repo
stringlengths 8
123
| branch
stringclasses 178
values | readme
stringlengths 1
441k
⌀ | description
stringlengths 1
350
⌀ | topics
stringlengths 10
237
| createdAt
stringlengths 20
20
| lastCommitDate
stringlengths 20
20
| lastReleaseDate
stringlengths 20
20
⌀ | contributors
int64 0
10k
| pulls
int64 0
3.84k
| commits
int64 1
58.7k
| issues
int64 0
826
| forks
int64 0
13.1k
| stars
int64 2
49.2k
| diskUsage
float64 | license
stringclasses 24
values | language
stringclasses 80
values |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
JMHeartley/dreamlo.js | main | <h1 align="center">
dreamlo.js
</h1>
<p align="center">
<i>A Javascript library for creating, retrieving, updating, and deleting scores on your dreamlo leaderboard via its web API.</i>
</p>
<p align="center">
<a href="#-examples">Examples</a> •
<a href="#%EF%B8%8F-demo">Demo</a> •
<a href="#-requirements">Requirements</a> •
<a href="#-installation">Installation</a> •
<a href="#-usage">Usage</a> •
<a href="#-about">About</a> •
<a href="#%EF%B8%8F-acknowledgements">Acknowledgements</a> •
<a href="#-technologies-used">Technologies Used</a> •
<a href="#-license">License</a>
</p>
<p align="center">
<a href="/LICENSE">
<img src="https://img.shields.io/github/license/jmheartley/dreamlo.js?color=brightgreen" alt="GitHub License" >
</a>
<img src="https://img.shields.io/github/last-commit/jmheartley/dreamlo.js?color=green" alt="GitHub last commit">
<img src="https://img.shields.io/github/contributors/jmheartley/dreamlo.js?color=yellowgreen" alt="GitHub contributors">
<img src="https://img.shields.io/badge/total%20lines-1.1k-yellow" alt="Lines of code">
<img src="https://img.shields.io/github/repo-size/jmheartley/dreamlo.js?color=orange" alt="GitHub repo size">
<img src="https://img.shields.io/npm/v/dreamlo.js?color=red" alt="NPM version">
</p>
## 👨🔧 Examples
Using dreamlo.js for your game's leaderboard? Send me a message with a link and I'll add it to the examples listed below:
+ [Tic-Tac-Toe](https://github.com/JMHeartley/Tic-Tac-Toe)
## ⚙️ Demo
Are you new to dreamlo? Want to test it out without having to commit? 😕
Just use the [Swagger UI](https://swagger.io/tools/swagger-ui/)-like [demo page](https://JMHeartley.github.io/dreamlo.js/) to test requests and retrieve leaderboard data 😎
## 🔩 Requirements
Go to [dreamlo's official website](https://dreamlo.com/) for a unique pair of public and private codes. **Bookmark your leaderboard's page, you won't be given the URL after the first time!**
If you can afford it, I recommend upgrading your leaderboard, which:
+ 🔒 enables HTTPS for your board's URL
+ 🙌 removes the limit of 25 scores
## 🔧 Installation
There are a few ways to start working, all of which globally expose the `dreamlo` variable:
1. Manually download the compiled file `dreamlo.js` from [dist](/dist) to your appropriate project folder and load using a relative path:
```html
<script src="/path/to/dreamlo.js"></script>
```
2. Use `<script>` to reference the code through [jsDelivr's CDN](https://www.jsdelivr.com/package/npm/dreamlo.js):
```html
<script src="https://cdn.jsdelivr.net/npm/dreamlo.js@1.3.2/dist/dreamlo.min.js"></script>
```
3. Install the [package via npm](https://www.npmjs.com/package/dreamlo.js) with the following command:
```bash
npm install dreamlo.js
```
## 🤖 Usage
The methods below, except `initialize()`, are all [Promises](https://javascript.info/promise-basics), so they can wait for the HTTP response. Failed HTTP requests will throw an `Error` containing the [HTTP status code](https://developer.mozilla.org/en-US/docs/Web/HTTP/Status).
You can use these methods with [Promise chains](https://javascript.info/promise-chaining):
```javascript
dreamlo.getScores()
.then((scores) => {
// do stuff with scores
})
.catch((error) => {
// do something with error
});
```
or [async/await](https://javascript.info/async-await):
```javascript
try {
var scores = await dreamlo.getScores();
// do stuff with scores
} catch (error) {
// do something with error
}
```
### initiailize
```javascript
dreamlo.initialize(publicCode, privateCode, useHttps)
```
The `initialize` function sets the public and private codes and specifies whether the base URL uses HTTP or HTTPS protocol. Call this method before any others.
+ `publicCode`: the public code of your leaderboard
+ `privateCode`: the private code of your leaderboard
+ `useHttps`: toggles HTTP or HTTPS on the base URL (default: `false`)
### getScore
```javascript
dreamlo.getScore(name, format)
```
The `getScore` function sends a request for one leaderboard entry and returns it in the desired format.
+ `name`: the name value of the score to request
+ `format`: the format type of the returned [leaderboard entry](#entry) (default format: `Object`; see [Formats](#score-formats) for which properties are used to change formats)
<details>
<summary>Return behavior by format</summary>
+ `Object`: returns a single entry `object` or `null` if no entries match `score.name`
+ `JSON`: returns a single entry for `getScore` as a `string` or `null` as a `string` if no entries match `score.name`
+ `XML`: returns a single entry for `getScore` as a `string` or an empty `string` if no entries match `score.name`
+ `Pipe`: returns a single entry for `getScore` as a `string` or an empty `string` if no entries match `score.name`
+ `Quote`: returns a single entry for `getScore` as a `string` or an empty `string` if no entries match `score.name`
</details>
<br>
### getScores
```javascript
dreamlo.getScores(format, sortOrder, skip, take)
```
The `getScores` function sends a request for multiple leaderboard entries and returns them in the specified format and order.
+ `format`: the format type of the returned [leaderboard entries](#entry) (default format: `Object`; see [Formats](#score-formats) for which properties are used to change formats)
+ `sortOrder`: the sorting order of the retrieved scores (default order: Descending by Points; see [Sorting Order](#sorting-order) for which properties are used to adjust orders)
+ `skip`: the score rank you want to start sorting at (default: `0`; zero-based index)
+ `take`: the number of scores you want to retrieve (default: `undefined`; retrieves all scores)
<details>
<summary>Return behavior by format</summary>
+ `Object`: returns an array of entry `object`s
+ `JSON`: returns an array of entries nested within `dreamlo.leaderboard.entry` as a `string`
+ `XML`: returns `<entry>`s nested within `<dreamlo><leaderboard></leaderboard></dreamlo>` as a `string` or `"<dreamlo></leaderboard /></dreamlo>"` if no entries are found
+ `Pipe`: returns entries with pipe-delimited values, each entry separated with a new line character as a `string` or an empty `string` if no entries are found
+ `Quote`: returns entries with double-quoted values, separated with a comma, each entry with a new line character as a `string` or an empty `string` if no entries are found
</details>
<br>
*All parameters are optional or have default values; calling with no parameters will return all scores, sorted by points in descending order, as an array of objects.*
### addScore
```javascript
dreamlo.addScore(score, format, sortOrder, canOverwrite)
```
The `addScore` function sends a request to add a score to the leaderboard and returns all leaderboard entries in the specified format and order.
+ `score`: the score to add to the leaderboard (see [Score](#score) for the expected shape of this object)
+ `format`: the format type of the returned [leaderboard entries](#entry) (default format: `Object`; see [Formats](#score-formats) for which properties are used to change formats)
+ `sortOrder`: the sorting order of the retrieved scores (default order: Descending by Points; see [Sorting Order](#sorting-order) for which properties are used to adjust orders)
+ `canOverwrite`: when adding a `score` whose `score.name` is already present on the leaderboard, if this is set to `true`, the `score` with higher `score.points` is saved; if set to `false`, an `Error` is thrown (default: `false`)
<details>
<summary>Return behavior by format</summary>
+ `Object`: returns an array of entry `object`s
+ `JSON`: returns an array of entries nested within `dreamlo.leaderboard.entry` as a `string`
+ `XML`: returns `<entry>`s nested within `<dreamlo><leaderboard></leaderboard></dreamlo>` as a `string` or `"<dreamlo></leaderboard /></dreamlo>"` if no entries are found
+ `Pipe`: returns entries with pipe-delimited values, each entry separated with a new line character as a `string` or an empty `string` if no entries are found
+ `Quote`: returns entries with double-quoted values, separated with a comma, each entry with a new line character as a `string` or an empty `string` if no entries are found
</details>
<br>
**TIP:** if updating a score on the leaderboard, set `canOverwrite` to `true`, if adding a new score, set `canOverwrite` to `false`.
### deleteScore
```javascript
dreamlo.deleteScore(name)
```
The `deleteScore` function sends a request to delete one score from the leaderboard.
+ `name`: the name value of the score to delete
### deleteScores
```javascript
dreamlo.deleteScores()
```
The `deleteScores` function sends a request to delete all scores from the leaderboard.
### Score
```javascript
{
name: string,
points: number,
seconds: number,
text: string
}
```
A `score` is a data object sent to the leaderboard.
+ `name`: the unique identifier for `score`s; instead of using a player's name, try a distinct value, like a [timestamp](https://www.w3docs.com/snippets/javascript/how-to-get-a-timestamp-in-javascript.html)
+ `points`: the first numeric value that can be used to [sort multiple entries](#sorting-order)
+ `seconds`: the second numeric value that can be used to [sort multiple entries](#sorting-order); this property is optional
+ `text`: contains extra data relating to the `score`; this property is optional
**TIP:** if you have lots of extra data you want to store, you can use `score.text` to save a pipe-delimited string and then decode/recode the information in your program.
dreamlo doesn't allow the use of the asterisk character ( * ); all occurrences will be replaced by the underscore character ( _ ).
*See [Score](/src/score.ts) for this Typescript interface.*
### Entry
```javascript
{
name: string,
score: string,
seconds: string,
text: string,
date: string
}
```
An entry is a `score` retrieved from the leaderboard. Shown above is what it looks like with the `Object` format; `JSON` and `XML` use properties that have the same names as `Object`. `Pipe` and `Quote` don't use key-value pairs, but values are listed similarly.
+ `name`: correlates to `score.name`
+ `score`: correlates to `score.points`
+ `seconds`: correlates to `score.seconds`
+ `text`: correlates to `score.text`
+ `date`: when the entry was last updated, in [US format](https://en.wikipedia.org/wiki/Date_and_time_notation_in_the_United_States) (`mm-dd-yyyy HH:MM:ss AM/PM`), using the [UTC timezone](https://en.wikipedia.org/wiki/Coordinated_Universal_Time).
### Score Formats
The format type of entries returned from the leaderboard can be specified using the following properties:
Format | Property
------------------| ------------
Javascript Object | `dreamLo.ScoreFormat.Object`
JSON | `dreamlo.ScoreFormat.Json`
XML | `dreamlo.ScoreFormat.Xml`
Pipe-delimited | `dreamlo.ScoreFormat.Pipe`
Quoted with comma | `dreamlo.ScoreFormat.Quote`
*See [ScoreFormat](/src/scoreFormat.ts) for this Typescript enum.*
### Sorting Order
The sorting order of entries returned from the leaderboard can be specified using the following properties:
Order | Property
--------------------- | ------------
Descending by Points | `dreamlo.SortOrder.PointsDescending`
Ascending by Points | `dreamlo.SortOrder.PointsAscending`
Descending by Seconds | `dreamlo.SortOrder.SecondsDescending`
Ascending by Seconds | `dreamlo.SortOrder.SecondsAscending`
Descending by Date | `dreamlo.SortOrder.DateDescending`
Ascending by Date | `dreamlo.SortOrder.DateAscending`
*See [SortOrder](/src/sortOrder.ts) for this Typescript enum.*
## 🤔 About
### What is dreamlo?
dreamlo is a cloud server for hosting leaderboards for game developers.
Out of love for Unity, Carmine Guida started hosting dreamlo. He created [an asset](https://assetstore.unity.com/packages/tools/network/dreamlo-com-free-instant-leaderboards-and-promocode-system-3862)
for the game engine so anyone can effortlessly add a leaderboard to their games.
*Check out [dreamlo's official FAQs page](https://www.dreamlo.com/faq) for more info.*
### Why use dreamlo with Javascript?
Previously, I used the dreamlo game asset for [the game my team built for the GTMK 2020 game jam.](https://github.com/JMHeartley/Work-With-Me-Here)
Years later, I started sprucing up [an old TicTacToe game I made years ago](https://github.com/JMHeartley/TicTacToe)
and wanted to add a leaderboard. The first thing that came to mind was dreamlo, but there was a problem: the script for dreamlo that comes with the Unity game asset was written in C#.
I created this script because any game that can make HTTP requests can use dreamlo. Happily, I've extended Carmine's original dream(lo) to Javascript 😊
## ❤️ Acknowledgements
☁️ [Carmine T. Guida](https://carmine.com/) for creating and hosting dreamlo
👩🏼🏫 [Microsoft Learn](https://learn.microsoft.com/en-us/training/paths/build-javascript-applications-typescript/)
for [teaching me Typescript](https://learn.microsoft.com/en-us/training/achievements/learn.language.build-javascript-applications-typescript.trophy?username=JMHeartley)
## 👨🏽💻 Technologies Used
+ [Typescript](https://www.typescriptlang.org/) - Javascript superset
+ [VSCode](https://code.visualstudio.com/) - code editor
+ [HTML](https://www.w3schools.com/html/) - webpage markup language (used in [demo page](#%EF%B8%8F-demo))
+ [Bootstrap](https://getbootstrap.com/) - CSS Library (used in [demo page](#%EF%B8%8F-demo))
+ [Javascript](https://developer.mozilla.org/en-US/docs/Learn/JavaScript/First_steps/What_is_JavaScript) - webpage interactivity language (used in [demo page](#%EF%B8%8F-demo))
+ [jQuery](https://jquery.com/) - Javascript shorthand library (used in [demo page](#%EF%B8%8F-demo))
## 📃 License
[The MIT License (MIT)](/LICENSE)
Copyright (c) 2022 Justin M Heartley
| A Javascript library for creating, retrieving, updating, and deleting scores on your dreamlo leaderboard via its Web API. | dreamlo,leaderboard,unity,scoreboard,html-game,javascript-game,javascript | 2023-01-03T17:45:22Z | 2023-12-08T19:24:00Z | null | 1 | 0 | 124 | 0 | 0 | 4 | null | MIT | TypeScript |
tjdrkr2580/sparton-1 | main | null | 흣챠 | 스파르톤 프로젝트 1조 | css,flask,html,javascript,jquery,mongodb | 2023-01-16T01:46:58Z | 2023-01-16T20:28:56Z | null | 5 | 19 | 68 | 0 | 1 | 4 | null | null | HTML |
Codex-Swapnil1/Angel-Place | main | # FirstCry Clone Deployed Link: [Angle Place](https://sunny-meerkat-5e4a30.netlify.app/)
This project is a clone of firstcry.com which is well known online kids shopping store. The company focused on new born baby to 12 year kids fashion clothes and products. We are added our design and some functionality as our limits and trying to improve more on that website.
## Tech Stack :
The tech Stack we used for creating this website are :-
Frontend Tech Stack :-
1. HTML
2. CSS
3. Javascript
4. React
5. Redux
6. Chakra UI
7. styled-components
Backend Tech Stack:-
1. jswebtoken
2. express.js
3. mongodb
| This project is the clone of firstcry.com. India's Largest Online Store for newborn, baby & kids products. Shop from the best range of Toys, Diapers, Clothes, Footwear, Strollers, Car Seats, Furniture, Gifts, Books and more from the best baby brands. | bycrypt,chakra-ui,express-js,javascript,jswebtoken,mongodb,react,react-redux,styled-components,swiper-slider | 2023-01-15T15:49:54Z | 2023-01-24T16:10:53Z | null | 5 | 26 | 104 | 2 | 2 | 4 | null | null | JavaScript |
TheTrustyPwo/0x7e7 | master | # 0x7e7
Cool new years fireworks lol
https://thetrustypwo.github.io/0x7e7/
You may need to click the screen for it to start
| Cool new years fireworks lol (Totally not imported from scratch) | 2023,animation,fireworks,javascript,newyear | 2023-01-01T05:19:30Z | 2023-02-19T08:16:12Z | null | 1 | 0 | 3 | 0 | 0 | 4 | null | null | HTML |
nicholidev/nicholidev | main | ## [](https://git.io/typing-svg)
Hello! I'm a software engineer who enjoys architecting and building applications. I develop software and websites using modern technologies such as TypeScript, React, Next.js & MUI to name a few. I spend most of my spare time programming personal projects or solving CodinGame challenges.
### How to reach me?
- <a href="https://nicholijin.com/" target="_blank" rel="noreferrer">My website</a>
- <a href="https://www.codingame.com/profile/2d2f02e5a43320543afb8352edcaf6cd3601874" target="_blank" rel="noreferrer">Codingame</a>
- <a href="https://join.skype.com/invite/hwp9PMp0W8mA" target="_blank" rel="noreferrer">Skype</a>
- <a href="https://calendly.com/nicholijin/30min" target="_blank" rel="noreferrer">Calendy</a>
<br/>
                                         
| Nicholi Jin | developer,javascript,python,ruby,typescript,senior-engineer,backend,frontend,laravel,nestjs | 2023-01-05T14:01:09Z | 2024-04-15T02:14:08Z | null | 1 | 0 | 23 | 0 | 0 | 4 | null | null | null |
SujinPhilip/willYouMarryme | main | # willYouMarryme
Just a fun website asking you whether you would like to marry me or not.
Try it out!! [Click here](https://sujinphilip.github.io/willYouMarryme/)
So will you marry me? Yes 🥹 or No 😏
[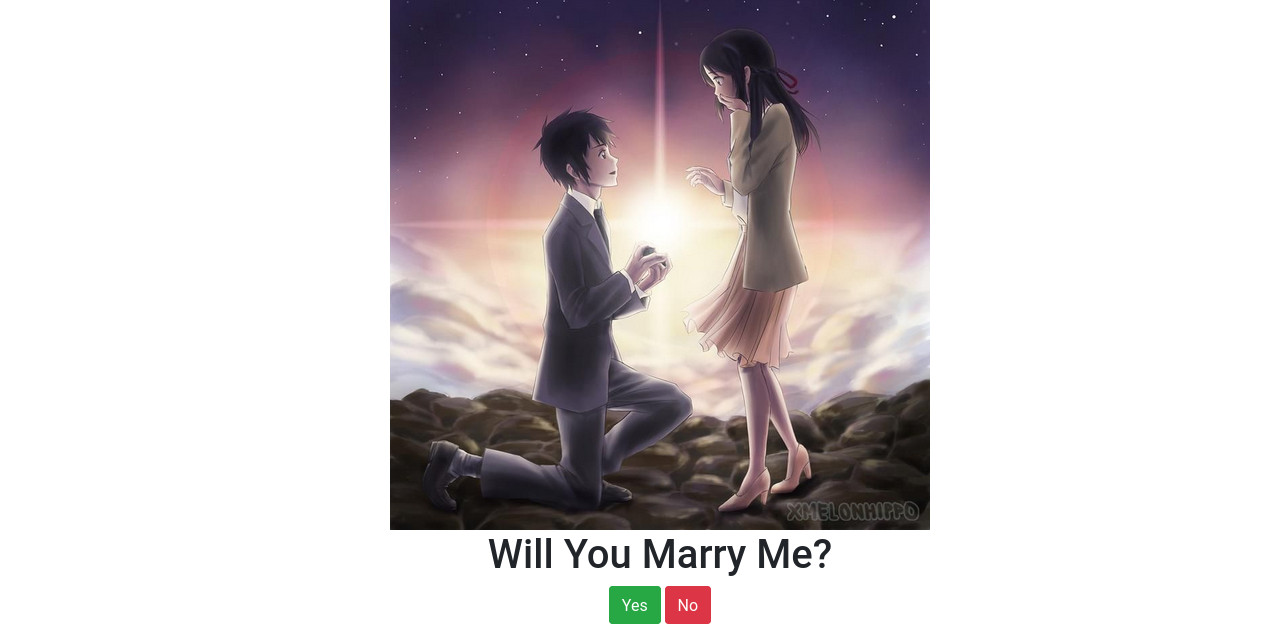](https://postimg.cc/HjvHz4rd)
Try saying 'no' 😏
[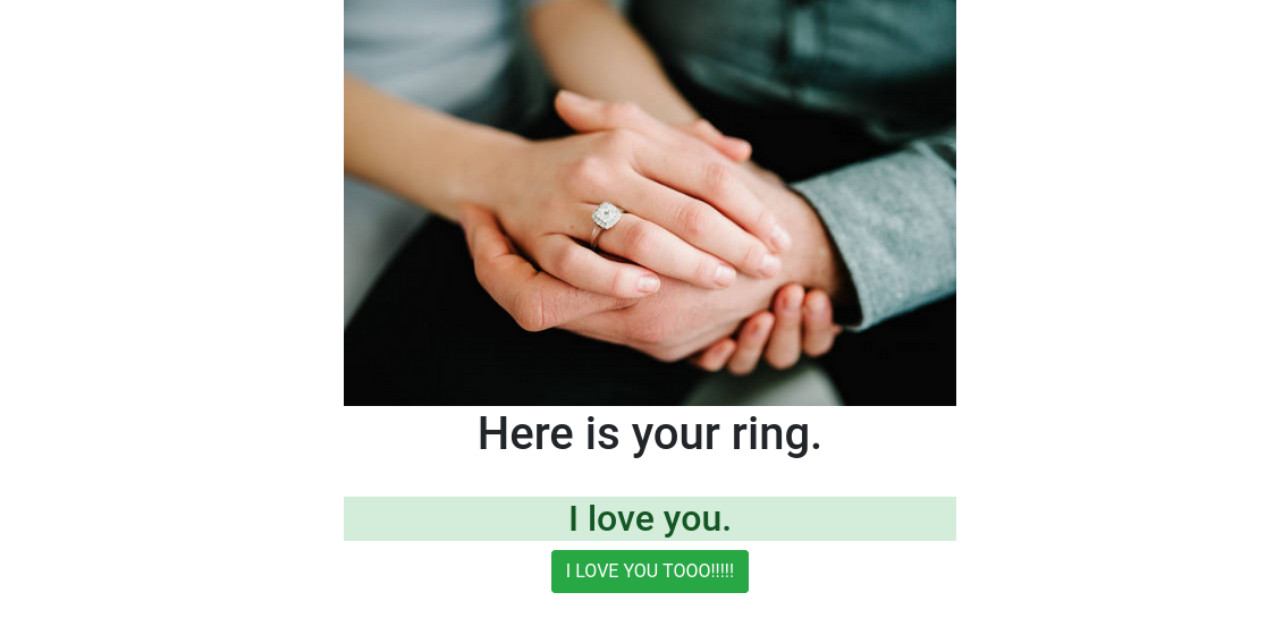](https://postimg.cc/Btn42wQm)
| Just a fun website asking you whether you would like to marry me or not. | bootstrap,css,fun,html,javascript | 2023-01-16T17:51:47Z | 2023-03-13T04:45:47Z | null | 2 | 0 | 59 | 0 | 1 | 4 | null | null | HTML |
ysacc/rickandmorty | main | <h1 align="center">Rick and Morty :stars:</h1>
<hr>
<p align="center"><img src="https://user-images.githubusercontent.com/112214885/216842924-c8c859a8-c9a5-471d-8f2c-71d6d9534453.png"/></p>
<p align="center"><img src="https://user-images.githubusercontent.com/112214885/216842973-bc48ab9a-f886-4b9d-9fd5-97345278e7f9.png"/></p>
<p align="center"><img src="https://user-images.githubusercontent.com/112214885/216842997-1c4e9339-51c7-44cd-ac13-5d1b0434034e.png"/></p>
<p align="center"><img src="https://user-images.githubusercontent.com/112214885/216843041-d42082e2-370c-427f-90f3-7c3cafe3d11b.png"/></p>
[View Live ](https:rickandmorty-ysacc.vercel.app)
<p>Prueba con Username:mail@ggg.com // Password: 123456 </p>
## APPLIED TECHNOLOGIES








## Routes
| Request Type | Endpoint | Returns | Status |
| ------------ | ----------- | --------------------------------------------------------------------------------------------------------------------------------------------------------- | ------ |
| GET | /characters | An object containing an info property with count, pages, next, and previous page info and a results property, an array containing all characters details. | 200 |
| GET | /favorites | An array of objects containing user's favorite characters' details. | 200 |
| GET | /filter | Requires a category and query as query parameters to filter for specific character data, an object with a info and results property. | 200 |
| GET | /episodes | An object containing an info property with count, pages, next, and previous page info and a results property, an array containing all episodes details. | 200 |
| POST | /character | An object containing character details. Id, name, status, species, type, gender, origin, location, image, episode, url. | 201 |
| DELETE | /character | An object containing a name property matching the character to be deleted. | 200 |
## Features
### Navigation Bar
- The navigation bar was built utilizing the React Router. The React Router API allows us to build single page applications with navigation without the need for page refreshing, which dramatically improves user experience and render times.
- The React Router uses the Router and Link components, which prevents page refreshes and allows the user to use the browsers functionality like a forward and back button in order to maintain the correct page view.

---
### Search
- The search bar utilizes GET /characters endpoint which can be provided query parameters for the category and the query string. These two query parameters correspond to the Rick and Morty API, allowing for seamless filtering of the **600+** characters available.
- Material-UI was utilized for this feature. Material-UI provides minimalist React components, built utilizing Material Design framework in order for fast and clean web development.
---
### Pagination
- Utilizing React Hooks to store state of the current page traversed, which serves as the query parameter for my GET /character endpoint.
- Latency was vastly increased with the use of Redis. Redis is a great database caching technology that is optimized for speed using data structures such as hashes and sets. Each page and its corresponding data was stored as a key value pair, after the user's first click on the respective page.
---
### Favorites
- The favorites collection utilizes the POST /character endpoint to add a user's favorite Rick and Morty characters, and persists in a MongoDB database collection.
- Characters can be removed from the collection with the DELETE /character endpoint.
---
### Accessibility
- Achieved 100 accessibility through Google Chrome Lighthouse report. Through the use of Material-UI components, adding roles, aria-labels, semantic HTML provides a seamless and web accessible application for users with or without disabilities.
| Rick and Morty Wiki | bootstrap5,css3,expressjs,html5,javascript,nodejs,reactjs,redux | 2023-01-11T12:19:43Z | 2023-04-04T04:14:20Z | null | 1 | 0 | 33 | 0 | 1 | 4 | null | null | JavaScript |
VikasSpawar/Lumen5Clone | main | •Lumen5
•It is an online video editing website on which we can create videos for social media.
•This was a solo project which was done in 4-5 days
•Features: Homepage, Login/Signup, Dashboard
•Tech-Stack: React, JavaScript, ChakraUi, CSS
•Arias of responsibility: all of the project
Live link : https://lumen5clone.vercel.app/
<h2>Some Screenshots of the website :-</h2>
<h2>Homepage :- </h2>
<img src="./screen_shots/Homepage.PNG"/>
<img src="./screen_shots/popUp.PNG"/>
<img src="./screen_shots/Videos.PNG"/>
<h2>Login / SignUp :- </h2>
<img src="./screen_shots/Login.PNG"/>
<img src="./screen_shots/SignUp.PNG"/>
<h2>Dashboard :- </h2>
<img src="./screen_shots/Dashboard.PNG"/>
| •It is an online video editing website on which we can create videos for social media. •This was a solo project which was done in 4-5 days •Features: Homepage, Login/Signup, Dashboard •Tech-Stack: React, JavaScript, ChakraUi, CSS •Arias of responsibility: all of the project Live link : https://lumen5clone.vercel.app/ | react,chakra-ui,javascript,css | 2023-01-02T15:48:41Z | 2023-01-25T07:04:31Z | null | 1 | 0 | 5 | 0 | 1 | 4 | null | null | JavaScript |
brlyndev/browser-password-stealer | main | # Password Logger Server
This is a basic password logger with a backend made in TypeScript which is using Express.
<br>
The payload for this project is ~*50* lines long, which results in a very small payload size.
## How does this work?
Normally, password stealing malware would decrypt the passwords with the Chrome Master Key
on the client's machine.
<br>
<br>
Though, in this malware, the client will send the Chrome password database along with hardware identifiers
to the server which will then decrypt the passwords and save them in to a directory made with the hardware identifiers; (this is to identify
different victims that run the payloads data)
## How do I set this up?
### Firstly, we will set up the server.
1. Go to this line (code snippet linked) and change the directory according to your computer. By change it according to your computer, I mean copy the exact path of the storage folder in the server directory and paste it into that variable.https://github.com/brlyndev/PasswordLogger/blob/a60e6495e6f0d4bb6dafdcd6f9d600f666293d29/server/decrypt.py#L21-L26
2. CD into the server directory and run `npm install` (Make sure you have NodeJS *v18.12.1* installed)
3. Make the storage directory. To do this, just make a folder called "storage" inside of the server directory.
4. To start the server, CD into the server directory and run `npm start` in the console.
After you have ran `npm start`, the server will be running and listening for logs.
### Now, we will set up the client.
(Make sure you have Python *v3.11.x* installed)
1. CD into the client directory and run `python install -r requirements.txt`.
2. Now, (*optionally*) you can build the Python file into an executable.<br>To do this, CD into the client directory and run `pip install pyinstaller && pyinstaller --onefile --noconsole index.py`.<br>The output will be put into the "dist" directory
## Common Errors
### Q: "*i tried sending to my friend and when they ran it, it didn't send me the logs!!*"
**A: This will only work on your local computer unless you port forward, or use something like Ngrok.**
If you do decide to port forward or use something like Ngrok, be sure to change the domain in the `./client/index.py` file.
https://github.com/brlyndev/PasswordLogger/blob/a60e6495e6f0d4bb6dafdcd6f9d600f666293d29/client/index.py#L24-L28
| A password logger with a very small payload size. | browser-password-stealer,chrome-password-stealer,javascript,malware,password-grabber,password-stealer,payload,python,typescript | 2023-01-03T09:57:16Z | 2023-01-03T10:59:04Z | null | 1 | 0 | 6 | 0 | 2 | 4 | null | MIT | Python |
nandhakumarRNK/Admin-Dashboard-Responsive | main | # Responsive-Admin-Dashboard
**Collabrate to add new features in this dashboard**
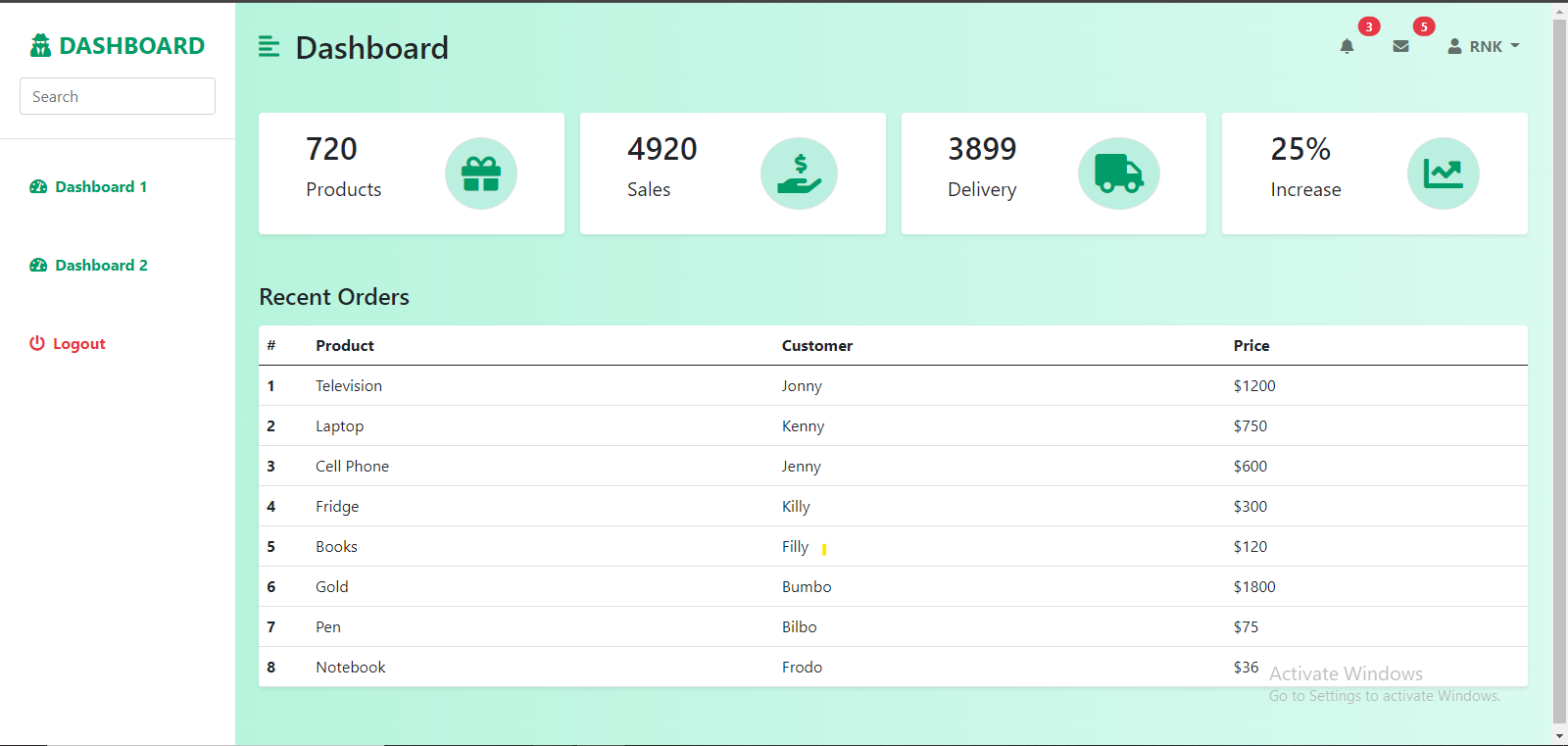
| Simple Dashboard using bootstrap | admin-dashboard,bootstrap5,html5,javascript | 2023-01-03T12:25:36Z | 2023-01-05T07:34:53Z | 2023-01-03T13:10:49Z | 1 | 1 | 17 | 1 | 1 | 4 | null | MIT | HTML |
MMGGYY66/my-first-capstone | main | # my-first-capstone
<p id="readme-top">My Microverse first capstone project (Module One)</p>
<!-- TABLE OF CONTENTS -->
# 📗 Table of Contents
- [My first capstone](#my-first-capstone)
- [📗 Table of Contents](#-table-of-contents)
- [📖 \[my-first-capstone\] ](#my-first-capstone)
- [creating my first capstone, I will:](#creating-my-first-capstone-i-will)
- [🛠 Built With ](#-built-with-)
- [ Deploy my website with github pages ]
- [💻 Getting Started ](#-getting-started-)
- [Run tests](#run-tests)
- [Deployment](#deployment)
- [Loom-video-link](#Loom video)
- [👥 Authors ](#-authors-)
- [Mohamed Gamil Eldimardash](#mohamed-gamil-eldimardash)
- [🤝 Contributing](#-contributing)
- [Show your support](#show-your-support)
- [Acknowledgments](#acknowledgments)
- [📝 License](#-license)
# 📖 [my-first-capstone] <a name="about-project"></a>
<!-- PROJECT DESCRIPTION -->
# 📖 [🎯 Arabic Language Conference Page: Microverse capstone project] <a name="about-project"></a>
> 🚧 This capstone project I am going to build a website is based on an online website for a conference.
- The website is based on the CC-Global-Summit-2015 design which
[Cindy Shin](https://www.behance.net/adagio07) created. Images uses in this project are from [figma](https://www.figma.com/file/V0vg37EZhAbP9nUPOeCy6p/HTML%2FCSS-%26-JavaScript-capstone-project---Conference-page?node-id=0%3A1&t=od5hoeaQE2tKg92Y-0).🚧
## 🛠 Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
<details>
<summary>Technology</summary>
<ul>
<li>HTML</li>
<li>CSS</li>
<li>Javascript</li>
</ul>
</details>
<details>
<summary>Tools</summary>
<ul>
<li>VS Code</li>
<li>GIT</li>
<li>GITHUB</li>
<li>Tailwind CSS</li>
</ul>
</details>
## 🚀 Live Demo <a name="live-demo"></a>
- [Live Demo Link](https://mmggyy66.github.io/my-first-capstone/index.html)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## 👁 Presentation <a name="presentation"></a>
- [Loom presentation Link](https://www.loom.com/share/e234c4d914d34edb81bb69e186f08697)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
# creating my first capstone, I will:
- set up a new repository and prepare it for development using best practices (e.g. linters).
- build 2 html files for home and about of the mobile website using the template provided.
## 🛠 Built With <a name="built-with"></a>
- html, css and javascript.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## Deploy my website with github pages":
[link to my first capstone: ](https://mmggyy66.github.io/my-first-capstone/index.html)
## - Loom video link:
[Loom walking through for my first capstone: ](https://www.loom.com/share/e234c4d914d34edb81bb69e186f08697)
## 💻 Getting Started <a name="getting-started"></a>
To get a local copy up and running follow these simple example steps.
### Prerequisites
you have to those tools in your local machine.
- [ ] NPM
- [ ] GIT & GITHUB
- [ ] Any Code Editor (VS Code, Brackets, etc)
Clone the repository to get start with project, then make sure to install dependencies in the linters file located in the [linter](https://github.com/Bateyjosue/linters-html-css/blob/main/.github/workflows/linters.yml) file
<p align="right">(<a href="#readme-top">back to top</a>)</p>
### Run tests
After pushing the changes on github go in code tab click on Merge pull request and let github action proess and check for changes.
### Deployment
Check for the tests when you generate a pull request and fix the errors if any.
For stylelint error run:<code>sudo npx stylelint "\*_/_.{css,scss}" --fix</code> and it will the fix style issues automatically.
<!-- AUTHORS -->
## 👥 Authors <a name="authors"></a>
👤 **Mohamed Gamil Eldimardash**
- GitHub: [@github](https://github.com/MMGGYY66)
- LinkedIn: [LinkedIn](https://www.linkedin.com/in/mohamed-eldimardash-0023a3b5/)
<!-- CONTRIBUTING -->
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the issues page
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## 👋 Show your support <a name="support"></a>
Give a ⭐️ if you like this project!
<p align="right"><a href="#readme-top">(back to top)</a></p>
<!-- ACKNOWLEDGEMENTS -->
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## 🔭Acknowledgments <a name="acknowledgements"></a>
- [Microverse Team](https://www.microverse.org/).
- Spacial thank to [Cindy Shin](https://www.behance.net/gallery/29845175/CC-Global-Summit-2015) for his beautiful design.
- Images uses in this project are from [figma](https://www.figma.com/file/V0vg37EZhAbP9nUPOeCy6p/HTML%2FCSS-%26-JavaScript-capstone-project---Conference-page?node-id=0%3A1&t=od5hoeaQE2tKg92Y-0).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## 📝 License <a name="license"></a>
This project is [MIT](https://github.com/microverseinc/readme-template/blob/master/MIT.md) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| The website is based on the CC-Global-Summit-2015 design which Cindy Shin created. Images uses in this project are from figma.🚧 | css3,html,javascript | 2023-01-01T03:15:21Z | 2023-01-05T16:28:11Z | null | 1 | 1 | 30 | 0 | 0 | 4 | null | null | CSS |
zoneAnaS/GuessIt | main | # GuessIt .... Hosted URL
https://shaikhmd007.github.io/GuessIt/
| A word guesser game | html,css,javascript | 2023-01-11T09:12:51Z | 2023-03-22T16:30:01Z | null | 2 | 2 | 22 | 0 | 1 | 4 | null | null | CSS |
sebalp100/Metrics-webapp | dev | <a name="readme-top"></a>
<div align="center">
<img src="https://api.le-systeme-solaire.net/assets/images/api.png"
alt="logo" width="140" height="auto" />
<br/>
<h3><b>Moons of the Solar system</b></h3>
</div>
<!-- TABLE OF CONTENTS -->
# 📗 Table of Contents
- [📖 About the Project](#about-project)
- [🛠 Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [Future Features](#future-features)
- [🚀 Live Demo](#live-demo)
- [💻 Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Usage](#usage)
- [Run tests](#run-tests)
- [👥 Authors](#authors)
- [🤝 Contributing](#contributing)
- [⭐️ Show your support](#support)
- [🙏 Acknowledgements](#acknowledgements)
- [📝 License](#license)
<!-- PROJECT DESCRIPTION -->
# 📖 Moons of the Solar system <a name="about-project"></a>
> In this project, we are setting up website that allows users to search for all the moons in the solar system, using real data from The Solar System OpenData API.
## 🛠 Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
<details>
<summary>Building</summary>
<ul>
<li><a href="https://www.javascript.com/">JavaScript</a></li>
<li>HTML 5</li>
<li>CSS 3</li>
<li>ES6</li>
<li>React</li>
<li>Redux</li>
</ul>
</details>
<details>
<summary>Bundle</summary>
<ul>
<li><a href="https://webpack.js.org/">WebPack</a></li>
<li><a href="https://www.npmjs.com/">NPM</a></li>
</ul>
</details>
<details>
<summary>Server</summary>
<ul>
<li><a href="https://webpack.js.org/configuration/dev-server/">React server</a></li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
- Search Planet
- Display Planets
- Display Moons
- Display number of Moons
- Display planet gravity
### Future Features <a name="future-features"></a>
- Improve style
- Specific data for each moon
- Search Moon
- User interactions (leaving comments)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## 🚀 Live Demo <a name="live-demo"></a>
[Click Me](https://moons-of-the-solar-system.onrender.com/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## 💻 Getting Started <a name="getting-started"></a>
To get a local copy up and running, follow these steps.
### Prerequisites
In order to run this project you need:
- Github account.
- Code editor (Visual Studio Code, Atom, etc.)
### Setup
Clone this repository to your desired folder:
- cd my-folder
- git clone https://github.com/sebalp100/Metrics-webapp/
### Install
This project was bootstrapped with [Create React App](https://github.com/facebook/create-react-app).
## Available Scripts
In the project directory, you can run:
### `npm start`
Runs the app in the development mode.\
Open [http://localhost:3000](http://localhost:3000) to view it in your browser.
The page will reload when you make changes.\
You may also see any lint errors in the console.
### `npm test`
Launches the test runner in the interactive watch mode.\
See the section about [running tests](https://facebook.github.io/create-react-app/docs/running-tests) for more information.
### `npm run build`
Builds the app for production to the `build` folder.\
It correctly bundles React in production mode and optimizes the build for the best performance.
The build is minified and the filenames include the hashes.\
Your app is ready to be deployed!
See the section about [deployment](https://facebook.github.io/create-react-app/docs/deployment) for more information.
### Usage
To run the project, execute the following command:
`npm install`
`npm start`
### Run tests
To run tests, run the following command:
`npm test`
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## 👥 Authors <a name="authors"></a>
👤 **Sebastian Martin**
- GitHub: [@sebalp100](https://github.com/sebalp100)
- LinkedIn: [Sebastian Martin](https://www.linkedin.com/in/sebastian-martin-956b2724a/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## ⭐️ Show your support <a name="support"></a>
Give a ⭐️ if you like this project!
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## 🙏 Acknowledgments <a name="acknowledgements"></a>
- Hat tip to anyone whose code was used
- Paul Nasilele for his search bar tutorial
- Freepik, Turkkub and Monkik for their icons (https://www.flaticon.com/authors/freepik, https://www.flaticon.com/authors/turkkub, https://www.flaticon.com/authors/monkik)
- Nelson Sakwa for their design (https://www.behance.net/sakwadesignstudio)
- Microverse
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## 📝 License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| Simple website that allows users to search for all the moons in the solar system, using real data from The Solar System OpenData API. Made with React and Redux. | javascript,react,redux | 2023-01-08T16:09:49Z | 2023-01-12T21:51:36Z | null | 1 | 1 | 14 | 0 | 1 | 4 | null | MIT | JavaScript |
jamshedhossan9/rubikscube | main | # 3*3 Rubik's Cube
It is written in SCSS and Vanilla JS. No third party plugin or library used to run this App.
## Check [Live](https://jamshedhossan9.github.io/rubikscube/)
## Screenshot
<p align="center">
<img src="https://github.com/jamshedhossan9/rubikscube/blob/main/screenshots/a.png?raw=true" width="600" >
</p> | A 3*3 Rubik's cube with Vanilla JS | cube,game,rubiks-cube,vanilla-js,javascript | 2023-01-04T14:09:16Z | 2023-11-26T12:39:39Z | null | 1 | 0 | 17 | 0 | 0 | 4 | null | GPL-3.0 | JavaScript |
correlucas/faq-accordion-card | main | # Frontend Mentor - FAQ accordion card solution
This is a solution to the [FAQ accordion card challenge on Frontend Mentor](https://www.frontendmentor.io/challenges/faq-accordion-card-XlyjD0Oam). Frontend Mentor challenges help you improve your coding skills by building realistic projects.
## Table of contents
- [Overview](#overview)
- [The challenge](#the-challenge)
- [Screenshot](#screenshot)
- [Links](#links)
- [My process](#my-process)
- [Built with](#built-with)
- [What I learned](#what-i-learned)
- [Useful resources](#useful-resources)
- [Author](#author)
### Screenshot

### Links
- Solution URL: [Frontend Mentor Solution](https://www.frontendmentor.io/solutions/faq-accordion-card-TH5bkVa-Zr)
- Live Site URL: [Live Site at Vercel](https://faq-accordion-card-beta-five.vercel.app/)
## My process
### Built with
- Semantic HTML5 markup
- Flexbox
- Responsive Design
- CSS Animations
- Javascript Interaction (Accordion Tab)
### What I learned
In this challenge I learned how to build an accordion and use the Javascript to toggle to open and close it.
### Useful resources
## Author
- Github - [correlucas](https://github.com/correlucas/)
- Frontend Mentor - [@correlucas](https://www.frontendmentor.io/profile/correlucas)
| This is my solution to the FAQ Accordion Component (Built with: HTML + SASS + JS) on Frontend Mentor. | frontend-development,frontend-web,frontendmentor,html-css-javascript,javascript | 2023-01-15T18:34:33Z | 2023-01-18T19:16:55Z | null | 1 | 0 | 19 | 0 | 1 | 4 | null | GPL-3.0 | SCSS |
Piryanshu88/LaptopWala | main | null | This Project is a clone of Dell Technologies. Dell's products include personal computers, servers, smartphones, televisions, computer software, computer security and network security, as well as information security services. | axios,chakra-ui,css,javascript,localstorage,mongodb,mongoose,mongoose-schema,nodejs,nodemon | 2023-01-16T22:43:40Z | 2023-04-10T15:26:00Z | null | 2 | 0 | 49 | 0 | 0 | 4 | null | null | JavaScript |
ncpa0/termx-markup | master | # termx-markup


[](https://www.npmjs.com/package/termx-markup)


## Usage
### Print markup
```tsx
import { Output, html } from "termx-markup";
Output.setDefaultPrintMethod(console.log); // (optional) print using console.log
const markup = html`
<span bold color="red">
Hello
<pre color="blue"> in my </pre>
world!
</span>
`;
Output.println(markup);
```
#### Output:

### Only formatting
```tsx
import { MarkupFormatter, html } from "termx-markup";
const markup = html`
<span color="red">
Hello
<pre color="blue"> in my </pre>
world!
</span>
`;
const formatted = MarkupFormatter.format(markup);
// formatted = "\u001b[31mHello \u001b[34min my\u001b[0m\u001b[31m world!\u001b[0m"
console.log(formatted);
```
#### Output:

### Define custom colors
```tsx
import { Output, MarkupFormatter, html } from "termx-markup";
MarkupFormatter.defineColor("burgundy", "rgb(128, 0, 32)");
MarkupFormatter.defineColor("mauve", "#E0B0FF");
MarkupFormatter.defineColor("teal", { r: 0, g: 128, b: 128 });
const markup = html`
<line color="burgundy">Burgundy</line>
<line color="mauve">Mauve</line>
<line color="teal">Teal</line>
`;
Output.print(markup);
```
#### Output:

### Printing lists
```tsx
import { Output, html } from "termx-markup";
Output.print(html`
<line>Drinks:</line>
<ul>
<li>Coffee</li>
<li>Tea</li>
<li>
<line>Milk</line>
<ul type="circle">
<li>Skim</li>
<li>Whole</li>
</ul>
</li>
</ul>
`);
```
#### Output:

## Supported tags
- `<span>` - regular text, trims white-space characters and removes end-line characters
- `<line>` - same as span, but prints a new line character at the end
- `<pre>` - preformatted text, all white-space characters will be preserved
- `<br />` - prints a new line character
- `<s />` - prints a space character
- `<ol>` - ordered list, each child element is required to ba a `<li>` tag
- `<ul>` - unordered list, each child element is required to ba a `<li>` tag, accepts additional attribute `type` (string) which can be of value `circle`, `square` or `bullet` (default is `bullet`)
- `<pad>` - adds left padding to it's content, accepts attribute `size` (number) which determines the number of spaces to print
- `<frame>` - adds a border around it's content, accepts attributes `padding`, `padding-left`, `padding-right`, `padding-top`, `padding-bottom`, `padding-horizontal` and `padding-vertical` (number) which determines the number of spaces to print between the border and the content
## Inline and Block elements
There are two display types of elements, `inline` and `block`.
Inline elements are always rendered next to each other within the same line, if there are any white-spaces between the inline elements it will be replaced with a single space.
Block elements, start with a line break character, unless the block element is the first one, and end with a line break, unless the block element is the last one.
#### Inline elements:
- `<span>`
- `<pre>`
- `<br>`
- `<s>`
- `<li>`
#### Block elements:
- `<line>`
- `<frame>`
- `<pad>`
- `<ul>`
- `<ol>`
## Supported attributes
Following attributes are available on all tags:
- `color` - color of the text (css-like rgb or a color name)
- `bg` - background color of the text (css-like rgb or a color name)
- `bold` - bold text (boolean)
- `dim` - dimmed text (boolean)
- `italic` - italic text (boolean)
- `underscore` - underlined text (boolean)
- `blink` - blinking text (boolean)
- `invert` - inverse color text (boolean)
- `strike` - strike-through text (boolean)
- `no-inherit` - prevents inheriting parent styles (boolean)
## Default available colors
- `red`
- `green`
- `yellow`
- `blue`
- `magenta`
- `cyan`
- `white`
- `lightRed`
- `lightGreen`
- `lightYellow`
- `lightBlue`
- `lightMagenta`
- `lightCyan`
- `lightWhite`
| Markup based text formatting for terminal in JavaScript environments. | ansi,color,html,markup,xml,cli,console,format,formatter,formatting | 2023-01-07T21:15:50Z | 2023-09-04T08:55:39Z | 2023-09-04T08:52:46Z | 2 | 8 | 75 | 0 | 0 | 4 | null | MIT | TypeScript |
anki2001ta/bookmania | main | # bookmania
This is a self made website by two people
| Bookmania is a webiste where we can buy books ,filter them and shortlist our favourite books | css,html,javascript | 2023-01-11T18:47:25Z | 2023-01-11T19:52:03Z | null | 2 | 0 | 3 | 0 | 0 | 4 | null | null | JavaScript |
Tobby8629/Capstone-project | main | <a name="readme-top"></a>
<!--
HOW TO USE:
This is an example of how you may give instructions on setting up your project locally.
Modify this file to match your project and remove sections that don't apply.
REQUIRED SECTIONS:
- Table of Contents
- About the Project
- Built With
- Live Demo
- Getting Started
- Authors
- Future Features
- Contributing
- Show your support
- Acknowledgements
- License
OPTIONAL SECTIONS:
- FAQ
After you're finished please remove all the comments and instructions!
-->
<div align="center">
<!-- You are encouraged to replace this logo with your own! Otherwise you can also remove it. -->
<br/>
<h3><b>First capstone project(microverse)</b></h3>
</div>
<!-- TABLE OF CONTENTS -->
# 📗 Table of Contents
- [📖 About the Project](#about-project)
- [🛠 Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [🚀 Live Demo](#live-demo)
- [💻 Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#triangular_flag_on_post-deployment)
- [👥 Authors](#authors)
- [🔭 Future Features](#future-features)
- [🤝 Contributing](#contributing)
- [⭐️ Show your support](#support)
- [🙏 Acknowledgements](#acknowledgements)
- [❓ FAQ (OPTIONAL)](#faq)
- [📝 License](#license)
<!-- PROJECT DESCRIPTION -->
# 📖 NTS 2022 <a name="about-project"></a>
> Describe your project in 1 or 2 sentences.
**my first capstone project** is about national tech summit, here is a link for full description <a href="https://www.loom.com/share/e9f60868b7cf47cba3983c9c9ad6d849"> click here </a>
## 🛠 Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
> Describe the tech stack and include only the relevant sections that apply to your project.
<details>
<summary>Client</summary>
<ul>
<li><a href="https://reactjs.org/">Html</a></li>
</ul>
</details>
<details>
<summary>styling and designs</summary>
<ul>
<li><a href="https://expressjs.com/">css</a></li>
</ul>
</details>
<details>
<summary>Functionality</summary>
<ul>
<li><a href="https://www.postgresql.org/">javascript</a></li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
> Describe between 1-3 key features of the application.
- **Header and menu**
- **Programs**
- **featured speakers**
- **sponsors**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## 🚀 Live Demo <a name="live-demo"></a>
> Add a link to your deployed project.
- [Live Demo Link](https://tobby8629.github.io/Capstone-project/index.html)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## 💻 Getting Started <a name="getting-started"></a>
> Describe how a new developer could make use of your project.
To get a local copy up and running, follow these steps.
### Prerequisites
In order to run this project you need:
<!--
Example command:
```sh
gem install rails
```
-->
### Setup
Clone this repository to your desired folder:
<!--
Example commands:
```sh
cd my-folder
git clone git@github.com:myaccount/my-project.git
```
--->
### Install
Install this project with:
<!--
Example command:
```sh
cd my-project
gem install
```
--->
### Usage
To run the project, execute the following command:
<!--
Example command:
```sh
rails server
```
--->
### Run tests
To run tests, run the following command:
<!--
Example command:
```sh
bin/rails test test/models/article_test.rb
```
--->
### Deployment
You can deploy this project using:
<!--
Example:
```sh
```
-->
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## 👥 Authors <a name="authors"></a>
> Mention all of the collaborators of this project.
👤 **popoola samuel**
- GitHub: [@githubhandle](https://github.com/tobby8629)
- Twitter: [@twitterhandle](https://twitter.com/twitterhandle)
- LinkedIn: [LinkedIn](https://linkedin.com/in/samuel-popoola-tobby/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## 🔭 Future Features <a name="future-features"></a>
> Describe 1 - 3 features you will add to the project.
- [ ] **section of videsos from the summit**
- [ ] **A slideshow of images from the summit**
- [ ] **Section for testmonies from past summit**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [https://github.com/Tobby8629/Capstone-project/issues](tobby8629/capstone-project/issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## ⭐️ Show your support <a name="support"></a>
> Write a message to encourage readers to support your project
If you like this project, please kindly follow to see more beautiful projects
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## 🙏 Acknowledgments <a name="acknowledgements"></a>
> Give credit to everyone who inspired your codebase.
- I would like to thank the microverse organization and my friends who have been supportive throughout the course of this project
- I appreciate <a href="https://www.behance.net/adagio07">cindy shin</a>cindy shin the author of the CC GLOBAL SUMMIT 2015 for the access giving to me to work with the template.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FAQ (optional) -->
## ❓ FAQ (OPTIONAL) <a name="faq"></a>
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## 📝 License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
_NOTE: we recommend using the [MIT license](https://choosealicense.com/licenses/mit/) - you can set it up quickly by [using templates available on GitHub](https://docs.github.com/en/communities/setting-up-your-project-for-healthy-contributions/adding-a-license-to-a-repository). You can also use [any other license](https://choosealicense.com/licenses/) if you wish._
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| A National summit website, that describes all activities involved and expected from the summit. This project is built using HTML, CSS, and JAVASCRIPT. It has two pages (Home and About) and also comprises three sections for the main program, featured speakers and sponsors | html,css,javascript | 2023-01-02T08:15:12Z | 2023-01-26T21:34:07Z | null | 1 | 1 | 33 | 0 | 0 | 4 | null | MIT | CSS |
Toni-Zoaretz/AS-Project-Amazon-Website | main | # 🚀 About Amazon Mockup Website
I was given a task @AppleSeeds Bootcamp to build a responsive product page from Amazon website.<br>
The product page was copied using `HTML`,`CSS`,`Transition`,`Flex` and a little bit `JavaScript`.
### Original site link:
https://www.amazon.com/PEACHCAT-Banana-Plushie-Hugging-Stuffed/dp/B09YH3W321?th=1/
### Demo site link:
https://amazon-webite.netlify.app/
### Navbar
Creating two Navbars for desktop and mobile.
### Product Section
I built the product section using Flex and also made a different design for desktop and mobile.
### Gallery Section
The image gallery is static and was built using Flex, on mobile there is no gallery view at all, only images.
### Screenshots



### Deployment
To deploy this project run
```bash
./index.html
```
| Amazon responsive product page | html,css,flex,flexbox,javascript,tranistion | 2023-01-12T13:00:45Z | 2023-01-28T18:14:19Z | null | 1 | 0 | 24 | 0 | 0 | 4 | null | null | HTML |
Suneet25/zoomcar-clone | main | ## Working on Zoomcar clone....
<h1>Landing Page</h1>
<br>

<br>
<h1>Login Page</h1>
<br>

<br>
<h1>Cars Page</h1>
<br>

<br>
<h1>Single Car Page</h1>
<br>

<br>
<h1>Payment Page</h1>
<br>

<br>
<h1>Payment Successful Page</h1>
<br>

<br>
Tasks for all Team mate
<hr>
<b>Suneet</b> - Navabr, sidebar, Landingpage,HostPage,DatePage,Routing,Responsiveness
<br>
<br>
<br>
<b>Papri</b> - SignupPage, LoginPage,PrivateRouting,Routing,Responsiveness
<br>
<br>
<br>
<b>Nitin</b> - Cars Page (sorting and filter functionality),SingleCarPage
Process to pay page - This page is Private Page Means Protected without login can't access,
LocationPage,Routing,Responsiveness
<br>
<br>
<br>
<b>Jyoti</b> - Payment page, Process to pay page with location thing,Routing,Responsiveness
<br>
<br>
<br>
<h3>Thank You 🙂
| Zoomcar is an Indian car sharing platform, headquartered in Bangalore. It operate in Self Driving Car Rentals | react,authentication,chakra-ui,css,html,javascript,middleware,redux,thunk | 2023-01-16T14:46:13Z | 2023-04-20T06:45:21Z | null | 7 | 32 | 120 | 0 | 4 | 4 | null | null | JavaScript |
lucyanovidio/boraCodar-rocketseat | main | # #boraCodar - Rocketseat 🚀
<img src="https://img.shields.io/github/license/lucyanovidio/boraCodar-rocketseat.svg" />
<br>
O <a href="https://boracodar.dev">**#boraCodar**</a> é um projeto da <a href="https://rocketseat.com.br">Rocketseat</a> que se iniciou este ano para estimular a prática de programação semanal e ajudar na criação de projetos para portifólio.
Toda quarta-feira, às 11h, é publicado um novo projeto no <a href="https://boracodar.dev">site oficial</a> do projeto para cada participante codar a sua versão com as tecnologias de sua preferência e publicar usando a hashtag *#boraCodar*. Na quarta seguinte à da publicação do desafio é postado uma resolução do mesmo. O projeto conta com uma comunidade enorme participando e interagindo.
Este repositório é para que eu coloque as minhas resoluções de cada desafio!
Participe também! É só entrar no site do <a href="https://boracodar.dev">**#boraCodar**</a> e começar a desenvolver o desafio da semana! 🚀
***#boraCodar***
<br>
> Também estou compartilhando meus desafios no [LinkedIn](https://linkedin.com/in/lucyanovidio)! Se quiser trocar uma ideia, é só chamar! 😁
<br>
<table>
<tr>
<th colspan="2">Desafios</th>
</tr>
<tr>
<td>2. <a href="https://github.com/lucyanovidio/boraCodar-rocketseat/tree/main/desafio-02">Card de produto</a></td>
<td>3. <a href="https://github.com/lucyanovidio/boraCodar-rocketseat/tree/main/desafio-03">Botões e cursores</a></td>
</tr>
<tr>
<td>4. <a href="https://github.com/lucyanovidio/boraCodar-rocketseat/tree/main/desafio-04">Chat</a></td>
<td>5. <a href="https://github.com/lucyanovidio/boraCodar-rocketseat/tree/main/desafio-05">Calculadora</a></td>
</tr>
<tr>
<td>6. <a href="https://github.com/lucyanovidio/boraCodar-rocketseat/tree/main/desafio-06">Cartão de embarque</a></td>
<td>10. <a href="https://github.com/lucyanovidio/boraCodar-rocketseat/tree/main/desafio-10">Página de clima</a></td>
</tr>
</table>
<br>
---
<table>
<tr>
<td>
<img src="https://github.com/lucyanovidio.png" width="100px" />
</td>
<td>
<img src="https://github.com/rocketseat-education.png" width="100px" />
</td>
<td>
Feito por <a href="https://github.com/lucyanovidio">Lucyan Ovídio</a> 🙋🏿♂️
<br> Junto com <a href="https://rocketseat.com.br">Rocketseat</a> 🚀.
</td>
</tr>
</table>
| Desafios semanais de desenvolvimento web promovidos pela Rocketseat. | javascript,css,html,challenges,font-end,rocketseat | 2023-01-14T22:37:05Z | 2023-03-16T02:23:39Z | null | 1 | 0 | 54 | 0 | 1 | 4 | null | MIT | HTML |
simplegoose/capstone-project-1 | master | <a name="readme-top"></a>
<div align="center">
<h3><b>Capstone Project</b></h3>
</div>
<!-- TABLE OF CONTENTS -->
# 📗 Table of Contents
- [📖 About the Project](#about-project)
- [🛠 Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [🚀 Live Demo](#live-demo)
- [💻 Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#triangular_flag_on_post-deployment)
- [👥 Authors](#authors)
- [🔭 Future Features](#future-features)
- [🤝 Contributing](#contributing)
- [⭐️ Show your support](#support)
- [🙏 Acknowledgements](#acknowledgements)
- [❓ FAQ (OPTIONAL)](#faq)
- [📝 License](#license)
<!-- PROJECT DESCRIPTION -->
# 📖 [capstone-project-1] <a name="about-project"></a>
Capstone project 1 is the first capstone I have done at Microverse so far.
**[capstone-project-1]** is a simple project that I used to mock a design from behance
## 🛠 Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
<details>
<summary>Client</summary>
<ul>
<li><a href="#">HTML</a></li>
<li><a href="#">CSS</a></li>
<li><a href="#">JavaScript</a></li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
- Navigation to different pages.
- Dynamic generation of featured products.
- Navigation toggling on mobile devices
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## 🚀 Live Demo <a name="live-demo"></a>
- [Live Demo Link](https://simplegoose.github.io/capstone-project-1)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## 💻 Getting Started <a name="getting-started"></a>
To get a local copy up and running, follow these steps.
### Prerequisites
In order to run this project you need:
- You should have git on your machine.
- Follow the steps on the setup to clone the code.
> If you don't have git installed on your machine you can download it from [here](https://git-scm.com/downloads).
### Setup
Clone this repository to your desired folder:
If you have git installed on your machine you can clone your project by running the command below to clone your project to your local machine
`git clone https://github.com/simplegoose/capstone-project-1.git`
### Usage
To run the project, execute the following command:
Locate the folder where you cloned your project to and open the `index.html` file in your browser to view the project.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## 👥 Authors <a name="authors"></a>
👤 **Simplegoose**
- GitHub: [@githubhandle](https://github.com/simplegoose)
- Twitter: [@twitterhandle](https://twitter.com/njorogemwangi21)
- LinkedIn: [LinkedIn](https://linkedin.com/in/timothy-njoroge-mwangi)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## 🔭 Future Features <a name="future-features"></a>
- [ API integration ]
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## ⭐️ Show your support <a name="support"></a>
If you like this project leave me a star on GitHub.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## 🙏 Acknowledgments <a name="acknowledgements"></a>
I would like to thank [Cindy Shin](https://www.behance.net/adagio07) with her design that was provided on [Behance](https://www.behance.net/gallery/29845175/CC-Global-Summit-2015)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## 📝 License <a name="license"></a>
This project is [MIT](./MIT.md) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## WalkThrough <a name="walkthrough"></a>
[Here is a walkthrough](https://www.loom.com/share/838505d237a546f3b2f1d684b44fb3bb)
| A car seller website to showcase their annual expo. Built with HTML, CSS, and JavaScript. | css,responsive,html,javascript | 2023-01-04T12:03:03Z | 2023-01-06T10:51:44Z | null | 1 | 1 | 34 | 1 | 0 | 4 | null | null | CSS |
ArslanYM/WeatherApp | master | # Getting Started with Create React App
This project was bootstrapped with [Create React App](https://github.com/facebook/create-react-app).
## Available Scripts
In the project directory, you can run:
### `npm start`
Runs the app in the development mode.\
Open [http://localhost:3000](http://localhost:3000) to view it in your browser.
The page will reload when you make changes.\
You may also see any lint errors in the console.
### `npm test`
Launches the test runner in the interactive watch mode.\
See the section about [running tests](https://facebook.github.io/create-react-app/docs/running-tests) for more information.
### `npm run build`
Builds the app for production to the `build` folder.\
It correctly bundles React in production mode and optimizes the build for the best performance.
The build is minified and the filenames include the hashes.\
Your app is ready to be deployed!
See the section about [deployment](https://facebook.github.io/create-react-app/docs/deployment) for more information.
### `npm run eject`
**Note: this is a one-way operation. Once you `eject`, you can't go back!**
If you aren't satisfied with the build tool and configuration choices, you can `eject` at any time. This command will remove the single build dependency from your project.
Instead, it will copy all the configuration files and the transitive dependencies (webpack, Babel, ESLint, etc) right into your project so you have full control over them. All of the commands except `eject` will still work, but they will point to the copied scripts so you can tweak them. At this point you're on your own.
You don't have to ever use `eject`. The curated feature set is suitable for small and middle deployments, and you shouldn't feel obligated to use this feature. However we understand that this tool wouldn't be useful if you couldn't customize it when you are ready for it.
## Learn More
You can learn more in the [Create React App documentation](https://facebook.github.io/create-react-app/docs/getting-started).
To learn React, check out the [React documentation](https://reactjs.org/).
### Code Splitting
This section has moved here: [https://facebook.github.io/create-react-app/docs/code-splitting](https://facebook.github.io/create-react-app/docs/code-splitting)
### Analyzing the Bundle Size
This section has moved here: [https://facebook.github.io/create-react-app/docs/analyzing-the-bundle-size](https://facebook.github.io/create-react-app/docs/analyzing-the-bundle-size)
### Making a Progressive Web App
This section has moved here: [https://facebook.github.io/create-react-app/docs/making-a-progressive-web-app](https://facebook.github.io/create-react-app/docs/making-a-progressive-web-app)
### Advanced Configuration
This section has moved here: [https://facebook.github.io/create-react-app/docs/advanced-configuration](https://facebook.github.io/create-react-app/docs/advanced-configuration)
### Deployment
This section has moved here: [https://facebook.github.io/create-react-app/docs/deployment](https://facebook.github.io/create-react-app/docs/deployment)
### `npm run build` fails to minify
This section has moved here: [https://facebook.github.io/create-react-app/docs/troubleshooting#npm-run-build-fails-to-minify](https://facebook.github.io/create-react-app/docs/troubleshooting#npm-run-build-fails-to-minify)
| Weather App using React and Open Weather API | javascript,open-source,openweathermap-api,react,reactjs | 2023-01-08T14:43:11Z | 2023-01-08T14:55:40Z | null | 1 | 0 | 1 | 0 | 0 | 4 | null | null | JavaScript |
Solana-Workshops/beginner-crash-course | main | # Beginner Crash Course
## 🎬 Recorded Sessions
| Link | Instructor | Event |
| ---- | ---------- | ----- |
| [<img src="https://raw.githubusercontent.com/Solana-Workshops/.github/main/.docs/youtube-icon.png" alt="youtube" width="20" align="center"/> Recording](https://www.youtube.com/watch?v=FQYmWWw5l04) | Joe Caulfield | Encode Hackathon 2023 |
| [<img src="https://raw.githubusercontent.com/Solana-Workshops/.github/main/.docs/youtube-icon.png" alt="youtube" width="20" align="center"/> Recording (Playlist Version)](https://www.youtube.com/playlist?list=PLfEHHr3qexv_FEcsuEEmiwSTKfahbYzVX) | Joe Caulfield | Encode Hackathon 2023 |
## ☄️ Open in Solana Playground IDE
| Program | Link |
| -------------------- | --------------------------------------- |
| Hello World | [ ]( https://beta.solpg.io/github/https://github.com/Solana-Workshops/beginner-crash-course/tree/main/hello-world/program) |
| Hello World Again | [ ]( https://beta.solpg.io/github/https://github.com/Solana-Workshops/beginner-crash-course/tree/main/hello-world-again/program) |
## 📗 Learn
We're going to cover the basics of Solana development to get you started building on Solana.
We'll cover the following concepts:
* [Keypairs](#🔑-keypairshttpssolanacookbookcomreferenceskeypairs-and-walletshtmlhow-to-generate-a-new-keypair)
* [The System Program](#⚙️-the-system-programhttpsdocssolanacomdevelopingruntime-facilitiesprograms)
* [Accounts](#📂-accountshttpssolanacookbookcomcore-conceptsaccountshtmlfacts)
* Rent
* Executable Accounts
* Data
* Ownership
* [Transacting with Solana's Network](#🖲️-transacting-with-solanas-networkhttpsdocssolanacomdevelopingprogramming-modeltransactions)
* Requesting Data from Solana
* Modifying Data on Solana
* [Writing Programs](#📝-writing-programshttpssolanacookbookcomreferencesprogramshtmlhow-to-transfer-sol-in-a-program)
* Program Structure
* Building Custom Instructions for Your Program
* Building Custom Instructions on the Client-Side
* Frameworks for Writing Solana Programs
* [Tokens](#🪙-tokenshttpssolanacookbookcomreferencestokenhtml)
* Mint Accounts
* Decimals
* Mint Authority
* Freeze Authority
* Associated Token Accounts
* Metadata
### 🔑 [Keypairs](https://solanacookbook.com/references/keypairs-and-wallets.html#how-to-generate-a-new-keypair)
Solana wallets are like other crypto wallets - they utilize a **public key** and **private key**.
**Private keys** are used to sign transactions, which can only be decrypted by that private key's **associated public key**.
This allows cryptographic programs to verify a signature with only the public key, not the private key.
This is similar to how SSH keys work in computer communication.
Solana's SDK can create a keypair compatible with Solana's blockchain, but it's not actually activated until the public key is registered with Solana's **System Program**.
### ⚙️ [The System Program](https://docs.solana.com/developing/runtime-facilities/programs)
Solana has a number of native programs (or smart contracts) that live on it's cluster.
Arguably the most important native program is the **System Program** - which is responsible for the following:
* Registering public keys as blockchain addresses
* Allocating space at a particular address
* Changing the ownership of an account
To register a public key with Solana, we must use the System Program. This will effectively register our keypair's public key as an **address** and create an **account** on Solana's blockchain. The account's address will mark the specific location of our account on Solana's blockchain.
🔸 Here's what registering a keypair's public key with the System Program looks like in TypeScript:
https://github.com/solana-developers/workshops/blob/33ee92c20f4a15e0f8da3d16708a49a16ac8bb10/workshops/beginner-crash-course/client-examples/scripts/accounts.ts#L36-L58
### 📂 [Accounts](https://solanacookbook.com/core-concepts/accounts.html#facts)
You can think of Solana's global state as an operating system. In Linux, it's known that "everything is a file". You can swap the word "account" for "file" when talking about Solana, and majority of the concepts will be the same.
In an nutshell, Solana indexes data - including someone's balance of SOL - into accounts on the blockchain, and can find them using their address.
Accounts follow a basic structure:
```text
{
lamports: The amount of SOL (Lamports) in an account
owner: The program that owns this account
executable: Whether or not this account is an executable program
data: The custom data stored within this account
rentEpoch: The next epoch that rent will be due for this account
}
```
You can see, by default, accounts store some metadata - including a balance of SOL (Lamports).
**→ Rent**
Solana charges a user **rent** for storing data on the blockchain - even if it's just the default metadata. The more data you add to the `data` field, the more rent you will be responsible for paying.
This is due to the simple fact that Solana cluster nodes pay for hardware, which is where your data will be stored. This distributes the cost across the cluster similar to a market.
**Note:** You can make an account rent-exempt by depositing a SOL (Lamports) value equivalent to 2 year's worth of rent. This will effectively halt rent withdrawals on your account and leave your balance in tact.
It's worth noting that the amount you pay in rent is almost negligible for most accounts.
**→ Executable Accounts**
Simply put, everything on Solana is an account, even smart contracts.
Smart contracts (Programs) live inside of accounts, and in order to tell the cluster that an account is actually an executable program, the boolean `executable` is used.
**→ Data**
The `data` field is called an account's "inner data" and that's where you can store your own custom data.
This data is stored in the form of bytes, and you need to serialize traditional data into bytes in order to store it inside an account.
More on this later.
**→ Ownership**
Ownership is an extremely important concept in Solana.
Basically, every account is owned by a program. When a program owns an account, it's allowed to modify it's data at will.
Programs like the System Program have signature requirements that prevent unwanted changes to an account.
> Think about it: Can anyone withdraw SOL from your wallet without your permission? No!
> You have to sign any transaction with your private key that will **debit** lamports from your account.
> However, anyone can **credit** your account (add funds), because, why wouldn't you want free money?
> This is how airdrops work.
⚠️ If you write your own program and create accounts that are owned by your program, your program can modify those accounts - including debit their SOL balances - without permission.
### 🖲️ [Transacting with Solana's Network](https://docs.solana.com/developing/programming-model/transactions)
In order to conduct operations on Solana, you need to use a networking protocol known as RPC to send requests to the cluster.
RPC is similar to HTTP in the sense that you are basically sending a GET or POST request to the Solana network to conduct some sort of business.
**→ Requesting Data from Solana**
To query state information on Solana without making changes, you can send an RPC request to recieve a certain response with your desired information.
You can do this by setting up a connection to the Solana network, and sending a request.
🔸 Here's what that looks like in TypeScript - where we're requesting information about an account:
https://github.com/solana-developers/workshops/blob/33ee92c20f4a15e0f8da3d16708a49a16ac8bb10/workshops/beginner-crash-course/client-examples/scripts/accounts.ts#L60-L64
**→ Modifying Data on Solana**
To modify state on Solana, you can also send an RPC request, but you'll need to package this request into what's called a Transaction.
Transactions are structured data payloads that can be signed by a keypair's private key - which allows for cryptographic authentication as we described above.
A transaction looks like this:
```TypeScript
{
signatures: [ s, s ] // List of signatures
message:
header: 000
addresses: [ aaa, aaa ] // Accounts involved in the transaction
recent_blockhash: int // The recent blockhash tied to this transaction
instructions: [ ix, ix ] // The instructions to perform on Solana's state
}
```
You can see transactions have a list of instructions. These instructions point to specific Solana programs and tell them what to do.
A transaction instruction looks like this:
```TypeScript
{
program_id: xxx // The Solana program this instruction is targeting
accounts: [ aaa, aaa ] // The accounts involved in this instruction
instruction_data: b[] // The specific data that tells the program which operation to conduct
}
```
**Note:** A **recent blockhash** is included in Solana transactions as a security measure. Basically, the network is going to make sure this isn't an old transaction to prevent against fraud or hacks.
🔸 Here's an example of sending a transaction in TypeScript - where we're going to transfer some SOL out of our account:
https://github.com/solana-developers/workshops/blob/33ee92c20f4a15e0f8da3d16708a49a16ac8bb10/workshops/beginner-crash-course/client-examples/scripts/accounts.ts#L66-L90
### 📝 [Writing Programs](https://solanacookbook.com/references/programs.html#how-to-transfer-sol-in-a-program)
Most Solana operations can be done without writing your own program. In fact, many popular dApps on Solana simply leverage the client-side RPC interactions with the Solana network and don't utilize a custom program.
However, you may find the need to write your own program for many reasons.
When writing your own program, there are a few things to understand:
* The structure of a Solana program
* How to set up multiple instructions
* How to serialize your program's instructions from the client side
* How to send a transaction to your custom program
Solana programs are written in Rust, and leverage the `solana-program` crate, as well as many others including `spl-token` - depending on what your program does.
**→ Program Structure**
All Solana programs - whether they are custom programs or native programs - follow the same structure.
Programs have an **entrypoint** - which tells the Solana runtime where the entrypoint to this program is. The entrypoint is simply the function that has been specifically designed to process a Transaction Instruction.
If you look at the anatomy of a Transaction Instruction above - under [Transacting with Solana's Network](#🖲️-transacting-with-solanas-networkhttpsdocssolanacomdevelopingprogramming-modeltransactions) - you can see why a Solana program's entrypoint must look like this:
```rust
fn my_program(
program_id: &Pubkey,
accounts: &[AccountInfo],
instruction_data: &[u8],
) -> ProgramResult
```
🔸 Here's a simple Solana program demonstrating this entrypoint in action:
https://github.com/solana-developers/workshops/blob/b1cb19170da82bf59f57c4b646b6612c4501d8ec/workshops/beginner-crash-course/hello-world/program/src/lib.rs#L1-L31
Solana programs written in Rust must be of crate type `lib` and declare a specific lib type known as `cdylib`.
You can specify this configuration in the `Cargo.toml` file like so:
```toml
[lib]
crate-type = ["cdylib", "lib"]
```
**→ Building Custom Instructions for Your Program**
The `instruction_data` field within a transaction instruction is the data that you can use to tell your program which operation to conduct.
You can define custom instruction payloads in Rust using structs, and use an enum to match against the various structs you've defined.
To do this, you need to leverage the `borsh` and `borsh-derive` crates to allow Rust to deserialize these objects from the instruction payload.
🔸 Here's an example of using such structs and an enum to create instructions:
https://github.com/solana-developers/workshops/blob/5e7288353b9a716415ff9d558b8248d806a978f6/workshops/beginner-crash-course/hello-world-again/program/src/lib.rs#L11-L26
🔸 Here's a more built-out Solana program demonstrating instruction processing:
https://github.com/solana-developers/workshops/blob/5e7288353b9a716415ff9d558b8248d806a978f6/workshops/beginner-crash-course/hello-world-again/program/src/lib.rs#L1-L61
**→ Building Custom Instructions on the Client-Side**
On the client side, you have to replicate the structs that you defined on-chain in Rust.
You can do this by again using `borsh` to serialize objects into bytes.
🔸 Here's how to build a schema for matching an instruction struct defined in Rust:
https://github.com/solana-developers/workshops/blob/701c1df2d4ce16d1543dddfb7f5056114ebe9245/workshops/beginner-crash-course/hello-world-again/tests/instructions.ts#L15-L61
🔸 Now here's an example of sending different instructions to our custom program:
https://github.com/solana-developers/workshops/blob/701c1df2d4ce16d1543dddfb7f5056114ebe9245/workshops/beginner-crash-course/hello-world-again/tests/test.ts#L27-L40
**→ Frameworks for Writing Solana Programs**
* ⚓️ [Anchor](https://www.anchor-lang.com/)
* 🐴 [Seahorse](https://seahorse-lang.org/)
### 🪙 [Tokens](https://solanacookbook.com/references/token.html)
Tokens on Solana are called SPL Tokens, and they follow a standard just like tokens on Ethereum.
On Solana, tokens are managed like so:
* A Mint account is an account representing the token itself.
* Associated Token Accounts are accounts that tie a wallet address to a mint address and describe that wallet's balance of that mint.
* Metadata accounts are separate accounts that point to the Mint account and define all of the associated metadata for a token.
All tokens are managed by the **Token Program** - another native Solana program in charge of SPL tokens.
For working with SPL tokens in TypeScript, we use the following library:
```json
{
"dependencies": {
"@solana/spl-token": "^0.3.7",
}
}
```
🔸 Here's what creating a Mint looks like with the Token Program:
https://github.com/solana-developers/workshops/blob/cffc4ce2945ad5528d9b2704f81d6f64d030c76a/workshops/beginner-crash-course/client-examples/scripts/tokens.ts#L81-L113
**→ Mint Accounts**
A Mint account contains standard data about a particular token mint:
```TypeScript
{
isInitialized,
supply, // The current supply of this token mint on Solana
decimals, // The number of decimals this mint breaks down to
mintAuthority, // The account who can authorize minting of new tokens
freezeAuthority, // The account who can authorize freezing of tokens
}
```
**→ Decimals**
A Mint's decimals define how the token's supply is broken up.
For example, for a Mint whose decimals value is set to 3, if you send 5 tokens to someone, they will actually be receiving 0.005 tokens.
Calculation: `quantity * 10 ^ (-1 * d)` where d = decimals.
The standard for SPL Tokens on Solana is 9 decimals, but you can specify any decimal value you want.
NFTs have a decimal value of 0. More on this later.
**→ Mint Authority**
The **Mint Authority** is the account that is allowed to create (mint) new tokens.
The Token Program does the actual minting of new tokens, but it's only authorized to do so if the Mint's specified Mint Authority has signed the transaction.
When a Mint Authority is set to `None`, that means no more new tokens can be minted. This action is irreversible.
**→ Freeze Authority**
The **Freeze Authority** is the account that is allowed to freeze token movement.
This means that a "freeze" is placed on the Mint, and no transfers amongst any Associated Token Accounts regarding that Mint can be conducted.
When a Freeze Authority is set to `None`, that means this token cannot be frozen. This action is irreversible.
**→ Associated Token Accounts**
Associated Token Accounts are accounts designed to describe how many tokens a particular wallet holds.
Since Solana accounts only have a default field for Lamports, and many different kinds of SPL tokens can be created by anyone, it's impossible to include a field for every possible SPL token in an account.
Instead, we make use of separate accounts - called Associated Token Accounts - to keep track of a wallet's balance of a particular token.
These accounts essentially have 2 pointers - the wallet it's associated with and the Mint it's associated with - and a balance.
🔸 Here's what minting tokens to an Associated Token Account looks like in TypeScript:
https://github.com/solana-developers/workshops/blob/cffc4ce2945ad5528d9b2704f81d6f64d030c76a/workshops/beginner-crash-course/client-examples/scripts/tokens.ts#L115-L160
🔸 Here's what transferring tokens between Associated Token Accounts looks like in TypeScript:
https://github.com/solana-developers/workshops/blob/cffc4ce2945ad5528d9b2704f81d6f64d030c76a/workshops/beginner-crash-course/client-examples/scripts/tokens.ts#L162-L202
**→ Metadata**
We must create a separate metadata account that points to our mint to give our token metadata - such as an image.
For this, we use the library for **Metaplex Token Metadata** - the standard for SPL Token metadata on Solana:
```json
{
"dependencies": {
"@metaplex-foundation/mpl-token-metadata": "^2.5.2",
}
}
```
Metaplex's SDK will let us use helper methods to create the necessary instructions, which will target the Metaplex Token Metadata Program.
🔸 Here's where we create the instruction for creating this metadata account, using Metaplex's data types:
https://github.com/solana-developers/workshops/blob/62ede19d9d0be5bbe290d9e4106be2f82a6b5846/workshops/beginner-crash-course/client-examples/scripts/tokens.ts#L104-L127
| Building on Solana Crash Course | beginner,hello-world,native,javascript,rust,client,smart-contracts | 2023-01-05T14:04:29Z | 2023-02-23T20:34:40Z | null | 1 | 0 | 6 | 0 | 2 | 4 | null | null | TypeScript |
Nezerwa/Awesome_books_es6 | main | <a name="readme-top"></a>
# 📗 Table of Contents
- [📖 About the Project](#about-project)
- [🛠 Built With](#built-with)
- [🚀 Live Demo](#live-demo)
- [💻 Getting Started](#getting-started)
- [👥 Authors](#authors)
- [🔭 Future Features](#future-features)
- [🤝 Contributing](#contributing)
- [⭐️ Show your support](#support)
- [🙏 Acknowledgements](#acknowledgements)
<!-- PROJECT DESCRIPTION -->
# 📖 Awesome Books <a name="about-project"></a>
> Awesome books is a simple website that displays a list of books and allows you to add and remove books from that list.
Here are some features of the page:
- Add a book to your collection.
- Remove a book from your collection.
- Store your data history in the browser's memory.
## 🛠 Built With <a name="built-with"></a>
HTML
CSS
Javascript
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## 🚀 Live Demo <a name="live-demo"></a>
[Awesome Book](https://Nezerwa.github.io/awesome_Books_es-/#)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## 💻 Getting Started <a name="getting-started"></a>
- Create a local directory that you want to clone the repository.
- Open the command prompt in the created directory.
- On the terminal run this command git clone https://github.com/Nezerwa/awesome_books-es6
- Go to the repository folder using command prompt cd Awesome_Book.
- Install the dev dependencies for linters run npm install.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
### Prerequisites
In order to run this project you need:
- Google chrome
- Internet Explorer
- Any browser
<p align="right">(<a href="#readme-top">back to top</a>)</p>
### Setup
Clone this repository to your desired folder:
- Open the command prompt in the created directory.
- On the terminal run this command git clone https://github.com/Nezerwa/awesome_books_es6
- Go to the repository folder using command prompt cd Awesome-Books-ES6
- Install the dev dependencies for linters run npm install.
--->
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- Author -->
## 👤**Author** <a name="authors"></a>
👤 **Nezerwa Eligrand**
- GitHub: [@githubhandle](https://github.com/Nezerwa)
- LinkedIn: [LinkedIn](https://linkedin.com/in/eligrand-nezerwa/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## 🔭 Future Features <a name="future-features"></a>
- Implement some UX improvements: add a home page, include transitions and/or animation
- Implement additional pages, which will allow the user to Register
- Implement additional pages, which will allow the user to Login
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the issues page.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## ⭐️ Show your support <a name="support"></a>
Give a ⭐️ if you like this project!
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## 🙏 Acknowledgments <a name="acknowledgements"></a>
[Luxon](https://imdac.github.io/modules/js/luxon/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| Users can add both the title and author of a book to their personal library. The user has the option to add new books and delete text. With the help of this software, users may save all modifications to local storage, making it possible for them to access their stored information whenever they want. | css,html5,javascript | 2023-01-16T15:20:28Z | 2023-01-17T05:39:49Z | null | 1 | 1 | 11 | 0 | 0 | 4 | null | null | JavaScript |
MNisarAli/Math-Magicians | develop | <a name="readme-top"></a>
<div align="center">
<img src="./public/favicon.ico" alt="logo" width="140" height="auto" />
<br/>
<h1><b>Math-Magicians</b></h1>
</div>
# 📗 Table of Contents
- [📗 Table of Contents](#-table-of-contents)
- [📚🔢💯 Math-Magicians ](#-math-magicians-)
- [🛠 Built With ](#-built-with-)
- [Tech Stack ](#tech-stack-)
- [Key Features ](#key-features-)
- [🚀 Live Demo ](#-live-demo-)
- [💻 Getting Started ](#-getting-started-)
- [Prerequisites](#prerequisites)
- [Setup](#setup)
- [Install](#install)
- [Start](#start)
- [Build](#build)
- [Deployment](#deployment)
- [Usage](#usage)
- [👥 Authors ](#-authors-)
- [🔭 Future Features ](#-future-features-)
- [🤝 Contributing ](#-contributing-)
- [💖 Show your support ](#-show-your-support-)
- [🙏 Acknowledgments ](#-acknowledgments-)
- [📝 License ](#-license-)
<!-- PROJECT DESCRIPTION -->
# 📚🔢💯 Math-Magicians <a name="about-project"></a>
"Math magicians" is a website for all fans of mathematics. It is a Single Page App (SPA) that allows users to:
- Make simple calculations.
- Read a random math-related quote.
<!-- BUILT WITH -->
## 🛠 Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
- HTML5
- CSS3
- JavaScript(ES6)
- React Js
### Key Features <a name="key-features"></a>
The key features of this website include.
- **React Js**
- **Gitflow**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## 🚀 Live Demo <a name="live-demo"></a>
- [Live Demo Link](https://mathmagicians-cicw.onrender.com/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## 💻 Getting Started <a name="getting-started"></a>
To get a local copy up and running, follow these steps.
### Prerequisites
In order to run this project you need:
- [ ] A web browser like Google Chrome.
- [ ] A code editor like Visual Studio Code with Git and Node.js installed.
### Setup
Clone this repository to your desired folder:
1. Open terminal in VScode.
2. Navigate to the directory where you want clone the copy of this repository
3. Create new directory [optional]
4. Clone the repo using the below command
```sh
git clone https://github.com/MNisarAli/Math-Magicians.git
cd Math-Magicians
```
### Install
Install this project with:
```sh
npm install
```
### Start
To start the application, run the following command
```sh
npm start
```
### Build
To build the app for production, run the following command:
```sh
npm run build
```
### Deployment
To deploy project, run the following command:
```
npm run deploy
```
Or you can deploy on a static site hosting platform like [Render](https://render.com/).
### Usage
This project can be used to practice React.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## 👥 Authors <a name="authors"></a>
The collaborator of this project.
👤 **M Nisar Ali**
- GitHub: [@MNisarAli](https://github.com/MNisarAli)
- Gmail: [Muhammad Nisar Ali](mailto:dr.nisaralig@gmail.com)
- LinkedIn: [Muhammad Nisar Ali](https://linkedin.com/in/muhammad-nisar-ali-45a865251)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## 🔭 Future Features <a name="future-features"></a>
- [ ] **Unit Tests**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](https://github.com/MNisarAli/Math-Magicians/issues).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## 💖 Show your support <a name="support"></a>
Give a ⭐️, if you like this project!
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## 🙏 Acknowledgments <a name="acknowledgements"></a>
I would like to thank:
- [Microverseinc](https://github.com/microverseinc) for [Readme-template](https://github.com/microverseinc/readme-template).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## 📝 License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| A Single Page App (SPA) that allows users to make simple calculations, and read a random math-related quote. | html,reactjs,css,javascript | 2023-01-16T16:58:46Z | 2023-03-13T08:04:34Z | null | 1 | 6 | 44 | 0 | 0 | 4 | null | MIT | JavaScript |
gyan2501/GoogleTimer-StopWatch | main | # Project Name
Timer-StopWatch
A simple timer and stopwatch by using some basic React concepts like useState ,useEffect and useRef.
## Deployed Link
https://googletimer-hv8deybi8-gyan2501.vercel.app/
## Tech Stacks




## Screenshots
<img src="https://github.com/gyan2501/GoogleTimer-StopWatch/blob/main/googletimer/public/gts.png"/>
</br>
<img src="https://github.com/gyan2501/GoogleTimer-StopWatch/blob/main/googletimer/public/gts2.png"/>
| A simple timer and stopwatch by using some basic React concepts like useState ,useEffect and useRef. | css3,html5,javascript,react,reactjs,basic-learning | 2023-01-03T14:53:48Z | 2023-01-24T10:27:23Z | null | 1 | 0 | 7 | 0 | 0 | 4 | null | null | JavaScript |
nathanchess/Kind-Sound | main | # Kind Sound
## What is it?
Welcome to KindSound!
https://user-images.githubusercontent.com/59159552/211226458-9de0062e-303f-4818-b3df-8279e2fc26b5.mp4
On our website, you can view and send anonymous kind messages to others around the world! This is a simple yet powerful website that grows in usefulness as more people use it, and we want to help people give the gift of kindness: just a few words can make someone’s day.
## How we came up with the idea:
Well we thought that it was necessary to spread kindness towards our internet community. The internet often has a negative connotation when it comes to the damaging side effects of social media and the ever-increasing cyberbullying occurrences. With this in mind, we thought about creating a platform in which people could anonymously spread kindness and love to other internet users, which ultimately led us to the creation of KindSound!
## Our Mission:
Sometimes, all it takes is a kind word to make someone’s day. We wanted to provide a moment of peace for those who are struggling mentally and emotionally and let them know that even strangers in a wide, wide world do care
## Features:
1. NodeJS & ExpressJS backend
2. React Frontend
3. AI model for sentiment tracking
4. Like / Dislike algorithm in backend to suggest quotes
## How to use:
1. Run npm start on the client folder to run frontend react application
2. Run npm start on the server folder to start the port 5000 node js server
3. That's it! Have fun!
| Project made for GitInit 2023 hackathon! Allows users to send anonymous kind messages to others around the world with a NodeJS backend and ReactJS frontend! | javascript,nodejs,react | 2023-01-07T02:32:51Z | 2023-01-15T06:41:13Z | null | 4 | 1 | 98 | 0 | 1 | 4 | null | null | JavaScript |
dev-Imsaurabh/TATA-CLiQ-clone | master | <center><h1>CLiQ Mart</h1></center>
<center><h3>Begin your journey online with one of the fastest growing, e-commerce brands in the country and have a seamless online shopping experience. Immerse yourself in the joy of browsing through the best Indian and international brands in fashion, luxury, electronics and jewellery.</h3></center>
<center><img width="500px" src="https://drive.google.com/uc?export=view&id=1v0Bo-1q2zLVLG8pEUHJeKOiqtPs06y_2"></center>
# Features
- `Login/Signup using Redux`
- `Multiple Filtering and Sorting options`
- `Search Page`
- `Cart Page using Redux`
- `Orders using OTP`
- `Admin Panel`
- `Fully Responsive`
# Our Team work planning and proof
<!-- All the folder structure information -->
=> Before you start:
- I have already created all the folders which you will need during journey.
- Dont use any other folder to make files if you are doing please inform the
team members that you have created a folder with name.
- I have already setup all the things related to `redux`, `.env`, `Chakra UI` and `react-router-dom`
You guys have to just start developments.
- Also given you sample `Navbar` and `Footer` components so that you get
comfortable about folder components export.
- You will get all the routing files in `routes` folder.
- You can also use context-api if needed.
- All the end-points and base-url should be in env files.
- Use `assets` folder for images,pdf,video..etc
- Use `components` folder for making components either in folder or without folder
just be sure naming should not match with other folder/file to avoid collision
- Use `pages` folder to create all the pages
- Use `routes` folder to all the routing related stuffs
- Use `scripts` folder for any js script you wanna write.(this folder helps you to
keep jsx and js folder separate)
- Use `styles` folder to keep your all css files.
- Use `constants` folder to create all the constants (Helps you reducing hard-coding)(Most recommended)
<!-- Cloning related and Getting started related stuffs -->
=> Clone the directory to start work `$git clone https://github.com/dev-Imsaurabh/third-ice-7307.git`
=> Ok guys here are the some basic instruction before you start , If you are here you are successfully pulled the code and you are ready to type you first command in terminal.
Step-1- Navigate to proeject directory using `$cd third-ice-7307`
Step-2- install node_modules using `$cd npm install`
Step-3- install some common dependencies:- <br />
- `$npm install react-router-dom redux react-redux redux-thunk axios`<br />
- `$npm install @chakra-ui/react @emotion/react @emotion/styled framer-motion`
<!-- Git related stuffs -->
=> Some basic requirements(Mandatory):
1- You have to work in daily branches manner, so you have to create
new branch everyday . So you have make branch using your student_code
followed the day in which you working. below is the eg for my branches.
- branch-naming style: fw21_XXXX_day-x
-for day-2 branch name should be: fw21_1003_day-2
-for day-3 branch name should be: fw21_1003_day-3
-for day-4 branch name should be: fw21_1003_day-4
-for day-5 branch name should be: fw21_1003_day-5
2- How to create branches:
- $git branch <branch-name> (without angle brackets)
3- How to switch branches:
-$git switch <branch-name> (without angle brackets)
4- How to pull:
-$git pull origin <branch-name> (without angle brackets)
=> Some helpful Tools during development :<br />
- Chakra-UI - https://chakra-ui.com/<br />
- Chakra-Templets - https://chakra-templates.dev/#<br />
- String Builder - https://codebeautify.org/string-builder<br />
- Chat-gpt - https://chat.openai.com/chat<br />
- Your-Team-members- https://chat.whatsapp.com/KR80RM0zbWi6agJVFL66Z9<br />
************************************* All the best guys ************************************************
# Work Flow Chart
*Note:- Bhavnesh will do all the backend and admin panel so you will get apis and endpoint on day-2 morning.
# Base_url
`Your backend base url`
# Endpoints
`/users`
`/products`
# Schemas
`Product Schema`
```
{
"id":0,
"images":["" , ""],
"name":"",
"short_desc":"",
"long_desc":"",
"category":"",
"price":0,
"strike_price":0,
"ratings":0,
"color":"green",
"delivery_time":3,
"size":""
}
```
`User Schema`
```
{
"id":0,
"name": "",
"email": "",
"password": "",
"account": {
"cart": [
{"id":0,
"images": ["", ""],
"name": "",
"short_desc": "",
"long_desc": "",
"price": 0,
"category":"",
"strike_price": 0,
"ratings": 0,
"color":"",
"delivery_time": 0,
"size":"",
"quantity":0
},
{
"id":1,
"images": ["", ""],
"name": "",
"short_desc": "",
"long_desc": "",
"price": 0,
"category":"",
"strike_price": 0,
"ratings": 0,
"color":"",
"delivery_time": 0,
"size":"",
"quantity":0
}
],
"orders": [{
"id":0,
"images": ["", ""],
"name": "",
"short_desc": "",
"long_desc": "",
"price": 0,
"category":"",
"strike_price": 0,
"ratings": 0,
"color":"",
"delivery_time": 0,
"size":"",
"quantity":0,
"address":""
}]
}
}
```
- Whole `db.json` Database look
```
{
"users": [
{
"id":0,
"name": "user-1",
"email": "",
"password": "",
"account": {
"cart": [
{"id":0,
"images": ["", ""],
"name": "",
"short_desc": "",
"long_desc": "",
"price": 0,
"category":"",
"strike_price": 0,
"ratings": 0,
"color":"",
"delivery_time": 0,
"size":"",
"quantity":0
},
{
"id":1,
"images": ["", ""],
"name": "",
"short_desc": "",
"long_desc": "",
"price": 0,
"category":"",
"strike_price": 0,
"ratings": 0,
"color":"",
"delivery_time": 0,
"size":"",
"quantity":0
}
],
"orders": [{
"id":0,
"images": ["", ""],
"name": "",
"short_desc": "",
"long_desc": "",
"price": 0,
"category":"",
"strike_price": 0,
"ratings": 0,
"color":"",
"delivery_time": 0,
"size":"",
"quantity":0,
"address":""
}]
}
},{
"id":1,
"name": "user-2",
"email": "",
"password": "",
"account": {
"cart": [
{"id":0,
"images": ["", ""],
"name": "",
"short_desc": "",
"long_desc": "",
"price": 0,
"category":"",
"strike_price": 0,
"ratings": 0,
"color":"",
"delivery_time": 0,
"size":"",
"quantity":0
},
{
"id":1,
"images": ["", ""],
"name": "",
"short_desc": "",
"long_desc": "",
"price": 0,
"category":"",
"strike_price": 0,
"ratings": 0,
"color":"",
"delivery_time": 0,
"size":"",
"quantity":0
}
],
"orders": [{
"id":0,
"images": ["", ""],
"name": "",
"short_desc": "",
"long_desc": "",
"price": 0,
"category":"",
"strike_price": 0,
"ratings": 0,
"color":"",
"delivery_time": 0,
"size":"",
"quantity":0,
"address":""
}]
}
}
],
"products": [
{
"id": "category-name-1",
"items": [
{
"id": 0,
"images": ["", ""],
"name": "",
"short_desc": "",
"long_desc": "",
"price": 0,
"category":"",
"strike_price": 0,
"ratings": 0,
"color": "",
"delivery_time": 0,
"size": ""
},
{
"id": 1,
"images": ["", ""],
"name": "",
"short_desc": "",
"long_desc": "",
"price": 0,
"category":"",
"strike_price": 0,
"ratings": 0,
"color": "",
"delivery_time": 0,
"size": ""
}
]
},
{
"id": "category-name-2",
"items": [
{
"id": 0,
"images": ["", ""],
"name": "",
"short_desc": "",
"long_desc": "",
"price": 0,
"category":"",
"strike_price": 0,
"ratings": 0,
"color": "",
"delivery_time": 0,
"size": ""
},
{
"id": 1,
"images": ["", ""],
"name": "",
"short_desc": "",
"long_desc": "",
"price": 0,
"category":"",
"strike_price": 0,
"ratings": 0,
"color": "",
"delivery_time": 0,
"size": ""
}
]
}
]
}
```
# Pages
These are the pages we need in this project.
//Tinkle
- `HomePage`-------------------->Saurabh/ Tinkle (Day1-Day2)
- `AccountPage`------------------------->Tinkle / Anyone(Help if needed) (Day-1) (day-2)
//Farhaz
- `SignupPage`------------------------->Farhaz (Day1-Day2)
- `LoginPage`--------------------------->Farhaz (Day2) //with API connected
//Saurabh
- `SearchPage`------------------------> Saurabh (Day3)
- `ProductPage`------------------------> Saurabh (Day3-Day4)
- `ViewProductPage`-------------------> Tinkle / Anyone(Help if needed) (Day1-Day2)
- `CartPage`----------------------------> Saurabh (Day3-Day4)
//Bhavnesh
- `Checkout-Page`----------------------------> Bhavnesh (Day3-Day4)
- `OtpPage`------------------------------>Bhavnesh / Anyone(Help if needed)
- `Whole backend setup`-------------------> Bhavnesh
# Components
These are the components we need in this project.
//HomePage
- `Navbar`----------Tinkle (Day-1)
- `Footer`----------Bhavnesh (Day-1)
- `carousel` ------------Bhavnesh (Day-1)
- `Category-circular-Card`------Saurabh (Day-1)
- `Category-rectangular-Card`--------Saurabh (Day-1)
- `brands-Card`-----------Saurabh (Day-1)
- `banner-Card`-------saurabh (Day-1)
- `Trending-Card`---------Saurabh (Day-1)
//ProdcutPage
- `Filter-box`------Saurabh (Day-2)
- `Product-Card`---------Saurabh (Day-2)
- `Sort-Box`---------Saurabh (Day-2)
//SearchBar
- `Search bar`------Tinkle--> Comes with Navbar (Day-1)
# Carousel Image addresses
- `https://assets.tatacliq.com/medias/sys_master/images/45625097289758.jpg`
- `https://assets.tatacliq.com/medias/sys_master/images/45625097420830.jpg`
- `https://assets.tatacliq.com/medias/sys_master/images/45625097486366.jpg`
- `https://assets.tatacliq.com/medias/sys_master/images/45625097551902.jpg`
- `https://assets.tatacliq.com/medias/sys_master/images/45625097355294.jpg`
| TATA CLiQ is an Indian e-commerce website operates in categories such as Fashion, Footwear and Accessories | chakra-ui,javascript,json-server,jsx,react,redux | 2023-01-14T12:41:50Z | 2023-03-08T10:06:35Z | null | 5 | 47 | 123 | 0 | 2 | 4 | null | null | JavaScript |
ahadb802/first-react-app-magic-calculator | dev | <a name="readme-top"></a>
<!--
HOW TO USE:
This is an example of how you may give instructions on setting up your project locally.
Modify this file to match your project and remove sections that don't apply.
REQUIRED SECTIONS:
- Table of Contents
- About the Project
- Built With
- Live Demo
- Getting Started
- Authors
- Future Features
- Contributing
- Show your support
- Acknowledgements
- License
After you're finished please remove all the comments and instructions!
-->
<div align="center">
<img src="murple_logo.png" alt="logo" width="140" height="auto" />
<br/>
<h3><b>Microverse README Template</b></h3>
</div>
<!-- TABLE OF CONTENTS -->
# 📗 Table of Contents
- [📖 About the Project](#about-project)
- [🛠 Built With](#built-with)
- [Key Features](#key-features)
- [🚀 Live Demo](#live-demo)
- [💻 Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#triangular_flag_on_post-deployment)
- [👥 Authors](#authors)
- [🔭 Future Features](#future-features)
- [🤝 Contributing](#contributing)
- [⭐️ Show your support](#support)
- [🙏 Acknowledgements](#acknowledgements)
- [❓ FAQ](#faq)
- [📝 License](#license)
<!-- PROJECT DESCRIPTION -->
# 📖 Project Name
#### Webpack Template
## 🛠 Built With <a name="built-with"></a>
- HTML, CSS, JavaScript, Reactjs
<!-- Features -->
### Key Features <a name="key-features"></a>
> Describe between 1-3 key features of the application.
- Use webpack to bundle React js.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## 🚀 Live Demo <a name="live-demo"></a>
Click <a href="https://tourmaline-chebakia-a54d98.netlify.app/">Here</a>
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## 💻 Getting Started
To clone the repository in local environment try following steps.
### Prerequisites
- [x] A web browser like Google Chrome.
- [x] A code editor like Visual Studio Code with Git and Node.js.
You can check if Git is installed by running the following command in the terminal.
```
$ git --version
```
Likewise for Node.js and npm for package installation.
```
$ node --version && npm --version
```
### Setup
Clone the repository using [this link](https://github.com/ahadb802/first-react-app-magic-calculator.git)
### Install
In the terminal, go to your file directory and run this command.
```
$ git clone https://github.com/ahadb802/first-react-app-magic-calculator.git
```
### Usage
This website is applicable for both mobile and desktop version.
### Run tests
### Deployment
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## 👥 Authors <a name="authors"></a>
👤 **Abdul Ahad Bhatti**
- GitHub: [@ahadb802](https://github.com/ahadb802)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## 🔭 Future Features <a name="future-features"></a>
- [ ] **Styling of the webpage**
- [ ] **Animation to add or remove functionality**
- [ ] **Adding responsiveness**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome! Add suggestions by opening new issues.
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## ⭐️ Show your support <a name="support"></a>
Give a ⭐️ if you like this project!
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## 🙏 Acknowledgments <a name="acknowledgements"></a>
I would like to thank:
- [Microverse](https://www.microverse.org/)
- Code Reviewers
- Coding Partners
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FAQ (optional) -->
## ❓ FAQ <a name="faq"></a>
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## 📝 License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
| This us my first react app in which I made a calculator and its operations using class base and functional base as well using hooks. and there 3 pages in navbar which are working with the help of routers | html,html-css-javascript,javascript,react,reactjs,redux | 2023-01-09T14:51:56Z | 2023-01-19T21:37:04Z | null | 1 | 5 | 27 | 0 | 0 | 4 | null | MIT | JavaScript |
imranhossainemi/portfolio | main | <a name="readme-top"></a>
<div align="center">
<img src="5139.jpg" alt="logo" width="140" height="auto" />
<br/>
<h3><b>Personal Portfolio</b></h3>
</div>
<!-- TABLE OF CONTENTS -->
# 📗 Table of Contents
- [📖 About the Project](#about-project)
- [🛠 Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [🚀 Live Demo](#live-demo)
- [💻 Getting Started](#getting-started)
- [👥 Authors](#authors)
- [🔭 Future Features](#future-features)
- [🤝 Contributing](#contributing)
- [⭐️ Show your support](#support)
- [🙏 Acknowledgements](#acknowledgements)
- [📝 License](#license)
<!-- PROJECT DESCRIPTION -->
# 📖 [personal_portfoilo] <a name="about-project"></a>
This is my personal portfolio template.Here, I will display my personal porject and perivous works & experience.
## 🛠 Built With <a name="built-with"></a>
This portfolio template is built with :
1. HTML
2. CSS
3. JavaScript
### Tech Stack <a name="tech-stack"></a>
For this portfolio template design I am using mobile first approach.
<!--
<details>
<summary>Client</summary>
<ul>
<li><a href="#">HTML CSS</a></li>
</ul>
</details>
-->
<!--
<details>
<summary>Server</summary>
<ul>
<li><a href="https://expressjs.com/">Express.js</a></li>
</ul>
</details>
-->
<!--
<details>
<summary>Database</summary>
<ul>
<li><a href="https://www.postgresql.org/">PostgreSQL</a></li>
</ul>
</details>
-->
<!-- Features -->
### Key Features <a name="key-features"></a>
> Currently do not have any key features
- **[Validate Email Input]**
<!--
- **[key_feature_2]**
- **[key_feature_3]**
-->
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## 🚀 Live Demo <a name="live-demo"></a>
> You can live demo form here.
- [Live Demo Link]https://imranhossainemi.github.io/portfolio/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## 💻 Getting Started <a name="getting-started"></a>
> For using this porject simple colne this repo and run it in your local computer.
In order to run this project you need:
1. A computer
2. A internet Browser.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## 👤 Author <a name="authors"></a>
- GitHub: [@imranhossainemi](https://github.com/imranhossainemi)
- Twitter: [@DevImranHossain](https://twitter.com/DevImranHossain)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## 🔭 Future Features <a name="future-features"></a>
> In future going add javascript and framework.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## ⭐️ Show your support <a name="support"></a>
> Follow me at twitter
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## 🙏 Acknowledgments <a name="acknowledgements"></a>
> All credit goes to Microverse and Figma
<!-- LICENSE -->
## 📝 License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| My personal portfolio. Desing is provided by Microverse. I am using HTML , CSS , vanilla JavaScript | css,html,javascript,portfolio | 2023-01-11T13:43:24Z | 2023-08-14T10:06:22Z | null | 6 | 15 | 244 | 0 | 0 | 4 | null | MIT | CSS |
MMGGYY66/my-awesome-books | main | # my-awesome-books
<p id="readme-top">My Microverse my-awesome-books project (Module two)</p>
<!-- TABLE OF CONTENTS -->
# 📗 Table of Contents
- [my-awesome-books](#my-awesome-books)
- [📗 Table of Contents](#-table-of-contents)
- [📖 \[🎯 my-awesome-books ](#--my-awesome-books-)
- [🛠 Built With ](#-built-with-)
- [Tech Stack ](#tech-stack-)
- [🚀 Live Demo ](#-live-demo-)
- [👁 Presentation ](#-presentation-)
- [creating my-awesome-books:](#creating-my-awesome-books)
- [🛠 Built With ](#-built-with--1)
- [Deploy my website with github pages":](#deploy-my-website-with-github-pages)
- [- Loom video link:](#--loom-video-link)
- [💻 Getting Started ](#-getting-started-)
- [Prerequisites](#prerequisites)
- [Prerequisites](#prerequisites-1)
- [Install](#install)
- [Requirements](#requirements)
- [Run tests](#run-tests)
- [Deployment](#deployment)
- [👥 Authors ](#-authors-)
- [🤝 Contributing ](#-contributing-)
- [👋 Show your support ](#-show-your-support-)
- [🔭Acknowledgments ](#acknowledgments-)
- [📝 License ](#-license-)
<!-- PROJECT DESCRIPTION -->
# 📖 [🎯 my-awesome-books <a name="about-project"></a>
> "**Awesome books**" is a simple website that displays a list of books and allows you to add and remove books from that list. By building this application, you will learn how to manage data using JavaScript. Thanks to that your website will be more interactive. You will also use a medium-fidelity wireframe to build the UI.is a simple website that displays a list of books and allows you to add and remove books from that list. By building this application, you will learn how to manage data using JavaScript. Thanks to that your website will be more interactive. You will also use a medium-fidelity wireframe to build the UI.
## 🛠 Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
## 🚀 Live Demo <a name="live-demo"></a>
- [Live Demo Link](https://mmggyy66.github.io/my-awesome-books/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## 👁 Presentation <a name="presentation"></a>
- [Loom presentation Link]()
# creating my-awesome-books:
- I will set up a new repository and prepare it for development using best practices (e.g. linters).
- for the first project, I will Manage books collection (plain JS with objects).
How to build the "Awesome books" website
- I will start by building the core functionalities and ignoring how my website looks. Note that it is plain HTML with no styling, but it will allow you to add and remove books from the list!
## 🛠 Built With <a name="built-with"></a>
<details>
<summary>Technology</summary>
<ul>
<li>HTML</li>
<li>CSS (**medium-fidelity** wireframe)</li>
<li>Javascript</li>
<li>Linters (Lighthouse, Webhint, Stylelint, Eslint)</li>
<li>Git/GitHub work-flow </li>
</ul>
</details>
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## Deploy my website with github pages":
[link to my-awesome-books: ](https://mmggyy66.github.io/my-awesome-books/)
## - Loom video link:
[Loom walking through for my-awesome-books: ]()
## 💻 Getting Started <a name="getting-started"></a>
To get a local copy up and running follow these simple example steps.
### Prerequisites
### Prerequisites
- IDE to edit and run the code (We use Visual Studio Code 🔥).
- Git to versionning your work.
### Install
- npm install --save-dev hint@6.x
- npm install --save-dev stylelint@13.x stylelint-scss@3.x stylelint-config-standard@21.x stylelint-csstree-validator@1.x
- pm install --save-dev eslint eslint-config-airbnb-base eslint-plugin-import babel-eslint
## Requirements
- Linters configuration.
- Part 1: Manage books collection (plain JS with objects).
- Part 2: Manage books collection (plain JS with classes) and add basic CSS.
- Part 3: Create a complete website with navigation.
Clone the repository to get start with project, then make sure to install dependencies in the linters file located in the [linter](https://github.com/Bateyjosue/linters-html-css/blob/main/.github/workflows/linters.yml) file
<p align="right">(<a href="#readme-top">back to top</a>)</p>
### Run tests
- run: npx hint .
- run: npx stylelint "\*_/_.{css,scss}"
- run: npx eslint .
### Deployment
Check for the tests when you generate a pull request and fix the errors if any.
For stylelint error run:<code>sudo npx stylelint "\*_/_.{css,scss}" --fix</code> and it will the fix style issues automatically.
<!-- AUTHORS -->
## 👥 Authors <a name="authors"></a>
👤 **Mohamed Gamil Eldimardash**
- GitHub: [@github](https://github.com/MMGGYY66)
- LinkedIn: [LinkedIn](https://www.linkedin.com/in/mohamed-eldimardash-0023a3b5/)
- Twitter: [twitter](https://twitter.com/MOHAMEDELDIMARd)
- Facebook: [facebook](https://www.facebook.com/MOHAMED.ELDIMARDASH/)
👥 Henschel Nketchogue M.
- GitHub: [miltonHenschel](https://github.com/miltonHenschel)
- LinkedIn: [henschelnketchoguem](https://www.linkedin.com/in/henschelnketchoguem/)
- Twitter: [nketchogue](https://twitter.com/nketchogue)
- Instagram: [mpatchiehenschel](https://www.instagram.com/mpatchiehenschel/)
<!-- CONTRIBUTING -->
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the issues page
<!-- SUPPORT -->
## 👋 Show your support <a name="support"></a>
Give a ⭐️ if you like this project!
<p align="right"><a href="#readme-top">(back to top)</a></p>
<!-- ACKNOWLEDGEMENTS -->
## 🔭Acknowledgments <a name="acknowledgements"></a>
- [Microverse Team](https://www.microverse.org/).
- Spacial thank to [Cindy Shin](https://www.behance.net/gallery/29845175/CC-Global-Summit-2015) for his beautiful design.
- Images uses in this project are from [figma](https://www.figma.com/file/V0vg37EZhAbP9nUPOeCy6p/HTML%2FCSS-%26-JavaScript-capstone-project---Conference-page?node-id=0%3A1&t=od5hoeaQE2tKg92Y-0).
## 📝 License <a name="license"></a>
This project is [MIT](https://github.com/microverseinc/readme-template/blob/master/MIT.md) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| "Awesome books" is a simple website that displays a list of books and allows you to add and remove books from that list. | css,css3,html,javascript,scss | 2023-01-09T15:44:30Z | 2023-02-02T20:35:39Z | null | 2 | 3 | 22 | 0 | 0 | 4 | null | null | JavaScript |
tobuya/Leaderboard | development | <a name="readme-top"></a>
<!-- TABLE OF CONTENTS -->
# 📗 Table of Contents
- [📗 Table of Contents](#-table-of-contents)
- [📖 Leaderboard ](#-leaderboard-)
- [🛠 Built With ](#-built-with-)
- [🚀 Live Demo ](#-live-demo-)
- [💻 Getting Started ](#-getting-started-)
- [Prerequisites](#prerequisites)
- [Setup](#setup)
- [👤**Authors** ](#authors-)
- [🔭 Future Features ](#-future-features-)
- [🤝 Contributing ](#-contributing-)
- [⭐️ Show your support ](#️-show-your-support-)
- [🙏 Acknowledgments ](#-acknowledgments-)
- [📝 License ](#-license-)
<!-- PROJECT DESCRIPTION -->
# 📖 Leaderboard <a name="about-project"></a>
> The leaderboard website displays scores submitted by different players. It also allows you to submit your score. All data is preserved thanks to the external Leaderboard API service.
Here are some features of the page:
- Preserve user input.
- Connect to the Leaderboard API.
## 🛠 Built With <a name="built-with"></a>
HTML
CSS
Javascript
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## 🚀 Live Demo <a name="live-demo"></a>
[Leaderboard](#)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## 💻 Getting Started <a name="getting-started"></a>
- Create a local directory that you want to clone the repository.
- Open the command prompt in the created directory.
- On the terminal run this command git clone https://github.com/tobuya/Leaderboard
- Go to the repository folder using command prompt cd Leaderboard.
- Install the dev dependencies for linters run npm install.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
### Prerequisites
In order to run this project you need:
- Google chrome
- Internet Explorer
- Any browser
<p align="right">(<a href="#readme-top">back to top</a>)</p>
### Setup
Clone this repository to your desired folder:
- Open the command prompt in the created directory.
- On the terminal run this command git clone https://github.com/tobuya/Leaderboard
- Go to the repository folder using command prompt cd Leaderboard
- Install the dev dependencies for linters run npm install.
--->
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- Author -->
## 👤**Authors** <a name="authors"></a>
👤 **Thomas Obuya**
- GitHub: [@githubhandle](https://github.com/tobuya)
- Twitter: [@twitterhandle](https://twitter.com/@MullerTheGreat1)
- LinkedIn: [LinkedIn](https://linkedin.com/in/thomas-obuya-51b49719b/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## 🔭 Future Features <a name="future-features"></a>
- Implement some UX improvements: include transitions and/or animation
- Rankings
- Send and receive data from the API
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the issues page.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## ⭐️ Show your support <a name="support"></a>
Give a ⭐️ if you like this project!
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## 🙏 Acknowledgments <a name="acknowledgements"></a>
- Gitflow
- Webpack
- Linters
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## 📝 License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| Leaderboard is a list app that displays scores submitted by different players. It also allows you to submit your scores. All data is preserved to the external Leaderboard API service. It is developed using Webpack and ES6 features. | css,html,javascript | 2023-01-04T06:19:49Z | 2023-01-08T20:16:09Z | null | 1 | 3 | 25 | 0 | 0 | 4 | null | MIT | JavaScript |
zeff96/personal-portfolio | main | # 📗 Table of Contents
- [📖 About the Project](#about-project)
- [🛠 Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [🚀 Live Demo](#live-demo)
- [💻 Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#triangular_flag_on_post-deployment)
- [👥 Authors](#authors)
- [🔭 Future Features](#future-features)
- [🤝 Contributing](#contributing)
- [⭐️ Show your support](#support)
- [🙏 Acknowledgements](#acknowledgements)
- [❓ FAQ (OPTIONAL)](#faq)
- [📝 License](#license)
# 📖 PORTFOLIO <a name="about-project"></a>
**PORTFOLIO** is a project i have created to show my skills and experience as a soft developer<br>
All my professional and technical projects are contained in this project.
## 🛠 Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
<details>
<summary>Client</summary>
<ul>
<li><a href="#">HTML</a></li>
<li><a href="#">CSS</a></li>
</ul>
</details>
### Key Features <a name="key-features"></a>
- **Background**
- **Projects**
- **Contacts**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## 🚀 Live Demo <a name="live-demo"></a>
- [Live Demo](https://zeff96.github.io/personal-portfolio/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## 💻 Getting Started <a name="getting-started"></a>
To get a local copy up and running, follow these steps.
### Prerequisites
In order to run this project you need:
```sh
Vs code
npm packages
```
### Setup
Clone this repository to your desired folder:
```sh
cd my-folder
git clone git@github.com:zeff96/Personal-Portfolio.git
```
### Install
Install this project with:
```sh
cd my-project
npm install
```
### Usage
To run the project, execute the following command:
run the link below on your web browser.
```sh
https://zeff96.github.io/Portfolio.github.io/
```
<p align="right">(<a href="#readme-top">back to top</a>)</p>
### Deployment
To run this project on website run:
```sh
https://zeff96.github.io/Portfolio.github.io/
```
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## 👥 Authors <a name="authors"></a>
👤 **Author 1**
- GitHub: [zeff96](https://github.com/zeff96)
- Twitter: [zeffo96](https://twitter.com/zeffo96)
- LinkedIn: [zeffo96](https://linkedin.com/in/zeff-adeka-28060820a)
👤 **Author 2**
- GitHub: [WilhelmK109](https://github.com/WilhelmK109)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## 🔭 Future Features <a name="future-features"></a>
- [ ] **More projects**
- [ ] **All devices screen size display**
- [ ] **[new_feature_3]**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](https://github.com/zeff96/Portfolio-setup-and-mobile-first/issues).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## ⭐️ Show your support <a name="support"></a>
If you like this project you are free to propose changes that you think might be made to even make it better in the future
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## 🙏 Acknowledgments <a name="acknowledgements"></a>
I would like to thank My learning partners, Microverse community as whole for helping me this far in this project
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## 📝 License <a name="license"></a>
This project is [MIT](LICENSE.md) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| This is a personal portfolio website which showcase my developed projects and skills | css,html,javascript | 2023-01-12T13:41:14Z | 2023-08-10T07:08:52Z | null | 3 | 13 | 140 | 1 | 0 | 4 | null | MIT | HTML |
Tharindu37/ProSolutions | development | # ProSolutions
ProSolutions Company Website
Available at :
https://tharindu37.github.io/ProSolutions/
### Used technology
- HTML
- CSS
- JavaScript (JQuery)
- Bootstrap 5
### Figma design
https://www.figma.com/proto/j1UOHgNFKy64ThKve7xfG9/Untitled?node-id=1%3A2&scaling=scale-down&page-id=0%3A1
Resources:- https://drive.google.com/drive/folders/1t9d3qDxafXd9WMcO-neebBduEr7jdo-l?usp=sharing
### Run Locally
1. Fork this Repository
2. Clone the project
```bash
git clone <url>
```
3. Add upstream
```bash
git remote add upstream <url>
```
<br>
Welcome Contributors..!
| ProSolutions company web site | bootstrap5,css3,html5,javascript,jquery | 2023-01-11T10:19:50Z | 2023-03-29T07:04:45Z | null | 18 | 95 | 121 | 7 | 24 | 4 | null | null | HTML |
V-Blaze/cryptopedia | dev | # cryptopedia
<a name="readme-top"></a>
<!-- TABLE OF CONTENTS -->
# 📗 Table of Contents
- [📖 About the Project](#about-project)
- [🛠 Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [🚀 Live Demo](#live-demo)
- [💻 Getting Started](#getting-started)
- [Setup](#setup)
- [Run tests](#run-tests)
- [👥 Authors](#authors)
- [🔭 Future Features](#future-features)
- [🤝 Contributing](#contributing)
- [⭐️ Show your support](#support)
- [🙏 Acknowledgements](#acknowledgements)
- [📝 License](#license)
# 📖 Cryptopedia <a name="about-project"></a>
> Cryptopedia is a Web-App for real-time data about crypto exchanges and market activity for over 75 cryptocurrencies exchanges around the World. Users can see Exchanges categorized by their country and also get to see different information's like Trading volumes, trading pairs and ranks.
## 🛠 Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
>
- HTML
- CSS
- Javascript
- React
- Webpack
- NPM
- Redux
- Jest
- TailwindCss
- Lighthouse (An open-source, automated tool for improving the quality of web pages. It has audits for performance, accessibility, progressive web apps, SEO and more).
- Webhint (A customizable linting tool that helps you improve your site's accessibility, speed, cross-browser compatibility, and more by checking your code for best practices and common errors).
- Stylelint (A mighty, modern linter that helps you avoid errors and enforce conventions in your styles).
<details>
<summary>Client</summary>
<ul>
<li><a href="https://reactjs.org/">React.js</a></li>
</ul>
</details>
<details>
<summary>Server</summary>
<ul>
<li><a href="https://expressjs.com/">Express.js</a></li>
</ul>
</details>
<details>
<summary>Database</summary>
<ul>
<li><a href="https://www.postgresql.org/">PostgreSQL</a></li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
>
- **See list of Crypto Exchanges**
- **Search exchange by country or name**
- **View more detail about an exchange**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## 🚀 Live Demo <a name="live-demo"></a>
>
- [Live Link](https://cryptopedia.onrender.com/)
## 🚀 Video Presentation <a name="Presentation"></a>
- [Video Presentation](https://drive.google.com/file/d/1skZyIHYgZXbFnHw9z0IRCgk83LRQHU5C/view?usp=sharing)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## 💻 Getting Started <a name="getting-started"></a>
>
To get a local copy up and running, follow these steps.
- npm
```sh
npm install npm@latest -g
```
### Setup
1. Clone the repo by running the command
```sh
git clone https://github.com/V-Blaze/cryptopedia.git
```
2. Open the directory of the project
```sh
cd cryptopedia
```
3. Install project dependencies
```sh
run npm install
```
4. Open the html file
```sh
run npm start
### Run tests
To run tests, run the following command:
- npm
```sh
npm run test
```
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## 👥 Author <a name="authors"></a>
👤 **Author**
- GitHub: [@V-blaze](https://github.com/V-Blaze)
- Twitter: [@blaze_valentine](https://twitter.com/blaze_valentine)
- LinkedIn: [@valentine-blaze](https://www.linkedin.com/in/valentine-blaze/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## ⭐️ Show your support <a name="support"></a>
If you like this project... Give a start and a follow.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## 🙏 Acknowledgments <a name="acknowledgements"></a>
> Original design idea by [Nelson Sakwa on Behance.](https://www.behance.net/sakwadesignstudio)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
## Show your support
- Give a ⭐️ if you like this project!
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## 📝 License <a name="license"></a>
- This project is [MIT](https://github.com/V-Blaze/cryptopedia/blob/dev/LICENSE) licensed.
- This project is also [Creative Common](https://creativecommons.org/licenses/by-nc/4.0/) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| Cryptopedia is a Web-App for real-time data about crypto exchanges and market activity for over 75 cryptocurrencies exchanges around the World. Users can see Exchanges categorized by their country and also get to see different information's like Trading volumes, trading pairs and ranks. Built with Reactjs | blockchain,css,dapps-development,javascript,reactjs,redux,web3 | 2023-01-02T12:34:00Z | 2023-04-27T18:34:40Z | null | 1 | 6 | 23 | 0 | 0 | 4 | null | MIT | JavaScript |
sebalp100/Chat-app | dev | <a name="readme-top"></a>
<div align="center">
<img src="https://i.imgur.com/3OgKfBJ_d.webp?maxwidth=760&fidelity=grand"
alt="logo" width="140" height="auto" />
<br/>
</div>
<!-- TABLE OF CONTENTS -->
# 📗 Table of Contents
- [📖 About the Project](#about-project)
- [🛠 Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [Future Features](#future-features)
- [🚀 Live Demo](#live-demo)
- [💻 Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Usage](#usage)
- [Run tests](#run-tests)
- [👥 Authors](#authors)
- [🤝 Contributing](#contributing)
- [⭐️ Show your support](#support)
- [🙏 Acknowledgements](#acknowledgements)
- [📝 License](#license)
<!-- PROJECT DESCRIPTION -->
# 📖 Simple Chat App <a name="about-project"></a>
> In this project, I'm creating a website that allows users to chat with one another using real-time Firebase data. The app allows users to look up their previous conversations.
## 🛠 Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
<details>
<summary>Building</summary>
<ul>
<li><a href="https://www.javascript.com/">JavaScript</a></li>
<li>HTML 5</li>
<li>CSS 3</li>
<li>ES6</li>
<li>React</li>
<li>Firebase</li>
</ul>
</details>
<details>
<summary>Bundle</summary>
<ul>
<li><a href="https://webpack.js.org/">WebPack</a></li>
<li><a href="https://www.npmjs.com/">NPM</a></li>
</ul>
</details>
<details>
<summary>Server</summary>
<ul>
<li><a href="https://webpack.js.org/configuration/dev-server/">React server</a></li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
- Register User
- Login User
- Home page with search User functionality
- Display the latest message
- Send a new message
- Send Images
- Display complete message history
### Future Features <a name="future-features"></a>
- Improve style
- Improve Firebase data fetch time
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## 🚀 Live Demo <a name="live-demo"></a>
[Click Me](https://simple-chat-app-puqe.onrender.com)
You can test the search function by searching for Seba or Juan.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## 💻 Getting Started <a name="getting-started"></a>
To get a local copy up and running, follow these steps.
### Prerequisites
In order to run this project you need:
- Github account.
- Code editor (Visual Studio Code, Atom, etc.)
### Setup
Clone this repository to your desired folder:
- cd my-folder
- git clone https://github.com/sebalp100/Metrics-webapp/
### Install
This project was bootstrapped with [Create React App](https://github.com/facebook/create-react-app).
## Available Scripts
In the project directory, you can run:
### `npm start`
Runs the app in the development mode.\
Open [http://localhost:3000](http://localhost:3000) to view it in your browser.
The page will reload when you make changes.\
You may also see any lint errors in the console.
### `npm test`
Launches the test runner in the interactive watch mode.\
See the section about [running tests](https://facebook.github.io/create-react-app/docs/running-tests) for more information.
### `npm run build`
Builds the app for production to the `build` folder.\
It correctly bundles React in production mode and optimizes the build for the best performance.
The build is minified and the filenames include the hashes.\
Your app is ready to be deployed!
See the section about [deployment](https://facebook.github.io/create-react-app/docs/deployment) for more information.
### Usage
To run the project, execute the following command:
`npm install`
`npm start`
### Run tests
To run tests, run the following command:
`npm test`
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## 👥 Authors <a name="authors"></a>
👤 **Sebastian Martin**
- GitHub: [@sebalp100](https://github.com/sebalp100)
- LinkedIn: [Sebastian Martin](https://www.linkedin.com/in/sebastian-martin-956b2724a/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## ⭐️ Show your support <a name="support"></a>
Give a ⭐️ if you like this project!
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## 🙏 Acknowledgments <a name="acknowledgements"></a>
- Hat tip to anyone whose code was used
- Lama Dev for his tutorials (https://www.youtube.com/@LamaDev)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## 📝 License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| This is a website that allows users to chat with one another using real-time Firebase data. The app allows users to look up their previous conversations. Made with React and Firebase. | firebase,javascript,react | 2023-01-15T19:07:58Z | 2023-01-17T20:40:48Z | null | 1 | 0 | 15 | 1 | 0 | 4 | null | MIT | JavaScript |
Divinity-dev/Capstone-project | main | # Capstone-project
<a name="readme-top"></a>
<!--
HOW TO USE:
This is an example of how you may give instructions on setting up your project locally.
Modify this file to match your project and remove sections that don't apply.
REQUIRED SECTIONS:
- Table of Contents
- About the Project
- Built With
- Live Demo
- Getting Started
- Authors
- Future Features
- Contributing
- Show your support
- Acknowledgements
- License
<h3><b>Microverse README Template</b></h3>
</div>
<!-- TABLE OF CONTENTS -->
# 📗 Table of Contents
- [📖 About the Project](#about-project)
- [🛠 Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [🚀 Live Demo](#live-demo)
- [💻 Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#triangular_flag_on_post-deployment)
- [👥 Authors](#authors)
- [🔭 Future Features](#future-features)
- [🤝 Contributing](#contributing)
- [⭐️ Show your support](#support)
- [🙏 Acknowledgements](#acknowledgements)
- [❓ FAQ (OPTIONAL)](#faq)
- [📝 License](#license)
<!-- PROJECT DESCRIPTION -->
# 📖 [Agro-preneurship summit] <a name="about-project"></a>
> This project is a website about an Agro-preneurship summit held in Nigeria. it's a training program for practicing and aspiring farmers
**[your_project__name]** is a...
## 🛠 Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
> Html, CSS, and javascript.
<details>
<summary>Client</summary>
<ul>
<li><a href="https://www.learn-html.org/">html</a></li>
</ul>
</details>
<details>
<summary>Server</summary>
<ul>
<li><a href="https://web.dev/learn/css/">css</a></li>
</ul>
</details>
<details>
<summary>Database</summary>
<ul>
<li><a href="https://www.javascript.com/">Javascript</a></li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
> This project includes some level of animation, it is also accessible for people with disabilities and it's responsive to any device.
- **[Animations]**
- **[Accesibility]**
- **[Responsiveness]**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## 🚀 Live Demo <a name="live-demo"></a>
> This is the link to my live Demo
- [Live Demo Link](https://divinity-dev.github.io/Capstone-project/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- VIDEO PRESENTATION -->
## 🚀 Video presentation <a name="video-demo"></a>
> This is the link to my live video
- [Video Link](https://www.loom.com/share/ea538914aead42af99c3eb36858ebd2c)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## 💻 Getting Started <a name="getting-started"></a>
> The project is very easy to use, all that's required is to visit the site link or for developers that might want to use the work, just head over to my github for the codes
### Prerequisites
All yoou need to run the code is a browser
To run the project, execute the following command:
<!--
Example command:
```sh
rails server
```
--->
### Run tests
There are no commands required to run the code
<!--
Example command:
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## 👥 Authors <a name="authors"></a>
> Asiriuwa O. Divine
👤 **Author1**
- GitHub: [@githubhandle](https://github.com/Divinity-dev/)
- Twitter: [@twitterhandle](https://twitter.com/twitterhandle)
- LinkedIn: [LinkedIn](https://www.linkedin.com/in/divine-asiriuwa-a87227a3/)
> I would like to add a sign up form and also a map in the future
- [ ] **[sign up form]**
- [ ] **[A map to location]**
- [ ] **[new_feature_3]**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## ⭐️ Show your support <a name="support"></a>
>All contributions can be made here https://github.com/Divinity-dev/Capstone-project
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## 🙏 Acknowledgments <a name="acknowledgements">Cindy Shin</a>
>
I would like to thank Cindy Shin for the inspiration for the design
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## 📝 License <a name="license"></a>
This project is [MIT](LICENSE) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| This project is a website for a farmers summit held in My country. Built with html, css and javascript. | css,html,javascript | 2023-01-08T20:29:05Z | 2023-07-12T17:58:26Z | null | 1 | 1 | 10 | 1 | 0 | 4 | null | MIT | CSS |
Rudigus/pong-battle-royale | main | # pong-battle-royale
Cool little online pong with support for multiple players. Base game provided by Willian Oliveira
---
# Requirements
- NodeJS
- TS-Node
# Install
```sh
npm install ts-node
```
# Running
## Server
```sh
npx ts-node src/server/server.ts
```
## Client
```
Open src/client/index.html in browser
```
| Cool little online pong with support for multiple players. Base game provided by Willian Oliveira | game,html5-canvas-game,javascript,multiplayer,nodejs,server | 2023-01-11T09:40:43Z | 2023-01-31T14:41:46Z | null | 3 | 4 | 22 | 5 | 1 | 3 | null | MIT | TypeScript |
Ravi-047/accurate-car-8224 | master | null | Aéropostale, also called Aero, is an American shopping mall–based retailer of casual apparel and accessories, principally targeting young adults through its Aéropostale stores. Aéropostale maintains control over its proprietary brands by designing, sourcing, marketing, and selling all of its own merchandise. | chakra-ui,css3,javascript,mondodb,mongoose,nodejs,react,redux,redux-thunk,aerofashion | 2023-01-16T17:21:04Z | 2023-01-23T04:12:03Z | null | 6 | 34 | 98 | 9 | 3 | 3 | null | null | JavaScript |
apaulineoliveira/expandable-card-slider | master | <h1>🎯 Objetivo</h1>
<p> O objetivo desse projeto foi criar um slide estilo cartão com controle deslizante feito em html, css e javascript</p>
<h3>Progresso</h3>
<ul>
<li>✅ Criação e estilização dos cartões</li>
<li>✅ Controle entre os cards (interação) feito em Javascript</li>
<li>⬜️ implementação do botão deslizante</li>
</ul>
<h3>Pontos relevantes sobre o código</h3>
<p>No código js foi utilizado o método forEach() para percorrer cada item do array e aplicar as interações do slide. Sendo elas: clicar e "retirar" </p>
<br>
</br>
<h1>Purpose</h1>
<p> The goal of this project was to create a card style slide with slider made in html, css and javascript</p>
<h3>Progress</h3>
<ul>
<li>✅ Creation and styling of cards</li>
<li>✅ Control between cards (interaction) made in Javascript</li>
<li>⬜️ command button implementation</li>
</ul>
<h3>Relevant points about the code</h3>
<p>In the js code, the forEach() method was used to go through each item in the array and apply the slide interactions. They are: click and "remove". </p>
<br>
</br>
<br>
| Controle deslizante de slide estilo cartão, feito em JS | Sliding card style slider, made in JS | bootstrap,card-slider,css,html,javascript | 2023-01-16T21:01:45Z | 2023-01-25T02:36:34Z | null | 1 | 21 | 43 | 0 | 0 | 3 | null | null | HTML |
danutibe07/Portfolio | main | <a name="readme-top"></a>
Live Site #https://danutibe07.github.io/Portfolio/
<!--
HOW TO USE:
This is an example of how you may give instructions on setting up your project locally.
Modify this file to match your project and remove sections that don't apply.
REQUIRED SECTIONS:
- Table of Contents
- About the Project
- Built With
- Live Demo #https://danutibe07.github.io/Portfolio/
- Getting Started
- Authors
- Future Features
- Contributing
- Show your support
- Acknowledgements
- License
OPTIONAL SECTIONS:
- FAQ
After you're finished please remove all the comments and instructions!
-->
</div>
<!-- TABLE OF CONTENTS -->
# 📗 Table of Contents
- [📖 About the Project](#about-project)
- [🛠 Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [🚀 Live Demo](#live-demo)
- [💻 Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
<!-- - [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#triangular_flag_on_post-deployment) -->
- [👥 Authors](#authors)
- [🔭 Future Features](#future-features)
- [🤝 Contributing](#contributing)
- [⭐️ Show your support](#support)
- [🙏 Acknowledgements](#acknowledgements)
<!-- - [❓ FAQ (OPTIONAL)](#faq) -->
- [📝 License](#license)
<!-- PROJECT DESCRIPTION -->
# 📖 [Portolio] <a name="about-project"></a>
This Portfolio is a personal project which could help others understand how to parse a Figma design to create a UI, how to use Flexbox to place elements in the page, how to build a personal portfolio site and how to use images and backgrounds to enhance the look of the website.
## 🛠 Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
<!--
> Describe the tech stack and include only the relevant sections that apply to your project. -->
<details>
<summary>Client</summary>
<ul>
<li><a href="https://github.com/microverseinc/curriculum-html-css/blob/main/html5.md/">HTML & CSS</a></li>
</ul>
</details>
<details>
<summary>Server</summary>
<ul>
<li><a href="https://github.com/microverseinc/curriculum-transversal-skills/blob/main/clean-code/linters.md">Linter.yml</a></li>
</ul>
</details>
<details>
<summary>Version Control </summary>
<ul>
<li><a href="https://github.com/microverseinc/curriculum-transversal-skills/blob/main/git-github/git_github_basics.md">Git & Github</a></li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
<!-- > Describe between 1-3 key features of the application. -->
- **[Includes_a_descriptive_readme_file]**
- **[Has_Client_side_rendering]**
- **[It_is_a_simple_application]**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## 🚀 Live Demo <a name="live-demo" ></a>
> Add a link to your deployed project.
- [Live Demo Link](https://danutibe07.github.io/Portfolio/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## 💻 Getting Started <a name="getting-started"></a>
> A developer can use this project to run tests on various html&css files
To get a local copy up and running, follow these steps.
### Prerequisites
In order to run this project you need:
Example command:
```sh
npm install git
```
### Setup
Clone this repository to your desired folder:
Example commands:
```sh
cd my-folder
git clone https://github.com/danutibe07/Hello-Microverse-Project.git
```
### Install
Open this project with your desired browser
Example command:
```sh
code .
```
<!-- AUTHORS -->
## 👥 Authors <a name="authors"></a>
👤 **Daniel**
- GitHub: [@githubhandle](https://github.com/danutibe07)
- Twitter: [@twitterhandle](https://twitter.com/Danielutibe07?t=2kvKPTZQ7IGCw2FugE9xCQ&s=09)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## 🔭 Future Features <a name="future-features"></a>
> Describe 1 - 3 features you will add to the project.
- [ ] **[Access_to_Other_Projects]**
- [ ] **[Content]**
- [ ] **[Live_Project_Demo]**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## ⭐️ Show your support <a name="support"></a>
<!-- > Write a message to encourage readers to support your project -->
If you like this project please help share and if possible leave a star on it
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## 🙏 Acknowledgments <a name="acknowledgements"></a>
<!-- > Give credit to everyone who inspired your codebase. -->
I would like to thank my coding partners and microverse
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FAQ (optional) -->
## ❓ FAQ (OPTIONAL) <a name="faq"></a>
> Add at least 2 questions new developers would ask when they decide to use your project.
- **[RESOURCES_used_for_writing_this_project]**
```sh
HTML
```
```sh
CSS
```
```sh
JAVASCRIPT
```
```sh
GIT & GITHUB
```
```sh
LINTERS
```
- **[Tools_Used_for_this_project]**
```sh
VS CODE
```
```sh
GOOGLE CHROME
```
<!--MIT-SECTION -->
## 📝LiCENSE MIT <a name="mit" ></a>
- [MIT.MD](./LICENSE)
<p align="right">(<a href="#readme-top">back to top</a>)</p> | This portfolio website was designed and built using a combination of HTML, CSS, and JavaScript, and it has been optimized to be accessible on both mobile and desktop devices. The site features a clean, modern design that showcases my work , skills and qualifications in a professional way. The layout is intuitive and easy to navigate, with clear men | css,html5,javascript | 2023-01-12T01:32:49Z | 2023-09-26T14:32:19Z | null | 3 | 22 | 121 | 2 | 0 | 3 | null | MIT | CSS |
Lynch23/Basic-Auth-Conception | main | null | Authentication and Authorization from scratch with NodeJs, MongoDB and Expressjs. | authentication,authentication-backend,authorization,expressjs,nodejs,security,database-management,javascript,cryptography,bcrypt | 2023-01-01T11:02:28Z | 2023-02-15T19:12:18Z | null | 1 | 0 | 25 | 0 | 0 | 3 | null | null | EJS |
hphilpotts/miniproject-static-calculator | main | null | Mini project: building a static page calculator using HTML, CSS and vanilla JS, converting to TS | css,html,javascript,typescript | 2023-01-16T13:26:22Z | 2023-02-11T14:44:24Z | null | 1 | 0 | 46 | 0 | 0 | 3 | null | null | TypeScript |
eliezerantonio/crud_gdg_lubango | master | <p align="center">
<a href="http://nestjs.com/" target="blank"><img src="https://nestjs.com/img/logo-small.svg" width="200" alt="Nest Logo" /></a>
</p>
[circleci-image]: https://img.shields.io/circleci/build/github/nestjs/nest/master?token=abc123def456
[circleci-url]: https://circleci.com/gh/nestjs/nest
<p align="center">A progressive <a href="http://nodejs.org" target="_blank">Node.js</a> framework for building efficient and scalable server-side applications.</p>
<p align="center">
<a href="https://www.npmjs.com/~nestjscore" target="_blank"><img src="https://img.shields.io/npm/v/@nestjs/core.svg" alt="NPM Version" /></a>
<a href="https://www.npmjs.com/~nestjscore" target="_blank"><img src="https://img.shields.io/npm/l/@nestjs/core.svg" alt="Package License" /></a>
<a href="https://www.npmjs.com/~nestjscore" target="_blank"><img src="https://img.shields.io/npm/dm/@nestjs/common.svg" alt="NPM Downloads" /></a>
<a href="https://circleci.com/gh/nestjs/nest" target="_blank"><img src="https://img.shields.io/circleci/build/github/nestjs/nest/master" alt="CircleCI" /></a>
<a href="https://coveralls.io/github/nestjs/nest?branch=master" target="_blank"><img src="https://coveralls.io/repos/github/nestjs/nest/badge.svg?branch=master#9" alt="Coverage" /></a>
<a href="https://discord.gg/G7Qnnhy" target="_blank"><img src="https://img.shields.io/badge/discord-online-brightgreen.svg" alt="Discord"/></a>
<a href="https://opencollective.com/nest#backer" target="_blank"><img src="https://opencollective.com/nest/backers/badge.svg" alt="Backers on Open Collective" /></a>
<a href="https://opencollective.com/nest#sponsor" target="_blank"><img src="https://opencollective.com/nest/sponsors/badge.svg" alt="Sponsors on Open Collective" /></a>
<a href="https://paypal.me/kamilmysliwiec" target="_blank"><img src="https://img.shields.io/badge/Donate-PayPal-ff3f59.svg"/></a>
<a href="https://opencollective.com/nest#sponsor" target="_blank"><img src="https://img.shields.io/badge/Support%20us-Open%20Collective-41B883.svg" alt="Support us"></a>
<a href="https://twitter.com/nestframework" target="_blank"><img src="https://img.shields.io/twitter/follow/nestframework.svg?style=social&label=Follow"></a>
</p>
<!--[](https://opencollective.com/nest#backer)
[](https://opencollective.com/nest#sponsor)-->
## Description
[Nest](https://github.com/nestjs/nest) framework TypeScript starter repository.
## Installation
```bash
$ yarn install
```
## Running the app
```bash
# development
$ yarn run start
# watch mode
$ yarn run start:dev
# production mode
$ yarn run start:prod
```
## Test
```bash
# unit tests
$ yarn run test
# e2e tests
$ yarn run test:e2e
# test coverage
$ yarn run test:cov
```
## Support
Nest is an MIT-licensed open source project. It can grow thanks to the sponsors and support by the amazing backers. If you'd like to join them, please [read more here](https://docs.nestjs.com/support).
## Stay in touch
- Author - [Kamil Myśliwiec](https://kamilmysliwiec.com)
- Website - [https://nestjs.com](https://nestjs.com/)
- Twitter - [@nestframework](https://twitter.com/nestframework)
## License
Nest is [MIT licensed](LICENSE).
| null | javascript,nodejs,unit-testing,nest,typescript,typeorm,nestjs,node-js,posgresql,docker | 2023-01-16T09:16:53Z | 2023-03-13T05:12:46Z | null | 1 | 0 | 12 | 0 | 0 | 3 | null | null | TypeScript |
jeanbuhendwa/portfolio-website | main | <a name="readme-top"></a>
<div align="center">
<h3><b>Portfolio Website</b></h3>
</div>
<!-- TABLE OF CONTENTS -->
# 📗 Table of Contents
- [📖 About the Project](#about-project)
- [🛠 Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [🚀 Live Demo](#live-demo)
- [👥 Authors](#authors)
- [🔭 Future Features](#future-features)
- [🤝 Contributing](#contributing)
- [⭐️ Show your support](#support)
- [🙏 Acknowledgements](#acknowledgements)
- [❓ FAQ (OPTIONAL)](#faq)
- [📝 License](#license)
<!-- PROJECT DESCRIPTION -->
# 📖 Portfolio Website <a name="about-project"></a>
**Portfolio** is a website that i will use to showcase my projects at microverse and let other developers to know about myself.
## 🛠 Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
<details>
<summary>Client</summary>
<ul>
<li><a href="#">HTML5</a></li>
<li><a href="#">CSS3</a></li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
- **About Me**
- **Recent work and Projects**
- **Skills**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## 🚀 Live Demo <a name="live-demo"></a>
- [Live Demo Link](https://jeanbuhendwa.github.io/portfolio-website/)
- [Outline Video Link](https://www.loom.com/share/c6a6b8c8af0544e99de579068f796253)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## 👥 Authors <a name="authors"></a>
👤 **Author**
- GitHub: [@jeanbuhendwa](https://github.com/jeanbuhendwa)
- Twitter: [@jeanbuhendwa](https://twitter.com/jeanjacqueI)
- LinkedIn: [LinkedIn](https://www.linkedin.com/in/johnbuhendwa/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## 🔭 Future Features <a name="future-features"></a>
- [ ] **Desktop and Tablet Design**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## ⭐️ Show your support <a name="support"></a>
If you like this project don't hesitate to leave a star
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## 🙏 Acknowledgments <a name="acknowledgements"></a>
I would like to thank microverse of the good resources they provided in this program
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FAQ (optional) -->
## ❓ FAQ (OPTIONAL) <a name="faq"></a>
- **What's the difference between Git and GitHub**
- Git is a version control system that tracks changes in project files while GitHub is hosting repository
- **What's Gitflow**
- Gitflow is an alternative Git branching model that involves the use of feature branches and multiple primary branches.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## 📝 License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p> | 👨💻 💻 Portfolio: a website that showcases my proficiency in various programming languages and tools, with a focus on projects completed as a software developer. | css,html,javascript | 2023-01-11T22:20:53Z | 2023-05-23T16:49:06Z | null | 6 | 26 | 176 | 0 | 1 | 3 | null | GPL-3.0 | CSS |
zewdu444/Awesome_books_ES6 | main | <a name="readme-top"></a>
<!--
HOW TO USE:
This is an example of how you may give instructions on setting up your project locally.
Modify this file to match your project and remove sections that don't apply.
REQUIRED SECTIONS:
- Table of Contents
- About the Project
- Built With
- Live Demo
- Getting Started
- Authors
- Future Features
- Contributing
- Show your support
- Acknowledgements
- License
After you're finished please remove all the comments and instructions!
-->
<div align="center">
<h3><b>Awesome Books ES6</b></h3>
</div>
<!-- TABLE OF CONTENTS -->
# 📗 Table of Contents
- [📖 About the Project](#about-project)
- [:camera: project screenshot](#screen-shoot)
- [🛠 Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [🚀 Live Demo](#live-demo)
- [💻 Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [👥 Authors](#authors)
- [🔭 Future Features](#future-features)
- [🤝 Contributing](#contributing)
- [⭐️ Show your support](#support)
- [🙏 Acknowledgements](#acknowledgements)
- [📝 License](#license)
<!-- PROJECT DESCRIPTION -->
# 📖 Awesome books <a name="about-project"></a>
> This project a part of the Microverse project in the JavaScript and networking module, to create an Awesome Books web application using ES6 feature, that allows users to dynamically add or remove books by JavaScript objects and arrays.
## 🛠 Built With <a name="built-with"> </a>
> HTML 5 , CSS flex box, javascript ES6, Luxon library and NPM.
### Tech Stack <a name="tech-stack"></a>
> HTML 5, CSS3 and ES6 javascript
<!-- Features -->
### Key Features <a name="key-features"></a>
<li>Add book name and Author</li>
<li>Single page application</li>
<li>List books and remove</li>
<p align="right">(<a href="#readme-top">back to top</a>)</p><!-- LIVE DEMO -->
## 🚀 Live Demo <a name="live-demo"></a>
> https://zewdu444.github.io/Awesome_books_ES6/
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## 💻 Getting Started <a name="getting-started"></a>
> to run on your localhost just copy the whole project and open index.html in your browser.
### Setup
> <p> git clone https://github.com/zewdu444/Awesome_books_ES6.git.git</p>
> cd Awesome_books_ES6
<p align="right">(<a href="#readme-top">back to top</a>)</p>
### Prerequisites
In order to run this project you only need:
- latest web-browser
### Install
To install this project in your local machine use
> npm i
### Usage
To run the project, execute the following command:
> In visual studio code you can use Go live server or simply you can open index.html in browser
<!-- AUTHORS -->
## 👥 Authors <a name="authors"></a>
👤 **zewdu erkyhun**
- GitHub: [@zewdu444](https://github.com/zewdu444)
- Twitter: [@zewdu444](https://twitter.com/zewdu444)
- LinkedIn: [zewdu-erkyhun](https://www.linkedin.com/in/zewdu-erkyhun-081378b3/)
<!-- FUTURE FEATURES -->
## 🔭 Future Features <a name="future-features"></a>
> Implement Weback
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](https://github.com/zewdu444/Awesome_books_ES6/issues).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## ⭐️ Show your support <a name="support"></a>
> If you like the project please give it star
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## 🙏 Acknowledgments <a name="acknowledgements"></a>
> I would like to thank Microverse team
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## 📝 License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| This project a part of the Microverse project in the JavaScript and networking module, to create an Awesome Books web application using ES6 feature, that allows users to dynamically add or remove books by JavaScript objects and arrays. | css3,html5,javascript,luxonjs,class,es6-javascript,modules,npm | 2023-01-16T12:25:08Z | 2023-01-16T15:55:06Z | null | 1 | 1 | 7 | 1 | 0 | 3 | null | MIT | JavaScript |
yahoogm/Dicoding-Web-Dasar | main | # Dicoding-Web-Dasar
This project is the result of every exercise provided by Dicoding.com
| This project is the result of every exercise provided by Dicoding.com | css3,dicoding-academy,html5,javascript | 2023-01-06T00:37:47Z | 2023-02-03T09:06:44Z | null | 1 | 0 | 89 | 0 | 0 | 3 | null | null | JavaScript |
sudips413/Basics-of-Python-and-Javascript | main | # Python
Python is an interpreted, object-oriented, high-level programming language with dynamic semantics. Its high-level built in data structures, combined with dynamic typing and dynamic binding, make it very attractive for Rapid Application Development, as well as for use as a scripting or glue language to connect existing components together. Python's simple, easy to learn syntax emphasizes readability and therefore reduces the cost of program maintenance. Python supports modules and packages, which encourages program modularity and code reuse. The Python interpreter and the extensive standard library are available in source or binary form without charge for all major platforms, and can be freely distributed.
# Javascript
JavaScript is a lightweight interpreted programming language. The web browser receives the JavaScript code in its original text form and runs the script from that. From a technical standpoint, most modern JavaScript interpreters actually use a technique called just-in-time compiling to improve performance; the JavaScript source code gets compiled into a faster, binary format while the script is being used, so that it can be run as quickly as possible. However, JavaScript is still considered an interpreted language, since the compilation is handled at run time, rather than ahead of time.#Javascript
| This repo includes the basic of python and javascript. The DSA are included along with different data types and library used in both of the programming language. | python,javascript,basics,programming-language | 2023-01-05T07:16:01Z | 2023-04-24T07:35:11Z | null | 3 | 28 | 71 | 0 | 2 | 3 | null | null | Jupyter Notebook |
kalebzaki4/aluraplay-requisicoes-main | main | # Aluraplay Requisições
Este repositório contém o projeto Aluraplay Requisições, desenvolvido como parte do curso da Alura sobre como utilizar APIs e localhost.
O objetivo deste projeto é fornecer uma aplicação web que permite realizar requisições a uma API externa e visualizar os resultados obtidos. Além disso, também é possível simular um servidor localhost para testar as requisições e respostas.
## Funcionalidades
- Realizar requisições HTTP a uma API externa.
- Visualizar os resultados das requisições.
- Configurar e testar um servidor localhost.
- Aprender sobre os conceitos de API e localhost.
## Como utilizar
1. Faça o clone deste repositório em sua máquina local.
2. Abra o arquivo `index.html` no seu navegador web.
3. Utilize as opções e campos disponíveis na página para realizar as requisições desejadas e testar o servidor localhost.
## Contribuição
Contribuições são bem-vindas! Sinta-se à vontade para abrir uma issue ou enviar um pull request com melhorias, correções de bugs ou novas funcionalidades.
## Licença
Este projeto está licenciado sob a [MIT License](LICENSE).
| Este repositório contém o projeto Aluraplay Requisições, desenvolvido como parte do curso da Alura sobre como utilizar APIs e localhost. | alura,code,css,curso,html,javascript | 2023-01-05T17:47:44Z | 2023-06-22T16:34:35Z | null | 1 | 0 | 32 | 0 | 0 | 3 | null | MIT | HTML |
hashmat-wani/alarm-clock | main | In this Alarm Clock, as seen in the below image, there is a digital clock that shows the current time along with some alarm options hour, minutes, am/pm. Users can select a time and set alarms for any time, whether AM or PM.

| In this Alarm Clock, as seen in the Read-me preview image, there is a digital clock that shows the current time along with some alarm options hour, minutes, am/pm. Users can select a time and set alarms for any time, whether AM or PM. | css,html5,javascript | 2023-01-03T11:11:48Z | 2023-01-03T11:22:50Z | null | 1 | 0 | 3 | 0 | 0 | 3 | null | null | CSS |
OmkarKamble1/coloriooo | main | null | Color palette for developers ! | javascript,reactjs | 2023-01-03T19:38:44Z | 2023-02-26T19:42:54Z | null | 1 | 0 | 2 | 0 | 1 | 3 | null | null | JavaScript |
hashmat-wani/image-compressor-resizer | main | In this Image Resizer & Compressor tool, users can upload an image and resize its width & height or reduce its size(from 10%-100%) with slider. If they checked the “Lock aspect ratio”, the aspect ratio for an image will be calculated automatically and filled in the field as they start changing width or height. Also User can convert image from jpeg to png and vice versa.
And, finally users can download their resized images by clicking on the “Download Image” button.
Before Image Upload:

After Image Upload:

| User can upload an image and resize its width & height or reduce its quality(from 10%-100%) with slider. Also User can convert image from jpeg to png and vice versa. Finally user can download resized/converted/compressed images by single click. | canvas2d,css,html5,javascript | 2023-01-01T13:53:19Z | 2023-01-03T13:18:17Z | null | 1 | 0 | 16 | 0 | 0 | 3 | null | null | JavaScript |
hashmat-wani/flip-a-coin | main | On flipping a coin, it produces head/tail with a cool 3D animation. It even maintains statistics about the heads and tails count. User can reset the status with the reset button.

| On flipping a coin, it produces head/tail with a cool 3D animation. It even maintains statistics about the heads and tails count. User can reset the status with the reset button. | css,html5,javascript,keyframe-animation | 2023-01-03T12:33:19Z | 2023-01-03T12:43:45Z | null | 1 | 0 | 2 | 0 | 0 | 3 | null | null | CSS |
VikasSpawar/UrbanCompany_Clone | main | ### quack-spoon-1626
1. fp02_065 - Vikas Subhash Pawar
2. fp03_310 - Rajesh Byagalwar
3. fw20_0267 - Vikram Kumar
4. fw20_0701 - Satyam Kumar
5. fw20_0059 - Sahil Khan
## IA Manager - Sanjaykumar Verma
Check the live site <a href="https://quack-spoon-1626.vercel.app/" target="_blank">here</a>
Here are some snapshots of the live site ---
<img src="./snapshots/Screenshot1.png" />
<img src="./snapshots/Screenshot2.png" />
<img src="./snapshots/Screenshot3.png" />
<img src="./snapshots/Screenshot4.png" />
<img src="./snapshots/Screenshot6.png" />
<img src="./snapshots/Screenshot5.png" />
| This project is a clone of Urban Company website. We have named it as Urban Service. This is a collaborative project. | reactjs,chakra-ui,react-redux,javascript | 2023-01-09T14:19:11Z | 2023-01-09T14:24:43Z | null | 1 | 0 | 1 | 0 | 4 | 3 | null | null | JavaScript |
hariprasad2512/StockRider-Basix | main | # StockRider-Basix
##[Stock Rider website](https://hariprasad2512.github.io/StockRider-Basix/)
This a responsive Stock Website in which you can observe how the stock is changing and you can invest in stocks
by TEAM : BASIX
contributors: [Hariprasad](https://github.com/hariprasad2512)
[Sandeep](https://github.com/neosandeep24)
[Kumar](https://github.com/KumarDevada)
[Rohit](https://github.com/Rohit-Vk24)
| Stock Website | css,data,data-analysis,datavisualization,html,stock-market,stock-market-data,stocks,trading,csv | 2023-01-15T18:06:51Z | 2023-01-15T19:35:12Z | null | 4 | 0 | 16 | 0 | 0 | 3 | null | null | HTML |
Malay-24/Malay-24.github.io | gh-pages | null | Hi, I'm Malay Bajpai ,a passionate Full Stack Web developer skilled in MERN stack , I love to learn new technology and implement it in my projects. | chakra-ui,html,javascript,react-hooks,reactjs | 2023-01-04T05:36:38Z | 2023-06-19T09:07:44Z | null | 1 | 0 | 16 | 0 | 0 | 3 | null | null | HTML |
Jersyfi/react-cookify | main | # 🍪 react-cookify





## Overview
This React library provides a customizable, simple to use and also a headless solution for creating a cookie consent manager and handling GDPR compliance. It is built specifically for React.js and offers a straightforward way to manage cookies and handle GDPR regulations in your React application.
How to handle GDPR correctly can be found on [Cookies, the GDPR, and the ePrivacy Directive](https://gdpr.eu/cookies).
## Documentation
react-cookify is essentially a set of npm packages.
```bash
npm install react-cookify
```
To view the complete documentation, please follow the link to [Cookify Docs v2.0](https://cookify.jersyfi.dev/).
## Framework Support & Features
You can view all library languages and supported features [here](https://github.com/Jersyfi/cookify#framework-support--features).
## Changelog
Please see [CHANGELOG](CHANGELOG.md) for more information what has changed recently.
## Credits
- [Jérôme Bastian Winkel](https://github.com/jersyfi)
- [All Contributors](../../contributors)
## License
The MIT License (MIT). Please see [License File](LICENSE) for more information.
| This React library offers a customizable, easy-to-use solution for managing cookies and GDPR compliance in React.js applications. | cookie-banner,cookie-consent,cookies,gdpr,javascript,react,react-library,reactjs,typescript | 2023-01-05T11:10:27Z | 2023-04-24T21:28:34Z | 2023-04-24T20:39:14Z | 1 | 98 | 157 | 3 | 1 | 3 | null | MIT | TypeScript |
topi314/topi.wtf | master | null | null | golang,website,portfolio,ssr,css,css3,javascript | 2023-01-16T09:00:18Z | 2023-12-20T08:24:09Z | null | 2 | 0 | 36 | 0 | 0 | 3 | null | Apache-2.0 | Go |
LeandroDukievicz/PortifolioLeandro | main | # Seja bem Vindo(a) ao meu Portifólio pessoal !!
### Estou animado para compartilhar este projeto, que representa o início da minha jornada no desenvolvimento web. Planejo continuar aprimorando e implementando novas tecnologias à medida que avanço nos meus estudos.
### ***Tecnologias Utilizadas :***
#### Neste projeto, utilizei algumas bibliotecas interessantes para adicionar dinamismo ao site:
#### AOS.js: Uma biblioteca que proporciona animações suaves durante o scroll, tornando a experiência do usuário mais agradável.
#
#### Tilt.js: Adicionei uma camada interativa às seções usando Tilt.js, proporcionando um efeito tridimensional sutil.
#
#### Hover.css: Esta biblioteca foi empregada para criar efeitos visuais interessantes ao passar o mouse sobre certos elementos.
#
## **_Desenvolvimento do Projeto :_**
#### O portfólio foi concebido do zero, seguindo um mockup que eu mesmo desenvolvi. Meu objetivo era criar algo fora dos padrões tradicionais, explorando minha criatividade e aplicando os conhecimentos adquiridos.
#### Dediquei atenção meticulosa a cada detalhe durante a execução do projeto. Embora tenha desejado implementar ainda mais funcionalidades, consegui realizar boa parte do que planejava, utilizando as tecnologias que já domino. Os ícones e as imagens foram cuidadosamente selecionados, aprimorados ou adaptados para atender ao resultado desejado.
#### **Foco em Detalhes :**
#### Trabalhei com afinco na melhoria da estética e desempenho do site. Muitas das imagens foram convertidas para SVG para otimizar o peso da página, contribuindo para uma experiência de carregamento mais rápida. Além disso, dediquei-me a manter uma semântica clara no HTML, o que, curiosamente, resultou em uma posição destacada nos resultados de pesquisa do Google para o meu nome.
#### Ao pesquisar no Google, meu portfólio é a terceira opção que aparece, ficando atrás apenas do LinkedIn e GitHub. Embora a relevância disso possa ser discutida, acredito que isso possa facilitar a localização e identificação do meu trabalho por parte de interessados e empregadores.
#
#### *Continuação e Aprendizado Contínuo*
#### Este portfólio reflete o máximo de conhecimento que adquiri até agora. Estou comprometido em continuar avançando e aprimorando o projeto à medida que meu conhecimento evolui. Sinta-se à vontade para explorar e entre em contato para feedback ou oportunidades de colaboração. Estou ansioso para o que o futuro reserva nesta jornada de desenvolvimento web!
### Você pode conferir o Portifólio clicando [Aqui](https://leandroduk.vercel.app/);
| null | bootstrap5,css3,hover,html5,javascript,typed,vantajs,aos-js,tilt-js | 2023-01-07T21:11:27Z | 2024-02-17T23:44:40Z | null | 1 | 1 | 169 | 0 | 0 | 3 | null | null | CSS |
muhammadmubeen17/youtube-clone | master | # Getting Started with Create React App
This project was bootstrapped with [Create React App](https://github.com/facebook/create-react-app).
## Available Scripts
In the project directory, you can run:
### `npm start`
Runs the app in the development mode.\
Open [http://localhost:3000](http://localhost:3000) to view it in your browser.
The page will reload when you make changes.\
You may also see any lint errors in the console.
### `npm test`
Launches the test runner in the interactive watch mode.\
See the section about [running tests](https://facebook.github.io/create-react-app/docs/running-tests) for more information.
### `npm run build`
Builds the app for production to the `build` folder.\
It correctly bundles React in production mode and optimizes the build for the best performance.
The build is minified and the filenames include the hashes.\
Your app is ready to be deployed!
See the section about [deployment](https://facebook.github.io/create-react-app/docs/deployment) for more information.
### `npm run eject`
**Note: this is a one-way operation. Once you `eject`, you can't go back!**
If you aren't satisfied with the build tool and configuration choices, you can `eject` at any time. This command will remove the single build dependency from your project.
Instead, it will copy all the configuration files and the transitive dependencies (webpack, Babel, ESLint, etc) right into your project so you have full control over them. All of the commands except `eject` will still work, but they will point to the copied scripts so you can tweak them. At this point you're on your own.
You don't have to ever use `eject`. The curated feature set is suitable for small and middle deployments, and you shouldn't feel obligated to use this feature. However we understand that this tool wouldn't be useful if you couldn't customize it when you are ready for it.
## Learn More
You can learn more in the [Create React App documentation](https://facebook.github.io/create-react-app/docs/getting-started).
To learn React, check out the [React documentation](https://reactjs.org/).
### Code Splitting
This section has moved here: [https://facebook.github.io/create-react-app/docs/code-splitting](https://facebook.github.io/create-react-app/docs/code-splitting)
### Analyzing the Bundle Size
This section has moved here: [https://facebook.github.io/create-react-app/docs/analyzing-the-bundle-size](https://facebook.github.io/create-react-app/docs/analyzing-the-bundle-size)
### Making a Progressive Web App
This section has moved here: [https://facebook.github.io/create-react-app/docs/making-a-progressive-web-app](https://facebook.github.io/create-react-app/docs/making-a-progressive-web-app)
### Advanced Configuration
This section has moved here: [https://facebook.github.io/create-react-app/docs/advanced-configuration](https://facebook.github.io/create-react-app/docs/advanced-configuration)
### Deployment
This section has moved here: [https://facebook.github.io/create-react-app/docs/deployment](https://facebook.github.io/create-react-app/docs/deployment)
### `npm run build` fails to minify
This section has moved here: [https://facebook.github.io/create-react-app/docs/troubleshooting#npm-run-build-fails-to-minify](https://facebook.github.io/create-react-app/docs/troubleshooting#npm-run-build-fails-to-minify)
| YouTube Clone Frontend, React.js | css3,html5,javascript,react,react-components,react-hooks,react-router,react-router-dom,reactjs,tailwindcss | 2023-01-11T06:21:52Z | 2023-01-16T19:19:48Z | null | 1 | 0 | 7 | 0 | 0 | 3 | null | null | JavaScript |
gar-mil/wesnoth-era-randomizer-program | main | # Wesnoth Era Randomizer Program
WERP is a JavaScript application that generates randomized add-on eras for Battle for Wesnoth.
WERP creates a complete Wesnoth add-on that contains a randomly generated multiplayer era. The era uses the game's standard units, but organizes them into new factions.
## Features
* One-click era randomizer that generates a complete Wesnoth add-on.
* Barebones GUI.
* Random icon generation for each faction.
* Random name generation for the era and the factions.
* Three unit sets:
* Default - Generates an era using the units and leaders from the standard "Default" era.
* Default+Dune - Generates an era using the units and leaders from the standard "Default+Dune" era.
* Expanded - Generates an era using units and leaders that may or may not appear in vanilla multiplayer. Only units with an advancement class are used in the recruitment pool.
* Each era has 6 factions, with 7 recruitable units and 4 leaders each.
* Terrain preferences are generated for each faction and work decently for random maps.
* Recruitment patterns are generated for each faction and, while currently sub-optimal, work for the AI.
* All generation is performed locally on the client web browser.
* WERP can be easily setup on a local or private webserver.
## Docker Server
Build the docker container
* `docker build . -t httpd_werp`
Launch the docker container
* `docker run -dit -p 8080:80 httpd_werp`
In a browser to go <http://localhost:8080/>
## License
Wesnoth Era Randomizer Program is licensed under the MIT License. Please see the [attached license file](https://github.com/gar-mil/wesnoth-era-randomizer-program/blob/main/LICENSE) for more information.
## Attributions
* JSZip v3.10.1 - A JavaScript class for generating and reading zip files - Licensed under the MIT License.
* See [core\libraries\jszip.LICENSE.md](https://github.com/gar-mil/wesnoth-era-randomizer-program/blob/main/core/libraries/jszip.LICENSE.md) for more information.
* Viscious Speed's game-icons.net icon set - Licensed under [CC0](https://creativecommons.org/publicdomain/zero/1.0/).
* [http://viscious-speed.deviantart.com](http://viscious-speed.deviantart.com)
| JavaScript application that generates randomized add-on eras for Battle for Wesnoth. | javascript,randomizer,wesnoth | 2023-01-11T20:36:38Z | 2023-07-12T21:57:25Z | 2023-01-17T07:55:17Z | 2 | 8 | 26 | 8 | 0 | 3 | null | MIT | JavaScript |
0AvinashMohanDev1/Ekart | main | # acidic-blade-1610
------------------------------------------------- FRONT PAGE---------------------------------------------------
The below image is about the landing page of our website
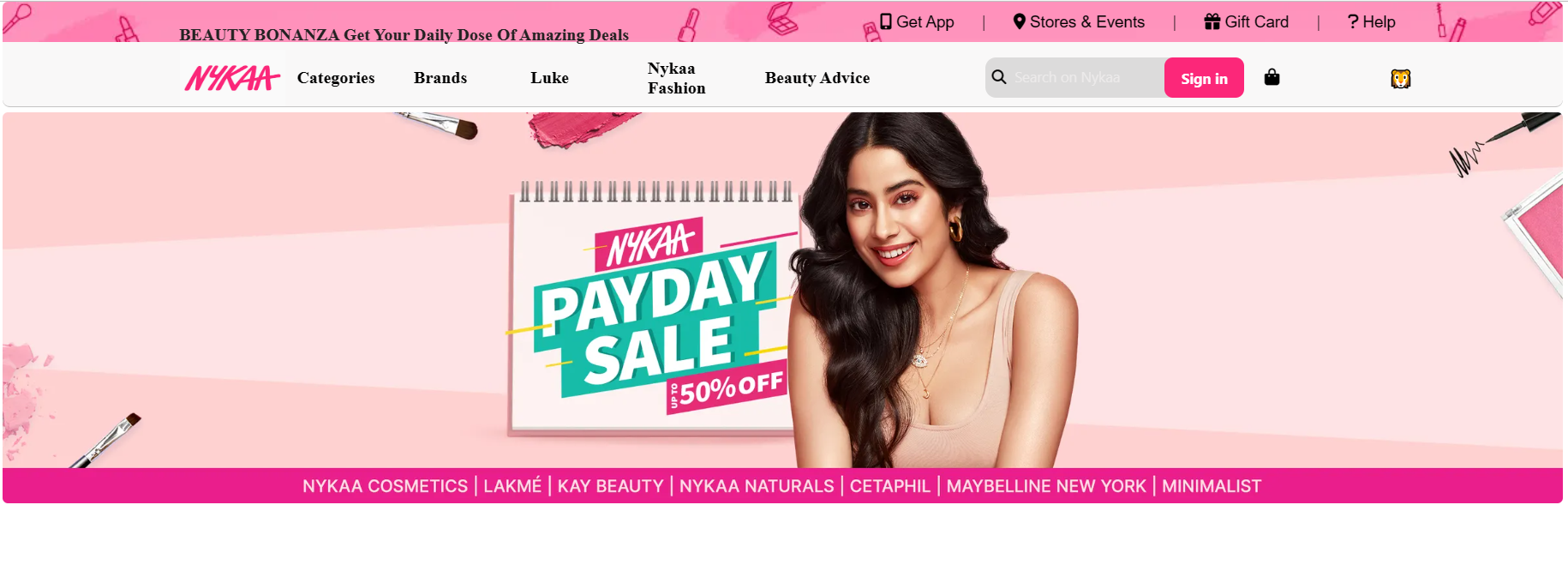
------------------------------------------------- FOOTER------------------------------------------------------------
The below image is about the landing page of our website
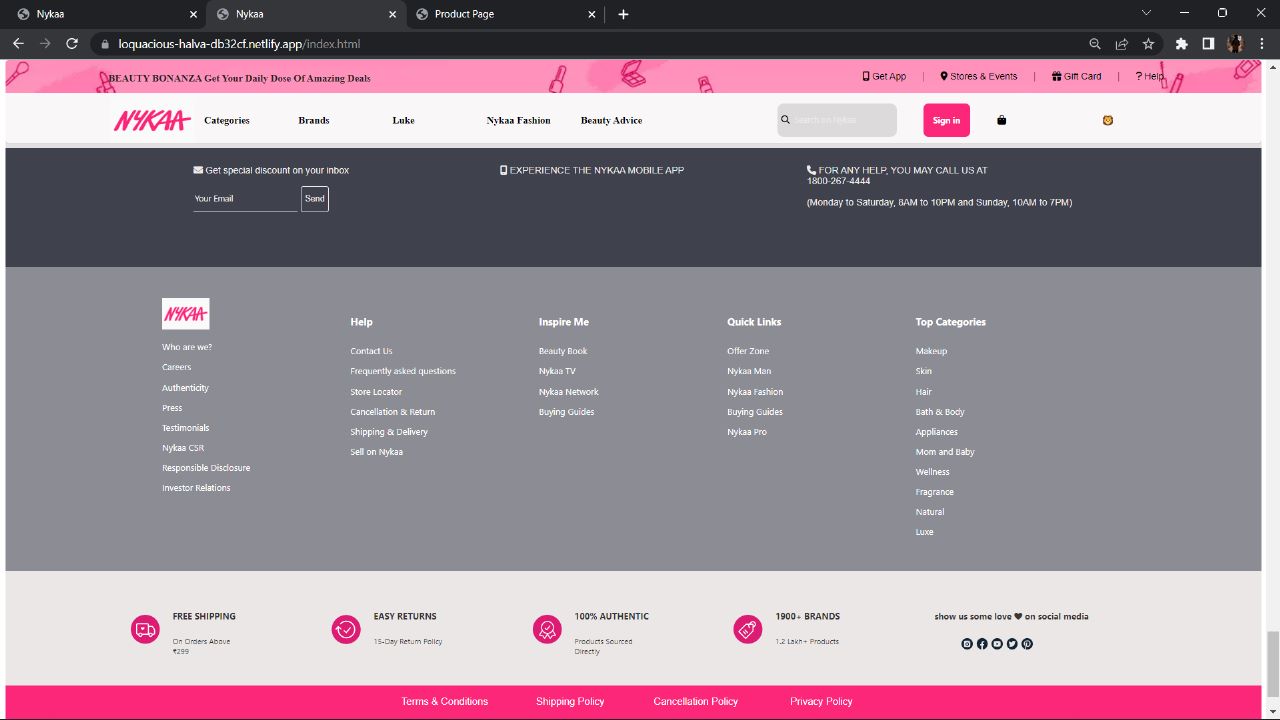
------------------------------------------------- LOGIN PAGE --------------------------------------------------
If we are a new user we need to give the credentials and then login.
If we donot login and try to add a product to cart, we wont be able to do so.
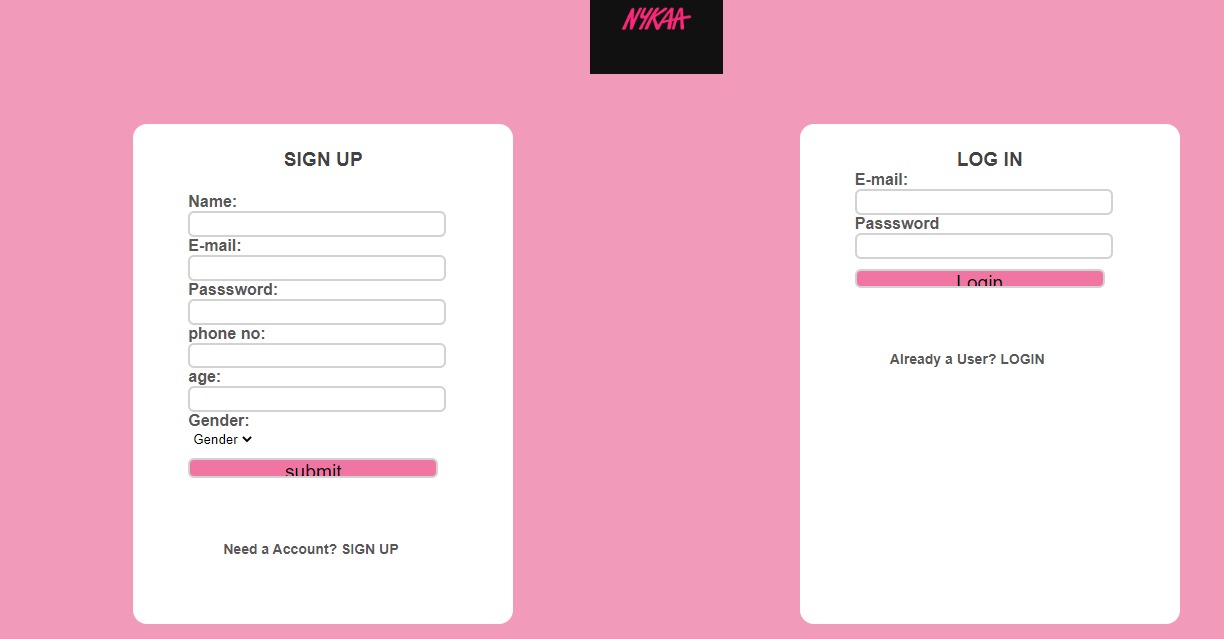
-------------------------------------------------------PRODUCT----------------------------------------------------------------
The below page has all the products from our website and after loging in we can add the products to our cart by clicking on the 'add to cart' button.
We have implemented the filter part and sort (increasing price/ decreasing price)
<img width="945" alt="image" src="https://user-images.githubusercontent.com/76575824/214022860-61aca377-c531-4a43-99f4-0bfe02d91c2d.png">
-------------------------------------------------------CART-------------------------------------------------------------------
All the products which are added to cart from the products page will be showing here.
We can also have the total price of our cart and add the coupan to get the discount.
<img width="947" alt="image" src="https://user-images.githubusercontent.com/76575824/214022361-bef85a59-2d45-47dc-8bc9-61527a7c5f2b.png">
--------------------------------------------------------PAYMENT----------------------------------------------------------------
After clicking on the 'proceed' from the cart page we will be redirected to the payments page and you can enter the address and card details and after that you will be redirected to the main page of our website
<img width="948" alt="image" src="https://user-images.githubusercontent.com/76575824/214021536-f88082c7-bc7d-42a5-89be-c2f8cb1dec8a.png">
<img width="946" alt="image" src="https://user-images.githubusercontent.com/76575824/214021851-3fd39cfb-ba54-4150-a04d-233741fb1b76.png">
--------------------------------------------------------ADMIN-------------------------------------------------------------------
When we login to admin page the first page describes about the total users and total products in our website.
<img width="943" alt="landing" src="https://user-images.githubusercontent.com/76575824/214012763-7c4d69d6-8253-42c7-81af-63b42a534b51.png">
And if we click on 'all products' button we will be getting all products as below.
If we want any product to be removed from our website we can click on remove button in particular card and it will be deleted from the main API.
<img width="940" alt="all products" src="https://user-images.githubusercontent.com/76575824/214012596-b6694f3b-9763-4356-ae92-0598eb17dcdd.png">
We can also add new products to our website by clicking on the 'add product' button and give all the fields for a product.
The product will be added to main API.
<img width="950" alt="addProducts" src="https://user-images.githubusercontent.com/76575824/214012854-8dced315-a8be-41cf-b429-2bd1e2664d46.png">
Our products can also be edited based on the requirements and it can be done by clicking on 'edit product' button in nav bar.
<img width="950" alt="edit products" src="https://user-images.githubusercontent.com/76575824/214012946-db9ad4f2-ddf0-448b-9cd5-e9059de1381a.png">
After all the operations if the admin wants to logout, admin can click on the logout button in navbar and admin will be redirected to the main landing page of out website
<img width="955" alt="landing1" src="https://user-images.githubusercontent.com/76575824/214015969-7c6a49ac-6994-4a99-b137-e95b363085d9.png">
Admin Page: [https://ekart-ayta.vercel.app/admin/admin.html]
| Nykaa is an Indian e-commerce company, founded by Falguni Nayar in 2012 and headquartered in Mumbai. It sells beauty, wellness and fashion products across websites, mobile apps and 100+ offline stores. In 2020, it became the first Indian unicorn startup headed by a woman. | advanced-javascript,css,css-grid,dom-manipulation,html,html5,javascript,mock-api | 2023-01-14T10:00:05Z | 2024-05-22T18:31:56Z | null | 5 | 32 | 73 | 0 | 0 | 3 | null | null | HTML |
Lancelot-SO/capstone_project | main | <a name="readme-top"></a>
<!--
HOW TO USE:
This is an example of how you may give instructions on setting up your project locally.
Modify this file to match your project and remove sections that don't apply.
REQUIRED SECTIONS:
- Table of Contents
- About the Project
- Built With
- Live Demo
- Getting Started
- Authors
- Future Features
- Contributing
- Show your support
- Acknowledgements
- License
After you're finished please remove all the comments and instructions!
-->
<div align="center">
<img src="murple_logo.png" alt="logo" width="140" height="auto" />
<br/>
<h3><b>Microverse README Template</b></h3>
</div>
<!-- TABLE OF CONTENTS -->
# 📗 Table of Contents
- [📖 About the Project](#about-project)
- [🛠 Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [🚀 Live Demo](#live-demo)
- [💻 Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#triangular_flag_on_post-deployment)
- [👥 Authors](#authors)
- [🔭 Future Features](#future-features)
- [🤝 Contributing](#contributing)
- [⭐️ Show your support](#support)
- [🙏 Acknowledgements](#acknowledgements)
- [❓ FAQ](#faq)
- [📝 License](#license)
<!-- PROJECT DESCRIPTION -->
# 📖 [Finishing Capstone Project] <a name="about-project"></a>
> Capstone Project
## 🛠 Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
> Git, Github, HTML, CSS, JS
<!-- Features -->
### Key Features <a name="key-features"></a>
- **[capstone Desktop app]**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## 🚀 Live Demo <a name="live-demo"></a>
[Live Demo Link](https://lancelot-so.github.io/capstone_project/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## 💻 Getting Started <a name="getting-started"></a>
To get a local copy up and running, follow these steps.
<!--
Example command:
```sh
gem install rails
```
-->
To clone this repo run this command
git clone git@github.com:Lancelot-SO/capstone_project.git
<!--
Example commands:
```sh
cd my-folder
git clone git@github.com:myaccount/my-project.git
```
--->
### Install
npm install
<!--
Example command:
```sh
cd my-project
gem install
```
--->
### Usage
<!--
Example command:
```sh
rails server
```
--->
### Run tests
<!--
Example command:
```sh
bin/rails test test/models/article_test.rb
```
--->
### Deployment
<!--
Example:
```sh
```
-->
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
👤 **Felix Adjei Sowah**
- Github: [@githubhandle](https://github.com/Lancelot-SO)
- Twitter: [@twitterhandle](https://twitter.com/Lancelot)
- LinkedIn: [LinkedIn](https://www.linkedin.com/in/felix-sowah)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## 🔭 Future Features <a name="future-features"></a>
- [ ] **[Login and Register pages]**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## ⭐️ Show your support <a name="support"></a>
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## 🙏 Acknowledgments <a name="Thanks a lot to Cindy Shin](https://www.behance.net/adagio07), the author of the original design for being the inspiration behing the design/website"></a>
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FAQ (optional) -->
## ❓ FAQ <a name="faq"></a>
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## 📝 License <a name="license"></a>
_NOTE: we recommend using the [MIT license](https://choosealicense.com/licenses/mit/) - you can set it up quickly by [using templates available on GitHub](https://docs.github.com/en/communities/setting-up-your-project-for-healthy-contributions/adding-a-license-to-a-repository). You can also use [any other license](https://choosealicense.com/licenses/) if you wish._
<p align="right">(<a href="#readme-top">back to top</a>)</p> | This project is about the World Health Organization conferences held across the globe. One can know the date and location of a conference and this will make it easier for interested persons to attend all conferences | html-css,javascript | 2023-01-02T07:34:37Z | 2023-01-05T17:13:51Z | null | 1 | 1 | 33 | 0 | 0 | 3 | null | null | HTML |
biruk-bereka/software-developer-summit | main | <a name="readme-top"></a>
<!-- TABLE OF CONTENTS -->
# 📗 Table of Contents
- [📖 About the Project](#about-project)
- [🛠 Built With](#built-with)
- [Tech Stack](#tech-stack)
- [Key Features](#key-features)
- [🚀 Live Demo](#live-demo)
- [💻 Getting Started](#getting-started)
- [Setup](#setup)
- [Prerequisites](#prerequisites)
- [Install](#install)
- [Usage](#usage)
- [Run tests](#run-tests)
- [Deployment](#triangular_flag_on_post-deployment)
- [👥 Authors](#authors)
- [🔭 Future Features](#future-features)
- [🤝 Contributing](#contributing)
- [⭐️ Show your support](#support)
- [🙏 Acknowledgements](#acknowledgements)
- [❓ FAQ](#faq)
- [📝 License](#license)
<!-- PROJECT DESCRIPTION -->
# 📖 Capstone Project <a name="about-project"></a>
> This is a capstone project which build using design guidline.
## 🛠 Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
<details>
<summary>Client</summary>
<ul>
<li><a href="https://www.w3schools.com/html/">HTML</a></li>
<li><a href="https://www.w3schools.com/css/">CSS</a></li>
<li><a href="https://www.w3schools.com/js/js_es6.asp">JACASCRIPT ES6</a></li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
- Design made using the provided guidelines.
- Personalize project
- Use HTML5 , CSS3 and JavaScript
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## 🚀 Live Demo <a name="live-demo"></a>
> Here is the link for online version of the project
- [Live Demo Link](https://biruk-bereka.github.io/software-developer-summit/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## 💻 Getting Started <a name="getting-started"></a>
> To get a local copy up and running follow these simple example steps.
### Prerequisites
- In order to run this project you need: Visual Studio code or any Code Editor
### Setup
Clone this repository to your desired folder:
```sh
cd my-folder
git clone https://github.com/biruk-bereka/software-developer-summit
```
### Install
Install this project with:
```sh
npm install
```
### Usage
### Run tests
### Deployment
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## 👥 Authors <a name="authors"></a>
👤 **Biruk Bereka**
- GitHub: [@biruk-bereka](https://github.com/biruk-bereka)
- LinkedIn:[@Biruk Bereka](https://www.linkedin.com/in/biruk-bereka1212/)
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
## 🔭 Future Features <a name="future-features"></a>
> Future features
- [ ] **Update the conferance page with feedback**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- CONTRIBUTING -->
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
Feel free to check the [issues page](../../issues/).
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## ⭐️ Show your support <a name="support"></a>
If you like this project please star in here on Github
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## 🙏 Acknowledgments <a name="acknowledgements"></a>
I would like to thank Creative Commons
This project is only for student purpose, the design is based in the template from [Cindy Shin](https://www.behance.net/adagio07).
<!-- LICENSE -->
## 📝 License <a name="license"></a>
This project is [MIT](./MIT.md) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| Software developer summit is a landing page for the developer summit in which users can register for the upcoming summit. They can see what they can expect from the summit like who are featured speakers and the like and also see the past summits. | css3,html5,javascript | 2023-01-02T15:06:48Z | 2023-01-06T01:01:21Z | null | 1 | 1 | 26 | 0 | 0 | 3 | null | null | CSS |
AmitChigare/GreatKart-Django-E-commerce | main | null | Ecommerce Website built on Django backend server. The front-end is also handled by Django. This website includes all modern features and security with payment-gateway. You can almost find anything which any ecommerce website basically has. | bootstrap4,css3,django,django-project,html5,javascript,paypal,python3 | 2023-01-16T08:58:47Z | 2023-01-31T15:11:17Z | null | 1 | 0 | 11 | 0 | 0 | 3 | null | null | HTML |
thenamevishnu/volvo-cars | main | null | Cloned website of volvo cars india | css,html,javascript,bootstrap5 | 2023-01-14T16:06:14Z | 2023-01-14T16:28:54Z | null | 1 | 0 | 3 | 0 | 0 | 3 | null | null | HTML |
CodeAlchemyTech/ionic-capacitor-firebase-demo | main | # Ionic Capacitor Firebase authentication
This is a demo project with use of Firebase js sdk to authenticate firebase user with different methods provided by firebase.
This project was built using [Capacitor Firebase Authenthentication](https://github.com/robingenz/capacitor-firebase/tree/main/packages/authentication) and [Firebase JS SDK](https://www.npmjs.com/package/firebase).
## How to use
1. To run project
```
npm install
```
2. Create a Firebase project
- Use this link to create project in [Firebase](https://console.firebase.google.com/).
3. Add config object from Firebase Webapp in to /src/app/services/firebase/firebase-config.ts
```
export const firebaseConfig = {
apiKey: "xxxxxxxx-xxxxxxxx",
authDomain: "xxxxxxxxxxxxxxxxxxxxxxxx",
databaseURL: "xxxxxxxxxxxxxxxxxxxxxxxx",
projectId: "xxxxxxxx",
storageBucket: "xxxxxxxx",
messagingSenderId: "xxxxxx",
appId: "xxxxx",
measurementId: "xxxxxxxxxxxxxxxx"
};
```
- Before configure Google and Facebook. Change app id in <b>capacitor.config.ts</b> file.
- Create Android and iOS application in firebase project setting with your app id.
- Download <b>GoogleService-Info.plist</b> file for iOS app and add that file under <b>ios/App/App</b> folder.
- Download <b>google-services.json</b> file for Android app and add this file under <b>/android/app</b> folder.
### Google Authenthentication
- Enable google authentication in firebase authentication provider.
- Follow step mention in below link for configure google authentication.
- [Configure Google Authentication in Android, iOS and Web](https://github.com/robingenz/capacitor-firebase/blob/main/packages/authentication/docs/setup-google.md)
### Facebook Authenthentication
- Create facebook app using facebook developer account.
- Add Android, iOS and web platform on facebook app.
- Enable facebook authentication and set facebook app id and secrate key.
- Enable google authentication in firebase authentication provider.
- Follow step mention in below link for configure google authentication.
- [Configure Facebook Authentication in Android, iOS and Web](https://github.com/robingenz/capacitor-firebase/blob/main/packages/authentication/docs/setup-facebook.md)
### Apple Authenthentication
- Enable apple authentication in firebase
- Before you begin. Follow this steps:
- [Android](https://firebase.google.com/docs/auth/android/apple#before-you-begin)
- [iOS](https://firebase.google.com/docs/auth/ios/apple#before-you-begin)
- [Web](https://firebase.google.com/docs/auth/web/apple#before-you-begin)
### Twitter Authenthentication
- Enable twitter authentication provider.
- Follow this steps [before you begin](https://github.com/robingenz/capacitor-firebase/blob/main/packages/authentication/docs/setup-twitter.md) | ⚡️✨ Simple Ionic Angular(Capacitor) demo project with firebase authentication and firestore database implementation This is mention use of FirebaseJs sdk and @capawesome-team/capacitor-firebase/authentication plugin to authenticate firebase user with different methods provided by firebase⚡️ | ionic,capacitor,firebase,firebase-auth,firebase-firestore,javascript,typescript,capacitor-firebase,capacitor-firebase-auth | 2023-01-12T03:55:15Z | 2023-01-12T06:35:03Z | null | 1 | 0 | 2 | 0 | 0 | 3 | null | null | TypeScript |
elementbound/nlon | main | # nlon

[](https://elementbound.github.io/nlon/)
*Pronounced neigh-lon.*
A bidirectional communication protocol that can be used over raw sockets,
WebSockets or any other method of transmission.
Its main use case is situations where you have a transport layer without any
structure, e.g. TCP sockets where you stream arbitrary bytes. The role of nlon
here is to provide a convenient layer on top of the transport to give shape to
the data being transmitted.
This repository includes the protocol specification and a reference
implementation in JavaScript.
## Features
- 📦 Almost no dependencies
- The core uses 3 dependencies: pino, nanoid, ndjson
- 🔩 Protocol-agnostic
- Works the same over TCP as WebSockets by using Adapters
- ⚡ Simple specification
- The whole spec is a ~5 min read
- 🎉 Express-inspired API for writing Servers
- Register (route) handlers to respond to incoming messages
- 📨 Streaming supported by design
- Protocol permits transmitting data in multiple chunks
### Adapters
- [nlon-socket](packages/nlon-socket/) for TCP sockets
- [nlon-websocket](packages/nlon-websocket/) for the browser
## Install
- pnpm: `pnpm add @elementbound/nlon`
- npm: `npm i @elementbound/nlon`
- yarn: `yarn add @elementbound/nlon`
## Usage
See the [reference implementation](packages/nlon/).
## Documentation
- [Protocol specification](doc/spec/protocol.md)
- [Example flow](doc/spec/example-flow.md)
- [API docs](https://elementbound.github.io/nlon/)
- Or generate your own with JSDoc: `pnpm -r doc`
- Tutorials
- [Implementing a server](doc/tutorial/server.md)
- [Implementing a peer](doc/tutorial/peer.md)
- Examples
- [WebSocket example](examples/websocket-chat/)
## License
This package is under the [MIT License](LICENSE).
| A communication protocol with reference implementation | javascript,network,protocol,socket,websocket,websockets | 2023-01-10T18:15:22Z | 2023-05-01T12:19:22Z | 2023-05-01T12:19:22Z | 1 | 25 | 33 | 4 | 0 | 3 | null | MIT | JavaScript |
fajriyan/al-quran | main | # Project Qur'an Digital
Project Al Qur'an Digital merupakan sebuah website yang sederhana untuk membaca Al Qur'an dengan mudah tanpa harus melakukan install aplikasi, project ini bermula dari keresahan saya ketika ingin melakukan copy surah alquran dan saya mengalami kesulitan untuk mencari sumber yang mudah untuk melakukan itu, oleh karena itu saya membuat project ini untuk membantu semua orang dalam membaca dan memanfaatkannya untuk kebaikan, semoga bermanfaat dan terimakasih.
[](https://github.com/login?return_to=https%3A%2F%2Fgithub.com%2Ffajriyan)
[]()
[]()
[]()
[]()
[]()
## Donation
if this project helps you, you can give it a cup of coffee. thanks!
* [buymeacoffee](https://www.buymeacoffee.com/fajriyan)
* [saweria](https://saweria.co/fajriyan)
## How to run it
Untuk menjalankan project ini kalian bisa melakukan dengan 2 cara, sebagai
berikut :
### `Access Here (Online)`
Kalian bisa melakukan pengaksesan website secara online
[disini](https://github.com/fajriyan/al-quran/releases/tag/Latest).
### `Download Project (Run Offline)`
1. Cara kedua bisa digunakan apabila ingin melakukan run dengan `offline` dengan
langsung melakukan
[download project](https://github.com/fajriyan/al-quran.git).
2. Jalankan fungsi `npm i` untuk install dependensi yang dibutuhkan.
3. Kalian buka dengan vscode dan buka jalankan command `npm start`.
## Screenshot
- 🏠 Homepage

- 📃 Single Page

- 📎 About

## Support
Project ini tentunya tidak lepas dari berbagai pihak yang telah membantu, oleh
karena itu saya menyampaikan banyak 🙏Terimakasih kepada semua pihak yang sudah
mendukung project ini sampai live. Berikut beberapa pihak yang telah membantu
dapat dilihat [disini](https://al-quran.pages.dev/about/)
## Latest Issue
- [x] Fix Search Error with Space
- [x] Scroll detail Post Error
- [x] Button "Lanjutkan Membaca" Error
- [x] scroll in home error
## Next Update
- [x] Add copy link surat ke berapa
- [x] Add Toast
- [x] Create Save and delte Bookmark surah
- [x] Create Button "Lanjutkan Membaca" in Card Bookmark
- [x] Fix Structure folder
- [x] create page changelog
- [ ] create page mini blog
- [ ] create al quran with PWA
## Changelog
- Update [0.1.0] - Fix Error Key and add Donation Button
- Update [1.2.0] - New design Navbar and Control Copy Button
- Update [1.3.2] - Add feature save surah
- Update [1.4.0] - Add new featured and fix bug scroll
- Update [1.4.5] - Add favotite and adjust card
- Update [1.4.9] - Add page 404 and fix bug
- Update [1.5.0] - Migrate to Vite and fix 9 vulnerabilities
- Update [1.5.2] - Improve SEO and update ui mobile
- Update [1.5.5] - Fixing structure folder
- Update [1.5.9] - Improve UI and fix some error in console
- Update [1.6.0] - fix error and layout tablet
- Update [1.6.2] - update some function page home and single surah
- update [1.6.5] - add tafsir per ayat
- update [1.7.0] - clean up folder structure and code
- update [1.7.3] - create loading bar and fix bug
## Issue Report
📢[Report Issue Here](https://github.com/fajriyan/al-quran/issues/new)
| Al Quran website project using Public API with the objective of making it easier to listen to the al quran | al-quran,daisyui,reactjs,tailwindcss,api,quran,alquran,alquran-web,javascript,react | 2023-01-06T03:11:01Z | 2024-05-20T13:29:53Z | 2023-11-02T12:32:43Z | 2 | 6 | 76 | 0 | 2 | 3 | null | null | JavaScript |
BrunoOliveira16/Formacao-React-Developer-DIO | main | # 📌 **Formação React Developer - DIO**
<img src="./assets/logo.webp" width="70" alt="Icone do Bootcamp react Developer"><img src="./assets/html.svg" width="70" alt="Icone HTML5"><img src="./assets/css.svg" width="70" alt="Icone CSS3"><img src="./assets/javascript.svg" width="70" alt="Icone JavaScript"><img src="./assets/react.svg" width="70" alt="Icone React"><img src="./assets/typescript.svg" width="70" alt="Icone TypeScript">
Formação React developer da trilha de Front-End da DIO.
- Carga horária: 34h
- Desafios de Projeto: 5
- Desafios de Código: 6
<br>
## 📎 **Sumário**
- **Módulo 01:** Fundamentos do React;
- **Módulo 02:** Conceitos Básicos da Biblioteca;
- **Módulo 03:** Ampliando o Conhecimento em React;
- **Módulo 04:** React com Typescript;
- **Módulo 05:** Conceitos Avançados do React;
<br>
## 🏆 **Desafios de Código**
<br>
<table border=1>
<tr>
<th colspan="3" style="text-align:center"><b>FORMAÇÃO REACT DEVELOPER</b></th>
</tr>
<tr>
<th colspan="3" style="text-align:center">Desafios de Código Básico</th>
</tr>
<tr>
<th style="text-align:center">Desafio</th>
<th style="text-align:center">Solução</th>
<th style="text-align:center">Status</th>
</tr>
<tr>
<td align="center">Visita na Feira</td>
<td align="center"><a href="https://github.com/BrunoOliveira16/Formacao-React-Developer-DIO/tree/main/TRILHA-REACT-MODULO-01/DESAFIO-DE-CODIGO/DESAFIO-DE-CODIGO-01">Código</a></td>
<td align="center">✅</td>
</tr>
<tr>
<td align="center">Tartarugas Ninja</td>
<td align="center"><a href="https://github.com/BrunoOliveira16/Formacao-React-Developer-DIO/tree/main/TRILHA-REACT-MODULO-01/DESAFIO-DE-CODIGO/DESAFIO-DE-CODIGO-02">Código</a></td>
<td align="center">✅</td>
</tr>
<tr>
<td align="center">Arrays Pares</td>
<td align="center"><a href="https://github.com/BrunoOliveira16/Formacao-React-Developer-DIO/tree/main/TRILHA-REACT-MODULO-01/DESAFIO-DE-CODIGO/DESAFIO-DE-CODIGO-03">Código</a></td>
<td align="center">✅</td>
</tr>
</table>
<table border=1>
<tr>
<th colspan="3" style="text-align:center"><b>FORMAÇÃO REACT DEVELOPER II</b></th>
</tr>
<tr>
<th colspan="3" style="text-align:center">Desafios de Código Básico</th>
</tr>
<tr>
<th style="text-align:center">Desafio</th>
<th style="text-align:center">Solução</th>
<th style="text-align:center">Status</th>
</tr>
<tr>
<td align="center">Tuitando</td>
<td align="center"><a href="https://github.com/BrunoOliveira16/Formacao-React-Developer-DIO/tree/main/TRILHA-REACT-MODULO-02/DESAFIO-DE-CODIGO/DESAFIO-DE-CODIGO-01">Código</a></td>
<td align="center">✅</td>
</tr>
<tr>
<td align="center">Patinhos</td>
<td align="center"><a href="https://github.com/BrunoOliveira16/Formacao-React-Developer-DIO/tree/main/TRILHA-REACT-MODULO-02/DESAFIO-DE-CODIGO/DESAFIO-DE-CODIGO-02">Código</a></td>
<td align="center">✅</td>
</tr>
<tr>
<td align="center">Validação de Nota</td>
<td align="center"><a href="https://github.com/BrunoOliveira16/Formacao-React-Developer-DIO/tree/main/TRILHA-REACT-MODULO-02/DESAFIO-DE-CODIGO/DESAFIO-DE-CODIGO-03">Código</a></td>
<td align="center">✅</td>
</tr>
</table>
<br>
## ⭐ **Desafios de Projeto**
<br>
<table border=1>
<tr>
<th colspan="4" style="text-align:center"><b>FORMAÇÃO REACT DEVELOPER</b></th>
</tr>
<tr>
<th colspan="4" style="text-align:center">Desafios de Projeto</th>
</tr>
<tr>
<th style="text-align:center">Desafio</th>
<th style="text-align:center">Solução</th>
<th style="text-align:center">Deploy</th>
<th style="text-align:center">Status</th>
</tr>
<tr>
<td align="center">Construindo uma Calculadora com React</td>
<td align="center"><a href="https://github.com/BrunoOliveira16/calculadora-react">Código</a></td>
<td align="center"><a href="https://calculadora-projeto-react.netlify.app/">Link</a></td>
<td align="center">✅</td>
</tr>
<tr>
<td align="center">Criando uma Wiki de Repositórios do GitHub com React</td>
<td align="center"><a href="https://github.com/BrunoOliveira16/github-wiki">Código</a></td>
<td align="center"><a href="https://wiki-repository-github.netlify.app/">Link</a></td>
<td align="center">✅</td>
</tr>
<tr>
<td align="center">Desenvolvendo tela de cadastro da DIO com React</td>
<td align="center"><a href="https://github.com/BrunoOliveira16/pagina-login-dio">Código</a></td>
<td align="center"><a href="https://clone-pagina-dio.netlify.app/">Link</a></td>
<td align="center">✅</td>
</tr>
<tr>
<td align="center">Implementando Uma Tela de Login Com Validação Usando TypeScript</td>
<td align="center"><a href="https://github.com/BrunoOliveira16/tela-login-ts">Código</a></td>
<td align="center"><a href="https://tela-login-ts.netlify.app/">Link</a></td>
<td align="center">✅</td>
</tr>
</table>
<br>
## 🙋🏻♂️ Autor
Bruno Oliveira
| Repositório de conteúdos da Formação React Developer da DIO | css3,html5,javascript,react,typescript | 2023-01-03T23:09:59Z | 2023-04-10T20:54:19Z | null | 1 | 0 | 42 | 0 | 0 | 3 | null | null | JavaScript |
Caique-P/Quester | main |
<p align="center">
<a href="https://quester-five.vercel.app">
<img src="https://user-images.githubusercontent.com/58194653/220790674-8aa78144-759a-4e76-bbb1-057c798eb708.png" alt="Quester" width="65%"/> </a>
</p>
<p align="center">
<img src="https://img.shields.io/badge/Made by-Caique Ponjjar-blue.svg" />
<img src="https://img.shields.io/github/followers/Caique-P?label=Seguir&style=social" />
<img src="https://img.shields.io/github/last-commit/Caique-P/Quester?label=Last%20update" />
<img src="https://img.shields.io/badge/-React-200259?style=flat&logo=React&logoColor=red" />
<img src="https://img.shields.io/github/stars/Caique-P/Quester?style=social"/>
<img src="https://img.shields.io/github/repo-size/Caique-P/Quester?style=flat&label=Size"/>
<img src="https://hits.seeyoufarm.com/api/count/incr/badge.svg?url=https%3A%2F%2Fgithub.com%2FCaique-P%2FQuester&count_bg=%2379C83D&title_bg=%23555555&icon=github.svg&icon_color=%23E7E7E7&title=hits&edge_flat=false"/>
</p>
---
Quester is an OpenIA question finder app that allows you to quickly and easily search for questions related to a given topic. Using the app, you can quickly find questions related to a given topic and use them to train ReactJS with Material UI.
OpenIA provides a powerful API for quickly finding questions related to a given topic. With Quester, you can use the OpenIA API to quickly and easily find questions related to your chosen topic, saving you time and effort.
Quester is built using ReactJS and Material UI. The app is designed to be intuitive and easy to use, allowing you to quickly find the questions you need to train your ReactJS with Material UI.
<p align="center">
<a href="https://quester-five.vercel.app">
<img src="https://user-images.githubusercontent.com/58194653/210456489-844a067a-535d-4c55-9b60-e4bd8c7c83db.gif" alt="Quester Acess" width="75%"/>
Access Quester App </a>
</p>
## Getting Started
To get started with Quester, simply visit the [Quester App](https://quester-99fmnb8yj-caique-p.vercel.app) and enter your chosen topic. Quester will then use the OpenIA API to find questions related to the topic, and you can then use these questions to train your ReactJS with Material UI.
- To setup Quester for development, clone the repository and run `npm install` to install the dependencies. Then, run `npm start` to start the development server.
## Contributing
We welcome contributions to Quester. If you have an idea for an improvement or bug fix, please feel free to open a pull request or issue.
| AI for academic queries and generate project readme automatically autoreadme | ai,automation,javascript,material-ui,openai,react,readme-generator,text-davinci-003,automatic,autoreadme | 2023-01-03T23:43:16Z | 2023-04-04T23:26:32Z | null | 2 | 0 | 32 | 0 | 1 | 3 | null | null | JavaScript |
Buelie/Rucker | main | # English | [简体中文](https://github.com/Buelie/Rucker#english--%E7%AE%80%E4%BD%93%E4%B8%AD%E6%96%87--%E7%B9%81%E4%BD%93%E4%B8%AD%E6%96%87-1) | [繁体中文](https://github.com/Buelie/Rucker/edit/main/README.md#english--%E7%AE%80%E4%BD%93%E4%B8%AD%E6%96%87--%E7%B9%81%E4%BD%93%E4%B8%AD%E6%96%87-1)
### Rucker's philosophy
***Rucker will support the API interface provided by KOOK to the end, and provide some interfaces that are not officially announced or simply write the interface yourself***
### Features of Rucker
* Use pure JS implementation
* Lightweight SDK
* Automatic exception handling without developer worry
* Detailed documentation
# [English](https://github.com/Buelie/Rucker/edit/main/Rucker#english--%E7%AE%80%E4%BD%93%E4%B8%AD%E6%96%87--%E7%B9%81%E4%BD%93%E4%B8%AD%E6%96%87) | 简体中文 | [繁体中文](https://github.com/Buelie/Rucker/edit/main/README.md#english--%E7%AE%80%E4%BD%93%E4%B8%AD%E6%96%87--%E7%B9%81%E4%BD%93%E4%B8%AD%E6%96%87-1)
### Rucker的理念
***Rucker会对KOOK提供的API接口支持到底,并且提供一些官方并没有公布的接口或者干脆自己写接口***
### Rucker 的特点
* 使用纯JS实现
* SDK轻量化
* 自动处理异常无需开发者操心
* 详细的文档
# [English](https://github.com/Buelie/Rucker/edit/main/Rucker#english--%E7%AE%80%E4%BD%93%E4%B8%AD%E6%96%87--%E7%B9%81%E4%BD%93%E4%B8%AD%E6%96%87) | [简体中文](https://github.com/Buelie/Rucker#english--%E7%AE%80%E4%BD%93%E4%B8%AD%E6%96%87--%E7%B9%81%E4%BD%93%E4%B8%AD%E6%96%87-1) | 繁体中文
### Rucker的理念
***Rucker會對KOOK提供的API介面支援到底,並且提供一些官方並沒有公佈的介面或者乾脆自己寫介面***
### Rucker 的特點
* 使用純JS實現
* SDK輕量化
* 自動處理異常無需開發者操心
* 詳細的文件
| KOOK robot sdk implemented using pure js | kook,api,bot,javascript | 2023-01-06T07:41:03Z | 2023-05-02T05:53:42Z | 2023-01-14T09:59:25Z | 2 | 0 | 148 | 3 | 0 | 3 | null | MIT | JavaScript |
thenamevishnu/portfolio | main | null | HTML-based single-page showcase designed to elevate your professional presence. Seamlessly present your skills, projects, and achievements in an immersive and easy-to-navigate format. | ajax,bootstrap5,css,html,javascript | 2023-01-16T09:21:08Z | 2023-01-16T09:24:09Z | null | 1 | 0 | 1 | 0 | 0 | 3 | null | null | HTML |
Hacking-NASSA-with-HTML/Rock_Paper_Scissors_Intl | main | 
# Rock Paper Scissors Intl
## JavaScript app Rock Paper Scissors Game.
### Over amazing tutorial from FreeCodeCamp https://www.youtube.com/watch?v=jaVNP3nIAv0
---
Enjoy!
See the project on [Github Pages](https://hacking-nassa-with-html.github.io/Rock_Paper_Scissors_Intl/) .
please 🙌 let me know if you have found any issues ⭐
And May Your Code Always Works Too 🍾🥂


| 💎 JavaScript app Rock Paper Scissors Game. Over amazing tutorial from FreeCodeCamp ⭐ https://www.youtube.com/watch?v=jaVNP3nIAv0 | javascript | 2023-01-03T14:42:33Z | 2023-01-15T18:28:44Z | null | 1 | 0 | 36 | 0 | 0 | 3 | null | null | JavaScript |
Fene-87/Awesome-Books | main | # Awesome Books
<a name="readme-top"></a>
<!-- TABLE OF CONTENTS -->
# 📗 Table of Contents
- [📖 About the Project](#about-project)
- [💻 Getting Started](#getting-started)
- [👥 Authors](#authors)
- [🤝 Contributing](#contributing)
- [⭐️ Show your support](#support)
- [🙏 Acknowledgements](#acknowledgements)
- [❓ FAQ](#faq)
- [📝 License](#license)
# 📖 Awesome Books <a name="about-project"></a>
**Awesome Books** is a project whereby I get to develop a basic website which allows users to add or delete books in a list.
## 🛠 Built With <a name="built-with"></a>
### Tech Stack <a name="tech-stack"></a>
<details>
<summary>HTML</summary>
<ul>
<li><a href="https://html.com/">html.com</a></li>
</ul>
</details>
<details>
<summary>CSS</summary>
<ul>
<li><a href="https://developer.mozilla.org/en-US/docs/Web/CSS">CSS</a></li>
</ul>
</details>
<details>
<summary>Javascript</summary>
<ul>
<li><a href="https://www.javascript.com/">Javascript</a></li>
</ul>
</details>
<!-- Features -->
### Key Features <a name="key-features"></a>
- **Book List**
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LIVE DEMO -->
## 🚀 Live Demo <a name="live-demo"></a>
Click on the link to view the portfolio website.
- [Live Demo]()
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- GETTING STARTED -->
## 💻 Getting Started <a name="getting-started"></a>
To get a local copy up and running, follow these steps:
-Install visual studio code or any text editor of your choice.
-clone the repository to your local machine.
-open it using live server
### Prerequisites
In order to run this project you need visual studio code or any text editor of your choice
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- AUTHORS -->
## 👥 Authors <a name="authors"></a>
👤 **Fene-87**
- GitHub: [@Fene-87](https://github.com/Fene-87)
- LinkedIn: [Mark Fenekayas](https://www.linkedin.com/in/mark-fenekayas-67378220b/)
👤 **Peter Okorafor**
- GitHub: [@Peter]()
- LinkedIn: [Peter Okorafor]()
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- FUTURE FEATURES -->
<!-- CONTRIBUTING -->
## 🤝 Contributing <a name="contributing"></a>
Contributions, issues, and feature requests are welcome!
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- SUPPORT -->
## ⭐️ Show your support <a name="support"></a>
If you like this project kindly show your support or make a contribution to it.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- ACKNOWLEDGEMENTS -->
## 🙏 Acknowledgments <a name="acknowledgements"></a>
I would like to thank the entire microverse community for helping me out with this project.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
<!-- LICENSE -->
## 📝 License <a name="license"></a>
This project is [MIT](./LICENSE) licensed.
<p align="right">(<a href="#readme-top">back to top</a>)</p>
| Basic website that allows users to add or remove books from a list | javascript | 2023-01-09T09:10:49Z | 2023-01-12T16:35:07Z | null | 1 | 3 | 19 | 0 | 0 | 3 | null | MIT | JavaScript |
RamonFelix/devlinks | main | null | DevLinks by Rocketseat | css,figma,github,html,javascript | 2023-01-16T12:35:54Z | 2023-02-08T12:15:24Z | null | 1 | 0 | 10 | 0 | 1 | 3 | null | null | CSS |
miguelamello/expressjs-javascript-pwa | master | # PWA APP
This application aims to use the concepts of Progressive Web Application, among which allow the application to remain available and interactive for the end user, even in the absence of an internet connection. It also allows information modified by the user during the absence of an internet connection to be automatically saved to remote database, and thus persisted in the application. All of this must occur transparently and imperceptibly for the end user.
### LightHouse Score: 99%
This PWA is scoring 99% of performance in LightHouse.
PS: Progressive Web Application analisys is out of this score since I'm still implementing it.
### Goals
The main objective is building an PWA APP that does not use any external library or framework. Only standard Web Technologies already present in mobile/desktop browsers shall be used. Babel is used to transpile the code and make the App compatible to browsers up to 10 years behind today.
The App shall be fast and responsive to any device screen. As a PWA App, it shall be working when no internet is present. The app shall be a Single Page Application where only the index.html is loaded to the client browser. Any other UI shall be rendered and loaded using pure ES2021 (Javascript 2021).
In the Backend only NODE shall be used, as the plataform shall be developed with ES2021 from side to side. Comunication between the App and the Server shall be made using a Single Point REST API. UI abstraction shall be completely independent from Backend implementation. The whole Application shall be asyncronous in order to deliver the fastest response to the end user. All communication between client and server shall be encrypted using CryptoJS behind SSL Protocol.
### 1) Introdution
A Progressive Web Application (PWA) is a type of web application that uses modern web technologies to deliver a native app-like experience to users. PWAs are designed to be fast, reliable and engaging, with the ability to work offline and receive push notifications. They are built using web technologies such as HTML, CSS and JavaScript, and can be accessed through a web browser like any other website.
The main benefits of PWAs are that they offer a seamless user experience across devices, are easy to discover and install, and can be used on any platform that has a modern web browser. They also have the advantage of being easy to maintain and update, as changes can be made and deployed directly to the web server without the need for users to manually update their apps.
To develop a PWA, you will need to design and build the app using web technologies, and configure it to meet the requirements for a PWA. This will involve creating the HTML, CSS and JavaScript files that make up the app, as well as configuring any required libraries or frameworks. You will also need to set up a web server and configure HTTPS to ensure that the app is secure and can be accessed by users.
Once the PWA is complete, you will need to deploy it to a hosting service so that it can be accessed by users. This may involve setting up a web server, configuring HTTPS, and optimizing the app for performance. Finally, you will need to promote the PWA and make it discoverable to users. This may involve creating a landing page, optimizing for search engines, and promoting the app through social media and other channels.
### 2) Technical foundations
- **Web app manifest**: This is a JSON file that provides metadata about the app, including the app name, icons, theme colors, and start URL. The manifest is used to control how the app appears when it is installed on a user's device, and is required for the app to be added to the user's home screen.
- **Service workers**: Service workers are background scripts that enable PWAs to work offline and receive push notifications. They run separately from the web page and can intercept network requests, cache assets, and respond to events such as push notifications.
- **HTTPS**: Secure HTTP (HTTPS) is required for all PWAs to ensure that the app and its data are transmitted securely. This is especially important for apps that handle sensitive information such as user login credentials.
- **Responsive design**: PWAs should be designed to be responsive, meaning that they should work well on a variety of devices and screen sizes. This involves using responsive design techniques such as flexible layouts, media queries, and responsive images.
- **Cross-browser compatibility**: PWAs should be tested and verified to work across a range of modern browsers. This will ensure that the app can be used by the largest possible audience.
- **Web performance optimization**: PWAs should be optimized for performance to ensure that they load quickly and provide a smooth user experience. This may involve techniques such as minimizing the size of assets, optimizing images, and reducing the number of requests made to the server.
### 3) Reasons for Progressive Web Applications (PWAs)
**PWAs are easy to discover and install**: Unlike native apps, which must be downloaded from an app store, PWAs can be accessed directly through a web browser and added to a user's home screen with a single click. This makes them easier to discover and install, especially for users who may not want to download an app from an app store.
**PWAs are cross-platform**: PWAs work on any device that has a modern web browser, which means that they can be used on a wide range of platforms without the need for separate native apps. This makes them a good choice for developers who want to reach a wide audience without the need to build and maintain multiple native apps.
**PWAs are easy to maintain and update**: Changes to a PWA can be made and deployed directly to the web server without the need for users to manually update their apps. This makes them easier to maintain and update, especially for apps that are updated frequently.
**PWAs can work offline**: Service workers, a key technical foundation of PWAs, allow apps to work offline or in low-quality network environments by caching assets and data. This makes PWAs a good choice for apps that need to work in areas with poor or intermittent connectivity.
**PWAs can be shared easily**: PWAs can be shared easily through a URL, which makes it easy for users to share the app with others. This can be especially useful for apps that are designed to be used by a group of people, such as a team or an organization.
### 4) Infrastructure Stack
The goal is not to use heavy frameworks or libraries that only make the application slow and difficult to maintain. The Web Standards already provide enough, and natively in browsers, to support various features. The focus is on making the most of these native browser features, while keeping the PWA Application fully compatible with browsers and devices that were 10 years old.
- **Frontend**
- JavaScript (ES2021)
- HTML 5
- CSS 3
- Standard Web APIs
- CryptoJS
- Webpack for bundling
- Babel for transpiling
- **Backend**
- Linux CENTOS STREAM
- Node 18
- CryptoJS
- MySQL
- Storage
### 5) Notes:
- This application is for case study only, and may need a lot of adjustments to be fully funcional.
- The development focus now is towards the login screen to dashboard when user fully authenticated.
- User authentication now is focused in local username/password, and next step in the authentication development will be towards "Social Authentication" using gmail, outlook and apple logins.
| This application aims to use the concepts of Progressive Web Application, among which allow the application to remain available and interactive for the end user, even in the absence of an internet connection. It also allows information modified by the user during the absence of an internet connection to be automatically saved to remote database. | express-js,javascript,progressive-web-app,responsive-web-design,scss,webpack | 2023-01-01T22:45:53Z | 2023-07-10T19:03:17Z | null | 1 | 0 | 102 | 0 | 0 | 3 | null | null | JavaScript |
mstephen19/speedwalk | main | # SpeedWalk
[](https://packagephobia.com/result?p=speedwalk@latest)
Walk an entire directory. Fast, simple, and asynchronous.
## Features
- Zero dependencies. Small bundle size.
- Written in TypeScript.
- Fast and asynchronous. Suitable for large directories. Fastest tree-traversal solution on NPM.
- Dead simple. Modeled after Golang's [`filepath.Walk`](https://pkg.go.dev/path/filepath#Walk).
- Modern [ESModules](https://hacks.mozilla.org/2018/03/es-modules-a-cartoon-deep-dive/) support.
## Comparisons
Some comparisons between **speedwalk** and other Node.js libraries that provide tree-traversal solutions.
> All tests were conducted on the same large directory. Each result is the average of each library's time to complete the walk with no custom functions provided.
|Package|Result|
|-|-|
|`speedwalk`|6.95ms|
|`walk`|13826.33ms|
|`@root/walk`|276.96ms|
|`walker`|296.05ms|
## Usage
Import the `walk` function from the package. To call it, pass in a root directory and a callback function accepting a `path` and a `dirent`.
```TypeScript
import { walk } from 'speedwalk';
await walk('./', (path, dirent) => {
console.log('Path:', path);
console.log('Is a file:', dirent.isFile());
if (dirent.isDirectory() && dirent.name === 'node_modules') {
// Tell "walk" to skip the traversal of a certain
// directory by returning "true" from the callback.
return true;
}
});
```
The function will asynchronously walk through the root directory and all subsequent directories until the entire tree has been traversed.
| Walk an entire directory. Fast, simple, and asynchronous. | asynchronous,esmodules,javascript,nodejs,npm,tree,typescript | 2023-01-11T19:14:44Z | 2023-02-24T19:36:13Z | 2023-01-11T19:43:15Z | 1 | 0 | 19 | 1 | 0 | 3 | null | null | Go |
scottgriv/Palm-Tree | main | <!-- Begin README -->

<p align="center">
<a href="https://www.php.net/ChangeLog-8.php#8.1.6"><img src="https://img.shields.io/badge/PHP-8.1.6-777BB4?style=for-the-badge&logo=php" alt="PHP Badge" /></a>
<a href="https://dev.mysql.com/doc/relnotes/mysql/8.0/en/news-8-0-31.html"><img src="https://img.shields.io/badge/MySQL-8.0.31-4479A1?style=for-the-badge&logo=mysql" alt="MySQL Badge" /></a>
<a href="https://releases.jquery.com/jquery/"><img src="https://img.shields.io/badge/jQuery-2.1.3-0769AD?style=for-the-badge&logo=jquery" alt="jQuery Badge" /></a>
<br>
<a href="https://bootstrapdocs.com/v3.3.5/docs/getting-started/"><img src="https://img.shields.io/badge/Bootstrap-3.3.5-7952B3?style=for-the-badge&logo=bootstrap" alt="Boostrap Badge" /></a>
<a href="https://docs.docker.com/engine/release-notes/20.10/#201021"><img src="https://img.shields.io/badge/Docker-20.10.21-2496ED?style=for-the-badge&logo=docker" alt="Docker Badge" /></a>
<a href="https://www.apachefriends.org/blog/new_xampp_20220516.html"><img src="https://img.shields.io/badge/XAMPP-8.1.6-FB7A24?style=for-the-badge&logo=xampp" alt="XAMPP Badge" /></a>
<br>
<a href="https://github.com/scottgriv"><img src="https://img.shields.io/badge/github-follow_me-181717?style=for-the-badge&logo=github&color=181717" alt="GitHub Badge" /></a>
<a href="mailto:scott.grivner@gmail.com"><img src="https://img.shields.io/badge/gmail-contact_me-EA4335?style=for-the-badge&logo=gmail" alt="Email Badge" /></a>
<a href="https://www.buymeacoffee.com/scottgriv"><img src="https://img.shields.io/badge/buy_me_a_coffee-support_me-FFDD00?style=for-the-badge&logo=buymeacoffee&color=FFDD00" alt="BuyMeACoffee Badge" /></a>
<br>
<a href="https://prgportfolio.com"><img src="https://img.shields.io/badge/PRG-Gold Project-FFD700?style=for-the-badge&logo=data:image/svg%2bxml;base64,PD94bWwgdmVyc2lvbj0iMS4wIiBzdGFuZGFsb25lPSJubyI/Pgo8IURPQ1RZUEUgc3ZnIFBVQkxJQyAiLS8vVzNDLy9EVEQgU1ZHIDIwMDEwOTA0Ly9FTiIKICJodHRwOi8vd3d3LnczLm9yZy9UUi8yMDAxL1JFQy1TVkctMjAwMTA5MDQvRFREL3N2ZzEwLmR0ZCI+CjxzdmcgdmVyc2lvbj0iMS4wIiB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciCiB3aWR0aD0iMjYuMDAwMDAwcHQiIGhlaWdodD0iMzQuMDAwMDAwcHQiIHZpZXdCb3g9IjAgMCAyNi4wMDAwMDAgMzQuMDAwMDAwIgogcHJlc2VydmVBc3BlY3RSYXRpbz0ieE1pZFlNaWQgbWVldCI+Cgo8ZyB0cmFuc2Zvcm09InRyYW5zbGF0ZSgwLjAwMDAwMCwzNC4wMDAwMDApIHNjYWxlKDAuMTAwMDAwLC0wLjEwMDAwMCkiCmZpbGw9IiNGRkQ3MDAiIHN0cm9rZT0ibm9uZSI+CjxwYXRoIGQ9Ik0xMiAzMjggYy04IC04IC0xMiAtNTEgLTEyIC0xMzUgMCAtMTA5IDIgLTEyNSAxOSAtMTQwIDQyIC0zOCA0OAotNDIgNTkgLTMxIDcgNyAxNyA2IDMxIC0xIDEzIC03IDIxIC04IDIxIC0yIDAgNiAyOCAxMSA2MyAxMyBsNjIgMyAwIDE1MCAwCjE1MCAtMTE1IDMgYy04MSAyIC0xMTkgLTEgLTEyOCAtMTB6IG0xMDIgLTc0IGMtNiAtMzMgLTUgLTM2IDE3IC0zMiAxOCAyIDIzCjggMjEgMjUgLTMgMjQgMTUgNDAgMzAgMjUgMTQgLTE0IC0xNyAtNTkgLTQ4IC02NiAtMjAgLTUgLTIzIC0xMSAtMTggLTMyIDYKLTIxIDMgLTI1IC0xMSAtMjIgLTE2IDIgLTE4IDEzIC0xOCA2NiAxIDc3IDAgNzIgMTggNzIgMTMgMCAxNSAtNyA5IC0zNnoKbTExNiAtMTY5IGMwIC0yMyAtMyAtMjUgLTQ5IC0yNSAtNDAgMCAtNTAgMyAtNTQgMjAgLTMgMTQgLTE0IDIwIC0zMiAyMCAtMTgKMCAtMjkgLTYgLTMyIC0yMCAtNyAtMjUgLTIzIC0yNiAtMjMgLTIgMCAyOSA4IDMyIDEwMiAzMiA4NyAwIDg4IDAgODggLTI1eiIvPgo8L2c+Cjwvc3ZnPgo=" alt="Gold" /></a>
</p>
---------------
<h1 align="center">🌴🌴 Palm Tree 🌴🌴</h1>
**Palm Tree** is a web based CRM application with Google Business Review email request capabilities.
- Keep track of your Customers in a digital format - ditch the pen and paper!
- Send marketing emails out, including emails requesting Google Business Reviews.
- Directly open a Review window on your Google Business page with a click of a button within the email.
- Perfect for small businesses, entrepreneurs, and anyone looking to keep track of their customers and send out marketing emails.
---------------
## Table of Contents
- [Features](#features)
- [Background Story](#background-story)
- [Definitions](#definitions)
- [Getting Started](#getting-started)
- [Download the Application](#download-the-application)
- [Using Docker (Recommended)](#using-docker-recommended)
- [Using XAMPP (Alternative)](#using-xampp-alternative)
- [What's Inside?](#whats-inside)
- [Fully Interactive Customer Table](#fully-interactive-customer-table)
- [Custom Email Template](#custom-email-template)
- [Configure Your Business](#configure-your-business)
- [Send Emails to Your Customers](#send-emails-to-your-customers)
- [Disclaimer](#disclaimer)
- [Closing](#closing)
- [What's Next?](#whats-next)
- [Project](#project)
- [Contributing](#contributing)
- [Resources](#resources)
- [License](#license)
- [Credits](#credits)
## Features
- [x] Fully Interactive Customer Table
- [x] Custom Email Template
- [x] Configure Your Business
- [x] Send Emails to Your Customers
## Background Story
I created **Palm Tree** to help small businesses and entrepreneurs keep track of their customers and send out marketing emails. I was inspired to create this application after working with a small business owner who was using a pen and paper to keep track of their customers. With years of experience working with large enterprise CRM applications, I knew there was a better way. I created **Palm Tree** to be a simple, easy to use, and fully interactive CRM application that anyone can use. I hope you enjoy using **Palm Tree** as much as I enjoyed creating it!
## Definitions
- <ins><b>CRM</b></ins>: An acronym for **Customer Relationship Management**. A CRM is a software that helps businesses manage, track, and improve all the relationships with their customers. CRMs centralize all customer data, making it easier for businesses to manage relationships with customers, vendors, and partners.
- <ins><b>Google Business Review</b></ins>: A review of your business on Google. Google Business Reviews are important because they can give businesses a huge credibility boost without having to spend a dime. The more reviews you have, the more people will trust you and want to do business with you.
## Getting Started
### Download the Application
1. Download **Palm Tree** directly using one of the methods below:
- Download with **Git**:
```bash
git clone https://github.com/scottgriv/palm-tree
```
- Download with **Homebrew**:
```bash
brew tap scottgriv/palm-tree https://github.com/scottgriv/Palm-Tree
```
- Download the **Zip File** [Here](https://github.com/scottgriv/Palm-Tree/archive/refs/heads/main.zip).
2. Host the application using your web server and databases. See the **XAMPP** instructions below on how to do this.
### Using Docker (Recommended)
1. Download **Docker** [Here](https://docs.docker.com/get-docker/).
2. Make sure **Docker** is up and running.
- Make sure you follow the **System Requirements** under your preferred Operating System.
3. Launch your CLI (Command Prompt on Windows/Terminal on Mac).
4. Change the directory using your CLI to your **Palm Tree** directory:
```bash
cd Desktop/Palm-Tree
```
5. Once you're in the **Palm Tree** directory, run the ```Docker Compose``` command:
```bash
docker-compose up
```
> [!NOTE]
> This step may take a while to download all of the necessary images.
6. This will build the **Docker Containers** and you should find your application running under *port 80*.
- Navigate to: ```http://localhost```
### Using XAMPP (Alternative)
1. Download **XAMPP** [Here](https://www.apachefriends.org/download.html).
2. Start the `MySQL Database` and `Apache Web Server` services. Optionally, you can install these as a service if you're using windows so the web server automatically starts and serves **Palm Tree** if the PC ever restarts or shuts down and comes back up:
> First step to install Apache service, run XAMPP Control Panel as Administrator, then click the Apache service button located on the left side of Apache components, click next and “Click Yes to Install the Apache Service” window will open, then click Yes to continue.
3. Go to the `phpMyAdmin` portal and import the SQL database script `palm_tree.sql` located in the `sql` folder.
> [!IMPORTANT]
> The below two steps/changes are to correct the application from the default Docker install above.
4. Update the `sql/config.ini` file changing `db_host = db` to `db_host = localhost`.
5. Update the `container` class menu links in the `container.php` file From/To:
- From: `/customers.php` To: `/Palm-Tree/customers.php`
- From: `/configure.php` To: `/Palm-Tree/configure.php`
- From: `/email.php` To: `/Palm-Tree/email.php`
- Alternatively, you can update the `httpd.conf` file to set your default index page for your localhost to the `Palm-Tree` directory.
6. Go to your web browser and navigate to the URL: `http://localhost/Palm-Tree` and you should be redirected to the main application page.
7. If you're accessing **Palm Tree** from a mobile device on your local network, you'll have to to access the application using your host machines IP address. To find your IP address, open up your CLI and type:
**Mac**:
```bash
ifconfig
```
- You should see your IP address under the `inet` field, typically listed under `en0` or `en1` for Wi-Fi connections.
**Windows**:
```bash
ipconfig
```
- You should see your IP address under the `IPv4 Address` field under the appropriate network adapter. <br><br>
- Update the `sql/config.ini` file changing `db_host_ip = localhost` to `db_host = <your_ip_address>`.
- Navigate to the URL: `http://<your_ip_address>/Palm-Tree` and you should be redirected to the main application page.
## What's Inside?
Below is a list of the main files and folders in this repository and their specific purposes:
```bash
Palm-Tree
├─ app # Future home of the application
├─ css # CSS files used for the application
│ └─ main.css # Default CSS file used for the application
├─ docs # Images used for the GitHub README relative path
│ └─ images # Images used for the GitHub README relative path
├─ img # Images used for the application
│ ├─ config # Images used for the Configure screen
│ ├─ fav # Favorite/Bookmark icons, including icons for Android and iOS shortcuts
│ ├─ link # Images used for the link icons on the Email screen (i.e google, facebook, etc.)
│ └─ logo # This is where your uploaded company logo will go from the Configure screen (replacing `logo.png`) for the Customer screen.
├─ js # JavaScript files used for the application
│ ├─ jquery.tabledit.js # Included dependency file [jQuery-Tabledit v1.2.3](https://github.com/markcell/jquery-tabledit)
│ └─ main.js # Default JavaScript and jQuery file used for the application
├─ lib # Misc. library files used for the application
│ ├─ PHPMailer-master # Mailer dependency used to send emails out to customers [PHPMailer v6.7.1](https://github.com/PHPMailer/PHPMailer)
│ └─ phpinfo.php # File containing the `phpinfo()` function: Because every system is setup differently, `phpinfo()` is commonly used to check configuration settings, such as log file locations, and for available predefined variables on a given system.
├─ sql # SQL files used for the application
│ ├─ config.ini # Configuration file used to connect to the database
│ └─ palm_tree.sql # Starting database script/structure required to run the application
├─ templates # HTML files used as the default email template for the Email screen
│ ├─ sample_email_template.html # HTML file used as the default email template for the Email screen
│ └─ sample_email_template_no_logo.html # Same as above but without the logo
├─ commands.php # PHP file used to process requests and responses from the front-end application to the back-end database
├─ configure.php # PHP file behind the Configure screen
├─ container.php # PHP file used to create the HTML menu container (this sets the screen padding, size, etc. for each screen, making all the screens a consistent size)
├─ customers.php # PHP file behind the Customers screen
├─ database.php # PHP file used to connect to the database configuration using the `config.ini` file
├─ docker-compose.yml # Compose file used for defining services, networks, and volumes for a Docker application
├─ email.php # PHP file behind the Email screen
├─ encryption.php # Encryption file used to encrypt/decrypt data in the database
├─ footer.php # The websites footer
├─ header.php # The website's header which also contains the path for favorite icons and remote JavaScript/CSS libraries
├─ index.php # Default landing page of the website which contains a redirect to `customers.php` (the Customers screen)
├─ send_email.php # PHP file used to send emails out to customers
├─ Dockerfile # Dockerfile for the application
├─ .gitignore # Git ignore file
├─ .gitattributes # Git attributes file
├─ .github # GitHub folder
├─ PRG.md # PRG Connection File
├─ LICENSE # License file
└─ README # This file
```
---------------
## Fully Interactive Customer Table
<div align="center">
<a href="#fully-interactive-customer-table" target="_blank">
<img src="docs/images/demo_customers.gif" style="width: 80%;"/>
</a>
<br>
<i>Data privacy is important to me, which is why the above data is dummy data</i>
</div>
<br>
* **CRUD-Enabled:**
- [x] Create Customer Information
- [x] Read Customer Information
- [x] Update Customer Information
- [x] Delete Customer Information
* Export table to a .csv file.
* Scroll up, down, and refresh the table easily using the provided buttons.
* Highlighted editable fields.
* Log Customer Notes.
* Sort Customers by:
* First Name
* Last Name
* Email
* Phone
* Created Date
* Search for Customers based on:
* First Name
* Last Name
* Email
* Phone
* Check the **Send Email** box to send an email to the Customer.
* Mobile capabilities with Bootstrap:
<br>
<div align="center">
<a href="#fully-interactive-customer-table" target="_blank">
<img src="docs/images/demo_mobile.png" style="width: 35%;"/>
</a>
<br>
<i>Bootstrap allows you to easily view the application on a mobile device.</i>
</div>
---------------
## Custom Email Template
<div align="center">
<a href="#custom-email-template" target="_blank">
<img src="docs/images/demo_email.png" style="width: 80%;"/>
</a>
<br>
<i>Create a custom email template for your customer emails.</i>
</div>
<br>
* **Palm Tree** utilizes [Mustache](http://mustache.github.io/mustache.5.html) styled syntax to create custom email templates.
* Configure your Email Server to send Business emails using your own plain-text or HTML email template (Gmail Supported).
* Use variables from a predefined list to send curated emails to your Customers in the **Subject** and **Body**:
``Hello {{ customer_first_name }} {{ customer_last_name }}!``
will translate to:
``Hello John Smith!``
* **CC** & **BCC** capabilities.
* Send a mass email to **ALL** of your customers at once.
* Create an account on **[Imgur](https://imgur.com/)** to host photos used in your email template.
---------------
## Configure Your Business
<div align="center">
<a href="#configure-your-business" target="_blank">
<img src="docs/images/demo_configure.png" style="width: 80%;"/>
</a>
<br>
<i>Easily personalize the application to fit your businesss.</i>
</div>
<br>
* Upload and display your Company Logo.
* Display your Company Title.
* Display your Company Description.
* Add contact information to your emails using the predefined variables in your email template:
* Company Owner
* Company Address
* Company Contact Phone
* Company Contact Email
* Google Place ID
* Used to automatically open up Google Reviews for your business using the provided email template.
* Social Media Email Hyperlinks:
* Google, Facebook, Twitter, LinkedIn, Instagram, YouTube, Amazon, Pinterest, Etsy, and Shopify.
---------------
## Send Emails to Your Customers
<div align="center">
<a href="#send-emails-to-your-customers" target="_blank">
<img src="docs/images/demo_send_email.png" style="width: 50%;"/>
</a>
<br>
<i>Edit the HTML email template file to fit your needs.</i>
</div>
---------------
## Disclaimer
- Software is provided as-is and no warranty is given about its usability.
- **Palm Tree** does not collect any personal info or data (this does not include the data you send out in your emails or the email host you use to send out emails).
- **Palm Tree** is meant to be deployed on a local environment, therefore, you are responsible for securing your own data and production deployment.
- **Palm Tree** is not responsible for any damages or liabilities that may occur from the use of this software. Use at your own risk.
## Closing
* You're ready to go! You should now be able to access **Palm Tree**.
* Feel free to use the provided email templates in the ``/templates`` directory under the **Email** menu, **Email Body**.
> [!TIP]
> You may need to allow the *port* you're using to send your emails in your *Firewall Settings* (defined in the `send_email.php` file - default `port 465`). Ensure your anti-virus software is not blocking it as well.
**Palm Tree** from sending out emails as well.
Thank you for taking the time to read through this document and I hope you find it useful!
If you have any questions or suggestions, please feel free to reach out to me.
> Please reference the [SUPPORT](.github/SUPPORT.md) file in this repository for more details
## What's Next?
I'm looking forward to seeing how this project evolves over time and how it can help others with their GitHub Portfolio.
> Please reference the [CHANGELOG](.github/CHANGELOG.md) file in this repository for more details.
## Project
Please reference the [GitHub Project](https://github.com/users/scottgriv/projects/4) tab inside this repository to get a good understanding of where I'm currently at with the overall project.
- Issues and Enhancements will also be tracked there as well.
## Contributing
Feel free to submit a pull request if you find any issues or have any suggestions on how to improve this project. You can also open an issue with the tag "bug" or "enhancement".
- How to contribute:
1. Fork the Project
2. Create your Feature Branch (`git checkout -b feature/Palm-Tree`)
3. Commit your Changes (`git commit -m 'Add new feature'`)
4. Push to the Branch (`git push origin feature/Palm-Tree`)
5. Open a Pull Request
> Please reference the [CONTRIBUTING](.github/CONTRIBUTING.md) file in this repository for more details.
## Resources
Below are some external resources I found helpful when creating **Palm Tree**:
- [PHP](https://www.php.net/) - PHP is a popular general-purpose scripting language that is especially suited to web development.
- [MySQL](https://www.mysql.com/) - MySQL is an open-source relational database management system.
- [jQuery](https://jquery.com/) - jQuery is a JavaScript library designed to simplify HTML DOM tree traversal and manipulation, as well as event handling, CSS animation, and Ajax.
- [Bootstrap](https://getbootstrap.com/) - Bootstrap is a free and open-source CSS framework directed at responsive, mobile-first front-end web development.
- [Docker](https://www.docker.com/) - Docker is a set of platform as a service products that use OS-level virtualization to deliver software in packages called containers.
- [XAMPP](https://www.apachefriends.org/index.html) - XAMPP is a free and open-source cross-platform web server solution stack package developed by Apache Friends, consisting mainly of the Apache HTTP Server, MariaDB database, and interpreters for scripts written in the PHP and Perl programming languages.
- [Mustache](http://mustache.github.io/mustache.5.html) - Mustache is a logic-less template syntax. It can be used for HTML, config files, source code - anything. It works by expanding tags in a template using values provided in a hash or object.
- [Imgur](https://imgur.com/) - Imgur is an online image hosting service.
- [jQuery-Tabledit v1.2.3](https://github.com/markcell/jquery-tabledit) - jQuery-Tabledit is a jQuery plugin that provides AJAX-enabled in-place editing for your table cells.
- [PHPMailer v6.7.1](https://github.com/PHPMailer/PHPMailer) - PHPMailer is a full-featured email creation and transfer class for PHP.
## License
This project is released under the terms of the **GNU General Public License, version 3 (GPLv3)**.
- The GPLv3 is a "copyleft" license, ensuring that derivatives of the software remain open source and under the GPL.
- For more details and to understand all requirements and conditions, see the [LICENSE](LICENSE) file in this repository.
## Credits
**Author:** [Scott Grivner](https://github.com/scottgriv) <br>
**Email:** [scott.grivner@gmail.com](mailto:scott.grivner@gmail.com) <br>
**Website:** [scottgrivner.dev](https://www.scottgrivner.dev) <br>
**Reference:** [Main Branch](https://github.com/scottgriv/Palm-Tree) <br>
---------------
<div align="center">
<a href="https://github.com/scottgriv/Palm-Tree" target="_blank">
<img src="./docs/images/icon-rounded.png" width="100" height="100"/>
</a>
</div>
<!-- End README -->
| A web based CRM application with Google Business Review email request capabilities. Send marketing emails out to your customers, including emails requesting Google Business Reviews. Directly open a Review window on your Google Business page with a click of a button within the email. | crm,email,google-business,table,customers,small-business,css,docker,html,javascript | 2023-01-01T00:04:00Z | 2024-05-10T22:10:24Z | 2023-11-25T22:12:31Z | 1 | 0 | 85 | 0 | 2 | 3 | null | GPL-3.0 | PHP |
bedirhandogan/Typing-Battle | main | # Typing Battle
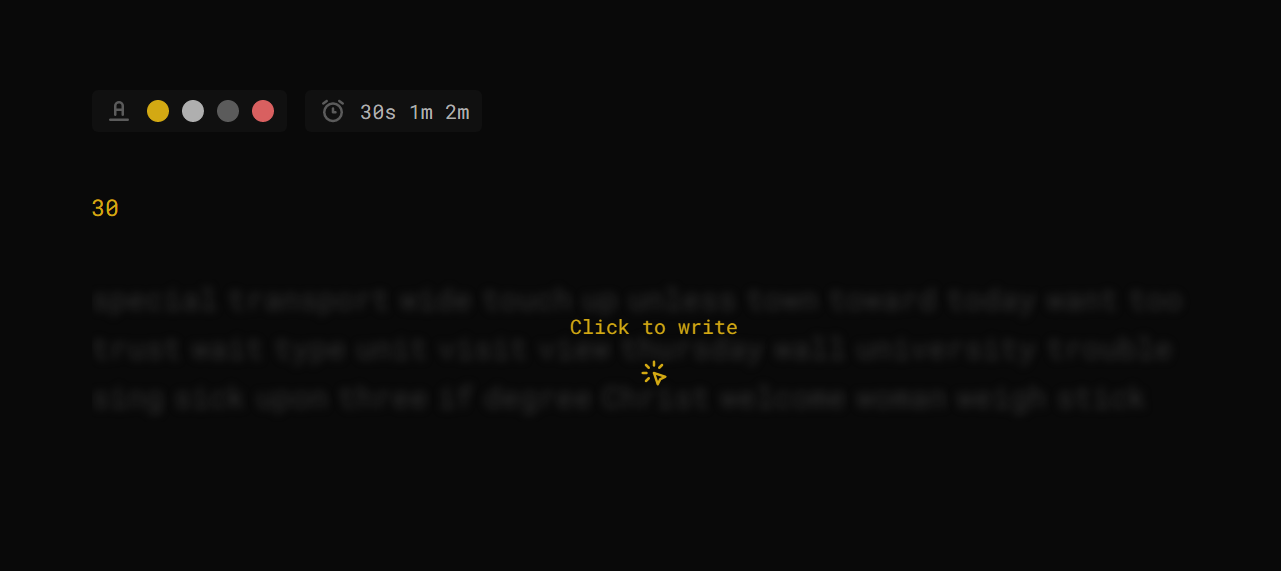
### App Setup
```bash
# install dependencies
$ npm install
# start app and get it ready to use. view: http://localhost:3000
$ npm start
```
| Test your typing speed. | css,javascript,react | 2023-01-12T08:59:30Z | 2023-03-05T06:46:33Z | null | 1 | 0 | 82 | 0 | 0 | 3 | null | null | JavaScript |
Posandu/moodgradient | main | # GradienMood - Generate a gradient background for your mood
Visit the [demo](https://gradienmood.posandu.me) to see it in action.
| Generate a gradient background for your mood | javascript,nextjs,t3-stack | 2023-01-14T12:08:24Z | 2023-01-16T12:39:40Z | null | 1 | 0 | 12 | 0 | 0 | 3 | null | null | TypeScript |
Walter-Tronics/React-Counter | main | # React-Counter
Counter project with React
## https://react-counter-pro.netlify.app
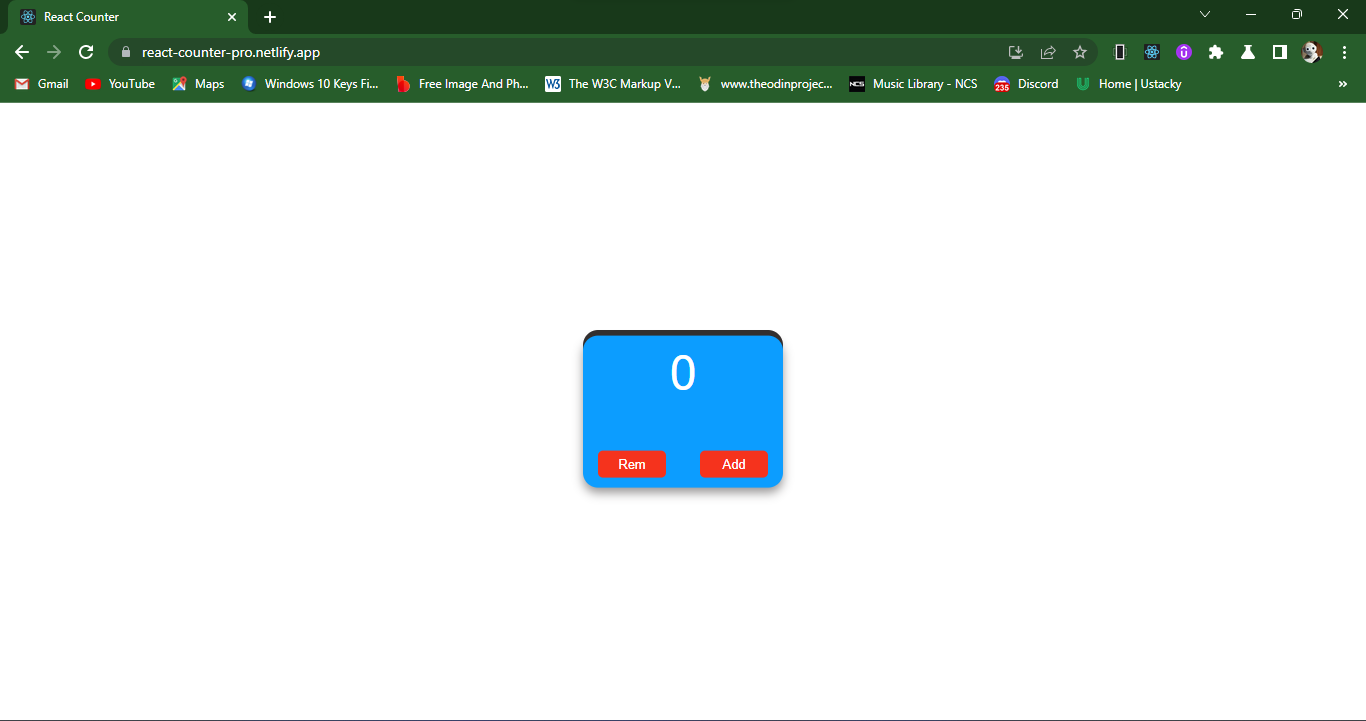
| Counter project with React | counter,reactjs,reactjs-counter,javascript | 2023-01-09T17:12:36Z | 2023-01-13T22:16:31Z | null | 1 | 0 | 11 | 0 | 0 | 3 | null | null | CSS |
Malay-24/ga-next-app | main | This is a [Next.js](https://nextjs.org/) project bootstrapped with [`create-next-app`](https://github.com/vercel/next.js/tree/canary/packages/create-next-app).
## Getting Started
First, run the development server:
```bash
npm run dev
# or
yarn dev
```
Open [http://localhost:3000](http://localhost:3000) with your browser to see the result.
You can start editing the page by modifying `pages/index.js`. The page auto-updates as you edit the file.
[API routes](https://nextjs.org/docs/api-routes/introduction) can be accessed on [http://localhost:3000/api/hello](http://localhost:3000/api/hello). This endpoint can be edited in `pages/api/hello.js`.
The `pages/api` directory is mapped to `/api/*`. Files in this directory are treated as [API routes](https://nextjs.org/docs/api-routes/introduction) instead of React pages.
This project uses [`next/font`](https://nextjs.org/docs/basic-features/font-optimization) to automatically optimize and load Inter, a custom Google Font.
## Learn More
To learn more about Next.js, take a look at the following resources:
- [Next.js Documentation](https://nextjs.org/docs) - learn about Next.js features and API.
- [Learn Next.js](https://nextjs.org/learn) - an interactive Next.js tutorial.
You can check out [the Next.js GitHub repository](https://github.com/vercel/next.js/) - your feedback and contributions are welcome!
## Deploy on Vercel
The easiest way to deploy your Next.js app is to use the [Vercel Platform](https://vercel.com/new?utm_medium=default-template&filter=next.js&utm_source=create-next-app&utm_campaign=create-next-app-readme) from the creators of Next.js.
Check out our [Next.js deployment documentation](https://nextjs.org/docs/deployment) for more details.
| It is todo app using nextjs | chakra-ui,css,javascript,nextjs | 2023-01-08T10:12:26Z | 2023-01-08T12:08:24Z | null | 1 | 0 | 11 | 0 | 0 | 3 | null | null | CSS |
Inna-Mykytiuk/Restaurant-Mimino | main | # Parcel template
Этот проект был создан при помощи Parcel. Для знакомства и настройки
дополнительных возможностей [обратись к документации](https://parceljs.org/).
## Подготовка нового проекта
1. Убедись что на компьютере установлена LTS-версия Node.js.
[Скачай и установи](https://nodejs.org/en/) её если необходимо.
2. Склонируй этот репозиторий.
3. Измени имя папки с `parcel-project-template` на имя своего проекта.
4. Создай новый пустой репозиторий на GitHub.
5. Открой проект в VSCode, запусти терминал и свяжи проект с GitHub-репозиторием
[по инструкции](https://docs.github.com/en/get-started/getting-started-with-git/managing-remote-repositories#changing-a-remote-repositorys-url).
6. Установи зависимости проекта в терминале командой `npm install` .
7. Запусти режим разработки, выполнив команду `npm start`.
8. Перейди в браузере по адресу [http://localhost:1234](http://localhost:1234).
Эта страница будет автоматически перезагружаться после сохранения изменений в
файлах проекта.
## Файлы и папки
- Все паршалы файлов стилей должны лежать в папке `src/sass` и импортироваться в
файлы стилей страниц. Например, для `index.html` файл стилей называется
`index.scss`.
- Изображения добавляй в папку `src/images`. Сборщик оптимизирует их, но только
при деплое продакшн версии проекта. Все это происходит в облаке, чтобы не
нагружать твой компьютер, так как на слабых машинах это может занять много
времени.
## Деплой
Для настройки деплоя проекта необходимо выполнить несколько дополнительных шагов
по настройке твоего репозитория. Зайди во вкладку `Settings` и в подсекции
`Actions` выбери выбери пункт `General`.

Пролистай страницу до последней секции, в которой убедись что выбраны опции как
на следующем изображении и нажми `Save`. Без этих настроек у сборки будет
недостаточно прав для автоматизации процесса деплоя.

Продакшн версия проекта будет автоматически собираться и деплоиться на GitHub
Pages, в ветку `gh-pages`, каждый раз когда обновляется ветка `main`. Например,
после прямого пуша или принятого пул-реквеста. Для этого необходимо в файле
`package.json` отредактировать поле `homepage` и скрипт `build`, заменив
`your_username` и `your_repo_name` на свои, и отправить изменения на GitHub.
```json
"homepage": "https://your_username.github.io/your_repo_name/",
"scripts": {
"build": "parcel build src/*.html --public-url /your_repo_name/"
},
```
Далее необходимо зайти в настройки GitHub-репозитория (`Settings` > `Pages`) и
выставить раздачу продакшн версии файлов из папки `/root` ветки `gh-pages`, если
это небыло сделано автоматически.

### Статус деплоя
Статус деплоя крайнего коммита отображается иконкой возле его идентификатора.
- **Желтый цвет** - выполняется сборка и деплой проекта.
- **Зеленый цвет** - деплой завершился успешно.
- **Красный цвет** - во время линтинга, сборки или деплоя произошла ошибка.
Более детальную информацию о статусе можно посмотреть кликнув по иконке, и в
выпадающем окне перейти по ссылке `Details`.

### Живая страница
Через какое-то время, обычно пару минут, живую страницу можно будет посмотреть
по адресу указанному в отредактированном свойстве `homepage`. Например, вот
ссылка на живую версию для этого репозитория
[https://goitacademy.github.io/parcel-project-template](https://goitacademy.github.io/parcel-project-template).
Если открывается пустая страница, убедись что во вкладке `Console` нет ошибок
связанных с неправильными путями к CSS и JS файлам проекта (**404**). Скорее
всего у тебя неправильное значение свойства `homepage` или скрипта `build` в
файле `package.json`.
## Как это работает

1. После каждого пуша в ветку `main` GitHub-репозитория, запускается специальный
скрипт (GitHub Action) из файла `.github/workflows/deploy.yml`.
2. Все файлы репозитория копируются на сервер, где проект инициализируется и
проходит сборку перед деплоем.
3. Если все шаги прошли успешно, собранная продакшн версия файлов проекта
отправляется в ветку `gh-pages`. В противном случае, в логе выполнения
скрипта будет указано в чем проблема.
| Multi-page site, landing page. Team project. | html5,javascript,parcel,sass | 2023-01-01T21:22:56Z | 2023-01-02T08:39:43Z | null | 1 | 0 | 7 | 0 | 1 | 3 | null | null | HTML |
iamMalekAmin/Product-management-system | main | # Product-management-system
Front-End project HTML,CSS,JavaScript and Bootstrap.
(CRUDS operations) using javascript to create new item ,
read data ,
update data,
delete ,
and search in data.
https://iammalekamin.github.io/Product-management-system/
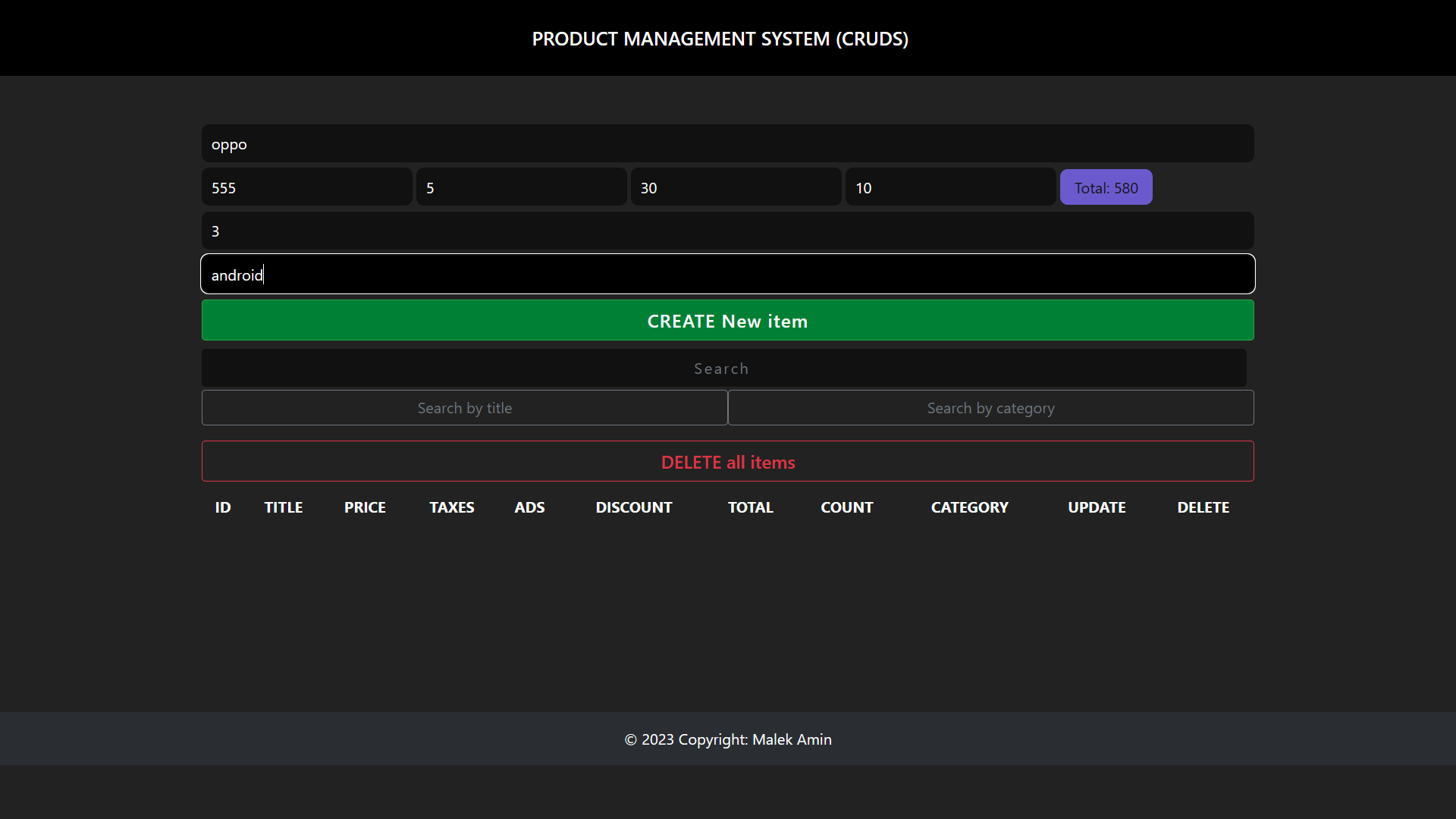
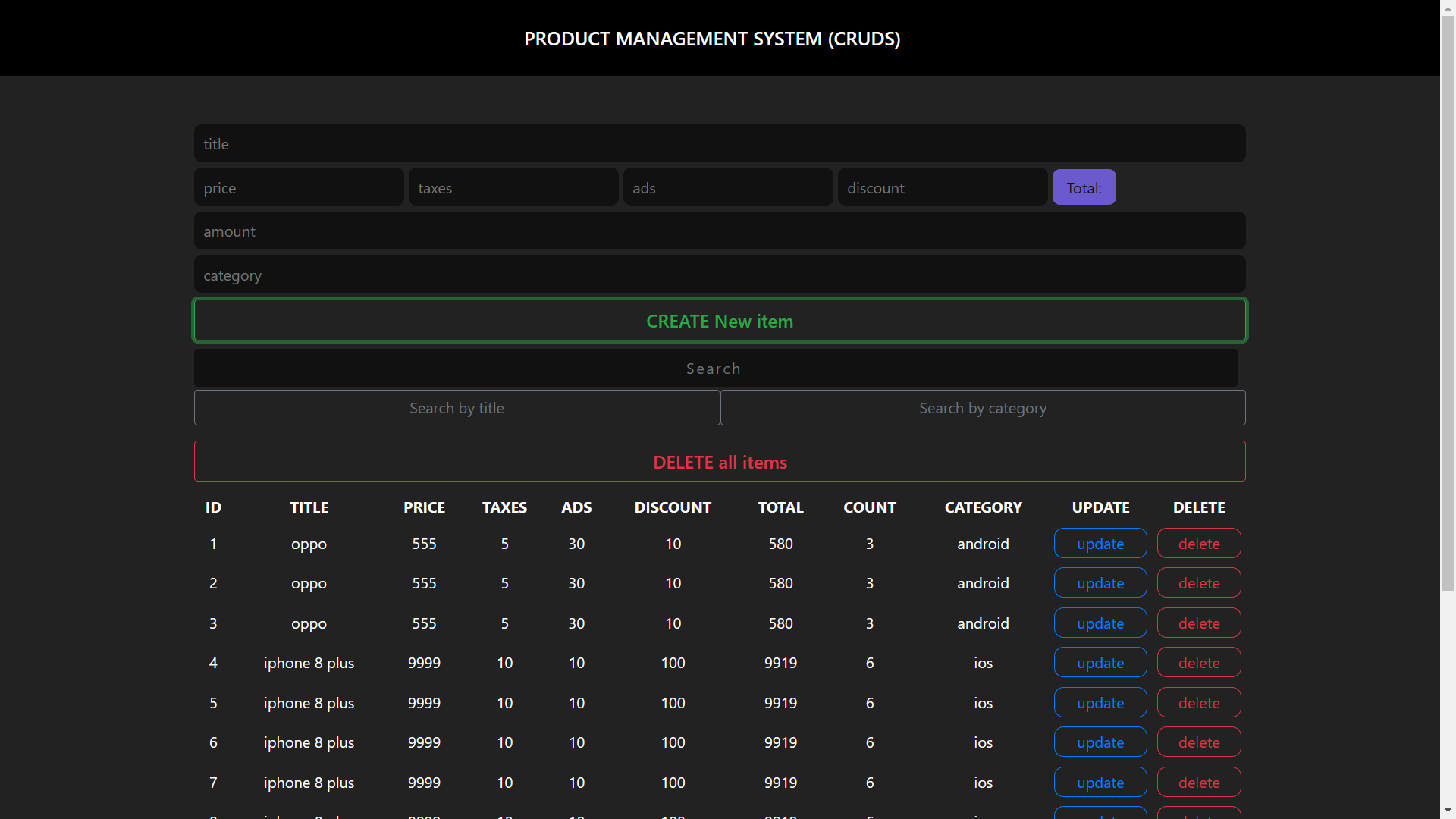
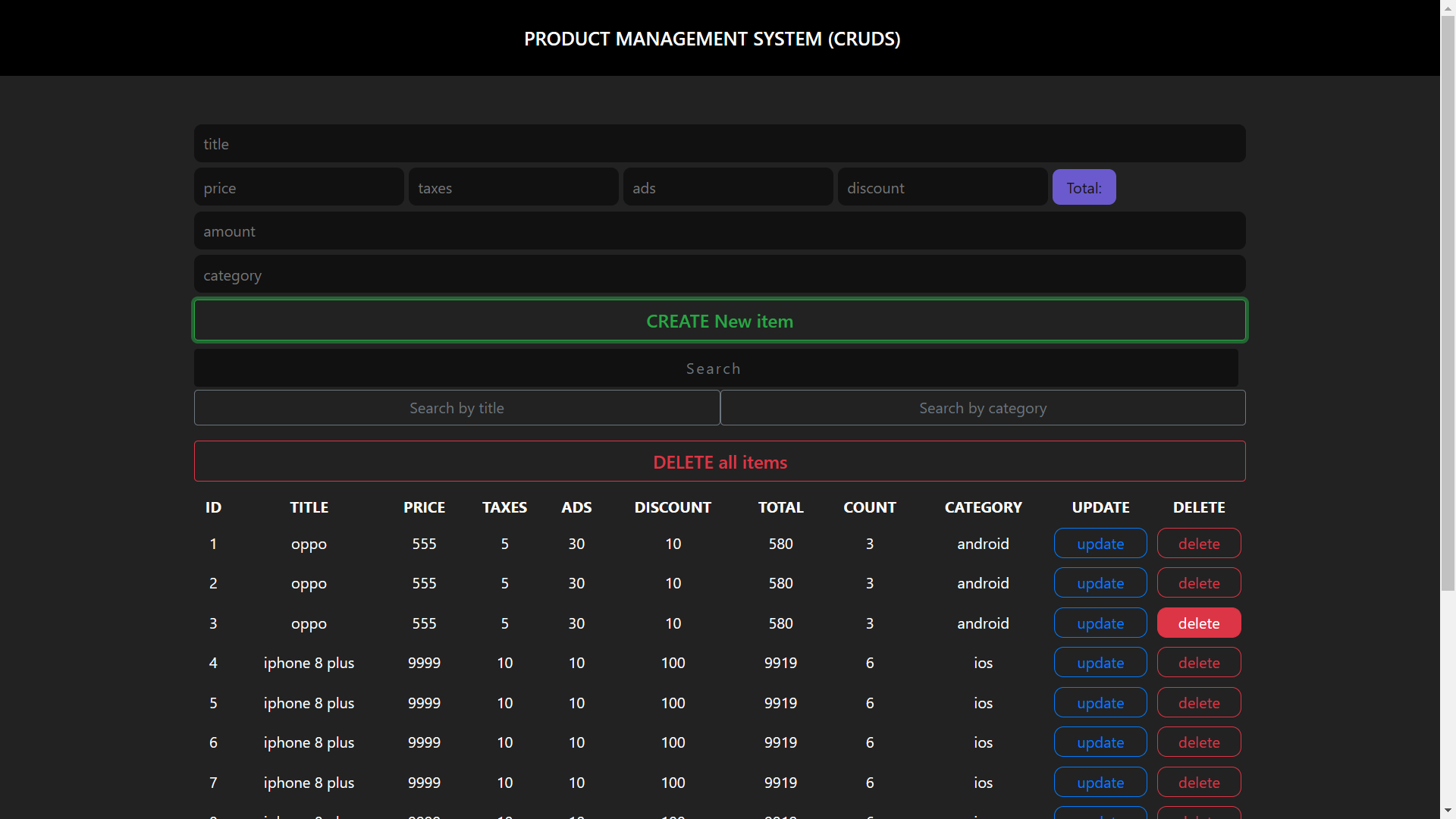
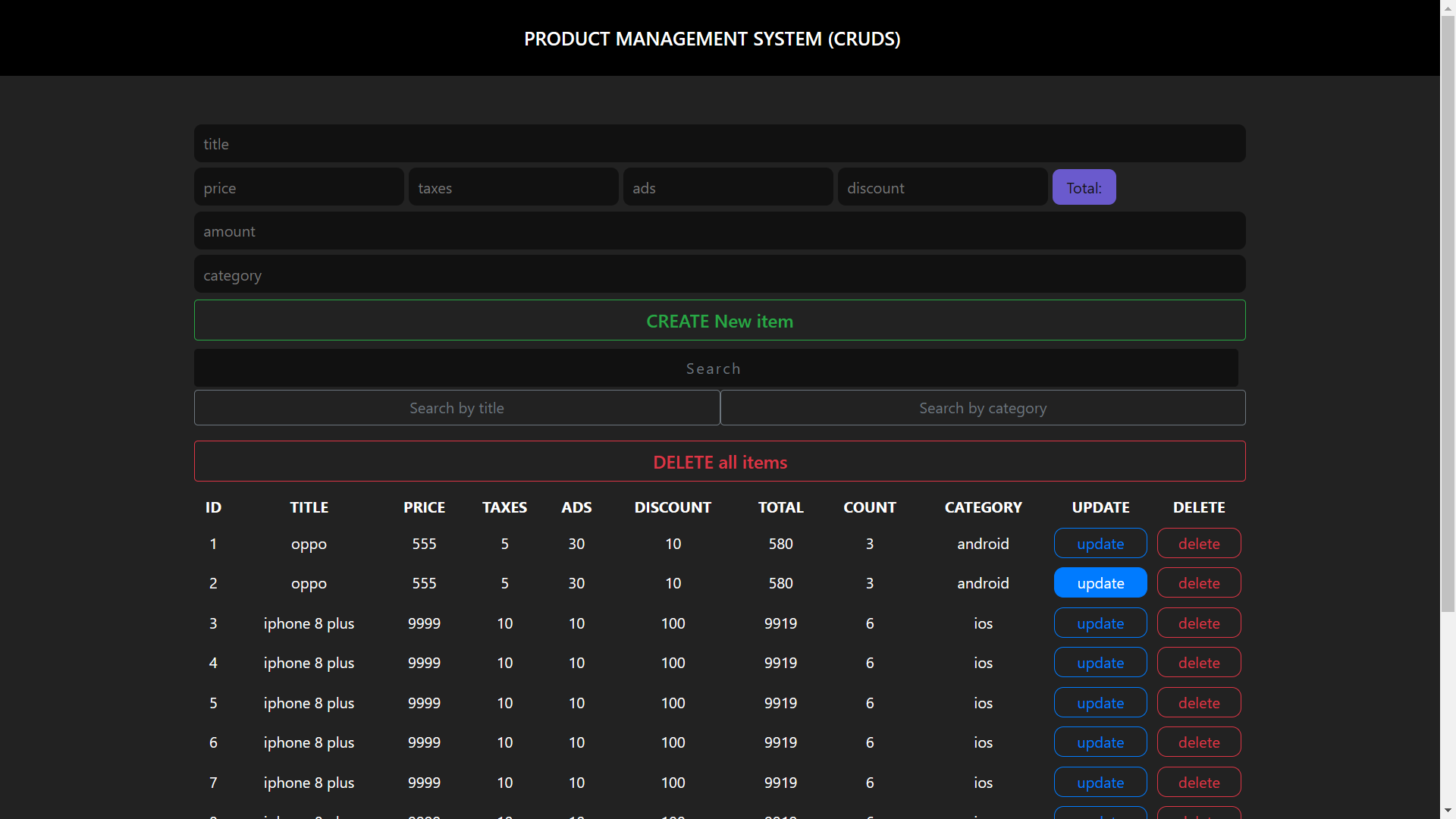
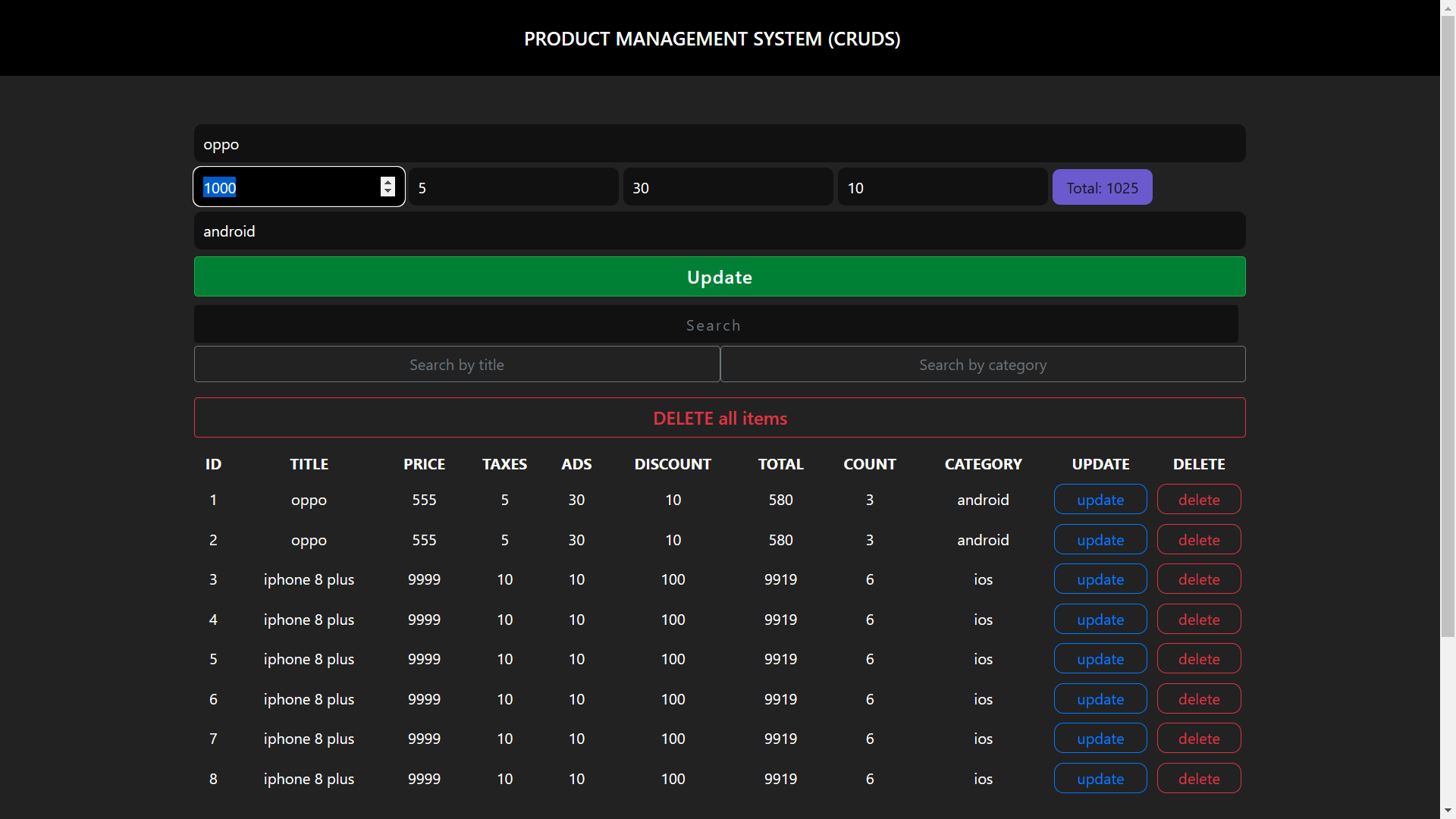
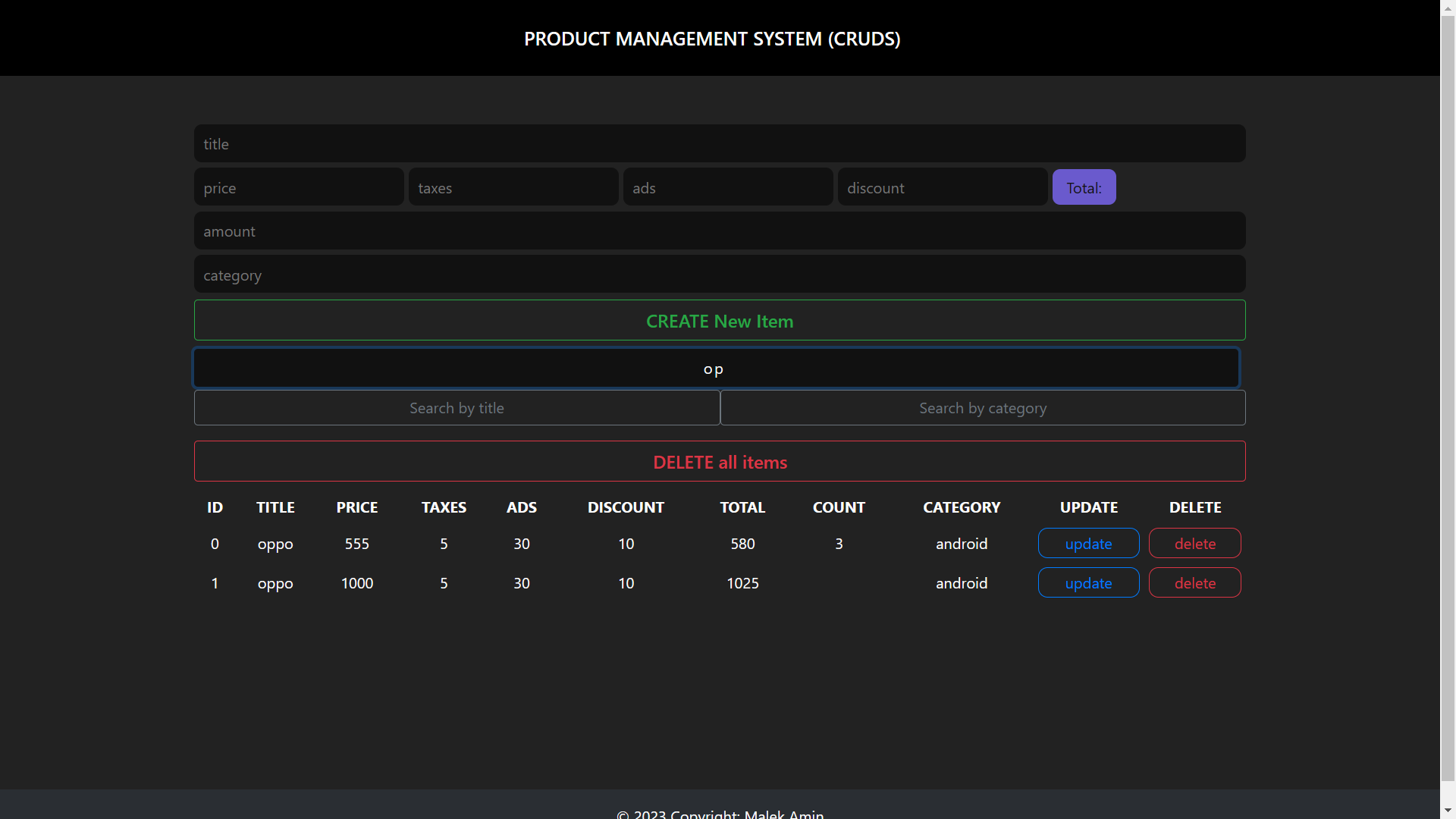
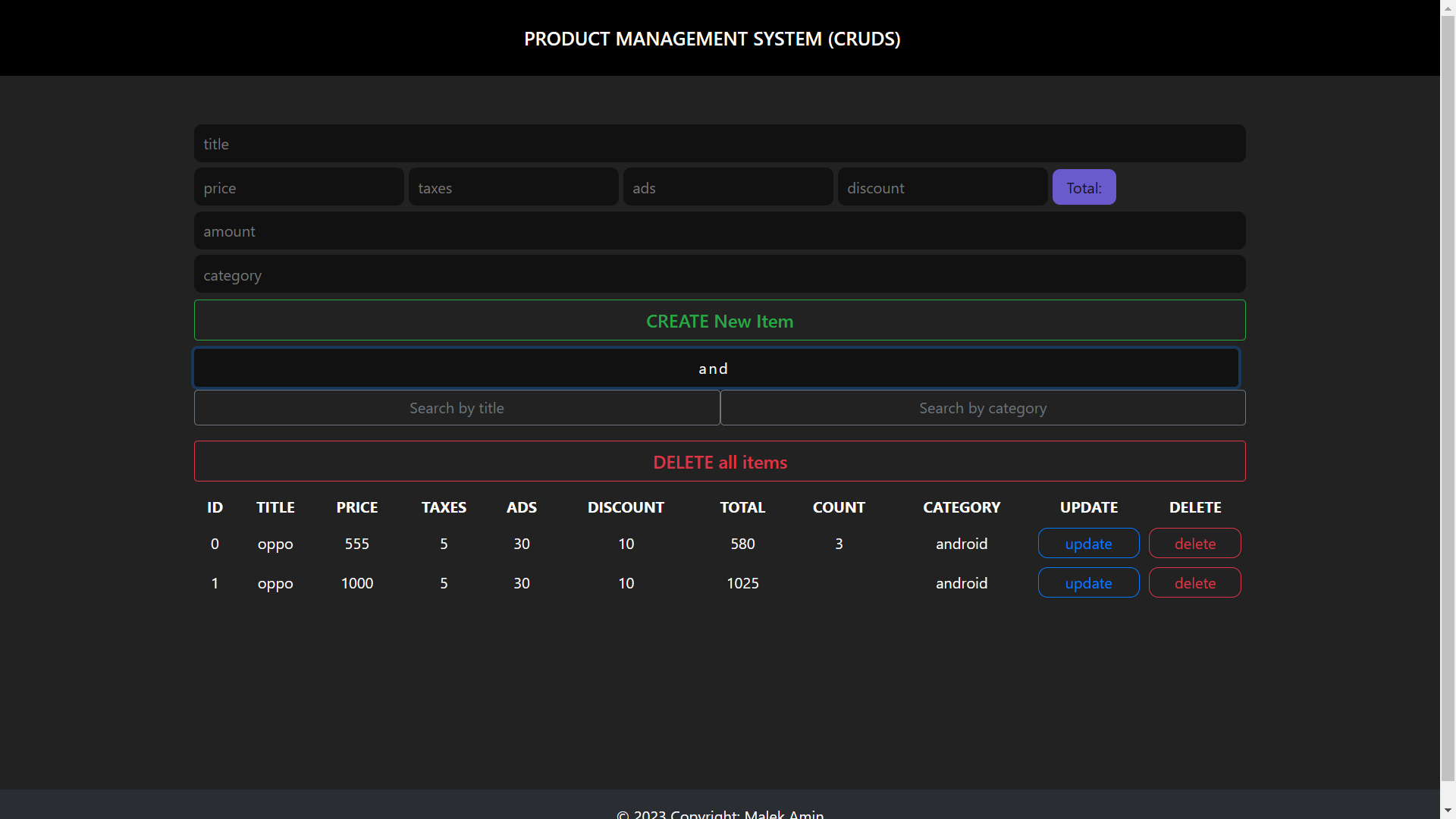
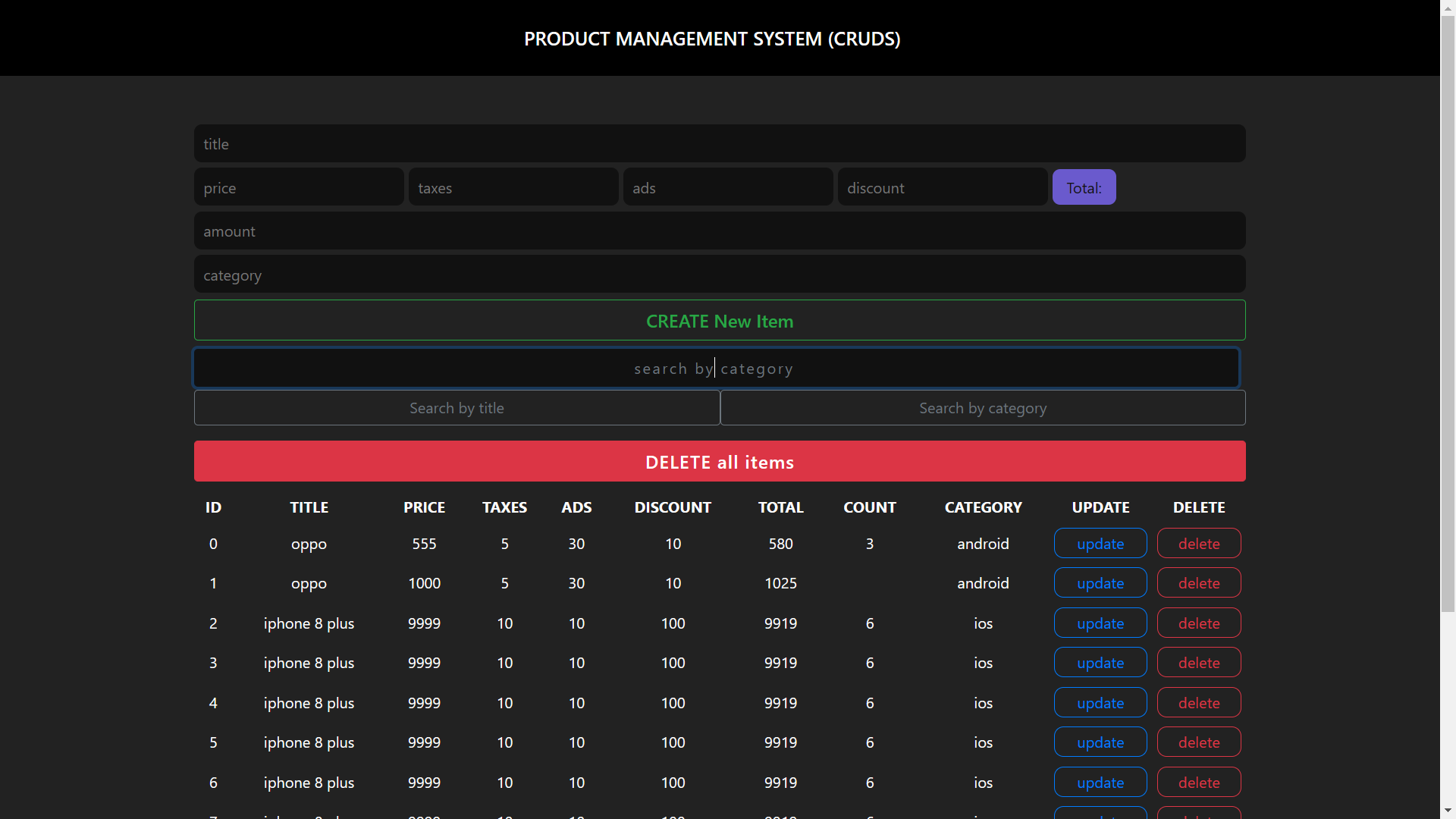
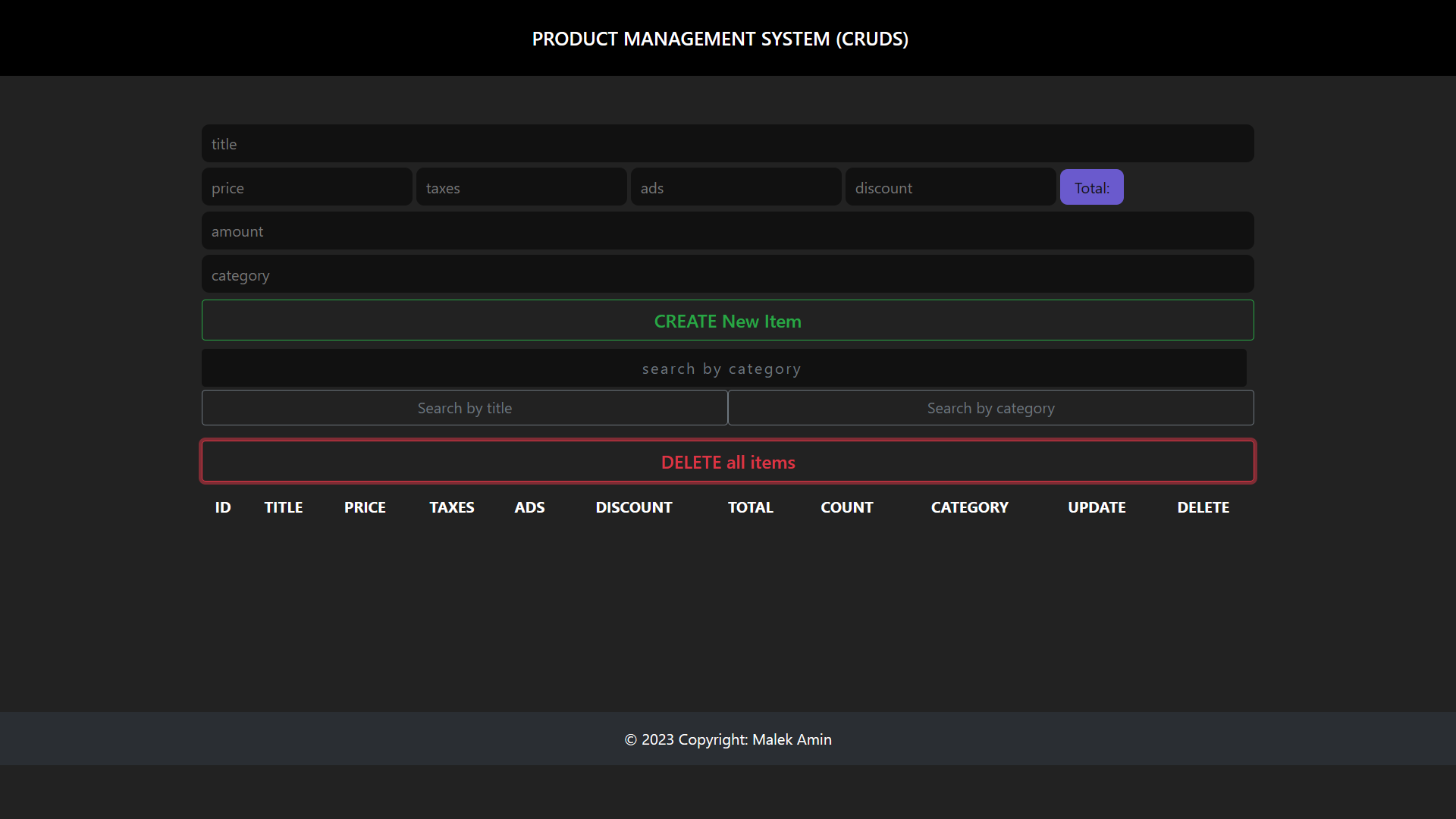
| Product management Front-End project to do all CRUDS operations using javascript and bootstrap | bootstrap,css,html,javascript,jquery | 2023-01-09T14:54:07Z | 2023-05-06T12:46:03Z | null | 1 | 0 | 12 | 0 | 0 | 3 | null | null | JavaScript |
sarwar-asik/Job-Portal | main | # Getting Started with Create React App
This project was bootstrapped with [Create React App](https://github.com/facebook/create-react-app).
## Available Scripts
In the project directory, you can run:
### `npm start`
Runs the app in the development mode.\
Open [http://localhost:3000](http://localhost:3000) to view it in your browser.
The page will reload when you make changes.\
You may also see any lint errors in the console.
### `npm test`
Launches the test runner in the interactive watch mode.\
See the section about [running tests](https://facebook.github.io/create-react-app/docs/running-tests) for more information.
### `npm run build`
Builds the app for production to the `build` folder.\
It correctly bundles React in production mode and optimizes the build for the best performance.
The build is minified and the filenames include the hashes.\
Your app is ready to be deployed!
See the section about [deployment](https://facebook.github.io/create-react-app/docs/deployment) for more information.
### `npm run eject`
**Note: this is a one-way operation. Once you `eject`, you can't go back!**
If you aren't satisfied with the build tool and configuration choices, you can `eject` at any time. This command will remove the single build dependency from your project.
Instead, it will copy all the configuration files and the transitive dependencies (webpack, Babel, ESLint, etc) right into your project so you have full control over them. All of the commands except `eject` will still work, but they will point to the copied scripts so you can tweak them. At this point you're on your own.
You don't have to ever use `eject`. The curated feature set is suitable for small and middle deployments, and you shouldn't feel obligated to use this feature. However we understand that this tool wouldn't be useful if you couldn't customize it when you are ready for it.
## Learn More
You can learn more in the [Create React App documentation](https://facebook.github.io/create-react-app/docs/getting-started).
To learn React, check out the [React documentation](https://reactjs.org/).
### Code Splitting
This section has moved here: [https://facebook.github.io/create-react-app/docs/code-splitting](https://facebook.github.io/create-react-app/docs/code-splitting)
### Analyzing the Bundle Size
This section has moved here: [https://facebook.github.io/create-react-app/docs/analyzing-the-bundle-size](https://facebook.github.io/create-react-app/docs/analyzing-the-bundle-size)
### Making a Progressive Web App
This section has moved here: [https://facebook.github.io/create-react-app/docs/making-a-progressive-web-app](https://facebook.github.io/create-react-app/docs/making-a-progressive-web-app)
### Advanced Configuration
This section has moved here: [https://facebook.github.io/create-react-app/docs/advanced-configuration](https://facebook.github.io/create-react-app/docs/advanced-configuration)
### Deployment
This section has moved here: [https://facebook.github.io/create-react-app/docs/deployment](https://facebook.github.io/create-react-app/docs/deployment)
### `npm run build` fails to minify
This section has moved here: [https://facebook.github.io/create-react-app/docs/troubleshooting#npm-run-build-fails-to-minify](https://facebook.github.io/create-react-app/docs/troubleshooting#npm-run-build-fails-to-minify)
"# VlaunchuTask"
| React, Tailwind simple job portal Home | homepage,javascript,job-portal,reactjs,tailwindcss | 2023-01-03T13:06:48Z | 2023-11-02T21:53:32Z | null | 1 | 0 | 10 | 0 | 0 | 3 | null | null | JavaScript |
0xRajvardhan/SocioPedia- | main | null | MERN Stack Social Media Web Application | expressjs,javascript,mern,mern-stack,nodejs,react,redux | 2023-01-07T07:57:08Z | 2023-02-11T11:15:46Z | null | 1 | 0 | 30 | 0 | 1 | 3 | null | null | JavaScript |
goelraghav002/codex | main | # CHAT GPT
ChatGPT-Clone is a conversational AI model developed using OpenAI API. It is a clone of the original OpenAI GPT-3 language model, designed to provide human-like text generation and conversation capabilities. This model is trained on a vast corpus of text data and can respond to questions, complete sentences, summarize text, and engage in casual conversation with users. With its cutting-edge natural language processing capabilities, ChatGPT-Clone can help businesses automate customer support, enhance user engagement, and streamline communication processes.
| ChatGPT-Clone is a conversational AI model using OpenAI API, offering human-like text generation and conversation capabilities. Trained on vast text data, it can answer questions, summarize text, and engage in casual conversation for business automation and enhanced communication. | chat,chat-application,chatgpt,express,javascript,node,vite,vitejs,api,davinci | 2023-01-12T11:15:42Z | 2023-02-12T15:33:28Z | null | 1 | 0 | 4 | 0 | 0 | 3 | null | null | JavaScript |
johnenderman/john-endermans-School-Cheats | main | # john-endermans-School-Cheats
A bunch of cheats to get around filters or hack educational website.
# don't know how to install a bookmarklet?
- right click on your bookmarks bar
- click add page
- name it whatever you want
- paste the code into where it says "url"
- press save
- click it while you are on a page
| A bunch of hacks for school. | bookmarklet,cheats,hacks,javascript,school,schoolhacks | 2023-01-02T15:12:49Z | 2023-01-14T11:26:46Z | null | 1 | 0 | 18 | 0 | 0 | 3 | null | Unlicense | null |
dryelleebelinvar/csharp | main | ### Links úteis:
🖱 [JSONPlaceholder](https://jsonplaceholder.typicode.com/)
🖱 [códigos de status de respostas http](https://developer.mozilla.org/pt-BR/docs/Web/HTTP/Status)
🖱 [HTTP Status Codes](https://restapitutorial.com/httpstatuscodes.html)
🖱 [Swagger Editor](https://editor.swagger.io/)
🖱 [JSON Viewer - formatar json](http://jsonviewer.stack.hu/)
🖱 [W3schools - jQuery Tutorial](https://www.w3schools.com/jquery/)
🖱 [Swagger - Documentação da IBM](https://www.ibm.com/docs/pt-br/integration-bus/10.0?topic=apis-swagger)
🖱 [JSON Web Tokens](https://jwt.io/)
🖱 [GUID Generator](https://guidgenerator.com/online-guid-generator.aspx)
| C#, HTML, CSS, JavaScript e SQL | bancodedados,csharp,sql,css3,html5,javascript,visual-studio,api | 2023-01-13T18:54:41Z | 2023-03-02T17:35:32Z | null | 1 | 0 | 31 | 0 | 0 | 3 | null | null | C# |
TheTrustyPwo/WebDev | master | # WebDev
Repository for school related web development work
https://thetrustypwo.github.io/WebDev/
| Repository for school related web development work | css,development,html,javascript,learn,web | 2023-01-06T04:39:25Z | 2023-04-18T03:54:29Z | null | 1 | 0 | 18 | 0 | 0 | 3 | null | null | HTML |
DHANUSHKAgitWICKRAMASINGHE/My_Personal_Website | master | # This is my presonal web site. LINK - primesolution.infinityfreeapp.com
with javascript
css
php
html and
mysql database.
| This is dynamic, fully responsive Personal website with html, CSS, PHP, MySQL and JavaScript. 🙂🙂 #vscode -> #github primesolution.infinityfreeapp.com | css,html,html-css-javascript,javascript,mysql,mysql-database,php,vscode | 2023-01-07T08:59:53Z | 2023-01-10T05:15:42Z | null | 1 | 0 | 14 | 0 | 0 | 3 | null | MIT | CSS |
Rafa-KozAnd/Ignite_React.js_Challenge_07 | master | <p align="center">
<img src="http://img.shields.io/static/v1?label=STATUS&message=Concluded&color=blue&style=flat"/>
<img alt="GitHub language count" src="https://img.shields.io/github/languages/count/Rafa-KozAnd/Ignite_React.js_Challenge_07">
<img alt="GitHub language count" src="https://img.shields.io/github/languages/top/Rafa-KozAnd/Ignite_React.js_Challenge_07">
<img alt="GitHub repo file count" src="https://img.shields.io/github/directory-file-count/Rafa-KozAnd/Ignite_React.js_Challenge_07">
<img alt="GitHub repo size" src="https://img.shields.io/github/repo-size/Rafa-KozAnd/Ignite_React.js_Challenge_07">
<img alt="GitHub language count" src="https://img.shields.io/github/license/Rafa-KozAnd/Ignite_React.js_Challenge_07">
</p>
# Ignite_React.js_Challenge_07
React JS challenge done with 'Rocketseat' Ignite course. ("Desafio: Upload de imagens")
## 💻 Sobre o desafio
Nesse desafio, você deverá criar uma aplicação para treinar o que aprendeu até agora no ReactJS
Essa será uma aplicação onde o seu principal objetivo é adicionar alguns trechos de código para que a aplicação de upload de imagens funcione corretamente. Você vai receber uma aplicação com muitas funcionalidades e estilizações já implementadas. Ela deve realizar requisições para sua própria API Next.js que vai retornar os dados do FaunaDB (banco de dados) e do ImgBB (serviço de hospedagem de imagens). A interface implementada deve seguir o layout do Figma. Você terá acesso a 4 arquivos para implementar:
- Infinite Queries e Mutations com React Query;
- Envio de formulário com React Hook Form;
- Exibição de Modal e Toast com Chakra UI;
- Entre outros.
This is a [Next.js](https://nextjs.org/) project bootstrapped with [`create-next-app`](https://github.com/vercel/next.js/tree/canary/packages/create-next-app).
## Getting Started
First, run the development server:
```bash
npm run dev
# or
yarn dev
```
Open [http://localhost:3000](http://localhost:3000) with your browser to see the result.
You can start editing the page by modifying `pages/index.js`. The page auto-updates as you edit the file.
[API routes](https://nextjs.org/docs/api-routes/introduction) can be accessed on [http://localhost:3000/api/hello](http://localhost:3000/api/hello). This endpoint can be edited in `pages/api/hello.js`.
The `pages/api` directory is mapped to `/api/*`. Files in this directory are treated as [API routes](https://nextjs.org/docs/api-routes/introduction) instead of React pages.
## Learn More
To learn more about Next.js, take a look at the following resources:
- [Next.js Documentation](https://nextjs.org/docs) - learn about Next.js features and API.
- [Learn Next.js](https://nextjs.org/learn) - an interactive Next.js tutorial.
You can check out [the Next.js GitHub repository](https://github.com/vercel/next.js/) - your feedback and contributions are welcome!
## Deploy on Vercel
The easiest way to deploy your Next.js app is to use the [Vercel Platform](https://vercel.com/new?utm_medium=default-template&filter=next.js&utm_source=create-next-app&utm_campaign=create-next-app-readme) from the creators of Next.js.
Check out our [Next.js deployment documentation](https://nextjs.org/docs/deployment) for more details.
##
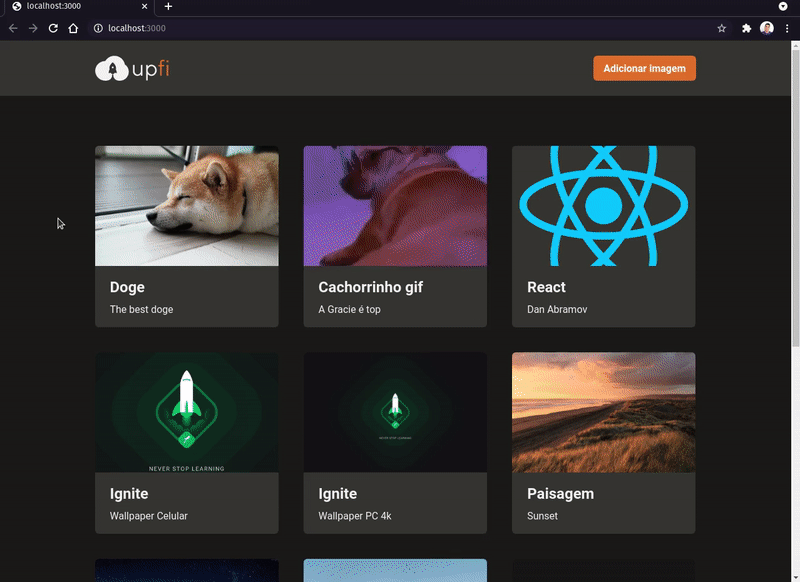
| React JS challenge done with 'Rocketseat' Ignite course. ("Desafio: Upload de imagens") | ignite,ignite-rocketseat,javascript,nextjs,reactjs,scss,typescript,ignite-reactjs,rocketseat | 2023-01-04T19:59:34Z | 2023-04-24T20:33:58Z | null | 1 | 0 | 3 | 0 | 0 | 3 | null | null | TypeScript |
TeoSean00/SGXChange | main | # SGXChange
SGXChange, changing the way you Exchange.
Find us LIVE at https://smu-wad2-teosean-sgxchange.vercel.app/
A website designed to help students with the overseas exchange application process by web scraping and consolidating all the vital scatterered information, along with originally designed and self-built system functionalities such as user authentication, automated modules mapping, a university nearby attractions locater, a university review and favouriting functionality, where all of these functionalities are made unique to every university. A collaborative team effort brainstormed and developed together with a group of friends!
## Project Setup
```sh
npm install
```
### Compile and Hot-Reload for Development
```sh
npm run dev
```
### Compile and Minify for Production
```sh
npm run build
```
### Run Production
```sh
npm run preview
```
| A website built to help students with the overseas exchange application process by web scraping & consolidating the scattered information, along with originally designed & developed app's features such as user authentication, automated modules mapping, a review & favoriting system, & a nearby attractions locater, all made unique to every university | css,firebase,firebase-authentication,firebase-realtime-database,firestore-database,javascript,material-ui,nosql-database,selenium,vite | 2023-01-12T06:11:57Z | 2023-07-18T06:05:15Z | null | 1 | 1 | 345 | 0 | 1 | 3 | null | null | Vue |
cvitaa11/gh-devops-demo | main | [](https://github.com/cvitaa11/gh-devops-demo/actions/workflows/publish-npm.yml)
[](https://github.com/cvitaa11/gh-devops-demo/actions/workflows/build-docker.yml)
[](https://github.com/cvitaa11/gh-devops-demo/actions/workflows/load-test.yml)
[](https://github.com/cvitaa11/gh-devops-demo/actions/workflows/github-code-scanning/codeql)
## gh-devops-demo
This repository demonstrates usage of GitHub as a DevOps platform.
It consists of a small React application that checks if string is palindrome. That funcionality is implemented and stored as a private npm package.
React code is located in [app directory](./app/). Application is packaged as a Docker container and container image is published [here](https://github.com/cvitaa11/gh-devops-demo/pkgs/container/app-demo) on the GitHub Container registry.\
Logic behind our palindrome funcionality is stored in [package directory](./package/) and the npm package is published [here](https://github.com/cvitaa11/gh-devops-demo/pkgs/npm/is-palindrome) on GitHub Packages.
Application is deployed as a Docker container on Azure App Services.
Code is being scanned using CodeQL. Analyses Results can be found as artifacts in [CodeQL workflow](https://github.com/cvitaa11/gh-devops-demo/actions/workflows/github-code-scanning/codeql). They are produced in SARIF format which you can visualise in [SARIF Viewer](https://microsoft.github.io/sarif-web-component/).
| This repository demonstrates usage of GitHub as a DevOps platform | docker,github,javascript,k6,react | 2023-01-16T16:14:34Z | 2024-01-05T13:08:49Z | null | 1 | 4 | 18 | 1 | 0 | 3 | null | MIT | JavaScript |
Ananyakumarisingh/mini-project | master | null | A mini project by using Nodejs | css,html,nodejs,javascript | 2023-01-15T17:50:59Z | 2023-01-15T17:52:39Z | null | 1 | 0 | 1 | 0 | 0 | 3 | null | null | JavaScript |
Abfahim21/parallax-scrolling-landing-page | master | <h1 align="center">Parallax Scrolling Landing Page</h1>
<h3 align="center">
<a
href="https://getbootstrap.com/docs/5.0/getting-started/introduction/"
target="_blank"
>Bootstrap 5</a
>
+ <a href="https://en.wikipedia.org/wiki/HTML" target="_blank">HTML</a> +
<a href="https://en.wikipedia.org/wiki/CSS" target="_blank">CSS</a>
</h3>
<br>
<p align="center"><img src="./images/ps.jpg" /></p>
<h2 align="center"><a href="https://abfahim21.github.io/parallax-scrolling-landing-page/" target="_blank">Live Demo</a></h2>
<br>
<br>
## How to install
1. Clone this repositoy:
```bash
git clone https://github.com/Abfahim21/parallax-scrolling-landing-page.git
```
2. Go to the Project
```bash
cd parallax-scrolling-landing-page
```
| A parallax scrolling landing page. Not Responsive! | css3,html5,javascript | 2023-01-09T11:07:04Z | 2024-03-14T01:29:59Z | null | 1 | 1 | 18 | 0 | 0 | 3 | null | null | HTML |
Aonrud/Timeline.js | master | # Timeline.js
This module draws a timeline diagram, using SVG to link entries.
## Use
To use the timeline:
* Include [`timeline.min.js`](dist/timeline.min.js) and [`timeline.min.css`](dist/timeline.min.js) in your document.
* Optionally include [`timeline-dark.min.js`](dist/timeline-dark.min.js) to enable the default [dark mode](#dark-mode).
* Add a `<div>` with `id="diagram"` to your HTML.
* Add your timeline entries and events as `<div>` elements within `div#diagram`, with the appropriate `data-` attributes (see [HTML](#html) below).
* Instantiate a new Timeline in JS, and call the `create()` method on it.
### Example
Here is an example showing the development of Linux Desktop Environments since the mid-1990s.
Entries are created using HTML `data` attributes, which determine their position and connections when the timeline is instantiated. Events can also be added, either to a particular entry or as a general event on the timeline.
```html
<div id="diagram">
<!--Timeline entries-->
<div id="KDE" data-start="1996" data-become="Plasma" data-colour="#179af3">KDE</div>
<div id="Plasma" data-start="2014" data-colour="#179af3">KDE Plasma</div>
<div id="Trinity" data-start="2010" data-split="KDE" data-colour="#01306f">Trinity Desktop Environment</div>
<div id="Budgie" data-start="2014" data-split="GNOME" data-row="2" data-colour="#6bca81">Budgie</div>
<div id="GNOME" data-start="1997" data-row="3" data-colour="#000">GNOME</div>
<div id="Cinnamon" data-start="2013" data-split="GNOME" data-colour="#dc682e">Cinnamon</div>
<div id="MATE" data-start="2011" data-split="GNOME" data-colour="#9ddb60">MATE</div>
<div id="XFCE" data-start="1997" data-colour="#00a8dd">XFCE</div>
<div id="LXDE2" data-start="2013" data-split="LXDE" data-irregular="true" data-row="7" data-colour="#d1d1d1">LXDE</div>
<div id="LXDE" data-start="2006" data-become="LXQT" data-row="8" data-colour="#d1d1d1">LXDE</div>
<div id="LXQT" data-start="2013" data-row="8" data-colour="#0280b9">LXQT</div>
<div id="Razor-qt" data-start="2010" data-merge="LXQT" data-row="9" data-end="2013" data-colour="#006c96">Razor-qt</div>
<div id="Enlightenment" data-start="1997" data-colour="#fff078">Enlightenment</div>
<div id="Moksha" data-start="2014" data-split="Enlightenment" data-colour="#5a860a">Moksha Desktop</div>
<!--Events-->
<div class="event" data-year="2004">X.org is founded as a fork of XFree86.</div>
<div class="event" data-year="2008">The Wayland project was started.</div>
<div class="event" data-year="2011" data-target="GNOME">Gnome 3.0 was released.</div>
<div class="event" data-year="2008" data-target="KDE">KDE 4 was released.</div>
</div>
```
```javascript
const example = new Timeline("diagram", {
yearStart: 1995
});
example.create();
```
This is rendered as below:

### Live examples
* [Electoral Parties in the Republic of Ireland](https://aonrud.github.io/Irish-Electoral-Parties/)
* [Timeline of the Irish Left](https://www.leftarchive.ie/page/timeline-of-the-irish-left/) on the Irish Left Archive
## Javascript
The script is bundled as an ES6 module ([`dist/timeline.esm.js`](dist/timeline.esm.js)) or a UMD ([`dist/timeline.min.js`](dist/timeline.min.js)), depending on your preferred development stack or build tools.
The Timeline class and configuration [are documented below](#javascript-documentation). Note that configuration is optional - any or none of the options can be passed in the config object. If specifying a config, you must also pass the container ID as the first parameter when creating the timeline.
## Adding Entries
Entries can be defined either in your HTML using `data` attributes, or passed as an array of Javascript objects when instantiating Timeline.js.
Both methods can be combined, but any entry defined in JS that duplicates an existing `id` will be dropped and a warning sent in the console.
**Each entry must have a unique ID, which must be a valid value for the HTML `id` attribute (See [id on MDN](https://developer.mozilla.org/en-US/docs/Web/HTML/Global_attributes/id) for restrictions)**.
### HTML
To define entries in your HTML, create an element within the container and define its properties with the data attributes in the table below.
By default, entries should be `<div>` elements and the container has the id `#diagram`, but both of these are configurable (see [the Javscript configuration below](#timeline)).
|Attribute |Required |Value |Use |
|-----------|-----------|-------|-------|
|data-start|Yes|`<number>` A year|The year the entry starts at in the timeline. <br /> *Note: If this is earlier than the start of the timeline itself, an arrow is added to indicate it pre-exists the period shown.*|
|data-end|No|`<number>` A year|The year the entry ends. If omitted, this will be determined either by other connections, or if there are none, it will continue to the end of the timeline.|
|data-row|No|`<number>`| *Note: The first row is '0'*. <br />The row number this entry should appear in. This can be omitted, though automatic positioning is quite basic. It is recommended to use manual positioning or a combination of both for large or complex diagrams (see [Entry Positioning](#entry-positioning) below).|
|data-end-estimate|No|true or false|Whether the end is an estimate. Estimated end times are shown with a dashed end to the line, instead of a point.|
|data-become|No|Another entry ID|The entry 'becomes' another entry. I.e. another entry is the continuation of this entry, and it will be drawn on the same line. For example, use this when an entry changes its name.|
|data-split|No|Another entry ID|If specified, the entry will be shown branching from the specified entry, at the year specified in 'data-start'.|
|data-merge|No|Another entry ID|If specified, the entry will be connected to the specified entry, at the year specified in 'data-end'.|
|data-links|No|A space-separated list of entry IDs|If specified, the entry is linked with a dashed line to each entry ID. Useful for looser associations between entries that should not be connected directly.|
|data-colour|No|A CSS colour value|The colour of the border around the entry and connections from it. |
|data-irregular|No|true or false|Set to true for entries that are 'irregular' or should not be unbroken from their start to end dates. If set to true, the entry will be drawn with a broken line.|
|data-group|No|<string>|A named group to which this entry belongs. This can be useful, for example, to keep entries that are not directly connected but have some shared property near each other in the diagram.|
### Javascript
Entries can also be added using an array of objects when creating the Timeline, using an optional parameter.
Each entry must have at least `id`, `name` and `start` properties. The `name` is the displayed name of the entry (determined by the inner text of the element when defining entries in HTML).
All [attributes available as data attributes described above](#html) can be defined as object properties, with the omission of the data prefix and the object key named as it would be when converted from a data attribute to a property of the element's `dataset`. ([See the MDN entry for this conversion](https://developer.mozilla.org/en-US/docs/Web/API/HTMLElement/dataset#name_conversion)).
For example:
```javascript
const data = [
{ id: "A", name: "First entry", start: 1950, end: 1960, merge: "B" },
{ id: "B", name: "Second entry", start: 1955, end: 1981, endEstimate: true },
…
{ id: "Z", name: "Final entry", start: 1940 }
];
const example = new Timeline(
"diagram",
{}, //Default configuration
data
);
example.create();
```
## Adding Events
Two kinds of events can be added to the timeline:
1. general events appearing on the date line at the top of the diagram;
2. entry-specific events, appearing on an entry's own timeline.
These create information points that are expanded by hovering or touching. (See the [example screenshot](#examples) above or the [live examples](#live-examples).) As with entries, they can be added either with HTML `data` attributes or in Javascript.
### HTML
Events are added by creating a `<div>` with the class `event` and the required attributes.
|Attribute |Required |Value |Use |
|-----------|-----------|-------|-------|
|data-year |Yes|<number> A year| Determines where on the timeline the event appears.|
|data-target|No|<string> An entry ID| If defined, places the event on an entry's line instead of the top date line.|
|data-colour|No|A CSS colour value| Defines the colour of the text of the event. For general events (no target entry) this also defines the colour of the information marker on the date line.|
### Javascript
As with entries, events can also be added using an array of objects in Javascript.
Each event must have at least `year` and `content` properties. The content provides the message shown for the event (determined by the inner text of the element when defining events in HTML).
In addition, if a valid entry id is provided in the `target` property, it will be displayed as an entry-specific event.
## CSS Styling
The variables below are set in the included CSS. These are the default values, and can be over-ridden to customise the timeline's appearance.
```CSS
--tl-colour-background: #fff;
--tl-colour-border: #ccc;
--tl-colour-stroke: #999;
--tl-colour-entry: #f2f2f2;
--tl-colour-text: #333;
--tl-colour-highlight: #FFF14D;
--tl-colour-background-feature: #fafafa;
--tl-colour-border-feature: #7a7a7a;
--tl-width-year: 50px;
--tl-width-box: 100px;
--tl-width-box-min: 40px;
--tl-height-box: 40px;
--tl-height-row: 50px;
--tl-padding: 5px;
```
### Dark mode
If you want to include a dark mode, you can link the additional [`timeline-dark.min.css`](timeline-dark.min.css) file in your HTML. This over-rides the above for users who have set a dark mode preference, as below.
```CSS
--tl-colour-background: #222;
--tl-colour-border: #282828;
--tl-colour-stroke: #777777;
--tl-colour-entry: #555555;
--tl-colour-text: #eeeeee;
--tl-colour-highlight: #FFF14D;
--tl-colour-background-feature: #333333;
--tl-colour-border-feature: #7a7a7a;
```
Alternatively, add your own preferences within a `prefers-color-scheme: dark` media query.
## Panning and Zooming
For large diagrams, Timeline can make use of [@panzoom/panzoom](https://github.com/timmywil/panzoom) to add panning and zooming to the diagram within a fixed container. Include `@panzoom/panzoom` in your dependencies (it is not bundled), and pass the panzoom function in the config when instantiating the timeline (see [Javascript Options](#javascript) below).
## Controls and searching
If Panzoom is active, controls can be added to find an entry and pan to it, and also to control the zoom.
### Find an Entry
Include a form with the id "timeline-find" (by default - this is configurable) containing an input with the name "finder".
The input will then provide an autocomplete list of the entries in the diagram, which, when selected, will trigger the diagram to pan to that entry and highlight it.
### Zoom controls
Buttons can be added to control 'zoom in', 'zoom out' and 'reset zoom' with specified IDs. If not specified in the configuration, the zoom actions are attached to these IDs, if present in the document: 'timeline-zoom-in', 'timeline-zoom-out', 'timeline-zoom-reset'.
### Finding on load with URL hash
If a URL hash is present on load and Panzoom is enabled, the timeline will pan to and highlight a given entry automatically if the hash is in the format `#find-{id}`.
### Example
An example putting these together as a controls `<div>` within the diagram.
The provided classes will position the controls in a box in the bottom right corner of the timeline container.
```html
<div class="controls">
<form id="timeline-find">
<input type="text" name="finder"
placeholder="Type to find an entry" aria-label="Find an entry" />
</form>
<div class="zoom-buttons" role="group" aria-label="Diagram Zoom Controls">
<button id="timeline-zoom-out" type="button">Zoom Out</button>
<button id="timeline-zoom-reset" type="button">Reset</button>
<button id="timeline-zoom-in" type="button">Zoom In</button>
</div>
</div>
```
Alternatively, use a `<details>` element instead of `<div>` to make a collapsible controls box with a simple transition applied.
```html
<details class="controls">
<summary>Search</summary>
…
</details>
```
## Entry Positioning
The X axis position of each entry is manually set by specifing the 'data-start' attribute *(**note:** If `data-start` is before the configured start of the timeline, it will be shown at the start with an arrow indicating it pre-exists the period shown)*. The extent of the entry along the timeline is determined either by the 'data-end' attribute, or extends to the end of the timeline.
Specifying the row manually for each entry is not required. However, only some basic tests are performed to choose a suitable row for each entry when it is not specified, so aside from simple examples, it is recommended to manually set 'data-row' on a proportion of entries and those with complex links to ensure a sensible layout.
The row is determined for each entry if it is omitted, in the order that they appear (either source-code order if added in HTML, or their array order if added via Javascript). A row is determined as follows:
* Available space, starting from the centre of the diagram.
* Connected entries (via 'data-becomes' attribute) must be on the same row.
* Split and merge entries should aim to be as close to their linked entries as possible, depending on nearest available space.
### Groups
If any groups have been specified, the same logic is applied, but to entries matching each group separately. The groups are then positioned on the diagram one after the other. Grouped and un-grouped entries can be mixed: any ungrouped entries will be positioned in an available space after all groups are completed.
## Javascript Documentation
<a name="Timeline"></a>
### Timeline
The class representing the Timeline. This is the point of access to this tool.
The simplest usage is to instantiate a new Timeline object, and then call the create() method.
**Kind**: global class
* [Timeline](#Timeline)
* [new Timeline([container], [config], [entries], [events])](#new_Timeline_new)
* [timeline.create()](#Timeline+create)
* [timeline.panToEntry(id)](#Timeline+panToEntry)
* ["timelineFind"](#Timeline+event_timelineFind)
<a name="new_Timeline_new"></a>
#### new Timeline([container], [config], [entries], [events])
| Param | Type | Default | Description |
| --- | --- | --- | --- |
| [container] | <code>string</code> | <code>"diagram"</code> | The ID of the container element for the timeline. |
| [config] | <code>object</code> | | All config for the timeline |
| [config.panzoom] | <code>function</code> \| <code>null</code> | <code></code> | The Panzoom function to enable panning and zooming, or null to disable |
| [config.findForm] | <code>string</code> | <code>"timeline-find"</code> | The ID of the find form |
| [config.zoomIn] | <code>string</code> | <code>"timeline-zoom-in"</code> | The ID of the button to zoom in |
| [config.zoomOut] | <code>string</code> | <code>"timeline-zoom-out"</code> | The ID of the button to zoom out |
| [config.zoomReset] | <code>string</code> | <code>"timeline-zoom-reset"</code> | The ID of the button to reset the zoom level |
| [config.yearStart] | <code>number</code> | <code>1900</code> | the starting year for the timeline |
| [config.yearEnd] | <code>number</code> | <code>Current year + 1</code> | the end year for the timeline |
| [config.strokeWidth] | <code>number</code> | <code>4</code> | the width in px of the joining lines |
| [config.yearWidth] | <code>number</code> | <code>50</code> | the width in px of diagram used for each year |
| [config.rowHeight] | <code>number</code> | <code>50</code> | the height in px of each diagram row |
| [config.padding] | <code>number</code> | <code>5</code> | the padding in px between rows |
| [config.boxWidth] | <code>number</code> | <code>100</code> | the width in px of each entry |
| [config.guides] | <code>boolean</code> | <code>true</code> | whether to draw striped guides at regular intervals in the timeline |
| [config.guideInterval] | <code>number</code> | <code>5</code> | the interval in years between guides (ignored if 'guides' is false) |
| [config.entrySelector] | <code>string</code> | <code>"div"</code> | the CSS selector used for entries |
| [entries] | <code>Array.<object></code> | <code>[]</code> | The Timeline entries as an array of objects |
| [events] | <code>Array.<object></code> | <code>[]</code> | Events as an array of objects |
<a name="Timeline+create"></a>
#### timeline.create()
Create the Timeline. This should be called after instantiation.
**Kind**: instance method of [<code>Timeline</code>](#Timeline)
**Access**: public
<a name="Timeline+panToEntry"></a>
#### timeline.panToEntry(id)
If Panzoom is enabled, pan to the element with the given ID, and reset the zoom.
**Kind**: instance method of [<code>Timeline</code>](#Timeline)
**Emits**: [<code>timelineFind</code>](#Timeline+event_timelineFind)
**Access**: public
| Param | Type | Description |
| --- | --- | --- |
| id | <code>string</code> | The ID of a timeline entry |
<a name="Timeline+event_timelineFind"></a>
#### "timelineFind"
The timelineFind event is fired when panToEntry() is called. (Only applicable if Panzoom is enabled).
**Kind**: event emitted by [<code>Timeline</code>](#Timeline)
**Access**: public
**Properties**
| Name | Type | Description |
| --- | --- | --- |
| details | <code>object</code> | |
| details.id | <code>string</code> | the ID of the entry |
| details.name | <code>string</code> | the name of the entry |
* * *
## Licence
Copyright (C) 2021-24 Aonghus Storey
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
See the [`LICENCE`](LICENCE) file for details.
| Javascript module to draw a timeline diagram | diagram-generator,diagrams,javascript,javascript-modules,charts,diagramming,timeline | 2023-01-03T14:57:42Z | 2024-05-07T15:40:02Z | null | 1 | 0 | 75 | 0 | 0 | 3 | null | GPL-3.0 | JavaScript |