input
stringlengths 50
13.9k
| output_program
stringlengths 5
655k
| output_answer
stringlengths 5
655k
| split
stringclasses 1
value | dataset
stringclasses 1
value |
---|---|---|---|---|
Decompose a number `num` into an array (tuple in Haskell, array of arrays `long[][]` in C# or Java) of the form `[[k1,k2,k3...], r]`, `([k1,k2,k3...], r)` in Haskell, `[[k1,k2,k3...], [r]]` in C# or Java) such that:
1. each kn is more than one
2. eack kn is maximized (first maximizing for 2 then 3 then 4 and so on)
3. and 2^(k1) + 3^(k2) + 4^(k3) + ... + n^(kn-1) + r = num
##Examples
```
# when there are no `k` more than 1:
3
[[], 3] =
3
# when the remainder is zero:
8330475
[[22, 13, 10, 8, 7, 6, 6, 5, 5, 5, 4, 4, 4, 3, 3, 3, 3, 3, 3, 2, 2, 2, 2], 0] =
2 ^ 22 + 3 ^ 13 + 4 ^ 10 + 5 ^ 8 + 6 ^ 7 + 7 ^ 6 + 8 ^ 6 + 9 ^ 5 + 10 ^ 5 + 11 ^ 5 + 12 ^ 4 + 13 ^ 4 + 14 ^ 4 + 15 ^ 3 + 16 ^ 3 + 17 ^ 3 + 18 ^ 3 + 19 ^ 3 + 20 ^ 3 + 21 ^ 2 + 22 ^ 2 + 23 ^ 2 + 24 ^ 2 + 0 = 8330475
# when there is both `k` and a remainder:
26
[[4, 2], 1] =
2 ^ 4 + 3 ^ 2 + 1 = 26
# when there is neither `k` nor a remainder:
0
[[], 0] =
0
```
As allways any feedback would be much appreciated | from math import log
def decompose(n):
i = 2
result = []
while n >= i*i:
k = int(log(n, i))
result.append(k)
n -= i ** k
i += 1
return [result, n]
| from math import log
def decompose(n):
i = 2
result = []
while n >= i*i:
k = int(log(n, i))
result.append(k)
n -= i ** k
i += 1
return [result, n]
| train | APPS_structured |
Create a function that takes a string as a parameter and does the following, in this order:
1. replaces every letter with the letter following it in the alphabet (see note below)
2. makes any vowels capital
3. makes any consonants lower case
**Note:** the alphabet should wrap around, so `Z` becomes `A`
So, for example the string `"Cat30"` would return `"dbU30"` (`Cat30 --> Dbu30 --> dbU30`) | def changer(s):
return s.lower().translate(str.maketrans('abcdefghijklmnopqrstuvwxyz', 'bcdEfghIjklmnOpqrstUvwxyzA')) | def changer(s):
return s.lower().translate(str.maketrans('abcdefghijklmnopqrstuvwxyz', 'bcdEfghIjklmnOpqrstUvwxyzA')) | train | APPS_structured |
# Task
We know that some numbers can be split into two primes. ie. `5 = 2 + 3, 10 = 3 + 7`. But some numbers are not. ie. `17, 27, 35`, etc..
Given a positive integer `n`. Determine whether it can be split into two primes. If yes, return the maximum product of two primes. If not, return `0` instead.
# Input/Output
`[input]` integer `n`
A positive integer.
`0 ≤ n ≤ 100000`
`[output]` an integer
The possible maximum product of two primes. or return `0` if it's impossible split into two primes.
# Example
For `n = 1`, the output should be `0`.
`1` can not split into two primes
For `n = 4`, the output should be `4`.
`4` can split into two primes `2 and 2`. `2 x 2 = 4`
For `n = 20`, the output should be `91`.
`20` can split into two primes `7 and 13` or `3 and 17`. The maximum product is `7 x 13 = 91` | def prime_product(n, sieve=[False, False, True, True]):
for x in range(len(sieve), n+1):
sieve.append(x % 2 and all(x % p for p in range(3, int(x ** .5) + 1, 2) if sieve[p]))
return next((p * (n - p) for p in range(n//2, 1, -1) if sieve[p] and sieve[n - p]), 0) | def prime_product(n, sieve=[False, False, True, True]):
for x in range(len(sieve), n+1):
sieve.append(x % 2 and all(x % p for p in range(3, int(x ** .5) + 1, 2) if sieve[p]))
return next((p * (n - p) for p in range(n//2, 1, -1) if sieve[p] and sieve[n - p]), 0) | train | APPS_structured |
When provided with a number between 0-9, return it in words.
Input :: 1
Output :: "One".
If your language supports it, try using a switch statement. | def switch_it_up(number):
#your code here
sayi={1:'One',2:'Two',3:'Three',4:'Four',5:'Five',6:'Six',7:'Seven',8:'Eight',9:'Nine',0:'Zero'}
return sayi[number] | def switch_it_up(number):
#your code here
sayi={1:'One',2:'Two',3:'Three',4:'Four',5:'Five',6:'Six',7:'Seven',8:'Eight',9:'Nine',0:'Zero'}
return sayi[number] | train | APPS_structured |
Little Nastya has a hobby, she likes to remove some letters from word, to obtain another word. But it turns out to be pretty hard for her, because she is too young. Therefore, her brother Sergey always helps her.
Sergey gives Nastya the word t and wants to get the word p out of it. Nastya removes letters in a certain order (one after another, in this order strictly), which is specified by permutation of letters' indices of the word t: a_1... a_{|}t|. We denote the length of word x as |x|. Note that after removing one letter, the indices of other letters don't change. For example, if t = "nastya" and a = [4, 1, 5, 3, 2, 6] then removals make the following sequence of words "nastya" $\rightarrow$ "nastya" $\rightarrow$ "nastya" $\rightarrow$ "nastya" $\rightarrow$ "nastya" $\rightarrow$ "nastya" $\rightarrow$ "nastya".
Sergey knows this permutation. His goal is to stop his sister at some point and continue removing by himself to get the word p. Since Nastya likes this activity, Sergey wants to stop her as late as possible. Your task is to determine, how many letters Nastya can remove before she will be stopped by Sergey.
It is guaranteed that the word p can be obtained by removing the letters from word t.
-----Input-----
The first and second lines of the input contain the words t and p, respectively. Words are composed of lowercase letters of the Latin alphabet (1 ≤ |p| < |t| ≤ 200 000). It is guaranteed that the word p can be obtained by removing the letters from word t.
Next line contains a permutation a_1, a_2, ..., a_{|}t| of letter indices that specifies the order in which Nastya removes letters of t (1 ≤ a_{i} ≤ |t|, all a_{i} are distinct).
-----Output-----
Print a single integer number, the maximum number of letters that Nastya can remove.
-----Examples-----
Input
ababcba
abb
5 3 4 1 7 6 2
Output
3
Input
bbbabb
bb
1 6 3 4 2 5
Output
4
-----Note-----
In the first sample test sequence of removing made by Nastya looks like this:
"ababcba" $\rightarrow$ "ababcba" $\rightarrow$ "ababcba" $\rightarrow$ "ababcba"
Nastya can not continue, because it is impossible to get word "abb" from word "ababcba".
So, Nastya will remove only three letters. | def check(x):
temp=[]
for i in t:
temp.append(i)
for i in range(x):
temp[a[i]-1]=''
#print(''.join(temp))
l=0;r=0;c=0
while r<len(s) and l<len(t):
if s[r]==temp[l]:
r+=1;c+=1
l+=1
#print(c)
return c==len(s)
t=input()
s=input()
a=list(map(int,input().split()))
lo=0;hi=len(a)
while lo<hi-1:
mid=lo+(hi-lo)//2
#print(lo,hi,mid)
if check(mid):
lo=mid
else:
hi=mid
print(lo)
| def check(x):
temp=[]
for i in t:
temp.append(i)
for i in range(x):
temp[a[i]-1]=''
#print(''.join(temp))
l=0;r=0;c=0
while r<len(s) and l<len(t):
if s[r]==temp[l]:
r+=1;c+=1
l+=1
#print(c)
return c==len(s)
t=input()
s=input()
a=list(map(int,input().split()))
lo=0;hi=len(a)
while lo<hi-1:
mid=lo+(hi-lo)//2
#print(lo,hi,mid)
if check(mid):
lo=mid
else:
hi=mid
print(lo)
| train | APPS_structured |
Today is Chocolate day and Kabir and Tara are visiting a Valentine fair. Upon arriving, they find a stall with an interesting game.
There are N$N$ jars having some chocolates in them. To win the game, one has to select the maximum number of consecutive jars such that the sum of count of chocolates in maximum and second maximum jar is less than or equal to k$k$ in that range.
Kabir wants to win the game so that he can gift the chocolates to Tara. You are a friend of Kabiir, help him win the game.
There will be at least one possible answer.
Note$Note$ :
- You have to select at least two jars.
- Count of chocolates in maximum and second maximum jar among selected consecutive jars may be equal.
-----Input:-----
- First line will contain T$T$, number of test cases.
- First line of each test case contains two space separated integers N,k$N, k$.
- Second line of each test case contains N$N$ space separated integer ai$a_i$ denotes number of chocolates in the jar.
-----Output:-----
For each test case print maximum number of jars.
-----Constraints:-----
- 1≤T≤10$1 \leq T \leq 10$
- 2≤N≤105$2 \leq N \leq 10^5$
- 1≤ai≤105$1 \leq a_i \leq 10^5$
-----Sample Input:-----
1
6 5
1 3 3 1 1 5
-----Sample Output:-----
3
-----EXPLANATION:-----
You can select 3rd$3^{rd}$, 4th$4^{th}$, and 5th$5^{th}$ jar as the sum of max and second max is equal to 4 which is less then 5. | # cook your dish here
for _ in range(int(input())):
n,k = list(map(int,input().split()))
x = [int(i)for i in input().split()]
a = []
count = 0
for i in x:
a.append(i)
t = a[:]
t.sort()
if len(t)>=2:
if t[-1]+t[-2]<=k and len(t)>count:
count = len(t)
else:
a.pop(0)
print(count)
| # cook your dish here
for _ in range(int(input())):
n,k = list(map(int,input().split()))
x = [int(i)for i in input().split()]
a = []
count = 0
for i in x:
a.append(i)
t = a[:]
t.sort()
if len(t)>=2:
if t[-1]+t[-2]<=k and len(t)>count:
count = len(t)
else:
a.pop(0)
print(count)
| train | APPS_structured |
Given a sequence of n integers a1, a2, ..., an, a 132 pattern is a subsequence ai, aj, ak such
that i < j < k and ai < ak < aj. Design an algorithm that takes a list of n numbers as input and checks whether there is a 132 pattern in the list.
Note: n will be less than 15,000.
Example 1:
Input: [1, 2, 3, 4]
Output: False
Explanation: There is no 132 pattern in the sequence.
Example 2:
Input: [3, 1, 4, 2]
Output: True
Explanation: There is a 132 pattern in the sequence: [1, 4, 2].
Example 3:
Input: [-1, 3, 2, 0]
Output: True
Explanation: There are three 132 patterns in the sequence: [-1, 3, 2], [-1, 3, 0] and [-1, 2, 0]. | class Solution:
def find132pattern(self, nums):
"""
:type nums: List[int]
:rtype: bool
"""
min_nums = [0] * len(nums)
for i, num in enumerate(nums):
min_nums[i] = min(min_nums[i-1], num) if i else num
stack = []
for i, num in reversed(list(enumerate(nums))):
while stack and stack[-1] <= min_nums[i]:
stack.pop()
if stack and num > stack[-1]:
return True
else:
stack.append(num)
return False | class Solution:
def find132pattern(self, nums):
"""
:type nums: List[int]
:rtype: bool
"""
min_nums = [0] * len(nums)
for i, num in enumerate(nums):
min_nums[i] = min(min_nums[i-1], num) if i else num
stack = []
for i, num in reversed(list(enumerate(nums))):
while stack and stack[-1] <= min_nums[i]:
stack.pop()
if stack and num > stack[-1]:
return True
else:
stack.append(num)
return False | train | APPS_structured |
My 5th kata, and 1st in a planned series of rock climbing themed katas.
In rock climbing ([bouldering](https://en.wikipedia.org/wiki/Bouldering) specifically), the V/Vermin (USA) climbing grades start at `'VB'` (the easiest grade), and then go `'V0'`, `'V0+'`, `'V1'`, `'V2'`, `'V3'`, `'V4'`, `'V5'` etc. up to `'V17'` (the hardest grade). You will be given a `list` (`lst`) of climbing grades and you have to write a function (`sort_grades`) to `return` a `list` of the grades sorted easiest to hardest.
If the input is an empty `list`, `return` an empty `list`; otherwise the input will always be a valid `list` of one or more grades.
Please do vote, rank, and provide any feedback on the kata. | def sort_grades(gs):
return sorted(gs, key=grade)
def grade(v):
if v == 'VB': return -2
if v == 'V0': return -1
if v == 'V0+': return 0
return int(v[1:]) | def sort_grades(gs):
return sorted(gs, key=grade)
def grade(v):
if v == 'VB': return -2
if v == 'V0': return -1
if v == 'V0+': return 0
return int(v[1:]) | train | APPS_structured |
The prime `149` has 3 permutations which are also primes: `419`, `491` and `941`.
There are 3 primes below `1000` with three prime permutations:
```python
149 ==> 419 ==> 491 ==> 941
179 ==> 197 ==> 719 ==> 971
379 ==> 397 ==> 739 ==> 937
```
But there are 9 primes below `1000` with two prime permutations:
```python
113 ==> 131 ==> 311
137 ==> 173 ==> 317
157 ==> 571 ==> 751
163 ==> 613 ==> 631
167 ==> 617 ==> 761
199 ==> 919 ==> 991
337 ==> 373 ==> 733
359 ==> 593 ==> 953
389 ==> 839 ==> 983
```
Finally, we can find 34 primes below `1000` with only one prime permutation:
```python
[13, 17, 37, 79, 107, 127, 139, 181, 191, 239, 241, 251, 277, 281, 283, 313, 347, 349, 367, 457, 461, 463, 467, 479, 563, 569, 577, 587, 619, 683, 709, 769, 787, 797]
```
Each set of permuted primes are represented by its smallest value, for example the set `149, 419, 491, 941` is represented by `149`, and the set has 3 permutations.
**Notes**
* the original number (`149` in the above example) is **not** counted as a permutation;
* permutations with leading zeros are **not valid**
## Your Task
Your task is to create a function that takes two arguments:
* an upper limit (`n_max`) and
* the number of prime permutations (`k_perms`) that the primes should generate **below** `n_max`
The function should return the following three values as a list:
* the number of permutational primes below the given limit,
* the smallest prime such prime,
* and the largest such prime
If no eligible primes were found below the limit, the output should be `[0, 0, 0]`
## Examples
Let's see how it would be with the previous cases:
```python
permutational_primes(1000, 3) ==> [3, 149, 379]
''' 3 primes with 3 permutations below 1000, smallest: 149, largest: 379 '''
permutational_primes(1000, 2) ==> [9, 113, 389]
''' 9 primes with 2 permutations below 1000, smallest: 113, largest: 389 '''
permutational_primes(1000, 1) ==> [34, 13, 797]
''' 34 primes with 1 permutation below 1000, smallest: 13, largest: 797 '''
```
Happy coding!! | def find_prime_kPerm(n, k):
sieve = n // 2 * [True]
for i in range(3, int(n ** .5) + 1, 2):
if sieve[i // 2]:
sieve[i*i // 2 :: i] = ((n - i*i - 1) // (2*i) + 1) * [False]
cycles = {}
for i in range(1, n // 2):
if sieve[i]:
cycles.setdefault(tuple(sorted(str(2*i + 1))), set()).add(2*i + 1)
k_perms = [min(cycle) for cycle in cycles.values() if len(cycle) == k + 1]
return [len(k_perms), min(k_perms, default=0), max(k_perms, default=0)] | def find_prime_kPerm(n, k):
sieve = n // 2 * [True]
for i in range(3, int(n ** .5) + 1, 2):
if sieve[i // 2]:
sieve[i*i // 2 :: i] = ((n - i*i - 1) // (2*i) + 1) * [False]
cycles = {}
for i in range(1, n // 2):
if sieve[i]:
cycles.setdefault(tuple(sorted(str(2*i + 1))), set()).add(2*i + 1)
k_perms = [min(cycle) for cycle in cycles.values() if len(cycle) == k + 1]
return [len(k_perms), min(k_perms, default=0), max(k_perms, default=0)] | train | APPS_structured |
The male gametes or sperm cells in humans and other mammals are heterogametic and contain one of two types of sex chromosomes. They are either X or Y. The female gametes or eggs however, contain only the X sex chromosome and are homogametic.
The sperm cell determines the sex of an individual in this case. If a sperm cell containing an X chromosome fertilizes an egg, the resulting zygote will be XX or female. If the sperm cell contains a Y chromosome, then the resulting zygote will be XY or male.
Determine if the sex of the offspring will be male or female based on the X or Y chromosome present in the male's sperm.
If the sperm contains the X chromosome, return "Congratulations! You're going to have a daughter.";
If the sperm contains the Y chromosome, return "Congratulations! You're going to have a son."; | def chromosome_check(sperm):
return f"Congratulations! You\'re going to have a {['daughter','son']['Y' in sperm]}."
| def chromosome_check(sperm):
return f"Congratulations! You\'re going to have a {['daughter','son']['Y' in sperm]}."
| train | APPS_structured |
Write a function that takes two arguments, and returns a new array populated with the elements **that only appear once, in either one array or the other, taken only once**; display order should follow what appears in arr1 first, then arr2:
```python
hot_singles([1, 2, 3, 3], [3, 2, 1, 4, 5]) # [4, 5]
hot_singles(["tartar", "blanket", "cinnamon"], ["cinnamon", "blanket", "domino"]) # ["tartar", "domino"]
hot_singles([77, "ciao"], [78, 42, "ciao"]) # [77, 78, 42]
hot_singles([1, 2, 3, 3], [3, 2, 1, 4, 5, 4]) # [4,5]
```
SPECIAL THANKS: @JulianKolbe ! | def ordered(l, arr1,arr2):
return [l,arr2.index(l) + len(arr1) if l in arr2 else arr1.index(l)]
def hot_singles(arr1, arr2):
L = [ ordered(l,arr1,arr2) for l in set(arr1) ^ set(arr2)]
return list(map(lambda l: l[0], sorted(L , key=lambda l: l[1]))) | def ordered(l, arr1,arr2):
return [l,arr2.index(l) + len(arr1) if l in arr2 else arr1.index(l)]
def hot_singles(arr1, arr2):
L = [ ordered(l,arr1,arr2) for l in set(arr1) ^ set(arr2)]
return list(map(lambda l: l[0], sorted(L , key=lambda l: l[1]))) | train | APPS_structured |
In this Kata, you will be given a ```number```, two indexes (```index1``` and ```index2```) and a ```digit``` to look for. Your task will be to check if the ```digit``` exists in the ```number```, within the ```indexes``` given.
Be careful, the ```index2``` is not necessarily more than the ```index1```.
```
index1 == 2 and index2 == 5 -> snippet from 2 to 5 positons;
index1 == 5 and index2 == 2 -> snippet from 2 to 5 positons;
number.length = 14;
0 <= index1 < 14;
0 <= index2 < 14;
index2 is inclusive in search snippet;
0 <= digit <= 9;
```
Find more details below:
```
checkDigit(12345678912345, 1, 0, 1) -> true, 1 exists in 12
checkDigit(12345678912345, 0, 1, 2) -> true, 2 exists in 12
checkDigit(67845123654000, 4, 2, 5) -> true, 4 exists in 845
checkDigit(66688445364856, 0, 0, 6) -> true, 6 exists in 6
checkDigit(87996599994565, 2, 5, 1) -> false, 1 doesn't exist in 9965
``` | def check_digit(number, index1, index2, digit):
number, digit = str(number), str(digit)
index1, index2 = sorted((index1, index2))
return digit in number[index1:index2+1] | def check_digit(number, index1, index2, digit):
number, digit = str(number), str(digit)
index1, index2 = sorted((index1, index2))
return digit in number[index1:index2+1] | train | APPS_structured |
Chef has recently been playing a lot of chess in preparation for the ICCT (International Chef Chess Tournament).
Since putting in long hours is not an easy task, Chef's mind wanders elsewhere. He starts counting the number of squares with odd side length on his chessboard..
However, Chef is not satisfied. He wants to know the number of squares of odd side length on a generic $N*N$ chessboard.
-----Input:-----
- The first line will contain a single integer $T$, the number of test cases.
- The next $T$ lines will have a single integer $N$, the size of the chess board.
-----Output:-----
For each test case, print a integer denoting the number of squares with odd length.
-----Constraints-----
- $1 \leq T \leq 100$
- $1 \leq N \leq 1000$
-----Sample Input:-----
2
3
8
-----Sample Output:-----
10
120 | # cook your dish here
for _ in range(int(input())):
n=int(input())
cnt=0
for i in range(1,n+1,2):
s=n+1-i
cnt=cnt+s*s
print(cnt)
| # cook your dish here
for _ in range(int(input())):
n=int(input())
cnt=0
for i in range(1,n+1,2):
s=n+1-i
cnt=cnt+s*s
print(cnt)
| train | APPS_structured |
Write a function generator that will generate the first `n` primes grouped in tuples of size `m`. If there are not enough primes for the last tuple it will have the remaining values as `None`.
## Examples
```python
For n = 11 and m = 2:
(2, 3), (5, 7), (11, 13), (17, 19), (23, 29), (31, None)
For n = 11 and m = 3:
(2, 3, 5), (7, 11, 13), (17, 19, 23), (29, 31, None)
For n = 11 and m = 5:
(2, 3, 5, 7, 11), (13, 17, 19, 23, 29), (31, None, None, None, None)]
For n = 3 and m = 1:
(2,), (3,), (5,)
```
Note: large numbers of `n` will be tested, up to 50000 | get_primes=lambda n,m:(tuple(g)for g in zip(*[iter([2]+[p for p in range(3,15*n,2)if all(p%d for d in range(3,int(p**.5)+1,2))][:n-1]+[None]*(m-1))]*m)) | get_primes=lambda n,m:(tuple(g)for g in zip(*[iter([2]+[p for p in range(3,15*n,2)if all(p%d for d in range(3,int(p**.5)+1,2))][:n-1]+[None]*(m-1))]*m)) | train | APPS_structured |
A group of rebels travelling on a square hoverboard is ambushed by Imperial Stormtroopers.Their big hoverboard is an easy target, so they decide to split the board into smaller square hoverboards so that they can bolt away easily.But they should also make sure they don't get too spread out.Help the rebels split the craft into minimum number of smaller crafts possible.
-----Input-----
A single integer N denoting the side length of the big hoverboard.
-----Output-----
In the first line, output the integer 'k' which is the minimum number of square boards into which the bigger board can be split up.
In the second line, output k space separated integers which denote the sizes of the smaller square hoverboards.This must be in increasing order of sizes.
-----Constraints-----
N ranges from 2 to 50.
-----Example-----
Input:
3
Output:
6
1 1 1 1 1 2
-----Explanation-----
A square of side length 3 can be split into smaller squares in two ways: Either into 9 squares of side1 or 5 squares of side 1 and 1 square of size 2.The second case is the favourable one. | a=int(input())
if(a%2==0):
print("4")
print(a/2,a/2,a/2,a/2)
else:
print("6")
print((a-1)/2,(a-1)/2,(a-1)/2,(a-1)/2,(a-1)/2,(a+1)/2) | a=int(input())
if(a%2==0):
print("4")
print(a/2,a/2,a/2,a/2)
else:
print("6")
print((a-1)/2,(a-1)/2,(a-1)/2,(a-1)/2,(a-1)/2,(a+1)/2) | train | APPS_structured |
You are given two positive integer lists with a random number of elements (1 <= n <= 100). Create a [GCD](https://en.wikipedia.org/wiki/Greatest_common_divisor) matrix and calculate the average of all values.
Return a float value rounded to 3 decimal places.
## Example
```
a = [1, 2, 3]
b = [4, 5, 6]
# a = 1 2 3 b =
gcd(a, b) = [ [1, 2, 1], # 4
[1, 1, 1], # 5
[1, 2, 3] ] # 6
average(gcd(a, b)) = 1.444
``` | from fractions import gcd
from itertools import product, starmap
from statistics import mean
def gcd_matrix(a, b):
return round(mean(starmap(gcd, product(a, b))), 3) | from fractions import gcd
from itertools import product, starmap
from statistics import mean
def gcd_matrix(a, b):
return round(mean(starmap(gcd, product(a, b))), 3) | train | APPS_structured |
A move consists of taking a point (x, y) and transforming it to either (x, x+y) or (x+y, y).
Given a starting point (sx, sy) and a target point (tx, ty), return True if and only if a sequence of moves exists to transform the point (sx, sy) to (tx, ty). Otherwise, return False.
Examples:
Input: sx = 1, sy = 1, tx = 3, ty = 5
Output: True
Explanation:
One series of moves that transforms the starting point to the target is:
(1, 1) -> (1, 2)
(1, 2) -> (3, 2)
(3, 2) -> (3, 5)
Input: sx = 1, sy = 1, tx = 2, ty = 2
Output: False
Input: sx = 1, sy = 1, tx = 1, ty = 1
Output: True
Note:
sx, sy, tx, ty will all be integers in the range [1, 10^9]. | class Solution:
def maxChunksToSorted(self, arr):
"""
:type arr: List[int]
:rtype: int
"""
cnt = 0
max_curr = -1
for i in range(len(arr)):
max_curr = max(max_curr, arr[i])
if max_curr == i:
cnt += 1
return cnt | class Solution:
def maxChunksToSorted(self, arr):
"""
:type arr: List[int]
:rtype: int
"""
cnt = 0
max_curr = -1
for i in range(len(arr)):
max_curr = max(max_curr, arr[i])
if max_curr == i:
cnt += 1
return cnt | train | APPS_structured |
You drop a ball from a given height. After each bounce, the ball returns to some fixed proportion of its previous height. If the ball bounces to height 1 or less, we consider it to have stopped bouncing. Return the number of bounces it takes for the ball to stop moving.
```
bouncingBall(initialHeight, bouncingProportion)
boucingBall(4, 0.5)
After first bounce, ball bounces to height 2
After second bounce, ball bounces to height 1
Therefore answer is 2 bounces
boucingBall(30, 0.3)
After first bounce, ball bounces to height 9
After second bounce, ball bounces to height 2.7
After third bounce, ball bounces to height 0.81
Therefore answer is 3 bounces
```
Initial height is an integer in range [2,1000]
Bouncing Proportion is a decimal in range [0, 1) | def bouncing_ball(h, bounce):
count = 0
while h > 1:
count += 1
h *= bounce
return count | def bouncing_ball(h, bounce):
count = 0
while h > 1:
count += 1
h *= bounce
return count | train | APPS_structured |
```if-not:swift
Write simple .camelCase method (`camel_case` function in PHP, `CamelCase` in C# or `camelCase` in Java) for strings. All words must have their first letter capitalized without spaces.
```
```if:swift
Write a simple `camelCase` function for strings. All words must have their first letter capitalized and all spaces removed.
```
For instance:
```python
camelcase("hello case") => HelloCase
camelcase("camel case word") => CamelCaseWord
```
```c#
using Kata;
"hello case".CamelCase(); // => "HelloCase"
"camel case word".CamelCase(); // => "CamelCaseWord"
```
Don't forget to rate this kata! Thanks :) | def camel_case(string):
return ''.join([i.capitalize() for i in string.split()]) | def camel_case(string):
return ''.join([i.capitalize() for i in string.split()]) | train | APPS_structured |
Another Fibonacci... yes but with other kinds of result.
The function is named `aroundFib` or `around_fib`, depending of the language.
Its parameter is `n` (positive integer).
First you have to calculate `f` the value of `fibonacci(n)` with `fibonacci(0) --> 0` and
`fibonacci(1) --> 1` (see: )
- 1) Find the count of each digit `ch` in `f` (`ch`: digit from 0 to 9), call this count `cnt` and find the maximum
value of `cnt`, call this maximum `maxcnt`. If there are ties, the digit `ch` to consider is the first one - in natural digit order - giving `maxcnt`.
- 2) Cut the value `f` into chunks of length at most `25`. The last chunk may be 25 long or less.
```
Example: for `n=100` you have only one chunk `354224848179261915075`
Example: for `n=180` f is `18547707689471986212190138521399707760` and you have two chunks
`1854770768947198621219013` and `8521399707760`. First length here is 25 and second one is 13.
```
- At last return a string in the following format:
"Last chunk ...; Max is ... for digit ..."
where Max is `maxcnt` and digit the first `ch` (in 0..9) leading to `maxcnt`.
```
Example: for `n=100` -> "Last chunk 354224848179261915075; Max is 3 for digit 1"
Example: for `n=180` -> "Last chunk 8521399707760; Max is 7 for digit 7"
Example: for `n=18000` -> "Last chunk 140258776000; Max is 409 for digit 1"
```
# Beware:
`fib(18000)` has `3762` digits. Values of `n` are between `500` and `25000`.
# Note
Translators are welcome for all languages, except for Ruby since the Bash tests needing Ruby a Ruby reference solution is already there though not yet published. | def around_fib(n):
f = str(fib(n))
ch = ['9', '8', '7', '6', '5', '4', '3', '2', '1', '0']
maxcnt = 0
chunk = ''
for digit in ch:
cnt = f.count(digit)
if cnt >= maxcnt:
maxcnt = cnt
maxdig = digit
l = len(f) % 25
if l == 0:
start = len(f) - 25
else:
start = len(f) - l
for i in range(start, len(f)):
chunk += f[i]
return 'Last chunk ' + chunk + '; Max is %d for digit ' % maxcnt + maxdig
def fib(n):
a = 0
b = 1
if n == 0:
return a
elif n == 1:
return b
else:
for i in range(2,n+1):
c = a + b
a = b
b = c
return b | def around_fib(n):
f = str(fib(n))
ch = ['9', '8', '7', '6', '5', '4', '3', '2', '1', '0']
maxcnt = 0
chunk = ''
for digit in ch:
cnt = f.count(digit)
if cnt >= maxcnt:
maxcnt = cnt
maxdig = digit
l = len(f) % 25
if l == 0:
start = len(f) - 25
else:
start = len(f) - l
for i in range(start, len(f)):
chunk += f[i]
return 'Last chunk ' + chunk + '; Max is %d for digit ' % maxcnt + maxdig
def fib(n):
a = 0
b = 1
if n == 0:
return a
elif n == 1:
return b
else:
for i in range(2,n+1):
c = a + b
a = b
b = c
return b | train | APPS_structured |
You are given a set of n pens, each of them can be red, blue, green, orange, and violet in color. Count a minimum number of pens that should be taken out from the set so that any two neighboring pens have different colors. Pens are considered to be neighboring if there are no other pens between them.
-----Input:-----
-
The first line contains t denoting the number of test cases.
-
The first line of each test case will contain a single integer n.
-
The second line of each test case will contain a string s.
(s contains only 'R', 'B', 'G', 'O', and 'V' characters denoting red, blue, green, orange, and violet respectively)
-----Output:-----
For each test case, print single line containing one integer - The minimum number of pens that need to be taken out.
-----Constraints-----
- $1 \leq T \leq 100$
- $1 \leq n \leq 1000$
-----Sample Input:-----
2
5
RBBRG
5
RBGOV
-----Sample Output:-----
1
0
-----EXPLANATION:-----
In first test case, two blue pens are neighboring each other, if we take out any one of them then the string will be RBRG in which each pen has different neighbors.
In second test case, no pen needs to be taken out as each pen has different neighbors. | # cook your dish here
from collections import deque
for i in range(int(input())):
n=int(input())
a=input()
c=0
for i in range(len(a)-1):
if a[i]==a[i+1]:
c+=1
print(c)
| # cook your dish here
from collections import deque
for i in range(int(input())):
n=int(input())
a=input()
c=0
for i in range(len(a)-1):
if a[i]==a[i+1]:
c+=1
print(c)
| train | APPS_structured |
My friend wants a new band name for her band. She like bands that use the formula: "The" + a noun with the first letter capitalized, for example:
`"dolphin" -> "The Dolphin"`
However, when a noun STARTS and ENDS with the same letter, she likes to repeat the noun twice and connect them together with the first and last letter, combined into one word (WITHOUT "The" in front), like this:
`"alaska" -> "Alaskalaska"`
Complete the function that takes a noun as a string, and returns her preferred band name written as a string. | def band_name_generator(s):
return (('The ' if s[0] != s[-1] else s[:-1]) + s).title() | def band_name_generator(s):
return (('The ' if s[0] != s[-1] else s[:-1]) + s).title() | train | APPS_structured |
# Rock Paper Scissors
Let's play! You have to return which player won! In case of a draw return `Draw!`.
Examples:
 | def rps(p1, p2):
if p1 == p2: return "Draw!"
if p1 == "rock":
if p2 == "paper": return "Player 2 won!"
else: return "Player 1 won!"
if p1 == "paper":
if p2 == "scissors": return "Player 2 won!"
else: return "Player 1 won!"
if p1 == "scissors":
if p2 == "rock": return "Player 2 won!"
else: return "Player 1 won!" | def rps(p1, p2):
if p1 == p2: return "Draw!"
if p1 == "rock":
if p2 == "paper": return "Player 2 won!"
else: return "Player 1 won!"
if p1 == "paper":
if p2 == "scissors": return "Player 2 won!"
else: return "Player 1 won!"
if p1 == "scissors":
if p2 == "rock": return "Player 2 won!"
else: return "Player 1 won!" | train | APPS_structured |
If you can't sleep, just count sheep!!
## Task:
Given a non-negative integer, `3` for example, return a string with a murmur: `"1 sheep...2 sheep...3 sheep..."`. Input will always be valid, i.e. no negative integers. | def count_sheep(n):
return "".join("%d sheep..." % (i + 1) for i in range(n)) | def count_sheep(n):
return "".join("%d sheep..." % (i + 1) for i in range(n)) | train | APPS_structured |
Your task in order to complete this Kata is to write a function which formats a duration, given as a number of seconds, in a human-friendly way.
The function must accept a non-negative integer. If it is zero, it just returns `"now"`. Otherwise, the duration is expressed as a combination of `years`, `days`, `hours`, `minutes` and `seconds`.
It is much easier to understand with an example:
```Fortran
formatDuration (62) // returns "1 minute and 2 seconds"
formatDuration (3662) // returns "1 hour, 1 minute and 2 seconds"
```
```python
format_duration(62) # returns "1 minute and 2 seconds"
format_duration(3662) # returns "1 hour, 1 minute and 2 seconds"
```
**For the purpose of this Kata, a year is 365 days and a day is 24 hours.**
Note that spaces are important.
### Detailed rules
The resulting expression is made of components like `4 seconds`, `1 year`, etc. In general, a positive integer and one of the valid units of time, separated by a space. The unit of time is used in plural if the integer is greater than 1.
The components are separated by a comma and a space (`", "`). Except the last component, which is separated by `" and "`, just like it would be written in English.
A more significant units of time will occur before than a least significant one. Therefore, `1 second and 1 year` is not correct, but `1 year and 1 second` is.
Different components have different unit of times. So there is not repeated units like in `5 seconds and 1 second`.
A component will not appear at all if its value happens to be zero. Hence, `1 minute and 0 seconds` is not valid, but it should be just `1 minute`.
A unit of time must be used "as much as possible". It means that the function should not return `61 seconds`, but `1 minute and 1 second` instead. Formally, the duration specified by of a component must not be greater than any valid more significant unit of time. | def format_duration(s):
dt = []
for b, w in [(60, 'second'), (60, 'minute'), (24, 'hour'), (365, 'day'), (s+1, 'year')]:
s, m = divmod(s, b)
if m: dt.append('%d %s%s' % (m, w, 's' * (m > 1)))
return ' and '.join(', '.join(dt[::-1]).rsplit(', ', 1)) or 'now' | def format_duration(s):
dt = []
for b, w in [(60, 'second'), (60, 'minute'), (24, 'hour'), (365, 'day'), (s+1, 'year')]:
s, m = divmod(s, b)
if m: dt.append('%d %s%s' % (m, w, 's' * (m > 1)))
return ' and '.join(', '.join(dt[::-1]).rsplit(', ', 1)) or 'now' | train | APPS_structured |
"Ring Ring!!"
Sherlock's phone suddenly started ringing. And it was none other than Jim Moriarty..
"Long time no see ! You miss me right ? Anyway we'll talk about it later . Let me first tell you something. Dr.Watson is with me . And you've got only one chance to save him . Here's your challenge:.
Given a number N and another number M, tell if the remainder of N%M is odd or even. If it's odd, then print "ODD" else print "EVEN"
If Sherlock can answer the query correctly, then Watson will be set free. He has approached you for help since you being a programmer.Can you help him?
-----Input-----
The first line contains, T, the number of test cases..
Each test case contains an integer, N and M
-----Output-----
Output the minimum value for each test case
-----Constraints-----
1 = T = 20
1 <= N <= 10^18
1 <= M<= 10^9
-----Subtasks-----
Subtask #1 : (20 points)
1 = T = 20
1 <= N <= 100
1 <= M<= 100
Subtask 2 : (80 points)
1 = T = 20
1 <= N <= 10^18
1 <= M<= 10^9
-----Example-----
Input:
2
4 4
6 5
Output:
EVEN
ODD | t=int(input())
for i in range(t):
a=input().split(" ")
n=int(a[0])
m=int(a[1])
if((n%m)%2==0):
print("EVEN")
else:
print("ODD") | t=int(input())
for i in range(t):
a=input().split(" ")
n=int(a[0])
m=int(a[1])
if((n%m)%2==0):
print("EVEN")
else:
print("ODD") | train | APPS_structured |
Write a comparator for a list of phonetic words for the letters of the [greek alphabet](https://en.wikipedia.org/wiki/Greek_alphabet).
A comparator is:
> *a custom comparison function of two arguments (iterable elements) which should return a negative, zero or positive number depending on whether the first argument is considered smaller than, equal to, or larger than the second argument*
*(source: https://docs.python.org/2/library/functions.html#sorted)*
The greek alphabet is preloded for you as `greek_alphabet`:
```python
greek_alphabet = (
'alpha', 'beta', 'gamma', 'delta', 'epsilon', 'zeta',
'eta', 'theta', 'iota', 'kappa', 'lambda', 'mu',
'nu', 'xi', 'omicron', 'pi', 'rho', 'sigma',
'tau', 'upsilon', 'phi', 'chi', 'psi', 'omega')
```
## Examples
```python
greek_comparator('alpha', 'beta') < 0
greek_comparator('psi', 'psi') == 0
greek_comparator('upsilon', 'rho') > 0
``` | def greek_comparator(l, r):
gt = (
'alpha', 'beta', 'gamma', 'delta', 'epsilon', 'zeta',
'eta', 'theta', 'iota', 'kappa', 'lambda', 'mu',
'nu', 'xi', 'omicron', 'pi', 'rho', 'sigma',
'tau', 'upsilon', 'phi', 'chi', 'psi', 'omega')
return gt.index(l)- gt.index(r) | def greek_comparator(l, r):
gt = (
'alpha', 'beta', 'gamma', 'delta', 'epsilon', 'zeta',
'eta', 'theta', 'iota', 'kappa', 'lambda', 'mu',
'nu', 'xi', 'omicron', 'pi', 'rho', 'sigma',
'tau', 'upsilon', 'phi', 'chi', 'psi', 'omega')
return gt.index(l)- gt.index(r) | train | APPS_structured |
Mia is working as a waitress at a breakfast diner. She can take up only one shift from 6 shifts a day i.e. from 10 am to 4 pm. She needs to save 300$ after completion of the month. She works only for $D$ days in the month. She estimates that she gets her highest tip in the first shift and the tip starts decreasing by 2% every hour as the day prolongs. She gets a minimum wage of $X$ $ for every shift. And her highest tip in the first shift is $Y$ $. Determine whether Mia will be able to save 300$ from her wages and tips after working $D$ days of the month. If she can, print YES, else print NO.
-----Constraints-----
- 8 <= D <=30
- 7 <= X <=30
- 4 <= Y <= 20
-----Input:-----
- First line has three parameters $D$, $X$ and $Y$ i.e. number of days worked, minimum wage and highest tip.
- Second line contains D integers indicating her shifts every $i$-th day she has worked.
-----Output:-----
- Print YES, if Mia has saved 300$, NO otherwise.
-----Sample Input:-----
9 17 5
1 3 2 4 5 6 1 2 2
-----Sample Output:-----
NO
-----Explanation:-----
No. of days Mia worked (D) is 9, so minimum wage she earns (X) is 17 dollars. Highest tip at first hour (Y) = 5 dollars, 1st day she took 1st shift and 2nd day she took 3rd shift and so on. Upon calculation we will find that Mia was not able to save 300 dollars. | # cook your dish here
d,x,y=[int(i) for i in list(input().split())]
l=[int(i) for i in list(input().split())]
sav=d*x
dec=(y*2)/100
for i in range(0,d):
temp=y
for j in range(1,l[i]):
temp-=dec
dec=(temp*2)/100
sav+=temp
if sav>=300:
print('YES')
else:
print("NO")
| # cook your dish here
d,x,y=[int(i) for i in list(input().split())]
l=[int(i) for i in list(input().split())]
sav=d*x
dec=(y*2)/100
for i in range(0,d):
temp=y
for j in range(1,l[i]):
temp-=dec
dec=(temp*2)/100
sav+=temp
if sav>=300:
print('YES')
else:
print("NO")
| train | APPS_structured |
Find the second-to-last element of a list.
Example:
```python
penultimate([1,2,3,4]) # => 3
penultimate(["Python is dynamic"]) # => 'i'
(courtesy of [haskell.org](http://www.haskell.org/haskellwiki/99_questions/1_to_10))
``` | def penultimate(a):
# implement here
try:
return a[-2]
except:
return None | def penultimate(a):
# implement here
try:
return a[-2]
except:
return None | train | APPS_structured |
It's March and you just can't seem to get your mind off brackets. However, it is not due to basketball. You need to extract statements within strings that are contained within brackets.
You have to write a function that returns a list of statements that are contained within brackets given a string. If the value entered in the function is not a string, well, you know where that variable should be sitting.
Good luck! | import re
def bracket_buster(string):
if not isinstance(string, str):
return "Take a seat on the bench."
return re.findall(r'\[(.*?)\]', string)
| import re
def bracket_buster(string):
if not isinstance(string, str):
return "Take a seat on the bench."
return re.findall(r'\[(.*?)\]', string)
| train | APPS_structured |
Mrs. Smith is trying to contact her husband, John Smith, but she forgot the secret phone number!
The only thing Mrs. Smith remembered was that any permutation of $n$ can be a secret phone number. Only those permutations that minimize secret value might be the phone of her husband.
The sequence of $n$ integers is called a permutation if it contains all integers from $1$ to $n$ exactly once.
The secret value of a phone number is defined as the sum of the length of the longest increasing subsequence (LIS) and length of the longest decreasing subsequence (LDS).
A subsequence $a_{i_1}, a_{i_2}, \ldots, a_{i_k}$ where $1\leq i_1 < i_2 < \ldots < i_k\leq n$ is called increasing if $a_{i_1} < a_{i_2} < a_{i_3} < \ldots < a_{i_k}$. If $a_{i_1} > a_{i_2} > a_{i_3} > \ldots > a_{i_k}$, a subsequence is called decreasing. An increasing/decreasing subsequence is called longest if it has maximum length among all increasing/decreasing subsequences.
For example, if there is a permutation $[6, 4, 1, 7, 2, 3, 5]$, LIS of this permutation will be $[1, 2, 3, 5]$, so the length of LIS is equal to $4$. LDS can be $[6, 4, 1]$, $[6, 4, 2]$, or $[6, 4, 3]$, so the length of LDS is $3$.
Note, the lengths of LIS and LDS can be different.
So please help Mrs. Smith to find a permutation that gives a minimum sum of lengths of LIS and LDS.
-----Input-----
The only line contains one integer $n$ ($1 \le n \le 10^5$) — the length of permutation that you need to build.
-----Output-----
Print a permutation that gives a minimum sum of lengths of LIS and LDS.
If there are multiple answers, print any.
-----Examples-----
Input
4
Output
3 4 1 2
Input
2
Output
2 1
-----Note-----
In the first sample, you can build a permutation $[3, 4, 1, 2]$. LIS is $[3, 4]$ (or $[1, 2]$), so the length of LIS is equal to $2$. LDS can be ony of $[3, 1]$, $[4, 2]$, $[3, 2]$, or $[4, 1]$. The length of LDS is also equal to $2$. The sum is equal to $4$. Note that $[3, 4, 1, 2]$ is not the only permutation that is valid.
In the second sample, you can build a permutation $[2, 1]$. LIS is $[1]$ (or $[2]$), so the length of LIS is equal to $1$. LDS is $[2, 1]$, so the length of LDS is equal to $2$. The sum is equal to $3$. Note that permutation $[1, 2]$ is also valid. | from math import sqrt
n = int(input())
step = int(sqrt(n))
assert step**2 <= n and (step + 1)**2 > n
ans = []
for start in range(0, n, step):
ans.extend(range(start, min(n, start + step))[::-1])
print(' '.join(str(v + 1) for v in ans)) | from math import sqrt
n = int(input())
step = int(sqrt(n))
assert step**2 <= n and (step + 1)**2 > n
ans = []
for start in range(0, n, step):
ans.extend(range(start, min(n, start + step))[::-1])
print(' '.join(str(v + 1) for v in ans)) | train | APPS_structured |
Nobody knows, but $N$ frogs live in Chef's garden.
Now they are siting on the X-axis and want to speak to each other. One frog can send a message to another one if the distance between them is less or equal to $K$.
Chef knows all $P$ pairs of frogs, which want to send messages. Help him to define can they or not!
Note : More than $1$ frog can be on the same point on the X-axis.
-----Input-----
- The first line contains three integers $N$, $K$ and $P$.
- The second line contains $N$ space-separated integers $A_1$, $A_2$, …, $A_N$ denoting the x-coordinates of frogs".
- Each of the next $P$ lines contains two integers $A$ and $B$ denoting the numbers of frogs according to the input.
-----Output-----
For each pair print "Yes" without a brackets if frogs can speak and "No" if they cannot.
-----Constraints-----
- $1 \le N, P \le 10^5$
- $0 \le A_i, K \le 10^9$
- $1 \le A, B \le N$
-----Example-----
-----Sample Input:-----
5 3 3
0 3 8 5 12
1 2
1 3
2 5
-----Sample Output:-----
Yes
Yes
No
-----Explanation-----
-
For pair $(1, 2)$ frog $1$ can directly speak to the frog $2$ as the distance between them is $3 - 0 = 3 \le K$ .
-
For pair $(1, 3)$ frog $1$ can send a message to frog $2$, frog $2$ can send it to frog $4$ and it can send it to frog $3$.
-
For pair $(2, 5)$ frogs can't send a message under current constraints. | # cook your dish here
from collections import defaultdict
def preCompute(arr, n, K):
nonlocal maxDistance
arr = list(enumerate(arr))
arr.sort(key = lambda x: -x[1])
# print(arr)
maxDistance[arr[0][0]] = arr[0][1] + K
for i in range(1, n):
if arr[i-1][1] - arr[i][1] <= K:
maxDistance[arr[i][0]] = maxDistance[arr[i-1][0]]
else:
maxDistance[arr[i][0]] = arr[i][1] + K
def answer(x, y):
# print(maxDistance)
if maxDistance[x-1] == maxDistance[y-1]:
return "Yes"
return "No"
maxDistance = defaultdict(int)
(n, k, p) = map(int, input().strip().split())
arr = list(map(int, input().strip().split()))
preCompute(arr, n, k)
for _ in range(p):
(x, y) = map(int, input().strip().split())
print(answer(x, y)) | # cook your dish here
from collections import defaultdict
def preCompute(arr, n, K):
nonlocal maxDistance
arr = list(enumerate(arr))
arr.sort(key = lambda x: -x[1])
# print(arr)
maxDistance[arr[0][0]] = arr[0][1] + K
for i in range(1, n):
if arr[i-1][1] - arr[i][1] <= K:
maxDistance[arr[i][0]] = maxDistance[arr[i-1][0]]
else:
maxDistance[arr[i][0]] = arr[i][1] + K
def answer(x, y):
# print(maxDistance)
if maxDistance[x-1] == maxDistance[y-1]:
return "Yes"
return "No"
maxDistance = defaultdict(int)
(n, k, p) = map(int, input().strip().split())
arr = list(map(int, input().strip().split()))
preCompute(arr, n, k)
for _ in range(p):
(x, y) = map(int, input().strip().split())
print(answer(x, y)) | train | APPS_structured |
Rohit has n empty boxes lying on the ground in a line. The size of the boxes is given in the form of an array $a$. The size of the ith box is denoted by $a[i]$. Since Rohit has a tiny room, there is a shortage of space. Therefore, he has to reduce the number of boxes on the ground by putting a box into another box that is at least twice the size of the current box i.e if we have to put the ith box into the jth box then $( 2*a[i] ) <= a[j]$.
Each box can contain a maximum of one box and the box which is kept in another box cannot
hold any box itself.
Find the minimum number of boxes that will remain on the ground after putting boxes into each other.
-----Input:-----
- The first line contains a single integer n.
- The next n lines contain the integer a[i] - the size of the i-th box.
-----Output:-----
Output a single integer denoting the minimum number of boxes remaining on the ground.
-----Constraints-----
- $1 \leq n \leq 5*10^5$
- $1 \leq a[i] \leq 10^5$
-----Subtasks-----
- 30 points : $1 \leq n \leq 10^2$
- 70 points : $1 \leq n \leq 5*10^5$
-----Sample Input:-----
5
16
1
4
8
2
-----Sample Output:-----
3 | # n=int(input())
# lst=[]
# for i in range(n):
# lst.append(int(input()))
# lst.sort()
# l=0
# r=1
# count=0
# while r<len(lst):
# if lst[l]*2<=lst[r]:
# count+=1
# l+=1
# r+=1
# else:
# r+=1
# print(len(lst)-count)
# cook your dish here
n = int(input())
lst = []
for i in range(n):
lst.append(int(input()))
lst.sort()
mini = abs(-n//2)
f_l,s_l = lst[:mini],lst[mini:]
ans = 0
j = 0
i = 0
extra=0
while(i<len(f_l) and j<len(s_l)):
if(f_l[i]*2<=s_l[j]):
ans+=1
i+=1
j+=1
else:
extra+=1
j+=1
# print(ans)
ans+=len(s_l)-j + len(f_l)-i + extra
# print(i,j,ans)
print(ans) | # n=int(input())
# lst=[]
# for i in range(n):
# lst.append(int(input()))
# lst.sort()
# l=0
# r=1
# count=0
# while r<len(lst):
# if lst[l]*2<=lst[r]:
# count+=1
# l+=1
# r+=1
# else:
# r+=1
# print(len(lst)-count)
# cook your dish here
n = int(input())
lst = []
for i in range(n):
lst.append(int(input()))
lst.sort()
mini = abs(-n//2)
f_l,s_l = lst[:mini],lst[mini:]
ans = 0
j = 0
i = 0
extra=0
while(i<len(f_l) and j<len(s_l)):
if(f_l[i]*2<=s_l[j]):
ans+=1
i+=1
j+=1
else:
extra+=1
j+=1
# print(ans)
ans+=len(s_l)-j + len(f_l)-i + extra
# print(i,j,ans)
print(ans) | train | APPS_structured |
Motu and Patlu are playing with a Magical Ball. Patlu find some interesting pattern in the motion of the ball that ball always bounce back from the ground after travelling a linear distance whose value is some power of $2$. Patlu gave Motu total distance $D$ travelled by the ball and ask him to calculate the minimum number of bounces that the ball makes before coming to rest.
-----Input:-----
- First line will contain $T$, number of testcases. Then the testcases follow.
- Each testcase contains of a single line of input, single integers $D$.
- Note : Power of $2$ must be a non-negative integer.
-----Output:-----
For each testcase, output in a single line answer, the minimum number of bounces the ball makes before coming to rest.
-----Constraints-----
- $1 \leq T \leq 10^5$
- $1$ $\leq$ $M$< $10$^18
-----Sample Input:-----
1
13
-----Sample Output:-----
2
-----EXPLANATION:----- | # cook your dish here
import math
t=int(input())
for i in range(t):
k=int(input())
count=0
temp=(int(math.log2(k)))
for j in range(temp,-1,-1):
if((k-(2**j))>=0):
k=k-(2**j)
count=count+1
print(count-1)
| # cook your dish here
import math
t=int(input())
for i in range(t):
k=int(input())
count=0
temp=(int(math.log2(k)))
for j in range(temp,-1,-1):
if((k-(2**j))>=0):
k=k-(2**j)
count=count+1
print(count-1)
| train | APPS_structured |
Bessie has way too many friends because she is everyone's favorite cow! Her new friend Rabbit is trying to hop over so they can play!
More specifically, he wants to get from $(0,0)$ to $(x,0)$ by making multiple hops. He is only willing to hop from one point to another point on the 2D plane if the Euclidean distance between the endpoints of a hop is one of its $n$ favorite numbers: $a_1, a_2, \ldots, a_n$. What is the minimum number of hops Rabbit needs to get from $(0,0)$ to $(x,0)$? Rabbit may land on points with non-integer coordinates. It can be proved that Rabbit can always reach his destination.
Recall that the Euclidean distance between points $(x_i, y_i)$ and $(x_j, y_j)$ is $\sqrt{(x_i-x_j)^2+(y_i-y_j)^2}$.
For example, if Rabbit has favorite numbers $1$ and $3$ he could hop from $(0,0)$ to $(4,0)$ in two hops as shown below. Note that there also exists other valid ways to hop to $(4,0)$ in $2$ hops (e.g. $(0,0)$ $\rightarrow$ $(2,-\sqrt{5})$ $\rightarrow$ $(4,0)$).
$1$ Here is a graphic for the first example. Both hops have distance $3$, one of Rabbit's favorite numbers.
In other words, each time Rabbit chooses some number $a_i$ and hops with distance equal to $a_i$ in any direction he wants. The same number can be used multiple times.
-----Input-----
The input consists of multiple test cases. The first line contains an integer $t$ ($1 \le t \le 1000$) — the number of test cases. Next $2t$ lines contain test cases — two lines per test case.
The first line of each test case contains two integers $n$ and $x$ ($1 \le n \le 10^5$, $1 \le x \le 10^9$) — the number of favorite numbers and the distance Rabbit wants to travel, respectively.
The second line of each test case contains $n$ integers $a_1, a_2, \ldots, a_n$ ($1 \le a_i \le 10^9$) — Rabbit's favorite numbers. It is guaranteed that the favorite numbers are distinct.
It is guaranteed that the sum of $n$ over all the test cases will not exceed $10^5$.
-----Output-----
For each test case, print a single integer — the minimum number of hops needed.
-----Example-----
Input
4
2 4
1 3
3 12
3 4 5
1 5
5
2 10
15 4
Output
2
3
1
2
-----Note-----
The first test case of the sample is shown in the picture above. Rabbit can hop to $(2,\sqrt{5})$, then to $(4,0)$ for a total of two hops. Each hop has a distance of $3$, which is one of his favorite numbers.
In the second test case of the sample, one way for Rabbit to hop $3$ times is: $(0,0)$ $\rightarrow$ $(4,0)$ $\rightarrow$ $(8,0)$ $\rightarrow$ $(12,0)$.
In the third test case of the sample, Rabbit can hop from $(0,0)$ to $(5,0)$.
In the fourth test case of the sample, Rabbit can hop: $(0,0)$ $\rightarrow$ $(5,10\sqrt{2})$ $\rightarrow$ $(10,0)$. | def solve():
n,d=list(map(int,input().split()))
a=list(map(int,input().split()))
a.sort()
for i in range(n):
if a[i]==d:
print("1")
return
for i in range(n):
if a[i]>=d:
print("2")
return
print(int((d-1)/(a[n-1])+1))
for _ in range(int(input())):
solve()
| def solve():
n,d=list(map(int,input().split()))
a=list(map(int,input().split()))
a.sort()
for i in range(n):
if a[i]==d:
print("1")
return
for i in range(n):
if a[i]>=d:
print("2")
return
print(int((d-1)/(a[n-1])+1))
for _ in range(int(input())):
solve()
| train | APPS_structured |
There are several cards arranged in a row, and each card has an associated number of points The points are given in the integer array cardPoints.
In one step, you can take one card from the beginning or from the end of the row. You have to take exactly k cards.
Your score is the sum of the points of the cards you have taken.
Given the integer array cardPoints and the integer k, return the maximum score you can obtain.
Example 1:
Input: cardPoints = [1,2,3,4,5,6,1], k = 3
Output: 12
Explanation: After the first step, your score will always be 1. However, choosing the rightmost card first will maximize your total score. The optimal strategy is to take the three cards on the right, giving a final score of 1 + 6 + 5 = 12.
Example 2:
Input: cardPoints = [2,2,2], k = 2
Output: 4
Explanation: Regardless of which two cards you take, your score will always be 4.
Example 3:
Input: cardPoints = [9,7,7,9,7,7,9], k = 7
Output: 55
Explanation: You have to take all the cards. Your score is the sum of points of all cards.
Example 4:
Input: cardPoints = [1,1000,1], k = 1
Output: 1
Explanation: You cannot take the card in the middle. Your best score is 1.
Example 5:
Input: cardPoints = [1,79,80,1,1,1,200,1], k = 3
Output: 202
Constraints:
1 <= cardPoints.length <= 10^5
1 <= cardPoints[i] <= 10^4
1 <= k <= cardPoints.length | class Solution:
def maxScore(self, cardPoints: List[int], k: int) -> int:
result = curr = 0
for i in range(-k, k):
curr += cardPoints[i]
if i >= 0:
curr -= cardPoints[i - k]
result = max(result, curr)
return result | class Solution:
def maxScore(self, cardPoints: List[int], k: int) -> int:
result = curr = 0
for i in range(-k, k):
curr += cardPoints[i]
if i >= 0:
curr -= cardPoints[i - k]
result = max(result, curr)
return result | train | APPS_structured |
Sam is an avid collector of numbers. Every time he finds a new number he throws it on the top of his number-pile. Help Sam organise his collection so he can take it to the International Number Collectors Conference in Cologne.
Given an array of numbers, your function should return an array of arrays, where each subarray contains all the duplicates of a particular number. Subarrays should be in the same order as the first occurence of the number they contain:
Assume the input is always going to be an array of numbers. If the input is an empty array, an empty array should be returned. | def group(arr):
answer, brac, counter, already = [], [], 0, []
for i in arr:
if arr.count(i) > 1 and i not in already:
already.append(i)
while counter < arr.count(i):
counter += 1
brac.append(i)
if counter == arr.count(i):
answer.append(brac)
brac = []
counter = 0
break
elif arr.count(i) == 1:
answer.append([i])
return answer | def group(arr):
answer, brac, counter, already = [], [], 0, []
for i in arr:
if arr.count(i) > 1 and i not in already:
already.append(i)
while counter < arr.count(i):
counter += 1
brac.append(i)
if counter == arr.count(i):
answer.append(brac)
brac = []
counter = 0
break
elif arr.count(i) == 1:
answer.append([i])
return answer | train | APPS_structured |
Help a fruit packer sort out the bad apples.
There are 7 varieties of apples, all packaged as pairs and stacked in a fruit box. Some of the apples are spoiled. The fruit packer will have to make sure the spoiled apples are either removed from the fruit box or replaced. Below is the breakdown:
Apple varieties are represented with numbers, `1 to 7`
A fruit package is represented with a 2 element array `[4,3]`
A fruit package with one bad apple, or a bad package, is represented with `[2,0]` or `[0,2]`
A fruit package with two bad apples, or a rotten package, is represented with `[0,0]`
A fruit box is represented with:
```
[ [ 1, 3 ],
[ 7, 6 ],
[ 7, 2 ],
[ 1, 3 ],
[ 0, 2 ],
[ 4, 5 ],
[ 0, 3 ],
[ 7, 6 ] ]
```
Write a program to clear the fruit box off bad apples.
The INPUT will be a fruit box represented with a 2D array: `[[1,3],[7,6],[7,2],[1,3],[0,2],[4,5],[0,3],[7,6]]`
The OUTPUT should be the fruit box void of bad apples: `[[1,3],[7,6],[7,2],[1,3],[2,3],[4,5],[7,6]]`
Conditions to be met:
1.A bad package should have the bad apple replaced if there is another bad package with a good apple to spare. Else, the bad package should be discarded.
2.The order of the packages in the fruit box should be preserved. Repackaging happens from the top of the fruit box `index = 0` to the bottom `nth index`. Also note how fruits in a package are ordered when repacking. Example shown in INPUT/OUPUT above.
3.Rotten packages should be discarded.
4.There can be packages with the same variety of apples, e.g `[1,1]`, this is not a problem. | def bad_apples(apples):
good_packages, bad_packages = [], []
for i, box in enumerate(apples):
if box[0] and box[1]:
good_packages.append([i, box])
elif box[0] or box[1]:
bad_packages.append([i, box[1] if box[1] else box[0]])
for a, b in zip(*[iter(bad_packages)]*2):
good_packages.append([a[0], [a[1], b[1]]])
return [box for _, box in sorted(good_packages)] | def bad_apples(apples):
good_packages, bad_packages = [], []
for i, box in enumerate(apples):
if box[0] and box[1]:
good_packages.append([i, box])
elif box[0] or box[1]:
bad_packages.append([i, box[1] if box[1] else box[0]])
for a, b in zip(*[iter(bad_packages)]*2):
good_packages.append([a[0], [a[1], b[1]]])
return [box for _, box in sorted(good_packages)] | train | APPS_structured |
Write a function that takes a string as input and reverse only the vowels of a string.
Example 1:
Given s = "hello", return "holle".
Example 2:
Given s = "leetcode", return "leotcede".
Note:
The vowels does not include the letter "y". | class Solution:
def reverseVowels(self, s):
"""
:type s: str
:rtype: str
"""
yuanyin=['a','e','i','o','u','A','E','I','O','U']
s=list(s)
i=0
j=len(s)-1
while i<=j:
while s[i] not in yuanyin and i<j:
i+=1
while s[j] not in yuanyin and i<j:
j-=1
temp=s[i]
s[i]=s[j]
s[j]=temp
i+=1
j-=1
return ''.join(s)
| class Solution:
def reverseVowels(self, s):
"""
:type s: str
:rtype: str
"""
yuanyin=['a','e','i','o','u','A','E','I','O','U']
s=list(s)
i=0
j=len(s)-1
while i<=j:
while s[i] not in yuanyin and i<j:
i+=1
while s[j] not in yuanyin and i<j:
j-=1
temp=s[i]
s[i]=s[j]
s[j]=temp
i+=1
j-=1
return ''.join(s)
| train | APPS_structured |
Your task is to Combine two Strings. But consider the rule...
By the way you don't have to check errors or incorrect input values, everything is ok without bad tricks, only two input strings and as result one output string;-)...
And here's the rule:
Input Strings `a` and `b`: For every character in string `a` swap the casing of every occurrence of the same character in string `b`. Then do the same casing swap with the inputs reversed. Return a single string consisting of the changed version of `a` followed by the changed version of `b`. A char of `a` is in `b` regardless if it's in upper or lower case - see the testcases too.
I think that's all;-)...
Some easy examples:
````
Input: "abc" and "cde" => Output: "abCCde"
Input: "ab" and "aba" => Output: "aBABA"
Input: "abab" and "bababa" => Output: "ABABbababa"
````
Once again for the last example - description from `KenKamau`, see discourse;-):
a) swap the case of characters in string `b` for every occurrence of that character in string `a`
char `'a'` occurs twice in string `a`, so we swap all `'a'` in string `b` twice. This means we start with `"bababa"` then `"bAbAbA"` => `"bababa"`
char `'b'` occurs twice in string `a` and so string `b` moves as follows: start with `"bababa"` then `"BaBaBa"` => `"bababa"`
b) then, swap the case of characters in string `a` for every occurrence in string `b`
char `'a'` occurs `3` times in string `b`. So string `a` swaps cases as follows: start with `"abab"` then => `"AbAb"` => `"abab"` => `"AbAb"`
char `'b'` occurs `3` times in string `b`. So string `a` swaps as follow: start with `"AbAb"` then => `"ABAB"` => `"AbAb"` => `"ABAB"`.
c) merge new strings `a` and `b`
return `"ABABbababa"`
There are some static tests at the beginning and many random tests if you submit your solution.
Hope you have fun:-)! | from collections import Counter
def swap_them(a, b):
cnt = Counter(b.lower())
return "".join(c.swapcase() if cnt[c.lower()] % 2 else c for c in a)
def work_on_strings(a, b):
return swap_them(a, b) + swap_them(b, a) | from collections import Counter
def swap_them(a, b):
cnt = Counter(b.lower())
return "".join(c.swapcase() if cnt[c.lower()] % 2 else c for c in a)
def work_on_strings(a, b):
return swap_them(a, b) + swap_them(b, a) | train | APPS_structured |
Given a non-negative integer N, find the largest number that is less than or equal to N with monotone increasing digits.
(Recall that an integer has monotone increasing digits if and only if each pair of adjacent digits x and y satisfy x .)
Example 1:
Input: N = 10
Output: 9
Example 2:
Input: N = 1234
Output: 1234
Example 3:
Input: N = 332
Output: 299
Note:
N is an integer in the range [0, 10^9]. | class Solution:
def monotoneIncreasingDigits(self, N):
"""
:type N: int
:rtype: int
"""
NN = ('000000000000000000' + str(N))[-11:]
chron = '0'
for i in range(10):
maxpossible = int(chron[-1])
for fill in range(int(chron[-1]),10):
if chron + str(fill)*(10-i) <= NN:
maxpossible = fill
chron = chron + str(maxpossible)
return int(chron)
| class Solution:
def monotoneIncreasingDigits(self, N):
"""
:type N: int
:rtype: int
"""
NN = ('000000000000000000' + str(N))[-11:]
chron = '0'
for i in range(10):
maxpossible = int(chron[-1])
for fill in range(int(chron[-1]),10):
if chron + str(fill)*(10-i) <= NN:
maxpossible = fill
chron = chron + str(maxpossible)
return int(chron)
| train | APPS_structured |
In telecomunications we use information coding to detect and prevent errors while sending data.
A parity bit is a bit added to a string of binary code that indicates whether the number of 1-bits in the string is even or odd. Parity bits are used as the simplest form of error detecting code, and can detect a 1 bit error.
In this case we are using even parity: the parity bit is set to `0` if the number of `1`-bits is even, and is set to `1` if odd.
We are using them for the transfer of ASCII characters in binary (7-bit strings): the parity is added to the end of the 7-bit string, forming the 8th bit.
In this Kata you are to test for 1-bit errors and return a new string consisting of all of the correct ASCII caracters **in 7 bit format** (removing the parity bit), or `"error"` in place of ASCII characters in which errors were detected.
For more information on parity bits: https://en.wikipedia.org/wiki/Parity_bit
## Examples
Correct 7 bit string with an even parity bit as the 8th bit:
```
"01011001" <-- The "1" on the right is the parity bit.
```
In this example, there are three 1-bits. Three is an odd number, and the parity bit is set to `1`. No errors are detected, so return `"0101100"` (7 bits).
Example of a string of ASCII characters:
```
"01011001 01101110 01100000 01010110 10001111 01100011"
```
This should return:
```
"0101100 error 0110000 0101011 error 0110001"
``` | def parity_bit(binary):
return ' '.join(map(lambda bits : 'error' if bits.count('1')%2 !=0 else bits[:-1] ,binary.split())) | def parity_bit(binary):
return ' '.join(map(lambda bits : 'error' if bits.count('1')%2 !=0 else bits[:-1] ,binary.split())) | train | APPS_structured |
#Adding values of arrays in a shifted way
You have to write a method, that gets two parameter:
```markdown
1. An array of arrays with int-numbers
2. The shifting value
```
#The method should add the values of the arrays to one new array.
The arrays in the array will all have the same size and this size will always be greater than 0.
The shifting value is always a value from 0 up to the size of the arrays.
There are always arrays in the array, so you do not need to check for null or empty.
#1. Example:
```
[[1,2,3,4,5,6], [7,7,7,7,7,-7]], 0
1,2,3,4,5,6
7,7,7,7,7,-7
--> [8,9,10,11,12,-1]
```
#2. Example
```
[[1,2,3,4,5,6], [7,7,7,7,7,7]], 3
1,2,3,4,5,6
7,7,7,7,7,7
--> [1,2,3,11,12,13,7,7,7]
```
#3. Example
```
[[1,2,3,4,5,6], [7,7,7,-7,7,7], [1,1,1,1,1,1]], 3
1,2,3,4,5,6
7,7,7,-7,7,7
1,1,1,1,1,1
--> [1,2,3,11,12,13,-6,8,8,1,1,1]
```
Have fun coding it and please don't forget to vote and rank this kata! :-)
I have created other katas. Have a look if you like coding and challenges. | from itertools import zip_longest as zl
def sum_arrays(arrays, shift):
shifted = [[0] * shift * i + arr for i, arr in enumerate(arrays)]
return [sum(t) for t in zl(*shifted, fillvalue=0)] | from itertools import zip_longest as zl
def sum_arrays(arrays, shift):
shifted = [[0] * shift * i + arr for i, arr in enumerate(arrays)]
return [sum(t) for t in zl(*shifted, fillvalue=0)] | train | APPS_structured |
Write a function that takes an array/list of numbers and returns a number such that
Explanation
total([1,2,3,4,5]) => 48
1+2=3--\ 3+5 => 8 \
2+3=5--/ \ == 8+12=>20\
==>5+7=> 12 / \ 20+28 => 48
3+4=7--\ / == 12+16=>28/
4+5=9--/ 7+9 => 16 /
if total([1,2,3]) => 8 then
first+second => 3 \
then 3+5 => 8
second+third => 5 /
### Examples
```python
total([-1,-1,-1]) => -4
total([1,2,3,4]) => 20
```
**Note:** each array/list will have at least an element and all elements will be valid numbers. | def total(arr):
while len(arr) > 1:
arr = [x+y for x,y in zip(arr,arr[1:])]
return arr[0] | def total(arr):
while len(arr) > 1:
arr = [x+y for x,y in zip(arr,arr[1:])]
return arr[0] | train | APPS_structured |
It's winter and taking a bath is a delicate matter. Chef has two buckets of water. The first bucket has $v_1$ volume of cold water at temperature $t_1$. The second has $v_2$ volume of hot water at temperature $t_2$. Chef wants to take a bath with at least $v_3$ volume of water which is at exactly $t_3$ temperature. To get the required amount of water, Chef can mix some water obtained from the first and second buckets.
Mixing $v_x$ volume of water at temperature $t_x$ with $v_y$ volume of water at temperature $t_y$ yields $v_x + v_y$ volume of water at temperature calculated as
vxtx+vytyvx+vyvxtx+vytyvx+vy\frac{v_x t_x + v_y t_y}{v_x + v_y}
Help Chef figure out if it is possible for him to take a bath with at least $v_3$ volume of water at temperature $t_3$.
Assume that Chef has no other source of water and that no heat is lost by the water in the buckets with time, so Chef cannot simply wait for the water to cool.
-----Input-----
- The first line contains $n$, the number of test cases. $n$ cases follow.
- Each testcase contains of a single line containing 6 integers $v_1, t_1, v_2, t_2, v_3, t_3$.
-----Output-----
- For each test case, print a single line containing "YES" if Chef can take a bath the way he wants and "NO" otherwise.
-----Constraints-----
- $1 \leq n \leq 10^5$
- $1 \leq v_1, v_2, v_3 \leq 10^6$
- $1 \leq t_1 < t_2 \leq 10^6$
- $1 \leq t_3 \leq 10^6$
-----Sample Input-----
3
5 10 5 20 8 15
5 10 5 20 1 30
5 10 5 20 5 20
-----Sample Output-----
YES
NO
YES
-----Explanation-----
- Case 1: Mixing all the water in both buckets yields 10 volume of water at temperature 15, which is more than the required 8.
- Case 2: It is not possible to get water at 30 temperature.
- Case 3: Chef can take a bath using only the water in the second bucket. | t = int(input())
for i in range(t):
v1,t1,v2,t2,v3,t3 = map(int,input().strip().split())
vx = t2 - t3
vy = t3 - t1
if(t1<=t3<=t2):
if((vx * v3 <= (vx + vy) * v1)and(vy * v3 <= (vx + vy) * v2)):
print("YES")
else:
print("NO")
else:
print("NO") | t = int(input())
for i in range(t):
v1,t1,v2,t2,v3,t3 = map(int,input().strip().split())
vx = t2 - t3
vy = t3 - t1
if(t1<=t3<=t2):
if((vx * v3 <= (vx + vy) * v1)and(vy * v3 <= (vx + vy) * v2)):
print("YES")
else:
print("NO")
else:
print("NO") | train | APPS_structured |
# Your task
Oh no... more lemmings!! And in Lemmings Planet a huge battle
is being fought between the two great rival races: the green
lemmings and the blue lemmings. Everybody was now assigned
to battle and they will fight until one of the races completely
dissapears: the Deadly War has begun!
Every single lemming has a power measure that describes its
ability to fight. When two single lemmings fight with each one,
the lemming with more power survives and the other one dies.
However, the power of the living lemming gets smaller after the
fight, exactly in the value of the power of the lemming that died.
For example, if we have a green lemming with power ``50`` and a
blue lemming with power ``40``, the blue one dies and the green one
survives, but having only power 10 after the battle ``(50-40=10)``.
If two lemmings have the same power when they fight, both of
them die.
In the fight between the two races, there are a certain number of battlefields. Each race assigns one
lemming for each battlefield, starting with the most powerful. So for example, if a race has 5 lemmings
with power ```{50, 50, 40, 40, 30}``` and we have `3` battlefields, then a lemming with power `50` will be assigned
to battlefield 1, another with `50` power will be assigned to battlefield 2 and last a lemming with power `40` will go to battlefield 3. The other race will do the same.
The Deadly War is processed by having each race send its best soldiers as described to the battle-
fields, making a battle round. Then, all battles process at the same time, and some of the lemmings
will emerge victorious (but with less power) and some of them will die. The surviving ones will return to their race’s army and then a new round will begin, with each race sending again its best remaining soldiers to the battlefields. If at some point a race does not have enough soldiers to fill all battlefields, then only the ones with soldiers from both races will have a fight.
The Deadly War ends when one of the races has no more lemmings or when both of them disappear
at the same time. For example, imagine a war with 2 battlefields and a green army with powers `{20,10}` and a blue army with powers `{10, 10, 15}`. The first round will have `20 vs 15` in battlefield 1 and `10 vs 10` in battlefield 2. After these battles, green race will still have a power `5` lemming (that won on battlefield 1) and blue race will have one with power 10 (that did not fight). The ones in battlefield 2
died, since they both had the same power. Afer that comes a second round, and only battlefield 1 will have a fight, being `5 vs 10`. The blue lemming wins, killing the last green soldier and giving the victory to the blue race!
But in the real battle, will victory be green or blue?
Given the number of battefields and the armies of both races, your task is to discover which race
will win the Deadly War and show the power of the surviving soldiers.
## Input
You are given B, SG and SB, representing respectively the number of battlefields available, a vector of integers size `n` of lemmings in the green army
and a vector of integers size `n` of lemmings in the blue army (1 ≤ B, SG, SB ≤ 100000).
The lemmings in each army do not need to come in any particular order.
## Output
For each test case you should return :
• "Green and Blue died" if both races died in the same round
• "Green wins : Each surviving soldier in descending order" if the green army won the Deadly War
• "Blue wins : Each surviving soldier in descending order" if the blue army won the Deadly War
## Example
```python
lemming_battle(5, [10], [10]) == "Green and Blue died"
lemming_battle(2, [20,10], [10,10,15]) == "Blue wins: 5"
lemming_battle(3, [50,40,30,40,50], [50,30,30,20,60]) == "Green wins: 10 10"
``` | def text(green, blue):
if green:
return "Green wins: {0}".format(' '.join(str(x) for x in green))
elif blue:
return "Blue wins: {0}".format(' '.join(str(x) for x in blue))
return 'Green and Blue died'
def lemming_battle(battlefield, green, blue):
green, blue = sorted(green, reverse=True), sorted(blue, reverse=True)
if not green or not blue:
return text(green, blue)
for i in range(battlefield):
try:
res = green[i] - blue[i]
if res == 0:
blue[i] = False
green[i] = False
elif res > 0:
green[i] = res
blue[i] = False
else:
blue[i] = abs(res)
green[i] = False
except IndexError:
break
return lemming_battle(battlefield, [x for x in green if x], [x for x in blue if x])
| def text(green, blue):
if green:
return "Green wins: {0}".format(' '.join(str(x) for x in green))
elif blue:
return "Blue wins: {0}".format(' '.join(str(x) for x in blue))
return 'Green and Blue died'
def lemming_battle(battlefield, green, blue):
green, blue = sorted(green, reverse=True), sorted(blue, reverse=True)
if not green or not blue:
return text(green, blue)
for i in range(battlefield):
try:
res = green[i] - blue[i]
if res == 0:
blue[i] = False
green[i] = False
elif res > 0:
green[i] = res
blue[i] = False
else:
blue[i] = abs(res)
green[i] = False
except IndexError:
break
return lemming_battle(battlefield, [x for x in green if x], [x for x in blue if x])
| train | APPS_structured |
Given an integer n, return a list of all simplified fractions between 0 and 1 (exclusive) such that the denominator is less-than-or-equal-to n. The fractions can be in any order.
Example 1:
Input: n = 2
Output: ["1/2"]
Explanation: "1/2" is the only unique fraction with a denominator less-than-or-equal-to 2.
Example 2:
Input: n = 3
Output: ["1/2","1/3","2/3"]
Example 3:
Input: n = 4
Output: ["1/2","1/3","1/4","2/3","3/4"]
Explanation: "2/4" is not a simplified fraction because it can be simplified to "1/2".
Example 4:
Input: n = 1
Output: []
Constraints:
1 <= n <= 100 | class Solution:
def simplifiedFractions(self, n: int) -> List[str]:
def gcd(a: int, b: int) -> int:
if a < b:
a, b = b, a
if b == 0:
return a
return gcd(b, a % b)
out = []
for denom in range(2, n + 1):
for num in range(1, denom):
if gcd(denom, num) == 1:
out.append(str(num) + '/' + str(denom))
return out | class Solution:
def simplifiedFractions(self, n: int) -> List[str]:
def gcd(a: int, b: int) -> int:
if a < b:
a, b = b, a
if b == 0:
return a
return gcd(b, a % b)
out = []
for denom in range(2, n + 1):
for num in range(1, denom):
if gcd(denom, num) == 1:
out.append(str(num) + '/' + str(denom))
return out | train | APPS_structured |
Given two strings s and t which consist of only lowercase letters.
String t is generated by random shuffling string s and then add one more letter at a random position.
Find the letter that was added in t.
Example:
Input:
s = "abcd"
t = "abcde"
Output:
e
Explanation:
'e' is the letter that was added. | class Solution:
def findTheDifference(self, s, t):
"""
:type s: str
:type t: str
:rtype: str
"""
for i in set(t):
if i not in set(s) or t.count(i)>s.count(i):
return i | class Solution:
def findTheDifference(self, s, t):
"""
:type s: str
:type t: str
:rtype: str
"""
for i in set(t):
if i not in set(s) or t.count(i)>s.count(i):
return i | train | APPS_structured |
# Task
Pero has been into robotics recently, so he decided to make a robot that checks whether a deck of poker cards is complete.
He’s already done a fair share of work - he wrote a programme that recognizes the suits of the cards. For simplicity’s sake, we can assume that all cards have a suit and a number.
The suit of the card is one of the characters `"P", "K", "H", "T"`, and the number of the card is an integer between `1` and `13`. The robot labels each card in the format `TXY` where `T` is the suit and `XY` is the number. If the card’s number consists of one digit, then X = 0. For example, the card of suit `"P"` and number `9` is labelled `"P09"`.
A complete deck has `52` cards in total. For each of the four suits there is exactly one card with a number between `1` and `13`.
The robot has read the labels of all the cards in the deck and combined them into the string `s`.
Your task is to help Pero finish the robot by writing a program that reads the string made out of card labels and returns the number of cards that are missing for each suit.
If there are two same cards in the deck, return array with `[-1, -1, -1, -1]` instead.
# Input/Output
- `[input]` string `s`
A correct string of card labels. 0 ≤ |S| ≤ 1000
- `[output]` an integer array
Array of four elements, representing the number of missing card of suits `"P", "K", "H", and "T"` respectively. If there are two same cards in the deck, return `[-1, -1, -1, -1]` instead.
# Example
For `s = "P01K02H03H04"`, the output should be `[12, 12, 11, 13]`.
`1` card from `"P"` suit, `1` card from `"K"` suit, `2` cards from `"H"` suit, no card from `"T"` suit.
For `s = "H02H10P11H02"`, the output should be `[-1, -1, -1, -1]`.
There are two same cards `"H02"` in the string `s`. | import re
from collections import Counter
def cards_and_pero(s):
cardDeck = re.findall(r'([PKHT])(\d{2})', s)
cnt = Counter(suit for suit,_ in cardDeck)
return [-1]*4 if len(set(cardDeck)) != len(cardDeck) else [ 13-cnt[suit] for suit in "PKHT"] | import re
from collections import Counter
def cards_and_pero(s):
cardDeck = re.findall(r'([PKHT])(\d{2})', s)
cnt = Counter(suit for suit,_ in cardDeck)
return [-1]*4 if len(set(cardDeck)) != len(cardDeck) else [ 13-cnt[suit] for suit in "PKHT"] | train | APPS_structured |
Doctor Kunj installed new software on cyborg Shresth.
This software introduced Shresth to range minimum queries.
Cyborg Shresth thought of T$T$ different problems in each of which you will be given
an array A$A$ of length N$N$ and an array B$B$ of length M$M$. In each of these problems, you have to calculate:
∑mi=1∑mj=irangeMin(B[i],B[j])$\sum_{i=1}^m \sum_{j=i}^m rangeMin(B[i],B[j])$
Where rangeMin(i,j)$rangeMin(i,j)$ returns the minimum element in the range of indices i$i$ to j$j$ (both included) in array A$A$.
It is given that array B$B$ consists of pairwise distinct elements and is in ascending order.
-----Input Format:-----
- First line will contain T$T$, the number of different problems Cyborg Shresth thought of.
- Each problem's input data will be given in three different lines.
- The first line will contain N$N$ and M$M$, the length of array A$A$ and B$B$ respectively.
- The second line will contain N$N$ space separated positive integers, which form the array A$A$. -
- The third line will contain M$M$ space separated positive integers, which form the array B$B$.
-----Output Format:-----
- For each different problem, print on a new line the answer to the problem, i.e. the value of ∑mi=1∑mj=irangeMin(B[i],B[j])$\sum_{i=1}^m \sum_{j=i}^m rangeMin(B[i],B[j])$ .
-----Constraints:-----
- 1≤T≤105$1 \leq T \leq {10}^5$
- 1≤N≤105$1 \leq N \leq {10}^5$
- 1≤M≤105$1 \leq M \leq {10}^5$
- 1≤A[i]≤109$1 \leq A[i] \leq 10^9$
- 1≤B[i]≤N$1 \leq B[i] \leq N$
It is guaranteed that the sum of N$N$ over all test cases does not exceed 106${10}^6$ .
-----Sample Input:-----
1
7 3
10 3 7 9 1 19 2
1 4 6
-----Sample Output:-----
43
-----Explanation:-----
For the given array A$A$, we have to calculate rangeMin(1,1)+rangeMin(1,4)+rangeMin(1,6)+rangeMin(4,4)+rangeMin(4,6)+rangeMin(6,6)$rangeMin(1,1) + rangeMin(1,4) + rangeMin(1,6) + rangeMin(4,4) + rangeMin(4,6) + rangeMin(6,6) $.
This is equal to 10+3+1+9+1+19=43$10 + 3 + 1 + 9 + 1 +19 = 43$. | def f(a,n):
l,r,s1,s2 = [0]*n, [0]*n, [], []
for i in range(n):
count = 1
while(len(s1)>0 and a[i]<s1[-1][0]):
count += s1[-1][1]
s1.pop()
s1.append((a[i],count))
l[i] = count
for i in range(n-1,-1,-1):
count = 1
while(len(s2)>0 and a[i]<=s2[-1][0]):
count += s2[-1][1]
s2.pop()
s2.append((a[i],count))
r[i] = count
count = 0
for i in range(n):
count += a[i]*l[i]*r[i]
return count
t = int(input()) | def f(a,n):
l,r,s1,s2 = [0]*n, [0]*n, [], []
for i in range(n):
count = 1
while(len(s1)>0 and a[i]<s1[-1][0]):
count += s1[-1][1]
s1.pop()
s1.append((a[i],count))
l[i] = count
for i in range(n-1,-1,-1):
count = 1
while(len(s2)>0 and a[i]<=s2[-1][0]):
count += s2[-1][1]
s2.pop()
s2.append((a[i],count))
r[i] = count
count = 0
for i in range(n):
count += a[i]*l[i]*r[i]
return count
t = int(input()) | train | APPS_structured |
Chef has a circular sequence $A$ of $N$ non-negative integers $A_1, A_2, \ldots, A_N$ where $A_i$ and $A_{i+1}$ are considered adjacent, and elements $A_1$ and $A_N$ are considered adjacent.
An operation on position $p$ in array $A$ is defined as replacing $A_p$ by the bitwise OR of elements adjacent to $A_p$. Formally, an operation is defined as:
- If $p = 1$, replace $A_1$ with $A_N | A_{2}$
- If $1 < p < N$, replace $A_p$ with $A_{p-1} | A_{p+1}$
- If $p = N$, replace $A_N$ with $A_{N-1} | A_1$
Here, '|' denotes the bitwise OR operation.
Now, Chef must apply operations at each position exactly once, but he may apply the operations in any order.
Being a friend of Chef, you are required to find a sequence of operations, such that after applying all the $N$ operations, the bitwise OR of the resulting array is $K$, or determine that no such sequence of operations exist.
-----Input:-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first line of each test case contains two space-separated integers $N$ and $K$ denoting the number of elements, and the required bitwise OR after operations.
- The second line contains $N$ space-separated integers $A_1, A_2, \ldots, A_N$.
-----Output:-----
For each test case, if no valid sequence of operations exist, print -1.
Otherwise, print $N$ space-separated integers in which $i$-th integer denote the position chosen in the $i$-th operation. If there are multiple valid sequences of operations, you may print any valid sequence.
-----Constraints-----
- $1 \le T \le 10^5$
- $3 \le N \le 2*10^5$
- $0 \le A_i, K \le 10^9$
- It's guaranteed that the total length of the arrays in one test file doesn't exceed $10^6$
-----Sample Input:-----
5
3 6
2 1 6
3 6
2 1 5
3 7
2 4 6
3 7
1 2 4
3 7
1 2 6
-----Sample Output:-----
2 1 3
-1
-1
-1
2 3 1
-----Explanation:-----
In the first test case, initially, the sequence is {2, 1, 6}.
- Chef applies the operation on the $2^{nd}$ index, so the sequence converts to {2, 6, 6}.
- Next, Chef applies the operation on the $1^{st}$ index, so the sequence converts to {6, 6, 6}.
- Next, Chef applies the operation on the $3^{rd}$ index, and this time sequence does not change.
The final sequence is {6, 6, 6}, and bitwise OR of this sequence is $6$ that is equal to given $K$. | # cook your dish here
tests = int(input())
for _ in range(tests):
n, k = [int(j) for j in input().split()]
nums = [int(j) for j in input().split()]
check = [(j - (j & k)) != 0 for j in nums]
impossible = check[0] and check[-1]
for i in range(n-1):
impossible = impossible or (check[i] and check[i+1])
if impossible:
print(-1)
elif sum(check) != 0:
start = -1
for i in range(-1,n-1):
if check[i]:
nums[i] = nums[i-1] | nums[i+1]
if start == -1:
start = i+1
for i in range(start,start+n):
if not check[i%n]:
nums[i%n] = nums[(i-1)%n] | nums[(i+1)%n]
answer = 0
for i in range(n):
answer = answer | nums[i]
if answer == k:
for i in range(n):
if check[i]:
print(i+1, end = " ")
for i in range(start,start+n):
if not check[i%n]:
print(i%n+1, end = " ")
print("")
else:
print(-1)
else:
for i in range(-1,n-1):
if nums[i] == nums[i] & (nums[i-1] | nums[i+1]):
for j in range(i,i+n):
nums[j%n] = nums[(j-1)%n] | nums[(j+1)%n]
answer = 0
for j in range(n):
answer = answer | nums[j]
if answer == k:
for j in range(i,i+n):
print((j+n)%n+1,end = " ")
print("")
else:
print(-1)
impossible = True
break
if not impossible:
for i in range(n):
ss = list(nums)
for j in range(i,i+n):
ss[j%n] = ss[(j+1)%n] | ss[(j-1)%n]
answer = 0
for j in range(n):
answer = answer | ss[j]
if answer == k:
for j in range(i,i+n):
print((j%n)+1,end=" ")
print("")
impossible = True
break
if not impossible:
print(-1)
| # cook your dish here
tests = int(input())
for _ in range(tests):
n, k = [int(j) for j in input().split()]
nums = [int(j) for j in input().split()]
check = [(j - (j & k)) != 0 for j in nums]
impossible = check[0] and check[-1]
for i in range(n-1):
impossible = impossible or (check[i] and check[i+1])
if impossible:
print(-1)
elif sum(check) != 0:
start = -1
for i in range(-1,n-1):
if check[i]:
nums[i] = nums[i-1] | nums[i+1]
if start == -1:
start = i+1
for i in range(start,start+n):
if not check[i%n]:
nums[i%n] = nums[(i-1)%n] | nums[(i+1)%n]
answer = 0
for i in range(n):
answer = answer | nums[i]
if answer == k:
for i in range(n):
if check[i]:
print(i+1, end = " ")
for i in range(start,start+n):
if not check[i%n]:
print(i%n+1, end = " ")
print("")
else:
print(-1)
else:
for i in range(-1,n-1):
if nums[i] == nums[i] & (nums[i-1] | nums[i+1]):
for j in range(i,i+n):
nums[j%n] = nums[(j-1)%n] | nums[(j+1)%n]
answer = 0
for j in range(n):
answer = answer | nums[j]
if answer == k:
for j in range(i,i+n):
print((j+n)%n+1,end = " ")
print("")
else:
print(-1)
impossible = True
break
if not impossible:
for i in range(n):
ss = list(nums)
for j in range(i,i+n):
ss[j%n] = ss[(j+1)%n] | ss[(j-1)%n]
answer = 0
for j in range(n):
answer = answer | ss[j]
if answer == k:
for j in range(i,i+n):
print((j%n)+1,end=" ")
print("")
impossible = True
break
if not impossible:
print(-1)
| train | APPS_structured |
Professor Chambouliard hast just discovered a new type of magnet material. He put particles of this material in a box made of small boxes arranged
in K rows and N columns as a kind of **2D matrix** `K x N` where `K` and `N` are postive integers.
He thinks that his calculations show that the force exerted by the particle in the small box `(k, n)` is:
The total force exerted by the first row with `k = 1` is:
We can go on with `k = 2` and then `k = 3` etc ... and consider:
## Task:
To help Professor Chambouliard can we calculate the function `doubles` that will take as parameter `maxk` and `maxn` such that `doubles(maxk, maxn) = S(maxk, maxn)`?
Experiences seems to show that this could be something around `0.7` when `maxk` and `maxn` are big enough.
### Examples:
```
doubles(1, 3) => 0.4236111111111111
doubles(1, 10) => 0.5580321939764581
doubles(10, 100) => 0.6832948559787737
```
### Notes:
- In `u(1, N)` the dot is the *multiplication operator*.
- Don't truncate or round:
Have a look in "RUN EXAMPLES" at "assertFuzzyEquals".
- [link to symbol Sigma](https://en.wikipedia.org/wiki/Summation) | def v(k,n):
return (1.0/k)*(n+1)**(-2*k)
def doubles(maxk, maxn):
total = 0.0
k = 1.0
while k <= maxk:
n = 1.0
while n <= maxn:
total += v(k,n)
n += 1
k += 1
return total | def v(k,n):
return (1.0/k)*(n+1)**(-2*k)
def doubles(maxk, maxn):
total = 0.0
k = 1.0
while k <= maxk:
n = 1.0
while n <= maxn:
total += v(k,n)
n += 1
k += 1
return total | train | APPS_structured |
This kata is part of the collection [Mary's Puzzle Books](https://www.codewars.com/collections/marys-puzzle-books).
Mary brought home a "spot the differences" book. The book is full of a bunch of problems, and each problem consists of two strings that are similar. However, in each string there are a few characters that are different. An example puzzle from her book is:
```
String 1: "abcdefg"
String 2: "abcqetg"
```
Notice how the "d" from String 1 has become a "q" in String 2, and "f" from String 1 has become a "t" in String 2.
It's your job to help Mary solve the puzzles. Write a program `spot_diff`/`Spot` that will compare the two strings and return a list with the positions where the two strings differ. In the example above, your program should return `[3, 5]` because String 1 is different from String 2 at positions 3 and 5.
NOTES:
```if-not:csharp
• If both strings are the same, return `[]`
```
```if:csharp
• If both strings are the same, return `new List()`
```
• Both strings will always be the same length
• Capitalization and punctuation matter | def spot_diff(s1, s2):
r=[]
for i in range (len(s1)):
if s1[i]!=s2[i]: r.append(i)
return r | def spot_diff(s1, s2):
r=[]
for i in range (len(s1)):
if s1[i]!=s2[i]: r.append(i)
return r | train | APPS_structured |
# Task
An `amazon` (also known as a queen+knight compound) is an imaginary chess piece that can move like a `queen` or a `knight` (or, equivalently, like a `rook`, `bishop`, or `knight`). The diagram below shows all squares which the amazon attacks from e4 (circles represent knight-like moves while crosses correspond to queen-like moves).
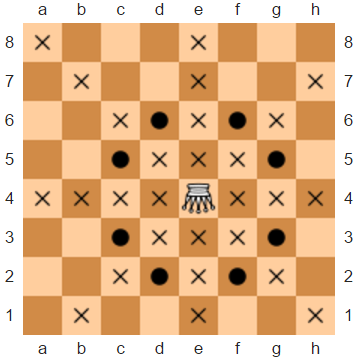
Recently you've come across a diagram with only three pieces left on the board: a `white amazon`, `white king` and `black king`.
It's black's move. You don't have time to determine whether the game is over or not, but you'd like to figure it out in your head.
Unfortunately, the diagram is smudged and you can't see the position of the `black king`, so it looks like you'll have to check them all.
Given the positions of white pieces on a standard chessboard, determine the number of possible black king's positions such that:
* It's a checkmate (i.e. black's king is under amazon's
attack and it cannot make a valid move);
* It's a check (i.e. black's king is under amazon's attack
but it can reach a safe square in one move);
* It's a stalemate (i.e. black's king is on a safe square
but it cannot make a valid move);
* Black's king is on a safe square and it can make a valid move.
Note that two kings cannot be placed on two adjacent squares (including two diagonally adjacent ones).
# Example
For `king = "d3" and amazon = "e4"`, the output should be `[5, 21, 0, 29]`.
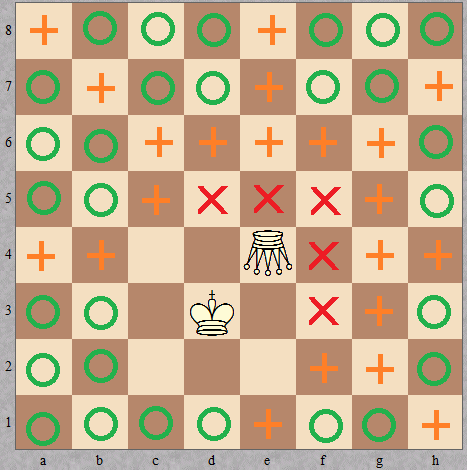
`Red crosses` correspond to the `checkmate` positions, `orange pluses` refer to `checks` and `green circles` denote `safe squares`.
For `king = "a1" and amazon = "g5"`, the output should be `[0, 29, 1, 29]`.
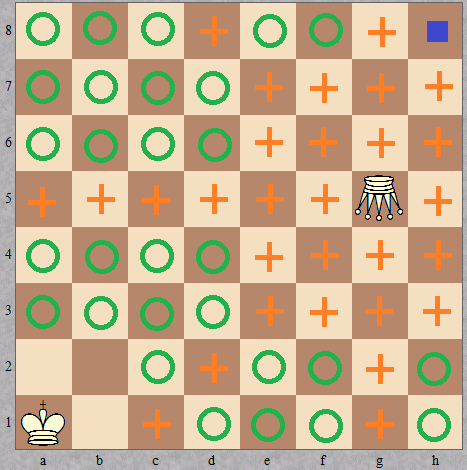
`Stalemate` position is marked by a `blue square`.
# Input
- String `king`
Position of white's king in chess notation.
- String `amazon`
Position of white's amazon in the same notation.
Constraints: `amazon ≠ king.`
# Output
An array of four integers, each equal to the number of black's king positions corresponding to a specific situation. The integers should be presented in the same order as the situations were described, `i.e. 0 for checkmates, 1 for checks, etc`. | from itertools import product
def get_pos(coord):
x = ord(coord[0]) - ord('a')
y = int(coord[1]) - 1
return [y, x]
def place_pieces(board, king, amazon, connected):
coord_king = get_pos(king)
board[coord_king[0]][coord_king[1]] = 8
coord_amazon = get_pos(amazon)
board[coord_amazon[0]][coord_amazon[1]] = 9
if coord_king[0] - 1 <= coord_amazon[0] <= coord_king[0] + 1 \
and coord_king[1] - 1 <= coord_amazon[1] <= coord_king[1] + 1:
connected[0] = 1
mark_attacked_squares(board, coord_king, coord_amazon)
def mark_attacked_squares(board, coord_king, coord_amazon):
mark_queen(board, coord_amazon)
mark_knight(board, coord_amazon)
mark_king(board, coord_king)
board[coord_amazon[0]][coord_amazon[1]] = 9
def mark_king(board, coord_king):
y = coord_king[0]
x = coord_king[1]
for i in product([-1, 0, 1], repeat=2):
if 0 <= y + i[0] < 8 and 0 <= x + i[1] < 8:
board[y + i[0]][x + i[1]] = 3
def mark_knight(board, coord_amazon):
y = coord_amazon[0]
x = coord_amazon[1]
for i in product([-2, -1, 1, 2], repeat=2):
if 0 <= y + i[0] < 8 and 0 <= x + i[1] < 8:
if board[y + i[0]][x + i[1]] == 0:
board[y + i[0]][x + i[1]] = 2
def mark_queen(board, coord_amazon):
y = coord_amazon[0]
x = coord_amazon[1]
while y >= 0:
if board[y][x] == 0:
board[y][x] = 2
if board[y][x] == 8:
break
y -= 1
y = coord_amazon[0]
while y < 8:
if board[y][x] == 0:
board[y][x] = 2
if board[y][x] == 8:
break
y += 1
y = coord_amazon[0]
while x >= 0:
if board[y][x] == 0:
board[y][x] = 2
if board[y][x] == 8:
break
x -= 1
x = coord_amazon[1]
while x < 8:
if board[y][x] == 0:
board[y][x] = 2
if board[y][x] == 8:
break
x += 1
x = coord_amazon[1]
while x >= 0 and y >= 0:
if board[y][x] == 0:
board[y][x] = 2
if board[y][x] == 8:
break
x -= 1
y -= 1
y = coord_amazon[0]
x = coord_amazon[1]
while x < 8 and y >= 0:
if board[y][x] == 0:
board[y][x] = 2
if board[y][x] == 8:
break
x += 1
y -= 1
y = coord_amazon[0]
x = coord_amazon[1]
while x >= 0 and y < 8:
if board[y][x] == 0:
board[y][x] = 2
if board[y][x] == 8:
break
x -= 1
y += 1
y = coord_amazon[0]
x = coord_amazon[1]
while x < 8 and y < 8:
if board[y][x] == 0:
board[y][x] = 2
if board[y][x] == 8:
break
x += 1
y += 1
y = coord_amazon[0]
x = coord_amazon[1]
def check_safe(board, y, x, connected):
for i in product([-1, 0, 1], repeat=2):
if 0 <= y + i[0] < 8 and 0 <= x + i[1] < 8:
if not (i[0] == 0 and i[1] == 0) and \
(board[y + i[0]][x + i[1]] == 0 or \
(connected[0] == 0 and board[y + i[0]][x + i[1]] == 9)):
return 1
return 0
def count_states(board, connected):
stalemate = check = checkmate = safe = 0
for y in range(8):
for x in range(8):
if board[y][x] == 0:
if check_safe(board, y, x, connected) == 1:
safe += 1
else:
stalemate += 1
elif board[y][x] == 2:
if check_safe(board, y, x, connected) == 0:
checkmate += 1
else:
check += 1
return [checkmate, check, stalemate, safe]
def amazon_check_mate(king, amazon):
board = [[0 for i in range(8)] for j in range(8)]
connected = [1]
connected[0] = 0
place_pieces(board, king, amazon, connected)
return count_states(board, connected)
# 0 = safe
# 8 = whiteking
# 9 = amazon
# 2 = attacked
# 3 = black king can't be here
| from itertools import product
def get_pos(coord):
x = ord(coord[0]) - ord('a')
y = int(coord[1]) - 1
return [y, x]
def place_pieces(board, king, amazon, connected):
coord_king = get_pos(king)
board[coord_king[0]][coord_king[1]] = 8
coord_amazon = get_pos(amazon)
board[coord_amazon[0]][coord_amazon[1]] = 9
if coord_king[0] - 1 <= coord_amazon[0] <= coord_king[0] + 1 \
and coord_king[1] - 1 <= coord_amazon[1] <= coord_king[1] + 1:
connected[0] = 1
mark_attacked_squares(board, coord_king, coord_amazon)
def mark_attacked_squares(board, coord_king, coord_amazon):
mark_queen(board, coord_amazon)
mark_knight(board, coord_amazon)
mark_king(board, coord_king)
board[coord_amazon[0]][coord_amazon[1]] = 9
def mark_king(board, coord_king):
y = coord_king[0]
x = coord_king[1]
for i in product([-1, 0, 1], repeat=2):
if 0 <= y + i[0] < 8 and 0 <= x + i[1] < 8:
board[y + i[0]][x + i[1]] = 3
def mark_knight(board, coord_amazon):
y = coord_amazon[0]
x = coord_amazon[1]
for i in product([-2, -1, 1, 2], repeat=2):
if 0 <= y + i[0] < 8 and 0 <= x + i[1] < 8:
if board[y + i[0]][x + i[1]] == 0:
board[y + i[0]][x + i[1]] = 2
def mark_queen(board, coord_amazon):
y = coord_amazon[0]
x = coord_amazon[1]
while y >= 0:
if board[y][x] == 0:
board[y][x] = 2
if board[y][x] == 8:
break
y -= 1
y = coord_amazon[0]
while y < 8:
if board[y][x] == 0:
board[y][x] = 2
if board[y][x] == 8:
break
y += 1
y = coord_amazon[0]
while x >= 0:
if board[y][x] == 0:
board[y][x] = 2
if board[y][x] == 8:
break
x -= 1
x = coord_amazon[1]
while x < 8:
if board[y][x] == 0:
board[y][x] = 2
if board[y][x] == 8:
break
x += 1
x = coord_amazon[1]
while x >= 0 and y >= 0:
if board[y][x] == 0:
board[y][x] = 2
if board[y][x] == 8:
break
x -= 1
y -= 1
y = coord_amazon[0]
x = coord_amazon[1]
while x < 8 and y >= 0:
if board[y][x] == 0:
board[y][x] = 2
if board[y][x] == 8:
break
x += 1
y -= 1
y = coord_amazon[0]
x = coord_amazon[1]
while x >= 0 and y < 8:
if board[y][x] == 0:
board[y][x] = 2
if board[y][x] == 8:
break
x -= 1
y += 1
y = coord_amazon[0]
x = coord_amazon[1]
while x < 8 and y < 8:
if board[y][x] == 0:
board[y][x] = 2
if board[y][x] == 8:
break
x += 1
y += 1
y = coord_amazon[0]
x = coord_amazon[1]
def check_safe(board, y, x, connected):
for i in product([-1, 0, 1], repeat=2):
if 0 <= y + i[0] < 8 and 0 <= x + i[1] < 8:
if not (i[0] == 0 and i[1] == 0) and \
(board[y + i[0]][x + i[1]] == 0 or \
(connected[0] == 0 and board[y + i[0]][x + i[1]] == 9)):
return 1
return 0
def count_states(board, connected):
stalemate = check = checkmate = safe = 0
for y in range(8):
for x in range(8):
if board[y][x] == 0:
if check_safe(board, y, x, connected) == 1:
safe += 1
else:
stalemate += 1
elif board[y][x] == 2:
if check_safe(board, y, x, connected) == 0:
checkmate += 1
else:
check += 1
return [checkmate, check, stalemate, safe]
def amazon_check_mate(king, amazon):
board = [[0 for i in range(8)] for j in range(8)]
connected = [1]
connected[0] = 0
place_pieces(board, king, amazon, connected)
return count_states(board, connected)
# 0 = safe
# 8 = whiteking
# 9 = amazon
# 2 = attacked
# 3 = black king can't be here
| train | APPS_structured |
# Do you speak retsec?
You and your friends want to play undercover agents. In order to exchange your *secret* messages, you've come up with the following system: you take the word, cut it in half, and place the first half behind the latter. If the word has an uneven number of characters, you leave the middle at its previous place:

That way, you'll be able to exchange your messages in private.
# Task
You're given a single word. Your task is to swap the halves. If the word has an uneven length, leave the character in the middle at that position and swap the chunks around it.
## Examples
```python
reverse_by_center("secret") == "retsec" # no center character
reverse_by_center("agent") == "nteag" # center character is "e"
```
## Remarks
Don't use this to actually exchange messages in private. | def reverse_by_center(s):
q, r = divmod(len(s), 2)
return s[-q:] + s[q:q+r] + s[:q] | def reverse_by_center(s):
q, r = divmod(len(s), 2)
return s[-q:] + s[q:q+r] + s[:q] | train | APPS_structured |
### Vaccinations for children under 5
You have been put in charge of administrating vaccinations for children in your local area. Write a function that will generate a list of vaccines for each child presented for vaccination, based on the child's age and vaccination history, and the month of the year.
#### The function takes three parameters: age, status and month
- The parameter 'age' will be given in weeks up to 16 weeks, and thereafter in months. You can assume that children presented will be scheduled for vaccination (eg '16 weeks', '12 months' etc).
- The parameter 'status' indicates if the child has missed a scheduled vaccination, and the argument will be a string that says 'up-to-date', or a scheduled stage (eg '8 weeks') that has been missed, in which case you need to add any missing shots to the list. Only one missed vaccination stage will be passed in per function call.
- If the month is 'september', 'october' or 'november' add 'offer fluVaccine' to the list.
- Make sure there are no duplicates in the returned list, and sort it alphabetically.
#### Example input and output
~~~~
input ('12 weeks', 'up-to-date', 'december')
output ['fiveInOne', 'rotavirus']
input ('12 months', '16 weeks', 'june')
output ['fiveInOne', 'hibMenC', 'measlesMumpsRubella', 'meningitisB', 'pneumococcal']
input ('40 months', '12 months', 'october')
output ['hibMenC', 'measlesMumpsRubella', 'meningitisB', 'offer fluVaccine', 'preSchoolBooster']
~~~~
#### To save you typing it up, here is the vaccinations list
~~~~
fiveInOne : ['8 weeks', '12 weeks', '16 weeks'],
//Protects against: diphtheria, tetanus, whooping cough, polio and Hib (Haemophilus influenzae type b)
pneumococcal : ['8 weeks', '16 weeks'],
//Protects against: some types of pneumococcal infection
rotavirus : ['8 weeks', '12 weeks'],
//Protects against: rotavirus infection, a common cause of childhood diarrhoea and sickness
meningitisB : ['8 weeks', '16 weeks', '12 months'],
//Protects against: meningitis caused by meningococcal type B bacteria
hibMenC : ['12 months'],
//Protects against: Haemophilus influenzae type b (Hib), meningitis caused by meningococcal group C bacteria
measlesMumpsRubella : ['12 months', '40 months'],
//Protects against: measles, mumps and rubella
fluVaccine : ['september','october','november'],
//Given at: annually in Sept/Oct
preSchoolBooster : ['40 months']
//Protects against: diphtheria, tetanus, whooping cough and polio
~~~~ | VACCINATIONS = {
"fiveInOne": ["8 weeks", "12 weeks", "16 weeks"],
"pneumococcal": ["8 weeks", "16 weeks"],
"rotavirus": ["8 weeks", "12 weeks"],
"meningitisB": ["8 weeks", "16 weeks", "12 months"],
"hibMenC": ["12 months"],
"measlesMumpsRubella": ["12 months", "40 months"],
"preSchoolBooster": ["40 months"],
}
def vaccine_list(age, status, month):
return sorted(
({"offer fluVaccine"} if month in ["september", "october", "november"] else set())
| {v for v, times in VACCINATIONS.items() if age in times or (status != "up-to-date" and status in times)}
) | VACCINATIONS = {
"fiveInOne": ["8 weeks", "12 weeks", "16 weeks"],
"pneumococcal": ["8 weeks", "16 weeks"],
"rotavirus": ["8 weeks", "12 weeks"],
"meningitisB": ["8 weeks", "16 weeks", "12 months"],
"hibMenC": ["12 months"],
"measlesMumpsRubella": ["12 months", "40 months"],
"preSchoolBooster": ["40 months"],
}
def vaccine_list(age, status, month):
return sorted(
({"offer fluVaccine"} if month in ["september", "october", "november"] else set())
| {v for v, times in VACCINATIONS.items() if age in times or (status != "up-to-date" and status in times)}
) | train | APPS_structured |
Give the summation of all even numbers in a Fibonacci sequence up to, but not including, the maximum value.
The Fibonacci sequence is a series of numbers where the next value is the addition of the previous two values. The series starts with 0 and 1:
0 1 1 2 3 5 8 13 21...
For example:
```python
eve_fib(0)==0
eve_fib(33)==10
eve_fib(25997544)==19544084
``` | def even_fib(m):
a, b = 0, 1
sum = 0
while a < m:
if a % 2 == 0:
sum += a
a, b = b, a + b
return sum | def even_fib(m):
a, b = 0, 1
sum = 0
while a < m:
if a % 2 == 0:
sum += a
a, b = b, a + b
return sum | train | APPS_structured |
You have been tasked with converting a number from base i (sqrt of -1) to base 10.
Recall how bases are defined:
abcdef = a * 10^5 + b * 10^4 + c * 10^3 + d * 10^2 + e * 10^1 + f * 10^0
Base i follows then like this:
... i^4 + i^3 + i^2 + i^1 + i^0
The only numbers in any place will be 1 or 0
Examples:
101 in base i would be:
1 * i^2 + 0 * i^1 + 1*i^0 = -1 + 0 + 1 = 0
10010 in base i would be:
1*i^4 + 0 * i^3 + 0 * i^2 + 1 * i^1 + 0 * i^0 = 1 + -i = 1-i
You must take some number, n, in base i and convert it into a number in base 10, in the format of a 2x1 vector.
[a,b] = a+b*i
The number will always be some combination of 0 and 1
In Python you will be given an integer.
In Javascript and C++ you will be given a string | from itertools import cycle
def convert(n):
c, i, val = cycle([1,1,-1,-1]), cycle([0,1]), [0, 0]
for l in str(n)[::-1]: val[next(i)] += next(c) * (l == '1')
return val | from itertools import cycle
def convert(n):
c, i, val = cycle([1,1,-1,-1]), cycle([0,1]), [0, 0]
for l in str(n)[::-1]: val[next(i)] += next(c) * (l == '1')
return val | train | APPS_structured |
Given a binary string $S$ consisting of only 1’s and 0’s where 1 represents a Square and 0 represents a Circle. The diameter of the circle and the side of the square must be any integer (obviously > 0) . You will have to perfectly inscribe (as shown in the example below) the respective geometric figure at $S$$i+1$ inside of $S$$i$ where $i$ $\epsilon$ $[0,N-2]$, if it is possible. Note that, it will not be possible to inscribe if the dimension of the geometric figure you are perfectly inscribing is not an integer and you will discard the rest of the string. Find the maximum number of circles we can inscribe in a square according to the given string.
For a given binary string there can be only one geometric figure and this figure is concentric.
For example : the string 1100 can be represented as the figure below, the first two squares have the same side length and the next two circles have the same diameter.
Another example : the string 0001 can be represented as the one given below
Again here, we have 3 circles of the same diameter and one square inscribed in them.
-----Input:-----
The first line contains $N$, the number of strings
Then each of the next $N$ lines contains a binary string $S$.
-----Output:-----
The $N$ lines of output should have $N$ integers in separate lines, the maximum number of circles we can inscribe in a square according to the given string $S$ .
-----Constraints-----
- 1 $\leq$ $N$ $\leq$ 103
- 1 $\leq$ length of string $S$ $\leq$ 104
-----Sample Input:-----
3
1110
0010
1001000
-----Sample Output:-----
1
0
2
-----Explanation:-----
In the first case, we can inscribe the string 1110 as : three squares of side length 4 units (on top of each other) and then we can inscribe one circle of diameter 4 units.
The answer is 1 since, there is 1 circle inscribed in a square.
In the second case 0010, Let the first two circles be of some diameter 10, we can see that we cannot inscribe another square of any integer dimension inside them.
So, the answer is 0.
In the third case 1001000, we can take the first square of size 10, then inscribe two circles of diameter 5, then we cannot inscribe another square in this since, it will not be of any possible integer dimension and we discard the rest of the string. | # cook your dish here
try:
t=int(input())
while(t):
n=input()
x=0
f=0
if(n[0]=='1'):
for i in range(1,len(n)):
if(n[i]=='1' and f==0):
continue
elif(n[i]=='0'):
x+=1
f=1
continue
elif(n[i]=='1' and f==1):
break
print(x)
t-=1
except:
pass | # cook your dish here
try:
t=int(input())
while(t):
n=input()
x=0
f=0
if(n[0]=='1'):
for i in range(1,len(n)):
if(n[i]=='1' and f==0):
continue
elif(n[i]=='0'):
x+=1
f=1
continue
elif(n[i]=='1' and f==1):
break
print(x)
t-=1
except:
pass | train | APPS_structured |
The XOR pair representation (XPR) of a positive integer $N$ is defined as a pair of integers $(A, B)$ such that:
- $1 \le A \le B \le N$
- $A \oplus B = N$
- if there is no way to choose $A$ and $B$ satisfying the above conditions, $A = B = -1$
- otherwise, the value of $A$ should be the smallest possible
These conditions uniquely define the XPR. Next, we define a function $F(N)$ = the value of $B$ in $XPR(N)$, and a function $G(L, R) = \sum\limits_{i=L}^R F(i)$.
You are given $L$ and $R$. Compute $G(L, R)$.
-----Input-----
- The first line of the input contains a single integer $T$ denoting the number of test cases. The description of $T$ test cases follows.
- The first and only line of each test case contains two space-separated integers $L$ and $R$.
-----Output-----
For each test case, print a single line containing one integer — the value of the function $G(L, R)$.
-----Constraints-----
- $1 \le T \le 10^5$
- $1 \le L \le R \le 10^9$
-----Example Input-----
5
1 10
3 6
4 10
10 17
100 159
-----Example Output-----
28
9
28
79
7485 | from math import *
def find(n):
if n==0:
return 0
if n==1:
return -1
if n==2:
return -2
a=1
ans=0
while a<=n:
ans+=(floor(n/a)-floor(n/(2*a)))*a
# print((floor(n/a)-floor(n/(2*a)))*a)
a*=2
k=int(log(n,2))
return n*(n+1)//2-int(ans)-k-1
#print(find(17))
t=int(input())
for _ in range(t):
l,r=list(map(int,input().split()))
print(find(r)-find(l-1))
| from math import *
def find(n):
if n==0:
return 0
if n==1:
return -1
if n==2:
return -2
a=1
ans=0
while a<=n:
ans+=(floor(n/a)-floor(n/(2*a)))*a
# print((floor(n/a)-floor(n/(2*a)))*a)
a*=2
k=int(log(n,2))
return n*(n+1)//2-int(ans)-k-1
#print(find(17))
t=int(input())
for _ in range(t):
l,r=list(map(int,input().split()))
print(find(r)-find(l-1))
| train | APPS_structured |
Write a program to determine if a string contains only unique characters.
Return true if it does and false otherwise.
The string may contain any of the 128 ASCII characters.
Characters are case-sensitive, e.g. 'a' and 'A' are considered different characters. | def has_unique_chars(str):
return len(set(str))==len(str) | def has_unique_chars(str):
return len(set(str))==len(str) | train | APPS_structured |
Algorithmic predicament - Bug Fixing #9
Oh no! Timmy's algorithim has gone wrong! help Timmy fix his algorithim!
Task
Your task is to fix timmy's algorithim so it returns the group name with the highest total age.
You will receive two groups of `people` objects, with two properties `name` and `age`. The name property is a string and the age property is a number.
Your goal is to make the total the age of all people having the same name through both groups and return the name of the one with the highest age. If two names have the same total age return the first alphabetical name. | def highest_age(group1,group2):
d = {}
lst = []
for i in group1 + group2:
name = i['name']
if name in d:
d[i['name']] += i['age']
else:
d[name] = i['age']
n = max(d.values())
for k, v in d.items():
if n == v:
lst.append(k)
return min(lst) | def highest_age(group1,group2):
d = {}
lst = []
for i in group1 + group2:
name = i['name']
if name in d:
d[i['name']] += i['age']
else:
d[name] = i['age']
n = max(d.values())
for k, v in d.items():
if n == v:
lst.append(k)
return min(lst) | train | APPS_structured |
Help Saurabh with his Chemistry Assignment.
Saurabh has been given a chemistry assignment by Ruby Mam. Though the assignment is simple but
Saurabh has to watch India vs Pakistan Match and he has no time to do the assignment by himself.
So Saurabh wants you to do his assignment so that he doesn’t get scolded by Ruby Mam . The assignment
is as follows , Suppose there are X particles initially at time t=0 in a box. At a time t the number of particles in
box becomes t times the number of particles at time t-1 . You will be given N and X where N is time at which the
number of particles in box is to be calculated and X is the number of particles at time t=0.
-----Input-----
The first line will contain the integer T, the number of test cases. Each test case consists of two space
separated integers N and X .
-----Output-----
For each test case, output the answer to the query. Since the output can be very large, output the answer modulo
10^6+3
-----Constraints-----
- 1 ≤ T ≤ 100000
- 1 ≤ N,X ≤ 10^18
-----Example-----
Input:
2
1 2
2 1
Output:
2
2
-----Explanation-----
Example case 2.At t=0 particles are 1 ,so at t=1 ,particles are 1*1 = 1 particles. At t=2, particles are 2*1 = 2 particles. | # your code goes here
mod=1000003
fact=[0]*mod
fact[0]=1
for i in range(1,mod):
fact[i]=(fact[i-1]*i)%mod
t=int(input())
while t:
t=t-1
s=input().split()
n=int(s[0])
x=int(s[1])
if n>=mod:
print(0)
else:
x=x%mod
ans=fact[n]
ans=(ans*x)%mod
print(ans) | # your code goes here
mod=1000003
fact=[0]*mod
fact[0]=1
for i in range(1,mod):
fact[i]=(fact[i-1]*i)%mod
t=int(input())
while t:
t=t-1
s=input().split()
n=int(s[0])
x=int(s[1])
if n>=mod:
print(0)
else:
x=x%mod
ans=fact[n]
ans=(ans*x)%mod
print(ans) | train | APPS_structured |
Check if given numbers are prime numbers.
If number N is prime ```return "Probable Prime"``` else ``` return "Composite"```.
HINT: Upper bount is really big so you should use an efficient algorithm.
Input
1 < N ≤ 10^(100)
Example
prime_or_composite(2) # should return Probable Prime
prime_or_composite(200) # should return Composite | _primes = [2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97]
def try_base(a, d, n, s):
if pow(a, d, n) == 1:
return False
for i in range(s):
if pow(a, 2**i * d, n) == n-1:
return False
return True
def miller_rabin(n):
if n in _primes:
return True
if any(not n % p for p in _primes):
return False
d, s = n - 1, 0
while not d & 1:
d, s = d >> 1, s + 1
return not any(try_base(a, d, n, s) for a in _primes)
def prime_or_composite(n):
return "Probable Prime" if miller_rabin(n) else "Composite" | _primes = [2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97]
def try_base(a, d, n, s):
if pow(a, d, n) == 1:
return False
for i in range(s):
if pow(a, 2**i * d, n) == n-1:
return False
return True
def miller_rabin(n):
if n in _primes:
return True
if any(not n % p for p in _primes):
return False
d, s = n - 1, 0
while not d & 1:
d, s = d >> 1, s + 1
return not any(try_base(a, d, n, s) for a in _primes)
def prime_or_composite(n):
return "Probable Prime" if miller_rabin(n) else "Composite" | train | APPS_structured |
Two sisters, A and B, play the piano every day. During the day, they can play in any order. That is, A might play first and then B, or it could be B first and then A. But each one of them plays the piano exactly once per day. They maintain a common log, in which they write their name whenever they play.
You are given the entries of the log, but you're not sure if it has been tampered with or not. Your task is to figure out whether these entries could be valid or not.
-----Input-----
- The first line of the input contains an integer $T$ denoting the number of test cases. The description of the test cases follows.
- The first line of each test case contains a string $s$ denoting the entries of the log.
-----Output-----
- For each test case, output yes or no according to the answer to the problem.
-----Constraints-----
- $1 \le T \le 500$
- $2 \le |s| \le 100$
- $|s|$ is even
- Each character of $s$ is either 'A' or 'B'
-----Example Input-----
4
AB
ABBA
ABAABB
AA
-----Example Output-----
yes
yes
no
no
-----Explanation-----
Testcase 1: There is only one day, and both A and B have played exactly once. So this is a valid log. Hence 'yes'.
Testcase 2: On the first day, A has played before B, and on the second day, B has played first. Hence, this is also a valid log.
Testcase 3: On the first day, A played before B, but on the second day, A seems to have played twice. This cannot happen, and hence this is 'no'. | def checkValidity(s):
count = 0
previous = ""
for x in s:
if count == 0:
previous = x
count += 1
elif count == 1:
count = 0
if previous == x:
return "no"
return "yes"
t = int(input())
for _ in range(t):
s = input()
print(checkValidity(s))
| def checkValidity(s):
count = 0
previous = ""
for x in s:
if count == 0:
previous = x
count += 1
elif count == 1:
count = 0
if previous == x:
return "no"
return "yes"
t = int(input())
for _ in range(t):
s = input()
print(checkValidity(s))
| train | APPS_structured |
The 26 letters of the English alphabets are randomly divided into 5 groups of 5 letters with the remaining letter being ignored. Each of the group is assigned a score of more than 0. The ignored letter always has a score of 0.
With this kata, write a function ```nameScore(name)``` to work out the score of a name that is passed to the function.
The output should be returned as an object:
Only letters have a score. Spaces do not.
You can safely assume that ```name``` does not contain any punctuations or symbols. There will also be no ```empty string``` or ```null``` value.
A static ```alpha``` object for testing has been preloaded for your convenience in the following format:
Note that the ```alpha``` object will be randomly generated each time you run the test.
#Example
In accordance to the above ```alpha``` object, the name ```Mary Jane``` will have a name score of ```20``` => M=3 + a=1 + r=4 + y=5 + J=2 + a=1 + n=3 + e=1 | ALPHA = {c:v for s,v in alpha.items() for c in s}
def name_score(name):
return {name: sum( ALPHA.get(c,0) for c in name.upper() )} | ALPHA = {c:v for s,v in alpha.items() for c in s}
def name_score(name):
return {name: sum( ALPHA.get(c,0) for c in name.upper() )} | train | APPS_structured |
Given a number **n**, return the number of positive odd numbers below **n**, EASY!
Expect large Inputs! | def odd_count(n):
# This work on test but takes too much time.
#counter = 0
#for num in range(0, n):
# if num % 2 != 0:
# counter += 1
return len(range(1, n,2)) | def odd_count(n):
# This work on test but takes too much time.
#counter = 0
#for num in range(0, n):
# if num % 2 != 0:
# counter += 1
return len(range(1, n,2)) | train | APPS_structured |
Given two non-negative integers low and high. Return the count of odd numbers between low and high (inclusive).
Example 1:
Input: low = 3, high = 7
Output: 3
Explanation: The odd numbers between 3 and 7 are [3,5,7].
Example 2:
Input: low = 8, high = 10
Output: 1
Explanation: The odd numbers between 8 and 10 are [9].
Constraints:
0 <= low <= high <= 10^9 | class Solution:
def countOdds(self, low: int, high: int) -> int:
return (high - low + 1) // 2 + (high % 2 and low % 2);
| class Solution:
def countOdds(self, low: int, high: int) -> int:
return (high - low + 1) // 2 + (high % 2 and low % 2);
| train | APPS_structured |
# Task
You are given a `moment` in time and space. What you must do is break it down into time and space, to determine if that moment is from the past, present or future.
`Time` is the sum of characters that increase time (i.e. numbers in range ['1'..'9'].
`Space` in the number of characters which do not increase time (i.e. all characters but those that increase time).
The moment of time is determined as follows:
```
If time is greater than space, than the moment is from the future.
If time is less than space, then the moment is from the past.
Otherwise, it is the present moment.```
You should return an array of three elements, two of which are false, and one is true. The true value should be at the `1st, 2nd or 3rd` place for `past, present and future` respectively.
# Examples
For `moment = "01:00 pm"`, the output should be `[true, false, false]`.
time equals 1, and space equals 7, so the moment is from the past.
For `moment = "12:02 pm"`, the output should be `[false, true, false]`.
time equals 5, and space equals 5, which means that it's a present moment.
For `moment = "12:30 pm"`, the output should be `[false, false, true]`.
time equals 6, space equals 5, so the moment is from the future.
# Input/Output
- `[input]` string `moment`
The moment of time and space that the input time came from.
- `[output]` a boolean array
Array of three elements, two of which are false, and one is true. The true value should be at the 1st, 2nd or 3rd place for past, present and future respectively. | def moment_of_time_in_space(moment):
t = sum(int(d) for d in moment if d.isdigit() and d!='0')
s = sum(c=='0' or not c.isdigit() for c in moment)
return [t<s, t==s, t>s] | def moment_of_time_in_space(moment):
t = sum(int(d) for d in moment if d.isdigit() and d!='0')
s = sum(c=='0' or not c.isdigit() for c in moment)
return [t<s, t==s, t>s] | train | APPS_structured |
=====Function Descriptions=====
.symmetric_difference()
The .symmetric_difference() operator returns a set with all the elements that are in the set and the iterable but not both.
Sometimes, a ^ operator is used in place of the .symmetric_difference() tool, but it only operates on the set of elements in set.
The set is immutable to the .symmetric_difference() operation (or ^ operation).
>>> s = set("Hacker")
>>> print s.symmetric_difference("Rank")
set(['c', 'e', 'H', 'n', 'R', 'r'])
>>> print s.symmetric_difference(set(['R', 'a', 'n', 'k']))
set(['c', 'e', 'H', 'n', 'R', 'r'])
>>> print s.symmetric_difference(['R', 'a', 'n', 'k'])
set(['c', 'e', 'H', 'n', 'R', 'r'])
>>> print s.symmetric_difference(enumerate(['R', 'a', 'n', 'k']))
set(['a', 'c', 'e', 'H', (0, 'R'), 'r', (2, 'n'), 'k', (1, 'a'), (3, 'k')])
>>> print s.symmetric_difference({"Rank":1})
set(['a', 'c', 'e', 'H', 'k', 'Rank', 'r'])
>>> s ^ set("Rank")
set(['c', 'e', 'H', 'n', 'R', 'r'])
=====Problem Statement=====
Students of District College have subscriptions to English and French newspapers. Some students have subscribed to English only, some have subscribed to French only, and some have subscribed to both newspapers.
You are given two sets of student roll numbers. One set has subscribed to the English newspaper, and one set has subscribed to the French newspaper. Your task is to find the total number of students who have subscribed to either the English or the French newspaper but not both.
=====Input Format=====
The first line contains the number of students who have subscribed to the English newspaper.
The second line contains the space separated list of student roll numbers who have subscribed to the English newspaper.
The third line contains the number of students who have subscribed to the French newspaper.
The fourth line contains the space separated list of student roll numbers who have subscribed to the French newspaper.
=====Constraints=====
0 < Total number of students in college < 1000
=====Output Format=====
Output total number of students who have subscriptions to the English or the French newspaper but not both. | e = int(input())
eng = set(map(int,input().split()))
f = int(input())
fre = set(map(int,input().split()))
print((len(eng ^ fre)))
| e = int(input())
eng = set(map(int,input().split()))
f = int(input())
fre = set(map(int,input().split()))
print((len(eng ^ fre)))
| train | APPS_structured |
You play a computer game. In this game, you lead a party of $m$ heroes, and you have to clear a dungeon with $n$ monsters. Each monster is characterized by its power $a_i$. Each hero is characterized by his power $p_i$ and endurance $s_i$.
The heroes clear the dungeon day by day. In the beginning of each day, you choose a hero (exactly one) who is going to enter the dungeon this day.
When the hero enters the dungeon, he is challenged by the first monster which was not defeated during the previous days (so, if the heroes have already defeated $k$ monsters, the hero fights with the monster $k + 1$). When the hero fights the monster, there are two possible outcomes:
if the monster's power is strictly greater than the hero's power, the hero retreats from the dungeon. The current day ends; otherwise, the monster is defeated.
After defeating a monster, the hero either continues fighting with the next monster or leaves the dungeon. He leaves the dungeon either if he has already defeated the number of monsters equal to his endurance during this day (so, the $i$-th hero cannot defeat more than $s_i$ monsters during each day), or if all monsters are defeated — otherwise, he fights with the next monster. When the hero leaves the dungeon, the current day ends.
Your goal is to defeat the last monster. What is the minimum number of days that you need to achieve your goal? Each day you have to use exactly one hero; it is possible that some heroes don't fight the monsters at all. Each hero can be used arbitrary number of times.
-----Input-----
The first line contains one integer $t$ ($1 \le t \le 10^5$) — the number of test cases. Then the test cases follow.
The first line of each test case contains one integer $n$ ($1 \le n \le 2 \cdot 10^5$) — the number of monsters in the dungeon.
The second line contains $n$ integers $a_1$, $a_2$, ..., $a_n$ ($1 \le a_i \le 10^9$), where $a_i$ is the power of the $i$-th monster.
The third line contains one integer $m$ ($1 \le m \le 2 \cdot 10^5$) — the number of heroes in your party.
Then $m$ lines follow, each describing a hero. Each line contains two integers $p_i$ and $s_i$ ($1 \le p_i \le 10^9$, $1 \le s_i \le n$) — the power and the endurance of the $i$-th hero.
It is guaranteed that the sum of $n + m$ over all test cases does not exceed $2 \cdot 10^5$.
-----Output-----
For each test case print one integer — the minimum number of days you have to spend to defeat all of the monsters (or $-1$ if it is impossible).
-----Example-----
Input
2
6
2 3 11 14 1 8
2
3 2
100 1
5
3 5 100 2 3
2
30 5
90 1
Output
5
-1 | 3
import os
import sys
def main():
T = read_int()
for _ in range(T):
N = read_int()
A = read_ints()
M = read_int()
H = [tuple(read_ints()) for _ in range(M)]
print(solve(N, A, M, H))
def solve(N, A, M, H):
H.sort(key=lambda h: (h[1], -h[0]))
spow = [0] * (N + 1)
s0 = 0
for p, s in H:
if s0 == s:
continue
spow[s] = p
s0 = s
maxp = 0
for d in range(N, -1, -1):
maxp = max(maxp, spow[d])
spow[d] = maxp
ans = 0
maxa = A[0]
if A[0] > spow[1]:
return -1
start = 0
for i, a in enumerate(A[1:]):
if a > spow[1]:
return -1
i += 1
days = i - start + 1
maxa = max(maxa, a)
if spow[days] < maxa:
ans += 1
maxa = a
start = i
return ans + 1
###############################################################################
# AUXILIARY FUNCTIONS
DEBUG = 'DEBUG' in os.environ
def inp():
return sys.stdin.readline().rstrip()
def read_int():
return int(inp())
def read_ints():
return [int(e) for e in inp().split()]
def dprint(*value, sep=' ', end='\n'):
if DEBUG:
print(*value, sep=sep, end=end)
def __starting_point():
main()
__starting_point() | 3
import os
import sys
def main():
T = read_int()
for _ in range(T):
N = read_int()
A = read_ints()
M = read_int()
H = [tuple(read_ints()) for _ in range(M)]
print(solve(N, A, M, H))
def solve(N, A, M, H):
H.sort(key=lambda h: (h[1], -h[0]))
spow = [0] * (N + 1)
s0 = 0
for p, s in H:
if s0 == s:
continue
spow[s] = p
s0 = s
maxp = 0
for d in range(N, -1, -1):
maxp = max(maxp, spow[d])
spow[d] = maxp
ans = 0
maxa = A[0]
if A[0] > spow[1]:
return -1
start = 0
for i, a in enumerate(A[1:]):
if a > spow[1]:
return -1
i += 1
days = i - start + 1
maxa = max(maxa, a)
if spow[days] < maxa:
ans += 1
maxa = a
start = i
return ans + 1
###############################################################################
# AUXILIARY FUNCTIONS
DEBUG = 'DEBUG' in os.environ
def inp():
return sys.stdin.readline().rstrip()
def read_int():
return int(inp())
def read_ints():
return [int(e) for e in inp().split()]
def dprint(*value, sep=' ', end='\n'):
if DEBUG:
print(*value, sep=sep, end=end)
def __starting_point():
main()
__starting_point() | train | APPS_structured |
Being a crewmate in the Among Us game, you will have to solve a task to win against the imposter.
The task : You are given a certain number of bulbs(Say n) which are initially in OFF position.
The bulbs are numbered from 1 to n. There will be n current fluctuations in such a way that in the 1st fluctuation all bulbs are toggled, in the 2nd fluctuation every 2nd bulb is toggled, in the 3rd fluctuation every 3rd bulb is toggled and so on.
Your job as a crewmate is to find the number of bulbs which are ON at the end of n fluctuations. But, the imposter has a special power which alerts it when a bulb with a number divisible by 3 is kept ON at the end of n fluctuations.
So, you will have to switch off the bulbs with numbers divisible by 3 and give the final result to complete the task and win the game.
-----Input:-----
- First line will contain $T$, number of testcases. Then the testcases follow.
- Each testcase contains of a single line of input, an integer $n$.
-----Output:-----
- For each testcase, output a single integer - the number of bulbs that will remain ON after $n$ fluctuations.
-----Constraints :-----
- $1 \leq T \leq 1000$
- $2 \leq n \leq 10^9$
-----Sample Input:-----
2
2
20
-----Sample Output:-----
1
3 | from sys import stdin
from math import ceil, gcd
# Input data
#stdin = open("input", "r")
def func():
return
for _ in range(int(stdin.readline())):
n = int(stdin.readline())
ans = 0
i = 1
while(i * i <= n):
if (i * i) % 3 != 0:
ans += 1
i += 1
print(ans)
| from sys import stdin
from math import ceil, gcd
# Input data
#stdin = open("input", "r")
def func():
return
for _ in range(int(stdin.readline())):
n = int(stdin.readline())
ans = 0
i = 1
while(i * i <= n):
if (i * i) % 3 != 0:
ans += 1
i += 1
print(ans)
| train | APPS_structured |
The string "PAYPALISHIRING" is written in a zigzag pattern on a given number of rows like this: (you may want to display this pattern in a fixed font for better legibility)
P A H N
A P L S I I G
Y I R
And then read line by line: "PAHNAPLSIIGYIR"
Write the code that will take a string and make this conversion given a number of rows:
string convert(string s, int numRows);
Example 1:
Input: s = "PAYPALISHIRING", numRows = 3
Output: "PAHNAPLSIIGYIR"
Example 2:
Input: s = "PAYPALISHIRING", numRows = 4
Output: "PINALSIGYAHRPI"
Explanation:
P I N
A L S I G
Y A H R
P I | class Solution:
def convert(self, s, numRows):
"""
:type s: str
:type numRows: int
:rtype: str
"""
if len(s) == 0 or numRows < 1:
return ''
if numRows == 1:
return s
maxStep = (numRows-1)*2
reStr = ''
for i in range(numRows):
j = i
step = maxStep-2*i
while j < len(s):
reStr += s[j]
if step == 0:
j += maxStep
else:
j += step
step = maxStep-step
if step == 0:
step = maxStep
return reStr | class Solution:
def convert(self, s, numRows):
"""
:type s: str
:type numRows: int
:rtype: str
"""
if len(s) == 0 or numRows < 1:
return ''
if numRows == 1:
return s
maxStep = (numRows-1)*2
reStr = ''
for i in range(numRows):
j = i
step = maxStep-2*i
while j < len(s):
reStr += s[j]
if step == 0:
j += maxStep
else:
j += step
step = maxStep-step
if step == 0:
step = maxStep
return reStr | train | APPS_structured |
*** Nova polynomial derivative***
This kata is from a series on polynomial handling. ( [#1](http://www.codewars.com/kata/nova-polynomial-1-add-1) [#2](http://www.codewars.com/kata/570eb07e127ad107270005fe) [#3](http://www.codewars.com/kata/5714041e8807940ff3001140 ) [#4](http://www.codewars.com/kata/571a2e2df24bdfd4e20001f5))
Consider a polynomial in a list where each element in the list element corresponds to the factors. The factor order is the position in the list. The first element is the zero order factor (the constant).
p = [a0, a1, a2, a3] signifies the polynomial a0 + a1x + a2x^2 + a3*x^3
In this kata return the derivative of a polynomial:
```python
poly_derivative([1, 2] ) = [2]
poly_derivative([9, 1, 3]) = [1, 6]
```
Previous Katas on Nova polynomial:
1. [poly_add](http://www.codewars.com/kata/nova-polynomial-1-add-1)
2. [poly_multiply](http://www.codewars.com/kata/570eb07e127ad107270005fe).
3. [poly_subtract](http://www.codewars.com/kata/5714041e8807940ff3001140 ) | def poly_derivative(p):
return [i * n for i, n in enumerate(p[1:], 1)] | def poly_derivative(p):
return [i * n for i, n in enumerate(p[1:], 1)] | train | APPS_structured |
You are a professional robber planning to rob houses along a street. Each house has a certain amount of money stashed, the only constraint stopping you from robbing each of them is that adjacent houses have security system connected and it will automatically contact the police if two adjacent houses were broken into on the same night.
Given a list of non-negative integers representing the amount of money of each house, determine the maximum amount of money you can rob tonight without alerting the police.
Example 1:
Input: nums = [1,2,3,1]
Output: 4
Explanation: Rob house 1 (money = 1) and then rob house 3 (money = 3).
Total amount you can rob = 1 + 3 = 4.
Example 2:
Input: nums = [2,7,9,3,1]
Output: 12
Explanation: Rob house 1 (money = 2), rob house 3 (money = 9) and rob house 5 (money = 1).
Total amount you can rob = 2 + 9 + 1 = 12.
Constraints:
0 <= nums.length <= 100
0 <= nums[i] <= 400 | class Solution:
def rob(self, nums: List[int]) -> int:
n = len(nums)
if n == 0:
return 0
if n == 1:
return nums[0]
max_to_i = [None] * n
max_to_i[0] = nums[0]
max_to_i[1] = max(nums[0], nums[1])
for i in range(2, n):
max_to_i[i] = max(nums[i] + max_to_i[i-2], max_to_i[i-1])
# max_to_i has len n, and contains max with the subarray [0:i].
# Total result is just max_to_i[n-1]
return max_to_i[-1] | class Solution:
def rob(self, nums: List[int]) -> int:
n = len(nums)
if n == 0:
return 0
if n == 1:
return nums[0]
max_to_i = [None] * n
max_to_i[0] = nums[0]
max_to_i[1] = max(nums[0], nums[1])
for i in range(2, n):
max_to_i[i] = max(nums[i] + max_to_i[i-2], max_to_i[i-1])
# max_to_i has len n, and contains max with the subarray [0:i].
# Total result is just max_to_i[n-1]
return max_to_i[-1] | train | APPS_structured |
Rakesh has built a model rocket and wants to test how stable it is. He usually uses a magic box which runs some tests on the rocket and tells if it is stable or not, but his friend broke it by trying to find out how stable he is (very delicate magic indeed). The box only gives a polynomial equation now which can help Rakesh find the stability (a failsafe by the manufacturers).
Rakesh reads the manual for the magic box and understands that in order to determine stability, he needs to take every other term and put them in two rows. Eg. If the polynomial is:
10 x^4 + 12 x^3 + 4 x^2 + 5 x + 3, the first two rows will be:
Row 1: 10 4 3
Row 2: 12 5 0
For all other rows, the nth element of the rth row is found recursively by multiplying the 1st element of the (r-1)th row and (n+1)th element of the (r-2)th row and subtracting it with 1st element of the (r-2)th row multiplied by (n+1)th element of the (r-1)th row.
So Row 3 will be (12 * 4 - 10 * 5) (12 * 3 - 10 * 0)
Row 3: -2 36 0
Row 4: -442 0 0
Row 5: -15912 0 0
There will not be any row six (number of rows = maximum power of x + 1)
The rocket is stable if there are no sign changes in the first column.
If all the elements of the rth row are 0, replace the nth element of the rth row by the nth element of the (r-1)th row multiplied by (maximum power of x + 4 - r - 2n).
If the first element of any row is 0 and some of the other elements are non zero, the rocket is unstable.
Can you help Rakesh check if his rocket is stable?
Input Format:
1. First row with number of test cases (T).
2. Next T rows with the coefficients of the polynomials for each case (10 12 4 5 3 for the case above).
Output Format:
1. "1" if stable (without "")
2. "0" if unstable (without "")
Sample Input:
1
10 12 4 5 3
Sample Output:
0 | import random
def sign(i):
if i>0:
return 1
elif i<=0:
return 0
bleh = []
for _ in range(int(input())):
p = list(map(int,input().rstrip().split()))
max_rows = len(p)
if all([x==0 for x in p]):
print(1)
continue
if max_rows <= 1:
bleh.append(max_rows)
continue
max_pow = max_rows-1
if len(p)%2 !=0 and len(p)>0:
p.append(0)
max_col = len(p)//2
rows = [[0 for _ in range(max_col)] for _ in range(max_rows)]
rows[0] = p[::2]
rows[1] = p[1::2]
if sign(rows[0][0]) != sign(rows[1][0]):
print(0)
continue
for r in range(2,max_rows):
for n in range(max_col-1):
rows[r][n] = rows[r-1][0]*rows[r-2][n+1]-rows[r-2][0]*rows[r-1][n+1]
last = sign(rows[0][0])
flag = 1
for i in range(1,len(rows)):
curr = sign(rows[i][0])
if rows[r] == [0 for _ in range(max_col)]:
for n in range(max_col):
rows[r][n] = rows[r-1][n]*(max_pow+4-(r+1)-2*(n+1))
elif rows[i][0] == 0:
if any([x != 0 for x in rows[i]]):
flag = 0
break
else:
curr = last
if curr != last:
flag = 0
break
last = curr
print(flag)
| import random
def sign(i):
if i>0:
return 1
elif i<=0:
return 0
bleh = []
for _ in range(int(input())):
p = list(map(int,input().rstrip().split()))
max_rows = len(p)
if all([x==0 for x in p]):
print(1)
continue
if max_rows <= 1:
bleh.append(max_rows)
continue
max_pow = max_rows-1
if len(p)%2 !=0 and len(p)>0:
p.append(0)
max_col = len(p)//2
rows = [[0 for _ in range(max_col)] for _ in range(max_rows)]
rows[0] = p[::2]
rows[1] = p[1::2]
if sign(rows[0][0]) != sign(rows[1][0]):
print(0)
continue
for r in range(2,max_rows):
for n in range(max_col-1):
rows[r][n] = rows[r-1][0]*rows[r-2][n+1]-rows[r-2][0]*rows[r-1][n+1]
last = sign(rows[0][0])
flag = 1
for i in range(1,len(rows)):
curr = sign(rows[i][0])
if rows[r] == [0 for _ in range(max_col)]:
for n in range(max_col):
rows[r][n] = rows[r-1][n]*(max_pow+4-(r+1)-2*(n+1))
elif rows[i][0] == 0:
if any([x != 0 for x in rows[i]]):
flag = 0
break
else:
curr = last
if curr != last:
flag = 0
break
last = curr
print(flag)
| train | APPS_structured |
Indian National Olympiad in Informatics 2013
N people live in Sequence Land. Instead of a name, each person is identified by a sequence of integers, called his or her id. Each id is a sequence with no duplicate elements. Two people are said to be each other’s relatives if their ids have at least K elements in common. The extended family of a resident of Sequence Land includes herself or himself, all relatives, relatives of relatives, relatives of relatives of relatives, and so on without any limit.
Given the ids of all residents of Sequence Land, including its President, and the number K, find the number of people in the extended family of the President of Sequence Land.
For example, suppose N = 4 and K = 2. Suppose the President has id (4, 6, 7, 8) and the other three residents have ids (8, 3, 0, 4), (0, 10), and (1, 2, 3, 0, 5, 8). Here, the President is directly related to (8, 3, 0, 4), who in turn is directly related to (1, 2, 3, 0, 5, 8). Thus, the President’s extended family consists of everyone other than (0, 10) and so has size 3.
-----Input format-----
• Line 1: Two space-separated integers, N followed by K.
• Lines 2 to N + 1: Each line describes an id of one of the residents of Sequence Land, beginning with the President on line 2. Each line consists of an integer p, followed by p distinct integers, the id.
-----Output format-----
The output consists of a single integer, the number of people in the extended family of the President.
-----Test Data-----
The testdata is grouped into two subtasks. In both subtasks, 1 ≤ N ≤ 300 and 1 ≤ K ≤ 300. Each number in each id is between 0 and 109 inclusive.
• Subtask 1 [30 points]: The number of elements in each id is between 1 and 10 inclusive.
• Subtask 2 [70 points]: The number of elements in each id is between 1 and 300 inclusive.
-----Example-----
Here is the sample input and output corresponding to the example above.
-----Sample input -----
4 2
4 4 6 7 8
4 8 3 0 4
2 0 10
6 1 2 3 0 5 8
-----Sample output-----
3
Note: Your program should not print anything other than what is specified in the output format. Please remove all diagnostic print statements before making your final submission. A program with extraneous output will be treated as incorrect! | # cook your dish here
from collections import defaultdict
n, k = list(map(int,input().split()))
b = []
drel = defaultdict(list)
ans = 0
for i in range(n):
a = list(map(int,input().split()))
b.append(set(a[1:]))
for i in range(n):
for j in range(n):
if i != j:
m = 0
for l in b[j]:
if l in b[i]:
m += 1
if m>=k:
drel[i].append(j)
break
visited = set()
def dfs(node):
global ans
visited.add(node)
for i in drel[node]:
if i not in visited:
ans += 1
dfs(i)
dfs(0)
print(ans+1)
| # cook your dish here
from collections import defaultdict
n, k = list(map(int,input().split()))
b = []
drel = defaultdict(list)
ans = 0
for i in range(n):
a = list(map(int,input().split()))
b.append(set(a[1:]))
for i in range(n):
for j in range(n):
if i != j:
m = 0
for l in b[j]:
if l in b[i]:
m += 1
if m>=k:
drel[i].append(j)
break
visited = set()
def dfs(node):
global ans
visited.add(node)
for i in drel[node]:
if i not in visited:
ans += 1
dfs(i)
dfs(0)
print(ans+1)
| train | APPS_structured |
# SpeedCode #2 - Array Madness
## Objective
Given two **integer arrays** ```a, b```, both of ```length >= 1```, create a program that returns ```true``` if the **sum of the squares** of each element in ```a``` is **strictly greater than** the **sum of the cubes** of each element in ```b```.
E.g.
```python
array_madness([4, 5, 6], [1, 2, 3]) => True #because 4 ** 2 + 5 ** 2 + 6 ** 2 > 1 ** 3 + 2 ** 3 + 3 ** 3
```
Get your timer out. Are you ready? Ready, get set, GO!!! | def array_madness(a,b):
return sum(_a**2 for _a in a) > sum(_b**3 for _b in b) | def array_madness(a,b):
return sum(_a**2 for _a in a) > sum(_b**3 for _b in b) | train | APPS_structured |
Gennady is one of the best child dentists in Berland. Today n children got an appointment with him, they lined up in front of his office.
All children love to cry loudly at the reception at the dentist. We enumerate the children with integers from 1 to n in the order they go in the line. Every child is associated with the value of his cofidence p_{i}. The children take turns one after another to come into the office; each time the child that is the first in the line goes to the doctor.
While Gennady treats the teeth of the i-th child, the child is crying with the volume of v_{i}. At that the confidence of the first child in the line is reduced by the amount of v_{i}, the second one — by value v_{i} - 1, and so on. The children in the queue after the v_{i}-th child almost do not hear the crying, so their confidence remains unchanged.
If at any point in time the confidence of the j-th child is less than zero, he begins to cry with the volume of d_{j} and leaves the line, running towards the exit, without going to the doctor's office. At this the confidence of all the children after the j-th one in the line is reduced by the amount of d_{j}.
All these events occur immediately one after the other in some order. Some cries may lead to other cries, causing a chain reaction. Once in the hallway it is quiet, the child, who is first in the line, goes into the doctor's office.
Help Gennady the Dentist to determine the numbers of kids, whose teeth he will cure. Print their numbers in the chronological order.
-----Input-----
The first line of the input contains a positive integer n (1 ≤ n ≤ 4000) — the number of kids in the line.
Next n lines contain three integers each v_{i}, d_{i}, p_{i} (1 ≤ v_{i}, d_{i}, p_{i} ≤ 10^6) — the volume of the cry in the doctor's office, the volume of the cry in the hall and the confidence of the i-th child.
-----Output-----
In the first line print number k — the number of children whose teeth Gennady will cure.
In the second line print k integers — the numbers of the children who will make it to the end of the line in the increasing order.
-----Examples-----
Input
5
4 2 2
4 1 2
5 2 4
3 3 5
5 1 2
Output
2
1 3
Input
5
4 5 1
5 3 9
4 1 2
2 1 8
4 1 9
Output
4
1 2 4 5
-----Note-----
In the first example, Gennady first treats the teeth of the first child who will cry with volume 4. The confidences of the remaining children will get equal to - 2, 1, 3, 1, respectively. Thus, the second child also cries at the volume of 1 and run to the exit. The confidence of the remaining children will be equal to 0, 2, 0. Then the third child will go to the office, and cry with volume 5. The other children won't bear this, and with a loud cry they will run to the exit.
In the second sample, first the first child goes into the office, he will cry with volume 4. The confidence of the remaining children will be equal to 5, - 1, 6, 8. Thus, the third child will cry with the volume of 1 and run to the exit. The confidence of the remaining children will be equal to 5, 5, 7. After that, the second child goes to the office and cry with the volume of 5. The confidences of the remaining children will be equal to 0, 3. Then the fourth child will go into the office and cry with the volume of 2. Because of this the confidence of the fifth child will be 1, and he will go into the office last. | n = int(input())
kids = []
for i in range(1, n+1):
kids.append([int(x) for x in input().split()] + [i])
kids.reverse()
ans = []
while kids:
v, d, p, k = kids.pop()
ans.append(k)
for i in range(max(-v,-len(kids)), 0):
kids[i][2] -= v+i+1
for i in range(len(kids)-1, -1, -1):
if kids[i][2] < 0:
for j in range(i):
kids[j][2] -= kids[i][1]
kids.pop(i)
print(len(ans))
for kid in ans: print(kid, end = ' ')
| n = int(input())
kids = []
for i in range(1, n+1):
kids.append([int(x) for x in input().split()] + [i])
kids.reverse()
ans = []
while kids:
v, d, p, k = kids.pop()
ans.append(k)
for i in range(max(-v,-len(kids)), 0):
kids[i][2] -= v+i+1
for i in range(len(kids)-1, -1, -1):
if kids[i][2] < 0:
for j in range(i):
kids[j][2] -= kids[i][1]
kids.pop(i)
print(len(ans))
for kid in ans: print(kid, end = ' ')
| train | APPS_structured |
A bracket sequence is a string containing only characters "(" and ")". A regular bracket sequence is a bracket sequence that can be transformed into a correct arithmetic expression by inserting characters "1" and "+" between the original characters of the sequence. For example, bracket sequences "()()" and "(())" are regular (the resulting expressions are: "(1)+(1)" and "((1+1)+1)"), and ")(", "(" and ")" are not.
Subsequence is a sequence that can be derived from another sequence by deleting some elements without changing the order of the remaining elements.
You are given a regular bracket sequence $s$ and an integer number $k$. Your task is to find a regular bracket sequence of length exactly $k$ such that it is also a subsequence of $s$.
It is guaranteed that such sequence always exists.
-----Input-----
The first line contains two integers $n$ and $k$ ($2 \le k \le n \le 2 \cdot 10^5$, both $n$ and $k$ are even) — the length of $s$ and the length of the sequence you are asked to find.
The second line is a string $s$ — regular bracket sequence of length $n$.
-----Output-----
Print a single string — a regular bracket sequence of length exactly $k$ such that it is also a subsequence of $s$.
It is guaranteed that such sequence always exists.
-----Examples-----
Input
6 4
()(())
Output
()()
Input
8 8
(()(()))
Output
(()(())) | n, k = map(int, input().split())
a = [0] * n
b = ['0'] * n
c = []
s = input()
for i in range(n):
if k != 0:
if s[i] == '(':
c.append(i)
else:
d = c.pop()
a[i] = 1
a[d] = 1
k -= 2
for i in range(n):
if a[i] == 1:
print(s[i], end = '')
| n, k = map(int, input().split())
a = [0] * n
b = ['0'] * n
c = []
s = input()
for i in range(n):
if k != 0:
if s[i] == '(':
c.append(i)
else:
d = c.pop()
a[i] = 1
a[d] = 1
k -= 2
for i in range(n):
if a[i] == 1:
print(s[i], end = '')
| train | APPS_structured |
Ayu loves distinct letter sequences ,a distinct letter sequence is defined by a sequence of small case english alphabets such that no character appears more then once.
But however there are two phrases that she doesn't like these phrases are "kar" and "shi" and she is given a sequence of distinct characters and she wonders how many such sequences she can form using all the characters such that these phrases don't occur.
Help her finding the number of such sequences.
New Year Gift - It is guaranteed that for sequences of length greater then 6 letters k,a,r,s,h,i will be present(we thought of being generous, thank us later :)).
-----Input:-----
- First line will contain $T$, number of testcases. Then the testcases follow.
- Each line consists of a string $S$ (3<=s.length<=18) of distinct characters.
-----Output:-----
Print the number of sequences that can be formed by permuting all the characters such that phrases "kar" and "shi" don't occur.
-----Constraints-----
- $1 \leq T \leq 10$
- $3 \leq S.length \leq 18$
-----Sample Input:-----
2
karp
abcd
-----Sample Output:-----
22
24 | # cook your dish here
from collections import deque, defaultdict
from math import sqrt, ceil,factorial
import sys
import copy
def get_array(): return list(map(int, sys.stdin.readline().strip().split()))
def get_ints(): return map(int, sys.stdin.readline().strip().split())
def input(): return sys.stdin.readline().strip()
for _ in range(int(input())):
s=input()
if len(s)>6:
ans=0
rem=len(s)-6
ans+=factorial(len(s))
ans-=2*(factorial(len(s)-2))
ans+=factorial(rem+2)
print(ans)
else:
if 'k' in s and 'r' in s and 'a' in s and 's' in s and 'h' in s and 'i' in s:
ans = 0
rem = len(s) - 6
ans += factorial(len(s))
ans -= 2 * (factorial(len(s) - 2))
ans += factorial(rem + 2)
print(ans)
else:
if 'k' in s and 'a' in s and 'r' in s:
ans=0
rem=len(s)-3
ans+=factorial(len(s))
ans-=factorial(rem+1)
print(ans)
continue
if 's' in s and 'h' in s and 'i' in s:
ans = 0
rem = len(s) - 3
ans += factorial(len(s))
ans -= factorial(rem + 1)
print(ans)
continue
print(factorial(len(s)))
| # cook your dish here
from collections import deque, defaultdict
from math import sqrt, ceil,factorial
import sys
import copy
def get_array(): return list(map(int, sys.stdin.readline().strip().split()))
def get_ints(): return map(int, sys.stdin.readline().strip().split())
def input(): return sys.stdin.readline().strip()
for _ in range(int(input())):
s=input()
if len(s)>6:
ans=0
rem=len(s)-6
ans+=factorial(len(s))
ans-=2*(factorial(len(s)-2))
ans+=factorial(rem+2)
print(ans)
else:
if 'k' in s and 'r' in s and 'a' in s and 's' in s and 'h' in s and 'i' in s:
ans = 0
rem = len(s) - 6
ans += factorial(len(s))
ans -= 2 * (factorial(len(s) - 2))
ans += factorial(rem + 2)
print(ans)
else:
if 'k' in s and 'a' in s and 'r' in s:
ans=0
rem=len(s)-3
ans+=factorial(len(s))
ans-=factorial(rem+1)
print(ans)
continue
if 's' in s and 'h' in s and 'i' in s:
ans = 0
rem = len(s) - 3
ans += factorial(len(s))
ans -= factorial(rem + 1)
print(ans)
continue
print(factorial(len(s)))
| train | APPS_structured |
Your car is old, it breaks easily. The shock absorbers are gone and you think it can handle about 15 more bumps before it dies totally.
Unfortunately for you, your drive is very bumpy! Given a string showing either flat road ("\_") or bumps ("n"), work out if you make it home safely. 15 bumps or under, return "Woohoo!", over 15 bumps return "Car Dead". | def bumps(road):
MAX_BUMPS = 15
SUCCESS_MESSAGE = 'Woohoo!'
ERROR_MESSAGE = 'Car Dead'
return SUCCESS_MESSAGE if road.count('n') <= MAX_BUMPS else ERROR_MESSAGE | def bumps(road):
MAX_BUMPS = 15
SUCCESS_MESSAGE = 'Woohoo!'
ERROR_MESSAGE = 'Car Dead'
return SUCCESS_MESSAGE if road.count('n') <= MAX_BUMPS else ERROR_MESSAGE | train | APPS_structured |
Given an array A, we may rotate it by a non-negative integer K so that the array becomes A[K], A[K+1], A{K+2], ... A[A.length - 1], A[0], A[1], ..., A[K-1]. Afterward, any entries that are less than or equal to their index are worth 1 point.
For example, if we have [2, 4, 1, 3, 0], and we rotate by K = 2, it becomes [1, 3, 0, 2, 4]. This is worth 3 points because 1 > 0 [no points], 3 > 1 [no points], 0 <= 2 [one point], 2 <= 3 [one point], 4 <= 4 [one point].
Over all possible rotations, return the rotation index K that corresponds to the highest score we could receive. If there are multiple answers, return the smallest such index K.
Example 1:
Input: [2, 3, 1, 4, 0]
Output: 3
Explanation:
Scores for each K are listed below:
K = 0, A = [2,3,1,4,0], score 2
K = 1, A = [3,1,4,0,2], score 3
K = 2, A = [1,4,0,2,3], score 3
K = 3, A = [4,0,2,3,1], score 4
K = 4, A = [0,2,3,1,4], score 3
So we should choose K = 3, which has the highest score.
Example 2:
Input: [1, 3, 0, 2, 4]
Output: 0
Explanation: A will always have 3 points no matter how it shifts.
So we will choose the smallest K, which is 0.
Note:
A will have length at most 20000.
A[i] will be in the range [0, A.length]. | class Solution:
def movesToChessboard(self, board):
"""
:type board: List[List[int]]
:rtype: int
"""
n = len(board)
for i in range(1, n):
for j in range(1, n):
if (board[0][0] + board[0][j] + board[i][0] + board[i][j]) % 2 != 0:
return -1
result = 0
x = 0
y = 0
for i in range(0, n):
if i % 2 == 0 and board[i][0] == 1:
x += 1
elif i % 2 == 1 and board[i][0] == 0:
y += 1
if n % 2 == 0:
if x != y:
return -1
result += min(x, n // 2 - x)
else:
if x == y:
result += x
elif (n + 1) // 2 - x == (n - 1) // 2 - y:
result += (n + 1) // 2 - x
else:
return -1
x = 0
y = 0
for i in range(0, n):
if i % 2 == 0 and board[0][i] == 1:
x += 1
elif i % 2 == 1 and board[0][i] == 0:
y += 1
if n % 2 == 0:
if x != y:
return -1
result += min(x, n // 2 - x)
else:
if x == y:
result += x
elif (n + 1) // 2 - x == (n - 1) // 2 - y:
result += (n + 1) // 2 - x
else:
return -1
return result
| class Solution:
def movesToChessboard(self, board):
"""
:type board: List[List[int]]
:rtype: int
"""
n = len(board)
for i in range(1, n):
for j in range(1, n):
if (board[0][0] + board[0][j] + board[i][0] + board[i][j]) % 2 != 0:
return -1
result = 0
x = 0
y = 0
for i in range(0, n):
if i % 2 == 0 and board[i][0] == 1:
x += 1
elif i % 2 == 1 and board[i][0] == 0:
y += 1
if n % 2 == 0:
if x != y:
return -1
result += min(x, n // 2 - x)
else:
if x == y:
result += x
elif (n + 1) // 2 - x == (n - 1) // 2 - y:
result += (n + 1) // 2 - x
else:
return -1
x = 0
y = 0
for i in range(0, n):
if i % 2 == 0 and board[0][i] == 1:
x += 1
elif i % 2 == 1 and board[0][i] == 0:
y += 1
if n % 2 == 0:
if x != y:
return -1
result += min(x, n // 2 - x)
else:
if x == y:
result += x
elif (n + 1) // 2 - x == (n - 1) // 2 - y:
result += (n + 1) // 2 - x
else:
return -1
return result
| train | APPS_structured |
# Task
let F(N) be the sum square of digits of N. So:
`F(1) = 1, F(3) = 9, F(123) = 14`
Choose a number A, the sequence {A0, A1, ...} is defined as followed:
```
A0 = A
A1 = F(A0)
A2 = F(A1) ...
```
if A = 123, we have:
```
123 → 14(1 x 1 + 2 x 2 + 3 x 3)
→ 17(1 x 1 + 4 x 4)
→ 50(1 x 1 + 7 x 7)
→ 25(5 x 5 + 0 x 0)
→ 29(2 x 2 + 5 x 5)
→ 85(2 x 2 + 9 x 9)
→ 89(8 x 8 + 5 x 5) ---
→ 145(8 x 8 + 9 x 9) |r
→ 42(1 x 1 + 4 x 4 + 5 x 5) |e
→ 20(4 x 4 + 2 x 2) |p
→ 4(2 x 2 + 0 x 0) |e
→ 16(4 x 4) |a
→ 37(1 x 1 + 6 x 6) |t
→ 58(3 x 3 + 7 x 7) |
→ 89(5 x 5 + 8 x 8) ---
→ ......
```
As you can see, the sequence repeats itself. Interestingly, whatever A is, there's an index such that from it, the sequence repeats again and again.
Let `G(A)` be the minimum length of the repeat sequence with A0 = A.
So `G(85) = 8` (8 number : `89,145,42, 20,4,16,37,58`)
Your task is to find G(A) and return it.
# Input/Output
- `[input]` integer `a0`
the A0 number
- `[output]` an integer
the length of the repeat sequence | def repeat_sequence_len(n):
s = []
while True:
n = sum(int(i) ** 2 for i in str(n))
if n not in s:
s.append(n)
else:
return len(s[s.index(n):]) | def repeat_sequence_len(n):
s = []
while True:
n = sum(int(i) ** 2 for i in str(n))
if n not in s:
s.append(n)
else:
return len(s[s.index(n):]) | train | APPS_structured |
Given an array `A` and an integer `x`, map each element in the array to `F(A[i],x)` then return the xor sum of the resulting array.
where F(n,x) is defined as follows:
F(n, x) = ^(x)Cx **+** ^(x+1)Cx **+** ^(x+2)Cx **+** ... **+** ^(n)Cx
and ^(n)Cx represents [Combination](https://en.m.wikipedia.org/wiki/Combination) in mathematics
### Example
```python
a = [7, 4, 11, 6, 5]
x = 3
# after mapping, `a` becomes [F(7,3), F(4,3), F(11,3), F(6,3), F(5,3)]
return F(7,3) ^ F(4,3) ^ F(11,3) ^ F(6,3) ^ F(5,3)
#=> 384
```
##### e.g
F(7, 3) = ^(3)C3 + ^(4)C3 + ^(5)C3 + ^(6)C3 + ^(7)C3
## Constraints
**1 <= x <= 10**
**x <= A[i] <= 10^(4)**
**5 <= |A| <= 10^(3)** | from gmpy2 import comb
from operator import xor
from functools import reduce
def transform(A, x):
return reduce( xor ,(comb(n+1,x+1) for n in A))
| from gmpy2 import comb
from operator import xor
from functools import reduce
def transform(A, x):
return reduce( xor ,(comb(n+1,x+1) for n in A))
| train | APPS_structured |
The chef is trying to decode some pattern problems, Chef wants your help to code it. Chef has one number K to form a new pattern. Help the chef to code this pattern problem.
-----Input:-----
- First-line will contain $T$, the number of test cases. Then the test cases follow.
- Each test case contains a single line of input, one integer $K$.
-----Output:-----
For each test case, output as the pattern.
-----Constraints-----
- $1 \leq T \leq 100$
- $1 \leq K \leq 100$
-----Sample Input:-----
4
1
2
3
4
-----Sample Output:-----
2
23
34
234
345
456
2345
3456
4567
5678
-----EXPLANATION:-----
No need, else pattern can be decode easily. | t=int(input())
for t in range(t):
n=int(input())
k=2
for i in range(1,n+1):
s=k
for j in range(1,n+1):
print(s,end="")
s+=1
k+=1
print() | t=int(input())
for t in range(t):
n=int(input())
k=2
for i in range(1,n+1):
s=k
for j in range(1,n+1):
print(s,end="")
s+=1
k+=1
print() | train | APPS_structured |
You start with a value in dollar form, e.g. $5.00. You must convert this value to a string in which the value is said, like '5 dollars' for example. This should account for ones, cents, zeroes, and negative values. Here are some examples:
```python
dollar_to_speech('$0.00') == '0 dollars.'
dollar_to_speech('$1.00') == '1 dollar.'
dollar_to_speech('$0.01') == '1 cent.'
dollar_to_speech('$5.00') == '5 dollars.'
dollar_to_speech('$20.18') == '20 dollars and 18 cents.'
dollar_to_speech('$-1.00') == 'No negative numbers are allowed!'
```
These examples account for pretty much everything. This kata has many specific outputs, so good luck! | def dollar_to_speech(s):
d, c = map(int, s[1:].split("."))
ds, cs = f"{d} dollar" + "s"*(d != 1), f"{c} cent" + "s"*(c != 1)
return "No negative numbers are allowed!" if d < 0 else f"{ds}." if c == 0 else f"{cs}." if d == 0 else f"{ds} and {cs}." | def dollar_to_speech(s):
d, c = map(int, s[1:].split("."))
ds, cs = f"{d} dollar" + "s"*(d != 1), f"{c} cent" + "s"*(c != 1)
return "No negative numbers are allowed!" if d < 0 else f"{ds}." if c == 0 else f"{cs}." if d == 0 else f"{ds} and {cs}." | train | APPS_structured |
Rani is teaching Raju maths via a game called N-Cube, which involves three sections involving N.
Rani gives Raju a number N, and Raju makes a list of Nth powers of integers in increasing order (1^N, 2^N, 3^N.. so on). This teaches him exponentiation.
Then Raju performs the following subtraction game N times : Take all pairs of consecutive numbers in the list and take their difference. These differences then form the new list for the next iteration of the game. Eg, if N was 6, the list proceeds as [1, 64, 729, 4096 ... ] to [63, 685, 3367 ...], and so on 5 more times.
After the subtraction game, Raju has to correctly tell Rani the Nth element of the list. This number is the value of the game.
After practice Raju became an expert in the game. To challenge him more, Rani will give two numbers M (where M is a prime) and R instead of just a single number N, and the game must start from M^(R - 1) instead of N. Since the value of the game can now become large, Raju just have to tell the largest integer K such that M^K divides this number. Since even K can be large, output K modulo 1000000007 (109+7).
-----Input-----
First line will contain T, number of testcases. Then the testcases follow
Each testcase contains of a single line of input, two integers M R
-----Output-----
For each testcase, output in a single line answer given by Raju to Rani modulo 1000000007.
-----Constraints-----
1<=T<=1000
2<=M<=109
30 points : 1<=R<=10000
70 points : 1<=R<=109
M is a prime number
-----Example-----
Input:
1
2 2
Output:
1 | tc=int(input())
for case in range(tc):
m,r=list(map(int,input().split()))
n=m**(r-1)
a=[i**n for i in range(1,2*n+1)]
tmp=2*n-1
for i in range(n):
for j in range(tmp-i):
a[j]=a[j+1]-a[j]
print((a[n-1]/m)%1000000007)
| tc=int(input())
for case in range(tc):
m,r=list(map(int,input().split()))
n=m**(r-1)
a=[i**n for i in range(1,2*n+1)]
tmp=2*n-1
for i in range(n):
for j in range(tmp-i):
a[j]=a[j+1]-a[j]
print((a[n-1]/m)%1000000007)
| train | APPS_structured |
Pig Latin is an English language game where the goal is to hide the meaning of a word from people not aware of the rules.
So, the goal of this kata is to wite a function that encodes a single word string to pig latin.
The rules themselves are rather easy:
1) The word starts with a vowel(a,e,i,o,u) -> return the original string plus "way".
2) The word starts with a consonant -> move consonants from the beginning of the word to the end of the word until the first vowel, then return it plus "ay".
3) The result must be lowercase, regardless of the case of the input. If the input string has any non-alpha characters, the function must return None, null, Nothing (depending on the language).
4) The function must also handle simple random strings and not just English words.
5) The input string has no vowels -> return the original string plus "ay".
For example, the word "spaghetti" becomes "aghettispay" because the first two letters ("sp") are consonants, so they are moved to the end of the string and "ay" is appended. | def pig_latin(s):
if s.isalpha():
s = s.lower()
if s[0] in 'aeiou':
return s + 'way'
for i in range(len(s)):
s = s[1:] + s[0]
if s[0] in 'aeiou':
break
return s + 'ay'
| def pig_latin(s):
if s.isalpha():
s = s.lower()
if s[0] in 'aeiou':
return s + 'way'
for i in range(len(s)):
s = s[1:] + s[0]
if s[0] in 'aeiou':
break
return s + 'ay'
| train | APPS_structured |
There is a country with $n$ citizens. The $i$-th of them initially has $a_{i}$ money. The government strictly controls the wealth of its citizens. Whenever a citizen makes a purchase or earns some money, they must send a receipt to the social services mentioning the amount of money they currently have.
Sometimes the government makes payouts to the poor: all citizens who have strictly less money than $x$ are paid accordingly so that after the payout they have exactly $x$ money. In this case the citizens don't send a receipt.
You know the initial wealth of every citizen and the log of all events: receipts and payouts. Restore the amount of money each citizen has after all events.
-----Input-----
The first line contains a single integer $n$ ($1 \le n \le 2 \cdot 10^{5}$) — the numer of citizens.
The next line contains $n$ integers $a_1$, $a_2$, ..., $a_n$ ($0 \le a_{i} \le 10^{9}$) — the initial balances of citizens.
The next line contains a single integer $q$ ($1 \le q \le 2 \cdot 10^{5}$) — the number of events.
Each of the next $q$ lines contains a single event. The events are given in chronological order.
Each event is described as either 1 p x ($1 \le p \le n$, $0 \le x \le 10^{9}$), or 2 x ($0 \le x \le 10^{9}$). In the first case we have a receipt that the balance of the $p$-th person becomes equal to $x$. In the second case we have a payoff with parameter $x$.
-----Output-----
Print $n$ integers — the balances of all citizens after all events.
-----Examples-----
Input
4
1 2 3 4
3
2 3
1 2 2
2 1
Output
3 2 3 4
Input
5
3 50 2 1 10
3
1 2 0
2 8
1 3 20
Output
8 8 20 8 10
-----Note-----
In the first example the balances change as follows: 1 2 3 4 $\rightarrow$ 3 3 3 4 $\rightarrow$ 3 2 3 4 $\rightarrow$ 3 2 3 4
In the second example the balances change as follows: 3 50 2 1 10 $\rightarrow$ 3 0 2 1 10 $\rightarrow$ 8 8 8 8 10 $\rightarrow$ 8 8 20 8 10 | n = int(input())
sp = list(map(int, input().split()))
m = int(input())
pos = [-1] * (n + 1)
m1 = 0
mem = []
for i in range(m):
sp1 = list(map(int, input().split()))
mem.append(sp1)
if sp1[0] == 1:
sp[sp1[1] - 1] = sp1[2]
pos[sp1[1] - 1] = i
else:
m1 = max(m1, sp1[1])
maxs = [-1] * (m + 1)
for i in range(m - 1, -1, -1):
sp1 = mem[i]
if (sp1[0] == 2):
if (i == m - 1 or sp1[1] > maxs[i + 1]):
maxs[i] = sp1[1]
else:
maxs[i] = maxs[i + 1]
else:
maxs[i] = maxs[i + 1]
for i in range(n):
if pos[i] != -1 and sp[i] < maxs[pos[i]]:
sp[i] = maxs[pos[i]]
elif pos[i] == -1:
sp[i] = max(sp[i], maxs[0])
print(*sp)
| n = int(input())
sp = list(map(int, input().split()))
m = int(input())
pos = [-1] * (n + 1)
m1 = 0
mem = []
for i in range(m):
sp1 = list(map(int, input().split()))
mem.append(sp1)
if sp1[0] == 1:
sp[sp1[1] - 1] = sp1[2]
pos[sp1[1] - 1] = i
else:
m1 = max(m1, sp1[1])
maxs = [-1] * (m + 1)
for i in range(m - 1, -1, -1):
sp1 = mem[i]
if (sp1[0] == 2):
if (i == m - 1 or sp1[1] > maxs[i + 1]):
maxs[i] = sp1[1]
else:
maxs[i] = maxs[i + 1]
else:
maxs[i] = maxs[i + 1]
for i in range(n):
if pos[i] != -1 and sp[i] < maxs[pos[i]]:
sp[i] = maxs[pos[i]]
elif pos[i] == -1:
sp[i] = max(sp[i], maxs[0])
print(*sp)
| train | APPS_structured |
In this kata, you will do addition and subtraction on a given string. The return value must be also a string.
**Note:** the input will not be empty.
## Examples
```
"1plus2plus3plus4" --> "10"
"1plus2plus3minus4" --> "2"
``` | def calculate(s):
# your code here
t=s.replace("plus","+")
y=t.replace("minus","-")
#print(y)
return str(eval(y))
| def calculate(s):
# your code here
t=s.replace("plus","+")
y=t.replace("minus","-")
#print(y)
return str(eval(y))
| train | APPS_structured |
Alice and Bob continue their games with piles of stones. There are several stones arranged in a row, and each stone has an associated value which is an integer given in the array stoneValue.
Alice and Bob take turns, with Alice starting first. On each player's turn, that player can take 1, 2 or 3 stones from the first remaining stones in the row.
The score of each player is the sum of values of the stones taken. The score of each player is 0 initially.
The objective of the game is to end with the highest score, and the winner is the player with the highest score and there could be a tie. The game continues until all the stones have been taken.
Assume Alice and Bob play optimally.
Return "Alice" if Alice will win, "Bob" if Bob will win or "Tie" if they end the game with the same score.
Example 1:
Input: values = [1,2,3,7]
Output: "Bob"
Explanation: Alice will always lose. Her best move will be to take three piles and the score become 6. Now the score of Bob is 7 and Bob wins.
Example 2:
Input: values = [1,2,3,-9]
Output: "Alice"
Explanation: Alice must choose all the three piles at the first move to win and leave Bob with negative score.
If Alice chooses one pile her score will be 1 and the next move Bob's score becomes 5. The next move Alice will take the pile with value = -9 and lose.
If Alice chooses two piles her score will be 3 and the next move Bob's score becomes 3. The next move Alice will take the pile with value = -9 and also lose.
Remember that both play optimally so here Alice will choose the scenario that makes her win.
Example 3:
Input: values = [1,2,3,6]
Output: "Tie"
Explanation: Alice cannot win this game. She can end the game in a draw if she decided to choose all the first three piles, otherwise she will lose.
Example 4:
Input: values = [1,2,3,-1,-2,-3,7]
Output: "Alice"
Example 5:
Input: values = [-1,-2,-3]
Output: "Tie"
Constraints:
1 <= values.length <= 50000
-1000 <= values[i] <= 1000 | class Solution:
def stoneGameIII(self, stoneValue: List[int]) -> str:
n = len(stoneValue)
memo = {}
def helper(i,total):
if i>=n:
return 0
elif (i) in memo:
return memo[i]
res = -math.inf
for x in range(1, min(4, n-i+1)):
s = sum(stoneValue[i:i+x])
res = max(res, total - helper(i+x,total-s))
memo[i] = res
return res
total = sum(stoneValue)
a = helper(0,total)
b = total - a
if a == b:
return 'Tie'
elif a > b:
return 'Alice'
else:
return 'Bob' | class Solution:
def stoneGameIII(self, stoneValue: List[int]) -> str:
n = len(stoneValue)
memo = {}
def helper(i,total):
if i>=n:
return 0
elif (i) in memo:
return memo[i]
res = -math.inf
for x in range(1, min(4, n-i+1)):
s = sum(stoneValue[i:i+x])
res = max(res, total - helper(i+x,total-s))
memo[i] = res
return res
total = sum(stoneValue)
a = helper(0,total)
b = total - a
if a == b:
return 'Tie'
elif a > b:
return 'Alice'
else:
return 'Bob' | train | APPS_structured |
You are given an array of N integers a1, a2, ..., aN and an integer K. Find the number of such unordered pairs {i, j} that
- i ≠ j
- |ai + aj - K| is minimal possible
Output the minimal possible value of |ai + aj - K| (where i ≠ j) and the number of such pairs for the given array and the integer K.
-----Input-----
The first line of the input contains an integer T denoting the number of test cases. The description of T test cases follows.
The first line of each test case consists of two space separated integers - N and K respectively.
The second line contains N single space separated integers - a1, a2, ..., aN respectively.
-----Output-----
For each test case, output a single line containing two single space separated integers - the minimal possible value of |ai + aj - K| and the number of unordered pairs {i, j} for which this minimal difference is reached.
-----Constraints-----
- 1 ≤ T ≤ 50
- 1 ≤ ai, K ≤ 109
- N = 2 - 31 point.
- 2 ≤ N ≤ 1000 - 69 points.
-----Example-----
Input:
1
4 9
4 4 2 6
Output:
1 4
-----Explanation:-----
The minimal possible absolute difference of 1 can be obtained by taking the pairs of a1 and a2, a1 and a4, a2 and a4, a3 and a4. | for _ in range(int(input())):
n,k=map(int,input().split())
l=list(map(int,input().split()))
aux=[]
for i in range(len(l)):
for j in range(i+1,len(l)):
aux.append(abs(l[i]+l[j]-k))
m=min(aux)
t_1=aux.count(m)
print(m,t_1) | for _ in range(int(input())):
n,k=map(int,input().split())
l=list(map(int,input().split()))
aux=[]
for i in range(len(l)):
for j in range(i+1,len(l)):
aux.append(abs(l[i]+l[j]-k))
m=min(aux)
t_1=aux.count(m)
print(m,t_1) | train | APPS_structured |
Serge came to the school dining room and discovered that there is a big queue here. There are $m$ pupils in the queue. He's not sure now if he wants to wait until the queue will clear, so he wants to know which dish he will receive if he does. As Serge is very tired, he asks you to compute it instead of him.
Initially there are $n$ dishes with costs $a_1, a_2, \ldots, a_n$. As you already know, there are the queue of $m$ pupils who have $b_1, \ldots, b_m$ togrogs respectively (pupils are enumerated by queue order, i.e the first pupil in the queue has $b_1$ togrogs and the last one has $b_m$ togrogs)
Pupils think that the most expensive dish is the most delicious one, so every pupil just buys the most expensive dish for which he has money (every dish has a single copy, so when a pupil has bought it nobody can buy it later), and if a pupil doesn't have money for any dish, he just leaves the queue (so brutal capitalism...)
But money isn't a problem at all for Serge, so Serge is buying the most expensive dish if there is at least one remaining.
Moreover, Serge's school has a very unstable economic situation and the costs of some dishes or number of togrogs of some pupils can change. More formally, you must process $q$ queries:
change $a_i$ to $x$. It means that the price of the $i$-th dish becomes $x$ togrogs. change $b_i$ to $x$. It means that the $i$-th pupil in the queue has $x$ togrogs now.
Nobody leaves the queue during those queries because a saleswoman is late.
After every query, you must tell Serge price of the dish which he will buy if he has waited until the queue is clear, or $-1$ if there are no dishes at this point, according to rules described above.
-----Input-----
The first line contains integers $n$ and $m$ ($1 \leq n, m \leq 300\ 000$) — number of dishes and pupils respectively. The second line contains $n$ integers $a_1, a_2, \ldots, a_n$ ($1 \leq a_i \leq 10^{6}$) — elements of array $a$. The third line contains $m$ integers $b_1, b_2, \ldots, b_{m}$ ($1 \leq b_i \leq 10^{6}$) — elements of array $b$. The fourth line conatins integer $q$ ($1 \leq q \leq 300\ 000$) — number of queries.
Each of the following $q$ lines contains as follows: if a query changes price of some dish, it contains $1$, and two integers $i$ and $x$ ($1 \leq i \leq n$, $1 \leq x \leq 10^{6}$), what means $a_i$ becomes $x$. if a query changes number of togrogs of some pupil, it contains $2$, and two integers $i$ and $x$ ($1 \leq i \leq m$, $1 \leq x \leq 10^{6}$), what means $b_i$ becomes $x$.
-----Output-----
For each of $q$ queries prints the answer as the statement describes, the answer of the $i$-th query in the $i$-th line (the price of the dish which Serge will buy or $-1$ if nothing remains)
-----Examples-----
Input
1 1
1
1
1
1 1 100
Output
100
Input
1 1
1
1
1
2 1 100
Output
-1
Input
4 6
1 8 2 4
3 3 6 1 5 2
3
1 1 1
2 5 10
1 1 6
Output
8
-1
4
-----Note-----
In the first sample after the first query, there is one dish with price $100$ togrogs and one pupil with one togrog, so Serge will buy the dish with price $100$ togrogs.
In the second sample after the first query, there is one dish with price one togrog and one pupil with $100$ togrogs, so Serge will get nothing.
In the third sample after the first query, nobody can buy the dish with price $8$, so Serge will take it. After the second query, all dishes will be bought, after the third one the third and fifth pupils will by the first and the second dishes respectively and nobody will by the fourth one. | import sys
from itertools import accumulate
class Lazysegtree:
#RAQ
def __init__(self, A, intv, initialize = True, segf = min):
#区間は 1-indexed で管理
self.N = len(A)
self.N0 = 2**(self.N-1).bit_length()
self.intv = intv
self.segf = segf
self.lazy = [0]*(2*self.N0)
if initialize:
self.data = [intv]*self.N0 + A + [intv]*(self.N0 - self.N)
for i in range(self.N0-1, 0, -1):
self.data[i] = self.segf(self.data[2*i], self.data[2*i+1])
else:
self.data = [intv]*(2*self.N0)
def _ascend(self, k):
k = k >> 1
c = k.bit_length()
for j in range(c):
idx = k >> j
self.data[idx] = self.segf(self.data[2*idx], self.data[2*idx+1]) \
+ self.lazy[idx]
def _descend(self, k):
k = k >> 1
idx = 1
c = k.bit_length()
for j in range(1, c+1):
idx = k >> (c - j)
ax = self.lazy[idx]
if not ax:
continue
self.lazy[idx] = 0
self.data[2*idx] += ax
self.data[2*idx+1] += ax
self.lazy[2*idx] += ax
self.lazy[2*idx+1] += ax
def query(self, l, r):
L = l+self.N0
R = r+self.N0
Li = L//(L & -L)
Ri = R//(R & -R)
self._descend(Li)
self._descend(Ri - 1)
s = self.intv
while L < R:
if R & 1:
R -= 1
s = self.segf(s, self.data[R])
if L & 1:
s = self.segf(s, self.data[L])
L += 1
L >>= 1
R >>= 1
return s
def add(self, l, r, x):
L = l+self.N0
R = r+self.N0
Li = L//(L & -L)
Ri = R//(R & -R)
while L < R :
if R & 1:
R -= 1
self.data[R] += x
self.lazy[R] += x
if L & 1:
self.data[L] += x
self.lazy[L] += x
L += 1
L >>= 1
R >>= 1
self._ascend(Li)
self._ascend(Ri-1)
def binsearch(self, l, r, check, reverse = False):
L = l+self.N0
R = r+self.N0
Li = L//(L & -L)
Ri = R//(R & -R)
self._descend(Li)
self._descend(Ri-1)
SL, SR = [], []
while L < R:
if R & 1:
R -= 1
SR.append(R)
if L & 1:
SL.append(L)
L += 1
L >>= 1
R >>= 1
if reverse:
for idx in (SR + SL[::-1]):
if check(self.data[idx]):
break
else:
return -1
while idx < self.N0:
ax = self.lazy[idx]
self.lazy[idx] = 0
self.data[2*idx] += ax
self.data[2*idx+1] += ax
self.lazy[2*idx] += ax
self.lazy[2*idx+1] += ax
idx = idx << 1
if check(self.data[idx+1]):
idx += 1
return idx - self.N0
else:
for idx in (SL + SR[::-1]):
if check(self.data[idx]):
break
else:
return -1
while idx < self.N0:
ax = self.lazy[idx]
self.lazy[idx] = 0
self.data[2*idx] += ax
self.data[2*idx+1] += ax
self.lazy[2*idx] += ax
self.lazy[2*idx+1] += ax
idx = idx << 1
if not check(self.data[idx]):
idx += 1
return idx - self.N0
def provfunc(self):
idx = 1
if self.data[1] >= 0:
return -1
while idx < self.N0:
ax = self.lazy[idx]
self.lazy[idx] = 0
self.data[2*idx] += ax
self.data[2*idx+1] += ax
self.lazy[2*idx] += ax
self.lazy[2*idx+1] += ax
idx = idx << 1
if self.data[idx+1] < 0:
idx += 1
return idx - self.N0
N, M = list(map(int, input().split()))
A = list(map(int, input().split()))
B = list(map(int, input().split()))
table = [0]*(10**6+1)
for a in A:
table[a] -= 1
for b in B:
table[b] += 1
table = list(accumulate(table[::-1]))[::-1]
T = Lazysegtree(table, 0, True, min)
Q = int(input())
Ans = [None]*Q
for q in range(Q):
t, i, x = list(map(int, sys.stdin.readline().split()))
i -= 1
if t == 1:
T.add(0, x+1, -1)
T.add(0, A[i]+1, 1)
A[i] = x
else:
T.add(0, x+1, 1)
T.add(0, B[i]+1, -1)
B[i] = x
Ans[q] = T.provfunc()
print('\n'.join(map(str, Ans)))
| import sys
from itertools import accumulate
class Lazysegtree:
#RAQ
def __init__(self, A, intv, initialize = True, segf = min):
#区間は 1-indexed で管理
self.N = len(A)
self.N0 = 2**(self.N-1).bit_length()
self.intv = intv
self.segf = segf
self.lazy = [0]*(2*self.N0)
if initialize:
self.data = [intv]*self.N0 + A + [intv]*(self.N0 - self.N)
for i in range(self.N0-1, 0, -1):
self.data[i] = self.segf(self.data[2*i], self.data[2*i+1])
else:
self.data = [intv]*(2*self.N0)
def _ascend(self, k):
k = k >> 1
c = k.bit_length()
for j in range(c):
idx = k >> j
self.data[idx] = self.segf(self.data[2*idx], self.data[2*idx+1]) \
+ self.lazy[idx]
def _descend(self, k):
k = k >> 1
idx = 1
c = k.bit_length()
for j in range(1, c+1):
idx = k >> (c - j)
ax = self.lazy[idx]
if not ax:
continue
self.lazy[idx] = 0
self.data[2*idx] += ax
self.data[2*idx+1] += ax
self.lazy[2*idx] += ax
self.lazy[2*idx+1] += ax
def query(self, l, r):
L = l+self.N0
R = r+self.N0
Li = L//(L & -L)
Ri = R//(R & -R)
self._descend(Li)
self._descend(Ri - 1)
s = self.intv
while L < R:
if R & 1:
R -= 1
s = self.segf(s, self.data[R])
if L & 1:
s = self.segf(s, self.data[L])
L += 1
L >>= 1
R >>= 1
return s
def add(self, l, r, x):
L = l+self.N0
R = r+self.N0
Li = L//(L & -L)
Ri = R//(R & -R)
while L < R :
if R & 1:
R -= 1
self.data[R] += x
self.lazy[R] += x
if L & 1:
self.data[L] += x
self.lazy[L] += x
L += 1
L >>= 1
R >>= 1
self._ascend(Li)
self._ascend(Ri-1)
def binsearch(self, l, r, check, reverse = False):
L = l+self.N0
R = r+self.N0
Li = L//(L & -L)
Ri = R//(R & -R)
self._descend(Li)
self._descend(Ri-1)
SL, SR = [], []
while L < R:
if R & 1:
R -= 1
SR.append(R)
if L & 1:
SL.append(L)
L += 1
L >>= 1
R >>= 1
if reverse:
for idx in (SR + SL[::-1]):
if check(self.data[idx]):
break
else:
return -1
while idx < self.N0:
ax = self.lazy[idx]
self.lazy[idx] = 0
self.data[2*idx] += ax
self.data[2*idx+1] += ax
self.lazy[2*idx] += ax
self.lazy[2*idx+1] += ax
idx = idx << 1
if check(self.data[idx+1]):
idx += 1
return idx - self.N0
else:
for idx in (SL + SR[::-1]):
if check(self.data[idx]):
break
else:
return -1
while idx < self.N0:
ax = self.lazy[idx]
self.lazy[idx] = 0
self.data[2*idx] += ax
self.data[2*idx+1] += ax
self.lazy[2*idx] += ax
self.lazy[2*idx+1] += ax
idx = idx << 1
if not check(self.data[idx]):
idx += 1
return idx - self.N0
def provfunc(self):
idx = 1
if self.data[1] >= 0:
return -1
while idx < self.N0:
ax = self.lazy[idx]
self.lazy[idx] = 0
self.data[2*idx] += ax
self.data[2*idx+1] += ax
self.lazy[2*idx] += ax
self.lazy[2*idx+1] += ax
idx = idx << 1
if self.data[idx+1] < 0:
idx += 1
return idx - self.N0
N, M = list(map(int, input().split()))
A = list(map(int, input().split()))
B = list(map(int, input().split()))
table = [0]*(10**6+1)
for a in A:
table[a] -= 1
for b in B:
table[b] += 1
table = list(accumulate(table[::-1]))[::-1]
T = Lazysegtree(table, 0, True, min)
Q = int(input())
Ans = [None]*Q
for q in range(Q):
t, i, x = list(map(int, sys.stdin.readline().split()))
i -= 1
if t == 1:
T.add(0, x+1, -1)
T.add(0, A[i]+1, 1)
A[i] = x
else:
T.add(0, x+1, 1)
T.add(0, B[i]+1, -1)
B[i] = x
Ans[q] = T.provfunc()
print('\n'.join(map(str, Ans)))
| train | APPS_structured |
Not everyone probably knows that Chef has younder brother Jeff. Currently Jeff learns to read.
He knows some subset of the letter of Latin alphabet. In order to help Jeff to study, Chef gave him a book with the text consisting of N words. Jeff can read a word iff it consists only of the letters he knows.
Now Chef is curious about which words his brother will be able to read, and which are not. Please help him!
-----Input-----
The first line of the input contains a lowercase Latin letter string S, consisting of the letters Jeff can read. Every letter will appear in S no more than once.
The second line of the input contains an integer N denoting the number of words in the book.
Each of the following N lines contains a single lowecase Latin letter string Wi, denoting the ith word in the book.
-----Output-----
For each of the words, output "Yes" (without quotes) in case Jeff can read it, and "No" (without quotes) otherwise.
-----Constraints-----
- 1 ≤ |S| ≤ 26
- 1 ≤ N ≤ 1000
- 1 ≤ |Wi| ≤ 12
- Each letter will appear in S no more than once.
- S, Wi consist only of lowercase Latin letters.
-----Subtasks-----
- Subtask #1 (31 point): |S| = 1, i.e. Jeff knows only one letter.
- Subtask #2 (69 point) : no additional constraints
-----Example-----
Input:act
2
cat
dog
Output:Yes
No
-----Explanation-----
The first word can be read.
The second word contains the letters d, o and g that aren't known by Jeff. | s1 = input()
for i in range(int(input())):
s2 = input()
a= ''.join(sorted(s1))
c= ''.join(set(s2))
b= ''.join(sorted(c))
if b in a:
print('Yes')
else:
print('No') | s1 = input()
for i in range(int(input())):
s2 = input()
a= ''.join(sorted(s1))
c= ''.join(set(s2))
b= ''.join(sorted(c))
if b in a:
print('Yes')
else:
print('No') | train | APPS_structured |
You want to build a temple for snakes. The temple will be built on a mountain range, which can be thought of as n blocks, where height of i-th block is given by hi. The temple will be made on a consecutive section of the blocks and its height should start from 1 and increase by exactly 1 each time till some height and then decrease by exactly 1 each time to height 1,
i.e. a consecutive section of 1, 2, 3, .. x-1, x, x-1, x-2, .., 1 can correspond to a temple. Also, heights of all the blocks other than of the temple should have zero height, so that the temple is visible to people who view it from the left side or right side.
You want to construct a temple. For that, you can reduce the heights of some of the blocks. In a single operation, you can reduce the height of a block by 1 unit. Find out minimum number of operations required to build a temple.
-----Input-----
The first line of the input contains an integer T denoting the number of test cases. The description of T test cases follows.
The first line of each test case contains an integer n.
The next line contains n integers, where the i-th integer denotes hi
-----Output-----
For each test case, output a new line with an integer corresponding to the answer of that testcase.
-----Constraints-----
- 1 ≤ T ≤ 10
- 2 ≤ n ≤ 105
- 1 ≤ hi ≤ 109
-----Example-----
Input
3
3
1 2 1
4
1 1 2 1
5
1 2 6 2 1
Output
0
1
3
-----Explanation-----
Example 1. The entire mountain range is already a temple. So, there is no need to make any operation.
Example 2. If you reduce the height of the first block to 0. You get 0 1 2 1. The blocks 1, 2, 1 form a temple. So, the answer is 1.
Example 3. One possible temple can be 1 2 3 2 1. It requires 3 operations to build. This is the minimum amount you have to spend in order to build a temple. | t=int(input())
for I in range(t):
n=int(input())
a=[0]+[int(i) for i in input().split()] + [0]
L=[0]*(n+2)
R=[0]*(n+2)
for i in range(1,n+1):
L[i] = min(1+L[i-1],a[i])
for i in range(n,0,-1):
R[i] = min(R[i+1]+1,a[i])
max_height=0
for i in range(1,n+1):
ht = min(L[i],R[i])
max_height = max(max_height,ht)
ans = sum(a) - max_height**2
print(ans)
| t=int(input())
for I in range(t):
n=int(input())
a=[0]+[int(i) for i in input().split()] + [0]
L=[0]*(n+2)
R=[0]*(n+2)
for i in range(1,n+1):
L[i] = min(1+L[i-1],a[i])
for i in range(n,0,-1):
R[i] = min(R[i+1]+1,a[i])
max_height=0
for i in range(1,n+1):
ht = min(L[i],R[i])
max_height = max(max_height,ht)
ans = sum(a) - max_height**2
print(ans)
| train | APPS_structured |
Once you complete this kata, there is a [15x15 Version](http://www.codewars.com/kata/15x15-nonogram-solver) that you can try.
And once you complete that, you can do the [Multisize Version](https://www.codewars.com/kata/5a5519858803853691000069) which goes up to 50x50.
# Description
For this kata, you will be making a Nonogram solver. :)
If you don't know what Nonograms are, you can [look at some instructions](https://www.puzzle-nonograms.com/faq.php) and also [try out some Nonograms here.](https://www.puzzle-nonograms.com/)
For this kata, you will only have to solve 5x5 Nonograms. :)
# Instructions
You need to complete the Nonogram class and the solve method:
```python
class Nonogram:
def __init__(self, clues):
pass
def solve(self):
pass
```
You will be given the clues and you should return the solved puzzle. All the puzzles will be solveable so you will not need to worry about that.
```if:python
The clues will be a tuple of the horizontal clues, then the vertical clues, which will contain the individual clues. For example, for the Nonogram:
```
```if:java
The clues will be a three dimensional array of the horizontal clues, then the vertical clues, which will contain the individual clues. For example, for the Nonogram:
```
```
| | | 1 | | |
| 1 | | 1 | | |
| 1 | 4 | 1 | 3 | 1 |
-------------------------
1 | | | | | |
-------------------------
2 | | | | | |
-------------------------
3 | | | | | |
-------------------------
2 1 | | | | | |
-------------------------
4 | | | | | |
-------------------------
```
The clues are on the top and the left of the puzzle, so in this case:
```if:python
The horizontal clues are: `((1, 1), (4,), (1, 1, 1), (3,), (1,))`, and the vertical clues are: `((1,), (2,), (3,), (2, 1), (4,))`. The horizontal clues are given from left to right. If there is more than one clue for the same column, the upper clue is given first. The vertical clues are given from top to bottom. If there is more than one clue for the same row, the leftmost clue is given first.
Therefore, the clue given to the `__init__` method would be `(((1, 1), (4,), (1, 1, 1), (3,), (1,)), ((1,), (2,), (3,), (2, 1), (4,)))`. You are given the horizontal clues first then the vertical clues second.
```
```if:java
The horizontal clues are: `{{1, 1}, {4}, {1, 1, 1}, {3}, {1}}`, and the vertical clues are: `{{1}, {2}, {3}, {2, 1}, {4}}`. The horizontal clues are given from left to right. If there is more than one clue for the same column, the upper clue is given first. The vertical clues are given from top to bottom. If there is more than one clue for the same row, the leftmost clue is given first.
Therefore, the clue given to the `solve` method would be `{{{1, 1}, {4}, {1, 1, 1}, {3}, {1}},
{{1}, {2}, {3}, {2, 1}, {4}}}`. You are given the horizontal clues first then the vertical clues second.
```
```if:python
You should return a tuple of the rows as your answer. In this case, the solved Nonogram looks like:
```
```if:java
You should return a two-dimensional array as your answer. In this case, the solved Nonogram looks like:
```
```
| | | 1 | | |
| 1 | | 1 | | |
| 1 | 4 | 1 | 3 | 1 |
-------------------------
1 | | | # | | |
-------------------------
2 | # | # | | | |
-------------------------
3 | | # | # | # | |
-------------------------
2 1 | # | # | | # | |
-------------------------
4 | | # | # | # | # |
-------------------------
```
```if:python
In the tuple, you should use 0 for a unfilled square and 1 for a filled square. Therefore, in this case, you should return:
~~~
((0, 0, 1, 0, 0),
(1, 1, 0, 0, 0),
(0, 1, 1, 1, 0),
(1, 1, 0, 1, 0),
(0, 1, 1, 1, 1))
~~~
```
```if:java
In the two-dimensional array, you should use 0 for a unfilled square and 1 for a filled square. Therefore, in this case, you should return:
~~~
{{0, 0, 1, 0, 0},
{1, 1, 0, 0, 0},
{0, 1, 1, 1, 0},
{1, 1, 0, 1, 0},
{0, 1, 1, 1, 1}}
~~~
```
Good Luck!!
If there is anything that is unclear or confusing, just let me know :) | import itertools
class Nonogram:
poss = {(1,1,1): set([(1,0,1,0,1)]),
(1,1): set([(0,0,1,0,1),(0,1,0,1,0),(1,0,1,0,0),(0,1,0,0,1),(1,0,0,1,0),(1,0,0,0,1)]),
(1,2): set([(1,0,1,1,0),(1,0,0,1,1),(0,1,0,1,1)]),
(1,3): set([(1,0,1,1,1)]),
(2,1): set([(1,1,0,1,0),(1,1,0,0,1),(0,1,1,0,1)]),
(2,2): set([(1,1,0,1,1)]),
(3,1): set([(1,1,1,0,1)]),
(1,): set([(0,0,0,0,1),(0,0,0,1,0),(0,0,1,0,0),(0,1,0,0,0),(1,0,0,0,0)]),
(2,): set([(0,0,0,1,1),(0,0,1,1,0),(0,1,1,0,0),(1,1,0,0,0)]),
(3,): set([(0,0,1,1,1),(0,1,1,1,0),(1,1,1,0,0)]),
(4,): set([(0,1,1,1,1),(1,1,1,1,0)]),
(5,): set([(1,1,1,1,1)])}
def __init__(self, clues):
self.h,self.w=(tuple(Nonogram.poss[clue] for clue in side) for side in clues)
def solve(self):
for r in itertools.product(*self.w):
if all(c in self.h[i] for i,c in enumerate(zip(*r))): return r
| import itertools
class Nonogram:
poss = {(1,1,1): set([(1,0,1,0,1)]),
(1,1): set([(0,0,1,0,1),(0,1,0,1,0),(1,0,1,0,0),(0,1,0,0,1),(1,0,0,1,0),(1,0,0,0,1)]),
(1,2): set([(1,0,1,1,0),(1,0,0,1,1),(0,1,0,1,1)]),
(1,3): set([(1,0,1,1,1)]),
(2,1): set([(1,1,0,1,0),(1,1,0,0,1),(0,1,1,0,1)]),
(2,2): set([(1,1,0,1,1)]),
(3,1): set([(1,1,1,0,1)]),
(1,): set([(0,0,0,0,1),(0,0,0,1,0),(0,0,1,0,0),(0,1,0,0,0),(1,0,0,0,0)]),
(2,): set([(0,0,0,1,1),(0,0,1,1,0),(0,1,1,0,0),(1,1,0,0,0)]),
(3,): set([(0,0,1,1,1),(0,1,1,1,0),(1,1,1,0,0)]),
(4,): set([(0,1,1,1,1),(1,1,1,1,0)]),
(5,): set([(1,1,1,1,1)])}
def __init__(self, clues):
self.h,self.w=(tuple(Nonogram.poss[clue] for clue in side) for side in clues)
def solve(self):
for r in itertools.product(*self.w):
if all(c in self.h[i] for i,c in enumerate(zip(*r))): return r
| train | APPS_structured |
A permutation p of size n is the sequence p_1, p_2, ..., p_{n}, consisting of n distinct integers, each of them is from 1 to n (1 ≤ p_{i} ≤ n).
A lucky permutation is such permutation p, that any integer i (1 ≤ i ≤ n) meets this condition p_{p}_{i} = n - i + 1.
You have integer n. Find some lucky permutation p of size n.
-----Input-----
The first line contains integer n (1 ≤ n ≤ 10^5) — the required permutation size.
-----Output-----
Print "-1" (without the quotes) if the lucky permutation p of size n doesn't exist.
Otherwise, print n distinct integers p_1, p_2, ..., p_{n} (1 ≤ p_{i} ≤ n) after a space — the required permutation.
If there are multiple answers, you can print any of them.
-----Examples-----
Input
1
Output
1
Input
2
Output
-1
Input
4
Output
2 4 1 3
Input
5
Output
2 5 3 1 4 | n=int(input())
L=[0]*(n+1)
X=[False]*(n+1)
if(n%4!=0 and n%4!=1):
print(-1)
else:
for i in range(1,n+1):
if(X[i]):
continue
X[i]=True
X[n-i+1]=True
for j in range(i+1,n+1):
if(X[j]):
continue
X[j]=True
X[n-j+1]=True
L[i]=j
L[n-i+1]=n-j+1
L[j]=n-i+1
L[n-j+1]=i
break
if(n%4==1):
L[n//2+1]=n//2+1
for i in range(1,n):
print(L[i],end=" ")
print(L[n])
| n=int(input())
L=[0]*(n+1)
X=[False]*(n+1)
if(n%4!=0 and n%4!=1):
print(-1)
else:
for i in range(1,n+1):
if(X[i]):
continue
X[i]=True
X[n-i+1]=True
for j in range(i+1,n+1):
if(X[j]):
continue
X[j]=True
X[n-j+1]=True
L[i]=j
L[n-i+1]=n-j+1
L[j]=n-i+1
L[n-j+1]=i
break
if(n%4==1):
L[n//2+1]=n//2+1
for i in range(1,n):
print(L[i],end=" ")
print(L[n])
| train | APPS_structured |
Given two strings: s1 and s2 with the same size, check if some permutation of string s1 can break some permutation of string s2 or vice-versa (in other words s2 can break s1).
A string x can break string y (both of size n) if x[i] >= y[i] (in alphabetical order) for all i between 0 and n-1.
Example 1:
Input: s1 = "abc", s2 = "xya"
Output: true
Explanation: "ayx" is a permutation of s2="xya" which can break to string "abc" which is a permutation of s1="abc".
Example 2:
Input: s1 = "abe", s2 = "acd"
Output: false
Explanation: All permutations for s1="abe" are: "abe", "aeb", "bae", "bea", "eab" and "eba" and all permutation for s2="acd" are: "acd", "adc", "cad", "cda", "dac" and "dca". However, there is not any permutation from s1 which can break some permutation from s2 and vice-versa.
Example 3:
Input: s1 = "leetcodee", s2 = "interview"
Output: true
Constraints:
s1.length == n
s2.length == n
1 <= n <= 10^5
All strings consist of lowercase English letters. | class Solution:
def checkIfCanBreak(self, s1: str, s2: str) -> bool:
from collections import Counter
def check_breakable(s1, s2):
s1_counter = Counter(s1)
s2_counter = Counter(s2)
cnt = 0
for char in 'abcdefghijklmnopqrstuvwxyz':
cnt += s1_counter[char] - s2_counter[char]
if cnt < 0:
return False
return True
return check_breakable(s1, s2) == True or check_breakable(s2,s1) == True | class Solution:
def checkIfCanBreak(self, s1: str, s2: str) -> bool:
from collections import Counter
def check_breakable(s1, s2):
s1_counter = Counter(s1)
s2_counter = Counter(s2)
cnt = 0
for char in 'abcdefghijklmnopqrstuvwxyz':
cnt += s1_counter[char] - s2_counter[char]
if cnt < 0:
return False
return True
return check_breakable(s1, s2) == True or check_breakable(s2,s1) == True | train | APPS_structured |
# Background
A spider web is defined by
* "rings" numbered out from the centre as `0`, `1`, `2`, `3`, `4`
* "radials" labelled clock-wise from the top as `A`, `B`, `C`, `D`, `E`, `F`, `G`, `H`
Here is a picture to help explain:
# Web Coordinates
As you can see, each point where the rings and the radials intersect can be described by a "web coordinate".
So in this example the spider is at `H3` and the fly is at `E2`
# Kata Task
Our friendly jumping spider is resting and minding his own spidery business at web-coordinate `spider`.
An inattentive fly bumbles into the web at web-coordinate `fly` and gets itself stuck.
Your task is to calculate and return **the
distance** the spider must jump to get to the fly.
# Example
The solution to the scenario described by the picture is ``4.63522``
# Notes
* The centre of the web will always be referred to as `A0`
* The rings intersect the radials at **evenly** spaced distances of **1 unit**
____
Good Luck!DM | from math import cos, radians, sin
def spider_to_fly(spider, fly):
(ts, rs), (tf, rf) = ((radians((ord(c) - 65) * 45), int(n)) for c, n in (spider, fly))
return ((rs*cos(ts) - rf*cos(tf))**2 + (rs*sin(ts) - rf*sin(tf))**2)**0.5
| from math import cos, radians, sin
def spider_to_fly(spider, fly):
(ts, rs), (tf, rf) = ((radians((ord(c) - 65) * 45), int(n)) for c, n in (spider, fly))
return ((rs*cos(ts) - rf*cos(tf))**2 + (rs*sin(ts) - rf*sin(tf))**2)**0.5
| train | APPS_structured |