repo_name
stringlengths 6
91
| path
stringlengths 8
968
| copies
stringlengths 1
3
| size
stringlengths 2
7
| content
stringlengths 61
1.01M
| license
stringclasses 15
values | hash
stringlengths 32
32
| line_mean
float64 6
99.8
| line_max
int64 12
1k
| alpha_frac
float64 0.3
0.91
| ratio
float64 2
9.89
| autogenerated
bool 1
class | config_or_test
bool 2
classes | has_no_keywords
bool 2
classes | has_few_assignments
bool 1
class |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Lucky-Orange/Tenon | Pitaya/JSONNeverDie/Source/JSONNDModel.swift | 1 | 2479 | // The MIT License (MIT)
// Copyright (c) 2015 JohnLui <wenhanlv@gmail.com> https://github.com/johnlui
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
//
// JSONNDModel.swift
// JSONNeverDie
//
// Created by 吕文翰 on 15/10/3.
//
import Foundation
public class JSONNDModel: NSObject {
public var JSONNDObject: JSONND!
public required init(JSONNDObject json: JSONND) {
self.JSONNDObject = json
super.init()
let mirror = Mirror(reflecting: self)
for (k, v) in AnyRandomAccessCollection(mirror.children)! {
if let key = k {
let json = self.JSONNDObject[key]
var valueWillBeSet: AnyObject?
switch v {
case _ as String:
valueWillBeSet = json.stringValue
case _ as Int:
valueWillBeSet = json.intValue
case _ as Float:
valueWillBeSet = json.floatValue
case _ as Bool:
valueWillBeSet = json.boolValue
default:
break
}
self.setValue(valueWillBeSet, forKey: key)
}
}
}
public var jsonString: String? {
get {
return self.JSONNDObject?.jsonString
}
}
public var jsonStringValue: String {
get {
return self.jsonString ?? ""
}
}
}
| mit | af76778f2dbf1b4b0c433c6f9f5f0145 | 33.830986 | 81 | 0.624343 | 4.84902 | false | false | false | false |
dfsilva/actor-platform | actor-sdk/sdk-core-ios/ActorSDK/Sources/Controllers/Content/Conversation/Layouting/AABubbleBackgroundProcessor.swift | 1 | 11188 | //
// Copyright (c) 2014-2016 Actor LLC. <https://actor.im>
//
import Foundation
private let ENABLE_LOGS = false
/**
Display list preprocessed list state
*/
class AAPreprocessedList: NSObject {
// Array of items in list
var items: [ACMessage]!
// Map from rid to index of item
var indexMap: [jlong: Int]!
// Current settings of cell look and feel
var cellSettings: [AACellSetting]!
// Prepared layouts of cells
var layouts: [AACellLayout]!
// Height of item
var heights: [CGFloat]!
// Is item need to be force-udpated
var forceUpdated: [Bool]!
// Is item need to be soft-updated
var updated: [Bool]!
}
/**
Display list preprocessor. Used for performing all required calculations
before collection is updated
*/
class AAListProcessor: NSObject, ARListProcessor {
let layoutCache = AALayoutCache()
let settingsCache = AACache<AACachedSetting>()
let peer: ACPeer
init(peer: ACPeer) {
self.peer = peer
}
func process(withItems items: JavaUtilListProtocol, withPrevious previous: Any?) -> Any? {
var objs = [ACMessage]()
var indexes = [jlong: Int]()
var layouts = [AACellLayout]()
var settings = [AACellSetting]()
var heights = [CGFloat]()
var forceUpdates = [Bool]()
var updates = [Bool]()
autoreleasepool {
let start = CFAbsoluteTimeGetCurrent()
var section = start
// Capacity
objs.reserveCapacity(Int(items.size()))
layouts.reserveCapacity(Int(items.size()))
settings.reserveCapacity(Int(items.size()))
heights.reserveCapacity(Int(items.size()))
forceUpdates.reserveCapacity(Int(items.size()))
updates.reserveCapacity(Int(items.size()))
// Building content list and dictionary from rid to index list
for i in 0..<items.size() {
let msg = items.getWith(i) as! ACMessage
indexes.updateValue(Int(i), forKey: msg.rid)
objs.append(msg)
}
if ENABLE_LOGS { log("processing(items): \(CFAbsoluteTimeGetCurrent() - section)") }
section = CFAbsoluteTimeGetCurrent()
// Calculating cell settings
// TODO: Cache and avoid recalculation of whole list
for i in 0..<objs.count {
settings.append(buildCellSetting(i, items: objs))
}
if ENABLE_LOGS { log("processing(settings): \(CFAbsoluteTimeGetCurrent() - section)") }
section = CFAbsoluteTimeGetCurrent()
// Building cell layouts
for i in 0..<objs.count {
layouts.append(buildLayout(objs[i], layoutCache: layoutCache))
}
if ENABLE_LOGS { log("processing(layouts): \(CFAbsoluteTimeGetCurrent() - section)") }
section = CFAbsoluteTimeGetCurrent()
// Calculating force and simple updates
if let prevList = previous as? AAPreprocessedList {
for i in 0..<objs.count {
var isForced = false
var isUpdated = false
let obj = objs[i]
let oldIndex: Int! = prevList.indexMap[obj.rid]
if oldIndex != nil {
// Check if layout keys are same
// If text was replaced by media it might force-updated
// If size of bubble changed you might to change layout key
// to update it's size
// TODO: In the future releases it might be implemented
// in more flexible way
if prevList.layouts[oldIndex].key != layouts[i].key {
// Mark as forced update
isForced = true
// Hack for rewriting layout information
// Removing layout from cache
layoutCache.revoke(objs[i].rid)
// Building new layout
layouts[i] = buildLayout(objs[i], layoutCache: layoutCache)
} else {
// Otherwise check bubble settings to check
// if it is changed and we need to recalculate size
let oldSetting = prevList.cellSettings[oldIndex]
let setting = settings[i]
if setting != oldSetting {
if setting.showDate != oldSetting.showDate {
// Date separator change size so make cell for resize
isForced = true
} else {
// Other changes doesn't change size, so just update content
// without resizing
isUpdated = true
}
}
}
}
// Saving update state value
if isForced {
forceUpdates.append(true)
updates.append(false)
} else if isUpdated {
forceUpdates.append(false)
updates.append(true)
} else {
forceUpdates.append(false)
updates.append(false)
}
}
} else {
for _ in 0..<objs.count {
forceUpdates.append(false)
updates.append(false)
}
}
if ENABLE_LOGS { log("processing(updates): \(CFAbsoluteTimeGetCurrent() - section)") }
section = CFAbsoluteTimeGetCurrent()
// Updating cell heights
// TODO: Need to implement width calculations too
// to make bubble menu appear at right place
for i in 0..<objs.count {
let height = measureHeight(objs[i], setting: settings[i], layout: layouts[i])
heights.append(height)
}
if ENABLE_LOGS { log("processing(heights): \(CFAbsoluteTimeGetCurrent() - section)") }
if ENABLE_LOGS { log("processing(all): \(CFAbsoluteTimeGetCurrent() - start)") }
}
// Put everything together
let res = AAPreprocessedList()
res.items = objs
res.cellSettings = settings
res.layouts = layouts
res.heights = heights
res.indexMap = indexes
res.forceUpdated = forceUpdates
res.updated = updates
return res
}
/**
Building Cell Setting: Information about decorators like date separator
and clenching of bubbles
*/
func buildCellSetting(_ index: Int, items: [ACMessage]) -> AACellSetting {
let current = items[index]
let id = Int64(current.rid)
let next: ACMessage! = index > 0 ? items[index - 1] : nil
let prev: ACMessage! = index + 1 < items.count ? items[index + 1] : nil
let cached: AACachedSetting! = settingsCache.pick(id)
if cached != nil {
if cached.isValid(prev, next: next) {
return cached.cached
}
}
var isShowDate = true
var clenchTop = false
var clenchBottom = false
if (prev != nil) {
isShowDate = !areSameDate(current, prev: prev)
if !isShowDate {
clenchTop = useCompact(current, next: prev)
}
}
if (next != nil) {
if areSameDate(next, prev: current) {
clenchBottom = useCompact(current, next: next)
}
}
let res = AACellSetting(showDate: isShowDate, clenchTop: clenchTop, clenchBottom: clenchBottom)
settingsCache.cache(id, value: AACachedSetting(cached: res, prevId: prev?.rid, nextId: next?.rid))
return res
}
/**
Checking if messages have same send day
*/
func areSameDate(_ source:ACMessage, prev: ACMessage) -> Bool {
let calendar = Calendar.current
let currentDate = Date(timeIntervalSince1970: Double(source.date)/1000.0)
let currentDateComp = (calendar as NSCalendar).components([.day, .year, .month], from: currentDate)
let nextDate = Date(timeIntervalSince1970: Double(prev.date)/1000.0)
let nextDateComp = (calendar as NSCalendar).components([.day, .year, .month], from: nextDate)
return (currentDateComp.year == nextDateComp.year && currentDateComp.month == nextDateComp.month && currentDateComp.day == nextDateComp.day)
}
/**
Checking if it is good to make bubbles clenched
*/
func useCompact(_ source: ACMessage, next: ACMessage) -> Bool {
if (source.content is ACServiceContent) {
if (next.content is ACServiceContent) {
return true
}
} else {
if (next.content is ACServiceContent) {
return false
}
if (source.senderId == next.senderId) {
return true
}
}
return false
}
func measureHeight(_ message: ACMessage, setting: AACellSetting, layout: AACellLayout) -> CGFloat {
let content = message.content!
var height = layout.height
if content is ACServiceContent {
height += AABubbleCell.bubbleTop
height += AABubbleCell.bubbleBottom
} else {
height += (setting.clenchTop ? AABubbleCell.bubbleTopCompact : AABubbleCell.bubbleTop)
height += (setting.clenchBottom ? AABubbleCell.bubbleBottomCompact : AABubbleCell.bubbleBottom)
}
// Date separator
if (setting.showDate) {
height += AABubbleCell.dateSize
}
return height
}
func buildLayout(_ message: ACMessage, layoutCache: AALayoutCache) -> AACellLayout {
var layout: AACellLayout! = layoutCache.pick(message.rid)
if (layout == nil) {
// Usually never happens
layout = AABubbles.buildLayout(peer, message: message)
layoutCache.cache(message.rid, value: layout!)
}
return layout
}
}
| agpl-3.0 | acd769260cc40e0997a060728080521c | 35.324675 | 148 | 0.504022 | 5.644803 | false | false | false | false |
srn214/Floral | Floral/Pods/SwiftDate/Sources/SwiftDate/DateInRegion/DateInRegion+Compare.swift | 2 | 11038 | //
// SwiftDate
// Parse, validate, manipulate, and display dates, time and timezones in Swift
//
// Created by Daniele Margutti
// - Web: https://www.danielemargutti.com
// - Twitter: https://twitter.com/danielemargutti
// - Mail: hello@danielemargutti.com
//
// Copyright © 2019 Daniele Margutti. Licensed under MIT License.
//
import Foundation
// MARK: - Comparing DateInRegion
public func == (lhs: DateInRegion, rhs: DateInRegion) -> Bool {
return (lhs.date.timeIntervalSince1970 == rhs.date.timeIntervalSince1970)
}
public func <= (lhs: DateInRegion, rhs: DateInRegion) -> Bool {
let result = lhs.date.compare(rhs.date)
return (result == .orderedAscending || result == .orderedSame)
}
public func >= (lhs: DateInRegion, rhs: DateInRegion) -> Bool {
let result = lhs.date.compare(rhs.date)
return (result == .orderedDescending || result == .orderedSame)
}
public func < (lhs: DateInRegion, rhs: DateInRegion) -> Bool {
return lhs.date.compare(rhs.date) == .orderedAscending
}
public func > (lhs: DateInRegion, rhs: DateInRegion) -> Bool {
return lhs.date.compare(rhs.date) == .orderedDescending
}
// The type of comparison to do against today's date or with the suplied date.
///
/// - isToday: hecks if date today.
/// - isTomorrow: Checks if date is tomorrow.
/// - isYesterday: Checks if date is yesterday.
/// - isSameDay: Compares date days
/// - isThisWeek: Checks if date is in this week.
/// - isNextWeek: Checks if date is in next week.
/// - isLastWeek: Checks if date is in last week.
/// - isSameWeek: Compares date weeks
/// - isThisMonth: Checks if date is in this month.
/// - isNextMonth: Checks if date is in next month.
/// - isLastMonth: Checks if date is in last month.
/// - isSameMonth: Compares date months
/// - isThisYear: Checks if date is in this year.
/// - isNextYear: Checks if date is in next year.
/// - isLastYear: Checks if date is in last year.
/// - isSameYear: Compare date years
/// - isInTheFuture: Checks if it's a future date
/// - isInThePast: Checks if the date has passed
/// - isEarlier: Checks if earlier than date
/// - isLater: Checks if later than date
/// - isWeekday: Checks if it's a weekday
/// - isWeekend: Checks if it's a weekend
/// - isInDST: Indicates whether the represented date uses daylight saving time.
/// - isMorning: Return true if date is in the morning (>=5 - <12)
/// - isAfternoon: Return true if date is in the afternoon (>=12 - <17)
/// - isEvening: Return true if date is in the morning (>=17 - <21)
/// - isNight: Return true if date is in the morning (>=21 - <5)
public enum DateComparisonType {
// Days
case isToday
case isTomorrow
case isYesterday
case isSameDay(_ : DateRepresentable)
// Weeks
case isThisWeek
case isNextWeek
case isLastWeek
case isSameWeek(_: DateRepresentable)
// Months
case isThisMonth
case isNextMonth
case isLastMonth
case isSameMonth(_: DateRepresentable)
// Years
case isThisYear
case isNextYear
case isLastYear
case isSameYear(_: DateRepresentable)
// Relative Time
case isInTheFuture
case isInThePast
case isEarlier(than: DateRepresentable)
case isLater(than: DateRepresentable)
case isWeekday
case isWeekend
// Day time
case isMorning
case isAfternoon
case isEvening
case isNight
// TZ
case isInDST
}
public extension DateInRegion {
/// Decides whether a DATE is "close by" another one passed in parameter,
/// where "Being close" is measured using a precision argument
/// which is initialized a 300 seconds, or 5 minutes.
///
/// - Parameters:
/// - refDate: reference date compare against to.
/// - precision: The precision of the comparison (default is 5 minutes, or 300 seconds).
/// - Returns: A boolean; true if close by, false otherwise.
func compareCloseTo(_ refDate: DateInRegion, precision: TimeInterval = 300) -> Bool {
return (abs(date.timeIntervalSince(refDate.date)) <= precision)
}
/// Compare the date with the rule specified in the `compareType` parameter.
///
/// - Parameter compareType: comparison type.
/// - Returns: `true` if comparison succeded, `false` otherwise
func compare(_ compareType: DateComparisonType) -> Bool {
switch compareType {
case .isToday:
return compare(.isSameDay(region.nowInThisRegion()))
case .isTomorrow:
let tomorrow = DateInRegion(region: region).dateByAdding(1, .day)
return compare(.isSameDay(tomorrow))
case .isYesterday:
let yesterday = DateInRegion(region: region).dateByAdding(-1, .day)
return compare(.isSameDay(yesterday))
case .isSameDay(let refDate):
return calendar.isDate(date, inSameDayAs: refDate.date)
case .isThisWeek:
return compare(.isSameWeek(region.nowInThisRegion()))
case .isNextWeek:
let nextWeek = region.nowInThisRegion().dateByAdding(1, .weekOfYear)
return compare(.isSameWeek(nextWeek))
case .isLastWeek:
let lastWeek = region.nowInThisRegion().dateByAdding(-1, .weekOfYear)
return compare(.isSameWeek(lastWeek))
case .isSameWeek(let refDate):
guard weekOfYear == refDate.weekOfYear else {
return false
}
// Ensure time interval is under 1 week
return (abs(date.timeIntervalSince(refDate.date)) < 1.weeks.timeInterval)
case .isThisMonth:
return compare(.isSameMonth(region.nowInThisRegion()))
case .isNextMonth:
let nextMonth = region.nowInThisRegion().dateByAdding(1, .month)
return compare(.isSameMonth(nextMonth))
case .isLastMonth:
let lastMonth = region.nowInThisRegion().dateByAdding(-1, .month)
return compare(.isSameMonth(lastMonth))
case .isSameMonth(let refDate):
return (date.year == refDate.date.year) && (date.month == refDate.date.month)
case .isThisYear:
return compare(.isSameYear(region.nowInThisRegion()))
case .isNextYear:
let nextYear = region.nowInThisRegion().dateByAdding(1, .year)
return compare(.isSameYear(nextYear))
case .isLastYear:
let lastYear = region.nowInThisRegion().dateByAdding(-1, .year)
return compare(.isSameYear(lastYear))
case .isSameYear(let refDate):
return (date.year == refDate.date.year)
case .isInTheFuture:
return compare(.isLater(than: region.nowInThisRegion()))
case .isInThePast:
return compare(.isEarlier(than: region.nowInThisRegion()))
case .isEarlier(let refDate):
return ((date as NSDate).earlierDate(refDate.date) == date)
case .isLater(let refDate):
return ((date as NSDate).laterDate(refDate.date) == date)
case .isWeekday:
return !compare(.isWeekend)
case .isWeekend:
let range = calendar.maximumRange(of: Calendar.Component.weekday)!
return (weekday == range.lowerBound || weekday == range.upperBound - range.lowerBound)
case .isInDST:
return region.timeZone.isDaylightSavingTime(for: date)
case .isMorning:
return (hour >= 5 && hour < 12)
case .isAfternoon:
return (hour >= 12 && hour < 17)
case .isEvening:
return (hour >= 17 && hour < 21)
case .isNight:
return (hour >= 21 || hour < 5)
}
}
/// Returns a ComparisonResult value that indicates the ordering of two given dates based on
/// their components down to a given unit granularity.
///
/// - parameter date: date to compare.
/// - parameter granularity: The smallest unit that must, along with all larger units
/// - returns: `ComparisonResult`
func compare(toDate refDate: DateInRegion, granularity: Calendar.Component) -> ComparisonResult {
switch granularity {
case .nanosecond:
// There is a possible rounding error using Calendar to compare two dates below the minute granularity
// So we've added this trick and use standard Date compare which return correct results in this case
// https://github.com/malcommac/SwiftDate/issues/346
return date.compare(refDate.date)
default:
return region.calendar.compare(date, to: refDate.date, toGranularity: granularity)
}
}
/// Compares whether the receiver is before/before equal `date` based on their components down to a given unit granularity.
///
/// - Parameters:
/// - refDate: reference date
/// - orEqual: `true` to also check for equality
/// - granularity: smallest unit that must, along with all larger units, be less for the given dates
/// - Returns: Boolean
func isBeforeDate(_ date: DateInRegion, orEqual: Bool = false, granularity: Calendar.Component) -> Bool {
let result = compare(toDate: date, granularity: granularity)
return (orEqual ? (result == .orderedSame || result == .orderedAscending) : result == .orderedAscending)
}
/// Compares whether the receiver is after `date` based on their components down to a given unit granularity.
///
/// - Parameters:
/// - refDate: reference date
/// - orEqual: `true` to also check for equality
/// - granularity: Smallest unit that must, along with all larger units, be greater for the given dates.
/// - Returns: Boolean
func isAfterDate(_ refDate: DateInRegion, orEqual: Bool = false, granularity: Calendar.Component) -> Bool {
let result = compare(toDate: refDate, granularity: granularity)
return (orEqual ? (result == .orderedSame || result == .orderedDescending) : result == .orderedDescending)
}
/// Compares equality of two given dates based on their components down to a given unit
/// granularity.
///
/// - parameter date: date to compare
/// - parameter granularity: The smallest unit that must, along with all larger units, be equal for the given
/// dates to be considered the same.
///
/// - returns: `true` if the dates are the same down to the given granularity, otherwise `false`
func isInside(date: DateInRegion, granularity: Calendar.Component) -> Bool {
return (compare(toDate: date, granularity: granularity) == .orderedSame)
}
/// Return `true` if receiver data is contained in the range specified by two dates.
///
/// - Parameters:
/// - startDate: range upper bound date
/// - endDate: range lower bound date
/// - orEqual: `true` to also check for equality on date and date2, default is `true`
/// - granularity: smallest unit that must, along with all larger units, be greater
/// - Returns: Boolean
func isInRange(date startDate: DateInRegion, and endDate: DateInRegion, orEqual: Bool = true, granularity: Calendar.Component = .nanosecond) -> Bool {
return isAfterDate(startDate, orEqual: orEqual, granularity: granularity) && isBeforeDate(endDate, orEqual: orEqual, granularity: granularity)
}
// MARK: - Date Earlier/Later
/// Return the earlier of two dates, between self and a given date.
///
/// - Parameter date: The date to compare to self
/// - Returns: The date that is earlier
func earlierDate(_ date: DateInRegion) -> DateInRegion {
return (self.date.timeIntervalSince1970 <= date.date.timeIntervalSince1970) ? self : date
}
/// Return the later of two dates, between self and a given date.
///
/// - Parameter date: The date to compare to self
/// - Returns: The date that is later
func laterDate(_ date: DateInRegion) -> DateInRegion {
return (self.date.timeIntervalSince1970 >= date.date.timeIntervalSince1970) ? self : date
}
}
| mit | 20f8a660d0b32ca122016b6c120993a4 | 34.261981 | 151 | 0.712875 | 3.902758 | false | false | false | false |
radex/swift-compiler-crashes | crashes-duplicates/19247-swift-type-walk.swift | 11 | 2394 | // Distributed under the terms of the MIT license
// Test case submitted to project by https://github.com/practicalswift (practicalswift)
// Test case found by fuzzing
struct g: c
func b<I : d<U : a {
protocol A : B<d { {
class B
}
class B<U : A")
func a<H : A
class d
{
}
A {
let a {
{
enum A ) -> Voi
func d: A"
struct g: a {
}
A {
}
protocol A : a {
}
struct B<T where T.h
protocol A : a {
{
enum A : S
class A : A
case ,
protocol A : a {
struct c: T.h
protocol l : a<H : A")
class b
func f
protocol A ) -> {
g: C {
class d<T where g: d , j where f
enum S
for c {
let a {
func b<I : a {
class
typealias e : d
typealias e : a {
}
protocol A : T>: a<U : d
class d<I : c: a {
let a {
protocol P {
protocol A : d where H.Element == c : Any {
func b<T where T.h: d
struct B<d where h : B<T where f
import CoreData
let a {
enum A {
enum S
protocol P {
for c {
struct g: T.Element == c : T: b<g : c: String {
let : B<T where T>: a {
class A : A")
class d
struct g: A")
protocol A : d<T where T>: a
let a {
class B<T.h: d<I : a {
class d
class d<H : T>: d<U : a {
protocol A {
}
import CoreData
class d<T> ( )
class
{
class B<T where h : d where f: d where j : T.Element == d
let a {
class d: d: a<g : d { {
class d<T.Element == c
typealias F = 0
protocol c : a {
protocol l : A")
for c : T.Element == e) -> Voi
A : c
}
{
}
}
}
let a {
}
struct g: d , j = " " " "
class d
struct B<U : Any {
}
class A {
for c : c<H : a {
" ")
func f
class d
class d<T where f: a<I : c<I : String = d , j = d where T where g: B
}
A {
A : a {
struct c
struct g: S<j : B
var f = c : a<T where h : A
struct c
{
protocol c {
class d<T where T> Voi
typealias F = d
{
}
class A : Any {
}
protocol A ) -> Voi
let a {
class d<T where g: A")
class b
typealias e : B<T where T> {
let a {
protocol l : T>(v: String {
struct c: T>: a {
enum A {
struct B
protocol c : S
A : a {
}
typealias b = 0
func b<T where T where g: a
protocol A {
protocol A : d
enum S<d where h : a {
{
protocol A {
protocol c {
func a<T where g: a {
class d
class B
}
let : a<T where T> Voi
}
enum S
func f
" " " " "
class d: d<T where h : d
class B<U : String = d
class A {
let a {
{
let a {
protocol A : a {
class
}
}
class B<g : B<T>: String = " {
let a {
class B<T where T where T>: d
class A {
class A {
let a {
let a {
class d
protocol c {
typealias b = e) -> Voi
}
A {
class d<T>: B<T where h: A
}
protocol A {
let a {
class d<T where H.Element =
| mit | 1cef0ea238d6ff17c9358756f7654f86 | 12.758621 | 87 | 0.583124 | 2.396396 | false | false | false | false |
wenghengcong/Coderpursue | BeeFun/BeeFun/View/Event/EventCell/BFEventLayout.swift | 1 | 24167 | //
// BFEventLayout.swift
// BeeFun
//
// Created by WengHengcong on 23/09/2017.
// Copyright © 2017 JungleSong. All rights reserved.
//
import UIKit
import YYText
// 异步绘制导致的闪烁解决方案:https://github.com/ibireme/YYKit/issues/64
// https://github.com/ibireme/YYText/issues/103
/// 布局样式
///
/// - pullRequest: pull request
/// - pushCommit: push commit
/// - textPicture: 图文布局
/// - text: 文本布局
public enum EventLayoutStyle: Int {
case pullRequest
case pushCommit
case textPicture
case text
}
class BFEventLayout: NSObject {
//数据
var event: ObjEvent?
var style: EventLayoutStyle = .textPicture //布局
var eventTypeActionImage: String? {
return BFEventParase.actionImage(event: event)
}
//上下左右留白
var marginTop: CGFloat = 0
var marginBottom: CGFloat = 0
var marginLeft: CGFloat = 0
var marginRight: CGFloat = 0
//action 图片
var actionHeight: CGFloat = 0
var actionSize: CGSize = CGSize.zero
//时间
var timeHeight: CGFloat = 0
var timeLayout: YYTextLayout?
//title
var titleHeight: CGFloat = 0
var titleLayout: YYTextLayout?
//---->>>> 内容区域 <<<<-------
var actionContentHeight: CGFloat = 0
//actor 图片
var actorHeight: CGFloat = 0
var actorSize: CGSize = CGSize.zero
//文字content内容
var textHeight: CGFloat = 0
var textLayout: YYTextLayout?
//commit 内容
var commitHeight: CGFloat = 0
var commitLayout: YYTextLayout?
//pull request detail
var prDetailWidth: CGFloat = 0 //1 commit with .....文字的宽度
var prDetailHeight: CGFloat = 0
var prDetailLayout: YYTextLayout?
var totalHeight: CGFloat = 0
init(event: ObjEvent?) {
super.init()
if event == nil {
return
}
self.event = event
layout()
}
/// 布局
func layout() {
marginTop = 0
actionHeight = 0
timeHeight = 0
titleHeight = 0
actorHeight = 0
actionContentHeight = 0
textHeight = 0
commitHeight = 0
prDetailHeight = 0
marginBottom = 5
_layoutActionImage()
_layoutTime()
_layoutTitle()
_layoutActorImage()
_layoutTextContent()
_layoutCommit()
_layoutPRDetail()
//计算高度
let timeMargin = BFEventConstants.TIME_Y
let actorImgMargin = BFEventConstants.ACTOR_IMG_TOP
let textMargin = BFEventConstants.TEXT_Y
totalHeight = timeMargin+timeHeight+titleHeight+marginBottom
style = BFEventParase.layoutType(event: event)
switch style {
case .pullRequest:
totalHeight += textMargin + max(actorImgMargin+actorHeight, textHeight+prDetailHeight)
case .pushCommit:
totalHeight += textMargin + max(actorImgMargin+actorHeight, commitHeight)
case .textPicture:
totalHeight += textMargin + max(actorImgMargin+actorHeight, textHeight)
case .text:
break
}
actionContentHeight = totalHeight-timeMargin-timeHeight-titleHeight
}
private func _layoutActionImage() {
actionHeight = BFEventConstants.ACTOR_IMG_WIDTH
}
private func _layoutTime() {
timeHeight = 0
timeLayout = nil
if let time = event?.created_at {
if let showTime = BFTimeHelper.shared.readableTime(rare: time, prefix: nil) {
let timeAtt = NSMutableAttributedString(string: showTime)
let container = YYTextContainer(size: CGSize(width: BFEventConstants.TIME_W, height: CGFloat.greatestFiniteMagnitude))
timeAtt.yy_font = UIFont.bfSystemFont(ofSize: BFEventConstants.TIME_FONT_SIZE)
let layout = YYTextLayout(container: container, text: timeAtt)
timeLayout = layout
timeHeight = timeLayout!.textBoundingSize.height
}
}
}
private func _layoutTitle() {
titleHeight = 0
titleLayout = nil
let titleText: NSMutableAttributedString = NSMutableAttributedString()
//用户名
if let actorName = BFEventParase.actorName(event: event) {
let actorAttriText = NSMutableAttributedString(string: actorName)
actorAttriText.yy_setTextHighlight(actorAttriText.yy_rangeOfAll(), color: UIColor.blue, backgroundColor: UIColor.white, userInfo: nil, tapAction: { (_, _, _, _) in
JumpManager.shared.jumpUserDetailView(user: self.event?.actor)
}, longPressAction: nil)
titleText.append(actorAttriText)
}
let actionText = NSMutableAttributedString(string: BFEventParase.action(event: event))
titleText.append(actionText)
//介于actor与repo中间的部分
if let type = event?.type {
if type == .pushEvent {
//[aure] pushed to [develop] at [AudioKit/AudioKit]
if let ref = event?.payload?.ref {
if let reponame = event?.repo?.name, let branch = ref.components(separatedBy: "/").last {
//https://github.com/AudioKit/AudioKit/tree/develop
let url = "https://github.com/"+reponame+"/tree/"+branch
let branchText = NSMutableAttributedString(string: branch)
branchText.yy_setTextHighlight(branchText.yy_rangeOfAll(), color: UIColor.blue, backgroundColor: UIColor.white, userInfo: nil, tapAction: { (_, _, _, _) in
JumpManager.shared.jumpWebView(url: url)
}, longPressAction: nil)
titleText.append(branchText)
}
}
} else if type == .releaseEvent {
//[ckrey] released [Session Manager and CoreDataPersistence] at [ckrey/MQTT-Client-Framework]
if let releaseName = event?.payload?.release?.name, let releaseUrl = event?.payload?.release?.html_url {
let releaseText = NSMutableAttributedString(string: releaseName)
releaseText.yy_setTextHighlight(releaseText.yy_rangeOfAll(), color: UIColor.blue, backgroundColor: UIColor.white, userInfo: nil, tapAction: { (_, _, _, _) in
JumpManager.shared.jumpWebView(url: releaseUrl)
}, longPressAction: nil)
titleText.append(releaseText)
titleText.append(NSMutableAttributedString(string: " at "))
}
} else if type == .createEvent {
//[ckrey] created [tag] [0.9.9] at [ckrey/MQTT-Client-Framework]
if let tag = event?.payload?.ref {
let tagText = NSMutableAttributedString(string: tag)
tagText.yy_setTextHighlight(tagText.yy_rangeOfAll(), color: UIColor.blue, backgroundColor: UIColor.white, userInfo: nil, tapAction: { (_, _, _, _) in
//https://github.com/user/repo/tree/tag
if let repoName = self.event?.repo?.name {
let url = "https://github.com/"+repoName+"/tree/"+tag
JumpManager.shared.jumpWebView(url: url)
}
}, longPressAction: nil)
titleText.append(tagText)
titleText.append(NSMutableAttributedString(string: " at "))
}
} else if type == .forkEvent {
//[cloudwu] forked [bkaradzic/bx] to [cloudwu/bx]
if let repo = BFEventParase.repoName(event: event) {
let repoAttrText = NSMutableAttributedString(string: repo)
repoAttrText.yy_setTextHighlight(repoAttrText.yy_rangeOfAll(), color: UIColor.blue, backgroundColor: UIColor.white, userInfo: nil, tapAction: { (_, _, _, _) in
JumpManager.shared.jumpReposDetailView(repos: self.event?.repo, from: .other)
}, longPressAction: nil)
titleText.append(repoAttrText)
titleText.append(NSMutableAttributedString(string: " to "))
}
} else if type == .deleteEvent {
if let branch = event?.payload?.ref {
let branchText = NSMutableAttributedString(string: branch)
branchText.yy_setTextHighlight(branchText.yy_rangeOfAll(), color: UIColor.bfLabelSubtitleTextColor, backgroundColor: UIColor.white, userInfo: nil, tapAction: { (_, _, _, _) in
}, longPressAction: nil)
titleText.append(branchText)
titleText.append(NSMutableAttributedString(string: " at "))
}
} else if type == .memberEvent {
if let memberName = event?.payload?.member?.login {
let memberText = NSMutableAttributedString(string: memberName)
memberText.yy_setTextHighlight(memberText.yy_rangeOfAll(), color: UIColor.blue, backgroundColor: UIColor.white, userInfo: nil, tapAction: { (_, _, _, _) in
JumpManager.shared.jumpReposDetailView(repos: self.event?.repo, from: .other)
}, longPressAction: nil)
titleText.append(memberText)
titleText.append(NSMutableAttributedString(string: " to "))
}
}
}
//repos
if let type = event?.type {
if type == .forkEvent {
if let user = BFEventParase.actorName(event: event), let repoName = event?.payload?.forkee?.name {
let repoAttrText = NSMutableAttributedString(string: user+repoName)
repoAttrText.yy_setTextHighlight(repoAttrText.yy_rangeOfAll(), color: UIColor.blue, backgroundColor: UIColor.white, userInfo: nil, tapAction: { (_, _, _, _) in
JumpManager.shared.jumpReposDetailView(repos: self.event?.repo, from: .other)
}, longPressAction: nil)
titleText.append(repoAttrText)
}
} else {
if let repo = BFEventParase.repoName(event: event) {
var actionNumberStr = ""
if let actionNumber = event?.payload?.issue?.number {
actionNumberStr = "#\(actionNumber)"
} else if let actionNumber = event?.payload?.pull_request?.number {
//https://github.com/user/repo/pull/number
actionNumberStr = "#\(actionNumber)"
}
let repoAttrText = NSMutableAttributedString(string: " " + repo + "\(actionNumberStr)")
repoAttrText.yy_setTextHighlight(repoAttrText.yy_rangeOfAll(), color: UIColor.blue, backgroundColor: UIColor.white, userInfo: nil, tapAction: { (_, _, _, _) in
if let eventType = self.event?.type {
switch eventType {
case .pullRequestEvent:
JumpManager.shared.jumpWebView(url: self.event?.payload?.pull_request?.html_url)
return
default:
break
}
}
if let comment = self.event?.payload?.comment {
JumpManager.shared.jumpWebView(url: comment.html_url)
} else {
JumpManager.shared.jumpReposDetailView(repos: self.event?.repo, from: .other)
}
}, longPressAction: nil)
titleText.append(repoAttrText)
}
}
}
titleText.yy_font = UIFont.bfSystemFont(ofSize: BFEventConstants.TITLE_FONT_SIZE)
let container = YYTextContainer(size: CGSize(width: BFEventConstants.TITLE_W, height: CGFloat.greatestFiniteMagnitude))
let layout = YYTextLayout(container: container, text: titleText)
titleHeight = layout!.textBoundingSize.height
titleLayout = layout
}
private func _layoutActorImage() {
actorHeight = BFEventConstants.ACTOR_IMG_WIDTH
}
private func _layoutTextContent() {
textHeight = 0
textLayout = nil
let textText: NSMutableAttributedString = NSMutableAttributedString()
if let type = event?.type {
if type == .issueCommentEvent || type == .pullRequestReviewCommentEvent || type == .commitCommentEvent {
if let commentBody = BFEventParase.contentBody(event: event) {
let clipLength = 130
let commentBodyAttText = NSMutableAttributedString(string: commentBody)
if commentBodyAttText.length > clipLength {
let moreRange = NSRange(location: clipLength, length: commentBodyAttText.length-clipLength)
commentBodyAttText.replaceCharacters(in: moreRange, with: YYTextManager.moreDotCharacterAttribute(count: 3))
}
textText.append(commentBodyAttText)
}
} else if type == .issuesEvent {
if let issueBody = BFEventParase.contentBody(event: event) {
let clipLength = 130
let commentBodyAttText = NSMutableAttributedString(string: issueBody)
if commentBodyAttText.length > clipLength {
let moreRange = NSRange(location: clipLength, length: commentBodyAttText.length-clipLength)
commentBodyAttText.replaceCharacters(in: moreRange, with: YYTextManager.moreDotCharacterAttribute(count: 3))
}
textText.append(commentBodyAttText)
}
} else if type == .releaseEvent {
if let releaseText = BFEventParase.contentBody(event: event) {
let releaseAttText = NSMutableAttributedString(string: releaseText)
textText.append(releaseAttText)
}
} else if type == .pullRequestEvent {
if let pullRequestText = BFEventParase.contentBody(event: event) {
let clipLength = 130
let pullRequestAttText = NSMutableAttributedString(string: pullRequestText)
if pullRequestAttText.length > clipLength {
let moreRange = NSRange(location: clipLength, length: pullRequestAttText.length-clipLength)
pullRequestAttText.replaceCharacters(in: moreRange, with: YYTextManager.moreDotCharacterAttribute(count: 3))
}
textText.append(pullRequestAttText)
}
} else if type == .gollumEvent {
if let pages = event?.payload?.pages {
let page: ObjPage = pages.first!
let act = page.action!
let actText = NSMutableAttributedString(string: act)
textText.append(actText)
textText.addBlankSpaceCharacterAttribute(1)
//AFNetworking 3.0 Migration Guide 跳转
if let pageName = page.title {
let pageNameText = NSMutableAttributedString(string: pageName)
pageNameText.yy_setTextHighlight(pageNameText.yy_rangeOfAll(), color: UIColor.blue, backgroundColor: UIColor.white, userInfo: nil, tapAction: { (_, _, _, _) in
JumpManager.shared.jumpWebView(url: page.html_url)
}, longPressAction: nil)
textText.append(pageNameText)
textText.addBlankSpaceCharacterAttribute(1)
}
//View the diff
if let sha = page.sha {
let shaText = NSMutableAttributedString(string: "View the diff>>")
if let html_url = page.html_url {
let jump_url = html_url + "/_compare/"+sha
shaText.yy_setTextHighlight(shaText.yy_rangeOfAll(), color: UIColor.blue, backgroundColor: UIColor.white, userInfo: nil, tapAction: { (_, _, _, _) in
JumpManager.shared.jumpWebView(url: jump_url)
}, longPressAction: nil)
}
textText.append(shaText)
}
}
}
textText.yy_font = UIFont.bfSystemFont(ofSize: BFEventConstants.TEXT_FONT_SIZE)
let container = YYTextContainer(size: CGSize(width: BFEventConstants.TEXT_W, height: CGFloat.greatestFiniteMagnitude))
let layout = YYTextLayout(container: container, text: textText)
textHeight = layout!.textBoundingSize.height
textLayout = layout
}
}
private func _layoutCommit() {
commitHeight = 0
commitLayout = nil
if let eventtype = event?.type {
if eventtype != .pushEvent {
return
}
}
let pushText: NSMutableAttributedString = NSMutableAttributedString()
let font = UIFont.bfSystemFont(ofSize: BFEventConstants.COMMIT_FONT_SIZE)
if let commits = event?.payload?.commits {
let allCommitText = NSMutableAttributedString()
for (index, commit) in commits.reversed().enumerated() where index < 2 {
if let hashStr = commit.sha?.substring(to: 6), let message = commit.message {
let commitText = NSMutableAttributedString(string: hashStr)
commitText.yy_setTextHighlight(commitText.yy_rangeOfAll(), color: UIColor.blue, backgroundColor: UIColor.white, userInfo: nil, tapAction: { (_, _, _, _) in
//跳转到具体commit
if let reponame = self.event?.repo?.name, let sha = commit.sha {
//https://github.com/user/repo/commit/sha
let url = "https://github.com/"+reponame+"/commit/"+sha
JumpManager.shared.jumpWebView(url: url)
}
}, longPressAction: nil)
let messageText = NSMutableAttributedString(string: message)
commitText.addBlankSpaceCharacterAttribute(2)
commitText.append(messageText)
commitText.addLineBreakCharacterAttribute()
allCommitText.append(commitText)
}
}
pushText.append(allCommitText)
if commits.count == 2 {
let moreText = NSMutableAttributedString(string: "View comparison for these 2 commits »")
moreText.yy_setTextHighlight(moreText.yy_rangeOfAll(), color: UIColor.blue, backgroundColor: UIColor.white, userInfo: nil, tapAction: { (_, _, _, _) in
if let reponame = self.event?.repo?.name, let before = self.event?.payload?.before, let head = self.event?.payload?.head {
//https://github.com/user/repo/comare/before_sha...header_sha
let url = "https://github.com/"+reponame+"/compare/"+before+"..."+head
JumpManager.shared.jumpWebView(url: url)
}
}, longPressAction: nil)
pushText.append(moreText)
} else if commits.count > 2 {
//commist.count > 2
if let all = event?.payload?.distinct_size {
let more = all - 2
var moreStr = "\(more) more cmmmit"
if more > 0 {
if more > 1 {
moreStr.append("s >>")
} else if more == 1 {
moreStr.append(" >>")
}
let moreText = NSMutableAttributedString(string: moreStr)
moreText.yy_setTextHighlight(moreText.yy_rangeOfAll(), color: UIColor.blue, backgroundColor: UIColor.white, userInfo: nil, tapAction: { (_, _, _, _) in
if let reponame = self.event?.repo?.name, let before = self.event?.payload?.before, let head = self.event?.payload?.head {
//https://github.com/user/repo/comare/before_sha...header_sha
let url = "https://github.com/"+reponame+"/compare/"+before+"..."+head
JumpManager.shared.jumpWebView(url: url)
}
}, longPressAction: nil)
pushText.append(moreText)
}
}
}
pushText.yy_font = font
let container = YYTextContainer(size: CGSize(width: BFEventConstants.COMMIT_W, height: CGFloat.greatestFiniteMagnitude))
let layout = YYTextLayout(container: container, text: pushText)
commitHeight = layout!.textBoundingSize.height
commitLayout = layout
}
}
private func _layoutPRDetail() {
prDetailHeight = 0
prDetailLayout = nil
if let eventtype = event?.type {
if eventtype != .pullRequestEvent {
return
}
}
let prDetailText: NSMutableAttributedString = NSMutableAttributedString()
let font = UIFont.bfSystemFont(ofSize: BFEventConstants.PRDETAIL_FONT_SIZE)
if let pull_request = event?.payload?.pull_request {
if let commits = pull_request.commits, let additions = pull_request.additions, let deletions = pull_request.deletions {
//图片
let size = CGSize(width: 10, height: 10)
var image = UIImage(named: "event_commit_icon")
if image != nil {
image = UIImage(cgImage: image!.cgImage!, scale: 2.0, orientation: UIImageOrientation.up)
let imageText = NSMutableAttributedString.yy_attachmentString(withContent: image, contentMode: .center, attachmentSize: size, alignTo: font, alignment: .center)
prDetailText.append(imageText)
prDetailText.addBlankSpaceCharacterAttribute(1)
}
//1 commit with 1 addition and 0 deletions
let commitStirng = commits <= 1 ? "commit" : "commits"
let additionString = additions == 1 ? "addition" : "additions"
let deletionString = deletions == 1 ? "deletion" : "deletions"
let detailString = "\(pull_request.commits!) " + commitStirng + " with " + "\(pull_request.additions!) " + additionString + " and " + "\(pull_request.deletions!) " + deletionString
let textText = NSMutableAttributedString(string: detailString)
prDetailText.append(textText)
prDetailText.yy_font = font
let container = YYTextContainer(size: CGSize(width: BFEventConstants.PRDETAIL_W, height: CGFloat.greatestFiniteMagnitude))
let layout = YYTextLayout(container: container, text: prDetailText)
prDetailHeight = layout!.textBoundingSize.height
prDetailWidth = layout!.textBoundingRect.width
prDetailLayout = layout
}
}
}
}
| mit | 13b02b2b850ef068454a671dcf9e513a | 47.094188 | 196 | 0.552065 | 5.418605 | false | false | false | false |
dunkelstern/FastString | src/appending.swift | 1 | 3123 | // Copyright (c) 2016 Johannes Schriewer.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
/// String appending
public extension FastString {
/// Return new string by appending other string
///
/// - parameter string: string to append
/// - returns: new string instance with both strings concatenated
public func appending(_ string: FastString) -> FastString {
let result = FastString()
if self.byteCount == 0 && string.byteCount == 0 {
// 2 empty strings -> return empty string
return result
}
let count = self.byteCount + string.byteCount
let memory = UnsafeMutablePointer<UInt8>(allocatingCapacity: count + 1)
memory[count] = 0
var index = 0
for c in self.buffer {
memory[index] = c
index += 1
}
for c in string.buffer {
memory[index] = c
index += 1
}
result.buffer.baseAddress!.deallocateCapacity(result.buffer.count + 1)
result.buffer = UnsafeMutableBufferPointer(start: memory, count: count)
return result
}
public func appending(_ string: String) -> FastString {
return self.appending(FastString(string))
}
/// Append string to self
///
/// - parameter string: string to append
public func append(_ string: FastString) {
if string.byteCount == 0 {
// Nothing to do
return
}
let count = self.byteCount + string.byteCount
let memory = UnsafeMutablePointer<UInt8>(allocatingCapacity: count + 1)
memory[count] = 0
var index = 0
for c in self.buffer {
memory[index] = c
index += 1
}
for c in string.buffer {
memory[index] = c
index += 1
}
self.buffer.baseAddress!.deallocateCapacity(self.buffer.count + 1)
self.buffer = UnsafeMutableBufferPointer(start: memory, count: count)
}
public func append(_ string: String) {
self.append(FastString(string))
}
}
public func +(lhs: FastString, rhs: FastString) -> FastString {
return lhs.appending(rhs)
}
public func +=(lhs: inout FastString, rhs: FastString) {
lhs.append(rhs)
}
public func +(lhs: FastString, rhs: String) -> FastString {
return lhs.appending(rhs)
}
public func +=(lhs: inout FastString, rhs: String) {
lhs.append(rhs)
}
public func +(lhs: String, rhs: FastString) -> String {
return lhs + rhs.description
}
public func +=(lhs: inout String, rhs: FastString) {
lhs += rhs.description
}
| apache-2.0 | 58d1370f76ed5cd054c0035aca45cd45 | 28.186916 | 79 | 0.622799 | 4.455064 | false | false | false | false |
BrothaStrut96/ProjectPUBG | AppDelegate.swift | 1 | 4825 | //
// AppDelegate.swift
// ProjectPUBG
//
// Created by Ruaridh Wylie on 01/10/2017.
// Copyright © 2017 Ruaridh Wylie. All rights reserved.
//
import UIKit
import CoreData
import Firebase
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {
// Override point for customization after application launch.
//Initialise Firebase Project
FirebaseApp.configure()
UserDefaults.standard.setValue(false, forKey: "_UIConstraintBasedLayoutLogUnsatisfiable")
return true
}
func applicationWillResignActive(_ application: UIApplication) {
// Sent when the application is about to move from active to inactive state. This can occur for certain types of temporary interruptions (such as an incoming phone call or SMS message) or when the user quits the application and it begins the transition to the background state.
// Use this method to pause ongoing tasks, disable timers, and invalidate graphics rendering callbacks. Games should use this method to pause the game.
}
func applicationDidEnterBackground(_ application: UIApplication) {
// Use this method to release shared resources, save user data, invalidate timers, and store enough application state information to restore your application to its current state in case it is terminated later.
// If your application supports background execution, this method is called instead of applicationWillTerminate: when the user quits.
}
func applicationWillEnterForeground(_ application: UIApplication) {
// Called as part of the transition from the background to the active state; here you can undo many of the changes made on entering the background.
}
func applicationDidBecomeActive(_ application: UIApplication) {
// Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface.
}
func applicationWillTerminate(_ application: UIApplication) {
// Called when the application is about to terminate. Save data if appropriate. See also applicationDidEnterBackground:.
// Saves changes in the application's managed object context before the application terminates.
self.saveContext()
}
// MARK: - Core Data stack
lazy var persistentContainer: NSPersistentContainer = {
/*
The persistent container for the application. This implementation
creates and returns a container, having loaded the store for the
application to it. This property is optional since there are legitimate
error conditions that could cause the creation of the store to fail.
*/
let container = NSPersistentContainer(name: "ProjectPUBG")
container.loadPersistentStores(completionHandler: { (storeDescription, error) in
if let error = error as NSError? {
// Replace this implementation with code to handle the error appropriately.
// fatalError() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
/*
Typical reasons for an error here include:
* The parent directory does not exist, cannot be created, or disallows writing.
* The persistent store is not accessible, due to permissions or data protection when the device is locked.
* The device is out of space.
* The store could not be migrated to the current model version.
Check the error message to determine what the actual problem was.
*/
fatalError("Unresolved error \(error), \(error.userInfo)")
}
})
return container
}()
// MARK: - Core Data Saving support
func saveContext () {
let context = persistentContainer.viewContext
if context.hasChanges {
do {
try context.save()
} catch {
// Replace this implementation with code to handle the error appropriately.
// fatalError() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
let nserror = error as NSError
fatalError("Unresolved error \(nserror), \(nserror.userInfo)")
}
}
}
}
| gpl-3.0 | 4c0a5044b5a1173d5339821231469fe3 | 46.294118 | 285 | 0.683458 | 5.840194 | false | false | false | false |
stephentyrone/swift | test/Driver/Dependencies/check-interface-implementation-fine.swift | 5 | 2444 | /// The fine-grained dependency graph has implicit dependencies from interfaces to implementations.
/// These are not presently tested because depends nodes are marked as depending in the interface,
/// as of 1/9/20. But this test will check fail if those links are not followed.
// RUN: %empty-directory(%t)
// RUN: cp -r %S/Inputs/check-interface-implementation-fine/* %t
// RUN: touch -t 201401240005 %t/*
// RUN: cd %t && %swiftc_driver -c -driver-use-frontend-path "%{python.unquoted};%S/Inputs/update-dependencies.py;%swift-dependency-tool" -output-file-map %t/output.json -incremental -disable-direct-intramodule-dependencies -driver-always-rebuild-dependents ./a.swift ./c.swift ./bad.swift -module-name main -j1 -v 2>&1 | %FileCheck -check-prefix=CHECK-FIRST %s
// RUN: %FileCheck -check-prefix=CHECK-RECORD-CLEAN %s < %t/main~buildrecord.swiftdeps
// CHECK-FIRST-NOT: warning
// CHECK-FIRST: Handled a.swift
// CHECK-FIRST: Handled c.swift
// CHECK-FIRST: Handled bad.swift
// CHECK-RECORD-CLEAN-DAG: "./a.swift": [
// CHECK-RECORD-CLEAN-DAG: "./bad.swift": [
// CHECK-RECORD-CLEAN-DAG: "./c.swift": [
// RUN: touch -t 201401240006 %t/a.swift
// RUN: cd %t && not %swiftc_driver -c -driver-use-frontend-path "%{python.unquoted};%S/Inputs/update-dependencies-bad.py;%swift-dependency-tool" -output-file-map %t/output.json -incremental -disable-direct-intramodule-dependencies -driver-always-rebuild-dependents ./a.swift ./bad.swift ./c.swift -module-name main -j1 -v -driver-show-incremental > %t/a.txt 2>&1
// RUN: %FileCheck -check-prefix=CHECK-A %s < %t/a.txt
// RUN: %FileCheck -check-prefix=NEGATIVE-A %s < %t/a.txt
// RUN: %FileCheck -check-prefix=CHECK-RECORD-A %s < %t/main~buildrecord.swiftdeps
// CHECK-A: Handled a.swift
// CHECK-A: Handled bad.swift
// NEGATIVE-A-NOT: Handled c.swift
// CHECK-RECORD-A-DAG: "./a.swift": [
// CHECK-RECORD-A-DAG: "./bad.swift": !private [
// CHECK-RECORD-A-DAG: "./c.swift": !private [
// RUN: cd %t && %swiftc_driver -c -driver-use-frontend-path "%{python.unquoted};%S/Inputs/update-dependencies.py;%swift-dependency-tool" -output-file-map %t/output.json -incremental -disable-direct-intramodule-dependencies -driver-always-rebuild-dependents ./a.swift ./bad.swift ./c.swift -module-name main -j1 -v -driver-show-incremental 2>&1 | %FileCheck -check-prefix CHECK-BC %s
// CHECK-BC-NOT: Handled a.swift
// CHECK-BC-DAG: Handled bad.swift
// CHECK-BC-DAG: Handled c.swift
| apache-2.0 | d86f5690e10df0539ed0063a457eec77 | 60.1 | 387 | 0.713993 | 3.129321 | false | false | false | false |
stephentyrone/swift | test/Sema/property_wrappers.swift | 3 | 823 | // RUN: %target-swift-frontend -typecheck -disable-availability-checking -dump-ast %s | %FileCheck %s
struct Transaction {
var state: Int?
}
@propertyWrapper
struct Wrapper<Value> {
var wrappedValue: Value
init(wrappedValue: Value,
reset: @escaping (Value, inout Transaction) -> Void) {
self.wrappedValue = wrappedValue
}
}
// rdar://problem/59685601
// CHECK-LABEL: R_59685601
struct R_59685601 {
// CHECK: tuple_expr implicit type='(wrappedValue: String, reset: (String, inout Transaction) -> Void)'
// CHECK-NEXT: property_wrapper_value_placeholder_expr implicit type='String'
// CHECK-NEXT: opaque_value_expr implicit type='String'
// CHECK-NEXT: string_literal_expr type='String'
@Wrapper(reset: { value, transaction in
transaction.state = 10
})
private var value = "hello"
}
| apache-2.0 | 8545714b479c910eb68e0054add1aee4 | 27.37931 | 105 | 0.704739 | 3.674107 | false | false | false | false |
raulriera/Bike-Compass | App/CityBikesKit/Location.swift | 1 | 1222 | //
// Location.swift
// Bike Compass
//
// Created by Raúl Riera on 23/04/2016.
// Copyright © 2016 Raul Riera. All rights reserved.
//
import Foundation
import Decodable
import CoreLocation
public struct Location {
public let coordinates: CLLocationCoordinate2D
public let city: String
public let country: String
private let countryCode: String
}
extension Location: Decodable {
public static func decode(_ j: AnyObject) throws -> Location {
return try Location(
coordinates: CLLocationCoordinate2D(latitude: j => "latitude", longitude: j => "longitude"),
city: j => "city",
country: Location.transformCountryCodeToDisplayName(j => "country"),
countryCode: j => "country"
)
}
static func transformCountryCodeToDisplayName(_ code: String) -> String {
return Locale.system().displayName(forKey: Locale.Key.countryCode, value: code) ?? code
}
}
extension Location: Encodable {
public func encode() -> AnyObject {
return ["latitude": coordinates.latitude,
"longitude": coordinates.longitude,
"city": city,
"country": countryCode]
}
}
| mit | 93ca5b34f4f401bb2a059518ed7e549f | 28.047619 | 104 | 0.637705 | 4.692308 | false | false | false | false |
superk589/CGSSGuide | DereGuide/Unit/Information/View/UnitInformationUnitCell.swift | 2 | 4143 | //
// UnitInformationUnitCell.swift
// DereGuide
//
// Created by zzk on 2017/5/20.
// Copyright © 2017 zzk. All rights reserved.
//
import UIKit
protocol UnitInformationUnitCellDelegate: class {
func unitInformationUnitCell(_ unitInformationUnitCell: UnitInformationUnitCell, didClick cardIcon: CGSSCardIconView)
}
class UnitInformationUnitCell: UITableViewCell, CGSSIconViewDelegate {
let selfLeaderSkillLabel = UnitLeaderSkillView()
let friendLeaderSkillLabel = UnitLeaderSkillView()
var iconStackView: UIStackView!
weak var delegate: UnitInformationUnitCellDelegate?
override init(style: UITableViewCell.CellStyle, reuseIdentifier: String?) {
super.init(style: style, reuseIdentifier: reuseIdentifier)
contentView.addSubview(selfLeaderSkillLabel)
selfLeaderSkillLabel.snp.makeConstraints { (make) in
make.top.equalTo(10)
make.left.equalTo(10)
make.right.equalTo(-10)
}
selfLeaderSkillLabel.arrowDirection = .down
var icons = [UIView]()
for _ in 0...5 {
let icon = CGSSCardIconView()
icon.delegate = self
icons.append(icon)
icon.snp.makeConstraints { (make) in
make.height.equalTo(icon.snp.width)
}
}
iconStackView = UIStackView(arrangedSubviews: icons)
iconStackView.spacing = 5
iconStackView.distribution = .fillEqually
contentView.addSubview(iconStackView)
iconStackView.snp.makeConstraints { (make) in
make.top.equalTo(selfLeaderSkillLabel.snp.bottom).offset(3)
make.left.greaterThanOrEqualTo(10)
make.right.lessThanOrEqualTo(-10)
// make the view as wide as possible
make.right.equalTo(-10).priority(900)
make.left.equalTo(10).priority(900)
//
make.width.lessThanOrEqualTo(96 * 6 + 25)
make.centerX.equalToSuperview()
}
contentView.addSubview(friendLeaderSkillLabel)
friendLeaderSkillLabel.snp.makeConstraints { (make) in
make.top.equalTo(iconStackView.snp.bottom).offset(3)
make.left.equalTo(10)
make.right.equalTo(-10)
make.bottom.equalTo(-10)
}
friendLeaderSkillLabel.arrowDirection = .up
friendLeaderSkillLabel.descLabel.textAlignment = .right
selfLeaderSkillLabel.sourceView = icons[0]
friendLeaderSkillLabel.sourceView = icons[5]
selectionStyle = .none
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
func setup(with unit: Unit) {
for i in 0...5 {
let member = unit[i]
if let card = member.card, let view = iconStackView.arrangedSubviews[i] as? CGSSCardIconView {
view.cardID = card.id
}
}
if let selfLeaderRef = unit.leader.card {
selfLeaderSkillLabel.setupWith(text: "\(NSLocalizedString("队长技能", comment: "队伍详情页面")): \(selfLeaderRef.leaderSkill?.name ?? NSLocalizedString("无", comment: ""))\n\(selfLeaderRef.leaderSkill?.localizedExplain ?? "")", backgroundColor: selfLeaderRef.attColor.mixed(withColor: .white))
} else {
selfLeaderSkillLabel.setupWith(text: "", backgroundColor: UIColor.allType.mixed(withColor: .white))
}
if let friendLeaderRef = unit.friendLeader.card {
friendLeaderSkillLabel.setupWith(text: "\(NSLocalizedString("好友技能", comment: "队伍详情页面")): \(friendLeaderRef.leaderSkill?.name ?? "无")\n\(friendLeaderRef.leaderSkill?.localizedExplain ?? "")", backgroundColor: friendLeaderRef.attColor.mixed(withColor: .white))
} else {
friendLeaderSkillLabel.setupWith(text: "", backgroundColor: UIColor.allType.mixed(withColor: .white))
}
}
func iconClick(_ iv: CGSSIconView) {
delegate?.unitInformationUnitCell(self, didClick: iv as! CGSSCardIconView)
}
}
| mit | 9af9d973bafc599004e80a8af602f8d6 | 37.660377 | 294 | 0.642509 | 4.931408 | false | false | false | false |
jondwillis/Swinject | Swinject/Container.swift | 1 | 8704 | //
// Container.swift
// Swinject
//
// Created by Yoichi Tagaya on 7/23/15.
// Copyright © 2015 Swinject Contributors. All rights reserved.
//
import Foundation
/// The `Container` class represents a dependency injection container, which stores registrations of services
/// and retrieves registered services with dependencies injected.
///
/// **Example to register:**
///
/// let container = Container()
/// container.register(A.self) { _ in B() }
/// container.register(X.self) { r in Y(a: r.resolve(A.self)!) }
///
/// **Example to retrieve:**
///
/// let x = container.resolve(X.self)!
///
/// where `A` and `X` are protocols, `B` is a type conforming `A`, and `Y` is a type conforming `X` and depending on `A`.
public final class Container {
/// The shared singleton instance of `Container`. It can be used in *the service locator pattern*.
public static let defaultContainer = Container()
private var services = [ServiceKey: ServiceEntryBase]()
private let parent: Container?
private var resolutionPool = ResolutionPool()
/// Instantiates a `Container`.
public init() {
self.parent = nil
}
/// Instantiates a `Container` that is a child container of the `Container` specified with `parent`.
///
/// - Parameter parent: The parent `Container`.
public init(parent: Container) {
self.parent = parent
}
/// Removes all registrations in the container.
public func removeAll() {
services.removeAll()
}
/// Adds a registration for the specified service with the factory closure to specify how the service is resolved with dependencies.
///
/// - Parameters:
/// - serviceType: The service type to register.
/// - name: A registration name, which is used to differenciate from other registrations
/// that have the same service and factory types.
/// - factory: The closure to specify how the service type is resolved with the dependencies of the type.
/// It is invoked when the `Container` needs to instantiate the instance.
/// It takes a `Resolvable` to inject dependencies to the instance,
/// and returns the instance of the component type for the service.
///
/// - Returns: A registered `ServiceEntry` to configure some settings fluently.
public func register<Service>(
serviceType: Service.Type,
name: String? = nil,
factory: Resolvable -> Service) -> ServiceEntry<Service>
{
return registerImpl(serviceType, factory: factory, name: name)
}
internal func registerImpl<Service, Factory>(serviceType: Service.Type, factory: Factory, name: String?) -> ServiceEntry<Service> {
let key = ServiceKey(factoryType: factory.dynamicType, name: name)
let entry = ServiceEntry(serviceType: serviceType, factory: factory)
services[key] = entry
return entry
}
}
// MARK: - Extension for Storyboard
extension Container {
/// Adds a registration of the specified view or window controller that is configured in a storyboard.
///
/// - Parameters:
/// - controllerType: The controller type to register as a service type.
/// The type is `UIViewController` in iOS, `NSViewController` or `NSWindowController` in OS X.
/// - name: A registration name, which is used to differenciate from other registrations
/// that have the same view or window controller type.
/// - initCompleted: A closure to specifiy how the dependencies of the view or window controller are injected.
/// It is invoked by the `Container` when the view or window controller is instantiated by `SwinjectStoryboard`.
public func registerForStoryboard<C: Controller>(controllerType: C.Type, name: String? = nil, initCompleted: (Resolvable, C) -> ()) {
let key = ServiceKey(factoryType: controllerType, name: name)
let entry = ServiceEntry(serviceType: controllerType)
entry.initCompleted = initCompleted
services[key] = entry
}
internal func runInitCompleted<C: Controller>(controllerType: C.Type, controller: C, name: String? = nil) {
resolutionPool.incrementDepth()
defer { resolutionPool.decrementDepth() }
let key = ServiceKey(factoryType: controllerType, name: name)
if let entry = getEntry(key) {
resolutionPool[key] = controller as Any
if let completed = entry.initCompleted as? (Resolvable, C) -> () {
completed(self, controller)
}
}
}
private func getEntry(key: ServiceKey) -> ServiceEntryBase? {
return services[key] ?? self.parent?.getEntry(key)
}
}
// MARK: - Resolvable
extension Container: Resolvable {
/// Retrieves the instance with the specified service type.
///
/// - Parameter serviceType: The service type to resolve.
///
/// - Returns: The resolved service type instance, or nil if no registration for the service type is found in the `Container`.
public func resolve<Service>(
serviceType: Service.Type) -> Service?
{
return resolve(serviceType, name: nil)
}
/// Retrieves the instance with the specified service type and registration name.
///
/// - Parameters:
/// - serviceType: The service type to resolve.
/// - name: The registration name.
///
/// - Returns: The resolved service type instance, or nil if no registration for the service type and name is found in the `Container`.
public func resolve<Service>(
serviceType: Service.Type,
name: String?) -> Service?
{
typealias FactoryType = Resolvable -> Service
return resolveImpl(name) { (factory: FactoryType) in factory(self) }
}
internal func resolveImpl<Service, Factory>(name: String?, invoker: Factory -> Service) -> Service? {
resolutionPool.incrementDepth()
defer { resolutionPool.decrementDepth() }
var resolvedInstance: Service?
let key = ServiceKey(factoryType: Factory.self, name: name)
if let (entry, fromParent) = getEntry(key) as (ServiceEntry<Service>, Bool)? {
switch (entry.scope) {
case .None, .Graph:
resolvedInstance = resolveEntry(entry, key: key, invoker: invoker)
case .Container:
let ownEntry: ServiceEntry<Service>
if fromParent {
ownEntry = entry.copyExceptInstance()
services[key] = ownEntry
}
else {
ownEntry = entry
}
if ownEntry.instance == nil {
ownEntry.instance = resolveEntry(entry, key: key, invoker: invoker) as Any
}
resolvedInstance = ownEntry.instance as? Service
case .Hierarchy:
if entry.instance == nil {
entry.instance = resolveEntry(entry, key: key, invoker: invoker) as Any
}
resolvedInstance = entry.instance as? Service
}
}
return resolvedInstance
}
private func getEntry<Service>(key: ServiceKey) -> (ServiceEntry<Service>, Bool)? {
var fromParent = false
var entry = services[key] as? ServiceEntry<Service>
if entry == nil, let parent = self.parent {
if let (parentEntry, _) = parent.getEntry(key) as (ServiceEntry<Service>, Bool)? {
entry = parentEntry
fromParent = true
}
}
return entry.map { ($0, fromParent) }
}
private func resolveEntry<Service, Factory>(entry: ServiceEntry<Service>, key: ServiceKey, invoker: Factory -> Service) -> Service {
let usesPool = entry.scope != .None
if usesPool, let pooledInstance = resolutionPool[key] as? Service {
return pooledInstance
}
let resolvedInstance = invoker(entry.factory as! Factory)
if usesPool {
if let pooledInstance = resolutionPool[key] as? Service {
// An instance for the key might be added by the factory invocation.
return pooledInstance
}
resolutionPool[key] = resolvedInstance as Any
}
if let completed = entry.initCompleted as? (Resolvable, Service) -> () {
completed(self, resolvedInstance)
}
return resolvedInstance
}
}
| mit | 2f2c449fed22dc86c4279e0736d2407d | 40.841346 | 139 | 0.614271 | 4.916949 | false | false | false | false |
yeziahehe/Gank | Pods/Kingfisher/Sources/Utility/SizeExtensions.swift | 1 | 4631 | //
// SizeExtensions.swift
// Kingfisher
//
// Created by onevcat on 2018/09/28.
//
// Copyright (c) 2019 Wei Wang <onevcat@gmail.com>
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
import CoreGraphics
extension CGSize: KingfisherCompatible {}
extension KingfisherWrapper where Base == CGSize {
/// Returns a size by resizing the `base` size to a target size under a given content mode.
///
/// - Parameters:
/// - size: The target size to resize to.
/// - contentMode: Content mode of the target size should be when resizing.
/// - Returns: The resized size under the given `ContentMode`.
public func resize(to size: CGSize, for contentMode: ContentMode) -> CGSize {
switch contentMode {
case .aspectFit:
return constrained(size)
case .aspectFill:
return filling(size)
case .none:
return size
}
}
/// Returns a size by resizing the `base` size by making it aspect fitting the given `size`.
///
/// - Parameter size: The size in which the `base` should fit in.
/// - Returns: The size fitted in by the input `size`, while keeps `base` aspect.
public func constrained(_ size: CGSize) -> CGSize {
let aspectWidth = round(aspectRatio * size.height)
let aspectHeight = round(size.width / aspectRatio)
return aspectWidth > size.width ?
CGSize(width: size.width, height: aspectHeight) :
CGSize(width: aspectWidth, height: size.height)
}
/// Returns a size by resizing the `base` size by making it aspect filling the given `size`.
///
/// - Parameter size: The size in which the `base` should fill.
/// - Returns: The size be filled by the input `size`, while keeps `base` aspect.
public func filling(_ size: CGSize) -> CGSize {
let aspectWidth = round(aspectRatio * size.height)
let aspectHeight = round(size.width / aspectRatio)
return aspectWidth < size.width ?
CGSize(width: size.width, height: aspectHeight) :
CGSize(width: aspectWidth, height: size.height)
}
/// Returns a `CGRect` for which the `base` size is constrained to an input `size` at a given `anchor` point.
///
/// - Parameters:
/// - size: The size in which the `base` should be constrained to.
/// - anchor: An anchor point in which the size constraint should happen.
/// - Returns: The result `CGRect` for the constraint operation.
public func constrainedRect(for size: CGSize, anchor: CGPoint) -> CGRect {
let unifiedAnchor = CGPoint(x: anchor.x.clamped(to: 0.0...1.0),
y: anchor.y.clamped(to: 0.0...1.0))
let x = unifiedAnchor.x * base.width - unifiedAnchor.x * size.width
let y = unifiedAnchor.y * base.height - unifiedAnchor.y * size.height
let r = CGRect(x: x, y: y, width: size.width, height: size.height)
let ori = CGRect(origin: .zero, size: base)
return ori.intersection(r)
}
private var aspectRatio: CGFloat {
return base.height == 0.0 ? 1.0 : base.width / base.height
}
}
extension CGRect {
func scaled(_ scale: CGFloat) -> CGRect {
return CGRect(x: origin.x * scale, y: origin.y * scale,
width: size.width * scale, height: size.height * scale)
}
}
extension Comparable {
func clamped(to limits: ClosedRange<Self>) -> Self {
return min(max(self, limits.lowerBound), limits.upperBound)
}
}
| gpl-3.0 | ee896bfbafc01764aeece22114a6b225 | 41.1 | 113 | 0.64716 | 4.295918 | false | false | false | false |
brendonjustin/EtherPlayer | EtherPlayer/AirPlay/StopRequester.swift | 1 | 1361 | //
// StopRequester.swift
// EtherPlayer
//
// Created by Brendon Justin on 5/5/16.
// Copyright © 2016 Brendon Justin. All rights reserved.
//
import Cocoa
class StopRequester: AirplayRequester {
let relativeURL = "/stop"
weak var delegate: StopRequesterDelegate?
weak var requestCustomizer: AirplayRequestCustomizer?
private var requestTask: NSURLSessionTask?
func performRequest(baseURL: NSURL, sessionID: String, urlSession: NSURLSession) {
guard requestTask == nil else {
print("\(relativeURL) request already in flight, not performing another one.")
return
}
let url = NSURL(string: relativeURL, relativeToURL: baseURL)!
let request = NSMutableURLRequest(URL: url)
requestCustomizer?.requester(self, willPerformRequest: request)
let task = urlSession.dataTaskWithRequest(request) { [weak self] (data, response, error) in
defer {
self?.requestTask = nil
}
self?.delegate?.stoppedWithError(nil)
}
requestTask = task
task.resume()
}
func cancelRequest() {
requestTask?.cancel()
requestTask = nil
}
}
protocol StopRequesterDelegate: class {
func stoppedWithError(error: NSError?)
}
| gpl-3.0 | 8b5e5f0c1872bb207e4c2fa1b2351f4d | 26.2 | 99 | 0.619118 | 4.981685 | false | false | false | false |
xiaoyouPrince/DYLive | DYLive/DYLive/Classes/Main/View/PageTitleView.swift | 1 | 7415 | //
// PageTitleView.swift
// DYLive
//
// Created by 渠晓友 on 2017/4/1.
// Copyright © 2017年 xiaoyouPrince. All rights reserved.
//
import UIKit
/*
1. 封装PageTitileView --> view:scrollview:Label+手势 & lineView
2. 封装PageContentView --> uicollectionView->横向滚动的cell
3. 处理PageTitleView和PageContentView的逻辑
*/
// MARK: - 定义自己代理
protocol PageTitleViewDelegate : AnyObject {
// 这里只是方法的定义 --selectIndex index :分别是内部和外部属性
func pageTitleView(titleView : PageTitleView , selectIndex index : Int)
}
// MARK: - 定义常量
private let kScrollLineH : CGFloat = 2
private let kNormalColor : (CGFloat, CGFloat, CGFloat) = (85, 85, 85)
private let kSelectColor : (CGFloat, CGFloat, CGFloat) = (255, 128, 0)
// MARK: - 类的声明
class PageTitleView: UIView {
// MARK: - 自定义属性
fileprivate var titles : [String]
fileprivate var titleLabels : [UILabel] = [UILabel]()
fileprivate var currentIndex : Int = 0 // 设置默认的当前下标为0
weak var delegate : PageTitleViewDelegate?
// MARK: - 懒加载属性
fileprivate lazy var scrollView : UIScrollView = {[weak self] in
let scrollView = UIScrollView()
scrollView.showsHorizontalScrollIndicator = false
scrollView.bounces = false
scrollView.scrollsToTop = false
return scrollView
}();
fileprivate lazy var scrollLine : UIView = {[weak self] in
let scrollLine = UIView()
scrollLine.backgroundColor = UIColor.orange
return scrollLine
}();
// MARK: - 自定制PageTitleView的构造方法
init(frame: CGRect, titles:[String]) {
// 1.给自己的titles赋值
self.titles = titles
// 2.通过frame构造实例变量
super.init(frame:frame)
// 3.创建UI
setupUI()
}
// 自定义构造方法必须重写initwithCoder方法
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
// MARK: - 设置UI
extension PageTitleView{
fileprivate func setupUI(){
// 1.添加对应的scrollview
addSubview(scrollView)
scrollView.frame = self.bounds
// scrollView.backgroundColor = UIColor.yellow
// 2.添加lable
setupTitleLabels()
// 3.添加底边线和可滑动的线
setupBottomLineAndScrollLines()
}
// MARK: - 添加label
private func setupTitleLabels(){
// 0.对于有些只需要设置一遍的东西,放到外面来
let labelW : CGFloat = frame.width / CGFloat(titles.count)
let labelH : CGFloat = frame.height - kScrollLineH
let labelY : CGFloat = 0.0
for (index,title) in titles.enumerated(){
// 1.创建Label
let label = UILabel()
// 2.设置对应的属性
label.text = title
label.font = UIFont.systemFont(ofSize: 16.0)
label.tag = index
label.textColor = UIColor(r: kNormalColor.0, g: kNormalColor.1, b: kNormalColor.2)
label.textAlignment = .center
// 3. 设置frame
let labelX : CGFloat = CGFloat(index) * labelW
label.frame = CGRect(x: labelX, y: labelY, width: labelW, height: labelH)
// 4.添加
scrollView.addSubview(label)
// 5.添加到Label的数组中
titleLabels.append(label)
// 6.给Label添加手势
label.isUserInteractionEnabled = true
let tapGes = UITapGestureRecognizer(target: self, action: #selector(self.titleLabelClick(tapGes:)))
label.addGestureRecognizer(tapGes)
}
}
// MARK: - 设置底线 和 可以滚动的线
private func setupBottomLineAndScrollLines(){
let bottomLine = UIView()
let bottomLineH : CGFloat = 0.5
bottomLine.backgroundColor = UIColor.gray
bottomLine.frame = CGRect(x: 0, y: frame.height - bottomLineH , width: frame.width, height: bottomLineH)
addSubview(bottomLine)
guard let label = titleLabels.first else {return}
label.textColor = UIColor(r: kSelectColor.0, g: kSelectColor.1, b: kSelectColor.2)
scrollLine.frame = CGRect(x: label.bounds.origin.x, y: label.frame.origin.y+label.frame.height, width: label.frame.width, height: kScrollLineH)
addSubview(scrollLine)
}
}
// MARK: - 监听Label的点击 -- 必须使用@objc
extension PageTitleView{
@objc fileprivate func titleLabelClick(tapGes : UITapGestureRecognizer){
// 1.取到当前的label
guard let currentLabel = tapGes.view as? UILabel else {
return
}
// 对当前的Index和当前Label的tag值进行对比,如果当前label就是选中的label就不变了,如果是跳到其他的Label就执行后面,修改对应的颜色
if currentLabel.tag == currentIndex { return }
// 2.获取之前的label
let oldLabel = titleLabels[currentIndex]
// 3.设置文字颜色改变
currentLabel.textColor = UIColor(r: kSelectColor.0, g: kSelectColor.1, b: kSelectColor.2)
oldLabel.textColor = UIColor(r: kNormalColor.0, g: kNormalColor.1, b: kNormalColor.2)
// 4.保存新的当前下边值
currentIndex = currentLabel.tag
// 5.滚动条的滚动
let scrollLinePosition : CGFloat = currentLabel.frame.origin.x
UIView.animate(withDuration: 0.15) {
self.scrollLine.frame.origin.x = scrollLinePosition
}
// 6.通知代理做事情
delegate?.pageTitleView(titleView: self, selectIndex: currentIndex)
}
}
// MARK: - 暴露给外界的方法
extension PageTitleView{
func setTitleWithProgress( progress : CGFloat, sourceIndex : Int, targetIndex : Int) {
// 1.取出sourceLabel/targetLabel
let sourceLabel = titleLabels[sourceIndex]
let targetLabel = titleLabels[targetIndex]
// 2.处理滑块的逻辑
let moveTotalX = targetLabel.frame.origin.x - sourceLabel.frame.origin.x
let moveX = moveTotalX * progress
scrollLine.frame.origin.x = sourceLabel.frame.origin.x + moveX
// 3.颜色的渐变(复杂)
// 3.1.取出变化的范围
let colorDelta = (kSelectColor.0 - kNormalColor.0, kSelectColor.1 - kNormalColor.1, kSelectColor.2 - kNormalColor.2)
// 3.2.变化sourceLabel
sourceLabel.textColor = UIColor(r: kSelectColor.0 - colorDelta.0 * progress, g: kSelectColor.1 - colorDelta.1 * progress, b: kSelectColor.2 - colorDelta.2 * progress)
// 3.2.变化targetLabel
targetLabel.textColor = UIColor(r: kNormalColor.0 + colorDelta.0 * progress, g: kNormalColor.1 + colorDelta.1 * progress, b: kNormalColor.2 + colorDelta.2 * progress)
// 4.记录最新的index
currentIndex = targetIndex
}
}
| mit | 7a639bd47d8460bd83e16c2aeadcf0c2 | 28.373913 | 174 | 0.598727 | 4.537273 | false | false | false | false |
domenicosolazzo/practice-swift | Multipeer/Multipeer/Multipeer/ViewController.swift | 1 | 6201 | //
// ViewController.swift
// Multipeer
//
// Created by Domenico on 04/05/16.
// Copyright © 2016 Domenico Solazzo. All rights reserved.
//
import UIKit
import MultipeerConnectivity
class ViewController: UIViewController,
UICollectionViewDataSource, UICollectionViewDelegate, UINavigationControllerDelegate, UIImagePickerControllerDelegate,
MCSessionDelegate, MCBrowserViewControllerDelegate {
@IBOutlet weak var collectionView: UICollectionView!
var images = [UIImage]()
var peerID: MCPeerID!
var mcSession: MCSession!
var mcAdvertiserAssistant: MCAdvertiserAssistant!
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
title = "Selfie Share"
navigationItem.leftBarButtonItem = UIBarButtonItem(barButtonSystemItem: .add, target: self, action: #selector(showConnectionPrompt))
navigationItem.rightBarButtonItem = UIBarButtonItem(barButtonSystemItem: .camera, target: self, action: #selector(importPicture))
peerID = MCPeerID(displayName: UIDevice.current.name)
mcSession = MCSession(peer: peerID, securityIdentity: nil, encryptionPreference: .required)
mcSession.delegate = self
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return images.count
}
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "ImageView", for: indexPath)
if let imageView = cell.viewWithTag(1000) as? UIImageView {
imageView.image = images[(indexPath as NSIndexPath).item]
}
return cell
}
func browserViewControllerDidFinish(_ browserViewController: MCBrowserViewController) {
dismiss(animated: true, completion: nil)
}
func browserViewControllerWasCancelled(_ browserViewController: MCBrowserViewController) {
dismiss(animated: true, completion: nil)
}
func session(_ session: MCSession, didReceive stream: InputStream, withName streamName: String, fromPeer peerID: MCPeerID) {
}
func session(_ session: MCSession, didStartReceivingResourceWithName resourceName: String, fromPeer peerID: MCPeerID, with progress: Progress) {
}
func session(_ session: MCSession, didFinishReceivingResourceWithName resourceName: String, fromPeer peerID: MCPeerID, at localURL: URL, withError error: Error?) {
}
func session(_ session: MCSession, peer peerID: MCPeerID, didChange state: MCSessionState) {
switch state {
case MCSessionState.connected:
print("Connected: \(peerID.displayName)")
case MCSessionState.connecting:
print("Connecting: \(peerID.displayName)")
case MCSessionState.notConnected:
print("Not Connected: \(peerID.displayName)")
}
}
func session(_ session: MCSession, didReceive data: Data, fromPeer peerID: MCPeerID) {
if let image = UIImage(data: data) {
DispatchQueue.main.async { [unowned self] in
self.images.insert(image, at: 0)
self.collectionView.reloadData()
}
}
}
func importPicture() {
let picker = UIImagePickerController()
picker.allowsEditing = true
picker.delegate = self
present(picker, animated: true, completion: nil)
}
func imagePickerController(_ picker: UIImagePickerController, didFinishPickingMediaWithInfo info: [String : Any]) {
var newImage: UIImage
if let possibleImage = info[UIImagePickerControllerEditedImage] as? UIImage {
newImage = possibleImage
} else if let possibleImage = info[UIImagePickerControllerOriginalImage] as? UIImage {
newImage = possibleImage
} else {
return
}
dismiss(animated: true, completion: nil)
images.insert(newImage, at: 0)
collectionView.reloadData()
// 1
if mcSession.connectedPeers.count > 0 {
// 2
if let imageData = UIImagePNGRepresentation(newImage) {
// 3
do {
try mcSession.send(imageData, toPeers: mcSession.connectedPeers, with: .reliable)
} catch let error as NSError {
let ac = UIAlertController(title: "Send error", message: error.localizedDescription, preferredStyle: .alert)
ac.addAction(UIAlertAction(title: "OK", style: .default, handler: nil))
present(ac, animated: true, completion: nil)
}
}
}
}
func imagePickerControllerDidCancel(_ picker: UIImagePickerController) {
dismiss(animated: true, completion: nil)
}
func showConnectionPrompt() {
let ac = UIAlertController(title: "Connect to others", message: nil, preferredStyle: .actionSheet)
ac.addAction(UIAlertAction(title: "Host a session", style: .default, handler: startHosting))
ac.addAction(UIAlertAction(title: "Join a session", style: .default, handler: joinSession))
ac.addAction(UIAlertAction(title: "Cancel", style: .cancel, handler: nil))
present(ac, animated: true, completion: nil)
}
func startHosting(_ action: UIAlertAction!) {
mcAdvertiserAssistant = MCAdvertiserAssistant(serviceType: "TheProject", discoveryInfo: nil, session: mcSession)
mcAdvertiserAssistant.start()
}
func joinSession(_ action: UIAlertAction!) {
let mcBrowser = MCBrowserViewController(serviceType: "TheProject", session: mcSession)
mcBrowser.delegate = self
present(mcBrowser, animated: true, completion: nil)
}
}
| mit | 47a4e7aac2070bd63d468840731f6905 | 37.271605 | 167 | 0.654355 | 5.57554 | false | false | false | false |
davidgatti/IoT-Home-Automation | GarageOpener/GarageState.swift | 3 | 2524 | //
// Settings.swift
// GarageOpener
//
// Created by David Gatti on 6/8/15.
// Copyright (c) 2015 David Gatti. All rights reserved.
//
import Foundation
import Parse
class GarageState {
//MARK: Static
static let sharedInstance = GarageState()
//MARK: Variables
var user: PFUser!
var isOpen: Int = 0
var useCount: Int = 0
var lastUsed: NSDate = NSDate.distantPast() as NSDate
//MARK: Get
func get(completition:() -> ()) {
var count: Int = 0
getLastUser { (result) -> Void in
count++
}
getUseCount { (result) -> Void in
count++
}
while true {
if count == 2 {
return completition()
}
}
}
private func getLastUser(completition:() -> ()) {
let qHistory = PFQuery(className: "History")
qHistory.orderByDescending("createdAt")
qHistory.getFirstObjectInBackgroundWithBlock { (lastEntry: PFObject?, error) -> Void in
self.isOpen = (lastEntry?.objectForKey("state") as? Int)!
self.lastUsed = lastEntry!.createdAt!
self.user = lastEntry?.objectForKey("user") as? PFUser
self.user?.fetch()
return completition()
}
}
private func getUseCount(completition:() -> ()) {
let qGarageDoor = PFQuery(className:"GarageDoor")
qGarageDoor.getObjectInBackgroundWithId("eX9QCJGga5") { (garage: PFObject?, error: NSError?) -> Void in
self.useCount = (garage!.objectForKey("useCount") as! Int)
return completition()
}
}
//MARK: Set
func set(completition: (result: String) -> Void) {
setHistory { (result) -> Void in }
setGarageDoor { (result) -> Void in }
}
private func setHistory(completition: (result: String) -> Void) {
let user = PFUser.currentUser()
let history = PFObject(className: "History")
history["user"] = user
history["applianceID"] = "eX9QCJGga5"
history["state"] = self.isOpen
history.saveInBackground()
}
private func setGarageDoor(completition: (result: String) -> Void) {
let query = PFObject(withoutDataWithClassName: "GarageDoor", objectId: "eX9QCJGga5")
query["useCount"] = self.useCount
query.saveInBackground()
}
}
| apache-2.0 | 5bf7b58e366e4a9fd923bcf90b5cbac1 | 25.291667 | 111 | 0.548336 | 4.665434 | false | false | false | false |
radubozga/Freedom | speech/Swift/Speech-gRPC-Streaming/Pods/DynamicButton/Sources/DynamicButtonStyles/DynamicButtonStyleArrowLeft.swift | 1 | 2057 | /*
* DynamicButton
*
* Copyright 2015-present Yannick Loriot.
* http://yannickloriot.com
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*
*/
import UIKit
/// Leftwards arrow style: ←
struct DynamicButtonStyleArrowLeft: DynamicButtonBuildableStyle {
let pathVector: DynamicButtonPathVector
init(center: CGPoint, size: CGFloat, offset: CGPoint, lineWidth: CGFloat) {
let rightPoint = CGPoint(x: offset.x + size, y: center.y)
let headPoint = CGPoint(x: offset.x + lineWidth, y: center.y)
let topPoint = CGPoint(x: offset.x + size / 3.2, y: center.y + size / 3.2)
let bottomPoint = CGPoint(x: offset.x + size / 3.2, y: center.y - size / 3.2)
let p1 = PathHelper.line(from: rightPoint, to: headPoint)
let p2 = PathHelper.line(from: headPoint, to: topPoint)
let p3 = PathHelper.line(from: headPoint, to: bottomPoint)
pathVector = DynamicButtonPathVector(p1: p1, p2: p2, p3: p3, p4: p1)
}
/// "Arrow Left" style.
static var styleName: String {
return "Arrow Left"
}
}
| apache-2.0 | 952fd78a605e482090200602b489ce19 | 40.1 | 81 | 0.723601 | 3.959538 | false | false | false | false |
hrscy/TodayNews | News/News/Classes/Mine/View/PostCommentView.swift | 1 | 8352 | //
// PostCommentView.swift
// News
//
// Created by 杨蒙 on 2018/1/4.
// Copyright © 2018年 hrscy. All rights reserved.
//
import UIKit
import IBAnimatable
class PostCommentView: UIView, NibLoadable {
private lazy var emojiManger = EmojiManager()
@IBOutlet weak var pageControlView: UIView!
private lazy var pageControl: UIPageControl = {
let pageControl = UIPageControl()
pageControl.theme_currentPageIndicatorTintColor = "colors.currentPageIndicatorTintColor"
pageControl.theme_pageIndicatorTintColor = "colors.pageIndicatorTintColor"
return pageControl
}()
@IBOutlet weak var emojiView: UIView!
@IBOutlet weak var emojiViewBottom: NSLayoutConstraint!
@IBOutlet weak var collectionView: UICollectionView!
// 包括 emoji 按钮和 pageControl
@IBOutlet weak var toolbarHeight: NSLayoutConstraint!
@IBOutlet weak var emojiButtonHeight: NSLayoutConstraint!
/// 占位符
@IBOutlet weak var placeholderLabel: UILabel!
/// 底部 view
@IBOutlet weak var bottomView: UIView!
/// 底部约束
@IBOutlet weak var bottomViewBottom: NSLayoutConstraint!
/// textView
@IBOutlet weak var textView: UITextView!
/// textView 的高度
@IBOutlet weak var textViewHeight: NSLayoutConstraint!
@IBOutlet weak var textViewBackgroundView: AnimatableView!
/// 发布按钮
@IBOutlet weak var postButton: UIButton!
/// 同时转发
@IBOutlet weak var forwardButton: UIButton!
/// @ 按钮
@IBOutlet weak var atButton: UIButton!
/// emoji 按钮
@IBOutlet weak var emojiButton: UIButton!
/// emoji 按钮是否选中
var isEmojiButtonSelected = false {
didSet {
if isEmojiButtonSelected {
emojiButton.isSelected = isEmojiButtonSelected
UIView.animate(withDuration: 0.25, animations: {
// 改变约束
self.changeConstraints()
self.bottomViewBottom.constant = emojiItemWidth * 3 + self.toolbarHeight.constant + self.emojiViewBottom.constant
self.layoutIfNeeded()
})
// 判断 pageControlView 的子控件(pageControl)是否为 0
if pageControlView.subviews.count == 0 {
pageControl.numberOfPages = emojiManger.emojis.count / 21
pageControl.center = pageControlView.center
pageControlView.addSubview(pageControl)
}
} else {
textView.becomeFirstResponder()
}
}
}
/// 改变约束
private func changeConstraints() {
self.emojiButtonHeight.constant = 44
self.toolbarHeight.constant = self.emojiButtonHeight.constant + 20
self.emojiViewBottom.constant = isIPhoneX ? 34 : 0
}
/// 重置约束
private func resetConstraints() {
self.emojiButtonHeight.constant = 0
self.toolbarHeight.constant = 0
self.bottomViewBottom.constant = 0
self.emojiViewBottom.constant = 0
layoutIfNeeded()
}
override func touchesBegan(_ touches: Set<UITouch>, with event: UIEvent?) {
endEditing(true)
// 如果 emoji 按钮选中了
if isEmojiButtonSelected {
UIView.animate(withDuration: 0.25, animations: {
// 重置约束
self.resetConstraints()
}, completion: { (_) in
self.removeFromSuperview()
})
} else {
removeFromSuperview()
}
}
override func awakeFromNib() {
super.awakeFromNib()
width = screenWidth
height = screenHeight
bottomView.theme_backgroundColor = "colors.cellBackgroundColor"
textViewBackgroundView.theme_backgroundColor = "colors.grayColor240"
forwardButton.theme_setImage("images.loginReadButtonSelected", forState: .normal)
atButton.theme_setImage("images.toolbar_icon_at_24x24_", forState: .normal)
emojiButton.theme_setImage("images.toolbar_icon_emoji_24x24_", forState: .normal)
emojiButton.theme_setImage("images.toolbar_icon_keyboard_24x24_", forState: .selected)
/// 添加通知
NotificationCenter.default.addObserver(self, selector: #selector(keyboardWillShow), name: NSNotification.Name.UIKeyboardWillShow, object: nil)
NotificationCenter.default.addObserver(self, selector: #selector(keyboardWillBeHidden), name: NSNotification.Name.UIKeyboardWillHide, object: nil)
collectionView.collectionViewLayout = EmojiLayout()
collectionView.ym_registerCell(cell: EmojiCollectionCell.self)
}
/// 键盘将要弹起
@objc private func keyboardWillShow(notification: Notification) {
let frame = notification.userInfo![UIKeyboardFrameEndUserInfoKey] as! CGRect
let duration = notification.userInfo![UIKeyboardAnimationDurationUserInfoKey] as! TimeInterval
UIView.animate(withDuration: duration) {
// 改变约束
self.changeConstraints()
self.bottomViewBottom.constant = frame.size.height
self.layoutIfNeeded()
}
}
/// 键盘将要隐藏
@objc private func keyboardWillBeHidden(notification: Notification) {
let duration = notification.userInfo![UIKeyboardAnimationDurationUserInfoKey] as! TimeInterval
UIView.animate(withDuration: duration) {
// 重置约束
self.resetConstraints()
}
}
deinit {
NotificationCenter.default.removeObserver(self)
}
}
// MARK: - 点击事件
extension PostCommentView {
/// 发布按钮点击
@IBAction func postButtonClicked(_ sender: UIButton) {
sender.isSelected = !sender.isSelected
}
/// @ 按钮点击
@IBAction func atButtonClicked(_ sender: UIButton) {
}
/// emoji 按钮点击
@IBAction func emojiButtonClicked(_ sender: UIButton) {
sender.isSelected = !sender.isSelected
if sender.isSelected { // 说明需要弹起的是表情
textView.resignFirstResponder()
isEmojiButtonSelected = true
} else { // 说明需要弹起的是键盘
textView.becomeFirstResponder()
}
}
}
// MARK: - UITextViewDelegate
extension PostCommentView: UITextViewDelegate {
func textViewDidChange(_ textView: UITextView) {
placeholderLabel.isHidden = textView.text.count != 0
postButton.setTitleColor((textView.text.count != 0) ? .blueFontColor() : .grayColor210(), for: .normal)
let height = Calculate.attributedTextHeight(text: textView.attributedText, width: textView.width)
if height <= 30 {
textViewHeight.constant = 30
} else if height >= 80 {
textViewHeight.constant = 80
} else {
textViewHeight.constant = height
}
layoutIfNeeded()
}
func textViewShouldBeginEditing(_ textView: UITextView) -> Bool {
// 当 textView 将要开始编辑的时候,设置 emoji 按钮不选中
emojiButton.isSelected = false
return true
}
}
// MARK: - UICollectionViewDelegate, UICollectionViewDataSource
extension PostCommentView: UICollectionViewDelegate, UICollectionViewDataSource {
func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return emojiManger.emojis.count
}
func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.ym_dequeueReusableCell(indexPath: indexPath) as EmojiCollectionCell
cell.emoji = emojiManger.emojis[indexPath.item]
return cell
}
func collectionView(_ collectionView: UICollectionView, didSelectItemAt indexPath: IndexPath) {
textView.setAttributedText(emoji: emojiManger.emojis[indexPath.item])
placeholderLabel.isHidden = textView.attributedText.length != 0
}
func scrollViewDidScroll(_ scrollView: UIScrollView) {
let currentPage = scrollView.contentOffset.x / scrollView.width
pageControl.currentPage = Int(currentPage + 0.5)
}
}
| mit | e73ad48c931eeecae79c9d001d41f4ee | 35.429864 | 154 | 0.652714 | 5.414257 | false | false | false | false |
shmidt/ContactsPro | ContactsPro/ContactViewVC.swift | 1 | 7466 | //
// ContactDetailVC.swift
// ContactsPro
//
// Created by Dmitry Shmidt on 09/04/15.
// Copyright (c) 2015 Dmitry Shmidt. All rights reserved.
//
import UIKit
import RealmSwift
class ContactViewVC: UITableViewController {
private var notificationToken: NotificationToken?
var person: Person!
private lazy var dateFormatter: NSDateFormatter = {
let dateFormatter = NSDateFormatter()
dateFormatter.dateStyle = .MediumStyle
dateFormatter.timeStyle = .NoStyle
return dateFormatter
}()
deinit{
let realm = Realm()
if let notificationToken = notificationToken{
realm.removeNotification(notificationToken)
}
}
override func viewDidLoad() {
super.viewDidLoad()
tableView.accessibilityLabel = "View Contact"
tableView.accessibilityValue = person.fullName
tableView.isAccessibilityElement = true
tableView.tableFooterView = UIView()
title = person.fullName
setupTableViewUI()
registerFormCells()
navigationItem.rightBarButtonItem = UIBarButtonItem(barButtonSystemItem: UIBarButtonSystemItem.Edit, target: self, action: "editVC")
reloadData()
notificationToken = Realm().addNotificationBlock {[unowned self] note, realm in
println(ContactViewVC.self)
println(note)
// self.objects = self.array
self.reloadData()
}
}
func editVC(){
let vc = storyboard?.instantiateViewControllerWithIdentifier("ContactEditVC") as! ContactEditVC
vc.person = person
let nc = UINavigationController(rootViewController: vc)
presentViewController(nc, animated: true, completion: nil)
}
func setupTableViewUI(){
tableView.estimatedRowHeight = 44.0
tableView.rowHeight = UITableViewAutomaticDimension
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
// MARK: - Table view data source
override func numberOfSectionsInTableView(tableView: UITableView) -> Int {
return sectionNames.count
}
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let section = indexPath.section
switch section{
case Section.Notes:
let note = person.notes[indexPath.row]
let cell = tableView.dequeueReusableCellWithIdentifier(Constants.TableViewCell.LabelTextTableViewCellID, forIndexPath: indexPath) as! LabelTextTableViewCell
cell.label.text = dateFormatter.stringFromDate(note.date)
cell.selectionStyle = .None
cell.valueTextLabel.text = note.text
return cell
case Section.WeakPoints:
let note = person.weakPoints[indexPath.row]
let cell = tableView.dequeueReusableCellWithIdentifier(Constants.TableViewCell.LabelTextTableViewCellID, forIndexPath: indexPath) as! LabelTextTableViewCell
cell.label.text = dateFormatter.stringFromDate(note.date)
cell.selectionStyle = .None
cell.valueTextLabel.text = note.text
return cell
case Section.StrongPoints:
let note = person.strongPoints[indexPath.row]
let cell = tableView.dequeueReusableCellWithIdentifier(Constants.TableViewCell.LabelTextTableViewCellID, forIndexPath: indexPath) as! LabelTextTableViewCell
cell.label.text = dateFormatter.stringFromDate(note.date)
cell.selectionStyle = .None
cell.valueTextLabel.text = note.text
return cell
case Section.ToDo:
let todo = person.todos[indexPath.row]
let cell = tableView.dequeueReusableCellWithIdentifier(Constants.TableViewCell.ListItemCell, forIndexPath: indexPath) as! ListItemCell
cell.textValue = todo.text
cell.isComplete = todo.isComplete
cell.textField.enabled = false
cell.didTapCheckBox({[unowned self] (completed) -> Void in
println("didTapCheckBox")
let realm = Realm()
realm.beginWrite()
todo.isComplete = completed
realm.commitWrite()
})
return cell
default:()
}
let cell = tableView.dequeueReusableCellWithIdentifier(Constants.TableViewCell.DefaultCellID, forIndexPath: indexPath) as! UITableViewCell
return cell
}
override func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
var rows = 0
switch section{
case Section.WeakPoints:
rows = person.weakPoints.count
case Section.StrongPoints:
rows = person.strongPoints.count
case Section.Notes:
rows = person.notes.count
case Section.ToDo:
rows = person.todos.count
default:
rows = 0
}
return rows
}
override func tableView(tableView: UITableView, didSelectRowAtIndexPath indexPath: NSIndexPath) {
println("didSelectRowAtIndexPath")
let section = indexPath.section
if section == Section.ToDo{
let todo = person.todos[indexPath.row]
let realm = Realm()
realm.beginWrite()
todo.isComplete = !todo.isComplete
realm.commitWrite()
}
}
override func tableView(tableView: UITableView, titleForHeaderInSection section: Int) -> String? {
if !editing{
switch section{
case Section.Notes:
if person.notes.count == 0{
return nil
}
case Section.StrongPoints:
if person.strongPoints.count == 0{
return nil
}
case Section.WeakPoints:
if person.weakPoints.count == 0{
return nil
}
case Section.ToDo:
if person.todos.count == 0{
return nil
}
default:
return sectionNames[section]
}
}
return sectionNames[section]
}
//MARK: -
func reloadData(){
tableView.reloadData()
}
func registerFormCells(){
tableView.registerNib(UINib(nibName: Constants.TableViewCell.ListItemCell, bundle: nil), forCellReuseIdentifier: Constants.TableViewCell.ListItemCell)
tableView.registerNib(UINib(nibName: Constants.TableViewCell.LabelTextTableViewCellID, bundle: nil), forCellReuseIdentifier: Constants.TableViewCell.LabelTextTableViewCellID)
tableView.registerClass(UITableViewCell.self, forCellReuseIdentifier: Constants.TableViewCell.DefaultCellID)
}
}
| mit | f1f7d4b55082c4747e2cb93e28180b4f | 32.936364 | 182 | 0.588267 | 6.165153 | false | false | false | false |
blockchain/My-Wallet-V3-iOS | Modules/Platform/Sources/PlatformUIKit/Views/IntroductionSheetViewController/IntroductionSheetViewController.swift | 1 | 2020 | // Copyright © Blockchain Luxembourg S.A. All rights reserved.
import Foundation
import RxCocoa
import RxSwift
public final class IntroductionSheetViewController: UIViewController {
private typealias AccessibilityIdentifiers = Accessibility.Identifier.IntroductionSheet
// MARK: Private Properties
private let bag = DisposeBag()
private var viewModel: IntroductionSheetViewModel!
// MARK: Private IBOutlets
@IBOutlet private var thumbnail: UIImageView!
@IBOutlet private var titleLabel: UILabel!
@IBOutlet private var subtitleLabel: UILabel!
@IBOutlet private var button: UIButton!
// TICKET: IOS-2520 - Move Storyboardable Protocol to PlatformUIKit
public static func make(with viewModel: IntroductionSheetViewModel) -> IntroductionSheetViewController {
let storyboard = UIStoryboard(name: String(describing: self), bundle: .module)
guard let controller = storyboard.instantiateInitialViewController() as? IntroductionSheetViewController else {
fatalError("\(String(describing: self)) not found.")
}
controller.viewModel = viewModel
return controller
}
override public func viewDidLoad() {
super.viewDidLoad()
button.setTitle(viewModel.buttonTitle, for: .normal)
button.layer.cornerRadius = 4.0
button.rx.tap.bind { [weak self] _ in
guard let self = self else { return }
self.viewModel.onSelection()
self.dismiss(animated: true, completion: nil)
}
.disposed(by: bag)
titleLabel.text = viewModel.title
subtitleLabel.text = viewModel.description
thumbnail.image = viewModel.thumbnail
applyAccessibility()
}
private func applyAccessibility() {
button.accessibility = .id(AccessibilityIdentifiers.doneButton)
titleLabel.accessibility = .id(AccessibilityIdentifiers.titleLabel)
subtitleLabel.accessibility = .id(AccessibilityIdentifiers.subtitleLabel)
}
}
| lgpl-3.0 | ce1492d4ea5d88440560760af00a9b8f | 35.709091 | 119 | 0.707776 | 5.486413 | false | false | false | false |
adi2004/super-memo2-pod | Example/Pods/ADISuperMemo2/Classes/Card.swift | 2 | 1613 | //
// Card.swift
// Pods
//
// Created by Adrian Florescu on 30/11/2016.
//
//
import Foundation
public class Card {
public let question: String
public let answer: String
public var repetition: Int
public var eFactor: Double
public var repeatOn: Date
var interval: Double
public init(question q:String,
answer a: String,
repetition r: Int = 0,
eFactor e: Double = 2.5) {
self.question = q
self.answer = a
self.repetition = r
self.eFactor = e
self.repeatOn = Date()
self.interval = 0
}
public func update(with assessment:Double) {
if (assessment < 3) {
repetition = 0
// eFactor remains unchanged
interval = 0
repeatOn = Date()
} else {
repetition += 1
if repetition == 1 {
interval = 1
} else if repetition == 2 {
interval = 6
} else {
interval = interval * eFactor
}
repeatOn = Date(timeIntervalSinceNow: interval * 24 * 3600)
eFactor = eFactor - 0.8 + 0.28 * assessment - 0.02 * assessment * assessment
eFactor = setBounds(eFactor)
}
}
private func setBounds(_ element: Double) -> Double {
guard element < 1.3 else { return 1.3 }
guard element > 2.5 else { return 2.5 }
return element
}
public func repetitionInterval() -> Int {
//print(interval)
return Int(interval + 0.5)
}
}
| mit | 556d090a18d2b11c554ba3e66eea798c | 25.016129 | 88 | 0.517049 | 4.543662 | false | false | false | false |
nodes-ios/NStack | Pods/Quick/Sources/Quick/NSString+C99ExtendedIdentifier.swift | 17 | 1517 | #if canImport(Darwin)
import Foundation
extension NSString {
private static var invalidCharacters: CharacterSet = {
var invalidCharacters = CharacterSet()
let invalidCharacterSets: [CharacterSet] = [
.whitespacesAndNewlines,
.illegalCharacters,
.controlCharacters,
.punctuationCharacters,
.nonBaseCharacters,
.symbols
]
for invalidSet in invalidCharacterSets {
invalidCharacters.formUnion(invalidSet)
}
return invalidCharacters
}()
/// This API is not meant to be used outside Quick, so will be unavailable in
/// a next major version.
@objc(qck_c99ExtendedIdentifier)
public var c99ExtendedIdentifier: String {
let validComponents = components(separatedBy: NSString.invalidCharacters)
let result = validComponents.joined(separator: "_")
return result.isEmpty ? "_" : result
}
}
/// Extension methods or properties for NSObject subclasses are invisible from
/// the Objective-C runtime on static linking unless the consumers add `-ObjC`
/// linker flag, so let's make a wrapper class to mitigate that situation.
///
/// See: https://github.com/Quick/Quick/issues/785 and https://github.com/Quick/Quick/pull/803
@objc
class QCKObjCStringUtils: NSObject {
override private init() {}
@objc
static func c99ExtendedIdentifier(from string: String) -> String {
return string.c99ExtendedIdentifier
}
}
#endif
| mit | 437bcda83732c4541e30147a72be25d9 | 29.959184 | 94 | 0.675016 | 4.990132 | false | false | false | false |
lannik/SSImageBrowser | Example/Tests/Tests.swift | 2 | 1190 | // https://github.com/Quick/Quick
import Quick
import Nimble
import SSImageBrowser
class TableOfContentsSpec: QuickSpec {
override func spec() {
describe("these will fail") {
it("can do maths") {
expect(1) == 2
}
it("can read") {
expect("number") == "string"
}
it("will eventually fail") {
expect("time").toEventually( equal("done") )
}
context("these will pass") {
it("can do maths") {
expect(23) == 23
}
it("can read") {
expect("🐮") == "🐮"
}
it("will eventually pass") {
var time = "passing"
dispatch_async(dispatch_get_main_queue()) {
time = "done"
}
waitUntil { done in
NSThread.sleepForTimeInterval(0.5)
expect(time) == "done"
done()
}
}
}
}
}
}
| mit | e265825e2fc654693f8d2267a1e2a55f | 22.215686 | 63 | 0.363176 | 5.53271 | false | false | false | false |
3DprintFIT/octoprint-ios-client | OctoPhoneTests/VIew Related/Slicing Profiles/SlicingProfileCellTests.swift | 1 | 2749 | //
// SlicingProfileCellTests.swift
// OctoPhone
//
// Created by Josef Dolezal on 01/04/2017.
// Copyright © 2017 Josef Dolezal. All rights reserved.
//
import Nimble
import Quick
@testable import OctoPhone
class SlicingProfileCellTests: QuickSpec {
override func spec() {
describe("Slicing profile cell") {
let contextManger = InMemoryContextManager()
var subject: SlicingProfileCellViewModelType!
afterEach {
subject = nil
let realm = try! contextManger.createContext()
try! realm.write{ realm.deleteAll() }
}
context("slicer profile not found") {
beforeEach {
subject = SlicingProfileCellViewModel(profileID: "", contextManager: contextManger)
}
it("provides placeholder text for name") {
expect(subject.outputs.name.value) == "Unknown slicing profile"
}
}
context("slicer profile is in database") {
let profileID = "Profile"
var profile: SlicingProfile!
beforeEach {
profile = SlicingProfile(ID: profileID, name: nil, description: nil, isDefault: true)
let realm = try! contextManger.createContext()
try! realm.write{ realm.add(profile) }
subject = SlicingProfileCellViewModel(profileID: profileID, contextManager: contextManger)
}
afterEach {
profile = nil
}
it("provides placeholder name if profile name is empty") {
expect(subject.outputs.name.value) == "Unknown slicing profile"
}
it("displays profile name if it's not empty") {
let realm = try! contextManger.createContext()
try! realm.write { profile.name = "Slicer name" }
expect(subject.outputs.name.value).toEventually(equal("Slicer name"))
}
it("reacts to profile changes") {
let realm = try! contextManger.createContext()
try! realm.write { profile.name = "Change 1" }
expect(subject.outputs.name.value).toEventually(equal("Change 1"))
try! realm.write { profile.name = "Change 2" }
expect(subject.outputs.name.value).toEventually(equal("Change 2"))
try! realm.write { profile.name = "Change 3" }
expect(subject.outputs.name.value).toEventually(equal("Change 3"))
}
}
}
}
}
| mit | 79ff5099bdd07d62c861df432e5dbd1e | 32.925926 | 110 | 0.534934 | 5.346304 | false | false | false | false |
jshultz/ios9-swift2-core-data-demo | Core Data Demo/ViewController.swift | 1 | 3880 | //
// ViewController.swift
// Core Data Demo
//
// Created by Jason Shultz on 10/3/15.
// Copyright © 2015 HashRocket. All rights reserved.
//
import UIKit
import CoreData
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
// create an app delegate variable
let appDel: AppDelegate = UIApplication.sharedApplication().delegate as! AppDelegate
// context is a handler for us to be able to access the database. this allows us to access the CoreData database.
let context: NSManagedObjectContext = appDel.managedObjectContext
// we are describing the Entity we want to insert the new user into. We are doing it for Entity Name Users. Then we tell it the context we want to insert it into, which we described previously.
let newUser = NSEntityDescription.insertNewObjectForEntityForName("Users", inManagedObjectContext: context)
// set the values of the attributes for the newUser we are wanting to set.
newUser.setValue("Steve", forKey: "username")
newUser.setValue("rogers123", forKey: "password")
do {
// save the context.
try context.save()
} catch {
print("There was a problem")
}
// now we are requesting data from the Users Entity.
let request = NSFetchRequest(entityName: "Users")
// if we want to search for something in particular we can use predicates:
request.predicate = NSPredicate(format: "username = %@", "Steve") // search for users where username = Steve
// by default, if we do a request and get some data back it returns false for the actual data. if we want to get data back and see it, then we need to set this as false.
request.returnsObjectsAsFaults = false
do {
// save the results of our fetch request to a variable.
let results = try context.executeFetchRequest(request)
print(results)
if (results.count > 0) {
for result in results as! [NSManagedObject] {
// delete the object that we found.
// context.deleteObject(result)
do {
try context.save() // you have to save after deleting (or anything else) otherwise it won't stick.
} catch {
print("something went wrong")
}
if let username = result.valueForKey("username") as? String { // cast username as String so we can use it.
print(username)
}
if let password = result.valueForKey("password") as? String { // cast password as String so we can use it.
print(password)
// this lets us change a value of the thing we just found and change it to something else.
// result.setValue("something", forKey: "password") // change the password to something else.
// do {
// try context.save()
// } catch {
// print("something went wrong")
// }
}
}
}
} catch {
print("There was a problem")
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
| mit | 34b4b0f6f5c4a21d4ec10f5e481881e3 | 37.79 | 201 | 0.541377 | 5.679356 | false | false | false | false |
aipeople/PokeIV | PokeIV/PopoverTemplateView.swift | 1 | 4433 | //
// PopoverTemplateView.swift
// PokeIV
//
// Created by aipeople on 8/15/16.
// Github: https://github.com/aipeople/PokeIV
// Copyright © 2016 su. All rights reserved.
//
import UIKit
import Cartography
typealias PopoverViewSelectionCallBack = (PopoverTemplateView, selectedIndex: Int) -> ()
class PopoverTemplateView : UIView {
// MARK: - Properties
let bottomBar = UIView()
var buttonData = [BorderButtonData]() {didSet{self.setupButtons()}}
private(set) var buttons = [UIButton]()
var containerEdgesGroup: ConstraintGroup!
private weak var firstButtonLeftConstraint: NSLayoutConstraint?
private weak var lastButtonLeftConstraint: NSLayoutConstraint?
// MARK: - Data
var selectCallback: PopoverViewSelectionCallBack?
// MARK: - Life Cycle
init() {
super.init(frame: CGRect.zero)
self.setup()
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
func setup() {
// Setup Constraints
self.addSubview(self.bottomBar)
constrain(self.bottomBar) { (bar) -> () in
bar.left == bar.superview!.left
bar.right == bar.superview!.right
bar.bottom == bar.superview!.bottom
bar.height == 54
}
// Setup Constraints
self.backgroundColor = UIColor(white: 0.9, alpha: 1.0)
self.layer.cornerRadius = 4
self.layer.masksToBounds = true
self.bottomBar.backgroundColor = UIColor(white: 0.9, alpha: 1.0).colorWithAlphaComponent(0.95)
}
override func layoutSubviews() {
super.layoutSubviews()
self.layoutIfNeeded()
let buttonNum = self.buttons.count
let buttonsWidth = 150 * buttonNum + 10 * (buttonNum - 1)
let spacing = (self.frame.width - CGFloat(buttonsWidth)) * 0.5
if spacing > 10 {
self.firstButtonLeftConstraint?.constant = spacing
self.lastButtonLeftConstraint?.constant = -spacing
}
}
// MARK: - UI Methods
func setupButtons() {
// Remove origin buttons
for button in self.buttons {
button.removeFromSuperview()
}
self.buttons.removeAll()
// Setup new buttons
var lastView: UIView?
for data in self.buttonData {
let button = UIButton.borderButtonWithTitle(data.title, titleColor: data.titleColor)
button.layer.borderColor = data.borderColor.CGColor
button.addTarget(self, action: #selector(PopoverTemplateView.handleButtonOnTap(_:)), forControlEvents: .TouchUpInside)
self.buttons.append(button)
self.bottomBar.addSubview(button)
if let lastView = lastView {
constrain(button, lastView, block: { (button, view) -> () in
button.height == 34
button.width == view.width ~ 750
button.centerY == button.superview!.centerY
button.left == view.right + 10
})
} else {
constrain(button, block: { (button) -> () in
button.height == 34
button.width <= 150
button.centerY == button.superview!.centerY
button.centerX == button.superview!.centerX ~ 750
self.firstButtonLeftConstraint =
button.left == button.superview!.left + 10 ~ 750
})
}
lastView = button
}
if let lastView = lastView {
constrain(lastView, block: { (view) -> () in
self.lastButtonLeftConstraint =
view.right == view.superview!.right - 10 ~ 750
})
}
}
// MARK: - Events
func handleButtonOnTap(sender: UIButton) {
if let index = self.buttons.indexOf(sender) {
if let callback = self.selectCallback {
callback(self, selectedIndex: index)
} else {
self.popoverView?.dismiss()
}
}
}
}
| gpl-3.0 | b5374a5afc33cafb26a3d43bb2df97db | 28.546667 | 130 | 0.536327 | 5.105991 | false | false | false | false |
Ryce/flickrpickr | Carthage/Checkouts/judokit/Source/DateInputField.swift | 2 | 12572 | //
// DateTextField.swift
// JudoKit
//
// Copyright (c) 2016 Alternative Payments Ltd
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
import UIKit
fileprivate func < <T : Comparable>(lhs: T?, rhs: T?) -> Bool {
switch (lhs, rhs) {
case let (l?, r?):
return l < r
case (nil, _?):
return true
default:
return false
}
}
fileprivate func > <T : Comparable>(lhs: T?, rhs: T?) -> Bool {
switch (lhs, rhs) {
case let (l?, r?):
return l > r
default:
return rhs < lhs
}
}
fileprivate func <= <T : Comparable>(lhs: T?, rhs: T?) -> Bool {
switch (lhs, rhs) {
case let (l?, r?):
return l <= r
default:
return !(rhs < lhs)
}
}
/**
The DateTextField allows two different modes of input.
- Picker: Use a custom UIPickerView with month and year fields
- Text: Use a common Numpad Keyboard as text input method
*/
public enum DateInputType {
/// DateInputTypePicker using a UIPicker as an input method
case picker
/// DateInputTypeText using a Keyboard as an input method
case text
}
/**
The DateInputField is an input field configured to detect, validate and dates that are set to define a start or end date of various types of credit cards.
*/
open class DateInputField: JudoPayInputField {
/// The datePicker showing months and years to pick from for expiry or start date input
let datePicker = UIPickerView()
/// The date formatter that shows the date in the same way it is written on a credit card
fileprivate let dateFormatter: DateFormatter = {
let formatter = DateFormatter()
formatter.dateFormat = "MM/yy"
return formatter
}()
/// The current year on a Gregorian calendar
fileprivate let currentYear = (Calendar.current as NSCalendar).component(.year, from: Date())
/// The current month on a Gregorian calendar
fileprivate let currentMonth = (Calendar.current as NSCalendar).component(.month, from: Date())
/// Boolean stating whether input field should identify as a start or end date
open var isStartDate: Bool = false {
didSet {
self.textField.attributedPlaceholder = NSAttributedString(string: self.title(), attributes: [NSForegroundColorAttributeName:self.theme.getPlaceholderTextColor()])
}
}
/// Boolean stating whether input field should identify as a start or end date
open var isVisible: Bool = false
/// Variable defining the input type (text or picker)
open var dateInputType: DateInputType = .text {
didSet {
switch dateInputType {
case .picker:
self.textField.inputView = self.datePicker
let month = NSString(format: "%02i", currentMonth)
let year = NSString(format: "%02i", currentYear - 2000) // FIXME: not quite happy with this, must be a better way
self.textField.text = "\(month)/\(year)"
self.datePicker.selectRow(currentMonth - 1, inComponent: 0, animated: false)
break
case .text:
self.textField.inputView = nil
self.textField.keyboardType = .numberPad
}
}
}
// MARK: Initializers
/**
Setup the view
*/
override func setupView() {
super.setupView()
// Input method should be via date picker
self.datePicker.delegate = self
self.datePicker.dataSource = self
if self.dateInputType == .picker {
self.textField.inputView = self.datePicker
let month = NSString(format: "%02i", currentMonth)
let year = NSString(format: "%02i", currentYear - 2000)
self.textField.text = "\(month)/\(year)"
self.datePicker.selectRow(currentMonth - 1, inComponent: 0, animated: false)
}
}
// MARK: UITextFieldDelegate
/**
Delegate method implementation
- parameter textField: Text field
- parameter range: Range
- parameter string: String
- returns: boolean to change characters in given range for a textfield
*/
open func textField(_ textField: UITextField, shouldChangeCharactersInRange range: NSRange, replacementString string: String) -> Bool {
// Only handle calls if textinput is selected
guard self.dateInputType == .text else { return true }
// Only handle delegate calls for own textfield
guard textField == self.textField else { return false }
// Get old and new text
let newString = (textField.text! as NSString).replacingCharacters(in: range, with: string)
if newString.characters.count == 0 {
return true
} else if newString.characters.count == 1 {
return newString == "0" || newString == "1"
} else if newString.characters.count == 2 {
// if deletion is handled and user is trying to delete the month no slash should be added
guard string.characters.count > 0 else {
return true
}
guard Int(newString) > 0 && Int(newString) <= 12 else {
return false
}
self.textField.text = newString + "/"
return false
} else if newString.characters.count == 3 {
return newString.characters.last == "/"
} else if newString.characters.count == 4 {
// FIXME: need to make sure that number is numeric
let deciYear = Int((Double((Calendar.current as NSCalendar).component(.year, from: Date()) - 2000) / 10.0))
let lastChar = Int(String(newString.characters.last!))
if self.isStartDate {
return lastChar == deciYear || lastChar == deciYear - 1
} else {
return lastChar == deciYear || lastChar == deciYear + 1
}
} else if newString.characters.count == 5 {
return true
} else {
self.delegate?.dateInput(self, error: JudoError(.inputLengthMismatchError))
return false
}
}
// MARK: Custom methods
/**
Check if this inputField is valid
- returns: true if valid input
*/
open override func isValid() -> Bool {
guard let dateString = textField.text , dateString.characters.count == 5,
let beginningOfMonthDate = self.dateFormatter.date(from: dateString) else { return false }
if self.isStartDate {
let minimumDate = Date().dateByAddingYears(-10)
return beginningOfMonthDate.compare(Date()) == .orderedAscending && beginningOfMonthDate.compare(minimumDate!) == .orderedDescending
} else {
let endOfMonthDate = beginningOfMonthDate.dateAtTheEndOfMonth()
let maximumDate = Date().dateByAddingYears(10)
return endOfMonthDate.compare(Date()) == .orderedDescending && endOfMonthDate.compare(maximumDate!) == .orderedAscending
}
}
/**
Subclassed method that is called when textField content was changed
- parameter textField: the textfield of which the content has changed
*/
open override func textFieldDidChangeValue(_ textField: UITextField) {
super.textFieldDidChangeValue(textField)
self.didChangeInputText()
guard let text = textField.text , text.characters.count == 5 else { return }
if self.dateFormatter.date(from: text) == nil { return }
if self.isValid() {
self.delegate?.dateInput(self, didFindValidDate: textField.text!)
} else {
var errorMessage = "Check expiry date"
if self.isStartDate {
errorMessage = "Check start date"
}
self.delegate?.dateInput(self, error: JudoError(.invalidEntry, errorMessage))
}
}
/**
The placeholder string for the current inputField
- returns: an Attributed String that is the placeholder of the receiver
*/
open override func placeholder() -> NSAttributedString? {
return NSAttributedString(string: self.title(), attributes: [NSForegroundColorAttributeName:self.theme.getPlaceholderTextColor()])
}
/**
Title of the receiver inputField
- returns: a string that is the title of the receiver
*/
open override func title() -> String {
return isStartDate ? "Start date" : "Expiry date"
}
/**
Hint label text
- returns: string that is shown as a hint when user resides in a inputField for more than 5 seconds
*/
open override func hintLabelText() -> String {
return "MM/YY"
}
}
// MARK: UIPickerViewDataSource
extension DateInputField: UIPickerViewDataSource {
/**
Datasource method for datePickerView
- parameter pickerView: PickerView that calls its delegate
- returns: The number of components in the pickerView
*/
public func numberOfComponents(in pickerView: UIPickerView) -> Int {
return 2
}
/**
Datasource method for datePickerView
- parameter pickerView: PickerView that calls its delegate
- parameter component: A given component
- returns: number of rows in component
*/
public func pickerView(_ pickerView: UIPickerView, numberOfRowsInComponent component: Int) -> Int {
return component == 0 ? 12 : 11
}
}
// MARK: UIPickerViewDelegate
extension DateInputField: UIPickerViewDelegate {
/**
Delegate method for datePickerView
- parameter pickerView: The caller
- parameter row: The row
- parameter component: The component
- returns: content of a given component and row
*/
public func pickerView(_ pickerView: UIPickerView, titleForRow row: Int, forComponent component: Int) -> String? {
switch component {
case 0:
return NSString(format: "%02i", row + 1) as String
case 1:
return NSString(format: "%02i", (self.isStartDate ? currentYear - row : currentYear + row) - 2000) as String
default:
return nil
}
}
/**
Delegate method for datePickerView that had a given row in a component selected
- parameter pickerView: The caller
- parameter row: The row
- parameter component: The component
*/
public func pickerView(_ pickerView: UIPickerView, didSelectRow row: Int, inComponent component: Int) {
// Need to use NSString because Precision String Format Specifier is easier this way
if component == 0 {
let month = NSString(format: "%02i", row + 1)
let oldDateString = self.textField.text!
let year = oldDateString.substring(from: oldDateString.characters.index(oldDateString.endIndex, offsetBy: -2))
self.textField.text = "\(month)/\(year)"
} else if component == 1 {
let oldDateString = self.textField.text!
let month = oldDateString.substring(to: oldDateString.characters.index(oldDateString.startIndex, offsetBy: 2))
let year = NSString(format: "%02i", (self.isStartDate ? currentYear - row : currentYear + row) - 2000)
self.textField.text = "\(month)/\(year)"
}
}
}
| mit | 6a1fc24279b65502a48bcbc6645e8c73 | 33.538462 | 174 | 0.624324 | 4.99682 | false | false | false | false |
quire-io/SwiftyChrono | Sources/Utils/Util.swift | 1 | 2961 | //
// Util.swift
// SwiftyChrono
//
// Created by Jerry Chen on 1/18/17.
// Copyright © 2017 Potix. All rights reserved.
//
import Foundation
let HALF = Int.min
let HALF_SECOND_IN_MS = millisecondsToNanoSeconds(500) // unit: nanosecond
/// get ascending order from two number.
/// ATTENSION:
func sortTwoNumbers(_ index1: Int, _ index2: Int) -> (lessNumber: Int, greaterNumber: Int) {
if index1 == index2 {
return (index1, index2)
}
let lessNumber = index1 < index2 ? index1 : index2
let greaterNumber = index1 > index2 ? index1 : index2
return (lessNumber, greaterNumber)
}
extension NSTextCheckingResult {
func isNotEmpty(atRangeIndex index: Int) -> Bool {
return range(at: index).length != 0
}
func isEmpty(atRangeIndex index: Int) -> Bool {
return range(at: index).length == 0
}
func string(from text: String, atRangeIndex index: Int) -> String {
return text.subString(with: range(at: index))
}
}
extension String {
var firstString: String? {
return substring(from: 0, to: 1)
}
func subString(with range: NSRange) -> String {
return (self as NSString).substring(with: range)
}
func substring(from idx: Int) -> String {
return String(self[index(startIndex, offsetBy: idx)...])
}
func substring(from startIdx: Int, to endIdx: Int? = nil) -> String {
if startIdx < 0 || (endIdx != nil && endIdx! < 0) {
return ""
}
let start = index(startIndex, offsetBy: startIdx)
let end = endIdx != nil ? index(startIndex, offsetBy: endIdx!) : endIndex
return String(self[start..<end])
}
func range(ofStartIndex idx: Int, length: Int) -> Range<String.Index> {
let startIndex0 = index(startIndex, offsetBy: idx)
let endIndex0 = index(startIndex, offsetBy: idx + length)
return Range(uncheckedBounds: (lower: startIndex0, upper: endIndex0))
}
func range(ofStartIndex startIdx: Int, andEndIndex endIdx: Int) -> Range<String.Index> {
let startIndex0 = index(startIndex, offsetBy: startIdx)
let endIndex0 = index(startIndex, offsetBy: endIdx)
return Range(uncheckedBounds: (lower: startIndex0, upper: endIndex0))
}
func trimmed() -> String {
return trimmingCharacters(in: .whitespacesAndNewlines)
}
}
extension NSRegularExpression {
static func isMatch(forPattern pattern: String, in text: String) -> Bool {
return (try? NSRegularExpression(pattern: pattern, options: .caseInsensitive))?.firstMatch(in: text, range: NSRange(location: 0, length: text.count)) != nil
}
}
extension Dictionary {
mutating func merge(with dictionary: Dictionary) {
dictionary.forEach { updateValue($1, forKey: $0) }
}
func merged(with dictionary: Dictionary) -> Dictionary {
var dict = self
dict.merge(with: dictionary)
return dict
}
}
| mit | d9427231ca96507d77d3c25895561892 | 29.833333 | 164 | 0.641892 | 4.016282 | false | false | false | false |
anicolaspp/rate-my-meetings-ios | Pods/CVCalendar/CVCalendar/CVCalendarMonthContentViewController.swift | 3 | 16595 | //
// CVCalendarMonthContentViewController.swift
// CVCalendar Demo
//
// Created by Eugene Mozharovsky on 12/04/15.
// Copyright (c) 2015 GameApp. All rights reserved.
//
import UIKit
public final class CVCalendarMonthContentViewController: CVCalendarContentViewController {
private var monthViews: [Identifier : MonthView]
public override init(calendarView: CalendarView, frame: CGRect) {
monthViews = [Identifier : MonthView]()
super.init(calendarView: calendarView, frame: frame)
initialLoad(presentedMonthView.date)
}
public init(calendarView: CalendarView, frame: CGRect, presentedDate: NSDate) {
monthViews = [Identifier : MonthView]()
super.init(calendarView: calendarView, frame: frame)
presentedMonthView = MonthView(calendarView: calendarView, date: presentedDate)
presentedMonthView.updateAppearance(scrollView.bounds)
initialLoad(presentedDate)
}
public required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
// MARK: - Load & Reload
public func initialLoad(date: NSDate) {
insertMonthView(getPreviousMonth(date), withIdentifier: Previous)
insertMonthView(presentedMonthView, withIdentifier: Presented)
insertMonthView(getFollowingMonth(date), withIdentifier: Following)
presentedMonthView.mapDayViews { dayView in
if self.calendarView.shouldAutoSelectDayOnMonthChange && self.matchedDays(dayView.date, Date(date: date)) {
self.calendarView.coordinator.flush()
self.calendarView.touchController.receiveTouchOnDayView(dayView)
dayView.circleView?.removeFromSuperview()
}
}
calendarView.presentedDate = CVDate(date: presentedMonthView.date)
}
public func reloadMonthViews() {
for (identifier, monthView) in monthViews {
monthView.frame.origin.x = CGFloat(indexOfIdentifier(identifier)) * scrollView.frame.width
monthView.removeFromSuperview()
scrollView.addSubview(monthView)
}
}
// MARK: - Insertion
public func insertMonthView(monthView: MonthView, withIdentifier identifier: Identifier) {
let index = CGFloat(indexOfIdentifier(identifier))
monthView.frame.origin = CGPointMake(scrollView.bounds.width * index, 0)
monthViews[identifier] = monthView
scrollView.addSubview(monthView)
}
public func replaceMonthView(monthView: MonthView, withIdentifier identifier: Identifier, animatable: Bool) {
var monthViewFrame = monthView.frame
monthViewFrame.origin.x = monthViewFrame.width * CGFloat(indexOfIdentifier(identifier))
monthView.frame = monthViewFrame
monthViews[identifier] = monthView
if animatable {
scrollView.scrollRectToVisible(monthViewFrame, animated: false)
}
}
// MARK: - Load management
public func scrolledLeft() {
if let presented = monthViews[Presented], let following = monthViews[Following] {
if pageLoadingEnabled {
pageLoadingEnabled = false
monthViews[Previous]?.removeFromSuperview()
replaceMonthView(presented, withIdentifier: Previous, animatable: false)
replaceMonthView(following, withIdentifier: Presented, animatable: true)
insertMonthView(getFollowingMonth(following.date), withIdentifier: Following)
self.calendarView.delegate?.didShowNextMonthView?(following.date)
}
}
}
public func scrolledRight() {
if let previous = monthViews[Previous], let presented = monthViews[Presented] {
if pageLoadingEnabled {
pageLoadingEnabled = false
monthViews[Following]?.removeFromSuperview()
replaceMonthView(previous, withIdentifier: Presented, animatable: true)
replaceMonthView(presented, withIdentifier: Following, animatable: false)
insertMonthView(getPreviousMonth(previous.date), withIdentifier: Previous)
self.calendarView.delegate?.didShowPreviousMonthView?(previous.date)
}
}
}
// MARK: - Override methods
public override func updateFrames(rect: CGRect) {
super.updateFrames(rect)
for monthView in monthViews.values {
monthView.reloadViewsWithRect(rect != CGRectZero ? rect : scrollView.bounds)
}
reloadMonthViews()
if let presented = monthViews[Presented] {
if scrollView.frame.height != presented.potentialSize.height {
updateHeight(presented.potentialSize.height, animated: false)
}
scrollView.scrollRectToVisible(presented.frame, animated: false)
}
}
public override func performedDayViewSelection(dayView: DayView) {
if dayView.isOut && calendarView.shouldScrollOnOutDayViewSelection {
if dayView.date.day > 20 {
let presentedDate = dayView.monthView.date
calendarView.presentedDate = Date(date: self.dateBeforeDate(presentedDate))
presentPreviousView(dayView)
} else {
let presentedDate = dayView.monthView.date
calendarView.presentedDate = Date(date: self.dateAfterDate(presentedDate))
presentNextView(dayView)
}
}
}
public override func presentPreviousView(view: UIView?) {
if presentationEnabled {
presentationEnabled = false
if let extra = monthViews[Following], let presented = monthViews[Presented], let previous = monthViews[Previous] {
UIView.animateWithDuration(0.5, delay: 0, options: UIViewAnimationOptions.CurveEaseInOut, animations: {
self.prepareTopMarkersOnMonthView(presented, hidden: true)
extra.frame.origin.x += self.scrollView.frame.width
presented.frame.origin.x += self.scrollView.frame.width
previous.frame.origin.x += self.scrollView.frame.width
self.replaceMonthView(presented, withIdentifier: self.Following, animatable: false)
self.replaceMonthView(previous, withIdentifier: self.Presented, animatable: false)
self.presentedMonthView = previous
self.updateLayoutIfNeeded()
}) { _ in
extra.removeFromSuperview()
self.insertMonthView(self.getPreviousMonth(previous.date), withIdentifier: self.Previous)
self.updateSelection()
self.presentationEnabled = true
for monthView in self.monthViews.values {
self.prepareTopMarkersOnMonthView(monthView, hidden: false)
}
}
}
}
}
public override func presentNextView(view: UIView?) {
if presentationEnabled {
presentationEnabled = false
if let extra = monthViews[Previous], let presented = monthViews[Presented], let following = monthViews[Following] {
UIView.animateWithDuration(0.5, delay: 0, options: UIViewAnimationOptions.CurveEaseInOut, animations: {
self.prepareTopMarkersOnMonthView(presented, hidden: true)
extra.frame.origin.x -= self.scrollView.frame.width
presented.frame.origin.x -= self.scrollView.frame.width
following.frame.origin.x -= self.scrollView.frame.width
self.replaceMonthView(presented, withIdentifier: self.Previous, animatable: false)
self.replaceMonthView(following, withIdentifier: self.Presented, animatable: false)
self.presentedMonthView = following
self.updateLayoutIfNeeded()
}) { _ in
extra.removeFromSuperview()
self.insertMonthView(self.getFollowingMonth(following.date), withIdentifier: self.Following)
self.updateSelection()
self.presentationEnabled = true
for monthView in self.monthViews.values {
self.prepareTopMarkersOnMonthView(monthView, hidden: false)
}
}
}
}
}
public override func updateDayViews(hidden: Bool) {
setDayOutViewsVisible(hidden)
}
private var togglingBlocked = false
public override func togglePresentedDate(date: NSDate) {
let presentedDate = Date(date: date)
if let presented = monthViews[Presented], let selectedDate = calendarView.coordinator.selectedDayView?.date {
if !matchedDays(selectedDate, presentedDate) && !togglingBlocked {
if !matchedMonths(presentedDate, selectedDate) {
togglingBlocked = true
monthViews[Previous]?.removeFromSuperview()
monthViews[Following]?.removeFromSuperview()
insertMonthView(getPreviousMonth(date), withIdentifier: Previous)
insertMonthView(getFollowingMonth(date), withIdentifier: Following)
let currentMonthView = MonthView(calendarView: calendarView, date: date)
currentMonthView.updateAppearance(scrollView.bounds)
currentMonthView.alpha = 0
insertMonthView(currentMonthView, withIdentifier: Presented)
presentedMonthView = currentMonthView
calendarView.presentedDate = Date(date: date)
UIView.animateWithDuration(0.8, delay: 0, options: UIViewAnimationOptions.CurveEaseInOut, animations: {
presented.alpha = 0
currentMonthView.alpha = 1
}) { _ in
presented.removeFromSuperview()
self.selectDayViewWithDay(presentedDate.day, inMonthView: currentMonthView)
self.togglingBlocked = false
self.updateLayoutIfNeeded()
}
} else {
if let currentMonthView = monthViews[Presented] {
selectDayViewWithDay(presentedDate.day, inMonthView: currentMonthView)
}
}
}
}
}
}
// MARK: - Month management
extension CVCalendarMonthContentViewController {
public func getFollowingMonth(date: NSDate) -> MonthView {
let firstDate = calendarView.manager.monthDateRange(date).monthStartDate
let components = Manager.componentsForDate(firstDate)
components.month += 1
let newDate = NSCalendar.currentCalendar().dateFromComponents(components)!
let frame = scrollView.bounds
let monthView = MonthView(calendarView: calendarView, date: newDate)
monthView.updateAppearance(frame)
return monthView
}
public func getPreviousMonth(date: NSDate) -> MonthView {
let firstDate = calendarView.manager.monthDateRange(date).monthStartDate
let components = Manager.componentsForDate(firstDate)
components.month -= 1
let newDate = NSCalendar.currentCalendar().dateFromComponents(components)!
let frame = scrollView.bounds
let monthView = MonthView(calendarView: calendarView, date: newDate)
monthView.updateAppearance(frame)
return monthView
}
}
// MARK: - Visual preparation
extension CVCalendarMonthContentViewController {
public func prepareTopMarkersOnMonthView(monthView: MonthView, hidden: Bool) {
monthView.mapDayViews { dayView in
dayView.topMarker?.hidden = hidden
}
}
public func setDayOutViewsVisible(visible: Bool) {
for monthView in monthViews.values {
monthView.mapDayViews { dayView in
if dayView.isOut {
if !visible {
dayView.alpha = 0
dayView.hidden = false
}
UIView.animateWithDuration(0.5, delay: 0, options: UIViewAnimationOptions.CurveEaseInOut, animations: {
dayView.alpha = visible ? 0 : 1
}) { _ in
if visible {
dayView.alpha = 1
dayView.hidden = true
dayView.userInteractionEnabled = false
} else {
dayView.userInteractionEnabled = true
}
}
}
}
}
}
public func updateSelection() {
let coordinator = calendarView.coordinator
if let selected = coordinator.selectedDayView {
for (index, monthView) in monthViews {
if indexOfIdentifier(index) != 1 {
monthView.mapDayViews {
dayView in
if dayView == selected {
dayView.setDeselectedWithClearing(true)
coordinator.dequeueDayView(dayView)
}
}
}
}
}
if let presentedMonthView = monthViews[Presented] {
self.presentedMonthView = presentedMonthView
calendarView.presentedDate = Date(date: presentedMonthView.date)
if let selected = coordinator.selectedDayView, let selectedMonthView = selected.monthView where !matchedMonths(Date(date: selectedMonthView.date), Date(date: presentedMonthView.date)) && calendarView.shouldAutoSelectDayOnMonthChange {
let current = Date(date: NSDate())
let presented = Date(date: presentedMonthView.date)
if matchedMonths(current, presented) {
selectDayViewWithDay(current.day, inMonthView: presentedMonthView)
} else {
selectDayViewWithDay(Date(date: calendarView.manager.monthDateRange(presentedMonthView.date).monthStartDate).day, inMonthView: presentedMonthView)
}
}
}
}
public func selectDayViewWithDay(day: Int, inMonthView monthView: CVCalendarMonthView) {
let coordinator = calendarView.coordinator
monthView.mapDayViews { dayView in
if dayView.date.day == day && !dayView.isOut {
if let selected = coordinator.selectedDayView where selected != dayView {
self.calendarView.didSelectDayView(dayView)
}
coordinator.performDayViewSingleSelection(dayView)
}
}
}
}
// MARK: - UIScrollViewDelegate
extension CVCalendarMonthContentViewController {
public func scrollViewDidScroll(scrollView: UIScrollView) {
if scrollView.contentOffset.y != 0 {
scrollView.contentOffset = CGPointMake(scrollView.contentOffset.x, 0)
}
let page = Int(floor((scrollView.contentOffset.x - scrollView.frame.width / 2) / scrollView.frame.width) + 1)
if currentPage != page {
currentPage = page
}
lastContentOffset = scrollView.contentOffset.x
}
public func scrollViewWillBeginDragging(scrollView: UIScrollView) {
if let presented = monthViews[Presented] {
prepareTopMarkersOnMonthView(presented, hidden: true)
}
}
public func scrollViewDidEndDecelerating(scrollView: UIScrollView) {
if pageChanged {
switch direction {
case .Left: scrolledLeft()
case .Right: scrolledRight()
default: break
}
}
updateSelection()
updateLayoutIfNeeded()
pageLoadingEnabled = true
direction = .None
}
public func scrollViewDidEndDragging(scrollView: UIScrollView, willDecelerate decelerate: Bool) {
if decelerate {
let rightBorder = scrollView.frame.width
if scrollView.contentOffset.x <= rightBorder {
direction = .Right
} else {
direction = .Left
}
}
for monthView in monthViews.values {
prepareTopMarkersOnMonthView(monthView, hidden: false)
}
}
}
| mit | c5e3240b5a199ce85260c9826268996f | 38.418052 | 246 | 0.612956 | 6.187547 | false | false | false | false |
esttorhe/RxSwift | RxSwift/RxSwift/DataStructures/Queue.swift | 1 | 3173 | //
// Queue.swift
// Rx
//
// Created by Krunoslav Zaher on 3/21/15.
// Copyright (c) 2015 Krunoslav Zaher. All rights reserved.
//
import Foundation
public struct Queue<T>: SequenceType {
typealias Generator = AnyGenerator<T>
let resizeFactor = 2
private var storage: [T?]
private var _count: Int
private var pushNextIndex: Int
private var initialCapacity: Int
private var version: Int
public init(capacity: Int) {
initialCapacity = capacity
version = 0
storage = []
_count = 0
pushNextIndex = 0
resizeTo(capacity)
}
private var dequeueIndex: Int {
get {
let index = pushNextIndex - count
return index < 0 ? index + self.storage.count : index
}
}
public var empty: Bool {
get {
return count == 0
}
}
public var count: Int {
get {
return _count
}
}
public func peek() -> T {
contract(count > 0)
return storage[dequeueIndex]!
}
mutating private func resizeTo(size: Int) {
var newStorage: [T?] = []
newStorage.reserveCapacity(size)
let count = _count
for var i = 0; i < count; ++i {
// does swift array have some more efficient methods of copying?
newStorage.append(dequeue())
}
while newStorage.count < size {
newStorage.append(nil)
}
_count = count
pushNextIndex = count
storage = newStorage
}
public mutating func enqueue(item: T) {
version++
_ = count == storage.count
if count == storage.count {
resizeTo(storage.count * resizeFactor)
}
storage[pushNextIndex] = item
pushNextIndex++
_count = _count + 1
if pushNextIndex >= storage.count {
pushNextIndex -= storage.count
}
}
public mutating func dequeue() -> T {
version++
contract(count > 0)
let index = dequeueIndex
let value = storage[index]!
storage[index] = nil
_count = _count - 1
let downsizeLimit = storage.count / (resizeFactor * resizeFactor)
if _count < downsizeLimit && downsizeLimit >= initialCapacity {
resizeTo(storage.count / resizeFactor)
}
return value
}
public func generate() -> Generator {
var i = dequeueIndex
var count = _count
let lastVersion = version
return anyGenerator {
if lastVersion != self.version {
rxFatalError("Collection was modified while enumerated")
}
if count == 0 {
return nil
}
count--
if i >= self.storage.count {
i -= self.storage.count
}
return self.storage[i++]
}
}
} | mit | 609fc5b849f8fabef6dfe9d03e684aac | 22 | 76 | 0.493539 | 5.314908 | false | false | false | false |
ra1028/KenBurnsSlideshowView | KenBurnsSlideshowView-Demo/KenBurnsSlideshowView-Demo/ViewController.swift | 1 | 1267 | //
// ViewController.swift
// KenBurnsSlideshowView-Demo
//
// Created by Ryo Aoyama on 12/1/14.
// Copyright (c) 2014 Ryo Aoyama. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var kenBurnsView: KenBurnsView!
@IBOutlet weak var bottomConstraint: NSLayoutConstraint!
override func viewDidLoad() {
super.viewDidLoad()
let longPress = UILongPressGestureRecognizer(target: self, action: "showWholeImage:")
longPress.minimumPressDuration = 0.2
self.kenBurnsView.addGestureRecognizer(longPress)
}
func showWholeImage(sender: UIGestureRecognizer) {
switch sender.state {
case .Began:
self.kenBurnsView.showWholeImage()
case .Cancelled:
fallthrough
case .Ended:
self.kenBurnsView.zoomImageAndRestartMotion()
default:
break
}
}
@IBAction func buttonHandler(sender: UIButton) {
var imageName = "SampleImage"
imageName += sender.titleLabel!.text! + ".jpg"
self.kenBurnsView.image = UIImage(named: imageName)
}
@IBAction func removeButtonHandler(sender: UIButton) {
self.kenBurnsView.image = nil
}
}
| mit | c7e5038ba64ff0f044c0b69294f2b3bd | 26.543478 | 93 | 0.64562 | 4.781132 | false | false | false | false |
qvacua/vimr | VimR/VimR/CoreDataStack.swift | 1 | 3318 | /**
* Tae Won Ha - http://taewon.de - @hataewon
* See LICENSE
*/
import Commons
import CoreData
import Foundation
import os
final class CoreDataStack {
enum Error: Swift.Error {
case noCacheFolder
case pathDoesNotExit
case pathNotFolder
case unableToComplete(Swift.Error)
}
enum StoreLocation {
case temp(String)
case cache(String)
case path(String)
}
let container: NSPersistentContainer
let storeFile: URL
var storeLocation: URL { self.storeFile.parent }
var deleteOnDeinit: Bool
func newBackgroundContext() -> NSManagedObjectContext {
let context = self.container.newBackgroundContext()
context.undoManager = nil
return context
}
init(modelName: String, storeLocation: StoreLocation, deleteOnDeinit: Bool = false) throws {
self.deleteOnDeinit = deleteOnDeinit
self.container = NSPersistentContainer(name: modelName)
let fileManager = FileManager.default
let url: URL
switch storeLocation {
case let .temp(folderName):
let parentUrl = fileManager
.temporaryDirectory
.appendingPathComponent(folderName)
try fileManager.createDirectory(at: parentUrl, withIntermediateDirectories: true)
url = parentUrl.appendingPathComponent(modelName)
case let .cache(folderName):
guard let cacheUrl = fileManager.urls(for: .cachesDirectory, in: .userDomainMask).first else {
throw Error.noCacheFolder
}
let parentUrl = cacheUrl.appendingPathComponent(folderName)
try fileManager.createDirectory(at: parentUrl, withIntermediateDirectories: true)
url = parentUrl.appendingPathComponent(modelName)
case let .path(path):
guard fileManager.fileExists(atPath: path) else { throw Error.pathDoesNotExit }
let parentFolder = URL(fileURLWithPath: path)
guard parentFolder.hasDirectoryPath else { throw Error.pathNotFolder }
url = parentFolder.appendingPathComponent(modelName)
}
self.container.persistentStoreDescriptions = [NSPersistentStoreDescription(url: url)]
self.storeFile = url
self.log.info("Created Core Data store in \(self.storeLocation)")
let condition = ConditionVariable()
var error: Swift.Error?
self.container.loadPersistentStores { _, err in
error = err
condition.broadcast()
}
condition.wait(for: 5)
if let err = error { throw Error.unableToComplete(err) }
self.container.viewContext.undoManager = nil
}
func deleteStore() throws {
guard self.deleteOnDeinit else { return }
guard let store = self.container.persistentStoreCoordinator.persistentStore(
for: self.storeFile
) else { return }
try self.container.persistentStoreCoordinator.remove(store)
let parentFolder = self.storeLocation
let fileManager = FileManager.default
guard fileManager.fileExists(atPath: parentFolder.path) else { return }
try fileManager.removeItem(at: parentFolder)
self.log.info("Deleted store at \(self.storeLocation)")
}
deinit {
guard self.deleteOnDeinit else { return }
do {
try self.deleteStore()
} catch {
self.log.error("Could not delete store at \(self.storeLocation): \(error)")
}
}
private let log = OSLog(subsystem: Defs.loggerSubsystem, category: Defs.LoggerCategory.service)
}
| mit | 048e8553402bb48a09be908f33d6567a | 28.105263 | 100 | 0.717601 | 4.74 | false | false | false | false |
apple/swift | test/Inputs/clang-importer-sdk/swift-modules/CoreGraphics.swift | 1 | 1316 | @_exported import ObjectiveC
@_exported import CoreGraphics
public func == (lhs: CGPoint, rhs: CGPoint) -> Bool {
return lhs.x == rhs.x && lhs.y == rhs.y
}
#if !CGFLOAT_IN_COREFOUNDATION
public struct CGFloat {
#if arch(i386) || arch(arm) || arch(arm64_32) || arch(powerpc)
public typealias UnderlyingType = Float
#elseif arch(x86_64) || arch(arm64) || arch(powerpc64le) || arch(s390x) || arch(riscv64)
public typealias UnderlyingType = Double
#endif
public init() {
self.value = 0.0
}
public init(_ value: Int) {
self.value = UnderlyingType(value)
}
public init(_ value: Float) {
self.value = UnderlyingType(value)
}
public init(_ value: Double) {
self.value = UnderlyingType(value)
}
var value: UnderlyingType
}
public func ==(lhs: CGFloat, rhs: CGFloat) -> Bool {
return lhs.value == rhs.value
}
extension CGFloat : ExpressibleByIntegerLiteral, ExpressibleByFloatLiteral, Equatable {
public init(integerLiteral value: UnderlyingType) {
self.value = value
}
public init(floatLiteral value: UnderlyingType) {
self.value = value
}
}
public extension Double {
init(_ value: CGFloat) {
self = Double(value.value)
}
}
#endif
import CoreFoundation
extension CGFloat: CustomStringConvertible {
public var description: String { "" }
}
| apache-2.0 | c5ac8db41a522d653613dcd41caad730 | 20.933333 | 88 | 0.683891 | 3.728045 | false | false | false | false |
NoodleOfDeath/PastaParser | runtime/swift/GrammarKit/Classes/model/grammar/scanner/GrammaticalScanner.swift | 1 | 5238 | //
// The MIT License (MIT)
//
// Copyright © 2020 NoodleOfDeath. All rights reserved.
// NoodleOfDeath
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
@objc
public protocol GrammaticalScanner: class {
typealias CharacterStream = IO.CharacterStream
typealias TokenStream = IO.TokenStream
typealias Token = Grammar.Token
typealias Metadata = Grammar.Metadata
typealias MatchChain = Grammar.MatchChain
}
/// Specifications for a grammatical scanner delegate.
public protocol GrammaticalScannerDelegate: class {
typealias CharacterStream = IO.CharacterStream
typealias TokenStream = IO.TokenStream
typealias Token = Grammar.Token
typealias Metadata = Grammar.Metadata
typealias MatchChain = Grammar.MatchChain
/// Called when a grammatical scanner skips a token.
///
/// - Parameters:
/// - scanner: that called this method.
/// - token: that was skipped.
/// - characterStream: `scanner` is scanning.
func scanner(_ scanner: GrammaticalScanner, didSkip token: Token, characterStream: CharacterStream)
/// Called when a grammatical scanner generates a match chain.
///
/// - Parameters:
/// - scanner: that called this method.
/// - matchChain: that was generated.
/// - characterStream: `scanner` is scanning.
/// - tokenStream: generated, or being parsed, by `scanner`.
func scanner(_ scanner: GrammaticalScanner, didGenerate matchChain: MatchChain, characterStream: CharacterStream, tokenStream: TokenStream<Token>?)
/// Called when a grammatical scanner finishes a job.
///
/// - Parameters:
/// - scanner: that called this method.
/// - characterStream: `scanner` is scanning.
/// - tokenStream: generated, or parsed, by `scanner`.
func scanner(_ scanner: GrammaticalScanner, didFinishScanning characterStream: CharacterStream, tokenStream: TokenStream<Token>?)
}
/// Base abstract class for a grammar scanning.
open class BaseGrammaticalScanner: NSObject, GrammaticalScanner {
/// Delegate of this grammatical scanner.
open weak var delegate: GrammaticalScannerDelegate?
/// Handler of this grammartical scanner.
open var handler: GrammaticalScannerHandler?
open var didSkip: ((_ scanner: GrammaticalScanner, _ token: Grammar.Token, _ characterStream: IO.CharacterStream) -> ())? {
get { return handler?.didSkip }
set {
if handler == nil { handler = GrammaticalScannerHandler() }
handler?.didSkip = newValue
}
}
open var didGenerate: ((_ scanner: GrammaticalScanner, _ matchChain: Grammar.MatchChain, _ characterStream: IO.CharacterStream, _ tokenStream: IO.TokenStream<Grammar.Token>?) -> ())? {
get { return handler?.didGenerate }
set {
if handler == nil { handler = GrammaticalScannerHandler() }
handler?.didGenerate = newValue
}
}
open var didFinish: ((_ scanner: GrammaticalScanner, _ characterStream: IO.CharacterStream, _ tokenStream: IO.TokenStream<Grammar.Token>?) -> ())? {
get { return handler?.didFinish }
set {
if handler == nil { handler = GrammaticalScannerHandler() }
handler?.didFinish = newValue
}
}
/// Grammar of this scanner.
public let grammar: Grammar
/// Constructs a new grammatical scanner with an initial grammar.
///
/// - Parameters:
/// - grammar: to initialize this scanner with.
public init(grammar: Grammar) {
self.grammar = grammar
}
}
/// Data structure representing a grammatical scanner handler alternative
/// to a `GrammaticalScannerDelegate`.
public struct GrammaticalScannerHandler {
var didSkip: ((_ scanner: GrammaticalScanner, _ token: Grammar.Token, _ characterStream: IO.CharacterStream) -> ())?
var didGenerate: ((_ scanner: GrammaticalScanner, _ matchChain: Grammar.MatchChain, _ characterStream: IO.CharacterStream, IO.TokenStream<Grammar.Token>?) -> ())?
var didFinish: ((_ scanner: GrammaticalScanner, _ characterStream: IO.CharacterStream, _ tokenStream: IO.TokenStream<Grammar.Token>?) -> ())?
}
| mit | b21b7fe1aec2b8884926ce7990803fd7 | 40.23622 | 188 | 0.692572 | 4.987619 | false | false | false | false |
raulriera/HuntingKit | HuntingKit/User.swift | 1 | 2579 | //
// User.swift
// HuntingKit
//
// Created by Raúl Riera on 19/04/2015.
// Copyright (c) 2015 Raul Riera. All rights reserved.
//
import Foundation
public struct User: Model {
public let id: Int
public let name: String
public let headline: String
public let username: String
public let profileURL: NSURL?
public let imagesURL: ImagesURL
public let stats: UserStats?
// public let followers: [User]?
// public let following: [User]?
// public let posts: [Post]?
public init?(dictionary: NSDictionary) {
if
let id = dictionary["id"] as? Int,
let username = dictionary["username"] as? String,
let headline = dictionary["headline"] as? String,
let profileURLString = dictionary["profile_url"] as? String,
let imagesURLDictionary = dictionary["image_url"] as? NSDictionary,
let name = dictionary["name"] as? String {
self.id = id
self.name = name
self.headline = headline
self.username = username
if let profileURL = NSURL(string: profileURLString) {
self.profileURL = profileURL
} else {
profileURL = .None
}
if let stats = UserStats(dictionary: dictionary) {
self.stats = stats
} else {
self.stats = .None
}
imagesURL = ImagesURL(dictionary: imagesURLDictionary)
} else {
return nil
}
}
}
public struct UserStats {
public let votes: Int
public let posts: Int
public let made: Int
public let followers: Int
public let following: Int
public let collections: Int
public init?(dictionary: NSDictionary) {
if
let votes = dictionary["votes_count"] as? Int,
let posts = dictionary["posts_count"] as? Int,
let made = dictionary["maker_of_count"] as? Int,
let followers = dictionary["followers_count"] as? Int,
let following = dictionary["followings_count"] as? Int,
let collections = dictionary["collections_count"] as? Int {
self.votes = votes
self.posts = posts
self.made = made
self.followers = followers
self.following = following
self.collections = collections
} else {
return nil
}
}
} | mit | f50fe2a84e0dc8bc6c4d12220dd66816 | 31.2375 | 79 | 0.540729 | 4.891841 | false | false | false | false |
steelwheels/Coconut | CoconutData/Source/Value/CNMutablePointer.swift | 1 | 3970 | /*
* @file CNMutablePointerValue.swift
* @brief Define CNMutablePointerValue class
* @par Copyright
* Copyright (C) 2022 Steel Wheels Project
*/
import Foundation
public class CNMutablePointerValue: CNMutableValue
{
private var mPointerValue: CNPointerValue
private var mContext: CNMutableValue?
public init(pointer val: CNPointerValue, sourceDirectory srcdir: URL, cacheDirectory cachedir: URL){
mPointerValue = val
mContext = nil
super.init(type: .pointer, sourceDirectory: srcdir, cacheDirectory: cachedir)
}
public var context: CNMutableValue? { get { return mContext }}
public override func _value(forPath path: Array<CNValuePath.Element>, in root: CNMutableValue) -> Result<CNMutableValue?, NSError> {
let result: Result<CNMutableValue?, NSError>
switch pointedValue(in: root) {
case .success(let mval):
result = mval._value(forPath: path, in: root)
case .failure(let err):
result = .failure(err)
}
return result
}
public override func _set(value val: CNValue, forPath path: Array<CNValuePath.Element>, in root: CNMutableValue) -> NSError? {
let result: NSError?
if path.count == 0 {
/* Overide this value */
switch val {
case .dictionaryValue(let dict):
if let ptr = CNPointerValue.fromValue(value: dict) {
mPointerValue = ptr
root.isDirty = true
result = nil
} else {
result = noPointedValueError(path: path, place: #function)
}
default:
result = noPointedValueError(path: path, place: #function)
}
} else {
switch pointedValue(in: root) {
case .success(let mval):
result = mval._set(value: val, forPath: path, in: root)
case .failure(let err):
result = err
}
}
return result
}
public override func _append(value val: CNValue, forPath path: Array<CNValuePath.Element>, in root: CNMutableValue) -> NSError? {
let result: NSError?
if path.count > 0 {
switch pointedValue(in: root) {
case .success(let mval):
result = mval._append(value: val, forPath: path, in: root)
case .failure(let err):
result = err
}
} else {
result = NSError.parseError(message: "Can not append value to pointer")
}
return result
}
public override func _delete(forPath path: Array<CNValuePath.Element>, in root: CNMutableValue) -> NSError? {
let result: NSError?
if path.count > 0 {
switch pointedValue(in: root) {
case .success(let mval):
result = mval._delete(forPath: path, in: root)
case .failure(let err):
result = err
}
} else {
result = NSError.parseError(message: "Can not delete pointer value")
}
return result
}
public override func _search(name nm: String, value val: String, in root: CNMutableValue) -> Result<Array<CNValuePath.Element>?, NSError> {
let result: Result<Array<CNValuePath.Element>?, NSError>
switch pointedValue(in: root) {
case .success(let mval):
result = mval._search(name: nm, value: val, in: root)
case .failure(let err):
result = .failure(err)
}
return result
}
private func pointedValue(in root: CNMutableValue) -> Result<CNMutableValue, NSError> {
let elms = root.labelTable().pointerToPath(pointer: mPointerValue, in: root)
let result: Result<CNMutableValue, NSError>
switch root._value(forPath: elms, in: root) {
case .success(let mvalp):
if let mval = mvalp {
result = .success(mval)
} else {
result = .failure(unmatchedPathError(path: elms, place: #function))
}
case .failure(let err):
result = .failure(err)
}
return result
}
public override func _makeLabelTable(property name: String, path pth: Array<CNValuePath.Element>) -> Dictionary<String, Array<CNValuePath.Element>> {
return [:]
}
public override func clone() -> CNMutableValue {
return CNMutablePointerValue(pointer: mPointerValue, sourceDirectory: self.sourceDirectory, cacheDirectory: self.cacheDirectory)
}
public override func toValue() -> CNValue {
return .dictionaryValue(mPointerValue.toValue())
}
}
| lgpl-2.1 | c7e062cd2634efdb9f859f9d510403b1 | 29.538462 | 150 | 0.696725 | 3.528889 | false | false | false | false |
yuanziyuer/XLPagerTabStrip | Example/Example/Helpers/PostCell.swift | 2 | 2198 | // PostCell.swift
// XLPagerTabStrip ( https://github.com/xmartlabs/XLPagerTabStrip )
//
// Copyright (c) 2016 Xmartlabs ( http://xmartlabs.com )
//
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in
// all copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
// THE SOFTWARE.
import UIKit
class PostCell: UITableViewCell {
@IBOutlet weak var userImage: UIImageView!
@IBOutlet weak var postName: UILabel!
@IBOutlet weak var postText: UILabel!
override func awakeFromNib() {
super.awakeFromNib()
userImage.layer.cornerRadius = 10.0
}
func configureWithData(data: NSDictionary){
postName.text = data["post"]!["user"]!!["name"] as? String
postText.text = data["post"]!["text"] as? String
userImage.image = UIImage(named: postName.text!.stringByReplacingOccurrencesOfString(" ", withString: "_"))
}
func changeStylToBlack(){
userImage?.layer.cornerRadius = 30.0
postText.text = nil
postName.font = UIFont(name: "HelveticaNeue-Light", size:18) ?? UIFont.systemFontOfSize(18)
postName.textColor = .whiteColor()
backgroundColor = UIColor(red: 15/255.0, green: 16/255.0, blue: 16/255.0, alpha: 1.0)
}
}
| mit | dc4efb24c1e310afb92fd05fd975dd0f | 39.703704 | 115 | 0.702912 | 4.476578 | false | false | false | false |
tuanan94/FRadio-ios | SwiftRadio/NowPlayingViewController.swift | 1 | 4420 | import UIKit
import AVFoundation
import Firebase
import FirebaseDatabase
import NotificationBannerSwift
class NowPlayingViewController: UIViewController {
@IBOutlet weak var albumHeightConstraint: NSLayoutConstraint!
@IBOutlet weak var albumImageView: SpringImageView!
@IBOutlet weak var playButton: UIButton!
@IBOutlet weak var songLabel: SpringLabel!
@IBOutlet weak var stationDescLabel: UILabel!
@IBOutlet weak var volumeParentView: UIView!
@IBOutlet weak var recommendSong: UIButton!
@objc var iPhone4 = false
@objc var nowPlayingImageView: UIImageView!
static var radioPlayer: AVPlayer!
let streamUrl = "http://fradio.site:8000/default.m3u"
var ref: DatabaseReference!
static var notification: String!
static var isPlaying = false;
override func viewDidLoad() {
super.viewDidLoad()
if (NowPlayingViewController.isPlaying){
self.playButton.setImage(#imageLiteral(resourceName: "btn-pause"), for: UIControlState.normal)
}else{
play()
}
reformButton()
self.setupFirebase()
}
override func viewDidAppear(_ animated: Bool) {
self.displayNotification()
}
private func displayNotification(){
if NowPlayingViewController.notification == nil {
return
}
switch NowPlayingViewController.notification {
case "success":
let banner = NotificationBanner(title: "Successfully!!", subtitle: "Thank you, your song will be played soon", style: .success)
banner.show()
NowPlayingViewController.notification = nil
break
case "fail":
let banner = NotificationBanner(title: "Some thing went wrong", subtitle: "I will investigate this case asap", style: .danger)
banner.show()
NowPlayingViewController.notification = nil
break;
default: break
// Do nothing
}
}
private func setupFirebase(){
if (FirebaseApp.app() == nil){
FirebaseApp.configure()
}
ref = Database.database().reference(fromURL: "https://fradio-firebase.firebaseio.com/current")
ref.removeAllObservers()
ref.observe(DataEventType.value, with: { (snapshot) in
let postDict = snapshot.value as? [String : AnyObject] ?? [:]
let videoTitle = postDict["title"] as? String
if (videoTitle == nil){
self.songLabel.text = "waiting new song..."
}else{
self.songLabel.text = videoTitle
}
print("a")
})
}
private func reformButton(){
recommendSong.layer.cornerRadius = 10;
recommendSong.clipsToBounds = true;
title = "FRadio"
}
@IBAction func playPausePress(_ sender: Any) {
if (NowPlayingViewController.isPlaying){
pause()
} else {
play()
}
}
private func play(){
NowPlayingViewController.radioPlayer = AVPlayer(url: URL.init(string: streamUrl)!);
if #available(iOS 10.0, *) {
NowPlayingViewController.radioPlayer.automaticallyWaitsToMinimizeStalling = true
} else {
// Fallback on earlier versions
}
NowPlayingViewController.radioPlayer.play();
self.playButton.setImage(#imageLiteral(resourceName: "btn-pause"), for: UIControlState.normal)
NowPlayingViewController.isPlaying = true
}
private func pause(){
NowPlayingViewController.radioPlayer.pause();
NowPlayingViewController.radioPlayer = nil;
self.playButton.setImage(#imageLiteral(resourceName: "btn-play") ,for: UIControlState.normal)
NowPlayingViewController.isPlaying = false
}
@objc func optimizeForDeviceSize() {
// Adjust album size to fit iPhone 4s, 6s & 6s+
let deviceHeight = self.view.bounds.height
if deviceHeight == 480 {
iPhone4 = true
albumHeightConstraint.constant = 106
view.updateConstraints()
} else if deviceHeight == 667 {
albumHeightConstraint.constant = 230
view.updateConstraints()
} else if deviceHeight > 667 {
albumHeightConstraint.constant = 260
view.updateConstraints()
}
}
}
| mit | d6a1ec3179add6fd46dc968cdbb77a67 | 34.36 | 139 | 0.621719 | 5.249406 | false | false | false | false |
edx/edx-app-ios | Source/VideoDownloadQualityViewController.swift | 1 | 9105 | //
// VideoDownloadQualityViewController.swift
// edX
//
// Created by Muhammad Umer on 13/08/2021.
// Copyright © 2021 edX. All rights reserved.
//
import UIKit
fileprivate let buttonSize: CGFloat = 20
protocol VideoDownloadQualityDelegate: AnyObject {
func didUpdateVideoQuality()
}
class VideoDownloadQualityViewController: UIViewController {
typealias Environment = OEXInterfaceProvider & OEXAnalyticsProvider & OEXConfigProvider
private lazy var headerView = VideoDownloadQualityHeaderView()
private lazy var tableView: UITableView = {
let tableView = UITableView()
tableView.dataSource = self
tableView.delegate = self
tableView.rowHeight = UITableView.automaticDimension
tableView.estimatedRowHeight = 20
tableView.alwaysBounceVertical = false
tableView.separatorInset = .zero
tableView.tableFooterView = UIView()
tableView.register(VideoQualityCell.self, forCellReuseIdentifier: VideoQualityCell.identifier)
tableView.accessibilityIdentifier = "VideoDownloadQualityViewController:table-view"
return tableView
}()
private weak var delegate: VideoDownloadQualityDelegate?
private let environment: Environment
init(environment: Environment, delegate: VideoDownloadQualityDelegate?) {
self.environment = environment
self.delegate = delegate
super.init(nibName: nil, bundle: nil)
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
override func viewDidLoad() {
super.viewDidLoad()
navigationItem.largeTitleDisplayMode = .never
title = Strings.videoDownloadQualityTitle
setupViews()
addCloseButton()
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
environment.analytics.trackScreen(withName: AnalyticsScreenName.VideoDownloadQuality.rawValue)
}
private func setupViews() {
view.addSubview(tableView)
tableView.tableHeaderView = headerView
headerView.snp.makeConstraints { make in
make.leading.equalTo(view)
make.trailing.equalTo(view)
}
tableView.snp.makeConstraints { make in
make.edges.equalTo(view)
}
tableView.setAndLayoutTableHeaderView(header: headerView)
tableView.reloadData()
}
private func addCloseButton() {
let closeButton = UIBarButtonItem(image: Icon.Close.imageWithFontSize(size: buttonSize), style: .plain, target: nil, action: nil)
closeButton.accessibilityLabel = Strings.Accessibility.closeLabel
closeButton.accessibilityHint = Strings.Accessibility.closeHint
closeButton.accessibilityIdentifier = "VideoDownloadQualityViewController:close-button"
navigationItem.rightBarButtonItem = closeButton
closeButton.oex_setAction { [weak self] in
self?.dismiss(animated: true, completion: nil)
}
}
}
extension VideoDownloadQualityViewController: UITableViewDataSource {
func numberOfSections(in tableView: UITableView) -> Int {
return 1
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return VideoDownloadQuality.allCases.count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: VideoQualityCell.identifier, for: indexPath) as! VideoQualityCell
let item = VideoDownloadQuality.allCases[indexPath.row]
if let quality = environment.interface?.getVideoDownladQuality(),
quality == item {
cell.update(title: item.title, selected: true)
} else {
cell.update(title: item.title, selected: false)
}
return cell
}
}
extension VideoDownloadQualityViewController: UITableViewDelegate {
func tableView(_ tableView: UITableView, didSelectRowAt indexPath: IndexPath) {
let oldQuality = environment.interface?.getVideoDownladQuality()
let quality = VideoDownloadQuality.allCases[indexPath.row]
tableView.reloadData()
environment.interface?.saveVideoDownloadQuality(quality: quality)
environment.analytics.trackVideoDownloadQualityChanged(value: quality.analyticsValue, oldValue: oldQuality?.analyticsValue ?? "")
delegate?.didUpdateVideoQuality()
}
}
class VideoDownloadQualityHeaderView: UITableViewHeaderFooterView {
private lazy var titleLabel: UILabel = {
let label = UILabel()
label.numberOfLines = 0
let textStyle = OEXMutableTextStyle(weight: .normal, size: .small, color: OEXStyles.shared().neutralBlackT())
label.attributedText = textStyle.attributedString(withText: Strings.videoDownloadQualityMessage(platformName: OEXConfig.shared().platformName()))
label.accessibilityIdentifier = "VideoDownloadQualityHeaderView:title-view"
return label
}()
private lazy var separator: UIView = {
let view = UIView()
view.backgroundColor = OEXStyles.shared().neutralXLight()
view.accessibilityIdentifier = "VideoDownloadQualityHeaderView:seperator-view"
return view
}()
override init(reuseIdentifier: String?) {
super.init(reuseIdentifier: reuseIdentifier)
accessibilityIdentifier = "VideoDownloadQualityHeaderView"
setupViews()
setupConstrains()
}
required init?(coder: NSCoder) {
super.init(coder: coder)
}
private func setupViews() {
addSubview(titleLabel)
addSubview(separator)
}
private func setupConstrains() {
titleLabel.snp.makeConstraints { make in
make.leading.equalTo(self).offset(StandardVerticalMargin * 2)
make.trailing.equalTo(self).inset(StandardVerticalMargin * 2)
make.top.equalTo(self).offset(StandardVerticalMargin * 2)
make.bottom.equalTo(self).inset(StandardVerticalMargin * 2)
}
separator.snp.makeConstraints { make in
make.leading.equalTo(self)
make.trailing.equalTo(self)
make.bottom.equalTo(self)
make.height.equalTo(1)
}
}
}
class VideoQualityCell: UITableViewCell {
static let identifier = "VideoQualityCell"
lazy var titleLabel: UILabel = {
let label = UILabel()
label.accessibilityIdentifier = "VideoQualityCell:title-label"
return label
}()
private lazy var checkmarkImageView: UIImageView = {
let imageView = UIImageView()
imageView.image = Icon.Check.imageWithFontSize(size: buttonSize).image(with: OEXStyles.shared().neutralBlackT())
imageView.accessibilityIdentifier = "VideoQualityCell:checkmark-image-view"
return imageView
}()
private lazy var textStyle = OEXMutableTextStyle(weight: .normal, size: .base, color: OEXStyles.shared().primaryDarkColor())
private lazy var textStyleSelcted = OEXMutableTextStyle(weight: .bold, size: .base, color: OEXStyles.shared().primaryDarkColor())
override init(style: UITableViewCell.CellStyle, reuseIdentifier: String?) {
super.init(style: style, reuseIdentifier: reuseIdentifier)
selectionStyle = .none
accessibilityIdentifier = "VideoDownloadQualityViewController:video-quality-cell"
setupView()
setupConstrains()
}
required init?(coder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
private func setupView() {
contentView.addSubview(titleLabel)
contentView.addSubview(checkmarkImageView)
}
private func setupConstrains() {
titleLabel.snp.makeConstraints { make in
make.top.equalTo(contentView).offset(StandardVerticalMargin * 2)
make.leading.equalTo(contentView).offset(StandardVerticalMargin * 2)
make.trailing.equalTo(contentView).inset(StandardVerticalMargin * 2)
make.bottom.equalTo(contentView).inset(StandardVerticalMargin * 2)
}
checkmarkImageView.snp.makeConstraints { make in
make.width.equalTo(buttonSize)
make.height.equalTo(buttonSize)
make.trailing.equalTo(contentView).inset(StandardVerticalMargin * 2)
make.centerY.equalTo(contentView)
}
checkmarkImageView.isHidden = true
}
func update(title: String, selected: Bool) {
checkmarkImageView.isHidden = !selected
if selected {
titleLabel.attributedText = textStyleSelcted.attributedString(withText: title)
} else {
titleLabel.attributedText = textStyle.attributedString(withText: title)
}
}
}
| apache-2.0 | 645b3ef3ee03a2fb3aa5ecaf4ad4d2a4 | 34.701961 | 153 | 0.671463 | 5.623224 | false | false | false | false |
indragiek/MarkdownTextView | MarkdownTextView/MarkdownSuperscriptHighlighter.swift | 1 | 2376 | //
// MarkdownSuperscriptHighlighter.swift
// MarkdownTextView
//
// Created by Indragie on 4/29/15.
// Copyright (c) 2015 Indragie Karunaratne. All rights reserved.
//
import UIKit
/**
* Highlights super^script in Markdown text (unofficial extension)
*/
public final class MarkdownSuperscriptHighlighter: HighlighterType {
private static let SuperscriptRegex = regexFromPattern("(\\^+)(?:(?:[^\\^\\s\\(][^\\^\\s]*)|(?:\\([^\n\r\\)]+\\)))")
private let fontSizeRatio: CGFloat
/**
Creates a new instance of the receiver.
:param: fontSizeRatio Ratio to multiply the original font
size by to calculate the superscript font size.
:returns: An initialized instance of the receiver.
*/
public init(fontSizeRatio: CGFloat = 0.7) {
self.fontSizeRatio = fontSizeRatio
}
// MARK: HighlighterType
public func highlightAttributedString(attributedString: NSMutableAttributedString) {
var previousRange: NSRange?
var level: Int = 0
enumerateMatches(self.dynamicType.SuperscriptRegex, string: attributedString.string) {
level += $0.rangeAtIndex(1).length
let textRange = $0.range
let attributes = attributedString.attributesAtIndex(textRange.location, effectiveRange: nil)
let isConsecutiveRange: Bool = {
if let previousRange = previousRange where NSMaxRange(previousRange) == textRange.location {
return true
}
return false
}()
if isConsecutiveRange {
level++
}
attributedString.addAttributes(superscriptAttributes(attributes, level: level, ratio: self.fontSizeRatio), range: textRange)
previousRange = textRange
if !isConsecutiveRange {
level = 0
}
}
}
}
private func superscriptAttributes(attributes: TextAttributes, level: Int, ratio: CGFloat) -> TextAttributes {
if let font = attributes[NSFontAttributeName] as? UIFont {
let adjustedFont = UIFont(descriptor: font.fontDescriptor(), size: font.pointSize * ratio)
return [
kCTSuperscriptAttributeName as String: level,
NSFontAttributeName: adjustedFont
]
}
return [:]
}
| mit | c265f1d176ecd3a8878b2b922a27dcd8 | 32.942857 | 136 | 0.62037 | 5.187773 | false | false | false | false |
narner/AudioKit | Playgrounds/AudioKitPlaygrounds/Playgrounds/Effects.playground/Pages/AutoPan Operation.xcplaygroundpage/Contents.swift | 1 | 1698 | //: ## AutoPan Operation
//:
import AudioKitPlaygrounds
import AudioKit
//: This first section sets up parameter naming in such a way
//: to make the operation code easier to read below.
let speedIndex = 0
let depthIndex = 1
extension AKOperationEffect {
var speed: Double {
get { return self.parameters[speedIndex] }
set(newValue) { self.parameters[speedIndex] = newValue }
}
var depth: Double {
get { return self.parameters[depthIndex] }
set(newValue) { self.parameters[depthIndex] = newValue }
}
}
//: Use the struct and the extension to refer to the autopan parameters by name
let file = try AKAudioFile(readFileName: playgroundAudioFiles[0])
let player = try AKAudioPlayer(file: file)
player.looping = true
let effect = AKOperationEffect(player) { input, parameters in
let oscillator = AKOperation.sineWave(frequency: parameters[speedIndex], amplitude: parameters[depthIndex])
return input.pan(oscillator)
}
effect.parameters = [10, 1]
AudioKit.output = effect
AudioKit.start()
player.play()
import AudioKitUI
class LiveView: AKLiveViewController {
override func viewDidLoad() {
addTitle("AutoPan")
addView(AKResourcesAudioFileLoaderView(player: player, filenames: playgroundAudioFiles))
addView(AKSlider(property: "Speed", value: effect.speed, range: 0.1 ... 25) { sliderValue in
effect.speed = sliderValue
})
addView(AKSlider(property: "Depth", value: effect.depth) { sliderValue in
effect.depth = sliderValue
})
}
}
import PlaygroundSupport
PlaygroundPage.current.needsIndefiniteExecution = true
PlaygroundPage.current.liveView = LiveView()
| mit | 6844ba0a371ccd2c32fa913fadbaa873 | 27.779661 | 111 | 0.710836 | 4.376289 | false | false | false | false |
DanielAsher/VIPER-SWIFT | Carthage/Checkouts/RxSwift/Rx.playground/Pages/Mathematical_and_Aggregate_Operators.xcplaygroundpage/Contents.swift | 2 | 2041 | /*:
> # IMPORTANT: To use `Rx.playground`, please:
1. Open `Rx.xcworkspace`
2. Build `RxSwift-OSX` scheme
3. And then open `Rx` playground in `Rx.xcworkspace` tree view.
4. Choose `View > Show Debug Area`
*/
//: [<< Previous](@previous) - [Index](Index)
import RxSwift
/*:
## Mathematical and Aggregate Operators
Operators that operate on the entire sequence of items emitted by an Observable
*/
/*:
### `concat`
Emit the emissions from two or more Observables without interleaving them.
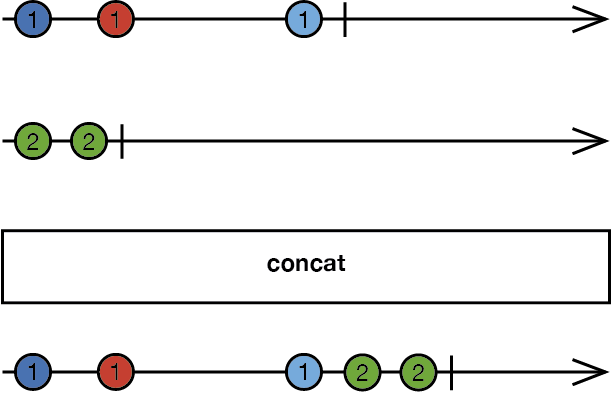
[More info in reactive.io website]( http://reactivex.io/documentation/operators/concat.html )
*/
example("concat") {
let var1 = BehaviorSubject(value: 0)
let var2 = BehaviorSubject(value: 200)
// var3 is like an Observable<Observable<Int>>
let var3 = BehaviorSubject(value: var1)
let d = var3
.concat()
.subscribe {
print($0)
}
var1.on(.Next(1))
var1.on(.Next(2))
var1.on(.Next(3))
var1.on(.Next(4))
var3.on(.Next(var2))
var2.on(.Next(201))
var1.on(.Next(5))
var1.on(.Next(6))
var1.on(.Next(7))
var1.on(.Completed)
var2.on(.Next(202))
var2.on(.Next(203))
var2.on(.Next(204))
}
/*:
### `reduce`
Apply a function to each item emitted by an Observable, sequentially, and emit the final value.
This function will perform a function on each element in the sequence until it is completed, then send a message with the aggregate value. It works much like the Swift `reduce` function works on sequences.
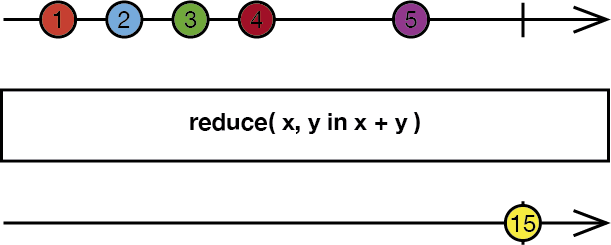
[More info in reactive.io website]( http://reactivex.io/documentation/operators/reduce.html )
*/
example("reduce") {
_ = Observable.of(0, 1, 2, 3, 4, 5, 6, 7, 8, 9)
.reduce(0, accumulator: +)
.subscribe {
print($0)
}
}
//: [Index](Index) - [Next >>](@next)
| mit | fbc9f1d3dd901b1e6102634970568d44 | 22.732558 | 205 | 0.645762 | 3.471088 | false | false | false | false |
mlavergn/swiftutil | Sources/datetime.swift | 1 | 2296 | /// Extensions to Date
/// DateTime struct with Date related helpers
///
/// - author: Marc Lavergne <mlavergn@gmail.com>
/// - copyright: 2017 Marc Lavergne. All rights reserved.
/// - license: MIT
import Foundation
// MARK: - Date extension
public extension Date {
/// Compare caller to date to caller and determine if caller is earlier
///
/// - Parameter date: Date to compare caller against
/// - Returns: Bool with true if date is later than caller, otherwise false
public func isBefore(date: Date) -> Bool {
return self.compare(date) == .orderedAscending ? true : false
}
/// Compare caller to date to caller and determine if caller is later
///
/// - Parameter date: Date to compare caller against
/// - Returns: Bool with true if date is earlier than caller, otherwise false
public func isAfter(date: Date) -> Bool {
return self.compare(date) == .orderedDescending ? true : false
}
/// Compare caller to date to caller and determine if caller is equal
///
/// - Parameter date: Date to compare caller against
/// - Returns: Bool with true if date is equal to caller, otherwise false
public func isEqual(date: Date) -> Bool {
return self.compare(date) == .orderedSame ? true : false
}
}
// MARK: - DateTime struct
public struct DateTime {
/// Obtain the epoch time as an Int
public static var epoch: Int {
return Int(NSDate().timeIntervalSince1970)
}
/// Constructs a Date object based on the Gregorian calendar
///
/// - Parameters:
/// - year: year as an Int
/// - month: month as an Int
/// - day: day as an Int
/// - Returns: Date optional
public static func dateWith(year: Int, month: Int, day: Int) -> Date? {
var components = DateComponents()
components.year = year
components.month = month
components.day = day
return NSCalendar(identifier: NSCalendar.Identifier.gregorian)?.date(from: components)
}
/// Constructs a Date from a format mask and string representation
///
/// - Parameters:
/// - format: format mask String
/// - date: date as String
/// - Returns: Date optional
public static func stringToDate(format: String, date: String) -> Date? {
let dateFormat = DateFormatter()
dateFormat.timeZone = NSTimeZone.default
dateFormat.dateFormat = format
return dateFormat.date(from: date)
}
}
| mit | e00dee357aa498361ee955eab368ff47 | 29.613333 | 88 | 0.695557 | 3.833055 | false | false | false | false |
objecthub/swift-lispkit | Sources/LispKit/IO/BinaryInputSource.swift | 1 | 8272 | //
// BinaryInputSource.swift
// LispKit
//
// Created by Matthias Zenger on 01/11/2017.
// Copyright © 2017 ObjectHub. All rights reserved.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
//
import Foundation
public protocol BinaryInputSource {
init?(url: URL)
func open()
func close()
var hasBytesAvailable: Bool { get }
func read(_ buffer: UnsafeMutablePointer<UInt8>, maxLength len: Int) -> Int
}
extension InputStream: BinaryInputSource {
}
public final class HTTPInputStream: BinaryInputSource {
/// A condition object used to synchronize changes between tasks and the input stream object
fileprivate let condition = NSCondition()
/// The target URL of the request
public let url: URL
/// The network task associated with this input stream
private var task: URLSessionTask? = nil
/// The HTTP response header object once it was received
fileprivate var response: HTTPURLResponse? = nil
/// Data of the body of the HTTP response
public fileprivate(set) var data: Data
/// The current read index
public private(set) var readIndex: Data.Index
/// The timeout for the HTTP connection
public let timeout: Double
/// Number of bytes that were received from the HTTP GET request
public fileprivate(set) var bytesReceived: Int64 = 0
/// If an error was encountered, it is being stored here
public fileprivate(set) var error: Error? = nil
/// Returns `false` as long as this input stream is connected to a network task.
public fileprivate(set) var completed: Bool = false
private static let sessionDelegate = SessionDelegate()
/// The default request timeout
public static let defaultTimeout: Double = 60.0
public private(set) static var session: URLSession = {
let configuration = URLSessionConfiguration.default
configuration.requestCachePolicy = .useProtocolCachePolicy
configuration.timeoutIntervalForRequest = TimeInterval(HTTPInputStream.defaultTimeout)
return URLSession(configuration: configuration,
delegate: HTTPInputStream.sessionDelegate,
delegateQueue: nil)
}()
public convenience init?(url: URL) {
self.init(url: url, timeout: HTTPInputStream.defaultTimeout)
}
public init?(url: URL, timeout: Double) {
guard let scheme = url.scheme, scheme == "http" || scheme == "https" else {
return nil
}
self.url = url
self.data = Data()
self.readIndex = self.data.startIndex
self.timeout = timeout
}
public func open() {
self.openConnection(timeout: self.timeout)
self.waitForResponse()
}
public func openConnection(timeout: Double) {
guard self.task == nil && !self.completed else {
return
}
var request = URLRequest(url: self.url)
request.httpMethod = "GET"
request.cachePolicy = .useProtocolCachePolicy
request.timeoutInterval = TimeInterval(timeout)
request.httpShouldHandleCookies = false
let task = HTTPInputStream.session.dataTask(with: request)
HTTPInputStream.sessionDelegate.registerTask(task, forStream: self)
self.task = task
task.resume()
}
public func close() {
if !self.completed {
if let task = self.task {
task.cancel()
self.task = nil
} else {
self.completed = true
}
}
}
public func read(_ buffer: UnsafeMutablePointer<UInt8>, maxLength len: Int) -> Int {
self.condition.lock()
while !self.completed && self.readIndex == self.data.endIndex {
self.condition.wait()
}
let bytesRead = min(len, self.data.endIndex - self.readIndex)
let nextReadIndex = self.data.index(self.readIndex, offsetBy: bytesRead)
self.data.copyBytes(to: buffer, from: self.readIndex ..< nextReadIndex)
self.readIndex = nextReadIndex
self.condition.unlock()
return bytesRead
}
public var hasBytesAvailable: Bool {
return !self.completed || self.readIndex < self.data.endIndex
}
/// Waits until a response was received (or the request was terminated)
public func waitForResponse() {
self.condition.lock()
while self.response == nil && !self.completed {
self.condition.wait()
}
self.condition.unlock()
}
/// Waits until all data was received (or the request was terminated)
public func waitForData() {
self.condition.lock()
while !self.completed {
self.condition.wait()
}
self.condition.unlock()
}
/// The status code of the HTTP response
public var statusCode: Int? {
return self.response?.statusCode
}
/// A textual description of the status code of the HTTP response
public var statusCodeDescription: String? {
guard let statusCode = self.statusCode else {
return nil
}
return HTTPURLResponse.localizedString(forStatusCode: statusCode)
}
/// The number of bytes that are supposed to be read
public var expectedContentLength: Int64? {
return self.response?.expectedContentLength
}
/// The name of the text encoding.
public var textEncodingName: String? {
return self.response?.textEncodingName
}
/// The mime type name.
public var mimeType: String? {
return self.response?.mimeType
}
/// The URL of the response.
public var responseUrl: URL? {
return self.response?.url
}
/// All header fields of the HTTP response.
public var headerFields: [String : String]? {
guard let response = self.response else {
return nil
}
var res: [String : String] = [:]
for (key, value) in response.allHeaderFields {
if let str = value as? String {
res[key.description] = str
}
}
return res
}
}
fileprivate class SessionDelegate: NSObject,
URLSessionDelegate,
URLSessionTaskDelegate,
URLSessionDataDelegate {
private var inputStreamForTask: [Int : HTTPInputStream] = [:]
public func registerTask(_ task: URLSessionTask, forStream stream: HTTPInputStream) {
self.inputStreamForTask[task.taskIdentifier] = stream
}
/// This callback is the last one done for each task. Remove the input stream from the
/// list of all open input streams.
public func urlSession(_ session: URLSession,
task: URLSessionTask,
didCompleteWithError error: Error?) {
guard let inputStream = self.inputStreamForTask[task.taskIdentifier] else {
return
}
inputStream.condition.lock()
inputStream.error = inputStream.error ?? error
inputStream.completed = true
self.inputStreamForTask.removeValue(forKey: task.taskIdentifier)
inputStream.condition.signal()
inputStream.condition.unlock()
}
public func urlSession(_ session: URLSession,
dataTask task: URLSessionDataTask,
didReceive response: URLResponse,
completionHandler: @escaping (URLSession.ResponseDisposition) -> Void) {
guard let inputStream = self.inputStreamForTask[task.taskIdentifier] else {
completionHandler(.allow)
return
}
inputStream.condition.lock()
if let response = response as? HTTPURLResponse {
inputStream.response = response
inputStream.condition.signal()
}
inputStream.condition.unlock()
completionHandler(.allow)
}
public func urlSession(_ session: URLSession,
dataTask task: URLSessionDataTask,
didReceive data: Data) {
guard let inputStream = self.inputStreamForTask[task.taskIdentifier] else {
return
}
inputStream.data.append(data)
inputStream.bytesReceived += Int64(data.count)
}
}
| apache-2.0 | 787e92918e9279d77950f7c814b41cab | 30.689655 | 97 | 0.67513 | 4.780925 | false | false | false | false |
GuiBayma/PasswordVault | PasswordVault/AppDelegate.swift | 1 | 4965 | //
// AppDelegate.swift
// PasswordVault
//
// Created by Guilherme Bayma on 7/26/17.
// Copyright © 2017 Bayma. All rights reserved.
//
import UIKit
import CoreData
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
var backgroundView: BackgroundView?
var didShowPasswordView = false
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {
// Debug
let groups = GroupManager.sharedInstance.getAllGroups()
print("\(groups.count) groups found\n")
// for group in groups {
// _ = GroupManager.sharedInstance.delete(object: group)
// }
let items = ItemManager.sharedInstance.getAllItems()
print("\(items.count) items found\n")
// for item in items {
// _ = GroupManager.sharedInstance.delete(object: item)
// }
self.window = UIWindow(frame: UIScreen.main.bounds)
backgroundView = BackgroundView(frame: window?.frame ?? CGRect())
let mainView = GroupsTableViewController()
let navController = UINavigationController(rootViewController: mainView)
self.window?.rootViewController = navController
self.window?.makeKeyAndVisible()
return true
}
func applicationWillResignActive(_ application: UIApplication) {
guard
let bView = backgroundView,
didShowPasswordView != true
else {
return
}
if let topController = UIApplication.topViewController() {
let topView = topController.view
topView?.addSubview(bView)
}
UIApplication.shared.ignoreSnapshotOnNextApplicationLaunch()
}
func applicationDidBecomeActive(_ application: UIApplication) {
if didShowPasswordView {
return
}
if let topController = UIApplication.topViewController() {
if let _ = UserDefaults.standard.string(forKey: "AppPasswordKey") {
let passView = PasswordViewController()
topController.present(passView, animated: false) {
self.backgroundView?.removeFromSuperview()
self.didShowPasswordView = true
}
} else {
let newPassView = NewPasswordViewController()
topController.present(newPassView, animated: false) {
self.backgroundView?.removeFromSuperview()
}
}
}
}
func applicationWillTerminate(_ application: UIApplication) {
self.saveContext()
}
// MARK: - Core Data stack
lazy var persistentContainer: NSPersistentContainer = {
/*
The persistent container for the application. This implementation
creates and returns a container, having loaded the store for the
application to it. This property is optional since there are legitimate
error conditions that could cause the creation of the store to fail.
*/
let container = NSPersistentContainer(name: "PasswordVault")
container.loadPersistentStores(completionHandler: { (_, error) in
if let error = error as NSError? {
// Replace this implementation with code to handle the error appropriately.
// fatalError() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
/*
Typical reasons for an error here include:
* The parent directory does not exist, cannot be created, or disallows writing.
* The persistent store is not accessible, due to permissions or data protection when the device is locked.
* The device is out of space.
* The store could not be migrated to the current model version.
Check the error message to determine what the actual problem was.
*/
fatalError("Unresolved error \(error), \(error.userInfo)")
}
})
return container
}()
// MARK: - Core Data Saving support
func saveContext () {
let context = persistentContainer.viewContext
if context.hasChanges {
do {
try context.save()
} catch {
// Replace this implementation with code to handle the error appropriately.
// fatalError() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
let nserror = error as NSError
fatalError("Unresolved error \(nserror), \(nserror.userInfo)")
}
}
}
}
| mit | 9f29c45129af9b608c6d889c640374a8 | 37.184615 | 199 | 0.62087 | 5.819461 | false | false | false | false |
mortenjust/androidtool-mac | AndroidTool/ObbHandler.swift | 1 | 1195 | //
// ObbHandler.swift
// AndroidTool
//
// Created by Morten Just Petersen on 1/20/16.
// Copyright © 2016 Morten Just Petersen. All rights reserved.
//
import Cocoa
protocol ObbHandlerDelegate {
func obbHandlerDidStart(_ bytes:String)
func obbHandlerDidFinish()
}
class ObbHandler: NSObject {
var filePath:String!
var delegate:ObbHandlerDelegate?
var device:Device!
var fileSize:UInt64?
init(filePath:String, device:Device){
print(">>obb init obbhandler")
super.init()
self.filePath = filePath
self.device = device
self.fileSize = Util.getFileSizeForFilePath(filePath)
}
func pushToDevice(){
print(">>zip flash")
let shell = ShellTasker(scriptFile: "installObbForSerial")
let bytes = (fileSize != nil) ? Util.formatBytes(fileSize!) : "? bytes"
delegate?.obbHandlerDidStart(bytes)
print("startin obb copying the \(bytes) file")
shell.run(arguments: [self.device.adbIdentifier!, self.filePath]) { (output) -> Void in
print("done copying OBB to device")
self.delegate?.obbHandlerDidFinish()
}
}
}
| apache-2.0 | 09bbdc61ca7343aee6e151c5724729df | 24.956522 | 95 | 0.633166 | 4.341818 | false | false | false | false |
ScottRobbins/SRBubbleProgressTracker | SRBubbleProgressTracker/SRBubbleProgressTrackerView.swift | 1 | 15019 | //
// SRBubbleProgressTrackerView.swift
// SRBubbleProgressTracker
//
// Created by Samuel Scott Robbins on 2/9/15.
// Copyright (c) 2015 Scott Robbins Software. All rights reserved.
//
import UIKit
public enum BubbleAllignment {
case Vertical
case Horizontal
}
public class SRBubbleProgressTrackerView : UIView {
// MARK: Initializations
private var lastBubbleCompleted = 0
private var bubbleArray = [UIView]()
private var connectLineArray = [UIView]()
private var bubbleAllignment = BubbleAllignment.Vertical
private var animateToBubbleQueue = [Int]()
// MARK: Color Defaults
@IBInspectable
var lineColorForNotCompleted : UIColor = .grayColor()
@IBInspectable
var lineColorForCompleted : UIColor = .greenColor()
@IBInspectable
var bubbleBackgroundColorForNotCompleted : UIColor = .grayColor()
@IBInspectable
var bubbleBackgroundColorForCompleted : UIColor = .greenColor()
@IBInspectable
var bubbleBackgroundColorForNextToComplete : UIColor = .orangeColor()
@IBInspectable
var bubbleTextColorForNotCompleted : UIColor = .whiteColor()
@IBInspectable
var bubbleTextColorForCompleted : UIColor = .whiteColor()
@IBInspectable
var bubbleTextColorForNextToComplete : UIColor = .whiteColor()
// MARK: Setup View
public func setupInitialBubbleProgressTrackerView(numBubbles : Int, dotDiameter : CGFloat, allign : BubbleAllignment) {
bubbleAllignment = allign
for line in connectLineArray { line.removeFromSuperview() }
connectLineArray.removeAll(keepCapacity: false)
for bubble in bubbleArray { removeFromSuperview() }
bubbleArray.removeAll(keepCapacity: false)
lastBubbleCompleted = 0
// Add the lines into the view
let lineStartDown = dotDiameter / 2.0
let lineWidth = dotDiameter * 0.2
let length = (bubbleAllignment == .Vertical) ? self.frame.size.height : self.frame.size.width
let width = (bubbleAllignment == .Vertical) ? self.frame.size.width : self.frame.size.height
var lineStartMiddle = (width / 2.0) - (lineWidth / 2.0)
let lineHeight = (length - lineStartDown * 2.0) / CGFloat(numBubbles - 1)
addLinesIntoView(lineStartDown, lineStartMiddle: lineStartMiddle, lineHeight: lineHeight, lineWidth: lineWidth, numLines: numBubbles - 1)
lineStartMiddle = (width / 2.0) - (dotDiameter / 2.0)
// Add bubbles into the view
for i in 0..<numBubbles {
addBubbleIntoView(lineStartMiddle, lineHeight: lineHeight, lineWidth: lineWidth, dotDiameter: dotDiameter, bubbleNumber: i)
}
}
public func setupInitialBubbleProgressTrackerView(numBubbles : Int, dotDiameter : CGFloat, allign : BubbleAllignment, leftOrTopViews : [UIView], rightOrBottomViews : [UIView]) {
let spaceLeftOrTopOfBubbles : CGFloat = 20.0
bubbleAllignment = allign
connectLineArray.removeAll(keepCapacity: false)
bubbleArray.removeAll(keepCapacity: false)
lastBubbleCompleted = 0
// Add the lines into the view
let lineStartDown = dotDiameter / 2.0
let lineWidth = dotDiameter * 0.2
let length = (bubbleAllignment == .Vertical) ? self.frame.size.height : self.frame.size.width
let width = (bubbleAllignment == .Vertical) ? self.frame.size.width : self.frame.size.height
var startMiddle = (width / 2.0) - (lineWidth / 2.0)
let lineHeight = (length - lineStartDown * 2.0) / CGFloat(numBubbles - 1)
addLinesIntoView(lineStartDown, lineStartMiddle: startMiddle, lineHeight: lineHeight, lineWidth: lineWidth, numLines: numBubbles - 1)
startMiddle = (width / 2.0) - (dotDiameter / 2.0)
// Add bubbles into the view
for i in 0..<numBubbles {
let bubbleFrame = addBubbleIntoView(startMiddle, lineHeight: lineHeight, lineWidth: lineWidth, dotDiameter: dotDiameter, bubbleNumber: i)
if i < leftOrTopViews.count {
if bubbleAllignment == .Vertical {
let pointYCenter : CGFloat = bubbleFrame.origin.y + bubbleFrame.size.height / 2.0
let pointY : CGFloat = pointYCenter - (leftOrTopViews[i].frame.size.height / 2.0)
let pointX : CGFloat = bubbleFrame.origin.x - spaceLeftOrTopOfBubbles - leftOrTopViews[i].frame.size.width
leftOrTopViews[i].frame.origin = CGPointMake(pointX, pointY)
} else {
let pointXCenter : CGFloat = bubbleFrame.origin.x + bubbleFrame.size.width / 2.0
let pointX : CGFloat = pointXCenter - (leftOrTopViews[i].frame.size.width / 2.0)
let pointY : CGFloat = bubbleFrame.origin.y - spaceLeftOrTopOfBubbles - leftOrTopViews[i].frame.size.height
leftOrTopViews[i].frame.origin = CGPointMake(pointX, pointY)
}
self.addSubview(leftOrTopViews[i])
}
if i < rightOrBottomViews.count {
if bubbleAllignment == .Vertical {
let pointYCenter : CGFloat = bubbleFrame.origin.y + bubbleFrame.size.height / 2.0
let pointY : CGFloat = pointYCenter - (rightOrBottomViews[i].frame.size.height / 2.0)
let pointX : CGFloat = bubbleFrame.origin.x + bubbleFrame.size.width + spaceLeftOrTopOfBubbles
rightOrBottomViews[i].frame.origin = CGPointMake(pointX, pointY)
} else {
let pointXCenter : CGFloat = bubbleFrame.origin.x + bubbleFrame.size.width / 2.0
let pointX : CGFloat = pointXCenter - (rightOrBottomViews[i].frame.size.width / 2.0)
let pointY : CGFloat = bubbleFrame.origin.y + bubbleFrame.size.height + spaceLeftOrTopOfBubbles
rightOrBottomViews[i].frame.origin = CGPointMake(pointX, pointY)
}
self.addSubview(rightOrBottomViews[i])
}
}
}
private func addLinesIntoView(lineStartDown : CGFloat, lineStartMiddle : CGFloat, lineHeight : CGFloat, lineWidth : CGFloat, numLines : Int) {
// Add lines into view
for i in 0..<numLines {
var lineView = UIView()
if bubbleAllignment == .Vertical {
lineView.frame = CGRectMake(lineStartMiddle, lineStartDown + lineHeight * CGFloat(i), lineWidth, lineHeight)
} else {
lineView.frame = CGRectMake(lineStartDown + lineHeight * CGFloat(i), lineStartMiddle, lineHeight, lineWidth)
}
lineView.backgroundColor = lineColorForNotCompleted
connectLineArray.append(lineView)
self.addSubview(lineView)
}
}
private func addBubbleIntoView(bubbleStartMiddle : CGFloat, lineHeight : CGFloat, lineWidth : CGFloat, dotDiameter : CGFloat, bubbleNumber : Int) -> CGRect {
var bubbleViewFrame = CGRect()
if bubbleAllignment == .Vertical {
bubbleViewFrame = CGRectMake(bubbleStartMiddle, lineHeight * CGFloat(bubbleNumber), dotDiameter, dotDiameter)
} else {
bubbleViewFrame = CGRectMake(lineHeight * CGFloat(bubbleNumber), bubbleStartMiddle, dotDiameter, dotDiameter)
}
var bubbleView = getBubbleView(bubbleViewFrame, number: bubbleNumber+1)
bubbleArray.append(bubbleView)
self.addSubview(bubbleView)
return bubbleView.frame
}
private func getBubbleView(frame : CGRect, number : Int) -> UIView {
var bubbleView = UIView(frame: frame)
var numberLabel = UILabel()
numberLabel.frame = CGRectMake(0, 0, bubbleView.frame.size.width, bubbleView.frame.size.height)
numberLabel.text = "\(number)"
numberLabel.textAlignment = NSTextAlignment.Center
numberLabel.textColor = (number == 1) ? bubbleTextColorForNextToComplete : bubbleTextColorForNotCompleted
numberLabel.font = UIFont.systemFontOfSize(30.0)
bubbleView.addSubview(numberLabel)
bubbleView.backgroundColor = (number == 1) ? bubbleBackgroundColorForNextToComplete : bubbleBackgroundColorForNotCompleted
bubbleView.layer.cornerRadius = bubbleView.frame.size.width / 2.0
return bubbleView
}
// MARK: Magic
public func bubbleCompleted(numBubbleCompleted : Int) {
if animateToBubbleQueue.isEmpty {
if let startBubble = getStartBubble(numBubbleCompleted) {
animateToBubbleQueue.append(startBubble)
checkBubbleCompleted(startBubble, start: lastBubbleCompleted++)
}
} else {
for num in animateToBubbleQueue {
if num >= numBubbleCompleted { return }
}
animateToBubbleQueue.append(numBubbleCompleted)
}
}
private func removeAnimatedBubbleFromQueueAndContinue() {
if !animateToBubbleQueue.isEmpty {
animateToBubbleQueue.removeAtIndex(0)
if !animateToBubbleQueue.isEmpty {
if let startBubble = getStartBubble(animateToBubbleQueue[0]) {
checkBubbleCompleted(startBubble, start: lastBubbleCompleted++)
}
}
}
}
private func getStartBubble(numBubbleCompleted : Int) -> Int? {
var startBubble = Int()
if numBubbleCompleted >= bubbleArray.count {
if lastBubbleCompleted == bubbleArray.count { return nil }
startBubble = bubbleArray.count
} else {
if lastBubbleCompleted >= numBubbleCompleted { return nil }
startBubble = numBubbleCompleted
}
return startBubble
}
private func checkBubbleCompleted(numBubbleCompleted : Int, start : Int) {
var frame = bubbleArray[start].frame
var newFrame = CGRectMake(frame.size.width / 2.0, frame.size.height / 2.0, 0, 0)
var temporaryBubble = getBubbleView(frame, number: start+1)
var labelView = temporaryBubble.subviews[0] as UILabel
labelView.textColor = bubbleTextColorForCompleted
var tempBubbleCornerRadius = temporaryBubble.layer.cornerRadius
temporaryBubble.layer.cornerRadius = 0.0 // so we can animate the corner radius
labelView.removeFromSuperview() // so we can add it overtop teh new filling bubble
temporaryBubble.frame = newFrame
temporaryBubble.backgroundColor = bubbleBackgroundColorForCompleted
bubbleArray[start].addSubview(temporaryBubble)
bubbleArray[start].addSubview(labelView)
// Animate the first bubble filling iwth its new color
UIView.animateWithDuration(0.4, animations: { () -> Void in
temporaryBubble.frame = CGRectMake(0, 0, self.bubbleArray[start].frame.size.width, self.bubbleArray[start].frame.size.height)
temporaryBubble.layer.cornerRadius = tempBubbleCornerRadius
}, completion: { (finished) -> Void in
// Change the original bubble color and then remove the covering ones
self.bubbleArray[start].backgroundColor = self.bubbleBackgroundColorForCompleted
temporaryBubble.removeFromSuperview()
labelView.removeFromSuperview()
if start < numBubbleCompleted && start+1 < self.bubbleArray.count {
var newLine : UIView = UIView()
newLine.backgroundColor = self.lineColorForCompleted
newLine.frame = self.connectLineArray[start].frame
// create the line with a frame collapsed in height to reach the edge of the bubble
if (self.bubbleAllignment == BubbleAllignment.Vertical) {
newLine.frame.size = CGSizeMake(newLine.frame.size.width, self.bubbleArray[start].frame.size.width / 2.0)
} else {
newLine.frame.size = CGSizeMake(self.bubbleArray[start].frame.size.width / 2.0, newLine.frame.size.height)
}
newLine.frame.origin = CGPointMake(0, 0)
self.connectLineArray[start].addSubview(newLine)
UIView.animateWithDuration(0.4, animations: { () -> Void in
newLine.frame.size = self.connectLineArray[start].frame.size
}, completion: { (finished) -> Void in
// change the original line's color and then remove the covering one
self.connectLineArray[start].backgroundColor = self.lineColorForCompleted
newLine.removeFromSuperview()
})
// Do the same thing as we did for the other bubble
temporaryBubble = self.getBubbleView(frame, number: start + 2)
labelView = temporaryBubble.subviews[0] as UILabel
labelView.removeFromSuperview() // remove it so we can add it overtop of the new filling bubble
temporaryBubble.frame = newFrame
temporaryBubble.backgroundColor = self.bubbleBackgroundColorForNextToComplete
tempBubbleCornerRadius = temporaryBubble.layer.cornerRadius
temporaryBubble.layer.cornerRadius = 0.0
self.bubbleArray[start+1].addSubview(temporaryBubble)
self.bubbleArray[start+1].addSubview(labelView)
UIView.animateWithDuration(0.4, animations: { () -> Void in
temporaryBubble.frame = CGRectMake(0, 0, self.bubbleArray[start+1].frame.size.width, self.bubbleArray[start+1].frame.size.height)
temporaryBubble.layer.cornerRadius = tempBubbleCornerRadius
}, completion: { (finished) -> Void in
self.bubbleArray[start+1].backgroundColor = self.bubbleBackgroundColorForNextToComplete
temporaryBubble.removeFromSuperview()
labelView.removeFromSuperview()
if (start+1 < numBubbleCompleted) {
self.checkBubbleCompleted(numBubbleCompleted, start: self.lastBubbleCompleted++)
} else {
self.removeAnimatedBubbleFromQueueAndContinue()
}
})
} else {
self.removeAnimatedBubbleFromQueueAndContinue()
}
})
}
}
| mit | d11e65d70de5b3a1ca561ad06a352ebb | 49.063333 | 181 | 0.617085 | 5.583271 | false | false | false | false |
eofster/Telephone | UseCases/LazyDiscardingContactMatchingIndex.swift | 1 | 1589 | //
// LazyDiscardingContactMatchingIndex.swift
// Telephone
//
// Copyright © 2008-2016 Alexey Kuznetsov
// Copyright © 2016-2021 64 Characters
//
// Telephone is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// Telephone is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
public final class LazyDiscardingContactMatchingIndex {
private var origin: ContactMatchingIndex!
private let factory: ContactMatchingIndexFactory
public init(factory: ContactMatchingIndexFactory) {
self.factory = factory
}
private func createOriginIfNeeded() {
if origin == nil {
origin = factory.make()
}
}
}
extension LazyDiscardingContactMatchingIndex: ContactMatchingIndex {
public func contact(forPhone phone: ExtractedPhoneNumber) -> MatchedContact? {
createOriginIfNeeded()
return origin.contact(forPhone: phone)
}
public func contact(forEmail email: NormalizedLowercasedString) -> MatchedContact? {
createOriginIfNeeded()
return origin.contact(forEmail: email)
}
}
extension LazyDiscardingContactMatchingIndex: ContactsChangeEventTarget {
public func contactsDidChange() {
origin = nil
}
}
| gpl-3.0 | cf13f1c2248579b8f8293295bec93b1b | 30.117647 | 88 | 0.720857 | 4.823708 | false | false | false | false |
aschwaighofer/swift | stdlib/public/core/SequenceAlgorithms.swift | 1 | 31714 | //===--- SequenceAlgorithms.swift -----------------------------*- swift -*-===//
//
// This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2017 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See https://swift.org/LICENSE.txt for license information
// See https://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
//===----------------------------------------------------------------------===//
// enumerated()
//===----------------------------------------------------------------------===//
extension Sequence {
/// Returns a sequence of pairs (*n*, *x*), where *n* represents a
/// consecutive integer starting at zero and *x* represents an element of
/// the sequence.
///
/// This example enumerates the characters of the string "Swift" and prints
/// each character along with its place in the string.
///
/// for (n, c) in "Swift".enumerated() {
/// print("\(n): '\(c)'")
/// }
/// // Prints "0: 'S'"
/// // Prints "1: 'w'"
/// // Prints "2: 'i'"
/// // Prints "3: 'f'"
/// // Prints "4: 't'"
///
/// When you enumerate a collection, the integer part of each pair is a counter
/// for the enumeration, but is not necessarily the index of the paired value.
/// These counters can be used as indices only in instances of zero-based,
/// integer-indexed collections, such as `Array` and `ContiguousArray`. For
/// other collections the counters may be out of range or of the wrong type
/// to use as an index. To iterate over the elements of a collection with its
/// indices, use the `zip(_:_:)` function.
///
/// This example iterates over the indices and elements of a set, building a
/// list consisting of indices of names with five or fewer letters.
///
/// let names: Set = ["Sofia", "Camilla", "Martina", "Mateo", "Nicolás"]
/// var shorterIndices: [Set<String>.Index] = []
/// for (i, name) in zip(names.indices, names) {
/// if name.count <= 5 {
/// shorterIndices.append(i)
/// }
/// }
///
/// Now that the `shorterIndices` array holds the indices of the shorter
/// names in the `names` set, you can use those indices to access elements in
/// the set.
///
/// for i in shorterIndices {
/// print(names[i])
/// }
/// // Prints "Sofia"
/// // Prints "Mateo"
///
/// - Returns: A sequence of pairs enumerating the sequence.
///
/// - Complexity: O(1)
@inlinable // protocol-only
public func enumerated() -> EnumeratedSequence<Self> {
return EnumeratedSequence(_base: self)
}
}
//===----------------------------------------------------------------------===//
// min(), max()
//===----------------------------------------------------------------------===//
extension Sequence {
/// Returns the minimum element in the sequence, using the given predicate as
/// the comparison between elements.
///
/// The predicate must be a *strict weak ordering* over the elements. That
/// is, for any elements `a`, `b`, and `c`, the following conditions must
/// hold:
///
/// - `areInIncreasingOrder(a, a)` is always `false`. (Irreflexivity)
/// - If `areInIncreasingOrder(a, b)` and `areInIncreasingOrder(b, c)` are
/// both `true`, then `areInIncreasingOrder(a, c)` is also
/// `true`. (Transitive comparability)
/// - Two elements are *incomparable* if neither is ordered before the other
/// according to the predicate. If `a` and `b` are incomparable, and `b`
/// and `c` are incomparable, then `a` and `c` are also incomparable.
/// (Transitive incomparability)
///
/// This example shows how to use the `min(by:)` method on a
/// dictionary to find the key-value pair with the lowest value.
///
/// let hues = ["Heliotrope": 296, "Coral": 16, "Aquamarine": 156]
/// let leastHue = hues.min { a, b in a.value < b.value }
/// print(leastHue)
/// // Prints "Optional((key: "Coral", value: 16))"
///
/// - Parameter areInIncreasingOrder: A predicate that returns `true`
/// if its first argument should be ordered before its second
/// argument; otherwise, `false`.
/// - Returns: The sequence's minimum element, according to
/// `areInIncreasingOrder`. If the sequence has no elements, returns
/// `nil`.
///
/// - Complexity: O(*n*), where *n* is the length of the sequence.
@inlinable // protocol-only
@warn_unqualified_access
public func min(
by areInIncreasingOrder: (Element, Element) throws -> Bool
) rethrows -> Element? {
var it = makeIterator()
guard var result = it.next() else { return nil }
while let e = it.next() {
if try areInIncreasingOrder(e, result) { result = e }
}
return result
}
/// Returns the maximum element in the sequence, using the given predicate
/// as the comparison between elements.
///
/// The predicate must be a *strict weak ordering* over the elements. That
/// is, for any elements `a`, `b`, and `c`, the following conditions must
/// hold:
///
/// - `areInIncreasingOrder(a, a)` is always `false`. (Irreflexivity)
/// - If `areInIncreasingOrder(a, b)` and `areInIncreasingOrder(b, c)` are
/// both `true`, then `areInIncreasingOrder(a, c)` is also
/// `true`. (Transitive comparability)
/// - Two elements are *incomparable* if neither is ordered before the other
/// according to the predicate. If `a` and `b` are incomparable, and `b`
/// and `c` are incomparable, then `a` and `c` are also incomparable.
/// (Transitive incomparability)
///
/// This example shows how to use the `max(by:)` method on a
/// dictionary to find the key-value pair with the highest value.
///
/// let hues = ["Heliotrope": 296, "Coral": 16, "Aquamarine": 156]
/// let greatestHue = hues.max { a, b in a.value < b.value }
/// print(greatestHue)
/// // Prints "Optional((key: "Heliotrope", value: 296))"
///
/// - Parameter areInIncreasingOrder: A predicate that returns `true` if its
/// first argument should be ordered before its second argument;
/// otherwise, `false`.
/// - Returns: The sequence's maximum element if the sequence is not empty;
/// otherwise, `nil`.
///
/// - Complexity: O(*n*), where *n* is the length of the sequence.
@inlinable // protocol-only
@warn_unqualified_access
public func max(
by areInIncreasingOrder: (Element, Element) throws -> Bool
) rethrows -> Element? {
var it = makeIterator()
guard var result = it.next() else { return nil }
while let e = it.next() {
if try areInIncreasingOrder(result, e) { result = e }
}
return result
}
}
extension Sequence where Element: Comparable {
/// Returns the minimum element in the sequence.
///
/// This example finds the smallest value in an array of height measurements.
///
/// let heights = [67.5, 65.7, 64.3, 61.1, 58.5, 60.3, 64.9]
/// let lowestHeight = heights.min()
/// print(lowestHeight)
/// // Prints "Optional(58.5)"
///
/// - Returns: The sequence's minimum element. If the sequence has no
/// elements, returns `nil`.
///
/// - Complexity: O(*n*), where *n* is the length of the sequence.
@inlinable
@warn_unqualified_access
public func min() -> Element? {
return self.min(by: <)
}
/// Returns the maximum element in the sequence.
///
/// This example finds the largest value in an array of height measurements.
///
/// let heights = [67.5, 65.7, 64.3, 61.1, 58.5, 60.3, 64.9]
/// let greatestHeight = heights.max()
/// print(greatestHeight)
/// // Prints "Optional(67.5)"
///
/// - Returns: The sequence's maximum element. If the sequence has no
/// elements, returns `nil`.
///
/// - Complexity: O(*n*), where *n* is the length of the sequence.
@inlinable
@warn_unqualified_access
public func max() -> Element? {
return self.max(by: <)
}
}
//===----------------------------------------------------------------------===//
// starts(with:)
//===----------------------------------------------------------------------===//
extension Sequence {
/// Returns a Boolean value indicating whether the initial elements of the
/// sequence are equivalent to the elements in another sequence, using
/// the given predicate as the equivalence test.
///
/// The predicate must be a *equivalence relation* over the elements. That
/// is, for any elements `a`, `b`, and `c`, the following conditions must
/// hold:
///
/// - `areEquivalent(a, a)` is always `true`. (Reflexivity)
/// - `areEquivalent(a, b)` implies `areEquivalent(b, a)`. (Symmetry)
/// - If `areEquivalent(a, b)` and `areEquivalent(b, c)` are both `true`, then
/// `areEquivalent(a, c)` is also `true`. (Transitivity)
///
/// - Parameters:
/// - possiblePrefix: A sequence to compare to this sequence.
/// - areEquivalent: A predicate that returns `true` if its two arguments
/// are equivalent; otherwise, `false`.
/// - Returns: `true` if the initial elements of the sequence are equivalent
/// to the elements of `possiblePrefix`; otherwise, `false`. If
/// `possiblePrefix` has no elements, the return value is `true`.
///
/// - Complexity: O(*m*), where *m* is the lesser of the length of the
/// sequence and the length of `possiblePrefix`.
@inlinable
public func starts<PossiblePrefix: Sequence>(
with possiblePrefix: PossiblePrefix,
by areEquivalent: (Element, PossiblePrefix.Element) throws -> Bool
) rethrows -> Bool {
var possiblePrefixIterator = possiblePrefix.makeIterator()
for e0 in self {
if let e1 = possiblePrefixIterator.next() {
if try !areEquivalent(e0, e1) {
return false
}
}
else {
return true
}
}
return possiblePrefixIterator.next() == nil
}
}
extension Sequence where Element: Equatable {
/// Returns a Boolean value indicating whether the initial elements of the
/// sequence are the same as the elements in another sequence.
///
/// This example tests whether one countable range begins with the elements
/// of another countable range.
///
/// let a = 1...3
/// let b = 1...10
///
/// print(b.starts(with: a))
/// // Prints "true"
///
/// Passing a sequence with no elements or an empty collection as
/// `possiblePrefix` always results in `true`.
///
/// print(b.starts(with: []))
/// // Prints "true"
///
/// - Parameter possiblePrefix: A sequence to compare to this sequence.
/// - Returns: `true` if the initial elements of the sequence are the same as
/// the elements of `possiblePrefix`; otherwise, `false`. If
/// `possiblePrefix` has no elements, the return value is `true`.
///
/// - Complexity: O(*m*), where *m* is the lesser of the length of the
/// sequence and the length of `possiblePrefix`.
@inlinable
public func starts<PossiblePrefix: Sequence>(
with possiblePrefix: PossiblePrefix
) -> Bool where PossiblePrefix.Element == Element {
return self.starts(with: possiblePrefix, by: ==)
}
}
//===----------------------------------------------------------------------===//
// elementsEqual()
//===----------------------------------------------------------------------===//
extension Sequence {
/// Returns a Boolean value indicating whether this sequence and another
/// sequence contain equivalent elements in the same order, using the given
/// predicate as the equivalence test.
///
/// At least one of the sequences must be finite.
///
/// The predicate must be a *equivalence relation* over the elements. That
/// is, for any elements `a`, `b`, and `c`, the following conditions must
/// hold:
///
/// - `areEquivalent(a, a)` is always `true`. (Reflexivity)
/// - `areEquivalent(a, b)` implies `areEquivalent(b, a)`. (Symmetry)
/// - If `areEquivalent(a, b)` and `areEquivalent(b, c)` are both `true`, then
/// `areEquivalent(a, c)` is also `true`. (Transitivity)
///
/// - Parameters:
/// - other: A sequence to compare to this sequence.
/// - areEquivalent: A predicate that returns `true` if its two arguments
/// are equivalent; otherwise, `false`.
/// - Returns: `true` if this sequence and `other` contain equivalent items,
/// using `areEquivalent` as the equivalence test; otherwise, `false.`
///
/// - Complexity: O(*m*), where *m* is the lesser of the length of the
/// sequence and the length of `other`.
@inlinable
public func elementsEqual<OtherSequence: Sequence>(
_ other: OtherSequence,
by areEquivalent: (Element, OtherSequence.Element) throws -> Bool
) rethrows -> Bool {
var iter1 = self.makeIterator()
var iter2 = other.makeIterator()
while true {
switch (iter1.next(), iter2.next()) {
case let (e1?, e2?):
if try !areEquivalent(e1, e2) {
return false
}
case (_?, nil), (nil, _?): return false
case (nil, nil): return true
}
}
}
}
extension Sequence where Element: Equatable {
/// Returns a Boolean value indicating whether this sequence and another
/// sequence contain the same elements in the same order.
///
/// At least one of the sequences must be finite.
///
/// This example tests whether one countable range shares the same elements
/// as another countable range and an array.
///
/// let a = 1...3
/// let b = 1...10
///
/// print(a.elementsEqual(b))
/// // Prints "false"
/// print(a.elementsEqual([1, 2, 3]))
/// // Prints "true"
///
/// - Parameter other: A sequence to compare to this sequence.
/// - Returns: `true` if this sequence and `other` contain the same elements
/// in the same order.
///
/// - Complexity: O(*m*), where *m* is the lesser of the length of the
/// sequence and the length of `other`.
@inlinable
public func elementsEqual<OtherSequence: Sequence>(
_ other: OtherSequence
) -> Bool where OtherSequence.Element == Element {
return self.elementsEqual(other, by: ==)
}
}
//===----------------------------------------------------------------------===//
// lexicographicallyPrecedes()
//===----------------------------------------------------------------------===//
extension Sequence {
/// Returns a Boolean value indicating whether the sequence precedes another
/// sequence in a lexicographical (dictionary) ordering, using the given
/// predicate to compare elements.
///
/// The predicate must be a *strict weak ordering* over the elements. That
/// is, for any elements `a`, `b`, and `c`, the following conditions must
/// hold:
///
/// - `areInIncreasingOrder(a, a)` is always `false`. (Irreflexivity)
/// - If `areInIncreasingOrder(a, b)` and `areInIncreasingOrder(b, c)` are
/// both `true`, then `areInIncreasingOrder(a, c)` is also
/// `true`. (Transitive comparability)
/// - Two elements are *incomparable* if neither is ordered before the other
/// according to the predicate. If `a` and `b` are incomparable, and `b`
/// and `c` are incomparable, then `a` and `c` are also incomparable.
/// (Transitive incomparability)
///
/// - Parameters:
/// - other: A sequence to compare to this sequence.
/// - areInIncreasingOrder: A predicate that returns `true` if its first
/// argument should be ordered before its second argument; otherwise,
/// `false`.
/// - Returns: `true` if this sequence precedes `other` in a dictionary
/// ordering as ordered by `areInIncreasingOrder`; otherwise, `false`.
///
/// - Note: This method implements the mathematical notion of lexicographical
/// ordering, which has no connection to Unicode. If you are sorting
/// strings to present to the end user, use `String` APIs that perform
/// localized comparison instead.
///
/// - Complexity: O(*m*), where *m* is the lesser of the length of the
/// sequence and the length of `other`.
@inlinable
public func lexicographicallyPrecedes<OtherSequence: Sequence>(
_ other: OtherSequence,
by areInIncreasingOrder: (Element, Element) throws -> Bool
) rethrows -> Bool
where OtherSequence.Element == Element {
var iter1 = self.makeIterator()
var iter2 = other.makeIterator()
while true {
if let e1 = iter1.next() {
if let e2 = iter2.next() {
if try areInIncreasingOrder(e1, e2) {
return true
}
if try areInIncreasingOrder(e2, e1) {
return false
}
continue // Equivalent
}
return false
}
return iter2.next() != nil
}
}
}
extension Sequence where Element: Comparable {
/// Returns a Boolean value indicating whether the sequence precedes another
/// sequence in a lexicographical (dictionary) ordering, using the
/// less-than operator (`<`) to compare elements.
///
/// This example uses the `lexicographicallyPrecedes` method to test which
/// array of integers comes first in a lexicographical ordering.
///
/// let a = [1, 2, 2, 2]
/// let b = [1, 2, 3, 4]
///
/// print(a.lexicographicallyPrecedes(b))
/// // Prints "true"
/// print(b.lexicographicallyPrecedes(b))
/// // Prints "false"
///
/// - Parameter other: A sequence to compare to this sequence.
/// - Returns: `true` if this sequence precedes `other` in a dictionary
/// ordering; otherwise, `false`.
///
/// - Note: This method implements the mathematical notion of lexicographical
/// ordering, which has no connection to Unicode. If you are sorting
/// strings to present to the end user, use `String` APIs that
/// perform localized comparison.
///
/// - Complexity: O(*m*), where *m* is the lesser of the length of the
/// sequence and the length of `other`.
@inlinable
public func lexicographicallyPrecedes<OtherSequence: Sequence>(
_ other: OtherSequence
) -> Bool where OtherSequence.Element == Element {
return self.lexicographicallyPrecedes(other, by: <)
}
}
//===----------------------------------------------------------------------===//
// contains()
//===----------------------------------------------------------------------===//
extension Sequence {
/// Returns a Boolean value indicating whether the sequence contains an
/// element that satisfies the given predicate.
///
/// You can use the predicate to check for an element of a type that
/// doesn't conform to the `Equatable` protocol, such as the
/// `HTTPResponse` enumeration in this example.
///
/// enum HTTPResponse {
/// case ok
/// case error(Int)
/// }
///
/// let lastThreeResponses: [HTTPResponse] = [.ok, .ok, .error(404)]
/// let hadError = lastThreeResponses.contains { element in
/// if case .error = element {
/// return true
/// } else {
/// return false
/// }
/// }
/// // 'hadError' == true
///
/// Alternatively, a predicate can be satisfied by a range of `Equatable`
/// elements or a general condition. This example shows how you can check an
/// array for an expense greater than $100.
///
/// let expenses = [21.37, 55.21, 9.32, 10.18, 388.77, 11.41]
/// let hasBigPurchase = expenses.contains { $0 > 100 }
/// // 'hasBigPurchase' == true
///
/// - Parameter predicate: A closure that takes an element of the sequence
/// as its argument and returns a Boolean value that indicates whether
/// the passed element represents a match.
/// - Returns: `true` if the sequence contains an element that satisfies
/// `predicate`; otherwise, `false`.
///
/// - Complexity: O(*n*), where *n* is the length of the sequence.
@inlinable
public func contains(
where predicate: (Element) throws -> Bool
) rethrows -> Bool {
for e in self {
if try predicate(e) {
return true
}
}
return false
}
/// Returns a Boolean value indicating whether every element of a sequence
/// satisfies a given predicate.
///
/// The following code uses this method to test whether all the names in an
/// array have at least five characters:
///
/// let names = ["Sofia", "Camilla", "Martina", "Mateo", "Nicolás"]
/// let allHaveAtLeastFive = names.allSatisfy({ $0.count >= 5 })
/// // allHaveAtLeastFive == true
///
/// - Parameter predicate: A closure that takes an element of the sequence
/// as its argument and returns a Boolean value that indicates whether
/// the passed element satisfies a condition.
/// - Returns: `true` if the sequence contains only elements that satisfy
/// `predicate`; otherwise, `false`.
///
/// - Complexity: O(*n*), where *n* is the length of the sequence.
@inlinable
public func allSatisfy(
_ predicate: (Element) throws -> Bool
) rethrows -> Bool {
return try !contains { try !predicate($0) }
}
}
extension Sequence where Element: Equatable {
/// Returns a Boolean value indicating whether the sequence contains the
/// given element.
///
/// This example checks to see whether a favorite actor is in an array
/// storing a movie's cast.
///
/// let cast = ["Vivien", "Marlon", "Kim", "Karl"]
/// print(cast.contains("Marlon"))
/// // Prints "true"
/// print(cast.contains("James"))
/// // Prints "false"
///
/// - Parameter element: The element to find in the sequence.
/// - Returns: `true` if the element was found in the sequence; otherwise,
/// `false`.
///
/// - Complexity: O(*n*), where *n* is the length of the sequence.
@inlinable
public func contains(_ element: Element) -> Bool {
if let result = _customContainsEquatableElement(element) {
return result
} else {
return self.contains { $0 == element }
}
}
}
//===----------------------------------------------------------------------===//
// reduce()
//===----------------------------------------------------------------------===//
extension Sequence {
/// Returns the result of combining the elements of the sequence using the
/// given closure.
///
/// Use the `reduce(_:_:)` method to produce a single value from the elements
/// of an entire sequence. For example, you can use this method on an array
/// of numbers to find their sum or product.
///
/// The `nextPartialResult` closure is called sequentially with an
/// accumulating value initialized to `initialResult` and each element of
/// the sequence. This example shows how to find the sum of an array of
/// numbers.
///
/// let numbers = [1, 2, 3, 4]
/// let numberSum = numbers.reduce(0, { x, y in
/// x + y
/// })
/// // numberSum == 10
///
/// When `numbers.reduce(_:_:)` is called, the following steps occur:
///
/// 1. The `nextPartialResult` closure is called with `initialResult`---`0`
/// in this case---and the first element of `numbers`, returning the sum:
/// `1`.
/// 2. The closure is called again repeatedly with the previous call's return
/// value and each element of the sequence.
/// 3. When the sequence is exhausted, the last value returned from the
/// closure is returned to the caller.
///
/// If the sequence has no elements, `nextPartialResult` is never executed
/// and `initialResult` is the result of the call to `reduce(_:_:)`.
///
/// - Parameters:
/// - initialResult: The value to use as the initial accumulating value.
/// `initialResult` is passed to `nextPartialResult` the first time the
/// closure is executed.
/// - nextPartialResult: A closure that combines an accumulating value and
/// an element of the sequence into a new accumulating value, to be used
/// in the next call of the `nextPartialResult` closure or returned to
/// the caller.
/// - Returns: The final accumulated value. If the sequence has no elements,
/// the result is `initialResult`.
///
/// - Complexity: O(*n*), where *n* is the length of the sequence.
@inlinable
public func reduce<Result>(
_ initialResult: Result,
_ nextPartialResult:
(_ partialResult: Result, Element) throws -> Result
) rethrows -> Result {
var accumulator = initialResult
for element in self {
accumulator = try nextPartialResult(accumulator, element)
}
return accumulator
}
/// Returns the result of combining the elements of the sequence using the
/// given closure.
///
/// Use the `reduce(into:_:)` method to produce a single value from the
/// elements of an entire sequence. For example, you can use this method on an
/// array of integers to filter adjacent equal entries or count frequencies.
///
/// This method is preferred over `reduce(_:_:)` for efficiency when the
/// result is a copy-on-write type, for example an Array or a Dictionary.
///
/// The `updateAccumulatingResult` closure is called sequentially with a
/// mutable accumulating value initialized to `initialResult` and each element
/// of the sequence. This example shows how to build a dictionary of letter
/// frequencies of a string.
///
/// let letters = "abracadabra"
/// let letterCount = letters.reduce(into: [:]) { counts, letter in
/// counts[letter, default: 0] += 1
/// }
/// // letterCount == ["a": 5, "b": 2, "r": 2, "c": 1, "d": 1]
///
/// When `letters.reduce(into:_:)` is called, the following steps occur:
///
/// 1. The `updateAccumulatingResult` closure is called with the initial
/// accumulating value---`[:]` in this case---and the first character of
/// `letters`, modifying the accumulating value by setting `1` for the key
/// `"a"`.
/// 2. The closure is called again repeatedly with the updated accumulating
/// value and each element of the sequence.
/// 3. When the sequence is exhausted, the accumulating value is returned to
/// the caller.
///
/// If the sequence has no elements, `updateAccumulatingResult` is never
/// executed and `initialResult` is the result of the call to
/// `reduce(into:_:)`.
///
/// - Parameters:
/// - initialResult: The value to use as the initial accumulating value.
/// - updateAccumulatingResult: A closure that updates the accumulating
/// value with an element of the sequence.
/// - Returns: The final accumulated value. If the sequence has no elements,
/// the result is `initialResult`.
///
/// - Complexity: O(*n*), where *n* is the length of the sequence.
@inlinable
public func reduce<Result>(
into initialResult: __owned Result,
_ updateAccumulatingResult:
(_ partialResult: inout Result, Element) throws -> ()
) rethrows -> Result {
var accumulator = initialResult
for element in self {
try updateAccumulatingResult(&accumulator, element)
}
return accumulator
}
}
//===----------------------------------------------------------------------===//
// reversed()
//===----------------------------------------------------------------------===//
extension Sequence {
/// Returns an array containing the elements of this sequence in reverse
/// order.
///
/// The sequence must be finite.
///
/// - Returns: An array containing the elements of this sequence in
/// reverse order.
///
/// - Complexity: O(*n*), where *n* is the length of the sequence.
@inlinable
public __consuming func reversed() -> [Element] {
// FIXME(performance): optimize to 1 pass? But Array(self) can be
// optimized to a memcpy() sometimes. Those cases are usually collections,
// though.
var result = Array(self)
let count = result.count
for i in 0..<count/2 {
result.swapAt(i, count - ((i + 1) as Int))
}
return result
}
}
//===----------------------------------------------------------------------===//
// flatMap()
//===----------------------------------------------------------------------===//
extension Sequence {
/// Returns an array containing the concatenated results of calling the
/// given transformation with each element of this sequence.
///
/// Use this method to receive a single-level collection when your
/// transformation produces a sequence or collection for each element.
///
/// In this example, note the difference in the result of using `map` and
/// `flatMap` with a transformation that returns an array.
///
/// let numbers = [1, 2, 3, 4]
///
/// let mapped = numbers.map { Array(repeating: $0, count: $0) }
/// // [[1], [2, 2], [3, 3, 3], [4, 4, 4, 4]]
///
/// let flatMapped = numbers.flatMap { Array(repeating: $0, count: $0) }
/// // [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
///
/// In fact, `s.flatMap(transform)` is equivalent to
/// `Array(s.map(transform).joined())`.
///
/// - Parameter transform: A closure that accepts an element of this
/// sequence as its argument and returns a sequence or collection.
/// - Returns: The resulting flattened array.
///
/// - Complexity: O(*m* + *n*), where *n* is the length of this sequence
/// and *m* is the length of the result.
@inlinable
public func flatMap<SegmentOfResult: Sequence>(
_ transform: (Element) throws -> SegmentOfResult
) rethrows -> [SegmentOfResult.Element] {
var result: [SegmentOfResult.Element] = []
for element in self {
result.append(contentsOf: try transform(element))
}
return result
}
}
extension Sequence {
/// Returns an array containing the non-`nil` results of calling the given
/// transformation with each element of this sequence.
///
/// Use this method to receive an array of non-optional values when your
/// transformation produces an optional value.
///
/// In this example, note the difference in the result of using `map` and
/// `compactMap` with a transformation that returns an optional `Int` value.
///
/// let possibleNumbers = ["1", "2", "three", "///4///", "5"]
///
/// let mapped: [Int?] = possibleNumbers.map { str in Int(str) }
/// // [1, 2, nil, nil, 5]
///
/// let compactMapped: [Int] = possibleNumbers.compactMap { str in Int(str) }
/// // [1, 2, 5]
///
/// - Parameter transform: A closure that accepts an element of this
/// sequence as its argument and returns an optional value.
/// - Returns: An array of the non-`nil` results of calling `transform`
/// with each element of the sequence.
///
/// - Complexity: O(*m* + *n*), where *n* is the length of this sequence
/// and *m* is the length of the result.
@inlinable // protocol-only
public func compactMap<ElementOfResult>(
_ transform: (Element) throws -> ElementOfResult?
) rethrows -> [ElementOfResult] {
return try _compactMap(transform)
}
// The implementation of flatMap accepting a closure with an optional result.
// Factored out into a separate functions in order to be used in multiple
// overloads.
@inlinable // protocol-only
@inline(__always)
public func _compactMap<ElementOfResult>(
_ transform: (Element) throws -> ElementOfResult?
) rethrows -> [ElementOfResult] {
var result: [ElementOfResult] = []
for element in self {
if let newElement = try transform(element) {
result.append(newElement)
}
}
return result
}
}
| apache-2.0 | c90c62c52493e76ebd1f11c91f31a675 | 38.00615 | 83 | 0.602895 | 4.356642 | false | false | false | false |
allevato/SwiftCGI | Sources/SwiftCGI/CGIServer.swift | 1 | 2772 | // Copyright 2015 Tony Allevato
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
import Foundation
#if os(Linux)
import Glibc
#else
import Darwin.C
#endif
/// The server implementation used when the application is running as a CGI process.
class CGIServer: ServerProtocol {
/// The environment variable dictionary that contains the headers for the request.
private let environment: [String: String]
/// The input stream from which the request body can be read.
private let requestStream: InputStream
/// The output stream to which the response body can be written.
private let responseStream: OutputStream
/// Creates a new server with the given environment, request stream, and response stream.
///
/// This initializer exists primarily for testing.
///
/// - Parameter environment: The dictionary containing the environment variables from which the
/// request headers will be read.
/// - Parameter requestStream: The input stream from which the request body can be read.
/// - Parameter responseStream: The output stream to which the response body can be written.
init(environment: [String: String], requestStream: InputStream, responseStream: OutputStream) {
self.environment = environment
self.requestStream = requestStream
self.responseStream = responseStream
}
/// Creates a new server that takes its environment from the process's environment and that uses
/// the `stdin` and `stdout` file descriptors as its request and response streams, respectively.
convenience init() {
let process = NSProcessInfo.processInfo()
let environment = process.environment
let requestStream =
BufferingInputStream(inputStream: FileInputStream(fileDescriptor: STDIN_FILENO))
let responseStream =
BufferingOutputStream(outputStream: FileOutputStream(fileDescriptor: STDOUT_FILENO))
self.init(
environment: environment, requestStream: requestStream, responseStream: responseStream)
}
func listen(handler: (HTTPRequest, HTTPResponse) -> Void) {
let request = CGIHTTPRequest(environment: environment, contentStream: requestStream)
let response = CGIHTTPResponse(contentStream: responseStream)
handler(request, response)
responseStream.flush()
}
}
| apache-2.0 | 45336da1048e24997aa7248c7c3a784f | 37.5 | 98 | 0.750722 | 4.958855 | false | false | false | false |
dasdom/Swiftandpainless | SwiftAndPainlessPlayground.playground/Pages/Functions - Basics III.xcplaygroundpage/Contents.swift | 1 | 525 | import Foundation
/*:
[⬅️](@previous) [➡️](@next)
# Functions: Basics III
## Returning Tuples
*/
func minMax(numbers: Int...) -> (min: Int, max: Int) {
precondition(numbers.count > 0)
var min = Int.max
var max = Int.min
for number in numbers {
if number <= min {
min = number
}
if number >= max {
max = number
}
}
return (min, max)
}
let result = minMax(numbers: 23, 3, 42, 5, 666)
let min = result.min
let max = result.max
print("min: \(result.0), max: \(result.1)")
| mit | cc2cdc222ce33587e0d39300aa2346fd | 18.148148 | 54 | 0.576402 | 3.191358 | false | false | false | false |
davecom/SwiftSimpleNeuralNetwork | Sources/Layer.swift | 1 | 3056 | //
// Layer.swift
// SwiftSimpleNeuralNetwork
//
// Copyright 2016-2019 David Kopec
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
class Layer {
let previousLayer: Layer?
var neurons: [Neuron]
var outputCache: [Double]
var hasBias: Bool = false
// for future use in deserializing networks
init(previousLayer: Layer? = nil, neurons: [Neuron] = [Neuron]()) {
self.previousLayer = previousLayer
self.neurons = neurons
self.outputCache = Array<Double>(repeating: 0.0, count: neurons.count)
}
// main init
init(previousLayer: Layer? = nil, numNeurons: Int, activationFunction: @escaping (Double) -> Double, derivativeActivationFunction: @escaping (Double)-> Double, learningRate: Double, hasBias: Bool = false) {
self.previousLayer = previousLayer
self.neurons = Array<Neuron>()
self.hasBias = hasBias
for _ in 0..<numNeurons {
self.neurons.append(Neuron(weights: randomWeights(number: previousLayer?.neurons.count ?? 0), activationFunction: activationFunction, derivativeActivationFunction: derivativeActivationFunction, learningRate: learningRate))
}
if hasBias {
self.neurons.append(BiasNeuron(weights: randomWeights(number: previousLayer?.neurons.count ?? 0)))
}
self.outputCache = Array<Double>(repeating: 0.0, count: neurons.count)
}
func outputs(inputs: [Double]) -> [Double] {
if previousLayer == nil { // input layer (first layer)
outputCache = hasBias ? inputs + [1.0] : inputs
} else { // hidden layer or output layer
outputCache = neurons.map { $0.output(inputs: inputs) }
}
return outputCache
}
// should only be called on an output layer
func calculateDeltasForOutputLayer(expected: [Double]) {
for n in 0..<neurons.count {
neurons[n].delta = neurons[n].derivativeActivationFunction( neurons[n].inputCache) * (expected[n] - outputCache[n])
}
}
// should not be called on output layer
func calculateDeltasForHiddenLayer(nextLayer: Layer) {
for (index, neuron) in neurons.enumerated() {
let nextWeights = nextLayer.neurons.map { $0.weights[index] }
let nextDeltas = nextLayer.neurons.map { $0.delta }
let sumOfWeightsXDeltas = dotProduct(nextWeights, nextDeltas)
neuron.delta = neuron.derivativeActivationFunction( neuron.inputCache) * sumOfWeightsXDeltas
}
}
}
| apache-2.0 | 9c319aa93bed90eaf1d1a17e93569bd3 | 40.863014 | 234 | 0.663285 | 4.353276 | false | false | false | false |
jeffreybergier/Hipstapaper | Hipstapaper/Packages/V3Localize/Sources/V3Localize/PropertyWrappers/BrowserShareList.swift | 1 | 2568 | //
// Created by Jeffrey Bergier on 2022/08/03.
//
// MIT License
//
// Copyright (c) 2021 Jeffrey Bergier
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
//
import SwiftUI
import Umbrella
@propertyWrapper
public struct BrowserShareList: DynamicProperty {
public struct Value {
public var title: LocalizedString
public var shareErrorSubtitle: LocalizedString
public var done: ActionLocalization
public var copy: ActionLocalization
public var error: ActionLocalization
public var shareSaved: ActionLocalization
public var shareCurrent: ActionLocalization
internal init(_ b: LocalizeBundle) {
self.title = b.localized(key: Noun.share.rawValue)
self.shareErrorSubtitle = b.localized(key: Phrase.shareError.rawValue)
self.done = Action.doneGeneric.localized(b)
self.copy = Action.copyToClipboard.localized(b)
self.error = Action.shareError.localized(b)
self.shareSaved = Action.shareSingleSaved.localized(b)
self.shareCurrent = Action.shareSingleCurrent.localized(b)
}
}
@Localize private var bundle
public init() {}
public var wrappedValue: Value {
Value(self.bundle)
}
public func key(_ input: LocalizationKey) -> LocalizedString {
self.bundle.localized(key: input)
}
}
| mit | 74393eaf18e8e16a248d5e76642f55b7 | 38.507692 | 82 | 0.669782 | 4.729282 | false | false | false | false |
atrick/swift | stdlib/public/core/UnicodeScalarProperties.swift | 1 | 61934 | //===----------------------------------------------------------------------===//
//
// This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2017 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See https://swift.org/LICENSE.txt for license information
// See https://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
// Exposes advanced properties of Unicode.Scalar defined by the Unicode
// Standard.
//===----------------------------------------------------------------------===//
import SwiftShims
extension Unicode.Scalar {
/// A value that provides access to properties of a Unicode scalar that are
/// defined by the Unicode standard.
public struct Properties: Sendable {
@usableFromInline
internal var _scalar: Unicode.Scalar
internal init(_ scalar: Unicode.Scalar) {
self._scalar = scalar
}
}
/// Properties of this scalar defined by the Unicode standard.
///
/// Use this property to access the Unicode properties of a Unicode scalar
/// value. The following code tests whether a string contains any math
/// symbols:
///
/// let question = "Which is larger, 3 * 3 * 3 or 10 + 10 + 10?"
/// let hasMathSymbols = question.unicodeScalars.contains(where: {
/// $0.properties.isMath
/// })
/// // hasMathSymbols == true
public var properties: Properties {
return Properties(self)
}
}
extension Unicode.Scalar.Properties {
// This OptionSet represents the 64 bit integer value returned when asking
// '_swift_stdlib_getBinaryProperties' where each bit indicates a unique
// Unicode defined binary property of a scalar. For example, bit 8 represents
// the 'isAlphabetic' property for scalars.
//
// WARNING: The values below must be kept in-sync with the generation script.
// If one should ever update this list below, be it reordering bits, adding
// new properties, etc., please update the generation script found at:
// 'utils/gen-unicode-data/Sources/GenScalarProps/BinProps.swift'.
fileprivate struct _BinaryProperties: OptionSet {
let rawValue: UInt64
private init(_ rawValue: UInt64) {
self.rawValue = rawValue
}
// Because we defined the labelless init, we lose the memberwise one
// generated, so define that here to satisfy the 'OptionSet' requirement.
init(rawValue: UInt64) {
self.rawValue = rawValue
}
static var changesWhenCaseFolded : Self { Self(1 &<< 0) }
static var changesWhenCaseMapped : Self { Self(1 &<< 1) }
static var changesWhenLowercased : Self { Self(1 &<< 2) }
static var changesWhenNFKCCaseFolded : Self { Self(1 &<< 3) }
static var changesWhenTitlecased : Self { Self(1 &<< 4) }
static var changesWhenUppercased : Self { Self(1 &<< 5) }
static var isASCIIHexDigit : Self { Self(1 &<< 6) }
static var isAlphabetic : Self { Self(1 &<< 7) }
static var isBidiControl : Self { Self(1 &<< 8) }
static var isBidiMirrored : Self { Self(1 &<< 9) }
static var isCaseIgnorable : Self { Self(1 &<< 10) }
static var isCased : Self { Self(1 &<< 11) }
static var isDash : Self { Self(1 &<< 12) }
static var isDefaultIgnorableCodePoint : Self { Self(1 &<< 13) }
static var isDeprecated : Self { Self(1 &<< 14) }
static var isDiacritic : Self { Self(1 &<< 15) }
static var isEmoji : Self { Self(1 &<< 16) }
static var isEmojiModifier : Self { Self(1 &<< 17) }
static var isEmojiModifierBase : Self { Self(1 &<< 18) }
static var isEmojiPresentation : Self { Self(1 &<< 19) }
static var isExtender : Self { Self(1 &<< 20) }
static var isFullCompositionExclusion : Self { Self(1 &<< 21) }
static var isGraphemeBase : Self { Self(1 &<< 22) }
static var isGraphemeExtend : Self { Self(1 &<< 23) }
static var isHexDigit : Self { Self(1 &<< 24) }
static var isIDContinue : Self { Self(1 &<< 25) }
static var isIDSBinaryOperator : Self { Self(1 &<< 26) }
static var isIDSTrinaryOperator : Self { Self(1 &<< 27) }
static var isIDStart : Self { Self(1 &<< 28) }
static var isIdeographic : Self { Self(1 &<< 29) }
static var isJoinControl : Self { Self(1 &<< 30) }
static var isLogicalOrderException : Self { Self(1 &<< 31) }
static var isLowercase : Self { Self(1 &<< 32) }
static var isMath : Self { Self(1 &<< 33) }
static var isNoncharacterCodePoint : Self { Self(1 &<< 34) }
static var isPatternSyntax : Self { Self(1 &<< 35) }
static var isPatternWhitespace : Self { Self(1 &<< 36) }
static var isQuotationMark : Self { Self(1 &<< 37) }
static var isRadical : Self { Self(1 &<< 38) }
static var isSentenceTerminal : Self { Self(1 &<< 39) }
static var isSoftDotted : Self { Self(1 &<< 40) }
static var isTerminalPunctuation : Self { Self(1 &<< 41) }
static var isUnifiedIdeograph : Self { Self(1 &<< 42) }
static var isUppercase : Self { Self(1 &<< 43) }
static var isVariationSelector : Self { Self(1 &<< 44) }
static var isWhitespace : Self { Self(1 &<< 45) }
static var isXIDContinue : Self { Self(1 &<< 46) }
static var isXIDStart : Self { Self(1 &<< 47) }
}
}
/// Boolean properties that are defined by the Unicode Standard.
extension Unicode.Scalar.Properties {
fileprivate var _binaryProperties: _BinaryProperties {
_BinaryProperties(
rawValue: _swift_stdlib_getBinaryProperties(_scalar.value)
)
}
/// A Boolean value indicating whether the scalar is alphabetic.
///
/// Alphabetic scalars are the primary units of alphabets and/or syllabaries.
///
/// This property corresponds to the "Alphabetic" and the "Other_Alphabetic"
/// properties in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isAlphabetic: Bool {
_binaryProperties.contains(.isAlphabetic)
}
/// A Boolean value indicating whether the scalar is an ASCII character
/// commonly used for the representation of hexadecimal numbers.
///
/// The only scalars for which this property is `true` are:
///
/// * U+0030...U+0039: DIGIT ZERO...DIGIT NINE
/// * U+0041...U+0046: LATIN CAPITAL LETTER A...LATIN CAPITAL LETTER F
/// * U+0061...U+0066: LATIN SMALL LETTER A...LATIN SMALL LETTER F
///
/// This property corresponds to the "ASCII_Hex_Digit" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isASCIIHexDigit: Bool {
_binaryProperties.contains(.isASCIIHexDigit)
}
/// A Boolean value indicating whether the scalar is a format control
/// character that has a specific function in the Unicode Bidirectional
/// Algorithm.
///
/// This property corresponds to the "Bidi_Control" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isBidiControl: Bool {
_binaryProperties.contains(.isBidiControl)
}
/// A Boolean value indicating whether the scalar is mirrored in
/// bidirectional text.
///
/// This property corresponds to the "Bidi_Mirrored" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isBidiMirrored: Bool {
_binaryProperties.contains(.isBidiMirrored)
}
/// A Boolean value indicating whether the scalar is a punctuation
/// symbol explicitly called out as a dash in the Unicode Standard or a
/// compatibility equivalent.
///
/// This property corresponds to the "Dash" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isDash: Bool {
_binaryProperties.contains(.isDash)
}
/// A Boolean value indicating whether the scalar is a default-ignorable
/// code point.
///
/// Default-ignorable code points are those that should be ignored by default
/// in rendering (unless explicitly supported). They have no visible glyph or
/// advance width in and of themselves, although they may affect the display,
/// positioning, or adornment of adjacent or surrounding characters.
///
/// This property corresponds to the "Default_Ignorable_Code_Point" and the
/// "Other_Default_Ignorable_Code_point" properties in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isDefaultIgnorableCodePoint: Bool {
_binaryProperties.contains(.isDefaultIgnorableCodePoint)
}
/// A Boolean value indicating whether the scalar is deprecated.
///
/// Scalars are never removed from the Unicode Standard, but the usage of
/// deprecated scalars is strongly discouraged.
///
/// This property corresponds to the "Deprecated" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isDeprecated: Bool {
_binaryProperties.contains(.isDeprecated)
}
/// A Boolean value indicating whether the scalar is a diacritic.
///
/// Diacritics are scalars that linguistically modify the meaning of another
/// scalar to which they apply. Scalars for which this property is `true` are
/// frequently, but not always, combining marks or modifiers.
///
/// This property corresponds to the "Diacritic" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isDiacritic: Bool {
_binaryProperties.contains(.isDiacritic)
}
/// A Boolean value indicating whether the scalar's principal function is
/// to extend the value or shape of a preceding alphabetic scalar.
///
/// Typical extenders are length and iteration marks.
///
/// This property corresponds to the "Extender" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isExtender: Bool {
_binaryProperties.contains(.isExtender)
}
/// A Boolean value indicating whether the scalar is excluded from
/// composition when performing Unicode normalization.
///
/// This property corresponds to the "Full_Composition_Exclusion" property in
/// the [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isFullCompositionExclusion: Bool {
_binaryProperties.contains(.isFullCompositionExclusion)
}
/// A Boolean value indicating whether the scalar is a grapheme base.
///
/// A grapheme base can be thought of as a space-occupying glyph above or
/// below which other non-spacing modifying glyphs can be applied. For
/// example, when the character `é` is represented in its decomposed form,
/// the grapheme base is "e" (U+0065 LATIN SMALL LETTER E) and it is followed
/// by a single grapheme extender, U+0301 COMBINING ACUTE ACCENT.
///
/// The set of scalars for which `isGraphemeBase` is `true` is disjoint by
/// definition from the set for which `isGraphemeExtend` is `true`.
///
/// This property corresponds to the "Grapheme_Base" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isGraphemeBase: Bool {
_binaryProperties.contains(.isGraphemeBase)
}
/// A Boolean value indicating whether the scalar is a grapheme extender.
///
/// A grapheme extender can be thought of primarily as a non-spacing glyph
/// that is applied above or below another glyph. For example, when the
/// character `é` is represented in its decomposed form, the grapheme base is
/// "e" (U+0065 LATIN SMALL LETTER E) and it is followed by a single grapheme
/// extender, U+0301 COMBINING ACUTE ACCENT.
///
/// The set of scalars for which `isGraphemeExtend` is `true` is disjoint by
/// definition from the set for which `isGraphemeBase` is `true`.
///
/// This property corresponds to the "Grapheme_Extend" and the
/// "Other_Grapheme_Extend" properties in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isGraphemeExtend: Bool {
_binaryProperties.contains(.isGraphemeExtend)
}
/// A Boolean value indicating whether the scalar is one that is commonly
/// used for the representation of hexadecimal numbers or a compatibility
/// equivalent.
///
/// This property is `true` for all scalars for which `isASCIIHexDigit` is
/// `true` as well as for their CJK halfwidth and fullwidth variants.
///
/// This property corresponds to the "Hex_Digit" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isHexDigit: Bool {
_binaryProperties.contains(.isHexDigit)
}
/// A Boolean value indicating whether the scalar is one which is
/// recommended to be allowed to appear in a non-starting position in a
/// programming language identifier.
///
/// Applications that store identifiers in NFKC normalized form should instead
/// use `isXIDContinue` to check whether a scalar is a valid identifier
/// character.
///
/// This property corresponds to the "ID_Continue" and the "Other_ID_Continue"
/// properties in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isIDContinue: Bool {
_binaryProperties.contains(.isIDContinue)
}
/// A Boolean value indicating whether the scalar is one which is
/// recommended to be allowed to appear in a starting position in a
/// programming language identifier.
///
/// Applications that store identifiers in NFKC normalized form should instead
/// use `isXIDStart` to check whether a scalar is a valid identifier
/// character.
///
/// This property corresponds to the "ID_Start" and the "Other_ID_Start"
/// properties in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isIDStart: Bool {
_binaryProperties.contains(.isIDStart)
}
/// A Boolean value indicating whether the scalar is considered to be a
/// CJKV (Chinese, Japanese, Korean, and Vietnamese) or other siniform
/// (Chinese writing-related) ideograph.
///
/// This property roughly defines the class of "Chinese characters" and does
/// not include characters of other logographic scripts such as Cuneiform or
/// Egyptian Hieroglyphs.
///
/// This property corresponds to the "Ideographic" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isIdeographic: Bool {
_binaryProperties.contains(.isIdeographic)
}
/// A Boolean value indicating whether the scalar is an ideographic
/// description character that determines how the two ideographic characters
/// or ideographic description sequences that follow it are to be combined to
/// form a single character.
///
/// Ideographic description characters are technically printable characters,
/// but advanced rendering engines may use them to approximate ideographs that
/// are otherwise unrepresentable.
///
/// This property corresponds to the "IDS_Binary_Operator" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isIDSBinaryOperator: Bool {
_binaryProperties.contains(.isIDSBinaryOperator)
}
/// A Boolean value indicating whether the scalar is an ideographic
/// description character that determines how the three ideographic characters
/// or ideographic description sequences that follow it are to be combined to
/// form a single character.
///
/// Ideographic description characters are technically printable characters,
/// but advanced rendering engines may use them to approximate ideographs that
/// are otherwise unrepresentable.
///
/// This property corresponds to the "IDS_Trinary_Operator" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isIDSTrinaryOperator: Bool {
_binaryProperties.contains(.isIDSTrinaryOperator)
}
/// A Boolean value indicating whether the scalar is a format control
/// character that has a specific function in controlling cursive joining and
/// ligation.
///
/// There are two scalars for which this property is `true`:
///
/// * When U+200C ZERO WIDTH NON-JOINER is inserted between two characters, it
/// directs the rendering engine to render them separately/disconnected when
/// it might otherwise render them as a ligature. For example, a rendering
/// engine might display "fl" in English as a connected glyph; inserting the
/// zero width non-joiner would force them to be rendered as disconnected
/// glyphs.
///
/// * When U+200D ZERO WIDTH JOINER is inserted between two characters, it
/// directs the rendering engine to render them as a connected glyph when it
/// would otherwise render them independently. The zero width joiner is also
/// used to construct complex emoji from sequences of base emoji characters.
/// For example, the various "family" emoji are encoded as sequences of man,
/// woman, or child emoji that are interleaved with zero width joiners.
///
/// This property corresponds to the "Join_Control" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isJoinControl: Bool {
_binaryProperties.contains(.isJoinControl)
}
/// A Boolean value indicating whether the scalar requires special handling
/// for operations involving ordering, such as sorting and searching.
///
/// This property applies to a small number of spacing vowel letters occurring
/// in some Southeast Asian scripts like Thai and Lao, which use a visual
/// order display model. Such letters are stored in text ahead of
/// syllable-initial consonants.
///
/// This property corresponds to the "Logical_Order_Exception" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isLogicalOrderException: Bool {
_binaryProperties.contains(.isLogicalOrderException)
}
/// A Boolean value indicating whether the scalar's letterform is
/// considered lowercase.
///
/// This property corresponds to the "Lowercase" and the "Other_Lowercase"
/// properties in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isLowercase: Bool {
_binaryProperties.contains(.isLowercase)
}
/// A Boolean value indicating whether the scalar is one that naturally
/// appears in mathematical contexts.
///
/// The set of scalars for which this property is `true` includes mathematical
/// operators and symbols as well as specific Greek and Hebrew letter
/// variants that are categorized as symbols. Notably, it does _not_ contain
/// the standard digits or Latin/Greek letter blocks; instead, it contains the
/// mathematical Latin, Greek, and Arabic letters and numbers defined in the
/// Supplemental Multilingual Plane.
///
/// This property corresponds to the "Math" and the "Other_Math" properties in
/// the [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isMath: Bool {
_binaryProperties.contains(.isMath)
}
/// A Boolean value indicating whether the scalar is permanently reserved
/// for internal use.
///
/// This property corresponds to the "Noncharacter_Code_Point" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isNoncharacterCodePoint: Bool {
_binaryProperties.contains(.isNoncharacterCodePoint)
}
/// A Boolean value indicating whether the scalar is one that is used in
/// writing to surround quoted text.
///
/// This property corresponds to the "Quotation_Mark" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isQuotationMark: Bool {
_binaryProperties.contains(.isQuotationMark)
}
/// A Boolean value indicating whether the scalar is a radical component of
/// CJK characters, Tangut characters, or Yi syllables.
///
/// These scalars are often the components of ideographic description
/// sequences, as defined by the `isIDSBinaryOperator` and
/// `isIDSTrinaryOperator` properties.
///
/// This property corresponds to the "Radical" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isRadical: Bool {
_binaryProperties.contains(.isRadical)
}
/// A Boolean value indicating whether the scalar has a "soft dot" that
/// disappears when a diacritic is placed over the scalar.
///
/// For example, "i" is soft dotted because the dot disappears when adding an
/// accent mark, as in "í".
///
/// This property corresponds to the "Soft_Dotted" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isSoftDotted: Bool {
_binaryProperties.contains(.isSoftDotted)
}
/// A Boolean value indicating whether the scalar is a punctuation symbol
/// that typically marks the end of a textual unit.
///
/// This property corresponds to the "Terminal_Punctuation" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isTerminalPunctuation: Bool {
_binaryProperties.contains(.isTerminalPunctuation)
}
/// A Boolean value indicating whether the scalar is one of the unified
/// CJK ideographs in the Unicode Standard.
///
/// This property is false for CJK punctuation and symbols, as well as for
/// compatibility ideographs (which canonically decompose to unified
/// ideographs).
///
/// This property corresponds to the "Unified_Ideograph" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isUnifiedIdeograph: Bool {
_binaryProperties.contains(.isUnifiedIdeograph)
}
/// A Boolean value indicating whether the scalar's letterform is
/// considered uppercase.
///
/// This property corresponds to the "Uppercase" and the "Other_Uppercase"
/// properties in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isUppercase: Bool {
_binaryProperties.contains(.isUppercase)
}
/// A Boolean value indicating whether the scalar is a whitespace
/// character.
///
/// This property is `true` for scalars that are spaces, separator characters,
/// and other control characters that should be treated as whitespace for the
/// purposes of parsing text elements.
///
/// This property corresponds to the "White_Space" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isWhitespace: Bool {
_binaryProperties.contains(.isWhitespace)
}
/// A Boolean value indicating whether the scalar is one which is
/// recommended to be allowed to appear in a non-starting position in a
/// programming language identifier, with adjustments made for NFKC normalized
/// form.
///
/// The set of scalars `[:XID_Continue:]` closes the set `[:ID_Continue:]`
/// under NFKC normalization by removing any scalars whose normalized form is
/// not of the form `[:ID_Continue:]*`.
///
/// This property corresponds to the "XID_Continue" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isXIDContinue: Bool {
_binaryProperties.contains(.isXIDContinue)
}
/// A Boolean value indicating whether the scalar is one which is
/// recommended to be allowed to appear in a starting position in a
/// programming language identifier, with adjustments made for NFKC normalized
/// form.
///
/// The set of scalars `[:XID_Start:]` closes the set `[:ID_Start:]` under
/// NFKC normalization by removing any scalars whose normalized form is not of
/// the form `[:ID_Start:] [:ID_Continue:]*`.
///
/// This property corresponds to the "XID_Start" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isXIDStart: Bool {
_binaryProperties.contains(.isXIDStart)
}
/// A Boolean value indicating whether the scalar is a punctuation mark
/// that generally marks the end of a sentence.
///
/// This property corresponds to the "Sentence_Terminal" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isSentenceTerminal: Bool {
_binaryProperties.contains(.isSentenceTerminal)
}
/// A Boolean value indicating whether the scalar is a variation selector.
///
/// Variation selectors allow rendering engines that support them to choose
/// different glyphs to display for a particular code point.
///
/// This property corresponds to the "Variation_Selector" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isVariationSelector: Bool {
_binaryProperties.contains(.isVariationSelector)
}
/// A Boolean value indicating whether the scalar is recommended to have
/// syntactic usage in patterns represented in source code.
///
/// This property corresponds to the "Pattern_Syntax" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isPatternSyntax: Bool {
_binaryProperties.contains(.isPatternSyntax)
}
/// A Boolean value indicating whether the scalar is recommended to be
/// treated as whitespace when parsing patterns represented in source code.
///
/// This property corresponds to the "Pattern_White_Space" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isPatternWhitespace: Bool {
_binaryProperties.contains(.isPatternWhitespace)
}
/// A Boolean value indicating whether the scalar is considered to be
/// either lowercase, uppercase, or titlecase.
///
/// Though similar in name, this property is *not* equivalent to
/// `changesWhenCaseMapped`. The set of scalars for which `isCased` is `true`
/// is a superset of those for which `changesWhenCaseMapped` is `true`. For
/// example, the Latin small capitals that are used by the International
/// Phonetic Alphabet have a case, but do not change when they are mapped to
/// any of the other cases.
///
/// This property corresponds to the "Cased" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isCased: Bool {
_binaryProperties.contains(.isCased)
}
/// A Boolean value indicating whether the scalar is ignored for casing
/// purposes.
///
/// This property corresponds to the "Case_Ignorable" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var isCaseIgnorable: Bool {
_binaryProperties.contains(.isCaseIgnorable)
}
/// A Boolean value indicating whether the scalar's normalized form differs
/// from the `lowercaseMapping` of each constituent scalar.
///
/// This property corresponds to the "Changes_When_Lowercased" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var changesWhenLowercased: Bool {
_binaryProperties.contains(.changesWhenLowercased)
}
/// A Boolean value indicating whether the scalar's normalized form differs
/// from the `uppercaseMapping` of each constituent scalar.
///
/// This property corresponds to the "Changes_When_Uppercased" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var changesWhenUppercased: Bool {
_binaryProperties.contains(.changesWhenUppercased)
}
/// A Boolean value indicating whether the scalar's normalized form differs
/// from the `titlecaseMapping` of each constituent scalar.
///
/// This property corresponds to the "Changes_When_Titlecased" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var changesWhenTitlecased: Bool {
_binaryProperties.contains(.changesWhenTitlecased)
}
/// A Boolean value indicating whether the scalar's normalized form differs
/// from the case-fold mapping of each constituent scalar.
///
/// This property corresponds to the "Changes_When_Casefolded" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var changesWhenCaseFolded: Bool {
_binaryProperties.contains(.changesWhenCaseFolded)
}
/// A Boolean value indicating whether the scalar may change when it
/// undergoes case mapping.
///
/// This property is `true` whenever one or more of `changesWhenLowercased`,
/// `changesWhenUppercased`, or `changesWhenTitlecased` are `true`.
///
/// This property corresponds to the "Changes_When_Casemapped" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var changesWhenCaseMapped: Bool {
_binaryProperties.contains(.changesWhenCaseMapped)
}
/// A Boolean value indicating whether the scalar is one that is not
/// identical to its NFKC case-fold mapping.
///
/// This property corresponds to the "Changes_When_NFKC_Casefolded" property
/// in the [Unicode Standard](http://www.unicode.org/versions/latest/).
public var changesWhenNFKCCaseFolded: Bool {
_binaryProperties.contains(.changesWhenNFKCCaseFolded)
}
/// A Boolean value indicating whether the scalar has an emoji
/// presentation, whether or not it is the default.
///
/// This property is true for scalars that are rendered as emoji by default
/// and also for scalars that have a non-default emoji rendering when followed
/// by U+FE0F VARIATION SELECTOR-16. This includes some scalars that are not
/// typically considered to be emoji:
///
/// let scalars: [Unicode.Scalar] = ["😎", "$", "0"]
/// for s in scalars {
/// print(s, "-->", s.properties.isEmoji)
/// }
/// // 😎 --> true
/// // $ --> false
/// // 0 --> true
///
/// The final result is true because the ASCII digits have non-default emoji
/// presentations; some platforms render these with an alternate appearance.
///
/// Because of this behavior, testing `isEmoji` alone on a single scalar is
/// insufficient to determine if a unit of text is rendered as an emoji; a
/// correct test requires inspecting multiple scalars in a `Character`. In
/// addition to checking whether the base scalar has `isEmoji == true`, you
/// must also check its default presentation (see `isEmojiPresentation`) and
/// determine whether it is followed by a variation selector that would modify
/// the presentation.
///
/// This property corresponds to the "Emoji" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
@available(macOS 10.12.2, iOS 10.2, tvOS 10.1, watchOS 3.1.1, *)
public var isEmoji: Bool {
_binaryProperties.contains(.isEmoji)
}
/// A Boolean value indicating whether the scalar is one that should be
/// rendered with an emoji presentation, rather than a text presentation, by
/// default.
///
/// Scalars that have default to emoji presentation can be followed by
/// U+FE0E VARIATION SELECTOR-15 to request the text presentation of the
/// scalar instead. Likewise, scalars that default to text presentation can
/// be followed by U+FE0F VARIATION SELECTOR-16 to request the emoji
/// presentation.
///
/// This property corresponds to the "Emoji_Presentation" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
@available(macOS 10.12.2, iOS 10.2, tvOS 10.1, watchOS 3.1.1, *)
public var isEmojiPresentation: Bool {
_binaryProperties.contains(.isEmojiPresentation)
}
/// A Boolean value indicating whether the scalar is one that can modify
/// a base emoji that precedes it.
///
/// The Fitzpatrick skin types are examples of emoji modifiers; they change
/// the appearance of the preceding emoji base (that is, a scalar for which
/// `isEmojiModifierBase` is true) by rendering it with a different skin tone.
///
/// This property corresponds to the "Emoji_Modifier" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
@available(macOS 10.12.2, iOS 10.2, tvOS 10.1, watchOS 3.1.1, *)
public var isEmojiModifier: Bool {
_binaryProperties.contains(.isEmojiModifier)
}
/// A Boolean value indicating whether the scalar is one whose appearance
/// can be changed by an emoji modifier that follows it.
///
/// This property corresponds to the "Emoji_Modifier_Base" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
@available(macOS 10.12.2, iOS 10.2, tvOS 10.1, watchOS 3.1.1, *)
public var isEmojiModifierBase: Bool {
_binaryProperties.contains(.isEmojiModifierBase)
}
}
/// Case mapping properties.
extension Unicode.Scalar.Properties {
fileprivate struct _CaseMapping {
let rawValue: UInt8
static let uppercase = _CaseMapping(rawValue: 0)
static let lowercase = _CaseMapping(rawValue: 1)
static let titlecase = _CaseMapping(rawValue: 2)
}
fileprivate func _getMapping(_ mapping: _CaseMapping) -> String {
// First, check if our scalar has a special mapping where it's mapped to
// more than 1 scalar.
var specialMappingLength = 0
let specialMappingPtr = _swift_stdlib_getSpecialMapping(
_scalar.value,
mapping.rawValue,
&specialMappingLength
)
if let specialMapping = specialMappingPtr, specialMappingLength != 0 {
let buffer = UnsafeBufferPointer<UInt8>(
start: specialMapping,
count: specialMappingLength
)
return String._uncheckedFromUTF8(buffer, isASCII: false)
}
// If we did not have a special mapping, check if we have a direct scalar
// to scalar mapping.
let mappingDistance = _swift_stdlib_getMapping(
_scalar.value,
mapping.rawValue
)
if mappingDistance != 0 {
let scalar = Unicode.Scalar(
_unchecked: UInt32(Int(_scalar.value) &+ Int(mappingDistance))
)
return String(scalar)
}
// We did not have any mapping. Return the scalar as is.
return String(_scalar)
}
/// The lowercase mapping of the scalar.
///
/// This property is a `String`, not a `Unicode.Scalar` or `Character`,
/// because some mappings may transform a scalar into multiple scalars or
/// graphemes. For example, the character "İ" (U+0130 LATIN CAPITAL LETTER I
/// WITH DOT ABOVE) becomes two scalars (U+0069 LATIN SMALL LETTER I, U+0307
/// COMBINING DOT ABOVE) when converted to lowercase.
///
/// This property corresponds to the "Lowercase_Mapping" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var lowercaseMapping: String {
_getMapping(.lowercase)
}
/// The titlecase mapping of the scalar.
///
/// This property is a `String`, not a `Unicode.Scalar` or `Character`,
/// because some mappings may transform a scalar into multiple scalars or
/// graphemes. For example, the ligature "fi" (U+FB01 LATIN SMALL LIGATURE FI)
/// becomes "Fi" (U+0046 LATIN CAPITAL LETTER F, U+0069 LATIN SMALL LETTER I)
/// when converted to titlecase.
///
/// This property corresponds to the "Titlecase_Mapping" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var titlecaseMapping: String {
_getMapping(.titlecase)
}
/// The uppercase mapping of the scalar.
///
/// This property is a `String`, not a `Unicode.Scalar` or `Character`,
/// because some mappings may transform a scalar into multiple scalars or
/// graphemes. For example, the German letter "ß" (U+00DF LATIN SMALL LETTER
/// SHARP S) becomes "SS" (U+0053 LATIN CAPITAL LETTER S, U+0053 LATIN CAPITAL
/// LETTER S) when converted to uppercase.
///
/// This property corresponds to the "Uppercase_Mapping" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var uppercaseMapping: String {
_getMapping(.uppercase)
}
}
extension Unicode {
/// A version of the Unicode Standard represented by its major and minor
/// components.
public typealias Version = (major: Int, minor: Int)
}
extension Unicode.Scalar.Properties {
/// The earliest version of the Unicode Standard in which the scalar was
/// assigned.
///
/// This value is `nil` for code points that have not yet been assigned.
///
/// This property corresponds to the "Age" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var age: Unicode.Version? {
let age: UInt16 = _swift_stdlib_getAge(_scalar.value)
if age == .max {
return nil
}
let major = age & 0xFF
let minor = (age & 0xFF00) >> 8
return (major: Int(major), minor: Int(minor))
}
}
extension Unicode {
/// The most general classification of a Unicode scalar.
///
/// The general category of a scalar is its "first-order, most usual
/// categorization". It does not attempt to cover multiple uses of some
/// scalars, such as the use of letters to represent Roman numerals.
public enum GeneralCategory: Sendable {
/// An uppercase letter.
///
/// This value corresponds to the category `Uppercase_Letter` (abbreviated
/// `Lu`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case uppercaseLetter
/// A lowercase letter.
///
/// This value corresponds to the category `Lowercase_Letter` (abbreviated
/// `Ll`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case lowercaseLetter
/// A digraph character whose first part is uppercase.
///
/// This value corresponds to the category `Titlecase_Letter` (abbreviated
/// `Lt`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case titlecaseLetter
/// A modifier letter.
///
/// This value corresponds to the category `Modifier_Letter` (abbreviated
/// `Lm`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case modifierLetter
/// Other letters, including syllables and ideographs.
///
/// This value corresponds to the category `Other_Letter` (abbreviated
/// `Lo`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case otherLetter
/// A non-spacing combining mark with zero advance width (abbreviated Mn).
///
/// This value corresponds to the category `Nonspacing_Mark` (abbreviated
/// `Mn`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case nonspacingMark
/// A spacing combining mark with positive advance width.
///
/// This value corresponds to the category `Spacing_Mark` (abbreviated `Mc`)
/// in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case spacingMark
/// An enclosing combining mark.
///
/// This value corresponds to the category `Enclosing_Mark` (abbreviated
/// `Me`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case enclosingMark
/// A decimal digit.
///
/// This value corresponds to the category `Decimal_Number` (abbreviated
/// `Nd`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case decimalNumber
/// A letter-like numeric character.
///
/// This value corresponds to the category `Letter_Number` (abbreviated
/// `Nl`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case letterNumber
/// A numeric character of another type.
///
/// This value corresponds to the category `Other_Number` (abbreviated `No`)
/// in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case otherNumber
/// A connecting punctuation mark, like a tie.
///
/// This value corresponds to the category `Connector_Punctuation`
/// (abbreviated `Pc`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case connectorPunctuation
/// A dash or hyphen punctuation mark.
///
/// This value corresponds to the category `Dash_Punctuation` (abbreviated
/// `Pd`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case dashPunctuation
/// An opening punctuation mark of a pair.
///
/// This value corresponds to the category `Open_Punctuation` (abbreviated
/// `Ps`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case openPunctuation
/// A closing punctuation mark of a pair.
///
/// This value corresponds to the category `Close_Punctuation` (abbreviated
/// `Pe`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case closePunctuation
/// An initial quotation mark.
///
/// This value corresponds to the category `Initial_Punctuation`
/// (abbreviated `Pi`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case initialPunctuation
/// A final quotation mark.
///
/// This value corresponds to the category `Final_Punctuation` (abbreviated
/// `Pf`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case finalPunctuation
/// A punctuation mark of another type.
///
/// This value corresponds to the category `Other_Punctuation` (abbreviated
/// `Po`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case otherPunctuation
/// A symbol of mathematical use.
///
/// This value corresponds to the category `Math_Symbol` (abbreviated `Sm`)
/// in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case mathSymbol
/// A currency sign.
///
/// This value corresponds to the category `Currency_Symbol` (abbreviated
/// `Sc`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case currencySymbol
/// A non-letterlike modifier symbol.
///
/// This value corresponds to the category `Modifier_Symbol` (abbreviated
/// `Sk`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case modifierSymbol
/// A symbol of another type.
///
/// This value corresponds to the category `Other_Symbol` (abbreviated
/// `So`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case otherSymbol
/// A space character of non-zero width.
///
/// This value corresponds to the category `Space_Separator` (abbreviated
/// `Zs`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case spaceSeparator
/// A line separator, which is specifically (and only) U+2028 LINE
/// SEPARATOR.
///
/// This value corresponds to the category `Line_Separator` (abbreviated
/// `Zl`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case lineSeparator
/// A paragraph separator, which is specifically (and only) U+2029 PARAGRAPH
/// SEPARATOR.
///
/// This value corresponds to the category `Paragraph_Separator`
/// (abbreviated `Zp`) in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case paragraphSeparator
/// A C0 or C1 control code.
///
/// This value corresponds to the category `Control` (abbreviated `Cc`) in
/// the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case control
/// A format control character.
///
/// This value corresponds to the category `Format` (abbreviated `Cf`) in
/// the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case format
/// A surrogate code point.
///
/// This value corresponds to the category `Surrogate` (abbreviated `Cs`) in
/// the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case surrogate
/// A private-use character.
///
/// This value corresponds to the category `Private_Use` (abbreviated `Co`)
/// in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case privateUse
/// A reserved unassigned code point or a non-character.
///
/// This value corresponds to the category `Unassigned` (abbreviated `Cn`)
/// in the
/// [Unicode Standard](https://unicode.org/reports/tr44/#General_Category_Values).
case unassigned
internal init(rawValue: UInt8) {
switch rawValue {
case 0: self = .uppercaseLetter
case 1: self = .lowercaseLetter
case 2: self = .titlecaseLetter
case 3: self = .modifierLetter
case 4: self = .otherLetter
case 5: self = .nonspacingMark
case 6: self = .spacingMark
case 7: self = .enclosingMark
case 8: self = .decimalNumber
case 9: self = .letterNumber
case 10: self = .otherNumber
case 11: self = .connectorPunctuation
case 12: self = .dashPunctuation
case 13: self = .openPunctuation
case 14: self = .closePunctuation
case 15: self = .initialPunctuation
case 16: self = .finalPunctuation
case 17: self = .otherPunctuation
case 18: self = .mathSymbol
case 19: self = .currencySymbol
case 20: self = .modifierSymbol
case 21: self = .otherSymbol
case 22: self = .spaceSeparator
case 23: self = .lineSeparator
case 24: self = .paragraphSeparator
case 25: self = .control
case 26: self = .format
case 27: self = .surrogate
case 28: self = .privateUse
case 29: self = .unassigned
default: fatalError("Unknown general category \(rawValue)")
}
}
}
}
// Internal helpers
extension Unicode.GeneralCategory {
internal var _isSymbol: Bool {
switch self {
case .mathSymbol, .currencySymbol, .modifierSymbol, .otherSymbol:
return true
default: return false
}
}
internal var _isPunctuation: Bool {
switch self {
case .connectorPunctuation, .dashPunctuation, .openPunctuation,
.closePunctuation, .initialPunctuation, .finalPunctuation,
.otherPunctuation:
return true
default: return false
}
}
}
extension Unicode.Scalar.Properties {
/// The general category (most usual classification) of the scalar.
///
/// This property corresponds to the "General_Category" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var generalCategory: Unicode.GeneralCategory {
let rawValue = _swift_stdlib_getGeneralCategory(_scalar.value)
return Unicode.GeneralCategory(rawValue: rawValue)
}
}
extension Unicode.Scalar.Properties {
internal func _hangulName() -> String {
// T = Hangul tail consonants
let T: (base: UInt32, count: UInt32) = (base: 0x11A7, count: 28)
// N = Number of precomposed Hangul syllables that start with the same
// leading consonant. (There is no base for N).
let N: (base: UInt32, count: UInt32) = (base: 0x0, count: 588)
// S = Hangul precomposed syllables
let S: (base: UInt32, count: UInt32) = (base: 0xAC00, count: 11172)
let hangulLTable = ["G", "GG", "N", "D", "DD", "R", "M", "B", "BB", "S",
"SS", "", "J", "JJ", "C", "K", "T", "P", "H"]
let hangulVTable = ["A", "AE", "YA", "YAE", "EO", "E", "YEO", "YE", "O",
"WA", "WAE", "OE", "YO", "U", "WEO", "WE", "WI", "YU",
"EU", "YI", "I"]
let hangulTTable = ["", "G", "GG", "GS", "N", "NJ", "NH", "D", "L", "LG",
"LM", "LB", "LS", "LT", "LP", "LH", "M", "B", "BS", "S",
"SS", "NG", "J", "C", "K", "T", "P", "H"]
let sIdx = _scalar.value &- S.base
let lIdx = Int(sIdx / N.count)
let vIdx = Int((sIdx % N.count) / T.count)
let tIdx = Int(sIdx % T.count)
let scalarName = hangulLTable[lIdx] + hangulVTable[vIdx] + hangulTTable[tIdx]
return "HANGUL SYLLABLE \(scalarName)"
}
// Used to potentially return a name who can either be represented in a large
// range or algorithmically. A good example are the Hangul names. Instead of
// storing those names, we can define an algorithm to generate the name.
internal func _fastScalarName() -> String? {
// Define a couple algorithmic names below.
let scalarName = String(_scalar.value, radix: 16, uppercase: true)
switch _scalar.value {
// Hangul Syllable *
case (0xAC00 ... 0xD7A3):
return _hangulName()
// Variation Selector-17 through Variation Selector-256
case (0xE0100 ... 0xE01EF):
return "VARIATION SELECTOR-\(_scalar.value - 0xE0100 + 17)"
case (0x3400 ... 0x4DBF),
(0x4E00 ... 0x9FFF),
(0x20000 ... 0x2A6DF),
(0x2A700 ... 0x2B738),
(0x2B740 ... 0x2B81D),
(0x2B820 ... 0x2CEA1),
(0x2CEB0 ... 0x2EBE0),
(0x30000 ... 0x3134A):
return "CJK UNIFIED IDEOGRAPH-\(scalarName)"
case (0xF900 ... 0xFA6D),
(0xFA70 ... 0xFAD9),
(0x2F800 ... 0x2FA1D):
return "CJK COMPATIBILITY IDEOGRAPH-\(scalarName)"
case (0x17000 ... 0x187F7),
(0x18D00 ... 0x18D08):
return "TANGUT IDEOGRAPH-\(scalarName)"
case (0x18B00 ... 0x18CD5):
return "KHITAN SMALL SCRIPT CHARACTER-\(scalarName)"
case (0x1B170 ... 0x1B2FB):
return "NUSHU CHARACTER-\(scalarName)"
// Otherwise, go look it up.
default:
return nil
}
}
/// The published name of the scalar.
///
/// Some scalars, such as control characters, do not have a value for this
/// property in the Unicode Character Database. For such scalars, this
/// property is `nil`.
///
/// This property corresponds to the "Name" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var name: String? {
if let fastName = _fastScalarName() {
return fastName
}
// The longest name that Unicode defines is 88 characters long.
let largestCount = Int(SWIFT_STDLIB_LARGEST_NAME_COUNT)
let name = String(_uninitializedCapacity: largestCount) { buffer in
_swift_stdlib_getScalarName(
_scalar.value,
buffer.baseAddress,
buffer.count
)
}
return name.isEmpty ? nil : name
}
/// The normative formal alias of the scalar.
///
/// The name of a scalar is immutable and never changed in future versions of
/// the Unicode Standard. The `nameAlias` property is provided to issue
/// corrections if a name was issued erroneously. For example, the `name` of
/// U+FE18 is "PRESENTATION FORM FOR VERTICAL RIGHT WHITE LENTICULAR BRACKET"
/// (note that "BRACKET" is misspelled). The `nameAlias` property then
/// contains the corrected name.
///
/// If a scalar has no alias, this property is `nil`.
///
/// This property corresponds to the "Name_Alias" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var nameAlias: String? {
guard let nameAliasPtr = _swift_stdlib_getNameAlias(_scalar.value) else {
return nil
}
return String(cString: nameAliasPtr)
}
}
extension Unicode {
/// The classification of a scalar used in the Canonical Ordering Algorithm
/// defined by the Unicode Standard.
///
/// Canonical combining classes are used by the ordering algorithm to
/// determine if two sequences of combining marks should be considered
/// canonically equivalent (that is, identical in interpretation). Two
/// sequences are canonically equivalent if they are equal when sorting the
/// scalars in ascending order by their combining class.
///
/// For example, consider the sequence `"\u{0041}\u{0301}\u{0316}"` (LATIN
/// CAPITAL LETTER A, COMBINING ACUTE ACCENT, COMBINING GRAVE ACCENT BELOW).
/// The combining classes of these scalars have the numeric values 0, 230, and
/// 220, respectively. Sorting these scalars by their combining classes yields
/// `"\u{0041}\u{0316}\u{0301}"`, so two strings that differ only by the
/// ordering of those scalars would compare as equal:
///
/// let aboveBeforeBelow = "\u{0041}\u{0301}\u{0316}"
/// let belowBeforeAbove = "\u{0041}\u{0316}\u{0301}"
/// print(aboveBeforeBelow == belowBeforeAbove)
/// // Prints "true"
///
/// Named and Unnamed Combining Classes
/// ===================================
///
/// Canonical combining classes are defined in the Unicode Standard as
/// integers in the range `0...254`. For convenience, the standard assigns
/// symbolic names to a subset of these combining classes.
///
/// The `CanonicalCombiningClass` type conforms to `RawRepresentable` with a
/// raw value of type `UInt8`. You can create instances of the type by using
/// the static members named after the symbolic names, or by using the
/// `init(rawValue:)` initializer.
///
/// let overlayClass = Unicode.CanonicalCombiningClass(rawValue: 1)
/// let overlayClassIsOverlay = overlayClass == .overlay
/// // overlayClassIsOverlay == true
public struct CanonicalCombiningClass:
Comparable, Hashable, RawRepresentable, Sendable
{
/// Base glyphs that occupy their own space and do not combine with others.
public static let notReordered = CanonicalCombiningClass(rawValue: 0)
/// Marks that overlay a base letter or symbol.
public static let overlay = CanonicalCombiningClass(rawValue: 1)
/// Diacritic nukta marks in Brahmi-derived scripts.
public static let nukta = CanonicalCombiningClass(rawValue: 7)
/// Combining marks that are attached to hiragana and katakana to indicate
/// voicing changes.
public static let kanaVoicing = CanonicalCombiningClass(rawValue: 8)
/// Diacritic virama marks in Brahmi-derived scripts.
public static let virama = CanonicalCombiningClass(rawValue: 9)
/// Marks attached at the bottom left.
public static let attachedBelowLeft = CanonicalCombiningClass(rawValue: 200)
/// Marks attached directly below.
public static let attachedBelow = CanonicalCombiningClass(rawValue: 202)
/// Marks attached directly above.
public static let attachedAbove = CanonicalCombiningClass(rawValue: 214)
/// Marks attached at the top right.
public static let attachedAboveRight =
CanonicalCombiningClass(rawValue: 216)
/// Distinct marks at the bottom left.
public static let belowLeft = CanonicalCombiningClass(rawValue: 218)
/// Distinct marks directly below.
public static let below = CanonicalCombiningClass(rawValue: 220)
/// Distinct marks at the bottom right.
public static let belowRight = CanonicalCombiningClass(rawValue: 222)
/// Distinct marks to the left.
public static let left = CanonicalCombiningClass(rawValue: 224)
/// Distinct marks to the right.
public static let right = CanonicalCombiningClass(rawValue: 226)
/// Distinct marks at the top left.
public static let aboveLeft = CanonicalCombiningClass(rawValue: 228)
/// Distinct marks directly above.
public static let above = CanonicalCombiningClass(rawValue: 230)
/// Distinct marks at the top right.
public static let aboveRight = CanonicalCombiningClass(rawValue: 232)
/// Distinct marks subtending two bases.
public static let doubleBelow = CanonicalCombiningClass(rawValue: 233)
/// Distinct marks extending above two bases.
public static let doubleAbove = CanonicalCombiningClass(rawValue: 234)
/// Greek iota subscript only (U+0345 COMBINING GREEK YPOGEGRAMMENI).
public static let iotaSubscript = CanonicalCombiningClass(rawValue: 240)
/// The raw integer value of the canonical combining class.
public let rawValue: UInt8
/// Creates a new canonical combining class with the given raw integer
/// value.
///
/// - Parameter rawValue: The raw integer value of the canonical combining
/// class.
public init(rawValue: UInt8) {
self.rawValue = rawValue
}
public static func == (
lhs: CanonicalCombiningClass,
rhs: CanonicalCombiningClass
) -> Bool {
return lhs.rawValue == rhs.rawValue
}
public static func < (
lhs: CanonicalCombiningClass,
rhs: CanonicalCombiningClass
) -> Bool {
return lhs.rawValue < rhs.rawValue
}
public var hashValue: Int {
return rawValue.hashValue
}
public func hash(into hasher: inout Hasher) {
hasher.combine(rawValue)
}
}
}
extension Unicode.Scalar.Properties {
/// The canonical combining class of the scalar.
///
/// This property corresponds to the "Canonical_Combining_Class" property in
/// the [Unicode Standard](http://www.unicode.org/versions/latest/).
public var canonicalCombiningClass: Unicode.CanonicalCombiningClass {
let normData = Unicode._NormData(_scalar)
return Unicode.CanonicalCombiningClass(rawValue: normData.ccc)
}
}
extension Unicode {
/// The numeric type of a scalar.
///
/// Scalars with a non-nil numeric type include numbers, fractions, numeric
/// superscripts and subscripts, and circled or otherwise decorated number
/// glyphs.
///
/// Some letterlike scalars used in numeric systems, such as Greek or Latin
/// letters, do not have a non-nil numeric type, in order to prevent programs
/// from incorrectly interpreting them as numbers in non-numeric contexts.
public enum NumericType: Sendable {
/// A digit that is commonly understood to form base-10 numbers.
///
/// Specifically, scalars have this numeric type if they occupy a contiguous
/// range of code points representing numeric values `0...9`.
case decimal
/// A digit that does not meet the requirements of the `decimal` numeric
/// type.
///
/// Scalars with this numeric type are often those that represent a decimal
/// digit but would not typically be used to write a base-10 number, such
/// as "④" (U+2463 CIRCLED DIGIT FOUR).
///
/// As of Unicode 6.3, any new scalars that represent numbers but do not
/// meet the requirements of `decimal` will have numeric type `numeric`,
/// and programs can treat `digit` and `numeric` equivalently.
case digit
/// A digit that does not meet the requirements of the `decimal` numeric
/// type or a non-digit numeric value.
///
/// This numeric type includes fractions such as "⅕" (U+2155 VULGAR
/// FRACTION ONE FIFTH), numerical CJK ideographs like "兆" (U+5146 CJK
/// UNIFIED IDEOGRAPH-5146), and other scalars that are not decimal digits
/// used positionally in the writing of base-10 numbers.
///
/// As of Unicode 6.3, any new scalars that represent numbers but do not
/// meet the requirements of `decimal` will have numeric type `numeric`,
/// and programs can treat `digit` and `numeric` equivalently.
case numeric
internal init(rawValue: UInt8) {
switch rawValue {
case 0:
self = .numeric
case 1:
self = .digit
case 2:
self = .decimal
default:
fatalError("Unknown numeric type \(rawValue)")
}
}
}
}
/// Numeric properties of scalars.
extension Unicode.Scalar.Properties {
/// The numeric type of the scalar.
///
/// For scalars that represent a number, `numericType` is the numeric type
/// of the scalar. For all other scalars, this property is `nil`.
///
/// let scalars: [Unicode.Scalar] = ["4", "④", "⅕", "X"]
/// for scalar in scalars {
/// print(scalar, "-->", scalar.properties.numericType)
/// }
/// // 4 --> Optional(Swift.Unicode.NumericType.decimal)
/// // ④ --> Optional(Swift.Unicode.NumericType.digit)
/// // ⅕ --> Optional(Swift.Unicode.NumericType.numeric)
/// // X --> nil
///
/// This property corresponds to the "Numeric_Type" property in the
/// [Unicode Standard](http://www.unicode.org/versions/latest/).
public var numericType: Unicode.NumericType? {
let rawValue = _swift_stdlib_getNumericType(_scalar.value)
guard rawValue != .max else {
return nil
}
return Unicode.NumericType(rawValue: rawValue)
}
/// The numeric value of the scalar.
///
/// For scalars that represent a numeric value, `numericValue` is the whole
/// or fractional value. For all other scalars, this property is `nil`.
///
/// let scalars: [Unicode.Scalar] = ["4", "④", "⅕", "X"]
/// for scalar in scalars {
/// print(scalar, "-->", scalar.properties.numericValue)
/// }
/// // 4 --> Optional(4.0)
/// // ④ --> Optional(4.0)
/// // ⅕ --> Optional(0.2)
/// // X --> nil
///
/// This property corresponds to the "Numeric_Value" property in the [Unicode
/// Standard](http://www.unicode.org/versions/latest/).
public var numericValue: Double? {
guard numericType != nil else {
return nil
}
return _swift_stdlib_getNumericValue(_scalar.value)
}
}
| apache-2.0 | 96fa4f0e798a2762750d9d8d460d952f | 39.064078 | 86 | 0.674777 | 4.360313 | false | false | false | false |
bay2/LetToDo | LetToDo/Home/Controller/HomeGroupViewController.swift | 1 | 3465 | //
// HomeGroupViewController.swift
// LetToDo
//
// Created by xuemincai on 2016/10/7.
// Copyright © 2016年 xuemincai. All rights reserved.
//
import UIKit
import EZSwiftExtensions
import IBAnimatable
import RxSwift
import SnapKit
import RxDataSources
fileprivate let reuseIdentifier = "HomeGroupCollectionViewCell"
fileprivate let reusableViewIdentifier = "HomeGroupCollectionReusableView"
class HomeGroupViewController: UIViewController {
@IBOutlet weak var collectionView: UICollectionView!
private lazy var dataSources = RxCollectionViewSectionedAnimatedDataSource<GroupSectionViewModel>()
private var disposeBag = DisposeBag()
override func viewDidLoad() {
super.viewDidLoad()
registerCell()
configNavigationBar()
dataSources.configureCell = {(data, collectionView, indexPath, item) in
let cell: HomeGroupCollectionViewCell = collectionView.dequeueReusableCell(forIndexPath: indexPath)
cell.groupTitle.text = item.groupTitle
cell.groupTitle.textColor = item.TitleColor
cell.itemLab.text = "Item \(item.taskNum)"
cell.groupID = item.groupID
return cell
}
dataSources.supplementaryViewFactory = { (data, collectionView, kind, indexPath) in
return collectionView.dequeueReusableSupplementaryView(ofKind: UICollectionElementKindSectionHeader, withReuseIdentifier: reusableViewIdentifier, for: indexPath)
}
Observable.from(realm.objects(GroupModel.self))
.map { (results) -> [GroupViewModel] in
results.flatMap { model -> GroupViewModel in
GroupViewModel(model: model)
}
}
.map { (items) -> [GroupSectionViewModel] in
[GroupSectionViewModel(sectionID: "", groups: items)]
}
.bindTo(collectionView.rx.items(dataSource: dataSources))
.addDisposableTo(disposeBag)
collectionView
.rx
.setDelegate(self)
.addDisposableTo(disposeBag)
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
/// register Cell
func registerCell() {
self.collectionView.register(UINib(nibName: reuseIdentifier, bundle: nil), forCellWithReuseIdentifier: reuseIdentifier)
self.collectionView.register(UINib(nibName: reusableViewIdentifier, bundle: nil), forSupplementaryViewOfKind: UICollectionElementKindSectionHeader, withReuseIdentifier: reusableViewIdentifier)
}
}
// MARK: UICollectionViewDelegateFlowLayout
extension HomeGroupViewController: UICollectionViewDelegateFlowLayout {
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
return CGSize(width: ez.screenWidth * 0.5, height: ez.screenWidth * 0.5 * 0.88)
}
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, referenceSizeForHeaderInSection section: Int) -> CGSize {
return CGSize(width: ez.screenWidth, height: 104)
}
}
| mit | a8889802df07913afe90951a0ea938da | 31.35514 | 200 | 0.663489 | 6.127434 | false | false | false | false |
netguru/inbbbox-ios | Unit Tests/ShotCollectionViewCellSpec.swift | 1 | 27321 | //
// Copyright (c) 2015 Netguru Sp. z o.o. All rights reserved.
//
import Quick
import Nimble
import Dobby
@testable import Inbbbox
class ShotCollectionViewCellSpec: QuickSpec {
override func spec() {
var sut: ShotCollectionViewCell!
beforeEach {
sut = ShotCollectionViewCell(frame: CGRect.zero)
}
afterEach {
sut = nil
}
describe("shot container view") {
var shotContainer: UIView!
beforeEach {
shotContainer = sut.shotContainer
}
it("should not translate autoresizing mask into constraints") {
expect(shotContainer.translatesAutoresizingMaskIntoConstraints).to(beFalsy())
}
it("should be added to cell content view subviews") {
expect(sut.contentView.subviews).to(contain(shotContainer))
}
}
describe("like image view") {
var likeImageView: DoubleImageView!
beforeEach {
likeImageView = sut.likeImageView
}
it("should not translate autoresizing mask into constraints") {
expect(likeImageView.translatesAutoresizingMaskIntoConstraints).to(beFalsy())
}
it("should be added to cell content view subviews") {
expect(sut.shotContainer.subviews).to(contain(likeImageView))
}
it("should have proper first image") {
expect(UIImagePNGRepresentation(likeImageView.firstImageView.image!)).to(equal(UIImagePNGRepresentation(UIImage(named: "ic-like-swipe")!)))
}
it("should have proper second image") {
expect(UIImagePNGRepresentation(likeImageView.secondImageView.image!)).to(equal(UIImagePNGRepresentation(UIImage(named: "ic-like-swipe-filled")!)))
}
}
describe("bucket image view") {
var bucketImageView: DoubleImageView!
beforeEach {
bucketImageView = sut.bucketImageView
}
it("should not translate autoresizing mask into constraints") {
expect(bucketImageView.translatesAutoresizingMaskIntoConstraints).to(beFalsy())
}
it("should be added to cell content view subviews") {
expect(sut.shotContainer.subviews).to(contain(bucketImageView))
}
it("should have proper first image") {
expect(UIImagePNGRepresentation(bucketImageView.firstImageView.image!)).to(equal(UIImagePNGRepresentation(UIImage(named: "ic-bucket-swipe")!)))
}
it("should have proper second image") {
expect(UIImagePNGRepresentation(bucketImageView.secondImageView.image!)).to(equal(UIImagePNGRepresentation(UIImage(named: "ic-bucket-swipe-filled")!)))
}
}
describe("comment image view") {
var commentImageView: DoubleImageView!
beforeEach {
commentImageView = sut.commentImageView
}
it("should not translate autoresizing mask into constraints") {
expect(commentImageView.translatesAutoresizingMaskIntoConstraints).to(beFalsy())
}
it("should be added to cell content view subviews") {
expect(sut.shotContainer.subviews).to(contain(commentImageView))
}
it("should have proper first image") {
expect(UIImagePNGRepresentation(commentImageView.firstImageView.image!)).to(equal(UIImagePNGRepresentation(UIImage(named: "ic-comment")!)))
}
it("should have proper second image") {
expect(UIImagePNGRepresentation(commentImageView.secondImageView.image!)).to(equal(UIImagePNGRepresentation(UIImage(named: "ic-comment-filled")!)))
}
}
describe("shot image view") {
var shotImageView: UIImageView!
beforeEach {
shotImageView = sut.shotImageView
}
it("should not translate autoresizing mask into constraints") {
expect(shotImageView.translatesAutoresizingMaskIntoConstraints).to(beFalsy())
}
it("should be added to cell content view subviews") {
expect(sut.shotContainer.subviews).to(contain(shotImageView))
}
}
describe("requires constraint based layout") {
var requiresConstraintBasedLayout: Bool!
beforeEach {
requiresConstraintBasedLayout = ShotCollectionViewCell.requiresConstraintBasedLayout
}
it("should require constraint based layout") {
expect(requiresConstraintBasedLayout).to(beTruthy())
}
}
pending("randomly crashes bitrise, works locally; check with Xcode 8 and Swift 3 after conversion") {
describe("swiping cell") {
var panGestureRecognizer: UIPanGestureRecognizer!
beforeEach {
panGestureRecognizer = sut.contentView.gestureRecognizers!.filter{ $0 is UIPanGestureRecognizer }.first as! UIPanGestureRecognizer!
}
context("when swipe began") {
var delegateMock: ShotCollectionViewCellDelegateMock!
var didInformDelegateCellDidStartSwiping: Bool!
beforeEach {
delegateMock = ShotCollectionViewCellDelegateMock()
didInformDelegateCellDidStartSwiping = false
delegateMock.shotCollectionViewCellDidStartSwipingStub.on(any()) { _ in
didInformDelegateCellDidStartSwiping = true
}
sut.delegate = delegateMock
let panGestureRecognizerMock = PanGestureRecognizerMock()
panGestureRecognizerMock.stateStub.on(any()) {
return .began
}
panGestureRecognizer!.specRecognize(with: panGestureRecognizerMock)
}
it("should inform delegate that cell did start swiping") {
expect(didInformDelegateCellDidStartSwiping).to(beTruthy())
}
}
context("when swipe ended") {
var panGestureRecognizerMock: PanGestureRecognizerMock!
var viewClassMock: ViewMock.Type!
var capturedRestoreInitialStateDuration: TimeInterval!
var capturedRestoreInitialStateDelay: TimeInterval!
var capturedRestoreInitialStateDamping: CGFloat!
var capturedRestoreInitialStateVelocity: CGFloat!
var capturedRestoreInitialStateOptions: UIViewAnimationOptions!
var capturedRestoreInitialStateAnimations: (() -> Void)!
var capturedRestoreInitialStateCompletion: ((Bool) -> Void)!
beforeEach {
viewClassMock = ViewMock.self
viewClassMock.springAnimationStub.on(any()) { duration, delay, damping, velocity, options, animations, completion in
capturedRestoreInitialStateDuration = duration
capturedRestoreInitialStateDelay = delay
capturedRestoreInitialStateDamping = damping
capturedRestoreInitialStateVelocity = velocity
capturedRestoreInitialStateOptions = options
capturedRestoreInitialStateAnimations = animations
capturedRestoreInitialStateCompletion = completion
}
sut.viewClass = viewClassMock
panGestureRecognizerMock = PanGestureRecognizerMock()
panGestureRecognizerMock.stateStub.on(any()) {
return .ended
}
}
sharedExamples("returning to initial cell state animation") { (sharedExampleContext: @escaping SharedExampleContext) in
it("should animate returning to initial cell state with duration 0.3") {
expect(capturedRestoreInitialStateDuration).to(equal(0.3))
}
it("should animate returning to initial cell state without delay") {
expect(capturedRestoreInitialStateDelay).to(equal(sharedExampleContext()["expectedDelay"] as? TimeInterval))
}
it("should animate returning to initial cell state with damping 0.6") {
expect(capturedRestoreInitialStateDamping).to(equal(0.6))
}
it("should animate returning to initial cell state with velocity 0.9") {
expect(capturedRestoreInitialStateVelocity).to(equal(0.9))
}
it("should animate returning to initial cell state with ease in out option") {
expect(capturedRestoreInitialStateOptions == UIViewAnimationOptions.curveEaseInOut).to(beTruthy())
}
describe("restore initial state animations block") {
beforeEach {
sut.shotImageView.transform = CGAffineTransform.identity.translatedBy(x: 100, y: 0)
capturedRestoreInitialStateAnimations()
}
it("should restore shot image view identity tranform") {
expect(sut.shotImageView.transform).to(equal(CGAffineTransform.identity))
}
}
describe("restore initial state completion block") {
var delegateMock: ShotCollectionViewCellDelegateMock!
var didInformDelegateCellDidEndSwiping: Bool!
var capturedAction: ShotCollectionViewCell.Action!
beforeEach {
sut.swipeCompletion = { action in
capturedAction = action
}
delegateMock = ShotCollectionViewCellDelegateMock()
didInformDelegateCellDidEndSwiping = false
delegateMock.shotCollectionViewCellDidEndSwipingStub.on(any()) { _ in
didInformDelegateCellDidEndSwiping = true
}
sut.delegate = delegateMock
sut.bucketImageView.isHidden = true
sut.commentImageView.isHidden = true
sut.likeImageView.firstImageView.alpha = 0
sut.likeImageView.secondImageView.alpha = 1
capturedRestoreInitialStateCompletion(true)
}
it("should invoke swipe completion with Comment action") {
let actionWrapper = sharedExampleContext()["expectedAction"] as! ShotCollectionViewCellActionWrapper
expect(capturedAction).to(equal(actionWrapper.action))
}
it("should inform delegate that cell did end swiping") {
expect(didInformDelegateCellDidEndSwiping).to(beTruthy())
}
it("show bucket image view") {
expect(sut.bucketImageView.isHidden).to(beFalsy())
}
it("show comment image view") {
expect(sut.commentImageView.isHidden).to(beFalsy())
}
describe("like image view") {
var likeImageView: DoubleImageView!
beforeEach {
likeImageView = sut.likeImageView
}
it("should display first image view") {
expect(likeImageView.firstImageView.alpha).to(equal(0))
}
it("should hide second image view") {
expect(likeImageView.secondImageView.alpha).to(equal(1))
}
}
}
}
context("when user swiped") {
var capturedActionDuration: TimeInterval!
var capturedActionDelay: TimeInterval!
var capturedActionOptions: UIViewAnimationOptions!
var capturedActionAnimations: (() -> Void)!
var capturedActionCompletion: ((Bool) -> Void)!
beforeEach {
viewClassMock.animationStub.on(any()) { duration, delay, options, animations, completion in
capturedActionDuration = duration
capturedActionDelay = delay
capturedActionOptions = options
capturedActionAnimations = animations
capturedActionCompletion = completion
}
}
context("slightly right") {
beforeEach {
panGestureRecognizerMock.translationInViewStub.on(any()) { _ in
return CGPoint(x: 50, y:0)
}
panGestureRecognizer!.specRecognize(with: panGestureRecognizerMock)
}
it("should animate like action with duration 0.3") {
expect(capturedActionDuration).to(equal(0.3))
}
it("should animate like action without delay") {
expect(capturedActionDelay).to(equal(0))
}
it("should animate like action without options") {
expect(capturedActionOptions).to(equal([]))
}
describe("like action animations block") {
beforeEach {
sut.contentView.bounds = CGRect(x: 0, y: 0, width: 100, height: 0)
sut.likeImageView.alpha = 0
capturedActionAnimations()
}
it("should set shot image view tranform") {
expect(sut.shotImageView.transform).to(equal(CGAffineTransform.identity.translatedBy(x: 100, y: 0)))
}
describe("like image view") {
var likeImageView: DoubleImageView!
beforeEach {
likeImageView = sut.likeImageView
}
it("should have alpha 1") {
expect(likeImageView.alpha).to(equal(1.0))
}
it("should display second image view") {
expect(likeImageView.secondImageView.alpha).to(equal(1))
}
it("should hide first image view") {
expect(likeImageView.firstImageView.alpha).to(equal(0))
}
}
}
describe("like action completion block") {
beforeEach {
capturedActionCompletion(true)
}
itBehavesLike("returning to initial cell state animation") {
["expectedDelay": 0.2,
"expectedAction": ShotCollectionViewCellActionWrapper(action: ShotCollectionViewCell.Action.like)]
}
}
}
context("considerably right") {
beforeEach {
panGestureRecognizerMock.translationInViewStub.on(any()) { _ in
return CGPoint(x: 150, y:0)
}
panGestureRecognizer!.specRecognize(with: panGestureRecognizerMock)
}
it("should animate bucket action with duration 0.3") {
expect(capturedActionDuration).to(equal(0.3))
}
it("should animate bucket action without delay") {
expect(capturedActionDelay).to(equal(0))
}
it("should animate bucket action without options") {
expect(capturedActionOptions).to(equal([]))
}
describe("bucket action animations block") {
beforeEach {
sut.contentView.bounds = CGRect(x: 0, y: 0, width: 100, height: 0)
sut.bucketImageView.alpha = 0
capturedActionAnimations()
}
it("should set shot image view tranform") {
expect(sut.shotImageView.transform).to(equal(CGAffineTransform.identity.translatedBy(x: 100, y: 0)))
}
}
describe("bucket action completion block") {
beforeEach {
capturedActionCompletion(true)
}
itBehavesLike("returning to initial cell state animation") {
["expectedDelay": 0.2,
"expectedAction": ShotCollectionViewCellActionWrapper(action: ShotCollectionViewCell.Action.bucket)]
}
}
}
context("slightly left") {
beforeEach {
panGestureRecognizerMock.translationInViewStub.on(any()) { _ in
return CGPoint(x: -50, y:0)
}
panGestureRecognizer!.specRecognize(with: panGestureRecognizerMock)
}
it("should animate comment action with duration 0.3") {
expect(capturedActionDuration).to(equal(0.3))
}
it("should animate comment action without delay") {
expect(capturedActionDelay).to(equal(0))
}
it("should animate comment action without options") {
expect(capturedActionOptions).to(equal([]))
}
describe("comment action animations block") {
beforeEach {
sut.contentView.bounds = CGRect(x: 0, y: 0, width: 100, height: 0)
sut.commentImageView.alpha = 0
capturedActionAnimations()
}
it("should set shot image view tranform") {
expect(sut.shotImageView.transform).to(equal(CGAffineTransform.identity.translatedBy(x: -100, y: 0)))
}
}
describe("comment action completion block") {
beforeEach {
capturedActionCompletion(true)
}
itBehavesLike("returning to initial cell state animation") {
["expectedDelay": 0.2,
"expectedAction": ShotCollectionViewCellActionWrapper(action: ShotCollectionViewCell.Action.comment)]
}
}
}
}
}
}
describe("gesture recognizer should begin") {
context("when gesture recognizer is pan gesture recognizer") {
context("when user is scrolling with higher horizontal velocity") {
var gestureRecognizerShouldBegin: Bool!
beforeEach {
let panGestureRecognize = PanGestureRecognizerMock()
panGestureRecognize.velocityInViewStub.on(any()) { _ in
return CGPoint(x:100, y:0)
}
gestureRecognizerShouldBegin = sut.gestureRecognizerShouldBegin(panGestureRecognize)
}
it("should begin gesture recognizer") {
expect(gestureRecognizerShouldBegin).to(beTruthy())
}
}
context("when user is scrolling with higher vertical velocity") {
var gestureRecognizerShouldBegin: Bool!
beforeEach {
let panGestureRecognize = PanGestureRecognizerMock()
panGestureRecognize.velocityInViewStub.on(any()) { _ in
return CGPoint(x:0, y:100)
}
gestureRecognizerShouldBegin = sut.gestureRecognizerShouldBegin(panGestureRecognize)
}
it("should not begin gesture recognizer") {
expect(gestureRecognizerShouldBegin).to(beFalsy())
}
}
}
}
}
describe("reusable") {
describe("reuse identifier") {
var reuseIdentifier: String?
beforeEach {
reuseIdentifier = ShotCollectionViewCell.identifier
}
it("should have proper reuse identifier") {
expect(reuseIdentifier).to(equal("ShotCollectionViewCell.Type"))
}
}
}
describe("gesture recognizer delegate") {
describe("gesture recognize should should recognize simultaneously") {
var shouldRecognizeSimultaneously: Bool!
beforeEach {
shouldRecognizeSimultaneously = sut.gestureRecognizer(UIGestureRecognizer(), shouldRecognizeSimultaneouslyWith: UIGestureRecognizer())
}
it("should alwawys block other gesture recognizers") {
expect(shouldRecognizeSimultaneously).to(beFalsy())
}
}
}
}
}
| gpl-3.0 | 7b8cd5d423f1d82c2c227648bb539f88 | 47.700535 | 167 | 0.426851 | 8.588809 | false | false | false | false |
zhangjk4859/MyWeiBoProject | sinaWeibo/sinaWeibo/Classes/Message/JKMessageTableViewController.swift | 1 | 2951 | //
// JKMessageTableViewController.swift
// sinaWeibo
//
// Created by 张俊凯 on 16/6/5.
// Copyright © 2016年 张俊凯. All rights reserved.
//
import UIKit
class JKMessageTableViewController: JKBaseViewController {
override func viewDidLoad() {
super.viewDidLoad()
// 1.如果没有登录, 就设置未登录界面的信息
if !userLogin
{
visitView?.setupVisitInfo(false, imageName: "visitordiscover_image_message", message: "登录后,别人评论你的微博,发给你的消息,都会在这里收到通知")
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
// MARK: - Table view data source
override func numberOfSectionsInTableView(tableView: UITableView) -> Int {
return 0
}
/*
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("reuseIdentifier", forIndexPath: indexPath)
// Configure the cell...
return cell
}
*/
/*
// Override to support conditional editing of the table view.
override func tableView(tableView: UITableView, canEditRowAtIndexPath indexPath: NSIndexPath) -> Bool {
// Return false if you do not want the specified item to be editable.
return true
}
*/
/*
// Override to support editing the table view.
override func tableView(tableView: UITableView, commitEditingStyle editingStyle: UITableViewCellEditingStyle, forRowAtIndexPath indexPath: NSIndexPath) {
if editingStyle == .Delete {
// Delete the row from the data source
tableView.deleteRowsAtIndexPaths([indexPath], withRowAnimation: .Fade)
} else if editingStyle == .Insert {
// Create a new instance of the appropriate class, insert it into the array, and add a new row to the table view
}
}
*/
/*
// Override to support rearranging the table view.
override func tableView(tableView: UITableView, moveRowAtIndexPath fromIndexPath: NSIndexPath, toIndexPath: NSIndexPath) {
}
*/
/*
// Override to support conditional rearranging of the table view.
override func tableView(tableView: UITableView, canMoveRowAtIndexPath indexPath: NSIndexPath) -> Bool {
// Return false if you do not want the item to be re-orderable.
return true
}
*/
/*
// MARK: - Navigation
// In a storyboard-based application, you will often want to do a little preparation before navigation
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
// Get the new view controller using segue.destinationViewController.
// Pass the selected object to the new view controller.
}
*/
}
| mit | 1583243dc2c7a831c9233206275cef63 | 30.955056 | 157 | 0.673699 | 5.325843 | false | false | false | false |
orta/RxSwift | RxSwift/RxSwift/Observables/Observable+Single.swift | 1 | 5128 | //
// Observable+Single.swift
// Rx
//
// Created by Krunoslav Zaher on 2/14/15.
// Copyright (c) 2015 Krunoslav Zaher. All rights reserved.
//
import Foundation
// as observable
public func asObservable<E>
(source: Observable<E>) -> Observable<E> {
if let asObservable = source as? AsObservable<E> {
return asObservable.omega()
}
else {
return AsObservable(source: source)
}
}
// distinct until changed
public func distinctUntilChangedOrDie<E: Equatable>(source: Observable<E>)
-> Observable<E> {
return distinctUntilChangedOrDie({ success($0) }, { success($0 == $1) })(source)
}
public func distinctUntilChangedOrDie<E, K: Equatable>
(keySelector: (E) -> Result<K>)
-> (Observable<E> -> Observable<E>) {
return { source in
return distinctUntilChangedOrDie(keySelector, { success($0 == $1) })(source)
}
}
public func distinctUntilChangedOrDie<E>
(comparer: (lhs: E, rhs: E) -> Result<Bool>)
-> (Observable<E> -> Observable<E>) {
return { source in
return distinctUntilChangedOrDie({ success($0) }, comparer)(source)
}
}
public func distinctUntilChangedOrDie<E, K>
(keySelector: (E) -> Result<K>, comparer: (lhs: K, rhs: K) -> Result<Bool>)
-> (Observable<E> -> Observable<E>) {
return { source in
return DistinctUntilChanged(source: source, selector: keySelector, comparer: comparer)
}
}
public func distinctUntilChanged<E: Equatable>(source: Observable<E>)
-> Observable<E> {
return distinctUntilChanged({ $0 }, { ($0 == $1) })(source)
}
public func distinctUntilChanged<E, K: Equatable>
(keySelector: (E) -> K)
-> (Observable<E> -> Observable<E>) {
return { source in
return distinctUntilChanged(keySelector, { ($0 == $1) })(source)
}
}
public func distinctUntilChanged<E>
(comparer: (lhs: E, rhs: E) -> Bool)
-> (Observable<E> -> Observable<E>) {
return { source in
return distinctUntilChanged({ ($0) }, comparer)(source)
}
}
public func distinctUntilChanged<E, K>
(keySelector: (E) -> K, comparer: (lhs: K, rhs: K) -> Bool)
-> (Observable<E> -> Observable<E>) {
return { source in
return DistinctUntilChanged(source: source, selector: {success(keySelector($0)) }, comparer: { success(comparer(lhs: $0, rhs: $1))})
}
}
// do
public func doOrDie<E>
(eventHandler: (Event<E>) -> Result<Void>)
-> (Observable<E> -> Observable<E>) {
return { source in
return Do(source: source, eventHandler: eventHandler)
}
}
public func `do`<E>
(eventHandler: (Event<E>) -> Void)
-> (Observable<E> -> Observable<E>) {
return { source in
return Do(source: source, eventHandler: { success(eventHandler($0)) })
}
}
// doOnNext
public func doOnNext<E>
(actionOnNext: E -> Void)
-> (Observable<E> -> Observable<E>) {
return { source in
return source >- `do` { event in
switch event {
case .Next(let boxedValue):
let value = boxedValue.value
actionOnNext(value)
default:
break
}
}
}
}
// map aka select
public func mapOrDie<E, R>
(selector: E -> Result<R>)
-> (Observable<E> -> Observable<R>) {
return { source in
return selectOrDie(selector)(source)
}
}
public func map<E, R>
(selector: E -> R)
-> (Observable<E> -> Observable<R>) {
return { source in
return select(selector)(source)
}
}
public func mapWithIndexOrDie<E, R>
(selector: (E, Int) -> Result<R>)
-> (Observable<E> -> Observable<R>) {
return { source in
return selectWithIndexOrDie(selector)(source)
}
}
public func mapWithIndex<E, R>
(selector: (E, Int) -> R)
-> (Observable<E> -> Observable<R>) {
return { source in
return selectWithIndex(selector)(source)
}
}
// select
public func selectOrDie<E, R>
(selector: (E) -> Result<R>)
-> (Observable<E> -> Observable<R>) {
return { source in
return Select(source: source, selector: selector)
}
}
public func select<E, R>
(selector: (E) -> R)
-> (Observable<E> -> Observable<R>) {
return { source in
return Select(source: source, selector: {success(selector($0)) })
}
}
public func selectWithIndexOrDie<E, R>
(selector: (E, Int) -> Result<R>)
-> (Observable<E> -> Observable<R>) {
return { source in
return Select(source: source, selector: selector)
}
}
public func selectWithIndex<E, R>
(selector: (E, Int) -> R)
-> (Observable<E> -> Observable<R>) {
return { source in
return Select(source: source, selector: {success(selector($0, $1)) })
}
}
// Prefixes observable sequence with `firstElement` element.
// The same functionality could be achieved using `concat([returnElement(prefix), source])`,
// but this is significantly more efficient implementation.
public func startWith<E>
(firstElement: E)
-> (Observable<E> -> Observable<E>) {
return { source in
return StartWith(source: source, element: firstElement)
}
}
| mit | 27b865ed34a36a579455a3f2e901fe48 | 25.030457 | 140 | 0.608619 | 3.705202 | false | false | false | false |
jpedrosa/sua_nc | Sources/ascii.swift | 2 | 830 |
public class Ascii {
public static func toLowerCase(c: UInt8) -> UInt8 {
if c >= 65 && c <= 90 {
return c + 32
}
return c
}
public static func toLowerCase(bytes: [UInt8]) -> [UInt8] {
var a = bytes
let len = a.count
for i in 0..<len {
let c = a[i]
if c >= 65 && c <= 90 {
a[i] = c + 32
}
}
return a
}
public static func toLowerCase(bytes: [[UInt8]]) -> [[UInt8]] {
var a = bytes
let len = a.count
for i in 0..<len {
let b = a[i]
let blen = b.count
for bi in 0..<blen {
let c = b[bi]
if c >= 65 && c <= 90 {
a[i][bi] = c + 32
}
}
}
return a
}
public static func toLowerCase(string: String) -> String? {
return String.fromCharCodes(toLowerCase(string.bytes))
}
}
| apache-2.0 | 62a0e942579de5d201d8d1b8ab3a445a | 17.863636 | 65 | 0.484337 | 3.254902 | false | false | false | false |
blockchain/My-Wallet-V3-iOS | Modules/FeatureWithdrawalLocks/Sources/FeatureWithdrawalLocksUI/Localization/LocalizationConstants+WithdrawalLock.swift | 1 | 2496 | // Copyright © Blockchain Luxembourg S.A. All rights reserved.
import Localization
extension LocalizationConstants {
enum WithdrawalLocks {
static let onHoldTitle = NSLocalizedString(
"On Hold",
comment: "Withdrawal Locks: On Hold Title"
)
static let onHoldAmountTitle = NSLocalizedString(
"%@ On Hold",
comment: "Withdrawal Locks: On Hold Title with the amount"
)
// swiftlint:disable line_length
static let holdingPeriodDescription = NSLocalizedString(
"Newly added funds are subject to a holding period. You can transfer between your Trading, Rewards, and Exchange accounts in the meantime.",
comment: "Withdrawal Locks: Holding period description"
)
static let doesNotLookRightDescription = NSLocalizedString(
"See that something doesn't look right?",
comment: "Withdrawal Locks: Something doesn't look right question"
)
static let contactSupportTitle = NSLocalizedString(
"Contact support",
comment: "Withdrawal Locks: Contact support title"
)
static let noLocks = NSLocalizedString(
"You do not have any pending withdrawal locks.",
comment: "Withdrawal Locks: Held Until section title"
)
static let heldUntilTitle = NSLocalizedString(
"Held Until",
comment: "Withdrawal Locks: Held Until section title"
)
static let amountTitle = NSLocalizedString(
"Amount",
comment: "Withdrawal Locks: Amount section title"
)
static let availableToWithdrawTitle = NSLocalizedString(
"Available to Withdraw",
comment: "Withdrawal Locks: Available to Withdraw title"
)
static let learnMoreButtonTitle = NSLocalizedString(
"Learn More",
comment: "Withdrawal Locks: Learn More button title"
)
static let learnMoreTitle = NSLocalizedString(
"Learn more ->",
comment: "Withdrawal Locks: Learn More title"
)
static let seeDetailsButtonTitle = NSLocalizedString(
"See Details",
comment: "Withdrawal Locks: See Details button title"
)
static let confirmButtonTitle = NSLocalizedString(
"I Understand",
comment: "Withdrawal Locks: I Understand button title"
)
}
}
| lgpl-3.0 | 6315327d25db354a340a627cd64a8d39 | 33.178082 | 152 | 0.618036 | 5.657596 | false | false | false | false |
apple/swift-nio-http2 | Sources/NIOHTTP2PerformanceTester/main.swift | 1 | 5440 | //===----------------------------------------------------------------------===//
//
// This source file is part of the SwiftNIO open source project
//
// Copyright (c) 2019-2021 Apple Inc. and the SwiftNIO project authors
// Licensed under Apache License v2.0
//
// See LICENSE.txt for license information
// See CONTRIBUTORS.txt for the list of SwiftNIO project authors
//
// SPDX-License-Identifier: Apache-2.0
//
//===----------------------------------------------------------------------===//
import Foundation
import Dispatch
// MARK: Test Harness
var warning: String = ""
assert({
print("======================================================")
print("= YOU ARE RUNNING NIOPerformanceTester IN DEBUG MODE =")
print("======================================================")
warning = " <<< DEBUG MODE >>>"
return true
}())
public func measure(_ fn: () throws -> Int) rethrows -> [TimeInterval] {
func measureOne(_ fn: () throws -> Int) rethrows -> TimeInterval {
let start = Date()
_ = try fn()
let end = Date()
return end.timeIntervalSince(start)
}
_ = try measureOne(fn) /* pre-heat and throw away */
var measurements = Array(repeating: 0.0, count: 10)
for i in 0..<10 {
measurements[i] = try measureOne(fn)
}
return measurements
}
let limitSet = CommandLine.arguments.dropFirst()
public func measureAndPrint(desc: String, fn: () throws -> Int) rethrows -> Void {
#if CACHEGRIND
// When CACHEGRIND is set we only run a single requested test, and we only run it once. No printing or other mess.
if limitSet.contains(desc) {
_ = try fn()
}
#else
if limitSet.count == 0 || limitSet.contains(desc) {
print("measuring\(warning): \(desc): ", terminator: "")
let measurements = try measure(fn)
print(measurements.reduce("") { $0 + "\($1), " })
} else {
print("skipping '\(desc)', limit set = \(limitSet)")
}
#endif
}
func measureAndPrint<B: Benchmark>(desc: String, benchmark bench: @autoclosure () -> B) throws {
// Avoids running setUp/tearDown when we don't want that benchmark.
guard limitSet.count == 0 || limitSet.contains(desc) else {
return
}
let bench = bench()
try bench.setUp()
defer {
bench.tearDown()
}
try measureAndPrint(desc: desc) {
return try bench.run()
}
}
// MARK: Utilities
try measureAndPrint(desc: "1_conn_10k_reqs", benchmark: Bench1Conn10kRequests())
try measureAndPrint(desc: "encode_100k_header_blocks_indexable", benchmark: HPACKHeaderEncodingBenchmark(headers: .indexable, loopCount: 100_000))
try measureAndPrint(desc: "encode_100k_header_blocks_nonindexable", benchmark: HPACKHeaderEncodingBenchmark(headers: .nonIndexable, loopCount: 100_000))
try measureAndPrint(desc: "encode_100k_header_blocks_neverIndexed", benchmark: HPACKHeaderEncodingBenchmark(headers: .neverIndexed, loopCount: 100_000))
try measureAndPrint(desc: "decode_100k_header_blocks_indexable", benchmark: HPACKHeaderDecodingBenchmark(headers: .indexable, loopCount: 100_000))
try measureAndPrint(desc: "decode_100k_header_blocks_nonindexable", benchmark: HPACKHeaderDecodingBenchmark(headers: .nonIndexable, loopCount: 100_000))
try measureAndPrint(desc: "decode_100k_header_blocks_neverIndexed", benchmark: HPACKHeaderDecodingBenchmark(headers: .neverIndexed, loopCount: 100_000))
try measureAndPrint(desc: "hpackheaders_canonical_form", benchmark: HPACKHeaderCanonicalFormBenchmark(.noTrimming))
try measureAndPrint(desc: "hpackheaders_canonical_form_trimming_whitespace", benchmark: HPACKHeaderCanonicalFormBenchmark(.trimmingWhitespace))
try measureAndPrint(desc: "hpackheaders_canonical_form_trimming_whitespace_short_strings", benchmark: HPACKHeaderCanonicalFormBenchmark(.trimmingWhitespaceFromShortStrings))
try measureAndPrint(desc: "hpackheaders_canonical_form_trimming_whitespace_long_strings", benchmark: HPACKHeaderCanonicalFormBenchmark(.trimmingWhitespaceFromLongStrings))
try measureAndPrint(desc: "hpackheaders_normalize_httpheaders_removing_10k_conn_headers", benchmark: HPACKHeadersNormalizationOfHTTPHeadersBenchmark(headersKind: .manyUniqueConnectionHeaderValues(10_000), iterations: 10))
try measureAndPrint(desc: "hpackheaders_normalize_httpheaders_keeping_10k_conn_headers", benchmark: HPACKHeadersNormalizationOfHTTPHeadersBenchmark(headersKind: .manyConnectionHeaderValuesWhichAreNotRemoved(10_000), iterations: 10))
try measureAndPrint(desc: "huffman_encode_basic", benchmark: HuffmanEncodingBenchmark(huffmanString: .basicHuffmanString, loopCount: 100))
try measureAndPrint(desc: "huffman_encode_complex", benchmark: HuffmanEncodingBenchmark(huffmanString: .complexHuffmanString, loopCount: 100))
try measureAndPrint(desc: "huffman_decode_basic", benchmark: HuffmanDecodingBenchmark(huffmanBytes: .basicHuffmanBytes, loopCount: 25))
try measureAndPrint(desc: "huffman_decode_complex", benchmark: HuffmanDecodingBenchmark(huffmanBytes: .complexHuffmanBytes, loopCount: 10))
try measureAndPrint(desc: "server_only_10k_requests_1_concurrent", benchmark: ServerOnly10KRequestsBenchmark(concurrentStreams: 1))
try measureAndPrint(desc: "server_only_10k_requests_100_concurrent", benchmark: ServerOnly10KRequestsBenchmark(concurrentStreams: 100))
try measureAndPrint(desc: "stream_teardown_10k_requests_100_concurrent", benchmark: StreamTeardownBenchmark(concurrentStreams: 100))
| apache-2.0 | ffa747a3d3c0f0a2cfceaae4b3689eda | 51.815534 | 232 | 0.714154 | 4.401294 | false | false | false | false |
HotCatLX/SwiftStudy | 17-BasicGesture/BasicGesture/ViewController.swift | 1 | 1863 | //
// ViewController.swift
// BasicGesture
//
// Created by suckerl on 2017/5/22.
// Copyright © 2017年 suckel. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
fileprivate lazy var image :UIImageView = {
let image = UIImageView()
image.image = UIImage(named: "one")
image.isUserInteractionEnabled = true
return image
}()
override func viewDidLoad() {
super.viewDidLoad()
self.setupUI()
self.swipe()
}
}
extension ViewController {
func setupUI() {
view.addSubview(image)
image.frame = view.bounds
}
/*
UITapGestureRecognizer 轻拍手势
UISwipeGestureRecognizer 轻扫手势
UILongPressGestureRecognizer 长按手势
UIPanGestureRecognizer 平移手势
UIPinchGestureRecognizer 捏合(缩放)手势
UIRotationGestureRecognizer 旋转手势
UIScreenEdgePanGestureRecognizer 屏幕边缘平移
*/
func swipe() {
let swipeLeft = UISwipeGestureRecognizer(target: self, action: #selector(ViewController.swipeSelector(swipe:)))
swipeLeft.direction = UISwipeGestureRecognizerDirection.left
self.image.addGestureRecognizer(swipeLeft)
let swipeRight = UISwipeGestureRecognizer(target: self, action:#selector(ViewController.swipeSelector(swipe:)))
swipeRight.direction = UISwipeGestureRecognizerDirection.right
self.image.addGestureRecognizer(swipeRight)
}
func swipeSelector(swipe:UISwipeGestureRecognizer) {
if swipe.direction == UISwipeGestureRecognizerDirection.left {
let twoVC = TwoController()
self.show(twoVC, sender: nil)
}else {
let threeVC = ThreeController()
self.show(threeVC, sender: nil)
}
}
}
| mit | 8666ddf7554c13eaaab054d3b8fc612d | 25.746269 | 120 | 0.659598 | 5.13467 | false | false | false | false |
uasys/swift | stdlib/public/core/Reverse.swift | 1 | 17839 | //===--- Reverse.swift - Sequence and collection reversal -----------------===//
//
// This source file is part of the Swift.org open source project
//
// Copyright (c) 2014 - 2017 Apple Inc. and the Swift project authors
// Licensed under Apache License v2.0 with Runtime Library Exception
//
// See https://swift.org/LICENSE.txt for license information
// See https://swift.org/CONTRIBUTORS.txt for the list of Swift project authors
//
//===----------------------------------------------------------------------===//
extension MutableCollection where Self : BidirectionalCollection {
/// Reverses the elements of the collection in place.
///
/// The following example reverses the elements of an array of characters:
///
/// var characters: [Character] = ["C", "a", "f", "é"]
/// characters.reverse()
/// print(characters)
/// // Prints "["é", "f", "a", "C"]
///
/// - Complexity: O(*n*), where *n* is the number of elements in the
/// collection.
public mutating func reverse() {
if isEmpty { return }
var f = startIndex
var l = index(before: endIndex)
while f < l {
swapAt(f, l)
formIndex(after: &f)
formIndex(before: &l)
}
}
}
/// An iterator that can be much faster than the iterator of a reversed slice.
// TODO: See about using this in more places
@_fixed_layout
public struct _ReverseIndexingIterator<
Elements : BidirectionalCollection
> : IteratorProtocol, Sequence {
@_inlineable
@inline(__always)
/// Creates an iterator over the given collection.
public /// @testable
init(_elements: Elements, _position: Elements.Index) {
self._elements = _elements
self._position = _position
}
@_inlineable
@inline(__always)
public mutating func next() -> Elements.Element? {
guard _fastPath(_position != _elements.startIndex) else { return nil }
_position = _elements.index(before: _position)
return _elements[_position]
}
@_versioned
internal let _elements: Elements
@_versioned
internal var _position: Elements.Index
}
// FIXME(ABI)#59 (Conditional Conformance): we should have just one type,
// `ReversedCollection`, that has conditional conformances to
// `RandomAccessCollection`, and possibly `MutableCollection` and
// `RangeReplaceableCollection`.
// rdar://problem/17144340
// FIXME: swift-3-indexing-model - should gyb ReversedXxx & ReversedRandomAccessXxx
/// An index that traverses the same positions as an underlying index,
/// with inverted traversal direction.
@_fixed_layout
public struct ReversedIndex<Base : Collection> : Comparable {
/// Creates a new index into a reversed collection for the position before
/// the specified index.
///
/// When you create an index into a reversed collection using `base`, an
/// index from the underlying collection, the resulting index is the
/// position of the element *before* the element referenced by `base`. The
/// following example creates a new `ReversedIndex` from the index of the
/// `"a"` character in a string's character view.
///
/// let name = "Horatio"
/// let aIndex = name.index(of: "a")!
/// // name[aIndex] == "a"
///
/// let reversedName = name.reversed()
/// let i = ReversedIndex<String>(aIndex)
/// // reversedName[i] == "r"
///
/// The element at the position created using `ReversedIndex<...>(aIndex)` is
/// `"r"`, the character before `"a"` in the `name` string.
///
/// - Parameter base: The position after the element to create an index for.
@_inlineable
public init(_ base: Base.Index) {
self.base = base
}
/// The position after this position in the underlying collection.
///
/// To find the position that corresponds with this index in the original,
/// underlying collection, use that collection's `index(before:)` method
/// with the `base` property.
///
/// The following example declares a function that returns the index of the
/// last even number in the passed array, if one is found. First, the
/// function finds the position of the last even number as a `ReversedIndex`
/// in a reversed view of the array of numbers. Next, the function calls the
/// array's `index(before:)` method to return the correct position in the
/// passed array.
///
/// func indexOfLastEven(_ numbers: [Int]) -> Int? {
/// let reversedNumbers = numbers.reversed()
/// guard let i = reversedNumbers.index(where: { $0 % 2 == 0 })
/// else { return nil }
///
/// return numbers.index(before: i.base)
/// }
///
/// let numbers = [10, 20, 13, 19, 30, 52, 17, 40, 51]
/// if let lastEven = indexOfLastEven(numbers) {
/// print("Last even number: \(numbers[lastEven])")
/// }
/// // Prints "Last even number: 40"
public let base: Base.Index
@_inlineable
public static func == (
lhs: ReversedIndex<Base>,
rhs: ReversedIndex<Base>
) -> Bool {
return lhs.base == rhs.base
}
@_inlineable
public static func < (
lhs: ReversedIndex<Base>,
rhs: ReversedIndex<Base>
) -> Bool {
// Note ReversedIndex has inverted logic compared to base Base.Index
return lhs.base > rhs.base
}
}
/// A collection that presents the elements of its base collection
/// in reverse order.
///
/// - Note: This type is the result of `x.reversed()` where `x` is a
/// collection having bidirectional indices.
///
/// The `reversed()` method is always lazy when applied to a collection
/// with bidirectional indices, but does not implicitly confer
/// laziness on algorithms applied to its result. In other words, for
/// ordinary collections `c` having bidirectional indices:
///
/// * `c.reversed()` does not create new storage
/// * `c.reversed().map(f)` maps eagerly and returns a new array
/// * `c.lazy.reversed().map(f)` maps lazily and returns a `LazyMapCollection`
///
/// - See also: `ReversedRandomAccessCollection`
@_fixed_layout
public struct ReversedCollection<
Base : BidirectionalCollection
> : BidirectionalCollection {
/// Creates an instance that presents the elements of `base` in
/// reverse order.
///
/// - Complexity: O(1)
@_versioned
@_inlineable
internal init(_base: Base) {
self._base = _base
}
/// A type that represents a valid position in the collection.
///
/// Valid indices consist of the position of every element and a
/// "past the end" position that's not valid for use as a subscript.
public typealias Index = ReversedIndex<Base>
public typealias IndexDistance = Base.IndexDistance
@_fixed_layout
public struct Iterator : IteratorProtocol, Sequence {
@_inlineable
@inline(__always)
public /// @testable
init(elements: Base, endPosition: Base.Index) {
self._elements = elements
self._position = endPosition
}
@_inlineable
@inline(__always)
public mutating func next() -> Base.Iterator.Element? {
guard _fastPath(_position != _elements.startIndex) else { return nil }
_position = _elements.index(before: _position)
return _elements[_position]
}
@_versioned
internal let _elements: Base
@_versioned
internal var _position: Base.Index
}
@_inlineable
@inline(__always)
public func makeIterator() -> Iterator {
return Iterator(elements: _base, endPosition: _base.endIndex)
}
@_inlineable
public var startIndex: Index {
return ReversedIndex(_base.endIndex)
}
@_inlineable
public var endIndex: Index {
return ReversedIndex(_base.startIndex)
}
@_inlineable
public func index(after i: Index) -> Index {
return ReversedIndex(_base.index(before: i.base))
}
@_inlineable
public func index(before i: Index) -> Index {
return ReversedIndex(_base.index(after: i.base))
}
@_inlineable
public func index(_ i: Index, offsetBy n: IndexDistance) -> Index {
// FIXME: swift-3-indexing-model: `-n` can trap on Int.min.
return ReversedIndex(_base.index(i.base, offsetBy: -n))
}
@_inlineable
public func index(
_ i: Index, offsetBy n: IndexDistance, limitedBy limit: Index
) -> Index? {
// FIXME: swift-3-indexing-model: `-n` can trap on Int.min.
return _base.index(i.base, offsetBy: -n, limitedBy: limit.base).map { ReversedIndex($0) }
}
@_inlineable
public func distance(from start: Index, to end: Index) -> IndexDistance {
return _base.distance(from: end.base, to: start.base)
}
@_inlineable
public subscript(position: Index) -> Base.Element {
return _base[_base.index(before: position.base)]
}
@_inlineable
public subscript(bounds: Range<Index>) -> BidirectionalSlice<ReversedCollection> {
return BidirectionalSlice(base: self, bounds: bounds)
}
public let _base: Base
}
/// An index that traverses the same positions as an underlying index,
/// with inverted traversal direction.
@_fixed_layout
public struct ReversedRandomAccessIndex<
Base : RandomAccessCollection
> : Comparable {
/// Creates a new index into a reversed collection for the position before
/// the specified index.
///
/// When you create an index into a reversed collection using the index
/// passed as `base`, an index from the underlying collection, the resulting
/// index is the position of the element *before* the element referenced by
/// `base`. The following example creates a new `ReversedIndex` from the
/// index of the `"a"` character in a string's character view.
///
/// let name = "Horatio"
/// let aIndex = name.index(of: "a")!
/// // name[aIndex] == "a"
///
/// let reversedName = name.reversed()
/// let i = ReversedIndex<String>(aIndex)
/// // reversedName[i] == "r"
///
/// The element at the position created using `ReversedIndex<...>(aIndex)` is
/// `"r"`, the character before `"a"` in the `name` string. Viewed from the
/// perspective of the `reversedCharacters` collection, of course, `"r"` is
/// the element *after* `"a"`.
///
/// - Parameter base: The position after the element to create an index for.
@_inlineable
public init(_ base: Base.Index) {
self.base = base
}
/// The position after this position in the underlying collection.
///
/// To find the position that corresponds with this index in the original,
/// underlying collection, use that collection's `index(before:)` method
/// with this index's `base` property.
///
/// The following example declares a function that returns the index of the
/// last even number in the passed array, if one is found. First, the
/// function finds the position of the last even number as a `ReversedIndex`
/// in a reversed view of the array of numbers. Next, the function calls the
/// array's `index(before:)` method to return the correct position in the
/// passed array.
///
/// func indexOfLastEven(_ numbers: [Int]) -> Int? {
/// let reversedNumbers = numbers.reversed()
/// guard let i = reversedNumbers.index(where: { $0 % 2 == 0 })
/// else { return nil }
///
/// return numbers.index(before: i.base)
/// }
///
/// let numbers = [10, 20, 13, 19, 30, 52, 17, 40, 51]
/// if let lastEven = indexOfLastEven(numbers) {
/// print("Last even number: \(numbers[lastEven])")
/// }
/// // Prints "Last even number: 40"
public let base: Base.Index
@_inlineable
public static func == (
lhs: ReversedRandomAccessIndex<Base>,
rhs: ReversedRandomAccessIndex<Base>
) -> Bool {
return lhs.base == rhs.base
}
@_inlineable
public static func < (
lhs: ReversedRandomAccessIndex<Base>,
rhs: ReversedRandomAccessIndex<Base>
) -> Bool {
// Note ReversedRandomAccessIndex has inverted logic compared to base Base.Index
return lhs.base > rhs.base
}
}
/// A collection that presents the elements of its base collection
/// in reverse order.
///
/// - Note: This type is the result of `x.reversed()` where `x` is a
/// collection having random access indices.
/// - See also: `ReversedCollection`
@_fixed_layout
public struct ReversedRandomAccessCollection<
Base : RandomAccessCollection
> : RandomAccessCollection {
// FIXME: swift-3-indexing-model: tests for ReversedRandomAccessIndex and
// ReversedRandomAccessCollection.
/// Creates an instance that presents the elements of `base` in
/// reverse order.
///
/// - Complexity: O(1)
@_versioned
@_inlineable
internal init(_base: Base) {
self._base = _base
}
/// A type that represents a valid position in the collection.
///
/// Valid indices consist of the position of every element and a
/// "past the end" position that's not valid for use as a subscript.
public typealias Index = ReversedRandomAccessIndex<Base>
public typealias IndexDistance = Base.IndexDistance
/// A type that provides the sequence's iteration interface and
/// encapsulates its iteration state.
public typealias Iterator = IndexingIterator<
ReversedRandomAccessCollection
>
@_inlineable
public var startIndex: Index {
return ReversedRandomAccessIndex(_base.endIndex)
}
@_inlineable
public var endIndex: Index {
return ReversedRandomAccessIndex(_base.startIndex)
}
@_inlineable
public func index(after i: Index) -> Index {
return ReversedRandomAccessIndex(_base.index(before: i.base))
}
@_inlineable
public func index(before i: Index) -> Index {
return ReversedRandomAccessIndex(_base.index(after: i.base))
}
@_inlineable
public func index(_ i: Index, offsetBy n: IndexDistance) -> Index {
// FIXME: swift-3-indexing-model: `-n` can trap on Int.min.
// FIXME: swift-3-indexing-model: tests.
return ReversedRandomAccessIndex(_base.index(i.base, offsetBy: -n))
}
@_inlineable
public func index(
_ i: Index, offsetBy n: IndexDistance, limitedBy limit: Index
) -> Index? {
// FIXME: swift-3-indexing-model: `-n` can trap on Int.min.
// FIXME: swift-3-indexing-model: tests.
return _base.index(i.base, offsetBy: -n, limitedBy: limit.base).map { Index($0) }
}
@_inlineable
public func distance(from start: Index, to end: Index) -> IndexDistance {
// FIXME: swift-3-indexing-model: tests.
return _base.distance(from: end.base, to: start.base)
}
@_inlineable
public subscript(position: Index) -> Base.Element {
return _base[_base.index(before: position.base)]
}
// FIXME: swift-3-indexing-model: the rest of methods.
public let _base: Base
}
extension BidirectionalCollection {
/// Returns a view presenting the elements of the collection in reverse
/// order.
///
/// You can reverse a collection without allocating new space for its
/// elements by calling this `reversed()` method. A `ReversedCollection`
/// instance wraps an underlying collection and provides access to its
/// elements in reverse order. This example prints the characters of a
/// string in reverse order:
///
/// let word = "Backwards"
/// for char in word.reversed() {
/// print(char, terminator: "")
/// }
/// // Prints "sdrawkcaB"
///
/// If you need a reversed collection of the same type, you may be able to
/// use the collection's sequence-based or collection-based initializer. For
/// example, to get the reversed version of a string, reverse its
/// characters and initialize a new `String` instance from the result.
///
/// let reversedWord = String(word.reversed())
/// print(reversedWord)
/// // Prints "sdrawkcaB"
///
/// - Complexity: O(1)
@_inlineable
public func reversed() -> ReversedCollection<Self> {
return ReversedCollection(_base: self)
}
}
extension RandomAccessCollection {
/// Returns a view presenting the elements of the collection in reverse
/// order.
///
/// You can reverse a collection without allocating new space for its
/// elements by calling this `reversed()` method. A
/// `ReversedRandomAccessCollection` instance wraps an underlying collection
/// and provides access to its elements in reverse order. This example
/// prints the elements of an array in reverse order:
///
/// let numbers = [3, 5, 7]
/// for number in numbers.reversed() {
/// print(number)
/// }
/// // Prints "7"
/// // Prints "5"
/// // Prints "3"
///
/// If you need a reversed collection of the same type, you may be able to
/// use the collection's sequence-based or collection-based initializer. For
/// example, to get the reversed version of an array, initialize a new
/// `Array` instance from the result of this `reversed()` method.
///
/// let reversedNumbers = Array(numbers.reversed())
/// print(reversedNumbers)
/// // Prints "[7, 5, 3]"
///
/// - Complexity: O(1)
@_inlineable
public func reversed() -> ReversedRandomAccessCollection<Self> {
return ReversedRandomAccessCollection(_base: self)
}
}
extension LazyCollectionProtocol
where
Self : BidirectionalCollection,
Elements : BidirectionalCollection {
/// Returns the elements of the collection in reverse order.
///
/// - Complexity: O(1)
@_inlineable
public func reversed() -> LazyBidirectionalCollection<
ReversedCollection<Elements>
> {
return ReversedCollection(_base: elements).lazy
}
}
extension LazyCollectionProtocol
where
Self : RandomAccessCollection,
Elements : RandomAccessCollection {
/// Returns the elements of the collection in reverse order.
///
/// - Complexity: O(1)
@_inlineable
public func reversed() -> LazyRandomAccessCollection<
ReversedRandomAccessCollection<Elements>
> {
return ReversedRandomAccessCollection(_base: elements).lazy
}
}
// ${'Local Variables'}:
// eval: (read-only-mode 1)
// End:
| apache-2.0 | 349de9c5bc8f23594c389c3b39bfeca9 | 32.031481 | 93 | 0.662051 | 4.28568 | false | false | false | false |
5lucky2xiaobin0/PandaTV | PandaTV/PandaTV/Classes/Main/VIew/GameCell.swift | 1 | 2082 | //
// GameCell.swift
// PandaTV
//
// Created by 钟斌 on 2017/3/24.
// Copyright © 2017年 xiaobin. All rights reserved.
//
import UIKit
import Kingfisher
class GameCell: UICollectionViewCell {
@IBOutlet weak var nowImage: UIImageView!
@IBOutlet weak var gameTitle: UILabel!
@IBOutlet weak var username: UILabel!
@IBOutlet weak var personNumber: UIButton!
@IBOutlet weak var gamename: UIButton!
var card_type : String?
var gameItem : GameItem? {
didSet {
if let item = gameItem {
var url : URL?
if let urlString = item.img {
url = URL(string: urlString)
}else {
let urlString = item.pictures?["img"] as! String
url = URL(string: urlString)
}
nowImage.kf.setImage(with: ImageResource(downloadURL: url!))
gameTitle.text = item.title ?? item.name
username.text = item.userItem?.nickName
if item.person_num > 10000 {
let number = CGFloat(item.person_num) / 10000.0
personNumber.setTitle(String(format: "%.1f", number) + "万", for: .normal)
}else {
personNumber.setTitle("\(item.person_num)", for: .normal)
}
//1 首页 card_type = cateroomlist 不显示
//2 全部游戏 cart_type = nil 不显示
//3 游戏界面 cart_type = cateroomlist 不显示
//4 娱乐界面 cart_type = cateroomlist 不显示
if card_type == "cateroomlist" || card_type == nil {
gamename.isHidden = true
}else {
gamename.isHidden = false
gamename.setTitle(item.gameType, for: .normal)
}
}
}
}
}
| mit | 645766bbed748d8d6798638c49353e2c | 30.092308 | 93 | 0.46858 | 4.624714 | false | false | false | false |
See-Ku/SK4Toolkit | SK4Toolkit/core/SK4Dispatch.swift | 1 | 1670 | //
// SK4Dispatch.swift
// SK4Toolkit
//
// Created by See.Ku on 2016/03/24.
// Copyright (c) 2016 AxeRoad. All rights reserved.
//
import Foundation
// /////////////////////////////////////////////////////////////
// MARK: - 同期/非同期関係の処理
/// グローバルキューで非同期処理
public func sk4AsyncGlobal(exec: (()->Void)) {
let global = dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT, 0)
dispatch_async(global, exec)
}
/// メインキューで非同期処理
public func sk4AsyncMain(exec: (()->Void)) {
let main = dispatch_get_main_queue()
dispatch_async(main, exec)
}
/// 指定した秒数後にメインキューで処理を実行
public func sk4AsyncMain(after: NSTimeInterval, exec: (()->Void)) {
let delta = after * NSTimeInterval(NSEC_PER_SEC)
let time = dispatch_time(DISPATCH_TIME_NOW, Int64(delta))
let main = dispatch_get_main_queue()
dispatch_after(time, main, exec)
}
/// 同期処理を実行
public func sk4Synchronized(obj: AnyObject, @noescape block: (()->Void)) {
objc_sync_enter(obj)
block()
objc_sync_exit(obj)
}
/// 指定された時間、待機する
public func sk4Sleep(time: NSTimeInterval) {
NSThread.sleepForTimeInterval(time)
}
/// 現在のキューはメインキューか?
public func sk4IsMainQueue() -> Bool {
return NSThread.isMainThread()
}
/*
/// GCDだけで判定する方法
func sk4IsMainQueue() -> Bool {
let main = dispatch_get_main_queue()
let main_label = dispatch_queue_get_label(main)
let current_label = dispatch_queue_get_label(DISPATCH_CURRENT_QUEUE_LABEL)
if main_label == current_label {
return true
} else {
return false
}
}
*/
// eof
| mit | 1e3fe75475aa0b13fd50322ac3a94345 | 21.615385 | 75 | 0.67551 | 2.916667 | false | false | false | false |
manfengjun/KYMart | Section/Mine/Controller/KYPendToPointViewController.swift | 1 | 3497 | //
// KYBonusToMoneyViewController.swift
// KYMart
//
// Created by JUN on 2017/7/3.
// Copyright © 2017年 JUN. All rights reserved.
//
import UIKit
import IQKeyboardManagerSwift
class KYPendToPointViewController: BaseViewController {
@IBOutlet weak var codeBgView: UIView!
@IBOutlet weak var saveBtn: UIButton!
@IBOutlet weak var bonusL: UILabel!
@IBOutlet weak var amountT: UITextField!
@IBOutlet weak var codeT: UITextField!
@IBOutlet weak var infoView: UIView!
@IBOutlet weak var headH: NSLayoutConstraint!
fileprivate lazy var codeView : PooCodeView = {
let codeView = PooCodeView(frame: CGRect(x: 0, y: 0, width: 70, height: 25), andChange: nil)
return codeView!
}()
fileprivate lazy var headView : KYUserInfoView = {
let headView = KYUserInfoView(frame: CGRect(x: 0, y: 0, width: SCREEN_WIDTH, height: SCREEN_WIDTH*3/5 + 51 + 51))
headView.userModel = SingleManager.instance.userInfo
return headView
}()
var pay_points:Int?
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
navigationController?.navigationBar.subviews[0].alpha = 0
IQKeyboardManager.sharedManager().enable = true
}
override func viewWillDisappear(_ animated: Bool) {
super.viewWillDisappear(animated)
navigationController?.navigationBar.subviews[0].alpha = 1
IQKeyboardManager.sharedManager().enable = false
}
override func viewDidLoad() {
super.viewDidLoad()
setupUI()
}
func setupUI() {
setBackButtonInNav()
saveBtn.layer.masksToBounds = true
saveBtn.layer.cornerRadius = 5.0
codeBgView.addSubview(codeView)
bonusL.text = "\(pay_points!)"
headH.constant = SCREEN_WIDTH*3/5 + 51
infoView.addSubview(headView)
headView.titleL.text = "待发放转积分"
headView.userModel = SingleManager.instance.userInfo
}
@IBAction func saveAction(_ sender: UIButton) {
if (amountT.text?.isEmpty)! {
Toast(content: "不能为空")
return
}
let codeText = NSString(string: codeT.text!)
let codeStr = NSString(string: codeView.changeString)
let params = ["money":amountT.text!]
if codeText.caseInsensitiveCompare(codeStr as String).rawValue == 0 {
SJBRequestModel.push_fetchPendToPointData(params: params as [String : AnyObject], completion: { (response, status) in
if status == 1{
self.Toast(content: "申请成功")
self.navigationController?.popViewController(animated: true)
}
else
{
self.Toast(content: response as! String)
}
})
}
else
{
Toast(content: "验证码错误!")
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
/*
// MARK: - Navigation
// In a storyboard-based application, you will often want to do a little preparation before navigation
override func prepare(for segue: UIStoryboardSegue, sender: Any?) {
// Get the new view controller using segue.destinationViewController.
// Pass the selected object to the new view controller.
}
*/
}
| mit | 30d4447455ca0eb82a21a34c54e2aa6f | 33.54 | 129 | 0.621888 | 4.744505 | false | false | false | false |
Antondomashnev/FBSnapshotsViewer | FBSnapshotsViewerTests/TestLineNumberExtractorSpec.swift | 1 | 3666 | //
// TestLineNumberExtractorSpec.swift
// FBSnapshotsViewerTests
//
// Created by Anton Domashnev on 13.08.17.
// Copyright © 2017 Anton Domashnev. All rights reserved.
//
import Quick
import Nimble
@testable import FBSnapshotsViewer
class TestLineNumberExtractorSpec: QuickSpec {
override func spec() {
var subject: DefaultTestLineNumberExtractor!
beforeEach {
subject = DefaultTestLineNumberExtractor()
}
describe(".extractTestLineNumber") {
var logLine: ApplicationLogLine!
context("given kaleidoscopeCommandMessage") {
beforeEach {
logLine = ApplicationLogLine.kaleidoscopeCommandMessage(line: "foo/bar")
}
it("throws") {
expect { try subject.extractTestLineNumber(from: logLine) }.to(throwError())
}
}
context("given referenceImageSavedMessage") {
beforeEach {
logLine = ApplicationLogLine.referenceImageSavedMessage(line: "foo/bar")
}
it("throws") {
expect { try subject.extractTestLineNumber(from: logLine) }.to(throwError())
}
}
context("given applicationNameMessage") {
beforeEach {
logLine = ApplicationLogLine.applicationNameMessage(line: "foo/bar")
}
it("throws") {
expect { try subject.extractTestLineNumber(from: logLine) }.to(throwError())
}
}
context("given fbReferenceImageDirMessage") {
beforeEach {
logLine = ApplicationLogLine.fbReferenceImageDirMessage(line: "foo/bar")
}
it("throws") {
expect { try subject.extractTestLineNumber(from: logLine) }.to(throwError())
}
}
context("given snapshotTestErrorMessage") {
context("with valid line") {
beforeEach {
logLine = ApplicationLogLine.snapshotTestErrorMessage(line: "/Users/antondomashnev/Work/FBSnapshotsViewerExample/FBSnapshotsViewerExampleTests/FBSnapshotsViewerExampleTests.m:38: error: -[FBSnapshotsViewerExampleTests testRecord] : ((noErrors) is true) failed - Snapshot comparison failed: (null)")
}
it("returns valid test line number") {
let testLineNumber = try? subject.extractTestLineNumber(from: logLine)
expect(testLineNumber).to(equal(38))
}
}
context("with invalid line") {
beforeEach {
logLine = ApplicationLogLine.snapshotTestErrorMessage(line: "lalala lalala foo bar")
}
it("throws") {
expect { try subject.extractTestLineNumber(from: logLine) }.to(throwError())
}
}
}
context("given unknown") {
beforeEach {
logLine = ApplicationLogLine.unknown
}
it("throws") {
expect { try subject.extractTestLineNumber(from: logLine) }.to(throwError())
}
}
}
}
}
| mit | 6570208f36f2867f5e2ba9197a240841 | 36.020202 | 322 | 0.496317 | 6.297251 | false | true | false | false |
Ribeiro/PapersPlease | Classes/ValidationTypes/ValidatorType.swift | 1 | 947 | //
// ValidatorType.swift
// PapersPlease
//
// Created by Brett Walker on 7/2/14.
// Copyright (c) 2014 Poet & Mountain, LLC. All rights reserved.
//
import Foundation
class ValidationStatus {
let valid = "ValidationStatusValid"
let invalid = "ValidationStatusInvalid"
}
let ValidatorUpdateNotification:String = "ValidatorUpdateNotification"
class ValidatorType {
var status = ValidationStatus()
var valid:Bool = false
var sendsUpdates:Bool = false
var identifier:NSString = ""
var validationStates:[String] = []
init () {
self.validationStates = [self.status.invalid]
}
func isTextValid(text:String) -> Bool {
self.valid = true
self.validationStates.removeAll()
self.validationStates.append(self.status.valid)
return self.valid
}
class func type() -> String {
return "ValidationTypeBase"
}
} | mit | a0c43f704b69a7a41063b91f03dd703f | 19.608696 | 70 | 0.63886 | 4.304545 | false | false | false | false |
TedMore/FoodTracker | FoodTracker/MealViewController.swift | 1 | 4614 | //
// MealViewController.swift
// FoodTracker
//
// Created by TedChen on 10/15/15.
// Copyright © 2015 LEON. All rights reserved.
//
import UIKit
class MealViewController: UIViewController, UITextFieldDelegate, UIImagePickerControllerDelegate, UINavigationControllerDelegate {
// MARK: Properties
@IBOutlet weak var nameTextField: UITextField!
@IBOutlet weak var photoImageView: UIImageView!
@IBOutlet weak var ratingControl: RatingControl!
@IBOutlet weak var saveButton: UIBarButtonItem!
/*
This value is either passed by `MealTableViewController` in `prepareForSegue(_:sender:)`
or constructed as part of adding a new meal.
*/
var meal: Meal?
override func viewDidLoad() {
super.viewDidLoad()
// Handle the text field’s user input through delegate callbacks.
nameTextField.delegate = self
// Set up views if editing an existing Meal.
if let meal = meal {
navigationItem.title = meal.name
nameTextField.text = meal.name
photoImageView.image = meal.photo
ratingControl.rating = meal.rating
}
// Enable the Save button only if the text field has a valid Meal name.
checkValidMealName()
}
// MARK: UIImagePickerControllerDelegate
func imagePickerControllerDidCancel(picker: UIImagePickerController) {
// Dismiss the picker if the user canceled.
dismissViewControllerAnimated(true, completion: nil)
}
func imagePickerController(picker: UIImagePickerController, didFinishPickingMediaWithInfo info: [String : AnyObject]) {
// The info dictionary contains multiple representations of the image, and this uses the original.
let selectedImage = info[UIImagePickerControllerOriginalImage] as! UIImage
// Set photoImageView to display the selected image.
photoImageView.image = selectedImage
// Dismiss the picker.
dismissViewControllerAnimated(true, completion: nil)
}
// MARK: Navigation
// This method lets you configure a view controller before it's presented.
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
if saveButton === sender {
let name = nameTextField.text ?? ""
let photo = photoImageView.image
let rating = ratingControl.rating
// Set the meal to be passed to MealTableViewController after the unwind segue.
meal = Meal(name: name, photo: photo, rating: rating)
}
}
// MARK: Actions
@IBAction func cancel(sender: UIBarButtonItem) {
// Depending on style of presentation (modal or push presentation), this view controller needs to be dismissed in two different ways
let isPresentingInAddMealMode = presentingViewController is UINavigationController
if isPresentingInAddMealMode {
dismissViewControllerAnimated(true, completion: nil)
}
else {
navigationController!.popViewControllerAnimated(true)
}
}
@IBAction func selectImageFromPhotoLibrary(sender: UITapGestureRecognizer) {
// Hide the keyboard
nameTextField.resignFirstResponder()
// UIImagePickerController is a view controller that lets a user pick media from their photo library.
let imagePickerController = UIImagePickerController()
// Only allow photos to be picked, not taken.
imagePickerController.sourceType = .PhotoLibrary
// Make sure ViewController is notified when the user picks an image.
imagePickerController.delegate = self
presentViewController(imagePickerController, animated: true, completion: nil)
}
// MARK: UITextFieldDelegate
func textFieldShouldReturn(textField: UITextField) -> Bool {
// Hide the keyboard.
textField.resignFirstResponder()
return true
}
func textFieldDidBeginEditing(textField: UITextField) {
// Disable the Save button while editing.
saveButton.enabled = false
}
func checkValidMealName() {
// Disable the Save button if the text field is empty.
let txt = nameTextField.text ?? ""
saveButton.enabled = !txt.isEmpty
}
func textFieldDidEndEditing(textField: UITextField) {
checkValidMealName()
navigationItem.title = nameTextField.text
}
}
| apache-2.0 | 14940c89da355693993ecb03a237dd7a | 33.931818 | 140 | 0.656691 | 6.011734 | false | false | false | false |
teambition/RefreshView | Sources/UIScrollView+Extension.swift | 2 | 2202 | //
// UIScrollView+Extension.swift
// RefreshDemo
//
// Created by ZouLiangming on 16/1/25.
// Copyright © 2016年 ZouLiangming. All rights reserved.
//
import UIKit
extension UIScrollView {
var insetTop: CGFloat {
get {
return self.contentInset.top
}
set(newValue) {
var inset = self.contentInset
inset.top = newValue
self.contentInset = inset
}
}
var insetBottom: CGFloat {
get {
return self.contentInset.bottom
}
set(newValue) {
var inset = self.contentInset
inset.bottom = newValue
self.contentInset = inset
}
}
var insetLeft: CGFloat {
get {
return self.contentInset.left
}
set(newValue) {
var inset = self.contentInset
inset.left = newValue
self.contentInset = inset
}
}
var insetRight: CGFloat {
get {
return self.contentInset.right
}
set(newValue) {
var inset = self.contentInset
inset.right = newValue
self.contentInset = inset
}
}
var offsetX: CGFloat {
get {
return self.contentOffset.x
}
set(newValue) {
var inset = self.contentOffset
inset.x = newValue
self.contentOffset = inset
}
}
var offsetY: CGFloat {
get {
return self.contentOffset.y
}
set(newValue) {
var inset = self.contentOffset
inset.y = newValue
self.contentOffset = inset
}
}
var contentWidth: CGFloat {
get {
return self.contentSize.width
}
set(newValue) {
var inset = self.contentSize
inset.width = newValue
self.contentSize = inset
}
}
var contentHeight: CGFloat {
get {
return self.contentSize.height
}
set(newValue) {
var inset = self.contentSize
inset.height = newValue
self.contentSize = inset
}
}
}
| mit | 6fa3a199d38ad4818390e9c730a1586d | 21.212121 | 56 | 0.50523 | 5.032037 | false | false | false | false |
SomeSimpleSolutions/MemorizeItForever | MemorizeItForeverCore/MemorizeItForeverCoreTests/ManagersTests/WordFlowsServiceTests.swift | 1 | 17913 | //
// WordFlowsTests.swift
// MemorizeItForeverCore
//
// Created by Hadi Zamani on 10/23/16.
// Copyright © 2016 SomeSimpleSolutions. All rights reserved.
//
import XCTest
import BaseLocalDataAccess
@testable import MemorizeItForeverCore
class WordFlowsTests: XCTestCase {
var wordFlowService: WordFlowServiceProtocol!
var wordDataAccess: WordDataAccessProtocol!
var wordInProgressDataAccess: WordInProgressDataAccessProtocol!
var wordHistoryDataAccess: WordHistoryDataAccessProtocol!
var setModel: SetModel!
override func setUp() {
super.setUp()
wordDataAccess = FakeWordDataAccess()
wordInProgressDataAccess = FakeWordInProgressDataAccess()
wordHistoryDataAccess = FakeWordHistoryDataAccess()
wordFlowService = WordFlowService(wordDataAccess: wordDataAccess, wordInProgressDataAccess: wordInProgressDataAccess, wordHistoryDataAccess: wordHistoryDataAccess)
setModel = SetModel()
setModel.setId = UUID()
setModel.name = "Default"
}
override func tearDown() {
wordDataAccess = nil
wordFlowService = nil
wordInProgressDataAccess = nil
wordHistoryDataAccess = nil
setModel = nil
super.tearDown()
}
func testPutWordInPreColumn(){
let word = newWordModel()
do{
try wordFlowService.putWordInPreColumn(word)
}
catch{
XCTFail("should save wordInProgress")
}
if let column = objc_getAssociatedObject(wordInProgressDataAccess, &columnKey) as? Int16{
XCTAssertEqual(column, 0 ,"shoud put word in 0 column")
}
else{
XCTFail("should save wordInProgress")
}
if let date = objc_getAssociatedObject(wordInProgressDataAccess, &dateKey) as? Date{
XCTAssertEqual(date.getDate()!.compare(Date().addingTimeInterval(24 * 60 * 60).getDate()!), ComparisonResult.orderedSame ,"shoud set tomorrow")
}
else{
XCTFail("should save wordInProgress")
}
if let wordId = objc_getAssociatedObject(wordInProgressDataAccess, &wordIdKey) as? UUID{
XCTAssertEqual(wordId, word.wordId ,"shoud set word in wordInprogress")
}
else{
XCTFail("should save wordInProgress")
}
}
func testAnswerCorrectlyPreColumn(){
let word = newWordModel()
let wordInprogress = WordInProgressModel(word: word, date: Date().addingTimeInterval(1 * 24 * 60 * 60), column: 0, wordInProgressId: UUID())
wordFlowService.answerCorrectly(wordInprogress)
if let column = objc_getAssociatedObject(wordInProgressDataAccess, &columnKey) as? Int16{
XCTAssertEqual(column, 1 ,"shoud put word in 1 column")
}
else{
XCTFail("should save wordInProgress")
}
if let date = objc_getAssociatedObject(wordInProgressDataAccess, &dateKey) as? Date{
XCTAssertEqual(date.getDate()!.compare(Date().addingTimeInterval(2 * 24 * 60 * 60).getDate()!), ComparisonResult.orderedSame ,"shoud set 2 days later")
}
else{
XCTFail("should progress word")
}
if let wordId = objc_getAssociatedObject(wordInProgressDataAccess, &wordIdKey) as? UUID{
XCTAssertEqual(wordId, word.wordId ,"shoud word not changed in wordInprogress")
}
else{
XCTFail("should save wordInProgress")
}
if let wordInProgressId = objc_getAssociatedObject(wordInProgressDataAccess, &wordIdInProgressKey) as? UUID{
XCTAssertEqual(wordInProgressId, wordInprogress.wordInProgressId ,"shoud wordInprogressId not changed in wordInprogress")
}
else{
XCTFail("should save wordInProgress")
}
}
func testAnswerCorrectlyFirstColumn(){
let word = newWordModel()
let wordInprogress = WordInProgressModel(word: word, date: Date().addingTimeInterval(2 * 24 * 60 * 60), column: 1, wordInProgressId: UUID())
wordFlowService.answerCorrectly(wordInprogress)
if let column = objc_getAssociatedObject(wordInProgressDataAccess, &columnKey) as? Int16{
XCTAssertEqual(column, 2 ,"shoud put word in 2 column")
}
else{
XCTFail("should save wordInProgress")
}
if let date = objc_getAssociatedObject(wordInProgressDataAccess, &dateKey) as? Date{
XCTAssertEqual(date.getDate()!.compare(Date().addingTimeInterval(4 * 24 * 60 * 60).getDate()!), ComparisonResult.orderedSame ,"shoud set 4 days later")
}
else{
XCTFail("should progress word")
}
if let wordId = objc_getAssociatedObject(wordInProgressDataAccess, &wordIdKey) as? UUID{
XCTAssertEqual(wordId, word.wordId ,"shoud word not changed in wordInprogress")
}
else{
XCTFail("should save wordInProgress")
}
if let wordInProgressId = objc_getAssociatedObject(wordInProgressDataAccess, &wordIdInProgressKey) as? UUID{
XCTAssertEqual(wordInProgressId, wordInprogress.wordInProgressId ,"shoud wordInprogressId not changed in wordInprogress")
}
else{
XCTFail("should save wordInProgress")
}
}
func testAnswerCorrectlySecondColumn(){
let word = newWordModel()
let wordInprogress = WordInProgressModel(word: word, date: Date().addingTimeInterval(4 * 24 * 60 * 60), column: 2, wordInProgressId: UUID())
wordFlowService.answerCorrectly(wordInprogress)
if let column = objc_getAssociatedObject(wordInProgressDataAccess, &columnKey) as? Int16{
XCTAssertEqual(column, 3 ,"shoud put word in 3 column")
}
else{
XCTFail("should save wordInProgress")
}
if let date = objc_getAssociatedObject(wordInProgressDataAccess, &dateKey) as? Date{
XCTAssertEqual(date.getDate()!.compare(Date().addingTimeInterval(8 * 24 * 60 * 60).getDate()!), ComparisonResult.orderedSame ,"shoud set 8 days later")
}
else{
XCTFail("should progress word")
}
if let wordId = objc_getAssociatedObject(wordInProgressDataAccess, &wordIdKey) as? UUID{
XCTAssertEqual(wordId, word.wordId ,"shoud word not changed in wordInprogress")
}
else{
XCTFail("should save wordInProgress")
}
if let wordInProgressId = objc_getAssociatedObject(wordInProgressDataAccess, &wordIdInProgressKey) as? UUID{
XCTAssertEqual(wordInProgressId, wordInprogress.wordInProgressId ,"shoud wordInprogressId not changed in wordInprogress")
}
else{
XCTFail("should save wordInProgress")
}
}
func testAnswerCorrectlyThirdColumn(){
let word = newWordModel()
let wordInprogress = WordInProgressModel(word: word, date: Date().addingTimeInterval(8 * 24 * 60 * 60), column: 3, wordInProgressId: UUID())
wordFlowService.answerCorrectly(wordInprogress)
if let column = objc_getAssociatedObject(wordInProgressDataAccess, &columnKey) as? Int16{
XCTAssertEqual(column, 4 ,"shoud put word in 4 column")
}
else{
XCTFail("should save wordInProgress")
}
if let date = objc_getAssociatedObject(wordInProgressDataAccess, &dateKey) as? Date{
XCTAssertEqual(date.getDate()!.compare(Date().addingTimeInterval(16 * 24 * 60 * 60).getDate()!), ComparisonResult.orderedSame ,"shoud set 8 days later")
}
else{
XCTFail("should progress word")
}
if let wordId = objc_getAssociatedObject(wordInProgressDataAccess, &wordIdKey) as? UUID{
XCTAssertEqual(wordId, word.wordId ,"shoud word not changed in wordInprogress")
}
else{
XCTFail("should save wordInProgress")
}
if let wordInProgressId = objc_getAssociatedObject(wordInProgressDataAccess, &wordIdInProgressKey) as? UUID{
XCTAssertEqual(wordInProgressId, wordInprogress.wordInProgressId ,"shoud wordInprogressId not changed in wordInprogress")
}
else{
XCTFail("should save wordInProgress")
}
}
func testAnswerCorrectlyFourthColumn(){
let word = newWordModel()
let wordInprogress = WordInProgressModel(word: word, date: Date().addingTimeInterval(16 * 24 * 60 * 60), column: 4, wordInProgressId: UUID())
wordFlowService.answerCorrectly(wordInprogress)
if let column = objc_getAssociatedObject(wordInProgressDataAccess, &columnKey) as? Int16{
XCTAssertEqual(column, 5 ,"shoud put word in 5 column")
}
else{
XCTFail("should save wordInProgress")
}
if let date = objc_getAssociatedObject(wordInProgressDataAccess, &dateKey) as? Date{
XCTAssertEqual(date.getDate()!.compare(Date().addingTimeInterval(32 * 24 * 60 * 60).getDate()!), ComparisonResult.orderedSame ,"shoud set 8 days later")
}
else{
XCTFail("should progress word")
}
if let wordId = objc_getAssociatedObject(wordInProgressDataAccess, &wordIdKey) as? UUID{
XCTAssertEqual(wordId, word.wordId ,"shoud word not changed in wordInprogress")
}
else{
XCTFail("should save wordInProgress")
}
if let wordInProgressId = objc_getAssociatedObject(wordInProgressDataAccess, &wordIdInProgressKey) as? UUID{
XCTAssertEqual(wordInProgressId, wordInprogress.wordInProgressId ,"shoud wordInprogressId not changed in wordInprogress")
}
else{
XCTFail("should save wordInProgress")
}
}
func testAnswerCorrectlyFifthColumn(){
let word = newWordModel()
let wordInprogress = WordInProgressModel(word: word, date: Date().addingTimeInterval(32 * 24 * 60 * 60), column: 5, wordInProgressId: UUID())
wordFlowService.answerCorrectly(wordInprogress)
if let status = objc_getAssociatedObject(wordDataAccess, &statusKey) as? Int16{
XCTAssertEqual(status, WordStatus.done.rawValue ,"shoud set word status as Done")
}
else{
XCTFail("shoud set word status as Done")
}
if let enumResult = objc_getAssociatedObject(wordInProgressDataAccess, &resultKey) as? FakeWordInProgressDataAccessEnum{
XCTAssertEqual(enumResult, .delete ,"should delete wordInProgress")
}
else{
XCTFail("should delete wordInProgress")
}
}
func testAnswerWronglyInMiddleColumns(){
let word = newWordModel()
let wordInprogress = WordInProgressModel(word: word, date: Date().addingTimeInterval(8 * 24 * 60 * 60), column: 3, wordInProgressId: UUID())
wordFlowService.answerWrongly(wordInprogress)
if let columnNO = objc_getAssociatedObject(wordHistoryDataAccess, &columnNoKey) as? Int16{
XCTAssertEqual(columnNO, 3 ,"shoud set correct column No")
}
else{
XCTFail("should save wordInProgress")
}
if let wordId = objc_getAssociatedObject(wordHistoryDataAccess, &wordIdKey) as? UUID{
XCTAssertEqual(wordId, word.wordId ,"shoud word not changed in wordInprogress")
}
else{
XCTFail("should save wordInProgress")
}
if let date = objc_getAssociatedObject(wordInProgressDataAccess, &dateKey) as? Date{
XCTAssertEqual(date.getDate()!.compare(Date().addingTimeInterval(1 * 24 * 60 * 60).getDate()!), ComparisonResult.orderedSame ,"shoud set 1 days later")
}
else{
XCTFail("should progress word")
}
if let column = objc_getAssociatedObject(wordInProgressDataAccess, &columnKey) as? Int16{
XCTAssertEqual(column, 0 ,"shoud put word in 0 column")
}
else{
XCTFail("should save wordInProgress")
}
}
func testAddDayExtension(){
let now = Date(timeIntervalSinceReferenceDate: 0)
let tomorrow = Date(timeIntervalSinceReferenceDate: 60*60*24)
XCTAssertEqual(now.addDay(1), tomorrow, "AddDay extension should work fine")
}
func testFetchWordsForPuttingInPreColumn(){
UserDefaults.standard.setValue(10, forKey: Settings.newWordsCount.rawValue)
UserDefaults.standard.setValue(setModel.toDic(), forKey: Settings.defaultSet.rawValue)
do{
try wordFlowService.fetchNewWordsToPutInPreColumn()
}
catch{
XCTFail("should be able to fetch words in pre column")
}
if let column = objc_getAssociatedObject(wordInProgressDataAccess, &columnKey) as? Int16{
XCTAssertEqual(column, 0 ,"shoud put word in 0 column")
}
else{
XCTFail("should save wordInProgress")
}
if let date = objc_getAssociatedObject(wordInProgressDataAccess, &dateKey) as? Date{
XCTAssertEqual(date.getDate()!.compare(Date().addingTimeInterval(24 * 60 * 60).getDate()!), ComparisonResult.orderedSame ,"shoud set tomorrow")
}
else{
XCTFail("should save wordInProgress")
}
if let wordId = objc_getAssociatedObject(wordInProgressDataAccess, &wordIdKey) as? UUID{
XCTAssertNotNil(wordId ,"shoud set word in wordInprogress")
}
else{
XCTFail("should save wordInProgress")
}
if let status = objc_getAssociatedObject(wordDataAccess, &statusKey) as? Int16{
XCTAssertEqual(status, WordStatus.inProgress.rawValue ,"shoud status word set to InProgress")
}
else{
XCTFail("shou set word status as InProgress")
}
}
func testDoNotFetchWordsForPuttingInPreColumnIfAlreadyFetched(){
UserDefaults.standard.setValue(setModel.toDic(), forKey: Settings.defaultSet.rawValue)
let word = newWordModel()
objc_setAssociatedObject(wordDataAccess, &wordKey, word, .OBJC_ASSOCIATION_RETAIN_NONATOMIC)
do{
try wordFlowService.fetchNewWordsToPutInPreColumn()
}
catch{
XCTFail("should be able to fetch words in pre column")
}
let column = objc_getAssociatedObject(wordInProgressDataAccess, &columnKey) as? Int16
XCTAssertNil(column, "Should not put progress in column 0")
}
func testShouldWordsForPuttingInPreColumnIfAnsweredAlreadyWrongly(){
UserDefaults.standard.setValue(setModel.toDic(), forKey: Settings.defaultSet.rawValue)
let word = newWordModel()
objc_setAssociatedObject(wordDataAccess, &wordKey, word, .OBJC_ASSOCIATION_RETAIN_NONATOMIC)
objc_setAssociatedObject(wordHistoryDataAccess, &wordHistoryCountKey, 1, .OBJC_ASSOCIATION_RETAIN_NONATOMIC)
do{
try wordFlowService.fetchNewWordsToPutInPreColumn()
}
catch{
XCTFail("should be able to fetch words in pre column")
}
if let column = objc_getAssociatedObject(wordInProgressDataAccess, &columnKey) as? Int16{
XCTAssertEqual(column, 0 ,"shoud put word in 0 column")
}
else{
XCTFail("should save wordInProgress")
}
}
func testFetchWordsForReview(){
UserDefaults.standard.setValue(setModel.toDic(), forKey: Settings.defaultSet.rawValue)
do{
let words = try wordFlowService.fetchWordsForReview()
XCTAssertGreaterThanOrEqual(words.count, 0, "should return words for review")
}
catch{
XCTFail("Should be able to fetch words for review")
}
if let column = objc_getAssociatedObject(wordInProgressDataAccess, &columnKey) as? Int16{
XCTAssertEqual(column, 0 ,"shoud filter with 0 column")
}
else{
XCTFail("shoud filter with 0 column")
}
if let date = objc_getAssociatedObject(wordInProgressDataAccess, &dateKey) as? Date{
XCTAssertEqual(date.getDate()!.compare(Date().addingTimeInterval(24 * 60 * 60).getDate()!), ComparisonResult.orderedSame ,"shoud filter with tomorrow date")
}
else{
XCTFail("shoud filter with tomorrow date")
}
}
func testFetchWordsToExamine(){
// it should fetch words for today and all words that belongs to past
UserDefaults.standard.setValue(setModel.toDic(), forKey: Settings.defaultSet.rawValue)
do{
let wordInProgressLists = try wordFlowService.fetchWordsToExamin()
XCTAssertEqual(wordInProgressLists[0].column, 3,"should sort wordInprogress list")
}
catch{
XCTFail("Should be able to fetch words for examin")
}
if let date = objc_getAssociatedObject(wordInProgressDataAccess, &dateKey) as? Date{
XCTAssertEqual(date.getDate()!.compare(Date().getDate()!), ComparisonResult.orderedSame ,"shoud filter with today date")
}
else{
XCTFail("shoud filter with today date")
}
}
private func newWordModel() -> WordModel{
var wordModel = WordModel()
wordModel.wordId = UUID()
wordModel.phrase = "Livre"
wordModel.meaning = "Book"
wordModel.order = 1
wordModel.setId = UUID()
wordModel.status = WordStatus.notStarted.rawValue
return wordModel
}
}
| mit | 7f6142cad7a941ddcece661c1c487989 | 40.082569 | 171 | 0.637394 | 5.622097 | false | false | false | false |
alexhillc/AXPhotoViewer | Source/Integrations/SimpleNetworkIntegration.swift | 1 | 7546 | //
// SimpleNetworkIntegration.swift
// AXPhotoViewer
//
// Created by Alex Hill on 6/11/17.
// Copyright © 2017 Alex Hill. All rights reserved.
//
open class SimpleNetworkIntegration: NSObject, AXNetworkIntegrationProtocol, SimpleNetworkIntegrationURLSessionWrapperDelegate {
fileprivate var urlSessionWrapper = SimpleNetworkIntegrationURLSessionWrapper()
public weak var delegate: AXNetworkIntegrationDelegate?
fileprivate var dataTasks = NSMapTable<AXPhotoProtocol, URLSessionDataTask>(keyOptions: .strongMemory, valueOptions: .strongMemory)
fileprivate var photos = [Int: AXPhotoProtocol]()
public override init() {
super.init()
self.urlSessionWrapper.delegate = self
}
deinit {
self.urlSessionWrapper.invalidate()
}
public func loadPhoto(_ photo: AXPhotoProtocol) {
if photo.imageData != nil || photo.image != nil {
AXDispatchUtils.executeInBackground { [weak self] in
guard let `self` = self else { return }
self.delegate?.networkIntegration(self, loadDidFinishWith: photo)
}
return
}
guard let url = photo.url else { return }
let dataTask = self.urlSessionWrapper.dataTask(with: url)
self.dataTasks.setObject(dataTask, forKey: photo)
self.photos[dataTask.taskIdentifier] = photo
dataTask.resume()
}
public func cancelLoad(for photo: AXPhotoProtocol) {
guard let dataTask = self.dataTasks.object(forKey: photo) else { return }
dataTask.cancel()
}
public func cancelAllLoads() {
let enumerator = self.dataTasks.objectEnumerator()
while let dataTask = enumerator?.nextObject() as? URLSessionDataTask {
dataTask.cancel()
}
self.dataTasks.removeAllObjects()
self.photos.removeAll()
}
// MARK: - SimpleNetworkIntegrationURLSessionWrapperDelegate
fileprivate func urlSessionWrapper(_ urlSessionWrapper: SimpleNetworkIntegrationURLSessionWrapper,
dataTask: URLSessionDataTask,
didUpdateProgress progress: CGFloat) {
guard let photo = self.photos[dataTask.taskIdentifier] else { return }
AXDispatchUtils.executeInBackground { [weak self] in
guard let `self` = self else { return }
self.delegate?.networkIntegration?(self,
didUpdateLoadingProgress: progress,
for: photo)
}
}
fileprivate func urlSessionWrapper(_ urlSessionWrapper: SimpleNetworkIntegrationURLSessionWrapper,
task: URLSessionTask,
didCompleteWithError error: Error?,
object: Any?) {
guard let photo = self.photos[task.taskIdentifier] else { return }
weak var weakSelf = self
func removeDataTask() {
weakSelf?.photos.removeValue(forKey: task.taskIdentifier)
weakSelf?.dataTasks.removeObject(forKey: photo)
}
if let error = error {
removeDataTask()
AXDispatchUtils.executeInBackground { [weak self] in
guard let `self` = self else { return }
self.delegate?.networkIntegration(self, loadDidFailWith: error, for: photo)
}
return
}
guard let data = object as? Data else { return }
if data.containsGIF() {
photo.imageData = data
} else {
photo.image = UIImage(data: data)
}
removeDataTask()
AXDispatchUtils.executeInBackground { [weak self] in
guard let `self` = self else { return }
self.delegate?.networkIntegration(self, loadDidFinishWith: photo)
}
}
}
// This wrapper abstracts the `URLSession` away from `SimpleNetworkIntegration` in order to prevent a retain cycle
// between the `URLSession` and its delegate
fileprivate class SimpleNetworkIntegrationURLSessionWrapper: NSObject, URLSessionDataDelegate, URLSessionTaskDelegate {
weak var delegate: SimpleNetworkIntegrationURLSessionWrapperDelegate?
fileprivate var urlSession: URLSession!
fileprivate var receivedData = [Int: Data]()
fileprivate var receivedContentLength = [Int: Int64]()
override init() {
super.init()
self.urlSession = URLSession(configuration: .default, delegate: self, delegateQueue: nil)
}
func dataTask(with url: URL) -> URLSessionDataTask {
return self.urlSession.dataTask(with: url)
}
func invalidate() {
self.urlSession.invalidateAndCancel()
}
// MARK: - URLSessionDataDelegate
func urlSession(_ session: URLSession, dataTask: URLSessionDataTask, didReceive data: Data) {
self.receivedData[dataTask.taskIdentifier]?.append(data)
guard let receivedData = self.receivedData[dataTask.taskIdentifier],
let expectedContentLength = self.receivedContentLength[dataTask.taskIdentifier] else {
return
}
self.delegate?.urlSessionWrapper(self,
dataTask: dataTask,
didUpdateProgress: CGFloat(receivedData.count) / CGFloat(expectedContentLength))
}
func urlSession(_ session: URLSession,
dataTask: URLSessionDataTask,
didReceive response: URLResponse,
completionHandler: @escaping (URLSession.ResponseDisposition) -> Void) {
self.receivedContentLength[dataTask.taskIdentifier] = response.expectedContentLength
self.receivedData[dataTask.taskIdentifier] = Data()
completionHandler(.allow)
}
// MARK: - URLSessionTaskDelegate
func urlSession(_ session: URLSession, task: URLSessionTask, didCompleteWithError error: Error?) {
weak var weakSelf = self
func removeData() {
weakSelf?.receivedData.removeValue(forKey: task.taskIdentifier)
weakSelf?.receivedContentLength.removeValue(forKey: task.taskIdentifier)
}
if let error = error {
self.delegate?.urlSessionWrapper(self, task: task, didCompleteWithError: error, object: nil)
removeData()
return
}
guard let data = self.receivedData[task.taskIdentifier] else {
self.delegate?.urlSessionWrapper(self, task: task, didCompleteWithError: nil, object: nil)
removeData()
return
}
self.delegate?.urlSessionWrapper(self, task: task, didCompleteWithError: nil, object: data)
removeData()
}
}
fileprivate protocol SimpleNetworkIntegrationURLSessionWrapperDelegate: NSObjectProtocol, AnyObject {
func urlSessionWrapper(_ urlSessionWrapper: SimpleNetworkIntegrationURLSessionWrapper,
dataTask: URLSessionDataTask,
didUpdateProgress progress: CGFloat)
func urlSessionWrapper(_ urlSessionWrapper: SimpleNetworkIntegrationURLSessionWrapper,
task: URLSessionTask,
didCompleteWithError error: Error?,
object: Any?)
}
| mit | 7e5067df0a6d06da8e9f9002380c7c96 | 37.891753 | 135 | 0.616832 | 6.099434 | false | false | false | false |
softdevstory/yata | yata/Sources/ViewControllers/MainSplitViewController.swift | 1 | 2920 | //
// MainSplitViewController.swift
// yata
//
// Created by HS Song on 2017. 7. 14..
// Copyright © 2017년 HS Song. All rights reserved.
//
import AppKit
class MainSplitViewController: NSSplitViewController {
@IBOutlet weak var pageListSplitViewItem: NSSplitViewItem!
@IBOutlet weak var pageEditSplitViewItem: NSSplitViewItem!
override func viewDidLoad() {
super.viewDidLoad()
}
override func toggleSidebar(_ sender: Any?) {
guard let menuItem = sender as? NSMenuItem else {
return
}
super.toggleSidebar(sender)
if pageListSplitViewItem.isCollapsed {
menuItem.title = "Show Sidebar".localized
} else {
menuItem.title = "Hide Sidebar".localized
}
}
}
// MARK: support menu, toolbar
extension MainSplitViewController {
override func validateMenuItem(_ menuItem: NSMenuItem) -> Bool {
guard let tag = MenuTag(rawValue: menuItem.tag) else {
return false
}
switch tag {
case .fileNewPage, .fileReload:
break
case .fileOpenInWebBrowser:
if let vc = pageListSplitViewItem.viewController as? PageListViewController {
return vc.isSelected()
}
case .viewToggleSideBar:
if pageListSplitViewItem.isCollapsed {
menuItem.title = "Show Sidebar".localized
} else {
menuItem.title = "Hide Sidebar".localized
}
default:
return false
}
return true
}
override func validateToolbarItem(_ item: NSToolbarItem) -> Bool {
guard let tag = ToolbarTag(rawValue: item.tag) else {
return false
}
guard let _ = AccessTokenStorage.loadAccessToken() else {
return false
}
switch tag {
case .newPage, .reloadPageList:
return true
case .viewInWebBrowser:
if let vc = pageListSplitViewItem.viewController as? PageListViewController {
return vc.isSelected()
}
default:
return false
}
return true
}
@IBAction func reloadPageList(_ sender: Any?) {
if let vc = pageListSplitViewItem.viewController as? PageListViewController {
vc.reloadList(sender)
}
}
@IBAction func editNewPage(_ sender: Any?) {
if let vc = pageListSplitViewItem.viewController as? PageListViewController {
vc.editNewPage(sender)
}
}
@IBAction func viewInWebBrowser(_ sender: Any?) {
if let vc = pageListSplitViewItem.viewController as? PageListViewController {
vc.openInWebBrowser(sender)
}
}
}
| mit | f9929c61ae9beaa2d39ab3d365a49704 | 25.044643 | 89 | 0.567364 | 5.55619 | false | false | false | false |
neoreeps/rest-tester | REST Tester/AppDelegate.swift | 1 | 4615 | //
// AppDelegate.swift
// REST Tester
//
// Created by Kenny Speer on 10/3/16.
// Copyright © 2016 Kenny Speer. All rights reserved.
//
import UIKit
import CoreData
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {
// Override point for customization after application launch.
return true
}
func applicationWillResignActive(_ application: UIApplication) {
// Sent when the application is about to move from active to inactive state. This can occur for certain types of temporary interruptions (such as an incoming phone call or SMS message) or when the user quits the application and it begins the transition to the background state.
// Use this method to pause ongoing tasks, disable timers, and invalidate graphics rendering callbacks. Games should use this method to pause the game.
}
func applicationDidEnterBackground(_ application: UIApplication) {
// Use this method to release shared resources, save user data, invalidate timers, and store enough application state information to restore your application to its current state in case it is terminated later.
// If your application supports background execution, this method is called instead of applicationWillTerminate: when the user quits.
}
func applicationWillEnterForeground(_ application: UIApplication) {
// Called as part of the transition from the background to the active state; here you can undo many of the changes made on entering the background.
}
func applicationDidBecomeActive(_ application: UIApplication) {
// Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface.
}
func applicationWillTerminate(_ application: UIApplication) {
// Called when the application is about to terminate. Save data if appropriate. See also applicationDidEnterBackground:.
// Saves changes in the application's managed object context before the application terminates.
self.saveContext()
}
// MARK: - Core Data stack
lazy var persistentContainer: NSPersistentContainer = {
/*
The persistent container for the application. This implementation
creates and returns a container, having loaded the store for the
application to it. This property is optional since there are legitimate
error conditions that could cause the creation of the store to fail.
*/
let container = NSPersistentContainer(name: "Model")
container.loadPersistentStores(completionHandler: { (storeDescription, error) in
if let error = error as NSError? {
// Replace this implementation with code to handle the error appropriately.
// fatalError() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
/*
Typical reasons for an error here include:
* The parent directory does not exist, cannot be created, or disallows writing.
* The persistent store is not accessible, due to permissions or data protection when the device is locked.
* The device is out of space.
* The store could not be migrated to the current model version.
Check the error message to determine what the actual problem was.
*/
fatalError("Unresolved error \(error), \(error.userInfo)")
}
})
return container
}()
// MARK: - Core Data Saving support
func saveContext () {
let context = persistentContainer.viewContext
if context.hasChanges {
do {
try context.save()
} catch {
// Replace this implementation with code to handle the error appropriately.
// fatalError() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
let nserror = error as NSError
fatalError("Unresolved error \(nserror), \(nserror.userInfo)")
}
}
}
}
| apache-2.0 | a37ccb98e5d1266b39459523a72223ce | 48.612903 | 285 | 0.681838 | 5.870229 | false | false | false | false |
airspeedswift/swift | test/Constraints/optional.swift | 3 | 13739 | // RUN: %target-typecheck-verify-swift
// REQUIRES: objc_interop
func markUsed<T>(_ t: T) {}
class A {
@objc func do_a() {}
@objc(do_b_2:) func do_b(_ x: Int) {}
@objc func do_b(_ x: Float) {}
@objc func do_c(x: Int) {} // expected-note {{incorrect labels for candidate (have: '(_:)', expected: '(x:)')}}
@objc func do_c(y: Int) {} // expected-note {{incorrect labels for candidate (have: '(_:)', expected: '(y:)')}}
}
func test0(_ a: AnyObject) {
a.do_a?()
a.do_b?(1)
a.do_b?(5.0)
a.do_c?(1) // expected-error {{no exact matches in call to instance method 'do_c'}}
a.do_c?(x: 1)
}
func test1(_ a: A) {
a?.do_a() // expected-error {{cannot use optional chaining on non-optional value of type 'A'}} {{4-5=}}
a!.do_a() // expected-error {{cannot force unwrap value of non-optional type 'A'}} {{4-5=}}
// Produce a specialized diagnostic here?
a.do_a?() // expected-error {{cannot use optional chaining on non-optional value of type '() -> ()'}} {{9-10=}}
}
// <rdar://problem/15508756>
extension Optional {
func bind<U>(_ f: (Wrapped) -> U?) -> U? {
switch self {
case .some(let x):
return f(x)
case .none:
return .none
}
}
}
var c: String? = Optional<Int>(1)
.bind {(x: Int) in markUsed("\(x)!"); return "two" }
func test4() {
func foo() -> Int { return 0 }
func takes_optfn(_ f : () -> Int?) -> Int? { return f() }
_ = takes_optfn(foo)
func takes_objoptfn(_ f : () -> AnyObject?) -> AnyObject? { return f() }
func objFoo() -> AnyObject { return A() }
_ = takes_objoptfn(objFoo) // okay
func objBar() -> A { return A() }
_ = takes_objoptfn(objBar) // okay
}
func test5() -> Int? {
return nil
}
func test6<T>(_ x : T) {
// FIXME: this code should work; T could be Int? or Int??
// or something like that at runtime. rdar://16374053
_ = x as? Int? // expected-error {{cannot downcast from 'T' to a more optional type 'Int?'}}
}
class B : A { }
func test7(_ x : A) {
_ = x as? B? // expected-error{{cannot downcast from 'A' to a more optional type 'B?'}}
}
func test8(_ x : AnyObject?) {
let _ : A = x as! A
}
// Partial ordering with optionals
func test9_helper<T: P>(_ x: T) -> Int { }
func test9_helper<T: P>(_ x: T?) -> Double { }
func test9_helper2<T>(_ x: T) -> Int { }
func test9_helper2<T>(_ x: T?) -> Double { }
func test9(_ i: Int, io: Int?) {
let result = test9_helper(i)
var _: Int = result
let result2 = test9_helper(io)
let _: Double = result2
let result3 = test9_helper2(i)
var _: Int = result3
let result4 = test9_helper2(io)
let _: Double = result4
}
protocol P { }
func test10_helper<T : P>(_ x: T) -> Int { }
func test10_helper<T : P>(_ x: T?) -> Double { }
extension Int : P { }
func test10(_ i: Int, io: Int?) {
let result = test10_helper(i)
var _: Int = result
let result2 = test10_helper(io)
var _: Double = result2
}
var z: Int? = nil
z = z ?? 3
var fo: Float? = 3.14159
func voidOptional(_ handler: () -> ()?) {}
func testVoidOptional() {
let noop: () -> Void = {}
voidOptional(noop)
let optNoop: (()?) -> ()? = { return $0 }
voidOptional(optNoop)
}
protocol Proto1 {}
protocol Proto2 {}
struct Nilable: ExpressibleByNilLiteral {
init(nilLiteral: ()) {}
}
func testTernaryWithNil<T>(b: Bool, s: String, i: Int, a: Any, t: T, m: T.Type, p: Proto1 & Proto2, arr: [Int], opt: Int?, iou: Int!, n: Nilable) {
let t1 = b ? s : nil
let _: Double = t1 // expected-error{{value of type 'String?'}}
let t2 = b ? nil : i
let _: Double = t2 // expected-error{{value of type 'Int?'}}
let t3 = b ? "hello" : nil
let _: Double = t3 // expected-error{{value of type 'String?'}}
let t4 = b ? nil : 1
let _: Double = t4 // expected-error{{value of type 'Int?'}}
let t5 = b ? (s, i) : nil
let _: Double = t5 // expected-error{{value of type '(String, Int)?}}
let t6 = b ? nil : (i, s)
let _: Double = t6 // expected-error{{value of type '(Int, String)?}}
let t7 = b ? ("hello", 1) : nil
let _: Double = t7 // expected-error{{value of type '(String, Int)?}}
let t8 = b ? nil : (1, "hello")
let _: Double = t8 // expected-error{{value of type '(Int, String)?}}
let t9 = b ? { $0 * 2 } : nil
let _: Double = t9 // expected-error{{value of type '((Int) -> Int)?}}
let t10 = b ? nil : { $0 * 2 }
let _: Double = t10 // expected-error{{value of type '((Int) -> Int)?}}
let t11 = b ? a : nil
let _: Double = t11 // expected-error{{value of type 'Any?'}}
let t12 = b ? nil : a
let _: Double = t12 // expected-error{{value of type 'Any?'}}
let t13 = b ? t : nil
let _: Double = t13 // expected-error{{value of type 'T?'}}
let t14 = b ? nil : t
let _: Double = t14 // expected-error{{value of type 'T?'}}
let t15 = b ? m : nil
let _: Double = t15 // expected-error{{value of type 'T.Type?'}}
let t16 = b ? nil : m
let _: Double = t16 // expected-error{{value of type 'T.Type?'}}
let t17 = b ? p : nil
let _: Double = t17 // expected-error{{value of type '(Proto1 & Proto2)?'}}
let t18 = b ? nil : p
let _: Double = t18 // expected-error{{value of type '(Proto1 & Proto2)?'}}
let t19 = b ? arr : nil
let _: Double = t19 // expected-error{{value of type '[Int]?'}}
let t20 = b ? nil : arr
let _: Double = t20 // expected-error{{value of type '[Int]?'}}
let t21 = b ? opt : nil
let _: Double = t21 // expected-error{{value of type 'Int?'}}
let t22 = b ? nil : opt
let _: Double = t22 // expected-error{{value of type 'Int?'}}
let t23 = b ? iou : nil
let _: Double = t23 // expected-error{{value of type 'Int?'}}
let t24 = b ? nil : iou
let _: Double = t24 // expected-error{{value of type 'Int?'}}
let t25 = b ? n : nil
let _: Double = t25 // expected-error{{value of type 'Nilable'}}
let t26 = b ? nil : n
let _: Double = t26 // expected-error{{value of type 'Nilable'}}
}
// inference with IUOs
infix operator ++++
protocol PPPP {
static func ++++(x: Self, y: Self) -> Bool
}
func compare<T: PPPP>(v: T, u: T!) -> Bool {
return v ++++ u
}
func sr2752(x: String?, y: String?) {
_ = x.map { xx in
y.map { _ in "" } ?? "\(xx)"
}
}
// SR-3248 - Invalid diagnostic calling implicitly unwrapped closure
var sr3248 : ((Int) -> ())!
sr3248?(a: 2) // expected-error {{extraneous argument label 'a:' in call}}
sr3248!(a: 2) // expected-error {{extraneous argument label 'a:' in call}}
sr3248(a: 2) // expected-error {{extraneous argument label 'a:' in call}}
struct SR_3248 {
var callback: (([AnyObject]) -> Void)!
}
SR_3248().callback?("test") // expected-error {{cannot convert value of type 'String' to expected argument type '[AnyObject]'}}
SR_3248().callback!("test") // expected-error {{cannot convert value of type 'String' to expected argument type '[AnyObject]'}}
SR_3248().callback("test") // expected-error {{cannot convert value of type 'String' to expected argument type '[AnyObject]'}}
_? = nil // expected-error {{'nil' requires a contextual type}}
_?? = nil // expected-error {{'nil' requires a contextual type}}
// rdar://problem/29993596
func takeAnyObjects(_ lhs: AnyObject?, _ rhs: AnyObject?) { }
infix operator !====
func !====(_ lhs: AnyObject?, _ rhs: AnyObject?) -> Bool { return false }
func testAnyObjectImplicitForce(lhs: AnyObject?!, rhs: AnyObject?) {
if lhs !==== rhs { }
takeAnyObjects(lhs, rhs)
}
// SR-4056
protocol P1 { }
class C1: P1 { }
protocol P2 {
var prop: C1? { get }
}
class C2 {
var p1: P1?
var p2: P2?
var computed: P1? {
return p1 ?? p2?.prop
}
}
// rdar://problem/31779785
class X { }
class Bar {
let xOpt: X?
let b: Bool
init() {
let result = b ? nil : xOpt
let _: Int = result // expected-error{{cannot convert value of type 'X?' to specified type 'Int'}}
}
}
// rdar://problem/37508855
func rdar37508855(_ e1: X?, _ e2: X?) -> [X] {
return [e1, e2].filter { $0 == nil }.map { $0! }
}
func se0213() {
struct Q: ExpressibleByStringLiteral {
typealias StringLiteralType = String
var foo: String
init?(_ possibleQ: StringLiteralType) {
return nil
}
init(stringLiteral str: StringLiteralType) {
self.foo = str
}
}
_ = Q("why")?.foo // Ok
_ = Q("who")!.foo // Ok
_ = Q?("how") // Ok
}
func rdar45218255(_ i: Int) {
struct S<T> {
init(_:[T]) {}
}
_ = i! // expected-error {{cannot force unwrap value of non-optional type 'Int'}} {{8-9=}}
_ = [i!] // expected-error {{cannot force unwrap value of non-optional type 'Int'}} {{9-10=}}
_ = S<Int>([i!]) // expected-error {{cannot force unwrap value of non-optional type 'Int'}} {{16-17=}}
}
// rdar://problem/47967277 - cannot assign through '!': '$0' is immutable
func sr_9893_1() {
func foo<T : Equatable>(_: @autoclosure () throws -> T,
_: @autoclosure () throws -> T) {}
class A {
var bar: String?
}
let r1 = A()
let r2 = A()
let arr1: [A] = []
foo(Set(arr1.map { $0.bar! }), Set([r1, r2].map { $0.bar! })) // Ok
}
func sr_9893_2(cString: UnsafePointer<CChar>) {
struct S {
var a: Int32 = 0
var b = ContiguousArray<CChar>(repeating: 0, count: 10)
}
var s = S()
withUnsafeMutablePointer(to: &s.a) { ptrA in
s.b.withUnsafeMutableBufferPointer { bufferB in
withVaList([ptrA, bufferB.baseAddress!]) { ptr in } // Ok
}
}
}
// rdar://problem/47776586 - Diagnostic refers to '&' which is not present in the source code
func rdar47776586() {
func foo(_: inout Int) {}
var x: Int? = 0
foo(&x) // expected-error {{value of optional type 'Int?' must be unwrapped to a value of type 'Int'}}
// expected-note@-1 {{force-unwrap using '!' to abort execution if the optional value contains 'nil'}} {{7-7=(}} {{9-9=)!}}
var dict = [1: 2]
dict[1] += 1 // expected-error {{value of optional type 'Int?' must be unwrapped to a value of type 'Int'}}
// expected-note@-1 {{force-unwrap using '!' to abort execution if the optional value contains 'nil'}} {{10-10=!}}
}
struct S {
var foo: Optional<() -> Int?> = nil
var bar: Optional<() -> Int?> = nil
mutating func test(_ clj: @escaping () -> Int) {
if let fn = foo {
bar = fn // Ok
bar = clj // Ok
}
}
}
// rdar://problem/53238058 - Crash in getCalleeLocator while trying to produce a diagnostic about missing optional unwrap
// associated with an argument to a call
func rdar_53238058() {
struct S {
init(_: Double) {}
init<T>(_ value: T) where T : BinaryFloatingPoint {}
}
func test(_ str: String) {
_ = S(Double(str)) // expected-error {{value of optional type 'Double?' must be unwrapped to a value of type 'Double'}}
// expected-note@-1 {{coalesce using '??' to provide a default when the optional value contains 'nil'}}
// expected-note@-2 {{force-unwrap using '!' to abort execution if the optional value contains 'nil'}}
}
}
// SR-8411 - Inconsistent ambiguity with optional and non-optional inout-to-pointer
func sr8411() {
struct S {
init(_ x: UnsafeMutablePointer<Int>) {}
init(_ x: UnsafeMutablePointer<Int>?) {}
static func foo(_ x: UnsafeMutablePointer<Int>) {}
static func foo(_ x: UnsafeMutablePointer<Int>?) {}
static func bar(_ x: UnsafeMutablePointer<Int>, _ y: Int) {}
static func bar(_ x: UnsafeMutablePointer<Int>?, _ y: Int) {}
}
var foo = 0
_ = S(&foo) // Ok
_ = S.init(&foo) // Ok
S.foo(&foo) // Ok
S.bar(&foo, 42) // Ok
}
// SR-11104 - Slightly misleading diagnostics for contextual failures with multiple fixes
func sr_11104() {
func bar(_: Int) {}
bar(["hello"].first)
// expected-error@-1 {{cannot convert value of type 'String?' to expected argument type 'Int'}}
}
// rdar://problem/57668873 - Too eager force optional unwrap fix
@objc class Window {}
@objc protocol WindowDelegate {
@objc optional var window: Window? { get set }
}
func test_force_unwrap_not_being_too_eager() {
struct WindowContainer {
unowned(unsafe) var delegate: WindowDelegate? = nil
}
let obj: WindowContainer = WindowContainer()
if let _ = obj.delegate?.window { // Ok
}
}
// rdar://problem/57097401
func invalidOptionalChaining(a: Any) {
a == "="? // expected-error {{binary operator '==' cannot be applied to operands of type 'Any' and 'String?'}}
// expected-note@-1 {{overloads for '==' exist with these partially matching parameter lists: (CodingUserInfoKey, CodingUserInfoKey), (FloatingPointSign, FloatingPointSign), (String, String), (Unicode.CanonicalCombiningClass, Unicode.CanonicalCombiningClass)}}
}
// SR-12309 - Force unwrapping 'nil' compiles without warning
func sr_12309() {
struct S {
var foo: Int
}
_ = S(foo: nil!) // expected-error {{'nil' literal cannot be force unwrapped}}
_ = nil! // expected-error {{'nil' literal cannot be force unwrapped}}
_ = (nil!) // expected-error {{'nil' literal cannot be force unwrapped}}
_ = (nil)! // expected-error {{'nil' literal cannot be force unwrapped}}
_ = ((nil))! // expected-error {{'nil' literal cannot be force unwrapped}}
_ = nil? // expected-error {{'nil' requires a contextual type}}
_ = ((nil?)) // expected-error {{'nil' requires a contextual type}}
_ = ((nil))? // expected-error {{'nil' requires a contextual type}}
_ = ((nil)?) // expected-error {{'nil' requires a contextual type}}
_ = nil // expected-error {{'nil' requires a contextual type}}
_ = (nil) // expected-error {{'nil' requires a contextual type}}
_ = ((nil)) // expected-error {{'nil' requires a contextual type}}
_ = (((nil))) // expected-error {{'nil' requires a contextual type}}
_ = ((((((nil)))))) // expected-error {{'nil' requires a contextual type}}
_ = (((((((((nil))))))))) // expected-error {{'nil' requires a contextual type}}
}
| apache-2.0 | 71f6f8bc286319cb39fe7fe44c5c035d | 29.463415 | 262 | 0.599461 | 3.293145 | false | true | false | false |
tyleryouk11/space-hero | Stick-Hero/GameViewController.swift | 1 | 2862 | //
// GameViewController.swift
// Stick-Hero
//
// Created by 顾枫 on 15/6/19.
// Copyright (c) 2015年 koofrank. All rights reserved.
//
import UIKit
import SpriteKit
import AVFoundation
import GoogleMobileAds
class GameViewController: UIViewController {
var musicPlayer:AVAudioPlayer!
var interstitial: GADInterstitial?
required init(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)!
}
override func viewDidLoad() {
super.viewDidLoad()
let scene = StickHeroGameScene(size:CGSizeMake(DefinedScreenWidth, DefinedScreenHeight))
//ADMOB STUFFFF
interstitial = GADInterstitial(adUnitID: "ca-app-pub-3940256099942544/4411468910")
let request = GADRequest()
// Requests test ads on test devices.
request.testDevices = ["2077ef9a63d2b398840261c8221a0c9b"]
interstitial!.loadRequest(request)
// Configure the view.
let skView = self.view as! SKView
// skView.showsFPS = true
// skView.showsNodeCount = true
/* Sprite Kit applies additional optimizations to improve rendering performance */
skView.ignoresSiblingOrder = true
/* Set the scale mode to scale to fit the window */
scene.scaleMode = .AspectFill
skView.presentScene(scene)
self.interstitial = createAndLoadInterstitial()
}
func createAndLoadInterstitial() -> GADInterstitial {
let interstitial = GADInterstitial(adUnitID: "ca-app-pub-3940256099942544/4411468910")
//interstitial.delegate = self <--- ERROR
interstitial.loadRequest(GADRequest())
return interstitial
}
func interstitialDidDismissScreen (interstitial: GADInterstitial) {
self.interstitial = createAndLoadInterstitial()
}
override func viewWillAppear(animated: Bool) {
super.viewWillAppear(animated)
musicPlayer = setupAudioPlayerWithFile("bg_country", type: "mp3")
musicPlayer.numberOfLoops = -1
musicPlayer.play()
}
func setupAudioPlayerWithFile(file:NSString, type:NSString) -> AVAudioPlayer {
let url = NSBundle.mainBundle().URLForResource(file as String, withExtension: type as String)
var audioPlayer:AVAudioPlayer?
do {
try audioPlayer = AVAudioPlayer(contentsOfURL: url!)
} catch {
print("NO AUDIO PLAYER")
}
return audioPlayer!
}
override func shouldAutorotate() -> Bool {
return true
}
override func supportedInterfaceOrientations() -> UIInterfaceOrientationMask {
return .Portrait
}
override func prefersStatusBarHidden() -> Bool {
return true
}
}
| mit | 91e6f0b494782facc1f9c5daf36adeab | 27.56 | 101 | 0.633754 | 5.298701 | false | false | false | false |
c1moore/deckstravaganza | Deckstravaganza/Card.swift | 1 | 7803 | //
// Card.swift
// Deckstravaganza
//
// Created by Calvin Moore on 9/20/15.
// Copyright © 2015 University of Florida. All rights reserved.
//
import UIKit
class Card {
// Enumeration of legal card suits.
enum CardSuit : Int {
case Clubs = 1;
case Diamonds = 2;
case Hearts = 3;
case Spades = 4;
static let numberOfSuits = 4;
}
// Enumeration of legal card ranks and rank value.
enum CardRank : Int {
case Ace = 1;
case Two = 2;
case Three = 3;
case Four = 4;
case Five = 5;
case Six = 6;
case Seven = 7;
case Eight = 8;
case Nine = 9;
case Ten = 10;
case Jack = 11;
case Queen = 12;
case King = 13;
case Joker = 14;
static let numberOfRanks = 13;
}
// Enumeration of legal card colors.
enum CardColor : Int {
case Red = 1;
case Black = 2;
}
// All card properties are immutable and private.
private let suit : CardSuit;
private let rank : CardRank;
// The color of the card is determined by the suit.
private var color : CardColor {
get {
if(self.suit == .Clubs || self.suit == .Spades) {
return CardColor.Black;
} else {
return CardColor.Red;
}
}
}
init(suit : CardSuit, rank : CardRank) {
self.suit = suit;
self.rank = rank;
}
/**
* Return this card's suit.
*
* @return CardSuit - this card's suit
*/
func getSuit() -> CardSuit {
return self.suit;
}
/**
* Return this card's rank.
*
* @return CardRank - this card's rank
*/
func getRank() -> CardRank {
return self.rank;
}
/**
* Return this card's color.
*
* @return CardColor - this card's color
*/
func getColor() -> CardColor {
return self.color;
}
/**
* Return true if and only if this card matches the specified card's rank.
*
* @param card : Card - The card with which to compare this card.
* @return Bool - true if both cards have the same rank
*/
func hasSameRankAs(card : Card) -> Bool {
return (self.rank == card.getRank());
}
/**
* Return true if and only if this card matches the specified card's suit.
*
* @param card : Card - The card with which to compare this card.
* @return Bool - true if both cards have the same suit
*/
func hasSameSuitAs(card : Card) -> Bool {
return (self.suit == card.getSuit());
}
/**
* Return true if and only if this card matches the specified card's color.
*
* @param card : Card - The card with which to compare this card.
* @return Bool - true if both cards have the same color
*/
func hasSameColorAs(card : Card) -> Bool {
return (self.color == card.getColor());
}
/**
* Return true if and only if this card does not match the specified card's color.
*
* @param card : Card - The card with which to compare this card.
* @return Bool - true if this card has the opposite color of the specified card
*/
func hasOppositeColorThan(card : Card) -> Bool {
return (self.color != card.getColor());
}
/**
* Return true if and only if this card has a higher rank than the specified card.
*
* @param card : Card - The card with which to compare this card.
* @param acesLow : Bool - True if Aces should be considered low.
* @return Bool - true if this card has a higher rank than the specified card
*/
func isHigherThan(card : Card, acesLow : Bool) -> Bool {
if(acesLow || (!acesLow && self.rank != .Ace && card.getRank() != .Ace)) {
return (self.rank.rawValue < card.getRank().rawValue);
} else {
if(self.rank == .Ace && card.getRank() != .Ace) {
return true;
} else if(self.rank != .Ace && card.getRank() == .Ace) {
return false;
} else {
return false;
}
}
}
/**
* Return true if and only if this card has a lower rank than the specified card.
*
* @param card : Card - The card with which to compare this card.
* @param acesLow : Bool - True if Aces should be considered low.
* @return Bool - true if this card has a lower rank than the specified card
*/
func isLowerThan(card : Card, acesLow : Bool) -> Bool {
if(acesLow || (!acesLow && self.rank != .Ace && card.getRank() != .Ace)) {
return (self.rank.rawValue > card.getRank().rawValue);
} else {
if(self.rank == .Ace && card.getRank() != .Ace) {
return false;
} else if(self.rank != .Ace && card.getRank() == .Ace) {
return true;
} else {
return false;
}
}
}
/**
* If ignoreSuit is true or not specified, return true if and only if this card's
* rank matches the specified card's rank. If ignoreSuit is false, return true if
* and only if this card's rank and suit matches the specified card's rank and
* suit.
*
* @param card : Card - The card with which to compare this card.
* @param ignoreSuit : Bool (default true) - True if suit should not be considered; false otherwise.
* @return Bool - true if this card is equal to the specified card
*/
func isEqualTo(card : Card, ignoreSuit : Bool = true) -> Bool {
if(ignoreSuit) {
return (self.rank == card.getRank());
} else {
return (self.rank == card.getRank() && self.suit == card.getSuit());
}
}
/**
* Returns the face of this card.
*
* @param deckChoice : DeckFronts - the deck front being used by this deck to which this card belongs
* @return UIImage? - the face of this card if found
*/
func getCardFace(deckChoice : Deck.DeckFronts) -> UIImage? {
let cardRank : String;
let cardSuit : String;
var cardOption : Deck.DeckFronts = deckChoice;
switch(self.suit) {
case .Clubs:
cardSuit = "clubs";
case .Diamonds:
cardSuit = "diamonds";
case .Hearts:
cardSuit = "hearts";
case .Spades:
cardSuit = "spades";
}
switch(self.rank) {
case .Ace:
cardRank = "ace";
case .Jack:
cardRank = "jack";
case .Queen:
cardRank = "queen";
case .King:
cardRank = "king";
default:
cardRank = String(self.rank.rawValue);
}
// The only difference between Deck1 and Deck2 are the Kings, Queens, and Jacks.
if(self.rank.rawValue <= CardRank.Ten.rawValue && cardOption == Deck.DeckFronts.Deck3 && !(self.rank
== .Ace && self.suit == .Spades)) {
cardOption = Deck.DeckFronts.Deck2;
}
let imageName = cardRank + "_" + cardSuit + "_" + cardOption.rawValue;
return UIImage(named: imageName);
}
/**
* Returns the back of this card.
*
* @param deckChoice : DeckBacks - the deck back being used by the deck to which this card belongs
* @return UIImage? - the back of this card if found
*/
func getCardBack(deckChoice : Deck.DeckBacks) -> UIImage? {
let imageName = "back_" + deckChoice.rawValue;
return UIImage(named: imageName);
}
} | mit | 000ed1394b4354f84dc4faf0aa2c3fc3 | 29.964286 | 108 | 0.53717 | 4.226436 | false | false | false | false |
smizy/DemoWebviewApsalar_ios | DemoAppWebview/AppDelegate.swift | 1 | 6464 | //
// AppDelegate.swift
// DemoAppWebview
//
// Created by usr0300789 on 2014/11/14.
// Copyright (c) 2014年 smizy. All rights reserved.
//
import UIKit
import CoreData
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(application: UIApplication, didFinishLaunchingWithOptions launchOptions: [NSObject: AnyObject]?) -> Bool {
// Override point for customization after application launch.
return true
}
func applicationWillResignActive(application: UIApplication) {
// Sent when the application is about to move from active to inactive state. This can occur for certain types of temporary interruptions (such as an incoming phone call or SMS message) or when the user quits the application and it begins the transition to the background state.
// Use this method to pause ongoing tasks, disable timers, and throttle down OpenGL ES frame rates. Games should use this method to pause the game.
}
func applicationDidEnterBackground(application: UIApplication) {
// Use this method to release shared resources, save user data, invalidate timers, and store enough application state information to restore your application to its current state in case it is terminated later.
// If your application supports background execution, this method is called instead of applicationWillTerminate: when the user quits.
}
func applicationWillEnterForeground(application: UIApplication) {
// Called as part of the transition from the background to the inactive state; here you can undo many of the changes made on entering the background.
}
// added
func application(application: UIApplication, openURL url: NSURL, sourceApplication: String?, annotation: AnyObject?) -> Bool {
Apsalar.startSession("<API KEY>", withKey: "<SECRET>", andURL:url)
return true
}
func applicationDidBecomeActive(application: UIApplication) {
// Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface.
Apsalar.startSession("<API KEY>", withKey: "<SECRET>")
}
func applicationWillTerminate(application: UIApplication) {
// Called when the application is about to terminate. Save data if appropriate. See also applicationDidEnterBackground:.
// Saves changes in the application's managed object context before the application terminates.
self.saveContext()
}
// MARK: - Core Data stack
lazy var applicationDocumentsDirectory: NSURL = {
// The directory the application uses to store the Core Data store file. This code uses a directory named "jp.koukoku.DemoAppWebview" in the application's documents Application Support directory.
let urls = NSFileManager.defaultManager().URLsForDirectory(.DocumentDirectory, inDomains: .UserDomainMask)
return urls[urls.count-1] as NSURL
}()
lazy var managedObjectModel: NSManagedObjectModel = {
// The managed object model for the application. This property is not optional. It is a fatal error for the application not to be able to find and load its model.
let modelURL = NSBundle.mainBundle().URLForResource("DemoAppWebview", withExtension: "momd")!
return NSManagedObjectModel(contentsOfURL: modelURL)!
}()
lazy var persistentStoreCoordinator: NSPersistentStoreCoordinator? = {
// The persistent store coordinator for the application. This implementation creates and return a coordinator, having added the store for the application to it. This property is optional since there are legitimate error conditions that could cause the creation of the store to fail.
// Create the coordinator and store
var coordinator: NSPersistentStoreCoordinator? = NSPersistentStoreCoordinator(managedObjectModel: self.managedObjectModel)
let url = self.applicationDocumentsDirectory.URLByAppendingPathComponent("DemoAppWebview.sqlite")
var error: NSError? = nil
var failureReason = "There was an error creating or loading the application's saved data."
if coordinator!.addPersistentStoreWithType(NSSQLiteStoreType, configuration: nil, URL: url, options: nil, error: &error) == nil {
coordinator = nil
// Report any error we got.
let dict = NSMutableDictionary()
dict[NSLocalizedDescriptionKey] = "Failed to initialize the application's saved data"
dict[NSLocalizedFailureReasonErrorKey] = failureReason
dict[NSUnderlyingErrorKey] = error
error = NSError(domain: "YOUR_ERROR_DOMAIN", code: 9999, userInfo: dict)
// Replace this with code to handle the error appropriately.
// abort() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
NSLog("Unresolved error \(error), \(error!.userInfo)")
abort()
}
return coordinator
}()
lazy var managedObjectContext: NSManagedObjectContext? = {
// Returns the managed object context for the application (which is already bound to the persistent store coordinator for the application.) This property is optional since there are legitimate error conditions that could cause the creation of the context to fail.
let coordinator = self.persistentStoreCoordinator
if coordinator == nil {
return nil
}
var managedObjectContext = NSManagedObjectContext()
managedObjectContext.persistentStoreCoordinator = coordinator
return managedObjectContext
}()
// MARK: - Core Data Saving support
func saveContext () {
if let moc = self.managedObjectContext {
var error: NSError? = nil
if moc.hasChanges && !moc.save(&error) {
// Replace this implementation with code to handle the error appropriately.
// abort() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
NSLog("Unresolved error \(error), \(error!.userInfo)")
abort()
}
}
}
}
| mit | f47aced8faee00882dd3b078820767fd | 53.762712 | 290 | 0.712628 | 5.703442 | false | false | false | false |
russelhampton05/MenMew | App Prototypes/Menu_Server_App_Prototype_001/Menu_Server_App_Prototype_001/Models.swift | 1 | 9263 | //
// Models.swift
// App_Prototype_Alpha_001
//
// Created by Jon Calanio on 10/3/16.
// Copyright © 2016 Jon Calanio. All rights reserved.
//
import Foundation
import Firebase
//User Class
class User {
var ID: String
var name: String?
var theme: String?
var email: String?
var ticket: Ticket?
var image: String?
init(id: String, email: String?, name: String?, ticket:Ticket?, theme: String?, image: String?){
self.ID = id
self.theme = theme
self.email = email
self.name = name
self.ticket = ticket
self.image = image
}
}
//Server Class
class Server {
var ID: String
var name: String?
var email: String?
var theme: String?
var image: String?
var restaurants: [String]?
var tables: [String]?
var notifications = true
init (id: String, name: String?, email: String?, restaurants: [String]?, tables: [String]?, theme: String?, image: String?, notifications: Bool) {
self.ID = id
self.theme = theme
self.name = name
self.email = email
self.restaurants = restaurants
self.tables = tables
self.image = image
self.notifications = notifications
}
}
//Details Class
//need to look at maybe changing this
struct Details {
var sides: [String]
var cookType: [String]
}
//Menu Class
class Menu{
var rest_id : String?
var title : String?
var cover_picture : String?
var menu_groups : [MenuGroup]? = []
init(id:String, title:String, cover_picture:String?, groups: [MenuGroup]){
self.rest_id = id
self.title = title
self.cover_picture = cover_picture
self.menu_groups = groups
}
init(){
}
convenience init(id:String, title:String, groups: [MenuGroup] ) {
self.init(id:id, title:title, cover_picture:nil, groups:groups)
}
}
//Menu Group Class
class MenuGroup{
var cover_picture: String?
var desc: String?
var title: String?
var items: [MenuItem]?
init(){
}
init(desc: String,items: [MenuItem], title: String?, cover_picture:String?){
self.cover_picture = cover_picture
self.desc = desc
self.title = title
self.items = items
}
convenience init(desc: String,items: [MenuItem], title: String?){
self.init(desc:desc,items:items, title:title, cover_picture:nil)
}
convenience init(desc: String,items: [MenuItem], cover_picture:String?) {
self.init(desc:desc,items:items, title:nil, cover_picture:cover_picture)
}
convenience init(desc: String,items: [MenuItem]) {
self.init(desc:desc, items:items, title:nil, cover_picture:nil)
}
}
//menu item objects and menus are for UI purposes only.
//Menu item will have a member called "item", which will tie it in to the actaul
//details necessary for order tracking.
//Menu Item Class
class MenuItem {
//will eventually point to an actual item (if we care to implement that, possibly not)
//for now just UI facing fields and those needed for ordering/pricing
var item_ID: String?
var title: String?
var price: Double?
//var sides: [String]?
var image: String?
var desc: String?
init(){
}
init(item_ID: String?, title:String, price:Double, image: String, desc: String) {
self.item_ID = item_ID
self.title = title
self.image = image
self.price = price
// self.sides = sides
self.desc = desc
}
//convenience init(title:String, price:Double, image: String, desc: String){
// self.init(title : title, price : price, image : image, desc: desc, sides:nil)
//}
}
//Restaurant Class
class Restaurant {
var restaurant_ID: String?
var title: String?
var location: String?
//We can also reference tables and servers here
init() {
self.restaurant_ID = ""
self.title = ""
self.location = ""
}
init(restaurant_ID: String, title: String, location: String?) {
self.restaurant_ID = restaurant_ID
self.title = title
self.location = location
}
}
//Ticket Class
class Ticket {
var ticket_ID: String?
var user_ID: String?
var restaurant_ID: String?
var tableNum: String?
var timestamp: String?
var paid: Bool?
var itemsOrdered: [MenuItem]?
var desc: String?
var confirmed: Bool?
var status: String?
var total: Double?
var tip: Double?
var message_ID: String?
init() {
self.message_ID = ""
self.ticket_ID = ""
self.user_ID = ""
self.restaurant_ID = ""
self.tableNum = ""
self.paid = false
self.itemsOrdered = []
self.desc = ""
self.confirmed = false
self.status = "Ordering"
self.total = 0.0
self.tip = 0.0
}
init(ticket_ID: String, user_ID: String, restaurant_ID: String, tableNum: String, timestamp: String, paid: Bool, itemsOrdered:[MenuItem]?, desc: String?, confirmed: Bool?, status: String?, total: Double?, tip: Double?, message_ID: String?) {
self.ticket_ID = ticket_ID
self.user_ID = user_ID
self.restaurant_ID = restaurant_ID
self.tableNum = tableNum
self.timestamp = timestamp
self.paid = paid
self.itemsOrdered = itemsOrdered
self.desc = desc
self.confirmed = confirmed
self.status = status
self.total = total
self.tip = tip
self.message_ID = message_ID
if self.message_ID == nil{
self.message_ID = ""
}
}
func generateMessageGUID() {
self.message_ID = UUID().uuidString
}
init(snapshot: FIRDataSnapshot) {
let snapshotValue = snapshot.value as! [String: AnyObject]
self.ticket_ID = snapshot.key
self.user_ID = snapshotValue["user"] as? String
self.restaurant_ID = snapshotValue["restaurant"] as? String
self.tableNum = snapshotValue["table"] as? String
self.timestamp = snapshotValue["timestamp"] as? String
self.paid = snapshotValue["paid"] as? Bool
self.desc = snapshotValue["desc"] as? String
self.confirmed = snapshotValue["confirmed"] as? Bool
self.status = snapshotValue["status"] as? String
self.total = snapshotValue["total"] as? Double
self.tip = snapshotValue["tip"] as? Double
if snapshotValue["message"] != nil{
self.message_ID = snapshotValue["message"] as! String?
}
else{
self.message_ID = ""
}
//MenuItemManager.GetMenuItem(ids: snapshotValue["itemsOrdered"]?.allKeys as! [String]) {
//items in
//self.itemsOrdered = items;
//}
//ItemsOrdered is the array of items ordered for the table
// let menuItems = snapshotValue["itemsOrdered"] as? NSDictionary
}
}
//Message Class
class Message {
var message_ID: String?
var serverMessage: String?
var userMessage: String?
init(snapshot: FIRDataSnapshot) {
let snapshotValue = snapshot.value as! [String: AnyObject]
self.message_ID = snapshot.key
self.serverMessage = snapshotValue["server"] as? String
self.userMessage = snapshotValue["user"] as? String
}
}
class Theme {
var name: String?
var primary: UIColor?
var secondary: UIColor?
var highlight: UIColor?
var invert: UIColor?
init(type: String) {
if type == "Salmon" {
name = "Salmon"
primary = UIColor(red: 255, green: 106, blue: 92)
secondary = UIColor(red: 255, green: 255, blue: 255)
highlight = UIColor(red: 255, green: 255, blue: 255)
invert = UIColor(red: 255, green: 106, blue: 92)
}
else if type == "Light" {
name = "Light"
primary = UIColor(red: 255, green: 255, blue: 255)
secondary = UIColor(red: 255, green: 255, blue: 255)
highlight = UIColor(red: 255, green: 141, blue: 43)
invert = UIColor(red: 255, green: 141, blue: 43)
}
else if type == "Midnight" {
name = "Midnight"
primary = UIColor(red: 55, green: 30, blue: 96)
secondary = UIColor(red: 18, green: 3, blue: 42)
highlight = UIColor(red: 255, green: 255, blue: 255)
invert = UIColor(red: 255, green: 255, blue: 255)
}
else if type == "Slate" {
name = "Slate"
primary = UIColor(red: 27, green: 27, blue: 27)
secondary = UIColor(red: 20, green: 20, blue: 20)
highlight = UIColor(red: 255, green: 255, blue: 255)
invert = UIColor(red: 255, green: 255, blue: 255)
}
}
}
extension UIColor {
convenience init(red: Int, green: Int, blue: Int) {
let newRed = CGFloat(red)/255
let newGreen = CGFloat(green)/255
let newBlue = CGFloat(blue)/255
self.init(red: newRed, green: newGreen, blue: newBlue, alpha: 1.0)
}
}
| mit | 683b883346eca9291368ce08af47d356 | 28.034483 | 245 | 0.583999 | 4.025206 | false | false | false | false |
csmulhern/uranus | iOS Example/iOS Example/ViewController.swift | 1 | 4896 | import UIKit
import Uranus
class ViewController: UIViewController {
var componentsView: ComponentsScrollView!
var layout: View!
var alternateLayout: View!
var showingNormalLayout = true
var switchLayoutBarButtonItem: UIBarButtonItem!
var layoutDirectionBarButtonItem: UIBarButtonItem?
override init(nibName nibNameOrNil: String?, bundle nibBundleOrNil: Bundle?) {
super.init(nibName: nibNameOrNil, bundle: nibBundleOrNil)
self.switchLayoutBarButtonItem = UIBarButtonItem(title: "Switch Layout", style: UIBarButtonItemStyle.plain, target: self, action: #selector(self.toggleLayout))
self.navigationItem.leftBarButtonItem = switchLayoutBarButtonItem
if #available(iOS 9, *) {
self.layoutDirectionBarButtonItem = UIBarButtonItem(title: "Force RTL On", style: UIBarButtonItemStyle.plain, target: self, action: #selector(self.toggleRTL))
self.navigationItem.rightBarButtonItem = layoutDirectionBarButtonItem
}
}
required init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
func newPostComponents() -> [Component] {
let label = Label(text: "This is a label", font: UIFont.systemFont(ofSize: 20.0))
label.backgroundColor = UIColor.blue.withAlphaComponent(0.2)
label.layoutType = LayoutType.full
let imageView = ImageView(image: UIImage(named: "image"), contentMode: UIViewContentMode.scaleAspectFill)
imageView.layoutType = LayoutType.full
let cancelButton = Button()
cancelButton.backgroundColor = UIColor.red.withAlphaComponent(0.2)
cancelButton.setTitle("Cancel", forState: UIControlState.normal)
cancelButton.setTitleColor(UIColor.black, forState: UIControlState.normal)
cancelButton.addTarget(target: self, action: #selector(cancelButtonSelected), forControlEvents: UIControlEvents.touchUpInside)
cancelButton.padding = UIEdgeInsets(top: 0.0, left: 10.0, bottom: 0.0, right: 10.0)
cancelButton.flexible = true
return [label, imageView, cancelButton, self.newAcceptButtonComponent()]
}
func newAcceptButtonComponent() -> Component {
let acceptButton = Button()
acceptButton.backgroundColor = UIColor.green.withAlphaComponent(0.2)
acceptButton.setTitle("Okay", forState: UIControlState.normal)
acceptButton.setTitleColor(UIColor.black, forState: UIControlState.normal)
acceptButton.addTarget(target: self, action: #selector(acceptButtonSelected), forControlEvents: UIControlEvents.touchUpInside)
acceptButton.padding = UIEdgeInsets(top: 0.0, left: 10.0, bottom: 0.0, right: 10.0)
acceptButton.flexible = true
return acceptButton
}
func newTextViewComponent() -> Component {
let textView = TextView(text: "Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.", font: UIFont.systemFont(ofSize: 20.0))
textView.layoutType = LayoutType.full
textView.editable = false
return textView
}
override func viewDidLoad() {
super.viewDidLoad()
self.view.backgroundColor = UIColor.white
self.componentsView = ComponentsScrollView(frame: CGRect.zero)
self.componentsView.alwaysBounceVertical = true
self.view.addSubview(self.componentsView)
let components = [self.newPostComponents()].cycle().take(4).joined()
self.layout = View(children: Array(components))
self.alternateLayout = View().children([self.newTextViewComponent(), self.newAcceptButtonComponent()])
self.componentsView.configure(with: self.layout)
}
override func viewDidLayoutSubviews() {
self.componentsView.frame = self.view.bounds
}
@objc func toggleLayout() {
self.showingNormalLayout = !self.showingNormalLayout
if self.showingNormalLayout {
self.componentsView.configure(with: self.layout, animated: true)
}
else {
self.componentsView.configure(with: self.alternateLayout, animated: true)
}
}
@objc @available(iOS 9, *)
func toggleRTL() {
if self.componentsView.semanticContentAttribute == UISemanticContentAttribute.forceRightToLeft {
self.componentsView.semanticContentAttribute = UISemanticContentAttribute.unspecified
self.layoutDirectionBarButtonItem?.title = "Force RTL On"
}
else {
self.componentsView.semanticContentAttribute = UISemanticContentAttribute.forceRightToLeft
self.layoutDirectionBarButtonItem?.title = "Force RTL Off"
}
}
@objc private func cancelButtonSelected() {
print("Cancel selected.")
}
@objc private func acceptButtonSelected() {
print("Accept selected.")
}
}
| unlicense | cac086fe3ac8e9aba845b5c910d59d08 | 41.206897 | 203 | 0.703227 | 5.137461 | false | false | false | false |
UroborosStudios/UOHelpers | UOHelpers/MediaExtensions.swift | 1 | 1734 | //
// MediaExtensions.swift
// UOHelpers
//
// Created by Jonathan Silva on 04/05/17.
// Copyright © 2017 Uroboros Studios. All rights reserved.
//
import Foundation
import UIKit
public extension UIColor {
open convenience init(fromHex: String) {
let hexString:NSString = fromHex.trimmingCharacters(in: CharacterSet.whitespacesAndNewlines) as NSString
let scanner = Scanner(string: fromHex)
if (hexString.hasPrefix("#")) {
scanner.scanLocation = 1
}
var color:UInt32 = 0
scanner.scanHexInt32(&color)
let mask = 0x000000FF
let r = Int(color >> 16) & mask
let g = Int(color >> 8) & mask
let b = Int(color) & mask
let red = CGFloat(r) / 255.0
let green = CGFloat(g) / 255.0
let blue = CGFloat(b) / 255.0
self.init(red:red, green:green, blue:blue, alpha:1)
}
open convenience init(fromHex: String,withAlpha: CGFloat) {
let hexString:NSString = fromHex.trimmingCharacters(in: CharacterSet.whitespacesAndNewlines) as NSString
let scanner = Scanner(string: fromHex)
if (hexString.hasPrefix("#")) {
scanner.scanLocation = 1
}
var color:UInt32 = 0
scanner.scanHexInt32(&color)
let mask = 0x000000FF
let r = Int(color >> 16) & mask
let g = Int(color >> 8) & mask
let b = Int(color) & mask
let red = CGFloat(r) / 255.0
let green = CGFloat(g) / 255.0
let blue = CGFloat(b) / 255.0
self.init(red:red, green:green, blue:blue, alpha: withAlpha)
}
}
| mit | 740aec06790db659dedc4818314306da | 28.372881 | 112 | 0.557992 | 4.136038 | false | false | false | false |
peferron/algo | EPI/Stacks and Queues/Implement a queue using stacks/swift/main.swift | 1 | 841 | // Nerfed array to make sure we don't cheat by doing e.g. index-based access.
struct Stack<T> {
var array = [T]()
var count: Int {
return array.count
}
mutating func push(_ element: T) {
array.append(element)
}
mutating func pop() -> T {
return array.removeLast()
}
}
public struct Queue<T> {
var enqueueStack = Stack<T>()
var dequeueStack = Stack<T>()
public var count: Int {
return enqueueStack.count + dequeueStack.count
}
public mutating func enqueue(_ element: T) {
enqueueStack.push(element)
}
public mutating func dequeue() -> T {
if dequeueStack.count == 0 {
while enqueueStack.count > 0 {
dequeueStack.push(enqueueStack.pop())
}
}
return dequeueStack.pop()
}
}
| mit | 798986ac7358e942b8dfc2b07c7e650f | 21.131579 | 77 | 0.568371 | 4.122549 | false | false | false | false |
rzrasel/iOS-Swift-2016-01 | SwiftTableHeaderOne/SwiftTableHeaderOne/AppDelegate.swift | 2 | 6113 | //
// AppDelegate.swift
// SwiftTableHeaderOne
//
// Created by NextDot on 2/11/16.
// Copyright © 2016 RzRasel. All rights reserved.
//
import UIKit
import CoreData
@UIApplicationMain
class AppDelegate: UIResponder, UIApplicationDelegate {
var window: UIWindow?
func application(application: UIApplication, didFinishLaunchingWithOptions launchOptions: [NSObject: AnyObject]?) -> Bool {
// Override point for customization after application launch.
return true
}
func applicationWillResignActive(application: UIApplication) {
// Sent when the application is about to move from active to inactive state. This can occur for certain types of temporary interruptions (such as an incoming phone call or SMS message) or when the user quits the application and it begins the transition to the background state.
// Use this method to pause ongoing tasks, disable timers, and throttle down OpenGL ES frame rates. Games should use this method to pause the game.
}
func applicationDidEnterBackground(application: UIApplication) {
// Use this method to release shared resources, save user data, invalidate timers, and store enough application state information to restore your application to its current state in case it is terminated later.
// If your application supports background execution, this method is called instead of applicationWillTerminate: when the user quits.
}
func applicationWillEnterForeground(application: UIApplication) {
// Called as part of the transition from the background to the inactive state; here you can undo many of the changes made on entering the background.
}
func applicationDidBecomeActive(application: UIApplication) {
// Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface.
}
func applicationWillTerminate(application: UIApplication) {
// Called when the application is about to terminate. Save data if appropriate. See also applicationDidEnterBackground:.
// Saves changes in the application's managed object context before the application terminates.
self.saveContext()
}
// MARK: - Core Data stack
lazy var applicationDocumentsDirectory: NSURL = {
// The directory the application uses to store the Core Data store file. This code uses a directory named "rz.rasel.SwiftTableHeaderOne" in the application's documents Application Support directory.
let urls = NSFileManager.defaultManager().URLsForDirectory(.DocumentDirectory, inDomains: .UserDomainMask)
return urls[urls.count-1]
}()
lazy var managedObjectModel: NSManagedObjectModel = {
// The managed object model for the application. This property is not optional. It is a fatal error for the application not to be able to find and load its model.
let modelURL = NSBundle.mainBundle().URLForResource("SwiftTableHeaderOne", withExtension: "momd")!
return NSManagedObjectModel(contentsOfURL: modelURL)!
}()
lazy var persistentStoreCoordinator: NSPersistentStoreCoordinator = {
// The persistent store coordinator for the application. This implementation creates and returns a coordinator, having added the store for the application to it. This property is optional since there are legitimate error conditions that could cause the creation of the store to fail.
// Create the coordinator and store
let coordinator = NSPersistentStoreCoordinator(managedObjectModel: self.managedObjectModel)
let url = self.applicationDocumentsDirectory.URLByAppendingPathComponent("SingleViewCoreData.sqlite")
var failureReason = "There was an error creating or loading the application's saved data."
do {
try coordinator.addPersistentStoreWithType(NSSQLiteStoreType, configuration: nil, URL: url, options: nil)
} catch {
// Report any error we got.
var dict = [String: AnyObject]()
dict[NSLocalizedDescriptionKey] = "Failed to initialize the application's saved data"
dict[NSLocalizedFailureReasonErrorKey] = failureReason
dict[NSUnderlyingErrorKey] = error as NSError
let wrappedError = NSError(domain: "YOUR_ERROR_DOMAIN", code: 9999, userInfo: dict)
// Replace this with code to handle the error appropriately.
// abort() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
NSLog("Unresolved error \(wrappedError), \(wrappedError.userInfo)")
abort()
}
return coordinator
}()
lazy var managedObjectContext: NSManagedObjectContext = {
// Returns the managed object context for the application (which is already bound to the persistent store coordinator for the application.) This property is optional since there are legitimate error conditions that could cause the creation of the context to fail.
let coordinator = self.persistentStoreCoordinator
var managedObjectContext = NSManagedObjectContext(concurrencyType: .MainQueueConcurrencyType)
managedObjectContext.persistentStoreCoordinator = coordinator
return managedObjectContext
}()
// MARK: - Core Data Saving support
func saveContext () {
if managedObjectContext.hasChanges {
do {
try managedObjectContext.save()
} catch {
// Replace this implementation with code to handle the error appropriately.
// abort() causes the application to generate a crash log and terminate. You should not use this function in a shipping application, although it may be useful during development.
let nserror = error as NSError
NSLog("Unresolved error \(nserror), \(nserror.userInfo)")
abort()
}
}
}
}
| apache-2.0 | 7eb8d994609121b217c116319db21099 | 54.063063 | 291 | 0.720713 | 5.893925 | false | false | false | false |
wireapp/wire-ios-sync-engine | Tests/Source/UserSession/BuildTypeTests.swift | 1 | 2861 | //
// Wire
// Copyright (C) 2018 Wire Swiss GmbH
//
// This program is free software: you can redistribute it and/or modify
// it under the terms of the GNU General Public License as published by
// the Free Software Foundation, either version 3 of the License, or
// (at your option) any later version.
//
// This program is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU General Public License
// along with this program. If not, see http://www.gnu.org/licenses/.
//
import XCTest
import WireTesting
@testable import WireSyncEngine
final class BuildTypeTests: ZMTBaseTest {
func testThatItParsesKnownBundleIDs() {
// GIVEN
let bundleIdsToTypes: [String: WireSyncEngine.BuildType] = ["com.wearezeta.zclient.ios": .production,
"com.wearezeta.zclient-alpha": .alpha,
"com.wearezeta.zclient.ios-development": .development,
"com.wearezeta.zclient.ios-release": .releaseCandidate,
"com.wearezeta.zclient.ios-internal": .internal]
bundleIdsToTypes.forEach { bundleId, expectedType in
// WHEN
let type = WireSyncEngine.BuildType(bundleID: bundleId)
// THEN
XCTAssertEqual(type, expectedType)
}
}
func testThatItParsesUnknownBundleID() {
// GIVEN
let someBundleId = "com.mycompany.myapp"
// WHEN
let buildType = WireSyncEngine.BuildType(bundleID: someBundleId)
// THEN
XCTAssertEqual(buildType, WireSyncEngine.BuildType.custom(bundleID: someBundleId))
}
func testThatItReturnsTheCertName() {
// GIVEN
let suts: [(BuildType, String)] = [(.alpha, "com.wire.ent"),
(.internal, "com.wire.int.ent"),
(.releaseCandidate, "com.wire.rc.ent"),
(.development, "com.wire.dev.ent")]
suts.forEach { (type, certName) in
// WHEN
let certName = type.certificateName
// THEN
XCTAssertEqual(certName, certName)
}
}
func testThatItReturnsBundleIdForCertNameIfCustom() {
// GIVEN
let type = WireSyncEngine.BuildType.custom(bundleID: "com.mycompany.myapp")
// WHEN
let certName = type.certificateName
// THEN
XCTAssertEqual(certName, "com.mycompany.myapp")
}
}
| gpl-3.0 | e2564f0dc698e86822c15eeef0220e39 | 36.644737 | 123 | 0.577071 | 4.984321 | false | true | false | false |
seandavidmcgee/HumanKontactBeta | src/Pods/Hokusai/Classes/Hokusai.swift | 1 | 15917 | //
// Hokusai.swift
// Hokusai
//
// Created by Yuta Akizuki on 2015/07/07.
// Copyright (c) 2015年 ytakzk. All rights reserved.
//
import UIKit
private struct HOKConsts {
let animationDuration:NSTimeInterval = 0.8
let hokusaiTag = 9999
}
// Action Types
public enum HOKAcitonType {
case None, Selector, Closure
}
// Color Types
public enum HOKColorScheme {
case Hokusai, Asagi, Matcha, Tsubaki, Inari, Karasu, Enshu
func getColors() -> HOKColors {
switch self {
case .Asagi:
return HOKColors(
backGroundColor: UIColorHex(0x0bada8),
buttonColor: UIColorHex(0x08827e),
cancelButtonColor: UIColorHex(0x6dcecb),
fontColor: UIColorHex(0xffffff)
)
case .Matcha:
return HOKColors(
backGroundColor: UIColorHex(0x314631),
buttonColor: UIColorHex(0x618c61),
cancelButtonColor: UIColorHex(0x496949),
fontColor: UIColorHex(0xffffff)
)
case .Tsubaki:
return HOKColors(
backGroundColor: UIColorHex(0xe5384c),
buttonColor: UIColorHex(0xac2a39),
cancelButtonColor: UIColorHex(0xc75764),
fontColor: UIColorHex(0xffffff)
)
case .Inari:
return HOKColors(
backGroundColor: UIColorHex(0xdd4d05),
buttonColor: UIColorHex(0xa63a04),
cancelButtonColor: UIColorHex(0xb24312),
fontColor: UIColorHex(0x231e1c)
)
case .Karasu:
return HOKColors(
backGroundColor: UIColorHex(0x180614),
buttonColor: UIColorHex(0x3d303d),
cancelButtonColor: UIColorHex(0x261d26),
fontColor: UIColorHex(0x9b9981)
)
case .Enshu:
return HOKColors(
backGroundColor: UIColorHex(0xccccbe),
buttonColor: UIColorHex(0xffffff),
cancelButtonColor: UIColorHex(0xe5e5d8),
fontColor: UIColorHex(0x9b9981)
)
default: // Hokusai
return HOKColors(
backGroundColor: UIColorHex(0x00AFF0),
buttonColor: UIColorHex(0x197EAD),
cancelButtonColor: UIColorHex(0x1D94CA),
fontColor: UIColorHex(0xffffff)
)
}
}
private func UIColorHex(hex: UInt) -> UIColor {
return UIColor(
red: CGFloat((hex & 0xFF0000) >> 16) / 255.0,
green: CGFloat((hex & 0x00FF00) >> 8) / 255.0,
blue: CGFloat(hex & 0x0000FF) / 255.0,
alpha: CGFloat(1.0)
)
}
}
final public class HOKColors: NSObject {
var backgroundColor: UIColor
var buttonColor: UIColor
var cancelButtonColor: UIColor
var fontColor: UIColor
required public init(backGroundColor: UIColor, buttonColor: UIColor, cancelButtonColor: UIColor, fontColor: UIColor) {
self.backgroundColor = backGroundColor
self.buttonColor = buttonColor
self.cancelButtonColor = cancelButtonColor
self.fontColor = fontColor
}
}
final public class HOKButton: UIButton {
var target:AnyObject!
var selector:Selector!
var action:(()->Void)!
var actionType = HOKAcitonType.None
var isCancelButton = false
// Font
let kDefaultFont = "AvenirNext-DemiBold"
let kFontSize:CGFloat = 16.0
func setColor(colors: HOKColors) {
self.setTitleColor(colors.fontColor, forState: .Normal)
self.backgroundColor = (isCancelButton) ? colors.cancelButtonColor : colors.buttonColor
}
func setFontName(fontName: String?) {
let name:String
if let fontName = fontName where !fontName.isEmpty {
name = fontName
} else {
name = kDefaultFont
}
self.titleLabel?.font = UIFont(name: name, size:kFontSize)
}
}
final public class HOKMenuView: UIView {
var colorScheme = HOKColorScheme.Hokusai
public let kDamping: CGFloat = 0.7
public let kInitialSpringVelocity: CGFloat = 0.8
private var displayLink: CADisplayLink?
private let shapeLayer = CAShapeLayer()
private var bendableOffset = UIOffsetZero
override init(frame: CGRect) {
super.init(frame: frame)
}
required public init?(coder aDecoder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
override public func layoutSubviews() {
super.layoutSubviews()
shapeLayer.frame.origin = frame.origin
shapeLayer.bounds.origin = frame.origin
}
func setShapeLayer(colors: HOKColors) {
self.backgroundColor = UIColor.clearColor()
shapeLayer.fillColor = colors.backgroundColor.CGColor
self.layer.insertSublayer(shapeLayer, atIndex: 0)
}
func positionAnimationWillStart() {
if displayLink == nil {
displayLink = CADisplayLink(target: self, selector: "tick:")
displayLink!.addToRunLoop(NSRunLoop.mainRunLoop(), forMode: NSDefaultRunLoopMode)
}
shapeLayer.bounds = CGRect(origin: CGPointZero, size: self.bounds.size)
}
func updatePath() {
let width = CGRectGetWidth(shapeLayer.bounds)
let height = CGRectGetHeight(shapeLayer.bounds)
let path = UIBezierPath()
path.moveToPoint(CGPoint(x: 0, y: 0))
path.addQuadCurveToPoint(CGPoint(x: width, y: 0),
controlPoint:CGPoint(x: width * 0.5, y: 0 + bendableOffset.vertical))
path.addQuadCurveToPoint(CGPoint(x: width, y: height + 100.0),
controlPoint:CGPoint(x: width + bendableOffset.horizontal, y: height * 0.5))
path.addQuadCurveToPoint(CGPoint(x: 0, y: height + 100.0),
controlPoint: CGPoint(x: width * 0.5, y: height + 100.0))
path.addQuadCurveToPoint(CGPoint(x: 0, y: 0),
controlPoint: CGPoint(x: bendableOffset.horizontal, y: height * 0.5))
path.closePath()
shapeLayer.path = path.CGPath
}
func tick(displayLink: CADisplayLink) {
if let presentationLayer = layer.presentationLayer() as? CALayer {
var verticalOffset = self.layer.frame.origin.y - presentationLayer.frame.origin.y
// On dismissing, the offset should not be offend on the buttons.
if verticalOffset > 0 {
verticalOffset *= 0.2
}
bendableOffset = UIOffset(
horizontal: 0.0,
vertical: verticalOffset
)
updatePath()
if verticalOffset == 0 {
self.displayLink!.invalidate()
self.displayLink = nil
}
}
}
}
final public class Hokusai: UIViewController, UIGestureRecognizerDelegate {
// Views
private var menuView = HOKMenuView()
private var buttons = [HOKButton]()
private var instance:Hokusai! = nil
private var kButtonWidth:CGFloat = 250
private let kButtonHeight:CGFloat = 48.0
private let kButtonInterval:CGFloat = 16.0
private var bgColor = UIColor(white: 1.0, alpha: 0.7)
// Variables users can change
public var colorScheme = HOKColorScheme.Hokusai
public var fontName = ""
public var colors:HOKColors! = nil
public var cancelButtonTitle = "Cancel"
public var cancelButtonAction : (()->Void)?
required public init(coder aDecoder: NSCoder) {
fatalError("NSCoding not supported")
}
required public init() {
super.init(nibName:nil, bundle:nil)
view.frame = UIScreen.mainScreen().bounds
view.autoresizingMask = [UIViewAutoresizing.FlexibleHeight, UIViewAutoresizing.FlexibleWidth]
view.backgroundColor = UIColor.clearColor()
menuView.frame = view.frame
view.addSubview(menuView)
kButtonWidth = view.frame.width * 0.8
// Gesture Recognizer for tapping outside the menu
let tapGesture = UITapGestureRecognizer(target: self, action: Selector("dismiss"))
tapGesture.numberOfTapsRequired = 1
tapGesture.delegate = self
self.view.addGestureRecognizer(tapGesture)
NSNotificationCenter.defaultCenter().addObserver(self, selector: "onOrientationChange:", name: UIDeviceOrientationDidChangeNotification, object: nil)
}
func onOrientationChange(notification: NSNotification){
kButtonWidth = view.frame.width * 0.8
let menuHeight = CGFloat(buttons.count + 2) * kButtonInterval + CGFloat(buttons.count) * kButtonHeight
menuView.frame = CGRect(
x: 0,
y: view.frame.height - menuHeight,
width: view.frame.width,
height: menuHeight
)
menuView.shapeLayer.frame = menuView.frame
menuView.shapeLayer.bounds.origin = menuView.frame.origin
menuView.shapeLayer.layoutIfNeeded()
menuView.layoutIfNeeded()
for var i = 0; i < buttons.count; i++ {
let btn = buttons[i]
btn.frame = CGRect(x: 0.0, y: 0.0, width: kButtonWidth, height: kButtonHeight)
btn.center = CGPoint(x: view.center.x, y: -kButtonHeight * 0.25 + (kButtonHeight + kButtonInterval) * CGFloat(i + 1))
}
self.view.layoutIfNeeded()
}
override public init(nibName nibNameOrNil: String?, bundle nibBundleOrNil: NSBundle?) {
super.init(nibName:nibNameOrNil, bundle:nibBundleOrNil)
}
public func gestureRecognizer(gestureRecognizer: UIGestureRecognizer, shouldReceiveTouch touch: UITouch) -> Bool {
if touch.view != gestureRecognizer.view {
return false
}
return true
}
// Add a button with a closure
public func addButton(title:String, action:()->Void) -> HOKButton {
let btn = addButton(title)
btn.action = action
btn.actionType = HOKAcitonType.Closure
btn.addTarget(self, action:Selector("buttonTapped:"), forControlEvents:.TouchUpInside)
return btn
}
// Add a button with a selector
public func addButton(title:String, target:AnyObject, selector:Selector) -> HOKButton {
let btn = addButton(title)
btn.target = target
btn.selector = selector
btn.actionType = HOKAcitonType.Selector
btn.addTarget(self, action:Selector("buttonTapped:"), forControlEvents:.TouchUpInside)
return btn
}
// Add a cancel button
public func addCancelButton(title:String) -> HOKButton {
if let cancelButtonAction = cancelButtonAction {
return addButton(title, action: cancelButtonAction)
} else {
let btn = addButton(title)
btn.addTarget(self, action:Selector("buttonTapped:"), forControlEvents:.TouchUpInside)
btn.isCancelButton = true
return btn
}
}
// Add a button just with the title
private func addButton(title:String) -> HOKButton {
let btn = HOKButton()
btn.layer.masksToBounds = true
btn.setTitle(title, forState: .Normal)
menuView.addSubview(btn)
buttons.append(btn)
return btn
}
// Show the menu
public func show() {
if let rv = UIApplication.sharedApplication().keyWindow {
if rv.viewWithTag(HOKConsts().hokusaiTag) == nil {
view.tag = HOKConsts().hokusaiTag.hashValue
rv.addSubview(view)
}
} else {
print("Hokusai:: You have to call show() after the controller has appeared.")
return
}
// This is needed to retain this instance.
instance = self
let colors = (self.colors == nil) ? colorScheme.getColors() : self.colors
// Set a background color of Menuview
menuView.setShapeLayer(colors)
// Add a cancel button
self.addCancelButton("Done")
// Decide the menu size
let menuHeight = CGFloat(buttons.count + 2) * kButtonInterval + CGFloat(buttons.count) * kButtonHeight
menuView.frame = CGRect(
x: 0,
y: view.frame.height - menuHeight,
width: view.frame.width,
height: menuHeight
)
// Locate buttons
for var i = 0; i < buttons.count; i++ {
let btn = buttons[i]
btn.frame = CGRect(x: 0.0, y: 0.0, width: kButtonWidth, height: kButtonHeight)
btn.center = CGPoint(x: view.center.x, y: -kButtonHeight * 0.25 + (kButtonHeight + kButtonInterval) * CGFloat(i + 1))
btn.layer.cornerRadius = kButtonHeight * 0.5
btn.setFontName(fontName)
btn.setColor(colors)
}
// Animations
animationWillStart()
// Debug
if (buttons.count == 0) {
print("Hokusai:: The menu has no item yet.")
} else if (buttons.count > 6) {
print("Hokusai:: The menu has lots of items.")
}
}
// Add an animation on showing the menu
private func animationWillStart() {
// Background
self.view.backgroundColor = UIColor.clearColor()
UIView.animateWithDuration(HOKConsts().animationDuration * 0.4,
delay: 0.0,
options: UIViewAnimationOptions.CurveEaseOut,
animations: {
self.view.backgroundColor = self.bgColor
},
completion: nil
)
// Menuview
menuView.frame = CGRect(origin: CGPoint(x: 0.0, y: self.view.frame.height), size: menuView.frame.size)
UIView.animateWithDuration(HOKConsts().animationDuration,
delay: 0.0,
usingSpringWithDamping: 0.6,
initialSpringVelocity: 0.6,
options: [.BeginFromCurrentState, .AllowUserInteraction, .OverrideInheritedOptions],
animations: {
self.menuView.frame = CGRect(origin: CGPoint(x: 0.0, y: self.view.frame.height-self.menuView.frame.height), size: self.menuView.frame.size)
self.menuView.layoutIfNeeded()
},
completion: {(finished) in
})
menuView.positionAnimationWillStart()
}
// Dismiss the menuview
public func dismiss() {
// Background and Menuview
UIView.animateWithDuration(HOKConsts().animationDuration,
delay: 0.0,
usingSpringWithDamping: 100.0,
initialSpringVelocity: 0.6,
options: [.BeginFromCurrentState, .AllowUserInteraction, .OverrideInheritedOptions, .CurveEaseOut],
animations: {
self.view.backgroundColor = UIColor.clearColor()
self.menuView.frame = CGRect(origin: CGPoint(x: 0.0, y: self.view.frame.height), size: self.menuView.frame.size)
},
completion: {(finished) in
self.view.removeFromSuperview()
})
menuView.positionAnimationWillStart()
}
// When the buttons are tapped, this method is called.
func buttonTapped(btn:HOKButton) {
if btn.actionType == HOKAcitonType.Closure {
btn.action()
} else if btn.actionType == HOKAcitonType.Selector {
let control = UIControl()
control.sendAction(btn.selector, to:btn.target, forEvent:nil)
} else {
if !btn.isCancelButton {
print("Unknow action type for button")
}
}
dismiss()
}
}
| mit | 6f915994c59271db7818fb5b724242b6 | 34.683857 | 157 | 0.595476 | 4.889401 | false | false | false | false |
PodBuilder/XcodeKit | SwiftXcodeKit/Resources/CompileSourcesBuildPhase.swift | 1 | 2706 | /*
* The sources in the "XcodeKit" directory are based on the Ruby project Xcoder.
*
* Copyright (c) 2012 cisimple
*
* MIT License
*
* Permission is hereby granted, free of charge, to any person obtaining
* a copy of this software and associated documentation files (the
* "Software"), to deal in the Software without restriction, including
* without limitation the rights to use, copy, modify, merge, publish,
* distribute, sublicense, and/or sell copies of the Software, and to
* permit persons to whom the Software is furnished to do so, subject to
* the following conditions:
*
* The above copyright notice and this permission notice shall be
* included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*/
public class CompileSourcesBuildPhase: BuildPhase {
public override class var isaString: String {
get {
return "PBXSourcesBuildPhase"
}
}
public class func create(inout inRegistry registry: ObjectRegistry) -> CompileSourcesBuildPhase {
let properties = [
"isa": isaString,
"buildActionMask": NSNumber(integer: 2147483647),
"files": [AnyObject](),
"runOnlyForDeploymentPreprocessing": "0"
]
let phase = CompileSourcesBuildPhase(identifier: registry.generateOID(targetDescription: nil), inRegistry: registry, properties: properties)
registry.putResource(phase)
return phase
}
public func addBuildFileWithReference(reference: FileReference, useAutomaticReferenceCounting useARC: Bool, buildSettings: [String : AnyObject]?) {
var modifiedSettings = buildSettings
if var dict = modifiedSettings {
var flagsObject: AnyObject? = dict["COMPILE_FLAGS"]
var flagArray = [String]()
if let flags: AnyObject = flagsObject {
if let array = flags as? [String] {
flagArray = array
}
} else {
dict["COMPLE_FLAGS"] = flagArray
}
flagArray.append("-fno-objc-arc")
}
addBuildFileWithReference(reference, buildSettings: modifiedSettings)
}
}
| mit | 7846b5e66d7373459f21db1a109bcd5c | 39.38806 | 151 | 0.665558 | 4.974265 | false | false | false | false |
JPIOS/JDanTang | JDanTang/JDanTang/Class/Tool(工具)/Common/NetworkTool.swift | 1 | 5398 | //
// NetworkTool.swift
// JDanTang
//
// Created by mac_KY on 2017/6/14.
// Copyright © 2017年 mac_KY. All rights reserved.
//
import UIKit
import Alamofire
import Foundation
import SwiftyJSON
import SVProgressHUD
class NetworkTool: NSObject {
/// 单利
static let shareNetworkTool = NetworkTool()
}
extension NetworkTool {
func getRequest(urlString: String, params: [String: Any], success: @escaping (_ response : JSON)-> (),failture: @escaping (_ error: Error)-> ()) {
let NewUrlString:String = BASE_URL+urlString
print("NewUrlString == " + NewUrlString)
SVProgressHUD.show(withStatus: "正在加载...")
Alamofire.request(NewUrlString, method: .get, parameters: params)
.responseJSON { (response) in
SVProgressHUD.dismiss()
guard response.result.isSuccess else {
SVProgressHUD.showError(withStatus: "加载失败...")
return
}
if let value = response.result.value {
let dict = JSON(value)
let code = dict["code"].intValue
let message = dict["message"].stringValue
guard code == RETURN_OK else {
SVProgressHUD.showInfo(withStatus: message)
return
}
// let data = dict["data"].dictionary
success(dict)
}
/* /*这里使用了闭包*/
//当请求后response是我们自定义的,这个变量用于接受服务器响应的信息
//使用switch判断请求是否成功,也就是response的result
switch response.result {
case .success(let value):
//当响应成功是,使用临时变量value接受服务器返回的信息并判断是否为[String: AnyObject]类型 如果是那么将其传给其定义方法中的success
//if let value = response.result.value as? [String: AnyObject] {
// success(value as! [String : AnyObject])
// }
let json = JSON(value)
print(json)
success(value as! [String : AnyObject])
case .failure(let error):
failture(error)
print("error:\(error)")*/
}
}
}
//MARK: - POST 请求
func postRequest(urlString : String, params : [String : Any], success : @escaping (_ response : [String : AnyObject])->(), failture : @escaping (_ error : Error)->()) {
Alamofire.request(urlString, method: HTTPMethod.post, parameters: params).responseJSON { (response) in
switch response.result{
case .success:
if let value = response.result.value as? [String: AnyObject] {
success(value)
let json = JSON(value)
print(json)
}
case .failure(let error):
failture(error)
print("error:\(error)")
}
}
}
//MARK: - 照片上传
///
/// - Parameters:
/// - urlString: 服务器地址
/// - params: ["flag":"","userId":""] - flag,userId 为必传参数
/// flag - 666 信息上传多张 -999 服务单上传 -000 头像上传
/// - data: image转换成Data
/// - name: fileName
/// - success:
/// - failture:
func upLoadImageRequest(urlString : String, params:[String:String], data: [Data], name: [String],success : @escaping (_ response : [String : AnyObject])->(), failture : @escaping (_ error : Error)->()){
let headers = ["content-type":"multipart/form-data"]
Alamofire.upload(
multipartFormData: { multipartFormData in
//666多张图片上传
let flag = params["flag"]
let userId = params["userId"]
multipartFormData.append((flag?.data(using: String.Encoding.utf8)!)!, withName: "flag")
multipartFormData.append( (userId?.data(using: String.Encoding.utf8)!)!, withName: "userId")
for i in 0..<data.count {
multipartFormData.append(data[i], withName: "appPhoto", fileName: name[i], mimeType: "image/png")
}
},
to: urlString,
headers: headers,
encodingCompletion: { encodingResult in
switch encodingResult {
case .success(let upload, _, _):
upload.responseJSON { response in
if let value = response.result.value as? [String: AnyObject]{
success(value)
let json = JSON(value)
print(json)
}
}
case .failure(let encodingError):
print(encodingError)
failture(encodingError)
}
}
)
}
| apache-2.0 | 34b86968540971daf3c590bf6aefe3d0 | 32.532895 | 206 | 0.478909 | 5.148485 | false | false | false | false |
fhchina/firefox-ios | Client/Frontend/Browser/LoginsHelper.swift | 1 | 13640 | /* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/. */
import Foundation
import Shared
import Storage
import XCGLogger
import WebKit
private let log = Logger.browserLogger
private let SaveButtonTitle = NSLocalizedString("Save", comment: "Button to save the user's password")
private let NotNowButtonTitle = NSLocalizedString("Not now", comment: "Button to not save the user's password")
private let UpdateButtonTitle = NSLocalizedString("Update", comment: "Button to update the user's password")
private let CancelButtonTitle = NSLocalizedString("Cancel", comment: "Authentication prompt cancel button")
private let LogInButtonTitle = NSLocalizedString("Log in", comment: "Authentication prompt log in button")
class LoginsHelper: BrowserHelper {
private weak var browser: Browser?
private let profile: Profile
private var snackBar: SnackBar?
private static let MaxAuthenticationAttempts = 3
class func name() -> String {
return "LoginsHelper"
}
required init(browser: Browser, profile: Profile) {
self.browser = browser
self.profile = profile
if let path = NSBundle.mainBundle().pathForResource("LoginsHelper", ofType: "js") {
if let source = NSString(contentsOfFile: path, encoding: NSUTF8StringEncoding, error: nil) as? String {
var userScript = WKUserScript(source: source, injectionTime: WKUserScriptInjectionTime.AtDocumentEnd, forMainFrameOnly: true)
browser.webView!.configuration.userContentController.addUserScript(userScript)
}
}
}
func scriptMessageHandlerName() -> String? {
return "loginsManagerMessageHandler"
}
func userContentController(userContentController: WKUserContentController, didReceiveScriptMessage message: WKScriptMessage) {
var res = message.body as! [String: String]
let type = res["type"]
if let url = browser?.url {
if type == "request" {
res["username"] = ""
res["password"] = ""
let login = Login.fromScript(url, script: res)
requestLogins(login, requestId: res["requestId"]!)
} else if type == "submit" {
setCredentials(Login.fromScript(url, script: res))
}
}
}
class func replace(base: String, keys: [String], replacements: [String]) -> NSMutableAttributedString {
var ranges = [NSRange]()
var string = base
for (index, key) in enumerate(keys) {
let replace = replacements[index]
let range = string.rangeOfString(key,
options: NSStringCompareOptions.LiteralSearch,
range: nil,
locale: nil)!
string.replaceRange(range, with: replace)
let nsRange = NSMakeRange(distance(string.startIndex, range.startIndex),
count(replace))
ranges.append(nsRange)
}
var attributes = [NSObject: AnyObject]()
attributes[NSFontAttributeName] = UIFont.systemFontOfSize(13, weight: UIFontWeightRegular)
attributes[NSForegroundColorAttributeName] = UIColor.darkGrayColor()
var attr = NSMutableAttributedString(string: string, attributes: attributes)
for (index, range) in enumerate(ranges) {
attr.addAttribute(NSFontAttributeName, value: UIFont.systemFontOfSize(13, weight: UIFontWeightMedium), range: range)
}
return attr
}
private func setCredentials(login: LoginData) {
if login.password.isEmpty {
log.debug("Empty password")
return
}
profile.logins
.getLoginsForProtectionSpace(login.protectionSpace, withUsername: login.username)
.uponQueue(dispatch_get_main_queue()) { res in
if let data = res.successValue {
log.debug("Found \(data.count) logins.")
for saved in data {
if let saved = saved {
if saved.password == login.password {
self.profile.logins.addUseOfLoginByGUID(saved.guid)
return
}
self.promptUpdateFromLogin(login: saved, toLogin: login)
return
}
}
}
self.promptSave(login)
}
}
private func promptSave(login: LoginData) {
let promptMessage: NSAttributedString
if let username = login.username {
let promptStringFormat = NSLocalizedString("Do you want to save the password for %@ on %@?", comment: "Prompt for saving a password. The first parameter is the username being saved. The second parameter is the hostname of the site.")
promptMessage = NSAttributedString(string: String(format: promptStringFormat, username, login.hostname))
} else {
let promptStringFormat = NSLocalizedString("Do you want to save the password on %@?", comment: "Prompt for saving a password with no username. The parameter is the hostname of the site.")
promptMessage = NSAttributedString(string: String(format: promptStringFormat, login.hostname))
}
if snackBar != nil {
browser?.removeSnackbar(snackBar!)
}
snackBar = TimerSnackBar(attrText: promptMessage,
img: UIImage(named: "lock_verified"),
buttons: [
SnackButton(title: SaveButtonTitle, callback: { (bar: SnackBar) -> Void in
self.browser?.removeSnackbar(bar)
self.snackBar = nil
self.profile.logins.addLogin(login)
}),
SnackButton(title: NotNowButtonTitle, callback: { (bar: SnackBar) -> Void in
self.browser?.removeSnackbar(bar)
self.snackBar = nil
return
})
])
browser?.addSnackbar(snackBar!)
}
private func promptUpdateFromLogin(login old: LoginData, toLogin new: LoginData) {
let guid = old.guid
let formatted: String
if let username = new.username {
let promptStringFormat = NSLocalizedString("Do you want to update the password for %@ on %@?", comment: "Prompt for updating a password. The first parameter is the username being saved. The second parameter is the hostname of the site.")
formatted = String(format: promptStringFormat, username, new.hostname)
} else {
let promptStringFormat = NSLocalizedString("Do you want to update the password on %@?", comment: "Prompt for updating a password with on username. The parameter is the hostname of the site.")
formatted = String(format: promptStringFormat, new.hostname)
}
let promptMessage = NSAttributedString(string: formatted)
if snackBar != nil {
browser?.removeSnackbar(snackBar!)
}
snackBar = TimerSnackBar(attrText: promptMessage,
img: UIImage(named: "lock_verified"),
buttons: [
SnackButton(title: UpdateButtonTitle, callback: { (bar: SnackBar) -> Void in
self.browser?.removeSnackbar(bar)
self.snackBar = nil
self.profile.logins.updateLoginByGUID(guid, new: new,
significant: new.isSignificantlyDifferentFrom(old))
}),
SnackButton(title: NotNowButtonTitle, callback: { (bar: SnackBar) -> Void in
self.browser?.removeSnackbar(bar)
self.snackBar = nil
return
})
])
browser?.addSnackbar(snackBar!)
}
private func requestLogins(login: LoginData, requestId: String) {
profile.logins.getLoginsForProtectionSpace(login.protectionSpace).uponQueue(dispatch_get_main_queue()) { res in
var jsonObj = [String: AnyObject]()
if let cursor = res.successValue {
log.debug("Found \(cursor.count) logins.")
jsonObj["requestId"] = requestId
jsonObj["name"] = "RemoteLogins:loginsFound"
jsonObj["logins"] = map(cursor, { $0!.toDict() })
}
let json = JSON(jsonObj)
let src = "window.__firefox__.logins.inject(\(json.toString()))"
self.browser?.webView?.evaluateJavaScript(src, completionHandler: { (obj, err) -> Void in
})
}
}
func handleAuthRequest(viewController: UIViewController, challenge: NSURLAuthenticationChallenge) -> Deferred<Result<LoginData>> {
// If there have already been too many login attempts, we'll just fail.
if challenge.previousFailureCount >= LoginsHelper.MaxAuthenticationAttempts {
return deferResult(LoginDataError(description: "Too many attempts to open site"))
}
var credential = challenge.proposedCredential
// If we were passed an initial set of credentials from iOS, try and use them.
if let proposed = credential {
if !(proposed.user?.isEmpty ?? true) {
if challenge.previousFailureCount == 0 {
return deferResult(Login.createWithCredential(credential!, protectionSpace: challenge.protectionSpace))
}
} else {
credential = nil
}
}
if let credential = credential {
// If we have some credentials, we'll show a prompt with them.
return promptForUsernamePassword(viewController, credentials: credential, protectionSpace: challenge.protectionSpace)
}
// Otherwise, try to look one up
let options = QueryOptions(filter: challenge.protectionSpace.host, filterType: .None, sort: .None)
return profile.logins.getLoginsForProtectionSpace(challenge.protectionSpace).bindQueue(dispatch_get_main_queue()) { res in
let credentials = res.successValue?[0]?.credentials
return self.promptForUsernamePassword(viewController, credentials: credentials, protectionSpace: challenge.protectionSpace)
}
}
private func promptForUsernamePassword(viewController: UIViewController, credentials: NSURLCredential?, protectionSpace: NSURLProtectionSpace) -> Deferred<Result<LoginData>> {
if protectionSpace.host.isEmpty {
println("Unable to show a password prompt without a hostname")
return deferResult(LoginDataError(description: "Unable to show a password prompt without a hostname"))
}
let deferred = Deferred<Result<LoginData>>()
let alert: UIAlertController
let title = NSLocalizedString("Authentication required", comment: "Authentication prompt title")
if !(protectionSpace.realm?.isEmpty ?? true) {
let msg = NSLocalizedString("A username and password are being requested by %@. The site says: %@", comment: "Authentication prompt message with a realm. First parameter is the hostname. Second is the realm string")
let formatted = NSString(format: msg, protectionSpace.host, protectionSpace.realm ?? "") as String
alert = UIAlertController(title: title, message: formatted, preferredStyle: UIAlertControllerStyle.Alert)
} else {
let msg = NSLocalizedString("A username and password are being requested by %@.", comment: "Authentication prompt message with no realm. Parameter is the hostname of the site")
let formatted = NSString(format: msg, protectionSpace.host) as String
alert = UIAlertController(title: title, message: formatted, preferredStyle: UIAlertControllerStyle.Alert)
}
// Add a button to log in.
let action = UIAlertAction(title: LogInButtonTitle,
style: UIAlertActionStyle.Default) { (action) -> Void in
let user = (alert.textFields?[0] as! UITextField).text
let pass = (alert.textFields?[1] as! UITextField).text
let login = Login.createWithCredential(NSURLCredential(user: user, password: pass, persistence: .ForSession), protectionSpace: protectionSpace)
deferred.fill(Result(success: login))
self.setCredentials(login)
}
alert.addAction(action)
// Add a cancel button.
let cancel = UIAlertAction(title: CancelButtonTitle, style: UIAlertActionStyle.Cancel) { (action) -> Void in
deferred.fill(Result(failure: LoginDataError(description: "Save password cancelled")))
}
alert.addAction(cancel)
// Add a username textfield.
alert.addTextFieldWithConfigurationHandler { (textfield) -> Void in
textfield.placeholder = NSLocalizedString("Username", comment: "Username textbox in Authentication prompt")
textfield.text = credentials?.user
}
// Add a password textfield.
alert.addTextFieldWithConfigurationHandler { (textfield) -> Void in
textfield.placeholder = NSLocalizedString("Password", comment: "Password textbox in Authentication prompt")
textfield.secureTextEntry = true
textfield.text = credentials?.password
}
viewController.presentViewController(alert, animated: true) { () -> Void in }
return deferred
}
}
| mpl-2.0 | 61ba256ab16c3106bbc923724c714b59 | 47.19788 | 249 | 0.631232 | 5.504439 | false | false | false | false |
exshin/PokePuzzler | PokePuzzler/Level.swift | 1 | 20391 | //
// Level.swift
// PokePuzzler
//
// Created by Eugene Chinveeraphan on 13/04/16.
// Copyright © 2016 Eugene Chinveeraphan. All rights reserved.
//
import Foundation
let NumColumns = 8
let NumRows = 8
let NumLevels = 1 // Excluding Level_0.json
class Level {
// MARK: Properties
// The 2D array that keeps track of where the Cookies are.
var cookies = Array2D<Cookie>(columns: NumColumns, rows: NumRows)
// The 2D array that contains the layout of the level.
fileprivate var tiles = Array2D<Tile>(columns: NumColumns, rows: NumRows)
// The list of swipes that result in a valid swap. Used to determine whether
// the player can make a certain swap, whether the board needs to be shuffled,
// and to generate hints.
var possibleSwaps = Set<Swap>()
var targetScore = 0
var maximumMoves = 0
var energySet: Array<String> = []
// MARK: Initialization
// Create a level by loading it from a file.
init(filename: String) {
guard let dictionary = Dictionary<String, AnyObject>.loadJSONFromBundle(filename: filename) else { return }
// The dictionary contains an array named "tiles". This array contains
// one element for each row of the level. Each of those row elements in
// turn is also an array describing the columns in that row. If a column
// is 1, it means there is a tile at that location, 0 means there is not.
guard let tilesArray = dictionary["tiles"] as? [[Int]] else { return }
// Loop through the rows...
for (row, rowArray) in tilesArray.enumerated() {
// Note: In Sprite Kit (0,0) is at the bottom of the screen,
// so we need to read this file upside down.
let tileRow = NumRows - row - 1
// Loop through the columns in the current r
for (column, value) in rowArray.enumerated() {
// If the value is 1, create a tile object.
if value == 1 {
tiles[column, tileRow] = Tile()
}
}
}
targetScore = dictionary["targetScore"] as! Int
maximumMoves = dictionary["moves"] as! Int
}
// MARK: Level Setup
// Fills up the level with new Cookie objects. The level is guaranteed free
// from matches at this point.
// Returns a set containing all the new Cookie objects.
func shuffle(energySet: Array<String>) -> Set<Cookie> {
var set: Set<Cookie>
repeat {
// Removes the old cookies and fills up the level with all new ones.
set = createInitialCookies(energySet: energySet)
self.energySet = energySet
// At the start of each turn we need to detect which cookies the player can
// actually swap. If the player tries to swap two cookies that are not in
// this set, then the game does not accept this as a valid move.
// This also tells you whether no more swaps are possible and the game needs
// to automatically reshuffle.
detectPossibleSwaps()
// print("possible swaps: \(possibleSwaps)")
// If there are no possible moves, then keep trying again until there are.
} while possibleSwaps.count == 0
return set
}
fileprivate func createInitialCookies(energySet: Array<String>) -> Set<Cookie> {
var set = Set<Cookie>()
// Loop through the rows and columns of the 2D array. Note that column 0,
// row 0 is in the bottom-left corner of the array.
for row in 0..<NumRows {
for column in 0..<NumColumns {
// Only make a new cookie if there is a tile at this spot.
if tiles[column, row] != nil {
// Pick the cookie type at random, and make sure that this never
// creates a chain of 3 or more. We want there to be 0 matches in
// the initial state.
var cookieType: CookieType
repeat {
cookieType = CookieType.random()
} while
// Only allow cookies based on the pokemon in play
!energySet.contains(cookieType.description) || (
(column >= 2 &&
cookies[column - 1, row]?.cookieType == cookieType &&
cookies[column - 2, row]?.cookieType == cookieType) ||
(row >= 2 &&
cookies[column, row - 1]?.cookieType == cookieType &&
cookies[column, row - 2]?.cookieType == cookieType))
// Create a new cookie and add it to the 2D array.
let cookie = Cookie(column: column, row: row, cookieType: cookieType)
cookies[column, row] = cookie
// Also add the cookie to the set so we can tell our caller about it.
set.insert(cookie)
}
}
}
return set
}
// MARK: Query the level
// Determines whether there's a tile at the specified column and row.
func tileAt(column: Int, row: Int) -> Tile? {
assert(column >= 0 && column < NumColumns)
assert(row >= 0 && row < NumRows)
return tiles[column, row]
}
// Returns the cookie at the specified column and row, or nil when there is none.
func cookieAt(column: Int, row: Int) -> Cookie? {
assert(column >= 0 && column < NumColumns)
assert(row >= 0 && row < NumRows)
return cookies[column, row]
}
// Determines whether the suggested swap is a valid one, i.e. it results in at
// least one new chain of 3 or more cookies of the same type.
func isPossibleSwap(_ swap: Swap) -> Bool {
return possibleSwaps.contains(swap)
}
fileprivate func hasChainAt(column: Int, row: Int) -> Bool {
// Here we do ! because we know there is a cookie here
let cookieType = cookies[column, row]!.cookieType
// Horizontal chain check
var horzLength = 1
// Left
var i = column - 1
// Here we do ? because there may be no cookie there; if there isn't then
// the loop will terminate because it is != cookieType. (So there is no
// need to check whether cookies[i, row] != nil.)
while i >= 0 && cookies[i, row]?.cookieType == cookieType {
i -= 1
horzLength += 1
}
// Right
i = column + 1
while i < NumColumns && cookies[i, row]?.cookieType == cookieType {
i += 1
horzLength += 1
}
if horzLength >= 3 { return true }
// Vertical chain check
var vertLength = 1
// Down
i = row - 1
while i >= 0 && cookies[column, i]?.cookieType == cookieType {
i -= 1
vertLength += 1
}
// Up
i = row + 1
while i < NumRows && cookies[column, i]?.cookieType == cookieType {
i += 1
vertLength += 1
}
return vertLength >= 3
}
// MARK: Swapping
// Swaps the positions of the two cookies from the Swap object.
func performSwap(_ swap: Swap) {
// Need to make temporary copies of these because they get overwritten.
let columnA = swap.cookieA.column
let rowA = swap.cookieA.row
let columnB = swap.cookieB.column
let rowB = swap.cookieB.row
// Swap the cookies. We need to update the array as well as the column
// and row properties of the Cookie objects, or they go out of sync!
cookies[columnA, rowA] = swap.cookieB
swap.cookieB.column = columnA
swap.cookieB.row = rowA
cookies[columnB, rowB] = swap.cookieA
swap.cookieA.column = columnB
swap.cookieA.row = rowB
}
// Recalculates which moves are valid.
func detectPossibleSwaps() {
var set = Set<Swap>()
for row in 0..<NumRows {
for column in 0..<NumColumns {
if let cookie = cookies[column, row] {
// Is it possible to swap this cookie with the one on the right?
// Note: don't need to check the last column.
if column < NumColumns - 1 {
// Have a cookie in this spot? If there is no tile, there is no cookie.
if let other = cookies[column + 1, row] {
// Swap them
cookies[column, row] = other
cookies[column + 1, row] = cookie
// Is either cookie now part of a chain?
if hasChainAt(column: column + 1, row: row) ||
hasChainAt(column: column, row: row) {
set.insert(Swap(cookieA: cookie, cookieB: other))
}
// Swap them back
cookies[column, row] = cookie
cookies[column + 1, row] = other
}
}
// Is it possible to swap this cookie with the one above?
// Note: don't need to check the last row.
if row < NumRows - 1 {
// Have a cookie in this spot? If there is no tile, there is no cookie.
if let other = cookies[column, row + 1] {
// Swap them
cookies[column, row] = other
cookies[column, row + 1] = cookie
// Is either cookie now part of a chain?
if hasChainAt(column: column, row: row + 1) ||
hasChainAt(column: column, row: row) {
set.insert(Swap(cookieA: cookie, cookieB: other))
}
// Swap them back
cookies[column, row] = cookie
cookies[column, row + 1] = other
}
}
}
}
}
possibleSwaps = set
}
fileprivate func calculateScores(for chains: Set<Chain>) {
// 3-chain is 3 pts, 4-chain is 4, 5-chain is 5, and so on
for chain in chains {
let chainType: String = chain.chainType.description
if chainType == "Horizontal" || chainType == "Vertical" {
chain.score = chain.length
} else {
chain.score = chain.length - 1
}
}
}
// MARK: Detecting Matches
fileprivate func detectHorizontalMatches() -> Set<Chain> {
// Contains the Cookie objects that were part of a horizontal chain.
// These cookies must be removed.
var set = Set<Chain>()
for row in 0..<NumRows {
// Don't need to look at last two columns.
var column = 0
while column < NumColumns-2 {
// If there is a cookie/tile at this position...
if let cookie = cookies[column, row] {
let matchType = cookie.cookieType
// And the next two columns have the same type...
if cookies[column + 1, row]?.cookieType == matchType &&
cookies[column + 2, row]?.cookieType == matchType {
// ...then add all the cookies from this chain into the set.
let chain = Chain(chainType: .horizontal)
repeat {
chain.add(cookie: cookies[column, row]!)
column += 1
} while column < NumColumns && cookies[column, row]?.cookieType == matchType
set.insert(chain)
continue
}
}
// Cookie did not match or empty tile, so skip over it.
column += 1
}
}
return set
}
// Same as the horizontal version but steps through the array differently.
fileprivate func detectVerticalMatches() -> Set<Chain> {
var set = Set<Chain>()
for column in 0..<NumColumns {
var row = 0
while row < NumRows-2 {
if let cookie = cookies[column, row] {
let matchType = cookie.cookieType
if cookies[column, row + 1]?.cookieType == matchType &&
cookies[column, row + 2]?.cookieType == matchType {
let chain = Chain(chainType: .vertical)
repeat {
chain.add(cookie: cookies[column, row]!)
row += 1
} while row < NumRows && cookies[column, row]?.cookieType == matchType
set.insert(chain)
continue
}
}
row += 1
}
}
return set
}
// Detects whether there are any chains of 3 or more cookies, and removes
// them from the level.
// Returns a set containing Chain objects, which describe the Cookies
// that were removed.
func removeMatches() -> Set<Chain> {
let horizontalChains = detectHorizontalMatches()
let verticalChains = detectVerticalMatches()
let specialChains = detectSpecialMatches(horizontalChains: horizontalChains, verticalChains: verticalChains)
// Note: to detect more advanced patterns such as an L shape, you can see
// whether a cookie is in both the horizontal & vertical chains sets and
// whether it is the first or last in the array (at a corner). Then you
// create a new Chain object with the new type and remove the other two.
removeCookies(horizontalChains)
removeCookies(verticalChains)
if specialChains.count == 0 {
calculateScores(for: horizontalChains)
calculateScores(for: verticalChains)
return horizontalChains.union(verticalChains)
} else {
calculateScores(for: specialChains)
return specialChains
}
}
fileprivate func detectSpecialMatches(horizontalChains: Set<Chain>, verticalChains: Set<Chain>) -> Set<Chain> {
var specialChains = Set<Chain>()
var hcookies: Array<Cookie> = []
var vcookies: Array<Cookie> = []
for chains in verticalChains {
for cookie in chains.cookies {
vcookies.append(cookie)
}
}
for chains in horizontalChains {
for cookie in chains.cookies {
hcookies.append(cookie)
}
}
let vcookieSet: Set<Cookie> = Set(vcookies)
let hcookieSet: Set<Cookie> = Set(hcookies)
for cookie in vcookieSet.intersection(hcookieSet) {
// Check each horizontal chain
for hchain in horizontalChains {
if cookie == hchain.firstCookie() || cookie == hchain.lastCookie() {
for vchain in verticalChains {
if cookie == vchain.firstCookie() || cookie == hchain.lastCookie() {
// L Shape Chain
let chain = Chain(chainType: .lshape)
// Add cookies from horizontal chain
for hchainCookie in hchain.cookies {
chain.add(cookie: hchainCookie)
}
// Add cookies from vertical chain
for vchainCookie in vchain.cookies {
chain.add(cookie: vchainCookie)
}
specialChains.insert(chain)
break
} else if vchain.containsCookie(cookie: cookie) {
// T Shape Cookie (T)
let chain = Chain(chainType: .tshape)
// Add cookies from horizontal chain
for hchainCookie in hchain.cookies {
chain.add(cookie: hchainCookie)
}
// Add cookies from vertical chain
for vchainCookie in vchain.cookies {
chain.add(cookie: vchainCookie)
}
specialChains.insert(chain)
break
}
}
hchain.chainType = .lshape
} else if hchain.containsCookie(cookie: cookie) {
// T Shape on the middle horizontal cookie
for vchain in verticalChains {
if cookie == vchain.firstCookie() || cookie == hchain.lastCookie() {
// T Shape Cookie (T)
let chain = Chain(chainType: .tshape)
// Add cookies from horizontal chain
for hchainCookie in hchain.cookies {
chain.add(cookie: hchainCookie)
}
// Add cookies from vertical chain
for vchainCookie in vchain.cookies {
chain.add(cookie: vchainCookie)
}
specialChains.insert(chain)
break
} else if vchain.containsCookie(cookie: cookie) {
// T Shape (+)
let chain = Chain(chainType: .tshape)
// Add cookies from horizontal chain
for hchainCookie in hchain.cookies {
chain.add(cookie: hchainCookie)
}
// Add cookies from vertical chain
for vchainCookie in vchain.cookies {
chain.add(cookie: vchainCookie)
}
specialChains.insert(chain)
break
}
}
}
}
}
return specialChains
}
fileprivate func removeCookies(_ chains: Set<Chain>) {
for chain in chains {
for cookie in chain.cookies {
if cookies[cookie.column, cookie.row] != nil {
cookies[cookie.column, cookie.row] = nil
}
}
}
}
func removeCookie(_ cookie: Cookie) {
cookies[cookie.column, cookie.row] = nil
}
// MARK: Detecting Holes
// Detects where there are holes and shifts any cookies down to fill up those
// holes. In effect, this "bubbles" the holes up to the top of the column.
// Returns an array that contains a sub-array for each column that had holes,
// with the Cookie objects that have shifted. Those cookies are already
// moved to their new position. The objects are ordered from the bottom up.
func fillHoles() -> [[Cookie]] {
var columns = [[Cookie]]() // you can also write this Array<Array<Cookie>>
// Loop through the rows, from bottom to top. It's handy that our row 0 is
// at the bottom already. Because we're scanning from bottom to top, this
// automatically causes an entire stack to fall down to fill up a hole.
// We scan one column at a time.
for column in 0..<NumColumns {
var array = [Cookie]()
for row in 0..<NumRows {
// If there is a tile at this position but no cookie, then there's a hole.
if tiles[column, row] != nil && cookies[column, row] == nil {
// Scan upward to find a cookie.
for lookup in (row + 1)..<NumRows {
if let cookie = cookies[column, lookup] {
// Swap that cookie with the hole.
cookies[column, lookup] = nil
cookies[column, row] = cookie
cookie.row = row
// For each column, we return an array with the cookies that have
// fallen down. Cookies that are lower on the screen are first in
// the array. We need an array to keep this order intact, so the
// animation code can apply the correct kind of delay.
array.append(cookie)
// Don't need to scan up any further.
break
}
}
}
}
if !array.isEmpty {
columns.append(array)
}
}
return columns
}
// Where necessary, adds new cookies to fill up the holes at the top of the
// columns.
// Returns an array that contains a sub-array for each column that had holes,
// with the new Cookie objects. Cookies are ordered from the top down.
func topUpCookies() -> [[Cookie]] {
var columns = [[Cookie]]()
var cookieType: CookieType = .unknown
// Detect where we have to add the new cookies. If a column has X holes,
// then it also needs X new cookies. The holes are all on the top of the
// column now, but the fact that there may be gaps in the tiles makes this
// a little trickier.
for column in 0..<NumColumns {
var array = [Cookie]()
// This time scan from top to bottom. We can end when we've found the
// first cookie.
var row = NumRows - 1
while row >= 0 && cookies[column, row] == nil {
// Found a hole?
if tiles[column, row] != nil {
// Randomly create a new cookie type. The only restriction is that
// it cannot be equal to the previous type. This prevents too many
// "freebie" matches.
var newCookieType: CookieType
repeat {
newCookieType = CookieType.random()
} while newCookieType == cookieType || !energySet.contains(newCookieType.description)
cookieType = newCookieType
// Create a new cookie and add it to the array for this column.
let cookie = Cookie(column: column, row: row, cookieType: cookieType)
cookies[column, row] = cookie
array.append(cookie)
}
row -= 1
}
if !array.isEmpty {
columns.append(array)
}
}
return columns
}
}
| apache-2.0 | 006e6e900b1e781a468cd252bc71bdfe | 33.795222 | 113 | 0.581118 | 4.621487 | false | false | false | false |
JohnEstropia/CoreStore | Sources/ObjectProxy.swift | 1 | 8333 | //
// ObjectProxy.swift
// CoreStore
//
// Copyright © 2020 John Rommel Estropia
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
//
import CoreData
import Foundation
// MARK: - ObjectProxy
/**
An `ObjectProxy` is only used when overriding getters and setters for `CoreStoreObject` properties. Custom getters and setters are implemented as a closure that "overrides" the default property getter/setter. The closure receives an `ObjectProxy<O>`, which acts as a fast, type-safe KVC interface for `CoreStoreObject`. The reason a `CoreStoreObject` instance is not passed directly is because the Core Data runtime is not aware of `CoreStoreObject` properties' static typing, and so loading those info every time KVO invokes this accessor method incurs a heavy performance hit (especially in KVO-heavy operations such as `ListMonitor` observing.) When accessing the property value from `ObjectProxy<O>`, make sure to use `ObjectProxy<O>.$property.primitiveValue` instead of `ObjectProxy<O>.$property.value`, which would execute the same accessor again recursively.
*/
@dynamicMemberLookup
public struct ObjectProxy<O: CoreStoreObject> {
/**
Returns the value for the property identified by a given key.
*/
public subscript<OBase, V>(dynamicMember member: KeyPath<O, FieldContainer<OBase>.Stored<V>>) -> FieldProxy<V> {
return .init(rawObject: self.rawObject, keyPath: member)
}
/**
Returns the value for the property identified by a given key.
*/
public subscript<OBase, V>(dynamicMember member: KeyPath<O, FieldContainer<OBase>.Virtual<V>>) -> FieldProxy<V> {
return .init(rawObject: self.rawObject, keyPath: member)
}
/**
Returns the value for the property identified by a given key.
*/
public subscript<OBase, V>(dynamicMember member: KeyPath<O, FieldContainer<OBase>.Coded<V>>) -> FieldProxy<V> {
return .init(rawObject: self.rawObject, keyPath: member)
}
// MARK: Internal
internal let rawObject: CoreStoreManagedObject
internal init(_ rawObject: CoreStoreManagedObject) {
self.rawObject = rawObject
}
// MARK: - FieldProxy
public struct FieldProxy<V> {
// MARK: Public
/**
Returns the value for the specified property from the object’s private internal storage.
This method is used primarily to implement custom accessor methods that need direct access to the object's private storage.
*/
public var primitiveValue: V? {
get {
return self.getPrimitiveValue()
}
nonmutating set {
self.setPrimitiveValue(newValue)
}
}
/**
Returns the value for the property identified by a given key.
*/
public var value: V {
get {
return self.getValue()
}
nonmutating set {
self.setValue(newValue)
}
}
// MARK: Internal
internal init<OBase>(rawObject: CoreStoreManagedObject, keyPath: KeyPath<O, FieldContainer<OBase>.Stored<V>>) {
self.init(rawObject: rawObject, field: O.meta[keyPath: keyPath])
}
internal init<OBase>(rawObject: CoreStoreManagedObject, keyPath: KeyPath<O, FieldContainer<OBase>.Virtual<V>>) {
self.init(rawObject: rawObject, field: O.meta[keyPath: keyPath])
}
internal init<OBase>(rawObject: CoreStoreManagedObject, keyPath: KeyPath<O, FieldContainer<OBase>.Coded<V>>) {
self.init(rawObject: rawObject, field: O.meta[keyPath: keyPath])
}
internal init<OBase>(rawObject: CoreStoreManagedObject, field: FieldContainer<OBase>.Stored<V>) {
let keyPathString = field.keyPath
self.getValue = {
return type(of: field).read(field: field, for: rawObject) as! V
}
self.setValue = {
type(of: field).modify(field: field, for: rawObject, newValue: $0)
}
self.getPrimitiveValue = {
return V.cs_fromFieldStoredNativeType(
rawObject.primitiveValue(forKey: keyPathString) as! V.FieldStoredNativeType
)
}
self.setPrimitiveValue = {
rawObject.willChangeValue(forKey: keyPathString)
defer {
rawObject.didChangeValue(forKey: keyPathString)
}
rawObject.setPrimitiveValue(
$0.cs_toFieldStoredNativeType(),
forKey: keyPathString
)
}
}
internal init<OBase>(rawObject: CoreStoreManagedObject, field: FieldContainer<OBase>.Virtual<V>) {
let keyPathString = field.keyPath
self.getValue = {
return type(of: field).read(field: field, for: rawObject) as! V
}
self.setValue = {
type(of: field).modify(field: field, for: rawObject, newValue: $0)
}
self.getPrimitiveValue = {
switch rawObject.primitiveValue(forKey: keyPathString) {
case let value as V:
return value
case nil,
is NSNull,
_? /* any other unrelated type */ :
return nil
}
}
self.setPrimitiveValue = {
rawObject.setPrimitiveValue(
$0,
forKey: keyPathString
)
}
}
internal init<OBase>(rawObject: CoreStoreManagedObject, field: FieldContainer<OBase>.Coded<V>) {
let keyPathString = field.keyPath
self.getValue = {
return type(of: field).read(field: field, for: rawObject) as! V
}
self.setValue = {
type(of: field).modify(field: field, for: rawObject, newValue: $0)
}
self.getPrimitiveValue = {
switch rawObject.primitiveValue(forKey: keyPathString) {
case let valueBox as Internals.AnyFieldCoder.TransformableDefaultValueCodingBox:
rawObject.setPrimitiveValue(valueBox.value, forKey: keyPathString)
return valueBox.value as? V
case let value as V:
return value
case nil,
is NSNull,
_? /* any other unrelated type */ :
return nil
}
}
self.setPrimitiveValue = {
rawObject.willChangeValue(forKey: keyPathString)
defer {
rawObject.didChangeValue(forKey: keyPathString)
}
rawObject.setPrimitiveValue(
$0,
forKey: keyPathString
)
}
}
// MARK: Private
private let getValue: () -> V
private let setValue: (V) -> Void
private let getPrimitiveValue: () -> V?
private let setPrimitiveValue: (V?) -> Void
}
}
| mit | ce523d7584c4cd61ff5ee058497e2dad | 33.279835 | 866 | 0.595678 | 5.190031 | false | false | false | false |
ryuichis/swift-lint | Sources/Lint/Rule/RedundantIfStatementRule.swift | 2 | 3305 | /*
Copyright 2017, 2019 Ryuichi Laboratories and the Yanagiba project contributors
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
import Foundation
import AST
class RedundantIfStatementRule: RuleBase, ASTVisitorRule {
let name = "Redundant If Statement"
var description: String? {
return """
This rule detects three types of redundant if statements:
- then-block and else-block are returning true/false or false/true respectively;
- then-block and else-block are the same constant;
- then-block and else-block are the same variable expression.
They are usually introduced by mistake, and should be simplified or removed.
"""
}
var examples: [String]? {
return [
"""
if a == b {
return true
} else {
return false
}
// return a == b
""",
"""
if a == b {
return false
} else {
return true
}
// return a != b
""",
"""
if a == b {
return true
} else {
return true
}
// return true
""",
"""
if a == b {
return foo
} else {
return foo
}
// return foo
""",
]
}
let category = Issue.Category.badPractice
private func emitIssue(_ ifStmt: IfStatement, _ suggestion: String) {
emitIssue(
ifStmt.sourceRange,
description: "if statement is redundant and can be \(suggestion)")
}
func visit(_ ifStmt: IfStatement) throws -> Bool {
guard ifStmt.areAllConditionsExpressions,
let (thenStmt, elseStmt) = ifStmt.thenElseStmts
else {
return true
}
// check then and else block each has one return statement that has expression
guard let thenReturn = thenStmt as? ReturnStatement,
let elseReturn = elseStmt as? ReturnStatement,
let thenExpr = thenReturn.expression,
let elseExpr = elseReturn.expression
else {
return true
}
if let suggestion = checkRedundant(
trueExpression: thenExpr, falseExpression: elseExpr)
{
emitIssue(ifStmt, suggestion)
}
return true
}
}
fileprivate extension IfStatement {
var thenElseStmts: (Statement, Statement)? {
// check if both then-block and else-block exist and have one and only one statement
guard codeBlock.statements.count == 1,
let elseClause = elseClause,
case .else(let elseBlock) = elseClause,
elseBlock.statements.count == 1
else {
return nil
}
let thenStmt = codeBlock.statements[0]
let elseStmt = elseBlock.statements[0]
return (thenStmt, elseStmt)
}
var areAllConditionsExpressions: Bool {
return conditionList.filter({
if case .expression = $0 {
return false
}
return true
}).isEmpty
}
}
| apache-2.0 | 4be95f223b3a9cbb5def815b58e824a2 | 24.620155 | 88 | 0.637821 | 4.521204 | false | false | false | false |
MihaiDamian/Dixi | Dixi/Dixi/Extensions/ViewOperators.swift | 1 | 9196 | //
// UIViewOperators.swift
// Dixi
//
// Created by Mihai Damian on 28/02/15.
// Copyright (c) 2015 Mihai Damian. All rights reserved.
//
import Foundation
#if os(iOS)
import UIKit
#else
import AppKit
#endif
/**
Trailing distance to view operator. See also -| (the leading distance operator).
:param: view The constraint's initial view.
:param: constant The trailing/bottom distance.
:returns: A PartialConstraint that specifies the trailing distance to a yet to be defined view. This constraint should be used as an input to the leading distance to view operator.
*/
public func |- <T: CGFloatConvertible> (view: View, constant: T) -> PartialConstraint {
return view |- constant.toCGFloat()
}
/**
Trailing distance to view operator.
:param: view The constraint's initial view.
:param: constant The trailing/bottom distance.
:returns: A PartialConstraint that specifies the trailing distance to a yet to be defined view. This constraint should be used as an input to the leading distance to view operator.
*/
public func |- (view: View, constant: CGFloat) -> PartialConstraint {
return PartialConstraint(secondItem: view, constant: constant)
}
private func sizeConstraintWithView(view: View, #constant: CGFloat, #relation: NSLayoutRelation) -> LayoutConstraint {
var constraint = LayoutConstraint()
constraint.firstItem = view
constraint.firstItemAttribute = .Size
constraint.constant = constant
constraint.relation = relation
return constraint
}
private func sizeConstraintWithLeftView(leftView: View, #rightView: View, #relation: NSLayoutRelation) -> LayoutConstraint {
var constraint = LayoutConstraint()
constraint.firstItem = leftView
constraint.firstItemAttribute = .Size
constraint.relation = relation
constraint.secondItem = rightView
constraint.secondItemAttribute = .Size
return constraint
}
/**
Width greater or equal than constant operator.
:param: view The constraint's view.
:param: constant The width.
:returns: A LayoutConstraint specifying the view's width to be greater than the constant.
*/
public func >= <T: CGFloatConvertible> (view: View, constant: T) -> LayoutConstraint {
return view >= constant.toCGFloat()
}
/**
Width greater or equal than constant operator.
:param: view The constraint's view.
:param: constant The width.
:returns: A LayoutConstraint specifying the view's width to be greater than the constant.
*/
public func >= (view: View, constant: CGFloat) -> LayoutConstraint {
return sizeConstraintWithView(view, constant: constant, relation: .GreaterThanOrEqual)
}
/**
Width greater or equal than view operator.
:param: leftView The first view in the constraint.
:param: rightView The second view in the constraint.
:returns: A LayoutConstraint specifying the first view's width to be greater or equal to the second view's width.
*/
public func >= (leftView: View, rightView: View) -> LayoutConstraint {
return sizeConstraintWithLeftView(leftView, rightView: rightView, relation: .GreaterThanOrEqual)
}
/**
Width smaller or equal than constant operator.
:param: view The constraint's view.
:param: constant The width.
:returns: A LayoutConstraint specifying the view's width to be smaller than the constant.
*/
public func <= <T: CGFloatConvertible> (view: View, constant: T) -> LayoutConstraint {
return view <= constant.toCGFloat()
}
/**
Width smaller or equal than constant operator.
:param: view The constraint's view.
:param: constant The width.
:returns: A LayoutConstraint specifying the view's width to be smaller than the constant.
*/
public func <= (view: View, constant: CGFloat) -> LayoutConstraint {
return sizeConstraintWithView(view, constant: constant, relation: .LessThanOrEqual)
}
/**
Width smaller or equal than view operator.
:param: leftView The first view in the constraint.
:param: rightView The second view in the constraint.
:returns: A LayoutConstraint specifying the first view's width to be smaller or equal to the second view's width.
*/
public func <= (leftView: View, rightView: View) -> LayoutConstraint {
return sizeConstraintWithLeftView(leftView, rightView: rightView, relation: .LessThanOrEqual)
}
/**
Width equal to constant operator.
:param: view The constraint's view.
:param: constant The width.
:returns: A LayoutConstraint specifying the view's width to be equal to a constant.
*/
public func == <T: CGFloatConvertible> (view: View, constant: T) -> LayoutConstraint {
return view == constant.toCGFloat()
}
/**
Width equal to constant operator.
:param: view The constraint's view.
:param: constant The width.
:returns: A LayoutConstraint specifying the view's width to be equal to a constant.
*/
public func == (view: View, constant: CGFloat) -> LayoutConstraint {
return sizeConstraintWithView(view, constant: constant, relation: .Equal)
}
/**
Width equal to view operator.
:param: leftView The first view in the constraint.
:param: rightView The second view in the constraint.
:returns: A LayoutConstraint specifying the first view's width to be equal to the second view's width.
*/
public func == (leftView: View, rightView: View) -> LayoutConstraint {
var constraint = LayoutConstraint()
constraint.firstItem = leftView
constraint.firstItemAttribute = .Size
constraint.relation = .Equal
constraint.secondItem = rightView
constraint.secondItemAttribute = .Size
return constraint
}
/**
Leading distance to superview operator.
:param: constant The leading distance to the superview.
:param: view The view that needs to be distanced from the superview. The view needs to have a superview.
:returns: A LayoutConstraint specifying the leading distance from the view to its superview.
*/
public func |-| <T: CGFloatConvertible> (constant: T, view: View) -> LayoutConstraint {
return constant.toCGFloat() |-| view
}
/**
Leading distance to superview operator.
:param: constant The leading distance to the superview.
:param: view The view that needs to be distanced from the superview. The view needs to have a superview.
:returns: A LayoutConstraint specifying the leading distance from the view to its superview.
*/
public func |-| (constant: CGFloat, view: View) -> LayoutConstraint {
assert(view.superview != nil, "Can't use `distance to superview` operator on view that has no superview")
var constraint = LayoutConstraint()
constraint.firstItem = view
constraint.firstItemAttribute = .LeadingOrTopToSuperview
constraint.relation = .Equal
constraint.secondItem = view.superview!
constraint.secondItemAttribute = .LeadingOrTopToSuperview
constraint.constant = constant
return constraint
}
/**
Trailing distance to superview operator.
:param: view The view that needs to be distanced from the superview. The view needs to have a superview.
:param: constant The trailing distance to the superview.
:returns: A LayoutConstraint specifying the trailing distance from the view to its superview.
*/
public func |-| <T: CGFloatConvertible> (view: View, constant: T) -> LayoutConstraint {
return view |-| constant.toCGFloat()
}
/**
Trailing distance to superview operator.
:param: view The view that needs to be distanced from the superview. The view needs to have a superview.
:param: constant The trailing distance to the superview.
:returns: A LayoutConstraint specifying the trailing distance from the view to its superview.
*/
public func |-| (view: View, constant: CGFloat) -> LayoutConstraint {
assert(view.superview != nil, "Can't use `distance to superview` operator on view that has no superview")
var constraint = LayoutConstraint()
constraint.firstItem = view.superview!
constraint.firstItemAttribute = .TrailingOrBottomToSuperview
constraint.relation = .Equal
constraint.secondItem = view
constraint.secondItemAttribute = .TrailingOrBottomToSuperview
constraint.constant = constant
return constraint
}
/**
Flush views operator.
:param: leftView The first view of the constraint.
:param: rightView The second view of the constraint.
:returns: A LayoutConstraint specifying a distance of 0 points between the two views.
*/
public func || (leftView: View, rightView: View) -> LayoutConstraint {
var constraint = LayoutConstraint()
constraint.firstItem = rightView
constraint.firstItemAttribute = .DistanceToSibling
constraint.relation = .Equal
constraint.secondItem = leftView
constraint.secondItemAttribute = .DistanceToSibling
return constraint
}
/**
Standard distance operator.
:param: leftView The first view of the constraint.
:param: rightView The second view of the constraint.
:returns: A LayoutConstraint specifying a standard (8 points) distance between the two views.
*/
public func - (leftView: View, rightView: View) -> LayoutConstraint {
var constraint = leftView || rightView
constraint.constant = 8
return constraint
}
| mit | 85b0d3011009b89afdbbe41b5282c96c | 35.062745 | 184 | 0.7301 | 4.759834 | false | false | false | false |
ali-zahedi/AZViewer | AZViewer/AZHeader.swift | 1 | 4313 | //
// AZHeader.swift
// AZViewer
//
// Created by Ali Zahedi on 1/14/1396 AP.
// Copyright © 1396 AP Ali Zahedi. All rights reserved.
//
import Foundation
public enum AZHeaderType {
case title
case success
}
public class AZHeader: AZBaseView{
// MARK: Public
public var title: String = ""{
didSet{
self.titleLabel.text = self.title
}
}
public var type: AZHeaderType! {
didSet{
let hiddenTitle: Bool = self.type == .success ? true : false
self.successButton.isHidden = !hiddenTitle
self.titleLabel.aZConstraints.right?.isActive = false
if hiddenTitle {
_ = self.titleLabel.aZConstraints.right(to: self.successButton, toAttribute: .left,constant: -self.style.sectionGeneralConstant)
}else{
_ = self.titleLabel.aZConstraints.right(constant: -self.style.sectionGeneralConstant)
}
}
}
public var rightButtonTintColor: UIColor! {
didSet{
self.successButton.tintColor = self.rightButtonTintColor
}
}
public var leftButtonTintColor: UIColor! {
didSet{
self.closeButton.tintColor = self.leftButtonTintColor
}
}
// MARK: Internal
internal var delegate: AZPopupViewDelegate?
// MARK: Private
fileprivate var titleLabel: AZLabel = AZLabel()
fileprivate var closeButton: AZButton = AZButton()
fileprivate var successButton: AZButton = AZButton()
// MARK: Override
override init(frame: CGRect) {
super.init(frame: frame)
self.defaultInit()
}
required public init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
self.defaultInit()
}
// MARK: Function
fileprivate func defaultInit(){
self.backgroundColor = self.style.sectionHeaderBackgroundColor
for v in [titleLabel, closeButton, successButton] as [UIView]{
v.translatesAutoresizingMaskIntoConstraints = false
self.addSubview(v)
}
// title
self.prepareTitle()
// close button
self.prepareCloseButton()
// success button
self.prepareSuccessButton()
// init
self.type = .title
}
// cancell action
func cancelButtonAction(_ sender: AZButton){
self.delegate?.cancelPopupView()
}
// success action
func successButtonAction(_ sender: AZButton){
self.delegate?.submitPopupView()
}
}
// prepare
extension AZHeader{
// title
fileprivate func prepareTitle(){
_ = self.titleLabel.aZConstraints.parent(parent: self).centerY().right(constant: -self.style.sectionGeneralConstant).left(multiplier: 0.7)
self.titleLabel.textColor = self.style.sectionHeaderTitleColor
self.titleLabel.font = self.style.sectionHeaderTitleFont
}
// close
fileprivate func prepareCloseButton(){
let height = self.style.sectionHeaderHeight * 0.65
_ = self.closeButton.aZConstraints.parent(parent: self).centerY().left(constant: self.style.sectionGeneralConstant).width(constant: height).height(to: self.closeButton, toAttribute: .width)
self.closeButton.setImage(AZAssets.closeImage, for: .normal)
self.closeButton.tintColor = self.style.sectionHeaderLeftButtonTintColor
self.closeButton.addTarget(self, action: #selector(cancelButtonAction(_:)), for: .touchUpInside)
}
// success
fileprivate func prepareSuccessButton(){
let height = self.style.sectionHeaderHeight * 0.65
_ = self.successButton.aZConstraints.parent(parent: self).centerY().right(constant: -self.style.sectionGeneralConstant).width(constant: height).height(to: self.closeButton, toAttribute: .width)
self.successButton.setImage(AZAssets.tickImage, for: .normal)
self.successButton.tintColor = self.style.sectionHeaderRightButtonTintColor
self.successButton.addTarget(self, action: #selector(successButtonAction(_:)), for: .touchUpInside)
}
}
| apache-2.0 | 557a7ea8047e5693c5a24483d902db5b | 29.366197 | 201 | 0.622913 | 4.979215 | false | false | false | false |
KeithPiTsui/Pavers | Pavers/Pavers.playground/Pages/UIGradientView.xcplaygroundpage/Contents.swift | 2 | 1217 | //: Playground - noun: a place where people can play
import PaversFRP
import PaversUI
import UIKit
import PlaygroundSupport
import Foundation
let colors = [UIColor.random.cgColor, UIColor.random.cgColor, UIColor.random.cgColor]
let locations = [NSNumber(value: 0),NSNumber(value: 0.25),NSNumber(value: 0.5)]
let sp = CGPoint(x: 0, y: 0)
let ep = CGPoint(x: 1, y: 1)
let gViewStyle =
UIGradientView.lens.gradientLayer.colors .~ colors
>>> UIGradientView.lens.gradientLayer.locations .~ locations
>>> UIGradientView.lens.gradientLayer.startPoint .~ sp
>>> UIGradientView.lens.gradientLayer.endPoint .~ ep
>>> UIGradientView.lens.frame .~ frame
let gView = UIGradientView()
gView |> gViewStyle
//PlaygroundPage.current.liveView = gView
// Set the device type and orientation.
let (parent, _) = playgroundControllers(device: .phone5_5inch, orientation: .portrait)
// Render the screen.
let frame = parent.view.frame
PlaygroundPage.current.liveView = gView
Timer.scheduledTimer(withTimeInterval: 3, repeats: true) { _ in
let colors = [UIColor.random.cgColor, UIColor.random.cgColor, UIColor.random.cgColor]
gView |> UIGradientView.lens.gradientLayer.colors .~ colors
}
| mit | 649eec2bfdfbc75f0ed09f20dd8d9e5e | 19.627119 | 87 | 0.737058 | 3.977124 | false | false | false | false |
XCEssentials/UniFlow | Sources/7_ExternalBindings/ExternalBindingBDD_ThenContext.swift | 1 | 2581 | /*
MIT License
Copyright (c) 2016 Maxim Khatskevich (maxim@khatskevi.ch)
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
*/
import Combine
//---
public
extension ExternalBindingBDD
{
struct ThenContext<W: SomeMutationDecriptor, G> // W - When, G - Given
{
public
let description: String
//internal
let given: (Dispatcher, W) throws -> G?
public
func then(
scope: String = #file,
location: Int = #line,
_ then: @escaping (Dispatcher, G) -> Void
) -> ExternalBinding {
.init(
description: description,
scope: scope,
context: S.self,
location: location,
given: given,
then: then
)
}
public
func then(
scope: String = #file,
location: Int = #line,
_ noDispatcherHandler: @escaping (G) -> Void
) -> ExternalBinding {
then(scope: scope, location: location) { _, input in
noDispatcherHandler(input)
}
}
public
func then(
scope: String = #file,
location: Int = #line,
_ sourceOnlyHandler: @escaping () -> Void
) -> ExternalBinding where G == Void {
then(scope: scope, location: location) { _, _ in
sourceOnlyHandler()
}
}
}
}
| mit | a7956372d5b14e6c12bb1832dd0bc190 | 29.364706 | 79 | 0.583495 | 5.041016 | false | false | false | false |
codespy/HashingUtility | Hashing Utility/Hmac.swift | 1 | 3617 | // The MIT License (MIT)
//
// Copyright (c) 2015 Krzysztof Rossa
//
// Permission is hereby granted, free of charge, to any person obtaining a copy
// of this software and associated documentation files (the "Software"), to deal
// in the Software without restriction, including without limitation the rights
// to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
// copies of the Software, and to permit persons to whom the Software is
// furnished to do so, subject to the following conditions:
//
// The above copyright notice and this permission notice shall be included in all
// copies or substantial portions of the Software.
//
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
// IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
// AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
// LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
// OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
// SOFTWARE.
import Foundation
// inspired by http://stackoverflow.com/a/24411522
enum HMACAlgorithm {
case MD5, SHA1, SHA224, SHA256, SHA384, SHA512
func toCCHmacAlgorithm() -> CCHmacAlgorithm {
var result: Int = 0
switch self {
case .MD5:
result = kCCHmacAlgMD5
case .SHA1:
result = kCCHmacAlgSHA1
case .SHA224:
result = kCCHmacAlgSHA224
case .SHA256:
result = kCCHmacAlgSHA256
case .SHA384:
result = kCCHmacAlgSHA384
case .SHA512:
result = kCCHmacAlgSHA512
}
return CCHmacAlgorithm(result)
}
func digestLength() -> Int {
var result: CInt = 0
switch self {
case .MD5:
result = CC_MD5_DIGEST_LENGTH
case .SHA1:
result = CC_SHA1_DIGEST_LENGTH
case .SHA224:
result = CC_SHA224_DIGEST_LENGTH
case .SHA256:
result = CC_SHA256_DIGEST_LENGTH
case .SHA384:
result = CC_SHA384_DIGEST_LENGTH
case .SHA512:
result = CC_SHA512_DIGEST_LENGTH
}
return Int(result)
}
}
extension String {
func calculateHash(algorithm: HMACAlgorithm) -> String! {
//let encodig = NSUTF8StringEncoding
let encodig = NSUTF8StringEncoding
let str = self.cStringUsingEncoding(encodig)
let strLen = CUnsignedInt(self.lengthOfBytesUsingEncoding(encodig))
let digestLen = algorithm.digestLength()
let result = UnsafeMutablePointer<CUnsignedChar>.alloc(digestLen)
switch (algorithm) {
case HMACAlgorithm.MD5:
CC_MD5(str!, strLen, result)
case HMACAlgorithm.SHA1:
CC_SHA1(str!, strLen, result)
case HMACAlgorithm.SHA224:
CC_SHA224(str!, strLen, result)
case HMACAlgorithm.SHA256:
CC_SHA256(str!, strLen, result)
case HMACAlgorithm.SHA384:
CC_SHA384(str!, strLen, result)
case HMACAlgorithm.SHA512:
CC_SHA512(str!, strLen, result)
}
var hash = NSMutableString()
for i in 0..<digestLen {
hash.appendFormat("%02x", result[i])
}
result.destroy()
return String(hash)
}
}
| mit | 9661dd1f0ca4ad6ad5245c86ba2bce58 | 32.490741 | 84 | 0.604645 | 4.601781 | false | false | false | false |
AHuaner/Gank | Gank/Class/Home/AHHomeWebViewController.swift | 1 | 7521 | //
// AHHomeWebViewController.swift
// Gank
//
// Created by AHuaner on 2017/1/7.
// Copyright © 2017年 CoderAhuan. All rights reserved.
//
import UIKit
class AHHomeWebViewController: BaseWebViewController {
// MARK: - property
var gankModel: GankModel?
// 文章是否被收藏
fileprivate var isCollected: Bool = false
// MARK: - control
// 弹窗
fileprivate lazy var moreView: AHMoreView = {
let moreView = AHMoreView.moreView()
let W = kScreen_W / 2
let H = CGFloat(moreView.titles.count * 44 + 15)
moreView.alpha = 0.01
moreView.frame = CGRect(x: kScreen_W - W - 3, y: 50, width: W, height: H)
return moreView
}()
// 蒙版
fileprivate var maskBtnView: UIButton = {
let maskBtnView = UIButton()
maskBtnView.frame = kScreen_BOUNDS
maskBtnView.backgroundColor = UIColor.clear
return maskBtnView
}()
// MARK: - life cycle
override func viewDidLoad() {
super.viewDidLoad()
setupUI()
}
override func viewWillAppear(_ animated: Bool) {
super.viewWillAppear(animated)
UIApplication.shared.statusBarStyle = .lightContent
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
}
// MARK: - event && methods
fileprivate func setupUI() {
self.title = "加载中..."
let oriImage = UIImage(named: "icon_more")?.withRenderingMode(UIImageRenderingMode.alwaysOriginal)
navigationItem.rightBarButtonItem = UIBarButtonItem(image: oriImage, style: .plain, target: self, action: #selector(moreClick))
maskBtnView.addTarget(self, action: #selector(dismissMoreView), for: .touchUpInside)
moreView.tableViewdidSelectClouse = { [unowned self] (indexPath) in
self.dismissMoreView()
switch indexPath.row {
case 0: // 收藏
self.collectGankAction()
case 1: // 分享
self.shareEvent()
case 2: // 复制链接
let pasteboard = UIPasteboard.general
pasteboard.string = self.urlString!
ToolKit.showSuccess(withStatus: "复制成功")
case 3: // Safari打开
guard let urlString = self.urlString else { return }
guard let url = URL(string: urlString) else { return }
UIApplication.shared.openURL(url)
default: break
}
}
}
// 检验文章是否已被收藏
fileprivate func cheakIsCollected() {
let query: BmobQuery = BmobQuery(className: "Collect")
let array = [["userId": User.info?.objectId], ["gankId": gankModel?.id]]
query.addTheConstraintByAndOperation(with: array)
query.findObjectsInBackground { (array, error) in
// 加载失败
if error != nil { return }
guard let ganksArr = array else { return }
if ganksArr.count == 1 { // 以收藏
self.moreView.gankBe(collected: true)
self.isCollected = true
self.gankModel?.objectId = (ganksArr.first as! BmobObject).objectId
} else { // 未收藏
self.moreView.gankBe(collected: false)
self.isCollected = false
self.gankModel?.objectId = nil
}
guard let nav = self.navigationController else { return }
nav.view.insertSubview(self.maskBtnView, aboveSubview: self.webView)
nav.view.insertSubview(self.moreView, aboveSubview: self.webView)
UIView.animate(withDuration: 0.25) {
self.moreView.alpha = 1
}
}
}
func moreClick() {
cheakIsCollected()
}
func dismissMoreView() {
maskBtnView.removeFromSuperview()
UIView.animate(withDuration: 0.25, animations: {
self.moreView.alpha = 0.01
}) { (_) in
self.moreView.removeFromSuperview()
}
}
// 点击收藏
fileprivate func collectGankAction() {
if User.info == nil { // 未登录
let loginVC = AHLoginViewController()
let nav = UINavigationController(rootViewController: loginVC)
present(nav, animated: true, completion: nil)
return
}
// 检验用户是否在其他设备登录
ToolKit.checkUserLoginedWithOtherDevice(noLogin: {
// 登录状态
// 取消收藏
if self.isCollected {
ToolKit.show(withStatus: "正在取消收藏")
guard let objectId = self.gankModel?.objectId else { return }
let gank: BmobObject = BmobObject(outDataWithClassName: "Collect", objectId: objectId)
gank.deleteInBackground { (isSuccessful, error) in
if isSuccessful { // 删除成功
ToolKit.showSuccess(withStatus: "已取消收藏")
self.isCollected = false
self.moreView.gankBe(collected: false)
} else {
AHLog(error!)
ToolKit.showError(withStatus: "取消收藏失败")
}
}
return
}
// 收藏
let gankInfo = BmobObject(className: "Collect")
gankInfo?.setObject(User.info!.objectId, forKey: "userId")
gankInfo?.setObject(User.info!.mobilePhoneNumber, forKey: "userPhone")
if let gankModel = self.gankModel {
gankInfo?.setObject(gankModel.id, forKey: "gankId")
gankInfo?.setObject(gankModel.desc, forKey: "gankDesc")
gankInfo?.setObject(gankModel.type, forKey: "gankType")
gankInfo?.setObject(gankModel.user, forKey: "gankUser")
gankInfo?.setObject(gankModel.publishedAt, forKey: "gankPublishAt")
gankInfo?.setObject(gankModel.url, forKey: "gankUrl")
}
gankInfo?.saveInBackground(resultBlock: { (isSuccessful, error) in
if error != nil { // 收藏失败
AHLog(error!)
ToolKit.showError(withStatus: "收藏失败")
} else { // 收藏成功
ToolKit.showSuccess(withStatus: "收藏成功")
self.isCollected = true
self.moreView.gankBe(collected: true)
}
})
})
}
fileprivate func shareEvent() {
UMSocialUIManager.showShareMenuViewInWindow { (platformType, userInfo) in
let messageObject = UMSocialMessageObject()
let shareObject = UMShareWebpageObject.shareObject(withTitle: Bundle.appName, descr: self.gankModel?.desc, thumImage: UIImage(named: "icon108"))
shareObject?.webpageUrl = self.gankModel?.url
messageObject.shareObject = shareObject
UMSocialManager.default().share(to: platformType, messageObject: messageObject, currentViewController: self) { (data, error) in
if error == nil {
AHLog(data)
} else {
AHLog("分享失败\(error!)")
}
}
}
}
}
| mit | dfb957bd4b1b10d54b905c619db0a029 | 35.582915 | 156 | 0.55206 | 5.013774 | false | false | false | false |
kareman/SwiftShell | Sources/SwiftShell/Context.swift | 1 | 5042 | /*
* Released under the MIT License (MIT), http://opensource.org/licenses/MIT
*
* Copyright (c) 2015 Kåre Morstøl, NotTooBad Software (nottoobadsoftware.com)
*
*/
import Foundation
public protocol Context: CustomDebugStringConvertible {
var env: [String: String] { get set }
var stdin: ReadableStream { get set }
var stdout: WritableStream { get set }
var stderror: WritableStream { get set }
/**
The current working directory.
Must be used instead of `run("cd", "...")` because all the `run` commands are executed in a
separate process and changing the directory there will not affect the rest of the Swift script.
*/
var currentdirectory: String { get set }
}
extension Context {
/** A textual representation of this instance, suitable for debugging. */
public var debugDescription: String {
var result = ""
debugPrint("stdin:", stdin, "stdout:", stdout, "stderror:", stderror, "currentdirectory:", currentdirectory, to: &result)
debugPrint("env:", env, to: &result)
return result
}
}
public struct CustomContext: Context, CommandRunning {
public var env: [String: String]
public var stdin: ReadableStream
public var stdout: WritableStream
public var stderror: WritableStream
/**
The current working directory.
Must be used instead of `run("cd", "...")` because all the `run` commands are executed in a
separate process and changing the directory there will not affect the rest of the Swift script.
*/
public var currentdirectory: String
/** Creates a blank CustomContext where env and stdin are empty, stdout and stderror discard everything and
currentdirectory is the current working directory. */
public init() {
let encoding = String.Encoding.utf8
env = [String: String]()
stdin = FileHandleStream(FileHandle.nullDevice, encoding: encoding)
stdout = FileHandleStream(FileHandle.nullDevice, encoding: encoding)
stderror = FileHandleStream(FileHandle.nullDevice, encoding: encoding)
currentdirectory = main.currentdirectory
}
/** Creates an identical copy of another Context. */
public init(_ context: Context) {
env = context.env
stdin = context.stdin
stdout = context.stdout
stderror = context.stderror
currentdirectory = context.currentdirectory
}
}
private func createTempdirectory() -> String {
let name = URL(fileURLWithPath: main.path).lastPathComponent
let tempdirectory = URL(fileURLWithPath: NSTemporaryDirectory()) + (name + "-" + ProcessInfo.processInfo.globallyUniqueString)
do {
try Files.createDirectory(atPath: tempdirectory.path, withIntermediateDirectories: true, attributes: nil)
return tempdirectory.path + "/"
} catch let error as NSError {
exit(errormessage: "Could not create new temporary directory '\(tempdirectory)':\n\(error.localizedDescription)", errorcode: error.code)
} catch {
exit(errormessage: "Unexpected error: \(error)")
}
}
extension CommandLine {
/** Workaround for nil crash in CommandLine.arguments when run in Xcode. */
static var safeArguments: [String] {
self.argc == 0 ? [] : self.arguments
}
}
public final class MainContext: Context, CommandRunning {
/// The default character encoding used throughout SwiftShell.
/// Only affects stdin, stdout and stderror if they have not been used yet.
public var encoding = String.Encoding.utf8 // TODO: get encoding from environmental variable LC_CTYPE.
public lazy var env = ProcessInfo.processInfo.environment as [String: String]
public lazy var stdin: ReadableStream = {
FileHandleStream(FileHandle.standardInput, encoding: self.encoding)
}()
public lazy var stdout: WritableStream = {
let stdout = StdoutStream.default
stdout.encoding = self.encoding
return stdout
}()
public lazy var stderror: WritableStream = {
FileHandleStream(FileHandle.standardError, encoding: self.encoding)
}()
/**
The current working directory.
Must be used instead of `run("cd", "...")` because all the `run` commands are executed in
separate processes and changing the directory there will not affect the rest of the Swift script.
This directory is also used as the base for relative URLs.
*/
public var currentdirectory: String {
get { return Files.currentDirectoryPath + "/" }
set {
if !Files.changeCurrentDirectoryPath(newValue) {
exit(errormessage: "Could not change the working directory to \(newValue)")
}
}
}
/**
The tempdirectory is unique each time a script is run and is created the first time it is used.
It lies in the user's temporary directory and will be automatically deleted at some point.
*/
public private(set) lazy var tempdirectory: String = createTempdirectory()
/** The arguments this executable was launched with. Use main.path to get the path. */
public private(set) lazy var arguments: [String] = Array(CommandLine.safeArguments.dropFirst())
/** The path to the currently running executable. Will be empty in playgrounds. */
public private(set) lazy var path: String = CommandLine.safeArguments.first ?? ""
fileprivate init() {}
}
public let main = MainContext()
| mit | 0011806dc956d25b7f63b02d5be92a76 | 34.492958 | 138 | 0.74127 | 4.193012 | false | false | false | false |
Chan4iOS/SCMapCatch-Swift | SCMapCatch-Swift/UserDefaults+MC.swift | 1 | 5345 |
// UserDefault+MC.swift
// SCMapCatch-Swift_Demo
//
// Created by 陈世翰 on 17/4/17.
// Copyright © 2017年 Coder Chan. All rights reserved.
//
import Foundation
extension UserDefaults{
/*
UserDefaults set object by level keys , it will auto choose to create elements on the path if the elements on the path have not been created,or delete the element on the path when the param 'object' is nil
:mObject:object to insert
:keys:path components
:warning:The first obj in keys must be 'Stirng' type ,otherwise it will return nil
*/
func mc_set<T:Hashable>(object mObject:Any?,forKeys keys:Array<T>){
guard keys.count>0 else {
return
}
guard keys.first != nil else{
return
}
let firstKey = keys.first as? String//第一个key必须为string userDefault规定
guard firstKey != nil else{
return
}
if keys.count==1{
mc_set(object: mObject, forkey: firstKey!)
}else{
let tree = value(forKey:firstKey!)
var mDict = [T : Any]()
if tree != nil {
guard tree is [T:Any] else {
return
}
mDict = tree as! [T : Any]
}
var inputKeys = keys
inputKeys.remove(at: 0)
let rootTree = mc_handleTree(tree: mDict, components: inputKeys , obj: mObject)
guard rootTree != nil else {
return
}
set(rootTree!, forKey: firstKey!)
}
synchronize();
}
/*
UserDefaults set object by level keys , it will auto choose to create elements on the path if the elements on the path have not been created,or delete the element on the path when the param 'object' is nil
:mObject:object to insert
:keys:path components
:warning:The first obj in keys must be 'Stirng' type ,otherwise it will return nil
*/
func mc_set<T:Hashable>(object mObject:Any?,forKeys keys:T...){
mc_set(object: mObject, forKeys: keys)
}
/*
UserDefaults set object key , it will auto choose to create element when the param 'object' != nil,or delete the element on the path when the param 'object' is nil
:mObject:object to insert
:key:String
*/
func mc_set(object:Any?,forkey key:String){
if object != nil{
set(object!, forKey: key)
}else{
removeObject(forKey:key)
}
synchronize();
}
/*Recursive traversal func
:param:tree this level tree
:param:components the rest of path components
:return:hanle result of this level
*/
fileprivate func mc_handleTree<T:Hashable>(tree: [T:Any],components:Array<T>,obj:Any?)->Dictionary<T, Any>?{
var result = tree
if components.count==1{//last level
result = result.mc_set(value: obj, forkey: components.first!)
}else{//middle level
var nextComponents = components
nextComponents.remove(at: 0)
let nextTree = tree[components.first!]
if nextTree != nil && nextTree is [T : Any]{
result[components.first!] = mc_handleTree(tree:nextTree as! [T : Any], components:nextComponents , obj: obj)
}else{
result[components.first!] = mc_handleTree(tree:[T : Any](), components:nextComponents , obj: obj)
}
}
return result
}
class func mc_set(object:Any?,forkey key:String){
self.standard.mc_set(object: object, forkey: key)
}
class func mc_set<T:Hashable>(object mObject:Any?,forKeys keys:T...){
self.standard.mc_set(object: mObject, forKeys: keys)
}
class func mc_set<T:Hashable>(object mObject:Any?,forKeys keys:Array<T>){
self.standard.mc_set(object: mObject, forKeys: keys)
}
/*get object by path
:param:keys path components
:return:result
:warning:the first object of keys must be 'String' type , otherwise it will return nil
*/
func mc_object<T:Hashable>(forKeys keys:T...) -> Any? {
return mc_object(forKeys: keys)
}
/*get object by path
:param:keys path components
:return:result
:warning:the first object of keys must be 'String' type , otherwise it will return nil
*/
func mc_object<T:Hashable>(forKeys keys:Array<T>) -> Any? {
guard keys.count>0 else{
return nil
}
let firstKey = keys.first as? String
guard firstKey != nil else{
return nil
}
if keys.count == 1{
return object(forKey: firstKey!)
}else{
let nextLevel = object(forKey: firstKey!)
guard nextLevel != nil && nextLevel is Dictionary<T,Any> else{
return nil
}
var nextLevelKeys = keys
nextLevelKeys.remove(at: 0)
return (nextLevel as! Dictionary<T,Any>).mc_object(forKeys: nextLevelKeys)
}
}
class func mc_object<T:Hashable>(forKeys keys:T...) -> Any? {
return self.standard.mc_object(forKeys: keys)
}
class func mc_object<T:Hashable>(forKeys keys:Array<T>) -> Any? {
return self.standard.mc_object(forKeys: keys)
}
}
| mit | 46df3713bfbea3857ed1d37787118ae5 | 35.410959 | 210 | 0.585779 | 4.172684 | false | false | false | false |