description
stringlengths 38
154k
| category
stringclasses 5
values | solutions
stringlengths 13
289k
| name
stringlengths 3
179
| id
stringlengths 24
24
| tags
sequencelengths 0
13
| url
stringlengths 54
54
| rank_name
stringclasses 8
values |
---|---|---|---|---|---|---|---|
Two red beads are placed between every two blue beads. There are N blue beads. After looking at the arrangement below work out the number of red beads.
<p>
<font color="blue">@</font>
<font color="red">@</font><font color="red">@</font>
<font color="blue">@</font>
<font color="red">@</font><font color="red">@</font>
<font color="blue">@</font>
<font color="red">@</font><font color="red">@</font>
<font color="blue">@</font>
<font color="red">@</font><font color="red">@</font>
<font color="blue">@</font>
<font color="red">@</font><font color="red">@</font>
<font color="blue">@</font>
</p>
<p>Implement <code>count_red_beads(n)</code> (in PHP <code>count_red_beads($n)</code>; in Java, Javascript, TypeScript, C, C++ <code>countRedBeads(n)</code>) so that it returns the number of <font color="red">red</font> beads.<br>
If there are less than 2 blue beads return 0.
</p> | reference | def count_red_beads(nb):
return max(0, 2 * (nb - 1))
| Simple beads count | 58712dfa5c538b6fc7000569 | [
"Fundamentals"
] | https://www.codewars.com/kata/58712dfa5c538b6fc7000569 | 7 kyu |
Count the number of divisors of a positive integer `n`.
Random tests go up to `n = 500000`.
### Examples (input --> output)
```
4 --> 3 // we have 3 divisors - 1, 2 and 4
5 --> 2 // we have 2 divisors - 1 and 5
12 --> 6 // we have 6 divisors - 1, 2, 3, 4, 6 and 12
30 --> 8 // we have 8 divisors - 1, 2, 3, 5, 6, 10, 15 and 30
```
Note you should only return a number, the count of divisors. The numbers between parentheses are shown only for you to see which numbers are counted in each case.
| reference | def divisors(n):
return len([l_div for l_div in range(1, n + 1) if n % l_div == 0])
| Count the divisors of a number | 542c0f198e077084c0000c2e | [
"Number Theory",
"Mathematics",
"Fundamentals"
] | https://www.codewars.com/kata/542c0f198e077084c0000c2e | 7 kyu |
An abundant number or excessive number is a number for which the sum of its proper divisors is greater than the number itself.
The integer 12 is the first abundant number. Its proper divisors are 1, 2, 3, 4 and 6 for a total of 16 (> 12).
Derive function `abundantNumber(num)/abundant_number(num)` which returns `true/True/.true.` if `num` is abundant, `false/False/.false.` if not. | reference | def abundant_number(num):
return (sum([e for e in range(1, num) if num % e == 0]) > num)
| Excessively Abundant Numbers | 56a75b91688b49ad94000015 | [
"Fundamentals",
"Mathematics",
"Algorithms"
] | https://www.codewars.com/kata/56a75b91688b49ad94000015 | 7 kyu |
Many people choose to obfuscate their email address when displaying it on the Web. One common way of doing this is by substituting the `@` and `.` characters for their literal equivalents in brackets.
Example 1:
```
user_name@example.com
=> user_name [at] example [dot] com
```
Example 2:
```
af5134@borchmore.edu
=> af5134 [at] borchmore [dot] edu
```
Example 3:
```
jim.kuback@ennerman-hatano.com
=> jim [dot] kuback [at] ennerman-hatano [dot] com
```
Using the examples above as a guide, write a function that takes an email address string and returns the obfuscated version as a string that replaces the characters `@` and `.` with `[at]` and `[dot]`, respectively.
>Notes
>* Input (`email`) will always be a string object. Your function should return a string.
>* Change only the `@` and `.` characters.
>* Email addresses may contain more than one `.` character.
>* Note the additional whitespace around the bracketed literals in the examples! | algorithms | def obfuscate(email):
return email . replace("@", " [at] "). replace(".", " [dot] ")
| Email Address Obfuscator | 562d8d4c434582007300004e | [
"Strings",
"Algorithms"
] | https://www.codewars.com/kata/562d8d4c434582007300004e | 7 kyu |
#### Task:
Your job here is to write a function (`keepOrder` in JS/CoffeeScript, `keep_order` in Ruby/Crystal/Python, `keeporder` in Julia), which takes a sorted array `ary` and a value `val`, and returns the lowest index where you could insert `val` to maintain the sorted-ness of the array. The input array will always be sorted in ascending order. It may contain duplicates.
_Do not modify the input._
#### Some examples:
```javascript
keepOrder([1, 2, 3, 4, 7], 5) //=> 4
^(index 4)
keepOrder([1, 2, 3, 4, 7], 0) //=> 0
^(index 0)
keepOrder([1, 1, 2, 2, 2], 2) //=> 2
^(index 2)
```
```coffeescript
keepOrder([1, 2, 3, 4, 7], 5) #=> 4
^(index 4)
keepOrder([1, 2, 3, 4, 7], 0) #=> 0
^(index 0)
keepOrder([1, 1, 2, 2, 2], 2) #=> 2
^(index 2)
```
```ruby
keep_order([1, 2, 3, 4, 7], 5) #=> 4
^(index 4)
keep_order([1, 2, 3, 4, 7], 0) #=> 0
^(index 0)
keep_order([1, 1, 2, 2, 2], 2) #=> 2
^(index 2)
```
```crystal
keep_order([1, 2, 3, 4, 7], 5) #=> 4
^(index 4)
keep_order([1, 2, 3, 4, 7], 0) #=> 0
^(index 0)
keep_order([1, 1, 2, 2, 2], 2) #=> 2
^(index 2)
```
```python
keep_order([1, 2, 3, 4, 7], 5) #=> 4
^(index 4)
keep_order([1, 2, 3, 4, 7], 0) #=> 0
^(index 0)
keep_order([1, 1, 2, 2, 2], 2) #=> 2
^(index 2)
```
```julia
# indexes start at 1!
keeporder([1, 2, 3, 4, 7], 5) #=> 5
^(index 5)
keeporder([1, 2, 3, 4, 7], 0) #=> 1
^(index 1)
keeporder([1, 1, 2, 2, 2], 2) #=> 3
^(index 3)
```
Also check out my other creations — [Naming Files](https://www.codewars.com/kata/naming-files), [Elections: Weighted Average](https://www.codewars.com/kata/elections-weighted-average), [Identify Case](https://www.codewars.com/kata/identify-case), [Split Without Loss](https://www.codewars.com/kata/split-without-loss), [Adding Fractions](https://www.codewars.com/kata/adding-fractions),
[Random Integers](https://www.codewars.com/kata/random-integers), [Implement String#transpose](https://www.codewars.com/kata/implement-string-number-transpose), [Implement Array#transpose!](https://www.codewars.com/kata/implement-array-number-transpose), [Arrays and Procs #1](https://www.codewars.com/kata/arrays-and-procs-number-1), and [Arrays and Procs #2](https://www.codewars.com/kata/arrays-and-procs-number-2).
If you notice any issues or have any suggestions/comments whatsoever, please don't hesitate to mark an issue or just comment. Thanks! | reference | from bisect import bisect_left as keep_order
| Keep the Order | 582aafca2d44a4a4560000e7 | [
"Fundamentals"
] | https://www.codewars.com/kata/582aafca2d44a4a4560000e7 | 7 kyu |
We all use 16:9, 16:10, 4:3 etc. ratios every day. Main task is to determine image ratio by its width and height dimensions.
Function should take width and height of an image and return a ratio string (ex."16:9").
If any of width or height entry is 0 function should throw an exception (or return `Nothing`). | algorithms | from fractions import Fraction
def calculate_ratio(w, h):
if w * h == 0:
raise ValueError
f = Fraction(w, h)
return f" { f . numerator } : { f . denominator } "
| Width-Height Ratio | 55486cb94c9d3251560000ff | [
"Algorithms"
] | https://www.codewars.com/kata/55486cb94c9d3251560000ff | 7 kyu |
Make a function that receives a value, ```val``` and outputs the smallest higher number than the given value, and this number belong to a set of positive integers that have the following properties:
- their digits occur only once
- they are odd
- they are multiple of three
```python
next_numb(12) == 15
next_numb(13) == 15
next_numb(99) == 105
next_numb(999999) == 1023459
next_number(9999999999) == "There is no possible number that
fulfills those requirements"
```
```javascript
nextNumb(12) == 15
nextNumb(13) == 15
nextNumb(99) == 105
nextNumb(999999) == 1023459
nextNumber(9999999999) == "There is no possible number that
fulfills those requirements"
```
```ruby
next_numb(12) == 15
next_numb(13) == 15
next_numb(99) == 105
next_numb(999999) == 1023459
next_number(9999999999) == "There is no possible number that fulfills those requirements"
```
```haskell
next 12 `shouldBe` Just 15
next 13 `shouldBe` Just 15
next 99 `shouldBe` Just 105
next 999999 `shouldBe` Just 1023459
next 9999999999 `shouldBe` Nothing
```
```cpp
nextNumb(12) == 15
nextNumb(13) == 15
nextNumb(99) == 105
nextNumb(999999) == 1023459
nextNumber(9999999999) == -1
```
Enjoy the kata!! | reference | def next_numb(val):
i = val + 1
while i <= 9999999999:
if i % 3 == 0 and i % 2 and len(str(i)) == len(set(str(i))):
return i
i += 1
return 'There is no possible number that fulfills those requirements'
| Next Featured Number Higher than a Given Value | 56abc5e63c91630882000057 | [
"Fundamentals"
] | https://www.codewars.com/kata/56abc5e63c91630882000057 | 7 kyu |
*`This kata is a tribute/fanwork to the TV-show: Supernatural`*
Balls!
Those wayward Winchester boys are in trouble again, hunting something down in New Orleans.
You are Bobby Singer, you know how "idjits" they can be, so you have to prepare.
They will call you any minute with the race of the thing, and you want to answer as soon as possible. By answer, I mean: tell them how to kill, or fight it.
You have something like a database (more like drunken doodling) to help them:
- werewolf : Silver knife or bullet to the heart
- vampire : Behead it with a machete
- wendigo : Burn it to death
- shapeshifter : Silver knife or bullet to the heart
- angel : Use the angelic blade
- demon : Use Ruby's knife, or some Jesus-juice
- ghost : Salt and iron, and don't forget to burn the corpse
- dragon : You have to find the excalibur for that
- djinn : Stab it with silver knife dipped in a lamb's blood
- pagan god : It depends on which one it is
- leviathan : Use some Borax, then kill Dick
- ghoul : Behead it
- jefferson starship : Behead it with a silver blade
- reaper : If it's nasty, you should gank who controls it
- rugaru : Burn it alive
- skinwalker : A silver bullet will do it
- phoenix : Use the colt
- witch : They are humans
- else : I have friggin no idea yet
You can access the database as `drunkenDoodling`/`drunken_doodling`/`DrunkenDoodling` depending on your language.
---
So a call would go down like this:
The guys call you:
```bob('rugaru')```
...and you reply (return) with the info, and your signature saying of yours!
```Burn it alive, idjits!```
<iframe src="//giphy.com/embed/G315RHN1G7cIw" width="480" height="234" frameBorder="0" style="max-width: 100%" class="giphy-embed" webkitAllowFullScreen mozallowfullscreen allowFullScreen></iframe>
| reference | def bob(what):
return "%s, idjits!" % drunkenDoodling . get(what, "I have friggin no idea yet")
| Supernatural | 55c9a8cda33889d69e00008b | [
"Games",
"Fundamentals"
] | https://www.codewars.com/kata/55c9a8cda33889d69e00008b | 7 kyu |
Punky loves wearing different colored socks, but Henry can't stand it.
Given an array of different colored socks, return a pair depending on who was picking them out.
Example:
```javascript
getSocks('Punky', ['red','blue','blue','green']) -> ['red', 'blue']
```
```coffeescript
getSocks('Punky', ['red','blue','blue','green']) -> ['red', 'blue']
```
```ruby
get_socks('Punky', ['red','blue','blue','green']) -> ['red', 'blue']
```
```python
get_socks('Punky', ['red','blue','blue','green']) -> ['red', 'blue']
```
```java
getSocks("Punky", ["red","blue","blue","green"]) -> ["red", "blue"]
```
Note that Punky can have any two colored socks, in any order, as long as they are different and both exist. Henry always picks a matching pair.
If there is no possible combination of socks, return an empty array.
<div style="width: 320px; text-align: center; color: white; border: white 1px solid;">
Check out my other 80's Kids Katas:
</div>
<div>
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-1-how-many-licks-does-it-take'><span style='color:#00C5CD'>80's Kids #1:</span> How Many Licks Does It Take</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-2-help-alf-find-his-spaceship'><span style='color:#00C5CD'>80's Kids #2:</span> Help Alf Find His Spaceship</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-3-punky-brewsters-socks'><span style='color:#00C5CD'>80's Kids #3:</span> Punky Brewster's Socks</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-4-legends-of-the-hidden-temple'><span style='color:#00C5CD'>80's Kids #4:</span> Legends of the Hidden Temple</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-5-you-cant-do-that-on-television'><span style='color:#00C5CD'>80's Kids #5:</span> You Can't Do That on Television</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-6-rock-em-sock-em-robots'><span style='color:#00C5CD'>80's Kids #6:</span> Rock 'Em, Sock 'Em Robots</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-7-shes-a-small-wonder'><span style='color:#00C5CD'>80's Kids #7:</span> She's a Small Wonder</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-8-the-secret-world-of-alex-mack'><span style='color:#00C5CD'>80's Kids #8:</span> The Secret World of Alex Mack</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-9-down-in-fraggle-rock'><span style='color:#00C5CD'>80's Kids #9:</span> Down in Fraggle Rock </a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-10-captain-planet'><span style='color:#00C5CD'>80's Kids #10:</span> Captain Planet </a><br />
</div>
| algorithms | def get_socks(name, socks):
if name == 'Punky':
if socks:
for s in socks[1:]:
if s != socks[0]:
return [socks[0], s]
elif name == 'Henry':
seen = set()
for s in socks:
if s in seen:
return [s, s]
seen . add(s)
else:
raise ValueError
return []
| 80's Kids #3: Punky Brewster's Socks | 5662292ee7e2da24e900012f | [
"Fundamentals",
"Algorithms",
"Arrays"
] | https://www.codewars.com/kata/5662292ee7e2da24e900012f | 7 kyu |
Change every letter in a given string to the next letter in the alphabet. The function takes a single parameter `s` (string).
Notes:
* Spaces and special characters should remain the same.
* Capital letters should transfer in the same way but remain capitilized.
## Examples
```
"Hello" --> "Ifmmp"
"What is your name?" --> "Xibu jt zpvs obnf?"
"zoo" --> "app"
"zzZAaa" --> "aaABbb"
```
| reference | def next_letter(string):
return "" . join(chr(ord(c) + (- 25 if c in 'zZ' else 1)) if c . isalpha() else c for c in string)
| Weird words | 57b2020eb69bfcbf64000375 | [
"Strings",
"Regular Expressions",
"Fundamentals"
] | https://www.codewars.com/kata/57b2020eb69bfcbf64000375 | 7 kyu |
Create a function that returns the total of a meal including tip and tax. You should not tip on the tax.
You will be given the subtotal, the tax as a percentage and the tip as a percentage. Please round your result to two decimal places. | games | def calculate_total(subtotal, tax, tip):
return round(subtotal * (1 + tax / 100.0 + tip / 100.0), 2)
| Calculate Meal Total | 58545549b45c01ccab00058c | [
"Fundamentals"
] | https://www.codewars.com/kata/58545549b45c01ccab00058c | 7 kyu |
Given a string, determine if it's a valid identifier.
## Here is the syntax for valid identifiers:
* Each identifier must have at least one character.
* The first character must be picked from: alpha, underscore, or dollar sign. The first character cannot be a digit.
* The rest of the characters (besides the first) can be from: alpha, digit, underscore, or dollar sign. In other words, it can be any valid identifier character.
### Examples of valid identifiers:
* i
* wo_rd
* b2h
### Examples of invalid identifiers:
* 1i
* wo rd
* !b2h | reference | import re
def is_valid(idn):
return re . compile('^[a-z_\$][a-z0-9_\$]*$', re . IGNORECASE). match(idn) != None
| Is valid identifier? | 563a8656d52a79f06c00001f | [
"Fundamentals",
"Regular Expressions",
"Strings"
] | https://www.codewars.com/kata/563a8656d52a79f06c00001f | 7 kyu |
Lucy loves to travel. Luckily she is a renowned computer scientist and gets to travel to international conferences using her department's budget.
Each year, Society for Exciting Computer Science Research (SECSR) organizes several conferences around the world. Lucy always picks one conference from that list that is hosted in a city she hasn't been to before, and if that leaves her with more than one option, she picks the conference that she thinks would be most relevant for her field of research.
Write a function `conferencePicker` that takes in two arguments:
- `citiesVisited`, a list of cities that Lucy has visited before, given as an array of strings.
- `citiesOffered`, a list of cities that will host SECSR conferences this year, given as an array of strings. `citiesOffered` will already be ordered in terms of the relevance of the conferences for Lucy's research (from the most to the least relevant).
The function should return the city that Lucy should visit, as a string.
Also note:
- You should allow for the possibility that Lucy hasn't visited any city before.
- SECSR organizes at least two conferences each year.
- If all of the offered conferences are hosted in cities that Lucy has visited before, the function should return `'No worthwhile conferences this year!'` (`Nothing` in Haskell)
Example:
```javascript
citiesVisited = ['Mexico City','Johannesburg','Stockholm','Osaka','Saint Petersburg','London'];
citiesOffered = ['Stockholm','Paris','Melbourne'];
conferencePicker (citiesVisited, citiesOffered);
// ---> 'Paris'
``` | reference | def conference_picker(cities_visited, cities_offered):
for city in cities_offered:
if city not in cities_visited:
return city
return 'No worthwhile conferences this year!'
| Conference Traveller | 56f5594a575d7d3c0e000ea0 | [
"Fundamentals",
"Arrays",
"Lists"
] | https://www.codewars.com/kata/56f5594a575d7d3c0e000ea0 | 7 kyu |
Create a function that takes two arguments:
1) An array of objects which feature the season, the team and the country of the Champions League winner.
2) Country (as a string, for example, 'Portugal')
You function should then return the number which represents the number of times a team from a given country has won. Return `0` if there have been no wins.
For example if the input array is as follows:
<img src=http://i.imgur.com/61bIUDY.png>
`countWins(winnerList1, 'Spain')` => should return 2<br>
`countWins(winnerList1, 'Portugal')` => should return 1<br>
`countWins(winnerList1, 'Sportland')` => should return 0<br>
| algorithms | def countWins(winnerList, country):
return sum(x . get('country') == country for x in winnerList)
| Find how many times did a team from a given country win the Champions League? | 581b30af1ef8ee6aea0015b9 | [
"Strings",
"Arrays",
"Data Structures",
"Algorithms"
] | https://www.codewars.com/kata/581b30af1ef8ee6aea0015b9 | 7 kyu |
Create a function that receives a (square) matrix and calculates the sum of both diagonals (main and secondary)
> Matrix = array of `n` length whose elements are `n` length arrays of integers.
3x3 example:
```javascript
diagonals( [
[ 1, 2, 3 ],
[ 4, 5, 6 ],
[ 7, 8, 9 ]
] );
returns -> 30 // 1 + 5 + 9 + 3 + 5 + 7
```
```python
sum_diagonals( [
[ 1, 2, 3 ],
[ 4, 5, 6 ],
[ 7, 8, 9 ]
] ) == 30 # 1 + 5 + 9 + 3 + 5 + 7
```
| algorithms | import numpy as np
def sum_diagonals(matrix):
# your code here
matrix = np . matrix(matrix)
return sum(np . diag(matrix, 0)) + sum(np . diag(np . flip(matrix, axis=1), 0))
| Diagonals sum | 5592fc599a7f40adac0000a8 | [
"Matrix",
"Algorithms"
] | https://www.codewars.com/kata/5592fc599a7f40adac0000a8 | 7 kyu |
Write a function that checks whether all elements in an array are square numbers. The function should be able to take any number of array elements.
Your function should return true if all elements in the array are square numbers and false if not.
An empty array should return `undefined` / `None` / `nil` /`false` (for C).
You can assume that all array elements will be positive integers.
Examples:
~~~if:coffeescript,javascript
```
isSquare([1, 4, 9, 16]) --> true
isSquare([3, 4, 7, 9]) --> false
isSquare([]) --> undefined
```
~~~
```python
is_square([1, 4, 9, 16]) --> True
is_square([3, 4, 7, 9]) --> False
is_square([]) --> None
```
```ruby
is_square([1, 4, 9, 16]) --> true
is_square([3, 4, 7, 9]) --> false
is_square([]) --> nil
```
```c
is_square({1, 4, 9, 16}) --> true
is_square({3, 4, 7, 9}) --> false
is_square({}) --> false
``` | reference | def is_square(arr):
if arr:
return all((a * * 0.5). is_integer() for a in arr)
| Are they square? | 56853c44b295170b73000007 | [
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/56853c44b295170b73000007 | 7 kyu |
You have two arrays in this kata, every array contains unique elements only. Your task is to calculate number of elements in the first array which are also present in the second array. | reference | def match_arrays(v, r):
return sum(x in r for x in v)
# DON'T remove
verbose = False # set to True to diplay arrays being tested in the random tests
| Array comparator | 561046a9f629a8aac000001d | [
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/561046a9f629a8aac000001d | 7 kyu |
## The Problem
James is a DJ at a local radio station. As it's getting to the top of the hour, he needs to find a song to play that will be short enough to fit in before the news block. He's got a database of songs that he'd like you to help him filter in order to do that.
## What To Do
Create `longestPossible`(`longest_possible` in python and ruby) helper function that takes 1 integer argument which is a maximum length of a song in seconds.
`songs` is an array of objects which are formatted as follows:
```javascript
{artist: 'Artist', title: 'Title String', playback: '04:30'}
```
```python
{'artist': 'Artist', 'title': 'Title String', 'playback': '04:30'}
```
```ruby
{artist: 'Artist', title: 'Title String', playback: '04:30'}
```
You can expect playback value to be formatted exactly like above.
Output should be a title of the longest song from the database that matches the criteria of not being longer than specified time. If there's no songs matching criteria in the database, return `false`.
| algorithms | def calculate_seconds(s):
minutes, seconds = [int(x) for x in s . split(':')]
return minutes * 60 + seconds
def longest_possible ( playback ):
candidates = [ song for song in songs if calculate_seconds ( song [ 'playback' ]) <= playback ]
return sorted ( candidates , key = lambda x : calculate_seconds ( x [ 'playback' ]), reverse = True )[ 0 ][ 'title' ] if len ( candidates ) > 0 else False | Radio DJ helper function | 561bbcb0fbbfb0f5010000ee | [
"Sorting",
"Filtering",
"Algorithms"
] | https://www.codewars.com/kata/561bbcb0fbbfb0f5010000ee | 7 kyu |
Your task is to write an update for a lottery machine. Its current version produces a sequence of random letters and integers (passed as a string to the function). Your code must filter out all letters and return **unique** integers as a string, in their order of first appearance. If there are no integers in the string return `"One more run!"`
## Examples
```
"hPrBKWDH8yc6Lt5NQZWQ" --> "865"
"ynMAisVpHEqpqHBqTrwH" --> "One more run!"
"555" --> "5"
```
| reference | def lottery(s):
return "" . join(dict . fromkeys(filter(str . isdigit, s))) or "One more run!"
| Lottery machine | 5832db03d5bafb7d96000107 | [
"Strings",
"Fundamentals"
] | https://www.codewars.com/kata/5832db03d5bafb7d96000107 | 7 kyu |
An array is given with palindromic and non-palindromic numbers. A *palindromic* number is a number that is the same from a reversed order. For example `122` is not a palindromic number, but `202` is one.
Your task is to write a function that returns an array with only `1`s and `0`s, where all palindromic numbers are replaced with a `1` and all non-palindromic numbers are replaced with a `0`.
For example:
```
[101, 2, 85, 33, 14014] ==> [1, 1, 0, 1, 0]
[45, 21, 303, 56] ==> [0, 0, 1, 0]
``` | algorithms | def convert_palindromes(numbers):
return [str(n) == str(n)[:: - 1] for n in numbers]
| Palindromes Here and There | 5838a66eaed8c259df000003 | [
"Algorithms"
] | https://www.codewars.com/kata/5838a66eaed8c259df000003 | 7 kyu |
Spoonerize... with numbers... numberize?... numboonerize?... noonerize? ...anyway! If you don't yet know what a spoonerism is and haven't yet tried my spoonerism kata, please do [check it out](http://www.codewars.com/kata/spoonerize-me) first.
You will create a function which takes an array of two positive integers, spoonerizes them, and returns the positive difference between them as a single number or ```0``` if the numbers are equal:
```
[123, 456] = 423 - 156 = 267
```
Your code must test that all array items are numbers and return ```"invalid array"``` if it finds that either item is not a number. The provided array will always contain 2 elements.
When the inputs are valid, they will always be integers, no floats will be passed. However, you must take into account that the numbers will be of varying magnitude, between and within test cases. | algorithms | def noonerize(numbers):
try:
num1 = int(str(numbers[1])[0] + str(numbers[0])[1:])
num2 = int(str(numbers[0])[0] + str(numbers[1])[1:])
except ValueError:
return "invalid array"
return abs(num1 - num2)
| Noonerize Me | 56dbed3a13c2f61ae3000bcd | [
"Mathematics",
"Arrays",
"Algorithms"
] | https://www.codewars.com/kata/56dbed3a13c2f61ae3000bcd | 7 kyu |
The [Chinese zodiac](https://en.wikipedia.org/wiki/Chinese_zodiac) is a repeating cycle of 12 years, with each year being represented by an animal and its reputed attributes. The lunar calendar is divided into cycles of 60 years each, and each year has a combination of an animal and an element. There are 12 animals and 5 elements; the animals change each year, and the elements change every 2 years. The current cycle was initiated in the year of 1984 which was the year of the Wood Rat.
Since the current calendar is Gregorian, I will only be using years from the epoch 1924.
*For simplicity I am counting the year as a whole year and not from January/February to the end of the year.*
##Task
Given a year, return the element and animal that year represents ("Element Animal").
For example I'm born in 1998 so I'm an "Earth Tiger".
```animals``` (or ```$animals``` in Ruby) is a preloaded array containing the animals in order:
```['Rat', 'Ox', 'Tiger', 'Rabbit', 'Dragon', 'Snake', 'Horse', 'Goat', 'Monkey', 'Rooster', 'Dog', 'Pig']```
```elements``` (or ```$elements``` in Ruby) is a preloaded array containing the elements in order:
```['Wood', 'Fire', 'Earth', 'Metal', 'Water']```
Tell me your zodiac sign and element in the comments. Happy coding :)
| reference | animals = ['Rat', 'Ox', 'Tiger', 'Rabbit', 'Dragon', 'Snake',
'Horse', 'Goat', 'Monkey', 'Rooster', 'Dog', 'Pig']
elements = ['Wood', 'Fire', 'Earth', 'Metal', 'Water']
def chinese_zodiac(year):
year -= 1984
return elements[year / / 2 % 5] + " " + animals[year % 12]
| Chinese Zodiac | 57a73e697cb1f31dd70000d2 | [
"Fundamentals",
"Arrays"
] | https://www.codewars.com/kata/57a73e697cb1f31dd70000d2 | 7 kyu |
When a warrior wants to talk with another one about peace or war he uses a smartphone. In one distinct country warriors who spent all time in training kata not always have enough money. So if they call some number they want to know which operator serves this number.
Write a function which **accepts number and return name of operator or string "no info"**, if operator can't be defined. number always looks like 8yyyxxxxxxx, where yyy corresponds to operator.
Here is short list of operators:
* 039 xxx xx xx - Golden Telecom
* 050 xxx xx xx - MTS
* 063 xxx xx xx - Life:)
* 066 xxx xx xx - MTS
* 067 xxx xx xx - Kyivstar
* 068 xxx xx xx - Beeline
* 093 xxx xx xx - Life:)
* 095 xxx xx xx - MTS
* 096 xxx xx xx - Kyivstar
* 097 xxx xx xx - Kyivstar
* 098 xxx xx xx - Kyivstar
* 099 xxx xx xx - MTS Test [Just return "MTS"] | reference | OPERATORS = {
'039': 'Golden Telecom', '050': 'MTS', '063': 'Life:)', '066': 'MTS',
'067': 'Kyivstar', '068': 'Beeline', '093': 'Life:)', '095': 'MTS',
'096': 'Kyivstar', '097': 'Kyivstar', '098': 'Kyivstar', '099': 'MTS'}
def detect_operator(num):
return OPERATORS . get(str(num)[1: 4], 'no info')
| Mobile operator detector | 55f8ba3be8d585b81e000080 | [
"Logic",
"Strings",
"Regular Expressions",
"Fundamentals"
] | https://www.codewars.com/kata/55f8ba3be8d585b81e000080 | 7 kyu |
Write function which will create a string from a list of strings, separated by space.
Example:
```["hello", "world"] -> "hello world"``` | reference | def words_to_sentence(words):
return ' ' . join(words)
| Words to sentence | 57a06005cf1fa5fbd5000216 | [
"Fundamentals"
] | https://www.codewars.com/kata/57a06005cf1fa5fbd5000216 | 7 kyu |
It's your birthday. Your colleagues buy you a cake. The numbers of candles on the cake is provided (`candles`). Please note this is not reality, and your age can be anywhere up to 1000. Yes, you would look a mess.
As a surprise, your colleagues have arranged for your friend to hide inside the cake and burst out. They pretend this is for your benefit, but likely it is just because they want to see you fall over covered in cake. Sounds fun!
When your friend jumps out of the cake, he/she will knock some of the candles to the floor. If the number of candles that fall is higher than 70% of total candles, the carpet will catch fire.
You will work out the number of candles that will fall from the provided lowercase string (`debris`). You must add up the character ASCII code of each *even indexed* (assume a 0 based indexing) character in the string, with the alphabetical position ("a" = 1, "b" = 2, etc.) of each *odd indexed* character to get the string's total.
For example:
```
"abc" --> "a" = 97, "b" = 2, "c" = 99 --> total = 198
```
If the carpet catches fire, return `"Fire!"`, if not, return `"That was close!"`.
**Notes**
* if there are no candles to begin with, the carpet cannot catch fire;
* as this is not reality, you may have more candles falling from the cake than the total... | reference | import string
def cake(candles: int, debris: str) - > str:
fallen_candles = sum(
string . ascii_letters . index(char) if index % 2 else ord(char)
for index, char in enumerate(debris)
)
return "Fire!" if candles and fallen_candles > candles * 0.7 else "That was close!"
| Birthday I - Cake | 5805ed25c2799821cb000005 | [
"Fundamentals",
"Arrays",
"Mathematics"
] | https://www.codewars.com/kata/5805ed25c2799821cb000005 | 7 kyu |
A spoonerism is a spoken phrase in which the first letters of two of the words are swapped around, often with amusing results.
In its most basic form a spoonerism is a two word phrase in which only the first letters of each word are swapped:
```"not picking" --> "pot nicking"```
Your task is to create a function that takes a string of two words, separated by a space: ```words``` and returns a spoonerism of those words in a string, as in the above example. A "word" in the context of this kata can contain any of the letters `A` through `Z` in upper or lower case, and the numbers `0` to `9`. Though spoonerisms are about letters in words in the domain of written and spoken language, numbers are included in the inputs for the random test cases and they require no special treatment.
NOTE: All input strings will contain only two words. Spoonerisms can be more complex. For example, three-word phrases in which the first letters of the first and last words are swapped: ```"pack of lies" --> "lack of pies"``` or more than one letter from a word is swapped: ```"flat battery --> "bat flattery"```
You are NOT expected to account for these, or any other nuances involved in spoonerisms.
Once you have completed this kata, a slightly more challenging take on the idea can be found here: http://www.codewars.com/kata/56dbed3a13c2f61ae3000bcd | algorithms | def spoonerize(words):
a, b = words . split()
return '{}{} {}{}' . format(b[0], a[1:], a[0], b[1:])
| Spoonerize Me | 56b8903933dbe5831e000c76 | [
"Strings",
"Algorithms"
] | https://www.codewars.com/kata/56b8903933dbe5831e000c76 | 7 kyu |
Write reverseList function that simply reverses lists. | reference | def reverse_list(lst):
return lst[:: - 1]
| Reverse list | 57a04da9e298a7ee43000111 | [
"Fundamentals",
"Lists"
] | https://www.codewars.com/kata/57a04da9e298a7ee43000111 | 7 kyu |
A grid is a perfect starting point for many games (Chess, battleships, Candy Crush!).
Making a digital chessboard I think is an interesting way of visualising how loops can work together.
Your task is to write a function that takes two integers `rows` and `columns` and returns a chessboard pattern as a two dimensional array.
So `chessBoard(6,4)` should return an array like this:
[
["O","X","O","X"],
["X","O","X","O"],
["O","X","O","X"],
["X","O","X","O"],
["O","X","O","X"],
["X","O","X","O"]
]
And `chessBoard(3,7)` should return this:
[
["O","X","O","X","O","X","O"],
["X","O","X","O","X","O","X"],
["O","X","O","X","O","X","O"]
]
The white spaces should be represented by an: `'O'`
and the black an: `'X'`
The first row should always start with a white space `'O'`
| algorithms | def chess_board(rows, columns):
return [["OX" [(row + col) % 2] for col in range(columns)] for row in range(rows)]
| draw me a chessboard | 56242b89689c35449b000059 | [
"Puzzles",
"Fundamentals",
"ASCII Art",
"Algorithms"
] | https://www.codewars.com/kata/56242b89689c35449b000059 | 7 kyu |
Write
```javascript
function remove(str, what)
```
```csharp
public static string Remove(string str, Dictionary<char,int> what)
```
```python
remove(text, what)
```
```ruby
remove(text, what)
```
that takes in a string ```str```(```text``` in Python) and an object/hash/dict/Dictionary ```what``` and returns a string with the chars removed in ```what```.
For example:
```javascript
remove('this is a string',{'t':1, 'i':2}) === 'hs s a string'
// remove from 'this is a string' the first 1 't' and the first 2 i's.
remove('hello world',{'x':5, 'i':2}) === 'hello world'
// there are no x's or i's, so nothing gets removed
remove('apples and bananas',{'a':50, 'n':1}) === 'pples d bnns'
// we don't have 50 a's, so just remove it till we hit end of string.
```
```csharp
Kata.Remove("this is a string", new Dictionary<char,int> { {'t', 1 }, {'i', 2 }}); // --> "hs s a string"
// remove from 'this is a string' the first 1 't' and the first 2 i's.
Kata.Remove("hello world", new Dictionary<char,int> { { 'x',5 }, {'i',2 }}); // --> "hello world"
// there are no x's or i's, so nothing gets removed
Kata.Remove("apples and bananas", new Dictionary<char,int> { {'a', 50 }, {'n', 1 }}); // --> "pples d bnns"
// we don't have 50 a's, so just remove it till we hit end of string.
```
```python
remove('this is a string',{'t':1, 'i':2}) == 'hs s a string'
# remove from 'this is a string' the first 1 't' and the first 2 i's.
remove('hello world',{'x':5, 'i':2}) == 'hello world'
# there are no x's or i's, so nothing gets removed
remove('apples and bananas',{'a':50, 'n':1}) == 'pples d bnns'
# we don't have 50 a's, so just remove it till we hit end of string.
```
```ruby
remove('this is a string',{'t'=>1, 'i'=>2}) == 'hs s a string'
# remove from 'this is a string' the first 1 't' and the first 2 i's.
remove('hello world',{'x'=>5, 'i'=>2}) == 'hello world'
# there are no x's or i's, so nothing gets removed
remove('apples and bananas',{'a'=>50, 'n'=>1}) == 'pples d bnns'
# we don't have 50 a's, so just remove it till we hit end of string.
``` | reference | def remove(text, what):
for char in what:
text = text . replace(char, '', what[char])
return text
| Not all but sometimes all | 564ab935de55a747d7000040 | [
"Strings",
"Fundamentals"
] | https://www.codewars.com/kata/564ab935de55a747d7000040 | 7 kyu |
There were and still are many problem in CW about palindrome numbers and palindrome strings. We suposse that you know which kind of numbers they are. If not, you may search about them using your favourite search engine.
In this kata you will be given a positive integer, ```val``` and you have to create the function ```next_pal()```(```nextPal``` Javascript) that will output the smallest palindrome number higher than ```val```.
Let's see:
```python
For Python
next_pal(11) == 22
next_pal(188) == 191
next_pal(191) == 202
next_pal(2541) == 2552
```
```javascript
For Javascript
nextPal(11) == 22
nextPal(188) == 191
nextPal(191) == 202
nextPal(2541) == 2552
```
```csharp
For C#
Kata.NextPal(11) == 22
Kata.NextPal(188) == 191
Kata.NextPal(191) == 202
Kata.NextPal(2541) == 2552
```
```ruby
For Ruby
next_pal(11) == 22
next_pal(188) == 191
next_pal(191) == 202
next_pal(2541) == 2552
```
```coffeescript
For CoffeeScript
nextPal(11) == 22
nextPal(188) == 191
nextPal(191) == 202
nextPal(2541) == 2552
```
```haskell
For Haskell
nextPal 11 == 22
nextPal 188 == 191
nextPal 191 == 202
nextPal 2541 == 2552
```
```cpp
For C++
next_pal(11) == 22
next_pal(188) == 191
next_pal(191) == 202
next_pal(2541) == 2552
```
You will be receiving values higher than 10, all valid.
Enjoy it!! | reference | def next_pal(val):
val += 1
while str(val) != str(val)[:: - 1]:
val += 1
return val
| Next Palindromic Number. | 56a6ce697c05fb4667000029 | [
"Fundamentals",
"Data Structures",
"Algorithms",
"Mathematics",
"Logic",
"Strings"
] | https://www.codewars.com/kata/56a6ce697c05fb4667000029 | 7 kyu |
The four bases found in DNA are adenine (A), cytosine (C), guanine (G) and thymine (T).
In genetics, GC-content is the percentage of Guanine (G) and Cytosine (C) bases on a DNA molecule that are either guanine or cytosine.
Given a DNA sequence (a string) return the GC-content in percent, rounded up to 2 decimal digits for Python, not rounded in all other languages.
For more information about GC-content you can take a look to [wikipedia GC-content](https://en.wikipedia.org/wiki/GC-content).
**For example**: the GC-content of the following DNA sequence is 50%:
"AAATTTCCCGGG".
**Note**: You can take a look to my others bio-info kata [here](http://www.codewars.com/users/nbeck/authored) | algorithms | def gc_content(seq):
if not seq:
return 0.0
else:
res = seq . count("C") + seq . count("G")
return round(res * 100 / len(seq), 2)
| DNA GC-content | 5747a9bbe2fab9a0c400012f | [
"Strings",
"Algorithms"
] | https://www.codewars.com/kata/5747a9bbe2fab9a0c400012f | 7 kyu |
<img src = http://images.wired.it/wp-content/uploads/2014/03/1394467702_chuck_norris_epic_split_3.jpg>
Chuck has lost count of how many asses he has kicked...
Chuck stopped counting at 100,000 because he prefers to kick things in the face instead of counting. That's just who he is.
To stop having to count like a mere mortal chuck developed his own special code using the hairs on his beard. You do not need to know the details of how it works, you simply need to know that the format is as follows: 'A8A8A8A8A8.-A%8.88.'
In Chuck's code, 'A' can be any capital letter and '8' can be any number 0-9 and any %, - or . symbols must not be changed.
Your task, to stop Chuck beating your ass with his little finger, is to use regex to verify if the number is a genuine Chuck score. If not it's probably some crap made up by his nemesis Bruce Lee. Return true if the provided count passes, and false if it does not.
```Javascript
Example:
'A8A8A8A8A8.-A%8.88.' <- don't forget final full stop :D\n
Tests:
'A2B8T1Q9W4.-F%5.34.' == true;
'a2B8T1Q9W4.-F%5.34.' == false; (small letter)
'A2B8T1Q9W4.-F%5.3B.' == false; (last char should be number)
'A2B8T1Q9W4.£F&5.34.' == false; (symbol changed from - and %)
```
The pattern only needs to appear within the text. The full input can be longer, i.e. the pattern can be surrounded by other characters... Chuck loves to be surrounded!
Ready, steady, VERIFY!! | algorithms | from re import search
def body_count(code):
return bool(search(r'(?:[A-Z]\d){5}\.-[A-Z]%\d\.\d{2}\.', code))
| Chuck Norris V - Body Count | 57066ad6cb72934c8400149e | [
"Regular Expressions",
"Algorithms"
] | https://www.codewars.com/kata/57066ad6cb72934c8400149e | 7 kyu |
Thanks to the effects of El Nino this year my holiday snorkelling trip was akin to being in a washing machine... Not fun at all.
Given a string made up of '~' and '\_' representing waves and calm respectively, your job is to check whether a person would become seasick.
Changes from calm to wave or wave to calm will add to the effect (really wave peak to trough but this will do). Find out how many changes in level the string has and if that number is more than 20% of the length of the string, return "Throw Up", else return "No Problem".
| reference | def sea_sick(sea):
return "Throw Up" if (sea . count("~_") + sea . count("_~")) / len(sea) > 0.2 else "No Problem"
| Holiday V - SeaSick Snorkelling | 57e90bcc97a0592126000064 | [
"Fundamentals",
"Strings",
"Arrays"
] | https://www.codewars.com/kata/57e90bcc97a0592126000064 | 7 kyu |
You've arrived at a carnival and head straight for the duck shooting tent. Why wouldn't you?
You will be given a set amount of ammo, and an aim rating of between 1 and 0. No your aim is not always perfect - hey maybe someone fiddled with the sights on the gun...
Anyway your task is to calculate how many successful shots you will be able to make given the available ammo and your aim score, then return a string representing the pool of ducks, with those ducks shot marked with 'X' and those that survived left unchanged. You will always shoot left to right.
Example of start and end duck string with two successful shots:
Start ---> |~~~~~22~2~~~~~|
**Bang!! Bang!!**
End ---> |~~~~~XX~2~~~~~|
All inputs will be correct type and never empty. | games | def duck_shoot(ammo, aim, ducks):
return ducks . replace('2', 'X', int(ammo * aim))
| Duck Shoot - Easy Version | 57d27a0a26427672b900046f | [
"Strings",
"Arrays"
] | https://www.codewars.com/kata/57d27a0a26427672b900046f | 7 kyu |
One of the first chain emails I ever received was about a supposed Cambridge University study that suggests your brain can read words no matter what order the letters are in, as long as the first and last letters of each word are correct.
Your task is to **create a function that can take any string and randomly jumble the letters within each word while leaving the first and last letters of the word in place.**
For example,
mixwords('Winter is coming') // returns 'Wntier is cminog' or 'Wtiner is conimg'
mixwords('Hey, friends!') // returns 'Hey, fierdns!' or 'Hey, fernids!'
* All punctuation should remain in place
* Words smaller than 3 letters should not change
* Letters must be randomly moved (and so calling the function multiple times with the same string should return different values
* Parameters that are not strings should return undefined
The tests do the following things to ensure a valid string is returned:
1. Check that the string returned is not equal to the string passed (you may have to revalidate the solution if your function randomly returns the same string)
2. Check that first and last letters of words remain in place
3. Check that punctuation remains in place
4. Checks string length remains the same
5. Checks the function returns `undefined` for non-strings
6. Checks that word interiors (the letters between the first and last) maintain the same letters, albeit in a different order
7. Checks that letters are randomly assigned. | reference | import re
from random import sample
def mix_words(string):
return re . sub(
r'(?<=[a-zA-Z])([a-zA-Z]{2,})(?=[a-zA-Z])',
lambda match: '' . join(
sample(match . group(1), len(match . group(1)))),
string)
| Cambridge Word Scramble | 564e48ebaaad20181e000024 | [
"Strings",
"Regular Expressions",
"Fundamentals"
] | https://www.codewars.com/kata/564e48ebaaad20181e000024 | 6 kyu |
Given a string and an array of index numbers, return the characters of the string rearranged to be in the order specified by the accompanying array.
Ex:
scramble('abcd', [0,3,1,2]) -> 'acdb'
The string that you will be returning back will have: 'a' at index 0, 'b' at index 3, 'c' at index 1, 'd' at index 2, because the order of those characters maps to their corresponding numbers in the index array.
In other words, put the first character in the string at the index described by the first element of the array
You can assume that you will be given a string and array of equal length and both containing valid characters (A-Z, a-z, or 0-9). | reference | def scramble(string, array):
return "" . join(v for _, v in sorted(zip(array, string)))
| String Scramble | 5822d89270ca28c85c0000f3 | [
"Fundamentals",
"Strings",
"Arrays"
] | https://www.codewars.com/kata/5822d89270ca28c85c0000f3 | 7 kyu |
There are just some things you can't do on television. In this case, you've just come back from having a "delicious" Barth burger and you're set to give an interview. The Barth burger has made you queezy, and you've forgotten some of the import rules of the "You Can't Do That on Television" set.
If you say any of the following words a large bucket of "water" will be dumped on you:
"water", "wet", "wash"
This is true for any form of those words, like "washing", "watered", etc.
If you say any of the following phrases you will be doused in "slime":
"I don't know", "slime"
If you say both in one sentence, a combination of water and slime, "sludge", will be dumped on you.
Write a function, bucketOf(str), that takes a string and determines what will be dumped on your head. If you haven't said anything you shouldn't have, the bucket should be filled with "air". The words should be tested regardless of case.
Examples:
```javascript
bucketOf("What is that, WATER?!?") -> "water"
bucketOf("I don't know if I'm doing this right.") -> "slime"
bucketOf("You won't get me!") -> "air"
```
```java
bucketOf("What is that, WATER?!?") -> "water"
bucketOf("I don't know if I'm doing this right.") -> "slime"
bucketOf("You won't get me!") -> "air"
```
```coffeescript
bucketOf("What is that, WATER?!?") -> "water"
bucketOf("I don't know if I'm doing this right.") -> "slime"
bucketOf("You won't get me!") -> "air"
```
```ruby
bucket_of("What is that, WATER?!?") -> "water"
bucket_of("I don't know if I'm doing this right.") -> "slime"
bucket_of("You won't get me!") -> "air"
```
```cpp
bucketOf("What is that, WATER?!?") -> "water"
bucketOf("I don't know if I'm doing this right.") -> "slime"
bucketOf("You won't get me!") -> "air"
```
<div style="width: 320px; text-align: center; color: white; border: white 1px solid;">
Check out my other 80's Kids Katas:
</div>
<div>
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-1-how-many-licks-does-it-take'><span style='color:#00C5CD'>80's Kids #1:</span> How Many Licks Does It Take</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-2-help-alf-find-his-spaceship'><span style='color:#00C5CD'>80's Kids #2:</span> Help Alf Find His Spaceship</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-3-punky-brewsters-socks'><span style='color:#00C5CD'>80's Kids #3:</span> Punky Brewster's Socks</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-4-legends-of-the-hidden-temple'><span style='color:#00C5CD'>80's Kids #4:</span> Legends of the Hidden Temple</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-5-you-cant-do-that-on-television'><span style='color:#00C5CD'>80's Kids #5:</span> You Can't Do That on Television</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-6-rock-em-sock-em-robots'><span style='color:#00C5CD'>80's Kids #6:</span> Rock 'Em, Sock 'Em Robots</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-7-shes-a-small-wonder'><span style='color:#00C5CD'>80's Kids #7:</span> She's a Small Wonder</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-8-the-secret-world-of-alex-mack'><span style='color:#00C5CD'>80's Kids #8:</span> The Secret World of Alex Mack</a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-9-down-in-fraggle-rock'><span style='color:#00C5CD'>80's Kids #9:</span> Down in Fraggle Rock </a><br />
<a style='text-decoration:none' href='http://www.codewars.com/kata/80-s-kids-number-10-captain-planet'><span style='color:#00C5CD'>80's Kids #10:</span> Captain Planet </a><br />
</div>
| algorithms | import re
WATER_PATTERN = re . compile(r"water|wet|wash", re . I)
SLIME_PATTERN = re . compile(r"\bI don't know\b|slime", re . I)
def bucket_of(said):
water = WATER_PATTERN . search(said)
slime = SLIME_PATTERN . search(said)
if water:
return 'sludge' if slime else 'water'
return 'slime' if slime else 'air'
| 80's Kids #5: You Can't Do That on Television | 5667525f0f157f7a0a000004 | [
"Fundamentals",
"Algorithms",
"Strings",
"Regular Expressions"
] | https://www.codewars.com/kata/5667525f0f157f7a0a000004 | 7 kyu |
You should write a function that takes a string and a positive integer `n`, splits the string into parts of length `n` and returns them in an array. It is ok for the last element to have less than `n` characters.
If `n` is not a number or not a valid size (`> 0`) (or is absent), you should return an empty array.
If `n` is greater than the length of the string, you should return an array with the only element being the same string.
Examples:
```javascript
stringChunk('codewars', 2) // ['co', 'de', 'wa', 'rs']
stringChunk('thiskataeasy', 4) // ['this', 'kata', 'easy']
stringChunk('hello world', 3) // ['hel', 'lo ', 'wor', 'ld']
stringChunk('sunny day', 0) // []
```
```coffeescript
stringChunk('codewars', 2) # ['co', 'de', 'wa', 'rs']
stringChunk('thiskataeasy', 4) # ['this', 'kata', 'easy']
stringChunk('hello world', 3) # ['hel', 'lo ', 'wor', 'ld']
stringChunk('sunny day', 0) # []
```
```python
string_chunk('codewars', 2) # ['co', 'de', 'wa', 'rs']
string_chunk('thiskataeasy', 4) # ['this', 'kata', 'easy']
string_chunk('hello world', 3) # ['hel', 'lo ', 'wor', 'ld']
string_chunk('sunny day', 0) # []
```
| reference | def string_chunk(xs, n=None):
try:
return [xs[i: i + n] for i in range(0, len(xs), n)]
except:
return []
| String chunks | 55b4f9906ac454650900007d | [
"Strings",
"Fundamentals"
] | https://www.codewars.com/kata/55b4f9906ac454650900007d | 7 kyu |
You are given a string of words and numbers. Extract the expression including:
1. the operator: either addition (`"gains"`) or subtraction (`"loses"`)
2. the two numbers that we are operating on
Return the result of the calculation.
**Notes:**
* `"loses"` and `"gains"` are the only two words describing operators
* No fruit debts nor bitten apples = The numbers are integers and no negatives
## Examples
```python
"Panda has 48 apples and loses 4" --> 44
"Jerry has 34 apples and gains 6" --> 40
```
Should be a nice little kata for you :)
| reference | def calculate(s):
x = [int(i) for i in s . split() if i . isdigit()]
return sum(x) if 'gains' in s . split() else x[0] - x[1]
| Fruit string calculator | 57b9fc5b8f5813384a000aa3 | [
"Strings",
"Regular Expressions",
"Fundamentals"
] | https://www.codewars.com/kata/57b9fc5b8f5813384a000aa3 | 7 kyu |
This series of katas will introduce you to basics of doing geometry with computers.
`Point` objects have `x`, `y` attributes. `Triangle` objects have attributes `a`, `b`, `c` describing their corners, each of them is a `Point`.
Write a function calculating area of a `Triangle` defined this way.
Tests round answers to 6 decimal places.
| reference | def triangle_area(t):
a, b, c = t . a, t . b, t . c
return round(abs(a . x * (b . y - c . y) + b . x * (c . y - a . y) + c . x * (a . y - b . y)) / 2, 6)
| Geometry Basics: Triangle Area in 2D | 58e4377c46e371aee7001262 | [
"Geometry",
"Fundamentals"
] | https://www.codewars.com/kata/58e4377c46e371aee7001262 | 6 kyu |
In computer science, a programming language is said to have first-class functions if it treats functions as first-class citizens. Specifically, this means the language supports passing functions as arguments to other functions, returning them as the values from other functions, and assigning them to variables or storing them in data structures.
First-class functions are a necessity for the functional programming style, in which the use of higher-order functions is a standard practice. A simple example of a higher-ordered function is the map function, which takes, as its arguments, a function and a list, and returns the list formed by applying the function to each member of the list. For a language to support map, it must support passing a function as an argument. See more on https://en.wikipedia.org/wiki/First-class_function
Your task is to rewrite your own `map` function which takes :
- an array
- a predicate function `func` which returns true (boolean) with even arguments
For example :
```
map([1,2,3,4],func)
produces
[ false, true, false, true ]
```
The code also has to perform input validation, return :
- 'given argument is not a function' if `func` is not a function
- 'array should contain only numbers' if any elements are not numbers | reference | def func(n):
return int(n) % 2 == 0
def map ( arr , somefunction ):
try :
return [ somefunction ( a ) for a in arr ]
except ValueError :
return 'array should contain only numbers'
except TypeError :
return 'given argument is not a function' | Map function issue | 560fbc2d636966b21e00009e | [
"Functional Programming",
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/560fbc2d636966b21e00009e | 7 kyu |
Given any number of boolean flags function should return true if and only if one of them is true while others are false. If function is called without arguments it should return false.
```javascript
onlyOne() --> false
onlyOne(true, false, false) --> true
onlyOne(true, false, false, true) --> false
onlyOne(false, false, false, false) --> false
```
```python
only_one() == False
only_one(True, False, False) == True
only_one(True, False, False, True) == False
only_one(False, False, False, False) == False
``` | algorithms | def only_one(* args):
return sum(args) == 1
| Only one | 5734c38da41454b7f700106e | [
"Algorithms"
] | https://www.codewars.com/kata/5734c38da41454b7f700106e | 7 kyu |
Chingel is practicing for a rowing competition to be held on this saturday. He is trying his best to win this tournament for which he needs to figure out how much time it takes to cover a certain distance.
**Input**
You will be provided with the total distance of the journey, speed of the boat and whether he is going downstream or upstream. The speed of the stream and direction of rowing will be given as a string. Check example test cases!
**Output**
The output returned should be the time taken to cover the distance. If the result has decimal places, round them to 2 fixed positions.
| reference | def time(distance, boat_speed, stream):
river_speed = int(stream . replace(
"Downstream ", '+'). replace("Upstream ", '-'))
return round(distance / (boat_speed + river_speed), 2)
| Upstream/Downstream | 58162692c2a518f03a000189 | [
"Fundamentals"
] | https://www.codewars.com/kata/58162692c2a518f03a000189 | 7 kyu |
Write function which takes a string and make an acronym of it.
Rule of making acronym in this kata:
1. split string to words by space char
2. take every first letter from word in given string
3. uppercase it
4. join them toghether
Eg:
```
Code wars -> C, w -> C W -> CW
```
**Note: There will be at least two words in the given string!**
| reference | def to_acronym(input):
# only call upper() once
return '' . join(word[0] for word in input . split()). upper()
| Make acronym | 57a60bad72292d3e93000a5a | [
"Fundamentals"
] | https://www.codewars.com/kata/57a60bad72292d3e93000a5a | 7 kyu |
You have stumbled across the divine pleasure that is owning a dog and a garden. Now time to pick up all the cr@p! :D
Given a 2D array to represent your garden, you must find and collect all of the dog cr@p - represented by '@'.
You will also be given the number of bags you have access to (bags), and the capactity of a bag (cap). If there are no bags then you can't pick anything up, so you can ignore cap.
You need to find out if you have enough capacity to collect all the cr@p and make your garden clean again.
If you do, return 'Clean', else return 'Cr@p'.
Watch out though - if your dog is out there ('D'), he gets very touchy about being watched. If he is there you need to return 'Dog!!'.
For example:
x=<br>
[[\_,\_,\_,\_,\_,\_]<br>
[\_,\_,\_,\_,@,\_]<br>
[@,\_,\_,\_,\_,\_]]
bags = 2, cap = 2
return --> 'Clean' | reference | from collections import Counter
from itertools import chain
def crap(garden, bags, cap):
c = Counter(chain(* garden))
return 'Dog!!' if c['D'] else ('Clean', 'Cr@p')[c['@'] > bags * cap]
| Clean up after your dog | 57faa6ff9610ce181b000028 | [
"Fundamentals",
"Strings",
"Arrays",
"Mathematics"
] | https://www.codewars.com/kata/57faa6ff9610ce181b000028 | 7 kyu |
Write function ```splitSentence``` which will create a list of strings from a string.
Example:
```"hello world" -> ["hello", "world"]``` | reference | def splitSentence(s):
return s . split()
| Sentence to words | 57a05e0172292dd8510001f7 | [
"Fundamentals"
] | https://www.codewars.com/kata/57a05e0172292dd8510001f7 | 7 kyu |
Imagine there's a big cube consisting of `$ n^3 $` small cubes. Calculate, how many small cubes are not visible from outside.
For example, if we have a cube which has 4 cubes in a row, then the function should return 8, because there are 8 cubes inside our cube (2 cubes in each dimension)
For a visual representation: --> https://imgur.com/a/AN8A5DJ | games | def not_visible_cubes(n):
return max(n - 2, 0) * * 3
| Invisible cubes | 560d6ebe7a8c737c52000084 | [
"Puzzles"
] | https://www.codewars.com/kata/560d6ebe7a8c737c52000084 | 7 kyu |
The notorious Captain Schneider has given you a very straight forward mission. Any data that comes through the system make sure that only non-special characters see the light of day.
Create a function that given a string, retains only the letters A-Z (upper and lowercase), 0-9 digits, and whitespace characters. Also, returns "Not a string!" if the entry type is not a string. | reference | import re
def nothing_special(s):
try:
return re . sub('[^a-z0-9\s]', '', s, flags=re . IGNORECASE)
except:
return 'Not a string!'
| Nothing special | 57029e77005264a3b9000eb5 | [
"Fundamentals",
"Regular Expressions"
] | https://www.codewars.com/kata/57029e77005264a3b9000eb5 | 7 kyu |
Bob is a chicken sexer. His job is to sort baby chicks into a Male(M) and Female(F) piles. When bob can't guess can throw his hands up and declare it with a '?'.
Bob's bosses don't trust Bob's ability just yet, so they have paired him with an expert sexer. All of Bob's decisions will be checked against the expert's choices to generate a correctness score.
## Scoring Rules
* When they agree, he gets 1 point.
* When they disagree but one has said '?', he gets 0.5 points.
* When they disagree completely, he gets 0 points. | reference | def correctness(bob, exp):
return sum(b == e or 0.5 * (b == '?' or e == '?') for b, e in zip(bob, exp))
| Chicken Sexing | 57ed40e3bd793e9c92000fcb | [
"Fundamentals"
] | https://www.codewars.com/kata/57ed40e3bd793e9c92000fcb | 7 kyu |
This just a simple physics problem:
A race car accelerates uniformly from `18.5 m/s` to `46.1 m/s` in `2.47 seconds`. Determine the acceleration of the car and the distance traveled.
Create a function `solveit(vi, vf, t)` that takes in all three values and outputs a list of acceleration and distance in format `[acceleration, distance]`.
Formula used:
```
a = (vf-vi)/t
d = vi*t + 0.5*a*(t**2)
```
If vi > vf then it should return empty list. The solution must be rounded off to double decimal place.
Example:
`[11.17, 79.78]`
| reference | def solveit(vi, vf, t):
a = (vf - vi) / t
d = vi * t + 0.5 * a * (t * * 2)
return [round(a, 2), round(d, 2)] if vi <= vf else []
| Simple Physics Problem | 57c3eb9fd6cf0ffd68000222 | [
"Fundamentals",
"Mathematics"
] | https://www.codewars.com/kata/57c3eb9fd6cf0ffd68000222 | 7 kyu |
Hey CodeWarrior,
we've got a lot to code today!
I hope you know the basic string manipulation methods, because this kata will be all about them.
Here we go...
## Background
We've got a very long string, containing a bunch of User IDs. This string is a listing, which seperates each user ID with a comma and a whitespace ("' "). Sometimes there are more than only one whitespace. Keep this in mind! Futhermore, some user Ids are written only in lowercase, others are mixed lowercase and uppercase characters. Each user ID starts with the same 3 letter "uid", e.g. "uid345edj". But that's not all! Some stupid student edited the string and added some hashtags (#). User IDs containing hashtags are invalid, so these hashtags should be removed!
## Task
1. Remove all hashtags
2. Remove the leading "uid" from each user ID
3. Return an array of strings --> split the string
4. Each user ID should be written in only lowercase characters
5. Remove leading and trailing whitespaces
---
## Note
```if:csharp
Even if this kata can be solved by using Regex or Linq, please try to find a solution by using only C#'s string class.
Some references for C#:
- [Microsoft MDSN: Trim](https://msdn.microsoft.com/de-de/library/t97s7bs3%28v=vs.110%29.aspx)
- [Microsoft MSDN: Split](https://msdn.microsoft.com/de-de/library/tabh47cf%28v=vs.110%29.aspx)
- [Microsoft MSDN: ToLower](https://msdn.microsoft.com/en-us/library/system.string.tolower%28v=vs.110%29.aspx)
- [Microsoft MSDN: Replace](https://msdn.microsoft.com/de-de/library/fk49wtc1%28v=vs.110%29.aspx)
- [Microsoft MSDN: Substring](https://msdn.microsoft.com/de-de/library/aka44szs%28v=vs.110%29.aspx)
```
```if:javascript
Even if this kata can be solved by using Regex, please try to find a solution by using only the built-in String methods.
Some references from MDN:
- [String.prototype.trim()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/Trim)
- [String.prototype.split()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/split)
- [String.prototype.toLowerCase()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/toLowerCase)
- [String.prototype.replace()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/replace)
- [String.prototype.substring()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/String/substring)
- [Array.prototype.map()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map)
```
```if-not:csharp,javascript
Even if this kata can be solved by using Regex, please try to find a solution by using only the built-in methods.
``` | reference | def get_users_ids(string):
return [w . replace("uid", "", 1). strip() for w in string . lower(). replace("#", ""). split(",")]
| String basics | 56326c13e63f90538d00004e | [
"Strings",
"Fundamentals",
"Regular Expressions"
] | https://www.codewars.com/kata/56326c13e63f90538d00004e | 7 kyu |
Write a function that adds from two invocations.
All inputs will be integers.
```
add(3)(4) // 7
add(12)(20) // 32
``` | reference | def add(a): return lambda b: a + b
| Test Your Knowledge Of Function Scope | 56d344c7fd3a52566700124b | [
"Fundamentals"
] | https://www.codewars.com/kata/56d344c7fd3a52566700124b | 7 kyu |
# Task
Write the processArray function, which takes an array and a callback function as parameters. The callback function can be, for example, a mathematical function that will be applied on each element of this array. Optionally, also write tests similar to the examples below.
# Examples
1) Array `[4, 8, 2, 7, 5]` after processing with function
```javascript
var myArray = [4, 8, 2, 7, 5];
myArray = processArray(myArray,function (a) {
return a * 2;
});
```
```python
function(a): return a*2;
```
```csharp
Kata.ProcessArray(new int[] {4, 8, 2, 7, 5}, (Func<int, int>)((v) => v * 2));
```
will be `[ 8, 16, 4, 14, 10 ]`.
2) Array `[7, 8, 9, 1, 2]` after processing with function
```javascript
var myArray = [7, 8, 9, 1, 2];
myArray = processArray(myArray, function (a) {
return a + 5;
});
```
```python
function(a): return a+5;
```
```csharp
Kata.ProcessArray(new int[] {7, 8, 9, 1, 2}, (Func<int, int>)((v) => v + 5));
```
will be `[ 12, 13, 14, 6, 7 ]`.
| algorithms | def process_array(arr, callback):
return list(map(callback, arr))
| Easy mathematical callback | 54b7c8d2cd7f51a839000ebf | [
"Algorithms"
] | https://www.codewars.com/kata/54b7c8d2cd7f51a839000ebf | 7 kyu |
<img src=http://www.myteespot.com/images/Images_d/d_7531.jpg>
It's Friday night, and Chuck is bored. He's already run 1,000 miles, stopping only to eat a family sized bag of Heatwave Doritos and a large fistful of M&Ms. He just can't stop thinking about kicking something!
There is only one thing for it, Chuck heads down to his local MMA gym and immediately challenges every fighter there to get in the cage and try and take him down... AT THE SAME TIME!
You are provided an array of strings that represent the cage and Chuck's opponents. Your task, in traditional Chuck style, is to take their heads off!! Throw punches, kicks, headbutts (or more likely - regex or iteration...) but whatever you do, remove their heads. Return the same array of strings, but with the heads ('O') removed and replaced with a space (' ').
If the provided array is empty, or is an empty string, return 'Gym is empty'. If you are given an array of numbers, return 'This isn't the gym!!'.
FIGHT!!
*Original design of this kata was a much more beautiful thing - the test cases illustrate the idea, and the intended output. I am unable to make the actual output go over multiple lines so for now at least you will have to imagine the beauty!* | algorithms | def head_smash(arr):
try:
return [a . replace('O', ' ') for a in arr] or 'Gym is empty'
except TypeError:
return "This isn't the gym!!"
| Chuck Norris III - Cage Match | 57061b6fcb7293901a000ac7 | [
"Fundamentals",
"Arrays",
"Regular Expressions",
"Algorithms"
] | https://www.codewars.com/kata/57061b6fcb7293901a000ac7 | 7 kyu |
Suzuki has a long list of chores required to keep the monastery in good order. Each chore can be completed independently of the others and assigned to any student. Knowing there will always be an even number of chores and that the number of students isn't limited, Suzuki needs to assign two chores to each student in a way which minimizes the total duration of the day's work.
For example, with the list of chores `[1, 5, 2, 8, 4, 9, 6, 4, 2, 2, 2, 9]`, he'll need 6 students whose total workload will be: `[7, 8, 8, 10, 10, 11]` (as for `[5+2, 4+4, 6+2, 8+2, 1+9, 9+2])`. In this case, the maximal workload is minimized to 11 (=9+2. Keep in mind two chores must be assigned to each student involved).
## Input/output
* Input: `10 ≤ chores length ≤ 30`, `chores length` is always even.
* Output: array of workloads, in ascending order.
Please also try the other Kata in this series..
* [Help Suzuki count his vegetables...](https://www.codewars.com/kata/56ff1667cc08cacf4b00171b)
* [Help Suzuki pack his coal basket!](https://www.codewars.com/kata/57f09d0bcedb892791000255)
* [Help Suzuki purchase his Tofu!](https://www.codewars.com/kata/57d4ecb8164a67b97c00003c)
* [Help Suzuki rake his garden!](https://www.codewars.com/kata/571c1e847beb0a8f8900153d)
* [Suzuki needs help lining up his students!](https://www.codewars.com/kata/5701800886306a876a001031)
* [How many stairs will Suzuki climb in 20 years?](https://www.codewars.com/kata/56fc55cd1f5a93d68a001d4e)
| algorithms | def chore_assignment(chores):
chores . sort()
return sorted([chores[i] + chores[- 1 - i] for i in range(len(chores) / / 2)])
| Help Suzuki complete his chores! | 584dc1b7766c2bb158000226 | [
"Algorithms"
] | https://www.codewars.com/kata/584dc1b7766c2bb158000226 | 7 kyu |
**This Kata is intended as a small challenge for my students**
All Star Code Challenge #22
Create a function that takes an integer argument of seconds and converts the value into a string describing how many hours and minutes comprise that many seconds.
Any remaining seconds left over are ignored.
Note:
The string output needs to be in the specific form - "X hour(s) and X minute(s)"
~~~ if:c
For C:
Memory must be allocated
~~~
For example:
```
3600 --> "1 hour(s) and 0 minute(s)"
3601 --> "1 hour(s) and 0 minute(s)"
3500 --> "0 hour(s) and 58 minute(s)"
323500 --> "89 hour(s) and 51 minute(s)"
```
| reference | def to_time(seconds):
return f' { seconds / / 3600 } hour(s) and {( seconds / / 60 ) % 60 } minute(s)'
| All Star Code Challenge #22 | 5865cff66b5699883f0001aa | [
"Fundamentals"
] | https://www.codewars.com/kata/5865cff66b5699883f0001aa | 7 kyu |
The 26 letters of the English alphabets are randomly divided into 5 groups of 5 letters with the remaining letter being ignored. Each of the group is assigned a score of more than 0. The ignored letter always has a score of 0.
With this kata, write a function ```nameScore(name)``` to work out the score of a name that is passed to the function.
The output should be returned as an object:
```javascript
{'Mary Jane':20}
```
Only letters have a score. Spaces do not.
You can safely assume that ```name``` does not contain any punctuations or symbols. There will also be no ```empty string``` or ```null``` value.
A static ```alpha``` object for testing has been preloaded for your convenience in the following format:
```
{'ABCDE':1, 'FGHIJ':2, 'KLMNO':3, 'PQRST':4, 'UVWXY':5}
'Z' is ignored
```
```if-not:python
Note that the ```alpha``` object will be randomly generated each time you run the test.
```
#Example
In accordance to the above ```alpha``` object, the name ```Mary Jane``` will have a name score of ```20``` => M=3 + a=1 + r=4 + y=5 + J=2 + a=1 + n=3 + e=1 | algorithms | def name_score(name):
return {name: sum(alpha[key] for c in name for key in alpha if c . upper() in key)}
| What is my name score? #1 | 576a29ab726f4bba4b000bb1 | [
"Strings",
"Arrays",
"Algorithms"
] | https://www.codewars.com/kata/576a29ab726f4bba4b000bb1 | 7 kyu |
# Backstory
<img src="https://images-eu.ssl-images-amazon.com/images/I/61FUCzdEinL._AA300_.jpg" align="middle">
To celebrate today's launch of my Hero's new book: `Alan Partridge: Nomad`, We have a new series of kata arranged around the great man himself.
# Task
Given an array of terms, if any of those terms relate to Alan Partridge, return `Mine's a Pint!`
The number of exclamation mark (`!`) after the `t` should be determined by the number of Alan related terms you find in the given array (`x`). The related terms are as follows:
```
Partridge
PearTree
Chat
Dan
Toblerone
Lynn
AlphaPapa
Nomad
```
If you don't find any related terms, return `Lynn, I've pierced my foot on a spike!!`
# Other katas in this series:
<a href="https://www.codewars.com/kata/alan-partridge-ii-apple-turnover">Alan Partridge II - Apple Turnover</a><br>
<a href=" https://www.codewars.com/kata/alan-partridge-iii-london
">Alan Partridge III - London</a><br>
| reference | def part(arr):
l = ["Partridge", "PearTree", "Chat", "Dan",
"Toblerone", "Lynn", "AlphaPapa", "Nomad"]
s = len([i for i in arr if i in l])
return "Mine's a Pint" + "!" * s if s > 0 else 'Lynn, I\'ve pierced my foot on a spike!!'
| Alan Partridge I - Partridge Watch | 5808c8eff0ed4210de000008 | [
"Fundamentals",
"Arrays",
"Strings"
] | https://www.codewars.com/kata/5808c8eff0ed4210de000008 | 7 kyu |
You are given an array including a list of basketball teams and their scores showing points scored vs points lost:
```javascript
const basketballResults = [
['Dallas Mavericks', '492:513'],
['Los Angeles Lakers', '641:637'],
['Houston Rockets', '494:458'],
['Los Angeles Clippers', '382:422'],
['New Orleans Pelicans', '433:454'],
['Oklahoma City Thunder', '315:318'],
['Golden State Warriors', '559:503'],
['Memphis Grizzlies', '550:511'],
['Portland Trail Blazers', '527:520'],
['Minnesota Timberwolves', '495:494'],
['Utah Jazz', '399:402'],
['Sacramento Kings', '420:431'],
['San Antonio Spurs', '469:460'],
['Phoenix Suns', '523:522'],
['Denver Nuggets', '646:658']
];
```
```python
basketball_results = [
["Dallas Mavericks", "492:513"],
["Los Angeles Lakers", "641:637"],
["Houston Rockets", "494:458"],
["Los Angeles Clippers", "382:422"],
["New Orleans Pelicans", "433:454"],
["Oklahoma City Thunder", "315:318"],
["Golden State Warriors", "559:503"],
["Memphis Grizzlies", "550:511"],
["Portland Trail Blazers", "527:520"],
["Minnesota Timberwolves", "495:494"],
["Utah Jazz", "399:402"],
["Sacramento Kings", "420:431"],
["San Antonio Spurs", "469:460"],
["Phoenix Suns", "523:522"],
["Denver Nuggets", "646:658"]
]
```
```ruby
basketball_results = [
["Dallas Mavericks", "492:513"],
["Los Angeles Lakers", "641:637"],
["Houston Rockets", "494:458"],
["Los Angeles Clippers", "382:422"],
["New Orleans Pelicans", "433:454"],
["Oklahoma City Thunder", "315:318"],
["Golden State Warriors", "559:503"],
["Memphis Grizzlies", "550:511"],
["Portland Trail Blazers", "527:520"],
["Minnesota Timberwolves", "495:494"],
["Utah Jazz", "399:402"],
["Sacramento Kings", "420:431"],
["San Antonio Spurs", "469:460"],
["Phoenix Suns", "523:522"],
["Denver Nuggets", "646:658"]
]
```
```crystal
basketball_results = [
["Dallas Mavericks", "492:513"],
["Los Angeles Lakers", "641:637"],
["Houston Rockets", "494:458"],
["Los Angeles Clippers", "382:422"],
["New Orleans Pelicans", "433:454"],
["Oklahoma City Thunder", "315:318"],
["Golden State Warriors", "559:503"],
["Memphis Grizzlies", "550:511"],
["Portland Trail Blazers", "527:520"],
["Minnesota Timberwolves", "495:494"],
["Utah Jazz", "399:402"],
["Sacramento Kings", "420:431"],
["San Antonio Spurs", "469:460"],
["Phoenix Suns", "523:522"],
["Denver Nuggets", "646:658"]
]
```
Your task it to return a number which represents the total number of points scored by a team from Los Angeles.
In the above example the correct result is a number of <b>1023</b>, as Los Angeles Lakers got 641 and Los Angeles Clippers got 382, so 641 + 382 = 1023.
Notes:
- The format of the Los Angeles team name will always start with 'Los Angeles' followed by one-word team name, for example, 'Los Angeles Name'.
- All letters will be in lower-case apart from first letters which will be in upper-case. Only letters will be used (no numbers, underscores, hyphens, special characters etc.)
- For example, the following strings do not represent a team from Los Angeles: 'Happy Los Angeles', 'Los Angeles', Los Angelesio Thunders', 'los angeles masters'.
- The number of teams from Los Angeles may vary.
- If there are no teams from Los Angeles, return the number 0.
- The order of the teams may vary.
- All values in the arrays will always be strings.
- Points will always be given in the following format '100:99' (points scored:points lost).
| algorithms | import re
def get_los_angeles_points(results):
pattern = re . compile('Los Angeles [A-Z][a-z]+')
return sum(int(k[1]. split(':')[0]) for k in results if pattern . fullmatch(k[0]))
| How many points did the teams from Los Angeles score? | 580559b17ab3396c58000abb | [
"Algorithms",
"Data Structures",
"Arrays",
"Regular Expressions"
] | https://www.codewars.com/kata/580559b17ab3396c58000abb | 7 kyu |
We have a matrix of integers with m rows and n columns.
```math
\begin{pmatrix}
a_{11} & a_{12} & \cdots &a_{1n} \\
a_{21} & a_{22} &\cdots &a_{2n} \\
\cdots & \cdots & \cdots & \cdots \\
\cdots & \cdots & \cdots & \cdots \\
a_{m1} & a_{m2} & \cdots &a_{mn}
\end{pmatrix}
```
We want to calculate the total sum for the matrix:
```math
\displaystyle\sum_{i=1}^{m} \sum_{j=1}^{n} (-1)^{i+j}a_{ij}
```
As you can see, the name "alternating sum" of the title is due to the sign of the terms that changes from one term to its contiguous one and so on.
Let's see an example:
```
matrix = [[1, 2, 3], [-3, -2, 1], [3, - 1, 2]]
total_sum = (1 - 2 + 3) + [-(-3) + (-2) - 1] + [3 - (-1) + 2] = 2 + 0 + 6 = 8
```
You may be given matrixes with their dimensions between these values:```10 < m < 300``` and ```10 < n < 300```.
More example cases in the Example Test Cases.
Enjoy it!!
| reference | def score_matrix(matrix):
return sum((- 1) * * (i + j) * matrix[i][j] for i in range(len(matrix)) for j in range(len(matrix[i])))
| #1 Matrices : Making an Alternating Sum | 5720eb05e8d6c5b24a0014c5 | [
"Fundamentals",
"Data Structures",
"Algorithms",
"Mathematics",
"Logic",
"Matrix"
] | https://www.codewars.com/kata/5720eb05e8d6c5b24a0014c5 | 7 kyu |
We need a method in the ```List Class``` that may count specific digits from a given list of integers. This marked digits will be given in a second list.
The method ```.count_spec_digits()/.countSpecDigits()``` will accept two arguments, a list of an uncertain amount of integers ```integers_lists/integersLists``` (and of an uncertain amount of digits, too) and a second list, ```digits_list/digitsList``` that has the specific digits to count which length cannot be be longer than 10 (It's obvious, we've got ten digits).
The method will output a list of tuples, each tuple having two elements, the first one will be a digit to count, and second one, its corresponding total frequency in all the integers of the first list. This list of tuples should be ordered with the same order that the digits have in digitsList
Let's see some cases:
```javascript
l = List()
integersList = [1, 1, 2 ,3 ,1 ,2 ,3 ,4]
digitsList = [1, 3]
l.count_spec_digits(integersList, digitsList) == [(1, 3), (3, 2)]
integersList = [-18, -31, 81, -19, 111, -888]
digitsList = [1, 8, 4]
l.count_spec_digits(integersList, digitsList) == [(1, 7), (8, 5), (4, 0)]
integersList = [-77, -65, 56, -79, 6666, 222]
digitsList = [1, 8, 4]
l.count_spec_digits(integersList, digitsList) == [(1, 0), (8, 0), (4, 0)]
```
```ruby
l = List()
integers_list = [1, 1, 2 ,3 ,1 ,2 ,3 ,4]
digits_list = [1, 3]
l.count_spec_digits(integers_list, digits_list) == [(1, 3), (3, 2)]
integers_list = [-18, -31, 81, -19, 111, -888]
digits_list = [1, 8, 4]
l.count_spec_digits(integers_list, digits_list) == [(1, 7), (8, 5), (4, 0)]
integers_list = [-77, -65, 56, -79, 6666, 222]
digits_list = [1, 8, 4]
l.count_spec_digits(integers_list, digits_list) == [(1, 0), (8, 0), (4, 0)]
```
```python
l = List()
integers_list = [1, 1, 2 ,3 ,1 ,2 ,3 ,4]
digits_list = [1, 3]
l.count_spec_digits(integers_list, digits_list) == [(1, 3), (3, 2)]
integers_list = [-18, -31, 81, -19, 111, -888]
digits_list = [1, 8, 4]
l.count_spec_digits(integers_list, digits_list) == [(1, 7), (8, 5), (4, 0)]
integers_list = [-77, -65, 56, -79, 6666, 222]
digits_list = [1, 8, 4]
l.count_spec_digits(integers_list, digits_list) == [(1, 0), (8, 0), (4, 0)]
```
```haskell
integersList = [1, 1, 2 ,3 ,1 ,2 ,3 ,4]
digitsList = [1, 3]
countSpecDigits integersList digitsList == [(1, 3), (3, 2)]
integersList = [(-18), (-31), 81, (-19), 111, (-888)]
digitsList = [1, 8, 4]
countSpecDigits integersList digitsList == [(1, 7), (8, 5), (4, 0)]
integersList = [(-77), (-65), 56, (-79), 6666, 222]
digitsList = [1, 8, 4]
countSpecDigits integersList digitsList == [(1, 0), (8, 0), (4, 0)]
```
```scala
val intsList = List(1, 1, 2, 3, 1, 2, 3, 4)
val digitsList = List(1, 3)
countSpecDigits(intsList, digitsList) == List((1, 3), (3, 2))
val intsList = List(-18, -31, 81, -19, 111, -888)
val digitsList = List(1, 8, 4)
countSpecDigits(intsList, digitsList) == List((1, 7), (8, 5), (4, 0))
val intsList = List(-77, -65, 56, -79, 6666, 222)
val digitsList = List(1, 8, 4)
countSpecDigits(intsList, digitsList) == List((1, 0), (8, 0), (4, 0))
```
```groovy
def integers = [1, 1, 2, 3, 1, 2, 3, 4]
def digits = [1, 3]
Kata.countSpecDigits(integers, digits) == [[1, 3], [3, 2]]
def integers = [-18, -31, 81, -19, 111, -888]
def digits = [1, 8, 4]
Kata.countSpecDigits(integers, digits) == [[1, 7], [8, 5], [4, 0]]
def integers = [-77, -65, 56, -79, 6666, 222]
def digits = [1, 8, 4]
Kata.countSpecDigits(integers, digits) == [[1, 0], [8, 0], [4, 0]]
```
Enjoy it!! | reference | from collections import Counter
class List (object):
@ staticmethod
def count_spec_digits(integers_list, digits_list):
counts = Counter('' . join(str(abs(a)) for a in integers_list))
return [(b, counts[str(b)]) for b in digits_list]
| Method For Counting Total Occurence Of Specific Digits | 56311e4fdd811616810000ce | [
"Fundamentals",
"Algorithms",
"Data Structures"
] | https://www.codewars.com/kata/56311e4fdd811616810000ce | 7 kyu |
In genetics, a sequence’s motif is a nucleotides (or amino-acid) sequence pattern. Sequence motifs have a biological significance. For more information you can take a look [here](https://en.wikipedia.org/wiki/Sequence_motif).
For this kata you need to complete the function `motif_locator`. This function receives 2 arguments - a sequence and a motif. Both arguments are strings.
You should return an array that contains all the start positions of the motif (in order). A sequence may contain 0 or more repetitions of the given motif. Note that the number of the first position is 1, not 0.
**Some examples:**
- For the `sequence` "ACGTGGGGACTAGGGG" and the `motif` "GGGG" the result should be [5, 13].
- For the `sequence` "ACCGTACCAAGGGACC" and the `motif` "AAT" the result should be []
- For the `sequence` "GGG" and the motif "GG" the result should be [1, 2]
**Note**: You can take a look to my others bio-info kata [here](http://www.codewars.com/users/nbeck/authored) | algorithms | def motif_locator(sequence, motif):
res, i = [], 0
while True:
i = sequence . find(motif, i) + 1
if not i:
return res
res . append(i)
| Find the motif in DNA sequence. | 5760c1c7f2717b91e20001a4 | [
"Algorithms",
"Strings"
] | https://www.codewars.com/kata/5760c1c7f2717b91e20001a4 | 7 kyu |
Quantum mechanics tells us that a molecule is only allowed to have specific, discrete amounts of internal energy. The 'rigid rotor model', a model for describing rotations, tells us that the amount of rotational energy a molecule can have is given by:
`E = B * J * (J + 1)`,
where J is the state the molecule is in, and B is the 'rotational constant' (specific to the molecular species).
Write a function that returns an array of allowed energies for levels between J<sub>min</sub> and J<sub>max</sub>.
Notes:
* return empty array if J<sub>min</sub> is greater than J<sub>max</sub> (as it make no sense).
* J<sub>min</sub>, J<sub>max</sub> are integers.
* physically B must be positive, so return empty array if B <= 0 | reference | def rot_energies(B, Jmin, Jmax):
return [B * J * (J + 1) for J in range(Jmin, Jmax + 1)] if B > 0 else []
| Rotational energy levels | 587b6a5e8726476f9b0000e7 | [
"Fundamentals"
] | https://www.codewars.com/kata/587b6a5e8726476f9b0000e7 | 7 kyu |
Polly is 8 years old. She is eagerly awaiting Christmas as she has a bone to pick with Santa Claus. Last year she asked for a horse, and he brought her a dolls house. Understandably she is livid.
The days seem to drag and drag so Polly asks her friend to help her keep count of how long it is until Christmas, in days. She will start counting from the first of December.
Your function should take 1 argument (a Date object) which will be the day of the year it is currently. The function should then work out how many days it is until Christmas.
Watch out for leap years! | algorithms | from datetime import date
def days_until_christmas(day):
christmas = date(day . year, 12, 25)
if day > christmas:
christmas = date(day . year + 1, 12, 25)
return (christmas - day). days
| Countdown to Christmas | 56f6b23c9400f5387d000d48 | [
"Date Time",
"Algorithms"
] | https://www.codewars.com/kata/56f6b23c9400f5387d000d48 | 7 kyu |
## Task
Create a function called `one` that accepts two params:
* a sequence
* a function
and returns `true` only if the function in the params returns `true` for exactly one (`1`) item in the sequence.
## Example
```
one([1, 3, 5, 6, 99, 1, 3], bigger_than_ten) -> true
one([1, 3, 5, 6, 99, 88, 3], bigger_than_ten) -> false
one([1, 3, 5, 6, 5, 1, 3], bigger_than_ten) -> false
```
If you need help, [here](http://www.rubycuts.com/enum-one) is a resource ( in Ruby ). | reference | def one(sq, fun):
return sum(map(fun, sq)) == 1
| Enumerable Magic #5- True for Just One? | 54599705cbae2aa60b0011a4 | [
"Fundamentals"
] | https://www.codewars.com/kata/54599705cbae2aa60b0011a4 | 7 kyu |
I'm sure you're familiar with factorials – that is, the product of an integer and all the integers below it.
For example, `5! = 120`, as `5 * 4 * 3 * 2 * 1 = 120`
Your challenge is to create a function that takes any number and returns the number that it is a factorial of. So, if your function receives `120`, it should return `"5!"` (as a string).
Of course, not every number is a factorial of another. In this case, your function would return `"None"` (as a string).
### Examples
* `120` will return `"5!"`
* `24` will return `"4!"`
* `150` will return `"None"` | reference | def reverse_factorial(num):
c = f = 1
while f < num:
c += 1
f *= c
return 'None' if f > num else "%d!" % c
| Reverse Factorials | 58067088c27998b119000451 | [
"Fundamentals"
] | https://www.codewars.com/kata/58067088c27998b119000451 | 7 kyu |
## Task
You need to implement two functions, `xor` and `or`, that replicate the behaviour of their respective operators:
- `xor` = Takes 2 values and returns `true` if, and only if, one of them is truthy.
- `or` = Takes 2 values and returns `true` if either one of them is truthy.
When doing so, **you cannot use the or operator: `||`**.
# Input
- Not all input will be booleans - there will be truthy and falsey values [the latter including also empty strings and empty arrays]
- There will always be 2 values provided
## Examples
- `xor(true, true)` should return `false`
- `xor(false, true)` should return `true`
- `or(true, false)` should return `true`
- `or(false, false)` should return `false` | reference | def func_or(a, b):
return not (bool(a) == bool(b) == False)
def func_xor(a, b):
return not (bool(a) == bool(b))
| Boolean logic from scratch | 584d2c19766c2b2f6a00004f | [
"Logic",
"Fundamentals"
] | https://www.codewars.com/kata/584d2c19766c2b2f6a00004f | 7 kyu |
Ready! Set! Fire... but where should you fire?
The battlefield is 3x3 wide grid. HQ has already provided you with an array for easier computing:
```javascript
var grid = ['top left', 'top middle', 'top right',
'middle left', 'center', 'middle right',
'bottom left', 'bottom middle', 'bottom right']
```
```python
grid = ['top left', 'top middle', 'top right',
'middle left', 'center', 'middle right',
'bottom left', 'bottom middle', 'bottom right']
```
```ruby
grid = ['top left', 'top middle', 'top right',
'middle left', 'center', 'middle right',
'bottom left', 'bottom middle', 'bottom right']
```
HQ radios you with 'x' and 'y' coordinates. x=0 y=0 being 'top left' part of the battlefield;
Your duty is to create a function that takes those Xs and Ys and return the correct grid sector to be hit.
```javascript
fire(0,0); => 'top left'
fire(1,2); => 'bottom middle'
etc.
```
```python
fire(0,0) # 'top left'
fire(1,2) # 'bottom middle'
etc.
```
```ruby
fire(0,0) # 'top left'
fire(1,2) # 'bottom middle'
etc.
```
Notice the grid is a monodimensional array, good luck! | reference | def fire(x, y):
return grid[x + 3 * y]
| Grid blast! | 54fdfe14762e2edf4a000a33 | [
"Fundamentals",
"Arrays"
] | https://www.codewars.com/kata/54fdfe14762e2edf4a000a33 | 7 kyu |
Given an Array and an Example-Array to sort to, write a function that sorts the Array following the Example-Array.
Assume Example Array catalogs all elements possibly seen in the input Array. However, the input Array does not necessarily have to have all elements seen in the Example.
Example:
Arr:
[1,3,4,4,4,4,5]
Example Arr:
[4,1,2,3,5]
Result:
[4,4,4,4,1,3,5] | reference | def example_sort(arr, example_arr):
return sorted(arr, key=example_arr . index)
| Sort by Example | 5747fcfce2fab91f43000697 | [
"Arrays",
"Sorting",
"Fundamentals"
] | https://www.codewars.com/kata/5747fcfce2fab91f43000697 | 7 kyu |
Challenge:
Given a two-dimensional array, return a new array which carries over only those arrays from the original, which were not empty and whose items are all of the same type (i.e. homogenous). For simplicity, the arrays inside the array will only contain characters and integers.
Example:
Given [[1, 5, 4], ['a', 3, 5], ['b'], [], ['1', 2, 3]], your function should return [[1, 5, 4], ['b']].
Addendum:
Please keep in mind that for this kata, we assume that empty arrays are not homogenous.
The resultant arrays should be in the order they were originally in and should not have its values changed.
No implicit type casting is allowed. A subarray [1, '2'] would be considered illegal and should be filtered out. | reference | def filter_homogenous(arrays):
return [a for a in arrays if len(set(map(type, a))) == 1]
| Homogenous arrays | 57ef016a7b45ef647a00002d | [
"Arrays",
"Fundamentals",
"Functional Programming"
] | https://www.codewars.com/kata/57ef016a7b45ef647a00002d | 7 kyu |
# Sports league table
Your local sports team manager wants to know how the team is doing in the league. You have been asked to write the manager a function that will allow them to update the league table.
## League details
The possible results in the league are `'draw'` and `'win'` with `3` points for a win and `1` point each for a draw.
The league table is stored in order from top to bottom in a 2-D array called `leagueTable` (Javascript) or `league_table` (Ruby and Python) with each element containing the team name, and the number of points that team has. For example:
```
[["teamC", 6], ["teamA", 4], ["teamB", 4], ["teamD", 3]]
```
## Function specification
Write a function which takes three parameters: first team's name, second team's name, and the result of the match (in Python version the `league_table` will be passed as an additional argument). The function must add the correct points to teams 1 and 2 based on the result of the match, and reorder the array based on new the league positions. League positions should be based first on the number of points then if points are equal, alphabetically. The function must also return the updated league table. | algorithms | def league_calculate(team1, team2, result, league_table):
for t in league_table:
if t[0] == team1:
if result == 'win':
t[1] += 3
elif result == 'draw':
t[1] += 1
elif t[0] == team2:
if result == 'draw':
t[1] += 1
return sorted(league_table, key=lambda x: (- x[1], x[0]))
| Sports league table - help your local team! | 566fd169d39cf89e1e000044 | [
"Fundamentals",
"Arrays",
"Algorithms"
] | https://www.codewars.com/kata/566fd169d39cf89e1e000044 | 7 kyu |
KISS stands for Keep It Simple Stupid.
It is a design principle for keeping things simple rather than complex.
You are the boss of Joe.
Joe is submitting words to you to publish to a blog. He likes to complicate things.
Define a function that determines if Joe's work is simple or complex.
Input will be non emtpy strings with no punctuation.
It is simple if:
``` the length of each word does not exceed the amount of words in the string ```
(See example test cases)
Otherwise it is complex.
If complex:
```python
return "Keep It Simple Stupid"
```
or if it was kept simple:
```python
return "Good work Joe!"
```
Note: Random test are random and nonsensical. Here is a silly example of a random test:
```python
"jump always mostly is touchy dancing choice is pineapples mostly"
``` | games | def is_kiss(words):
wordsL = words . split(' ')
l = len(wordsL)
for word in wordsL:
if len(word) > l:
return "Keep It Simple Stupid"
return "Good work Joe!"
| KISS - Keep It Simple Stupid | 57eeb8cc5f79f6465a0015c1 | [
"Strings",
"Fundamentals"
] | https://www.codewars.com/kata/57eeb8cc5f79f6465a0015c1 | 7 kyu |
Jenny is 9 years old. She is the youngest detective in North America. Jenny is a 3rd grader student, so when a new mission comes up, she gets a code to decipher in a form of a sticker (with numbers) in her math notebook and a comment (a sentence) in her writing notebook. All she needs to do is to figure out one word, from there she already knows what to do.
And here comes your role - you can help Jenny find out what the word is!
In order to find out what the word is, you should use the sticker (array of 3 numbers) to retrive 3 letters from the comment (string) that create the word.
- Each of the numbers in the array refers to the position of a letter in the string, in increasing order.
- Spaces are not places, you need the actual letters. No spaces.
- The returned word should be all lowercase letters.
- if you can't find one of the letters using the index numbers, return "No mission today". Jenny would be very sad, but that's life... :(
Example:
input: [5, 0, 3], "I Love You"
output: "ivy"
(0 = "i", 3 = "v", 5 = "y")
| reference | def missing(nums, s):
ans = []
s = s . replace(' ', '')
try:
for i in sorted(nums):
ans . append(s[i])
return '' . join(ans). lower()
except IndexError:
return ("No mission today")
| Jenny the youngest detective | 58b972cae826b960a300003e | [
"Strings",
"Arrays",
"Algorithms",
"Fundamentals"
] | https://www.codewars.com/kata/58b972cae826b960a300003e | 7 kyu |
# Two samurai generals are discussing dinner plans after a battle, but they can't seem to agree.
The discussion gets heated and you are cannot risk favoring either of them as this might damage your political standing with either of the two clans the samurai generals belong to. Thus, the only thing left to do is find what the common ground of what they are saying is.
Compare the proposals with the following function:
```javascript
function commonGround(s1, s2)
```
```python
def common_ground(s1,s2)
```
```ruby
def common_ground(s1,s2)
```
The parameters ```s1``` and ```s2``` are the strings representing what each of the generals said. You should output a string containing the words in ```s1``` that also occur in ```s2```.
Each word in the resulting string shall occur once, and the order of the words need to follow the order of the first occurence of each word in ```s2```.
If they are saying nothing in common, kill both samurai and blame a ninja. (output "death") | reference | def common_ground(s1, s2):
lst = []
for w in s2 . split():
if w in s1 . split() and w not in lst:
lst . append(w)
return ' ' . join(lst) if lst else "death"
| Dinner Plans | 57212c55b6fa235edc0002a2 | [
"Strings",
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/57212c55b6fa235edc0002a2 | 7 kyu |
# Description
Write a function that checks whether a credit card number is correct or not, using the Luhn algorithm.
The algorithm is as follows:
* From the rightmost digit, which is the check digit, moving left, double the value of every second digit; if the product of this doubling operation is greater than 9 (e.g., 8 × 2 = 16), then sum the digits of the products (e.g., 16: 1 + 6 = 7, 18: 1 + 8 = 9) or alternatively subtract 9 from the product (e.g., 16: 16 - 9 = 7, 18: 18 - 9 = 9).
* Take the sum of all the digits.
* If the total modulo 10 is equal to 0 (if the total ends in zero) then the number is valid according to the Luhn formula; else it is not valid.
The input is a string with the full credit card number, in groups of 4 digits separated by spaces, i.e. "1234 5678 9012 3456"
Don´t worry about wrong inputs, they will always be a string with 4 groups of 4 digits each separated by space.
# Examples
`valid_card?("5457 6238 9823 4311") # True`
`valid_card?("5457 6238 9323 4311") # False`
for reference check: https://en.wikipedia.org/wiki/Luhn_algorithm | algorithms | def valid_card(card):
s = list(map(int, str(card . replace(' ', ''))))
s[0:: 2] = [d * 2 - 9 if d * 2 > 9 else d * 2 for d in s[0:: 2]]
return sum(s) % 10 == 0
| Credit Card Checker | 56d55dcdc87df58c81000605 | [
"Fundamentals",
"Algorithms"
] | https://www.codewars.com/kata/56d55dcdc87df58c81000605 | 7 kyu |
Create a function that will return true if all numbers in the sequence follow the same counting pattern. If the sequence of numbers does not follow the same pattern, the function should return false.
Sequences will be presented in an array of varied length. Each array will have a minimum of 3 numbers in it.
The sequences are all simple and will not step up in varying increments such as a Fibonacci sequence.
JavaScript examples:
```javascript
validateSequence([1,2,3,4,5,6,7,8,9]) === true
validateSequence([1,2,3,4,5,8,7,8,9]) === false
validateSequence([2,4,6,8,10]) === true
validateSequence([0,2,4,6,8]) === true
validateSequence([1,3,5,7,9]) === true
validateSequence([1,2,4,8,16,32,64]) === false
validateSequence([0,1,1,2,3,5,8,13,21,34]) === false
```
| reference | def validate_sequence(seq):
return len({a - b for a, b in zip(seq, seq[1:])}) == 1
| Simple Sequence Validator | 553f01db29490a69ff000049 | [
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/553f01db29490a69ff000049 | 7 kyu |
We have the number ```12385```. We want to know the value of the closest cube but higher than 12385. The answer will be ```13824```.
Now, another case. We have the number ```1245678```. We want to know the 5th power, closest and higher than that number. The value will be ```1419857```.
We need a function ```find_next_power``` ( ```findNextPower``` in JavaScript, CoffeeScript and Haskell), that receives two arguments, a value ```val```, and the exponent of the power,``` pow_```, and outputs the value that we want to find.
Let'see some cases:
```python
find_next_power(12385, 3) == 13824
find_next_power(1245678, 5) == 1419857
```
```javascript
findNextPower(12385, 3) == 13824
findNextPower(1245678, 5) == 1419857
```
```ruby
find_next_power(12385, 3) == 13824
find_next_power(1245678, 5) == 1419857
```
```haskell
findNextPower 12385 3 `shouldBe` 13824
findNextPower 1245678 5 `shouldBe` 1419857
```
```coffeescript
findNextPower(12385, 3) == 13824
findNextPower(1245678, 5) == 1419857
```
```ocaml
find_next_power 12385 3 = 13824
find_next_power 1245678 5 = 1419857
```
```cpp
findNextPower(12385, 3) == 13824
findNextPower(1245678, 5) == 1419857
```
The value, ```val``` will be always a positive integer.
The power, ```pow_```, always higher than ```1```.
Happy coding!! | reference | def find_next_power(val, pow_):
return int(val * * (1.0 / pow_) + 1) * * pow_
| Find the smallest power higher than a given a value | 56ba65c6a15703ac7e002075 | [
"Fundamentals",
"Mathematics",
"Logic"
] | https://www.codewars.com/kata/56ba65c6a15703ac7e002075 | 7 kyu |
Given a list of rows of a square matrix, find the product of the main diagonal.
Examples:
```
[[1, 0], [0, 1]] should return 1
[[1, 2, 3], [4, 5, 6], [7, 8, 9]] should return 45
```
http://en.wikipedia.org/wiki/Main_diagonal
| algorithms | def main_diagonal_product(mat):
prod = 1
for i in range(0, len(mat)):
prod *= mat[i][i]
return prod
| Product of the main diagonal of a square matrix. | 551204b7509063d9ba000b45 | [
"Matrix",
"Linear Algebra",
"Algorithms"
] | https://www.codewars.com/kata/551204b7509063d9ba000b45 | 7 kyu |
<h2>Description</h2>
In English we often use "neutral vowel sounds" such as "umm", "err", "ahh" as fillers in conversations to help them run smoothly.
Bob always finds himself saying "err". Infact he adds an "err" to every single word he says that ends in a consonant! Because Bob is odd, he likes to stick to this habit even when emailing.
<h2>Task</h2>
Bob is begging you to write a function that adds "err" to the end of every word whose last letter is a consonant (not a vowel, y counts as a consonant).
The input is a string that can contain upper and lowercase characters, some punctuation but no numbers. The solution should be returned as a string.
NOTE: If the word ends with an uppercase consonant, the following "err" will be uppercase --> "ERR".
eg:
```
"Hello, I am Mr Bob" --> "Hello, I amerr Mrerr Boberr"
"THIS IS CRAZY!" --> "THISERR ISERR CRAZYERR!"
```
Good luck!
| reference | def err_bob(s):
res = ""
for i, c in enumerate(s):
res += c
if i == len(s) - 1 or s[i + 1] in " .,:;!?":
if c . islower() and c not in "aeiou":
res += "err"
if c . isupper() and c not in "AEIOU":
res += "ERR"
return res
| Please help Bob | 5751fef5dcc1079ac5001cff | [
"Fundamentals"
] | https://www.codewars.com/kata/5751fef5dcc1079ac5001cff | 7 kyu |
You're in ancient Greece and giving Philoctetes a hand in preparing a training exercise for Hercules! You've filled a pit with two different ferocious mythical creatures for Hercules to battle!
**Orthus**

**Hydra**

The formidable **"Orthus"** is a 2 headed dog with 1 tail. The mighty **"Hydra"** has 5 heads and 1 tail.
Before Hercules goes in, he asks you "How many of each beast am I up against!?".
You know the total number of heads and the total number of tails, that's the dangerous parts, right? But you didn't consider how many of each beast.
## Task
Given the number of heads and the number of tails, work out the number of each mythical beast!
The data is given as two parameters. Your answer should be returned as an array:
```javascript
VALID -> [24 , 15] INVALID -> "No solutions"
[Orthus, Hydra]
```
```python
VALID -> [24 , 15] INVALID -> "No solutions"
```
```csharp
VALID -> [24 , 15] INVALID -> null
```
If there aren't any cases for the given amount of heads and tails - return "No solutions" or null (C#).
| reference | def beasts(h, t):
out = [(5 * t - h) / 3, (h - 2 * t) / 3]
return all(x . is_integer() and x >= 0 for x in out) and out or 'No solutions'
| Mythical Heads and Tails | 5751aa92f2dac7695d000fb0 | [
"Mathematics",
"Fundamentals"
] | https://www.codewars.com/kata/5751aa92f2dac7695d000fb0 | 7 kyu |
Create a function that takes a number and returns an array of strings containing the number cut off at each digit.
### Examples
* `420` should return ``["4", "42", "420"]``
* `2017` should return `["2", "20", "201", "2017"]`
* `2010` should return `["2", "20", "201", "2010"]`
* `4020` should return `["4", "40", "402", "4020"]`
* `80200` should return `["8", "80", "802", "8020", "80200"]`
PS: The input is guaranteed to be an integer in the range `[0, 1000000]` | reference | def create_array_of_tiers(n):
n = str(n)
return [n[: i] for i in range(1, len(n) + 1)]
| Number to digit tiers | 586bca7fa44cfc833e00005c | [
"Strings",
"Fundamentals"
] | https://www.codewars.com/kata/586bca7fa44cfc833e00005c | 7 kyu |
You will be given an array which will include both integers and characters.
Return an array of length 2 with a[0] representing the mean of the ten integers as a floating point number. There will always be 10 integers and 10 characters. Create a single string with the characters and return it as a[1] while maintaining the original order.
```
lst = ['u', '6', 'd', '1', 'i', 'w', '6', 's', 't', '4', 'a', '6', 'g', '1', '2', 'w', '8', 'o', '2', '0']
```
Here is an example of your return
```
[3.6, "udiwstagwo"]
```
In C# and Java the mean return is a double. | reference | def mean(lst):
return [sum(int(n) for n in lst if n . isdigit()) / 10.0, "" . join(c for c in lst if c . isalpha())]
| Calculate mean and concatenate string | 56f7493f5d7c12d1690000b6 | [
"Fundamentals"
] | https://www.codewars.com/kata/56f7493f5d7c12d1690000b6 | 7 kyu |
Write a function that takes an array of strings as an argument and returns a sorted array containing the same strings, ordered from shortest to longest.
For example, if this array were passed as an argument:
``` ["Telescopes", "Glasses", "Eyes", "Monocles"] ```
Your function would return the following array:
``` ["Eyes", "Glasses", "Monocles", "Telescopes"] ```
All of the strings in the array passed to your function will be different lengths, so you will not have to decide how to order multiple strings of the same length. | reference | def sort_by_length(arr):
return sorted(arr, key=len)
| Sort array by string length | 57ea5b0b75ae11d1e800006c | [
"Sorting",
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/57ea5b0b75ae11d1e800006c | 7 kyu |
Write a function that takes an array of **unique** integers and returns the minimum number of integers needed to make the values of the array consecutive from the lowest number to the highest number.
## Example
```
[4, 8, 6] --> 2
Because 5 and 7 need to be added to have [4, 5, 6, 7, 8]
[-1, -5] --> 3
Because -2, -3, -4 need to be added to have [-5, -4, -3, -2, -1]
[1] --> 0
[] --> 0
``` | reference | def consecutive(arr):
return max(arr) - min(arr) + 1 - len(arr) if arr else 0
| How many consecutive numbers are needed? | 559cc2d2b802a5c94700000c | [
"Arrays",
"Fundamentals"
] | https://www.codewars.com/kata/559cc2d2b802a5c94700000c | 7 kyu |
The Cat In The Hat has cat A under his hat, cat A has cat B under his hat and so on until Z
The Cat In The Hat is 2,000,000 cat units tall.
Each cat is 2.5 times bigger than the cat underneath their hat.
Find the total height of the cats if they are standing on top of one another.
Counting starts from the Cat In The Hat
n = the number of cats
fix to 3 decimal places.
| reference | def height(n):
height = cat = 2000000
for i in range(n):
cat /= 2.5
height += cat
return f' { height : .3 f } '
| Cats in hats | 57b5907920b104772c00002a | [
"Fundamentals"
] | https://www.codewars.com/kata/57b5907920b104772c00002a | 7 kyu |
Removed due to copyright infringement.
<!---
### Task
Given two cells on the standard chess board, determine whether they have the same color or not.
### Example
For `cell1 = "A1" and cell2 = "C3"`, the output should be `true`.
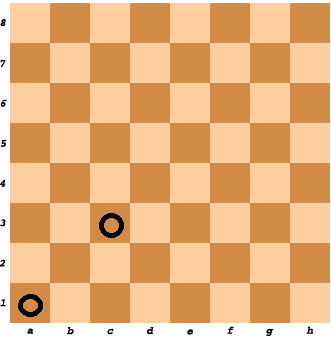
For `cell1 = "A1" and cell2 = "H3"`, the output should be `false`.
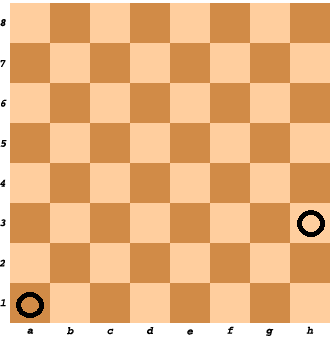
### Input/Output
- `[input]` string `cell1`
- `[input]` string `cell2`
- `[output]` a boolean value
`true` if both cells have the same color, `false` otherwise.
---> | games | def chess_board_cell_color(a, b):
return (ord(a[0]) + int(a[1])) % 2 == (ord(b[0]) + int(b[1])) % 2
| Chess Fun #1: Chess Board Cell Color | 5894134c8afa3618c9000146 | [
"Puzzles",
"Games"
] | https://www.codewars.com/kata/5894134c8afa3618c9000146 | 6 kyu |
This is the first part of this kata series. Second part is [here](https://www.codewars.com/kata/assembler-interpreter-part-ii/).
We want to create a simple interpreter of assembler which will support the following instructions:
- `mov x y` - copies `y` (either a constant value or the content of a register) into register `x`
- `inc x` - increases the content of the register `x` by one
- `dec x` - decreases the content of the register `x` by one
- `jnz x y` - jumps to an instruction `y` steps away (positive means forward, negative means backward, y can be a register or a constant), but only if `x` (a constant or a register) is not zero
Register names are alphabetical (letters only). Constants are always integers (positive or negative).
Note: the `jnz` instruction moves relative to itself. For example, an offset of `-1` would continue at the previous instruction, while an offset of `2` would skip over the next instruction.
The function will take an input list with the sequence of the program instructions and will execute them. The program ends when there are no more instructions to execute, then it returns a dictionary (a table in COBOL) with the contents of the registers.
Also, every `inc`/`dec`/`jnz` on a register will always be preceeded by a `mov` on the register first, so you don't need to worry about uninitialized registers.
## Example
```
["mov a 5"; "inc a"; "dec a"; "dec a"; "jnz a -1"; "inc a"]
visualized:
mov a 5
inc a
dec a
dec a
jnz a -1
inc a
```
The above code will:
* set register `a` to `5`,
* increase its value by `1`,
* decrease its value by `2`,
* then decrease its value until it is zero (`jnz a -1` jumps to the previous instruction if `a` is not zero)
* and then increase its value by `1`, leaving register `a` at `1`
So, the function should return:
```scala
Map("a"->1)
```
```python
{'a': 1}
```
```java
{a=1}
```
```csharp
new Dictionary<string, int> { { "a" , 1 } };
```
```haskell
fromList [("a", 1)]
```
```julia
Dict{String,Number}("a"=>1)
```
```rust
{"a": 1}
```
```clojure
{:a 1}
```
```go
map[string]int{"a": 1}
```
```crystal
{"a" => 1}
```
```cobol
[["a", 1]]
```
```c
(int[]){['a'] = 1}
```
This kata is based on the [Advent of Code 2016 - day 12](https://adventofcode.com/2016/day/12)
| algorithms | def simple_assembler(program):
d, i = {}, 0
while i < len(program):
cmd, r, v = (program[i] + ' 0'). split()[: 3]
if cmd == 'inc':
d[r] += 1
if cmd == 'dec':
d[r] -= 1
if cmd == 'mov':
d[r] = d[v] if v in d else int(v)
if cmd == 'jnz' and (d[r] if r in d else int(r)):
i += int(v) - 1
i += 1
return d
| Simple assembler interpreter | 58e24788e24ddee28e000053 | [
"Interpreters",
"Algorithms"
] | https://www.codewars.com/kata/58e24788e24ddee28e000053 | 5 kyu |
# Task
You are given two strings s and t of the same length, consisting of uppercase English letters. Your task is to find the minimum number of "replacement operations" needed to get some `anagram` of the string t from the string s. A replacement operation is performed by picking exactly one character from the string s and replacing it by some other character.
About `anagram`: А string x is an anagram of a string y if one can get y by rearranging the letters of x. For example, the strings "MITE" and "TIME" are anagrams, so are "BABA" and "AABB", but "ABBAC" and "CAABA" are not.
# Example
For s = "AABAA" and t = "BBAAA", the output should be 1;
For s = "OVGHK" and t = "RPGUC", the output should be 4.
# Input/Output
- `[input]` string `s`
Constraints: `5 ≤ s.length ≤ 35`.
- `[input]` string `t`
Constraints: `t.length = s.length`.
- `[output]` an integer
The minimum number of replacement operations needed to get an anagram of the string t from the string s. | games | from collections import Counter
def create_anagram(s, t):
return sum((Counter(s) - Counter(t)). values())
| Simple Fun #32: Create Anagram | 5887099cc815166a960000c6 | [
"Puzzles"
] | https://www.codewars.com/kata/5887099cc815166a960000c6 | 7 kyu |
# Task
Given some points(array `A`) on the same line, determine the minimum number of line segments with length `L` needed to cover all of the given points. A point is covered if it is located inside some segment or on its bounds.
# Example
For `A = [1, 3, 4, 5, 8]` and `L = 3`, the output should be `2`.
Check out the image below for better understanding:
<div style="background-color: white">
<img src="https://codefightsuserpics.s3.amazonaws.com/tasks/segmentCover/img/example.png?_tm=1474900035857">
</div>
For `A = [1, 5, 2, 4, 3]` and `L = 1`, the output should be `3`.
segment1: `1-2`(covered points 1,2),
segment2: `3-4`(covered points 3,4),
segment3: `5-6`(covered point 5)
For `A = [1, 10, 100, 1000]` and `L = 1`, the output should be `4`.
segment1: `1-2`(covered point 1),
segment2: `10-11`(covered point 10),
segment3: `100-101`(covered point 100),
segment4: `1000-1001`(covered point 1000)
# Input/Output
- `[input]` integer array A
Array of point coordinates on the line (all points are different).
Constraints:
`1 ≤ A.length ≤ 50,`
`-5000 ≤ A[i] ≤ 5000.`
- `[input]` integer `L`
Segment length, a positive integer.
Constraints: `1 ≤ L ≤ 100.`
- `[output]` an integer
The minimum number of line segments that can cover all of the given points. | games | def segment_cover(A, L):
n = 1
s = min(A)
for i in sorted(A):
if s + L < i:
s = i
n += 1
return n
| Simple Fun #109: Segment Cover | 589ac16a0cccbff11d000115 | [
"Puzzles"
] | https://www.codewars.com/kata/589ac16a0cccbff11d000115 | 7 kyu |
If you're faced with an input box, like this:
+--------------+
Enter the price of the item, in dollars: | |
+--------------+
do you put the $ sign in, or not? Inevitably, some people will type a $ sign and others will leave it out. The instructions could be made clearer - but that won't help those annoying people who never read instructions anyway.
A better solution is to write code that can handle the input whether it includes a $ sign or not.
So, we need a simple function that converts a string representing a number (possibly with a $ sign in front of it) into the number itself.
Remember to consider negative numbers (the - sign may come either before or after the $ sign, if there is one), and any extraneous space characters (leading, trailing, or around the $ sign) that the users might put in. You do not need to handle input with trailing characters other than spaces (e.g. "1.2 3" or "1$"). If the given string does not represent a number, (either with or without a $ sign), return 0.0 .
| reference | def money_value(s):
try:
return float(s . replace("$", ""). replace(" ", ""))
except:
return 0.0
| Unwanted dollars | 587309155cfd6b9fb60000a0 | [
"Fundamentals"
] | https://www.codewars.com/kata/587309155cfd6b9fb60000a0 | 6 kyu |
**This Kata is intended as a small challenge for my students**
All Star Code Challenge #29
Your friend Nhoj has dislexia, but can easily read messages if the words are written backwards.
Create a function called `reverseSentence()/reverse_sentence()` that accepts a string argument. The function returns a string of the same length with each word reversed, but still in their original order.
```javascript
reverseSentence("Hello !Nhoj Want to have lunch?"); // => 'olleH johN! tnaW ot evah ?hcnul'
```
```python
reverse_sentence("Hello !Nhoj Want to have lunch?") # "olleH johN! tnaW ot evah ?hcnul"
```
```ruby
reverse_sentence("Hello !Nhoj Want to have lunch?") # "olleH johN! tnaW ot evah ?hcnul"
```
```crystal
reverse_sentence("Hello !Nhoj Want to have lunch?") # "olleH johN! tnaW ot evah ?hcnul"
```
Note:
A "word" should be considered a string split by a space character, " "
Letter capitalization should be maintained. | reference | def reverse_sentence(sentence):
return ' ' . join(w[:: - 1] for w in sentence . split())
| All Star Code Challenge #29 | 5866ec8b2e8d9cec7e0000bb | [
"Fundamentals"
] | https://www.codewars.com/kata/5866ec8b2e8d9cec7e0000bb | 7 kyu |
# Task
You have some people who are betting money, and they all start with the same amount of money (this number>0).
Find out if the given end-state of amounts is possible after the betting is over and money is redistributed.
# Input/Output
- `[input]` integer array arr
the proposed end-state showing final amounts for each player
- `[output]` a boolean value
`true` if this is a possible end-state and `false` otherwise
# Examples
- For `arr = [0, 56, 100]`, the output should be `true`.
Three players start with the same amount of money 52.
At the end of game, player 1 lose `52`, player2 win `4`, and player3 win `48`.
- For `arr = [0, 0, 0]`, the output should be `false`.
Players should start with a positive number of of money.
- For `arr = [11]`, the output should be `true`.
One player always keep his money at the end of game.
- For `arr = [100, 100, 100, 90, 1, 0, 0]`, the output should be `false`.
These players can not start with the same amount of money.
| games | def learn_charitable_game(arr):
return sum(arr) % len(arr) == 0 and sum(arr) > 0
| Simple Fun #131: Learn Charitable Game | 58a651ff27f95429f80000d0 | [
"Puzzles"
] | https://www.codewars.com/kata/58a651ff27f95429f80000d0 | 7 kyu |
Program a function `sumAverage(arr)` where `arr` is an array containing arrays full of numbers, for example:
```javascript
sumAverage([[1, 2, 2, 1], [2, 2, 2, 1]]);
```
```python
sum_average([[1, 2, 2, 1], [2, 2, 2, 1]]);
```
```ruby
sum_average([[1, 2, 2, 1], [2, 2, 2, 1]]);
```
```php
sumAverage([[1, 2, 2, 1], [2, 2, 2, 1]]);
```
```julia
sumaverage([[1, 2, 2, 1], [2, 2, 2, 1]]);
```
First, determine the average of each array. Then, return the sum of all the averages.
- All numbers will be less than 100 and greater than -100.
- `arr` will contain a maximum of 50 arrays.
- After calculating all the averages, add them **all** together, **then** round down, as shown in the example below:
The example given: `sumAverage([[3, 4, 1, 3, 5, 1, 4], [21, 54, 33, 21, 77]])`, the answer being 44.
1. Calculate the average of each individual array:
```
[3, 4, 1, 3, 5, 1, 4] = (3 + 4 + 1 + 3 + 5 + 1 + 4) / 7 = 3
[21, 54, 33, 21, 77] = (21 + 54 + 33 + 21 + 77) / 5 = 41.2
```
2. Add the average of each array together:
```
3 + 41.2 = 44.2
```
3. Round the final average down:
```javascript
Math.floor(44.2) = 44
```
```php
floor(44.2) = 44
```
```julia
floor(44.2) = 44
```
~~~if:julia
In Julia, the `Statistics` package is preloaded.
~~~
```python
import math
math.floor(44.2) = 44
``` | algorithms | from statistics import mean
from math import floor
def sum_average(arr):
return floor(sum(map(mean, arr)))
| Sum of Array Averages | 56d5166ec87df55dbe000063 | [
"Arrays",
"Mathematics",
"Algorithms"
] | https://www.codewars.com/kata/56d5166ec87df55dbe000063 | 7 kyu |
This series of katas will introduce you to basics of doing geometry with computers.
`Point` objects have `x`, `y` attributes. `Triangle` objects have attributes `a`, `b`, `c` describing their corners, each of them is a `Point`.
Write a function calculating perimeter of a `Triangle` defined this way.
Tests round answers to 6 decimal places.
| reference | from math import hypot
def triangle_perimeter(t):
return sum(
hypot(p1 . x - p2 . x, p1 . y - p2 . y)
for p1, p2 in [(t . a, t . b), (t . b, t . c), (t . c, t . a)]
)
| Geometry Basics: Triangle Perimeter in 2D | 58e3e62f20617b6d7700120a | [
"Geometry",
"Fundamentals"
] | https://www.codewars.com/kata/58e3e62f20617b6d7700120a | 7 kyu |
You have to create a function that converts integer given as string into ASCII uppercase letters or spaces.
All ASCII characters have their numerical order in table.
For example,
```
from ASCII table, character of number 65 is "A".
```
Numbers will be next to each other, So you have to split given number to two digit long integers.
### Examples (input -> output)
```
'658776' -> 'AWL' (because in ASCII table 'A' is 65, 'W' is 87, 'L' is 76)
'73327673756932858080698267658369' ->'I LIKE UPPERCASE'
```
Good Luck!
| reference | def convert(number):
return '' . join(chr(int(number[a: a + 2])) for a in range(0, len(number), 2))
| ASCII letters from Number | 589ebcb9926baae92e000001 | [
"Fundamentals"
] | https://www.codewars.com/kata/589ebcb9926baae92e000001 | 7 kyu |
# Task
Alireza and Ali have a `3×3 table` and playing on that. They have 4 tables (2×2) A,B,C and D in this table.
At beginning all of 9 numbers in 3×3 table is zero.
Alireza in each move choose a 2×2 table from A, B, C and D and increase all of 4 numbers in that by one.
He asks Ali, how much he increase table A, B, C and D. (if he cheats you should return `[-1]`)
Your task is to help Ali.
# Example
For
```
table=
[[1,2,1],
[2,4,2],
[1,2,1]]```
The result should be `[1,1,1,1]`
For:
```
Table=
[[3,7,4],
[5,16,11],
[2,9,7]]```
The result should be `[3,4,2,7]`
# Input/Output
- `[input]` 2D integer array `table`
`3×3` table.
- `[output]` an integer array | algorithms | def table_game(table):
(a, ab, b), (ac, abcd, bd), (c, cd, d) = table
if (a + b == ab) and (c + d == cd) and (a + c == ac) and (b + d == bd) and (a + b + c + d == abcd):
return [a, b, c, d]
return [- 1]
| Simple Fun #145: Table Game | 58aa7f18821a769a7d000190 | [
"Algorithms"
] | https://www.codewars.com/kata/58aa7f18821a769a7d000190 | 7 kyu |
Complete the function which takes a non-zero integer as its argument.
If the integer is divisible by 3, return the string `"Java"`.
If the integer is divisible by 3 and divisible by 4, return the string `"Coffee"`
If one of the condition above is true and the integer is even, add `"Script"` to the end of the string.
If none of the condition is true, return the string `"mocha_missing!"`
## Examples
```python
1 --> "mocha_missing!"
3 --> "Java"
6 --> "JavaScript"
12 --> "CoffeeScript"
``` | reference | def caffeineBuzz(n):
buzz = "mocha_missing!"
if n % 3 == 0:
buzz = "Java"
if n % 4 == 0:
buzz = "Coffee"
if n % 2 == 0:
buzz += "Script"
return buzz
| Caffeine Script | 5434283682b0fdb0420000e6 | [
"Fundamentals",
"Strings"
] | https://www.codewars.com/kata/5434283682b0fdb0420000e6 | 7 kyu |
# Task
We have a N×N `matrix` (N<10) and a robot.
We wrote in each point of matrix x and y coordinates of a point of matrix.
When robot goes to a point of matrix, reads x and y and transfer to point with x and y coordinates.
For each point in the matrix we want to know if robot returns back to it after `EXACTLY k` moves. So your task is to count points to which Robot returns in `EXACTLY k` moves.
You should stop counting moves as soon as the robot returns to the starting point. That is, if the robot returns to the starting point in fewer than k moves, that point should not count as a valid point.
# example
For:
```
matrix=[
["0,1","0,0","1,2"],
["1,1","1,0","0,2"],
["2,1","2,0","0,0"]]
k= 2
```
The result should be `8`
```
Robot start at (0,0) --> (0,1) --> (0,0), total 2 moves
Robot start at (0,1) --> (0,0) --> (0,1), total 2 moves
Robot start at (0,2) --> (1,2) --> (0,2), total 2 moves
Robot start at (1,2) --> (0,2) --> (1,2), total 2 moves
Robot start at (1,0) --> (1,1) --> (1,0), total 2 moves
Robot start at (1,1) --> (1,0) --> (1,1), total 2 moves
Robot start at (2,0) --> (2,1) --> (2,0), total 2 moves
Robot start at (2,1) --> (2,0) --> (2,1), total 2 moves
Robot start at (2,2) --> (0,0) --> (0,1) --> (0,0) --> (0,1) ....
(Robot can not transfer back to 2,2)
```
So the result is 8.
# Input/Output
- `[input]` 2D integer array matrix
n x n matrix. 3 <= n <=9
- `[input]` integer `k`
`2 <= k <= 5`
- `[output]` an integer | games | def robot_transfer(matrix, k):
mt = {(i, j): tuple(map(int, c . split(',')))
for i, r in enumerate(matrix) for j, c in enumerate(r)}
res = 0
for m in mt:
cur = m
for j in range(k):
cur = mt[cur]
if cur == m:
break
res += (cur == m and j == k - 1)
return res
| Simple Fun #150: Robot Transfer | 58aaa3ca821a767300000017 | [
"Puzzles"
] | https://www.codewars.com/kata/58aaa3ca821a767300000017 | 6 kyu |
# Task
Given string `s`, which contains only letters from `a to z` in lowercase.
A set of alphabet is given by `abcdefghijklmnopqrstuvwxyz`.
2 sets of alphabets mean 2 or more alphabets.
Your task is to find the missing letter(s). You may need to output them by the order a-z. It is possible that there is more than one missing letter from more than one set of alphabet.
If the string contains all of the letters in the alphabet, return an empty string `""`
# Example
For `s='abcdefghijklmnopqrstuvwxy'`
The result should be `'z'`
For `s='aabbccddeeffgghhiijjkkllmmnnooppqqrrssttuuvvwwxxyy'`
The result should be `'zz'`
For `s='abbccddeeffgghhiijjkkllmmnnooppqqrrssttuuvvwwxxy'`
The result should be `'ayzz'`
For `s='codewars'`
The result should be `'bfghijklmnpqtuvxyz'`
# Input/Output
- `[input]` string `s`
Given string(s) contains one or more set of alphabets in lowercase.
- `[output]` a string
Find the letters contained in each alphabet but not in the string(s). Output them by the order `a-z`. If missing alphabet is repeated, please repeat them like `"bbccdd"`, not `"bcdbcd"` | games | from collections import Counter
from string import ascii_lowercase
def missing_alphabets(s):
c = Counter(s)
m = max(c . values())
return '' . join(letter * (m - c[letter]) for letter in ascii_lowercase)
| Simple Fun #135: Missing Alphabets | 58a664bb586e986c940001d5 | [
"Puzzles"
] | https://www.codewars.com/kata/58a664bb586e986c940001d5 | 6 kyu |
To introduce the problem think to my neighbor who drives a tanker truck.
The level indicator is down and he is worried
because he does not know if he will be able to make deliveries.
We put the truck on a horizontal ground and measured the height of the liquid in the tank.
Fortunately the tank is a perfect cylinder and the vertical walls on each end are flat.
The height of the remaining liquid is `h`, the diameter of the cylinder base is `d`,
the total volume is `vt` (h, d, vt are positive or null integers).
You can assume that `h` <= `d`.
Could you calculate the remaining volume of the liquid?
Your function `tankvol(h, d, vt)` returns an integer which is the truncated result (e.g floor)
of your float calculation.
#### Examples:
```
tankvol(40,120,3500) should return 1021 (calculation gives about: 1021.26992027)
tankvol(60,120,3500) should return 1750
tankvol(80,120,3500) should return 2478 (calculation gives about: 2478.73007973)
```
#### Tank vertical section:
 | reference | import math
def tankvol(h, d, vt):
r = d / 2
theta = math . acos((r - h) / r)
return int(vt * (theta - math . sin(theta) * (r - h) / r) / math . pi)
| Tank Truck | 55f3da49e83ca1ddae0000ad | [
"Mathematics",
"Geometry"
] | https://www.codewars.com/kata/55f3da49e83ca1ddae0000ad | 6 kyu |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.