instruction
stringlengths 0
30k
⌀ |
---|
I get "Bad PCD format" error.
I would like to know what's wrong with PCD file.
This is the header to the PCD file:
header = "# .PCD v.7 - Point Cloud Data file format
VERSION .7
FIELDS x y z data
SIZE 4 4 4
TYPE F F F
COUNT 1 1 1
WIDTH 0
HEIGHT 1
VIEWPOINT 0 0 0 1 0 0 0
POINTS 0
DATA binary" |
step 1: delete the 'node_modules' folder
step 2: yarn (or npm) install
I couldn't get the Node Modules folder back after 'yarn'
and I needed to add a file.yarnrc.yml containing " nodeLinker: node-modules "
After that again yarn (or npm) install
That worked for me.
|
I'm trying to fetch apis and combine the json objects into a single variable array that I can loop through.
using .push my variable array ends up as..
```
[
[
{"a","1"}
],
[
{"b","2"}
]
]
```
when i want this..
```
[
{"a","1"}
{"b","2"}
]
```
Here's my trimmed down code..
```
var combinedJson = [];
const r1 = fetch(firstJson).then(response => response.json());
const r2 = fetch(secondJson).then(response => response.json());
Promise.all([r1, r2])
.then(([d1, d2]) => {
combinedJson.push(d1, d2);
console.log(combinedJson);
})
.catch(error => {
console.error(error);
});
``` |
With JS, it's a known problem that `\b` does not work well with strings containing special chars (JS engine believes chars like `ç` are word bondaries).
So I have this code:
"aaa aabb cc ccc abba".replace(/\b((.)\2+)\b|(.)\3+/g,"$1$3");
It correctly returns `aaa ab cc ccc aba`. However, if the input string has special chars, it does not work anymore, for example:
"ááá áább çç ççç ábbá".replace(/\b((.)\2+)\b|(.)\3+/g,"$1$3");
The code above returns `á ább ç ç ábbá` which is not expecetd, it should have been `ááá áb çç ççç ábá`.
So I decided I didnt want to use `\b` anymore because I will only accept word boundaries as ` ` (space) and begginig/end of string (^ or $). So I tried this regex:
"ááá áább çç ççç ábbá".replace(new RegExp("(^| )((.)\\3+)( |$)|(.)\\5+","g"),"$1$2$4$5");
It returned `ááá áb çç ç ábá` which is almost correct, it should have returned `ááá áb çç ççç ábá`.
How can I make the last regex work without using lookheads, lookbehind, lookaround... Is there an easy fix to the last regex? Or, is there a fix to the `\b` that makes `\b` work as expected? |
`Backward slash + b` does not work as expected on regex |
|javascript|regex| |
This is considered as anti pattern in modern React ecosystem.
**According to single responsibility principle.**
Keep your business logic in a simple way with custom hooks (Use prototype inheritance in it, if required), To store API calls use Tanstack Query and to store global data use Jotai (Atoms). This libraries are very easy to learn and maintain.
You don't need to write Redux (action, reducers and store), Redux toolkit and other boilerplate codes today. Even you learn this concepts it was not so useful in other stacks.
Even today many job requirements asks for Redux questions, I hope they will update their projects with the mentioned best practices soon. |
Namespace error while doing R CMD check --as-cran with "foreach" package functions |
|r|foreach|namespaces| |
null |
In Visual Studio Code, how can you automatically add `const` when you press (<kbd>Ctrl</kbd> + <kbd>S</kbd>)?
|
i am trying to get application context for showToast but not able to get it here is my code, always getting error :- lateinit property appContext has not been initialized, kindly help
```
package com.coding.APPNAVIGATION.MenuAndNavigationDrawer
import android.app.Application
import android.content.Context
open class MainApplication : Application() {
override fun onCreate() {
super.onCreate()
MainApplication.appContext = applicationContext
}
companion object {
lateinit var appContext : Context
}
}
```
```
package com.coding.APPNAVIGATION.MenuAndNavigationDrawer.Utils
import android.widget.Toast
object Utils{
fun showToast(message : String){
Toast.makeText(MainApplication.appContext, message, Toast.LENGTH_LONG).show()
}
}
```
i want to get application context to run showToast anywhere within the project |
`template<>
inline bool
_Sp_counted_base<_S_atomic>::
_M_add_ref_lock_nothrow() noexcept
{
// Perform lock-free add-if-not-zero operation.
_Atomic_word __count = _M_get_use_count();
do
{
if (__count == 0)
return false;
// Replace the current counter value with the old value + 1, as
// long as it's not changed meanwhile.
}
while (!__atomic_compare_exchange_n(&_M_use_count, &__count, __count + 1,
true, __ATOMIC_ACQ_REL,
__ATOMIC_RELAXED));
return true;
}`
this is in c++/11/bits/shared_ptr_base.h: _M_add_ref_lock_nothrow()
Question:
"__count" only get value at first(not in loop)
Is it possible for this loop function to enter an infinite loop?
hope answer!
thank you! |
Is it possible for this loop function to enter an infinite loop in c++11 source code? |
|c++11| |
null |
How can I auto add 'const' in Flutter in Visual Studio Code? |
if(tid\<n)
{
gain = in_degree[neigh]*out_degree[tid] + out_degree[neighbour]*in_degree[tid]/total_weight
//here let say node 0 moves to 2
atomicExch(&node_community[0, node_community[2] // because node 0 is in node 2 now
atomicAdd(&in_degree[2],in_degree[0] // because node 0 is in node 2 now
atomicAdd(&out_degree[2],out_degree[0] // because node 0 is in node 2 now }
}
this is the process, in this problem during calculation of gain all the thread should see the update value of 2 which values of 2+values 0 but threads see only previous value of 2.
how to solve that ? here is the output:
node is: 0
node is: 1
node is: 2
node is: 3
node is: 4
node is: 5
//HERE IS THS PROBLEM (UPDATED VALUES ARE NOT VISIBLE TO THE REST OF THREDS WHO EXECUTED BEFORE THE ATOMIC WRITE)
updated_node is: 0 // this should be 2
updated_values are: 48,37. // this should be(48+15(values of 2))+(37+12(values of 0))
comm. in out
0 shifted to ->> 2 - 15 - 12
1 shifted to ->> 1 - 8 - 10
2 shifted to ->> 2 - 48 - 37
I have tried using , __syncthreads(), _threadfence() and shared memory foe reading writing values can any one tell what could be the issue ?? |
I did a comparison of the messages generated by Service Bus Explorer and those generated by my code. Service Bus Explorer wrapped the subject in double quotes where my code did not.
Updating the correlation rule to subject/label equals jobCosts instead of "jobCosts" did the trick and the subscription started picking up the messages I send from my code. |
1. Please refer to a similar case, it answers admin access is required.
[Cannot install mongoose using npm install][1]
The diagnostic output displayed in your own case has some useful hints as well.
2. **Try it with admin account** : npm ERR! If you believe this might be a permissions issue, please double-check the
npm ERR! permissions of the file and its containing directories, or try running
npm ERR! the command again as root/Administrator.
3. **Check log about the issue** : npm ERR! C:\Users\Lab\AppData\Local\npm-cache\_logs\2024-03-28T11_14_21_900Z-debug-0.log
PS F:\SCALER\NODE JS\Module1\mongoose>
[1]: https://stackoverflow.com/questions/33884609/cannot-install-mongoose-using-npm-install
Thanks
WeDoTheBest4You |
I have some python code:
```
class Meta(type):
def __new__(cls, name, base, attrs):
attrs.update({'name': '', 'email': ''})
return super().__new__(cls, name, base, attrs)
def __init__(cls, name, base, attrs):
super().__init__(name, base, attrs)
cls.__init__ = Meta.func
def func(self, *args, **kwargs):
setattr(self, 'LOCAL', True)
class Man(metaclass=Meta):
login = 'user'
psw = '12345'
```
How do I write this statement as OOP-true (Meta.func)? In the row: `cls.__init__ = Meta.func`
If someone wants to change the name of the metaclass, it will stop working. Because it will be necessary to make changes inside this metaclass. Because I explicitly specified its name in the code. I think this is not right. But I do not know how to express it in another way.
I tried `cls.__init__ = cls.func`, but it doesn't create local variables for the Man class object. |
You should add **overflow: hidden;** or remove **max-height** from parent.
<!-- begin snippet: js hide: false console: true babel: false -->
<!-- language: lang-css -->
.parent {
margin: 5px;
max-width: 400px;
max-height: 100px;
background: pink;
}
.details {
width: 100%;
text-align: center;
font-size: 14pt;
display: grid;
grid-template-rows: 1fr 1fr;
}
.detail1 {
grid-row: 1;
overflow-y: hidden;
}
.detail2 {
grid-row: 2;
overflow-y: hidden;
}
<!-- language: lang-html -->
<div class="parent">
<div class="details">
<div class="detail1">
lotsoftext lotsoftext lotsoftext lotsoftext lotsoftext lotsoftext lotsoftext lotsoftext lotsoftext lotsoftext lotsoftext lotsoftext
</div>
<div class="detail2">
lotsmoretext lotsmoretext lotsmoretext lotsmoretext lotsmoretext lotsmoretext
lotsmoretext lotsmoretext lotsmoretext lotsmoretext lotsmoretext lotsmoretext
</div>
</div>
</div>
<!-- end snippet -->
|
**Sample Input:**
> var sampleInput={
> name: {
> firstName: "sangram",
> midddleName: "shivaji",
> lastName: "Desai",
> },
> address: {
> street: "Naradawe Road",
> line1: "near railway station",
> line2: "behind annapurna hotel",
> city: "kankavali",
> pin: "416602",
> },
> };
**Sample Output:**
> var sampleOutput= [
> ["name",[
> ["firstName","sangram"],
> ["midddleName","shivaji"],
> ["lastName","Desai"]
> ]
> ],
> ["address",[
> ["street","Naradawe Road"],
> ["line1","near railway station"],
> ["line2","behind annapurna hotel"],
> ["city","kankavali"],
> ["pin","416602"]
> ]
> ]
> ]
**Solution:**
> const person = {
> name: {
> firstName: "sangram",
> midddleName: "shivaji",
> lastName: "Desai",
> },
> address: {
> street: "Naradawe Road",
> line1: "near railway station",
> line2: "behind annapurna hotel",
> city: "kankavali",
> pin: "416602",
> },
> };
>
> function convertObjectTwoArray(person) {
> let resultArray = [];
> for (let key in person) {
> if ((typeof person[key]).toString() === "object") {
> resultArray.push([key, convertObjectTwoArray(person[key])]);
> } else {
> resultArray.push([key, person[key]]);
> }
> }
> return resultArray;
> }
>
> //find result & print
> let resultArray = convertObjectTwoArray(person);
> console.log( JSON.stringify(resultArray));
**Output:**
> [
> ["name",[
> ["firstName","sangram"],
> ["midddleName","shivaji"],
> ["lastName","Desai"]
> ]
> ],
> ["address",[
> ["street","Naradawe Road"],
> ["line1","near railway station"],
> ["line2","behind annapurna hotel"],
> ["city","kankavali"],
> ["pin","416602"]
> ]
> ]
> ] |
1. delete the 'node_modules' folder
2. yarn (or npm) install
I couldn't get the Node Modules folder back after 'yarn'
and I needed to add a file.yarnrc.yml containing " nodeLinker: node-modules "
After that again yarn (or npm) install
That worked for me.
|
I get "Bad PCD format" error.
I would like to know what's wrong with the PCD file.
This is the header to the PCD file:
header = "# .PCD v.7 - Point Cloud Data file format
VERSION .7
FIELDS x y z data
SIZE 4 4 4
TYPE F F F
COUNT 1 1 1
WIDTH 0
HEIGHT 1
VIEWPOINT 0 0 0 1 0 0 0
POINTS 0
DATA binary" |
1. delete the 'node_modules' folder
2. yarn (or npm) install
I couldn't get the Node Modules folder back after 'yarn'
and I needed to add a file .yarnrc.yml containing " nodeLinker: node-modules "
After that again yarn (or npm) install
That worked for me.
|
Maybe try this it would work.
function validateEmail(email) {
const emailPattern = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return emailPattern.test(email);
} |
I started to learn WireMock. My first experience is not very positive. Here's a failing MRE:
```java
import com.github.tomakehurst.wiremock.WireMockServer;
import org.junit.jupiter.api.Test;
public class GenericTest {
@Test
void test() {
new WireMockServer(8090);
}
}
```
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.wiremock</groupId>
<artifactId>wiremock</artifactId>
<version>3.5.1</version>
<scope>test</scope>
</dependency>
```
```lang-none
java.lang.NoClassDefFoundError: org/eclipse/jetty/util/thread/ThreadPool
```
I debugged it a little:
```java
public WireMockServer(int port) {
this(/* -> this */ wireMockConfig() /* <- throws */.port(port));
}
```
```java
// WireMockConfiguration
// ↓ throwing inline
private ThreadPoolFactory threadPoolFactory = new QueuedThreadPoolFactory();
public static WireMockConfiguration wireMockConfig() {
return /* implicit no-args constructor */ new WireMockConfiguration();
}
```
```java
package com.github.tomakehurst.wiremock.jetty;
import com.github.tomakehurst.wiremock.core.Options;
import com.github.tomakehurst.wiremock.http.ThreadPoolFactory;
// ↓ package org.eclipse does not exist, these lines are in red
import org.eclipse.jetty.util.thread.QueuedThreadPool;
import org.eclipse.jetty.util.thread.ThreadPool;
public class QueuedThreadPoolFactory implements ThreadPoolFactory {
@Override
public ThreadPool buildThreadPool(Options options) {
return new QueuedThreadPool(options.containerThreads());
}
}
```
My conclusion:
1. WireMock has a dependency on `org.eclipse`
2. WireMock doesn't include this dependency in its artifact
3. I have to provide it manually
```xml
<!-- like so -->
<dependency>
<groupId>org.eclipse.jetty</groupId>
<artifactId>jetty-util</artifactId>
<version>12.0.7</version>
</dependency>
```
I even visited their [GitHub][1] to see for myself if the dependency is marked as `provided`, but they use Gradle, and I don't know Gradle
But that's not all! You'll also have to include (at least) `com.github.jknack.handlebars` and `com.google.common.cache` (see `com.github.tomakehurst.wiremock.extension.responsetemplating.TemplateEngine`)
My question:
1. What is the rationale behind the decision to exclude multiple dependencies from the WireMock artifact?
[1]: https://github.com/wiremock/wiremock/tree/master |
importing React solved my problem
import React from 'react'; |
I have a large dataset that is difficult to investigate without analysis tools. It's general form is this, but with 16 "ItemType0" columns and 16 "ItemType1", "ItemType2", etc columns.
It represents the properties (many of them) of up to 16 different items recorded at a single timestep, then properties of that timestep.
|Time|ItemType0[0].property|ItemType0[1].property|Property|
|:--:|:-------------------:|:-------------------:|:------:|
|1 |1 |0 |2 |
|2 |0 |1 |2 |
|3 |3 |3 |2 |
I'd like to receive:
|Time|ItemType0.property|Property|
|:--:|:----------------:|:------:|
|1 |1 |2 |
|2 |0 |2 |
|3 |3 |2 |
|1 |0 |2 |
|2 |1 |2 |
|3 |3 |2 |
```
import pandas as pd
wide_df = pd.DataFrame({
"Time": [1,2,3],
"ItemType0[0].property": [1,0,3],
"ItemType0[1].property": [0,1,3],
"Property": [2,2,2]})
```
What I've tried:
1. Melt:
```
ids = [col for col in wide_df.columns if "[" not in col]
inter_df = pd.melt(wide_df, id_vars=ids, var_name="Source")
```
MemoryError: Unable to allocate 28.3 GiB for an array with shape (15,506831712) and data type uint32
2. I wouldn't even know where to begin with `pd.wide_to_long` as everything doesn't start with the same. |
I have a react native app with an android native java module that accesses my local Google Fit healthstore using the Java Google Fit API:
DataReadRequest readRequest = new DataReadRequest.Builder()
.enableServerQueries()
.aggregate(DataType.AGGREGATE_STEP_COUNT_DELTA)
.bucketByTime(interval, TimeUnit.SECONDS)
.setTimeRange(start, end, TimeUnit.MILLISECONDS)
.build();
Fitness.getHistoryClient(getReactContext(), getGoogleAccount())
.readData(readRequest)
.addOnSuccessListener(response -> {
for (Bucket bucket : response.getBuckets()) {
for (DataSet dataSet : bucket.getDataSets()) {
readDataSet(dataSet);
}
}
try {
getCallback().onComplete(getReport().toMap());
} catch (JSONException e) {
getCallback().onFailure(e);
}
})
.addOnFailureListener(e -> getCallback().onFailure(e));
My problem is that for some `start` and `end` intervals for a particular user, the code gets stuck in the `HistoryClient`'s `.readData(readRequest)`, never resolving to the `onSuccessListener` or `onFailureListener` callbacks. In one particular case, to correct this, I can vary the `start` or `end` date to reduce the range, and suddenly the history client returns a data response. There doesn't seem to be any pattern of this bug relative to the `start` and `end` of the `readRequest`. In this case, the range was only over a week or so.
I initially thought that some data samples in Google Fit may be corrupt, thus reducing the range of the request would miss these samples, hence explaining why it may suddenly work by tinkering with `start` and `end`. However, by repositioning the `start` and `end` to explicitly cover these suspected samples, Google Fit works normally and a response is returned. I can timeout the async call using a `CompletableFuture`, therefore I know there is a `.readData` thread spinning in there somewhere! No exception is thrown.
I have set up all relevant read permissions in my google account's oAuth credentials - I can verify in my user account settings that the connected app indeed has these health data read permissions. I am using
'com.google.android.gms:play-services-fitness:21.1.0'
'com.google.android.gms:play-services-auth:21.0.0'
in my android build file. I have noticed the problem for both `react-native 0.65.3` (android `targetSdkVersion 31`, `compileSdkVersion 31`) and `react-native 0.73.2` (android `targetSdkVersion 34`, `compileSdkVersion 34`).
Are there any further steps I can take to diagnose the bug? When viewing the date range in Google Fit app, I see no problem and the step counts are there. |
|arrays|matlab|genetic-algorithm|optim| |
In my case, simply restarting the application wasn't enough to access the environment variables. What worked for me was:
1. Stopping the application (using Ctrl+C in the terminal)
2. Rebuilding it with **npm run build**
3. Starting it again with **npm start**
After this env vars were successfully loaded. |
|android|android-jetpack-compose|google-signin|credentials|android-credential-manager| |
I've a OpenID connection in place who was working yesterday and today i'm unable to connect when i'm on my local server i've also the same openID online and it works perfectly fine. it throw me the error 'You must have either https wrappers or curl enabled.' when I click on the login button
I've saw on another post this:
'Have you tried adding or uncommenting extension=php_curl.dll or extension=php_openssl.dll to your php.ini?'
I've tried it but didn't worked for me.
BTW my phpmyadmin return also :
'The mysqli extension is missing. Please check your PHP configuration. See our documentation for more information.' |
My openID Authentication return 'You must have either https wrappers or curl enabled.' |
|php|authentication|openid|steam| |
null |
I have an existing map in Firebase that I'm trying to update with Flutter. Executing the code 'await documentReference.update()' causes the simulator to lose connection.
Network connectivity is good.
Rules are good.[![The map/list that I'm trying to update the existing map/list with.][1]][1]
'''await documentReference.update(outerMap);'''
[![Existing Firebase Collection][2]][2]
[1]: https://i.stack.imgur.com/8VGwA.png
[2]: https://i.stack.imgur.com/GOEOH.png |
Updating existing document in Firebase using Flutter causes Lost Of Connection to the Simulator |
|flutter|firebase|google-cloud-firestore| |
I im needing to detect a pc or/and get bytes from USB, i was testing and nor my own application in kotlin or play store apps can detect my pc, there is a way to make this work?
The connection i meant is PC:Host - Cellphone:Client
(PC sends data to an Android phone, and the phone retrieves it)
USB: Type-C 2.0 (not OTG)
Android code:
```
val DeviceMan = getSystemService(Context.USB_SERVICE) as UsbManager
val Accesoryls = DeviceMan.accessoryList //Gives null
val Devicels = DeviceMan.deviceList //Gives size == 0
```
The manifest XML already has the feature and permissions
The cellphone is in USB depuration mode
The PC detects the cellphone (But when sending raw data to the USB, the access is denied)
PC code (Minimal):
std::cout << "Opening device..." << std::endl;
CelHandle = libusb_open_device_with_vid_pid(contexto, CelularDesc.idVendor, CelularDesc.idProduct);
std::cout << "Detaching device..." << std::endl;
libusb_set_auto_detach_kernel_driver(CelHandle, 0);
std::cout << "Claiming device..." << std::endl;
libusb_claim_interface(CelHandle, 0);
int endsize;
std::cout << "Transfering device..." << std::endl;
std::vector<uchar> buf = CaptureScreen();
libusb_config_descriptor* Cdescriptor;
for(int i = 0; i < CelularDesc.bNumConfigurations; i++){
libusb_get_config_descriptor(Celular, i,&Cdescriptor);
if(Cdescriptor->bNumInterfaces == 1){
break;
}
}
int ENDPOINT;
std::cout << Cdescriptor->bNumInterfaces << std::endl;
for (int i = 0; i < Cdescriptor->bNumInterfaces; i++){
libusb_interface interface = Cdescriptor->interface[i];
for (int j = 0; j < interface.num_altsetting; j++){
libusb_interface_descriptor LIdescriptor = interface.altsetting[j];
for (int k = 0; k < LIdescriptor.bNumEndpoints; k++){
libusb_endpoint_descriptor Edescriptor = LIdescriptor.endpoint[k];
if (Edescriptor.bEndpointAddress == 0x81){
std::cout << Edescriptor.bEndpointAddress << std::endl;
ENDPOINT = Edescriptor.bEndpointAddress;
std::cout << "Encontro endpoint address" << Edescriptor.bDescriptorType << std::endl;
break;
}
else{
ENDPOINT = Edescriptor.bEndpointAddress;
}
}
}
}
for (int i = 0; i < buf.size(); i++){
int r = libusb_bulk_transfer(CelHandle, ENDPOINT, &buf[i], 1, &endsize, 0);
std::cout << int(buf[i]) << std::endl;
if (r < 0){
std::cout << "Transfer error " << r << std::endl;
system("PAUSE");
}
}
std::cout << "BYTES: " << endsize << std::endl;
Always is transfer error (if having timeout, the error is a timeout error, else, it gets stuck)
PC Operative system: Windows 11
Physical cellphone Operative system: Android 9
Deprecated function mentioned in a comment:
val accessory = intent.getParcelableExtra(UsbManager.EXTRA_ACCESSORY) as UsbAccessory
In the intent file:
@Deprecated
public @Nullable <T extends Parcelable> T getParcelableExtra(String name) {
return mExtras == null ? null : mExtras.<T>getParcelable(name);
}
/**
* Retrieve extended data from the intent.
*
* @param name The name of the desired item.
* @param clazz The type of the object expected.
*
* @return the value of an item previously added with putExtra(),
* or null if no Parcelable value was found.
*
* @see #putExtra(String, Parcelable)
*/
public @Nullable <T> T getParcelableExtra(@Nullable String name, @NonNull Class<T> clazz) {
return mExtras == null ? null : mExtras.getParcelable(name, clazz);
}
Also tried changing the minimum android version to the API 12, like one post said, but didn't work |
Going with the code you provided (and with defining the CSS variables which were not defined in your code), you can do it like this. The comments are within the code (CSS), an they begin with `this is new`.
<!-- begin snippet: js hide: false console: true babel: false -->
<!-- language: lang-css -->
/* this is new - I'm guessing you have your CSS variables defined, just not posted in your
example code. I've added them here for testing purposes
*/
:root {
--medium: 75px;
--med-ub: 150px;
--med-small-lb: 37px;
--med-large: 90px;
--med-large-ub: 120px;
--med-large-lb: 25px;
--large: 80px;
--primary_colour: rgba(37,168,219,1);
--tirtiary_colour: rgba(37,100,219,1);
}
.content_container {
display: flex;
justify-content: space-evenly;
}
.tablet {
display: inline-flex;
margin-top: 5vh;
/*Height restraints*/
height: var(--medium);
max-height: var(--med-ub);
min-height: var(--med-small-lb);
/*Width restraints*/
width: var(--med-large);
max-width: var(--med-large-ub);
min-width: var(--med-large-lb);
/*Margins*/
margin-right: 1vw;
margin-left: 1vw;
background-color: var(--primary_colour);
border: solid 1px var(--tirtiary_colour);
border-radius: 1vw;
transition: 1s;
/* this is new - needed for centering the #text */
position:relative;
text-align: center;
}
#code_block {
opacity: 1;
/* this is new - provided an image link for testing purposes, you will use your own */
background-image: url("https://placehold.co/600x400/F23/99FFAC");
/* this is new - provided to prevent the bg img from spilling out due to .tablet border radius */
border-radius: 1vw;
/* this is not needed */
/*background-color: rgba(0,0,0,0.5);*/
background-size: cover;
background-repeat: no-repeat;
/* this is new - just a personal preference */
background-position: center;
transition: opacity 1s ease;
/* this is new - added because your #code_block will not be showing, as it has no content */
height: 100%;
width: 100%;
}
/* this is new - you are hovering over the .tablet, and that should trigger 0 opacity for #code_block */
.tablet:hover #code_block {
opacity: 0;
transition: all 1s ease;
}
.tablet:hover {
width: var(--large);
height: var(--medium);
transition: 1s;
/* this is new - background color is added to fit with your requirements,
the cursor was my addition - simply to indicate clickability */
background-color: black;
cursor: pointer;
}
/* this is new - I went with 0 opacity instead of display block. Also, additional CSS was added:
• transition
• centering horizontally and vertically (left, right, margin, top, translate)
*/
#text {
position: absolute;
color: white;
opacity: 0;
transition: all 1s ease;
left: 0;
right: 0;
margin: auto;
top: 50%;
transform: translateY(-50%);
}
/* this is new - instead of hovering exactly on #text, the effect is triggered when you hover
over .tablet, and that effect is applied to #text
*/
.tablet:hover #text {
opacity: 1;
transition: all 1s ease;
}
<!-- language: lang-html -->
<div class="content_container">
<div class="tablet">
<p id="text">Text</p>
<div id="code_block"></div>
</div>
<div class="tablet"></div>
<div class="tablet"></div>
<div class="tablet"></div>
</div>
<!-- end snippet --> |
As mentioned in the comments, you can use the `excluded` parameter of [`np.vectorize`][1]:
```python3
import numpy as np
def f(x, y):
assert np.shape(x) == ()
assert np.shape(y) != ()
return x*len(y)
f2 = np.vectorize(f, excluded=[1, 'y'])
x = np.arange(3)
y = ['a', 'b', 'c']
f2(x, y) # array([0, 3, 6])
f2(x, y=y) # array([0, 3, 6])
```
[1]: https://numpy.org/doc/stable/reference/generated/numpy.vectorize.html |
> My question was not about browser requests (i know how to handle them) but about requsts maded from server side, for example if you put some import in the first code block of your answer.
In general you can use [`inotify`](https://man7.org/linux/man-pages/man7/inotify.7.html).
If the file exists you can use [`Deno.watchFs()`](https://deno.land/api@v1.41.3?s=Deno.watchFs).
Some of my recent file system observer experiments https://github.com/guest271314/fs, and some I did a while ago https://github.com/guest271314/captureSystemAudio?tab=readme-ov-file#usage. |
Same issue for me.
Try to use
return navigateTo("/sign-in", { external: true });
instead
return navigateTo("/sign-in"); |
I have a Javafx program that I'm trying to connect to a SQL Database through Windows Authentication mode. I have put the mssql-jdbc_auth-12.6.1.x64.dll file in the - C:\Program Files\Java\jdk-17\bin. I have set the -Djava.library.path="C:\Program Files\Java\jdk-17\bin" within Intellij run configurations. The connection file looks like the following:
try{
Class.forName("com.microsoft.sqlserver.jdbc.SQLServerDriver");
return DriverManager.getConnection("jdbc:sqlserver://NEMGLASQL001\\SQLSERVER:1433;database=gaitData; integratedSecurity = true; authenticationScheme=NativeAuthentication;");
} catch (ClassNotFoundException | SQLException e) {
throw new RuntimeException(e);
}
}
The code that I'm running to show all results in a specific table is as following:
try{
DatabaseConnection db = new DatabaseConnection();
Connection cn1 = db.getDBConnection();
String sql = "SELECT * FROM DiagnosisCode";
Statement statement = cn1.createStatement();
ResultSet resultSet = statement.executeQuery(sql);
} catch (Exception e) {
throw new RuntimeException(e);
}
The errors showing are -
Mar 19, 2024 9:39:48 AM com.microsoft.sqlserver.jdbc.AuthenticationJNI <clinit>
WARNING: Failed to load the sqljdbc_auth.dll cause : no sqljdbc_auth in java.library.path: C:\Program Files\Java\jdk-17\bin;C:\WINDOWS\Sun\Java\bin;C:\WINDOWS\system32;C:\WINDOWS;C:\Program Files\Common Files\Oracle\Java\javapath;C:\WINDOWS\system32;C:\WINDOWS;C:\WINDOWS\System32\Wbem;C:\WINDOWS\System32\WindowsPowerShell\v1.0\;C:\WINDOWS\System32\OpenSSH\;C:\Program Files (x86)\Sennheiser\SenncomSDK\;C:\Program Files (x86)\PuTTY\;C:\Program Files (x86)\Microsoft SQL Server\160\Tools\Binn\;C:\Program Files\Microsoft SQL Server\160\Tools\Binn\;C:\Program Files\Microsoft SQL Server\Client SDK\ODBC\170\Tools\Binn\;C:\Program Files\Microsoft SQL Server\160\DTS\Binn\;C:\Program Files (x86)\Microsoft SQL Server\160\DTS\Binn\;C:\Users\sh0184\AppData\Local\Microsoft\WindowsApps;C:\Users\sh0184\AppData\Local\Programs\Microsoft VS Code\bin;C:\Users\sh0184\AppData\Local\JetBrains\IntelliJ IDEA Community Edition 2023.3\bin;;C:\Users\sh0184\AppData\Local\Programs\Git\cmd;C:\Users\sh0184\AppData\Local\JetBrains\IntelliJ IDEA 2023.3.2\bin;;.
does the sqlauth dll need to go somewhere else? |
Google Fit SDK API gets stuck executing historyClient.readData |
|android|react-native|google-fit|google-fit-sdk|google-fitness-api| |
For some reason I've made some change either in whitespace somewhere, that it makes it fail.
```
public function test() {
$multiLineSring = <<<EOD
this awesome person
has become something else
entirely
EOD;
}
```
I get the following error: `PHP Parse error: syntax error, unexpected '' (T_ENCAPSED_AND_WHITESPACE), expecting identifier (T_STRING) or variable (T_VARIABLE) or number (T_NUM_STRING) in` |
In which bizarre scenario can multiline strings fail to work? |
|php| |
I am trying to adopt [Swift Charts][1] but after adding it to my project and importing it in the file, I am still seeing warning messages in Xcode. I've read [other threads][2] and tried suggestions routes like clearing the cache, resetting Xcode, removing/re-adding the framework, etc. Nothing works.
import UIKit
import SwiftUI
import Charts
class MainQuickMenu: UIViewController {
... skipping IBOutlets, variables, lifecycle, etc...
// First error thrown below "Cannot find type 'BarChartView' in scope"
func createBarChart(data: [NSObject], containerView: UIView) -> BarChartView {
let startDate = Calendar.current.date(byAdding: .day, value: -90, to: Date()) ?? Date()
var dateCounts = [String: Int]()
for task in data {
if let createdDate = task.value(forKey: "createdDate") as? Date, createdDate >= startDate {
let formatter = DateFormatter()
formatter.dateFormat = "yyyy-MM-dd" // You can adjust the date format as needed
let dateString = formatter.string(from: createdDate)
dateCounts[dateString, default: 0] += 1
}
}
let chartView = BarChartView()
var dataEntries: [BarChartDataEntry] = []
var index = 0
....
Tried so far:
- I've tried with and without Charts.framework added to "Frameworks, libraries..."
- I updated my entire project and code to conform to an iOS16 deployment target, as that's a requirement for Swift Charts.
- I added SwiftUI to the file, even though the project is UIKit based, as I was willing to learn SwiftUI and adopt it for this view but still the errors persist.
- I also tried importing SwiftUI and adding a standalone (outside of the class) struct using Chart components, to see if it was my class, but Chart classes still got errors.
Adding images below of configs as well.
Errors
[![enter image description here][3]][3]
Swift Charts Framework Imported
[![enter image description here][4]][4]
iOS16 deployment target
[![enter image description here][5]][5]
My goal here is just to understand how to begin to work with these Charts classes without the errors. Am I not importing Swift Charts correctly? To be clear, I'm not looking for an actual solution to the charts themselves.
[1]: https://developer.apple.com/documentation/charts
[2]: https://stackoverflow.com/questions/48263413/import-swift-charts
[3]: https://i.stack.imgur.com/uvVAr.png
[4]: https://i.stack.imgur.com/UQY7w.png
[5]: https://i.stack.imgur.com/YgGny.png |
Swift Charts not importing into project correctly |
I'm trying to create a test to check the correct behaviout of my service object,
```ruby
def api_call_post(connection, message, url)
pp message
response = connection.post do |conn|
conn.url url
conn.body = message
conn.headers = @headers
end
check_response(response)
end
...
```
this is the test :
```ruby
test "create a title" do
body = { "name" => 'some name',
"external_id" =>'004',
"title_type" =>'feature',
"tags" => 'some tag' }.to_json
puts body
stub_request(:post, "some web")
.with(body: body )
.to_return(status: 201, body: "Created", headers: {})
response = MovidaApi.new(payload).create_title
assert response.success?
assert_equal "Created", response.body
end
...
```
The problem comes when I include the `.with` in the stub, (without it's working ok),
I printed statements and the output are exactly the same.
The error is:
Error:
MovidaApiTest#test_create_a_title:
WebMock::NetConnectNotAllowedError: Real HTTP connections are disabled. Unregistered request: POST https://staging-movida.bebanjo.net/api/titles with headers
{'Accept'=>'application/json', 'Accept-Encoding'=>'gzip;q=1.0,deflate;q=0.6,identity;q=0.3', 'Content-Length'=>'0', 'Content-Type'=>'application/json', 'User-Agent'=>'Ruby'}
What i'm doing wrong?
I tried to use the snippet suggested but still don't work.
I expect the test to pass |
I have created a Python script to deploy in Google Cloud Functions, in order to manage the creation of new documents on my Firestore database (Firestore triggers). I am deploying the function in the Google Cloud console, not locally. The functions is implemented correctly without any error. However, when I create a new document, an error occurs that is related with Flask, which is nosense as my Python script does not require Flask. This is all the setup of the function : [![enter image description here][1]][1] [![enter image description here][2]][2] [![enter image description here][3]][3], this is the error : [![enter image description here][4]][4], and this is the Firestore section, document, and value created : [![enter image description here][5]][5]. Why am I having this error? Thanks in advance!
[1]: https://i.stack.imgur.com/ivIgy.png
[2]: https://i.stack.imgur.com/iQJDU.png
[3]: https://i.stack.imgur.com/KSwQX.png
[4]: https://i.stack.imgur.com/lWDHL.png
[5]: https://i.stack.imgur.com/E0EDa.png |
Nonsense error using a Python Google Cloud Function |
|python|google-cloud-platform|google-cloud-firestore|google-cloud-functions| |
'use client'
import { useEffect, useState, useRef } from 'react'
type CredentialRequestOptions = {
otp: OTPOptions
signal: AbortSignal
}
type OTPOptions = {
transport: string[]
}
const InputConfirmCode = () => {
const [value, setValue] = useState<string>()
const hiddenOTPRef = useRef<HTMLInputElement>(null)
useEffect(() => {
if ('OTPCredential' in window) {
if (!hiddenOTPRef.current) return
const ac = new AbortController()
const o: CredentialRequestOptions = {
otp: { transport: ['sms'] },
signal: ac.signal,
}
navigator.credentials
.get(o)
.then((otp: any) => {
if (hiddenOTPRef.current) {
hiddenOTPRef.current.value = otp.code
autoFill()
}
})
.catch((err) => {
console.error(err)
})
}
}, [])
const autoFill = () => {
if (hiddenOTPRef.current && hiddenOTPRef.current.value.length === 5) {
setValue(hiddenOTPRef.current.value)
}
}
return (
<input
style={{ padding: '14px' }}
type="hidden"
value={value}
autoComplete="one-time-code"
inputMode="numeric"
ref={hiddenOTPRef}
/>
)
}
export default InputConfirmCode
|
I have the following problem:
I have the URL to a picture 'HTTP://WWW.ROLANDSCHWAIGER.AT/DURCHBLICK.JPG' saved in my database. I think you see the problem here: The URL is in uppercase. Now I want to display the picture in the SAP GUI, but for that, I have to convert it to lowercase.
I have the following code from a tutorial, but without the conversion:
*&---------------------------------------------------------------------
*
*& Report ZDURCHBLICK_24035
*&---------------------------------------------------------------------
*
*&
*&---------------------------------------------------------------------
*
REPORT zdurchblick_24035.
TABLES: zproject_24035.
PARAMETERS pa_proj TYPE zproject_24035-projekt OBLIGATORY.
DATA gs_project TYPE zproject_24035.
*Controls
DATA: go_container TYPE REF TO cl_gui_custom_container.
DATA: go_picture TYPE REF TO cl_gui_picture.
START-OF-SELECTION.
WRITE: / 'Durchblick 3.0'.
SELECT SINGLE * FROM zproject_24035 INTO @gs_project WHERE projekt =
@pa_proj.
WRITE gs_project.
IF sy-subrc = 0.
WRITE 'Wert im System gefunden'.
ELSE.
WRITE 'Kein Wert gefunden'.
ENDIF.
WRITE : /'Es wurden', sy-dbcnt, 'Werte gefunden'.
AT LINE-SELECTION.
zproject_24035 = gs_project.
CALL SCREEN 9100.
*&---------------------------------------------------------------------
*
*& Module CREATE_CONROLS OUTPUT
*&---------------------------------------------------------------------
*
*&
*&---------------------------------------------------------------------
*
MODULE create_conrols OUTPUT.
* SET PF-STATUS 'xxxxxxxx'.
* SET TITLEBAR 'xxx'.
IF go_container IS NOT BOUND.
CREATE OBJECT go_container
EXPORTING
container_name = 'BILD'.
CREATE OBJECT go_picture
EXPORTING
parent = go_container.
CALL METHOD go_picture->load_picture_from_url
EXPORTING
url = gs_project-bild.
ENDIF.
ENDMODULE.
|
I am creating an operating system and I created a child process using c with this code:
```
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <unistd.h>
#include <windows.h>
int main() {
FILE *fptr;
// Open a file in read mode
fptr = fopen("filename.txt", "r");
// Store the content of the file
char myString[100];
fgets(myString, 100, fptr);
fclose(fptr);
PROCESS_INFORMATION ni;
STARTUPINFO li;
ZeroMemory(&li, sizeof(li));
li.cb = sizeof(li);
if (CreateProcess(NULL, "child_process.exe", NULL, NULL, FALSE, 0, NULL, NULL, &li, &ni)) {
// Parent process
WaitForSingleObject(ni.hProcess, INFINITE);
CloseHandle(ni.hProcess);
CloseHandle(ni.hThread);
} else {
// Child process
}
pid_t pid = getpid();
printf("(%d) WARNING: These processes are vital for the OS:\n", pid);
printf("(%d) %d\n", pid, pid);
printf("(%d) %s\n\n\n", pid, myString);
return 0;
}
```
And I could not end the child process. I do not want to use signals as they are too complex and I am a beginner.
I tried using ` return 0;` and it did not work the process was still running |
Is there a function to end a child process? |
|c|operating-system| |
i am trying to get application context for showToast but not able to get it here is my code, always getting error :- lateinit property appContext has not been initialized, kindly help
```
package com.coding.APPNAVIGATION.MenuAndNavigationDrawer
import android.app.Application
import android.content.Context
open class MainApplication : Application() {
override fun onCreate() {
super.onCreate()
MainApplication.appContext = applicationContext
}
companion object {
lateinit var appContext : Context
}
}
```
```
package com.coding.APPNAVIGATION.MenuAndNavigationDrawer.Utils
import android.widget.Toast
import com.coding.APPNAVIGATION.MenuAndNavigationDrawer.MainApplication
object Utils{
fun showToast(message : String){
Toast.makeText(MainApplication.appContext, message, Toast.LENGTH_LONG).show()
}
}
```
i want to get application context to run showToast anywhere within the project |
I am trying to create a directory and a file inside it in Java (spring) and it working fine when I execute it on Windows, but after deploying the war file on Wildfly on linux it's throwing the error:
**code snippet:**
```
String filePath = "reports/";
File f = new File(filePath);
if (!f.exists()) {
boolean b=f.mkdir();
if(b) {
File xlFile = new File(filePath+ File.separator + "report.xlsx");
}
}
```
Error after deploying it on wildfly that is runnig on Ubuntu:
```
{
"data": "java.io.FileNotFoundException: reports/Attendance.xlsx (No such file or directory)",
"message": "Error while writing file",
"status": 400,
"isSuccess": true
}
```
Please guide.
I tried many ways to do this but nothing works. |
How can I remove a kernel from Jupyter Notebook?
I have an R kernel on my Jupyter Notebook. Recently, the kernel always dies right after I open a new notebook.
|
Remove the kernel on a Jupyter Notebook |
I cant show a pdf document save it in a database table direcly to a WebBrowser using a MemoryStream.
I have a DataBase with pfd documents saved in a table filed, and I want to show the pdf document saved in a table field over a WebBrowser. I have this code:
```
<WebBrowser x:Name="pdfWebViewer" Grid.Row="1" Margin="0,0,439,0"/>
<WebBrowser x:Name="pdfWebViewer2" Grid.Row="1" Margin="425,0,0,0"/>
```
and
```
pdfWebViewer.Navigate(new Uri("about:blank"));
if (_record != null)
{
_bytes = _record.PDF_DOC; // It's a BLOB Database field ;
MemoryStream outstream = new MemoryStream(_record.PDF_DOC.ToArray());
System.IO.File.WriteAllBytes(_path + "borar.pdf", _record.PDF_DOC.ToArray());
pdfWebViewer.Navigate(_path + "borar.pdf");
pdfWebViewer2.NavigateToStream(outstream);
}
```
The result is :
[](https://i.stack.imgur.com/15BoW.png)
Document on the left is pdfWebViewer and on the right is pdfWebViewer2 |
WPF How to show a MemoryStream PDF document to a WebBrowser |
null |
Change this line of code
chain = cl.user_session.set("chain")
to
chain = cl.user_session.get("chain")
Because we are already setting the chain variable above, here we have to get that |
How to create a logic that when I logged in as a seller, each of them have different set of products.
NOTE: I temporarily hardcoded "2" for the parameters to add seller id in the Database.GetProductDetails() and Database.AddProduct().
```
**FROM THE DATABASE.CS**
//Initializing for Seller Accounts Database
public async static void InitializeDB_SELLERACCOUNTS()
{
await ApplicationData.Current.LocalFolder.CreateFileAsync("MyDatabase.db", CreationCollisionOption.OpenIfExists);
string pathtoDB = Path.Combine(ApplicationData.Current.LocalFolder.Path, "MyDatabase.db");
using (SqliteConnection con = new SqliteConnection($"Filename={pathtoDB}"))
{
con.Open();
string initCMD = @"CREATE TABLE IF NOT EXISTS Sellers (
SELLER_ID INTEGER PRIMARY KEY AUTOINCREMENT,
BusinessName TEXT NOT NULL,
Email TEXT NOT NULL,
Username TEXT NOT NULL,
LastName TEXT NOT NULL,
FirstName TEXT NOT NULL,
MiddleName TEXT NOT NULL,
Password TEXT NOT NULL,
PhoneNumber TEXT NOT NULL,
AddressLine1 TEXT NOT NULL,
AddressLine2 TEXT NOT NULL
)";
SqliteCommand CMDcreateTable = new SqliteCommand(initCMD, con);
CMDcreateTable.ExecuteReader();
con.Close();
}
}
//Initializing for Product Details Database
public async static void InitializeDB_PRODUCTDETAILS()
{
await ApplicationData.Current.LocalFolder.CreateFileAsync("MyDatabase.db", CreationCollisionOption.OpenIfExists);
string pathtoDB = Path.Combine(ApplicationData.Current.LocalFolder.Path, "MyDatabase.db");
using (SqliteConnection con = new SqliteConnection($"Filename={pathtoDB}"))
{
con.Open();
string initCMD = @"CREATE TABLE IF NOT EXISTS ProductDetails (
PRODUCTDETAILS_ID INTEGER PRIMARY KEY AUTOINCREMENT,
Seller_ID INTEGER NOT NULL,
ProductName TEXT NOT NULL,
ProductCategory TEXT,
ProductPrice REAL,
ProductDescription TEXT,
ProductQuantity INTEGER,
ProductPicture BLOB,
FOREIGN KEY(Seller_ID) REFERENCES Sellers(SELLER_ID)
)";
SqliteCommand CMDcreateTable = new SqliteCommand(initCMD, con);
CMDcreateTable.ExecuteReader();
con.Close();
}
}
// Query to Retreive Seller's specific product details
public static List<ProductDetails> GetProductDetails(int seller_id)
{
List<ProductDetails> productList = new List<ProductDetails>();
string pathtoDB = Path.Combine(ApplicationData.Current.LocalFolder.Path, "MyDatabase.db");
using (SqliteConnection con = new SqliteConnection($"Filename={pathtoDB}"))
{
con.Open();
string selectCMD = "SELECT * FROM ProductDetails WHERE Seller_ID = @Seller_ID";
SqliteCommand cmdSelectRecords = new SqliteCommand(selectCMD, con);
cmdSelectRecords.Parameters.AddWithValue("@Seller_ID", seller_id);
SqliteDataReader reader = cmdSelectRecords.ExecuteReader();
while (reader.Read())
{
ProductDetails product = new ProductDetails();
product.PRODUCTDETAILS_ID = reader.GetInt32(0);
product.Seller_ID = reader.GetInt32(1);
product.ProductName = reader.GetString(2);
product.ProductCategory = reader.IsDBNull(3) ? null : reader.GetString(3);
product.ProductPrice = reader.GetDouble(4);
product.ProductDescription = reader.GetString(5);
product.ProductQuantity = reader.GetInt32(6);
product.ProductPicture = reader.IsDBNull(7) ? null : GetByteArrayFromBlob(reader, 7); // Retrieve image data as byte array
productList.Add(product);
}
reader.Close();
con.Close();
}
return productList;
}
**FROM THE YOURWATERPRODUCTS.CS**
public sealed partial class YourWaterProducts : Page
{
private StorageFile selectedFile;
public YourWaterProducts()
{
this.InitializeComponent();
PopulateProductList();
}
private void PopulateProductList()
{
List<ProductDetails> productDetailsList = Database.GetProductDetails(2);
// Bind the list of product details to the ListView
ListProducts.ItemsSource = productDetailsList;
}
**FROM THE ADDPRODUCTS.CS**
if (result == ContentDialogResult.Primary)
{
// If the user confirms, proceed to add the product
string productName = tbxProductName.Text;
string productCategory = (cbxProductCategory.SelectedItem as ComboBoxItem)?.Content.ToString();
string productDescription = tbxProductDescription.Text;
byte[] productPicture = await ConvertImageToByteArray(selectedFile);
// Call AddProduct with SellerId
Database.AddProduct(2, productName, productCategory, productPrice, productDescription, productQuantity, productPicture);
```
I really hope that when I try to logged in as a seller, each of them will show up different sets of products based on their seller's id. |
How to make that each seller has its own different set of products using sqlite and uwp |
|c#|sqlite|uwp| |
null |
i am trying to make a uart code for nuvorton m0516LAN but i am facing this issue
RTE/Device/M0516LAN/retarget.c(337): error: call to undeclared function 'asm'; ISO C99 and later do not support implicit function declarations [-Wimplicit-function-declaration]
here is the handler function
void HardFault_Handler(void)
{
asm("MOVS r0, #4 \n"
"MOV r1, LR \n"
"TST r0, r1 \n" /*; check LR bit 2 */
"BEQ 1f \n" /*; stack use MSP */
"MRS R0, PSP \n" /*; stack use PSP, read PSP */
"MOV R1, LR \n" /*; LR current value */
"B Hard_Fault_Handler \n"
"1: \n"
"MRS R0, MSP \n" /*; LR current value */
"B Hard_Fault_Handler \n"
::[Hard_Fault_Handler] "r" (Hard_Fault_Handler) // input
);
while(1);
} |
(V8 developer here.)
It's not the hashing (or lack thereof), it's the flattening (or lack thereof) of strings that have been built up with thousands of `+= 'x'` single-character concatenations.
With a couple of exceptions, V8 usually handles string concatenations by creating a "cons string" (sometimes also called "rope"), which is essentially a pair of pointers at its two halves; with the `+= 'x'` strategy you're hence creating a very long linked list of single-character strings. Some operations require (and therefore cause) flattening of such strings, others don't. Always flattening cons strings automatically (doesn't matter whether it's in the background or not) would have undesirable costs in terms of CPU and memory.
Here's a way to speed up the test massively by constructing the strings more efficiently:
<!-- begin snippet: js hide: false console: true babel: null -->
<!-- language: lang-js -->
(()=>{
// making 10k character strings
const big_strings = [];
const palette = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789";
for (let i = 0; i < 100; i++) {
// CHANGED: [], .push(...), .join("")
let big_string = [];
for (let i = 0; i < 10_000; i++) {
big_string.push(palette[Math.floor(Math.random() * palette.length)]);
}
big_strings.push(big_string.join(""));
// End of changes, everything below is unchanged.
}
// comparison functions
function cmp1(a, b) {
return a === b;
}
function cmp2(a, b) {
const lol = new Set();
lol.add(a);
lol.add(b);
return a === b;
}
// tests
let sorted_1, sorted_2;
console.time("Test 1");
for (let i = 0; i < 100; i++) {
sorted_1 = big_strings.slice();
sorted_1.sort(cmp1);
}
console.timeEnd("Test 1");
console.time("Test 1");
for (let i = 0; i < 100; i++) {
sorted_1 = big_strings.slice();
sorted_1.sort(cmp1);
}
console.timeEnd("Test 1");
console.time("Test 1");
for (let i = 0; i < 100; i++) {
sorted_1 = big_strings.slice();
sorted_1.sort(cmp1);
}
console.timeEnd("Test 1");
console.time("Test 2");
for (let i = 0; i < 100; i++) {
sorted_2 = big_strings.slice();
sorted_2.sort(cmp2);
}
console.timeEnd("Test 2");
console.time("Test 1");
for (let i = 0; i < 100; i++) {
sorted_1 = big_strings.slice();
sorted_1.sort(cmp1);
}
console.timeEnd("Test 1");
// sanity check
let good = 0;
const total = Math.max(sorted_1.length, sorted_2.length);
for (let i = 0; i < total; i++) {
if (sorted_1[i] === sorted_2[i]) {
good++;
} else {
throw("Results do not match.");
}
}
console.log(`Sanity check OK ${good} / ${total}`);
})();
<!-- end snippet -->
**Beware of microbenchmarks, because they will mislead you!** If the strings in your real application are created differently, you will (quite likely) take away incorrect conclusions from your original test. |
|swift|swiftcharts| |
I'm implementing a drag&drop in JS, it works but the detection of my file is quite bad (when the file is drag over the dropzone it isn't always detected).
Also i would like to click anywhere in the dropzone to activate my input it doesn't work either.
Here is my code and a link to my codePen to test it: https://codepen.io/ChloeMarieDom/pen/QWPLqNz?editors=0010
<!-- begin snippet: js hide: false console: true babel: false -->
<!-- language: lang-js -->
// Variables
const dropzone = document.querySelector('.dropzone');
const filenameDisplay = document.querySelector('.filename');
const input = document.querySelector('.input');
const uploadBtn = document.querySelector('.upload-btn');
const syncing = document.querySelector('.syncing');
const done = document.querySelector('.done');
const upload = document.querySelector('.upload');
const line = document.querySelector('.line');
dropzone.addEventListener("dragover", (e) => {
e.preventDefault();
e.stopPropagation();
dropzone.classList.add("dragover");
});
dropzone.addEventListener("dragleave", () => {
dropzone.classList.remove("dragover");
});
dropzone.addEventListener("drop", (e) => {
e.preventDefault();
e.stopPropagation();
dropzone.classList.remove("dragover");
handleFiles(e.dataTransfer.files);
});
dropzone.addEventListener("dragenter", (e) => {
e.preventDefault();
e.stopPropagation();
dropzone.classList.add("dragover");
});
dropzone.addEventListener("click", () => {
input.click();
});
input.addEventListener('change', (event) => {
const files = event.target.files;
if (files.length > 0) {
const fileName = files[0].name;
document.querySelector('.filename').textContent = fileName;
upload.style.display = 'none';
}
});
dropzone.addEventListener('click', () => {
input.click();
})
uploadBtn.addEventListener('click', () => {
const files = document.querySelector('.input').files;
if (files.length > 0) {
dropzone.style.transition = 'opacity 0.5s ease';
syncing.style.transition = 'opacity 1s ease';
dropzone.style.opacity = '0';
syncing.style.opacity = '0.3';
line.classList.add('active');
uploadBtn.textContent = 'Uploading...';
setTimeout(() => {
done.style.transition = 'opacity 1s ease';
syncing.style.transition = 'opacity 0.5s ease';
syncing.style.opacity = '0';
done.style.opacity = '0.3';
uploadBtn.textContent = 'Done!';
input.value = '';
}, 5000);
}
});
<!-- language: lang-css -->
@import url(https://fonts.googleapis.com/css?family=Open+Sans:400);
.frame {
position: absolute;
display: flex;
justify-content: center;
align-items: center;
top: 50%;
left: 50%;
width: 400px;
height: 400px;
margin-top: -200px;
margin-left: -200px;
border-radius: 2px;
box-shadow: 4px 8px 16px 0 rgba(0, 0, 0, 0.1);
background: linear-gradient(to top right, #3A92AF 0%, #5CA05A 100%);
color: #676767;
font-family: "Open Sans", Helvetica, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
}
.container {
position: absolute;
width: 300px;
height: 260px;
background: #fff;
border-radius: 3px;
box-shadow: 8px 10px 15px 0 rgba(0, 0, 0, 0.2);
}
.title {
position: absolute;
top: 0;
width: 100%;
font-size: 16px;
text-align: center;
border-bottom: 1px solid #676767;
line-height: 50px;
}
.line {
position: relative;
width: 0px;
height: 3px;
top: 49px;
left: 0;
background: #6ECE3B;
}
.line.active {
animation: progressFill 5s ease-out forwards;
}
@keyframes progressFill {
from {
width: 0;
}
to {
width: 100%;
}
}
.dropzone {
visibility: show;
position: absolute;
display: flex;
justify-content: center;
align-items: center;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 100px;
height: 80px;
border: 1px dashed #676767;
border-radius: 3px;
}
.dropzone.dragover {
background-color: rgba(0, 0, 0, 0.1);
}
.upload {
position: absolute;
width: 60px;
opacity: 0.3;
}
.input {
position: absolute;
inset: 0;
opacity: 0;
}
.filename {
overflow: hidden;
}
.syncing {
opacity: 0;
position: absolute;
top: calc(50% - 25px);
left: calc(50% - 25px);
width: 50px;
height: 50px;
animation: rotate 2s linear infinite;
}
@keyframes rotate {
from {
transform: rotate(0deg);
}
to {
transform: rotate(360deg);
}
}
.done {
opacity: 0;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 50px;
height: 50px;
}
.upload-btn {
position: relative;
top: 180px;
left: 80px;
width: 140px;
height: 40px;
line-height: 40px;
text-align: center;
background: #6ECE3B;
border-radius: 3px;
cursor: pointer;
color: #fff;
font-size: 14px;
box-shadow: 0 2px 0 0 #498C25;
transition: all 0.2s ease-in-out;
}
.upload-btn:hover {
box-shadow: 0 2px 0 0 #498C25, 0 2px 10px 0 #6ECE3B;
}
<!-- language: lang-html -->
<div class="frame">
<div class="container">
<div class="title">Drop file to upload</div>
<div class="line"></div>
<div class="dropzone">
<svg class="upload" viewBox="0 0 640 512" width="100" title="cloud-upload-alt">
<path d="M537.6 226.6c4.1-10.7 6.4-22.4 6.4-34.6 0-53-43-96-96-96-19.7 0-38.1 6-53.3 16.2C367 64.2 315.3 32 256 32c-88.4 0-160 71.6-160 160 0 2.7.1 5.4.2 8.1C40.2 219.8 0 273.2 0 336c0 79.5 64.5 144 144 144h368c70.7 0 128-57.3 128-128 0-61.9-44-113.6-102.4-125.4zM393.4 288H328v112c0 8.8-7.2 16-16 16h-48c-8.8 0-16-7.2-16-16V288h-65.4c-14.3 0-21.4-17.2-11.3-27.3l105.4-105.4c6.2-6.2 16.4-6.2 22.6 0l105.4 105.4c10.1 10.1 2.9 27.3-11.3 27.3z" />
</svg>
<span class="filename"></span>
<input type="file" class="input">
</div>
<div class="upload-btn">Upload file</div>
<svg class="syncing" viewBox="0 0 512 512" width="100" title="circle-notch">
<path d="M288 39.056v16.659c0 10.804 7.281 20.159 17.686 23.066C383.204 100.434 440 171.518 440 256c0 101.689-82.295 184-184 184-101.689 0-184-82.295-184-184 0-84.47 56.786-155.564 134.312-177.219C216.719 75.874 224 66.517 224 55.712V39.064c0-15.709-14.834-27.153-30.046-23.234C86.603 43.482 7.394 141.206 8.003 257.332c.72 137.052 111.477 246.956 248.531 246.667C393.255 503.711 504 392.788 504 256c0-115.633-79.14-212.779-186.211-240.236C302.678 11.889 288 23.456 288 39.056z" />
</svg>
<svg class="done" viewBox="0 0 512 512" width="100" title="check-circle">
<path d="M504 256c0 136.967-111.033 248-248 248S8 392.967 8 256 119.033 8 256 8s248 111.033 248 248zM227.314 387.314l184-184c6.248-6.248 6.248-16.379 0-22.627l-22.627-22.627c-6.248-6.249-16.379-6.249-22.628 0L216 308.118l-70.059-70.059c-6.248-6.248-16.379-6.248-22.628 0l-22.627 22.627c-6.248 6.248-6.248 16.379 0 22.627l104 104c6.249 6.249 16.379 6.249 22.628.001z" />
</svg>
</div>
</div>
<!-- end snippet -->
this is what i tried to click everywhere in the dropzone :
`dropzone.addEventListener("click", () => {
input.click();
});`
|
Using the `keyboard` module, we can block every key on the keyboard:
import keyboard
for key in range(150): #150 should be enough for all the keys on the keyboard
keyboard.block_key(key) #block the key until unblocked
#do stuff
for key in range(150):
keyboard.unblock_key(key) #unblocks all the keys
To make this a little easier and readable in your program, you could create two simple functions for it:
import keyboard
def block():
for key in range(150):
keyboard.block_key(key)
def unblock():
for key in range(150):
keyboard.unblock_key(key)
block()
#do stuff
unblock() |
How to Silent Login with NextAuth? |
I have the following tables that are connect via "NBR":
````
Process
````
[![Process table][1]][1]
````
Service
````
[![Service table][2]][2]
[1]: https://i.stack.imgur.com/RZzIn.png
[2]: https://i.stack.imgur.com/fBNAS.png
````
create table service(
DIV CHAR(4 BYTE),
PAID CHAR(2 BYTE),
NBR CHAR(3 BYTE),
MODEL CHAR(1 BYTE),
LINE CHAR(1 BYTE),
YMD NUMBER(8,0)
);
create table process(
DIV CHAR(4 BYTE),
PAID CHAR(2 BYTE),
NBR CHAR(3 BYTE),
BANK CHAR(2 BYTE),
UNIT CHAR(2 BYTE),
CAR CHAR(2 BYTE)
);
INSERT INTO SERVICE (NBR, LINE, PAID, DIV, MODEL, YMD) values ('555', '1', 'NY', 'AR91', '6', 20240311);
INSERT INTO SERVICE (NBR, LINE, PAID, DIV, MODEL, YMD) values ('555', '2', 'NY', 'AR91', '6', 20240311);
INSERT INTO SERVICE (NBR, LINE, PAID, DIV, MODEL, YMD) values ('555', '3', 'NY', 'ZZ1', '6', 20240311);
INSERT INTO SERVICE (NBR, LINE, PAID, DIV, MODEL, YMD) values ('556', '1', 'NY', 'AR10', '8', 20240311);
INSERT INTO SERVICE (NBR, LINE, PAID, DIV, MODEL, YMD) values ('556', '2', 'NY', 'ZZ1', '8', 20240311);
INSERT INTO SERVICE (NBR, LINE, PAID, DIV, MODEL, YMD) values ('557', '1', 'NY', 'AR10', '9', 20240311);
INSERT INTO SERVICE (NBR, LINE, PAID, DIV, MODEL, YMD) values ('600', '1', 'NY', 'ZZ1', '5', 20240311);
INSERT INTO SERVICE (NBR, LINE, PAID, DIV, MODEL, YMD) values ('601', '1', 'NY', 'ZZ1', '7', 20240311);
INSERT INTO PROCESS (NBR, DIV, CAR, BANK, UNIT, PAID) values ('555','AR91','M','1','MC','NY');
INSERT INTO PROCESS (NBR, DIV, CAR, BANK, UNIT, PAID) values ('556','AR91','M','1','MC','NY');
INSERT INTO PROCESS (NBR, DIV, CAR, BANK, UNIT, PAID) values ('557','AR10','M','1','MC','NY');
INSERT INTO PROCESS (NBR, DIV, CAR, BANK, UNIT, PAID) values ('600','ZZ1','M','1','MC','NY');
INSERT INTO PROCESS (NBR, DIV, CAR, BANK, UNIT, PAID) values ('601','ZZ1','M','1','MC','NY');
````
I want to filter out records from "Service" table so that if any "NBR" has a "DIV" matching the following, it will not be included:
('AR91', 'AR10', 'AG55', 'AZ56', 'CZ12')
I want the results of my query to be:
````
NBR | MODEL
600 5
601 7
````
This is my attempt but it is still returning records that have a matching "DIV":
````
select distinct
s.nbr,
s.model
from
process p
left outer join service s on p.nbr = s.nbr
where
p.unit = 'MC' and p.car = 'M' and p.bank = '1'
and p.paid in ('NY', 'NJ')
and s.paid = 'NY' and length(s.ymd) = 8 and s.ymd between to_number('20240303', '99999999') and to_number( '20240311', '99999999')
AND not exists (select 1 from service s2 where s2.div IN ('AR91', 'AR10', 'AG55', 'AZ56', 'CZ12') and s2.nbr = s.nbr )
```` |
> So each `a[n]` is a `int*` type variable.
No. The type of `a[n]` is `int [3]`, which is an array of 3 `int`.
`int *` is a pointer to three `int`.
These are different things. In memory, an array of 3 `int` has bytes for 3 `int` objects, and those bytes contain the values of the `int`. In memory, an `int *` has bytes for an address, and those bytes contain the values of the address.
In an expression, `a[n]` will be automatically converted to the address of its first element except when it is the operand of `sizeof` or of unary `&`. This conversion is performed by taking the address of `a[n][0]`, and then the result will be an `int *`. But, to work with C properly, you must remember that `a[n]` is actually an array, even though this conversion is automatically performed.
> And since array name `a` is originally `&a[0]`, it will be a `int**` type variable.
No. The type of `a` is `int [3][3]`. As with `a[n]`, in an expression, it will be automatically converted to a pointer to its first element except when it is the operand of `sizeof` or unary `&`. The result will be `&a[0]`, and the type of that will be `int (*)[3]`, which is a pointer to an array of 3 `int`.
This will **not** be further converted to `int **`. The result of the automatic conversion will be a pointer to a place in memory where there are bytes representing 3 `int`. An `int **` would point to a place in memory where there are bytes representing an address.
> `p = a; //possible error, from int** to int*`
Since the result of the automatic conversion of `a` has type `int (*)[3]` and `p` has type `int *`, this attempts to assign an `int (*)[3]` to `int *`. This violates the constraints for simple assignment in C 2018 6.5.16.1 1, so the C implementation is required to issue a diagnostic message for it.
Then the behavior is not defined by the C standard. However, most C implementations will convert the `int (*)[3]` to `int *` and produce a pointer to the first `int` in `a`. |
This can be handled in Angular on the component side instead of the html by using this routine.
- The reference to the collection is made
- A subscription to that reference is created.
- we then check each document to see if the field we are looking for is empty
- Once the empty field is found, we store that document id in a class variable.
First, create a service .ts component to handle the backend work (this component can be useful to many other components: Firestoreservice.
This component will contain these exported routines (see this post for that component firestore.service.ts:
https://stackoverflow.com/questions/51336131/how-to-retrieve-document-ids-for-collections-in-angularfire2s-firestore
In your component, import this service and make a private firestoreservice: Firestoreservice; reference in your constructor.
Done!
This component would need to import the Firestoreservice
<!-- begin snippet: js hide: false console: true babel: false -->
<!-- language: lang-html -->
this.firestoreData = this.firestore.getCollection(this.dataPath); //where dataPath = the collection name
this.firestoreData.subscribe(firestoreData => {
for (let dID of firestoreData ) {
if (dID.field1 == this.ClassVariable1) {
if (dID.field2 == '') {
this.ClassVariable2 = dID.id; //assign the Firestore documentID to a class variable for use later
}
}
}
} );
<!-- end snippet -->
|
{"Voters":[{"Id":472495,"DisplayName":"halfer"},{"Id":466862,"DisplayName":"Mark Rotteveel"},{"Id":354577,"DisplayName":"Chris"}],"SiteSpecificCloseReasonIds":[18]} |
You can use Docker [Volumes](https://docs.docker.com/storage/volumes/)
> Volumes can be more safely shared among multiple containers.
You can define Volumes on `dockerfile`, `docker-compose` file or on `cli`
But to share volumes, you should define named volumes.
You can do so using `cli` or `docker-compose` file.
docker-compose file
```docker
services:
py_app:
image: python
volumes:
- myapp:/home/python/lib
volumes:
myapp:
```
A named volume is declared for the path /home/python/lib.
Docker will create a volume, named myapp, (folder on your pc) and mount it to /home/python/lib inside the container. This means that any data written to /home/python/lib inside the container will be stored in the volume and persist even after the container is stopped or removed. And you can hook (mount) this volume to other containers too.
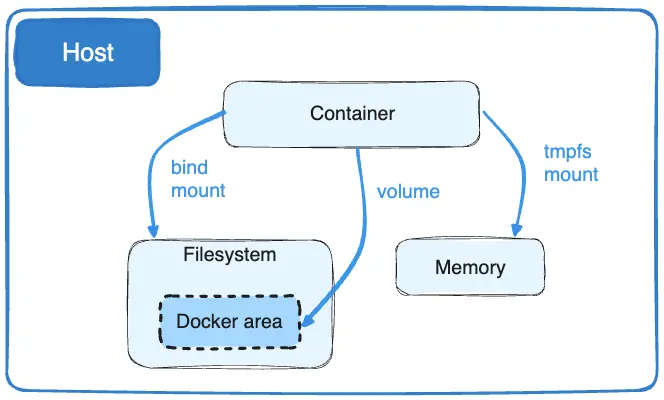
_image from docker docs [link](https://docs.docker.com/storage/volumes/)_
Beware containers might overwrite each other's data stored on the volume. |
Given a graph G with V nodes and V different colors, I would like to assign colors to these nodes such that the color assigned to a node is as visually distinct as it can get from all of its neighbors. All V colors must be assigned to the n
V nodes.
I have tried using a greedy approach in the CIELAB color space to calculate distances between the colors as follows:
1. Traverse the graph in a breadth first manner
2. Process a node. While processing a node, check which neighbors have been assigned a color. The first node gets assigned a random color.
3. Find a color from the existing V colors that is maximally distinct from the neighboring colors.
4. Assign that color to the current node.
5. Iterate through steps 2-4
This algorithm does not yield good results. What I wish to have is all colors being used in the graph such that every node is distinct from its neighbors. Maybe this can be thought of as an optimization problem where we are trying to maximize the difference between two nodes along an edge. Any guidance on this will be appreciated! Thanks! |
|android|save-image| |
I want the command to run every minute after 9pm.
What should I do?
--------------------------------------------------
For testing, below schedule is not working.
1) not working
*/1 21 * * * date >> /opt/cron.log 2>&1
2) working
*/1 * * * * daet >> /opt/cron.log 2>&1
I want the command to run every minute after 9pm. |
crontab run every minute after 9pm |
|cron| |
null |
Given a graph G with V nodes and V different colors, I would like to assign colors to these nodes such that the color assigned to a node is as visually distinct as it can get from all of its neighbors. All V colors must be assigned to the
V nodes.
I have tried using a greedy approach in the CIELAB color space to calculate distances between the colors as follows:
1. Traverse the graph in a breadth first manner
2. Process a node. While processing a node, check which neighbors have been assigned a color. The first node gets assigned a random color.
3. Find a color from the existing V colors that is maximally distinct from the neighboring colors.
4. Assign that color to the current node.
5. Iterate through steps 2-4
This algorithm does not yield good results. What I wish to have is all colors being used in the graph such that every node is distinct from its neighbors. Maybe this can be thought of as an optimization problem where we are trying to maximize the difference between two nodes along an edge. Any guidance on this will be appreciated! Thanks! |
Failed to load the sqljdbc_auth.dll cause : no sqljdbc_auth in java.library.path |
|sql-authentication|javafx-17| |
I'm trying to figure out how to configure Firefox such that all `console.log()` calls by visited websites are written to Firefox's `stdout` or `stderr`.
I already achieved this for Chrome using the CLI flags `--enable-logging`, `--v=1` and `--log-level=0`.
According to [Firefox documentation][1], the environment variables `MOZ_LOG` and `MOZ_LOG_FILE` should be set to configure logging.
However, I have not found a setting where `console.log()` calls are logged.
I've already tried setting these variables as indicated below, which are examples found in the aforementioned documentation and in a [StackExchange answer][2].
1) `MOZ_LOG=timestamp,rotate:200,nsHttp:5,cache2:5,nsSocketTransport:5,nsHostResolver:5`
2) `MOZ_LOG=all:5`
None of these seem to log `console.log()` calls however.
So I guess my questions are:
- Is it even possible to log `console.log()` calls to Firefox's `stdout` or `stderr`?
- And if so, how can this be configured?
[1]: https://firefox-source-docs.mozilla.org/networking/http/logging.html
[2]: https://superuser.com/a/1642781 |
How to write `console.log()` calls to Firefox's `stdout` or `stderr`? |
|logging|firefox|browser| |
You're looking at implementation details, and then summarizing them. You left out one critical detail: for small sets, the hash table size is multiplied by 4.
https://stackoverflow.com/questions/75291343/what-is-the-internal-load-factor-of-a-sets-in-python
Note that this is all subject to change. As the linked question notes, the load factor has changed from 2/3 to 3/5 in 2017. Reasonable code does not depend on this. |
You can use a flag, a rookie mistake is to exit a single loop, remember the double break, another practice that is not recommended but necessary in very high performance processes is to use goto and my favorite, a function with a return.
#include <iostream>
// Dont clone vars, use references, for performance
int calc(int &row, int &cols, int &max) {
int sum = 0;
for (int i = 0; i < row; i++) {
for (int j = 0; j < cols; j++) {
sum += i + j;
if (sum > max) {
return sum;
}
}
}
return sum;
}
int main() {
int rows = 1000;
int cols = 1000;
int max = 1000;
int result = calc(rows, cols, max);
std::cout << "Result: " << result;
return 0;
}
Alternative version, a little more modular:
#include <iostream>
// Dont clone vars, use references, for performance
void calc(int &row, int &cols, int &max, int &result) {
int sum = 0;
for (int i = 0; i < row; i++) {
for (int j = 0; j < cols; j++) {
sum += i + j;
if (sum > max) {
result = sum;
return;
}
}
}
result = sum;
}
int main() {
int rows = 1000;
int cols = 1000;
int max = 1000;
int result = 0;
calc(rows, cols, max, result);
std::cout << "Result: " << result;
return 0;
}
GOTO example, completely discouraged today:
#include <iostream>
int main() {
int rows = 1000;
int cols = 1000;
int max = 1000;
int result = 0;
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result += i + j;
if (result > max) {
goto end;
}
}
}
end:
std::cout << "Result: " << result;
return 0;
}
|
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.