description
stringlengths 38
154k
| category
stringclasses 5
values | solutions
stringlengths 13
289k
| name
stringlengths 3
179
| id
stringlengths 24
24
| tags
sequencelengths 0
13
| url
stringlengths 54
54
| rank_name
stringclasses 8
values |
---|---|---|---|---|---|---|---|
This series of katas will introduce you to basics of doing geometry with computers.
Point objects have `x`, `y` attributes.
Circle objects have `center` which is a `Point`, and `radius` which is a number.
Write a function calculating distance between `Circle a` and `Circle b`.
If they're overlapping or one is completely within the other, just return zero.
Tests round answers to 6 decimal places, so you don't need to round them yourselves. | reference | def distance_between_circles(a, b):
dis = ((a . center . x - b . center . x) * * 2 + (a . center . y - b . center . y) * * 2) * * 0.5
return max(0, dis - a . radius - b . radius)
| Geometry Basics: Distance between circles in 2D | 58e3031ce265671f6a000542 | [
"Geometry",
"Fundamentals"
] | https://www.codewars.com/kata/58e3031ce265671f6a000542 | 7 kyu |
You get a "text" and have to shift the vowels by "n" positions to the right.<br/>
(Negative value for n should shift to the left.)<br/>
"Position" means the vowel's position if taken as one item in a list of all vowels within the string.<br>
A shift by 1 would mean, that every vowel shifts to the place of the next vowel.<br><br>
Shifting over the edges of the text should continue at the other edge.<br/>
<br/>
Example:<br/>
<br/>
text = "This is a test!"<br/>
n = 1<br/>
output = "Thes is i tast!"<br/>
<br/>
text = "This is a test!"<br/>
n = 3<br/>
output = "This as e tist!"<br/>
<br/>
If text is null or empty return exactly this value.<br>
Vowels are "a,e,i,o,u".
<br>
<br>
<br>
Have fun coding it and please don't forget to vote and rank this kata! :-)<br>
I have created other katas. Have a look if you like coding and challenges. | algorithms | import re
from collections import deque
def vowel_shift(text, n):
try:
tokens = re . split(r'([aeiouAEIOU])', text)
if len(tokens) > 1:
vowels = deque(tokens[1:: 2])
vowels . rotate(n)
tokens[1:: 2] = vowels
return '' . join(tokens)
except TypeError:
return None
| Vowel Shifting | 577e277c9fb2a5511c00001d | [
"Strings",
"Regular Expressions",
"Algorithms"
] | https://www.codewars.com/kata/577e277c9fb2a5511c00001d | 6 kyu |
#Make them bark
You have been hired by a dogbreeder to write a program to keep record of his dogs.
You've already made a constructor `Dog`, so no one has to hardcode every puppy.
The work seems to be done. It's high time to collect the payment.
..hold on! The dogbreeder says he wont pay you, until he can make every dog object `.bark()`. Even the ones already done with your constructor.
"Every dog barks" he says. He also refuses to rewrite them, lazy as he is.
You can't even count how much objects that bastard client of yours already made. He has a lot of dogs, and none of them can `.bark()`.
Can you solve this problem, or will you let this client outsmart you for good?
### Practical info:
- The `.bark()` method of a dog should return the string `'Woof!'`.
- The contructor you made (it is preloaded) looks like this:
```javascript
function Dog(name, breed, sex, age){
this.name = name;
this.breed = breed;
this.sex = sex;
this.age = age;
}
```
```coffeescript
class Dog
constructor: (@name, @breed, @sex, @age) ->
```
```python
class Dog(object):
def __init__(self, name, breed, sex, age):
self.name = name
self.breed = breed
self.sex = sex
self.age = age
```
```ruby
class Dog
def initialize(name, breed, sex, age)
@name = name
@breed = breed
@sex = sex
@age = age
end
end
```
>**Hint:** A friend of yours just told you about how javascript handles classes diferently from other programming languages. He couldn't stop ranting about *"prototypes"*, or something like that. Maybe that could help you... | reference | def bark(self):
return "Woof!"
Dog . bark = bark
| Make them bark! | 5535572c1de94ba2db0000f6 | [
"Fundamentals"
] | https://www.codewars.com/kata/5535572c1de94ba2db0000f6 | 7 kyu |
A "Lucky Seven" is the number seven surrounded by numbers that add up to form a perfect cube. The surrounding numbers will be described as the numbers directly above, below, and next to (not diagonally) the number 7. You will be given a 2D array containing at least 1 lucky seven. Your function should return the number of lucky sevens in the array.
Here are some "Lucky Sevens" for example:
```
[ [ 'x', 513, 'x', 'x', 'x' ],
[ 82, 7, 32, 'x', 'x' ],
[ 'x', 102, 'x', 'x', 'x' ],
[ 'x', 'x', 'x', 'x', 'x' ],
[ 'x', 'x', 'x', 'x', 'x' ] ]
[ [ 'x', 'x', 'x', 'x', 'x' ],
[ 'x', 'x', 'x', 'x', 'x' ],
[ 'x', 'x', 'x', 'x', 'x' ],
[ 'x', 'x', 'x', 'x', 9 ],
[ 'x', 'x', 'x', 55, 7 ] ]
[ [ 'x', 'x', 'x', 'x', 'x' ],
[ 'x', 1, 'x', 'x', 'x' ],
[ 0, 7, 0, 'x', 'x' ],
[ 'x', 0, 'x', 'x', 'x' ],
[ 'x', 'x', 'x', 'x', 'x' ] ]
```
Good luck! | reference | def luckySevens(arr):
def check(x, y):
t = sum(arr[x + dx][y + dy]
for dx, dy in dIdx if 0 <= x + dx < lX and 0 <= y + dy < lY)
return abs(t * * (1 / 3) - round(t * * (1 / 3))) <= 1e-8
dIdx = ((1, 0), (- 1, 0), (0, 1), (0, - 1))
lX, lY = len(arr), len(arr[0])
return sum(check(x, y) for x in range(lX) for y in range(lY) if arr[x][y] == 7)
| Lucky Sevens | 58275b63083e128edb00098d | [
"Fundamentals"
] | https://www.codewars.com/kata/58275b63083e128edb00098d | 6 kyu |
# Task
You are given a string `s`. Every letter in `s` appears once.
Consider all strings formed by rearranging the letters in `s`. After ordering these strings in dictionary order, return the middle term. (If the sequence has a even length `n`, define its middle term to be the `(n/2)`th term.)
# Example
For `s = "abc"`, the result should be `"bac"`.
```
The permutations in order are:
"abc", "acb", "bac", "bca", "cab", "cba"
So, The middle term is "bac".```
# Input/Output
- `[input]` string `s`
unique letters (`2 <= length <= 26`)
- `[output]` a string
middle permutation. | games | def middle_permutation(string):
s = sorted(string)
if len(s) % 2 == 0:
return s . pop(len(s) / / 2 - 1) + '' . join(s[:: - 1])
else:
return s . pop(len(s) / / 2) + middle_permutation(s)
| Simple Fun #159: Middle Permutation | 58ad317d1541651a740000c5 | [
"Puzzles"
] | https://www.codewars.com/kata/58ad317d1541651a740000c5 | 4 kyu |
You have to sort the inner content of every word of a string in descending order.
The inner content is the content of a word without first and the last char.
Some examples:
```
"sort the inner content in descending order" --> "srot the inner ctonnet in dsnnieedcg oredr"
"wait for me" --> "wiat for me"
"this kata is easy" --> "tihs ktaa is esay"
```
Words are made up of lowercase letters.
The string will never be null and will never be empty.
In C/C++ the string is always nul-terminated.
Have fun coding it and please don't forget to vote and rank this kata! :-)
I have also created other katas. Take a look if you enjoyed this kata! | algorithms | def sort_the_inner_content(str):
words = str . split()
output = []
for word in words:
if len(word) > 2:
output . append(
word[0] + '' . join(sorted(word[1: - 1], reverse=True)) + word[- 1])
else:
output . append(word)
return ' ' . join(output)
| Srot the inner ctonnet in dsnnieedcg oredr | 5898b4b71d298e51b600014b | [
"Strings",
"Logic",
"Algorithms"
] | https://www.codewars.com/kata/5898b4b71d298e51b600014b | 6 kyu |
<p>You are given an array of unique numbers. The numbers represent points. The higher the number the higher the points.
In the array [1,3,2] 3 is the highest point value so it gets 1st place. 2 is the second highest so it gets second place. 1 is the 3rd highest so it gets 3rd place.
Your task is to return an array giving each number its rank in the array. </p>
input // [1,3,2] <br>
output // [3,1,2]<br>
```rankings([1,2,3,4,5]) // [5,4,3,2,1]``` <br>
```rankings([3,4,1,2,5])// [3,2,5,4,1]``` <br>
```rankings([10,20,40,50,30]) // [5, 4, 2, 1, 3]``` <br>
```rankings([1, 10]) // [2, 1]``` <br>
```rankings([22, 33, 18, 9, 110, 4, 1, 88, 6, 50]) //```
```[5, 4, 6, 7, 1, 9, 10, 2, 8, 3]``` | reference | def rankings(arr):
dct = {v: i for i, v in enumerate(sorted(arr, reverse=True), 1)}
return [dct[v] for v in arr]
| Ranking System | 58e16de3a312d34d000000bd | [
"Fundamentals"
] | https://www.codewars.com/kata/58e16de3a312d34d000000bd | 6 kyu |
# Task
Consider the following operation:
We take a positive integer `n` and replace it with the sum of its `prime factors` (if a prime number is presented multiple times in the factorization of `n`, then it's counted the same number of times in the sum).
This operation is applied sequentially first to the given number, then to the first result, then to the second result and so on.., until the result remains the same.
Given number `n`, find the final result of the operation.
# Example
For `n = 24`, the output should be `5`.
```
24 -> (2 + 2 + 2 + 3) = 9 -> (3 + 3) = 6 -> (2 + 3) = 5 -> 5.
So the answer for n = 24 is 5.```
# Input/Output
- `[input]` integer `n`
Constraints: `2 β€ n β€ 10000.`
- `[output]` an integer | games | def factor_sum(n):
fs = sum(factors(n))
return fs if fs == n else factor_sum(fs)
def factors(n, p=2):
while p * p <= n:
while not n % p:
yield p
n / /= p
p += 2 - (p == 2)
if n > 1 and p > 2:
yield n
| Simple Fun #115: Factor Sum | 589d2bf7dfdef0817e0001aa | [
"Puzzles"
] | https://www.codewars.com/kata/589d2bf7dfdef0817e0001aa | 6 kyu |
Scheduling is how the processor decides which jobs(processes) get to use the processor and for how long. This can cause a lot of problems. Like a really long process taking the entire CPU and freezing all the other processes. One solution is Shortest Job First(SJF), which today you will be implementing.
SJF works by, well, letting the shortest jobs take the CPU first. If the jobs are the same size then it is First In First Out (FIFO). The idea is that the shorter jobs will finish quicker, so theoretically jobs won't get frozen because of large jobs. (In practice they're frozen because of small jobs).
You will be implementing:
```javascript
function SJF(jobs, index)
```
```python
def SJF(jobs, index)
```
```ruby
def SJF(jobs, index)
```
```haskell
sjf :: [Integer] -> Int -> Integer
```
```rust
fn sjf(jobs: &[usize], index: usize) -> usize
```
It takes in:
1. "jobs" a non-empty array of positive integers. They represent the clock-cycles(cc) needed to finish the job.
2. "index" a positive integer. That represents the job we're interested in.
SJF returns:
1. A positive integer representing the cc it takes to complete the job at index.
Here's an example:
```
SJF([3, 10, 20, 1, 2], 0)
at 0cc [3, 10, 20, 1, 2] jobs[3] starts
at 1cc [3, 10, 20, 0, 2] jobs[3] finishes, jobs[4] starts
at 3cc [3, 10, 20, 0, 0] jobs[4] finishes, jobs[0] starts
at 6cc [0, 10, 20, 0, 0] jobs[0] finishes
```
so:
```
SJF([3,10,20,1,2], 0) == 6
``` | algorithms | def SJF(jobs, index):
return sum(j for i, j in enumerate(jobs)
if j < jobs[index] or (j == jobs[index] and i <= index))
| Scheduling (Shortest Job First or SJF) | 550cc572b9e7b563be00054f | [
"Scheduling",
"Queues",
"Algorithms"
] | https://www.codewars.com/kata/550cc572b9e7b563be00054f | 6 kyu |
You are the "computer expert" of a local Athletic Association (C.A.A.).
Many teams of runners come to compete. Each time you get a string of
all race results of every team who has run.
For example here is a string showing the individual results of a team of 5 runners:
` "01|15|59, 1|47|6, 01|17|20, 1|32|34, 2|3|17" `
Each part of the string is of the form: ` h|m|s `
where h, m, s (h for hour, m for minutes, s for seconds) are positive or null integer (represented as strings) with one or two digits. Substrings in the input string are separated by `, ` or `,`.
To compare the results of the teams you are asked for giving
three statistics; **range, average and median**.
- `Range` : difference between the lowest and highest values.
In {4, 6, 9, 3, 7} the lowest value is 3, and the highest is 9,
so the range is 9 β 3 = 6.
- `Mean or Average` : To calculate mean, add together all of the numbers
and then divide the sum by the total count of numbers.
- `Median` : In statistics, the median is the number separating the higher half
of a data sample from the lower half.
The median of a finite list of numbers can be found by arranging all
the observations from lowest value to highest value and picking the middle one
(e.g., the median of {3, 3, 5, 9, 11} is 5) when there is an odd number of observations.
If there is an even number of observations, then there is no single middle value;
the median is then defined to be the mean of the two middle values
(the median of {3, 5, 6, 9} is (5 + 6) / 2 = 5.5).
Your task is to return a string giving these 3 values. For the example given above,
the string result will be
`"Range: 00|47|18 Average: 01|35|15 Median: 01|32|34"`
of the form:
"Range: hh|mm|ss Average: hh|mm|ss Median: hh|mm|ss"`
where hh, mm, ss are integers (represented by strings) with *each 2 digits*.
#### *Remarks*:
1. if a result in seconds is ab.xy... it will be given **truncated** as ab.
2. if the given string is "" you will return ""
| reference | def stat(strg):
def get_time(s):
'''Returns the time, in seconds, represented by s.'''
hh, mm, ss = [int(v) for v in s . split('|')]
return hh * 3600 + mm * 60 + ss
def format_time(time):
'''Returns the given time as a string in the form "hh|mm|ss".'''
hh = time / / 3600
mm = time / / 60 % 60
ss = time % 60
return '{hh:02d}|{mm:02d}|{ss:02d}' . format(* * locals())
def get_range(times):
return times[- 1] - times[0]
def get_average(times):
return sum(times) / / len(times)
def get_median(times):
middle = len(times) >> 1
return (times[middle] if len(times) & 1 else
(times[middle - 1] + times[middle]) / / 2)
if strg == '':
return strg
times = [get_time(s) for s in strg . split(', ')]
times . sort()
rng = format_time(get_range(times))
avg = format_time(get_average(times))
mdn = format_time(get_median(times))
return 'Range: {rng} Average: {avg} Median: {mdn}' . format(* * locals())
| Statistics for an Athletic Association | 55b3425df71c1201a800009c | [
"Fundamentals",
"Strings",
"Statistics",
"Mathematics",
"Data Science"
] | https://www.codewars.com/kata/55b3425df71c1201a800009c | 6 kyu |
Some people just have a first name; some people have first and last names and some people have first, middle and last names.
You task is to initialize the middle names (if there is any).
## Examples
```
'Jack Ryan' => 'Jack Ryan'
'Lois Mary Lane' => 'Lois M. Lane'
'Dimitri' => 'Dimitri'
'Alice Betty Catherine Davis' => 'Alice B. C. Davis'
``` | reference | def initializeNames(name):
names = name . split()
names[1: - 1] = map(lambda n: n[0] + '.', names[1: - 1])
return ' ' . join(names)
| Initialize my name | 5768a693a3205e1cc100071f | [
"Strings",
"Fundamentals"
] | https://www.codewars.com/kata/5768a693a3205e1cc100071f | 7 kyu |
*You are a composer who just wrote an awesome piece of music. Now it's time to present it to a band that will perform your piece, but there's a problem! The singers vocal range doesn't stretch as your piece requires, and you have to transpose the whole piece.*
# Your task
Given a list of notes (represented as strings) and an interval, output a list of transposed notes in *sharp* notation.
**Input notes may be represented both in flat and sharp notations (more on that below).**
**For this kata, assume that input is always valid and the song is *at least* 1 note long.**
**Assume that interval is an integer between -12 and 12.**
# Short intro to musical notation
Transposing a single note means shifting its value by a certain interval.
The notes are as following:
A, A#, B, C, C#, D, D#, E, F, F#, G, G#.
This is using *sharp* notation, where '#' after a note means that it is one step higher than the note. So A# is one step higher than A.
An alternative to sharp notation is the *flat* notation:
A, Bb, B, C, Db, D, Eb, E, F, Gb, G, Ab.
The 'b' after a note means that it is one step lower than the note.
# Examples
['G'] -> 5 steps -> ['C']
['Db'] -> -4 steps -> ['A']
['E', 'F'] -> 1 step -> ['F', 'F#']
| algorithms | def transpose(song, interval):
altern = {"Bb": "A#", "Db": "C#", "Eb": "D#", "Gb": "F#", "Ab": "G#"}
notes = ['A', 'A#', 'B', 'C', 'C#', 'D', 'D#', 'E', 'F', 'F#', 'G', 'G#']
return [notes[(notes . index(altern . get(i, i)) + interval) % 12] for i in song]
| Transposing a song | 55b6a3a3c776ce185c000021 | [
"Fundamentals",
"Algorithms",
"Strings",
"Lists"
] | https://www.codewars.com/kata/55b6a3a3c776ce185c000021 | 7 kyu |
- Input : an array of integers.
- Output : this array, but sorted in such a way that there are two wings:
- the left wing with numbers decreasing,
- the right wing with numbers increasing.
- the two wings have the same length. If the length of the array is odd
the wings are around the bottom, if the length is even the bottom is
considered to be part of the right wing.
- each integer `l` of the left wing must be greater or equal to its counterpart `r` in the right wing, the
difference `l - r` being as small as possible.
In other words the right wing must be nearly as steep as the left wing.
The function is `make_valley or makeValley or make-valley`.
```
a = [79, 35, 54, 19, 35, 25]
make_valley(a) --> [79, 35, 25, *19*, 35, 54]
The bottom is 19, left wing is [79, 35, 25], right wing is [*19*, 35, 54].
79..................54
35..........35
25.
..19
a = [67, 93, 100, -16, 65, 97, 92]
make_valley(a) --> [100, 93, 67, *-16*, 65, 92, 97]
The bottom is -16, left wing [100, 93, 67] and right wing [65, 92, 97] have same length.
100.........................97
93..........
.........92
67......
.....65
-16
a = [66, 55, 100, 68, 46, -82, 12, 72, 12, 38]
make_valley(a) --> [100, 68, 55, 38, 12, *-82*, 12, 46, 66, 72]
The bottom is -82, left wing is [100, 68, 55, 38, 12]], right wing is [*-82*, 12, 46, 66, 72].
a = [14,14,14,14,7,14]
make_valley(a) => [14, 14, 14, *7*, 14, 14]
a = [14,14,14,14,14]
make_valley(a) => [14, 14, *14*, 14, 14]
```
#### A counter-example:
```
a = [17, 17, 15, 14, 8, 7, 7, 5, 4, 4, 1]
A solution could be [17, 17, 15, 14, 8, 1, 4, 4, 5, 7, 7]
but the right wing [4, 4, 5, 7, 7] is much flatter than the left one
[17, 17, 15, 14, 8].
Summing the differences between left and right wing:
(17-7)+(17-7)+(15-5)+(14-4)+(8-4) = 44
Consider the following solution:
[17, 15, 8, 7, 4, 1, 4, 5, 7, 14, 17]
Summing the differences between left and right wing:
(17-17)+(15-14)+(8-7)+(7-5)+(4-4) = 4
The right wing is nearly as steep as the right one.
```
| reference | def make_valley(arr):
arr = sorted(arr, reverse=True)
return arr[:: 2] + arr[1:: 2][:: - 1]
| How Green Is My Valley? | 56e3cd1d93c3d940e50006a4 | [
"Fundamentals"
] | https://www.codewars.com/kata/56e3cd1d93c3d940e50006a4 | 7 kyu |
There's a waiting room with N chairs set in single row. Chairs are consecutively numbered from 1 to N. First is closest to the entrance (which is exit as well).
For some reason people choose a chair in the following way
1. Find a place as far from other people as possible
2. Find a place as close to exit as possible
All chairs must be occupied before the first person will be served
So it looks like this for 10 chairs and 10 patients
<table>
<thead>
<tr>
<th>Chairs</th>
<th>1</th>
<th>2</th>
<th>3</th>
<th>4</th>
<th>5</th>
<th>6</th>
<th>7</th>
<th>8</th>
<th>9</th>
<th>10</th>
<tr>
</thead>
<tbody>
<tr>
<td>Patients</td>
<td>1</td>
<td>7</td>
<td>5</td>
<td>8</td>
<td>3</td>
<td>9</td>
<td>4</td>
<td>6</td>
<td>10</td>
<td>2</td>
</tr>
</tbody>
</table>
Your task is to find last patient's chair's number.
```
Input: number of chairs N, an integer greater than 2.
Output: a positive integer, the last patient's chair number.
```
Have fun :) | algorithms | def last_chair(n):
# Propn:
# Given there are n seats, n >= 2. The (n-1)th seat is always the
# last to be taken (taken in iteration n).
# Proof:
# Suppose that, for some n >= 2, the (n-1)th seat is taken on
# iteration i > 2. The nth seat is already taken, since the 2nd
# iteration will always claim it. Therefore i must sit beside
# at least one person (the occupant of seat n).
# Additionally, the (n-2)th seat must also already be taken.
# If it were not taken, (n-2) would have a free seat at (n-1)
# and would be closer to the exit, and so would rank higher
# than (n-1) in the choice algorithm.
# Therefore (n-1) will only be chosen when (n-2) and (n) are
# both taken.
# Also, all other seats must also be taken, since
# otherwise i would taken them, having at most as many people
# around as seat (n-1) and being closer to the exit.
# Therefore (n-1) is the last seat to be taken.
return n - 1
| Waiting room | 542f0c36d002f8cd8a0005e5 | [
"Sorting",
"Puzzles",
"Algorithms"
] | https://www.codewars.com/kata/542f0c36d002f8cd8a0005e5 | 7 kyu |
A number is simply made up of digits.
The number 1256 is made up of the digits 1, 2, 5, and 6.
For 1256 there are 24 distinct permutations of the digits:
1256, 1265, 1625, 1652, 1562, 1526, 2156, 2165, 2615, 2651, 2561, 2516,
5126, 5162, 5216, 5261, 5621, 5612, 6125, 6152, 6251, 6215, 6521, 6512.
Your goal is to write a program that takes a number, n, and returns the average value of all distinct permutations of the digits in n. Your answer should be rounded to the nearest integer. For the example above the return value would be 3889. *
n will never be negative
A few examples:
```python
permutation_average(2)
return 2
permutation_average(25)
>>> 25 + 52 = 77
>>> 77 / 2 = 38.5
return 39 *
permutation_average(20)
>>> 20 + 02 = 22
>>> 22 / 2 = 11
return 11
permutation_average(737)
>>> 737 + 377 + 773 = 1887
>>> 1887 / 3 = 629
return 629
```
Note: Your program should be able to handle numbers up to 6 digits long
~~~if:python
\* Python version 3 and above uses Banker Rounding so the expected values for those tests would be 3888 and 38 respectively
~~~
~~~if-not:python
\* ignore these marks, they're for Python only
~~~ | algorithms | from itertools import permutations
def permutation_average(n):
perms = [float('' . join(e)) for e in permutations(str(n))]
return int(round(sum(perms) / len(perms)))
| Permutation Average | 56b18992240660a97c00000a | [
"Algorithms"
] | https://www.codewars.com/kata/56b18992240660a97c00000a | 7 kyu |
Write a function that returns the index of the first occurence of the word "Wally". "Wally" must not be part of another word, but it can be directly followed by a punctuation mark. If no such "Wally" exists, return -1.
Examples:
"Wally" => 0
"Where's Wally" => 8
"Where's Waldo" => -1
"DWally Wallyd .Wally" => -1
"Hi Wally." => 3
"It's Wally's." => 5
"Wally Wally" => 0
"'Wally Wally" => 7
| reference | from re import compile
def wheres_wally(string):
m = compile('(^|.*[\s])(Wally)([\.,\s\']|$)'). match(string)
return m . start(2) if m else - 1
| Where's Wally | 55f91a98db47502cfc00001b | [
"Regular Expressions",
"Fundamentals"
] | https://www.codewars.com/kata/55f91a98db47502cfc00001b | 7 kyu |
Write a function that takes an array/list of numbers and returns a number such that
Explanation
total([1,2,3,4,5]) => 48
<pre>
1+2=3--\ 3+5 => 8 \
2+3=5--/ \ == 8+12=>20\
==>5+7=> 12 / \ 20+28 => 48
3+4=7--\ / == 12+16=>28/
4+5=9--/ 7+9 => 16 /
</pre><br/>
if total([1,2,3]) => 8 then
<pre>
first+second => 3 \
then 3+5 => 8
second+third => 5 /
</pre><br/>
### Examples
```javascript
total([-1,-1,-1]) => -4
total([1,2,3,4]) => 20
```
```python
total([-1,-1,-1]) => -4
total([1,2,3,4]) => 20
```
```ruby
total([-1,-1,-1]) => -4
total([1,2,3,4]) => 20
```
```haskell
total [1..5] `shouldBe` (48)
total [5,4..1] `shouldBe` (48)
total [-1,-1,-1] `shouldBe` (-4)
total [1,2,3,4] `shouldBe` (20)
```
**Note:** each array/list will have at least an element and all elements will be valid numbers. | reference | def total(arr):
while len(arr) > 1:
arr = [x + y for x, y in zip(arr, arr[1:])]
return arr[0]
| sum2total | 559fed8454b12433ff0000a2 | [
"Logic",
"Mathematics",
"Arrays",
"Lists",
"Fundamentals"
] | https://www.codewars.com/kata/559fed8454b12433ff0000a2 | 7 kyu |
Every day we can send from the server a certain limit of e-mails.
## Task:
Write a function that will return the integer number of e-mails sent in the percentage of the limit.
Example:
```
limit - 1000;
emails sent - 101;
return - 10%; // because integer from 10,1 = 10
```
### Arguments:
- `sent`: number of e-mails sent today (integer)
- `limit`: number of e-mails you can send today (integer)
### When:
- the argument `sent = 0`, then return the message: `"No e-mails sent"`;
- the argument `sent >= limit`, then return the message: `"Daily limit is reached";`
- the argument `limit is empty`, then default `limit = 1000` emails;
### Good luck! | reference | def get_percentage(sent, limit=1000):
if not sent:
return "No e-mails sent"
elif sent >= limit:
return "Daily limit is reached"
return "{}%" . format(int(sent * 100 / limit))
| How many e-mails we sent today? | 58a369fa5b3daf464200006c | [
"Fundamentals",
"Logic",
"Mathematics"
] | https://www.codewars.com/kata/58a369fa5b3daf464200006c | 7 kyu |
Yet another staple for the functional programmer. You have a sequence of values and some predicate for those values. You want to remove the longest prefix of elements such that the predicate is true for each element. We'll call this the dropWhile function. It accepts two arguments. The first is the sequence of values, and the second is the predicate function. The function does not change the value of the original sequence.
```python
def isEven(num):
return num % 2 == 0
arr = [2,4,6,8,1,2,5,4,3,2]
dropWhile(arr, isEven) == [1,2,5,4,3,2] # True
```
```javascript
function isEven(num) {
return num % 2 === 0;
}
var seq = [2,4,6,8,1,2,5,4,3,2];
dropWhile(seq, isEven) // -> [1,2,5,4,3,2]
```
```haskell
dropWhile [2,4,6,8,1,2,5,4,3,2] even -- -> [1,2,5,4,3,2]
```
```cpp
auto isEven = [](int value) -> bool { return abs(value) % 2 == 0; };
dropWhile({ 2, 4, 6, 8, 1, 2, 5, 4, 3, 2 }, isEven) // -> { 1, 2, 5, 4, 3, 2 }
```
```csharp
Func<int, bool> isEven = (value) => value % 2 == 0;
dropWhile(new int[] { 2, 4, 6, 8, 1, 2, 5, 4, 3, 2 }, isEven) // -> { 1, 2, 5, 4, 3, 2 }
```
```lambdacalc
drop-while [2,4,6,8,1,2,5,4,3,2] even --> [1,2,5,4,3,2]
```
```lua
local function is_even(x)
return x%2==0
end
drop_while({2,4,6,8,1,2,5,4,3,2}, is_even) --> [1,2,5,4,3,2]
```
```ocaml
let is_even n = n mod 2 = 0 in
drop_while [2; 4; 6; 8; 1; 2; 5; 4; 3; 2] is_even
(* -> [1; 2; 5; 4; 3; 2] *)
```
```c
bool isEven (int n) { return (n % 2) == 0; }
size_t count;
int *result = dropWhile((int[]) {2, 4, 5, 7, 8, 10 }, 6, isEven, &count);
// result = { 5, 7, 8, 10 }; count = 4
```
Your task is to implement the dropWhile function. If you've got a [span function](http://www.codewars.com/kata/the-span-function) lying around, this is a one-liner! Alternatively, if you have a [takeWhile function](http://www.codewars.com/kata/the-takewhile-function) on your hands, then combined with the dropWhile function, you can implement the span function in one line. This is the beauty of functional programming: there are a whole host of useful functions, many of which can be implemented in terms of each other.
~~~if:lambdacalc
### Encodings
purity: `LetRec`
numEncoding: `BinaryScott`
export constructors `nil, cons` and deconstructor `foldl` for your `List` encoding
export constructors `False, True` for your `Boolean` encoding
~~~ | algorithms | from itertools import dropwhile
def drop_while(arr, pred):
return list(dropwhile(pred, arr))
| The dropWhile Function | 54f9c37106098647f400080a | [
"Functional Programming",
"Arrays",
"Algorithms"
] | https://www.codewars.com/kata/54f9c37106098647f400080a | 7 kyu |
Write a function that appends the items from sequence 2 onto sequence 1, returning the newly formed sequence. Your function should also be able to handle nested sequences.
All inputs will be arrays/nested arrays.
For example:
```
['a','b','c'], [1,2,3] --> ['a','b','c',1,2,3]
[['x','x'],'B'], ['c','D'] --> [['x','x'],'B','c','D']
``` | reference | def append_arrays(seq1, seq2):
return seq1 + seq2
| Array Appender | 53a8a476947277a3020001cc | [
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/53a8a476947277a3020001cc | 7 kyu |
Write a function that takes two array arguments, and returns a new array populated with the elements **that appear in either array, but not in both. Elements should only appear once in the returned array**.
The order of the elements in the result should follow what appears in the first array, then the second one.
## Examples
```
[1, 2, 3, 3], [3, 2, 1, 4, 5]) --> [4, 5]
["tartar", "blanket", "cinnamon"], ["cinnamon", "blanket", "domino"] --> ["tartar", "domino"]
[77, "ciao"], [78, 42, "ciao"] --> [77, 78, 42]
[1, 2, 3, 3], [3, 2, 1, 4, 5, 4] --> [4,5]
[1, 2, 3] , [3, 3, 2, 1] --> []
```
SPECIAL THANKS: @JulianKolbe ! | algorithms | def hot_singles(arr1, arr2):
a = []
for x in arr1 + arr2:
if x in set(arr1) ^ set(arr2) and x not in a:
a . append(x)
return a
| noobCode 04: HOT SINGLES...compare two arrays, return the unpaired items ! | 57475353facb0e7431000651 | [
"Arrays",
"Lists",
"Algorithms"
] | https://www.codewars.com/kata/57475353facb0e7431000651 | 7 kyu |
How many licks does it take to get to the tootsie roll center of a tootsie pop?
A group of engineering students from Purdue University reported that its licking machine, modeled after a human tongue, took an average of 364 licks to get to the center of a Tootsie Pop. Twenty of the group's volunteers assumed the licking challenge-unassisted by machinery-and averaged 252 licks each to the center.
Your task, if you choose to accept it, is to write a function that will return the number of licks it took to get to the tootsie roll center of a tootsie pop, given some environmental variables.
Everyone knows it's harder to lick a tootsie pop in cold weather but it's easier if the sun is out. You will be given an object of environmental conditions for each trial paired with a value that will increase or decrease the number of licks. The environmental conditions all apply to the same trial.
Assuming that it would normally take 252 licks to get to the tootsie roll center of a tootsie pop, return the new total of licks along with the condition that proved to be most challenging (causing the most added licks) in that trial.
Example:
```
totalLicks({ "freezing temps": 10, "clear skies": -2 });
```
Should return:
```
"It took 260 licks to get to the tootsie roll center of a tootsie pop. The toughest challenge was freezing temps."
```
Other cases:
If there are no challenges, the toughest challenge sentence should be omitted. If there are multiple challenges with the highest toughest amount, the first one presented will be the toughest.
If an environment variable is present, it will be either a positive or negative integer. No need to validate.
<div style="width: 320px; text-align: center; color: white; border: white 1px solid;">
Check out my other 80's Kids Katas:
</div>
<div>
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-1-how-many-licks-does-it-take'><span style='color:#00C5CD'>80's Kids #1:</span> How Many Licks Does It Take</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-2-help-alf-find-his-spaceship'><span style='color:#00C5CD'>80's Kids #2:</span> Help Alf Find His Spaceship</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-3-punky-brewsters-socks'><span style='color:#00C5CD'>80's Kids #3:</span> Punky Brewster's Socks</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-4-legends-of-the-hidden-temple'><span style='color:#00C5CD'>80's Kids #4:</span> Legends of the Hidden Temple</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-5-you-cant-do-that-on-television'><span style='color:#00C5CD'>80's Kids #5:</span> You Can't Do That on Television</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-6-rock-em-sock-em-robots'><span style='color:#00C5CD'>80's Kids #6:</span> Rock 'Em, Sock 'Em Robots</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-7-shes-a-small-wonder'><span style='color:#00C5CD'>80's Kids #7:</span> She's a Small Wonder</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-8-the-secret-world-of-alex-mack'><span style='color:#00C5CD'>80's Kids #8:</span> The Secret World of Alex Mack</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-9-down-in-fraggle-rock'><span style='color:#00C5CD'>80's Kids #9:</span> Down in Fraggle Rock </a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-10-captain-planet'><span style='color:#00C5CD'>80's Kids #10:</span> Captain Planet </a><br />
</div>
| algorithms | def total_licks(env):
d = 252
vm = 0
for k, v in env . items():
d += v
if v > vm:
vm, km = v, k
return 'It took ' + str(d) + ' licks to get to the tootsie roll center of a tootsie pop.' + (' The toughest challenge was ' + km + '.' if vm > 0 else '')
| 80's Kids #1: How Many Licks Does it Take? | 566091b73e119a073100003a | [
"Algorithms"
] | https://www.codewars.com/kata/566091b73e119a073100003a | 7 kyu |
You have recently discovered that horses travel in a unique pattern - they're either running (at top speed) or resting (standing still).
Here's an example of how one particular horse might travel:
```
The horse Blaze can run at 14 metres/second for 60 seconds, but must then rest for 45 seconds.
After 500 seconds Blaze will have traveled 4200 metres.
```
Your job is to write a function that returns how long a horse will have traveled after a given time.
####Input:
* totalTime - How long the horse will be traveling (in seconds)
* runTime - How long the horse can run for before having to rest (in seconds)
* restTime - How long the horse have to rest for after running (in seconds)
* speed - The max speed of the horse (in metres/second)
| games | def travel(total_time, run_time, rest_time, speed):
q, r = divmod(total_time, run_time + rest_time)
return (q * run_time + min(r, run_time)) * speed
| How far will I go? | 56d46b8fda159582e100001b | [
"Puzzles"
] | https://www.codewars.com/kata/56d46b8fda159582e100001b | 7 kyu |
_Friday 13th or Black Friday is considered as unlucky day. Calculate how many unlucky days are in the given year._
Find the number of Friday 13th in the given year.
__Input:__ Year [*in Gregorian calendar*](https://en.wikipedia.org/wiki/Gregorian_calendar) as integer.
__Output:__ Number of Black Fridays in the year as an integer.
__Examples:__
unluckyDays(2015) == 3
unluckyDays(1986) == 1 | reference | from datetime import date
def unlucky_days(year):
return sum(date(year, m, 13). weekday() == 4 for m in range(1, 13))
| Unlucky Days | 56eb0be52caf798c630013c0 | [
"Date Time"
] | https://www.codewars.com/kata/56eb0be52caf798c630013c0 | 7 kyu |
Did you ever want to know how many days old are you? Complete the function which returns your age in days. The birthday is given in the following order: `year, month, day`.
For example if today is 30 November 2015 then
```
ageInDays(2015, 11, 1) returns "You are 29 days old"
```
The birthday is expected to be in the past.
Suggestions on how to improve the kata are welcome!
| reference | from datetime import date
def ageInDays(year, month, day):
return 'You are {} days old' . format((date . today() - date(year, month, day)). days)
| Age in days | 5803753aab6c2099e600000e | [
"Date Time",
"Fundamentals"
] | https://www.codewars.com/kata/5803753aab6c2099e600000e | 7 kyu |
We all want to climb the leaderboard. Even given some of the massive scores on there, it's nice to know how close you are...
In this kata you will be given a username and their score, your score (not your real score) and you need to calculate how many kata you need to complete to *beat* their score, by 1 point exactly.
As this is the easy version (harder one to folow at some point in the future), let's assume only Beta kata and 8kyu kata are available. Worth 3 and 1 point respectively.
Return a string in this format: `"To beat <user>'s score, I must complete <x> Beta kata and <y> 8kyu kata."`
If the total number of kata you need to complete >1000, add `"Dammit!"` to the end of the string, like so: `"To beat <user>'s score, I must complete <x> Beta kata and <y> 8kyu kata. Dammit!"`
If your score is higher than the user's already, return `"Winning!"` and if they are equal, return `"Only need one!"`
**Note:** You are looking to complete as few kata as possible to get to your target. | reference | def leader_b(user, user_score, your_score):
if your_score > user_score:
return "Winning!"
if your_score == user_score:
return "Only need one!"
beta, eight = divmod(user_score - your_score, 3)
dammit = " Dammit!" if beta + eight > 1000 else ""
return f"To beat { user } 's score, I must complete { beta } Beta kata and { eight } 8kyu kata. { dammit } "
| Codewars Leaderboard Climber | 57d28215264276ea010002cf | [
"Fundamentals",
"Strings",
"Mathematics"
] | https://www.codewars.com/kata/57d28215264276ea010002cf | 7 kyu |
A palindrome is a word, phrase, number, or other sequence of characters which reads the same backward as forward. Examples of numerical palindromes are:
<p>2332
<br>110011
<br>54322345
For a given number ```num```, return its closest numerical palindrome which can either be smaller or larger than ```num```. If there are 2 possible values, the larger value should be returned. If ```num``` is a numerical palindrome itself, return it.
For this kata, single digit numbers will <u>NOT</u> be considered numerical palindromes.
Also, you know the drill - be sure to return "Not valid" if the input is not an integer or is less than 0.
```
palindrome(8) => 11
palindrome(281) => 282
palindrome(1029) => 1001
palindrome(1221) => 1221
palindrome("1221") => "Not valid"
```
~~~if:haskell
In Haskell the function should return a Maybe Int with Nothing for cases where the argument is less than zero.
~~~
~~~if:cobol
In COBOL, `n` will always be an integer. Assign `-1` to `result` when `n` is strictly negative.
~~~
<h2><u>Other Kata in this Series:</u></h2>
<a href="https://www.codewars.com/kata/58ba6fece3614ba7c200017f">Numerical Palindrome #1</a>
<br><a href="https://www.codewars.com/kata/numerical-palindrome-number-1-dot-5">Numerical Palindrome #1.5</a>
<br><a href="https://www.codewars.com/kata/58de819eb76cf778fe00005c">Numerical Palindrome #2</a>
<br><a href="https://www.codewars.com/kata/58df62fe95923f7a7f0000cc">Numerical Palindrome #3</a>
<br><a href="https://www.codewars.com/kata/58e2708f9bd67fee17000080">Numerical Palindrome #3.5</a>
<br><b>Numerical Palindrome #4</b>
<br><a href="https://www.codewars.com/kata/58e26b5d92d04c7a4f00020a">Numerical Palindrome #5</a>
| reference | def palindrome(num):
def is_palindrome(chunk): return int(chunk) > 9 and chunk == chunk[:: - 1]
if not isinstance(num, int) or num < 0:
return 'Not valid'
i = 0
while (True):
if is_palindrome(str(num + i)):
return num + i
if is_palindrome(str(num - i)):
return num - i
i += 1
| Numerical Palindrome #4 | 58df8b4d010a9456140000c7 | [
"Fundamentals"
] | https://www.codewars.com/kata/58df8b4d010a9456140000c7 | 6 kyu |
# Story
<div style='float:right; width: 240px'>

</div>
You are a cook in an alien restaurant at a distant planet called Kahumo.
These aliens have some specific physiology features:
* Aliens eat only one type of food in one day. Each food has a special factor called `flavour` (let's designate it as *k* hereafter).
* Each alien has several mouths of different size. The amount of food each mouth eats depends on mouth number. Second mouth must eat exactly *k* times more than the first, third mouth must eat exactly *k* times more than the second, and so on.
> For example, if *k* is 2, and the first mouth ate 1 kg of food, second mouth must eat `2 * 1 = 2` kg of food, third eats `2 * 2 = 4` kg of food, fourth eats `2 * 4 = 8` kg and so on.
Food on Kahumo is very valuable and spoils fast. This means that if a client orders a certain amount of food, you have to serve it all in the exact proportions required for each of your client's mouths. If any of client's mouths eats less or more than requested, client will feel pain in his stomach, and may spray your eyes with his mildly acidic emotion fluid (which also is kahumoling saliva) as a sign of disrespect. (Please, not again, this juice in my eyes makes me sick!)
Kahumolings have very sensitive tongues, but not absolutely sensitive. They can feel deviations within 9 grams. This means that if you serve your client 100.005 kg of food instead of 100.000, kahumoling will probably not notice.
# Function features
## Arguments
* `food` is the amount of food your client has ordered.
* `flavour` is the *k* factor of the food your client ordered.
* `mouths` is the amount of mouths of your client.
## Return format
* Serve the result in the following format: `[x, y, z, ...]`, where values correspond to the amount of food served to a certain mouth (float; you can round it to 3 decimal places) in ascending order (low to high).
# Tests
* Number of served mouths must correspond to the number of mouths your client has.
* Total amount of food must correspond to the amount ordered by your client.
* Each next mouth must receive exactly *k* times more food than the previous.
# Examples
```csharp
serve( 31, 2, 5) returns [1.0, 2.0, 4.0, 8.0, 16.0]
serve(728, 3, 6) returns [2.0, 6.0, 18.0, 54.0, 162.0, 486.0]
serve(100, 1.5, 3) returns [21.053, 31.579, 47.368]
```
```ruby
serve( 31, 2, 5) returns [1.0, 2.0, 4.0, 8.0, 16.0]
serve(728, 3, 6) returns [2.0, 6.0, 18.0, 54.0, 162.0, 486.0]
serve(100, 1.5, 3) returns [21.053, 31.579, 47.368]
```
```javascript
serve( 31, 2, 5) returns [1.0, 2.0, 4.0, 8.0, 16.0]
serve(728, 3, 6) returns [2.0, 6.0, 18.0, 54.0, 162.0, 486.0]
serve(100, 1.5, 3) returns [21.053, 31.579, 47.368]
```
```coffeescript
serve( 31, 2, 5) returns [1.0, 2.0, 4.0, 8.0, 16.0]
serve(728, 3, 6) returns [2.0, 6.0, 18.0, 54.0, 162.0, 486.0]
serve(100, 1.5, 3) returns [21.053, 31.579, 47.368]
```
```python
serve(31, 2, 5) returns {1, 2, 4, 8, 16}
serve(728, 3, 6) returns {2, 6, 18, 54, 162, 486}
serve(100, 1.5, 3) returns {21.053, 31.579, 47.368}
```
```java
serve(31, 2, 5) returns {1.0, 2.0, 4.0, 8.0, 16.0}
serve(728, 3, 6) returns {2.0, 6.0, 18.0, 54.0, 162.0, 486.0}
serve(100, 1.5, 3) returns {21.053, 31.579, 47.368}
```
| algorithms | def serve(f, k, m):
s = f / m if k == 1 else f / ((k * * m - 1) / (k - 1))
return [s * k * * i for i in range(m)]
| Feed Kahumolings! | 557b75579b03996942000061 | [
"Algorithms"
] | https://www.codewars.com/kata/557b75579b03996942000061 | 6 kyu |
# Sort an array by value and index
Your task is to sort an array of integer numbers by the product of the value and the index of the positions.
<br><br>
For sorting the index starts at 1, NOT at 0!<br>
The sorting has to be ascending.<br>
The array will never be null and will always contain numbers.
<br><br>
Example:
```
Input: 23, 2, 3, 4, 5
Product of value and index:
23 => 23 * 1 = 23 -> Output-Pos 4
2 => 2 * 2 = 4 -> Output-Pos 1
3 => 3 * 3 = 9 -> Output-Pos 2
4 => 4 * 4 = 16 -> Output-Pos 3
5 => 5 * 5 = 25 -> Output-Pos 5
Output: 2, 3, 4, 23, 5
```
<br><br><br>
Have fun coding it and please don't forget to vote and rank this kata! :-)
I have also created other katas. Take a look if you enjoyed this kata! | algorithms | def sort_by_value_and_index(arr):
return [y[1] for y in sorted(enumerate(arr), key=lambda x: (x[0] + 1) * x[1])]
| Sort an array by value and index | 58e0cb3634a3027180000040 | [
"Arrays",
"Algorithms",
"Sorting"
] | https://www.codewars.com/kata/58e0cb3634a3027180000040 | 7 kyu |
Write a function that returns a sequence (index begins with 1) of all the even characters from a string. If the string is smaller than two characters or longer than 100 characters, the function should return "invalid string".
For example:
`````
"abcdefghijklm" --> ["b", "d", "f", "h", "j", "l"]
"a" --> "invalid string"
````` | reference | def even_chars(st):
if len(st) < 2 or len(st) > 100:
return 'invalid string'
else:
return [st[i] for i in range(1, len(st), 2)]
| Return a string's even characters. | 566044325f8fddc1c000002c | [
"Fundamentals",
"Strings",
"Arrays"
] | https://www.codewars.com/kata/566044325f8fddc1c000002c | 7 kyu |
Implement a function which filters out array values which satisfy the given predicate.
```javascript
reject([1, 2, 3, 4, 5, 6], (n) => n % 2 === 0) => [1, 3, 5]
```
```csharp
Kata.Reject(new int[] {1, 2, 3, 4, 5, 6}, (n) => n % 2 == 0) => new int[] {1, 3, 5}
```
```php
reject([1, 2, 3, 4, 5, 6], function ($n) {return $n % 2 == 0;}) => [1, 3, 5]
```
```python
reject([1, 2, 3, 4, 5, 6], lambda x: x % 2 == 0) => [1, 3, 5]
``` | reference | def reject(seq, predicate):
return [item for item in seq if not predicate(item)]
| The reject() function | 52988f3f7edba9839c00037d | [
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/52988f3f7edba9839c00037d | 7 kyu |
Write a program that, given a word, computes the scrabble score for that word.
## Letter Values
You'll need these:
```
Letter Value
A, E, I, O, U, L, N, R, S, T 1
D, G 2
B, C, M, P 3
F, H, V, W, Y 4
K 5
J, X 8
Q, Z 10
```
```if:ruby,javascript,cfml
There will be a preloaded hashtable `$dict` with all these values: `$dict["E"] == 1`.
```
```if:haskell
There will be a preloaded list of `(Char, Int)` tuples called `dict` with all these values.
```
```if:python
There will be a preloaded dictionary `dict_scores` with all these values: `dict_scores["E"] == 1`
```
```if:go
There will be a preloaded map `DictScores` with all these values: `DictScores["E"] == 1`
```
## Examples
```
"cabbage" --> 14
```
"cabbage" should be scored as worth 14 points:
- 3 points for C
- 1 point for A, twice
- 3 points for B, twice
- 2 points for G
- 1 point for E
And to total:
`3 + 2*1 + 2*3 + 2 + 1` = `3 + 2 + 6 + 3` = `14`
Empty string should return `0`. The string can contain spaces and letters (upper and lower case), you should calculate the scrabble score only of the letters in that string.
```
"" --> 0
"STREET" --> 6
"st re et" --> 6
"ca bba g e" --> 14
```
| reference | def scrabble_score(stg):
return sum(dict_scores . get(c, 0) for c in stg . upper())
| Scrabble Score | 558fa34727c2d274c10000ae | [
"Fundamentals",
"Strings"
] | https://www.codewars.com/kata/558fa34727c2d274c10000ae | 7 kyu |
You'll be passed an array of objects (`list`) - you must sort them in descending order based on the value of the specified property (`sortBy`).
## Example
When sorted by `"a"`, this:
```python
[
{"a": 1, "b": 3},
{"a": 3, "b": 2},
{"a": 2, "b": 40},
{"a": 4, "b": 12}
]
```
should return:
```python
[
{"a": 4, "b": 12},
{"a": 3, "b": 2},
{"a": 2, "b": 40},
{"a": 1, "b": 3}
]
```
The values will always be numbers, and the properties will always exist. | reference | def sort_list(sort_key, l):
return sorted(l, key=lambda x: x[sort_key], reverse=True)
| Return a sorted list of objects | 52705ed65de62b733f000064 | [
"Arrays",
"Sorting",
"Fundamentals"
] | https://www.codewars.com/kata/52705ed65de62b733f000064 | 7 kyu |
Write a method that will search an array of strings for all strings that contain another string, ignoring capitalization. Then return an array of the found strings.
The method takes two parameters, the query string and the array of strings to search, and returns an array.
If the string isn't contained in any of the strings in the array, the method returns an array containing a single string: "Empty" (or `Nothing` in Haskell, or "None" in Python and C)
### Examples
If the string to search for is "me", and the array to search is ["home", "milk", "Mercury", "fish"], the method should return ["home", "Mercury"].
| reference | def word_search(query, seq):
return [x for x in seq if query . lower() in x . lower()] or ["None"]
| Partial Word Searching | 54b81566cd7f51408300022d | [
"Arrays",
"Strings",
"Fundamentals"
] | https://www.codewars.com/kata/54b81566cd7f51408300022d | 7 kyu |
Implement a function which behaves like the 'uniq -c' command in UNIX.
It takes as input a sequence and returns a sequence in which all duplicate elements following each other have been reduced to one instance together with the number of times a duplicate elements occurred in the original array.
## Example:
```javascript
['a','a','b','b','c','a','b','c'] --> [['a',2],['b',2],['c',1],['a',1],['b',1],['c',1]]
```
```python
['a','a','b','b','c','a','b','c'] --> [('a',2),('b',2),('c',1),('a',1),('b',1),('c',1)]
``` | algorithms | from itertools import groupby
def uniq_c(seq):
return [(k, sum(1 for _ in g)) for k, g in groupby(seq)]
| uniq -c (UNIX style) | 52250aca906b0c28f80003a1 | [
"Arrays",
"Algorithms"
] | https://www.codewars.com/kata/52250aca906b0c28f80003a1 | 5 kyu |
*It seemed a good idea at the time...*
# <span style='color:red'>Why I did it?</span>
After a year on **Codewars** I really needed a holiday...
But not wanting to drift backwards in the honour rankings while I was away, I hatched a cunning plan!
# <span style='color:red'>The Cunning Plan</spac>
So I borrowed my friend's "Clone Machine" and cloned myself :-)
Now my clone can do my Kata solutions for me and I can relax!
Brilliant!!
<hr>
Furthermore, at the end of the day my clone can re-clone herself...
Double brilliant!!
I wonder why I didn't think to do this earlier?
<hr>
So as I left for the airport I gave my clone instructions to:
* do my Kata solutions for me
* feed the cat
* try to keep the house tidy and not eat too much
* sleep
* clone yourself
* repeat same next day
# <span style='color:red'>The Flaw</span>
Well, how was I supposed to know that cloned DNA is faulty?
:-(
Every time they sleep they wake up with decreased ability - they get slower... they get dumber... they are only able to solve 1 less Kata than they could the previous day.
For example, if they can solve 10 Kata today, then tomorrow they can solve only 9 Kata, then 8, 7, 6... Eventually they can't do much more than sit around all day playing video games.
And (unlike me), when the clone cannot solve any more Kata they are no longer clever enough to operate the clone machine either!
# <span style='color:red'>The Return Home</span>
I suspected something was wrong when I noticed my **Codewars** honour had stopped rising.
I made a hasty return home...
...and found 100s of clones scattered through the house. Mostly they sit harmlessly mumbling to themselves. The largest group have made a kind of nest in my loungeroom where they sit catatonic in front of the PlayStation.
The whole place needs fumigating.
The fridge and pantry are empty.
And I can't find the cat.
# <span style='color:red'>Kata Task</span>
Write a method to predict the final outcome where:
Input:
* `kata-per-day` is the number of Kata I can solve per day
Output:
* ```[number-of-clones, number-of-kata-solved-by-clones]```
| algorithms | def clonewars(k):
return [2 * * max(k - 1, 0), 2 * * (k + 1) - k - 2]
| Send in the Clones | 58ddffda929dfc2cae0000a5 | [
"Algorithms"
] | https://www.codewars.com/kata/58ddffda929dfc2cae0000a5 | 7 kyu |
Implement the function which should return `true` if given object is a vowel (meaning `a, e, i, o, u`, uppercase or lowercase), and `false` otherwise. | reference | def is_vowel(s):
return s . lower() in set("aeiou")
| Regexp Basics - is it a vowel? | 567bed99ee3451292c000025 | [
"Regular Expressions",
"Fundamentals"
] | https://www.codewars.com/kata/567bed99ee3451292c000025 | 7 kyu |
Create the function `prefill` that returns an array of `n` elements that all have the same value `v`. See if you can do this *without* using a loop.
You have to validate input:
* `v` can be *anything* (primitive or otherwise)
* if `v` is ommited, fill the array with `undefined`
* if `n` is 0, return an empty array
* if `n` is anything other than an **integer** or **integer-formatted** string (e.g. `'123'`) that is `>=0`, throw a `TypeError`
When throwing a `TypeError`, the message should be `n is invalid`, where you replace `n` for the actual value passed to the function.
Code Examples
```javascript
prefill(3,1) --> [1,1,1]
prefill(2,"abc") --> ['abc','abc']
prefill("1", 1) --> [1]
prefill(3, prefill(2,'2d'))
--> [['2d','2d'],['2d','2d'],['2d','2d']]
prefill("xyz", 1)
--> throws TypeError with message "xyz is invalid"
```
```ruby
prefill(3,1) --> [1,1,1]
prefill(2,"abc") --> ['abc','abc']
prefill("1", 1) --> [1]
prefill(3, prefill(2,'2d'))
--> [['2d','2d'],['2d','2d'],['2d','2d']]
prefill("xyz", 1)
--> throws TypeError with message "xyz is invalid"
```
```python
prefill(3,1) --> [1,1,1]
prefill(2,"abc") --> ['abc','abc']
prefill("1", 1) --> [1]
prefill(3, prefill(2,'2d'))
--> [['2d','2d'],['2d','2d'],['2d','2d']]
prefill("xyz", 1)
--> throws TypeError with message "xyz is invalid"
```
```coffeescript
prefill 3, 1 #returns [1, 1, 1]
prefill 2, "abc" #returns ["abc","abc"]
prefill "1", 1 #returns [1]
prefill 3, prefill(2, "2d")
#returns [['2d','2d'],['2d','2d'],['2d','2d']]
prefill "xyz", 1
#throws TypeError with message "xyz is invalid"
```
| reference | def prefill(n=0, v=None):
try:
return [v] * int(n)
except:
raise TypeError(str(n) + ' is invalid')
| Prefill an Array | 54129112fb7c188740000162 | [
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/54129112fb7c188740000162 | 6 kyu |
Complete the function to determine the number of bits required to convert integer `A` to integer `B` (where `A` and `B` >= 0)
The upper limit for `A` and `B` is 2<sup>16</sup>, `int.MaxValue` or similar.
For example, you can change 31 to 14 by flipping the 4th and 0th bit:
```
31 0 0 0 1 1 1 1 1
14 0 0 0 0 1 1 1 0
--- ---------------
bit 7 6 5 4 3 2 1 0
```
Thus `31` and `14` should return `2`.
| algorithms | def convert_bits(a, b):
return bin(a ^ b). count("1")
| Delta Bits | 538948d4daea7dc4d200023f | [
"Bits",
"Binary",
"Algorithms"
] | https://www.codewars.com/kata/538948d4daea7dc4d200023f | 7 kyu |
Write a module Converter that can take ASCII text and convert it to hexadecimal. The class should also be able to take hexadecimal and convert it to ASCII text.
To make the conversion well defined, each ASCII character is represented by exactly two hex digits, left-padding with a `0` if needed.
The conversion from ascii to hex should produce lowercase strings (i.e. `f6` instead of `F6`).
### Example
```ruby
Converter.to_hex("Look mom, no hands")
=> "4c6f6f6b206d6f6d2c206e6f2068616e6473"
Converter.to_ascii("4c6f6f6b206d6f6d2c206e6f2068616e6473")
=> "Look mom, no hands"
```
```python
Converter.to_hex("Look mom, no hands")
=> "4c6f6f6b206d6f6d2c206e6f2068616e6473"
Converter.to_ascii("4c6f6f6b206d6f6d2c206e6f2068616e6473")
=> "Look mom, no hands"
```
```javascript
Converter.toHex("Look mom, no hands")
=> "4c6f6f6b206d6f6d2c206e6f2068616e6473"
Converter.toAscii("4c6f6f6b206d6f6d2c206e6f2068616e6473")
=> "Look mom, no hands"
``` | reference | class Converter ():
@ staticmethod
def to_ascii(h):
return '' . join(chr(int(h[i: i + 2], 16)) for i in range(0, len(h), 2))
@ staticmethod
def to_hex(s):
return '' . join(hex(ord(char))[2:] for char in s)
| ASCII hex converter | 52fea6fd158f0576b8000089 | [
"Fundamentals",
"Bits",
"Strings",
"Object-oriented Programming"
] | https://www.codewars.com/kata/52fea6fd158f0576b8000089 | 6 kyu |
Every Turkish citizen has an identity number whose validity can be checked by these set of rules:
- It is an 11 digit number
- First digit can't be zero
- Take the sum of 1st, 3rd, 5th, 7th and 9th digit and multiply it by 7.
Then subtract the sum of 2nd, 4th, 6th and 8th digits from this value.
Modulus 10 of the result should be equal to 10th digit.
- Sum of first ten digits' modulus 10 should be equal to eleventh digit.
Example:
10167994524
// 1+1+7+9+5= 23 // "Take the sum of 1st, 3rd, 5th, 7th and 9th digit..."
// 23 * 7= 161 // "...and multiply it by 7"
// 0+6+9+4 = 19 // "Take the sum of 2nd, 4th, 6th and 8th digits..."
// 161 - 19 = 142 // "...and subtract from first value"
// "Modulus 10 of the result should be equal to 10th digit"
10167994524
^ = 2 = 142 % 10
// 1+0+1+6+7+9+9+4+5+2 = 44
// "Sum of first ten digits' modulus 10 should be equal to eleventh digit"
10167994524
^ = 4 = 44 % 10
Your task is to write a function to check the validity of a given number.
Return `true` or `false` accordingly.
Note: The input can be a string in some cases. | algorithms | def check_valid_tr_number(n):
return type(n) == int and len(str(n)) == 11 and \
8 * sum(map(int, str(n)[: - 1: 2])
) % 10 == sum(map(int, str(n)[: - 1])) % 10 == n % 10
| Turkish National Identity Number | 556021360863a1708900007b | [
"Algorithms"
] | https://www.codewars.com/kata/556021360863a1708900007b | 6 kyu |
Return the century of the input year. The input will always be a 4 digit string, so there is no need for validation.
### Examples
```
"1999" --> "20th"
"2011" --> "21st"
"2154" --> "22nd"
"2259" --> "23rd"
"1124" --> "12th"
"2000" --> "20th"
``` | algorithms | def what_century(year):
n = (int(year) - 1) / / 100 + 1
return str(n) + ("th" if n < 20 else {1: "st", 2: "nd", 3: "rd"}. get(n % 10, "th"))
| What century is it? | 52fb87703c1351ebd200081f | [
"Strings",
"Algorithms",
"Date Time"
] | https://www.codewars.com/kata/52fb87703c1351ebd200081f | 6 kyu |
Write a function that takes a piece of text in the form of a string and returns the letter frequency count for the text. This count excludes numbers, spaces and all punctuation marks. Upper and lower case versions of a character are equivalent and the result should all be in lowercase.
The function should return a list of tuples (in Python and Haskell) or arrays (in other languages) sorted by the most frequent letters first. The Rust implementation should return an ordered BTreeMap.
Letters with the same frequency are ordered alphabetically.
For example:
```python
letter_frequency('aaAabb dddDD hhcc')
```
```haskell
letterFrequency "aaAabb dddDD hhcc"
```
```javascript
letterFrequency('aaAabb dddDD hhcc')
```
```ruby
letter_frequency('aaAabb dddDD hhcc')
```
```rust
letter_frequency("aaAabb dddDD hhcc")
```
```C++
letter_frequency("aaAabb dddDD hhcc")
```
will return
```python
[('d',5), ('a',4), ('b',2), ('c',2), ('h',2)]
```
```haskell
[('d',5), ('a',4), ('b',2), ('c',2), ('h',2)]
```
```javascript
[['d',5], ['a',4], ['b',2], ['c',2], ['h',2]]
```
```ruby
[['d',5], ['a',4], ['b',2], ['c',2], ['h',2]]
```
```rust
[('d',5), ('a',4), ('b',2), ('c',2), ('h',2)]
```
```C++
std::vector<std::pair<char, size_t>>{{'d',5}, {'a',4}, {'b',2}, {'c',2}, {'h',2}}
```
Letter frequency analysis is often used to analyse simple substitution cipher texts like those created by the Caesar cipher.
| algorithms | from collections import Counter
from operator import itemgetter
def letter_frequency(text):
items = Counter(c for c in text . lower() if c . isalpha()). items()
return sorted(
sorted(items, key=itemgetter(0)),
key=itemgetter(1),
reverse=True
)
| Character frequency | 53e895e28f9e66a56900011a | [
"Strings",
"Algorithms"
] | https://www.codewars.com/kata/53e895e28f9e66a56900011a | 6 kyu |
The year is 2070 and intelligent connected machines have replaced humans in most things. There are still a few critical jobs for mankind including machine software developer, for writing and maintaining the AI software, and machine forward deployed engineer for controlling the intelligent machines in the field. Lorimar is a forward deployed machine engineer and at any given time he controls thousands of BOT robots through real time interfaces and intelligent automation software.
While Lorimar remains highly skilled in the art of computer programming the people in general have fallen away from their technical roots. Much of the innovation leading to the development of the machines was done by the early pioneers. Today the people are relying on past success to prop up their machine dependant society. There are bugs, flaws, vulnerabilities and BOT robots in need of update, patching and maintenance. In some cases the BOT is isolated and siloed with no human interaction. When this happens it must be located and disabled. Lorimar is on the hunt and tracking an infected non-responsive and dangerous BOT originally commissioned for automated security services in highly hostile urban battlefield settings. This particular BOT is very unpredictable as its operating environment is not understood by modern developers who have become reliant on extreme abstraction without fully understanding the inner workings of the system.
Your task is to search through a string of integers related to BOT system internals found during a recent scan of communication systems searching for a pattern.
```
100 β€ log β€ 100000
1 β€ n β€ 10000
Integer is Prime
Integer is 4 digits long such as 1031
Integer third digit is either 2 or 3 such as 1021 or 1031
^ ^
| |
The count of the integer in the log is > 3
If there are > 50 integers in the log matching this pattern the BOT at this location should be disabled
Here is an example
All the integers below are prime:
log= ['8923', '5639', '2423', '3929', '7723',
'8923', '5639', '2423', '3929', '7723',
'8923', '5639', '2423', '3929', '7723',
'8923', '5639', '2423', '3929', '7723',
'8923', '5639', '2423', '3929', '7723',
'8923', '5639', '2423', '3929', '7723',
'8923', '5639', '2423', '3929', '7723',
'8923', '5639', '2423', '3929', '7723',
'8923', '5639', '2423', '3929', '7723',
'8923', '5639', '2423', '3929', '7723',
'8923']
Here is the Count
({'2423': 10, '3929': 10, '5639': 10, '7723': 10, '8923': 11})
Count = 51
Count > 50
Return "match disable bot"
If these rules do not hold
Return "no match continue"
```
Please also try the other Kata in this series..
* [AD2070:Help Lorimar troubleshoot his robots- ultrasonic distance analysis](https://www.codewars.com/kata/57102bbfd860a3369300089c)
| algorithms | from collections import Counter
def is_prime(n): return n > 1 and all(n % d for d in range(2, int(n * * .5) + 1))
PRIMES = {str(n) for n in range(10 * * 3, 10 * * 4) if str(n)[2] in '23' and is_prime(n)}
def search_disable(log): return ['no match continue', 'match disable bot'][
sum(c for n, c in Counter(log . split()). items() if c > 3 and n in PRIMES) > 50]
| AD2070: Help Lorimar troubleshoot his robots-Search and Disable | 57dc0ffed8f92982af0000f6 | [
"Data Structures",
"Mathematics",
"Algorithms",
"Data Science"
] | https://www.codewars.com/kata/57dc0ffed8f92982af0000f6 | 6 kyu |
Modify the `kebabize` function so that it converts a camel case string into a kebab case.
```
"camelsHaveThreeHumps" --> "camels-have-three-humps"
"camelsHave3Humps" --> "camels-have-humps"
"CAMEL" --> "c-a-m-e-l"
```
Notes:
- the returned string should only contain lowercase letters | reference | def kebabize(s):
return '' . join(c if c . islower() else '-' + c . lower() for c in s if c . isalpha()). strip('-')
| Kebabize | 57f8ff867a28db569e000c4a | [
"Fundamentals",
"Strings",
"Regular Expressions"
] | https://www.codewars.com/kata/57f8ff867a28db569e000c4a | 6 kyu |
> In information theory and computer science, the Levenshtein distance is a string metric for measuring the difference between two sequences. Informally, the Levenshtein distance between two words is the minimum number of single-character edits (i.e. insertions, deletions or substitutions) required to change one word into the other.
(http://en.wikipedia.org/wiki/Levenshtein_distance)
Your task is to implement a function which calculates the Levenshtein distance for two arbitrary strings. | algorithms | def levenshtein(a, b):
d = [[0] * (len(b) + 1) for _ in range(len(a) + 1)]
d[0][:] = range(len(b) + 1)
for i in range(1, len(a) + 1):
d[i][0] = i
for i, x in enumerate(a):
for j, y in enumerate(b):
d[i + 1][j + 1] = min(1 + d[i][j + 1], 1 + d[i + 1]
[j], d[i][j] + (1 if x != y else 0))
return d[- 1][- 1]
| Levenshtein Distance | 545cdb4f61778e52810003a2 | [
"Strings",
"Algorithms"
] | https://www.codewars.com/kata/545cdb4f61778e52810003a2 | 6 kyu |
<b>Linked lists</b> are data structures composed of nested or chained objects, each containing a single value and a reference to the next object.
Here's an example of a list:
```javascript
{value: 1, next: {value: 2, next: {value: 3, next: null}}}
```
```python
class LinkedList:
def __init__(self, value=0, next=None):
self.value = value
self.next = next
LinkedList(1, LinkedList(2, LinkedList(3)))
```
```ruby
{value: 1, next: {value: 2, next: {value: 3, next: null}}}
```
Write a function listToArray (or list\_to\_array in Python) that converts a list to an array, like this:
```
[1, 2, 3]
```
Assume all inputs are valid lists with at least one value. For the purpose of simplicity, all values will be either numbers, strings, or Booleans. | reference | def list_to_array(lst):
arr = []
while lst != None:
arr . append(lst . value)
lst = lst . next
return arr
| LinkedList -> Array | 557dd2a061f099504a000088 | [
"Data Structures",
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/557dd2a061f099504a000088 | 7 kyu |
# Story
Peter lives on a hill, and he always moans about the way to his home. "It's always just up. I never get a rest". But you're pretty sure that at least at one point Peter's altitude doesn't rise, but fall. To get him, you use a nefarious plan: you attach an altimeter to his backpack and you read the data from his way back at the next day.
# Task
You're given a list of compareable elements:
```c
double heights[n]
```
```csharp
heights = new int[0 ... 100]
```
```haskell
Ord a => [a]
```
```javascript
heights = [h1, h2, h3, β¦, hn]
```
```python
heights = [Integers or Floats]
```
```ruby
heights = [Integers or Floats]
```
```cobol
heights = [pic s9(2)]
```
Your job is to check whether for any `x` all successors are greater or equal to `x`.
```c
is_monotone(3, {1, 2, 3}) == true
is_monotone(3, {1, 1, 2}) == true
is_monotone(1, {1}) == true
is_monotone(3, {3, 2, 1}) == false
is_monotone(3, {3, 2, 2}) == false
```
```csharp
Kata.IsMonotone(new int[] {1,2,3}) => true
Kata.IsMonotone(new int[] {1,1,2}) => true
Kata.IsMonotone(new int[] {1}) => true
Kata.IsMonotone(new int[] {3,2,1}) => false
Kata.IsMonotone(new int[] {3,2,2}) => false
```
```haskell
isMonotone [1,2,3] == True
isMonotone [1,1,2] == True
isMonotone [1] == True
isMonotone [3,2,2] == False
```
```javascript
isMonotone([1,2,3]) == true
isMonotone([1,1,2]) == true
isMonotone([1]) == true
isMonotone([3,2,1]) == false
isMonotone([3,2,2]) == false
```
```python
is_monotone([1,2,3]) == True
is_monotone([1,1,2]) == True
is_monotone([1]) == True
is_monotone([3,2,1]) == False
is_monotone([3,2,2]) == False
```
```ruby
is_monotone([1,2,3]) == True
is_monotone([1,1,2]) == True
is_monotone([1]) == True
is_monotone([3,2,1]) == False
is_monotone([3,2,2]) == False
```
```cobol
IsMonotone([1,2,3]) = 1
IsMonotone([1,1,2]) = 1
IsMonotone([1]) = 1
IsMonotone([3,2,1]) = 0
IsMonotone([3,2,2]) = 0
```
If the list is empty, Peter has probably removed your altimeter, so we cannot prove him wrong and he's still right:
```c
is_monotone(0, NULL) => true
```
```csharp
Kata.IsMonotone(new int[] {}) => true
```
```haskell
isMonotone [] == True
```
```javascript
isMonotone([]) == True
```
```python
is_monotone([]) == True
```
```ruby
isMonotone([]) == True
```
Such a sequence is also called *monotone* or [*monotonic* sequence](https://en.wikipedia.org/wiki/Monotonic_function), hence the name `isMonotone`. | reference | def is_monotone(heights):
return sorted(heights) == heights
| Monotone travel | 54466996990c921f90000d61 | [
"Lists",
"Fundamentals"
] | https://www.codewars.com/kata/54466996990c921f90000d61 | 7 kyu |
Dave has a lot of data he is required to apply filters to, which are simple enough, but he wants a shorter way of doing so.
He wants the following functions to work as expected:
```ruby
even # [1,2,3,4,5].even should return [2,4]
odd # [1,2,3,4,5].odd should return [1,3,5]
under # [1,2,3,4,5].under(4) should return [1,2,3]
over # [1,2,3,4,5].over(4) should return [5]
in_range # [1,2,3,4,5].in_range(1..3) should return [1,2,3]
```
```javascript
even // [1,2,3,4,5].even() should return [2,4]
odd // [1,2,3,4,5].odd() should return [1,3,5]
under // [1,2,3,4,5].under(4) should return [1,2,3]
over // [1,2,3,4,5].over(4) should return [5]
inRange // [1,2,3,4,5].inRange(1,3) should return [1,2,3]
```
```python
even # list([1,2,3,4,5]).even() should return [2,4]
odd # list([1,2,3,4,5]).odd() should return [1,3,5]
under # list([1,2,3,4,5]).under(4) should return [1,2,3]
over # list([1,2,3,4,5]).over(4) should return [5]
in_range # list([1,2,3,4,5]).in_range(1, 3) should return [1,2,3]
```
They should also work when used together, for example:
```ruby
(1..100).to_a.even.in_range(18..30) # should return [18, 20, 22, 24, 26, 28, 30]
```
```javascript
[1,2,18,19,20,21,22,30,40,50,100].even().inRange(18,30) // should return [18, 20, 22, 30]
```
```python
list(list([1,2,3,4,5,6,7,8,9,10]).even()).under(5) # should return [2,4]
```
And finally the filters should only accept integer values from an array, for example:
```ruby
["a", 1, "b", 300, "x", "q", 63, 122, 181, "z", 0.83, 0.11].even # should return [300, 122]
```
```javascript
["a", 1, "b", 300, "x", "q", 63, 122, 181, "z", 0.83, 0.11].even() // should return [300, 122]
```
```python
list(["a", 1, "b", 300, "x", "q", 63, 122, 181, "z", 0.83, 0.11]).even() // should return [300, 122]
```
Note: List with non-numbers will be tested as well. | reference | import __builtin__
class CustomList (list):
def ints(self):
return [x for x in self if isinstance(x, int)]
def even(self):
return [x for x in self . ints() if x % 2 == 0]
def odd(self):
return [x for x in self . ints() if x % 2 == 1]
def under(self, ceil):
return [x for x in self . ints() if x < ceil]
def over(self, floor):
return [x for x in self . ints() if x > floor]
def in_range(self, min, max):
return [x for x in self . ints() if min <= x <= max]
__builtin__ . list = CustomList
| Custom Array Filters | 53fc954904a45eda6b00097f | [
"Arrays",
"Fundamentals",
"Object-oriented Programming"
] | https://www.codewars.com/kata/53fc954904a45eda6b00097f | 6 kyu |
To pass the series of gates guarded by the owls, Kenneth needs to present them each with a highly realistic portrait of one. Unfortunately, he is absolutely rubbish at drawing, and needs some code to return a brand new portrait with a moment's notice.
All owl heads look like this:
''0v0''
Such beautiful eyes!
However, they differ in their plumage, which is always symmetrical, eg.:
VVHVAV''0v0''VAVHVV
or
YYAYAH8XH''0v0''HX8HAYAYY
So Kenneth needs a method that will take a garble of text generated by mashing at his keyboard (numbers and letters, but he knows how to avoid punctuation etc.) for a bit and give him a symmetrical owl with a lovely little face, with a truly symmetrical plumage made of uppercase letters and numbers.
(To be clear, the acceptable characters for the plumage are 8,W,T,Y,U,I,O,A,H,X,V and M.) | reference | import re
def owl_pic(text):
str = re . sub('[^ 8,W,T,Y,U,I,O,A,H,X,V,M]', '', text . upper())
return str + "''0v0''" + str[:: - 1]
| The Owls Are Not What They Seem | 55aa7acc42216b3dd6000022 | [
"Regular Expressions",
"Strings",
"Fundamentals"
] | https://www.codewars.com/kata/55aa7acc42216b3dd6000022 | 7 kyu |
**This Kata is intended as a small challenge for my students**
All Star Code Challenge #24
Your friend David is an architect who is working on a triangle-focused design. He needs a quick way for knowing the measurement of a right triangle's side, only knowing two of the sides.
He knows about the Pythagorean Theorem, but is too lazy to do the math. Help him by making 2 functions to ease his troubles.
Create 2 functions:
1) hypotenuse(), which takes 2 integer arguments, the length of two regular sides of a right triangle, and returns the length of the missing side, the hypotenuse, as a number.
2) leg(), which takes 2 integer arguments, the first being the length of the hypotenuse, and the second being the length of a regular side of a right triangle. This function should return the length of the missing regular side, as a number.
```javascript
hypotenuse(3,4) // => 5
leg(5,3) // => 4
```
https://en.wikipedia.org/wiki/Pythagorean_theorem
Note:
Do NOT round the ouput number
You do not have to verify if the input values are "proper", assume them to be non-zero integers | reference | import math
def hypotenuse(a, b):
return math . hypot(a, b)
def leg(c, a):
return (c * * 2 - a * * 2) * * .5
| All Star Code Challenge #24 | 5866c6cf442e3f16f9000089 | [
"Fundamentals"
] | https://www.codewars.com/kata/5866c6cf442e3f16f9000089 | 7 kyu |
A very easy task for you!
You have to create a method, that corrects a given date string.
There was a problem in addition, so many of the date strings are broken.
Date-Format is european. That means "DD.MM.YYYY".
<br>
<br>
Some examples:
"30.02.2016" -> "01.03.2016"<br>
"40.06.2015" -> "10.07.2015"<br>
"11.13.2014" -> "11.01.2015"<br>
"99.11.2010" -> "07.02.2011"<br>
If the input-string is null or empty return exactly this value!<br/>
If the date-string-format is invalid, return null.
Hint: Correct first the month and then the day!
Have fun coding it and please don't forget to vote and rank this kata! :-)
I have created other katas. Have a look if you like coding and challenges. | reference | import re
from datetime import date, timedelta
def date_correct(text):
if not text:
return text
try:
d, m, y = map(int, re . match(
r'^(\d{2})\.(\d{2})\.(\d{4})$', text). groups())
mo, m = divmod(m - 1, 12)
return (date(y + mo, m + 1, 1) + timedelta(days=d - 1)). strftime('%d.%m.%Y')
except AttributeError:
return None
| Correct the date-string | 5787628de55533d8ce000b84 | [
"Parsing",
"Strings",
"Fundamentals"
] | https://www.codewars.com/kata/5787628de55533d8ce000b84 | 7 kyu |
Hello! Your are given x and y and 2D array size tuple (width, height) and you have to:
<ol><li>Calculate the according index in 1D space (zero-based). </li>
<li>Do reverse operation.</li>
</ol>
<pre>Implement:
to_1D(x, y, size):
--returns index in 1D space
to_2D(n, size)
--returns x and y in 2D space</pre>
<pre>
1D array: [0, 1, 2, 3, 4, 5, 6, 7, 8]
2D array: [[<b>0</b> -> (0,0), <b>1</b> -> (1,0), <b>2</b> -> (2,0)],
[<b>3</b> -> (0,1), <b>4</b> -> (1,1), <b>5</b> -> (2,1)],
[<b>6</b> -> (0,2), <b>7</b> -> (1,2), <b>8</b> -> (2,2)]]
</pre>
<hr>
<code>to_1D(0, 0, (3,3)) returns 0</code>
<code>to_1D(1, 1, (3,3)) returns 4</code>
<code>to_1D(2, 2, (3,3)) returns 8</code>
<code>to_2D(5, (3,3)) returns (2,1)</code>
<code>to_2D(3, (3,3)) returns (0,1)</code>
Assume all input are valid:
<code>
1 < width < 500;
1 < height < 500
</code> | reference | def to_1D(x, y, size):
return y * size[0] + x
def to_2D(n, size):
return (n % size[0], n / / size[0])
| 2D / 1D array coordinates mapping | 588dd9c3dc49de0bd400016d | [
"Arrays",
"Mathematics",
"Fundamentals"
] | https://www.codewars.com/kata/588dd9c3dc49de0bd400016d | 7 kyu |
Given a number `n`, make a down arrow shaped pattern.
For example, when `n = 5`, the output would be:
123454321
1234321
12321
121
1
and for `n = 11`, it would be:
123456789010987654321
1234567890987654321
12345678987654321
123456787654321
1234567654321
12345654321
123454321
1234321
12321
121
1
An important thing to note in the above example is that the numbers greater than 9 still stay single digit, like after 9 it would be 0 - 9 again instead of 10 - 19.
Note:
- There are spaces for the indentation on the left of each line and no spaces on the right.
- Return ```""``` if given n<1.
Have fun!
| algorithms | def half(i, n):
return "" . join(str(d % 10) for d in range(1, n - i + 1))
def line(i, n):
h = half(i, n)
return " " * i + h + h[- 2:: - 1]
def get_a_down_arrow_of(n):
return "\n" . join(line(i, n) for i in range(n))
| Down Arrow With Numbers | 5645b24e802c6326f7000049 | [
"Algorithms"
] | https://www.codewars.com/kata/5645b24e802c6326f7000049 | 7 kyu |
# Story&Task
The capital of Berland has n multifloor buildings. The architect who built up the capital was very creative, so all houses in the city were built in one row.
Let's enumerate all the houses from left to right, starting with 0. A house is considered to be luxurious if the number of floors in it is strictly greater than in each house with larger number. In other words, a house is luxurious if the number of floors in it is strictly greater than in all houses, located to the right from it.
The new architect is interested in n questions, the ith of them is the following: "how many floors should be added to the ith house to make it luxurious?" (For each i from 1 to n, inclusive). You need to help him cope with this task.
Note that all these questions are independent from each other β the answer to the question for house i does not affect other answers (i.e., the floors to the houses are not actually added).
# Input/Output
- `[input]` integer array `houses`
Array of positive integers, representing the number of floors in each house.
The ith element is the number of floors in the ith house.
`1 β€ houses.length β€ 1000`
- `[output]` an integer array
An array has the same length as input array, the ith element represents the number of floors that should be added to the ith house to make it luxurious.
# Example
For `houses = [1,2,3,1,2]`, the output should be `[3,2,0,2,0]`.
```
For houses[0], 3 floors should be added,
then its floors is strictly greater than all houses of right side.
For houses[1], 2 floors should be added.
For houses[2], no need to add floor
because it's already the luxurious.
For houses[3], 2 floors need to added
For houses[4], no house on the right,
so no need to add any floor.
``` | algorithms | def luxhouse(houses):
return [max(0, max(houses[i:]) - h + 1) for i, h in enumerate(houses[: - 1], 1)] + [0]
| Simple Fun #200: Luxurious House | 58c8af49fd407dea5f000042 | [
"Algorithms"
] | https://www.codewars.com/kata/58c8af49fd407dea5f000042 | 6 kyu |
# Introduction
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
Good one Shaggy! We all love to watch Scooby Doo, Shaggy Rogers, Fred Jones, Daphne Blake and Velma Dinkley solve the clues and figure out who was the villain. The story plot rarely differed from one episode to the next. Scooby and his team followed the clue then unmasked the villain at the end.
</pre>
# Task
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
Your task is to initially solve the clues and then use those clues to unmask the villain. You will be given a string of letters that you must manipulate in a way that the clues guide you. You must then output the villain.
</pre>
You will be given an Array of potential villains and you must only return the correct masked villain.
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
<b><u>Potential Villains for the example test cases</u></b>
`Black Knights, Puppet Master, Ghost Clowner, Witch Doctors, Waxed Phantom, Manor Phantom, Ghost Bigfoot, Haunted Horse, Davy Crockett, Captain Injun, Greens Gloobs, Ghostly Manor, Netty Crabbes, King Katazuma, Gators Ghouls, Headless Jack, Mambas Wambas, Medicines Man, Demon Sharker, Kelpy Monster, Gramps Vamper, Phantom Racer, Skeletons Men, Moon Monsters`
</pre>
There will be different villains for the main test cases!
<pre style="white-space: pre-wrap;white-space: -moz-pre-wrap;white-space: -pre-wrap;white-space: -o-pre-wrap;word-wrap: break-word;">
<b>Clue 1:</b> The first clue is in a 'house' on 'String Class' Avenue.
</pre>
Good luck!
# Kata Series
If you enjoyed this, then please try one of my other Katas. Any feedback, translations and grading of beta Katas are greatly appreciated. Thank you.
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58663693b359c4a6560001d6" target="_blank">Maze Runner</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58693bbfd7da144164000d05" target="_blank">Scooby Doo Puzzle</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/7KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586a1af1c66d18ad81000134" target="_blank">Driving License</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586c0909c1923fdb89002031" target="_blank">Connect 4</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/586e6d4cb98de09e3800014f" target="_blank">Vending Machine</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/587136ba2eefcb92a9000027" target="_blank">Snakes and Ladders</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58a848258a6909dd35000003" target="_blank">Mastermind</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58b2c5de4cf8b90723000051" target="_blank">Guess Who?</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58f5c63f1e26ecda7e000029" target="_blank">Am I safe to drive?</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58f5c63f1e26ecda7e000029" target="_blank">Mexican Wave</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/58fdcc51b4f81a0b1e00003e" target="_blank">Pigs in a Pen</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/590300eb378a9282ba000095" target="_blank">Hungry Hippos</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/5904be220881cb68be00007d" target="_blank">Plenty of Fish in the Pond</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/590adadea658017d90000039" target="_blank">Fruit Machine</a></span>
<span style="display: flex !important;"><img style="margin:0px;" src="https://raw.githubusercontent.com/adrianeyre/codewars/master/Ruby/Authored/6KYU.png" alt="Rank"/> <a href="https://www.codewars.com/kata/591eab1d192fe0435e000014" target="_blank">Car Park Escape</a></span> | games | import difflib
from string import ascii_lowercase, ascii_uppercase
tbl = str . maketrans(ascii_lowercase + ascii_uppercase,
(ascii_lowercase[5:] + ascii_lowercase[: 5]) * 2)
def scoobydoo(villian, villians):
villian = villian . replace(' ', '')
villian = villian[- 6:: - 1] + villian[: - 6: - 1]
villian = '' . join(v . translate(tbl) if i %
2 else v for i, v in enumerate(villian))
return difflib . get_close_matches(villian, villians, n=1)[0]
| Scooby Doo Puzzle | 58693bbfd7da144164000d05 | [
"Puzzles",
"Cryptography",
"Algorithms"
] | https://www.codewars.com/kata/58693bbfd7da144164000d05 | 6 kyu |
# Task
Given an array `arr` and a number `n`. Call a pair of numbers from the array a `Perfect Pair` if their sum is equal to `n`.
Find all of the perfect pairs and return the sum of their **indices**.
Note that any element of the array can only be counted in one Perfect Pair. If there are multiple correct answers, return the smallest one.
# Example
For `arr = [1, 4, 2, 3, 0, 5] and n = 7`, the result should be `11`.
Since the Perfect Pairs are `(4, 3)` and `(2, 5)` with indices `1 + 3 + 2 + 5 = 11`.
For `arr = [1, 3, 2, 4] and n = 4`, the result should be `1`.
Since the element at `index 0` (i.e. 1) and the element at `index 1` (i.e. 3) form the only Perfect Pair.
# Input/Output
- `[input]` integer array `arr`
array of non-negative integers.
- `[input]` integer `n`
positive integer
- `[output]` integer
sum of indices, `0` if no Perfect Pair exists. | algorithms | def pairwise(arr, n):
s = []
for i in range(len(arr) - 1):
for j in range(i + 1, len(arr)):
if j in s or i in s:
continue
if arr[i] + arr[j] == n:
s . append(i)
s . append(j)
return sum(s)
| Simple Fun #162: Pair Wise | 58afa8185eb02ea2a7000094 | [
"Algorithms"
] | https://www.codewars.com/kata/58afa8185eb02ea2a7000094 | 6 kyu |
# Task
You are given two string `a` an `s`. Starting with an empty string we can do the following two operations:
```
append the given string a to the end of the current string.
erase one symbol of the current string.```
Your task is to find the least number of operations needed to construct the given string s. Assume that all the letters from `s` appear in `a` at least once.
# Example
For `a = "aba", s = "abbaab"`, the result should be `6`.
Coinstruction:
`"" -> "aba" -> "ab" -> "ababa" -> "abba" -> "abbaaba" -> "abbaab".`
So, the result is 6.
For `a = "aba", s = "a"`, the result should be `3`.
Coinstruction:
`"" -> "aba" -> "ab" -> "a".`
So, the result is 3.
For `a = "aba", s = "abaa"`, the result should be `4`.
Coinstruction:
`"" -> "aba" -> "abaaba" -> "abaab" -> "abaa".`
So, the result is 4.
# Input/Output
- `[input]` string `a`
string to be appended. Contains only lowercase English letters.
1 <= a.length <= 20
- `[input]` string `s`
desired string containing only lowercase English letters.
1 <= s.length < 1000
- `[output]` an integer
minimum number of operations | games | import re
def string_constructing(a, s):
# "-1" because one empty string is always found at the end
n = len(re . findall('?' . join(list(a)) + '?', s)) - 1
return n + len(a) * n - len(s)
| Simple Fun #122: String Constructing | 58a3a735cebc0630830000c0 | [
"Puzzles"
] | https://www.codewars.com/kata/58a3a735cebc0630830000c0 | 6 kyu |
## Task
An `ATM` ran out of 10 dollar bills and only has `100, 50 and 20` dollar bills.
Given an amount between `40 and 10000 dollars (inclusive)` and assuming that the ATM wants to use as few bills as possible, determinate the minimal number of 100, 50 and 20 dollar bills the ATM needs to dispense (in that order).
## Example
For `n = 250`, the result should be `[2, 1, 0]`.
For `n = 260`, the result should be `[2, 0, 3]`.
For `n = 370`, the result should be `[3, 1, 1]`.
## Input/Output
- `[input]` integer `n`
Amount of money to withdraw. Assume that `n` is always exchangeable with `[100, 50, 20]` bills.
- `[output]` integer array
An array of number of `100, 50 and 20` dollar bills needed to complete the withdraw (in that order). | games | def withdraw(n):
n50 = 0
n20, r = divmod(n, 20)
if r == 10:
n20 -= 2
n50 += 1
n100, n20 = divmod(n20, 5)
return [n100, n50, n20]
| Simple Fun #165: Withdraw | 58afce23b0e8046a960000eb | [
"Puzzles"
] | https://www.codewars.com/kata/58afce23b0e8046a960000eb | 6 kyu |
# Task
John is new to spreadsheets. He is well aware of rows and columns, but he is not comfortable with spreadsheets numbering system.
```
Spreadsheet Row Column
A1 R1C1
D5 R5C4
AA48 R48C27
BK12 R12C63```
Since John has a lot of work to do both in row-column and spreadsheet systems, he needs a program that converts cell numbers from one system to another.
# Example
For `s = "A1"`, the result should be `"R1C1"`.
For `s = "R48C27"`, the result should be `"AA48"`.
- Input/Output
- `[input]` string `s`
The position (in spreadsheet or row-column numbering system).
`Spreadsheet : A1 to CRXO65535`
`Row-Column: R1C1 to R65535C65535`
- `[output]` a string
The position (in the opposite format; if input was in spreadsheet system, the output should be int row-column system, and vise versa). | algorithms | import re
def spreadsheet(s):
nums = re . findall(r'(\d+)', s)
if len(nums) == 2:
n, cStr = int(nums[1]), ''
while n:
n, r = divmod(n - 1, 26)
cStr += chr(r + 65)
return "{}{}" . format(cStr[:: - 1], nums[0])
else:
return "R{}C{}" . format(nums[0], sum(26 * * i * (ord(c) - 64) for i, c in enumerate(re . sub(r'\d', '', s)[:: - 1])))
| Simple Fun #167: Spreadsheet | 58b38f24c723bf6b660000d8 | [
"Algorithms"
] | https://www.codewars.com/kata/58b38f24c723bf6b660000d8 | 5 kyu |
# Task
Given an array of integers, sum consecutive even numbers and consecutive odd numbers. Repeat the process while it can be done and return the length of the final array.
# Example
For `arr = [2, 1, 2, 2, 6, 5, 0, 2, 0, 5, 5, 7, 7, 4, 3, 3, 9]`
The result should be `6`.
```
[2, 1, 2, 2, 6, 5, 0, 2, 0, 5, 5, 7, 7, 4, 3, 3, 9] -->
2+2+6 0+2+0 5+5+7+7 3+3+9
[2, 1, 10, 5, 2, 24, 4, 15 ] -->
2+24+4
[2, 1, 10, 5, 30, 15 ]
The length of final array is 6
```
# Input/Output
- `[input]` integer array `arr`
A non-empty array,
`1 β€ arr.length β€ 1000`
`0 β€ arr[i] β€ 1000`
- `[output]` an integer
The length of the final array
~~~if:lambdacalc
# Encodings
purity: `LetRec`
numEncoding: `BinaryScott`
export constructors `nil, cons` for your `List` encoding
~~~ | games | from itertools import groupby
def sum_groups(arr):
newarr = [sum(j) for i, j in groupby(arr, key=lambda x: x % 2 == 0)]
return len(newarr) if newarr == arr else sum_groups(newarr)
| Simple Fun #170: Sum Groups | 58b3c2bd917a5caec0000017 | [
"Arrays",
"Algorithms",
"Lists",
"Data Structures"
] | https://www.codewars.com/kata/58b3c2bd917a5caec0000017 | 6 kyu |
# Task
You are given a regular array `arr`. Let's call a `step` the difference between two adjacent elements.
Your task is to sum the elements which belong to the sequence of consecutive elements of length `at least 3 (but as long as possible)`, such that the steps between the elements in this sequence are the same.
Note that some elements belong to two sequences and have to be counted twice.
# Example
For `arr = [54, 70, 86, 1, -2, -5, 0, 5, 78, 145, 212, 15]`, the output should be `639`.
```
There are 4 sequences of equal steps in the given array:
{54, 70, 86} => step +16
{1, -2, -5} => step -3
{-5, 0, 5} => step +5
{78, 145, 212} => step +67
So the answer is
(54 + 70 + 86) +
(1 - 2 - 5) +
(-5 + 0 + 5) +
(78 + 145 + 212) = 639.
The last element 15 was not be counted.
```
For `arr = [7, 2, 3, 2, -2, 400, 802]`, the output should be `1200`.
```
There is only 1 sequence in arr:
{-2, 400, 802} => step +402
So the answer is: -2 + 400 + 802 = 1200
```
For `arr = [1, 2, 3, 4, 5]`, the output should be `15`.
Note that we should only count {1, 2, 3, 4, 5} as a whole, any other small subset such as {1, 2, 3},{2, 3, 4},{3, 4, 5} are belong to {1, 2, 3, 4, 5}.
# Input/Output
- `[input]` array.integer `arr`
`3 β€ arr.length β€ 100`
- `[output]` an integer
The sum of sequences. | algorithms | def sum_of_regular_numbers(arr):
result = []
new = []
for i in range(len(arr)):
if len(new) < 2 or (len(new) >= 2 and arr[i] - new[- 1] == new[- 1] - new[- 2]):
new . append(arr[i])
else:
if len(new) >= 3:
result += new
new = [new[- 1], arr[i]]
if len(new) > 2:
result += new
return sum(result)
| Simple Fun #191: Sum Of Regular Numbers | 58c20c8d61aefc518f0000fd | [
"Algorithms"
] | https://www.codewars.com/kata/58c20c8d61aefc518f0000fd | 6 kyu |
# Task
Given an integer `n`, find the maximal number you can obtain by deleting exactly one digit of the given number.
# Example
For `n = 152`, the output should be `52`;
For `n = 1001`, the output should be `101`.
# Input/Output
- `[input]` integer `n`
Constraints: `10 β€ n β€ 1000000.`
- `[output]` an integer | games | def delete_digit(n):
s = str(n)
return int(max(s[: i] + s[i + 1:] for i in range(len(s))))
| Simple Fun #79: Delete a Digit | 5894318275f2c75695000146 | [
"Puzzles"
] | https://www.codewars.com/kata/5894318275f2c75695000146 | 6 kyu |
# Task
After becoming famous, CodeBots decided to move to a new building and live together. The building is represented by a rectangular matrix of rooms, each cell containing an integer - the price of the room. Some rooms are free (their cost is 0), but that's probably because they are haunted, so all the bots are afraid of them. That is why any room that is free or is located anywhere below a free room in the same column is not considered suitable for the bots.
Help the bots calculate the total price of all the rooms that are suitable for them.
# Example
For
```
matrix = [[0, 1, 1, 2],
[0, 5, 0, 0],
[2, 0, 3, 3]]```
the output should be `9`.
Here's the rooms matrix with unsuitable rooms marked with 'x':
```
[[x, 1, 1, 2],
[x, 5, x, x],
[x, x, x, x]]```
Thus, the answer is `1 + 5 + 1 + 2 = 9`.
# Input/Output
- `[input]` 2D integer array `matrix`
2-dimensional array of integers representing a rectangular matrix of the building.
Constraints:
`1 β€ matrix.length β€ 10,`
`1 β€ matrix[0].length β€ 10,`
`0 β€ matrix[i][j] β€ 100.`
- `[output]` an integer | games | def matrix_elements_sum(m):
return sum(sum(e for i, e in enumerate(c) if 0 not in c or i < c . index(0)) for c in zip(* m))
| Simple Fun #65: Matrix Elements Sum | 5893eb36779ce5faab0000da | [
"Puzzles",
"Matrix",
"Algorithms"
] | https://www.codewars.com/kata/5893eb36779ce5faab0000da | 6 kyu |
Modify the spacify function so that it returns the given string with spaces inserted between each character.
```coffeescript
spacify=("hello world") -> # returns "h e l l o w o r l d
```
```crystal
spacify("hello world") # returns "h e l l o w o r l d"
```
```haskell
spacify "hello world" -- returns "h e l l o w o r l d"
```
```java
spacify("hello world") // returns "h e l l o w o r l d"
```
```javascript
spacify("hello world") // returns "h e l l o w o r l d"
```
```python
spacify("hello world") # returns "h e l l o w o r l d"
```
```ruby
spacify("hello world") # returns "h e l l o w o r l d"
```
```c
spacify("hello world") // returns "h e l l o w o r l d"
```
```php
spacify("hello world") // "h e l l o w o r l d"
```
```swift
spacify("hello world") // "h e l l o w o r l d"
``` | reference | def spacify(string):
return " " . join(string)
| Spacify | 57f8ee485cae443c4d000127 | [
"Fundamentals",
"Strings",
"Arrays"
] | https://www.codewars.com/kata/57f8ee485cae443c4d000127 | 7 kyu |
Is every value in the array an array?
This should only test the second array dimension of the array. The values of the nested arrays don't have to be arrays.
Examples:
```javascript
[[1],[2]] => true
['1','2'] => false
[{1:1},{2:2}] => false
```
```python
[[1],[2]] => true
['1','2'] => false
[{1:1},{2:2}] => false
```
```ruby
[[1],[2]] => true
['1','2'] => false
[{1:1},{2:2}] => false
```
```c
[[1],[2]] => true
['1','2'] => false
[{1:1},{2:2}] => false
```
```php
[[1], [2]] => true
["1", "2"] => false
[
new class {
public $one = 1;
},
new class {
public $two = 2;
}
] => false
``` | reference | def arr_check(arr):
return all(isinstance(el, list) for el in arr)
| Is every value in the array an array? | 582c81d982a0a65424000201 | [
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/582c81d982a0a65424000201 | 7 kyu |
Write a function that can return the smallest value of an array or the index of that value. The function's 2nd parameter will tell whether it should return the value or the index.
Assume the first parameter will always be an array filled with at least 1 number and no duplicates. Assume the second parameter will be a string holding one of two values: 'value' and 'index'.
```javascript
min([1,2,3,4,5], 'value') // => 1
min([1,2,3,4,5], 'index') // => 0
```
```java
Arrays.findSmallest(new int[]{1,2,3,4,5}, 'value') // => 1
Arrays.findSmallest(new int[]{1,2,3,4,5}, 'index') // => 0
```
```C#
Kata.FindSmallest(new int[]{ 1, 2 , 3, 4, 5}, "value") // => 1
Kata.FindSmallest(new int[]{ 1, 2 , 3, 4, 5}, "index") // => 0
``` | reference | def find_smallest(numbers, to_return):
return min(numbers) if to_return == 'value' else numbers . index(min(numbers))
| Smallest value of an array | 544a54fd18b8e06d240005c0 | [
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/544a54fd18b8e06d240005c0 | 7 kyu |
# Task
In the popular `Minesweeper` game you have a board with some mines and those cells that don't contain a mine have a number in it that indicates the total number of mines in the neighboring cells. Starting off with some arrangement of mines we want to create a `Minesweeper` game setup.
# Example
For
```
matrix = [[true, false, false],
[false, true, false],
[false, false, false]]```
the output should be
```
minesweeper(matrix) = [[1, 2, 1],
[2, 1, 1],
[1, 1, 1]]```
Check out the image below for better understanding:
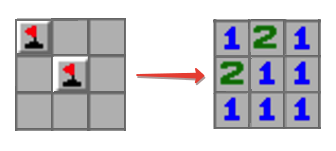
# Input/Output
- `[input]` 2D boolean array `matrix`
A non-empty rectangular matrix consisting of boolean values - `true` if the corresponding cell contains a mine, `false` otherwise.
Constraints:
`2 β€ matrix.length β€ 10,`
`2 β€ matrix[0].length β€ 10.`
- `[output]` 2D integer array
Rectangular matrix of the same size as matrix each cell of which contains an integer equal to the number of mines in the neighboring cells. Two cells are called neighboring if they share at least one corner. | games | import numpy as np
from scipy import signal
def minesweeper(matrix):
return signal . convolve2d(np . array(matrix), np . array([[1, 1, 1], [1, 0, 1], [1, 1, 1]]), mode='same'). tolist()
| Simple Fun #83: MineSweeper | 58952e29f0902eae8b0000cb | [
"Puzzles"
] | https://www.codewars.com/kata/58952e29f0902eae8b0000cb | 6 kyu |
You are given two strings (st1, st2) as inputs. Your task is to return a string containing the numbers in st2 which are not in str1. Make sure the numbers are returned in ascending order.
All inputs will be a string of numbers.
Here are some examples:
```javascript
findAdded('4455446', '447555446666'); // '56667'
findAdded('44554466', '447554466'); // '7'
findAdded('9876521', '9876543211'); // '134'
findAdded('678', '876'); // ''
findAdded('678', '6'); // ''
```
| reference | from collections import Counter
def findAdded(st1, st2):
return '' . join(sorted((Counter(st2) - Counter(st1)). elements()))
| Find Added | 58de77a2c19f096a5a00013f | [
"Fundamentals",
"Strings"
] | https://www.codewars.com/kata/58de77a2c19f096a5a00013f | 6 kyu |
This series of katas will introduce you to basics of doing geometry with computers.
`Point` objects have `x`, `y`, and `z` attributes. For Haskell there are `Point` data types described with record syntax with fields `x`, `y`, and `z`.
Write a function calculating distance between `Point a` and `Point b`.
Tests round answers to 6 decimal places. Tests in Haskell will not round. | reference | def distance_between_points(a, b):
return ((a . x - b . x) * * 2 + (a . y - b . y) * * 2 + (a . z - b . z) * * 2) * * 0.5
| Geometry Basics: Distance between points in 3D | 58dceee2c9613aacb40000b9 | [
"Geometry",
"Fundamentals"
] | https://www.codewars.com/kata/58dceee2c9613aacb40000b9 | 7 kyu |
The aim of the kata is to try to show how difficult it can be to calculate decimals of an irrational number with a certain precision. We have chosen to get a few decimals of the number "pi" using
the following infinite series (Leibniz 1646β1716):
PI / 4 = 1 - 1/3 + 1/5 - 1/7 + ... which gives an approximation of PI / 4.
http://en.wikipedia.org/wiki/Leibniz_formula_for_%CF%80
To have a measure of the difficulty we will count how many iterations are needed to calculate PI with a given precision of `epsilon`.
There are several ways to determine the precision of the calculus but to keep things easy we will calculate `PI` within epsilon of your language Math::PI constant.
*In other words*, given as input a precision of `epsilon` we will stop the iterative process when the absolute value of the difference between our calculation using the Leibniz series and the Math::PI constant of your language is less than `epsilon`.
Your function returns an array or a string or a tuple depending on the language (See sample tests) with
- your number of iterations
- your approximation of PI with 10 decimals
```if:clojure
Clojure : You can use
"round" (see "Your solution") to return PI with 10 decimals
```
```if:haskell
Haskell : You can use
"trunc10Dble" (see "Your solution") to return PI with 10 decimals
```
```if:ocaml
OCaml : You can use
"rnd10" (see "Your solution") to return PI with 10 decimals
```
```if:rust
Rust : You can use
"rnd10" (see "Your solution") to return PI with 10 decimals
```
```if:scala
Scala :
Approximation of PI with 10 or less decimals without trailing zeroes
```
#### Example :
Given epsilon = 0.001 your function gets an approximation of 3.140592653839794 for PI at the end of 1000 iterations : since you are within `epsilon` of `Math::PI` you return
```
iter_pi(0.001) --> [1000, 3.1405926538]
```
#### Notes :
Unfortunately, this series converges too slowly to be useful,
as it takes over 300 terms to obtain a 2 decimal place precision.
To obtain 100 decimal places of PI, it was calculated that
one would need to use at least 10^50 terms of this expansion!
About PI : http://www.geom.uiuc.edu/~huberty/math5337/groupe/expresspi.html | reference | from math import pi
def iter_pi(epsilon):
n = 1
approx = 4
while abs(approx - pi) > epsilon:
n += 1
approx += (- 4, 4)[n % 2] / (n * 2 - 1.0)
return [n, round(approx, 10)]
| PI approximation | 550527b108b86f700000073f | [
"Arrays",
"Mathematics",
"Fundamentals"
] | https://www.codewars.com/kata/550527b108b86f700000073f | 6 kyu |
A [sequence or a series](http://world.mathigon.org/Sequences), in mathematics, is a string of objects, like numbers, that follow a particular pattern. The individual elements in a sequence are called terms. A simple example is `3, 6, 9, 12, 15, 18, 21, ...`, where the pattern is: _"add 3 to the previous term"_.
In this kata, we will be using a more complicated sequence: `0, 1, 3, 6, 10, 15, 21, 28, ...`
This sequence is generated with the pattern: _"the nth term is the sum of numbers from 0 to n, inclusive"_.
```
[ 0, 1, 3, 6, ...]
0 0+1 0+1+2 0+1+2+3
```
## Your Task
Complete the function that takes an integer `n` and returns a list/array of length `abs(n) + 1` of the arithmetic series explained above. When`n < 0` return the sequence with negative terms.
## Examples
```
5 --> [0, 1, 3, 6, 10, 15]
-5 --> [0, -1, -3, -6, -10, -15]
7 --> [0, 1, 3, 6, 10, 15, 21, 28]
```
| reference | def sum_of_n(n):
return [(- 1 if n < 0 else 1) * sum(xrange(i + 1)) for i in xrange(abs(n) + 1)]
| Basic Sequence Practice | 5436f26c4e3d6c40e5000282 | [
"Fundamentals"
] | https://www.codewars.com/kata/5436f26c4e3d6c40e5000282 | 7 kyu |
[Harshad numbers](http://en.wikipedia.org/wiki/Harshad_number) (also called Niven numbers) are positive numbers that can be divided (without remainder) by the sum of their digits.
For example, the following numbers are Harshad numbers:
* 10, because 1 + 0 = 1 and 10 is divisible by 1
* 27, because 2 + 7 = 9 and 27 is divisible by 9
* 588, because 5 + 8 + 8 = 21 and 588 is divisible by 21
While these numbers are not:
* 19, because 1 + 9 = 10 and 19 is not divisible by 10
* 589, because 5 + 8 + 9 = 22 and 589 is not divisible by 22
* 1001, because 1 + 1 = 2 and 1001 is not divisible by 2
Harshad numbers can be found in any number base, but we are going to focus on base 10 exclusively.
## Your task
Your task is to complete the skeleton Harshad object ("static class") which has 3 functions:
* ```isValid()``` that checks if `n` is a Harshad number or not
* ```getNext()``` that returns the next Harshad number > `n`
* ```getSerie()``` that returns a series of `n` Harshad numbers, optional `start` value not included
You do not need to care about the passed parameters in the test cases, they will always be valid integers (except for the start argument in `getSerie()` which is optional and should default to `0`).
**Note:** only the first 2000 Harshad numbers will be checked in the tests.
## Examples
```javascript
Harshad.isValid(1) ==> true
Harshad.getNext(0) ==> 1
Harshad.getSerie(3) ==> [ 1, 2, 3 ]
Harshad.getSerie(3, 1000) ==> [ 1002, 1008, 1010 ]
```
```ruby
Harshad.is_valid(1) ==> true
Harshad.get_next(0) ==> 1
Harshad.get_serie(3) ==> [ 1, 2, 3 ]
Harshad.get_serie(3, 1000) ==> [ 1002, 1008, 1010 ]
```
```python
Harshad.is_valid(1) ==> True
Harshad.get_next(0) ==> 1
Harshad.get_series(3) ==> [ 1, 2, 3 ]
Harshad.get_series(3, 1000) ==> [ 1002, 1008, 1010 ]
``` | reference | from itertools import count, islice
class Harshad:
@ staticmethod
def is_valid(number):
return number % sum(map(int, str(number))) == 0
@ classmethod
def get_next(cls, number):
return next(i for i in count(number + 1) if cls . is_valid(i))
@ classmethod
def get_series(cls, c, start=0):
return list(islice(filter(cls . is_valid, (i for i in count(start + 1))), c))
| Harshad or Niven numbers | 54a0689443ab7271a90000c6 | [
"Fundamentals",
"Mathematics",
"Object-oriented Programming"
] | https://www.codewars.com/kata/54a0689443ab7271a90000c6 | 6 kyu |
<h3>Description:</h3>
Given a string, you need to write a method that order every letter in this string in ascending order.
Also, you should validate that the given string is not empty or null. If so, the method should return:
```
"Invalid String!"
```
<h4>Notes</h4>
β’ the given string can be lowercase and uppercase.<br />
β’ the string could include spaces or other special characters like '# ! or ,'. Sort them based on their ASCII codes
<h3>Examples</h3>
```
"hello world" => " dehllloorw"
"bobby" => "bbboy"
"" => "Invalid String!"
"!Hi You!" => " !!HYiou"
```
<strong>Good luck! Hope you enjoy it</strong> | reference | def order_word(s):
return "" . join(sorted(s)) if s else "Invalid String!"
| Ordering the words! | 55d7e5aa7b619a86ed000070 | [
"Strings",
"Sorting",
"Fundamentals"
] | https://www.codewars.com/kata/55d7e5aa7b619a86ed000070 | 7 kyu |
Everybody knows a little german, right? But remembering the correct articles is a tough job. Write yourself a little helper, that returns the noun with the matching article:
- each noun containing less than 2 vowels has the article "das"
- each noun containing 2/3 vowels has the article "die"
- each noun containing more than 3 vowels has the article "der"
Caution: Vowels are "a,e,i,o,u". Umlaute (Γ€ ΓΆ ΓΌ) are also being counted!
(This Kata is a joke, there is no such grammar rule!) | reference | def der_die_das(wort):
a = sum(x in "aAeEiIoOuUÀâü" for x in wort)
return f' { "das" if a < 2 else "die" if a < 4 else "der" } { wort } '
| Deutschstunde | 552cd8624a414ec2b2000086 | [
"Fundamentals"
] | https://www.codewars.com/kata/552cd8624a414ec2b2000086 | 7 kyu |
Naming multiple files can be a pain sometimes.
#### Task:
Your job here is to create a function that will take three parameters, `fmt`, `nbr` and `start`, and create an array of `nbr` elements formatted according to `frm` with the starting index `start`. `fmt` will have `<index_no>` inserted at various locations; this is where the file index number goes in each file.
#### Description of edge cases:
1. If `nbr` is less than or equal to 0, or not whole, return an empty array.
2. If `fmt` does not contain `'<index_no>'`, just return an array with `nbr` elements that are all equal to `fmt`.
3. If `start` is not an integer, return an empty array.
#### What each parameter looks like:
```ruby
frm.class #=> String
: "text_to_stay_constant_from_file_to_file <index_no>"
nbr.class #=> Fixnum
: number_of_files
start.class #=> Fixnum
: index_no_of_first_file
name_file(frm, nbr, start).class #=> Array(Fixnum)
```
```crystal
frm.class #=> String
: "text_to_stay_constant_from_file_to_file <index_no>"
nbr.class #=> Int32
: number_of_files
start.class #=> Int32
: index_no_of_first_file
name_file(frm, nbr, start).class #=> Array(Int32)
```
```python
type(frm) #=> str
: "text_to_stay_constant_from_file_to_file <index_no>"
type(nbr) #=> int
: number_of_files
type(start) #=> int
: index_no_of_first_file
type(name_file(frm, nbr, start)) #=> list
```
```javascript
typeof frm #=> 'string'
: "text_to_stay_constant_from_file_to_file <index_no>"
typeof nbr #=> 'number'
: number_of_files
typeof start #=> 'number'
: index_no_of_first_file
typeof (nameFile(frm, nbr, start)) #=> 'array'
```
```coffeescript
typeof frm #=> 'string'
: "text_to_stay_constant_from_file_to_file <index_no>"
typeof nbr #=> 'number'
: number_of_files
typeof start #=> 'number'
: index_no_of_first_file
typeof (nameFile(frm, nbr, start)) #=> 'array'
```
#### Some examples:
```ruby
name_file('IMG <index_no>', 4, 1)
#=> ['IMG 1', 'IMG 2', 'IMG 3', 'IMG 4']
name_file('image #<index_no>.jpg', 3, 7)
#=> ['image #7.jpg', 'image #8.jpg', 'image #9.jpg']
name_file('#<index_no> #<index_no>', 3, -2)
#=> ['#-2 #-2', '#-1 #-1', '#0 #0']
```
```crystal
name_file("IMG <index_no>", 4, 1)
#=> ["IMG 1", "IMG 2", "IMG 3", "IMG 4"])
name_file("image #<index_no>.jpg", 3, 7)
#=> ["image #7.jpg", "image #8.jpg", "image #9.jpg"]
name_file("#<index_no> #<index_no>", 3, -2)
#=> ["#-2 #-2", "#-1 #-1", "#0 #0"]
```
```python
name_file("IMG <index_no>", 4, 1)
#=> ["IMG 1", "IMG 2", "IMG 3", "IMG 4"])
name_file("image #<index_no>.jpg", 3, 7)
#=> ["image #7.jpg", "image #8.jpg", "image #9.jpg"]
name_file("#<index_no> #<index_no>", 3, -2)
#=> ["#-2 #-2", "#-1 #-1", "#0 #0"]
```
```javascript
nameFile("IMG <index_no>", 4, 1)
#=> ["IMG 1", "IMG 2", "IMG 3", "IMG 4"])
nameFile("image #<index_no>.jpg", 3, 7)
#=> ["image #7.jpg", "image #8.jpg", "image #9.jpg"]
nameFile("#<index_no> #<index_no>", 3, -2)
#=> ["#-2 #-2", "#-1 #-1", "#0 #0"]
```
```coffeescript
nameFile("IMG <index_no>", 4, 1)
#=> ["IMG 1", "IMG 2", "IMG 3", "IMG 4"])
nameFile("image #<index_no>.jpg", 3, 7)
#=> ["image #7.jpg", "image #8.jpg", "image #9.jpg"]
nameFile("#<index_no> #<index_no>", 3, -2)
#=> ["#-2 #-2", "#-1 #-1", "#0 #0"]
```
Also check out my other creations β [Elections: Weighted Average](https://www.codewars.com/kata/elections-weighted-average), [Identify Case](https://www.codewars.com/kata/identify-case), [Split Without Loss](https://www.codewars.com/kata/split-without-loss), [Adding Fractions](https://www.codewars.com/kata/adding-fractions),
[Random Integers](https://www.codewars.com/kata/random-integers), [Implement String#transpose](https://www.codewars.com/kata/implement-string-number-transpose), [Implement Array#transpose!](https://www.codewars.com/kata/implement-array-number-transpose), [Arrays and Procs #1](https://www.codewars.com/kata/arrays-and-procs-number-1), and [Arrays and Procs #2](https://www.codewars.com/kata/arrays-and-procs-number-2).
If you notice any issues or have any suggestions/comments whatsoever, please don't hesitate to mark an issue or just comment. Thanks! | reference | def name_file(fmt, nbr, start):
try:
return [fmt . replace('<index_no>', '{0}'). format(i)
for i in range(start, start + nbr)]
except TypeError:
return []
| Naming Files | 5829994cd04efd4373000468 | [
"Fundamentals"
] | https://www.codewars.com/kata/5829994cd04efd4373000468 | 7 kyu |
# Reducing by rules to get the result
Your task is to reduce a list of numbers to one number.<br>
For this you get a list of rules, how you have to reduce the numbers.<br>
You have to use these rules consecutively. So when you get to the end of the list of rules, you start again at the beginning.
An example is clearer than more words...
```
numbers: [ 2.0, 2.0, 3.0, 4.0 ]
rules: [ (a,b) => a + b, (a,b) => a - b ]
result: 5.0
You get a list of four numbers.
There are two rules. First rule says: Sum the two numbers a and b. Second rule says: Subtract b from a.
The steps in progressing:
1. Rule 1: First number + second number -> 2.0 + 2.0 = 4.0
2. Rule 2: result from step before - third number -> 4.0 - 3.0 = 1.0
3. Rule 1: result from step before + forth number -> 1.0 + 4.0 = 5.0
```
Both lists/arrays are never null and will always contain valid elements.<br>
The list of numbers will always contain more than 1 numbers.<br>
In the list of numbers will only be values greater than 0.<br>
Every rule takes always two input parameter.
<br><br><br>
Have fun coding it and please don't forget to vote and rank this kata! :-)
I have also created other katas. Take a look if you enjoyed this kata! | algorithms | from functools import reduce
from itertools import cycle
def reduce_by_rules(lst, rules):
rs = cycle(rules)
return reduce(lambda x, y: next(rs)(x, y), lst)
| Reducing by rules to get the result | 585ba6dff59b3cef3f000132 | [
"Arrays",
"Logic",
"Algorithms"
] | https://www.codewars.com/kata/585ba6dff59b3cef3f000132 | 6 kyu |
Write a function that will randomly upper and lower characters in a string - `randomCase()` (`random_case()` for Python).
A few examples:
```
randomCase("Lorem ipsum dolor sit amet, consectetur adipiscing elit") == "lOReM ipSum DOloR SiT AmeT, cOnsEcTEtuR aDiPiSciNG eLIt"
randomCase("Donec eleifend cursus lobortis") == "DONeC ElEifEnD CuRsuS LoBoRTIs"
```
Notes:
1. This function will work within the basic ASCII character set to make this kata easier - so no need to make the function multibyte safe.
2. The letters MUST be selected randomly - filters are set to make sure there is no cheating! | reference | import random
def random_case(x):
return "" . join([random . choice([c . lower(), c . upper()]) for c in x])
| RaNDoM CAsE | 57073869924f34185100036d | [
"Fundamentals"
] | https://www.codewars.com/kata/57073869924f34185100036d | 7 kyu |
Marcus was spending his last summer day playing chess with his friend Rose.
Surprisingly, they had a lot of pieces (we suspect Marcus is a part-time thief, but we will leave that aside), and Marcus wondered in how many different positions could 8 towers (rooks) be in the board, without threatening themselves.
Rose (who was smarter) was wondering if there was any relation between the size of the board, and the number of positions.
So, you should help!
Write a function that, given N (positive-only integer) the size of the board , returns the number of different combinations in which these towers can be.
Example:
```javascript
towerCombination(2) returns 2, because only the following possibilities can be achieved.
| x 0 |
| 0 x |
| 0 x |
| x 0 |
```
```ruby
tower_combination(2) returns 2, because only the following possibilities can be achieved.
| x 0 |
| 0 x |
| 0 x |
| x 0 |
```
```javascript
towerCombination(3) returns 6, because only the following possibilities can be achieved.
| x 0 0 |
| 0 x 0 |
| 0 0 x |
| x 0 0 |
| 0 0 x |
| 0 x 0 |
| 0 x 0 |
| x 0 0 |
| 0 0 x |
| 0 x 0 |
| 0 0 x |
| x 0 0 |
| 0 0 x |
| x 0 0 |
| 0 x 0 |
| 0 0 x |
| 0 x 0 |
| x 0 0 |
```
```ruby
tower_combination(3) returns 6, because only the following possibilities can be achieved.
| x 0 0 |
| 0 x 0 |
| 0 0 x |
| x 0 0 |
| 0 0 x |
| 0 x 0 |
| 0 x 0 |
| x 0 0 |
| 0 0 x |
| 0 x 0 |
| 0 0 x |
| x 0 0 |
| 0 0 x |
| x 0 0 |
| 0 x 0 |
| 0 0 x |
| 0 x 0 |
| x 0 0 |
```
| algorithms | import math
def tower_combination(n):
return math . factorial(n)
| 8 towers | 535bea76cdbf50281a00004c | [
"Algorithms"
] | https://www.codewars.com/kata/535bea76cdbf50281a00004c | 7 kyu |
## Task
Given a number, return the maximum value by rearranging its digits.
Examples:
`(123) => 321` `(786) => 876` `("001") => 100` `(999) => 999` `(10543) => 54310`
*^Note the number may be given as a string* | reference | def rotate_to_max(n):
return int('' . join(sorted(str(n), reverse=True)))
| Rotate to the max | 579fa665bb9944f9340005d2 | [
"Fundamentals",
"Numbers",
"Data Types"
] | https://www.codewars.com/kata/579fa665bb9944f9340005d2 | 7 kyu |
Write a function that accepts two parameters, i) a string (containing a list of words) and ii) an integer (n). The function should alphabetize the list based on the nth letter of each word.
The letters should be compared case-insensitive. If both letters are the same, order them normally (lexicographically), again, case-insensitive.
Example:
```javascript
function sortIt('bid, zag', 2) //=> 'zag, bid'
```
```ruby
function sortIt('bid, zag', 2) //=> 'zag, bid'
```
```python
sort_it('bid, zag', 2) #=> 'zag, bid'
```
```haskell
sortIt ["bid", "zag"] 2 `shouldBe` ["zag", "bid"]
```
The length of all words provided in the list will be >= n. The format will be "x, x, x". In Haskell you'll get a list of `String`s instead. | reference | def sort_it(list_, n):
return ', ' . join(sorted(list_ . split(', '), key=lambda i: i[n - 1]))
| Alphabetize a list by the nth character | 54eea36b7f914221eb000e2f | [
"Lists",
"Strings",
"Sorting",
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/54eea36b7f914221eb000e2f | 7 kyu |
<img src = https://teamfisticuffs.files.wordpress.com/2011/09/beardfist.jpg>
It has long been rumoured that behind Chuck's beard is not a chin, but another fist!
When shaving, Chuck accidentally punched himself in the face. He is the only man that could take that punch without dying, but that doesn't mean it didn't sting! Chuck can't remember a thing - he needs your help!!
Hidden within the provided sequence of sequences are numbers that represent the letters of the words for some of Chuck's favourite things! Your job is to translate them, and return the words so that Chuck can get back to the business of punching and kicking things. | algorithms | from itertools import chain
def fist_beard(arr):
return '' . join(map(chr, chain(* arr)))
| Chuck Norris IV - Bearded Fist | 57066708cb7293901a0013a1 | [
"Fundamentals",
"Algorithms",
"Arrays",
"Puzzles"
] | https://www.codewars.com/kata/57066708cb7293901a0013a1 | 7 kyu |
Your non-profit company has assigned you the task of calculating some simple statistics on donations. You have an array of integers, representing various amounts of donations your company has been given. In particular, you're interested in the median value for donations.
The median is the middle number of a **sorted list of numbers**. If the list is of even length, the 2 middle values are averaged.
Write a function that takes an array of integers as an argument and returns the median of those integers.
Notes:
- The sorting step is vital.
- Input arrays are non-empty.
### Examples
Median of `[33,99,100,30,29,50]` is 41.5.
Median of `[3,2,1]` is 2.
| reference | from statistics import median
| All Star Code Challenge #14 - Find the median | 5864eb8039c5ab9cd400005c | [
"Fundamentals"
] | https://www.codewars.com/kata/5864eb8039c5ab9cd400005c | 7 kyu |
## Task
The string is called `prime` if it cannot be constructed by concatenating some (more than one) equal strings together.
For example, "abac" is prime, but "xyxy" is not("xyxy"="xy"+"xy").
Given a string determine if it is prime or not.
## Input/Output
- `[input]` string `s`
string containing only lowercase English letters
- `[output]` a boolean value
`true` if the string is prime, `false` otherwise | games | def prime_string(s):
return (s + s). find(s, 1) == len(s)
| Simple Fun #116: Prime String | 589d36bbb6c071f7c20000f7 | [
"Puzzles"
] | https://www.codewars.com/kata/589d36bbb6c071f7c20000f7 | 6 kyu |
# Task
You are given an array of integers `a` and a non-negative number of operations `k`, applied to the array. Each operation consists of two parts:
```
find the maximum element value of the array;
replace each element a[i] with (maximum element value - a[i]).```
How will the array look like after `k` such operations?
# Example
For `a = [-4, 0, -1, 0]` and `k = 2`, the output should be `[0, 4, 3, 4]`.
```
initial array: [-4, 0, -1, 0]
1st operation:
find the maximum value --> 0
replace each element: --> [(0 - -4), (0 - 0), (0 - -1), (0 - 0)]
--> [4, 0, 1, 0]
2nd operation:
find the maximum value --> 4
replace each element: --> [(4 - 4), (4 - 0), (4 - 1), (4 - 0)]
--> [0, 4, 3, 4]
```
For `a = [0, -1, 0, 0, -1, -1, -1, -1, 1, -1]` and `k = 1`,
the output should be `[1, 2, 1, 1, 2, 2, 2, 2, 0, 2]`.
```
initial array: [0, -1, 0, 0, -1, -1, -1, -1, 1, -1]
1st operation:
find the maximum value --> 1
replace each element: -->
[(1-0),(1- -1),(1-0),(1-0),(1- -1),(1- -1),(1- -1),(1- -1),(1-1),(1- -1)]
--> [1, 2, 1, 1, 2, 2, 2, 2, 0, 2]
```
# Input/Output
- `[input]` integer array a
The initial array.
Constraints:
`1 <= a.length <= 100`
`-100 <= a[i] <= 100`
- `[input]` integer `k`
non-negative number of operations.
Constraints: `0 <= k <= 100000`
- [output] an integer array
The array after `k` operations. | games | def array_operations(a, n):
li = []
for i in range(n):
m = max(a)
a = [m - i for i in a]
if a in li:
if not n & 1:
return li[- 1]
return a
li . append(a)
return a
| Simple Fun #110: Array Operations | 589aca25fef1a81a10000057 | [
"Puzzles"
] | https://www.codewars.com/kata/589aca25fef1a81a10000057 | 6 kyu |
No description!!!
Input :: [10,20,25,0]
Output :: ["+0", "+10", "+15", "-10"]
`Show some love, rank and upvote!` | games | def equalize(arr):
return ["{:+d}" . format(i - arr[0]) for i in arr]
| Equalize the array! | 580a1a4af195dbc9ed00006c | [
"Fundamentals",
"Puzzles",
"Arrays"
] | https://www.codewars.com/kata/580a1a4af195dbc9ed00006c | 7 kyu |
You will be given a string.
You need to return an array of three strings by gradually pulling apart the string.
You should repeat the following steps until the string length is 1:
a) remove the final character from the original string, add to solution string 1.
b) remove the first character from the original string, add to solution string 2.
The final solution string value is made up of the remaining character from the original string, once originalstring.length == 1.
Example:
"exampletesthere"
becomes:
["erehtse","example","t"]
The Kata title gives a hint of one technique to solve.
| reference | def pop_shift(s):
il, ir = len(s) / / 2, (len(s) + 1) / / 2
return [s[: ir - 1: - 1], s[: il], s[il: ir]]
| PopShift | 57cec34272f983e17800001e | [
"Fundamentals",
"Strings",
"Arrays"
] | https://www.codewars.com/kata/57cec34272f983e17800001e | 7 kyu |
The function takes _cents_ value (int) and needs to return the minimum number of coins combination of the same value.
The function should return ***an array*** where
coins[0] = pennies ==> $00.01
coins[1] = nickels ==> $00.05
coins[2] = dimes ==> $00.10
coins[3] = quarters ==> $00.25
So for example:
``coinCombo(6) --> [1, 1, 0, 0]``
| algorithms | def coin_combo(c):
R = []
for p in (25, 10, 5, 1):
R . append(c / / p)
c = c % p
return R[:: - 1]
| Calculator: Coin Combination | 564d0490e96393fc5c000029 | [
"Fundamentals",
"Algorithms"
] | https://www.codewars.com/kata/564d0490e96393fc5c000029 | 7 kyu |
In Star Labs we use password system to unlock the lab doors and only Team Flash is given the password for these labs. The password system is made of a `n x n` keypad (`n > 0`).
One day Zoom saw Cisco using the password. He figured out that the password is symmetric about the centre point (the centre point for a `n x n` keypad will be the point with co-ordinates `(n / 2, n / 2)`). Now he has to try all possible symmetric combinations to unlock the door. Since he does not have enough time, he asks you to determine whether a given password is symmetric or not.
You will be given a flattened `n x n` binary grid containing elements as `0` if that key is not used and `1` if that key is used. You need to tell whether the given binary grid is symmetric according to the centre point. The grid might not be square; in that case you should return `"No"`.
## Your Task
Write a function that takes an one-dimensional array of integers containing `0` and `1` as input and returning `"Yes"` or `"No"` depending on whether the given password is symmetric or not.
## Examples
* ```
[1, 1, 0, 0, 0, 0, 0, 1, 1] => "Yes"
```
The given password is symmetric about the centre point. For ease of understanding, the above 1D array can be represented as follows:
```
1 1 0
0 0 0
0 1 1
```
* ```
[1, 1, 0, 0, 1, 0, 0, 1] => "No"
```
This array cannot be represented as a `n x n` matrix, so it's invalid.
* ```
[1, 0, 1, 1, 0, 0, 0, 0, 0] => "No"
```
This password is not symmetric along the centre point. | reference | def help_zoom(key):
return 'Yes' if key == key[:: - 1] else 'No'
| Password System | 57a23e3753ba332b8e0008da | [
"Fundamentals"
] | https://www.codewars.com/kata/57a23e3753ba332b8e0008da | 7 kyu |
Given an array of integers (x), and a target (t), you must find out if any two consecutive numbers in the array sum to t. If so, remove the second number.
Example:
x = [1, 2, 3, 4, 5]<br>
t = 3 <br>
1+2 = t, so remove 2. No other pairs = t, so rest of array remains:
[1, 3, 4, 5]
Work through the array from left to right.
Return the resulting array. | reference | def trouble(x, t):
arr = [x[0]]
for c in x[1:]:
if c + arr[- 1] != t:
arr . append(c)
return arr
| Double Trouble | 57f7796697d62fc93d0001b8 | [
"Fundamentals",
"Arrays"
] | https://www.codewars.com/kata/57f7796697d62fc93d0001b8 | 7 kyu |
Given value of sine,implement function which will return sine,cosine,tangent,and cotangent in list. order must be same as in the description and every number must be rounded to 2 decimal places.If tangent or cotangent can not be calculated just don't contain them in result list.
Trygonometry - https://en.wikipedia.org/wiki/Trigonometry | reference | def sctc(sin):
cos = (1 - sin * * 2) * * 0.5
if 0 in (sin, cos):
res = [sin, cos, 0.0]
else:
res = [sin, cos, sin / cos, cos / sin]
return list(map(lambda x: round(x, 2), res))
| Sine,cosine and others | 57d52a7f76da830e43000188 | [
"Mathematics",
"Fundamentals"
] | https://www.codewars.com/kata/57d52a7f76da830e43000188 | 7 kyu |
Implement function which will return sum of roots of a quadratic equation rounded to 2 decimal places, if there are any possible roots, else return **None/null/nil/nothing**. If you use discriminant,when discriminant = 0, x1 = x2 = root => return sum of both roots. There will always be valid arguments.
Quadratic equation - https://en.wikipedia.org/wiki/Quadratic_equation | reference | def roots(a, b, c):
if b * * 2 >= 4 * a * c:
return round(- b / a, 2)
| Find the sum of the roots of a quadratic equation | 57d448c6ba30875437000138 | [
"Mathematics",
"Fundamentals"
] | https://www.codewars.com/kata/57d448c6ba30875437000138 | 7 kyu |
<img src='http://images.huffingtonpost.com/2016-07-13-1468421604-4642908-IronmanLogo.jpg'>
An Ironman Triathlon is one of a series of long-distance triathlon races organized by the World Triathlon Corporaion (WTC).
It consists of a 2.4-mile swim, a 112-mile bicycle ride and a marathon (26.2-mile) (run, raced in that order and without a break. It hurts... trust me.
Your task is to take a distance that an athlete is through the race, and return one of the following:
If the distance is zero, return `'Starting Line... Good Luck!'`.
If the athlete will be swimming, return an object with `'Swim'` as the key, and the remaining race distance as the value.
If the athlete will be riding their bike, return an object with `'Bike'` as the key, and the remaining race distance as the value.
If the athlete will be running, and has more than 10 miles to go, return an object with `'Run'` as the key, and the remaining race distance as the value.
If the athlete has 10 miles or less to go, return return an object with `'Run'` as the key, and `'Nearly there!'` as the value.
Finally, if the athlete has completed te distance, return `"You're done! Stop running!"`.
All distance should be calculated to two decimal places.
| reference | def i_tri(s):
total = 2.4 + 112 + 26.2
to_go = '%.2f to go!' % (total - s)
return ('Starting Line... Good Luck!' if s == 0 else
{'Swim': to_go} if s < 2.4 else
{'Bike': to_go} if s < 2.4 + 112 else
{'Run': to_go} if s < total - 10 else
{'Run': 'Nearly there!'} if s < total else
"You're done! Stop running!")
| Ironman Triathlon | 57d001b405c186ccb6000304 | [
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/57d001b405c186ccb6000304 | 7 kyu |
Welcome to the Mathematics gameshow. I'm your host, Apex Rhombus, and it's time for the lightning round!
Today we'll talk about a hypothetical bottle. This entire bottle weighs 120 grams. Its contents weigh twice as much as the bottle itself. What, may I ask, do the contents weigh?
...Did you guess 80 grams? Correct! Now that you've got that idea, I'm gonna ask you that question in 10 different ways so you'd better get ready!
----------------------------------------------------------------------
Let's make a `contentWeight` function that takes in two parameters: `bottleWeight` and `scale`. This function will return the weight of the contents inside the bottle.
`bottleWeight` will be an integer representing the weight of the entire bottle (contents included).
`scale` will be a string that you will need to parse. It will tell you how the content weight compares to the weight of the bottle by itself. `2 times larger`, `6 times larger`, and `15 times smaller` would all be valid strings (smaller and larger are the only comparison words).
The first test case has been filled out for you. Good luck!
| algorithms | def content_weight(bottle_weight, scale):
a, _, c = scale . split(" ")
return bottle_weight * int(a) / (int(a) + 1) if c == "larger" else bottle_weight / (int(a) + 1)
| Weight of its Contents | 53921994d8f00b93df000bea | [
"Algorithms",
"Mathematics"
] | https://www.codewars.com/kata/53921994d8f00b93df000bea | 7 kyu |
Paul is an excellent coder and sits high on the CW leaderboard. He solves kata like a banshee but would also like to lead a normal life, with other activities. But he just can't stop solving all the kata!!
Given an array (x) you need to calculate the Paul Misery Score. The values are worth the following points:
```
kata = 5
Petes kata = 10
life = 0
eating = 1
```
The Misery Score is the total points gained from the array. Once you have the total, return as follows:
```
< 40 = 'Super happy!'
< 70 >= 40 = 'Happy!'
< 100 >= 70 = 'Sad!'
> 100 = 'Miserable!'
``` | reference | def paul(x):
points = {'life': 0, 'eating': 1, 'kata': 5, 'Petes kata': 10}
misery = sum(map(points . get, x))
return ['Miserable!', 'Sad!', 'Happy!', 'Super happy!'][(misery < 40) + (misery < 70) + (misery < 100)]
| Paul's Misery | 57ee31c5e77282c24d000024 | [
"Fundamentals",
"Arrays"
] | https://www.codewars.com/kata/57ee31c5e77282c24d000024 | 7 kyu |
**A number is self-descriptive when the n'th digit describes the amount n appears in the number.**
**Example:**
For the number 21200:
- There are two 0's in the number, so the first digit is 2.
- There is one 1 in the number, so the second digit is 1.
- There are two 2's in the number, so the third digit is 2.
- There are no 3's in the number, so the fourth digit is 0.
- There are no 4's in the number, so the fifth digit is 0.
**Numbers can be of any length up to 9 digits and are only full integers. For a given number, derive a function ```selfDescriptive(num)``` that returns:**
- ```True``` if the number is self-descriptive
- ```False``` if the number is not. | reference | from collections import Counter
def self_descriptive(num):
s = [int(a) for a in str(num)]
cnt = Counter(s)
return all(cnt[i] == b for i, b in enumerate(s))
| Self-Descriptive Numbers | 56a628758f8d06b59800000f | [
"Fundamentals",
"Mathematics",
"Number Theory"
] | https://www.codewars.com/kata/56a628758f8d06b59800000f | 7 kyu |
In genetic the reverse complement of a sequence is formed by **reversing** the sequence and then taking the complement of each symbol.
The four nucleotides in DNA is Adenine (A), Cytosine (C), Guanine (G) and Thymine (Thymine).
- A is the complement of T
- C is the complement of G.
This is a bi-directional relation so:
- T is the complement of A
- G is the complement of C.
For this kata you need to complete the reverse complement function that take a DNA string and return the reverse complement string.
**Note**: You need to take care of lower and upper case. And if a sequence conatains some invalid characters you need to return "Invalid sequence".
This kata is based on the following [one](http://www.codewars.com/kata/complementary-dna/ruby) but with a little step in addition.
| algorithms | def reverse_complement(dna):
base_pair = {'A': 'T', 'T': 'A', 'C': 'G', 'G': 'C'}
return "" . join(base_pair[base] for base in dna[:: - 1]. upper()) if set(dna . upper()). issubset({'A', 'T', 'C', 'G', ""}) else "Invalid sequence"
| Reverse complement (DNA ) | 574a7d0dca4a8a0fbe000100 | [
"Strings",
"Algorithms"
] | https://www.codewars.com/kata/574a7d0dca4a8a0fbe000100 | 7 kyu |
# Task
let F(N) be the sum square of digits of N. So:
`F(1) = 1, F(3) = 9, F(123) = 14`
Choose a number A, the sequence {A0, A1, ...} is defined as followed:
```
A0 = A
A1 = F(A0)
A2 = F(A1) ...
```
if A = 123, we have:
```
123 β 14(1 x 1 + 2 x 2 + 3 x 3)
β 17(1 x 1 + 4 x 4)
β 50(1 x 1 + 7 x 7)
β 25(5 x 5 + 0 x 0)
β 29(2 x 2 + 5 x 5)
β 85(2 x 2 + 9 x 9)
β 89(8 x 8 + 5 x 5) ---
β 145(8 x 8 + 9 x 9) |r
β 42(1 x 1 + 4 x 4 + 5 x 5) |e
β 20(4 x 4 + 2 x 2) |p
β 4(2 x 2 + 0 x 0) |e
β 16(4 x 4) |a
β 37(1 x 1 + 6 x 6) |t
β 58(3 x 3 + 7 x 7) |
β 89(5 x 5 + 8 x 8) ---
β ......
```
As you can see, the sequence repeats itself. Interestingly, whatever A is, there's an index such that from it, the sequence repeats again and again.
Let `G(A)` be the minimum length of the repeat sequence with A0 = A.
So `G(85) = 8` (8 number : `89,145,42, 20,4,16,37,58`)
Your task is to find G(A) and return it.
# Input/Output
- `[input]` integer `a0`
the A0 number
- `[output]` an integer
the length of the repeat sequence | games | def repeat_sequence_len(n):
s = []
while True:
n = sum(int(i) * * 2 for i in str(n))
if n not in s:
s . append(n)
else:
return len(s[s . index(n):])
| Simple Fun #129: Repeat Sequence Length | 58a3f57ecebc06bfcb00009c | [
"Puzzles"
] | https://www.codewars.com/kata/58a3f57ecebc06bfcb00009c | 7 kyu |
In this kata, you will write a function that returns the positions and the values of the "peaks" (or local maxima) of a numeric array.
For example, the array `arr = [0, 1, 2, 5, 1, 0]` has a peak at position `3` with a value of `5` (since `arr[3]` equals `5`).
~~~if-not:php,cpp,java,csharp,fsharp
The output will be returned as an object with two properties: pos and peaks. Both of these properties should be arrays. If there is no peak in the given array, then the output should be `{pos: [], peaks: []}`.
~~~
~~~if:php
The output will be returned as an associative array with two key-value pairs: `'pos'` and `'peaks'`. Both of them should be (non-associative) arrays. If there is no peak in the given array, simply return `['pos' => [], 'peaks' => []]`.
~~~
~~~if:cpp
The output will be returned as an object of type `PeakData` which has two members: `pos` and `peaks`. Both of these members should be `vector<int>`s. If there is no peak in the given array then the output should be a `PeakData` with an empty vector for both the `pos` and `peaks` members.
`PeakData` is defined in Preloaded as follows:
```cpp
struct PeakData {
vector<int> pos, peaks;
};
```
~~~
~~~if:java
The output will be returned as a ``Map<String,List<integer>>` with two key-value pairs: `"pos"` and `"peaks"`. If there is no peak in the given array, simply return `{"pos" => [], "peaks" => []}`.
~~~
~~~if:csharp
The output will be returned as a `Dictionary<string, List<int>>` with two key-value pairs: `"pos"` and `"peaks"`.
If there is no peak in the given array, simply return `{"pos" => new List<int>(), "peaks" => new List<int>()}`.
~~~
~~~if:fsharp
The output will be returned as a record type `PeakPositionInfo` with two fields: `Pos` and `Peaks`.
If there is no peak in the given array, simply return `{ Pos = []; Peaks = [] }`.
~~~
~~~if:cobol
For COBOL, the answer will be stored in the two tables `positions` and `peaks` provided in the linkage section.
If there is no peak in the given array, simply set the length of both tables to 0.
Since COBOL is a 1-indexed language, the indexes stored in `peaks` will be shifted by 1 in comparison with other languages.
~~~
Example: `pickPeaks([3, 2, 3, 6, 4, 1, 2, 3, 2, 1, 2, 3])` should return `{pos: [3, 7], peaks: [6, 3]}` (or equivalent in other languages)
All input arrays will be valid integer arrays (although it could still be empty), so you won't need to validate the input.
The first and last elements of the array will not be considered as peaks (in the context of a mathematical function, we don't know what is after and before and therefore, we don't know if it is a peak or not).
Also, beware of plateaus !!! `[1, 2, 2, 2, 1]` has a peak while `[1, 2, 2, 2, 3]` and `[1, 2, 2, 2, 2]` do not. In case of a plateau-peak, please only return the position and value of the beginning of the plateau. For example:
`pickPeaks([1, 2, 2, 2, 1])` returns `{pos: [1], peaks: [2]}` (or equivalent in other languages)
Have fun! | algorithms | def pick_peaks(arr):
pos = []
prob_peak = False
for i in range(1, len(arr)):
if arr[i] > arr[i-1]:
prob_peak = i
elif arr[i] < arr[i-1] and prob_peak:
pos.append(prob_peak)
prob_peak = False
return {'pos':pos, 'peaks':[arr[i] for i in pos]} | Pick peaks | 5279f6fe5ab7f447890006a7 | [
"Arrays",
"Algorithms"
] | https://www.codewars.com/kata/5279f6fe5ab7f447890006a7 | 5 kyu |
This is the simple version of [Fastest Code : Equal to 24](http://www.codewars.com/kata/574e890e296e412a0400149c).
## Task
A game I played when I was young: Draw 4 cards from playing cards, use ```+ - * / and ()``` to make the final results equal to 24.
You will coding in function ```equalTo24```. Function accept 4 parameters ```a b c d```(4 cards), value range is 1-13.
The result is a string such as ```"2*2*2*3"``` ,```(4+2)*(5-1)```; If it is not possible to calculate the 24, please return "It's not possible!"
All four cards are to be used, only use three or two cards are incorrect; Use a card twice or more is incorrect too.
You just need to return one correct solution, don't need to find out all the possibilities.
## Examples
```javascript
equalTo24(1,2,3,4) // can return "(1+3)*(2+4)" or "1*2*3*4"
equalTo24(2,3,4,5) // can return "(5+3-2)*4" or "(3+4+5)*2"
equalTo24(3,4,5,6) // can return "(3-4+5)*6"
equalTo24(1,1,1,1) // should return "It's not possible!"
equalTo24(13,13,13,13) // should return "It's not possible!"
```
```coffeescript
equalTo24(1,2,3,4) # can return "(1+3)*(2+4)" or "1*2*3*4"
equalTo24(2,3,4,5) # can return "(5+3-2)*4" or "(3+4+5)*2"
equalTo24(3,4,5,6) # can return "(3-4+5)*6"
equalTo24(1,1,1,1) # should return "It's not possible!"
equalTo24(13,13,13,13) # should return "It's not possible!"
``` | games | from itertools import permutations
def equal_to_24(*aceg):
ops = '+-*/'
for b in ops:
for d in ops:
for f in ops:
for (a,c,e,g) in permutations(aceg):
for s in make_string(a,b,c,d,e,f,g):
try:
if eval(s + '== 24'):
return s
except:
pass
return "It's not possible!"
def make_string(a,b,c,d,e,f,g):
return [f"(({a} {b} {c}) {d} {e}) {f} {g}",
f"({a} {b} {c}) {d} ({e} {f} {g})",
f"{a} {b} ({c} {d} ({e} {f} {g}))"] | T.T.T.#2: Equal to 24 | 574be65a974b95eaf40008da | [
"Puzzles",
"Games"
] | https://www.codewars.com/kata/574be65a974b95eaf40008da | 4 kyu |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.