</h3>
<h3><img align="center" src="https://user-images.githubusercontent.com/16562078/211368692-db0438c1-9080-4032-9bc3-771c54914adc.png"> Parameter "y" = 100</h3>
## Notes
This is my first project using javascript so maybe there are ways to improve the quality of the code.
Let me know if you have any ideas or recommendations to improve. | Numeric keyboard for amazfit band 7, gts 4, gts 4 mini and gts 3 with zepp os v1 | amazfit,zeppos,band7,javascript,keyboard,zepp,gts3,gts4,gts4mini | 2023-01-09T15:51:11Z | 2023-02-12T03:26:45Z | null | 2 | 0 | 9 | 0 | 0 | 4 | null | null | JavaScript |
piyush-agrawal6/ChatAI-Bot | master |
## To Run Locally
Clone the project
```bash
git clone https://github.com/piyush-agrawal6/ChatAI-Bot.git
```
Install dependencies
```bash
npm install
```
Start the server
```bash
npm run dev
```
# Chat AI
Chat AI is a large language model chatbot made by using OpenAI based on text-davinci-003 API. It has a remarkable ability to interact in conversational dialogue form and provide responses that can appear surprisingly human.
<!-- <img src="https://i.ibb.co/4WkwWc2/logo.png" width="500" height="190px"> -->
## Tech Stack
**Client:**
- **JavaScript**
- **HTML**
- **CSS**
- **Fetch API**
**Server:**
- **Node Js**
- **OpenAI API**
- **CYCLIC**
## ๐ Links
Client - https://chat-ai-bot.netlify.app/
Server - https://github.com/piyush-agrawal6/ChatAI---Backend
## Features
- API Request
- Responsive design
- Error handling
## Screenshots
Main page

## Blog / Presentation
Presentation -
Blog -
## Contributors
- [@Piyush Agrawal](https://github.com/piyush-agrawal6)
| Chat AI is a large language model chatbot developed by using OpenAI based on text-davinci-003 API. It has a remarkable ability to interact in conversational dialogue form and provide responses that can appear surprisingly human. | api,chatbot,javascript,openapi,text-davinci-003 | 2023-01-01T02:25:00Z | 2023-01-01T06:53:28Z | null | 1 | 0 | 19 | 0 | 1 | 4 | null | null | CSS |
jackseg80/cryptoBot_2023 | master | # backtest_tools
## Set up du projet
----
Prรฉrequis: Python, Node, Git
Mise en place du projet:
>git clone https://github.com/jackseg80/cryptoBot_2023.git
>cd backtest_tools
Mise en place de l'environnement virtuel (trรจs recommandรฉ):
>python -m venv .venv
>.venv\Scripts\activate
>pip install -r .\requirements.txt
Installation des dรฉpendances python (Bien vรฉrifier que vous avez (.venv) au dรฉbut de votre ligne de commande):
>pip install -r requirements.txt
Installation des dรฉpendances node:
>npm install
Le projet est prรชt ร รชtre utilisรฉ. | Robot Trading (analysis, backtest) | javascript,python,analysis,backtesting,crypto,trading-bot | 2023-01-11T17:07:58Z | 2024-01-17T18:01:43Z | null | 3 | 0 | 150 | 0 | 1 | 4 | null | null | Jupyter Notebook |
D4Community/d4-website | master |
<p align="center">
<img src="https://user-images.githubusercontent.com/85388413/220329457-fad38629-4100-4703-843c-125ef2bd1c3d.png" height="120">
</p>
<h1 align= "center"><a href="https://d4community.netlify.app/">D4-Community Website</a></h1>
Website for the D4 Community that helps in keeping engagement with members and keeps them updated for the latest community updates/events.
### ๐ ๏ธ Installation
1. Fork the repo
2. Clone the repository `git clone https://github.com/D4Community/d4-website.git `
3. Run `npm i` Or `npm install`to install all dependencies
4. Run `npm start` to start the application
5. Visit `https://localhost:3000` to view the application
## โ๏ธ Tech Stack/ Libraries used:
1. [React](https://reactjs.org/)
2. [HTML5](https://developer.mozilla.org/en-US/docs/Glossary/HTML5)
3. [CSS3](https://developer.mozilla.org/en-US/docs/Web/CSS)
4. [JavaScript](https://developer.mozilla.org/en-US/docs/Web/JavaScript)
### ๐งพ Prerequisites
Before starting out, you'll need to install the following on your computer.
1. [NodeJS](https://nodejs.org/en/download/)
2. [Git](https://git-scm.com/downloads)
3. [NPM](https://www.npmjs.com/)
# ๐ Contribute
Contributions make the open source community an amazing place to learn, inspire, and create.
Any contributions you make are **truly appreciated**.<br>
Before contributing, see <a href="https://github.com/D4Community/d4-website/blob/master/CONTRIBUTING.md">Contribution guide</a> for more information.
# Code of Conduct
Check out <a href="https://github.com/D4Community/d4-website/blob/master/CODE_OF_CONDUCT.md">Code of Conduct</a> to know inclusive environment that respects all contributions.
## Thanks to all the contributors โค๏ธ
<img src="https://contrib.rocks/image?repo=D4Community/d4-website"/>
| This Repository contains the Codebase for D4-Community website developed using React. | react,css,html,open-source,website,javascript,hacktoberfest | 2023-01-12T23:08:54Z | 2023-11-01T18:15:44Z | null | 9 | 12 | 69 | 4 | 6 | 4 | null | Apache-2.0 | JavaScript |
ADITYAGABA1322/LeetCode-Soluion-in-cpp-Java | main | # LeetCode-Soluion-in-C++ && JAVA && Python && Python3 && C && C# && JavaScript && Swift && Dart ๐ซถ
Hi Everyone. It's me Aditya and Here I'm providing you with Top Companies(MAANG) ask LeetCode Question Solutions with full Explanation(Line to Line)
In Nine Languages:
C++๐ซถ && JAVA && Python && Python3 && C && C# && JavaScript && Swift && Dart ๐ฅ
<img align="right" src="https://fastly.jsdelivr.net/gh/doocs/leetcode@main/images/Knight.gif" width="20%" />
<img src="https://user-images.githubusercontent.com/73097560/115834477-dbab4500-a447-11eb-908a-139a6edaec5c.gif" width = "70%" >
<img align="bottom" src="https://th.bing.com/th/id/OIP.fL9ebmsw89Pfx_DWsgLDkAHaB7?pid=ImgDet&rs=1" width="70%" />
<img src="https://user-images.githubusercontent.com/73097560/115834477-dbab4500-a447-11eb-908a-139a6edaec5c.gif" width="70%">
<img align="bottom" src="https://assets.leetcode.com/lc_store/leetcode_kit/leetcode_kit_1640301367.png" width="100%" />
| MISSION CRACK MAANG ๐ | leetcode,consistency,cpp,java,c,csharp,javascript,python,python3,swift | 2023-01-10T09:44:03Z | 2024-05-21T18:29:08Z | null | 1 | 0 | 6,401 | 0 | 2 | 4 | null | GPL-3.0 | Java |
t-bello7/micro_budget_app | dev | <a name="readme-top"></a>
# ๐ Table of Contents
- [๐ About the Project](#about-project)
- [๐ Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [๐ Live Demo](#live-demo)
- [๐ป Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#triangular_flag_on_post-deployment)
- [๐ฅ Authors](#authors)
- [๐ญ Future Features](#future-features)
- [๐ค Contributing](#contributing)
- [โญ๏ธ Show your support](#support)
- [๐ Acknowledgements](#acknowledgements)
- [โ FAQ](#faq)
- [๐ License](#license)
<!-- PROJECT DESCRIPTION -->
# ๐ MyBudget <a name="about-project"></a>
> MyBudget application
** Mybudget ** is a ruby project where you can manage your budget: you have a list of transactions associated with a category, so that you can see how much money you spent and on what.
<!--
<div style="position: relative; padding-bottom: 56.25%; height: 0;"><iframe src="https://www.loom.com/embed/18895e5ca97a4224b5b5add9f127a99c" frameborder="0" webkitallowfullscreen mozallowfullscreen allowfullscreen style="position: absolute; top: 0; left: 0; width: 100%; height: 100%;"></iframe></div> -->
[](https://www.loom.com/embed/18895e5ca97a4224b5b5add9f127a99c)
## ๐ Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
<details>
<summary>Client</summary>
<ul>
<li><a href="https://sass-lang.com/">SCSS</a></li>
</ul>
</details>
<details>
<summary>Server</summary>
<ul>
<li><a href="https://rubyonrails.org/">Ruby on Rails</a></li>
</ul>
</details>
<details>
<summary>Database</summary>
<ul>
<li><a href="https://www.postgresql.org/">PostgreSQL</a></li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
> Describe between 1-3 key features of the application.
- **Users can register**
- **Users can Login**
- **Users can create budget transactions based on categories**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## ๐ Live Demo <a name="live-demo"></a>
> Add a link to your deployed project.
- [Live Demo Link](https://budget-app-uy9a.onrender.com)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## ๐ป Getting Started <a name="getting-started"></a>
> Describe how a new developer could make use of your project.
To get a local copy up and running, follow these steps.
### Prerequisites
In order to run this project you need:
- Ruby
- Knowledge of Gems, Rails and Ruby
<!--
Example command:
```sh
gem install rails
```
-->
### Setup
Clone this repository to your desired folder:
```
git clone https://github.com/t-bello7/micro-budget_app.git
```
### Install
Install this project with:
```
cd micro-budget
gem install
```
### Usage
To run the project, execute the following command:
```
bin/rails server
```
### Run tests
To run tests, run the following command:
Example command:
```
bin/rails rspec spec
```
### Deployment
You can deploy this project using:
<!--
Example:
```sh
```
-->
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## ๐ฅ Authors <a name="authors"></a>
๐ค **Author1**
- GitHub: [@t-bello7](https://github.com/t-bello7)
- Twitter: [@__tbello](https://twitter.com/__tbello)
- LinkedIn: [Bello Oluwatomisin](https://linkedin.com/in/tbello7)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## ๐ค Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## โญ๏ธ Show your support <a name="support"></a>
If you like this project you can show support by giving a star to the project
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## ๐ Acknowledgments <a name="acknowledgements"></a>
- I would like to thank microverse and Gorails community
- Snapscan - iOs design and branding Design Guidelies by Gregoire Vella https://www.behance.net/gallery/19759151/Snapscan-iOs-design-and-branding?tracking_source=
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FAQ (optional) -->
<!--
## โ FAQ <a name="faq"></a>
> Add at least 2 questions new developers would ask when they decide to use your project.
- **[Question_1]**
- [Answer_1]
- **[Question_2]**
- [Answer_2]
<p align="right">(<a href="#readme-top">back to top</a>)</p> -->
<!-- LICENSE -->
## ๐ License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p> | A mobile web application where you can manage your budget: you have a list of transactions associated with a category, so that you can see how much money you spent and on what. Built with Ruby on Rails, JavaScript, SCSS, and PostgreSQL | javascript,postgresql,ruby,scss,scss-mixins | 2023-01-02T07:39:28Z | 2023-01-07T21:06:20Z | null | 1 | 1 | 18 | 0 | 0 | 4 | null | MIT | Ruby |
idlework/react-native-media-query-hook | main | # react-native-media-query-hook
A simplified version of media queries in the browser. Currently supports minWidth, maxWidth, minHeight, maxHeight, and orientation to detect landscape or portrait modus. The hook is typescript first.
## Installation
_npm_
```shell
$ npm i react-native-media-query-hook
```
_yarn_
```shell
$ yarn add react-native-media-query-hook
```
## How to use
_import_
```typescript
import useMediaQuery from 'react-native-media-query-hook';
```
_basic_
```typescript
// The outcome results in a boolean statement.
const isSmallScreen = useMediaQuery({
maxWidth: 480,
});
```
_more sophisticated queries_
```typescript
// Detect different screen sizes
const isSmallScreen = useMediaQuery({
maxWidth: 480,
});
const isMediumScreen = useMediaQuery({
minWidth: 481,
maxWidth: 1024,
});
const islargeScreen = useMediaQuery({
minWidth: 1025,
});
// Detect portrait / landscape mode
const isPortrait = useMediaQuery({
orientation: 'portrait',
});
const isLandscape = useMediaQuery({
orientation: 'landscape',
});
// Put it all together
const isMediumPortraitScreen = useMediaQuery({
minWidth: 481,
maxWidth: 1024,
minHeight: 481,
maxHeight: 1200,
orientation: 'portrait',
});
```
## Contributing
[Issues reports](https://github.com/idlework/react-native-media-query-hook/issues) are more than welcome. The best way to report a problem is to add a reproduction of the error with a code example.
[Pull requests](https://github.com/idlework/react-native-media-query-hook/pulls) are also very welcome. This way we can extend the library quicker with everybody's needs. If you have ideas about the interface, lets discuss it in an [issue ticket](https://github.com/idlework/react-native-media-query-hook/issues).
## License
react-native-media-query-hook is [MIT licensed](./LICENSE).
| A simplified version of the media queries in css. Currently supports minWidth, maxWidth, minHeight, maxHeight, and orientation to detect landscape or portrait modus. | hook,hooks,media-queries,package,react-native,typescript,javascript,javascript-hook-library,javascript-hooks,javascript-library | 2023-01-14T19:09:15Z | 2023-01-15T18:05:03Z | 2023-01-15T17:59:23Z | 1 | 0 | 4 | 0 | 0 | 4 | null | MIT | TypeScript |
gosiacodes/React-PD-Meter-App | main | # :sparkles: React PD Meter App :sparkles:
React PD-Meter App (measures distance between pupils) with MediaPipe Face Mesh - internship project (2023)
https://gosiacodes.github.io/React-PD-Meter-App/
## :pushpin: _How does the application work?_
Application uses your device camera.
In this project I used Googles AI solution MediaPipe Face Mesh that estimates 468 3D face landmarks in real-time.
It uses machine learning (ML) to infer the 3D surface geometry, requiring only a single camera input.
AI finds your pupils and measures distance between them.
It is not that correct when you take multiple shots, but it is fun anyway.
### _Instruction:_
Best results can be achieved when the face is about 40 cm from the camera.
Make sure there is good light and the camera is clean.
Sit still and do not move your head.
Look straight into the camera or at the red dot at the top and take a picture.
The result on the left is the approximate distance between pupils.
The result on the right is the PD average if you take multiple shots.
You can retake the picture as many times as you like (wait for the video to reload).
## :pushpin: _GUI example_

************************************************************************************
## Getting Started with Create React App
This project was bootstrapped with [Create React App](https://github.com/facebook/create-react-app).
## Available Scripts
In the project directory, you can run:
### `npm start`
Runs the app in the development mode.\
Open [http://localhost:3000](http://localhost:3000) to view it in your browser.
The page will reload when you make changes.\
You may also see any lint errors in the console.
### `npm test`
Launches the test runner in the interactive watch mode.\
See the section about [running tests](https://facebook.github.io/create-react-app/docs/running-tests) for more information.
### `npm run build`
Builds the app for production to the `build` folder.\
It correctly bundles React in production mode and optimizes the build for the best performance.
The build is minified and the filenames include the hashes.\
Your app is ready to be deployed!
See the section about [deployment](https://facebook.github.io/create-react-app/docs/deployment) for more information.
### `npm run eject`
**Note: this is a one-way operation. Once you `eject`, you can't go back!**
If you aren't satisfied with the build tool and configuration choices, you can `eject` at any time. This command will remove the single build dependency from your project.
Instead, it will copy all the configuration files and the transitive dependencies (webpack, Babel, ESLint, etc) right into your project so you have full control over them. All of the commands except `eject` will still work, but they will point to the copied scripts so you can tweak them. At this point you're on your own.
You don't have to ever use `eject`. The curated feature set is suitable for small and middle deployments, and you shouldn't feel obligated to use this feature. However we understand that this tool wouldn't be useful if you couldn't customize it when you are ready for it.
## Learn More
You can learn more in the [Create React App documentation](https://facebook.github.io/create-react-app/docs/getting-started).
To learn React, check out the [React documentation](https://reactjs.org/).
### Code Splitting
This section has moved here: [https://facebook.github.io/create-react-app/docs/code-splitting](https://facebook.github.io/create-react-app/docs/code-splitting)
### Analyzing the Bundle Size
This section has moved here: [https://facebook.github.io/create-react-app/docs/analyzing-the-bundle-size](https://facebook.github.io/create-react-app/docs/analyzing-the-bundle-size)
### Making a Progressive Web App
This section has moved here: [https://facebook.github.io/create-react-app/docs/making-a-progressive-web-app](https://facebook.github.io/create-react-app/docs/making-a-progressive-web-app)
### Advanced Configuration
This section has moved here: [https://facebook.github.io/create-react-app/docs/advanced-configuration](https://facebook.github.io/create-react-app/docs/advanced-configuration)
### Deployment
This section has moved here: [https://facebook.github.io/create-react-app/docs/deployment](https://facebook.github.io/create-react-app/docs/deployment)
### `npm run build` fails to minify
This section has moved here: [https://facebook.github.io/create-react-app/docs/troubleshooting#npm-run-build-fails-to-minify](https://facebook.github.io/create-react-app/docs/troubleshooting#npm-run-build-fails-to-minify)
| React PD-Meter App (measures distance between pupils) with MediaPipe Face Mesh - internship project (2023) | react,react-components,react-js,reactjs,facemesh,mediapipe,mediapipe-face-detection,mediapipe-facemesh,pupil-detection,ai | 2023-01-04T13:10:31Z | 2023-07-27T17:49:55Z | null | 1 | 0 | 14 | 0 | 2 | 4 | null | null | JavaScript |
akash-singh8/Time-Analytics | main | null | Brower extension helps you track and analyze your website usage. | chrome-extension,extension,javascript,timer | 2023-01-10T14:40:51Z | 2023-01-10T19:42:35Z | null | 1 | 0 | 12 | 0 | 1 | 4 | null | null | JavaScript |