language
stringlengths 0
24
| filename
stringlengths 9
214
| code
stringlengths 99
9.93M
|
---|---|---|
Go | bettercap/session/session_json.go | package session
import (
"encoding/json"
"net"
"runtime"
"time"
"github.com/bettercap/bettercap/caplets"
"github.com/bettercap/bettercap/core"
"github.com/bettercap/bettercap/network"
"github.com/bettercap/bettercap/packets"
)
var flagNames = []string{
"UP",
"BROADCAST",
"LOOPBACK",
"POINT2POINT",
"MULTICAST",
}
type addrJSON struct {
Address string `json:"address"`
Type string `json:"type"`
}
type ifaceJSON struct {
Index int `json:"index"`
MTU int `json:"mtu"`
Name string `json:"name"`
MAC string `json:"mac"`
Vendor string `json:"vendor"`
Flags []string `json:"flags"`
Addresses []addrJSON `json:"addresses"`
}
type resourcesJSON struct {
NumCPU int `json:"cpus"`
MaxCPU int `json:"max_cpus"`
NumGoroutine int `json:"goroutines"`
Alloc uint64 `json:"alloc"`
Sys uint64 `json:"sys"`
NumGC uint32 `json:"gcs"`
}
type SessionJSON struct {
Version string `json:"version"`
OS string `json:"os"`
Arch string `json:"arch"`
GoVersion string `json:"goversion"`
Resources resourcesJSON `json:"resources"`
Interfaces []ifaceJSON `json:"interfaces"`
Options core.Options `json:"options"`
Interface *network.Endpoint `json:"interface"`
Gateway *network.Endpoint `json:"gateway"`
Env *Environment `json:"env"`
Lan *network.LAN `json:"lan"`
WiFi *network.WiFi `json:"wifi"`
BLE *network.BLE `json:"ble"`
HID *network.HID `json:"hid"`
Queue *packets.Queue `json:"packets"`
StartedAt time.Time `json:"started_at"`
PolledAt time.Time `json:"polled_at"`
Active bool `json:"active"`
GPS GPS `json:"gps"`
Modules ModuleList `json:"modules"`
Caplets []*caplets.Caplet `json:"caplets"`
}
func (s *Session) MarshalJSON() ([]byte, error) {
var m runtime.MemStats
runtime.ReadMemStats(&m)
doc := SessionJSON{
Version: core.Version,
OS: runtime.GOOS,
Arch: runtime.GOARCH,
GoVersion: runtime.Version(),
Resources: resourcesJSON{
NumCPU: runtime.NumCPU(),
MaxCPU: runtime.GOMAXPROCS(0),
NumGoroutine: runtime.NumGoroutine(),
Alloc: m.Alloc,
Sys: m.Sys,
NumGC: m.NumGC,
},
Interfaces: make([]ifaceJSON, 0),
Options: s.Options,
Interface: s.Interface,
Gateway: s.Gateway,
Env: s.Env,
Lan: s.Lan,
WiFi: s.WiFi,
BLE: s.BLE,
HID: s.HID,
Queue: s.Queue,
StartedAt: s.StartedAt,
PolledAt: time.Now(),
Active: s.Active,
GPS: s.GPS,
Modules: s.Modules,
Caplets: caplets.List(),
}
ifaces, err := net.Interfaces()
if err != nil {
return nil, err
}
for _, iface := range ifaces {
mac := network.NormalizeMac(iface.HardwareAddr.String())
ij := ifaceJSON{
Index: iface.Index,
MTU: iface.MTU,
Name: iface.Name,
MAC: mac,
Vendor: network.ManufLookup(mac),
Flags: make([]string, 0),
Addresses: make([]addrJSON, 0),
}
if addrs, err := iface.Addrs(); err == nil {
for _, addr := range addrs {
ij.Addresses = append(ij.Addresses, addrJSON{
Address: addr.String(),
Type: addr.Network(),
})
}
}
for bit, name := range flagNames {
if iface.Flags&(1<<uint(bit)) != 0 {
ij.Flags = append(ij.Flags, name)
}
}
doc.Interfaces = append(doc.Interfaces, ij)
}
return json.Marshal(doc)
} |
Go | bettercap/session/session_parse.go | package session
import (
"fmt"
"strings"
"github.com/evilsocket/islazy/str"
)
func ParseCommands(line string) []string {
args := []string{}
buf := ""
singleQuoted := false
doubleQuoted := false
finish := false
line = strings.Replace(line, `""`, `"<empty>"`, -1)
line = strings.Replace(line, `''`, `"<empty>"`, -1)
for _, c := range line {
switch c {
case ';':
if !singleQuoted && !doubleQuoted {
finish = true
} else {
buf += string(c)
}
case '"':
if doubleQuoted {
// finish of quote
doubleQuoted = false
} else if singleQuoted {
// quote initiated with ', so we ignore it
buf += string(c)
} else {
// quote init here
doubleQuoted = true
}
case '\'':
if singleQuoted {
singleQuoted = false
} else if doubleQuoted {
buf += string(c)
} else {
singleQuoted = true
}
default:
buf += string(c)
}
if finish {
buf = strings.Replace(buf, `<empty>`, `""`, -1)
args = append(args, buf)
finish = false
buf = ""
}
}
if len(buf) > 0 {
buf = strings.Replace(buf, `<empty>`, `""`, -1)
args = append(args, buf)
}
cmds := make([]string, 0)
for _, cmd := range args {
cmd = str.Trim(cmd)
if cmd != "" || (len(cmd) > 0 && cmd[0] != '#') {
cmds = append(cmds, cmd)
}
}
return cmds
}
func (s *Session) parseEnvTokens(str string) (string, error) {
for _, m := range reEnvVarCapture.FindAllString(str, -1) {
varName := strings.Trim(strings.Replace(m, "env.", "", -1), "{}")
if found, value := s.Env.Get(varName); found {
str = strings.Replace(str, m, value, -1)
} else {
return "", fmt.Errorf("variable '%s' is not defined", varName)
}
}
return str, nil
} |
Go | bettercap/session/session_routing.go | package session
import (
"github.com/bettercap/bettercap/network"
"github.com/bettercap/bettercap/routing"
"github.com/evilsocket/islazy/log"
"time"
)
type gateway struct {
IP string `json:"ip"`
MAC string `json:"mac"`
}
type GatewayChange struct {
Type string `json:"type"`
Prev gateway `json:"prev"`
New gateway `json:"new"`
}
func (s *Session) routeMon() {
var err error
var gw4 *network.Endpoint
var gwIP6, gwMAC6 string
s.Events.Log(log.INFO, "gateway monitor started ...")
if gw4 = s.Gateway; gw4 == nil {
gw4 = &network.Endpoint{}
}
gwIP6, err = routing.Gateway(routing.IPv6, s.Interface.Name())
if err != nil {
s.Events.Log(log.ERROR, "error getting ipv6 gateway: %v", err)
} else if gwIP6 != "" {
gwMAC6, err = network.ArpLookup(s.Interface.Name(), gwIP6, true)
if err != nil {
s.Events.Log(log.DEBUG, "error getting %s ipv6 gateway mac: %v", gwIP6, err)
}
}
for {
s.Events.Log(log.DEBUG, "[gw] ipv4=%s(%s) ipv6=%s(%s)", gw4.IP, gw4.HwAddress, gwIP6, gwMAC6)
time.Sleep(5 * time.Second)
gw4now, err := network.FindGateway(s.Interface)
if gw4now == nil {
gw4now = &network.Endpoint{}
}
if err != nil {
s.Events.Log(log.ERROR, "error getting ipv4 gateway: %v", err)
} else {
if gw4now.IpAddress != gw4.IpAddress || gw4now.HwAddress != gw4.HwAddress {
s.Events.Add("gateway.change", GatewayChange{
Type: string(routing.IPv4),
Prev: gateway{
IP: gw4.IpAddress,
MAC: gw4.HwAddress,
},
New: gateway{
IP: gw4now.IpAddress,
MAC: gw4now.HwAddress,
},
})
}
}
gw4 = gw4now
gwMAC6now := ""
gwIP6now, err := routing.Gateway(routing.IPv6, s.Interface.Name())
if err != nil {
s.Events.Log(log.ERROR, "error getting ipv6 gateway: %v", err)
} else if gwIP6now != "" {
gwMAC6now, err = network.ArpLookup(s.Interface.Name(), gwIP6now, true)
if err != nil {
s.Events.Log(log.DEBUG, "error getting %s ipv6 gateway mac: %v", gwIP6now, err)
}
}
if gwIP6now != gwIP6 || gwMAC6now != gwMAC6 {
s.Events.Add("gateway.change", GatewayChange{
Type: string(routing.IPv6),
Prev: gateway{
IP: gwIP6,
MAC: gwMAC6,
},
New: gateway{
IP: gwIP6now,
MAC: gwMAC6now,
},
})
}
gwIP6 = gwIP6now
gwMAC6 = gwMAC6now
}
} |
Go | bettercap/session/session_setup.go | package session
import (
"fmt"
"os"
"os/signal"
"strings"
"syscall"
"time"
"github.com/bettercap/bettercap/caplets"
"github.com/bettercap/readline"
"github.com/evilsocket/islazy/fs"
"github.com/evilsocket/islazy/log"
)
func containsCapitals(s string) bool {
for _, ch := range s {
if ch < 133 && ch > 101 {
return false
}
}
return true
}
func (s *Session) setupReadline() (err error) {
prefixCompleters := make([]readline.PrefixCompleterInterface, 0)
for _, h := range s.CoreHandlers {
if h.Completer == nil {
prefixCompleters = append(prefixCompleters, readline.PcItem(h.Name))
} else {
prefixCompleters = append(prefixCompleters, h.Completer)
}
}
tree := make(map[string][]string)
for _, m := range s.Modules {
for _, h := range m.Handlers() {
if h.Completer == nil {
parts := strings.Split(h.Name, " ")
name := parts[0]
if _, found := tree[name]; !found {
tree[name] = []string{}
}
var appendedOption = strings.Join(parts[1:], " ")
if len(appendedOption) > 0 && !containsCapitals(appendedOption) {
tree[name] = append(tree[name], appendedOption)
}
} else {
prefixCompleters = append(prefixCompleters, h.Completer)
}
}
}
for _, caplet := range caplets.List() {
tree[caplet.Name] = []string{}
}
for root, subElems := range tree {
item := readline.PcItem(root)
item.Children = []readline.PrefixCompleterInterface{}
for _, child := range subElems {
item.Children = append(item.Children, readline.PcItem(child))
}
prefixCompleters = append(prefixCompleters, item)
}
history := ""
if !*s.Options.NoHistory {
history, _ = fs.Expand(HistoryFile)
}
cfg := readline.Config{
HistoryFile: history,
InterruptPrompt: "^C",
EOFPrompt: "^D",
AutoComplete: readline.NewPrefixCompleter(prefixCompleters...),
}
s.Input, err = readline.NewEx(&cfg)
return err
}
func (s *Session) startNetMon() {
// keep reading network events in order to add / update endpoints
go func() {
for event := range s.Queue.Activities {
if !s.Active {
return
}
if s.IsOn("net.recon") && event.Source {
addr := event.IP.String()
mac := event.MAC.String()
existing := s.Lan.AddIfNew(addr, mac)
if existing != nil {
existing.LastSeen = time.Now()
} else {
existing, _ = s.Lan.Get(mac)
}
if existing != nil && event.Meta != nil {
existing.OnMeta(event.Meta)
}
}
}
}()
}
func (s *Session) setupSignals() {
c := make(chan os.Signal)
signal.Notify(c, os.Interrupt, syscall.SIGTERM)
go func() {
<-c
fmt.Println()
s.Events.Log(log.WARNING, "Got SIGTERM")
s.Close()
os.Exit(0)
}()
}
func (s *Session) setupEnv() {
s.Env.Set("iface.index", fmt.Sprintf("%d", s.Interface.Index))
s.Env.Set("iface.name", s.Interface.Name())
s.Env.Set("iface.ipv4", s.Interface.IpAddress)
s.Env.Set("iface.ipv6", s.Interface.Ip6Address)
s.Env.Set("iface.mac", s.Interface.HwAddress)
s.Env.Set("gateway.address", s.Gateway.IpAddress)
s.Env.Set("gateway.mac", s.Gateway.HwAddress)
if found, v := s.Env.Get(PromptVariable); !found || v == "" {
if s.Interface.IsMonitor() {
s.Env.Set(PromptVariable, DefaultPromptMonitor)
} else {
s.Env.Set(PromptVariable, DefaultPrompt)
}
}
dbg := "false"
if *s.Options.Debug {
dbg = "true"
}
s.Env.WithCallback("log.debug", dbg, func(newValue string) {
newDbg := false
if newValue == "true" {
newDbg = true
}
s.Events.SetDebug(newDbg)
})
silent := "false"
if *s.Options.Silent {
silent = "true"
}
s.Env.WithCallback("log.silent", silent, func(newValue string) {
newSilent := false
if newValue == "true" {
newSilent = true
}
s.Events.SetSilent(newSilent)
})
} |
Go | bettercap/session/session_setup_test.go | package session
import "testing"
func Test_containsCapitals(t *testing.T) {
type args struct {
s string
}
tests := []struct {
name string
args args
want bool
}{
{
name: "Test all alpha lowercase",
args: args{s: "abcdefghijklmnopqrstuvwxyz"},
want: false,
},
{
name: "Test all alpha uppercase",
args: args{s: "ABCDEFGHIJKLMNOPQRSTUVWXYZ"},
want: true,
},
{
name: "Test special chars",
args: args{s: "!\"#$%&'()*+,-./:;<=>?@[\\]^_`{|}~"},
want: false,
},
// Add test for UTF8 ?
// {
// name: "Test special UTF-8 chars",
// args: args{s: "€©¶αϚϴЈ"},
// want: false,
// },
}
for _, tt := range tests {
t.Run(tt.name, func(t *testing.T) {
if got := containsCapitals(tt.args.s); got != tt.want {
t.Errorf("containsCapitals() = %v, want %v", got, tt.want)
}
})
}
} |
Go | bettercap/session/session_test.go | package session
import (
"fmt"
"testing"
)
func TestParseCommands(t *testing.T) {
//commands := ParseCommands("wifi.recon on; asdf; \"asdf;\" asdf")
t.Run("handles a semicolon as a delimiter", func(t *testing.T) {
first := "wifi.recon on"
second := "wifi.ap"
cmd := fmt.Sprintf("%s; %s", first, second)
commands := ParseCommands(cmd)
if l := len(commands); l != 2 {
t.Fatalf("Expected 2 commands, got %d", l)
}
if got := commands[0]; got != first {
t.Fatalf("expected %s got %s", first, got)
}
if got := commands[1]; got != second {
t.Fatalf("expected %s got %s", second, got)
}
})
t.Run("handles semicolon inside quotes", func(t *testing.T) {
cmd := "set ticker.commands \"clear; net.show\""
commands := ParseCommands(cmd)
if l := len(commands); l != 1 {
t.Fatalf("expected 1 command, got %d", l)
}
// Expect double-quotes stripped
expected := "set ticker.commands clear; net.show"
if got := commands[0]; got != expected {
fmt.Println(got)
t.Fatalf("expected %s got %s", cmd, got)
}
})
t.Run("handles semicolon inside single quotes", func(t *testing.T) {
cmd := "set ticker.commands 'clear; net.show'"
commands := ParseCommands(cmd)
if l := len(commands); l != 1 {
t.Fatalf("expected 1 command, got %d", l)
}
// Expect double-quotes stripped
expected := "set ticker.commands clear; net.show"
if got := commands[0]; got != expected {
fmt.Println(got)
t.Fatalf("expected %s got %s", cmd, got)
}
})
t.Run("handles semicolon inside single quotes inside quote", func(t *testing.T) {
cmd := "set ticker.commands \"'clear; net.show'\""
commands := ParseCommands(cmd)
if l := len(commands); l != 1 {
t.Fatalf("expected 1 command, got %d", l)
}
// Expect double-quotes stripped
expected := "set ticker.commands 'clear; net.show'"
if got := commands[0]; got != expected {
fmt.Println(got)
t.Fatalf("expected %s got %s", cmd, got)
}
})
t.Run("handles semicolon inside quotes inside single quote", func(t *testing.T) {
cmd := "set ticker.commands '\"clear; net.show\"'"
commands := ParseCommands(cmd)
if l := len(commands); l != 1 {
t.Fatalf("expected 1 command, got %d", l)
}
// Expect double-quotes stripped
expected := "set ticker.commands \"clear; net.show\""
if got := commands[0]; got != expected {
fmt.Println(got)
t.Fatalf("expected %s got %s", cmd, got)
}
})
t.Run("handle mismatching quote", func(t *testing.T) {
cmd := "set ticker.commands \"clear; echo it's working ?\""
commands := ParseCommands(cmd)
if l := len(commands); l != 1 {
t.Fatalf("expected 1 command, got %d", l)
}
// Expect double-quotes stripped
expected := "set ticker.commands clear; echo it's working ?"
if got := commands[0]; got != expected {
fmt.Println(got)
t.Fatalf("expected %s got %s", cmd, got)
}
})
} |
Go | bettercap/tls/cert.go | package tls
import (
"crypto/rand"
"crypto/rsa"
"crypto/x509"
"crypto/x509/pkix"
"encoding/pem"
"math/big"
"os"
"strconv"
"time"
"github.com/bettercap/bettercap/session"
)
type CertConfig struct {
Bits int
Country string
Locality string
Organization string
OrganizationalUnit string
CommonName string
}
var (
DefaultLegitConfig = CertConfig{
Bits: 4096,
Country: "US",
Locality: "",
Organization: "bettercap devteam",
OrganizationalUnit: "https://bettercap.org/",
CommonName: "bettercap",
}
DefaultSpoofConfig = CertConfig{
Bits: 4096,
Country: "US",
Locality: "Scottsdale",
Organization: "GoDaddy.com, Inc.",
OrganizationalUnit: "https://certs.godaddy.com/repository/",
CommonName: "Go Daddy Secure Certificate Authority - G2",
}
)
func CertConfigToModule(prefix string, m *session.SessionModule, defaults CertConfig) {
m.AddParam(session.NewIntParameter(prefix+".certificate.bits", strconv.Itoa(defaults.Bits),
"Number of bits of the RSA private key of the generated HTTPS certificate."))
m.AddParam(session.NewStringParameter(prefix+".certificate.country", defaults.Country, ".*",
"Country field of the generated HTTPS certificate."))
m.AddParam(session.NewStringParameter(prefix+".certificate.locality", defaults.Locality, ".*",
"Locality field of the generated HTTPS certificate."))
m.AddParam(session.NewStringParameter(prefix+".certificate.organization", defaults.Organization, ".*",
"Organization field of the generated HTTPS certificate."))
m.AddParam(session.NewStringParameter(prefix+".certificate.organizationalunit", defaults.OrganizationalUnit, ".*",
"Organizational Unit field of the generated HTTPS certificate."))
m.AddParam(session.NewStringParameter(prefix+".certificate.commonname", defaults.CommonName, ".*",
"Common Name field of the generated HTTPS certificate."))
}
func CertConfigFromModule(prefix string, m session.SessionModule) (cfg CertConfig, err error) {
if err, cfg.Bits = m.IntParam(prefix + ".certificate.bits"); err != nil {
return cfg, err
} else if err, cfg.Country = m.StringParam(prefix + ".certificate.country"); err != nil {
return cfg, err
} else if err, cfg.Locality = m.StringParam(prefix + ".certificate.locality"); err != nil {
return cfg, err
} else if err, cfg.Organization = m.StringParam(prefix + ".certificate.organization"); err != nil {
return cfg, err
} else if err, cfg.OrganizationalUnit = m.StringParam(prefix + ".certificate.organizationalunit"); err != nil {
return cfg, err
} else if err, cfg.CommonName = m.StringParam(prefix + ".certificate.commonname"); err != nil {
return cfg, err
}
return cfg, err
}
func CreateCertificate(cfg CertConfig, ca bool) (*rsa.PrivateKey, []byte, error) {
priv, err := rsa.GenerateKey(rand.Reader, cfg.Bits)
if err != nil {
return nil, nil, err
}
notBefore := time.Now()
aYear := time.Duration(365*24) * time.Hour
notAfter := notBefore.Add(aYear)
serialNumberLimit := new(big.Int).Lsh(big.NewInt(1), 128)
serialNumber, err := rand.Int(rand.Reader, serialNumberLimit)
if err != nil {
return nil, nil, err
}
template := x509.Certificate{
SerialNumber: serialNumber,
Subject: pkix.Name{
Country: []string{cfg.Country},
Locality: []string{cfg.Locality},
Organization: []string{cfg.Organization},
OrganizationalUnit: []string{cfg.OrganizationalUnit},
CommonName: cfg.CommonName,
},
NotBefore: notBefore,
NotAfter: notAfter,
KeyUsage: x509.KeyUsageKeyEncipherment | x509.KeyUsageDigitalSignature | x509.KeyUsageCertSign,
ExtKeyUsage: []x509.ExtKeyUsage{x509.ExtKeyUsageClientAuth, x509.ExtKeyUsageServerAuth},
BasicConstraintsValid: true,
IsCA: ca,
}
cert, err := x509.CreateCertificate(rand.Reader, &template, &template, &priv.PublicKey, priv)
if err != nil {
return nil, nil, err
}
return priv, cert, err
}
func Generate(cfg CertConfig, certPath string, keyPath string, ca bool) error {
keyFile, err := os.Create(keyPath)
if err != nil {
return err
}
defer keyFile.Close()
certFile, err := os.Create(certPath)
if err != nil {
return err
}
defer certFile.Close()
priv, cert, err := CreateCertificate(cfg, ca)
if err != nil {
return err
}
if err := pem.Encode(keyFile, &pem.Block{Type: "RSA PRIVATE KEY", Bytes: x509.MarshalPKCS1PrivateKey(priv)}); err != nil {
return err
}
return pem.Encode(certFile, &pem.Block{Type: "CERTIFICATE", Bytes: cert})
} |
Go | bettercap/tls/sign.go | package tls
import (
"crypto/rand"
"crypto/rsa"
"crypto/tls"
"crypto/x509"
"crypto/x509/pkix"
"fmt"
"math/big"
"net"
"time"
"github.com/bettercap/bettercap/log"
)
func getServerCertificate(host string, port int) *x509.Certificate {
log.Debug("Fetching TLS certificate from %s:%d ...", host, port)
config := tls.Config{InsecureSkipVerify: true}
conn, err := tls.Dial("tcp", fmt.Sprintf("%s:%d", host, port), &config)
if err != nil {
log.Warning("Could not fetch TLS certificate from %s:%d: %s", host, port, err)
return nil
}
defer conn.Close()
state := conn.ConnectionState()
return state.PeerCertificates[0]
}
func SignCertificateForHost(ca *tls.Certificate, host string, port int) (cert *tls.Certificate, err error) {
var x509ca *x509.Certificate
var template x509.Certificate
if x509ca, err = x509.ParseCertificate(ca.Certificate[0]); err != nil {
return
}
srvCert := getServerCertificate(host, port)
if srvCert == nil {
log.Debug("Could not fetch TLS certificate, falling back to default template.")
notBefore := time.Now()
aYear := time.Duration(365*24) * time.Hour
notAfter := notBefore.Add(aYear)
serialNumberLimit := new(big.Int).Lsh(big.NewInt(1), 128)
serialNumber, err := rand.Int(rand.Reader, serialNumberLimit)
if err != nil {
return nil, err
}
template = x509.Certificate{
SerialNumber: serialNumber,
Issuer: x509ca.Subject,
Subject: pkix.Name{
Country: []string{"US"},
Locality: []string{"Scottsdale"},
Organization: []string{"GoDaddy.com, Inc."},
OrganizationalUnit: []string{"https://certs.godaddy.com/repository/"},
CommonName: "Go Daddy Secure Certificate Authority - G2",
},
NotBefore: notBefore,
NotAfter: notAfter,
KeyUsage: x509.KeyUsageKeyEncipherment | x509.KeyUsageDigitalSignature | x509.KeyUsageCertSign,
ExtKeyUsage: []x509.ExtKeyUsage{x509.ExtKeyUsageClientAuth, x509.ExtKeyUsageServerAuth},
BasicConstraintsValid: true,
}
if ip := net.ParseIP(host); ip != nil {
template.IPAddresses = append(template.IPAddresses, ip)
} else {
template.DNSNames = append(template.DNSNames, host)
}
} else {
template = x509.Certificate{
SerialNumber: srvCert.SerialNumber,
Issuer: x509ca.Subject,
Subject: srvCert.Subject,
NotBefore: srvCert.NotBefore,
NotAfter: srvCert.NotAfter,
KeyUsage: srvCert.KeyUsage,
ExtKeyUsage: srvCert.ExtKeyUsage,
IPAddresses: srvCert.IPAddresses,
DNSNames: srvCert.DNSNames,
BasicConstraintsValid: true,
}
}
var certpriv *rsa.PrivateKey
if certpriv, err = rsa.GenerateKey(rand.Reader, 1024); err != nil {
return
}
var derBytes []byte
if derBytes, err = x509.CreateCertificate(rand.Reader, &template, x509ca, &certpriv.PublicKey, ca.PrivateKey); err != nil {
return
}
return &tls.Certificate{
Certificate: [][]byte{derBytes, ca.Certificate[0]},
PrivateKey: certpriv,
}, nil
} |
HTML | BurpBounty/BappDescription.html | <p>This BurpSuite extension allows you, in a quick and simple way, to improve
the active and passive BurpSuite scanner by means of personalized rules
through a very intuitive graphical interface. Through an advanced search of
patterns and an improvement of the payload to send, we can create our own
issue profiles both in the active scanner and in the passive.</p>
<p>* Usage manual: <a href="https://github.com/wagiro/BurpBounty/wiki/usage">Wiki</a>.</p>
<p>* You can download profile from: <a href="https://github.com/wagiro/BurpBounty/tree/master/profiles">Github</a>.</p>
<p>* Author's on Twitter: <a href="https://twitter.com/BurpBounty">@BurpBounty</a> <a href="https://twitter.com/egarme">@egarme</a></p>
<p>* More information at: <a href="https://burpbounty.net">https://burpbounty.net</a></p>
<p>* If you need more power, I invite you to try the new <b>Burp Bounty Pro</b>, which gives you more power and automation during your manual pentests.</p> |
BurpBounty/BappManifest.bmf | Uuid: 618f0b2489564607825e93eeed8b9e0a
ExtensionType: 1
Name: Burp Bounty, Scan Check Builder
RepoName: scan-check-builder
ScreenVersion: 4.0
SerialVersion: 21
MinPlatformVersion: 0
ProOnly: True
Author: Eduardo Garcia
ShortDescription: Extend the Burp active and passive scanner by creating custom scan checks with an intuitive graphical interface.
EntryPoint: build/libs/scan-check-builder-all.jar
BuildCommand: gradle fatJar |
|
BurpBounty/build.gradle | apply plugin: 'java'
repositories {
mavenCentral()
}
dependencies {
compile 'net.portswigger.burp.extender:burp-extender-api:1.7.13'
compile group: 'com.google.code.gson', name: 'gson', version: '2.8.5'
}
targetCompatibility = '1.8'
sourceCompatibility = '1.8'
sourceSets {
main {
java {
srcDir 'src'
}
}
}
task fatJar(type: Jar) {
baseName = project.name + '-all'
from { configurations.compile.collect { it.isDirectory() ? it : zipTree(it) } }
with jar
} |
|
Markdown | BurpBounty/CHANGELOG.md | ## Changelog
**4.0.0 20210902**
* Burp Bounty Pro 1.6 core
* Quick issue alert
* More options for create profiles
**3.6.0 20201005**
* Fixed bug with Match And Replace
* Fixed bug with content-type detection
* Fixed regex bug that contains commas
**3.5.0 20201001**
* Fixed bug with Payload and Payload without encode match type
* Changed the default directory from user.dir to user.home
* Added <payload> and <grep> variables for printing issue details in Advisory.
* Fixed regex grep case sensitive
* Better redirection performance
**3.4.0 20200621**
* Fixed bug with delete button on windows systems
* Fixed bug with Java array
**3.3.0 20200607**
* Fixed bug with windows systems
* New look and feel
* A lot of code rewritten for further optimization
**3.2.0 20200513**
* You can filter profiles by type
* Better and faster scroll
* New look and feel
* A lot of code rewritten for further optimization
* BurpCollaborator has been improved to use fewer hosts
**3.1.0 20200407**
* Fixed 12 issues from github
* Better performance of the match algorithm
* "Path discovery" option has been improved
* Profiles adapted to the new version
**3.0.6beta 20190819**
* Fixed error with tab
* Fixed error with status code
**3.0.5beta 20190612**
* Choose insertion points type for one profile
* Fixed error with redirections
**3.0.4beta 20190217**
* "Path discovery" feature add new insertion points
* New tags for extract matches and better issue documentation
* Variations/Invariations match type feature
* Algorithm optimization
* New profiles added
**3.0.3beta 20190206**
* Add Match and Replace feature
* Delete Collaborator button (now start automatically)
* Improve Collaborator Thread
* Some minor improvements
**3.0.2beta 20181217**
* Fixed error with comma separated
**3.0.1beta 20181207**
* Fixed error with timeout
**3.0.0beta 20181204**
* New multi-tab look and feel
* Passive scanner for requests
* Content-length comparer (for blindSQLi, etc.)
* Tags system for organize your profiles
* New BurpCollaborator Interaction
* New Profile Manager
* Deleted “not in cookie” functionality
* Some minor improvements
**2.3 20181029**
* Improved profile manager
* Fixed some minor problems
**2.2 20181026**
* Fixed some minor problems
**2.1 20181024**
* Replace strings in payloads
* Field to put profile authors information
* Timeout option for blind vulns
* Multiple lines bb json file
**2.0 20181020**
* Add the burpcollaborator support
* Follow redirects and how many to follow
* Payload append or replace
* Space encoding choose
* Response codes to avoid
* Content type to avoid
**1.2 20180607**
- Solved bug with payload space
- Add "Exclude HTTP Headers" feature
- Add "Only in HTTP Headers" feature
**1.1 20180606**
- Some improvements for integrate with the BApps Store
**1.0 20180531**
- First public release |
Markdown | BurpBounty/Changelog_3.0.4beta.md | # Improvements Burp Bounty 3.0.4beta:
### "Path discovery" feature add new insertion points
New insertion points are added to the requests (To discover hidden files and directories), when you check the "Path Discovery" option in "Payload Options" section. For example in the request:
GET /dir1/dir2/file.php?param=value HTTP/1.1
Generate three new Insertion points:
1- GET {HERE} HTTP/1.1<br>
2- GET /dir1{HERE} HTTP/1.1<br>
3- GET /dir1/dir2{HERE} HTTP/1.1<br>
Then, if you put in payload /.git/HEAD, the three new request are:
1- GET /.git/HEAD HTTP/1.1<br>
2- GET /dir1/.git/HEAD HTTP/1.1<br>
3- GET /dir1/dir2/.git/HEAD HTTP/1.1<br>
without param=value.
Another example, in request:
GET / HTTP/1.1<br>
Generate one new insertion point:
1- GET {HERE} HTTP/1.1<br>
Then, if you put in payload "/assets../static/app.js", the one new request are:
1- GET /assets../static/app.js HTTP/1.1<br>
<br>
For discover some useful files or directories:

### New tags for extract matches and better issue documentation
All the matches of the requests and responses are highlighted. You can extract the matches of the requests and responses to the issuedetail, through the <payload> tags for the payloads and <grep> for the greps. It's useful for example, for extract endpoint from regex through passive scanner:

### Variations/Invariations match type feature
You can add issues by checking Variations/Invariations between the base response, and each payoad response. I have 31 different attributes for this(the names of the attributes are quite descriptive):

### Algorithm optimization
Improved some algorithms for better performance.
### New profiles added
Various profiles was added in profiles directory |
Markdown | BurpBounty/Changelog_3.0.5beta.md | # Improvements Burp Bounty 3.0.5beta:
### Choose insertion points type for one profile
For better optimization, now you can choose the insertion point type for one profile. For example, for discover new application paths, you only will choose the "Path discover" insertion point type, avoiding other innecesaries requests.

### Fixed error with redirections
In some cases the regex for redirection can cause 100% of the CPU usage. |
BurpBounty/LICENCE | Apache License
Version 2.0, January 2004
http://www.apache.org/licenses/
TERMS AND CONDITIONS FOR USE, REPRODUCTION, AND DISTRIBUTION
1. Definitions.
"License" shall mean the terms and conditions for use, reproduction,
and distribution as defined by Sections 1 through 9 of this document.
"Licensor" shall mean the copyright owner or entity authorized by
the copyright owner that is granting the License.
"Legal Entity" shall mean the union of the acting entity and all
other entities that control, are controlled by, or are under common
control with that entity. For the purposes of this definition,
"control" means (i) the power, direct or indirect, to cause the
direction or management of such entity, whether by contract or
otherwise, or (ii) ownership of fifty percent (50%) or more of the
outstanding shares, or (iii) beneficial ownership of such entity.
"You" (or "Your") shall mean an individual or Legal Entity
exercising permissions granted by this License.
"Source" form shall mean the preferred form for making modifications,
including but not limited to software source code, documentation
source, and configuration files.
"Object" form shall mean any form resulting from mechanical
transformation or translation of a Source form, including but
not limited to compiled object code, generated documentation,
and conversions to other media types.
"Work" shall mean the work of authorship, whether in Source or
Object form, made available under the License, as indicated by a
copyright notice that is included in or attached to the work
(an example is provided in the Appendix below).
"Derivative Works" shall mean any work, whether in Source or Object
form, that is based on (or derived from) the Work and for which the
editorial revisions, annotations, elaborations, or other modifications
represent, as a whole, an original work of authorship. For the purposes
of this License, Derivative Works shall not include works that remain
separable from, or merely link (or bind by name) to the interfaces of,
the Work and Derivative Works thereof.
"Contribution" shall mean any work of authorship, including
the original version of the Work and any modifications or additions
to that Work or Derivative Works thereof, that is intentionally
submitted to Licensor for inclusion in the Work by the copyright owner
or by an individual or Legal Entity authorized to submit on behalf of
the copyright owner. For the purposes of this definition, "submitted"
means any form of electronic, verbal, or written communication sent
to the Licensor or its representatives, including but not limited to
communication on electronic mailing lists, source code control systems,
and issue tracking systems that are managed by, or on behalf of, the
Licensor for the purpose of discussing and improving the Work, but
excluding communication that is conspicuously marked or otherwise
designated in writing by the copyright owner as "Not a Contribution."
"Contributor" shall mean Licensor and any individual or Legal Entity
on behalf of whom a Contribution has been received by Licensor and
subsequently incorporated within the Work.
2. Grant of Copyright License. Subject to the terms and conditions of
this License, each Contributor hereby grants to You a perpetual,
worldwide, non-exclusive, no-charge, royalty-free, irrevocable
copyright license to reproduce, prepare Derivative Works of,
publicly display, publicly perform, sublicense, and distribute the
Work and such Derivative Works in Source or Object form.
3. Grant of Patent License. Subject to the terms and conditions of
this License, each Contributor hereby grants to You a perpetual,
worldwide, non-exclusive, no-charge, royalty-free, irrevocable
(except as stated in this section) patent license to make, have made,
use, offer to sell, sell, import, and otherwise transfer the Work,
where such license applies only to those patent claims licensable
by such Contributor that are necessarily infringed by their
Contribution(s) alone or by combination of their Contribution(s)
with the Work to which such Contribution(s) was submitted. If You
institute patent litigation against any entity (including a
cross-claim or counterclaim in a lawsuit) alleging that the Work
or a Contribution incorporated within the Work constitutes direct
or contributory patent infringement, then any patent licenses
granted to You under this License for that Work shall terminate
as of the date such litigation is filed.
4. Redistribution. You may reproduce and distribute copies of the
Work or Derivative Works thereof in any medium, with or without
modifications, and in Source or Object form, provided that You
meet the following conditions:
(a) You must give any other recipients of the Work or
Derivative Works a copy of this License; and
(b) You must cause any modified files to carry prominent notices
stating that You changed the files; and
(c) You must retain, in the Source form of any Derivative Works
that You distribute, all copyright, patent, trademark, and
attribution notices from the Source form of the Work,
excluding those notices that do not pertain to any part of
the Derivative Works; and
(d) If the Work includes a "NOTICE" text file as part of its
distribution, then any Derivative Works that You distribute must
include a readable copy of the attribution notices contained
within such NOTICE file, excluding those notices that do not
pertain to any part of the Derivative Works, in at least one
of the following places: within a NOTICE text file distributed
as part of the Derivative Works; within the Source form or
documentation, if provided along with the Derivative Works; or,
within a display generated by the Derivative Works, if and
wherever such third-party notices normally appear. The contents
of the NOTICE file are for informational purposes only and
do not modify the License. You may add Your own attribution
notices within Derivative Works that You distribute, alongside
or as an addendum to the NOTICE text from the Work, provided
that such additional attribution notices cannot be construed
as modifying the License.
You may add Your own copyright statement to Your modifications and
may provide additional or different license terms and conditions
for use, reproduction, or distribution of Your modifications, or
for any such Derivative Works as a whole, provided Your use,
reproduction, and distribution of the Work otherwise complies with
the conditions stated in this License.
5. Submission of Contributions. Unless You explicitly state otherwise,
any Contribution intentionally submitted for inclusion in the Work
by You to the Licensor shall be under the terms and conditions of
this License, without any additional terms or conditions.
Notwithstanding the above, nothing herein shall supersede or modify
the terms of any separate license agreement you may have executed
with Licensor regarding such Contributions.
6. Trademarks. This License does not grant permission to use the trade
names, trademarks, service marks, or product names of the Licensor,
except as required for reasonable and customary use in describing the
origin of the Work and reproducing the content of the NOTICE file.
7. Disclaimer of Warranty. Unless required by applicable law or
agreed to in writing, Licensor provides the Work (and each
Contributor provides its Contributions) on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
implied, including, without limitation, any warranties or conditions
of TITLE, NON-INFRINGEMENT, MERCHANTABILITY, or FITNESS FOR A
PARTICULAR PURPOSE. You are solely responsible for determining the
appropriateness of using or redistributing the Work and assume any
risks associated with Your exercise of permissions under this License.
8. Limitation of Liability. In no event and under no legal theory,
whether in tort (including negligence), contract, or otherwise,
unless required by applicable law (such as deliberate and grossly
negligent acts) or agreed to in writing, shall any Contributor be
liable to You for damages, including any direct, indirect, special,
incidental, or consequential damages of any character arising as a
result of this License or out of the use or inability to use the
Work (including but not limited to damages for loss of goodwill,
work stoppage, computer failure or malfunction, or any and all
other commercial damages or losses), even if such Contributor
has been advised of the possibility of such damages.
9. Accepting Warranty or Additional Liability. While redistributing
the Work or Derivative Works thereof, You may choose to offer,
and charge a fee for, acceptance of support, warranty, indemnity,
or other liability obligations and/or rights consistent with this
License. However, in accepting such obligations, You may act only
on Your own behalf and on Your sole responsibility, not on behalf
of any other Contributor, and only if You agree to indemnify,
defend, and hold each Contributor harmless for any liability
incurred by, or claims asserted against, such Contributor by reason
of your accepting any such warranty or additional liability.
END OF TERMS AND CONDITIONS
APPENDIX: How to apply the Apache License to your work.
To apply the Apache License to your work, attach the following
boilerplate notice, with the fields enclosed by brackets "[]"
replaced with your own identifying information. (Don't include
the brackets!) The text should be enclosed in the appropriate
comment syntax for the file format. We also recommend that a
file or class name and description of purpose be included on the
same "printed page" as the copyright notice for easier
identification within third-party archives.
Copyright 2018 Eduardo Garcia Melia
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License. |
|
Markdown | BurpBounty/README.md | [](https://github.com/wagiro/BurpBounty/releases)
[](https://github.com/wagiro/BurpBounty/issues)
[]()
[](https://github.com/wagiro/BurpBounty/)
[](https://github.com/wagiro/BurpBounty/)
[](https://twitter.com/intent/follow?screen_name=burpbounty)
# Burp Bounty Free - Scan Check Builder (BApp Store)
This Burp Suite extension allows you, in a quick and simple way, to improve the active and passive burpsuite scanner by means of personalized rules through a very intuitive graphical interface. Through an advanced search of patterns and an improvement of the payload to send, we can create our own issue profiles both in the active scanner and in the passive.<br/>
Download releases:
* https://github.com/wagiro/BurpBounty/releases/
<br/>If you need more power, I invite you to try the new <b>Burp Bounty Pro</b>, which gives you more power and automation during your manual pentests.
<br/>More information at: [https://burpbounty.net](https://burpbounty.net) and [Burp Bounty Pro vs Free](https://burpbounty.net/burp-bounty-pro-vs-free/).
## Usage
* Go to [Usage](https://github.com/wagiro/BurpBounty/wiki/usage) section or the slides of [Ekoparty Security Conference](https://burpbounty.net/burp-bounty-ekoparty-2020/).
## Profiles
* Thanks to [Six2dez1](https://github.com/six2dez) for collect all of the Burp Bounty profiles and also share their own. You can find the collection [HERE](https://github.com/wagiro/BurpBounty/tree/master/profiles/)
* Also thanks to:
- [Xer0Days](https://twitter.com/Xer0Days)
- [Gocha](https://twitter.com/GochaOqradze)
- [Sy3Omda](https://twitter.com/Sy3Omda)
- [Syed](https://twitter.com/syed__umar)
- [n00py1](https://twitter.com/n00py1)
- [legik](https://github.com/legik)
All of them have contributed by sharing their Burp Bounty profiles
### For example videos please visit our youtube channel:
* [YouTube](https://www.youtube.com/channel/UCSq4R2o9_nGIMHWZ4H98GkQ/videos)
<br/>
**Blind RCE with BurpBounty using Burp Collaborator<br/>**
[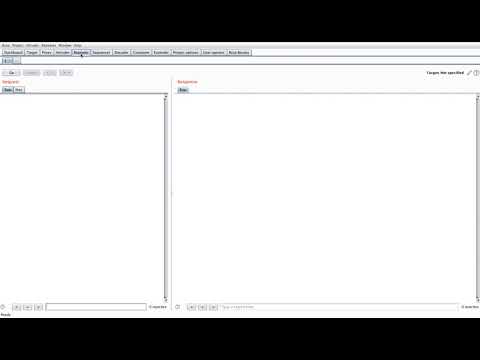](https://www.youtube.com/watch?v=kcyUueb56aM)
<br/>
## Special Thanks
* Thanks to [Nexsus](https://twitter.com/Nexsus1985) for helping me to detect errors in the extension and thus be able to solve them. |
Java | BurpBounty/main/java/burp/BurpExtender.java | package burp;
import burpbountyfree.BurpBountyExtension;
public class BurpExtender extends BurpBountyExtension {
} |
Java | BurpBounty/main/java/burp/IBurpCollaboratorClientContext.java | package burp;
/*
* @(#)IBurpCollaboratorClientContext.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
import java.util.List;
/**
* This interface represents an instance of a Burp Collaborator client context,
* which can be used to generate Burp Collaborator payloads and poll the
* Collaborator server for any network interactions that result from using those
* payloads. Extensions can obtain new instances of this class by calling
* <code>IBurpExtenderCallbacks.createBurpCollaboratorClientContext()</code>.
* Note that each Burp Collaborator client context is tied to the Collaborator
* server configuration that was in place at the time the context was created.
*/
public interface IBurpCollaboratorClientContext
{
/**
* This method is used to generate new Burp Collaborator payloads.
*
* @param includeCollaboratorServerLocation Specifies whether to include the
* Collaborator server location in the generated payload.
* @return The payload that was generated.
*/
String generatePayload(boolean includeCollaboratorServerLocation);
/**
* This method is used to retrieve all interactions received by the
* Collaborator server resulting from payloads that were generated for this
* context.
*
* @return The Collaborator interactions that have occurred resulting from
* payloads that were generated for this context.
*/
List<IBurpCollaboratorInteraction> fetchAllCollaboratorInteractions();
/**
* This method is used to retrieve interactions received by the Collaborator
* server resulting from a single payload that was generated for this
* context.
*
* @param payload The payload for which interactions will be retrieved.
* @return The Collaborator interactions that have occurred resulting from
* the given payload.
*/
List<IBurpCollaboratorInteraction> fetchCollaboratorInteractionsFor(String payload);
/**
* This method is used to retrieve all interactions made by Burp Infiltrator
* instrumentation resulting from payloads that were generated for this
* context.
*
* @return The interactions triggered by the Burp Infiltrator
* instrumentation that have occurred resulting from payloads that were
* generated for this context.
*/
List<IBurpCollaboratorInteraction> fetchAllInfiltratorInteractions();
/**
* This method is used to retrieve interactions made by Burp Infiltrator
* instrumentation resulting from a single payload that was generated for
* this context.
*
* @param payload The payload for which interactions will be retrieved.
* @return The interactions triggered by the Burp Infiltrator
* instrumentation that have occurred resulting from the given payload.
*/
List<IBurpCollaboratorInteraction> fetchInfiltratorInteractionsFor(String payload);
/**
* This method is used to retrieve the network location of the Collaborator
* server.
*
* @return The hostname or IP address of the Collaborator server.
*/
String getCollaboratorServerLocation();
} |
Java | BurpBounty/main/java/burp/IBurpCollaboratorInteraction.java | package burp;
/*
* @(#)IBurpCollaboratorInteraction.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
import java.util.Map;
/**
* This interface represents a network interaction that occurred with the Burp
* Collaborator server.
*/
public interface IBurpCollaboratorInteraction
{
/**
* This method is used to retrieve a property of the interaction. Properties
* of all interactions are: interaction_id, type, client_ip, and time_stamp.
* Properties of DNS interactions are: query_type and raw_query. The
* raw_query value is Base64-encoded. Properties of HTTP interactions are:
* protocol, request, and response. The request and response values are
* Base64-encoded.
*
* @param name The name of the property to retrieve.
* @return A string representing the property value, or null if not present.
*/
String getProperty(String name);
/**
* This method is used to retrieve a map containing all properties of the
* interaction.
*
* @return A map containing all properties of the interaction.
*/
Map<String, String> getProperties();
} |
Java | BurpBounty/main/java/burp/IBurpExtender.java | package burp;
/*
* @(#)IBurpExtender.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* All extensions must implement this interface.
*
* Implementations must be called BurpExtender, in the package burp, must be
* declared public, and must provide a default (public, no-argument)
* constructor.
*/
public interface IBurpExtender
{
/**
* This method is invoked when the extension is loaded. It registers an
* instance of the
* <code>IBurpExtenderCallbacks</code> interface, providing methods that may
* be invoked by the extension to perform various actions.
*
* @param callbacks An
* <code>IBurpExtenderCallbacks</code> object.
*/
void registerExtenderCallbacks(IBurpExtenderCallbacks callbacks);
} |
Java | BurpBounty/main/java/burp/IBurpExtenderCallbacks.java | package burp;
/*
* @(#)IBurpExtenderCallbacks.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
import java.awt.Component;
import java.io.OutputStream;
import java.util.List;
import java.util.Map;
/**
* This interface is used by Burp Suite to pass to extensions a set of callback
* methods that can be used by extensions to perform various actions within
* Burp.
*
* When an extension is loaded, Burp invokes its
* <code>registerExtenderCallbacks()</code> method and passes an instance of the
* <code>IBurpExtenderCallbacks</code> interface. The extension may then invoke
* the methods of this interface as required in order to extend Burp's
* functionality.
*/
public interface IBurpExtenderCallbacks
{
/**
* Flag used to identify Burp Suite as a whole.
*/
int TOOL_SUITE = 0x00000001;
/**
* Flag used to identify the Burp Target tool.
*/
int TOOL_TARGET = 0x00000002;
/**
* Flag used to identify the Burp Proxy tool.
*/
int TOOL_PROXY = 0x00000004;
/**
* Flag used to identify the Burp Spider tool.
*/
int TOOL_SPIDER = 0x00000008;
/**
* Flag used to identify the Burp Scanner tool.
*/
int TOOL_SCANNER = 0x00000010;
/**
* Flag used to identify the Burp Intruder tool.
*/
int TOOL_INTRUDER = 0x00000020;
/**
* Flag used to identify the Burp Repeater tool.
*/
int TOOL_REPEATER = 0x00000040;
/**
* Flag used to identify the Burp Sequencer tool.
*/
int TOOL_SEQUENCER = 0x00000080;
/**
* Flag used to identify the Burp Decoder tool.
*/
int TOOL_DECODER = 0x00000100;
/**
* Flag used to identify the Burp Comparer tool.
*/
int TOOL_COMPARER = 0x00000200;
/**
* Flag used to identify the Burp Extender tool.
*/
int TOOL_EXTENDER = 0x00000400;
/**
* This method is used to set the display name for the current extension,
* which will be displayed within the user interface for the Extender tool.
*
* @param name The extension name.
*/
void setExtensionName(String name);
/**
* This method is used to obtain an <code>IExtensionHelpers</code> object,
* which can be used by the extension to perform numerous useful tasks.
*
* @return An object containing numerous helper methods, for tasks such as
* building and analyzing HTTP requests.
*/
IExtensionHelpers getHelpers();
/**
* This method is used to obtain the current extension's standard output
* stream. Extensions should write all output to this stream, allowing the
* Burp user to configure how that output is handled from within the UI.
*
* @return The extension's standard output stream.
*/
OutputStream getStdout();
/**
* This method is used to obtain the current extension's standard error
* stream. Extensions should write all error messages to this stream,
* allowing the Burp user to configure how that output is handled from
* within the UI.
*
* @return The extension's standard error stream.
*/
OutputStream getStderr();
/**
* This method prints a line of output to the current extension's standard
* output stream.
*
* @param output The message to print.
*/
void printOutput(String output);
/**
* This method prints a line of output to the current extension's standard
* error stream.
*
* @param error The message to print.
*/
void printError(String error);
/**
* This method is used to register a listener which will be notified of
* changes to the extension's state. <b>Note:</b> Any extensions that start
* background threads or open system resources (such as files or database
* connections) should register a listener and terminate threads / close
* resources when the extension is unloaded.
*
* @param listener An object created by the extension that implements the
* <code>IExtensionStateListener</code> interface.
*/
void registerExtensionStateListener(IExtensionStateListener listener);
/**
* This method is used to retrieve the extension state listeners that are
* registered by the extension.
*
* @return A list of extension state listeners that are currently registered
* by this extension.
*/
List<IExtensionStateListener> getExtensionStateListeners();
/**
* This method is used to remove an extension state listener that has been
* registered by the extension.
*
* @param listener The extension state listener to be removed.
*/
void removeExtensionStateListener(IExtensionStateListener listener);
/**
* This method is used to register a listener which will be notified of
* requests and responses made by any Burp tool. Extensions can perform
* custom analysis or modification of these messages by registering an HTTP
* listener.
*
* @param listener An object created by the extension that implements the
* <code>IHttpListener</code> interface.
*/
void registerHttpListener(IHttpListener listener);
/**
* This method is used to retrieve the HTTP listeners that are registered by
* the extension.
*
* @return A list of HTTP listeners that are currently registered by this
* extension.
*/
List<IHttpListener> getHttpListeners();
/**
* This method is used to remove an HTTP listener that has been registered
* by the extension.
*
* @param listener The HTTP listener to be removed.
*/
void removeHttpListener(IHttpListener listener);
/**
* This method is used to register a listener which will be notified of
* requests and responses being processed by the Proxy tool. Extensions can
* perform custom analysis or modification of these messages, and control
* in-UI message interception, by registering a proxy listener.
*
* @param listener An object created by the extension that implements the
* <code>IProxyListener</code> interface.
*/
void registerProxyListener(IProxyListener listener);
/**
* This method is used to retrieve the Proxy listeners that are registered
* by the extension.
*
* @return A list of Proxy listeners that are currently registered by this
* extension.
*/
List<IProxyListener> getProxyListeners();
/**
* This method is used to remove a Proxy listener that has been registered
* by the extension.
*
* @param listener The Proxy listener to be removed.
*/
void removeProxyListener(IProxyListener listener);
/**
* This method is used to register a listener which will be notified of new
* issues that are reported by the Scanner tool. Extensions can perform
* custom analysis or logging of Scanner issues by registering a Scanner
* listener.
*
* @param listener An object created by the extension that implements the
* <code>IScannerListener</code> interface.
*/
void registerScannerListener(IScannerListener listener);
/**
* This method is used to retrieve the Scanner listeners that are registered
* by the extension.
*
* @return A list of Scanner listeners that are currently registered by this
* extension.
*/
List<IScannerListener> getScannerListeners();
/**
* This method is used to remove a Scanner listener that has been registered
* by the extension.
*
* @param listener The Scanner listener to be removed.
*/
void removeScannerListener(IScannerListener listener);
/**
* This method is used to register a listener which will be notified of
* changes to Burp's suite-wide target scope.
*
* @param listener An object created by the extension that implements the
* <code>IScopeChangeListener</code> interface.
*/
void registerScopeChangeListener(IScopeChangeListener listener);
/**
* This method is used to retrieve the scope change listeners that are
* registered by the extension.
*
* @return A list of scope change listeners that are currently registered by
* this extension.
*/
List<IScopeChangeListener> getScopeChangeListeners();
/**
* This method is used to remove a scope change listener that has been
* registered by the extension.
*
* @param listener The scope change listener to be removed.
*/
void removeScopeChangeListener(IScopeChangeListener listener);
/**
* This method is used to register a factory for custom context menu items.
* When the user invokes a context menu anywhere within Burp, the factory
* will be passed details of the invocation event, and asked to provide any
* custom context menu items that should be shown.
*
* @param factory An object created by the extension that implements the
* <code>IContextMenuFactory</code> interface.
*/
void registerContextMenuFactory(IContextMenuFactory factory);
/**
* This method is used to retrieve the context menu factories that are
* registered by the extension.
*
* @return A list of context menu factories that are currently registered by
* this extension.
*/
List<IContextMenuFactory> getContextMenuFactories();
/**
* This method is used to remove a context menu factory that has been
* registered by the extension.
*
* @param factory The context menu factory to be removed.
*/
void removeContextMenuFactory(IContextMenuFactory factory);
/**
* This method is used to register a factory for custom message editor tabs.
* For each message editor that already exists, or is subsequently created,
* within Burp, the factory will be asked to provide a new instance of an
* <code>IMessageEditorTab</code> object, which can provide custom rendering
* or editing of HTTP messages.
*
* @param factory An object created by the extension that implements the
* <code>IMessageEditorTabFactory</code> interface.
*/
void registerMessageEditorTabFactory(IMessageEditorTabFactory factory);
/**
* This method is used to retrieve the message editor tab factories that are
* registered by the extension.
*
* @return A list of message editor tab factories that are currently
* registered by this extension.
*/
List<IMessageEditorTabFactory> getMessageEditorTabFactories();
/**
* This method is used to remove a message editor tab factory that has been
* registered by the extension.
*
* @param factory The message editor tab factory to be removed.
*/
void removeMessageEditorTabFactory(IMessageEditorTabFactory factory);
/**
* This method is used to register a provider of Scanner insertion points.
* For each base request that is actively scanned, Burp will ask the
* provider to provide any custom scanner insertion points that are
* appropriate for the request.
*
* @param provider An object created by the extension that implements the
* <code>IScannerInsertionPointProvider</code> interface.
*/
void registerScannerInsertionPointProvider(
IScannerInsertionPointProvider provider);
/**
* This method is used to retrieve the Scanner insertion point providers
* that are registered by the extension.
*
* @return A list of Scanner insertion point providers that are currently
* registered by this extension.
*/
List<IScannerInsertionPointProvider> getScannerInsertionPointProviders();
/**
* This method is used to remove a Scanner insertion point provider that has
* been registered by the extension.
*
* @param provider The Scanner insertion point provider to be removed.
*/
void removeScannerInsertionPointProvider(
IScannerInsertionPointProvider provider);
/**
* This method is used to register a custom Scanner check. When performing
* scanning, Burp will ask the check to perform active or passive scanning
* on the base request, and report any Scanner issues that are identified.
*
* @param check An object created by the extension that implements the
* <code>IScannerCheck</code> interface.
*/
void registerScannerCheck(IScannerCheck check);
/**
* This method is used to retrieve the Scanner checks that are registered by
* the extension.
*
* @return A list of Scanner checks that are currently registered by this
* extension.
*/
List<IScannerCheck> getScannerChecks();
/**
* This method is used to remove a Scanner check that has been registered by
* the extension.
*
* @param check The Scanner check to be removed.
*/
void removeScannerCheck(IScannerCheck check);
/**
* This method is used to register a factory for Intruder payloads. Each
* registered factory will be available within the Intruder UI for the user
* to select as the payload source for an attack. When this is selected, the
* factory will be asked to provide a new instance of an
* <code>IIntruderPayloadGenerator</code> object, which will be used to
* generate payloads for the attack.
*
* @param factory An object created by the extension that implements the
* <code>IIntruderPayloadGeneratorFactory</code> interface.
*/
void registerIntruderPayloadGeneratorFactory(
IIntruderPayloadGeneratorFactory factory);
/**
* This method is used to retrieve the Intruder payload generator factories
* that are registered by the extension.
*
* @return A list of Intruder payload generator factories that are currently
* registered by this extension.
*/
List<IIntruderPayloadGeneratorFactory>
getIntruderPayloadGeneratorFactories();
/**
* This method is used to remove an Intruder payload generator factory that
* has been registered by the extension.
*
* @param factory The Intruder payload generator factory to be removed.
*/
void removeIntruderPayloadGeneratorFactory(
IIntruderPayloadGeneratorFactory factory);
/**
* This method is used to register a custom Intruder payload processor. Each
* registered processor will be available within the Intruder UI for the
* user to select as the action for a payload processing rule.
*
* @param processor An object created by the extension that implements the
* <code>IIntruderPayloadProcessor</code> interface.
*/
void registerIntruderPayloadProcessor(IIntruderPayloadProcessor processor);
/**
* This method is used to retrieve the Intruder payload processors that are
* registered by the extension.
*
* @return A list of Intruder payload processors that are currently
* registered by this extension.
*/
List<IIntruderPayloadProcessor> getIntruderPayloadProcessors();
/**
* This method is used to remove an Intruder payload processor that has been
* registered by the extension.
*
* @param processor The Intruder payload processor to be removed.
*/
void removeIntruderPayloadProcessor(IIntruderPayloadProcessor processor);
/**
* This method is used to register a custom session handling action. Each
* registered action will be available within the session handling rule UI
* for the user to select as a rule action. Users can choose to invoke an
* action directly in its own right, or following execution of a macro.
*
* @param action An object created by the extension that implements the
* <code>ISessionHandlingAction</code> interface.
*/
void registerSessionHandlingAction(ISessionHandlingAction action);
/**
* This method is used to retrieve the session handling actions that are
* registered by the extension.
*
* @return A list of session handling actions that are currently registered
* by this extension.
*/
List<ISessionHandlingAction> getSessionHandlingActions();
/**
* This method is used to remove a session handling action that has been
* registered by the extension.
*
* @param action The extension session handling action to be removed.
*/
void removeSessionHandlingAction(ISessionHandlingAction action);
/**
* This method is used to unload the extension from Burp Suite.
*/
void unloadExtension();
/**
* This method is used to add a custom tab to the main Burp Suite window.
*
* @param tab An object created by the extension that implements the
* <code>ITab</code> interface.
*/
void addSuiteTab(ITab tab);
/**
* This method is used to remove a previously-added tab from the main Burp
* Suite window.
*
* @param tab An object created by the extension that implements the
* <code>ITab</code> interface.
*/
void removeSuiteTab(ITab tab);
/**
* This method is used to customize UI components in line with Burp's UI
* style, including font size, colors, table line spacing, etc. The action
* is performed recursively on any child components of the passed-in
* component.
*
* @param component The UI component to be customized.
*/
void customizeUiComponent(Component component);
/**
* This method is used to create a new instance of Burp's HTTP message
* editor, for the extension to use in its own UI.
*
* @param controller An object created by the extension that implements the
* <code>IMessageEditorController</code> interface. This parameter is
* optional and may be <code>null</code>. If it is provided, then the
* message editor will query the controller when required to obtain details
* about the currently displayed message, including the
* <code>IHttpService</code> for the message, and the associated request or
* response message. If a controller is not provided, then the message
* editor will not support context menu actions, such as sending requests to
* other Burp tools.
* @param editable Indicates whether the editor created should be editable,
* or used only for message viewing.
* @return An object that implements the <code>IMessageEditor</code>
* interface, and which the extension can use in its own UI.
*/
IMessageEditor createMessageEditor(IMessageEditorController controller,
boolean editable);
/**
* This method returns the command line arguments that were passed to Burp
* on startup.
*
* @return The command line arguments that were passed to Burp on startup.
*/
String[] getCommandLineArguments();
/**
* This method is used to save configuration settings for the extension in a
* persistent way that survives reloads of the extension and of Burp Suite.
* Saved settings can be retrieved using the method
* <code>loadExtensionSetting()</code>.
*
* @param name The name of the setting.
* @param value The value of the setting. If this value is <code>null</code>
* then any existing setting with the specified name will be removed.
*/
void saveExtensionSetting(String name, String value);
/**
* This method is used to load configuration settings for the extension that
* were saved using the method <code>saveExtensionSetting()</code>.
*
* @param name The name of the setting.
* @return The value of the setting, or <code>null</code> if no value is
* set.
*/
String loadExtensionSetting(String name);
/**
* This method is used to create a new instance of Burp's plain text editor,
* for the extension to use in its own UI.
*
* @return An object that implements the <code>ITextEditor</code> interface,
* and which the extension can use in its own UI.
*/
ITextEditor createTextEditor();
/**
* This method can be used to send an HTTP request to the Burp Repeater
* tool. The request will be displayed in the user interface, but will not
* be issued until the user initiates this action.
*
* @param host The hostname of the remote HTTP server.
* @param port The port of the remote HTTP server.
* @param useHttps Flags whether the protocol is HTTPS or HTTP.
* @param request The full HTTP request.
* @param tabCaption An optional caption which will appear on the Repeater
* tab containing the request. If this value is <code>null</code> then a
* default tab index will be displayed.
*/
void sendToRepeater(
String host,
int port,
boolean useHttps,
byte[] request,
String tabCaption);
/**
* This method can be used to send an HTTP request to the Burp Intruder
* tool. The request will be displayed in the user interface, and markers
* for attack payloads will be placed into default locations within the
* request.
*
* @param host The hostname of the remote HTTP server.
* @param port The port of the remote HTTP server.
* @param useHttps Flags whether the protocol is HTTPS or HTTP.
* @param request The full HTTP request.
*/
void sendToIntruder(
String host,
int port,
boolean useHttps,
byte[] request);
/**
* This method can be used to send an HTTP request to the Burp Intruder
* tool. The request will be displayed in the user interface, and markers
* for attack payloads will be placed into the specified locations within
* the request.
*
* @param host The hostname of the remote HTTP server.
* @param port The port of the remote HTTP server.
* @param useHttps Flags whether the protocol is HTTPS or HTTP.
* @param request The full HTTP request.
* @param payloadPositionOffsets A list of index pairs representing the
* payload positions to be used. Each item in the list must be an int[2]
* array containing the start and end offsets for the payload position.
*/
void sendToIntruder(
String host,
int port,
boolean useHttps,
byte[] request,
List<int[]> payloadPositionOffsets);
/**
* This method can be used to send data to the Comparer tool.
*
* @param data The data to be sent to Comparer.
*/
void sendToComparer(byte[] data);
/**
* This method can be used to send a seed URL to the Burp Spider tool. If
* the URL is not within the current Spider scope, the user will be asked if
* they wish to add the URL to the scope. If the Spider is not currently
* running, it will be started. The seed URL will be requested, and the
* Spider will process the application's response in the normal way.
*
* @param url The new seed URL to begin spidering from.
*/
void sendToSpider(
java.net.URL url);
/**
* This method can be used to send an HTTP request to the Burp Scanner tool
* to perform an active vulnerability scan. If the request is not within the
* current active scanning scope, the user will be asked if they wish to
* proceed with the scan.
*
* @param host The hostname of the remote HTTP server.
* @param port The port of the remote HTTP server.
* @param useHttps Flags whether the protocol is HTTPS or HTTP.
* @param request The full HTTP request.
* @return The resulting scan queue item.
*/
IScanQueueItem doActiveScan(
String host,
int port,
boolean useHttps,
byte[] request);
/**
* This method can be used to send an HTTP request to the Burp Scanner tool
* to perform an active vulnerability scan, based on a custom list of
* insertion points that are to be scanned. If the request is not within the
* current active scanning scope, the user will be asked if they wish to
* proceed with the scan.
*
* @param host The hostname of the remote HTTP server.
* @param port The port of the remote HTTP server.
* @param useHttps Flags whether the protocol is HTTPS or HTTP.
* @param request The full HTTP request.
* @param insertionPointOffsets A list of index pairs representing the
* positions of the insertion points that should be scanned. Each item in
* the list must be an int[2] array containing the start and end offsets for
* the insertion point.
* @return The resulting scan queue item.
*/
IScanQueueItem doActiveScan(
String host,
int port,
boolean useHttps,
byte[] request,
List<int[]> insertionPointOffsets);
/**
* This method can be used to send an HTTP request to the Burp Scanner tool
* to perform a passive vulnerability scan.
*
* @param host The hostname of the remote HTTP server.
* @param port The port of the remote HTTP server.
* @param useHttps Flags whether the protocol is HTTPS or HTTP.
* @param request The full HTTP request.
* @param response The full HTTP response.
*/
void doPassiveScan(
String host,
int port,
boolean useHttps,
byte[] request,
byte[] response);
/**
* This method can be used to issue HTTP requests and retrieve their
* responses.
*
* @param httpService The HTTP service to which the request should be sent.
* @param request The full HTTP request.
* @return An object that implements the <code>IHttpRequestResponse</code>
* interface, and which the extension can query to obtain the details of the
* response.
*/
IHttpRequestResponse makeHttpRequest(IHttpService httpService,
byte[] request);
/**
* This method can be used to issue HTTP requests and retrieve their
* responses.
*
* @param host The hostname of the remote HTTP server.
* @param port The port of the remote HTTP server.
* @param useHttps Flags whether the protocol is HTTPS or HTTP.
* @param request The full HTTP request.
* @return The full response retrieved from the remote server.
*/
byte[] makeHttpRequest(
String host,
int port,
boolean useHttps,
byte[] request);
/**
* This method can be used to query whether a specified URL is within the
* current Suite-wide scope.
*
* @param url The URL to query.
* @return Returns <code>true</code> if the URL is within the current
* Suite-wide scope.
*/
boolean isInScope(java.net.URL url);
/**
* This method can be used to include the specified URL in the Suite-wide
* scope.
*
* @param url The URL to include in the Suite-wide scope.
*/
void includeInScope(java.net.URL url);
/**
* This method can be used to exclude the specified URL from the Suite-wide
* scope.
*
* @param url The URL to exclude from the Suite-wide scope.
*/
void excludeFromScope(java.net.URL url);
/**
* This method can be used to display a specified message in the Burp Suite
* alerts tab.
*
* @param message The alert message to display.
*/
void issueAlert(String message);
/**
* This method returns details of all items in the Proxy history.
*
* @return The contents of the Proxy history.
*/
IHttpRequestResponse[] getProxyHistory();
/**
* This method returns details of items in the site map.
*
* @param urlPrefix This parameter can be used to specify a URL prefix, in
* order to extract a specific subset of the site map. The method performs a
* simple case-sensitive text match, returning all site map items whose URL
* begins with the specified prefix. If this parameter is null, the entire
* site map is returned.
*
* @return Details of items in the site map.
*/
IHttpRequestResponse[] getSiteMap(String urlPrefix);
/**
* This method returns all of the current scan issues for URLs matching the
* specified literal prefix.
*
* @param urlPrefix This parameter can be used to specify a URL prefix, in
* order to extract a specific subset of scan issues. The method performs a
* simple case-sensitive text match, returning all scan issues whose URL
* begins with the specified prefix. If this parameter is null, all issues
* are returned.
* @return Details of the scan issues.
*/
IScanIssue[] getScanIssues(String urlPrefix);
/**
* This method is used to generate a report for the specified Scanner
* issues. The report format can be specified. For all other reporting
* options, the default settings that appear in the reporting UI wizard are
* used.
*
* @param format The format to be used in the report. Accepted values are
* HTML and XML.
* @param issues The Scanner issues to be reported.
* @param file The file to which the report will be saved.
*/
void generateScanReport(String format, IScanIssue[] issues,
java.io.File file);
/**
* This method is used to retrieve the contents of Burp's session handling
* cookie jar. Extensions that provide an
* <code>ISessionHandlingAction</code> can query and update the cookie jar
* in order to handle unusual session handling mechanisms.
*
* @return A list of <code>ICookie</code> objects representing the contents
* of Burp's session handling cookie jar.
*/
List<ICookie> getCookieJarContents();
/**
* This method is used to update the contents of Burp's session handling
* cookie jar. Extensions that provide an
* <code>ISessionHandlingAction</code> can query and update the cookie jar
* in order to handle unusual session handling mechanisms.
*
* @param cookie An <code>ICookie</code> object containing details of the
* cookie to be updated. If the cookie jar already contains a cookie that
* matches the specified domain and name, then that cookie will be updated
* with the new value and expiration, unless the new value is
* <code>null</code>, in which case the cookie will be removed. If the
* cookie jar does not already contain a cookie that matches the specified
* domain and name, then the cookie will be added.
*/
void updateCookieJar(ICookie cookie);
/**
* This method can be used to add an item to Burp's site map with the
* specified request/response details. This will overwrite the details of
* any existing matching item in the site map.
*
* @param item Details of the item to be added to the site map
*/
void addToSiteMap(IHttpRequestResponse item);
/**
* This method can be used to restore Burp's state from a specified saved
* state file. This method blocks until the restore operation is completed,
* and must not be called from the event dispatch thread.
*
* @param file The file containing Burp's saved state.
* @deprecated State files have been replaced with Burp project files.
*/
@Deprecated
void restoreState(java.io.File file);
/**
* This method can be used to save Burp's state to a specified file. This
* method blocks until the save operation is completed, and must not be
* called from the event dispatch thread.
*
* @param file The file to save Burp's state in.
* @deprecated State files have been replaced with Burp project files.
*/
@Deprecated
void saveState(java.io.File file);
/**
* This method is no longer supported. Please use saveConfigAsJson() instead.
*
* @return A Map of name/value Strings reflecting Burp's current
* configuration.
* @deprecated Use <code>saveConfigAsJson()</code> instead.
*/
@Deprecated
Map<String, String> saveConfig();
/**
* This method is no longer supported. Please use loadConfigFromJson() instead.
*
* @param config A map of name/value Strings to use as Burp's new
* configuration.
* @deprecated Use <code>loadConfigFromJson()</code> instead.
*/
@Deprecated
void loadConfig(Map<String, String> config);
/**
* This method causes Burp to save its current project-level configuration
* in JSON format. This is the same format that can be saved and loaded via
* the Burp user interface. To include only certain sections of the
* configuration, you can optionally supply the path to each section that
* should be included, for example: "project_options.connections". If no
* paths are provided, then the entire configuration will be saved.
*
* @param configPaths A list of Strings representing the path to each
* configuration section that should be included.
* @return A String representing the current configuration in JSON format.
*/
String saveConfigAsJson(String... configPaths);
/**
* This method causes Burp to load a new project-level configuration from
* the JSON String provided. This is the same format that can be saved and
* loaded via the Burp user interface. Partial configurations are
* acceptable, and any settings not specified will be left unmodified.
*
* Any user-level configuration options contained in the input will be
* ignored.
*
* @param config A JSON String containing the new configuration.
*/
void loadConfigFromJson(String config);
/**
* This method sets the master interception mode for Burp Proxy.
*
* @param enabled Indicates whether interception of Proxy messages should be
* enabled.
*/
void setProxyInterceptionEnabled(boolean enabled);
/**
* This method retrieves information about the version of Burp in which the
* extension is running. It can be used by extensions to dynamically adjust
* their behavior depending on the functionality and APIs supported by the
* current version.
*
* @return An array of Strings comprised of: the product name (e.g. Burp
* Suite Professional), the major version (e.g. 1.5), the minor version
* (e.g. 03)
*/
String[] getBurpVersion();
/**
* This method retrieves the absolute path name of the file from which the
* current extension was loaded.
*
* @return The absolute path name of the file from which the current
* extension was loaded.
*/
String getExtensionFilename();
/**
* This method determines whether the current extension was loaded as a BApp
* (a Burp App from the BApp Store).
*
* @return Returns true if the current extension was loaded as a BApp.
*/
boolean isExtensionBapp();
/**
* This method can be used to shut down Burp programmatically, with an
* optional prompt to the user. If the method returns, the user canceled the
* shutdown prompt.
*
* @param promptUser Indicates whether to prompt the user to confirm the
* shutdown.
*/
void exitSuite(boolean promptUser);
/**
* This method is used to create a temporary file on disk containing the
* provided data. Extensions can use temporary files for long-term storage
* of runtime data, avoiding the need to retain that data in memory.
*
* @param buffer The data to be saved to a temporary file.
* @return An object that implements the <code>ITempFile</code> interface.
*/
ITempFile saveToTempFile(byte[] buffer);
/**
* This method is used to save the request and response of an
* <code>IHttpRequestResponse</code> object to temporary files, so that they
* are no longer held in memory. Extensions can used this method to convert
* <code>IHttpRequestResponse</code> objects into a form suitable for
* long-term storage.
*
* @param httpRequestResponse The <code>IHttpRequestResponse</code> object
* whose request and response messages are to be saved to temporary files.
* @return An object that implements the
* <code>IHttpRequestResponsePersisted</code> interface.
*/
IHttpRequestResponsePersisted saveBuffersToTempFiles(
IHttpRequestResponse httpRequestResponse);
/**
* This method is used to apply markers to an HTTP request or response, at
* offsets into the message that are relevant for some particular purpose.
* Markers are used in various situations, such as specifying Intruder
* payload positions, Scanner insertion points, and highlights in Scanner
* issues.
*
* @param httpRequestResponse The <code>IHttpRequestResponse</code> object
* to which the markers should be applied.
* @param requestMarkers A list of index pairs representing the offsets of
* markers to be applied to the request message. Each item in the list must
* be an int[2] array containing the start and end offsets for the marker.
* The markers in the list should be in sequence and not overlapping. This
* parameter is optional and may be <code>null</code> if no request markers
* are required.
* @param responseMarkers A list of index pairs representing the offsets of
* markers to be applied to the response message. Each item in the list must
* be an int[2] array containing the start and end offsets for the marker.
* The markers in the list should be in sequence and not overlapping. This
* parameter is optional and may be <code>null</code> if no response markers
* are required.
* @return An object that implements the
* <code>IHttpRequestResponseWithMarkers</code> interface.
*/
IHttpRequestResponseWithMarkers applyMarkers(
IHttpRequestResponse httpRequestResponse,
List<int[]> requestMarkers,
List<int[]> responseMarkers);
/**
* This method is used to obtain the descriptive name for the Burp tool
* identified by the tool flag provided.
*
* @param toolFlag A flag identifying a Burp tool ( <code>TOOL_PROXY</code>,
* <code>TOOL_SCANNER</code>, etc.). Tool flags are defined within this
* interface.
* @return The descriptive name for the specified tool.
*/
String getToolName(int toolFlag);
/**
* This method is used to register a new Scanner issue. <b>Note:</b>
* Wherever possible, extensions should implement custom Scanner checks
* using <code>IScannerCheck</code> and report issues via those checks, so
* as to integrate with Burp's user-driven workflow, and ensure proper
* consolidation of duplicate reported issues. This method is only designed
* for tasks outside of the normal testing workflow, such as importing
* results from other scanning tools.
*
* @param issue An object created by the extension that implements the
* <code>IScanIssue</code> interface.
*/
void addScanIssue(IScanIssue issue);
/**
* This method is used to create a new Burp Collaborator client context,
* which can be used to generate Burp Collaborator payloads and poll the
* Collaborator server for any network interactions that result from using
* those payloads.
*
* @return A new instance of <code>IBurpCollaboratorClientContext</code>
* that can be used to generate Collaborator payloads and retrieve
* interactions.
*/
IBurpCollaboratorClientContext createBurpCollaboratorClientContext();
/**
* This method parses the specified request and returns details of each
* request parameter.
*
* @param request The request to be parsed.
* @return An array of: <code>String[] { name, value, type }</code>
* containing details of the parameters contained within the request.
* @deprecated Use <code>IExtensionHelpers.analyzeRequest()</code> instead.
*/
@Deprecated
String[][] getParameters(byte[] request);
/**
* This method parses the specified request and returns details of each HTTP
* header.
*
* @param message The request to be parsed.
* @return An array of HTTP headers.
* @deprecated Use <code>IExtensionHelpers.analyzeRequest()</code> or
* <code>IExtensionHelpers.analyzeResponse()</code> instead.
*/
@Deprecated
String[] getHeaders(byte[] message);
/**
* This method can be used to register a new menu item which will appear on
* the various context menus that are used throughout Burp Suite to handle
* user-driven actions.
*
* @param menuItemCaption The caption to be displayed on the menu item.
* @param menuItemHandler The handler to be invoked when the user clicks on
* the menu item.
* @deprecated Use <code>registerContextMenuFactory()</code> instead.
*/
@Deprecated
void registerMenuItem(
String menuItemCaption,
IMenuItemHandler menuItemHandler);
} |
Java | BurpBounty/main/java/burp/IContextMenuFactory.java | package burp;
/*
* @(#)IContextMenuFactory.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
import javax.swing.JMenuItem;
import java.util.List;
/**
* Extensions can implement this interface and then call
* <code>IBurpExtenderCallbacks.registerContextMenuFactory()</code> to register
* a factory for custom context menu items.
*/
public interface IContextMenuFactory
{
/**
* This method will be called by Burp when the user invokes a context menu
* anywhere within Burp. The factory can then provide any custom context
* menu items that should be displayed in the context menu, based on the
* details of the menu invocation.
*
* @param invocation An object that implements the
* <code>IContextMenuInvocation</code> interface, which the extension can
* query to obtain details of the context menu invocation.
* @return A list of custom menu items (which may include sub-menus,
* checkbox menu items, etc.) that should be displayed. Extensions may
* return
* <code>null</code> from this method, to indicate that no menu items are
* required.
*/
List<JMenuItem> createMenuItems(IContextMenuInvocation invocation);
} |
Java | BurpBounty/main/java/burp/IContextMenuInvocation.java | package burp;
/*
* @(#)IContextMenuInvocation.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
import java.awt.event.InputEvent;
/**
* This interface is used when Burp calls into an extension-provided
* <code>IContextMenuFactory</code> with details of a context menu invocation.
* The custom context menu factory can query this interface to obtain details of
* the invocation event, in order to determine what menu items should be
* displayed.
*/
public interface IContextMenuInvocation
{
/**
* Used to indicate that the context menu is being invoked in a request
* editor.
*/
static final byte CONTEXT_MESSAGE_EDITOR_REQUEST = 0;
/**
* Used to indicate that the context menu is being invoked in a response
* editor.
*/
static final byte CONTEXT_MESSAGE_EDITOR_RESPONSE = 1;
/**
* Used to indicate that the context menu is being invoked in a non-editable
* request viewer.
*/
static final byte CONTEXT_MESSAGE_VIEWER_REQUEST = 2;
/**
* Used to indicate that the context menu is being invoked in a non-editable
* response viewer.
*/
static final byte CONTEXT_MESSAGE_VIEWER_RESPONSE = 3;
/**
* Used to indicate that the context menu is being invoked in the Target
* site map tree.
*/
static final byte CONTEXT_TARGET_SITE_MAP_TREE = 4;
/**
* Used to indicate that the context menu is being invoked in the Target
* site map table.
*/
static final byte CONTEXT_TARGET_SITE_MAP_TABLE = 5;
/**
* Used to indicate that the context menu is being invoked in the Proxy
* history.
*/
static final byte CONTEXT_PROXY_HISTORY = 6;
/**
* Used to indicate that the context menu is being invoked in the Scanner
* results.
*/
static final byte CONTEXT_SCANNER_RESULTS = 7;
/**
* Used to indicate that the context menu is being invoked in the Intruder
* payload positions editor.
*/
static final byte CONTEXT_INTRUDER_PAYLOAD_POSITIONS = 8;
/**
* Used to indicate that the context menu is being invoked in an Intruder
* attack results.
*/
static final byte CONTEXT_INTRUDER_ATTACK_RESULTS = 9;
/**
* Used to indicate that the context menu is being invoked in a search
* results window.
*/
static final byte CONTEXT_SEARCH_RESULTS = 10;
/**
* This method can be used to retrieve the native Java input event that was
* the trigger for the context menu invocation.
*
* @return The <code>InputEvent</code> that was the trigger for the context
* menu invocation.
*/
InputEvent getInputEvent();
/**
* This method can be used to retrieve the Burp tool within which the
* context menu was invoked.
*
* @return A flag indicating the Burp tool within which the context menu was
* invoked. Burp tool flags are defined in the
* <code>IBurpExtenderCallbacks</code> interface.
*/
int getToolFlag();
/**
* This method can be used to retrieve the context within which the menu was
* invoked.
*
* @return An index indicating the context within which the menu was
* invoked. The indices used are defined within this interface.
*/
byte getInvocationContext();
/**
* This method can be used to retrieve the bounds of the user's selection
* into the current message, if applicable.
*
* @return An int[2] array containing the start and end offsets of the
* user's selection in the current message. If the user has not made any
* selection in the current message, both offsets indicate the position of
* the caret within the editor. If the menu is not being invoked from a
* message editor, the method returns <code>null</code>.
*/
int[] getSelectionBounds();
/**
* This method can be used to retrieve details of the HTTP requests /
* responses that were shown or selected by the user when the context menu
* was invoked.
*
* <b>Note:</b> For performance reasons, the objects returned from this
* method are tied to the originating context of the messages within the
* Burp UI. For example, if a context menu is invoked on the Proxy intercept
* panel, then the
* <code>IHttpRequestResponse</code> returned by this method will reflect
* the current contents of the interception panel, and this will change when
* the current message has been forwarded or dropped. If your extension
* needs to store details of the message for which the context menu has been
* invoked, then you should query those details from the
* <code>IHttpRequestResponse</code> at the time of invocation, or you
* should use
* <code>IBurpExtenderCallbacks.saveBuffersToTempFiles()</code> to create a
* persistent read-only copy of the
* <code>IHttpRequestResponse</code>.
*
* @return An array of <code>IHttpRequestResponse</code> objects
* representing the items that were shown or selected by the user when the
* context menu was invoked. This method returns <code>null</code> if no
* messages are applicable to the invocation.
*/
IHttpRequestResponse[] getSelectedMessages();
/**
* This method can be used to retrieve details of the Scanner issues that
* were selected by the user when the context menu was invoked.
*
* @return An array of <code>IScanIssue</code> objects representing the
* issues that were selected by the user when the context menu was invoked.
* This method returns <code>null</code> if no Scanner issues are applicable
* to the invocation.
*/
IScanIssue[] getSelectedIssues();
} |
Java | BurpBounty/main/java/burp/ICookie.java | package burp;
/*
* @(#)ICookie.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
import java.util.Date;
/**
* This interface is used to hold details about an HTTP cookie.
*/
public interface ICookie
{
/**
* This method is used to retrieve the domain for which the cookie is in
* scope.
*
* @return The domain for which the cookie is in scope. <b>Note:</b> For
* cookies that have been analyzed from responses (by calling
* <code>IExtensionHelpers.analyzeResponse()</code> and then
* <code>IResponseInfo.getCookies()</code>, the domain will be
* <code>null</code> if the response did not explicitly set a domain
* attribute for the cookie.
*/
String getDomain();
/**
* This method is used to retrieve the path for which the cookie is in
* scope.
*
* @return The path for which the cookie is in scope or null if none is set.
*/
String getPath();
/**
* This method is used to retrieve the expiration time for the cookie.
*
* @return The expiration time for the cookie, or
* <code>null</code> if none is set (i.e., for non-persistent session
* cookies).
*/
Date getExpiration();
/**
* This method is used to retrieve the name of the cookie.
*
* @return The name of the cookie.
*/
String getName();
/**
* This method is used to retrieve the value of the cookie.
* @return The value of the cookie.
*/
String getValue();
} |
Java | BurpBounty/main/java/burp/IExtensionHelpers.java | package burp;
/*
* @(#)IExtensionHelpers.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
import java.net.URL;
import java.util.List;
/**
* This interface contains a number of helper methods, which extensions can use
* to assist with various common tasks that arise for Burp extensions.
*
* Extensions can call <code>IBurpExtenderCallbacks.getHelpers</code> to obtain
* an instance of this interface.
*/
public interface IExtensionHelpers
{
/**
* This method can be used to analyze an HTTP request, and obtain various
* key details about it.
*
* @param request An <code>IHttpRequestResponse</code> object containing the
* request to be analyzed.
* @return An <code>IRequestInfo</code> object that can be queried to obtain
* details about the request.
*/
IRequestInfo analyzeRequest(IHttpRequestResponse request);
/**
* This method can be used to analyze an HTTP request, and obtain various
* key details about it.
*
* @param httpService The HTTP service associated with the request. This is
* optional and may be <code>null</code>, in which case the resulting
* <code>IRequestInfo</code> object will not include the full request URL.
* @param request The request to be analyzed.
* @return An <code>IRequestInfo</code> object that can be queried to obtain
* details about the request.
*/
IRequestInfo analyzeRequest(IHttpService httpService, byte[] request);
/**
* This method can be used to analyze an HTTP request, and obtain various
* key details about it. The resulting <code>IRequestInfo</code> object will
* not include the full request URL. To obtain the full URL, use one of the
* other overloaded <code>analyzeRequest()</code> methods.
*
* @param request The request to be analyzed.
* @return An <code>IRequestInfo</code> object that can be queried to obtain
* details about the request.
*/
IRequestInfo analyzeRequest(byte[] request);
/**
* This method can be used to analyze an HTTP response, and obtain various
* key details about it.
*
* @param response The response to be analyzed.
* @return An <code>IResponseInfo</code> object that can be queried to
* obtain details about the response.
*/
IResponseInfo analyzeResponse(byte[] response);
/**
* This method can be used to retrieve details of a specified parameter
* within an HTTP request. <b>Note:</b> Use <code>analyzeRequest()</code> to
* obtain details of all parameters within the request.
*
* @param request The request to be inspected for the specified parameter.
* @param parameterName The name of the parameter to retrieve.
* @return An <code>IParameter</code> object that can be queried to obtain
* details about the parameter, or <code>null</code> if the parameter was
* not found.
*/
IParameter getRequestParameter(byte[] request, String parameterName);
/**
* This method can be used to URL-decode the specified data.
*
* @param data The data to be decoded.
* @return The decoded data.
*/
String urlDecode(String data);
/**
* This method can be used to URL-encode the specified data. Any characters
* that do not need to be encoded within HTTP requests are not encoded.
*
* @param data The data to be encoded.
* @return The encoded data.
*/
String urlEncode(String data);
/**
* This method can be used to URL-decode the specified data.
*
* @param data The data to be decoded.
* @return The decoded data.
*/
byte[] urlDecode(byte[] data);
/**
* This method can be used to URL-encode the specified data. Any characters
* that do not need to be encoded within HTTP requests are not encoded.
*
* @param data The data to be encoded.
* @return The encoded data.
*/
byte[] urlEncode(byte[] data);
/**
* This method can be used to Base64-decode the specified data.
*
* @param data The data to be decoded.
* @return The decoded data.
*/
byte[] base64Decode(String data);
/**
* This method can be used to Base64-decode the specified data.
*
* @param data The data to be decoded.
* @return The decoded data.
*/
byte[] base64Decode(byte[] data);
/**
* This method can be used to Base64-encode the specified data.
*
* @param data The data to be encoded.
* @return The encoded data.
*/
String base64Encode(String data);
/**
* This method can be used to Base64-encode the specified data.
*
* @param data The data to be encoded.
* @return The encoded data.
*/
String base64Encode(byte[] data);
/**
* This method can be used to convert data from String form into an array of
* bytes. The conversion does not reflect any particular character set, and
* a character with the hex representation 0xWXYZ will always be converted
* into a byte with the representation 0xYZ. It performs the opposite
* conversion to the method <code>bytesToString()</code>, and byte-based
* data that is converted to a String and back again using these two methods
* is guaranteed to retain its integrity (which may not be the case with
* conversions that reflect a given character set).
*
* @param data The data to be converted.
* @return The converted data.
*/
byte[] stringToBytes(String data);
/**
* This method can be used to convert data from an array of bytes into
* String form. The conversion does not reflect any particular character
* set, and a byte with the representation 0xYZ will always be converted
* into a character with the hex representation 0x00YZ. It performs the
* opposite conversion to the method <code>stringToBytes()</code>, and
* byte-based data that is converted to a String and back again using these
* two methods is guaranteed to retain its integrity (which may not be the
* case with conversions that reflect a given character set).
*
* @param data The data to be converted.
* @return The converted data.
*/
String bytesToString(byte[] data);
/**
* This method searches a piece of data for the first occurrence of a
* specified pattern. It works on byte-based data in a way that is similar
* to the way the native Java method <code>String.indexOf()</code> works on
* String-based data.
*
* @param data The data to be searched.
* @param pattern The pattern to be searched for.
* @param caseSensitive Flags whether or not the search is case-sensitive.
* @param from The offset within <code>data</code> where the search should
* begin.
* @param to The offset within <code>data</code> where the search should
* end.
* @return The offset of the first occurrence of the pattern within the
* specified bounds, or -1 if no match is found.
*/
int indexOf(byte[] data,
byte[] pattern,
boolean caseSensitive,
int from,
int to);
/**
* This method builds an HTTP message containing the specified headers and
* message body. If applicable, the Content-Length header will be added or
* updated, based on the length of the body.
*
* @param headers A list of headers to include in the message.
* @param body The body of the message, of <code>null</code> if the message
* has an empty body.
* @return The resulting full HTTP message.
*/
byte[] buildHttpMessage(List<String> headers, byte[] body);
/**
* This method creates a GET request to the specified URL. The headers used
* in the request are determined by the Request headers settings as
* configured in Burp Spider's options.
*
* @param url The URL to which the request should be made.
* @return A request to the specified URL.
*/
byte[] buildHttpRequest(URL url);
/**
* This method adds a new parameter to an HTTP request, and if appropriate
* updates the Content-Length header.
*
* @param request The request to which the parameter should be added.
* @param parameter An <code>IParameter</code> object containing details of
* the parameter to be added. Supported parameter types are:
* <code>PARAM_URL</code>, <code>PARAM_BODY</code> and
* <code>PARAM_COOKIE</code>.
* @return A new HTTP request with the new parameter added.
*/
byte[] addParameter(byte[] request, IParameter parameter);
/**
* This method removes a parameter from an HTTP request, and if appropriate
* updates the Content-Length header.
*
* @param request The request from which the parameter should be removed.
* @param parameter An <code>IParameter</code> object containing details of
* the parameter to be removed. Supported parameter types are:
* <code>PARAM_URL</code>, <code>PARAM_BODY</code> and
* <code>PARAM_COOKIE</code>.
* @return A new HTTP request with the parameter removed.
*/
byte[] removeParameter(byte[] request, IParameter parameter);
/**
* This method updates the value of a parameter within an HTTP request, and
* if appropriate updates the Content-Length header. <b>Note:</b> This
* method can only be used to update the value of an existing parameter of a
* specified type. If you need to change the type of an existing parameter,
* you should first call <code>removeParameter()</code> to remove the
* parameter with the old type, and then call <code>addParameter()</code> to
* add a parameter with the new type.
*
* @param request The request containing the parameter to be updated.
* @param parameter An <code>IParameter</code> object containing details of
* the parameter to be updated. Supported parameter types are:
* <code>PARAM_URL</code>, <code>PARAM_BODY</code> and
* <code>PARAM_COOKIE</code>.
* @return A new HTTP request with the parameter updated.
*/
byte[] updateParameter(byte[] request, IParameter parameter);
/**
* This method can be used to toggle a request's method between GET and
* POST. Parameters are relocated between the URL query string and message
* body as required, and the Content-Length header is created or removed as
* applicable.
*
* @param request The HTTP request whose method should be toggled.
* @return A new HTTP request using the toggled method.
*/
byte[] toggleRequestMethod(byte[] request);
/**
* This method constructs an <code>IHttpService</code> object based on the
* details provided.
*
* @param host The HTTP service host.
* @param port The HTTP service port.
* @param protocol The HTTP service protocol.
* @return An <code>IHttpService</code> object based on the details
* provided.
*/
IHttpService buildHttpService(String host, int port, String protocol);
/**
* This method constructs an <code>IHttpService</code> object based on the
* details provided.
*
* @param host The HTTP service host.
* @param port The HTTP service port.
* @param useHttps Flags whether the HTTP service protocol is HTTPS or HTTP.
* @return An <code>IHttpService</code> object based on the details
* provided.
*/
IHttpService buildHttpService(String host, int port, boolean useHttps);
/**
* This method constructs an <code>IParameter</code> object based on the
* details provided.
*
* @param name The parameter name.
* @param value The parameter value.
* @param type The parameter type, as defined in the <code>IParameter</code>
* interface.
* @return An <code>IParameter</code> object based on the details provided.
*/
IParameter buildParameter(String name, String value, byte type);
/**
* This method constructs an <code>IScannerInsertionPoint</code> object
* based on the details provided. It can be used to quickly create a simple
* insertion point based on a fixed payload location within a base request.
*
* @param insertionPointName The name of the insertion point.
* @param baseRequest The request from which to build scan requests.
* @param from The offset of the start of the payload location.
* @param to The offset of the end of the payload location.
* @return An <code>IScannerInsertionPoint</code> object based on the
* details provided.
*/
IScannerInsertionPoint makeScannerInsertionPoint(
String insertionPointName,
byte[] baseRequest,
int from,
int to);
/**
* This method analyzes one or more responses to identify variations in a
* number of attributes and returns an <code>IResponseVariations</code>
* object that can be queried to obtain details of the variations.
*
* @param responses The responses to analyze.
* @return An <code>IResponseVariations</code> object representing the
* variations in the responses.
*/
IResponseVariations analyzeResponseVariations(byte[]... responses);
/**
* This method analyzes one or more responses to identify the number of
* occurrences of the specified keywords and returns an
* <code>IResponseKeywords</code> object that can be queried to obtain
* details of the number of occurrences of each keyword.
*
* @param keywords The keywords to look for.
* @param responses The responses to analyze.
* @return An <code>IResponseKeywords</code> object representing the counts
* of the keywords appearing in the responses.
*/
IResponseKeywords analyzeResponseKeywords(List<String> keywords, byte[]... responses);
} |
Java | BurpBounty/main/java/burp/IExtensionStateListener.java | package burp;
/*
* @(#)IExtensionStateListener.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* Extensions can implement this interface and then call
* <code>IBurpExtenderCallbacks.registerExtensionStateListener()</code> to
* register an extension state listener. The listener will be notified of
* changes to the extension's state. <b>Note:</b> Any extensions that start
* background threads or open system resources (such as files or database
* connections) should register a listener and terminate threads / close
* resources when the extension is unloaded.
*/
public interface IExtensionStateListener
{
/**
* This method is called when the extension is unloaded.
*/
void extensionUnloaded();
} |
Java | BurpBounty/main/java/burp/IHttpListener.java | package burp;
/*
* @(#)IHttpListener.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* Extensions can implement this interface and then call
* <code>IBurpExtenderCallbacks.registerHttpListener()</code> to register an
* HTTP listener. The listener will be notified of requests and responses made
* by any Burp tool. Extensions can perform custom analysis or modification of
* these messages by registering an HTTP listener.
*/
public interface IHttpListener
{
/**
* This method is invoked when an HTTP request is about to be issued, and
* when an HTTP response has been received.
*
* @param toolFlag A flag indicating the Burp tool that issued the request.
* Burp tool flags are defined in the
* <code>IBurpExtenderCallbacks</code> interface.
* @param messageIsRequest Flags whether the method is being invoked for a
* request or response.
* @param messageInfo Details of the request / response to be processed.
* Extensions can call the setter methods on this object to update the
* current message and so modify Burp's behavior.
*/
void processHttpMessage(int toolFlag,
boolean messageIsRequest,
IHttpRequestResponse messageInfo);
} |
Java | BurpBounty/main/java/burp/IHttpRequestResponse.java | package burp;
/*
* @(#)IHttpRequestResponse.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* This interface is used to retrieve and update details about HTTP messages.
*
* <b>Note:</b> The setter methods generally can only be used before the message
* has been processed, and not in read-only contexts. The getter methods
* relating to response details can only be used after the request has been
* issued.
*/
public interface IHttpRequestResponse
{
/**
* This method is used to retrieve the request message.
*
* @return The request message.
*/
byte[] getRequest();
/**
* This method is used to update the request message.
*
* @param message The new request message.
*/
void setRequest(byte[] message);
/**
* This method is used to retrieve the response message.
*
* @return The response message.
*/
byte[] getResponse();
/**
* This method is used to update the response message.
*
* @param message The new response message.
*/
void setResponse(byte[] message);
/**
* This method is used to retrieve the user-annotated comment for this item,
* if applicable.
*
* @return The user-annotated comment for this item, or null if none is set.
*/
String getComment();
/**
* This method is used to update the user-annotated comment for this item.
*
* @param comment The comment to be assigned to this item.
*/
void setComment(String comment);
/**
* This method is used to retrieve the user-annotated highlight for this
* item, if applicable.
*
* @return The user-annotated highlight for this item, or null if none is
* set.
*/
String getHighlight();
/**
* This method is used to update the user-annotated highlight for this item.
*
* @param color The highlight color to be assigned to this item. Accepted
* values are: red, orange, yellow, green, cyan, blue, pink, magenta, gray,
* or a null String to clear any existing highlight.
*/
void setHighlight(String color);
/**
* This method is used to retrieve the HTTP service for this request /
* response.
*
* @return An
* <code>IHttpService</code> object containing details of the HTTP service.
*/
IHttpService getHttpService();
/**
* This method is used to update the HTTP service for this request /
* response.
*
* @param httpService An
* <code>IHttpService</code> object containing details of the new HTTP
* service.
*/
void setHttpService(IHttpService httpService);
} |
Java | BurpBounty/main/java/burp/IHttpRequestResponsePersisted.java | package burp;
/*
* @(#)IHttpRequestResponsePersisted.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* This interface is used for an
* <code>IHttpRequestResponse</code> object whose request and response messages
* have been saved to temporary files using
* <code>IBurpExtenderCallbacks.saveBuffersToTempFiles()</code>.
*/
public interface IHttpRequestResponsePersisted extends IHttpRequestResponse
{
/**
* This method is deprecated and no longer performs any action.
*/
@Deprecated
void deleteTempFiles();
} |
Java | BurpBounty/main/java/burp/IHttpRequestResponseWithMarkers.java | package burp;
/*
* @(#)IHttpRequestResponseWithMarkers.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
import java.util.List;
/**
* This interface is used for an
* <code>IHttpRequestResponse</code> object that has had markers applied.
* Extensions can create instances of this interface using
* <code>IBurpExtenderCallbacks.applyMarkers()</code>, or provide their own
* implementation. Markers are used in various situations, such as specifying
* Intruder payload positions, Scanner insertion points, and highlights in
* Scanner issues.
*/
public interface IHttpRequestResponseWithMarkers extends IHttpRequestResponse
{
/**
* This method returns the details of the request markers.
*
* @return A list of index pairs representing the offsets of markers for the
* request message. Each item in the list is an int[2] array containing the
* start and end offsets for the marker. The method may return
* <code>null</code> if no request markers are defined.
*/
List<int[]> getRequestMarkers();
/**
* This method returns the details of the response markers.
*
* @return A list of index pairs representing the offsets of markers for the
* response message. Each item in the list is an int[2] array containing the
* start and end offsets for the marker. The method may return
* <code>null</code> if no response markers are defined.
*/
List<int[]> getResponseMarkers();
} |
Java | BurpBounty/main/java/burp/IHttpService.java | package burp;
/*
* @(#)IHttpService.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* This interface is used to provide details about an HTTP service, to which
* HTTP requests can be sent.
*/
public interface IHttpService
{
/**
* This method returns the hostname or IP address for the service.
*
* @return The hostname or IP address for the service.
*/
String getHost();
/**
* This method returns the port number for the service.
*
* @return The port number for the service.
*/
int getPort();
/**
* This method returns the protocol for the service.
*
* @return The protocol for the service. Expected values are "http" or
* "https".
*/
String getProtocol();
} |
Java | BurpBounty/main/java/burp/IInterceptedProxyMessage.java | package burp;
/*
* @(#)IInterceptedProxyMessage.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
import java.net.InetAddress;
/**
* This interface is used to represent an HTTP message that has been intercepted
* by Burp Proxy. Extensions can register an
* <code>IProxyListener</code> to receive details of proxy messages using this
* interface. *
*/
public interface IInterceptedProxyMessage
{
/**
* This action causes Burp Proxy to follow the current interception rules to
* determine the appropriate action to take for the message.
*/
static final int ACTION_FOLLOW_RULES = 0;
/**
* This action causes Burp Proxy to present the message to the user for
* manual review or modification.
*/
static final int ACTION_DO_INTERCEPT = 1;
/**
* This action causes Burp Proxy to forward the message to the remote server
* or client, without presenting it to the user.
*/
static final int ACTION_DONT_INTERCEPT = 2;
/**
* This action causes Burp Proxy to drop the message.
*/
static final int ACTION_DROP = 3;
/**
* This action causes Burp Proxy to follow the current interception rules to
* determine the appropriate action to take for the message, and then make a
* second call to processProxyMessage.
*/
static final int ACTION_FOLLOW_RULES_AND_REHOOK = 0x10;
/**
* This action causes Burp Proxy to present the message to the user for
* manual review or modification, and then make a second call to
* processProxyMessage.
*/
static final int ACTION_DO_INTERCEPT_AND_REHOOK = 0x11;
/**
* This action causes Burp Proxy to skip user interception, and then make a
* second call to processProxyMessage.
*/
static final int ACTION_DONT_INTERCEPT_AND_REHOOK = 0x12;
/**
* This method retrieves a unique reference number for this
* request/response.
*
* @return An identifier that is unique to a single request/response pair.
* Extensions can use this to correlate details of requests and responses
* and perform processing on the response message accordingly.
*/
int getMessageReference();
/**
* This method retrieves details of the intercepted message.
*
* @return An <code>IHttpRequestResponse</code> object containing details of
* the intercepted message.
*/
IHttpRequestResponse getMessageInfo();
/**
* This method retrieves the currently defined interception action. The
* default action is
* <code>ACTION_FOLLOW_RULES</code>. If multiple proxy listeners are
* registered, then other listeners may already have modified the
* interception action before it reaches the current listener. This method
* can be used to determine whether this has occurred.
*
* @return The currently defined interception action. Possible values are
* defined within this interface.
*/
int getInterceptAction();
/**
* This method is used to update the interception action.
*
* @param interceptAction The new interception action. Possible values are
* defined within this interface.
*/
void setInterceptAction(int interceptAction);
/**
* This method retrieves the name of the Burp Proxy listener that is
* processing the intercepted message.
*
* @return The name of the Burp Proxy listener that is processing the
* intercepted message. The format is the same as that shown in the Proxy
* Listeners UI - for example, "127.0.0.1:8080".
*/
String getListenerInterface();
/**
* This method retrieves the client IP address from which the request for
* the intercepted message was received.
*
* @return The client IP address from which the request for the intercepted
* message was received.
*/
InetAddress getClientIpAddress();
} |
Java | BurpBounty/main/java/burp/IIntruderAttack.java | package burp;
/*
* @(#)IIntruderAttack.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* This interface is used to hold details about an Intruder attack.
*/
public interface IIntruderAttack
{
/**
* This method is used to retrieve the HTTP service for the attack.
*
* @return The HTTP service for the attack.
*/
IHttpService getHttpService();
/**
* This method is used to retrieve the request template for the attack.
*
* @return The request template for the attack.
*/
byte[] getRequestTemplate();
} |
Java | BurpBounty/main/java/burp/IIntruderPayloadGenerator.java | package burp;
/*
* @(#)IIntruderPayloadGenerator.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* This interface is used for custom Intruder payload generators. Extensions
* that have registered an
* <code>IIntruderPayloadGeneratorFactory</code> must return a new instance of
* this interface when required as part of a new Intruder attack.
*/
public interface IIntruderPayloadGenerator
{
/**
* This method is used by Burp to determine whether the payload generator is
* able to provide any further payloads.
*
* @return Extensions should return
* <code>false</code> when all the available payloads have been used up,
* otherwise
* <code>true</code>.
*/
boolean hasMorePayloads();
/**
* This method is used by Burp to obtain the value of the next payload.
*
* @param baseValue The base value of the current payload position. This
* value may be
* <code>null</code> if the concept of a base value is not applicable (e.g.
* in a battering ram attack).
* @return The next payload to use in the attack.
*/
byte[] getNextPayload(byte[] baseValue);
/**
* This method is used by Burp to reset the state of the payload generator
* so that the next call to
* <code>getNextPayload()</code> returns the first payload again. This
* method will be invoked when an attack uses the same payload generator for
* more than one payload position, for example in a sniper attack.
*/
void reset();
} |
Java | BurpBounty/main/java/burp/IIntruderPayloadGeneratorFactory.java | package burp;
/*
* @(#)IIntruderPayloadGeneratorFactory.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* Extensions can implement this interface and then call
* <code>IBurpExtenderCallbacks.registerIntruderPayloadGeneratorFactory()</code>
* to register a factory for custom Intruder payloads.
*/
public interface IIntruderPayloadGeneratorFactory
{
/**
* This method is used by Burp to obtain the name of the payload generator.
* This will be displayed as an option within the Intruder UI when the user
* selects to use extension-generated payloads.
*
* @return The name of the payload generator.
*/
String getGeneratorName();
/**
* This method is used by Burp when the user starts an Intruder attack that
* uses this payload generator.
*
* @param attack An
* <code>IIntruderAttack</code> object that can be queried to obtain details
* about the attack in which the payload generator will be used.
* @return A new instance of
* <code>IIntruderPayloadGenerator</code> that will be used to generate
* payloads for the attack.
*/
IIntruderPayloadGenerator createNewInstance(IIntruderAttack attack);
} |
Java | BurpBounty/main/java/burp/IIntruderPayloadProcessor.java | package burp;
/*
* @(#)IIntruderPayloadProcessor.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* Extensions can implement this interface and then call
* <code>IBurpExtenderCallbacks.registerIntruderPayloadProcessor()</code> to
* register a custom Intruder payload processor.
*/
public interface IIntruderPayloadProcessor
{
/**
* This method is used by Burp to obtain the name of the payload processor.
* This will be displayed as an option within the Intruder UI when the user
* selects to use an extension-provided payload processor.
*
* @return The name of the payload processor.
*/
String getProcessorName();
/**
* This method is invoked by Burp each time the processor should be applied
* to an Intruder payload.
*
* @param currentPayload The value of the payload to be processed.
* @param originalPayload The value of the original payload prior to
* processing by any already-applied processing rules.
* @param baseValue The base value of the payload position, which will be
* replaced with the current payload.
* @return The value of the processed payload. This may be
* <code>null</code> to indicate that the current payload should be skipped,
* and the attack will move directly to the next payload.
*/
byte[] processPayload(
byte[] currentPayload,
byte[] originalPayload,
byte[] baseValue);
} |
Java | BurpBounty/main/java/burp/IMenuItemHandler.java | package burp;
/*
* @(#)IMenuItemHandler.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* Extensions can implement this interface and then call
* <code>IBurpExtenderCallbacks.registerMenuItem()</code> to register a custom
* context menu item.
*
* @deprecated Use
* <code>IContextMenuFactory</code> instead.
*/
@Deprecated
public interface IMenuItemHandler
{
/**
* This method is invoked by Burp Suite when the user clicks on a custom
* menu item which the extension has registered with Burp.
*
* @param menuItemCaption The caption of the menu item which was clicked.
* This parameter enables extensions to provide a single implementation
* which handles multiple different menu items.
* @param messageInfo Details of the HTTP message(s) for which the context
* menu was displayed.
*/
void menuItemClicked(
String menuItemCaption,
IHttpRequestResponse[] messageInfo);
} |
Java | BurpBounty/main/java/burp/IMessageEditor.java | package burp;
/*
* @(#)IMessageEditor.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
import java.awt.Component;
/**
* This interface is used to provide extensions with an instance of Burp's HTTP
* message editor, for the extension to use in its own UI. Extensions should
* call <code>IBurpExtenderCallbacks.createMessageEditor()</code> to obtain an
* instance of this interface.
*/
public interface IMessageEditor
{
/**
* This method returns the UI component of the editor, for extensions to add
* to their own UI.
*
* @return The UI component of the editor.
*/
Component getComponent();
/**
* This method is used to display an HTTP message in the editor.
*
* @param message The HTTP message to be displayed.
* @param isRequest Flags whether the message is an HTTP request or
* response.
*/
void setMessage(byte[] message, boolean isRequest);
/**
* This method is used to retrieve the currently displayed message, which
* may have been modified by the user.
*
* @return The currently displayed HTTP message.
*/
byte[] getMessage();
/**
* This method is used to determine whether the current message has been
* modified by the user.
*
* @return An indication of whether the current message has been modified by
* the user since it was first displayed.
*/
boolean isMessageModified();
/**
* This method returns the data that is currently selected by the user.
*
* @return The data that is currently selected by the user, or
* <code>null</code> if no selection is made.
*/
byte[] getSelectedData();
/**
* This method can be used to retrieve the bounds of the user's selection
* into the displayed message, if applicable.
*
* @return An int[2] array containing the start and end offsets of the
* user's selection within the displayed message. If the user has not made
* any selection in the current message, both offsets indicate the position
* of the caret within the editor. For some editor views, the concept of
* selection within the message does not apply, in which case this method
* returns null.
*/
int[] getSelectionBounds();
} |
Java | BurpBounty/main/java/burp/IMessageEditorController.java | package burp;
/*
* @(#)IMessageEditorController.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* This interface is used by an
* <code>IMessageEditor</code> to obtain details about the currently displayed
* message. Extensions that create instances of Burp's HTTP message editor can
* optionally provide an implementation of
* <code>IMessageEditorController</code>, which the editor will invoke when it
* requires further information about the current message (for example, to send
* it to another Burp tool). Extensions that provide custom editor tabs via an
* <code>IMessageEditorTabFactory</code> will receive a reference to an
* <code>IMessageEditorController</code> object for each tab instance they
* generate, which the tab can invoke if it requires further information about
* the current message.
*/
public interface IMessageEditorController
{
/**
* This method is used to retrieve the HTTP service for the current message.
*
* @return The HTTP service for the current message.
*/
IHttpService getHttpService();
/**
* This method is used to retrieve the HTTP request associated with the
* current message (which may itself be a response).
*
* @return The HTTP request associated with the current message.
*/
byte[] getRequest();
/**
* This method is used to retrieve the HTTP response associated with the
* current message (which may itself be a request).
*
* @return The HTTP response associated with the current message.
*/
byte[] getResponse();
} |
Java | BurpBounty/main/java/burp/IMessageEditorTab.java | package burp;
/*
* @(#)IMessageEditorTab.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
import java.awt.Component;
/**
* Extensions that register an
* <code>IMessageEditorTabFactory</code> must return instances of this
* interface, which Burp will use to create custom tabs within its HTTP message
* editors.
*/
public interface IMessageEditorTab
{
/**
* This method returns the caption that should appear on the custom tab when
* it is displayed. <b>Note:</b> Burp invokes this method once when the tab
* is first generated, and the same caption will be used every time the tab
* is displayed.
*
* @return The caption that should appear on the custom tab when it is
* displayed.
*/
String getTabCaption();
/**
* This method returns the component that should be used as the contents of
* the custom tab when it is displayed. <b>Note:</b> Burp invokes this
* method once when the tab is first generated, and the same component will
* be used every time the tab is displayed.
*
* @return The component that should be used as the contents of the custom
* tab when it is displayed.
*/
Component getUiComponent();
/**
* The hosting editor will invoke this method before it displays a new HTTP
* message, so that the custom tab can indicate whether it should be enabled
* for that message.
*
* @param content The message that is about to be displayed, or a zero-length
* array if the existing message is to be cleared.
* @param isRequest Indicates whether the message is a request or a
* response.
* @return The method should return
* <code>true</code> if the custom tab is able to handle the specified
* message, and so will be displayed within the editor. Otherwise, the tab
* will be hidden while this message is displayed.
*/
boolean isEnabled(byte[] content, boolean isRequest);
/**
* The hosting editor will invoke this method to display a new message or to
* clear the existing message. This method will only be called with a new
* message if the tab has already returned
* <code>true</code> to a call to
* <code>isEnabled()</code> with the same message details.
*
* @param content The message that is to be displayed, or
* <code>null</code> if the tab should clear its contents and disable any
* editable controls.
* @param isRequest Indicates whether the message is a request or a
* response.
*/
void setMessage(byte[] content, boolean isRequest);
/**
* This method returns the currently displayed message.
*
* @return The currently displayed message.
*/
byte[] getMessage();
/**
* This method is used to determine whether the currently displayed message
* has been modified by the user. The hosting editor will always call
* <code>getMessage()</code> before calling this method, so any pending
* edits should be completed within
* <code>getMessage()</code>.
*
* @return The method should return
* <code>true</code> if the user has modified the current message since it
* was first displayed.
*/
boolean isModified();
/**
* This method is used to retrieve the data that is currently selected by
* the user.
*
* @return The data that is currently selected by the user. This may be
* <code>null</code> if no selection is currently made.
*/
byte[] getSelectedData();
} |
Java | BurpBounty/main/java/burp/IMessageEditorTabFactory.java | package burp;
/*
* @(#)IMessageEditorTabFactory.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* Extensions can implement this interface and then call
* <code>IBurpExtenderCallbacks.registerMessageEditorTabFactory()</code> to
* register a factory for custom message editor tabs. This allows extensions to
* provide custom rendering or editing of HTTP messages, within Burp's own HTTP
* editor.
*/
public interface IMessageEditorTabFactory
{
/**
* Burp will call this method once for each HTTP message editor, and the
* factory should provide a new instance of an
* <code>IMessageEditorTab</code> object.
*
* @param controller An
* <code>IMessageEditorController</code> object, which the new tab can query
* to retrieve details about the currently displayed message. This may be
* <code>null</code> for extension-invoked message editors where the
* extension has not provided an editor controller.
* @param editable Indicates whether the hosting editor is editable or
* read-only.
* @return A new
* <code>IMessageEditorTab</code> object for use within the message editor.
*/
IMessageEditorTab createNewInstance(IMessageEditorController controller,
boolean editable);
} |
Java | BurpBounty/main/java/burp/IParameter.java | package burp;
/*
* @(#)IParameter.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* This interface is used to hold details about an HTTP request parameter.
*/
public interface IParameter
{
/**
* Used to indicate a parameter within the URL query string.
*/
static final byte PARAM_URL = 0;
/**
* Used to indicate a parameter within the message body.
*/
static final byte PARAM_BODY = 1;
/**
* Used to indicate an HTTP cookie.
*/
static final byte PARAM_COOKIE = 2;
/**
* Used to indicate an item of data within an XML structure.
*/
static final byte PARAM_XML = 3;
/**
* Used to indicate the value of a tag attribute within an XML structure.
*/
static final byte PARAM_XML_ATTR = 4;
/**
* Used to indicate the value of a parameter attribute within a multi-part
* message body (such as the name of an uploaded file).
*/
static final byte PARAM_MULTIPART_ATTR = 5;
/**
* Used to indicate an item of data within a JSON structure.
*/
static final byte PARAM_JSON = 6;
/**
* This method is used to retrieve the parameter type.
*
* @return The parameter type. The available types are defined within this
* interface.
*/
byte getType();
/**
* This method is used to retrieve the parameter name.
*
* @return The parameter name.
*/
String getName();
/**
* This method is used to retrieve the parameter value.
*
* @return The parameter value.
*/
String getValue();
/**
* This method is used to retrieve the start offset of the parameter name
* within the HTTP request.
*
* @return The start offset of the parameter name within the HTTP request,
* or -1 if the parameter is not associated with a specific request.
*/
int getNameStart();
/**
* This method is used to retrieve the end offset of the parameter name
* within the HTTP request.
*
* @return The end offset of the parameter name within the HTTP request, or
* -1 if the parameter is not associated with a specific request.
*/
int getNameEnd();
/**
* This method is used to retrieve the start offset of the parameter value
* within the HTTP request.
*
* @return The start offset of the parameter value within the HTTP request,
* or -1 if the parameter is not associated with a specific request.
*/
int getValueStart();
/**
* This method is used to retrieve the end offset of the parameter value
* within the HTTP request.
*
* @return The end offset of the parameter value within the HTTP request, or
* -1 if the parameter is not associated with a specific request.
*/
int getValueEnd();
} |
Java | BurpBounty/main/java/burp/IProxyListener.java | package burp;
/*
* @(#)IProxyListener.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* Extensions can implement this interface and then call
* <code>IBurpExtenderCallbacks.registerProxyListener()</code> to register a
* Proxy listener. The listener will be notified of requests and responses being
* processed by the Proxy tool. Extensions can perform custom analysis or
* modification of these messages, and control in-UI message interception, by
* registering a proxy listener.
*/
public interface IProxyListener
{
/**
* This method is invoked when an HTTP message is being processed by the
* Proxy.
*
* @param messageIsRequest Indicates whether the HTTP message is a request
* or a response.
* @param message An
* <code>IInterceptedProxyMessage</code> object that extensions can use to
* query and update details of the message, and control whether the message
* should be intercepted and displayed to the user for manual review or
* modification.
*/
void processProxyMessage(
boolean messageIsRequest,
IInterceptedProxyMessage message);
} |
Java | BurpBounty/main/java/burp/IRequestInfo.java | package burp;
/*
* @(#)IRequestInfo.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
import java.net.URL;
import java.util.List;
/**
* This interface is used to retrieve key details about an HTTP request.
* Extensions can obtain an
* <code>IRequestInfo</code> object for a given request by calling
* <code>IExtensionHelpers.analyzeRequest()</code>.
*/
public interface IRequestInfo
{
/**
* Used to indicate that there is no content.
*/
static final byte CONTENT_TYPE_NONE = 0;
/**
* Used to indicate URL-encoded content.
*/
static final byte CONTENT_TYPE_URL_ENCODED = 1;
/**
* Used to indicate multi-part content.
*/
static final byte CONTENT_TYPE_MULTIPART = 2;
/**
* Used to indicate XML content.
*/
static final byte CONTENT_TYPE_XML = 3;
/**
* Used to indicate JSON content.
*/
static final byte CONTENT_TYPE_JSON = 4;
/**
* Used to indicate AMF content.
*/
static final byte CONTENT_TYPE_AMF = 5;
/**
* Used to indicate unknown content.
*/
static final byte CONTENT_TYPE_UNKNOWN = -1;
/**
* This method is used to obtain the HTTP method used in the request.
*
* @return The HTTP method used in the request.
*/
String getMethod();
/**
* This method is used to obtain the URL in the request.
*
* @return The URL in the request.
*/
URL getUrl();
/**
* This method is used to obtain the HTTP headers contained in the request.
*
* @return The HTTP headers contained in the request.
*/
List<String> getHeaders();
/**
* This method is used to obtain the parameters contained in the request.
*
* @return The parameters contained in the request.
*/
List<IParameter> getParameters();
/**
* This method is used to obtain the offset within the request where the
* message body begins.
*
* @return The offset within the request where the message body begins.
*/
int getBodyOffset();
/**
* This method is used to obtain the content type of the message body.
*
* @return An indication of the content type of the message body. Available
* types are defined within this interface.
*/
byte getContentType();
} |
Java | BurpBounty/main/java/burp/IResponseInfo.java | package burp;
/*
* @(#)IResponseInfo.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
import java.util.List;
/**
* This interface is used to retrieve key details about an HTTP response.
* Extensions can obtain an
* <code>IResponseInfo</code> object for a given response by calling
* <code>IExtensionHelpers.analyzeResponse()</code>.
*/
public interface IResponseInfo
{
/**
* This method is used to obtain the HTTP headers contained in the response.
*
* @return The HTTP headers contained in the response.
*/
List<String> getHeaders();
/**
* This method is used to obtain the offset within the response where the
* message body begins.
*
* @return The offset within the response where the message body begins.
*/
int getBodyOffset();
/**
* This method is used to obtain the HTTP status code contained in the
* response.
*
* @return The HTTP status code contained in the response.
*/
short getStatusCode();
/**
* This method is used to obtain details of the HTTP cookies set in the
* response.
*
* @return A list of <code>ICookie</code> objects representing the cookies
* set in the response, if any.
*/
List<ICookie> getCookies();
/**
* This method is used to obtain the MIME type of the response, as stated in
* the HTTP headers.
*
* @return A textual label for the stated MIME type, or an empty String if
* this is not known or recognized. The possible labels are the same as
* those used in the main Burp UI.
*/
String getStatedMimeType();
/**
* This method is used to obtain the MIME type of the response, as inferred
* from the contents of the HTTP message body.
*
* @return A textual label for the inferred MIME type, or an empty String if
* this is not known or recognized. The possible labels are the same as
* those used in the main Burp UI.
*/
String getInferredMimeType();
} |
Java | BurpBounty/main/java/burp/IResponseKeywords.java | package burp;
/*
* @(#)IResponseKeywords.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
import java.util.List;
/**
* This interface is used to represent the counts of keywords appearing in a
* number of HTTP responses.
*/
public interface IResponseKeywords
{
/**
* This method is used to obtain the list of keywords whose counts vary
* between the analyzed responses.
*
* @return The keywords whose counts vary between the analyzed responses.
*/
List<String> getVariantKeywords();
/**
* This method is used to obtain the list of keywords whose counts do not
* vary between the analyzed responses.
*
* @return The keywords whose counts do not vary between the analyzed
* responses.
*/
List<String> getInvariantKeywords();
/**
* This method is used to obtain the number of occurrences of an individual
* keyword in a response.
*
* @param keyword The keyword whose count will be retrieved.
* @param responseIndex The index of the response. Note responses are
* indexed from zero in the order they were originally supplied to the
* <code>IExtensionHelpers.analyzeResponseKeywords()</code> and
* <code>IResponseKeywords.updateWith()</code> methods.
* @return The number of occurrences of the specified keyword for the
* specified response.
*/
int getKeywordCount(String keyword, int responseIndex);
/**
* This method is used to update the analysis based on additional responses.
*
* @param responses The new responses to include in the analysis.
*/
void updateWith(byte[]... responses);
} |
Java | BurpBounty/main/java/burp/IResponseVariations.java | package burp;
/*
* @(#)IResponseVariations.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
import java.util.List;
/**
* This interface is used to represent variations between a number HTTP
* responses, according to various attributes.
*/
public interface IResponseVariations
{
/**
* This method is used to obtain the list of attributes that vary between
* the analyzed responses.
*
* @return The attributes that vary between the analyzed responses.
*/
List<String> getVariantAttributes();
/**
* This method is used to obtain the list of attributes that do not vary
* between the analyzed responses.
*
* @return The attributes that do not vary between the analyzed responses.
*/
List<String> getInvariantAttributes();
/**
* This method is used to obtain the value of an individual attribute in a
* response. Note that the values of some attributes are intrinsically
* meaningful (e.g. a word count) while the values of others are less so
* (e.g. a checksum of the HTML tag names).
*
* @param attributeName The name of the attribute whose value will be
* retrieved. Extension authors can obtain the list of supported attributes
* by generating an <code>IResponseVariations</code> object for a single
* response and calling
* <code>IResponseVariations.getInvariantAttributes()</code>.
* @param responseIndex The index of the response. Note that responses are
* indexed from zero in the order they were originally supplied to the
* <code>IExtensionHelpers.analyzeResponseVariations()</code> and
* <code>IResponseVariations.updateWith()</code> methods.
* @return The value of the specified attribute for the specified response.
*/
int getAttributeValue(String attributeName, int responseIndex);
/**
* This method is used to update the analysis based on additional responses.
*
* @param responses The new responses to include in the analysis.
*/
void updateWith(byte[]... responses);
} |
Java | BurpBounty/main/java/burp/IScanIssue.java | package burp;
/*
* @(#)IScanIssue.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* This interface is used to retrieve details of Scanner issues. Extensions can
* obtain details of issues by registering an <code>IScannerListener</code> or
* by calling <code>IBurpExtenderCallbacks.getScanIssues()</code>. Extensions
* can also add custom Scanner issues by registering an
* <code>IScannerCheck</code> or calling
* <code>IBurpExtenderCallbacks.addScanIssue()</code>, and providing their own
* implementations of this interface. Note that issue descriptions and other
* text generated by extensions are subject to an HTML whitelist that allows
* only formatting tags and simple hyperlinks.
*/
public interface IScanIssue
{
/**
* This method returns the URL for which the issue was generated.
*
* @return The URL for which the issue was generated.
*/
java.net.URL getUrl();
/**
* This method returns the name of the issue type.
*
* @return The name of the issue type (e.g. "SQL injection").
*/
String getIssueName();
/**
* This method returns a numeric identifier of the issue type. See the Burp
* Scanner help documentation for a listing of all the issue types.
*
* @return A numeric identifier of the issue type.
*/
int getIssueType();
/**
* This method returns the issue severity level.
*
* @return The issue severity level. Expected values are "High", "Medium",
* "Low", "Information" or "False positive".
*
*/
String getSeverity();
/**
* This method returns the issue confidence level.
*
* @return The issue confidence level. Expected values are "Certain", "Firm"
* or "Tentative".
*/
String getConfidence();
/**
* This method returns a background description for this type of issue.
*
* @return A background description for this type of issue, or
* <code>null</code> if none applies. A limited set of HTML tags may be
* used.
*/
String getIssueBackground();
/**
* This method returns a background description of the remediation for this
* type of issue.
*
* @return A background description of the remediation for this type of
* issue, or <code>null</code> if none applies. A limited set of HTML tags
* may be used.
*/
String getRemediationBackground();
/**
* This method returns detailed information about this specific instance of
* the issue.
*
* @return Detailed information about this specific instance of the issue,
* or <code>null</code> if none applies. A limited set of HTML tags may be
* used.
*/
String getIssueDetail();
/**
* This method returns detailed information about the remediation for this
* specific instance of the issue.
*
* @return Detailed information about the remediation for this specific
* instance of the issue, or <code>null</code> if none applies. A limited
* set of HTML tags may be used.
*/
String getRemediationDetail();
/**
* This method returns the HTTP messages on the basis of which the issue was
* generated.
*
* @return The HTTP messages on the basis of which the issue was generated.
* <b>Note:</b> The items in this array should be instances of
* <code>IHttpRequestResponseWithMarkers</code> if applicable, so that
* details of the relevant portions of the request and response messages are
* available.
*/
IHttpRequestResponse[] getHttpMessages();
/**
* This method returns the HTTP service for which the issue was generated.
*
* @return The HTTP service for which the issue was generated.
*/
IHttpService getHttpService();
} |
Java | BurpBounty/main/java/burp/IScannerCheck.java | package burp;
/*
* @(#)IScannerCheck.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
import java.util.List;
/**
* Extensions can implement this interface and then call
* <code>IBurpExtenderCallbacks.registerScannerCheck()</code> to register a
* custom Scanner check. When performing scanning, Burp will ask the check to
* perform active or passive scanning on the base request, and report any
* Scanner issues that are identified.
*/
public interface IScannerCheck
{
/**
* The Scanner invokes this method for each base request / response that is
* passively scanned. <b>Note:</b> Extensions should only analyze the
* HTTP messages provided during passive scanning, and should not make any
* new HTTP requests of their own.
*
* @param baseRequestResponse The base HTTP request / response that should
* be passively scanned.
* @return A list of <code>IScanIssue</code> objects, or <code>null</code>
* if no issues are identified.
*/
List<IScanIssue> doPassiveScan(IHttpRequestResponse baseRequestResponse);
/**
* The Scanner invokes this method for each insertion point that is actively
* scanned. Extensions may issue HTTP requests as required to carry out
* active scanning, and should use the
* <code>IScannerInsertionPoint</code> object provided to build scan
* requests for particular payloads.
* <b>Note:</b>
* Scan checks should submit raw non-encoded payloads to insertion points,
* and the insertion point has responsibility for performing any data
* encoding that is necessary given the nature and location of the insertion
* point.
*
* @param baseRequestResponse The base HTTP request / response that should
* be actively scanned.
* @param insertionPoint An <code>IScannerInsertionPoint</code> object that
* can be queried to obtain details of the insertion point being tested, and
* can be used to build scan requests for particular payloads.
* @return A list of <code>IScanIssue</code> objects, or <code>null</code>
* if no issues are identified.
*/
List<IScanIssue> doActiveScan(
IHttpRequestResponse baseRequestResponse,
IScannerInsertionPoint insertionPoint);
/**
* The Scanner invokes this method when the custom Scanner check has
* reported multiple issues for the same URL path. This can arise either
* because there are multiple distinct vulnerabilities, or because the same
* (or a similar) request has been scanned more than once. The custom check
* should determine whether the issues are duplicates. In most cases, where
* a check uses distinct issue names or descriptions for distinct issues,
* the consolidation process will simply be a matter of comparing these
* features for the two issues.
*
* @param existingIssue An issue that was previously reported by this
* Scanner check.
* @param newIssue An issue at the same URL path that has been newly
* reported by this Scanner check.
* @return An indication of which issue(s) should be reported in the main
* Scanner results. The method should return <code>-1</code> to report the
* existing issue only, <code>0</code> to report both issues, and
* <code>1</code> to report the new issue only.
*/
int consolidateDuplicateIssues(
IScanIssue existingIssue,
IScanIssue newIssue);
} |
Java | BurpBounty/main/java/burp/IScannerInsertionPoint.java | package burp;
/*
* @(#)IScannerInsertionPoint.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* This interface is used to define an insertion point for use by active Scanner
* checks. Extensions can obtain instances of this interface by registering an
* <code>IScannerCheck</code>, or can create instances for use by Burp's own
* scan checks by registering an
* <code>IScannerInsertionPointProvider</code>.
*/
public interface IScannerInsertionPoint
{
/**
* Used to indicate where the payload is inserted into the value of a URL
* parameter.
*/
static final byte INS_PARAM_URL = 0x00;
/**
* Used to indicate where the payload is inserted into the value of a body
* parameter.
*/
static final byte INS_PARAM_BODY = 0x01;
/**
* Used to indicate where the payload is inserted into the value of an HTTP
* cookie.
*/
static final byte INS_PARAM_COOKIE = 0x02;
/**
* Used to indicate where the payload is inserted into the value of an item
* of data within an XML data structure.
*/
static final byte INS_PARAM_XML = 0x03;
/**
* Used to indicate where the payload is inserted into the value of a tag
* attribute within an XML structure.
*/
static final byte INS_PARAM_XML_ATTR = 0x04;
/**
* Used to indicate where the payload is inserted into the value of a
* parameter attribute within a multi-part message body (such as the name of
* an uploaded file).
*/
static final byte INS_PARAM_MULTIPART_ATTR = 0x05;
/**
* Used to indicate where the payload is inserted into the value of an item
* of data within a JSON structure.
*/
static final byte INS_PARAM_JSON = 0x06;
/**
* Used to indicate where the payload is inserted into the value of an AMF
* parameter.
*/
static final byte INS_PARAM_AMF = 0x07;
/**
* Used to indicate where the payload is inserted into the value of an HTTP
* request header.
*/
static final byte INS_HEADER = 0x20;
/**
* Used to indicate where the payload is inserted into a URL path folder.
*/
static final byte INS_URL_PATH_FOLDER = 0x21;
/**
* Used to indicate where the payload is inserted into a URL path folder.
* This is now deprecated; use <code>INS_URL_PATH_FOLDER</code> instead.
*/
@Deprecated
static final byte INS_URL_PATH_REST = INS_URL_PATH_FOLDER;
/**
* Used to indicate where the payload is inserted into the name of an added
* URL parameter.
*/
static final byte INS_PARAM_NAME_URL = 0x22;
/**
* Used to indicate where the payload is inserted into the name of an added
* body parameter.
*/
static final byte INS_PARAM_NAME_BODY = 0x23;
/**
* Used to indicate where the payload is inserted into the body of the HTTP
* request.
*/
static final byte INS_ENTIRE_BODY = 0x24;
/**
* Used to indicate where the payload is inserted into the URL path
* filename.
*/
static final byte INS_URL_PATH_FILENAME = 0x25;
/**
* Used to indicate where the payload is inserted at a location manually
* configured by the user.
*/
static final byte INS_USER_PROVIDED = 0x40;
/**
* Used to indicate where the insertion point is provided by an
* extension-registered
* <code>IScannerInsertionPointProvider</code>.
*/
static final byte INS_EXTENSION_PROVIDED = 0x41;
/**
* Used to indicate where the payload is inserted at an unknown location
* within the request.
*/
static final byte INS_UNKNOWN = 0x7f;
/**
* This method returns the name of the insertion point.
*
* @return The name of the insertion point (for example, a description of a
* particular request parameter).
*/
String getInsertionPointName();
/**
* This method returns the base value for this insertion point.
*
* @return the base value that appears in this insertion point in the base
* request being scanned, or <code>null</code> if there is no value in the
* base request that corresponds to this insertion point.
*/
String getBaseValue();
/**
* This method is used to build a request with the specified payload placed
* into the insertion point. There is no requirement for extension-provided
* insertion points to adjust the Content-Length header in requests if the
* body length has changed, although Burp-provided insertion points will
* always do this and will return a request with a valid Content-Length
* header.
* <b>Note:</b>
* Scan checks should submit raw non-encoded payloads to insertion points,
* and the insertion point has responsibility for performing any data
* encoding that is necessary given the nature and location of the insertion
* point.
*
* @param payload The payload that should be placed into the insertion
* point.
* @return The resulting request.
*/
byte[] buildRequest(byte[] payload);
/**
* This method is used to determine the offsets of the payload value within
* the request, when it is placed into the insertion point. Scan checks may
* invoke this method when reporting issues, so as to highlight the relevant
* part of the request within the UI.
*
* @param payload The payload that should be placed into the insertion
* point.
* @return An int[2] array containing the start and end offsets of the
* payload within the request, or null if this is not applicable (for
* example, where the insertion point places a payload into a serialized
* data structure, the raw payload may not literally appear anywhere within
* the resulting request).
*/
int[] getPayloadOffsets(byte[] payload);
/**
* This method returns the type of the insertion point.
*
* @return The type of the insertion point. Available types are defined in
* this interface.
*/
byte getInsertionPointType();
} |
Java | BurpBounty/main/java/burp/IScannerInsertionPointProvider.java | package burp;
/*
* @(#)IScannerInsertionPointProvider.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
import java.util.List;
/**
* Extensions can implement this interface and then call
* <code>IBurpExtenderCallbacks.registerScannerInsertionPointProvider()</code>
* to register a factory for custom Scanner insertion points.
*/
public interface IScannerInsertionPointProvider
{
/**
* When a request is actively scanned, the Scanner will invoke this method,
* and the provider should provide a list of custom insertion points that
* will be used in the scan. <b>Note:</b> these insertion points are used in
* addition to those that are derived from Burp Scanner's configuration, and
* those provided by any other Burp extensions.
*
* @param baseRequestResponse The base request that will be actively
* scanned.
* @return A list of
* <code>IScannerInsertionPoint</code> objects that should be used in the
* scanning, or
* <code>null</code> if no custom insertion points are applicable for this
* request.
*/
List<IScannerInsertionPoint> getInsertionPoints(
IHttpRequestResponse baseRequestResponse);
} |
Java | BurpBounty/main/java/burp/IScannerListener.java | package burp;
/*
* @(#)IScannerListener.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* Extensions can implement this interface and then call
* <code>IBurpExtenderCallbacks.registerScannerListener()</code> to register a
* Scanner listener. The listener will be notified of new issues that are
* reported by the Scanner tool. Extensions can perform custom analysis or
* logging of Scanner issues by registering a Scanner listener.
*/
public interface IScannerListener
{
/**
* This method is invoked when a new issue is added to Burp Scanner's
* results.
*
* @param issue An
* <code>IScanIssue</code> object that the extension can query to obtain
* details about the new issue.
*/
void newScanIssue(IScanIssue issue);
} |
Java | BurpBounty/main/java/burp/IScanQueueItem.java | package burp;
/*
* @(#)IScanQueueItem.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* This interface is used to retrieve details of items in the Burp Scanner
* active scan queue. Extensions can obtain references to scan queue items by
* calling
* <code>IBurpExtenderCallbacks.doActiveScan()</code>.
*/
public interface IScanQueueItem
{
/**
* This method returns a description of the status of the scan queue item.
*
* @return A description of the status of the scan queue item.
*/
String getStatus();
/**
* This method returns an indication of the percentage completed for the
* scan queue item.
*
* @return An indication of the percentage completed for the scan queue
* item.
*/
byte getPercentageComplete();
/**
* This method returns the number of requests that have been made for the
* scan queue item.
*
* @return The number of requests that have been made for the scan queue
* item.
*/
int getNumRequests();
/**
* This method returns the number of network errors that have occurred for
* the scan queue item.
*
* @return The number of network errors that have occurred for the scan
* queue item.
*/
int getNumErrors();
/**
* This method returns the number of attack insertion points being used for
* the scan queue item.
*
* @return The number of attack insertion points being used for the scan
* queue item.
*/
int getNumInsertionPoints();
/**
* This method allows the scan queue item to be canceled.
*/
void cancel();
/**
* This method returns details of the issues generated for the scan queue
* item. <b>Note:</b> different items within the scan queue may contain
* duplicated versions of the same issues - for example, if the same request
* has been scanned multiple times. Duplicated issues are consolidated in
* the main view of scan results. Extensions can register an
* <code>IScannerListener</code> to get details only of unique, newly
* discovered Scanner issues post-consolidation.
*
* @return Details of the issues generated for the scan queue item.
*/
IScanIssue[] getIssues();
} |
Java | BurpBounty/main/java/burp/IScopeChangeListener.java | package burp;
/*
* @(#)IScopeChangeListener.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* Extensions can implement this interface and then call
* <code>IBurpExtenderCallbacks.registerScopeChangeListener()</code> to register
* a scope change listener. The listener will be notified whenever a change
* occurs to Burp's suite-wide target scope.
*/
public interface IScopeChangeListener
{
/**
* This method is invoked whenever a change occurs to Burp's suite-wide
* target scope.
*/
void scopeChanged();
} |
Java | BurpBounty/main/java/burp/ISessionHandlingAction.java | package burp;
/*
* @(#)ISessionHandlingAction.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* Extensions can implement this interface and then call
* <code>IBurpExtenderCallbacks.registerSessionHandlingAction()</code> to
* register a custom session handling action. Each registered action will be
* available within the session handling rule UI for the user to select as a
* rule action. Users can choose to invoke an action directly in its own right,
* or following execution of a macro.
*/
public interface ISessionHandlingAction
{
/**
* This method is used by Burp to obtain the name of the session handling
* action. This will be displayed as an option within the session handling
* rule editor when the user selects to execute an extension-provided
* action.
*
* @return The name of the action.
*/
String getActionName();
/**
* This method is invoked when the session handling action should be
* executed. This may happen as an action in its own right, or as a
* sub-action following execution of a macro.
*
* @param currentRequest The base request that is currently being processed.
* The action can query this object to obtain details about the base
* request. It can issue additional requests of its own if necessary, and
* can use the setter methods on this object to update the base request.
* @param macroItems If the action is invoked following execution of a
* macro, this parameter contains the result of executing the macro.
* Otherwise, it is
* <code>null</code>. Actions can use the details of the macro items to
* perform custom analysis of the macro to derive values of non-standard
* session handling tokens, etc.
*/
void performAction(
IHttpRequestResponse currentRequest,
IHttpRequestResponse[] macroItems);
} |
Java | BurpBounty/main/java/burp/ITab.java | package burp;
/*
* @(#)ITab.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
import java.awt.Component;
/**
* This interface is used to provide Burp with details of a custom tab that will
* be added to Burp's UI, using a method such as
* <code>IBurpExtenderCallbacks.addSuiteTab()</code>.
*/
public interface ITab
{
/**
* Burp uses this method to obtain the caption that should appear on the
* custom tab when it is displayed.
*
* @return The caption that should appear on the custom tab when it is
* displayed.
*/
String getTabCaption();
/**
* Burp uses this method to obtain the component that should be used as the
* contents of the custom tab when it is displayed.
*
* @return The component that should be used as the contents of the custom
* tab when it is displayed.
*/
Component getUiComponent();
} |
Java | BurpBounty/main/java/burp/ITempFile.java | package burp;
/*
* @(#)ITempFile.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
/**
* This interface is used to hold details of a temporary file that has been
* created via a call to
* <code>IBurpExtenderCallbacks.saveToTempFile()</code>.
*
*/
public interface ITempFile
{
/**
* This method is used to retrieve the contents of the buffer that was saved
* in the temporary file.
*
* @return The contents of the buffer that was saved in the temporary file.
*/
byte[] getBuffer();
/**
* This method is deprecated and no longer performs any action.
*/
@Deprecated
void delete();
} |
Java | BurpBounty/main/java/burp/ITextEditor.java | package burp;
/*
* @(#)ITextEditor.java
*
* Copyright PortSwigger Ltd. All rights reserved.
*
* This code may be used to extend the functionality of Burp Suite Community Edition
* and Burp Suite Professional, provided that this usage does not violate the
* license terms for those products.
*/
import java.awt.Component;
/**
* This interface is used to provide extensions with an instance of Burp's raw
* text editor, for the extension to use in its own UI. Extensions should call
* <code>IBurpExtenderCallbacks.createTextEditor()</code> to obtain an instance
* of this interface.
*/
public interface ITextEditor
{
/**
* This method returns the UI component of the editor, for extensions to add
* to their own UI.
*
* @return The UI component of the editor.
*/
Component getComponent();
/**
* This method is used to control whether the editor is currently editable.
* This status can be toggled on and off as required.
*
* @param editable Indicates whether the editor should be currently
* editable.
*/
void setEditable(boolean editable);
/**
* This method is used to update the currently displayed text in the editor.
*
* @param text The text to be displayed.
*/
void setText(byte[] text);
/**
* This method is used to retrieve the currently displayed text.
*
* @return The currently displayed text.
*/
byte[] getText();
/**
* This method is used to determine whether the user has modified the
* contents of the editor.
*
* @return An indication of whether the user has modified the contents of
* the editor since the last call to
* <code>setText()</code>.
*/
boolean isTextModified();
/**
* This method is used to obtain the currently selected text.
*
* @return The currently selected text, or
* <code>null</code> if the user has not made any selection.
*/
byte[] getSelectedText();
/**
* This method can be used to retrieve the bounds of the user's selection
* into the displayed text, if applicable.
*
* @return An int[2] array containing the start and end offsets of the
* user's selection within the displayed text. If the user has not made any
* selection in the current message, both offsets indicate the position of
* the caret within the editor.
*/
int[] getSelectionBounds();
/**
* This method is used to update the search expression that is shown in the
* search bar below the editor. The editor will automatically highlight any
* regions of the displayed text that match the search expression.
*
* @param expression The search expression.
*/
void setSearchExpression(String expression);
} |
BurpBounty/main/java/burpbountyfree/ActiveProfile.form | <?xml version="1.0" encoding="UTF-8" ?>
<Form version="1.8" maxVersion="1.9" type="org.netbeans.modules.form.forminfo.JPanelFormInfo">
<NonVisualComponents>
<Component class="javax.swing.ButtonGroup" name="buttonGroup1">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup2">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup3">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup4">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup5">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup6">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</NonVisualComponents>
<Properties>
<Property name="preferredSize" type="java.awt.Dimension" editor="org.netbeans.beaninfo.editors.DimensionEditor">
<Dimension value="[831, 664]"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="FormSettings_autoResourcing" type="java.lang.Integer" value="0"/>
<AuxValue name="FormSettings_autoSetComponentName" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_generateFQN" type="java.lang.Boolean" value="true"/>
<AuxValue name="FormSettings_generateMnemonicsCode" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_i18nAutoMode" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_layoutCodeTarget" type="java.lang.Integer" value="1"/>
<AuxValue name="FormSettings_listenerGenerationStyle" type="java.lang.Integer" value="0"/>
<AuxValue name="FormSettings_variablesLocal" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_variablesModifier" type="java.lang.Integer" value="2"/>
</AuxValues>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel12" min="-2" max="-2" attributes="0"/>
<EmptySpace type="unrelated" max="-2" attributes="0"/>
<Component id="text1" max="32767" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jLabel18" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="textauthor" min="-2" pref="228" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
</Group>
<Component id="headerstab" alignment="0" pref="831" max="32767" attributes="0"/>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="text1" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="jLabel12" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="textauthor" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="jLabel18" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="unrelated" max="-2" attributes="0"/>
<Component id="headerstab" pref="606" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JTextField" name="text1">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="0"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel18">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="0"/>
</Property>
<Property name="text" type="java.lang.String" value="Author:"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel12">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="0"/>
</Property>
<Property name="text" type="java.lang.String" value="Name:"/>
</Properties>
</Component>
<Component class="javax.swing.JTextField" name="textauthor">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="0"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Container class="javax.swing.JTabbedPane" name="headerstab">
<Properties>
<Property name="autoscrolls" type="boolean" value="true"/>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="0"/>
</Property>
<Property name="preferredSize" type="java.awt.Dimension" editor="org.netbeans.beaninfo.editors.DimensionEditor">
<Dimension value="[750, 500]"/>
</Property>
</Properties>
<Events>
<EventHandler event="stateChanged" listener="javax.swing.event.ChangeListener" parameters="javax.swing.event.ChangeEvent" handler="headerstabStateChanged"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout"/>
<SubComponents>
<Container class="javax.swing.JScrollPane" name="jScrollPane5">
<Properties>
<Property name="preferredSize" type="java.awt.Dimension" editor="org.netbeans.beaninfo.editors.DimensionEditor">
<Dimension value="[0, 0]"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_CreateCodeCustom" type="java.lang.String" value="new javax.swing.JScrollPane()"/>
<AuxValue name="JavaCodeGenerator_InitCodePost" type="java.lang.String" value="jScrollPane5.getVerticalScrollBar().setUnitIncrement(20);"/>
</AuxValues>
<Constraints>
<Constraint layoutClass="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout" value="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout$JTabbedPaneConstraintsDescription">
<JTabbedPaneConstraints tabName=" Request ">
<Property name="tabTitle" type="java.lang.String" value=" Request "/>
</JTabbedPaneConstraints>
</Constraint>
</Constraints>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Container class="javax.swing.JPanel" name="jPanel10">
<Properties>
<Property name="maximumSize" type="java.awt.Dimension" editor="org.netbeans.beaninfo.editors.DimensionEditor">
<Dimension value="[0, 0]"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="6" pref="6" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="jLabel53" alignment="0" min="-2" pref="704" max="-2" attributes="0"/>
<Component id="jLabel52" alignment="0" min="-2" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<Component id="check8" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="text5" max="32767" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="jButton9" alignment="1" max="32767" attributes="0"/>
<Component id="jButton6" alignment="1" max="32767" attributes="0"/>
<Component id="jButton8" alignment="0" max="32767" attributes="0"/>
<Component id="jButton7" alignment="0" min="-2" pref="93" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="jScrollPane4" max="32767" attributes="0"/>
<Component id="combo2" pref="670" max="32767" attributes="0"/>
</Group>
</Group>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="button18" alignment="0" min="-2" pref="89" max="-2" attributes="0"/>
<Component id="button19" alignment="0" min="-2" pref="89" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel1" min="-2" max="-2" attributes="0"/>
<Component id="jScrollPane14" min="-2" pref="673" max="-2" attributes="0"/>
<Component id="jLabel20" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
</Group>
<Component id="jLabel19" alignment="0" min="-2" pref="704" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="47" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel17" alignment="0" min="-2" max="-2" attributes="0"/>
<Group type="103" alignment="0" groupAlignment="1" attributes="0">
<Component id="jLabel10" min="-2" max="-2" attributes="0"/>
<Component id="jLabel11" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
<Component id="jLabel5" alignment="0" min="-2" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="1" attributes="0">
<Component id="button6" min="-2" pref="89" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="12" pref="12" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="103" alignment="0" groupAlignment="0" attributes="0">
<Component id="button3" alignment="0" min="-2" pref="89" max="-2" attributes="0"/>
<Component id="button4" alignment="1" min="-2" pref="89" max="-2" attributes="0"/>
</Group>
<Component id="button5" alignment="1" min="-2" pref="89" max="-2" attributes="0"/>
</Group>
</Group>
<Component id="button2" alignment="1" min="-2" pref="89" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="textpayloads" pref="670" max="32767" attributes="0"/>
<Component id="jScrollPane16" max="32767" attributes="0"/>
</Group>
</Group>
</Group>
<EmptySpace pref="15" max="32767" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel22" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel23" alignment="0" min="-2" pref="704" max="-2" attributes="0"/>
<Component id="jLabel54" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel55" alignment="0" min="-2" pref="704" max="-2" attributes="0"/>
</Group>
<EmptySpace min="0" pref="0" max="32767" attributes="0"/>
</Group>
</Group>
</Group>
<Component id="jSeparator2" alignment="0" max="32767" attributes="0"/>
<Component id="jSeparator4" alignment="0" max="32767" attributes="0"/>
<Component id="jSeparator3" alignment="1" max="32767" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="183" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="extensionprovided" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="header" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="urlpathfilename" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="entirebody" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="paramxml" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="All" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="30" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="paramjson" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="parambody" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="paramcookie" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="urlpathfolder" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="paramamf" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="paramxmlattr" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="51" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="unknown" min="-2" max="-2" attributes="0"/>
<Component id="parammultipartattr" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="paramnamebody" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="paramnameurl" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="userprovided" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="paramurl" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<Group type="102" attributes="0">
<Component id="replace" min="-2" max="-2" attributes="0"/>
<EmptySpace type="unrelated" max="-2" attributes="0"/>
<Component id="append" min="-2" max="-2" attributes="0"/>
<EmptySpace type="unrelated" max="-2" attributes="0"/>
<Component id="insert" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace min="0" pref="0" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="10" max="-2" attributes="0"/>
<Component id="jLabel5" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel19" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="textpayloads" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="button3" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<Component id="button6" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="button2" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="button4" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="button5" min="-2" max="-2" attributes="0"/>
<EmptySpace min="0" pref="96" max="32767" attributes="0"/>
</Group>
<Component id="jScrollPane16" pref="0" max="32767" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jSeparator2" min="-2" pref="10" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel54" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel55" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="23" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel10" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="append" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="replace" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="insert" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="23" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<Component id="jLabel11" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="151" max="-2" attributes="0"/>
<Component id="jLabel17" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Component id="jSeparator4" min="-2" pref="10" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel52" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel53" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jLabel1" min="-2" max="-2" attributes="0"/>
<EmptySpace type="unrelated" min="-2" max="-2" attributes="0"/>
<Component id="jLabel20" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<Component id="button19" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="button18" min="-2" max="-2" attributes="0"/>
</Group>
<Component id="jScrollPane14" min="-2" pref="119" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Component id="jSeparator3" min="-2" pref="10" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel22" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel23" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Component id="jButton9" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jButton8" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jButton7" min="-2" max="-2" attributes="0"/>
</Group>
<Component id="jScrollPane4" alignment="0" min="-2" pref="99" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="combo2" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="jButton6" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="19" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="check8" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="text5" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<Group type="102" attributes="0">
<Group type="103" groupAlignment="3" attributes="0">
<Component id="paramamf" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="parammultipartattr" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="All" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="parambody" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="paramnamebody" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="urlpathfilename" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="extensionprovided" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="paramcookie" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="paramurl" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="header" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="paramjson" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="paramnameurl" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="entirebody" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="urlpathfolder" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="userprovided" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="paramxml" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="paramxmlattr" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="unknown" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
<EmptySpace max="-2" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JCheckBox" name="parambody">
<Properties>
<Property name="text" type="java.lang.String" value="Param body"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator2">
</Component>
<Component class="javax.swing.JTextField" name="text5">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JButton" name="jButton9">
<Properties>
<Property name="text" type="java.lang.String" value="Remove"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="jButton9removeEncoder"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="button6">
<Properties>
<Property name="text" type="java.lang.String" value="Add"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button6setToPayload"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JButton" name="jButton8">
<Properties>
<Property name="text" type="java.lang.String" value="Up"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="jButton8upEncoder"/>
</Events>
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane14">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTable" name="table4">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="13" style="0"/>
</Property>
<Property name="model" type="javax.swing.table.TableModel" editor="org.netbeans.modules.form.RADConnectionPropertyEditor">
<Connection code="model4" type="code"/>
</Property>
<Property name="columnModel" type="javax.swing.table.TableColumnModel" editor="org.netbeans.modules.form.editors2.TableColumnModelEditor">
<TableColumnModel selectionModel="0"/>
</Property>
<Property name="showGrid" type="boolean" value="false"/>
<Property name="tableHeader" type="javax.swing.table.JTableHeader" editor="org.netbeans.modules.form.editors2.JTableHeaderEditor">
<TableHeader reorderingAllowed="true" resizingAllowed="true"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_SerializeTo" type="java.lang.String" value="BurpBountyGui_table4"/>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JLabel" name="jLabel22">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Payload Encoding"/>
</Properties>
</Component>
<Component class="javax.swing.JCheckBox" name="urlpathfolder">
<Properties>
<Property name="text" type="java.lang.String" value="Url path folder"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane4">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JList" name="list3">
<Properties>
<Property name="model" type="javax.swing.ListModel" editor="org.netbeans.modules.form.RADConnectionPropertyEditor">
<Connection code="encoder" type="code"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_TypeParameters" type="java.lang.String" value="<String>"/>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JCheckBox" name="header">
<Properties>
<Property name="text" type="java.lang.String" value="Header"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator3">
</Component>
<Component class="javax.swing.JCheckBox" name="paramurl">
<Properties>
<Property name="text" type="java.lang.String" value="Param url"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JButton" name="button3">
<Properties>
<Property name="text" type="java.lang.String" value="Load File"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button3loadPayloads"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel55">
<Properties>
<Property name="text" type="java.lang.String" value="You can define the payload options."/>
</Properties>
</Component>
<Component class="javax.swing.JCheckBox" name="paramcookie">
<Properties>
<Property name="text" type="java.lang.String" value="Param cookie"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel52">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Match and Replace"/>
</Properties>
</Component>
<Component class="javax.swing.JCheckBox" name="paramnamebody">
<Properties>
<Property name="text" type="java.lang.String" value="Param name body"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JButton" name="button2">
<Properties>
<Property name="text" type="java.lang.String" value="Paste"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button2pastePayload"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="paramamf">
<Properties>
<Property name="text" type="java.lang.String" value="Param AMF"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="urlpathfilename">
<Properties>
<Property name="text" type="java.lang.String" value="Url path filename"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="unknown">
<Properties>
<Property name="text" type="java.lang.String" value="Unknown"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel11">
<Properties>
<Property name="text" type="java.lang.String" value="Insertion point type:"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel17">
<Properties>
<Property name="text" type="java.lang.String" value="<html> * More info at <a href=\u005c"\u005c">Burp Suite Extender API</a></html>" containsInvalidXMLChars="true"/>
</Properties>
<Events>
<EventHandler event="mouseClicked" listener="java.awt.event.MouseListener" parameters="java.awt.event.MouseEvent" handler="jLabel17goWeb"/>
</Events>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator4">
</Component>
<Component class="javax.swing.JButton" name="button4">
<Properties>
<Property name="text" type="java.lang.String" value="Remove"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button4removePayload"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JButton" name="button18">
<Properties>
<Property name="text" type="java.lang.String" value="Remove"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button18removeMatchReplace"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="button19">
<Properties>
<Property name="text" type="java.lang.String" value="Add"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="addMatchReplace"/>
</Events>
</Component>
<Component class="javax.swing.JComboBox" name="combo2">
<Properties>
<Property name="model" type="javax.swing.ComboBoxModel" editor="org.netbeans.modules.form.editors2.ComboBoxModelEditor">
<StringArray count="6">
<StringItem index="0" value="URL-encode key characters"/>
<StringItem index="1" value="URL-encode all characters"/>
<StringItem index="2" value="URL-encode all characters (Unicode)"/>
<StringItem index="3" value="HTML-encode key characters"/>
<StringItem index="4" value="HTML-encode all characters"/>
<StringItem index="5" value="Base64-encode"/>
</StringArray>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_TypeParameters" type="java.lang.String" value="<String>"/>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="extensionprovided">
<Properties>
<Property name="text" type="java.lang.String" value="Path discovery"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="parammultipartattr">
<Properties>
<Property name="text" type="java.lang.String" value="Param multipart attr"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="paramjson">
<Properties>
<Property name="text" type="java.lang.String" value="Param json"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="paramxmlattr">
<Properties>
<Property name="text" type="java.lang.String" value="Param xml attr"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="paramnameurl">
<Properties>
<Property name="text" type="java.lang.String" value="Param name url"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JTextField" name="textpayloads">
<Properties>
<Property name="toolTipText" type="java.lang.String" value=""/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="userprovided">
<Properties>
<Property name="text" type="java.lang.String" value="User provided"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel54">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Payload Options"/>
</Properties>
</Component>
<Component class="javax.swing.JButton" name="jButton6">
<Properties>
<Property name="text" type="java.lang.String" value="Add"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="jButton6addEncoder"/>
</Events>
</Component>
<Component class="javax.swing.JLabel" name="jLabel19">
<Properties>
<Property name="text" type="java.lang.String" value="You can define one or more payloads. Each payload of this section will be sent at each insertion point."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel10">
<Properties>
<Property name="text" type="java.lang.String" value="Payload position:"/>
</Properties>
</Component>
<Component class="javax.swing.JButton" name="button5">
<Properties>
<Property name="text" type="java.lang.String" value="Clear"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button5removeAllPayloads"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="replace">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup1"/>
</Property>
<Property name="text" type="java.lang.String" value="Replace"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel5">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Payload "/>
</Properties>
</Component>
<Component class="javax.swing.JCheckBox" name="check8">
<Properties>
<Property name="text" type="java.lang.String" value="URL-Encode these characters:"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="entirebody">
<Properties>
<Property name="text" type="java.lang.String" value="Entire body"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="All">
<Properties>
<Property name="text" type="java.lang.String" value="All "/>
</Properties>
<Events>
<EventHandler event="itemStateChanged" listener="java.awt.event.ItemListener" parameters="java.awt.event.ItemEvent" handler="AllItemStateChanged"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="paramxml">
<Properties>
<Property name="text" type="java.lang.String" value="Param xml"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="paramxmlActionPerformed"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel23">
<Properties>
<Property name="text" type="java.lang.String" value="You can define the encoding of payloads. You can encode each payload multiple times."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel53">
<Properties>
<Property name="text" type="java.lang.String" value="These settings are used to automatically replace part of request when the active scanner run."/>
</Properties>
</Component>
<Component class="javax.swing.JRadioButton" name="append">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup1"/>
</Property>
<Property name="text" type="java.lang.String" value="Append"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JButton" name="jButton7">
<Properties>
<Property name="text" type="java.lang.String" value="Down"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="jButton7downEncoder"/>
</Events>
</Component>
<Component class="javax.swing.JLabel" name="jLabel1">
<Properties>
<Property name="text" type="java.lang.String" value="- {PAYLOAD} token will be replaced by your payload"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel20">
<Properties>
<Property name="text" type="java.lang.String" value="- {BC} token will be replaced by burpcollaborator host"/>
</Properties>
</Component>
<Component class="javax.swing.JRadioButton" name="insert">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup1"/>
</Property>
<Property name="text" type="java.lang.String" value="Insert"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane16">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTable" name="table6">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="13" style="0"/>
</Property>
<Property name="model" type="javax.swing.table.TableModel" editor="org.netbeans.modules.form.RADConnectionPropertyEditor">
<Connection code="modelpayload" type="code"/>
</Property>
<Property name="columnModel" type="javax.swing.table.TableColumnModel" editor="org.netbeans.modules.form.editors2.TableColumnModelEditor">
<TableColumnModel selectionModel="0"/>
</Property>
<Property name="showGrid" type="boolean" value="false"/>
<Property name="tableHeader" type="javax.swing.table.JTableHeader" editor="org.netbeans.modules.form.editors2.JTableHeaderEditor">
<TableHeader reorderingAllowed="true" resizingAllowed="true"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_SerializeTo" type="java.lang.String" value="BurpBountyGui_table4"/>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
</SubComponents>
</Container>
</SubComponents>
</Container>
<Container class="javax.swing.JScrollPane" name="jScrollPane6">
<Properties>
<Property name="horizontalScrollBarPolicy" type="int" value="31"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_InitCodePost" type="java.lang.String" value="jScrollPane6.getVerticalScrollBar().setUnitIncrement(20);"/>
</AuxValues>
<Constraints>
<Constraint layoutClass="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout" value="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout$JTabbedPaneConstraintsDescription">
<JTabbedPaneConstraints tabName=" Response ">
<Property name="tabTitle" type="java.lang.String" value=" Response "/>
</JTabbedPaneConstraints>
</Constraint>
</Constraints>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Container class="javax.swing.JPanel" name="jPanel11">
<Properties>
<Property name="autoscrolls" type="boolean" value="true"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_LayoutCodePost" type="java.lang.String" value="JScrollPane responseresScroll = new JScrollPane(jPanel11, 
 JScrollPane.VERTICAL_SCROLLBAR_ALWAYS, JScrollPane.HORIZONTAL_SCROLLBAR_NEVER);"/>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jSeparator5" alignment="0" max="32767" attributes="0"/>
<Component id="jSeparator6" alignment="1" max="32767" attributes="0"/>
<Component id="jSeparator11" alignment="1" max="32767" attributes="0"/>
<Group type="102" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="20" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel6" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel2" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="unrelated" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="rb1" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="rb2" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="rb3" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="rb4" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="sp1" alignment="0" min="-2" pref="56" max="-2" attributes="0"/>
</Group>
</Group>
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel31" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel30" alignment="0" min="-2" pref="769" max="-2" attributes="0"/>
<Component id="onlyhttp" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="check4" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="check1" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="excludehttp" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel29" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel28" alignment="0" min="-2" pref="769" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="check72" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="check71" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="15" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<Component id="text73" min="-2" pref="441" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="negativeURL" min="-2" max="-2" attributes="0"/>
</Group>
<Group type="102" attributes="0">
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="text71" pref="441" max="32767" attributes="0"/>
<Component id="text72" max="32767" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="negativeCT" min="-2" max="-2" attributes="0"/>
<Component id="negativeRC" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
</Group>
</Group>
</Group>
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="1" attributes="0">
<Group type="102" alignment="1" attributes="0">
<Component id="button8" min="-2" pref="89" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="textgreps" min="-2" pref="662" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="1" max="-2" attributes="0">
<Component id="button20" alignment="0" min="-2" pref="89" max="-2" attributes="0"/>
<Component id="button10" alignment="0" max="32767" attributes="0"/>
<Component id="button7" alignment="0" min="-2" pref="89" max="-2" attributes="0"/>
<Component id="button21" alignment="1" min="-2" pref="89" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jScrollPane15" min="-2" pref="662" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
<Group type="102" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Component id="check73" min="-2" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel25" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel24" alignment="0" min="-2" pref="769" max="-2" attributes="0"/>
<Component id="jLabel26" alignment="0" min="-2" pref="769" max="-2" attributes="0"/>
<Component id="Attributes" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel27" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Component id="jPanel1" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace pref="50" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="10" max="-2" attributes="0"/>
<Component id="jLabel27" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel26" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jPanel1" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="18" max="-2" attributes="0"/>
<Component id="Attributes" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Component id="jSeparator11" min="-2" pref="3" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel25" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel24" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="textgreps" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="button8" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="32767" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jScrollPane15" min="-2" pref="206" max="-2" attributes="0"/>
<Group type="102" attributes="0">
<Component id="button21" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="button7" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="button20" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="button10" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jSeparator6" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel31" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel30" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Component id="check4" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="check1" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="excludehttp" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="onlyhttp" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="check71" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="text71" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="negativeCT" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="check72" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="text72" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="negativeRC" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="check73" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="text73" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="negativeURL" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Component id="jSeparator5" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel29" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel28" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="rb1" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="jLabel6" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Component id="rb2" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="rb3" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="rb4" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel2" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="sp1" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JCheckBox" name="check4">
<Properties>
<Property name="text" type="java.lang.String" value="Negative match"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="check1">
<Properties>
<Property name="text" type="java.lang.String" value="Case sensitive"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="excludehttp">
<Properties>
<Property name="text" type="java.lang.String" value="Exclude HTTP headers"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="onlyhttp">
<Properties>
<Property name="text" type="java.lang.String" value="Only in HTTP headers"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="check71">
<Properties>
<Property name="text" type="java.lang.String" value="Content type"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="check72">
<Properties>
<Property name="text" type="java.lang.String" value="Status code"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JTextField" name="text72">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JTextField" name="text71">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="negativeCT">
<Properties>
<Property name="text" type="java.lang.String" value="Negative match"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="negativeRC">
<Properties>
<Property name="text" type="java.lang.String" value="Negative match"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel24">
<Properties>
<Property name="text" type="java.lang.String" value="You can define one or more greps. For each payload response, each grep will be searched with specific grep options."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel25">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Grep"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel26">
<Properties>
<Property name="text" type="java.lang.String" value="You can define grep type."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel27">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Match Type"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel28">
<Properties>
<Property name="text" type="java.lang.String" value="You can define how your profile handles redirections."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel29">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Redirections"/>
</Properties>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator5">
</Component>
<Component class="javax.swing.JLabel" name="jLabel30">
<Properties>
<Property name="text" type="java.lang.String" value="These settings can be used to specify grep options of your profile."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel31">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Grep Options"/>
</Properties>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator6">
</Component>
<Component class="javax.swing.JRadioButton" name="rb1">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup4"/>
</Property>
<Property name="text" type="java.lang.String" value="Never"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="rb2">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup4"/>
</Property>
<Property name="text" type="java.lang.String" value="On-site only"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="rb3">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup4"/>
</Property>
<Property name="text" type="java.lang.String" value="In-scope only"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="rb4">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup4"/>
</Property>
<Property name="text" type="java.lang.String" value="Always"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel2">
<Properties>
<Property name="text" type="java.lang.String" value="Max redirections:"/>
</Properties>
</Component>
<Component class="javax.swing.JSpinner" name="sp1">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel6">
<Properties>
<Property name="text" type="java.lang.String" value="Follow redirections: "/>
</Properties>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator11">
</Component>
<Container class="javax.swing.JPanel" name="Attributes">
<Properties>
<Property name="border" type="javax.swing.border.Border" editor="org.netbeans.modules.form.RADConnectionPropertyEditor">
<Connection code="javax.swing.BorderFactory.createTitledBorder(null, "Attributes", javax.swing.border.TitledBorder.CENTER, javax.swing.border.TitledBorder.TOP)" type="code"/>
</Property>
</Properties>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="page_title" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="non_hidden_form_input_types" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="input_image_labels" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="status_code" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="visible_text" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="word_count" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="div_ids" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="button_submit_labels" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="content_type" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="outbound_edge_tag_names" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="anchor_labels" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="etag_header" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="whole_body_content" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="content_length" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="visible_word_count" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="header_tags" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="input_submit_labels" alignment="0" min="-2" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="tag_names" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="first_header_tag" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="set_cookie_names" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="line_count" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="comments" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="tag_ids" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="last_modified_header" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="outbound_edge_count" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="initial_body_content" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="css_classes" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="canonical_link" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="limited_body_content" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="content_location" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="location" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Component id="outbound_edge_count" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="initial_body_content" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="content_location" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="limited_body_content" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="canonical_link" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="css_classes" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="location" min="-2" max="-2" attributes="0"/>
<EmptySpace min="0" pref="0" max="32767" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Component id="content_type" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="outbound_edge_tag_names" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="anchor_labels" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="whole_body_content" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="etag_header" min="-2" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Component id="tag_ids" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="comments" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="line_count" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="set_cookie_names" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="last_modified_header" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Component id="visible_word_count" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="content_length" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="header_tags" min="-2" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Component id="first_header_tag" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="tag_names" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="input_submit_labels" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
<Group type="102" alignment="0" attributes="0">
<Component id="status_code" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="input_image_labels" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="non_hidden_form_input_types" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="page_title" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="visible_text" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="button_submit_labels" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="div_ids" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="word_count" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JCheckBox" name="status_code">
<Properties>
<Property name="text" type="java.lang.String" value="status_code"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="input_image_labels">
<Properties>
<Property name="text" type="java.lang.String" value="input_image_labels"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="non_hidden_form_input_types">
<Properties>
<Property name="text" type="java.lang.String" value="non_hidden_form_input_types"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="page_title">
<Properties>
<Property name="text" type="java.lang.String" value="page_title"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="visible_text">
<Properties>
<Property name="text" type="java.lang.String" value="visible_text"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="button_submit_labels">
<Properties>
<Property name="text" type="java.lang.String" value="button_submit_labels"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="div_ids">
<Properties>
<Property name="text" type="java.lang.String" value="div_ids"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="word_count">
<Properties>
<Property name="text" type="java.lang.String" value="word_count"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="content_type">
<Properties>
<Property name="text" type="java.lang.String" value="content_type"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="outbound_edge_tag_names">
<Properties>
<Property name="text" type="java.lang.String" value="outbound_edge_tag_names"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="location">
<Properties>
<Property name="text" type="java.lang.String" value="location"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="css_classes">
<Properties>
<Property name="text" type="java.lang.String" value="css_classes"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="last_modified_header">
<Properties>
<Property name="text" type="java.lang.String" value="last_modified_header"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="set_cookie_names">
<Properties>
<Property name="text" type="java.lang.String" value="set_cookie_names"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="line_count">
<Properties>
<Property name="text" type="java.lang.String" value="line_count"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="comments">
<Properties>
<Property name="text" type="java.lang.String" value="comments"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="tag_ids">
<Properties>
<Property name="text" type="java.lang.String" value="tag_ids"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="header_tags">
<Properties>
<Property name="text" type="java.lang.String" value="header_tags"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="content_length">
<Properties>
<Property name="text" type="java.lang.String" value="content_length"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="visible_word_count">
<Properties>
<Property name="text" type="java.lang.String" value="visible_word_count"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="whole_body_content">
<Properties>
<Property name="text" type="java.lang.String" value="whole_body_content"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="etag_header">
<Properties>
<Property name="text" type="java.lang.String" value="etag_header"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="first_header_tag">
<Properties>
<Property name="text" type="java.lang.String" value="first_header_tag"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="tag_names">
<Properties>
<Property name="text" type="java.lang.String" value="tag_names"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="input_submit_labels">
<Properties>
<Property name="text" type="java.lang.String" value="input_submit_labels"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="outbound_edge_count">
<Properties>
<Property name="text" type="java.lang.String" value="outbound_edge_count"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="content_location">
<Properties>
<Property name="text" type="java.lang.String" value="content_location"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="initial_body_content">
<Properties>
<Property name="text" type="java.lang.String" value="initial_body_content"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="limited_body_content">
<Properties>
<Property name="text" type="java.lang.String" value="limited_body_content"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="canonical_link">
<Properties>
<Property name="text" type="java.lang.String" value="canonical_link"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="anchor_labels">
<Properties>
<Property name="text" type="java.lang.String" value="anchor_labels"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Container class="javax.swing.JScrollPane" name="jScrollPane15">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTable" name="table5">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="13" style="0"/>
</Property>
<Property name="model" type="javax.swing.table.TableModel" editor="org.netbeans.modules.form.RADConnectionPropertyEditor">
<Connection code="modelgrep" type="code"/>
</Property>
<Property name="showGrid" type="boolean" value="false"/>
<Property name="tableHeader" type="javax.swing.table.JTableHeader" editor="org.netbeans.modules.form.editors2.JTableHeaderEditor">
<TableHeader reorderingAllowed="true" resizingAllowed="true"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_SerializeTo" type="java.lang.String" value="BurpBountyGui_table4"/>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JButton" name="button20">
<Properties>
<Property name="text" type="java.lang.String" value="Remove"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button20removeMatchReplace"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="button10">
<Properties>
<Property name="text" type="java.lang.String" value="Clear"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button10removeAllGrep"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="button7">
<Properties>
<Property name="text" type="java.lang.String" value="Paste"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button7pasteGrep"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="button21">
<Properties>
<Property name="text" type="java.lang.String" value="Add"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button21addGrep"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="button8">
<Properties>
<Property name="text" type="java.lang.String" value="Load File"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button8loadGrep"/>
</Events>
</Component>
<Component class="javax.swing.JTextField" name="textgreps">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="negativeURL">
<Properties>
<Property name="text" type="java.lang.String" value="Negative match"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JTextField" name="text73">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="check73">
<Properties>
<Property name="text" type="java.lang.String" value="URL Extension"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Container class="javax.swing.JPanel" name="jPanel1">
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="1" attributes="0">
<Group type="102" alignment="1" attributes="0">
<Component id="radio4" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="58" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="1" attributes="0">
<Component id="radio12" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="radio3" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
</Group>
</Group>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="variationsRadio" min="-2" max="-2" attributes="0"/>
<Component id="invariationsRadio" min="-2" max="-2" attributes="0"/>
<Component id="radio22" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="36" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="radiotime" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="radiohttp" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="30" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Component id="texttime1" min="-2" pref="100" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel21" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="texttime2" min="-2" pref="100" max="-2" attributes="0"/>
</Group>
<Component id="resposecode" min="-2" pref="226" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel16" min="-2" pref="62" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Component id="radiocl" min="-2" max="-2" attributes="0"/>
<EmptySpace type="unrelated" max="-2" attributes="0"/>
<Component id="textcl" min="-2" pref="100" max="-2" attributes="0"/>
<EmptySpace type="unrelated" max="-2" attributes="0"/>
<Component id="jLabel42" min="-2" pref="62" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace max="-2" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="17" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<Group type="103" groupAlignment="3" attributes="0">
<Component id="radiocl" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="textcl" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="jLabel42" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="radio22" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="radiohttp" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="resposecode" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="texttime2" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="texttime1" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="jLabel21" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="jLabel16" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="radiotime" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<Group type="102" attributes="0">
<Component id="radio4" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="radio3" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="variationsRadio" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="radio12" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="invariationsRadio" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
<EmptySpace max="-2" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JRadioButton" name="radiocl">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup3"/>
</Property>
<Property name="text" type="java.lang.String" value="Content Length difference"/>
</Properties>
<Events>
<EventHandler event="itemStateChanged" listener="java.awt.event.ItemListener" parameters="java.awt.event.ItemEvent" handler="radioclSelect"/>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="radioclActionPerformed"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JTextField" name="textcl">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio12">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup3"/>
</Property>
<Property name="text" type="java.lang.String" value="Payload"/>
</Properties>
<Events>
<EventHandler event="itemStateChanged" listener="java.awt.event.ItemListener" parameters="java.awt.event.ItemEvent" handler="radio12payloadMatchType"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio22">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup3"/>
</Property>
<Property name="text" type="java.lang.String" value="Payload without encode"/>
</Properties>
<Events>
<EventHandler event="itemStateChanged" listener="java.awt.event.ItemListener" parameters="java.awt.event.ItemEvent" handler="radio22payloadencodeMatchType"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel42">
<Properties>
<Property name="text" type="java.lang.String" value="Bytes"/>
</Properties>
</Component>
<Component class="javax.swing.JRadioButton" name="invariationsRadio">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup3"/>
</Property>
<Property name="text" type="java.lang.String" value="Invariations"/>
</Properties>
<Events>
<EventHandler event="itemStateChanged" listener="java.awt.event.ItemListener" parameters="java.awt.event.ItemEvent" handler="invariationsRadioinvariations"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radiotime">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup3"/>
</Property>
<Property name="text" type="java.lang.String" value="Timeout between"/>
</Properties>
<Events>
<EventHandler event="itemStateChanged" listener="java.awt.event.ItemListener" parameters="java.awt.event.ItemEvent" handler="radiotimeTimeoutSelect"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radiohttp">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup3"/>
</Property>
<Property name="text" type="java.lang.String" value="HTTP response codes"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio3">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup3"/>
</Property>
<Property name="text" type="java.lang.String" value="Regex"/>
</Properties>
<Events>
<EventHandler event="itemStateChanged" listener="java.awt.event.ItemListener" parameters="java.awt.event.ItemEvent" handler="radio3regexMatchType"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JTextField" name="texttime1">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel21">
<Properties>
<Property name="text" type="java.lang.String" value="and"/>
</Properties>
</Component>
<Component class="javax.swing.JRadioButton" name="radio4">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup3"/>
</Property>
<Property name="text" type="java.lang.String" value="Simple string"/>
</Properties>
<Events>
<EventHandler event="itemStateChanged" listener="java.awt.event.ItemListener" parameters="java.awt.event.ItemEvent" handler="radio4stringMatchType"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JTextField" name="texttime2">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel16">
<Properties>
<Property name="text" type="java.lang.String" value="Seconds"/>
</Properties>
</Component>
<Component class="javax.swing.JRadioButton" name="variationsRadio">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup3"/>
</Property>
<Property name="text" type="java.lang.String" value="Variations"/>
</Properties>
<Events>
<EventHandler event="itemStateChanged" listener="java.awt.event.ItemListener" parameters="java.awt.event.ItemEvent" handler="variationsRadiovariations"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JTextField" name="resposecode">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
</SubComponents>
</Container>
</SubComponents>
</Container>
<Container class="javax.swing.JScrollPane" name="jScrollPane10">
<AuxValues>
<AuxValue name="JavaCodeGenerator_InitCodePre" type="java.lang.String" value="jScrollPane10.getVerticalScrollBar().setUnitIncrement(20);"/>
</AuxValues>
<Constraints>
<Constraint layoutClass="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout" value="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout$JTabbedPaneConstraintsDescription">
<JTabbedPaneConstraints tabName=" Issue ">
<Property name="tabTitle" type="java.lang.String" value=" Issue "/>
</JTabbedPaneConstraints>
</Constraint>
</Constraints>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Container class="javax.swing.JPanel" name="jPanel12">
<Properties>
<Property name="autoscrolls" type="boolean" value="true"/>
</Properties>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jSeparator7" max="32767" attributes="0"/>
<Component id="jSeparator8" alignment="1" max="32767" attributes="0"/>
<Component id="jSeparator9" alignment="1" max="32767" attributes="0"/>
<Component id="jSeparator10" alignment="1" max="32767" attributes="0"/>
<Group type="102" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel33" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel35" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel34" alignment="0" min="-2" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel13" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jScrollPane7" min="-2" pref="612" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel9" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jScrollPane1" min="-2" pref="612" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel15" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jScrollPane9" min="-2" pref="612" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel14" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jScrollPane8" min="-2" pref="612" max="-2" attributes="0"/>
</Group>
<Component id="jLabel37" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel36" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel39" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel38" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel32" alignment="0" min="-2" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel3" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel4" min="-2" pref="73" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Component id="radio8" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="189" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="radio6" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="radio7" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="radio5" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="32767" attributes="0"/>
<Component id="jLabel7" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="radio9" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="radio11" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="radio10" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
</Group>
<Component id="text4" alignment="0" min="-2" pref="419" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
</Group>
<Component id="jLabel41" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel40" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace pref="93" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="10" max="-2" attributes="0"/>
<Component id="jLabel33" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel32" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel3" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="text4" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel7" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="radio9" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Component id="radio10" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="radio11" min="-2" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel4" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="radio5" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Component id="radio6" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="radio7" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="radio8" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jSeparator7" min="-2" pref="10" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel35" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel34" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel9" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jScrollPane1" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Component id="jSeparator8" min="-2" pref="10" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel37" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel36" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel13" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jScrollPane7" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jSeparator9" min="-2" pref="10" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel39" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel38" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel15" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jScrollPane9" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Component id="jSeparator10" min="-2" pref="10" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel41" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel40" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel14" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jScrollPane8" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JLabel" name="jLabel32">
<Properties>
<Property name="text" type="java.lang.String" value="You can define the issue properties."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel33">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Issue Properties"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel3">
<Properties>
<Property name="text" type="java.lang.String" value="Issue Name:"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel4">
<Properties>
<Property name="text" type="java.lang.String" value="Severity:"/>
</Properties>
</Component>
<Component class="javax.swing.JRadioButton" name="radio5">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup5"/>
</Property>
<Property name="text" type="java.lang.String" value="High"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio6">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup5"/>
</Property>
<Property name="text" type="java.lang.String" value="Medium"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio7">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup5"/>
</Property>
<Property name="text" type="java.lang.String" value="Low"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio8">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup5"/>
</Property>
<Property name="text" type="java.lang.String" value="Information"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel7">
<Properties>
<Property name="text" type="java.lang.String" value="Confidence:"/>
</Properties>
</Component>
<Component class="javax.swing.JRadioButton" name="radio9">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup6"/>
</Property>
<Property name="text" type="java.lang.String" value="Certain"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio10">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup6"/>
</Property>
<Property name="text" type="java.lang.String" value="Firm"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio11">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup6"/>
</Property>
<Property name="text" type="java.lang.String" value="Tentative"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JTextField" name="text4">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator7">
</Component>
<Component class="javax.swing.JLabel" name="jLabel34">
<Properties>
<Property name="text" type="java.lang.String" value="You can define the issue details."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel35">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Issue Detail"/>
</Properties>
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane7">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTextArea" name="textarea2">
<Properties>
<Property name="columns" type="int" value="20"/>
<Property name="rows" type="int" value="5"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JLabel" name="jLabel13">
<Properties>
<Property name="text" type="java.lang.String" value="Description:"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel36">
<Properties>
<Property name="text" type="java.lang.String" value="You can define the issue background."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel37">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Issue Background"/>
</Properties>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator8">
</Component>
<Component class="javax.swing.JLabel" name="jLabel38">
<Properties>
<Property name="text" type="java.lang.String" value="You can define the remediation detail."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel39">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Remediation Detail"/>
</Properties>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator9">
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane1">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTextArea" name="textarea1">
<Properties>
<Property name="columns" type="int" value="20"/>
<Property name="rows" type="int" value="5"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JLabel" name="jLabel9">
<Properties>
<Property name="text" type="java.lang.String" value="Description:"/>
</Properties>
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane8">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTextArea" name="textarea3">
<Properties>
<Property name="columns" type="int" value="20"/>
<Property name="rows" type="int" value="5"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JLabel" name="jLabel14">
<Properties>
<Property name="text" type="java.lang.String" value="Description:"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel40">
<Properties>
<Property name="text" type="java.lang.String" value="You can define the remediation background."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel41">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Remediation Background"/>
</Properties>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator10">
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane9">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTextArea" name="textarea4">
<Properties>
<Property name="columns" type="int" value="20"/>
<Property name="rows" type="int" value="5"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JLabel" name="jLabel15">
<Properties>
<Property name="text" type="java.lang.String" value="Description:"/>
</Properties>
</Component>
</SubComponents>
</Container>
</SubComponents>
</Container>
<Container class="javax.swing.JPanel" name="jPanel3">
<Constraints>
<Constraint layoutClass="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout" value="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout$JTabbedPaneConstraintsDescription">
<JTabbedPaneConstraints tabName=" Tags ">
<Property name="tabTitle" type="java.lang.String" value=" Tags "/>
</JTabbedPaneConstraints>
</Constraint>
</Constraints>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel47" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel46" alignment="0" min="-2" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="newTagb" alignment="0" max="32767" attributes="0"/>
<Component id="addTag" alignment="0" min="-2" pref="93" max="-2" attributes="0"/>
<Component id="removetag" alignment="0" max="32767" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="jScrollPane11" alignment="0" max="32767" attributes="0"/>
<Component id="newTagCombo" alignment="0" min="-2" pref="468" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="10" max="-2" attributes="0"/>
<Component id="jLabel47" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel46" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jScrollPane11" alignment="0" min="-2" pref="99" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<Component id="newTagb" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="removetag" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="newTagCombo" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="addTag" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JButton" name="removetag">
<Properties>
<Property name="text" type="java.lang.String" value="Remove"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="removetag"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="addTag">
<Properties>
<Property name="text" type="java.lang.String" value="Add"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="addTag"/>
</Events>
</Component>
<Component class="javax.swing.JComboBox" name="newTagCombo">
<AuxValues>
<AuxValue name="JavaCodeGenerator_SerializeTo" type="java.lang.String" value="BurpBountyGui_newTagCombo"/>
<AuxValue name="JavaCodeGenerator_TypeParameters" type="java.lang.String" value="<String>"/>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane11">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JList" name="listtag">
<Properties>
<Property name="model" type="javax.swing.ListModel" editor="org.netbeans.modules.form.RADConnectionPropertyEditor">
<Connection code="tag" type="code"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_TypeParameters" type="java.lang.String" value="<String>"/>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JLabel" name="jLabel46">
<Properties>
<Property name="text" type="java.lang.String" value="You can define one or multiple tags for this profile."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel47">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Set Tags"/>
</Properties>
</Component>
<Component class="javax.swing.JButton" name="newTagb">
<Properties>
<Property name="text" type="java.lang.String" value="New Tag"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="newTagbnewTag"/>
</Events>
</Component>
</SubComponents>
</Container>
</SubComponents>
</Container>
</SubComponents>
</Form> |
|
Java | BurpBounty/main/java/burpbountyfree/ActiveProfile.java | /*
Copyright 2018 Eduardo Garcia Melia <wagiro@gmail.com>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package burpbountyfree;
import burp.IBurpExtenderCallbacks;
import java.awt.Desktop;
import java.awt.Toolkit;
import java.awt.datatransfer.Clipboard;
import java.awt.datatransfer.DataFlavor;
import java.awt.datatransfer.Transferable;
import java.awt.datatransfer.UnsupportedFlavorException;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.io.PrintWriter;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import javax.swing.DefaultCellEditor;
import javax.swing.DefaultListModel;
import javax.swing.JComboBox;
import javax.swing.JDialog;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JScrollPane;
import javax.swing.RowSorter;
import javax.swing.SortOrder;
import javax.swing.table.DefaultTableModel;
import javax.swing.table.TableModel;
import javax.swing.table.TableRowSorter;
/**
*
* @author eduardogarcia
*/
public class ActiveProfile extends javax.swing.JPanel {
/**
* Creates new form ActiveProfile
*/
DefaultListModel payload;
DefaultListModel grep;
DefaultListModel encoder;
DefaultListModel tag;
DefaultListModel tagmanager;
List<Headers> headers;
List<String> variationAttributes;
List<Integer> insertionPointType;
List<String> Tags;
Boolean pathDiscovery;
DefaultTableModel model;
DefaultTableModel model1;
DefaultTableModel model2;
DefaultTableModel model4;
DefaultTableModel model9;
DefaultTableModel model10;
DefaultTableModel rulemodel;
DefaultTableModel modelgrep;
DefaultTableModel modelpayload;
IBurpExtenderCallbacks callbacks;
String filename;
JComboBox operator;
public ActiveProfile(IBurpExtenderCallbacks callbacks) {
payload = new DefaultListModel();
grep = new DefaultListModel();
encoder = new DefaultListModel();
tag = new DefaultListModel();
tagmanager = new DefaultListModel();
model4 = new DefaultTableModel();
model9 = new DefaultTableModel();
model10 = new DefaultTableModel();
modelgrep = new DefaultTableModel();
modelpayload = new DefaultTableModel();
headers = new ArrayList();
variationAttributes = new ArrayList();
insertionPointType = new ArrayList();
this.callbacks = callbacks;
operator = new JComboBox();
modelgrep = new DefaultTableModel() {
@Override
public Class<?> getColumnClass(int columnIndex) {
Class clazz = String.class;
switch (columnIndex) {
case 0:
clazz = Boolean.class;
break;
}
return clazz;
}
@Override
public boolean isCellEditable(int row, int column) {
if (row == 0 && column == 1) {
return false;
} else {
return true;
}
}
};
modelpayload = new DefaultTableModel() {
@Override
public Class<?> getColumnClass(int columnIndex) {
Class clazz = String.class;
switch (columnIndex) {
case 0:
clazz = Boolean.class;
break;
}
return clazz;
}
};
initComponents();
if (callbacks.loadExtensionSetting("filename") != null) {
filename = callbacks.loadExtensionSetting("filename") + File.separator;
} else {
filename = System.getProperty("user.home") + File.separator;
}
showHeaders(headers);
showGrepsTable();
showPayloadsTable();
}
public void showGrepsTable() {
modelgrep.setNumRows(0);
modelgrep.setColumnCount(0);
modelgrep.addColumn("Enabled");
modelgrep.addColumn("Operator");
modelgrep.addColumn("Value");
operator.addItem("Or");
operator.addItem("And");
table5.getColumnModel().getColumn(0).setPreferredWidth(5);
table5.getColumnModel().getColumn(1).setPreferredWidth(15);
table5.getColumnModel().getColumn(2).setPreferredWidth(400);
table5.getColumnModel().getColumn(1).setCellEditor(new DefaultCellEditor(operator));
TableRowSorter<TableModel> sorter = new TableRowSorter<>(table5.getModel());
table5.setRowSorter(sorter);
List<RowSorter.SortKey> sortKeys = new ArrayList<>();
sorter.setSortKeys(sortKeys);
sorter.sort();
}
public void showPayloadsTable() {
modelpayload.setNumRows(0);
modelpayload.setColumnCount(0);
modelpayload.addColumn("Enabled");
modelpayload.addColumn("Value");
table6.getColumnModel().getColumn(0).setPreferredWidth(5);
table6.getColumnModel().getColumn(1).setPreferredWidth(415);
TableRowSorter<TableModel> sorter = new TableRowSorter<>(table6.getModel());
table6.setRowSorter(sorter);
List<RowSorter.SortKey> sortKeys = new ArrayList<>();
sorter.setSortKeys(sortKeys);
sorter.sort();
}
public void showGreps(List<String> greps) {
for (String grepline : greps) {
if(grepline.startsWith("true,") || grepline.startsWith("false,") ){
List<String> array = Arrays.asList(grepline.split(",", 3));
if (modelgrep.getRowCount() == 0) {
if (array.get(0).equals("true")) {
modelgrep.addRow(new Object[]{true, "", array.get(2)});
} else {
modelgrep.addRow(new Object[]{false, "", array.get(2)});
}
} else {
if (array.get(0).equals("true")) {
modelgrep.addRow(new Object[]{true, array.get(1), array.get(2)});
} else {
modelgrep.addRow(new Object[]{false, array.get(1), array.get(2)});
}
}
} else {
if (modelgrep.getRowCount() == 0) {
modelgrep.addRow(new Object[]{true, "", grepline});
} else {
modelgrep.addRow(new Object[]{true, "Or", grepline});
}
}
}
}
public void showPayloads(List<String> payloads) {
for (String payloadline : payloads) {
if(payloadline.startsWith("true,") || payloadline.startsWith("false,") ){
List<String> array = Arrays.asList(payloadline.split(",", 2));
if (array.get(0).equals("true")) {
modelpayload.addRow(new Object[]{true, array.get(1)});
} else {
modelpayload.addRow(new Object[]{false, array.get(1)});
}
} else {
modelpayload.addRow(new Object[]{true, payloadline});
}
}
}
public void loadGrepsFile(DefaultTableModel model) {
//Load file for implement payloads and match load button
List<String> grep = new ArrayList();
String line;
JFrame parentFrame = new JFrame();
JFileChooser fileChooser = new JFileChooser();
fileChooser.setDialogTitle("Specify a file to load");
int userSelection = fileChooser.showOpenDialog(parentFrame);
if (userSelection == JFileChooser.APPROVE_OPTION) {
File fileload = fileChooser.getSelectedFile();
textgreps.setText(fileload.getAbsolutePath());
try {
BufferedReader bufferreader = new BufferedReader(new FileReader(fileload.getAbsolutePath()));
line = bufferreader.readLine();
while (line != null) {
grep.add(line);
line = bufferreader.readLine();
}
bufferreader.close();
showGreps(grep);
} catch (FileNotFoundException ex) {
System.out.println("ActiveProfile line 213:" + ex.getMessage());
} catch (IOException ex) {
System.out.println("ActiveProfile line 215:" + ex.getMessage());
}
}
}
public void loadPayloadFile(DefaultTableModel model) {
//Load file for implement payloads and match load button
List<String> payloads = new ArrayList();
String line;
JFrame parentFrame = new JFrame();
JFileChooser fileChooser = new JFileChooser();
fileChooser.setDialogTitle("Specify a file to load");
int userSelection = fileChooser.showOpenDialog(parentFrame);
if (userSelection == JFileChooser.APPROVE_OPTION) {
File fileload = fileChooser.getSelectedFile();
textpayloads.setText(fileload.getAbsolutePath());
try {
BufferedReader bufferreader = new BufferedReader(new FileReader(fileload.getAbsolutePath()));
line = bufferreader.readLine();
while (line != null) {
payloads.add(line);
line = bufferreader.readLine();
}
bufferreader.close();
showPayloads(payloads);
} catch (FileNotFoundException ex) {
System.out.println("ActiveProfile line 309:" + ex.getMessage());
} catch (IOException ex) {
System.out.println("ActiveProfile line 308:" + ex.getMessage());
}
}
}
public void showHeaders(List<Headers> Header) {
JComboBox jcb = new JComboBox();
JComboBox jcb1 = new JComboBox();
//model for active profiles
model4.setNumRows(0);
model4.setColumnCount(0);
model4.addColumn("Item");
model4.addColumn("Match");
model4.addColumn("Replace");
model4.addColumn("Type");
jcb.addItem("Payload");
jcb.addItem("Request");
jcb1.addItem("String");
jcb1.addItem("Regex");
table4.getColumnModel().getColumn(0).setPreferredWidth(140);
table4.getColumnModel().getColumn(1).setPreferredWidth(400);
table4.getColumnModel().getColumn(2).setPreferredWidth(450);
table4.getColumnModel().getColumn(3).setPreferredWidth(120);
table4.getColumnModel().getColumn(0).setCellEditor(new DefaultCellEditor(jcb));
table4.getColumnModel().getColumn(3).setCellEditor(new DefaultCellEditor(jcb1));
TableRowSorter<TableModel> sorter = new TableRowSorter<>(table4.getModel());
table4.setRowSorter(sorter);
List<RowSorter.SortKey> sortKeys = new ArrayList<>();
sortKeys.add(new RowSorter.SortKey(0, SortOrder.DESCENDING));
sorter.setSortKeys(sortKeys);
sorter.sort();
for (int i = 0; i < Header.size(); i++) {
model4.addRow(new Object[]{Header.get(i).type, Header.get(i).match, Header.get(i).replace, Header.get(i).regex});
}
}
public void setEnabledVariations(boolean state) {
Attributes.setEnabled(state);
status_code.setEnabled(state);
input_image_labels.setEnabled(state);
non_hidden_form_input_types.setEnabled(state);
page_title.setEnabled(state);
visible_text.setEnabled(state);
button_submit_labels.setEnabled(state);
div_ids.setEnabled(state);
word_count.setEnabled(state);
content_type.setEnabled(state);
outbound_edge_tag_names.setEnabled(state);
whole_body_content.setEnabled(state);
etag_header.setEnabled(state);
visible_word_count.setEnabled(state);
content_length.setEnabled(state);
header_tags.setEnabled(state);
tag_ids.setEnabled(state);
comments.setEnabled(state);
line_count.setEnabled(state);
set_cookie_names.setEnabled(state);
last_modified_header.setEnabled(state);
first_header_tag.setEnabled(state);
tag_names.setEnabled(state);
input_submit_labels.setEnabled(state);
outbound_edge_count.setEnabled(state);
initial_body_content.setEnabled(state);
content_location.setEnabled(state);
limited_body_content.setEnabled(state);
canonical_link.setEnabled(state);
css_classes.setEnabled(state);
location.setEnabled(state);
anchor_labels.setEnabled(state);
}
public String getClipboardContents() {
//Get clipboard contents for implement grep and match paste button
String result = "";
Clipboard clipboard = Toolkit.getDefaultToolkit().getSystemClipboard();
Transferable contents = clipboard.getContents(null);
boolean hasTransferableText = (contents != null) && contents.isDataFlavorSupported(DataFlavor.stringFlavor);
if (hasTransferableText) {
try {
result = (String) contents.getTransferData(DataFlavor.stringFlavor);
} catch (UnsupportedFlavorException | IOException ex) {
System.out.println("ActiveProfile line 304:" + ex.getMessage());
}
}
return result;
}
public void setSelectedInsertionPointType(boolean state) {
All.setSelected(state);
extensionprovided.setSelected(state);
header.setSelected(state);
entirebody.setSelected(state);
paramamf.setSelected(state);
parambody.setSelected(state);
paramcookie.setSelected(state);
paramjson.setSelected(state);
urlpathfolder.setSelected(state);
parammultipartattr.setSelected(state);
paramnamebody.setSelected(state);
paramnameurl.setSelected(state);
userprovided.setSelected(state);
paramurl.setSelected(state);
paramxml.setSelected(state);
paramxmlattr.setSelected(state);
urlpathfilename.setSelected(state);
unknown.setSelected(state);
}
public void swap(int a, int b) {
Object aObject = encoder.getElementAt(a);
Object bObject = encoder.getElementAt(b);
encoder.set(a, bObject);
encoder.set(b, aObject);
}
public void loadPayloadsFile(DefaultListModel list) {
//Load file for implement payloads and match load button
DefaultListModel List = list;
String line;
JFrame parentFrame = new JFrame();
JFileChooser fileChooser = new JFileChooser();
fileChooser.setDialogTitle("Specify a file to load");
int userSelection = fileChooser.showOpenDialog(parentFrame);
if (userSelection == JFileChooser.APPROVE_OPTION) {
File fileload = fileChooser.getSelectedFile();
textpayloads.setText(fileload.getAbsolutePath());
try {
BufferedReader bufferreader = new BufferedReader(new FileReader(fileload.getAbsolutePath()));
line = bufferreader.readLine();
while (line != null) {
List.addElement(line);
line = bufferreader.readLine();
}
bufferreader.close();
} catch (FileNotFoundException ex) {
System.out.println("ActiveProfile line 361:" + ex.getMessage());
} catch (IOException ex) {
System.out.println("ActiveProfile line 363:" + ex.getMessage());
}
}
}
public void loadGrepsFile(DefaultListModel list) {
//Load file for implement payloads and match load button
DefaultListModel List = list;
String line;
JFrame parentFrame = new JFrame();
JFileChooser fileChooser = new JFileChooser();
fileChooser.setDialogTitle("Specify a file to load");
int userSelection = fileChooser.showOpenDialog(parentFrame);
if (userSelection == JFileChooser.APPROVE_OPTION) {
File fileload = fileChooser.getSelectedFile();
textgreps.setText(fileload.getAbsolutePath());
try {
BufferedReader bufferreader = new BufferedReader(new FileReader(fileload.getAbsolutePath()));
line = bufferreader.readLine();
while (line != null) {
List.addElement(line);
line = bufferreader.readLine();
}
bufferreader.close();
} catch (FileNotFoundException ex) {
System.out.println("ActiveProfile line 391:" + ex.getMessage());
} catch (IOException ex) {
System.out.println("ActiveProfile line 393:" + ex.getMessage());
}
}
}
public void addNewTag(String str) {
if (!str.isEmpty()) {
try {
BufferedWriter out = new BufferedWriter(new FileWriter(filename.concat("tags.txt"), true));
out.write(str.concat("\n"));
out.close();
} catch (IOException e) {
System.out.println("ActiveProfile line 405:" + e.getMessage());
}
}
}
public void removeTag(String tag) {
String file = filename.concat("tags.txt");
try {
File inFile = new File(file);
if (!inFile.isFile()) {
System.out.println("ActiveProfile line 417:");
return;
}
//Construct the new file that will later be renamed to the original filename.
File tempFile = new File(inFile.getAbsolutePath().concat(".tmp"));
BufferedReader br = new BufferedReader(new FileReader(file));
PrintWriter pw = new PrintWriter(new FileWriter(tempFile));
String line = null;
//Read from the original file and write to the new
//unless content matches data to be removed.
while ((line = br.readLine()) != null) {
if (!line.trim().equals(tag)) {
pw.println(line);
pw.flush();
}
}
pw.close();
br.close();
//Delete the original file
if (!inFile.delete()) {
System.out.println("Activeprofile line 443 Could not delete file");
return;
}
//Rename the new file to the filename the original file had.
if (!tempFile.renameTo(inFile)) {
System.out.println("ActiveProfile line 449 Could not rename file");
}
} catch (FileNotFoundException ex) {
System.out.println("ActiveProfile line 453:" + ex.getMessage());
} catch (IOException ex) {
System.out.println("ActiveProfile line 455:" + ex.getMessage());
}
}
public void showTags() {
List<String> tags = readFile(filename.concat("tags.txt"));
newTagCombo.removeAllItems();
tagmanager.removeAllElements();
for (String tag : tags) {
newTagCombo.addItem(tag);
tagmanager.addElement(tag);
}
}
private List<String> readFile(String filename) {
List<String> records = new ArrayList();
try {
BufferedReader reader = new BufferedReader(new FileReader(filename));
String line;
while ((line = reader.readLine()) != null) {
records.add(line);
}
reader.close();
} catch (Exception e) {
System.out.println("ActiveProfile line 494:" + e.getMessage());
}
return records;
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
buttonGroup1 = new javax.swing.ButtonGroup();
buttonGroup2 = new javax.swing.ButtonGroup();
buttonGroup3 = new javax.swing.ButtonGroup();
buttonGroup4 = new javax.swing.ButtonGroup();
buttonGroup5 = new javax.swing.ButtonGroup();
buttonGroup6 = new javax.swing.ButtonGroup();
text1 = new javax.swing.JTextField();
jLabel18 = new javax.swing.JLabel();
jLabel12 = new javax.swing.JLabel();
textauthor = new javax.swing.JTextField();
headerstab = new javax.swing.JTabbedPane();
jScrollPane5 = new javax.swing.JScrollPane();
jPanel10 = new javax.swing.JPanel();
parambody = new javax.swing.JCheckBox();
jSeparator2 = new javax.swing.JSeparator();
text5 = new javax.swing.JTextField();
jButton9 = new javax.swing.JButton();
button6 = new javax.swing.JButton();
jButton8 = new javax.swing.JButton();
jScrollPane14 = new javax.swing.JScrollPane();
table4 = new javax.swing.JTable();
jLabel22 = new javax.swing.JLabel();
urlpathfolder = new javax.swing.JCheckBox();
jScrollPane4 = new javax.swing.JScrollPane();
list3 = new javax.swing.JList<>();
header = new javax.swing.JCheckBox();
jSeparator3 = new javax.swing.JSeparator();
paramurl = new javax.swing.JCheckBox();
button3 = new javax.swing.JButton();
jLabel55 = new javax.swing.JLabel();
paramcookie = new javax.swing.JCheckBox();
jLabel52 = new javax.swing.JLabel();
paramnamebody = new javax.swing.JCheckBox();
button2 = new javax.swing.JButton();
paramamf = new javax.swing.JCheckBox();
urlpathfilename = new javax.swing.JCheckBox();
unknown = new javax.swing.JCheckBox();
jLabel11 = new javax.swing.JLabel();
jLabel17 = new javax.swing.JLabel();
jSeparator4 = new javax.swing.JSeparator();
button4 = new javax.swing.JButton();
button18 = new javax.swing.JButton();
button19 = new javax.swing.JButton();
combo2 = new javax.swing.JComboBox<>();
extensionprovided = new javax.swing.JCheckBox();
parammultipartattr = new javax.swing.JCheckBox();
paramjson = new javax.swing.JCheckBox();
paramxmlattr = new javax.swing.JCheckBox();
paramnameurl = new javax.swing.JCheckBox();
textpayloads = new javax.swing.JTextField();
userprovided = new javax.swing.JCheckBox();
jLabel54 = new javax.swing.JLabel();
jButton6 = new javax.swing.JButton();
jLabel19 = new javax.swing.JLabel();
jLabel10 = new javax.swing.JLabel();
button5 = new javax.swing.JButton();
replace = new javax.swing.JRadioButton();
jLabel5 = new javax.swing.JLabel();
check8 = new javax.swing.JCheckBox();
entirebody = new javax.swing.JCheckBox();
All = new javax.swing.JCheckBox();
paramxml = new javax.swing.JCheckBox();
jLabel23 = new javax.swing.JLabel();
jLabel53 = new javax.swing.JLabel();
append = new javax.swing.JRadioButton();
jButton7 = new javax.swing.JButton();
jLabel1 = new javax.swing.JLabel();
jLabel20 = new javax.swing.JLabel();
insert = new javax.swing.JRadioButton();
jScrollPane16 = new javax.swing.JScrollPane();
table6 = new javax.swing.JTable();
jScrollPane6 = new javax.swing.JScrollPane();
jPanel11 = new javax.swing.JPanel();
check4 = new javax.swing.JCheckBox();
check1 = new javax.swing.JCheckBox();
excludehttp = new javax.swing.JCheckBox();
onlyhttp = new javax.swing.JCheckBox();
check71 = new javax.swing.JCheckBox();
check72 = new javax.swing.JCheckBox();
text72 = new javax.swing.JTextField();
text71 = new javax.swing.JTextField();
negativeCT = new javax.swing.JCheckBox();
negativeRC = new javax.swing.JCheckBox();
jLabel24 = new javax.swing.JLabel();
jLabel25 = new javax.swing.JLabel();
jLabel26 = new javax.swing.JLabel();
jLabel27 = new javax.swing.JLabel();
jLabel28 = new javax.swing.JLabel();
jLabel29 = new javax.swing.JLabel();
jSeparator5 = new javax.swing.JSeparator();
jLabel30 = new javax.swing.JLabel();
jLabel31 = new javax.swing.JLabel();
jSeparator6 = new javax.swing.JSeparator();
rb1 = new javax.swing.JRadioButton();
rb2 = new javax.swing.JRadioButton();
rb3 = new javax.swing.JRadioButton();
rb4 = new javax.swing.JRadioButton();
jLabel2 = new javax.swing.JLabel();
sp1 = new javax.swing.JSpinner();
jLabel6 = new javax.swing.JLabel();
jSeparator11 = new javax.swing.JSeparator();
Attributes = new javax.swing.JPanel();
status_code = new javax.swing.JCheckBox();
input_image_labels = new javax.swing.JCheckBox();
non_hidden_form_input_types = new javax.swing.JCheckBox();
page_title = new javax.swing.JCheckBox();
visible_text = new javax.swing.JCheckBox();
button_submit_labels = new javax.swing.JCheckBox();
div_ids = new javax.swing.JCheckBox();
word_count = new javax.swing.JCheckBox();
content_type = new javax.swing.JCheckBox();
outbound_edge_tag_names = new javax.swing.JCheckBox();
location = new javax.swing.JCheckBox();
css_classes = new javax.swing.JCheckBox();
last_modified_header = new javax.swing.JCheckBox();
set_cookie_names = new javax.swing.JCheckBox();
line_count = new javax.swing.JCheckBox();
comments = new javax.swing.JCheckBox();
tag_ids = new javax.swing.JCheckBox();
header_tags = new javax.swing.JCheckBox();
content_length = new javax.swing.JCheckBox();
visible_word_count = new javax.swing.JCheckBox();
whole_body_content = new javax.swing.JCheckBox();
etag_header = new javax.swing.JCheckBox();
first_header_tag = new javax.swing.JCheckBox();
tag_names = new javax.swing.JCheckBox();
input_submit_labels = new javax.swing.JCheckBox();
outbound_edge_count = new javax.swing.JCheckBox();
content_location = new javax.swing.JCheckBox();
initial_body_content = new javax.swing.JCheckBox();
limited_body_content = new javax.swing.JCheckBox();
canonical_link = new javax.swing.JCheckBox();
anchor_labels = new javax.swing.JCheckBox();
jScrollPane15 = new javax.swing.JScrollPane();
table5 = new javax.swing.JTable();
button20 = new javax.swing.JButton();
button10 = new javax.swing.JButton();
button7 = new javax.swing.JButton();
button21 = new javax.swing.JButton();
button8 = new javax.swing.JButton();
textgreps = new javax.swing.JTextField();
negativeURL = new javax.swing.JCheckBox();
text73 = new javax.swing.JTextField();
check73 = new javax.swing.JCheckBox();
jPanel1 = new javax.swing.JPanel();
radiocl = new javax.swing.JRadioButton();
textcl = new javax.swing.JTextField();
radio12 = new javax.swing.JRadioButton();
radio22 = new javax.swing.JRadioButton();
jLabel42 = new javax.swing.JLabel();
invariationsRadio = new javax.swing.JRadioButton();
radiotime = new javax.swing.JRadioButton();
radiohttp = new javax.swing.JRadioButton();
radio3 = new javax.swing.JRadioButton();
texttime1 = new javax.swing.JTextField();
jLabel21 = new javax.swing.JLabel();
radio4 = new javax.swing.JRadioButton();
texttime2 = new javax.swing.JTextField();
jLabel16 = new javax.swing.JLabel();
variationsRadio = new javax.swing.JRadioButton();
resposecode = new javax.swing.JTextField();
jScrollPane10 = new javax.swing.JScrollPane();
jPanel12 = new javax.swing.JPanel();
jLabel32 = new javax.swing.JLabel();
jLabel33 = new javax.swing.JLabel();
jLabel3 = new javax.swing.JLabel();
jLabel4 = new javax.swing.JLabel();
radio5 = new javax.swing.JRadioButton();
radio6 = new javax.swing.JRadioButton();
radio7 = new javax.swing.JRadioButton();
radio8 = new javax.swing.JRadioButton();
jLabel7 = new javax.swing.JLabel();
radio9 = new javax.swing.JRadioButton();
radio10 = new javax.swing.JRadioButton();
radio11 = new javax.swing.JRadioButton();
text4 = new javax.swing.JTextField();
jSeparator7 = new javax.swing.JSeparator();
jLabel34 = new javax.swing.JLabel();
jLabel35 = new javax.swing.JLabel();
jScrollPane7 = new javax.swing.JScrollPane();
textarea2 = new javax.swing.JTextArea();
jLabel13 = new javax.swing.JLabel();
jLabel36 = new javax.swing.JLabel();
jLabel37 = new javax.swing.JLabel();
jSeparator8 = new javax.swing.JSeparator();
jLabel38 = new javax.swing.JLabel();
jLabel39 = new javax.swing.JLabel();
jSeparator9 = new javax.swing.JSeparator();
jScrollPane1 = new javax.swing.JScrollPane();
textarea1 = new javax.swing.JTextArea();
jLabel9 = new javax.swing.JLabel();
jScrollPane8 = new javax.swing.JScrollPane();
textarea3 = new javax.swing.JTextArea();
jLabel14 = new javax.swing.JLabel();
jLabel40 = new javax.swing.JLabel();
jLabel41 = new javax.swing.JLabel();
jSeparator10 = new javax.swing.JSeparator();
jScrollPane9 = new javax.swing.JScrollPane();
textarea4 = new javax.swing.JTextArea();
jLabel15 = new javax.swing.JLabel();
jPanel3 = new javax.swing.JPanel();
removetag = new javax.swing.JButton();
addTag = new javax.swing.JButton();
newTagCombo = new javax.swing.JComboBox<>();
jScrollPane11 = new javax.swing.JScrollPane();
listtag = new javax.swing.JList<>();
jLabel46 = new javax.swing.JLabel();
jLabel47 = new javax.swing.JLabel();
newTagb = new javax.swing.JButton();
setPreferredSize(new java.awt.Dimension(831, 664));
text1.setFont(new java.awt.Font("Lucida Grande", 0, 14)); // NOI18N
jLabel18.setFont(new java.awt.Font("Lucida Grande", 0, 14)); // NOI18N
jLabel18.setText("Author:");
jLabel12.setFont(new java.awt.Font("Lucida Grande", 0, 14)); // NOI18N
jLabel12.setText("Name:");
textauthor.setFont(new java.awt.Font("Lucida Grande", 0, 14)); // NOI18N
headerstab.setAutoscrolls(true);
headerstab.setFont(new java.awt.Font("Lucida Grande", 0, 14)); // NOI18N
headerstab.setPreferredSize(new java.awt.Dimension(750, 500));
headerstab.addChangeListener(new javax.swing.event.ChangeListener() {
public void stateChanged(javax.swing.event.ChangeEvent evt) {
headerstabStateChanged(evt);
}
});
jScrollPane5.setPreferredSize(new java.awt.Dimension(0, 0));
jScrollPane5.getVerticalScrollBar().setUnitIncrement(20);
jPanel10.setMaximumSize(new java.awt.Dimension(0, 0));
parambody.setText("Param body");
jButton9.setText("Remove");
jButton9.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton9removeEncoder(evt);
}
});
button6.setText("Add");
button6.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button6setToPayload(evt);
}
});
jButton8.setText("Up");
jButton8.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton8upEncoder(evt);
}
});
table4.setFont(new java.awt.Font("Lucida Grande", 0, 13)); // NOI18N
table4.setModel(model4);
table4.setShowGrid(false);
jScrollPane14.setViewportView(table4);
jLabel22.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel22.setForeground(new java.awt.Color(255, 102, 51));
jLabel22.setText("Payload Encoding");
urlpathfolder.setText("Url path folder");
list3.setModel(encoder);
jScrollPane4.setViewportView(list3);
header.setText("Header");
paramurl.setText("Param url");
button3.setText("Load File");
button3.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button3loadPayloads(evt);
}
});
jLabel55.setText("You can define the payload options.");
paramcookie.setText("Param cookie");
jLabel52.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel52.setForeground(new java.awt.Color(255, 102, 51));
jLabel52.setText("Match and Replace");
paramnamebody.setText("Param name body");
button2.setText("Paste");
button2.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button2pastePayload(evt);
}
});
paramamf.setText("Param AMF");
urlpathfilename.setText("Url path filename");
unknown.setText("Unknown");
jLabel11.setText("Insertion point type:");
jLabel17.setText("<html> * More info at <a href=\\\"\\\">Burp Suite Extender API</a></html>");
jLabel17.addMouseListener(new java.awt.event.MouseAdapter() {
public void mouseClicked(java.awt.event.MouseEvent evt) {
jLabel17goWeb(evt);
}
});
button4.setText("Remove");
button4.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button4removePayload(evt);
}
});
button18.setText("Remove");
button18.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button18removeMatchReplace(evt);
}
});
button19.setText("Add");
button19.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
addMatchReplace(evt);
}
});
combo2.setModel(new javax.swing.DefaultComboBoxModel<>(new String[] { "URL-encode key characters", "URL-encode all characters", "URL-encode all characters (Unicode)", "HTML-encode key characters", "HTML-encode all characters", "Base64-encode" }));
extensionprovided.setText("Path discovery");
parammultipartattr.setText("Param multipart attr");
paramjson.setText("Param json");
paramxmlattr.setText("Param xml attr");
paramnameurl.setText("Param name url");
textpayloads.setToolTipText("");
userprovided.setText("User provided");
jLabel54.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel54.setForeground(new java.awt.Color(255, 102, 51));
jLabel54.setText("Payload Options");
jButton6.setText("Add");
jButton6.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton6addEncoder(evt);
}
});
jLabel19.setText("You can define one or more payloads. Each payload of this section will be sent at each insertion point.");
jLabel10.setText("Payload position:");
button5.setText("Clear");
button5.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button5removeAllPayloads(evt);
}
});
buttonGroup1.add(replace);
replace.setText("Replace");
jLabel5.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel5.setForeground(new java.awt.Color(255, 102, 51));
jLabel5.setText("Payload ");
check8.setText("URL-Encode these characters:");
entirebody.setText("Entire body");
All.setText("All ");
All.addItemListener(new java.awt.event.ItemListener() {
public void itemStateChanged(java.awt.event.ItemEvent evt) {
AllItemStateChanged(evt);
}
});
paramxml.setText("Param xml");
paramxml.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
paramxmlActionPerformed(evt);
}
});
jLabel23.setText("You can define the encoding of payloads. You can encode each payload multiple times.");
jLabel53.setText("These settings are used to automatically replace part of request when the active scanner run.");
buttonGroup1.add(append);
append.setText("Append");
jButton7.setText("Down");
jButton7.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jButton7downEncoder(evt);
}
});
jLabel1.setText("- {PAYLOAD} token will be replaced by your payload");
jLabel20.setText("- {BC} token will be replaced by burpcollaborator host");
buttonGroup1.add(insert);
insert.setText("Insert");
table6.setFont(new java.awt.Font("Lucida Grande", 0, 13)); // NOI18N
table6.setModel(modelpayload);
table6.setShowGrid(false);
jScrollPane16.setViewportView(table6);
javax.swing.GroupLayout jPanel10Layout = new javax.swing.GroupLayout(jPanel10);
jPanel10.setLayout(jPanel10Layout);
jPanel10Layout.setHorizontalGroup(
jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel10Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel10Layout.createSequentialGroup()
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel10Layout.createSequentialGroup()
.addGap(6, 6, 6)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jLabel53, javax.swing.GroupLayout.PREFERRED_SIZE, 704, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel52)
.addGroup(jPanel10Layout.createSequentialGroup()
.addComponent(check8)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(text5))
.addGroup(jPanel10Layout.createSequentialGroup()
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jButton9, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jButton6, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jButton8, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jButton7, javax.swing.GroupLayout.PREFERRED_SIZE, 93, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jScrollPane4)
.addComponent(combo2, 0, 670, Short.MAX_VALUE)))
.addGroup(jPanel10Layout.createSequentialGroup()
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(button18, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(button19, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel1)
.addComponent(jScrollPane14, javax.swing.GroupLayout.PREFERRED_SIZE, 673, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel20)))))
.addComponent(jLabel19, javax.swing.GroupLayout.PREFERRED_SIZE, 704, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(jPanel10Layout.createSequentialGroup()
.addGap(47, 47, 47)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel17, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jLabel10)
.addComponent(jLabel11))))
.addComponent(jLabel5)
.addGroup(jPanel10Layout.createSequentialGroup()
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(button6, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel10Layout.createSequentialGroup()
.addGap(12, 12, 12)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(button3, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(button4, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE))
.addComponent(button5, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addComponent(button2, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addGap(18, 18, 18)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(textpayloads, javax.swing.GroupLayout.DEFAULT_SIZE, 670, Short.MAX_VALUE)
.addComponent(jScrollPane16))))
.addContainerGap(15, Short.MAX_VALUE))
.addGroup(jPanel10Layout.createSequentialGroup()
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel22)
.addComponent(jLabel23, javax.swing.GroupLayout.PREFERRED_SIZE, 704, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel54)
.addComponent(jLabel55, javax.swing.GroupLayout.PREFERRED_SIZE, 704, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(0, 0, Short.MAX_VALUE))))
.addComponent(jSeparator2)
.addComponent(jSeparator4)
.addComponent(jSeparator3, javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(jPanel10Layout.createSequentialGroup()
.addGap(183, 183, 183)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel10Layout.createSequentialGroup()
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(extensionprovided)
.addComponent(header)
.addComponent(urlpathfilename)
.addComponent(entirebody)
.addComponent(paramxml)
.addComponent(All))
.addGap(30, 30, 30)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(paramjson)
.addComponent(parambody)
.addComponent(paramcookie)
.addComponent(urlpathfolder)
.addComponent(paramamf)
.addComponent(paramxmlattr))
.addGap(51, 51, 51)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(unknown)
.addComponent(parammultipartattr)
.addComponent(paramnamebody)
.addComponent(paramnameurl)
.addComponent(userprovided)
.addComponent(paramurl)))
.addGroup(jPanel10Layout.createSequentialGroup()
.addComponent(replace)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(append)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(insert)))
.addGap(0, 0, Short.MAX_VALUE))
);
jPanel10Layout.setVerticalGroup(
jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel10Layout.createSequentialGroup()
.addGap(10, 10, 10)
.addComponent(jLabel5)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel19)
.addGap(25, 25, 25)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(textpayloads, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(button3))
.addGap(18, 18, 18)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel10Layout.createSequentialGroup()
.addComponent(button6)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(button2)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(button4)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(button5)
.addGap(0, 96, Short.MAX_VALUE))
.addComponent(jScrollPane16, javax.swing.GroupLayout.PREFERRED_SIZE, 0, Short.MAX_VALUE))
.addGap(18, 18, 18)
.addComponent(jSeparator2, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel54)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel55)
.addGap(23, 23, 23)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel10)
.addComponent(append)
.addComponent(replace)
.addComponent(insert))
.addGap(23, 23, 23)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel10Layout.createSequentialGroup()
.addComponent(jLabel11)
.addGap(151, 151, 151)
.addComponent(jLabel17, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(jSeparator4, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel52)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel53)
.addGap(18, 18, 18)
.addComponent(jLabel1)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(jLabel20)
.addGap(18, 18, 18)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel10Layout.createSequentialGroup()
.addComponent(button19)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(button18))
.addComponent(jScrollPane14, javax.swing.GroupLayout.PREFERRED_SIZE, 119, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addComponent(jSeparator3, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel22)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel23)
.addGap(25, 25, 25)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addGroup(jPanel10Layout.createSequentialGroup()
.addComponent(jButton9)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jButton8)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jButton7))
.addComponent(jScrollPane4, javax.swing.GroupLayout.PREFERRED_SIZE, 99, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(combo2, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jButton6))
.addGap(19, 19, 19)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(check8)
.addComponent(text5, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addGroup(jPanel10Layout.createSequentialGroup()
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(paramamf)
.addComponent(parammultipartattr)
.addComponent(All))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(parambody)
.addComponent(paramnamebody)
.addComponent(urlpathfilename))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(extensionprovided)
.addComponent(paramcookie)
.addComponent(paramurl))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(header)
.addComponent(paramjson)
.addComponent(paramnameurl))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(entirebody)
.addComponent(urlpathfolder)
.addComponent(userprovided))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(paramxml)
.addComponent(paramxmlattr)
.addComponent(unknown))))
.addContainerGap())
);
jScrollPane5.setViewportView(jPanel10);
headerstab.addTab(" Request ", jScrollPane5);
jScrollPane6.setHorizontalScrollBarPolicy(javax.swing.ScrollPaneConstants.HORIZONTAL_SCROLLBAR_NEVER);
jScrollPane6.getVerticalScrollBar().setUnitIncrement(20);
jPanel11.setAutoscrolls(true);
check4.setText("Negative match");
check1.setText("Case sensitive");
excludehttp.setText("Exclude HTTP headers");
onlyhttp.setText("Only in HTTP headers");
check71.setText("Content type");
check72.setText("Status code");
negativeCT.setText("Negative match");
negativeRC.setText("Negative match");
jLabel24.setText("You can define one or more greps. For each payload response, each grep will be searched with specific grep options.");
jLabel25.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel25.setForeground(new java.awt.Color(255, 102, 51));
jLabel25.setText("Grep");
jLabel26.setText("You can define grep type.");
jLabel27.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel27.setForeground(new java.awt.Color(255, 102, 51));
jLabel27.setText("Match Type");
jLabel28.setText("You can define how your profile handles redirections.");
jLabel29.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel29.setForeground(new java.awt.Color(255, 102, 51));
jLabel29.setText("Redirections");
jLabel30.setText("These settings can be used to specify grep options of your profile.");
jLabel31.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel31.setForeground(new java.awt.Color(255, 102, 51));
jLabel31.setText("Grep Options");
buttonGroup4.add(rb1);
rb1.setText("Never");
buttonGroup4.add(rb2);
rb2.setText("On-site only");
buttonGroup4.add(rb3);
rb3.setText("In-scope only");
buttonGroup4.add(rb4);
rb4.setText("Always");
jLabel2.setText("Max redirections:");
jLabel6.setText("Follow redirections: ");
Attributes.setBorder(javax.swing.BorderFactory.createTitledBorder(null, "Attributes", javax.swing.border.TitledBorder.CENTER, javax.swing.border.TitledBorder.TOP));
status_code.setText("status_code");
input_image_labels.setText("input_image_labels");
non_hidden_form_input_types.setText("non_hidden_form_input_types");
page_title.setText("page_title");
visible_text.setText("visible_text");
button_submit_labels.setText("button_submit_labels");
div_ids.setText("div_ids");
word_count.setText("word_count");
content_type.setText("content_type");
outbound_edge_tag_names.setText("outbound_edge_tag_names");
location.setText("location");
css_classes.setText("css_classes");
last_modified_header.setText("last_modified_header");
set_cookie_names.setText("set_cookie_names");
line_count.setText("line_count");
comments.setText("comments");
tag_ids.setText("tag_ids");
header_tags.setText("header_tags");
content_length.setText("content_length");
visible_word_count.setText("visible_word_count");
whole_body_content.setText("whole_body_content");
etag_header.setText("etag_header");
first_header_tag.setText("first_header_tag");
tag_names.setText("tag_names");
input_submit_labels.setText("input_submit_labels");
outbound_edge_count.setText("outbound_edge_count");
content_location.setText("content_location");
initial_body_content.setText("initial_body_content");
limited_body_content.setText("limited_body_content");
canonical_link.setText("canonical_link");
anchor_labels.setText("anchor_labels");
javax.swing.GroupLayout AttributesLayout = new javax.swing.GroupLayout(Attributes);
Attributes.setLayout(AttributesLayout);
AttributesLayout.setHorizontalGroup(
AttributesLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(AttributesLayout.createSequentialGroup()
.addContainerGap()
.addGroup(AttributesLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(page_title)
.addComponent(non_hidden_form_input_types)
.addComponent(input_image_labels)
.addComponent(status_code)
.addComponent(visible_text)
.addComponent(word_count)
.addComponent(div_ids)
.addComponent(button_submit_labels))
.addGap(18, 18, 18)
.addGroup(AttributesLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(content_type)
.addComponent(outbound_edge_tag_names)
.addComponent(anchor_labels)
.addComponent(etag_header)
.addComponent(whole_body_content)
.addComponent(content_length)
.addComponent(visible_word_count)
.addComponent(header_tags))
.addGap(18, 18, 18)
.addGroup(AttributesLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(input_submit_labels)
.addGroup(AttributesLayout.createSequentialGroup()
.addGroup(AttributesLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(tag_names)
.addComponent(first_header_tag)
.addComponent(set_cookie_names)
.addComponent(line_count)
.addComponent(comments)
.addComponent(tag_ids)
.addComponent(last_modified_header))
.addGap(18, 18, 18)
.addGroup(AttributesLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(outbound_edge_count)
.addComponent(initial_body_content)
.addComponent(css_classes)
.addComponent(canonical_link)
.addComponent(limited_body_content)
.addComponent(content_location)
.addComponent(location))))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
AttributesLayout.setVerticalGroup(
AttributesLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(AttributesLayout.createSequentialGroup()
.addContainerGap()
.addGroup(AttributesLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(AttributesLayout.createSequentialGroup()
.addComponent(outbound_edge_count)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(initial_body_content)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(content_location)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(limited_body_content)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(canonical_link)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(css_classes)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(location)
.addGap(0, 0, Short.MAX_VALUE))
.addGroup(AttributesLayout.createSequentialGroup()
.addGroup(AttributesLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(AttributesLayout.createSequentialGroup()
.addGroup(AttributesLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(AttributesLayout.createSequentialGroup()
.addComponent(content_type)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(outbound_edge_tag_names)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(anchor_labels)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(whole_body_content)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(etag_header))
.addGroup(AttributesLayout.createSequentialGroup()
.addComponent(tag_ids)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(comments)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(line_count)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(set_cookie_names)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(last_modified_header)))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(AttributesLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(AttributesLayout.createSequentialGroup()
.addComponent(visible_word_count)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(content_length)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(header_tags))
.addGroup(AttributesLayout.createSequentialGroup()
.addComponent(first_header_tag)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(tag_names)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(input_submit_labels))))
.addGroup(AttributesLayout.createSequentialGroup()
.addComponent(status_code)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(input_image_labels)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(non_hidden_form_input_types)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(page_title)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(visible_text)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(button_submit_labels)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(div_ids)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(word_count)))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))))
);
table5.setFont(new java.awt.Font("Lucida Grande", 0, 13)); // NOI18N
table5.setModel(modelgrep);
table5.setShowGrid(false);
jScrollPane15.setViewportView(table5);
button20.setText("Remove");
button20.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button20removeMatchReplace(evt);
}
});
button10.setText("Clear");
button10.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button10removeAllGrep(evt);
}
});
button7.setText("Paste");
button7.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button7pasteGrep(evt);
}
});
button21.setText("Add");
button21.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button21addGrep(evt);
}
});
button8.setText("Load File");
button8.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button8loadGrep(evt);
}
});
negativeURL.setText("Negative match");
check73.setText("URL Extension");
buttonGroup3.add(radiocl);
radiocl.setText("Content Length difference");
radiocl.addItemListener(new java.awt.event.ItemListener() {
public void itemStateChanged(java.awt.event.ItemEvent evt) {
radioclSelect(evt);
}
});
radiocl.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
radioclActionPerformed(evt);
}
});
buttonGroup3.add(radio12);
radio12.setText("Payload");
radio12.addItemListener(new java.awt.event.ItemListener() {
public void itemStateChanged(java.awt.event.ItemEvent evt) {
radio12payloadMatchType(evt);
}
});
buttonGroup3.add(radio22);
radio22.setText("Payload without encode");
radio22.addItemListener(new java.awt.event.ItemListener() {
public void itemStateChanged(java.awt.event.ItemEvent evt) {
radio22payloadencodeMatchType(evt);
}
});
jLabel42.setText("Bytes");
buttonGroup3.add(invariationsRadio);
invariationsRadio.setText("Invariations");
invariationsRadio.addItemListener(new java.awt.event.ItemListener() {
public void itemStateChanged(java.awt.event.ItemEvent evt) {
invariationsRadioinvariations(evt);
}
});
buttonGroup3.add(radiotime);
radiotime.setText("Timeout between");
radiotime.addItemListener(new java.awt.event.ItemListener() {
public void itemStateChanged(java.awt.event.ItemEvent evt) {
radiotimeTimeoutSelect(evt);
}
});
buttonGroup3.add(radiohttp);
radiohttp.setText("HTTP response codes");
buttonGroup3.add(radio3);
radio3.setText("Regex");
radio3.addItemListener(new java.awt.event.ItemListener() {
public void itemStateChanged(java.awt.event.ItemEvent evt) {
radio3regexMatchType(evt);
}
});
jLabel21.setText("and");
buttonGroup3.add(radio4);
radio4.setText("Simple string");
radio4.addItemListener(new java.awt.event.ItemListener() {
public void itemStateChanged(java.awt.event.ItemEvent evt) {
radio4stringMatchType(evt);
}
});
jLabel16.setText("Seconds");
buttonGroup3.add(variationsRadio);
variationsRadio.setText("Variations");
variationsRadio.addItemListener(new java.awt.event.ItemListener() {
public void itemStateChanged(java.awt.event.ItemEvent evt) {
variationsRadiovariations(evt);
}
});
javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1);
jPanel1.setLayout(jPanel1Layout);
jPanel1Layout.setHorizontalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(radio4)
.addGap(58, 58, 58))
.addGroup(javax.swing.GroupLayout.Alignment.LEADING, jPanel1Layout.createSequentialGroup()
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(radio12, javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(radio3, javax.swing.GroupLayout.Alignment.LEADING))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)))
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(variationsRadio)
.addComponent(invariationsRadio)
.addComponent(radio22))
.addGap(36, 36, 36)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(radiotime)
.addComponent(radiohttp))
.addGap(30, 30, 30)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(texttime1, javax.swing.GroupLayout.PREFERRED_SIZE, 100, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel21)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(texttime2, javax.swing.GroupLayout.PREFERRED_SIZE, 100, javax.swing.GroupLayout.PREFERRED_SIZE))
.addComponent(resposecode, javax.swing.GroupLayout.PREFERRED_SIZE, 226, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel16, javax.swing.GroupLayout.PREFERRED_SIZE, 62, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(radiocl)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(textcl, javax.swing.GroupLayout.PREFERRED_SIZE, 100, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(jLabel42, javax.swing.GroupLayout.PREFERRED_SIZE, 62, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addContainerGap())
);
jPanel1Layout.setVerticalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addGap(17, 17, 17)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(radiocl)
.addComponent(textcl, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel42)
.addComponent(radio22))
.addGap(18, 18, 18)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(radiohttp)
.addComponent(resposecode, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(texttime2, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(texttime1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel21)
.addComponent(jLabel16)
.addComponent(radiotime)))
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(radio4)
.addGap(18, 18, 18)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(radio3)
.addComponent(variationsRadio))
.addGap(18, 18, 18)
.addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(radio12)
.addComponent(invariationsRadio))))
.addContainerGap())
);
javax.swing.GroupLayout jPanel11Layout = new javax.swing.GroupLayout(jPanel11);
jPanel11.setLayout(jPanel11Layout);
jPanel11Layout.setHorizontalGroup(
jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jSeparator5)
.addComponent(jSeparator6, javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jSeparator11, javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(jPanel11Layout.createSequentialGroup()
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel11Layout.createSequentialGroup()
.addGap(20, 20, 20)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel6)
.addComponent(jLabel2))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(rb1)
.addComponent(rb2)
.addComponent(rb3)
.addComponent(rb4)
.addComponent(sp1, javax.swing.GroupLayout.PREFERRED_SIZE, 56, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addGroup(jPanel11Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel31)
.addComponent(jLabel30, javax.swing.GroupLayout.PREFERRED_SIZE, 769, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(onlyhttp)
.addComponent(check4)
.addComponent(check1)
.addComponent(excludehttp)
.addComponent(jLabel29)
.addComponent(jLabel28, javax.swing.GroupLayout.PREFERRED_SIZE, 769, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(jPanel11Layout.createSequentialGroup()
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(check72)
.addComponent(check71))
.addGap(15, 15, 15)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel11Layout.createSequentialGroup()
.addComponent(text73, javax.swing.GroupLayout.PREFERRED_SIZE, 441, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(negativeURL))
.addGroup(jPanel11Layout.createSequentialGroup()
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(text71, javax.swing.GroupLayout.DEFAULT_SIZE, 441, Short.MAX_VALUE)
.addComponent(text72))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(negativeCT)
.addComponent(negativeRC)))))))
.addGroup(jPanel11Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(jPanel11Layout.createSequentialGroup()
.addComponent(button8, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(textgreps, javax.swing.GroupLayout.PREFERRED_SIZE, 662, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(javax.swing.GroupLayout.Alignment.LEADING, jPanel11Layout.createSequentialGroup()
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING, false)
.addComponent(button20, javax.swing.GroupLayout.Alignment.LEADING, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(button10, javax.swing.GroupLayout.Alignment.LEADING, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(button7, javax.swing.GroupLayout.Alignment.LEADING, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(button21, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addComponent(jScrollPane15, javax.swing.GroupLayout.PREFERRED_SIZE, 662, javax.swing.GroupLayout.PREFERRED_SIZE))))
.addGroup(jPanel11Layout.createSequentialGroup()
.addContainerGap()
.addComponent(check73))
.addGroup(jPanel11Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel25)
.addComponent(jLabel24, javax.swing.GroupLayout.PREFERRED_SIZE, 769, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel26, javax.swing.GroupLayout.PREFERRED_SIZE, 769, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(Attributes, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel27)))
.addGroup(jPanel11Layout.createSequentialGroup()
.addContainerGap()
.addComponent(jPanel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addContainerGap(50, Short.MAX_VALUE))
);
jPanel11Layout.setVerticalGroup(
jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel11Layout.createSequentialGroup()
.addGap(10, 10, 10)
.addComponent(jLabel27)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel26)
.addGap(18, 18, 18)
.addComponent(jPanel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(Attributes, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(jSeparator11, javax.swing.GroupLayout.PREFERRED_SIZE, 3, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel25)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel24)
.addGap(18, 18, 18)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(textgreps, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(button8))
.addGap(18, 18, Short.MAX_VALUE)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jScrollPane15, javax.swing.GroupLayout.PREFERRED_SIZE, 206, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(jPanel11Layout.createSequentialGroup()
.addComponent(button21)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(button7)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(button20)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(button10)))
.addGap(18, 18, 18)
.addComponent(jSeparator6, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel31)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel30)
.addGap(25, 25, 25)
.addComponent(check4)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(check1)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(excludehttp)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(onlyhttp)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(check71)
.addComponent(text71, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(negativeCT))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(check72)
.addComponent(text72, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(negativeRC))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(check73)
.addComponent(text73, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(negativeURL))
.addGap(18, 18, 18)
.addComponent(jSeparator5, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel29)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel28)
.addGap(25, 25, 25)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(rb1)
.addComponent(jLabel6))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(rb2)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(rb3)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(rb4)
.addGap(18, 18, 18)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel2)
.addComponent(sp1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
JScrollPane responseresScroll = new JScrollPane(jPanel11,
JScrollPane.VERTICAL_SCROLLBAR_ALWAYS, JScrollPane.HORIZONTAL_SCROLLBAR_NEVER);
jScrollPane6.setViewportView(jPanel11);
headerstab.addTab(" Response ", jScrollPane6);
jScrollPane10.getVerticalScrollBar().setUnitIncrement(20);
jPanel12.setAutoscrolls(true);
jLabel32.setText("You can define the issue properties.");
jLabel33.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel33.setForeground(new java.awt.Color(255, 102, 51));
jLabel33.setText("Issue Properties");
jLabel3.setText("Issue Name:");
jLabel4.setText("Severity:");
buttonGroup5.add(radio5);
radio5.setText("High");
buttonGroup5.add(radio6);
radio6.setText("Medium");
buttonGroup5.add(radio7);
radio7.setText("Low");
buttonGroup5.add(radio8);
radio8.setText("Information");
jLabel7.setText("Confidence:");
buttonGroup6.add(radio9);
radio9.setText("Certain");
buttonGroup6.add(radio10);
radio10.setText("Firm");
buttonGroup6.add(radio11);
radio11.setText("Tentative");
jLabel34.setText("You can define the issue details.");
jLabel35.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel35.setForeground(new java.awt.Color(255, 102, 51));
jLabel35.setText("Issue Detail");
textarea2.setColumns(20);
textarea2.setRows(5);
jScrollPane7.setViewportView(textarea2);
jLabel13.setText("Description:");
jLabel36.setText("You can define the issue background.");
jLabel37.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel37.setForeground(new java.awt.Color(255, 102, 51));
jLabel37.setText("Issue Background");
jLabel38.setText("You can define the remediation detail.");
jLabel39.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel39.setForeground(new java.awt.Color(255, 102, 51));
jLabel39.setText("Remediation Detail");
textarea1.setColumns(20);
textarea1.setRows(5);
jScrollPane1.setViewportView(textarea1);
jLabel9.setText("Description:");
textarea3.setColumns(20);
textarea3.setRows(5);
jScrollPane8.setViewportView(textarea3);
jLabel14.setText("Description:");
jLabel40.setText("You can define the remediation background.");
jLabel41.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel41.setForeground(new java.awt.Color(255, 102, 51));
jLabel41.setText("Remediation Background");
textarea4.setColumns(20);
textarea4.setRows(5);
jScrollPane9.setViewportView(textarea4);
jLabel15.setText("Description:");
javax.swing.GroupLayout jPanel12Layout = new javax.swing.GroupLayout(jPanel12);
jPanel12.setLayout(jPanel12Layout);
jPanel12Layout.setHorizontalGroup(
jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jSeparator7)
.addComponent(jSeparator8, javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jSeparator9, javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jSeparator10, javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(jPanel12Layout.createSequentialGroup()
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel12Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel33)
.addComponent(jLabel35)
.addComponent(jLabel34)
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(jLabel13)
.addGap(18, 18, 18)
.addComponent(jScrollPane7, javax.swing.GroupLayout.PREFERRED_SIZE, 612, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(jLabel9)
.addGap(18, 18, 18)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 612, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(jLabel15)
.addGap(18, 18, 18)
.addComponent(jScrollPane9, javax.swing.GroupLayout.PREFERRED_SIZE, 612, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(jLabel14)
.addGap(18, 18, 18)
.addComponent(jScrollPane8, javax.swing.GroupLayout.PREFERRED_SIZE, 612, javax.swing.GroupLayout.PREFERRED_SIZE))
.addComponent(jLabel37)
.addComponent(jLabel36)
.addComponent(jLabel39)
.addComponent(jLabel38)
.addComponent(jLabel32)
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(jLabel3)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(jLabel4, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(radio8)
.addGap(189, 189, 189))
.addGroup(jPanel12Layout.createSequentialGroup()
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(radio6)
.addComponent(radio7)
.addComponent(radio5))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jLabel7)
.addGap(18, 18, 18)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(radio9)
.addComponent(radio11)
.addComponent(radio10)))))
.addComponent(text4, javax.swing.GroupLayout.PREFERRED_SIZE, 419, javax.swing.GroupLayout.PREFERRED_SIZE)))))
.addComponent(jLabel41)
.addComponent(jLabel40))
.addContainerGap(93, Short.MAX_VALUE))
);
jPanel12Layout.setVerticalGroup(
jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel12Layout.createSequentialGroup()
.addGap(10, 10, 10)
.addComponent(jLabel33)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel32)
.addGap(25, 25, 25)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel3)
.addComponent(text4, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel12Layout.createSequentialGroup()
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel7)
.addComponent(radio9))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(radio10)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(radio11))
.addGroup(jPanel12Layout.createSequentialGroup()
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel4)
.addComponent(radio5))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(radio6)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(radio7)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(radio8)))
.addGap(18, 18, 18)
.addComponent(jSeparator7, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel35)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel34)
.addGap(25, 25, 25)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel9)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addComponent(jSeparator8, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel37)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel36)
.addGap(25, 25, 25)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel13)
.addComponent(jScrollPane7, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addComponent(jSeparator9, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel39)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel38)
.addGap(25, 25, 25)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel15)
.addComponent(jScrollPane9, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addComponent(jSeparator10, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel41)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel40)
.addGap(25, 25, 25)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel14)
.addComponent(jScrollPane8, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
jScrollPane10.setViewportView(jPanel12);
headerstab.addTab(" Issue ", jScrollPane10);
removetag.setText("Remove");
removetag.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
removetag(evt);
}
});
addTag.setText("Add");
addTag.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
addTag(evt);
}
});
listtag.setModel(tag);
jScrollPane11.setViewportView(listtag);
jLabel46.setText("You can define one or multiple tags for this profile.");
jLabel47.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel47.setForeground(new java.awt.Color(255, 102, 51));
jLabel47.setText("Set Tags");
newTagb.setText("New Tag");
newTagb.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
newTagbnewTag(evt);
}
});
javax.swing.GroupLayout jPanel3Layout = new javax.swing.GroupLayout(jPanel3);
jPanel3.setLayout(jPanel3Layout);
jPanel3Layout.setHorizontalGroup(
jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel3Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel47)
.addComponent(jLabel46)
.addGroup(jPanel3Layout.createSequentialGroup()
.addGroup(jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(newTagb, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(addTag, javax.swing.GroupLayout.PREFERRED_SIZE, 93, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(removetag, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addGap(18, 18, 18)
.addGroup(jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jScrollPane11)
.addComponent(newTagCombo, javax.swing.GroupLayout.PREFERRED_SIZE, 468, javax.swing.GroupLayout.PREFERRED_SIZE))))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
jPanel3Layout.setVerticalGroup(
jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel3Layout.createSequentialGroup()
.addGap(10, 10, 10)
.addComponent(jLabel47)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel46)
.addGap(25, 25, 25)
.addGroup(jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jScrollPane11, javax.swing.GroupLayout.PREFERRED_SIZE, 99, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(jPanel3Layout.createSequentialGroup()
.addComponent(newTagb)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(removetag)))
.addGap(18, 18, 18)
.addGroup(jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(newTagCombo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(addTag))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
headerstab.addTab(" Tags ", jPanel3);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jLabel12)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(text1)
.addGap(18, 18, 18)
.addComponent(jLabel18)
.addGap(18, 18, 18)
.addComponent(textauthor, javax.swing.GroupLayout.PREFERRED_SIZE, 228, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap())
.addComponent(headerstab, javax.swing.GroupLayout.DEFAULT_SIZE, 831, Short.MAX_VALUE)
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(text1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel12)
.addComponent(textauthor, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel18))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(headerstab, javax.swing.GroupLayout.DEFAULT_SIZE, 606, Short.MAX_VALUE))
);
}// </editor-fold>//GEN-END:initComponents
private void jButton9removeEncoder(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton9removeEncoder
int selectedIndex = list3.getSelectedIndex();
if (selectedIndex != -1) {
encoder.remove(selectedIndex);
}
}//GEN-LAST:event_jButton9removeEncoder
private void button6setToPayload(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button6setToPayload
modelpayload.addRow(new Object[]{true, "Value"});
}//GEN-LAST:event_button6setToPayload
private void jButton8upEncoder(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton8upEncoder
int selectedIndex = list3.getSelectedIndex();
if (selectedIndex != 0) {
swap(selectedIndex, selectedIndex - 1);
list3.setSelectedIndex(selectedIndex - 1);
list3.ensureIndexIsVisible(selectedIndex - 1);
}
}//GEN-LAST:event_jButton8upEncoder
private void button3loadPayloads(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button3loadPayloads
loadPayloadsFile(payload);
}//GEN-LAST:event_button3loadPayloads
private void button2pastePayload(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button2pastePayload
String element = getClipboardContents();
List<String> lines = Arrays.asList(element.split("\n"));
showPayloads(lines);
}//GEN-LAST:event_button2pastePayload
private void jLabel17goWeb(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_jLabel17goWeb
try {
Desktop.getDesktop().browse(new URI("https://portswigger.net/burp/extender/api/burp/IScannerInsertionPoint.html"));
} catch (URISyntaxException | IOException e) {
System.out.println("Active profile line 2109 Help web not opened: " + e);
}
}//GEN-LAST:event_jLabel17goWeb
private void button4removePayload(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button4removePayload
int[] rows = table6.getSelectedRows();
Arrays.sort(rows);
for (int i = rows.length - 1; i >= 0; i--) {
int row = rows[i];
int modelRow = table6.convertRowIndexToModel(row);
modelpayload.removeRow(modelRow);
}
}//GEN-LAST:event_button4removePayload
private void button18removeMatchReplace(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button18removeMatchReplace
int[] rows = table4.getSelectedRows();
Arrays.sort(rows);
for (int i = rows.length - 1; i >= 0; i--) {
int row = rows[i];
int modelRow = table4.convertRowIndexToModel(row);
model4.removeRow(modelRow);
}
}//GEN-LAST:event_button18removeMatchReplace
private void addMatchReplace(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_addMatchReplace
model4.addRow(new Object[]{"Payload", "Leave blank to add a new header", "Leave blank to remove a matched header", "String"});
}//GEN-LAST:event_addMatchReplace
private void jButton6addEncoder(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton6addEncoder
if (!encoder.isEmpty() && encoder.firstElement().equals(" ")) {
encoder.removeElementAt(0);
encoder.addElement(combo2.getSelectedItem().toString());
} else {
encoder.addElement(combo2.getSelectedItem().toString());
}
}//GEN-LAST:event_jButton6addEncoder
private void button5removeAllPayloads(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button5removeAllPayloads
int rowCount = modelpayload.getRowCount();
for (int i = rowCount - 1; i >= 0; i--) {
modelpayload.removeRow(i);
}
}//GEN-LAST:event_button5removeAllPayloads
private void AllItemStateChanged(java.awt.event.ItemEvent evt) {//GEN-FIRST:event_AllItemStateChanged
if (evt.getStateChange() == java.awt.event.ItemEvent.SELECTED) {
extensionprovided.setSelected(true);
header.setSelected(true);
entirebody.setSelected(true);
paramamf.setSelected(true);
parambody.setSelected(true);
paramcookie.setSelected(true);
paramjson.setSelected(true);
urlpathfolder.setSelected(true);
parammultipartattr.setSelected(true);
paramnamebody.setSelected(true);
paramnameurl.setSelected(true);
userprovided.setSelected(true);
paramurl.setSelected(true);
paramxml.setSelected(true);
paramxmlattr.setSelected(true);
urlpathfilename.setSelected(true);
unknown.setSelected(true);
} else {
extensionprovided.setSelected(false);
header.setSelected(false);
entirebody.setSelected(false);
paramamf.setSelected(false);
parambody.setSelected(false);
paramcookie.setSelected(false);
paramjson.setSelected(false);
urlpathfolder.setSelected(false);
parammultipartattr.setSelected(false);
paramnamebody.setSelected(false);
paramnameurl.setSelected(false);
userprovided.setSelected(false);
paramurl.setSelected(false);
paramxml.setSelected(false);
paramxmlattr.setSelected(false);
urlpathfilename.setSelected(false);
unknown.setSelected(false);
}
}//GEN-LAST:event_AllItemStateChanged
private void paramxmlActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_paramxmlActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_paramxmlActionPerformed
private void jButton7downEncoder(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton7downEncoder
int selectedIndex = list3.getSelectedIndex();
if (selectedIndex != encoder.getSize() - 1) {
swap(selectedIndex, selectedIndex + 1);
list3.setSelectedIndex(selectedIndex + 1);
list3.ensureIndexIsVisible(selectedIndex + 1);
}
}//GEN-LAST:event_jButton7downEncoder
private void removetag(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_removetag
int selectedIndex = listtag.getSelectedIndex();
if (selectedIndex != -1) {
tag.remove(selectedIndex);
}
}//GEN-LAST:event_removetag
private void addTag(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_addTag
tag.addElement(newTagCombo.getSelectedItem());
}//GEN-LAST:event_addTag
private void newTagbnewTag(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_newTagbnewTag
Integer result;
NewTag nt = new NewTag();
JOptionPane jopane1 = new JOptionPane(nt, JOptionPane.PLAIN_MESSAGE, JOptionPane.OK_CANCEL_OPTION);
JDialog dialog = jopane1.createDialog(this, "New Tag");
dialog.setLocationRelativeTo(null);
dialog.setVisible(true);
Object selectedValue = jopane1.getValue();
if (selectedValue != null) {
result = ((Integer) selectedValue).intValue();
if (result == JOptionPane.OK_OPTION) {
addNewTag(nt.newTagtext.getText());
showTags();
}
}
}//GEN-LAST:event_newTagbnewTag
private void headerstabStateChanged(javax.swing.event.ChangeEvent evt) {//GEN-FIRST:event_headerstabStateChanged
int activePane = headerstab.getSelectedIndex();
if (activePane == 3) {
showTags();
}
}//GEN-LAST:event_headerstabStateChanged
private void button8loadGrep(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button8loadGrep
loadGrepsFile(modelgrep);
}//GEN-LAST:event_button8loadGrep
private void button21addGrep(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button21addGrep
if (modelgrep.getRowCount() == 0) {
modelgrep.addRow(new Object[]{true, "", "Value"});
} else {
modelgrep.addRow(new Object[]{true, "Or", "Value"});
}
}//GEN-LAST:event_button21addGrep
private void button7pasteGrep(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button7pasteGrep
String element = getClipboardContents();
List<String> lines = Arrays.asList(element.split("\n"));
showGreps(lines);
}//GEN-LAST:event_button7pasteGrep
private void button10removeAllGrep(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button10removeAllGrep
int rowCount = modelgrep.getRowCount();
for (int i = rowCount - 1; i >= 0; i--) {
modelgrep.removeRow(i);
}
}//GEN-LAST:event_button10removeAllGrep
private void button20removeMatchReplace(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button20removeMatchReplace
int[] rows = table5.getSelectedRows();
Arrays.sort(rows);
for (int i = rows.length - 1; i >= 0; i--) {
int row = rows[i];
int modelRow = table5.convertRowIndexToModel(row);
modelgrep.removeRow(modelRow);
}
}//GEN-LAST:event_button20removeMatchReplace
private void invariationsRadioinvariations(java.awt.event.ItemEvent evt) {//GEN-FIRST:event_invariationsRadioinvariations
if (evt.getStateChange() == java.awt.event.ItemEvent.DESELECTED) {
setEnabledVariations(false);
} else if (evt.getStateChange() == java.awt.event.ItemEvent.SELECTED) {
setEnabledVariations(true);
}
}//GEN-LAST:event_invariationsRadioinvariations
private void variationsRadiovariations(java.awt.event.ItemEvent evt) {//GEN-FIRST:event_variationsRadiovariations
if (evt.getStateChange() == java.awt.event.ItemEvent.DESELECTED) {
setEnabledVariations(false);
} else if (evt.getStateChange() == java.awt.event.ItemEvent.SELECTED) {
setEnabledVariations(true);
}
}//GEN-LAST:event_variationsRadiovariations
private void radioclActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_radioclActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_radioclActionPerformed
private void radioclSelect(java.awt.event.ItemEvent evt) {//GEN-FIRST:event_radioclSelect
if (evt.getStateChange() == java.awt.event.ItemEvent.SELECTED) {
setEnabledVariations(false);
} else if (evt.getStateChange() == java.awt.event.ItemEvent.DESELECTED) {
setEnabledVariations(true);
}
}//GEN-LAST:event_radioclSelect
private void radiotimeTimeoutSelect(java.awt.event.ItemEvent evt) {//GEN-FIRST:event_radiotimeTimeoutSelect
if (evt.getStateChange() == java.awt.event.ItemEvent.SELECTED) {
setEnabledVariations(false);
} else if (evt.getStateChange() == java.awt.event.ItemEvent.DESELECTED) {
setEnabledVariations(true);
}
}//GEN-LAST:event_radiotimeTimeoutSelect
private void radio22payloadencodeMatchType(java.awt.event.ItemEvent evt) {//GEN-FIRST:event_radio22payloadencodeMatchType
if (evt.getStateChange() == java.awt.event.ItemEvent.SELECTED) {
setEnabledVariations(false);
}
}//GEN-LAST:event_radio22payloadencodeMatchType
private void radio3regexMatchType(java.awt.event.ItemEvent evt) {//GEN-FIRST:event_radio3regexMatchType
if (evt.getStateChange() == java.awt.event.ItemEvent.SELECTED) {
setEnabledVariations(false);
}
}//GEN-LAST:event_radio3regexMatchType
private void radio4stringMatchType(java.awt.event.ItemEvent evt) {//GEN-FIRST:event_radio4stringMatchType
if (evt.getStateChange() == java.awt.event.ItemEvent.SELECTED) {
setEnabledVariations(false);
}
}//GEN-LAST:event_radio4stringMatchType
private void radio12payloadMatchType(java.awt.event.ItemEvent evt) {//GEN-FIRST:event_radio12payloadMatchType
if (evt.getStateChange() == java.awt.event.ItemEvent.SELECTED) {
setEnabledVariations(false);
}
}//GEN-LAST:event_radio12payloadMatchType
// Variables declaration - do not modify//GEN-BEGIN:variables
public javax.swing.JCheckBox All;
private javax.swing.JPanel Attributes;
private javax.swing.JButton addTag;
public javax.swing.JCheckBox anchor_labels;
public javax.swing.JRadioButton append;
private javax.swing.JButton button10;
private javax.swing.JButton button18;
private javax.swing.JButton button19;
public javax.swing.JButton button2;
private javax.swing.JButton button20;
private javax.swing.JButton button21;
public javax.swing.JButton button3;
public javax.swing.JButton button4;
public javax.swing.JButton button5;
public javax.swing.JButton button6;
private javax.swing.JButton button7;
private javax.swing.JButton button8;
public javax.swing.ButtonGroup buttonGroup1;
public javax.swing.ButtonGroup buttonGroup2;
public javax.swing.ButtonGroup buttonGroup3;
public javax.swing.ButtonGroup buttonGroup4;
public javax.swing.ButtonGroup buttonGroup5;
public javax.swing.ButtonGroup buttonGroup6;
public javax.swing.JCheckBox button_submit_labels;
public javax.swing.JCheckBox canonical_link;
public javax.swing.JCheckBox check1;
public javax.swing.JCheckBox check4;
public javax.swing.JCheckBox check71;
public javax.swing.JCheckBox check72;
public javax.swing.JCheckBox check73;
public javax.swing.JCheckBox check8;
public javax.swing.JComboBox<String> combo2;
public javax.swing.JCheckBox comments;
public javax.swing.JCheckBox content_length;
public javax.swing.JCheckBox content_location;
public javax.swing.JCheckBox content_type;
public javax.swing.JCheckBox css_classes;
public javax.swing.JCheckBox div_ids;
public javax.swing.JCheckBox entirebody;
public javax.swing.JCheckBox etag_header;
public javax.swing.JCheckBox excludehttp;
public javax.swing.JCheckBox extensionprovided;
public javax.swing.JCheckBox first_header_tag;
public javax.swing.JCheckBox header;
public javax.swing.JCheckBox header_tags;
public javax.swing.JTabbedPane headerstab;
public javax.swing.JCheckBox initial_body_content;
public javax.swing.JCheckBox input_image_labels;
public javax.swing.JCheckBox input_submit_labels;
public javax.swing.JRadioButton insert;
public javax.swing.JRadioButton invariationsRadio;
private javax.swing.JButton jButton6;
private javax.swing.JButton jButton7;
private javax.swing.JButton jButton8;
private javax.swing.JButton jButton9;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel10;
private javax.swing.JLabel jLabel11;
private javax.swing.JLabel jLabel12;
private javax.swing.JLabel jLabel13;
private javax.swing.JLabel jLabel14;
private javax.swing.JLabel jLabel15;
private javax.swing.JLabel jLabel16;
private javax.swing.JLabel jLabel17;
private javax.swing.JLabel jLabel18;
private javax.swing.JLabel jLabel19;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel20;
private javax.swing.JLabel jLabel21;
private javax.swing.JLabel jLabel22;
private javax.swing.JLabel jLabel23;
private javax.swing.JLabel jLabel24;
private javax.swing.JLabel jLabel25;
private javax.swing.JLabel jLabel26;
private javax.swing.JLabel jLabel27;
private javax.swing.JLabel jLabel28;
private javax.swing.JLabel jLabel29;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel30;
private javax.swing.JLabel jLabel31;
private javax.swing.JLabel jLabel32;
private javax.swing.JLabel jLabel33;
private javax.swing.JLabel jLabel34;
private javax.swing.JLabel jLabel35;
private javax.swing.JLabel jLabel36;
private javax.swing.JLabel jLabel37;
private javax.swing.JLabel jLabel38;
private javax.swing.JLabel jLabel39;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel40;
private javax.swing.JLabel jLabel41;
private javax.swing.JLabel jLabel42;
private javax.swing.JLabel jLabel46;
private javax.swing.JLabel jLabel47;
private javax.swing.JLabel jLabel5;
private javax.swing.JLabel jLabel52;
private javax.swing.JLabel jLabel53;
private javax.swing.JLabel jLabel54;
private javax.swing.JLabel jLabel55;
private javax.swing.JLabel jLabel6;
private javax.swing.JLabel jLabel7;
private javax.swing.JLabel jLabel9;
private javax.swing.JPanel jPanel1;
public javax.swing.JPanel jPanel10;
public javax.swing.JPanel jPanel11;
private javax.swing.JPanel jPanel12;
private javax.swing.JPanel jPanel3;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JScrollPane jScrollPane10;
private javax.swing.JScrollPane jScrollPane11;
private javax.swing.JScrollPane jScrollPane14;
private javax.swing.JScrollPane jScrollPane15;
private javax.swing.JScrollPane jScrollPane16;
private javax.swing.JScrollPane jScrollPane4;
private javax.swing.JScrollPane jScrollPane5;
private javax.swing.JScrollPane jScrollPane6;
private javax.swing.JScrollPane jScrollPane7;
private javax.swing.JScrollPane jScrollPane8;
private javax.swing.JScrollPane jScrollPane9;
private javax.swing.JSeparator jSeparator10;
private javax.swing.JSeparator jSeparator11;
private javax.swing.JSeparator jSeparator2;
private javax.swing.JSeparator jSeparator3;
private javax.swing.JSeparator jSeparator4;
private javax.swing.JSeparator jSeparator5;
private javax.swing.JSeparator jSeparator6;
private javax.swing.JSeparator jSeparator7;
private javax.swing.JSeparator jSeparator8;
private javax.swing.JSeparator jSeparator9;
public javax.swing.JCheckBox last_modified_header;
public javax.swing.JCheckBox limited_body_content;
public javax.swing.JCheckBox line_count;
public javax.swing.JList<String> list3;
public javax.swing.JList<String> listtag;
public javax.swing.JCheckBox location;
public javax.swing.JCheckBox negativeCT;
public javax.swing.JCheckBox negativeRC;
public javax.swing.JCheckBox negativeURL;
public javax.swing.JComboBox<String> newTagCombo;
private javax.swing.JButton newTagb;
public javax.swing.JCheckBox non_hidden_form_input_types;
public javax.swing.JCheckBox onlyhttp;
public javax.swing.JCheckBox outbound_edge_count;
public javax.swing.JCheckBox outbound_edge_tag_names;
public javax.swing.JCheckBox page_title;
public javax.swing.JCheckBox paramamf;
public javax.swing.JCheckBox parambody;
public javax.swing.JCheckBox paramcookie;
public javax.swing.JCheckBox paramjson;
public javax.swing.JCheckBox parammultipartattr;
public javax.swing.JCheckBox paramnamebody;
public javax.swing.JCheckBox paramnameurl;
public javax.swing.JCheckBox paramurl;
public javax.swing.JCheckBox paramxml;
public javax.swing.JCheckBox paramxmlattr;
public javax.swing.JRadioButton radio10;
public javax.swing.JRadioButton radio11;
public javax.swing.JRadioButton radio12;
public javax.swing.JRadioButton radio22;
public javax.swing.JRadioButton radio3;
public javax.swing.JRadioButton radio4;
public javax.swing.JRadioButton radio5;
public javax.swing.JRadioButton radio6;
public javax.swing.JRadioButton radio7;
public javax.swing.JRadioButton radio8;
public javax.swing.JRadioButton radio9;
public javax.swing.JRadioButton radiocl;
public javax.swing.JRadioButton radiohttp;
public javax.swing.JRadioButton radiotime;
public javax.swing.JRadioButton rb1;
public javax.swing.JRadioButton rb2;
public javax.swing.JRadioButton rb3;
public javax.swing.JRadioButton rb4;
private javax.swing.JButton removetag;
public javax.swing.JRadioButton replace;
public javax.swing.JTextField resposecode;
public javax.swing.JCheckBox set_cookie_names;
public javax.swing.JSpinner sp1;
public javax.swing.JCheckBox status_code;
public javax.swing.JTable table4;
public javax.swing.JTable table5;
public javax.swing.JTable table6;
public javax.swing.JCheckBox tag_ids;
public javax.swing.JCheckBox tag_names;
public javax.swing.JTextField text1;
public javax.swing.JTextField text4;
public javax.swing.JTextField text5;
public javax.swing.JTextField text71;
public javax.swing.JTextField text72;
public javax.swing.JTextField text73;
public javax.swing.JTextArea textarea1;
public javax.swing.JTextArea textarea2;
public javax.swing.JTextArea textarea3;
public javax.swing.JTextArea textarea4;
public javax.swing.JTextField textauthor;
public javax.swing.JTextField textcl;
public javax.swing.JTextField textgreps;
public javax.swing.JTextField textpayloads;
public javax.swing.JTextField texttime1;
public javax.swing.JTextField texttime2;
public javax.swing.JCheckBox unknown;
public javax.swing.JCheckBox urlpathfilename;
public javax.swing.JCheckBox urlpathfolder;
public javax.swing.JCheckBox userprovided;
public javax.swing.JRadioButton variationsRadio;
public javax.swing.JCheckBox visible_text;
public javax.swing.JCheckBox visible_word_count;
public javax.swing.JCheckBox whole_body_content;
public javax.swing.JCheckBox word_count;
// End of variables declaration//GEN-END:variables
} |
Java | BurpBounty/main/java/burpbountyfree/ActiveScanner.java | /*
Copyright 2018 Eduardo Garcia Melia <wagiro@gmail.com>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package burpbountyfree;
import burp.IBurpCollaboratorClientContext;
import burp.IBurpExtenderCallbacks;
import burp.IExtensionHelpers;
import burp.IHttpRequestResponse;
import burp.IHttpService;
import burp.IRequestInfo;
import burp.IResponseInfo;
import burp.IResponseVariations;
import burp.IScanIssue;
import burp.IScannerInsertionPoint;
import com.google.gson.Gson;
import com.google.gson.JsonArray;
import java.net.URL;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.UUID;
/**
*
* @author wagiro
*/
public class ActiveScanner {
private IBurpExtenderCallbacks callbacks;
private IExtensionHelpers helpers;
JsonArray active_profiles;
BurpBountyExtension bbe;
Gson gson;
CollaboratorData burpCollaboratorData;
BurpBountyGui bbg;
JsonArray allprofiles;
Integer redirtype;
List<String> rules_done = new ArrayList<>();
Integer smartscandelay;
Utils utils;
String issuename;
String name;
String issuedetail;
String issuebackground;
String remediationdetail;
String remediationbackground;
String charstourlencode;
int matchtype;
String issueseverity;
String issueconfidence;
boolean excludeHTTP;
boolean onlyHTTP;
boolean notresponse;
boolean iscontenttype;
boolean isresponsecode;
boolean negativect;
boolean negativerc;
String contenttype;
String responsecode;
String httpresponsecode;
boolean casesensitive;
boolean urlencode;
Integer maxredirect;
int payloadposition;
String timeout1;
String timeout2;
String contentLength;
List<String> payloads;
List<String> payloadsEncoded;
List<String> greps;
List<String> encoders;
ProfilesProperties profile_property;
List<Headers> headers;
List<String> variationAttributes;
List<Integer> insertionPointType;
String urlextension;
Boolean isurlextension;
Boolean NegativeUrlExtension;
GrepMatch gm;
Boolean passive;
List<Integer> responseCodes = new ArrayList<>(Arrays.asList(300, 301, 303, 302, 307, 308));
int limitredirect = 30;
public ActiveScanner(BurpBountyExtension bbe, IBurpExtenderCallbacks callbacks, CollaboratorData burpCollaboratorData, JsonArray allprofiles, BurpBountyGui bbg) {
this.callbacks = callbacks;
helpers = callbacks.getHelpers();
this.burpCollaboratorData = burpCollaboratorData;
this.allprofiles = allprofiles;
this.bbe = bbe;
this.bbg = bbg;
utils = new Utils(bbe, callbacks, burpCollaboratorData, allprofiles, bbg);
gm = new GrepMatch(this.callbacks);
}
public void runAScan(IHttpRequestResponse baseRequestResponse, IScannerInsertionPoint insertionPoint, JsonArray activeprofiles, Boolean come_from_passive, Boolean reqpassive, String rule_name, Boolean passive) {
try {
this.passive = passive;
Boolean collaborator = true;
IBurpCollaboratorClientContext CollaboratorClientContext2 = null;
gson = new Gson();
try {
CollaboratorClientContext2 = callbacks.createBurpCollaboratorClientContext();
burpCollaboratorData.setCollaboratorClientContext(CollaboratorClientContext2);
} catch (Exception ex) {
System.out.println("ActiveScanner line 115: " + ex.getMessage());
collaborator = false;
}
for (int i = 0; i < activeprofiles.size(); i++) {
Object idata = activeprofiles.get(i);
profile_property = gson.fromJson(idata.toString(), ProfilesProperties.class);
//initialized the profile values
setProfilesValues(profile_property);
if (!come_from_passive && !insertionPointType.contains(insertionPoint.getInsertionPointType() & 0xFF)) {
continue;
}
if (come_from_passive && !reqpassive) {
Boolean continue_or_not = false;
for (int ip : insertionPointType) {
if (insertionPoint.getInsertionPointName().endsWith("_" + String.valueOf(ip))) {
continue_or_not = true;
}
}
if (!continue_or_not) {
continue;//COMPROBAR ESTE RETURN
}
}
//inicializa valores de payload, payloadencode y grep
processPayloads();
for (String payload : payloads) {
if (payload.startsWith("false,")) {
continue;
} else if (payload.startsWith("true,")) {
payload = payload.replace("true,", "");
}
Thread.sleep(60);
String bchost = "";
if (urlencode) {
payload = utils.encodeTheseURL(payload, charstourlencode);
}
if (payloadposition == 2) {
payload = insertionPoint.getBaseValue().concat(payload);
}
if (payloadposition == 3) {
payload = insertPayload(insertionPoint.getBaseValue(), payload);
}
//processHeaders
try {
if (!headers.isEmpty()) {
for (int x = 0; x < headers.size(); x++) {
if (headers.get(x).type.equals("Payload")) {
if (headers.get(x).regex.equals("String")) {
payload = payload.replace(headers.get(x).match, headers.get(x).replace);
} else {
payload = payload.replaceAll(headers.get(x).match, headers.get(x).replace);
}
}
if (headers.get(x).type.equals("Request") && collaborator) {
if (headers.get(x).regex.equals("String")) {
String a = headers.get(x).replace;
if (headers.get(x).replace.contains("{BC}")) {
bchost = CollaboratorClientContext2.generatePayload(true);
String subdomain = generateString();
bchost = subdomain + "." + bchost;
}
}
}
}
}
} catch (Exception ex) {
//escondido porque muestra mucho
bchost = "";
}
if (payload.contains(" ") && utils.encode(insertionPoint, reqpassive)) {//for avoid space in payload
payload = payload.replace(" ", "%20");
}
if (matchtype == 5)//Timeout match type
{
timeoutMatchType(baseRequestResponse, insertionPoint, payload, rule_name);
} else if (matchtype == 7 || matchtype == 8)//Variations match type//Invariation match type
{
variationsInvariationsMatchType(baseRequestResponse, insertionPoint, payload, rule_name);
} else if (matchtype == 6)//Content Length difference match type
{
contentLengthMatchType(baseRequestResponse, insertionPoint, payload, rule_name);
} else if (matchtype == 9)//HTTP Response Code
{
httpCodeMatchType(baseRequestResponse, insertionPoint, payload, rule_name);
} else if (payload.contains("{BC}") && collaborator || !bchost.equals("") && collaborator)//Burp Collaborator
{
CollaboratorMatchType(baseRequestResponse, insertionPoint, payload, bchost, rule_name, CollaboratorClientContext2);
} else {//String, Regex, Payload, Payload without encode match types
stringRegexMatchType(baseRequestResponse, insertionPoint, payload, rule_name,CollaboratorClientContext2,bchost);
}
}
}
} catch (Exception ex) {
System.out.println("ActiveScanner line 175: " + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
}
}
public static String insertPayload(String original, String payload) {
String bagBegin = original.substring(0, original.length() / 2);
String bagEnd = original.substring(original.length() / 2, original.length());
return bagBegin + payload + bagEnd;
}
public void processPayloads() {
//If encoders exist...
if (!encoders.isEmpty()) {
switch (matchtype) {
case 1:
payloadsEncoded = utils.processPayload(payloads, encoders);
payloads = new ArrayList(payloadsEncoded);
break;
case 2:
payloadsEncoded = utils.processPayload(payloads, encoders);
payloads = new ArrayList(payloadsEncoded);
break;
case 3:
payloadsEncoded = utils.processPayload(payloads, encoders);
greps = new ArrayList();
for (String p : payloads) {
greps.add("true,Or," + p);
}
payloads = payloadsEncoded;
break;
case 4:
greps = new ArrayList();
payloadsEncoded = utils.processPayload(payloads, encoders);
for (String p : payloads) {
greps.add("true,Or," + p);
}
payloads = new ArrayList(payloadsEncoded);
break;
default:
payloadsEncoded = utils.processPayload(payloads, encoders);
payloads = new ArrayList(payloadsEncoded);
break;
}
} else {
if (matchtype == 3) {
for (String p : payloads) {
greps.add("true,Or," + p);
}
}
}
}
public void setProfilesValues(ProfilesProperties profile_property) {
payloads = profile_property.getPayloads();
name = profile_property.getProfileName();
greps = profile_property.getGreps();
issuename = profile_property.getIssueName();
issueseverity = profile_property.getIssueSeverity();
issueconfidence = profile_property.getIssueConfidence();
issuedetail = profile_property.getIssueDetail();
issuebackground = profile_property.getIssueBackground();
remediationdetail = profile_property.getRemediationDetail();
remediationbackground = profile_property.getRemediationBackground();
matchtype = profile_property.getMatchType();
notresponse = profile_property.getNotResponse();
casesensitive = profile_property.getCaseSensitive();
encoders = profile_property.getEncoder();
urlencode = profile_property.getUrlEncode();
charstourlencode = profile_property.getCharsToUrlEncode();
iscontenttype = profile_property.getIsContentType();
isresponsecode = profile_property.getIsResponseCode();
contenttype = profile_property.getContentType();
responsecode = profile_property.getResponseCode();
httpresponsecode = profile_property.getHttpResponseCode();
excludeHTTP = profile_property.getExcludeHTTP();
onlyHTTP = profile_property.getOnlyHTTP();
negativect = profile_property.getNegativeCT();
negativerc = profile_property.getNegativeRC();
maxredirect = profile_property.getMaxRedir();
redirtype = profile_property.getRedirection();
payloadposition = profile_property.getPayloadPosition();
timeout1 = profile_property.getTime1();
timeout2 = profile_property.getTime2();
contentLength = profile_property.getContentLength();
headers = profile_property.getHeader() != null ? profile_property.getHeader() : new ArrayList();
variationAttributes = profile_property.getVariationAttributes() != null ? profile_property.getVariationAttributes() : new ArrayList();
insertionPointType = profile_property.getInsertionPointType() != null ? profile_property.getInsertionPointType() : new ArrayList(Arrays.asList(0));
isurlextension = profile_property.getIsURLExtension();
urlextension = profile_property.getURLExtension();
NegativeUrlExtension = profile_property.getNegativeURLExtension();
}
public void timeoutMatchType(IHttpRequestResponse baseRequestResponse, IScannerInsertionPoint insertionPoint, String payload, String rule_name) {
long startTime, endTime, difference = 0;
try {
Boolean isduplicated = utils.checkDuplicated(baseRequestResponse, issuename, insertionPoint);
if (!isduplicated) {
IHttpService httpService = baseRequestResponse.getHttpService();
startTime = System.currentTimeMillis();
IHttpRequestResponse payloadRequestResponse;
try {
payloadRequestResponse = callbacks.makeHttpRequest(httpService, new BuildUnencodeRequest(helpers).buildUnencodedRequest(insertionPoint, helpers.stringToBytes(payload), headers, ""));
} catch (Exception e) {
return;
}
if (payloadRequestResponse != null) {
endTime = System.currentTimeMillis();
difference = (endTime - startTime);
IResponseInfo r;
IResponseInfo rbase;
try {
if (payloadRequestResponse.getResponse() == null || baseRequestResponse.getResponse() == null) {
return;
}
r = helpers.analyzeResponse(payloadRequestResponse.getResponse());
rbase = helpers.analyzeResponse(baseRequestResponse.getResponse());
} catch (Exception ex) {
System.out.println("ActiveScanner line 378: " + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
return;
}
Integer responseCode = new Integer(r.getStatusCode());
Integer responseCodeBase = new Integer(rbase.getStatusCode());
IRequestInfo requestInfo = helpers.analyzeRequest(payloadRequestResponse);
if ((!isresponsecode || isresponsecode && utils.isResponseCode(responsecode, negativerc, responseCode) && utils.isResponseCode(responsecode, negativerc, responseCodeBase)) && (!iscontenttype || iscontenttype && utils.isContentType(contenttype, negativect, r))) {
try {
Integer time1 = Integer.parseInt(timeout1);
Integer time2 = Integer.parseInt(timeout2);
if (time2 * 1000 >= difference && difference >= time1 * 1000) {
callbacks.addScanIssue(new CustomScanIssue(payloadRequestResponse.getHttpService(), helpers.analyzeRequest(payloadRequestResponse).getUrl(),
new IHttpRequestResponse[]{callbacks.applyMarkers(payloadRequestResponse, null, null)},
"BurpBounty - " + issuename, "Vulnerable parameter: " + insertionPoint.getInsertionPointName() + ".<br/>" + issuedetail.replace("<payload>", payload), issueseverity,
issueconfidence, remediationdetail.replace("<payload>", payload), issuebackground.replace("<payload>", payload),
remediationbackground.replace("<payload>", payload)));
return;
}
} catch (NumberFormatException e) {
}
}
}
}
} catch (Exception ex) {
System.out.println("ActiveScanner line 410: " + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
}
}
public void httpCodeMatchType(IHttpRequestResponse baseRequestResponse, IScannerInsertionPoint insertionPoint, String payload, String rule_name) {
try {
Boolean isduplicated = utils.checkDuplicated(baseRequestResponse, issuename, insertionPoint);
if (!isduplicated) {
IHttpService httpService = baseRequestResponse.getHttpService();
IHttpRequestResponse payloadRequestResponse;
try {
payloadRequestResponse = callbacks.makeHttpRequest(httpService, new BuildUnencodeRequest(helpers).buildUnencodedRequest(insertionPoint, helpers.stringToBytes(payload), headers, ""));
} catch (Exception e) {
return;
}
if (payloadRequestResponse != null) {
IResponseInfo r;
try {
if (payloadRequestResponse.getResponse() == null) {
return;
}
r = helpers.analyzeResponse(payloadRequestResponse.getResponse());
} catch (Exception ex) {
System.out.println("ActiveScanner line 440: " + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
return;
}
Integer responseCode = new Integer(r.getStatusCode());
IRequestInfo requestInfo = helpers.analyzeRequest(payloadRequestResponse);
if ((!iscontenttype || iscontenttype && utils.isContentType(contenttype, negativect, r))) {
if (utils.isResponseCode(httpresponsecode, negativerc, responseCode)) {
List responseMarkers = new ArrayList(1);
List requestMarkers = new ArrayList(1);
requestMarkers.add(new int[]{helpers.bytesToString(payloadRequestResponse.getRequest()).indexOf(payload),
helpers.bytesToString(payloadRequestResponse.getRequest()).indexOf(payload) + payload.length()});
String grep = "HTTP/";
responseMarkers.add(new int[]{helpers.bytesToString(payloadRequestResponse.getResponse()).toUpperCase().indexOf(grep) + 9,
helpers.bytesToString(payloadRequestResponse.getResponse()).toUpperCase().indexOf(grep) + 12});
callbacks.addScanIssue(new CustomScanIssue(payloadRequestResponse.getHttpService(), helpers.analyzeRequest(payloadRequestResponse).getUrl(),
new IHttpRequestResponse[]{callbacks.applyMarkers(payloadRequestResponse, requestMarkers, responseMarkers)},
"BurpBounty - " + issuename, "Vulnerable parameter: " + insertionPoint.getInsertionPointName() + ".<br/>" + issuedetail, issueseverity,
issueconfidence, remediationdetail, issuebackground, remediationbackground));
return;
}
}
}
}
} catch (Exception ex) {
System.out.println("ActiveScanner line 475: " + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
}
}
public void variationsInvariationsMatchType(IHttpRequestResponse baseRequestResponse, IScannerInsertionPoint insertionPoint, String payload, String rule_name) {
Integer responseCode;
IResponseInfo r;
try {
Boolean isduplicated = utils.checkDuplicated(baseRequestResponse, issuename, insertionPoint);
if (!isduplicated) {
IHttpService httpService = baseRequestResponse.getHttpService();
IHttpRequestResponse requestResponse;
try {
requestResponse = callbacks.makeHttpRequest(httpService, new BuildUnencodeRequest(helpers).buildUnencodedRequest(insertionPoint, helpers.stringToBytes(payload), headers, ""));
} catch (Exception e) {
return;
}
if (requestResponse == null) {
return;
}
try {
if (requestResponse.getResponse() == null) {
return;
}
r = helpers.analyzeResponse(requestResponse.getResponse());
} catch (Exception ex) {
System.out.println("ActiveScanner line 509: " + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
return;
}
IResponseVariations ipv = helpers.analyzeResponseVariations(baseRequestResponse.getResponse(), requestResponse.getResponse());
List<String> var;
List<String> var2;
if (matchtype == 7) {
var = ipv.getVariantAttributes();
var2 = ipv.getInvariantAttributes();
} else {
var = ipv.getInvariantAttributes();
var2 = ipv.getVariantAttributes();
}
List requestMarkers = new ArrayList();
byte[] request;
try {
request = requestResponse.getRequest();
} catch (Exception e) {
return;
}
responseCode = new Integer(r.getStatusCode());
IRequestInfo requestInfo = helpers.analyzeRequest(requestResponse);
if ((!isresponsecode || isresponsecode && utils.isResponseCode(responsecode, negativerc, responseCode)) && (!iscontenttype || iscontenttype && utils.isContentType(contenttype, negativect, r))) {
if (var.containsAll(variationAttributes) && !var2.containsAll(variationAttributes)) {
int start = 0;
byte[] match = helpers.stringToBytes(payload);
int end = 0;
while (start < request.length) {
end = end + 1;
if (end == 30) {
break;
}
start = helpers.indexOf(request, match, false, start, request.length);
if (start == -1) {
break;
}
requestMarkers.add(new int[]{start, start + match.length});
start += match.length;
}
callbacks.addScanIssue(new CustomScanIssue(requestResponse.getHttpService(), helpers.analyzeRequest(requestResponse).getUrl(),
new IHttpRequestResponse[]{callbacks.applyMarkers(requestResponse, requestMarkers, null)},
"BurpBounty - " + issuename, "Vulnerable parameter: " + insertionPoint.getInsertionPointName() + ".<br/>" + issuedetail.replace("<payload>", helpers.urlEncode(payload)), issueseverity,
issueconfidence, remediationdetail.replace("<payload>", helpers.urlEncode(payload)), issuebackground.replace("<payload>", helpers.urlEncode(payload)),
remediationbackground.replace("<payload>", helpers.urlEncode(payload))));
return;
}
}
}
} catch (Exception ex) {
System.out.println("ActiveScanner line 566: " + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
}
}
public void contentLengthMatchType(IHttpRequestResponse baseRequestResponse, IScannerInsertionPoint insertionPoint, String payload, String rule_name) {
try {
Boolean isduplicated = utils.checkDuplicated(baseRequestResponse, issuename, insertionPoint);
if (!isduplicated) {
IHttpService httpService = baseRequestResponse.getHttpService();
IHttpRequestResponse requestResponse;
try {
requestResponse = callbacks.makeHttpRequest(httpService, new BuildUnencodeRequest(helpers).buildUnencodedRequest(insertionPoint, helpers.stringToBytes(payload), headers, ""));
} catch (Exception e) {
return;
}
if (requestResponse == null) {
return;
}
IResponseInfo r;
IResponseInfo rbase;
try {
r = helpers.analyzeResponse(requestResponse.getResponse());
rbase = helpers.analyzeResponse(baseRequestResponse.getResponse());
} catch (Exception e) {
return;
}
try {
if (requestResponse.getResponse() == null || baseRequestResponse.getResponse() == null) {
return;
}
r = helpers.analyzeResponse(requestResponse.getResponse());
rbase = helpers.analyzeResponse(baseRequestResponse.getResponse());
} catch (Exception ex) {
System.out.println("ActiveScanner line 608: " + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
return;
}
Integer responseCode = new Integer(r.getStatusCode());
Integer responseCodeBase = new Integer(rbase.getStatusCode());
IRequestInfo requestInfo = helpers.analyzeRequest(requestResponse);
if ((!isresponsecode || isresponsecode && utils.isResponseCode(responsecode, negativerc, responseCode) && utils.isResponseCode(responsecode, negativerc, responseCodeBase)) && (!iscontenttype || iscontenttype && utils.isContentType(contenttype, negativect, r))) {
int baseResponseContentLength = utils.getContentLength(baseRequestResponse);
int currentResponseContentLength = utils.getContentLength(requestResponse);
if (Math.abs(baseResponseContentLength - currentResponseContentLength) > Integer.parseInt(contentLength)) {
List responseMarkers = new ArrayList(1);
String grep = "CONTENT-LENGTH:";
responseMarkers.add(new int[]{helpers.bytesToString(requestResponse.getResponse()).toUpperCase().indexOf(grep),
helpers.bytesToString(requestResponse.getResponse()).toUpperCase().indexOf(grep) + grep.length()});
callbacks.addScanIssue(new CustomScanIssue(requestResponse.getHttpService(), helpers.analyzeRequest(requestResponse).getUrl(),
new IHttpRequestResponse[]{callbacks.applyMarkers(requestResponse, null, responseMarkers)},
"BurpBounty - " + issuename, "Vulnerable parameter: " + insertionPoint.getInsertionPointName() + ".<br/>" + issuedetail.replace("<payload>", helpers.urlEncode(payload)).replace("<grep>", helpers.urlEncode(grep)), issueseverity,
issueconfidence, remediationdetail.replace("<payload>", helpers.urlEncode(payload)).replace("<grep>", helpers.urlEncode(grep)), issuebackground.replace("<payload>", helpers.urlEncode(payload)).replace("<grep>", helpers.urlEncode(grep)),
remediationbackground.replace("<payload>", helpers.urlEncode(payload)).replace("<grep>", helpers.urlEncode(grep))));
return;
}
}
}
} catch (Exception ex) {
System.out.println("ActiveScanner line 502: " + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
}
}
public void CollaboratorMatchType(IHttpRequestResponse baseRequestResponse, IScannerInsertionPoint insertionPoint, String payload, String bchost, String rule_name, IBurpCollaboratorClientContext CollaboratorClientContext2) {
int loop = 0;
Boolean redirect = true;
try {
if (bchost.isEmpty()) {
bchost = CollaboratorClientContext2.generatePayload(true);
String subdomain = generateString();
bchost = subdomain + "." + bchost;
}
} catch (Exception ex) {
return;
}
try {
Boolean isduplicated = utils.checkDuplicated(baseRequestResponse, issuename, insertionPoint);
if (!isduplicated) {
IHttpService httpService = baseRequestResponse.getHttpService();
payload = payload.replace("{BC}", bchost);
IHttpRequestResponse requestResponse;
try {
requestResponse = callbacks.makeHttpRequest(httpService, new BuildUnencodeRequest(helpers).buildUnencodedRequest(insertionPoint, helpers.stringToBytes(payload), headers, bchost));
} catch (Exception e) {
return;
}
if (requestResponse == null) {
return;
}
IResponseInfo r;
try {
if (requestResponse.getResponse() == null) {
return;
}
r = helpers.analyzeResponse(requestResponse.getResponse());
} catch (Exception ex) {
System.out.println("ActiveScanner line 685: " + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
return;
}
burpCollaboratorData.setIssueProperties(requestResponse, bchost, issuename, issuedetail.replace("<payload>", helpers.urlEncode(payload)), issueseverity.replace("<payload>", helpers.urlEncode(payload)), issueconfidence.replace("<payload>", (payload)), remediationdetail.replace("<payload>", (payload)), issuebackground.replace("<payload>", (payload)), remediationbackground.replace("<payload>", (payload)));
Integer responseCode = new Integer(r.getStatusCode());
do {
if (responseCodes.contains(responseCode) && loop < limitredirect) {
httpService = requestResponse.getHttpService();
URL url = null;
try {
url = utils.getRedirection(requestResponse, httpService, redirtype);
} catch (NullPointerException e) {
redirect = false;
}
if (url != null) {
try {
byte[] checkRequest = helpers.buildHttpRequest(url);
checkRequest = utils.getMatchAndReplace(headers, checkRequest, payload, bchost);
int port = 0;
if (url.getPort() == -1) {
port = url.getDefaultPort();
}
IHttpService newrequest = helpers.buildHttpService(url.getHost(), port, url.getProtocol());
requestResponse = callbacks.makeHttpRequest(newrequest, checkRequest);
} catch (Exception e) {
return;
}
if (requestResponse == null) {
return;
}
try {
if (requestResponse.getResponse() == null) {
return;
}
r = helpers.analyzeResponse(requestResponse.getResponse());
} catch (Exception ex) {
System.out.println("ActiveScanner line 735: " + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
return;
}
responseCode = new Integer(r.getStatusCode());
}
loop += 1;
} else {
redirect = false;
}
} while (redirect);
}
} catch (Exception ex) {
System.out.println("ActiveScanner line 589: " + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
}
}
public void stringRegexMatchType(IHttpRequestResponse baseRequestResponse, IScannerInsertionPoint insertionPoint, String payload, String rule_name,IBurpCollaboratorClientContext CollaboratorClientContext2, String bchost) {
int loop = 0;
Boolean redirect = true;
try {
if (bchost.isEmpty()) {
bchost = CollaboratorClientContext2.generatePayload(true);
String subdomain = generateString();
bchost = subdomain + "." + bchost;
}
} catch (Exception ex) {
bchost = "";
}
//multiarray
int grep_index = 0;
ArrayList<ArrayList<String>> greps_final = new ArrayList<>();
IScanIssue matches = null;
try {
Boolean isduplicated = utils.checkDuplicated(baseRequestResponse, issuename, insertionPoint);
if (!isduplicated) {
IHttpService httpService = baseRequestResponse.getHttpService();
greps_final.add(new ArrayList());
for (String grep : greps) {
String[] tokens = grep.split(",", 3);
if (tokens[0].equals("true")) {
if (tokens[1].equals("And") || tokens[1].equals("")) {
if (!tokens[2].equals("")) {
greps_final.get(grep_index).add(tokens[2]);
}
} else {
if (!tokens[2].equals("")) {
if (!greps_final.get(0).isEmpty()) {
greps_final.add(new ArrayList());
grep_index = grep_index + 1;
greps_final.get(grep_index).add(tokens[2]);
} else {
greps_final.get(grep_index).add(tokens[2]);
}
}
}
}
}
IHttpRequestResponse requestResponse;
try {
requestResponse = callbacks.makeHttpRequest(httpService, new BuildUnencodeRequest(helpers).buildUnencodedRequest(insertionPoint, helpers.stringToBytes(payload), headers, bchost));
} catch (NullPointerException e) {
return;
}
if (requestResponse == null) {
return;
}
IHttpRequestResponse redirectRequestResponse = requestResponse;
IResponseInfo r;
try {
if (redirectRequestResponse.getResponse() == null) {
return;
}
r = helpers.analyzeResponse(redirectRequestResponse.getResponse());
} catch (Exception ex) {
System.out.println("ActiveScanner line 816: " + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
return;
}
Integer responseCode = new Integer(r.getStatusCode());
IRequestInfo requestInfo = helpers.analyzeRequest(requestResponse);
if ((!isresponsecode || isresponsecode && utils.isResponseCode(responsecode, negativerc, responseCode)) && (!iscontenttype || iscontenttype && utils.isContentType(contenttype, negativect, r))) {
for (int x = 0; x <= grep_index; x++) {
if (!greps_final.get(x).isEmpty()) {
matches = gm.getResponseMatches(requestResponse, payload, greps_final.get(x), issuename, "Vulnerable parameter: " + insertionPoint.getInsertionPointName() + ".<br/>" + issuedetail, issuebackground, remediationdetail, remediationbackground, charstourlencode, matchtype,
issueseverity, issueconfidence, notresponse, casesensitive, urlencode, excludeHTTP, onlyHTTP);
//mirar si peta asi
if (matches != null) {
callbacks.addScanIssue(matches);
return;
}
}
}
}
do {
if (responseCodes.contains(responseCode) && redirtype != 1 && loop < maxredirect && maxredirect < limitredirect) {
httpService = requestResponse.getHttpService();
URL url = null;
try {
url = utils.getRedirection(requestResponse, httpService, redirtype);
} catch (NullPointerException e) {
redirect = false;
}
if (url != null) {
try {
byte[] checkRequest = helpers.buildHttpRequest(url);
checkRequest = utils.getMatchAndReplace(headers, checkRequest, payload, "");
int port = 0;
if (url.getPort() == -1) {
port = url.getDefaultPort();
}
IHttpService newrequest = helpers.buildHttpService(url.getHost(), port, url.getProtocol());
requestResponse = callbacks.makeHttpRequest(newrequest, checkRequest);
} catch (Exception e) {
return;
}
if (requestResponse == null) {
return;
}
try {
if (requestResponse.getResponse() == null) {
return;
}
r = helpers.analyzeResponse(requestResponse.getResponse());
} catch (Exception ex) {
System.out.println("ActiveScanner line 880: " + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
return;
}
responseCode = new Integer(r.getStatusCode());
requestInfo = helpers.analyzeRequest(baseRequestResponse);
if ((!isresponsecode || isresponsecode && utils.isResponseCode(responsecode, negativerc, responseCode)) && (!iscontenttype || iscontenttype && utils.isContentType(contenttype, negativect, r))) {
for (int x = 0; x <= grep_index; x++) {
if (!greps_final.get(x).isEmpty()) {
matches = gm.getResponseMatches(requestResponse, payload, greps_final.get(x), issuename, "Vulnerable parameter: " + insertionPoint.getInsertionPointName() + ".<br/>" + issuedetail, issuebackground, remediationdetail, remediationbackground, charstourlencode, matchtype,
issueseverity, issueconfidence, notresponse, casesensitive, urlencode, excludeHTTP, onlyHTTP);
//mirar si peta asi
if (matches != null) {
callbacks.addScanIssue(matches);
return;
}
}
}
}
} else {
redirect = false;
}
} else {
redirect = false;
}
loop += 1;
} while (redirect);
}
} catch (Exception ex) {
System.out.println("ActiveScanner line 729: " + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
}
}
public static String generateString() {
String uuid = UUID.randomUUID().toString().replace("-", "").replace("7", "");
return "7"+uuid+"7";
}
} |
Java | BurpBounty/main/java/burpbountyfree/BuildUnencodeRequest.java | package burpbountyfree;
import burp.IExtensionHelpers;
import burp.IRequestInfo;
import burp.IScannerInsertionPoint;
import java.util.ArrayList;
import java.util.List;
public class BuildUnencodeRequest {
private IExtensionHelpers helpers;
BuildUnencodeRequest(IExtensionHelpers helpers) {
this.helpers = helpers;
}
byte[] buildUnencodedRequest(IScannerInsertionPoint iScannerInsertionPoint, byte[] payload, List<Headers> headers, String bchost) {
byte[] canary = buildCanary(payload.length);
byte[] request = iScannerInsertionPoint.buildRequest(canary);
int canaryPos = findCanary(canary, request);
System.arraycopy(payload, 0, request, canaryPos, payload.length);
byte[] finalRequest = request;
String tempRequest = helpers.bytesToString(request);
String stringpayload = helpers.bytesToString(payload);
if (!headers.isEmpty()) {
try {
for (int x = 0; x < headers.size(); x++) {
String replace = headers.get(x).replace;
if (headers.get(x).type.equals("Request")) {
if (headers.get(x).regex.equals("String")) {
if (replace.contains("{PAYLOAD}")) {
replace = replace.replace("{PAYLOAD}", stringpayload);
}
if (replace.contains("{BC}")) {
replace = replace.replace("{BC}", bchost);
}
if (headers.get(x).match.isEmpty()) {
tempRequest = tempRequest.replace("\r\n\r\n", "\r\n" + replace + "\r\n\r\n");
} else {
tempRequest = tempRequest.replace(headers.get(x).match, replace);
}
} else {
if (replace.contains("{PAYLOAD}")) {
replace = replace.replaceAll("\\{PAYLOAD\\}", stringpayload);
}
if (replace.contains("{BC}")) {
replace = replace.replaceAll("\\{BC\\}", bchost);
}
if (headers.get(x).match.isEmpty()) {
tempRequest = tempRequest.replaceAll("\\r\\n\\r\\n", "\r\n" + replace + "\r\n\r\n");
} else {
tempRequest = tempRequest.replaceAll(headers.get(x).match, replace);
}
}
}
}
} catch (Exception e) {
// System.out.println("BuildUnencodeRequest line 898: " + e.getMessage());
// for (StackTraceElement element : e.getStackTrace()) {
// System.out.println(element);
// }
}
}
if (tempRequest.toLowerCase().contains("Content-Length: ".toLowerCase())) {
byte[] byteRequest = helpers.stringToBytes(tempRequest);
IRequestInfo messageInfo = helpers.analyzeRequest(byteRequest);
int bodyOffset = messageInfo.getBodyOffset();
List<String> newheaders = messageInfo.getHeaders();
int actualBody = request.length - bodyOffset;
for (String header : newheaders) {
if (header.toLowerCase().startsWith("Content-Length: ".toLowerCase())) {
header = "Content-Length: " + actualBody;
}
}
byte[] body = helpers.stringToBytes(tempRequest.substring(bodyOffset, byteRequest.length));
finalRequest = helpers.buildHttpMessage(newheaders, body);
}else{
finalRequest = helpers.stringToBytes(tempRequest);
}
return finalRequest;
}
private byte[] buildCanary(int payloadLength) {
byte[] canary = new byte[payloadLength];
for (int i = 0; i < payloadLength; i++) {
canary[i] = '$';
}
return canary;
}
private int findCanary(byte[] canary, byte[] request) {
int canaryPos = helpers.indexOf(request, canary, false, 0, request.length);
return canaryPos;
}
} |
Java | BurpBounty/main/java/burpbountyfree/BurpBountyExtension.java | /*
Copyright 2018 Eduardo Garcia Melia <wagiro@gmail.com>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package burpbountyfree;
import burp.IBurpExtender;
import burp.IBurpExtenderCallbacks;
import burp.IExtensionHelpers;
import burp.IExtensionStateListener;
import burp.IHttpRequestResponse;
import burp.IHttpService;
import burp.IMessageEditor;
import burp.IMessageEditorController;
import burp.IRequestInfo;
import burp.IScanIssue;
import burp.IScannerCheck;
import burp.IScannerInsertionPoint;
import burp.IScannerInsertionPointProvider;
import burp.ITab;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import java.awt.Dimension;
import javax.swing.ScrollPaneConstants;
import java.awt.Component;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JScrollPane;
import javax.swing.SwingUtilities;
public class BurpBountyExtension implements IBurpExtender, ITab, IScannerCheck, IExtensionStateListener, IScannerInsertionPointProvider, IMessageEditorController {
public static IBurpExtenderCallbacks callbacks;
private IExtensionHelpers helpers;
private JScrollPane optionsTab;
private BurpBountyGui panel;
ProfilesProperties issue;
BurpCollaboratorThread BurpCollaborator;
BurpCollaboratorThread bct;
CollaboratorData burpCollaboratorData;
List<byte[]> responses;
List<String[]> urls;
JsonArray profiles;
JsonArray rules;
Integer bchost_number = 0;
Boolean settext = false;
public IMessageEditor requestViewer;
public IMessageEditor responseViewer;
public IHttpRequestResponse currentlyDisplayedItem;
JsonArray allprofiles = new JsonArray();
JsonArray activeprofiles = new JsonArray();
JsonArray passiveresprofiles = new JsonArray();
JsonArray passivereqprofiles = new JsonArray();
List<IScanIssue> issues = new ArrayList();
List<String> params = new ArrayList();
int scanner = 0;
Boolean enabled = false;
List<Integer> insertion_point_type = new ArrayList();
JsonArray allrules = new JsonArray();
@Override
public void registerExtenderCallbacks(IBurpExtenderCallbacks callbacks) {
this.callbacks = callbacks;
this.helpers = callbacks.getHelpers();
callbacks.setExtensionName("Burp Bounty Free");
responses = new ArrayList();
urls = new ArrayList();
urls.add(new String[]{"testXXYY", "test"});
try {
burpCollaboratorData = new CollaboratorData(helpers);
bct = new BurpCollaboratorThread(callbacks, burpCollaboratorData);
bct.start();
} catch (Exception e) {
System.out.println("BurpBountyExtension line 108:" + e.getMessage());
}
SwingUtilities.invokeLater(new Runnable() {
@Override
public void run() {
panel = new BurpBountyGui(BurpBountyExtension.this);
optionsTab = new JScrollPane(panel, ScrollPaneConstants.VERTICAL_SCROLLBAR_AS_NEEDED, ScrollPaneConstants.HORIZONTAL_SCROLLBAR_AS_NEEDED);
optionsTab.setPreferredSize(new Dimension(600, 600));
optionsTab.getVerticalScrollBar().setUnitIncrement(20);
callbacks.registerScannerCheck(BurpBountyExtension.this);
callbacks.registerExtensionStateListener(BurpBountyExtension.this);
callbacks.registerScannerInsertionPointProvider(BurpBountyExtension.this);
requestViewer = callbacks.createMessageEditor(BurpBountyExtension.this, false);
responseViewer = callbacks.createMessageEditor(BurpBountyExtension.this, false);
callbacks.addSuiteTab(BurpBountyExtension.this);
callbacks.printOutput("- Burp Bounty Free v4.0");
callbacks.printOutput("- For bugs please on the official github: https://github.com/wagiro/BurpBounty/");
callbacks.printOutput("- Created and developed by Eduardo Garcia Melia <egarcia@burpbounty.net>");
callbacks.printOutput("\nBurp Bounty team:");
callbacks.printOutput("- Eduardo Garcia Melia <egarcia@burpbounty.net>");
callbacks.printOutput("- Jaime Restrepo <jrestrepo@burpbounty.net>");
}
});
}
@Override
public void extensionUnloaded() {
bct.doStop();
callbacks.printOutput("- Burp Bounty extension was unloaded");
}
@Override
public List<IScannerInsertionPoint> getInsertionPoints(IHttpRequestResponse baseRequestResponse) {
List<IScannerInsertionPoint> insertionPoints = new ArrayList();
Gson gson = new Gson();
Boolean exist_insertion_point_in_profiles = false;
try {
if (baseRequestResponse == null || baseRequestResponse.getRequest() == null) {
return insertionPoints;
}
for (int i = 0; i < activeprofiles.size(); i++) {
Object idata = activeprofiles.get(i);
ProfilesProperties profile_property = gson.fromJson(idata.toString(), ProfilesProperties.class);
for (int insertionPoint : profile_property.getInsertionPointType()) {
if (insertionPoint == 65) {
exist_insertion_point_in_profiles = true;
}
}
}
if (!exist_insertion_point_in_profiles) {
return insertionPoints;
}
IRequestInfo request = helpers.analyzeRequest(baseRequestResponse);
String url = request.getUrl().getHost();
byte[] match = helpers.stringToBytes("/");
byte[] req = baseRequestResponse.getRequest();
int len = helpers.bytesToString(baseRequestResponse.getRequest()).indexOf(" HTTP");
int firstSlash = helpers.bytesToString(baseRequestResponse.getRequest()).indexOf(" /");
int beginAt = 0;
while (beginAt < len) {
beginAt = helpers.indexOf(req, match, false, beginAt, len);
if (beginAt == -1) {
break;
}
String mark = helpers.bytesToString(baseRequestResponse.getRequest()).substring(firstSlash, beginAt);
if (!params.contains(url + ":p4r4m" + mark)) {
insertionPoints.add(helpers.makeScannerInsertionPoint("p4r4m" + mark, baseRequestResponse.getRequest(), beginAt, len));
params.add(url + ":p4r4m" + mark);
}
beginAt += match.length;
}
} catch (NullPointerException ex) {
System.out.println("BurpBountyExtension line 167: " + ex.getMessage());//Da Number Format Exception
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
return insertionPoints;
}
return insertionPoints;
}
@Override
public List<IScanIssue> doActiveScan(IHttpRequestResponse baseRequestResponse, IScannerInsertionPoint insertionPoint) {
if (activeprofiles.size() == 0) {
return issues;
}
try {
ActiveScanner as = new ActiveScanner(this, callbacks, burpCollaboratorData, allprofiles, panel);
as.runAScan(baseRequestResponse, insertionPoint, activeprofiles, false, false, "", false);
} catch (Exception ex) {
System.out.println("BurpBountyExtension line 189: " + ex.getMessage());//Da Number Format Exception
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
}
return issues;
}
@Override
public List<IScanIssue> doPassiveScan(IHttpRequestResponse baseRequestResponse) {
if (passiveresprofiles.size() > 0) {
try {
PassiveResponseScanner prs = new PassiveResponseScanner(this, callbacks, burpCollaboratorData, allprofiles, activeprofiles, panel);
prs.runResPScan(baseRequestResponse, passiveresprofiles, allrules, urls, false);
} catch (Exception ex) {
System.out.println("BurpBountyExtension line 206: " + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
}
}
if (passivereqprofiles.size() > 0) {
try {
PassiveRequestScanner pqs = new PassiveRequestScanner(this, callbacks, burpCollaboratorData, allprofiles, activeprofiles, panel);
pqs.runReqPScan(baseRequestResponse, passivereqprofiles, allrules, urls, false);
} catch (Exception ex) {
System.out.println("BurpBountyExtension line 219: " + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
}
}
return issues;
}
@Override
public int consolidateDuplicateIssues(IScanIssue existingIssue, IScanIssue newIssue) {
if (existingIssue.getIssueName().equals(newIssue.getIssueName())) {
return -1;
} else {
return 0;
}
}
@Override
public String getTabCaption() {
return "Burp Bounty Free";
}
@Override
public Component getUiComponent() {
return optionsTab;
}
public void setTest(Boolean test) {
settext = test;
}
public void setAllProfiles(JsonArray allProfiles) {
allprofiles = allProfiles;
setActiveProfiles(allprofiles);
setPassiveProfiles(allprofiles);
}
public void setActiveProfiles(JsonArray allprofiles) {
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
int scanner = 0;
ProfilesProperties issue;
List<Integer> insertion_point_type = new ArrayList();
Boolean enabled = false;
activeprofiles = new JsonArray();
for (int i = 0; i < allprofiles.size(); i++) {
try {
Object idata = allprofiles.get(i);
issue = gson.fromJson(idata.toString(), ProfilesProperties.class);
scanner = issue.getScanner();
enabled = issue.getEnabled();
insertion_point_type = issue.getInsertionPointType();
} catch (Exception ex) {
System.out.println("BurpBountyExtension line 399: " + ex.getMessage());
continue;
}
if (scanner == 1 && enabled) {
activeprofiles.add(allprofiles.get(i));
}
}
}
public void setPassiveProfiles(JsonArray allprofiles) {
passiveresprofiles = new JsonArray();
passivereqprofiles = new JsonArray();
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
int scanner = 0;
Boolean enabled = false;
ProfilesProperties issue;
for (int i = 0; i < allprofiles.size(); i++) {
try {
Object idata = allprofiles.get(i);
issue = gson.fromJson(idata.toString(), ProfilesProperties.class);
scanner = issue.getScanner();
enabled = issue.getEnabled();
} catch (Exception ex) {
System.out.println("BurpBountyExtension line 341: " + ex.getMessage());
continue;
}
if (enabled && scanner == 2) {
passiveresprofiles.add(allprofiles.get(i));
} else if (enabled && scanner == 3) {
passivereqprofiles.add(allprofiles.get(i));
}
}
}
public void setRules(JsonArray allRules) {
allrules = allRules;
}
@Override
public byte[] getRequest() {
return currentlyDisplayedItem.getRequest();
}
@Override
public byte[] getResponse() {
return currentlyDisplayedItem.getResponse();
}
@Override
public IHttpService getHttpService() {
return currentlyDisplayedItem.getHttpService();
}
} |
BurpBounty/main/java/burpbountyfree/BurpBountyGui.form | <?xml version="1.0" encoding="UTF-8" ?>
<Form version="1.8" maxVersion="1.9" type="org.netbeans.modules.form.forminfo.JPanelFormInfo">
<NonVisualComponents>
<Component class="javax.swing.ButtonGroup" name="buttonGroup1">
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup2">
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup3">
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup4">
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup5">
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup6">
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup7">
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup8">
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup9">
</Component>
<Component class="javax.swing.JCheckBoxMenuItem" name="jCheckBoxMenuItem1">
<Properties>
<Property name="selected" type="boolean" value="true"/>
<Property name="text" type="java.lang.String" value="jCheckBoxMenuItem1"/>
</Properties>
</Component>
<Component class="javax.swing.JMenuItem" name="jMenuItem1">
<Properties>
<Property name="text" type="java.lang.String" value="jMenuItem1"/>
</Properties>
</Component>
<Container class="javax.swing.JPopupMenu" name="jPopupMenu1">
<Layout class="org.netbeans.modules.form.compat2.layouts.DesignAbsoluteLayout">
<Property name="useNullLayout" type="boolean" value="true"/>
</Layout>
<SubComponents>
<MenuItem class="javax.swing.JMenuItem" name="jMenuItem2">
<Properties>
<Property name="text" type="java.lang.String" value="Enable"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="jMenuItem2ActionPerformed"/>
</Events>
</MenuItem>
<MenuItem class="javax.swing.JMenuItem" name="jMenuItem3">
<Properties>
<Property name="text" type="java.lang.String" value="Disable"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="jMenuItem3ActionPerformed"/>
</Events>
</MenuItem>
</SubComponents>
</Container>
<Container class="javax.swing.JPopupMenu" name="jPopupMenu2">
<Layout class="org.netbeans.modules.form.compat2.layouts.DesignAbsoluteLayout">
<Property name="useNullLayout" type="boolean" value="true"/>
</Layout>
<SubComponents>
<MenuItem class="javax.swing.JMenuItem" name="jMenuItem4">
<Properties>
<Property name="text" type="java.lang.String" value="Enable"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="jMenuItem4ActionPerformed"/>
</Events>
</MenuItem>
<MenuItem class="javax.swing.JMenuItem" name="jMenuItem5">
<Properties>
<Property name="text" type="java.lang.String" value="Disable"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="jMenuItem5ActionPerformed"/>
</Events>
</MenuItem>
</SubComponents>
</Container>
<Container class="javax.swing.JPopupMenu" name="jPopupMenu3">
<Layout class="org.netbeans.modules.form.compat2.layouts.DesignAbsoluteLayout">
<Property name="useNullLayout" type="boolean" value="true"/>
</Layout>
<SubComponents>
<MenuItem class="javax.swing.JMenuItem" name="jMenuItem6">
<Properties>
<Property name="text" type="java.lang.String" value="Enable"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="jMenuItem6ActionPerformed"/>
</Events>
</MenuItem>
<MenuItem class="javax.swing.JMenuItem" name="jMenuItem7">
<Properties>
<Property name="text" type="java.lang.String" value="Disable"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="jMenuItem7ActionPerformed"/>
</Events>
</MenuItem>
</SubComponents>
</Container>
<Container class="javax.swing.JPopupMenu" name="jPopupMenu4">
<Layout class="org.netbeans.modules.form.compat2.layouts.DesignAbsoluteLayout">
<Property name="useNullLayout" type="boolean" value="true"/>
</Layout>
<SubComponents>
<MenuItem class="javax.swing.JMenuItem" name="jMenuItem8">
<Properties>
<Property name="text" type="java.lang.String" value="Enable"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="jMenuItem8ActionPerformed"/>
</Events>
</MenuItem>
<MenuItem class="javax.swing.JMenuItem" name="jMenuItem9">
<Properties>
<Property name="text" type="java.lang.String" value="Disable"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="jMenuItem9ActionPerformed"/>
</Events>
</MenuItem>
</SubComponents>
</Container>
<Container class="javax.swing.JSplitPane" name="jSplitPane1">
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JSplitPaneSupportLayout"/>
</Container>
</NonVisualComponents>
<Properties>
<Property name="autoscrolls" type="boolean" value="true"/>
</Properties>
<AuxValues>
<AuxValue name="FormSettings_autoResourcing" type="java.lang.Integer" value="0"/>
<AuxValue name="FormSettings_autoSetComponentName" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_generateFQN" type="java.lang.Boolean" value="true"/>
<AuxValue name="FormSettings_generateMnemonicsCode" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_i18nAutoMode" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_layoutCodeTarget" type="java.lang.Integer" value="1"/>
<AuxValue name="FormSettings_listenerGenerationStyle" type="java.lang.Integer" value="0"/>
<AuxValue name="FormSettings_variablesLocal" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_variablesModifier" type="java.lang.Integer" value="2"/>
</AuxValues>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jTabbedPane2" alignment="0" max="32767" attributes="0"/>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jTabbedPane2" alignment="0" max="32767" attributes="0"/>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Container class="javax.swing.JTabbedPane" name="jTabbedPane2">
<Events>
<EventHandler event="stateChanged" listener="javax.swing.event.ChangeListener" parameters="javax.swing.event.ChangeEvent" handler="showprofiles"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout"/>
<SubComponents>
<Container class="javax.swing.JPanel" name="jPanel1">
<Properties>
<Property name="autoscrolls" type="boolean" value="true"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
<Constraints>
<Constraint layoutClass="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout" value="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout$JTabbedPaneConstraintsDescription">
<JTabbedPaneConstraints tabName=" Profiles ">
<Property name="tabTitle" type="java.lang.String" value=" Profiles "/>
</JTabbedPaneConstraints>
</Constraint>
</Constraints>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Component id="jPanel6" max="32767" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Component id="jPanel6" max="32767" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Container class="javax.swing.JPanel" name="jPanel6">
<Properties>
<Property name="enabled" type="boolean" value="false"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jtabpane" alignment="0" pref="0" max="32767" attributes="0"/>
<Group type="102" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel43" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel44" alignment="0" min="-2" pref="575" max="-2" attributes="0"/>
</Group>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</Group>
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="380" max="-2" attributes="0"/>
<Component id="jLabel45" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="newTagCombo2" min="-2" pref="325" max="-2" attributes="0"/>
<EmptySpace min="0" pref="0" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="10" max="-2" attributes="0"/>
<Component id="jLabel43" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel44" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="newTagCombo2" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="jLabel45" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="18" max="-2" attributes="0"/>
<Component id="jtabpane" pref="0" max="32767" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JLabel" name="jLabel43">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Profile Manager"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel44">
<Properties>
<Property name="text" type="java.lang.String" value="In this section you can manage the profiles. "/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel45">
<Properties>
<Property name="text" type="java.lang.String" value="Filter by Tag"/>
</Properties>
</Component>
<Component class="javax.swing.JComboBox" name="newTagCombo2">
<Properties>
<Property name="model" type="javax.swing.ComboBoxModel" editor="org.netbeans.modules.form.editors2.ComboBoxModelEditor">
<StringArray count="0"/>
</Property>
</Properties>
<Events>
<EventHandler event="itemStateChanged" listener="java.awt.event.ItemListener" parameters="java.awt.event.ItemEvent" handler="selectTag"/>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="newTagCombo2ActionPerformed"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_TypeParameters" type="java.lang.String" value="<String>"/>
</AuxValues>
</Component>
<Container class="javax.swing.JTabbedPane" name="jtabpane">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="0"/>
</Property>
</Properties>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout"/>
<SubComponents>
<Container class="javax.swing.JPanel" name="jPanel3">
<Constraints>
<Constraint layoutClass="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout" value="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout$JTabbedPaneConstraintsDescription">
<JTabbedPaneConstraints tabName=" Active Profiles ">
<Property name="tabTitle" type="java.lang.String" value=" Active Profiles "/>
</JTabbedPaneConstraints>
</Constraint>
</Constraints>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="jButton2" alignment="0" max="32767" attributes="0"/>
<Component id="button13" alignment="0" max="32767" attributes="0"/>
<Component id="jButton16" alignment="0" min="-2" pref="103" max="-2" attributes="0"/>
</Group>
<EmptySpace pref="860" max="32767" attributes="0"/>
</Group>
<Group type="103" rootIndex="1" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="133" max="-2" attributes="0"/>
<Component id="jScrollPane5" pref="830" max="32767" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Component id="jButton16" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jButton2" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="button13" min="-2" max="-2" attributes="0"/>
<EmptySpace pref="743" max="32767" attributes="0"/>
</Group>
<Group type="103" rootIndex="1" groupAlignment="0" attributes="0">
<Component id="jScrollPane5" alignment="0" pref="835" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Container class="javax.swing.JScrollPane" name="jScrollPane5">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTable" name="table3">
<Properties>
<Property name="autoCreateRowSorter" type="boolean" value="true"/>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Arial" size="14" style="0"/>
</Property>
<Property name="model" type="javax.swing.table.TableModel" editor="org.netbeans.modules.form.RADConnectionPropertyEditor">
<Connection code="model" type="code"/>
</Property>
<Property name="columnModel" type="javax.swing.table.TableColumnModel" editor="org.netbeans.modules.form.editors2.TableColumnModelEditor">
<TableColumnModel selectionModel="0"/>
</Property>
<Property name="componentPopupMenu" type="javax.swing.JPopupMenu" editor="org.netbeans.modules.form.ComponentChooserEditor">
<ComponentRef name="jPopupMenu1"/>
</Property>
<Property name="tableHeader" type="javax.swing.table.JTableHeader" editor="org.netbeans.modules.form.editors2.JTableHeaderEditor">
<TableHeader reorderingAllowed="false" resizingAllowed="true"/>
</Property>
</Properties>
<Events>
<EventHandler event="mousePressed" listener="java.awt.event.MouseListener" parameters="java.awt.event.MouseEvent" handler="table3MousePressed"/>
<EventHandler event="mouseReleased" listener="java.awt.event.MouseListener" parameters="java.awt.event.MouseEvent" handler="table3MouseReleased"/>
</Events>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JButton" name="jButton16">
<Properties>
<Property name="text" type="java.lang.String" value="Add"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="addActiveProfile"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="jButton2">
<Properties>
<Property name="text" type="java.lang.String" value="Edit"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="editActiveProfile"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="button13">
<Properties>
<Property name="text" type="java.lang.String" value="Remove"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="removeProfiles"/>
</Events>
</Component>
</SubComponents>
</Container>
<Container class="javax.swing.JPanel" name="jPanel5">
<Constraints>
<Constraint layoutClass="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout" value="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout$JTabbedPaneConstraintsDescription">
<JTabbedPaneConstraints tabName=" Passive Request Profiles ">
<Property name="tabTitle" type="java.lang.String" value=" Passive Request Profiles "/>
</JTabbedPaneConstraints>
</Constraint>
</Constraints>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="jButton3" alignment="0" max="32767" attributes="0"/>
<Component id="button14" alignment="0" max="32767" attributes="0"/>
<Component id="jButton17" alignment="0" min="-2" pref="103" max="-2" attributes="0"/>
</Group>
<EmptySpace pref="860" max="32767" attributes="0"/>
</Group>
<Group type="103" rootIndex="1" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="133" max="-2" attributes="0"/>
<Component id="jScrollPane6" pref="830" max="32767" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Component id="jButton17" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jButton3" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="button14" min="-2" max="-2" attributes="0"/>
<EmptySpace pref="743" max="32767" attributes="0"/>
</Group>
<Group type="103" rootIndex="1" groupAlignment="0" attributes="0">
<Component id="jScrollPane6" alignment="0" pref="835" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Container class="javax.swing.JScrollPane" name="jScrollPane6">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTable" name="table1">
<Properties>
<Property name="autoCreateRowSorter" type="boolean" value="true"/>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Arial" size="14" style="0"/>
</Property>
<Property name="model" type="javax.swing.table.TableModel" editor="org.netbeans.modules.form.RADConnectionPropertyEditor">
<Connection code="model1" type="code"/>
</Property>
<Property name="rowSorter" type="javax.swing.RowSorter" editor="org.netbeans.modules.form.ComponentChooserEditor">
<ComponentRef name="null"/>
</Property>
<Property name="tableHeader" type="javax.swing.table.JTableHeader" editor="org.netbeans.modules.form.editors2.JTableHeaderEditor">
<TableHeader reorderingAllowed="false" resizingAllowed="true"/>
</Property>
</Properties>
<Events>
<EventHandler event="mousePressed" listener="java.awt.event.MouseListener" parameters="java.awt.event.MouseEvent" handler="table1MousePressed"/>
<EventHandler event="mouseReleased" listener="java.awt.event.MouseListener" parameters="java.awt.event.MouseEvent" handler="table1MouseReleased"/>
</Events>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JButton" name="jButton17">
<Properties>
<Property name="text" type="java.lang.String" value="Add"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="addRequestProfile"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="jButton3">
<Properties>
<Property name="text" type="java.lang.String" value="Edit"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="editRequestProfile"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="button14">
<Properties>
<Property name="text" type="java.lang.String" value="Remove"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="removeProfiles"/>
</Events>
</Component>
</SubComponents>
</Container>
<Container class="javax.swing.JPanel" name="jPanel7">
<Constraints>
<Constraint layoutClass="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout" value="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout$JTabbedPaneConstraintsDescription">
<JTabbedPaneConstraints tabName=" Passive Response Profiles ">
<Property name="tabTitle" type="java.lang.String" value=" Passive Response Profiles "/>
</JTabbedPaneConstraints>
</Constraint>
</Constraints>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="jButton4" alignment="0" max="32767" attributes="0"/>
<Component id="button15" alignment="0" max="32767" attributes="0"/>
<Component id="jButton18" alignment="0" min="-2" pref="103" max="-2" attributes="0"/>
</Group>
<EmptySpace pref="860" max="32767" attributes="0"/>
</Group>
<Group type="103" rootIndex="1" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="133" max="-2" attributes="0"/>
<Component id="jScrollPane10" pref="830" max="32767" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Component id="jButton18" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jButton4" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="button15" min="-2" max="-2" attributes="0"/>
<EmptySpace pref="743" max="32767" attributes="0"/>
</Group>
<Group type="103" rootIndex="1" groupAlignment="0" attributes="0">
<Component id="jScrollPane10" alignment="0" pref="835" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Container class="javax.swing.JScrollPane" name="jScrollPane10">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTable" name="table2">
<Properties>
<Property name="autoCreateRowSorter" type="boolean" value="true"/>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Arial" size="14" style="0"/>
</Property>
<Property name="model" type="javax.swing.table.TableModel" editor="org.netbeans.modules.form.RADConnectionPropertyEditor">
<Connection code="model2" type="code"/>
</Property>
<Property name="rowSorter" type="javax.swing.RowSorter" editor="org.netbeans.modules.form.ComponentChooserEditor">
<ComponentRef name="null"/>
</Property>
<Property name="tableHeader" type="javax.swing.table.JTableHeader" editor="org.netbeans.modules.form.editors2.JTableHeaderEditor">
<TableHeader reorderingAllowed="false" resizingAllowed="true"/>
</Property>
</Properties>
<Events>
<EventHandler event="mousePressed" listener="java.awt.event.MouseListener" parameters="java.awt.event.MouseEvent" handler="table2MousePressed"/>
<EventHandler event="mouseReleased" listener="java.awt.event.MouseListener" parameters="java.awt.event.MouseEvent" handler="table2MouseReleased"/>
</Events>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JButton" name="jButton18">
<Properties>
<Property name="text" type="java.lang.String" value="Add"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="addResponseProfile"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="jButton4">
<Properties>
<Property name="text" type="java.lang.String" value="Edit"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="editResponseProfile"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="button15">
<Properties>
<Property name="text" type="java.lang.String" value="Remove"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="removeProfiles"/>
</Events>
</Component>
</SubComponents>
</Container>
</SubComponents>
</Container>
</SubComponents>
</Container>
</SubComponents>
</Container>
<Container class="javax.swing.JPanel" name="jPanel4">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
<Constraints>
<Constraint layoutClass="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout" value="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout$JTabbedPaneConstraintsDescription">
<JTabbedPaneConstraints tabName=" Options ">
<Property name="tabTitle" type="java.lang.String" value=" Options "/>
</JTabbedPaneConstraints>
</Constraint>
</Constraints>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jSeparator13" alignment="0" max="32767" attributes="0"/>
<Group type="102" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel50" alignment="0" min="-2" pref="575" max="-2" attributes="0"/>
<Component id="jLabel51" alignment="0" min="-2" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="jButton5" pref="108" max="32767" attributes="0"/>
<Component id="jButton1" max="32767" attributes="0"/>
</Group>
<EmptySpace type="unrelated" max="-2" attributes="0"/>
<Component id="text11" min="-2" pref="700" max="-2" attributes="0"/>
</Group>
<Component id="jLabel48" alignment="0" min="-2" pref="575" max="-2" attributes="0"/>
<Component id="jLabel49" min="-2" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="jButton11" max="32767" attributes="0"/>
<Component id="jButton12" min="-2" pref="105" max="-2" attributes="0"/>
</Group>
<EmptySpace type="unrelated" max="-2" attributes="0"/>
<Component id="jScrollPane13" min="-2" pref="700" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace min="0" pref="160" max="32767" attributes="0"/>
</Group>
</Group>
<EmptySpace max="-2" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="10" max="-2" attributes="0"/>
<Component id="jLabel51" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel50" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jButton5" linkSize="24" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="text11" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Component id="jButton1" linkSize="24" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jSeparator13" min="-2" pref="10" max="-2" attributes="0"/>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Component id="jLabel49" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel48" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Component id="jButton11" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jButton12" min="-2" max="-2" attributes="0"/>
</Group>
<Component id="jScrollPane13" min="-2" pref="205" max="-2" attributes="0"/>
</Group>
<EmptySpace pref="544" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JLabel" name="jLabel50">
<Properties>
<Property name="text" type="java.lang.String" value="In this section specify the base profiles directory. "/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel51">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Directory"/>
</Properties>
</Component>
<Component class="javax.swing.JButton" name="jButton5">
<Properties>
<Property name="text" type="java.lang.String" value="Directory"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="loadConfigFile"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="jButton1">
<Properties>
<Property name="text" type="java.lang.String" value="Reload"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="profilesReload"/>
</Events>
</Component>
<Component class="javax.swing.JTextField" name="text11">
<Properties>
<Property name="toolTipText" type="java.lang.String" value=""/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator13">
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane13">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JList" name="listtagmanager">
<Properties>
<Property name="model" type="javax.swing.ListModel" editor="org.netbeans.modules.form.RADConnectionPropertyEditor">
<Connection code="tagmanager" type="code"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_TypeParameters" type="java.lang.String" value="<String>"/>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JButton" name="jButton12">
<Properties>
<Property name="text" type="java.lang.String" value="Remove"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="removeTagManager"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="jButton11">
<Properties>
<Property name="text" type="java.lang.String" value="Add"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="newTag"/>
</Events>
</Component>
<Component class="javax.swing.JLabel" name="jLabel48">
<Properties>
<Property name="text" type="java.lang.String" value="In this section you can manage the tags."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel49">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Tags Manager"/>
</Properties>
</Component>
</SubComponents>
</Container>
<Container class="javax.swing.JPanel" name="jPanel10">
<Events>
<EventHandler event="mouseClicked" listener="java.awt.event.MouseListener" parameters="java.awt.event.MouseEvent" handler="goWebBurp"/>
</Events>
<Constraints>
<Constraint layoutClass="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout" value="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout$JTabbedPaneConstraintsDescription">
<JTabbedPaneConstraints tabName=" About ">
<Property name="tabTitle" type="java.lang.String" value=" About "/>
</JTabbedPaneConstraints>
</Constraint>
</Constraints>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<EmptySpace min="-2" pref="14" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="jLabel58" min="-2" max="-2" attributes="0"/>
<Component id="jLabel57" alignment="0" min="-2" max="-2" attributes="0"/>
<Group type="102" alignment="1" attributes="0">
<Component id="jLabel23" pref="428" max="32767" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel7" min="-2" pref="262" max="-2" attributes="0"/>
</Group>
<Component id="jLabel1" alignment="0" pref="0" max="32767" attributes="0"/>
<Component id="jLabel3" alignment="0" pref="0" max="32767" attributes="0"/>
<Component id="jLabel12" alignment="0" pref="0" max="32767" attributes="0"/>
<Group type="102" alignment="1" attributes="0">
<Component id="jLabel6" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="202" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="206" max="-2" attributes="0"/>
<Component id="jLabel22" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel24" min="-2" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="131" max="-2" attributes="0"/>
<Component id="jLabel10" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace pref="1138" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="23" max="-2" attributes="0"/>
<Group type="103" groupAlignment="1" attributes="0">
<Component id="jLabel23" alignment="1" min="-2" pref="115" max="-2" attributes="0"/>
<Component id="jLabel7" alignment="1" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="27" max="-2" attributes="0"/>
<Component id="jLabel58" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel1" min="-2" pref="93" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jLabel57" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Component id="jLabel12" min="-2" pref="237" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jLabel6" min="-2" pref="32" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jLabel3" min="-2" pref="61" max="-2" attributes="0"/>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Component id="jLabel10" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel22" alignment="3" min="-2" pref="27" max="-2" attributes="0"/>
<Component id="jLabel24" alignment="3" min="-2" pref="27" max="-2" attributes="0"/>
</Group>
<EmptySpace pref="133" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JLabel" name="jLabel57">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="36" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="3a" green="5c" red="e5" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="About Pro."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel12">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Arial" size="14" style="0"/>
</Property>
<Property name="text" type="java.lang.String" value="<html><p style="text-align: justify;"> Burp Bounty Pro is a Burp Suite Pro extension that improves the active and passive scanner by means of advanced and customized vulnerability profiles through a very intuitive graphical interface. <br><br> On the one hand, it acts as a the most advanced and flexible web application vulnerability scanner, being able to add your own vulnerability profiles, or add your own custom payloads/requests to the existing vulnerability profiles. <br><br> On the other hand, it can simulate a manual pentest in search of maximum efficiency, without making unnecessary requests, it scans the targets only for those potentially vulnerable parameters, with the most effective payloads. <br><br> Finally, this extension also helps you by collecting valuable information when performing the manual pentest, such as possible vulnerable parameters, versions detection and more.</p></html>"/>
<Property name="horizontalTextPosition" type="int" value="4"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel6">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Tahoma" size="18" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="70" green="4e" red="0" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="You are using Burp Bounty Free 4.0"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel22">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Tahoma" size="12" style="0"/>
</Property>
<Property name="text" type="java.lang.String" value="By using this software your are accepting the"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel7">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Tahoma" size="30" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="3a" green="63" red="e5" type="rgb"/>
</Property>
<Property name="horizontalAlignment" type="int" value="4"/>
<Property name="text" type="java.lang.String" value="<html>Ride First<br> on Bug Hunting.</html>"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel23">
<Properties>
<Property name="horizontalAlignment" type="int" value="0"/>
<Property name="icon" type="javax.swing.Icon" editor="org.netbeans.modules.form.editors2.IconEditor">
<Image iconType="3" name="/logo_free.png"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel24">
<Properties>
<Property name="text" type="java.lang.String" value="<html><a href=\u005c"\u005c">EULA</a></html>" containsInvalidXMLChars="true"/>
</Properties>
<Events>
<EventHandler event="mouseClicked" listener="java.awt.event.MouseListener" parameters="java.awt.event.MouseEvent" handler="jLabel24goWeb"/>
</Events>
</Component>
<Component class="javax.swing.JLabel" name="jLabel1">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Arial" size="14" style="0"/>
</Property>
<Property name="text" type="java.lang.String" value="<html><p style="text-align: justify;"> Burp Bounty Free is a Burp Suite extension that allows you, in a quick and simple way, to improve the active and passive Burp Suite scanner by means of personalized profiles through a very intuitive graphical interface. Through an advanced search of patterns and an improvement of the payload to send, we can create our own issue profiles both in the active scanner and in the passive.</p></html>"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel58">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="36" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="3a" green="5c" red="e5" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="About Free."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel3">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Arial" size="14" style="0"/>
</Property>
<Property name="text" type="java.lang.String" value="<html><p style="text-align: justify;">If you need more power, I invite you to try the new Burp Bounty Pro, which gives you more power and automation during your manual pentests.</p></html>"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel10">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Arial" size="24" style="0"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="70" green="4e" red="0" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="<html>More information at: <a href=\u005c"\u005c">https://burpbounty.net</a></html>" containsInvalidXMLChars="true"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_SerializeTo" type="java.lang.String" value="BurpBountyGui_jLabel3"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Container class="javax.swing.JPanel" name="jPanel8">
<Constraints>
<Constraint layoutClass="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout" value="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout$JTabbedPaneConstraintsDescription">
<JTabbedPaneConstraints tabName=" Burp Bounty Pro ">
<Property name="tabTitle" type="java.lang.String" value=" Burp Bounty Pro "/>
</JTabbedPaneConstraints>
</Constraint>
</Constraints>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="19" max="-2" attributes="0"/>
<Component id="jLabel5" min="-2" max="-2" attributes="0"/>
<EmptySpace pref="789" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel5" min="-2" pref="714" max="-2" attributes="0"/>
<EmptySpace min="0" pref="271" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JLabel" name="jLabel5">
<Properties>
<Property name="icon" type="javax.swing.Icon" editor="org.netbeans.modules.form.editors2.IconEditor">
<Image iconType="3" name="/Tabla.png"/>
</Property>
</Properties>
<Events>
<EventHandler event="mouseClicked" listener="java.awt.event.MouseListener" parameters="java.awt.event.MouseEvent" handler="goImageWeb"/>
</Events>
</Component>
</SubComponents>
</Container>
</SubComponents>
</Container>
</SubComponents>
</Form> |
|
Java | BurpBounty/main/java/burpbountyfree/BurpBountyGui.java | /*
Copyright 2018 Eduardo Garcia Melia <wagiro@gmail.com>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package burpbountyfree;
import burp.IBurpExtenderCallbacks;
import burp.IExtensionHelpers;
import burp.IHttpRequestResponse;
import burp.IResponseInfo;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
import com.google.gson.reflect.TypeToken;
import com.google.gson.stream.JsonReader;
import java.awt.Desktop;
import java.awt.Dimension;
import java.awt.Toolkit;
import java.awt.datatransfer.Clipboard;
import java.awt.datatransfer.DataFlavor;
import java.awt.datatransfer.Transferable;
import java.awt.datatransfer.UnsupportedFlavorException;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.FilenameFilter;
import java.io.IOException;
import java.io.OutputStreamWriter;
import java.io.PrintWriter;
import java.net.URI;
import java.net.URISyntaxException;
import java.nio.file.Files;
import java.util.ArrayList;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
import javax.swing.DefaultListModel;
import javax.swing.JDialog;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JTable;
import javax.swing.RowSorter;
import javax.swing.SortOrder;
import javax.swing.event.TableModelEvent;
import javax.swing.event.TableModelListener;
import javax.swing.table.DefaultTableModel;
import javax.swing.table.TableModel;
import javax.swing.table.TableRowSorter;
public class BurpBountyGui extends javax.swing.JPanel {
private IBurpExtenderCallbacks callbacks;
private IExtensionHelpers helpers;
public String filename;
Boolean pathDiscovery;
JsonArray allrules;
JsonArray allprofiles;
JsonArray activeprofiles;
JsonArray passiveresprofiles;
JsonArray passivereqprofiles;
DefaultTableModel model;
DefaultTableModel model1;
DefaultTableModel model2;
DefaultTableModel model4;
DefaultTableModel model9;
DefaultTableModel model10;
DefaultTableModel rulemodel;
DefaultTableModel modeltagmanager;
DefaultTableModel dashboardmodel;
DefaultListModel tagmanager;
String profiles_directory;
BurpBountyExtension parent;
public BurpBountyGui(BurpBountyExtension parent) {
try {
this.callbacks = parent.callbacks;
this.helpers = callbacks.getHelpers();
this.parent = parent;
filename = "";
model4 = new DefaultTableModel();
model9 = new DefaultTableModel();
model10 = new DefaultTableModel();
modeltagmanager = new DefaultTableModel();
dashboardmodel = new DefaultTableModel();
rulemodel = new DefaultTableModel();
allprofiles = new JsonArray();
allrules = new JsonArray();
activeprofiles = new JsonArray();
passiveresprofiles = new JsonArray();
passivereqprofiles = new JsonArray();
tagmanager = new DefaultListModel();
if (callbacks.loadExtensionSetting("filename") != null) {
filename = callbacks.loadExtensionSetting("filename");
if (filename.endsWith(File.separator)) {
profiles_directory = filename ;
} else {
profiles_directory = filename + File.separator ;
}
} else {
filename = System.getProperty("user.home");
if (filename.endsWith(File.separator)) {
profiles_directory = filename ;
} else {
profiles_directory = filename + File.separator;
}
}
createDirectories(profiles_directory);
model = new DefaultTableModel() {
@Override
public Class<?> getColumnClass(int columnIndex) {
Class clazz = String.class;
switch (columnIndex) {
case 0:
clazz = Boolean.class;
break;
}
return clazz;
}
@Override
public boolean isCellEditable(int row, int column) {
return column == 0;
}
};
model1 = new DefaultTableModel() {
@Override
public Class<?> getColumnClass(int columnIndex) {
Class clazz = String.class;
switch (columnIndex) {
case 0:
clazz = Boolean.class;
break;
}
return clazz;
}
@Override
public boolean isCellEditable(int row, int column) {
return column == 0;
}
};
model2 = new DefaultTableModel() {
@Override
public Class<?> getColumnClass(int columnIndex) {
Class clazz = String.class;
switch (columnIndex) {
case 0:
clazz = Boolean.class;
break;
}
return clazz;
}
@Override
public boolean isCellEditable(int row, int column) {
return column == 0;
}
};
//main
initComponents();
text11.setText(filename);
checkProfilesProperties(profiles_directory);
makeTagsFile();
showTags();
showProfiles("All");
} catch (Exception e) {
System.out.println("BurpBountyGui: line 461");
for (StackTraceElement element : e.getStackTrace()) {
System.out.println(element);
}
}
}
public void createDirectories(String profiles_directory) {
File profiles = new File(profiles_directory);
if (!profiles.exists()) {
profiles.mkdir();
}
}
public void updateGreps(String file, ProfilesProperties issue) {
//Load file for implement payloads
List greps = new ArrayList();
String line;
File fileload = new File(file);
try {
BufferedReader bufferreader = new BufferedReader(new FileReader(fileload.getAbsolutePath()));
line = bufferreader.readLine();
while (line != null) {
greps.add(line);
line = bufferreader.readLine();
}
bufferreader.close();
issue.setGreps(greps);
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
String strJson = gson.toJson(issue);
FileWriter writer = null;
writer = new FileWriter(profiles_directory + File.separator + issue.getProfileName().concat(".bb"));
writer.write("[" + strJson + "]");
writer.close();
} catch (FileNotFoundException ex) {
System.out.println("BurpBountyGui line 1675:" + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
} catch (IOException ex) {
System.out.println("BurpBountyGui line 1078:" + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
}
}
private List<String> readFile(String filename) {
List<String> records = new ArrayList();
try {
FileReader reader2 = new FileReader(filename);
BufferedReader reader = new BufferedReader(reader2);
String line;
while ((line = reader.readLine()) != null) {
records.add(line);
}
reader2.close();
reader.close();
} catch (Exception e) {
System.out.println("BurpBountyGui line 1882:" + e.getMessage());
for (StackTraceElement element : e.getStackTrace()) {
System.out.println(element);
}
}
return records;
}
public void checkProfilesProperties(String profiles_directory) {
FileReader fr;
JsonArray alldata = new JsonArray();
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
File f = new File(profiles_directory);
if (f.exists() && f.isDirectory()) {
for (File file : f.listFiles()) {
if (file.getName().endsWith(".bb")) {
try {
fr = new FileReader(file.getAbsolutePath());
} catch (IOException ex) {
System.out.println("BurpBountyGui line 1796:" + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
continue;
}
JsonParser parser = new JsonParser();
JsonArray data = new JsonArray();
ProfilesProperties profile_property;
try {
JsonReader json = new JsonReader((fr));
data.addAll(parser.parse(json).getAsJsonArray());
Object idata = data.get(0);
profile_property = gson.fromJson(idata.toString(), ProfilesProperties.class);
String name = "";
name = profile_property.getProfileName();
JsonObject bbObj = data.get(0).getAsJsonObject();
if (name == null) {
name = profile_property.getName();
if (name == null) {
System.out.println("Profile name corrupted");
continue;
} else {
bbObj.remove("Name");
bbObj.addProperty("ProfileName", name);
}
}
data = new JsonArray();
data.add(bbObj);
alldata.addAll(data);
} catch (Exception e) {
System.out.println("BurpBountyGui line 1939");
for (StackTraceElement element : e.getStackTrace()) {
System.out.println(element);
}
continue;
}
try {
fr.close();
} catch (IOException ex) {
System.out.println("BurpBountyGui line 1825:" + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
continue;
}
}
}
}
parent.setAllProfiles(alldata);
allprofiles = alldata;
setActiveProfiles(allprofiles);
setPassiveProfiles(allprofiles);
}
public void setActiveProfiles(JsonArray allprofiles) {
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
int scanner = 0;
ProfilesProperties issue;
Boolean enabled = false;
activeprofiles = new JsonArray();
for (int i = 0; i < allprofiles.size(); i++) {
try {
Object idata = allprofiles.get(i);
issue = gson.fromJson(idata.toString(), ProfilesProperties.class);
scanner = issue.getScanner();
enabled = issue.getEnabled();
} catch (Exception ex) {
System.out.println("BurpBountyExtension line 399: " + ex.getMessage());
continue;
}
if (scanner == 1 && enabled) {
activeprofiles.add(allprofiles.get(i));
}
}
}
public void setPassiveProfiles(JsonArray allprofiles) {
passiveresprofiles = new JsonArray();
passivereqprofiles = new JsonArray();
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
int scanner = 0;
Boolean enabled = false;
ProfilesProperties issue;
for (int i = 0; i < allprofiles.size(); i++) {
try {
Object idata = allprofiles.get(i);
issue = gson.fromJson(idata.toString(), ProfilesProperties.class);
scanner = issue.getScanner();
enabled = issue.getEnabled();
} catch (Exception ex) {
System.out.println("BurpBountyExtension line 341: " + ex.getMessage());
continue;
}
if (enabled && scanner == 2) {
passiveresprofiles.add(allprofiles.get(i));
} else if (enabled && scanner == 3) {
passivereqprofiles.add(allprofiles.get(i));
}
}
}
public JsonArray getProfiles() {
parent.setAllProfiles(allprofiles);
return allprofiles;
}
public String getClipboardContents() {
//Get clipboard contents for implement grep and match paste button
String result = "";
Clipboard clipboard = Toolkit.getDefaultToolkit().getSystemClipboard();
Transferable contents = clipboard.getContents(null);
boolean hasTransferableText = (contents != null) && contents.isDataFlavorSupported(DataFlavor.stringFlavor);
if (hasTransferableText) {
try {
result = (String) contents.getTransferData(DataFlavor.stringFlavor);
} catch (UnsupportedFlavorException | IOException ex) {
System.out.println("BurpBountyGui line 1866:" + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
}
}
return result;
}
public void loadConfigFile() {
JFrame parentFrame = new JFrame();
JFileChooser fileChooser = new JFileChooser();
fileChooser.setDialogTitle("Specify a base directory to load");
fileChooser.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
int userSelection = fileChooser.showOpenDialog(parentFrame);
if (userSelection == JFileChooser.APPROVE_OPTION) {
File fileload = fileChooser.getSelectedFile();
profiles_directory = fileload.toString() + File.separator;
String file = fileload.getAbsolutePath() + File.separator;
text11.setText(file);
checkProfilesProperties(profiles_directory);
makeTagsFile();
showTags();
showProfiles("All");
filename = file;
this.callbacks.saveExtensionSetting("filename", file);
}
}
public void setEnableDisableProfile(String enable, JTable table) {
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
JsonArray json2 = new JsonArray();
List<ProfilesProperties> newjson = gson.fromJson(json2, new TypeToken<List<ProfilesProperties>>() {
}.getType());
int[] rows = table.getSelectedRows();
for (Integer row : rows) {
try {
String profile_name = table.getValueAt(row, 1).toString();
JsonArray data = new JsonArray();
FileReader reader = new FileReader(profiles_directory + File.separator + profile_name.concat(".bb"));
JsonReader json = new JsonReader(reader);
JsonParser parser = new JsonParser();
data.addAll(parser.parse(json).getAsJsonArray());
Object idata = data.get(0);
ProfilesProperties profile_properties = gson.fromJson(idata.toString(), ProfilesProperties.class
);
if (enable.contains("Yes")) {
profile_properties.setEnabled(true);
} else {
profile_properties.setEnabled(false);
}
newjson.clear();
newjson.add(profile_properties);
FileOutputStream fileStream = new FileOutputStream(profiles_directory + File.separator + profile_name.concat(".bb"));
String fjson = gson.toJson(newjson);
OutputStreamWriter writer = new OutputStreamWriter(fileStream, "UTF-8");
writer.write(fjson);
reader.close();
json.close();
writer.close();
} catch (IOException e) {
System.out.println("BurpBountyGui line 1956:" + e.getMessage());
for (StackTraceElement element : e.getStackTrace()) {
System.out.println(element);
}
}
}
checkProfilesProperties(profiles_directory);
showProfiles("All");
}
public void deleteTagProfiles(String tag) {
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
File f = new File(profiles_directory);
JsonArray json2 = new JsonArray();
List<ProfilesProperties> newjson = gson.fromJson(json2, new TypeToken<List<ProfilesProperties>>() {
}.getType());
File[] files = f.listFiles(new FilenameFilter() {
@Override
public boolean accept(File dir, String name) {
if (name.toLowerCase().endsWith(".bb")) {
return true;
} else {
return false;
}
}
});
if (f.exists() && f.isDirectory()) {
for (File file : files) {
try {
JsonArray data = new JsonArray();
FileReader reader = new FileReader(file.getAbsolutePath());
JsonReader json = new JsonReader(reader);
JsonParser parser = new JsonParser();
data.addAll(parser.parse(json).getAsJsonArray());
Object idata = data.get(0);
ProfilesProperties profile_properties = gson.fromJson(idata.toString(), ProfilesProperties.class);
List<String> tags = profile_properties.getTags();
List<String> finaltags = new ArrayList();
if (tags != null) {
for (String dtag : tags) {
if (!dtag.equals(tag)) {
finaltags.add(dtag);
}
}
}
profile_properties.setTags(finaltags);
newjson.clear();
newjson.add(profile_properties);
FileOutputStream fileStream = new FileOutputStream(file.getAbsoluteFile());
String fjson = gson.toJson(newjson);
OutputStreamWriter writer = new OutputStreamWriter(fileStream, "UTF-8");
writer.write(fjson);
reader.close();
writer.close();
json.close();
} catch (IOException e) {
System.out.println("BurpBountyGui line 2065:" + e.getMessage());
for (StackTraceElement element : e.getStackTrace()) {
System.out.println(element);
}
}
}
}
checkProfilesProperties(profiles_directory);
showProfiles("All");
}
public void makeTagsFile() {
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
allprofiles = getProfiles();
List<String> tags = new ArrayList();
for (int i = 0; i < allprofiles.size(); i++) {
Object idata = allprofiles.get(0);
ProfilesProperties profile_properties;
try {
profile_properties = gson.fromJson(idata.toString(), ProfilesProperties.class);
tags.addAll(profile_properties.getTags());
} catch (IllegalStateException e) {
for (StackTraceElement element : e.getStackTrace()) {
System.out.println(element);
}
continue;
}
}
Set<String> singles = new TreeSet<>();
Set<String> multiples = new TreeSet<>();
for (String x : tags) {
if (!multiples.contains(x)) {
if (singles.contains(x)) {
singles.remove(x);
multiples.add(x);
} else {
singles.add(x);
}
}
}
tags.clear();
tags.addAll(singles);
tags.addAll(multiples);
File file = new File(profiles_directory + File.separator + "tags.txt");
if (!file.exists()) {
file.getParentFile().mkdirs();
}
List<String> existenttags = readFile(profiles_directory + File.separator + "tags.txt");
for (String tag : tags) {
if (!existenttags.contains(tag)) {
addNewTag(tag);
}
}
}
public class profilesModelListener implements TableModelListener {
@Override
public void tableChanged(TableModelEvent e) {
int row = e.getFirstRow();
int column = e.getColumn();
TableModel model = (TableModel) e.getSource();
if (column == 0) {
Boolean checked = (Boolean) model.getValueAt(row, column);
if (checked) {
try {
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
JsonArray json2 = new JsonArray();
List<ProfilesProperties> newjson = gson.fromJson(json2, new TypeToken<List<ProfilesProperties>>() {
}.getType());
String profile_name = model.getValueAt(row, 1).toString();
JsonArray data = new JsonArray();
JsonReader json;
OutputStreamWriter writer;
try ( FileReader reader = new FileReader(profiles_directory + File.separator + profile_name.concat(".bb"))) {
json = new JsonReader(reader);
JsonParser parser = new JsonParser();
data.addAll(parser.parse(json).getAsJsonArray());
Object idata = data.get(0);
ProfilesProperties profile_properties = gson.fromJson(idata.toString(), ProfilesProperties.class
);
profile_properties.setEnabled(true);
newjson.clear();
newjson.add(profile_properties);
FileOutputStream fileStream = new FileOutputStream(profiles_directory + File.separator + profile_name.concat(".bb"));
String fjson = gson.toJson(newjson);
writer = new OutputStreamWriter(fileStream, "UTF-8");
writer.write(fjson);
}
writer.close();
json.close();
checkProfilesProperties(profiles_directory);
} catch (Exception ex) {
System.out.println("BurpBountyGui line 1956:" + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
}
} else {
try {
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
JsonArray json2 = new JsonArray();
List<ProfilesProperties> newjson = gson.fromJson(json2, new TypeToken<List<ProfilesProperties>>() {
}.getType());
String profile_name = model.getValueAt(row, 1).toString();
JsonArray data = new JsonArray();
JsonReader json;
try ( FileReader reader = new FileReader(profiles_directory + File.separator + profile_name.concat(".bb"))) {
json = new JsonReader(reader);
JsonParser parser = new JsonParser();
data.addAll(parser.parse(json).getAsJsonArray());
Object idata = data.get(0);
ProfilesProperties profile_properties = gson.fromJson(idata.toString(), ProfilesProperties.class
);
profile_properties.setEnabled(false);
newjson.clear();
newjson.add(profile_properties);
FileOutputStream fileStream = new FileOutputStream(profiles_directory + File.separator + profile_name.concat(".bb"));
String fjson = gson.toJson(newjson);
try ( OutputStreamWriter writer = new OutputStreamWriter(fileStream, "UTF-8")) {
writer.write(fjson);
}
}
json.close();
checkProfilesProperties(profiles_directory);
} catch (Exception ex) {
System.out.println("BurpBountyGui line 1956:" + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
}
}
}
}
}
public int getContentLength(IHttpRequestResponse response) {
IResponseInfo response_info;
try {
response_info = helpers.analyzeResponse(response.getResponse());
} catch (NullPointerException ex) {
System.out.println("Utils line 1279: " + ex.getMessage());
return 0;
}
int ContentLength = 0;
for (String headers : response_info.getHeaders()) {
if (headers.toUpperCase().startsWith("CONTENT-LENGTH:")) {
ContentLength = Integer.parseInt(headers.split("\\s+")[1]);
break;
}
}
return ContentLength;
}
public void showProfiles(String Tag) {
JsonArray json = getProfiles();
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
ProfilesProperties profile_property;
//model for active profiles
model.setNumRows(0);
model.setColumnCount(0);
model.addColumn("Enabled");
model.addColumn("Profile Name");
model.addColumn("Author's Twitter");
table3.getColumnModel().getColumn(0).setPreferredWidth(75);
table3.getColumnModel().getColumn(0).setMaxWidth(75);
table3.getColumnModel().getColumn(2).setPreferredWidth(150);
table3.getColumnModel().getColumn(2).setMaxWidth(150);
table3.getColumnModel().getColumn(1).setPreferredWidth(850);
TableRowSorter<TableModel> sorter3 = new TableRowSorter<>(table3.getModel());
table3.setRowSorter(sorter3);
List<RowSorter.SortKey> sortKeys3 = new ArrayList<>();
sortKeys3.add(new RowSorter.SortKey(1, SortOrder.ASCENDING));
sorter3.setSortKeys(sortKeys3);
sorter3.sort();
table3.setAutoResizeMode(JTable.AUTO_RESIZE_ALL_COLUMNS);
table3.getModel().addTableModelListener(new profilesModelListener());
//model for passive response
model1.setNumRows(0);
model1.setColumnCount(0);
model1.addColumn("Enabled");
model1.addColumn("Profile Name");
model1.addColumn("Author's Twitter");
table1.getColumnModel().getColumn(0).setPreferredWidth(75);
table1.getColumnModel().getColumn(0).setMaxWidth(75);
table1.getColumnModel().getColumn(2).setPreferredWidth(150);
table1.getColumnModel().getColumn(2).setMaxWidth(150);
table1.getColumnModel().getColumn(1).setPreferredWidth(850);
TableRowSorter<TableModel> sorter1 = new TableRowSorter<>(table1.getModel());
table1.setRowSorter(sorter1);
List<RowSorter.SortKey> sortKeys1 = new ArrayList<>();
sortKeys1.add(new RowSorter.SortKey(1, SortOrder.ASCENDING));
sorter1.setSortKeys(sortKeys1);
sorter1.sort();
table1.setAutoResizeMode(JTable.AUTO_RESIZE_ALL_COLUMNS);
table1.getModel().addTableModelListener(new profilesModelListener());
//model for passive request
model2.setNumRows(0);
model2.setColumnCount(0);
model2.addColumn("Enabled");
model2.addColumn("Profile Name");
model2.addColumn("Author's Twitter");
table2.getColumnModel().getColumn(0).setPreferredWidth(75);
table2.getColumnModel().getColumn(0).setMaxWidth(75);
table2.getColumnModel().getColumn(2).setPreferredWidth(150);
table2.getColumnModel().getColumn(2).setMaxWidth(150);
table2.getColumnModel().getColumn(1).setPreferredWidth(850);
TableRowSorter<TableModel> sorter2 = new TableRowSorter<>(table2.getModel());
table2.setRowSorter(sorter2);
List<RowSorter.SortKey> sortKeys2 = new ArrayList<>();
sortKeys2.add(new RowSorter.SortKey(1, SortOrder.ASCENDING));
sorter2.setSortKeys(sortKeys1);
sorter2.sort();
table2.setAutoResizeMode(JTable.AUTO_RESIZE_ALL_COLUMNS);
table2.getModel().addTableModelListener(new profilesModelListener());
if (json != null) {
for (JsonElement pa : json) {
JsonObject bbObj = pa.getAsJsonObject();
profile_property = gson.fromJson(bbObj.toString(), ProfilesProperties.class
);
if (Tag.equals("All")) {
if (profile_property.getScanner() == 1) {
model.addRow(new Object[]{profile_property.getEnabled(), profile_property.getProfileName(), profile_property.getAuthor()});
} else if (profile_property.getScanner() == 2) {
model2.addRow(new Object[]{profile_property.getEnabled(), profile_property.getProfileName(), profile_property.getAuthor()});
} else if (profile_property.getScanner() == 3) {
model1.addRow(new Object[]{profile_property.getEnabled(), profile_property.getProfileName(), profile_property.getAuthor()});
}
} else {
try {
for (String tag : profile_property.getTags()) {
if (tag.equals(Tag) || Tag.isEmpty() || Tag.equals("All")) {
if (profile_property.getScanner() == 1) {
model.addRow(new Object[]{profile_property.getEnabled(), profile_property.getProfileName(), profile_property.getAuthor()});
} else if (profile_property.getScanner() == 2) {
model2.addRow(new Object[]{profile_property.getEnabled(), profile_property.getProfileName(), profile_property.getAuthor()});
} else if (profile_property.getScanner() == 3) {
model1.addRow(new Object[]{profile_property.getEnabled(), profile_property.getProfileName(), profile_property.getAuthor()});
}
}
}
} catch (NullPointerException e) {
for (StackTraceElement element : e.getStackTrace()) {
System.out.println(element);
}
if (profile_property.getScanner() == 1) {
model.addRow(new Object[]{profile_property.getEnabled(), profile_property.getProfileName(), profile_property.getAuthor()});
} else if (profile_property.getScanner() == 2) {
model2.addRow(new Object[]{profile_property.getEnabled(), profile_property.getProfileName(), profile_property.getAuthor()});
} else if (profile_property.getScanner() == 3) {
model1.addRow(new Object[]{profile_property.getEnabled(), profile_property.getProfileName(), profile_property.getAuthor()});
}
}
}
}
}
}
public void deleteProfile(JTable table) {
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
File f = new File(profiles_directory);
File[] files = f.listFiles(new FilenameFilter() {
@Override
public boolean accept(File dir, String name) {
if (name.toLowerCase().endsWith(".bb")) {
return true;
} else {
return false;
}
}
});
int[] rows = table.getSelectedRows();
if (f.exists() && f.isDirectory()) {
for (File file : files) {
for (Integer row : rows) {
try {
JsonArray data = new JsonArray();
FileReader reader = new FileReader(file.getAbsolutePath());
JsonReader json = new JsonReader(reader);
JsonParser parser = new JsonParser();
data.addAll(parser.parse(json).getAsJsonArray());
Object idata = data.get(0);
ProfilesProperties i = gson.fromJson(idata.toString(), ProfilesProperties.class
);
String pname = table.getValueAt(row, 1).toString();
if (pname.equals(i.getProfileName())) {
reader.close();
json.close();
Files.delete(file.toPath());
break;
}
reader.close();
} catch (IOException e) {
System.out.println("BurpBountyGui line 2490:" + e.getMessage());
for (StackTraceElement element : e.getStackTrace()) {
System.out.println(element);
}
}
}
}
}
checkProfilesProperties(profiles_directory);
showProfiles("All");
}
public String getProfilesFilename() {
return profiles_directory;
}
public String getFilename() {
return filename;
}
public void addNewTag(String str) {
if (!str.isEmpty()) {
try {
BufferedWriter out = new BufferedWriter(new FileWriter(profiles_directory + File.separator + "tags.txt", true));
out.write(str.concat("\n"));
out.close();
} catch (IOException e) {
System.out.println("BurpBountyGui line 2497:" + e.getMessage());
for (StackTraceElement element : e.getStackTrace()) {
System.out.println(element);
}
}
}
}
public void removeTag(String tag) {
String file = profiles_directory + File.separator + "tags.txt";
try {
File inFile = new File(file);
if (!inFile.isFile()) {
System.out.println("BurpBountyGui line 2509:");
return;
}
//Construct the new file that will later be renamed to the original filename.
File tempFile = new File(inFile.getAbsolutePath().concat(".tmp"));
BufferedReader br = new BufferedReader(new FileReader(file));
PrintWriter pw = new PrintWriter(new FileWriter(tempFile));
String line = null;
//Read from the original file and write to the new
//unless content matches data to be removed.
while ((line = br.readLine()) != null) {
if (!line.trim().equals(tag)) {
pw.println(line);
pw.flush();
}
}
pw.close();
br.close();
//Delete the original file
if (!inFile.delete()) {
System.out.println("Could not delete file, line 2535");
return;
}
//Rename the new file to the filename the original file had.
if (!tempFile.renameTo(inFile)) {
System.out.println("Could not rename file line 2541");
}
} catch (FileNotFoundException ex) {
System.out.println("BurpBountyGui line 2559:" + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
} catch (IOException ex) {
System.out.println("BurpBountyGui line 2562:" + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
}
}
public void showTags() {
List<String> tags = readFile(profiles_directory + File.separator + "tags.txt");
newTagCombo2.removeAllItems();
tagmanager.removeAllElements();
for (String tag : tags) {
newTagCombo2.addItem(tag);
tagmanager.addElement(tag);
}
newTagCombo2.setSelectedItem("All");
}
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
buttonGroup1 = new javax.swing.ButtonGroup();
buttonGroup2 = new javax.swing.ButtonGroup();
buttonGroup3 = new javax.swing.ButtonGroup();
buttonGroup4 = new javax.swing.ButtonGroup();
buttonGroup5 = new javax.swing.ButtonGroup();
buttonGroup6 = new javax.swing.ButtonGroup();
buttonGroup7 = new javax.swing.ButtonGroup();
buttonGroup8 = new javax.swing.ButtonGroup();
buttonGroup9 = new javax.swing.ButtonGroup();
jCheckBoxMenuItem1 = new javax.swing.JCheckBoxMenuItem();
jMenuItem1 = new javax.swing.JMenuItem();
jPopupMenu1 = new javax.swing.JPopupMenu();
jMenuItem2 = new javax.swing.JMenuItem();
jMenuItem3 = new javax.swing.JMenuItem();
jPopupMenu2 = new javax.swing.JPopupMenu();
jMenuItem4 = new javax.swing.JMenuItem();
jMenuItem5 = new javax.swing.JMenuItem();
jPopupMenu3 = new javax.swing.JPopupMenu();
jMenuItem6 = new javax.swing.JMenuItem();
jMenuItem7 = new javax.swing.JMenuItem();
jPopupMenu4 = new javax.swing.JPopupMenu();
jMenuItem8 = new javax.swing.JMenuItem();
jMenuItem9 = new javax.swing.JMenuItem();
jSplitPane1 = new javax.swing.JSplitPane();
jTabbedPane2 = new javax.swing.JTabbedPane();
jPanel1 = new javax.swing.JPanel();
jPanel6 = new javax.swing.JPanel();
jLabel43 = new javax.swing.JLabel();
jLabel44 = new javax.swing.JLabel();
jLabel45 = new javax.swing.JLabel();
newTagCombo2 = new javax.swing.JComboBox<>();
jtabpane = new javax.swing.JTabbedPane();
jPanel3 = new javax.swing.JPanel();
jScrollPane5 = new javax.swing.JScrollPane();
table3 = new javax.swing.JTable();
jButton16 = new javax.swing.JButton();
jButton2 = new javax.swing.JButton();
button13 = new javax.swing.JButton();
jPanel5 = new javax.swing.JPanel();
jScrollPane6 = new javax.swing.JScrollPane();
table1 = new javax.swing.JTable();
jButton17 = new javax.swing.JButton();
jButton3 = new javax.swing.JButton();
button14 = new javax.swing.JButton();
jPanel7 = new javax.swing.JPanel();
jScrollPane10 = new javax.swing.JScrollPane();
table2 = new javax.swing.JTable();
jButton18 = new javax.swing.JButton();
jButton4 = new javax.swing.JButton();
button15 = new javax.swing.JButton();
jPanel4 = new javax.swing.JPanel();
jLabel50 = new javax.swing.JLabel();
jLabel51 = new javax.swing.JLabel();
jButton5 = new javax.swing.JButton();
jButton1 = new javax.swing.JButton();
text11 = new javax.swing.JTextField();
jSeparator13 = new javax.swing.JSeparator();
jScrollPane13 = new javax.swing.JScrollPane();
listtagmanager = new javax.swing.JList<>();
jButton12 = new javax.swing.JButton();
jButton11 = new javax.swing.JButton();
jLabel48 = new javax.swing.JLabel();
jLabel49 = new javax.swing.JLabel();
jPanel10 = new javax.swing.JPanel();
jLabel57 = new javax.swing.JLabel();
jLabel12 = new javax.swing.JLabel();
jLabel6 = new javax.swing.JLabel();
jLabel22 = new javax.swing.JLabel();
jLabel7 = new javax.swing.JLabel();
jLabel23 = new javax.swing.JLabel();
jLabel24 = new javax.swing.JLabel();
jLabel1 = new javax.swing.JLabel();
jLabel58 = new javax.swing.JLabel();
jLabel3 = new javax.swing.JLabel();
jLabel10 = new javax.swing.JLabel();
jPanel8 = new javax.swing.JPanel();
jLabel5 = new javax.swing.JLabel();
jCheckBoxMenuItem1.setSelected(true);
jCheckBoxMenuItem1.setText("jCheckBoxMenuItem1");
jMenuItem1.setText("jMenuItem1");
jMenuItem2.setText("Enable");
jMenuItem2.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jMenuItem2ActionPerformed(evt);
}
});
jPopupMenu1.add(jMenuItem2);
jMenuItem3.setText("Disable");
jMenuItem3.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jMenuItem3ActionPerformed(evt);
}
});
jPopupMenu1.add(jMenuItem3);
jMenuItem4.setText("Enable");
jMenuItem4.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jMenuItem4ActionPerformed(evt);
}
});
jPopupMenu2.add(jMenuItem4);
jMenuItem5.setText("Disable");
jMenuItem5.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jMenuItem5ActionPerformed(evt);
}
});
jPopupMenu2.add(jMenuItem5);
jMenuItem6.setText("Enable");
jMenuItem6.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jMenuItem6ActionPerformed(evt);
}
});
jPopupMenu3.add(jMenuItem6);
jMenuItem7.setText("Disable");
jMenuItem7.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jMenuItem7ActionPerformed(evt);
}
});
jPopupMenu3.add(jMenuItem7);
jMenuItem8.setText("Enable");
jMenuItem8.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jMenuItem8ActionPerformed(evt);
}
});
jPopupMenu4.add(jMenuItem8);
jMenuItem9.setText("Disable");
jMenuItem9.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
jMenuItem9ActionPerformed(evt);
}
});
jPopupMenu4.add(jMenuItem9);
setAutoscrolls(true);
jTabbedPane2.addChangeListener(new javax.swing.event.ChangeListener() {
public void stateChanged(javax.swing.event.ChangeEvent evt) {
showprofiles(evt);
}
});
jPanel1.setAutoscrolls(true);
jPanel6.setEnabled(false);
jLabel43.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel43.setForeground(new java.awt.Color(255, 102, 51));
jLabel43.setText("Profile Manager");
jLabel44.setText("In this section you can manage the profiles. ");
jLabel45.setText("Filter by Tag");
newTagCombo2.addItemListener(new java.awt.event.ItemListener() {
public void itemStateChanged(java.awt.event.ItemEvent evt) {
selectTag(evt);
}
});
newTagCombo2.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
newTagCombo2ActionPerformed(evt);
}
});
jtabpane.setFont(new java.awt.Font("Lucida Grande", 0, 14)); // NOI18N
table3.setAutoCreateRowSorter(true);
table3.setFont(new java.awt.Font("Arial", 0, 14)); // NOI18N
table3.setModel(model);
table3.setComponentPopupMenu(jPopupMenu1);
table3.getTableHeader().setReorderingAllowed(false);
table3.addMouseListener(new java.awt.event.MouseAdapter() {
public void mousePressed(java.awt.event.MouseEvent evt) {
table3MousePressed(evt);
}
public void mouseReleased(java.awt.event.MouseEvent evt) {
table3MouseReleased(evt);
}
});
jScrollPane5.setViewportView(table3);
jButton16.setText("Add");
jButton16.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
addActiveProfile(evt);
}
});
jButton2.setText("Edit");
jButton2.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
editActiveProfile(evt);
}
});
button13.setText("Remove");
button13.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
removeProfiles(evt);
}
});
javax.swing.GroupLayout jPanel3Layout = new javax.swing.GroupLayout(jPanel3);
jPanel3.setLayout(jPanel3Layout);
jPanel3Layout.setHorizontalGroup(
jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel3Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jButton2, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(button13, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jButton16, javax.swing.GroupLayout.PREFERRED_SIZE, 103, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(860, Short.MAX_VALUE))
.addGroup(jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel3Layout.createSequentialGroup()
.addGap(133, 133, 133)
.addComponent(jScrollPane5, javax.swing.GroupLayout.DEFAULT_SIZE, 830, Short.MAX_VALUE)
.addContainerGap()))
);
jPanel3Layout.setVerticalGroup(
jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel3Layout.createSequentialGroup()
.addContainerGap()
.addComponent(jButton16)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jButton2)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(button13)
.addContainerGap(743, Short.MAX_VALUE))
.addGroup(jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jScrollPane5, javax.swing.GroupLayout.DEFAULT_SIZE, 835, Short.MAX_VALUE))
);
jtabpane.addTab(" Active Profiles ", jPanel3);
table1.setAutoCreateRowSorter(true);
table1.setFont(new java.awt.Font("Arial", 0, 14)); // NOI18N
table1.setModel(model1);
table1.setRowSorter(null);
table1.getTableHeader().setReorderingAllowed(false);
table1.addMouseListener(new java.awt.event.MouseAdapter() {
public void mousePressed(java.awt.event.MouseEvent evt) {
table1MousePressed(evt);
}
public void mouseReleased(java.awt.event.MouseEvent evt) {
table1MouseReleased(evt);
}
});
jScrollPane6.setViewportView(table1);
jButton17.setText("Add");
jButton17.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
addRequestProfile(evt);
}
});
jButton3.setText("Edit");
jButton3.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
editRequestProfile(evt);
}
});
button14.setText("Remove");
button14.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
removeProfiles(evt);
}
});
javax.swing.GroupLayout jPanel5Layout = new javax.swing.GroupLayout(jPanel5);
jPanel5.setLayout(jPanel5Layout);
jPanel5Layout.setHorizontalGroup(
jPanel5Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel5Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel5Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jButton3, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(button14, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jButton17, javax.swing.GroupLayout.PREFERRED_SIZE, 103, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(860, Short.MAX_VALUE))
.addGroup(jPanel5Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel5Layout.createSequentialGroup()
.addGap(133, 133, 133)
.addComponent(jScrollPane6, javax.swing.GroupLayout.DEFAULT_SIZE, 830, Short.MAX_VALUE)
.addContainerGap()))
);
jPanel5Layout.setVerticalGroup(
jPanel5Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel5Layout.createSequentialGroup()
.addContainerGap()
.addComponent(jButton17)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jButton3)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(button14)
.addContainerGap(743, Short.MAX_VALUE))
.addGroup(jPanel5Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jScrollPane6, javax.swing.GroupLayout.DEFAULT_SIZE, 835, Short.MAX_VALUE))
);
jtabpane.addTab(" Passive Request Profiles ", jPanel5);
table2.setAutoCreateRowSorter(true);
table2.setFont(new java.awt.Font("Arial", 0, 14)); // NOI18N
table2.setModel(model2);
table2.setRowSorter(null);
table2.getTableHeader().setReorderingAllowed(false);
table2.addMouseListener(new java.awt.event.MouseAdapter() {
public void mousePressed(java.awt.event.MouseEvent evt) {
table2MousePressed(evt);
}
public void mouseReleased(java.awt.event.MouseEvent evt) {
table2MouseReleased(evt);
}
});
jScrollPane10.setViewportView(table2);
jButton18.setText("Add");
jButton18.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
addResponseProfile(evt);
}
});
jButton4.setText("Edit");
jButton4.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
editResponseProfile(evt);
}
});
button15.setText("Remove");
button15.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
removeProfiles(evt);
}
});
javax.swing.GroupLayout jPanel7Layout = new javax.swing.GroupLayout(jPanel7);
jPanel7.setLayout(jPanel7Layout);
jPanel7Layout.setHorizontalGroup(
jPanel7Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel7Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel7Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jButton4, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(button15, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jButton18, javax.swing.GroupLayout.PREFERRED_SIZE, 103, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(860, Short.MAX_VALUE))
.addGroup(jPanel7Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel7Layout.createSequentialGroup()
.addGap(133, 133, 133)
.addComponent(jScrollPane10, javax.swing.GroupLayout.DEFAULT_SIZE, 830, Short.MAX_VALUE)
.addContainerGap()))
);
jPanel7Layout.setVerticalGroup(
jPanel7Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel7Layout.createSequentialGroup()
.addContainerGap()
.addComponent(jButton18)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jButton4)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(button15)
.addContainerGap(743, Short.MAX_VALUE))
.addGroup(jPanel7Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jScrollPane10, javax.swing.GroupLayout.DEFAULT_SIZE, 835, Short.MAX_VALUE))
);
jtabpane.addTab(" Passive Response Profiles ", jPanel7);
javax.swing.GroupLayout jPanel6Layout = new javax.swing.GroupLayout(jPanel6);
jPanel6.setLayout(jPanel6Layout);
jPanel6Layout.setHorizontalGroup(
jPanel6Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel6Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel6Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jtabpane, javax.swing.GroupLayout.PREFERRED_SIZE, 0, Short.MAX_VALUE)
.addGroup(jPanel6Layout.createSequentialGroup()
.addGroup(jPanel6Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel43)
.addComponent(jLabel44, javax.swing.GroupLayout.PREFERRED_SIZE, 575, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))))
.addGroup(jPanel6Layout.createSequentialGroup()
.addGap(380, 380, 380)
.addComponent(jLabel45)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(newTagCombo2, javax.swing.GroupLayout.PREFERRED_SIZE, 325, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(0, 0, Short.MAX_VALUE))
);
jPanel6Layout.setVerticalGroup(
jPanel6Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel6Layout.createSequentialGroup()
.addGap(10, 10, 10)
.addComponent(jLabel43)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel44)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel6Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(newTagCombo2, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel45))
.addGap(18, 18, 18)
.addComponent(jtabpane, javax.swing.GroupLayout.PREFERRED_SIZE, 0, Short.MAX_VALUE)
.addContainerGap())
);
javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1);
jPanel1.setLayout(jPanel1Layout);
jPanel1Layout.setHorizontalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(jPanel6, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addContainerGap())
);
jPanel1Layout.setVerticalGroup(
jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel1Layout.createSequentialGroup()
.addComponent(jPanel6, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addContainerGap())
);
jTabbedPane2.addTab(" Profiles ", jPanel1);
jLabel50.setText("In this section specify the base profiles directory. ");
jLabel51.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel51.setForeground(new java.awt.Color(255, 102, 51));
jLabel51.setText("Directory");
jButton5.setText("Directory");
jButton5.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
loadConfigFile(evt);
}
});
jButton1.setText("Reload");
jButton1.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
profilesReload(evt);
}
});
text11.setToolTipText("");
listtagmanager.setModel(tagmanager);
jScrollPane13.setViewportView(listtagmanager);
jButton12.setText("Remove");
jButton12.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
removeTagManager(evt);
}
});
jButton11.setText("Add");
jButton11.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
newTag(evt);
}
});
jLabel48.setText("In this section you can manage the tags.");
jLabel49.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel49.setForeground(new java.awt.Color(255, 102, 51));
jLabel49.setText("Tags Manager");
javax.swing.GroupLayout jPanel4Layout = new javax.swing.GroupLayout(jPanel4);
jPanel4.setLayout(jPanel4Layout);
jPanel4Layout.setHorizontalGroup(
jPanel4Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel4Layout.createSequentialGroup()
.addGroup(jPanel4Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jSeparator13)
.addGroup(jPanel4Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel4Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel50, javax.swing.GroupLayout.PREFERRED_SIZE, 575, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel51)
.addGroup(jPanel4Layout.createSequentialGroup()
.addGroup(jPanel4Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jButton5, javax.swing.GroupLayout.DEFAULT_SIZE, 108, Short.MAX_VALUE)
.addComponent(jButton1, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(text11, javax.swing.GroupLayout.PREFERRED_SIZE, 700, javax.swing.GroupLayout.PREFERRED_SIZE))
.addComponent(jLabel48, javax.swing.GroupLayout.PREFERRED_SIZE, 575, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel49)
.addGroup(jPanel4Layout.createSequentialGroup()
.addGroup(jPanel4Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jButton11, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jButton12, javax.swing.GroupLayout.PREFERRED_SIZE, 105, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(jScrollPane13, javax.swing.GroupLayout.PREFERRED_SIZE, 700, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addGap(0, 160, Short.MAX_VALUE)))
.addContainerGap())
);
jPanel4Layout.setVerticalGroup(
jPanel4Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel4Layout.createSequentialGroup()
.addGap(10, 10, 10)
.addComponent(jLabel51)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel50)
.addGap(25, 25, 25)
.addGroup(jPanel4Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jButton5)
.addComponent(text11, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jButton1)
.addGap(18, 18, 18)
.addComponent(jSeparator13, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(jLabel49)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel48)
.addGap(25, 25, 25)
.addGroup(jPanel4Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel4Layout.createSequentialGroup()
.addComponent(jButton11)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jButton12))
.addComponent(jScrollPane13, javax.swing.GroupLayout.PREFERRED_SIZE, 205, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(544, Short.MAX_VALUE))
);
jPanel4Layout.linkSize(javax.swing.SwingConstants.VERTICAL, new java.awt.Component[] {jButton1, jButton5});
jTabbedPane2.addTab(" Options ", jPanel4);
jPanel10.addMouseListener(new java.awt.event.MouseAdapter() {
public void mouseClicked(java.awt.event.MouseEvent evt) {
goWebBurp(evt);
}
});
jLabel57.setFont(new java.awt.Font("Lucida Grande", 1, 36)); // NOI18N
jLabel57.setForeground(new java.awt.Color(229, 92, 58));
jLabel57.setText("About Pro.");
jLabel12.setFont(new java.awt.Font("Arial", 0, 14)); // NOI18N
jLabel12.setText("<html><p style=\"text-align: justify;\"> Burp Bounty Pro is a Burp Suite Pro extension that improves the active and passive scanner by means of advanced and customized vulnerability profiles through a very intuitive graphical interface. <br><br> On the one hand, it acts as a the most advanced and flexible web application vulnerability scanner, being able to add your own vulnerability profiles, or add your own custom payloads/requests to the existing vulnerability profiles. <br><br> On the other hand, it can simulate a manual pentest in search of maximum efficiency, without making unnecessary requests, it scans the targets only for those potentially vulnerable parameters, with the most effective payloads. <br><br> Finally, this extension also helps you by collecting valuable information when performing the manual pentest, such as possible vulnerable parameters, versions detection and more.</p></html>");
jLabel12.setHorizontalTextPosition(javax.swing.SwingConstants.RIGHT);
jLabel6.setFont(new java.awt.Font("Tahoma", 1, 18)); // NOI18N
jLabel6.setForeground(new java.awt.Color(0, 78, 112));
jLabel6.setText("You are using Burp Bounty Free 4.0");
jLabel22.setFont(new java.awt.Font("Tahoma", 0, 12)); // NOI18N
jLabel22.setText("By using this software your are accepting the");
jLabel7.setFont(new java.awt.Font("Tahoma", 1, 30)); // NOI18N
jLabel7.setForeground(new java.awt.Color(229, 99, 58));
jLabel7.setHorizontalAlignment(javax.swing.SwingConstants.RIGHT);
jLabel7.setText("<html>Ride First<br> on Bug Hunting.</html>");
jLabel23.setHorizontalAlignment(javax.swing.SwingConstants.CENTER);
jLabel23.setIcon(new javax.swing.ImageIcon(getClass().getResource("/logo_free.png"))); // NOI18N
jLabel24.setText("<html><a href=\\\"\\\">EULA</a></html>");
jLabel24.addMouseListener(new java.awt.event.MouseAdapter() {
public void mouseClicked(java.awt.event.MouseEvent evt) {
jLabel24goWeb(evt);
}
});
jLabel1.setFont(new java.awt.Font("Arial", 0, 14)); // NOI18N
jLabel1.setText("<html><p style=\"text-align: justify;\"> Burp Bounty Free is a Burp Suite extension that allows you, in a quick and simple way, to improve the active and passive Burp Suite scanner by means of personalized profiles through a very intuitive graphical interface. Through an advanced search of patterns and an improvement of the payload to send, we can create our own issue profiles both in the active scanner and in the passive.</p></html>");
jLabel58.setFont(new java.awt.Font("Lucida Grande", 1, 36)); // NOI18N
jLabel58.setForeground(new java.awt.Color(229, 92, 58));
jLabel58.setText("About Free.");
jLabel3.setFont(new java.awt.Font("Arial", 0, 14)); // NOI18N
jLabel3.setText("<html><p style=\"text-align: justify;\">If you need more power, I invite you to try the new Burp Bounty Pro, which gives you more power and automation during your manual pentests.</p></html>");
jLabel10.setFont(new java.awt.Font("Arial", 0, 24)); // NOI18N
jLabel10.setForeground(new java.awt.Color(0, 78, 112));
jLabel10.setText("<html>More information at: <a href=\\\"\\\">https://burpbounty.net</a></html>");
javax.swing.GroupLayout jPanel10Layout = new javax.swing.GroupLayout(jPanel10);
jPanel10.setLayout(jPanel10Layout);
jPanel10Layout.setHorizontalGroup(
jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel10Layout.createSequentialGroup()
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel10Layout.createSequentialGroup()
.addGap(14, 14, 14)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jLabel58)
.addComponent(jLabel57)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, jPanel10Layout.createSequentialGroup()
.addComponent(jLabel23, javax.swing.GroupLayout.DEFAULT_SIZE, 428, Short.MAX_VALUE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel7, javax.swing.GroupLayout.PREFERRED_SIZE, 262, javax.swing.GroupLayout.PREFERRED_SIZE))
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 0, Short.MAX_VALUE)
.addComponent(jLabel3, javax.swing.GroupLayout.PREFERRED_SIZE, 0, Short.MAX_VALUE)
.addComponent(jLabel12, javax.swing.GroupLayout.PREFERRED_SIZE, 0, Short.MAX_VALUE)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, jPanel10Layout.createSequentialGroup()
.addComponent(jLabel6)
.addGap(202, 202, 202))))
.addGroup(jPanel10Layout.createSequentialGroup()
.addGap(206, 206, 206)
.addComponent(jLabel22)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel24, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel10Layout.createSequentialGroup()
.addGap(131, 131, 131)
.addComponent(jLabel10, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addContainerGap(1138, Short.MAX_VALUE))
);
jPanel10Layout.setVerticalGroup(
jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel10Layout.createSequentialGroup()
.addGap(23, 23, 23)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jLabel23, javax.swing.GroupLayout.PREFERRED_SIZE, 115, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel7, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(27, 27, 27)
.addComponent(jLabel58)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 93, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(jLabel57)
.addGap(18, 18, 18)
.addComponent(jLabel12, javax.swing.GroupLayout.PREFERRED_SIZE, 237, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(jLabel6, javax.swing.GroupLayout.PREFERRED_SIZE, 32, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(jLabel3, javax.swing.GroupLayout.PREFERRED_SIZE, 61, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(jLabel10, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addGroup(jPanel10Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel22, javax.swing.GroupLayout.PREFERRED_SIZE, 27, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel24, javax.swing.GroupLayout.PREFERRED_SIZE, 27, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(133, Short.MAX_VALUE))
);
jTabbedPane2.addTab(" About ", jPanel10);
jLabel5.setIcon(new javax.swing.ImageIcon(getClass().getResource("/Tabla.png"))); // NOI18N
jLabel5.addMouseListener(new java.awt.event.MouseAdapter() {
public void mouseClicked(java.awt.event.MouseEvent evt) {
goImageWeb(evt);
}
});
javax.swing.GroupLayout jPanel8Layout = new javax.swing.GroupLayout(jPanel8);
jPanel8.setLayout(jPanel8Layout);
jPanel8Layout.setHorizontalGroup(
jPanel8Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel8Layout.createSequentialGroup()
.addGap(19, 19, 19)
.addComponent(jLabel5)
.addContainerGap(789, Short.MAX_VALUE))
);
jPanel8Layout.setVerticalGroup(
jPanel8Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel8Layout.createSequentialGroup()
.addComponent(jLabel5, javax.swing.GroupLayout.PREFERRED_SIZE, 714, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(0, 271, Short.MAX_VALUE))
);
jTabbedPane2.addTab(" Burp Bounty Pro ", jPanel8);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jTabbedPane2)
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jTabbedPane2)
);
}// </editor-fold>//GEN-END:initComponents
private void showprofiles(javax.swing.event.ChangeEvent evt) {//GEN-FIRST:event_showprofiles
}//GEN-LAST:event_showprofiles
private void profilesReload(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_profilesReload
String fileload = text11.getText();
profiles_directory = fileload;
checkProfilesProperties(profiles_directory);
makeTagsFile();
showTags();
showProfiles("All");
this.callbacks.saveExtensionSetting("filename", fileload);
}//GEN-LAST:event_profilesReload
private void loadConfigFile(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_loadConfigFile
loadConfigFile();
}//GEN-LAST:event_loadConfigFile
private void removeTagManager(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_removeTagManager
int selectedIndex = listtagmanager.getSelectedIndex();
String tag = "";
if (selectedIndex != -1) {
tag = tagmanager.get(selectedIndex).toString();
if (!tag.equals("All")) {
tagmanager.remove(selectedIndex);
deleteTagProfiles(tag);
removeTag(tag);
showTags();
}
}
}//GEN-LAST:event_removeTagManager
private void removeProfiles(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_removeProfiles
int activePane = jtabpane.getSelectedIndex();
if (activePane == 0) {
deleteProfile(table3);
} else if (activePane == 1) {
deleteProfile(table1);
} else if (activePane == 2) {
deleteProfile(table2);
}
}//GEN-LAST:event_removeProfiles
private void newTagCombo2ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_newTagCombo2ActionPerformed
// TODO add your handling code here:
}//GEN-LAST:event_newTagCombo2ActionPerformed
private void selectTag(java.awt.event.ItemEvent evt) {//GEN-FIRST:event_selectTag
if ((evt.getStateChange() == java.awt.event.ItemEvent.SELECTED)) {
showProfiles(newTagCombo2.getItemAt(newTagCombo2.getSelectedIndex()));
}
}//GEN-LAST:event_selectTag
private void newTag(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_newTag
Integer result;
NewTag nt = new NewTag();
JOptionPane jopane1 = new JOptionPane(nt, JOptionPane.PLAIN_MESSAGE, JOptionPane.OK_CANCEL_OPTION);
JDialog dialog = jopane1.createDialog(this, "New Tag");
dialog.setLocationRelativeTo(null);
dialog.setVisible(true);
Object selectedValue = jopane1.getValue();
if (selectedValue != null) {
result = ((Integer) selectedValue).intValue();
if (result == JOptionPane.OK_OPTION) {
addNewTag(nt.newTagtext.getText());
showTags();
}
}
}//GEN-LAST:event_newTag
private void addActiveProfile(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_addActiveProfile
Integer result;
ActiveProfile profile = new ActiveProfile(callbacks);
JOptionPane jopane1 = new JOptionPane(profile, JOptionPane.PLAIN_MESSAGE, JOptionPane.OK_CANCEL_OPTION);
JDialog dialog = jopane1.createDialog(jopane1, "Add New Active Profile");
dialog.setSize(new Dimension(900, 760));
dialog.setResizable(true);
dialog.setLocationRelativeTo(null);
dialog.setVisible(true);
Object selectedValue = jopane1.getValue();
if (selectedValue != null) {
result = ((Integer) selectedValue).intValue();
if (result == JOptionPane.OK_OPTION) {
if (!profile.text1.getText().isEmpty()) {
ProfilesManager profile_manager = new ProfilesManager(profiles_directory);
profile_manager.saveActiveAttackValues(profile);
checkProfilesProperties(profiles_directory);
showProfiles("All");
showTags();
}
}
}
}//GEN-LAST:event_addActiveProfile
private void table3MouseReleased(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_table3MouseReleased
if (evt.isPopupTrigger()) {
jPopupMenu1.show(table3, evt.getX(), evt.getY());
}
}//GEN-LAST:event_table3MouseReleased
private void table3MousePressed(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_table3MousePressed
if (evt.isPopupTrigger()) {
jPopupMenu1.show(table3, evt.getX(), evt.getY());
}
}//GEN-LAST:event_table3MousePressed
private void jMenuItem2ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jMenuItem2ActionPerformed
setEnableDisableProfile("Yes", table3);
}//GEN-LAST:event_jMenuItem2ActionPerformed
private void jMenuItem3ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jMenuItem3ActionPerformed
setEnableDisableProfile("No", table3);
}//GEN-LAST:event_jMenuItem3ActionPerformed
private void jMenuItem4ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jMenuItem4ActionPerformed
setEnableDisableProfile("Yes", table1);
}//GEN-LAST:event_jMenuItem4ActionPerformed
private void jMenuItem5ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jMenuItem5ActionPerformed
setEnableDisableProfile("No", table1);
}//GEN-LAST:event_jMenuItem5ActionPerformed
private void jMenuItem6ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jMenuItem6ActionPerformed
setEnableDisableProfile("Yes", table2);
}//GEN-LAST:event_jMenuItem6ActionPerformed
private void jMenuItem7ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jMenuItem7ActionPerformed
setEnableDisableProfile("No", table2);
}//GEN-LAST:event_jMenuItem7ActionPerformed
private void table1MouseReleased(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_table1MouseReleased
if (evt.isPopupTrigger()) {
jPopupMenu2.show(table1, evt.getX(), evt.getY());
}
}//GEN-LAST:event_table1MouseReleased
private void table1MousePressed(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_table1MousePressed
if (evt.isPopupTrigger()) {
jPopupMenu2.show(table1, evt.getX(), evt.getY());
}
}//GEN-LAST:event_table1MousePressed
private void table2MouseReleased(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_table2MouseReleased
if (evt.isPopupTrigger()) {
jPopupMenu3.show(table2, evt.getX(), evt.getY());
}
}//GEN-LAST:event_table2MouseReleased
private void table2MousePressed(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_table2MousePressed
if (evt.isPopupTrigger()) {
jPopupMenu3.show(table2, evt.getX(), evt.getY());
}
}//GEN-LAST:event_table2MousePressed
private void editActiveProfile(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_editActiveProfile
Integer result;
String profile_name = table3.getValueAt(table3.getSelectedRow(), 1).toString();
ActiveProfile profile = new ActiveProfile(callbacks);
JOptionPane jopane1 = new JOptionPane(profile, JOptionPane.PLAIN_MESSAGE, JOptionPane.OK_CANCEL_OPTION);
JDialog dialog = jopane1.createDialog(jopane1, "Edit Active Profile");
dialog.setSize(new Dimension(920, 760));
dialog.setResizable(true);
dialog.setLocationRelativeTo(null);
ProfilesManager profile_manager = new ProfilesManager(profiles_directory);
profile_manager.setActiveAttackValues(profile_name, allprofiles, profile);
dialog.setVisible(true);
Object selectedValue = jopane1.getValue();
if (selectedValue != null) {
result = ((Integer) selectedValue).intValue();
if (result == JOptionPane.OK_OPTION) {
profile_manager.saveActiveAttackValues(profile);
checkProfilesProperties(profiles_directory);
showProfiles("All");
showTags();
}
}
}//GEN-LAST:event_editActiveProfile
private void addRequestProfile(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_addRequestProfile
Integer result;
RequestProfile profile = new RequestProfile(callbacks);
JOptionPane jopane1 = new JOptionPane(profile, JOptionPane.PLAIN_MESSAGE, JOptionPane.OK_CANCEL_OPTION);
JDialog dialog = jopane1.createDialog(jopane1, "Add New Passive Request Profile");
dialog.setSize(new Dimension(900, 760));
dialog.setResizable(true);
dialog.setLocationRelativeTo(null);
dialog.setVisible(true);
Object selectedValue = jopane1.getValue();
if (selectedValue != null) {
result = ((Integer) selectedValue).intValue();
if (result == JOptionPane.OK_OPTION) {
if (!profile.text1.getText().isEmpty()) {
ProfilesManager profile_manager = new ProfilesManager(profiles_directory);
profile_manager.saveRequestAttackValues(profile);
checkProfilesProperties(profiles_directory);
showProfiles("All");
showTags();
}
}
}
}//GEN-LAST:event_addRequestProfile
private void editRequestProfile(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_editRequestProfile
Integer result;
String profile_name = table1.getValueAt(table1.getSelectedRow(), 1).toString();
RequestProfile profile = new RequestProfile(callbacks);
JOptionPane jopane1 = new JOptionPane(profile, JOptionPane.PLAIN_MESSAGE, JOptionPane.OK_CANCEL_OPTION);
JDialog dialog = jopane1.createDialog(jopane1, "Edit Passive Request Profile");
dialog.setSize(new Dimension(920, 760));
dialog.setResizable(true);
dialog.setLocationRelativeTo(null);
ProfilesManager profile_manager = new ProfilesManager(profiles_directory);
profile_manager.setRequestAttackValues(profile_name, allprofiles, profile);
dialog.setVisible(true);
Object selectedValue = jopane1.getValue();
if (selectedValue != null) {
result = ((Integer) selectedValue).intValue();
if (result == JOptionPane.OK_OPTION) {
profile_manager.saveRequestAttackValues(profile);
checkProfilesProperties(profiles_directory);
showProfiles("All");
showTags();
}
}
}//GEN-LAST:event_editRequestProfile
private void addResponseProfile(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_addResponseProfile
Integer result;
ResponseProfile profile = new ResponseProfile(callbacks);
JOptionPane jopane1 = new JOptionPane(profile, JOptionPane.PLAIN_MESSAGE, JOptionPane.OK_CANCEL_OPTION);
JDialog dialog = jopane1.createDialog(jopane1, "Add New Passive Response Profile");
dialog.setSize(new Dimension(900, 760));
dialog.setResizable(true);
dialog.setLocationRelativeTo(null);
dialog.setVisible(true);
Object selectedValue = jopane1.getValue();
if (selectedValue != null) {
result = ((Integer) selectedValue).intValue();
if (result == JOptionPane.OK_OPTION) {
if (!profile.text1.getText().isEmpty()) {
ProfilesManager profile_manager = new ProfilesManager(profiles_directory);
profile_manager.saveResponseAttackValues(profile);
checkProfilesProperties(profiles_directory);
showProfiles("All");
showTags();
}
}
}
}//GEN-LAST:event_addResponseProfile
private void editResponseProfile(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_editResponseProfile
Integer result;
String profile_name = table2.getValueAt(table2.getSelectedRow(), 1).toString();
ResponseProfile profile = new ResponseProfile(callbacks);
JOptionPane jopane1 = new JOptionPane(profile, JOptionPane.PLAIN_MESSAGE, JOptionPane.OK_CANCEL_OPTION);
JDialog dialog = jopane1.createDialog(jopane1, "Edit Passive Response Profile");
dialog.setSize(new Dimension(920, 760));
dialog.setResizable(true);
dialog.setLocationRelativeTo(null);
ProfilesManager profile_manager = new ProfilesManager(profiles_directory);
profile_manager.setResponseAttackValues(profile_name, allprofiles, profile);
dialog.setVisible(true);
Object selectedValue = jopane1.getValue();
if (selectedValue != null) {
result = ((Integer) selectedValue).intValue();
if (result == JOptionPane.OK_OPTION) {
profile_manager.saveResponseAttackValues(profile);
checkProfilesProperties(profiles_directory);
showProfiles("All");
showTags();
}
}
}//GEN-LAST:event_editResponseProfile
private void jMenuItem8ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jMenuItem8ActionPerformed
}//GEN-LAST:event_jMenuItem8ActionPerformed
private void jMenuItem9ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jMenuItem9ActionPerformed
}//GEN-LAST:event_jMenuItem9ActionPerformed
private void jLabel24goWeb(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_jLabel24goWeb
try {
Desktop.getDesktop().browse(new URI("https://burpbounty.net/legal"));
} catch (URISyntaxException | IOException e) {
callbacks.printError("Burp Bounty Gui 4383 Help web not opened: " + e);
}
}//GEN-LAST:event_jLabel24goWeb
private void goWebBurp(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_goWebBurp
try {
Desktop.getDesktop().browse(new URI("https://burpbounty.net"));
} catch (URISyntaxException | IOException e) {
callbacks.printError("Active profile line 2109 Help web not opened: " + e);
}
}//GEN-LAST:event_goWebBurp
private void goImageWeb(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_goImageWeb
try {
Desktop.getDesktop().browse(new URI("https://burpbounty.net"));
} catch (URISyntaxException | IOException e) {
callbacks.printError("Burp Bounty Gui 4383 Help web not opened: " + e);
}
}//GEN-LAST:event_goImageWeb
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton button13;
private javax.swing.JButton button14;
private javax.swing.JButton button15;
private javax.swing.ButtonGroup buttonGroup1;
private javax.swing.ButtonGroup buttonGroup2;
private javax.swing.ButtonGroup buttonGroup3;
private javax.swing.ButtonGroup buttonGroup4;
private javax.swing.ButtonGroup buttonGroup5;
private javax.swing.ButtonGroup buttonGroup6;
private javax.swing.ButtonGroup buttonGroup7;
private javax.swing.ButtonGroup buttonGroup8;
private javax.swing.ButtonGroup buttonGroup9;
private javax.swing.JButton jButton1;
private javax.swing.JButton jButton11;
private javax.swing.JButton jButton12;
private javax.swing.JButton jButton16;
private javax.swing.JButton jButton17;
private javax.swing.JButton jButton18;
private javax.swing.JButton jButton2;
private javax.swing.JButton jButton3;
private javax.swing.JButton jButton4;
private javax.swing.JButton jButton5;
private javax.swing.JCheckBoxMenuItem jCheckBoxMenuItem1;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel10;
private javax.swing.JLabel jLabel12;
private javax.swing.JLabel jLabel22;
public javax.swing.JLabel jLabel23;
private javax.swing.JLabel jLabel24;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel43;
private javax.swing.JLabel jLabel44;
private javax.swing.JLabel jLabel45;
private javax.swing.JLabel jLabel48;
private javax.swing.JLabel jLabel49;
private javax.swing.JLabel jLabel5;
private javax.swing.JLabel jLabel50;
private javax.swing.JLabel jLabel51;
private javax.swing.JLabel jLabel57;
private javax.swing.JLabel jLabel58;
private javax.swing.JLabel jLabel6;
private javax.swing.JLabel jLabel7;
private javax.swing.JMenuItem jMenuItem1;
private javax.swing.JMenuItem jMenuItem2;
private javax.swing.JMenuItem jMenuItem3;
private javax.swing.JMenuItem jMenuItem4;
private javax.swing.JMenuItem jMenuItem5;
private javax.swing.JMenuItem jMenuItem6;
private javax.swing.JMenuItem jMenuItem7;
private javax.swing.JMenuItem jMenuItem8;
private javax.swing.JMenuItem jMenuItem9;
public javax.swing.JPanel jPanel1;
private javax.swing.JPanel jPanel10;
private javax.swing.JPanel jPanel3;
public javax.swing.JPanel jPanel4;
private javax.swing.JPanel jPanel5;
public javax.swing.JPanel jPanel6;
private javax.swing.JPanel jPanel7;
private javax.swing.JPanel jPanel8;
private javax.swing.JPopupMenu jPopupMenu1;
private javax.swing.JPopupMenu jPopupMenu2;
private javax.swing.JPopupMenu jPopupMenu3;
private javax.swing.JPopupMenu jPopupMenu4;
private javax.swing.JScrollPane jScrollPane10;
private javax.swing.JScrollPane jScrollPane13;
private javax.swing.JScrollPane jScrollPane5;
private javax.swing.JScrollPane jScrollPane6;
private javax.swing.JSeparator jSeparator13;
private javax.swing.JSplitPane jSplitPane1;
public javax.swing.JTabbedPane jTabbedPane2;
private javax.swing.JTabbedPane jtabpane;
public javax.swing.JList<String> listtagmanager;
private javax.swing.JComboBox<String> newTagCombo2;
private javax.swing.JTable table1;
private javax.swing.JTable table2;
private javax.swing.JTable table3;
public javax.swing.JTextField text11;
// End of variables declaration//GEN-END:variables
} |
Java | BurpBounty/main/java/burpbountyfree/BurpCollaboratorThread.java | /*
Copyright 2018 Eduardo Garcia Melia <wagiro@gmail.com>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package burpbountyfree;
import burp.IBurpCollaboratorClientContext;
import burp.IBurpCollaboratorInteraction;
import burp.IBurpExtenderCallbacks;
import burp.IExtensionHelpers;
import burp.IHttpRequestResponse;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Properties;
public class BurpCollaboratorThread extends Thread {
private IBurpExtenderCallbacks callbacks;
private IExtensionHelpers helpers;
public List<IBurpCollaboratorClientContext> CollaboratorClientContext;
HashMap<String, IHttpRequestResponse> ccrequestResponse;
HashMap<String, Properties> issues;
public boolean doStop;
Properties issueProperties;
private String issuename;
private String issuedetail;
private String issuebackground;
private String remediationdetail;
private String remediationbackground;
private String issueseverity;
private String issueconfidence;
CollaboratorData burpCollaboratorData;
public BurpCollaboratorThread(IBurpExtenderCallbacks callbacks, CollaboratorData burpCollaboratorData)
{
this.callbacks = callbacks;
helpers = callbacks.getHelpers();
this.burpCollaboratorData = burpCollaboratorData;
CollaboratorClientContext = new ArrayList();
ccrequestResponse = new HashMap();
issues = new HashMap();
doStop = false;
issueProperties = new Properties();
issuename = "";
issuedetail = "";
issuebackground = "";
remediationdetail = "";
remediationbackground = "";
issueseverity = "";
issueconfidence = "";
this.callbacks = callbacks;
helpers = callbacks.getHelpers();
this.burpCollaboratorData = burpCollaboratorData;
}
public void doStop() {
doStop = true;
}
public boolean keepRunning() {
return doStop == false;
}
@Override
public void run() {
while (keepRunning()) {
CollaboratorClientContext = burpCollaboratorData.getCollaboratorClientContext();
try {
for (int client = 0; client < CollaboratorClientContext.size(); client++) {
List<IBurpCollaboratorInteraction> CollaboratorInteraction = CollaboratorClientContext.get(client).fetchAllCollaboratorInteractions();
if (CollaboratorInteraction != null && !CollaboratorInteraction.isEmpty()) {
for (int interaction = 0; interaction < CollaboratorInteraction.size(); interaction++) {
addIssue(CollaboratorClientContext.get(client), CollaboratorInteraction.get(interaction));
}
}
}
BurpCollaboratorThread.sleep(10000);
} catch (NullPointerException | InterruptedException e) {
System.out.println("Thread error: " + e);
} catch (IllegalStateException ex) {
this.doStop();
}
}
}
public void addIssue(IBurpCollaboratorClientContext cc, IBurpCollaboratorInteraction interactions) {
String interaction_id = interactions.getProperty("interaction_id");
String bchost = interaction_id + ".burpcollaborator.net";
String type = interactions.getProperty("type");
String client_ip = interactions.getProperty("client_ip");
String time_stamp = interactions.getProperty("time_stamp");
String query_type = interactions.getProperty("query_type");
try {
if (type.equals("DNS")) {
String raw_query = interactions.getProperty("raw_query");
if (raw_query != null) {
String query = new String(helpers.base64Decode(raw_query));
String subdomain = query.split("7")[1];
subdomain = "7"+subdomain+"7";
bchost = subdomain + "." + bchost;
}
} else if (type.equals("HTTP")) {
String query = interactions.getProperty("request");
String request = new String(helpers.base64Decode(query));
int sub_ini = request.indexOf("Host: ");
int sub_end = request.indexOf("\r\n", sub_ini);
bchost = request.substring(sub_ini+6, sub_end);
}
} catch (Exception e) {
System.out.println("Thread2 error: " + e);
}
issueProperties = burpCollaboratorData.getIssueProperties(bchost);
if (issueProperties == null) {
return;
}
issuename = issueProperties.getProperty("issuename");
issuedetail = issueProperties.getProperty("issuedetail");
issuebackground = issueProperties.getProperty("issuebackground");
remediationdetail = issueProperties.getProperty("remediationdetail");
remediationbackground = issueProperties.getProperty("remediationbackground");
issueseverity = issueProperties.getProperty("issueseverity");
issueconfidence = issueProperties.getProperty("issueconfidence");
issuedetail = issuedetail + "<br><br><strong>BurpCollaborator data:</strong><br><br><strong>Interaction id: </strong>" + interaction_id + "<br><strong>type: </strong>" + type
+ "<br><strong>client_ip: </strong>" + client_ip + "<br><strong>time_stamp: </strong>" + time_stamp + "<br><strong>query_type: </strong>" + query_type + "<br>";
List requestMarkers = new ArrayList();
int start = 0;
IHttpRequestResponse requestResponse = burpCollaboratorData.getRequestResponse(bchost);
byte[] match = helpers.stringToBytes(bchost);
byte[] request = requestResponse.getRequest();
while (start < request.length) {
start = helpers.indexOf(request, match, false, start, request.length);
if (start == -1) {
break;
}
requestMarkers.add(new int[]{start, start + match.length});
start += match.length;
}
callbacks.addScanIssue(new CustomScanIssue(requestResponse.getHttpService(), helpers.analyzeRequest(requestResponse).getUrl(),
new IHttpRequestResponse[]{callbacks.applyMarkers(requestResponse, requestMarkers, null)}, "BurpBounty - " + issuename,
issuedetail, issueseverity, issueconfidence, remediationdetail, issuebackground, remediationbackground));
}
} |
Java | BurpBounty/main/java/burpbountyfree/CollaboratorData.java | /*
Copyright 2018 Eduardo Garcia Melia <wagiro@gmail.com>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package burpbountyfree;
import burp.IBurpCollaboratorClientContext;
import burp.IExtensionHelpers;
import burp.IHttpRequestResponse;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Properties;
public class CollaboratorData {
private IExtensionHelpers helpers;
private List<IBurpCollaboratorClientContext> CollaboratorClientContext;
HashMap<String, IHttpRequestResponse> ccrequestResponse;
HashMap<String, Properties> issues;
Properties issueProperties;
public CollaboratorData(IExtensionHelpers helpers) {
this.helpers = helpers;
CollaboratorClientContext = new ArrayList();
ccrequestResponse = new HashMap();
issues = new HashMap();
issueProperties = new Properties();
}
public synchronized void setIssueProperties(IHttpRequestResponse requestResponse, String bchost, String issuename, String issuedetail, String issueseverity, String issueconfidence,
String issuebackground, String remediationdetail, String remediationbackground) {
issueProperties = new Properties();
issueProperties.put("issuename", issuename);
issueProperties.put("issuedetail", issuedetail);
issueProperties.put("issueseverity", issueseverity);
issueProperties.put("issueconfidence", issueconfidence);
issueProperties.put("issuebackground", issuebackground);
issueProperties.put("remediationdetail", remediationdetail);
issueProperties.put("remediationbackground", remediationbackground);
issues.put(bchost, issueProperties);
ccrequestResponse.put(bchost, requestResponse);
}
public synchronized Properties getIssueProperties(String bchost) {
return issues.get(bchost);
}
public synchronized List<IBurpCollaboratorClientContext> getCollaboratorClientContext() {
return CollaboratorClientContext;
}
public synchronized void setCollaboratorClientContext(IBurpCollaboratorClientContext bccc) {
CollaboratorClientContext.add(bccc);
}
public synchronized IHttpRequestResponse getRequestResponse(String bchost) {
return ccrequestResponse.get(bchost);
}
} |
Java | BurpBounty/main/java/burpbountyfree/CustomScanIssue.java | /*
Copyright 2018 Eduardo Garcia Melia <wagiro@gmail.com>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package burpbountyfree;
import burp.IHttpRequestResponse;
import burp.IHttpService;
import burp.IScanIssue;
import java.net.URL;
class CustomScanIssue implements IScanIssue {
private final IHttpService httpService;
private final URL url;
private final IHttpRequestResponse[] httpMessages;
private final String name;
private final String detail;
private final String severity;
private final String issueConfidence;
private final String issueRemediation;
private final String issueBackground;
private final String issueClassification;
public CustomScanIssue(
IHttpService httpService,
URL url,
IHttpRequestResponse[] httpMessages,
String name,
String detail,
String severity,
String issueConfidence,
String issueRemediation,
String issueBackground,
String issueClassification) {
this.httpService = httpService;
this.url = url;
this.httpMessages = httpMessages;
this.name = name;
this.detail = detail;
this.severity = severity;
this.issueConfidence = issueConfidence;
this.issueRemediation = issueRemediation;
this.issueBackground = issueBackground;
this.issueClassification = issueClassification;
}
@Override
public URL getUrl() {
return url;
}
@Override
public String getIssueName() {
return name;
}
@Override
public int getIssueType() {
return 0x08000000;
}
@Override
public String getSeverity() {
return severity;
}
@Override
public String getConfidence() {
return issueConfidence;
}
@Override
public String getIssueBackground() {
return issueBackground;
}
@Override
public String getRemediationBackground() {
return issueRemediation;
}
@Override
public String getIssueDetail() {
return detail;
}
@Override
public String getRemediationDetail() {
return issueClassification;
}
@Override
public IHttpRequestResponse[] getHttpMessages() {
return httpMessages;
}
@Override
public IHttpService getHttpService() {
return httpService;
}
} |
Java | BurpBounty/main/java/burpbountyfree/GrepMatch.java | /*
Copyright 2018 Eduardo Garcia Melia <wagiro@gmail.com>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package burpbountyfree;
import burp.IBurpExtenderCallbacks;
import burp.IExtensionHelpers;
import burp.IHttpRequestResponse;
import burp.IResponseInfo;
import burp.IRequestInfo;
import burp.IScanIssue;
import burp.IScannerInsertionPoint;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import java.util.regex.PatternSyntaxException;
public class GrepMatch {
private IBurpExtenderCallbacks callbacks;
private IExtensionHelpers helpers;
List<String> greps;
public GrepMatch(IBurpExtenderCallbacks callbacks) {
this.callbacks = callbacks;
this.helpers = callbacks.getHelpers();
greps = new ArrayList();
}
public IScanIssue getResponseMatches(IHttpRequestResponse requestResponse, String payload, List<String> greps, String issuename, String issuedetail, String issuebackground,
String remediationdetail, String remediationbackground, String charstourlencode, int matchtype, String issueseverity, String issueconfidence, boolean notresponse,
boolean casesensitive, boolean urlencode, boolean excludeHTTP, boolean onlyHTTP) {
String responseString;
String headers = "";
Pattern p;
Matcher m;
IResponseInfo responseInfo = helpers.analyzeResponse(requestResponse.getResponse());
byte[] request = requestResponse.getRequest();
List<int[]> responseMarkers = new ArrayList();
List<int[]> requestMarkers = new ArrayList();
for (String grep : greps) {
Boolean vuln = false;
if (casesensitive || matchtype == 2) {
responseString = helpers.bytesToString(requestResponse.getResponse());
for (String header : responseInfo.getHeaders()) {
headers += header + "\r\n";
}
} else {
responseString = helpers.bytesToString(requestResponse.getResponse()).toUpperCase();
grep = grep.toUpperCase();
for (String header : responseInfo.getHeaders()) {
headers += header.toUpperCase() + "\r\n";
}
}
if (matchtype == 2) {
String matches = "<br>";
//Start regex grep
int beginAt = 0;
try {
if (excludeHTTP && !onlyHTTP) {
beginAt = responseInfo.getBodyOffset();
p = Pattern.compile(grep, Pattern.CASE_INSENSITIVE);
m = p.matcher(responseString);
} else if (!excludeHTTP && onlyHTTP) {
p = Pattern.compile(grep, Pattern.CASE_INSENSITIVE);
m = p.matcher(headers);
} else {
p = Pattern.compile(grep, Pattern.CASE_INSENSITIVE);
m = p.matcher(responseString);
}
} catch (PatternSyntaxException pse) {
System.out.println("GrepMatch line 93 Incorrect regex: " + pse.getPattern());
return null;
}
if (!payload.equals("")) {
int start = 0;
byte[] match = helpers.stringToBytes(payload);
while (start < request.length) {
start = helpers.indexOf(request, match, false, start, request.length);
if (start == -1) {
break;
}
requestMarkers.add(new int[]{start, start + match.length});
start += match.length;
}
}
if (notresponse) {
if (m.find(beginAt)) {
return null;
}
} else {
int end = 0;
while (m.find(beginAt)) {
end = end + 1;
if (end == 30) {
break;
}
responseMarkers.add(new int[]{m.start(), m.end()});
matches = matches + m.group().toLowerCase() + "<br>";
beginAt = m.end();
vuln = true;
}
if (!vuln) {
return null;
}
}
//End regex grep
//Start Simple String, payload in response and payload without encode
} else {
int beginAt = 0;
byte[] response = helpers.stringToBytes(responseString);
if (excludeHTTP && !onlyHTTP) {
beginAt = responseInfo.getBodyOffset();
} else if (!excludeHTTP && onlyHTTP) {
response = helpers.stringToBytes(headers);
}
if (!payload.equals("")) {
int start = 0;
byte[] match = helpers.stringToBytes(payload);
int end = 0;
while (start < request.length) {
end = end + 1;
if (end == 30) {
break;
}
start = helpers.indexOf(request, match, false, start, request.length);
if (start == -1) {
break;
}
requestMarkers.add(new int[]{start, start + match.length});
start += match.length;
}
}
if (notresponse) {
if (responseString.contains(grep)) {
return null;
}
} else {
byte[] match = helpers.stringToBytes(grep);
int end = 0;
while (beginAt < response.length) {
end = end + 1;
if (end == 30) {
break;
}
beginAt = helpers.indexOf(response, match, false, beginAt, response.length);
if (beginAt == -1) {
break;
}
responseMarkers.add(new int[]{beginAt, beginAt + match.length});
beginAt += match.length;
vuln = true;
}
if (!vuln) {
return null;
}
}
//End Simple String, payload in response and payload without encode
}
}
Collections.sort(responseMarkers, new Comparator<int[]>() {
private static final int INDEX = 0;
@Override
public int compare(int[] o1, int[] o2) {
return Integer.compare(o1[INDEX], o2[INDEX]);
}
});
Collections.sort(requestMarkers, new Comparator<int[]>() {
private static final int INDEX = 0;
@Override
public int compare(int[] o1, int[] o2) {
return Integer.compare(o1[INDEX], o2[INDEX]);
}
});
String results_req = "";
String results_resp = "";
for (int[] res : responseMarkers) {
results_resp = results_resp + "<br/> " + helpers.urlDecode(helpers.bytesToString(requestResponse.getResponse()).substring(res[0], res[1]));
}
for (int[] req : requestMarkers) {
results_req = results_req + "<br/> " + helpers.urlDecode(helpers.bytesToString(requestResponse.getRequest()).substring(req[0], req[1]));
}
return new CustomScanIssue(requestResponse.getHttpService(), helpers.analyzeRequest(requestResponse).getUrl(),
new IHttpRequestResponse[]{callbacks.applyMarkers(requestResponse, requestMarkers, responseMarkers)
}, "BurpBounty - " + issuename, issuedetail.replace("<payload>", results_req).replace("<grep>", results_resp), issueseverity.replace("<payload>", results_req).replace("<grep>", results_resp), issueconfidence.replace("<payload>", results_req).replace("<grep>", results_resp), remediationdetail.replace("<payload>", results_req).replace("<grep>", results_resp), issuebackground.replace("<payload>", results_req).replace("<grep>", results_resp), remediationbackground.replace("<payload>", results_req).replace("<grep>", results_resp));
}
public Object[] getRequestMatches(IHttpRequestResponse requestResponse, List<String> greps, String issuename, String issuedetail, String issuebackground,
String remediationdetail, String remediationbackground, int matchtype, String issueseverity, String issueconfidence, Boolean casesensitive, Boolean notresponse, Boolean scanas, int scantype) {
String data = "";
Pattern p;
Matcher m;
byte[] request = requestResponse.getRequest();
List<int[]> requestMarkers = new ArrayList();
IRequestInfo requestInfo = helpers.analyzeRequest(requestResponse.getRequest());
List<IScannerInsertionPoint> insertionPoints = new ArrayList();
int matches = 0;
List<int[]> requestMarkersInsertionPoints = new ArrayList();
Boolean isInsertionPoint = true;
for (String grep : greps) {
String[] tokens = grep.split(",", 3);
String insertionPoint = tokens[0];
String where = tokens[1];
String value = tokens[2];
grep = value;
if (casesensitive && matchtype == 1) {
switch (insertionPoint) {
case "All Request":
data = helpers.bytesToString(requestResponse.getRequest());
isInsertionPoint = false;
break;
default:
break;
}
} else if (matchtype == 1) {
switch (insertionPoint) {
case "All Request":
data = helpers.bytesToString(requestResponse.getRequest()).toUpperCase();
grep = grep.toUpperCase();
isInsertionPoint = false;
break;
default:
break;
}
} else if (matchtype == 2) {
Matcher matcher;
try {
p = Pattern.compile(grep, Pattern.CASE_INSENSITIVE);
} catch (PatternSyntaxException pse) {
System.out.println("Grep Match line 251 Incorrect regex: " + pse.getPattern());
return null;
}
switch (insertionPoint) {
case "All Request":
matcher = p.matcher(helpers.bytesToString(requestResponse.getRequest()));
if (matcher.find()) {
data = helpers.bytesToString(requestResponse.getRequest());
}
isInsertionPoint = false;
break;
default:
break;
}
}
try {
if (!isInsertionPoint) {
if (matchtype == 2) {
//Start regex grep
int beginAt = 0;
if (!data.isEmpty()) {
try {
p = Pattern.compile(grep, Pattern.CASE_INSENSITIVE);
m = p.matcher(data);
} catch (Exception e) {
System.out.println("Grep Match line 251 Incorrect regex: " + e.getMessage());
return null;
}
int end = 0;
while (m.find(beginAt)) {
end = end + 1;
if (end == 30) {
break;
}
if (notresponse) {
return null;
} else {
matches = matches + 1;
requestMarkers.add(new int[]{m.start(), m.end()});
beginAt = m.end();
}
}
}
//End regex grep
//Start Simple String, payload in response and payload without encode
} else {
int beginAt = 0;
if (data.contains(grep.toUpperCase())) {
if (notresponse) {
return null;
} else {
matches = matches + 1;
//if (grep.length() >= 3) {
byte[] match = helpers.stringToBytes(grep);
int end = 1;
while (beginAt < request.length) {
end = end + 1;
if (end == 30) {
break;
}
beginAt = helpers.indexOf(request, match, false, beginAt, request.length);
if (beginAt == -1) {
break;
}
requestMarkers.add(new int[]{beginAt, beginAt + match.length});
beginAt += match.length;
}
//}
}
}
//End Simple String, payload and payload without encode
}
} else {
if (matchtype == 2) {
//Start regex grep
int beginAt = 0;
String[] match = data.split(",", 3);
for (String single : match) {
if (!single.isEmpty()) {
try {
p = Pattern.compile(grep, Pattern.CASE_INSENSITIVE);
m = p.matcher(single);
} catch (PatternSyntaxException pse) {
System.out.println("Grep Match line 251 Incorrect regex: " + pse.getPattern());
return null;
}
if (m.find(beginAt)) {
if (notresponse) {
return null;
} else {
matches = matches + 1;
requestMarkers.addAll(requestMarkersInsertionPoints);
}
}
}
}
} else {
if (data.contains(grep.toUpperCase())) {
if (notresponse) {
return null;
} else {
matches = matches + 1;
requestMarkers.addAll(requestMarkersInsertionPoints);
}
}
}
}
} catch (Exception e) {
System.out.println("Grep Match line 898: " + e.getMessage());
return null;
}
}
try {
if (matches >= greps.size()) {
Collections.sort(requestMarkers, new Comparator<int[]>() {
private static final int INDEX = 0;
@Override
public int compare(int[] o1, int[] o2) {
return Integer.compare(o1[INDEX], o2[INDEX]);
}
});
List<int[]> requestMarkers_noduplicates = new ArrayList();
if (requestMarkers.size() == 1) {
requestMarkers_noduplicates = requestMarkers;
} else {
for (int i = 0; i <= requestMarkers.size() - 1; i++) {
if (i == requestMarkers.size() - 1) {
requestMarkers_noduplicates.add(requestMarkers.get(i));
} else if (requestMarkers.get(i)[0] != requestMarkers.get(i + 1)[0]) {
requestMarkers_noduplicates.add(requestMarkers.get(i));
}
}
}
String results_req = "";
for (int[] req : requestMarkers) {
results_req = results_req + "<br/>- " + helpers.urlDecode(helpers.bytesToString(requestResponse.getRequest()).substring(req[0], req[1]).replace("\"", "").replace("'", ""));
}
return new Object[]{new CustomScanIssue(requestResponse.getHttpService(), helpers.analyzeRequest(requestResponse).getUrl(),
new IHttpRequestResponse[]{callbacks.applyMarkers(requestResponse, requestMarkers_noduplicates, null)},
"BurpBounty - " + issuename, "It may be that the detected parameter is highlighted in red more times through the request. "
+ "This is because of the marking system the burpsuite uses.<br/><br/>" + issuedetail.replace("<payload>", results_req), issueseverity.replace("<payload>", results_req), issueconfidence.replace("<payload>", results_req), remediationdetail.replace("<payload>", results_req), issuebackground.replace("<payload>", results_req), remediationbackground.replace("<payload>", results_req)), insertionPoints};
} else {
return null;
}
} catch (Exception e) {
System.out.println("Grep Match line 898: " + e.getMessage());
return null;
}
}
} |
Java | BurpBounty/main/java/burpbountyfree/Headers.java | /*
Copyright 2018 Eduardo Garcia Melia <wagiro@gmail.com>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package burpbountyfree;
public class Headers {
String type = "";
String match = "";
String replace = "";
String regex = "";
Headers(String type, String match, String replace, String regex) {
this.type = type;
this.match = match;
this.replace = replace;
this.regex = regex;
}
} |
BurpBounty/main/java/burpbountyfree/NewTag.form | <?xml version="1.0" encoding="UTF-8" ?>
<Form version="1.5" maxVersion="1.9" type="org.netbeans.modules.form.forminfo.JPanelFormInfo">
<Properties>
<Property name="alignmentX" type="float" editor="org.netbeans.modules.form.RADConnectionPropertyEditor">
<Connection code="300" type="code"/>
</Property>
<Property name="alignmentY" type="float" editor="org.netbeans.modules.form.RADConnectionPropertyEditor">
<Connection code="300" type="code"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="FormSettings_autoResourcing" type="java.lang.Integer" value="0"/>
<AuxValue name="FormSettings_autoSetComponentName" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_generateFQN" type="java.lang.Boolean" value="true"/>
<AuxValue name="FormSettings_generateMnemonicsCode" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_i18nAutoMode" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_layoutCodeTarget" type="java.lang.Integer" value="1"/>
<AuxValue name="FormSettings_listenerGenerationStyle" type="java.lang.Integer" value="0"/>
<AuxValue name="FormSettings_variablesLocal" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_variablesModifier" type="java.lang.Integer" value="2"/>
</AuxValues>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="newTagtext" alignment="0" min="-2" pref="267" max="-2" attributes="0"/>
<Component id="jLabel47" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel47" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="newTagtext" min="-2" max="-2" attributes="0"/>
<EmptySpace pref="13" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JTextField" name="newTagtext">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel47">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Set new tag"/>
</Properties>
</Component>
</SubComponents>
</Form> |
|
Java | BurpBounty/main/java/burpbountyfree/NewTag.java | /*
Copyright 2018 Eduardo Garcia Melia <wagiro@gmail.com>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package burpbountyfree;
public class NewTag extends javax.swing.JPanel {
public NewTag() {
initComponents();
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
newTagtext = new javax.swing.JTextField();
jLabel47 = new javax.swing.JLabel();
setAlignmentX(300);
setAlignmentY(300);
jLabel47.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel47.setForeground(new java.awt.Color(255, 102, 51));
jLabel47.setText("Set new tag");
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(newTagtext, javax.swing.GroupLayout.PREFERRED_SIZE, 267, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel47))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jLabel47)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(newTagtext, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(13, Short.MAX_VALUE))
);
}// </editor-fold>//GEN-END:initComponents
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JLabel jLabel47;
public javax.swing.JTextField newTagtext;
// End of variables declaration//GEN-END:variables
} |
Java | BurpBounty/main/java/burpbountyfree/PassiveRequestScanner.java | /*
Copyright 2018 Eduardo Garcia Melia <wagiro@gmail.com>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package burpbountyfree;
import burp.IBurpExtenderCallbacks;
import burp.IExtensionHelpers;
import burp.IHttpRequestResponse;
import burp.IRequestInfo;
import burp.IScanIssue;
import com.google.gson.Gson;
import com.google.gson.JsonArray;
import java.util.ArrayList;
import java.util.List;
/**
*
* @author wagiro
*/
public class PassiveRequestScanner {
private IBurpExtenderCallbacks callbacks;
private IExtensionHelpers helpers;
JsonArray active_profiles;
BurpBountyExtension bbe;
Gson gson;
CollaboratorData burpCollaboratorData;
BurpBountyGui bbg;
JsonArray allprofiles;
JsonArray activeprofiles;
Integer redirtype;
Integer smartscandelay;
ActiveScanner active_scanner;
Utils utils;
String issuename;
String issuedetail;
String issuebackground;
String remediationdetail;
String remediationbackground;
int matchtype;
String issueseverity;
String issueconfidence;
boolean notresponse;
boolean casesensitive;
List<String> greps;
ProfilesProperties profile_property;
String urlextension;
Boolean isurlextension;
Boolean NegativeUrlExtension;
Boolean scanas;
int scantype;
GrepMatch gm;
public PassiveRequestScanner(BurpBountyExtension bbe, IBurpExtenderCallbacks callbacks, CollaboratorData burpCollaboratorData, JsonArray allprofiles, JsonArray activeprofiles, BurpBountyGui bbg) {
this.callbacks = callbacks;
helpers = callbacks.getHelpers();
this.burpCollaboratorData = burpCollaboratorData;
gson = new Gson();
this.allprofiles = allprofiles;
this.activeprofiles = activeprofiles;
this.bbe = bbe;
this.bbg = bbg;
gm = new GrepMatch(callbacks);
utils = new Utils(bbe, callbacks, burpCollaboratorData, allprofiles, bbg);
}
public void runReqPScan(IHttpRequestResponse baseRequestResponse, JsonArray passivereqprofiles, JsonArray rules, List<String[]> urls, Boolean passive) {
try {
if (baseRequestResponse.getRequest() == null) {
return;
}
for (int i = 0; i < passivereqprofiles.size(); i++) {
int grep_index = 0;
ArrayList<ArrayList<String>> greps_final = new ArrayList<>();
Object idata = passivereqprofiles.get(i);
profile_property = gson.fromJson(idata.toString(), ProfilesProperties.class);
setProfilesValues(profile_property);
Boolean isduplicated = utils.checkDuplicatedPassive(baseRequestResponse, issuename);
if (!isduplicated || passive) {
IRequestInfo requestInfo = helpers.analyzeRequest(baseRequestResponse);
greps_final.add(new ArrayList());
for (String grep : greps) {
String[] tokens = grep.split(",", 5);
if (tokens[0].equals("true")) {
if (tokens[1].equals("And") || tokens[1].equals("")) {
if (!tokens[4].equals("")) {
greps_final.get(grep_index).add(tokens[2] + "," + tokens[3] + "," + tokens[4]);
}
} else {
if (!tokens[4].equals("")) {
if (!greps_final.get(0).isEmpty()) {
greps_final.add(new ArrayList());
grep_index = grep_index + 1;
greps_final.get(grep_index).add(tokens[2] + "," + tokens[3] + "," + tokens[4]);
} else {
greps_final.get(grep_index).add(tokens[2] + "," + tokens[3] + "," + tokens[4]);
}
}
}
}
}
for (int x = 0; x <= grep_index; x++) {
Object[] matches = null;
try {
if (!greps_final.get(x).isEmpty()) {
matches = gm.getRequestMatches(baseRequestResponse, greps_final.get(x), issuename, issuedetail, issuebackground, remediationdetail, remediationbackground, matchtype,
issueseverity, issueconfidence, casesensitive, notresponse, scanas, scantype);
}
if (matches != null) {
callbacks.addScanIssue((IScanIssue) matches[0]);
}
} catch (Exception e) {
System.out.println("PassiveRequestScanner line 153: " + e.getMessage());
for (StackTraceElement element : e.getStackTrace()) {
System.out.println(element);
}
System.out.println(issuename);
continue;
}
}
}
}
} catch (Exception e) {
System.out.println("PassiveRequestScanner line 164: " + e.getMessage());
for (StackTraceElement element : e.getStackTrace()) {
System.out.println(element);
}
}
}
public void setProfilesValues(ProfilesProperties profile_property) {
greps = profile_property.getGreps();
issuename = profile_property.getIssueName();
issueseverity = profile_property.getIssueSeverity();
issueconfidence = profile_property.getIssueConfidence();
issuedetail = profile_property.getIssueDetail();
issuebackground = profile_property.getIssueBackground();
remediationdetail = profile_property.getRemediationDetail();
remediationbackground = profile_property.getRemediationBackground();
matchtype = profile_property.getMatchType();
notresponse = profile_property.getNotResponse();
casesensitive = profile_property.getCaseSensitive();
isurlextension = profile_property.getIsURLExtension();
urlextension = profile_property.getURLExtension();
NegativeUrlExtension = profile_property.getNegativeURLExtension();
scantype = profile_property.getScanType();
scanas = profile_property.getScanAs();
}
} |
Java | BurpBounty/main/java/burpbountyfree/PassiveResponseScanner.java | /*
Copyright 2018 Eduardo Garcia Melia <wagiro@gmail.com>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package burpbountyfree;
import burp.IBurpExtenderCallbacks;
import burp.IExtensionHelpers;
import burp.IHttpRequestResponse;
import burp.IParameter;
import burp.IRequestInfo;
import burp.IResponseInfo;
import burp.IScanIssue;
import burp.IScannerInsertionPoint;
import com.google.gson.Gson;
import com.google.gson.JsonArray;
import java.util.ArrayList;
import java.util.List;
/**
*
* @author wagiro
*/
public class PassiveResponseScanner {
private IBurpExtenderCallbacks callbacks;
private IExtensionHelpers helpers;
JsonArray active_profiles;
BurpBountyExtension bbe;
Gson gson;
CollaboratorData burpCollaboratorData;
BurpBountyGui bbg;
JsonArray allprofiles;
Integer redirtype;
Integer smartscandelay;
String issuename;
String issuedetail;
String issuebackground;
String remediationdetail;
String remediationbackground;
int matchtype;
int scope;
String issueseverity;
String issueconfidence;
boolean excludeHTTP;
boolean onlyHTTP;
boolean notresponse;
boolean iscontenttype;
boolean isresponsecode;
boolean negativect;
boolean negativerc;
String contenttype;
String responsecode;
boolean casesensitive;
List<String> greps;
ProfilesProperties profile_property;
String urlextension;
Boolean isurlextension;
Boolean NegativeUrlExtension;
ActiveScanner active_scanner;
Utils utils;
JsonArray activeprofiles;
GrepMatch gm;
public PassiveResponseScanner(BurpBountyExtension bbe, IBurpExtenderCallbacks callbacks, CollaboratorData burpCollaboratorData, JsonArray allprofiles, JsonArray activeprofiles, BurpBountyGui bbg) {
this.callbacks = callbacks;
helpers = callbacks.getHelpers();
this.burpCollaboratorData = burpCollaboratorData;
gson = new Gson();
this.allprofiles = allprofiles;
this.activeprofiles = activeprofiles;
this.bbe = bbe;
this.bbg = bbg;
utils = new Utils(bbe, callbacks, burpCollaboratorData, allprofiles, bbg);
gm = new GrepMatch(callbacks);
}
public void runResPScan(IHttpRequestResponse baseRequestResponse, JsonArray passiveresprofiles, JsonArray rules, List<String[]> urls, Boolean passive) {
try {
if (baseRequestResponse.getResponse() == null) {
return;
}
for (int i = 0; i < passiveresprofiles.size(); i++) {
IResponseInfo r;
int grep_index = 0;
ArrayList<ArrayList<String>> greps_final = new ArrayList<>();
Object idata = passiveresprofiles.get(i);
profile_property = gson.fromJson(idata.toString(), ProfilesProperties.class);
setProfilesValues(profile_property);
try {
r = helpers.analyzeResponse(baseRequestResponse.getResponse());
} catch (NullPointerException e) {
System.out.println("PassiveResponseScanner line 616: " + e.getMessage());
return;
}
Boolean isduplicated = utils.checkDuplicatedPassiveResponse(baseRequestResponse, issuename);
if (!isduplicated || passive) {
Integer responseCode = new Integer(r.getStatusCode());
IRequestInfo requestInfo = helpers.analyzeRequest(baseRequestResponse);
if ((isresponsecode && !utils.isResponseCode(responsecode, negativerc, responseCode)) || (iscontenttype && !utils.isContentType(contenttype, negativect, r))) {
continue;
}
greps_final.add(new ArrayList());
for (String grep : greps) {
String[] tokens;
try {
tokens = grep.split(",", 3);
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("PassiveResponseScanner line 140: " + e.getMessage());
continue;
}
if (tokens[0].equals("true")) {
if (tokens[1].equals("And") || tokens[1].equals("")) {
if (!tokens[2].equals("")) {
greps_final.get(grep_index).add(tokens[2]);
}
} else {
if (!tokens[2].equals("")) {
if (!greps_final.get(0).isEmpty()) {
greps_final.add(new ArrayList());
grep_index = grep_index + 1;
greps_final.get(grep_index).add(tokens[2]);
} else {
greps_final.get(grep_index).add(tokens[2]);
}
}
}
}
}
for (int x = 0; x <= grep_index; x++) {
IScanIssue matches = null;
if (!greps_final.get(x).isEmpty()) {
matches = gm.getResponseMatches(baseRequestResponse, "", greps_final.get(x), issuename, issuedetail, issuebackground, remediationdetail, remediationbackground, "", matchtype,
issueseverity, issueconfidence, notresponse, casesensitive, false, excludeHTTP, onlyHTTP);
}
if (matches != null) {
if (scope != 1) {//porperty.equals("All Matches")) {
callbacks.addScanIssue(matches);
} else {
Boolean isUrlScanned = true;
for (String url[] : urls) {
if (url[1].contains(baseRequestResponse.getHttpService().getHost())) {
if (url[0].contains(issuename)) {
isUrlScanned = false;
}
}
}
if (isUrlScanned) {
callbacks.addScanIssue(matches);
urls.add(new String[]{issuename, baseRequestResponse.getHttpService().getHost()});
}
}
}
}
}
}
} catch (Exception e) {
System.out.println("PassiveResponseScanner line 210: " + e.getMessage());
for (StackTraceElement element : e.getStackTrace()) {
System.out.println(element);
}
}
}
public void setProfilesValues(ProfilesProperties profile_property) {
greps = profile_property.getGreps();
issuename = profile_property.getIssueName();
issueseverity = profile_property.getIssueSeverity();
issueconfidence = profile_property.getIssueConfidence();
issuedetail = profile_property.getIssueDetail();
issuebackground = profile_property.getIssueBackground();
remediationdetail = profile_property.getRemediationDetail();
remediationbackground = profile_property.getRemediationBackground();
matchtype = profile_property.getMatchType();
scope = profile_property.getScope();
notresponse = profile_property.getNotResponse();
casesensitive = profile_property.getCaseSensitive();
iscontenttype = profile_property.getIsContentType();
isresponsecode = profile_property.getIsResponseCode();
contenttype = profile_property.getContentType();
responsecode = profile_property.getResponseCode();
excludeHTTP = profile_property.getExcludeHTTP();
onlyHTTP = profile_property.getOnlyHTTP();
negativect = profile_property.getNegativeCT();
negativerc = profile_property.getNegativeRC();
isurlextension = profile_property.getIsURLExtension();
urlextension = profile_property.getURLExtension();
NegativeUrlExtension = profile_property.getNegativeURLExtension();
}
} |
Java | BurpBounty/main/java/burpbountyfree/ProfilesManager.java | /*
Copyright 2018 Eduardo Garcia Melia <wagiro@gmail.com>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package burpbountyfree;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonArray;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.reflect.TypeToken;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.io.OutputStreamWriter;
import java.util.ArrayList;
import java.util.List;
import javax.swing.DefaultListModel;
/**
*
* @author wagiro
*/
public class ProfilesManager {
private String name;
private String issuename;
private String issuedetail;
private String issuebackground;
private String remediationdetail;
private String remediationbackground;
private String charstourlencode;
private int matchtype;
private int scope;
private String issueseverity;
private String issueconfidence;
private String responsecode;
private String contenttype;
private boolean negativect;
private boolean negativerc;
private boolean notresponse;
private boolean casesensitive;
private boolean excludeHTTP;
private boolean onlyHTTP;
private boolean urlencode;
private boolean isresponsecode;
private boolean iscontenttype;
private String urlextension;
private boolean isurlextension;
private boolean NegativeUrlExtension;
private boolean Scanas;
private int Scantype;
private int redirtype;
private int maxRedir;
private int payloadPosition;
private String payloadsfile;
private String grepsfile;
private String timeOut1;
private String timeOut2;
private String contentLength;
private String httpResponseCode;
private String author;
private String profiles_directory;
private List<Headers> headers;
private List<String> variationAttributes;
private List<Integer> insertionPointType;
public ProfilesManager(String profiles_directory) {
this.profiles_directory = profiles_directory;
name = "";
issuename = "";
issuedetail = "";
issuebackground = "";
remediationdetail = "";
remediationbackground = "";
charstourlencode = "";
matchtype = 0;
scope = 0;
issueseverity = "";
issueconfidence = "";
responsecode = "";
contenttype = "";
httpResponseCode = "";
negativect = false;
negativerc = false;
notresponse = false;
casesensitive = false;
excludeHTTP = false;
onlyHTTP = false;
urlencode = false;
isresponsecode = false;
iscontenttype = false;
redirtype = 0;
maxRedir = 0;
payloadPosition = 0;
Scantype = 0;
payloadsfile = "";
grepsfile = "";
timeOut1 = "";
timeOut2 = "";
contentLength = "";
author = "";
urlextension = "";
isurlextension = false;
NegativeUrlExtension = false;
Scanas = false;
headers = new ArrayList();
variationAttributes = new ArrayList();
insertionPointType = new ArrayList();
}
public void setActiveAttackValues(String profile_name, JsonArray activeprofiles, ActiveProfile profile) {
//Set Attack values when select from main combobox
try {
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
JsonArray json = activeprofiles;
ProfilesProperties profile_property = new ProfilesProperties();
if (json != null) {
for (JsonElement pa : json) {
JsonObject bbObj = pa.getAsJsonObject();
if (bbObj.get("ProfileName").getAsString().equals(profile_name)) {
profile_property = gson.fromJson(bbObj.toString(), ProfilesProperties.class
);
}
}
}
name = profile_property.getProfileName();
casesensitive = profile_property.getCaseSensitive();
notresponse = profile_property.getNotResponse();
matchtype = profile_property.getMatchType();
issuename = profile_property.getIssueName();
issueseverity = profile_property.getIssueSeverity();
issueconfidence = profile_property.getIssueConfidence();
issuedetail = profile_property.getIssueDetail();
issuebackground = profile_property.getIssueBackground();
remediationdetail = profile_property.getRemediationDetail();
remediationbackground = profile_property.getRemediationBackground();
urlencode = profile_property.getUrlEncode();
charstourlencode = profile_property.getCharsToUrlEncode();
iscontenttype = profile_property.getIsContentType();
isresponsecode = profile_property.getIsResponseCode();
contenttype = profile_property.getContentType();
responsecode = profile_property.getResponseCode();
excludeHTTP = profile_property.getExcludeHTTP();
onlyHTTP = profile_property.getOnlyHTTP();
negativect = profile_property.getNegativeCT();
negativerc = profile_property.getNegativeRC();
isurlextension = profile_property.getIsURLExtension();
urlextension = profile_property.getURLExtension();
NegativeUrlExtension = profile_property.getNegativeURLExtension();
redirtype = profile_property.getRedirection();
maxRedir = profile_property.getMaxRedir();
payloadsfile = profile_property.getpayloadsFile();
grepsfile = profile_property.getgrepsFile();
payloadPosition = profile_property.getPayloadPosition();
timeOut1 = profile_property.getTime1();
timeOut2 = profile_property.getTime2();
author = profile_property.getAuthor();
contentLength = profile_property.getContentLength();
httpResponseCode = profile_property.getHttpResponseCode();
headers = profile_property.getHeader();
variationAttributes = profile_property.getVariationAttributes();
insertionPointType = profile_property.getInsertionPointType();
profile.textauthor.setText(author);
profile.text1.setText(name);
if (payloadPosition == 1) {
profile.buttonGroup1.setSelected(profile.replace.getModel(), true);
} else if (payloadPosition == 2) {
profile.buttonGroup1.setSelected(profile.append.getModel(), true);
}else if (payloadPosition == 3) {
profile.buttonGroup1.setSelected(profile.insert.getModel(), true);
}
profile.grep.removeAllElements();
profile.payload.removeAllElements();
profile.encoder.removeAllElements();
profile.tag.removeAllElements();
profile.textpayloads.setText(payloadsfile);
profile.textgreps.setText(grepsfile);
profile.showGreps(profile_property.getGreps());
profile.showPayloads(profile_property.getPayloads());
// if (!payloadsfile.isEmpty()) {
// loadPath(payloadsfile, profile.payload);
// updatePayloads(payloadsfile, profile_property);
//
// } else {
// for (String pay : profile_property.getPayloads()) {
// profile.payload.addElement(pay);
// }
// }
if (profile_property.getTags() != null) {
for (String t : profile_property.getTags()) {
profile.tag.addElement(t);
}
}
for (String enc : profile_property.getEncoder()) {
profile.encoder.addElement(enc);
}
profile.text71.setText(contenttype);
profile.text72.setText(responsecode);
profile.text73.setText(urlextension);
profile.check8.setSelected(urlencode);
profile.text5.setText(charstourlencode);
profile.excludehttp.setSelected(excludeHTTP);
profile.onlyhttp.setSelected(onlyHTTP);
if (timeOut2.equals("0")) {
profile.texttime2.setText("");
} else {
profile.texttime2.setText(timeOut2);
}
if (timeOut1.equals("0")) {
profile.texttime1.setText("");
} else {
profile.texttime1.setText(timeOut1);
}
profile.textcl.setText(contentLength);
profile.resposecode.setText(httpResponseCode);
switch (matchtype) {
case 1:
profile.buttonGroup3.setSelected(profile.radio4.getModel(), true);
break;
case 2:
profile.buttonGroup3.setSelected(profile.radio3.getModel(), true);
break;
case 3:
profile.buttonGroup3.setSelected(profile.radio12.getModel(), true);
break;
case 4:
profile.buttonGroup3.setSelected(profile.radio22.getModel(), true);
break;
case 5:
profile.buttonGroup3.setSelected(profile.radiotime.getModel(), true);
break;
case 6:
profile.buttonGroup3.setSelected(profile.radiocl.getModel(), true);
break;
case 7:
profile.buttonGroup3.setSelected(profile.variationsRadio.getModel(), true);
break;
case 8:
profile.buttonGroup3.setSelected(profile.invariationsRadio.getModel(), true);
break;
case 9:
profile.buttonGroup3.setSelected(profile.radiohttp.getModel(), true);
break;
default:
profile.buttonGroup3.clearSelection();
break;
}
switch (redirtype) {
case 1:
profile.buttonGroup4.setSelected(profile.rb1.getModel(), true);
break;
case 2:
profile.buttonGroup4.setSelected(profile.rb2.getModel(), true);
break;
case 3:
profile.buttonGroup4.setSelected(profile.rb3.getModel(), true);
break;
case 4:
profile.buttonGroup4.setSelected(profile.rb4.getModel(), true);
break;
default:
profile.buttonGroup4.clearSelection();
break;
}
profile.showHeaders(headers);
setSelectedVariations(false, profile);
if (variationAttributes.contains("status_code")) {
profile.status_code.setSelected(true);
}
if (variationAttributes.contains("input_image_labels")) {
profile.input_image_labels.setSelected(true);
}
if (variationAttributes.contains("non_hidden_form_input_types")) {
profile.non_hidden_form_input_types.setSelected(true);
}
if (variationAttributes.contains("page_title")) {
profile.page_title.setSelected(true);
}
if (variationAttributes.contains("visible_text")) {
profile.visible_text.setSelected(true);
}
if (variationAttributes.contains("button_submit_labels")) {
profile.button_submit_labels.setSelected(true);
}
if (variationAttributes.contains("div_ids")) {
profile.div_ids.setSelected(true);
}
if (variationAttributes.contains("word_count")) {
profile.word_count.setSelected(true);
}
if (variationAttributes.contains("content_type")) {
profile.content_type.setSelected(true);
}
if (variationAttributes.contains("outbound_edge_tag_names")) {
profile.outbound_edge_tag_names.setSelected(true);
}
if (variationAttributes.contains("whole_body_content")) {
profile.whole_body_content.setSelected(true);
}
if (variationAttributes.contains("etag_header")) {
profile.etag_header.setSelected(true);
}
if (variationAttributes.contains("visible_word_count")) {
profile.visible_word_count.setSelected(true);
}
if (variationAttributes.contains("content_length")) {
profile.content_length.setSelected(true);
}
if (variationAttributes.contains("header_tags")) {
profile.header_tags.setSelected(true);
}
if (variationAttributes.contains("tag_ids")) {
profile.tag_ids.setSelected(true);
}
if (variationAttributes.contains("comments")) {
profile.comments.setSelected(true);
}
if (variationAttributes.contains("line_count")) {
profile.line_count.setSelected(true);
}
if (variationAttributes.contains("set_cookie_names")) {
profile.set_cookie_names.setSelected(true);
}
if (variationAttributes.contains("last_modified_header")) {
profile.last_modified_header.setSelected(true);
}
if (variationAttributes.contains("first_header_tag")) {
profile.first_header_tag.setSelected(true);
}
if (variationAttributes.contains("tag_names")) {
profile.tag_names.setSelected(true);
}
if (variationAttributes.contains("input_submit_labels")) {
profile.input_submit_labels.setSelected(true);
}
if (variationAttributes.contains("outbound_edge_count")) {
profile.outbound_edge_count.setSelected(true);
}
if (variationAttributes.contains("initial_body_content")) {
profile.initial_body_content.setSelected(true);
}
if (variationAttributes.contains("content_location")) {
profile.content_location.setSelected(true);
}
if (variationAttributes.contains("limited_body_content")) {
profile.limited_body_content.setSelected(true);
}
if (variationAttributes.contains("canonical_link")) {
profile.canonical_link.setSelected(true);
}
if (variationAttributes.contains("css_classes")) {
profile.css_classes.setSelected(true);
}
if (variationAttributes.contains("location")) {
profile.location.setSelected(true);
}
if (variationAttributes.contains("anchor_labels")) {
profile.anchor_labels.setSelected(true);
}
profile.setSelectedInsertionPointType(false);
if (insertionPointType.contains(18)) {
profile.All.setSelected(true);
}
if (insertionPointType.contains(65)) {
profile.extensionprovided.setSelected(true);
}
if (insertionPointType.contains(32)) {
profile.header.setSelected(true);
}
if (insertionPointType.contains(36)) {
profile.entirebody.setSelected(true);
}
if (insertionPointType.contains(7)) {
profile.paramamf.setSelected(true);
}
if (insertionPointType.contains(1)) {
profile.parambody.setSelected(true);
}
if (insertionPointType.contains(2)) {
profile.paramcookie.setSelected(true);
}
if (insertionPointType.contains(6)) {
profile.paramjson.setSelected(true);
}
if (insertionPointType.contains(33)) {
profile.urlpathfolder.setSelected(true);
}
if (insertionPointType.contains(5)) {
profile.parammultipartattr.setSelected(true);
}
if (insertionPointType.contains(35)) {
profile.paramnamebody.setSelected(true);
}
if (insertionPointType.contains(34)) {
profile.paramnameurl.setSelected(true);
}
if (insertionPointType.contains(64)) {
profile.userprovided.setSelected(true);
}
if (insertionPointType.contains(0)) {
profile.paramurl.setSelected(true);
}
if (insertionPointType.contains(3)) {
profile.paramxml.setSelected(true);
}
if (insertionPointType.contains(4)) {
profile.paramxmlattr.setSelected(true);
}
if (insertionPointType.contains(37)) {
profile.urlpathfilename.setSelected(true);
}
if (insertionPointType.contains(127)) {
profile.unknown.setSelected(true);
}
profile.check1.setSelected(casesensitive);
profile.check4.setSelected(notresponse);
profile.check71.setSelected(iscontenttype);
profile.check72.setSelected(isresponsecode);
profile.check73.setSelected(isurlextension);
profile.negativeCT.setSelected(negativect);
profile.negativeRC.setSelected(negativerc);
profile.negativeURL.setSelected(NegativeUrlExtension);
profile.text4.setText(issuename);
profile.textarea1.setText(issuedetail);
profile.textarea2.setText(issuebackground);
profile.textarea3.setText(remediationdetail);
profile.textarea4.setText(remediationbackground);
profile.sp1.setValue(maxRedir);
switch (issueseverity) {
case "High":
profile.buttonGroup5.setSelected(profile.radio5.getModel(), true);
break;
case "Medium":
profile.buttonGroup5.setSelected(profile.radio6.getModel(), true);
break;
case "Low":
profile.buttonGroup5.setSelected(profile.radio7.getModel(), true);
break;
case "Information":
profile.buttonGroup5.setSelected(profile.radio8.getModel(), true);
break;
default:
break;
}
switch (issueconfidence) {
case "Certain":
profile.buttonGroup6.setSelected(profile.radio9.getModel(), true);
break;
case "Firm":
profile.buttonGroup6.setSelected(profile.radio10.getModel(), true);
break;
case "Tentative":
profile.buttonGroup6.setSelected(profile.radio11.getModel(), true);
break;
default:
break;
}
} catch (Exception e) {
System.out.println("PofilesManager line 499:" + e.getMessage());
for (StackTraceElement element : e.getStackTrace()) {
System.out.println(element);
}
}
}
public void saveActiveAttackValues(ActiveProfile profile) {
headers = new ArrayList();
variationAttributes = new ArrayList();
insertionPointType = new ArrayList();
//Save attack with fields values
try {
//get GUI values
ProfilesProperties newfile = new ProfilesProperties();
newfile.setProfileName(profile.text1.getText());
newfile.setAuthor(profile.textauthor.getText());
newfile.setScanner(1);
if (profile.replace.isSelected()) {
newfile.setPayloadPosition(1);
} else if (profile.append.isSelected()) {
newfile.setPayloadPosition(2);
} else if (profile.insert.isSelected()) {
newfile.setPayloadPosition(3);
}
newfile.setEnabled(true);
List encoders = new ArrayList();
List payloads = new ArrayList();
List greps = new ArrayList();
List tags = new ArrayList();
newfile.setPayloadsFile(profile.textpayloads.getText());
for (int i = 0; i < profile.modelpayload.getRowCount(); i++) {
if (!profile.modelpayload.getValueAt(i, 1).toString().isEmpty()) {
payloads.add(profile.modelpayload.getValueAt(i, 0).toString() + "," + profile.modelpayload.getValueAt(i, 1).toString());
}
}
newfile.setPayloads(payloads);
newfile.setGrepsFile(profile.textgreps.getText());
for (int i = 0; i < profile.modelgrep.getRowCount(); i++) {
if (!profile.modelgrep.getValueAt(i, 2).toString().isEmpty()) {
greps.add(profile.modelgrep.getValueAt(i, 0).toString() + "," + profile.modelgrep.getValueAt(i, 1).toString() + "," + profile.modelgrep.getValueAt(i, 2).toString());
}
}
newfile.setGreps(greps);
for (int row = 0; row < profile.model4.getRowCount(); row++) {
headers.add(new Headers((String) profile.model4.getValueAt(row, 0), (String) profile.model4.getValueAt(row, 1), (String) profile.model4.getValueAt(row, 2), (String) profile.model4.getValueAt(row, 3)));
}
newfile.setHeader(headers);
for (int i = 0; i < profile.listtag.getModel().getSize(); i++) {
Object item = profile.listtag.getModel().getElementAt(i);
if (!item.toString().isEmpty()) {
tags.add(item.toString().replaceAll("\r", "").replaceAll("\n", ""));
}
}
if (!tags.contains("All")) {
tags.add("All");
newfile.setTags(tags);
} else {
newfile.setTags(tags);
}
for (int i = 0; i < profile.list3.getModel().getSize(); i++) {
Object item = profile.list3.getModel().getElementAt(i);
if (!item.toString().isEmpty()) {
encoders.add(item.toString().replaceAll("\r", "").replaceAll("\n", ""));
}
}
newfile.setEncoder(encoders);
newfile.setCharsToUrlEncode(profile.text5.getText());
newfile.setUrlEncode(profile.check8.isSelected());
newfile.setExcludeHTTP(profile.excludehttp.isSelected());
newfile.setOnlyHTTP(profile.onlyhttp.isSelected());
newfile.setContentType(profile.text71.getText());
newfile.setResponseCode(profile.text72.getText());
newfile.setURLExtension(profile.text73.getText());
newfile.setTime2(profile.texttime2.getText());
newfile.setTime1(profile.texttime1.getText());
newfile.setContentLength(profile.textcl.getText());
newfile.setHttpResponseCode(profile.resposecode.getText());
if (profile.radio4.isSelected()) {
newfile.setMatchType(1);
} else if (profile.radio3.isSelected()) {
newfile.setMatchType(2);
} else if (profile.radio12.isSelected()) {
newfile.setMatchType(3);
} else if (profile.radio22.isSelected()) {
newfile.setMatchType(4);
} else if (profile.radiotime.isSelected()) {
newfile.setMatchType(5);
} else if (profile.radiocl.isSelected()) {
newfile.setMatchType(6);
} else if (profile.variationsRadio.isSelected()) {
newfile.setMatchType(7);
} else if (profile.invariationsRadio.isSelected()) {
newfile.setMatchType(8);
} else if (profile.radiohttp.isSelected()) {
newfile.setMatchType(9);
} else {
newfile.setMatchType(0);
}
if (profile.rb1.isSelected()) {
newfile.setRedirType(1);
} else if (profile.rb2.isSelected()) {
newfile.setRedirType(2);
} else if (profile.rb3.isSelected()) {
newfile.setRedirType(3);
} else if (profile.rb4.isSelected()) {
newfile.setRedirType(4);
} else {
newfile.setRedirType(0);
}
if (profile.status_code.isSelected()) {
variationAttributes.add("status_code");
}
if (profile.input_image_labels.isSelected()) {
variationAttributes.add("input_image_labels");
}
if (profile.non_hidden_form_input_types.isSelected()) {
variationAttributes.add("non_hidden_form_input_types");
}
if (profile.page_title.isSelected()) {
variationAttributes.add("page_title");
}
if (profile.visible_text.isSelected()) {
variationAttributes.add("visible_text");
}
if (profile.button_submit_labels.isSelected()) {
variationAttributes.add("button_submit_labels");
}
if (profile.div_ids.isSelected()) {
variationAttributes.add("div_ids");
}
if (profile.word_count.isSelected()) {
variationAttributes.add("word_count");
}
if (profile.content_type.isSelected()) {
variationAttributes.add("content_type");
}
if (profile.outbound_edge_tag_names.isSelected()) {
variationAttributes.add("outbound_edge_tag_names");
}
if (profile.whole_body_content.isSelected()) {
variationAttributes.add("whole_body_content");
}
if (profile.etag_header.isSelected()) {
variationAttributes.add("etag_header");
}
if (profile.visible_word_count.isSelected()) {
variationAttributes.add("visible_word_count");
}
if (profile.content_length.isSelected()) {
variationAttributes.add("content_length");
}
if (profile.header_tags.isSelected()) {
variationAttributes.add("header_tags");
}
if (profile.tag_ids.isSelected()) {
variationAttributes.add("tag_ids");
}
if (profile.comments.isSelected()) {
variationAttributes.add("comments");
}
if (profile.line_count.isSelected()) {
variationAttributes.add("line_count");
}
if (profile.set_cookie_names.isSelected()) {
variationAttributes.add("set_cookie_names");
}
if (profile.last_modified_header.isSelected()) {
variationAttributes.add("last_modified_header");
}
if (profile.first_header_tag.isSelected()) {
variationAttributes.add("first_header_tag");
}
if (profile.tag_names.isSelected()) {
variationAttributes.add("tag_names");
}
if (profile.input_submit_labels.isSelected()) {
variationAttributes.add("input_submit_labels");
}
if (profile.outbound_edge_count.isSelected()) {
variationAttributes.add("outbound_edge_count");
}
if (profile.initial_body_content.isSelected()) {
variationAttributes.add("initial_body_content");
}
if (profile.content_location.isSelected()) {
variationAttributes.add("content_location");
}
if (profile.limited_body_content.isSelected()) {
variationAttributes.add("limited_body_content");
}
if (profile.canonical_link.isSelected()) {
variationAttributes.add("canonical_link");
}
if (profile.css_classes.isSelected()) {
variationAttributes.add("css_classes");
}
if (profile.location.isSelected()) {
variationAttributes.add("location");
}
if (profile.anchor_labels.isSelected()) {
variationAttributes.add("anchor_labels");
}
newfile.setVariationAttributes(variationAttributes);
if (profile.All.isSelected()) {
insertionPointType.add(18);
insertionPointType.add(65);
insertionPointType.add(32);
insertionPointType.add(36);
insertionPointType.add(7);
insertionPointType.add(1);
insertionPointType.add(2);
insertionPointType.add(6);
insertionPointType.add(33);
insertionPointType.add(5);
insertionPointType.add(35);
insertionPointType.add(34);
insertionPointType.add(64);
insertionPointType.add(0);
insertionPointType.add(3);
insertionPointType.add(4);
insertionPointType.add(37);
insertionPointType.add(127);
}
if (profile.extensionprovided.isSelected()) {
insertionPointType.add(65);
}
if (profile.header.isSelected()) {
insertionPointType.add(32);
}
if (profile.entirebody.isSelected()) {
insertionPointType.add(36);
}
if (profile.paramamf.isSelected()) {
insertionPointType.add(7);
}
if (profile.parambody.isSelected()) {
insertionPointType.add(1);
}
if (profile.paramcookie.isSelected()) {
insertionPointType.add(2);
}
if (profile.paramjson.isSelected()) {
insertionPointType.add(6);
}
if (profile.urlpathfolder.isSelected()) {
insertionPointType.add(33);
}
if (profile.parammultipartattr.isSelected()) {
insertionPointType.add(5);
}
if (profile.paramnamebody.isSelected()) {
insertionPointType.add(35);
}
if (profile.paramnameurl.isSelected()) {
insertionPointType.add(34);
}
if (profile.userprovided.isSelected()) {
insertionPointType.add(64);
}
if (profile.paramurl.isSelected()) {
insertionPointType.add(0);
}
if (profile.paramxml.isSelected()) {
insertionPointType.add(3);
}
if (profile.paramxmlattr.isSelected()) {
insertionPointType.add(4);
}
if (profile.urlpathfilename.isSelected()) {
insertionPointType.add(37);
}
if (profile.unknown.isSelected()) {
insertionPointType.add(127);
}
newfile.setInsertionPointType(insertionPointType);
newfile.setCaseSensitive(profile.check1.isSelected());
newfile.setNotResponse(profile.check4.isSelected());
newfile.setIsContentType(profile.check71.isSelected());
newfile.setIsResponseCode(profile.check72.isSelected());
newfile.setIsURLExtension(profile.check73.isSelected());
newfile.setNegativeCT(profile.negativeCT.isSelected());
newfile.setNegativeRC(profile.negativeRC.isSelected());
newfile.setNegativeURLExtension(profile.negativeURL.isSelected());
newfile.setIssueName(profile.text4.getText());
newfile.setIssueDetail(profile.textarea1.getText());
newfile.setIssueBackground(profile.textarea2.getText());
newfile.setRemediationDetail(profile.textarea3.getText());
newfile.setRemediationBackground(profile.textarea4.getText());
newfile.setMaxRedir((Integer) profile.sp1.getValue());
if (profile.radio5.isSelected()) {
newfile.setIssueSeverity("High");
} else if (profile.radio6.isSelected()) {
newfile.setIssueSeverity("Medium");
} else if (profile.radio7.isSelected()) {
newfile.setIssueSeverity("Low");
} else if (profile.radio8.isSelected()) {
newfile.setIssueSeverity("Information");
} else {
newfile.setIssueSeverity("");
}
if (profile.radio9.isSelected()) {
newfile.setIssueConfidence("Certain");
} else if (profile.radio10.isSelected()) {
newfile.setIssueConfidence("Firm");
} else if (profile.radio11.isSelected()) {
newfile.setIssueConfidence("Tentative");
} else {
newfile.setIssueConfidence("");
}
//Save start
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
JsonArray ijson = new JsonArray();
List<ProfilesProperties> newjson = gson.fromJson(ijson, new TypeToken<List<ProfilesProperties>>() {
}.getType());
newjson.add(newfile);
String json = gson.toJson(newjson);
//Write JSON String to file
FileOutputStream fileStream;
fileStream = new FileOutputStream(new File(profiles_directory + File.separator + profile.text1.getText().concat(".bb")));
OutputStreamWriter writer = new OutputStreamWriter(fileStream, "UTF-8");
writer.write(json);
writer.close();
} catch (IOException e) {
System.out.println("ProfilesManager line 852:");
for (StackTraceElement element : e.getStackTrace()) {
System.out.println(element);
}
}
}
public void setResponseAttackValues(String profile_name, JsonArray passiveresprofiles, ResponseProfile profile) {
//Set Attack values when select from main combobox
try {
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
JsonArray json = passiveresprofiles;
ProfilesProperties profile_property = new ProfilesProperties();
if (json != null) {
for (JsonElement pa : json) {
JsonObject bbObj = pa.getAsJsonObject();
if (bbObj.get("ProfileName").getAsString().equals(profile_name)) {
profile_property = gson.fromJson(bbObj.toString(), ProfilesProperties.class
);
}
}
}
name = profile_property.getProfileName();
casesensitive = profile_property.getCaseSensitive();
notresponse = profile_property.getNotResponse();
matchtype = profile_property.getMatchType();
scope = profile_property.getScope();
issuename = profile_property.getIssueName();
issueseverity = profile_property.getIssueSeverity();
issueconfidence = profile_property.getIssueConfidence();
issuedetail = profile_property.getIssueDetail();
issuebackground = profile_property.getIssueBackground();
remediationdetail = profile_property.getRemediationDetail();
remediationbackground = profile_property.getRemediationBackground();
iscontenttype = profile_property.getIsContentType();
isresponsecode = profile_property.getIsResponseCode();
contenttype = profile_property.getContentType();
responsecode = profile_property.getResponseCode();
excludeHTTP = profile_property.getExcludeHTTP();
onlyHTTP = profile_property.getOnlyHTTP();
negativect = profile_property.getNegativeCT();
negativerc = profile_property.getNegativeRC();
redirtype = profile_property.getRedirection();
isurlextension = profile_property.getIsURLExtension();
urlextension = profile_property.getURLExtension();
NegativeUrlExtension = profile_property.getNegativeURLExtension();
maxRedir = profile_property.getMaxRedir();
grepsfile = profile_property.getgrepsFile();
payloadPosition = profile_property.getPayloadPosition();
author = profile_property.getAuthor();
contentLength = profile_property.getContentLength();
profile.textauthor.setText(author);
profile.text1.setText(name);
if (profile_property.getTags() != null) {
for (String t : profile_property.getTags()) {
profile.tag.addElement(t);
}
}
profile.showGreps(profile_property.getGreps());
profile.text71.setText(contenttype);
profile.text72.setText(responsecode);
profile.excludehttp.setSelected(excludeHTTP);
profile.onlyhttp.setSelected(onlyHTTP);
switch (matchtype) {
case 1:
profile.buttonGroup3.setSelected(profile.radio4.getModel(), true);
break;
case 2:
profile.buttonGroup3.setSelected(profile.radio3.getModel(), true);
break;
default:
profile.buttonGroup3.clearSelection();
break;
}
switch (scope) {
case 1:
profile.buttonGroup7.setSelected(profile.first_match.getModel(), true);
break;
case 2:
profile.buttonGroup7.setSelected(profile.all_matches.getModel(), true);
break;
default:
profile.buttonGroup7.clearSelection();
break;
}
switch (redirtype) {
case 1:
profile.buttonGroup4.setSelected(profile.rb1.getModel(), true);
break;
case 2:
profile.buttonGroup4.setSelected(profile.rb2.getModel(), true);
break;
case 3:
profile.buttonGroup4.setSelected(profile.rb3.getModel(), true);
break;
case 4:
profile.buttonGroup4.setSelected(profile.rb4.getModel(), true);
break;
default:
profile.buttonGroup4.clearSelection();
break;
}
profile.check1.setSelected(casesensitive);
profile.check4.setSelected(notresponse);
profile.check71.setSelected(iscontenttype);
profile.check72.setSelected(isresponsecode);
profile.negativeCT.setSelected(negativect);
profile.negativeRC.setSelected(negativerc);
profile.text4.setText(issuename);
profile.textarea1.setText(issuedetail);
profile.textarea2.setText(issuebackground);
profile.textarea3.setText(remediationdetail);
profile.textarea4.setText(remediationbackground);
profile.sp1.setValue(maxRedir);
profile.text73.setText(urlextension);
profile.check73.setSelected(isurlextension);
profile.negativeURL.setSelected(NegativeUrlExtension);
switch (issueseverity) {
case "High":
profile.buttonGroup5.setSelected(profile.radio5.getModel(), true);
break;
case "Medium":
profile.buttonGroup5.setSelected(profile.radio6.getModel(), true);
break;
case "Low":
profile.buttonGroup5.setSelected(profile.radio7.getModel(), true);
break;
case "Information":
profile.buttonGroup5.setSelected(profile.radio8.getModel(), true);
break;
default:
break;
}
switch (issueconfidence) {
case "Certain":
profile.buttonGroup6.setSelected(profile.radio9.getModel(), true);
break;
case "Firm":
profile.buttonGroup6.setSelected(profile.radio10.getModel(), true);
break;
case "Tentative":
profile.buttonGroup6.setSelected(profile.radio11.getModel(), true);
break;
default:
break;
}
} catch (Exception e) {
System.out.println("ProfilesManager line 1015:" + e.getMessage());
for (StackTraceElement element : e.getStackTrace()) {
System.out.println(element);
}
}
}
public void saveResponseAttackValues(ResponseProfile profile) {
//Save attack with fields values
try {
//get GUI values
ProfilesProperties newfile = new ProfilesProperties();
newfile.setProfileName(profile.text1.getText());
newfile.setAuthor(profile.textauthor.getText());
newfile.setScanner(2);
newfile.setEnabled(true);
List greps = new ArrayList();
List tags = new ArrayList();
newfile.setGrepsFile(profile.textgreps.getText());
for (int i = 0; i < profile.modelgrep.getRowCount(); i++) {
if (!profile.modelgrep.getValueAt(i, 2).toString().isEmpty()) {
greps.add(profile.modelgrep.getValueAt(i, 0).toString() + "," + profile.modelgrep.getValueAt(i, 1).toString() + "," + profile.modelgrep.getValueAt(i, 2).toString());
}
}
newfile.setGreps(greps);
for (int i = 0; i < profile.listtag.getModel().getSize(); i++) {
Object item = profile.listtag.getModel().getElementAt(i);
if (!item.toString().isEmpty()) {
tags.add(item.toString().replaceAll("\r", "").replaceAll("\n", ""));
}
}
if (!tags.contains("All")) {
tags.add("All");
newfile.setTags(tags);
} else {
newfile.setTags(tags);
}
if (profile.radio4.isSelected()) {
newfile.setMatchType(1);
} else if (profile.radio3.isSelected()) {
newfile.setMatchType(2);
} else {
newfile.setMatchType(0);
}
if (profile.first_match.isSelected()) {
newfile.setScope(1);
} else if (profile.all_matches.isSelected()) {
newfile.setScope(2);
}
newfile.setExcludeHTTP(profile.excludehttp.isSelected());
newfile.setOnlyHTTP(profile.onlyhttp.isSelected());
newfile.setContentType(profile.text71.getText());
newfile.setResponseCode(profile.text72.getText());
newfile.setURLExtension(profile.text73.getText());
newfile.setIsURLExtension(profile.check73.isSelected());
newfile.setNegativeURLExtension(profile.negativeURL.isSelected());
if (profile.rb1.isSelected()) {
newfile.setRedirType(1);
} else if (profile.rb2.isSelected()) {
newfile.setRedirType(2);
} else if (profile.rb3.isSelected()) {
newfile.setRedirType(3);
} else if (profile.rb4.isSelected()) {
newfile.setRedirType(4);
} else {
newfile.setRedirType(0);
}
newfile.setCaseSensitive(profile.check1.isSelected());
newfile.setNotResponse(profile.check4.isSelected());
newfile.setIsContentType(profile.check71.isSelected());
newfile.setIsResponseCode(profile.check72.isSelected());
newfile.setNegativeCT(profile.negativeCT.isSelected());
newfile.setNegativeRC(profile.negativeRC.isSelected());
newfile.setIssueName(profile.text4.getText());
newfile.setIssueDetail(profile.textarea1.getText());
newfile.setIssueBackground(profile.textarea2.getText());
newfile.setRemediationDetail(profile.textarea3.getText());
newfile.setRemediationBackground(profile.textarea4.getText());
newfile.setMaxRedir((Integer) profile.sp1.getValue());
if (profile.radio5.isSelected()) {
newfile.setIssueSeverity("High");
} else if (profile.radio6.isSelected()) {
newfile.setIssueSeverity("Medium");
} else if (profile.radio7.isSelected()) {
newfile.setIssueSeverity("Low");
} else if (profile.radio8.isSelected()) {
newfile.setIssueSeverity("Information");
} else {
newfile.setIssueSeverity("");
}
if (profile.radio9.isSelected()) {
newfile.setIssueConfidence("Certain");
} else if (profile.radio10.isSelected()) {
newfile.setIssueConfidence("Firm");
} else if (profile.radio11.isSelected()) {
newfile.setIssueConfidence("Tentative");
} else {
newfile.setIssueConfidence("");
}
//Save start
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
JsonArray ijson = new JsonArray();
List<ProfilesProperties> newjson = gson.fromJson(ijson, new TypeToken<List<ProfilesProperties>>() {
}.getType());
newjson.add(newfile);
String json = gson.toJson(newjson);
//Write JSON String to file
FileOutputStream fileStream;
fileStream = new FileOutputStream(new File(profiles_directory + File.separator + profile.text1.getText().concat(".bb")));
OutputStreamWriter writer = new OutputStreamWriter(fileStream, "UTF-8");
writer.write(json);
writer.close();
} catch (IOException e) {
System.out.println("ProfilesManager line 1149:");
for (StackTraceElement element : e.getStackTrace()) {
System.out.println(element);
}
}
}
public void setRequestAttackValues(String profile_name,JsonArray passivereqprofiles, RequestProfile profile) {
//Set Attack values when select from main combobox
try {
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
JsonArray json = passivereqprofiles;
ProfilesProperties profile_property = new ProfilesProperties();
if (json != null) {
for (JsonElement pa : json) {
JsonObject bbObj = pa.getAsJsonObject();
if (bbObj.get("ProfileName").getAsString().equals(profile_name)) {
profile_property = gson.fromJson(bbObj.toString(), ProfilesProperties.class
);
}
}
}
name = profile_property.getProfileName();
casesensitive = profile_property.getCaseSensitive();
notresponse = profile_property.getNotResponse();
matchtype = profile_property.getMatchType();
issuename = profile_property.getIssueName();
issueseverity = profile_property.getIssueSeverity();
issueconfidence = profile_property.getIssueConfidence();
issuedetail = profile_property.getIssueDetail();
issuebackground = profile_property.getIssueBackground();
remediationdetail = profile_property.getRemediationDetail();
remediationbackground = profile_property.getRemediationBackground();
iscontenttype = profile_property.getIsContentType();
isresponsecode = profile_property.getIsResponseCode();
contenttype = profile_property.getContentType();
responsecode = profile_property.getResponseCode();
excludeHTTP = profile_property.getExcludeHTTP();
onlyHTTP = profile_property.getOnlyHTTP();
negativect = profile_property.getNegativeCT();
negativerc = profile_property.getNegativeRC();
redirtype = profile_property.getRedirection();
maxRedir = profile_property.getMaxRedir();
grepsfile = profile_property.getgrepsFile();
payloadPosition = profile_property.getPayloadPosition();
author = profile_property.getAuthor();
contentLength = profile_property.getContentLength();
isurlextension = profile_property.getIsURLExtension();
urlextension = profile_property.getURLExtension();
NegativeUrlExtension = profile_property.getNegativeURLExtension();
Scanas = profile_property.getScanAs();
Scantype = profile_property.getScanType();
profile.textauthor.setText(author);
profile.text1.setText(name);
profile.grep.removeAllElements();
profile.tag.removeAllElements();
profile.textgreps.setText(grepsfile);
profile.showGreps(profile_property.getGreps());
if (profile_property.getTags() != null) {
for (String t : profile_property.getTags()) {
profile.tag.addElement(t);
}
}
switch (matchtype) {
case 1:
profile.buttonGroup3.setSelected(profile.radio4.getModel(), true);
break;
case 2:
profile.buttonGroup3.setSelected(profile.radio3.getModel(), true);
break;
default:
profile.buttonGroup3.clearSelection();
break;
}
profile.check1.setSelected(casesensitive);
profile.check4.setSelected(notresponse);
profile.text4.setText(issuename);
profile.textarea1.setText(issuedetail);
profile.textarea2.setText(issuebackground);
profile.textarea3.setText(remediationdetail);
profile.textarea4.setText(remediationbackground);
profile.text73.setText(urlextension);
profile.check73.setSelected(isurlextension);
profile.negativeURL.setSelected(NegativeUrlExtension);
switch (issueseverity) {
case "High":
profile.buttonGroup5.setSelected(profile.radio5.getModel(), true);
break;
case "Medium":
profile.buttonGroup5.setSelected(profile.radio6.getModel(), true);
break;
case "Low":
profile.buttonGroup5.setSelected(profile.radio7.getModel(), true);
break;
case "Information":
profile.buttonGroup5.setSelected(profile.radio8.getModel(), true);
break;
default:
break;
}
switch (issueconfidence) {
case "Certain":
profile.buttonGroup6.setSelected(profile.radio9.getModel(), true);
break;
case "Firm":
profile.buttonGroup6.setSelected(profile.radio10.getModel(), true);
break;
case "Tentative":
profile.buttonGroup6.setSelected(profile.radio11.getModel(), true);
break;
default:
break;
}
} catch (Exception e) {
System.out.println("ProfilesManager line 1286:" + e.getMessage());
for (StackTraceElement element : e.getStackTrace()) {
System.out.println(element);
}
}
}
public void saveRequestAttackValues(RequestProfile profile) {
//Save attack with fields values
try {
//get GUI values
ProfilesProperties newfile = new ProfilesProperties();
newfile.setProfileName(profile.text1.getText());
newfile.setAuthor(profile.textauthor.getText());
newfile.setScanner(3);
newfile.setEnabled(true);
List greps = new ArrayList();
List tags = new ArrayList();
newfile.setGrepsFile(profile.textgreps.getText());
for (int i = 0; i < profile.modelgrep.getRowCount(); i++) {
if (!profile.modelgrep.getValueAt(i, 4).toString().isEmpty()) {
greps.add(profile.modelgrep.getValueAt(i, 0).toString() + "," + profile.modelgrep.getValueAt(i, 1).toString() + "," + profile.modelgrep.getValueAt(i, 2).toString() + "," + profile.modelgrep.getValueAt(i, 3).toString() + "," + profile.modelgrep.getValueAt(i, 4).toString());
}
}
newfile.setGreps(greps);
for (int i = 0; i < profile.listtag.getModel().getSize(); i++) {
Object item = profile.listtag.getModel().getElementAt(i);
if (!item.toString().isEmpty()) {
tags.add(item.toString().replaceAll("\r", "").replaceAll("\n", ""));
}
}
if (!tags.contains("All")) {
tags.add("All");
newfile.setTags(tags);
} else {
newfile.setTags(tags);
}
newfile.setURLExtension(profile.text73.getText());
if (profile.radio4.isSelected()) {
newfile.setMatchType(1);
} else if (profile.radio3.isSelected()) {
newfile.setMatchType(2);
} else {
newfile.setMatchType(0);
}
newfile.setVariationAttributes(variationAttributes);
newfile.setCaseSensitive(profile.check1.isSelected());
newfile.setNotResponse(profile.check4.isSelected());
newfile.setIsURLExtension(profile.check73.isSelected());
newfile.setNegativeURLExtension(profile.negativeURL.isSelected());
newfile.setIssueName(profile.text4.getText());
newfile.setIssueDetail(profile.textarea1.getText());
newfile.setIssueBackground(profile.textarea2.getText());
newfile.setRemediationDetail(profile.textarea3.getText());
newfile.setRemediationBackground(profile.textarea4.getText());
if (profile.radio5.isSelected()) {
newfile.setIssueSeverity("High");
} else if (profile.radio6.isSelected()) {
newfile.setIssueSeverity("Medium");
} else if (profile.radio7.isSelected()) {
newfile.setIssueSeverity("Low");
} else if (profile.radio8.isSelected()) {
newfile.setIssueSeverity("Information");
} else {
newfile.setIssueSeverity("");
}
if (profile.radio9.isSelected()) {
newfile.setIssueConfidence("Certain");
} else if (profile.radio10.isSelected()) {
newfile.setIssueConfidence("Firm");
} else if (profile.radio11.isSelected()) {
newfile.setIssueConfidence("Tentative");
} else {
newfile.setIssueConfidence("");
}
//Save start
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
JsonArray ijson = new JsonArray();
List<ProfilesProperties> newjson = gson.fromJson(ijson, new TypeToken<List<ProfilesProperties>>() {
}.getType());
newjson.add(newfile);
String json = gson.toJson(newjson);
//Write JSON String to file
FileOutputStream fileStream;
fileStream = new FileOutputStream(new File(profiles_directory + File.separator + profile.text1.getText().concat(".bb")));
OutputStreamWriter writer = new OutputStreamWriter(fileStream, "UTF-8");
writer.write(json);
writer.close();
} catch (IOException e) {
System.out.println("ProfilesManager line 1403:");
for (StackTraceElement element : e.getStackTrace()) {
System.out.println(element);
}
}
}
public void setSelectedVariations(boolean state, ActiveProfile profile) {
profile.status_code.setSelected(state);
profile.input_image_labels.setSelected(state);
profile.non_hidden_form_input_types.setSelected(state);
profile.page_title.setSelected(state);
profile.visible_text.setSelected(state);
profile.button_submit_labels.setSelected(state);
profile.div_ids.setSelected(state);
profile.word_count.setSelected(state);
profile.content_type.setSelected(state);
profile.outbound_edge_tag_names.setSelected(state);
profile.whole_body_content.setSelected(state);
profile.etag_header.setSelected(state);
profile.visible_word_count.setSelected(state);
profile.content_length.setSelected(state);
profile.header_tags.setSelected(state);
profile.tag_ids.setSelected(state);
profile.comments.setSelected(state);
profile.line_count.setSelected(state);
profile.set_cookie_names.setSelected(state);
profile.last_modified_header.setSelected(state);
profile.first_header_tag.setSelected(state);
profile.tag_names.setSelected(state);
profile.input_submit_labels.setSelected(state);
profile.outbound_edge_count.setSelected(state);
profile.initial_body_content.setSelected(state);
profile.content_location.setSelected(state);
profile.limited_body_content.setSelected(state);
profile.canonical_link.setSelected(state);
profile.css_classes.setSelected(state);
profile.location.setSelected(state);
profile.anchor_labels.setSelected(state);
}
public void loadPath(String file, DefaultListModel list) {
//Load file for implement payloads
DefaultListModel List = list;
String line;
File fileload = new File(file);
try {
BufferedReader bufferreader = new BufferedReader(new FileReader(fileload.getAbsolutePath()));
line = bufferreader.readLine();
while (line != null) {
List.addElement(line);
line = bufferreader.readLine();
}
bufferreader.close();
} catch (FileNotFoundException ex) {
System.out.println("ProfilesManager line 1912:" + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
} catch (IOException ex) {
System.out.println("ProfilesManager line 1815:" + ex.getMessage());
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
}
}
public void updatePayloads(String file, ProfilesProperties issue) {
//Load file for implement payloads
List payloads = new ArrayList();
String line;
File fileload = new File(file);
try {
BufferedReader bufferreader = new BufferedReader(new FileReader(fileload.getAbsolutePath()));
line = bufferreader.readLine();
while (line != null) {
payloads.add(line);
line = bufferreader.readLine();
}
bufferreader.close();
issue.setPayloads(payloads);
GsonBuilder builder = new GsonBuilder().setPrettyPrinting();
Gson gson = builder.create();
String strJson = gson.toJson(issue);
FileWriter writer = null;
writer = new FileWriter(profiles_directory + File.separator + issue.getProfileName().concat(".bb"));
writer.write("[" + strJson + "]");
writer.close();
} catch (FileNotFoundException ex) {
System.out.println("ProfilesManager line 1639:");
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
} catch (IOException ex) {
System.out.println("ProfilesManager line 1042:");
for (StackTraceElement element : ex.getStackTrace()) {
System.out.println(element);
}
}
}
} |
Java | BurpBounty/main/java/burpbountyfree/ProfilesProperties.java | /*
Copyright 2018 Eduardo Garcia Melia <wagiro@gmail.com>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package burpbountyfree;
import java.util.ArrayList;
import java.util.List;
public class ProfilesProperties {
private String ProfileName;
private String Name = "";
private boolean Enabled = false;
private int Scanner = 0;
private String Author = "";
private List<String> Payloads = new ArrayList();
private List<String> Encoder = new ArrayList();
private boolean UrlEncode = false;
private String CharsToUrlEncode = "";
private List<String> Grep = new ArrayList();
private List<String> Tags = new ArrayList();
private boolean PayloadResponse = false;
private boolean NotResponse = false;
private String TimeOut1 = "";
private String TimeOut2 = "";
private boolean isTime = false;
private String contentLength = "";
private boolean iscontentLength = false;
private boolean CaseSensitive = false;
private boolean ExcludeHTTP = false;
private boolean OnlyHTTP = false;
private boolean IsContentType = false;
private String ContentType = "";
private String HttpResponseCode = "";
private boolean NegativeCT = false;
private boolean IsResponseCode = false;
private String ResponseCode = "";
private boolean NegativeRC = false;
private String urlextension = "";
private boolean isurlextension = false;
private boolean NegativeUrlExtension = false;
private int MatchType = 0;
private int Scope = 0;
private int RedirType = 0;
private int MaxRedir = 0;
private int payloadPosition;
private String payloadsFile = "";
private String grepsFile = "";
private String IssueName = "";
private String IssueSeverity = "";
private String IssueConfidence = "";
private String IssueDetail = "";
private String RemediationDetail = "";
private String IssueBackground = "";
private String RemediationBackground = "";
private List<Headers> Header = new ArrayList();
private List<String> VariationAttributes = new ArrayList();
private List<Integer> InsertionPointType = new ArrayList();
private Boolean Scanas = false;
private int Scantype = 0;
private boolean pathDiscovery = false;
public ProfilesProperties() {
super();
}
public String getProfileName() {
return ProfileName;
}
public String getName() {
return Name;
}
public List<Headers> getHeader() {
return Header;
}
public List<String> getVariationAttributes() {
return VariationAttributes;
}
public List<Integer> getInsertionPointType() {
return InsertionPointType;
}
public String getAuthor() {
return Author;
}
public boolean getEnabled() {
return Enabled;
}
public boolean getScanAs() {
return Scanas;
}
public int getScanner() {
return Scanner;
}
public int getScanType() {
return Scantype;
}
public int getPayloadPosition() {
return payloadPosition;
}
public List<String> getPayloads() {
return Payloads;
}
public List<String> getEncoder() {
return Encoder;
}
public String getCharsToUrlEncode() {
return CharsToUrlEncode;
}
public String getpayloadsFile() {
return payloadsFile;
}
public String getgrepsFile() {
return grepsFile;
}
public List<String> getGreps() {
return Grep;
}
public List<String> getTags() {
return Tags;
}
public boolean getCaseSensitive() {
return CaseSensitive;
}
public boolean getPayloadResponse() {
return PayloadResponse;
}
public boolean getNotResponse() {
return NotResponse;
}
public boolean getExcludeHTTP() {
return ExcludeHTTP;
}
public boolean getOnlyHTTP() {
return OnlyHTTP;
}
public boolean getIsContentType() {
return IsContentType;
}
public String getContentType() {
return ContentType;
}
public String getHttpResponseCode() {
return HttpResponseCode;
}
public String getTime1() {
return TimeOut1;
}
public String getTime2() {
return TimeOut2;
}
public boolean getIsTime() {
return isTime;
}
public boolean getPathDiscover() {
return pathDiscovery;
}
public String getContentLength() {
return contentLength;
}
public boolean getIsContentLength() {
return iscontentLength;
}
public boolean getNegativeCT() {
return NegativeCT;
}
public boolean getIsResponseCode() {
return IsResponseCode;
}
public String getResponseCode() {
return ResponseCode;
}
public boolean getNegativeRC() {
return NegativeRC;
}
public boolean getIsURLExtension() {
return isurlextension;
}
public String getURLExtension() {
return urlextension;
}
public boolean getNegativeURLExtension() {
return NegativeUrlExtension;
}
public boolean getUrlEncode() {
return UrlEncode;
}
public int getMatchType() {
return MatchType;
}
public int getScope() {
return Scope;
}
public int getRedirection() {
return RedirType;
}
public int getMaxRedir() {
return MaxRedir;
}
public String getIssueName() {
return IssueName;
}
public String getIssueSeverity() {
return IssueSeverity;
}
public String getIssueConfidence() {
return IssueConfidence;
}
public String getIssueDetail() {
return IssueDetail;
}
public String getIssueBackground() {
return IssueBackground;
}
public String getRemediationDetail() {
return RemediationDetail;
}
public String getRemediationBackground() {
return RemediationBackground;
}
//Set functions
public void setProfileName(String profilename) {
ProfileName = profilename;
}
public void setHeader(List<Headers> header) {
Header = header;
}
public void setVariationAttributes(List<String> variationAttributes) {
VariationAttributes = variationAttributes;
}
public void setInsertionPointType(List<Integer> insertionPointType) {
InsertionPointType = insertionPointType;
}
public void setAuthor(String author) {
Author = author;
}
public void setEnabled(boolean enabled) {
Enabled = enabled;
}
public void setScanAs(boolean scanas) {
Scanas = scanas;
}
public void setScanner(int scanner) {
Scanner = scanner;
}
public void setScanType(int scantype) {
Scantype = scantype;
}
public void setPayloadPosition(int payloadposition) {
payloadPosition = payloadposition;
}
public void setPayloads(List<String> payloads) {
Payloads = payloads;
}
public void setEncoder(List<String> encoder) {
Encoder = encoder;
}
public void setCharsToUrlEncode(String charstourlencode) {
CharsToUrlEncode = charstourlencode;
}
public void setPayloadsFile(String payloadsfile) {
payloadsFile = payloadsfile;
}
public void setGrepsFile(String grepsfile) {
grepsFile = grepsfile;
}
public void setGreps(List<String> grep) {
Grep = grep;
}
public void setTags(List<String> tags) {
Tags = tags;
}
public void setPathDiscovery(boolean pathdiscovery) {
pathDiscovery = pathdiscovery;
}
public void setCaseSensitive(boolean casesensitive) {
CaseSensitive = casesensitive;
}
public void setPayloadResponse(boolean payloadresponse) {
PayloadResponse = payloadresponse;
}
public void setNotResponse(boolean notresponse) {
NotResponse = notresponse;
}
public void setOnlyHTTP(boolean onlyHTTP) {
OnlyHTTP = onlyHTTP;
}
public void setExcludeHTTP(boolean excludeHTTP) {
ExcludeHTTP = excludeHTTP;
}
public void setIsContentType(boolean iscontenttype) {
IsContentType = iscontenttype;
}
public void setTime1(String timeout1) {
TimeOut1 = timeout1;
}
public void setTime2(String timeout2) {
TimeOut2 = timeout2;
}
public void setIsTime(boolean istime) {
isTime = istime;
}
public void setContentLength(String contentlength) {
contentLength = contentlength;
}
public void setHttpResponseCode(String httpresponsecode) {
HttpResponseCode = httpresponsecode;
}
public void setIsContentLength(boolean iscontentlength) {
iscontentLength = iscontentlength;
}
public void setURLExtension(String urlExtension) {
urlextension = urlExtension;
}
public void setIsURLExtension(boolean isurlExtension) {
isurlextension = isurlExtension;
}
public void setNegativeURLExtension(boolean negativeurlextension) {
NegativeUrlExtension = negativeurlextension;
}
public void setContentType(String contenttype) {
ContentType = contenttype;
}
public void setNegativeCT(boolean negativect) {
NegativeCT = negativect;
}
public void setIsResponseCode(boolean isresponsecode) {
IsResponseCode = isresponsecode;
}
public void setResponseCode(String responsecode) {
ResponseCode = responsecode;
}
public void setNegativeRC(boolean negativerc) {
NegativeRC = negativerc;
}
public void setUrlEncode(boolean urlencode) {
UrlEncode = urlencode;
}
public void setMatchType(int matchtype) {
MatchType = matchtype;
}
public void setScope(int scope) {
Scope = scope;
}
public void setRedirType(int redirtype) {
RedirType = redirtype;
}
public void setMaxRedir(int maxredir) {
MaxRedir = maxredir;
}
public void setIssueName(String issuename) {
IssueName = issuename;
}
public void setIssueSeverity(String issueseverity) {
IssueSeverity = issueseverity;
}
public void setIssueConfidence(String issueconfidence) {
IssueConfidence = issueconfidence;
}
public void setIssueDetail(String issuedetail) {
IssueDetail = issuedetail;
}
public void setIssueBackground(String issuebackground) {
IssueBackground = issuebackground;
}
public void setRemediationDetail(String remediationdetail) {
RemediationDetail = remediationdetail;
}
public void setRemediationBackground(String remediationbackground) {
RemediationBackground = remediationbackground;
}
} |
BurpBounty/main/java/burpbountyfree/RequestProfile.form | <?xml version="1.0" encoding="UTF-8" ?>
<Form version="1.8" maxVersion="1.9" type="org.netbeans.modules.form.forminfo.JPanelFormInfo">
<NonVisualComponents>
<Component class="javax.swing.ButtonGroup" name="buttonGroup1">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup2">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup3">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup4">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup5">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup6">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup7">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</NonVisualComponents>
<AuxValues>
<AuxValue name="FormSettings_autoResourcing" type="java.lang.Integer" value="0"/>
<AuxValue name="FormSettings_autoSetComponentName" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_generateFQN" type="java.lang.Boolean" value="true"/>
<AuxValue name="FormSettings_generateMnemonicsCode" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_i18nAutoMode" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_layoutCodeTarget" type="java.lang.Integer" value="1"/>
<AuxValue name="FormSettings_listenerGenerationStyle" type="java.lang.Integer" value="0"/>
<AuxValue name="FormSettings_variablesLocal" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_variablesModifier" type="java.lang.Integer" value="2"/>
</AuxValues>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel12" min="-2" max="-2" attributes="0"/>
<EmptySpace type="unrelated" max="-2" attributes="0"/>
<Component id="text1" max="32767" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jLabel18" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="textauthor" min="-2" pref="165" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="64" max="-2" attributes="0"/>
</Group>
<Component id="headerstab" alignment="0" pref="831" max="32767" attributes="0"/>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="text1" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="jLabel12" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="textauthor" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="jLabel18" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="headerstab" min="-2" max="-2" attributes="0"/>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JTextField" name="text1">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="0"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel18">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="0"/>
</Property>
<Property name="text" type="java.lang.String" value="Author:"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel12">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="0"/>
</Property>
<Property name="text" type="java.lang.String" value="Name:"/>
</Properties>
</Component>
<Component class="javax.swing.JTextField" name="textauthor">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="0"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Container class="javax.swing.JTabbedPane" name="headerstab">
<Properties>
<Property name="autoscrolls" type="boolean" value="true"/>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="0"/>
</Property>
<Property name="preferredSize" type="java.awt.Dimension" editor="org.netbeans.beaninfo.editors.DimensionEditor">
<Dimension value="[800, 600]"/>
</Property>
</Properties>
<Events>
<EventHandler event="stateChanged" listener="javax.swing.event.ChangeListener" parameters="javax.swing.event.ChangeEvent" handler="headerstabStateChanged"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout"/>
<SubComponents>
<Container class="javax.swing.JScrollPane" name="jScrollPane6">
<Properties>
<Property name="horizontalScrollBarPolicy" type="int" value="31"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_InitCodePost" type="java.lang.String" value="jScrollPane6.getVerticalScrollBar().setUnitIncrement(20);"/>
</AuxValues>
<Constraints>
<Constraint layoutClass="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout" value="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout$JTabbedPaneConstraintsDescription">
<JTabbedPaneConstraints tabName=" Request ">
<Property name="tabTitle" type="java.lang.String" value=" Request "/>
</JTabbedPaneConstraints>
</Constraint>
</Constraints>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Container class="javax.swing.JPanel" name="jPanel11">
<Properties>
<Property name="autoscrolls" type="boolean" value="true"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_LayoutCodePost" type="java.lang.String" value="JScrollPane responseresScroll = new JScrollPane(jPanel11, 
 JScrollPane.VERTICAL_SCROLLBAR_ALWAYS, JScrollPane.HORIZONTAL_SCROLLBAR_NEVER);"/>
</AuxValues>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jSeparator6" alignment="1" max="32767" attributes="0"/>
<Group type="102" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="103" alignment="0" groupAlignment="1" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Component id="button8" min="-2" pref="89" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="textgreps" min="-2" pref="598" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="9" max="-2" attributes="0"/>
<Component id="jLabel5" min="-2" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="1" attributes="0">
<Group type="103" groupAlignment="1" max="-2" attributes="0">
<Component id="button18" alignment="0" min="-2" pref="89" max="-2" attributes="0"/>
<Component id="button10" alignment="0" max="32767" attributes="0"/>
<Component id="button7" alignment="0" min="-2" pref="89" max="-2" attributes="0"/>
<Component id="button19" alignment="1" min="-2" pref="89" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<Component id="jLabel1" min="-2" max="-2" attributes="0"/>
<EmptySpace type="unrelated" min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="radio4" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="radio3" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<Component id="jScrollPane14" min="-2" pref="598" max="-2" attributes="0"/>
</Group>
<EmptySpace pref="132" max="32767" attributes="0"/>
</Group>
</Group>
<Group type="102" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Component id="check73" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="text73" min="-2" pref="579" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="negativeURL" min="-2" max="-2" attributes="0"/>
</Group>
<Component id="jLabel31" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel30" alignment="0" min="-2" pref="769" max="-2" attributes="0"/>
<Component id="check4" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="check1" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel25" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel24" alignment="0" min="-2" pref="769" max="-2" attributes="0"/>
</Group>
<EmptySpace pref="42" max="32767" attributes="0"/>
</Group>
</Group>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel25" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel24" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="textgreps" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="button8" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="32767" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jScrollPane14" min="-2" pref="206" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<Component id="button19" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="button7" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="button10" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="button18" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="radio4" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="jLabel1" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="radio3" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Component id="jLabel5" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jSeparator6" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel31" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel30" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Component id="check4" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="check1" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="check73" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="text73" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="negativeURL" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace pref="58" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JRadioButton" name="radio4">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup3"/>
</Property>
<Property name="text" type="java.lang.String" value="Simple string"/>
</Properties>
<Events>
<EventHandler event="itemStateChanged" listener="java.awt.event.ItemListener" parameters="java.awt.event.ItemEvent" handler="radio4stringMatchType"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio3">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup3"/>
</Property>
<Property name="text" type="java.lang.String" value="Regex"/>
</Properties>
<Events>
<EventHandler event="itemStateChanged" listener="java.awt.event.ItemListener" parameters="java.awt.event.ItemEvent" handler="radio3regexMatchType"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="check4">
<Properties>
<Property name="text" type="java.lang.String" value="Negative match"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="check1">
<Properties>
<Property name="text" type="java.lang.String" value="Case sensitive"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel24">
<Properties>
<Property name="text" type="java.lang.String" value="You can define one or more greps."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel25">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Grep"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel30">
<Properties>
<Property name="text" type="java.lang.String" value="These settings can be used to specify grep options of your profile."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel31">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Grep Options"/>
</Properties>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator6">
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane14">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTable" name="table4">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="13" style="0"/>
</Property>
<Property name="model" type="javax.swing.table.TableModel" editor="org.netbeans.modules.form.RADConnectionPropertyEditor">
<Connection code="modelgrep" type="code"/>
</Property>
<Property name="showGrid" type="boolean" value="false"/>
<Property name="tableHeader" type="javax.swing.table.JTableHeader" editor="org.netbeans.modules.form.editors2.JTableHeaderEditor">
<TableHeader reorderingAllowed="true" resizingAllowed="true"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_SerializeTo" type="java.lang.String" value="BurpBountyGui_table4"/>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JButton" name="button18">
<Properties>
<Property name="text" type="java.lang.String" value="Remove"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button18removeMatchReplace"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="button10">
<Properties>
<Property name="text" type="java.lang.String" value="Clear"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button10removeAllGrep"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="button7">
<Properties>
<Property name="text" type="java.lang.String" value="Paste"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button7pasteGrep"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="button19">
<Properties>
<Property name="text" type="java.lang.String" value="Add"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button19addGrep"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="button8">
<Properties>
<Property name="text" type="java.lang.String" value="Load File"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button8loadGrep"/>
</Events>
</Component>
<Component class="javax.swing.JTextField" name="textgreps">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel1">
<Properties>
<Property name="text" type="java.lang.String" value="Match Type: "/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel5">
<Properties>
<Property name="text" type="java.lang.String" value="<html> * More info about insertion points at <a href=\u005c\u005c\u005c"\u005c\u005c\u005c">Burp Suite Extender API</a></html>" containsInvalidXMLChars="true"/>
</Properties>
<Events>
<EventHandler event="mouseClicked" listener="java.awt.event.MouseListener" parameters="java.awt.event.MouseEvent" handler="goweb"/>
</Events>
</Component>
<Component class="javax.swing.JCheckBox" name="check73">
<Properties>
<Property name="text" type="java.lang.String" value="URL Extension"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JTextField" name="text73">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="negativeURL">
<Properties>
<Property name="text" type="java.lang.String" value="Negative match"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
</SubComponents>
</Container>
<Container class="javax.swing.JScrollPane" name="jScrollPane10">
<AuxValues>
<AuxValue name="JavaCodeGenerator_InitCodePre" type="java.lang.String" value="jScrollPane10.getVerticalScrollBar().setUnitIncrement(20);"/>
</AuxValues>
<Constraints>
<Constraint layoutClass="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout" value="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout$JTabbedPaneConstraintsDescription">
<JTabbedPaneConstraints tabName=" Issue ">
<Property name="tabTitle" type="java.lang.String" value=" Issue "/>
</JTabbedPaneConstraints>
</Constraint>
</Constraints>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Container class="javax.swing.JPanel" name="jPanel12">
<Properties>
<Property name="autoscrolls" type="boolean" value="true"/>
</Properties>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jSeparator7" max="32767" attributes="0"/>
<Component id="jSeparator8" alignment="1" max="32767" attributes="0"/>
<Component id="jSeparator9" alignment="1" max="32767" attributes="0"/>
<Component id="jSeparator10" alignment="1" max="32767" attributes="0"/>
<Group type="102" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel33" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel35" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel34" alignment="0" min="-2" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel13" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jScrollPane7" min="-2" pref="612" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel9" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jScrollPane1" min="-2" pref="612" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel15" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jScrollPane9" min="-2" pref="612" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel14" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jScrollPane8" min="-2" pref="612" max="-2" attributes="0"/>
</Group>
<Component id="jLabel37" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel36" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel39" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel38" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel32" alignment="0" min="-2" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel3" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel4" min="-2" pref="73" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Component id="radio8" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="189" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="radio6" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="radio7" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="radio5" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="32767" attributes="0"/>
<Component id="jLabel7" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="radio9" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="radio11" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="radio10" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
</Group>
<Component id="text4" alignment="0" min="-2" pref="419" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
</Group>
<Component id="jLabel41" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel40" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace pref="93" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="10" max="-2" attributes="0"/>
<Component id="jLabel33" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel32" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel3" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="text4" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel7" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="radio9" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Component id="radio10" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="radio11" min="-2" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel4" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="radio5" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Component id="radio6" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="radio7" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="radio8" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jSeparator7" min="-2" pref="10" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel35" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel34" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel9" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jScrollPane1" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Component id="jSeparator8" min="-2" pref="10" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel37" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel36" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel13" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jScrollPane7" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jSeparator9" min="-2" pref="10" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel39" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel38" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel15" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jScrollPane9" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Component id="jSeparator10" min="-2" pref="10" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel41" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel40" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel14" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jScrollPane8" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JLabel" name="jLabel32">
<Properties>
<Property name="text" type="java.lang.String" value="You can define the issue properties."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel33">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Issue Properties"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel3">
<Properties>
<Property name="text" type="java.lang.String" value="Issue Name:"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel4">
<Properties>
<Property name="text" type="java.lang.String" value="Severity:"/>
</Properties>
</Component>
<Component class="javax.swing.JRadioButton" name="radio5">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup5"/>
</Property>
<Property name="text" type="java.lang.String" value="High"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio6">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup5"/>
</Property>
<Property name="text" type="java.lang.String" value="Medium"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio7">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup5"/>
</Property>
<Property name="text" type="java.lang.String" value="Low"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio8">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup5"/>
</Property>
<Property name="text" type="java.lang.String" value="Information"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel7">
<Properties>
<Property name="text" type="java.lang.String" value="Confidence:"/>
</Properties>
</Component>
<Component class="javax.swing.JRadioButton" name="radio9">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup6"/>
</Property>
<Property name="text" type="java.lang.String" value="Certain"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio10">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup6"/>
</Property>
<Property name="text" type="java.lang.String" value="Firm"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio11">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup6"/>
</Property>
<Property name="text" type="java.lang.String" value="Tentative"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JTextField" name="text4">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator7">
</Component>
<Component class="javax.swing.JLabel" name="jLabel34">
<Properties>
<Property name="text" type="java.lang.String" value="You can define the issue details."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel35">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Issue Detail"/>
</Properties>
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane7">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTextArea" name="textarea2">
<Properties>
<Property name="columns" type="int" value="20"/>
<Property name="rows" type="int" value="5"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JLabel" name="jLabel13">
<Properties>
<Property name="text" type="java.lang.String" value="Description:"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel36">
<Properties>
<Property name="text" type="java.lang.String" value="You can define the issue background."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel37">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Issue Background"/>
</Properties>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator8">
</Component>
<Component class="javax.swing.JLabel" name="jLabel38">
<Properties>
<Property name="text" type="java.lang.String" value="You can define the remediation detail."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel39">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Remediation Detail"/>
</Properties>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator9">
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane1">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTextArea" name="textarea1">
<Properties>
<Property name="columns" type="int" value="20"/>
<Property name="rows" type="int" value="5"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JLabel" name="jLabel9">
<Properties>
<Property name="text" type="java.lang.String" value="Description:"/>
</Properties>
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane8">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTextArea" name="textarea3">
<Properties>
<Property name="columns" type="int" value="20"/>
<Property name="rows" type="int" value="5"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JLabel" name="jLabel14">
<Properties>
<Property name="text" type="java.lang.String" value="Description:"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel40">
<Properties>
<Property name="text" type="java.lang.String" value="You can define the remediation background."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel41">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Remediation Background"/>
</Properties>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator10">
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane9">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTextArea" name="textarea4">
<Properties>
<Property name="columns" type="int" value="20"/>
<Property name="rows" type="int" value="5"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JLabel" name="jLabel15">
<Properties>
<Property name="text" type="java.lang.String" value="Description:"/>
</Properties>
</Component>
</SubComponents>
</Container>
</SubComponents>
</Container>
<Container class="javax.swing.JPanel" name="jPanel3">
<Constraints>
<Constraint layoutClass="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout" value="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout$JTabbedPaneConstraintsDescription">
<JTabbedPaneConstraints tabName=" Tags ">
<Property name="tabTitle" type="java.lang.String" value=" Tags "/>
</JTabbedPaneConstraints>
</Constraint>
</Constraints>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel47" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel46" alignment="0" min="-2" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="newTagb" alignment="0" max="32767" attributes="0"/>
<Component id="addTag" alignment="0" min="-2" pref="93" max="-2" attributes="0"/>
<Component id="removetag" alignment="0" max="32767" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="jScrollPane11" alignment="0" max="32767" attributes="0"/>
<Component id="newTagCombo" alignment="0" min="-2" pref="468" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="10" max="-2" attributes="0"/>
<Component id="jLabel47" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel46" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jScrollPane11" alignment="0" min="-2" pref="99" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<Component id="newTagb" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="removetag" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="newTagCombo" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="addTag" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JButton" name="removetag">
<Properties>
<Property name="text" type="java.lang.String" value="Remove"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="removetag"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="addTag">
<Properties>
<Property name="text" type="java.lang.String" value="Add"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="addTag"/>
</Events>
</Component>
<Component class="javax.swing.JComboBox" name="newTagCombo">
<AuxValues>
<AuxValue name="JavaCodeGenerator_SerializeTo" type="java.lang.String" value="BurpBountyGui_newTagCombo"/>
<AuxValue name="JavaCodeGenerator_TypeParameters" type="java.lang.String" value="<String>"/>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane11">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JList" name="listtag">
<Properties>
<Property name="model" type="javax.swing.ListModel" editor="org.netbeans.modules.form.RADConnectionPropertyEditor">
<Connection code="tag" type="code"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_TypeParameters" type="java.lang.String" value="<String>"/>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JLabel" name="jLabel46">
<Properties>
<Property name="text" type="java.lang.String" value="You can define one or multiple tags for this profile."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel47">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Set Tags"/>
</Properties>
</Component>
<Component class="javax.swing.JButton" name="newTagb">
<Properties>
<Property name="text" type="java.lang.String" value="New Tag"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="newTagbnewTag"/>
</Events>
</Component>
</SubComponents>
</Container>
</SubComponents>
</Container>
</SubComponents>
</Form> |
|
Java | BurpBounty/main/java/burpbountyfree/RequestProfile.java | /*
Copyright 2018 Eduardo Garcia Melia <wagiro@gmail.com>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package burpbountyfree;
import burp.IBurpExtenderCallbacks;
import java.awt.Desktop;
import java.awt.Toolkit;
import java.awt.datatransfer.Clipboard;
import java.awt.datatransfer.DataFlavor;
import java.awt.datatransfer.Transferable;
import java.awt.datatransfer.UnsupportedFlavorException;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.io.PrintWriter;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import javax.swing.DefaultCellEditor;
import javax.swing.DefaultListModel;
import javax.swing.JComboBox;
import javax.swing.JDialog;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JScrollPane;
import javax.swing.RowSorter;
import javax.swing.table.DefaultTableModel;
import javax.swing.table.TableModel;
import javax.swing.table.TableRowSorter;
/**
*
* @author eduardogarcia
*/
public class RequestProfile extends javax.swing.JPanel {
/**
* Creates new form RequestProfile
*/
DefaultListModel payload;
DefaultListModel grep;
DefaultListModel encoder;
DefaultListModel tag;
DefaultListModel tagmanager;
List<Headers> headers;
List<String> variationAttributes;
List<Integer> insertionPointType;
List<String> Tags;
Boolean pathDiscovery;
DefaultTableModel model;
DefaultTableModel model1;
DefaultTableModel model2;
DefaultTableModel model4;
DefaultTableModel model9;
DefaultTableModel model10;
DefaultTableModel rulemodel;
DefaultTableModel modelgrep;
IBurpExtenderCallbacks callbacks;
String filename;
public RequestProfile(IBurpExtenderCallbacks callbacks) {
payload = new DefaultListModel();
grep = new DefaultListModel();
encoder = new DefaultListModel();
tag = new DefaultListModel();
tagmanager = new DefaultListModel();
model4 = new DefaultTableModel();
model9 = new DefaultTableModel();
model10 = new DefaultTableModel();
modelgrep = new DefaultTableModel();
headers = new ArrayList();
variationAttributes = new ArrayList();
insertionPointType = new ArrayList();
this.callbacks = callbacks;
modelgrep = new DefaultTableModel() {
@Override
public Class<?> getColumnClass(int columnIndex) {
Class clazz = String.class;
switch (columnIndex) {
case 0:
clazz = Boolean.class;
break;
}
return clazz;
}
@Override
public boolean isCellEditable(int row, int column) {
if (row == 0 && column == 1) {
return false;
} else {
return true;
}
}
};
initComponents();
if (callbacks.loadExtensionSetting("filename") != null) {
filename = callbacks.loadExtensionSetting("filename") + File.separator;
} else {
filename = System.getProperty("user.home") + File.separator;
}
showTags();
showGrepsTable();
}
public String getClipboardContents() {
//Get clipboard contents for implement grep and match paste button
String result = "";
Clipboard clipboard = Toolkit.getDefaultToolkit().getSystemClipboard();
Transferable contents = clipboard.getContents(null);
boolean hasTransferableText = (contents != null) && contents.isDataFlavorSupported(DataFlavor.stringFlavor);
if (hasTransferableText) {
try {
result = (String) contents.getTransferData(DataFlavor.stringFlavor);
} catch (UnsupportedFlavorException | IOException ex) {
System.out.println("RequestProfile line 147: " + ex.getMessage());
}
}
return result;
}
public void swap(int a, int b) {
Object aObject = encoder.getElementAt(a);
Object bObject = encoder.getElementAt(b);
encoder.set(a, bObject);
encoder.set(b, aObject);
}
public void showGrepsTable() {
JComboBox operator = new JComboBox();
JComboBox ipt = new JComboBox();
JComboBox param = new JComboBox();
modelgrep.setNumRows(0);
modelgrep.setColumnCount(0);
modelgrep.addColumn("Enabled");
modelgrep.addColumn("Operator");
modelgrep.addColumn("Insertion point type");
modelgrep.addColumn("Parameter");
modelgrep.addColumn("Grep value");
table4.getColumnModel().getColumn(0).setPreferredWidth(25);
table4.getColumnModel().getColumn(1).setPreferredWidth(30);
table4.getColumnModel().getColumn(2).setPreferredWidth(100);
table4.getColumnModel().getColumn(3).setPreferredWidth(40);
table4.getColumnModel().getColumn(4).setPreferredWidth(200);
operator.addItem("Or");
operator.addItem("And");
ipt.addItem("All Request");
param.addItem("Value");
table4.getColumnModel().getColumn(1).setCellEditor(new DefaultCellEditor(operator));
table4.getColumnModel().getColumn(2).setCellEditor(new DefaultCellEditor(ipt));
table4.getColumnModel().getColumn(3).setCellEditor(new DefaultCellEditor(param));
TableRowSorter<TableModel> sorter = new TableRowSorter<>(table4.getModel());
table4.setRowSorter(sorter);
List<RowSorter.SortKey> sortKeys = new ArrayList<>();
sorter.setSortKeys(sortKeys);
sorter.sort();
}
public void showGreps(List<String> greps) {
for (String grepline : greps) {
List<String> array = Arrays.asList(grepline.split(",",5));
if (array.size() > 1) {
if (modelgrep.getRowCount() == 0) {
if (array.get(0).equals("true")) {
modelgrep.addRow(new Object[]{true, "", array.get(2), array.get(3), array.get(4)});
} else {
modelgrep.addRow(new Object[]{false, "", array.get(2), array.get(3), array.get(4)});
}
} else {
if (array.get(0).equals("true")) {
modelgrep.addRow(new Object[]{true, array.get(1), array.get(2), array.get(3), array.get(4)});
} else {
modelgrep.addRow(new Object[]{false, array.get(1), array.get(2), array.get(3), array.get(4)});
}
}
} else {
if (modelgrep.getRowCount() == 0) {
modelgrep.addRow(new Object[]{true, "", "All Request", "Value", grepline});
} else {
modelgrep.addRow(new Object[]{true, "Or", "All Request", "Value", grepline});
}
}
}
}
public void loadGrepsFile(DefaultTableModel model) {
//Load file for implement payloads and match load button
List<String> grep = new ArrayList();
String line;
JFrame parentFrame = new JFrame();
JFileChooser fileChooser = new JFileChooser();
fileChooser.setDialogTitle("Specify a file to load");
int userSelection = fileChooser.showOpenDialog(parentFrame);
if (userSelection == JFileChooser.APPROVE_OPTION) {
File fileload = fileChooser.getSelectedFile();
textgreps.setText(fileload.getAbsolutePath());
try {
BufferedReader bufferreader = new BufferedReader(new FileReader(fileload.getAbsolutePath()));
line = bufferreader.readLine();
while (line != null) {
grep.add(line);
line = bufferreader.readLine();
}
bufferreader.close();
showGreps(grep);
} catch (FileNotFoundException ex) {
System.out.println("RequestProfile line 263:");
} catch (IOException ex) {
System.out.println("RequestProfile line 267:");
}
}
}
public void addNewTag(String str) {
if (!str.isEmpty()) {
try {
BufferedWriter out = new BufferedWriter(new FileWriter(filename.concat("tags.txt"), true));
out.write(str.concat("\n"));
out.close();
} catch (IOException e) {
System.out.println("RequestProfile line 278: " + e.getMessage());
}
}
}
public void removeTag(String tag) {
String file = filename.concat("tags.txt");
try {
File inFile = new File(file);
if (!inFile.isFile()) {
System.out.println("RequestProfile line 289: " + "Parameter is not an existing file");
return;
}
//Construct the new file that will later be renamed to the original filename.
File tempFile = new File(inFile.getAbsolutePath().concat(".tmp"));
BufferedReader br = new BufferedReader(new FileReader(file));
PrintWriter pw = new PrintWriter(new FileWriter(tempFile));
String line = null;
//Read from the original file and write to the new
//unless content matches data to be removed.
while ((line = br.readLine()) != null) {
if (!line.trim().equals(tag)) {
pw.println(line);
pw.flush();
}
}
pw.close();
br.close();
//Delete the original file
if (!inFile.delete()) {
System.out.println("RequestProfile line 315: " + "Could not delete file");
return;
}
//Rename the new file to the filename the original file had.
if (!tempFile.renameTo(inFile)) {
System.out.println("RequestProfile line 321: " + "Could not rename file");
}
} catch (FileNotFoundException ex) {
System.out.println("RequestProfile line 325:");
} catch (IOException ex) {
System.out.println("RequestProfile line 329:");
}
}
public void showTags() {
List<String> tags = readFile(filename.concat("tags.txt"));
newTagCombo.removeAllItems();
tagmanager.removeAllElements();
for (String tag : tags) {
newTagCombo.addItem(tag);
tagmanager.addElement(tag);
}
}
private List<String> readFile(String filename) {
List<String> records = new ArrayList();
try {
BufferedReader reader = new BufferedReader(new FileReader(filename));
String line;
while ((line = reader.readLine()) != null) {
records.add(line);
}
reader.close();
} catch (Exception e) {
System.out.println("RequestPorfile line 363:" + e.getMessage());
}
return records;
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
buttonGroup1 = new javax.swing.ButtonGroup();
buttonGroup2 = new javax.swing.ButtonGroup();
buttonGroup3 = new javax.swing.ButtonGroup();
buttonGroup4 = new javax.swing.ButtonGroup();
buttonGroup5 = new javax.swing.ButtonGroup();
buttonGroup6 = new javax.swing.ButtonGroup();
buttonGroup7 = new javax.swing.ButtonGroup();
text1 = new javax.swing.JTextField();
jLabel18 = new javax.swing.JLabel();
jLabel12 = new javax.swing.JLabel();
textauthor = new javax.swing.JTextField();
headerstab = new javax.swing.JTabbedPane();
jScrollPane6 = new javax.swing.JScrollPane();
jPanel11 = new javax.swing.JPanel();
radio4 = new javax.swing.JRadioButton();
radio3 = new javax.swing.JRadioButton();
check4 = new javax.swing.JCheckBox();
check1 = new javax.swing.JCheckBox();
jLabel24 = new javax.swing.JLabel();
jLabel25 = new javax.swing.JLabel();
jLabel30 = new javax.swing.JLabel();
jLabel31 = new javax.swing.JLabel();
jSeparator6 = new javax.swing.JSeparator();
jScrollPane14 = new javax.swing.JScrollPane();
table4 = new javax.swing.JTable();
button18 = new javax.swing.JButton();
button10 = new javax.swing.JButton();
button7 = new javax.swing.JButton();
button19 = new javax.swing.JButton();
button8 = new javax.swing.JButton();
textgreps = new javax.swing.JTextField();
jLabel1 = new javax.swing.JLabel();
jLabel5 = new javax.swing.JLabel();
check73 = new javax.swing.JCheckBox();
text73 = new javax.swing.JTextField();
negativeURL = new javax.swing.JCheckBox();
jScrollPane10 = new javax.swing.JScrollPane();
jPanel12 = new javax.swing.JPanel();
jLabel32 = new javax.swing.JLabel();
jLabel33 = new javax.swing.JLabel();
jLabel3 = new javax.swing.JLabel();
jLabel4 = new javax.swing.JLabel();
radio5 = new javax.swing.JRadioButton();
radio6 = new javax.swing.JRadioButton();
radio7 = new javax.swing.JRadioButton();
radio8 = new javax.swing.JRadioButton();
jLabel7 = new javax.swing.JLabel();
radio9 = new javax.swing.JRadioButton();
radio10 = new javax.swing.JRadioButton();
radio11 = new javax.swing.JRadioButton();
text4 = new javax.swing.JTextField();
jSeparator7 = new javax.swing.JSeparator();
jLabel34 = new javax.swing.JLabel();
jLabel35 = new javax.swing.JLabel();
jScrollPane7 = new javax.swing.JScrollPane();
textarea2 = new javax.swing.JTextArea();
jLabel13 = new javax.swing.JLabel();
jLabel36 = new javax.swing.JLabel();
jLabel37 = new javax.swing.JLabel();
jSeparator8 = new javax.swing.JSeparator();
jLabel38 = new javax.swing.JLabel();
jLabel39 = new javax.swing.JLabel();
jSeparator9 = new javax.swing.JSeparator();
jScrollPane1 = new javax.swing.JScrollPane();
textarea1 = new javax.swing.JTextArea();
jLabel9 = new javax.swing.JLabel();
jScrollPane8 = new javax.swing.JScrollPane();
textarea3 = new javax.swing.JTextArea();
jLabel14 = new javax.swing.JLabel();
jLabel40 = new javax.swing.JLabel();
jLabel41 = new javax.swing.JLabel();
jSeparator10 = new javax.swing.JSeparator();
jScrollPane9 = new javax.swing.JScrollPane();
textarea4 = new javax.swing.JTextArea();
jLabel15 = new javax.swing.JLabel();
jPanel3 = new javax.swing.JPanel();
removetag = new javax.swing.JButton();
addTag = new javax.swing.JButton();
newTagCombo = new javax.swing.JComboBox<>();
jScrollPane11 = new javax.swing.JScrollPane();
listtag = new javax.swing.JList<>();
jLabel46 = new javax.swing.JLabel();
jLabel47 = new javax.swing.JLabel();
newTagb = new javax.swing.JButton();
text1.setFont(new java.awt.Font("Lucida Grande", 0, 14)); // NOI18N
jLabel18.setFont(new java.awt.Font("Lucida Grande", 0, 14)); // NOI18N
jLabel18.setText("Author:");
jLabel12.setFont(new java.awt.Font("Lucida Grande", 0, 14)); // NOI18N
jLabel12.setText("Name:");
textauthor.setFont(new java.awt.Font("Lucida Grande", 0, 14)); // NOI18N
headerstab.setAutoscrolls(true);
headerstab.setFont(new java.awt.Font("Lucida Grande", 0, 14)); // NOI18N
headerstab.setPreferredSize(new java.awt.Dimension(800, 600));
headerstab.addChangeListener(new javax.swing.event.ChangeListener() {
public void stateChanged(javax.swing.event.ChangeEvent evt) {
headerstabStateChanged(evt);
}
});
jScrollPane6.setHorizontalScrollBarPolicy(javax.swing.ScrollPaneConstants.HORIZONTAL_SCROLLBAR_NEVER);
jScrollPane6.getVerticalScrollBar().setUnitIncrement(20);
jPanel11.setAutoscrolls(true);
buttonGroup3.add(radio4);
radio4.setText("Simple string");
radio4.addItemListener(new java.awt.event.ItemListener() {
public void itemStateChanged(java.awt.event.ItemEvent evt) {
radio4stringMatchType(evt);
}
});
buttonGroup3.add(radio3);
radio3.setText("Regex");
radio3.addItemListener(new java.awt.event.ItemListener() {
public void itemStateChanged(java.awt.event.ItemEvent evt) {
radio3regexMatchType(evt);
}
});
check4.setText("Negative match");
check1.setText("Case sensitive");
jLabel24.setText("You can define one or more greps.");
jLabel25.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel25.setForeground(new java.awt.Color(255, 102, 51));
jLabel25.setText("Grep");
jLabel30.setText("These settings can be used to specify grep options of your profile.");
jLabel31.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel31.setForeground(new java.awt.Color(255, 102, 51));
jLabel31.setText("Grep Options");
table4.setFont(new java.awt.Font("Lucida Grande", 0, 13)); // NOI18N
table4.setModel(modelgrep);
table4.setShowGrid(false);
jScrollPane14.setViewportView(table4);
button18.setText("Remove");
button18.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button18removeMatchReplace(evt);
}
});
button10.setText("Clear");
button10.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button10removeAllGrep(evt);
}
});
button7.setText("Paste");
button7.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button7pasteGrep(evt);
}
});
button19.setText("Add");
button19.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button19addGrep(evt);
}
});
button8.setText("Load File");
button8.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button8loadGrep(evt);
}
});
jLabel1.setText("Match Type: ");
jLabel5.setText("<html> * More info about insertion points at <a href=\\\\\\\"\\\\\\\">Burp Suite Extender API</a></html>");
jLabel5.addMouseListener(new java.awt.event.MouseAdapter() {
public void mouseClicked(java.awt.event.MouseEvent evt) {
goweb(evt);
}
});
check73.setText("URL Extension");
negativeURL.setText("Negative match");
javax.swing.GroupLayout jPanel11Layout = new javax.swing.GroupLayout(jPanel11);
jPanel11.setLayout(jPanel11Layout);
jPanel11Layout.setHorizontalGroup(
jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jSeparator6, javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(jPanel11Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(javax.swing.GroupLayout.Alignment.LEADING, jPanel11Layout.createSequentialGroup()
.addComponent(button8, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(textgreps, javax.swing.GroupLayout.PREFERRED_SIZE, 598, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(javax.swing.GroupLayout.Alignment.LEADING, jPanel11Layout.createSequentialGroup()
.addGap(9, 9, 9)
.addComponent(jLabel5, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel11Layout.createSequentialGroup()
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING, false)
.addComponent(button18, javax.swing.GroupLayout.Alignment.LEADING, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(button10, javax.swing.GroupLayout.Alignment.LEADING, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(button7, javax.swing.GroupLayout.Alignment.LEADING, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(button19, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel11Layout.createSequentialGroup()
.addComponent(jLabel1)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(radio4)
.addComponent(radio3)))
.addComponent(jScrollPane14, javax.swing.GroupLayout.PREFERRED_SIZE, 598, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(132, Short.MAX_VALUE)))
.addGroup(jPanel11Layout.createSequentialGroup()
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel11Layout.createSequentialGroup()
.addComponent(check73)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(text73, javax.swing.GroupLayout.PREFERRED_SIZE, 579, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(negativeURL))
.addComponent(jLabel31)
.addComponent(jLabel30, javax.swing.GroupLayout.PREFERRED_SIZE, 769, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(check4)
.addComponent(check1)
.addComponent(jLabel25)
.addComponent(jLabel24, javax.swing.GroupLayout.PREFERRED_SIZE, 769, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(42, Short.MAX_VALUE))))
);
jPanel11Layout.setVerticalGroup(
jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel11Layout.createSequentialGroup()
.addContainerGap()
.addComponent(jLabel25)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel24)
.addGap(18, 18, 18)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(textgreps, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(button8))
.addGap(18, 18, Short.MAX_VALUE)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jScrollPane14, javax.swing.GroupLayout.PREFERRED_SIZE, 206, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(jPanel11Layout.createSequentialGroup()
.addComponent(button19)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(button7)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(button10)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(button18)))
.addGap(18, 18, 18)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(radio4)
.addComponent(jLabel1))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(radio3)
.addGap(18, 18, 18)
.addComponent(jLabel5, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(jSeparator6, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel31)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel30)
.addGap(18, 18, 18)
.addComponent(check4)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(check1)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(check73)
.addComponent(text73, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(negativeURL))
.addContainerGap(58, Short.MAX_VALUE))
);
JScrollPane responseresScroll = new JScrollPane(jPanel11,
JScrollPane.VERTICAL_SCROLLBAR_ALWAYS, JScrollPane.HORIZONTAL_SCROLLBAR_NEVER);
jScrollPane6.setViewportView(jPanel11);
headerstab.addTab(" Request ", jScrollPane6);
jScrollPane10.getVerticalScrollBar().setUnitIncrement(20);
jPanel12.setAutoscrolls(true);
jLabel32.setText("You can define the issue properties.");
jLabel33.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel33.setForeground(new java.awt.Color(255, 102, 51));
jLabel33.setText("Issue Properties");
jLabel3.setText("Issue Name:");
jLabel4.setText("Severity:");
buttonGroup5.add(radio5);
radio5.setText("High");
buttonGroup5.add(radio6);
radio6.setText("Medium");
buttonGroup5.add(radio7);
radio7.setText("Low");
buttonGroup5.add(radio8);
radio8.setText("Information");
jLabel7.setText("Confidence:");
buttonGroup6.add(radio9);
radio9.setText("Certain");
buttonGroup6.add(radio10);
radio10.setText("Firm");
buttonGroup6.add(radio11);
radio11.setText("Tentative");
jLabel34.setText("You can define the issue details.");
jLabel35.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel35.setForeground(new java.awt.Color(255, 102, 51));
jLabel35.setText("Issue Detail");
textarea2.setColumns(20);
textarea2.setRows(5);
jScrollPane7.setViewportView(textarea2);
jLabel13.setText("Description:");
jLabel36.setText("You can define the issue background.");
jLabel37.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel37.setForeground(new java.awt.Color(255, 102, 51));
jLabel37.setText("Issue Background");
jLabel38.setText("You can define the remediation detail.");
jLabel39.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel39.setForeground(new java.awt.Color(255, 102, 51));
jLabel39.setText("Remediation Detail");
textarea1.setColumns(20);
textarea1.setRows(5);
jScrollPane1.setViewportView(textarea1);
jLabel9.setText("Description:");
textarea3.setColumns(20);
textarea3.setRows(5);
jScrollPane8.setViewportView(textarea3);
jLabel14.setText("Description:");
jLabel40.setText("You can define the remediation background.");
jLabel41.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel41.setForeground(new java.awt.Color(255, 102, 51));
jLabel41.setText("Remediation Background");
textarea4.setColumns(20);
textarea4.setRows(5);
jScrollPane9.setViewportView(textarea4);
jLabel15.setText("Description:");
javax.swing.GroupLayout jPanel12Layout = new javax.swing.GroupLayout(jPanel12);
jPanel12.setLayout(jPanel12Layout);
jPanel12Layout.setHorizontalGroup(
jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jSeparator7)
.addComponent(jSeparator8, javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jSeparator9, javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jSeparator10, javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(jPanel12Layout.createSequentialGroup()
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel12Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel33)
.addComponent(jLabel35)
.addComponent(jLabel34)
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(jLabel13)
.addGap(18, 18, 18)
.addComponent(jScrollPane7, javax.swing.GroupLayout.PREFERRED_SIZE, 612, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(jLabel9)
.addGap(18, 18, 18)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 612, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(jLabel15)
.addGap(18, 18, 18)
.addComponent(jScrollPane9, javax.swing.GroupLayout.PREFERRED_SIZE, 612, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(jLabel14)
.addGap(18, 18, 18)
.addComponent(jScrollPane8, javax.swing.GroupLayout.PREFERRED_SIZE, 612, javax.swing.GroupLayout.PREFERRED_SIZE))
.addComponent(jLabel37)
.addComponent(jLabel36)
.addComponent(jLabel39)
.addComponent(jLabel38)
.addComponent(jLabel32)
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(jLabel3)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(jLabel4, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(radio8)
.addGap(189, 189, 189))
.addGroup(jPanel12Layout.createSequentialGroup()
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(radio6)
.addComponent(radio7)
.addComponent(radio5))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jLabel7)
.addGap(18, 18, 18)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(radio9)
.addComponent(radio11)
.addComponent(radio10)))))
.addComponent(text4, javax.swing.GroupLayout.PREFERRED_SIZE, 419, javax.swing.GroupLayout.PREFERRED_SIZE)))))
.addComponent(jLabel41)
.addComponent(jLabel40))
.addContainerGap(93, Short.MAX_VALUE))
);
jPanel12Layout.setVerticalGroup(
jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel12Layout.createSequentialGroup()
.addGap(10, 10, 10)
.addComponent(jLabel33)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel32)
.addGap(25, 25, 25)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel3)
.addComponent(text4, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel12Layout.createSequentialGroup()
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel7)
.addComponent(radio9))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(radio10)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(radio11))
.addGroup(jPanel12Layout.createSequentialGroup()
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel4)
.addComponent(radio5))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(radio6)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(radio7)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(radio8)))
.addGap(18, 18, 18)
.addComponent(jSeparator7, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel35)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel34)
.addGap(25, 25, 25)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel9)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addComponent(jSeparator8, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel37)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel36)
.addGap(25, 25, 25)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel13)
.addComponent(jScrollPane7, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addComponent(jSeparator9, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel39)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel38)
.addGap(25, 25, 25)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel15)
.addComponent(jScrollPane9, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addComponent(jSeparator10, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel41)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel40)
.addGap(25, 25, 25)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel14)
.addComponent(jScrollPane8, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
jScrollPane10.setViewportView(jPanel12);
headerstab.addTab(" Issue ", jScrollPane10);
removetag.setText("Remove");
removetag.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
removetag(evt);
}
});
addTag.setText("Add");
addTag.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
addTag(evt);
}
});
listtag.setModel(tag);
jScrollPane11.setViewportView(listtag);
jLabel46.setText("You can define one or multiple tags for this profile.");
jLabel47.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel47.setForeground(new java.awt.Color(255, 102, 51));
jLabel47.setText("Set Tags");
newTagb.setText("New Tag");
newTagb.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
newTagbnewTag(evt);
}
});
javax.swing.GroupLayout jPanel3Layout = new javax.swing.GroupLayout(jPanel3);
jPanel3.setLayout(jPanel3Layout);
jPanel3Layout.setHorizontalGroup(
jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel3Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel47)
.addComponent(jLabel46)
.addGroup(jPanel3Layout.createSequentialGroup()
.addGroup(jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(newTagb, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(addTag, javax.swing.GroupLayout.PREFERRED_SIZE, 93, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(removetag, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addGap(18, 18, 18)
.addGroup(jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jScrollPane11)
.addComponent(newTagCombo, javax.swing.GroupLayout.PREFERRED_SIZE, 468, javax.swing.GroupLayout.PREFERRED_SIZE))))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
jPanel3Layout.setVerticalGroup(
jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel3Layout.createSequentialGroup()
.addGap(10, 10, 10)
.addComponent(jLabel47)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel46)
.addGap(25, 25, 25)
.addGroup(jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jScrollPane11, javax.swing.GroupLayout.PREFERRED_SIZE, 99, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(jPanel3Layout.createSequentialGroup()
.addComponent(newTagb)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(removetag)))
.addGap(18, 18, 18)
.addGroup(jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(newTagCombo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(addTag))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
headerstab.addTab(" Tags ", jPanel3);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jLabel12)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(text1)
.addGap(18, 18, 18)
.addComponent(jLabel18)
.addGap(18, 18, 18)
.addComponent(textauthor, javax.swing.GroupLayout.PREFERRED_SIZE, 165, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(64, 64, 64))
.addComponent(headerstab, javax.swing.GroupLayout.DEFAULT_SIZE, 831, Short.MAX_VALUE)
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(text1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel12)
.addComponent(textauthor, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel18))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(headerstab, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
}// </editor-fold>//GEN-END:initComponents
private void radio4stringMatchType(java.awt.event.ItemEvent evt) {//GEN-FIRST:event_radio4stringMatchType
}//GEN-LAST:event_radio4stringMatchType
private void radio3regexMatchType(java.awt.event.ItemEvent evt) {//GEN-FIRST:event_radio3regexMatchType
}//GEN-LAST:event_radio3regexMatchType
private void removetag(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_removetag
int selectedIndex = listtag.getSelectedIndex();
if (selectedIndex != -1) {
tag.remove(selectedIndex);
}
}//GEN-LAST:event_removetag
private void addTag(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_addTag
tag.addElement(newTagCombo.getSelectedItem());
}//GEN-LAST:event_addTag
private void newTagbnewTag(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_newTagbnewTag
Integer result;
NewTag nt = new NewTag();
JOptionPane jopane1 = new JOptionPane(nt, JOptionPane.PLAIN_MESSAGE, JOptionPane.OK_CANCEL_OPTION);
JDialog dialog = jopane1.createDialog(this, "New Tag");
dialog.setLocationRelativeTo(null);
dialog.setVisible(true);
Object selectedValue = jopane1.getValue();
if (selectedValue != null) {
result = ((Integer) selectedValue).intValue();
if (result == JOptionPane.OK_OPTION) {
addNewTag(nt.newTagtext.getText());
showTags();
}
}
}//GEN-LAST:event_newTagbnewTag
private void headerstabStateChanged(javax.swing.event.ChangeEvent evt) {//GEN-FIRST:event_headerstabStateChanged
int activePane = headerstab.getSelectedIndex();
if (activePane == 3) {
showTags();
}
}//GEN-LAST:event_headerstabStateChanged
private void button18removeMatchReplace(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button18removeMatchReplace
int[] rows = table4.getSelectedRows();
Arrays.sort(rows);
for (int i = rows.length - 1; i >= 0; i--) {
int row = rows[i];
int modelRow = table4.convertRowIndexToModel(row);
modelgrep.removeRow(modelRow);
}
}//GEN-LAST:event_button18removeMatchReplace
private void button10removeAllGrep(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button10removeAllGrep
int rowCount = modelgrep.getRowCount();
for (int i = rowCount - 1; i >= 0; i--) {
modelgrep.removeRow(i);
}
}//GEN-LAST:event_button10removeAllGrep
private void button7pasteGrep(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button7pasteGrep
String element = getClipboardContents();
List<String> lines = Arrays.asList(element.split("\n"));
showGreps(lines);
}//GEN-LAST:event_button7pasteGrep
private void button19addGrep(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button19addGrep
if (modelgrep.getRowCount() == 0) {
modelgrep.addRow(new Object[]{true, "", "All Request", "Value", "Change me"});
} else {
modelgrep.addRow(new Object[]{true, "Or", "All Request", "Value", "Change me"});
}
}//GEN-LAST:event_button19addGrep
private void button8loadGrep(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button8loadGrep
loadGrepsFile(modelgrep);
}//GEN-LAST:event_button8loadGrep
private void goweb(java.awt.event.MouseEvent evt) {//GEN-FIRST:event_goweb
try {
Desktop.getDesktop().browse(new URI("https://portswigger.net/burp/extender/api/burp/IParameter.html"));
} catch (URISyntaxException | IOException e) {
System.out.println("RequestProfile line 1094: " + "Help web not opened: " + e);
}
}//GEN-LAST:event_goweb
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton addTag;
private javax.swing.JButton button10;
private javax.swing.JButton button18;
private javax.swing.JButton button19;
private javax.swing.JButton button7;
private javax.swing.JButton button8;
public javax.swing.ButtonGroup buttonGroup1;
public javax.swing.ButtonGroup buttonGroup2;
public javax.swing.ButtonGroup buttonGroup3;
public javax.swing.ButtonGroup buttonGroup4;
public javax.swing.ButtonGroup buttonGroup5;
public javax.swing.ButtonGroup buttonGroup6;
public javax.swing.ButtonGroup buttonGroup7;
public javax.swing.JCheckBox check1;
public javax.swing.JCheckBox check4;
public javax.swing.JCheckBox check73;
public javax.swing.JTabbedPane headerstab;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel12;
private javax.swing.JLabel jLabel13;
private javax.swing.JLabel jLabel14;
private javax.swing.JLabel jLabel15;
private javax.swing.JLabel jLabel18;
private javax.swing.JLabel jLabel24;
private javax.swing.JLabel jLabel25;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel30;
private javax.swing.JLabel jLabel31;
private javax.swing.JLabel jLabel32;
private javax.swing.JLabel jLabel33;
private javax.swing.JLabel jLabel34;
private javax.swing.JLabel jLabel35;
private javax.swing.JLabel jLabel36;
private javax.swing.JLabel jLabel37;
private javax.swing.JLabel jLabel38;
private javax.swing.JLabel jLabel39;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel40;
private javax.swing.JLabel jLabel41;
private javax.swing.JLabel jLabel46;
private javax.swing.JLabel jLabel47;
private javax.swing.JLabel jLabel5;
private javax.swing.JLabel jLabel7;
private javax.swing.JLabel jLabel9;
private javax.swing.JPanel jPanel11;
private javax.swing.JPanel jPanel12;
private javax.swing.JPanel jPanel3;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JScrollPane jScrollPane10;
private javax.swing.JScrollPane jScrollPane11;
private javax.swing.JScrollPane jScrollPane14;
private javax.swing.JScrollPane jScrollPane6;
private javax.swing.JScrollPane jScrollPane7;
private javax.swing.JScrollPane jScrollPane8;
private javax.swing.JScrollPane jScrollPane9;
private javax.swing.JSeparator jSeparator10;
private javax.swing.JSeparator jSeparator6;
private javax.swing.JSeparator jSeparator7;
private javax.swing.JSeparator jSeparator8;
private javax.swing.JSeparator jSeparator9;
public javax.swing.JList<String> listtag;
public javax.swing.JCheckBox negativeURL;
public javax.swing.JComboBox<String> newTagCombo;
private javax.swing.JButton newTagb;
public javax.swing.JRadioButton radio10;
public javax.swing.JRadioButton radio11;
public javax.swing.JRadioButton radio3;
public javax.swing.JRadioButton radio4;
public javax.swing.JRadioButton radio5;
public javax.swing.JRadioButton radio6;
public javax.swing.JRadioButton radio7;
public javax.swing.JRadioButton radio8;
public javax.swing.JRadioButton radio9;
private javax.swing.JButton removetag;
public javax.swing.JTable table4;
public javax.swing.JTextField text1;
public javax.swing.JTextField text4;
public javax.swing.JTextField text73;
public javax.swing.JTextArea textarea1;
public javax.swing.JTextArea textarea2;
public javax.swing.JTextArea textarea3;
public javax.swing.JTextArea textarea4;
public javax.swing.JTextField textauthor;
public javax.swing.JTextField textgreps;
// End of variables declaration//GEN-END:variables
} |
BurpBounty/main/java/burpbountyfree/ResponseProfile.form | <?xml version="1.0" encoding="UTF-8" ?>
<Form version="1.8" maxVersion="1.9" type="org.netbeans.modules.form.forminfo.JPanelFormInfo">
<NonVisualComponents>
<Component class="javax.swing.ButtonGroup" name="buttonGroup1">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup2">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup3">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup4">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup5">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup6">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.ButtonGroup" name="buttonGroup7">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</NonVisualComponents>
<AuxValues>
<AuxValue name="FormSettings_autoResourcing" type="java.lang.Integer" value="0"/>
<AuxValue name="FormSettings_autoSetComponentName" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_generateFQN" type="java.lang.Boolean" value="true"/>
<AuxValue name="FormSettings_generateMnemonicsCode" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_i18nAutoMode" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_layoutCodeTarget" type="java.lang.Integer" value="1"/>
<AuxValue name="FormSettings_listenerGenerationStyle" type="java.lang.Integer" value="0"/>
<AuxValue name="FormSettings_variablesLocal" type="java.lang.Boolean" value="false"/>
<AuxValue name="FormSettings_variablesModifier" type="java.lang.Integer" value="2"/>
</AuxValues>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel12" min="-2" max="-2" attributes="0"/>
<EmptySpace type="unrelated" max="-2" attributes="0"/>
<Component id="text1" max="32767" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jLabel18" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="textauthor" min="-2" pref="165" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="70" max="-2" attributes="0"/>
</Group>
<Component id="headerstab" alignment="0" pref="831" max="32767" attributes="0"/>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="text1" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="jLabel12" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="textauthor" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="jLabel18" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="headerstab" min="-2" max="-2" attributes="0"/>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JTextField" name="text1">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="0"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel18">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="0"/>
</Property>
<Property name="text" type="java.lang.String" value="Author:"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel12">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="0"/>
</Property>
<Property name="text" type="java.lang.String" value="Name:"/>
</Properties>
</Component>
<Component class="javax.swing.JTextField" name="textauthor">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="0"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Container class="javax.swing.JTabbedPane" name="headerstab">
<Properties>
<Property name="autoscrolls" type="boolean" value="true"/>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="0"/>
</Property>
<Property name="preferredSize" type="java.awt.Dimension" editor="org.netbeans.beaninfo.editors.DimensionEditor">
<Dimension value="[800, 600]"/>
</Property>
</Properties>
<Events>
<EventHandler event="stateChanged" listener="javax.swing.event.ChangeListener" parameters="javax.swing.event.ChangeEvent" handler="headerstabStateChanged"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout"/>
<SubComponents>
<Container class="javax.swing.JScrollPane" name="jScrollPane6">
<Properties>
<Property name="horizontalScrollBarPolicy" type="int" value="31"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_InitCodePost" type="java.lang.String" value="jScrollPane6.getVerticalScrollBar().setUnitIncrement(20);"/>
</AuxValues>
<Constraints>
<Constraint layoutClass="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout" value="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout$JTabbedPaneConstraintsDescription">
<JTabbedPaneConstraints tabName=" Response ">
<Property name="tabTitle" type="java.lang.String" value=" Response "/>
</JTabbedPaneConstraints>
</Constraint>
</Constraints>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Container class="javax.swing.JPanel" name="jPanel11">
<Properties>
<Property name="autoscrolls" type="boolean" value="true"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_LayoutCodePost" type="java.lang.String" value="JScrollPane responseresScroll = new JScrollPane(jPanel11, 
 JScrollPane.VERTICAL_SCROLLBAR_ALWAYS, JScrollPane.HORIZONTAL_SCROLLBAR_NEVER);"/>
</AuxValues>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jSeparator5" alignment="0" max="32767" attributes="0"/>
<Component id="jSeparator6" alignment="1" max="32767" attributes="0"/>
<Group type="102" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="20" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel6" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel2" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="unrelated" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="rb1" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="rb2" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="rb3" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="rb4" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="sp1" alignment="0" min="-2" pref="56" max="-2" attributes="0"/>
</Group>
</Group>
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel31" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="onlyhttp" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="check4" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="check1" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="excludehttp" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel29" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel28" alignment="0" min="-2" pref="769" max="-2" attributes="0"/>
<Group type="103" alignment="0" groupAlignment="1" max="-2" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="check72" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="check71" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="15" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="text71" max="32767" attributes="0"/>
<Component id="text72" max="32767" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="negativeCT" min="-2" max="-2" attributes="0"/>
<Component id="negativeRC" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<Group type="102" attributes="0">
<Component id="text73" min="-2" pref="547" max="-2" attributes="0"/>
<EmptySpace max="32767" attributes="0"/>
<Component id="negativeURL" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
<Component id="jLabel30" alignment="0" min="-2" pref="769" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel25" alignment="0" min="-2" max="-2" attributes="0"/>
<Group type="103" alignment="0" groupAlignment="1" attributes="0">
<Group type="102" alignment="1" attributes="0">
<Component id="button8" linkSize="1" min="-2" pref="89" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="textgreps" min="-2" pref="662" max="-2" attributes="0"/>
</Group>
<Group type="103" alignment="0" groupAlignment="1" attributes="0">
<Component id="jLabel24" alignment="0" min="-2" pref="769" max="-2" attributes="0"/>
<Group type="102" alignment="1" attributes="0">
<Group type="103" groupAlignment="1" max="-2" attributes="0">
<Component id="button18" linkSize="1" alignment="0" min="-2" pref="89" max="-2" attributes="0"/>
<Component id="button10" linkSize="1" alignment="0" max="32767" attributes="0"/>
<Component id="button7" linkSize="1" alignment="0" min="-2" pref="89" max="-2" attributes="0"/>
<Component id="button19" linkSize="1" min="-2" pref="89" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" attributes="0">
<Component id="jLabel1" min="-2" max="-2" attributes="0"/>
<EmptySpace type="unrelated" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="radio3" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="radio4" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="85" max="-2" attributes="0"/>
<Component id="jLabel5" min="-2" max="-2" attributes="0"/>
<EmptySpace type="unrelated" min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="all_matches" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="first_match" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<Component id="jScrollPane14" min="-2" pref="662" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
</Group>
</Group>
</Group>
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Component id="check73" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace pref="42" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel25" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel24" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="textgreps" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="button8" linkSize="2" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace pref="18" max="32767" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jScrollPane14" min="-2" pref="206" max="-2" attributes="0"/>
<Group type="102" attributes="0">
<Component id="button19" linkSize="2" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="button7" linkSize="2" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="button10" linkSize="2" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="button18" linkSize="2" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel1" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="radio4" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="1" max="-2" attributes="0"/>
<Component id="radio3" min="-2" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel5" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="first_match" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="1" max="-2" attributes="0"/>
<Component id="all_matches" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace min="-2" pref="18" max="-2" attributes="0"/>
<Component id="jSeparator6" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel31" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel30" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Component id="check4" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="check1" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="excludehttp" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="onlyhttp" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="check71" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="text71" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="negativeCT" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="check72" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="text72" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="negativeRC" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="check73" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="text73" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="negativeURL" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace min="-2" pref="26" max="-2" attributes="0"/>
<Component id="jSeparator5" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel29" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel28" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="rb1" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="jLabel6" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Component id="rb2" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="rb3" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="rb4" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel2" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="sp1" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace pref="22" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JButton" name="button10">
<Properties>
<Property name="text" type="java.lang.String" value="Clear"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button10removeAllGrep"/>
</Events>
</Component>
<Component class="javax.swing.JRadioButton" name="radio4">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup3"/>
</Property>
<Property name="text" type="java.lang.String" value="Simple string"/>
</Properties>
<Events>
<EventHandler event="itemStateChanged" listener="java.awt.event.ItemListener" parameters="java.awt.event.ItemEvent" handler="radio4stringMatchType"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio3">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup3"/>
</Property>
<Property name="text" type="java.lang.String" value="Regex"/>
</Properties>
<Events>
<EventHandler event="itemStateChanged" listener="java.awt.event.ItemListener" parameters="java.awt.event.ItemEvent" handler="radio3regexMatchType"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="check4">
<Properties>
<Property name="text" type="java.lang.String" value="Negative match"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="check1">
<Properties>
<Property name="text" type="java.lang.String" value="Case sensitive"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="excludehttp">
<Properties>
<Property name="text" type="java.lang.String" value="Exclude HTTP headers"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="onlyhttp">
<Properties>
<Property name="text" type="java.lang.String" value="Only in HTTP headers"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="check71">
<Properties>
<Property name="text" type="java.lang.String" value="Content type"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="check72">
<Properties>
<Property name="text" type="java.lang.String" value="Status code"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JTextField" name="text72">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JTextField" name="text71">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="negativeCT">
<Properties>
<Property name="text" type="java.lang.String" value="Negative match"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="negativeRC">
<Properties>
<Property name="text" type="java.lang.String" value="Negative match"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel24">
<Properties>
<Property name="text" type="java.lang.String" value="You can define one or more greps."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel25">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Grep"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel28">
<Properties>
<Property name="text" type="java.lang.String" value="You can define how your profile handles redirections."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel29">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Redirections"/>
</Properties>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator5">
</Component>
<Component class="javax.swing.JLabel" name="jLabel30">
<Properties>
<Property name="text" type="java.lang.String" value="These settings can be used to specify grep options of your profile."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel31">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Grep Options"/>
</Properties>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator6">
</Component>
<Component class="javax.swing.JRadioButton" name="rb1">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup4"/>
</Property>
<Property name="text" type="java.lang.String" value="Never"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="rb2">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup4"/>
</Property>
<Property name="text" type="java.lang.String" value="On-site only"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="rb3">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup4"/>
</Property>
<Property name="text" type="java.lang.String" value="In-scope only"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="rb4">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup4"/>
</Property>
<Property name="text" type="java.lang.String" value="Always"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel2">
<Properties>
<Property name="text" type="java.lang.String" value="Max redirections:"/>
</Properties>
</Component>
<Component class="javax.swing.JSpinner" name="sp1">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel6">
<Properties>
<Property name="text" type="java.lang.String" value="Follow redirections: "/>
</Properties>
</Component>
<Component class="javax.swing.JButton" name="button19">
<Properties>
<Property name="text" type="java.lang.String" value="Add"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="addGrep"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="button18">
<Properties>
<Property name="text" type="java.lang.String" value="Remove"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button18removeMatchReplace"/>
</Events>
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane14">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTable" name="table4">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="13" style="0"/>
</Property>
<Property name="model" type="javax.swing.table.TableModel" editor="org.netbeans.modules.form.RADConnectionPropertyEditor">
<Connection code="modelgrep" type="code"/>
</Property>
<Property name="columnModel" type="javax.swing.table.TableColumnModel" editor="org.netbeans.modules.form.editors2.TableColumnModelEditor">
<TableColumnModel selectionModel="0"/>
</Property>
<Property name="showGrid" type="boolean" value="false"/>
<Property name="tableHeader" type="javax.swing.table.JTableHeader" editor="org.netbeans.modules.form.editors2.JTableHeaderEditor">
<TableHeader reorderingAllowed="true" resizingAllowed="true"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_SerializeTo" type="java.lang.String" value="BurpBountyGui_table4"/>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JTextField" name="textgreps">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JButton" name="button8">
<Properties>
<Property name="text" type="java.lang.String" value="Load File"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button8loadGrep"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="button7">
<Properties>
<Property name="text" type="java.lang.String" value="Paste"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="button7pasteGrep"/>
</Events>
</Component>
<Component class="javax.swing.JLabel" name="jLabel1">
<Properties>
<Property name="text" type="java.lang.String" value="Match Type: "/>
</Properties>
</Component>
<Component class="javax.swing.JCheckBox" name="check73">
<Properties>
<Property name="text" type="java.lang.String" value="URL Extension"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JTextField" name="text73">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JCheckBox" name="negativeURL">
<Properties>
<Property name="text" type="java.lang.String" value="Negative match"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="first_match">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup7"/>
</Property>
<Property name="text" type="java.lang.String" value="Only once per domain"/>
</Properties>
<Events>
<EventHandler event="itemStateChanged" listener="java.awt.event.ItemListener" parameters="java.awt.event.ItemEvent" handler="first_matchstringMatchType"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel5">
<Properties>
<Property name="text" type="java.lang.String" value="Show issue:"/>
</Properties>
</Component>
<Component class="javax.swing.JRadioButton" name="all_matches">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup7"/>
</Property>
<Property name="text" type="java.lang.String" value="All times in a domain"/>
</Properties>
<Events>
<EventHandler event="itemStateChanged" listener="java.awt.event.ItemListener" parameters="java.awt.event.ItemEvent" handler="all_matchesregexMatchType"/>
</Events>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
</SubComponents>
</Container>
<Container class="javax.swing.JScrollPane" name="jScrollPane10">
<AuxValues>
<AuxValue name="JavaCodeGenerator_InitCodePre" type="java.lang.String" value="jScrollPane10.getVerticalScrollBar().setUnitIncrement(20);"/>
</AuxValues>
<Constraints>
<Constraint layoutClass="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout" value="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout$JTabbedPaneConstraintsDescription">
<JTabbedPaneConstraints tabName=" Issue ">
<Property name="tabTitle" type="java.lang.String" value=" Issue "/>
</JTabbedPaneConstraints>
</Constraint>
</Constraints>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Container class="javax.swing.JPanel" name="jPanel12">
<Properties>
<Property name="autoscrolls" type="boolean" value="true"/>
</Properties>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jSeparator7" max="32767" attributes="0"/>
<Component id="jSeparator8" alignment="1" max="32767" attributes="0"/>
<Component id="jSeparator9" alignment="1" max="32767" attributes="0"/>
<Component id="jSeparator10" alignment="1" max="32767" attributes="0"/>
<Group type="102" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel33" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel35" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel34" alignment="0" min="-2" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel13" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jScrollPane7" min="-2" pref="612" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel9" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jScrollPane1" min="-2" pref="612" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel15" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jScrollPane9" min="-2" pref="612" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel14" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jScrollPane8" min="-2" pref="612" max="-2" attributes="0"/>
</Group>
<Component id="jLabel37" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel36" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel39" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel38" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel32" alignment="0" min="-2" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel3" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Component id="jLabel4" min="-2" pref="73" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Component id="radio8" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="189" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="0" attributes="0">
<Component id="radio6" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="radio7" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="radio5" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="32767" attributes="0"/>
<Component id="jLabel7" min="-2" max="-2" attributes="0"/>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="radio9" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="radio11" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="radio10" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
</Group>
<Component id="text4" alignment="0" min="-2" pref="419" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
</Group>
<Component id="jLabel41" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel40" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace pref="93" max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="10" max="-2" attributes="0"/>
<Component id="jLabel33" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel32" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel3" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="text4" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel7" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="radio9" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Component id="radio10" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="radio11" min="-2" max="-2" attributes="0"/>
</Group>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="3" attributes="0">
<Component id="jLabel4" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="radio5" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="-2" attributes="0"/>
<Component id="radio6" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="radio7" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="radio8" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jSeparator7" min="-2" pref="10" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel35" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel34" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel9" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jScrollPane1" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Component id="jSeparator8" min="-2" pref="10" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel37" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel36" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel13" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jScrollPane7" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Component id="jSeparator9" min="-2" pref="10" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel39" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel38" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel15" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jScrollPane9" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace type="separate" min="-2" max="-2" attributes="0"/>
<Component id="jSeparator10" min="-2" pref="10" max="-2" attributes="0"/>
<EmptySpace min="-2" max="-2" attributes="0"/>
<Component id="jLabel41" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel40" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel14" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jScrollPane8" alignment="0" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JLabel" name="jLabel32">
<Properties>
<Property name="text" type="java.lang.String" value="You can define the issue properties."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel33">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Issue Properties"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel3">
<Properties>
<Property name="text" type="java.lang.String" value="Issue Name:"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel4">
<Properties>
<Property name="text" type="java.lang.String" value="Severity:"/>
</Properties>
</Component>
<Component class="javax.swing.JRadioButton" name="radio5">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup5"/>
</Property>
<Property name="text" type="java.lang.String" value="High"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio6">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup5"/>
</Property>
<Property name="text" type="java.lang.String" value="Medium"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio7">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup5"/>
</Property>
<Property name="text" type="java.lang.String" value="Low"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio8">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup5"/>
</Property>
<Property name="text" type="java.lang.String" value="Information"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JLabel" name="jLabel7">
<Properties>
<Property name="text" type="java.lang.String" value="Confidence:"/>
</Properties>
</Component>
<Component class="javax.swing.JRadioButton" name="radio9">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup6"/>
</Property>
<Property name="text" type="java.lang.String" value="Certain"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio10">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup6"/>
</Property>
<Property name="text" type="java.lang.String" value="Firm"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JRadioButton" name="radio11">
<Properties>
<Property name="buttonGroup" type="javax.swing.ButtonGroup" editor="org.netbeans.modules.form.RADComponent$ButtonGroupPropertyEditor">
<ComponentRef name="buttonGroup6"/>
</Property>
<Property name="text" type="java.lang.String" value="Tentative"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JTextField" name="text4">
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator7">
</Component>
<Component class="javax.swing.JLabel" name="jLabel34">
<Properties>
<Property name="text" type="java.lang.String" value="You can define the issue details."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel35">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Issue Detail"/>
</Properties>
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane7">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTextArea" name="textarea2">
<Properties>
<Property name="columns" type="int" value="20"/>
<Property name="rows" type="int" value="5"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JLabel" name="jLabel13">
<Properties>
<Property name="text" type="java.lang.String" value="Description:"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel36">
<Properties>
<Property name="text" type="java.lang.String" value="You can define the issue background."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel37">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Issue Background"/>
</Properties>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator8">
</Component>
<Component class="javax.swing.JLabel" name="jLabel38">
<Properties>
<Property name="text" type="java.lang.String" value="You can define the remediation detail."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel39">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Remediation Detail"/>
</Properties>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator9">
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane1">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTextArea" name="textarea1">
<Properties>
<Property name="columns" type="int" value="20"/>
<Property name="rows" type="int" value="5"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JLabel" name="jLabel9">
<Properties>
<Property name="text" type="java.lang.String" value="Description:"/>
</Properties>
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane8">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTextArea" name="textarea3">
<Properties>
<Property name="columns" type="int" value="20"/>
<Property name="rows" type="int" value="5"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JLabel" name="jLabel14">
<Properties>
<Property name="text" type="java.lang.String" value="Description:"/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel40">
<Properties>
<Property name="text" type="java.lang.String" value="You can define the remediation background."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel41">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Remediation Background"/>
</Properties>
</Component>
<Component class="javax.swing.JSeparator" name="jSeparator10">
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane9">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JTextArea" name="textarea4">
<Properties>
<Property name="columns" type="int" value="20"/>
<Property name="rows" type="int" value="5"/>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JLabel" name="jLabel15">
<Properties>
<Property name="text" type="java.lang.String" value="Description:"/>
</Properties>
</Component>
</SubComponents>
</Container>
</SubComponents>
</Container>
<Container class="javax.swing.JPanel" name="jPanel3">
<Constraints>
<Constraint layoutClass="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout" value="org.netbeans.modules.form.compat2.layouts.support.JTabbedPaneSupportLayout$JTabbedPaneConstraintsDescription">
<JTabbedPaneConstraints tabName=" Tags ">
<Property name="tabTitle" type="java.lang.String" value=" Tags "/>
</JTabbedPaneConstraints>
</Constraint>
</Constraints>
<Layout>
<DimensionLayout dim="0">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jLabel47" alignment="0" min="-2" max="-2" attributes="0"/>
<Component id="jLabel46" alignment="0" min="-2" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="newTagb" alignment="0" max="32767" attributes="0"/>
<Component id="addTag" alignment="0" min="-2" pref="93" max="-2" attributes="0"/>
<Component id="removetag" alignment="0" max="32767" attributes="0"/>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" max="-2" attributes="0">
<Component id="jScrollPane11" alignment="0" max="32767" attributes="0"/>
<Component id="newTagCombo" alignment="0" min="-2" pref="468" max="-2" attributes="0"/>
</Group>
</Group>
</Group>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
<DimensionLayout dim="1">
<Group type="103" groupAlignment="0" attributes="0">
<Group type="102" alignment="0" attributes="0">
<EmptySpace min="-2" pref="10" max="-2" attributes="0"/>
<Component id="jLabel47" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="jLabel46" min="-2" max="-2" attributes="0"/>
<EmptySpace min="-2" pref="25" max="-2" attributes="0"/>
<Group type="103" groupAlignment="0" attributes="0">
<Component id="jScrollPane11" alignment="0" min="-2" pref="99" max="-2" attributes="0"/>
<Group type="102" alignment="0" attributes="0">
<Component id="newTagb" min="-2" max="-2" attributes="0"/>
<EmptySpace max="-2" attributes="0"/>
<Component id="removetag" min="-2" max="-2" attributes="0"/>
</Group>
</Group>
<EmptySpace type="separate" max="-2" attributes="0"/>
<Group type="103" groupAlignment="3" attributes="0">
<Component id="newTagCombo" alignment="3" min="-2" max="-2" attributes="0"/>
<Component id="addTag" alignment="3" min="-2" max="-2" attributes="0"/>
</Group>
<EmptySpace max="32767" attributes="0"/>
</Group>
</Group>
</DimensionLayout>
</Layout>
<SubComponents>
<Component class="javax.swing.JButton" name="removetag">
<Properties>
<Property name="text" type="java.lang.String" value="Remove"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="removetag"/>
</Events>
</Component>
<Component class="javax.swing.JButton" name="addTag">
<Properties>
<Property name="text" type="java.lang.String" value="Add"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="addTag"/>
</Events>
</Component>
<Component class="javax.swing.JComboBox" name="newTagCombo">
<AuxValues>
<AuxValue name="JavaCodeGenerator_SerializeTo" type="java.lang.String" value="BurpBountyGui_newTagCombo"/>
<AuxValue name="JavaCodeGenerator_TypeParameters" type="java.lang.String" value="<String>"/>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
<Container class="javax.swing.JScrollPane" name="jScrollPane11">
<AuxValues>
<AuxValue name="autoScrollPane" type="java.lang.Boolean" value="true"/>
</AuxValues>
<Layout class="org.netbeans.modules.form.compat2.layouts.support.JScrollPaneSupportLayout"/>
<SubComponents>
<Component class="javax.swing.JList" name="listtag">
<Properties>
<Property name="model" type="javax.swing.ListModel" editor="org.netbeans.modules.form.RADConnectionPropertyEditor">
<Connection code="tag" type="code"/>
</Property>
</Properties>
<AuxValues>
<AuxValue name="JavaCodeGenerator_TypeParameters" type="java.lang.String" value="<String>"/>
<AuxValue name="JavaCodeGenerator_VariableModifier" type="java.lang.Integer" value="1"/>
</AuxValues>
</Component>
</SubComponents>
</Container>
<Component class="javax.swing.JLabel" name="jLabel46">
<Properties>
<Property name="text" type="java.lang.String" value="You can define one or multiple tags for this profile."/>
</Properties>
</Component>
<Component class="javax.swing.JLabel" name="jLabel47">
<Properties>
<Property name="font" type="java.awt.Font" editor="org.netbeans.beaninfo.editors.FontEditor">
<Font name="Lucida Grande" size="14" style="1"/>
</Property>
<Property name="foreground" type="java.awt.Color" editor="org.netbeans.beaninfo.editors.ColorEditor">
<Color blue="33" green="66" red="ff" type="rgb"/>
</Property>
<Property name="text" type="java.lang.String" value="Set Tags"/>
</Properties>
</Component>
<Component class="javax.swing.JButton" name="newTagb">
<Properties>
<Property name="text" type="java.lang.String" value="New Tag"/>
</Properties>
<Events>
<EventHandler event="actionPerformed" listener="java.awt.event.ActionListener" parameters="java.awt.event.ActionEvent" handler="newTagbnewTag"/>
</Events>
</Component>
</SubComponents>
</Container>
</SubComponents>
</Container>
</SubComponents>
</Form> |
|
Java | BurpBounty/main/java/burpbountyfree/ResponseProfile.java | /*
Copyright 2018 Eduardo Garcia Melia <wagiro@gmail.com>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package burpbountyfree;
import burp.IBurpExtenderCallbacks;
import com.google.gson.JsonArray;
import com.google.gson.JsonParser;
import com.google.gson.stream.JsonReader;
import java.awt.Toolkit;
import java.awt.datatransfer.Clipboard;
import java.awt.datatransfer.DataFlavor;
import java.awt.datatransfer.Transferable;
import java.awt.datatransfer.UnsupportedFlavorException;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import javax.swing.DefaultCellEditor;
import javax.swing.DefaultListModel;
import javax.swing.JComboBox;
import javax.swing.JDialog;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JScrollPane;
import javax.swing.RowSorter;
import javax.swing.table.DefaultTableModel;
import javax.swing.table.TableModel;
import javax.swing.table.TableRowSorter;
/**
*
* @author eduardogarcia
*/
public class ResponseProfile extends javax.swing.JPanel {
/**
* Creates new form RequestProfile
*/
DefaultListModel payload;
DefaultTableModel grep;
DefaultListModel encoder;
DefaultListModel tag;
DefaultListModel tagmanager;
List<Headers> headers;
List<String> variationAttributes;
List<Integer> insertionPointType;
List<String> Tags;
Boolean pathDiscovery;
DefaultTableModel model;
DefaultTableModel model1;
DefaultTableModel model2;
DefaultTableModel model4;
DefaultTableModel model9;
DefaultTableModel model10;
DefaultTableModel modelgrep;
DefaultTableModel rulemodel;
IBurpExtenderCallbacks callbacks;
String filename;
JComboBox operator;
public ResponseProfile(IBurpExtenderCallbacks callbacks) {
payload = new DefaultListModel();
grep = new DefaultTableModel();
encoder = new DefaultListModel();
tag = new DefaultListModel();
tagmanager = new DefaultListModel();
model4 = new DefaultTableModel();
model9 = new DefaultTableModel();
model10 = new DefaultTableModel();
modelgrep = new DefaultTableModel();
headers = new ArrayList();
variationAttributes = new ArrayList();
insertionPointType = new ArrayList();
operator = new JComboBox();
this.callbacks = callbacks;
modelgrep = new DefaultTableModel() {
@Override
public Class<?> getColumnClass(int columnIndex) {
Class clazz = String.class;
switch (columnIndex) {
case 0:
clazz = Boolean.class;
break;
}
return clazz;
}
@Override
public boolean isCellEditable(int row, int column) {
if(row == 0 && column == 1){
return false;
}else{
return true;
}
}
};
initComponents();
if (callbacks.loadExtensionSetting("filename") != null) {
filename = callbacks.loadExtensionSetting("filename") + File.separator;
} else {
filename = System.getProperty("user.home") + File.separator;
}
showTags();
showGrepsTable();
}
public String getClipboardContents() {
//Get clipboard contents for implement grep and match paste button
String result = "";
Clipboard clipboard = Toolkit.getDefaultToolkit().getSystemClipboard();
Transferable contents = clipboard.getContents(null);
boolean hasTransferableText = (contents != null) && contents.isDataFlavorSupported(DataFlavor.stringFlavor);
if (hasTransferableText) {
try {
result = (String) contents.getTransferData(DataFlavor.stringFlavor);
} catch (UnsupportedFlavorException | IOException ex) {
System.out.println("ResponseProfile line 151: "+ex.getMessage());
}
}
return result;
}
public void swap(int a, int b) {
Object aObject = encoder.getElementAt(a);
Object bObject = encoder.getElementAt(b);
encoder.set(a, bObject);
encoder.set(b, aObject);
}
public void loadGrepsFile(DefaultTableModel model) {
//Load file for implement payloads and match load button
List<String> grep = new ArrayList();
String line;
JFrame parentFrame = new JFrame();
JFileChooser fileChooser = new JFileChooser();
fileChooser.setDialogTitle("Specify a file to load");
int userSelection = fileChooser.showOpenDialog(parentFrame);
if (userSelection == JFileChooser.APPROVE_OPTION) {
File fileload = fileChooser.getSelectedFile();
textgreps.setText(fileload.getAbsolutePath());
try {
BufferedReader bufferreader = new BufferedReader(new FileReader(fileload.getAbsolutePath()));
line = bufferreader.readLine();
while (line != null) {
grep.add(line);
line = bufferreader.readLine();
}
bufferreader.close();
showGreps(grep);
} catch (FileNotFoundException ex) {
System.out.println("ResponseProfile line 189:");
} catch (IOException ex) {
System.out.println("ResponseProfile line 193:");
}
}
}
public JsonArray initJson() {
//Init json form filename
FileReader fr;
try {
JsonArray data = new JsonArray();
File f = new File(filename);
if (f.exists() && f.isDirectory()) {
for (File file : f.listFiles()) {
if (file.getName().endsWith(".bb")) {
fr = new FileReader(file.getAbsolutePath());
JsonReader json = new JsonReader((fr));
JsonParser parser = new JsonParser();
data.addAll(parser.parse(json).getAsJsonArray());
fr.close();
}
}
}
return data;
} catch (Exception e) {
System.out.println("ResponseProfile line 216: "+e.getMessage());
return null;
}
}
public void showGrepsTable() {
modelgrep.setNumRows(0);
modelgrep.setColumnCount(0);
modelgrep.addColumn("Enabled");
modelgrep.addColumn("Operator");
modelgrep.addColumn("Value");
operator.addItem("Or");
operator.addItem("And");
table4.getColumnModel().getColumn(0).setPreferredWidth(7);
table4.getColumnModel().getColumn(1).setPreferredWidth(15);
table4.getColumnModel().getColumn(2).setPreferredWidth(460);
table4.getColumnModel().getColumn(1).setCellEditor(new DefaultCellEditor(operator));
TableRowSorter<TableModel> sorter = new TableRowSorter<>(table4.getModel());
table4.setRowSorter(sorter);
List<RowSorter.SortKey> sortKeys = new ArrayList<>();
sorter.setSortKeys(sortKeys);
sorter.sort();
}
public void showGreps(List<String> greps) {
for (String grepline : greps) {
List<String> array = Arrays.asList(grepline.split(",",3));
if (array.size() > 1) {
if (modelgrep.getRowCount() == 0) {
if (array.get(0).equals("true")) {
modelgrep.addRow(new Object[]{true, "", array.get(2)});
} else {
modelgrep.addRow(new Object[]{false, "", array.get(2)});
}
} else {
if (array.get(0).equals("true")) {
modelgrep.addRow(new Object[]{true, array.get(1), array.get(2)});
} else {
modelgrep.addRow(new Object[]{false, array.get(1), array.get(2)});
}
}
} else {
if (modelgrep.getRowCount() == 0) {
modelgrep.addRow(new Object[]{true, "", grepline});
} else {
modelgrep.addRow(new Object[]{true, "Or", grepline});
}
}
}
}
public void addNewTag(String str) {
if (!str.isEmpty()) {
try {
BufferedWriter out = new BufferedWriter(new FileWriter(filename.concat("tags.txt"), true));
out.write(str.concat("\n"));
out.close();
} catch (IOException e) {
System.out.println("ResponseProfile line 281: "+"exception occoured" + e.getMessage());
}
}
}
public void removeTag(String tag) {
String file = filename.concat("tags.txt");
try {
File inFile = new File(file);
if (!inFile.isFile()) {
System.out.println("ResponseProfile line 293: "+"Parameter is not an existing file");
return;
}
//Construct the new file that will later be renamed to the original filename.
File tempFile = new File(inFile.getAbsolutePath().concat(".tmp"));
BufferedReader br = new BufferedReader(new FileReader(file));
PrintWriter pw = new PrintWriter(new FileWriter(tempFile));
String line = null;
//Read from the original file and write to the new
//unless content matches data to be removed.
while ((line = br.readLine()) != null) {
if (!line.trim().equals(tag)) {
pw.println(line);
pw.flush();
}
}
pw.close();
br.close();
//Delete the original file
if (!inFile.delete()) {
System.out.println("ResponseProfile line 319: "+"Could not delete file");
return;
}
//Rename the new file to the filename the original file had.
if (!tempFile.renameTo(inFile)) {
System.out.println("ResponseProfile line 325: "+"Could not rename file");
}
} catch (FileNotFoundException ex) {
System.out.println("ResponseProfile line 329:");
} catch (IOException ex) {
System.out.println("ResponseProfile line 333:");
}
}
public void showTags() {
List<String> tags = readFile(filename.concat("tags.txt"));
newTagCombo.removeAllItems();
tagmanager.removeAllElements();
for (String tag : tags) {
newTagCombo.addItem(tag);
tagmanager.addElement(tag);
}
}
private List<String> readFile(String filename) {
List<String> records = new ArrayList();
try {
BufferedReader reader = new BufferedReader(new FileReader(filename));
String line;
while ((line = reader.readLine()) != null) {
records.add(line);
}
reader.close();
} catch (Exception e) {
System.out.println("ResponsePorfile line 372:"+e.getMessage());
}
return records;
}
/**
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always
* regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">//GEN-BEGIN:initComponents
private void initComponents() {
buttonGroup1 = new javax.swing.ButtonGroup();
buttonGroup2 = new javax.swing.ButtonGroup();
buttonGroup3 = new javax.swing.ButtonGroup();
buttonGroup4 = new javax.swing.ButtonGroup();
buttonGroup5 = new javax.swing.ButtonGroup();
buttonGroup6 = new javax.swing.ButtonGroup();
buttonGroup7 = new javax.swing.ButtonGroup();
text1 = new javax.swing.JTextField();
jLabel18 = new javax.swing.JLabel();
jLabel12 = new javax.swing.JLabel();
textauthor = new javax.swing.JTextField();
headerstab = new javax.swing.JTabbedPane();
jScrollPane6 = new javax.swing.JScrollPane();
jPanel11 = new javax.swing.JPanel();
button10 = new javax.swing.JButton();
radio4 = new javax.swing.JRadioButton();
radio3 = new javax.swing.JRadioButton();
check4 = new javax.swing.JCheckBox();
check1 = new javax.swing.JCheckBox();
excludehttp = new javax.swing.JCheckBox();
onlyhttp = new javax.swing.JCheckBox();
check71 = new javax.swing.JCheckBox();
check72 = new javax.swing.JCheckBox();
text72 = new javax.swing.JTextField();
text71 = new javax.swing.JTextField();
negativeCT = new javax.swing.JCheckBox();
negativeRC = new javax.swing.JCheckBox();
jLabel24 = new javax.swing.JLabel();
jLabel25 = new javax.swing.JLabel();
jLabel28 = new javax.swing.JLabel();
jLabel29 = new javax.swing.JLabel();
jSeparator5 = new javax.swing.JSeparator();
jLabel30 = new javax.swing.JLabel();
jLabel31 = new javax.swing.JLabel();
jSeparator6 = new javax.swing.JSeparator();
rb1 = new javax.swing.JRadioButton();
rb2 = new javax.swing.JRadioButton();
rb3 = new javax.swing.JRadioButton();
rb4 = new javax.swing.JRadioButton();
jLabel2 = new javax.swing.JLabel();
sp1 = new javax.swing.JSpinner();
jLabel6 = new javax.swing.JLabel();
button19 = new javax.swing.JButton();
button18 = new javax.swing.JButton();
jScrollPane14 = new javax.swing.JScrollPane();
table4 = new javax.swing.JTable();
textgreps = new javax.swing.JTextField();
button8 = new javax.swing.JButton();
button7 = new javax.swing.JButton();
jLabel1 = new javax.swing.JLabel();
check73 = new javax.swing.JCheckBox();
text73 = new javax.swing.JTextField();
negativeURL = new javax.swing.JCheckBox();
first_match = new javax.swing.JRadioButton();
jLabel5 = new javax.swing.JLabel();
all_matches = new javax.swing.JRadioButton();
jScrollPane10 = new javax.swing.JScrollPane();
jPanel12 = new javax.swing.JPanel();
jLabel32 = new javax.swing.JLabel();
jLabel33 = new javax.swing.JLabel();
jLabel3 = new javax.swing.JLabel();
jLabel4 = new javax.swing.JLabel();
radio5 = new javax.swing.JRadioButton();
radio6 = new javax.swing.JRadioButton();
radio7 = new javax.swing.JRadioButton();
radio8 = new javax.swing.JRadioButton();
jLabel7 = new javax.swing.JLabel();
radio9 = new javax.swing.JRadioButton();
radio10 = new javax.swing.JRadioButton();
radio11 = new javax.swing.JRadioButton();
text4 = new javax.swing.JTextField();
jSeparator7 = new javax.swing.JSeparator();
jLabel34 = new javax.swing.JLabel();
jLabel35 = new javax.swing.JLabel();
jScrollPane7 = new javax.swing.JScrollPane();
textarea2 = new javax.swing.JTextArea();
jLabel13 = new javax.swing.JLabel();
jLabel36 = new javax.swing.JLabel();
jLabel37 = new javax.swing.JLabel();
jSeparator8 = new javax.swing.JSeparator();
jLabel38 = new javax.swing.JLabel();
jLabel39 = new javax.swing.JLabel();
jSeparator9 = new javax.swing.JSeparator();
jScrollPane1 = new javax.swing.JScrollPane();
textarea1 = new javax.swing.JTextArea();
jLabel9 = new javax.swing.JLabel();
jScrollPane8 = new javax.swing.JScrollPane();
textarea3 = new javax.swing.JTextArea();
jLabel14 = new javax.swing.JLabel();
jLabel40 = new javax.swing.JLabel();
jLabel41 = new javax.swing.JLabel();
jSeparator10 = new javax.swing.JSeparator();
jScrollPane9 = new javax.swing.JScrollPane();
textarea4 = new javax.swing.JTextArea();
jLabel15 = new javax.swing.JLabel();
jPanel3 = new javax.swing.JPanel();
removetag = new javax.swing.JButton();
addTag = new javax.swing.JButton();
newTagCombo = new javax.swing.JComboBox<>();
jScrollPane11 = new javax.swing.JScrollPane();
listtag = new javax.swing.JList<>();
jLabel46 = new javax.swing.JLabel();
jLabel47 = new javax.swing.JLabel();
newTagb = new javax.swing.JButton();
text1.setFont(new java.awt.Font("Lucida Grande", 0, 14)); // NOI18N
jLabel18.setFont(new java.awt.Font("Lucida Grande", 0, 14)); // NOI18N
jLabel18.setText("Author:");
jLabel12.setFont(new java.awt.Font("Lucida Grande", 0, 14)); // NOI18N
jLabel12.setText("Name:");
textauthor.setFont(new java.awt.Font("Lucida Grande", 0, 14)); // NOI18N
headerstab.setAutoscrolls(true);
headerstab.setFont(new java.awt.Font("Lucida Grande", 0, 14)); // NOI18N
headerstab.setPreferredSize(new java.awt.Dimension(800, 600));
headerstab.addChangeListener(new javax.swing.event.ChangeListener() {
public void stateChanged(javax.swing.event.ChangeEvent evt) {
headerstabStateChanged(evt);
}
});
jScrollPane6.setHorizontalScrollBarPolicy(javax.swing.ScrollPaneConstants.HORIZONTAL_SCROLLBAR_NEVER);
jScrollPane6.getVerticalScrollBar().setUnitIncrement(20);
jPanel11.setAutoscrolls(true);
button10.setText("Clear");
button10.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button10removeAllGrep(evt);
}
});
buttonGroup3.add(radio4);
radio4.setText("Simple string");
radio4.addItemListener(new java.awt.event.ItemListener() {
public void itemStateChanged(java.awt.event.ItemEvent evt) {
radio4stringMatchType(evt);
}
});
buttonGroup3.add(radio3);
radio3.setText("Regex");
radio3.addItemListener(new java.awt.event.ItemListener() {
public void itemStateChanged(java.awt.event.ItemEvent evt) {
radio3regexMatchType(evt);
}
});
check4.setText("Negative match");
check1.setText("Case sensitive");
excludehttp.setText("Exclude HTTP headers");
onlyhttp.setText("Only in HTTP headers");
check71.setText("Content type");
check72.setText("Status code");
negativeCT.setText("Negative match");
negativeRC.setText("Negative match");
jLabel24.setText("You can define one or more greps.");
jLabel25.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel25.setForeground(new java.awt.Color(255, 102, 51));
jLabel25.setText("Grep");
jLabel28.setText("You can define how your profile handles redirections.");
jLabel29.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel29.setForeground(new java.awt.Color(255, 102, 51));
jLabel29.setText("Redirections");
jLabel30.setText("These settings can be used to specify grep options of your profile.");
jLabel31.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel31.setForeground(new java.awt.Color(255, 102, 51));
jLabel31.setText("Grep Options");
buttonGroup4.add(rb1);
rb1.setText("Never");
buttonGroup4.add(rb2);
rb2.setText("On-site only");
buttonGroup4.add(rb3);
rb3.setText("In-scope only");
buttonGroup4.add(rb4);
rb4.setText("Always");
jLabel2.setText("Max redirections:");
jLabel6.setText("Follow redirections: ");
button19.setText("Add");
button19.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
addGrep(evt);
}
});
button18.setText("Remove");
button18.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button18removeMatchReplace(evt);
}
});
table4.setFont(new java.awt.Font("Lucida Grande", 0, 13)); // NOI18N
table4.setModel(modelgrep);
table4.setShowGrid(false);
jScrollPane14.setViewportView(table4);
button8.setText("Load File");
button8.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button8loadGrep(evt);
}
});
button7.setText("Paste");
button7.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
button7pasteGrep(evt);
}
});
jLabel1.setText("Match Type: ");
check73.setText("URL Extension");
negativeURL.setText("Negative match");
buttonGroup7.add(first_match);
first_match.setText("Only once per domain");
first_match.addItemListener(new java.awt.event.ItemListener() {
public void itemStateChanged(java.awt.event.ItemEvent evt) {
first_matchstringMatchType(evt);
}
});
jLabel5.setText("Show issue:");
buttonGroup7.add(all_matches);
all_matches.setText("All times in a domain");
all_matches.addItemListener(new java.awt.event.ItemListener() {
public void itemStateChanged(java.awt.event.ItemEvent evt) {
all_matchesregexMatchType(evt);
}
});
javax.swing.GroupLayout jPanel11Layout = new javax.swing.GroupLayout(jPanel11);
jPanel11.setLayout(jPanel11Layout);
jPanel11Layout.setHorizontalGroup(
jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jSeparator5)
.addComponent(jSeparator6, javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(jPanel11Layout.createSequentialGroup()
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel11Layout.createSequentialGroup()
.addGap(20, 20, 20)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel6)
.addComponent(jLabel2))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(rb1)
.addComponent(rb2)
.addComponent(rb3)
.addComponent(rb4)
.addComponent(sp1, javax.swing.GroupLayout.PREFERRED_SIZE, 56, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addGroup(jPanel11Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel31)
.addComponent(onlyhttp)
.addComponent(check4)
.addComponent(check1)
.addComponent(excludehttp)
.addComponent(jLabel29)
.addComponent(jLabel28, javax.swing.GroupLayout.PREFERRED_SIZE, 769, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING, false)
.addGroup(javax.swing.GroupLayout.Alignment.LEADING, jPanel11Layout.createSequentialGroup()
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(check72)
.addComponent(check71))
.addGap(15, 15, 15)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel11Layout.createSequentialGroup()
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(text71)
.addComponent(text72))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(negativeCT)
.addComponent(negativeRC)))
.addGroup(jPanel11Layout.createSequentialGroup()
.addComponent(text73, javax.swing.GroupLayout.PREFERRED_SIZE, 547, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(negativeURL))))
.addComponent(jLabel30, javax.swing.GroupLayout.Alignment.LEADING, javax.swing.GroupLayout.PREFERRED_SIZE, 769, javax.swing.GroupLayout.PREFERRED_SIZE))))
.addGroup(jPanel11Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel25)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(jPanel11Layout.createSequentialGroup()
.addComponent(button8, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(18, 18, 18)
.addComponent(textgreps, javax.swing.GroupLayout.PREFERRED_SIZE, 662, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(javax.swing.GroupLayout.Alignment.LEADING, jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jLabel24, javax.swing.GroupLayout.Alignment.LEADING, javax.swing.GroupLayout.PREFERRED_SIZE, 769, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(jPanel11Layout.createSequentialGroup()
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING, false)
.addComponent(button18, javax.swing.GroupLayout.Alignment.LEADING, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(button10, javax.swing.GroupLayout.Alignment.LEADING, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(button7, javax.swing.GroupLayout.Alignment.LEADING, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(button19, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel11Layout.createSequentialGroup()
.addComponent(jLabel1)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(radio3)
.addComponent(radio4))
.addGap(85, 85, 85)
.addComponent(jLabel5)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(all_matches)
.addComponent(first_match)))
.addComponent(jScrollPane14, javax.swing.GroupLayout.PREFERRED_SIZE, 662, javax.swing.GroupLayout.PREFERRED_SIZE)))))))
.addGroup(jPanel11Layout.createSequentialGroup()
.addContainerGap()
.addComponent(check73)))
.addContainerGap(42, Short.MAX_VALUE))
);
jPanel11Layout.linkSize(javax.swing.SwingConstants.HORIZONTAL, new java.awt.Component[] {button10, button18, button19, button7, button8});
jPanel11Layout.setVerticalGroup(
jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel11Layout.createSequentialGroup()
.addContainerGap()
.addComponent(jLabel25)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel24)
.addGap(25, 25, 25)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(textgreps, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(button8))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 18, Short.MAX_VALUE)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jScrollPane14, javax.swing.GroupLayout.PREFERRED_SIZE, 206, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(jPanel11Layout.createSequentialGroup()
.addComponent(button19)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(button7)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(button10)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(button18)))
.addGap(18, 18, 18)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel11Layout.createSequentialGroup()
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel1)
.addComponent(radio4))
.addGap(1, 1, 1)
.addComponent(radio3))
.addGroup(jPanel11Layout.createSequentialGroup()
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel5)
.addComponent(first_match))
.addGap(1, 1, 1)
.addComponent(all_matches)))
.addGap(18, 18, 18)
.addComponent(jSeparator6, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel31)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel30)
.addGap(25, 25, 25)
.addComponent(check4)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(check1)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(excludehttp)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(onlyhttp)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(check71)
.addComponent(text71, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(negativeCT))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(check72)
.addComponent(text72, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(negativeRC))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(check73)
.addComponent(text73, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(negativeURL))
.addGap(26, 26, 26)
.addComponent(jSeparator5, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel29)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel28)
.addGap(25, 25, 25)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(rb1)
.addComponent(jLabel6))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(rb2)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(rb3)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(rb4)
.addGap(18, 18, 18)
.addGroup(jPanel11Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel2)
.addComponent(sp1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(22, Short.MAX_VALUE))
);
jPanel11Layout.linkSize(javax.swing.SwingConstants.VERTICAL, new java.awt.Component[] {button10, button18, button19, button7, button8});
JScrollPane responseresScroll = new JScrollPane(jPanel11,
JScrollPane.VERTICAL_SCROLLBAR_ALWAYS, JScrollPane.HORIZONTAL_SCROLLBAR_NEVER);
jScrollPane6.setViewportView(jPanel11);
headerstab.addTab(" Response ", jScrollPane6);
jScrollPane10.getVerticalScrollBar().setUnitIncrement(20);
jPanel12.setAutoscrolls(true);
jLabel32.setText("You can define the issue properties.");
jLabel33.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel33.setForeground(new java.awt.Color(255, 102, 51));
jLabel33.setText("Issue Properties");
jLabel3.setText("Issue Name:");
jLabel4.setText("Severity:");
buttonGroup5.add(radio5);
radio5.setText("High");
buttonGroup5.add(radio6);
radio6.setText("Medium");
buttonGroup5.add(radio7);
radio7.setText("Low");
buttonGroup5.add(radio8);
radio8.setText("Information");
jLabel7.setText("Confidence:");
buttonGroup6.add(radio9);
radio9.setText("Certain");
buttonGroup6.add(radio10);
radio10.setText("Firm");
buttonGroup6.add(radio11);
radio11.setText("Tentative");
jLabel34.setText("You can define the issue details.");
jLabel35.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel35.setForeground(new java.awt.Color(255, 102, 51));
jLabel35.setText("Issue Detail");
textarea2.setColumns(20);
textarea2.setRows(5);
jScrollPane7.setViewportView(textarea2);
jLabel13.setText("Description:");
jLabel36.setText("You can define the issue background.");
jLabel37.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel37.setForeground(new java.awt.Color(255, 102, 51));
jLabel37.setText("Issue Background");
jLabel38.setText("You can define the remediation detail.");
jLabel39.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel39.setForeground(new java.awt.Color(255, 102, 51));
jLabel39.setText("Remediation Detail");
textarea1.setColumns(20);
textarea1.setRows(5);
jScrollPane1.setViewportView(textarea1);
jLabel9.setText("Description:");
textarea3.setColumns(20);
textarea3.setRows(5);
jScrollPane8.setViewportView(textarea3);
jLabel14.setText("Description:");
jLabel40.setText("You can define the remediation background.");
jLabel41.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel41.setForeground(new java.awt.Color(255, 102, 51));
jLabel41.setText("Remediation Background");
textarea4.setColumns(20);
textarea4.setRows(5);
jScrollPane9.setViewportView(textarea4);
jLabel15.setText("Description:");
javax.swing.GroupLayout jPanel12Layout = new javax.swing.GroupLayout(jPanel12);
jPanel12.setLayout(jPanel12Layout);
jPanel12Layout.setHorizontalGroup(
jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jSeparator7)
.addComponent(jSeparator8, javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jSeparator9, javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(jSeparator10, javax.swing.GroupLayout.Alignment.TRAILING)
.addGroup(jPanel12Layout.createSequentialGroup()
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel12Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel33)
.addComponent(jLabel35)
.addComponent(jLabel34)
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(jLabel13)
.addGap(18, 18, 18)
.addComponent(jScrollPane7, javax.swing.GroupLayout.PREFERRED_SIZE, 612, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(jLabel9)
.addGap(18, 18, 18)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, 612, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(jLabel15)
.addGap(18, 18, 18)
.addComponent(jScrollPane9, javax.swing.GroupLayout.PREFERRED_SIZE, 612, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(jLabel14)
.addGap(18, 18, 18)
.addComponent(jScrollPane8, javax.swing.GroupLayout.PREFERRED_SIZE, 612, javax.swing.GroupLayout.PREFERRED_SIZE))
.addComponent(jLabel37)
.addComponent(jLabel36)
.addComponent(jLabel39)
.addComponent(jLabel38)
.addComponent(jLabel32)
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(jLabel3)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(jLabel4, javax.swing.GroupLayout.PREFERRED_SIZE, 73, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel12Layout.createSequentialGroup()
.addComponent(radio8)
.addGap(189, 189, 189))
.addGroup(jPanel12Layout.createSequentialGroup()
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(radio6)
.addComponent(radio7)
.addComponent(radio5))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(jLabel7)
.addGap(18, 18, 18)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(radio9)
.addComponent(radio11)
.addComponent(radio10)))))
.addComponent(text4, javax.swing.GroupLayout.PREFERRED_SIZE, 419, javax.swing.GroupLayout.PREFERRED_SIZE)))))
.addComponent(jLabel41)
.addComponent(jLabel40))
.addContainerGap(93, Short.MAX_VALUE))
);
jPanel12Layout.setVerticalGroup(
jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel12Layout.createSequentialGroup()
.addGap(10, 10, 10)
.addComponent(jLabel33)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel32)
.addGap(25, 25, 25)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel3)
.addComponent(text4, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel12Layout.createSequentialGroup()
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel7)
.addComponent(radio9))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(radio10)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(radio11))
.addGroup(jPanel12Layout.createSequentialGroup()
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel4)
.addComponent(radio5))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(radio6)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(radio7)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(radio8)))
.addGap(18, 18, 18)
.addComponent(jSeparator7, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel35)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel34)
.addGap(25, 25, 25)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel9)
.addComponent(jScrollPane1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addComponent(jSeparator8, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel37)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel36)
.addGap(25, 25, 25)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel13)
.addComponent(jScrollPane7, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addComponent(jSeparator9, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel39)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel38)
.addGap(25, 25, 25)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel15)
.addComponent(jScrollPane9, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addComponent(jSeparator10, javax.swing.GroupLayout.PREFERRED_SIZE, 10, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel41)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel40)
.addGap(25, 25, 25)
.addGroup(jPanel12Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel14)
.addComponent(jScrollPane8, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
jScrollPane10.setViewportView(jPanel12);
headerstab.addTab(" Issue ", jScrollPane10);
removetag.setText("Remove");
removetag.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
removetag(evt);
}
});
addTag.setText("Add");
addTag.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
addTag(evt);
}
});
listtag.setModel(tag);
jScrollPane11.setViewportView(listtag);
jLabel46.setText("You can define one or multiple tags for this profile.");
jLabel47.setFont(new java.awt.Font("Lucida Grande", 1, 14)); // NOI18N
jLabel47.setForeground(new java.awt.Color(255, 102, 51));
jLabel47.setText("Set Tags");
newTagb.setText("New Tag");
newTagb.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
newTagbnewTag(evt);
}
});
javax.swing.GroupLayout jPanel3Layout = new javax.swing.GroupLayout(jPanel3);
jPanel3.setLayout(jPanel3Layout);
jPanel3Layout.setHorizontalGroup(
jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel3Layout.createSequentialGroup()
.addContainerGap()
.addGroup(jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jLabel47)
.addComponent(jLabel46)
.addGroup(jPanel3Layout.createSequentialGroup()
.addGroup(jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(newTagb, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)
.addComponent(addTag, javax.swing.GroupLayout.PREFERRED_SIZE, 93, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(removetag, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
.addGap(18, 18, 18)
.addGroup(jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(jScrollPane11)
.addComponent(newTagCombo, javax.swing.GroupLayout.PREFERRED_SIZE, 468, javax.swing.GroupLayout.PREFERRED_SIZE))))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
jPanel3Layout.setVerticalGroup(
jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(jPanel3Layout.createSequentialGroup()
.addGap(10, 10, 10)
.addComponent(jLabel47)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(jLabel46)
.addGap(25, 25, 25)
.addGroup(jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(jScrollPane11, javax.swing.GroupLayout.PREFERRED_SIZE, 99, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(jPanel3Layout.createSequentialGroup()
.addComponent(newTagb)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(removetag)))
.addGap(18, 18, 18)
.addGroup(jPanel3Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(newTagCombo, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(addTag))
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
headerstab.addTab(" Tags ", jPanel3);
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addComponent(jLabel12)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addComponent(text1)
.addGap(18, 18, 18)
.addComponent(jLabel18)
.addGap(18, 18, 18)
.addComponent(textauthor, javax.swing.GroupLayout.PREFERRED_SIZE, 165, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGap(70, 70, 70))
.addComponent(headerstab, javax.swing.GroupLayout.DEFAULT_SIZE, 831, Short.MAX_VALUE)
);
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addContainerGap()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(text1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel12)
.addComponent(textauthor, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(jLabel18))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(headerstab, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE))
);
}// </editor-fold>//GEN-END:initComponents
private void button10removeAllGrep(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button10removeAllGrep
int rowCount = modelgrep.getRowCount();
for (int i = rowCount - 1; i >= 0; i--) {
modelgrep.removeRow(i);
}
}//GEN-LAST:event_button10removeAllGrep
private void radio4stringMatchType(java.awt.event.ItemEvent evt) {//GEN-FIRST:event_radio4stringMatchType
}//GEN-LAST:event_radio4stringMatchType
private void radio3regexMatchType(java.awt.event.ItemEvent evt) {//GEN-FIRST:event_radio3regexMatchType
}//GEN-LAST:event_radio3regexMatchType
private void removetag(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_removetag
int selectedIndex = listtag.getSelectedIndex();
if (selectedIndex != -1) {
tag.remove(selectedIndex);
}
}//GEN-LAST:event_removetag
private void addTag(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_addTag
tag.addElement(newTagCombo.getSelectedItem());
}//GEN-LAST:event_addTag
private void newTagbnewTag(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_newTagbnewTag
Integer result;
NewTag nt = new NewTag();
JOptionPane jopane1 = new JOptionPane(nt, JOptionPane.PLAIN_MESSAGE, JOptionPane.OK_CANCEL_OPTION);
JDialog dialog = jopane1.createDialog(this, "New Tag");
dialog.setLocationRelativeTo(null);
dialog.setVisible(true);
Object selectedValue = jopane1.getValue();
if (selectedValue != null) {
result = ((Integer) selectedValue).intValue();
if (result == JOptionPane.OK_OPTION) {
addNewTag(nt.newTagtext.getText());
showTags();
}
}
}//GEN-LAST:event_newTagbnewTag
private void headerstabStateChanged(javax.swing.event.ChangeEvent evt) {//GEN-FIRST:event_headerstabStateChanged
int activePane = headerstab.getSelectedIndex();
if (activePane == 3) {
showTags();
}
}//GEN-LAST:event_headerstabStateChanged
private void addGrep(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_addGrep
if (modelgrep.getRowCount() == 0) {
modelgrep.addRow(new Object[]{true, "", "Value"});
} else {
modelgrep.addRow(new Object[]{true, "Or", "Value"});
}
}//GEN-LAST:event_addGrep
private void button18removeMatchReplace(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button18removeMatchReplace
int[] rows = table4.getSelectedRows();
Arrays.sort(rows);
for (int i = rows.length - 1; i >= 0; i--) {
int row = rows[i];
int modelRow = table4.convertRowIndexToModel(row);
modelgrep.removeRow(modelRow);
}
}//GEN-LAST:event_button18removeMatchReplace
private void button8loadGrep(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button8loadGrep
loadGrepsFile(modelgrep);
}//GEN-LAST:event_button8loadGrep
private void button7pasteGrep(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_button7pasteGrep
String element = getClipboardContents();
List<String> lines = Arrays.asList(element.split("\n"));
showGreps(lines);
}//GEN-LAST:event_button7pasteGrep
private void first_matchstringMatchType(java.awt.event.ItemEvent evt) {//GEN-FIRST:event_first_matchstringMatchType
// TODO add your handling code here:
}//GEN-LAST:event_first_matchstringMatchType
private void all_matchesregexMatchType(java.awt.event.ItemEvent evt) {//GEN-FIRST:event_all_matchesregexMatchType
// TODO add your handling code here:
}//GEN-LAST:event_all_matchesregexMatchType
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JButton addTag;
public javax.swing.JRadioButton all_matches;
private javax.swing.JButton button10;
private javax.swing.JButton button18;
private javax.swing.JButton button19;
private javax.swing.JButton button7;
private javax.swing.JButton button8;
public javax.swing.ButtonGroup buttonGroup1;
public javax.swing.ButtonGroup buttonGroup2;
public javax.swing.ButtonGroup buttonGroup3;
public javax.swing.ButtonGroup buttonGroup4;
public javax.swing.ButtonGroup buttonGroup5;
public javax.swing.ButtonGroup buttonGroup6;
public javax.swing.ButtonGroup buttonGroup7;
public javax.swing.JCheckBox check1;
public javax.swing.JCheckBox check4;
public javax.swing.JCheckBox check71;
public javax.swing.JCheckBox check72;
public javax.swing.JCheckBox check73;
public javax.swing.JCheckBox excludehttp;
public javax.swing.JRadioButton first_match;
public javax.swing.JTabbedPane headerstab;
private javax.swing.JLabel jLabel1;
private javax.swing.JLabel jLabel12;
private javax.swing.JLabel jLabel13;
private javax.swing.JLabel jLabel14;
private javax.swing.JLabel jLabel15;
private javax.swing.JLabel jLabel18;
private javax.swing.JLabel jLabel2;
private javax.swing.JLabel jLabel24;
private javax.swing.JLabel jLabel25;
private javax.swing.JLabel jLabel28;
private javax.swing.JLabel jLabel29;
private javax.swing.JLabel jLabel3;
private javax.swing.JLabel jLabel30;
private javax.swing.JLabel jLabel31;
private javax.swing.JLabel jLabel32;
private javax.swing.JLabel jLabel33;
private javax.swing.JLabel jLabel34;
private javax.swing.JLabel jLabel35;
private javax.swing.JLabel jLabel36;
private javax.swing.JLabel jLabel37;
private javax.swing.JLabel jLabel38;
private javax.swing.JLabel jLabel39;
private javax.swing.JLabel jLabel4;
private javax.swing.JLabel jLabel40;
private javax.swing.JLabel jLabel41;
private javax.swing.JLabel jLabel46;
private javax.swing.JLabel jLabel47;
private javax.swing.JLabel jLabel5;
private javax.swing.JLabel jLabel6;
private javax.swing.JLabel jLabel7;
private javax.swing.JLabel jLabel9;
private javax.swing.JPanel jPanel11;
private javax.swing.JPanel jPanel12;
private javax.swing.JPanel jPanel3;
private javax.swing.JScrollPane jScrollPane1;
private javax.swing.JScrollPane jScrollPane10;
private javax.swing.JScrollPane jScrollPane11;
private javax.swing.JScrollPane jScrollPane14;
private javax.swing.JScrollPane jScrollPane6;
private javax.swing.JScrollPane jScrollPane7;
private javax.swing.JScrollPane jScrollPane8;
private javax.swing.JScrollPane jScrollPane9;
private javax.swing.JSeparator jSeparator10;
private javax.swing.JSeparator jSeparator5;
private javax.swing.JSeparator jSeparator6;
private javax.swing.JSeparator jSeparator7;
private javax.swing.JSeparator jSeparator8;
private javax.swing.JSeparator jSeparator9;
public javax.swing.JList<String> listtag;
public javax.swing.JCheckBox negativeCT;
public javax.swing.JCheckBox negativeRC;
public javax.swing.JCheckBox negativeURL;
public javax.swing.JComboBox<String> newTagCombo;
private javax.swing.JButton newTagb;
public javax.swing.JCheckBox onlyhttp;
public javax.swing.JRadioButton radio10;
public javax.swing.JRadioButton radio11;
public javax.swing.JRadioButton radio3;
public javax.swing.JRadioButton radio4;
public javax.swing.JRadioButton radio5;
public javax.swing.JRadioButton radio6;
public javax.swing.JRadioButton radio7;
public javax.swing.JRadioButton radio8;
public javax.swing.JRadioButton radio9;
public javax.swing.JRadioButton rb1;
public javax.swing.JRadioButton rb2;
public javax.swing.JRadioButton rb3;
public javax.swing.JRadioButton rb4;
private javax.swing.JButton removetag;
public javax.swing.JSpinner sp1;
public javax.swing.JTable table4;
public javax.swing.JTextField text1;
public javax.swing.JTextField text4;
public javax.swing.JTextField text71;
public javax.swing.JTextField text72;
public javax.swing.JTextField text73;
public javax.swing.JTextArea textarea1;
public javax.swing.JTextArea textarea2;
public javax.swing.JTextArea textarea3;
public javax.swing.JTextArea textarea4;
public javax.swing.JTextField textauthor;
public javax.swing.JTextField textgreps;
// End of variables declaration//GEN-END:variables
} |
Java | BurpBounty/main/java/burpbountyfree/Utils.java | /*
Copyright 2018 Eduardo Garcia Melia <wagiro@gmail.com>
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package burpbountyfree;
import burp.IBurpExtenderCallbacks;
import burp.IExtensionHelpers;
import burp.IHttpRequestResponse;
import burp.IHttpService;
import burp.IRequestInfo;
import burp.IResponseInfo;
import burp.IScanIssue;
import burp.IScannerInsertionPoint;
import com.google.gson.Gson;
import com.google.gson.JsonArray;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
/**
*
* @author wagiro
*/
public class Utils {
private IBurpExtenderCallbacks callbacks;
private IExtensionHelpers helpers;
JsonArray active_profiles;
BurpBountyExtension bbe;
Gson gson;
CollaboratorData burpCollaboratorData;
BurpBountyGui bbg;
JsonArray allprofiles;
Integer redirtype;
List<String> rules_done = new ArrayList<>();
Integer smartscandelay;
public Utils(BurpBountyExtension bbe, IBurpExtenderCallbacks callbacks, CollaboratorData burpCollaboratorData, JsonArray allprofiles, BurpBountyGui bbg) {
this.callbacks = callbacks;
helpers = callbacks.getHelpers();
this.burpCollaboratorData = burpCollaboratorData;
gson = new Gson();
this.allprofiles = allprofiles;
this.bbe = bbe;
this.bbg = bbg;
}
public Boolean checkDuplicated(IHttpRequestResponse requestResponse, String issuename, IScannerInsertionPoint insertionPoint) {
IRequestInfo request = helpers.analyzeRequest(requestResponse);
String host = request.getUrl().getProtocol() + "://" + request.getUrl().getHost();
IScanIssue[] issues = callbacks.getScanIssues(host);
Boolean duplicated = false;
for (IScanIssue issue : issues) {
String path = issue.getUrl().getPath();
String is = issue.getIssueName();
if (is.equals("BurpBounty - " + issuename) && path.equals(request.getUrl().getPath())) {
String details = issue.getIssueDetail();
if (details.contains("Vulnerable parameter")) {
String param = details.substring(details.indexOf(": ") + 2, details.indexOf("."));
if (param.equals(insertionPoint.getInsertionPointName())) {
duplicated = true;
}
// String param = details.substring(details.indexOf(": ") + 2, details.indexOf("."));
// String param2 = insertionPoint.getInsertionPointName()+"_"+String.valueOf(insertionPoint.getInsertionPointType());
// //Revisar prque posa el param2 com a artist_0_65 y el param es artist
// if (param.equals(insertionPoint.getInsertionPointName()+"_"+String.valueOf(insertionPoint.getInsertionPointType()))) {
// duplicated = true;
// }
}
}
}
if (duplicated) {
return true;
} else {
return false;
}
}
public Boolean checkDuplicatedPassiveResponse(IHttpRequestResponse requestResponse, String issuename) {
IRequestInfo request = helpers.analyzeRequest(requestResponse);
String host = request.getUrl().getProtocol() + "://" + request.getUrl().getHost();
IScanIssue[] issues = callbacks.getScanIssues(host);
Boolean duplicated = false;
for (IScanIssue issue : issues) {
String path = issue.getUrl().getPath();
String is = issue.getIssueName();
if (is.equals("BurpBounty - " + issuename) && path.equals(request.getUrl().getPath())) {
duplicated = true;
}
}
if (duplicated) {
return true;
} else {
return false;
}
}
public Boolean checkDuplicatedPassive(IHttpRequestResponse requestResponse, String issuename) {
IRequestInfo request = helpers.analyzeRequest(requestResponse);
String host = request.getUrl().getProtocol() + "://" + request.getUrl().getHost();
IScanIssue[] issues = callbacks.getScanIssues(host);
Boolean duplicated = false;
for (IScanIssue issue : issues) {
String path = issue.getUrl().getPath();
String is = issue.getIssueName();
if (is.equals("BurpBounty - " + issuename) && path.equals(request.getUrl().getPath())) {
duplicated = true;
}
}
if (duplicated) {
return true;
} else {
return false;
}
}
public List<IScannerInsertionPoint> getInsertionPoints(IHttpRequestResponse baseRequestResponse) {
List<IScannerInsertionPoint> insertionPoints = new ArrayList();
try {
if (baseRequestResponse == null || baseRequestResponse.getRequest() == null || baseRequestResponse.getResponse() == null) {
return insertionPoints;
}
IRequestInfo request = helpers.analyzeRequest(baseRequestResponse);
byte[] req = baseRequestResponse.getRequest();
if (request.getMethod().equals("GET")) {
String url = request.getUrl().getHost();
byte[] match = helpers.stringToBytes("/");
int len = helpers.bytesToString(baseRequestResponse.getRequest()).indexOf("HTTP");
int beginAt = 0;
List<String> params = new ArrayList();
while (beginAt < len) {
beginAt = helpers.indexOf(req, match, false, beginAt, len);
if (beginAt == -1) {
break;
}
if (!params.contains(url + ":p4r4m" + beginAt)) {
insertionPoints.add(helpers.makeScannerInsertionPoint("p4r4m" + beginAt + "_65", baseRequestResponse.getRequest(), beginAt, helpers.bytesToString(baseRequestResponse.getRequest()).indexOf(" HTTP")));
params.add(url + ":p4r4m" + beginAt);
}
beginAt += match.length;
}
}
} catch (NullPointerException e) {
return null;
}
return insertionPoints;
}
public boolean encode(IScannerInsertionPoint insertionPoint, boolean request) {
byte value = insertionPoint.getInsertionPointType();
String value2 = insertionPoint.getInsertionPointName();
if ((value == 0 || value == 34 || value == 33 || value == 37 || value == 65) && !request) {
return true;
} else if (value2.endsWith("_0") || value2.endsWith("_34") || value2.endsWith("_33") || value2.endsWith("_37") || value2.endsWith("_65")) {
return true;
} else {
return false;
}
}
public URL getRedirection(IHttpRequestResponse response, IHttpService httpService, Integer redirtype) {
try {
URL url = getLocation(httpService, response);
if (url.toString().contains("burpcollaborator.net")) {
return url;
} else if (redirtype == 2) {
if (url.getHost().contains(httpService.getHost())) {
return url;
}
} else if (redirtype == 3) {
boolean isurl = callbacks.isInScope(url);
if (isurl) {
return url;
}
} else if (redirtype == 4) {
return url;
} else {
return null;
}
return null;
} catch (NullPointerException | ArrayIndexOutOfBoundsException ex) {
//Mirar esto genera muchos erroresSystem.out.println("Utils line 1207: " + ex.getMessage());
return null;
}
}
public URL getLocation(IHttpService httpService, IHttpRequestResponse response) {
String[] host = null;
String Location = "";
URL url;
try {
IResponseInfo response_info = helpers.analyzeResponse(response.getResponse());
for (String header : response_info.getHeaders()) {
if (header.toUpperCase().startsWith("LOCATION:")) {
host = header.split("\\s+");
Location = host[1];
break;
}
}
if (Location.startsWith("http://") || Location.startsWith("https://")) {
url = new URL(Location);
return url;
} else if (Location.startsWith("/")) {
url = new URL(httpService.getProtocol() + "://" + httpService.getHost() + Location);
return url;
} else {
url = new URL(httpService.getProtocol() + "://" + httpService.getHost() + "/" + Location);
return url;
}
} catch (MalformedURLException | NullPointerException | ArrayIndexOutOfBoundsException ex) {
System.out.println("Utils line 1237: " + ex.getMessage());
return null;
}
}
public byte[] getMatchAndReplace(List<Headers> headers, byte[] checkRequest, String payload, String bchost) {
String tempRequest = helpers.bytesToString(checkRequest);
if (!headers.isEmpty()) {
for (int x = 0; x < headers.size(); x++) {
String replace = headers.get(x).replace;
if (headers.get(x).type.equals("Request")) {
if (headers.get(x).regex.equals("String")) {
if (replace.contains("{PAYLOAD}")) {
replace = replace.replace("{PAYLOAD}", payload);
}
if (replace.contains("{BC}")) {
replace = replace.replace("{BC}", bchost);
}
if (headers.get(x).match.isEmpty()) {
tempRequest = tempRequest.replace("\r\n\r\n", "\r\n" + replace + "\r\n\r\n");
} else {
tempRequest = tempRequest.replace(headers.get(x).match, replace);
}
} else {
if (replace.contains("{PAYLOAD}")) {
replace = replace.replaceAll("\\{PAYLOAD\\}", payload);
}
if (replace.contains("{BC}")) {
replace = replace.replaceAll("\\{BC\\}", bchost);
}
if (headers.get(x).match.isEmpty()) {
tempRequest = tempRequest.replaceAll("\\r\\n\\r\\n", "\r\n" + replace + "\r\n\r\n");
} else {
tempRequest = tempRequest.replaceAll(headers.get(x).match, replace);
}
}
}
}
}
return helpers.stringToBytes(tempRequest);
}
public int getContentLength(IHttpRequestResponse response) {
IResponseInfo response_info;
try {
response_info = helpers.analyzeResponse(response.getResponse());
} catch (NullPointerException ex) {
System.out.println("Utils line 1279: " + ex.getMessage());
return 0;
}
int ContentLength = 0;
for (String headers : response_info.getHeaders()) {
if (headers.toUpperCase().startsWith("CONTENT-LENGTH:")) {
ContentLength = Integer.parseInt(headers.split("\\s+")[1]);
break;
}
}
return ContentLength;
}
public boolean isResponseCode(String responsecodes, boolean negativerc, Integer responsecode) {
if (responsecodes.isEmpty()) {
return false;
}
List<String> items = Arrays.asList(responsecodes.split("\\s*,\\s*"));
String code = Integer.toString(responsecode);
if (items.contains(code)) {
if (!negativerc) {
return true;
} else {
return false;
}
} else {
if (negativerc) {
return true;
} else {
return false;
}
}
}
public boolean isContentType(String contenttype, boolean negativect, IResponseInfo r) {
List<String> HEADERS = r.getHeaders();
if (contenttype.isEmpty()) {
return false;
}
List<String> items = Arrays.asList(contenttype.toUpperCase().split("\\s*,\\s*"));
for (String header : HEADERS) {
if (header.toUpperCase().startsWith("CONTENT-TYPE:")) {
String content_type = header.substring(header.lastIndexOf(":") + 2).split(";")[0].toUpperCase();
if (items.contains(content_type)) {
if (negativect) {
return false;
}
break;
} else {
if (!negativect) {
return false;
}
}
}
}
return true;
}
public List processPayload(List<String> payloads, List<String> encoders) {
List pay = new ArrayList();
for (String payload : payloads) {
payload = payload.replace("true,", "");
for (String p : encoders) {
switch (p) {
case "URL-encode key characters":
payload = encodeKeyURL(payload);
break;
case "URL-encode all characters":
payload = encodeURL(payload);
break;
case "URL-encode all characters (Unicode)":
payload = encodeUnicodeURL(payload);
break;
case "HTML-encode key characters":
payload = encodeKeyHTML(payload);
break;
case "HTML-encode all characters":
payload = encodeHTML(payload);
break;
case "Base64-encode":
payload = helpers.base64Encode(payload);
default:
break;
}
}
pay.add(payload);
}
return pay;
}
public static String encodeURL(String s) {
StringBuffer out = new StringBuffer();
for (int i = 0; i < s.length(); i++) {
char c = s.charAt(i);
out.append("%" + Integer.toHexString((int) c));
}
return out.toString();
}
public static String encodeUnicodeURL(String s) {
StringBuffer out = new StringBuffer();
for (int i = 0; i < s.length(); i++) {
char c = s.charAt(i);
out.append("%u00" + Integer.toHexString((int) c));
}
return out.toString();
}
public static String encodeHTML(String s) {
StringBuffer out = new StringBuffer();
for (int i = 0; i < s.length(); i++) {
char c = s.charAt(i);
out.append("&#x" + Integer.toHexString((int) c) + ";");
}
return out.toString();
}
public static String encodeKeyHTML(String s) {
StringBuffer out = new StringBuffer();
String key = "\\<\\(\\[\\\\\\^\\-\\=\\$\\!\\|\\]\\)\\?\\*\\+\\.\\>]\\&\\%\\:\\@ ";
for (int i = 0; i < s.length(); i++) {
char c = s.charAt(i);
if (key.contains(s.substring(i, i + 1))) {
out.append("&#x" + Integer.toHexString((int) c) + ";");
} else {
out.append(c);
}
}
return out.toString();
}
public static String encodeKeyURL(String s) {
StringBuffer out = new StringBuffer();
String key = "\\<\\(\\[\\\\\\^\\-\\=\\$\\!\\|\\]\\)\\?\\*\\+\\.\\>]\\&\\%\\:\\@ ";
for (int i = 0; i < s.length(); i++) {
char c = s.charAt(i);
if (key.contains(s.substring(i, i + 1))) {
out.append("%" + Integer.toHexString((int) c));
} else {
out.append(c);
}
}
return out.toString();
}
public static String encodeTheseURL(String s, String characters) {
StringBuffer out = new StringBuffer();
for (int i = 0; i < s.length(); i++) {
char c = s.charAt(i);
if (characters.indexOf(c) >= 0) {
out.append("%" + Integer.toHexString((int) c));
} else {
out.append(c);
}
}
return out.toString();
}
} |
BitBake | BurpBounty/profiles/AccessToken.bb | [
{
"ProfileName": "AccessToken",
"Name": "",
"Enabled": true,
"Scanner": 2,
"Author": "@egarme",
"Payloads": [],
"Encoder": [],
"UrlEncode": false,
"CharsToUrlEncode": "",
"Grep": [
"true,,access_token"
],
"Tags": [
"All"
],
"PayloadResponse": false,
"NotResponse": false,
"TimeOut1": "",
"TimeOut2": "",
"isTime": false,
"contentLength": "",
"iscontentLength": false,
"CaseSensitive": false,
"ExcludeHTTP": false,
"OnlyHTTP": false,
"IsContentType": false,
"ContentType": "",
"HttpResponseCode": "",
"NegativeCT": false,
"IsResponseCode": false,
"ResponseCode": "",
"NegativeRC": false,
"urlextension": "",
"isurlextension": false,
"NegativeUrlExtension": false,
"MatchType": 1,
"Scope": 2,
"RedirType": 0,
"MaxRedir": 0,
"payloadPosition": 0,
"payloadsFile": "",
"grepsFile": "",
"IssueName": "AccessToken",
"IssueSeverity": "Information",
"IssueConfidence": "Firm",
"IssueDetail": "Access Token Found",
"RemediationDetail": "",
"IssueBackground": "",
"RemediationBackground": "",
"Header": [],
"VariationAttributes": [],
"InsertionPointType": [],
"Scanas": false,
"Scantype": 0,
"pathDiscovery": false
}
] |
BitBake | BurpBounty/profiles/AmazonAWS.bb | [
{
"ProfileName": "AmazonAWS",
"Name": "",
"Enabled": true,
"Scanner": 2,
"Author": "@egarme",
"Payloads": [],
"Encoder": [],
"UrlEncode": false,
"CharsToUrlEncode": "",
"Grep": [
"true,,s3..*amazonaws.com"
],
"Tags": [
"All"
],
"PayloadResponse": false,
"NotResponse": false,
"TimeOut1": "",
"TimeOut2": "",
"isTime": false,
"contentLength": "",
"iscontentLength": false,
"CaseSensitive": false,
"ExcludeHTTP": false,
"OnlyHTTP": false,
"IsContentType": false,
"ContentType": "",
"HttpResponseCode": "",
"NegativeCT": false,
"IsResponseCode": false,
"ResponseCode": "",
"NegativeRC": false,
"urlextension": "",
"isurlextension": false,
"NegativeUrlExtension": false,
"MatchType": 2,
"Scope": 2,
"RedirType": 0,
"MaxRedir": 0,
"payloadPosition": 0,
"payloadsFile": "",
"grepsFile": "",
"IssueName": "AmazonAWS",
"IssueSeverity": "Information",
"IssueConfidence": "Firm",
"IssueDetail": "Amazon AWS found: \u003cbr\u003e\u003cgrep\u003e",
"RemediationDetail": "",
"IssueBackground": "",
"RemediationBackground": "",
"Header": [],
"VariationAttributes": [],
"InsertionPointType": [],
"Scanas": false,
"Scantype": 0,
"pathDiscovery": false
}
] |
BitBake | BurpBounty/profiles/AmazonAWSRequest.bb | [
{
"ProfileName": "AmazonAWSRequest",
"Name": "",
"Enabled": true,
"Scanner": 3,
"Author": "@egarme",
"Payloads": [],
"Encoder": [],
"UrlEncode": false,
"CharsToUrlEncode": "",
"Grep": [
"true,,All Request,Name,s3..*amazonaws.com"
],
"Tags": [
"All"
],
"PayloadResponse": false,
"NotResponse": false,
"TimeOut1": "",
"TimeOut2": "",
"isTime": false,
"contentLength": "",
"iscontentLength": false,
"CaseSensitive": false,
"ExcludeHTTP": false,
"OnlyHTTP": false,
"IsContentType": false,
"ContentType": "",
"HttpResponseCode": "",
"NegativeCT": false,
"IsResponseCode": false,
"ResponseCode": "",
"NegativeRC": false,
"urlextension": "",
"isurlextension": false,
"NegativeUrlExtension": false,
"MatchType": 2,
"Scope": 0,
"RedirType": 0,
"MaxRedir": 0,
"payloadPosition": 0,
"payloadsFile": "",
"grepsFile": "",
"IssueName": "AmazonAWS",
"IssueSeverity": "Information",
"IssueConfidence": "Firm",
"IssueDetail": "Amazon AWS found: \u003cbr\u003e\u003cgrep\u003e",
"RemediationDetail": "",
"IssueBackground": "",
"RemediationBackground": "",
"Header": [],
"VariationAttributes": [],
"InsertionPointType": [],
"Scanas": false,
"Scantype": 0,
"pathDiscovery": false
}
] |
BitBake | BurpBounty/profiles/Amazon_AWS_S3_Url.bb | [
{
"ProfileName": "Amazon_AWS_S3_Url",
"Name": "",
"Enabled": true,
"Scanner": 2,
"Author": "@six2dez1",
"Payloads": [],
"Encoder": [],
"UrlEncode": false,
"CharsToUrlEncode": "",
"Grep": [
"true,,[a-zA-Z0-9-\\.\\_]+\\.s3\\.amazonaws\\.com",
"true,Or,s3:\\/\\/[a-zA-Z0-9-\\.\\_]+",
"true,Or,s3.amazonaws.com\\/[a-zA-Z0-9-\\.\\_]+",
"true,Or,s3.console.aws.amazon.com\\/s3\\/buckets\\/[a-zA-Z0-9-\\.\\_]+",
"true,Or,s3\\\\.amazonaws.com[/]+|[a-zA-Z0-9_-]*\\\\.s3\\\\.amazonaws.com"
],
"Tags": [
"Cloud",
"All"
],
"PayloadResponse": false,
"NotResponse": false,
"TimeOut1": "",
"TimeOut2": "",
"isTime": false,
"contentLength": "",
"iscontentLength": false,
"CaseSensitive": false,
"ExcludeHTTP": false,
"OnlyHTTP": false,
"IsContentType": true,
"ContentType": "text/css,image/jpeg,image/png,image/svg+xml,image/gif,image/tiff,image/webp,image/x-icon,application/font-woff,image/vnd.microsoft.icon,font/ttf,font/woff2",
"HttpResponseCode": "",
"NegativeCT": true,
"IsResponseCode": false,
"ResponseCode": "",
"NegativeRC": false,
"urlextension": "",
"isurlextension": false,
"NegativeUrlExtension": false,
"MatchType": 2,
"Scope": 2,
"RedirType": 0,
"MaxRedir": 0,
"payloadPosition": 0,
"payloadsFile": "",
"grepsFile": "",
"IssueName": "AWS Url Detected",
"IssueSeverity": "Information",
"IssueConfidence": "Certain",
"IssueDetail": "",
"RemediationDetail": "",
"IssueBackground": "",
"RemediationBackground": "",
"Header": [],
"VariationAttributes": [],
"InsertionPointType": [],
"Scanas": false,
"Scantype": 0,
"pathDiscovery": false
}
] |
BitBake | BurpBounty/profiles/Amazon_MWS_Auth_Token.bb | [
{
"ProfileName": "Amazon_MWS_Auth_Token",
"Name": "",
"Enabled": true,
"Scanner": 2,
"Author": "@six2dez1",
"Payloads": [],
"Encoder": [],
"UrlEncode": false,
"CharsToUrlEncode": "",
"Grep": [
"true,,amzn\\\\.mws\\\\.[0-9a-f]{8}-[0-9a-f]{4}-[0-9a-f]{4}-[0-9a-f]{4}-[0-9a-f]{12}"
],
"Tags": [
"All"
],
"PayloadResponse": false,
"NotResponse": false,
"TimeOut1": "",
"TimeOut2": "",
"isTime": false,
"contentLength": "",
"iscontentLength": false,
"CaseSensitive": false,
"ExcludeHTTP": false,
"OnlyHTTP": false,
"IsContentType": true,
"ContentType": "text/css,image/jpeg,image/png,image/svg+xml,image/gif,image/tiff,image/webp,image/x-icon,application/font-woff,image/vnd.microsoft.icon,font/ttf,font/woff2",
"HttpResponseCode": "",
"NegativeCT": true,
"IsResponseCode": false,
"ResponseCode": "",
"NegativeRC": false,
"urlextension": "",
"isurlextension": false,
"NegativeUrlExtension": false,
"MatchType": 2,
"Scope": 2,
"RedirType": 0,
"MaxRedir": 0,
"payloadPosition": 0,
"payloadsFile": "",
"grepsFile": "",
"IssueName": "Amazon MWS url found",
"IssueSeverity": "Information",
"IssueConfidence": "Certain",
"IssueDetail": "Amazon MWS url found",
"RemediationDetail": "",
"IssueBackground": "",
"RemediationBackground": "",
"Header": [],
"VariationAttributes": [],
"InsertionPointType": [],
"Scanas": false,
"Scantype": 0,
"pathDiscovery": false
}
] |
BitBake | BurpBounty/profiles/Android_WebView_JS.bb | [
{
"ProfileName": "Android_WebView_JS",
"Name": "",
"Enabled": true,
"Scanner": 2,
"Author": "@six2dez1",
"Payloads": [],
"Encoder": [],
"UrlEncode": false,
"CharsToUrlEncode": "",
"Grep": [
"true,,setJavaScriptEnabled\\(true\\)"
],
"Tags": [
"All",
"Mobile"
],
"PayloadResponse": false,
"NotResponse": false,
"TimeOut1": "",
"TimeOut2": "",
"isTime": false,
"contentLength": "",
"iscontentLength": false,
"CaseSensitive": false,
"ExcludeHTTP": false,
"OnlyHTTP": false,
"IsContentType": true,
"ContentType": "text/css,image/jpeg,image/png,image/svg+xml,image/gif,image/tiff,image/webp,image/x-icon,application/font-woff,image/vnd.microsoft.icon,font/ttf,font/woff2",
"HttpResponseCode": "",
"NegativeCT": true,
"IsResponseCode": false,
"ResponseCode": "",
"NegativeRC": false,
"urlextension": "",
"isurlextension": false,
"NegativeUrlExtension": false,
"MatchType": 2,
"Scope": 2,
"RedirType": 0,
"MaxRedir": 0,
"payloadPosition": 0,
"payloadsFile": "",
"grepsFile": "",
"IssueName": "Possible Android Webview JS enabled",
"IssueSeverity": "Information",
"IssueConfidence": "Certain",
"IssueDetail": "Possible Android Webview JS enabled",
"RemediationDetail": "",
"IssueBackground": "",
"RemediationBackground": "",
"Header": [],
"VariationAttributes": [],
"InsertionPointType": [],
"Scanas": false,
"Scantype": 0,
"pathDiscovery": false
}
] |
BitBake | BurpBounty/profiles/ApiKeyRequest.bb | [
{
"ProfileName": "ApiKeyRequest",
"Name": "",
"Enabled": true,
"Scanner": 3,
"Author": "@egarme",
"Payloads": [],
"Encoder": [],
"UrlEncode": false,
"CharsToUrlEncode": "",
"Grep": [
"true,,All Request,Value,api_key",
"true,Or,All Request,Value,api-key",
"true,Or,All Request,Value,api key",
"true,Or,All Request,Value,apikey"
],
"Tags": [
"API",
"All"
],
"PayloadResponse": false,
"NotResponse": false,
"TimeOut1": "",
"TimeOut2": "",
"isTime": false,
"contentLength": "",
"iscontentLength": false,
"CaseSensitive": false,
"ExcludeHTTP": false,
"OnlyHTTP": false,
"IsContentType": false,
"ContentType": "",
"HttpResponseCode": "",
"NegativeCT": false,
"IsResponseCode": false,
"ResponseCode": "",
"NegativeRC": false,
"urlextension": "",
"isurlextension": false,
"NegativeUrlExtension": false,
"MatchType": 1,
"Scope": 0,
"RedirType": 0,
"MaxRedir": 0,
"payloadPosition": 0,
"payloadsFile": "",
"grepsFile": "",
"IssueName": "ApiKeyRequest",
"IssueSeverity": "Information",
"IssueConfidence": "Firm",
"IssueDetail": "Api Key found",
"RemediationDetail": "",
"IssueBackground": "",
"RemediationBackground": "",
"Header": [],
"VariationAttributes": [],
"InsertionPointType": [],
"Scanas": false,
"Scantype": 0,
"pathDiscovery": false
}
] |
BitBake | BurpBounty/profiles/ApiKeyResponse.bb | [
{
"ProfileName": "ApiKeyResponse",
"Name": "",
"Enabled": true,
"Scanner": 2,
"Author": "@egarme",
"Payloads": [],
"Encoder": [],
"UrlEncode": false,
"CharsToUrlEncode": "",
"Grep": [
"true,,api_key",
"true,Or,api-key",
"true,Or,api key",
"true,Or,apikey"
],
"Tags": [
"All"
],
"PayloadResponse": false,
"NotResponse": false,
"TimeOut1": "",
"TimeOut2": "",
"isTime": false,
"contentLength": "",
"iscontentLength": false,
"CaseSensitive": false,
"ExcludeHTTP": false,
"OnlyHTTP": false,
"IsContentType": false,
"ContentType": "",
"HttpResponseCode": "",
"NegativeCT": false,
"IsResponseCode": false,
"ResponseCode": "",
"NegativeRC": false,
"urlextension": "",
"isurlextension": false,
"NegativeUrlExtension": false,
"MatchType": 1,
"Scope": 2,
"RedirType": 0,
"MaxRedir": 0,
"payloadPosition": 0,
"payloadsFile": "",
"grepsFile": "",
"IssueName": "ApiKeyResponse",
"IssueSeverity": "Information",
"IssueConfidence": "Firm",
"IssueDetail": "Api Key found",
"RemediationDetail": "",
"IssueBackground": "",
"RemediationBackground": "",
"Header": [],
"VariationAttributes": [],
"InsertionPointType": [],
"Scanas": false,
"Scantype": 0,
"pathDiscovery": false
}
] |
BitBake | BurpBounty/profiles/ApiPath.bb | [
{
"ProfileName": "ApiPath",
"Name": "",
"Enabled": true,
"Scanner": 3,
"Author": "@egarme",
"Payloads": [],
"Encoder": [],
"UrlEncode": false,
"CharsToUrlEncode": "",
"Grep": [
"true,,All Request,Value,/api/",
"true,Or,All Request,Value,/internal_api"
],
"Tags": [
"API",
"All"
],
"PayloadResponse": false,
"NotResponse": false,
"TimeOut1": "",
"TimeOut2": "",
"isTime": false,
"contentLength": "",
"iscontentLength": false,
"CaseSensitive": false,
"ExcludeHTTP": false,
"OnlyHTTP": false,
"IsContentType": false,
"ContentType": "",
"HttpResponseCode": "",
"NegativeCT": false,
"IsResponseCode": false,
"ResponseCode": "",
"NegativeRC": false,
"urlextension": "",
"isurlextension": false,
"NegativeUrlExtension": false,
"MatchType": 1,
"Scope": 0,
"RedirType": 0,
"MaxRedir": 0,
"payloadPosition": 0,
"payloadsFile": "",
"grepsFile": "",
"IssueName": "ApiPath",
"IssueSeverity": "Information",
"IssueConfidence": "Firm",
"IssueDetail": "Api Path found",
"RemediationDetail": "",
"IssueBackground": "",
"RemediationBackground": "",
"Header": [],
"VariationAttributes": [],
"InsertionPointType": [],
"Scanas": false,
"Scantype": 0,
"pathDiscovery": false
}
] |
BitBake | BurpBounty/profiles/API_Keys.bb | [
{
"ProfileName": "API_Keys",
"Name": "",
"Enabled": true,
"Scanner": 2,
"Author": "@six2dez1",
"Payloads": [],
"Encoder": [],
"UrlEncode": false,
"CharsToUrlEncode": "",
"Grep": [
"true,,(?:\\s|\u003d|:|\"|^)AKC[a-zA-Z0-9]{10,}",
"true,Or,^(AAAA[A-Za-z0-9_-]{7}:[A-Za-z0-9_-]{140})",
"true,Or,[^a-zA-Z0-9](AAAA[A-Za-z0-9_-]{7}:[A-Za-z0-9_-]{140})",
"true,Or,(?i)github(.{0,20})?(?-i)[\u0027\\\"][0-9a-zA-Z]{35,40}",
"true,Or,^(EAACEdEose0cBA[0-9A-Za-z]+)",
"true,Or,[^a-zA-Z0-9](EAACEdEose0cBA[0-9A-Za-z]+)",
"true,Or,(?i)(facebook|fb)(.{0,20})?(?-i)[\u0027\\\"][0-9a-f]{32}",
"true,Or,[^a-zA-Z0-9](AIza[0-9A-Za-z-_]{35})",
"true,Or,^(AIza[0-9A-Za-z-_]{35})",
"true,Or,(?i)(google|gcp|youtube|drive|yt)(.{0,20})?[\u0027\\\"][AIza[0-9a-z\\\\-_]{35}][\u0027\\\"]",
"true,Or,^([0-9a-fA-F]{8}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{12})",
"true,Or,[^a-zA-Z0-9]([0-9a-fA-F]{8}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{4}-[0-9a-fA-F]{12})",
"true,Or,(?i)heroku.{0,30}[0-9A-F]{8}-[0-9A-F]{4}-[0-9A-F]{4}-[0-9A-F]{4}-[0-9A-F]{12}",
"true,Or,(?i)heroku(.{0,20})?[\u0027\"][0-9a-f]{8}-[0-9a-f]{4}-[0-9a-f]{4}-[0-9a-f]{12}[\u0027\"]",
"true,Or,^([0-9a-f]{32}-us[0-9])",
"true,Or,[^a-zA-Z0-9]([0-9a-f]{32}-us[0-9])",
"true,Or,^(key-[0-9a-zA-Z]{32})",
"true,Or,[^a-zA-Z0-9](key-[0-9a-zA-Z]{32})",
"true,Or,SG\\.[0-9A-Za-z\\-_]{22}\\.[0-9A-Za-z\\-_]{43}",
"true,Or,(?i)sauce.{0,50}(\\\\\\\"|\u0027|`)?[0-9a-f-]{36}(\\\\\\\"|\u0027|`)?",
"true,Or,xox.-[0-9]{12}-[0-9]{12}-[0-9a-zA-Z]{24}",
"true,Or,(xox[p|b|o|a]-[0-9]{12}-[0-9]{12}-[0-9]{12}-[a-z0-9]{32})",
"true,Or,(xox[baprs]-([0-9a-zA-Z]{10,48})?)",
"true,Or,https:\\/\\/hooks.slack.com\\/services\\/T[a-zA-Z0-9_]{8}\\/B[a-zA-Z0-9_]{8}\\/[a-zA-Z0-9_]{24}",
"true,Or,sq0[a-z]{3}-[0-9A-Za-z\\-_]{22,43}",
"true,Or,(?:r|s)k_live_[0-9a-zA-Z]{24}",
"true,Or,^(SK[0-9a-fA-F]{32})",
"true,Or,[^a-zA-Z0-9](SK[0-9a-fA-F]{32})",
"true,Or,^(R_[0-9a-f]{32})",
"true,Or,[^a-zA-Z0-9](R_[0-9a-f]{32})",
"true,Or,(?i)nr-internal-api-key",
"true,Or,(?i)NRAK-[A-Z0-9]{27}",
"true,Or,(?i)NRRA-[a-f0-9]{42}",
"true,Or,(?i)NRAA-[a-f0-9]{27}"
],
"Tags": [
"All"
],
"PayloadResponse": false,
"NotResponse": false,
"TimeOut1": "",
"TimeOut2": "",
"isTime": false,
"contentLength": "",
"iscontentLength": false,
"CaseSensitive": false,
"ExcludeHTTP": false,
"OnlyHTTP": false,
"IsContentType": true,
"ContentType": "text/css,image/jpeg,image/png,image/svg+xml,image/gif,image/tiff,image/webp,image/x-icon,application/font-woff,image/vnd.microsoft.icon,font/ttf,font/woff2",
"HttpResponseCode": "",
"NegativeCT": true,
"IsResponseCode": false,
"ResponseCode": "",
"NegativeRC": false,
"urlextension": "",
"isurlextension": false,
"NegativeUrlExtension": false,
"MatchType": 2,
"Scope": 2,
"RedirType": 0,
"MaxRedir": 0,
"payloadPosition": 0,
"payloadsFile": "",
"grepsFile": "",
"IssueName": "API Key or Token discovered",
"IssueSeverity": "Medium",
"IssueConfidence": "Firm",
"IssueDetail": "API Key or Token discovered, check how to take advantage of it here https://github.com/streaak/keyhacks",
"RemediationDetail": "",
"IssueBackground": "",
"RemediationBackground": "",
"Header": [],
"VariationAttributes": [],
"InsertionPointType": [],
"Scanas": false,
"Scantype": 0,
"pathDiscovery": false
}
] |
BitBake | BurpBounty/profiles/Artifactory_API_Token.bb | [
{
"ProfileName": "Artifactory_API_Token",
"Name": "",
"Enabled": true,
"Scanner": 2,
"Author": "@six2dez1",
"Payloads": [],
"Encoder": [],
"UrlEncode": false,
"CharsToUrlEncode": "",
"Grep": [
"true,,(?:\\s|\u003d|:|\"|^)AKC[a-zA-Z0-9]{10,}"
],
"Tags": [
"All"
],
"PayloadResponse": false,
"NotResponse": false,
"TimeOut1": "",
"TimeOut2": "",
"isTime": false,
"contentLength": "",
"iscontentLength": false,
"CaseSensitive": false,
"ExcludeHTTP": false,
"OnlyHTTP": false,
"IsContentType": true,
"ContentType": "text/css,image/jpeg,image/png,image/svg+xml,image/gif,image/tiff,image/webp,image/x-icon,application/font-woff,image/vnd.microsoft.icon,font/ttf,font/woff2",
"HttpResponseCode": "",
"NegativeCT": true,
"IsResponseCode": false,
"ResponseCode": "",
"NegativeRC": false,
"urlextension": "",
"isurlextension": false,
"NegativeUrlExtension": false,
"MatchType": 2,
"Scope": 2,
"RedirType": 0,
"MaxRedir": 0,
"payloadPosition": 0,
"payloadsFile": "",
"grepsFile": "",
"IssueName": "Artifactory API Token",
"IssueSeverity": "Information",
"IssueConfidence": "Certain",
"IssueDetail": "",
"RemediationDetail": "",
"IssueBackground": "",
"RemediationBackground": "",
"Header": [],
"VariationAttributes": [],
"InsertionPointType": [],
"Scanas": false,
"Scantype": 0,
"pathDiscovery": false
}
] |
BitBake | BurpBounty/profiles/Artifactory_Password.bb | [
{
"ProfileName": "Artifactory_Password",
"Name": "",
"Enabled": true,
"Scanner": 2,
"Author": "@six2dez1",
"Payloads": [],
"Encoder": [],
"UrlEncode": false,
"CharsToUrlEncode": "",
"Grep": [
"true,,(?:\\s|\u003d|:|\"|^)AP[\\dABCDEF][a-zA-Z0-9]{8,}"
],
"Tags": [
"All"
],
"PayloadResponse": false,
"NotResponse": false,
"TimeOut1": "",
"TimeOut2": "",
"isTime": false,
"contentLength": "",
"iscontentLength": false,
"CaseSensitive": false,
"ExcludeHTTP": false,
"OnlyHTTP": false,
"IsContentType": true,
"ContentType": "text/css,image/jpeg,image/png,image/svg+xml,image/gif,image/tiff,image/webp,image/x-icon,application/font-woff,image/vnd.microsoft.icon,font/ttf,font/woff2",
"HttpResponseCode": "",
"NegativeCT": true,
"IsResponseCode": false,
"ResponseCode": "",
"NegativeRC": false,
"urlextension": "",
"isurlextension": false,
"NegativeUrlExtension": false,
"MatchType": 2,
"Scope": 2,
"RedirType": 0,
"MaxRedir": 0,
"payloadPosition": 0,
"payloadsFile": "",
"grepsFile": "",
"IssueName": "Artifactory Password",
"IssueSeverity": "Information",
"IssueConfidence": "Certain",
"IssueDetail": "",
"RemediationDetail": "",
"IssueBackground": "",
"RemediationBackground": "",
"Header": [],
"VariationAttributes": [],
"InsertionPointType": [],
"Scanas": false,
"Scantype": 0,
"pathDiscovery": false
}
] |
BitBake | BurpBounty/profiles/AuthorizationBearerToken.bb | [
{
"ProfileName": "AuthorizationBearerToken",
"Name": "",
"Enabled": true,
"Scanner": 3,
"Author": "@egarme",
"Payloads": [],
"Encoder": [],
"UrlEncode": false,
"CharsToUrlEncode": "",
"Grep": [
"true,,All Request,Value,Authorization: Bearer"
],
"Tags": [
"JWT",
"All"
],
"PayloadResponse": false,
"NotResponse": false,
"TimeOut1": "",
"TimeOut2": "",
"isTime": false,
"contentLength": "",
"iscontentLength": false,
"CaseSensitive": false,
"ExcludeHTTP": false,
"OnlyHTTP": false,
"IsContentType": false,
"ContentType": "",
"HttpResponseCode": "",
"NegativeCT": false,
"IsResponseCode": false,
"ResponseCode": "",
"NegativeRC": false,
"urlextension": "",
"isurlextension": false,
"NegativeUrlExtension": false,
"MatchType": 1,
"Scope": 0,
"RedirType": 0,
"MaxRedir": 0,
"payloadPosition": 0,
"payloadsFile": "",
"grepsFile": "",
"IssueName": "AuthorizationBearerToken",
"IssueSeverity": "Information",
"IssueConfidence": "Firm",
"IssueDetail": "Authorization Bearer Token Found",
"RemediationDetail": "",
"IssueBackground": "",
"RemediationBackground": "",
"Header": [],
"VariationAttributes": [],
"InsertionPointType": [],
"Scanas": false,
"Scantype": 0,
"pathDiscovery": false
}
] |
BitBake | BurpBounty/profiles/Authorization_Bearer.bb | [
{
"ProfileName": "Authorization_Bearer",
"Name": "",
"Enabled": true,
"Scanner": 2,
"Author": "@six2dez1",
"Payloads": [],
"Encoder": [],
"UrlEncode": false,
"CharsToUrlEncode": "",
"Grep": [
"true,,bearer\\s*[a-zA-Z0-9_\\-\\.\u003d:_\\+\\/]+"
],
"Tags": [
"All"
],
"PayloadResponse": false,
"NotResponse": false,
"TimeOut1": "",
"TimeOut2": "",
"isTime": false,
"contentLength": "",
"iscontentLength": false,
"CaseSensitive": false,
"ExcludeHTTP": false,
"OnlyHTTP": false,
"IsContentType": true,
"ContentType": "text/css,image/jpeg,image/png,image/svg+xml,image/gif,image/tiff,image/webp,image/x-icon,application/font-woff,image/vnd.microsoft.icon,font/ttf,font/woff2,application/javascript",
"HttpResponseCode": "",
"NegativeCT": true,
"IsResponseCode": false,
"ResponseCode": "",
"NegativeRC": false,
"urlextension": "",
"isurlextension": false,
"NegativeUrlExtension": false,
"MatchType": 2,
"Scope": 2,
"RedirType": 0,
"MaxRedir": 0,
"payloadPosition": 0,
"payloadsFile": "",
"grepsFile": "",
"IssueName": "Authorization Bearer",
"IssueSeverity": "Information",
"IssueConfidence": "Certain",
"IssueDetail": "Authorization Bearer",
"RemediationDetail": "",
"IssueBackground": "",
"RemediationBackground": "",
"Header": [],
"VariationAttributes": [],
"InsertionPointType": [],
"Scanas": false,
"Scantype": 0,
"pathDiscovery": false
}
] |
BitBake | BurpBounty/profiles/AWS_Access_Key_ID.bb | [
{
"ProfileName": "AWS_Access_Key_ID",
"Name": "",
"Enabled": true,
"Scanner": 2,
"Author": "@six2dez1",
"Payloads": [],
"Encoder": [],
"UrlEncode": false,
"CharsToUrlEncode": "",
"Grep": [
"true,,[^a-zA-Z0-9](AKIA[a-zA-Z0-9]{16})",
"true,Or,(AccessKeyId|aws_access_key_id)",
"true,Or,^(AKIA[a-zA-Z0-9]{16})",
"true,Or,^((A3T[A-Z0-9]|AKIA|AGPA|AIDA|AROA|AIPA|ANPA|ANVA|ASIA)[A-Z0-9]{16})",
"true,Or,[^a-zA-Z0-9]((A3T[A-Z0-9]|AKIA|AGPA|AIDA|AROA|AIPA|ANPA|ANVA|ASIA)[A-Z0-9]{16})"
],
"Tags": [
"All",
"Cloud"
],
"PayloadResponse": false,
"NotResponse": false,
"TimeOut1": "",
"TimeOut2": "",
"isTime": false,
"contentLength": "",
"iscontentLength": false,
"CaseSensitive": false,
"ExcludeHTTP": false,
"OnlyHTTP": false,
"IsContentType": true,
"ContentType": "text/css,image/jpeg,image/png,image/svg+xml,image/gif,image/tiff,image/webp,image/x-icon,application/font-woff,image/vnd.microsoft.icon,font/ttf,font/woff2",
"HttpResponseCode": "",
"NegativeCT": true,
"IsResponseCode": false,
"ResponseCode": "",
"NegativeRC": false,
"urlextension": "",
"isurlextension": false,
"NegativeUrlExtension": false,
"MatchType": 2,
"Scope": 2,
"RedirType": 0,
"MaxRedir": 0,
"payloadPosition": 0,
"payloadsFile": "",
"grepsFile": "",
"IssueName": "AWS Access Key ID",
"IssueSeverity": "Information",
"IssueConfidence": "Certain",
"IssueDetail": "",
"RemediationDetail": "",
"IssueBackground": "",
"RemediationBackground": "",
"Header": [],
"VariationAttributes": [],
"InsertionPointType": [],
"Scanas": false,
"Scantype": 0,
"pathDiscovery": false
}
] |
BitBake | BurpBounty/profiles/AWS_Client_Secret.bb | [
{
"ProfileName": "AWS_Client_Secret",
"Name": "",
"Enabled": true,
"Scanner": 2,
"Author": "@six2dez1",
"Payloads": [],
"Encoder": [],
"UrlEncode": false,
"CharsToUrlEncode": "",
"Grep": [
"true,,(SecretAccessKey|aws_secret_access_key)"
],
"Tags": [
"All",
"Cloud"
],
"PayloadResponse": false,
"NotResponse": false,
"TimeOut1": "",
"TimeOut2": "",
"isTime": false,
"contentLength": "",
"iscontentLength": false,
"CaseSensitive": false,
"ExcludeHTTP": false,
"OnlyHTTP": false,
"IsContentType": true,
"ContentType": "text/css,image/jpeg,image/png,image/svg+xml,image/gif,image/tiff,image/webp,image/x-icon,application/font-woff,image/vnd.microsoft.icon,font/ttf,font/woff2",
"HttpResponseCode": "",
"NegativeCT": true,
"IsResponseCode": false,
"ResponseCode": "",
"NegativeRC": false,
"urlextension": "",
"isurlextension": false,
"NegativeUrlExtension": false,
"MatchType": 2,
"Scope": 2,
"RedirType": 0,
"MaxRedir": 0,
"payloadPosition": 0,
"payloadsFile": "",
"grepsFile": "",
"IssueName": "AWS Client Secret",
"IssueSeverity": "Information",
"IssueConfidence": "Certain",
"IssueDetail": "AWS Client Secret",
"RemediationDetail": "",
"IssueBackground": "",
"RemediationBackground": "",
"Header": [],
"VariationAttributes": [],
"InsertionPointType": [],
"Scanas": false,
"Scantype": 0,
"pathDiscovery": false
}
] |
BitBake | BurpBounty/profiles/AWS_Creds_File.bb | [
{
"ProfileName": "AWS_Creds_File",
"Name": "",
"Enabled": true,
"Scanner": 2,
"Author": "@six2dez1",
"Payloads": [],
"Encoder": [],
"UrlEncode": false,
"CharsToUrlEncode": "",
"Grep": [
"true,,(?i)(aws_access_key_id|aws_secret_access_key)(.{0,20})?\u003d.[0-9a-zA-Z\\/+]{20,40}"
],
"Tags": [
"All"
],
"PayloadResponse": false,
"NotResponse": false,
"TimeOut1": "",
"TimeOut2": "",
"isTime": false,
"contentLength": "",
"iscontentLength": false,
"CaseSensitive": false,
"ExcludeHTTP": false,
"OnlyHTTP": false,
"IsContentType": true,
"ContentType": "text/css,image/jpeg,image/png,image/svg+xml,image/gif,image/tiff,image/webp,image/x-icon,application/font-woff,image/vnd.microsoft.icon,font/ttf,font/woff2",
"HttpResponseCode": "",
"NegativeCT": true,
"IsResponseCode": false,
"ResponseCode": "",
"NegativeRC": false,
"urlextension": "",
"isurlextension": false,
"NegativeUrlExtension": false,
"MatchType": 2,
"Scope": 2,
"RedirType": 0,
"MaxRedir": 0,
"payloadPosition": 0,
"payloadsFile": "",
"grepsFile": "",
"IssueName": "AWS Credential Files",
"IssueSeverity": "Information",
"IssueConfidence": "Certain",
"IssueDetail": "",
"RemediationDetail": "",
"IssueBackground": "",
"RemediationBackground": "",
"Header": [],
"VariationAttributes": [],
"InsertionPointType": [],
"Scanas": false,
"Scantype": 0,
"pathDiscovery": false
}
] |
BitBake | BurpBounty/profiles/AWS_EC2_Url.bb | [
{
"ProfileName": "AWS_EC2_Url",
"Name": "",
"Enabled": true,
"Scanner": 2,
"Author": "@six2dez1",
"Payloads": [],
"Encoder": [],
"UrlEncode": false,
"CharsToUrlEncode": "",
"Grep": [
"true,,ec2-[0-9-]+.cd-[a-z0-9-]+.compute.amazonaws.com"
],
"Tags": [
"All"
],
"PayloadResponse": false,
"NotResponse": false,
"TimeOut1": "",
"TimeOut2": "",
"isTime": false,
"contentLength": "",
"iscontentLength": false,
"CaseSensitive": false,
"ExcludeHTTP": false,
"OnlyHTTP": false,
"IsContentType": true,
"ContentType": "text/css,image/jpeg,image/png,image/svg+xml,image/gif,image/tiff,image/webp,image/x-icon,application/font-woff,image/vnd.microsoft.icon,font/ttf,font/woff2",
"HttpResponseCode": "",
"NegativeCT": true,
"IsResponseCode": false,
"ResponseCode": "",
"NegativeRC": false,
"urlextension": "",
"isurlextension": false,
"NegativeUrlExtension": false,
"MatchType": 2,
"Scope": 2,
"RedirType": 0,
"MaxRedir": 0,
"payloadPosition": 0,
"payloadsFile": "",
"grepsFile": "",
"IssueName": "AWS EC2 Url detected",
"IssueSeverity": "Information",
"IssueConfidence": "Certain",
"IssueDetail": "",
"RemediationDetail": "",
"IssueBackground": "",
"RemediationBackground": "",
"Header": [],
"VariationAttributes": [],
"InsertionPointType": [],
"Scanas": false,
"Scantype": 0,
"pathDiscovery": false
}
] |
BitBake | BurpBounty/profiles/AWS_Region.bb | [
{
"ProfileName": "AWS_Region",
"Name": "",
"Enabled": true,
"Scanner": 2,
"Author": "@six2dez1",
"Payloads": [],
"Encoder": [],
"UrlEncode": false,
"CharsToUrlEncode": "",
"Grep": [
"true,,(us(-gov)?|ap|ca|cn|eu|sa)-(central|(north|south)?(east|west)?)-\\d"
],
"Tags": [
"All"
],
"PayloadResponse": false,
"NotResponse": false,
"TimeOut1": "",
"TimeOut2": "",
"isTime": false,
"contentLength": "",
"iscontentLength": false,
"CaseSensitive": false,
"ExcludeHTTP": false,
"OnlyHTTP": false,
"IsContentType": true,
"ContentType": "text/css,image/jpeg,image/png,image/svg+xml,image/gif,image/tiff,image/webp,image/x-icon,application/font-woff,image/vnd.microsoft.icon,font/ttf,font/woff2",
"HttpResponseCode": "",
"NegativeCT": true,
"IsResponseCode": false,
"ResponseCode": "",
"NegativeRC": false,
"urlextension": "",
"isurlextension": false,
"NegativeUrlExtension": false,
"MatchType": 2,
"Scope": 2,
"RedirType": 0,
"MaxRedir": 0,
"payloadPosition": 0,
"payloadsFile": "",
"grepsFile": "",
"IssueName": "AWS Region detected",
"IssueSeverity": "Information",
"IssueConfidence": "Certain",
"IssueDetail": "",
"RemediationDetail": "",
"IssueBackground": "",
"RemediationBackground": "",
"Header": [],
"VariationAttributes": [],
"InsertionPointType": [],
"Scanas": false,
"Scantype": 0,
"pathDiscovery": false
}
] |