text
stringlengths 2
104M
| meta
dict |
---|---|
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Union Find by size supporting undos
// Indices are 0-indexed
// Constructor Arguments:
// N: the number of elements in the set
// Fields:
// UF: a vector of integers representing the parent of each element in the
// tree, or the negative of the size of the set if that element is a root
// cnt: the current number of disjoint sets
// history: a vector of tuples storing the history of all join calls
// Functions:
// find(v): finds the root of the set containing element v
// join(v, w): merges the sets containing v and w, returns true if they
// were originally disjoint before the merge, false otherwise
// undo(): undoes the last call to join regardless of its return value
// by popping from the history stack
// connected(v, w): returns true if v and w are in the same set,
// false otherwise
// getSize(v): returns the size of the set containing element v
// In practice, has a small constant
// Time Complexity:
// constructor: O(N)
// find, join, connected, getSize: O(log N)
// undo: O(1)
// Memory Complexity: O(N + Q) for Q calls to join
// Tested:
// https://dmoj.ca/problem/apio19p2
struct UnionFindUndo {
vector<int> UF; int cnt; vector<tuple<int, int, int>> history;
UnionFindUndo(int N) : UF(N, -1), cnt(N) { history.reserve(N); }
int find(int v) { return UF[v] < 0 ? v : find(UF[v]); }
bool join(int v, int w) {
if ((v = find(v)) == (w = find(w))) {
history.emplace_back(v, w, 0); return false;
}
if (UF[v] > UF[w]) swap(v, w);
history.emplace_back(v, w, UF[w]);
UF[v] += UF[w]; UF[w] = v; cnt--; return true;
}
void undo() {
int v, w, ufw; tie(v, w, ufw) = history.back(); history.pop_back();
if (ufw == 0) return;
UF[w] = ufw; UF[v] -= UF[w]; cnt++;
}
bool connected(int v, int w) { return find(v) == find(w); }
int getSize(int v) { return -UF[find(v)]; }
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Weighted Union Find by size with path compression
// Indices are 0-indexed
// Template Arguments:
// T: the type of the weight
// Op: a struct with the cumulative operation to combine weights
// Required Functions:
// operator (l, r): combines the values l and r, must be associative and
// commutative
// Constructor Arguments:
// W: a vector of type T of the weights of each element in the set
// op: an instance of the Op struct
// Fields:
// UF: a vector of integers representing the parent of each element in the
// tree, or the negative of the size of the set if that element is a root
// W: a vector of type T representing the weight of all elements in the
// connected component if and only if that element is a root
// cnt: the current number of disjoint sets
// Functions:
// find(v): finds the root of the set containing element v
// join(v, w): merges the sets containing v and w, returns true if they
// were originally disjoint before the merge, false otherwise
// connected(v, w): returns true if v and w are in the same set,
// false otherwise
// getSize(v): returns the size of the set containing element v
// getWeight(v): returns the weight of the set containing element v
// In practice, has a small constant
// Time Complexity:
// constructor: O(N)
// find, join, connected: O(alpha N) amortized, O(log N) worse case
// getSize, getWeight: O(alpha N) amortized, O(log N) worse case
// Memory Complexity: O(N)
// Tested:
// https://dmoj.ca/problem/noi15p5
template <class T, class Op> struct WeightedUnionFind {
vector<int> UF; vector<T> W; int cnt; Op op;
WeightedUnionFind(vector<T> W, Op op = Op())
: UF(W.size(), -1), W(move(W)), cnt(UF.size()), op(op) {}
int find(int v) { return UF[v] < 0 ? v : UF[v] = find(UF[v]); }
bool join(int v, int w) {
if ((v = find(v)) == (w = find(w))) return false;
if (UF[v] > UF[w]) swap(v, w);
UF[v] += UF[w]; UF[w] = v; W[v] = op(move(W[v]), move(W[w])); cnt--;
return true;
}
bool connected(int v, int w) { return find(v) == find(w); }
int getSize(int v) { return -UF[find(v)]; }
T &getWeight(int v) { return W[find(v)]; }
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Union Find by size with path compression
// Indices are 0-indexed
// Constructor Arguments:
// N: the number of elements in the set
// Fields:
// UF: a vector of integers representing the parent of each element in the
// tree, or the negative of the size of the set if that element is a root
// cnt: the current number of disjoint sets
// Functions:
// find(v): finds the root of the set containing element v
// join(v, w): merges the sets containing v and w, returns true if they
// were originally disjoint before the merge, false otherwise
// connected(v, w): returns true if v and w are in the same set,
// false otherwise
// getSize(v): returns the size of the set containing element v
// In practice, has a small constant
// Time Complexity:
// constructor: O(N)
// find, join, connected, getSize: O(alpha N) amortized, O(log N) worse case
// Memory Complexity: O(N)
// Tested:
// Fuzz and Stress Tested
// https://judge.yosupo.jp/problem/unionfind
// https://dmoj.ca/problem/ds2
// https://atcoder.jp/contests/abc177/tasks/abc177_d
struct UnionFind {
vector<int> UF; int cnt; UnionFind(int N) : UF(N, -1), cnt(N) {}
int find(int v) { return UF[v] < 0 ? v : UF[v] = find(UF[v]); }
bool join(int v, int w) {
if ((v = find(v)) == (w = find(w))) return false;
if (UF[v] > UF[w]) swap(v, w);
UF[v] += UF[w]; UF[w] = v; cnt--; return true;
}
bool connected(int v, int w) { return find(v) == find(w); }
int getSize(int v) { return -UF[find(v)]; }
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Weighted Union Find by size supporting undos
// Indices are 0-indexed
// Template Arguments:
// T: the type of the weight
// Op: a struct with the cumulative operation to combine weights
// Required Functions:
// operator (l, r): combines the values l and r, must be associative and
// commutative
// Constructor Arguments:
// W: a vector of type T of the weights of each element in the set
// op: an instance of the Op struct
// Fields:
// UF: a vector of integers representing the parent of each element in the
// tree, or the negative of the size of the set if that element is a root
// W: a vector of type T representing the weight of all elements in the
// connected component if and only if that element is a root
// cnt: the current number of disjoint sets
// history: a vector of tuples storing the history of all join calls
// Functions:
// find(v): finds the root of the set containing element v
// join(v, w): merges the sets containing v and w, returns true if they
// were originally disjoint before the merge, false otherwise
// undo(): undoes the last call to join regardless of its return value
// by popping from the history stack
// connected(v, w): returns true if v and w are in the same set,
// false otherwise
// getSize(v): returns the size of the set containing element v
// getWeight(v): returns the weight of the set containing element v
// In practice, has a small constant
// Time Complexity:
// constructor: O(N)
// find, join, connected, getSize, getWeight: O(log N)
// undo: O(1)
// Memory Complexity: O(N + Q) for Q calls to join
// Tested:
// https://judge.yosupo.jp/problem/dynamic_graph_vertex_add_component_sum
template <class T, class Op> struct WeightedUnionFindUndo {
vector<int> UF; vector<T> W; int cnt;
vector<tuple<int, int, int, T, T>> history; Op op;
WeightedUnionFindUndo(vector<T> W, Op op = Op())
: UF(W.size(), -1), W(move(W)), cnt(UF.size()), op(op) {
history.reserve(W.size());
}
int find(int v) { return UF[v] < 0 ? v : find(UF[v]); }
bool join(int v, int w) {
if ((v = find(v)) == (w = find(w))) {
history.emplace_back(v, w, 0, W[v], W[w]); return false;
}
if (UF[v] > UF[w]) swap(v, w);
history.emplace_back(v, w, UF[w], W[v], W[w]);
UF[v] += UF[w]; UF[w] = v; W[v] = op(move(W[v]), move(W[w])); cnt--;
return true;
}
void undo() {
int v, w, ufw; T wv, ww; tie(v, w, ufw, wv, ww) = history.back();
history.pop_back(); if (ufw == 0) return;
UF[w] = ufw; UF[v] -= UF[w]; W[v] = wv; W[w] = ww; cnt++;
}
bool connected(int v, int w) { return find(v) == find(w); }
int getSize(int v) { return -UF[find(v)]; }
T &getWeight(int v) { return W[find(v)]; }
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
#include "../../search/BinarySearch.h"
using namespace std;
// Union Find by size supporting queries for a past version of
// the data structure but only allowed for updates to the latest version
// Constructor Arguments:
// N: the number of elements in the set
// Fields:
// UF: a vector of vectors of pairs containing the time that the parent or
// size has changed for an element, and the parent or size of the element
// at that time; the second element in the pair represents the parent of
// that element in the tree, or the negative of the size of the set if that
// element is a root
// cnt: a vector of pairs containing the time that the number of disjoint
// sets changes and the number of disjoint sets at that time
// curTime: the current time, begins at -1 before any joins, and increases
// by 1 after every join call
// Functions:
// find(t, v): finds the root of the set containing element v at time t
// join(v, w): merges the sets containing v and w, returns true if they
// were originally disjoint before the merge, false otherwise
// connected(t, v, w): returns true if v and w are in the same set,
// at time t, false otherwise
// getSize(t, v): returns the size of the set containing element v at time t
// getCnt(t): returns the number of disjoint sets at time t
// getFirst(v, w): returns the first time that elements v and w are in the
// same set, -1 if v == w and curTime + 1 if they are never in the
// same set
// In practice, find, join, connected, getSize, getCnt have a small constant,
// getFirst has a very small constant
// Time Complexity:
// constructor: O(N)
// find, join, connected, getSize, getCnt: O(log N)
// getFirst: O(log N log Q) after Q join operations
// Memory Complexity: O(N)
// Tested:
// https://codeforces.com/contest/1253/problem/F
struct PartiallyPersistentUnionFind {
vector<vector<pair<int, int>>> UF; vector<pair<int, int>> cnt; int curTime;
PartiallyPersistentUnionFind(int N)
: UF(N, vector<pair<int, int>>{make_pair(curTime, -1)}),
cnt{make_pair(curTime, N)}, curTime(-1) {}
int find(int t, int v) {
return UF[v].back().second < 0 || UF[v].back().first > t
? v : find(t, UF[v].back().second);
}
bool join(int v, int w) {
curTime++;
if ((v = find(curTime, v)) == (w = find(curTime, w))) return false;
int ufv = UF[v].back().second, ufw = UF[w].back().second;
if (ufv > ufw) { swap(v, w); swap(ufv, ufw); }
UF[v].emplace_back(curTime, ufv + ufw); UF[w].emplace_back(curTime, v);
cnt.emplace_back(curTime, cnt.back().second - 1); return true;
}
bool connected(int t, int v, int w) { return find(t, v) == find(t, w); }
int getSize(int t, int v) {
v = find(t, v);
return -prev(upper_bound(UF[v].begin(), UF[v].end(),
make_pair(t, INT_MAX)))->second;
}
int getCnt(int t) {
return prev(upper_bound(cnt.begin(), cnt.end(),
make_pair(t, INT_MAX)))->second;
}
int getFirst(int v, int w) {
return bsearch<FIRST>(-1, curTime + 1, [&] (int x) {
return connected(x, v, w);
});
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Persistent Array where copy assignment/constructor creates
// a new version of the data structure
// Indices are 0-indexed
// Template Arguments:
// T: the type of each element
// Constructor Arguments:
// N: the size of the array
// A: a vector of type T
// Functions:
// get(k): returns a constant reference to the kth element in the
// current version
// set(k, v): sets the kth element to index v in the current version
// size(): returns the size of the array
// In practice, has a moderate constant
// Time Complexity:
// constructor, get, set: O(log N)
// size: O(1)
// Memory Complexity: O(N + Q log N) for Q operations
// Tested:
// https://judge.yosupo.jp/problem/persistent_queue
template <class T> struct PersistentArray {
struct Node; using ptr = shared_ptr<Node>; int N; ptr root;
struct Node {
T v; ptr l, r;
Node(const T &v, const ptr &l = ptr(), const ptr &r = ptr())
: v(v), l(l), r(r) {}
};
ptr build(const vector<T> &A, int l, int r) {
if (l >= r) return l == r ? make_shared<Node>(A[l]) : ptr();
int m = l + (r - l) / 2;
ptr left = build(A, l, m - 1); ptr right = build(A, m + 1, r);
return make_shared<Node>(A[m], left, right);
}
ptr dfs(const ptr &x, int l, int r, int k, const T &v) {
int m = l + (r - l) / 2;
if (k < m) return make_shared<Node>(x->v, dfs(x->l, l, m - 1, k, v), x->r);
else if (k > m)
return make_shared<Node>(x->v, x->l, dfs(x->r, m + 1, r, k, v));
else return make_shared<Node>(v, x->l, x->r);
}
PersistentArray(const vector<T> &A)
: N(A.size()), root(build(A, 0, N - 1)) {}
PersistentArray(int N, const T &v = T())
: PersistentArray(vector<T>(N, v)) {}
const T &get(int k) const {
ptr x = root; for (int l = 0, r = N - 1, m; k != (m = l + (r - l) / 2);) {
if (k < m) { x = x->l; r = m - 1; }
else { x = x->r; l = m + 1; }
}
return x->v;
}
void set(int k, const T &v) { root = dfs(root, 0, N - 1, k, v); }
int size() const { return N; }
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Builds a cartesian tree where all vertices j in vertex i's subtree have
// !cmp(A[i], A[j]) and (cmp(A[j], A[i]) or j < i)
// Indices are 0-indexed
// Template Arguments:
// T: the type of each element in the array
// Cmp: the comparator to compare two values,
// convention is same as std::priority_queue in STL
// Required Functions:
// operator (a, b): returns true if and only if a compares less than b
// Function Arguments:
// A: a vector of type T
// cmp: an instance of the Cmp struct
// Return Value: a vector of integers representing the parent of each vertex
// in the cartesian tree, or -1 if that vertex is the root
// In practice, has a moderate constant
// Time Complexity: O(N)
// Memory Complexity: O(N)
// Tested:
// https://judge.yosupo.jp/problem/cartesian_tree
template <class T, class Cmp = less<T>>
vector<int> cartesianTree(const vector<T> &A, Cmp cmp = Cmp()) {
int N = A.size(), top = 0; vector<int> stk(N), P(N, -1);
for (int i = 0; i < N; stk[top++] = i++) {
int last = -1; while (top > 0 && cmp(A[stk[top - 1]], A[i])) {
if (last != -1) P[last] = stk[top - 1];
last = stk[--top];
}
if (last != -1) P[last] = i;
if (top > 0) P[i] = stk[top - 1];
}
return P;
}
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Binary Trie
// Template Arguments:
// T: the type of the values being inserted and queried
// Constructor Arguments:
// MX: the maximum value of v for any function call
// Functions:
// empty(): returns whether the trie is empty or not
// insert(v): inserts the value v into the trie if it doesn't already exist,
// returns whether it was inserted or not
// contains(v): returns whether the trie contains the value v
// erase(v): erases the value v into the trie if it exists,
// returns whether it was erased or not
// minXor(v): returns the minimum value of v xor any value in the trie, trie
// cannot be empty
// maxXor(v): returns the maximum value of v xor any value in the trie, trie
// cannot be empty
// Time Complexity:
// constructor: O(1)
// insert, contains, erase, minXor, maxXor: O(log MX)
// Memory Complexity: O(Q log MX) for Q insertions
// Tested:
// Fuzz Tested
// https://judge.yosupo.jp/problem/set_xor_min
// https://www.acmicpc.net/problem/16903
template <class T> struct BinaryTrie {
struct Node : public array<int, 2> {
Node() : array<int, 2>() { fill(-1); }
};
vector<Node> TR; vector<int> deleted; int root, lg;
int makeNode() {
if (deleted.empty()) { TR.emplace_back(); return int(TR.size()) - 1; }
int i = deleted.back(); deleted.pop_back(); TR[i] = Node(); return i;
}
BinaryTrie(T MX)
: root(-1), lg(MX == 0 ? 0 : __lg(MX)) {}
bool empty() { return root == -1; }
bool insert(T v) {
bool ret = false; if (root == -1) root = makeNode();
for (int cur = root, i = lg; i >= 0; i--) {
bool b = (v >> i) & 1;
if (TR[cur][b] == -1) { int n = makeNode(); ret = true; TR[cur][b] = n; }
cur = TR[cur][b];
}
return ret;
}
bool contains(T v) {
if (root == -1) return false;
for (int cur = root, i = lg; i >= 0; i--) {
bool b = (v >> i) & 1; if (TR[cur][b] == -1) return false;
cur = TR[cur][b];
}
return true;
}
bool erase(T v) {
bool ret = false;
function<int(int, int)> rec = [&] (int cur, int i) {
if (i == -1) { ret = true; deleted.push_back(cur); return -1; }
bool b = (v >> i) & 1; if (TR[cur][b] == -1) return cur;
TR[cur][b] = rec(TR[cur][b], i - 1);
if (TR[cur][0] == -1 && TR[cur][1] == -1) {
assert(ret); deleted.push_back(cur); return -1;
} else return cur;
};
if (root != -1) root = rec(root, lg);
return ret;
}
T minXor(T v) {
T ret = 0; for (int cur = root, i = lg; i >= 0; i--) {
bool b = (v >> i) & 1; if (TR[cur][b] == -1) {
ret ^= T(1) << i; cur = TR[cur][b ^ 1];
} else cur = TR[cur][b];
}
return ret;
}
T maxXor(T v) {
T ret = 0; for (int cur = root, i = lg; i >= 0; i--) {
bool b = (v >> i) & 1; if (TR[cur][b ^ 1] == -1) cur = TR[cur][b];
else { ret ^= T(1) << i; cur = TR[cur][b ^ 1]; }
}
return ret;
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
#include "Splay.h"
using namespace std;
// Operations on ranges of a dynamic array, backed by a splay tree
// Supports point/range updates/queries, range reversals,
// and binary searching as long as Node contains the appropriate flags
// Also supports insertion and erasing at an index or with a comparator
// (as long as all values in the tree are sorted by the same comparator),
// with insert_at inserting an element or range before the specified index
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// Node: typedef/using of the node class containing information about
// each node in the tree
// Required Fields:
// Data: the data type
// Lazy: the lazy type
// static const RANGE_UPDATES: a boolean indicating whether
// range updates are permitted
// static const RANGE_QUERIES: a boolean indicating whether
// range queries are permitted
// static const RANGE_REVERSALS: a boolean indicating whether
// range reversals are permitted
// l: a pointer to the left child
// r: a pointer to the right child
// val: the value of type Data being stored
// lz: only required if RANGE_UPDATES is true, the value of type
// Lazy to be propagated
// sbtr: only required if RANGE_QUERIES is true, the aggregate
// value of type Data for the subtree
// Required Functions:
// constructor(v): initializes a node with the value v
// propagate(): propagates the current node's lazy information
// (including rev) to its children
// apply(v): applies the lazy value v to the node
// reverse(): only required if RANGE_REVERSALS is true, reverse
// this node's subtree (aggregate data and any lazy
// flags should be reversed)
// static qdef(): only required if RANGE_QUERIES is true, returns the
// query default value
// Constructor Arguments:
// N: the size of the array
// A: the array
// Functions:
// insert_at(i, v): inserts a node before index i by passing v to the
// node constructor
// insert_at(i, A): inserts the elements in the vector A before index i,
// where the elements are passed to the node constructor in the order
// they appear
// insert(v, cmp): inserts a node before the first of node y where
// cmp(y->val, v) returns false, by passing v to the node constructor
// erase_at(i): erases the node at index i
// erase_at(j): erases the nodes between index i and j inclusive
// erase(v, cmp): erases the first node y where both cmp(y->val, v) and
// cmp(v, y->val) returns false
// update(i, v): updates the node at index i with the lazy value v
// update(i, j, v): only valid if Node::RANGE_UPDATES is true, updates the
// nodes between index i and j inclusive with the lazy value v
// reverse(i, j): only valid if Node::RANGE_REVERSALS is true, reverses the
// nodes between index i and j inclusive
// size(): returns the number of nodes in the trees
// at(i): returns the value of the node at index i
// lower_bound(v, cmp): returns the index and pointer to the value of the
// first node y such that cmp(y->val, v) returns false, returns
// {size(), nullptr} if none exist
// upper_bound(v, cmp): returns the index and pointer to the value of the
// first node y such that cmp(v, y->val) returns true, returns
// {size(), nullptr} if none exist
// find(v, cmp): returns the index and pointer to the value of the
// first node y such that both cmp(y->val, v) and cmp(v, y->val) return
// false, returns {size(), nullptr} if none exist
// query(i, j): only valid if Node::RANGE_QUERIES is true, returns the
// aggregate value of the nodes between index i and j inclusive,
// Node::qdef() if empty
// values(): returns a vector of the values of all nodes in the tree
// Time Complexity:
// constructor: O(N)
// insert, insert_at: O(log N + M) amortized for M inserted elements
// erase: erase_at: O(log N + M) amortized for M deleted elements
// update, reverse, at, lower_bound, upper_bound, find, query:
// O(log N) amortized
// values: O(N)
// size: O(1)
// Memory Complexity: O(N)
// Tested:
// https://dmoj.ca/problem/ds4 (insert, erase, at, find, values)
// https://codeforces.com/contest/863/problem/D (reverse)
// https://dmoj.ca/problem/acc1p1 (reverse, range queries)
// https://dmoj.ca/problem/noi05p2
// (insert_at, erase_at, range update, reverse, range queries)
// https://judge.yosupo.jp/problem/dynamic_sequence_range_affine_range_sum
// (insert_at, erase_at, range update, reverse, range queries)
template <class Node> struct DynamicRangeOperations : public Splay<Node> {
using Data = typename Node::Data; using Lazy = typename Node::Lazy;
Node *root; using Tree = Splay<Node>;
using Tree::makeNode; using Tree::applyToRange; using Tree::select;
using Tree::getFirst; using Tree::build; using Tree::clear;
template <class T>
DynamicRangeOperations(const vector<T> &A) : root(build(A)) {}
DynamicRangeOperations() : root(nullptr) {}
template <class T> void insert_at(int i, const T &v) {
applyToRange(root, i, i - 1, [&] (Node *&x) { x = makeNode(v); });
}
template <class T> void insert_at(int i, const vector<T> &A) {
applyToRange(root, i, i - 1, [&] (Node *&x) { x = build(A); });
}
template <class T, class Comp> void insert(const T &v, Comp cmp) {
insert_at(getFirst(root, v, cmp).first, v);
}
void erase_at(int i) {
applyToRange(root, i, i, [&] (Node *&x) { clear(x); x = nullptr; });
}
void erase_at(int i, int j) {
if (i <= j)
applyToRange(root, i, j, [&] (Node *&x) { clear(x); x = nullptr; });
}
template <class Comp> void erase(const Data &v, Comp cmp) {
pair<int, Node *> p = getFirst(root, v, cmp);
if (p.second && !cmp(p.second->val, v) && !cmp(v, p.second->val))
erase_at(p.first);
}
void update(int i, const Lazy &v) {
applyToRange(root, i, i, [&] (Node *&x) { x->apply(v); });
}
template <const int _ = Node::RANGE_UPDATES>
typename enable_if<_>::type update(int i, int j, const Lazy &v) {
if (i <= j) applyToRange(root, i, j, [&] (Node *&x) { x->apply(v); });
}
template <const int _ = Node::RANGE_REVERSALS>
typename enable_if<_>::type reverse(int i, int j) {
if (i <= j) applyToRange(root, i, j, [&] (Node *&x) { x->reverse(); });
}
int size() { return root ? root->sz : 0; }
Data at(int i) { return select(root, i)->val; }
template <class Comp>
pair<int, Data *> lower_bound(const Data &v, Comp cmp) {
pair<int, Node *> p = getFirst(root, v, cmp);
return make_pair(p.first, p.second ? &p.second->val : nullptr);
}
template <class Comp>
pair<int, Data *> upper_bound(const Data &v, Comp cmp) {
return lower_bound(v, [&] (const Data &a, const Data &b) {
return !cmp(b, a);
});
}
template <class Comp> pair<int, Data *> find(const Data &v, Comp cmp) {
pair<int, Data *> ret = lower_bound(v, cmp);
if (!ret.second || cmp(v, *(ret.second)) || cmp(*(ret.second), v))
return make_pair(size(), nullptr);
return ret;
}
template <const int _ = Node::RANGE_QUERIES>
typename enable_if<_, Data>::type query(int i, int j) {
Data ret = Node::qdef();
if (i <= j) applyToRange(root, i, j, [&] (Node *&x) { ret = x->sbtr; });
return ret;
}
vector<Data> values() {
vector<Data> ret; ret.reserve(size());
function<void(Node *)> dfs = [&] (Node *x) {
if (!x) return;
x->propagate(); dfs(x->l); ret.push_back(x->val); dfs(x->r);
};
dfs(root); return ret;
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Sample node structs for Splay, DynamicRangeOperations,
// and LinkCutTree with different required fields required for each
// Sample node class for a single value of type T, supporting range reversals,
// and point assignment
// Template Arguments:
// T: the type of value to store
// Constructor Arguments:
// v: the value of type Data to store
// Fields:
// Data: the data type, equivalent to T
// Lazy: the lazy type, equivalent to T
// static const RANGE_UPDATES: a boolean indicating whether range updates
// are permitted
// static const RANGE_QUERIES: a boolean indicating whether range queries
// are permitted
// static const RANGE_REVERSALS: a boolean indicating whether range reversals
// are permitted
// rev: a boolean indicating whether the subtree needs to be reversed
// sz: the number of nodes in the subtree
// l: a pointer to the left child
// r: a pointer to the right child
// p: a pointer to the parent
// val: the value of type Data being stored
// Functions:
// update(): updates the current node's information based on its children
// propagate(): propagates the current node's lazy information (including
// rev) to its children
// apply(v): applies the lazy value v to the node
// reverse(): reverse this node's subtree (lazy flags
// should be reversed)
// Tested:
// https://dmoj.ca/problem/ds4
// https://codeforces.com/contest/1288/problem/E
// https://codeforces.com/contest/863/problem/D
// https://codeforces.com/contest/13/problem/E
// https://oj.uz/problem/view/JOI13_synchronization
template <class T> struct NodeVal {
using Data = T; using Lazy = Data;
static const bool RANGE_UPDATES = false, RANGE_QUERIES = false;
static const bool RANGE_REVERSALS = true;
bool rev; int sz; NodeVal *l, *r, *p; Data val;
NodeVal(const Data &v)
: rev(false), sz(1), l(nullptr), r(nullptr), p(nullptr), val(v) {}
void update() {
sz = 1;
if (l) sz += l->sz;
if (r) sz += r->sz;
}
void propagate() {
if (rev) {
if (l) l->reverse();
if (r) r->reverse();
rev = false;
}
}
void apply(const Lazy &v) { val = v; }
void reverse() { rev = !rev; swap(l, r); }
};
// Sample node class for aggregate range queries using a struct to combine
// Data and Lazy, along with range reversals, and point assignment
// Template Arguments:
// C: struct to combine data and lazy values
// Required Fields:
// Data: the data type
// Lazy: the lazy type
// Required Functions:
// static qdef(): returns the query default value of type Data
// static merge(l, r): returns the values l of type Data merged with
// r of type Data, must be associative
// static applyLazy(l, r): returns the value r of type Lazy applied to
// l of type Data, must be associative
// static revData(v): reverses the value v of type Data
// Sample Struct: supporting point increments and range max queries
// struct C {
// using Data = int;
// using Lazy = int;
// static Data qdef() { return numeric_limits<int>::min(); }
// static Data merge(const Data &l, const Data &r) {
// return max(l, r);
// }
// static Data applyLazy(const Data &l, const Lazy &r) {
// return l + r;
// }
// static void revData(Data &v) {}
// };
// Constructor Arguments:
// v: the value of type Data to store
// Fields:
// Data: the data type, equivalent to C::Data
// Lazy: the lazy type, equivalent to C::Lazy
// static const RANGE_UPDATES: a boolean indicating whether range updates
// are permitted
// static const RANGE_QUERIES: a boolean indicating whether range queries
// are permitted
// static const RANGE_REVERSALS: a boolean indicating whether range reversals
// are permitted
// rev: a boolean flag indicating whether the subtree needs to be reversed
// sz: the number of nodes in the subtree
// l: a pointer to the left child
// r: a pointer to the right child
// p: a pointer to the parent
// val: the value of type Data being stored
// sbtr: the aggregate value of type Data for the subtree
// Functions:
// update(): updates the current node's information based on its children
// propagate(): propagates the current node's lazy information (including
// rev) to its children
// apply(v): applies the lazy value v to the node
// reverse(): reverse this node's subtree (aggregate data and any
// lazy flags should be reversed)
// static qdef(): returns the query default value for the struct C
// Tested:
// https://dmoj.ca/problem/dmpg17g2
// https://dmoj.ca/problem/coi08p2
// https://www.spoj.com/problems/QTREE2/
// https://judge.yosupo.jp/problem/dynamic_tree_vertex_set_path_composite
template <class C> struct NodeAgg {
using Data = typename C::Data; using Lazy = typename C::Lazy;
static const bool RANGE_UPDATES = false, RANGE_QUERIES = true;
static const bool RANGE_REVERSALS = true;
bool rev; int sz; NodeAgg *l, *r, *p; Data val, sbtr;
NodeAgg(const Data &v)
: rev(false), sz(1), l(nullptr), r(nullptr), p(nullptr),
val(v), sbtr(v) {}
void update() {
sz = 1; sbtr = val;
if (l) { sz += l->sz; sbtr = C::merge(l->sbtr, sbtr); }
if (r) { sz += r->sz; sbtr = C::merge(sbtr, r->sbtr); }
}
void propagate() {
if (rev) {
if (l) l->reverse();
if (r) r->reverse();
rev = false;
}
}
void apply(const Lazy &v) {
val = C::applyLazy(val, v); sbtr = C::applyLazy(sbtr, v);
}
void reverse() { rev = !rev; swap(l, r); C::revData(sbtr); }
static Data qdef() { return C::qdef(); }
};
// Sample node class for aggregate range queries and lazy range updates
// using a struct to combine Data and Lazy, along with range reversals
// Template Arguments:
// C: struct to combine data and lazy values
// Required Fields:
// Data: the data type
// Lazy: the lazy type
// Required Functions:
// static qdef(): returns the query default value of type Data
// static merge(l, r): returns the values l of type Data merged with
// r of type Data, must be associative
// static applyLazy(l, r): returns the value r of type Lazy applied to
// l of type Data, must be associative
// static applyLazy(l, r, k): returns the value r of type Lazy applied to
// l of type Data over a segment of length k, must be associative
// static mergeLazy(l, r): returns the values l of type Lazy merged with
// r of type Lazy, must be associative
// static revData(v): reverses the value v of type Data
// Sample Struct: supporting range assignments and range sum queries
// struct C {
// using Data = int;
// using Lazy = int;
// static Data qdef() { return 0; }
// static Lazy ldef() { return numeric_limits<int>::min(); }
// static Data merge(const Data &l, const Data &r) { return l + r; }
// static Data applyLazy(const Data &l, const Lazy &r, int k) {
// return r * k;
// }
// static Lazy mergeLazy(const Lazy &l, const Lazy &r) { return r; }
// static void revData(Data &v) {}
// };
// Constructor Arguments:
// v: the value of type Data to store
// Fields:
// Data: the data type, equivalent to C::Data
// Lazy: the lazy type, equivalent to C::Lazy
// static const RANGE_UPDATES: a boolean indicating whether range updates
// are permitted
// static const RANGE_QUERIES: a boolean indicating whether range queries
// are permitted
// static const RANGE_REVERSALS: a boolean indicating whether range reversals
// are permitted
// rev: a boolean flag indicating whether the subtree needs to be reversed
// sz: the number of nodes in the subtree
// l: a pointer to the left child
// r: a pointer to the right child
// p: a pointer to the parent
// lz: the value of type Lazy to be propagated
// val: the value of type Data being stored
// sbtr: the aggregate value of type Data for the subtree
// Functions:
// update(): updates the current node's information based on its children
// propagate(): propagates the current node's lazy information (including
// rev) to its children
// apply(v): applies the lazy value v to the node
// reverse(): reverse this node's subtree (aggregate data and any
// lazy flags should be reversed)
// static qdef(): returns the query default value for the struct C
// Tested:
// https://dmoj.ca/problem/acc1p1
// https://dmoj.ca/problem/noi05p2
// https://dmoj.ca/problem/ds5easy
// https://judge.yosupo.jp/problem/dynamic_sequence_range_affine_range_sum
template <class C> struct NodeLazyAgg {
using Data = typename C::Data; using Lazy = typename C::Lazy;
static const bool RANGE_UPDATES = true, RANGE_QUERIES = true;
static const bool RANGE_REVERSALS = true;
bool rev; int sz; NodeLazyAgg *l, *r, *p; Lazy lz; Data val, sbtr;
NodeLazyAgg(const Data &v)
: rev(false), sz(1), l(nullptr), r(nullptr), p(nullptr),
lz(C::ldef()), val(v), sbtr(v) {}
void update() {
sz = 1; sbtr = val;
if (l) { sz += l->sz; sbtr = C::merge(l->sbtr, sbtr); }
if (r) { sz += r->sz; sbtr = C::merge(sbtr, r->sbtr); }
}
void propagate() {
if (rev) {
if (l) l->reverse();
if (r) r->reverse();
rev = false;
}
if (lz != C::ldef()) {
if (l) l->apply(lz);
if (r) r->apply(lz);
lz = C::ldef();
}
}
void apply(const Lazy &v) {
lz = C::mergeLazy(lz, v); val = C::applyLazy(val, v, 1);
sbtr = C::applyLazy(sbtr, v, sz);
}
void reverse() { rev = !rev; swap(l, r); C::revData(sbtr); }
static Data qdef() { return C::qdef(); }
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Generic Splay Tree node operations supporting a generic node class (such
// as the structs in BSTNode)
// Can be used in conjunction with DynamicRangeOperations to support
// range operations using splay node operations
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// Node: a generic node class (sample structs are in BSTNode)
// Required Fields:
// sz: the number of nodes in the subtree
// l: a pointer to the left child
// r: a pointer to the right child
// p: a pointer to the parent
// val: only required if getFirst is called, the value being stored
// Required Functions:
// constructor(v): initializes a node with the value v
// update(): updates the current node's information based on its children
// propagate(): propagates the current node's lazy information (including
// rev) to its children
// Container: a container to contain Node objects (or some child class of
// Node), recommended to use deque<Node> or vector<Node> if there is
// a fixed number of nodes and TR can be reserved beforehand
// Fields:
// TR: a container of type Container to store the nodes (including
// potentially deleted nodes)
// deleted: a deque of node pointers to store deleted nodes
// Functions:
// splay(x): splays the node x to the root of the tree
// makeNode(v): creates a new node passing v to the node constructor
// applyToRange(root, i, j, f): applies the function f (accepting a
// node pointer or reference to a node pointer) to a node x where x
// is the disconnected subtree with indices in the range [i, j] for the
// tree rooted at root (passed by reference)
// select(root, k): returns the kth node (0-indexed) in the subtree of root,
// and makes it the new root (or the last node accessed if null)
// index(root, x): returns the index of node x in the tree rooted at root,
// and makes it the new root
// getFirst(root, v, cmp): returns the first node y (and its index) in the
// tree rooted at root where cmp(y->val, v) returns false, and makes it the
// new root (or the last node accessed if null)
// build(A): builds a splay tree on the array A
// clear(x): adds all nodes in the subtree of x to the deleted buffer
// In practice, has a moderate constant, not as fast as segment trees
// Time Complexity:
// build, clear: O(N)
// makeNode: O(1)
// splay, applyToRange, select, index, getFirst: O(log N) amortized
// Memory Complexity: O(N)
// Tested:
// https://dmoj.ca/problem/ds4 (applyToRange, select, getFirst)
// https://codeforces.com/contest/1288/problem/E (applyToRange, index)
// https://codeforces.com/contest/863/problem/D (applyToRange)
// https://dmoj.ca/problem/dmpg17g2 (applyToRange)
// https://dmoj.ca/problem/acc1p1 (applyToRange)
// https://dmoj.ca/problem/noi05p2 (applyToRange)
// https://judge.yosupo.jp/problem/dynamic_sequence_range_affine_range_sum
// (applyToRange)
template <class Node, class Container = deque<Node>> struct Splay {
Container TR; deque<Node *> deleted;
template <class T> Node *makeNode(const T &v) {
if (deleted.empty()) { TR.emplace_back(v); return &TR.back(); }
Node *x = deleted.back(); deleted.pop_back();
*x = typename Container::value_type(v); return x;
}
bool isRoot(Node *x) { return !x->p || (x != x->p->l && x != x->p->r); }
void connect(Node *x, Node *p, bool hasCh, bool isL) {
if (x) x->p = p;
if (hasCh) (isL ? p->l : p->r) = x;
}
void rotate(Node *x) {
Node *p = x->p, *g = p->p; bool isRootP = isRoot(p), isL = x == p->l;
connect(isL ? x->r : x->l, p, true, isL); connect(p, x, true, !isL);
connect(x, g, !isRootP, !isRootP && p == g->l); p->update();
}
void splay(Node *x) {
while (!isRoot(x)) {
Node *p = x->p, *g = p->p; if (!isRoot(p)) g->propagate();
p->propagate(); x->propagate();
if (!isRoot(p)) rotate((x == p->l) == (p == g->l) ? p : x);
rotate(x);
}
x->propagate(); x->update();
}
template <class F> void applyToRange(Node *&root, int i, int j, F f) {
if (i == 0 && j == (root ? root->sz : 0) - 1) {
f(root); if (root) { root->propagate(); root->update(); }
} else {
Node *t = i ? select(root, i - 1)->r : select(root, j + 1)->l;
connect(nullptr, root, true, !i); root->update();
connect(t, nullptr, false, !i); applyToRange(t, 0, j - i, f);
connect(t, root, true, !i); root->update();
}
}
Node *select(Node *&root, int k) {
Node *last = nullptr; while (root) {
(last = root)->propagate(); int t = root->l ? root->l->sz : 0;
if (t > k) root = root->l;
else if (t < k) { root = root->r; k -= t + 1; }
else break;
}
if (last) splay(root = last);
return root;
}
int index(Node *&root, Node *x) {
root = x; if (!root) return -1;
splay(root); return root->l ? root->l->sz : 0;
}
template <class T, class Comp>
pair<int, Node *> getFirst(Node *&root, const T &v, Comp cmp) {
pair<int, Node *> ret(0, nullptr); Node *last = nullptr; while (root) {
(last = root)->propagate();
if (!cmp(root->val, v)) { ret.second = root; root = root->l; }
else { ret.first += 1 + (root->l ? root->l->sz : 0); root = root->r; }
}
if (last) splay(root = last);
return ret;
}
template <class T> Node *buildRec(const vector<T> &A, int l, int r) {
if (l > r) return nullptr;
int m = l + (r - l) / 2; Node *left = buildRec(A, l, m - 1);
Node *ret = makeNode(A[m]), *right = buildRec(A, m + 1, r);
connect(left, ret, ret, true); connect(right, ret, ret, false);
ret->update(); return ret;
}
template <class T>
Node *build(const vector<T> &A) { return buildRec(A, 0, int(A.size()) - 1); }
void clear(Node *x) {
if (!x) return;
clear(x->l); deleted.push_back(x); clear(x->r);
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Bottom up 2D segment tree supporting point updates and range queries
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// C: struct to combine data and lazy values
// Required Fields:
// Data: the data type
// Lazy: the lazy type
// Required Functions:
// static qdef(): returns the query default value of type Data
// static merge(l, r): returns the values l of type Data merged with
// r of type Data, must be associative
// static applyLazy(l, r): returns the value r of type Lazy applied to
// l of type Data, must be associative
// Sample Struct: supporting point increments and range max queries
// struct C {
// using Data = int;
// using Lazy = int;
// static Data qdef() { return numeric_limits<int>::min(); }
// static Data merge(const Data &l, const Data &r) {
// return max(l, r);
// }
// static Data applyLazy(const Data &l, const Lazy &r) {
// return l + r;
// }
// };
// Constructor Arguments:
// N: the size of the first dimension of the array
// M: the size of the second dimension of the array
// A: a 2D vector of elements of type Data
// vdef: the default value to fill the array with
// Functions:
// update(i, j, v): updates the value A[i][j] with the lazy value v
// query(u, d, l, r): queries the range [u, d] in the first dimension and
// [l, r] in the second dimension and returns the aggregate value
// In practice, has a small constant, not quite as fast as fenwick trees
// Time Complexity:
// constructor: O(NM)
// update, query: O(log N log M)
// Memory Complexity: O(NM)
// Tested:
// Fuzz and Stress Tested
template <class C> struct SegmentTreeBottomUp2D {
using Data = typename C::Data; using Lazy = typename C::Lazy;
int N, M; vector<vector<Data>> TR;
void build() {
for (int i = 0; i < N; i++) for (int j = M - 1; j > 0; j--)
TR[N + i][j] = C::merge(TR[N + i][j * 2], TR[N + i][j * 2 + 1]);
for (int i = N - 1; i > 0; i--) {
for (int j = 0; j < M; j++)
TR[i][M + j] = C::merge(TR[i * 2][M + j], TR[i * 2 + 1][M + j]);
for (int j = M - 1; j > 0; j--)
TR[i][j] = C::merge(TR[i][j * 2], TR[i][j * 2 + 1]);
}
}
SegmentTreeBottomUp2D(const vector<vector<Data>> &A)
: N(A.size()), M(N == 0 ? 0 : A[0].size()),
TR(N * 2, vector<Data>(M * 2, C::qdef())) {
for (int i = 0; i < N; i++)
copy(A[i].begin(), A[i].end(), TR[N + i].begin() + M);
build();
}
SegmentTreeBottomUp2D(int N, int M, const Data &vdef)
: N(N), M(M), TR(N * 2, vector<Data>(M * 2, C::qdef())) {
for (int i = 0; i < N; i++)
fill(TR[N + i].begin() + M, TR[N + i].end(), vdef);
build();
}
void update(int i, int j, const Lazy &v) {
for (int ii = N + i; ii > 0; ii /= 2) {
if (ii == N + i) TR[N + i][M + j] = C::applyLazy(TR[N + i][M + j], v);
else TR[ii][M + j] = C::merge(TR[ii * 2][M + j], TR[ii * 2 + 1][M + j]);
for (int jj = M + j; jj /= 2;)
TR[ii][jj] = C::merge(TR[ii][jj * 2], TR[ii][jj * 2 + 1]);
}
}
Data query(int u, int d, int l, int r) {
auto query = [&] (int i, int l, int r) {
Data ql = C::qdef(), qr = C::qdef();
for (l += M, r += M; l <= r; l /= 2, r /= 2) {
if (l % 2) ql = C::merge(ql, TR[i][l++]);
if (!(r % 2)) qr = C::merge(TR[i][r--], qr);
}
return C::merge(ql, qr);
};
Data qu = C::qdef(), qd = C::qdef();
for (u += N, d += N; u <= d; u /= 2, d /= 2) {
if (u % 2) qu = C::merge(qu, query(u++, l, r));
if (!(d % 2)) qd = C::merge(query(d--, l, r), qd);
}
return C::merge(qu, qd);
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Top down segment tree supporting point updates and range queries,
// with a lazy template argument to enable range updates
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// LAZY: boolean to indicate whether or not range updates are enabled
// C: struct to combine data and lazy values
// Required Fields:
// Data: the data type
// Lazy: the lazy type
// Required Functions:
// static qdef(): returns the query default value of type Data
// static ldef(): only required if LAZY is true, returns the lazy
// default value of type Lazy
// static merge(l, r): returns the values l of type Data merged with
// r of type Data, must be associative
// static applyLazy(l, r): only required if LAZY is false, returns the
// value r of type Lazy applied to l of type Data, must be associative
// static applyLazy(l, r, k): only required if LAZY is true, returns the
// value r of type Lazy applied to l of type Data over a segment of
// length k, must be associative
// static mergeLazy(l, r): only required if LAZY is true, returns
// the values l of type Lazy merged with r of type Lazy,
// must be associative
// Sample Struct: C1 supports point increments and range max queries,
// while C2 supports range assignments and range sum queries
// struct C1 {
// using Data = int;
// using Lazy = int;
// static Data qdef() { return numeric_limits<int>::min(); }
// static Data merge(const Data &l, const Data &r) {
// return max(l, r);
// }
// static Data applyLazy(const Data &l, const Lazy &r) {
// return l + r;
// }
// };
// struct C2 {
// using Data = int;
// using Lazy = int;
// static Data qdef() { return 0; }
// static Lazy ldef() { return numeric_limits<int>::min(); }
// static Data merge(const Data &l, const Data &r) { return l + r; }
// static Data applyLazy(const Data &l, const Lazy &r, int k) {
// return r * k;
// }
// static Lazy mergeLazy(const Lazy &l, const Lazy &r) { return r; }
// };
// Constructor Arguments:
// N: the size of the array
// A: a vector of type C::Data
// Functions:
// update(i, v): updates the index i with the lazy value v
// update(l, r, v): update the range [l, r] with the lazy value v
// query(l, r): queries the range [l, r] and returns the aggregate value
// bsearchPrefix(l, r, f): returns the smallest index i in the range [l, r]
// such that f(query(l, i)) returns true or r + 1 if none exist
// bsearchSuffix(l, r, f): returns the largest index i in the range [l, r]
// such that f(query(i, r)) returns true or l - 1 if none exist
// In practice, has a small constant, not quite as fast as fenwick trees
// or bottom up non lazy segment trees,
// and similar performance as bottom up lazy segment trees
// Time Complexity:
// constructor: O(N)
// update, query, bsearchPrefix, bsearchSuffix: O(log N)
// Memory Complexity: O(N)
// Tested:
// https://dmoj.ca/problem/ds3 (LAZY = false)
// https://dmoj.ca/problem/dmpg17g2 (LAZY = false)
// https://dmoj.ca/problem/coci17c1p5 (LAZY = false, bsearchPrefix)
// https://judge.yosupo.jp/problem/point_set_range_composite (LAZY = false)
// https://dmoj.ca/problem/lazy (LAZY = true)
// https://mcpt.ca/problem/seq3 (LAZY = true)
// https://judge.yosupo.jp/problem/range_affine_range_sum (LAZY = true)
// https://dmoj.ca/problem/dmopc17c4p6 (LAZY = true)
template <const bool LAZY, class C> struct SegmentTreeTopDown {
#define lazy_def template <const bool _ = LAZY> typename enable_if<_>::type
#define agg_def template <const bool _ = LAZY> typename enable_if<!_>::type
using Data = typename C::Data; using Lazy = typename C::Lazy;
int N; vector<Data> TR; vector<Lazy> LZ;
lazy_def apply(int x, int tl, int tr, const Lazy &v) {
TR[x] = C::applyLazy(TR[x], v, tr - tl + 1);
if (x < int(LZ.size())) LZ[x] = C::mergeLazy(LZ[x], v);
}
agg_def apply(int x, int, int, const Lazy &v) {
TR[x] = C::applyLazy(TR[x], v);
}
lazy_def propagate(int x, int tl, int tr) {
if (LZ[x] != C::ldef()) {
int m = tl + (tr - tl) / 2; apply(x * 2, tl, m, LZ[x]);
apply(x * 2 + 1, m + 1, tr, LZ[x]); LZ[x] = C::ldef();
}
}
agg_def propagate(int, int, int) {}
void build(const vector<Data> &A, int x, int tl, int tr) {
if (tl == tr) { TR[x] = A[tl]; return; }
int m = tl + (tr - tl) / 2;
build(A, x * 2, tl, m); build(A, x * 2 + 1, m + 1, tr);
TR[x] = C::merge(TR[x * 2], TR[x * 2 + 1]);
}
void update(int x, int tl, int tr, int l, int r, const Lazy &v) {
if (l <= tl && tr <= r) { apply(x, tl, tr, v); return; }
propagate(x, tl, tr); int m = tl + (tr - tl) / 2;
if (tl <= r && l <= m) update(x * 2, tl, m, l, r, v);
if (m + 1 <= r && l <= tr) update(x * 2 + 1, m + 1, tr, l, r, v);
TR[x] = C::merge(TR[x * 2], TR[x * 2 + 1]);
}
Data query(int x, int tl, int tr, int l, int r) {
if (r < tl || tr < l) return C::qdef();
if (l <= tl && tr <= r) return TR[x];
propagate(x, tl, tr); int m = tl + (tr - tl) / 2;
return C::merge(query(x * 2, tl, m, l, r),
query(x * 2 + 1, m + 1, tr, l, r));
}
template <class F>
int bsearchPrefix(int x, int tl, int tr, int l, int r, Data &agg, F f) {
if (r < tl || tr < l) return r + 1;
if (tl != tr) propagate(x, tl, tr);
if (l <= tl && tr <= r) {
Data v = C::merge(agg, TR[x]); if (!f(v)) { agg = v; return r + 1; }
}
if (tl == tr) return tl;
int m = tl + (tr - tl) / 2;
int ret = bsearchPrefix(x * 2, tl, m, l, r, agg, f);
return ret <= r ? ret : bsearchPrefix(x * 2 + 1, m + 1, tr, l, r, agg, f);
}
template <class F>
int bsearchSuffix(int x, int tl, int tr, int l, int r, Data &agg, F f) {
if (r < tl || tr < l) return l - 1;
if (tl != tr) propagate(x, tl, tr);
if (l <= tl && tr <= r) {
Data v = C::merge(TR[x], agg); if (!f(v)) { agg = v; return l - 1; }
}
if (tl == tr) return tl;
int m = tl + (tr - tl) / 2;
int ret = bsearchSuffix(x * 2 + 1, m + 1, tr, l, r, agg, f);
return l <= ret ? ret : bsearchSuffix(x * 2, tl, m, l, r, agg, f);
}
SegmentTreeTopDown(int N)
: N(N), TR(N == 0 ? 0 : 1 << __lg(N * 4 - 1), C::qdef()) {
if (LAZY) LZ.resize(N == 0 ? 0 : 1 << __lg(N * 2 - 1), C::ldef());
}
SegmentTreeTopDown(const vector<Data> &A) : SegmentTreeTopDown(A.size()) {
if (N > 0) { build(A, 1, 0, N - 1); }
}
lazy_def update(int l, int r, const Lazy &v) {
update(1, 0, N - 1, l, r, v);
}
void update(int i, const Lazy &v) { update(1, 0, N - 1, i, i, v); }
Data query(int l, int r) { return query(1, 0, N - 1, l, r); }
template <class F> int bsearchPrefix(int l, int r, F f) {
Data agg = C::qdef(); return bsearchPrefix(1, 0, N - 1, l, r, agg, f);
}
template <class F> int bsearchSuffix(int l, int r, F f) {
Data agg = C::qdef(); return bsearchSuffix(1, 0, N - 1, l, r, agg, f);
}
#undef lazy_def
#undef agg_def
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Top down segment tree supporting advanced range updates and queries
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// C: struct to combine data and lazy values
// Required Fields:
// Data: the data type
// Lazy: the lazy type
// Arg: the argument type
// Required Functions:
// static qdef(): returns the query default value of type Data
// static ldef(): returns the lazy default value of type Lazy
// static makeLazy(d, a): returns a lazy value created from the Data d
// and Arg a
// static breakCond(d, a): returns the condition for when to stop the
// recusion based on Data d and Arg a
// static tagCond(d, a): returns the condition for when to tag
// based on Data d and Arg a
// static merge(l, r): returns the values l of type Data merged with
// r of type Data, must be associative
// static applyLazy(l, r, k): returns the value r of type Lazy applied to
// l of type Data over a segment of length k, must be associative
// static mergeLazy(l, r): returns the values l of type Lazy merged with
// r of type Lazy, must be associative
// Sample Struct: supporting range modulo and range sum queries
// struct C {
// struct Data { int sm, mx, mn; };
// using Lazy = int;
// using Arg = int;
// static Data qdef() {
// Data ret; ret.sm = 0;
// ret.mx = numeric_limits<int>::min();
// ret.mn = numeric_limits<int>::max();
// return ret;
// }
// static Lazy ldef() { return -1; }
// static Lazy makeLazy(const Data &d, const Arg &a) { return a; }
// static bool breakCond(const Data &d, const Arg &a) {
// return d.mx < a;
// }
// static bool tagCond(const Data &d, const Arg &a) {
// return d.mn == d.mx;
// }
// static Data merge(const Data &l, const Data &r) {
// Data ret; ret.sm = l.sm + r.sm;
// ret.mx = max(l.mx, r.mx); ret.mn = min(l.mn, r.mn); return ret;
// }
// static Data applyLazy(const Data &l, const Lazy &r, int k) {
// Data ret; ret.sm = r * k; ret.mx = ret.mn = r; return ret;
// }
// static Lazy mergeLazy(const Lazy &l, const Lazy &r) { return r; }
// };
// Constructor Arguments:
// N: the size of the array
// A: a vector of type C::Data
// Functions:
// update(l, r, v): update the range [l, r] with the Arg v
// query(l, r): queries the range [l, r] and returns the aggregate value
// bsearchPrefix(l, r, f): returns the smallest index i in the range [l, r]
// such that f(query(l, i)) returns true or r + 1 if none exist
// bsearchSuffix(l, r, f): returns the largest index i in the range [l, r]
// such that f(query(i, r)) returns true of l - 1 if none exist
// In practice, has a small constant
// Time Complexity:
// constructor: O(N)
// update: dependent on C
// query, bsearchPrefix, bsearchSuffix: O(log N)
// Memory Complexity: O(N)
// Tested:
// https://codeforces.com/contest/438/problem/D
// https://judge.yosupo.jp/problem/range_chmin_chmax_add_range_sum
template <class C> struct SegmentTreeBeats {
using Data = typename C::Data; using Lazy = typename C::Lazy;
using Arg = typename C::Arg;
struct Node {
Data val; Lazy lz; Node() : val(C::qdef()), lz(C::ldef()) {}
};
int N; vector<Node> TR;
void apply(int x, int tl, int tr, const Lazy &v) {
TR[x].val = C::applyLazy(TR[x].val, v, tr - tl + 1);
TR[x].lz = C::mergeLazy(TR[x].lz, v);
}
void propagate(int x, int tl, int tr) {
if (TR[x].lz != C::ldef()) {
int m = tl + (tr - tl) / 2; apply(x * 2, tl, m, TR[x].lz);
apply(x * 2 + 1, m + 1, tr, TR[x].lz); TR[x].lz = C::ldef();
}
}
void build(const vector<Data> &A, int x, int tl, int tr) {
if (tl == tr) { TR[x].val = A[tl]; return; }
int m = tl + (tr - tl) / 2;
build(A, x * 2, tl, m); build(A, x * 2 + 1, m + 1, tr);
TR[x].val = C::merge(TR[x * 2].val, TR[x * 2 + 1].val);
}
void update(int x, int tl, int tr, int l, int r, const Arg &v) {
if (r < tl || tr < l || C::breakCond(TR[x].val, v)) return;
if (l <= tl && tr <= r && C::tagCond(TR[x].val, v)) {
Lazy lz = C::makeLazy(TR[x].val, v);
TR[x].val = C::applyLazy(TR[x].val, lz, tr - tl + 1);
TR[x].lz = C::mergeLazy(TR[x].lz, lz); return;
}
propagate(x, tl, tr); int m = tl + (tr - tl) / 2;
update(x * 2, tl, m, l, r, v); update(x * 2 + 1, m + 1, tr, l, r, v);
TR[x].val = C::merge(TR[x * 2].val, TR[x * 2 + 1].val);
}
Data query(int x, int tl, int tr, int l, int r) {
if (r < tl || tr < l) return C::qdef();
if (l <= tl && tr <= r) return TR[x].val;
propagate(x, tl, tr); int m = tl + (tr - tl) / 2;
return C::merge(query(x * 2, tl, m, l, r),
query(x * 2 + 1, m + 1, tr, l, r));
}
template <class F>
int bsearchPrefix(int x, int tl, int tr, int l, int r, Data &agg, F f) {
if (r < tl || tr < l) return r + 1;
if (tl != tr) propagate(x, tl, tr);
if (l <= tl && tr <= r) {
Data v = C::merge(agg, TR[x].val); if (!f(v)) { agg = v; return r + 1; }
}
if (tl == tr) return tl;
int m = tl + (tr - tl) / 2;
int ret = bsearchPrefix(x * 2, tl, m, l, r, agg, f);
return ret <= r ? ret : bsearchPrefix(x * 2 + 1, m + 1, tr, l, r, agg, f);
}
template <class F>
int bsearchSuffix(int x, int tl, int tr, int l, int r, Data &agg, F f) {
if (r < tl || tr < l) return l - 1;
if (tl != tr) propagate(x, tl, tr);
if (l <= tl && tr <= r) {
Data v = C::merge(agg, TR[x].val); if (!f(v)) { agg = v; return l - 1; }
}
if (tl == tr) return tl;
int m = tl + (tr - tl) / 2;
int ret = bsearchSuffix(x * 2 + 1, m + 1, tr, l, r, agg, f);
return l <= ret ? ret : bsearchSuffix(x * 2, tl, m, l, r, agg, f);
}
SegmentTreeBeats(int N) : N(N), TR(N == 0 ? 0 : 1 << __lg(N * 4 - 1)) {}
SegmentTreeBeats(const vector<Data> &A) : SegmentTreeBeats(A.size()) {
if (N > 0) { build(A, 1, 0, N - 1); }
}
void update(int l, int r, const Arg &v) { update(1, 0, N - 1, l, r, v); }
Data query(int l, int r) { return query(1, 0, N - 1, l, r); }
template <class F> int bsearchPrefix(int l, int r, F f) {
Data agg = C::qdef(); return bsearchPrefix(1, 0, N - 1, l, r, agg, f);
}
template <class F> int bsearchSuffix(int l, int r, F f) {
Data agg = C::qdef(); return bsearchSuffix(1, 0, N - 1, l, r, agg, f);
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Bottom up segment tree supporting range updates and range queries
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// C: struct to combine data and lazy values
// Required Fields:
// Data: the data type
// Lazy: the lazy type
// Required Functions:
// static qdef(): returns the query default value of type Data
// static ldef(): returns the lazy default value of type Lazy
// static merge(l, r): returns the values l of type Data merged with
// r of type Data, must be associative
// static applyLazy(l, r, k): returns the value r of type Lazy applied to
// l of type Data over a segment of length k, must be associative
// static mergeLazy(l, r): returns the values l of type Lazy merged with
// r of type Lazy, must be associative
// Sample Struct: supporting range assignments and range sum queries
// struct C {
// using Data = int;
// using Lazy = int;
// static Data qdef() { return 0; }
// static Lazy ldef() { return numeric_limits<int>::min(); }
// static Data merge(const Data &l, const Data &r) { return l + r; }
// static Data applyLazy(const Data &l, const Lazy &r, int k) {
// return r * k;
// }
// static Lazy mergeLazy(const Lazy &l, const Lazy &r) { return r; }
// };
// Constructor Arguments:
// N: the size of the array
// A: a vector of type C::Data, memory is saved if this is moved and has
// a capacity of 2N
// Functions:
// update(l, r, v): update the range [l, r] with the lazy value v
// query(l, r): queries the range [l, r] and returns the aggregate value
// In practice, has a small constant, not quite as fast as fenwick trees,
// and similar performance as top down segment trees
// Time Complexity:
// constructor: O(N)
// update, query: O(log N)
// Memory Complexity: O(N)
// Tested:
// https://dmoj.ca/problem/lazy
// https://mcpt.ca/problem/seq3
// https://judge.yosupo.jp/problem/range_affine_range_sum
// https://dmoj.ca/problem/dmopc17c4p6
template <class C> struct SegmentTreeLazyBottomUp {
using Data = typename C::Data; using Lazy = typename C::Lazy;
int N, lgN; vector<Data> TR; vector<Lazy> LZ;
void apply(int i, const Lazy &v, int k) {
TR[i] = C::applyLazy(TR[i], v, k);
if (i < N) LZ[i] = C::mergeLazy(LZ[i], v);
}
void eval(int i, int k) {
TR[i] = C::merge(TR[i * 2], TR[i * 2 + 1]);
if (LZ[i] != C::ldef()) TR[i] = C::applyLazy(TR[i], LZ[i], k);
}
void propagate(int i) {
int h = lgN + 1, k = 1 << lgN, ii = i >> h;
for (; h > 0; ii = i >> --h, k /= 2) if (LZ[ii] != C::ldef()) {
apply(ii * 2, LZ[ii], k); apply(ii * 2 + 1, LZ[ii], k);
LZ[ii] = C::ldef();
}
}
SegmentTreeLazyBottomUp(int N)
: N(N), lgN(N == 0 ? 0 : __lg(N)),
TR(N * 2, C::qdef()), LZ(N, C::ldef()) {}
SegmentTreeLazyBottomUp(vector<Data> A)
: N(A.size()), lgN(N == 0 ? 0 : __lg(N)), TR(move(A)), LZ(N, C::ldef()) {
TR.resize(N * 2, C::qdef()); copy_n(TR.begin(), N, TR.begin() + N);
for (int i = N - 1; i > 0; i--) TR[i] = C::merge(TR[i * 2], TR[i * 2 + 1]);
}
void update(int l, int r, const Lazy &v) {
propagate(l += N); propagate(r += N); bool bl = 0, br = 0; int k = 1;
for (; l <= r; l /= 2, r /= 2, k *= 2) {
if (bl) eval(l - 1, k);
if (br) eval(r + 1, k);
if (l % 2) { apply(l++, v, k); bl = 1; }
if (!(r % 2)) { apply(r--, v, k); br = 1; }
}
for (l--, r++; r; l /= 2, r /= 2, k *= 2) {
if (bl) eval(l, k);
if (br && (!bl || l != r)) eval(r, k);
}
}
Data query(int l, int r) {
propagate(l += N); propagate(r += N); Data ql = C::qdef(), qr = C::qdef();
for (; l <= r; l /= 2, r /= 2) {
if (l % 2) ql = C::merge(ql, TR[l++]);
if (!(r % 2)) qr = C::merge(TR[r--], qr);
}
return C::merge(ql, qr);
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Bottom up segment tree supporting point updates and range queries
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// C: struct to combine data and lazy values
// Required Fields:
// Data: the data type
// Lazy: the lazy type
// Required Functions:
// static qdef(): returns the query default value of type Data
// static merge(l, r): returns the values l of type Data merged with
// r of type Data, must be associative
// static applyLazy(l, r): returns the value r of type Lazy applied to
// l of type Data, must be associative
// Sample Struct: supporting point increments and range max queries
// struct C {
// using Data = int;
// using Lazy = int;
// static Data qdef() { return numeric_limits<int>::min(); }
// static Data merge(const Data &l, const Data &r) {
// return max(l, r);
// }
// static Data applyLazy(const Data &l, const Lazy &r) {
// return l + r;
// }
// };
// Constructor Arguments:
// N: the size of the array
// A: a vector of type C::Data, memory is saved if this is moved and has
// a capacity of 2N
// Functions:
// update(i, v): updates the index i with the lazy value v
// query(l, r): queries the range [l, r] and returns the aggregate value
// In practice, has a small constant, not quite as fast as fenwick trees,
// but faster than top down segment trees
// Time Complexity:
// constructor: O(N)
// update, query: O(log N)
// Memory Complexity: O(N)
// Tested:
// https://dmoj.ca/problem/ds3
// https://dmoj.ca/problem/dmpg17g2
// https://dmoj.ca/problem/coci17c1p5
// https://judge.yosupo.jp/problem/point_set_range_composite
template <class C> struct SegmentTreeBottomUp {
using Data = typename C::Data; using Lazy = typename C::Lazy;
int N; vector<Data> TR;
SegmentTreeBottomUp(int N) : N(N), TR(N * 2, C::qdef()) {}
SegmentTreeBottomUp(vector<Data> A) : N(A.size()), TR(move(A)) {
TR.resize(N * 2, C::qdef()); copy_n(TR.begin(), N, TR.begin() + N);
for (int i = N - 1; i > 0; i--) TR[i] = C::merge(TR[i * 2], TR[i * 2 + 1]);
}
void update(int i, const Lazy &v) {
for (i += N, TR[i] = C::applyLazy(TR[i], v); i /= 2;)
TR[i] = C::merge(TR[i * 2], TR[i * 2 + 1]);
}
Data query(int l, int r) {
Data ql = C::qdef(), qr = C::qdef();
for (l += N, r += N; l <= r; l /= 2, r /= 2) {
if (l % 2) ql = C::merge(ql, TR[l++]);
if (!(r % 2)) qr = C::merge(TR[r--], qr);
}
return C::merge(ql, qr);
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Top down dynamic segment tree supporting range updates and range queries
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// IndexType: the type of the index of the array
// LAZY: boolean to indicate whether or not range updates are enabled
// PERSISTENT: boolean to indicate whether or not updates are persistent
// C: struct to combine data and lazy values
// Required Fields:
// Data: the data type
// Lazy: the lazy type
// Required Functions:
// static qdef(): returns the query default value of type Data
// static ldef(): only required if LAZY is true, returns the lazy
// default value of type Lazy
// static merge(l, r): returns the values l of type Data merged with
// r of type Data, must be associative
// static applyLazy(l, r): only required if LAZY is false, returns the
// value r of type Lazy applied to l of type Data, must be associative
// static applyLazy(l, r, k): only required if LAZY is true, returns the
// value r of type Lazy applied to l of type Data over a segment of
// length k, must be associative
// static mergeLazy(l, r): only required if LAZY is true, returns
// the values l of type Lazy merged with r of type Lazy,
// must be associative
// static getSegmentVdef(k): returns the default value over a segment of
// length k
// Sample Struct: C1 supports point assignments and range sum queries,
// where the default value of each index is 1, C2 supports
// range assignments and range sum queries, where the default value of
// each index is 1
// struct C1 {
// using Data = int;
// using Lazy = int;
// static Data qdef() { return 0; }
// static Data merge(const Data &l, const Data &r) { return l + r; }
// static Data applyLazy(const Data &l, const Lazy &r) { return r; }
// template <class IndexType> static Data getSegmentVdef(IndexType k) {
// return k;
// }
// };
// struct C2 {
// using Data = int;
// using Lazy = int;
// static Data qdef() { return 0; }
// static Lazy ldef() { return numeric_limits<int>::min(); }
// static Data merge(const Data &l, const Data &r) { return l + r; }
// template <class IndexType>
// static Data applyLazy(const Data &l, const Lazy &r, int k) {
// return r * k;
// }
// static Lazy mergeLazy(const Lazy &l, const Lazy &r) { return r; }
// template <class IndexType> static Data getSegmentVdef(IndexType k) {
// return k;
// }
// };
// Constructor Arguments:
// N: the size of the array
// A: a vector of type C::Data
// Functions:
// update(i, v, newRoot): updates the index i with the lazy value v
// and creates a new version if newRoot is true
// update(i, v, newRoot): only valid if LAZY is true, updates
// the range [l, r] with the lazy value v and creates
// a new version if newRoot is true
// query(l, r, rootInd): queries the range [l, r] for the version rootInd (or
// the latest version of rootInd is -1), and returns the aggregate value
// bsearchPrefix(l, r, f, rootInd): returns the smallest index i in the
// range [l, r] for the version rootInd (or the latest version of rootInd
// is -1) such that f(query(l, i)) returns true or r + 1 if none exist
// bsearchSuffix(l, r, f, rootInd): returns the largest index i in the
// range [l, r] for the version rootInd (or the latest version of rootInd
// is -1) such that f(query(i, r)) returns true of l - 1 if none exist
// revert(rootInd): creates a new version based off of version rootInd
// reserveNodes(k): reserves space for k nodes in the dynamic segment tree
// In practice, has a moderate constant
// Time Complexity:
// constructor: O(1) for size constructor,
// O(N) for iteartor and generating function constructors
// update, query, bsearchPrefix, bsearchSuffix: O(log N) amortized unless
// reserveNodes is called beforehand
// Memory Complexity: O(U log N) for U updates for single argument constructor,
// O(N + U log N) for two argument constructor
// Tested:
// https://dmoj.ca/problem/ccc05s5 (LAZY = false, PERSISTENT = false)
// https://dmoj.ca/problem/wc18c4s4 (LAZY = false, PERSISTENT = true,
// bsearchPrefix, bsearchSuffix)
// https://codeforces.com/contest/1080/problem/F
// (LAZY = false, PERSISTENT = true)
// https://codeforces.com/contest/915/problem/E
// (LAZY = true, PERSISTENT = false)
// https://www.spoj.com/problems/TTM/ (LAZY = true, PERSISTENT = true)
template <class IndexType, const bool LAZY, const bool PERSISTENT, class C>
struct DynamicSegmentTree {
#define lazy_def template <const bool _ = LAZY> typename enable_if<_>::type
#define agg_def template <const bool _ = LAZY> typename enable_if<!_>::type
static_assert(is_integral<IndexType>::value, "IndexType must be integeral");
using Data = typename C::Data; using Lazy = typename C::Lazy;
template <const bool _, const int __ = 0> struct Node {
Data val; int l, r; Node(const Data &val) : val(val), l(-1), r(-1) {}
};
template <const int __> struct Node<true, __> {
Data val; Lazy lz; int l, r;
Node(const Data &val) : val(val), lz(C::ldef()), l(-1), r(-1) {}
};
IndexType N; vector<int> roots; vector<Node<LAZY>> TR;
int makeNode(int cp, IndexType tl, IndexType tr) {
if (~cp) TR.push_back(TR[cp]);
else TR.emplace_back(C::getSegmentVdef(tr - tl + 1));
return int(TR.size()) - 1;
}
lazy_def apply(int x, IndexType tl, IndexType tr, const Lazy &v) {
TR[x].val = C::applyLazy(TR[x].val, v, tr - tl + 1);
TR[x].lz = C::mergeLazy(TR[x].lz, v);
}
agg_def apply(int x, IndexType, IndexType, const Lazy &v) {
TR[x].val = C::applyLazy(TR[x].val, v);
}
lazy_def propagate(int x, IndexType tl, IndexType tr) {
if (TR[x].lz != C::ldef()) {
IndexType m = tl + (tr - tl) / 2; if (!~TR[x].l || PERSISTENT) {
int nl = makeNode(TR[x].l, tl, m); TR[x].l = nl;
}
if (!~TR[x].r || PERSISTENT) {
int nr = makeNode(TR[x].r, m + 1, tr); TR[x].r = nr;
}
apply(TR[x].l, tl, m, TR[x].lz); apply(TR[x].r, m + 1, tr, TR[x].lz);
TR[x].lz = C::ldef();
}
}
agg_def propagate(int, IndexType, IndexType) {}
int build(const vector<Data> &A, IndexType tl, IndexType tr) {
int x = makeNode(-1, tl, tr);
if (tl == tr) { TR[x].val = A[tl]; return x; }
IndexType m = tl + (tr - tl) / 2; int nl = build(A, tl, m); TR[x].l = nl;
int nr = build(A, m + 1, tr); TR[x].r = nr;
TR[x].val = C::merge(TR[TR[x].l].val, TR[TR[x].r].val); return x;
}
int update(int y, IndexType tl, IndexType tr, IndexType l, IndexType r,
const Lazy &v, int trSz) {
int x = !~y || (PERSISTENT && y < trSz) ? makeNode(y, tl, tr) : y;
if (l <= tl && tr <= r) { apply(x, tl, tr, v); return x; }
propagate(x, tl, tr); IndexType m = tl + (tr - tl) / 2;
if (tl <= r && l <= m) {
int nl = update(TR[x].l, tl, m, l, r, v, trSz); TR[x].l = nl;
}
if (m + 1 <= r && l <= tr) {
int nr = update(TR[x].r, m + 1, tr, l, r, v, trSz); TR[x].r = nr;
}
TR[x].val = C::merge(~TR[x].l ? TR[TR[x].l].val
: C::getSegmentVdef(m - tl + 1),
~TR[x].r ? TR[TR[x].r].val
: C::getSegmentVdef(tr - m));
return x;
}
Data query(int x, IndexType tl, IndexType tr, IndexType l, IndexType r) {
if (r < tl || tr < l) return C::qdef();
if (!~x) return C::getSegmentVdef(min(r, tr) - max(l, tl) + 1);
if (l <= tl && tr <= r) return TR[x].val;
propagate(x, tl, tr); IndexType m = tl + (tr - tl) / 2;
return C::merge(query(TR[x].l, tl, m, l, r),
query(TR[x].r, m + 1, tr, l, r));
}
template <class F>
int bsearchPrefix(int y, IndexType tl, IndexType tr,
IndexType l, IndexType r, Data &agg, F f, IndexType &ret) {
if (r < tl || tr < l) { ret = r + 1; return y; }
int x = !~y ? makeNode(y, tl, tr) : y; if (tl != tr) propagate(x, tl, tr);
if (l <= tl && tr <= r) {
Data v = C::merge(agg, TR[x].val);
if (!f(v)) { agg = v; ret = r + 1; return x; }
}
if (tl == tr) { ret = tl; return x; }
int m = tl + (tr - tl) / 2;
int nl = bsearchPrefix(TR[x].l, tl, m, l, r, agg, f, ret);
TR[x].l = nl; if (ret <= r) return x;
int nr = bsearchPrefix(TR[x].r, m + 1, tr, l, r, agg, f, ret);
TR[x].r = nr; return x;
}
template <class F>
int bsearchSuffix(int y, IndexType tl, IndexType tr,
IndexType l, IndexType r, Data &agg, F f, IndexType &ret) {
if (r < tl || tr < l) { ret = l - 1; return y; }
int x = !~y ? makeNode(y, tl, tr) : y; if (tl != tr) propagate(x, tl, tr);
if (l <= tl && tr <= r) {
Data v = C::merge(agg, TR[x].val);
if (!f(v)) { agg = v; ret = l - 1; return x; }
}
if (tl == tr) { ret = tl; return x; }
int m = tl + (tr - tl) / 2;
int nr = bsearchSuffix(TR[x].r, m + 1, tr, l, r, agg, f, ret);
TR[x].r = nr; if (l <= ret) return x;
int nl = bsearchSuffix(TR[x].l, tl, m, l, r, agg, f, ret);
TR[x].l = nl; return x;
}
DynamicSegmentTree(const vector<Data> &A) : N(A.size()) {
if (N > 0) {
reserveNodes(N * 2 - 1); roots.push_back(build(A, 0, N - 1));
}
}
DynamicSegmentTree(IndexType N) : N(N) { roots.push_back(-1); }
lazy_def update(IndexType l, IndexType r, const Lazy &v, bool newRoot) {
int nr = update(roots.back(), 0, N - 1, l, r, v, TR.size());
if (newRoot) roots.push_back(nr);
else roots.back() = nr;
}
void update(IndexType i, const Lazy &v, bool newRoot) {
int nr = update(roots.back(), 0, N - 1, i, i, v, TR.size());
if (newRoot) roots.push_back(nr);
else roots.back() = nr;
}
Data query(IndexType l, IndexType r, int rootInd = -1) {
return query(~rootInd ? roots[rootInd] : roots.back(), 0, N - 1, l, r);
}
template <class F>
IndexType bsearchPrefix(int l, int r, F f, int rootInd = -1) {
Data agg = C::qdef(); IndexType ret = r + 1;
int &root = ~rootInd ? roots[rootInd] : roots.back();
root = bsearchPrefix(root, 0, N - 1, l, r, agg, f, ret);
return ret;
}
template <class F>
IndexType bsearchSuffix(int l, int r, F f, int rootInd = -1) {
Data agg = C::qdef(); IndexType ret = l - 1;
int &root = ~rootInd ? roots[rootInd] : roots.back();
root = bsearchSuffix(root, 0, N - 1, l, r, agg, f, ret);
return ret;
}
void revert(int rootInd) { roots.push_back(roots[rootInd]); }
void reserveNodes(int k) { TR.reserve(k); }
#undef lazy_def
#undef agg_def
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Segment Tree supporting range updates with updates in the form of
// adding m + b, 2m + b, 3m + b, ... to the interval [l, r],
// and range sum queries
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// T: the type of the element of the array
// Constructor Arguments:
// N: the size of the array
// A: a vector of type T
// Functions:
// update(l, r, m, b): update the range [l, r] with
// m + b, 2m + b, 3m + b, ...
// query(l, r): returns the sum of elements in the range [l, r]
// In practice, has a small constant, but slower than FenwickTreeAffine
// Time Complexity:
// constructor: O(N)
// update, query: O(log N)
// Memory Complexity: O(N)
// Tested:
// https://dmoj.ca/problem/acc3p4
template <class T> struct SegmentTreeAffine {
using Pair = pair<T, T>;
Pair add(const Pair &l, const Pair &r) {
return Pair(l.first + r.first, l.second + r.second);
}
Pair mul(const Pair &l, const Pair &r) {
return Pair(l.first * r.first, l.second * r.second);
}
int N; vector<Pair> TR, LZ;
T sumTo(T k) { return k * (k + 1) / 2; }
Pair sumBet(T l, T r) { return Pair(r - l + 1, sumTo(r) - sumTo(l - 1)); }
void apply(int x, int tl, int tr, const Pair &v) {
TR[x] = add(TR[x], mul(v, sumBet(tl, tr)));
if (x < int(LZ.size())) LZ[x] = add(LZ[x], v);
}
void propagate(int x, int tl, int tr) {
if (LZ[x] != Pair()) {
int m = tl + (tr - tl) / 2; apply(x * 2, tl, m, LZ[x]);
apply(x * 2 + 1, m + 1, tr, LZ[x]); LZ[x] = Pair();
}
}
void build(const vector<T> &A, int x, int tl, int tr) {
if (tl == tr) { TR[x] = Pair(A[tl], T()); return; }
int m = tl + (tr - tl) / 2; build(A, x * 2, tl, m);
build(A, x * 2 + 1, m + 1, tr); TR[x] = add(TR[x * 2], TR[x * 2 + 1]);
}
void update(int x, int tl, int tr, int l, int r, const Pair &v) {
if (l <= tl && tr <= r) { apply(x, tl, tr, v); return; }
propagate(x, tl, tr); int m = tl + (tr - tl) / 2;
if (tl <= r && l <= m) update(x * 2, tl, m, l, r, v);
if (m + 1 <= r && l <= tr) update(x * 2 + 1, m + 1, tr, l, r, v);
TR[x] = add(TR[x * 2], TR[x * 2 + 1]);
}
Pair query(int x, int tl, int tr, int l, int r) {
if (r < tl || tr < l) return Pair();
if (l <= tl && tr <= r) return TR[x];
propagate(x, tl, tr); int m = tl + (tr - tl) / 2;
return add(query(x * 2, tl, m, l, r), query(x * 2 + 1, m + 1, tr, l, r));
}
SegmentTreeAffine(int N)
: N(N), TR(N == 0 ? 0 : 1 << __lg(N * 4 - 1)),
LZ(N == 0 ? 0 : 1 << __lg(N * 2 - 1)) {}
SegmentTreeAffine(const vector<T> &A) : SegmentTreeAffine(A.size()) {
if (N > 0) build(A, 1, 0, N - 1);
}
void update(int l, int r, T m, T b) {
update(1, 0, N - 1, l, r, Pair((1 - l) * m + b, m));
}
T query(int l, int r) {
Pair q = query(1, 0, N - 1, l, r); return q.first + q.second;
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Fenwick Tree or Binary Indexed Tree supporting point updates
// and range queries over a cumulative functor, such as sum, max, and min,
// in any number of dimensions
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// D: the number of dimensions of the fenwick tree
// T: the type of each element
// Op: a struct with the cumulative operation (plus<T> by default)
// Required Functions:
// operator (l, r): combines the values l and r
// Inv: a struct with the inverse cumulative operation (minus<T> by default),
// if Op has no inverse, some dummy struct may need to be provided
// Required Functions:
// operator (l, r): performs the inverse operation on values l and r
// Constructor Arguments:
// N: the size of the first dimension
// ...args: the sizes of the second, third, fourth, etc. dimensions, with
// the last value being the identity element (T() by default)
// Functions:
// update(i, ...args): updates the index i in the first dimension,
// and ...args for the next dimensions, with the last argument
// being the value to update the index
// queryPrefix(r, ...args): queries the cumulative value of the range [0, r]
// in the first dimension, with the remaining arguments for the
// next dimensions
// query(l, r, ...args): queries the cumulative value of the range [l, r]
// in the first dimension, and alternating arguments in args for beginning
// and ending ranges for the next dimensions
// In practice, has a small constant, faster than segment trees and comparable
// to the 1D version
// Time Complexity:
// constructor: O(PI(N_i))
// update, queryPrefix: O(PI(log(N_i)))
// query: O(2^D * PI(log(N_i)))
// Memory Complexity: O(PI(N_i))
// PI is the product function, N_i is the size in the ith dimension
// Tested:
// Fuzz and Stress Tested
// https://dmoj.ca/problem/ioi01p1
// https://dmoj.ca/problem/gfssoc1s4
// https://dmoj.ca/problem/ccc19s5
template <const int D, class T, class Op = plus<T>, class Inv = minus<T>>
struct FenwickTree {
int N; vector<FenwickTree<D - 1, T, Op, Inv>> BIT; T val;
template <class ...Args> FenwickTree(int N, Args &&...args)
: N(N),
BIT(N + 1, FenwickTree<D - 1, T, Op, Inv>(forward<Args>(args)...)),
val(BIT[0].val) {}
template <class ...Args> void update(int i, Args &&...args) {
for (i++; i <= N; i += i & -i) BIT[i].update(forward<Args>(args)...);
}
template <class ...Args> T queryPrefix(int r, Args &&...args) {
T ret = val; for (r++; r > 0; r -= r & -r)
ret = Op()(ret, BIT[r].queryPrefix(forward<Args>(args)...));
return ret;
}
template <class ...Args> T query(int l, int r, Args &&...args) {
T ret = val; for (; l > 0; l -= l & -l)
ret = Inv()(ret, BIT[l].query(forward<Args>(args)...));
for (r++; r > 0; r -= r & -r)
ret = Op()(ret, BIT[r].query(forward<Args>(args)...));
return ret;
}
};
template <class T, class Op, class Inv> struct FenwickTree<0, T, Op, Inv> {
T val; FenwickTree(T qdef = T()) : val(qdef) {}
void update(T v) { val = Op()(val, v); }
T queryPrefix() { return val; }
T query() { return val; }
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Fenwick Tree or Binary Indexed Tree supporting range updates
// and point queries, over a cumulative functor, such as sum, max, and min,
// in any number of dimensions
// Indices are 0-indexed and ranges are inclusive
// Order of arguments for update differs slightly from FenwickTreeRangePoint1D
// Template Arguments:
// D: the number of dimensions of the fenwick tree
// T: the type of each element
// Op: a struct with the cumulative operation (plus<T> by default)
// Required Functions:
// operator (l, r): combines the values l and r, must be associative and
// commutatitve
// Inv: a struct with the inverse cumulative operation (minus<T> by default),
// if Op has no inverse, some dummy struct may need to be provided
// Required Functions:
// operator (l, r): performs the inverse operation on values l and r
// Constructor Arguments:
// N: the size of the first dimension
// ...args: the sizes of the second, third, fourth, etc. dimensions, with
// the last value being the identity element (T() by default)
// Functions:
// updateSuffix(v, l, ...args): updates the suffix starting from index l in
// the first dimension, and ...args the next dimensions with the value a
// being updated to op(a, v)
// update(v, l, r, ...args): updates the range [l, r] in the first
// dimension, and alternating arguments in args for beginning and
// ending ranges for the next dimensions with the value a being updated to
// op(a, v)
// get(i, ...args): queries the value of index i in the first
// dimension, and ...args for the next dimensions
// In practice, has a small constant, faster than segment trees and comparable
// to the 1D version
// Time Complexity:
// constructor: O(PI(N_i))
// update: O(2^D * PI(log(N_i)))
// updateSuffix, getValue: O(PI(log(N_i)))
// Memory Complexity: O(PI(N_i))
// PI is the product function, N_i is the size in the ith dimension,
// and D is the number of dimensions
// Tested:
// Fuzz and Stress Tested
// https://mcpt.ca/problem/adifferenceproblem
template <const int D, class T, class Op = plus<T>, class Inv = minus<T>>
struct FenwickTreeRangePoint {
int N; vector<FenwickTreeRangePoint<D - 1, T, Op, Inv>> BIT; T val;
template <class ...Args> FenwickTreeRangePoint(int N, Args &&...args)
: N(N), BIT(N + 1, FenwickTreeRangePoint<D - 1, T, Op, Inv>(
forward<Args>(args)...)),
val(BIT[0].val) {}
template <class ...Args> void updateSuffix(T v, int l, Args &&...args) {
for (l++; l <= N; l += l & -l)
BIT[l].updateSuffix(v, forward<Args>(args)...);
}
template <class ...Args> void update(T v, int l, int r, Args &&...args) {
for (l++; l <= N; l += l & -l) BIT[l].update(v, forward<Args>(args)...);
for (r += 2; r <= N; r += r & -r)
BIT[r].update(Inv()(val, v), forward<Args>(args)...);
}
template <class ...Args> T get(int i, Args &&...args) {
T ret = val; for (i++; i > 0; i -= i & -i)
ret = Op()(ret, BIT[i].get(forward<Args>(args)...));
return ret;
}
};
template <class T, class Op, class Inv>
struct FenwickTreeRangePoint<0, T, Op, Inv> {
T val; FenwickTreeRangePoint(T qdef = T()) : val(qdef) {}
void updateSuffix(T v) { val = Op()(val, v); }
void update(T v) { val = Op()(val, v); }
T get() { return val; }
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
#include "../../PolicyBasedDataStructures.h"
#include "../../sqrt/SqrtBufferSimple.h"
#include "../../sqrt/SqrtBuffer.h"
using namespace std;
using namespace __gnu_pbds;
// A collection of sparse fenwick trees implemented in various methods
// Some are only sparse in 1 dimension, others are sparse in 2 dimensions
// Certain implementations only allow for increments and decrements of 1
// In general, offline fenwick trees are faster than sqrt implementations,
// which are faster than the pbds implementations
// Sparse Fenwick Tree supporting point updates (with any value)
// and range queries over a cumulative function or functor,
// such as sum, max, and min, in 2 dimensions (sparse in 1 dimension)
// All update indices must be known beforehand
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// T: the type of each element
// IndexType: the type of the index of the second dimension of the array
// Op: a struct with the cumulative operation (plus<T> by default)
// Required Functions:
// operator (l, r): combines the values l and r, must be associative and
// commutatitve
// Constructor Arguments:
// N: the size of the first dimension of the array
// updateInds: a vector of pairs of ints and IndexType containing the indices
// for both dimensions for each update
// qdef: the identity element of the operation
// op: an instance of the Op struct
// Functions:
// update(i, j, v): modifies the value A[i][j] to op(A[i][j], v), (i, j)
// must be an element of updateInds
// query(d, r): queries the cumulative value of the range [0, d] in the
// first dimension and [0, r] in the second dimension
// query(d, l, r, inv): queries the cumulative value of the range [0, d]
// in the first dimension and [l, r] in the second dimension, where inv is
// the inverse of op (minus<T>() by default)
// query(u, d, l, r, inv): queries the cumulative value of the range [u, d]
// in the first dimension and [l, r] in the second dimension, where inv is
// the inverse of op (minus<T>() by default)
// In practice, has a small constant
// Time Complexity:
// constructor: O(Q (log Q + log N) + N) for U updates
// update, query: O(log N log U) for U updates
// Memory Complexity: O(N + U log N) for U updates
// Tested:
// https://codeforces.com/contest/1093/problem/E
// https://codeforces.com/contest/1523/problem/G
template <class T, class IndexType, class Op = plus<T>>
struct OfflineSemiSparseFenwickTree2D {
static_assert(is_integral<IndexType>::value, "IndexType must be integeral");
int N; vector<int> st, cnt; vector<IndexType> inds; vector<T> BIT;
T qdef; Op op;
int getInd(int i, IndexType j) {
return upper_bound(inds.begin() + st[i], inds.begin() + st[i] + cnt[i], j)
- inds.begin() - st[i];
}
OfflineSemiSparseFenwickTree2D(int N,
vector<pair<int, IndexType>> updateInds,
T qdef = T(), Op op = Op())
: N(N), st(N + 1, 0), cnt(N + 1, 0), qdef(qdef), op(op) {
sort(updateInds.begin(), updateInds.end(),
[&] (const pair<int, IndexType> &a, const pair<int, IndexType> &b) {
return a.second < b.second;
});
vector<IndexType> last(N + 1, IndexType());
for (auto &&u : updateInds) for (int i = u.first + 1; i <= N; i += i & -i)
if (cnt[i] == 0 || u.second != last[i]) { cnt[i]++; last[i] = u.second; }
for (int i = 1; i <= N; i++) st[i] = st[i - 1] + cnt[i - 1];
inds.resize(st[N] + cnt[N]); BIT.resize(st[N] + cnt[N], qdef);
fill(cnt.begin(), cnt.end(), 0); for (auto &&u : updateInds)
for (int i = u.first + 1; i <= N; i += i & -i)
if (cnt[i] == 0 || u.second != inds[st[i] + cnt[i] - 1])
inds[st[i] + cnt[i]++] = u.second;
}
void update(int i, IndexType j, T v) {
for (i++; i <= N; i += i & -i)
for (int s = st[i], c = cnt[i], y = getInd(i, j); y <= c; y += y & -y)
BIT[s + y - 1] = op(BIT[s + y - 1], v);
}
T query(int d, IndexType r) {
T ret = qdef; for (d++; d > 0; d -= d & -d)
for (int s = st[d], y = getInd(d, r); y > 0; y -= y & -y)
ret = op(ret, BIT[s + y - 1]);
return ret;
}
template <class Inv = minus<T>>
T query(int d, IndexType l, IndexType r, Inv inv = Inv()) {
return inv(query(d, r), query(d, l - 1));
}
template <class Inv = minus<T>>
T query(int u, int d, IndexType l, IndexType r, Inv inv = Inv()) {
return inv(query(d, l, r), query(u - 1, l, r));
}
};
// Sparse Fenwick Tree supporting point updates (with any value)
// and range queries over a cumulative function or functor,
// such as sum, max, and min, in 2 dimensions (sparse in 2 dimensions)
// All update indices must be known beforehand
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// T: the type of each element
// IndexType1: the type of the index of the first dimension of the array
// IndexType2: the type of the index of the second dimension of the array
// Op: a struct with the cumulative operation (plus<T> by default)
// Required Functions:
// operator (l, r): combines the values l and r, must be associative and
// commutatitve
// Constructor Arguments:
// updateInds: a vector of pairs of IndexType1 and IndexType2 containing
// the indices for both dimensions for each update
// qdef: the identity element of the operation
// op: an instance of the Op struct
// Functions:
// update(i, j, v): modifies the value A[i][j] to op(A[i][j], v), (i, j)
// must be an element of updateInds
// query(d, r): queries the cumulative value of the range [0, d] in the
// first dimension and [0, r] in the second dimension
// query(d, l, r, inv): queries the cumulative value of the range [0, d]
// in the first dimension and [l, r] in the second dimension, where inv is
// the inverse of op (minus<T>() by default)
// query(u, d, l, r, inv): queries the cumulative value of the range [u, d]
// in the first dimension and [l, r] in the second dimension, where inv is
// the inverse of op (minus<T>() by default)
// In practice, has a small constant
// Time Complexity:
// constructor: O(U log U) for U updates
// update, query: O((log U)^2) for U updates
// Memory Complexity: O(U log U) for U updates
// Tested:
// https://judge.yosupo.jp/problem/point_add_rectangle_sum
// https://codeforces.com/contest/1093/problem/E
// https://codeforces.com/contest/1523/problem/G
template <class T, class IndexType1, class IndexType2, class Op = plus<T>>
struct OfflineSparseFenwickTree2D {
static_assert(is_integral<IndexType1>::value,
"IndexType1 must be integeral");
static_assert(is_integral<IndexType2>::value,
"IndexType2 must be integeral");
int U; vector<int> st, cnt; vector<IndexType1> inds1;
vector<IndexType2> inds2; vector<T> BIT; T qdef; Op op;
int getInd1(IndexType1 i) {
return upper_bound(inds1.begin(), inds1.end(), i) - inds1.begin();
}
int getInd2(int i, IndexType2 j) {
return upper_bound(inds2.begin() + st[i],
inds2.begin() + st[i] + cnt[i], j)
- inds2.begin() - st[i];
}
OfflineSparseFenwickTree2D(vector<pair<IndexType1, IndexType2>> updateInds,
T qdef = T(), Op op = Op())
: inds1(updateInds.size()), qdef(qdef), op(op) {
sort(updateInds.begin(), updateInds.end(),
[&] (const pair<IndexType1, IndexType2> &a,
const pair<IndexType1, IndexType2> &b) {
return a.second < b.second;
});
for (int i = 0; i < int(updateInds.size()); i++)
inds1[i] = updateInds[i].first;
sort(inds1.begin(), inds1.end());
inds1.erase(unique(inds1.begin(), inds1.end()), inds1.end());
U = int(inds1.size()); st.assign(U + 1, 0); cnt.assign(U + 1, 0);
vector<IndexType2> last(U + 1, IndexType2()); for (auto &&u : updateInds)
for (int i = getInd1(u.first); i <= U; i += i & -i)
if (cnt[i] == 0 || u.second != last[i]) {
cnt[i]++; last[i] = u.second;
}
for (int i = 1; i <= U; i++) st[i] = st[i - 1] + cnt[i - 1];
inds2.resize(st[U] + cnt[U]); BIT.resize(st[U] + cnt[U], qdef);
fill(cnt.begin(), cnt.end(), 0); for (auto &&u : updateInds)
for (int i = getInd1(u.first); i <= U; i += i & -i)
if (cnt[i] == 0 || u.second != inds2[st[i] + cnt[i] - 1])
inds2[st[i] + cnt[i]++] = u.second;
}
void update(IndexType1 i, IndexType2 j, T v) {
for (int x = getInd1(i); x <= U; x += x & -x)
for (int s = st[x], c = cnt[x], y = getInd2(x, j); y <= c; y += y & -y)
BIT[s + y - 1] = op(BIT[s + y - 1], v);
}
T query(IndexType1 d, IndexType2 r) {
T ret = qdef; for (int x = getInd1(d); x > 0; x -= x & -x)
for (int s = st[x], y = getInd2(x, r); y > 0; y -= y & -y)
ret = op(ret, BIT[s + y - 1]);
return ret;
}
template <class Inv = minus<T>>
T query(IndexType1 d, IndexType2 l, IndexType2 r, Inv inv = Inv()) {
return inv(query(d, r), query(d, l - 1));
}
template <class Inv = minus<T>>
T query(IndexType1 u, IndexType1 d, IndexType2 l, IndexType2 r,
Inv inv = Inv()) {
return inv(query(d, l, r), query(u - 1, l, r));
}
};
// Sparse Fenwick Tree supporting point updates (with value 1 and -1)
// and range sum queries in 2 dimensions
// using SqrtBufferSimple (sparse in 1 dimension)
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// IndexType: the type of the index of the second dimension of the array
// Constructor Arguments:
// N: the size of the first dimension of the array
// SCALE: the value to scale sqrt by (for SqrtBufferSimple)
// Functions:
// add(i, j): add 1 to the value A[i][j]
// rem(i, j): subtract 1 from the value A[i][j]
// query(d, r): queries the sum of the range [0, d] in the first dimension
// and [0, r] in the second dimension
// query(d, l, r): queries the sum of the range [0, d] in the first dimension
// and [l, r] in the second dimension
// query(u, d, l, r): queries the sum of the range [u, d] in the first
// dimension and [l, r] in the second dimension
// In practice, has a very small constant
// Time Complexity:
// constructor: O(N)
// add, rem: O(log N) amortized
// query: O(log N sqrt U) amortized for U updates
// Memory Complexity: O(N + U log N) for U updates
// Tested:
// https://codeforces.com/contest/1093/problem/E
// https://dmoj.ca/problem/dmopc19c7p5
template <class IndexType> struct SemiSparseFenwickTree2DSimple {
static_assert(is_integral<IndexType>::value, "IndexType must be integeral");
int N; vector<SqrtBufferSimple<IndexType>> IN, OUT;
SemiSparseFenwickTree2DSimple(int N, double SCALE = 1)
: N(N), IN(N + 1, SqrtBufferSimple<IndexType>(SCALE)),
OUT(N + 1, SqrtBufferSimple<IndexType>(SCALE)) {}
void add(int i, IndexType j) {
for (i++; i <= N; i += i & -i) IN[i].insert(j);
}
void rem(int i, IndexType j) {
for (i++; i <= N; i += i & -i) OUT[i].insert(j);
}
int query(int d, IndexType r) {
int ret = 0; for (d++; d > 0; d -= d & -d)
ret += IN[d].aboveInd(r) - OUT[d].aboveInd(r);
return ret;
}
int query(int d, IndexType l, IndexType r) {
int ret = 0; for (d++; d > 0; d -= d & -d)
ret += IN[d].count(l, r) - OUT[d].count(l, r);
return ret;
}
int query(int u, int d, IndexType l, IndexType r) {
return query(d, l, r) - query(u - 1, l, r);
}
};
// Sparse Fenwick Tree supporting point updates (with any value)
// and range sum queries in 2 dimensions using SqrtBuffer (sparse in
// 1 dimension)
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// T: the type of each element
// IndexType: the type of the index of the second dimension of the array
// Constructor Arguments:
// N: the size of the first dimension of the array
// SCALE: the value to scale sqrt by (for SqrtBuffer)
// Functions:
// update(i, j, v): add v to the value A[i][j]
// query(d, r): queries the sum of the range [0, d] in the first dimension
// and [0, r] in the second dimension
// query(d, l, r): queries the sum of the range [0, d] in the first dimension
// and [l, r] in the second dimension
// query(u, d, l, r): queries the sum of the range [u, d] in the first
// dimension and [l, r] in the second dimension
// In practice, has a very small constant
// Time Complexity:
// constructor: O(N)
// update: O(log N) amortized
// query: O(log N sqrt U) amortized for U updates
// Memory Complexity: O(N + U log N) for U updates
// Tested:
// https://dmoj.ca/problem/apio19p3
// https://dmoj.ca/problem/ioi01p1
template <class T, class IndexType> struct SemiSparseFenwickTree2D {
static_assert(is_integral<IndexType>::value, "IndexType must be integeral");
int N; vector<SqrtBuffer<T, IndexType>> BIT;
SemiSparseFenwickTree2D(int N, double SCALE = 1)
: N(N), BIT(N + 1, SqrtBuffer<T, IndexType>(SCALE)) {}
void update(int i, IndexType j, T v) {
for (i++; i <= N; i += i & -i) BIT[i].emplace(j, v);
}
T query(int d, IndexType r) {
T ret = T(); for (d++; d > 0; d -= d & -d) ret += BIT[d].aboveInd(r);
return ret;
}
T query(int d, IndexType l, IndexType r) {
T ret = T(); for (d++; d > 0; d -= d & -d) ret += BIT[d].count(l, r);
return ret;
}
T query(int u, int d, IndexType l, IndexType r) {
return query(d, l, r) - query(u - 1, l, r);
}
};
// Sparse Fenwick Tree supporting point updates (with value 1 and -1)
// and range sum queries in 2 dimensions
// using pbds hash_table for the first dimension,
// and SqrtBufferSimple for the second dimension (sparse in 2 dimensions)
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// IndexType1: the type of the index of the first dimension of the array
// IndexType2: the type of the index of the second dimension of the array
// Container: the container to represent an unordered mapping from
// IndexType1 to pair of SqrtBufferSimple<IndexType2>
// Constructor Arguments:
// N: the size of the first dimension of the array
// SCALE: the value to scale sqrt by (for SqrtBufferSimple)
// Functions:
// add(i, j): add 1 to the value A[i][j]
// rem(i, j): subtract 1 from the value A[i][j]
// query(d, r): queries the sum of the range [0, d] in the first dimension
// and [0, r] in the second dimension
// query(d, l, r): queries the sum of the range [0, d] in the first dimension
// and [l, r] in the second dimension
// query(u, d, l, r): queries the sum of the range [u, d] in the first
// dimension and [l, r] in the second dimension
// In practice, has a very small constant
// Time Complexity:
// constructor: O(1)
// add, rem: O(log N) amortized
// query: O(log N sqrt U) amortized for U updates
// Memory Complexity: O(U log N) for U updates
// Tested:
// https://codeforces.com/contest/1093/problem/E
template <class IndexType1, class IndexType2,
class Container = hashmap<
IndexType1, pair<SqrtBufferSimple<IndexType2>,
SqrtBufferSimple<IndexType2>>>>
struct SparseFenwickTree2DSimple {
static_assert(is_integral<IndexType1>::value,
"IndexType1 must be integeral");
static_assert(is_integral<IndexType2>::value,
"IndexType2 must be integeral");
IndexType1 N; double SCALE; Container BIT;
SparseFenwickTree2DSimple(IndexType1 N, double SCALE = 1)
: N(N), SCALE(SCALE) {}
void add(IndexType1 i, IndexType2 j) {
i++; for (IndexType1 x = i; x <= N; x += x & -x) {
auto it = BIT.find(x); if (it == BIT.end()) {
BIT[x] = make_pair(SqrtBufferSimple<IndexType2>(SCALE),
SqrtBufferSimple<IndexType2>(SCALE));
}
BIT[x].first.insert(j);
}
}
void rem(IndexType1 i, IndexType2 j) {
i++; for (IndexType1 x = i; x <= N; x += x & -x) {
auto it = BIT.find(x); if (it == BIT.end()) {
BIT[x] = make_pair(SqrtBufferSimple<IndexType2>(SCALE),
SqrtBufferSimple<IndexType2>(SCALE));
}
BIT[x].second.insert(j);
}
}
int query(IndexType1 d, IndexType2 r) {
d++; int ret = 0; for (IndexType1 x = d; x > 0; x -= x & -x) {
auto it = BIT.find(x);
ret += it->second.first.aboveInd(r) - it->second.second.aboveInd(r);
}
return ret;
}
int query(IndexType1 d, IndexType2 l, IndexType2 r) {
d++; int ret = 0; for (IndexType1 x = d; x > 0; x -= x & -x) {
auto it = BIT.find(x);
ret += it->second.first.count(l, r) - it->second.second.count(l, r);
}
return ret;
}
int query(IndexType1 u, IndexType1 d, IndexType2 l, IndexType2 r) {
return query(d, l, r) - query(u - 1, l, r);
}
};
// Sparse Fenwick Tree supporting point updates (with any value)
// and range sum queries in 2 dimensions
// using pbds hash_table for the first dimension,
// and SqrtBuffer for the second dimension (sparse in 2 dimensions)
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// T: the type of each element
// IndexType1: the type of the index of the first dimension of the array
// IndexType2: the type of the index of the second dimension of the array
// Container: the container to represent an unordered mapping from
// IndexType1 to SqrtBuffer<IndexType2, T>
// Constructor Arguments:
// N: the size of the first dimension of the array
// SCALE: the value to scale sqrt by (for SqrtBuffer)
// Functions:
// update(i, j, v): add v to the value A[i][j]
// query(d, r): queries the sum of the range [0, d] in the first dimension
// and [0, r] in the second dimension
// query(d, l, r): queries the sum of the range [0, d] in the first dimension
// and [l, r] in the second dimension
// query(u, d, l, r): queries the sum of the range [u, d] in the first
// dimension and [l, r] in the second dimension
// In practice, has a very small constant
// Time Complexity:
// constructor: O(1)
// update: O(log N) amortized
// query: O(log N sqrt U) amortized for U updates
// Memory Complexity: O(U log N) for U update
// Tested:
// https://dmoj.ca/problem/fallingsnowflakes
template <class T, class IndexType1, class IndexType2,
class Container = hashmap<IndexType1, SqrtBuffer<IndexType2, T>>>
struct SparseFenwickTree2D {
static_assert(is_integral<IndexType1>::value,
"IndexType1 must be integeral");
static_assert(is_integral<IndexType2>::value,
"IndexType2 must be integeral");
IndexType1 N; double SCALE; Container BIT;
SparseFenwickTree2D(IndexType1 N, double SCALE = 1) : N(N), SCALE(SCALE) {}
void update(IndexType1 i, IndexType2 j, T v) {
i++; for (IndexType1 x = i; x <= N; x += x & -x) {
auto it = BIT.find(x); if (it == BIT.end()) {
(BIT[x] = SqrtBuffer<IndexType2, T>(SCALE)).emplace(j, v);
} else it->second.emplace(j, v);
}
}
T query(IndexType1 d, IndexType2 r) {
d++; T ret = T(); for (IndexType1 x = d; x > 0; x -= x & -x) {
auto it = BIT.find(x);
if (it != BIT.end()) ret += it->second.aboveInd(r);
}
return ret;
}
T query(IndexType1 d, IndexType2 l, IndexType2 r) {
d++; T ret = T(); for (IndexType1 x = d; x > 0; x -= x & -x) {
auto it = BIT.find(x);
if (it != BIT.end()) ret += it->second.count(l, r);
}
return ret;
}
T query(IndexType1 u, IndexType1 d, IndexType2 l, IndexType2 r) {
return query(d, l, r) - query(u - 1, l, r);
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
#include "FenwickTree1D.h"
#include "../../../math/LagrangePolynomialInterpolation.h"
using namespace std;
// FenwickTree supporting range updates with updates in the form of
// adding v, 2^k v, 3^k v, ... to the range [l, r], and range sum queries
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// T: the type of each element
// Constructor Arguments:
// N: the size of the array
// Functions:
// update(l, r, v, k): add v, 2^k v, 3^k v, ... to the range [l, r]
// query(r): queries the sum of the range [0, r]
// query(l, r): queries the sum of the range [l, r]
// In practice, has a small constant, faster than segment trees
// Time Complexity:
// constructor: O(N + K^3)
// update: O(K (K + log N))
// rsq: O(K log N)
// Memory Complexity: O(KN + K^3)
// Tested:
// https://dmoj.ca/problem/tle17c3p6
template <class T> struct FenwickTreePowerSum {
int N, K; vector<FenwickTree1D<T>> FT; vector<T> fact;
vector<vector<T>> cof, pascal; vector<vector<vector<T>>> sumDiff;
FenwickTreePowerSum(int N, int K)
: N(N), K(K), FT(K + 2, FenwickTree1D<T>(N)), fact(K + 2, T(1)),
cof(K + 1), pascal(K + 2), sumDiff(K + 1, vector<vector<T>>(K + 2)) {
for (int i = 1; i < K + 2; i++) fact.push_back(fact.back() * T(i));
for (int i = 0; i < K + 1; i++) {
vector<pair<T, T>> P; P.reserve(i + 2); P.emplace_back(T(), T());
for (int j = 1; j < i + 2; j++) {
T p = T(1); for (int k = 1; k <= i; k++) p *= T(j);
P.emplace_back(T(j), P.back().second + fact[i + 1] * p);
}
cof[i] = lagrangePolynomialInterpolation(P);
}
for (int k = 0; k < K + 1; k++) for (int i = 0; i < K + 2; i++)
sumDiff[k][i].resize(K + 2 - i, T());
for (int i = 0; i < K + 2; i++) {
pascal[i].resize(i + 1, T(1)); for (int j = 1; j < i; j++)
pascal[i][j] = pascal[i - 1][j - 1] + pascal[i - 1][j];
}
for (int k = 0; k < K + 1; k++) for (int i = 0; i < k + 2; i++) {
T sign = T(1); for (int j = 0; j <= i; j++, sign = -sign) {
T f = fact[K + 1] / fact[k + 1];
sumDiff[k][i - j][j] += cof[k][i] * pascal[i][j] * f * sign;
}
}
}
void update(int l, int r, T v, int k) {
assert(k <= K); T s = T(l - 1), len = T(r - l + 1);
for (int i = 0; i < k + 2; i++) {
T mul = sumDiff[k][i][k + 1 - i];
for (int j = k - i; j >= 0; j--) mul = mul * s + sumDiff[k][i][j];
FT[i].update(l, v * mul); FT[i].update(r + 1, -v * mul);
}
T mul = cof[k][k + 1];
for (int i = k; i >= 0; i--) mul = mul * len + cof[k][i];
mul = mul * (fact[K + 1] / fact[k + 1]); FT[0].update(r + 1, v * mul);
}
T query(int r) {
T ret = FT[K + 1].query(r);
for (int i = K; i >= 0; i--) ret = ret * r + FT[i].query(r);
return ret / fact[K + 1];
}
T query(int l, int r) { return query(r) - query(l - 1); }
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
#include "FenwickTreeRangePoint.h"
using namespace std;
// Supports range additions and range sum queries in 2D where
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// T: the type of the element of the grid
// Constructor Arguments:
// N: the size of the first dimension of the array
// M: the size of the second dimension of the array
// Functions:
// update(u, d, l, r, v): adds v to all elements in the range [u, d] in the
// first dimension and [l, r] in the second dimension
// query(d, r): queries the sum of the range [0, d] in the
// first dimension and [0, r] in the second dimension
// query(d, l, r): queries the sum of the range [0, d] in the
// first dimension and [l, r] in the second dimension
// query(u, d, l, r): queries the sum of the range [u, d] in the
// first dimension and [l, r] in the second dimension
// In practice, has a moderate constant
// Time Complexity:
// constructor: O(NM)
// update, query: O(log N log M)
// Memory Complexity: O(NM)
// Tested:
// Fuzz and Stress Tested
template <class T> struct RangeAddRangeSum2D {
int N, M; vector<FenwickTreeRangePoint<2, T>> FT;
RangeAddRangeSum2D(int N, int M)
: N(N), M(M), FT(4, FenwickTreeRangePoint<2, T>(N, M)) {}
void update(int u, int d, int l, int r, const T &v) {
FT[0].update(v, u, d, l, r);
FT[1].update(-v * T(l - 1), u, d, l, r);
FT[1].update(v * T(r - l + 1), u, d, r + 1, M - 1);
FT[2].update(-v * T(u - 1), u, d, l, r);
FT[2].update(v * T(d - u + 1), d + 1, N - 1, l, r);
FT[3].update(v * T(u - 1) * T(l - 1), u, d, l, r);
FT[3].update(-v * T(u - 1) * T(r - l + 1), u, d, r + 1, M - 1);
FT[3].update(-v * T(l - 1) * T(d - u + 1), d + 1, N - 1, l, r);
FT[3].updateSuffix(v * T(d - u + 1) * T(r - l + 1), d + 1, r + 1);
}
T query(int d, int r) {
return FT[0].get(d, r) * T(d) * T(r) + FT[1].get(d, r) * T(d)
+ FT[2].get(d, r) * T(r) + FT[3].get(d, r);
}
T query(int d, int l, int r) { return query(d, r) - query(d, l - 1); }
T query(int u, int d, int l, int r) {
return query(d, l, r) - query(u - 1, l, r);
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Fenwick Tree or Binary Indexed Tree supporting point updates
// and range queries over a cumulative function or functor,
// such as max and min, in K dimensions, even if K is not known at
// compile time
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// T: the type of each element
// Op: a struct with the cumulative operation (plus<T> by default)
// Required Functions:
// operator (l, r): combines the values l and r, must be associative and
// commutatitve
// Constructor Arguments:
// D: a vector of length K with the size of each dimension
// A: the flattened array, represented by a vector of type T
// Functions:
// values(inv): returns a vector of the fenwick tree decomposed into a
// flattened array, where inv is the inverse of op (minus<T>() by default)
// update(ind, v): updates the indices specified by the vector ind holding
// the index for each dimension, with the value a being modified to
// op(a, v)
// query(ind): queries the cumulative value of the prefix bounded by the
// indices specified by the vector ind holding the index for each
// dimension, where inv is the inverse of Op (minus<T> by default)
// query(ind, inv): queries the cumulative value of the indices specified by
// the vector ind holding a pair specifying the range for each dimension,
// where inv is the inverse of Op (minus<T> by default)
// In practice, has a small constant, slower than FenwickTree.h
// Time Complexity:
// constructor: O(PI(N_i))
// update, query(ind): O(PI(log(N_i)))
// query(ind, inv): O(2^K * PI(log(N_i)))
// Memory Complexity: O(PI(N_i))
// PI is the product function, N_i is the size in the ith dimension
// Tested:
// https://dmoj.ca/problem/inaho2
// https://dmoj.ca/problem/inaho7
template <class T, class Op = plus<T>> struct FenwickTreeKD {
int K; vector<int> D, suf; vector<T> BIT; T qdef; Op op;
int dig(int i, int d) { return i % suf[d] / suf[d + 1]; }
int nxt(int i) { i++; i += i & -i; return --i; }
FenwickTreeKD(const vector<int> &D, T qdef = T(), Op op = Op())
: K(D.size()), D(D), suf(K + 1, 1), qdef(qdef), op(op) {
for (int i = K - 1; i >= 0; i--) suf[i] = suf[i + 1] * D[i];
BIT.resize(suf[0], qdef);
}
FenwickTreeKD(const vector<int> &D, vector<T> A, T qdef = T(), Op op = Op())
: K(D.size()), D(D), suf(K + 1, 1), BIT(move(A)), qdef(qdef), op(op) {
for (int i = K - 1; i >= 0; i--) suf[i] = suf[i + 1] * D[i];
assert(int(BIT.size()) == suf[0]);
for (int d = 0; d < K; d++) for (int i = 0; i < suf[0]; i++)
if (nxt(dig(i, d)) < D[d]) {
int j = i + (nxt(dig(i, d)) - dig(i, d)) * suf[d + 1];
BIT[j] = op(BIT[j], BIT[i]);
}
}
template <class Inv = minus<T>> vector<T> values(Inv inv = Inv()) {
vector<T> ret = BIT; for (int d = K - 1; d >= 0; d--)
for (int i = suf[0] - 1; i >= 0; i--) if (nxt(dig(i, d)) < D[d]) {
int j = i + (nxt(dig(i, d)) - dig(i, d)) * suf[d + 1];
ret[j] = inv(ret[j], ret[i]);
}
return ret;
}
void update(const vector<int> &ind, const T &v, int d = 0, int pos = 0) {
if (d == K) BIT[pos] = op(BIT[pos], v);
else for (int i = ind[d] + 1; i <= D[d]; i += i & -i)
update(ind, v, d + 1, pos + (i - 1) * suf[d + 1]);
}
T query(const vector<int> &ind, int d = 0, int pos = 0) {
T ret = qdef; if (d == K) ret = op(ret, BIT[pos]);
else for (int r = ind[d] + 1; r > 0; r -= r & r)
ret = op(ret, query(ind, d + 1, pos + (r - 1) * suf[d + 1]));
return ret;
}
template <class Inv = minus<T>>
T query(const vector<pair<int, int>> &ind, Inv inv = Inv(),
int d = 0, int pos = 0) {
T ret = qdef; if (d == K) ret = op(ret, BIT[pos]);
else {
for (int l = ind[d].first; l > 0; l -= l & -l)
ret = inv(ret, query(ind, inv, d + 1, pos + (l - 1) * suf[d + 1]));
for (int r = ind[d].second + 1; r > 0; r -= r & -r)
ret = op(ret, query(ind, inv, d + 1, pos + (r - 1) * suf[d + 1]));
}
return ret;
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
#include "FenwickTreeRangePoint1D.h"
using namespace std;
// Fenwick Tree supporting range updates with updates in the form of
// adding m + b, 2m + b, 3m + b, ... to the range [l, r],
// and range sum queries
// Indices are 0-indexed and ranges are inclusive
// numeric_limits<T>::max() * N * N must not overflow
// Template Arguments:
// T: the type of each element
// A: a vector of type T, memory is saved if this is moved and has
// a capacity of N + 1
// Constructor Arguments:
// N: the size of the array
// Functions:
// update(l, r, m, b): add m + b, 2m + b, 3m + b, ... to the range [l, r]
// query(r): queries the sum of the range [0, r]
// query(l, r): queries the sum of the range [l, r]
// In practice, has a small constant, faster than SegmentTreeAffine
// Time Complexity:
// constructor: O(N)
// update, query: O(log N)
// Memory Complexity: O(N)
// Tested:
// https://dmoj.ca/problem/acc3p4
template <class T> struct FenwickTreeAffine {
vector<FenwickTreeRangePoint1D<T>> FT;
FenwickTreeAffine(int N) : FT(3, FenwickTreeRangePoint1D<T>(N)) {}
FenwickTreeAffine(vector<T> A) {
for (auto &&a : A) a *= T(2);
FT.reserve(3); FT.emplace_back(move(A));
FT.emplace_back(FT[0].N); FT.emplace_back(FT[0].N);
}
T query(int r) {
return (FT[2].get(r) * T(r) * T(r) + FT[1].get(r) * T(r)
+ FT[0].get(r)) / T(2);
}
T query(int l, int r) { return query(r) - query(l - 1); }
void update(int l, int r, T m, T b) {
FT[2].update(l, r, m);
FT[1].update(l, r, m * (T(1) - T(l - 1) * T(2)) + b * T(2));
T con1 = m * T(l - 1) * T(l - 2) + b * T(1 - l) * T(2);
T con2 = m * T(r - l + 1) * T(r - l + 2) + b * (r - l + 1) * T(2);
FT[0].update(l, con1); FT[0].update(r + 1, con2 - con1);
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Fenwick Tree or Binary Indexed Tree supporting range updates
// and point queries, over a cumulative functor, such as sum, max, and min,
// in 1 dimension
// Indices are 0-indexed and ranges are inclusive
// Order of arguments for update differs slightly from FenwickTreeRangePoint
// Template Arguments:
// T: the type of each element
// Op: a struct with the cumulative operation (plus<T> by default)
// Required Functions:
// operator (l, r): combines the values l and r, must be associative and
// commutatitve
// Constructor Arguments:
// N: the size of the array
// A: a vector of type T, memory is saved if this is moved and has
// a capacity of N + 1
// qdef: the identity element of the operation
// op: an instance of the Op struct
// inv(l, r): a functor or function that performs the inverse operation of
// op (minus<T>() by default)
// Functions:
// values(inv): returns a vector of the fenwick tree decomposed into an
// array, where inv is the inverse of op (minus<T>() by default)
// update(l, v): sets A[i] to op(A[i], v) for the range [l..N - 1]
// update(l, r, v, inv): add A[i] to op(A[i], v) for the range [l..r]
// get(i): queries the value at index i, where inv is the inverse of
// op (minus<T>() by default)
// bsearch(v, cmp): returns the first index where cmp(A[i], v)
// returns false, or N if no such index exists
// In practice, this version performs as well as the multidimensional version
// In practice, has a small constant, faster than segment trees
// Time Complexity:
// constructor, values: O(N)
// update, get: O(log N)
// Memory Complexity: O(N)
// Tested:
// Fuzz and Stress Tested
// https://mcpt.ca/problem/asquirrelproblem
// https://codeforces.com/contest/1404/problem/C
template <class T, class Op = plus<T>> struct FenwickTreeRangePoint1D {
int N; vector<T> BIT; Op op;
FenwickTreeRangePoint1D(int N, T qdef = T(), Op op = Op())
: N(N), BIT(N + 1, qdef), op(op) {}
template <class Inv = minus<T>>
FenwickTreeRangePoint1D(vector<T> A, T qdef = T(),
Op op = Op(), Inv inv = Inv())
: N(A.size()), BIT(move(A)), op(op) {
adjacent_difference(BIT.begin(), BIT.end(), BIT.begin(), inv);
BIT.reserve(N + 1); BIT.insert(BIT.begin(), qdef);
for (int i = 1; i <= N; i++) {
int j = i + (i & -i); if (j <= N) BIT[j] = op(BIT[j], BIT[i]);
}
}
template <class Inv = minus<T>> vector<T> values(Inv inv = Inv()) {
vector<T> ret(BIT.begin() + 1, BIT.end()); for (int i = N; i >= 1; i--) {
int j = i + (i & -i);
if (j <= N) ret[j - 1] = inv(ret[j - 1], ret[i - 1]);
}
for (int i = 1; i < N; i++) ret[i] = op(ret[i - 1], ret[i]);
return ret;
}
void update(int l, T v) {
for (l++; l <= N; l += l & -l) BIT[l] = op(BIT[l], v);
}
template <class Inv = minus<T>>
void update(int l, int r, T v, Inv inv = Inv()) {
update(l, v); update(r + 1, inv(BIT[0], v));
}
T get(int i) {
T ret = BIT[0]; for (i++; i > 0; i -= i & -i) ret = op(ret, BIT[i]);
return ret;
}
template <class F> int bsearch(T v, F cmp) {
T val = BIT[0]; int ind = 0; for (int j = __lg(N + 1); j >= 0; j--) {
int i = ind + (1 << j);
if (i <= N && cmp(op(val, BIT[i]), v)) val = op(val, BIT[ind = i]);
}
return ind;
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
#include "../../PolicyBasedDataStructures.h"
using namespace std;
using namespace __gnu_pbds;
// A collection of sparse fenwick trees implemented in various methods
// If bsearch is not required and online is enforced, then SqrtBufferSimple and
// SqrtBuffer should be used instead
// If updates only involve values 1 and -1, and online is enforced,
// then RootArray should be used instead
// In general, offline fenwick tree is faster than SqrtBufferSimple,
// SqrtBuffer, and RootArray, which are faster than the pbds implementation
// Sparse Fenwick Tree supporting point updates (with any value)
// and range queries over a cumulative function or functor,
// such as sum, max, and min, in 1 dimension
// All update indices must be known beforehand
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// T: the type of each element
// IndexType: the type of the index of the array
// Op: a struct with the cumulative operation (plus<T> by default)
// Required Functions:
// operator (l, r): combines the values l and r, must be associative and
// commutatitve
// Constructor Arguments:
// N: the size of the array
// updateInds: a vector of IndexType containing the indices for each update
// qdef: the identity element of the operation
// op: an instance of the Op struct
// Functions:
// update(i, v): modifies the value A[i] to op(A[i], v), i must be an element
// in updateInds
// query(r): queries the cumulative value of the range [0, r]
// query(l, r, inv): queries the cumulative value of the range [l, r], where
// inv is the inverse of op (minus<T>() by default)
// bsearch(v, cmp): returns the first index where cmp(op(A[0..i]), v)
// returns false, or N if no such index exists, assumes A is sorted by cmp
// In practice, has a small constant
// Time Complexity:
// constructor: O(Q log U) for U updates
// update, query, bsearch: O(log U) for U updates
// Memory Complexity: O(U) for U updates
// Tested:
// https://codeforces.com/contest/903/problem/D
// https://dmoj.ca/problem/cco10p3
// https://atcoder.jp/contests/dp/tasks/dp_q
template <class T, class IndexType, class Op = plus<T>>
struct OfflineSparseFenwickTree1D {
static_assert(is_integral<IndexType>::value, "IndexType must be integeral");
IndexType N; vector<T> BIT; vector<IndexType> inds; T qdef; Op op;
int getInd(IndexType i) {
return std::upper_bound(inds.begin(), inds.end(), i) - inds.begin();
}
OfflineSparseFenwickTree1D(IndexType N, const vector<IndexType> &updateInds,
T qdef = T(), Op op = Op())
: N(N), inds(updateInds), qdef(qdef), op(op) {
sort(inds.begin(), inds.end());
inds.erase(unique(inds.begin(), inds.end()), inds.end());
BIT.assign(int(inds.size()) + 1, qdef);
}
void update(IndexType i, T v) {
for (int x = getInd(i); x <= int(inds.size()); x += x & -x)
BIT[x] = op(BIT[x], v);
}
T query(IndexType r) {
T ret = qdef;
for (int x = getInd(r); x > 0; x -= x & -x) ret = op(ret, BIT[x]);
return ret;
}
template <class Inv = minus<T>>
T query(IndexType l, IndexType r, Inv inv = Inv()) {
return inv(query(r), query(l - 1));
}
template <class F> IndexType bsearch(T v, F cmp) {
T agg = qdef; int ind = 0;
for (int j = __lg(int(inds.size()) + 1); j >= 0; j--) {
int i = ind + (1 << j);
if (i < int(inds.size()) && cmp(op(agg, BIT[i]), v))
agg = op(agg, BIT[ind = i]);
}
return ind == int(inds.size()) ? N : inds[ind];
}
};
// Sparse Fenwick Tree supporting point updates (with any value)
// and range queries over a cumulative function or functor,
// such as sum, max, and min, in 1 dimension using pbds hash_table
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// T: the type of each element
// IndexType: the type of the index of the array
// Op: a struct with the cumulative operation (plus<T> by default)
// Required Functions:
// operator (l, r): combines the values l and r, must be associative and
// commutatitve
// Constructor Arguments:
// N: the size of the array
// qdef: the identity element of the operation
// op: an instance of the Op struct
// Functions:
// update(i, v): modifies the value A[i] to op(A[i], v)
// query(r): queries the cumulative value of the range [0, r]
// query(l, r, inv): queries the cumulative value of the range [l, r], where
// inv is the inverse of op (minus<T>() by default)
// bsearch(v, cmp): returns the first index where cmp(op(A[0..i]), v)
// returns false, or N if no such index exists
// In practice, has a moderate constant
// Time Complexity:
// constructor: O(1)
// update, query, bsearch: O(log N) on average
// Memory Complexity: O(U log N) for U updates
// Tested:
// https://codeforces.com/contest/903/problem/D
// https://dmoj.ca/problem/cco10p3
// https://atcoder.jp/contests/dp/tasks/dp_q
template <class T, class IndexType, class Container = hashmap<IndexType, T>,
class Op = plus<T>>
struct SparseFenwickTree1D {
static_assert(is_integral<IndexType>::value, "IndexType must be integeral");
IndexType N; Container BIT; T qdef; Op op;
SparseFenwickTree1D(IndexType N, T qdef = T(), Op op = Op())
: N(N), qdef(qdef), op(op) {}
void update(IndexType i, T v) {
for (i++; i <= N; i += i & -i) BIT[i] = op(BIT[i], v);
}
T query(IndexType r) {
T ret = qdef; for (r++; r > 0; r -= r & -r) {
auto it = BIT.find(r); if (it != BIT.end()) ret = op(ret, it->second);
}
return ret;
}
template <class Inv = minus<T>>
T query(IndexType l, IndexType r, Inv inv = Inv()) {
return inv(query(r), query(l - 1));
}
template <class F> IndexType bsearch(T v, F cmp) {
T agg = qdef; IndexType ind = 0;
for (IndexType j = __lg(N + 1); j >= 0; j--) {
IndexType i = ind + (1 << j); if (i <= N) {
auto it = BIT.find(i); T val = it == BIT.end() ? 0 : it->second;
if (cmp(op(agg, val), v)) { agg = op(agg, val); ind = i; }
}
}
return ind;
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Fenwick Tree or Binary Indexed Tree supporting point updates
// and range queries over a cumulative function or functor,
// such as sum, max, and min, in 1 dimension
// Indices are 0-indexed and ranges are inclusive
// Template Arguments:
// T: the type of each element
// Op: a struct with the cumulative operation (plus<T> by default)
// Required Functions:
// operator (l, r): combines the values l and r, must be associative and
// commutatitve
// Constructor Arguments:
// N: the size of the array
// A: a vector of type T, memory is saved if this is moved and has
// a capacity of N + 1
// qdef: the identity element of the operation
// op: an instance of the Op struct
// Functions:
// values(inv): returns a vector of the fenwick tree decomposed into an
// array, where inv is the inverse of op (minus<T>() by default)
// update(i, v): modifies the value A[i] to op(A[i], v)
// query(r): queries the cumulative value of the range [0, r]
// query(l, r, inv): queries the cumulative value of the range [l, r], where
// inv is the inverse of op (minus<T>() by default)
// bsearch(v, cmp): returns the first index where cmp(op(A[0..i]), v)
// returns false, or N if no such index exists
// In practice, has a small constant, faster than segment trees
// Time Complexity:
// constructor, values: O(N)
// update, query, bsearch: O(log N)
// Memory Complexity: O(N)
// Tested:
// Fuzz and Stress Tested
// https://dmoj.ca/problem/ds1
// https://judge.yosupo.jp/problem/point_add_range_sum
// https://atcoder.jp/contests/dp/tasks/dp_q
template <class T, class Op = plus<T>> struct FenwickTree1D {
int N; vector<T> BIT; Op op;
FenwickTree1D(int N, T qdef = T(), Op op = Op())
: N(N), BIT(N + 1, qdef), op(op) {}
FenwickTree1D(vector<T> A, T qdef = T(), Op op = Op())
: N(A.size()), BIT(move(A)), op(op) {
BIT.reserve(N + 1); BIT.insert(BIT.begin(), qdef);
for (int i = 1; i <= N; i++) {
int j = i + (i & -i); if (j <= N) BIT[j] = op(BIT[j], BIT[i]);
}
}
template <class Inv = minus<T>> vector<T> values(Inv inv = Inv()) {
vector<T> ret(BIT.begin() + 1, BIT.end()); for (int i = N; i >= 1; i--) {
int j = i + (i & -i);
if (j <= N) ret[j - 1] = inv(ret[j - 1], ret[i - 1]);
}
return ret;
}
void update(int i, T v) {
for (i++; i <= N; i += i & -i) BIT[i] = op(BIT[i], v);
}
T query(int r) {
T ret = BIT[0]; for (r++; r > 0; r -= r & -r) ret = op(ret, BIT[r]);
return ret;
}
template <class Inv = minus<T>> T query(int l, int r, Inv inv = Inv()) {
return inv(query(r), query(l - 1));
}
template <class F> int bsearch(T v, F cmp) {
T agg = BIT[0]; int ind = 0; for (int j = __lg(N + 1); j >= 0; j--) {
int i = ind + (1 << j);
if (i <= N && cmp(op(agg, BIT[i]), v)) agg = op(agg, BIT[ind = i]);
}
return ind;
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
#include "FenwickTree1D.h"
using namespace std;
// Fenwick Tree or Binary Indexed Tree supporting range updates
// and range sum queries in 1 dimension
// Indices are 0-indexed and ranges are inclusive
// numeric_limits<T>::max() * N must not overflow
// Template Arguments:
// T: the type of each element
// Constructor Arguments:
// N: the size of the array
// A: a vector of type T, memory is saved if this is moved and has
// a capacity of N + 1
// Functions:
// update(l, r, v): add v to the range [l..r]
// query(r): queries the sum of the range [0, r]
// query(l, r): queries the sum of the range [l, r]
// In practice, has a small constant, faster than segment trees
// Time Complexity:
// constructor: O(N)
// update, query: O(log N)
// Memory Complexity: O(N)
// Tested:
// Fuzz and Stress Tested
// http://www.usaco.org/index.php?page=viewproblem2&cpid=973
template <class T> struct FenwickTreeRange1D {
vector<FenwickTree1D<T>> FT;
FenwickTreeRange1D(int N) : FT(2, FenwickTree1D<T>(N)) {}
FenwickTreeRange1D(vector<T> A) {
FT.reserve(2); FT.emplace_back(move(A)); FT.emplace_back(FT[0].N);
}
T query(int r) { return FT[1].query(r) * T(r) + FT[0].query(r); }
T query(int l, int r) { return query(r) - query(l - 1); }
void update(int l, int r, T v) {
FT[1].update(l, v); FT[1].update(r + 1, -v);
FT[0].update(l, v * T(1 - l)); FT[0].update(r + 1, v * T(r));
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
using namespace std;
// Fenwick Tree supporting range sum queries where all values are 0 or 1,
// and values can be updated after they are set
// Indices are 0-indexed and ranges are inclusive
// Constructor Arguments:
// N: the size of the array
// Functions:
// set(i, v): sets the initial value of index i with the boolean value v
// get(i): gets the current value of index i
// build(): builds the fenwick tree, set should not be called afterwards,
// does not need to be called if there are no calls to set
// update(i, v): updates the value of index i with the boolean value v,
// should not be called before build unless there were no calls to set
// query(r): queries the sum of the range [0, r]
// query(l, r): queries the sum of the range [l, r]
// bsearch(v, cmp): returns the first index where cmp(sum(A[0..i]), v)
// returns false, or N if no such index exists
// In practice, has a moderate constant, performs faster than a regular fenwick
// tree and uses less memory
// Time Complexity:
// constructor, set, get: O(1)
// update, query, bsearch, lower_bound, upper_bound: O(log(N / 64))
// build: O(N / 64)
// Memory Complexity: O(N / 64)
// Tested:
// Fuzz and Stress Tested
// https://judge.yosupo.jp/problem/predecessor_problem
struct BitFenwickTree {
int N, M; vector<uint64_t> mask; vector<int> BIT;
BitFenwickTree(int N) : N(N), M((N >> 6) + 1), mask(M, 0), BIT(M + 1, 0) {}
void set(int i, bool v) {
int j = i >> 6, k = i & 63;
mask[j] = (mask[j] & ~(uint64_t(1) << k)) | (uint64_t(v) << k);
}
bool get(int i) { return (mask[i >> 6] >> (i & 63)) & 1; }
void build() {
for (int i = 1; i <= M; i++) {
BIT[i] += __builtin_popcountll(mask[i - 1]); int j = i + (i & -i);
if (j <= M) BIT[j] += BIT[i];
}
}
void update(int i, bool v) {
int j = i >> 6, k = i & 63; if (((mask[j] >> k) & 1) != v) {
mask[j] ^= uint64_t(1) << k; int delta = v ? 1 : -1;
for (j++; j <= M; j += j & -j) BIT[j] += delta;
}
}
int query(int r) {
r++; int j = r >> 6, k = r & 63, ret = 0;
for (int i = j; i > 0; i -= i & -i) ret += BIT[i];
return ret + __builtin_popcountll(mask[j] & ((uint64_t(1) << k) - 1));
}
int query(int l, int r) { return query(r) - query(l - 1); }
template <class F> int bsearch(int v, F cmp) {
int sum = 0, ind = 0; for (int j = __lg(M + 1); j >= 0; j--) {
int i = ind + (1 << j);
if (i <= M && cmp(sum + BIT[i], v)) sum += BIT[ind = i];
}
if (ind == M) return N;
uint64_t m = mask[ind]; ind <<= 6;
for (;; ind++, m >>= 1) if (!cmp(sum += m & 1, v)) break;
return ind;
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
#if __cplusplus < 201402L
#include "../../../utils/MakeUnique.h"
#endif
using namespace std;
// Skew Heap supporting merges
// Template Arguments:
// T: the type of each element
// Cmp: the comparator to compare two values of type T,
// convention is same as std::priority_queue in STL
// Required Functions:
// operator (a, b): returns true if and only if a compares less than b
// Functions:
// empty(): returns whether the heap is empty
// size(): returns the number of elements in the heap
// top(): returns the largest element in the heap based on the comparator
// pop(): pops the largest element in the heap based on the comparator,
// and returns that element
// push(key): pushes val onto the heap
// merge(h): merges the heap h into this heap, destroys heap h
// In practice, has a moderate constant
// Time Complexity:
// constructor, empty, size, top: O(1)
// pop, push: O(log N) amortized
// merge: O(log (N + M)) amortized where M is the size of heap h
// Memory Complexity: O(N)
// Tested:
// https://dmoj.ca/problem/apio16p2
template <class T, class Cmp = less<T>> struct SkewHeap {
struct Node { T val; unique_ptr<Node> l, r; Node(const T &v) : val(v) {} };
Cmp cmp; int cnt; unique_ptr<Node> root;
unique_ptr<Node> merge(unique_ptr<Node> a, unique_ptr<Node> b) {
if (!a || !b) return a ? move(a) : move(b);
if (cmp(a->val, b->val)) a.swap(b);
a->l.swap(a->r); a->r = merge(move(b), move(a->r)); return move(a);
}
SkewHeap() : cnt(0) {}
bool empty() const { return !root; }
int size() const { return cnt; }
T top() const { return root->val; }
T pop() {
T ret = root->val; root = merge(move(root->l), move(root->r)); cnt--;
return ret;
}
void push(const T &val) {
root = merge(move(root), make_unique<Node>(val)); cnt++;
}
void merge(SkewHeap &h) {
root = merge(move(root), move(h.root)); cnt += h.cnt;
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
#include "../../../utils/Random.h"
using namespace std;
// Persistent Heap supporting merges where copy assignment/constructor creates
// a new version of the data structure
// Template Arguments:
// T: the type of each element
// Cmp: the comparator to compare two values of type T,
// convention is same as std::priority_queue in STL
// Required Functions:
// operator (a, b): returns true if and only if a compares less than b
// Functions:
// empty(): returns whether the current heap is empty
// size(): returns the number of elements in the current heap
// top(): returns the largest element in the current heap based
// on the comparator
// pop(): pops the largest element in the current heap based
// on the comparator, and returns that element
// push(key): pushes val onto the current heap
// merge(h): merges the heap h into the current heap
// In practice, has a moderate constant
// Time Complexity:
// constructor, empty, size, top: O(1)
// pop, push: O(log N) expected
// merge: O(log (N + M)) expected where M is the size of heap h
// Memory Complexity: O(N + Q log N) for Q operations
// Tested:
// https://dmoj.ca/problem/wac4p5
template <class T, class Cmp = less<T>> struct PersistentRandomizedHeap {
struct Node; using ptr = shared_ptr<Node>;
struct Node {
T val; ptr l, r;
Node(const T &v, const ptr &l = ptr(), const ptr &r = ptr())
: val(v), l(l), r(r) {}
};
Cmp cmp; int cnt; ptr root;
ptr merge(ptr a, ptr b) {
if (!a || !b) return a ? a : b;
if (cmp(a->val, b->val)) a.swap(b);
return rng() % 2 ? make_shared<Node>(a->val, a->l, merge(a->r, b))
: make_shared<Node>(a->val, merge(a->l, b), a->r);
}
PersistentRandomizedHeap() : cnt(0) {}
bool empty() const { return !root; }
int size() const { return cnt; }
T top() { return root->val; }
T pop() {
T ret = root->val; root = merge(root->l, root->r); cnt--; return ret;
}
void push(const T &val) {
root = merge(root, make_shared<Node>(val)); cnt++;
}
void merge(const PersistentRandomizedHeap &h) {
root = merge(root, h.root); cnt += h.cnt;
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
#include "../../../utils/Random.h"
using namespace std;
// Persistent Heap supporting merges and increments where
// copy assignment/constructor creates a new version of the data structure
// Template Arguments:
// T: the type of each element
// Cmp: the comparator to compare two values of type T,
// convention is same as std::priority_queue in STL
// Required Functions:
// operator (a, b): returns true if and only if a compares less than b
// Delta: the type of the incremental element
// Functions:
// empty(): returns whether the current heap is empty
// size(): returns the number of elements in the current heap
// top(): returns the largest element in the current heap based
// on the comparator
// pop(): pops the largest element in the current heap based
// on the comparator, and returns that element
// push(key): pushes val onto the current heap
// increment(delta): increments all elements in the current heap by delta
// merge(h): merges the heap h into the current heap
// In practice, has a moderate constant
// Time Complexity:
// constructor, empty, size, top, increment: O(1)
// pop, push: O(log N) expected
// merge: O(log (N + M)) expected where M is the size of heap h
// Memory Complexity: O(N + Q log N) for Q operations
// Tested:
// https://dmoj.ca/problem/wac4p5
template <class T, class Cmp = less<T>, class Delta = T>
struct PersistentRandomizedHeapIncremental {
struct Node; using ptr = shared_ptr<Node>;
struct Node {
T val; Delta delta; ptr l, r;
Node(const T &v, const Delta &d,
const ptr &l = ptr(), const ptr &r = ptr())
: val(v), delta(d), l(l), r(r) {}
};
Cmp cmp; Delta ddef; int cnt; ptr root;
void propagate(ptr &a) {
a->val += a->delta;
if (a->l) { a->l = make_shared<Node>(*a->l); a->l->delta += a->delta; }
if (a->r) { a->r = make_shared<Node>(*a->r); a->r->delta += a->delta; }
a->delta = ddef;
}
ptr merge(ptr a, ptr b) {
if (!a || !b) return a ? a : b;
propagate(a); propagate(b); if (cmp(a->val, b->val)) a.swap(b);
return rng() % 2
? make_shared<Node>(a->val, a->delta, a->l, merge(a->r, b))
: make_shared<Node>(a->val, a->delta, merge(a->l, b), a->r);
}
PersistentRandomizedHeapIncremental(const Delta &ddef = Delta())
: ddef(ddef), cnt(0) {}
bool empty() const { return !root; }
int size() const { return cnt; }
T top() { propagate(root); return root->val; }
T pop() {
propagate(root); T ret = root->val;
root = merge(root->l, root->r); cnt--; return ret;
}
void push(const T &val) {
root = merge(root, make_shared<Node>(val, ddef)); cnt++;
}
void increment(const Delta &delta) {
if (root) { root = make_shared<Node>(*root); root->delta += delta; }
}
void merge(const PersistentRandomizedHeapIncremental &h) {
root = merge(root, h.root); cnt += h.cnt;
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
#pragma once
#include <bits/stdc++.h>
#if __cplusplus < 201402L
#include "../../../utils/MakeUnique.h"
#endif
using namespace std;
// Skew Heap supporting merges and increments
// Template Arguments:
// T: the type of each element
// Cmp: the comparator to compare two values of type T,
// convention is same as std::priority_queue in STL
// Required Functions:
// operator (a, b): returns true if and only if a compares less than b
// Delta: the type of the incremental element
// Functions:
// empty(): returns whether the heap is empty
// size(): returns the number of elements in the heap
// top(): returns the largest element in the heap based on the comparator
// pop(): pops the largest element in the heap based on the comparator,
// and returns that element
// push(key): pushes val onto the heap
// increment(delta): increments all elements in the heap by delta
// merge(h): merges the heap h into this heap, destroys heap h
// In practice, has a moderate constant
// Time Complexity:
// constructor, empty, size, top, increment: O(1)
// pop, push: O(log N) amortized
// merge: O(log (N + M)) amortized
// Memory Complexity: O(N)
// Tested:
// https://codeforces.com/contest/1041/problem/D
template <class T, class Cmp = less<T>, class Delta = T>
struct SkewHeapIncremental {
Cmp cmp; Delta ddef;
struct Node {
T val; Delta delta; unique_ptr<Node> l, r;
Node(const T &v, const Delta &d) : val(v), delta(d) {}
};
int cnt; unique_ptr<Node> root;
void propagate(unique_ptr<Node> &a) {
a->val += a->delta;
if (a->l) a->l->delta += a->delta;
if (a->r) a->r->delta += a->delta;
a->delta = ddef;
}
unique_ptr<Node> merge(unique_ptr<Node> a, unique_ptr<Node> b) {
if (!a || !b) return a ? move(a) : move(b);
propagate(a); propagate(b); if (cmp(a->val, b->val)) a.swap(b);
a->l.swap(a->r); a->r = merge(move(b), move(a->r)); return move(a);
}
SkewHeapIncremental(const Delta &ddef = Delta()) : ddef(ddef), cnt(0) {}
bool empty() const { return !root; }
int size() const { return cnt; }
T top() { propagate(root); return root->val; }
T pop() {
propagate(root); T ret = root->val;
root = merge(move(root->l), move(root->r)); cnt--; return ret;
}
void push(const T &val) {
root = merge(move(root), make_unique<Node>(val, ddef)); cnt++;
}
void increment(const Delta &delta) { if (root) root->delta += delta; }
void merge(SkewHeapIncremental &h) {
root = merge(move(root), move(h.root)); cnt += h.cnt;
}
};
| {
"repo_name": "wesley-a-leung/Resources",
"stars": "34",
"repo_language": "C++",
"file_name": "SkewHeapIncremental.h",
"mime_type": "text/x-c++"
} |
cmake_minimum_required(VERSION 3.4)
project(FeatureDetector CXX)
add_subdirectory(src/x86)
target_include_directories(FeatureDetector PUBLIC src)
if(CMAKE_PROJECT_NAME STREQUAL PROJECT_NAME)
add_executable(FeatureDetector_main src/Main.cpp)
target_link_libraries(FeatureDetector_main PUBLIC FeatureDetector)
endif()
| {
"repo_name": "Mysticial/FeatureDetector",
"stars": "246",
"repo_language": "C++",
"file_name": "FeatureDetector.vcxproj.filters",
"mime_type": "text/xml"
} |
Program('bin/FeatureDetector', ['src/Main.cpp', 'src/x86/cpu_x86.cpp'])
| {
"repo_name": "Mysticial/FeatureDetector",
"stars": "246",
"repo_language": "C++",
"file_name": "FeatureDetector.vcxproj.filters",
"mime_type": "text/xml"
} |
Microsoft Visual Studio Solution File, Format Version 12.00
# Visual Studio 2013
VisualStudioVersion = 12.0.31101.0
MinimumVisualStudioVersion = 10.0.40219.1
Project("{8BC9CEB8-8B4A-11D0-8D11-00A0C91BC942}") = "FeatureDetector", "FeatureDetector\FeatureDetector.vcxproj", "{5AF4062D-386F-48AA-B824-2C10A4B1BA97}"
EndProject
Global
GlobalSection(SolutionConfigurationPlatforms) = preSolution
Debug|Win32 = Debug|Win32
Release|Win32 = Release|Win32
EndGlobalSection
GlobalSection(ProjectConfigurationPlatforms) = postSolution
{5AF4062D-386F-48AA-B824-2C10A4B1BA97}.Debug|Win32.ActiveCfg = Debug|Win32
{5AF4062D-386F-48AA-B824-2C10A4B1BA97}.Debug|Win32.Build.0 = Debug|Win32
{5AF4062D-386F-48AA-B824-2C10A4B1BA97}.Release|Win32.ActiveCfg = Release|Win32
{5AF4062D-386F-48AA-B824-2C10A4B1BA97}.Release|Win32.Build.0 = Release|Win32
EndGlobalSection
GlobalSection(SolutionProperties) = preSolution
HideSolutionNode = FALSE
EndGlobalSection
EndGlobal
| {
"repo_name": "Mysticial/FeatureDetector",
"stars": "246",
"repo_language": "C++",
"file_name": "FeatureDetector.vcxproj.filters",
"mime_type": "text/xml"
} |
# FeatureDetector
A simple app that detects what hardware features are supported by the host CPU and operating system.
-----
Sample output for a Haswell processor:
````
CPU Vendor String: GenuineIntel
CPU Vendor:
AMD = No
Intel = Yes
OS Features:
64-bit = Yes
OS AVX = Yes
OS AVX512 = No
Hardware Features:
MMX = Yes
x64 = Yes
ABM = Yes
RDRAND = Yes
BMI1 = Yes
BMI2 = Yes
ADX = No
MPX = No
PREFETCHWT1 = No
SIMD: 128-bit
SSE = Yes
SSE2 = Yes
SSE3 = Yes
SSSE3 = Yes
SSE4a = No
SSE4.1 = Yes
SSE4.2 = Yes
AES-NI = Yes
SHA = No
SIMD: 256-bit
AVX = Yes
XOP = No
FMA3 = Yes
FMA4 = No
AVX2 = Yes
SIMD: 512-bit
AVX512-F = No
AVX512-CD = No
AVX512-PF = No
AVX512-ER = No
AVX512-VL = No
AVX512-BW = No
AVX512-DQ = No
AVX512-IFMA = No
AVX512-VBMI = No
Summary:
Safe to use AVX: Yes
Safe to use AVX512: No
| {
"repo_name": "Mysticial/FeatureDetector",
"stars": "246",
"repo_language": "C++",
"file_name": "FeatureDetector.vcxproj.filters",
"mime_type": "text/xml"
} |
/* Main.cpp
*
* Author : Alexander J. Yee
* Date Created : 04/17/2015
* Last Modified : 04/17/2015
*
*/
#include <iostream>
using std::cout;
using std::endl;
#include "x86/cpu_x86.h"
using namespace FeatureDetector;
int main(){
cout << "CPU Vendor String: " << cpu_x86::get_vendor_string() << endl;
cout << endl;
cpu_x86::print_host();
#if _WIN32
system("pause");
#endif
}
| {
"repo_name": "Mysticial/FeatureDetector",
"stars": "246",
"repo_language": "C++",
"file_name": "FeatureDetector.vcxproj.filters",
"mime_type": "text/xml"
} |
/* cpu_x86_Linux.ipp
*
* Author : Alexander J. Yee
* Date Created : 04/12/2014
* Last Modified : 04/12/2014
*
*/
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
// Dependencies
#include <cpuid.h>
#include "cpu_x86.h"
namespace FeatureDetector{
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
void cpu_x86::cpuid(int32_t out[4], int32_t eax, int32_t ecx){
__cpuid_count(eax, ecx, out[0], out[1], out[2], out[3]);
}
uint64_t xgetbv(unsigned int index){
uint32_t eax, edx;
__asm__ __volatile__("xgetbv" : "=a"(eax), "=d"(edx) : "c"(index));
return ((uint64_t)edx << 32) | eax;
}
#define _XCR_XFEATURE_ENABLED_MASK 0
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
// Detect 64-bit
bool cpu_x86::detect_OS_x64(){
// We only support x64 on Linux.
return true;
}
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
}
| {
"repo_name": "Mysticial/FeatureDetector",
"stars": "246",
"repo_language": "C++",
"file_name": "FeatureDetector.vcxproj.filters",
"mime_type": "text/xml"
} |
/* cpu_x86.h
*
* Author : Alexander J. Yee
* Date Created : 04/12/2014
* Last Modified : 04/12/2014
*
*/
#pragma once
#ifndef _cpu_x86_H
#define _cpu_x86_H
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
// Dependencies
#include <stdint.h>
#include <string>
namespace FeatureDetector{
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
struct cpu_x86{
// Vendor
bool Vendor_AMD;
bool Vendor_Intel;
// OS Features
bool OS_x64;
bool OS_AVX;
bool OS_AVX512;
// Misc.
bool HW_MMX;
bool HW_x64;
bool HW_ABM;
bool HW_RDRAND;
bool HW_RDSEED;
bool HW_BMI1;
bool HW_BMI2;
bool HW_ADX;
bool HW_MPX;
bool HW_PREFETCHW;
bool HW_PREFETCHWT1;
bool HW_RDPID;
// SIMD: 128-bit
bool HW_SSE;
bool HW_SSE2;
bool HW_SSE3;
bool HW_SSSE3;
bool HW_SSE41;
bool HW_SSE42;
bool HW_SSE4a;
bool HW_AES;
bool HW_SHA;
// SIMD: 256-bit
bool HW_AVX;
bool HW_XOP;
bool HW_FMA3;
bool HW_FMA4;
bool HW_AVX2;
// SIMD: 512-bit
bool HW_AVX512_F;
bool HW_AVX512_CD;
// Knights Landing
bool HW_AVX512_PF;
bool HW_AVX512_ER;
// Skylake Purley
bool HW_AVX512_VL;
bool HW_AVX512_BW;
bool HW_AVX512_DQ;
// Cannon Lake
bool HW_AVX512_IFMA;
bool HW_AVX512_VBMI;
// Knights Mill
bool HW_AVX512_VPOPCNTDQ;
bool HW_AVX512_4VNNIW;
bool HW_AVX512_4FMAPS;
// Cascade Lake
bool HW_AVX512_VNNI;
// Cooper Lake
bool HW_AVX512_BF16;
// Ice Lake
bool HW_AVX512_VBMI2;
bool HW_GFNI;
bool HW_VAES;
bool HW_AVX512_VPCLMUL;
bool HW_AVX512_BITALG;
public:
cpu_x86();
void detect_host();
void print() const;
static void print_host();
static void cpuid(int32_t out[4], int32_t eax, int32_t ecx);
static std::string get_vendor_string();
private:
static void print(const char* label, bool yes);
static bool detect_OS_x64();
static bool detect_OS_AVX();
static bool detect_OS_AVX512();
};
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
}
#endif
| {
"repo_name": "Mysticial/FeatureDetector",
"stars": "246",
"repo_language": "C++",
"file_name": "FeatureDetector.vcxproj.filters",
"mime_type": "text/xml"
} |
/* cpu_x86.cpp
*
* Author : Alexander J. Yee
* Date Created : 04/12/2014
* Last Modified : 04/12/2014
*
*/
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
// Dependencies
#include <cstring>
#include <iostream>
#include "cpu_x86.h"
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
#if defined(__x86_64__) || defined(_M_X64) || defined(__i386) || defined(_M_IX86)
# if _WIN32
# include "cpu_x86_Windows.ipp"
# elif defined(__GNUC__) || defined(__clang__)
# include "cpu_x86_Linux.ipp"
# else
# error "No cpuid intrinsic defined for compiler."
# endif
#else
# error "No cpuid intrinsic defined for processor architecture."
#endif
namespace FeatureDetector{
using std::cout;
using std::endl;
using std::memcpy;
using std::memset;
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
void cpu_x86::print(const char* label, bool yes){
cout << label;
cout << (yes ? "Yes" : "No") << endl;
}
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
cpu_x86::cpu_x86(){
memset(this, 0, sizeof(*this));
}
bool cpu_x86::detect_OS_AVX(){
// Copied from: http://stackoverflow.com/a/22521619/922184
bool avxSupported = false;
int32_t cpuInfo[4];
cpuid(cpuInfo, 1, 0);
bool osUsesXSAVE_XRSTORE = (cpuInfo[2] & (1 << 27)) != 0;
bool cpuAVXSuport = (cpuInfo[2] & (1 << 28)) != 0;
if (osUsesXSAVE_XRSTORE && cpuAVXSuport)
{
uint64_t xcrFeatureMask = xgetbv(_XCR_XFEATURE_ENABLED_MASK);
avxSupported = (xcrFeatureMask & 0x6) == 0x6;
}
return avxSupported;
}
bool cpu_x86::detect_OS_AVX512(){
if (!detect_OS_AVX())
return false;
uint64_t xcrFeatureMask = xgetbv(_XCR_XFEATURE_ENABLED_MASK);
return (xcrFeatureMask & 0xe6) == 0xe6;
}
std::string cpu_x86::get_vendor_string(){
int32_t CPUInfo[4];
char name[13];
cpuid(CPUInfo, 0, 0);
memcpy(name + 0, &CPUInfo[1], 4);
memcpy(name + 4, &CPUInfo[3], 4);
memcpy(name + 8, &CPUInfo[2], 4);
name[12] = '\0';
return name;
}
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
void cpu_x86::detect_host(){
// OS Features
OS_x64 = detect_OS_x64();
OS_AVX = detect_OS_AVX();
OS_AVX512 = detect_OS_AVX512();
// Vendor
std::string vendor(get_vendor_string());
if (vendor == "GenuineIntel"){
Vendor_Intel = true;
}else if (vendor == "AuthenticAMD"){
Vendor_AMD = true;
}
int32_t info[4];
cpuid(info, 0, 0);
int nIds = info[0];
cpuid(info, 0x80000000, 0);
uint32_t nExIds = info[0];
// Detect Features
if (nIds >= 0x00000001){
cpuid(info, 0x00000001, 0);
HW_MMX = (info[3] & ((int)1 << 23)) != 0;
HW_SSE = (info[3] & ((int)1 << 25)) != 0;
HW_SSE2 = (info[3] & ((int)1 << 26)) != 0;
HW_SSE3 = (info[2] & ((int)1 << 0)) != 0;
HW_SSSE3 = (info[2] & ((int)1 << 9)) != 0;
HW_SSE41 = (info[2] & ((int)1 << 19)) != 0;
HW_SSE42 = (info[2] & ((int)1 << 20)) != 0;
HW_AES = (info[2] & ((int)1 << 25)) != 0;
HW_AVX = (info[2] & ((int)1 << 28)) != 0;
HW_FMA3 = (info[2] & ((int)1 << 12)) != 0;
HW_RDRAND = (info[2] & ((int)1 << 30)) != 0;
}
if (nIds >= 0x00000007){
cpuid(info, 0x00000007, 0);
HW_AVX2 = (info[1] & ((int)1 << 5)) != 0;
HW_BMI1 = (info[1] & ((int)1 << 3)) != 0;
HW_BMI2 = (info[1] & ((int)1 << 8)) != 0;
HW_ADX = (info[1] & ((int)1 << 19)) != 0;
HW_MPX = (info[1] & ((int)1 << 14)) != 0;
HW_SHA = (info[1] & ((int)1 << 29)) != 0;
HW_RDSEED = (info[1] & ((int)1 << 18)) != 0;
HW_PREFETCHWT1 = (info[2] & ((int)1 << 0)) != 0;
HW_RDPID = (info[2] & ((int)1 << 22)) != 0;
HW_AVX512_F = (info[1] & ((int)1 << 16)) != 0;
HW_AVX512_CD = (info[1] & ((int)1 << 28)) != 0;
HW_AVX512_PF = (info[1] & ((int)1 << 26)) != 0;
HW_AVX512_ER = (info[1] & ((int)1 << 27)) != 0;
HW_AVX512_VL = (info[1] & ((int)1 << 31)) != 0;
HW_AVX512_BW = (info[1] & ((int)1 << 30)) != 0;
HW_AVX512_DQ = (info[1] & ((int)1 << 17)) != 0;
HW_AVX512_IFMA = (info[1] & ((int)1 << 21)) != 0;
HW_AVX512_VBMI = (info[2] & ((int)1 << 1)) != 0;
HW_AVX512_VPOPCNTDQ = (info[2] & ((int)1 << 14)) != 0;
HW_AVX512_4VNNIW = (info[3] & ((int)1 << 2)) != 0;
HW_AVX512_4FMAPS = (info[3] & ((int)1 << 3)) != 0;
HW_AVX512_VNNI = (info[2] & ((int)1 << 11)) != 0;
HW_AVX512_VBMI2 = (info[2] & ((int)1 << 6)) != 0;
HW_GFNI = (info[2] & ((int)1 << 8)) != 0;
HW_VAES = (info[2] & ((int)1 << 9)) != 0;
HW_AVX512_VPCLMUL = (info[2] & ((int)1 << 10)) != 0;
HW_AVX512_BITALG = (info[2] & ((int)1 << 12)) != 0;
cpuid(info, 0x00000007, 1);
HW_AVX512_BF16 = (info[0] & ((int)1 << 5)) != 0;
}
if (nExIds >= 0x80000001){
cpuid(info, 0x80000001, 0);
HW_x64 = (info[3] & ((int)1 << 29)) != 0;
HW_ABM = (info[2] & ((int)1 << 5)) != 0;
HW_SSE4a = (info[2] & ((int)1 << 6)) != 0;
HW_FMA4 = (info[2] & ((int)1 << 16)) != 0;
HW_XOP = (info[2] & ((int)1 << 11)) != 0;
HW_PREFETCHW = (info[2] & ((int)1 << 8)) != 0;
}
}
void cpu_x86::print() const{
cout << "CPU Vendor:" << endl;
print(" AMD = ", Vendor_AMD);
print(" Intel = ", Vendor_Intel);
cout << endl;
cout << "OS Features:" << endl;
#ifdef _WIN32
print(" 64-bit = ", OS_x64);
#endif
print(" OS AVX = ", OS_AVX);
print(" OS AVX512 = ", OS_AVX512);
cout << endl;
cout << "Hardware Features:" << endl;
print(" MMX = ", HW_MMX);
print(" x64 = ", HW_x64);
print(" ABM = ", HW_ABM);
print(" RDRAND = ", HW_RDRAND);
print(" RDSEED = ", HW_RDSEED);
print(" BMI1 = ", HW_BMI1);
print(" BMI2 = ", HW_BMI2);
print(" ADX = ", HW_ADX);
print(" MPX = ", HW_MPX);
print(" PREFETCHW = ", HW_PREFETCHW);
print(" PREFETCHWT1 = ", HW_PREFETCHWT1);
print(" RDPID = ", HW_RDPID);
print(" GFNI = ", HW_GFNI);
print(" VAES = ", HW_VAES);
cout << endl;
cout << "SIMD: 128-bit" << endl;
print(" SSE = ", HW_SSE);
print(" SSE2 = ", HW_SSE2);
print(" SSE3 = ", HW_SSE3);
print(" SSSE3 = ", HW_SSSE3);
print(" SSE4a = ", HW_SSE4a);
print(" SSE4.1 = ", HW_SSE41);
print(" SSE4.2 = ", HW_SSE42);
print(" AES-NI = ", HW_AES);
print(" SHA = ", HW_SHA);
cout << endl;
cout << "SIMD: 256-bit" << endl;
print(" AVX = ", HW_AVX);
print(" XOP = ", HW_XOP);
print(" FMA3 = ", HW_FMA3);
print(" FMA4 = ", HW_FMA4);
print(" AVX2 = ", HW_AVX2);
cout << endl;
cout << "SIMD: 512-bit" << endl;
print(" AVX512-F = ", HW_AVX512_F);
print(" AVX512-CD = ", HW_AVX512_CD);
print(" AVX512-PF = ", HW_AVX512_PF);
print(" AVX512-ER = ", HW_AVX512_ER);
print(" AVX512-VL = ", HW_AVX512_VL);
print(" AVX512-BW = ", HW_AVX512_BW);
print(" AVX512-DQ = ", HW_AVX512_DQ);
print(" AVX512-IFMA = ", HW_AVX512_IFMA);
print(" AVX512-VBMI = ", HW_AVX512_VBMI);
print(" AVX512-VPOPCNTDQ = ", HW_AVX512_VPOPCNTDQ);
print(" AVX512-4VNNIW = ", HW_AVX512_4VNNIW);
print(" AVX512-4FMAPS = ", HW_AVX512_4FMAPS);
print(" AVX512-VBMI2 = ", HW_AVX512_VBMI2);
print(" AVX512-VPCLMUL = ", HW_AVX512_VPCLMUL);
print(" AVX512-VNNI = ", HW_AVX512_VNNI);
print(" AVX512-BITALG = ", HW_AVX512_BITALG);
print(" AVX512-BF16 = ", HW_AVX512_BF16);
cout << endl;
cout << "Summary:" << endl;
print(" Safe to use AVX: ", HW_AVX && OS_AVX);
print(" Safe to use AVX512: ", HW_AVX512_F && OS_AVX512);
cout << endl;
}
void cpu_x86::print_host(){
cpu_x86 features;
features.detect_host();
features.print();
}
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
}
| {
"repo_name": "Mysticial/FeatureDetector",
"stars": "246",
"repo_language": "C++",
"file_name": "FeatureDetector.vcxproj.filters",
"mime_type": "text/xml"
} |
/* cpu_x86_Windows.ipp
*
* Author : Alexander J. Yee
* Date Created : 04/12/2014
* Last Modified : 04/12/2014
*
*/
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
// Dependencies
#include <Windows.h>
#include <intrin.h>
#include "cpu_x86.h"
namespace FeatureDetector{
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
void cpu_x86::cpuid(int32_t out[4], int32_t eax, int32_t ecx){
__cpuidex(out, eax, ecx);
}
__int64 xgetbv(unsigned int x){
return _xgetbv(x);
}
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
// Detect 64-bit - Note that this snippet of code for detecting 64-bit has been copied from MSDN.
typedef BOOL (WINAPI *LPFN_ISWOW64PROCESS) (HANDLE, PBOOL);
BOOL IsWow64()
{
BOOL bIsWow64 = FALSE;
LPFN_ISWOW64PROCESS fnIsWow64Process = (LPFN_ISWOW64PROCESS) GetProcAddress(
GetModuleHandle(TEXT("kernel32")), "IsWow64Process");
if (NULL != fnIsWow64Process)
{
if (!fnIsWow64Process(GetCurrentProcess(), &bIsWow64))
{
printf("Error Detecting Operating System.\n");
printf("Defaulting to 32-bit OS.\n\n");
bIsWow64 = FALSE;
}
}
return bIsWow64;
}
bool cpu_x86::detect_OS_x64(){
#ifdef _M_X64
return true;
#else
return IsWow64() != 0;
#endif
}
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
}
| {
"repo_name": "Mysticial/FeatureDetector",
"stars": "246",
"repo_language": "C++",
"file_name": "FeatureDetector.vcxproj.filters",
"mime_type": "text/xml"
} |
add_library(FeatureDetector cpu_x86.cpp cpu_x86.h cpu_x86_Linux.ipp cpu_x86_Windows.ipp)
| {
"repo_name": "Mysticial/FeatureDetector",
"stars": "246",
"repo_language": "C++",
"file_name": "FeatureDetector.vcxproj.filters",
"mime_type": "text/xml"
} |
<?xml version="1.0" encoding="utf-8"?>
<Project DefaultTargets="Build" ToolsVersion="12.0" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
<ItemGroup Label="ProjectConfigurations">
<ProjectConfiguration Include="Debug|Win32">
<Configuration>Debug</Configuration>
<Platform>Win32</Platform>
</ProjectConfiguration>
<ProjectConfiguration Include="Release|Win32">
<Configuration>Release</Configuration>
<Platform>Win32</Platform>
</ProjectConfiguration>
</ItemGroup>
<ItemGroup>
<ClInclude Include="..\src\x86\cpu_x86.h" />
</ItemGroup>
<ItemGroup>
<None Include="..\src\x86\cpu_x86_Linux.ipp" />
<None Include="..\src\x86\cpu_x86_Windows.ipp" />
</ItemGroup>
<ItemGroup>
<ClCompile Include="..\src\Main.cpp" />
<ClCompile Include="..\src\x86\cpu_x86.cpp" />
</ItemGroup>
<PropertyGroup Label="Globals">
<ProjectGuid>{5AF4062D-386F-48AA-B824-2C10A4B1BA97}</ProjectGuid>
<Keyword>Win32Proj</Keyword>
<RootNamespace>FeatureDetector</RootNamespace>
</PropertyGroup>
<Import Project="$(VCTargetsPath)\Microsoft.Cpp.Default.props" />
<PropertyGroup Condition="'$(Configuration)|$(Platform)'=='Debug|Win32'" Label="Configuration">
<ConfigurationType>Application</ConfigurationType>
<UseDebugLibraries>true</UseDebugLibraries>
<PlatformToolset>v142</PlatformToolset>
<CharacterSet>Unicode</CharacterSet>
</PropertyGroup>
<PropertyGroup Condition="'$(Configuration)|$(Platform)'=='Release|Win32'" Label="Configuration">
<ConfigurationType>Application</ConfigurationType>
<UseDebugLibraries>false</UseDebugLibraries>
<PlatformToolset>v142</PlatformToolset>
<WholeProgramOptimization>true</WholeProgramOptimization>
<CharacterSet>Unicode</CharacterSet>
</PropertyGroup>
<Import Project="$(VCTargetsPath)\Microsoft.Cpp.props" />
<ImportGroup Label="ExtensionSettings">
</ImportGroup>
<ImportGroup Label="PropertySheets" Condition="'$(Configuration)|$(Platform)'=='Debug|Win32'">
<Import Project="$(UserRootDir)\Microsoft.Cpp.$(Platform).user.props" Condition="exists('$(UserRootDir)\Microsoft.Cpp.$(Platform).user.props')" Label="LocalAppDataPlatform" />
</ImportGroup>
<ImportGroup Label="PropertySheets" Condition="'$(Configuration)|$(Platform)'=='Release|Win32'">
<Import Project="$(UserRootDir)\Microsoft.Cpp.$(Platform).user.props" Condition="exists('$(UserRootDir)\Microsoft.Cpp.$(Platform).user.props')" Label="LocalAppDataPlatform" />
</ImportGroup>
<PropertyGroup Label="UserMacros" />
<PropertyGroup Condition="'$(Configuration)|$(Platform)'=='Debug|Win32'">
<LinkIncremental>true</LinkIncremental>
</PropertyGroup>
<PropertyGroup Condition="'$(Configuration)|$(Platform)'=='Release|Win32'">
<LinkIncremental>false</LinkIncremental>
</PropertyGroup>
<ItemDefinitionGroup Condition="'$(Configuration)|$(Platform)'=='Debug|Win32'">
<ClCompile>
<PrecompiledHeader>
</PrecompiledHeader>
<WarningLevel>Level3</WarningLevel>
<Optimization>Disabled</Optimization>
<PreprocessorDefinitions>WIN32;_DEBUG;_CONSOLE;_LIB;%(PreprocessorDefinitions)</PreprocessorDefinitions>
<SDLCheck>true</SDLCheck>
</ClCompile>
<Link>
<SubSystem>Console</SubSystem>
<GenerateDebugInformation>true</GenerateDebugInformation>
</Link>
</ItemDefinitionGroup>
<ItemDefinitionGroup Condition="'$(Configuration)|$(Platform)'=='Release|Win32'">
<ClCompile>
<WarningLevel>Level3</WarningLevel>
<PrecompiledHeader>
</PrecompiledHeader>
<Optimization>MaxSpeed</Optimization>
<FunctionLevelLinking>true</FunctionLevelLinking>
<IntrinsicFunctions>true</IntrinsicFunctions>
<PreprocessorDefinitions>WIN32;NDEBUG;_CONSOLE;_LIB;%(PreprocessorDefinitions)</PreprocessorDefinitions>
<SDLCheck>true</SDLCheck>
</ClCompile>
<Link>
<SubSystem>Console</SubSystem>
<GenerateDebugInformation>true</GenerateDebugInformation>
<EnableCOMDATFolding>true</EnableCOMDATFolding>
<OptimizeReferences>true</OptimizeReferences>
</Link>
</ItemDefinitionGroup>
<Import Project="$(VCTargetsPath)\Microsoft.Cpp.targets" />
<ImportGroup Label="ExtensionTargets">
</ImportGroup>
</Project> | {
"repo_name": "Mysticial/FeatureDetector",
"stars": "246",
"repo_language": "C++",
"file_name": "FeatureDetector.vcxproj.filters",
"mime_type": "text/xml"
} |
<?xml version="1.0" encoding="utf-8"?>
<Project ToolsVersion="12.0" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
<PropertyGroup />
</Project> | {
"repo_name": "Mysticial/FeatureDetector",
"stars": "246",
"repo_language": "C++",
"file_name": "FeatureDetector.vcxproj.filters",
"mime_type": "text/xml"
} |
<?xml version="1.0" encoding="utf-8"?>
<Project ToolsVersion="4.0" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
<ItemGroup>
<Filter Include="Source Files">
<UniqueIdentifier>{4FC737F1-C7A5-4376-A066-2A32D752A2FF}</UniqueIdentifier>
<Extensions>cpp;c;cc;cxx;def;odl;idl;hpj;bat;asm;asmx</Extensions>
</Filter>
<Filter Include="Header Files">
<UniqueIdentifier>{93995380-89BD-4b04-88EB-625FBE52EBFB}</UniqueIdentifier>
<Extensions>h;hh;hpp;hxx;hm;inl;inc;xsd</Extensions>
</Filter>
<Filter Include="Resource Files">
<UniqueIdentifier>{67DA6AB6-F800-4c08-8B7A-83BB121AAD01}</UniqueIdentifier>
<Extensions>rc;ico;cur;bmp;dlg;rc2;rct;bin;rgs;gif;jpg;jpeg;jpe;resx;tiff;tif;png;wav;mfcribbon-ms</Extensions>
</Filter>
<Filter Include="Header Files\x86">
<UniqueIdentifier>{7fdc0f8e-15b1-4062-a100-fb566c7e9c8a}</UniqueIdentifier>
</Filter>
</ItemGroup>
<ItemGroup>
<None Include="..\src\x86\cpu_x86_Windows.ipp">
<Filter>Header Files\x86</Filter>
</None>
<None Include="..\src\x86\cpu_x86_Linux.ipp">
<Filter>Header Files\x86</Filter>
</None>
</ItemGroup>
<ItemGroup>
<ClCompile Include="..\src\Main.cpp">
<Filter>Source Files</Filter>
</ClCompile>
<ClCompile Include="..\src\x86\cpu_x86.cpp">
<Filter>Header Files\x86</Filter>
</ClCompile>
</ItemGroup>
<ItemGroup>
<ClInclude Include="..\src\x86\cpu_x86.h">
<Filter>Header Files\x86</Filter>
</ClInclude>
</ItemGroup>
</Project> | {
"repo_name": "Mysticial/FeatureDetector",
"stars": "246",
"repo_language": "C++",
"file_name": "FeatureDetector.vcxproj.filters",
"mime_type": "text/xml"
} |
## Contributor License Agreement
By contributing you agree to the [LICENSE](https://github.com/simonebrunozzi/open-guide-to-learning-tech-geek-nerdy-stuff/blob/master/LICENSE) of this repository.
## Contributor Code of Conduct
By contributing you agree to respect the [Code of Conduct](https://github.com/simonebrunozzi/open-guide-to-learning-tech-geek-nerdy-stuff/blob/master/CODE-OF-CONDUCT.md) of this repository.
## Information on how to contribute
We prefer free material, although there might be non-free material that is so good that we still want to include it in here.
The principles behind adding a new link (or a new category) are the following:
1. You can propose a new category if it belongs to the same tech/geek/nerd stuff.
2. You can propose a new "link", by actually simply adding
```
[$succint_description]($link)
```
Example:
```
[Video games and AI](http://togelius.blogspot.com/2016/01/why-video-games-are-essential-for.html)
```
If your link points to a discussion, which then points to the actual resource, such as in the case of a Hacker News discussion, you can simply write:
```
[$succint_description]($link-to-resource) ([HN discussion]($link-to-discussion))
```
Example:
```
[Berkeley AI Materials](http://ai.berkeley.edu/project_overview.html) ([HN discussion](https://news.ycombinator.com/item?id=10929985))
```
If you want to specify the file format (e.g. PDF), you can add it after the `$succint_description`.
## Editing/formatting Guidelines
- We typically don't like files hosted on "temporary" locations, e.g. google drive, dropbox, mega, etc.
- We always prefer a `https` link over a `http` one -- as long as they are on the same domain and serve the same content
- On root domains, strip the trailing slash: use `http://example.com` instead of `http://example.com/`
- Always prefer the shortest link: `http://example.com/dir/` is better than `http://example.com/dir/index.html`
- Do not include URL shortener links
- Most files are `.md` files. Try to learn [Markdown](https://guides.github.com/features/mastering-markdown/) to be a more effective contributor.
| {
"repo_name": "simonebrunozzi-zz/open-guide-to-learning-tech-geek-nerdy-stuff",
"stars": "51",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
# Contributor Code of Conduct
As contributors and maintainers of this project, and in the interest of
fostering an open and welcoming community, we pledge to respect all people who
contribute through reporting issues, posting feature requests, updating
documentation, submitting pull requests or patches, and other activities.
We are committed to making participation in this project a harassment-free
experience for everyone, regardless of level of experience, gender, gender
identity and expression, sexual orientation, disability, personal appearance,
body size, race, ethnicity, age, religion, or nationality.
Examples of unacceptable behavior by participants include:
* The use of sexualized language or imagery
* Personal attacks
* Trolling or insulting/derogatory comments
* Public or private harassment
* Publishing other's private information, such as physical or electronic
addresses, without explicit permission
* Other unethical or unprofessional conduct
Project maintainers have the right and responsibility to remove, edit, or
reject comments, commits, code, wiki edits, issues, and other contributions
that are not aligned to this Code of Conduct, or to ban temporarily or
permanently any contributor for other behaviors that they deem inappropriate,
threatening, offensive, or harmful.
By adopting this Code of Conduct, project maintainers commit themselves to
fairly and consistently applying these principles to every aspect of managing
this project. Project maintainers who do not follow or enforce the Code of
Conduct may be permanently removed from the project team.
This code of conduct applies both within project spaces and in public spaces
when an individual is representing the project or its community.
Instances of abusive, harassing, or otherwise unacceptable behavior may be
reported by contacting a project maintainer at victorfelder at gmail.com. All
complaints will be reviewed and investigated and will result in a response that
is deemed necessary and appropriate to the circumstances. Maintainers are
obligated to maintain confidentiality with regard to the reporter of an
incident.
This Code of Conduct is adapted from the [Contributor Covenant][homepage],
version 1.3.0, available at
[http://contributor-covenant.org/version/1/3/0/][version]
[homepage]: http://contributor-covenant.org
[version]: http://contributor-covenant.org/version/1/3/0/
| {
"repo_name": "simonebrunozzi-zz/open-guide-to-learning-tech-geek-nerdy-stuff",
"stars": "51",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
# Open Guide To Learning Tech/Geek/Nerdy Stuff
A list of resources to learn tech/geek/nerdy related topics, e.g. programming languages, android development, math, korean language and the like.
## Introduction
Over time I've built a decent list of links to web resources which contain good material to learn stuff.
What stuff? "Tech" stuff (like Python, BSD, Vim, Security, Javascript, Unix), or other geeky/nerdy stuff (math, how to learn the Korean language, Philosophy, etc).
Two quick examples of how one of these entries look like:
```
BSD: https://hardenedbsd.org/
```
```
Learn Korean: https://news.ycombinator.com/item?id=9935042
```
To avoid confusion, links are grouped into categories.
### Making this list publicly available, and letting everyone collaborate
I think it is valuable to make this list available to everyone, and most importantly, open to anyone's contribution.
If you know how to use Github, it should be easy for you to add your own links.
Over time, my hope is that these contribution will make this list of resources very useful as a good "filter" to find just the good stuff.
Want to contribute? [Go here to read how to](https://github.com/simonebrunozzi/open-guide-to-learning-tech-geek-nerdy-stuff/blob/master/CONTRIBUTING.md), and make sure you respect the [code of conduct](https://github.com/simonebrunozzi/open-guide-to-learning-tech-geek-nerdy-stuff/blob/master/CODE-OF-CONDUCT.md).
Any contribution will be released under the same [license](https://github.com/simonebrunozzi/open-guide-to-learning-tech-geek-nerdy-stuff/blob/master/LICENSE).
**Table of Contents**
- [Android](#android)
- [Artificial Intelligence](#artificial-intelligence)
- [Assembler](#assembler)
- [Cloud Computing](#cloud-computing)
- [Command Line](#command-line)
- [Compilers](#compilers)
- [Computer science](#computer-science)
- [Containers](#containers)
- [DevOps](#devops)
- [Education](#education)
- [Java](#java)
- [Javascript](#javascript)
- [PostgreSQL](#postgresql)
- [Python](#python)
- [Unix](#unix)
- [Vim](#vim)
- [Miscellaneous](#miscellaneous)
- [Temporary or unorganized](#temporary-or-unorganized)
- [Further Reading](#further-reading)
- [Credits](#credits)
- [Disclaimer](#disclaimer)
- [License](#license)
## Android
- [Android apps](https://www.youtube.com/playlist?list=PLGLfVvz_LVvSPjWpLPFEfOCbezi6vATIh)
- [Android tutorial](http://www.vogella.com/tutorials/Android/article.html)
- [Android training](https://developer.android.com/training/index.html)
- [Android development for beginners](https://www.udacity.com/course/android-development-for-beginners--ud837)
- [Developing Android apps](https://www.udacity.com/course/developing-android-apps--ud853)
- [Writing Android apps](http://lifehacker.com/i-want-to-write-android-apps-where-do-i-start-1643818268)
- [Sketch (design a mobile app)](https://www.youtube.com/watch?v=YjsQJShFewI)
## Artificial Intelligence
- [Berkeley AI Materials](http://ai.berkeley.edu/project_overview.html) ([HN discussion](https://news.ycombinator.com/item?id=10929985))
- [Video games and AI](http://togelius.blogspot.com/2016/01/why-video-games-are-essential-for.html)
- [Machine Learning](https://www.udacity.com/course/machine-learning-engineer-nanodegree--nd009)
- [Torch, a scientific computing framework for luajit](http://torch.ch/)
- [Neural networks](http://neuralnetworksanddeeplearning.com/chap6.html) ([HN discussion](https://news.ycombinator.com/item?id=9951352))
- [Artificial Neural Networks for beginners](http://blogs.mathworks.com/loren/2015/08/04/artificial-neural-networks-for-beginners/)
- [Machine learning](http://www.computervisiontalks.com/tag/machine-learning-class-10-701/) ([HN discussion](https://news.ycombinator.com/item?id=9920529))
- [Machine Learning](https://www.cs.ox.ac.uk/people/nando.defreitas/machinelearning/)
- [Google's free Deep Learning course](https://www.udacity.com/course/deep-learning--ud730)
## Assembler
- [CROBOTS (1985) (like CoreWar)](http://tpoindex.github.io/crobots/) ([HN discussion](https://news.ycombinator.com/item?id=10331849))
## Cloud Computing
- [Learn Mesos](http://open.mesosphere.com/intro-course/)
- [Learn cloud computing](https://cloudacademy.com)
- [Dynamo: Amazon's Highly Available Key-value Store](http://www.allthingsdistributed.com/files/amazon-dynamo-sosp2007.pdf)
## Command Line
- [Learn code the hard way](http://cli.learncodethehardway.org/book/)
- [Bash / Sh](https://news.ycombinator.com/item?id=10068567)
- [Art of CLI](https://github.com/jlevy/the-art-of-command-line)
- [Command line course](https://www.codecademy.com/en/courses/learn-the-command-line)
## Compilers
- [Build a simple interpreter](http://ruslanspivak.com/lsbasi-part5/)
- [Compiler construction](https://news.ycombinator.com/item?id=10785164)
- [Compiler construction](https://www.inf.ethz.ch/personal/wirth/CompilerConstruction/index.html)
- [Papers on compilers](https://news.ycombinator.com/item?id=10786842)
- [Let's Build a Compiler, by Jack Crenshaw](http://compilers.iecc.com/crenshaw/)
- [Logic, Languages, Compilation, and Verification](http://www.cs.uoregon.edu/research/summerschool/summer12/curriculum.html)
## Computer Science
- [OSS University](https://github.com/open-source-society/computer-science-and-engineering)
- [Computer Science for all](http://www.cs.hmc.edu/csforall/)
- [Math for Computer Science](http://ocw.mit.edu/courses/electrical-engineering-and-computer-science/6-042j-mathematics-for-computer-science-fall-2010/video-lectures/) ([HN discussion](https://news.ycombinator.com/item?id=9311752))
- [What is code - http](//www.bloomberg.com/graphics/2015-paul-ford-what-is-code/)
- [A plain english introduction to CAP Theorem](http://ksat.me/a-plain-english-introduction-to-cap-theorem/)
- [Critique to the CAP Theorem](http://markburgess.org/blog_cap.html)
## Containers
- [Docker for beginners](http://prakhar.me/docker-curriculum/)
- [Containers resources](https://github.com/borntorock/awesome-containers/blob/master/awesome-containers.md)
- [Network containers](https://www.zerotier.com/blog/?p=490)
## DevOps
- [Ops School](http://www.opsschool.org/en/latest/)
## Education
- [Lumiar (Ricardo Semler)](http://lumiar.org.br/index.php/a-escola/?lang=en)
- [International Baccalaureate](http://www.ibo.org/)
- [European Graduate School](http://www.egs.edu/department/philosophy-art-critical-thought/admissions-pact)
- [21st century education](https://minerva.kgi.edu/)
## Java
- [Learn Java the hard way](https://learnjavathehardway.org/book/ex00.html)
- [Course on Java](https://www.udemy.com/course/subscribe/?courseId=24823&dtcode=jPSadG83nzV3)
- [Tutorial on Java](https://www.udemy.com/java-tutorial/learn/#/lecture/131404)
- [Java basics](http://teamtreehouse.com/library/java-basics)
- [Intro to Java Programming](https://www.udacity.com/course/intro-to-java-programming--cs046)
- [Course on Eclipse](https://www.udemy.com/course/subscribe/?courseId=354422&dtcode=hxFBwbI3nzVe)
## Javascript
- [Grand Javascript school](http://raganwald.com/2015/04/24/hilberts-school.html) ([HN discussion](https://news.ycombinator.com/item?id=9439479))
- [Javascript game development](http://codeincomplete.com/games/)
- [Write Pacman in Javascript](http://www.masswerk.at/JavaPac/pacman-howto.html)
- [You don't know Javascript](https://github.com/getify/You-Dont-Know-JS)
## Postgresql
- [Postgres guide](http://www.postgresguide.com/) ([HN discussion](https://news.ycombinator.com/item?id=9993237))
- [Postgres CLI](http://pgcli.com/)
- [MySQL CLI (for comparison)](http://mycli.net/)
- [PostgreSQL command line](http://phili.pe/posts/postgresql-on-the-command-line/) ([HN discussion](https://news.ycombinator.com/item?id=10458866))
- [SQL tabs (PostgreSQL)](http://www.sqltabs.com/) ([HN discussion](https://news.ycombinator.com/item?id=10802631))
- [Embracing SQL in PostgreSQL](http://rob.conery.io/2015/02/24/embracing-sql-in-postgres/)
- [Curated list of great PostgreSQL resources](https://github.com/dhamaniasad/awesome-postgres)
## Python
- [Learn Python](http://aysinoruz.com/i-taught-myself-python-on-the-internet-and-so-can-you/)
- [ML + Python](https://news.ycombinator.com/item?id=9902524)
- [Intermediate python](http://book.pythontips.com/en/latest/) ([HN discussion](https://news.ycombinator.com/item?id=10075078))
- [Tutorials to learn Python](https://news.ycombinator.com/item?id=10135935)
- [Python Sudoku](http://norvig.com/sudoku.html)
- [Python IDE](http://pybee.org/)
- [Interactive Python](http://interactivepython.org/runestone/static/pythonds/index.html)
- [Python the hard way](http://learnpythonthehardway.org/book/) (3rd edition)
- [Norvig's python stuff](http://nbviewer.ipython.org/url/norvig.com/ipython/Probability.ipynb)
- [IDLE Python](http://www.tkdocs.com/tutorial/idle.html)
- [The Zen of Python](https://www.python.org/dev/peps/pep-0020/)
## Unix
- [Bro pages](http://bropages.org/browse)
- [Unix toolbox](http://cb.vu/unixtoolbox.xhtml)
- [Hardened BSD](https://hardenedbsd.org/)
## Vim
- [VIM as IDE](https://github.com/jez/vim-as-an-ide)
- [Learn VIM while playing a game](http://vim-adventures.com/) ([HN discussion](https://news.ycombinator.com/item?id=9250190))
- [Vim takeaways](http://sankho.github.io/web_log/2015/08/02/vim-8-takeaways-from-one-year-of-typing.html) ([HN discussion](https://news.ycombinator.com/item?id=9996088))
- [Study Vim](https://danielmiessler.com/study/vim/)
- [Vim galore](https://github.com/mhinz/vim-galore)
## Working in the US
- Calculating the federal income tax: https://www.khanacademy.org/economics-finance-domain/core-finance/taxes-topic/taxes/v/calculating-federal-taxes-and-take-home-pay
- 2016 federal tax rates: http://www.forbes.com/sites/kellyphillipserb/2015/10/21/irs-announces-2016-tax-rates-standard-deductions-exemption-amounts-and-more/
- California standard deductions: https://www.ftb.ca.gov/individuals/faq/net/902.shtml
- California tax brackets: http://www.tax-brackets.org/californiataxtable
## Miscellaneous
- [How to minimize procrastination](https://myelin.io/how-to-minimize-procrastination)
- [How to learn efficiently](http://lemire.me/blog/2014/12/30/how-to-learn-efficiently/) ([HN discussion](https://news.ycombinator.com/item?id=8815148))
- [Learn to read Korean](http://ryanestradadotcom.tumblr.com/post/20461267965/learn-to-read-korean-in-15-minutes) ([HN discussion](https://news.ycombinator.com/item?id=9935042))
- [Thinking about thinking](http://edge.org/events/the-edge-master-class-2007-a-short-course-in-thinking-about-thinking)
- [Philosophy](http://plato.stanford.edu/)
- [Math](http://www.math.ucla.edu/~tao/preprints/problem.ps) ([HN discussion](https://news.ycombinator.com/item?id=9942638))
- [React.js fundamentals](http://courses.reactjsprogram.com/courses/reactjsfundamentals)
- [Learning Paths by O'Reilly](http://shop.oreilly.com/category/learning-path.do)
- [DevOps Wiki](https://github.com/Leo-G/DevopsWiki/blob/master/README.md)
- [Computer Science video lectures](http://csvideolectures.com/)
## Temporary or unorganized
- [Entrepreneur](https://www.udacity.com/course/tech-entrepreneur-nanodegree--nd007)
- [Spinnaker, deploy artifacts to the cloud](http://spinnaker.io/)
- [Flux / React](https://medium.com/code-cartoons/a-cartoon-guide-to-flux-6157355ab207)
- [Mapzen search](https://news.ycombinator.com/item?id=10318541)
- [Book "Architecture of open source applications"](http://aosabook.org/en/index.html)
- [Kali Linux](https://www.kali.org/downloads/)
- [APIs](https://news.ycombinator.com/item?id=9987838)
- [Swift](http://books.aidanf.net/learn-swift/introduction)
- [Security](http://99percentinvisible.org/episode/perfect-security/) ([HN discussion](https://news.ycombinator.com/item?id=9545972))
- [Rust](http://blog.rust-lang.org/2015/04/24/Rust-Once-Run-Everywhere.html)
- [Erlang / Elixir](https://news.ycombinator.com/item?id=9426103)
- [Competence Matrix](http://sijinjoseph.com/programmer-competency-matrix/)
- [Data Science masters](http://datasciencemasters.org/)
- [Bitcoin](https://bitcoin.org/bitcoin.pdf)
- [RegEx crossword](https://regexcrossword.com/)
- [Scala](https://scala.epfl.ch/)
## Further reading
n.a.
# Content ends here
## Credits
Many thanks to [all contributors](https://github.com/simonebrunozzi/open-guide-to-learning-tech-geek-nerdy-stuff/graphs/contributors) to this project.
Thanks also to those who have given detailed feedback - they will be listed below here.
Example:
[anonymous coward](https://twitter.com/anonymous-coward),
The original author is Simone Brunozzi [Twitter](https://twitter.com/simon) [blog](http://www.brunozzi.com) [medium](https://medium.com/simone-brunozzi)
## Disclaimer
*This guide and all associated comments and discussion do not constitute any advice in any respect, and they do not represent an endorsement of any product or service.
No reader should act or refrain from acting on the basis of any information presented herein without seeking the advice of counsel in the relevant jurisdiction.
The author(s) expressly disclaim all liability in respect of any actions taken or not taken based on any contents of this guide or associated content.*
## License
[](https://creativecommons.org/publicdomain/zero/1.0/)
This work is licensed under a Creative Commons CC0 1.0 Universal (CC0 1.0) license.
License details [are available here](https://github.com/simonebrunozzi/open-guide-to-learning-tech-geek-nerdy-stuff/blob/master/LICENSE).
| {
"repo_name": "simonebrunozzi-zz/open-guide-to-learning-tech-geek-nerdy-stuff",
"stars": "51",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
Microsoft Visual Studio Solution File, Format Version 12.00
# Visual Studio Express 2013 for Windows Desktop
VisualStudioVersion = 12.0.21005.1
MinimumVisualStudioVersion = 10.0.40219.1
Project("{8BC9CEB8-8B4A-11D0-8D11-00A0C91BC942}") = "tetris-win32", "tetris-win32.vcxproj", "{278AC16E-53B1-4910-BAD7-5B146DAF9B4E}"
EndProject
Global
GlobalSection(SolutionConfigurationPlatforms) = preSolution
Debug|Win32 = Debug|Win32
Release|Win32 = Release|Win32
EndGlobalSection
GlobalSection(ProjectConfigurationPlatforms) = postSolution
{278AC16E-53B1-4910-BAD7-5B146DAF9B4E}.Debug|Win32.ActiveCfg = Debug|Win32
{278AC16E-53B1-4910-BAD7-5B146DAF9B4E}.Debug|Win32.Build.0 = Debug|Win32
{278AC16E-53B1-4910-BAD7-5B146DAF9B4E}.Release|Win32.ActiveCfg = Release|Win32
{278AC16E-53B1-4910-BAD7-5B146DAF9B4E}.Release|Win32.Build.0 = Release|Win32
EndGlobalSection
GlobalSection(SolutionProperties) = preSolution
HideSolutionNode = FALSE
EndGlobalSection
EndGlobal
| {
"repo_name": "eliangcs/tetris-win32",
"stars": "30",
"repo_language": "C++",
"file_name": "PieceSet.h",
"mime_type": "text/x-c++"
} |
<?xml version="1.0" encoding="utf-8"?>
<Project DefaultTargets="Build" ToolsVersion="12.0" xmlns="http://schemas.microsoft.com/developer/msbuild/2003">
<ItemGroup Label="ProjectConfigurations">
<ProjectConfiguration Include="Debug|Win32">
<Configuration>Debug</Configuration>
<Platform>Win32</Platform>
</ProjectConfiguration>
<ProjectConfiguration Include="Release|Win32">
<Configuration>Release</Configuration>
<Platform>Win32</Platform>
</ProjectConfiguration>
</ItemGroup>
<PropertyGroup Label="Globals">
<ProjectGuid>{278AC16E-53B1-4910-BAD7-5B146DAF9B4E}</ProjectGuid>
<Keyword>Win32Proj</Keyword>
<RootNamespace>tetriswin32</RootNamespace>
</PropertyGroup>
<Import Project="$(VCTargetsPath)\Microsoft.Cpp.Default.props" />
<PropertyGroup Condition="'$(Configuration)|$(Platform)'=='Debug|Win32'" Label="Configuration">
<ConfigurationType>Application</ConfigurationType>
<UseDebugLibraries>true</UseDebugLibraries>
<PlatformToolset>v120</PlatformToolset>
<CharacterSet>Unicode</CharacterSet>
</PropertyGroup>
<PropertyGroup Condition="'$(Configuration)|$(Platform)'=='Release|Win32'" Label="Configuration">
<ConfigurationType>Application</ConfigurationType>
<UseDebugLibraries>false</UseDebugLibraries>
<PlatformToolset>v120</PlatformToolset>
<WholeProgramOptimization>true</WholeProgramOptimization>
<CharacterSet>Unicode</CharacterSet>
</PropertyGroup>
<Import Project="$(VCTargetsPath)\Microsoft.Cpp.props" />
<ImportGroup Label="ExtensionSettings">
</ImportGroup>
<ImportGroup Label="PropertySheets" Condition="'$(Configuration)|$(Platform)'=='Debug|Win32'">
<Import Project="$(UserRootDir)\Microsoft.Cpp.$(Platform).user.props" Condition="exists('$(UserRootDir)\Microsoft.Cpp.$(Platform).user.props')" Label="LocalAppDataPlatform" />
</ImportGroup>
<ImportGroup Label="PropertySheets" Condition="'$(Configuration)|$(Platform)'=='Release|Win32'">
<Import Project="$(UserRootDir)\Microsoft.Cpp.$(Platform).user.props" Condition="exists('$(UserRootDir)\Microsoft.Cpp.$(Platform).user.props')" Label="LocalAppDataPlatform" />
</ImportGroup>
<PropertyGroup Label="UserMacros" />
<PropertyGroup Condition="'$(Configuration)|$(Platform)'=='Debug|Win32'">
<LinkIncremental>true</LinkIncremental>
</PropertyGroup>
<PropertyGroup Condition="'$(Configuration)|$(Platform)'=='Release|Win32'">
<LinkIncremental>false</LinkIncremental>
</PropertyGroup>
<ItemDefinitionGroup Condition="'$(Configuration)|$(Platform)'=='Debug|Win32'">
<ClCompile>
<PrecompiledHeader>
</PrecompiledHeader>
<WarningLevel>Level3</WarningLevel>
<Optimization>Disabled</Optimization>
<PreprocessorDefinitions>WIN32;_DEBUG;_WINDOWS;%(PreprocessorDefinitions)</PreprocessorDefinitions>
</ClCompile>
<Link>
<SubSystem>Windows</SubSystem>
<GenerateDebugInformation>true</GenerateDebugInformation>
<AdditionalDependencies>Winmm.lib;%(AdditionalDependencies)</AdditionalDependencies>
</Link>
</ItemDefinitionGroup>
<ItemDefinitionGroup Condition="'$(Configuration)|$(Platform)'=='Release|Win32'">
<ClCompile>
<WarningLevel>Level3</WarningLevel>
<PrecompiledHeader>
</PrecompiledHeader>
<Optimization>MaxSpeed</Optimization>
<FunctionLevelLinking>true</FunctionLevelLinking>
<IntrinsicFunctions>true</IntrinsicFunctions>
<PreprocessorDefinitions>WIN32;NDEBUG;_WINDOWS;%(PreprocessorDefinitions)</PreprocessorDefinitions>
</ClCompile>
<Link>
<SubSystem>Windows</SubSystem>
<GenerateDebugInformation>true</GenerateDebugInformation>
<EnableCOMDATFolding>true</EnableCOMDATFolding>
<OptimizeReferences>true</OptimizeReferences>
<AdditionalDependencies>Winmm.lib;%(AdditionalDependencies)</AdditionalDependencies>
</Link>
</ItemDefinitionGroup>
<ItemGroup>
<ClCompile Include="src\DrawEngine.cpp" />
<ClCompile Include="src\Game.cpp" />
<ClCompile Include="src\Level.cpp" />
<ClCompile Include="src\main.cpp" />
<ClCompile Include="src\Piece.cpp" />
<ClCompile Include="src\PieceSet.cpp" />
</ItemGroup>
<ItemGroup>
<ClInclude Include="src\DrawEngine.h" />
<ClInclude Include="src\Game.h" />
<ClInclude Include="src\Level.h" />
<ClInclude Include="src\Piece.h" />
<ClInclude Include="src\PieceSet.h" />
</ItemGroup>
<Import Project="$(VCTargetsPath)\Microsoft.Cpp.targets" />
<ImportGroup Label="ExtensionTargets">
</ImportGroup>
</Project> | {
"repo_name": "eliangcs/tetris-win32",
"stars": "30",
"repo_language": "C++",
"file_name": "PieceSet.h",
"mime_type": "text/x-c++"
} |
Classic tetris game implemented with Win32 API.

| {
"repo_name": "eliangcs/tetris-win32",
"stars": "30",
"repo_language": "C++",
"file_name": "PieceSet.h",
"mime_type": "text/x-c++"
} |
#ifndef GAME_H
#define GAME_H
#include "Level.h"
#include "PieceSet.h"
#include "Piece.h"
//
// Game flow.
// Only Game and DrawEngine are exposed to main().
//
class Game
{
public:
Game(DrawEngine &de);
~Game();
// Restarts the game
void restart();
// Handles player's key press
bool keyPress(int vk);
// Updates data or graphics
void timerUpdate();
// Pass true to pause, pass false to resume
void pause(bool paused);
// Called on WM_PAINT
void repaint() const;
bool isGameOver() const;
protected:
// Shows GAME OVER message
inline void drawGameOver() const
{
de.drawText(TEXT("GAME OVER"), 3, 10);
de.drawText(TEXT("Press ENTER to restart"), 2, 9);
}
// Shows PAUSE message
inline void drawPause() const
{
de.drawText(TEXT("PAUSE"), 4, 10);
de.drawText(TEXT("Press PAUSE again to continue"), 1, 9);
}
Level *level;
DrawEngine &de;
// Is game currently paused?
bool isPaused;
};
#endif // GAME_H
| {
"repo_name": "eliangcs/tetris-win32",
"stars": "30",
"repo_language": "C++",
"file_name": "PieceSet.h",
"mime_type": "text/x-c++"
} |
#include "PieceSet.h"
PieceSet::PieceSet()
{
for (int i = 0; i < NUM_PIECES; i++)
for (int j = 0; j < NUM_ROTATIONS; j++)
pieces[i][j] = 0;
POINT apt[NUM_ROTATIONS];
// 0, I piece, red
apt[0].x = 0; apt[0].y = 0;
apt[1].x = 0; apt[1].y = 1;
apt[2].x = 0; apt[2].y = 2;
apt[3].x = 0; apt[3].y = 3;
pieces[0][0] = new Piece(0, 0, RGB(255,0,0), apt);
// 1, L piece, orange
apt[0].x = 0; apt[0].y = 0;
apt[1].x = 1; apt[1].y = 0;
apt[2].x = 0; apt[2].y = 1;
apt[3].x = 0; apt[3].y = 2;
pieces[1][0] = new Piece(1, 0, RGB(230,130,24), apt);
// 2, counter-L piece, yellow
apt[0].x = 0; apt[0].y = 0;
apt[1].x = 1; apt[1].y = 0;
apt[2].x = 1; apt[2].y = 1;
apt[3].x = 1; apt[3].y = 2;
pieces[2][0] = new Piece(2, 0, RGB(255,255,0), apt);
// 3, S piece, green
apt[0].x = 0; apt[0].y = 0;
apt[1].x = 1; apt[1].y = 0;
apt[2].x = 1; apt[2].y = 1;
apt[3].x = 2; apt[3].y = 1;
pieces[3][0] = new Piece(3, 0, RGB(120,200,80), apt);
// 4, Z piece, blue
apt[0].x = 1; apt[0].y = 0;
apt[1].x = 2; apt[1].y = 0;
apt[2].x = 0; apt[2].y = 1;
apt[3].x = 1; apt[3].y = 1;
pieces[4][0] = new Piece(4, 0, RGB(100,180,255), apt);
// 5, Square piece, dark blue
apt[0].x = 0; apt[0].y = 0;
apt[1].x = 1; apt[1].y = 0;
apt[2].x = 0; apt[2].y = 1;
apt[3].x = 1; apt[3].y = 1;
pieces[5][0] = new Piece(5, 0, RGB(20,100,200), apt);
// 6, T piece, purple
apt[0].x = 0; apt[0].y = 0;
apt[1].x = 1; apt[1].y = 0;
apt[2].x = 2; apt[2].y = 0;
apt[3].x = 1; apt[3].y = 1;
pieces[6][0] = new Piece(6, 0, RGB(220,180,255), apt);
// Create piece rotations
rotateAll();
}
PieceSet::~PieceSet()
{
for (int i = 0; i < NUM_PIECES; i++)
for (int j = 0; j < NUM_ROTATIONS; j++)
if (pieces[i][j] != 0)
delete pieces[i][j];
}
Piece *PieceSet::getPiece(int id, int rotation) const
{
if (id >= NUM_PIECES || id < 0 || rotation >= NUM_ROTATIONS || rotation < 0)
return NULL;
return pieces[id][rotation];
}
void PieceSet::rotateAll()
{
POINT apt[NUM_ROTATIONS];
for (int i = 0; i < NUM_PIECES; i++)
{
pieces[i][0]->getBody(apt);
for (int j = 1; j < NUM_ROTATIONS; j++)
{
rotate(apt);
if (pieces[i][j] != 0)
delete pieces[i][j];
pieces[i][j] = new Piece(i, j, pieces[i][0]->getColor(), apt);
}
}
}
void PieceSet::rotate(POINT* apt, int numPoints)
{
int tmp;
// X' = -Y
// Y' = X
for (int i = 0; i < numPoints; i++)
{
tmp = apt[i].x;
apt[i].x = -apt[i].y;
apt[i].y = tmp;
}
}
| {
"repo_name": "eliangcs/tetris-win32",
"stars": "30",
"repo_language": "C++",
"file_name": "PieceSet.h",
"mime_type": "text/x-c++"
} |
#include "Piece.h"
#include <iostream>
using namespace std;
Piece::Piece(int pieceId, int pieceRotation, COLORREF pieceColor,
const POINT *apt, int numPoints) :
id(pieceId), rotation(pieceRotation), nPoints(numPoints),
color(pieceColor), width(0), height(0)
{
POINT bottomLeft = apt[0];
for (int i = 1; i < nPoints; i++)
{
bottomLeft.x = min(apt[i].x, bottomLeft.x);
bottomLeft.y = min(apt[i].y, bottomLeft.y);
}
body = new POINT[nPoints];
for (int i = 0; i < nPoints; i++)
{
body[i].x = apt[i].x - bottomLeft.x;
body[i].y = apt[i].y - bottomLeft.y;
width = max((int)body[i].x + 1, width);
height = max((int)body[i].y + 1, height);
}
}
Piece::~Piece()
{
if (body)
delete [] body;
}
void Piece::getBody(POINT *apt) const
{
for (int i = 0; i < nPoints; i++)
apt[i] = body[i];
}
int Piece::getSkirt(POINT *apt) const
{
int i = 0;
for (int x = 0; x < width; x++)
{
for (int y = 0; y < height; y++)
{
if (isPointExists(x, y))
{
apt[i].x = x;
apt[i].y = y;
i++;
break;
}
}
}
return i;
}
int Piece::getLeftSide(POINT *apt) const
{
int i = 0;
for (int y = 0; y < height; y++)
{
for (int x = 0; x < height; x++)
{
if (isPointExists(x, y))
{
apt[i].x = x;
apt[i].y = y;
i++;
break;
}
}
}
return i;
}
int Piece::getRightSide(POINT *apt) const
{
int i = 0;
for (int y = 0; y < height; y++)
{
for (int x = width - 1; x >= 0; x--)
{
if (isPointExists(x, y))
{
apt[i].x = x;
apt[i].y = y;
i++;
break;
}
}
}
return i;
}
void Piece::print() const
{
cout << "width = " << width << endl;
cout << "height = " << height << endl;
cout << "nPoints = " << nPoints << endl;
cout << "color = " << hex << color << endl;
for (int y = height - 1; y >= 0; y--)
{
for (int x = 0; x < width; x++)
{
if (isPointExists(x, y))
cout << "#";
else
cout << " ";
}
cout << endl;
}
}
bool Piece::isPointExists(int x, int y) const
{
for (int i = 0; i < 4; i++)
{
if (body[i].x == x && body[i].y == y)
return true;
}
return false;
}
| {
"repo_name": "eliangcs/tetris-win32",
"stars": "30",
"repo_language": "C++",
"file_name": "PieceSet.h",
"mime_type": "text/x-c++"
} |
#ifndef DRAW_ENGINE_H
#define DRAW_ENGINE_H
#include "Piece.h"
#include <windows.h>
//
// Draw engine implemented with Win32 API GDI.
//
class DrawEngine
{
public:
// hdc: handle to DC
// hwnd: handle to window
// pxPerBlock: cell size in pixels
// width & height: level width and height
DrawEngine(HDC hdc, HWND hwnd, int pxPerBlock = 25,
int width = 10, int height = 20);
~DrawEngine();
// Fills a cell
void drawBlock(int x, int y, COLORREF color);
// Draws UI (gray area)
void drawInterface();
// Draws a text message
void drawText(TCHAR *szText, int x, int y) const;
// Draws all kinds of info
void drawScore(int score, int x, int y) const;
void drawSpeed(int speed, int x, int y) const;
void drawNextPiece(Piece &piece, int x, int y);
protected:
HDC hdc; // Handle to DC
HWND hwnd; // Handle to window
RECT rect; // For internal temporary usage
int width; // Level width in cells
int height; // Level height
};
#endif // DRAW_ENGINE_H
| {
"repo_name": "eliangcs/tetris-win32",
"stars": "30",
"repo_language": "C++",
"file_name": "PieceSet.h",
"mime_type": "text/x-c++"
} |
#include "Level.h"
#include <ctime>
Level::Level(DrawEngine &de, int width, int height) :
de(de), width(width), height(height), lastTime(0.0), speed(500), score(-1)
{
srand(time(0));
// Allocate the drawing board
board = new COLORREF*[width];
for (int i = 0; i < width; i++)
{
board[i] = new COLORREF[height];
for (int j = 0; j < height; j++)
board[i][j] = RGB(0, 0, 0);
}
// 初始化目前和下一個方塊
current = 0;
next = pieceSet.getRandomPiece();
}
Level::~Level()
{
for (int i = 0; i < width; i++)
delete [] board[i];
delete [] board;
}
void Level::drawBoard() const
{
for (int i = 0; i < width; i++)
for (int j = 0; j < height; j++)
de.drawBlock(i, j, board[i][j]);
}
void Level::timerUpdate()
{
// If the time isn't up, don't drop nor update
currentTime = timeGetTime();
if (currentTime - lastTime < speed)
return;
// Time's up, drop
// If the piece hits the bottom, check if player gets score, drop the next
// piece, increase speed, redraw info
// If player gets score, increase more speed
if (current == NULL || !move(0, -1))
{
int lines = clearRows();
speed = max(speed - 2 * lines, 100);
score += 1 + lines * lines * 5;
dropRandomPiece();
drawScore();
drawSpeed();
drawNextPiece();
}
lastTime = timeGetTime();
}
bool Level::place(int x, int y, const Piece &piece)
{
// Out of boundary or the position has been filled
if (x + piece.getWidth() > width || isCovered(piece, x, y))
return false;
posX = x;
posY = y;
POINT apt[4];
piece.getBody(apt);
COLORREF color = piece.getColor();
for (int i = 0; i < 4; i++)
{
if (y + apt[i].y > height - 1)
continue;
board[x+apt[i].x][y+apt[i].y] = color;
}
return true;
}
bool Level::rotate()
{
Piece *tmp = current;
// Move the piece if it needs some space to rotate
int disX = max(posX + current->getHeight() - width, 0);
// Go to next rotation state (0-3)
int rotation = (current->getRotation() + 1) % PieceSet::NUM_ROTATIONS;
clear(*current);
current = pieceSet.getPiece(current->getId(), rotation);
// Rotate successfully
if (place(posX - disX, posY, *current))
return true;
// If the piece cannot rotate due to insufficient space, undo it
current = tmp;
place(posX, posY, *current);
return false;
}
bool Level::move(int cxDistance, int cyDistance)
{
if (posX + cxDistance < 0 || posY + cyDistance < 0 ||
posX + current->getWidth() + cxDistance > width)
return false;
if (cxDistance < 0 && isHitLeft())
return false;
if (cxDistance > 0 && isHitRight())
return false;
if (cyDistance < 0 && isHitBottom())
return false;
clear(*current);
return place(posX + cxDistance, posY + cyDistance, *current);
}
void Level::clear(const Piece &piece)
{
POINT apt[4];
piece.getBody(apt);
int x, y;
for (int i = 0; i < 4; i++) {
x = posX + apt[i].x;
y = posY + apt[i].y;
if (x > width -1 || y > height - 1)
continue;
board[posX+apt[i].x][posY+apt[i].y] = RGB(0,0,0);
}
}
void Level::dropRandomPiece()
{
current = next;
next = pieceSet.getRandomPiece();
place(3, height - 1, *current);
}
bool Level::isHitBottom() const
{
POINT apt[4];
int n = current->getSkirt(apt);
int x, y;
for (int i = 0; i < n; i++)
{
x = posX + apt[i].x;
y = posY + apt[i].y;
if (y < height && (y == 0 || board[x][y-1] != RGB(0,0,0)))
return true;
}
return false;
}
bool Level::isHitLeft() const
{
POINT apt[4];
int n = current->getLeftSide(apt);
int x, y;
for (int i = 0; i < n; i++)
{
x = posX + apt[i].x;
y = posY + apt[i].y;
if (y > height - 1)
continue;
if (x == 0 || board[x-1][y] != RGB(0,0,0))
return true;
}
return false;
}
bool Level::isHitRight() const
{
POINT apt[4];
int n = current->getRightSide(apt);
int x, y;
for (int i = 0; i < n; i++)
{
x = posX + apt[i].x;
y = posY + apt[i].y;
if (y > height - 1)
continue;
if (x == width - 1 || board[x+1][y] != RGB(0,0,0))
return true;
}
return false;
}
bool Level::isCovered(const Piece &piece, int x, int y) const
{
POINT apt[4];
piece.getBody(apt);
int tmpX, tmpY;
for (int i = 0; i < 4; i++)
{
tmpX = apt[i].x + x;
tmpY = apt[i].y + y;
if (tmpX > width - 1 || tmpY > height -1)
continue;
if (board[tmpX][tmpY] != RGB(0,0,0))
return true;
}
return false;
}
int Level::clearRows()
{
bool isComplete;
int rows = 0;
for (int i = 0; i < height; i++)
{
for (int j = 0; j < width; j++)
{
if (board[j][i] == RGB(0,0,0))
{
isComplete = false;
break;
}
// The row is full
if (j == width - 1)
isComplete = true;
}
// If the row is full, clear it (fill with black)
if (isComplete)
{
for (int j = 0; j < width; j++)
board[j][i] = RGB(0,0,0);
// Move rows down
for (int k = i; k < height - 1; k++)
{
for (int m = 0; m < width; m++)
board[m][k] = board[m][k+1];
}
i = -1;
rows++;
}
}
return rows;
}
bool Level::isGameOver()
{
// Exclude the current piece
if (current)
clear(*current);
// If there's a piece on the top, game over
for (int i = 0; i < width; i++) {
if (board[i][height-1]) {
if (current)
place(posX, posY, *current);
return true;
}
}
// Put the current piece back
if (current != 0)
place(posX, posY, *current);
return false;
}
void Level::drawSpeed() const
{
de.drawSpeed((500 - speed) / 2, width + 1, 12);
}
void Level::drawScore() const
{
de.drawScore(score, width + 1, 13);
}
void Level::drawNextPiece() const
{
de.drawNextPiece(*next, width + 1, 14);
}
| {
"repo_name": "eliangcs/tetris-win32",
"stars": "30",
"repo_language": "C++",
"file_name": "PieceSet.h",
"mime_type": "text/x-c++"
} |
#include "Game.h"
#include <iostream>
using namespace std;
Game::Game(DrawEngine &de) :
de(de), isPaused(false)
{
level = new Level(de, 10, 20);
}
Game::~Game()
{
delete level;
}
void Game::restart()
{
delete level;
level = new Level(de, 10, 20);
isPaused = false;
repaint();
}
bool Game::keyPress(int vk)
{
// When pausing, ignore keys other than PAUSE and ENTER
if (vk != VK_PAUSE && vk != VK_RETURN && isPaused)
return false;
switch (vk)
{
case VK_UP:
level->rotate();
break;
case VK_DOWN:
level->move(0, -1);
break;
case VK_LEFT:
level->move(-1, 0);
break;
case VK_RIGHT:
level->move(1, 0);
break;
case VK_SPACE:
level->rotate();
break;
case VK_PAUSE:
pause(!isPaused);
break;
case VK_RETURN:
// You can only restart on game over
if (level->isGameOver())
restart();
default:
return false;
}
return true;
}
void Game::timerUpdate()
{
// Don't update game when pausing
if (isPaused)
return;
// If game is over, show GAME OVER
if (level->isGameOver()) {
isPaused = true;
drawGameOver();
return;
}
// Update game data
level->timerUpdate();
// Redraw
level->drawBoard();
}
void Game::pause(bool paused)
{
// Don't pause if game is over
if (isGameOver())
return;
isPaused = paused;
if (paused)
drawPause();
level->drawScore();
level->drawSpeed();
}
void Game::repaint() const
{
de.drawInterface();
level->drawScore();
level->drawSpeed();
level->drawNextPiece();
level->drawBoard();
if (level->isGameOver())
drawGameOver();
else if (isPaused)
drawPause();
}
bool Game::isGameOver() const
{
return level->isGameOver();
}
| {
"repo_name": "eliangcs/tetris-win32",
"stars": "30",
"repo_language": "C++",
"file_name": "PieceSet.h",
"mime_type": "text/x-c++"
} |
#include "Piece.h"
#include "Game.h"
#include <windows.h>
#include <iostream>
using namespace std;
const int PX_PER_BLOCK = 25; // Cell size in pixels
const int SCREEN_WIDTH = 10; // Level width in cells
const int SCREEN_HEIGHT = 20; // Level height in cells
const int GAME_SPEED = 33; // Update the game every GAME_SPEED millisecs (= 1/fps)
const int TIMER_ID = 1;
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance,
PSTR szCmdLine, int iCmdShow)
{
static TCHAR szAppName[] = TEXT("tetris");
HWND hwnd;
MSG msg;
WNDCLASSEX wc;
// We need to repaint a lot, using CS_OWNDC is more efficient
wc.cbSize = sizeof(WNDCLASSEX);
wc.style = CS_HREDRAW | CS_VREDRAW | CS_OWNDC;
wc.lpfnWndProc = WndProc;
wc.cbClsExtra = 0;
wc.cbWndExtra = 0;
wc.hInstance = hInstance;
wc.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wc.hCursor = LoadCursor(NULL, IDC_ARROW);
wc.hbrBackground = (HBRUSH)GetStockObject(BLACK_BRUSH);
wc.lpszMenuName = NULL;
wc.lpszClassName = szAppName;
wc.hIconSm = NULL;
if (!RegisterClassEx(&wc))
{
MessageBox(NULL, TEXT("Program requires Windows NT!"),
szAppName, MB_ICONERROR);
return 0;
}
hwnd = CreateWindow(szAppName,
TEXT("Eliang's Tetris"),
WS_MINIMIZEBOX | WS_SYSMENU, // No window resizing
CW_USEDEFAULT,
CW_USEDEFAULT,
SCREEN_WIDTH * PX_PER_BLOCK + 156,
SCREEN_HEIGHT * PX_PER_BLOCK + 25,
NULL,
NULL,
hInstance,
NULL);
ShowWindow(hwnd, iCmdShow);
UpdateWindow(hwnd);
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam)
{
HDC hdc;
PAINTSTRUCT ps;
static Game *game;
static DrawEngine *de;
switch (message)
{
case WM_CREATE:
hdc = GetDC(hwnd);
de = new DrawEngine(hdc, hwnd, PX_PER_BLOCK);
game = new Game(*de);
SetTimer(hwnd, TIMER_ID, GAME_SPEED, NULL);
ReleaseDC(hwnd, hdc);
return 0;
case WM_KEYDOWN:
game->keyPress(wParam);
return 0;
case WM_TIMER:
game->timerUpdate();
return 0;
case WM_KILLFOCUS:
KillTimer(hwnd, TIMER_ID);
game->pause(true);
return 0;
case WM_SETFOCUS:
SetTimer(hwnd, TIMER_ID, GAME_SPEED, NULL);
return 0;
case WM_PAINT:
hdc = BeginPaint(hwnd, &ps);
game->repaint();
EndPaint(hwnd, &ps);
return 0;
case WM_DESTROY:
delete de;
delete game;
KillTimer(hwnd, TIMER_ID);
PostQuitMessage(0);
return 0;
}
return DefWindowProc(hwnd, message, wParam, lParam);
}
| {
"repo_name": "eliangcs/tetris-win32",
"stars": "30",
"repo_language": "C++",
"file_name": "PieceSet.h",
"mime_type": "text/x-c++"
} |
#ifndef LEVEL_H
#define LEVEL_H
#include "DrawEngine.h"
#include "PieceSet.h"
#include <windows.h>
//
// Level stores current snapshot of the game, including the dropping piece,
// the next piece, the piece generator (PieceSet), the position of the
// dropping piece, the width and height of the level, the dropping speed,
// and a two-dimentional color array that is used to represent the canvas.
//
class Level
{
public:
// de: used to draw the level
// width & height: level size in cells
Level(DrawEngine &de, int width = 10, int height = 20);
~Level();
// Draws the level
void drawBoard() const;
// Rotates the dropping piece, returns true if successful
bool rotate();
// Moves the dropping piece, returns true if successful
// cxDistance is horizontal movement, positive value is right
// cyDistance is vertical movement, positive value is up (normally it's
// negaive)
bool move(int cxDistance, int cyDistance);
// Updates the level based on the current speed
void timerUpdate();
bool isGameOver();
// Draw different kinds of info
void drawSpeed() const;
void drawScore() const;
void drawNextPiece() const;
protected:
// Places a piece somewhere
// If there isn't enough space, does nothing and returns false
bool place(int x, int y, const Piece &piece);
// Clears a piece on the canvas
void clear(const Piece& piece);
// Releases the next dropping piece
void dropRandomPiece();
// Checks if the piece hits the boundary
bool isHitBottom() const;
bool isHitLeft() const;
bool isHitRight() const;
// Checks if a piece can move to a position
bool isCovered(const Piece &piece, int x, int y) const;
// Clears "full" rows, returns the number of rows being cleared
int clearRows();
COLORREF **board; // The cavnas, the drawing board
DrawEngine &de; // Does graphics stuffs
PieceSet pieceSet; // Piece generator
Piece *current; // Current dropping piece
Piece *next; // Next piece
int width; // Level width (in cells)
int height; // Level height
int posX; // X coordinate of dropping piece (Cartesian system)
int posY; // Y coordinate of dropping piece
int speed; // Drop a cell every _speed_ millisecs
double lastTime; // Last time updated
double currentTime; // Current update time
int score; // Player's score
};
#endif // LEVEL_H
| {
"repo_name": "eliangcs/tetris-win32",
"stars": "30",
"repo_language": "C++",
"file_name": "PieceSet.h",
"mime_type": "text/x-c++"
} |
#include "DrawEngine.h"
DrawEngine::DrawEngine(HDC hdc, HWND hwnd, int pxPerBlock,
int width, int height) :
hdc(hdc), hwnd(hwnd), width(width), height(height)
{
GetClientRect(hwnd, &rect);
SaveDC(hdc);
// Set up coordinate system
SetMapMode(hdc, MM_ISOTROPIC);
SetViewportExtEx(hdc, pxPerBlock, pxPerBlock, 0);
SetWindowExtEx(hdc, 1, -1, 0);
SetViewportOrgEx(hdc, 0, rect.bottom, 0);
// Set default colors
SetTextColor(hdc, RGB(255,255,255));
SetBkColor(hdc, RGB(70,70,70));
SetBkMode(hdc, TRANSPARENT);
}
DrawEngine::~DrawEngine()
{
// Restore coordinate system
RestoreDC(hdc, -1);
}
void DrawEngine::drawBlock(int x, int y, COLORREF color)
{
HBRUSH hBrush = CreateSolidBrush(color);
rect.left = x;
rect.right = x + 1;
rect.top = y;
rect.bottom = y + 1;
FillRect(hdc, &rect, hBrush);
// Draw left and bottom black border
MoveToEx(hdc, x, y + 1, NULL);
LineTo(hdc, x, y);
LineTo(hdc, x + 1, y);
DeleteObject(hBrush);
}
void DrawEngine::drawInterface()
{
// Draw a gray area at the right
HBRUSH hBrush = CreateSolidBrush(RGB(70,70,70));
rect.top = height;
rect.left = width;
rect.bottom = 0;
rect.right = width + 8;
FillRect(hdc, &rect, hBrush);
DeleteObject(hBrush);
}
void DrawEngine::drawText(TCHAR *szText, int x, int y) const
{
TextOut(hdc, x, y, szText, lstrlen(szText));
}
void DrawEngine::drawScore(int score, int x, int y) const
{
TCHAR szBuffer[20];
int len = wsprintf(szBuffer, TEXT("Score: %6d"), score);
SetBkMode(hdc, OPAQUE);
TextOut(hdc, x, y, szBuffer, len);
SetBkMode(hdc, TRANSPARENT);
}
void DrawEngine::drawSpeed(int speed, int x, int y) const
{
TCHAR szBuffer[20];
int len = wsprintf(szBuffer, TEXT("Speed: %6d"), speed);
SetBkMode(hdc, OPAQUE);
TextOut(hdc, x, y, szBuffer, len);
SetBkMode(hdc, TRANSPARENT);
}
void DrawEngine::drawNextPiece(Piece &piece, int x, int y)
{
TCHAR szBuffer[] = TEXT("Next:");
TextOut(hdc, x, y + 5, szBuffer, lstrlen(szBuffer));
COLORREF color = piece.getColor();
// Draw the piece in a 4x4 square area
for (int i = 0; i < 4; i++)
{
for (int j = 0; j < 4; j++)
{
if (piece.isPointExists(i, j))
drawBlock(i + x, j + y, color);
else
drawBlock(i + x, j + y, RGB(0,0,0));
}
}
}
| {
"repo_name": "eliangcs/tetris-win32",
"stars": "30",
"repo_language": "C++",
"file_name": "PieceSet.h",
"mime_type": "text/x-c++"
} |
#ifndef PIECE_H
#define PIECE_H
#include <windows.h>
//
// A piece in Teris game. This class is only used by PieceSet. Other classes
// access Piece through PieceSet.
//
// Every piece is composed by 4 POINTs, using Cartesian coordinate system.
// That is, the most bottom left point is (0, 0), the values on x-axis
// increase to the right, and values on y-axis increase to the top.
//
// To represent a piece, it is snapped to the bottom-left corner. For example,
// when the 'I' piece stands vertically, the point array stores:
// (0,0) (0,1) (0,2) (0,3)
//
class Piece
{
public:
// pieceId: piece type ID
// pieceRotation: how many time is the piece rotated (0-3)
// pieceColor: piece color in RGB
// apt: array of points of which the piece is composed. This constructor
// moves these points automatically to snap the piece to bottom-left
// corner (0,0)
// numPoints: number of points in apt
Piece(int pieceId, int pieceRotation, COLORREF pieceColor,
const POINT *apt, int numPoints = 4);
~Piece();
// Getters for piece properties
inline int getWidth() const { return width; }
inline int getHeight() const { return height; }
inline int getNPoints() const { return nPoints; }
inline int getId() const { return id; }
inline int getRotation() const { return rotation; }
inline COLORREF getColor() const { return color; }
// If any of the following methods accepts a POINT pointer, make sure the
// size of the array is big enough
// Gets the points of the piece
void getBody(POINT *apt) const;
// Gets the bottom, left, or right part of points of the piece
int getSkirt(POINT *apt) const;
int getLeftSide(POINT *apt) const;
int getRightSide(POINT *apt) const;
// Determines if the piece has a point (x, y)
bool isPointExists(int x, int y) const;
// Prints a piece (for debugging)
void print() const;
protected:
// POINT array of which the piece is composed
POINT *body;
// Number of points in body
int nPoints;
// Make rotation more faster
int width;
int height;
// Piece type ID and rotation
int id;
int rotation;
// Piece color in RGB
COLORREF color;
};
#endif // PIECE_H
| {
"repo_name": "eliangcs/tetris-win32",
"stars": "30",
"repo_language": "C++",
"file_name": "PieceSet.h",
"mime_type": "text/x-c++"
} |
#ifndef PIECESET_H
#define PIECESET_H
#include "Piece.h"
//
// PieceSet generates 7 types of pieces. Each piece has 4 rotations, so there
// are 7 x 4 = 28 configurations in total.
//
// All 28 configurations are kept in memory after you new PieceSet(). To get
// get piece, use getPiece() or getRandomPiece().
//
class PieceSet
{
public:
// Constants
enum { NUM_ROTATIONS = 4, NUM_PIECES = 7 };
PieceSet();
~PieceSet();
// Gets a specific piece
// id: one of 7 piece types (0-6)
// rotation: one of 4 rotations (0-3)
Piece *getPiece(int id, int rotation = 0) const;
// Gets a random piece
inline Piece *getRandomPiece() const
{
return getPiece(rand() % NUM_PIECES, rand() % NUM_ROTATIONS);
}
protected:
// Stores all 28 configurations of pieces
Piece *pieces[NUM_PIECES][NUM_ROTATIONS];
// Initializes piece rotations
void rotateAll();
// Rotates points by 90 degrees counterclockwise
void rotate(POINT *apt, int numPoints = 4);
};
#endif // PIECESET_H
| {
"repo_name": "eliangcs/tetris-win32",
"stars": "30",
"repo_language": "C++",
"file_name": "PieceSet.h",
"mime_type": "text/x-c++"
} |
---
title: "Fundamentals of RMarkdown"
author: "Mike Frank & Chris Hartgerink"
date: "July 31st, 2017"
output:
revealjs::revealjs_presentation:
theme: black
highlight: pygments
incremental: true
center: true
transition: slide
reveal_options:
slideNumber: true
fig_caption: false
bibliography: library.bib
---
## Workshop
1. Short intro to Markdown (this!)
2. A document you can work through to get started
3. Exercises along the way where we'll highlight how to make useful documents (e.g. APA manuscript)
4. No need to stay until end if you feel you've got what you wanted
## Learning goals
By the end of this workshop you should:
* Know what Markdown is and how the syntax works,
* See how to integrate code and data with RMarkdown,
* Understand the different output formats from RMarkdown and how to generate them
* Know about generating APA format files with [`papaja`](https://github.com/crsh/papaja) and bibtex.
## RMarkdown = R + Markdown
1. It combines the magic of R with the simplicity of Markdown documents
1. The purpose: write your manuscripts and automatically insert results!
1. Use cases (amongst others):
* prevent rounding errors
* promote computational reproducibility
* encourage error correction by minimizing manual work
## The Markdown
- Markdown is the simplest document language!
- Open standard, convertable into `.tex`, `.docx`, `.html`, `.pdf`, etc
- You can [learn Markdown in five (!) minutes](https://learnxinyminutes.com/docs/markdown/)
- Despite being simple, very powerful and used on many web platforms
## Basic Markdown: headings
- `# Header 1` creates a heading of the first level
- `## Header 2` creates a heading of the _second_ level
- `### Header 3` ...
- etc
## Basic Markdown: letter styles
- `**bold**` creates **bold**
- `*italic*` creates *italic*
- `***bold and italic!***` creates ***bold and italic!***
## The R
- If you're here, you probably know R
- Programming language for statistics
- Packages to add functionality
- Everything open-source!
## Combining R and Rmarkdown
- Can write text, include code
- We can include both code and its outputs, or just one of the two
- "Literate programming": mix code and explanation so that it's easier to figure out what's going on.
## Example code chunk
```{r}
x <- rnorm(100, 50, 20)
y <- rnorm(143, 51, 20)
t.test(x, y)
```
## Example in line
1. However, we can also evaluate code in text, and replace text with output
1. For example, let's round $\pi$ to four decimal places, using the code `round(pi, 4)`
* `r round(pi, 4)`
<!-- ## RMarkdown is more powerful -->
<!-- - It's not just about individual results. -->
<!-- - Ensures data preparation, analysis code is IN the manuscript -->
<!-- - Plus: Don't make all those tables and figures manually! :-) -->
## Output formats
- HTML - easy to share results on the web
- PDF - for manuscripts/reports (requires installing `latex`)
- Word - for editing with non-R collaborators, journals
## Templates
- Nicely formatted handouts / reports
- APA manuscripts using `papaja` (we'll get into this in depth)
- Specialized styles like PNAS or Cognitive Science
- Don't waste time reformatting - just change the template
## RMarkdown presentations
- If you want to go overboard, you can render slides from code too
- This is not always perfect, but it's great for course notes, especially teaching stats.
- This presentation also written in Rmarkdown!
## Workshop
Work through the handout accompanying these slides, which you can find at [http://bit.ly/rmarkdown-pdf](https://bit.ly/rmarkdown-pdf) and [http://bit.ly/rmarkdown-html](https://bit.ly/rmarkdown-html)
| {
"repo_name": "libscie/rmarkdown-workshop",
"stars": "27",
"repo_language": "HTML",
"file_name": "README.md",
"mime_type": "text/plain"
} |
%% This BibTeX bibliography file was created using BibDesk.
%% http://bibdesk.sourceforge.net/
%% Created for Michael Frank at 2017-07-31 09:31:00 -0400
%% Saved with string encoding Unicode (UTF-8)
@article{nuijten2016,
Author = {Nuijten, Mich{\`e}le B and Hartgerink, Chris HJ and van Assen, Marcel ALM and Epskamp, Sacha and Wicherts, Jelte M},
Date-Added = {2017-07-25 01:33:07 +0000},
Date-Modified = {2017-07-25 01:33:09 +0000},
Journal = {Behavior research methods},
Number = {4},
Pages = {1205--1226},
Publisher = {Springer},
Title = {The prevalence of statistical reporting errors in psychology (1985--2013)},
Volume = {48},
Year = {2016}}
@book{xie2013,
Author = {Xie, Yihui},
Date-Added = {2015-11-25 22:42:30 +0000},
Date-Modified = {2017-07-25 01:33:09 +0000},
Publisher = {CRC Press},
Title = {Dynamic Documents with R and knitr},
Volume = {29},
Year = {2013}}
| {
"repo_name": "libscie/rmarkdown-workshop",
"stars": "27",
"repo_language": "HTML",
"file_name": "README.md",
"mime_type": "text/plain"
} |
Version: 1.0
RestoreWorkspace: Default
SaveWorkspace: Default
AlwaysSaveHistory: Default
EnableCodeIndexing: Yes
UseSpacesForTab: Yes
NumSpacesForTab: 2
Encoding: UTF-8
RnwWeave: Sweave
LaTeX: pdfLaTeX
| {
"repo_name": "libscie/rmarkdown-workshop",
"stars": "27",
"repo_language": "HTML",
"file_name": "README.md",
"mime_type": "text/plain"
} |
---
title: "RMarkdown for writing reproducible scientific papers"
author: '[Mike Frank](mailto:mcfrank@stanford.edu) & [Chris Hartgerink](mailto:chris@libscie.org)'
date: '`r Sys.Date()`'
output:
tufte::tufte_html:
toc: yes
toc_depth: 1
tufte::tufte_handout:
citation_package: natbib
latex_engine: pdflatex
tufte::tufte_book:
citation_package: natbib
latex_engine: pdflatex
link-citations: yes
bibliography: library.bib
---
```{r, echo=FALSE}
library(knitr)
opts_chunk$set(echo=TRUE,
warning=FALSE, message=FALSE,
cache=FALSE)
```
# Introduction
This document is a short tutorial on using RMarkdown to mix prose and code together for creating reproducible scientific documents.^[There is also a slidedeck that goes along with this handout available **[here](https://libscie.github.io/rmarkdown-workshop)**, which is worth looking at if you don't know what you're doing on this page and what to look at. Going through this document takes at most two hours, assuming you already know some basic `R` programming. If you find any errors and have a Github account, **[please suggest changes here](https://github.com/libscie/rmarkdown-workshop/edit/master/handout.Rmd)**. All **[content](https://github.com/libscie/rmarkdown-workshop)** is CC 0 licensed, so feel free to remix and reuse!]
The tutorial is based on documents that both Chris and Mike wrote independently (see [here](https://elifesciences.org/labs/cad57bcf/composing-reproducible-manuscripts-using-r-markdown) and [here](http://babieslearninglanguage.blogspot.com/2015/11/preventing-statistical-reporting-errors.html) if you're interested). In short: RMarkdown allows you to create documents that are compiled with code, producing your next scientific paper.^[Note: this is also possible for Python and other open-source data analysis languages, but we focus on R.]
Now we're together trying to help spread the word, because it can make writing manuscripts so much easier! We wrote this handout in RMarkdown as well (looks really sweet, no?).
## Who is this aimed at?
We aim this document at anyone writing manuscripts and using R, including those who...
1. ...collaborate with people who use Word
2. ...want to write complex equations
3. ...want to be able to change bibliography styles with less hassle
4. ...want to spend more time actually doing research!
## Why write reproducible papers?
Cool, thanks for sticking with us and reading up through here!
There are three reasons to write reproducible papers. To be right, to be reproducible, and to be efficient. There are more, but these are convincing to us. In more depth:
1. To avoid errors. Using an automated method for scraping APA-formatted stats out of PDFs, @nuijten2016 found that over 10% of p-values in published papers were inconsistent with the reported details of the statistical test, and 1.6% were what they called "grossly" inconsistent, e.g. difference between the p-value and the test statistic meant that one implied statistical significance and the other did not. Nearly half of all papers had errors in them.
2. To promote computational reproducibility. Computational reproducibility means that other people can take your data and get the same numbers that are in your paper. Even if you don't have errors, it can still be very hard to recover the numbers from published papers because of ambiguities in analysis. Creating a document that literally specifies where all the numbers come from in terms of code that operates over the data removes all this ambiguity.
3. To create spiffy documents that can be revised easily. This is actually a really big neglected one for us. At least one of us used to tweak tables and figures by hand constantly, leading to a major incentive *never to rerun analyses* because it would mean re-pasting and re-illustratoring all the numbers and figures in a paper. That's a bad thing! It means you have an incentive to be lazy and to avoid redoing your stuff. And you waste tons of time when you do. In contrast, with a reproducible document, you can just rerun with a tweak to the code. You can even specify what you want the figures and tables to look like before you're done with all the data collection (e.g., for purposes of preregistraion or a registered report).
## Learning goals
By the end of this class you should:
* Know what Markdown is and how the syntax works,
* See how to integrate code and data in RMarkdown,
* Understand the different output formats from RMarkdown and how to generate them, and
* Know about generating APA format files with `papaja` and bibtex.
# Getting Started
## Installation
Before we get started with running everything, make sure to have `R` installed (download [here](https://cran.r-project.org/)) and `Rstudio` (download [here](https://www.rstudio.com/products/rstudio/download/#download)). If you haven't yet, go do that first and we'll be here when you get back!
## Exercise
Great, you installed both `R` and `Rstudio`! Next up, making your first RMarkdown file.
Fire up Rstudio and create a new RMarkdown file. Don't worry about the settings, we'll get to that later.
```{r, echo = FALSE}
knitr::include_graphics('figures/newfile.png')
```
If you click on "Knit" (or hit `CTRL+SHIFT+K`) the RMarkdown file will run and generate all results and present you with a PDF file, HTML file, or a Word file. If RStudio requests you to install packages, click yes and see whether everything works to begin with.
We need that before we teach you more about RMarkdown. But you should feel good if you get here already, because honestly, you're about 80% of the way to being able to write basic RMarkdown files. It's _that_ easy.
# Structure of an RMarkdown file
An RMarkdown file contains several parts. Most essential are the header, the body text, and code chunks.
## Header
Headers in RMarkdown files contain some metadata about your document, which you can customize to your liking. Below is a simple example that purely states the title, author name(s), date^[Pro-tip: you can use the `Sys.Date()` function to have that use the current date when creating the document.], and output format.
```yaml
---
title: "Untitled"
author: "NAME"
date: "July 28, 2017"
output: html_document
---
```
For now, go ahead and set `html_document` to `word_document`, except if you have strong preferences for `HTML` or `PDF`.^[Note: to create PDF documents you also need a TeX installation. Don't know what that is? You probably don't have it then. More info below.]
## Body text
The body of the document is where you actually write your reports. This is primarily written in the Markdown format, which is explained in the [Markdown syntax](#markdown-syntax) section.
The beauty of RMarkdown is, however, that you can evaluate `R` code right in the text. To do this, you start inline code with \`r, type the code you want to run, and close it again with a \`. Usually, this key is below the escape (`ESC`) key or next to the left SHIFT button.
For example, if you want to have the result of 48 times 35 in your text, you type \` r 48-35\`, which returns `r 48 - 35`. Please note that if you return a value with many decimals, it will also print these depending on your settings (for example, `r pi`).
## Code chunks
In the section above we introduced you to running code inside text, but often you need to take several steps in order to get to the result you need. And you don't want to do data cleaning in the text! This is why there are code chunks. A simple example is a code chunk loading packages.
First, insert a code chunk by going to `Code->Insert code chunk` or by pressing `CTRL+ALT+I`. Inside this code chunk you can then type for example, `library(ggplot2)` and create an object `x`.
```{r}
library(ggplot2)
x <- 1 + 1
```
If you do not want to have the contents of the code chunk to be put into your document, you include `echo=FALSE` at the start of the code chunk. We can now use the contents from the above code chunk to print results (e.g., $x=`r x`$).
These code chunks can contain whatever you need, including tables, and figures (which we will go into more later). Note that all code chunks regard the location of the RMarkdown as the working directory, so when you try to read in data use the relative path in.
## Exercises
These exercises are all about failure modes to RMarkdown. Go ahead and switch over to your new RMarkdown file.
1. Create a code chunk. In it, use `write.csv(USArrests, "USArrests.csv")` to write out some data to your hard drive. Subsequently, find that file on your computer. Where is it?
2. Load the `ggplot2` package (or any other that you use frequently) in a code chunk and regenerate the document. Now try to load some packages you've never heard of, like `adehabitatHS` or `QCA`. What happens?
## Solutions
1. RMarkdown files operate out of the directory in which they are located. If you read in data, it's important to write paths relatively starting from that "project root" directory, instead of absolute -- otherwise when you share the file the paths will all be wrong. Similarly, if you write out data they go in the root directory.
2. If you don't have packages installed, the knitting process will fail. How do you head this off? We like to load all our packages at the top of the document so it's easy to know what you need to install to make it run; it's also easy for users to see what packages they need. Some folks `install.packages` *in the script*, though we don't advocate for that here.
# Markdown syntax
Markdown is one of the simplest document languages around, that is an open standard and can be converted into `.tex`, `.docx`, `.html`, `.pdf`, etc. This is the main workhorse of RMarkdown and is very powerful. You can [learn Markdown in five (!) minutes](https://learnxinyminutes.com/docs/markdown/) Other resources include [http://rmarkdown.rstudio.com/authoring_basics.html](), and [this cheat sheet](https://www.rstudio.com/wp-content/uploads/2015/02/rmarkdown-cheatsheet.pdf).
You can do some pretty cool tricks with Markdown, but these are the basics:
* It's easy to get `*italic*` or `**bold**`.
* You can get headings using `# heading1` for first level, `## heading2` for second-level, and `### heading3` for third level.
* Lists are delimited with `*` for each entry.
* You can write links by writing `[here's my link](http://foo.com)`.
If you want a more extensive description of all the potential of Markdown, [this introduction to Markdown](https://daringfireball.net/projects/markdown/) is highly detailed.
## Exercises
Swap over to your new sample markdown.
1. Outlining using headings is a really great way to keep things organized! Try making a bunch of headings, and then recompiling your document.
2. Add a table of contents. This will involve going to the header of the document (the `YAML`), and adding some options to the `html document` bit. You want it to look like this (indentation must to be correct):
```yaml
output:
html_document:
toc: true
```
Now recompile. Looks nice, right?^[Pro-tip: you can specify how deep the TOC should go by adding `toc_depth: 2` to go two levels deep]
3. Try adding another option: `toc_float: true`. Recompile -- super cool. There are plenty more great output options that you can modify. [Here is a link to the documentation.](http://rmarkdown.rstudio.com/html_document_format.html)
# Headers, Tables, and Graphs
## Headers
We're going to want more libraries loaded (for now we're loading them inline).
```{r}
library(knitr)
library(ggplot2)
library(broom)
library(devtools)
```
We often also add `chunk options` to each code chunk so that, for example:
- code does or doesn't display inline (`echo` setting)
- figures are shown at various sizes (`fig.width` and `fig.height` settings)
- warnings and messages are suppressed (`warning` and `message` settings)
- computations are cached (`cache` setting)
There are many others available as well. Caching can be very helpful for large files, but can also cause problems when there are external dependencies that change. An example that is useful for manuscripts is:
```{r eval=FALSE}
opts_chunk$set(fig.width=8, fig.height=5,
echo=TRUE,
warning=FALSE, message=FALSE,
cache=TRUE)
```
## Graphs
It's really easy to include graphs, like this one. (Using the `mtcars` dataset that comes with `ggplot2`).
```{r}
qplot(hp, mpg, col = factor(cyl), data = mtcars)
```
All you have to do is make the plot and it will render straight into the text.
External graphics can also be included, as follows:
```{r eval = FALSE}
knitr::include_graphics("path/to/file")
```
## Tables
There are many ways to make good-looking tables using RMarkdown, depending on your display purpose.
- The `knitr` package (which powers RMarkdown) comes with the `kable` function. It's versatile and makes perfectly reasonable tables. It also has a `digits` argument for controlling rounding.
- For HTML tables, there is the `DT` package, which provides `datatable` -- these are pretty and interactive javascript-based tables that you can click on and search in. Not great for static documents though.
- For APA manuscripts, it can also be helpful to use the `xtable` package, which creates very flexible LaTeX tables. These can be tricky to get right but they are completely customizable provided you want to google around and learn a bit about tex.
We recommend starting with `kable`:
```{r}
kable(head(mtcars), digits = 1)
```
## Statistics
It's also really easy to include statistical tests of various types.
For this, an option is the `broom` package, which formats the outputs of various tests really nicely. Paired with knitr's `kable` you can make very simple tables in just a few lines of code.
```{r}
mod <- lm(mpg ~ hp + cyl, data = mtcars)
kable(tidy(mod), digits = 3)
```
Of course, cleaning these up can take some work. For example, we'd need to rename a bunch of fields to make this table have the labels we wanted (e.g., to turn `hp` into `Horsepower`).
We often need APA-formatted statistics. We can compute them first, and then print them inline.
```{r}
ts <- with(mtcars,t.test(hp[cyl==4], hp[cyl==6]))
```
> There's a statistically-significant difference in horsepower for 4- and 6-cylinder cars ($t(`r round(ts$parameter,2)`) = `r round(ts$statistic,2)`$, $p = `r round(ts$p.value,3)`$).
To insert these stats inline I wrote e.g. `round(ts$parameter, 2)` inside an inline code block.^[APA would require omission of the leading zero. `papaja::printp()` will let you do that, see below.]
Note that rounding can occasionally get you in trouble here, because it's very easy to have an output of $p = 0$ when in fact $p$ can never be exactly equal to 0. Nonetheless, this can help you prevent rounding errors and the wrath of `statcheck`.
## Exercises
1. Using the `mtcars` dataset, insert a table and a graph of your choice into the document.^[If you're feeling uninspired, try `hist(mtcars$mpg)`.]
# Writing APA-format papers
(Thanks to [Frederick Aust](http://github.com/crsh) for contributing this section!)
The end-game of reproducible research is to knit your entire paper. We'll focus on APA-style writeups. Managing APA format is a pain in the best of times. Isn't it nice to get it done for you?
We're going to use the `papaja` package. `papaja` is a R-package including a R Markdown template that can be used to produce documents that adhere to the American Psychological Association (APA) manuscript guidelines (6th Edition).
## Software requirements
To use `papaja`, make sure you are using the latest versions of R and RStudio. If you want to create PDF- in addition to DOCX-files you need **[TeX](http://de.wikipedia.org/wiki/TeX) 2013 or later**. Try [MikTeX](http://miktex.org/) for Windows, [MacTeX](https://tug.org/mactex/) for Mac, or [TeX Live](http://www.tug.org/texlive/) for Linux. Some Linux users may need a few additional TeX packages for the LaTeX document class `apa6` to work.^[For Ubuntu, we suggest running: `sudo apt-get install texlive texlive-publishers texlive-fonts-extra texlive-latex-extra texlive-humanities lmodern`.]
## Installing `papaja`
`papaja` has not yet been released on CRAN but you can install it from GitHub.
```{r install_papapja, eval = FALSE}
# Install devtools package if necessary
if(!"devtools" %in% rownames(installed.packages())) install.packages("devtools")
# Install papaja
devtools::install_github("crsh/papaja")
```
## Creating a document
The APA manuscript template should now be available through the RStudio menus when creating a new R Markdown file.
```{r template-selection, echo = FALSE, fig.cap = "papaja's APA6 template is available through the RStudio menues."}
knitr::include_graphics("figures/template_selection.png")
```
When you click RStudio's *Knit* button `papaja`, `rmarkdown,` and `knitr` work together to create an APA conform manuscript that includes both your manuscript text and the results of any embedded R code.
```{r knit-button, echo = FALSE, fig.cap = "The *Knit* button in the RStudio."}
knitr::include_graphics("figures/knitting.png")
```
Note, if you don't have TeX installed on your computer, or if you would like to create a Word document replace `output: papaja::apa6_pdf` with `output: papaja::apa6_word` in the document YAML header.
`papaja` provides some rendering options that only work if you use `output: papaja::apa6_pdf`.
`figsintext` indicates whether figures and tables should be included at the end of the document---as required by APA guidelines---or rendered in the body of the document.
If `figurelist`, `tablelist`, or `footnotelist` are set to `yes` a list of figure captions, table captions, or footnotes is given following the reference section.
`lineno` indicates whether lines should be continuously numbered through out the manuscript.
## Reporting statistical analyses
`apa_print()` facilitates reporting of statistical analyses.
The function formats the contents of R objects and produces readily reportable text.
```{r eval=FALSE}
recall_anova <- afex::aov_car(
Recall ~ (Task * Valence * Dosage) + Error(Subject/(Task * Valence)) + Dosage
, data = mixed_data
, type = 3
)
recall_anova_results <- apa_print(recall_anova, es = "pes")
recall_anova_results_p <- apa_print(recall_anova, es = "pes", in_paren = TRUE)
```
Now, you can report the results of your analyses like so:
> Item valence (\` r anova_results_p$full$Valence\`) and the task affected recall performance, \` r anova_results$full$Task\`; the dosage, however, had no effect on recall, \` r anova_results$full$Dosage\`. There was no significant interaction.
`apa_print()` also creates reportable tables---in this case a complete ANOVA table.
You can include the table into the document by passing `recall_anova_results$table` to `apa_table()`.
Remeber to include the `results = "asis"` argument in the chunk options of the chunk that generates the table.
```{r eval=FALSE}
apa_table(
recall_anova_results$table
, align = c("lrcrrr")
, caption = "ANOVA table for the analyis of the example data set."
, note = "This is a table created using R and papaja."
)
```
## Cross-referencing
`papaja` enables the use of `bookdown` cross-referencing syntax as detailed in the [bookdown documentation](https://bookdown.org/yihui/bookdown/cross-references.html).
Generally, a cross-reference to a figure, table, or document section can be done by using the syntax `\@ref(label)`.
If you set a figure caption in a code chunk via the chunk option `fig.cap = "This is my figure caption."`, the label for that figure is based on the label of the code chunk, e.g., if the chunk label is `foo`, the figure label will be `fig:foo`.
If you used `knitr::kable()` or `apa_table()` to create a table, the label for that table is, again, based on the label of the code chunk, e.g., if the chunk label is `foo`, the figure label will be `tab:foo`.
## Bibiographic management
It's also possible to include references using `bibtex`, by using `@ref` syntax. An option for managing references is [bibdesk](http://bibdesk.sourceforge.net/), which integrates with google scholar.^[But many other options are possible.]
With a bibtex file included, you can refer to papers. As an example, `@nuijten2016` results in the in text citation "@nuijten2016", or cite them parenthetically with `[@nuijten2016]` [@nuijten2016]. Take a look at the `papaja` APA example to see how this works.
`citr` is an R package that provides an easy-to-use [RStudio addin](https://rstudio.github.io/rstudioaddins/) that facilitates inserting citations.
The addin will automatically look up the Bib(La)TeX-file(s) specified in the YAML front matter.
The references for the inserted citations are automatically added to the documents reference section.
<!-- # ```{r citr-gif, echo = FALSE, fig.align = "center", fig.cap = "Demonstration of the RStudio addin from the `citr` package that inserts R Markdown citations."} -->
<!-- # knitr::include_graphics("figures/addin_demo.gif") -->
<!-- # ``` -->
Once `citr` is installed (`install.packages("citr")`) and you have restarted your R session, the addin appears in the menus and you can define a [keyboard shortcut](https://rstudio.github.io/rstudioaddins/#keyboard-shorcuts) to call the addin.
## Exercise
Make sure you've got `papaja`, then open a new template file. Compile this document, and look at how awesome it is. (To compile you need `texlive`, a library for compiling markdown to PDF, so you may need to wait and install this later if it's not working).
Try pasting in your figure and table from your other RMarkdown (don't forget any libraries you need to make it compile). Presto, ready to submit!
For a bit more on `papaja`, check out **[this guide](https://rpubs.com/YaRrr/papaja_guide)**.
# Further Frontiers
## Other dissemination methods
We didn't spend as much time on it here, but one of the biggest strengths of RMarkdown is how you can easily switch between formats. For example, we made **[our slides in RMarkdown](https://libscie.github.io/rmarkdown-workshop)** using `revealjs` (`ioslides` is also a good option).
We also like to share HTML RMarkdown reports with collaborators using the [RPubs service](http://rpubs.com) or hosting of your own choice. It's incredibly easy to share your work publicly this way -- you just push the "publish" button in the upper right hand corner of the RStudio viewer window. Then you set up an account, and you are on your way. We find this is a great method for sending analysis writeups -- no more pasting figures into email! Hosting HTML files on your own page is also easy if you know how that works.
And of course because not everyone uses R, you can simply click on the knit options at the top of the code window to switch to a Word output format. Then your collaborators can edit the text in Word (or upload to Google docs) and you can re-merge the changes with your reproducible code. This isn't a perfect workflow yet, but it's not too bad.
## Computational reproducibility concerns
Once you provide code and data for your RMarkdown, you are most of the way to full computational reproducibility. Other people should be able to get the same numbers as you for your paper!
But there's still one wrinkle. What if you use linear mixed effect models from `lme4` and then the good people developing that package make it better -- but that changes your numbers? Package versioning (and versioning on `R` itself) is a real concern. To combat this issue, there are a number of tools out there.
* The `packrat` package is a solution for package versioning for `R`. It downloads local copies of all your packages, and that can ensure that you have all the right stuff to distribute with your work.^[We've found that this package is a bit tricky to use so we don't always recommend it, but it may improve in future.]
* Some other groups are using Docker, a system for making virtual machines that have everything for your project inside them and can be unpacked on other machines to reproduce your *exact* environment. We haven't worked with these but they seem like a good option for full reproducibility.
* The `checkpoint` package allows you to install packages exactly as they were on CRAN (the main repository for packages), so you can "freeze" your package versions ([more info here](https://cran.r-project.org/web/packages/checkpoint/index.html)).
If you don't want to pursue these heavier-weight options, one simple thing you *can* do is end your RMarkdown with a printout of all the packages you used. Do this by running `sessionInfo`.
```{r}
sessionInfo()
```
| {
"repo_name": "libscie/rmarkdown-workshop",
"stars": "27",
"repo_language": "HTML",
"file_name": "README.md",
"mime_type": "text/plain"
} |
# A workshop for rmarkdown
Content for RMarkdown workshop at SIPS 2017.
`handout.Rmd` is the tutorial content. You can find the HTML version [here](https://libscie.github.io/rmarkdown-workshop/handout.html) or the PDF version [here](https://libscie.github.io/rmarkdown-workshop/handout.pdf)
You'll need [RStudio](http://www.rstudio.com) for this workshop. Also, to get the full APA functionality working, you'll need to install `papaja` and follow all the texlive installation instructions on the [papaja page](https://github.com/crsh/papaja). | {
"repo_name": "libscie/rmarkdown-workshop",
"stars": "27",
"repo_language": "HTML",
"file_name": "README.md",
"mime_type": "text/plain"
} |
[color_theme]
background_color="2e3440ff"
base_type_color="8fbcbbff"
bookmark_color="147dfaff"
brace_mismatch_color="ff786bff"
breakpoint_color="ff786bff"
caret_background_color="000000ff"
caret_color="ffffffff"
code_folding_color="ffffffb3"
comment_color="63708aff"
completion_background_color="333b4fff"
completion_existing_color="ffffff24"
completion_font_color="ccced3ff"
completion_scroll_color="ffffff12"
completion_selected_color="ffffff12"
current_line_color="434c5e6a"
engine_type_color="8fbcbbff"
executing_line_color="33cc3366"
function_color="88c0d0ff"
gdscript/function_definition_color="88c0d0ff"
gdscript/node_path_color="ebcb8bff"
keyword_color="81a1c1ff"
line_length_guideline_color="4d576b80"
line_number_color="4c566aff"
mark_color="bf626b60"
member_variable_color="d8dee9ff"
number_color="b48eadff"
safe_line_number_color="507358ff"
search_result_border_color="699ce861"
search_result_color="ffffff12"
selection_color="434c5eff"
string_color="a3be8cff"
symbol_color="81a1c1ff"
text_color="eceff4ff"
text_selected_color="1a1d28ff"
user_type_color="8fbcbbff"
word_highlighted_color="4c566aff"
| {
"repo_name": "godotengine/godot-syntax-themes",
"stars": "267",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
[color_theme]
background_color="002b36ff"
completion_background_color="3d3d3dff"
completion_selected_color="ffffff11"
completion_existing_color="ffffff23"
completion_scroll_color="ffffff11"
completion_font_color="cececeff"
caret_color="ffffffff"
caret_background_color="000000ff"
line_number_color="586e75ff"
text_color="839496ff"
text_selected_color="eee8d5ff"
keyword_color="b58900ff"
base_type_color="dc322fff"
engine_type_color="d33682ff"
function_color="268bd2ff"
member_variable_color="268bd2ff"
comment_color="586e75ff"
string_color="2aa198ff"
number_color="d33682ff"
symbol_color="839496ff"
selection_color="274642ff"
brace_mismatch_color="ff3333ff"
current_line_color="073642ff"
line_length_guideline_color="103b46ff"
mark_color="ff323238"
breakpoint_color="ff4d56ff"
code_folding_color="808080ff"
word_highlighted_color="004a5cff"
search_result_color="4d3b24ff"
search_result_border_color="00000000"
gdscript/function_definition_color="2aa198ff"
gdscript/node_path_color="d33682ff"
| {
"repo_name": "godotengine/godot-syntax-themes",
"stars": "267",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
[color_theme]
background_color="242424ff"
completion_background_color="343434ff"
completion_selected_color="464646ff"
completion_existing_color="dfdfdf21"
completion_scroll_color="ffffffff"
completion_font_color="aaaaaaff"
caret_color="aaaaaaff"
caret_background_color="2c2c2cff"
line_number_color="666666ff"
text_color="ccccccff"
text_selected_color="ccccccff"
keyword_color="cc8242ff"
base_type_color="a5c261ff"
engine_type_color="ffc66dff"
function_color="ffc66dff"
member_variable_color="9e7bb0ff"
comment_color="707070ff"
string_color="6a8759ff"
number_color="7a9ec2ff"
symbol_color="ccccccff"
selection_color="00c3ff30"
brace_mismatch_color="ff3333ff"
current_line_color="ffffff0b"
line_length_guideline_color="333333ff"
mark_color="ff323238"
breakpoint_color="cccc6633"
code_folding_color="808080ff"
word_highlighted_color="00c2ff14"
search_result_color="653618ff"
search_result_border_color="00000000"
gdscript/function_definition_color="ffc66dff"
gdscript/node_path_color="bbb529ff"
| {
"repo_name": "godotengine/godot-syntax-themes",
"stars": "267",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
[color_theme]
background_color="282c34ff"
completion_background_color="21252bff"
completion_selected_color="2c313aff"
completion_existing_color="314365ff"
completion_scroll_color="2c313aff"
completion_font_color="abb2bfff"
caret_color="f8f8f0ff"
caret_background_color="000000ff"
line_number_color="495162ff"
safe_line_number_color="808080ff"
text_color="abb2bfff"
text_selected_color="abb2bfff"
keyword_color="e06c75ff"
base_type_color="d19a66ff"
engine_type_color="61afefff"
user_type_color="56b6c2ff"
function_color="98c379ff"
member_variable_color="61afefff"
comment_color="5c6370ff"
string_color="e5c07bff"
number_color="c678ddff"
symbol_color="808080ff"
selection_color="3e4451ff"
brace_mismatch_color="c24038ff"
current_line_color="383e4aff"
line_length_guideline_color="3b4048ff"
mark_color="ff323238"
breakpoint_color="c24038ff"
code_folding_color="808080ff"
word_highlighted_color="3e4141ff"
search_result_color="314365ff"
search_result_border_color="00000000"
gdscript/function_definition_color="98c379ff"
gdscript/node_path_color="d19a66ff"
| {
"repo_name": "godotengine/godot-syntax-themes",
"stars": "267",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
[color_theme]
background_color="282828ff"
completion_background_color="3d3d3dff"
completion_selected_color="ffffff11"
completion_existing_color="ffffff23"
completion_scroll_color="ffffff11"
completion_font_color="cececeff"
caret_color="a89984ff"
caret_background_color="000000ff"
line_number_color="928374ff"
text_color="fbf1c7ff"
text_selected_color="ebdbb2ff"
keyword_color="fb4934ff"
base_type_color="fabd2fff"
engine_type_color="83a598ff"
function_color="8ec07cff"
member_variable_color="e64e59ff"
comment_color="928374ff"
string_color="b8bb26ff"
number_color="d3869bff"
symbol_color="fbf1c7ff"
selection_color="504945ff"
brace_mismatch_color="ff3333ff"
current_line_color="3c3836ff"
line_length_guideline_color="383838ff"
mark_color="ff323238"
breakpoint_color="ff4d56ff"
code_folding_color="808080ff"
word_highlighted_color="2f2c2bff"
search_result_color="68391bff"
search_result_border_color="ffffff00"
gdscript/function_definition_color="b8bb26ff"
gdscript/node_path_color="d3869bff"
| {
"repo_name": "godotengine/godot-syntax-themes",
"stars": "267",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
[color_theme]
symbol_color="bcbcbcff"
keyword_color="8fafd7ff"
base_type_color="8787afff"
engine_type_color="8787afff"
user_type_color="8787afff"
comment_color="6c6c6cff"
string_color="87af87ff"
background_color="262626ff"
completion_background_color="444444ff"
completion_selected_color="6c6c6cff"
completion_existing_color="5fafaf59"
completion_scroll_color="6c6c6cff"
completion_font_color="bcbcbcff"
text_color="bcbcbcff"
line_number_color="444444ff"
safe_line_number_color="5f875f59"
caret_color="5fafafff"
caret_background_color="444444ff"
text_selected_color="ccccccff"
selection_color="00c3ff30"
brace_mismatch_color="ff3333ff"
current_line_color="ffffff0b"
line_length_guideline_color="444444ff"
word_highlighted_color="ffffff1a"
number_color="ffffafff"
function_color="ffffffff"
member_variable_color="bcbcbcff"
mark_color="ff323238"
bookmark_color="87af87ff"
breakpoint_color="ff8700ff"
code_folding_color="6c6c6cff"
search_result_color="5fafaf59"
search_result_border_color="00000000"
gdscript/function_definition_color="ffffafff"
gdscript/node_path_color="5fafafff"
| {
"repo_name": "godotengine/godot-syntax-themes",
"stars": "267",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
[color_theme]
background_color="25252aff"
completion_background_color="2c2a32ff"
completion_selected_color="434244ff"
completion_existing_color="dfdfdf21"
completion_scroll_color="ffffffff"
completion_font_color="aaaaaaff"
caret_color="ffffffff"
caret_background_color="000000ff"
line_number_color="aaaaaa66"
text_color="ffffffff"
text_selected_color="ffffffff"
keyword_color="668ad1ff"
base_type_color="4da941ff"
engine_type_color="cf7d34ff"
function_color="e36b48ff"
member_variable_color="d16060ff"
comment_color="767f8cff"
string_color="ffc35aff"
number_color="ff9292ff"
symbol_color="9b859dff"
selection_color="333539ff"
brace_mismatch_color="ff3333ff"
current_line_color="1e1e23ff"
line_length_guideline_color="35353aff"
mark_color="ff323238"
breakpoint_color="cccc6633"
code_folding_color="808080ff"
word_highlighted_color="cce5e526"
search_result_color="0c3f0cff"
search_result_border_color="197219ff"
gdscript/function_definition_color="ffc35aff"
gdscript/node_path_color="ff9292ff"
| {
"repo_name": "godotengine/godot-syntax-themes",
"stars": "267",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
[color_theme]
background_color="ffffffff"
base_type_color="af00dbff"
bookmark_color="147dfaff"
brace_mismatch_color="ff3333ff"
breakpoint_color="cccc6633"
caret_background_color="000000ff"
caret_color="000000ff"
code_folding_color="808080ff"
comment_color="008000ff"
completion_background_color="eeeeeeff"
completion_existing_color="dfdfdf21"
completion_font_color="333333ff"
completion_scroll_color="aaaaaaff"
completion_selected_color="ccccccff"
control_flow_keyword_color="a70edcff"
current_line_color="0000000c"
engine_type_color="267f99ff"
executing_line_color="33cc3366"
function_color="795e26ff"
gdscript/function_definition_color="795e26ff"
gdscript/node_path_color="2f6bbdff"
keyword_color="0000ffff"
line_length_guideline_color="eeeeeeff"
line_number_color="397591ff"
mark_color="ff323230"
member_variable_color="001080ff"
number_color="09885aff"
safe_line_number_color="77a35dff"
search_result_border_color="00000000"
search_result_color="f8c9abff"
selection_color="add6ffff"
string_color="a31515ff"
symbol_color="000000ff"
text_color="000000ff"
text_selected_color="000000ff"
user_type_color="09885aff"
word_highlighted_color="d9e9feff"
| {
"repo_name": "godotengine/godot-syntax-themes",
"stars": "267",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
[color_theme]
background_color="282828ff"
completion_background_color="3d3d3dff"
completion_selected_color="ffffff11"
completion_existing_color="ffffff23"
completion_scroll_color="ffffff11"
completion_font_color="cececeff"
caret_color="f8f8f0ff"
caret_background_color="000000ff"
line_number_color="aaaaaa66"
text_color="f8f8f2ff"
text_selected_color="f8f8f2ff"
keyword_color="f92672ff"
base_type_color="a6e22eff"
engine_type_color="ae81ffff"
function_color="66d9efff"
member_variable_color="66d9efff"
comment_color="75715eff"
string_color="e6db74ff"
number_color="ae81ffff"
symbol_color="f8f8f2ff"
selection_color="49483eff"
brace_mismatch_color="ff3333ff"
current_line_color="323232ff"
line_length_guideline_color="393939ff"
mark_color="ff323238"
breakpoint_color="ff4d56ff"
code_folding_color="808080ff"
word_highlighted_color="3e4141ff"
search_result_color="673917ff"
search_result_border_color="00000000"
gdscript/function_definition_color="a6e22eff"
gdscript/node_path_color="fd971fff"
| {
"repo_name": "godotengine/godot-syntax-themes",
"stars": "267",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
[color_theme]
background_color="282b34ff"
completion_background_color="3d3d3dff"
completion_selected_color="ffffff11"
completion_existing_color="ffffff23"
completion_scroll_color="ffffff11"
completion_font_color="cececeff"
caret_color="528affff"
caret_background_color="000000ff"
line_number_color="6a717eff"
text_color="aab1bfff"
text_selected_color="aab1bfff"
keyword_color="c677ddff"
base_type_color="e6bf7bff"
engine_type_color="56b6c1ff"
function_color="60aeedff"
member_variable_color="e06c75ff"
comment_color="6f6f6fff"
string_color="97c379ff"
number_color="d19a66ff"
symbol_color="aab1bfff"
selection_color="404859ff"
brace_mismatch_color="ff3333ff"
current_line_color="30353eff"
line_length_guideline_color="383b44ff"
mark_color="ff323238"
breakpoint_color="ff4d56ff"
code_folding_color="808080ff"
word_highlighted_color="353840ff"
search_result_color="314365ff"
search_result_border_color="4982ffff"
gdscript/function_definition_color="60aeedff"
gdscript/node_path_color="d19a66ff"
| {
"repo_name": "godotengine/godot-syntax-themes",
"stars": "267",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
# Theme previews
## Atom Dark
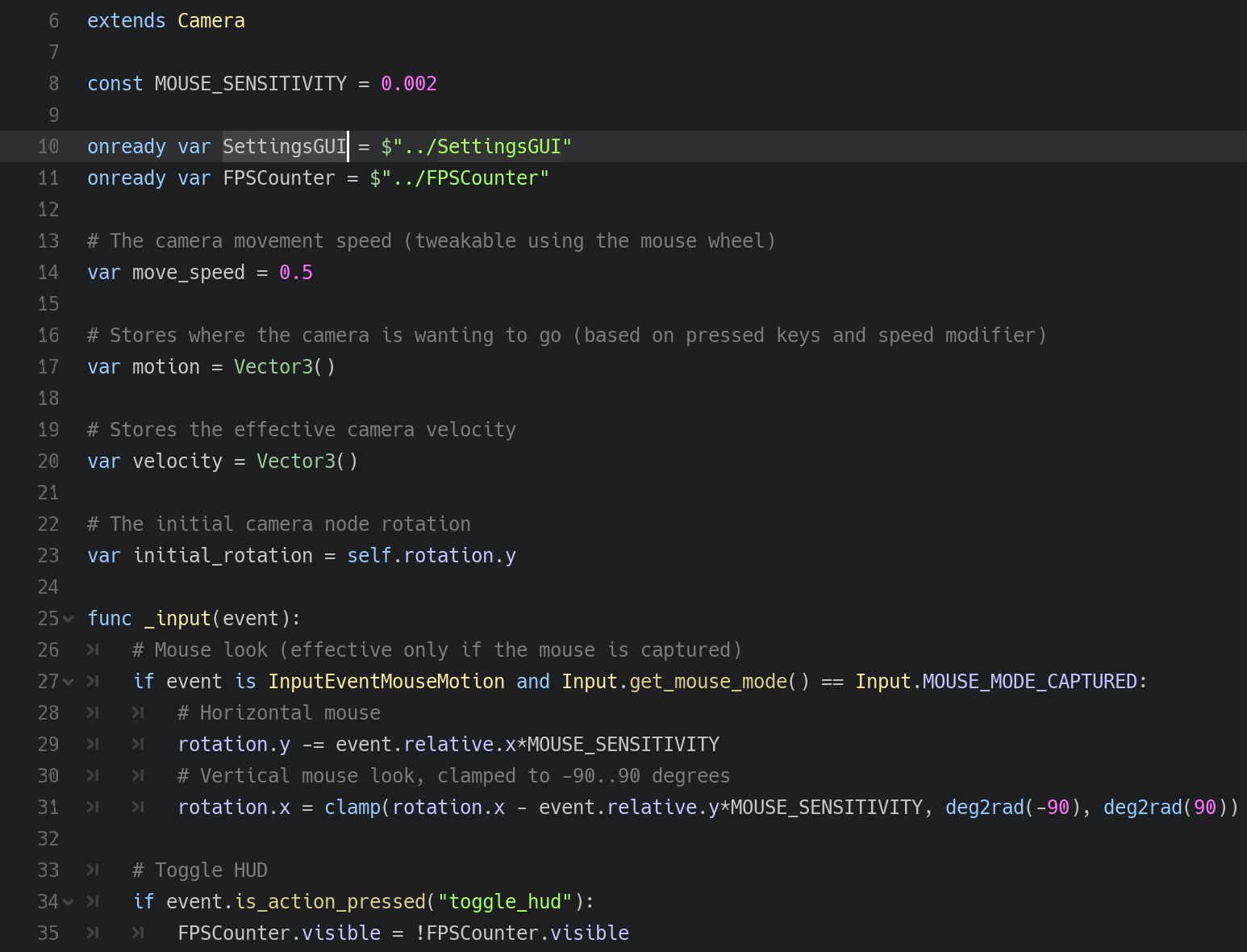
## Darcula
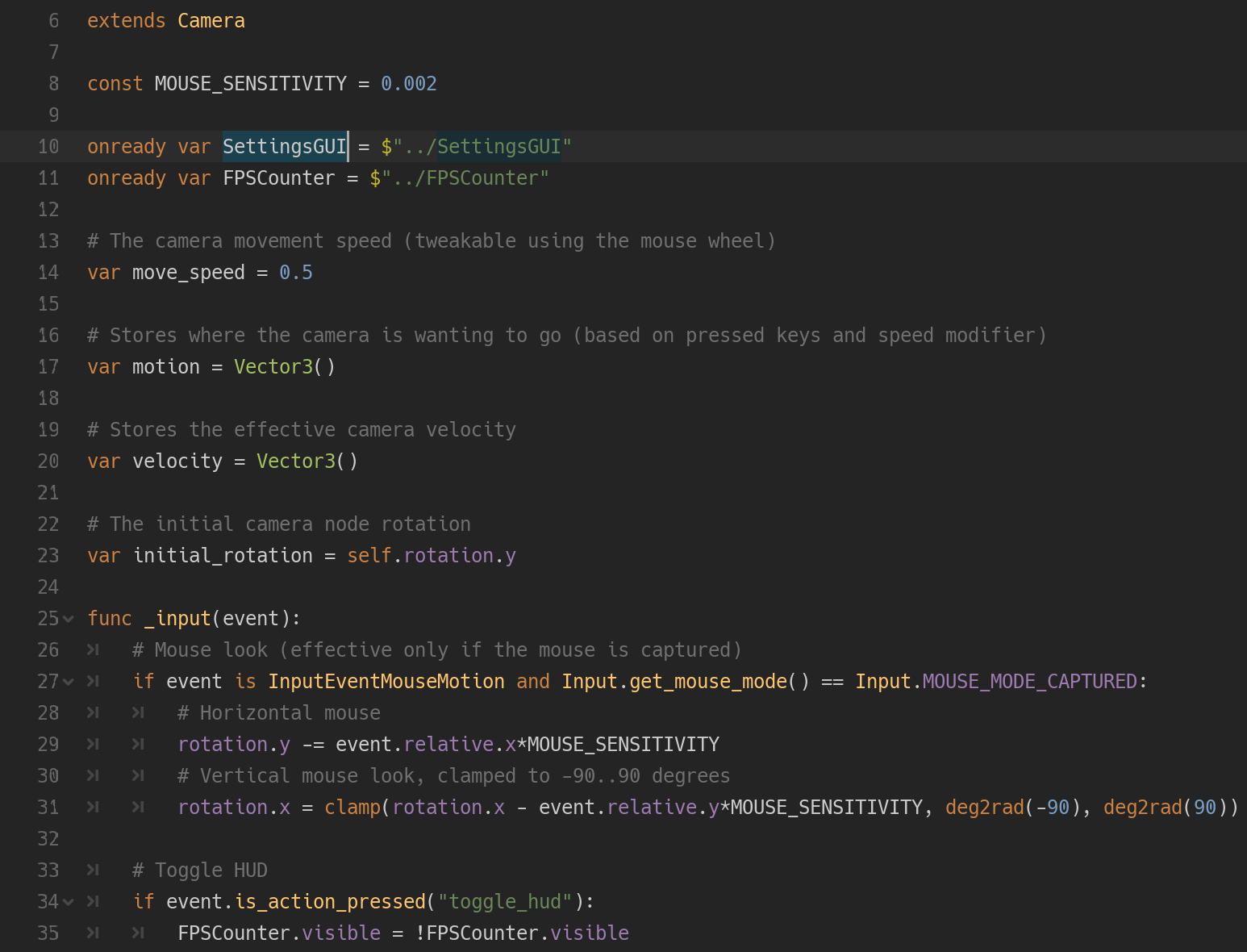
## Dracula
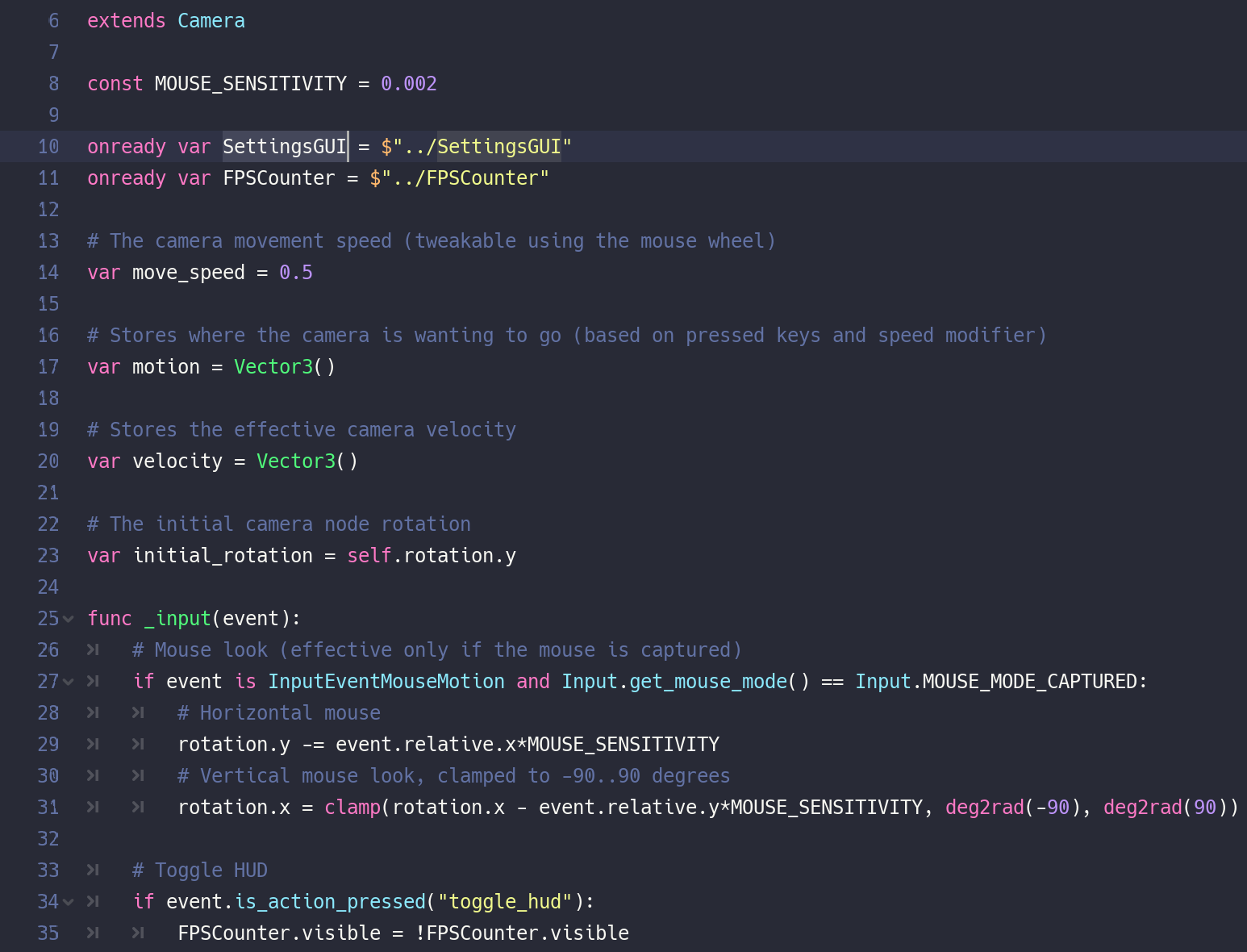
## Gruvbox Dark
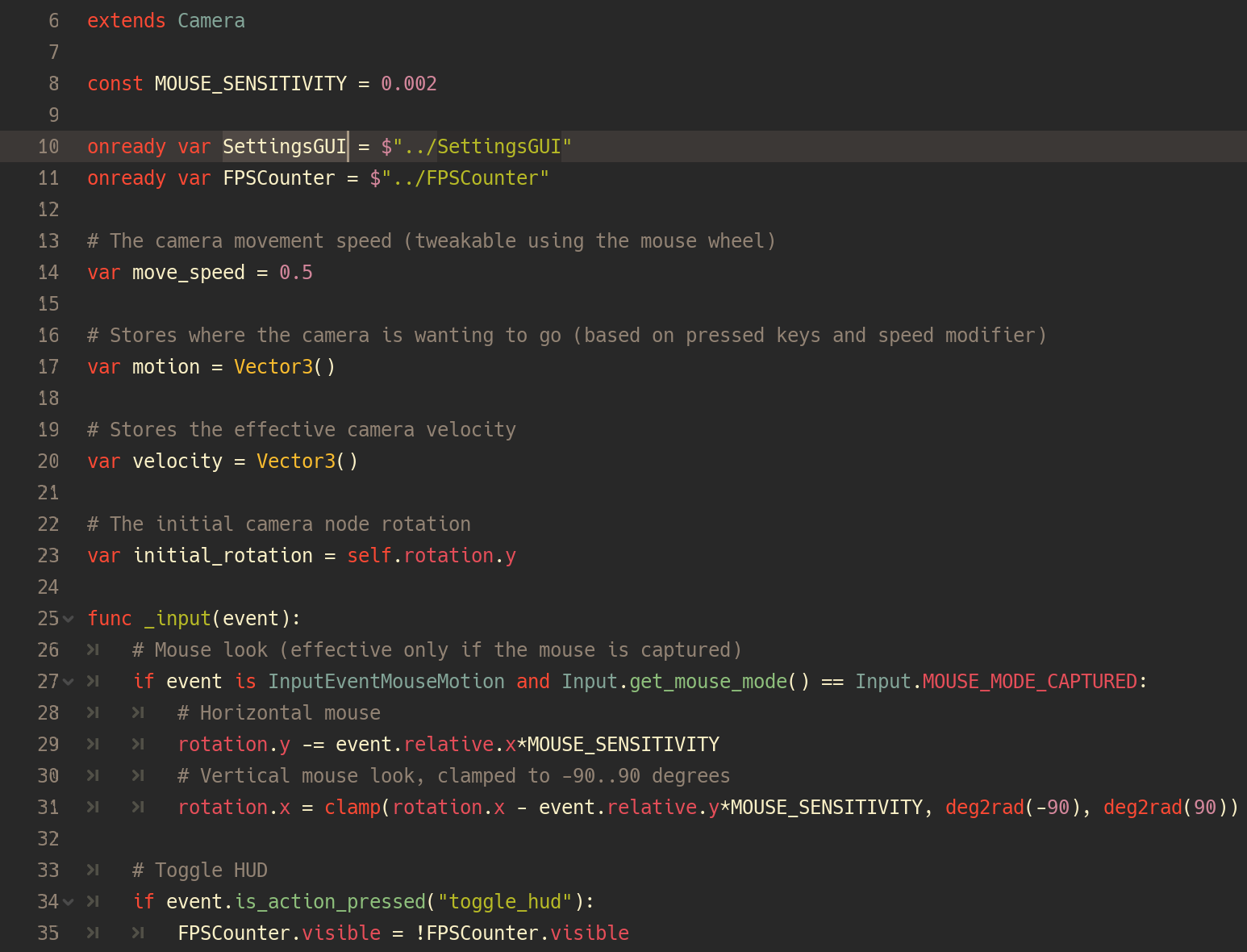
## Metro
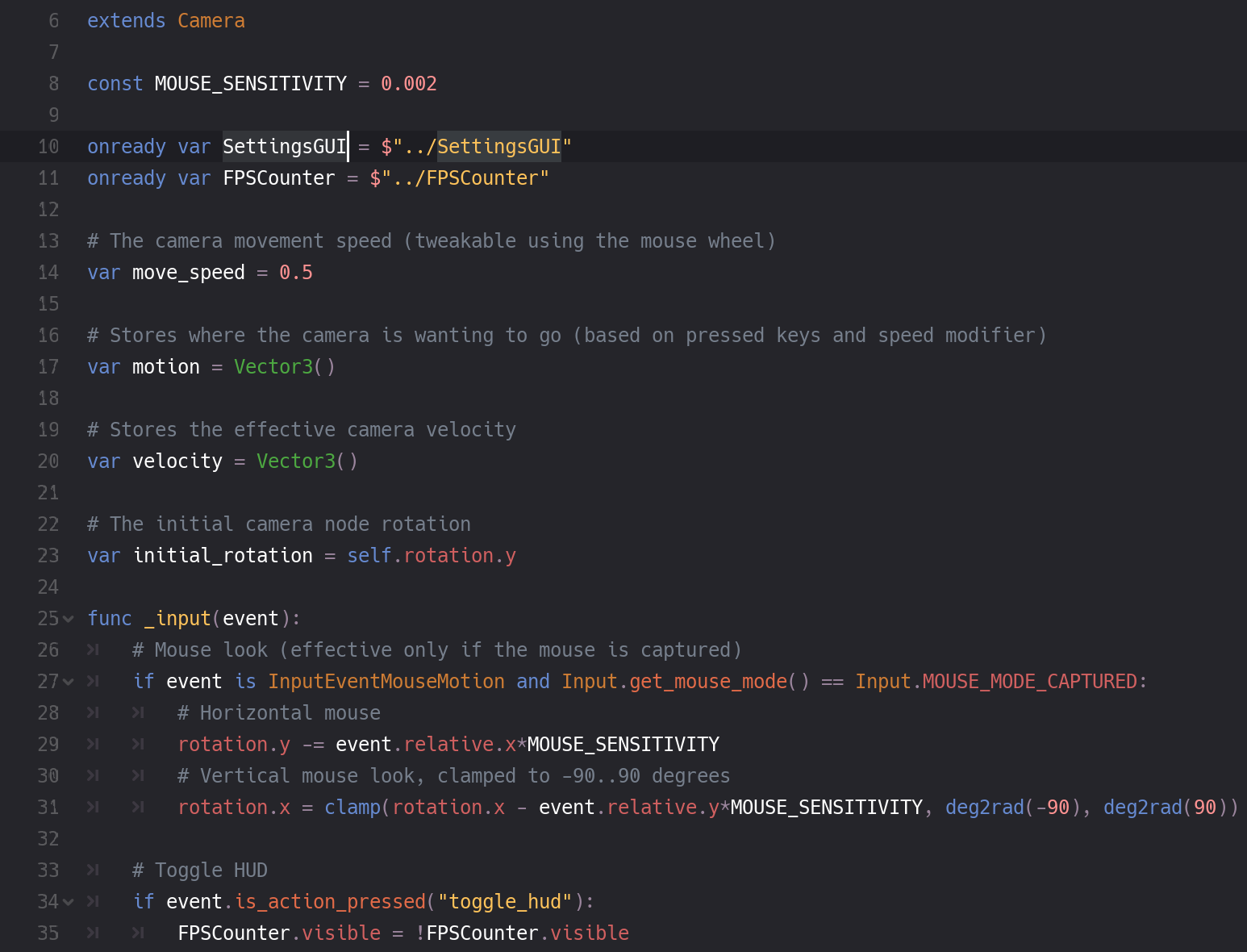
## Monokai
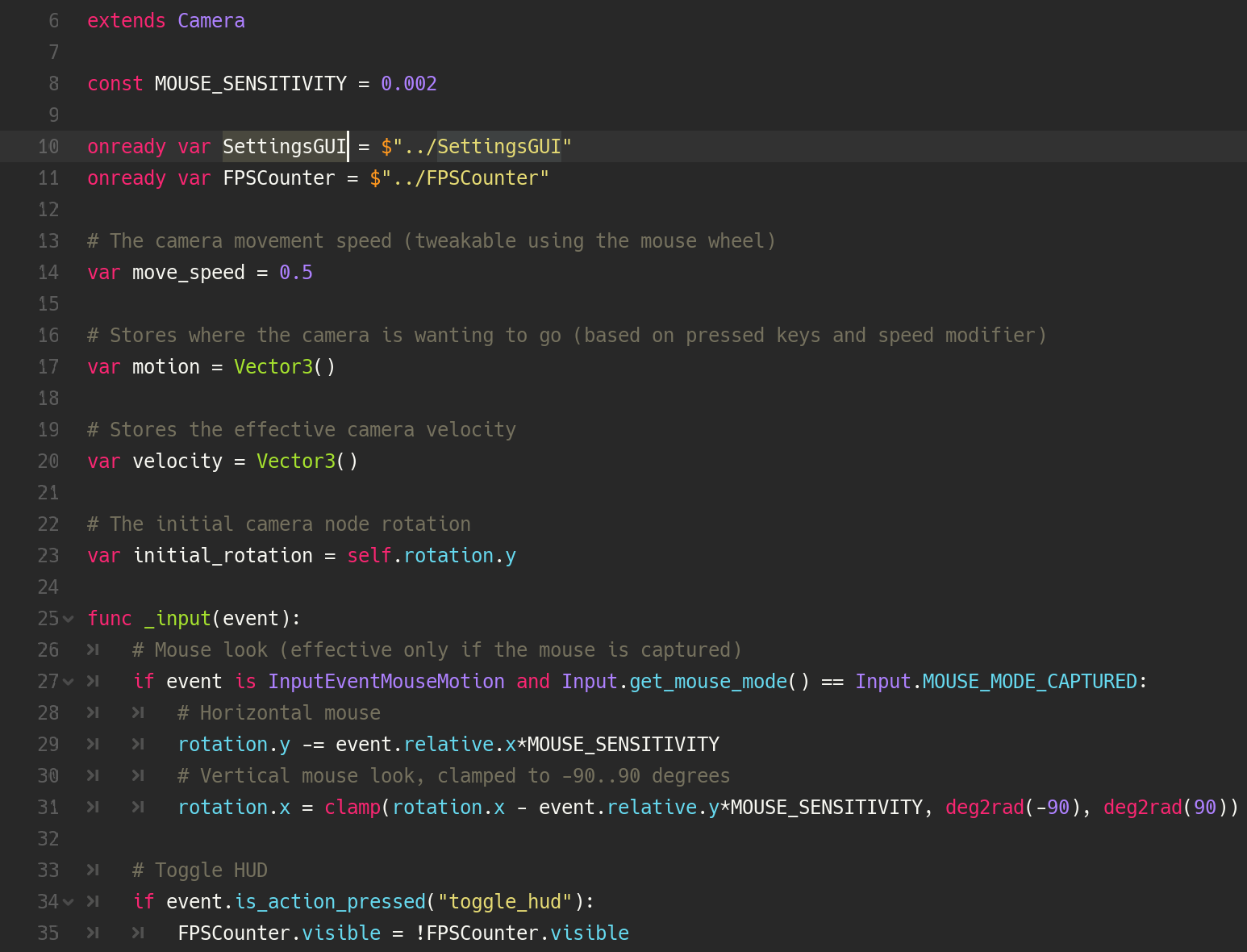
## One Dark
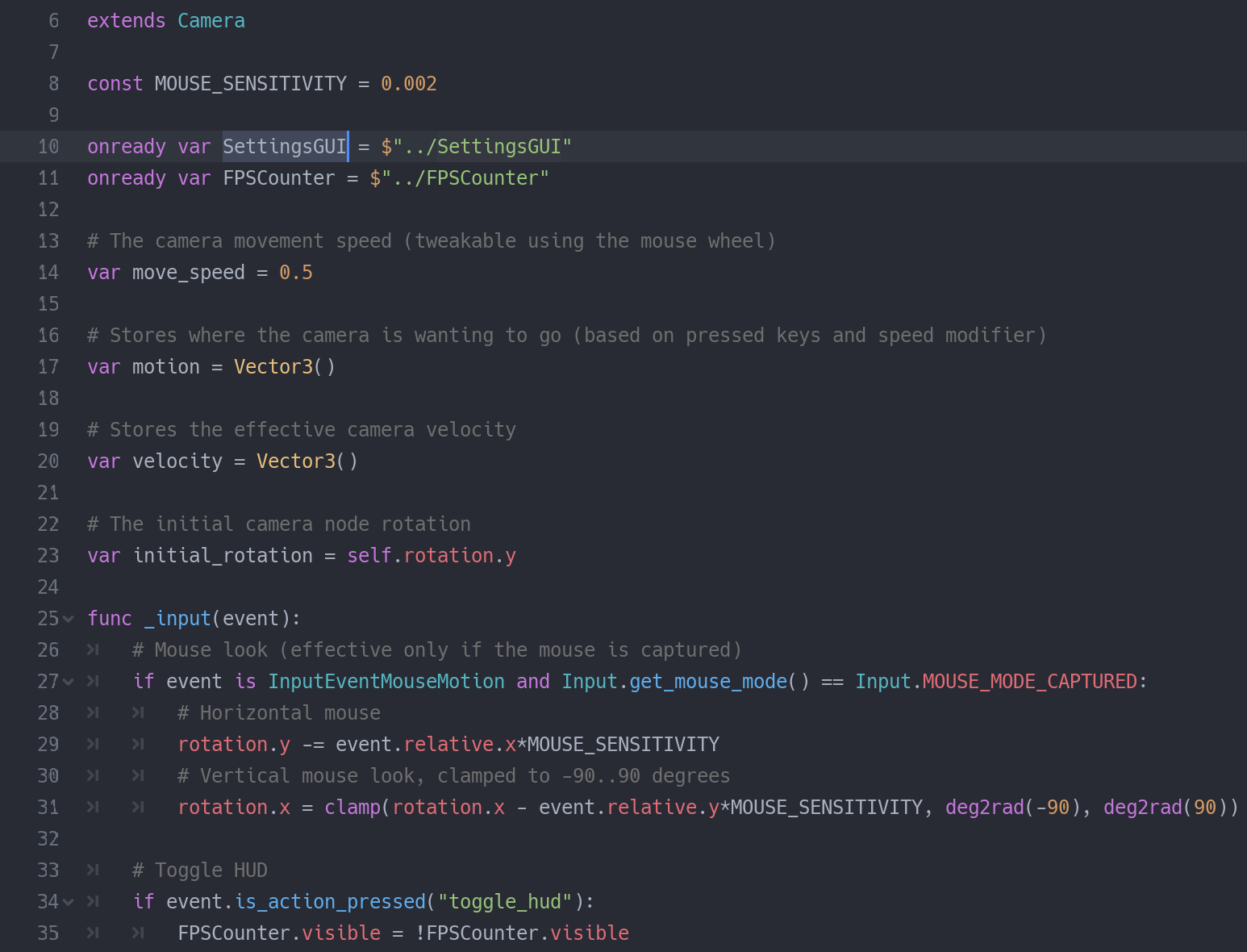
## Quiet Light
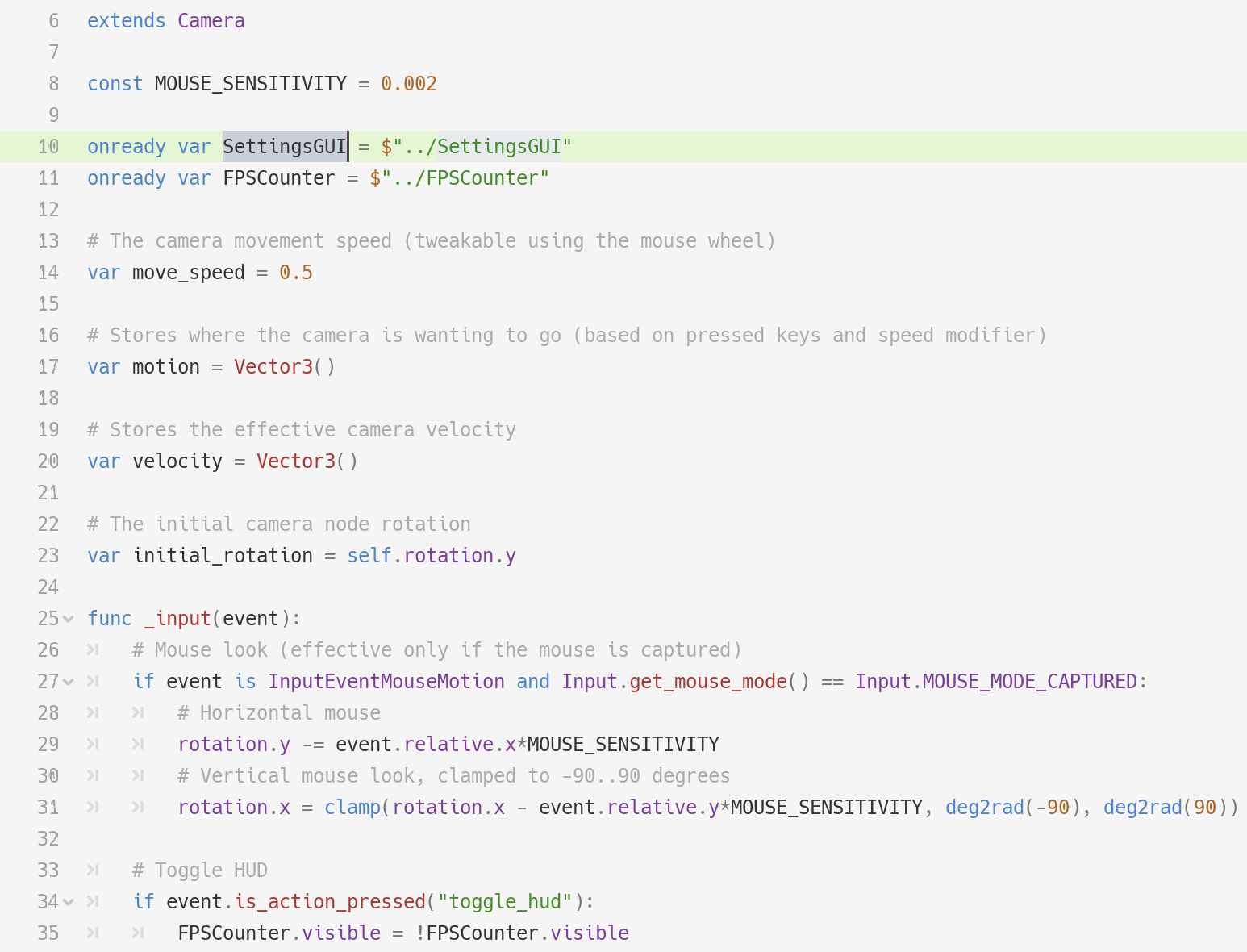
## Solarized Dark
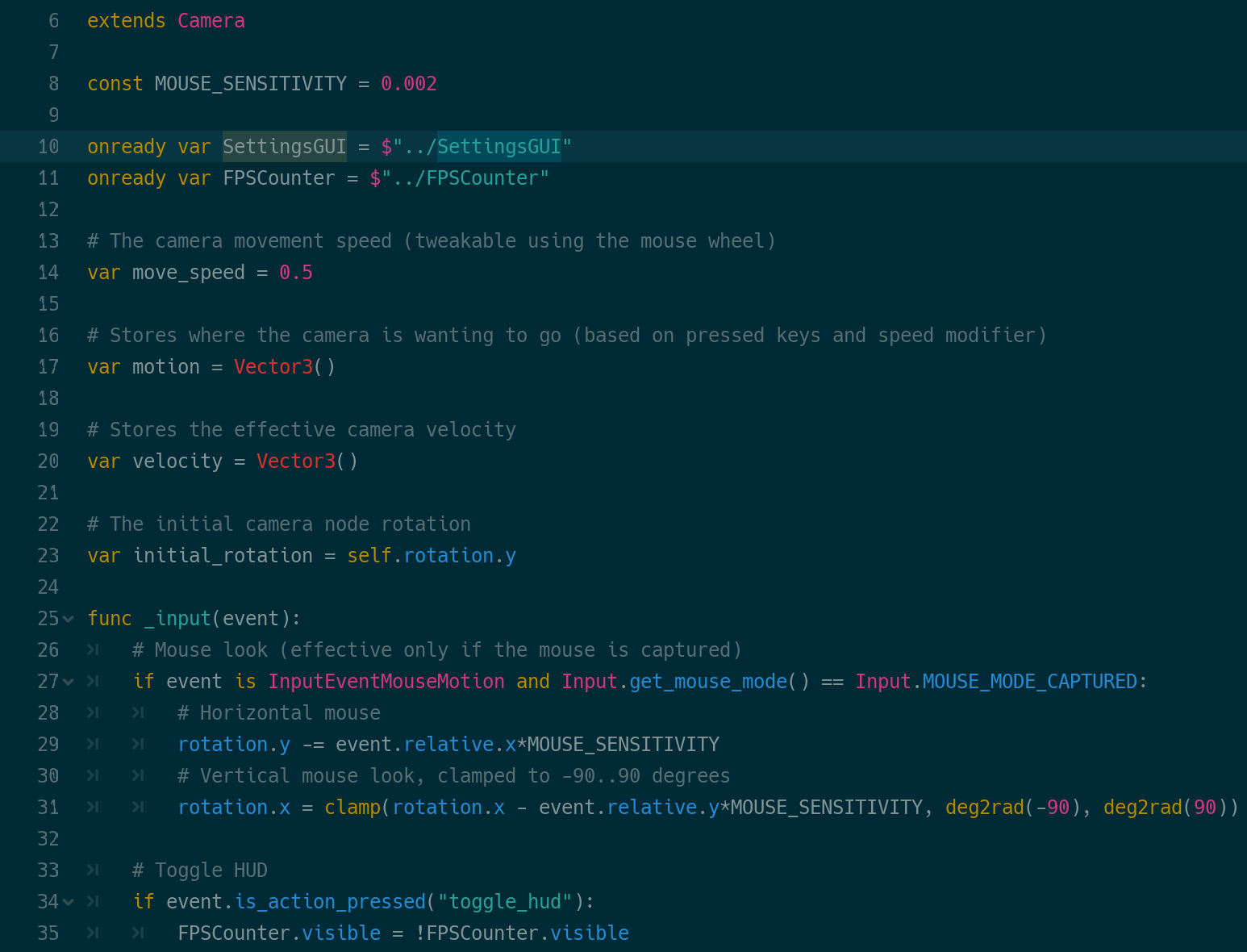
## Solarized Light
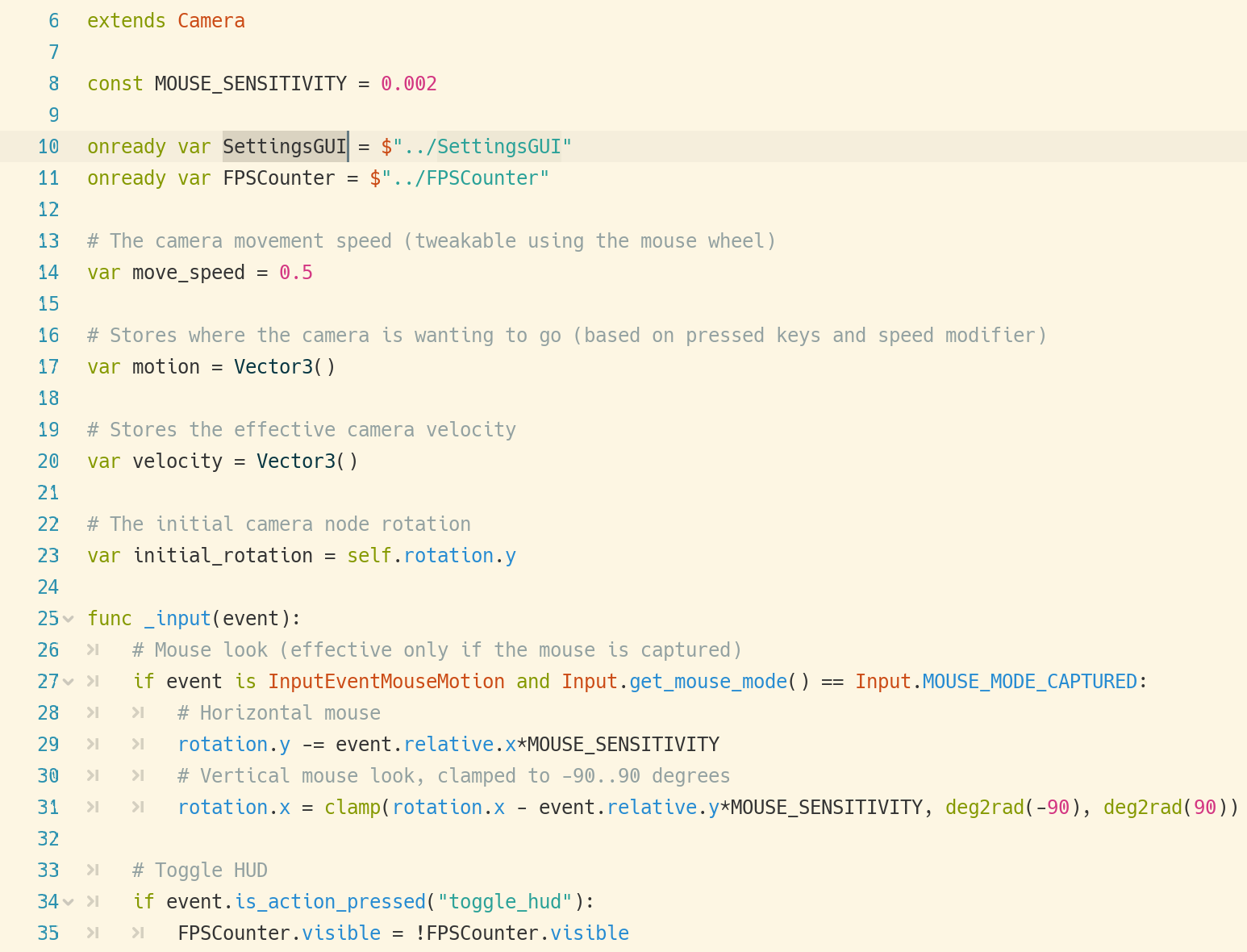
## VS Code Dark
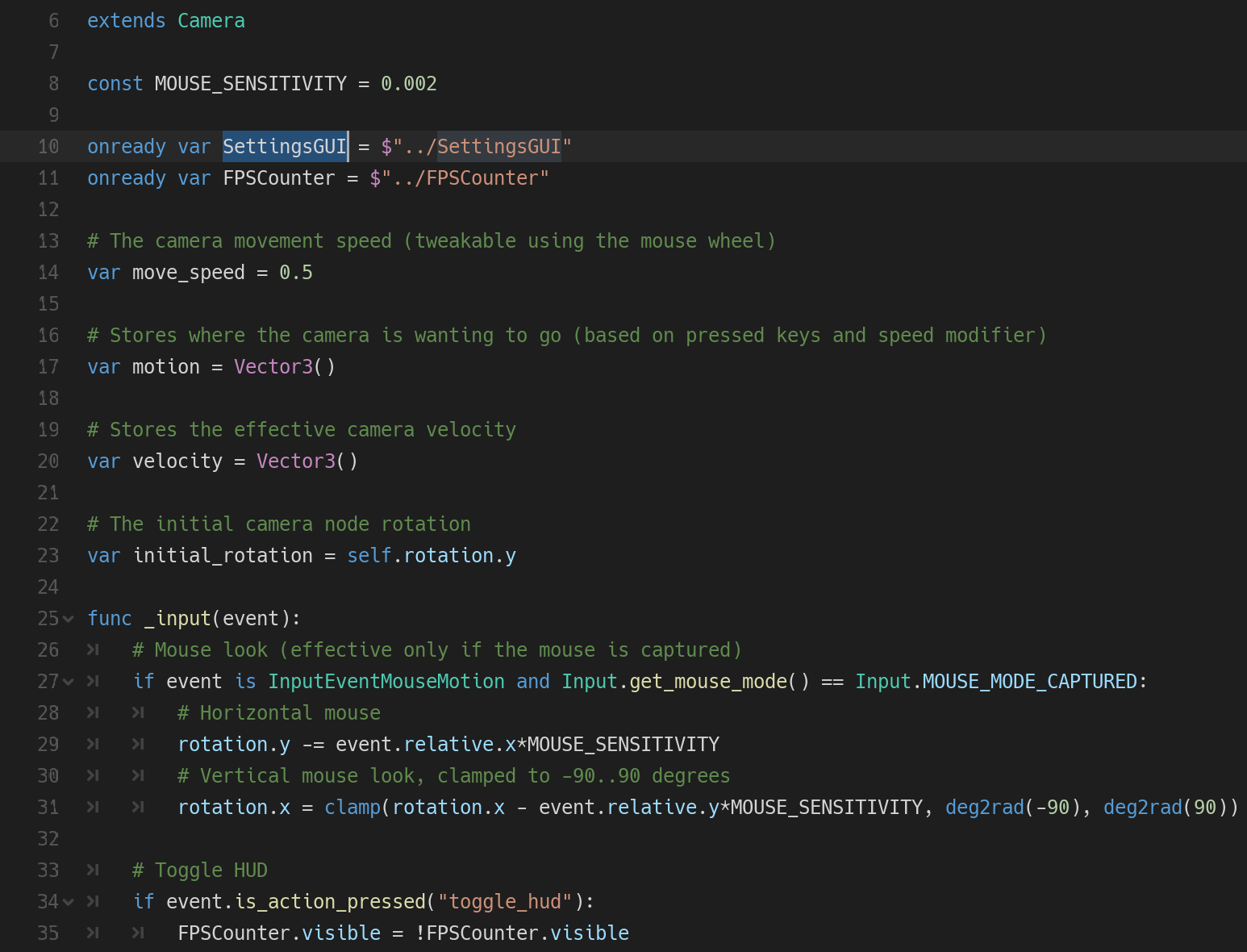
## VS Code Light
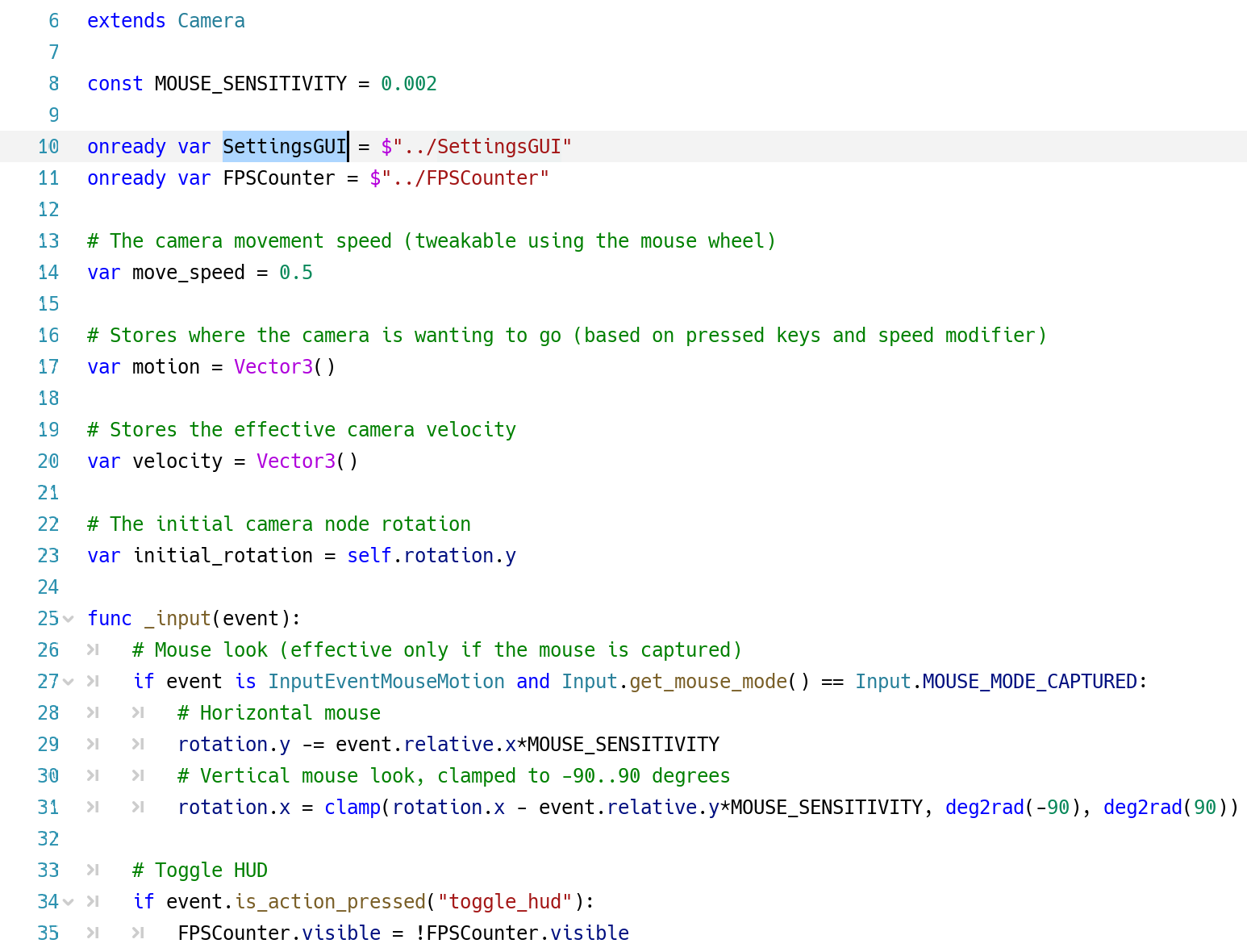
| {
"repo_name": "godotengine/godot-syntax-themes",
"stars": "267",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
[color_theme]
background_color="1f2430ff"
base_type_color="5ce6ccff"
bookmark_color="73d0ffff"
brace_mismatch_color="ff786bff"
breakpoint_color="f28779ff"
caret_background_color="1f2430ff"
caret_color="ffcc66ff"
code_folding_color="ffffffb3"
comment_color="5c6773ff"
completion_background_color="333b4fff"
completion_existing_color="ffffff24"
completion_font_color="ccced3ff"
completion_scroll_color="ffffff12"
completion_selected_color="ffffff12"
current_line_color="0000002b"
engine_type_color="5ccfe6ff"
executing_line_color="33cc3366"
function_color="f28779ff"
gdscript/function_definition_color="f29e74ff"
gdscript/node_path_color="73b7ffff"
keyword_color="ffa759ff"
line_length_guideline_color="2e3547ff"
line_number_color="3f4654ff"
mark_color="80231b83"
member_variable_color="ffcc66ff"
number_color="ffcc66ff"
safe_line_number_color="33664dff"
search_result_border_color="ffcc66ff"
search_result_color="25272dff"
selection_color="2a3546ff"
string_color="bae67eff"
symbol_color="f29e74ff"
text_color="cbccc6ff"
text_selected_color="cbccc6ff"
user_type_color="73d0ffff"
word_highlighted_color="262f3eff"
| {
"repo_name": "godotengine/godot-syntax-themes",
"stars": "267",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
[color_theme]
background_color="282a36ff"
completion_background_color="2c2a32ff"
completion_selected_color="434244ff"
completion_existing_color="dfdfdf21"
completion_scroll_color="ffffffff"
completion_font_color="aaaaaaff"
caret_color="aeafadff"
caret_background_color="000000ff"
line_number_color="6272a4ff"
text_color="f8f8f2ff"
text_selected_color="f8f8f2ff"
keyword_color="ff79c6ff"
base_type_color="50fa7bff"
engine_type_color="8be9fdff"
function_color="8be9fdff"
member_variable_color="f8f8f2ff"
comment_color="6272a4ff"
string_color="f1fa8cff"
number_color="bd93f9ff"
symbol_color="f8f8f2ff"
selection_color="44475aff"
brace_mismatch_color="ff3333ff"
current_line_color="2f3245ff"
line_length_guideline_color="393c4fff"
mark_color="ff323238"
breakpoint_color="cccc6633"
code_folding_color="808080ff"
word_highlighted_color="424450ff"
search_result_color="5e6069ff"
search_result_border_color="00000000"
gdscript/function_definition_color="50fa7bff"
gdscript/node_path_color="ffb86cff"
| {
"repo_name": "godotengine/godot-syntax-themes",
"stars": "267",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
[color_theme]
background_color="f5f5f5ff"
completion_background_color="e5e5e5ff"
completion_selected_color="d5d5d5ff"
completion_existing_color="dfdfdf21"
completion_scroll_color="959595ff"
completion_font_color="454545ff"
caret_color="54494bff"
caret_background_color="000000ff"
line_number_color="9da39aff"
text_color="333333ff"
text_selected_color="333333ff"
keyword_color="4b83cdff"
base_type_color="aa3731ff"
engine_type_color="7a3e9dff"
function_color="aa3731ff"
member_variable_color="7a3e9dff"
comment_color="aaaaaaff"
string_color="448c27ff"
number_color="ab6526ff"
symbol_color="777777ff"
selection_color="c9d0d9ff"
brace_mismatch_color="ff3333ff"
current_line_color="e4f6d4ff"
line_length_guideline_color="e4e4e4ff"
mark_color="ff323230"
breakpoint_color="cccc6633"
code_folding_color="808080ff"
word_highlighted_color="e8ebeeff"
search_result_color="edc9d8ff"
search_result_border_color="00000000"
gdscript/function_definition_color="aa3731ff"
gdscript/node_path_color="ab6526ff"
| {
"repo_name": "godotengine/godot-syntax-themes",
"stars": "267",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
[color_theme]
background_color="1d1f21ff"
base_type_color="99cc99ff"
bookmark_color="147dfaff"
brace_mismatch_color="ff3333ff"
breakpoint_color="ff4d56ff"
caret_background_color="000000ff"
caret_color="ffffffff"
code_folding_color="808080ff"
comment_color="7c7c7cff"
completion_background_color="3d3d3dff"
completion_existing_color="ffffff23"
completion_font_color="cececeff"
completion_scroll_color="ffffff11"
completion_selected_color="ffffff11"
current_line_color="ffffff12"
engine_type_color="f9ee98ff"
executing_line_color="33cc3366"
function_color="dad085ff"
gdscript/function_definition_color="f9ee98ff"
gdscript/node_path_color="99cc99ff"
keyword_color="96cbfeff"
line_length_guideline_color="2d2f31ff"
line_number_color="656866ff"
mark_color="ff323238"
member_variable_color="c6c5feff"
number_color="ff73fdff"
safe_line_number_color="ccf7d3bf"
search_result_border_color="ffffffb2"
search_result_color="ffffff11"
selection_color="444444ff"
string_color="a8ff60ff"
symbol_color="c5c8c6ff"
text_color="c5c8c6ff"
text_selected_color="eeeeeeff"
user_type_color="c6ffedff"
word_highlighted_color="343434ff"
| {
"repo_name": "godotengine/godot-syntax-themes",
"stars": "267",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
[color_theme]
background_color="1C1E26ff"
completion_background_color="2E303E80"
completion_selected_color="6C6F9380"
completion_existing_color="D5D8DA20"
completion_scroll_color="232530ff"
completion_font_color="D5D8DAff"
caret_color="e95378ff"
caret_background_color="1C1E26ff"
line_number_color="D5D8DA80"
text_color="D5D8DAff"
text_selected_color="6C6F9380"
keyword_color="B877DBE6"
base_type_color="FAC29Aff"
engine_type_color="FAC29Aff"
user_type_color="FAC29Aff"
function_color="25B2BCff"
member_variable_color="E95678ff"
comment_color="D5D8DA80"
string_color="FAB795ff"
number_color="FAB795ff"
symbol_color="D5D8DAff"
selection_color="6C6F9380"
brace_mismatch_color="E95678B3"
current_line_color="1C1E2680"
line_length_guideline_color="ffffffff"
mark_color="E9567820"
breakpoint_color="ff0000ff"
code_folding_color="ffffffff"
word_highlighted_color="6C6F9330"
search_result_color="6C6F9380"
search_result_border_color="6C6F9380"
gdscript/function_definition_color="25B2BCff"
gdscript/node_path_color="F09383ff"
| {
"repo_name": "godotengine/godot-syntax-themes",
"stars": "267",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
[color_theme]
background_color="fdf6e3ff"
completion_background_color="ede6d3ff"
completion_selected_color="ddd6b3ff"
completion_existing_color="dfdfdf21"
completion_scroll_color="657b83ff"
completion_font_color="444444ff"
caret_color="657b83ff"
caret_background_color="000000ff"
line_number_color="2b91afff"
text_color="333333ff"
text_selected_color="333333ff"
keyword_color="859900ff"
base_type_color="073642ff"
engine_type_color="cb4b16ff"
function_color="268bd2ff"
member_variable_color="268bd2ff"
comment_color="93a1a1ff"
string_color="2aa198ff"
number_color="d33682ff"
symbol_color="333333ff"
selection_color="dad3c1ff"
brace_mismatch_color="ff3333ff"
current_line_color="f5eedcff"
line_length_guideline_color="e5deccff"
mark_color="ff323230"
breakpoint_color="cccc6633"
code_folding_color="808080ff"
word_highlighted_color="eee8d5ff"
search_result_color="f7c399ff"
search_result_border_color="00000000"
gdscript/function_definition_color="268bd2ff"
gdscript/node_path_color="cb4b16ff"
| {
"repo_name": "godotengine/godot-syntax-themes",
"stars": "267",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
[color_theme]
background_color="1e1e1eff"
base_type_color="c586c0ff"
bookmark_color="147dfaff"
brace_mismatch_color="ff3333ff"
breakpoint_color="cccc6633"
caret_background_color="000000ff"
caret_color="aeafadff"
code_folding_color="808080ff"
comment_color="608b4eff"
completion_background_color="2c2a32ff"
completion_existing_color="dfdfdf21"
completion_font_color="aaaaaaff"
completion_scroll_color="ffffffff"
completion_selected_color="434244ff"
control_flow_keyword_color="bf88beff"
current_line_color="ffffff0b"
engine_type_color="4ec9b0ff"
executing_line_color="33cc3366"
function_color="dcdcaaff"
gdscript/function_definition_color="dcdcaaff"
gdscript/node_path_color="6bbcfbff"
keyword_color="569cd6ff"
line_length_guideline_color="333333ff"
line_number_color="aaaaaa66"
mark_color="ff323238"
member_variable_color="9cdcfeff"
number_color="b5cea8ff"
safe_line_number_color="ccf7d3bf"
search_result_border_color="00000000"
search_result_color="613214ff"
selection_color="264f78ff"
string_color="ce9178ff"
symbol_color="d4d4d4ff"
text_color="d4d4d4ff"
text_selected_color="d4d4d4ff"
user_type_color="c6ffedff"
word_highlighted_color="343a40ff"
| {
"repo_name": "godotengine/godot-syntax-themes",
"stars": "267",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
# Godot syntax themes for Godot 4.x
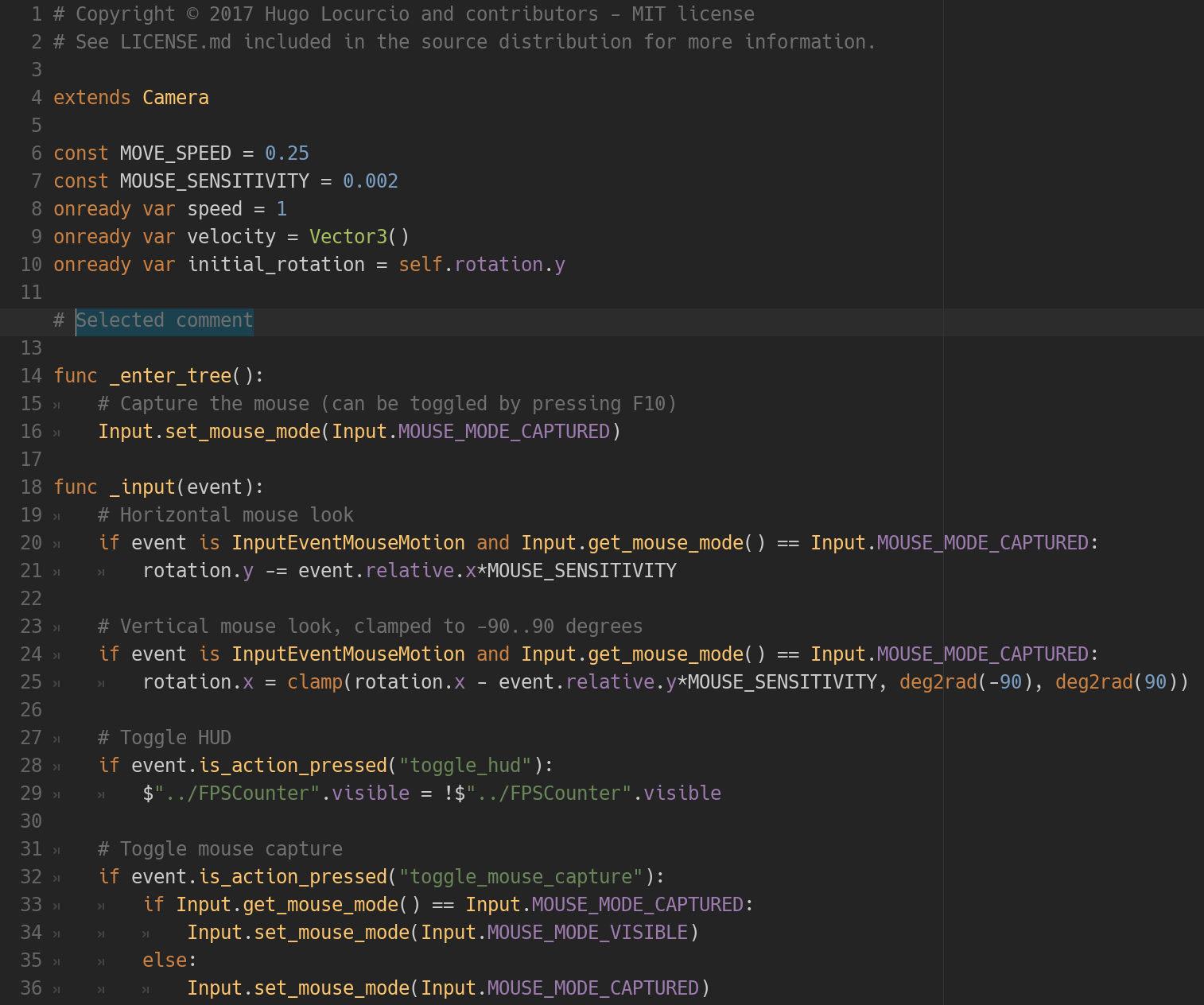
*The Darcula theme in action.*
This repository contains many syntax themes for Godot, for use in the built-in
script editor.
**This branch contains themes *only* compatible with Godot 4.x.**
These themes will not display correctly in Godot 3.x.
[Browse the `3.x` branch](https://github.com/godotengine/godot-syntax-themes/tree/3.x)
to find themes compatible with Godot 3.x.
*Want even more themes? Check out
[base16-godot](https://github.com/Calinou/base16-godot) for automatically
generated themes.*
## Available themes
**See [THEMES.md](/THEMES.md) for preview images.**
| Dark | Light |
|-------------------------|--------------------------|
| Apprentice | Quiet Light |
| Atom Dark | Solarized Light |
| Ayu Mirage | Visual Studio Code Light |
| Darcula | |
| Dracula | |
| Gruvbox Dark | |
| Horizon | |
| Metro | |
| Monokai | |
| Nord | |
| One Dark | |
| One Monokai | |
| Solarized Dark | |
| Visual Studio Code Dark | |
## Installation
Place the `.tet` files in your Godot text editor theme directory:
- On Linux: `~/.config/godot/text_editor_themes/`
- On macOS: `~/Library/Application Support/Godot/text_editor_themes/`
- On Windows: `%APPDATA%\Godot\text_editor_themes\`
**Note:** If you installed Godot using Steam, your Godot text editor theme
folder should be placed in `steamapps/common/Godot Engine/editor_data/text_editor_themes/`
in your Steam installation folder.
To change the theme, open a project in the editor, click on **Editor** in the
top menu, then go to the **Editor Settings** then **Text Editor**. You should
now be able to choose the desired theme.
**Tip:** You can clone this Git repository directly into the text editor themes
path (if the destination folder does not exist) using the following command:
```bash
# On Linux:
git clone https://github.com/godotengine/godot-syntax-themes.git ~/.config/godot/text_editor_themes
# On macOS:
git clone https://github.com/godotengine/godot-syntax-themes.git "~/Library/Application Support/Godot/text_editor_themes"
# On Windows:
git clone https://github.com/godotengine/godot-syntax-themes.git "%APPDATA%\Godot\text_editor_themes"
```
## License
Copyright © 2016-present Hugo Locurcio and contributors
Files in this repository are licensed under CC0 1.0 Universal,
see [LICENSE.md](/LICENSE.md) for more information.
| {
"repo_name": "godotengine/godot-syntax-themes",
"stars": "267",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/plain"
} |
status
======
[](https://discord.gg/vu6Vt9d)
My name is r-lyeh and I code videogames :neckbeard:
This repo is meant to be a semi-conclusive list of projects in which the author is / has participated in.
- The list below is archived. A newer list can be [found here](https://github.com/r-lyeh/statvs).
- The tools below are Public Domain.
- The specifications below are Public Domain (or CC0 licensed), written in Markdown.
- The libraries below are Public Domain (or _ZLIB/LibPNG licensed_), written in C++.
- Most of my source code is (where possible) _cross-platform_, _header-only_ and _self-contained_, and have been used in _commercial games_. It is therefore pretty much battle-tested, but use it at your own risk. No warranties provided.
- Extension convention: `.md` for documents, `.hpp/.cpp` for sources, `.cc` for samples and `.cxx` for tests and benchmarks.
## Released
Fully released / tested; more features may be incoming, but these are considered to be in a 'stable' state.
Tags|Repository
:----------------:|:------------
io|[Apathy (C++03)](http://github.com/r-lyeh/apathy) <a href="https://travis-ci.org/r-lyeh-archived/apathy"><img src="https://api.travis-ci.org/r-lyeh-archived/apathy.svg?branch=master" align="right" /></a> <br/> lightweight *path/file/mstream/mmap IO library*.
debug|[Assume (C++03)](http://github.com/r-lyeh/assume) <a href="https://travis-ci.org/r-lyeh-archived/assume"><img src="https://api.travis-ci.org/r-lyeh-archived/assume.svg?branch=master" align="right" /></a> <br/> smarter *assert replacement* for LHS/RHS values.
tool, image|[Attila (Win32) :star2:](https://github.com/r-lyeh/attila) <a href="https://travis-ci.org/r-lyeh-archived/attila"><img src="https://api.travis-ci.org/r-lyeh-archived/attila.svg?branch=master" align="right" /></a> <br/> tiny *atlas texture/image packer tool*.
security|[Auth (C++03)](http://github.com/r-lyeh/auth) <a href="https://travis-ci.org/r-lyeh-archived/auth"><img src="https://api.travis-ci.org/r-lyeh-archived/auth.svg?branch=master" align="right" /></a> <br/> simple, lightweight and safe client-server *authentication system*.
encoding|[Base (C++03)](http://github.com/r-lyeh/base) <a href="https://travis-ci.org/r-lyeh-archived/base"><img src="https://api.travis-ci.org/r-lyeh-archived/base.svg?branch=master" align="right" /></a> <br/> *base91/base85/base64* de/encoders.
serial|[Bourne (C++11)](http://github.com/r-lyeh/bourne) <a href="https://travis-ci.org/r-lyeh-archived/bourne"><img src="https://api.travis-ci.org/r-lyeh-archived/bourne.svg?branch=master" align="right" /></a> <br/> lightweight *JSON de/serializer*.
user-interface|[Bubble (C++11)](https://github.com/r-lyeh/bubble) <br/> simple and lightweight *dialog library for Windows*.
compression|[Bundle (C++03,C++11):star2:](https://github.com/r-lyeh/bundle) <a href="https://travis-ci.org/r-lyeh-archived/bundle"><img src="https://api.travis-ci.org/r-lyeh-archived/bundle.svg?branch=master" align="right" /></a> <br/> an embeddable *compression library* that supports 23 algorithms and 2 archivers.
compression,tool|[Bundler (Win32,OSX)](https://github.com/r-lyeh/bundler) <br/> *command-line archiver* that supports 23 compression algorithms.
data-structures|[Burg (C++11)](https://github.com/r-lyeh/burg) <a href="https://travis-ci.org/r-lyeh-archived/burg"><img src="https://api.travis-ci.org/r-lyeh-archived/burg.svg?branch=master" align="right" /></a> <br/> simple burg *linear predictor*.
hashing|[Cocoa (C++11):star2:](http://github.com/r-lyeh/cocoa) <a href="https://travis-ci.org/r-lyeh-archived/cocoa"><img src="https://api.travis-ci.org/r-lyeh-archived/cocoa.svg?branch=master" align="right" /></a> <br/> *hashing library* that provides interface for CRC32, CRC64, GCRC, RS, JS, PJW, ELF, BKDR, SBDM, DJB, DJB2, BP, FNV, AP, BJ1, MH2, SHA1, SFH.
encoding|[Collage (C++03)](https://github.com/r-lyeh/collage) <a href="https://travis-ci.org/r-lyeh-archived/collage"><img src="https://api.travis-ci.org/r-lyeh-archived/collage.svg?branch=master" align="right" /></a> <br/> lightweight library to *diff and patch* arbitrary data.
unit-testing|[Dessert (C++11):star2:](http://github.com/r-lyeh/dessert) <a href="https://travis-ci.org/r-lyeh-archived/dessert"><img src="https://api.travis-ci.org/r-lyeh-archived/dessert.svg?branch=master" align="right" /></a> <br/> lightweight *unit-testing framework*.
debug|[Dollar (C++11)](https://github.com/r-lyeh/dollar) <a href="https://goo.gl/nR594V"><img src="https://goo.gl/OtOjg4" align="right" /></a> <br> portable/generic *CPU profiler* with chrome://tracing support.
debug|[DrEcho (C++11)](http://github.com/r-lyeh/DrEcho) <a href="https://travis-ci.org/r-lyeh-archived/DrEcho"><img src="https://api.travis-ci.org/r-lyeh-archived/DrEcho.svg?branch=master" align="right" /></a> <br/> DrEcho *spices your terminal up*.
debug|[Error64 (C,C++)](http://github.com/r-lyeh/error64) <a href="https://travis-ci.org/r-lyeh-archived/error64"><img src="https://api.travis-ci.org/r-lyeh-archived/error64.svg?branch=master" align="right" /></a> <br/> Handle custom 64-bit error codes, with extended meta-info
gameplay|[Flare (C++03)](https://github.com/r-lyeh/flare) <a href="https://travis-ci.org/r-lyeh-archived/flare"><img src="https://api.travis-ci.org/r-lyeh-archived/flare.svg?branch=master" align="right" /></a> <br/> lightweight C++ API to deal with *digital signals/logical buttons*.
network|[Flow (C++11)](https://github.com/r-lyeh/flow) <a href="https://travis-ci.org/r-lyeh-archived/flow"><img src="https://api.travis-ci.org/r-lyeh-archived/flow.svg?branch=master" align="right" /></a> <br/> lightweight network downloader with native fallbacks aimed to gamedev.
text|[Fmt11 (C++11)](http://github.com/r-lyeh/fmt11) <a href="https://travis-ci.org/r-lyeh-archived/fmt11"><img src="https://api.travis-ci.org/r-lyeh-archived/fmt11.svg?branch=master" align="right" /></a> <br/> tiny *format/mustache templating* library.
gameplay|[Frodo (C++11)](https://github.com/r-lyeh/frodo) <a href="https://travis-ci.org/r-lyeh-archived/frodo"><img src="https://api.travis-ci.org/r-lyeh-archived/frodo.svg?branch=master" align="right" /></a> <br/> lightweight *ring dependency framework*.
gameplay|[FSM (C++11)](http://github.com/r-lyeh/fsm) <a href="https://travis-ci.org/r-lyeh-archived/fsm"><img src="https://api.travis-ci.org/r-lyeh-archived/fsm.svg?branch=master" align="right" /></a> <br/> lightweight Hierarchical/*Finite-State Machine* (H/FSM) class.
io|[GetOpt (C++11)](http://github.com/r-lyeh/getopt) <a href="https://travis-ci.org/r-lyeh-archived/getopt"><img src="https://api.travis-ci.org/r-lyeh-archived/getopt.svg?branch=master" align="right" /></a> <br/> simple *command-line options handler*.
io|[Giant (C++11)](http://github.com/r-lyeh/giant) <a href="https://travis-ci.org/r-lyeh-archived/giant"><img src="https://api.travis-ci.org/r-lyeh-archived/giant.svg?branch=master" align="right" /></a> <br/> tiny library to *handle little/big endianness*.
debug|[Heal (C++03)](http://github.com/r-lyeh/heal) <a href="https://travis-ci.org/r-lyeh-archived/heal"><img src="https://api.travis-ci.org/r-lyeh-archived/heal.svg?branch=master" align="right" /></a> <br/> lightweight library to aid and *debug applications*.
render|[Hertz (C++11)](https://github.com/r-lyeh/Hertz)<a href="https://travis-ci.org/r-lyeh-archived/Hertz"><img src="https://api.travis-ci.org/r-lyeh-archived/hertz.svg?branch=master" align="right" /></a> <br/> simple *framerate locker*.
input|[Hyde (C++03)](http://github.com/r-lyeh/hyde) <br/> lightweight and simple Human *Inferface Device* (HID) library.
hashing|[ID (C++11)](http://github.com/r-lyeh/id) <a href="https://travis-ci.org/r-lyeh-archived/id"><img src="https://api.travis-ci.org/r-lyeh-archived/id.svg?branch=master" align="right" /></a> <br/> simple *compile-time hasher* and sequential ID generator.
mesh, tool|[Img2Sky (Win32)](http://github.com/r-lyeh/img2sky) <br/> *vertex-color mesh builder tool* for skyboxes and static geometry.
io|[Journey (C++11)](http://github.com/r-lyeh/journey) <a href="https://travis-ci.org/r-lyeh-archived/journey"><img src="https://api.travis-ci.org/r-lyeh-archived/journey.svg?branch=master" align="right" /></a> <br/> lightweight *append-only, header-less, journaling file format*.
spec|[JXML](http://github.com/r-lyeh/JXML) <br/> loss-less representation of *JSON in XML*.
spec|[JXMLex](http://github.com/r-lyeh/JXMLex) <br/> expressive representation of *JSON in XML*.
network|[Knot (C++03)](http://github.com/r-lyeh/knot) <a href="https://travis-ci.org/r-lyeh-archived/knot"><img src="https://api.travis-ci.org/r-lyeh-archived/knot.svg?branch=master" align="right" /></a> <br/> lightweight and simple *TCP networking* C++ library.
gameplay|[Kult (C++11):star2:](https://github.com/r-lyeh/kult) <a href="https://travis-ci.org/r-lyeh-archived/kult"><img src="https://api.travis-ci.org/r-lyeh-archived/kult.svg?branch=master" align="right" /></a> <br/> lightweight *entity/component/system* library.
gameplay|[Live (C++11)](http://github.com/r-lyeh/live) <a href="https://travis-ci.org/r-lyeh-archived/live"><img src="https://api.travis-ci.org/r-lyeh-archived/live.svg?branch=master" align="right" /></a> <br/> *automatic reloader* of variables, featuring type inference.
data-structures|[LRU (C++11)](https://github.com/r-lyeh/lru) <a href="https://travis-ci.org/r-lyeh-archived/LRU"><img src="https://api.travis-ci.org/r-lyeh-archived/LRU.svg?branch=master" align="right" /></a> <br/> lightweight *LRU cache structure* for list<T> and map<K,V> containers.
gameplay|[Memo (C++11)](http://github.com/r-lyeh/memo) <a href="https://travis-ci.org/r-lyeh-archived/memo"><img src="https://api.travis-ci.org/r-lyeh-archived/memo.svg?branch=master" align="right" /></a> <br/> simple and lightweight *factory class*, featuring automatic type casting.
stats|[Metrics (C++11)](https://github.com/r-lyeh/metrics) <a href="https://goo.gl/l0C5wv"><img src="https://goo.gl/bn24Kq" align="right" /></a> <br/> table metrics w/ benchmarking, unit conversions in CSV,TSV,ASCII,markdown.
io|[mINI (C++11)](https://github.com/r-lyeh/mINI)<a href="https://travis-ci.org/r-lyeh-archived/mINI"><img src="https://api.travis-ci.org/r-lyeh-archived/mINI.svg?branch=master" align="right" /></a> <br/> very minimal *.INI reader/writer*.
data-structures|[Oak (C++03)](http://github.com/r-lyeh/oak) <a href="https://goo.gl/l2IoOI"><img src="https://goo.gl/8YevhJ" align="right" /></a> <br/> simple and lightweight *tree container*.
design|[Pitch (Markdown)](https://github.com/r-lyeh/pitch) <br/> forkable game *pitch template*.
spec,tool,image|[Pug](https://github.com/r-lyeh/pug) <br/> *pug, png with a twist*: lossy image format.
network|[Route66 (C++03)](https://github.com/r-lyeh/route66) <a href="https://goo.gl/rE1dM1"><img src="https://goo.gl/TD7UpH" align="right" /></a> <br/> lightweight *embeddable HTTP server*.
io|[Quant (C++03)](https://github.com/r-lyeh/quant) <a href="https://goo.gl/gSHXKv"><img src="https://goo.gl/Kuv8pn" align="right" /></a> <br/> *quantization suite* to/from half-floats, s/unorm bytes, quats and vec3s.
time|[Sand (C++11)](http://github.com/r-lyeh/sand) <a href="https://travis-ci.org/r-lyeh-archived/sand"><img src="https://api.travis-ci.org/r-lyeh-archived/sand.svg?branch=master" align="right" /></a> <br/> lightweight *timer controller*.
script|[Scriptorium:star:](https://github.com/r-lyeh/scriptorium) <br/> Game *Scripting Languages benchmarked*.
data|[Sentry (C++11)](https://github.com/r-lyeh/sentry) <a href="https://travis-ci.org/r-lyeh-archived/sentry"><img src="https://api.travis-ci.org/r-lyeh-archived/sentry.svg?branch=master" align="right" /></a> <br/> lightweight *data monitor*.
hashing|[Sole (C++11):star2:](http://github.com/r-lyeh/sole) <a href="https://travis-ci.org/r-lyeh-archived/sole"><img src="https://api.travis-ci.org/r-lyeh-archived/sole.svg?branch=master" align="right" /></a> <br/> lightweight library to generate universally *unique identificators* (UUID) both v1 and v4.
image|[Spot (C++11):star2:](http://github.com/r-lyeh/spot) <a href="https://travis-ci.org/r-lyeh-archived/spot"><img src="https://api.travis-ci.org/r-lyeh-archived/spot.svg?branch=master" align="right" /></a> <br/> compact and embeddable RGBA/HSLA library that supports *WEBP, JPG, progressive JPG, PNG, TGA, DDS DXT1/2/3/4/5, BMP, PSD, GIF, PVR2/3 (ETC1/PVRTC), KTX (ETC1/PVRTC), PKM (ETC1), HDR, PIC, PNM (PPM/PGM), CRN, PUG, FLIF, CCZ, EXR and vectorial SVG files*.
databases |[SQLight (C++11)](http://github.com/r-lyeh/sqlight) <a href="https://travis-ci.org/r-lyeh-archived/sqlight"><img src="https://api.travis-ci.org/r-lyeh-archived/sqlight.svg?branch=master" align="right" /></a> <br/> lightweight *MySQL client*.
misc |[TinyBits (C,C++)](http://github.com/r-lyeh/tinybits) <br/> tiny bits and *useful snippets*.
debug|[Tracey (C++11):star2:](http://github.com/r-lyeh/tracey) <a href="https://travis-ci.org/r-lyeh-archived/tracey"><img src="https://api.travis-ci.org/r-lyeh-archived/tracey.svg?branch=master" align="right" /></a> <br/> lightweight and simple C++ memory *leak finder* with no dependencies.
data-structures|[Trie (C++11)](http://github.com/r-lyeh/trie) <a href="https://travis-ci.org/r-lyeh-archived/trie"><img src="https://api.travis-ci.org/r-lyeh-archived/trie.svg?branch=master" align="right" /></a> <br/> lightweight and simple *autocompletion* data structure.
animation |[Tween (C++03)](https://github.com/r-lyeh/tween) <a href="https://travis-ci.org/r-lyeh-archived/tween"><img src="https://api.travis-ci.org/r-lyeh-archived/tween.svg?branch=master" align="right" /></a> <br/> lightweight *easing library*.
data-structures|[Unordered_map (C++11)](http://github.com/r-lyeh/unordered_map) <a href="https://travis-ci.org/r-lyeh-archived/unordered_map"><img src="https://api.travis-ci.org/r-lyeh-archived/unordered_map.svg?branch=master" align="right" /></a> <br/> *portable header* for std::unordered_map<K,V> template.
debug|[Unifont (C++11)](http://github.com/r-lyeh/unifont) <a href="https://travis-ci.org/r-lyeh-archived/unifont"><img src="https://api.travis-ci.org/r-lyeh-archived/unifont.svg?branch=master" align="right" /></a> <br/> *embeddable console 1bpp font*.
spec, io|[Unify (C++11)](http://github.com/r-lyeh/Unify) <a href="https://travis-ci.org/r-lyeh-archived/unify"><img src="https://api.travis-ci.org/r-lyeh-archived/unify.svg?branch=master" align="right" /></a> <br/> a function to *normalize resouce identificators*.
network|[VLE (C99, C++03)](https://github.com/r-lyeh/vle) <a href="https://travis-ci.org/r-lyeh-archived/vle"><img src="https://api.travis-ci.org/r-lyeh-archived/vle.svg?branch=master" align="right" /></a> <br/> simple *variable-length encoder/decoder*.
audio|[Wave (C++11)](http://github.com/r-lyeh/wave) <br/> lightweight *3D sound wrapper* for OpenAL that supports OGG and MusePack decoding.
utils|[Warp (C++11):star2:](http://github.com/r-lyeh/warp) <a href="https://travis-ci.org/r-lyeh-archived/warp"><img src="https://api.travis-ci.org/r-lyeh-archived/warp.svg?branch=master" align="right" /></a> <br/> a handy *string interpolator*.
text|[Wire (C++11):star2:](http://github.com/r-lyeh/wire) <a href="https://travis-ci.org/r-lyeh-archived/wire"><img src="https://api.travis-ci.org/r-lyeh-archived/wire.svg?branch=master" align="right" /></a> <br/> a drop-in *std::string replacement* with extended functionality and safe C/C++ formatters.
## Contributions
Forked projects or in collaboration with other coders.
* [jsonxx](http://github.com/hjiang/jsonxx) -- lightweight *JSON parser* written in C++.
* [IMGUI](http://github.com/r-lyeh/imgui) -- OpenGL 2/3 *Immediate Mode GUI* toolkit.
* [units](http://github.com/r-lyeh/units) -- numerical quantities with units (C++03).
* [unlzma](http://github.com/r-lyeh/unlzma) -- A very compact LZMA decoder (C++03).
* [eval](http://github.com/r-lyeh/eval) -- very simple expression evaluator using shunting-yard algorithm and RPN (C++11).
## Personal
These are mostly released experiments, but are geared towards personal use; as such, they may be of very limited use during development.
* [Bridge](https://github.com/r-lyeh/bridge) -- a standard C++/boost *compatibility layer*, plus a few utils (C++11/C++03).
* [Cash-of-clans](https://github.com/r-lyeh/cash-of-clans) -- a free re-implementation of a working *game economy system*.
* [Crawler](https://github.com/r-lyeh/crawler) -- a quick prototiping platform for Windows (C++11).
* [CLDR](https://github.com/r-lyeh/cldr) -- compact data from the Unicode Common Locale Data Repository.
* [Codex](https://github.com/r-lyeh/codex) -- lightweight and simple C++ library to escape, unescape, read, write and convert from/to different *encoding charsets*.
* [Duty](https://github.com/r-lyeh/duty) -- lightweight *task manager* for parallel coroutines and serial jobs (C++11).
* [Emojis](https://github.com/r-lyeh/emojis) -- emojis, atlased and indexed.
* [RGB332 (tool)](https://github.com/r-lyeh/rgb332) -- custom uniform *RGB332 palette*.
* [Variant](https://github.com/r-lyeh/variant) -- *varying* class that clones javascript behaviour as much as possible (C++11).
* [Malloc-survey](https://github.com/r-lyeh/malloc-survey) -- benchmark for different *memory allocators*.
* [Test-physics](https://github.com/r-lyeh/test-physics) -- benchmark for different *physics integrators*.
## In Progress
Proof-of-concepts until stabilised, currently in mid/active development.
* [AVA](https://github.com/r-lyeh/AVA) -- tiny *3D game engine* in C++, with C and Lua interfaces.
* [Jabba](https://github.com/r-lyeh/jabba) -- (C++11).
* [Play](https://github.com/r-lyeh/play) -- easy *alternative to scripting* in gameplay code.
* [Solace](https://github.com/r-lyeh/solace) -- modern *console replacement*.
* [Wake](https://github.com/r-lyeh/wake) -- a no-brainer *asset build system*. Designed for zombies.
* [Watchmen](https://github.com/r-lyeh/watchmen) -- *asset dependency system* for games in run-time.
* [Juice]() --
* [Graybox]() --
* [Drop]() --
* [Checklist]() --
## Planned
Yet to be committed, removed and/or finished. Someday.
* [Blender](http://github.com/r-lyeh/blender)
* [Gate](https://github.com/r-lyeh/gate)
* [Geo](https://github.com/r-lyeh/geo)
* [Grog](https://github.com/r-lyeh/grog)
* [Ling](https://github.com/r-lyeh/ling)
* [Metatracker](http://github.com/r-lyeh/metatracker)
* [Realm](https://github.com/r-lyeh/realm)
* [Silo](https://github.com/r-lyeh/silo)
* [Skull](https://github.com/r-lyeh/skull)
* [Social](https://github.com/r-lyeh/social)
* [Tint](http://github.com/r-lyeh/tint)
* [Unposix](https://github.com/r-lyeh/unposix)
* [Wrapp](https://github.com/r-lyeh/wrapp)
## On Hold / Abandoned
These are either fully abandoned, or are suffering from an existential crisis.
* [FortFont](http://github.com/r-lyeh/fortfont) -- collection of *western, CJK and iconographic fonts* free for commercial usage.
* [Eve](https://github.com/r-lyeh/eve) -- deprecated game engine where graphics are low priority (C++11).
* [Moon9](https://github.com/r-lyeh/moon9) -- old game engine.
* [Stringbase](http://github.com/r-lyeh/stringbase) -- collaborative effort aimed to *translate common texts* found in videogames and regular apps.
* [Wood](https://github.com/r-lyeh/wood) -- old tree data structure.
## Resume
For completeness.
* [Vitae](http://github.com/r-lyeh/vitae) -- my self-compilable *C++ resume*.
| {
"repo_name": "r-lyeh-archived/statvs",
"stars": "343",
"repo_language": "None",
"file_name": "README.md",
"mime_type": "text/html"
} |
@echo off
git fetch origin
git reset --hard main
"libs/pythonlib/python.exe" -m pip install --force-reinstall -r requirements.txt
| {
"repo_name": "Rubiksman78/RenAI-Chat",
"stars": "46",
"repo_language": "Python",
"file_name": "parsing.py",
"mime_type": "text/x-script.python"
} |
# Contributor Covenant Code of Conduct
## Our Pledge
We as members, contributors, and leaders pledge to make participation in our
community a harassment-free experience for everyone, regardless of age, body
size, visible or invisible disability, ethnicity, sex characteristics, gender
identity and expression, level of experience, education, socio-economic status,
nationality, personal appearance, race, religion, or sexual identity
and orientation.
We pledge to act and interact in ways that contribute to an open, welcoming,
diverse, inclusive, and healthy community.
## Our Standards
Examples of behavior that contributes to a positive environment for our
community include:
* Demonstrating empathy and kindness toward other people
* Being respectful of differing opinions, viewpoints, and experiences
* Giving and gracefully accepting constructive feedback
* Accepting responsibility and apologizing to those affected by our mistakes,
and learning from the experience
* Focusing on what is best not just for us as individuals, but for the
overall community
Examples of unacceptable behavior include:
* The use of sexualized language or imagery, and sexual attention or
advances of any kind
* Trolling, insulting or derogatory comments, and personal or political attacks
* Public or private harassment
* Publishing others' private information, such as a physical or email
address, without their explicit permission
* Other conduct which could reasonably be considered inappropriate in a
professional setting
## Enforcement Responsibilities
Community leaders are responsible for clarifying and enforcing our standards of
acceptable behavior and will take appropriate and fair corrective action in
response to any behavior that they deem inappropriate, threatening, offensive,
or harmful.
Community leaders have the right and responsibility to remove, edit, or reject
comments, commits, code, wiki edits, issues, and other contributions that are
not aligned to this Code of Conduct, and will communicate reasons for moderation
decisions when appropriate.
## Scope
This Code of Conduct applies within all community spaces, and also applies when
an individual is officially representing the community in public spaces.
Examples of representing our community include using an official e-mail address,
posting via an official social media account, or acting as an appointed
representative at an online or offline event.
## Enforcement
Instances of abusive, harassing, or otherwise unacceptable behavior may be
reported to the community leaders responsible for enforcement at
.
All complaints will be reviewed and investigated promptly and fairly.
All community leaders are obligated to respect the privacy and security of the
reporter of any incident.
## Enforcement Guidelines
Community leaders will follow these Community Impact Guidelines in determining
the consequences for any action they deem in violation of this Code of Conduct:
### 1. Correction
**Community Impact**: Use of inappropriate language or other behavior deemed
unprofessional or unwelcome in the community.
**Consequence**: A private, written warning from community leaders, providing
clarity around the nature of the violation and an explanation of why the
behavior was inappropriate. A public apology may be requested.
### 2. Warning
**Community Impact**: A violation through a single incident or series
of actions.
**Consequence**: A warning with consequences for continued behavior. No
interaction with the people involved, including unsolicited interaction with
those enforcing the Code of Conduct, for a specified period of time. This
includes avoiding interactions in community spaces as well as external channels
like social media. Violating these terms may lead to a temporary or
permanent ban.
### 3. Temporary Ban
**Community Impact**: A serious violation of community standards, including
sustained inappropriate behavior.
**Consequence**: A temporary ban from any sort of interaction or public
communication with the community for a specified period of time. No public or
private interaction with the people involved, including unsolicited interaction
with those enforcing the Code of Conduct, is allowed during this period.
Violating these terms may lead to a permanent ban.
### 4. Permanent Ban
**Community Impact**: Demonstrating a pattern of violation of community
standards, including sustained inappropriate behavior, harassment of an
individual, or aggression toward or disparagement of classes of individuals.
**Consequence**: A permanent ban from any sort of public interaction within
the community.
## Attribution
This Code of Conduct is adapted from the [Contributor Covenant][homepage],
version 2.0, available at
https://www.contributor-covenant.org/version/2/0/code_of_conduct.html.
Community Impact Guidelines were inspired by [Mozilla's code of conduct
enforcement ladder](https://github.com/mozilla/diversity).
[homepage]: https://www.contributor-covenant.org
For answers to common questions about this code of conduct, see the FAQ at
https://www.contributor-covenant.org/faq. Translations are available at
https://www.contributor-covenant.org/translations.
| {
"repo_name": "Rubiksman78/RenAI-Chat",
"stars": "46",
"repo_language": "Python",
"file_name": "parsing.py",
"mime_type": "text/x-script.python"
} |
@echo off
"libs/pythonlib/python.exe" -m pip install numpy==1.23.0
"libs/pythonlib/python.exe" main.py
| {
"repo_name": "Rubiksman78/RenAI-Chat",
"stars": "46",
"repo_language": "Python",
"file_name": "parsing.py",
"mime_type": "text/x-script.python"
} |
import os,sys
import tkinter as tk
import json
import yaml
# Configuration
args = sys.argv[1:]
save_ids = os.path.exists("save_text.txt")
root = tk.Tk()
root.title("RenAI Chat Login")
root.geometry("700x250")
root.configure(background='#333333')
def get_input():
global GAME_PATH
global USE_TTS
global CHAT_MODEL
global LAUNCH_YOURSELF
global TTS_MODEL
global USE_SPEECH_RECOGNITION
global VOICE_SAMPLE_TORTOISE
global VOICE_SAMPLE_COQUI
global CHARACTER_JSON
USE_TTS = use_tts.get()
GAME_PATH = game_path.get()
LAUNCH_YOURSELF = launch_yourself.get()
CHAT_MODEL = chat_model.get()
TTS_MODEL = tts_model.get()
USE_SPEECH_RECOGNITION = use_speech_recognition.get()
VOICE_SAMPLE_TORTOISE = voice_sample_tortoise.get()
VOICE_SAMPLE_COQUI = voice_sample_coqui.get()
CHARACTER_JSON =character_json.get()
root.destroy()
other_frame = tk.LabelFrame(root,bg='#333333',text="General Settings",fg='white',font=("Helvetica", 16))
other_frame.grid(row=5, column=0)
use_tts = tk.StringVar()
game_path = tk.StringVar()
launch_yourself = tk.StringVar()
chat_model = tk.StringVar()
tts_model = tk.StringVar()
use_speech_recognition = tk.StringVar()
voice_sample_tortoise = tk.StringVar()
voice_sample_coqui = tk.StringVar()
character_json = tk.StringVar()
#General Settings
tk.Label(other_frame, text="Game Path",bg='#333333',fg='white').grid(row=1, column=0)
tk.Label(other_frame, text="Launch Yourself",bg='#333333',fg='white').grid(row=1, column=3)
tk.Label(other_frame, text="Use TTS",bg='#333333',fg='white').grid(row=3, column=0)
tk.Label(other_frame, text="TTS model",bg='#333333',fg='white').grid(row=3, column=3)
tk.Label(other_frame, text="Chatbot Model",bg='#333333',fg='white').grid(row=4, column=0)
tk.Label(other_frame, text="Use Speech Recognition",bg='#333333',fg='white').grid(row=6, column=0)
tk.Label(other_frame, text="Voice Sample (Tortoise)",bg='#333333',fg='white').grid(row=7, column=0)
tk.Label(other_frame, text="Voice Sample (Your TTS)",bg='#333333',fg='white').grid(row=7, column=3)
tk.Label(other_frame, text="Character JSON",bg='#333333',fg='white').grid(row=4, column=2)
#Textual Inputs
tk.Entry(other_frame, textvariable=game_path,width=25).grid(row=1, column=1)
#Scrollable Inputs
all_models = os.listdir("chatbot_models")
all_models = [x for x in all_models if not x.endswith(".txt")]
if len(all_models) == 0:
all_models = ["No models found"]
chat_menu = tk.OptionMenu(other_frame, chat_model, *all_models)
chat_menu.config(bg='white',fg='black')
chat_menu.grid(row=4, column=1)
tts_menu = tk.OptionMenu(other_frame, tts_model, "Your TTS", "Tortoise TTS")
tts_menu.config( bg='white',fg='black')
tts_menu.grid(row=3, column=4)
all_voices_tortoise = os.listdir("tortoise_audios")
all_voices_tortoise = [x for x in all_voices_tortoise if not x.endswith(".txt")]
voice_menu = tk.OptionMenu(other_frame, voice_sample_tortoise, *all_voices_tortoise)
voice_menu.config( bg='white',fg='black')
voice_menu.grid(row=7, column=1)
all_voices_coquiai = os.listdir("coquiai_audios")
all_voices_coquiai = [x for x in all_voices_coquiai if x.endswith(".wav")]
if len(all_voices_coquiai) == 0:
all_voices_coquiai = ["No voices found"]
voice_menu = tk.OptionMenu(other_frame, voice_sample_coqui, *all_voices_coquiai)
voice_menu.config( bg='white',fg='black')
voice_menu.grid(row=7, column=4)
all_characters = os.listdir("char_json")
all_characters = [x for x in all_characters if not x.endswith(".txt")]
character_menu = tk.OptionMenu(other_frame, character_json, *all_characters)
character_menu.config( bg='white',fg='black')
character_menu.grid(row=4, column=3)
#Yes/No Inputs
tk.Radiobutton(other_frame, text="Yes", variable=launch_yourself, value=True,bg='#333333',activeforeground='white',fg='white',activebackground="#333333",selectcolor='#333333').grid(row=1, column=4)
tk.Radiobutton(other_frame, text="No", variable=launch_yourself, value=False,bg='#333333',activeforeground='white',fg='white',activebackground="#333333",selectcolor='#333333').grid(row=1, column=5)
tk.Radiobutton(other_frame, text="Yes", variable=use_tts, value=True,bg='#333333',activeforeground='white',fg='white',activebackground="#333333",selectcolor='#333333').grid(row=3, column=1)
tk.Radiobutton(other_frame, text="No", variable=use_tts, value=False,bg='#333333',activeforeground='white',fg='white',activebackground="#333333",selectcolor='#333333').grid(row=3, column=2)
tk.Radiobutton(other_frame, text="Yes", variable=use_speech_recognition, value=True,bg='#333333',activeforeground='white',fg='white',activebackground="#333333",selectcolor='#333333').grid(row=6, column=1)
tk.Radiobutton(other_frame, text="No", variable=use_speech_recognition, value=False,bg='#333333',activeforeground='white',fg='white',activebackground="#333333",selectcolor='#333333').grid(row=6, column=2)
button = tk.Button(root, text="Submit", command=get_input,bg='#FF3399',fg='white')
button.place(relx=0.5, rely=0.95, anchor=tk.CENTER)
#KEEP_CONFIG = int(KEEP_CONFIG)
if not os.path.exists("config.json"):
#Set default values
launch_yourself.set(0)
use_tts.set(0)
chat_model.set("NO_MODEL_SET")
tts_model.set("Your TTS")
use_speech_recognition.set(0)
voice_sample_tortoise.set("SELECT_A_VOICE")
voice_sample_coqui.set("talk_13.wav")
character_json.set("SELECT_A_CHARACTER")
else:
with open("config.json", "r") as f:
config = json.load(f)
GAME_PATH = config["GAME_PATH"]
USE_TTS = config["USE_TTS"]
LAUNCH_YOURSELF = config["LAUNCH_YOURSELF"]
TTS_MODEL = config["TTS_MODEL"]
CHAT_MODEL = config["CHAT_MODEL"]
USE_SPEECH_RECOGNITION = config["USE_SPEECH_RECOGNITION"]
VOICE_SAMPLE_TORTOISE = config["VOICE_SAMPLE_TORTOISE"]
VOICE_SAMPLE_COQUI = config["VOICE_SAMPLE_COQUI"]
CHARACTER_JSON = config["CHARACTER_JSON"]
#Set saved values
launch_yourself.set(LAUNCH_YOURSELF)
use_tts.set(USE_TTS)
chat_model.set(CHAT_MODEL)
tts_model.set(TTS_MODEL)
use_speech_recognition.set(USE_SPEECH_RECOGNITION)
voice_sample_tortoise.set(VOICE_SAMPLE_TORTOISE)
voice_sample_coqui.set(VOICE_SAMPLE_COQUI)
character_json.set(CHARACTER_JSON)
root.mainloop()
#Convert string to int (0 or 1, False or True)
USE_TTS = int(USE_TTS)
LAUNCH_YOURSELF = int(LAUNCH_YOURSELF)
USE_SPEECH_RECOGNITION = int(USE_SPEECH_RECOGNITION)
#Save model chosen in chatbot config
with open("chatbot/chatbot_config.yml", "r") as f:
config_chat = yaml.safe_load(f)
config_chat["model_name"] = "chatbot_models/" + CHAT_MODEL
with open("chatbot/chatbot_config.yml", "w") as f:
yaml.dump(config_chat, f)
#Save config to a json file
CONFIG = {
"GAME_PATH": GAME_PATH,
"USE_TTS": USE_TTS,
"LAUNCH_YOURSELF": LAUNCH_YOURSELF,
"TTS_MODEL": TTS_MODEL,
"CHAT_MODEL": CHAT_MODEL,
"USE_SPEECH_RECOGNITION": USE_SPEECH_RECOGNITION,
"VOICE_SAMPLE_TORTOISE": VOICE_SAMPLE_TORTOISE,
"VOICE_SAMPLE_COQUI": VOICE_SAMPLE_COQUI,
"CHARACTER_JSON": CHARACTER_JSON
}
with open("config.json", "w") as f:
json.dump(CONFIG, f)
| {
"repo_name": "Rubiksman78/RenAI-Chat",
"stars": "46",
"repo_language": "Python",
"file_name": "parsing.py",
"mime_type": "text/x-script.python"
} |
from chatbot.prompting import build_prompt_for
from chatbot.model import run_raw_inference
import typing as t
import logging
logger = logging.getLogger(__name__)
logging.basicConfig(level=logging.INFO)
def inference_fn(model,tokenizer,history: str, user_input: str,
generation_settings: t.Dict[str, t.Any],
char_settings: t.Dict[str,t.Any],
history_length = 8,
count = 0,
) -> str:
char_name = char_settings["char_name"]
char_persona = char_settings["char_persona"]
char_greeting = char_settings["char_greeting"]
world_scenario = char_settings["world_scenario"]
example_dialogue = char_settings["example_dialogue"]
#print(char_persona)
if count == 0 and char_greeting is not None:
return f"{char_greeting}"
prompt = build_prompt_for(history=history,
user_message=user_input,
char_name=char_name,
char_persona=char_persona,
example_dialogue=example_dialogue,
world_scenario=world_scenario,
history_lenght=history_length)
model_output = run_raw_inference(model, tokenizer, prompt,
user_input, **generation_settings)
#remove last line and keep the last line before
last_line = model_output.splitlines()[-1]
list_lines = model_output.splitlines()
if last_line.startswith("You:"):
bot_message = list_lines[-2]
else:
bot_message = last_line
#remove the char name at the beginning of the line
bot_message = bot_message.replace(f"{char_name}: ","")
return bot_message | {
"repo_name": "Rubiksman78/RenAI-Chat",
"stars": "46",
"repo_language": "Python",
"file_name": "parsing.py",
"mime_type": "text/x-script.python"
} |
from pathlib import Path
from TTS.utils.manage import ModelManager
from TTS.utils.synthesizer import Synthesizer
class TTS:
"""TODO: Add voice conversion and Capacitron support."""
def __init__(self, model_name: str = None, progress_bar: bool = True, gpu=False):
"""🐸TTS python interface that allows to load and use the released models.
Example with a multi-speaker model:
>>> from TTS.api import TTS
>>> tts = TTS(TTS.list_models()[0])
>>> wav = tts.tts("This is a test! This is also a test!!", speaker=tts.speakers[0], language=tts.languages[0])
>>> tts.tts_to_file(text="Hello world!", speaker=tts.speakers[0], language=tts.languages[0], file_path="output.wav")
Example with a single-speaker model:
>>> tts = TTS(model_name="tts_models/de/thorsten/tacotron2-DDC", progress_bar=False, gpu=False)
>>> tts.tts_to_file(text="Ich bin eine Testnachricht.", file_path="output.wav")
Args:
model_name (str, optional): Model name to load. You can list models by ```tts.models```. Defaults to None.
progress_bar (bool, optional): Whether to pring a progress bar while downloading a model. Defaults to True.
gpu (bool, optional): Enable/disable GPU. Some models might be too slow on CPU. Defaults to False.
"""
self.manager = ModelManager(models_file=self.get_models_file_path(), progress_bar=progress_bar, verbose=False)
self.synthesizer = None
if model_name:
self.load_model_by_name(model_name, gpu)
@property
def models(self):
return self.manager.list_tts_models()
@property
def is_multi_speaker(self):
if hasattr(self.synthesizer.tts_model, "speaker_manager") and self.synthesizer.tts_model.speaker_manager:
return self.synthesizer.tts_model.speaker_manager.num_speakers > 1
return False
@property
def is_multi_lingual(self):
if hasattr(self.synthesizer.tts_model, "language_manager") and self.synthesizer.tts_model.language_manager:
return self.synthesizer.tts_model.language_manager.num_languages > 1
return False
@property
def speakers(self):
if not self.is_multi_speaker:
return None
return self.synthesizer.tts_model.speaker_manager.speaker_names
@property
def languages(self):
if not self.is_multi_lingual:
return None
return self.synthesizer.tts_model.language_manager.language_names
@staticmethod
def get_models_file_path():
return Path(__file__).parent / ".models.json"
@staticmethod
def list_models():
manager = ModelManager(models_file=TTS.get_models_file_path(), progress_bar=False, verbose=False)
return manager.list_tts_models()
def download_model_by_name(self, model_name: str):
model_path, config_path, model_item = self.manager.download_model(model_name)
if model_item["default_vocoder"] is None:
return model_path, config_path, None, None
vocoder_path, vocoder_config_path, _ = self.manager.download_model(model_item["default_vocoder"])
return model_path, config_path, vocoder_path, vocoder_config_path
def load_model_by_name(self, model_name: str, gpu: bool = False):
model_path, config_path, vocoder_path, vocoder_config_path = self.download_model_by_name(model_name)
# init synthesizer
# None values are fetch from the model
self.synthesizer = Synthesizer(
tts_checkpoint=model_path,
tts_config_path=config_path,
tts_speakers_file=None,
tts_languages_file=None,
vocoder_checkpoint=vocoder_path,
vocoder_config=vocoder_config_path,
encoder_checkpoint=None,
encoder_config=None,
use_cuda=gpu,
)
def tts(self, text: str, speaker: str = None, language: str = None):
"""Convert text to speech.
Args:
text (str):
Input text to synthesize.
speaker (str, optional):
Speaker name for multi-speaker. You can check whether loaded model is multi-speaker by
`tts.is_multi_speaker` and list speakers by `tts.speakers`. Defaults to None.
language (str, optional):
Language code for multi-lingual models. You can check whether loaded model is multi-lingual
`tts.is_multi_lingual` and list available languages by `tts.languages`. Defaults to None.
"""
wav = self.synthesizer.tts(
text=text,
speaker_name=speaker,
language_name=language,
speaker_wav=None,
reference_wav=None,
style_wav=None,
style_text=None,
reference_speaker_name=None,
)
return wav
def tts_to_file(self, text: str, speaker: str = None, language: str = None, file_path: str = "output.wav"):
"""Convert text to speech.
Args:
text (str):
Input text to synthesize.
speaker (str, optional):
Speaker name for multi-speaker. You can check whether loaded model is multi-speaker by
`tts.is_multi_speaker` and list speakers by `tts.speakers`. Defaults to None.
language (str, optional):
Language code for multi-lingual models. You can check whether loaded model is multi-lingual
`tts.is_multi_lingual` and list available languages by `tts.languages`. Defaults to None.
file_path (str, optional):
Output file path. Defaults to "output.wav".
"""
wav = self.tts(text=text, speaker=speaker, language=language)
self.synthesizer.save_wav(wav=wav, path=file_path)
class my_TTS(TTS):
def __init__(self, *args, **kwargs):
super(my_TTS, self).__init__(*args, **kwargs)
def tts(self, text: str, speaker: str = None, language: str = None,speaker_wav: str = None, reference_wav: str = None, style_wav: str = None, style_text: str = None, reference_speaker_name: str = None):
"""Synthesize text to speech."""
wav = self.synthesizer.tts(
text=text,
speaker_name=speaker,
language_name=language,
speaker_wav=speaker_wav,
reference_wav=reference_wav,
style_wav=style_wav,
style_text=style_text,
reference_speaker_name=reference_speaker_name,
)
return wav
def tts_to_file(self, text: str, speaker: str = None, language: str = None, file_path: str = "output.wav", speaker_wav: str = None, reference_wav: str = None, style_wav: str = None, style_text: str = None, reference_speaker_name: str = None):
wav = self.tts(text=text, speaker=speaker, language=language,speaker_wav=speaker_wav, reference_wav=reference_wav, style_wav=style_wav, style_text=style_text, reference_speaker_name=reference_speaker_name)
self.synthesizer.save_wav(wav=wav, path=file_path) | {
"repo_name": "Rubiksman78/RenAI-Chat",
"stars": "46",
"repo_language": "Python",
"file_name": "parsing.py",
"mime_type": "text/x-script.python"
} |
import torch
from pathlib import Path
def infer(spec_gen_model, vocoder_model, str_input, speaker=None):
"""
Synthesizes spectrogram and audio from a text string given a spectrogram synthesis and vocoder model.
Args:
spec_gen_model: Spectrogram generator model (FastPitch in our case)
vocoder_model: Vocoder model (HiFiGAN in our case)
str_input: Text input for the synthesis
speaker: Speaker ID
Returns:
spectrogram and waveform of the synthesized audio.
"""
with torch.no_grad():
parsed = spec_gen_model.parse(str_input)
if speaker is not None:
speaker = torch.tensor([speaker]).long().to(device=spec_gen_model.device)
spectrogram = spec_gen_model.generate_spectrogram(tokens=parsed, speaker=speaker)
audio = vocoder_model.convert_spectrogram_to_audio(spec=spectrogram)
if spectrogram is not None:
if isinstance(spectrogram, torch.Tensor):
spectrogram = spectrogram.to('cpu').numpy()
if len(spectrogram.shape) == 3:
spectrogram = spectrogram[0]
if isinstance(audio, torch.Tensor):
audio = audio.to('cpu').numpy()
return spectrogram, audio
def get_best_ckpt_from_last_run(
base_dir,
new_speaker_id,
duration_mins,
mixing_enabled,
original_speaker_id,
model_name="FastPitch"
):
mixing = "no_mixing" if not mixing_enabled else "mixing"
d = f"{original_speaker_id}_to_{new_speaker_id}_{mixing}_{duration_mins}_mins"
exp_dirs = list([i for i in (Path(base_dir) / d / model_name).iterdir() if i.is_dir()])
last_exp_dir = sorted(exp_dirs)[-1]
last_checkpoint_dir = last_exp_dir / "checkpoints"
last_ckpt = list(last_checkpoint_dir.glob('*-last.ckpt'))
if len(last_ckpt) == 0:
raise ValueError(f"There is no last checkpoint in {last_checkpoint_dir}.")
return str(last_ckpt[0])
| {
"repo_name": "Rubiksman78/RenAI-Chat",
"stars": "46",
"repo_language": "Python",
"file_name": "parsing.py",
"mime_type": "text/x-script.python"
} |
from socket import AF_INET, socket, SOCK_STREAM
from threading import Thread
import os,sys
import subprocess
import time
import simpleaudio as sa
try:
from tts_api import my_TTS
except:
my_TTS = None
import torch
import numpy as np
import IPython.display as ipd
from login_screen import CONFIG
import re
import yaml
import json
GAME_PATH = CONFIG["GAME_PATH"]
USE_TTS = CONFIG["USE_TTS"]
LAUNCH_YOURSELF = CONFIG["LAUNCH_YOURSELF"]
TTS_MODEL = CONFIG["TTS_MODEL"]
USE_SPEECH_RECOGNITION = CONFIG["USE_SPEECH_RECOGNITION"]
VOICE_SAMPLE_TORTOISE = CONFIG["VOICE_SAMPLE_TORTOISE"]
VOICE_SAMPLE_COQUI = CONFIG["VOICE_SAMPLE_COQUI"]
CHARACTER_JSON = CONFIG["CHARACTER_JSON"]
#Disable print from TTS Coqui AI
class HiddenPrints:
def __enter__(self):
self._original_stdout = sys.stdout
sys.stdout = open(os.devnull, 'w')
def __exit__(self, exc_type, exc_val, exc_tb):
sys.stdout.close()
sys.stdout = self._original_stdout
######LOAD CHATBOT CONFIG######
from chatbot.model import build_model_and_tokenizer_for
from run_chatbot import inference_fn
import gc
with open("chatbot/chatbot_config.yml", "r") as f:
CHAT_CONFIG = yaml.safe_load(f)
with open(f"char_json/{CHARACTER_JSON}", "r") as f:
char_settings = json.load(f)
f.close()
model_name = CHAT_CONFIG["model_name"]
gc.collect()
torch.cuda.empty_cache()
chat_model, tokenizer = build_model_and_tokenizer_for(model_name)
generation_settings = {
"max_new_tokens": CHAT_CONFIG["max_new_tokens"],
"temperature": CHAT_CONFIG["temperature"],
"repetition_penalty": CHAT_CONFIG["repetition_penalty"],
"top_p": CHAT_CONFIG["top_p"],
"top_k": CHAT_CONFIG["top_k"],
"do_sample": CHAT_CONFIG["do_sample"],
"typical_p":CHAT_CONFIG["typical_p"],
}
context_size = CHAT_CONFIG["context_size"]
with open("chat_history.txt", "a") as chat_history:
chat_history.write("Conversation started at: " + time.strftime("%Y-%m-%d %H:%M:%S", time.localtime()) + "\n")
#################################
#########Load the TTS model##########
with HiddenPrints():
if USE_TTS:
from tortoise.api import TextToSpeech,MODELS_DIR
from tortoise.utils.audio import load_voices
from voicefixer import VoiceFixer
if TTS_MODEL == "Your TTS":
tts_model = my_TTS(model_name="tts_models/multilingual/multi-dataset/your_tts")
sampling_rate = 16000
elif TTS_MODEL == "Tortoise TTS":
tts_model = TextToSpeech(
models_dir=MODELS_DIR,
high_vram=False,
kv_cache=True,
)
voice_samples, conditioning_latents = load_voices([VOICE_SAMPLE_TORTOISE], ["tortoise_audios"])
vfixer = VoiceFixer()
sampling_rate = 24000
else:
print("No TTS model selected")
def play_TTS(step,msg,play_obj):
if USE_TTS:
print("Using TTS")
if step > 0:
play_obj.stop()
msg_audio = msg.replace("\n"," ")
msg_audio = msg_audio.replace("{i}","")
msg_audio = msg_audio.replace("{/i}",".")
msg_audio = msg_audio.replace("~","!")
msg_audio = emoji_pattern.sub(r'', msg_audio)
msg_audio = uni_chr_re.sub(r'', msg_audio)
with HiddenPrints():
if TTS_MODEL == "Your TTS":
audio = tts_model.tts(text=msg_audio,speaker_wav=f'coquiai_audios/{VOICE_SAMPLE_COQUI}', language='en')
elif TTS_MODEL == "Tortoise TTS":
gen, _ = tts_model.tts(
text=msg_audio,
k=1,
voice_samples=voice_samples,
conditioning_latents=conditioning_latents,
num_autoregressive_samples=8,
diffusion_iterations=20,
sampler="ddim",
return_deterministic_state=True,
latent_averaging_mode=1,
length_penalty=1.8,
max_mel_tokens=500,
cond_free_k=2,
top_p=0.85,
repetition_penalty=2.,
)
audio = gen.squeeze(0).cpu().numpy()
audio = ipd.Audio(audio, rate=sampling_rate)
play_obj = sa.play_buffer(audio.data, 1, 2, sampling_rate)
return play_obj
#####################################
####Load the speech recognizer#####
if USE_SPEECH_RECOGNITION:
import speech_recognition as sr
import whisper
english = True
def init_stt(model="base", english=True,energy=300, pause=0.8, dynamic_energy=False):
if model != "large" and english:
model = model + ".en"
audio_model = whisper.load_model(model)
r = sr.Recognizer()
r.energy_threshold = energy
r.pause_threshold = pause
r.dynamic_energy_threshold = dynamic_energy
return r,audio_model
r,audio_model = init_stt()
#####################################
GAME_PATH = GAME_PATH.replace("\\", "/")
clients = {}
addresses = {}
HOST = '127.0.0.1'
PORT = 12346
BUFSIZE = 1024
ADDRESS = (HOST, PORT)
SERVER = socket(AF_INET, SOCK_STREAM)
SERVER.bind(ADDRESS)
queued = False
os.environ['TF_CPP_MIN_LOG_LEVEL'] = '2'
emoji_pattern = re.compile("["
u"\U0001F600-\U0001F64F" # emoticons
u"\U0001F300-\U0001F5FF" # symbols & pictographs
u"\U0001F680-\U0001F6FF" # transport & map symbols
u"\U0001F1E0-\U0001F1FF" # flags (iOS)
u"\U00002500-\U00002BEF" # chinese char
u"\U00002702-\U000027B0"
u"\U00002702-\U000027B0"
u"\U000024C2-\U0001F251"
u"\U0001f926-\U0001f937"
u"\U00010000-\U0010ffff"
u"\u2640-\u2642"
u"\u2600-\u2B55"
u"\u200d"
u"\u23cf"
u"\u23e9"
u"\u231a"
u"\ufe0f" # dingbats
u"\u3030"
u"\u2014"
"]+", flags=re.UNICODE)
uni_chr_re = re.compile(r'\\u[0-9a-fA-F]{4}')
#Launch the game
if not LAUNCH_YOURSELF:
subprocess.Popen(GAME_PATH+'/RenAIChat.exe')
def listen():
""" Wait for incoming connections """
print("Waiting for connection...")
while True:
client, client_address = SERVER.accept()
print("%s:%s has connected." % client_address)
addresses[client] = client_address
Thread(target = call, args = (client,)).start()
def call(client):
thread = Thread(target=listenToClient, args=(client,), daemon=True)
thread.start()
def sendMessage(msg, name=""):
""" send message to all users present in
the chat room"""
for client in clients:
client.send(bytes(name, "utf8") + msg)
def send_answer(received_msg,msg):
action_to_take = "none"
action_to_take = action_to_take.encode("utf-8")
emotion = "".encode("utf-8")
msg = msg.encode("utf-8")
msg_to_send = msg + b"/g" + emotion + b"/g" + action_to_take
sendMessage(msg_to_send)
def listenToClient(client):
""" Get client username """
name = "User"
clients[client] = name
launched = False
chat_count = 0
play_obj = None
if os.path.exists("char_history.txt"):
history = open("char_history.txt","r").read()
#Remove lines with the pattern "Conversation started at: 2023-02-14 14:14:17"
history = re.sub(r"Conversation started at: \d{4}-\d{2}-\d{2} \d{2}:\d{2}:\d{2}","",history)
else:
history = ""
while True:
received_msg = client.recv(BUFSIZE).decode("utf-8") #Message indicating the mode used (chatbot,camera_int or camera)
received_msg = received_msg.split("/m")
rest_msg = received_msg[1]
received_msg = received_msg[0]
if received_msg == "chatbot":
if '/g' in rest_msg:
received_msg , step = rest_msg.split("/g")
else:
received_msg = client.recv(BUFSIZE).decode("utf-8") #Message containing the user input
received_msg , step = received_msg.split("/g")
step = int(step)
#Speech to text
if received_msg == "begin_record":
if USE_SPEECH_RECOGNITION:
with sr.Microphone(sample_rate=16000) as source:
sendMessage("yes".encode("utf-8"))
#get and save audio to wav file
audio = r.listen(source)
torch_audio = torch.from_numpy(np.frombuffer(audio.get_raw_data(), np.int16).flatten().astype(np.float32) / 32768.0)
audio_data = torch_audio
if english:
result = audio_model.transcribe(audio_data,language='english')
else:
result = audio_model.transcribe(audio_data)
received_msg = result['text']
else:
sendMessage("no".encode("utf-8"))
continue
print("User: "+received_msg)
while True:
if chat_count == 0:
sendMessage("server_ok".encode("utf-8"))
ok_ready = client.recv(BUFSIZE).decode("utf-8")
bot_message = inference_fn(chat_model,tokenizer,history, "",generation_settings,char_settings,history_length=context_size,count=chat_count)
else:
bot_message = inference_fn(chat_model,tokenizer,history, received_msg,generation_settings,char_settings,history_length=context_size,count=chat_count)
history = history + "\n" + f"You: {received_msg}" + "\n" + f"{bot_message}"
if received_msg != "QUIT":
if received_msg == "REGEN":
history.replace("\n" + f"You: {received_msg}" + "\n" + f"{bot_message}","")
bot_message = inference_fn(chat_model,tokenizer,history, received_msg,generation_settings,char_settings,history_length=context_size,count=chat_count)
bot_message = bot_message.replace("<USER>","Player")
play_obj = play_TTS(step,bot_message,play_obj)
print("Sent: "+ bot_message)
send_answer(received_msg,bot_message)
chat_count += 1
if chat_count > 1:
with open("chat_history.txt", "a",encoding="utf-8") as f:
f.write(f"You: {received_msg}" + "\n" + f'{char_settings["char_name"]}: {bot_message}' + "\n")
break
if __name__ == "__main__":
SERVER.listen(5)
ACCEPT_THREAD = Thread(target=listen)
ACCEPT_THREAD.start()
ACCEPT_THREAD.join()
SERVER.close()
| {
"repo_name": "Rubiksman78/RenAI-Chat",
"stars": "46",
"repo_language": "Python",
"file_name": "parsing.py",
"mime_type": "text/x-script.python"
} |
playwright~=1.31.0
simpleaudio~=1.0.4
IPython~=8.10.0
tensorflow-macos~=2.11.0
opencv-python~=4.7.0.72
transformers~=4.26.1
Pillow~=9.4.0
SpeechRecognition~=3.9.0
git+https://github.com/openai/whisper.git
tk~=0.1.0
bitsandbytes~=0.37.0
numpy==1.23.0
| {
"repo_name": "Rubiksman78/RenAI-Chat",
"stars": "46",
"repo_language": "Python",
"file_name": "parsing.py",
"mime_type": "text/x-script.python"
} |
playwright~=1.31.0
#simpleaudio-1.0.4-cp38-cp38-win_amd64.whl
IPython~=8.10.0
tensorflow~=2.11.0
opencv-python~=4.7.0.72
transformers~=4.26.1
Pillow~=9.4.0
SpeechRecognition~=3.9.0
git+https://github.com/openai/whisper.git
tk~=0.1.0
bitsandbytes~=0.37.0
numpy==1.23.0
PyAudio
| {
"repo_name": "Rubiksman78/RenAI-Chat",
"stars": "46",
"repo_language": "Python",
"file_name": "parsing.py",
"mime_type": "text/x-script.python"
} |
<h1 align="center"> :email: RenAI Chat </h1>
<p align="center">
<a href="https://github.com/Rubiksman78/RenAI-Chat/releases/latest">
<img alt="Latest release" src="https://img.shields.io/github/v/release/Rubiksman78/RenAI-Chat">
</a>
</p>
This project aims to create a fun interface to use conversational models in the form of a Visual Novel in Renpy.
It's using multiple AI models:
- [Pygmalion](https://huggingface.co/PygmalionAI) conversational AI or other Huggingface models
- [TTS Coqui-AI](https://github.com/coqui-ai/TTS) and [Tortoise-TTS](https://github.com/152334H/tortoise-tts-fast) for Text to Speech
- [OpenAI Whisper](https://github.com/openai/whisper) with [microphone option](https://github.com/mallorbc/whisper_mic) for Speech to Text
# Overview
## :boom: Installation
Check out the wiki [page](https://github.com/Rubiksman78/RenAI-Chat/wiki).
## :fire: Features
- Speak without scripted text using the latest chatbots
- Clone your character voice with a Text to Speech module using extracts of voiced dialogues
- Talk with your own voice thanks to Speech recognition
## :eyeglasses: How to add chatbot models
- Check the [wiki](https://github.com/Rubiksman78/RenAI-Chat/wiki/Chatbots-info) to setup your GPU and for pratical info about chatbots to use
- On Huggingface website go to the model page, for example `https://huggingface.co/PygmalionAI/pygmalion-2.7b`
- Follow the instructions to download the model like this on your Command Prompt or Powershell opened wherever you want:
```
git lfs install
git clone https://huggingface.co/PygmalionAI/pygmalion-2.7b
```
- Once the download is finished, put the folder created in `chatbot_models` in the RenAIChat folder. You will be able to choose it in your next login !
## :microphone: Customize voice
For Your TTS model (worse but faster, ~5s per turn):
You can change the voice used by placing extracts in the `coquiai_audios` folder. The longer the extract, the longer the TTS will take to generate the audio at each turn (~1min is good). It has to be `.wav` audio files, use an online converter if you have `.mp3` files.
For Tortoise TTS model (better but slower, ~40s per turn): You can change the voice samples in `tortoise_voices_` folder. Create your own character by adding a folder with the name of your character and put the audio samples in it. The samples must be around 10 seconds long and at the number of 5 maximum. Prefer `.wav` files too.
On CPU, it can take 10x more time to generate the voice (Tortoise TTS can have unexpected behaviour on CPU)
## :paperclip: Customize character json
Put the json of your character in the folder `char_json` in the format:
```
{
"char_name":...,
"char_persona":...,
"char_greeting":...,
"world_scenario":...,
"example_dialogue":"<START>\nYou:... \nCharName:...\n..."
}
```
## :camera: Customize game appearance
In your RenAIChat folder with the Renpy files (so the other zip file `RenAIChat-version-pc/mac`), add a transparent sprite of your character with the filename `char.png` and add a background named `bg.png` in the folder `game/images`. Don't forget to resize your BG to 1920x1080 and your character to the size you want (without being more than 1920x1080).
# Python installation
## ❓Installation
- Clone the repository or download the latest release (`source code.zip`)
- Go to the project folder with your favorite IDE
- Be sure to have Python installed (3.8 or 3.9), it is not tested and functional before 3.7 and after 3.10.
To setup all the libraries:
- Run these commands in a terminal opened within the project folder to install the packages:
```
pip install -r requirements.txt
```
- If you have a CUDA compatible GPU, run this too:
```
pip install --force torch torchvision torchaudio --extra-index-url https://download.pytorch.org/whl/cu117
```
- If there was an error returned during the installation of the packages, delete the corresponding line in `requirements.txt` and dowload the package concerned manually
- To download TTS (with Coqui AI TTS), run these commands:
```
git clone https://github.com/coqui-ai/TTS
cd TTS
pip install -e .
cd ../
```
- To download TTS (with Tortoise-TTS), run these commands:
```
git clone https://github.com/152334H/tortoise-tts-fast
cd tortoise-tts-fast
pip install -e .
cd ../
```
- `simpleaudio` or other packages might need to install Visual Studio C++ Tools too (see tutorial [here](https://stackoverflow.com/questions/64261546/how-to-solve-error-microsoft-visual-c-14-0-or-greater-is-required-when-inst)), for `simpleaudio` follow [this](https://stackoverflow.com/questions/67312738/error-command-errored-out-with-exit-status-1-python-when-installing-simple)
- If you want to use Huggingface models with int8, follow these intructions:
- Download these 2 dll files from [here](https://github.com/DeXtmL/bitsandbytes-win-prebuilt). Move those files in your python packages folder, on Windows it is something like `C:\Users\MyName\AppData\Local\Programs\Python\Python39\Lib\site-packages\bitsandbytes`
- Edit `bitsandbytes\cuda_setup\main.py`:
- Change `ct.cdll.LoadLibrary(binary_path)` to `ct.cdll.LoadLibrary(str(binary_path))` two times in the file.
- Replace the this line ```if not torch.cuda.is_available(): return 'libsbitsandbytes_cpu.so', None, None, None, None``` with ```if torch.cuda.is_available(): return 'libbitsandbytes_cuda116.dll', None, None, None, None```
- Run the script with `python main.py`
- For troubleshooting and other issues, don't hesitate to submit an issue
| {
"repo_name": "Rubiksman78/RenAI-Chat",
"stars": "46",
"repo_language": "Python",
"file_name": "parsing.py",
"mime_type": "text/x-script.python"
} |