code
stringlengths 2
1.05M
| repo_name
stringlengths 4
116
| path
stringlengths 3
991
| language
stringclasses 30
values | license
stringclasses 15
values | size
int32 3
1.05M
|
---|---|---|---|---|---|
use std::collections::{HashMap};
use std::path::{Path};
use std::sync::{Arc};
use crossbeam::sync::{MsQueue};
use glium::backend::{Facade};
use debug::{gnomon, indicator};
use inverse_kinematics::{Chain};
use model::{Model};
use unlit_model::{UnlitModel};
use render::render_frame::{RenderFrame};
pub const DEPTH_DIMENSION: u32 = 2048;
#[derive(Eq, PartialEq, Hash, Copy, Clone)]
pub enum ModelId {
Player,
Scene,
IKModel, // TODO: we are going to need more of these / a dynamic way to generate ids and load at a later time
Tree,
// DEBUG
Gnomon,
Indicator,
}
pub struct RenderContext {
pub q: Arc<MsQueue<RenderFrame>>, // TODO: make private and provide minimal decent api
window_size: (u32, u32), // TODO: maybe this should be a per RenderFrame parameter
pub models: HashMap<ModelId, Arc<Model>>,
// DEBUG
pub unlit_models: HashMap<ModelId, Arc<UnlitModel>>,
}
impl RenderContext {
pub fn new<F: Facade>(facade: &F, q: Arc<MsQueue<RenderFrame>>, window_size: (u32, u32), ik_chains: &[Chain]) -> RenderContext {
let model_map = load_initial_models(facade, ik_chains);
// DEBUG
let mut unlit_models = HashMap::new();
unlit_models.insert(ModelId::Gnomon, Arc::new(gnomon::model(facade)));
unlit_models.insert(ModelId::Indicator, Arc::new(indicator::model(facade)));
RenderContext {
q: q,
window_size: window_size,
models: model_map,
// DEBUG
unlit_models: unlit_models,
}
}
pub fn aspect_ratio(&self) -> f32 {
(self.window_size.0 as f32) / (self.window_size.1 as f32)
}
}
// TODO: don't pass in chains but make something like IntoModel
//
fn load_initial_models<F: Facade>(facade: &F, ik_chains: &[Chain]) -> HashMap<ModelId, Arc<Model>> {
let mut map = HashMap::new();
const MODEL_PATH_STRINGS: [(ModelId, &'static str); 3] = [
(ModelId::Player, "./data/player.obj"),
(ModelId::Scene, "./data/level.obj"),
(ModelId::Tree, "./data/tree.obj")
];
for &(model_id, path) in &MODEL_PATH_STRINGS {
let model = Arc::new(Model::new(facade, &Path::new(path)));
map.insert(model_id, model);
}
for chain in ik_chains {
map.insert(ModelId::IKModel, Arc::new(chain.model(facade)));
}
map
}
unsafe impl Send for RenderContext {}
unsafe impl Sync for RenderContext {}
| AnthonyMl/sg-rs | src/render/render_context.rs | Rust | mit | 2,238 |
var ratio = require('ratio')
function error(actual, expected) {
return Math.abs(actual - expected) / expected
}
function approx(target, max) {
max = (max || 10)
// find a good approximation
var best = 1, j, e, result
for (var i = 1; i < max; i++) {
j = Math.round(i * target)
e = error(j / i, target)
if (e >= best) continue
best = e
result = ratio(j, i)
}
return result
}
module.exports = approx
| agnoster/approx | index.js | JavaScript | mit | 474 |
// -------------------------------------------------------------------------------------
// Author: Sourabh S Joshi (cbrghostrider); Copyright - All rights reserved.
// For email, run on linux (perl v5.8.5):
// perl -e 'print pack "H*","736f75726162682e732e6a6f73686940676d61696c2e636f6d0a"'
// -------------------------------------------------------------------------------------
// Author - Sourabh S Joshi
#ifndef POKER_HAND_H_
#define POKER_HAND_H_
#include <iostream>
#include <array>
#include <vector>
#include <string>
#include <sstream>
using std::vector;
using std::ostream;
using std::pair;
class Card {
public:
enum CardSuit {
Clubs, Diamonds, Hearts, Spades, NUM_SUITS
};
enum CardVal {
Two, Three, Four, Five, Six, Seven, Eight, Nine, Ten, Jack, Queen, King, Ace, NUM_VALS
};
Card(CardSuit suit, CardVal val) : suit_(suit), val_(val) {}
~Card() {}
//comparison operators only compare on value of card
bool operator==(const Card& rhs) const { return (val_ == rhs.val_);}
bool operator<(const Card& rhs) const { return (val_ < rhs.val_);}
bool operator>(const Card& rhs) const { return (val_ > rhs.val_);}
CardVal GetVal() const {return val_;}
CardSuit GetSuit() const {return suit_;}
friend ostream& operator<<(ostream& os, const Card& cd);
private:
CardSuit suit_;
CardVal val_;
};
class Pokerhand {
public:
enum HandType {
PHHighCard, PHPair, PHTwoPair, PHThreeOfAKind, PHStraight, PHFlush, PHFullHouse, PHFourOfAKind, PHStraightFlush, PH_NUM_TYPES
};
Pokerhand(std::string istr);
virtual ~Pokerhand() {}
vector<Card> AllCards() const {return hand_;}
vector<Card> TieBreakCards() const {return tiebreak_cards_;}
HandType GetType() const {return type_;}
std::string GetTypeStr() const;
//comparison to compare 2 poker hands
bool operator>(const Pokerhand& rhs) const {return (Compare_(rhs) == PH_GT);}
bool operator<(const Pokerhand& rhs) const {return (Compare_(rhs) == PH_LT);}
bool operator==(const Pokerhand& rhs) const {return (Compare_(rhs) == PH_EQ);}
friend ostream& operator<<(ostream& os, const Pokerhand& ph);
void ProcessPokerHand();
private:
//each of these methods returns a bool, saying whether the poker hand is of that type
//also returns a vector of cards in order, so that tie-breakers can be resolved
Card* XOfAKind__(unsigned int num);
bool XOfAKind_(unsigned int num);
bool MNOfAKind_(unsigned int m, unsigned int n);
bool HighCard_();
bool Pair_();
bool TwoPair_();
bool ThreeOfAKind_();
bool Straight_();
bool Flush_();
bool FullHouse_();
bool FourOfAKind_();
bool StraightFlush_();
//since C++ STL lacks an ordering type, I am forced to create one
enum Ordering {
PH_LT, PH_EQ, PH_GT
};
Ordering Compare_(const Pokerhand& rhs) const;
private:
vector<Card> hand_;
HandType type_;
vector<Card> tiebreak_cards_;
};
int PlayPokerHands(vector<std::pair<int, Pokerhand> >& hands);
bool DetectCheating(vector<std::pair<int, Pokerhand> >& hands);
#endif /* POKER_HAND_H_ */
| cbrghostrider/Hacking | cpp-katas/Poker-Hand/poker-hand.h | C | mit | 3,193 |
/*
* Copyright (c) 2015 by Rafael Angel Aznar Aparici (rafaaznar at gmail dot com)
*
* openAUSIAS: The stunning micro-library that helps you to develop easily
* AJAX web applications by using Java and jQuery
* openAUSIAS is distributed under the MIT License (MIT)
* Sources at https://github.com/rafaelaznar/
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*
*/
'use strict';
/* Controllers */
moduloUsuariocursoxusuario.controller('UsuariocursoxusuarioPListController', ['$scope', '$routeParams', 'serverService', '$location', 'redirectService', 'sharedSpaceService', 'checkSessionStorageService',
function ($scope, $routeParams, serverService, $location, redirectService, sharedSpaceService, checkSessionStorageService) {
checkSessionStorageService.isSessionSessionStoraged();
$scope.visibles = {};
$scope.visibles.id = true;
$scope.visibles.titulo = true;
$scope.visibles.descripcion = true;
$scope.visibles.nota = true;
$scope.ob = "usuariocursoxusuario";
$scope.op = "plist";
$scope.title = "Listado de usuariocursoxusuario";
$scope.icon = "fa-file-text-o";
$scope.neighbourhood = 2;
if (!$routeParams.page) {
$routeParams.page = 1;
}
if (!$routeParams.rpp) {
$routeParams.rpp = 999;
}
$scope.numpage = $routeParams.page;
$scope.rpp = $routeParams.rpp;
$scope.predicate = 'id';
$scope.reverse = false;
$scope.orderCliente = function (predicate) {
$scope.predicate = predicate;
$scope.reverse = ($scope.predicate === predicate) ? !$scope.reverse : false;
};
$scope.getListaCursos = function () {
serverService.getDataFromPromise(serverService.promise_getListaCursos()).then(function (data) {
redirectService.checkAndRedirect(data);
if (data.status != 200) {
$scope.statusListaCursos = "Error en la recepción de datos del servidor";
} else {
$scope.listaCursos = data.message;
for (var i = 0; i < $scope.listaCursos.length; i++) {
if (i > 0) {
if ($scope.listaCursos[i].obj_curso.id == $scope.listaCursos[i - 1].obj_curso.id) {
$scope.listaCursos[i - 1].alProfesores.push($scope.listaCursos[i].alProfesores[0]);
$scope.listaCursos.splice(i, 1);
i = i - 1;
}
}
}
}
});
};
//$scope.rppPad = serverService.getNrppBar($scope.ob, $scope.op, $scope.numpage, $scope.rpp);
// $scope.order = $routeParams.order;
// $scope.ordervalue = $routeParams.value;
//
// $scope.filter = $routeParams.filter;
// $scope.filteroperator = $routeParams.filteroperator;
// $scope.filtervalue = $routeParams.filtervalue;
//
// $scope.systemfilter = $routeParams.systemfilter;
// $scope.systemfilteroperator = $routeParams.systemfilteroperator;
// $scope.systemfiltervalue = $routeParams.systemfiltervalue;
$scope.order = "";
$scope.ordervalue = "";
$scope.filter = "id";
$scope.filteroperator = "like";
$scope.filtervalue = "";
$scope.systemfilter = "";
$scope.systemfilteroperator = "";
$scope.systemfiltervalue = "";
$scope.params = "";
$scope.paramsWithoutOrder = "";
$scope.paramsWithoutFilter = "";
$scope.paramsWithoutSystemFilter = "";
if ($routeParams.order && $routeParams.ordervalue) {
$scope.order = $routeParams.order;
$scope.ordervalue = $routeParams.ordervalue;
$scope.orderParams = "&order=" + $routeParams.order + "&ordervalue=" + $routeParams.ordervalue;
$scope.paramsWithoutFilter += $scope.orderParams;
$scope.paramsWithoutSystemFilter += $scope.orderParams;
} else {
$scope.orderParams = "";
}
if ($routeParams.filter && $routeParams.filteroperator && $routeParams.filtervalue) {
$scope.filter = $routeParams.filter;
$scope.filteroperator = $routeParams.filteroperator;
$scope.filtervalue = $routeParams.filtervalue;
$scope.filterParams = "&filter=" + $routeParams.filter + "&filteroperator=" + $routeParams.filteroperator + "&filtervalue=" + $routeParams.filtervalue;
$scope.paramsWithoutOrder += $scope.filterParams;
$scope.paramsWithoutSystemFilter += $scope.filterParams;
} else {
$scope.filterParams = "";
}
if ($routeParams.systemfilter && $routeParams.systemfilteroperator && $routeParams.systemfiltervalue) {
$scope.systemFilterParams = "&systemfilter=" + $routeParams.systemfilter + "&systemfilteroperator=" + $routeParams.systemfilteroperator + "&systemfiltervalue=" + $routeParams.systemfiltervalue;
$scope.paramsWithoutOrder += $scope.systemFilterParams;
$scope.paramsWithoutFilter += $scope.systemFilterParams;
} else {
$scope.systemFilterParams = "";
}
$scope.params = ($scope.orderParams + $scope.filterParams + $scope.systemFilterParams);
//$scope.paramsWithoutOrder = $scope.paramsWithoutOrder.replace('&', '?');
//$scope.paramsWithoutFilter = $scope.paramsWithoutFilter.replace('&', '?');
//$scope.paramsWithoutSystemFilter = $scope.paramsWithoutSystemFilter.replace('&', '?');
$scope.params = $scope.params.replace('&', '?');
$scope.getCursosPage = function () {
serverService.getDataFromPromise(serverService.promise_getSomeUsuariocursoXUsuario($scope.ob, $scope.rpp, $scope.numpage, $scope.filterParams, $scope.orderParams, $scope.systemFilterParams)).then(function (data) {
redirectService.checkAndRedirect(data);
if (data.status != 200) {
$scope.status = "Error en la recepción de datos del servidor";
} else {
$scope.pages = data.message.pages.message;
if (parseInt($scope.numpage) > parseInt($scope.pages))
$scope.numpage = $scope.pages;
$scope.page = data.message.page.message;
$scope.registers = data.message.registers.message;
$scope.status = "";
}
});
}
$scope.getCursosPage();
// $scope.pages = serverService.getPages($scope.ob, $scope.rpp, null, null, null, null, null, null).then(function (datos5) {
// $scope.pages = data['data'];
// if (parseInt($scope.page) > parseInt($scope.pages))
// $scope.page = $scope.pages;
// //$location.path( "#/clientes/" +$scope.pages + "/" + $scope.pages);
// });
// $scope.$watch('pages', function () {
// $scope.$broadcast('myApp.construirBotoneraPaginas');
// }, true)
//
$scope.getRangeArray = function (lowEnd, highEnd) {
var rangeArray = [];
for (var i = lowEnd; i <= highEnd; i++) {
rangeArray.push(i);
}
return rangeArray;
};
$scope.evaluateMin = function (lowEnd, highEnd) {
return Math.min(lowEnd, highEnd);
};
$scope.evaluateMax = function (lowEnd, highEnd) {
return Math.max(lowEnd, highEnd);
};
$scope.dofilter = function () {
if ($scope.filter != "" && $scope.filteroperator != "" && $scope.filtervalue != "") {
//console.log('#/' + $scope.ob + '/' + $scope.op + '/' + $scope.numpage + '/' + $scope.rpp + '?filter=' + $scope.filter + '&filteroperator=' + $scope.filteroperator + '&filtervalue=' + $scope.filtervalue + $scope.paramsWithoutFilter);
if ($routeParams.order && $routeParams.ordervalue) {
if ($routeParams.systemfilter && $routeParams.systemfilteroperator) {
$location.path($scope.ob + '/' + $scope.op + '/' + $scope.numpage + '/' + $scope.rpp).search('filter', $scope.filter).search('filteroperator', $scope.filteroperator).search('filtervalue', $scope.filtervalue).search('order', $routeParams.order).search('ordervalue', $routeParams.ordervalue).search('systemfilter', $routeParams.systemfilter).search('systemfilteroperator', $routeParams.systemfilteroperator).search('systemfiltervalue', $routeParams.systemfiltervalue);
} else {
$location.path($scope.ob + '/' + $scope.op + '/' + $scope.numpage + '/' + $scope.rpp).search('filter', $scope.filter).search('filteroperator', $scope.filteroperator).search('filtervalue', $scope.filtervalue).search('order', $routeParams.order).search('ordervalue', $routeParams.ordervalue);
}
} else {
$location.path($scope.ob + '/' + $scope.op + '/' + $scope.numpage + '/' + $scope.rpp).search('filter', $scope.filter).search('filteroperator', $scope.filteroperator).search('filtervalue', $scope.filtervalue);
}
}
return false;
};
//$scope.$on('myApp.construirBotoneraPaginas', function () {
// $scope.botoneraPaginas = serverService.getPaginationBar($scope.ob, $scope.op, $scope.page, $scope.pages, 2, $scope.rpp);
//})
//
// $scope.prettyFieldNames = serverService.getPrettyFieldNames($scope.ob).then(function (datos4) {
// datos4['data'].push('acciones');
// $scope.prettyFieldNames = datos4['data'];
// });
//
// $scope.clientes = serverService.getPage($scope.ob, $scope.page, null, null, $scope.rpp, null, null, null, null, null, null).then(function (datos3) {
// $scope.clientes = datos3['list'];
//
// });
//
// $scope.fieldNames = serverService.getFieldNames($scope.ob).then(function (datos6) {
// $scope.fieldNames = datos6['data'];
// $scope.selectedFilterFieldName = null;
// });
//
//
// $scope.$watch('numPagina', function () {
// $scope.$broadcast('myApp.construirPagina');
// }, true)
//
// $scope.$on('myApp.construirPagina', function () {
//
// $scope.clientes = serverService.getPage($scope.ob, $scope.page, null, null, $scope.rpp, null, null, null, null, null, null).then(function (datos3) {
// $scope.clientes = datos3['list'];
//
// });
//
// })
//
// $scope.filtrar = function () {
// alert("f")
//
//
// };
// $scope.$watch('filteroperator', function () {
// console.log($scope.filter);
// console.log($scope.filteroperator);
// console.log($scope.filtervalue);
// }, true)
$scope.newObj = function (args) {
$scope.objEdit = {};
$scope.position = undefined;
switch (args.strClass) {
case 'curso':
$scope.objEdit.id = 0;
break;
case 'inscribirse':
$scope.mostrarPass={};
if (sharedSpaceService.getFase() == 0) {
serverService.getDataFromPromise(serverService.promise_getOne('curso', args.id)).then(function (data) {
$scope.objEdit = data.message;
$scope.objEdit.password;
$scope.mostrarPass.mostrar=true;
$scope.mostrarPass.idCurso=args.id;
});
} else {
$scope.objEdit = sharedSpaceService.getObject();
sharedSpaceService.setFase(0);
}
break;
}
;
};
$scope.edit = function (args) {
$scope.objEdit = {};
switch (args.strClass) {
case 'curso':
$scope.position = $scope.page.alUsuariocursos.indexOf(args.levelOne);
if (sharedSpaceService.getFase() == 0) {
serverService.getDataFromPromise(serverService.promise_getOne(args.strClass, args.id)).then(function (data) {
$scope.objEdit = data.message;
//date conversion
});
} else {
$scope.objEdit = sharedSpaceService.getObject();
sharedSpaceService.setFase(0);
}
break;
}
;
};
$scope.save = function (type) {
switch (type) {
case 'curso':
serverService.getDataFromPromise(serverService.promise_setCurso('curso', {json: JSON.stringify(serverService.array_identificarArray($scope.objEdit))})).then(function (data) {
redirectService.checkAndRedirect(data);
if (data.status = 200) {
$scope.result = data;
if ($scope.position !== undefined) {
$scope.page.alUsuariocursos[$scope.position].obj_curso = $scope.objEdit;
$scope.position = undefined;
} else {
$scope.objFinal = {};
$scope.objFinal.id = $scope.result.message.idUsuarioCurso;
$scope.objFinal.obj_curso = {id: $scope.result.message.idCurso};
$scope.objFinal.obj_curso.titulo = $scope.objEdit.titulo;
$scope.objFinal.obj_curso.descripcion = $scope.objEdit.descripcion;
$scope.page.alUsuariocursos.push($scope.objFinal);
}
}
});
break;
case 'inscribirse':
serverService.getDataFromPromise(serverService.promise_setUsuariocurso('usuariocurso', {json: JSON.stringify(serverService.array_identificarArray($scope.objEdit))})).then(function (data) {
redirectService.checkAndRedirect(data);
if (data.status = 200) {
$scope.getCursosPage();
$scope.mostrarContraseña.mostrar=undefined;
$scope.mostrarContraseña.idCurso=undefined;
}
});
break;
}
;
};
$scope.remove = function (type, id, idUsuarioCurso) {
switch (type) {
case 'curso':
serverService.getDataFromPromise(serverService.promise_removeCurso(type, id, idUsuarioCurso)).then(function (data) {
redirectService.checkAndRedirect(data);
if (data.status = 200) {
$scope.result = data;
$scope.page.alUsuariocursos.splice($scope.position, 1);
$scope.position = undefined;
}
});
break;
}
};
}]); | Ilthur/boletinweb | src/main/webapp/js/usuariocursoxusuario/plist.js | JavaScript | mit | 16,663 |
.yan-github {
position: fixed;
bottom:0;
right:0;
min-width:350px;
outline:1px solid rgba(0, 0, 0, 0.3);
min-height:200px;
background: #FFFFFF;
border-radius:3px;
box-shadow: 0 3px 7px rgba(0, 0, 0, 0.3);
z-index: 10000
}
.yan-github ul{
padding:0
}
.yan-github li {
list-style-type: none
}
.yan-header {
margin: 10px;
border-bottom: 1px solid #2299DD;
padding-bottom: 5px;
}
.yan-header h2 {
margin:0;
}
.yan-header .yan-close {
float: right;
font-size: 0.8em;
cursor: pointer
}
.yan-github form {
margin: 10px
}
.yan-github fieldset {
border: 0;
margin: 5px;
margin-bottom: 20px
}
.yan-github fieldset::after {
content: "";
display: block;
clear: both
}
.yan-github legend {
font-size: 1.2em;
margin-bottom: 10px;
border-bottom: 1px solid #e5e5e5;
width: 100%;
}
.yan-label-block{
display: block;
margin: 5px
}
.yan-btn {
display: inline-block;
padding: 6px 12px;
margin-bottom: 0;
font-size: 14px;
font-weight: normal;
line-height: 1.428571429;
text-align: center;
white-space: nowrap;
vertical-align: middle;
cursor: pointer;
background-image: none;
border: 1px solid transparent;
border-radius: 4px;
}
.yan-btn:hover {
color: #333;
}
| yanhaijing/githubFansTool | src/css/index.css | CSS | mit | 1,340 |
/**
* Write the input to the paramsified file
*
* ---
* INPUTS:
*
* - FILES
* Write the list of files to the paramsified file (line feed after each filename).
*
* - STRINGS
* Write the strings (concatenated) to the paramsified file.
*
* - STRING
* Write the string to the paramsified file.
*
* - UNDEFINED
* Touch the file.
*
* OUTPUT:
*
* - FILES
* The filename that was written.
* ---
*
* @module tasks
* @submodule write
*/
var State = require('../state'),
path = require('path'),
mkdirp = require('mkdirp').mkdirp,
fs = require('fs');
/**
* Write out the input to the destination filename.
*
* This can only apply to single string inputs.
*
* @method writeTask
* @param options {Object} Write task options
* @param options.name {String} Filename to write to.
* @param options.encoding {String} [default='utf8'] Encoding to use when writing the file
* @param options.mkdir {Boolean} [default=true] Make destination directory if it does not exist
* @param options.dirmode {String} [default='0755'] Create new directories with this mode (chmod)
* @param status {EventEmitter} Status object, handles 'complete' and 'failed' task exit statuses.
* @param logger {winston.Logger} Logger instance, if additional logging required (other than task exit status)
* @return {undefined}
* @public
*/
function writeTask(options, status, logger) {
var self = this,
pathname = path.resolve(path.dirname(options.name)),
name = options.name,
encoding = options && options.encoding || 'utf8',
mkdir = options && options.mkdir || true,
dirmode = options && options.dirmode || '0755';
// Write the content to the specified file.
function writeFile(filename, data) {
fs.writeFile(filename, data, encoding, function(err) {
if (err) {
status.emit('failed', 'write', 'error writing destination file: ' + err);
} else {
self._state.set(State.TYPES.FILES, [ filename ]);
status.emit('complete', 'write', 'wrote ' + filename);
}
});
}
// Create the paramsified path if it does not exist.
function mkdirIfNotExist(filename, callback) {
path.exists(filename, function(exists) {
if (!exists) {
if (mkdir === true) {
mkdirp(filename, dirmode, function(err) {
if (err) {
callback(err);
} else {
callback(null);
}
});
} else {
callback('tried to write a file to a non-existing directory and mkdir is false');
}
} else {
callback(null);
}
});
}
switch (this._state.get().type) {
case State.TYPES.FILES:
mkdirIfNotExist(pathname, function(err) {
if (err) {
status.emit('failed', 'write', 'error creating destination directory: ' + err);
} else {
writeFile(name, self._state.get().value.join("\n"));
}
});
break;
case State.TYPES.STRING:
mkdirIfNotExist(pathname, function(err) {
if (err) {
status.emit('failed', 'write', 'error creating destination directory: ' + err);
} else {
writeFile(name, self._state.get().value);
}
});
break;
case State.TYPES.STRINGS:
mkdirIfNotExist(pathname, function(err) {
if (err) {
status.emit('failed', 'write', 'error creating destination directory: ' + err);
} else {
writeFile(name, self._state.get().value.join(""));
}
});
break;
case State.TYPES.UNDEFINED:
mkdirIfNotExist(pathname, function(err) {
if (err) {
status.emit('failed', 'write', 'error creating destination directory: ' + err);
} else {
writeFile(name, "");
}
});
break;
default:
status.emit('failed', 'write', 'unrecognised input type: ' + this._type);
break;
}
}
exports.tasks = {
'write' : {
callback: writeTask
}
}; | mosen/buildy | lib/buildy/tasks/write.js | JavaScript | mit | 4,521 |
# frozen_string_literal: true
require 'grape/router'
require 'grape/api/instance'
module Grape
# The API class is the primary entry point for creating Grape APIs. Users
# should subclass this class in order to build an API.
class API
# Class methods that we want to call on the API rather than on the API object
NON_OVERRIDABLE = (Class.new.methods + %i[call call! configuration compile! inherited]).freeze
class << self
attr_accessor :base_instance, :instances
# Rather than initializing an object of type Grape::API, create an object of type Instance
def new(*args, &block)
base_instance.new(*args, &block)
end
# When inherited, will create a list of all instances (times the API was mounted)
# It will listen to the setup required to mount that endpoint, and replicate it on any new instance
def inherited(api, base_instance_parent = Grape::API::Instance)
api.initial_setup(base_instance_parent)
api.override_all_methods!
make_inheritable(api)
end
# Initialize the instance variables on the remountable class, and the base_instance
# an instance that will be used to create the set up but will not be mounted
def initial_setup(base_instance_parent)
@instances = []
@setup = Set.new
@base_parent = base_instance_parent
@base_instance = mount_instance
end
# Redefines all methods so that are forwarded to add_setup and be recorded
def override_all_methods!
(base_instance.methods - NON_OVERRIDABLE).each do |method_override|
define_singleton_method(method_override) do |*args, &block|
add_setup(method_override, *args, &block)
end
end
end
# Configure an API from the outside. If a block is given, it'll pass a
# configuration hash to the block which you can use to configure your
# API. If no block is given, returns the configuration hash.
# The configuration set here is accessible from inside an API with
# `configuration` as normal.
def configure
config = @base_instance.configuration
if block_given?
yield config
self
else
config
end
end
# This is the interface point between Rack and Grape; it accepts a request
# from Rack and ultimately returns an array of three values: the status,
# the headers, and the body. See [the rack specification]
# (http://www.rubydoc.info/github/rack/rack/master/file/SPEC) for more.
# NOTE: This will only be called on an API directly mounted on RACK
def call(*args, &block)
instance_for_rack.call(*args, &block)
end
# Allows an API to itself be inheritable:
def make_inheritable(api)
# When a child API inherits from a parent API.
def api.inherited(child_api)
# The instances of the child API inherit from the instances of the parent API
Grape::API.inherited(child_api, base_instance)
end
end
# Alleviates problems with autoloading by tring to search for the constant
def const_missing(*args)
if base_instance.const_defined?(*args)
base_instance.const_get(*args)
else
super
end
end
# The remountable class can have a configuration hash to provide some dynamic class-level variables.
# For instance, a description could be done using: `desc configuration[:description]` if it may vary
# depending on where the endpoint is mounted. Use with care, if you find yourself using configuration
# too much, you may actually want to provide a new API rather than remount it.
def mount_instance(**opts)
instance = Class.new(@base_parent)
instance.configuration = Grape::Util::EndpointConfiguration.new(opts[:configuration] || {})
instance.base = self
replay_setup_on(instance)
instance
end
# Replays the set up to produce an API as defined in this class, can be called
# on classes that inherit from Grape::API
def replay_setup_on(instance)
@setup.each do |setup_step|
replay_step_on(instance, setup_step)
end
end
def respond_to?(method, include_private = false)
super(method, include_private) || base_instance.respond_to?(method, include_private)
end
def respond_to_missing?(method, include_private = false)
base_instance.respond_to?(method, include_private)
end
def method_missing(method, *args, &block)
# If there's a missing method, it may be defined on the base_instance instead.
if respond_to_missing?(method)
base_instance.send(method, *args, &block)
else
super
end
end
def compile!
require 'grape/eager_load'
instance_for_rack.compile! # See API::Instance.compile!
end
private
def instance_for_rack
if never_mounted?
base_instance
else
mounted_instances.first
end
end
# Adds a new stage to the set up require to get a Grape::API up and running
def add_setup(method, *args, &block)
setup_step = { method: method, args: args, block: block }
@setup += [setup_step]
last_response = nil
@instances.each do |instance|
last_response = replay_step_on(instance, setup_step)
end
last_response
end
def replay_step_on(instance, setup_step)
return if skip_immediate_run?(instance, setup_step[:args])
args = evaluate_arguments(instance.configuration, *setup_step[:args])
response = instance.send(setup_step[:method], *args, &setup_step[:block])
if skip_immediate_run?(instance, [response])
response
else
evaluate_arguments(instance.configuration, response).first
end
end
# Skips steps that contain arguments to be lazily executed (on re-mount time)
def skip_immediate_run?(instance, args)
instance.base_instance? &&
(any_lazy?(args) || args.any? { |arg| arg.is_a?(Hash) && any_lazy?(arg.values) })
end
def any_lazy?(args)
args.any? { |argument| argument.respond_to?(:lazy?) && argument.lazy? }
end
def evaluate_arguments(configuration, *args)
args.map do |argument|
if argument.respond_to?(:lazy?) && argument.lazy?
argument.evaluate_from(configuration)
elsif argument.is_a?(Hash)
argument.transform_values { |value| evaluate_arguments(configuration, value).first }
elsif argument.is_a?(Array)
evaluate_arguments(configuration, *argument)
else
argument
end
end
end
def never_mounted?
mounted_instances.empty?
end
def mounted_instances
instances - [base_instance]
end
end
end
end
| dblock/grape | lib/grape/api.rb | Ruby | mit | 7,030 |
(function () {
"use strict";
/**
* This module have an ability to contain constants.
* No one can reinitialize any of already initialized constants
*/
var module = (function () {
var constants = {},
hasOwnProperty = Object.prototype.hasOwnProperty,
prefix = (Math.random() + "_").slice(2),
isAllowed;
/**
* This private method checks if value is acceptable
* @param value {String|Number|Boolean} - the value of the constant
* @returns {boolean}
* @private
*/
isAllowed = function (value) {
switch (typeof value) {
case "number":
return true;
case "string":
return true;
case "boolean":
return true;
default:
return false;
}
};
return {
/**
* Constant getter
* @param name {String} - the name of the constant
* @returns {String|Number|Boolean|null}
*/
getConstant: function (name) {
if (this.isDefined(name) === true) {
return constants[prefix + name];
}
return undefined;
},
/**
* Setter
* @param name {String} - the name of the constant
* @param value {String|Number|Boolean} - the value of the constant
* @returns {boolean}
*/
setConstant: function (name, value) {
if (isAllowed(value) !== true) {
return false;
}
if (this.isDefined(name) === true) {
return false;
}
constants[prefix + name] = value;
return true;
},
/**
* This method checks if constant is already defined
* @param name {String} - the name of the constant
* @returns {boolean}
*/
isDefined: function (name) {
return hasOwnProperty.call(constants, prefix + name);
}
};
})();
/**Testing*/
module.setConstant("test", 123);
print("test == " + module.getConstant("test"));
print("idDefined(\"test\") == " + module.isDefined("test"));
print("test2 == " + module.getConstant("test2"));
print("idDefined(\"test2\") == " + module.isDefined("test2"));
print("");
module.setConstant("test", 321);
print("test == " + module.getConstant("test"));
print("");
module.setConstant("test3", {a: 123});
print("test3 == " + module.getConstant("test3"));
})(); | AlexeyPopovUA/JavaScript-design-patterns | js/samples/object-constants/ObjectConstants.js | JavaScript | mit | 2,762 |
# frozen_string_literal: true
module RuboCop
module Formatter
# This formatter formats report data in clang style.
# The precise location of the problem is shown together with the
# relevant source code.
class ClangStyleFormatter < SimpleTextFormatter
ELLIPSES = '...'
def report_file(file, offenses)
offenses.each { |offense| report_offense(file, offense) }
end
private
def report_offense(file, offense)
output.printf(
"%<path>s:%<line>d:%<column>d: %<severity>s: %<message>s\n",
path: cyan(smart_path(file)),
line: offense.line,
column: offense.real_column,
severity: colored_severity_code(offense),
message: message(offense)
)
begin
return unless valid_line?(offense)
report_line(offense.location)
report_highlighted_area(offense.highlighted_area)
rescue IndexError # rubocop:disable Lint/SuppressedException
# range is not on a valid line; perhaps the source file is empty
end
end
def valid_line?(offense)
!offense.location.source_line.blank?
end
def report_line(location)
source_line = location.source_line
if location.first_line == location.last_line
output.puts(source_line)
else
output.puts("#{source_line} #{yellow(ELLIPSES)}")
end
end
def report_highlighted_area(highlighted_area)
output.puts("#{' ' * highlighted_area.begin_pos}" \
"#{'^' * highlighted_area.size}")
end
end
end
end
| smakagon/rubocop | lib/rubocop/formatter/clang_style_formatter.rb | Ruby | mit | 1,635 |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="description" content="An approach to developing modern web applications">
<meta name="author" content="Marc J. Greenberg">
<title>ElectricDiscoTech</title>
<link rel="shortcut icon" href="favicon.ico" type="image/x-icon">
<link rel="icon" href="favicon.ico" type="image/x-icon">
<link rel="stylesheet" href="css/style.min.css" type="text/css">
<script src="js/jquery.min.js"></script>
<script src="js/uikit.min.js"></script>
<script src="js/clipboard.min.js"></script>
<script src="js/edt.min.js"></script>
</head>
<body class="edt-background">
<div class="inc:site/header.html"></div>
<div class="uk-grid" data-uk-grid-margin>
<div class="uk-width-medium-1-1">
<div class="edt-front2"></div>
</div>
</div>
<div class="edt-middle">
<div class="uk-container uk-container-center">
<div class="uk-grid" data-uk-grid-margin>
<div class="edt-sidebar uk-width-medium-1-4 uk-hidden-small">
<ul class="edt-nav uk-nav" data-uk-nav>
<li class="uk-nav-header">Conceptual</li>
<li><a href="readme.html">Dance Floor</a></li>
<li><a href="source.html">First Steps</a></li>
<li><a href="tools.html">Do the Hustle</a></li>
<ul class="uk-nav uk-margin-left">
<li><a href="winivp.html"><i class="uk-icon-windows uk-margin-right"></i>Windows (ivp)</a></li>
<li><a href="linuxivp.html"><i class="uk-icon-linux uk-margin-right"></i>Linux (ivp)</a></li>
<li class="uk-active"><a href="osxivp.html"><i class="uk-icon-apple uk-margin-right"></i>Mac OS X (ivp)</a></li>
</ul>
<li><a href="bower.html">Disco Duck</a></li>
<li><a href="tasks.html">Disco Pig</a></li>
<li class="uk-nav-header"></li>
<li><a href=""></a></li>
</ul>
</div>
<div class="edt-main uk-width-medium-3-4">
<article class="uk-article">
<h2 id="osxivp">
<img src="img/osx.png" width="48"/>
<a href="#osxivp" class="uk-link-reset"> Mac OS X ivp for <i class="edt-tla2">EDT</i></a>
</h2>
<hr class="uk-article-divider">
<div class="uk-grid">
<div class="uk-width-medium-1-2">
<p>
<a href="http://www.apple.com/osx/">Mac OS X</a> is currently my favorite dance partner.
It is a common practice among dance instructors, to have the students
switch partners frequently during the class in order to get them used to
dancing with different partners. Using OS X as a base platform I can seamlessly
run virtualized windows and/or linux on demand.
</p>
<p>
While I mentioned this before, a good code editor is the hammer in a modern web application developers toolbox.
For this project I am using one of Microsoft's latest concoctions, <a href=https://code.visualstudio.com/>
Visual Studio Code</a>. It runs on windows, linux and os x. It's open source and
<a href="https://github.com/Microsoft/vscode">forkable on github</a> and I really like.
</p>
</div>
<div class="uk-width-medium-1-4">
<img src="img/discothink.png" width="80%"></a>
</div>
</div>
</article>
</div>
<div class="uk-width-medium-1-4">
</div>
</div>
<!-- Git -->
<div class="uk-grid">
<div class="uk-width-medium-1-4">
<a href="https://github.com/explore">
<img style="margin-top:2.5em;margin-left:-18px;" src="img/github-octocat.png">
</a>
</div>
<div class="uk-width-medium-2-4">
<hr class="uk-article-divider" style="margin-top:-48px;">
<h4><a href="http://git-scm.com/">Git</a></h4>
<!--//http://git-scm.com/-->
<p>Let's start a shell and validate the ability to run git from the command prompt:</p>
<button class="edt-clip" data-clipboard-text="git --version"><i class="uk-icon-clipboard"></i></button>
<pre class="edt-border uk-margin-bottom">$ git --version
git version 2.4.9 (Apple Git-60)
</pre>
<p>
If for some reason you don't have git installed, you can download the
<a href="http://git-scm.com/download/mac">git-osx-installer</a>
from <a href="http://git-scm.com/">get-scm.com</a> If you can use use git then
clone a copy of <span class="edt-tla2">EDT</span>:
</p>
<button class="edt-clip" data-clipboard-text="git clone https://github.com/codemarc/ElectricDiscoTech"><i class="uk-icon-clipboard"></i></button>
<pre class="edt-border">$ git clone https://github.com/codemarc/ElectricDiscoTech
Cloning into 'ElectricDiscoTech'...
remote: Counting objects: 254, done.
remote: Compressing objects: 100% (30/30), done.
remote: Total 254 (delta 10), reused 0 (delta 0), pack-reused 223
Receiving objects: 100% (254/254), 5.69 MiB | 3.31 MiB/s, done.
Resolving deltas: 100% (101/101), done.
Checking connectivity... done.
</pre>
</div>
</div>
<!-- NODE/NPM -->
<div class="uk-grid">
<div class="uk-width-medium-1-4">
<a href="https://nodejs.org/">
<img style="margin-top:3.5em" src="img/nodejs-npm.png" width="70%">
</a>
</div>
<div class="uk-width-medium-2-4">
<hr class="uk-article-divider">
<h4><a href="https://www.npmjs.com/">Npm</a></h4>
<p>Now let's see which version of npm is installed:</p>
<button class="edt-clip" data-clipboard-text="npm -v"><i class="uk-icon-clipboard"></i></button>
<pre>$ npm -v
-bash: npm: command not found
</pre>
<p>
Oops, forgot to install node. <a href="https://www.npmjs.com/">npm</a> is part of <a href="https://nodejs.org">Node.js</a>.
On the Node.js website you can quickly find the download for installable package for OS X (x64).
</p>
<button class="edt-clip" data-clipboard-text="npm -v"><i class="uk-icon-clipboard"></i></button>
<pre class="edt-border">$ npm -v
2.14.7
</pre>
<p>
You can use npm to update npm.
</p>
<button class="edt-clip" data-clipboard-text="sudo npm install -g npm"><i class="uk-icon-clipboard"></i></button>
<pre class="edt-border uk-margin-large-bottom">$ sudo npm install -g npm
⋮
$ npm -v
3.5.0
</pre>
</div>
</div>
<!-- BOWER -->
<div class="uk-grid">
<div class="uk-width-medium-1-4">
<a href="http://bower.io/">
<img class="uk-margin-left" src="img/bower.png" width="45%">
</a>
</div>
<div class="uk-width-medium-2-4">
<hr class="uk-article-divider" style="margin-top:-36px;">
<h4><a href="http://bower.io/">Bower</a></h4>
<p><a href="http://bower.io/">bower</a> is billed as a package manager for the web. What it saves you from is endless hours
of searching for source components. If you know the name of a component and its author has created a package for it (most
popular components have the by now) then all you need to do to grab a copy using:
<span class="uk-margin-left uk-text-primary">bower install
<package></span>
</p>
<p>To install or update bower run:</p>
<button class="edt-clip" data-clipboard-text="npm install -g bower"><i class="uk-icon-clipboard"></i></button>
<pre>$ npm install -g bower
⋮
$ bower -version
1.6.5
</pre>
</div>
</div>
</div>
<div class="inc:site/footer.html"></div>
</body>
<script>new Clipboard('.edt-clip');</script>
</html> | codemarc/ElectricDiscoTech | dev/docs/osxivp.html | HTML | mit | 10,042 |
package me.nereo.multi_image_selector;
import android.app.Activity;
import android.content.Context;
import android.content.Intent;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.graphics.Matrix;
import android.graphics.RectF;
import android.net.Uri;
import android.os.Bundle;
import android.os.Handler;
import android.os.Looper;
import android.support.annotation.Nullable;
import android.text.TextUtils;
import android.util.DisplayMetrics;
import android.util.Log;
import android.view.Gravity;
import android.view.MotionEvent;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.FrameLayout;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
import com.facebook.common.executors.CallerThreadExecutor;
import com.facebook.common.references.CloseableReference;
import com.facebook.datasource.DataSource;
import com.facebook.drawee.backends.pipeline.Fresco;
import com.facebook.drawee.controller.AbstractDraweeController;
import com.facebook.drawee.drawable.ProgressBarDrawable;
import com.facebook.drawee.drawable.ScalingUtils;
import com.facebook.drawee.generic.GenericDraweeHierarchy;
import com.facebook.drawee.generic.GenericDraweeHierarchyBuilder;
import com.facebook.drawee.interfaces.DraweeController;
import com.facebook.imagepipeline.core.ImagePipeline;
import com.facebook.imagepipeline.datasource.BaseBitmapDataSubscriber;
import com.facebook.imagepipeline.image.CloseableImage;
import com.facebook.imagepipeline.request.ImageRequest;
import com.facebook.imagepipeline.request.ImageRequestBuilder;
import java.io.File;
import me.nereo.multi_image_selector.view.ClipImageBorderView;
import me.nereo.multi_image_selector.view.zoomable.DefaultZoomableController;
import me.nereo.multi_image_selector.view.zoomable.ZoomableDraweeView;
/**
* Created by sunny on 2015/12/22.
* 图片裁剪
*/
public class ClipPhotoActivity extends Activity implements OnClickListener, IBitmapShow {
public static final String TAG = ClipPhotoActivity.class.getSimpleName();
private String imgUrl;
private TextView mTitle;
private Button mCommit;
private ImageView mBack;
private ZoomableDraweeView mGestureImageView;
private ClipImageBorderView clip_image_borderview;
//图片的平移与缩放
float mCurrentScale;
float last_x = -1;
float last_y = -1;
boolean move = false;
public static void startClipPhotoActivity(Context context, String uri) {
Intent targetIntent = new Intent(context, ClipPhotoActivity.class);
targetIntent.putExtra(TAG, uri);
context.startActivity(targetIntent);
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_clip_photo);
setTitle("图片裁剪");
initIntentParams();
}
@Override
protected void onStart() {
super.onStart();
}
@Override
protected void onResume() {
super.onResume();
/* new Handler(Looper.getMainLooper()).postDelayed(new Runnable() {
@Override
public void run() {
getBitmap();
}
},100);*/
getBitmap();
}
private void getBitmap() {
int width = clip_image_borderview.getWidth();
int height = clip_image_borderview.getHeight();
Log.e("with", "with===" + width + "\nheight===" + height);
DisplayMetrics displayMetrics = new DisplayMetrics();
getWindowManager().getDefaultDisplay().getMetrics(displayMetrics);
int screenWidth = displayMetrics.widthPixels;
int screenHeight = displayMetrics.heightPixels;
/* FrameLayout.LayoutParams layoutParams = new FrameLayout.LayoutParams(screenWidth - 40, screenWidth - 40);
layoutParams.gravity = Gravity.CENTER;
mGestureImageView.setLayoutParams(layoutParams);*/
if (!TextUtils.isEmpty(imgUrl)) {
// ImageLoaderUtils.load(imgUrl,mGestureImageView);
//解决图片倒置
ImageRequest imageRequest = ImageRequestBuilder
.newBuilderWithSource(Uri.parse("file://" + imgUrl))
.setAutoRotateEnabled(true).build();
//解决图片多指缩放
DraweeController controller = Fresco.newDraweeControllerBuilder()
.setImageRequest(imageRequest)
.setTapToRetryEnabled(true)
.build();
/* DefaultZoomableController controller = DefaultZoomableController.newInstance();
controller.setEnabled(true);*/
GenericDraweeHierarchy hierarchy = new GenericDraweeHierarchyBuilder(getResources())
.setActualImageScaleType(ScalingUtils.ScaleType.FIT_CENTER)
.setProgressBarImage(new ProgressBarDrawable())
.build();
mGestureImageView.setController(controller);
mGestureImageView.setHierarchy(hierarchy);
//图片等比例缩放
// getAvatarBitmap(imageRequest, controller, hierarchy, ClipPhotoActivity.this);
}
}
@Override
public void onContentChanged() {
mTitle = (TextView) findViewById(R.id.photo_title);
mGestureImageView = (ZoomableDraweeView) findViewById(R.id.gesture_iv);
mBack = (ImageView) findViewById(R.id.btn_back);
mBack.setOnClickListener(this);
clip_image_borderview = (ClipImageBorderView) findViewById(R.id.clip_image_borderview);
mCommit = (Button) findViewById(R.id.commit);
mCommit.setOnClickListener(this);
}
@Override
public void setTitle(CharSequence title) {
mTitle.setText(title);
}
private void initIntentParams() {
Intent mIntent = getIntent();
if (mIntent != null) {
imgUrl = mIntent.getStringExtra(TAG);
}
}
@Override
public void onClick(View v) {
int id = v.getId();
if (id == R.id.btn_back) {
finish();
} else if (id == R.id.commit) {
if (mGestureImageView != null) {
Bitmap bm = mGestureImageView.clip();
Log.e("bm", "bm:" + bm.toString());
Intent resultIntent = new Intent();
Bundle mBundle = new Bundle();
mBundle.putParcelable(TAG, bm);
resultIntent.putExtras(mBundle);
setResult(101, resultIntent);
finish();
}
}
}
private void getAvatarBitmap(ImageRequest imageRequest,
final DefaultZoomableController controller, final GenericDraweeHierarchy hierarchy, final IBitmapShow callback) {
ImagePipeline imagePipeline = Fresco.getImagePipeline();
DataSource<CloseableReference<CloseableImage>> dataSource =
imagePipeline.fetchDecodedImage(imageRequest, ClipPhotoActivity.this);
dataSource.subscribe(new BaseBitmapDataSubscriber() {
@Override
protected void onNewResultImpl(@Nullable Bitmap bitmap) {
if (bitmap != null) {
if (callback != null) {
callback.onBitmapLoadedSuccess(bitmap, controller, hierarchy);
}
}
}
@Override
protected void onFailureImpl(DataSource<CloseableReference<CloseableImage>> dataSource) {
}
}, CallerThreadExecutor.getInstance());
}
@Override
public void onBitmapLoadedSuccess(Bitmap bm, DefaultZoomableController controller, GenericDraweeHierarchy hierarchy) {
int bmWidth = bm.getWidth();
int bmHeight = bm.getHeight();
int newWidth = clip_image_borderview.getWidth() == 0 ? 200 : clip_image_borderview.getWidth();
int newHeight = clip_image_borderview.getHeight() == 0 ? 200 : clip_image_borderview.getHeight();
Log.e("bmWidth", "bmWidth:" + bmWidth + ",\nbmHeight:" + bmHeight +
",\nnewWidth:" + newWidth + ",\nnewHeight:" + newHeight);
float scaleWidth = ((float) newWidth) / bmWidth;
float scaleHeight = ((float) newHeight) / bmHeight;
Matrix matrix = new Matrix();
matrix.postScale(scaleWidth, scaleHeight);
Bitmap newBm = Bitmap.createBitmap(bm, 0, 0, bmWidth, bmHeight, matrix, true);
mGestureImageView.setZoomableController(controller);
mGestureImageView.setHierarchy(hierarchy);
mGestureImageView.setImageBitmap(newBm);
mGestureImageView.setOnTouchListener(new View.OnTouchListener() {
float baseValue = 0;
@Override
public boolean onTouch(View v, MotionEvent event) {
switch (event.getAction()) {
case MotionEvent.ACTION_DOWN:
float x = last_x = event.getRawX();
float y = last_y = event.getRawY();
move = false;
break;
case MotionEvent.ACTION_MOVE:
if (event.getPointerCount() == 2) {
//双指
float x1 = event.getX(0) - event.getX(1);
float y1 = event.getY(0) - event.getY(1);
//计算2点之间的距离
float value = (float) Math.sqrt(x1 * x1 + y1 * y1);
if (baseValue == 0) {
baseValue = value;
} else {
//由2点之间的距离来计算缩放比例
if ((value - baseValue) >= 10 || (baseValue - value) >= 10) {
float scale = value / baseValue;
img_scale(scale);
}
}
} else if (event.getPointerCount() == 1) {
//单指
float x2 = event.getRawX();
float y2 = event.getRawY();
x2 -= last_x;
y2 -= last_y;
if (x2 >= 10 || y2 >= 10 || x2 <= -10 || y2 <= -10) {
img_translate(x2, y2);
last_x = event.getRawX();
last_y = event.getRawY();
}
}
break;
}
return false;
}
});
}
/**
* 平移
*
* @param x2
* @param y2
*/
private void img_translate(float x2, float y2) {
if (mGestureImageView != null)
mGestureImageView.img_translate(x2, y2);
}
/**
* 缩放
*
* @param scale
*/
private void img_scale(float scale) {
if (mGestureImageView != null) {
mGestureImageView.img_scale(scale);
}
}
}
| SunnyLy/LocalImageChoose | multi-image-selector/src/main/java/me/nereo/multi_image_selector/ClipPhotoActivity.java | Java | mit | 11,258 |
# NS
Provides a Ruby wrapper for the Dutch NS API.
## Installation
Add this line to your application's Gemfile:
gem 'ns'
And then execute:
$ bundle
Or install it yourself as:
$ gem install ns
## Usage
ns_client = Ns::Client.new(api_key, api_password)
available_stations = ns_client.get_stations
advice = ns_client.get_travel_advice(available_stations[0],
available_stations[1])
Should return a travel advice based on the first and second available station.
## Contributing
1. Fork it
2. Create your feature branch (`git checkout -b my-new-feature`)
3. Commit your changes (`git commit -am 'Added some feature'`)
4. Push to the branch (`git push origin my-new-feature`)
5. Create new Pull Request
| jaimie-van-santen/ns | README.md | Markdown | mit | 777 |
#include <onyxudp/udpclient.h>
#include <stdio.h>
#include <string.h>
#include <assert.h>
/* This test just makes sure the client library compiles and doens't crash on start. */
void on_error(udp_client_params_t *client, UDPERR code, char const *name) {
fprintf(stderr, "on_error: code %d: %s\n", code, name);
assert(!"should not happen");
}
int main() {
udp_client_params_t params;
memset(¶ms, 0, sizeof(params));
params.app_id = 123;
params.app_version = 321;
params.on_error = on_error;
params.on_idle = NULL;
params.on_payload = NULL;
params.on_disconnect = NULL;
udp_client_t *client = udp_client_initialize(¶ms);
assert(client != NULL);
udp_client_terminate(client);
return 0;
}
| jwatte/onyxnet | test/client/client.cpp | C++ | mit | 756 |
# alsa-switch
Switching of Alsa audio devices
the purpose of alsa-switch is to control the flow of multiple audio-streams going through a system while supporting priority streams
You invoke audio-switch like this:
auido-switch <input-device> <output-device> <control-file>
for example
audio-switch microphone speaker control1
This creates a named pipe named "control1", it is ready to connect "microphone" audio device to "speaker" audio device once the control pipe is telling it to do so.
Writing a character to the control channel does this
commands to send:
'1'...'9' priority 1...9 announcement
'0' end of announcement (channel muted)
typical use case
you have audio source1...9
you have virtual speakers 1...9
you mix virtual speaker 1...9 to physical speaker in alsa (/etc/asound.conf)
you run alsa-switch and it will create control files 1...9
now all sound sources are muted
if source 2 now has a level 8 priority annoucement it would write '8' into "control2"
this would mute all audio streams which have no announcement or a priority announcement of level '9'
1 is the highest priority
9 is the lowest priority
0 is channel is muted
At startup all channels are muted.
Should a higher priority annoucement come in now on source 3, then source 2 would become muted too.
Once source 3 is finished and source 2 is still in priority level 8, the audio from source 2 would be heard again.
| andreasfink/alsa-switch | README.md | Markdown | mit | 1,452 |
package wanghaisheng.com.yakerweather;
import org.junit.Test;
import static org.junit.Assert.*;
/**
* To work on unit tests, switch the Test Artifact in the Build Variants view.
*/
public class ExampleUnitTest {
@Test
public void addition_isCorrect() throws Exception {
assertEquals(4, 2 + 2);
}
} | sheng-xiaoya/YakerWeather | app/src/test/java/wanghaisheng/com/yakerweather/ExampleUnitTest.java | Java | mit | 322 |
//
// 2016-01-21, jjuiddong
//
// »ç°¢ÇüÀ» ã´Â´Ù.
//
#pragma once
namespace cvproc
{
class cDetectRect
{
public:
cDetectRect();
virtual ~cDetectRect();
bool Init();
bool Detect(const cv::Mat &src);
void UpdateParam(const cRecognitionEdgeConfig &recogConfig);
public:
bool m_show;
cRectContour m_rect;
int m_threshold1;
int m_threshold2;
int m_minArea;
double m_minCos;
double m_maxCos;
vector<vector<cv::Point> > m_contours;
cv::Mat m_gray;
cv::Mat m_edges;
cv::Mat m_binMat;
};
}
| jjuiddong/Common | CamCommon/detectrect.h | C | mit | 529 |
<?php
/**
* Copyright 2012 Klarna AB
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* This file demonstrates the use of the Klarna library to complete
* the purchase and display the thank you page snippet.
*
* PHP version 5.3.4
*
* @category Payment
* @package Payment_Klarna
* @subpackage Examples
* @author Klarna <support@klarna.com>
* @copyright 2012 Klarna AB
* @license http://www.apache.org/licenses/LICENSE-2.0 Apache license v2.0
* @link http://developers.klarna.com/
*/
require_once 'src/Klarna/Checkout.php';
session_start();
Klarna_Checkout_Order::$contentType
= "application/vnd.klarna.checkout.aggregated-order-v2+json";
$sharedSecret = 'sharedSecret';
@$checkoutId = $_GET['klarna_order'];
$connector = Klarna_Checkout_Connector::create($sharedSecret);
$order = new Klarna_Checkout_Order($connector, $checkoutId);
$order->fetch();
if ($order['status'] == "checkout_complete") {
// At this point make sure the order is created in your system and send a
// confirmation email to the customer
$update['status'] = 'created';
$update['merchant_reference'] = array(
'orderid1' => uniqid()
);
$order->update($update);
}
| chrisVdd/Time2web | vendor/klarna/checkout/docs/examples/push.php | PHP | mit | 1,718 |
import * as React from 'react';
import { AppliedFilter, DataTypes, GridFilters, numberWithCommas, ReactPowerTable, withInternalPaging, withInternalSorting } from '../../src/';
import { defaultColumns, partyList, sampledata } from './shared';
// //if coming in from DTO
// const availDTO = [
// { fieldName: 'number', dataType: 'int' },
// { fieldName: 'president', dataType: 'string' },
// { fieldName: 'birth_year', dataType: 'int' },
// { fieldName: 'death_year', dataType: 'int', canBeNull: true },
// { fieldName: 'took_office', dataType: 'date' },
// { fieldName: 'left_office', dataType: 'date', canBeNull: true },
// { fieldName: 'party', dataType: 'string' },
// ];
// const availableFiltersMap = createKeyedMap(availDTO.map(m => new DataTypes[m.dataType](m)), m=>m.fieldName);
//availableFilters.party = new DataTypes.list(availableFilters.party, partyList);
const partyListOptions = partyList.map(m => ({ label: m.label, value: m.label }));
//if building in JS
const availableFilters = [
new DataTypes.int('number'),
new DataTypes.string('president'),
new DataTypes.int('birth_year'),
new DataTypes.decimal({ fieldName: 'death_year', canBeNull: true }),
new DataTypes.date({ fieldName: 'took_office' }),
new DataTypes.date({ fieldName: 'left_office', canBeNull: true }),
new DataTypes.list('party', partyListOptions),
new DataTypes.boolean({ fieldName: 'assasinated', displayName: 'was assasinated' }),
new DataTypes.timespan({ fieldName: 'timeBorn', displayName: 'time born' }),
];
//const columns = [...defaultColumns, { field: m => m.timeBorn, width: 80, formatter: formatTimeValue }];
const assasinatedPresidents = [16, 20, 25, 35];
function padStart(str: string, targetLength: number, padString: string) {
// tslint:disable-next-line:no-bitwise
targetLength = targetLength >> 0; //truncate if number, or convert non-number to 0;
padString = String(typeof padString !== 'undefined' ? padString : ' ');
if (str.length >= targetLength) {
return String(str);
} else {
targetLength = targetLength - str.length;
if (targetLength > padString.length) {
padString += padString.repeat(targetLength / padString.length); //append to original to ensure we are longer than needed
}
return padString.slice(0, targetLength) + str;
}
}
const data = sampledata.map(m => ({ ...m, assasinated: assasinatedPresidents.indexOf(m.number) > -1, timeBorn: padStart(Math.floor((Math.random() * 24)).toString(), 2, '0') + ':00' }));
//const availableFiltersMap = createKeyedMap(availableFilters, m => m.fieldName);
//availableFiltersMap.number.operations.gt.displayName = 'greater than TEST';
availableFilters.find(m => m.fieldName === 'death_year').operations['null'].displayName = 'still alive';
interface FiltersExampleState {
appliedFilters: Array<AppliedFilter<any>>;
}
const Table = withInternalSorting(withInternalPaging(ReactPowerTable));
export class FiltersExample extends React.Component<never, FiltersExampleState> {
constructor(props: never) {
super(props);
this.state = { appliedFilters: [] };
this.handleFiltersChange = this.handleFiltersChange.bind(this);
}
handleFiltersChange(newFilters: Array<AppliedFilter<any>>) {
console.log('onFiltersChange', newFilters);
this.setState({ appliedFilters: newFilters });
}
render() {
let filteredData = data;
this.state.appliedFilters.forEach(m => {
filteredData = m.filter.applyFilter(filteredData, m.operation, m.value);
});
return (
<div className="row">
<div className="col-md-3">
<div className="grid-filters">
<div className="small">
{numberWithCommas(filteredData.length) + ' Presidents'}
</div>
<div style={{ marginTop: 10 }} />
<GridFilters availableFilters={availableFilters} appliedFilters={this.state.appliedFilters} onFiltersChange={this.handleFiltersChange} />
</div>
</div>
<div className="col-md-9">
<Table columns={defaultColumns} keyColumn="number" rows={filteredData} sorting={{ column: 'number' }} />
</div>
</div>
);
}
}
| coolkev/react-power-table | examples/src/filters.tsx | TypeScript | mit | 4,479 |
<section id="content">
<!--start container-->
<div id="breadcrumbs-wrapper" class="" style="width:100%;">
<div class="header-search-wrapper grey hide-on-large-only">
<i class="mdi-action-search active"></i>
<input type="text" name="Search" class="header-search-input z-depth-2" placeholder="Explore Materialize">
</div>
<div class="container">
<div class="row">
<div class="col s12 m12 l12">
<h5 class="breadcrumbs-title">New Progres perunit</h5>
<ol class="breadcrumb">
<li><a href="<?php echo site_url('administrator'); ?>">Dashboard</a></li>
<li><a href="<?php echo site_url('administrator/main/progres_unit');?>">Progres perUnit</a></li>
<li class="active">New Progres perUnit</li>
</ol>
</div>
</div>
</div>
</div>
<div class="col s12 m12 l6">
<div class="card-panel">
<div class="col s12 m12 l6" style="width:100%;">
<div class="card-panel" style="width:100%;">
<h4 class="header2">New Progres perUnit</h4>
<div class="row">
<div class="row">
<?=form_open('action/save_progresunit');?>
<div class="input-field col s12">
<label for="spk" class="active">SPK</label>
<select name="spk" id="spk">
<?php $query = $this->db->query("SELECT DISTINCT spk FROM tbl_resume");
foreach ($query->result() as $datap) { ?>
<option value="<?=$datap->spk;?>"><?=$datap->spk;?></option>
<?php } ?>
</select>
</div>
</div>
<div class="row">
<div class="input-field col s12">
<input type="hidden" name="nama_kavling_hidden" id="nama_kavling_hidden">
<label for="nama_kavling" class="active">Kavling</label>
<select id="nama_kavling" name="nama_kavling">
<option></option>
</select>
</div>
</div>
<div class="row">
<div class="input-field col s12">
<input placeholder="" id="sdmingguini" type="text" name="sdmingguini">
<label for="sdmingguini" class="active">Progres</label>
</div>
</div>
<div class="input-field col s12">
<button class="btn cyan waves-effect waves-light right" type="submit" name="action" style="margin-left:10px;">Submit
</button>
<button onclick="goBack()" class="btn cyan waves-effect waves-light right" type="submit" name="action">Cancel
</button>
<?=form_close();?>
</div>
</div>
</div>
</div>
</div>
</div>
</form>
<!--start container-->
</section>
<!--end container-->
<!-- END CONTENT -->
<!--end container-->
<script>
function goBack() {
window.history.go(-1);
}
</script>
<script>
$("#spk").on('change', function(){
$('#spk_hidden').val($(this).val());
$("#nama_kavling").empty();
$.ajax({
url: '<?php echo site_url("action/get_rsm")?>',
type: 'POST',
data: {spk: $(this).val()},
dataType:'json',
success : function(data){
console.log(data);
for(var index in data){
$("#nama_kavling").append('<option value='+data[index].nama_kavling+'>'+data[index].nama_kavling+'</option>');
}
$("#nama_kavling").material_select();
}
})
.done(function(response) {
$("#nama_kavling").append('<option>'+response+'</option>')
});
$("#nama_kavling").on('change', function(){
$('#nama_kavling_hidden').val($(this).val());
$.ajax({
url: '<?php echo site_url("action/getdata")?>',
type: 'POST',
data: {nama_kavling : $(this).val()},
dataType : 'json',
success : function(data){
$("#sdmingguini").val(data.sdmingguini)
console.log(data);
}
})
})
})
$("#nama_kavling").on('change', function(){
$('#nama_kavling_hidden').val($(this).val());
$.ajax({
url: '<?php echo site_url("action/getdata")?>',
type: 'POST',
data: {nama_kavling : $(this).val()},
dataType : 'json',
success : function(data){
$("#sdmingguini").val(data.sdmingguini)
console.log(data);
}
})
})
</script>
| MiftahulxHuda/rekon | application/views/page/new_progresunit.php | PHP | mit | 5,595 |
foscam-android-sdk
==================
Foscam Android SDK for H.264 IP Cameras (FI9821W)
05/12/2015: THIS PROJECT IS DEPRECATED. Please refer to http://foscam.us/forum/technical-support-f3.html for future updates.
| quatanium/foscam-android-sdk | README.md | Markdown | mit | 215 |
package network
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License. See License.txt in the project root for license information.
//
// Code generated by Microsoft (R) AutoRest Code Generator.
// Changes may cause incorrect behavior and will be lost if the code is regenerated.
import (
"context"
"github.com/Azure/go-autorest/autorest"
"github.com/Azure/go-autorest/autorest/azure"
"github.com/Azure/go-autorest/tracing"
"net/http"
)
// HubVirtualNetworkConnectionsClient is the network Client
type HubVirtualNetworkConnectionsClient struct {
BaseClient
}
// NewHubVirtualNetworkConnectionsClient creates an instance of the HubVirtualNetworkConnectionsClient client.
func NewHubVirtualNetworkConnectionsClient(subscriptionID string) HubVirtualNetworkConnectionsClient {
return NewHubVirtualNetworkConnectionsClientWithBaseURI(DefaultBaseURI, subscriptionID)
}
// NewHubVirtualNetworkConnectionsClientWithBaseURI creates an instance of the HubVirtualNetworkConnectionsClient
// client using a custom endpoint. Use this when interacting with an Azure cloud that uses a non-standard base URI
// (sovereign clouds, Azure stack).
func NewHubVirtualNetworkConnectionsClientWithBaseURI(baseURI string, subscriptionID string) HubVirtualNetworkConnectionsClient {
return HubVirtualNetworkConnectionsClient{NewWithBaseURI(baseURI, subscriptionID)}
}
// Get retrieves the details of a HubVirtualNetworkConnection.
// Parameters:
// resourceGroupName - the resource group name of the VirtualHub.
// virtualHubName - the name of the VirtualHub.
// connectionName - the name of the vpn connection.
func (client HubVirtualNetworkConnectionsClient) Get(ctx context.Context, resourceGroupName string, virtualHubName string, connectionName string) (result HubVirtualNetworkConnection, err error) {
if tracing.IsEnabled() {
ctx = tracing.StartSpan(ctx, fqdn+"/HubVirtualNetworkConnectionsClient.Get")
defer func() {
sc := -1
if result.Response.Response != nil {
sc = result.Response.Response.StatusCode
}
tracing.EndSpan(ctx, sc, err)
}()
}
req, err := client.GetPreparer(ctx, resourceGroupName, virtualHubName, connectionName)
if err != nil {
err = autorest.NewErrorWithError(err, "network.HubVirtualNetworkConnectionsClient", "Get", nil, "Failure preparing request")
return
}
resp, err := client.GetSender(req)
if err != nil {
result.Response = autorest.Response{Response: resp}
err = autorest.NewErrorWithError(err, "network.HubVirtualNetworkConnectionsClient", "Get", resp, "Failure sending request")
return
}
result, err = client.GetResponder(resp)
if err != nil {
err = autorest.NewErrorWithError(err, "network.HubVirtualNetworkConnectionsClient", "Get", resp, "Failure responding to request")
return
}
return
}
// GetPreparer prepares the Get request.
func (client HubVirtualNetworkConnectionsClient) GetPreparer(ctx context.Context, resourceGroupName string, virtualHubName string, connectionName string) (*http.Request, error) {
pathParameters := map[string]interface{}{
"connectionName": autorest.Encode("path", connectionName),
"resourceGroupName": autorest.Encode("path", resourceGroupName),
"subscriptionId": autorest.Encode("path", client.SubscriptionID),
"virtualHubName": autorest.Encode("path", virtualHubName),
}
const APIVersion = "2018-11-01"
queryParameters := map[string]interface{}{
"api-version": APIVersion,
}
preparer := autorest.CreatePreparer(
autorest.AsGet(),
autorest.WithBaseURL(client.BaseURI),
autorest.WithPathParameters("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/virtualHubs/{virtualHubName}/hubVirtualNetworkConnections/{connectionName}", pathParameters),
autorest.WithQueryParameters(queryParameters))
return preparer.Prepare((&http.Request{}).WithContext(ctx))
}
// GetSender sends the Get request. The method will close the
// http.Response Body if it receives an error.
func (client HubVirtualNetworkConnectionsClient) GetSender(req *http.Request) (*http.Response, error) {
return client.Send(req, azure.DoRetryWithRegistration(client.Client))
}
// GetResponder handles the response to the Get request. The method always
// closes the http.Response Body.
func (client HubVirtualNetworkConnectionsClient) GetResponder(resp *http.Response) (result HubVirtualNetworkConnection, err error) {
err = autorest.Respond(
resp,
azure.WithErrorUnlessStatusCode(http.StatusOK),
autorest.ByUnmarshallingJSON(&result),
autorest.ByClosing())
result.Response = autorest.Response{Response: resp}
return
}
// List retrieves the details of all HubVirtualNetworkConnections.
// Parameters:
// resourceGroupName - the resource group name of the VirtualHub.
// virtualHubName - the name of the VirtualHub.
func (client HubVirtualNetworkConnectionsClient) List(ctx context.Context, resourceGroupName string, virtualHubName string) (result ListHubVirtualNetworkConnectionsResultPage, err error) {
if tracing.IsEnabled() {
ctx = tracing.StartSpan(ctx, fqdn+"/HubVirtualNetworkConnectionsClient.List")
defer func() {
sc := -1
if result.lhvncr.Response.Response != nil {
sc = result.lhvncr.Response.Response.StatusCode
}
tracing.EndSpan(ctx, sc, err)
}()
}
result.fn = client.listNextResults
req, err := client.ListPreparer(ctx, resourceGroupName, virtualHubName)
if err != nil {
err = autorest.NewErrorWithError(err, "network.HubVirtualNetworkConnectionsClient", "List", nil, "Failure preparing request")
return
}
resp, err := client.ListSender(req)
if err != nil {
result.lhvncr.Response = autorest.Response{Response: resp}
err = autorest.NewErrorWithError(err, "network.HubVirtualNetworkConnectionsClient", "List", resp, "Failure sending request")
return
}
result.lhvncr, err = client.ListResponder(resp)
if err != nil {
err = autorest.NewErrorWithError(err, "network.HubVirtualNetworkConnectionsClient", "List", resp, "Failure responding to request")
return
}
if result.lhvncr.hasNextLink() && result.lhvncr.IsEmpty() {
err = result.NextWithContext(ctx)
return
}
return
}
// ListPreparer prepares the List request.
func (client HubVirtualNetworkConnectionsClient) ListPreparer(ctx context.Context, resourceGroupName string, virtualHubName string) (*http.Request, error) {
pathParameters := map[string]interface{}{
"resourceGroupName": autorest.Encode("path", resourceGroupName),
"subscriptionId": autorest.Encode("path", client.SubscriptionID),
"virtualHubName": autorest.Encode("path", virtualHubName),
}
const APIVersion = "2018-11-01"
queryParameters := map[string]interface{}{
"api-version": APIVersion,
}
preparer := autorest.CreatePreparer(
autorest.AsGet(),
autorest.WithBaseURL(client.BaseURI),
autorest.WithPathParameters("/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Network/virtualHubs/{virtualHubName}/hubVirtualNetworkConnections", pathParameters),
autorest.WithQueryParameters(queryParameters))
return preparer.Prepare((&http.Request{}).WithContext(ctx))
}
// ListSender sends the List request. The method will close the
// http.Response Body if it receives an error.
func (client HubVirtualNetworkConnectionsClient) ListSender(req *http.Request) (*http.Response, error) {
return client.Send(req, azure.DoRetryWithRegistration(client.Client))
}
// ListResponder handles the response to the List request. The method always
// closes the http.Response Body.
func (client HubVirtualNetworkConnectionsClient) ListResponder(resp *http.Response) (result ListHubVirtualNetworkConnectionsResult, err error) {
err = autorest.Respond(
resp,
azure.WithErrorUnlessStatusCode(http.StatusOK),
autorest.ByUnmarshallingJSON(&result),
autorest.ByClosing())
result.Response = autorest.Response{Response: resp}
return
}
// listNextResults retrieves the next set of results, if any.
func (client HubVirtualNetworkConnectionsClient) listNextResults(ctx context.Context, lastResults ListHubVirtualNetworkConnectionsResult) (result ListHubVirtualNetworkConnectionsResult, err error) {
req, err := lastResults.listHubVirtualNetworkConnectionsResultPreparer(ctx)
if err != nil {
return result, autorest.NewErrorWithError(err, "network.HubVirtualNetworkConnectionsClient", "listNextResults", nil, "Failure preparing next results request")
}
if req == nil {
return
}
resp, err := client.ListSender(req)
if err != nil {
result.Response = autorest.Response{Response: resp}
return result, autorest.NewErrorWithError(err, "network.HubVirtualNetworkConnectionsClient", "listNextResults", resp, "Failure sending next results request")
}
result, err = client.ListResponder(resp)
if err != nil {
err = autorest.NewErrorWithError(err, "network.HubVirtualNetworkConnectionsClient", "listNextResults", resp, "Failure responding to next results request")
}
return
}
// ListComplete enumerates all values, automatically crossing page boundaries as required.
func (client HubVirtualNetworkConnectionsClient) ListComplete(ctx context.Context, resourceGroupName string, virtualHubName string) (result ListHubVirtualNetworkConnectionsResultIterator, err error) {
if tracing.IsEnabled() {
ctx = tracing.StartSpan(ctx, fqdn+"/HubVirtualNetworkConnectionsClient.List")
defer func() {
sc := -1
if result.Response().Response.Response != nil {
sc = result.page.Response().Response.Response.StatusCode
}
tracing.EndSpan(ctx, sc, err)
}()
}
result.page, err = client.List(ctx, resourceGroupName, virtualHubName)
return
}
| Azure/azure-sdk-for-go | services/network/mgmt/2018-11-01/network/hubvirtualnetworkconnections.go | GO | mit | 9,593 |
var address = '';
var config = {};
var requestGroupId = 0;
var readRequestsTodo = 0;
var readRequestsDone = 0;
var scanRequestsTodo = [0, 0, 0];
var scanRequestsDone = [0, 0, 0];
var scanResults = [];
var requestSentCounter = 0;
var requestSuccessCounter = 0;
var requestFailureCounter = 0;
var requestInProgress = false;
var requestQueue = [];
var socket = io({
transports: ['websocket'],
timeout: 10000,
reconnectionDelay: 500,
autoConnect: true
});
socket.on('reconnect', function()
{
window.location.reload();
});
var READ_RESPONSE_HANDLERS = {
in: handleReadInputResponse,
out: handleReadOutputResponse,
anal: handleReadAnalogResponse,
func: handleReadFunctionResponse
};
$(function()
{
$('#config-address').on('change', function(e)
{
updateAddress(e.target.value);
reset();
});
$('#config-scan').on('click', scan);
$('#config-file').on('change', function(e)
{
var file = e.target.files[0];
if (!file || !/\.conf/.test(file.name))
{
updateInput('');
reset();
return;
}
var reader = new FileReader();
reader.onload = function(e)
{
updateInput(e.target.result);
reset();
};
reader.readAsText(file);
});
$('#config-input').on('change', function(e)
{
$('#config-file').val('');
updateInput(e.target.value);
reset();
});
$('#outputs').on('click', '.output', function(e)
{
toggleOutput(config[e.currentTarget.dataset.id]);
});
$('#analogs')
.on('change', '.form-control', function(e)
{
setAnalog(config[$(e.currentTarget).closest('.analog')[0].dataset.id], e.currentTarget.value);
})
.on('keyup', '.form-control', function(e)
{
if (e.keyCode === 13)
{
setAnalog(config[$(e.currentTarget).closest('.analog')[0].dataset.id], e.currentTarget.value);
}
});
$('#functions')
.on('change', '.form-control', function(e)
{
setFunction(config[$(e.currentTarget).closest('.function')[0].dataset.id], e.currentTarget.value);
})
.on('keyup', '.form-control', function(e)
{
if (e.keyCode === 13)
{
setFunction(config[$(e.currentTarget).closest('.function')[0].dataset.id], e.currentTarget.value);
}
});
$(window).on('resize', resizeInput);
resizeInput();
updateAddress(localStorage.ADDRESS || '');
updateInput(localStorage.INPUT || '');
reset();
});
function resizeInput()
{
var height = window.innerHeight - 90 - 54 - 20 - 53 - 15 - 53 - 15 - 30;
$('#config-input').css('height', height + 'px');
}
function updateAddress(newAddress)
{
newAddress = newAddress.trim();
localStorage.ADDRESS = newAddress;
$('#config-address').val(newAddress);
if (newAddress.length && newAddress.indexOf('.') === -1)
{
newAddress = '[' + newAddress + ']';
}
address = newAddress;
}
function updateInput(newInput)
{
parseInput(newInput);
if (!Object.keys(config).length)
{
newInput = 'Nieprawidłowy lub pusty plik!';
}
else
{
localStorage.INPUT = newInput;
}
$('#config-input').val(newInput);
}
function parseInput(input)
{
config = {};
var re = /([A-Z0-9_-]+)\s+([0-9]+)\s+([0-9]+)\s+(in|out|anal|func)/ig;
var matches;
while (matches = re.exec(input))
{
var name = matches[1];
config[name] = {
type: matches[4],
name: name,
tim: parseInt(matches[2], 10),
channel: parseInt(matches[3], 10),
writing: false,
value: null
};
}
}
function renderIo()
{
var html = {
inputs: [],
outputs: [],
analogs: [],
functions: []
};
Object.keys(config).forEach(function(key)
{
var io = config[key];
if (io.type === 'in')
{
renderInput(html.inputs, io);
}
else if (io.type === 'out')
{
renderOutput(html.outputs, io);
}
else if (io.type === 'anal')
{
renderAnalog(html.analogs, io);
}
else if (io.type === 'func')
{
renderFunction(html.functions, io);
}
});
Object.keys(html).forEach(function(ioType)
{
$('#' + ioType).html(html[ioType].join(''));
});
}
function renderInput(html, io)
{
html.push(
'<span class="input label label-default" data-id="', io.name, '">',
io.name,
'</span>'
);
}
function renderOutput(html, io)
{
html.push(
'<button class="output btn btn-default" data-id="', io.name, '">',
io.name,
'</button>'
);
}
function renderAnalog(html, io)
{
html.push(
'<div class="analog" data-id="', io.name, '"><div class="input-group"><span class="input-group-addon">',
io.name,
'</span><input type="number" class="form-control" min="0" max="65535" step="1"></div></div>'
);
}
function renderFunction(html, io)
{
html.push(
'<div class="function" data-id="', io.name, '"><div class="input-group"><span class="input-group-addon">',
io.name,
'</span><input type="text" class="form-control"></div></div>'
);
}
function reset()
{
++requestGroupId;
Object.keys(config).forEach(function(key)
{
config[key].value = null;
});
readRequestsDone = 0;
readRequestsTodo = 0;
requestSentCounter = 0;
requestSuccessCounter = 0;
requestFailureCounter = 0;
updateRequestCounter();
renderIo();
readAll();
}
function updateRequestCounter()
{
$('#requestCounter-success').text(requestSuccessCounter);
$('#requestCounter-failure').text(requestFailureCounter);
$('#requestCounter-sent').text(requestSentCounter);
}
function readAll()
{
if (readRequestsDone !== readRequestsTodo)
{
return;
}
if (!address.length)
{
return;
}
var keys = Object.keys(config);
readRequestsDone = 0;
readRequestsTodo = keys.length;
keys.forEach(function(key)
{
read(config[key]);
});
requestSentCounter += readRequestsTodo;
updateRequestCounter();
}
function read(io)
{
var reqGroupId = requestGroupId;
var req = {
type: 'NON',
code: 'GET',
uri: 'coap://' + address + '/io/RD?tim=' + io.tim + '&ch=' + io.channel + '&t=' + io.type
};
socket.emit('request', req, function(err, res)
{
if (requestGroupId !== reqGroupId)
{
return;
}
++readRequestsDone;
if (err)
{
++requestFailureCounter;
//console.error('Error reading %s: %s', io.name, err.message);
}
else if (res.payload.indexOf('0') !== 0)
{
++requestFailureCounter;
console.error('Error reading %s (%s): %d', io.name, res.code, res.payload.split('\n')[0]);
}
else
{
++requestSuccessCounter;
READ_RESPONSE_HANDLERS[io.type](io, res);
}
updateRequestCounter();
if (readRequestsDone === readRequestsTodo)
{
setTimeout(readAll, 333);
}
});
}
function handleReadInputResponse(io, res)
{
var $input = $('.input[data-id="' + io.name + '"]');
if (!$input.length)
{
return;
}
$input.removeClass('label-default label-success label-danger');
io.value = res.payload.indexOf('ON') !== -1
? true
: res.payload.indexOf('OFF') !== -1
? false
: null;
if (io.value === true)
{
$input.addClass('label-success');
}
else if (io.value === false)
{
$input.addClass('label-danger');
}
else
{
$input.addClass('label-default');
}
}
function handleReadOutputResponse(io, res)
{
if (io.writing)
{
return;
}
var $output = $('.output[data-id="' + io.name + '"]');
if (!$output.length)
{
return;
}
io.value = res.payload.indexOf('ON') !== -1
? true
: res.payload.indexOf('OFF') !== -1
? false
: null;
$output
.removeClass('btn-default btn-success btn-danger')
.addClass(io.value === null ? 'btn-default' : io.value ? 'btn-success' : 'btn-danger');
}
function handleReadAnalogResponse(io, res)
{
if (io.writing)
{
return;
}
var $analog = $('.analog[data-id="' + io.name + '"]');
var $input = $analog.find('.form-control');
if (!$analog.length || document.activeElement === $input[0])
{
return;
}
io.value = parseInt(res.payload.split('\n')[3], 10) || 0;
$input.val(io.value);
}
function handleReadFunctionResponse(io, res)
{
if (io.writing)
{
return;
}
var $function = $('.function[data-id="' + io.name + '"]');
var $input = $function.find('.form-control');
if (!$function.length || document.activeElement === $input[0])
{
return;
}
io.value = res.payload.trim().split('\n')[3];
if (io.value === undefined)
{
io.value = '';
}
$input.val(io.value);
}
function toggleOutput(io)
{
if (io.writing)
{
return;
}
io.writing = true;
var $output = $('.output[data-id="' + io.name + '"]');
$output
.removeClass('btn-default btn-success btn-danger')
.addClass('btn-warning');
++requestSentCounter;
updateRequestCounter();
var reqGroupId = requestGroupId;
var req = {
type: 'NON',
code: 'POST',
uri: 'coap://' + address + '/io/WD?tim=' + io.tim
+ '&ch=' + io.channel
+ '&t=' + io.type
+ '&tD=' + (io.value ? 'OFF' : 'ON')
};
socket.emit('request', req, function(err, res)
{
if (requestGroupId !== reqGroupId)
{
return;
}
io.writing = false;
if (err)
{
++requestFailureCounter;
console.error('Error writing %s: %s', io.name, err.message);
}
else if (res.payload.indexOf('0') !== 0)
{
++requestFailureCounter;
console.error('Error writing %s (%s): %d', io.name, res.code, res.payload.split('\n')[0]);
}
else
{
++requestSuccessCounter;
io.value = !io.value;
}
$output
.removeClass('btn-warning')
.addClass(io.value === null ? 'btn-default' : io.value ? 'btn-success' : 'btn-danger');
updateRequestCounter();
});
}
function setAnalog(io, value)
{
value = parseInt(value, 10);
if (io.writing || isNaN(value) || value < 0 || value > 0xFFFF)
{
return;
}
io.writing = true;
var $analog = $('.analog[data-id="' + io.name + '"]');
var $input = $analog.find('.form-control');
$analog.addClass('is-writing');
$input.attr('readonly', true);
++requestSentCounter;
updateRequestCounter();
var reqGroupId = requestGroupId;
var req = {
type: 'NON',
code: 'POST',
uri: 'coap://' + address + '/io/WD?tim=' + io.tim
+ '&ch=' + io.channel
+ '&t=' + io.type
+ '&tD=' + value
};
socket.emit('request', req, function(err, res)
{
if (requestGroupId !== reqGroupId)
{
return;
}
$analog.removeClass('is-writing');
io.writing = false;
if (err)
{
++requestFailureCounter;
console.error('Error writing %s: %s', io.name, err.message);
}
else if (res.payload.indexOf('0') !== 0)
{
++requestFailureCounter;
console.error('Error writing %s (%s): %d', io.name, res.code, res.payload.split('\n')[0]);
}
else
{
++requestSuccessCounter;
io.value = value;
$input.val(value).attr('readonly', false);
}
updateRequestCounter();
});
}
function setFunction(io, value)
{
if (io.writing)
{
return;
}
io.writing = true;
var $function = $('.function[data-id="' + io.name + '"]');
var $input = $function.find('.form-control');
$function.addClass('is-writing');
$input.attr('readonly', true);
++requestSentCounter;
updateRequestCounter();
var reqGroupId = requestGroupId;
var req = {
type: 'NON',
code: 'POST',
uri: 'coap://' + address + '/io/WD?tim=' + io.tim
+ '&ch=' + io.channel
+ '&t=' + io.type
+ '&tD=' + value
};
socket.emit('request', req, function(err, res)
{
if (requestGroupId !== reqGroupId)
{
return;
}
$function.removeClass('is-writing');
io.writing = false;
if (err)
{
++requestFailureCounter;
console.error('Error writing %s: %s', io.name, err.message);
}
else if (res.payload.indexOf('0') !== 0)
{
++requestFailureCounter;
console.error('Error writing %s (%s): %d', io.name, res.code, res.payload.split('\n')[0]);
}
else
{
++requestSuccessCounter;
io.value = value;
$input.val(value).attr('readonly', false);
}
updateRequestCounter();
});
}
function unlockAfterScan()
{
scanRequestsDone = [0, 0];
scanRequestsTodo = [0, 0];
scanResults = [];
$('#config').find('.form-control').prop('disabled', false);
}
function scan()
{
if (!address.length || scanRequestsTodo[0] !== 0)
{
return;
}
updateInput('');
reset();
$('#config').find('.form-control').prop('disabled', true);
var req = {
type: 'CON',
code: 'GET',
uri: 'coap://' + address + '/io/TDisc'
};
var options = {
exchangeTimeout: 10000,
transactionTimeout: 1000,
maxRetransmit: 3,
blockSize: 64
};
updateRequestCounter(++requestSentCounter);
queueRequest(req, options, function(err, res)
{
if (err)
{
updateRequestCounter(++requestFailureCounter);
unlockAfterScan();
return console.error("Error scanning: %s", err.message);
}
updateRequestCounter(++requestSuccessCounter);
var tims = res.payload.split('\n')[1].split(',');
scanRequestsDone = [0, 0];
scanRequestsTodo = [tims.length, 0];
scanResults = [];
tims.forEach(function(timId)
{
scanTim(parseInt(timId.trim(), 10));
});
});
}
function scanTim(timId)
{
var req = {
type: 'CON',
code: 'GET',
uri: 'coap://' + address + '/io/CDisc?tim=' + timId
};
var options = {
exchangeTimeout: 10000,
transactionTimeout: 1000,
maxRetransmit: 3,
blockSize: 64
};
updateRequestCounter(++requestSentCounter);
queueRequest(req, options, function(err, res)
{
if (err)
{
updateRequestCounter(++requestFailureCounter);
unlockAfterScan();
return console.error("Error scanning TIM %d: %s", timId, err.message);
}
updateRequestCounter(++requestSuccessCounter);
++scanRequestsDone[0];
var lines = res.payload.split('\n');
var channelIds = lines[2].split(',');
var transducerNames = lines[3].replace(/"/g, '').split(',');
scanRequestsTodo[1] += channelIds.length;
channelIds.forEach(function(channelId, i)
{
setTimeout(
scanChannel,
Math.round(10 + Math.random() * 200),
timId,
parseInt(channelId.trim(), 10),
transducerNames[i]
);
});
});
}
function scanChannel(timId, channelId, transducerName)
{
var req = {
type: 'CON',
code: 'GET',
uri: 'coap://' + address + '/io/RTeds?tim=' + timId + '&ch=' + channelId + '&TT=4'
};
var options = {
exchangeTimeout: 10000,
transactionTimeout: 1000,
maxRetransmit: 3,
blockSize: 64
};
updateRequestCounter(++requestSentCounter);
queueRequest(req, options, function(err, res)
{
if (err)
{
updateRequestCounter(++requestFailureCounter);
unlockAfterScan();
return console.error("Error scanning channel %d of TIM %d: %s", channelId, timId, err.message);
}
updateRequestCounter(++requestSuccessCounter);
++scanRequestsDone[1];
var type = null;
if (/Digital Input/i.test(res.payload))
{
type = 'in';
}
else if (/Digital Output/i.test(res.payload))
{
type = 'out';
}
else if (/(Digit.*?|Analog|Step g) (Input|Output)/i.test(res.payload))
{
type = 'anal';
}
if (type)
{
scanResults.push({
timId: timId,
channelId: channelId,
name: (transducerName || ('T_' + timId + '_' + channelId)).trim(),
type: type
});
}
if (scanRequestsDone[1] === scanRequestsTodo[1])
{
buildInputFromScanResults();
}
});
}
function buildInputFromScanResults()
{
var input = [];
scanResults.sort(function(a, b)
{
var r = a.timId - b.timId;
if (r === 0)
{
r = a.channelId - b.channelId;
}
return r;
});
scanResults.forEach(function(io)
{
input.push(io.name + ' ' + io.timId + ' ' + io.channelId + ' ' + io.type);
});
unlockAfterScan();
updateInput(input.join('\n'));
renderIo();
readAll();
}
function queueRequest(req, options, callback)
{
requestQueue.push(req, options, callback);
if (!requestInProgress)
{
sendNextRequest();
}
}
function sendNextRequest()
{
var req = requestQueue.shift();
var options = requestQueue.shift();
var callback = requestQueue.shift();
if (!callback)
{
return;
}
requestInProgress = true;
socket.emit('request', req, options, function(err, res)
{
requestInProgress = false;
callback(err, res);
sendNextRequest();
});
}
| morkai/walkner-iovis | frontend/main.js | JavaScript | mit | 16,664 |
<ul class="breadcrumb">
{% for url, label in breadcrumbs %}
<li>
{% ifnotequal forloop.counter breadcrumbs_total %}
<a href="{{ url }}">{{ label|safe }}</a>
{% else %}
{{ label|safe }}
{% endifnotequal %}
{% if not forloop.last %}
<span class="divider">/</span>
{% endif %}
</li>
{% endfor %}
</ul>
| WhySoGeeky/DroidPot | venv/lib/python2.7/site-packages/django_bootstrap_breadcrumbs/templates/django_bootstrap_breadcrumbs/bootstrap2.html | HTML | mit | 431 |
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>metacoq-erasure: 3 m 5 s</title>
<link rel="shortcut icon" type="image/png" href="../../../../../favicon.png" />
<link href="../../../../../bootstrap.min.css" rel="stylesheet">
<link href="../../../../../bootstrap-custom.css" rel="stylesheet">
<link href="//maxcdn.bootstrapcdn.com/font-awesome/4.2.0/css/font-awesome.min.css" rel="stylesheet">
<script src="../../../../../moment.min.js"></script>
<!-- HTML5 Shim and Respond.js IE8 support of HTML5 elements and media queries -->
<!-- WARNING: Respond.js doesn't work if you view the page via file:// -->
<!--[if lt IE 9]>
<script src="https://oss.maxcdn.com/html5shiv/3.7.2/html5shiv.min.js"></script>
<script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script>
<![endif]-->
</head>
<body>
<div class="container">
<div class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" href="../../../../.."><i class="fa fa-lg fa-flag-checkered"></i> Coq bench</a>
</div>
<div id="navbar" class="collapse navbar-collapse">
<ul class="nav navbar-nav">
<li><a href="../..">clean / released</a></li>
<li class="active"><a href="">8.9.0 / metacoq-erasure - 1.0~alpha+8.9</a></li>
</ul>
</div>
</div>
</div>
<div class="article">
<div class="row">
<div class="col-md-12">
<a href="../..">« Up</a>
<h1>
metacoq-erasure
<small>
1.0~alpha+8.9
<span class="label label-success">3 m 5 s</span>
</small>
</h1>
<p><em><script>document.write(moment("2020-07-26 11:43:01 +0000", "YYYY-MM-DD HH:mm:ss Z").fromNow());</script> (2020-07-26 11:43:01 UTC)</em><p>
<h2>Context</h2>
<pre># Packages matching: installed
# Name # Installed # Synopsis
base-bigarray base
base-num base Num library distributed with the OCaml compiler
base-threads base
base-unix base
camlp5 7.12 Preprocessor-pretty-printer of OCaml
conf-findutils 1 Virtual package relying on findutils
conf-m4 1 Virtual package relying on m4
coq 8.9.0 Formal proof management system
num 0 The Num library for arbitrary-precision integer and rational arithmetic
ocaml 4.04.2 The OCaml compiler (virtual package)
ocaml-base-compiler 4.04.2 Official 4.04.2 release
ocaml-config 1 OCaml Switch Configuration
ocamlfind 1.8.1 A library manager for OCaml
# opam file:
opam-version: "2.0"
maintainer: "matthieu.sozeau@inria.fr"
homepage: "https://metacoq.github.io/metacoq"
dev-repo: "git+https://github.com/MetaCoq/metacoq.git#coq-8.8"
bug-reports: "https://github.com/MetaCoq/metacoq/issues"
authors: ["Abhishek Anand <aa755@cs.cornell.edu>"
"Simon Boulier <simon.boulier@inria.fr>"
"Cyril Cohen <cyril.cohen@inria.fr>"
"Yannick Forster <forster@ps.uni-saarland.de>"
"Fabian Kunze <fkunze@fakusb.de>"
"Gregory Malecha <gmalecha@gmail.com>"
"Matthieu Sozeau <matthieu.sozeau@inria.fr>"
"Nicolas Tabareau <nicolas.tabareau@inria.fr>"
"Théo Winterhalter <theo.winterhalter@inria.fr>"
]
license: "MIT"
build: [
["sh" "./configure.sh"]
[make "-j%{jobs}%" "-C" "erasure"]
[make "test-suite"] {with-test}
]
install: [
[make "-C" "erasure" "install"]
]
depends: [
"ocaml" {> "4.02.3"}
"coq" {>= "8.9" & < "8.10~"}
"coq-metacoq-template" {= version}
"coq-metacoq-checker" {= version}
"coq-metacoq-pcuic" {= version}
"coq-metacoq-safechecker" {= version}
]
synopsis: "Implementation and verification of an erasure procedure for Coq"
description: """
MetaCoq is a meta-programming framework for Coq.
The Erasure module provides a complete specification of Coq's so-called
\"extraction\" procedure, starting from the PCUIC calculus and targeting
untyped call-by-value lambda-calculus.
The `erasure` function translates types and proofs in well-typed terms
into a dummy `tBox` constructor, following closely P. Letouzey's PhD
thesis.
"""
url {
src: "https://github.com/MetaCoq/metacoq/archive/1.0-alpha+8.9.tar.gz"
checksum: "sha256=899ef4ee73b1684a0f1d2e37ab9ab0f9b24424f6d8a10a10efd474c0ed93488e"
}</pre>
<h2>Lint</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Dry install</h2>
<p>Dry install with the current Coq version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam install -y --show-action coq-metacoq-erasure.1.0~alpha+8.9 coq.8.9.0</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<p>Dry install without Coq/switch base, to test if the problem was incompatibility with the current Coq/OCaml version:</p>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>true</code></dd>
<dt>Return code</dt>
<dd>0</dd>
</dl>
<h2>Install dependencies</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam list; echo; ulimit -Sv 4000000; timeout 2h opam install -y --deps-only coq-metacoq-erasure.1.0~alpha+8.9 coq.8.9.0</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>25 m 27 s</dd>
</dl>
<h2>Install</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam list; echo; ulimit -Sv 16000000; timeout 2h opam install -y -v coq-metacoq-erasure.1.0~alpha+8.9 coq.8.9.0</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Duration</dt>
<dd>3 m 5 s</dd>
</dl>
<h2>Installation size</h2>
<p>Total: 13 M</p>
<ul>
<li>3 M <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/ErasureFunction.vo</code></li>
<li>1 M <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/ErasureCorrectness.vo</code></li>
<li>1 M <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/SafeErasureFunction.vo</code></li>
<li>980 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/ESubstitution.vo</code></li>
<li>897 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/metacoq_erasure_plugin.cmxs</code></li>
<li>866 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/SafeTemplateErasure.vo</code></li>
<li>824 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/Prelim.vo</code></li>
<li>794 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EArities.vo</code></li>
<li>793 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/Extraction.vo</code></li>
<li>756 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EInversion.vo</code></li>
<li>364 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/ELiftSubst.vo</code></li>
<li>177 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/metacoq_erasure_plugin.cmi</code></li>
<li>172 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/Extract.vo</code></li>
<li>158 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/ErasureCorrectness.glob</code></li>
<li>123 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/ELiftSubst.glob</code></li>
<li>114 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EAll.vo</code></li>
<li>114 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EWcbvEval.vo</code></li>
<li>108 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/ErasureFunction.glob</code></li>
<li>97 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EAstUtils.vo</code></li>
<li>79 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EArities.glob</code></li>
<li>77 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/SafeErasureFunction.glob</code></li>
<li>70 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/metacoq_erasure_plugin.cmx</code></li>
<li>68 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EWcbvEval.glob</code></li>
<li>66 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/ESubstitution.glob</code></li>
<li>63 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EAst.vo</code></li>
<li>63 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EPretty.vo</code></li>
<li>53 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/ETyping.vo</code></li>
<li>49 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/Prelim.glob</code></li>
<li>49 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/ErasureCorrectness.v</code></li>
<li>42 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EInduction.vo</code></li>
<li>38 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/Extract.glob</code></li>
<li>37 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EAstUtils.glob</code></li>
<li>36 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EWndEval.vo</code></li>
<li>34 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/Loader.vo</code></li>
<li>30 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/ErasureFunction.v</code></li>
<li>26 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/SafeErasureFunction.v</code></li>
<li>26 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/SafeTemplateErasure.glob</code></li>
<li>26 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EPretty.glob</code></li>
<li>23 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EWcbvEval.v</code></li>
<li>22 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EArities.v</code></li>
<li>22 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EInversion.glob</code></li>
<li>17 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/ELiftSubst.v</code></li>
<li>15 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/ESubstitution.v</code></li>
<li>13 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EAst.glob</code></li>
<li>12 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/Prelim.v</code></li>
<li>11 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/ETyping.glob</code></li>
<li>9 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/SafeTemplateErasure.v</code></li>
<li>8 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EAstUtils.v</code></li>
<li>8 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EInduction.glob</code></li>
<li>7 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/Extract.v</code></li>
<li>6 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EPretty.v</code></li>
<li>6 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/metacoq_erasure_plugin.cmxa</code></li>
<li>6 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EAst.v</code></li>
<li>3 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/ETyping.v</code></li>
<li>3 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EWndEval.v</code></li>
<li>3 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EInversion.v</code></li>
<li>3 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EInduction.v</code></li>
<li>2 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EWndEval.glob</code></li>
<li>1 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/Extraction.v</code></li>
<li>1 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/Extraction.glob</code></li>
<li>1 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EAll.glob</code></li>
<li>1 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/Loader.glob</code></li>
<li>1 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/Loader.v</code></li>
<li>1 K <code>../ocaml-base-compiler.4.04.2/lib/coq/user-contrib/MetaCoq/Erasure/EAll.v</code></li>
</ul>
<h2>Uninstall</h2>
<dl class="dl-horizontal">
<dt>Command</dt>
<dd><code>opam remove -y coq-metacoq-erasure.1.0~alpha+8.9</code></dd>
<dt>Return code</dt>
<dd>0</dd>
<dt>Missing removes</dt>
<dd>
none
</dd>
<dt>Wrong removes</dt>
<dd>
none
</dd>
</dl>
</div>
</div>
</div>
<hr/>
<div class="footer">
<p class="text-center">
<small>Sources are on <a href="https://github.com/coq-bench">GitHub</a>. © Guillaume Claret.</small>
</p>
</div>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script>
<script src="../../../../../bootstrap.min.js"></script>
</body>
</html>
| coq-bench/coq-bench.github.io | clean/Linux-x86_64-4.04.2-2.0.5/released/8.9.0/metacoq-erasure/1.0~alpha+8.9.html | HTML | mit | 15,736 |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Matrix
{
public class Matrix<T>
where T : struct
{
private T[,] matrix;
public Matrix(int x, int y)
{
matrix = new T[x, y];
}
public int LengthX
{
get
{
return this.matrix.GetLength(0);
}
}
public int LengthY
{
get
{
return this.matrix.GetLength(1);
}
}
public T this [int x, int y]
{
get
{
if (matrix.GetLength(0) > x && matrix.GetLength(1) > y)
{
return this.matrix[x, y];
}
else
{
throw new IndexOutOfRangeException();
}
}
set
{
if (matrix.GetLength(0) > x && matrix.GetLength(1) > y)
{
this.matrix[x, y] = value;
}
else
{
throw new IndexOutOfRangeException();
}
}
}
private static bool IsMatricesTheSameSize(Matrix<T> matrix1, Matrix<T> matrix2)
{
if (matrix1.matrix.GetLength(0) == matrix2.matrix.GetLength(0) && matrix1.matrix.GetLength(1) == matrix2.matrix.GetLength(1))
{
return true;
}
else
{
return false;
}
}
private static bool IsMultiplicationPossible(Matrix<T> matrix1, Matrix<T> matrix2)
{
if (matrix1.LengthX == matrix2.LengthY && matrix1.LengthY == matrix2.LengthX)
{
return true;
}
else
{
return false;
}
}
public static Matrix<T> operator +(Matrix<T> matrix1, Matrix<T> matrix2)
{
Matrix<T> newMatrix = new Matrix<T>(matrix1.LengthX, matrix1.LengthY);
dynamic matrice1 = matrix1;
dynamic matrice2 = matrix2;
if (Matrix<T>.IsMatricesTheSameSize(matrix1, matrix2))
{
for (int i = 0; i < matrix1.LengthX; i++)
{
for (int j = 0; j < matrix1.LengthY; j++)
{
newMatrix[i, j] = matrice1[i, j] + matrice2[i, j];
}
}
}
else
{
throw new InvalidOperationException();
}
return newMatrix;
}
public static Matrix<T> operator -(Matrix<T> matrix1, Matrix<T> matrix2)
{
Matrix<T> newMatrix = new Matrix<T>(matrix1.LengthX, matrix1.LengthY);
dynamic matrice1 = matrix1;
dynamic matrice2 = matrix2;
if (Matrix<T>.IsMatricesTheSameSize(matrix1, matrix2))
{
for (int i = 0; i < matrix1.LengthX; i++)
{
for (int j = 0; j < matrix1.LengthY; j++)
{
newMatrix[i, j] = matrice1[i, j] - matrice2[i, j];
}
}
}
else
{
throw new InvalidOperationException();
}
return newMatrix;
}
public static Matrix<T> operator *(Matrix<T> matrix1, Matrix<T> matrix2)
{
Matrix<T> newMatrix = new Matrix<T>(matrix1.LengthX, matrix2.LengthY);
dynamic matrice1 = matrix1;
dynamic matrice2 = matrix2;
if (Matrix<T>.IsMultiplicationPossible(matrix1, matrix2))
{
for (int i = 0; i < matrix1.LengthX; i++)
{
for (int j = 0; j < matrix1.LengthY; j++)
{
newMatrix[i, j] = matrice1[i, j] * matrice2[j, i];
}
}
}
else
{
throw new InvalidOperationException();
}
return newMatrix;
}
public static bool operator true(T elementOfMetrix)
{
return elementOfMetrix.Equals(default(T));
}
}
}
| dirk-dagger-667/telerik-c--OOP-lectures | DefineClassPartTwo1/Matrix/Matrix.cs | C# | mit | 4,419 |
// Licensed to the .NET Foundation under one or more agreements.
// The .NET Foundation licenses this file to you under the MIT license.
// See the LICENSE file in the project root for more information.
#nullable disable
using System;
using System.Collections.Generic;
using System.Collections.Immutable;
using System.ComponentModel;
using System.Globalization;
using System.IO;
using System.IO.MemoryMappedFiles;
using System.Linq;
using System.Reflection;
using System.Reflection.Metadata;
using System.Reflection.PortableExecutable;
using System.Runtime.InteropServices;
using System.Security.Cryptography;
using System.Text;
using System.Text.RegularExpressions;
using System.Threading;
using Microsoft.CodeAnalysis;
using Microsoft.CodeAnalysis.CSharp.Syntax;
using Microsoft.CodeAnalysis.CSharp.Test.Utilities;
using Microsoft.CodeAnalysis.Diagnostics;
using Microsoft.CodeAnalysis.Emit;
using Microsoft.CodeAnalysis.PooledObjects;
using Microsoft.CodeAnalysis.Test.Resources.Proprietary;
using Microsoft.CodeAnalysis.Test.Utilities;
using Microsoft.CodeAnalysis.Text;
using Microsoft.DiaSymReader;
using Roslyn.Test.PdbUtilities;
using Roslyn.Test.Utilities;
using Roslyn.Test.Utilities.TestGenerators;
using Roslyn.Utilities;
using Xunit;
using Basic.Reference.Assemblies;
using static Microsoft.CodeAnalysis.CommonDiagnosticAnalyzers;
using static Roslyn.Test.Utilities.SharedResourceHelpers;
using static Roslyn.Test.Utilities.TestMetadata;
namespace Microsoft.CodeAnalysis.CSharp.CommandLine.UnitTests
{
public class CommandLineTests : CommandLineTestBase
{
#if NETCOREAPP
private static readonly string s_CSharpCompilerExecutable;
private static readonly string s_DotnetCscRun;
#else
private static readonly string s_CSharpCompilerExecutable = Path.Combine(
Path.GetDirectoryName(typeof(CommandLineTests).GetTypeInfo().Assembly.Location),
Path.Combine("dependency", "csc.exe"));
private static readonly string s_DotnetCscRun = ExecutionConditionUtil.IsMono ? "mono" : string.Empty;
#endif
private static readonly string s_CSharpScriptExecutable;
private static readonly string s_compilerVersion = CommonCompiler.GetProductVersion(typeof(CommandLineTests));
static CommandLineTests()
{
#if NETCOREAPP
var cscDllPath = Path.Combine(
Path.GetDirectoryName(typeof(CommandLineTests).GetTypeInfo().Assembly.Location),
Path.Combine("dependency", "csc.dll"));
var dotnetExe = DotNetCoreSdk.ExePath;
var netStandardDllPath = AppDomain.CurrentDomain.GetAssemblies()
.FirstOrDefault(assembly => !assembly.IsDynamic && assembly.Location.EndsWith("netstandard.dll")).Location;
var netStandardDllDir = Path.GetDirectoryName(netStandardDllPath);
// Since we are using references based on the UnitTest's runtime, we need to use
// its runtime config when executing out program.
var runtimeConfigPath = Path.ChangeExtension(Assembly.GetExecutingAssembly().Location, "runtimeconfig.json");
s_CSharpCompilerExecutable = $@"""{dotnetExe}"" ""{cscDllPath}"" /r:""{netStandardDllPath}"" /r:""{netStandardDllDir}/System.Private.CoreLib.dll"" /r:""{netStandardDllDir}/System.Console.dll"" /r:""{netStandardDllDir}/System.Runtime.dll""";
s_DotnetCscRun = $@"""{dotnetExe}"" exec --runtimeconfig ""{runtimeConfigPath}""";
s_CSharpScriptExecutable = s_CSharpCompilerExecutable.Replace("csc.dll", Path.Combine("csi", "csi.dll"));
#else
s_CSharpScriptExecutable = s_CSharpCompilerExecutable.Replace("csc.exe", Path.Combine("csi", "csi.exe"));
#endif
}
private class TestCommandLineParser : CSharpCommandLineParser
{
private readonly Dictionary<string, string> _responseFiles;
private readonly Dictionary<string, string[]> _recursivePatterns;
private readonly Dictionary<string, string[]> _patterns;
public TestCommandLineParser(
Dictionary<string, string> responseFiles = null,
Dictionary<string, string[]> patterns = null,
Dictionary<string, string[]> recursivePatterns = null,
bool isInteractive = false)
: base(isInteractive)
{
_responseFiles = responseFiles;
_recursivePatterns = recursivePatterns;
_patterns = patterns;
}
internal override IEnumerable<string> EnumerateFiles(string directory,
string fileNamePattern,
SearchOption searchOption)
{
var key = directory + "|" + fileNamePattern;
if (searchOption == SearchOption.TopDirectoryOnly)
{
return _patterns[key];
}
else
{
return _recursivePatterns[key];
}
}
internal override TextReader CreateTextFileReader(string fullPath)
{
return new StringReader(_responseFiles[fullPath]);
}
}
private CSharpCommandLineArguments ScriptParse(IEnumerable<string> args, string baseDirectory)
{
return CSharpCommandLineParser.Script.Parse(args, baseDirectory, SdkDirectory);
}
private CSharpCommandLineArguments FullParse(string commandLine, string baseDirectory, string sdkDirectory = null, string additionalReferenceDirectories = null)
{
sdkDirectory = sdkDirectory ?? SdkDirectory;
var args = CommandLineParser.SplitCommandLineIntoArguments(commandLine, removeHashComments: true);
return CSharpCommandLineParser.Default.Parse(args, baseDirectory, sdkDirectory, additionalReferenceDirectories);
}
[ConditionalFact(typeof(WindowsDesktopOnly))]
[WorkItem(34101, "https://github.com/dotnet/roslyn/issues/34101")]
public void SuppressedWarnAsErrorsStillEmit()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
#pragma warning disable 1591
public class P {
public static void Main() {}
}");
const string docName = "doc.xml";
var cmd = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "/errorlog:errorlog", $"/doc:{docName}", "/warnaserror", src.Path });
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = cmd.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("", outWriter.ToString());
string exePath = Path.Combine(dir.Path, "temp.exe");
Assert.True(File.Exists(exePath));
var result = ProcessUtilities.Run(exePath, arguments: "");
Assert.Equal(0, result.ExitCode);
}
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = ConditionalSkipReason.TestExecutionNeedsWindowsTypes)]
public void XmlMemoryMapped()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText("class C {}");
const string docName = "doc.xml";
var cmd = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "/t:library", "/preferreduilang:en", $"/doc:{docName}", src.Path });
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = cmd.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("", outWriter.ToString());
var xmlPath = Path.Combine(dir.Path, docName);
using (var fileStream = new FileStream(xmlPath, FileMode.Open, FileAccess.Read, FileShare.ReadWrite))
using (var mmf = MemoryMappedFile.CreateFromFile(fileStream, "xmlMap", 0, MemoryMappedFileAccess.Read, HandleInheritability.None, leaveOpen: true))
{
exitCode = cmd.Run(outWriter);
Assert.StartsWith($"error CS0016: Could not write to output file '{xmlPath}' -- ", outWriter.ToString());
Assert.Equal(1, exitCode);
}
}
[Fact]
public void SimpleAnalyzerConfig()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("test.cs").WriteAllText(@"
class C
{
int _f;
}");
var analyzerConfig = dir.CreateFile(".editorconfig").WriteAllText(@"
[*.cs]
dotnet_diagnostic.cs0169.severity = none");
var cmd = CreateCSharpCompiler(null, dir.Path, new[] {
"/nologo",
"/t:library",
"/preferreduilang:en",
"/analyzerconfig:" + analyzerConfig.Path,
src.Path });
Assert.Equal(analyzerConfig.Path, Assert.Single(cmd.Arguments.AnalyzerConfigPaths));
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = cmd.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("", outWriter.ToString());
Assert.Null(cmd.AnalyzerOptions);
}
[Fact]
public void AnalyzerConfigWithOptions()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("test.cs").WriteAllText(@"
class C
{
int _f;
}");
var additionalFile = dir.CreateFile("file.txt");
var analyzerConfig = dir.CreateFile(".editorconfig").WriteAllText(@"
[*.cs]
dotnet_diagnostic.cs0169.severity = none
dotnet_diagnostic.Warning01.severity = none
my_option = my_val
[*.txt]
dotnet_diagnostic.cs0169.severity = none
my_option2 = my_val2");
var cmd = CreateCSharpCompiler(null, dir.Path, new[] {
"/nologo",
"/t:library",
"/analyzerconfig:" + analyzerConfig.Path,
"/analyzer:" + Assembly.GetExecutingAssembly().Location,
"/nowarn:8032",
"/additionalfile:" + additionalFile.Path,
src.Path });
Assert.Equal(analyzerConfig.Path, Assert.Single(cmd.Arguments.AnalyzerConfigPaths));
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = cmd.Run(outWriter);
Assert.Equal("", outWriter.ToString());
Assert.Equal(0, exitCode);
var comp = cmd.Compilation;
var tree = comp.SyntaxTrees.Single();
var compilerTreeOptions = comp.Options.SyntaxTreeOptionsProvider;
Assert.True(compilerTreeOptions.TryGetDiagnosticValue(tree, "cs0169", CancellationToken.None, out var severity));
Assert.Equal(ReportDiagnostic.Suppress, severity);
Assert.True(compilerTreeOptions.TryGetDiagnosticValue(tree, "warning01", CancellationToken.None, out severity));
Assert.Equal(ReportDiagnostic.Suppress, severity);
var analyzerOptions = cmd.AnalyzerOptions.AnalyzerConfigOptionsProvider;
var options = analyzerOptions.GetOptions(tree);
Assert.NotNull(options);
Assert.True(options.TryGetValue("my_option", out string val));
Assert.Equal("my_val", val);
Assert.False(options.TryGetValue("my_option2", out _));
Assert.False(options.TryGetValue("dotnet_diagnostic.cs0169.severity", out _));
options = analyzerOptions.GetOptions(cmd.AnalyzerOptions.AdditionalFiles.Single());
Assert.NotNull(options);
Assert.True(options.TryGetValue("my_option2", out val));
Assert.Equal("my_val2", val);
Assert.False(options.TryGetValue("my_option", out _));
Assert.False(options.TryGetValue("dotnet_diagnostic.cs0169.severity", out _));
}
[Fact]
public void AnalyzerConfigBadSeverity()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("test.cs").WriteAllText(@"
class C
{
int _f;
}");
var analyzerConfig = dir.CreateFile(".editorconfig").WriteAllText(@"
[*.cs]
dotnet_diagnostic.cs0169.severity = garbage");
var cmd = CreateCSharpCompiler(null, dir.Path, new[] {
"/nologo",
"/t:library",
"/preferreduilang:en",
"/analyzerconfig:" + analyzerConfig.Path,
src.Path });
Assert.Equal(analyzerConfig.Path, Assert.Single(cmd.Arguments.AnalyzerConfigPaths));
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = cmd.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal(
$@"warning InvalidSeverityInAnalyzerConfig: The diagnostic 'cs0169' was given an invalid severity 'garbage' in the analyzer config file at '{analyzerConfig.Path}'.
test.cs(4,9): warning CS0169: The field 'C._f' is never used
", outWriter.ToString());
Assert.Null(cmd.AnalyzerOptions);
}
[Fact]
public void AnalyzerConfigsInSameDir()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("test.cs").WriteAllText(@"
class C
{
int _f;
}");
var configText = @"
[*.cs]
dotnet_diagnostic.cs0169.severity = suppress";
var analyzerConfig1 = dir.CreateFile("analyzerconfig1").WriteAllText(configText);
var analyzerConfig2 = dir.CreateFile("analyzerconfig2").WriteAllText(configText);
var cmd = CreateCSharpCompiler(null, dir.Path, new[] {
"/nologo",
"/t:library",
"/preferreduilang:en",
"/analyzerconfig:" + analyzerConfig1.Path,
"/analyzerconfig:" + analyzerConfig2.Path,
src.Path
});
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = cmd.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal(
$"error CS8700: Multiple analyzer config files cannot be in the same directory ('{dir.Path}').",
outWriter.ToString().TrimEnd());
}
// This test should only run when the machine's default encoding is shift-JIS
[ConditionalFact(typeof(WindowsDesktopOnly), typeof(HasShiftJisDefaultEncoding), Reason = "https://github.com/dotnet/roslyn/issues/30321")]
public void CompileShiftJisOnShiftJis()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("sjis.cs").WriteAllBytes(TestResources.General.ShiftJisSource);
var cmd = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", src.Path });
Assert.Null(cmd.Arguments.Encoding);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = cmd.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("", outWriter.ToString());
var result = ProcessUtilities.Run(Path.Combine(dir.Path, "sjis.exe"), arguments: "", workingDirectory: dir.Path);
Assert.Equal(0, result.ExitCode);
Assert.Equal("星野 八郎太", File.ReadAllText(Path.Combine(dir.Path, "output.txt"), Encoding.GetEncoding(932)));
}
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30321")]
public void RunWithShiftJisFile()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("sjis.cs").WriteAllBytes(TestResources.General.ShiftJisSource);
var cmd = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "/codepage:932", src.Path });
Assert.Equal(932, cmd.Arguments.Encoding?.WindowsCodePage);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = cmd.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("", outWriter.ToString());
var result = ProcessUtilities.Run(Path.Combine(dir.Path, "sjis.exe"), arguments: "", workingDirectory: dir.Path);
Assert.Equal(0, result.ExitCode);
Assert.Equal("星野 八郎太", File.ReadAllText(Path.Combine(dir.Path, "output.txt"), Encoding.GetEncoding(932)));
}
[WorkItem(946954, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/946954")]
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30321")]
public void CompilerBinariesAreAnyCPU()
{
Assert.Equal(ProcessorArchitecture.MSIL, AssemblyName.GetAssemblyName(s_CSharpCompilerExecutable).ProcessorArchitecture);
}
[Fact]
public void ResponseFiles1()
{
string rsp = Temp.CreateFile().WriteAllText(@"
/r:System.dll
/nostdlib
# this is ignored
System.Console.WriteLine(""*?""); # this is error
a.cs
").Path;
var cmd = CreateCSharpCompiler(rsp, WorkingDirectory, new[] { "b.cs" });
cmd.Arguments.Errors.Verify(
// error CS2001: Source file 'System.Console.WriteLine(*?);' could not be found
Diagnostic(ErrorCode.ERR_FileNotFound).WithArguments("System.Console.WriteLine(*?);"));
AssertEx.Equal(new[] { "System.dll" }, cmd.Arguments.MetadataReferences.Select(r => r.Reference));
AssertEx.Equal(new[] { Path.Combine(WorkingDirectory, "a.cs"), Path.Combine(WorkingDirectory, "b.cs") }, cmd.Arguments.SourceFiles.Select(file => file.Path));
CleanupAllGeneratedFiles(rsp);
}
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = ConditionalSkipReason.TestExecutionNeedsWindowsTypes)]
public void ResponseFiles_RelativePaths()
{
var parentDir = Temp.CreateDirectory();
var baseDir = parentDir.CreateDirectory("temp");
var dirX = baseDir.CreateDirectory("x");
var dirAB = baseDir.CreateDirectory("a b");
var dirSubDir = baseDir.CreateDirectory("subdir");
var dirGoo = parentDir.CreateDirectory("goo");
var dirBar = parentDir.CreateDirectory("bar");
string basePath = baseDir.Path;
Func<string, string> prependBasePath = fileName => Path.Combine(basePath, fileName);
var parser = new TestCommandLineParser(responseFiles: new Dictionary<string, string>()
{
{ prependBasePath(@"a.rsp"), @"
""@subdir\b.rsp""
/r:..\v4.0.30319\System.dll
/r:.\System.Data.dll
a.cs @""..\c.rsp"" @\d.rsp
/libpaths:..\goo;../bar;""a b""
"
},
{ Path.Combine(dirSubDir.Path, @"b.rsp"), @"
b.cs
"
},
{ prependBasePath(@"..\c.rsp"), @"
c.cs /lib:x
"
},
{ Path.Combine(Path.GetPathRoot(basePath), @"d.rsp"), @"
# comment
d.cs
"
}
}, isInteractive: false);
var args = parser.Parse(new[] { "first.cs", "second.cs", "@a.rsp", "last.cs" }, basePath, SdkDirectory);
args.Errors.Verify();
Assert.False(args.IsScriptRunner);
string[] resolvedSourceFiles = args.SourceFiles.Select(f => f.Path).ToArray();
string[] references = args.MetadataReferences.Select(r => r.Reference).ToArray();
AssertEx.Equal(new[] { "first.cs", "second.cs", "b.cs", "a.cs", "c.cs", "d.cs", "last.cs" }.Select(prependBasePath), resolvedSourceFiles);
AssertEx.Equal(new[] { typeof(object).Assembly.Location, @"..\v4.0.30319\System.dll", @".\System.Data.dll" }, references);
AssertEx.Equal(new[] { RuntimeEnvironment.GetRuntimeDirectory() }.Concat(new[] { @"x", @"..\goo", @"../bar", @"a b" }.Select(prependBasePath)), args.ReferencePaths.ToArray());
Assert.Equal(basePath, args.BaseDirectory);
}
#nullable enable
[ConditionalFact(typeof(WindowsOnly))]
public void NullBaseDirectoryNotAddedToKeyFileSearchPaths()
{
var parser = CSharpCommandLineParser.Default.Parse(new[] { "c:/test.cs" }, baseDirectory: null, SdkDirectory);
AssertEx.Equal(ImmutableArray.Create<string>(), parser.KeyFileSearchPaths);
Assert.Null(parser.OutputDirectory);
parser.Errors.Verify(
// error CS8762: Output directory could not be determined
Diagnostic(ErrorCode.ERR_NoOutputDirectory).WithLocation(1, 1)
);
}
[ConditionalFact(typeof(WindowsOnly))]
public void NullBaseDirectoryWithAdditionalFiles()
{
var parser = CSharpCommandLineParser.Default.Parse(new[] { "/additionalfile:web.config", "c:/test.cs" }, baseDirectory: null, SdkDirectory);
AssertEx.Equal(ImmutableArray.Create<string>(), parser.KeyFileSearchPaths);
Assert.Null(parser.OutputDirectory);
parser.Errors.Verify(
// error CS2021: File name 'web.config' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments("web.config").WithLocation(1, 1),
// error CS8762: Output directory could not be determined
Diagnostic(ErrorCode.ERR_NoOutputDirectory).WithLocation(1, 1)
);
}
[ConditionalFact(typeof(WindowsOnly))]
public void NullBaseDirectoryWithAdditionalFiles_Wildcard()
{
var parser = CSharpCommandLineParser.Default.Parse(new[] { "/additionalfile:*", "c:/test.cs" }, baseDirectory: null, SdkDirectory);
AssertEx.Equal(ImmutableArray.Create<string>(), parser.KeyFileSearchPaths);
Assert.Null(parser.OutputDirectory);
parser.Errors.Verify(
// error CS2001: Source file '*' could not be found.
Diagnostic(ErrorCode.ERR_FileNotFound).WithArguments("*").WithLocation(1, 1),
// error CS8762: Output directory could not be determined
Diagnostic(ErrorCode.ERR_NoOutputDirectory).WithLocation(1, 1)
);
}
#nullable disable
[Fact, WorkItem(29252, "https://github.com/dotnet/roslyn/issues/29252")]
public void NoSdkPath()
{
var parentDir = Temp.CreateDirectory();
var parser = CSharpCommandLineParser.Default.Parse(new[] { "file.cs", $"-out:{parentDir.Path}", "/noSdkPath" }, parentDir.Path, null);
AssertEx.Equal(ImmutableArray<string>.Empty, parser.ReferencePaths);
}
[Fact, WorkItem(29252, "https://github.com/dotnet/roslyn/issues/29252")]
public void NoSdkPathReferenceSystemDll()
{
string source = @"
class C
{
}
";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile("a.cs");
file.WriteAllText(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "/preferreduilang:en", "/nosdkpath", "/r:System.dll", "a.cs" });
var exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal("error CS0006: Metadata file 'System.dll' could not be found", outWriter.ToString().Trim());
}
[ConditionalFact(typeof(WindowsOnly))]
public void SourceFiles_Patterns()
{
var parser = new TestCommandLineParser(
patterns: new Dictionary<string, string[]>()
{
{ @"C:\temp|*.cs", new[] { "a.cs", "b.cs", "c.cs" } }
},
recursivePatterns: new Dictionary<string, string[]>()
{
{ @"C:\temp\a|*.cs", new[] { @"a\x.cs", @"a\b\b.cs", @"a\c.cs" } },
});
var args = parser.Parse(new[] { @"*.cs", @"/recurse:a\*.cs" }, @"C:\temp", SdkDirectory);
args.Errors.Verify();
string[] resolvedSourceFiles = args.SourceFiles.Select(f => f.Path).ToArray();
AssertEx.Equal(new[] { @"C:\temp\a.cs", @"C:\temp\b.cs", @"C:\temp\c.cs", @"C:\temp\a\x.cs", @"C:\temp\a\b\b.cs", @"C:\temp\a\c.cs" }, resolvedSourceFiles);
}
[Fact]
public void ParseQuotedMainType()
{
// Verify the main switch are unquoted when used because of the issue with
// MSBuild quoting some usages and not others. A quote character is not valid in either
// these names.
CSharpCommandLineArguments args;
var folder = Temp.CreateDirectory();
CreateFile(folder, "a.cs");
args = DefaultParse(new[] { "/main:Test", "a.cs" }, folder.Path);
args.Errors.Verify();
Assert.Equal("Test", args.CompilationOptions.MainTypeName);
args = DefaultParse(new[] { "/main:\"Test\"", "a.cs" }, folder.Path);
args.Errors.Verify();
Assert.Equal("Test", args.CompilationOptions.MainTypeName);
args = DefaultParse(new[] { "/main:\"Test.Class1\"", "a.cs" }, folder.Path);
args.Errors.Verify();
Assert.Equal("Test.Class1", args.CompilationOptions.MainTypeName);
args = DefaultParse(new[] { "/m:Test", "a.cs" }, folder.Path);
args.Errors.Verify();
Assert.Equal("Test", args.CompilationOptions.MainTypeName);
args = DefaultParse(new[] { "/m:\"Test\"", "a.cs" }, folder.Path);
args.Errors.Verify();
Assert.Equal("Test", args.CompilationOptions.MainTypeName);
args = DefaultParse(new[] { "/m:\"Test.Class1\"", "a.cs" }, folder.Path);
args.Errors.Verify();
Assert.Equal("Test.Class1", args.CompilationOptions.MainTypeName);
// Use of Cyrillic namespace
args = DefaultParse(new[] { "/m:\"решения.Class1\"", "a.cs" }, folder.Path);
args.Errors.Verify();
Assert.Equal("решения.Class1", args.CompilationOptions.MainTypeName);
}
[Fact]
[WorkItem(21508, "https://github.com/dotnet/roslyn/issues/21508")]
public void ArgumentStartWithDashAndContainingSlash()
{
CSharpCommandLineArguments args;
var folder = Temp.CreateDirectory();
args = DefaultParse(new[] { "-debug+/debug:portable" }, folder.Path);
args.Errors.Verify(
// error CS2007: Unrecognized option: '-debug+/debug:portable'
Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("-debug+/debug:portable").WithLocation(1, 1),
// warning CS2008: No source files specified.
Diagnostic(ErrorCode.WRN_NoSources).WithLocation(1, 1),
// error CS1562: Outputs without source must have the /out option specified
Diagnostic(ErrorCode.ERR_OutputNeedsName).WithLocation(1, 1)
);
}
[WorkItem(546009, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546009")]
[WorkItem(545991, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/545991")]
[ConditionalFact(typeof(WindowsOnly))]
public void SourceFiles_Patterns2()
{
var folder = Temp.CreateDirectory();
CreateFile(folder, "a.cs");
CreateFile(folder, "b.vb");
CreateFile(folder, "c.cpp");
var folderA = folder.CreateDirectory("A");
CreateFile(folderA, "A_a.cs");
CreateFile(folderA, "A_b.cs");
CreateFile(folderA, "A_c.vb");
var folderB = folder.CreateDirectory("B");
CreateFile(folderB, "B_a.cs");
CreateFile(folderB, "B_b.vb");
CreateFile(folderB, "B_c.cpx");
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, folder.Path, new[] { "/nologo", "/preferreduilang:en", "/t:library", @"/recurse:.", "/out:abc.dll" }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("warning CS2008: No source files specified.", outWriter.ToString().Trim());
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, folder.Path, new[] { "/nologo", "/preferreduilang:en", "/t:library", @"/recurse:. ", "/out:abc.dll" }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("warning CS2008: No source files specified.", outWriter.ToString().Trim());
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, folder.Path, new[] { "/nologo", "/preferreduilang:en", "/t:library", @"/recurse: . ", "/out:abc.dll" }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("warning CS2008: No source files specified.", outWriter.ToString().Trim());
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, folder.Path, new[] { "/nologo", "/preferreduilang:en", "/t:library", @"/recurse:././.", "/out:abc.dll" }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("warning CS2008: No source files specified.", outWriter.ToString().Trim());
CSharpCommandLineArguments args;
string[] resolvedSourceFiles;
args = DefaultParse(new[] { @"/recurse:*.cp*", @"/recurse:a\*.c*", @"/out:a.dll" }, folder.Path);
args.Errors.Verify();
resolvedSourceFiles = args.SourceFiles.Select(f => f.Path).ToArray();
AssertEx.Equal(new[] { folder.Path + @"\c.cpp", folder.Path + @"\B\B_c.cpx", folder.Path + @"\a\A_a.cs", folder.Path + @"\a\A_b.cs", }, resolvedSourceFiles);
args = DefaultParse(new[] { @"/recurse:.\\\\\\*.cs", @"/out:a.dll" }, folder.Path);
args.Errors.Verify();
resolvedSourceFiles = args.SourceFiles.Select(f => f.Path).ToArray();
Assert.Equal(4, resolvedSourceFiles.Length);
args = DefaultParse(new[] { @"/recurse:.////*.cs", @"/out:a.dll" }, folder.Path);
args.Errors.Verify();
resolvedSourceFiles = args.SourceFiles.Select(f => f.Path).ToArray();
Assert.Equal(4, resolvedSourceFiles.Length);
}
[ConditionalFact(typeof(WindowsOnly))]
public void SourceFile_BadPath()
{
var args = DefaultParse(new[] { @"e:c:\test\test.cs", "/t:library" }, WorkingDirectory);
Assert.Equal(3, args.Errors.Length);
Assert.Equal((int)ErrorCode.FTL_InvalidInputFileName, args.Errors[0].Code);
Assert.Equal((int)ErrorCode.WRN_NoSources, args.Errors[1].Code);
Assert.Equal((int)ErrorCode.ERR_OutputNeedsName, args.Errors[2].Code);
}
private void CreateFile(TempDirectory folder, string file)
{
var f = folder.CreateFile(file);
f.WriteAllText("");
}
[Fact, WorkItem(546023, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546023")]
public void Win32ResourceArguments()
{
string[] args = new string[]
{
@"/win32manifest:..\here\there\everywhere\nonexistent"
};
var parsedArgs = DefaultParse(args, WorkingDirectory);
var compilation = CreateCompilation(new SyntaxTree[0]);
IEnumerable<DiagnosticInfo> errors;
CSharpCompiler.GetWin32ResourcesInternal(StandardFileSystem.Instance, MessageProvider.Instance, parsedArgs, compilation, out errors);
Assert.Equal(1, errors.Count());
Assert.Equal((int)ErrorCode.ERR_CantOpenWin32Manifest, errors.First().Code);
Assert.Equal(2, errors.First().Arguments.Count());
args = new string[]
{
@"/Win32icon:\bogus"
};
parsedArgs = DefaultParse(args, WorkingDirectory);
CSharpCompiler.GetWin32ResourcesInternal(StandardFileSystem.Instance, MessageProvider.Instance, parsedArgs, compilation, out errors);
Assert.Equal(1, errors.Count());
Assert.Equal((int)ErrorCode.ERR_CantOpenIcon, errors.First().Code);
Assert.Equal(2, errors.First().Arguments.Count());
args = new string[]
{
@"/Win32Res:\bogus"
};
parsedArgs = DefaultParse(args, WorkingDirectory);
CSharpCompiler.GetWin32ResourcesInternal(StandardFileSystem.Instance, MessageProvider.Instance, parsedArgs, compilation, out errors);
Assert.Equal(1, errors.Count());
Assert.Equal((int)ErrorCode.ERR_CantOpenWin32Res, errors.First().Code);
Assert.Equal(2, errors.First().Arguments.Count());
args = new string[]
{
@"/Win32Res:goo.win32data:bar.win32data2"
};
parsedArgs = DefaultParse(args, WorkingDirectory);
CSharpCompiler.GetWin32ResourcesInternal(StandardFileSystem.Instance, MessageProvider.Instance, parsedArgs, compilation, out errors);
Assert.Equal(1, errors.Count());
Assert.Equal((int)ErrorCode.ERR_CantOpenWin32Res, errors.First().Code);
Assert.Equal(2, errors.First().Arguments.Count());
args = new string[]
{
@"/Win32icon:goo.win32data:bar.win32data2"
};
parsedArgs = DefaultParse(args, WorkingDirectory);
CSharpCompiler.GetWin32ResourcesInternal(StandardFileSystem.Instance, MessageProvider.Instance, parsedArgs, compilation, out errors);
Assert.Equal(1, errors.Count());
Assert.Equal((int)ErrorCode.ERR_CantOpenIcon, errors.First().Code);
Assert.Equal(2, errors.First().Arguments.Count());
args = new string[]
{
@"/Win32manifest:goo.win32data:bar.win32data2"
};
parsedArgs = DefaultParse(args, WorkingDirectory);
CSharpCompiler.GetWin32ResourcesInternal(StandardFileSystem.Instance, MessageProvider.Instance, parsedArgs, compilation, out errors);
Assert.Equal(1, errors.Count());
Assert.Equal((int)ErrorCode.ERR_CantOpenWin32Manifest, errors.First().Code);
Assert.Equal(2, errors.First().Arguments.Count());
}
[Fact]
public void Win32ResConflicts()
{
var parsedArgs = DefaultParse(new[] { "/win32res:goo", "/win32icon:goob", "a.cs" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal((int)ErrorCode.ERR_CantHaveWin32ResAndIcon, parsedArgs.Errors.First().Code);
parsedArgs = DefaultParse(new[] { "/win32res:goo", "/win32manifest:goob", "a.cs" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal((int)ErrorCode.ERR_CantHaveWin32ResAndManifest, parsedArgs.Errors.First().Code);
parsedArgs = DefaultParse(new[] { "/win32res:", "a.cs" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal((int)ErrorCode.ERR_NoFileSpec, parsedArgs.Errors.First().Code);
Assert.Equal(1, parsedArgs.Errors.First().Arguments.Count);
parsedArgs = DefaultParse(new[] { "/win32Icon: ", "a.cs" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal((int)ErrorCode.ERR_NoFileSpec, parsedArgs.Errors.First().Code);
Assert.Equal(1, parsedArgs.Errors.First().Arguments.Count);
parsedArgs = DefaultParse(new[] { "/win32Manifest:", "a.cs" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal((int)ErrorCode.ERR_NoFileSpec, parsedArgs.Errors.First().Code);
Assert.Equal(1, parsedArgs.Errors.First().Arguments.Count);
parsedArgs = DefaultParse(new[] { "/win32Manifest:goo", "/noWin32Manifest", "a.cs" }, WorkingDirectory);
Assert.Equal(0, parsedArgs.Errors.Length);
Assert.True(parsedArgs.NoWin32Manifest);
Assert.Null(parsedArgs.Win32Manifest);
}
[Fact]
public void Win32ResInvalid()
{
var parsedArgs = DefaultParse(new[] { "/win32res", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("/win32res"));
parsedArgs = DefaultParse(new[] { "/win32res+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/win32res+"));
parsedArgs = DefaultParse(new[] { "/win32icon", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("/win32icon"));
parsedArgs = DefaultParse(new[] { "/win32icon+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/win32icon+"));
parsedArgs = DefaultParse(new[] { "/win32manifest", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("/win32manifest"));
parsedArgs = DefaultParse(new[] { "/win32manifest+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/win32manifest+"));
}
[Fact]
public void Win32IconContainsGarbage()
{
string tmpFileName = Temp.CreateFile().WriteAllBytes(new byte[] { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 }).Path;
var parsedArgs = DefaultParse(new[] { "/win32icon:" + tmpFileName, "a.cs" }, WorkingDirectory);
var compilation = CreateCompilation(new SyntaxTree[0]);
IEnumerable<DiagnosticInfo> errors;
CSharpCompiler.GetWin32ResourcesInternal(StandardFileSystem.Instance, MessageProvider.Instance, parsedArgs, compilation, out errors);
Assert.Equal(1, errors.Count());
Assert.Equal((int)ErrorCode.ERR_ErrorBuildingWin32Resources, errors.First().Code);
Assert.Equal(1, errors.First().Arguments.Count());
CleanupAllGeneratedFiles(tmpFileName);
}
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30289")]
public void Win32ResQuotes()
{
string[] responseFile = new string[] {
@" /win32res:d:\\""abc def""\a""b c""d\a.res",
};
CSharpCommandLineArguments args = DefaultParse(CSharpCommandLineParser.ParseResponseLines(responseFile), @"c:\");
Assert.Equal(@"d:\abc def\ab cd\a.res", args.Win32ResourceFile);
responseFile = new string[] {
@" /win32icon:d:\\""abc def""\a""b c""d\a.ico",
};
args = DefaultParse(CSharpCommandLineParser.ParseResponseLines(responseFile), @"c:\");
Assert.Equal(@"d:\abc def\ab cd\a.ico", args.Win32Icon);
responseFile = new string[] {
@" /win32manifest:d:\\""abc def""\a""b c""d\a.manifest",
};
args = DefaultParse(CSharpCommandLineParser.ParseResponseLines(responseFile), @"c:\");
Assert.Equal(@"d:\abc def\ab cd\a.manifest", args.Win32Manifest);
}
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30289")]
public void ParseResources()
{
var diags = new List<Diagnostic>();
ResourceDescription desc = CSharpCommandLineParser.ParseResourceDescription("", @"\somepath\someFile.goo.bar", WorkingDirectory, diags, embedded: false);
Assert.Equal(0, diags.Count);
Assert.Equal(@"someFile.goo.bar", desc.FileName);
Assert.Equal("someFile.goo.bar", desc.ResourceName);
desc = CSharpCommandLineParser.ParseResourceDescription("", @"\somepath\someFile.goo.bar,someName", WorkingDirectory, diags, embedded: false);
Assert.Equal(0, diags.Count);
Assert.Equal(@"someFile.goo.bar", desc.FileName);
Assert.Equal("someName", desc.ResourceName);
desc = CSharpCommandLineParser.ParseResourceDescription("", @"\somepath\s""ome Fil""e.goo.bar,someName", WorkingDirectory, diags, embedded: false);
Assert.Equal(0, diags.Count);
Assert.Equal(@"some File.goo.bar", desc.FileName);
Assert.Equal("someName", desc.ResourceName);
desc = CSharpCommandLineParser.ParseResourceDescription("", @"\somepath\someFile.goo.bar,""some Name"",public", WorkingDirectory, diags, embedded: false);
Assert.Equal(0, diags.Count);
Assert.Equal(@"someFile.goo.bar", desc.FileName);
Assert.Equal("some Name", desc.ResourceName);
Assert.True(desc.IsPublic);
// Use file name in place of missing resource name.
desc = CSharpCommandLineParser.ParseResourceDescription("", @"\somepath\someFile.goo.bar,,private", WorkingDirectory, diags, embedded: false);
Assert.Equal(0, diags.Count);
Assert.Equal(@"someFile.goo.bar", desc.FileName);
Assert.Equal("someFile.goo.bar", desc.ResourceName);
Assert.False(desc.IsPublic);
// Quoted accessibility is fine.
desc = CSharpCommandLineParser.ParseResourceDescription("", @"\somepath\someFile.goo.bar,,""private""", WorkingDirectory, diags, embedded: false);
Assert.Equal(0, diags.Count);
Assert.Equal(@"someFile.goo.bar", desc.FileName);
Assert.Equal("someFile.goo.bar", desc.ResourceName);
Assert.False(desc.IsPublic);
// Leading commas are not ignored...
desc = CSharpCommandLineParser.ParseResourceDescription("", @",,\somepath\someFile.goo.bar,,private", WorkingDirectory, diags, embedded: false);
diags.Verify(
// error CS1906: Invalid option '\somepath\someFile.goo.bar'; Resource visibility must be either 'public' or 'private'
Diagnostic(ErrorCode.ERR_BadResourceVis).WithArguments(@"\somepath\someFile.goo.bar"));
diags.Clear();
Assert.Null(desc);
// ...even if there's whitespace between them.
desc = CSharpCommandLineParser.ParseResourceDescription("", @", ,\somepath\someFile.goo.bar,,private", WorkingDirectory, diags, embedded: false);
diags.Verify(
// error CS1906: Invalid option '\somepath\someFile.goo.bar'; Resource visibility must be either 'public' or 'private'
Diagnostic(ErrorCode.ERR_BadResourceVis).WithArguments(@"\somepath\someFile.goo.bar"));
diags.Clear();
Assert.Null(desc);
// Trailing commas are ignored...
desc = CSharpCommandLineParser.ParseResourceDescription("", @"\somepath\someFile.goo.bar,,private", WorkingDirectory, diags, embedded: false);
diags.Verify();
diags.Clear();
Assert.Equal("someFile.goo.bar", desc.FileName);
Assert.Equal("someFile.goo.bar", desc.ResourceName);
Assert.False(desc.IsPublic);
// ...even if there's whitespace between them.
desc = CSharpCommandLineParser.ParseResourceDescription("", @"\somepath\someFile.goo.bar,,private, ,", WorkingDirectory, diags, embedded: false);
diags.Verify();
diags.Clear();
Assert.Equal("someFile.goo.bar", desc.FileName);
Assert.Equal("someFile.goo.bar", desc.ResourceName);
Assert.False(desc.IsPublic);
desc = CSharpCommandLineParser.ParseResourceDescription("", @"\somepath\someFile.goo.bar,someName,publi", WorkingDirectory, diags, embedded: false);
diags.Verify(Diagnostic(ErrorCode.ERR_BadResourceVis).WithArguments("publi"));
Assert.Null(desc);
diags.Clear();
desc = CSharpCommandLineParser.ParseResourceDescription("", @"D:rive\relative\path,someName,public", WorkingDirectory, diags, embedded: false);
diags.Verify(Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(@"D:rive\relative\path"));
Assert.Null(desc);
diags.Clear();
desc = CSharpCommandLineParser.ParseResourceDescription("", @"inva\l*d?path,someName,public", WorkingDirectory, diags, embedded: false);
diags.Verify(Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(@"inva\l*d?path"));
Assert.Null(desc);
diags.Clear();
desc = CSharpCommandLineParser.ParseResourceDescription("", (string)null, WorkingDirectory, diags, embedded: false);
diags.Verify(Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments(""));
Assert.Null(desc);
diags.Clear();
desc = CSharpCommandLineParser.ParseResourceDescription("", "", WorkingDirectory, diags, embedded: false);
diags.Verify(Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments(""));
Assert.Null(desc);
diags.Clear();
desc = CSharpCommandLineParser.ParseResourceDescription("", " ", WorkingDirectory, diags, embedded: false);
diags.Verify(
// error CS2005: Missing file specification for '' option
Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("").WithLocation(1, 1));
diags.Clear();
Assert.Null(desc);
desc = CSharpCommandLineParser.ParseResourceDescription("", " , ", WorkingDirectory, diags, embedded: false);
diags.Verify(
// error CS2005: Missing file specification for '' option
Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("").WithLocation(1, 1));
diags.Clear();
Assert.Null(desc);
desc = CSharpCommandLineParser.ParseResourceDescription("", "path, ", WorkingDirectory, diags, embedded: false);
diags.Verify();
diags.Clear();
Assert.Equal("path", desc.FileName);
Assert.Equal("path", desc.ResourceName);
Assert.True(desc.IsPublic);
desc = CSharpCommandLineParser.ParseResourceDescription("", " ,name", WorkingDirectory, diags, embedded: false);
diags.Verify(
// error CS2005: Missing file specification for '' option
Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("").WithLocation(1, 1));
diags.Clear();
Assert.Null(desc);
desc = CSharpCommandLineParser.ParseResourceDescription("", " , , ", WorkingDirectory, diags, embedded: false);
diags.Verify(
// error CS1906: Invalid option ' '; Resource visibility must be either 'public' or 'private'
Diagnostic(ErrorCode.ERR_BadResourceVis).WithArguments(" "));
diags.Clear();
Assert.Null(desc);
desc = CSharpCommandLineParser.ParseResourceDescription("", "path, , ", WorkingDirectory, diags, embedded: false);
diags.Verify(
// error CS1906: Invalid option ' '; Resource visibility must be either 'public' or 'private'
Diagnostic(ErrorCode.ERR_BadResourceVis).WithArguments(" "));
diags.Clear();
Assert.Null(desc);
desc = CSharpCommandLineParser.ParseResourceDescription("", " ,name, ", WorkingDirectory, diags, embedded: false);
diags.Verify(
// error CS1906: Invalid option ' '; Resource visibility must be either 'public' or 'private'
Diagnostic(ErrorCode.ERR_BadResourceVis).WithArguments(" "));
diags.Clear();
Assert.Null(desc);
desc = CSharpCommandLineParser.ParseResourceDescription("", " , ,private", WorkingDirectory, diags, embedded: false);
diags.Verify(
// error CS2005: Missing file specification for '' option
Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("").WithLocation(1, 1));
diags.Clear();
Assert.Null(desc);
desc = CSharpCommandLineParser.ParseResourceDescription("", "path,name,", WorkingDirectory, diags, embedded: false);
diags.Verify(
// CONSIDER: Dev10 actually prints "Invalid option '|'" (note the pipe)
// error CS1906: Invalid option ''; Resource visibility must be either 'public' or 'private'
Diagnostic(ErrorCode.ERR_BadResourceVis).WithArguments(""));
diags.Clear();
Assert.Null(desc);
desc = CSharpCommandLineParser.ParseResourceDescription("", "path,name,,", WorkingDirectory, diags, embedded: false);
diags.Verify(
// CONSIDER: Dev10 actually prints "Invalid option '|'" (note the pipe)
// error CS1906: Invalid option ''; Resource visibility must be either 'public' or 'private'
Diagnostic(ErrorCode.ERR_BadResourceVis).WithArguments(""));
diags.Clear();
Assert.Null(desc);
desc = CSharpCommandLineParser.ParseResourceDescription("", "path,name, ", WorkingDirectory, diags, embedded: false);
diags.Verify(
// error CS1906: Invalid option ''; Resource visibility must be either 'public' or 'private'
Diagnostic(ErrorCode.ERR_BadResourceVis).WithArguments(" "));
diags.Clear();
Assert.Null(desc);
desc = CSharpCommandLineParser.ParseResourceDescription("", "path, ,private", WorkingDirectory, diags, embedded: false);
diags.Verify();
diags.Clear();
Assert.Equal("path", desc.FileName);
Assert.Equal("path", desc.ResourceName);
Assert.False(desc.IsPublic);
desc = CSharpCommandLineParser.ParseResourceDescription("", " ,name,private", WorkingDirectory, diags, embedded: false);
diags.Verify(
// error CS2005: Missing file specification for '' option
Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("").WithLocation(1, 1));
diags.Clear();
Assert.Null(desc);
var longE = new String('e', 1024);
desc = CSharpCommandLineParser.ParseResourceDescription("", String.Format("path,{0},private", longE), WorkingDirectory, diags, embedded: false);
diags.Verify(); // Now checked during emit.
diags.Clear();
Assert.Equal("path", desc.FileName);
Assert.Equal(longE, desc.ResourceName);
Assert.False(desc.IsPublic);
var longI = new String('i', 260);
desc = CSharpCommandLineParser.ParseResourceDescription("", String.Format("{0},e,private", longI), WorkingDirectory, diags, embedded: false);
diags.Verify(
// error CS2021: File name 'iiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiii' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments("iiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiiii").WithLocation(1, 1));
}
[Fact]
public void ManagedResourceOptions()
{
CSharpCommandLineArguments parsedArgs;
ResourceDescription resourceDescription;
parsedArgs = DefaultParse(new[] { "/resource:a", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.DisplayHelp);
resourceDescription = parsedArgs.ManifestResources.Single();
Assert.Null(resourceDescription.FileName); // since embedded
Assert.Equal("a", resourceDescription.ResourceName);
parsedArgs = DefaultParse(new[] { "/res:b", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.DisplayHelp);
resourceDescription = parsedArgs.ManifestResources.Single();
Assert.Null(resourceDescription.FileName); // since embedded
Assert.Equal("b", resourceDescription.ResourceName);
parsedArgs = DefaultParse(new[] { "/linkresource:c", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.DisplayHelp);
resourceDescription = parsedArgs.ManifestResources.Single();
Assert.Equal("c", resourceDescription.FileName);
Assert.Equal("c", resourceDescription.ResourceName);
parsedArgs = DefaultParse(new[] { "/linkres:d", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.DisplayHelp);
resourceDescription = parsedArgs.ManifestResources.Single();
Assert.Equal("d", resourceDescription.FileName);
Assert.Equal("d", resourceDescription.ResourceName);
}
[Fact]
public void ManagedResourceOptions_SimpleErrors()
{
var parsedArgs = DefaultParse(new[] { "/resource:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("/resource:"));
parsedArgs = DefaultParse(new[] { "/resource: ", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("/resource:"));
parsedArgs = DefaultParse(new[] { "/res", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/res"));
parsedArgs = DefaultParse(new[] { "/RES+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/RES+"));
parsedArgs = DefaultParse(new[] { "/res-:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/res-:"));
parsedArgs = DefaultParse(new[] { "/linkresource:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("/linkresource:"));
parsedArgs = DefaultParse(new[] { "/linkresource: ", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("/linkresource:"));
parsedArgs = DefaultParse(new[] { "/linkres", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/linkres"));
parsedArgs = DefaultParse(new[] { "/linkRES+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/linkRES+"));
parsedArgs = DefaultParse(new[] { "/linkres-:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/linkres-:"));
}
[Fact]
public void Link_SimpleTests()
{
var parsedArgs = DefaultParse(new[] { "/link:a", "/link:b,,,,c", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
AssertEx.Equal(new[] { "a", "b", "c" },
parsedArgs.MetadataReferences.
Where((res) => res.Properties.EmbedInteropTypes).
Select((res) => res.Reference));
parsedArgs = DefaultParse(new[] { "/Link: ,,, b ,,", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
AssertEx.Equal(new[] { " b " },
parsedArgs.MetadataReferences.
Where((res) => res.Properties.EmbedInteropTypes).
Select((res) => res.Reference));
parsedArgs = DefaultParse(new[] { "/l:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("/l:"));
parsedArgs = DefaultParse(new[] { "/L", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "/L"));
parsedArgs = DefaultParse(new[] { "/l+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/l+"));
parsedArgs = DefaultParse(new[] { "/link-:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/link-:"));
}
[ConditionalFact(typeof(WindowsOnly))]
public void Recurse_SimpleTests()
{
var dir = Temp.CreateDirectory();
var file1 = dir.CreateFile("a.cs");
var file2 = dir.CreateFile("b.cs");
var file3 = dir.CreateFile("c.txt");
var file4 = dir.CreateDirectory("d1").CreateFile("d.txt");
var file5 = dir.CreateDirectory("d2").CreateFile("e.cs");
file1.WriteAllText("");
file2.WriteAllText("");
file3.WriteAllText("");
file4.WriteAllText("");
file5.WriteAllText("");
var parsedArgs = DefaultParse(new[] { "/recurse:" + dir.ToString() + "\\*.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
AssertEx.Equal(new[] { "{DIR}\\a.cs", "{DIR}\\b.cs", "{DIR}\\d2\\e.cs" },
parsedArgs.SourceFiles.Select((file) => file.Path.Replace(dir.ToString(), "{DIR}")));
parsedArgs = DefaultParse(new[] { "*.cs" }, dir.ToString());
parsedArgs.Errors.Verify();
AssertEx.Equal(new[] { "{DIR}\\a.cs", "{DIR}\\b.cs" },
parsedArgs.SourceFiles.Select((file) => file.Path.Replace(dir.ToString(), "{DIR}")));
parsedArgs = DefaultParse(new[] { "/reCURSE:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("/reCURSE:"));
parsedArgs = DefaultParse(new[] { "/RECURSE: ", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("/RECURSE:"));
parsedArgs = DefaultParse(new[] { "/recurse", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/recurse"));
parsedArgs = DefaultParse(new[] { "/recurse+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/recurse+"));
parsedArgs = DefaultParse(new[] { "/recurse-:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/recurse-:"));
CleanupAllGeneratedFiles(file1.Path);
CleanupAllGeneratedFiles(file2.Path);
CleanupAllGeneratedFiles(file3.Path);
CleanupAllGeneratedFiles(file4.Path);
CleanupAllGeneratedFiles(file5.Path);
}
[Fact]
public void Reference_SimpleTests()
{
var parsedArgs = DefaultParse(new[] { "/nostdlib", "/r:a", "/REFERENCE:b,,,,c", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
AssertEx.Equal(new[] { "a", "b", "c" },
parsedArgs.MetadataReferences.
Where((res) => !res.Properties.EmbedInteropTypes).
Select((res) => res.Reference));
parsedArgs = DefaultParse(new[] { "/Reference: ,,, b ,,", "/nostdlib", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
AssertEx.Equal(new[] { " b " },
parsedArgs.MetadataReferences.
Where((res) => !res.Properties.EmbedInteropTypes).
Select((res) => res.Reference));
parsedArgs = DefaultParse(new[] { "/Reference:a=b,,,", "/nostdlib", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal("a", parsedArgs.MetadataReferences.Single().Properties.Aliases.Single());
Assert.Equal("b", parsedArgs.MetadataReferences.Single().Reference);
parsedArgs = DefaultParse(new[] { "/r:a=b,,,c", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_OneAliasPerReference).WithArguments("b,,,c"));
parsedArgs = DefaultParse(new[] { "/r:1=b", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadExternIdentifier).WithArguments("1"));
parsedArgs = DefaultParse(new[] { "/r:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("/r:"));
parsedArgs = DefaultParse(new[] { "/R", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "/R"));
parsedArgs = DefaultParse(new[] { "/reference+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/reference+"));
parsedArgs = DefaultParse(new[] { "/reference-:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/reference-:"));
}
[Fact]
public void Target_SimpleTests()
{
var parsedArgs = DefaultParse(new[] { "/target:exe", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(OutputKind.ConsoleApplication, parsedArgs.CompilationOptions.OutputKind);
parsedArgs = DefaultParse(new[] { "/t:module", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(OutputKind.NetModule, parsedArgs.CompilationOptions.OutputKind);
parsedArgs = DefaultParse(new[] { "/target:library", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(OutputKind.DynamicallyLinkedLibrary, parsedArgs.CompilationOptions.OutputKind);
parsedArgs = DefaultParse(new[] { "/TARGET:winexe", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(OutputKind.WindowsApplication, parsedArgs.CompilationOptions.OutputKind);
parsedArgs = DefaultParse(new[] { "/target:appcontainerexe", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(OutputKind.WindowsRuntimeApplication, parsedArgs.CompilationOptions.OutputKind);
parsedArgs = DefaultParse(new[] { "/target:winmdobj", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(OutputKind.WindowsRuntimeMetadata, parsedArgs.CompilationOptions.OutputKind);
parsedArgs = DefaultParse(new[] { "/target:winexe", "/T:exe", "/target:module", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(OutputKind.NetModule, parsedArgs.CompilationOptions.OutputKind);
parsedArgs = DefaultParse(new[] { "/t", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/t"));
parsedArgs = DefaultParse(new[] { "/target:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.FTL_InvalidTarget));
parsedArgs = DefaultParse(new[] { "/target:xyz", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.FTL_InvalidTarget));
parsedArgs = DefaultParse(new[] { "/T+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/T+"));
parsedArgs = DefaultParse(new[] { "/TARGET-:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/TARGET-:"));
}
[Fact]
public void Target_SimpleTestsNoSource()
{
var parsedArgs = DefaultParse(new[] { "/target:exe" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// warning CS2008: No source files specified.
Diagnostic(ErrorCode.WRN_NoSources).WithLocation(1, 1),
// error CS1562: Outputs without source must have the /out option specified
Diagnostic(ErrorCode.ERR_OutputNeedsName).WithLocation(1, 1));
Assert.Equal(OutputKind.ConsoleApplication, parsedArgs.CompilationOptions.OutputKind);
parsedArgs = DefaultParse(new[] { "/t:module" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// warning CS2008: No source files specified.
Diagnostic(ErrorCode.WRN_NoSources).WithLocation(1, 1),
// error CS1562: Outputs without source must have the /out option specified
Diagnostic(ErrorCode.ERR_OutputNeedsName).WithLocation(1, 1));
Assert.Equal(OutputKind.NetModule, parsedArgs.CompilationOptions.OutputKind);
parsedArgs = DefaultParse(new[] { "/target:library" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// warning CS2008: No source files specified.
Diagnostic(ErrorCode.WRN_NoSources).WithLocation(1, 1),
// error CS1562: Outputs without source must have the /out option specified
Diagnostic(ErrorCode.ERR_OutputNeedsName).WithLocation(1, 1));
Assert.Equal(OutputKind.DynamicallyLinkedLibrary, parsedArgs.CompilationOptions.OutputKind);
parsedArgs = DefaultParse(new[] { "/TARGET:winexe" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// warning CS2008: No source files specified.
Diagnostic(ErrorCode.WRN_NoSources).WithLocation(1, 1),
// error CS1562: Outputs without source must have the /out option specified
Diagnostic(ErrorCode.ERR_OutputNeedsName).WithLocation(1, 1));
Assert.Equal(OutputKind.WindowsApplication, parsedArgs.CompilationOptions.OutputKind);
parsedArgs = DefaultParse(new[] { "/target:appcontainerexe" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// warning CS2008: No source files specified.
Diagnostic(ErrorCode.WRN_NoSources).WithLocation(1, 1),
// error CS1562: Outputs without source must have the /out option specified
Diagnostic(ErrorCode.ERR_OutputNeedsName).WithLocation(1, 1));
Assert.Equal(OutputKind.WindowsRuntimeApplication, parsedArgs.CompilationOptions.OutputKind);
parsedArgs = DefaultParse(new[] { "/target:winmdobj" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// warning CS2008: No source files specified.
Diagnostic(ErrorCode.WRN_NoSources).WithLocation(1, 1),
// error CS1562: Outputs without source must have the /out option specified
Diagnostic(ErrorCode.ERR_OutputNeedsName).WithLocation(1, 1));
Assert.Equal(OutputKind.WindowsRuntimeMetadata, parsedArgs.CompilationOptions.OutputKind);
parsedArgs = DefaultParse(new[] { "/target:winexe", "/T:exe", "/target:module" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// warning CS2008: No source files specified.
Diagnostic(ErrorCode.WRN_NoSources).WithLocation(1, 1),
// error CS1562: Outputs without source must have the /out option specified
Diagnostic(ErrorCode.ERR_OutputNeedsName).WithLocation(1, 1));
Assert.Equal(OutputKind.NetModule, parsedArgs.CompilationOptions.OutputKind);
parsedArgs = DefaultParse(new[] { "/t" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2007: Unrecognized option: '/t'
Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/t").WithLocation(1, 1),
// warning CS2008: No source files specified.
Diagnostic(ErrorCode.WRN_NoSources).WithLocation(1, 1),
// error CS1562: Outputs without source must have the /out option specified
Diagnostic(ErrorCode.ERR_OutputNeedsName).WithLocation(1, 1));
parsedArgs = DefaultParse(new[] { "/target:" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2019: Invalid target type for /target: must specify 'exe', 'winexe', 'library', or 'module'
Diagnostic(ErrorCode.FTL_InvalidTarget).WithLocation(1, 1),
// warning CS2008: No source files specified.
Diagnostic(ErrorCode.WRN_NoSources).WithLocation(1, 1),
// error CS1562: Outputs without source must have the /out option specified
Diagnostic(ErrorCode.ERR_OutputNeedsName).WithLocation(1, 1));
parsedArgs = DefaultParse(new[] { "/target:xyz" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2019: Invalid target type for /target: must specify 'exe', 'winexe', 'library', or 'module'
Diagnostic(ErrorCode.FTL_InvalidTarget).WithLocation(1, 1),
// warning CS2008: No source files specified.
Diagnostic(ErrorCode.WRN_NoSources).WithLocation(1, 1),
// error CS1562: Outputs without source must have the /out option specified
Diagnostic(ErrorCode.ERR_OutputNeedsName).WithLocation(1, 1));
parsedArgs = DefaultParse(new[] { "/T+" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2007: Unrecognized option: '/T+'
Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/T+").WithLocation(1, 1),
// warning CS2008: No source files specified.
Diagnostic(ErrorCode.WRN_NoSources).WithLocation(1, 1),
// error CS1562: Outputs without source must have the /out option specified
Diagnostic(ErrorCode.ERR_OutputNeedsName).WithLocation(1, 1));
parsedArgs = DefaultParse(new[] { "/TARGET-:" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2007: Unrecognized option: '/TARGET-:'
Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/TARGET-:").WithLocation(1, 1),
// warning CS2008: No source files specified.
Diagnostic(ErrorCode.WRN_NoSources).WithLocation(1, 1),
// error CS1562: Outputs without source must have the /out option specified
Diagnostic(ErrorCode.ERR_OutputNeedsName).WithLocation(1, 1));
}
[Fact]
public void ModuleManifest()
{
CSharpCommandLineArguments args = DefaultParse(new[] { "/win32manifest:blah", "/target:module", "a.cs" }, WorkingDirectory);
args.Errors.Verify(
// warning CS1927: Ignoring /win32manifest for module because it only applies to assemblies
Diagnostic(ErrorCode.WRN_CantHaveManifestForModule));
// Illegal, but not clobbered.
Assert.Equal("blah", args.Win32Manifest);
}
// The following test is failing in the Linux Debug test leg of CI.
// This issus is being tracked by https://github.com/dotnet/roslyn/issues/58077
[ConditionalFact(typeof(WindowsOrMacOSOnly))]
public void ArgumentParsing()
{
var sdkDirectory = SdkDirectory;
var parsedArgs = CSharpCommandLineParser.Script.Parse(new[] { "a + b" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.DisplayHelp);
Assert.True(parsedArgs.SourceFiles.Any());
parsedArgs = CSharpCommandLineParser.Script.Parse(new[] { "a + b; c" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.DisplayHelp);
Assert.True(parsedArgs.SourceFiles.Any());
parsedArgs = CSharpCommandLineParser.Script.Parse(new[] { "/help" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.DisplayHelp);
Assert.False(parsedArgs.SourceFiles.Any());
parsedArgs = CSharpCommandLineParser.Script.Parse(new[] { "/version" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.DisplayVersion);
Assert.False(parsedArgs.SourceFiles.Any());
parsedArgs = CSharpCommandLineParser.Script.Parse(new[] { "/langversion:?" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.DisplayLangVersions);
Assert.False(parsedArgs.SourceFiles.Any());
parsedArgs = CSharpCommandLineParser.Script.Parse(new[] { "//langversion:?" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify(
// error CS2001: Source file '//langversion:?' could not be found.
Diagnostic(ErrorCode.ERR_FileNotFound).WithArguments("//langversion:?").WithLocation(1, 1)
);
parsedArgs = CSharpCommandLineParser.Script.Parse(new[] { "/version", "c.csx" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.DisplayVersion);
Assert.True(parsedArgs.SourceFiles.Any());
parsedArgs = CSharpCommandLineParser.Script.Parse(new[] { "/version:something" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.DisplayVersion);
Assert.False(parsedArgs.SourceFiles.Any());
parsedArgs = CSharpCommandLineParser.Script.Parse(new[] { "/?" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.DisplayHelp);
Assert.False(parsedArgs.SourceFiles.Any());
parsedArgs = CSharpCommandLineParser.Script.Parse(new[] { "c.csx /langversion:6" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.DisplayHelp);
Assert.True(parsedArgs.SourceFiles.Any());
parsedArgs = CSharpCommandLineParser.Script.Parse(new[] { "/langversion:-1", "c.csx", }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify(
// error CS1617: Invalid option '-1' for /langversion. Use '/langversion:?' to list supported values.
Diagnostic(ErrorCode.ERR_BadCompatMode).WithArguments("-1").WithLocation(1, 1));
Assert.False(parsedArgs.DisplayHelp);
Assert.Equal(1, parsedArgs.SourceFiles.Length);
parsedArgs = CSharpCommandLineParser.Script.Parse(new[] { "c.csx /r:s=d /r:d.dll" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.DisplayHelp);
Assert.True(parsedArgs.SourceFiles.Any());
parsedArgs = CSharpCommandLineParser.Script.Parse(new[] { "@roslyn_test_non_existing_file" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify(
// error CS2011: Error opening response file 'D:\R0\Main\Binaries\Debug\dd'
Diagnostic(ErrorCode.ERR_OpenResponseFile).WithArguments(Path.Combine(WorkingDirectory, @"roslyn_test_non_existing_file")));
Assert.False(parsedArgs.DisplayHelp);
Assert.False(parsedArgs.SourceFiles.Any());
parsedArgs = CSharpCommandLineParser.Script.Parse(new[] { "c /define:DEBUG" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.DisplayHelp);
Assert.True(parsedArgs.SourceFiles.Any());
parsedArgs = CSharpCommandLineParser.Script.Parse(new[] { "\\" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.DisplayHelp);
Assert.True(parsedArgs.SourceFiles.Any());
parsedArgs = CSharpCommandLineParser.Script.Parse(new[] { "/r:d.dll", "c.csx" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.DisplayHelp);
Assert.True(parsedArgs.SourceFiles.Any());
parsedArgs = CSharpCommandLineParser.Script.Parse(new[] { "/define:goo", "c.csx" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify(
// error CS2007: Unrecognized option: '/define:goo'
Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/define:goo"));
Assert.False(parsedArgs.DisplayHelp);
Assert.True(parsedArgs.SourceFiles.Any());
parsedArgs = CSharpCommandLineParser.Script.Parse(new[] { "\"/r d.dll\"" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.DisplayHelp);
Assert.True(parsedArgs.SourceFiles.Any());
parsedArgs = CSharpCommandLineParser.Script.Parse(new[] { "/r: d.dll", "a.cs" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.DisplayHelp);
Assert.True(parsedArgs.SourceFiles.Any());
}
[Theory]
[InlineData("iso-1", LanguageVersion.CSharp1)]
[InlineData("iso-2", LanguageVersion.CSharp2)]
[InlineData("1", LanguageVersion.CSharp1)]
[InlineData("1.0", LanguageVersion.CSharp1)]
[InlineData("2", LanguageVersion.CSharp2)]
[InlineData("2.0", LanguageVersion.CSharp2)]
[InlineData("3", LanguageVersion.CSharp3)]
[InlineData("3.0", LanguageVersion.CSharp3)]
[InlineData("4", LanguageVersion.CSharp4)]
[InlineData("4.0", LanguageVersion.CSharp4)]
[InlineData("5", LanguageVersion.CSharp5)]
[InlineData("5.0", LanguageVersion.CSharp5)]
[InlineData("6", LanguageVersion.CSharp6)]
[InlineData("6.0", LanguageVersion.CSharp6)]
[InlineData("7", LanguageVersion.CSharp7)]
[InlineData("7.0", LanguageVersion.CSharp7)]
[InlineData("7.1", LanguageVersion.CSharp7_1)]
[InlineData("7.2", LanguageVersion.CSharp7_2)]
[InlineData("7.3", LanguageVersion.CSharp7_3)]
[InlineData("8", LanguageVersion.CSharp8)]
[InlineData("8.0", LanguageVersion.CSharp8)]
[InlineData("9", LanguageVersion.CSharp9)]
[InlineData("9.0", LanguageVersion.CSharp9)]
[InlineData("preview", LanguageVersion.Preview)]
public void LangVersion_CanParseCorrectVersions(string value, LanguageVersion expectedVersion)
{
var parsedArgs = DefaultParse(new[] { $"/langversion:{value}", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(expectedVersion, parsedArgs.ParseOptions.LanguageVersion);
Assert.Equal(expectedVersion, parsedArgs.ParseOptions.SpecifiedLanguageVersion);
var scriptParsedArgs = ScriptParse(new[] { $"/langversion:{value}" }, WorkingDirectory);
scriptParsedArgs.Errors.Verify();
Assert.Equal(expectedVersion, scriptParsedArgs.ParseOptions.LanguageVersion);
Assert.Equal(expectedVersion, scriptParsedArgs.ParseOptions.SpecifiedLanguageVersion);
}
[Theory]
[InlineData("6", "7", LanguageVersion.CSharp7)]
[InlineData("7", "6", LanguageVersion.CSharp6)]
[InlineData("7", "1", LanguageVersion.CSharp1)]
[InlineData("6", "iso-1", LanguageVersion.CSharp1)]
[InlineData("6", "iso-2", LanguageVersion.CSharp2)]
[InlineData("6", "default", LanguageVersion.Default)]
[InlineData("7", "default", LanguageVersion.Default)]
[InlineData("iso-2", "6", LanguageVersion.CSharp6)]
public void LangVersion_LatterVersionOverridesFormerOne(string formerValue, string latterValue, LanguageVersion expectedVersion)
{
var parsedArgs = DefaultParse(new[] { $"/langversion:{formerValue}", $"/langversion:{latterValue}", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(expectedVersion, parsedArgs.ParseOptions.SpecifiedLanguageVersion);
}
[Fact]
public void LangVersion_DefaultMapsCorrectly()
{
LanguageVersion defaultEffectiveVersion = LanguageVersion.Default.MapSpecifiedToEffectiveVersion();
Assert.NotEqual(LanguageVersion.Default, defaultEffectiveVersion);
var parsedArgs = DefaultParse(new[] { "/langversion:default", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(LanguageVersion.Default, parsedArgs.ParseOptions.SpecifiedLanguageVersion);
Assert.Equal(defaultEffectiveVersion, parsedArgs.ParseOptions.LanguageVersion);
}
[Fact]
public void LangVersion_LatestMapsCorrectly()
{
LanguageVersion latestEffectiveVersion = LanguageVersion.Latest.MapSpecifiedToEffectiveVersion();
Assert.NotEqual(LanguageVersion.Latest, latestEffectiveVersion);
var parsedArgs = DefaultParse(new[] { "/langversion:latest", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(LanguageVersion.Latest, parsedArgs.ParseOptions.SpecifiedLanguageVersion);
Assert.Equal(latestEffectiveVersion, parsedArgs.ParseOptions.LanguageVersion);
}
[Fact]
public void LangVersion_NoValueSpecified()
{
var parsedArgs = DefaultParse(new[] { "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(LanguageVersion.Default, parsedArgs.ParseOptions.SpecifiedLanguageVersion);
}
[Theory]
[InlineData("iso-3")]
[InlineData("iso1")]
[InlineData("8.1")]
[InlineData("10.1")]
[InlineData("11")]
[InlineData("1000")]
public void LangVersion_BadVersion(string value)
{
DefaultParse(new[] { $"/langversion:{value}", "a.cs" }, WorkingDirectory).Errors.Verify(
// error CS1617: Invalid option 'XXX' for /langversion. Use '/langversion:?' to list supported values.
Diagnostic(ErrorCode.ERR_BadCompatMode).WithArguments(value).WithLocation(1, 1)
);
}
[Theory]
[InlineData("0")]
[InlineData("05")]
[InlineData("07")]
[InlineData("07.1")]
[InlineData("08")]
[InlineData("09")]
public void LangVersion_LeadingZeroes(string value)
{
DefaultParse(new[] { $"/langversion:{value}", "a.cs" }, WorkingDirectory).Errors.Verify(
// error CS8303: Specified language version 'XXX' cannot have leading zeroes
Diagnostic(ErrorCode.ERR_LanguageVersionCannotHaveLeadingZeroes).WithArguments(value).WithLocation(1, 1));
}
[Theory]
[InlineData("/langversion")]
[InlineData("/langversion:")]
[InlineData("/LANGversion:")]
public void LangVersion_NoVersion(string option)
{
DefaultParse(new[] { option, "a.cs" }, WorkingDirectory).Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for '/langversion:' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "/langversion:").WithLocation(1, 1));
}
[Fact]
public void LangVersion_LangVersions()
{
var args = DefaultParse(new[] { "/langversion:?" }, WorkingDirectory);
args.Errors.Verify(
// warning CS2008: No source files specified.
Diagnostic(ErrorCode.WRN_NoSources).WithLocation(1, 1),
// error CS1562: Outputs without source must have the /out option specified
Diagnostic(ErrorCode.ERR_OutputNeedsName).WithLocation(1, 1)
);
Assert.True(args.DisplayLangVersions);
}
[Fact]
public void LanguageVersionAdded_Canary()
{
// When a new version is added, this test will break. This list must be checked:
// - update the "UpgradeProject" codefixer
// - update all the tests that call this canary
// - update MaxSupportedLangVersion (a relevant test should break when new version is introduced)
// - email release management to add to the release notes (see old example: https://github.com/dotnet/core/pull/1454)
AssertEx.SetEqual(new[] { "default", "1", "2", "3", "4", "5", "6", "7.0", "7.1", "7.2", "7.3", "8.0", "9.0", "10.0", "latest", "latestmajor", "preview" },
Enum.GetValues(typeof(LanguageVersion)).Cast<LanguageVersion>().Select(v => v.ToDisplayString()));
// For minor versions and new major versions, the format should be "x.y", such as "7.1"
}
[Fact]
public void LanguageVersion_GetErrorCode()
{
var versions = Enum.GetValues(typeof(LanguageVersion))
.Cast<LanguageVersion>()
.Except(new[] {
LanguageVersion.Default,
LanguageVersion.Latest,
LanguageVersion.LatestMajor,
LanguageVersion.Preview
})
.Select(v => v.GetErrorCode());
var errorCodes = new[]
{
ErrorCode.ERR_FeatureNotAvailableInVersion1,
ErrorCode.ERR_FeatureNotAvailableInVersion2,
ErrorCode.ERR_FeatureNotAvailableInVersion3,
ErrorCode.ERR_FeatureNotAvailableInVersion4,
ErrorCode.ERR_FeatureNotAvailableInVersion5,
ErrorCode.ERR_FeatureNotAvailableInVersion6,
ErrorCode.ERR_FeatureNotAvailableInVersion7,
ErrorCode.ERR_FeatureNotAvailableInVersion7_1,
ErrorCode.ERR_FeatureNotAvailableInVersion7_2,
ErrorCode.ERR_FeatureNotAvailableInVersion7_3,
ErrorCode.ERR_FeatureNotAvailableInVersion8,
ErrorCode.ERR_FeatureNotAvailableInVersion9,
ErrorCode.ERR_FeatureNotAvailableInVersion10,
};
AssertEx.SetEqual(versions, errorCodes);
// The canary check is a reminder that this test needs to be updated when a language version is added
LanguageVersionAdded_Canary();
}
[Theory,
InlineData(LanguageVersion.CSharp1, LanguageVersion.CSharp1),
InlineData(LanguageVersion.CSharp2, LanguageVersion.CSharp2),
InlineData(LanguageVersion.CSharp3, LanguageVersion.CSharp3),
InlineData(LanguageVersion.CSharp4, LanguageVersion.CSharp4),
InlineData(LanguageVersion.CSharp5, LanguageVersion.CSharp5),
InlineData(LanguageVersion.CSharp6, LanguageVersion.CSharp6),
InlineData(LanguageVersion.CSharp7, LanguageVersion.CSharp7),
InlineData(LanguageVersion.CSharp7_1, LanguageVersion.CSharp7_1),
InlineData(LanguageVersion.CSharp7_2, LanguageVersion.CSharp7_2),
InlineData(LanguageVersion.CSharp7_3, LanguageVersion.CSharp7_3),
InlineData(LanguageVersion.CSharp8, LanguageVersion.CSharp8),
InlineData(LanguageVersion.CSharp9, LanguageVersion.CSharp9),
InlineData(LanguageVersion.CSharp10, LanguageVersion.CSharp10),
InlineData(LanguageVersion.CSharp10, LanguageVersion.LatestMajor),
InlineData(LanguageVersion.CSharp10, LanguageVersion.Latest),
InlineData(LanguageVersion.CSharp10, LanguageVersion.Default),
InlineData(LanguageVersion.Preview, LanguageVersion.Preview),
]
public void LanguageVersion_MapSpecifiedToEffectiveVersion(LanguageVersion expectedMappedVersion, LanguageVersion input)
{
Assert.Equal(expectedMappedVersion, input.MapSpecifiedToEffectiveVersion());
Assert.True(expectedMappedVersion.IsValid());
// The canary check is a reminder that this test needs to be updated when a language version is added
LanguageVersionAdded_Canary();
}
[Theory,
InlineData("iso-1", true, LanguageVersion.CSharp1),
InlineData("ISO-1", true, LanguageVersion.CSharp1),
InlineData("iso-2", true, LanguageVersion.CSharp2),
InlineData("1", true, LanguageVersion.CSharp1),
InlineData("1.0", true, LanguageVersion.CSharp1),
InlineData("2", true, LanguageVersion.CSharp2),
InlineData("2.0", true, LanguageVersion.CSharp2),
InlineData("3", true, LanguageVersion.CSharp3),
InlineData("3.0", true, LanguageVersion.CSharp3),
InlineData("4", true, LanguageVersion.CSharp4),
InlineData("4.0", true, LanguageVersion.CSharp4),
InlineData("5", true, LanguageVersion.CSharp5),
InlineData("5.0", true, LanguageVersion.CSharp5),
InlineData("05", false, LanguageVersion.Default),
InlineData("6", true, LanguageVersion.CSharp6),
InlineData("6.0", true, LanguageVersion.CSharp6),
InlineData("7", true, LanguageVersion.CSharp7),
InlineData("7.0", true, LanguageVersion.CSharp7),
InlineData("07", false, LanguageVersion.Default),
InlineData("7.1", true, LanguageVersion.CSharp7_1),
InlineData("7.2", true, LanguageVersion.CSharp7_2),
InlineData("7.3", true, LanguageVersion.CSharp7_3),
InlineData("8", true, LanguageVersion.CSharp8),
InlineData("8.0", true, LanguageVersion.CSharp8),
InlineData("9", true, LanguageVersion.CSharp9),
InlineData("9.0", true, LanguageVersion.CSharp9),
InlineData("10", true, LanguageVersion.CSharp10),
InlineData("10.0", true, LanguageVersion.CSharp10),
InlineData("08", false, LanguageVersion.Default),
InlineData("07.1", false, LanguageVersion.Default),
InlineData("default", true, LanguageVersion.Default),
InlineData("latest", true, LanguageVersion.Latest),
InlineData("latestmajor", true, LanguageVersion.LatestMajor),
InlineData("preview", true, LanguageVersion.Preview),
InlineData("latestpreview", false, LanguageVersion.Default),
InlineData(null, true, LanguageVersion.Default),
InlineData("bad", false, LanguageVersion.Default)]
public void LanguageVersion_TryParseDisplayString(string input, bool success, LanguageVersion expected)
{
Assert.Equal(success, LanguageVersionFacts.TryParse(input, out var version));
Assert.Equal(expected, version);
// The canary check is a reminder that this test needs to be updated when a language version is added
LanguageVersionAdded_Canary();
}
[Fact]
public void LanguageVersion_TryParseTurkishDisplayString()
{
var originalCulture = Thread.CurrentThread.CurrentCulture;
Thread.CurrentThread.CurrentCulture = new CultureInfo("tr-TR", useUserOverride: false);
Assert.True(LanguageVersionFacts.TryParse("ISO-1", out var version));
Assert.Equal(LanguageVersion.CSharp1, version);
Thread.CurrentThread.CurrentCulture = originalCulture;
}
[Fact]
public void LangVersion_ListLangVersions()
{
var dir = Temp.CreateDirectory();
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { "/langversion:?" });
int exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
var expected = Enum.GetValues(typeof(LanguageVersion)).Cast<LanguageVersion>()
.Select(v => v.ToDisplayString());
var actual = outWriter.ToString();
var acceptableSurroundingChar = new[] { '\r', '\n', '(', ')', ' ' };
foreach (var version in expected)
{
if (version == "latest")
continue;
var foundIndex = actual.IndexOf(version);
Assert.True(foundIndex > 0, $"Missing version '{version}'");
Assert.True(Array.IndexOf(acceptableSurroundingChar, actual[foundIndex - 1]) >= 0);
Assert.True(Array.IndexOf(acceptableSurroundingChar, actual[foundIndex + version.Length]) >= 0);
}
}
[Fact]
[WorkItem(546961, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546961")]
public void Define()
{
var parsedArgs = DefaultParse(new[] { "a.cs" }, WorkingDirectory);
Assert.Equal(0, parsedArgs.ParseOptions.PreprocessorSymbolNames.Count());
Assert.False(parsedArgs.Errors.Any());
parsedArgs = DefaultParse(new[] { "/d:GOO", "a.cs" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.ParseOptions.PreprocessorSymbolNames.Count());
Assert.Contains("GOO", parsedArgs.ParseOptions.PreprocessorSymbolNames);
Assert.False(parsedArgs.Errors.Any());
parsedArgs = DefaultParse(new[] { "/d:GOO;BAR,ZIP", "a.cs" }, WorkingDirectory);
Assert.Equal(3, parsedArgs.ParseOptions.PreprocessorSymbolNames.Count());
Assert.Contains("GOO", parsedArgs.ParseOptions.PreprocessorSymbolNames);
Assert.Contains("BAR", parsedArgs.ParseOptions.PreprocessorSymbolNames);
Assert.Contains("ZIP", parsedArgs.ParseOptions.PreprocessorSymbolNames);
Assert.False(parsedArgs.Errors.Any());
parsedArgs = DefaultParse(new[] { "/d:GOO;4X", "a.cs" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.ParseOptions.PreprocessorSymbolNames.Count());
Assert.Contains("GOO", parsedArgs.ParseOptions.PreprocessorSymbolNames);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal((int)ErrorCode.WRN_DefineIdentifierRequired, parsedArgs.Errors.First().Code);
Assert.Equal("4X", parsedArgs.Errors.First().Arguments[0]);
IEnumerable<Diagnostic> diagnostics;
// The docs say /d:def1[;def2]
string compliant = "def1;def2;def3";
var expected = new[] { "def1", "def2", "def3" };
var parsed = CSharpCommandLineParser.ParseConditionalCompilationSymbols(compliant, out diagnostics);
diagnostics.Verify();
Assert.Equal<string>(expected, parsed);
// Bug 17360: Dev11 allows for a terminating semicolon
var dev11Compliant = "def1;def2;def3;";
parsed = CSharpCommandLineParser.ParseConditionalCompilationSymbols(dev11Compliant, out diagnostics);
diagnostics.Verify();
Assert.Equal<string>(expected, parsed);
// And comma
dev11Compliant = "def1,def2,def3,";
parsed = CSharpCommandLineParser.ParseConditionalCompilationSymbols(dev11Compliant, out diagnostics);
diagnostics.Verify();
Assert.Equal<string>(expected, parsed);
// This breaks everything
var nonCompliant = "def1;;def2;";
parsed = CSharpCommandLineParser.ParseConditionalCompilationSymbols(nonCompliant, out diagnostics);
diagnostics.Verify(
// warning CS2029: Invalid name for a preprocessing symbol; '' is not a valid identifier
Diagnostic(ErrorCode.WRN_DefineIdentifierRequired).WithArguments(""));
Assert.Equal(new[] { "def1", "def2" }, parsed);
// Bug 17360
parsedArgs = DefaultParse(new[] { "/d:public1;public2;", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
}
[Fact]
public void Debug()
{
var platformPdbKind = PathUtilities.IsUnixLikePlatform ? DebugInformationFormat.PortablePdb : DebugInformationFormat.Pdb;
var parsedArgs = DefaultParse(new[] { "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.DebugPlusMode);
Assert.False(parsedArgs.EmitPdb);
Assert.False(parsedArgs.EmitPdbFile);
Assert.Equal(parsedArgs.EmitOptions.DebugInformationFormat, platformPdbKind);
parsedArgs = DefaultParse(new[] { "/debug-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.DebugPlusMode);
Assert.False(parsedArgs.EmitPdb);
Assert.False(parsedArgs.EmitPdbFile);
Assert.Equal(parsedArgs.EmitOptions.DebugInformationFormat, platformPdbKind);
parsedArgs = DefaultParse(new[] { "/debug", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.DebugPlusMode);
Assert.True(parsedArgs.EmitPdb);
Assert.True(parsedArgs.EmitPdbFile);
Assert.Equal(parsedArgs.EmitOptions.DebugInformationFormat, platformPdbKind);
parsedArgs = DefaultParse(new[] { "/debug+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.CompilationOptions.DebugPlusMode);
Assert.True(parsedArgs.EmitPdb);
Assert.True(parsedArgs.EmitPdbFile);
Assert.Equal(parsedArgs.EmitOptions.DebugInformationFormat, platformPdbKind);
parsedArgs = DefaultParse(new[] { "/debug+", "/debug-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.DebugPlusMode);
Assert.False(parsedArgs.EmitPdb);
Assert.False(parsedArgs.EmitPdbFile);
Assert.Equal(parsedArgs.EmitOptions.DebugInformationFormat, platformPdbKind);
parsedArgs = DefaultParse(new[] { "/debug:full", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.DebugPlusMode);
Assert.True(parsedArgs.EmitPdb);
Assert.Equal(parsedArgs.EmitOptions.DebugInformationFormat, platformPdbKind);
parsedArgs = DefaultParse(new[] { "/debug:FULL", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.DebugPlusMode);
Assert.True(parsedArgs.EmitPdb);
Assert.Equal(parsedArgs.EmitOptions.DebugInformationFormat, platformPdbKind);
Assert.Equal(Path.Combine(WorkingDirectory, "a.pdb"), parsedArgs.GetPdbFilePath("a.dll"));
parsedArgs = DefaultParse(new[] { "/debug:pdbonly", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.DebugPlusMode);
Assert.True(parsedArgs.EmitPdb);
Assert.Equal(parsedArgs.EmitOptions.DebugInformationFormat, platformPdbKind);
parsedArgs = DefaultParse(new[] { "/debug:portable", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.DebugPlusMode);
Assert.True(parsedArgs.EmitPdb);
Assert.Equal(DebugInformationFormat.PortablePdb, parsedArgs.EmitOptions.DebugInformationFormat);
Assert.Equal(Path.Combine(WorkingDirectory, "a.pdb"), parsedArgs.GetPdbFilePath("a.dll"));
parsedArgs = DefaultParse(new[] { "/debug:embedded", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.DebugPlusMode);
Assert.True(parsedArgs.EmitPdb);
Assert.Equal(DebugInformationFormat.Embedded, parsedArgs.EmitOptions.DebugInformationFormat);
Assert.Equal(Path.Combine(WorkingDirectory, "a.pdb"), parsedArgs.GetPdbFilePath("a.dll"));
parsedArgs = DefaultParse(new[] { "/debug:PDBONLY", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.DebugPlusMode);
Assert.True(parsedArgs.EmitPdb);
Assert.Equal(parsedArgs.EmitOptions.DebugInformationFormat, platformPdbKind);
parsedArgs = DefaultParse(new[] { "/debug:full", "/debug:pdbonly", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.DebugPlusMode);
Assert.True(parsedArgs.EmitPdb);
Assert.Equal(parsedArgs.EmitOptions.DebugInformationFormat, platformPdbKind);
parsedArgs = DefaultParse(new[] { "/debug:pdbonly", "/debug:full", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.DebugPlusMode);
Assert.True(parsedArgs.EmitPdb);
Assert.Equal(platformPdbKind, parsedArgs.EmitOptions.DebugInformationFormat);
parsedArgs = DefaultParse(new[] { "/debug:pdbonly", "/debug-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.DebugPlusMode);
Assert.False(parsedArgs.EmitPdb);
Assert.Equal(platformPdbKind, parsedArgs.EmitOptions.DebugInformationFormat);
parsedArgs = DefaultParse(new[] { "/debug:pdbonly", "/debug-", "/debug", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.DebugPlusMode);
Assert.True(parsedArgs.EmitPdb);
Assert.Equal(platformPdbKind, parsedArgs.EmitOptions.DebugInformationFormat);
parsedArgs = DefaultParse(new[] { "/debug:pdbonly", "/debug-", "/debug+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.CompilationOptions.DebugPlusMode);
Assert.True(parsedArgs.EmitPdb);
Assert.Equal(platformPdbKind, parsedArgs.EmitOptions.DebugInformationFormat);
parsedArgs = DefaultParse(new[] { "/debug:embedded", "/debug-", "/debug+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.CompilationOptions.DebugPlusMode);
Assert.True(parsedArgs.EmitPdb);
Assert.Equal(DebugInformationFormat.Embedded, parsedArgs.EmitOptions.DebugInformationFormat);
parsedArgs = DefaultParse(new[] { "/debug:embedded", "/debug-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.DebugPlusMode);
Assert.False(parsedArgs.EmitPdb);
Assert.Equal(DebugInformationFormat.Embedded, parsedArgs.EmitOptions.DebugInformationFormat);
parsedArgs = DefaultParse(new[] { "/debug:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "debug"));
parsedArgs = DefaultParse(new[] { "/debug:+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadDebugType).WithArguments("+"));
parsedArgs = DefaultParse(new[] { "/debug:invalid", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadDebugType).WithArguments("invalid"));
parsedArgs = DefaultParse(new[] { "/debug-:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/debug-:"));
}
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30289")]
public void Pdb()
{
var parsedArgs = DefaultParse(new[] { "/pdb:something", "a.cs" }, WorkingDirectory);
Assert.Equal(Path.Combine(WorkingDirectory, "something.pdb"), parsedArgs.PdbPath);
Assert.Equal(Path.Combine(WorkingDirectory, "something.pdb"), parsedArgs.GetPdbFilePath("a.dll"));
Assert.False(parsedArgs.EmitPdbFile);
parsedArgs = DefaultParse(new[] { "/pdb:something", "/debug:embedded", "a.cs" }, WorkingDirectory);
Assert.Equal(Path.Combine(WorkingDirectory, "something.pdb"), parsedArgs.PdbPath);
Assert.Equal(Path.Combine(WorkingDirectory, "something.pdb"), parsedArgs.GetPdbFilePath("a.dll"));
Assert.False(parsedArgs.EmitPdbFile);
parsedArgs = DefaultParse(new[] { "/debug", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Null(parsedArgs.PdbPath);
Assert.True(parsedArgs.EmitPdbFile);
Assert.Equal(Path.Combine(WorkingDirectory, "a.pdb"), parsedArgs.GetPdbFilePath("a.dll"));
parsedArgs = DefaultParse(new[] { "/pdb", "/debug", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("/pdb"));
Assert.Equal(Path.Combine(WorkingDirectory, "a.pdb"), parsedArgs.GetPdbFilePath("a.dll"));
parsedArgs = DefaultParse(new[] { "/pdb:", "/debug", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("/pdb:"));
parsedArgs = DefaultParse(new[] { "/pdb:something", "/debug", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
// temp: path changed
//parsedArgs = DefaultParse(new[] { "/debug", "/pdb:.x", "a.cs" }, baseDirectory);
//parsedArgs.Errors.Verify(
// // error CS2021: File name '.x' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
// Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(".x"));
parsedArgs = DefaultParse(new[] { @"/pdb:""""", "/debug", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2005: Missing file specification for '/pdb:""' option
Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments(@"/pdb:""""").WithLocation(1, 1));
parsedArgs = DefaultParse(new[] { "/pdb:C:\\", "/debug", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments("C:\\"));
// Should preserve fully qualified paths
parsedArgs = DefaultParse(new[] { @"/pdb:C:\MyFolder\MyPdb.pdb", "/debug", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"C:\MyFolder\MyPdb.pdb", parsedArgs.PdbPath);
// Should preserve fully qualified paths
parsedArgs = DefaultParse(new[] { @"/pdb:c:\MyPdb.pdb", "/debug", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"c:\MyPdb.pdb", parsedArgs.PdbPath);
parsedArgs = DefaultParse(new[] { @"/pdb:\MyFolder\MyPdb.pdb", "/debug", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(Path.Combine(Path.GetPathRoot(WorkingDirectory), @"MyFolder\MyPdb.pdb"), parsedArgs.PdbPath);
// Should handle quotes
parsedArgs = DefaultParse(new[] { @"/pdb:""C:\My Folder\MyPdb.pdb""", "/debug", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"C:\My Folder\MyPdb.pdb", parsedArgs.PdbPath);
// Should expand partially qualified paths
parsedArgs = DefaultParse(new[] { @"/pdb:MyPdb.pdb", "/debug", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(FileUtilities.ResolveRelativePath("MyPdb.pdb", WorkingDirectory), parsedArgs.PdbPath);
// Should expand partially qualified paths
parsedArgs = DefaultParse(new[] { @"/pdb:..\MyPdb.pdb", "/debug", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
// Temp: Path info changed
// Assert.Equal(FileUtilities.ResolveRelativePath("MyPdb.pdb", "..\\", baseDirectory), parsedArgs.PdbPath);
parsedArgs = DefaultParse(new[] { @"/pdb:\\b", "/debug", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2021: File name '.x' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(@"\\b"));
Assert.Null(parsedArgs.PdbPath);
parsedArgs = DefaultParse(new[] { @"/pdb:\\b\OkFileName.pdb", "/debug", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2021: File name '.x' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(@"\\b\OkFileName.pdb"));
Assert.Null(parsedArgs.PdbPath);
parsedArgs = DefaultParse(new[] { @"/pdb:\\server\share\MyPdb.pdb", "/debug", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"\\server\share\MyPdb.pdb", parsedArgs.PdbPath);
// invalid name:
parsedArgs = DefaultParse(new[] { "/pdb:a.b\0b", "/debug", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments("a.b\0b"));
Assert.Null(parsedArgs.PdbPath);
parsedArgs = DefaultParse(new[] { "/pdb:a\uD800b.pdb", "/debug", "a.cs" }, WorkingDirectory);
//parsedArgs.Errors.Verify(
// Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments("a\uD800b.pdb"));
Assert.Null(parsedArgs.PdbPath);
// Dev11 reports CS0016: Could not write to output file 'd:\Temp\q\a<>.z'
parsedArgs = DefaultParse(new[] { @"/pdb:""a<>.pdb""", "a.vb" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2021: File name 'a<>.pdb' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments("a<>.pdb"));
Assert.Null(parsedArgs.PdbPath);
parsedArgs = DefaultParse(new[] { "/pdb:.x", "/debug", "a.cs" }, WorkingDirectory);
//parsedArgs.Errors.Verify(
// // error CS2021: File name '.x' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
// Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(".x"));
Assert.Null(parsedArgs.PdbPath);
}
[Fact]
public void SourceLink()
{
var parsedArgs = DefaultParse(new[] { "/sourcelink:sl.json", "/debug:portable", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(Path.Combine(WorkingDirectory, "sl.json"), parsedArgs.SourceLink);
parsedArgs = DefaultParse(new[] { "/sourcelink:sl.json", "/debug:embedded", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(Path.Combine(WorkingDirectory, "sl.json"), parsedArgs.SourceLink);
parsedArgs = DefaultParse(new[] { @"/sourcelink:""s l.json""", "/debug:embedded", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(Path.Combine(WorkingDirectory, "s l.json"), parsedArgs.SourceLink);
parsedArgs = DefaultParse(new[] { "/sourcelink:sl.json", "/debug:full", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new[] { "/sourcelink:sl.json", "/debug:pdbonly", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new[] { "/sourcelink:sl.json", "/debug-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SourceLinkRequiresPdb));
parsedArgs = DefaultParse(new[] { "/sourcelink:sl.json", "/debug+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new[] { "/sourcelink:sl.json", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SourceLinkRequiresPdb));
}
[Fact]
public void SourceLink_EndToEnd_EmbeddedPortable()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("a.cs");
src.WriteAllText(@"class C { public static void Main() {} }");
var sl = dir.CreateFile("sl.json");
sl.WriteAllText(@"{ ""documents"" : {} }");
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "/debug:embedded", "/sourcelink:sl.json", "a.cs" });
int exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
var peStream = File.OpenRead(Path.Combine(dir.Path, "a.exe"));
using (var peReader = new PEReader(peStream))
{
var entry = peReader.ReadDebugDirectory().Single(e => e.Type == DebugDirectoryEntryType.EmbeddedPortablePdb);
using (var mdProvider = peReader.ReadEmbeddedPortablePdbDebugDirectoryData(entry))
{
var blob = mdProvider.GetMetadataReader().GetSourceLinkBlob();
AssertEx.Equal(File.ReadAllBytes(sl.Path), blob);
}
}
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
}
[Fact]
public void SourceLink_EndToEnd_Portable()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("a.cs");
src.WriteAllText(@"class C { public static void Main() {} }");
var sl = dir.CreateFile("sl.json");
sl.WriteAllText(@"{ ""documents"" : {} }");
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "/debug:portable", "/sourcelink:sl.json", "a.cs" });
int exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
var pdbStream = File.OpenRead(Path.Combine(dir.Path, "a.pdb"));
using (var mdProvider = MetadataReaderProvider.FromPortablePdbStream(pdbStream))
{
var blob = mdProvider.GetMetadataReader().GetSourceLinkBlob();
AssertEx.Equal(File.ReadAllBytes(sl.Path), blob);
}
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
}
[Fact]
public void SourceLink_EndToEnd_Windows()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("a.cs");
src.WriteAllText(@"class C { public static void Main() {} }");
var sl = dir.CreateFile("sl.json");
byte[] slContent = Encoding.UTF8.GetBytes(@"{ ""documents"" : {} }");
sl.WriteAllBytes(slContent);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "/debug:full", "/sourcelink:sl.json", "a.cs" });
int exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
var pdbStream = File.OpenRead(Path.Combine(dir.Path, "a.pdb"));
var actualData = PdbValidation.GetSourceLinkData(pdbStream);
AssertEx.Equal(slContent, actualData);
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
}
[Fact]
public void Embed()
{
var parsedArgs = DefaultParse(new[] { "a.cs " }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Empty(parsedArgs.EmbeddedFiles);
parsedArgs = DefaultParse(new[] { "/embed", "/debug:portable", "a.cs", "b.cs", "c.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
AssertEx.Equal(parsedArgs.SourceFiles, parsedArgs.EmbeddedFiles);
AssertEx.Equal(
new[] { "a.cs", "b.cs", "c.cs" }.Select(f => Path.Combine(WorkingDirectory, f)),
parsedArgs.EmbeddedFiles.Select(f => f.Path));
parsedArgs = DefaultParse(new[] { "/embed:a.cs", "/embed:b.cs", "/debug:embedded", "a.cs", "b.cs", "c.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
AssertEx.Equal(
new[] { "a.cs", "b.cs" }.Select(f => Path.Combine(WorkingDirectory, f)),
parsedArgs.EmbeddedFiles.Select(f => f.Path));
parsedArgs = DefaultParse(new[] { "/embed:a.cs;b.cs", "/debug:portable", "a.cs", "b.cs", "c.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
AssertEx.Equal(
new[] { "a.cs", "b.cs" }.Select(f => Path.Combine(WorkingDirectory, f)),
parsedArgs.EmbeddedFiles.Select(f => f.Path));
parsedArgs = DefaultParse(new[] { "/embed:a.cs,b.cs", "/debug:portable", "a.cs", "b.cs", "c.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
AssertEx.Equal(
new[] { "a.cs", "b.cs" }.Select(f => Path.Combine(WorkingDirectory, f)),
parsedArgs.EmbeddedFiles.Select(f => f.Path));
parsedArgs = DefaultParse(new[] { @"/embed:""a,b.cs""", "/debug:portable", "a,b.cs", "c.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
AssertEx.Equal(
new[] { "a,b.cs" }.Select(f => Path.Combine(WorkingDirectory, f)),
parsedArgs.EmbeddedFiles.Select(f => f.Path));
parsedArgs = DefaultParse(new[] { "/embed:a.txt", "/embed", "/debug:portable", "a.cs", "b.cs", "c.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(); ;
AssertEx.Equal(
new[] { "a.txt", "a.cs", "b.cs", "c.cs" }.Select(f => Path.Combine(WorkingDirectory, f)),
parsedArgs.EmbeddedFiles.Select(f => f.Path));
parsedArgs = DefaultParse(new[] { "/embed", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_CannotEmbedWithoutPdb));
parsedArgs = DefaultParse(new[] { "/embed:a.txt", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_CannotEmbedWithoutPdb));
parsedArgs = DefaultParse(new[] { "/embed", "/debug-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_CannotEmbedWithoutPdb));
parsedArgs = DefaultParse(new[] { "/embed:a.txt", "/debug-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_CannotEmbedWithoutPdb));
parsedArgs = DefaultParse(new[] { "/embed", "/debug:full", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new[] { "/embed", "/debug:pdbonly", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new[] { "/embed", "/debug+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
}
[Theory]
[InlineData("/debug:portable", "/embed", new[] { "embed.cs", "embed2.cs", "embed.xyz" })]
[InlineData("/debug:portable", "/embed:embed.cs", new[] { "embed.cs", "embed.xyz" })]
[InlineData("/debug:portable", "/embed:embed2.cs", new[] { "embed2.cs" })]
[InlineData("/debug:portable", "/embed:embed.xyz", new[] { "embed.xyz" })]
[InlineData("/debug:embedded", "/embed", new[] { "embed.cs", "embed2.cs", "embed.xyz" })]
[InlineData("/debug:embedded", "/embed:embed.cs", new[] { "embed.cs", "embed.xyz" })]
[InlineData("/debug:embedded", "/embed:embed2.cs", new[] { "embed2.cs" })]
[InlineData("/debug:embedded", "/embed:embed.xyz", new[] { "embed.xyz" })]
public void Embed_EndToEnd_Portable(string debugSwitch, string embedSwitch, string[] expectedEmbedded)
{
// embed.cs: large enough to compress, has #line directives
const string embed_cs =
@"///////////////////////////////////////////////////////////////////////////////
class Program {
static void Main() {
#line 1 ""embed.xyz""
System.Console.WriteLine(""Hello, World"");
#line 3
System.Console.WriteLine(""Goodbye, World"");
}
}
///////////////////////////////////////////////////////////////////////////////";
// embed2.cs: small enough to not compress, no sequence points
const string embed2_cs =
@"class C
{
}";
// target of #line
const string embed_xyz =
@"print Hello, World
print Goodbye, World";
Assert.True(embed_cs.Length >= EmbeddedText.CompressionThreshold);
Assert.True(embed2_cs.Length < EmbeddedText.CompressionThreshold);
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("embed.cs");
var src2 = dir.CreateFile("embed2.cs");
var txt = dir.CreateFile("embed.xyz");
src.WriteAllText(embed_cs);
src2.WriteAllText(embed2_cs);
txt.WriteAllText(embed_xyz);
var expectedEmbeddedMap = new Dictionary<string, string>();
if (expectedEmbedded.Contains("embed.cs"))
{
expectedEmbeddedMap.Add(src.Path, embed_cs);
}
if (expectedEmbedded.Contains("embed2.cs"))
{
expectedEmbeddedMap.Add(src2.Path, embed2_cs);
}
if (expectedEmbedded.Contains("embed.xyz"))
{
expectedEmbeddedMap.Add(txt.Path, embed_xyz);
}
var output = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", debugSwitch, embedSwitch, "embed.cs", "embed2.cs" });
int exitCode = csc.Run(output);
Assert.Equal("", output.ToString().Trim());
Assert.Equal(0, exitCode);
switch (debugSwitch)
{
case "/debug:embedded":
ValidateEmbeddedSources_Portable(expectedEmbeddedMap, dir, isEmbeddedPdb: true);
break;
case "/debug:portable":
ValidateEmbeddedSources_Portable(expectedEmbeddedMap, dir, isEmbeddedPdb: false);
break;
case "/debug:full":
ValidateEmbeddedSources_Windows(expectedEmbeddedMap, dir);
break;
}
Assert.Empty(expectedEmbeddedMap);
CleanupAllGeneratedFiles(src.Path);
}
private static void ValidateEmbeddedSources_Portable(Dictionary<string, string> expectedEmbeddedMap, TempDirectory dir, bool isEmbeddedPdb)
{
using (var peReader = new PEReader(File.OpenRead(Path.Combine(dir.Path, "embed.exe"))))
{
var entry = peReader.ReadDebugDirectory().SingleOrDefault(e => e.Type == DebugDirectoryEntryType.EmbeddedPortablePdb);
Assert.Equal(isEmbeddedPdb, entry.DataSize > 0);
using (var mdProvider = isEmbeddedPdb ?
peReader.ReadEmbeddedPortablePdbDebugDirectoryData(entry) :
MetadataReaderProvider.FromPortablePdbStream(File.OpenRead(Path.Combine(dir.Path, "embed.pdb"))))
{
var mdReader = mdProvider.GetMetadataReader();
foreach (var handle in mdReader.Documents)
{
var doc = mdReader.GetDocument(handle);
var docPath = mdReader.GetString(doc.Name);
SourceText embeddedSource = mdReader.GetEmbeddedSource(handle);
if (embeddedSource == null)
{
continue;
}
Assert.Equal(expectedEmbeddedMap[docPath], embeddedSource.ToString());
Assert.True(expectedEmbeddedMap.Remove(docPath));
}
}
}
}
private static void ValidateEmbeddedSources_Windows(Dictionary<string, string> expectedEmbeddedMap, TempDirectory dir)
{
ISymUnmanagedReader5 symReader = null;
try
{
symReader = SymReaderFactory.CreateReader(File.OpenRead(Path.Combine(dir.Path, "embed.pdb")));
foreach (var doc in symReader.GetDocuments())
{
var docPath = doc.GetName();
var sourceBlob = doc.GetEmbeddedSource();
if (sourceBlob.Array == null)
{
continue;
}
var sourceStr = Encoding.UTF8.GetString(sourceBlob.Array, sourceBlob.Offset, sourceBlob.Count);
Assert.Equal(expectedEmbeddedMap[docPath], sourceStr);
Assert.True(expectedEmbeddedMap.Remove(docPath));
}
}
catch
{
symReader?.Dispose();
}
}
private static void ValidateWrittenSources(Dictionary<string, Dictionary<string, string>> expectedFilesMap, Encoding encoding = null)
{
foreach ((var dirPath, var fileMap) in expectedFilesMap.ToArray())
{
foreach (var file in Directory.GetFiles(dirPath))
{
var name = Path.GetFileName(file);
var content = File.ReadAllText(file, encoding ?? Encoding.UTF8);
Assert.Equal(fileMap[name], content);
Assert.True(fileMap.Remove(name));
}
Assert.Empty(fileMap);
Assert.True(expectedFilesMap.Remove(dirPath));
}
Assert.Empty(expectedFilesMap);
}
[Fact]
public void Optimize()
{
var parsedArgs = DefaultParse(new[] { "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(new CSharpCompilationOptions(OutputKind.ConsoleApplication).OptimizationLevel, parsedArgs.CompilationOptions.OptimizationLevel);
parsedArgs = DefaultParse(new[] { "/optimize-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(OptimizationLevel.Debug, parsedArgs.CompilationOptions.OptimizationLevel);
parsedArgs = DefaultParse(new[] { "/optimize", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(OptimizationLevel.Release, parsedArgs.CompilationOptions.OptimizationLevel);
parsedArgs = DefaultParse(new[] { "/optimize+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(OptimizationLevel.Release, parsedArgs.CompilationOptions.OptimizationLevel);
parsedArgs = DefaultParse(new[] { "/optimize+", "/optimize-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(OptimizationLevel.Debug, parsedArgs.CompilationOptions.OptimizationLevel);
parsedArgs = DefaultParse(new[] { "/optimize:+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/optimize:+"));
parsedArgs = DefaultParse(new[] { "/optimize:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/optimize:"));
parsedArgs = DefaultParse(new[] { "/optimize-:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/optimize-:"));
parsedArgs = DefaultParse(new[] { "/o-", "a.cs" }, WorkingDirectory);
Assert.Equal(OptimizationLevel.Debug, parsedArgs.CompilationOptions.OptimizationLevel);
parsedArgs = DefaultParse(new string[] { "/o", "a.cs" }, WorkingDirectory);
Assert.Equal(OptimizationLevel.Release, parsedArgs.CompilationOptions.OptimizationLevel);
parsedArgs = DefaultParse(new string[] { "/o+", "a.cs" }, WorkingDirectory);
Assert.Equal(OptimizationLevel.Release, parsedArgs.CompilationOptions.OptimizationLevel);
parsedArgs = DefaultParse(new string[] { "/o+", "/optimize-", "a.cs" }, WorkingDirectory);
Assert.Equal(OptimizationLevel.Debug, parsedArgs.CompilationOptions.OptimizationLevel);
parsedArgs = DefaultParse(new string[] { "/o:+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/o:+"));
parsedArgs = DefaultParse(new string[] { "/o:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/o:"));
parsedArgs = DefaultParse(new string[] { "/o-:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/o-:"));
}
[Fact]
public void Deterministic()
{
var parsedArgs = DefaultParse(new[] { "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.Deterministic);
parsedArgs = DefaultParse(new[] { "/deterministic+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.CompilationOptions.Deterministic);
parsedArgs = DefaultParse(new[] { "/deterministic", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.CompilationOptions.Deterministic);
parsedArgs = DefaultParse(new[] { "/deterministic-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.Deterministic);
}
[Fact]
public void ParseReferences()
{
var parsedArgs = DefaultParse(new string[] { "/r:goo.dll", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(2, parsedArgs.MetadataReferences.Length);
parsedArgs = DefaultParse(new string[] { "/r:goo.dll;", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(2, parsedArgs.MetadataReferences.Length);
Assert.Equal(MscorlibFullPath, parsedArgs.MetadataReferences[0].Reference);
Assert.Equal(MetadataReferenceProperties.Assembly, parsedArgs.MetadataReferences[0].Properties);
Assert.Equal("goo.dll", parsedArgs.MetadataReferences[1].Reference);
Assert.Equal(MetadataReferenceProperties.Assembly, parsedArgs.MetadataReferences[1].Properties);
parsedArgs = DefaultParse(new string[] { @"/l:goo.dll", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(2, parsedArgs.MetadataReferences.Length);
Assert.Equal(MscorlibFullPath, parsedArgs.MetadataReferences[0].Reference);
Assert.Equal(MetadataReferenceProperties.Assembly, parsedArgs.MetadataReferences[0].Properties);
Assert.Equal("goo.dll", parsedArgs.MetadataReferences[1].Reference);
Assert.Equal(MetadataReferenceProperties.Assembly.WithEmbedInteropTypes(true), parsedArgs.MetadataReferences[1].Properties);
parsedArgs = DefaultParse(new string[] { @"/addmodule:goo.dll", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(2, parsedArgs.MetadataReferences.Length);
Assert.Equal(MscorlibFullPath, parsedArgs.MetadataReferences[0].Reference);
Assert.Equal(MetadataReferenceProperties.Assembly, parsedArgs.MetadataReferences[0].Properties);
Assert.Equal("goo.dll", parsedArgs.MetadataReferences[1].Reference);
Assert.Equal(MetadataReferenceProperties.Module, parsedArgs.MetadataReferences[1].Properties);
parsedArgs = DefaultParse(new string[] { @"/r:a=goo.dll", "/l:b=bar.dll", "/addmodule:c=mod.dll", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(4, parsedArgs.MetadataReferences.Length);
Assert.Equal(MscorlibFullPath, parsedArgs.MetadataReferences[0].Reference);
Assert.Equal(MetadataReferenceProperties.Assembly, parsedArgs.MetadataReferences[0].Properties);
Assert.Equal("goo.dll", parsedArgs.MetadataReferences[1].Reference);
Assert.Equal(MetadataReferenceProperties.Assembly.WithAliases(new[] { "a" }), parsedArgs.MetadataReferences[1].Properties);
Assert.Equal("bar.dll", parsedArgs.MetadataReferences[2].Reference);
Assert.Equal(MetadataReferenceProperties.Assembly.WithAliases(new[] { "b" }).WithEmbedInteropTypes(true), parsedArgs.MetadataReferences[2].Properties);
Assert.Equal("c=mod.dll", parsedArgs.MetadataReferences[3].Reference);
Assert.Equal(MetadataReferenceProperties.Module, parsedArgs.MetadataReferences[3].Properties);
// TODO: multiple files, quotes, etc.
}
[Fact]
public void ParseAnalyzers()
{
var parsedArgs = DefaultParse(new string[] { @"/a:goo.dll", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(1, parsedArgs.AnalyzerReferences.Length);
Assert.Equal("goo.dll", parsedArgs.AnalyzerReferences[0].FilePath);
parsedArgs = DefaultParse(new string[] { @"/analyzer:goo.dll", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(1, parsedArgs.AnalyzerReferences.Length);
Assert.Equal("goo.dll", parsedArgs.AnalyzerReferences[0].FilePath);
parsedArgs = DefaultParse(new string[] { "/analyzer:\"goo.dll\"", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(1, parsedArgs.AnalyzerReferences.Length);
Assert.Equal("goo.dll", parsedArgs.AnalyzerReferences[0].FilePath);
parsedArgs = DefaultParse(new string[] { @"/a:goo.dll;bar.dll", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(2, parsedArgs.AnalyzerReferences.Length);
Assert.Equal("goo.dll", parsedArgs.AnalyzerReferences[0].FilePath);
Assert.Equal("bar.dll", parsedArgs.AnalyzerReferences[1].FilePath);
parsedArgs = DefaultParse(new string[] { @"/a:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("/a:"));
parsedArgs = DefaultParse(new string[] { "/a", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "/a"));
}
[Fact]
public void Analyzers_Missing()
{
string source = @"
class C
{
}
";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile("a.cs");
file.WriteAllText(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "/preferreduilang:en", "/a:missing.dll", "a.cs" });
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal("error CS0006: Metadata file 'missing.dll' could not be found", outWriter.ToString().Trim());
// Clean up temp files
CleanupAllGeneratedFiles(file.Path);
}
[Fact]
public void Analyzers_Empty()
{
string source = @"
class C
{
}
";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile("a.cs");
file.WriteAllText(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "/preferreduilang:en", "/t:library", "/a:" + typeof(object).Assembly.Location, "a.cs" });
int exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.DoesNotContain("warning", outWriter.ToString());
CleanupAllGeneratedFiles(file.Path);
}
private TempFile CreateRuleSetFile(string source)
{
var dir = Temp.CreateDirectory();
var file = dir.CreateFile("a.ruleset");
file.WriteAllText(source);
return file;
}
[Fact]
public void RuleSetSwitchPositive()
{
string source = @"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""12.0"">
<IncludeAll Action=""Warning"" />
<Rules AnalyzerId=""Microsoft.Analyzers.ManagedCodeAnalysis"" RuleNamespace=""Microsoft.Rules.Managed"">
<Rule Id=""CA1012"" Action=""Error"" />
<Rule Id=""CA1013"" Action=""Warning"" />
<Rule Id=""CA1014"" Action=""None"" />
</Rules>
</RuleSet>
";
var file = CreateRuleSetFile(source);
var parsedArgs = DefaultParse(new string[] { @"/ruleset:" + file.Path, "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(expected: file.Path, actual: parsedArgs.RuleSetPath);
Assert.True(parsedArgs.CompilationOptions.SpecificDiagnosticOptions.ContainsKey("CA1012"));
Assert.True(parsedArgs.CompilationOptions.SpecificDiagnosticOptions["CA1012"] == ReportDiagnostic.Error);
Assert.True(parsedArgs.CompilationOptions.SpecificDiagnosticOptions.ContainsKey("CA1013"));
Assert.True(parsedArgs.CompilationOptions.SpecificDiagnosticOptions["CA1013"] == ReportDiagnostic.Warn);
Assert.True(parsedArgs.CompilationOptions.SpecificDiagnosticOptions.ContainsKey("CA1014"));
Assert.True(parsedArgs.CompilationOptions.SpecificDiagnosticOptions["CA1014"] == ReportDiagnostic.Suppress);
Assert.True(parsedArgs.CompilationOptions.GeneralDiagnosticOption == ReportDiagnostic.Warn);
}
[Fact]
public void RuleSetSwitchQuoted()
{
string source = @"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""12.0"">
<IncludeAll Action=""Warning"" />
<Rules AnalyzerId=""Microsoft.Analyzers.ManagedCodeAnalysis"" RuleNamespace=""Microsoft.Rules.Managed"">
<Rule Id=""CA1012"" Action=""Error"" />
<Rule Id=""CA1013"" Action=""Warning"" />
<Rule Id=""CA1014"" Action=""None"" />
</Rules>
</RuleSet>
";
var file = CreateRuleSetFile(source);
var parsedArgs = DefaultParse(new string[] { @"/ruleset:" + "\"" + file.Path + "\"", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(expected: file.Path, actual: parsedArgs.RuleSetPath);
}
[Fact]
public void RuleSetSwitchParseErrors()
{
var parsedArgs = DefaultParse(new string[] { @"/ruleset", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "ruleset"));
Assert.Null(parsedArgs.RuleSetPath);
parsedArgs = DefaultParse(new string[] { @"/ruleset:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "ruleset"));
Assert.Null(parsedArgs.RuleSetPath);
parsedArgs = DefaultParse(new string[] { @"/ruleset:blah", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
Diagnostic(ErrorCode.ERR_CantReadRulesetFile).WithArguments(Path.Combine(TempRoot.Root, "blah"), "File not found."));
Assert.Equal(expected: Path.Combine(TempRoot.Root, "blah"), actual: parsedArgs.RuleSetPath);
parsedArgs = DefaultParse(new string[] { @"/ruleset:blah;blah.ruleset", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
Diagnostic(ErrorCode.ERR_CantReadRulesetFile).WithArguments(Path.Combine(TempRoot.Root, "blah;blah.ruleset"), "File not found."));
Assert.Equal(expected: Path.Combine(TempRoot.Root, "blah;blah.ruleset"), actual: parsedArgs.RuleSetPath);
var file = CreateRuleSetFile("Random text");
parsedArgs = DefaultParse(new string[] { @"/ruleset:" + file.Path, "a.cs" }, WorkingDirectory);
//parsedArgs.Errors.Verify(
// Diagnostic(ErrorCode.ERR_CantReadRulesetFile).WithArguments(file.Path, "Data at the root level is invalid. Line 1, position 1."));
Assert.Equal(expected: file.Path, actual: parsedArgs.RuleSetPath);
var err = parsedArgs.Errors.Single();
Assert.Equal((int)ErrorCode.ERR_CantReadRulesetFile, err.Code);
Assert.Equal(2, err.Arguments.Count);
Assert.Equal(file.Path, (string)err.Arguments[0]);
var currentUICultureName = Thread.CurrentThread.CurrentUICulture.Name;
if (currentUICultureName.Length == 0 || currentUICultureName.StartsWith("en", StringComparison.OrdinalIgnoreCase))
{
Assert.Equal("Data at the root level is invalid. Line 1, position 1.", (string)err.Arguments[1]);
}
}
[WorkItem(892467, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/892467")]
[Fact]
public void Analyzers_Found()
{
string source = @"
class C
{
}
";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile("a.cs");
file.WriteAllText(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
// This assembly has a MockAbstractDiagnosticAnalyzer type which should get run by this compilation.
var csc = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "/preferreduilang:en", "/t:library", "/a:" + Assembly.GetExecutingAssembly().Location, "a.cs" });
int exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
// Diagnostic thrown
Assert.True(outWriter.ToString().Contains("a.cs(2,7): warning Warning01: Throwing a diagnostic for types declared"));
// Diagnostic cannot be instantiated
Assert.True(outWriter.ToString().Contains("warning CS8032"));
CleanupAllGeneratedFiles(file.Path);
}
[Fact]
public void Analyzers_WithRuleSet()
{
string source = @"
class C
{
int x;
}
";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile("a.cs");
file.WriteAllText(source);
string rulesetSource = @"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""12.0"">
<Rules AnalyzerId=""Microsoft.Analyzers.ManagedCodeAnalysis"" RuleNamespace=""Microsoft.Rules.Managed"">
<Rule Id=""Warning01"" Action=""Error"" />
</Rules>
</RuleSet>
";
var ruleSetFile = CreateRuleSetFile(rulesetSource);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
// This assembly has a MockAbstractDiagnosticAnalyzer type which should get run by this compilation.
var csc = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "/preferreduilang:en", "/t:library", "/a:" + Assembly.GetExecutingAssembly().Location, "a.cs", "/ruleset:" + ruleSetFile.Path });
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
// Diagnostic thrown as error.
Assert.True(outWriter.ToString().Contains("a.cs(2,7): error Warning01: Throwing a diagnostic for types declared"));
// Clean up temp files
CleanupAllGeneratedFiles(file.Path);
}
[WorkItem(912906, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/912906")]
[Fact]
public void Analyzers_CommandLineOverridesRuleset1()
{
string source = @"
class C
{
}
";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile("a.cs");
file.WriteAllText(source);
string rulesetSource = @"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""12.0"">
<IncludeAll Action=""Warning"" />
</RuleSet>
";
var ruleSetFile = CreateRuleSetFile(rulesetSource);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
// This assembly has a MockAbstractDiagnosticAnalyzer type which should get run by this compilation.
var csc = CreateCSharpCompiler(null, dir.Path,
new[] {
"/nologo", "/preferreduilang:en", "/t:library",
"/a:" + Assembly.GetExecutingAssembly().Location, "a.cs",
"/ruleset:" + ruleSetFile.Path, "/warnaserror+", "/nowarn:8032" });
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
// Diagnostic thrown as error: command line always overrides ruleset.
Assert.Contains("a.cs(2,7): error Warning01: Throwing a diagnostic for types declared", outWriter.ToString(), StringComparison.Ordinal);
outWriter = new StringWriter(CultureInfo.InvariantCulture);
csc = CreateCSharpCompiler(null, dir.Path,
new[] {
"/nologo", "/preferreduilang:en", "/t:library",
"/a:" + Assembly.GetExecutingAssembly().Location, "a.cs",
"/warnaserror+", "/ruleset:" + ruleSetFile.Path, "/nowarn:8032" });
exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
// Diagnostic thrown as error: command line always overrides ruleset.
Assert.Contains("a.cs(2,7): error Warning01: Throwing a diagnostic for types declared", outWriter.ToString(), StringComparison.Ordinal);
// Clean up temp files
CleanupAllGeneratedFiles(file.Path);
}
[Fact]
[WorkItem(468, "https://github.com/dotnet/roslyn/issues/468")]
public void RuleSet_GeneralCommandLineOptionOverridesGeneralRuleSetOption()
{
var dir = Temp.CreateDirectory();
string ruleSetSource = @"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""12.0"">
<IncludeAll Action=""Warning"" />
</RuleSet>
";
var ruleSetFile = dir.CreateFile("Rules.ruleset").WriteAllText(ruleSetSource);
var arguments = DefaultParse(
new[]
{
"/nologo",
"/t:library",
"/ruleset:Rules.ruleset",
"/warnaserror+",
"a.cs"
},
dir.Path);
var errors = arguments.Errors;
Assert.Empty(errors);
Assert.Equal(actual: arguments.CompilationOptions.GeneralDiagnosticOption, expected: ReportDiagnostic.Error);
}
[Fact]
[WorkItem(468, "https://github.com/dotnet/roslyn/issues/468")]
public void RuleSet_GeneralWarnAsErrorPromotesWarningFromRuleSet()
{
var dir = Temp.CreateDirectory();
string ruleSetSource = @"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""12.0"">
<Rules AnalyzerId=""Microsoft.Analyzers.ManagedCodeAnalysis"" RuleNamespace=""Microsoft.Rules.Managed"">
<Rule Id=""Test001"" Action=""Warning"" />
</Rules>
</RuleSet>
";
var ruleSetFile = dir.CreateFile("Rules.ruleset").WriteAllText(ruleSetSource);
var arguments = DefaultParse(
new[]
{
"/nologo",
"/t:library",
"/ruleset:Rules.ruleset",
"/warnaserror+",
"a.cs"
},
dir.Path);
var errors = arguments.Errors;
Assert.Empty(errors);
Assert.Equal(actual: arguments.CompilationOptions.GeneralDiagnosticOption, expected: ReportDiagnostic.Error);
Assert.Equal(actual: arguments.CompilationOptions.SpecificDiagnosticOptions["Test001"], expected: ReportDiagnostic.Error);
}
[Fact]
[WorkItem(468, "https://github.com/dotnet/roslyn/issues/468")]
public void RuleSet_GeneralWarnAsErrorDoesNotPromoteInfoFromRuleSet()
{
var dir = Temp.CreateDirectory();
string ruleSetSource = @"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""12.0"">
<Rules AnalyzerId=""Microsoft.Analyzers.ManagedCodeAnalysis"" RuleNamespace=""Microsoft.Rules.Managed"">
<Rule Id=""Test001"" Action=""Info"" />
</Rules>
</RuleSet>
";
var ruleSetFile = dir.CreateFile("Rules.ruleset").WriteAllText(ruleSetSource);
var arguments = DefaultParse(
new[]
{
"/nologo",
"/t:library",
"/ruleset:Rules.ruleset",
"/warnaserror+",
"a.cs"
},
dir.Path);
var errors = arguments.Errors;
Assert.Empty(errors);
Assert.Equal(actual: arguments.CompilationOptions.GeneralDiagnosticOption, expected: ReportDiagnostic.Error);
Assert.Equal(actual: arguments.CompilationOptions.SpecificDiagnosticOptions["Test001"], expected: ReportDiagnostic.Info);
}
[Fact]
[WorkItem(468, "https://github.com/dotnet/roslyn/issues/468")]
public void RuleSet_SpecificWarnAsErrorPromotesInfoFromRuleSet()
{
var dir = Temp.CreateDirectory();
string ruleSetSource = @"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""12.0"">
<Rules AnalyzerId=""Microsoft.Analyzers.ManagedCodeAnalysis"" RuleNamespace=""Microsoft.Rules.Managed"">
<Rule Id=""Test001"" Action=""Info"" />
</Rules>
</RuleSet>
";
var ruleSetFile = dir.CreateFile("Rules.ruleset").WriteAllText(ruleSetSource);
var arguments = DefaultParse(
new[]
{
"/nologo",
"/t:library",
"/ruleset:Rules.ruleset",
"/warnaserror+:Test001",
"a.cs"
},
dir.Path);
var errors = arguments.Errors;
Assert.Empty(errors);
Assert.Equal(actual: arguments.CompilationOptions.GeneralDiagnosticOption, expected: ReportDiagnostic.Default);
Assert.Equal(actual: arguments.CompilationOptions.SpecificDiagnosticOptions["Test001"], expected: ReportDiagnostic.Error);
}
[Fact]
[WorkItem(468, "https://github.com/dotnet/roslyn/issues/468")]
public void RuleSet_GeneralWarnAsErrorMinusResetsRules()
{
var dir = Temp.CreateDirectory();
string ruleSetSource = @"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""12.0"">
<Rules AnalyzerId=""Microsoft.Analyzers.ManagedCodeAnalysis"" RuleNamespace=""Microsoft.Rules.Managed"">
<Rule Id=""Test001"" Action=""Warning"" />
</Rules>
</RuleSet>
";
var ruleSetFile = dir.CreateFile("Rules.ruleset").WriteAllText(ruleSetSource);
var arguments = DefaultParse(
new[]
{
"/nologo",
"/t:library",
"/ruleset:Rules.ruleset",
"/warnaserror+",
"/warnaserror-",
"a.cs"
},
dir.Path);
var errors = arguments.Errors;
Assert.Empty(errors);
Assert.Equal(actual: arguments.CompilationOptions.GeneralDiagnosticOption, expected: ReportDiagnostic.Default);
Assert.Equal(actual: arguments.CompilationOptions.SpecificDiagnosticOptions["Test001"], expected: ReportDiagnostic.Warn);
}
[Fact]
[WorkItem(468, "https://github.com/dotnet/roslyn/issues/468")]
public void RuleSet_SpecificWarnAsErrorMinusResetsRules()
{
var dir = Temp.CreateDirectory();
string ruleSetSource = @"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""12.0"">
<Rules AnalyzerId=""Microsoft.Analyzers.ManagedCodeAnalysis"" RuleNamespace=""Microsoft.Rules.Managed"">
<Rule Id=""Test001"" Action=""Warning"" />
</Rules>
</RuleSet>
";
var ruleSetFile = dir.CreateFile("Rules.ruleset").WriteAllText(ruleSetSource);
var arguments = DefaultParse(
new[]
{
"/nologo",
"/t:library",
"/ruleset:Rules.ruleset",
"/warnaserror+",
"/warnaserror-:Test001",
"a.cs"
},
dir.Path);
var errors = arguments.Errors;
Assert.Empty(errors);
Assert.Equal(actual: arguments.CompilationOptions.GeneralDiagnosticOption, expected: ReportDiagnostic.Error);
Assert.Equal(actual: arguments.CompilationOptions.SpecificDiagnosticOptions["Test001"], expected: ReportDiagnostic.Warn);
}
[Fact]
[WorkItem(468, "https://github.com/dotnet/roslyn/issues/468")]
public void RuleSet_SpecificWarnAsErrorMinusDefaultsRuleNotInRuleSet()
{
var dir = Temp.CreateDirectory();
string ruleSetSource = @"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""12.0"">
<Rules AnalyzerId=""Microsoft.Analyzers.ManagedCodeAnalysis"" RuleNamespace=""Microsoft.Rules.Managed"">
<Rule Id=""Test001"" Action=""Warning"" />
</Rules>
</RuleSet>
";
var ruleSetFile = dir.CreateFile("Rules.ruleset").WriteAllText(ruleSetSource);
var arguments = DefaultParse(
new[]
{
"/nologo",
"/t:library",
"/ruleset:Rules.ruleset",
"/warnaserror+:Test002",
"/warnaserror-:Test002",
"a.cs"
},
dir.Path);
var errors = arguments.Errors;
Assert.Empty(errors);
Assert.Equal(actual: arguments.CompilationOptions.GeneralDiagnosticOption, expected: ReportDiagnostic.Default);
Assert.Equal(actual: arguments.CompilationOptions.SpecificDiagnosticOptions["Test001"], expected: ReportDiagnostic.Warn);
Assert.Equal(actual: arguments.CompilationOptions.SpecificDiagnosticOptions["Test002"], expected: ReportDiagnostic.Default);
}
[Fact]
[WorkItem(468, "https://github.com/dotnet/roslyn/issues/468")]
public void NoWarn_SpecificNoWarnOverridesRuleSet()
{
var dir = Temp.CreateDirectory();
string ruleSetSource = @"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""12.0"">
<Rules AnalyzerId=""Microsoft.Analyzers.ManagedCodeAnalysis"" RuleNamespace=""Microsoft.Rules.Managed"">
<Rule Id=""Test001"" Action=""Warning"" />
</Rules>
</RuleSet>
";
var ruleSetFile = dir.CreateFile("Rules.ruleset").WriteAllText(ruleSetSource);
var arguments = DefaultParse(
new[]
{
"/nologo",
"/t:library",
"/ruleset:Rules.ruleset",
"/nowarn:Test001",
"a.cs"
},
dir.Path);
var errors = arguments.Errors;
Assert.Empty(errors);
Assert.Equal(expected: ReportDiagnostic.Default, actual: arguments.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(expected: 1, actual: arguments.CompilationOptions.SpecificDiagnosticOptions.Count);
Assert.Equal(expected: ReportDiagnostic.Suppress, actual: arguments.CompilationOptions.SpecificDiagnosticOptions["Test001"]);
}
[Fact]
[WorkItem(468, "https://github.com/dotnet/roslyn/issues/468")]
public void NoWarn_SpecificNoWarnOverridesGeneralWarnAsError()
{
var dir = Temp.CreateDirectory();
string ruleSetSource = @"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""12.0"">
<Rules AnalyzerId=""Microsoft.Analyzers.ManagedCodeAnalysis"" RuleNamespace=""Microsoft.Rules.Managed"">
<Rule Id=""Test001"" Action=""Warning"" />
</Rules>
</RuleSet>
";
var ruleSetFile = dir.CreateFile("Rules.ruleset").WriteAllText(ruleSetSource);
var arguments = DefaultParse(
new[]
{
"/nologo",
"/t:library",
"/ruleset:Rules.ruleset",
"/warnaserror+",
"/nowarn:Test001",
"a.cs"
},
dir.Path);
var errors = arguments.Errors;
Assert.Empty(errors);
Assert.Equal(expected: ReportDiagnostic.Error, actual: arguments.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(expected: 1, actual: arguments.CompilationOptions.SpecificDiagnosticOptions.Count);
Assert.Equal(expected: ReportDiagnostic.Suppress, actual: arguments.CompilationOptions.SpecificDiagnosticOptions["Test001"]);
}
[Fact]
[WorkItem(468, "https://github.com/dotnet/roslyn/issues/468")]
public void NoWarn_SpecificNoWarnOverridesSpecificWarnAsError()
{
var dir = Temp.CreateDirectory();
string ruleSetSource = @"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""12.0"">
<Rules AnalyzerId=""Microsoft.Analyzers.ManagedCodeAnalysis"" RuleNamespace=""Microsoft.Rules.Managed"">
<Rule Id=""Test001"" Action=""Warning"" />
</Rules>
</RuleSet>
";
var ruleSetFile = dir.CreateFile("Rules.ruleset").WriteAllText(ruleSetSource);
var arguments = DefaultParse(
new[]
{
"/nologo",
"/t:library",
"/ruleset:Rules.ruleset",
"/nowarn:Test001",
"/warnaserror+:Test001",
"a.cs"
},
dir.Path);
var errors = arguments.Errors;
Assert.Empty(errors);
Assert.Equal(expected: ReportDiagnostic.Default, actual: arguments.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(expected: 1, actual: arguments.CompilationOptions.SpecificDiagnosticOptions.Count);
Assert.Equal(expected: ReportDiagnostic.Suppress, actual: arguments.CompilationOptions.SpecificDiagnosticOptions["Test001"]);
}
[Fact]
[WorkItem(35748, "https://github.com/dotnet/roslyn/issues/35748")]
public void NoWarn_Nullable()
{
var dir = Temp.CreateDirectory();
var arguments = DefaultParse(
new[]
{
"/nologo",
"/t:library",
"/nowarn:nullable",
"a.cs"
},
dir.Path);
var errors = arguments.Errors;
Assert.Empty(errors);
Assert.Equal(expected: ReportDiagnostic.Default, actual: arguments.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(expected: ErrorFacts.NullableWarnings.Count + 2, actual: arguments.CompilationOptions.SpecificDiagnosticOptions.Count);
foreach (string warning in ErrorFacts.NullableWarnings)
{
Assert.Equal(expected: ReportDiagnostic.Suppress, actual: arguments.CompilationOptions.SpecificDiagnosticOptions[warning]);
}
Assert.Equal(expected: ReportDiagnostic.Suppress,
actual: arguments.CompilationOptions.SpecificDiagnosticOptions[MessageProvider.Instance.GetIdForErrorCode((int)ErrorCode.WRN_MissingNonNullTypesContextForAnnotation)]);
Assert.Equal(expected: ReportDiagnostic.Suppress,
actual: arguments.CompilationOptions.SpecificDiagnosticOptions[MessageProvider.Instance.GetIdForErrorCode((int)ErrorCode.WRN_MissingNonNullTypesContextForAnnotationInGeneratedCode)]);
}
[Fact]
[WorkItem(35748, "https://github.com/dotnet/roslyn/issues/35748")]
public void NoWarn_Nullable_Capitalization()
{
var dir = Temp.CreateDirectory();
var arguments = DefaultParse(
new[]
{
"/nologo",
"/t:library",
"/nowarn:NullABLE",
"a.cs"
},
dir.Path);
var errors = arguments.Errors;
Assert.Empty(errors);
Assert.Equal(expected: ReportDiagnostic.Default, actual: arguments.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(expected: ErrorFacts.NullableWarnings.Count + 2, actual: arguments.CompilationOptions.SpecificDiagnosticOptions.Count);
foreach (string warning in ErrorFacts.NullableWarnings)
{
Assert.Equal(expected: ReportDiagnostic.Suppress, actual: arguments.CompilationOptions.SpecificDiagnosticOptions[warning]);
}
Assert.Equal(expected: ReportDiagnostic.Suppress,
actual: arguments.CompilationOptions.SpecificDiagnosticOptions[MessageProvider.Instance.GetIdForErrorCode((int)ErrorCode.WRN_MissingNonNullTypesContextForAnnotation)]);
Assert.Equal(expected: ReportDiagnostic.Suppress,
actual: arguments.CompilationOptions.SpecificDiagnosticOptions[MessageProvider.Instance.GetIdForErrorCode((int)ErrorCode.WRN_MissingNonNullTypesContextForAnnotationInGeneratedCode)]);
}
[Fact]
[WorkItem(35748, "https://github.com/dotnet/roslyn/issues/35748")]
public void NoWarn_Nullable_MultipleArguments()
{
var dir = Temp.CreateDirectory();
var arguments = DefaultParse(
new[]
{
"/nologo",
"/t:library",
"/nowarn:nullable,Test001",
"a.cs"
},
dir.Path);
var errors = arguments.Errors;
Assert.Empty(errors);
Assert.Equal(expected: ReportDiagnostic.Default, actual: arguments.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(expected: ErrorFacts.NullableWarnings.Count + 3, actual: arguments.CompilationOptions.SpecificDiagnosticOptions.Count);
foreach (string warning in ErrorFacts.NullableWarnings)
{
Assert.Equal(expected: ReportDiagnostic.Suppress, actual: arguments.CompilationOptions.SpecificDiagnosticOptions[warning]);
}
Assert.Equal(expected: ReportDiagnostic.Suppress, actual: arguments.CompilationOptions.SpecificDiagnosticOptions["Test001"]);
Assert.Equal(expected: ReportDiagnostic.Suppress,
actual: arguments.CompilationOptions.SpecificDiagnosticOptions[MessageProvider.Instance.GetIdForErrorCode((int)ErrorCode.WRN_MissingNonNullTypesContextForAnnotation)]);
Assert.Equal(expected: ReportDiagnostic.Suppress,
actual: arguments.CompilationOptions.SpecificDiagnosticOptions[MessageProvider.Instance.GetIdForErrorCode((int)ErrorCode.WRN_MissingNonNullTypesContextForAnnotationInGeneratedCode)]);
}
[Fact]
[WorkItem(35748, "https://github.com/dotnet/roslyn/issues/35748")]
public void WarnAsError_Nullable()
{
var dir = Temp.CreateDirectory();
var arguments = DefaultParse(
new[]
{
"/nologo",
"/t:library",
"/warnaserror:nullable",
"a.cs"
},
dir.Path);
var errors = arguments.Errors;
Assert.Empty(errors);
Assert.Equal(expected: ReportDiagnostic.Default, actual: arguments.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(expected: ErrorFacts.NullableWarnings.Count + 2, actual: arguments.CompilationOptions.SpecificDiagnosticOptions.Count);
foreach (string warning in ErrorFacts.NullableWarnings)
{
Assert.Equal(expected: ReportDiagnostic.Error, actual: arguments.CompilationOptions.SpecificDiagnosticOptions[warning]);
}
Assert.Equal(expected: ReportDiagnostic.Error,
actual: arguments.CompilationOptions.SpecificDiagnosticOptions[MessageProvider.Instance.GetIdForErrorCode((int)ErrorCode.WRN_MissingNonNullTypesContextForAnnotation)]);
Assert.Equal(expected: ReportDiagnostic.Error,
actual: arguments.CompilationOptions.SpecificDiagnosticOptions[MessageProvider.Instance.GetIdForErrorCode((int)ErrorCode.WRN_MissingNonNullTypesContextForAnnotationInGeneratedCode)]);
}
[WorkItem(912906, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/912906")]
[Fact]
public void Analyzers_CommandLineOverridesRuleset2()
{
string source = @"
class C
{
}
";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile("a.cs");
file.WriteAllText(source);
string rulesetSource = @"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""12.0"">
<Rules AnalyzerId=""Microsoft.Analyzers.ManagedCodeAnalysis"" RuleNamespace=""Microsoft.Rules.Managed"">
<Rule Id=""Warning01"" Action=""Error"" />
</Rules>
</RuleSet>
";
var ruleSetFile = CreateRuleSetFile(rulesetSource);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
// This assembly has a MockAbstractDiagnosticAnalyzer type which should get run by this compilation.
var csc = CreateCSharpCompiler(null, dir.Path,
new[] {
"/nologo", "/t:library",
"/a:" + Assembly.GetExecutingAssembly().Location, "a.cs",
"/ruleset:" + ruleSetFile.Path, "/warn:0" });
int exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
// Diagnostic suppressed: commandline always overrides ruleset.
Assert.DoesNotContain("Warning01", outWriter.ToString(), StringComparison.Ordinal);
outWriter = new StringWriter(CultureInfo.InvariantCulture);
csc = CreateCSharpCompiler(null, dir.Path,
new[] {
"/nologo", "/t:library",
"/a:" + Assembly.GetExecutingAssembly().Location, "a.cs",
"/warn:0", "/ruleset:" + ruleSetFile.Path });
exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
// Diagnostic suppressed: commandline always overrides ruleset.
Assert.DoesNotContain("Warning01", outWriter.ToString(), StringComparison.Ordinal);
// Clean up temp files
CleanupAllGeneratedFiles(file.Path);
}
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30289")]
public void DiagnosticFormatting()
{
string source = @"
using System;
class C
{
public static void Main()
{
Goo(0);
#line 10 ""c:\temp\a\1.cs""
Goo(1);
#line 20 ""C:\a\..\b.cs""
Goo(2);
#line 30 ""C:\a\../B.cs""
Goo(3);
#line 40 ""../b.cs""
Goo(4);
#line 50 ""..\b.cs""
Goo(5);
#line 60 ""C:\X.cs""
Goo(6);
#line 70 ""C:\x.cs""
Goo(7);
#line 90 "" ""
Goo(9);
#line 100 ""C:\*.cs""
Goo(10);
#line 110 """"
Goo(11);
#line hidden
Goo(12);
#line default
Goo(13);
#line 140 ""***""
Goo(14);
}
}
";
var dir = Temp.CreateDirectory();
dir.CreateFile("a.cs").WriteAllText(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "/preferreduilang:en", "/t:library", "a.cs" });
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
// with /fullpaths off
string expected = @"
a.cs(8,13): error CS0103: The name 'Goo' does not exist in the current context
c:\temp\a\1.cs(10,13): error CS0103: The name 'Goo' does not exist in the current context
C:\b.cs(20,13): error CS0103: The name 'Goo' does not exist in the current context
C:\B.cs(30,13): error CS0103: The name 'Goo' does not exist in the current context
" + Path.GetFullPath(Path.Combine(dir.Path, @"..\b.cs")) + @"(40,13): error CS0103: The name 'Goo' does not exist in the current context
" + Path.GetFullPath(Path.Combine(dir.Path, @"..\b.cs")) + @"(50,13): error CS0103: The name 'Goo' does not exist in the current context
C:\X.cs(60,13): error CS0103: The name 'Goo' does not exist in the current context
C:\x.cs(70,13): error CS0103: The name 'Goo' does not exist in the current context
(90,7): error CS0103: The name 'Goo' does not exist in the current context
C:\*.cs(100,7): error CS0103: The name 'Goo' does not exist in the current context
(110,7): error CS0103: The name 'Goo' does not exist in the current context
(112,13): error CS0103: The name 'Goo' does not exist in the current context
a.cs(32,13): error CS0103: The name 'Goo' does not exist in the current context
***(140,13): error CS0103: The name 'Goo' does not exist in the current context";
AssertEx.Equal(
expected.Split(new[] { "\r\n", "\n" }, StringSplitOptions.RemoveEmptyEntries),
outWriter.ToString().Split(new[] { "\r\n", "\n" }, StringSplitOptions.RemoveEmptyEntries),
itemSeparator: "\r\n");
// with /fullpaths on
outWriter = new StringWriter(CultureInfo.InvariantCulture);
csc = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "/preferreduilang:en", "/t:library", "/fullpaths", "a.cs" });
exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
expected = @"
" + Path.Combine(dir.Path, @"a.cs") + @"(8,13): error CS0103: The name 'Goo' does not exist in the current context
c:\temp\a\1.cs(10,13): error CS0103: The name 'Goo' does not exist in the current context
C:\b.cs(20,13): error CS0103: The name 'Goo' does not exist in the current context
C:\B.cs(30,13): error CS0103: The name 'Goo' does not exist in the current context
" + Path.GetFullPath(Path.Combine(dir.Path, @"..\b.cs")) + @"(40,13): error CS0103: The name 'Goo' does not exist in the current context
" + Path.GetFullPath(Path.Combine(dir.Path, @"..\b.cs")) + @"(50,13): error CS0103: The name 'Goo' does not exist in the current context
C:\X.cs(60,13): error CS0103: The name 'Goo' does not exist in the current context
C:\x.cs(70,13): error CS0103: The name 'Goo' does not exist in the current context
(90,7): error CS0103: The name 'Goo' does not exist in the current context
C:\*.cs(100,7): error CS0103: The name 'Goo' does not exist in the current context
(110,7): error CS0103: The name 'Goo' does not exist in the current context
(112,13): error CS0103: The name 'Goo' does not exist in the current context
" + Path.Combine(dir.Path, @"a.cs") + @"(32,13): error CS0103: The name 'Goo' does not exist in the current context
***(140,13): error CS0103: The name 'Goo' does not exist in the current context";
AssertEx.Equal(
expected.Split(new[] { "\r\n", "\n" }, StringSplitOptions.RemoveEmptyEntries),
outWriter.ToString().Split(new[] { "\r\n", "\n" }, StringSplitOptions.RemoveEmptyEntries),
itemSeparator: "\r\n");
}
[WorkItem(540891, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/540891")]
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30289")]
public void ParseOut()
{
const string baseDirectory = @"C:\abc\def\baz";
var parsedArgs = DefaultParse(new[] { @"/out:""""", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2021: File name '' contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(""));
parsedArgs = DefaultParse(new[] { @"/out:", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2005: Missing file specification for '/out:' option
Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("/out:"));
parsedArgs = DefaultParse(new[] { @"/refout:", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2005: Missing file specification for '/refout:' option
Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("/refout:"));
parsedArgs = DefaultParse(new[] { @"/refout:ref.dll", "/refonly", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS8301: Do not use refout when using refonly.
Diagnostic(ErrorCode.ERR_NoRefOutWhenRefOnly).WithLocation(1, 1));
parsedArgs = DefaultParse(new[] { @"/refout:ref.dll", "/link:b", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new[] { "/refonly", "/link:b", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new[] { "/refonly:incorrect", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2007: Unrecognized option: '/refonly:incorrect'
Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/refonly:incorrect").WithLocation(1, 1)
);
parsedArgs = DefaultParse(new[] { @"/refout:ref.dll", "/target:module", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS8302: Cannot compile net modules when using /refout or /refonly.
Diagnostic(ErrorCode.ERR_NoNetModuleOutputWhenRefOutOrRefOnly).WithLocation(1, 1)
);
parsedArgs = DefaultParse(new[] { @"/refonly", "/target:module", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS8302: Cannot compile net modules when using /refout or /refonly.
Diagnostic(ErrorCode.ERR_NoNetModuleOutputWhenRefOutOrRefOnly).WithLocation(1, 1)
);
// Dev11 reports CS2007: Unrecognized option: '/out'
parsedArgs = DefaultParse(new[] { @"/out", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2005: Missing file specification for '/out' option
Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("/out"));
parsedArgs = DefaultParse(new[] { @"/out+", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/out+"));
// Should preserve fully qualified paths
parsedArgs = DefaultParse(new[] { @"/out:C:\MyFolder\MyBinary.dll", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal("MyBinary", parsedArgs.CompilationName);
Assert.Equal("MyBinary.dll", parsedArgs.OutputFileName);
Assert.Equal("MyBinary.dll", parsedArgs.CompilationOptions.ModuleName);
Assert.Equal(@"C:\MyFolder", parsedArgs.OutputDirectory);
Assert.Equal(@"C:\MyFolder\MyBinary.dll", parsedArgs.GetOutputFilePath(parsedArgs.OutputFileName));
// Should handle quotes
parsedArgs = DefaultParse(new[] { @"/out:""C:\My Folder\MyBinary.dll""", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"MyBinary", parsedArgs.CompilationName);
Assert.Equal("MyBinary.dll", parsedArgs.OutputFileName);
Assert.Equal("MyBinary.dll", parsedArgs.CompilationOptions.ModuleName);
Assert.Equal(@"C:\My Folder", parsedArgs.OutputDirectory);
// Should expand partially qualified paths
parsedArgs = DefaultParse(new[] { @"/out:MyBinary.dll", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal("MyBinary", parsedArgs.CompilationName);
Assert.Equal("MyBinary.dll", parsedArgs.OutputFileName);
Assert.Equal("MyBinary.dll", parsedArgs.CompilationOptions.ModuleName);
Assert.Equal(baseDirectory, parsedArgs.OutputDirectory);
Assert.Equal(Path.Combine(baseDirectory, "MyBinary.dll"), parsedArgs.GetOutputFilePath(parsedArgs.OutputFileName));
// Should expand partially qualified paths
parsedArgs = DefaultParse(new[] { @"/out:..\MyBinary.dll", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal("MyBinary", parsedArgs.CompilationName);
Assert.Equal("MyBinary.dll", parsedArgs.OutputFileName);
Assert.Equal("MyBinary.dll", parsedArgs.CompilationOptions.ModuleName);
Assert.Equal(@"C:\abc\def", parsedArgs.OutputDirectory);
// not specified: exe
parsedArgs = DefaultParse(new[] { @"a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Null(parsedArgs.CompilationName);
Assert.Null(parsedArgs.OutputFileName);
Assert.Null(parsedArgs.CompilationOptions.ModuleName);
Assert.Equal(baseDirectory, parsedArgs.OutputDirectory);
// not specified: dll
parsedArgs = DefaultParse(new[] { @"/target:library", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal("a", parsedArgs.CompilationName);
Assert.Equal("a.dll", parsedArgs.OutputFileName);
Assert.Equal("a.dll", parsedArgs.CompilationOptions.ModuleName);
Assert.Equal(baseDirectory, parsedArgs.OutputDirectory);
// not specified: module
parsedArgs = DefaultParse(new[] { @"/target:module", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Null(parsedArgs.CompilationName);
Assert.Equal("a.netmodule", parsedArgs.CompilationOptions.ModuleName);
Assert.Equal(baseDirectory, parsedArgs.OutputDirectory);
// not specified: appcontainerexe
parsedArgs = DefaultParse(new[] { @"/target:appcontainerexe", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Null(parsedArgs.CompilationName);
Assert.Null(parsedArgs.OutputFileName);
Assert.Null(parsedArgs.CompilationOptions.ModuleName);
Assert.Equal(baseDirectory, parsedArgs.OutputDirectory);
// not specified: winmdobj
parsedArgs = DefaultParse(new[] { @"/target:winmdobj", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal("a", parsedArgs.CompilationName);
Assert.Equal("a.winmdobj", parsedArgs.OutputFileName);
Assert.Equal("a.winmdobj", parsedArgs.CompilationOptions.ModuleName);
Assert.Equal(baseDirectory, parsedArgs.OutputDirectory);
// drive-relative path:
char currentDrive = Directory.GetCurrentDirectory()[0];
parsedArgs = DefaultParse(new[] { currentDrive + @":a.cs", "b.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2021: File name 'D:a.cs' is contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(currentDrive + ":a.cs"));
Assert.Null(parsedArgs.CompilationName);
Assert.Null(parsedArgs.OutputFileName);
Assert.Null(parsedArgs.CompilationOptions.ModuleName);
Assert.Equal(baseDirectory, parsedArgs.OutputDirectory);
// UNC
parsedArgs = DefaultParse(new[] { @"/out:\\b", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2021: File name '.x' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(@"\\b"));
Assert.Null(parsedArgs.OutputFileName);
Assert.Null(parsedArgs.CompilationName);
Assert.Null(parsedArgs.CompilationOptions.ModuleName);
parsedArgs = DefaultParse(new[] { @"/out:\\server\share\file.exe", "a.vb" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"\\server\share", parsedArgs.OutputDirectory);
Assert.Equal("file.exe", parsedArgs.OutputFileName);
Assert.Equal("file", parsedArgs.CompilationName);
Assert.Equal("file.exe", parsedArgs.CompilationOptions.ModuleName);
// invalid name:
parsedArgs = DefaultParse(new[] { "/out:a.b\0b", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2021: File name '.x' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments("a.b\0b"));
Assert.Null(parsedArgs.OutputFileName);
Assert.Null(parsedArgs.CompilationName);
Assert.Null(parsedArgs.CompilationOptions.ModuleName);
// Temporary skip following scenarios because of the error message changed (path)
//parsedArgs = DefaultParse(new[] { "/out:a\uD800b.dll", "a.cs" }, baseDirectory);
//parsedArgs.Errors.Verify(
// // error CS2021: File name '.x' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
// Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments("a\uD800b.dll"));
// Dev11 reports CS0016: Could not write to output file 'd:\Temp\q\a<>.z'
parsedArgs = DefaultParse(new[] { @"/out:""a<>.dll""", "a.vb" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2021: File name 'a<>.dll' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments("a<>.dll"));
Assert.Null(parsedArgs.OutputFileName);
Assert.Null(parsedArgs.CompilationName);
Assert.Null(parsedArgs.CompilationOptions.ModuleName);
parsedArgs = DefaultParse(new[] { @"/out:.exe", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2021: File name '.exe' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(".exe")
);
Assert.Null(parsedArgs.OutputFileName);
Assert.Null(parsedArgs.CompilationName);
Assert.Null(parsedArgs.CompilationOptions.ModuleName);
parsedArgs = DefaultParse(new[] { @"/t:exe", @"/out:.exe", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2021: File name '.exe' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(".exe")
);
Assert.Null(parsedArgs.OutputFileName);
Assert.Null(parsedArgs.CompilationName);
Assert.Null(parsedArgs.CompilationOptions.ModuleName);
parsedArgs = DefaultParse(new[] { @"/t:library", @"/out:.dll", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2021: File name '.dll' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(".dll")
);
Assert.Null(parsedArgs.OutputFileName);
Assert.Null(parsedArgs.CompilationName);
Assert.Null(parsedArgs.CompilationOptions.ModuleName);
parsedArgs = DefaultParse(new[] { @"/t:module", @"/out:.netmodule", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2021: File name '.netmodule' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(".netmodule")
);
Assert.Null(parsedArgs.OutputFileName);
Assert.Null(parsedArgs.CompilationName);
Assert.Null(parsedArgs.CompilationOptions.ModuleName);
parsedArgs = DefaultParse(new[] { ".cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Null(parsedArgs.OutputFileName);
Assert.Null(parsedArgs.CompilationName);
Assert.Null(parsedArgs.CompilationOptions.ModuleName);
parsedArgs = DefaultParse(new[] { @"/t:exe", ".cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Null(parsedArgs.OutputFileName);
Assert.Null(parsedArgs.CompilationName);
Assert.Null(parsedArgs.CompilationOptions.ModuleName);
parsedArgs = DefaultParse(new[] { @"/t:library", ".cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2021: File name '.dll' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(".dll")
);
Assert.Null(parsedArgs.OutputFileName);
Assert.Null(parsedArgs.CompilationName);
Assert.Null(parsedArgs.CompilationOptions.ModuleName);
parsedArgs = DefaultParse(new[] { @"/t:module", ".cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(".netmodule", parsedArgs.OutputFileName);
Assert.Null(parsedArgs.CompilationName);
Assert.Equal(".netmodule", parsedArgs.CompilationOptions.ModuleName);
}
[WorkItem(546012, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546012")]
[WorkItem(546007, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546007")]
[Fact]
public void ParseOut2()
{
var parsedArgs = DefaultParse(new[] { "/out:.x", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2021: File name '.x' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(".x"));
Assert.Null(parsedArgs.OutputFileName);
Assert.Null(parsedArgs.CompilationName);
Assert.Null(parsedArgs.CompilationOptions.ModuleName);
parsedArgs = DefaultParse(new[] { "/out:.x", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2021: File name '.x' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(".x"));
Assert.Null(parsedArgs.OutputFileName);
Assert.Null(parsedArgs.CompilationName);
Assert.Null(parsedArgs.CompilationOptions.ModuleName);
}
[Fact]
public void ParseInstrumentTestNames()
{
var parsedArgs = DefaultParse(SpecializedCollections.EmptyEnumerable<string>(), WorkingDirectory);
Assert.True(parsedArgs.EmitOptions.InstrumentationKinds.SequenceEqual(ImmutableArray<InstrumentationKind>.Empty));
parsedArgs = DefaultParse(new[] { @"/instrument", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for 'instrument' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "instrument"));
Assert.True(parsedArgs.EmitOptions.InstrumentationKinds.SequenceEqual(ImmutableArray<InstrumentationKind>.Empty));
parsedArgs = DefaultParse(new[] { @"/instrument:""""", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for 'instrument' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "instrument"));
Assert.True(parsedArgs.EmitOptions.InstrumentationKinds.SequenceEqual(ImmutableArray<InstrumentationKind>.Empty));
parsedArgs = DefaultParse(new[] { @"/instrument:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for 'instrument' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "instrument"));
Assert.True(parsedArgs.EmitOptions.InstrumentationKinds.SequenceEqual(ImmutableArray<InstrumentationKind>.Empty));
parsedArgs = DefaultParse(new[] { "/instrument:", "Test.Flag.Name", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for 'instrument' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "instrument"));
Assert.True(parsedArgs.EmitOptions.InstrumentationKinds.SequenceEqual(ImmutableArray<InstrumentationKind>.Empty));
parsedArgs = DefaultParse(new[] { "/instrument:InvalidOption", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
Diagnostic(ErrorCode.ERR_InvalidInstrumentationKind).WithArguments("InvalidOption"));
Assert.True(parsedArgs.EmitOptions.InstrumentationKinds.SequenceEqual(ImmutableArray<InstrumentationKind>.Empty));
parsedArgs = DefaultParse(new[] { "/instrument:None", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
Diagnostic(ErrorCode.ERR_InvalidInstrumentationKind).WithArguments("None"));
Assert.True(parsedArgs.EmitOptions.InstrumentationKinds.SequenceEqual(ImmutableArray<InstrumentationKind>.Empty));
parsedArgs = DefaultParse(new[] { "/instrument:TestCoverage,InvalidOption", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
Diagnostic(ErrorCode.ERR_InvalidInstrumentationKind).WithArguments("InvalidOption"));
Assert.True(parsedArgs.EmitOptions.InstrumentationKinds.SequenceEqual(ImmutableArray.Create(InstrumentationKind.TestCoverage)));
parsedArgs = DefaultParse(new[] { "/instrument:TestCoverage", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.EmitOptions.InstrumentationKinds.SequenceEqual(ImmutableArray.Create(InstrumentationKind.TestCoverage)));
parsedArgs = DefaultParse(new[] { @"/instrument:""TestCoverage""", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.EmitOptions.InstrumentationKinds.SequenceEqual(ImmutableArray.Create(InstrumentationKind.TestCoverage)));
parsedArgs = DefaultParse(new[] { @"/instrument:""TESTCOVERAGE""", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.EmitOptions.InstrumentationKinds.SequenceEqual(ImmutableArray.Create(InstrumentationKind.TestCoverage)));
parsedArgs = DefaultParse(new[] { "/instrument:TestCoverage,TestCoverage", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.EmitOptions.InstrumentationKinds.SequenceEqual(ImmutableArray.Create(InstrumentationKind.TestCoverage)));
parsedArgs = DefaultParse(new[] { "/instrument:TestCoverage", "/instrument:TestCoverage", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.EmitOptions.InstrumentationKinds.SequenceEqual(ImmutableArray.Create(InstrumentationKind.TestCoverage)));
}
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30289")]
public void ParseDoc()
{
const string baseDirectory = @"C:\abc\def\baz";
var parsedArgs = DefaultParse(new[] { @"/doc:""""", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for '/doc:' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "/doc:"));
Assert.Null(parsedArgs.DocumentationPath);
parsedArgs = DefaultParse(new[] { @"/doc:", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for '/doc:' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "/doc:"));
Assert.Null(parsedArgs.DocumentationPath);
// NOTE: no colon in error message '/doc'
parsedArgs = DefaultParse(new[] { @"/doc", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for '/doc' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "/doc"));
Assert.Null(parsedArgs.DocumentationPath);
parsedArgs = DefaultParse(new[] { @"/doc+", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/doc+"));
Assert.Null(parsedArgs.DocumentationPath);
// Should preserve fully qualified paths
parsedArgs = DefaultParse(new[] { @"/doc:C:\MyFolder\MyBinary.xml", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"C:\MyFolder\MyBinary.xml", parsedArgs.DocumentationPath);
Assert.Equal(DocumentationMode.Diagnose, parsedArgs.ParseOptions.DocumentationMode);
// Should handle quotes
parsedArgs = DefaultParse(new[] { @"/doc:""C:\My Folder\MyBinary.xml""", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"C:\My Folder\MyBinary.xml", parsedArgs.DocumentationPath);
Assert.Equal(DocumentationMode.Diagnose, parsedArgs.ParseOptions.DocumentationMode);
// Should expand partially qualified paths
parsedArgs = DefaultParse(new[] { @"/doc:MyBinary.xml", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(Path.Combine(baseDirectory, "MyBinary.xml"), parsedArgs.DocumentationPath);
Assert.Equal(DocumentationMode.Diagnose, parsedArgs.ParseOptions.DocumentationMode);
// Should expand partially qualified paths
parsedArgs = DefaultParse(new[] { @"/doc:..\MyBinary.xml", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"C:\abc\def\MyBinary.xml", parsedArgs.DocumentationPath);
Assert.Equal(DocumentationMode.Diagnose, parsedArgs.ParseOptions.DocumentationMode);
// drive-relative path:
char currentDrive = Directory.GetCurrentDirectory()[0];
parsedArgs = DefaultParse(new[] { "/doc:" + currentDrive + @":a.xml", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2021: File name 'D:a.xml' is contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(currentDrive + ":a.xml"));
Assert.Null(parsedArgs.DocumentationPath);
Assert.Equal(DocumentationMode.Diagnose, parsedArgs.ParseOptions.DocumentationMode); //Even though the format was incorrect
// UNC
parsedArgs = DefaultParse(new[] { @"/doc:\\b", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(@"\\b"));
Assert.Null(parsedArgs.DocumentationPath);
Assert.Equal(DocumentationMode.Diagnose, parsedArgs.ParseOptions.DocumentationMode); //Even though the format was incorrect
parsedArgs = DefaultParse(new[] { @"/doc:\\server\share\file.xml", "a.vb" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"\\server\share\file.xml", parsedArgs.DocumentationPath);
Assert.Equal(DocumentationMode.Diagnose, parsedArgs.ParseOptions.DocumentationMode);
// invalid name:
parsedArgs = DefaultParse(new[] { "/doc:a.b\0b", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments("a.b\0b"));
Assert.Null(parsedArgs.DocumentationPath);
Assert.Equal(DocumentationMode.Diagnose, parsedArgs.ParseOptions.DocumentationMode); //Even though the format was incorrect
// Temp
// parsedArgs = DefaultParse(new[] { "/doc:a\uD800b.xml", "a.cs" }, baseDirectory);
// parsedArgs.Errors.Verify(
// Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments("a\uD800b.xml"));
// Assert.Null(parsedArgs.DocumentationPath);
// Assert.Equal(DocumentationMode.Diagnose, parsedArgs.ParseOptions.DocumentationMode); //Even though the format was incorrect
parsedArgs = DefaultParse(new[] { @"/doc:""a<>.xml""", "a.vb" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2021: File name 'a<>.xml' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments("a<>.xml"));
Assert.Null(parsedArgs.DocumentationPath);
Assert.Equal(DocumentationMode.Diagnose, parsedArgs.ParseOptions.DocumentationMode); //Even though the format was incorrect
}
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30289")]
public void ParseErrorLog()
{
const string baseDirectory = @"C:\abc\def\baz";
var parsedArgs = DefaultParse(new[] { @"/errorlog:""""", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<(error log option format>' for '/errorlog:' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments(CSharpCommandLineParser.ErrorLogOptionFormat, "/errorlog:"));
Assert.Null(parsedArgs.ErrorLogOptions);
Assert.False(parsedArgs.CompilationOptions.ReportSuppressedDiagnostics);
parsedArgs = DefaultParse(new[] { @"/errorlog:", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<(error log option format>' for '/errorlog:' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments(CSharpCommandLineParser.ErrorLogOptionFormat, "/errorlog:"));
Assert.Null(parsedArgs.ErrorLogOptions);
Assert.False(parsedArgs.CompilationOptions.ReportSuppressedDiagnostics);
parsedArgs = DefaultParse(new[] { @"/errorlog", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<(error log option format>' for '/errorlog' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments(CSharpCommandLineParser.ErrorLogOptionFormat, "/errorlog"));
Assert.Null(parsedArgs.ErrorLogOptions);
Assert.False(parsedArgs.CompilationOptions.ReportSuppressedDiagnostics);
// Should preserve fully qualified paths
parsedArgs = DefaultParse(new[] { @"/errorlog:C:\MyFolder\MyBinary.xml", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"C:\MyFolder\MyBinary.xml", parsedArgs.ErrorLogOptions.Path);
Assert.True(parsedArgs.CompilationOptions.ReportSuppressedDiagnostics);
// Escaped quote in the middle is an error
parsedArgs = DefaultParse(new[] { @"/errorlog:C:\""My Folder""\MyBinary.xml", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(@"C:""My Folder\MyBinary.xml").WithLocation(1, 1));
// Should handle quotes
parsedArgs = DefaultParse(new[] { @"/errorlog:""C:\My Folder\MyBinary.xml""", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"C:\My Folder\MyBinary.xml", parsedArgs.ErrorLogOptions.Path);
Assert.True(parsedArgs.CompilationOptions.ReportSuppressedDiagnostics);
// Should expand partially qualified paths
parsedArgs = DefaultParse(new[] { @"/errorlog:MyBinary.xml", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(Path.Combine(baseDirectory, "MyBinary.xml"), parsedArgs.ErrorLogOptions.Path);
Assert.True(parsedArgs.CompilationOptions.ReportSuppressedDiagnostics);
// Should expand partially qualified paths
parsedArgs = DefaultParse(new[] { @"/errorlog:..\MyBinary.xml", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"C:\abc\def\MyBinary.xml", parsedArgs.ErrorLogOptions.Path);
Assert.True(parsedArgs.CompilationOptions.ReportSuppressedDiagnostics);
// drive-relative path:
char currentDrive = Directory.GetCurrentDirectory()[0];
parsedArgs = DefaultParse(new[] { "/errorlog:" + currentDrive + @":a.xml", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2021: File name 'D:a.xml' is contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(currentDrive + ":a.xml"));
Assert.Null(parsedArgs.ErrorLogOptions);
Assert.False(parsedArgs.CompilationOptions.ReportSuppressedDiagnostics);
// UNC
parsedArgs = DefaultParse(new[] { @"/errorlog:\\b", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(@"\\b"));
Assert.Null(parsedArgs.ErrorLogOptions);
Assert.False(parsedArgs.CompilationOptions.ReportSuppressedDiagnostics);
parsedArgs = DefaultParse(new[] { @"/errorlog:\\server\share\file.xml", "a.vb" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"\\server\share\file.xml", parsedArgs.ErrorLogOptions.Path);
// invalid name:
parsedArgs = DefaultParse(new[] { "/errorlog:a.b\0b", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments("a.b\0b"));
Assert.Null(parsedArgs.ErrorLogOptions);
Assert.False(parsedArgs.CompilationOptions.ReportSuppressedDiagnostics);
parsedArgs = DefaultParse(new[] { @"/errorlog:""a<>.xml""", "a.vb" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2021: File name 'a<>.xml' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments("a<>.xml"));
Assert.Null(parsedArgs.ErrorLogOptions);
Assert.False(parsedArgs.CompilationOptions.ReportSuppressedDiagnostics);
// Parses SARIF version.
parsedArgs = DefaultParse(new[] { @"/errorlog:C:\MyFolder\MyBinary.xml,version=2", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"C:\MyFolder\MyBinary.xml", parsedArgs.ErrorLogOptions.Path);
Assert.Equal(SarifVersion.Sarif2, parsedArgs.ErrorLogOptions.SarifVersion);
Assert.True(parsedArgs.CompilationOptions.ReportSuppressedDiagnostics);
// Invalid SARIF version.
string[] invalidSarifVersions = new string[] { @"C:\MyFolder\MyBinary.xml,version=1.0.0", @"C:\MyFolder\MyBinary.xml,version=2.1.0", @"C:\MyFolder\MyBinary.xml,version=42" };
foreach (string invalidSarifVersion in invalidSarifVersions)
{
parsedArgs = DefaultParse(new[] { $"/errorlog:{invalidSarifVersion}", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2046: Command-line syntax error: 'C:\MyFolder\MyBinary.xml,version=42' is not a valid value for the '/errorlog:' option. The value must be of the form '<file>[,version={1|1.0|2|2.1}]'.
Diagnostic(ErrorCode.ERR_BadSwitchValue).WithArguments(invalidSarifVersion, "/errorlog:", CSharpCommandLineParser.ErrorLogOptionFormat));
Assert.Null(parsedArgs.ErrorLogOptions);
Assert.False(parsedArgs.CompilationOptions.ReportSuppressedDiagnostics);
}
// Invalid errorlog qualifier.
const string InvalidErrorLogQualifier = @"C:\MyFolder\MyBinary.xml,invalid=42";
parsedArgs = DefaultParse(new[] { $"/errorlog:{InvalidErrorLogQualifier}", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2046: Command-line syntax error: 'C:\MyFolder\MyBinary.xml,invalid=42' is not a valid value for the '/errorlog:' option. The value must be of the form '<file>[,version={1|1.0|2|2.1}]'.
Diagnostic(ErrorCode.ERR_BadSwitchValue).WithArguments(InvalidErrorLogQualifier, "/errorlog:", CSharpCommandLineParser.ErrorLogOptionFormat));
Assert.Null(parsedArgs.ErrorLogOptions);
Assert.False(parsedArgs.CompilationOptions.ReportSuppressedDiagnostics);
// Too many errorlog qualifiers.
const string TooManyErrorLogQualifiers = @"C:\MyFolder\MyBinary.xml,version=2,version=2";
parsedArgs = DefaultParse(new[] { $"/errorlog:{TooManyErrorLogQualifiers}", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2046: Command-line syntax error: 'C:\MyFolder\MyBinary.xml,version=2,version=2' is not a valid value for the '/errorlog:' option. The value must be of the form '<file>[,version={1|1.0|2|2.1}]'.
Diagnostic(ErrorCode.ERR_BadSwitchValue).WithArguments(TooManyErrorLogQualifiers, "/errorlog:", CSharpCommandLineParser.ErrorLogOptionFormat));
Assert.Null(parsedArgs.ErrorLogOptions);
Assert.False(parsedArgs.CompilationOptions.ReportSuppressedDiagnostics);
}
[ConditionalFact(typeof(WindowsOnly))]
public void AppConfigParse()
{
const string baseDirectory = @"C:\abc\def\baz";
var parsedArgs = DefaultParse(new[] { @"/appconfig:""""", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing ':<text>' for '/appconfig:' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments(":<text>", "/appconfig:"));
Assert.Null(parsedArgs.AppConfigPath);
parsedArgs = DefaultParse(new[] { "/appconfig:", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing ':<text>' for '/appconfig:' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments(":<text>", "/appconfig:"));
Assert.Null(parsedArgs.AppConfigPath);
parsedArgs = DefaultParse(new[] { "/appconfig", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing ':<text>' for '/appconfig' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments(":<text>", "/appconfig"));
Assert.Null(parsedArgs.AppConfigPath);
parsedArgs = DefaultParse(new[] { "/appconfig:a.exe.config", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"C:\abc\def\baz\a.exe.config", parsedArgs.AppConfigPath);
// If ParseDoc succeeds, all other possible AppConfig paths should succeed as well -- they both call ParseGenericFilePath
}
[Fact]
public void AppConfigBasic()
{
var srcFile = Temp.CreateFile().WriteAllText(@"class A { static void Main(string[] args) { } }");
var srcDirectory = Path.GetDirectoryName(srcFile.Path);
var appConfigFile = Temp.CreateFile().WriteAllText(
@"<?xml version=""1.0"" encoding=""utf-8"" ?>
<configuration>
<runtime>
<assemblyBinding xmlns=""urn:schemas-microsoft-com:asm.v1"">
<supportPortability PKT=""7cec85d7bea7798e"" enable=""false""/>
</assemblyBinding>
</runtime>
</configuration>");
var silverlight = Temp.CreateFile().WriteAllBytes(ProprietaryTestResources.silverlight_v5_0_5_0.System_v5_0_5_0_silverlight).Path;
var net4_0dll = Temp.CreateFile().WriteAllBytes(ResourcesNet451.System).Path;
// Test linking two appconfig dlls with simple src
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = CreateCSharpCompiler(null, srcDirectory,
new[] { "/nologo",
"/r:" + silverlight,
"/r:" + net4_0dll,
"/appconfig:" + appConfigFile.Path,
srcFile.Path }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("", outWriter.ToString().Trim());
CleanupAllGeneratedFiles(srcFile.Path);
CleanupAllGeneratedFiles(appConfigFile.Path);
}
[ConditionalFact(typeof(WindowsOnly))]
public void AppConfigBasicFail()
{
var srcFile = Temp.CreateFile().WriteAllText(@"class A { static void Main(string[] args) { } }");
var srcDirectory = Path.GetDirectoryName(srcFile.Path);
string root = Path.GetPathRoot(srcDirectory); // Make sure we pick a drive that exists and is plugged in to avoid 'Drive not ready'
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = CreateCSharpCompiler(null, srcDirectory,
new[] { "/nologo", "/preferreduilang:en",
$@"/appconfig:{root}DoesNotExist\NOwhere\bonobo.exe.config" ,
srcFile.Path }).Run(outWriter);
Assert.NotEqual(0, exitCode);
Assert.Equal($@"error CS7093: Cannot read config file '{root}DoesNotExist\NOwhere\bonobo.exe.config' -- 'Could not find a part of the path '{root}DoesNotExist\NOwhere\bonobo.exe.config'.'", outWriter.ToString().Trim());
CleanupAllGeneratedFiles(srcFile.Path);
}
[ConditionalFact(typeof(WindowsOnly))]
public void ParseDocAndOut()
{
const string baseDirectory = @"C:\abc\def\baz";
// Can specify separate directories for binary and XML output.
var parsedArgs = DefaultParse(new[] { @"/doc:a\b.xml", @"/out:c\d.exe", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"C:\abc\def\baz\a\b.xml", parsedArgs.DocumentationPath);
Assert.Equal(@"C:\abc\def\baz\c", parsedArgs.OutputDirectory);
Assert.Equal("d.exe", parsedArgs.OutputFileName);
// XML does not fall back on output directory.
parsedArgs = DefaultParse(new[] { @"/doc:b.xml", @"/out:c\d.exe", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"C:\abc\def\baz\b.xml", parsedArgs.DocumentationPath);
Assert.Equal(@"C:\abc\def\baz\c", parsedArgs.OutputDirectory);
Assert.Equal("d.exe", parsedArgs.OutputFileName);
}
[ConditionalFact(typeof(WindowsOnly))]
public void ParseErrorLogAndOut()
{
const string baseDirectory = @"C:\abc\def\baz";
// Can specify separate directories for binary and error log output.
var parsedArgs = DefaultParse(new[] { @"/errorlog:a\b.xml", @"/out:c\d.exe", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"C:\abc\def\baz\a\b.xml", parsedArgs.ErrorLogOptions.Path);
Assert.Equal(@"C:\abc\def\baz\c", parsedArgs.OutputDirectory);
Assert.Equal("d.exe", parsedArgs.OutputFileName);
// XML does not fall back on output directory.
parsedArgs = DefaultParse(new[] { @"/errorlog:b.xml", @"/out:c\d.exe", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(@"C:\abc\def\baz\b.xml", parsedArgs.ErrorLogOptions.Path);
Assert.Equal(@"C:\abc\def\baz\c", parsedArgs.OutputDirectory);
Assert.Equal("d.exe", parsedArgs.OutputFileName);
}
[Fact]
public void ModuleAssemblyName()
{
var parsedArgs = DefaultParse(new[] { @"/target:module", "/moduleassemblyname:goo", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal("goo", parsedArgs.CompilationName);
Assert.Equal("a.netmodule", parsedArgs.CompilationOptions.ModuleName);
parsedArgs = DefaultParse(new[] { @"/target:library", "/moduleassemblyname:goo", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS0734: The /moduleassemblyname option may only be specified when building a target type of 'module'
Diagnostic(ErrorCode.ERR_AssemblyNameOnNonModule));
parsedArgs = DefaultParse(new[] { @"/target:exe", "/moduleassemblyname:goo", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS0734: The /moduleassemblyname option may only be specified when building a target type of 'module'
Diagnostic(ErrorCode.ERR_AssemblyNameOnNonModule));
parsedArgs = DefaultParse(new[] { @"/target:winexe", "/moduleassemblyname:goo", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS0734: The /moduleassemblyname option may only be specified when building a target type of 'module'
Diagnostic(ErrorCode.ERR_AssemblyNameOnNonModule));
}
[Fact]
public void ModuleName()
{
var parsedArgs = DefaultParse(new[] { @"/target:module", "/modulename:goo", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal("goo", parsedArgs.CompilationOptions.ModuleName);
parsedArgs = DefaultParse(new[] { @"/target:library", "/modulename:bar", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal("bar", parsedArgs.CompilationOptions.ModuleName);
parsedArgs = DefaultParse(new[] { @"/target:exe", "/modulename:CommonLanguageRuntimeLibrary", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal("CommonLanguageRuntimeLibrary", parsedArgs.CompilationOptions.ModuleName);
parsedArgs = DefaultParse(new[] { @"/target:winexe", "/modulename:goo", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal("goo", parsedArgs.CompilationOptions.ModuleName);
parsedArgs = DefaultParse(new[] { @"/target:exe", "/modulename:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for 'modulename' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "modulename").WithLocation(1, 1)
);
}
[Fact]
public void ModuleName001()
{
var dir = Temp.CreateDirectory();
var file1 = dir.CreateFile("a.cs");
file1.WriteAllText(@"
class c1
{
public static void Main(){}
}
");
var exeName = "aa.exe";
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { "/modulename:hocusPocus ", "/out:" + exeName + " ", file1.Path });
int exitCode = csc.Run(outWriter);
if (exitCode != 0)
{
Console.WriteLine(outWriter.ToString());
Assert.Equal(0, exitCode);
}
Assert.Equal(1, Directory.EnumerateFiles(dir.Path, exeName).Count());
using (var metadata = ModuleMetadata.CreateFromImage(File.ReadAllBytes(Path.Combine(dir.Path, "aa.exe"))))
{
var peReader = metadata.Module.GetMetadataReader();
Assert.True(peReader.IsAssembly);
Assert.Equal("aa", peReader.GetString(peReader.GetAssemblyDefinition().Name));
Assert.Equal("hocusPocus", peReader.GetString(peReader.GetModuleDefinition().Name));
}
if (System.IO.File.Exists(exeName))
{
System.IO.File.Delete(exeName);
}
CleanupAllGeneratedFiles(file1.Path);
}
[Fact]
public void ParsePlatform()
{
var parsedArgs = DefaultParse(new[] { @"/platform:x64", "a.cs" }, WorkingDirectory);
Assert.False(parsedArgs.Errors.Any());
Assert.Equal(Platform.X64, parsedArgs.CompilationOptions.Platform);
parsedArgs = DefaultParse(new[] { @"/platform:X86", "a.cs" }, WorkingDirectory);
Assert.False(parsedArgs.Errors.Any());
Assert.Equal(Platform.X86, parsedArgs.CompilationOptions.Platform);
parsedArgs = DefaultParse(new[] { @"/platform:itanum", "a.cs" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal((int)ErrorCode.ERR_BadPlatformType, parsedArgs.Errors.First().Code);
Assert.Equal(Platform.AnyCpu, parsedArgs.CompilationOptions.Platform);
parsedArgs = DefaultParse(new[] { "/platform:itanium", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(Platform.Itanium, parsedArgs.CompilationOptions.Platform);
parsedArgs = DefaultParse(new[] { "/platform:anycpu", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(Platform.AnyCpu, parsedArgs.CompilationOptions.Platform);
parsedArgs = DefaultParse(new[] { "/platform:anycpu32bitpreferred", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(Platform.AnyCpu32BitPreferred, parsedArgs.CompilationOptions.Platform);
parsedArgs = DefaultParse(new[] { "/platform:arm", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(Platform.Arm, parsedArgs.CompilationOptions.Platform);
parsedArgs = DefaultParse(new[] { "/platform", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<string>' for 'platform' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<string>", "/platform"));
Assert.Equal(Platform.AnyCpu, parsedArgs.CompilationOptions.Platform); //anycpu is default
parsedArgs = DefaultParse(new[] { "/platform:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<string>' for 'platform' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<string>", "/platform:"));
Assert.Equal(Platform.AnyCpu, parsedArgs.CompilationOptions.Platform); //anycpu is default
}
[WorkItem(546016, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546016")]
[WorkItem(545997, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/545997")]
[WorkItem(546019, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546019")]
[WorkItem(546029, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546029")]
[Fact]
public void ParseBaseAddress()
{
var parsedArgs = DefaultParse(new[] { @"/baseaddress:x64", "a.cs" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal((int)ErrorCode.ERR_BadBaseNumber, parsedArgs.Errors.First().Code);
parsedArgs = DefaultParse(new[] { @"/platform:x64", @"/baseaddress:0x8000000000011111", "a.cs" }, WorkingDirectory);
Assert.False(parsedArgs.Errors.Any());
Assert.Equal(0x8000000000011111ul, parsedArgs.EmitOptions.BaseAddress);
parsedArgs = DefaultParse(new[] { @"/platform:x86", @"/baseaddress:0x8000000000011111", "a.cs" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal((int)ErrorCode.ERR_BadBaseNumber, parsedArgs.Errors.First().Code);
parsedArgs = DefaultParse(new[] { @"/baseaddress:", "a.cs" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal((int)ErrorCode.ERR_SwitchNeedsNumber, parsedArgs.Errors.First().Code);
parsedArgs = DefaultParse(new[] { @"/baseaddress:-23", "a.cs" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal((int)ErrorCode.ERR_BadBaseNumber, parsedArgs.Errors.First().Code);
parsedArgs = DefaultParse(new[] { @"/platform:x64", @"/baseaddress:01777777777777777777777", "a.cs" }, WorkingDirectory);
Assert.Equal(ulong.MaxValue, parsedArgs.EmitOptions.BaseAddress);
parsedArgs = DefaultParse(new[] { @"/platform:x64", @"/baseaddress:0x0000000100000000", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new[] { @"/platform:x64", @"/baseaddress:0xffff8000", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new[] { "test.cs", "/platform:x86", "/baseaddress:0xffffffff" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadBaseNumber).WithArguments("0xFFFFFFFF"));
parsedArgs = DefaultParse(new[] { "test.cs", "/platform:x86", "/baseaddress:0xffff8000" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadBaseNumber).WithArguments("0xFFFF8000"));
parsedArgs = DefaultParse(new[] { "test.cs", "/baseaddress:0xffff8000" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadBaseNumber).WithArguments("0xFFFF8000"));
parsedArgs = DefaultParse(new[] { "C:\\test.cs", "/platform:x86", "/baseaddress:0xffff7fff" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new[] { "C:\\test.cs", "/platform:x64", "/baseaddress:0xffff8000" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new[] { "C:\\test.cs", "/platform:x64", "/baseaddress:0x100000000" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new[] { "test.cs", "/baseaddress:0xFFFF0000FFFF0000" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadBaseNumber).WithArguments("0xFFFF0000FFFF0000"));
parsedArgs = DefaultParse(new[] { "C:\\test.cs", "/platform:x64", "/baseaddress:0x10000000000000000" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadBaseNumber).WithArguments("0x10000000000000000"));
parsedArgs = DefaultParse(new[] { "C:\\test.cs", "/baseaddress:0xFFFF0000FFFF0000" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadBaseNumber).WithArguments("0xFFFF0000FFFF0000"));
}
[Fact]
public void ParseFileAlignment()
{
var parsedArgs = DefaultParse(new[] { @"/filealign:x64", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2024: Invalid file section alignment number 'x64'
Diagnostic(ErrorCode.ERR_InvalidFileAlignment).WithArguments("x64"));
parsedArgs = DefaultParse(new[] { @"/filealign:0x200", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(0x200, parsedArgs.EmitOptions.FileAlignment);
parsedArgs = DefaultParse(new[] { @"/filealign:512", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(512, parsedArgs.EmitOptions.FileAlignment);
parsedArgs = DefaultParse(new[] { @"/filealign:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2035: Command-line syntax error: Missing ':<number>' for 'filealign' option
Diagnostic(ErrorCode.ERR_SwitchNeedsNumber).WithArguments("filealign"));
parsedArgs = DefaultParse(new[] { @"/filealign:-23", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2024: Invalid file section alignment number '-23'
Diagnostic(ErrorCode.ERR_InvalidFileAlignment).WithArguments("-23"));
parsedArgs = DefaultParse(new[] { @"/filealign:020000", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(8192, parsedArgs.EmitOptions.FileAlignment);
parsedArgs = DefaultParse(new[] { @"/filealign:0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2024: Invalid file section alignment number '0'
Diagnostic(ErrorCode.ERR_InvalidFileAlignment).WithArguments("0"));
parsedArgs = DefaultParse(new[] { @"/filealign:123", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2024: Invalid file section alignment number '123'
Diagnostic(ErrorCode.ERR_InvalidFileAlignment).WithArguments("123"));
}
[ConditionalFact(typeof(WindowsOnly))]
public void SdkPathAndLibEnvVariable()
{
var dir = Temp.CreateDirectory();
var lib1 = dir.CreateDirectory("lib1");
var lib2 = dir.CreateDirectory("lib2");
var lib3 = dir.CreateDirectory("lib3");
var sdkDirectory = SdkDirectory;
var parsedArgs = DefaultParse(new[] { @"/lib:lib1", @"/libpath:lib2", @"/libpaths:lib3", "a.cs" }, dir.Path, sdkDirectory: sdkDirectory);
AssertEx.Equal(new[]
{
sdkDirectory,
lib1.Path,
lib2.Path,
lib3.Path
}, parsedArgs.ReferencePaths);
}
[ConditionalFact(typeof(WindowsOnly))]
public void SdkPathAndLibEnvVariable_Errors()
{
var parsedArgs = DefaultParse(new[] { @"/lib:c:lib2", @"/lib:o:\sdk1", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// warning CS1668: Invalid search path 'c:lib2' specified in '/LIB option' -- 'path is too long or invalid'
Diagnostic(ErrorCode.WRN_InvalidSearchPathDir).WithArguments(@"c:lib2", "/LIB option", "path is too long or invalid"),
// warning CS1668: Invalid search path 'o:\sdk1' specified in '/LIB option' -- 'directory does not exist'
Diagnostic(ErrorCode.WRN_InvalidSearchPathDir).WithArguments(@"o:\sdk1", "/LIB option", "directory does not exist"));
parsedArgs = DefaultParse(new[] { @"/lib:c:\Windows,o:\Windows;e:;", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// warning CS1668: Invalid search path 'o:\Windows' specified in '/LIB option' -- 'directory does not exist'
Diagnostic(ErrorCode.WRN_InvalidSearchPathDir).WithArguments(@"o:\Windows", "/LIB option", "directory does not exist"),
// warning CS1668: Invalid search path 'e:' specified in '/LIB option' -- 'path is too long or invalid'
Diagnostic(ErrorCode.WRN_InvalidSearchPathDir).WithArguments(@"e:", "/LIB option", "path is too long or invalid"));
parsedArgs = DefaultParse(new[] { @"/lib:c:\Windows,.\Windows;e;", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// warning CS1668: Invalid search path '.\Windows' specified in '/LIB option' -- 'directory does not exist'
Diagnostic(ErrorCode.WRN_InvalidSearchPathDir).WithArguments(@".\Windows", "/LIB option", "directory does not exist"),
// warning CS1668: Invalid search path 'e' specified in '/LIB option' -- 'directory does not exist'
Diagnostic(ErrorCode.WRN_InvalidSearchPathDir).WithArguments(@"e", "/LIB option", "directory does not exist"));
parsedArgs = DefaultParse(new[] { @"/lib:c:\Windows,o:\Windows;e:; ; ; ; ", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// warning CS1668: Invalid search path 'o:\Windows' specified in '/LIB option' -- 'directory does not exist'
Diagnostic(ErrorCode.WRN_InvalidSearchPathDir).WithArguments(@"o:\Windows", "/LIB option", "directory does not exist"),
// warning CS1668: Invalid search path 'e:' specified in '/LIB option' -- 'path is too long or invalid'
Diagnostic(ErrorCode.WRN_InvalidSearchPathDir).WithArguments("e:", "/LIB option", "path is too long or invalid"),
// warning CS1668: Invalid search path ' ' specified in '/LIB option' -- 'path is too long or invalid'
Diagnostic(ErrorCode.WRN_InvalidSearchPathDir).WithArguments(" ", "/LIB option", "path is too long or invalid"),
// warning CS1668: Invalid search path ' ' specified in '/LIB option' -- 'path is too long or invalid'
Diagnostic(ErrorCode.WRN_InvalidSearchPathDir).WithArguments(" ", "/LIB option", "path is too long or invalid"),
// warning CS1668: Invalid search path ' ' specified in '/LIB option' -- 'path is too long or invalid'
Diagnostic(ErrorCode.WRN_InvalidSearchPathDir).WithArguments(" ", "/LIB option", "path is too long or invalid"));
parsedArgs = DefaultParse(new[] { @"/lib", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<path list>", "lib"));
parsedArgs = DefaultParse(new[] { @"/lib:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<path list>", "lib"));
parsedArgs = DefaultParse(new[] { @"/lib+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/lib+"));
parsedArgs = DefaultParse(new[] { @"/lib: ", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<path list>", "lib"));
}
[Fact, WorkItem(546005, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546005")]
public void SdkPathAndLibEnvVariable_Relative_csc()
{
var tempFolder = Temp.CreateDirectory();
var baseDirectory = tempFolder.ToString();
var subFolder = tempFolder.CreateDirectory("temp");
var subDirectory = subFolder.ToString();
var src = Temp.CreateFile("a.cs");
src.WriteAllText("public class C{}");
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, subDirectory, new[] { "/nologo", "/t:library", "/out:abc.xyz", src.ToString() }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("", outWriter.ToString().Trim());
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, baseDirectory, new[] { "/nologo", "/lib:temp", "/r:abc.xyz", "/t:library", src.ToString() }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("", outWriter.ToString().Trim());
CleanupAllGeneratedFiles(src.Path);
}
[Fact]
public void UnableWriteOutput()
{
var tempFolder = Temp.CreateDirectory();
var baseDirectory = tempFolder.ToString();
var subFolder = tempFolder.CreateDirectory("temp");
var src = Temp.CreateFile("a.cs");
src.WriteAllText("public class C{}");
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, baseDirectory, new[] { "/nologo", "/preferreduilang:en", "/t:library", "/out:" + subFolder.ToString(), src.ToString() }).Run(outWriter);
Assert.Equal(1, exitCode);
Assert.True(outWriter.ToString().Trim().StartsWith("error CS2012: Cannot open '" + subFolder.ToString() + "' for writing -- '", StringComparison.Ordinal)); // Cannot create a file when that file already exists.
CleanupAllGeneratedFiles(src.Path);
}
[Fact]
public void ParseHighEntropyVA()
{
var parsedArgs = DefaultParse(new[] { @"/highentropyva", "a.cs" }, WorkingDirectory);
Assert.False(parsedArgs.Errors.Any());
Assert.True(parsedArgs.EmitOptions.HighEntropyVirtualAddressSpace);
parsedArgs = DefaultParse(new[] { @"/highentropyva+", "a.cs" }, WorkingDirectory);
Assert.False(parsedArgs.Errors.Any());
Assert.True(parsedArgs.EmitOptions.HighEntropyVirtualAddressSpace);
parsedArgs = DefaultParse(new[] { @"/highentropyva-", "a.cs" }, WorkingDirectory);
Assert.False(parsedArgs.Errors.Any());
Assert.False(parsedArgs.EmitOptions.HighEntropyVirtualAddressSpace);
parsedArgs = DefaultParse(new[] { @"/highentropyva:-", "a.cs" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal(EmitOptions.Default.HighEntropyVirtualAddressSpace, parsedArgs.EmitOptions.HighEntropyVirtualAddressSpace);
parsedArgs = DefaultParse(new[] { @"/highentropyva:", "a.cs" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal(EmitOptions.Default.HighEntropyVirtualAddressSpace, parsedArgs.EmitOptions.HighEntropyVirtualAddressSpace);
//last one wins
parsedArgs = DefaultParse(new[] { @"/highenTROPyva+", @"/HIGHentropyva-", "a.cs" }, WorkingDirectory);
Assert.False(parsedArgs.Errors.Any());
Assert.False(parsedArgs.EmitOptions.HighEntropyVirtualAddressSpace);
}
[Fact]
public void Checked()
{
var parsedArgs = DefaultParse(new[] { @"/checked+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.CompilationOptions.CheckOverflow);
parsedArgs = DefaultParse(new[] { @"/checked-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.CheckOverflow);
parsedArgs = DefaultParse(new[] { @"/checked", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.CompilationOptions.CheckOverflow);
parsedArgs = DefaultParse(new[] { @"/checked-", @"/checked", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.CompilationOptions.CheckOverflow);
parsedArgs = DefaultParse(new[] { @"/checked:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/checked:"));
}
[Fact]
public void Nullable()
{
var parsedArgs = DefaultParse(new[] { "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable+", "/langversion:7.0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8630: Invalid 'nullable' value: 'Enabled' for C# 7.0. Please use language version '8.0' or greater.
Diagnostic(ErrorCode.ERR_NullableOptionNotAvailable).WithArguments("nullable", "Enable", "7.0", "8.0").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for 'nullable' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "nullable").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:yes", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'yes' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("yes").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:enable", "/langversion:7.0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8630: Invalid 'nullable' value: 'Enable' for C# 7.0. Please use language version '8.0' or greater.
Diagnostic(ErrorCode.ERR_NullableOptionNotAvailable).WithArguments("nullable", "Enable", "7.0", "8.0").WithLocation(1, 1));
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:disable", "/langversion:7.0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:safeonly", "/langversion:7.0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'safeonly' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("safeonly").WithLocation(1, 1));
parsedArgs = DefaultParse(new[] { @"/nullable+", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable-", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for 'nullable' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "nullable").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:yes", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'yes' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("yes").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:eNable", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:disablE", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Safeonly", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'Safeonly' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("Safeonly").WithLocation(1, 1)
);
parsedArgs = DefaultParse(new[] { @"/nullable-", @"/nullable-", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable-", @"/nullable", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable-", @"/nullable+", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable-", @"/nullable:", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for 'nullable' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "nullable").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable-", @"/nullable:YES", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'YES' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("YES").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable-", @"/nullable:disable", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable-", @"/nullable:enable", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable-", @"/nullable:safeonly", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'safeonly' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("safeonly").WithLocation(1, 1)
);
parsedArgs = DefaultParse(new[] { @"/nullable+", @"/nullable-", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable+", @"/nullable", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable+", @"/nullable+", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable+", @"/nullable:", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for 'nullable' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "nullable").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable+", @"/nullable:YES", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'YES' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("YES").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable+", @"/nullable:disable", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable+", @"/nullable:enable", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable+", @"/nullable:safeonly", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'safeonly' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("safeonly").WithLocation(1, 1)
);
parsedArgs = DefaultParse(new[] { @"/nullable:safeonly", @"/nullable-", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'safeonly' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("safeonly").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:safeonly", @"/nullable", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'safeonly' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("safeonly").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:safeonly", @"/nullable+", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'safeonly' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("safeonly").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:safeonly", @"/nullable:", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'safeonly' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("safeonly").WithLocation(1, 1),
// error CS2006: Command-line syntax error: Missing '<text>' for 'nullable' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "nullable").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:safeonly", @"/nullable:YES", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'safeonly' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("safeonly").WithLocation(1, 1),
// error CS8636: Invalid option 'YES' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("YES").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:safeonly", @"/nullable:disable", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'safeonly' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("safeonly").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:safeonly", @"/nullable:enable", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'safeonly' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("safeonly").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:safeonly", @"/nullable:safeonly", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'safeonly' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("safeonly").WithLocation(1, 1),
// error CS8636: Invalid option 'safeonly' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("safeonly").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:", "/langversion:7.3", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for 'nullable' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "nullable").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:yeS", "/langversion:7.0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'yeS' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("yeS").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable+", "/langversion:7.3", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8630: Invalid 'nullable' value: 'Enable' for C# 7.3. Please use language version '8.0' or greater.
Diagnostic(ErrorCode.ERR_NullableOptionNotAvailable).WithArguments("nullable", "Enable", "7.3", "8.0").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable-", "/langversion:7.0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable", "/langversion:7.3", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8630: Invalid 'nullable' value: 'Enabled' for C# 7.3. Please use language version '8.0' or greater.
Diagnostic(ErrorCode.ERR_NullableOptionNotAvailable).WithArguments("nullable", "Enable", "7.3", "8.0").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:enable", "/langversion:7.3", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8630: Invalid 'nullable' value: 'Enabled' for C# 7.3. Please use language version '8.0' or greater.
Diagnostic(ErrorCode.ERR_NullableOptionNotAvailable).WithArguments("nullable", "Enable", "7.3", "8.0").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:disable", "/langversion:7.0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:safeonly", "/langversion:7.3", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'safeonly' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("safeonly").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { "a.cs", "/langversion:8" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { "a.cs", "/langversion:7.3" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:""safeonly""", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'safeonly' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("safeonly").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:\""enable\""", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option '"enable"' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("\"enable\"").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:\\disable\\", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option '\\disable\\' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("\\\\disable\\\\").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:\\""enable\\""", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option '\enable\' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("\\enable\\").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:safeonlywarnings", "/langversion:7.0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'safeonlywarnings' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("safeonlywarnings").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:SafeonlyWarnings", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'SafeonlyWarnings' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("SafeonlyWarnings").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable-", @"/nullable:safeonlyWarnings", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'safeonlyWarnings' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("safeonlyWarnings").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:warnings", "/langversion:7.0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8630: Invalid 'nullable' value: 'Warnings' for C# 7.0. Please use language version '8.0' or greater.
Diagnostic(ErrorCode.ERR_NullableOptionNotAvailable).WithArguments("nullable", "Warnings", "7.0", "8.0").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Warnings, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Warnings", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Warnings, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable-", @"/nullable:Warnings", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Warnings, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable+", @"/nullable:Warnings", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Warnings, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Warnings", @"/nullable-", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Warnings", @"/nullable", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Warnings", @"/nullable+", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Warnings", @"/nullable:", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for 'nullable' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "nullable").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Warnings, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Warnings", @"/nullable:YES", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'YES' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("YES").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Warnings, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Warnings", @"/nullable:disable", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Warnings", @"/nullable:enable", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Warnings", @"/nullable:Warnings", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Warnings, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Warnings", "/langversion:7.3", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8630: Invalid 'nullable' value: 'Annotations' for C# 7.3. Please use language version '8.0' or greater.
Diagnostic(ErrorCode.ERR_NullableOptionNotAvailable).WithArguments("nullable", "Warnings", "7.3", "8.0").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Warnings, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:annotations", "/langversion:7.0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8630: Invalid 'nullable' value: 'Annotations' for C# 7.0. Please use language version '8.0' or greater.
Diagnostic(ErrorCode.ERR_NullableOptionNotAvailable).WithArguments("nullable", "Annotations", "7.0", "8.0").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Annotations, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Annotations", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Annotations, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable-", @"/nullable:Annotations", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Annotations, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable+", @"/nullable:Annotations", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Annotations, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Annotations", @"/nullable-", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Annotations", @"/nullable", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Annotations", @"/nullable+", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Annotations", @"/nullable:", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for 'nullable' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "nullable").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Annotations, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Annotations", @"/nullable:YES", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8636: Invalid option 'YES' for /nullable; must be 'disable', 'enable', 'warnings' or 'annotations'
Diagnostic(ErrorCode.ERR_BadNullableContextOption).WithArguments("YES").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Annotations, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Annotations", @"/nullable:disable", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Disable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Annotations", @"/nullable:enable", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Enable, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Annotations", @"/nullable:Annotations", "/langversion:8", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(NullableContextOptions.Annotations, parsedArgs.CompilationOptions.NullableContextOptions);
parsedArgs = DefaultParse(new[] { @"/nullable:Annotations", "/langversion:7.3", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS8630: Invalid 'nullable' value: 'Annotations' for C# 7.3. Please use language version '8.0' or greater.
Diagnostic(ErrorCode.ERR_NullableOptionNotAvailable).WithArguments("nullable", "Annotations", "7.3", "8.0").WithLocation(1, 1)
);
Assert.Equal(NullableContextOptions.Annotations, parsedArgs.CompilationOptions.NullableContextOptions);
}
[Fact]
public void Usings()
{
CSharpCommandLineArguments parsedArgs;
var sdkDirectory = SdkDirectory;
parsedArgs = CSharpCommandLineParser.Script.Parse(new string[] { "/u:Goo.Bar" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify();
AssertEx.Equal(new[] { "Goo.Bar" }, parsedArgs.CompilationOptions.Usings.AsEnumerable());
parsedArgs = CSharpCommandLineParser.Script.Parse(new string[] { "/u:Goo.Bar;Baz", "/using:System.Core;System" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify();
AssertEx.Equal(new[] { "Goo.Bar", "Baz", "System.Core", "System" }, parsedArgs.CompilationOptions.Usings.AsEnumerable());
parsedArgs = CSharpCommandLineParser.Script.Parse(new string[] { "/u:Goo;;Bar" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify();
AssertEx.Equal(new[] { "Goo", "Bar" }, parsedArgs.CompilationOptions.Usings.AsEnumerable());
parsedArgs = CSharpCommandLineParser.Script.Parse(new string[] { "/u:" }, WorkingDirectory, sdkDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<namespace>' for '/u:' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<namespace>", "/u:"));
}
[Fact]
public void WarningsErrors()
{
var parsedArgs = DefaultParse(new string[] { "/nowarn", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2035: Command-line syntax error: Missing ':<number>' for 'nowarn' option
Diagnostic(ErrorCode.ERR_SwitchNeedsNumber).WithArguments("nowarn"));
parsedArgs = DefaultParse(new string[] { "/nowarn:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2035: Command-line syntax error: Missing ':<number>' for 'nowarn' option
Diagnostic(ErrorCode.ERR_SwitchNeedsNumber).WithArguments("nowarn"));
// Previous versions of the compiler used to report a warning (CS1691)
// whenever an unrecognized warning code was supplied via /nowarn or /warnaserror.
// We no longer generate a warning in such cases.
parsedArgs = DefaultParse(new string[] { "/nowarn:-1", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new string[] { "/nowarn:abc", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new string[] { "/warnaserror:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2035: Command-line syntax error: Missing ':<number>' for 'warnaserror' option
Diagnostic(ErrorCode.ERR_SwitchNeedsNumber).WithArguments("warnaserror"));
parsedArgs = DefaultParse(new string[] { "/warnaserror:-1", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new string[] { "/warnaserror:70000", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new string[] { "/warnaserror:abc", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new string[] { "/warnaserror+:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2035: Command-line syntax error: Missing ':<number>' for '/warnaserror+:' option
Diagnostic(ErrorCode.ERR_SwitchNeedsNumber).WithArguments("warnaserror+"));
parsedArgs = DefaultParse(new string[] { "/warnaserror-:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2035: Command-line syntax error: Missing ':<number>' for '/warnaserror-:' option
Diagnostic(ErrorCode.ERR_SwitchNeedsNumber).WithArguments("warnaserror-"));
parsedArgs = DefaultParse(new string[] { "/w", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2035: Command-line syntax error: Missing ':<number>' for '/w' option
Diagnostic(ErrorCode.ERR_SwitchNeedsNumber).WithArguments("w"));
parsedArgs = DefaultParse(new string[] { "/w:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2035: Command-line syntax error: Missing ':<number>' for '/w:' option
Diagnostic(ErrorCode.ERR_SwitchNeedsNumber).WithArguments("w"));
parsedArgs = DefaultParse(new string[] { "/warn:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2035: Command-line syntax error: Missing ':<number>' for '/warn:' option
Diagnostic(ErrorCode.ERR_SwitchNeedsNumber).WithArguments("warn"));
parsedArgs = DefaultParse(new string[] { "/w:-1", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS1900: Warning level must be zero or greater
Diagnostic(ErrorCode.ERR_BadWarningLevel).WithArguments("w"));
parsedArgs = DefaultParse(new string[] { "/w:5", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new string[] { "/warn:-1", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS1900: Warning level must be zero or greater
Diagnostic(ErrorCode.ERR_BadWarningLevel).WithArguments("warn"));
parsedArgs = DefaultParse(new string[] { "/warn:5", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
// Previous versions of the compiler used to report a warning (CS1691)
// whenever an unrecognized warning code was supplied via /nowarn or /warnaserror.
// We no longer generate a warning in such cases.
parsedArgs = DefaultParse(new string[] { "/warnaserror:1,2,3", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new string[] { "/nowarn:1,2,3", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new string[] { "/nowarn:1;2;;3", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
}
private static void AssertSpecificDiagnostics(int[] expectedCodes, ReportDiagnostic[] expectedOptions, CSharpCommandLineArguments args)
{
var actualOrdered = args.CompilationOptions.SpecificDiagnosticOptions.OrderBy(entry => entry.Key);
AssertEx.Equal(
expectedCodes.Select(i => MessageProvider.Instance.GetIdForErrorCode(i)),
actualOrdered.Select(entry => entry.Key));
AssertEx.Equal(expectedOptions, actualOrdered.Select(entry => entry.Value));
}
[Fact]
public void WarningsParse()
{
var parsedArgs = DefaultParse(new string[] { "/warnaserror", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(ReportDiagnostic.Error, parsedArgs.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(4, parsedArgs.CompilationOptions.WarningLevel);
Assert.Equal(0, parsedArgs.CompilationOptions.SpecificDiagnosticOptions.Count);
parsedArgs = DefaultParse(new string[] { "/warnaserror:1062,1066,1734", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(ReportDiagnostic.Default, parsedArgs.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(4, parsedArgs.CompilationOptions.WarningLevel);
AssertSpecificDiagnostics(new[] { 1062, 1066, 1734 }, new[] { ReportDiagnostic.Error, ReportDiagnostic.Error, ReportDiagnostic.Error }, parsedArgs);
parsedArgs = DefaultParse(new string[] { "/warnaserror:+1062,+1066,+1734", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(ReportDiagnostic.Default, parsedArgs.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(4, parsedArgs.CompilationOptions.WarningLevel);
AssertSpecificDiagnostics(new[] { 1062, 1066, 1734 }, new[] { ReportDiagnostic.Error, ReportDiagnostic.Error, ReportDiagnostic.Error }, parsedArgs);
parsedArgs = DefaultParse(new string[] { "/warnaserror+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(ReportDiagnostic.Error, parsedArgs.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(4, parsedArgs.CompilationOptions.WarningLevel);
AssertSpecificDiagnostics(new int[0], new ReportDiagnostic[0], parsedArgs);
parsedArgs = DefaultParse(new string[] { "/warnaserror+:1062,1066,1734", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(ReportDiagnostic.Default, parsedArgs.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(4, parsedArgs.CompilationOptions.WarningLevel);
AssertSpecificDiagnostics(new[] { 1062, 1066, 1734 }, new[] { ReportDiagnostic.Error, ReportDiagnostic.Error, ReportDiagnostic.Error }, parsedArgs);
parsedArgs = DefaultParse(new string[] { "/warnaserror-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(ReportDiagnostic.Default, parsedArgs.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(4, parsedArgs.CompilationOptions.WarningLevel);
AssertSpecificDiagnostics(new int[0], new ReportDiagnostic[0], parsedArgs);
parsedArgs = DefaultParse(new string[] { "/warnaserror-:1062,1066,1734", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(ReportDiagnostic.Default, parsedArgs.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(4, parsedArgs.CompilationOptions.WarningLevel);
AssertSpecificDiagnostics(new[] { 1062, 1066, 1734 }, new[] { ReportDiagnostic.Default, ReportDiagnostic.Default, ReportDiagnostic.Default }, parsedArgs);
parsedArgs = DefaultParse(new string[] { "/warnaserror+:1062,1066,1734", "/warnaserror-:1762,1974", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(ReportDiagnostic.Default, parsedArgs.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(4, parsedArgs.CompilationOptions.WarningLevel);
AssertSpecificDiagnostics(
new[] { 1062, 1066, 1734, 1762, 1974 },
new[] { ReportDiagnostic.Error, ReportDiagnostic.Error, ReportDiagnostic.Error, ReportDiagnostic.Default, ReportDiagnostic.Default },
parsedArgs);
parsedArgs = DefaultParse(new string[] { "/warnaserror+:1062,1066,1734", "/warnaserror-:1062,1974", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(ReportDiagnostic.Default, parsedArgs.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(4, parsedArgs.CompilationOptions.WarningLevel);
Assert.Equal(4, parsedArgs.CompilationOptions.SpecificDiagnosticOptions.Count);
AssertSpecificDiagnostics(new[] { 1062, 1066, 1734, 1974 }, new[] { ReportDiagnostic.Default, ReportDiagnostic.Error, ReportDiagnostic.Error, ReportDiagnostic.Default }, parsedArgs);
parsedArgs = DefaultParse(new string[] { "/warnaserror-:1062,1066,1734", "/warnaserror+:1062,1974", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(ReportDiagnostic.Default, parsedArgs.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(4, parsedArgs.CompilationOptions.WarningLevel);
AssertSpecificDiagnostics(new[] { 1062, 1066, 1734, 1974 }, new[] { ReportDiagnostic.Error, ReportDiagnostic.Default, ReportDiagnostic.Default, ReportDiagnostic.Error }, parsedArgs);
parsedArgs = DefaultParse(new string[] { "/w:1", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(ReportDiagnostic.Default, parsedArgs.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(1, parsedArgs.CompilationOptions.WarningLevel);
AssertSpecificDiagnostics(new int[0], new ReportDiagnostic[0], parsedArgs);
parsedArgs = DefaultParse(new string[] { "/warn:1", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(ReportDiagnostic.Default, parsedArgs.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(1, parsedArgs.CompilationOptions.WarningLevel);
AssertSpecificDiagnostics(new int[0], new ReportDiagnostic[0], parsedArgs);
parsedArgs = DefaultParse(new string[] { "/warn:1", "/warnaserror+:1062,1974", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(ReportDiagnostic.Default, parsedArgs.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(1, parsedArgs.CompilationOptions.WarningLevel);
AssertSpecificDiagnostics(new[] { 1062, 1974 }, new[] { ReportDiagnostic.Error, ReportDiagnostic.Error }, parsedArgs);
parsedArgs = DefaultParse(new string[] { "/nowarn:1062,1066,1734", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(ReportDiagnostic.Default, parsedArgs.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(4, parsedArgs.CompilationOptions.WarningLevel);
AssertSpecificDiagnostics(new[] { 1062, 1066, 1734 }, new[] { ReportDiagnostic.Suppress, ReportDiagnostic.Suppress, ReportDiagnostic.Suppress }, parsedArgs);
parsedArgs = DefaultParse(new string[] { @"/nowarn:""1062 1066 1734""", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(ReportDiagnostic.Default, parsedArgs.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(4, parsedArgs.CompilationOptions.WarningLevel);
AssertSpecificDiagnostics(new[] { 1062, 1066, 1734 }, new[] { ReportDiagnostic.Suppress, ReportDiagnostic.Suppress, ReportDiagnostic.Suppress }, parsedArgs);
parsedArgs = DefaultParse(new string[] { "/nowarn:1062,1066,1734", "/warnaserror:1066,1762", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(ReportDiagnostic.Default, parsedArgs.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(4, parsedArgs.CompilationOptions.WarningLevel);
AssertSpecificDiagnostics(new[] { 1062, 1066, 1734, 1762 }, new[] { ReportDiagnostic.Suppress, ReportDiagnostic.Suppress, ReportDiagnostic.Suppress, ReportDiagnostic.Error }, parsedArgs);
parsedArgs = DefaultParse(new string[] { "/warnaserror:1066,1762", "/nowarn:1062,1066,1734", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(ReportDiagnostic.Default, parsedArgs.CompilationOptions.GeneralDiagnosticOption);
Assert.Equal(4, parsedArgs.CompilationOptions.WarningLevel);
AssertSpecificDiagnostics(new[] { 1062, 1066, 1734, 1762 }, new[] { ReportDiagnostic.Suppress, ReportDiagnostic.Suppress, ReportDiagnostic.Suppress, ReportDiagnostic.Error }, parsedArgs);
}
[Fact]
public void AllowUnsafe()
{
CSharpCommandLineArguments parsedArgs = DefaultParse(new[] { "/unsafe", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.CompilationOptions.AllowUnsafe);
parsedArgs = DefaultParse(new[] { "/unsafe+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.CompilationOptions.AllowUnsafe);
parsedArgs = DefaultParse(new[] { "/UNSAFE-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.AllowUnsafe);
parsedArgs = DefaultParse(new[] { "/unsafe-", "/unsafe+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.CompilationOptions.AllowUnsafe);
parsedArgs = DefaultParse(new[] { "a.cs" }, WorkingDirectory); // default
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.AllowUnsafe);
parsedArgs = DefaultParse(new[] { "/unsafe:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/unsafe:"));
parsedArgs = DefaultParse(new[] { "/unsafe:+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/unsafe:+"));
parsedArgs = DefaultParse(new[] { "/unsafe-:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/unsafe-:"));
}
[Fact]
public void DelaySign()
{
CSharpCommandLineArguments parsedArgs;
parsedArgs = DefaultParse(new[] { "/delaysign", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.NotNull(parsedArgs.CompilationOptions.DelaySign);
Assert.True((bool)parsedArgs.CompilationOptions.DelaySign);
parsedArgs = DefaultParse(new[] { "/delaysign+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.NotNull(parsedArgs.CompilationOptions.DelaySign);
Assert.True((bool)parsedArgs.CompilationOptions.DelaySign);
parsedArgs = DefaultParse(new[] { "/DELAYsign-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.NotNull(parsedArgs.CompilationOptions.DelaySign);
Assert.False((bool)parsedArgs.CompilationOptions.DelaySign);
parsedArgs = DefaultParse(new[] { "/delaysign:-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2007: Unrecognized option: '/delaysign:-'
Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/delaysign:-"));
Assert.Null(parsedArgs.CompilationOptions.DelaySign);
}
[Fact]
public void PublicSign()
{
var parsedArgs = DefaultParse(new[] { "/publicsign", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.CompilationOptions.PublicSign);
parsedArgs = DefaultParse(new[] { "/publicsign+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.CompilationOptions.PublicSign);
parsedArgs = DefaultParse(new[] { "/PUBLICsign-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.CompilationOptions.PublicSign);
parsedArgs = DefaultParse(new[] { "/publicsign:-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2007: Unrecognized option: '/publicsign:-'
Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/publicsign:-").WithLocation(1, 1));
Assert.False(parsedArgs.CompilationOptions.PublicSign);
}
[WorkItem(8360, "https://github.com/dotnet/roslyn/issues/8360")]
[Fact]
public void PublicSign_KeyFileRelativePath()
{
var parsedArgs = DefaultParse(new[] { "/publicsign", "/keyfile:test.snk", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(Path.Combine(WorkingDirectory, "test.snk"), parsedArgs.CompilationOptions.CryptoKeyFile);
}
[Fact]
[WorkItem(11497, "https://github.com/dotnet/roslyn/issues/11497")]
public void PublicSignWithEmptyKeyPath()
{
DefaultParse(new[] { "/publicsign", "/keyfile:", "a.cs" }, WorkingDirectory).Errors.Verify(
// error CS2005: Missing file specification for 'keyfile' option
Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("keyfile").WithLocation(1, 1));
}
[Fact]
[WorkItem(11497, "https://github.com/dotnet/roslyn/issues/11497")]
public void PublicSignWithEmptyKeyPath2()
{
DefaultParse(new[] { "/publicsign", "/keyfile:\"\"", "a.cs" }, WorkingDirectory).Errors.Verify(
// error CS2005: Missing file specification for 'keyfile' option
Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("keyfile").WithLocation(1, 1));
}
[WorkItem(546301, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546301")]
[Fact]
public void SubsystemVersionTests()
{
CSharpCommandLineArguments parsedArgs = DefaultParse(new[] { "/subsystemversion:4.0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(SubsystemVersion.Create(4, 0), parsedArgs.EmitOptions.SubsystemVersion);
// wrongly supported subsystem version. CompilationOptions data will be faithful to the user input.
// It is normalized at the time of emit.
parsedArgs = DefaultParse(new[] { "/subsystemversion:0.0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(); // no error in Dev11
Assert.Equal(SubsystemVersion.Create(0, 0), parsedArgs.EmitOptions.SubsystemVersion);
parsedArgs = DefaultParse(new[] { "/subsystemversion:0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(); // no error in Dev11
Assert.Equal(SubsystemVersion.Create(0, 0), parsedArgs.EmitOptions.SubsystemVersion);
parsedArgs = DefaultParse(new[] { "/subsystemversion:3.99", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(); // no error in Dev11
Assert.Equal(SubsystemVersion.Create(3, 99), parsedArgs.EmitOptions.SubsystemVersion);
parsedArgs = DefaultParse(new[] { "/subsystemversion:4.0", "/SUBsystemversion:5.333", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(SubsystemVersion.Create(5, 333), parsedArgs.EmitOptions.SubsystemVersion);
parsedArgs = DefaultParse(new[] { "/subsystemversion:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "subsystemversion"));
parsedArgs = DefaultParse(new[] { "/subsystemversion", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "subsystemversion"));
parsedArgs = DefaultParse(new[] { "/subsystemversion-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/subsystemversion-"));
parsedArgs = DefaultParse(new[] { "/subsystemversion: ", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "subsystemversion"));
parsedArgs = DefaultParse(new[] { "/subsystemversion: 4.1", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_InvalidSubsystemVersion).WithArguments(" 4.1"));
parsedArgs = DefaultParse(new[] { "/subsystemversion:4 .0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_InvalidSubsystemVersion).WithArguments("4 .0"));
parsedArgs = DefaultParse(new[] { "/subsystemversion:4. 0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_InvalidSubsystemVersion).WithArguments("4. 0"));
parsedArgs = DefaultParse(new[] { "/subsystemversion:.", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_InvalidSubsystemVersion).WithArguments("."));
parsedArgs = DefaultParse(new[] { "/subsystemversion:4.", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_InvalidSubsystemVersion).WithArguments("4."));
parsedArgs = DefaultParse(new[] { "/subsystemversion:.0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_InvalidSubsystemVersion).WithArguments(".0"));
parsedArgs = DefaultParse(new[] { "/subsystemversion:4.2 ", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new[] { "/subsystemversion:4.65536", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_InvalidSubsystemVersion).WithArguments("4.65536"));
parsedArgs = DefaultParse(new[] { "/subsystemversion:65536.0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_InvalidSubsystemVersion).WithArguments("65536.0"));
parsedArgs = DefaultParse(new[] { "/subsystemversion:-4.0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_InvalidSubsystemVersion).WithArguments("-4.0"));
// TODO: incompatibilities: versions lower than '6.2' and 'arm', 'winmdobj', 'appcontainer'
}
[Fact]
public void MainType()
{
CSharpCommandLineArguments parsedArgs = DefaultParse(new[] { "/m:A.B.C", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal("A.B.C", parsedArgs.CompilationOptions.MainTypeName);
parsedArgs = DefaultParse(new[] { "/m: ", "a.cs" }, WorkingDirectory); // Mimicking Dev11
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "m"));
Assert.Null(parsedArgs.CompilationOptions.MainTypeName);
// overriding the value
parsedArgs = DefaultParse(new[] { "/m:A.B.C", "/MAIN:X.Y.Z", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal("X.Y.Z", parsedArgs.CompilationOptions.MainTypeName);
// error
parsedArgs = DefaultParse(new[] { "/maiN:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "main"));
parsedArgs = DefaultParse(new[] { "/MAIN+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/MAIN+"));
parsedArgs = DefaultParse(new[] { "/M", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "m"));
// incompatible values /main && /target
parsedArgs = DefaultParse(new[] { "/main:a", "/t:library", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_NoMainOnDLL));
parsedArgs = DefaultParse(new[] { "/main:a", "/t:module", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_NoMainOnDLL));
}
[Fact]
public void Codepage()
{
CSharpCommandLineArguments parsedArgs = DefaultParse(new[] { "/CodePage:1200", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal("Unicode", parsedArgs.Encoding.EncodingName);
parsedArgs = DefaultParse(new[] { "/CodePage:1200", "/codePAGE:65001", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal("Unicode (UTF-8)", parsedArgs.Encoding.EncodingName);
// error
parsedArgs = DefaultParse(new[] { "/codepage:0", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.FTL_BadCodepage).WithArguments("0"));
parsedArgs = DefaultParse(new[] { "/codepage:abc", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.FTL_BadCodepage).WithArguments("abc"));
parsedArgs = DefaultParse(new[] { "/codepage:-5", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.FTL_BadCodepage).WithArguments("-5"));
parsedArgs = DefaultParse(new[] { "/codepage: ", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.FTL_BadCodepage).WithArguments(""));
parsedArgs = DefaultParse(new[] { "/codepage:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.FTL_BadCodepage).WithArguments(""));
parsedArgs = DefaultParse(new[] { "/codepage", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "codepage"));
parsedArgs = DefaultParse(new[] { "/codepage+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/codepage+"));
}
[Fact, WorkItem(24735, "https://github.com/dotnet/roslyn/issues/24735")]
public void ChecksumAlgorithm()
{
CSharpCommandLineArguments parsedArgs = DefaultParse(new[] { "/checksumAlgorithm:sHa1", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(SourceHashAlgorithm.Sha1, parsedArgs.ChecksumAlgorithm);
Assert.Equal(HashAlgorithmName.SHA256, parsedArgs.EmitOptions.PdbChecksumAlgorithm);
parsedArgs = DefaultParse(new[] { "/checksumAlgorithm:sha256", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(SourceHashAlgorithm.Sha256, parsedArgs.ChecksumAlgorithm);
Assert.Equal(HashAlgorithmName.SHA256, parsedArgs.EmitOptions.PdbChecksumAlgorithm);
parsedArgs = DefaultParse(new[] { "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(SourceHashAlgorithm.Sha256, parsedArgs.ChecksumAlgorithm);
Assert.Equal(HashAlgorithmName.SHA256, parsedArgs.EmitOptions.PdbChecksumAlgorithm);
// error
parsedArgs = DefaultParse(new[] { "/checksumAlgorithm:256", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.FTL_BadChecksumAlgorithm).WithArguments("256"));
parsedArgs = DefaultParse(new[] { "/checksumAlgorithm:sha-1", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.FTL_BadChecksumAlgorithm).WithArguments("sha-1"));
parsedArgs = DefaultParse(new[] { "/checksumAlgorithm:sha", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.FTL_BadChecksumAlgorithm).WithArguments("sha"));
parsedArgs = DefaultParse(new[] { "/checksumAlgorithm: ", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "checksumalgorithm"));
parsedArgs = DefaultParse(new[] { "/checksumAlgorithm:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "checksumalgorithm"));
parsedArgs = DefaultParse(new[] { "/checksumAlgorithm", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "checksumalgorithm"));
parsedArgs = DefaultParse(new[] { "/checksumAlgorithm+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/checksumAlgorithm+"));
}
[Fact]
public void AddModule()
{
CSharpCommandLineArguments parsedArgs = DefaultParse(new[] { "/noconfig", "/nostdlib", "/addmodule:abc.netmodule", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(1, parsedArgs.MetadataReferences.Length);
Assert.Equal("abc.netmodule", parsedArgs.MetadataReferences[0].Reference);
Assert.Equal(MetadataImageKind.Module, parsedArgs.MetadataReferences[0].Properties.Kind);
parsedArgs = DefaultParse(new[] { "/noconfig", "/nostdlib", "/aDDmodule:c:\\abc;c:\\abc;d:\\xyz", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(3, parsedArgs.MetadataReferences.Length);
Assert.Equal("c:\\abc", parsedArgs.MetadataReferences[0].Reference);
Assert.Equal(MetadataImageKind.Module, parsedArgs.MetadataReferences[0].Properties.Kind);
Assert.Equal("c:\\abc", parsedArgs.MetadataReferences[1].Reference);
Assert.Equal(MetadataImageKind.Module, parsedArgs.MetadataReferences[1].Properties.Kind);
Assert.Equal("d:\\xyz", parsedArgs.MetadataReferences[2].Reference);
Assert.Equal(MetadataImageKind.Module, parsedArgs.MetadataReferences[2].Properties.Kind);
// error
parsedArgs = DefaultParse(new[] { "/ADDMODULE", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "/addmodule:"));
parsedArgs = DefaultParse(new[] { "/ADDMODULE+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/ADDMODULE+"));
parsedArgs = DefaultParse(new[] { "/ADDMODULE:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("/ADDMODULE:"));
}
[Fact, WorkItem(530751, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/530751")]
public void CS7061fromCS0647_ModuleWithCompilationRelaxations()
{
string source1 = Temp.CreateFile(prefix: "", extension: ".cs").WriteAllText(@"
using System.Runtime.CompilerServices;
[assembly: CompilationRelaxations(CompilationRelaxations.NoStringInterning)]
public class Mod { }").Path;
string source2 = Temp.CreateFile(prefix: "", extension: ".cs").WriteAllText(@"
using System.Runtime.CompilerServices;
[assembly: CompilationRelaxations(4)]
public class Mod { }").Path;
string source = Temp.CreateFile(prefix: "", extension: ".cs").WriteAllText(@"
using System.Runtime.CompilerServices;
[assembly: CompilationRelaxations(CompilationRelaxations.NoStringInterning)]
class Test { static void Main() {} }").Path;
var baseDir = Path.GetDirectoryName(source);
// === Scenario 1 ===
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, baseDir, new[] { "/nologo", "/t:module", source1 }).Run(outWriter);
Assert.Equal(0, exitCode);
var modfile = source1.Substring(0, source1.Length - 2) + "netmodule";
outWriter = new StringWriter(CultureInfo.InvariantCulture);
var parsedArgs = DefaultParse(new[] { "/nologo", "/addmodule:" + modfile, source }, WorkingDirectory);
parsedArgs.Errors.Verify();
exitCode = CreateCSharpCompiler(null, baseDir, new[] { "/nologo", "/addmodule:" + modfile, source }).Run(outWriter);
Assert.Empty(outWriter.ToString());
// === Scenario 2 ===
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, baseDir, new[] { "/nologo", "/t:module", source2 }).Run(outWriter);
Assert.Equal(0, exitCode);
modfile = source2.Substring(0, source2.Length - 2) + "netmodule";
outWriter = new StringWriter(CultureInfo.InvariantCulture);
parsedArgs = DefaultParse(new[] { "/nologo", "/addmodule:" + modfile, source }, WorkingDirectory);
parsedArgs.Errors.Verify();
exitCode = CreateCSharpCompiler(null, baseDir, new[] { "/nologo", "/preferreduilang:en", "/addmodule:" + modfile, source }).Run(outWriter);
Assert.Equal(1, exitCode);
// Dev11: CS0647 (Emit)
Assert.Contains("error CS7061: Duplicate 'CompilationRelaxationsAttribute' attribute in", outWriter.ToString(), StringComparison.Ordinal);
CleanupAllGeneratedFiles(source1);
CleanupAllGeneratedFiles(source2);
CleanupAllGeneratedFiles(source);
}
[Fact, WorkItem(530780, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/530780")]
public void AddModuleWithExtensionMethod()
{
string source1 = Temp.CreateFile(prefix: "", extension: ".cs").WriteAllText(@"public static class Extensions { public static bool EB(this bool b) { return b; } }").Path;
string source2 = Temp.CreateFile(prefix: "", extension: ".cs").WriteAllText(@"class C { static void Main() {} }").Path;
var baseDir = Path.GetDirectoryName(source2);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, baseDir, new[] { "/nologo", "/t:module", source1 }).Run(outWriter);
Assert.Equal(0, exitCode);
var modfile = source1.Substring(0, source1.Length - 2) + "netmodule";
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, baseDir, new[] { "/nologo", "/addmodule:" + modfile, source2 }).Run(outWriter);
Assert.Equal(0, exitCode);
CleanupAllGeneratedFiles(source1);
CleanupAllGeneratedFiles(source2);
}
[Fact, WorkItem(546297, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546297")]
public void OLDCS0013FTL_MetadataEmitFailureSameModAndRes()
{
string source1 = Temp.CreateFile(prefix: "", extension: ".cs").WriteAllText(@"class Mod { }").Path;
string source2 = Temp.CreateFile(prefix: "", extension: ".cs").WriteAllText(@"class C { static void Main() {} }").Path;
var baseDir = Path.GetDirectoryName(source2);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, baseDir, new[] { "/nologo", "/t:module", source1 }).Run(outWriter);
Assert.Equal(0, exitCode);
var modfile = source1.Substring(0, source1.Length - 2) + "netmodule";
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, baseDir, new[] { "/nologo", "/preferreduilang:en", "/addmodule:" + modfile, "/linkres:" + modfile, source2 }).Run(outWriter);
Assert.Equal(1, exitCode);
// Native gives CS0013 at emit stage
Assert.Equal("error CS7041: Each linked resource and module must have a unique filename. Filename '" + Path.GetFileName(modfile) + "' is specified more than once in this assembly", outWriter.ToString().Trim());
CleanupAllGeneratedFiles(source1);
CleanupAllGeneratedFiles(source2);
}
[Fact]
public void Utf8Output()
{
CSharpCommandLineArguments parsedArgs = DefaultParse(new[] { "/utf8output", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True((bool)parsedArgs.Utf8Output);
parsedArgs = DefaultParse(new[] { "/utf8output", "/utf8output", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True((bool)parsedArgs.Utf8Output);
parsedArgs = DefaultParse(new[] { "/utf8output:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/utf8output:"));
}
[Fact]
public void CscCompile_WithSourceCodeRedirectedViaStandardInput_ProducesRunnableProgram()
{
string tempDir = Temp.CreateDirectory().Path;
ProcessResult result = RuntimeInformation.IsOSPlatform(OSPlatform.Windows) ?
ProcessUtilities.Run("cmd", $@"/C echo ^
class A ^
{{ ^
public static void Main() =^^^> ^
System.Console.WriteLine(""Hello World!""); ^
}} | {s_CSharpCompilerExecutable} /nologo /t:exe -"
.Replace(Environment.NewLine, string.Empty), workingDirectory: tempDir) :
ProcessUtilities.Run("/usr/bin/env", $@"sh -c ""echo \
class A \
{{ \
public static void Main\(\) =\> \
System.Console.WriteLine\(\\\""Hello World\!\\\""\)\; \
}} | {s_CSharpCompilerExecutable} /nologo /t:exe -""", workingDirectory: tempDir,
// we are testing shell's piped/redirected stdin behavior explicitly
// instead of using Process.StandardInput.Write(), so we set
// redirectStandardInput to true, which implies that isatty of child
// process is false and thereby Console.IsInputRedirected will return
// true in csc code.
redirectStandardInput: true);
Assert.False(result.ContainsErrors, $"Compilation error(s) occurred: {result.Output} {result.Errors}");
string output = RuntimeInformation.IsOSPlatform(OSPlatform.Windows) ?
ProcessUtilities.RunAndGetOutput("cmd.exe", $@"/C ""{s_DotnetCscRun} -.exe""", expectedRetCode: 0, startFolder: tempDir) :
ProcessUtilities.RunAndGetOutput("sh", $@"-c ""{s_DotnetCscRun} -.exe""", expectedRetCode: 0, startFolder: tempDir);
Assert.Equal("Hello World!", output.Trim());
}
[Fact]
public void CscCompile_WithSourceCodeRedirectedViaStandardInput_ProducesLibrary()
{
var name = Guid.NewGuid().ToString() + ".dll";
string tempDir = Temp.CreateDirectory().Path;
ProcessResult result = RuntimeInformation.IsOSPlatform(OSPlatform.Windows) ?
ProcessUtilities.Run("cmd", $@"/C echo ^
class A ^
{{ ^
public A Get() =^^^> default; ^
}} | {s_CSharpCompilerExecutable} /nologo /t:library /out:{name} -"
.Replace(Environment.NewLine, string.Empty), workingDirectory: tempDir) :
ProcessUtilities.Run("/usr/bin/env", $@"sh -c ""echo \
class A \
{{ \
public A Get\(\) =\> default\; \
}} | {s_CSharpCompilerExecutable} /nologo /t:library /out:{name} -""", workingDirectory: tempDir,
// we are testing shell's piped/redirected stdin behavior explicitly
// instead of using Process.StandardInput.Write(), so we set
// redirectStandardInput to true, which implies that isatty of child
// process is false and thereby Console.IsInputRedirected will return
// true in csc code.
redirectStandardInput: true);
Assert.False(result.ContainsErrors, $"Compilation error(s) occurred: {result.Output} {result.Errors}");
var assemblyName = AssemblyName.GetAssemblyName(Path.Combine(tempDir, name));
Assert.Equal(name.Replace(".dll", ", Version=0.0.0.0, Culture=neutral, PublicKeyToken=null"),
assemblyName.ToString());
}
[Fact(Skip = "https://github.com/dotnet/roslyn/issues/55727")]
public void CsiScript_WithSourceCodeRedirectedViaStandardInput_ExecutesNonInteractively()
{
string tempDir = Temp.CreateDirectory().Path;
ProcessResult result = RuntimeInformation.IsOSPlatform(OSPlatform.Windows) ?
ProcessUtilities.Run("cmd", $@"/C echo Console.WriteLine(""Hello World!"") | {s_CSharpScriptExecutable} -") :
ProcessUtilities.Run("/usr/bin/env", $@"sh -c ""echo Console.WriteLine\(\\\""Hello World\!\\\""\) | {s_CSharpScriptExecutable} -""",
workingDirectory: tempDir,
// we are testing shell's piped/redirected stdin behavior explicitly
// instead of using Process.StandardInput.Write(), so we set
// redirectStandardInput to true, which implies that isatty of child
// process is false and thereby Console.IsInputRedirected will return
// true in csc code.
redirectStandardInput: true);
Assert.False(result.ContainsErrors, $"Compilation error(s) occurred: {result.Output} {result.Errors}");
Assert.Equal("Hello World!", result.Output.Trim());
}
[Fact]
public void CscCompile_WithRedirectedInputIndicatorAndStandardInputNotRedirected_ReportsCS8782()
{
if (Console.IsInputRedirected)
{
// [applicable to both Windows and Unix]
// if our parent (xunit) process itself has input redirected, we cannot test this
// error case because our child process will inherit it and we cannot achieve what
// we are aiming for: isatty(0):true and thereby Console.IsInputerRedirected:false in
// child. running this case will make StreamReader to hang (waiting for input, that
// we do not propagate: parent.In->child.In).
//
// note: in Unix we can "close" fd0 by appending `0>&-` in the `sh -c` command below,
// but that will also not impact the result of isatty(), and in turn causes a different
// compiler error.
return;
}
string tempDir = Temp.CreateDirectory().Path;
ProcessResult result = RuntimeInformation.IsOSPlatform(OSPlatform.Windows) ?
ProcessUtilities.Run("cmd", $@"/C ""{s_CSharpCompilerExecutable} /nologo /t:exe -""", workingDirectory: tempDir) :
ProcessUtilities.Run("/usr/bin/env", $@"sh -c ""{s_CSharpCompilerExecutable} /nologo /t:exe -""", workingDirectory: tempDir);
Assert.True(result.ContainsErrors);
Assert.Contains(((int)ErrorCode.ERR_StdInOptionProvidedButConsoleInputIsNotRedirected).ToString(), result.Output);
}
[Fact]
public void CscCompile_WithMultipleStdInOperators_WarnsCS2002()
{
string tempDir = Temp.CreateDirectory().Path;
ProcessResult result = RuntimeInformation.IsOSPlatform(OSPlatform.Windows) ?
ProcessUtilities.Run("cmd", $@"/C echo ^
class A ^
{{ ^
public static void Main() =^^^> ^
System.Console.WriteLine(""Hello World!""); ^
}} | {s_CSharpCompilerExecutable} /nologo - /t:exe -"
.Replace(Environment.NewLine, string.Empty)) :
ProcessUtilities.Run("/usr/bin/env", $@"sh -c ""echo \
class A \
{{ \
public static void Main\(\) =\> \
System.Console.WriteLine\(\\\""Hello World\!\\\""\)\; \
}} | {s_CSharpCompilerExecutable} /nologo - /t:exe -""", workingDirectory: tempDir,
// we are testing shell's piped/redirected stdin behavior explicitly
// instead of using Process.StandardInput.Write(), so we set
// redirectStandardInput to true, which implies that isatty of child
// process is false and thereby Console.IsInputRedirected will return
// true in csc code.
redirectStandardInput: true);
Assert.Contains(((int)ErrorCode.WRN_FileAlreadyIncluded).ToString(), result.Output);
}
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30321")]
public void CscUtf8Output_WithRedirecting_Off()
{
var srcFile = Temp.CreateFile().WriteAllText("\u265A").Path;
var tempOut = Temp.CreateFile();
var output = ProcessUtilities.RunAndGetOutput("cmd", "/C \"" + s_CSharpCompilerExecutable + "\" /nologo /preferreduilang:en /t:library " + srcFile + " > " + tempOut.Path, expectedRetCode: 1);
Assert.Equal("", output.Trim());
Assert.Equal("SRC.CS(1,1): error CS1056: Unexpected character '?'", tempOut.ReadAllText().Trim().Replace(srcFile, "SRC.CS"));
CleanupAllGeneratedFiles(srcFile);
CleanupAllGeneratedFiles(tempOut.Path);
}
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30321")]
public void CscUtf8Output_WithRedirecting_On()
{
var srcFile = Temp.CreateFile().WriteAllText("\u265A").Path;
var tempOut = Temp.CreateFile();
var output = ProcessUtilities.RunAndGetOutput("cmd", "/C \"" + s_CSharpCompilerExecutable + "\" /utf8output /nologo /preferreduilang:en /t:library " + srcFile + " > " + tempOut.Path, expectedRetCode: 1);
Assert.Equal("", output.Trim());
Assert.Equal("SRC.CS(1,1): error CS1056: Unexpected character '♚'", tempOut.ReadAllText().Trim().Replace(srcFile, "SRC.CS"));
CleanupAllGeneratedFiles(srcFile);
CleanupAllGeneratedFiles(tempOut.Path);
}
[WorkItem(546653, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546653")]
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30321")]
public void NoSourcesWithModule()
{
var folder = Temp.CreateDirectory();
var aCs = folder.CreateFile("a.cs");
aCs.WriteAllText("public class C {}");
var output = ProcessUtilities.RunAndGetOutput(s_CSharpCompilerExecutable, $"/nologo /t:module /out:a.netmodule \"{aCs}\"", startFolder: folder.ToString());
Assert.Equal("", output.Trim());
output = ProcessUtilities.RunAndGetOutput(s_CSharpCompilerExecutable, "/nologo /t:library /out:b.dll /addmodule:a.netmodule ", startFolder: folder.ToString());
Assert.Equal("", output.Trim());
output = ProcessUtilities.RunAndGetOutput(s_CSharpCompilerExecutable, "/nologo /preferreduilang:en /t:module /out:b.dll /addmodule:a.netmodule ", startFolder: folder.ToString());
Assert.Equal("warning CS2008: No source files specified.", output.Trim());
CleanupAllGeneratedFiles(aCs.Path);
}
[WorkItem(546653, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546653")]
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30321")]
public void NoSourcesWithResource()
{
var folder = Temp.CreateDirectory();
var aCs = folder.CreateFile("a.cs");
aCs.WriteAllText("public class C {}");
var output = ProcessUtilities.RunAndGetOutput(s_CSharpCompilerExecutable, "/nologo /t:library /out:b.dll /resource:a.cs", startFolder: folder.ToString());
Assert.Equal("", output.Trim());
CleanupAllGeneratedFiles(aCs.Path);
}
[WorkItem(546653, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546653")]
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30321")]
public void NoSourcesWithLinkResource()
{
var folder = Temp.CreateDirectory();
var aCs = folder.CreateFile("a.cs");
aCs.WriteAllText("public class C {}");
var output = ProcessUtilities.RunAndGetOutput(s_CSharpCompilerExecutable, "/nologo /t:library /out:b.dll /linkresource:a.cs", startFolder: folder.ToString());
Assert.Equal("", output.Trim());
CleanupAllGeneratedFiles(aCs.Path);
}
[Fact]
public void KeyContainerAndKeyFile()
{
// KEYCONTAINER
CSharpCommandLineArguments parsedArgs = DefaultParse(new[] { "/keycontainer:RIPAdamYauch", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal("RIPAdamYauch", parsedArgs.CompilationOptions.CryptoKeyContainer);
parsedArgs = DefaultParse(new[] { "/keycontainer", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for 'keycontainer' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "keycontainer"));
Assert.Null(parsedArgs.CompilationOptions.CryptoKeyContainer);
parsedArgs = DefaultParse(new[] { "/keycontainer-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2007: Unrecognized option: '/keycontainer-'
Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/keycontainer-"));
Assert.Null(parsedArgs.CompilationOptions.CryptoKeyContainer);
parsedArgs = DefaultParse(new[] { "/keycontainer:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for 'keycontainer' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "keycontainer"));
Assert.Null(parsedArgs.CompilationOptions.CryptoKeyContainer);
parsedArgs = DefaultParse(new[] { "/keycontainer: ", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "keycontainer"));
Assert.Null(parsedArgs.CompilationOptions.CryptoKeyContainer);
// KEYFILE
parsedArgs = DefaultParse(new[] { @"/keyfile:\somepath\s""ome Fil""e.goo.bar", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
//EDMAURER let's not set the option in the event that there was an error.
//Assert.Equal(@"\somepath\some File.goo.bar", parsedArgs.CompilationOptions.CryptoKeyFile);
parsedArgs = DefaultParse(new[] { "/keyFile", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2005: Missing file specification for 'keyfile' option
Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("keyfile"));
Assert.Null(parsedArgs.CompilationOptions.CryptoKeyFile);
parsedArgs = DefaultParse(new[] { "/keyFile: ", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(Diagnostic(ErrorCode.ERR_NoFileSpec).WithArguments("keyfile"));
Assert.Null(parsedArgs.CompilationOptions.CryptoKeyFile);
parsedArgs = DefaultParse(new[] { "/keyfile-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS2007: Unrecognized option: '/keyfile-'
Diagnostic(ErrorCode.ERR_BadSwitch).WithArguments("/keyfile-"));
Assert.Null(parsedArgs.CompilationOptions.CryptoKeyFile);
// DEFAULTS
parsedArgs = DefaultParse(new[] { "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Null(parsedArgs.CompilationOptions.CryptoKeyFile);
Assert.Null(parsedArgs.CompilationOptions.CryptoKeyContainer);
// KEYFILE | KEYCONTAINER conflicts
parsedArgs = DefaultParse(new[] { "/keyFile:a", "/keyContainer:b", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal("a", parsedArgs.CompilationOptions.CryptoKeyFile);
Assert.Equal("b", parsedArgs.CompilationOptions.CryptoKeyContainer);
parsedArgs = DefaultParse(new[] { "/keyContainer:b", "/keyFile:a", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal("a", parsedArgs.CompilationOptions.CryptoKeyFile);
Assert.Equal("b", parsedArgs.CompilationOptions.CryptoKeyContainer);
}
[Fact, WorkItem(554551, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/554551")]
public void CS1698WRN_AssumedMatchThis()
{
// compile with: /target:library /keyfile:mykey.snk
var text1 = @"[assembly:System.Reflection.AssemblyVersion(""2"")]
public class CS1698_a {}
";
// compile with: /target:library /reference:CS1698_a.dll /keyfile:mykey.snk
var text2 = @"public class CS1698_b : CS1698_a {}
";
//compile with: /target:library /out:cs1698_a.dll /reference:cs1698_b.dll /keyfile:mykey.snk
var text = @"[assembly:System.Reflection.AssemblyVersion(""3"")]
public class CS1698_c : CS1698_b {}
public class CS1698_a {}
";
var folder = Temp.CreateDirectory();
var cs1698a = folder.CreateFile("CS1698a.cs");
cs1698a.WriteAllText(text1);
var cs1698b = folder.CreateFile("CS1698b.cs");
cs1698b.WriteAllText(text2);
var cs1698 = folder.CreateFile("CS1698.cs");
cs1698.WriteAllText(text);
var snkFile = Temp.CreateFile().WriteAllBytes(TestResources.General.snKey);
var kfile = "/keyfile:" + snkFile.Path;
CSharpCommandLineArguments parsedArgs = DefaultParse(new[] { "/t:library", kfile, "CS1698a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new[] { "/t:library", kfile, "/r:" + cs1698a.Path, "CS1698b.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
parsedArgs = DefaultParse(new[] { "/t:library", kfile, "/r:" + cs1698b.Path, "/out:" + cs1698a.Path, "CS1698.cs" }, WorkingDirectory);
// Roslyn no longer generates a warning for this...since this was only a warning, we're not really
// saving anyone...does not provide high value to implement...
// warning CS1698: Circular assembly reference 'CS1698a, Version=2.0.0.0, Culture=neutral,PublicKeyToken = 9e9d6755e7bb4c10'
// does not match the output assembly name 'CS1698a, Version = 3.0.0.0, Culture = neutral, PublicKeyToken = 9e9d6755e7bb4c10'.
// Try adding a reference to 'CS1698a, Version = 2.0.0.0, Culture = neutral, PublicKeyToken = 9e9d6755e7bb4c10' or changing the output assembly name to match.
parsedArgs.Errors.Verify();
CleanupAllGeneratedFiles(snkFile.Path);
CleanupAllGeneratedFiles(cs1698a.Path);
CleanupAllGeneratedFiles(cs1698b.Path);
CleanupAllGeneratedFiles(cs1698.Path);
}
[ConditionalFact(typeof(ClrOnly), Reason = "https://github.com/dotnet/roslyn/issues/30926")]
public void BinaryFileErrorTest()
{
var binaryPath = Temp.CreateFile().WriteAllBytes(ResourcesNet451.mscorlib).Path;
var csc = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/preferreduilang:en", binaryPath });
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal(
"error CS2015: '" + binaryPath + "' is a binary file instead of a text file",
outWriter.ToString().Trim());
CleanupAllGeneratedFiles(binaryPath);
}
#if !NETCOREAPP
[WorkItem(530221, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/530221")]
[WorkItem(5660, "https://github.com/dotnet/roslyn/issues/5660")]
[ConditionalFact(typeof(WindowsOnly), typeof(IsEnglishLocal))]
public void Bug15538()
{
// Several Jenkins VMs are still running with local systems permissions. This suite won't run properly
// in that environment. Removing this check is being tracked by issue #79.
using (var identity = System.Security.Principal.WindowsIdentity.GetCurrent())
{
if (identity.IsSystem)
{
return;
}
// The icacls command fails on our Helix machines and it appears to be related to the use of the $ in
// the username.
// https://github.com/dotnet/roslyn/issues/28836
if (StringComparer.OrdinalIgnoreCase.Equals(Environment.UserDomainName, "WORKGROUP"))
{
return;
}
}
var folder = Temp.CreateDirectory();
var source = folder.CreateFile("src.vb").WriteAllText("").Path;
var _ref = folder.CreateFile("ref.dll").WriteAllText("").Path;
try
{
var output = ProcessUtilities.RunAndGetOutput("cmd", "/C icacls " + _ref + " /inheritance:r /Q");
Assert.Equal("Successfully processed 1 files; Failed processing 0 files", output.Trim());
output = ProcessUtilities.RunAndGetOutput("cmd", "/C icacls " + _ref + @" /deny %USERDOMAIN%\%USERNAME%:(r,WDAC) /Q");
Assert.Equal("Successfully processed 1 files; Failed processing 0 files", output.Trim());
output = ProcessUtilities.RunAndGetOutput("cmd", "/C \"" + s_CSharpCompilerExecutable + "\" /nologo /preferreduilang:en /r:" + _ref + " /t:library " + source, expectedRetCode: 1);
Assert.Equal("error CS0009: Metadata file '" + _ref + "' could not be opened -- Access to the path '" + _ref + "' is denied.", output.Trim());
}
finally
{
var output = ProcessUtilities.RunAndGetOutput("cmd", "/C icacls " + _ref + " /reset /Q");
Assert.Equal("Successfully processed 1 files; Failed processing 0 files", output.Trim());
File.Delete(_ref);
}
CleanupAllGeneratedFiles(source);
}
#endif
[WorkItem(545832, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/545832")]
[Fact]
public void ResponseFilesWithEmptyAliasReference()
{
string source = Temp.CreateFile("a.cs").WriteAllText(@"
// <Area> ExternAlias - command line alias</Area>
// <Title>
// negative test cases: empty file name ("""")
// </Title>
// <Description>
// </Description>
// <RelatedBugs></RelatedBugs>
//<Expects Status=error>CS1680:.*myAlias=</Expects>
// <Code>
class myClass
{
static int Main()
{
return 1;
}
}
// </Code>
").Path;
string rsp = Temp.CreateFile().WriteAllText(@"
/nologo
/r:myAlias=""""
").Path;
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
// csc errors_whitespace_008.cs @errors_whitespace_008.cs.rsp
var csc = CreateCSharpCompiler(rsp, WorkingDirectory, new[] { source, "/preferreduilang:en" });
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal("error CS1680: Invalid reference alias option: 'myAlias=' -- missing filename", outWriter.ToString().Trim());
CleanupAllGeneratedFiles(source);
CleanupAllGeneratedFiles(rsp);
}
[Fact]
public void ResponseFileOrdering()
{
var rspFilePath1 = Temp.CreateFile().WriteAllText(@"
/b
/c
").Path;
assertOrder(
new[] { "/a", "/b", "/c", "/d" },
new[] { "/a", @$"@""{rspFilePath1}""", "/d" });
var rspFilePath2 = Temp.CreateFile().WriteAllText(@"
/c
/d
").Path;
rspFilePath1 = Temp.CreateFile().WriteAllText(@$"
/b
@""{rspFilePath2}""
").Path;
assertOrder(
new[] { "/a", "/b", "/c", "/d", "/e" },
new[] { "/a", @$"@""{rspFilePath1}""", "/e" });
rspFilePath1 = Temp.CreateFile().WriteAllText(@$"
/b
").Path;
rspFilePath2 = Temp.CreateFile().WriteAllText(@"
# this will be ignored
/c
/d
").Path;
assertOrder(
new[] { "/a", "/b", "/c", "/d", "/e" },
new[] { "/a", @$"@""{rspFilePath1}""", $@"@""{rspFilePath2}""", "/e" });
void assertOrder(string[] expected, string[] args)
{
var flattenedArgs = ArrayBuilder<string>.GetInstance();
var diagnostics = new List<Diagnostic>();
CSharpCommandLineParser.Default.FlattenArgs(
args,
diagnostics,
flattenedArgs,
scriptArgsOpt: null,
baseDirectory: Path.DirectorySeparatorChar == '\\' ? @"c:\" : "/");
Assert.Empty(diagnostics);
Assert.Equal(expected, flattenedArgs);
flattenedArgs.Free();
}
}
[WorkItem(545832, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/545832")]
[Fact]
public void ResponseFilesWithEmptyAliasReference2()
{
string source = Temp.CreateFile("a.cs").WriteAllText(@"
// <Area> ExternAlias - command line alias</Area>
// <Title>
// negative test cases: empty file name ("""")
// </Title>
// <Description>
// </Description>
// <RelatedBugs></RelatedBugs>
//<Expects Status=error>CS1680:.*myAlias=</Expects>
// <Code>
class myClass
{
static int Main()
{
return 1;
}
}
// </Code>
").Path;
string rsp = Temp.CreateFile().WriteAllText(@"
/nologo
/r:myAlias="" ""
").Path;
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
// csc errors_whitespace_008.cs @errors_whitespace_008.cs.rsp
var csc = CreateCSharpCompiler(rsp, WorkingDirectory, new[] { source, "/preferreduilang:en" });
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal("error CS1680: Invalid reference alias option: 'myAlias=' -- missing filename", outWriter.ToString().Trim());
CleanupAllGeneratedFiles(source);
CleanupAllGeneratedFiles(rsp);
}
[WorkItem(1784, "https://github.com/dotnet/roslyn/issues/1784")]
[Fact]
public void QuotedDefineInRespFile()
{
string source = Temp.CreateFile("a.cs").WriteAllText(@"
#if NN
class myClass
{
#endif
static int Main()
#if DD
{
return 1;
#endif
#if AA
}
#endif
#if BB
}
#endif
").Path;
string rsp = Temp.CreateFile().WriteAllText(@"
/d:""DD""
/d:""AA;BB""
/d:""N""N
").Path;
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
// csc errors_whitespace_008.cs @errors_whitespace_008.cs.rsp
var csc = CreateCSharpCompiler(rsp, WorkingDirectory, new[] { source, "/preferreduilang:en" });
int exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
CleanupAllGeneratedFiles(source);
CleanupAllGeneratedFiles(rsp);
}
[WorkItem(1784, "https://github.com/dotnet/roslyn/issues/1784")]
[Fact]
public void QuotedDefineInRespFileErr()
{
string source = Temp.CreateFile("a.cs").WriteAllText(@"
#if NN
class myClass
{
#endif
static int Main()
#if DD
{
return 1;
#endif
#if AA
}
#endif
#if BB
}
#endif
").Path;
string rsp = Temp.CreateFile().WriteAllText(@"
/d:""DD""""
/d:""AA;BB""
/d:""N"" ""N
").Path;
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
// csc errors_whitespace_008.cs @errors_whitespace_008.cs.rsp
var csc = CreateCSharpCompiler(rsp, WorkingDirectory, new[] { source, "/preferreduilang:en" });
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
CleanupAllGeneratedFiles(source);
CleanupAllGeneratedFiles(rsp);
}
[Fact]
public void ResponseFileSplitting()
{
string[] responseFile;
responseFile = new string[] {
@"a.cs b.cs ""c.cs e.cs""",
@"hello world # this is a comment"
};
IEnumerable<string> args = CSharpCommandLineParser.ParseResponseLines(responseFile);
AssertEx.Equal(new[] { "a.cs", "b.cs", @"c.cs e.cs", "hello", "world" }, args);
// Check comment handling; comment character only counts at beginning of argument
responseFile = new string[] {
@" # ignore this",
@" # ignore that ""hello""",
@" a.cs #3.cs",
@" b#.cs c#d.cs #e.cs",
@" ""#f.cs""",
@" ""#g.cs #h.cs"""
};
args = CSharpCommandLineParser.ParseResponseLines(responseFile);
AssertEx.Equal(new[] { "a.cs", "b#.cs", "c#d.cs", "#f.cs", "#g.cs #h.cs" }, args);
// Check backslash escaping
responseFile = new string[] {
@"a\b\c d\\e\\f\\ \\\g\\\h\\\i \\\\ \\\\\k\\\\\",
};
args = CSharpCommandLineParser.ParseResponseLines(responseFile);
AssertEx.Equal(new[] { @"a\b\c", @"d\\e\\f\\", @"\\\g\\\h\\\i", @"\\\\", @"\\\\\k\\\\\" }, args);
// More backslash escaping and quoting
responseFile = new string[] {
@"a\""a b\\""b c\\\""c d\\\\""d e\\\\\""e f"" g""",
};
args = CSharpCommandLineParser.ParseResponseLines(responseFile);
AssertEx.Equal(new[] { @"a\""a", @"b\\""b c\\\""c d\\\\""d", @"e\\\\\""e", @"f"" g""" }, args);
// Quoting inside argument is valid.
responseFile = new string[] {
@" /o:""goo.cs"" /o:""abc def""\baz ""/o:baz bar""bing",
};
args = CSharpCommandLineParser.ParseResponseLines(responseFile);
AssertEx.Equal(new[] { @"/o:""goo.cs""", @"/o:""abc def""\baz", @"""/o:baz bar""bing" }, args);
}
[ConditionalFact(typeof(WindowsOnly))]
private void SourceFileQuoting()
{
string[] responseFile = new string[] {
@"d:\\""abc def""\baz.cs ab""c d""e.cs",
};
CSharpCommandLineArguments args = DefaultParse(CSharpCommandLineParser.ParseResponseLines(responseFile), @"c:\");
AssertEx.Equal(new[] { @"d:\abc def\baz.cs", @"c:\abc de.cs" }, args.SourceFiles.Select(file => file.Path));
}
[WorkItem(544441, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/544441")]
[Fact]
public void OutputFileName1()
{
string source1 = @"
class A
{
}
";
string source2 = @"
class B
{
static void Main() { }
}
";
// Name comes from first input (file, not class) name, since DLL.
CheckOutputFileName(
source1, source2,
inputName1: "p.cs", inputName2: "q.cs",
commandLineArguments: new[] { "/target:library" },
expectedOutputName: "p.dll");
}
[WorkItem(544441, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/544441")]
[Fact]
public void OutputFileName2()
{
string source1 = @"
class A
{
}
";
string source2 = @"
class B
{
static void Main() { }
}
";
// Name comes from command-line option.
CheckOutputFileName(
source1, source2,
inputName1: "p.cs", inputName2: "q.cs",
commandLineArguments: new[] { "/target:library", "/out:r.dll" },
expectedOutputName: "r.dll");
}
[WorkItem(544441, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/544441")]
[Fact]
public void OutputFileName3()
{
string source1 = @"
class A
{
}
";
string source2 = @"
class B
{
static void Main() { }
}
";
// Name comes from name of file containing entrypoint, since EXE.
CheckOutputFileName(
source1, source2,
inputName1: "p.cs", inputName2: "q.cs",
commandLineArguments: new[] { "/target:exe" },
expectedOutputName: "q.exe");
}
[WorkItem(544441, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/544441")]
[Fact]
public void OutputFileName4()
{
string source1 = @"
class A
{
}
";
string source2 = @"
class B
{
static void Main() { }
}
";
// Name comes from command-line option.
CheckOutputFileName(
source1, source2,
inputName1: "p.cs", inputName2: "q.cs",
commandLineArguments: new[] { "/target:exe", "/out:r.exe" },
expectedOutputName: "r.exe");
}
[WorkItem(544441, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/544441")]
[Fact]
public void OutputFileName5()
{
string source1 = @"
class A
{
static void Main() { }
}
";
string source2 = @"
class B
{
static void Main() { }
}
";
// Name comes from name of file containing entrypoint - affected by /main, since EXE.
CheckOutputFileName(
source1, source2,
inputName1: "p.cs", inputName2: "q.cs",
commandLineArguments: new[] { "/target:exe", "/main:A" },
expectedOutputName: "p.exe");
}
[WorkItem(544441, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/544441")]
[Fact]
public void OutputFileName6()
{
string source1 = @"
class A
{
static void Main() { }
}
";
string source2 = @"
class B
{
static void Main() { }
}
";
// Name comes from name of file containing entrypoint - affected by /main, since EXE.
CheckOutputFileName(
source1, source2,
inputName1: "p.cs", inputName2: "q.cs",
commandLineArguments: new[] { "/target:exe", "/main:B" },
expectedOutputName: "q.exe");
}
[WorkItem(544441, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/544441")]
[Fact]
public void OutputFileName7()
{
string source1 = @"
partial class A
{
static partial void Main() { }
}
";
string source2 = @"
partial class A
{
static partial void Main();
}
";
// Name comes from name of file containing entrypoint, since EXE.
CheckOutputFileName(
source1, source2,
inputName1: "p.cs", inputName2: "q.cs",
commandLineArguments: new[] { "/target:exe" },
expectedOutputName: "p.exe");
}
[WorkItem(544441, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/544441")]
[Fact]
public void OutputFileName8()
{
string source1 = @"
partial class A
{
static partial void Main();
}
";
string source2 = @"
partial class A
{
static partial void Main() { }
}
";
// Name comes from name of file containing entrypoint, since EXE.
CheckOutputFileName(
source1, source2,
inputName1: "p.cs", inputName2: "q.cs",
commandLineArguments: new[] { "/target:exe" },
expectedOutputName: "q.exe");
}
[Fact]
public void OutputFileName9()
{
string source1 = @"
class A
{
}
";
string source2 = @"
class B
{
static void Main() { }
}
";
// Name comes from first input (file, not class) name, since winmdobj.
CheckOutputFileName(
source1, source2,
inputName1: "p.cs", inputName2: "q.cs",
commandLineArguments: new[] { "/target:winmdobj" },
expectedOutputName: "p.winmdobj");
}
[Fact]
public void OutputFileName10()
{
string source1 = @"
class A
{
}
";
string source2 = @"
class B
{
static void Main() { }
}
";
// Name comes from name of file containing entrypoint, since appcontainerexe.
CheckOutputFileName(
source1, source2,
inputName1: "p.cs", inputName2: "q.cs",
commandLineArguments: new[] { "/target:appcontainerexe" },
expectedOutputName: "q.exe");
}
[Fact]
public void OutputFileName_Switch()
{
string source1 = @"
class A
{
}
";
string source2 = @"
class B
{
static void Main() { }
}
";
// Name comes from name of file containing entrypoint, since EXE.
CheckOutputFileName(
source1, source2,
inputName1: "p.cs", inputName2: "q.cs",
commandLineArguments: new[] { "/target:exe", "/out:r.exe" },
expectedOutputName: "r.exe");
}
[Fact]
public void OutputFileName_NoEntryPoint()
{
string source = @"
class C
{
}
";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile("a.cs");
file.WriteAllText(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "/preferreduilang:en", "/target:exe", "a.cs" });
int exitCode = csc.Run(outWriter);
Assert.NotEqual(0, exitCode);
Assert.Equal("error CS5001: Program does not contain a static 'Main' method suitable for an entry point", outWriter.ToString().Trim());
CleanupAllGeneratedFiles(file.Path);
}
[Fact, WorkItem(1093063, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/1093063")]
public void VerifyDiagnosticSeverityNotLocalized()
{
string source = @"
class C
{
}
";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile("a.cs");
file.WriteAllText(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "/target:exe", "a.cs" });
int exitCode = csc.Run(outWriter);
Assert.NotEqual(0, exitCode);
// If "error" was localized, below assert will fail on PLOC builds. The output would be something like: "!pTCvB!vbc : !FLxft!error 表! CS5001:"
Assert.Contains("error CS5001:", outWriter.ToString().Trim());
CleanupAllGeneratedFiles(file.Path);
}
[Fact]
public void NoLogo_1()
{
string source = @"
class C
{
}
";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile("a.cs");
file.WriteAllText(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "/target:library", "a.cs" });
int exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal(@"",
outWriter.ToString().Trim());
CleanupAllGeneratedFiles(file.Path);
}
[Fact]
public void NoLogo_2()
{
string source = @"
class C
{
}
";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile("a.cs");
file.WriteAllText(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { "/target:library", "/preferreduilang:en", "a.cs" });
int exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
var patched = Regex.Replace(outWriter.ToString().Trim(), "version \\d+\\.\\d+\\.\\d+(-[\\w\\d]+)*", "version A.B.C-d");
patched = ReplaceCommitHash(patched);
Assert.Equal(@"
Microsoft (R) Visual C# Compiler version A.B.C-d (HASH)
Copyright (C) Microsoft Corporation. All rights reserved.".Trim(),
patched);
CleanupAllGeneratedFiles(file.Path);
}
[Theory,
InlineData("Microsoft (R) Visual C# Compiler version A.B.C-d (<developer build>)",
"Microsoft (R) Visual C# Compiler version A.B.C-d (HASH)"),
InlineData("Microsoft (R) Visual C# Compiler version A.B.C-d (ABCDEF01)",
"Microsoft (R) Visual C# Compiler version A.B.C-d (HASH)"),
InlineData("Microsoft (R) Visual C# Compiler version A.B.C-d (abcdef90)",
"Microsoft (R) Visual C# Compiler version A.B.C-d (HASH)"),
InlineData("Microsoft (R) Visual C# Compiler version A.B.C-d (12345678)",
"Microsoft (R) Visual C# Compiler version A.B.C-d (HASH)")]
public void TestReplaceCommitHash(string orig, string expected)
{
Assert.Equal(expected, ReplaceCommitHash(orig));
}
private static string ReplaceCommitHash(string s)
{
// open paren, followed by either <developer build> or 8 hex, followed by close paren
return Regex.Replace(s, "(\\((<developer build>|[a-fA-F0-9]{8})\\))", "(HASH)");
}
[Fact]
public void ExtractShortCommitHash()
{
Assert.Null(CommonCompiler.ExtractShortCommitHash(null));
Assert.Equal("", CommonCompiler.ExtractShortCommitHash(""));
Assert.Equal("<", CommonCompiler.ExtractShortCommitHash("<"));
Assert.Equal("<developer build>", CommonCompiler.ExtractShortCommitHash("<developer build>"));
Assert.Equal("1", CommonCompiler.ExtractShortCommitHash("1"));
Assert.Equal("1234567", CommonCompiler.ExtractShortCommitHash("1234567"));
Assert.Equal("12345678", CommonCompiler.ExtractShortCommitHash("12345678"));
Assert.Equal("12345678", CommonCompiler.ExtractShortCommitHash("123456789"));
}
private void CheckOutputFileName(string source1, string source2, string inputName1, string inputName2, string[] commandLineArguments, string expectedOutputName)
{
var dir = Temp.CreateDirectory();
var file1 = dir.CreateFile(inputName1);
file1.WriteAllText(source1);
var file2 = dir.CreateFile(inputName2);
file2.WriteAllText(source2);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, commandLineArguments.Concat(new[] { inputName1, inputName2 }).ToArray());
int exitCode = csc.Run(outWriter);
if (exitCode != 0)
{
Console.WriteLine(outWriter.ToString());
Assert.Equal(0, exitCode);
}
Assert.Equal(1, Directory.EnumerateFiles(dir.Path, "*" + PathUtilities.GetExtension(expectedOutputName)).Count());
Assert.Equal(1, Directory.EnumerateFiles(dir.Path, expectedOutputName).Count());
using (var metadata = ModuleMetadata.CreateFromImage(File.ReadAllBytes(Path.Combine(dir.Path, expectedOutputName))))
{
var peReader = metadata.Module.GetMetadataReader();
Assert.True(peReader.IsAssembly);
Assert.Equal(PathUtilities.RemoveExtension(expectedOutputName), peReader.GetString(peReader.GetAssemblyDefinition().Name));
Assert.Equal(expectedOutputName, peReader.GetString(peReader.GetModuleDefinition().Name));
}
if (System.IO.File.Exists(expectedOutputName))
{
System.IO.File.Delete(expectedOutputName);
}
CleanupAllGeneratedFiles(file1.Path);
CleanupAllGeneratedFiles(file2.Path);
}
[Fact]
public void MissingReference()
{
string source = @"
class C
{
}
";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile("a.cs");
file.WriteAllText(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "/preferreduilang:en", "/r:missing.dll", "a.cs" });
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal("error CS0006: Metadata file 'missing.dll' could not be found", outWriter.ToString().Trim());
CleanupAllGeneratedFiles(file.Path);
}
[WorkItem(545025, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/545025")]
[ConditionalFact(typeof(WindowsOnly))]
public void CompilationWithWarnAsError_01()
{
string source = @"
public class C
{
public static void Main()
{
}
}";
// Baseline without warning options (expect success)
int exitCode = GetExitCode(source, "a.cs", new String[] { });
Assert.Equal(0, exitCode);
// The case with /warnaserror (expect to be success, since there will be no warning)
exitCode = GetExitCode(source, "b.cs", new[] { "/warnaserror" });
Assert.Equal(0, exitCode);
// The case with /warnaserror and /nowarn:1 (expect success)
// Note that even though the command line option has a warning, it is not going to become an error
// in order to avoid the halt of compilation.
exitCode = GetExitCode(source, "c.cs", new[] { "/warnaserror", "/nowarn:1" });
Assert.Equal(0, exitCode);
}
[WorkItem(545025, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/545025")]
[ConditionalFact(typeof(WindowsOnly))]
public void CompilationWithWarnAsError_02()
{
string source = @"
public class C
{
public static void Main()
{
int x; // CS0168
}
}";
// Baseline without warning options (expect success)
int exitCode = GetExitCode(source, "a.cs", new String[] { });
Assert.Equal(0, exitCode);
// The case with /warnaserror (expect failure)
exitCode = GetExitCode(source, "b.cs", new[] { "/warnaserror" });
Assert.NotEqual(0, exitCode);
// The case with /warnaserror:168 (expect failure)
exitCode = GetExitCode(source, "c.cs", new[] { "/warnaserror:168" });
Assert.NotEqual(0, exitCode);
// The case with /warnaserror:219 (expect success)
exitCode = GetExitCode(source, "c.cs", new[] { "/warnaserror:219" });
Assert.Equal(0, exitCode);
// The case with /warnaserror and /nowarn:168 (expect success)
exitCode = GetExitCode(source, "d.cs", new[] { "/warnaserror", "/nowarn:168" });
Assert.Equal(0, exitCode);
}
private int GetExitCode(string source, string fileName, string[] commandLineArguments)
{
var dir = Temp.CreateDirectory();
var file = dir.CreateFile(fileName);
file.WriteAllText(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, commandLineArguments.Concat(new[] { fileName }).ToArray());
int exitCode = csc.Run(outWriter);
return exitCode;
}
[WorkItem(545247, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/545247")]
[ConditionalFact(typeof(WindowsOnly))]
public void CompilationWithNonExistingOutPath()
{
string source = @"
public class C
{
public static void Main()
{
}
}";
var fileName = "a.cs";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile(fileName);
file.WriteAllText(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { fileName, "/preferreduilang:en", "/target:exe", "/out:sub\\a.exe" });
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Contains("error CS2012: Cannot open '" + dir.Path + "\\sub\\a.exe' for writing", outWriter.ToString(), StringComparison.Ordinal);
CleanupAllGeneratedFiles(file.Path);
}
[WorkItem(545247, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/545247")]
[Fact]
public void CompilationWithWrongOutPath_01()
{
string source = @"
public class C
{
public static void Main()
{
}
}";
var fileName = "a.cs";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile(fileName);
file.WriteAllText(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { fileName, "/preferreduilang:en", "/target:exe", "/out:sub\\" });
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
var message = outWriter.ToString();
Assert.Contains("error CS2021: File name", message, StringComparison.Ordinal);
Assert.Contains("sub", message, StringComparison.Ordinal);
CleanupAllGeneratedFiles(file.Path);
}
[WorkItem(545247, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/545247")]
[Fact]
public void CompilationWithWrongOutPath_02()
{
string source = @"
public class C
{
public static void Main()
{
}
}";
var fileName = "a.cs";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile(fileName);
file.WriteAllText(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { fileName, "/preferreduilang:en", "/target:exe", "/out:sub\\ " });
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
var message = outWriter.ToString();
Assert.Contains("error CS2021: File name", message, StringComparison.Ordinal);
Assert.Contains("sub", message, StringComparison.Ordinal);
CleanupAllGeneratedFiles(file.Path);
}
[WorkItem(545247, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/545247")]
[ConditionalFact(typeof(WindowsDesktopOnly))]
public void CompilationWithWrongOutPath_03()
{
string source = @"
public class C
{
public static void Main()
{
}
}";
var fileName = "a.cs";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile(fileName);
file.WriteAllText(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { fileName, "/preferreduilang:en", "/target:exe", "/out:aaa:\\a.exe" });
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Contains(@"error CS2021: File name 'aaa:\a.exe' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long", outWriter.ToString(), StringComparison.Ordinal);
CleanupAllGeneratedFiles(file.Path);
}
[WorkItem(545247, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/545247")]
[Fact]
public void CompilationWithWrongOutPath_04()
{
string source = @"
public class C
{
public static void Main()
{
}
}";
var fileName = "a.cs";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile(fileName);
file.WriteAllText(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, new[] { fileName, "/preferreduilang:en", "/target:exe", "/out: " });
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Contains("error CS2005: Missing file specification for '/out:' option", outWriter.ToString(), StringComparison.Ordinal);
CleanupAllGeneratedFiles(file.Path);
}
[Fact]
public void EmittedSubsystemVersion()
{
var compilation = CSharpCompilation.Create("a.dll", references: new[] { MscorlibRef }, options: TestOptions.ReleaseDll);
var peHeaders = new PEHeaders(compilation.EmitToStream(options: new EmitOptions(subsystemVersion: SubsystemVersion.Create(5, 1))));
Assert.Equal(5, peHeaders.PEHeader.MajorSubsystemVersion);
Assert.Equal(1, peHeaders.PEHeader.MinorSubsystemVersion);
}
[Fact]
public void CreateCompilationWithKeyFile()
{
string source = @"
public class C
{
public static void Main()
{
}
}";
var fileName = "a.cs";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile(fileName);
file.WriteAllText(source);
var cmd = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "a.cs", "/keyfile:key.snk", });
var comp = cmd.CreateCompilation(TextWriter.Null, new TouchedFileLogger(), NullErrorLogger.Instance);
Assert.IsType<DesktopStrongNameProvider>(comp.Options.StrongNameProvider);
}
[Fact]
public void CreateCompilationWithKeyContainer()
{
string source = @"
public class C
{
public static void Main()
{
}
}";
var fileName = "a.cs";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile(fileName);
file.WriteAllText(source);
var cmd = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "a.cs", "/keycontainer:bbb", });
var comp = cmd.CreateCompilation(TextWriter.Null, new TouchedFileLogger(), NullErrorLogger.Instance);
Assert.Equal(typeof(DesktopStrongNameProvider), comp.Options.StrongNameProvider.GetType());
}
[Fact]
public void CreateCompilationFallbackCommand()
{
string source = @"
public class C
{
public static void Main()
{
}
}";
var fileName = "a.cs";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile(fileName);
file.WriteAllText(source);
var cmd = CreateCSharpCompiler(null, dir.Path, new[] { "/nologo", "a.cs", "/keyFile:key.snk", "/features:UseLegacyStrongNameProvider" });
var comp = cmd.CreateCompilation(TextWriter.Null, new TouchedFileLogger(), NullErrorLogger.Instance);
Assert.Equal(typeof(DesktopStrongNameProvider), comp.Options.StrongNameProvider.GetType());
}
[Fact]
public void CreateCompilation_MainAndTargetIncompatibilities()
{
string source = @"
public class C
{
public static void Main()
{
}
}";
var fileName = "a.cs";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile(fileName);
file.WriteAllText(source);
var compilation = CSharpCompilation.Create("a.dll", options: TestOptions.ReleaseDll);
var options = compilation.Options;
Assert.Equal(0, options.Errors.Length);
options = options.WithMainTypeName("a");
options.Errors.Verify(
// error CS2017: Cannot specify /main if building a module or library
Diagnostic(ErrorCode.ERR_NoMainOnDLL)
);
var comp = CSharpCompilation.Create("a.dll", options: options);
comp.GetDiagnostics().Verify(
// error CS2017: Cannot specify /main if building a module or library
Diagnostic(ErrorCode.ERR_NoMainOnDLL)
);
options = options.WithOutputKind(OutputKind.WindowsApplication);
options.Errors.Verify();
comp = CSharpCompilation.Create("a.dll", options: options);
comp.GetDiagnostics().Verify(
// error CS1555: Could not find 'a' specified for Main method
Diagnostic(ErrorCode.ERR_MainClassNotFound).WithArguments("a")
);
options = options.WithOutputKind(OutputKind.NetModule);
options.Errors.Verify(
// error CS2017: Cannot specify /main if building a module or library
Diagnostic(ErrorCode.ERR_NoMainOnDLL)
);
comp = CSharpCompilation.Create("a.dll", options: options);
comp.GetDiagnostics().Verify(
// error CS2017: Cannot specify /main if building a module or library
Diagnostic(ErrorCode.ERR_NoMainOnDLL)
);
options = options.WithMainTypeName(null);
options.Errors.Verify();
comp = CSharpCompilation.Create("a.dll", options: options);
comp.GetDiagnostics().Verify();
CleanupAllGeneratedFiles(file.Path);
}
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30328")]
public void SpecifyProperCodePage()
{
byte[] source = {
0x63, // c
0x6c, // l
0x61, // a
0x73, // s
0x73, // s
0x20, //
0xd0, 0x96, // Utf-8 Cyrillic character
0x7b, // {
0x7d, // }
};
var fileName = "a.cs";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile(fileName);
file.WriteAllBytes(source);
var output = ProcessUtilities.RunAndGetOutput(s_CSharpCompilerExecutable, $"/nologo /t:library \"{file}\"", startFolder: dir.Path);
Assert.Equal("", output); // Autodetected UTF8, NO ERROR
output = ProcessUtilities.RunAndGetOutput(s_CSharpCompilerExecutable, $"/nologo /preferreduilang:en /t:library /codepage:20127 \"{file}\"", expectedRetCode: 1, startFolder: dir.Path); // 20127: US-ASCII
// 0xd0, 0x96 ==> ERROR
Assert.Equal(@"
a.cs(1,7): error CS1001: Identifier expected
a.cs(1,7): error CS1514: { expected
a.cs(1,7): error CS1513: } expected
a.cs(1,7): error CS8803: Top-level statements must precede namespace and type declarations.
a.cs(1,7): error CS1525: Invalid expression term '??'
a.cs(1,9): error CS1525: Invalid expression term '{'
a.cs(1,9): error CS1002: ; expected
".Trim(),
Regex.Replace(output, "^.*a.cs", "a.cs", RegexOptions.Multiline).Trim());
CleanupAllGeneratedFiles(file.Path);
}
[ConditionalFact(typeof(WindowsOnly))]
public void DefaultWin32ResForExe()
{
var source = @"
class C
{
static void Main() { }
}
";
CheckManifestString(source, OutputKind.ConsoleApplication, explicitManifest: null, expectedManifest:
@"<?xml version=""1.0"" encoding=""utf-16""?>
<ManifestResource Size=""490"">
<Contents><![CDATA[<?xml version=""1.0"" encoding=""UTF-8"" standalone=""yes""?>
<assembly xmlns=""urn:schemas-microsoft-com:asm.v1"" manifestVersion=""1.0"">
<assemblyIdentity version=""1.0.0.0"" name=""MyApplication.app""/>
<trustInfo xmlns=""urn:schemas-microsoft-com:asm.v2"">
<security>
<requestedPrivileges xmlns=""urn:schemas-microsoft-com:asm.v3"">
<requestedExecutionLevel level=""asInvoker"" uiAccess=""false""/>
</requestedPrivileges>
</security>
</trustInfo>
</assembly>]]></Contents>
</ManifestResource>");
}
[ConditionalFact(typeof(WindowsOnly))]
public void DefaultManifestForDll()
{
var source = @"
class C
{
}
";
CheckManifestString(source, OutputKind.DynamicallyLinkedLibrary, explicitManifest: null, expectedManifest: null);
}
[ConditionalFact(typeof(WindowsOnly))]
public void DefaultManifestForWinExe()
{
var source = @"
class C
{
static void Main() { }
}
";
CheckManifestString(source, OutputKind.WindowsApplication, explicitManifest: null, expectedManifest:
@"<?xml version=""1.0"" encoding=""utf-16""?>
<ManifestResource Size=""490"">
<Contents><![CDATA[<?xml version=""1.0"" encoding=""UTF-8"" standalone=""yes""?>
<assembly xmlns=""urn:schemas-microsoft-com:asm.v1"" manifestVersion=""1.0"">
<assemblyIdentity version=""1.0.0.0"" name=""MyApplication.app""/>
<trustInfo xmlns=""urn:schemas-microsoft-com:asm.v2"">
<security>
<requestedPrivileges xmlns=""urn:schemas-microsoft-com:asm.v3"">
<requestedExecutionLevel level=""asInvoker"" uiAccess=""false""/>
</requestedPrivileges>
</security>
</trustInfo>
</assembly>]]></Contents>
</ManifestResource>");
}
[ConditionalFact(typeof(WindowsOnly))]
public void DefaultManifestForAppContainerExe()
{
var source = @"
class C
{
static void Main() { }
}
";
CheckManifestString(source, OutputKind.WindowsRuntimeApplication, explicitManifest: null, expectedManifest:
@"<?xml version=""1.0"" encoding=""utf-16""?>
<ManifestResource Size=""490"">
<Contents><![CDATA[<?xml version=""1.0"" encoding=""UTF-8"" standalone=""yes""?>
<assembly xmlns=""urn:schemas-microsoft-com:asm.v1"" manifestVersion=""1.0"">
<assemblyIdentity version=""1.0.0.0"" name=""MyApplication.app""/>
<trustInfo xmlns=""urn:schemas-microsoft-com:asm.v2"">
<security>
<requestedPrivileges xmlns=""urn:schemas-microsoft-com:asm.v3"">
<requestedExecutionLevel level=""asInvoker"" uiAccess=""false""/>
</requestedPrivileges>
</security>
</trustInfo>
</assembly>]]></Contents>
</ManifestResource>");
}
[ConditionalFact(typeof(WindowsOnly))]
public void DefaultManifestForWinMD()
{
var source = @"
class C
{
}
";
CheckManifestString(source, OutputKind.WindowsRuntimeMetadata, explicitManifest: null, expectedManifest: null);
}
[ConditionalFact(typeof(WindowsOnly))]
public void DefaultWin32ResForModule()
{
var source = @"
class C
{
}
";
CheckManifestString(source, OutputKind.NetModule, explicitManifest: null, expectedManifest: null);
}
[ConditionalFact(typeof(WindowsOnly))]
public void ExplicitWin32ResForExe()
{
var source = @"
class C
{
static void Main() { }
}
";
var explicitManifest =
@"<?xml version=""1.0"" encoding=""UTF-8"" standalone=""yes""?>
<assembly xmlns=""urn:schemas-microsoft-com:asm.v1"" manifestVersion=""1.0"">
<assemblyIdentity version=""1.0.0.0"" name=""Test.app""/>
<trustInfo xmlns=""urn:schemas-microsoft-com:asm.v2"">
<security>
<requestedPrivileges xmlns=""urn:schemas-microsoft-com:asm.v3"">
<requestedExecutionLevel level=""asInvoker"" uiAccess=""false""/>
</requestedPrivileges>
</security>
</trustInfo>
</assembly>";
var explicitManifestStream = new MemoryStream(Encoding.UTF8.GetBytes(explicitManifest));
var expectedManifest =
@"<?xml version=""1.0"" encoding=""utf-16""?>
<ManifestResource Size=""476"">
<Contents><![CDATA[" +
explicitManifest +
@"]]></Contents>
</ManifestResource>";
CheckManifestString(source, OutputKind.ConsoleApplication, explicitManifest, expectedManifest);
}
// DLLs don't get the default manifest, but they do respect explicitly set manifests.
[ConditionalFact(typeof(WindowsOnly))]
public void ExplicitWin32ResForDll()
{
var source = @"
class C
{
static void Main() { }
}
";
var explicitManifest =
@"<?xml version=""1.0"" encoding=""UTF-8"" standalone=""yes""?>
<assembly xmlns=""urn:schemas-microsoft-com:asm.v1"" manifestVersion=""1.0"">
<assemblyIdentity version=""1.0.0.0"" name=""Test.app""/>
<trustInfo xmlns=""urn:schemas-microsoft-com:asm.v2"">
<security>
<requestedPrivileges xmlns=""urn:schemas-microsoft-com:asm.v3"">
<requestedExecutionLevel level=""asInvoker"" uiAccess=""false""/>
</requestedPrivileges>
</security>
</trustInfo>
</assembly>";
var expectedManifest =
@"<?xml version=""1.0"" encoding=""utf-16""?>
<ManifestResource Size=""476"">
<Contents><![CDATA[" +
explicitManifest +
@"]]></Contents>
</ManifestResource>";
CheckManifestString(source, OutputKind.DynamicallyLinkedLibrary, explicitManifest, expectedManifest);
}
// Modules don't have manifests, even if one is explicitly specified.
[ConditionalFact(typeof(WindowsOnly))]
public void ExplicitWin32ResForModule()
{
var source = @"
class C
{
}
";
var explicitManifest =
@"<?xml version=""1.0"" encoding=""UTF-8"" standalone=""yes""?>
<assembly xmlns=""urn:schemas-microsoft-com:asm.v1"" manifestVersion=""1.0"">
<assemblyIdentity version=""1.0.0.0"" name=""Test.app""/>
<trustInfo xmlns=""urn:schemas-microsoft-com:asm.v2"">
<security>
<requestedPrivileges xmlns=""urn:schemas-microsoft-com:asm.v3"">
<requestedExecutionLevel level=""asInvoker"" uiAccess=""false""/>
</requestedPrivileges>
</security>
</trustInfo>
</assembly>";
CheckManifestString(source, OutputKind.NetModule, explicitManifest, expectedManifest: null);
}
[DllImport("kernel32.dll", SetLastError = true)]
private static extern IntPtr LoadLibraryEx(string lpFileName, IntPtr hFile, uint dwFlags);
[DllImport("kernel32.dll", SetLastError = true)]
private static extern bool FreeLibrary([In] IntPtr hFile);
private void CheckManifestString(string source, OutputKind outputKind, string explicitManifest, string expectedManifest)
{
var dir = Temp.CreateDirectory();
var sourceFile = dir.CreateFile("Test.cs").WriteAllText(source);
string outputFileName;
string target;
switch (outputKind)
{
case OutputKind.ConsoleApplication:
outputFileName = "Test.exe";
target = "exe";
break;
case OutputKind.WindowsApplication:
outputFileName = "Test.exe";
target = "winexe";
break;
case OutputKind.DynamicallyLinkedLibrary:
outputFileName = "Test.dll";
target = "library";
break;
case OutputKind.NetModule:
outputFileName = "Test.netmodule";
target = "module";
break;
case OutputKind.WindowsRuntimeMetadata:
outputFileName = "Test.winmdobj";
target = "winmdobj";
break;
case OutputKind.WindowsRuntimeApplication:
outputFileName = "Test.exe";
target = "appcontainerexe";
break;
default:
throw TestExceptionUtilities.UnexpectedValue(outputKind);
}
MockCSharpCompiler csc;
if (explicitManifest == null)
{
csc = CreateCSharpCompiler(null, dir.Path, new[]
{
string.Format("/target:{0}", target),
string.Format("/out:{0}", outputFileName),
Path.GetFileName(sourceFile.Path),
});
}
else
{
var manifestFile = dir.CreateFile("Test.config").WriteAllText(explicitManifest);
csc = CreateCSharpCompiler(null, dir.Path, new[]
{
string.Format("/target:{0}", target),
string.Format("/out:{0}", outputFileName),
string.Format("/win32manifest:{0}", Path.GetFileName(manifestFile.Path)),
Path.GetFileName(sourceFile.Path),
});
}
int actualExitCode = csc.Run(new StringWriter(CultureInfo.InvariantCulture));
Assert.Equal(0, actualExitCode);
//Open as data
IntPtr lib = LoadLibraryEx(Path.Combine(dir.Path, outputFileName), IntPtr.Zero, 0x00000002);
if (lib == IntPtr.Zero)
throw new Win32Exception(Marshal.GetLastWin32Error());
const string resourceType = "#24";
var resourceId = outputKind == OutputKind.DynamicallyLinkedLibrary ? "#2" : "#1";
uint manifestSize;
if (expectedManifest == null)
{
Assert.Throws<Win32Exception>(() => Win32Res.GetResource(lib, resourceId, resourceType, out manifestSize));
}
else
{
IntPtr manifestResourcePointer = Win32Res.GetResource(lib, resourceId, resourceType, out manifestSize);
string actualManifest = Win32Res.ManifestResourceToXml(manifestResourcePointer, manifestSize);
Assert.Equal(expectedManifest, actualManifest);
}
FreeLibrary(lib);
}
[WorkItem(544926, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/544926")]
[ConditionalFact(typeof(WindowsOnly), Reason = "https://github.com/dotnet/roslyn/issues/30289")]
public void ResponseFilesWithNoconfig_01()
{
string source = Temp.CreateFile("a.cs").WriteAllText(@"
public class C
{
public static void Main()
{
int x; // CS0168
}
}").Path;
string rsp = Temp.CreateFile().WriteAllText(@"
/warnaserror
").Path;
// Checks the base case without /noconfig (expect to see error)
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(rsp, WorkingDirectory, new[] { source, "/preferreduilang:en" });
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Contains("error CS0168: The variable 'x' is declared but never used\r\n", outWriter.ToString(), StringComparison.Ordinal);
// Checks the case with /noconfig (expect to see warning, instead of error)
outWriter = new StringWriter(CultureInfo.InvariantCulture);
csc = CreateCSharpCompiler(rsp, WorkingDirectory, new[] { source, "/noconfig", "/preferreduilang:en" });
exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Contains("warning CS0168: The variable 'x' is declared but never used\r\n", outWriter.ToString(), StringComparison.Ordinal);
// Checks the case with /NOCONFIG (expect to see warning, instead of error)
outWriter = new StringWriter(CultureInfo.InvariantCulture);
csc = CreateCSharpCompiler(rsp, WorkingDirectory, new[] { source, "/NOCONFIG", "/preferreduilang:en" });
exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Contains("warning CS0168: The variable 'x' is declared but never used\r\n", outWriter.ToString(), StringComparison.Ordinal);
// Checks the case with -noconfig (expect to see warning, instead of error)
outWriter = new StringWriter(CultureInfo.InvariantCulture);
csc = CreateCSharpCompiler(rsp, WorkingDirectory, new[] { source, "-noconfig", "/preferreduilang:en" });
exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Contains("warning CS0168: The variable 'x' is declared but never used\r\n", outWriter.ToString(), StringComparison.Ordinal);
CleanupAllGeneratedFiles(source);
CleanupAllGeneratedFiles(rsp);
}
[WorkItem(544926, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/544926")]
[ConditionalFact(typeof(WindowsOnly))]
public void ResponseFilesWithNoconfig_02()
{
string source = Temp.CreateFile("a.cs").WriteAllText(@"
public class C
{
public static void Main()
{
}
}").Path;
string rsp = Temp.CreateFile().WriteAllText(@"
/noconfig
").Path;
// Checks the case with /noconfig inside the response file (expect to see warning)
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(rsp, WorkingDirectory, new[] { source, "/preferreduilang:en" });
int exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Contains("warning CS2023: Ignoring /noconfig option because it was specified in a response file\r\n", outWriter.ToString(), StringComparison.Ordinal);
// Checks the case with /noconfig inside the response file as along with /nowarn (expect to see warning)
// to verify that this warning is not suppressed by the /nowarn option (See MSDN).
outWriter = new StringWriter(CultureInfo.InvariantCulture);
csc = CreateCSharpCompiler(rsp, WorkingDirectory, new[] { source, "/preferreduilang:en", "/nowarn:2023" });
exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Contains("warning CS2023: Ignoring /noconfig option because it was specified in a response file\r\n", outWriter.ToString(), StringComparison.Ordinal);
CleanupAllGeneratedFiles(source);
CleanupAllGeneratedFiles(rsp);
}
[WorkItem(544926, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/544926")]
[ConditionalFact(typeof(WindowsOnly), Reason = "https://github.com/dotnet/roslyn/issues/30289")]
public void ResponseFilesWithNoconfig_03()
{
string source = Temp.CreateFile("a.cs").WriteAllText(@"
public class C
{
public static void Main()
{
}
}").Path;
string rsp = Temp.CreateFile().WriteAllText(@"
/NOCONFIG
").Path;
// Checks the case with /noconfig inside the response file (expect to see warning)
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(rsp, WorkingDirectory, new[] { source, "/preferreduilang:en" });
int exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Contains("warning CS2023: Ignoring /noconfig option because it was specified in a response file\r\n", outWriter.ToString(), StringComparison.Ordinal);
// Checks the case with /NOCONFIG inside the response file as along with /nowarn (expect to see warning)
// to verify that this warning is not suppressed by the /nowarn option (See MSDN).
outWriter = new StringWriter(CultureInfo.InvariantCulture);
csc = CreateCSharpCompiler(rsp, WorkingDirectory, new[] { source, "/preferreduilang:en", "/nowarn:2023" });
exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Contains("warning CS2023: Ignoring /noconfig option because it was specified in a response file\r\n", outWriter.ToString(), StringComparison.Ordinal);
CleanupAllGeneratedFiles(source);
CleanupAllGeneratedFiles(rsp);
}
[WorkItem(544926, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/544926")]
[ConditionalFact(typeof(WindowsOnly))]
public void ResponseFilesWithNoconfig_04()
{
string source = Temp.CreateFile("a.cs").WriteAllText(@"
public class C
{
public static void Main()
{
}
}").Path;
string rsp = Temp.CreateFile().WriteAllText(@"
-noconfig
").Path;
// Checks the case with /noconfig inside the response file (expect to see warning)
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(rsp, WorkingDirectory, new[] { source, "/preferreduilang:en" });
int exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Contains("warning CS2023: Ignoring /noconfig option because it was specified in a response file\r\n", outWriter.ToString(), StringComparison.Ordinal);
// Checks the case with -noconfig inside the response file as along with /nowarn (expect to see warning)
// to verify that this warning is not suppressed by the /nowarn option (See MSDN).
outWriter = new StringWriter(CultureInfo.InvariantCulture);
csc = CreateCSharpCompiler(rsp, WorkingDirectory, new[] { source, "/preferreduilang:en", "/nowarn:2023" });
exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Contains("warning CS2023: Ignoring /noconfig option because it was specified in a response file\r\n", outWriter.ToString(), StringComparison.Ordinal);
CleanupAllGeneratedFiles(source);
CleanupAllGeneratedFiles(rsp);
}
[Fact, WorkItem(530024, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/530024")]
public void NoStdLib()
{
var src = Temp.CreateFile("a.cs");
src.WriteAllText("public class C{}");
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/t:library", src.ToString() }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("", outWriter.ToString().Trim());
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/preferreduilang:en", "/nostdlib", "/t:library", src.ToString() }).Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal("{FILE}(1,14): error CS0518: Predefined type 'System.Object' is not defined or imported",
outWriter.ToString().Replace(Path.GetFileName(src.Path), "{FILE}").Trim());
// Bug#15021: breaking change - empty source no error with /nostdlib
src.WriteAllText("namespace System { }");
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/nostdlib", "/t:library", "/runtimemetadataversion:v4.0.30319", "/langversion:8", src.ToString() }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("", outWriter.ToString().Trim());
CleanupAllGeneratedFiles(src.Path);
}
private string GetDefaultResponseFilePath()
{
var cscRsp = global::TestResources.ResourceLoader.GetResourceBlob("csc.rsp");
return Temp.CreateFile().WriteAllBytes(cscRsp).Path;
}
[Fact, WorkItem(530359, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/530359")]
public void NoStdLib02()
{
#region "source"
var source = @"
// <Title>A collection initializer can be declared with a user-defined IEnumerable that is declared in a user-defined System.Collections</Title>
using System.Collections;
class O<T> where T : new()
{
public T list = new T();
}
class C
{
static StructCollection sc = new StructCollection { 1 };
public static int Main()
{
ClassCollection cc = new ClassCollection { 2 };
var o1 = new O<ClassCollection> { list = { 5 } };
var o2 = new O<StructCollection> { list = sc };
return 0;
}
}
struct StructCollection : IEnumerable
{
public int added;
#region IEnumerable Members
public void Add(int t)
{
added = t;
}
#endregion
}
class ClassCollection : IEnumerable
{
public int added;
#region IEnumerable Members
public void Add(int t)
{
added = t;
}
#endregion
}
namespace System.Collections
{
public interface IEnumerable
{
void Add(int t);
}
}
";
#endregion
#region "mslib"
var mslib = @"
namespace System
{
public class Object {}
public struct Byte { }
public struct Int16 { }
public struct Int32 { }
public struct Int64 { }
public struct Single { }
public struct Double { }
public struct SByte { }
public struct UInt32 { }
public struct UInt64 { }
public struct Char { }
public struct Boolean { }
public struct UInt16 { }
public struct UIntPtr { }
public struct IntPtr { }
public class Delegate { }
public class String {
public int Length { get { return 10; } }
}
public class MulticastDelegate { }
public class Array { }
public class Exception { public Exception(string s){} }
public class Type { }
public class ValueType { }
public class Enum { }
public interface IEnumerable { }
public interface IDisposable { }
public class Attribute { }
public class ParamArrayAttribute { }
public struct Void { }
public struct RuntimeFieldHandle { }
public struct RuntimeTypeHandle { }
public class Activator
{
public static T CreateInstance<T>(){return default(T);}
}
namespace Collections
{
public interface IEnumerator { }
}
namespace Runtime
{
namespace InteropServices
{
public class OutAttribute { }
}
namespace CompilerServices
{
public class RuntimeHelpers { }
}
}
namespace Reflection
{
public class DefaultMemberAttribute { }
}
}
";
#endregion
var src = Temp.CreateFile("NoStdLib02.cs");
src.WriteAllText(source + mslib);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/noconfig", "/nostdlib", "/runtimemetadataversion:v4.0.30319", "/nowarn:8625", src.ToString() }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("", outWriter.ToString().Trim());
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/nostdlib", "/runtimemetadataversion:v4.0.30319", "/nowarn:8625", src.ToString() }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("", outWriter.ToString().Trim());
string OriginalSource = src.Path;
src = Temp.CreateFile("NoStdLib02b.cs");
src.WriteAllText(mslib);
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(GetDefaultResponseFilePath(), WorkingDirectory, new[] { "/nologo", "/noconfig", "/nostdlib", "/t:library", "/runtimemetadataversion:v4.0.30319", "/nowarn:8625", src.ToString() }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("", outWriter.ToString().Trim());
CleanupAllGeneratedFiles(OriginalSource);
CleanupAllGeneratedFiles(src.Path);
}
[Fact, WorkItem(546018, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546018"), WorkItem(546020, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546020"), WorkItem(546024, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546024"), WorkItem(546049, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546049")]
public void InvalidDefineSwitch()
{
var src = Temp.CreateFile("a.cs");
src.WriteAllText("public class C{}");
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/preferreduilang:en", src.ToString(), "/define" }).Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal("error CS2006: Command-line syntax error: Missing '<text>' for '/define' option", outWriter.ToString().Trim());
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/preferreduilang:en", "/t:library", src.ToString(), @"/define:""""" }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("warning CS2029: Invalid name for a preprocessing symbol; '' is not a valid identifier", outWriter.ToString().Trim());
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/preferreduilang:en", "/t:library", src.ToString(), "/define: " }).Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal("error CS2006: Command-line syntax error: Missing '<text>' for '/define:' option", outWriter.ToString().Trim());
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/preferreduilang:en", "/t:library", src.ToString(), "/define:" }).Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal("error CS2006: Command-line syntax error: Missing '<text>' for '/define:' option", outWriter.ToString().Trim());
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/preferreduilang:en", "/t:library", src.ToString(), "/define:,,," }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("warning CS2029: Invalid name for a preprocessing symbol; '' is not a valid identifier", outWriter.ToString().Trim());
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/preferreduilang:en", "/t:library", src.ToString(), "/define:,blah,Blah" }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("warning CS2029: Invalid name for a preprocessing symbol; '' is not a valid identifier", outWriter.ToString().Trim());
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/preferreduilang:en", "/t:library", src.ToString(), "/define:a;;b@" }).Run(outWriter);
Assert.Equal(0, exitCode);
var errorLines = outWriter.ToString().Trim().Split(new string[] { Environment.NewLine }, StringSplitOptions.None);
Assert.Equal("warning CS2029: Invalid name for a preprocessing symbol; '' is not a valid identifier", errorLines[0]);
Assert.Equal("warning CS2029: Invalid name for a preprocessing symbol; 'b@' is not a valid identifier", errorLines[1]);
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/preferreduilang:en", "/t:library", src.ToString(), "/define:a,b@;" }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("warning CS2029: Invalid name for a preprocessing symbol; 'b@' is not a valid identifier", outWriter.ToString().Trim());
//Bug 531612 - Native would normally not give the 2nd warning
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/preferreduilang:en", "/t:library", src.ToString(), @"/define:OE_WIN32=-1:LANG_HOST_EN=-1:LANG_OE_EN=-1:LANG_PRJ_EN=-1:HOST_COM20SDKEVERETT=-1:EXEMODE=-1:OE_NT5=-1:Win32=-1", @"/d:TRACE=TRUE,DEBUG=TRUE" }).Run(outWriter);
Assert.Equal(0, exitCode);
errorLines = outWriter.ToString().Trim().Split(new string[] { Environment.NewLine }, StringSplitOptions.None);
Assert.Equal(@"warning CS2029: Invalid name for a preprocessing symbol; 'OE_WIN32=-1:LANG_HOST_EN=-1:LANG_OE_EN=-1:LANG_PRJ_EN=-1:HOST_COM20SDKEVERETT=-1:EXEMODE=-1:OE_NT5=-1:Win32=-1' is not a valid identifier", errorLines[0]);
Assert.Equal(@"warning CS2029: Invalid name for a preprocessing symbol; 'TRACE=TRUE' is not a valid identifier", errorLines[1]);
CleanupAllGeneratedFiles(src.Path);
}
[WorkItem(733242, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/733242")]
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30321")]
public void Bug733242()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("a.cs");
src.WriteAllText(
@"
/// <summary>ABC...XYZ</summary>
class C {} ");
var xml = dir.CreateFile("a.xml");
xml.WriteAllText("EMPTY");
using (var xmlFileHandle = File.Open(xml.ToString(), FileMode.Open, FileAccess.Read, FileShare.Delete | FileShare.ReadWrite))
{
var output = ProcessUtilities.RunAndGetOutput(s_CSharpCompilerExecutable, String.Format("/nologo /t:library /doc:\"{1}\" \"{0}\"", src.ToString(), xml.ToString()), startFolder: dir.ToString());
Assert.Equal("", output.Trim());
Assert.True(File.Exists(Path.Combine(dir.ToString(), "a.xml")));
using (var reader = new StreamReader(xmlFileHandle))
{
var content = reader.ReadToEnd();
Assert.Equal(
@"<?xml version=""1.0""?>
<doc>
<assembly>
<name>a</name>
</assembly>
<members>
<member name=""T:C"">
<summary>ABC...XYZ</summary>
</member>
</members>
</doc>".Trim(), content.Trim());
}
}
CleanupAllGeneratedFiles(src.Path);
CleanupAllGeneratedFiles(xml.Path);
}
[WorkItem(768605, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/768605")]
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30321")]
public void Bug768605()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("a.cs");
src.WriteAllText(
@"
/// <summary>ABC</summary>
class C {}
/// <summary>XYZ</summary>
class E {}
");
var xml = dir.CreateFile("a.xml");
xml.WriteAllText("EMPTY");
var output = ProcessUtilities.RunAndGetOutput(s_CSharpCompilerExecutable, String.Format("/nologo /t:library /doc:\"{1}\" \"{0}\"", src.ToString(), xml.ToString()), startFolder: dir.ToString());
Assert.Equal("", output.Trim());
using (var reader = new StreamReader(xml.ToString()))
{
var content = reader.ReadToEnd();
Assert.Equal(
@"<?xml version=""1.0""?>
<doc>
<assembly>
<name>a</name>
</assembly>
<members>
<member name=""T:C"">
<summary>ABC</summary>
</member>
<member name=""T:E"">
<summary>XYZ</summary>
</member>
</members>
</doc>".Trim(), content.Trim());
}
src.WriteAllText(
@"
/// <summary>ABC</summary>
class C {}
");
output = ProcessUtilities.RunAndGetOutput(s_CSharpCompilerExecutable, String.Format("/nologo /t:library /doc:\"{1}\" \"{0}\"", src.ToString(), xml.ToString()), startFolder: dir.ToString());
Assert.Equal("", output.Trim());
using (var reader = new StreamReader(xml.ToString()))
{
var content = reader.ReadToEnd();
Assert.Equal(
@"<?xml version=""1.0""?>
<doc>
<assembly>
<name>a</name>
</assembly>
<members>
<member name=""T:C"">
<summary>ABC</summary>
</member>
</members>
</doc>".Trim(), content.Trim());
}
CleanupAllGeneratedFiles(src.Path);
CleanupAllGeneratedFiles(xml.Path);
}
[Fact]
public void ParseFullpaths()
{
var parsedArgs = DefaultParse(new[] { "a.cs" }, WorkingDirectory);
Assert.False(parsedArgs.PrintFullPaths);
parsedArgs = DefaultParse(new[] { "a.cs", "/fullpaths" }, WorkingDirectory);
Assert.True(parsedArgs.PrintFullPaths);
parsedArgs = DefaultParse(new[] { "a.cs", "/fullpaths:" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal((int)ErrorCode.ERR_BadSwitch, parsedArgs.Errors.First().Code);
parsedArgs = DefaultParse(new[] { "a.cs", "/fullpaths: " }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal((int)ErrorCode.ERR_BadSwitch, parsedArgs.Errors.First().Code);
parsedArgs = DefaultParse(new[] { "a.cs", "/fullpaths+" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal((int)ErrorCode.ERR_BadSwitch, parsedArgs.Errors.First().Code);
parsedArgs = DefaultParse(new[] { "a.cs", "/fullpaths+:" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal((int)ErrorCode.ERR_BadSwitch, parsedArgs.Errors.First().Code);
}
[Fact]
public void CheckFullpaths()
{
string source = Temp.CreateFile(prefix: "", extension: ".cs").WriteAllText(@"
public class C
{
public static void Main()
{
string x;
}
}").Path;
var baseDir = Path.GetDirectoryName(source);
var fileName = Path.GetFileName(source);
// Checks the base case without /fullpaths (expect to see relative path name)
// c:\temp> csc.exe c:\temp\a.cs
// a.cs(6,16): warning CS0168: The variable 'x' is declared but never used
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, baseDir, new[] { source, "/preferreduilang:en" });
int exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Contains(fileName + "(6,16): warning CS0168: The variable 'x' is declared but never used", outWriter.ToString(), StringComparison.Ordinal);
// Checks the base case without /fullpaths when the file is located in the sub-folder (expect to see relative path name)
// c:\temp> csc.exe c:\temp\example\a.cs
// example\a.cs(6,16): warning CS0168: The variable 'x' is declared but never used
outWriter = new StringWriter(CultureInfo.InvariantCulture);
csc = CreateCSharpCompiler(null, Directory.GetParent(baseDir).FullName, new[] { source, "/preferreduilang:en" });
exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Contains(fileName + "(6,16): warning CS0168: The variable 'x' is declared but never used", outWriter.ToString(), StringComparison.Ordinal);
Assert.DoesNotContain(source, outWriter.ToString(), StringComparison.Ordinal);
// Checks the base case without /fullpaths when the file is not located under the base directory (expect to see the full path name)
// c:\temp> csc.exe c:\test\a.cs
// c:\test\a.cs(6,16): warning CS0168: The variable 'x' is declared but never used
outWriter = new StringWriter(CultureInfo.InvariantCulture);
csc = CreateCSharpCompiler(null, Temp.CreateDirectory().Path, new[] { source, "/preferreduilang:en" });
exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Contains(source + "(6,16): warning CS0168: The variable 'x' is declared but never used", outWriter.ToString(), StringComparison.Ordinal);
// Checks the case with /fullpaths (expect to see the full paths)
// c:\temp> csc.exe c:\temp\a.cs /fullpaths
// c:\temp\a.cs(6,16): warning CS0168: The variable 'x' is declared but never used
outWriter = new StringWriter(CultureInfo.InvariantCulture);
csc = CreateCSharpCompiler(null, baseDir, new[] { source, "/fullpaths", "/preferreduilang:en" });
exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Contains(source + @"(6,16): warning CS0168: The variable 'x' is declared but never used", outWriter.ToString(), StringComparison.Ordinal);
// Checks the base case without /fullpaths when the file is located in the sub-folder (expect to see the full path name)
// c:\temp> csc.exe c:\temp\example\a.cs /fullpaths
// c:\temp\example\a.cs(6,16): warning CS0168: The variable 'x' is declared but never used
outWriter = new StringWriter(CultureInfo.InvariantCulture);
csc = CreateCSharpCompiler(null, Directory.GetParent(baseDir).FullName, new[] { source, "/preferreduilang:en", "/fullpaths" });
exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Contains(source + "(6,16): warning CS0168: The variable 'x' is declared but never used", outWriter.ToString(), StringComparison.Ordinal);
// Checks the base case without /fullpaths when the file is not located under the base directory (expect to see the full path name)
// c:\temp> csc.exe c:\test\a.cs /fullpaths
// c:\test\a.cs(6,16): warning CS0168: The variable 'x' is declared but never used
outWriter = new StringWriter(CultureInfo.InvariantCulture);
csc = CreateCSharpCompiler(null, Temp.CreateDirectory().Path, new[] { source, "/preferreduilang:en", "/fullpaths" });
exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Contains(source + "(6,16): warning CS0168: The variable 'x' is declared but never used", outWriter.ToString(), StringComparison.Ordinal);
CleanupAllGeneratedFiles(source);
CleanupAllGeneratedFiles(Path.Combine(Path.GetDirectoryName(Path.GetDirectoryName(source)), Path.GetFileName(source)));
}
[Fact]
public void DefaultResponseFile()
{
var sdkDirectory = SdkDirectory;
MockCSharpCompiler csc = new MockCSharpCompiler(
GetDefaultResponseFilePath(),
RuntimeUtilities.CreateBuildPaths(WorkingDirectory, sdkDirectory),
new string[0]);
AssertEx.Equal(csc.Arguments.MetadataReferences.Select(r => r.Reference), new string[]
{
MscorlibFullPath,
"Accessibility.dll",
"Microsoft.CSharp.dll",
"System.Configuration.dll",
"System.Configuration.Install.dll",
"System.Core.dll",
"System.Data.dll",
"System.Data.DataSetExtensions.dll",
"System.Data.Linq.dll",
"System.Data.OracleClient.dll",
"System.Deployment.dll",
"System.Design.dll",
"System.DirectoryServices.dll",
"System.dll",
"System.Drawing.Design.dll",
"System.Drawing.dll",
"System.EnterpriseServices.dll",
"System.Management.dll",
"System.Messaging.dll",
"System.Runtime.Remoting.dll",
"System.Runtime.Serialization.dll",
"System.Runtime.Serialization.Formatters.Soap.dll",
"System.Security.dll",
"System.ServiceModel.dll",
"System.ServiceModel.Web.dll",
"System.ServiceProcess.dll",
"System.Transactions.dll",
"System.Web.dll",
"System.Web.Extensions.Design.dll",
"System.Web.Extensions.dll",
"System.Web.Mobile.dll",
"System.Web.RegularExpressions.dll",
"System.Web.Services.dll",
"System.Windows.Forms.dll",
"System.Workflow.Activities.dll",
"System.Workflow.ComponentModel.dll",
"System.Workflow.Runtime.dll",
"System.Xml.dll",
"System.Xml.Linq.dll",
}, StringComparer.OrdinalIgnoreCase);
}
[Fact]
public void DefaultResponseFileNoConfig()
{
MockCSharpCompiler csc = CreateCSharpCompiler(GetDefaultResponseFilePath(), WorkingDirectory, new[] { "/noconfig" });
Assert.Equal(csc.Arguments.MetadataReferences.Select(r => r.Reference), new string[]
{
MscorlibFullPath,
}, StringComparer.OrdinalIgnoreCase);
}
[Fact, WorkItem(545954, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/545954")]
public void TestFilterParseDiagnostics()
{
string source = Temp.CreateFile(prefix: "", extension: ".cs").WriteAllText(@"
#pragma warning disable 440
using global = A; // CS0440
class A
{
static void Main() {
#pragma warning suppress 440
}
}").Path;
var baseDir = Path.GetDirectoryName(source);
var fileName = Path.GetFileName(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, baseDir, new[] { "/nologo", "/preferreduilang:en", source.ToString() }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal(Path.GetFileName(source) + "(7,17): warning CS1634: Expected 'disable' or 'restore'", outWriter.ToString().Trim());
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, baseDir, new[] { "/nologo", "/nowarn:1634", source.ToString() }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("", outWriter.ToString().Trim());
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, baseDir, new[] { "/nologo", "/preferreduilang:en", Path.Combine(baseDir, "nonexistent.cs"), source.ToString() }).Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal("error CS2001: Source file '" + Path.Combine(baseDir, "nonexistent.cs") + "' could not be found.", outWriter.ToString().Trim());
CleanupAllGeneratedFiles(source);
}
[Fact, WorkItem(546058, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546058")]
public void TestNoWarnParseDiagnostics()
{
string source = Temp.CreateFile(prefix: "", extension: ".cs").WriteAllText(@"
class Test
{
static void Main()
{
//Generates warning CS1522: Empty switch block
switch (1) { }
//Generates warning CS0642: Possible mistaken empty statement
while (false) ;
{ }
}
}
").Path;
var baseDir = Path.GetDirectoryName(source);
var fileName = Path.GetFileName(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/nowarn:1522,642", source.ToString() }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("", outWriter.ToString().Trim());
CleanupAllGeneratedFiles(source);
}
[Fact, WorkItem(41610, "https://github.com/dotnet/roslyn/issues/41610")]
public void TestWarnAsError_CS8632()
{
string source = Temp.CreateFile(prefix: "", extension: ".cs").WriteAllText(@"
public class C
{
public string? field;
public static void Main()
{
}
}
").Path;
var baseDir = Path.GetDirectoryName(source);
var fileName = Path.GetFileName(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, baseDir, new[] { "/nologo", "/preferreduilang:en", "/warn:3", "/warnaserror:nullable", source.ToString() }).Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal(
$@"{fileName}(4,18): error CS8632: The annotation for nullable reference types should only be used in code within a '#nullable' annotations context.", outWriter.ToString().Trim());
CleanupAllGeneratedFiles(source);
}
[Fact, WorkItem(546076, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546076")]
public void TestWarnAsError_CS1522()
{
string source = Temp.CreateFile(prefix: "", extension: ".cs").WriteAllText(@"
public class Test
{
// CS0169 (level 3)
private int x;
// CS0109 (level 4)
public new void Method() { }
public static int Main()
{
int i = 5;
// CS1522 (level 1)
switch (i) { }
return 0;
// CS0162 (level 2)
i = 6;
}
}
").Path;
var baseDir = Path.GetDirectoryName(source);
var fileName = Path.GetFileName(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, baseDir, new[] { "/nologo", "/preferreduilang:en", "/warn:3", "/warnaserror", source.ToString() }).Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal(
$@"{fileName}(12,20): error CS1522: Empty switch block
{fileName}(15,9): error CS0162: Unreachable code detected
{fileName}(5,17): error CS0169: The field 'Test.x' is never used", outWriter.ToString().Trim());
CleanupAllGeneratedFiles(source);
}
[Fact(), WorkItem(546025, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546025")]
public void TestWin32ResWithBadResFile_CS1583ERR_BadWin32Res_01()
{
string source = Temp.CreateFile(prefix: "", extension: ".cs").WriteAllText(@"class Test { static void Main() {} }").Path;
string badres = Temp.CreateFile().WriteAllBytes(TestResources.DiagnosticTests.badresfile).Path;
var baseDir = Path.GetDirectoryName(source);
var fileName = Path.GetFileName(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, baseDir, new[]
{
"/nologo",
"/preferreduilang:en",
"/win32res:" + badres,
source
}).Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal("error CS1583: Error reading Win32 resources -- Image is too small.", outWriter.ToString().Trim());
CleanupAllGeneratedFiles(source);
CleanupAllGeneratedFiles(badres);
}
[Fact(), WorkItem(217718, "https://devdiv.visualstudio.com/DevDiv/_workitems?id=217718")]
public void TestWin32ResWithBadResFile_CS1583ERR_BadWin32Res_02()
{
string source = Temp.CreateFile(prefix: "", extension: ".cs").WriteAllText(@"class Test { static void Main() {} }").Path;
string badres = Temp.CreateFile().WriteAllBytes(new byte[] { 0, 0 }).Path;
var baseDir = Path.GetDirectoryName(source);
var fileName = Path.GetFileName(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, baseDir, new[]
{
"/nologo",
"/preferreduilang:en",
"/win32res:" + badres,
source
}).Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal("error CS1583: Error reading Win32 resources -- Unrecognized resource file format.", outWriter.ToString().Trim());
CleanupAllGeneratedFiles(source);
CleanupAllGeneratedFiles(badres);
}
[Fact, WorkItem(546114, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546114")]
public void TestFilterCommandLineDiagnostics()
{
string source = Temp.CreateFile(prefix: "", extension: ".cs").WriteAllText(@"
class A
{
static void Main() { }
}").Path;
var baseDir = Path.GetDirectoryName(source);
var fileName = Path.GetFileName(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/target:library", "/out:goo.dll", "/nowarn:2008" }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("", outWriter.ToString().Trim());
System.IO.File.Delete(System.IO.Path.Combine(baseDir, "goo.dll"));
CleanupAllGeneratedFiles(source);
}
[Fact, WorkItem(546452, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546452")]
public void CS1691WRN_BadWarningNumber_Bug15905()
{
string source = Temp.CreateFile(prefix: "", extension: ".cs").WriteAllText(@"
class Program
{
#pragma warning disable 1998
public static void Main() { }
#pragma warning restore 1998
} ").Path;
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
// Repro case 1
int exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/warnaserror", source.ToString() }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("", outWriter.ToString().Trim());
// Repro case 2
exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/nowarn:1998", source.ToString() }).Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("", outWriter.ToString().Trim());
CleanupAllGeneratedFiles(source);
}
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = ConditionalSkipReason.NativePdbRequiresDesktop)]
public void ExistingPdb()
{
var dir = Temp.CreateDirectory();
var source1 = dir.CreateFile("program1.cs").WriteAllText(@"
class " + new string('a', 10000) + @"
{
public static void Main()
{
}
}");
var source2 = dir.CreateFile("program2.cs").WriteAllText(@"
class Program2
{
public static void Main() { }
}");
var source3 = dir.CreateFile("program3.cs").WriteAllText(@"
class Program3
{
public static void Main() { }
}");
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int oldSize = 16 * 1024;
var exe = dir.CreateFile("Program.exe");
using (var stream = File.OpenWrite(exe.Path))
{
byte[] buffer = new byte[oldSize];
stream.Write(buffer, 0, buffer.Length);
}
var pdb = dir.CreateFile("Program.pdb");
using (var stream = File.OpenWrite(pdb.Path))
{
byte[] buffer = new byte[oldSize];
stream.Write(buffer, 0, buffer.Length);
}
int exitCode1 = CreateCSharpCompiler(null, dir.Path, new[] { "/debug:full", "/out:Program.exe", source1.Path }).Run(outWriter);
Assert.NotEqual(0, exitCode1);
ValidateZeroes(exe.Path, oldSize);
ValidateZeroes(pdb.Path, oldSize);
int exitCode2 = CreateCSharpCompiler(null, dir.Path, new[] { "/debug:full", "/out:Program.exe", source2.Path }).Run(outWriter);
Assert.Equal(0, exitCode2);
using (var peFile = File.OpenRead(exe.Path))
{
PdbValidation.ValidateDebugDirectory(peFile, null, pdb.Path, hashAlgorithm: default, hasEmbeddedPdb: false, isDeterministic: false);
}
Assert.True(new FileInfo(exe.Path).Length < oldSize);
Assert.True(new FileInfo(pdb.Path).Length < oldSize);
int exitCode3 = CreateCSharpCompiler(null, dir.Path, new[] { "/debug:full", "/out:Program.exe", source3.Path }).Run(outWriter);
Assert.Equal(0, exitCode3);
using (var peFile = File.OpenRead(exe.Path))
{
PdbValidation.ValidateDebugDirectory(peFile, null, pdb.Path, hashAlgorithm: default, hasEmbeddedPdb: false, isDeterministic: false);
}
}
private static void ValidateZeroes(string path, int count)
{
using (var stream = File.OpenRead(path))
{
byte[] buffer = new byte[count];
stream.Read(buffer, 0, buffer.Length);
for (int i = 0; i < buffer.Length; i++)
{
if (buffer[i] != 0)
{
Assert.True(false);
}
}
}
}
/// <summary>
/// When the output file is open with <see cref="FileShare.Read"/> | <see cref="FileShare.Delete"/>
/// the compiler should delete the file to unblock build while allowing the reader to continue
/// reading the previous snapshot of the file content.
///
/// On Windows we can read the original data directly from the stream without creating a memory map.
/// </summary>
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = ConditionalSkipReason.NativePdbRequiresDesktop)]
public void FileShareDeleteCompatibility_Windows()
{
var dir = Temp.CreateDirectory();
var libSrc = dir.CreateFile("Lib.cs").WriteAllText("class C { }");
var libDll = dir.CreateFile("Lib.dll").WriteAllText("DLL");
var libPdb = dir.CreateFile("Lib.pdb").WriteAllText("PDB");
var fsDll = new FileStream(libDll.Path, FileMode.Open, FileAccess.Read, FileShare.Read | FileShare.Delete);
var fsPdb = new FileStream(libPdb.Path, FileMode.Open, FileAccess.Read, FileShare.Read | FileShare.Delete);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, dir.Path, new[] { "/target:library", "/debug:full", libSrc.Path }).Run(outWriter);
if (exitCode != 0)
{
AssertEx.AssertEqualToleratingWhitespaceDifferences("", outWriter.ToString());
}
Assert.Equal(0, exitCode);
AssertEx.Equal(new byte[] { 0x4D, 0x5A }, ReadBytes(libDll.Path, 2));
AssertEx.Equal(new[] { (byte)'D', (byte)'L', (byte)'L' }, ReadBytes(fsDll, 3));
AssertEx.Equal(new byte[] { 0x4D, 0x69 }, ReadBytes(libPdb.Path, 2));
AssertEx.Equal(new[] { (byte)'P', (byte)'D', (byte)'B' }, ReadBytes(fsPdb, 3));
fsDll.Dispose();
fsPdb.Dispose();
AssertEx.Equal(new[] { "Lib.cs", "Lib.dll", "Lib.pdb" }, Directory.GetFiles(dir.Path).Select(p => Path.GetFileName(p)).Order());
}
/// <summary>
/// On Linux/Mac <see cref="FileShare.Delete"/> on its own doesn't do anything.
/// We need to create the actual memory map. This works on Windows as well.
/// </summary>
[WorkItem(8896, "https://github.com/dotnet/roslyn/issues/8896")]
[ConditionalFact(typeof(WindowsDesktopOnly), typeof(IsEnglishLocal), Reason = "https://github.com/dotnet/roslyn/issues/30321")]
public void FileShareDeleteCompatibility_Xplat()
{
var bytes = TestResources.MetadataTests.InterfaceAndClass.CSClasses01;
var mvid = ReadMvid(new MemoryStream(bytes));
var dir = Temp.CreateDirectory();
var libSrc = dir.CreateFile("Lib.cs").WriteAllText("class C { }");
var libDll = dir.CreateFile("Lib.dll").WriteAllBytes(bytes);
var libPdb = dir.CreateFile("Lib.pdb").WriteAllBytes(bytes);
var fsDll = new FileStream(libDll.Path, FileMode.Open, FileAccess.Read, FileShare.Read | FileShare.Delete);
var fsPdb = new FileStream(libPdb.Path, FileMode.Open, FileAccess.Read, FileShare.Read | FileShare.Delete);
var peDll = new PEReader(fsDll);
var pePdb = new PEReader(fsPdb);
// creates memory map view:
var imageDll = peDll.GetEntireImage();
var imagePdb = pePdb.GetEntireImage();
var output = ProcessUtilities.RunAndGetOutput(s_CSharpCompilerExecutable, $"/target:library /debug:portable \"{libSrc.Path}\"", startFolder: dir.ToString());
AssertEx.AssertEqualToleratingWhitespaceDifferences($@"
Microsoft (R) Visual C# Compiler version {s_compilerVersion}
Copyright (C) Microsoft Corporation. All rights reserved.", output);
// reading original content from the memory map:
Assert.Equal(mvid, ReadMvid(new MemoryStream(imageDll.GetContent().ToArray())));
Assert.Equal(mvid, ReadMvid(new MemoryStream(imagePdb.GetContent().ToArray())));
// reading original content directly from the streams:
fsDll.Position = 0;
fsPdb.Position = 0;
Assert.Equal(mvid, ReadMvid(fsDll));
Assert.Equal(mvid, ReadMvid(fsPdb));
// reading new content from the file:
using (var fsNewDll = File.OpenRead(libDll.Path))
{
Assert.NotEqual(mvid, ReadMvid(fsNewDll));
}
// Portable PDB metadata signature:
AssertEx.Equal(new[] { (byte)'B', (byte)'S', (byte)'J', (byte)'B' }, ReadBytes(libPdb.Path, 4));
// dispose PEReaders (they dispose the underlying file streams)
peDll.Dispose();
pePdb.Dispose();
AssertEx.Equal(new[] { "Lib.cs", "Lib.dll", "Lib.pdb" }, Directory.GetFiles(dir.Path).Select(p => Path.GetFileName(p)).Order());
// files can be deleted now:
File.Delete(libSrc.Path);
File.Delete(libDll.Path);
File.Delete(libPdb.Path);
// directory can be deleted (should be empty):
Directory.Delete(dir.Path, recursive: false);
}
private static Guid ReadMvid(Stream stream)
{
using (var peReader = new PEReader(stream, PEStreamOptions.LeaveOpen))
{
var mdReader = peReader.GetMetadataReader();
return mdReader.GetGuid(mdReader.GetModuleDefinition().Mvid);
}
}
// Seems like File.SetAttributes(libDll.Path, FileAttributes.ReadOnly) doesn't restrict access to the file on Mac (Linux passes).
[ConditionalFact(typeof(WindowsOnly)), WorkItem(8939, "https://github.com/dotnet/roslyn/issues/8939")]
public void FileShareDeleteCompatibility_ReadOnlyFiles()
{
var dir = Temp.CreateDirectory();
var libSrc = dir.CreateFile("Lib.cs").WriteAllText("class C { }");
var libDll = dir.CreateFile("Lib.dll").WriteAllText("DLL");
File.SetAttributes(libDll.Path, FileAttributes.ReadOnly);
var fsDll = new FileStream(libDll.Path, FileMode.Open, FileAccess.Read, FileShare.Read | FileShare.Delete);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, dir.Path, new[] { "/target:library", "/preferreduilang:en", libSrc.Path }).Run(outWriter);
Assert.Contains($"error CS2012: Cannot open '{libDll.Path}' for writing", outWriter.ToString());
AssertEx.Equal(new[] { (byte)'D', (byte)'L', (byte)'L' }, ReadBytes(libDll.Path, 3));
AssertEx.Equal(new[] { (byte)'D', (byte)'L', (byte)'L' }, ReadBytes(fsDll, 3));
fsDll.Dispose();
AssertEx.Equal(new[] { "Lib.cs", "Lib.dll" }, Directory.GetFiles(dir.Path).Select(p => Path.GetFileName(p)).Order());
}
[Fact]
public void FileShareDeleteCompatibility_ExistingDirectory()
{
var dir = Temp.CreateDirectory();
var libSrc = dir.CreateFile("Lib.cs").WriteAllText("class C { }");
var libDll = dir.CreateDirectory("Lib.dll");
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, dir.Path, new[] { "/target:library", "/preferreduilang:en", libSrc.Path }).Run(outWriter);
Assert.Contains($"error CS2012: Cannot open '{libDll.Path}' for writing", outWriter.ToString());
}
private byte[] ReadBytes(Stream stream, int count)
{
var buffer = new byte[count];
stream.Read(buffer, 0, count);
return buffer;
}
private byte[] ReadBytes(string path, int count)
{
using (var stream = File.OpenRead(path))
{
return ReadBytes(stream, count);
}
}
[Fact]
public void IOFailure_DisposeOutputFile()
{
var srcPath = MakeTrivialExe(Temp.CreateDirectory().Path);
var exePath = Path.Combine(Path.GetDirectoryName(srcPath), "test.exe");
var csc = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/preferreduilang:en", $"/out:{exePath}", srcPath });
csc.FileSystem = TestableFileSystem.CreateForStandard(openFileFunc: (file, mode, access, share) =>
{
if (file == exePath)
{
return new TestStream(backingStream: new MemoryStream(),
dispose: () => { throw new IOException("Fake IOException"); });
}
return File.Open(file, mode, access, share);
});
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
Assert.Equal(1, csc.Run(outWriter));
Assert.Contains($"error CS0016: Could not write to output file '{exePath}' -- 'Fake IOException'{Environment.NewLine}", outWriter.ToString());
}
[Fact]
public void IOFailure_DisposePdbFile()
{
var srcPath = MakeTrivialExe(Temp.CreateDirectory().Path);
var exePath = Path.Combine(Path.GetDirectoryName(srcPath), "test.exe");
var pdbPath = Path.ChangeExtension(exePath, "pdb");
var csc = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/preferreduilang:en", "/debug", $"/out:{exePath}", srcPath });
csc.FileSystem = TestableFileSystem.CreateForStandard(openFileFunc: (file, mode, access, share) =>
{
if (file == pdbPath)
{
return new TestStream(backingStream: new MemoryStream(),
dispose: () => { throw new IOException("Fake IOException"); });
}
return File.Open(file, mode, access, share);
});
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
Assert.Equal(1, csc.Run(outWriter));
Assert.Contains($"error CS0016: Could not write to output file '{pdbPath}' -- 'Fake IOException'{Environment.NewLine}", outWriter.ToString());
}
[Fact]
public void IOFailure_DisposeXmlFile()
{
var srcPath = MakeTrivialExe(Temp.CreateDirectory().Path);
var xmlPath = Path.Combine(Path.GetDirectoryName(srcPath), "test.xml");
var csc = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/preferreduilang:en", $"/doc:{xmlPath}", srcPath });
csc.FileSystem = TestableFileSystem.CreateForStandard(openFileFunc: (file, mode, access, share) =>
{
if (file == xmlPath)
{
return new TestStream(backingStream: new MemoryStream(),
dispose: () => { throw new IOException("Fake IOException"); });
}
return File.Open(file, mode, access, share);
});
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
Assert.Equal(1, csc.Run(outWriter));
Assert.Equal($"error CS0016: Could not write to output file '{xmlPath}' -- 'Fake IOException'{Environment.NewLine}", outWriter.ToString());
}
[Theory]
[InlineData("portable")]
[InlineData("full")]
public void IOFailure_DisposeSourceLinkFile(string format)
{
var srcPath = MakeTrivialExe(Temp.CreateDirectory().Path);
var sourceLinkPath = Path.Combine(Path.GetDirectoryName(srcPath), "test.json");
var csc = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/preferreduilang:en", "/debug:" + format, $"/sourcelink:{sourceLinkPath}", srcPath });
csc.FileSystem = TestableFileSystem.CreateForStandard(openFileFunc: (file, mode, access, share) =>
{
if (file == sourceLinkPath)
{
return new TestStream(backingStream: new MemoryStream(Encoding.UTF8.GetBytes(@"
{
""documents"": {
""f:/build/*"" : ""https://raw.githubusercontent.com/my-org/my-project/1111111111111111111111111111111111111111/*""
}
}
")),
dispose: () => { throw new IOException("Fake IOException"); });
}
return File.Open(file, mode, access, share);
});
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
Assert.Equal(1, csc.Run(outWriter));
Assert.Equal($"error CS0016: Could not write to output file '{sourceLinkPath}' -- 'Fake IOException'{Environment.NewLine}", outWriter.ToString());
}
[Fact]
public void IOFailure_OpenOutputFile()
{
string sourcePath = MakeTrivialExe();
string exePath = Path.Combine(Path.GetDirectoryName(sourcePath), "test.exe");
var csc = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/preferreduilang:en", $"/out:{exePath}", sourcePath });
csc.FileSystem = TestableFileSystem.CreateForStandard(openFileFunc: (file, mode, access, share) =>
{
if (file == exePath)
{
throw new IOException();
}
return File.Open(file, mode, access, share);
});
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
Assert.Equal(1, csc.Run(outWriter));
Assert.Contains($"error CS2012: Cannot open '{exePath}' for writing", outWriter.ToString());
System.IO.File.Delete(sourcePath);
System.IO.File.Delete(exePath);
CleanupAllGeneratedFiles(sourcePath);
}
[Fact]
public void IOFailure_OpenPdbFileNotCalled()
{
string sourcePath = MakeTrivialExe();
string exePath = Path.Combine(Path.GetDirectoryName(sourcePath), "test.exe");
string pdbPath = Path.ChangeExtension(exePath, ".pdb");
var csc = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/debug-", $"/out:{exePath}", sourcePath });
csc.FileSystem = TestableFileSystem.CreateForStandard(openFileFunc: (file, mode, access, share) =>
{
if (file == pdbPath)
{
throw new IOException();
}
return File.Open(file, (FileMode)mode, (FileAccess)access, (FileShare)share);
});
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
Assert.Equal(0, csc.Run(outWriter));
System.IO.File.Delete(sourcePath);
System.IO.File.Delete(exePath);
System.IO.File.Delete(pdbPath);
CleanupAllGeneratedFiles(sourcePath);
}
[Fact]
public void IOFailure_OpenXmlFinal()
{
string sourcePath = MakeTrivialExe();
string xmlPath = Path.Combine(WorkingDirectory, "Test.xml");
var csc = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/preferreduilang:en", "/doc:" + xmlPath, sourcePath });
csc.FileSystem = TestableFileSystem.CreateForStandard(openFileFunc: (file, mode, access, share) =>
{
if (file == xmlPath)
{
throw new IOException();
}
else
{
return File.Open(file, (FileMode)mode, (FileAccess)access, (FileShare)share);
}
});
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = csc.Run(outWriter);
var expectedOutput = string.Format("error CS0016: Could not write to output file '{0}' -- 'I/O error occurred.'", xmlPath);
Assert.Equal(expectedOutput, outWriter.ToString().Trim());
Assert.NotEqual(0, exitCode);
System.IO.File.Delete(xmlPath);
System.IO.File.Delete(sourcePath);
CleanupAllGeneratedFiles(sourcePath);
}
private string MakeTrivialExe(string directory = null)
{
return Temp.CreateFile(directory: directory, prefix: "", extension: ".cs").WriteAllText(@"
class Program
{
public static void Main() { }
} ").Path;
}
[Fact, WorkItem(546452, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546452")]
public void CS1691WRN_BadWarningNumber_AllErrorCodes()
{
const int jump = 200;
for (int i = 0; i < 8000; i += (8000 / jump))
{
int startErrorCode = (int)i * jump;
int endErrorCode = startErrorCode + jump;
string source = ComputeSourceText(startErrorCode, endErrorCode);
// Previous versions of the compiler used to report a warning (CS1691)
// whenever an unrecognized warning code was supplied in a #pragma directive
// (or via /nowarn /warnaserror flags on the command line).
// Going forward, we won't generate any warning in such cases. This will make
// maintenance of backwards compatibility easier (we no longer need to worry
// about breaking existing projects / command lines if we deprecate / remove
// an old warning code).
Test(source, startErrorCode, endErrorCode);
}
}
private static string ComputeSourceText(int startErrorCode, int endErrorCode)
{
string pragmaDisableWarnings = String.Empty;
for (int errorCode = startErrorCode; errorCode < endErrorCode; errorCode++)
{
string pragmaDisableStr = @"#pragma warning disable " + errorCode.ToString() + @"
";
pragmaDisableWarnings += pragmaDisableStr;
}
return pragmaDisableWarnings + @"
public class C
{
public static void Main() { }
}";
}
private void Test(string source, int startErrorCode, int endErrorCode)
{
string sourcePath = Temp.CreateFile(prefix: "", extension: ".cs").WriteAllText(source).Path;
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", sourcePath }).Run(outWriter);
Assert.Equal(0, exitCode);
var cscOutput = outWriter.ToString().Trim();
for (int errorCode = startErrorCode; errorCode < endErrorCode; errorCode++)
{
Assert.True(cscOutput == string.Empty, "Failed at error code: " + errorCode);
}
CleanupAllGeneratedFiles(sourcePath);
}
[Fact]
public void WriteXml()
{
var source = @"
/// <summary>
/// A subtype of <see cref=""object""/>.
/// </summary>
public class C { }
";
var sourcePath = Temp.CreateFile(directory: WorkingDirectory, extension: ".cs").WriteAllText(source).Path;
string xmlPath = Path.Combine(WorkingDirectory, "Test.xml");
var csc = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/target:library", "/out:Test.dll", "/doc:" + xmlPath, sourcePath });
var writer = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = csc.Run(writer);
if (exitCode != 0)
{
Console.WriteLine(writer.ToString());
Assert.Equal(0, exitCode);
}
var bytes = File.ReadAllBytes(xmlPath);
var actual = new string(Encoding.UTF8.GetChars(bytes));
var expected = @"
<?xml version=""1.0""?>
<doc>
<assembly>
<name>Test</name>
</assembly>
<members>
<member name=""T:C"">
<summary>
A subtype of <see cref=""T:System.Object""/>.
</summary>
</member>
</members>
</doc>
";
Assert.Equal(expected.Trim(), actual.Trim());
System.IO.File.Delete(xmlPath);
System.IO.File.Delete(sourcePath);
CleanupAllGeneratedFiles(sourcePath);
CleanupAllGeneratedFiles(xmlPath);
}
[WorkItem(546468, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/546468")]
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30289")]
public void CS2002WRN_FileAlreadyIncluded()
{
const string cs2002 = @"warning CS2002: Source file '{0}' specified multiple times";
TempDirectory tempParentDir = Temp.CreateDirectory();
TempDirectory tempDir = tempParentDir.CreateDirectory("tmpDir");
TempFile tempFile = tempDir.CreateFile("a.cs").WriteAllText(@"public class A { }");
// Simple case
var commandLineArgs = new[] { "a.cs", "a.cs" };
// warning CS2002: Source file 'a.cs' specified multiple times
string aWrnString = String.Format(cs2002, "a.cs");
TestCS2002(commandLineArgs, tempDir.Path, 0, aWrnString);
// Multiple duplicates
commandLineArgs = new[] { "a.cs", "a.cs", "a.cs" };
// warning CS2002: Source file 'a.cs' specified multiple times
var warnings = new[] { aWrnString };
TestCS2002(commandLineArgs, tempDir.Path, 0, warnings);
// Case-insensitive
commandLineArgs = new[] { "a.cs", "A.cs" };
// warning CS2002: Source file 'A.cs' specified multiple times
string AWrnString = String.Format(cs2002, "A.cs");
TestCS2002(commandLineArgs, tempDir.Path, 0, AWrnString);
// Different extensions
tempDir.CreateFile("a.csx");
commandLineArgs = new[] { "a.cs", "a.csx" };
// No errors or warnings
TestCS2002(commandLineArgs, tempDir.Path, 0, String.Empty);
// Absolute vs Relative
commandLineArgs = new[] { @"tmpDir\a.cs", tempFile.Path };
// warning CS2002: Source file 'tmpDir\a.cs' specified multiple times
string tmpDiraString = String.Format(cs2002, @"tmpDir\a.cs");
TestCS2002(commandLineArgs, tempParentDir.Path, 0, tmpDiraString);
// Both relative
commandLineArgs = new[] { @"tmpDir\..\tmpDir\a.cs", @"tmpDir\a.cs" };
// warning CS2002: Source file 'tmpDir\a.cs' specified multiple times
TestCS2002(commandLineArgs, tempParentDir.Path, 0, tmpDiraString);
// With wild cards
commandLineArgs = new[] { tempFile.Path, @"tmpDir\*.cs" };
// warning CS2002: Source file 'tmpDir\a.cs' specified multiple times
TestCS2002(commandLineArgs, tempParentDir.Path, 0, tmpDiraString);
// "/recurse" scenarios
commandLineArgs = new[] { @"/recurse:a.cs", @"tmpDir\a.cs" };
// warning CS2002: Source file 'tmpDir\a.cs' specified multiple times
TestCS2002(commandLineArgs, tempParentDir.Path, 0, tmpDiraString);
commandLineArgs = new[] { @"/recurse:a.cs", @"/recurse:tmpDir\..\tmpDir\*.cs" };
// warning CS2002: Source file 'tmpDir\a.cs' specified multiple times
TestCS2002(commandLineArgs, tempParentDir.Path, 0, tmpDiraString);
// Invalid file/path characters
const string cs1504 = @"error CS1504: Source file '{0}' could not be opened -- {1}";
commandLineArgs = new[] { "/preferreduilang:en", tempFile.Path, "tmpDir\a.cs" };
// error CS1504: Source file '{0}' could not be opened: Illegal characters in path.
var formattedcs1504Str = String.Format(cs1504, PathUtilities.CombineAbsoluteAndRelativePaths(tempParentDir.Path, "tmpDir\a.cs"), "Illegal characters in path.");
TestCS2002(commandLineArgs, tempParentDir.Path, 1, formattedcs1504Str);
commandLineArgs = new[] { tempFile.Path, @"tmpDi\r*a?.cs" };
var parseDiags = new[] {
// error CS2021: File name 'tmpDi\r*a?.cs' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(@"tmpDi\r*a?.cs"),
// error CS2001: Source file 'tmpDi\r*a?.cs' could not be found.
Diagnostic(ErrorCode.ERR_FileNotFound).WithArguments(@"tmpDi\r*a?.cs")};
TestCS2002(commandLineArgs, tempParentDir.Path, 1, (string[])null, parseDiags);
char currentDrive = Directory.GetCurrentDirectory()[0];
commandLineArgs = new[] { tempFile.Path, currentDrive + @":a.cs" };
parseDiags = new[] {
// error CS2021: File name 'e:a.cs' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Diagnostic(ErrorCode.FTL_InvalidInputFileName).WithArguments(currentDrive + @":a.cs")};
TestCS2002(commandLineArgs, tempParentDir.Path, 1, (string[])null, parseDiags);
commandLineArgs = new[] { "/preferreduilang:en", tempFile.Path, @":a.cs" };
// error CS1504: Source file '{0}' could not be opened: {1}
var formattedcs1504 = String.Format(cs1504, PathUtilities.CombineAbsoluteAndRelativePaths(tempParentDir.Path, @":a.cs"), @"The given path's format is not supported.");
TestCS2002(commandLineArgs, tempParentDir.Path, 1, formattedcs1504);
CleanupAllGeneratedFiles(tempFile.Path);
System.IO.Directory.Delete(tempParentDir.Path, true);
}
private void TestCS2002(string[] commandLineArgs, string baseDirectory, int expectedExitCode, string compileDiagnostic, params DiagnosticDescription[] parseDiagnostics)
{
TestCS2002(commandLineArgs, baseDirectory, expectedExitCode, new[] { compileDiagnostic }, parseDiagnostics);
}
private void TestCS2002(string[] commandLineArgs, string baseDirectory, int expectedExitCode, string[] compileDiagnostics, params DiagnosticDescription[] parseDiagnostics)
{
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var allCommandLineArgs = new[] { "/nologo", "/preferreduilang:en", "/t:library" }.Concat(commandLineArgs).ToArray();
// Verify command line parser diagnostics.
DefaultParse(allCommandLineArgs, baseDirectory).Errors.Verify(parseDiagnostics);
// Verify compile.
int exitCode = CreateCSharpCompiler(null, baseDirectory, allCommandLineArgs).Run(outWriter);
Assert.Equal(expectedExitCode, exitCode);
if (parseDiagnostics.IsEmpty())
{
// Verify compile diagnostics.
string outString = String.Empty;
for (int i = 0; i < compileDiagnostics.Length; i++)
{
if (i != 0)
{
outString += @"
";
}
outString += compileDiagnostics[i];
}
Assert.Equal(outString, outWriter.ToString().Trim());
}
else
{
Assert.Null(compileDiagnostics);
}
}
[Fact]
public void ErrorLineEnd()
{
var tree = SyntaxFactory.ParseSyntaxTree("class C public { }", path: "goo");
var comp = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/errorendlocation" });
var loc = new SourceLocation(tree.GetCompilationUnitRoot().FindToken(6));
var diag = new CSDiagnostic(new DiagnosticInfo(MessageProvider.Instance, (int)ErrorCode.ERR_MetadataNameTooLong), loc);
var text = comp.DiagnosticFormatter.Format(diag);
string stringStart = "goo(1,7,1,8)";
Assert.Equal(stringStart, text.Substring(0, stringStart.Length));
}
[Fact]
public void ReportAnalyzer()
{
var parsedArgs1 = DefaultParse(new[] { "a.cs", "/reportanalyzer" }, WorkingDirectory);
Assert.True(parsedArgs1.ReportAnalyzer);
var parsedArgs2 = DefaultParse(new[] { "a.cs", "" }, WorkingDirectory);
Assert.False(parsedArgs2.ReportAnalyzer);
}
[Fact]
public void ReportAnalyzerOutput()
{
var srcFile = Temp.CreateFile().WriteAllText(@"class C {}");
var srcDirectory = Path.GetDirectoryName(srcFile.Path);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, srcDirectory, new[] { "/reportanalyzer", "/t:library", "/a:" + Assembly.GetExecutingAssembly().Location, srcFile.Path });
var exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
var output = outWriter.ToString();
Assert.Contains(CodeAnalysisResources.AnalyzerExecutionTimeColumnHeader, output, StringComparison.Ordinal);
Assert.Contains("WarningDiagnosticAnalyzer (Warning01)", output, StringComparison.Ordinal);
CleanupAllGeneratedFiles(srcFile.Path);
}
[Fact]
[WorkItem(40926, "https://github.com/dotnet/roslyn/issues/40926")]
public void SkipAnalyzersParse()
{
var parsedArgs = DefaultParse(new[] { "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.SkipAnalyzers);
parsedArgs = DefaultParse(new[] { "/skipanalyzers+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.SkipAnalyzers);
parsedArgs = DefaultParse(new[] { "/skipanalyzers", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.SkipAnalyzers);
parsedArgs = DefaultParse(new[] { "/SKIPANALYZERS+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.SkipAnalyzers);
parsedArgs = DefaultParse(new[] { "/skipanalyzers-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.SkipAnalyzers);
parsedArgs = DefaultParse(new[] { "/skipanalyzers-", "/skipanalyzers+", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.True(parsedArgs.SkipAnalyzers);
parsedArgs = DefaultParse(new[] { "/skipanalyzers", "/skipanalyzers-", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.False(parsedArgs.SkipAnalyzers);
}
[Theory, CombinatorialData]
[WorkItem(40926, "https://github.com/dotnet/roslyn/issues/40926")]
public void SkipAnalyzersSemantics(bool skipAnalyzers)
{
var srcFile = Temp.CreateFile().WriteAllText(@"class C {}");
var srcDirectory = Path.GetDirectoryName(srcFile.Path);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var skipAnalyzersFlag = "/skipanalyzers" + (skipAnalyzers ? "+" : "-");
var csc = CreateCSharpCompiler(null, srcDirectory, new[] { skipAnalyzersFlag, "/reportanalyzer", "/t:library", "/a:" + Assembly.GetExecutingAssembly().Location, srcFile.Path });
var exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
var output = outWriter.ToString();
if (skipAnalyzers)
{
Assert.DoesNotContain(CodeAnalysisResources.AnalyzerExecutionTimeColumnHeader, output, StringComparison.Ordinal);
Assert.DoesNotContain(new WarningDiagnosticAnalyzer().ToString(), output, StringComparison.Ordinal);
}
else
{
Assert.Contains(CodeAnalysisResources.AnalyzerExecutionTimeColumnHeader, output, StringComparison.Ordinal);
Assert.Contains(new WarningDiagnosticAnalyzer().ToString(), output, StringComparison.Ordinal);
}
CleanupAllGeneratedFiles(srcFile.Path);
}
[Fact]
[WorkItem(24835, "https://github.com/dotnet/roslyn/issues/24835")]
public void TestCompilationSuccessIfOnlySuppressedDiagnostics()
{
var srcFile = Temp.CreateFile().WriteAllText(@"
#pragma warning disable Warning01
class C { }
");
var errorLog = Temp.CreateFile();
var csc = CreateCSharpCompiler(
null,
workingDirectory: Path.GetDirectoryName(srcFile.Path),
args: new[] { "/errorlog:" + errorLog.Path, "/warnaserror+", "/nologo", "/t:library", srcFile.Path },
analyzers: ImmutableArray.Create<DiagnosticAnalyzer>(new WarningDiagnosticAnalyzer()));
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = csc.Run(outWriter);
// Previously, the compiler would return error code 1 without printing any diagnostics
Assert.Empty(outWriter.ToString());
Assert.Equal(0, exitCode);
CleanupAllGeneratedFiles(srcFile.Path);
CleanupAllGeneratedFiles(errorLog.Path);
}
[Fact]
[WorkItem(1759, "https://github.com/dotnet/roslyn/issues/1759")]
public void AnalyzerDiagnosticThrowsInGetMessage()
{
var srcFile = Temp.CreateFile().WriteAllText(@"class C {}");
var srcDirectory = Path.GetDirectoryName(srcFile.Path);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/t:library", srcFile.Path },
analyzers: ImmutableArray.Create<DiagnosticAnalyzer>(new AnalyzerThatThrowsInGetMessage()));
var exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
var output = outWriter.ToString();
// Verify that the diagnostic reported by AnalyzerThatThrowsInGetMessage is reported, though it doesn't have the message.
Assert.Contains(AnalyzerThatThrowsInGetMessage.Rule.Id, output, StringComparison.Ordinal);
// Verify that the analyzer exception diagnostic for the exception throw in AnalyzerThatThrowsInGetMessage is also reported.
Assert.Contains(AnalyzerExecutor.AnalyzerExceptionDiagnosticId, output, StringComparison.Ordinal);
Assert.Contains(nameof(NotImplementedException), output, StringComparison.Ordinal);
CleanupAllGeneratedFiles(srcFile.Path);
}
[Fact]
[WorkItem(3707, "https://github.com/dotnet/roslyn/issues/3707")]
public void AnalyzerExceptionDiagnosticCanBeConfigured()
{
var srcFile = Temp.CreateFile().WriteAllText(@"class C {}");
var srcDirectory = Path.GetDirectoryName(srcFile.Path);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/t:library", $"/warnaserror:{AnalyzerExecutor.AnalyzerExceptionDiagnosticId}", srcFile.Path },
analyzers: ImmutableArray.Create<DiagnosticAnalyzer>(new AnalyzerThatThrowsInGetMessage()));
var exitCode = csc.Run(outWriter);
Assert.NotEqual(0, exitCode);
var output = outWriter.ToString();
// Verify that the analyzer exception diagnostic for the exception throw in AnalyzerThatThrowsInGetMessage is also reported.
Assert.Contains(AnalyzerExecutor.AnalyzerExceptionDiagnosticId, output, StringComparison.Ordinal);
Assert.Contains(nameof(NotImplementedException), output, StringComparison.Ordinal);
CleanupAllGeneratedFiles(srcFile.Path);
}
[Fact]
[WorkItem(4589, "https://github.com/dotnet/roslyn/issues/4589")]
public void AnalyzerReportsMisformattedDiagnostic()
{
var srcFile = Temp.CreateFile().WriteAllText(@"class C {}");
var srcDirectory = Path.GetDirectoryName(srcFile.Path);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/t:library", srcFile.Path },
analyzers: ImmutableArray.Create<DiagnosticAnalyzer>(new AnalyzerReportingMisformattedDiagnostic()));
var exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
var output = outWriter.ToString();
// Verify that the diagnostic reported by AnalyzerReportingMisformattedDiagnostic is reported with the message format string, instead of the formatted message.
Assert.Contains(AnalyzerThatThrowsInGetMessage.Rule.Id, output, StringComparison.Ordinal);
Assert.Contains(AnalyzerThatThrowsInGetMessage.Rule.MessageFormat.ToString(CultureInfo.InvariantCulture), output, StringComparison.Ordinal);
CleanupAllGeneratedFiles(srcFile.Path);
}
[Fact]
public void ErrorPathsFromLineDirectives()
{
string sampleProgram = @"
#line 10 "".."" //relative path
using System*
";
var syntaxTree = SyntaxFactory.ParseSyntaxTree(sampleProgram, path: "filename.cs");
var comp = CreateCSharpCompiler(null, WorkingDirectory, new string[] { });
var text = comp.DiagnosticFormatter.Format(syntaxTree.GetDiagnostics().First());
//Pull off the last segment of the current directory.
var expectedPath = Path.GetDirectoryName(WorkingDirectory);
//the end of the diagnostic's "file" portion should be signaled with the '(' of the line/col info.
Assert.Equal('(', text[expectedPath.Length]);
sampleProgram = @"
#line 10 "".>"" //invalid path character
using System*
";
syntaxTree = SyntaxFactory.ParseSyntaxTree(sampleProgram, path: "filename.cs");
text = comp.DiagnosticFormatter.Format(syntaxTree.GetDiagnostics().First());
Assert.True(text.StartsWith(".>", StringComparison.Ordinal));
sampleProgram = @"
#line 10 ""http://goo.bar/baz.aspx"" //URI
using System*
";
syntaxTree = SyntaxFactory.ParseSyntaxTree(sampleProgram, path: "filename.cs");
text = comp.DiagnosticFormatter.Format(syntaxTree.GetDiagnostics().First());
Assert.True(text.StartsWith("http://goo.bar/baz.aspx", StringComparison.Ordinal));
}
[WorkItem(1119609, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/1119609")]
[Fact]
public void PreferredUILang()
{
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/preferreduilang" }).Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Contains("CS2006", outWriter.ToString(), StringComparison.Ordinal);
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/preferreduilang:" }).Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Contains("CS2006", outWriter.ToString(), StringComparison.Ordinal);
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/preferreduilang:zz" }).Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Contains("CS2038", outWriter.ToString(), StringComparison.Ordinal);
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/preferreduilang:en-zz" }).Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Contains("CS2038", outWriter.ToString(), StringComparison.Ordinal);
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/preferreduilang:en-US" }).Run(outWriter);
Assert.Equal(1, exitCode);
Assert.DoesNotContain("CS2038", outWriter.ToString(), StringComparison.Ordinal);
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/preferreduilang:de" }).Run(outWriter);
Assert.Equal(1, exitCode);
Assert.DoesNotContain("CS2038", outWriter.ToString(), StringComparison.Ordinal);
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/preferreduilang:de-AT" }).Run(outWriter);
Assert.Equal(1, exitCode);
Assert.DoesNotContain("CS2038", outWriter.ToString(), StringComparison.Ordinal);
}
[WorkItem(531263, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/531263")]
[Fact]
public void EmptyFileName()
{
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = CreateCSharpCompiler(null, WorkingDirectory, new[] { "" }).Run(outWriter);
Assert.NotEqual(0, exitCode);
// error CS2021: File name '' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long
Assert.Contains("CS2021", outWriter.ToString(), StringComparison.Ordinal);
}
[WorkItem(747219, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/747219")]
[Fact]
public void NoInfoDiagnostics()
{
string filePath = Temp.CreateFile().WriteAllText(@"
using System.Diagnostics; // Unused.
").Path;
var cmd = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/nologo", "/target:library", filePath });
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = cmd.Run(outWriter);
Assert.Equal(0, exitCode);
Assert.Equal("", outWriter.ToString().Trim());
CleanupAllGeneratedFiles(filePath);
}
[Fact]
public void RuntimeMetadataVersion()
{
var parsedArgs = DefaultParse(new[] { "a.cs", "/runtimemetadataversion" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal((int)ErrorCode.ERR_SwitchNeedsString, parsedArgs.Errors.First().Code);
parsedArgs = DefaultParse(new[] { "a.cs", "/runtimemetadataversion:" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal((int)ErrorCode.ERR_SwitchNeedsString, parsedArgs.Errors.First().Code);
parsedArgs = DefaultParse(new[] { "a.cs", "/runtimemetadataversion: " }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal((int)ErrorCode.ERR_SwitchNeedsString, parsedArgs.Errors.First().Code);
parsedArgs = DefaultParse(new[] { "a.cs", "/runtimemetadataversion:v4.0.30319" }, WorkingDirectory);
Assert.Equal(0, parsedArgs.Errors.Length);
Assert.Equal("v4.0.30319", parsedArgs.EmitOptions.RuntimeMetadataVersion);
parsedArgs = DefaultParse(new[] { "a.cs", "/runtimemetadataversion:-_+@%#*^" }, WorkingDirectory);
Assert.Equal(0, parsedArgs.Errors.Length);
Assert.Equal("-_+@%#*^", parsedArgs.EmitOptions.RuntimeMetadataVersion);
var comp = CreateEmptyCompilation(string.Empty);
Assert.Equal("v4.0.30319", ModuleMetadata.CreateFromImage(comp.EmitToArray(new EmitOptions(runtimeMetadataVersion: "v4.0.30319"))).Module.MetadataVersion);
comp = CreateEmptyCompilation(string.Empty);
Assert.Equal("_+@%#*^", ModuleMetadata.CreateFromImage(comp.EmitToArray(new EmitOptions(runtimeMetadataVersion: "_+@%#*^"))).Module.MetadataVersion);
}
[WorkItem(715339, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/715339")]
[ConditionalFact(typeof(WindowsOnly))]
public void WRN_InvalidSearchPathDir()
{
var baseDir = Temp.CreateDirectory();
var sourceFile = baseDir.CreateFile("Source.cs");
var invalidPath = "::";
var nonExistentPath = "DoesNotExist";
// lib switch
DefaultParse(new[] { "/lib:" + invalidPath, sourceFile.Path }, WorkingDirectory).Errors.Verify(
// warning CS1668: Invalid search path '::' specified in '/LIB option' -- 'path is too long or invalid'
Diagnostic(ErrorCode.WRN_InvalidSearchPathDir).WithArguments("::", "/LIB option", "path is too long or invalid"));
DefaultParse(new[] { "/lib:" + nonExistentPath, sourceFile.Path }, WorkingDirectory).Errors.Verify(
// warning CS1668: Invalid search path 'DoesNotExist' specified in '/LIB option' -- 'directory does not exist'
Diagnostic(ErrorCode.WRN_InvalidSearchPathDir).WithArguments("DoesNotExist", "/LIB option", "directory does not exist"));
// LIB environment variable
DefaultParse(new[] { sourceFile.Path }, WorkingDirectory, additionalReferenceDirectories: invalidPath).Errors.Verify(
// warning CS1668: Invalid search path '::' specified in 'LIB environment variable' -- 'path is too long or invalid'
Diagnostic(ErrorCode.WRN_InvalidSearchPathDir).WithArguments("::", "LIB environment variable", "path is too long or invalid"));
DefaultParse(new[] { sourceFile.Path }, WorkingDirectory, additionalReferenceDirectories: nonExistentPath).Errors.Verify(
// warning CS1668: Invalid search path 'DoesNotExist' specified in 'LIB environment variable' -- 'directory does not exist'
Diagnostic(ErrorCode.WRN_InvalidSearchPathDir).WithArguments("DoesNotExist", "LIB environment variable", "directory does not exist"));
CleanupAllGeneratedFiles(sourceFile.Path);
}
[WorkItem(650083, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/650083")]
[InlineData("a.cs /t:library /appconfig:.\\aux.config")]
[InlineData("a.cs /out:com1.dll")]
[InlineData("a.cs /doc:..\\lpt2.xml")]
[InlineData("a.cs /pdb:..\\prn.pdb")]
[Theory]
public void ReservedDeviceNameAsFileName(string commandLine)
{
var parsedArgs = DefaultParse(commandLine.Split(new[] { ' ' }, StringSplitOptions.RemoveEmptyEntries), WorkingDirectory);
if (ExecutionConditionUtil.OperatingSystemRestrictsFileNames)
{
Assert.Equal(1, parsedArgs.Errors.Length);
Assert.Equal((int)ErrorCode.FTL_InvalidInputFileName, parsedArgs.Errors.First().Code);
}
else
{
Assert.Equal(0, parsedArgs.Errors.Length);
}
}
[Fact]
public void ReservedDeviceNameAsFileName2()
{
string filePath = Temp.CreateFile().WriteAllText(@"class C {}").Path;
// make sure reserved device names don't
var cmd = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/r:com2.dll", "/target:library", "/preferreduilang:en", filePath });
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = cmd.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Contains("error CS0006: Metadata file 'com2.dll' could not be found", outWriter.ToString(), StringComparison.Ordinal);
cmd = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/link:..\\lpt8.dll", "/target:library", "/preferreduilang:en", filePath });
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = cmd.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Contains("error CS0006: Metadata file '..\\lpt8.dll' could not be found", outWriter.ToString(), StringComparison.Ordinal);
cmd = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/lib:aux", "/preferreduilang:en", filePath });
outWriter = new StringWriter(CultureInfo.InvariantCulture);
exitCode = cmd.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Contains("warning CS1668: Invalid search path 'aux' specified in '/LIB option' -- 'directory does not exist'", outWriter.ToString(), StringComparison.Ordinal);
CleanupAllGeneratedFiles(filePath);
}
[Fact]
public void ParseFeatures()
{
var args = DefaultParse(new[] { "/features:Test", "a.vb" }, WorkingDirectory);
args.Errors.Verify();
Assert.Equal("Test", args.ParseOptions.Features.Single().Key);
args = DefaultParse(new[] { "/features:Test", "a.vb", "/Features:Experiment" }, WorkingDirectory);
args.Errors.Verify();
Assert.Equal(2, args.ParseOptions.Features.Count);
Assert.True(args.ParseOptions.Features.ContainsKey("Test"));
Assert.True(args.ParseOptions.Features.ContainsKey("Experiment"));
args = DefaultParse(new[] { "/features:Test=false,Key=value", "a.vb" }, WorkingDirectory);
args.Errors.Verify();
Assert.True(args.ParseOptions.Features.SetEquals(new Dictionary<string, string> { { "Test", "false" }, { "Key", "value" } }));
args = DefaultParse(new[] { "/features:Test,", "a.vb" }, WorkingDirectory);
args.Errors.Verify();
Assert.True(args.ParseOptions.Features.SetEquals(new Dictionary<string, string> { { "Test", "true" } }));
}
[ConditionalFact(typeof(WindowsOnly), Reason = "https://github.com/dotnet/roslyn/issues/30289")]
public void ParseAdditionalFile()
{
var args = DefaultParse(new[] { "/additionalfile:web.config", "a.cs" }, WorkingDirectory);
args.Errors.Verify();
Assert.Equal(Path.Combine(WorkingDirectory, "web.config"), args.AdditionalFiles.Single().Path);
args = DefaultParse(new[] { "/additionalfile:web.config", "a.cs", "/additionalfile:app.manifest" }, WorkingDirectory);
args.Errors.Verify();
Assert.Equal(2, args.AdditionalFiles.Length);
Assert.Equal(Path.Combine(WorkingDirectory, "web.config"), args.AdditionalFiles[0].Path);
Assert.Equal(Path.Combine(WorkingDirectory, "app.manifest"), args.AdditionalFiles[1].Path);
args = DefaultParse(new[] { "/additionalfile:web.config", "a.cs", "/additionalfile:web.config" }, WorkingDirectory);
args.Errors.Verify();
Assert.Equal(2, args.AdditionalFiles.Length);
Assert.Equal(Path.Combine(WorkingDirectory, "web.config"), args.AdditionalFiles[0].Path);
Assert.Equal(Path.Combine(WorkingDirectory, "web.config"), args.AdditionalFiles[1].Path);
args = DefaultParse(new[] { "/additionalfile:..\\web.config", "a.cs" }, WorkingDirectory);
args.Errors.Verify();
Assert.Equal(Path.Combine(WorkingDirectory, "..\\web.config"), args.AdditionalFiles.Single().Path);
var baseDir = Temp.CreateDirectory();
baseDir.CreateFile("web1.config");
baseDir.CreateFile("web2.config");
baseDir.CreateFile("web3.config");
args = DefaultParse(new[] { "/additionalfile:web*.config", "a.cs" }, baseDir.Path);
args.Errors.Verify();
Assert.Equal(3, args.AdditionalFiles.Length);
Assert.Equal(Path.Combine(baseDir.Path, "web1.config"), args.AdditionalFiles[0].Path);
Assert.Equal(Path.Combine(baseDir.Path, "web2.config"), args.AdditionalFiles[1].Path);
Assert.Equal(Path.Combine(baseDir.Path, "web3.config"), args.AdditionalFiles[2].Path);
args = DefaultParse(new[] { "/additionalfile:web.config;app.manifest", "a.cs" }, WorkingDirectory);
args.Errors.Verify();
Assert.Equal(2, args.AdditionalFiles.Length);
Assert.Equal(Path.Combine(WorkingDirectory, "web.config"), args.AdditionalFiles[0].Path);
Assert.Equal(Path.Combine(WorkingDirectory, "app.manifest"), args.AdditionalFiles[1].Path);
args = DefaultParse(new[] { "/additionalfile:web.config,app.manifest", "a.cs" }, WorkingDirectory);
args.Errors.Verify();
Assert.Equal(2, args.AdditionalFiles.Length);
Assert.Equal(Path.Combine(WorkingDirectory, "web.config"), args.AdditionalFiles[0].Path);
Assert.Equal(Path.Combine(WorkingDirectory, "app.manifest"), args.AdditionalFiles[1].Path);
args = DefaultParse(new[] { "/additionalfile:web.config,app.manifest", "a.cs" }, WorkingDirectory);
args.Errors.Verify();
Assert.Equal(2, args.AdditionalFiles.Length);
Assert.Equal(Path.Combine(WorkingDirectory, "web.config"), args.AdditionalFiles[0].Path);
Assert.Equal(Path.Combine(WorkingDirectory, "app.manifest"), args.AdditionalFiles[1].Path);
args = DefaultParse(new[] { @"/additionalfile:""web.config,app.manifest""", "a.cs" }, WorkingDirectory);
args.Errors.Verify();
Assert.Equal(1, args.AdditionalFiles.Length);
Assert.Equal(Path.Combine(WorkingDirectory, "web.config,app.manifest"), args.AdditionalFiles[0].Path);
args = DefaultParse(new[] { "/additionalfile:web.config:app.manifest", "a.cs" }, WorkingDirectory);
args.Errors.Verify();
Assert.Equal(1, args.AdditionalFiles.Length);
Assert.Equal(Path.Combine(WorkingDirectory, "web.config:app.manifest"), args.AdditionalFiles[0].Path);
args = DefaultParse(new[] { "/additionalfile", "a.cs" }, WorkingDirectory);
args.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<file list>", "additionalfile"));
Assert.Equal(0, args.AdditionalFiles.Length);
args = DefaultParse(new[] { "/additionalfile:", "a.cs" }, WorkingDirectory);
args.Errors.Verify(Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<file list>", "additionalfile"));
Assert.Equal(0, args.AdditionalFiles.Length);
}
[Fact]
public void ParseEditorConfig()
{
var args = DefaultParse(new[] { "/analyzerconfig:.editorconfig", "a.cs" }, WorkingDirectory);
args.Errors.Verify();
Assert.Equal(Path.Combine(WorkingDirectory, ".editorconfig"), args.AnalyzerConfigPaths.Single());
args = DefaultParse(new[] { "/analyzerconfig:.editorconfig", "a.cs", "/analyzerconfig:subdir\\.editorconfig" }, WorkingDirectory);
args.Errors.Verify();
Assert.Equal(2, args.AnalyzerConfigPaths.Length);
Assert.Equal(Path.Combine(WorkingDirectory, ".editorconfig"), args.AnalyzerConfigPaths[0]);
Assert.Equal(Path.Combine(WorkingDirectory, "subdir\\.editorconfig"), args.AnalyzerConfigPaths[1]);
args = DefaultParse(new[] { "/analyzerconfig:.editorconfig", "a.cs", "/analyzerconfig:.editorconfig" }, WorkingDirectory);
args.Errors.Verify();
Assert.Equal(2, args.AnalyzerConfigPaths.Length);
Assert.Equal(Path.Combine(WorkingDirectory, ".editorconfig"), args.AnalyzerConfigPaths[0]);
Assert.Equal(Path.Combine(WorkingDirectory, ".editorconfig"), args.AnalyzerConfigPaths[1]);
args = DefaultParse(new[] { "/analyzerconfig:..\\.editorconfig", "a.cs" }, WorkingDirectory);
args.Errors.Verify();
Assert.Equal(Path.Combine(WorkingDirectory, "..\\.editorconfig"), args.AnalyzerConfigPaths.Single());
args = DefaultParse(new[] { "/analyzerconfig:.editorconfig;subdir\\.editorconfig", "a.cs" }, WorkingDirectory);
args.Errors.Verify();
Assert.Equal(2, args.AnalyzerConfigPaths.Length);
Assert.Equal(Path.Combine(WorkingDirectory, ".editorconfig"), args.AnalyzerConfigPaths[0]);
Assert.Equal(Path.Combine(WorkingDirectory, "subdir\\.editorconfig"), args.AnalyzerConfigPaths[1]);
args = DefaultParse(new[] { "/analyzerconfig", "a.cs" }, WorkingDirectory);
args.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<file list>' for 'analyzerconfig' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<file list>", "analyzerconfig").WithLocation(1, 1));
Assert.Equal(0, args.AnalyzerConfigPaths.Length);
args = DefaultParse(new[] { "/analyzerconfig:", "a.cs" }, WorkingDirectory);
args.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<file list>' for 'analyzerconfig' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<file list>", "analyzerconfig").WithLocation(1, 1));
Assert.Equal(0, args.AnalyzerConfigPaths.Length);
}
[Fact]
public void NullablePublicOnly()
{
string source =
@"namespace System.Runtime.CompilerServices
{
public sealed class NullableAttribute : Attribute { } // missing constructor
}
public class Program
{
private object? F = null;
}";
string errorMessage = "error CS0656: Missing compiler required member 'System.Runtime.CompilerServices.NullableAttribute..ctor'";
string filePath = Temp.CreateFile().WriteAllText(source).Path;
int exitCode;
string output;
// No /feature
(exitCode, output) = compileAndRun(featureOpt: null);
Assert.Equal(1, exitCode);
Assert.Contains(errorMessage, output, StringComparison.Ordinal);
// /features:nullablePublicOnly
(exitCode, output) = compileAndRun("/features:nullablePublicOnly");
Assert.Equal(0, exitCode);
Assert.DoesNotContain(errorMessage, output, StringComparison.Ordinal);
// /features:nullablePublicOnly=true
(exitCode, output) = compileAndRun("/features:nullablePublicOnly=true");
Assert.Equal(0, exitCode);
Assert.DoesNotContain(errorMessage, output, StringComparison.Ordinal);
// /features:nullablePublicOnly=false (the value is ignored)
(exitCode, output) = compileAndRun("/features:nullablePublicOnly=false");
Assert.Equal(0, exitCode);
Assert.DoesNotContain(errorMessage, output, StringComparison.Ordinal);
CleanupAllGeneratedFiles(filePath);
(int, string) compileAndRun(string featureOpt)
{
var args = new[] { "/target:library", "/preferreduilang:en", "/langversion:8", "/nullable+", filePath };
if (featureOpt != null) args = args.Concat(featureOpt).ToArray();
var compiler = CreateCSharpCompiler(null, WorkingDirectory, args);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = compiler.Run(outWriter);
return (exitCode, outWriter.ToString());
};
}
// See also NullableContextTests.NullableAnalysisFlags_01().
[Fact]
public void NullableAnalysisFlags()
{
string source =
@"class Program
{
#nullable enable
static object F1() => null;
#nullable disable
static object F2() => null;
}";
string filePath = Temp.CreateFile().WriteAllText(source).Path;
string fileName = Path.GetFileName(filePath);
string[] expectedWarningsAll = new[] { fileName + "(4,27): warning CS8603: Possible null reference return." };
string[] expectedWarningsNone = Array.Empty<string>();
AssertEx.Equal(expectedWarningsAll, compileAndRun(featureOpt: null));
AssertEx.Equal(expectedWarningsAll, compileAndRun("/features:run-nullable-analysis"));
AssertEx.Equal(expectedWarningsAll, compileAndRun("/features:run-nullable-analysis=always"));
AssertEx.Equal(expectedWarningsNone, compileAndRun("/features:run-nullable-analysis=never"));
AssertEx.Equal(expectedWarningsAll, compileAndRun("/features:run-nullable-analysis=ALWAYS")); // unrecognized value (incorrect case) ignored
AssertEx.Equal(expectedWarningsAll, compileAndRun("/features:run-nullable-analysis=NEVER")); // unrecognized value (incorrect case) ignored
AssertEx.Equal(expectedWarningsAll, compileAndRun("/features:run-nullable-analysis=true")); // unrecognized value ignored
AssertEx.Equal(expectedWarningsAll, compileAndRun("/features:run-nullable-analysis=false")); // unrecognized value ignored
AssertEx.Equal(expectedWarningsAll, compileAndRun("/features:run-nullable-analysis=unknown")); // unrecognized value ignored
CleanupAllGeneratedFiles(filePath);
string[] compileAndRun(string featureOpt)
{
var args = new[] { "/target:library", "/preferreduilang:en", "/nologo", filePath };
if (featureOpt != null) args = args.Concat(featureOpt).ToArray();
var compiler = CreateCSharpCompiler(null, WorkingDirectory, args);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
int exitCode = compiler.Run(outWriter);
return outWriter.ToString().Split(new[] { '\n', '\r' }, StringSplitOptions.RemoveEmptyEntries);
};
}
[Fact]
public void Compiler_Uses_DriverCache()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
}");
int sourceCallbackCount = 0;
var generator = new PipelineCallbackGenerator((ctx) =>
{
ctx.RegisterSourceOutput(ctx.ParseOptionsProvider, (spc, po) =>
{
sourceCallbackCount++;
});
});
// with no cache, we'll see the callback execute multiple times
RunWithNoCache();
Assert.Equal(1, sourceCallbackCount);
RunWithNoCache();
Assert.Equal(2, sourceCallbackCount);
RunWithNoCache();
Assert.Equal(3, sourceCallbackCount);
// now re-run with a cache
GeneratorDriverCache cache = new GeneratorDriverCache();
sourceCallbackCount = 0;
RunWithCache();
Assert.Equal(1, sourceCallbackCount);
RunWithCache();
Assert.Equal(1, sourceCallbackCount);
RunWithCache();
Assert.Equal(1, sourceCallbackCount);
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
Directory.Delete(dir.Path, true);
void RunWithNoCache() => VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/langversion:preview" }, generators: new[] { generator.AsSourceGenerator() }, analyzers: null);
void RunWithCache() => VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/langversion:preview", "/features:enable-generator-cache" }, generators: new[] { generator.AsSourceGenerator() }, driverCache: cache, analyzers: null);
}
[Fact]
public void Compiler_Doesnt_Use_Cache_From_Other_Compilation()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
}");
int sourceCallbackCount = 0;
var generator = new PipelineCallbackGenerator((ctx) =>
{
ctx.RegisterSourceOutput(ctx.ParseOptionsProvider, (spc, po) =>
{
sourceCallbackCount++;
});
});
// now re-run with a cache
GeneratorDriverCache cache = new GeneratorDriverCache();
sourceCallbackCount = 0;
RunWithCache("1.dll");
Assert.Equal(1, sourceCallbackCount);
RunWithCache("1.dll");
Assert.Equal(1, sourceCallbackCount);
// now emulate a new compilation, and check we were invoked, but only once
RunWithCache("2.dll");
Assert.Equal(2, sourceCallbackCount);
RunWithCache("2.dll");
Assert.Equal(2, sourceCallbackCount);
// now re-run our first compilation
RunWithCache("1.dll");
Assert.Equal(2, sourceCallbackCount);
// a new one
RunWithCache("3.dll");
Assert.Equal(3, sourceCallbackCount);
// and another old one
RunWithCache("2.dll");
Assert.Equal(3, sourceCallbackCount);
RunWithCache("1.dll");
Assert.Equal(3, sourceCallbackCount);
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
Directory.Delete(dir.Path, true);
void RunWithCache(string outputPath) => VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/langversion:preview", "/out:" + outputPath, "/features:enable-generator-cache" }, generators: new[] { generator.AsSourceGenerator() }, driverCache: cache, analyzers: null);
}
[Fact]
public void Compiler_Can_Enable_DriverCache()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
}");
int sourceCallbackCount = 0;
var generator = new PipelineCallbackGenerator((ctx) =>
{
ctx.RegisterSourceOutput(ctx.ParseOptionsProvider, (spc, po) =>
{
sourceCallbackCount++;
});
});
// run with the cache
GeneratorDriverCache cache = new GeneratorDriverCache();
sourceCallbackCount = 0;
RunWithCache();
Assert.Equal(1, sourceCallbackCount);
RunWithCache();
Assert.Equal(1, sourceCallbackCount);
RunWithCache();
Assert.Equal(1, sourceCallbackCount);
// now re-run with the cache disabled
sourceCallbackCount = 0;
RunWithCacheDisabled();
Assert.Equal(1, sourceCallbackCount);
RunWithCacheDisabled();
Assert.Equal(2, sourceCallbackCount);
RunWithCacheDisabled();
Assert.Equal(3, sourceCallbackCount);
// now clear the cache as well as disabling, and verify we don't put any entries into it either
cache = new GeneratorDriverCache();
sourceCallbackCount = 0;
RunWithCacheDisabled();
Assert.Equal(1, sourceCallbackCount);
Assert.Equal(0, cache.CacheSize);
RunWithCacheDisabled();
Assert.Equal(2, sourceCallbackCount);
Assert.Equal(0, cache.CacheSize);
RunWithCacheDisabled();
Assert.Equal(3, sourceCallbackCount);
Assert.Equal(0, cache.CacheSize);
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
Directory.Delete(dir.Path, true);
void RunWithCache() => VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/langversion:preview", "/features:enable-generator-cache" }, generators: new[] { generator.AsSourceGenerator() }, driverCache: cache, analyzers: null);
void RunWithCacheDisabled() => VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/langversion:preview" }, generators: new[] { generator.AsSourceGenerator() }, driverCache: cache, analyzers: null);
}
[Fact]
public void Adding_Or_Removing_A_Generator_Invalidates_Cache()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
}");
int sourceCallbackCount = 0;
int sourceCallbackCount2 = 0;
var generator = new PipelineCallbackGenerator((ctx) =>
{
ctx.RegisterSourceOutput(ctx.ParseOptionsProvider, (spc, po) =>
{
sourceCallbackCount++;
});
});
var generator2 = new PipelineCallbackGenerator2((ctx) =>
{
ctx.RegisterSourceOutput(ctx.ParseOptionsProvider, (spc, po) =>
{
sourceCallbackCount2++;
});
});
// run with the cache
GeneratorDriverCache cache = new GeneratorDriverCache();
RunWithOneGenerator();
Assert.Equal(1, sourceCallbackCount);
Assert.Equal(0, sourceCallbackCount2);
RunWithOneGenerator();
Assert.Equal(1, sourceCallbackCount);
Assert.Equal(0, sourceCallbackCount2);
RunWithTwoGenerators();
Assert.Equal(2, sourceCallbackCount);
Assert.Equal(1, sourceCallbackCount2);
RunWithTwoGenerators();
Assert.Equal(2, sourceCallbackCount);
Assert.Equal(1, sourceCallbackCount2);
// this seems counterintuitive, but when the only thing to change is the generator, we end up back at the state of the project when
// we just ran a single generator. Thus we already have an entry in the cache we can use (the one created by the original call to
// RunWithOneGenerator above) meaning we can use the previously cached results and not run.
RunWithOneGenerator();
Assert.Equal(2, sourceCallbackCount);
Assert.Equal(1, sourceCallbackCount2);
void RunWithOneGenerator() => VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/langversion:preview", "/features:enable-generator-cache" }, generators: new[] { generator.AsSourceGenerator() }, driverCache: cache, analyzers: null);
void RunWithTwoGenerators() => VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/langversion:preview", "/features:enable-generator-cache" }, generators: new[] { generator.AsSourceGenerator(), generator2.AsSourceGenerator() }, driverCache: cache, analyzers: null);
}
[Fact]
public void Compiler_Updates_Cached_Driver_AdditionalTexts()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText("class C { }");
var additionalFile = dir.CreateFile("additionalFile.txt").WriteAllText("some text");
int sourceCallbackCount = 0;
int additionalFileCallbackCount = 0;
var generator = new PipelineCallbackGenerator((ctx) =>
{
ctx.RegisterSourceOutput(ctx.ParseOptionsProvider, (spc, po) =>
{
sourceCallbackCount++;
});
ctx.RegisterSourceOutput(ctx.AdditionalTextsProvider, (spc, po) =>
{
additionalFileCallbackCount++;
});
});
GeneratorDriverCache cache = new GeneratorDriverCache();
RunWithCache();
Assert.Equal(1, sourceCallbackCount);
Assert.Equal(1, additionalFileCallbackCount);
RunWithCache();
Assert.Equal(1, sourceCallbackCount);
Assert.Equal(1, additionalFileCallbackCount);
additionalFile.WriteAllText("some new content");
RunWithCache();
Assert.Equal(1, sourceCallbackCount);
Assert.Equal(2, additionalFileCallbackCount); // additional file was updated
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
Directory.Delete(dir.Path, true);
void RunWithCache() => VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/langversion:preview", "/features:enable-generator-cache", "/additionalFile:" + additionalFile.Path }, generators: new[] { generator.AsSourceGenerator() }, driverCache: cache, analyzers: null);
}
[Fact]
public void Compiler_DoesNotCache_Driver_ConfigProvider()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText("class C { }");
var editorconfig = dir.CreateFile(".editorconfig").WriteAllText(@"
[temp.cs]
a = localA
");
var globalconfig = dir.CreateFile(".globalconfig").WriteAllText(@"
is_global = true
a = globalA");
int sourceCallbackCount = 0;
int configOptionsCallbackCount = 0;
int filteredGlobalCallbackCount = 0;
int filteredLocalCallbackCount = 0;
string globalA = "";
string localA = "";
var generator = new PipelineCallbackGenerator((ctx) =>
{
ctx.RegisterSourceOutput(ctx.ParseOptionsProvider, (spc, po) =>
{
sourceCallbackCount++;
});
ctx.RegisterSourceOutput(ctx.AnalyzerConfigOptionsProvider, (spc, po) =>
{
configOptionsCallbackCount++;
po.GlobalOptions.TryGetValue("a", out globalA);
});
ctx.RegisterSourceOutput(ctx.AnalyzerConfigOptionsProvider.Select((p, _) => { p.GlobalOptions.TryGetValue("a", out var value); return value; }), (spc, value) =>
{
filteredGlobalCallbackCount++;
globalA = value;
});
var syntaxTreeInput = ctx.CompilationProvider.Select((c, _) => c.SyntaxTrees.First());
ctx.RegisterSourceOutput(ctx.AnalyzerConfigOptionsProvider.Combine(syntaxTreeInput).Select((p, _) => { p.Left.GetOptions(p.Right).TryGetValue("a", out var value); return value; }), (spc, value) =>
{
filteredLocalCallbackCount++;
localA = value;
});
});
GeneratorDriverCache cache = new GeneratorDriverCache();
RunWithCache();
Assert.Equal(1, sourceCallbackCount);
Assert.Equal(1, configOptionsCallbackCount);
Assert.Equal(1, filteredGlobalCallbackCount);
Assert.Equal(1, filteredLocalCallbackCount);
Assert.Equal("globalA", globalA);
Assert.Equal("localA", localA);
RunWithCache();
Assert.Equal(1, sourceCallbackCount);
Assert.Equal(2, configOptionsCallbackCount); // we can't compare the provider directly, so we consider it modified
Assert.Equal(1, filteredGlobalCallbackCount); // however, the values in it will cache out correctly.
Assert.Equal(1, filteredLocalCallbackCount);
editorconfig.WriteAllText(@"
[temp.cs]
a = diffLocalA
");
RunWithCache();
Assert.Equal(1, sourceCallbackCount);
Assert.Equal(3, configOptionsCallbackCount);
Assert.Equal(1, filteredGlobalCallbackCount); // the provider changed, but only the local value changed
Assert.Equal(2, filteredLocalCallbackCount);
Assert.Equal("globalA", globalA);
Assert.Equal("diffLocalA", localA);
globalconfig.WriteAllText(@"
is_global = true
a = diffGlobalA
");
RunWithCache();
Assert.Equal(1, sourceCallbackCount);
Assert.Equal(4, configOptionsCallbackCount);
Assert.Equal(2, filteredGlobalCallbackCount); // only the global value was changed
Assert.Equal(2, filteredLocalCallbackCount);
Assert.Equal("diffGlobalA", globalA);
Assert.Equal("diffLocalA", localA);
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
Directory.Delete(dir.Path, true);
void RunWithCache() => VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/langversion:preview", "/features:enable-generator-cache", "/analyzerConfig:" + editorconfig.Path, "/analyzerConfig:" + globalconfig.Path }, generators: new[] { generator.AsSourceGenerator() }, driverCache: cache, analyzers: null);
}
[Fact]
public void Compiler_DoesNotCache_Compilation()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
}");
int sourceCallbackCount = 0;
var generator = new PipelineCallbackGenerator((ctx) =>
{
ctx.RegisterSourceOutput(ctx.CompilationProvider, (spc, po) =>
{
sourceCallbackCount++;
});
});
// now re-run with a cache
GeneratorDriverCache cache = new GeneratorDriverCache();
RunWithCache();
Assert.Equal(1, sourceCallbackCount);
RunWithCache();
Assert.Equal(2, sourceCallbackCount);
RunWithCache();
Assert.Equal(3, sourceCallbackCount);
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
Directory.Delete(dir.Path, true);
void RunWithCache() => VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/langversion:preview", "/features:enable-generator-cache" }, generators: new[] { generator.AsSourceGenerator() }, driverCache: cache, analyzers: null);
}
private static int OccurrenceCount(string source, string word)
{
var n = 0;
var index = source.IndexOf(word, StringComparison.Ordinal);
while (index >= 0)
{
++n;
index = source.IndexOf(word, index + word.Length, StringComparison.Ordinal);
}
return n;
}
private string VerifyOutput(TempDirectory sourceDir, TempFile sourceFile,
bool includeCurrentAssemblyAsAnalyzerReference = true,
string[] additionalFlags = null,
int expectedInfoCount = 0,
int expectedWarningCount = 0,
int expectedErrorCount = 0,
int? expectedExitCode = null,
bool errorlog = false,
bool skipAnalyzers = false,
IEnumerable<ISourceGenerator> generators = null,
GeneratorDriverCache driverCache = null,
params DiagnosticAnalyzer[] analyzers)
{
var args = new[] {
"/nologo", "/preferreduilang:en", "/t:library",
sourceFile.Path
};
if (includeCurrentAssemblyAsAnalyzerReference)
{
args = args.Append("/a:" + Assembly.GetExecutingAssembly().Location);
}
if (errorlog)
{
args = args.Append("/errorlog:errorlog");
}
if (skipAnalyzers)
{
args = args.Append("/skipAnalyzers");
}
if (additionalFlags != null)
{
args = args.Append(additionalFlags);
}
var csc = CreateCSharpCompiler(null, sourceDir.Path, args, analyzers: analyzers.ToImmutableArrayOrEmpty(), generators: generators.ToImmutableArrayOrEmpty(), driverCache: driverCache);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = csc.Run(outWriter);
var output = outWriter.ToString();
expectedExitCode ??= expectedErrorCount > 0 ? 1 : 0;
Assert.True(
expectedExitCode == exitCode,
string.Format("Expected exit code to be '{0}' was '{1}'.{2} Output:{3}{4}",
expectedExitCode, exitCode, Environment.NewLine, Environment.NewLine, output));
Assert.DoesNotContain("hidden", output, StringComparison.Ordinal);
if (expectedInfoCount == 0)
{
Assert.DoesNotContain("info", output, StringComparison.Ordinal);
}
else
{
// Info diagnostics are only logged with /errorlog.
Assert.True(errorlog);
Assert.Equal(expectedInfoCount, OccurrenceCount(output, "info"));
}
if (expectedWarningCount == 0)
{
Assert.DoesNotContain("warning", output, StringComparison.Ordinal);
}
else
{
Assert.Equal(expectedWarningCount, OccurrenceCount(output, "warning"));
}
if (expectedErrorCount == 0)
{
Assert.DoesNotContain("error", output, StringComparison.Ordinal);
}
else
{
Assert.Equal(expectedErrorCount, OccurrenceCount(output, "error"));
}
return output;
}
[WorkItem(899050, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/899050")]
[Fact]
public void NoWarnAndWarnAsError_AnalyzerDriverWarnings()
{
// This assembly has an abstract MockAbstractDiagnosticAnalyzer type which should cause
// compiler warning CS8032 to be produced when compilations created in this test try to load it.
string source = @"using System;";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile("a.cs");
file.WriteAllText(source);
var output = VerifyOutput(dir, file, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that compiler warning CS8032 can be suppressed via /warn:0.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warn:0" });
Assert.True(string.IsNullOrEmpty(output));
// TEST: Verify that compiler warning CS8032 can be individually suppressed via /nowarn:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/nowarn:CS8032" });
Assert.True(string.IsNullOrEmpty(output));
// TEST: Verify that compiler warning CS8032 can be promoted to an error via /warnaserror.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror" }, expectedErrorCount: 1);
Assert.Contains("error CS8032", output, StringComparison.Ordinal);
// TEST: Verify that compiler warning CS8032 can be individually promoted to an error via /warnaserror:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror:8032" }, expectedErrorCount: 1);
Assert.Contains("error CS8032", output, StringComparison.Ordinal);
CleanupAllGeneratedFiles(file.Path);
}
[WorkItem(899050, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/899050")]
[WorkItem(981677, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/981677")]
[WorkItem(1021115, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/1021115")]
[Fact]
public void NoWarnAndWarnAsError_HiddenDiagnostic()
{
// This assembly has a HiddenDiagnosticAnalyzer type which should produce custom hidden
// diagnostics for #region directives present in the compilations created in this test.
var source = @"using System;
#region Region
#endregion";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile("a.cs");
file.WriteAllText(source);
var output = VerifyOutput(dir, file, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that /warn:0 has no impact on custom hidden diagnostic Hidden01.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warn:0" });
Assert.True(string.IsNullOrEmpty(output));
// TEST: Verify that /nowarn: has no impact on custom hidden diagnostic Hidden01.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/nowarn:Hidden01" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that /warnaserror+ has no impact on custom hidden diagnostic Hidden01.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror+", "/nowarn:8032" });
Assert.True(string.IsNullOrEmpty(output));
// TEST: Verify that /warnaserror- has no impact on custom hidden diagnostic Hidden01.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that /warnaserror: promotes custom hidden diagnostic Hidden01 to an error.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror:Hidden01" }, expectedWarningCount: 1, expectedErrorCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
Assert.Contains("a.cs(2,1): error Hidden01: Throwing a diagnostic for #region", output, StringComparison.Ordinal);
// TEST: Verify that /warnaserror-: has no impact on custom hidden diagnostic Hidden01.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-:Hidden01" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify /nowarn: overrides /warnaserror:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror:Hidden01", "/nowarn:Hidden01" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify /nowarn: overrides /warnaserror:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/nowarn:Hidden01", "/warnaserror:Hidden01" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify /nowarn: overrides /warnaserror-:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-:Hidden01", "/nowarn:Hidden01" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify /nowarn: overrides /warnaserror-:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/nowarn:Hidden01", "/warnaserror-:Hidden01" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that /warn:0 has no impact on custom hidden diagnostic Hidden01.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warn:0", "/warnaserror:Hidden01" });
Assert.True(string.IsNullOrEmpty(output));
// TEST: Verify that /warn:0 has no impact on custom hidden diagnostic Hidden01.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror:Hidden01", "/warn:0" });
Assert.True(string.IsNullOrEmpty(output));
// TEST: Verify that last /warnaserror[+/-]: flag on command line wins.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror+:Hidden01", "/warnaserror-:Hidden01" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that last /warnaserror[+/-]: flag on command line wins.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-:Hidden01", "/warnaserror+:Hidden01" }, expectedWarningCount: 1, expectedErrorCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
Assert.Contains("a.cs(2,1): error Hidden01: Throwing a diagnostic for #region", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-] and /warnaserror[+/-]:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-", "/warnaserror+:Hidden01" }, expectedWarningCount: 1, expectedErrorCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
Assert.Contains("a.cs(2,1): error Hidden01: Throwing a diagnostic for #region", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-]: and /warnaserror[+/-].
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-:Hidden01", "/warnaserror+" }, expectedErrorCount: 1);
Assert.Contains("error CS8032", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-] and /warnaserror[+/-]:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror+", "/warnaserror+:Hidden01", "/nowarn:8032" }, expectedErrorCount: 1);
Assert.Contains("a.cs(2,1): error Hidden01: Throwing a diagnostic for #region", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-]: and /warnaserror[+/-].
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror+:Hidden01", "/warnaserror+", "/nowarn:8032" });
Assert.True(string.IsNullOrEmpty(output));
// TEST: Verify that last one wins between /warnaserror[+/-]: and /warnaserror[+/-].
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror+:Hidden01", "/warnaserror-" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-] and /warnaserror[+/-]:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror+", "/warnaserror-:Hidden01", "/nowarn:8032" });
Assert.True(string.IsNullOrEmpty(output));
// TEST: Verify that last one wins between /warnaserror[+/-]: and /warnaserror[+/-].
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-:Hidden01", "/warnaserror-" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-] and /warnaserror[+/-]:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-", "/warnaserror-:Hidden01" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
CleanupAllGeneratedFiles(file.Path);
}
[WorkItem(899050, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/899050")]
[WorkItem(981677, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/981677")]
[WorkItem(1021115, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/1021115")]
[WorkItem(42166, "https://github.com/dotnet/roslyn/issues/42166")]
[CombinatorialData, Theory]
public void NoWarnAndWarnAsError_InfoDiagnostic(bool errorlog)
{
// NOTE: Info diagnostics are only logged on command line when /errorlog is specified. See https://github.com/dotnet/roslyn/issues/42166 for details.
// This assembly has an InfoDiagnosticAnalyzer type which should produce custom info
// diagnostics for the #pragma warning restore directives present in the compilations created in this test.
var source = @"using System;
#pragma warning restore";
var name = "a.cs";
string output;
output = GetOutput(name, source, expectedWarningCount: 1, expectedInfoCount: errorlog ? 1 : 0, errorlog: errorlog);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
if (errorlog)
Assert.Contains("a.cs(2,1): info Info01: Throwing a diagnostic for #pragma restore", output, StringComparison.Ordinal);
// TEST: Verify that /warn:0 suppresses custom info diagnostic Info01.
output = GetOutput(name, source, additionalFlags: new[] { "/warn:0" }, errorlog: errorlog);
// TEST: Verify that custom info diagnostic Info01 can be individually suppressed via /nowarn:.
output = GetOutput(name, source, additionalFlags: new[] { "/nowarn:Info01" }, expectedWarningCount: 1, errorlog: errorlog);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that custom info diagnostic Info01 can never be promoted to an error via /warnaserror+.
output = GetOutput(name, source, additionalFlags: new[] { "/warnaserror+", "/nowarn:8032" }, expectedInfoCount: errorlog ? 1 : 0, errorlog: errorlog);
if (errorlog)
Assert.Contains("a.cs(2,1): info Info01: Throwing a diagnostic for #pragma restore", output, StringComparison.Ordinal);
// TEST: Verify that custom info diagnostic Info01 is still reported as an info when /warnaserror- is used.
output = GetOutput(name, source, additionalFlags: new[] { "/warnaserror-" }, expectedWarningCount: 1, expectedInfoCount: errorlog ? 1 : 0, errorlog: errorlog);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
if (errorlog)
Assert.Contains("a.cs(2,1): info Info01: Throwing a diagnostic for #pragma restore", output, StringComparison.Ordinal);
// TEST: Verify that custom info diagnostic Info01 can be individually promoted to an error via /warnaserror:.
output = GetOutput(name, source, additionalFlags: new[] { "/warnaserror:Info01" }, expectedWarningCount: 1, expectedErrorCount: 1, errorlog: errorlog);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
Assert.Contains("a.cs(2,1): error Info01: Throwing a diagnostic for #pragma restore", output, StringComparison.Ordinal);
// TEST: Verify that custom info diagnostic Info01 is still reported as an info when passed to /warnaserror-:.
output = GetOutput(name, source, additionalFlags: new[] { "/warnaserror-:Info01" }, expectedWarningCount: 1, expectedInfoCount: errorlog ? 1 : 0, errorlog: errorlog);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
if (errorlog)
Assert.Contains("a.cs(2,1): info Info01: Throwing a diagnostic for #pragma restore", output, StringComparison.Ordinal);
// TEST: Verify /nowarn overrides /warnaserror.
output = GetOutput(name, source, additionalFlags: new[] { "/warnaserror:Info01", "/nowarn:Info01" }, expectedWarningCount: 1, errorlog: errorlog);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify /nowarn overrides /warnaserror.
output = GetOutput(name, source, additionalFlags: new[] { "/nowarn:Info01", "/warnaserror:Info01" }, expectedWarningCount: 1, errorlog: errorlog);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify /nowarn overrides /warnaserror-.
output = GetOutput(name, source, additionalFlags: new[] { "/warnaserror-:Info01", "/nowarn:Info01" }, expectedWarningCount: 1, errorlog: errorlog);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify /nowarn overrides /warnaserror-.
output = GetOutput(name, source, additionalFlags: new[] { "/nowarn:Info01", "/warnaserror-:Info01" }, expectedWarningCount: 1, errorlog: errorlog);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that /warn:0 has no impact on custom info diagnostic Info01.
output = GetOutput(name, source, additionalFlags: new[] { "/warn:0", "/warnaserror:Info01" }, errorlog: errorlog);
// TEST: Verify that /warn:0 has no impact on custom info diagnostic Info01.
output = GetOutput(name, source, additionalFlags: new[] { "/warnaserror:Info01", "/warn:0" });
// TEST: Verify that last /warnaserror[+/-]: flag on command line wins.
output = GetOutput(name, source, additionalFlags: new[] { "/warnaserror+:Info01", "/warnaserror-:Info01" }, expectedWarningCount: 1, expectedInfoCount: errorlog ? 1 : 0, errorlog: errorlog);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
if (errorlog)
Assert.Contains("a.cs(2,1): info Info01: Throwing a diagnostic for #pragma restore", output, StringComparison.Ordinal);
// TEST: Verify that last /warnaserror[+/-]: flag on command line wins.
output = GetOutput(name, source, additionalFlags: new[] { "/warnaserror-:Info01", "/warnaserror+:Info01" }, expectedWarningCount: 1, expectedErrorCount: 1, errorlog: errorlog);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
Assert.Contains("a.cs(2,1): error Info01: Throwing a diagnostic for #pragma restore", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-] and /warnaserror[+/-]:.
output = GetOutput(name, source, additionalFlags: new[] { "/warnaserror-", "/warnaserror+:Info01" }, expectedWarningCount: 1, expectedErrorCount: 1, errorlog: errorlog);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
Assert.Contains("a.cs(2,1): error Info01: Throwing a diagnostic for #pragma restore", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-]: and /warnaserror[+/-].
output = GetOutput(name, source, additionalFlags: new[] { "/warnaserror-:Info01", "/warnaserror+", "/nowarn:8032" }, expectedInfoCount: errorlog ? 1 : 0, errorlog: errorlog);
if (errorlog)
Assert.Contains("a.cs(2,1): info Info01: Throwing a diagnostic for #pragma restore", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-]: and /warnaserror[+/-].
output = GetOutput(name, source, additionalFlags: new[] { "/warnaserror+:Info01", "/warnaserror+", "/nowarn:8032" }, expectedInfoCount: errorlog ? 1 : 0, errorlog: errorlog);
if (errorlog)
Assert.Contains("a.cs(2,1): info Info01: Throwing a diagnostic for #pragma restore", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-] and /warnaserror[+/-]:.
output = GetOutput(name, source, additionalFlags: new[] { "/warnaserror+", "/warnaserror+:Info01", "/nowarn:8032" }, expectedErrorCount: 1, errorlog: errorlog);
Assert.Contains("a.cs(2,1): error Info01: Throwing a diagnostic for #pragma restore", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-]: and /warnaserror[+/-].
output = GetOutput(name, source, additionalFlags: new[] { "/warnaserror+:Info01", "/warnaserror-" }, expectedWarningCount: 1, expectedInfoCount: errorlog ? 1 : 0, errorlog: errorlog);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
if (errorlog)
Assert.Contains("a.cs(2,1): info Info01: Throwing a diagnostic for #pragma restore", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-] and /warnaserror[+/-]:.
output = GetOutput(name, source, additionalFlags: new[] { "/warnaserror+", "/warnaserror-:Info01", "/nowarn:8032" }, expectedInfoCount: errorlog ? 1 : 0, errorlog: errorlog);
if (errorlog)
Assert.Contains("a.cs(2,1): info Info01: Throwing a diagnostic for #pragma restore", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-]: and /warnaserror[+/-].
output = GetOutput(name, source, additionalFlags: new[] { "/warnaserror-:Info01", "/warnaserror-" }, expectedWarningCount: 1, expectedInfoCount: errorlog ? 1 : 0, errorlog: errorlog);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
if (errorlog)
Assert.Contains("a.cs(2,1): info Info01: Throwing a diagnostic for #pragma restore", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-] and /warnaserror[+/-]:.
output = GetOutput(name, source, additionalFlags: new[] { "/warnaserror-", "/warnaserror-:Info01" }, expectedWarningCount: 1, expectedInfoCount: errorlog ? 1 : 0, errorlog: errorlog);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
if (errorlog)
Assert.Contains("a.cs(2,1): info Info01: Throwing a diagnostic for #pragma restore", output, StringComparison.Ordinal);
}
private string GetOutput(
string name,
string source,
bool includeCurrentAssemblyAsAnalyzerReference = true,
string[] additionalFlags = null,
int expectedInfoCount = 0,
int expectedWarningCount = 0,
int expectedErrorCount = 0,
bool errorlog = false)
{
var dir = Temp.CreateDirectory();
var file = dir.CreateFile(name);
file.WriteAllText(source);
var output = VerifyOutput(dir, file, includeCurrentAssemblyAsAnalyzerReference, additionalFlags, expectedInfoCount, expectedWarningCount, expectedErrorCount, null, errorlog);
CleanupAllGeneratedFiles(file.Path);
return output;
}
[WorkItem(11368, "https://github.com/dotnet/roslyn/issues/11368")]
[WorkItem(899050, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/899050")]
[WorkItem(981677, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/981677")]
[WorkItem(998069, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/998069")]
[WorkItem(998724, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/998724")]
[WorkItem(1021115, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/1021115")]
[Fact]
public void NoWarnAndWarnAsError_WarningDiagnostic()
{
// This assembly has a WarningDiagnosticAnalyzer type which should produce custom warning
// diagnostics for source types present in the compilations created in this test.
string source = @"
class C
{
static void Main()
{
int i;
}
}
";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile("a.cs");
file.WriteAllText(source);
var output = VerifyOutput(dir, file, expectedWarningCount: 3);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
Assert.Contains("a.cs(2,7): warning Warning01: Throwing a diagnostic for types declared", output, StringComparison.Ordinal);
Assert.Contains("a.cs(6,13): warning CS0168: The variable 'i' is declared but never used", output, StringComparison.Ordinal);
// TEST: Verify that compiler warning CS0168 as well as custom warning diagnostic Warning01 can be suppressed via /warn:0.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warn:0" });
Assert.True(string.IsNullOrEmpty(output));
// TEST: Verify that compiler warning CS0168 as well as custom warning diagnostic Warning01 can be individually suppressed via /nowarn:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/nowarn:0168,Warning01,58000" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that diagnostic ids are processed in case-sensitive fashion inside /nowarn:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/nowarn:cs0168,warning01,700000" }, expectedWarningCount: 3);
Assert.Contains("a.cs(2,7): warning Warning01: Throwing a diagnostic for types declared", output, StringComparison.Ordinal);
Assert.Contains("a.cs(6,13): warning CS0168: The variable 'i' is declared but never used", output, StringComparison.Ordinal);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that compiler warning CS0168 as well as custom warning diagnostic Warning01 can be promoted to errors via /warnaserror.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror", "/nowarn:8032" }, expectedErrorCount: 2);
Assert.Contains("a.cs(2,7): error Warning01: Throwing a diagnostic for types declared", output, StringComparison.Ordinal);
Assert.Contains("a.cs(6,13): error CS0168: The variable 'i' is declared but never used", output, StringComparison.Ordinal);
// TEST: Verify that compiler warning CS0168 as well as custom warning diagnostic Warning01 can be promoted to errors via /warnaserror+.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror+", "/nowarn:8032" }, expectedErrorCount: 2);
Assert.Contains("a.cs(2,7): error Warning01: Throwing a diagnostic for types declared", output, StringComparison.Ordinal);
Assert.Contains("a.cs(6,13): error CS0168: The variable 'i' is declared but never used", output, StringComparison.Ordinal);
// TEST: Verify that /warnaserror- keeps compiler warning CS0168 as well as custom warning diagnostic Warning01 as warnings.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-" }, expectedWarningCount: 3);
Assert.Contains("a.cs(2,7): warning Warning01: Throwing a diagnostic for types declared", output, StringComparison.Ordinal);
Assert.Contains("a.cs(6,13): warning CS0168: The variable 'i' is declared but never used", output, StringComparison.Ordinal);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that custom warning diagnostic Warning01 can be individually promoted to an error via /warnaserror:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror:Something,Warning01" }, expectedWarningCount: 2, expectedErrorCount: 1);
Assert.Contains("a.cs(2,7): error Warning01: Throwing a diagnostic for types declared", output, StringComparison.Ordinal);
Assert.Contains("a.cs(6,13): warning CS0168: The variable 'i' is declared but never used", output, StringComparison.Ordinal);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that compiler warning CS0168 can be individually promoted to an error via /warnaserror+:.
// This doesn't work correctly currently - promoting compiler warning CS0168 to an error causes us to no longer report any custom warning diagnostics as errors (Bug 998069).
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror+:CS0168" }, expectedWarningCount: 2, expectedErrorCount: 1);
Assert.Contains("a.cs(2,7): warning Warning01: Throwing a diagnostic for types declared", output, StringComparison.Ordinal);
Assert.Contains("a.cs(6,13): error CS0168: The variable 'i' is declared but never used", output, StringComparison.Ordinal);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that diagnostic ids are processed in case-sensitive fashion inside /warnaserror.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror:cs0168,warning01,58000" }, expectedWarningCount: 3);
Assert.Contains("a.cs(2,7): warning Warning01: Throwing a diagnostic for types declared", output, StringComparison.Ordinal);
Assert.Contains("a.cs(6,13): warning CS0168: The variable 'i' is declared but never used", output, StringComparison.Ordinal);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that custom warning diagnostic Warning01 as well as compiler warning CS0168 can be promoted to errors via /warnaserror:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror:CS0168,Warning01" }, expectedWarningCount: 1, expectedErrorCount: 2);
Assert.Contains("a.cs(2,7): error Warning01: Throwing a diagnostic for types declared", output, StringComparison.Ordinal);
Assert.Contains("a.cs(6,13): error CS0168: The variable 'i' is declared but never used", output, StringComparison.Ordinal);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that /warn:0 overrides /warnaserror+.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warn:0", "/warnaserror+" });
// TEST: Verify that /warn:0 overrides /warnaserror.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror", "/warn:0" });
// TEST: Verify that /warn:0 overrides /warnaserror-.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-", "/warn:0" });
// TEST: Verify that /warn:0 overrides /warnaserror-.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warn:0", "/warnaserror-" });
// TEST: Verify that /nowarn: overrides /warnaserror:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror:Something,CS0168,Warning01", "/nowarn:0168,Warning01,58000" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that /nowarn: overrides /warnaserror:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/nowarn:0168,Warning01,58000", "/warnaserror:Something,CS0168,Warning01" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that /nowarn: overrides /warnaserror-:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-:Something,CS0168,Warning01", "/nowarn:0168,Warning01,58000" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that /nowarn: overrides /warnaserror-:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/nowarn:0168,Warning01,58000", "/warnaserror-:Something,CS0168,Warning01" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that /nowarn: overrides /warnaserror+.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror+", "/nowarn:0168,Warning01,58000,8032" });
// TEST: Verify that /nowarn: overrides /warnaserror+.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/nowarn:0168,Warning01,58000,8032", "/warnaserror+" });
// TEST: Verify that /nowarn: overrides /warnaserror-.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-", "/nowarn:0168,Warning01,58000,8032" });
// TEST: Verify that /nowarn: overrides /warnaserror-.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/nowarn:0168,Warning01,58000,8032", "/warnaserror-" });
// TEST: Verify that /warn:0 overrides /warnaserror:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror:Something,CS0168,Warning01", "/warn:0" });
// TEST: Verify that /warn:0 overrides /warnaserror:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warn:0", "/warnaserror:Something,CS0168,Warning01" });
// TEST: Verify that last /warnaserror[+/-] flag on command line wins.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-", "/warnaserror+" }, expectedErrorCount: 1);
Assert.Contains("error CS8032", output, StringComparison.Ordinal);
// TEST: Verify that last /warnaserror[+/-] flag on command line wins.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror", "/warnaserror-" }, expectedWarningCount: 3);
Assert.Contains("a.cs(2,7): warning Warning01: Throwing a diagnostic for types declared", output, StringComparison.Ordinal);
Assert.Contains("a.cs(6,13): warning CS0168: The variable 'i' is declared but never used", output, StringComparison.Ordinal);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that last /warnaserror[+/-]: flag on command line wins.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-:Warning01", "/warnaserror+:Warning01" }, expectedWarningCount: 2, expectedErrorCount: 1);
Assert.Contains("a.cs(2,7): error Warning01: Throwing a diagnostic for types declared", output, StringComparison.Ordinal);
Assert.Contains("a.cs(6,13): warning CS0168: The variable 'i' is declared but never used", output, StringComparison.Ordinal);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that last /warnaserror[+/-]: flag on command line wins.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror+:Warning01", "/warnaserror-:Warning01" }, expectedWarningCount: 3);
Assert.Contains("a.cs(2,7): warning Warning01: Throwing a diagnostic for types declared", output, StringComparison.Ordinal);
Assert.Contains("a.cs(6,13): warning CS0168: The variable 'i' is declared but never used", output, StringComparison.Ordinal);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-]: and /warnaserror[+/-].
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-:Warning01,CS0168,58000,8032", "/warnaserror+" }, expectedErrorCount: 1);
Assert.Contains("error CS8032", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-] and /warnaserror[+/-]:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror", "/warnaserror-:Warning01,CS0168,58000,8032" }, expectedWarningCount: 3);
Assert.Contains("a.cs(2,7): warning Warning01: Throwing a diagnostic for types declared", output, StringComparison.Ordinal);
Assert.Contains("a.cs(6,13): warning CS0168: The variable 'i' is declared but never used", output, StringComparison.Ordinal);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-]: and /warnaserror[+/-].
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror:Warning01,58000,8032", "/warnaserror-" }, expectedWarningCount: 3);
Assert.Contains("a.cs(2,7): warning Warning01: Throwing a diagnostic for types declared", output, StringComparison.Ordinal);
Assert.Contains("a.cs(6,13): warning CS0168: The variable 'i' is declared but never used", output, StringComparison.Ordinal);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-] and /warnaserror[+/-]:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-", "/warnaserror+:Warning01" }, expectedWarningCount: 2, expectedErrorCount: 1);
Assert.Contains("a.cs(2,7): error Warning01: Throwing a diagnostic for types declared", output, StringComparison.Ordinal);
Assert.Contains("a.cs(6,13): warning CS0168: The variable 'i' is declared but never used", output, StringComparison.Ordinal);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-]: and /warnaserror[+/-].
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror:Warning01,CS0168,58000", "/warnaserror+" }, expectedErrorCount: 1);
Assert.Contains("error CS8032", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-] and /warnaserror[+/-]:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror", "/warnaserror+:Warning01,CS0168,58000" }, expectedErrorCount: 1);
Assert.Contains("error CS8032", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-]: and /warnaserror[+/-].
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-:Warning01,58000,8032", "/warnaserror-" }, expectedWarningCount: 3);
Assert.Contains("a.cs(2,7): warning Warning01: Throwing a diagnostic for types declared", output, StringComparison.Ordinal);
Assert.Contains("a.cs(6,13): warning CS0168: The variable 'i' is declared but never used", output, StringComparison.Ordinal);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that last one wins between /warnaserror[+/-] and /warnaserror[+/-]:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-", "/warnaserror-:Warning01,58000,8032" }, expectedWarningCount: 3);
Assert.Contains("a.cs(2,7): warning Warning01: Throwing a diagnostic for types declared", output, StringComparison.Ordinal);
Assert.Contains("a.cs(6,13): warning CS0168: The variable 'i' is declared but never used", output, StringComparison.Ordinal);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
CleanupAllGeneratedFiles(file.Path);
}
[WorkItem(899050, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/899050")]
[WorkItem(981677, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/981677")]
[Fact]
public void NoWarnAndWarnAsError_ErrorDiagnostic()
{
// This assembly has an ErrorDiagnosticAnalyzer type which should produce custom error
// diagnostics for #pragma warning disable directives present in the compilations created in this test.
string source = @"using System;
#pragma warning disable";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile("a.cs");
file.WriteAllText(source);
var output = VerifyOutput(dir, file, expectedErrorCount: 1, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
Assert.Contains("a.cs(2,1): error Error01: Throwing a diagnostic for #pragma disable", output, StringComparison.Ordinal);
// TEST: Verify that custom error diagnostic Error01 can't be suppressed via /warn:0.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warn:0" }, expectedErrorCount: 1);
Assert.Contains("a.cs(2,1): error Error01: Throwing a diagnostic for #pragma disable", output, StringComparison.Ordinal);
// TEST: Verify that custom error diagnostic Error01 can be suppressed via /nowarn:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/nowarn:Error01" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that /nowarn: overrides /warnaserror+.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror+", "/nowarn:Error01" }, expectedErrorCount: 1);
Assert.Contains("error CS8032", output, StringComparison.Ordinal);
// TEST: Verify that /nowarn: overrides /warnaserror.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/nowarn:Error01", "/warnaserror" }, expectedErrorCount: 1);
Assert.Contains("error CS8032", output, StringComparison.Ordinal);
// TEST: Verify that /nowarn: overrides /warnaserror+:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/nowarn:Error01", "/warnaserror+:Error01" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that /nowarn: overrides /warnaserror:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror:Error01", "/nowarn:Error01" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that /nowarn: overrides /warnaserror-.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-", "/nowarn:Error01" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that /nowarn: overrides /warnaserror-.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/nowarn:Error01", "/warnaserror-" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that /nowarn: overrides /warnaserror-.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-:Error01", "/nowarn:Error01" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that /nowarn: overrides /warnaserror-.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/nowarn:Error01", "/warnaserror-:Error01" }, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
// TEST: Verify that nothing bad happens when using /warnaserror[+/-] when custom error diagnostic Error01 is present.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror" }, expectedErrorCount: 1);
Assert.Contains("error CS8032", output, StringComparison.Ordinal);
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror+" }, expectedErrorCount: 1);
Assert.Contains("error CS8032", output, StringComparison.Ordinal);
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-" }, expectedErrorCount: 1, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
Assert.Contains("a.cs(2,1): error Error01: Throwing a diagnostic for #pragma disable", output, StringComparison.Ordinal);
// TEST: Verify that nothing bad happens if someone passes custom error diagnostic Error01 to /warnaserror[+/-]:.
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror:Error01" }, expectedErrorCount: 1, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
Assert.Contains("a.cs(2,1): error Error01: Throwing a diagnostic for #pragma disable", output, StringComparison.Ordinal);
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror+:Error01" }, expectedErrorCount: 1, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
Assert.Contains("a.cs(2,1): error Error01: Throwing a diagnostic for #pragma disable", output, StringComparison.Ordinal);
output = VerifyOutput(dir, file, additionalFlags: new[] { "/warnaserror-:Error01" }, expectedErrorCount: 1, expectedWarningCount: 1);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
Assert.Contains("a.cs(2,1): error Error01: Throwing a diagnostic for #pragma disable", output, StringComparison.Ordinal);
CleanupAllGeneratedFiles(file.Path);
}
[Fact]
[WorkItem(11497, "https://github.com/dotnet/roslyn/issues/11497")]
public void ConsistentErrorMessageWhenProvidingNoKeyFile()
{
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/keyfile:", "/target:library", "/nologo", "/preferreduilang:en", "a.cs" });
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal("error CS2005: Missing file specification for 'keyfile' option", outWriter.ToString().Trim());
}
[Fact]
[WorkItem(11497, "https://github.com/dotnet/roslyn/issues/11497")]
public void ConsistentErrorMessageWhenProvidingEmptyKeyFile()
{
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/keyfile:\"\"", "/target:library", "/nologo", "/preferreduilang:en", "a.cs" });
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal("error CS2005: Missing file specification for 'keyfile' option", outWriter.ToString().Trim());
}
[Fact]
[WorkItem(11497, "https://github.com/dotnet/roslyn/issues/11497")]
public void ConsistentErrorMessageWhenProvidingNoKeyFile_PublicSign()
{
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/keyfile:", "/publicsign", "/target:library", "/nologo", "/preferreduilang:en", "a.cs" });
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal("error CS2005: Missing file specification for 'keyfile' option", outWriter.ToString().Trim());
}
[Fact]
[WorkItem(11497, "https://github.com/dotnet/roslyn/issues/11497")]
public void ConsistentErrorMessageWhenProvidingEmptyKeyFile_PublicSign()
{
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, WorkingDirectory, new[] { "/keyfile:\"\"", "/publicsign", "/target:library", "/nologo", "/preferreduilang:en", "a.cs" });
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Equal("error CS2005: Missing file specification for 'keyfile' option", outWriter.ToString().Trim());
}
[WorkItem(981677, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/981677")]
[Fact]
public void NoWarnAndWarnAsError_CompilerErrorDiagnostic()
{
string source = @"using System;
class C
{
static void Main()
{
int i = new Exception();
}
}";
var dir = Temp.CreateDirectory();
var file = dir.CreateFile("a.cs");
file.WriteAllText(source);
var output = VerifyOutput(dir, file, includeCurrentAssemblyAsAnalyzerReference: false, expectedErrorCount: 1);
Assert.Contains("a.cs(6,17): error CS0029: Cannot implicitly convert type 'System.Exception' to 'int'", output, StringComparison.Ordinal);
// TEST: Verify that compiler error CS0029 can't be suppressed via /warn:0.
output = VerifyOutput(dir, file, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/warn:0" }, expectedErrorCount: 1);
Assert.Contains("a.cs(6,17): error CS0029: Cannot implicitly convert type 'System.Exception' to 'int'", output, StringComparison.Ordinal);
// TEST: Verify that compiler error CS0029 can't be suppressed via /nowarn:.
output = VerifyOutput(dir, file, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/nowarn:29" }, expectedErrorCount: 1);
Assert.Contains("a.cs(6,17): error CS0029: Cannot implicitly convert type 'System.Exception' to 'int'", output, StringComparison.Ordinal);
output = VerifyOutput(dir, file, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/nowarn:CS0029" }, expectedErrorCount: 1);
Assert.Contains("a.cs(6,17): error CS0029: Cannot implicitly convert type 'System.Exception' to 'int'", output, StringComparison.Ordinal);
// TEST: Verify that nothing bad happens when using /warnaserror[+/-] when compiler error CS0029 is present.
output = VerifyOutput(dir, file, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/warnaserror" }, expectedErrorCount: 1);
Assert.Contains("a.cs(6,17): error CS0029: Cannot implicitly convert type 'System.Exception' to 'int'", output, StringComparison.Ordinal);
output = VerifyOutput(dir, file, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/warnaserror+" }, expectedErrorCount: 1);
Assert.Contains("a.cs(6,17): error CS0029: Cannot implicitly convert type 'System.Exception' to 'int'", output, StringComparison.Ordinal);
output = VerifyOutput(dir, file, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/warnaserror-" }, expectedErrorCount: 1);
Assert.Contains("a.cs(6,17): error CS0029: Cannot implicitly convert type 'System.Exception' to 'int'", output, StringComparison.Ordinal);
// TEST: Verify that nothing bad happens if someone passes compiler error CS0029 to /warnaserror[+/-]:.
output = VerifyOutput(dir, file, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/warnaserror:0029" }, expectedErrorCount: 1);
Assert.Contains("a.cs(6,17): error CS0029: Cannot implicitly convert type 'System.Exception' to 'int'", output, StringComparison.Ordinal);
output = VerifyOutput(dir, file, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/warnaserror+:CS0029" }, expectedErrorCount: 1);
Assert.Contains("a.cs(6,17): error CS0029: Cannot implicitly convert type 'System.Exception' to 'int'", output, StringComparison.Ordinal);
output = VerifyOutput(dir, file, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/warnaserror-:29" }, expectedErrorCount: 1);
Assert.Contains("a.cs(6,17): error CS0029: Cannot implicitly convert type 'System.Exception' to 'int'", output, StringComparison.Ordinal);
output = VerifyOutput(dir, file, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/warnaserror-:CS0029" }, expectedErrorCount: 1);
Assert.Contains("a.cs(6,17): error CS0029: Cannot implicitly convert type 'System.Exception' to 'int'", output, StringComparison.Ordinal);
CleanupAllGeneratedFiles(file.Path);
}
[WorkItem(1021115, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/1021115")]
[Fact]
public void WarnAsError_LastOneWins1()
{
var arguments = DefaultParse(new[] { "/warnaserror-:3001", "/warnaserror" }, null);
var options = arguments.CompilationOptions;
var comp = CreateCompilation(@"[assembly: System.CLSCompliant(true)]
public class C
{
public void M(ushort i)
{
}
public static void Main(string[] args) {}
}", options: options);
comp.VerifyDiagnostics(
// (4,26): warning CS3001: Argument type 'ushort' is not CLS-compliant
// public void M(ushort i)
Diagnostic(ErrorCode.WRN_CLS_BadArgType, "i")
.WithArguments("ushort")
.WithLocation(4, 26)
.WithWarningAsError(true));
}
[WorkItem(1021115, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/1021115")]
[Fact]
public void WarnAsError_LastOneWins2()
{
var arguments = DefaultParse(new[] { "/warnaserror", "/warnaserror-:3001" }, null);
var options = arguments.CompilationOptions;
var comp = CreateCompilation(@"[assembly: System.CLSCompliant(true)]
public class C
{
public void M(ushort i)
{
}
public static void Main(string[] args) {}
}", options: options);
comp.VerifyDiagnostics(
// (4,26): warning CS3001: Argument type 'ushort' is not CLS-compliant
// public void M(ushort i)
Diagnostic(ErrorCode.WRN_CLS_BadArgType, "i")
.WithArguments("ushort")
.WithLocation(4, 26)
.WithWarningAsError(false));
}
[WorkItem(1091972, "http://vstfdevdiv:8080/DevDiv2/DevDiv/_workitems/edit/1091972")]
[WorkItem(444, "CodePlex")]
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30321")]
public void Bug1091972()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("a.cs");
src.WriteAllText(
@"
/// <summary>ABC...XYZ</summary>
class C {
static void Main()
{
var textStreamReader = new System.IO.StreamReader(typeof(C).Assembly.GetManifestResourceStream(""doc.xml""));
System.Console.WriteLine(textStreamReader.ReadToEnd());
}
} ");
var output = ProcessUtilities.RunAndGetOutput(s_CSharpCompilerExecutable, String.Format("/nologo /doc:doc.xml /out:out.exe /resource:doc.xml \"{0}\"", src.ToString()), startFolder: dir.ToString());
Assert.Equal("", output.Trim());
Assert.True(File.Exists(Path.Combine(dir.ToString(), "doc.xml")));
var expected =
@"<?xml version=""1.0""?>
<doc>
<assembly>
<name>out</name>
</assembly>
<members>
<member name=""T:C"">
<summary>ABC...XYZ</summary>
</member>
</members>
</doc>".Trim();
using (var reader = new StreamReader(Path.Combine(dir.ToString(), "doc.xml")))
{
var content = reader.ReadToEnd();
Assert.Equal(expected, content.Trim());
}
output = ProcessUtilities.RunAndGetOutput(Path.Combine(dir.ToString(), "out.exe"), startFolder: dir.ToString());
Assert.Equal(expected, output.Trim());
CleanupAllGeneratedFiles(src.Path);
}
[ConditionalFact(typeof(WindowsOnly))]
public void CommandLineMisc()
{
CSharpCommandLineArguments args = null;
string baseDirectory = @"c:\test";
Func<string, CSharpCommandLineArguments> parse = (x) => FullParse(x, baseDirectory);
args = parse(@"/out:""a.exe""");
Assert.Equal(@"a.exe", args.OutputFileName);
args = parse(@"/pdb:""a.pdb""");
Assert.Equal(Path.Combine(baseDirectory, @"a.pdb"), args.PdbPath);
// The \ here causes " to be treated as a quote, not as an escaping construct
args = parse(@"a\""b c""\d.cs");
Assert.Equal(
new[] { @"c:\test\a""b", @"c:\test\c\d.cs" },
args.SourceFiles.Select(x => x.Path));
args = parse(@"a\\""b c""\d.cs");
Assert.Equal(
new[] { @"c:\test\a\b c\d.cs" },
args.SourceFiles.Select(x => x.Path));
args = parse(@"/nostdlib /r:""a.dll"",""b.dll"" c.cs");
Assert.Equal(
new[] { @"a.dll", @"b.dll" },
args.MetadataReferences.Select(x => x.Reference));
args = parse(@"/nostdlib /r:""a-s.dll"",""b-s.dll"" c.cs");
Assert.Equal(
new[] { @"a-s.dll", @"b-s.dll" },
args.MetadataReferences.Select(x => x.Reference));
args = parse(@"/nostdlib /r:""a,;s.dll"",""b,;s.dll"" c.cs");
Assert.Equal(
new[] { @"a,;s.dll", @"b,;s.dll" },
args.MetadataReferences.Select(x => x.Reference));
}
[Fact]
public void CommandLine_ScriptRunner1()
{
var args = ScriptParse(new[] { "--", "script.csx", "b", "c" }, baseDirectory: WorkingDirectory);
AssertEx.Equal(new[] { Path.Combine(WorkingDirectory, "script.csx") }, args.SourceFiles.Select(f => f.Path));
AssertEx.Equal(new[] { "b", "c" }, args.ScriptArguments);
args = ScriptParse(new[] { "--", "@script.csx", "b", "c" }, baseDirectory: WorkingDirectory);
AssertEx.Equal(new[] { Path.Combine(WorkingDirectory, "@script.csx") }, args.SourceFiles.Select(f => f.Path));
AssertEx.Equal(new[] { "b", "c" }, args.ScriptArguments);
args = ScriptParse(new[] { "--", "-script.csx", "b", "c" }, baseDirectory: WorkingDirectory);
AssertEx.Equal(new[] { Path.Combine(WorkingDirectory, "-script.csx") }, args.SourceFiles.Select(f => f.Path));
AssertEx.Equal(new[] { "b", "c" }, args.ScriptArguments);
args = ScriptParse(new[] { "script.csx", "--", "b", "c" }, baseDirectory: WorkingDirectory);
AssertEx.Equal(new[] { Path.Combine(WorkingDirectory, "script.csx") }, args.SourceFiles.Select(f => f.Path));
AssertEx.Equal(new[] { "--", "b", "c" }, args.ScriptArguments);
args = ScriptParse(new[] { "script.csx", "a", "b", "c" }, baseDirectory: WorkingDirectory);
AssertEx.Equal(new[] { Path.Combine(WorkingDirectory, "script.csx") }, args.SourceFiles.Select(f => f.Path));
AssertEx.Equal(new[] { "a", "b", "c" }, args.ScriptArguments);
args = ScriptParse(new[] { "script.csx", "a", "--", "b", "c" }, baseDirectory: WorkingDirectory);
AssertEx.Equal(new[] { Path.Combine(WorkingDirectory, "script.csx") }, args.SourceFiles.Select(f => f.Path));
AssertEx.Equal(new[] { "a", "--", "b", "c" }, args.ScriptArguments);
args = ScriptParse(new[] { "-i", "script.csx", "a", "b", "c" }, baseDirectory: WorkingDirectory);
Assert.True(args.InteractiveMode);
AssertEx.Equal(new[] { Path.Combine(WorkingDirectory, "script.csx") }, args.SourceFiles.Select(f => f.Path));
AssertEx.Equal(new[] { "a", "b", "c" }, args.ScriptArguments);
args = ScriptParse(new[] { "-i", "--", "script.csx", "a", "b", "c" }, baseDirectory: WorkingDirectory);
Assert.True(args.InteractiveMode);
AssertEx.Equal(new[] { Path.Combine(WorkingDirectory, "script.csx") }, args.SourceFiles.Select(f => f.Path));
AssertEx.Equal(new[] { "a", "b", "c" }, args.ScriptArguments);
args = ScriptParse(new[] { "-i", "--", "--", "--" }, baseDirectory: WorkingDirectory);
Assert.True(args.InteractiveMode);
AssertEx.Equal(new[] { Path.Combine(WorkingDirectory, "--") }, args.SourceFiles.Select(f => f.Path));
Assert.True(args.SourceFiles[0].IsScript);
AssertEx.Equal(new[] { "--" }, args.ScriptArguments);
// TODO: fails on Linux (https://github.com/dotnet/roslyn/issues/5904)
// Result: C:\/script.csx
//args = ScriptParse(new[] { "-i", "script.csx", "--", "--" }, baseDirectory: @"C:\");
//Assert.True(args.InteractiveMode);
//AssertEx.Equal(new[] { @"C:\script.csx" }, args.SourceFiles.Select(f => f.Path));
//Assert.True(args.SourceFiles[0].IsScript);
//AssertEx.Equal(new[] { "--" }, args.ScriptArguments);
}
[WorkItem(127403, "https://devdiv.visualstudio.com:443/defaultcollection/DevDiv/_workitems/edit/127403")]
[Fact]
public void ParseSeparatedPaths_QuotedComma()
{
var paths = CSharpCommandLineParser.ParseSeparatedPaths(@"""a, b""");
Assert.Equal(
new[] { @"a, b" },
paths);
}
[Fact]
[CompilerTrait(CompilerFeature.Determinism)]
public void PathMapParser()
{
var s = PathUtilities.DirectorySeparatorStr;
var parsedArgs = DefaultParse(new[] { "/pathmap:", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(ImmutableArray.Create<KeyValuePair<string, string>>(), parsedArgs.PathMap);
parsedArgs = DefaultParse(new[] { "/pathmap:K1=V1", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(KeyValuePairUtil.Create("K1" + s, "V1" + s), parsedArgs.PathMap[0]);
parsedArgs = DefaultParse(new[] { $"/pathmap:abc{s}=/", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(KeyValuePairUtil.Create("abc" + s, "/"), parsedArgs.PathMap[0]);
parsedArgs = DefaultParse(new[] { "/pathmap:K1=V1,K2=V2", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(KeyValuePairUtil.Create("K1" + s, "V1" + s), parsedArgs.PathMap[0]);
Assert.Equal(KeyValuePairUtil.Create("K2" + s, "V2" + s), parsedArgs.PathMap[1]);
parsedArgs = DefaultParse(new[] { "/pathmap:,", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(ImmutableArray.Create<KeyValuePair<string, string>>(), parsedArgs.PathMap);
parsedArgs = DefaultParse(new[] { "/pathmap:,,", "a.cs" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Count());
Assert.Equal((int)ErrorCode.ERR_InvalidPathMap, parsedArgs.Errors[0].Code);
parsedArgs = DefaultParse(new[] { "/pathmap:,,,", "a.cs" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Count());
Assert.Equal((int)ErrorCode.ERR_InvalidPathMap, parsedArgs.Errors[0].Code);
parsedArgs = DefaultParse(new[] { "/pathmap:k=,=v", "a.cs" }, WorkingDirectory);
Assert.Equal(2, parsedArgs.Errors.Count());
Assert.Equal((int)ErrorCode.ERR_InvalidPathMap, parsedArgs.Errors[0].Code);
Assert.Equal((int)ErrorCode.ERR_InvalidPathMap, parsedArgs.Errors[1].Code);
parsedArgs = DefaultParse(new[] { "/pathmap:k=v=bad", "a.cs" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Count());
Assert.Equal((int)ErrorCode.ERR_InvalidPathMap, parsedArgs.Errors[0].Code);
parsedArgs = DefaultParse(new[] { "/pathmap:k=", "a.cs" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Count());
Assert.Equal((int)ErrorCode.ERR_InvalidPathMap, parsedArgs.Errors[0].Code);
parsedArgs = DefaultParse(new[] { "/pathmap:=v", "a.cs" }, WorkingDirectory);
Assert.Equal(1, parsedArgs.Errors.Count());
Assert.Equal((int)ErrorCode.ERR_InvalidPathMap, parsedArgs.Errors[0].Code);
parsedArgs = DefaultParse(new[] { "/pathmap:\"supporting spaces=is hard\"", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(KeyValuePairUtil.Create("supporting spaces" + s, "is hard" + s), parsedArgs.PathMap[0]);
parsedArgs = DefaultParse(new[] { "/pathmap:\"K 1=V 1\",\"K 2=V 2\"", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(KeyValuePairUtil.Create("K 1" + s, "V 1" + s), parsedArgs.PathMap[0]);
Assert.Equal(KeyValuePairUtil.Create("K 2" + s, "V 2" + s), parsedArgs.PathMap[1]);
parsedArgs = DefaultParse(new[] { "/pathmap:\"K 1\"=\"V 1\",\"K 2\"=\"V 2\"", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(KeyValuePairUtil.Create("K 1" + s, "V 1" + s), parsedArgs.PathMap[0]);
Assert.Equal(KeyValuePairUtil.Create("K 2" + s, "V 2" + s), parsedArgs.PathMap[1]);
parsedArgs = DefaultParse(new[] { "/pathmap:\"a ==,,b\"=\"1,,== 2\",\"x ==,,y\"=\"3 4\",", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(KeyValuePairUtil.Create("a =,b" + s, "1,= 2" + s), parsedArgs.PathMap[0]);
Assert.Equal(KeyValuePairUtil.Create("x =,y" + s, "3 4" + s), parsedArgs.PathMap[1]);
parsedArgs = DefaultParse(new[] { @"/pathmap:C:\temp\=/_1/,C:\temp\a\=/_2/,C:\temp\a\b\=/_3/", "a.cs", @"a\b.cs", @"a\b\c.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(KeyValuePairUtil.Create(@"C:\temp\a\b\", "/_3/"), parsedArgs.PathMap[0]);
Assert.Equal(KeyValuePairUtil.Create(@"C:\temp\a\", "/_2/"), parsedArgs.PathMap[1]);
Assert.Equal(KeyValuePairUtil.Create(@"C:\temp\", "/_1/"), parsedArgs.PathMap[2]);
}
[Theory]
[InlineData("", new string[0])]
[InlineData(",", new[] { "", "" })]
[InlineData(",,", new[] { "," })]
[InlineData(",,,", new[] { ",", "" })]
[InlineData(",,,,", new[] { ",," })]
[InlineData("a,", new[] { "a", "" })]
[InlineData("a,b", new[] { "a", "b" })]
[InlineData(",,a,,,,,b,,", new[] { ",a,,", "b," })]
public void SplitWithDoubledSeparatorEscaping(string str, string[] expected)
{
AssertEx.Equal(expected, CommandLineParser.SplitWithDoubledSeparatorEscaping(str, ','));
}
[ConditionalFact(typeof(WindowsOnly), Reason = "https://github.com/dotnet/roslyn/issues/30289")]
[CompilerTrait(CompilerFeature.Determinism)]
public void PathMapPdbParser()
{
var dir = Path.Combine(WorkingDirectory, "a");
var parsedArgs = DefaultParse(new[] { $@"/pathmap:{dir}=b:\", "a.cs", @"/pdb:a\data.pdb", "/debug:full" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(Path.Combine(dir, @"data.pdb"), parsedArgs.PdbPath);
// This value is calculate during Emit phases and should be null even in the face of a pathmap targeting it.
Assert.Null(parsedArgs.EmitOptions.PdbFilePath);
}
[ConditionalFact(typeof(WindowsOnly), Reason = ConditionalSkipReason.NativePdbRequiresDesktop)]
[CompilerTrait(CompilerFeature.Determinism)]
public void PathMapPdbEmit()
{
void AssertPdbEmit(TempDirectory dir, string pdbPath, string pePdbPath, params string[] extraArgs)
{
var source = @"class Program { static void Main() { } }";
var src = dir.CreateFile("a.cs").WriteAllText(source);
var defaultArgs = new[] { "/nologo", "a.cs", "/out:a.exe", "/debug:full", $"/pdb:{pdbPath}" };
var isDeterministic = extraArgs.Contains("/deterministic");
var args = defaultArgs.Concat(extraArgs).ToArray();
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, args);
int exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
var exePath = Path.Combine(dir.Path, "a.exe");
Assert.True(File.Exists(exePath));
Assert.True(File.Exists(pdbPath));
using (var peStream = File.OpenRead(exePath))
{
PdbValidation.ValidateDebugDirectory(peStream, null, pePdbPath, hashAlgorithm: default, hasEmbeddedPdb: false, isDeterministic);
}
}
// Case with no mappings
using (var dir = new DisposableDirectory(Temp))
{
var pdbPath = Path.Combine(dir.Path, "a.pdb");
AssertPdbEmit(dir, pdbPath, pdbPath);
}
// Simple mapping
using (var dir = new DisposableDirectory(Temp))
{
var pdbPath = Path.Combine(dir.Path, "a.pdb");
AssertPdbEmit(dir, pdbPath, @"q:\a.pdb", $@"/pathmap:{dir.Path}=q:\");
}
// Simple mapping deterministic
using (var dir = new DisposableDirectory(Temp))
{
var pdbPath = Path.Combine(dir.Path, "a.pdb");
AssertPdbEmit(dir, pdbPath, @"q:\a.pdb", $@"/pathmap:{dir.Path}=q:\", "/deterministic");
}
// Partial mapping
using (var dir = new DisposableDirectory(Temp))
{
dir.CreateDirectory("pdb");
var pdbPath = Path.Combine(dir.Path, @"pdb\a.pdb");
AssertPdbEmit(dir, pdbPath, @"q:\pdb\a.pdb", $@"/pathmap:{dir.Path}=q:\");
}
// Legacy feature flag
using (var dir = new DisposableDirectory(Temp))
{
var pdbPath = Path.Combine(dir.Path, "a.pdb");
AssertPdbEmit(dir, pdbPath, @"a.pdb", $@"/features:pdb-path-determinism");
}
// Unix path map
using (var dir = new DisposableDirectory(Temp))
{
var pdbPath = Path.Combine(dir.Path, "a.pdb");
AssertPdbEmit(dir, pdbPath, @"/a.pdb", $@"/pathmap:{dir.Path}=/");
}
// Multi-specified path map with mixed slashes
using (var dir = new DisposableDirectory(Temp))
{
var pdbPath = Path.Combine(dir.Path, "a.pdb");
AssertPdbEmit(dir, pdbPath, "/goo/a.pdb", $"/pathmap:{dir.Path}=/goo,{dir.Path}{PathUtilities.DirectorySeparatorChar}=/bar");
}
}
[CompilerTrait(CompilerFeature.Determinism)]
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30321")]
public void DeterministicPdbsRegardlessOfBitness()
{
var dir = Temp.CreateDirectory();
var dir32 = dir.CreateDirectory("32");
var dir64 = dir.CreateDirectory("64");
var programExe32 = dir32.CreateFile("Program.exe");
var programPdb32 = dir32.CreateFile("Program.pdb");
var programExe64 = dir64.CreateFile("Program.exe");
var programPdb64 = dir64.CreateFile("Program.pdb");
var sourceFile = dir.CreateFile("Source.cs").WriteAllText(@"
using System;
using System.Linq;
using System.Collections.Generic;
namespace N
{
using I4 = System.Int32;
class Program
{
public static IEnumerable<int> F()
{
I4 x = 1;
yield return 1;
yield return x;
}
public static void Main(string[] args)
{
dynamic x = 1;
const int a = 1;
F().ToArray();
Console.WriteLine(x + a);
}
}
}");
var csc32src = $@"
using System;
using System.Reflection;
class Runner
{{
static int Main(string[] args)
{{
var assembly = Assembly.LoadFrom(@""{s_CSharpCompilerExecutable}"");
var program = assembly.GetType(""Microsoft.CodeAnalysis.CSharp.CommandLine.Program"");
var main = program.GetMethod(""Main"");
return (int)main.Invoke(null, new object[] {{ args }});
}}
}}
";
var csc32 = CreateCompilationWithMscorlib46(csc32src, options: TestOptions.ReleaseExe.WithPlatform(Platform.X86), assemblyName: "csc32");
var csc32exe = dir.CreateFile("csc32.exe").WriteAllBytes(csc32.EmitToArray());
dir.CopyFile(Path.ChangeExtension(s_CSharpCompilerExecutable, ".exe.config"), "csc32.exe.config");
dir.CopyFile(Path.Combine(Path.GetDirectoryName(s_CSharpCompilerExecutable), "csc.rsp"));
var output = ProcessUtilities.RunAndGetOutput(csc32exe.Path, $@"/nologo /debug:full /deterministic /out:Program.exe /pathmap:""{dir32.Path}""=X:\ ""{sourceFile.Path}""", expectedRetCode: 0, startFolder: dir32.Path);
Assert.Equal("", output);
output = ProcessUtilities.RunAndGetOutput(s_CSharpCompilerExecutable, $@"/nologo /debug:full /deterministic /out:Program.exe /pathmap:""{dir64.Path}""=X:\ ""{sourceFile.Path}""", expectedRetCode: 0, startFolder: dir64.Path);
Assert.Equal("", output);
AssertEx.Equal(programExe32.ReadAllBytes(), programExe64.ReadAllBytes());
AssertEx.Equal(programPdb32.ReadAllBytes(), programPdb64.ReadAllBytes());
}
[WorkItem(7588, "https://github.com/dotnet/roslyn/issues/7588")]
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30321")]
public void Version()
{
var folderName = Temp.CreateDirectory().ToString();
var argss = new[]
{
"/version",
"a.cs /version /preferreduilang:en",
"/version /nologo",
"/version /help",
};
foreach (var args in argss)
{
var output = ProcessUtilities.RunAndGetOutput(s_CSharpCompilerExecutable, args, startFolder: folderName);
Assert.Equal(s_compilerVersion, output.Trim());
}
}
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30321")]
public void RefOut()
{
var dir = Temp.CreateDirectory();
var refDir = dir.CreateDirectory("ref");
var src = dir.CreateFile("a.cs");
src.WriteAllText(@"
public class C
{
/// <summary>Main method</summary>
public static void Main()
{
System.Console.Write(""Hello"");
}
/// <summary>Private method</summary>
private static void PrivateMethod()
{
System.Console.Write(""Private"");
}
}");
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path,
new[] { "/nologo", "/out:a.exe", "/refout:ref/a.dll", "/doc:doc.xml", "/deterministic", "/langversion:7", "a.cs" });
int exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
var exe = Path.Combine(dir.Path, "a.exe");
Assert.True(File.Exists(exe));
MetadataReaderUtils.VerifyPEMetadata(exe,
new[] { "TypeDefinition:<Module>", "TypeDefinition:C" },
new[] { "MethodDefinition:Void C.Main()", "MethodDefinition:Void C.PrivateMethod()", "MethodDefinition:Void C..ctor()" },
new[] { "CompilationRelaxationsAttribute", "RuntimeCompatibilityAttribute", "DebuggableAttribute" }
);
var doc = Path.Combine(dir.Path, "doc.xml");
Assert.True(File.Exists(doc));
var content = File.ReadAllText(doc);
var expectedDoc =
@"<?xml version=""1.0""?>
<doc>
<assembly>
<name>a</name>
</assembly>
<members>
<member name=""M:C.Main"">
<summary>Main method</summary>
</member>
<member name=""M:C.PrivateMethod"">
<summary>Private method</summary>
</member>
</members>
</doc>";
Assert.Equal(expectedDoc, content.Trim());
var output = ProcessUtilities.RunAndGetOutput(exe, startFolder: dir.Path);
Assert.Equal("Hello", output.Trim());
var refDll = Path.Combine(refDir.Path, "a.dll");
Assert.True(File.Exists(refDll));
// The types and members that are included needs further refinement.
// See issue https://github.com/dotnet/roslyn/issues/17612
MetadataReaderUtils.VerifyPEMetadata(refDll,
new[] { "TypeDefinition:<Module>", "TypeDefinition:C" },
new[] { "MethodDefinition:Void C.Main()", "MethodDefinition:Void C..ctor()" },
new[] { "CompilationRelaxationsAttribute", "RuntimeCompatibilityAttribute", "DebuggableAttribute", "ReferenceAssemblyAttribute" }
);
// Clean up temp files
CleanupAllGeneratedFiles(dir.Path);
CleanupAllGeneratedFiles(refDir.Path);
}
[Fact]
public void RefOutWithError()
{
var dir = Temp.CreateDirectory();
dir.CreateDirectory("ref");
var src = dir.CreateFile("a.cs");
src.WriteAllText(@"class C { public static void Main() { error(); } }");
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path,
new[] { "/nologo", "/out:a.dll", "/refout:ref/a.dll", "/deterministic", "/preferreduilang:en", "a.cs" });
int exitCode = csc.Run(outWriter);
Assert.Equal(1, exitCode);
var dll = Path.Combine(dir.Path, "a.dll");
Assert.False(File.Exists(dll));
var refDll = Path.Combine(dir.Path, Path.Combine("ref", "a.dll"));
Assert.False(File.Exists(refDll));
Assert.Equal("a.cs(1,39): error CS0103: The name 'error' does not exist in the current context", outWriter.ToString().Trim());
// Clean up temp files
CleanupAllGeneratedFiles(dir.Path);
}
[Fact]
public void RefOnly()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("a.cs");
src.WriteAllText(@"
using System;
class C
{
/// <summary>Main method</summary>
public static void Main()
{
error(); // semantic error in method body
}
private event Action E1
{
add { }
remove { }
}
private event Action E2;
/// <summary>Private Class Field</summary>
private int field;
/// <summary>Private Struct</summary>
private struct S
{
/// <summary>Private Struct Field</summary>
private int field;
}
}");
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path,
new[] { "/nologo", "/out:a.dll", "/refonly", "/debug", "/deterministic", "/langversion:7", "/doc:doc.xml", "a.cs" });
int exitCode = csc.Run(outWriter);
Assert.Equal("", outWriter.ToString());
Assert.Equal(0, exitCode);
var refDll = Path.Combine(dir.Path, "a.dll");
Assert.True(File.Exists(refDll));
// The types and members that are included needs further refinement.
// See issue https://github.com/dotnet/roslyn/issues/17612
MetadataReaderUtils.VerifyPEMetadata(refDll,
new[] { "TypeDefinition:<Module>", "TypeDefinition:C", "TypeDefinition:S" },
new[] { "MethodDefinition:Void C.Main()", "MethodDefinition:Void C..ctor()" },
new[] { "CompilationRelaxationsAttribute", "RuntimeCompatibilityAttribute", "DebuggableAttribute", "ReferenceAssemblyAttribute" }
);
var pdb = Path.Combine(dir.Path, "a.pdb");
Assert.False(File.Exists(pdb));
var doc = Path.Combine(dir.Path, "doc.xml");
Assert.True(File.Exists(doc));
var content = File.ReadAllText(doc);
var expectedDoc =
@"<?xml version=""1.0""?>
<doc>
<assembly>
<name>a</name>
</assembly>
<members>
<member name=""M:C.Main"">
<summary>Main method</summary>
</member>
<member name=""F:C.field"">
<summary>Private Class Field</summary>
</member>
<member name=""T:C.S"">
<summary>Private Struct</summary>
</member>
<member name=""F:C.S.field"">
<summary>Private Struct Field</summary>
</member>
</members>
</doc>";
Assert.Equal(expectedDoc, content.Trim());
// Clean up temp files
CleanupAllGeneratedFiles(dir.Path);
}
[Fact]
public void CompilingCodeWithInvalidPreProcessorSymbolsShouldProvideDiagnostics()
{
var parsedArgs = DefaultParse(new[] { "/define:1", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// warning CS2029: Invalid name for a preprocessing symbol; '1' is not a valid identifier
Diagnostic(ErrorCode.WRN_DefineIdentifierRequired).WithArguments("1").WithLocation(1, 1));
}
[Fact]
public void WhitespaceInDefine()
{
var parsedArgs = DefaultParse(new[] { "/define:\" a\"", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify();
Assert.Equal("a", parsedArgs.ParseOptions.PreprocessorSymbols.Single());
}
[Fact]
public void WhitespaceInDefine_OnlySpaces()
{
var parsedArgs = DefaultParse(new[] { "/define:\" \"", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
Diagnostic(ErrorCode.WRN_DefineIdentifierRequired).WithArguments(" ").WithLocation(1, 1)
);
Assert.True(parsedArgs.ParseOptions.PreprocessorSymbols.IsEmpty);
}
[Fact]
public void CompilingCodeWithInvalidLanguageVersionShouldProvideDiagnostics()
{
var parsedArgs = DefaultParse(new[] { "/langversion:1000", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// error CS1617: Invalid option '1000' for /langversion. Use '/langversion:?' to list supported values.
Diagnostic(ErrorCode.ERR_BadCompatMode).WithArguments("1000").WithLocation(1, 1));
}
[Fact, WorkItem(16913, "https://github.com/dotnet/roslyn/issues/16913")]
public void CompilingCodeWithMultipleInvalidPreProcessorSymbolsShouldErrorOut()
{
var parsedArgs = DefaultParse(new[] { "/define:valid1,2invalid,valid3", "/define:4,5,valid6", "a.cs" }, WorkingDirectory);
parsedArgs.Errors.Verify(
// warning CS2029: Invalid value for '/define'; '2invalid' is not a valid identifier
Diagnostic(ErrorCode.WRN_DefineIdentifierRequired).WithArguments("2invalid"),
// warning CS2029: Invalid value for '/define'; '4' is not a valid identifier
Diagnostic(ErrorCode.WRN_DefineIdentifierRequired).WithArguments("4"),
// warning CS2029: Invalid value for '/define'; '5' is not a valid identifier
Diagnostic(ErrorCode.WRN_DefineIdentifierRequired).WithArguments("5"));
}
[WorkItem(406649, "https://devdiv.visualstudio.com/DevDiv/_workitems?id=406649")]
[ConditionalFact(typeof(WindowsDesktopOnly), typeof(IsEnglishLocal), Reason = "https://github.com/dotnet/roslyn/issues/30321")]
public void MissingCompilerAssembly()
{
var dir = Temp.CreateDirectory();
var cscPath = dir.CopyFile(s_CSharpCompilerExecutable).Path;
dir.CopyFile(typeof(Compilation).Assembly.Location);
// Missing Microsoft.CodeAnalysis.CSharp.dll.
var result = ProcessUtilities.Run(cscPath, arguments: "/nologo /t:library unknown.cs", workingDirectory: dir.Path);
Assert.Equal(1, result.ExitCode);
Assert.Equal(
$"Could not load file or assembly '{typeof(CSharpCompilation).Assembly.FullName}' or one of its dependencies. The system cannot find the file specified.",
result.Output.Trim());
// Missing System.Collections.Immutable.dll.
dir.CopyFile(typeof(CSharpCompilation).Assembly.Location);
result = ProcessUtilities.Run(cscPath, arguments: "/nologo /t:library unknown.cs", workingDirectory: dir.Path);
Assert.Equal(1, result.ExitCode);
Assert.Equal(
$"Could not load file or assembly '{typeof(ImmutableArray).Assembly.FullName}' or one of its dependencies. The system cannot find the file specified.",
result.Output.Trim());
}
#if NET472
[ConditionalFact(typeof(WindowsDesktopOnly), typeof(IsEnglishLocal), Reason = "https://github.com/dotnet/roslyn/issues/30321")]
public void LoadinganalyzerNetStandard13()
{
var analyzerFileName = "AnalyzerNS13.dll";
var srcFileName = "src.cs";
var analyzerDir = Temp.CreateDirectory();
var analyzerFile = analyzerDir.CreateFile(analyzerFileName).WriteAllBytes(CreateCSharpAnalyzerNetStandard13(Path.GetFileNameWithoutExtension(analyzerFileName)));
var srcFile = analyzerDir.CreateFile(srcFileName).WriteAllText("public class C { }");
var result = ProcessUtilities.Run(s_CSharpCompilerExecutable, arguments: $"/nologo /t:library /analyzer:{analyzerFileName} {srcFileName}", workingDirectory: analyzerDir.Path);
var outputWithoutPaths = Regex.Replace(result.Output, " in .*", "");
AssertEx.AssertEqualToleratingWhitespaceDifferences(
$@"warning AD0001: Analyzer 'TestAnalyzer' threw an exception of type 'System.NotImplementedException' with message '28'.
System.NotImplementedException: 28
at TestAnalyzer.get_SupportedDiagnostics()
at Microsoft.CodeAnalysis.Diagnostics.AnalyzerManager.AnalyzerExecutionContext.<>c__DisplayClass20_0.<ComputeDiagnosticDescriptors>b__0(Object _)
at Microsoft.CodeAnalysis.Diagnostics.AnalyzerExecutor.ExecuteAndCatchIfThrows_NoLock[TArg](DiagnosticAnalyzer analyzer, Action`1 analyze, TArg argument, Nullable`1 info)
-----", outputWithoutPaths);
Assert.Equal(0, result.ExitCode);
}
#endif
private static ImmutableArray<byte> CreateCSharpAnalyzerNetStandard13(string analyzerAssemblyName)
{
var minSystemCollectionsImmutableSource = @"
[assembly: System.Reflection.AssemblyVersion(""1.2.3.0"")]
namespace System.Collections.Immutable
{
public struct ImmutableArray<T>
{
}
}
";
var minCodeAnalysisSource = @"
using System;
[assembly: System.Reflection.AssemblyVersion(""2.0.0.0"")]
namespace Microsoft.CodeAnalysis.Diagnostics
{
[AttributeUsage(AttributeTargets.Class)]
public sealed class DiagnosticAnalyzerAttribute : Attribute
{
public DiagnosticAnalyzerAttribute(string firstLanguage, params string[] additionalLanguages) {}
}
public abstract class DiagnosticAnalyzer
{
public abstract System.Collections.Immutable.ImmutableArray<DiagnosticDescriptor> SupportedDiagnostics { get; }
public abstract void Initialize(AnalysisContext context);
}
public abstract class AnalysisContext
{
}
}
namespace Microsoft.CodeAnalysis
{
public sealed class DiagnosticDescriptor
{
}
}
";
var minSystemCollectionsImmutableImage = CSharpCompilation.Create(
"System.Collections.Immutable",
new[] { SyntaxFactory.ParseSyntaxTree(minSystemCollectionsImmutableSource) },
new MetadataReference[] { NetStandard13.SystemRuntime },
new CSharpCompilationOptions(OutputKind.DynamicallyLinkedLibrary, cryptoPublicKey: TestResources.TestKeys.PublicKey_b03f5f7f11d50a3a)).EmitToArray();
var minSystemCollectionsImmutableRef = MetadataReference.CreateFromImage(minSystemCollectionsImmutableImage);
var minCodeAnalysisImage = CSharpCompilation.Create(
"Microsoft.CodeAnalysis",
new[] { SyntaxFactory.ParseSyntaxTree(minCodeAnalysisSource) },
new MetadataReference[]
{
NetStandard13.SystemRuntime,
minSystemCollectionsImmutableRef
},
new CSharpCompilationOptions(OutputKind.DynamicallyLinkedLibrary, cryptoPublicKey: TestResources.TestKeys.PublicKey_31bf3856ad364e35)).EmitToArray();
var minCodeAnalysisRef = MetadataReference.CreateFromImage(minCodeAnalysisImage);
var analyzerSource = @"
using System;
using System.Collections.ObjectModel;
using System.Collections.Immutable;
using System.ComponentModel;
using System.Diagnostics;
using System.Globalization;
using System.IO;
using System.IO.Compression;
using System.Net.Http;
using System.Net.Security;
using System.Net.Sockets;
using System.Reflection;
using System.Runtime.InteropServices;
using System.Runtime.Serialization;
using System.Security.AccessControl;
using System.Security.Cryptography;
using System.Security.Principal;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Xml;
using System.Xml.Serialization;
using System.Xml.XPath;
using Microsoft.CodeAnalysis;
using Microsoft.CodeAnalysis.Diagnostics;
using Microsoft.Win32.SafeHandles;
[DiagnosticAnalyzer(""C#"")]
public class TestAnalyzer : DiagnosticAnalyzer
{
public override ImmutableArray<DiagnosticDescriptor> SupportedDiagnostics => throw new NotImplementedException(new[]
{
typeof(Win32Exception), // Microsoft.Win32.Primitives
typeof(AppContext), // System.AppContext
typeof(Console), // System.Console
typeof(ValueTuple), // System.ValueTuple
typeof(FileVersionInfo), // System.Diagnostics.FileVersionInfo
typeof(Process), // System.Diagnostics.Process
typeof(ChineseLunisolarCalendar), // System.Globalization.Calendars
typeof(ZipArchive), // System.IO.Compression
typeof(ZipFile), // System.IO.Compression.ZipFile
typeof(FileOptions), // System.IO.FileSystem
typeof(FileAttributes), // System.IO.FileSystem.Primitives
typeof(HttpClient), // System.Net.Http
typeof(AuthenticatedStream), // System.Net.Security
typeof(IOControlCode), // System.Net.Sockets
typeof(RuntimeInformation), // System.Runtime.InteropServices.RuntimeInformation
typeof(SerializationException), // System.Runtime.Serialization.Primitives
typeof(GenericIdentity), // System.Security.Claims
typeof(Aes), // System.Security.Cryptography.Algorithms
typeof(CspParameters), // System.Security.Cryptography.Csp
typeof(AsnEncodedData), // System.Security.Cryptography.Encoding
typeof(AsymmetricAlgorithm), // System.Security.Cryptography.Primitives
typeof(SafeX509ChainHandle), // System.Security.Cryptography.X509Certificates
typeof(IXmlLineInfo), // System.Xml.ReaderWriter
typeof(XmlNode), // System.Xml.XmlDocument
typeof(XPathDocument), // System.Xml.XPath
typeof(XDocumentExtensions), // System.Xml.XPath.XDocument
typeof(CodePagesEncodingProvider),// System.Text.Encoding.CodePages
typeof(ValueTask<>), // System.Threading.Tasks.Extensions
// csc doesn't ship with facades for the following assemblies.
// Analyzers can't use them unless they carry the facade with them.
// typeof(SafePipeHandle), // System.IO.Pipes
// typeof(StackFrame), // System.Diagnostics.StackTrace
// typeof(BindingFlags), // System.Reflection.TypeExtensions
// typeof(AccessControlActions), // System.Security.AccessControl
// typeof(SafeAccessTokenHandle), // System.Security.Principal.Windows
// typeof(Thread), // System.Threading.Thread
}.Length.ToString());
public override void Initialize(AnalysisContext context)
{
}
}";
var references =
new MetadataReference[]
{
minCodeAnalysisRef,
minSystemCollectionsImmutableRef
};
references = references.Concat(NetStandard13.All).ToArray();
var analyzerImage = CSharpCompilation.Create(
analyzerAssemblyName,
new SyntaxTree[] { SyntaxFactory.ParseSyntaxTree(analyzerSource) },
references: references,
new CSharpCompilationOptions(OutputKind.DynamicallyLinkedLibrary)).EmitToArray();
return analyzerImage;
}
[WorkItem(406649, "https://devdiv.visualstudio.com/DevDiv/_workitems?id=484417")]
[ConditionalFact(typeof(WindowsDesktopOnly), typeof(IsEnglishLocal), Reason = "https://github.com/dotnet/roslyn/issues/30321")]
public void MicrosoftDiaSymReaderNativeAltLoadPath()
{
var dir = Temp.CreateDirectory();
var cscDir = Path.GetDirectoryName(s_CSharpCompilerExecutable);
// copy csc and dependencies except for DSRN:
foreach (var filePath in Directory.EnumerateFiles(cscDir))
{
var fileName = Path.GetFileName(filePath);
if (fileName.StartsWith("csc") ||
fileName.StartsWith("System.") ||
fileName.StartsWith("Microsoft.") && !fileName.StartsWith("Microsoft.DiaSymReader.Native"))
{
dir.CopyFile(filePath);
}
}
dir.CreateFile("Source.cs").WriteAllText("class C { void F() { } }");
var cscCopy = Path.Combine(dir.Path, "csc.exe");
var arguments = "/nologo /t:library /debug:full Source.cs";
// env variable not set (deterministic) -- DSRN is required:
var result = ProcessUtilities.Run(
cscCopy,
arguments + " /deterministic",
workingDirectory: dir.Path,
additionalEnvironmentVars: new[] { KeyValuePairUtil.Create("MICROSOFT_DIASYMREADER_NATIVE_ALT_LOAD_PATH", "") });
AssertEx.AssertEqualToleratingWhitespaceDifferences(
"error CS0041: Unexpected error writing debug information -- 'Unable to load DLL 'Microsoft.DiaSymReader.Native.amd64.dll': " +
"The specified module could not be found. (Exception from HRESULT: 0x8007007E)'", result.Output.Trim());
// env variable not set (non-deterministic) -- globally registered SymReader is picked up:
result = ProcessUtilities.Run(cscCopy, arguments, workingDirectory: dir.Path);
AssertEx.AssertEqualToleratingWhitespaceDifferences("", result.Output.Trim());
// env variable set:
result = ProcessUtilities.Run(
cscCopy,
arguments + " /deterministic",
workingDirectory: dir.Path,
additionalEnvironmentVars: new[] { KeyValuePairUtil.Create("MICROSOFT_DIASYMREADER_NATIVE_ALT_LOAD_PATH", cscDir) });
Assert.Equal("", result.Output.Trim());
}
[ConditionalFact(typeof(WindowsOnly))]
[WorkItem(21935, "https://github.com/dotnet/roslyn/issues/21935")]
public void PdbPathNotEmittedWithoutPdb()
{
var dir = Temp.CreateDirectory();
var source = @"class Program { static void Main() { } }";
var src = dir.CreateFile("a.cs").WriteAllText(source);
var args = new[] { "/nologo", "a.cs", "/out:a.exe", "/debug-" };
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(null, dir.Path, args);
int exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
var exePath = Path.Combine(dir.Path, "a.exe");
Assert.True(File.Exists(exePath));
using (var peStream = File.OpenRead(exePath))
using (var peReader = new PEReader(peStream))
{
var debugDirectory = peReader.PEHeaders.PEHeader.DebugTableDirectory;
Assert.Equal(0, debugDirectory.Size);
Assert.Equal(0, debugDirectory.RelativeVirtualAddress);
}
}
[Fact]
public void StrongNameProviderWithCustomTempPath()
{
var tempDir = Temp.CreateDirectory();
var workingDir = Temp.CreateDirectory();
workingDir.CreateFile("a.cs");
var buildPaths = new BuildPaths(clientDir: "", workingDir: workingDir.Path, sdkDir: null, tempDir: tempDir.Path);
var csc = new MockCSharpCompiler(null, buildPaths, args: new[] { "/features:UseLegacyStrongNameProvider", "/nostdlib", "a.cs" });
var comp = csc.CreateCompilation(new StringWriter(), new TouchedFileLogger(), errorLogger: null);
Assert.True(!comp.SignUsingBuilder);
}
public class QuotedArgumentTests : CommandLineTestBase
{
private static readonly string s_rootPath = ExecutionConditionUtil.IsWindows
? @"c:\"
: "/";
private void VerifyQuotedValid<T>(string name, string value, T expected, Func<CSharpCommandLineArguments, T> getValue)
{
var args = DefaultParse(new[] { $"/{name}:{value}", "a.cs" }, s_rootPath);
Assert.Equal(0, args.Errors.Length);
Assert.Equal(expected, getValue(args));
args = DefaultParse(new[] { $@"/{name}:""{value}""", "a.cs" }, s_rootPath);
Assert.Equal(0, args.Errors.Length);
Assert.Equal(expected, getValue(args));
}
private void VerifyQuotedInvalid<T>(string name, string value, T expected, Func<CSharpCommandLineArguments, T> getValue)
{
var args = DefaultParse(new[] { $"/{name}:{value}", "a.cs" }, s_rootPath);
Assert.Equal(0, args.Errors.Length);
Assert.Equal(expected, getValue(args));
args = DefaultParse(new[] { $@"/{name}:""{value}""", "a.cs" }, s_rootPath);
Assert.True(args.Errors.Length > 0);
}
[WorkItem(12427, "https://github.com/dotnet/roslyn/issues/12427")]
[Fact]
public void DebugFlag()
{
var platformPdbKind = PathUtilities.IsUnixLikePlatform ? DebugInformationFormat.PortablePdb : DebugInformationFormat.Pdb;
var list = new List<Tuple<string, DebugInformationFormat>>()
{
Tuple.Create("portable", DebugInformationFormat.PortablePdb),
Tuple.Create("full", platformPdbKind),
Tuple.Create("pdbonly", platformPdbKind),
Tuple.Create("embedded", DebugInformationFormat.Embedded)
};
foreach (var tuple in list)
{
VerifyQuotedValid("debug", tuple.Item1, tuple.Item2, x => x.EmitOptions.DebugInformationFormat);
}
}
[WorkItem(12427, "https://github.com/dotnet/roslyn/issues/12427")]
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30328")]
public void CodePage()
{
VerifyQuotedValid("codepage", "1252", 1252, x => x.Encoding.CodePage);
}
[WorkItem(12427, "https://github.com/dotnet/roslyn/issues/12427")]
[Fact]
public void Target()
{
var list = new List<Tuple<string, OutputKind>>()
{
Tuple.Create("exe", OutputKind.ConsoleApplication),
Tuple.Create("winexe", OutputKind.WindowsApplication),
Tuple.Create("library", OutputKind.DynamicallyLinkedLibrary),
Tuple.Create("module", OutputKind.NetModule),
Tuple.Create("appcontainerexe", OutputKind.WindowsRuntimeApplication),
Tuple.Create("winmdobj", OutputKind.WindowsRuntimeMetadata)
};
foreach (var tuple in list)
{
VerifyQuotedInvalid("target", tuple.Item1, tuple.Item2, x => x.CompilationOptions.OutputKind);
}
}
[WorkItem(12427, "https://github.com/dotnet/roslyn/issues/12427")]
[Fact]
public void PlatformFlag()
{
var list = new List<Tuple<string, Platform>>()
{
Tuple.Create("x86", Platform.X86),
Tuple.Create("x64", Platform.X64),
Tuple.Create("itanium", Platform.Itanium),
Tuple.Create("anycpu", Platform.AnyCpu),
Tuple.Create("anycpu32bitpreferred",Platform.AnyCpu32BitPreferred),
Tuple.Create("arm", Platform.Arm)
};
foreach (var tuple in list)
{
VerifyQuotedValid("platform", tuple.Item1, tuple.Item2, x => x.CompilationOptions.Platform);
}
}
[WorkItem(12427, "https://github.com/dotnet/roslyn/issues/12427")]
[Fact]
public void WarnFlag()
{
VerifyQuotedValid("warn", "1", 1, x => x.CompilationOptions.WarningLevel);
}
[WorkItem(12427, "https://github.com/dotnet/roslyn/issues/12427")]
[Fact]
public void LangVersionFlag()
{
VerifyQuotedValid("langversion", "2", LanguageVersion.CSharp2, x => x.ParseOptions.LanguageVersion);
}
}
[Fact]
[WorkItem(23525, "https://github.com/dotnet/roslyn/issues/23525")]
public void InvalidPathCharacterInPathMap()
{
string filePath = Temp.CreateFile().WriteAllText("").Path;
var compiler = CreateCSharpCompiler(null, WorkingDirectory, new[]
{
filePath,
"/debug:embedded",
"/pathmap:test\\=\"",
"/target:library",
"/preferreduilang:en"
});
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = compiler.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Contains("error CS8101: The pathmap option was incorrectly formatted.", outWriter.ToString(), StringComparison.Ordinal);
}
[WorkItem(23525, "https://github.com/dotnet/roslyn/issues/23525")]
[ConditionalFact(typeof(WindowsDesktopOnly), Reason = "https://github.com/dotnet/roslyn/issues/30289")]
public void InvalidPathCharacterInPdbPath()
{
string filePath = Temp.CreateFile().WriteAllText("").Path;
var compiler = CreateCSharpCompiler(null, WorkingDirectory, new[]
{
filePath,
"/debug:embedded",
"/pdb:test\\?.pdb",
"/target:library",
"/preferreduilang:en"
});
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = compiler.Run(outWriter);
Assert.Equal(1, exitCode);
Assert.Contains("error CS2021: File name 'test\\?.pdb' is empty, contains invalid characters, has a drive specification without an absolute path, or is too long", outWriter.ToString(), StringComparison.Ordinal);
}
[WorkItem(20242, "https://github.com/dotnet/roslyn/issues/20242")]
[ConditionalFact(typeof(IsEnglishLocal))]
public void TestSuppression_CompilerParserWarningAsError()
{
string source = @"
class C
{
long M(int i)
{
// warning CS0078 : The 'l' suffix is easily confused with the digit '1' -- use 'L' for clarity
return 0l;
}
}
";
var srcDirectory = Temp.CreateDirectory();
var srcFile = srcDirectory.CreateFile("a.cs");
srcFile.WriteAllText(source);
// Verify that parser warning CS0078 is reported.
var output = VerifyOutput(srcDirectory, srcFile, expectedWarningCount: 1, includeCurrentAssemblyAsAnalyzerReference: false);
Assert.Contains("warning CS0078", output, StringComparison.Ordinal);
// Verify that parser warning CS0078 is reported as error for /warnaserror.
output = VerifyOutput(srcDirectory, srcFile, expectedErrorCount: 1,
additionalFlags: new[] { "/warnAsError" }, includeCurrentAssemblyAsAnalyzerReference: false);
Assert.Contains("error CS0078", output, StringComparison.Ordinal);
// Verify that parser warning CS0078 is suppressed with diagnostic suppressor even with /warnaserror
// and info diagnostic is logged with programmatic suppression information.
var suppressor = new DiagnosticSuppressorForId("CS0078");
output = VerifyOutput(srcDirectory, srcFile, expectedInfoCount: 1, expectedWarningCount: 0, expectedErrorCount: 0,
additionalFlags: new[] { "/warnAsError" },
includeCurrentAssemblyAsAnalyzerReference: false,
errorlog: true,
analyzers: suppressor);
Assert.DoesNotContain($"error CS0078", output, StringComparison.Ordinal);
Assert.DoesNotContain($"warning CS0078", output, StringComparison.Ordinal);
// Diagnostic '{0}: {1}' was programmatically suppressed by a DiagnosticSuppressor with suppression ID '{2}' and justification '{3}'
var suppressionMessage = string.Format(CodeAnalysisResources.SuppressionDiagnosticDescriptorMessage,
suppressor.SuppressionDescriptor.SuppressedDiagnosticId,
new CSDiagnostic(new CSDiagnosticInfo(ErrorCode.WRN_LowercaseEllSuffix, "l"), Location.None).GetMessage(CultureInfo.InvariantCulture),
suppressor.SuppressionDescriptor.Id,
suppressor.SuppressionDescriptor.Justification);
Assert.Contains("info SP0001", output, StringComparison.Ordinal);
Assert.Contains(suppressionMessage, output, StringComparison.Ordinal);
CleanupAllGeneratedFiles(srcFile.Path);
}
[WorkItem(20242, "https://github.com/dotnet/roslyn/issues/20242")]
[ConditionalTheory(typeof(IsEnglishLocal)), CombinatorialData]
public void TestSuppression_CompilerSyntaxWarning(bool skipAnalyzers)
{
// warning CS1522: Empty switch block
// NOTE: Empty switch block warning is reported by the C# language parser
string source = @"
class C
{
void M(int i)
{
switch (i)
{
}
}
}";
var srcDirectory = Temp.CreateDirectory();
var srcFile = srcDirectory.CreateFile("a.cs");
srcFile.WriteAllText(source);
// Verify that compiler warning CS1522 is reported.
var output = VerifyOutput(srcDirectory, srcFile, expectedWarningCount: 1, includeCurrentAssemblyAsAnalyzerReference: false, skipAnalyzers: skipAnalyzers);
Assert.Contains("warning CS1522", output, StringComparison.Ordinal);
// Verify that compiler warning CS1522 is suppressed with diagnostic suppressor
// and info diagnostic is logged with programmatic suppression information.
var suppressor = new DiagnosticSuppressorForId("CS1522");
// Diagnostic '{0}: {1}' was programmatically suppressed by a DiagnosticSuppressor with suppression ID '{2}' and justification '{3}'
var suppressionMessage = string.Format(CodeAnalysisResources.SuppressionDiagnosticDescriptorMessage,
suppressor.SuppressionDescriptor.SuppressedDiagnosticId,
new CSDiagnostic(new CSDiagnosticInfo(ErrorCode.WRN_EmptySwitch), Location.None).GetMessage(CultureInfo.InvariantCulture),
suppressor.SuppressionDescriptor.Id,
suppressor.SuppressionDescriptor.Justification);
output = VerifyOutput(srcDirectory, srcFile, expectedInfoCount: 1, expectedWarningCount: 0, includeCurrentAssemblyAsAnalyzerReference: false,
analyzers: suppressor, errorlog: true, skipAnalyzers: skipAnalyzers);
Assert.DoesNotContain($"warning CS1522", output, StringComparison.Ordinal);
Assert.Contains($"info SP0001", output, StringComparison.Ordinal);
Assert.Contains(suppressionMessage, output, StringComparison.Ordinal);
// Verify that compiler warning CS1522 is reported as error for /warnaserror.
output = VerifyOutput(srcDirectory, srcFile, expectedErrorCount: 1,
additionalFlags: new[] { "/warnAsError" }, includeCurrentAssemblyAsAnalyzerReference: false, skipAnalyzers: skipAnalyzers);
Assert.Contains("error CS1522", output, StringComparison.Ordinal);
// Verify that compiler warning CS1522 is suppressed with diagnostic suppressor even with /warnaserror
// and info diagnostic is logged with programmatic suppression information.
output = VerifyOutput(srcDirectory, srcFile, expectedInfoCount: 1, expectedWarningCount: 0, expectedErrorCount: 0,
additionalFlags: new[] { "/warnAsError" },
includeCurrentAssemblyAsAnalyzerReference: false,
errorlog: true,
skipAnalyzers: skipAnalyzers,
analyzers: suppressor);
Assert.DoesNotContain($"error CS1522", output, StringComparison.Ordinal);
Assert.DoesNotContain($"warning CS1522", output, StringComparison.Ordinal);
Assert.Contains("info SP0001", output, StringComparison.Ordinal);
Assert.Contains(suppressionMessage, output, StringComparison.Ordinal);
CleanupAllGeneratedFiles(srcFile.Path);
}
[WorkItem(20242, "https://github.com/dotnet/roslyn/issues/20242")]
[ConditionalTheory(typeof(IsEnglishLocal)), CombinatorialData]
public void TestSuppression_CompilerSemanticWarning(bool skipAnalyzers)
{
string source = @"
class C
{
// warning CS0169: The field 'C.f' is never used
private readonly int f;
}";
var srcDirectory = Temp.CreateDirectory();
var srcFile = srcDirectory.CreateFile("a.cs");
srcFile.WriteAllText(source);
// Verify that compiler warning CS0169 is reported.
var output = VerifyOutput(srcDirectory, srcFile, expectedWarningCount: 1, includeCurrentAssemblyAsAnalyzerReference: false, skipAnalyzers: skipAnalyzers);
Assert.Contains("warning CS0169", output, StringComparison.Ordinal);
// Verify that compiler warning CS0169 is suppressed with diagnostic suppressor
// and info diagnostic is logged with programmatic suppression information.
var suppressor = new DiagnosticSuppressorForId("CS0169");
// Diagnostic '{0}: {1}' was programmatically suppressed by a DiagnosticSuppressor with suppression ID '{2}' and justification '{3}'
var suppressionMessage = string.Format(CodeAnalysisResources.SuppressionDiagnosticDescriptorMessage,
suppressor.SuppressionDescriptor.SuppressedDiagnosticId,
new CSDiagnostic(new CSDiagnosticInfo(ErrorCode.WRN_UnreferencedField, "C.f"), Location.None).GetMessage(CultureInfo.InvariantCulture),
suppressor.SuppressionDescriptor.Id,
suppressor.SuppressionDescriptor.Justification);
output = VerifyOutput(srcDirectory, srcFile, expectedInfoCount: 1, expectedWarningCount: 0, includeCurrentAssemblyAsAnalyzerReference: false,
analyzers: suppressor, errorlog: true, skipAnalyzers: skipAnalyzers);
Assert.DoesNotContain($"warning CS0169", output, StringComparison.Ordinal);
Assert.Contains("info SP0001", output, StringComparison.Ordinal);
Assert.Contains(suppressionMessage, output, StringComparison.Ordinal);
// Verify that compiler warning CS0169 is reported as error for /warnaserror.
output = VerifyOutput(srcDirectory, srcFile, expectedErrorCount: 1,
additionalFlags: new[] { "/warnAsError" }, includeCurrentAssemblyAsAnalyzerReference: false, skipAnalyzers: skipAnalyzers);
Assert.Contains("error CS0169", output, StringComparison.Ordinal);
// Verify that compiler warning CS0169 is suppressed with diagnostic suppressor even with /warnaserror
// and info diagnostic is logged with programmatic suppression information.
output = VerifyOutput(srcDirectory, srcFile, expectedInfoCount: 1, expectedWarningCount: 0, expectedErrorCount: 0,
additionalFlags: new[] { "/warnAsError" },
includeCurrentAssemblyAsAnalyzerReference: false,
errorlog: true,
skipAnalyzers: skipAnalyzers,
analyzers: suppressor);
Assert.DoesNotContain($"error CS0169", output, StringComparison.Ordinal);
Assert.DoesNotContain($"warning CS0169", output, StringComparison.Ordinal);
Assert.Contains("info SP0001", output, StringComparison.Ordinal);
Assert.Contains(suppressionMessage, output, StringComparison.Ordinal);
CleanupAllGeneratedFiles(srcFile.Path);
}
[WorkItem(20242, "https://github.com/dotnet/roslyn/issues/20242")]
[Fact]
public void TestNoSuppression_CompilerSyntaxError()
{
// error CS1001: Identifier expected
string source = @"
class { }";
var srcDirectory = Temp.CreateDirectory();
var srcFile = srcDirectory.CreateFile("a.cs");
srcFile.WriteAllText(source);
// Verify that compiler syntax error CS1001 is reported.
var output = VerifyOutput(srcDirectory, srcFile, expectedErrorCount: 1, includeCurrentAssemblyAsAnalyzerReference: false);
Assert.Contains("error CS1001", output, StringComparison.Ordinal);
// Verify that compiler syntax error CS1001 cannot be suppressed with diagnostic suppressor.
output = VerifyOutput(srcDirectory, srcFile, expectedErrorCount: 1, includeCurrentAssemblyAsAnalyzerReference: false,
analyzers: new DiagnosticSuppressorForId("CS1001"));
Assert.Contains("error CS1001", output, StringComparison.Ordinal);
CleanupAllGeneratedFiles(srcFile.Path);
}
[WorkItem(20242, "https://github.com/dotnet/roslyn/issues/20242")]
[Fact]
public void TestNoSuppression_CompilerSemanticError()
{
// error CS0246: The type or namespace name 'UndefinedType' could not be found (are you missing a using directive or an assembly reference?)
string source = @"
class C
{
void M(UndefinedType x) { }
}";
var srcDirectory = Temp.CreateDirectory();
var srcFile = srcDirectory.CreateFile("a.cs");
srcFile.WriteAllText(source);
// Verify that compiler error CS0246 is reported.
var output = VerifyOutput(srcDirectory, srcFile, expectedErrorCount: 1, includeCurrentAssemblyAsAnalyzerReference: false);
Assert.Contains("error CS0246", output, StringComparison.Ordinal);
// Verify that compiler error CS0246 cannot be suppressed with diagnostic suppressor.
output = VerifyOutput(srcDirectory, srcFile, expectedErrorCount: 1, includeCurrentAssemblyAsAnalyzerReference: false,
analyzers: new DiagnosticSuppressorForId("CS0246"));
Assert.Contains("error CS0246", output, StringComparison.Ordinal);
CleanupAllGeneratedFiles(srcFile.Path);
}
[WorkItem(20242, "https://github.com/dotnet/roslyn/issues/20242")]
[ConditionalFact(typeof(IsEnglishLocal))]
public void TestSuppression_AnalyzerWarning()
{
string source = @"
class C { }";
var srcDirectory = Temp.CreateDirectory();
var srcFile = srcDirectory.CreateFile("a.cs");
srcFile.WriteAllText(source);
// Verify that analyzer warning is reported.
var analyzer = new CompilationAnalyzerWithSeverity(DiagnosticSeverity.Warning, configurable: true);
var output = VerifyOutput(srcDirectory, srcFile, expectedWarningCount: 1,
includeCurrentAssemblyAsAnalyzerReference: false,
analyzers: analyzer);
Assert.Contains($"warning {analyzer.Descriptor.Id}", output, StringComparison.Ordinal);
// Verify that analyzer warning is suppressed with diagnostic suppressor
// and info diagnostic is logged with programmatic suppression information.
var suppressor = new DiagnosticSuppressorForId(analyzer.Descriptor.Id);
// Diagnostic '{0}: {1}' was programmatically suppressed by a DiagnosticSuppressor with suppression ID '{2}' and justification '{3}'
var suppressionMessage = string.Format(CodeAnalysisResources.SuppressionDiagnosticDescriptorMessage,
suppressor.SuppressionDescriptor.SuppressedDiagnosticId,
analyzer.Descriptor.MessageFormat,
suppressor.SuppressionDescriptor.Id,
suppressor.SuppressionDescriptor.Justification);
var analyzerAndSuppressor = new DiagnosticAnalyzer[] { analyzer, suppressor };
output = VerifyOutput(srcDirectory, srcFile, expectedInfoCount: 1, expectedWarningCount: 0,
includeCurrentAssemblyAsAnalyzerReference: false,
errorlog: true,
analyzers: analyzerAndSuppressor);
Assert.DoesNotContain($"warning {analyzer.Descriptor.Id}", output, StringComparison.Ordinal);
Assert.Contains("info SP0001", output, StringComparison.Ordinal);
Assert.Contains(suppressionMessage, output, StringComparison.Ordinal);
// Verify that analyzer warning is reported as error for /warnaserror.
output = VerifyOutput(srcDirectory, srcFile, expectedErrorCount: 1,
additionalFlags: new[] { "/warnAsError" },
includeCurrentAssemblyAsAnalyzerReference: false,
analyzers: analyzer);
Assert.Contains($"error {analyzer.Descriptor.Id}", output, StringComparison.Ordinal);
// Verify that analyzer warning is suppressed with diagnostic suppressor even with /warnaserror
// and info diagnostic is logged with programmatic suppression information.
output = VerifyOutput(srcDirectory, srcFile, expectedInfoCount: 1, expectedWarningCount: 0,
additionalFlags: new[] { "/warnAsError" },
includeCurrentAssemblyAsAnalyzerReference: false,
errorlog: true,
analyzers: analyzerAndSuppressor);
Assert.DoesNotContain($"warning {analyzer.Descriptor.Id}", output, StringComparison.Ordinal);
Assert.Contains("info SP0001", output, StringComparison.Ordinal);
Assert.Contains(suppressionMessage, output, StringComparison.Ordinal);
// Verify that "NotConfigurable" analyzer warning cannot be suppressed with diagnostic suppressor.
analyzer = new CompilationAnalyzerWithSeverity(DiagnosticSeverity.Warning, configurable: false);
suppressor = new DiagnosticSuppressorForId(analyzer.Descriptor.Id);
analyzerAndSuppressor = new DiagnosticAnalyzer[] { analyzer, suppressor };
output = VerifyOutput(srcDirectory, srcFile, expectedWarningCount: 1,
includeCurrentAssemblyAsAnalyzerReference: false,
analyzers: analyzerAndSuppressor);
Assert.Contains($"warning {analyzer.Descriptor.Id}", output, StringComparison.Ordinal);
CleanupAllGeneratedFiles(srcFile.Path);
}
[WorkItem(20242, "https://github.com/dotnet/roslyn/issues/20242")]
[Fact]
public void TestNoSuppression_AnalyzerError()
{
string source = @"
class C { }";
var srcDirectory = Temp.CreateDirectory();
var srcFile = srcDirectory.CreateFile("a.cs");
srcFile.WriteAllText(source);
// Verify that analyzer error is reported.
var analyzer = new CompilationAnalyzerWithSeverity(DiagnosticSeverity.Error, configurable: true);
var output = VerifyOutput(srcDirectory, srcFile, expectedErrorCount: 1,
includeCurrentAssemblyAsAnalyzerReference: false,
analyzers: analyzer);
Assert.Contains($"error {analyzer.Descriptor.Id}", output, StringComparison.Ordinal);
// Verify that analyzer error cannot be suppressed with diagnostic suppressor.
var suppressor = new DiagnosticSuppressorForId(analyzer.Descriptor.Id);
var analyzerAndSuppressor = new DiagnosticAnalyzer[] { analyzer, suppressor };
output = VerifyOutput(srcDirectory, srcFile, expectedErrorCount: 1,
includeCurrentAssemblyAsAnalyzerReference: false,
analyzers: analyzerAndSuppressor);
Assert.Contains($"error {analyzer.Descriptor.Id}", output, StringComparison.Ordinal);
CleanupAllGeneratedFiles(srcFile.Path);
}
[WorkItem(38674, "https://github.com/dotnet/roslyn/issues/38674")]
[InlineData(DiagnosticSeverity.Warning, false)]
[InlineData(DiagnosticSeverity.Info, true)]
[InlineData(DiagnosticSeverity.Info, false)]
[InlineData(DiagnosticSeverity.Hidden, false)]
[Theory]
public void TestCategoryBasedBulkAnalyzerDiagnosticConfiguration(DiagnosticSeverity defaultSeverity, bool errorlog)
{
var analyzer = new NamedTypeAnalyzerWithConfigurableEnabledByDefault(isEnabledByDefault: true, defaultSeverity);
var diagnosticId = analyzer.Descriptor.Id;
var category = analyzer.Descriptor.Category;
// Verify category based configuration without any diagnostic ID configuration is respected.
var analyzerConfigText = $@"
[*.cs]
dotnet_analyzer_diagnostic.category-{category}.severity = error";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Error);
// Verify category based configuration does not get applied for suppressed diagnostic.
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Suppress, noWarn: true);
// Verify category based configuration does not get applied for diagnostic configured in ruleset.
var rulesetText = $@"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""12.0"">
<Rules AnalyzerId=""Microsoft.CodeAnalysis"" RuleNamespace=""Microsoft.CodeAnalysis"">
<Rule Id=""{diagnosticId}"" Action=""Warning"" />
</Rules>
</RuleSet>";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Warn, rulesetText: rulesetText);
// Verify category based configuration before diagnostic ID configuration is not respected.
analyzerConfigText = $@"
[*.cs]
dotnet_analyzer_diagnostic.category-{category}.severity = error
dotnet_diagnostic.{diagnosticId}.severity = warning";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Warn);
// Verify category based configuration after diagnostic ID configuration is not respected.
analyzerConfigText = $@"
[*.cs]
dotnet_diagnostic.{diagnosticId}.severity = warning
dotnet_analyzer_diagnostic.category-{category}.severity = error";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Warn);
// Verify global config based configuration before diagnostic ID configuration is not respected.
analyzerConfigText = $@"
is_global = true
dotnet_analyzer_diagnostic.category-{category}.severity = error
dotnet_diagnostic.{diagnosticId}.severity = warning";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Warn);
// Verify global config based configuration after diagnostic ID configuration is not respected.
analyzerConfigText = $@"
is_global = true
dotnet_diagnostic.{diagnosticId}.severity = warning
dotnet_analyzer_diagnostic.category-{category}.severity = error";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Warn);
// Verify category based configuration to warning + /warnaserror reports errors.
analyzerConfigText = $@"
[*.cs]
dotnet_analyzer_diagnostic.category-{category}.severity = warning";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, warnAsError: true, expectedDiagnosticSeverity: ReportDiagnostic.Error);
// Verify disabled by default analyzer is not enabled by category based configuration.
analyzer = new NamedTypeAnalyzerWithConfigurableEnabledByDefault(isEnabledByDefault: false, defaultSeverity);
analyzerConfigText = $@"
[*.cs]
dotnet_analyzer_diagnostic.category-{category}.severity = error";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Suppress);
// Verify disabled by default analyzer is not enabled by category based configuration in global config
analyzer = new NamedTypeAnalyzerWithConfigurableEnabledByDefault(isEnabledByDefault: false, defaultSeverity);
analyzerConfigText = $@"
is_global=true
dotnet_analyzer_diagnostic.category-{category}.severity = error";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Suppress);
if (defaultSeverity == DiagnosticSeverity.Hidden ||
defaultSeverity == DiagnosticSeverity.Info && !errorlog)
{
// Verify analyzer with Hidden severity OR Info severity + no /errorlog is not executed.
analyzer = new NamedTypeAnalyzerWithConfigurableEnabledByDefault(isEnabledByDefault: true, defaultSeverity, throwOnAllNamedTypes: true);
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText: string.Empty, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Suppress);
// Verify that bulk configuration 'none' entry does not enable this analyzer.
analyzerConfigText = $@"
[*.cs]
dotnet_analyzer_diagnostic.category-{category}.severity = none";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Suppress);
// Verify that bulk configuration 'none' entry does not enable this analyzer via global config
analyzerConfigText = $@"
[*.cs]
dotnet_analyzer_diagnostic.category-{category}.severity = none";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Suppress);
}
}
[WorkItem(38674, "https://github.com/dotnet/roslyn/issues/38674")]
[InlineData(DiagnosticSeverity.Warning, false)]
[InlineData(DiagnosticSeverity.Info, true)]
[InlineData(DiagnosticSeverity.Info, false)]
[InlineData(DiagnosticSeverity.Hidden, false)]
[Theory]
public void TestBulkAnalyzerDiagnosticConfiguration(DiagnosticSeverity defaultSeverity, bool errorlog)
{
var analyzer = new NamedTypeAnalyzerWithConfigurableEnabledByDefault(isEnabledByDefault: true, defaultSeverity);
var diagnosticId = analyzer.Descriptor.Id;
// Verify bulk configuration without any diagnostic ID configuration is respected.
var analyzerConfigText = $@"
[*.cs]
dotnet_analyzer_diagnostic.severity = error";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Error);
// Verify bulk configuration does not get applied for suppressed diagnostic.
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Suppress, noWarn: true);
// Verify bulk configuration does not get applied for diagnostic configured in ruleset.
var rulesetText = $@"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""12.0"">
<Rules AnalyzerId=""Microsoft.CodeAnalysis"" RuleNamespace=""Microsoft.CodeAnalysis"">
<Rule Id=""{diagnosticId}"" Action=""Warning"" />
</Rules>
</RuleSet>";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Warn, rulesetText: rulesetText);
// Verify bulk configuration before diagnostic ID configuration is not respected.
analyzerConfigText = $@"
[*.cs]
dotnet_analyzer_diagnostic.severity = error
dotnet_diagnostic.{diagnosticId}.severity = warning";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Warn);
// Verify bulk configuration after diagnostic ID configuration is not respected.
analyzerConfigText = $@"
[*.cs]
dotnet_diagnostic.{diagnosticId}.severity = warning
dotnet_analyzer_diagnostic.severity = error";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Warn);
// Verify bulk configuration to warning + /warnaserror reports errors.
analyzerConfigText = $@"
[*.cs]
dotnet_analyzer_diagnostic.severity = warning";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, warnAsError: true, expectedDiagnosticSeverity: ReportDiagnostic.Error);
// Verify disabled by default analyzer is not enabled by bulk configuration.
analyzer = new NamedTypeAnalyzerWithConfigurableEnabledByDefault(isEnabledByDefault: false, defaultSeverity);
analyzerConfigText = $@"
[*.cs]
dotnet_analyzer_diagnostic.severity = error";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Suppress);
if (defaultSeverity == DiagnosticSeverity.Hidden ||
defaultSeverity == DiagnosticSeverity.Info && !errorlog)
{
// Verify analyzer with Hidden severity OR Info severity + no /errorlog is not executed.
analyzer = new NamedTypeAnalyzerWithConfigurableEnabledByDefault(isEnabledByDefault: true, defaultSeverity, throwOnAllNamedTypes: true);
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText: string.Empty, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Suppress);
// Verify that bulk configuration 'none' entry does not enable this analyzer.
analyzerConfigText = $@"
[*.cs]
dotnet_analyzer_diagnostic.severity = none";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Suppress);
}
}
[WorkItem(38674, "https://github.com/dotnet/roslyn/issues/38674")]
[InlineData(DiagnosticSeverity.Warning, false)]
[InlineData(DiagnosticSeverity.Info, true)]
[InlineData(DiagnosticSeverity.Info, false)]
[InlineData(DiagnosticSeverity.Hidden, false)]
[Theory]
public void TestMixedCategoryBasedAndBulkAnalyzerDiagnosticConfiguration(DiagnosticSeverity defaultSeverity, bool errorlog)
{
var analyzer = new NamedTypeAnalyzerWithConfigurableEnabledByDefault(isEnabledByDefault: true, defaultSeverity);
var diagnosticId = analyzer.Descriptor.Id;
var category = analyzer.Descriptor.Category;
// Verify category based configuration before bulk analyzer diagnostic configuration is respected.
var analyzerConfigText = $@"
[*.cs]
dotnet_analyzer_diagnostic.category-{category}.severity = error
dotnet_analyzer_diagnostic.severity = warning";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Error);
// Verify category based configuration after bulk analyzer diagnostic configuration is respected.
analyzerConfigText = $@"
[*.cs]
dotnet_analyzer_diagnostic.severity = warning
dotnet_analyzer_diagnostic.category-{category}.severity = error";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Error);
// Verify neither category based nor bulk diagnostic configuration is respected when specific diagnostic ID is configured in analyzer config.
analyzerConfigText = $@"
[*.cs]
dotnet_diagnostic.{diagnosticId}.severity = warning
dotnet_analyzer_diagnostic.category-{category}.severity = none
dotnet_analyzer_diagnostic.severity = suggestion";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Warn);
// Verify neither category based nor bulk diagnostic configuration is respected when specific diagnostic ID is configured in ruleset.
analyzerConfigText = $@"
[*.cs]
dotnet_analyzer_diagnostic.category-{category}.severity = none
dotnet_analyzer_diagnostic.severity = suggestion";
var rulesetText = $@"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""12.0"">
<Rules AnalyzerId=""Microsoft.CodeAnalysis"" RuleNamespace=""Microsoft.CodeAnalysis"">
<Rule Id=""{diagnosticId}"" Action=""Warning"" />
</Rules>
</RuleSet>";
TestBulkAnalyzerConfigurationCore(analyzer, analyzerConfigText, errorlog, expectedDiagnosticSeverity: ReportDiagnostic.Warn, rulesetText);
}
private void TestBulkAnalyzerConfigurationCore(
NamedTypeAnalyzerWithConfigurableEnabledByDefault analyzer,
string analyzerConfigText,
bool errorlog,
ReportDiagnostic expectedDiagnosticSeverity,
string rulesetText = null,
bool noWarn = false,
bool warnAsError = false)
{
var diagnosticId = analyzer.Descriptor.Id;
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("test.cs").WriteAllText(@"class C { }");
var analyzerConfig = dir.CreateFile(".editorconfig").WriteAllText(analyzerConfigText);
var arguments = new[] {
"/nologo",
"/t:library",
"/preferreduilang:en",
"/analyzerconfig:" + analyzerConfig.Path,
src.Path };
if (noWarn)
{
arguments = arguments.Append($"/nowarn:{diagnosticId}");
}
if (warnAsError)
{
arguments = arguments.Append($"/warnaserror");
}
if (errorlog)
{
arguments = arguments.Append($"/errorlog:errorlog");
}
if (rulesetText != null)
{
var rulesetFile = CreateRuleSetFile(rulesetText);
arguments = arguments.Append($"/ruleset:{rulesetFile.Path}");
}
var cmd = CreateCSharpCompiler(null, dir.Path, arguments,
analyzers: ImmutableArray.Create<DiagnosticAnalyzer>(analyzer));
Assert.Equal(analyzerConfig.Path, Assert.Single(cmd.Arguments.AnalyzerConfigPaths));
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = cmd.Run(outWriter);
var expectedErrorCode = expectedDiagnosticSeverity == ReportDiagnostic.Error ? 1 : 0;
Assert.Equal(expectedErrorCode, exitCode);
var prefix = expectedDiagnosticSeverity switch
{
ReportDiagnostic.Error => "error",
ReportDiagnostic.Warn => "warning",
ReportDiagnostic.Info => errorlog ? "info" : null,
ReportDiagnostic.Hidden => null,
ReportDiagnostic.Suppress => null,
_ => throw ExceptionUtilities.UnexpectedValue(expectedDiagnosticSeverity)
};
if (prefix == null)
{
Assert.DoesNotContain(diagnosticId, outWriter.ToString());
}
else
{
Assert.Contains($"{prefix} {diagnosticId}: {analyzer.Descriptor.MessageFormat}", outWriter.ToString());
}
}
[Theory]
[InlineData(true)]
[InlineData(false)]
[WorkItem(37779, "https://github.com/dotnet/roslyn/issues/37779")]
public void CompilerWarnAsErrorDoesNotEmit(bool warnAsError)
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
int _f; // CS0169: unused field
}");
var docName = "temp.xml";
var pdbName = "temp.pdb";
var additionalArgs = new[] { $"/doc:{docName}", $"/pdb:{pdbName}", "/debug" };
if (warnAsError)
{
additionalArgs = additionalArgs.Append("/warnaserror").AsArray();
}
var expectedErrorCount = warnAsError ? 1 : 0;
var expectedWarningCount = !warnAsError ? 1 : 0;
var output = VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false,
additionalArgs,
expectedErrorCount: expectedErrorCount,
expectedWarningCount: expectedWarningCount);
var expectedOutput = warnAsError ? "error CS0169" : "warning CS0169";
Assert.Contains(expectedOutput, output);
string binaryPath = Path.Combine(dir.Path, "temp.dll");
Assert.True(File.Exists(binaryPath) == !warnAsError);
string pdbPath = Path.Combine(dir.Path, pdbName);
Assert.True(File.Exists(pdbPath) == !warnAsError);
string xmlDocFilePath = Path.Combine(dir.Path, docName);
Assert.True(File.Exists(xmlDocFilePath) == !warnAsError);
}
[Theory]
[InlineData(true)]
[InlineData(false)]
[WorkItem(37779, "https://github.com/dotnet/roslyn/issues/37779")]
public void AnalyzerConfigSeverityEscalationToErrorDoesNotEmit(bool analyzerConfigSetToError)
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
int _f; // CS0169: unused field
}");
var docName = "temp.xml";
var pdbName = "temp.pdb";
var additionalArgs = new[] { $"/doc:{docName}", $"/pdb:{pdbName}", "/debug" };
if (analyzerConfigSetToError)
{
var analyzerConfig = dir.CreateFile(".editorconfig").WriteAllText(@"
[*.cs]
dotnet_diagnostic.cs0169.severity = error");
additionalArgs = additionalArgs.Append("/analyzerconfig:" + analyzerConfig.Path).ToArray();
}
var expectedErrorCount = analyzerConfigSetToError ? 1 : 0;
var expectedWarningCount = !analyzerConfigSetToError ? 1 : 0;
var output = VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false,
additionalArgs,
expectedErrorCount: expectedErrorCount,
expectedWarningCount: expectedWarningCount);
var expectedOutput = analyzerConfigSetToError ? "error CS0169" : "warning CS0169";
Assert.Contains(expectedOutput, output);
string binaryPath = Path.Combine(dir.Path, "temp.dll");
Assert.True(File.Exists(binaryPath) == !analyzerConfigSetToError);
string pdbPath = Path.Combine(dir.Path, pdbName);
Assert.True(File.Exists(pdbPath) == !analyzerConfigSetToError);
string xmlDocFilePath = Path.Combine(dir.Path, docName);
Assert.True(File.Exists(xmlDocFilePath) == !analyzerConfigSetToError);
}
[Theory]
[InlineData(true)]
[InlineData(false)]
[WorkItem(37779, "https://github.com/dotnet/roslyn/issues/37779")]
public void RulesetSeverityEscalationToErrorDoesNotEmit(bool rulesetSetToError)
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
int _f; // CS0169: unused field
}");
var docName = "temp.xml";
var pdbName = "temp.pdb";
var additionalArgs = new[] { $"/doc:{docName}", $"/pdb:{pdbName}", "/debug" };
if (rulesetSetToError)
{
string source = @"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""12.0"">
<Rules AnalyzerId=""Microsoft.CodeAnalysis"" RuleNamespace=""Microsoft.CodeAnalysis"">
<Rule Id=""CS0169"" Action=""Error"" />
</Rules>
</RuleSet>
";
var rulesetFile = CreateRuleSetFile(source);
additionalArgs = additionalArgs.Append("/ruleset:" + rulesetFile.Path).ToArray();
}
var expectedErrorCount = rulesetSetToError ? 1 : 0;
var expectedWarningCount = !rulesetSetToError ? 1 : 0;
var output = VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false,
additionalArgs,
expectedErrorCount: expectedErrorCount,
expectedWarningCount: expectedWarningCount);
var expectedOutput = rulesetSetToError ? "error CS0169" : "warning CS0169";
Assert.Contains(expectedOutput, output);
string binaryPath = Path.Combine(dir.Path, "temp.dll");
Assert.True(File.Exists(binaryPath) == !rulesetSetToError);
string pdbPath = Path.Combine(dir.Path, pdbName);
Assert.True(File.Exists(pdbPath) == !rulesetSetToError);
string xmlDocFilePath = Path.Combine(dir.Path, docName);
Assert.True(File.Exists(xmlDocFilePath) == !rulesetSetToError);
}
[Theory]
[InlineData(true)]
[InlineData(false)]
[WorkItem(37779, "https://github.com/dotnet/roslyn/issues/37779")]
public void AnalyzerWarnAsErrorDoesNotEmit(bool warnAsError)
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText("class C { }");
var additionalArgs = warnAsError ? new[] { "/warnaserror" } : null;
var expectedErrorCount = warnAsError ? 1 : 0;
var expectedWarningCount = !warnAsError ? 1 : 0;
var output = VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false,
additionalArgs,
expectedErrorCount: expectedErrorCount,
expectedWarningCount: expectedWarningCount,
analyzers: new[] { new WarningDiagnosticAnalyzer() });
var expectedDiagnosticSeverity = warnAsError ? "error" : "warning";
Assert.Contains($"{expectedDiagnosticSeverity} {WarningDiagnosticAnalyzer.Warning01.Id}", output);
string binaryPath = Path.Combine(dir.Path, "temp.dll");
Assert.True(File.Exists(binaryPath) == !warnAsError);
}
// Currently, configuring no location diagnostics through editorconfig is not supported.
[Theory(Skip = "https://github.com/dotnet/roslyn/issues/38042")]
[CombinatorialData]
public void AnalyzerConfigRespectedForNoLocationDiagnostic(ReportDiagnostic reportDiagnostic, bool isEnabledByDefault, bool noWarn, bool errorlog)
{
var analyzer = new AnalyzerWithNoLocationDiagnostics(isEnabledByDefault);
TestAnalyzerConfigRespectedCore(analyzer, analyzer.Descriptor, reportDiagnostic, noWarn, errorlog);
}
[WorkItem(37876, "https://github.com/dotnet/roslyn/issues/37876")]
[Theory]
[CombinatorialData]
public void AnalyzerConfigRespectedForDisabledByDefaultDiagnostic(ReportDiagnostic analyzerConfigSeverity, bool isEnabledByDefault, bool noWarn, bool errorlog)
{
var analyzer = new NamedTypeAnalyzerWithConfigurableEnabledByDefault(isEnabledByDefault, defaultSeverity: DiagnosticSeverity.Warning);
TestAnalyzerConfigRespectedCore(analyzer, analyzer.Descriptor, analyzerConfigSeverity, noWarn, errorlog);
}
private void TestAnalyzerConfigRespectedCore(DiagnosticAnalyzer analyzer, DiagnosticDescriptor descriptor, ReportDiagnostic analyzerConfigSeverity, bool noWarn, bool errorlog)
{
if (analyzerConfigSeverity == ReportDiagnostic.Default)
{
// "dotnet_diagnostic.ID.severity = default" is not supported.
return;
}
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("test.cs").WriteAllText(@"class C { }");
var analyzerConfig = dir.CreateFile(".editorconfig").WriteAllText($@"
[*.cs]
dotnet_diagnostic.{descriptor.Id}.severity = {analyzerConfigSeverity.ToAnalyzerConfigString()}");
var arguments = new[] {
"/nologo",
"/t:library",
"/preferreduilang:en",
"/analyzerconfig:" + analyzerConfig.Path,
src.Path };
if (noWarn)
{
arguments = arguments.Append($"/nowarn:{descriptor.Id}");
}
if (errorlog)
{
arguments = arguments.Append($"/errorlog:errorlog");
}
var cmd = CreateCSharpCompiler(null, dir.Path, arguments,
analyzers: ImmutableArray.Create<DiagnosticAnalyzer>(analyzer));
Assert.Equal(analyzerConfig.Path, Assert.Single(cmd.Arguments.AnalyzerConfigPaths));
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = cmd.Run(outWriter);
var expectedErrorCode = !noWarn && analyzerConfigSeverity == ReportDiagnostic.Error ? 1 : 0;
Assert.Equal(expectedErrorCode, exitCode);
// NOTE: Info diagnostics are only logged on command line when /errorlog is specified. See https://github.com/dotnet/roslyn/issues/42166 for details.
if (!noWarn &&
(analyzerConfigSeverity == ReportDiagnostic.Error ||
analyzerConfigSeverity == ReportDiagnostic.Warn ||
(analyzerConfigSeverity == ReportDiagnostic.Info && errorlog)))
{
var prefix = analyzerConfigSeverity == ReportDiagnostic.Error ? "error" : analyzerConfigSeverity == ReportDiagnostic.Warn ? "warning" : "info";
Assert.Contains($"{prefix} {descriptor.Id}: {descriptor.MessageFormat}", outWriter.ToString());
}
else
{
Assert.DoesNotContain(descriptor.Id.ToString(), outWriter.ToString());
}
}
[Fact]
[WorkItem(3705, "https://github.com/dotnet/roslyn/issues/3705")]
public void IsUserConfiguredGeneratedCodeInAnalyzerConfig()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
void M(C? c)
{
_ = c.ToString(); // warning CS8602: Dereference of a possibly null reference.
}
}");
var output = VerifyOutput(dir, src, additionalFlags: new[] { "/nullable" }, expectedWarningCount: 1, includeCurrentAssemblyAsAnalyzerReference: false);
// warning CS8602: Dereference of a possibly null reference.
Assert.Contains("warning CS8602", output, StringComparison.Ordinal);
// generated_code = true
var analyzerConfigFile = dir.CreateFile(".editorconfig");
var analyzerConfig = analyzerConfigFile.WriteAllText(@"
[*.cs]
generated_code = true");
output = VerifyOutput(dir, src, additionalFlags: new[] { "/nullable", "/analyzerconfig:" + analyzerConfig.Path }, expectedWarningCount: 1, includeCurrentAssemblyAsAnalyzerReference: false);
Assert.DoesNotContain("warning CS8602", output, StringComparison.Ordinal);
// warning CS8669: The annotation for nullable reference types should only be used in code within a '#nullable' annotations context. Auto-generated code requires an explicit '#nullable' directive in source.
Assert.Contains("warning CS8669", output, StringComparison.Ordinal);
// generated_code = false
analyzerConfig = analyzerConfigFile.WriteAllText(@"
[*.cs]
generated_code = false");
output = VerifyOutput(dir, src, additionalFlags: new[] { "/nullable", "/analyzerconfig:" + analyzerConfig.Path }, expectedWarningCount: 1, includeCurrentAssemblyAsAnalyzerReference: false);
// warning CS8602: Dereference of a possibly null reference.
Assert.Contains("warning CS8602", output, StringComparison.Ordinal);
// generated_code = auto
analyzerConfig = analyzerConfigFile.WriteAllText(@"
[*.cs]
generated_code = auto");
output = VerifyOutput(dir, src, additionalFlags: new[] { "/nullable", "/analyzerconfig:" + analyzerConfig.Path }, expectedWarningCount: 1, includeCurrentAssemblyAsAnalyzerReference: false);
// warning CS8602: Dereference of a possibly null reference.
Assert.Contains("warning CS8602", output, StringComparison.Ordinal);
}
[WorkItem(42166, "https://github.com/dotnet/roslyn/issues/42166")]
[CombinatorialData, Theory]
public void TestAnalyzerFilteringBasedOnSeverity(DiagnosticSeverity defaultSeverity, bool errorlog)
{
// This test verifies that analyzer execution is skipped at build time for the following:
// 1. Analyzer reporting Hidden diagnostics
// 2. Analyzer reporting Info diagnostics, when /errorlog is not specified
var analyzerShouldBeSkipped = defaultSeverity == DiagnosticSeverity.Hidden ||
defaultSeverity == DiagnosticSeverity.Info && !errorlog;
// We use an analyzer that throws an exception on every analyzer callback.
// So an AD0001 analyzer exception diagnostic is reported if analyzer executed, otherwise not.
var analyzer = new NamedTypeAnalyzerWithConfigurableEnabledByDefault(isEnabledByDefault: true, defaultSeverity, throwOnAllNamedTypes: true);
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("test.cs").WriteAllText(@"class C { }");
var args = new[] { "/nologo", "/t:library", "/preferreduilang:en", src.Path };
if (errorlog)
args = args.Append("/errorlog:errorlog");
var cmd = CreateCSharpCompiler(null, dir.Path, args, analyzers: ImmutableArray.Create<DiagnosticAnalyzer>(analyzer));
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = cmd.Run(outWriter);
Assert.Equal(0, exitCode);
var output = outWriter.ToString();
if (analyzerShouldBeSkipped)
{
Assert.Empty(output);
}
else
{
Assert.Contains("warning AD0001: Analyzer 'Microsoft.CodeAnalysis.CommonDiagnosticAnalyzers+NamedTypeAnalyzerWithConfigurableEnabledByDefault' threw an exception of type 'System.NotImplementedException'",
output, StringComparison.Ordinal);
}
}
[WorkItem(47017, "https://github.com/dotnet/roslyn/issues/47017")]
[CombinatorialData, Theory]
public void TestWarnAsErrorMinusDoesNotEnableDisabledByDefaultAnalyzers(DiagnosticSeverity defaultSeverity, bool isEnabledByDefault)
{
// This test verifies that '/warnaserror-:DiagnosticId' does not affect if analyzers are executed or skipped..
// Setup the analyzer to always throw an exception on analyzer callbacks for cases where we expect analyzer execution to be skipped:
// 1. Disabled by default analyzer, i.e. 'isEnabledByDefault == false'.
// 2. Default severity Hidden/Info: We only execute analyzers reporting Warning/Error severity diagnostics on command line builds.
var analyzerShouldBeSkipped = !isEnabledByDefault ||
defaultSeverity == DiagnosticSeverity.Hidden ||
defaultSeverity == DiagnosticSeverity.Info;
var analyzer = new NamedTypeAnalyzerWithConfigurableEnabledByDefault(isEnabledByDefault, defaultSeverity, throwOnAllNamedTypes: analyzerShouldBeSkipped);
var diagnosticId = analyzer.Descriptor.Id;
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("test.cs").WriteAllText(@"class C { }");
// Verify '/warnaserror-:DiagnosticId' behavior.
var args = new[] { "/warnaserror+", $"/warnaserror-:{diagnosticId}", "/nologo", "/t:library", "/preferreduilang:en", src.Path };
var cmd = CreateCSharpCompiler(null, dir.Path, args, analyzers: ImmutableArray.Create<DiagnosticAnalyzer>(analyzer));
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = cmd.Run(outWriter);
var expectedExitCode = !analyzerShouldBeSkipped && defaultSeverity == DiagnosticSeverity.Error ? 1 : 0;
Assert.Equal(expectedExitCode, exitCode);
var output = outWriter.ToString();
if (analyzerShouldBeSkipped)
{
Assert.Empty(output);
}
else
{
var prefix = defaultSeverity == DiagnosticSeverity.Warning ? "warning" : "error";
Assert.Contains($"{prefix} {diagnosticId}: {analyzer.Descriptor.MessageFormat}", output);
}
}
[WorkItem(49446, "https://github.com/dotnet/roslyn/issues/49446")]
[Theory]
// Verify '/warnaserror-:ID' prevents escalation to 'Error' when config file bumps severity to 'Warning'
[InlineData(false, DiagnosticSeverity.Info, DiagnosticSeverity.Warning, DiagnosticSeverity.Error)]
[InlineData(true, DiagnosticSeverity.Info, DiagnosticSeverity.Warning, DiagnosticSeverity.Warning)]
// Verify '/warnaserror-:ID' prevents escalation to 'Error' when default severity is 'Warning' and no config file setting is specified.
[InlineData(false, DiagnosticSeverity.Warning, null, DiagnosticSeverity.Error)]
[InlineData(true, DiagnosticSeverity.Warning, null, DiagnosticSeverity.Warning)]
// Verify '/warnaserror-:ID' prevents escalation to 'Error' when default severity is 'Warning' and config file bumps severity to 'Error'
[InlineData(false, DiagnosticSeverity.Warning, DiagnosticSeverity.Error, DiagnosticSeverity.Error)]
[InlineData(true, DiagnosticSeverity.Warning, DiagnosticSeverity.Error, DiagnosticSeverity.Warning)]
// Verify '/warnaserror-:ID' has no effect when default severity is 'Info' and config file bumps severity to 'Error'
[InlineData(false, DiagnosticSeverity.Info, DiagnosticSeverity.Error, DiagnosticSeverity.Error)]
[InlineData(true, DiagnosticSeverity.Info, DiagnosticSeverity.Error, DiagnosticSeverity.Error)]
public void TestWarnAsErrorMinusDoesNotNullifyEditorConfig(
bool warnAsErrorMinus,
DiagnosticSeverity defaultSeverity,
DiagnosticSeverity? severityInConfigFile,
DiagnosticSeverity expectedEffectiveSeverity)
{
var analyzer = new NamedTypeAnalyzerWithConfigurableEnabledByDefault(isEnabledByDefault: true, defaultSeverity, throwOnAllNamedTypes: false);
var diagnosticId = analyzer.Descriptor.Id;
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("test.cs").WriteAllText(@"class C { }");
var additionalFlags = new[] { "/warnaserror+" };
if (severityInConfigFile.HasValue)
{
var severityString = DiagnosticDescriptor.MapSeverityToReport(severityInConfigFile.Value).ToAnalyzerConfigString();
var analyzerConfig = dir.CreateFile(".editorconfig").WriteAllText($@"
[*.cs]
dotnet_diagnostic.{diagnosticId}.severity = {severityString}");
additionalFlags = additionalFlags.Append($"/analyzerconfig:{analyzerConfig.Path}").ToArray();
}
if (warnAsErrorMinus)
{
additionalFlags = additionalFlags.Append($"/warnaserror-:{diagnosticId}").ToArray();
}
int expectedWarningCount = 0, expectedErrorCount = 0;
switch (expectedEffectiveSeverity)
{
case DiagnosticSeverity.Warning:
expectedWarningCount = 1;
break;
case DiagnosticSeverity.Error:
expectedErrorCount = 1;
break;
default:
throw ExceptionUtilities.UnexpectedValue(expectedEffectiveSeverity);
}
VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false,
expectedWarningCount: expectedWarningCount,
expectedErrorCount: expectedErrorCount,
additionalFlags: additionalFlags,
analyzers: new[] { analyzer });
}
[Fact]
public void SourceGenerators_EmbeddedSources()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
}");
var generatedSource = "public class D { }";
var generator = new SingleFileTestGenerator(generatedSource, "generatedSource.cs");
VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/debug:embedded", "/out:embed.exe" }, generators: new[] { generator }, analyzers: null);
var generatorPrefix = GeneratorDriver.GetFilePathPrefixForGenerator(generator);
ValidateEmbeddedSources_Portable(new Dictionary<string, string> { { Path.Combine(dir.Path, generatorPrefix, $"generatedSource.cs"), generatedSource } }, dir, true);
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
Directory.Delete(dir.Path, true);
}
[Theory, CombinatorialData]
[WorkItem(40926, "https://github.com/dotnet/roslyn/issues/40926")]
public void TestSourceGeneratorsWithAnalyzers(bool includeCurrentAssemblyAsAnalyzerReference, bool skipAnalyzers)
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
}");
var generatedSource = "public class D { }";
var generator = new SingleFileTestGenerator(generatedSource, "generatedSource.cs");
// 'skipAnalyzers' should have no impact on source generator execution, but should prevent analyzer execution.
var skipAnalyzersFlag = "/skipAnalyzers" + (skipAnalyzers ? "+" : "-");
// Verify analyzers were executed only if both the following conditions were satisfied:
// 1. Current assembly was added as an analyzer reference, i.e. "includeCurrentAssemblyAsAnalyzerReference = true" and
// 2. We did not explicitly request skipping analyzers, i.e. "skipAnalyzers = false".
var expectedAnalyzerExecution = includeCurrentAssemblyAsAnalyzerReference && !skipAnalyzers;
// 'WarningDiagnosticAnalyzer' generates a warning for each named type.
// We expect two warnings for this test: type "C" defined in source and the source generator defined type.
// Additionally, we also have an analyzer that generates "warning CS8032: An instance of analyzer cannot be created"
// CS8032 is generated with includeCurrentAssemblyAsAnalyzerReference even when we are skipping analyzers as we will instantiate all analyzers, just not execute them.
var expectedWarningCount = expectedAnalyzerExecution ? 3 : (includeCurrentAssemblyAsAnalyzerReference ? 1 : 0);
var output = VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference,
expectedWarningCount: expectedWarningCount,
additionalFlags: new[] { "/debug:embedded", "/out:embed.exe", skipAnalyzersFlag },
generators: new[] { generator });
// Verify source generator was executed, regardless of the value of 'skipAnalyzers'.
var generatorPrefix = GeneratorDriver.GetFilePathPrefixForGenerator(generator);
ValidateEmbeddedSources_Portable(new Dictionary<string, string> { { Path.Combine(dir.Path, generatorPrefix, "generatedSource.cs"), generatedSource } }, dir, true);
if (expectedAnalyzerExecution)
{
Assert.Contains("warning Warning01", output, StringComparison.Ordinal);
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
}
else if (includeCurrentAssemblyAsAnalyzerReference)
{
Assert.Contains("warning CS8032", output, StringComparison.Ordinal);
}
else
{
Assert.Empty(output);
}
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
}
[Theory]
[InlineData("partial class D {}", "file1.cs", "partial class E {}", "file2.cs")] // different files, different names
[InlineData("partial class D {}", "file1.cs", "partial class E {}", "file1.cs")] // different files, same names
[InlineData("partial class D {}", "file1.cs", "partial class D {}", "file2.cs")] // same files, different names
[InlineData("partial class D {}", "file1.cs", "partial class D {}", "file1.cs")] // same files, same names
[InlineData("partial class D {}", "file1.cs", "", "file2.cs")] // empty second file
public void SourceGenerators_EmbeddedSources_MultipleFiles(string source1, string source1Name, string source2, string source2Name)
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
}");
var generator = new SingleFileTestGenerator(source1, source1Name);
var generator2 = new SingleFileTestGenerator2(source2, source2Name);
VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/debug:embedded", "/out:embed.exe" }, generators: new[] { generator, generator2 }, analyzers: null);
var generator1Prefix = GeneratorDriver.GetFilePathPrefixForGenerator(generator);
var generator2Prefix = GeneratorDriver.GetFilePathPrefixForGenerator(generator2);
ValidateEmbeddedSources_Portable(new Dictionary<string, string>
{
{ Path.Combine(dir.Path, generator1Prefix, source1Name), source1},
{ Path.Combine(dir.Path, generator2Prefix, source2Name), source2},
}, dir, true);
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
Directory.Delete(dir.Path, true);
}
[Fact]
public void SourceGenerators_WriteGeneratedSources()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
}");
var generatedDir = dir.CreateDirectory("generated");
var generatedSource = "public class D { }";
var generator = new SingleFileTestGenerator(generatedSource, "generatedSource.cs");
VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/generatedfilesout:" + generatedDir.Path, "/langversion:preview", "/out:embed.exe" }, generators: new[] { generator }, analyzers: null);
var generatorPrefix = GeneratorDriver.GetFilePathPrefixForGenerator(generator);
ValidateWrittenSources(new() { { Path.Combine(generatedDir.Path, generatorPrefix), new() { { "generatedSource.cs", generatedSource } } } });
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
Directory.Delete(dir.Path, true);
}
[Fact]
public void SourceGenerators_OverwriteGeneratedSources()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
}");
var generatedDir = dir.CreateDirectory("generated");
var generatedSource1 = "class D { } class E { }";
var generator1 = new SingleFileTestGenerator(generatedSource1, "generatedSource.cs");
VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/generatedfilesout:" + generatedDir.Path, "/langversion:preview", "/out:embed.exe" }, generators: new[] { generator1 }, analyzers: null);
var generatorPrefix = GeneratorDriver.GetFilePathPrefixForGenerator(generator1);
ValidateWrittenSources(new() { { Path.Combine(generatedDir.Path, generatorPrefix), new() { { "generatedSource.cs", generatedSource1 } } } });
var generatedSource2 = "public class D { }";
var generator2 = new SingleFileTestGenerator(generatedSource2, "generatedSource.cs");
VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/generatedfilesout:" + generatedDir.Path, "/langversion:preview", "/out:embed.exe" }, generators: new[] { generator2 }, analyzers: null);
ValidateWrittenSources(new() { { Path.Combine(generatedDir.Path, generatorPrefix), new() { { "generatedSource.cs", generatedSource2 } } } });
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
Directory.Delete(dir.Path, true);
}
[Theory]
[InlineData("partial class D {}", "file1.cs", "partial class E {}", "file2.cs")] // different files, different names
[InlineData("partial class D {}", "file1.cs", "partial class E {}", "file1.cs")] // different files, same names
[InlineData("partial class D {}", "file1.cs", "partial class D {}", "file2.cs")] // same files, different names
[InlineData("partial class D {}", "file1.cs", "partial class D {}", "file1.cs")] // same files, same names
[InlineData("partial class D {}", "file1.cs", "", "file2.cs")] // empty second file
public void SourceGenerators_WriteGeneratedSources_MultipleFiles(string source1, string source1Name, string source2, string source2Name)
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
}");
var generatedDir = dir.CreateDirectory("generated");
var generator = new SingleFileTestGenerator(source1, source1Name);
var generator2 = new SingleFileTestGenerator2(source2, source2Name);
VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/generatedfilesout:" + generatedDir.Path, "/langversion:preview", "/out:embed.exe" }, generators: new[] { generator, generator2 }, analyzers: null);
var generator1Prefix = GeneratorDriver.GetFilePathPrefixForGenerator(generator);
var generator2Prefix = GeneratorDriver.GetFilePathPrefixForGenerator(generator2);
ValidateWrittenSources(new()
{
{ Path.Combine(generatedDir.Path, generator1Prefix), new() { { source1Name, source1 } } },
{ Path.Combine(generatedDir.Path, generator2Prefix), new() { { source2Name, source2 } } }
});
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
Directory.Delete(dir.Path, true);
}
[ConditionalFact(typeof(DesktopClrOnly))] //CoreCLR doesn't support SxS loading
[WorkItem(47990, "https://github.com/dotnet/roslyn/issues/47990")]
public void SourceGenerators_SxS_AssemblyLoading()
{
// compile the generators
var dir = Temp.CreateDirectory();
var snk = Temp.CreateFile("TestKeyPair_", ".snk", dir.Path).WriteAllBytes(TestResources.General.snKey);
var src = dir.CreateFile("generator.cs");
var virtualSnProvider = new DesktopStrongNameProvider(ImmutableArray.Create(dir.Path));
string createGenerator(string version)
{
var generatorSource = $@"
using Microsoft.CodeAnalysis;
[assembly:System.Reflection.AssemblyVersion(""{version}"")]
[Generator]
public class TestGenerator : ISourceGenerator
{{
public void Execute(GeneratorExecutionContext context) {{ context.AddSource(""generatedSource.cs"", ""//from version {version}""); }}
public void Initialize(GeneratorInitializationContext context) {{ }}
}}";
var path = Path.Combine(dir.Path, Guid.NewGuid().ToString() + ".dll");
var comp = CreateEmptyCompilation(source: generatorSource,
references: TargetFrameworkUtil.NetStandard20References.Add(MetadataReference.CreateFromAssemblyInternal(typeof(ISourceGenerator).Assembly)),
options: TestOptions.DebugDll.WithCryptoKeyFile(Path.GetFileName(snk.Path)).WithStrongNameProvider(virtualSnProvider),
assemblyName: "generator");
comp.VerifyDiagnostics();
comp.Emit(path);
return path;
}
var gen1 = createGenerator("1.0.0.0");
var gen2 = createGenerator("2.0.0.0");
var generatedDir = dir.CreateDirectory("generated");
VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/generatedfilesout:" + generatedDir.Path, "/analyzer:" + gen1, "/analyzer:" + gen2 }.ToArray());
// This is wrong! Both generators are writing the same file out, over the top of each other
// See https://github.com/dotnet/roslyn/issues/47990
ValidateWrittenSources(new()
{
// { Path.Combine(generatedDir.Path, "generator", "TestGenerator"), new() { { "generatedSource.cs", "//from version 1.0.0.0" } } },
{ Path.Combine(generatedDir.Path, "generator", "TestGenerator"), new() { { "generatedSource.cs", "//from version 2.0.0.0" } } }
});
}
[Fact]
public void SourceGenerators_DoNotWriteGeneratedSources_When_No_Directory_Supplied()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
}");
var generatedDir = dir.CreateDirectory("generated");
var generatedSource = "public class D { }";
var generator = new SingleFileTestGenerator(generatedSource, "generatedSource.cs");
VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/langversion:preview", "/out:embed.exe" }, generators: new[] { generator }, analyzers: null);
ValidateWrittenSources(new() { { generatedDir.Path, new() } });
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
Directory.Delete(dir.Path, true);
}
[Fact]
public void SourceGenerators_Error_When_GeneratedDir_NotExist()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
}");
var generatedDirPath = Path.Combine(dir.Path, "noexist");
var generatedSource = "public class D { }";
var generator = new SingleFileTestGenerator(generatedSource, "generatedSource.cs");
var output = VerifyOutput(dir, src, expectedErrorCount: 1, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/generatedfilesout:" + generatedDirPath, "/langversion:preview", "/out:embed.exe" }, generators: new[] { generator }, analyzers: null);
Assert.Contains("CS0016:", output);
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
Directory.Delete(dir.Path, true);
}
[Fact]
public void SourceGenerators_GeneratedDir_Has_Spaces()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
}");
var generatedDir = dir.CreateDirectory("generated files");
var generatedSource = "public class D { }";
var generator = new SingleFileTestGenerator(generatedSource, "generatedSource.cs");
VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/generatedfilesout:" + generatedDir.Path, "/langversion:preview", "/out:embed.exe" }, generators: new[] { generator }, analyzers: null);
var generatorPrefix = GeneratorDriver.GetFilePathPrefixForGenerator(generator);
ValidateWrittenSources(new() { { Path.Combine(generatedDir.Path, generatorPrefix), new() { { "generatedSource.cs", generatedSource } } } });
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
Directory.Delete(dir.Path, true);
}
[Fact]
public void ParseGeneratedFilesOut()
{
string root = PathUtilities.IsUnixLikePlatform ? "/" : "c:\\";
string baseDirectory = Path.Combine(root, "abc", "def");
var parsedArgs = DefaultParse(new[] { @"/generatedfilesout:", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for '/generatedfilesout:' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "/generatedfilesout:"));
Assert.Null(parsedArgs.GeneratedFilesOutputDirectory);
parsedArgs = DefaultParse(new[] { @"/generatedfilesout:""""", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify(
// error CS2006: Command-line syntax error: Missing '<text>' for '/generatedfilesout:' option
Diagnostic(ErrorCode.ERR_SwitchNeedsString).WithArguments("<text>", "/generatedfilesout:\"\""));
Assert.Null(parsedArgs.GeneratedFilesOutputDirectory);
parsedArgs = DefaultParse(new[] { @"/generatedfilesout:outdir", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(Path.Combine(baseDirectory, "outdir"), parsedArgs.GeneratedFilesOutputDirectory);
parsedArgs = DefaultParse(new[] { @"/generatedfilesout:""outdir""", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(Path.Combine(baseDirectory, "outdir"), parsedArgs.GeneratedFilesOutputDirectory);
parsedArgs = DefaultParse(new[] { @"/generatedfilesout:out dir", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(Path.Combine(baseDirectory, "out dir"), parsedArgs.GeneratedFilesOutputDirectory);
parsedArgs = DefaultParse(new[] { @"/generatedfilesout:""out dir""", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(Path.Combine(baseDirectory, "out dir"), parsedArgs.GeneratedFilesOutputDirectory);
var absPath = Path.Combine(root, "outdir");
parsedArgs = DefaultParse(new[] { $@"/generatedfilesout:{absPath}", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(absPath, parsedArgs.GeneratedFilesOutputDirectory);
parsedArgs = DefaultParse(new[] { $@"/generatedfilesout:""{absPath}""", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(absPath, parsedArgs.GeneratedFilesOutputDirectory);
absPath = Path.Combine(root, "generated files");
parsedArgs = DefaultParse(new[] { $@"/generatedfilesout:{absPath}", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(absPath, parsedArgs.GeneratedFilesOutputDirectory);
parsedArgs = DefaultParse(new[] { $@"/generatedfilesout:""{absPath}""", "a.cs" }, baseDirectory);
parsedArgs.Errors.Verify();
Assert.Equal(absPath, parsedArgs.GeneratedFilesOutputDirectory);
}
[Fact]
public void SourceGenerators_Error_When_NoDirectoryArgumentGiven()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
}");
var output = VerifyOutput(dir, src, expectedErrorCount: 2, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/generatedfilesout:", "/langversion:preview", "/out:embed.exe" });
Assert.Contains("error CS2006: Command-line syntax error: Missing '<text>' for '/generatedfilesout:' option", output);
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
Directory.Delete(dir.Path, true);
}
[Fact]
public void SourceGenerators_ReportedWrittenFiles_To_TouchedFilesLogger()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
}");
var generatedDir = dir.CreateDirectory("generated");
var generatedSource = "public class D { }";
var generator = new SingleFileTestGenerator(generatedSource, "generatedSource.cs");
VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/generatedfilesout:" + generatedDir.Path, $"/touchedfiles:{dir.Path}/touched", "/langversion:preview", "/out:embed.exe" }, generators: new[] { generator }, analyzers: null);
var touchedFiles = Directory.GetFiles(dir.Path, "touched*");
Assert.Equal(2, touchedFiles.Length);
string[] writtenText = File.ReadAllLines(Path.Combine(dir.Path, "touched.write"));
Assert.Equal(2, writtenText.Length);
Assert.EndsWith("EMBED.EXE", writtenText[0], StringComparison.OrdinalIgnoreCase);
Assert.EndsWith("GENERATEDSOURCE.CS", writtenText[1], StringComparison.OrdinalIgnoreCase);
// Clean up temp files
CleanupAllGeneratedFiles(src.Path);
Directory.Delete(dir.Path, true);
}
[Fact]
[WorkItem(44087, "https://github.com/dotnet/roslyn/issues/44087")]
public void SourceGeneratorsAndAnalyzerConfig()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
}");
var analyzerConfig = dir.CreateFile(".editorconfig").WriteAllText(@"
[*.cs]
key = value");
var generator = new SingleFileTestGenerator("public class D {}", "generated.cs");
VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/analyzerconfig:" + analyzerConfig.Path }, generators: new[] { generator }, analyzers: null);
}
[Fact]
public void SourceGeneratorsCanReadAnalyzerConfig()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
}");
var analyzerConfig1 = dir.CreateFile(".globaleditorconfig").WriteAllText(@"
is_global = true
key1 = value1
[*.cs]
key2 = value2
[*.vb]
key3 = value3");
var analyzerConfig2 = dir.CreateFile(".editorconfig").WriteAllText(@"
[*.cs]
key4 = value4
[*.vb]
key5 = value5");
var subDir = dir.CreateDirectory("subDir");
var analyzerConfig3 = subDir.CreateFile(".editorconfig").WriteAllText(@"
[*.cs]
key6 = value6
[*.vb]
key7 = value7");
var generator = new CallbackGenerator((ic) => { }, (gc) =>
{
// can get the global options
var globalOptions = gc.AnalyzerConfigOptions.GlobalOptions;
Assert.True(globalOptions.TryGetValue("key1", out var keyValue));
Assert.Equal("value1", keyValue);
Assert.False(globalOptions.TryGetValue("key2", out _));
Assert.False(globalOptions.TryGetValue("key3", out _));
Assert.False(globalOptions.TryGetValue("key4", out _));
Assert.False(globalOptions.TryGetValue("key5", out _));
Assert.False(globalOptions.TryGetValue("key6", out _));
Assert.False(globalOptions.TryGetValue("key7", out _));
// can get the options for class C
var classOptions = gc.AnalyzerConfigOptions.GetOptions(gc.Compilation.SyntaxTrees.First());
Assert.True(classOptions.TryGetValue("key1", out keyValue));
Assert.Equal("value1", keyValue);
Assert.False(classOptions.TryGetValue("key2", out _));
Assert.False(classOptions.TryGetValue("key3", out _));
Assert.True(classOptions.TryGetValue("key4", out keyValue));
Assert.Equal("value4", keyValue);
Assert.False(classOptions.TryGetValue("key5", out _));
Assert.False(classOptions.TryGetValue("key6", out _));
Assert.False(classOptions.TryGetValue("key7", out _));
});
var args = new[] {
"/analyzerconfig:" + analyzerConfig1.Path,
"/analyzerconfig:" + analyzerConfig2.Path,
"/analyzerconfig:" + analyzerConfig3.Path,
"/t:library",
src.Path
};
var cmd = CreateCSharpCompiler(null, dir.Path, args, generators: ImmutableArray.Create<ISourceGenerator>(generator));
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = cmd.Run(outWriter);
Assert.Equal(0, exitCode);
// test for both the original tree and the generated one
var provider = cmd.AnalyzerOptions.AnalyzerConfigOptionsProvider;
// get the global options
var globalOptions = provider.GlobalOptions;
Assert.True(globalOptions.TryGetValue("key1", out var keyValue));
Assert.Equal("value1", keyValue);
Assert.False(globalOptions.TryGetValue("key2", out _));
Assert.False(globalOptions.TryGetValue("key3", out _));
Assert.False(globalOptions.TryGetValue("key4", out _));
Assert.False(globalOptions.TryGetValue("key5", out _));
Assert.False(globalOptions.TryGetValue("key6", out _));
Assert.False(globalOptions.TryGetValue("key7", out _));
// get the options for class C
var classOptions = provider.GetOptions(cmd.Compilation.SyntaxTrees.First());
Assert.True(classOptions.TryGetValue("key1", out keyValue));
Assert.Equal("value1", keyValue);
Assert.False(classOptions.TryGetValue("key2", out _));
Assert.False(classOptions.TryGetValue("key3", out _));
Assert.True(classOptions.TryGetValue("key4", out keyValue));
Assert.Equal("value4", keyValue);
Assert.False(classOptions.TryGetValue("key5", out _));
Assert.False(classOptions.TryGetValue("key6", out _));
Assert.False(classOptions.TryGetValue("key7", out _));
// get the options for generated class D
var generatedOptions = provider.GetOptions(cmd.Compilation.SyntaxTrees.Last());
Assert.True(generatedOptions.TryGetValue("key1", out keyValue));
Assert.Equal("value1", keyValue);
Assert.False(generatedOptions.TryGetValue("key2", out _));
Assert.False(generatedOptions.TryGetValue("key3", out _));
Assert.True(classOptions.TryGetValue("key4", out keyValue));
Assert.Equal("value4", keyValue);
Assert.False(generatedOptions.TryGetValue("key5", out _));
Assert.False(generatedOptions.TryGetValue("key6", out _));
Assert.False(generatedOptions.TryGetValue("key7", out _));
}
[Theory]
[CombinatorialData]
public void SourceGeneratorsRunRegardlessOfLanguageVersion(LanguageVersion version)
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"class C {}");
var generator = new CallbackGenerator(i => { }, e => throw null);
var output = VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/langversion:" + version.ToDisplayString() }, generators: new[] { generator }, expectedWarningCount: 1, expectedErrorCount: 1, expectedExitCode: 0);
Assert.Contains("CS8785: Generator 'CallbackGenerator' failed to generate source.", output);
}
[DiagnosticAnalyzer(LanguageNames.CSharp)]
private sealed class FieldAnalyzer : DiagnosticAnalyzer
{
private static readonly DiagnosticDescriptor _rule = new DiagnosticDescriptor("Id", "Title", "Message", "Category", DiagnosticSeverity.Warning, isEnabledByDefault: true);
public override ImmutableArray<DiagnosticDescriptor> SupportedDiagnostics => ImmutableArray.Create(_rule);
public override void Initialize(AnalysisContext context)
{
context.RegisterSyntaxNodeAction(AnalyzeFieldDeclaration, SyntaxKind.FieldDeclaration);
}
private static void AnalyzeFieldDeclaration(SyntaxNodeAnalysisContext context)
{
}
}
[Fact]
[WorkItem(44000, "https://github.com/dotnet/roslyn/issues/44000")]
public void TupleField_ForceComplete()
{
var source =
@"namespace System
{
public struct ValueTuple<T1>
{
public T1 Item1;
public ValueTuple(T1 item1)
{
Item1 = item1;
}
}
}";
var srcFile = Temp.CreateFile().WriteAllText(source);
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var csc = CreateCSharpCompiler(
null,
WorkingDirectory,
new[] { "/nologo", "/t:library", srcFile.Path },
analyzers: ImmutableArray.Create<DiagnosticAnalyzer>(new FieldAnalyzer())); // at least one analyzer required
var exitCode = csc.Run(outWriter);
Assert.Equal(0, exitCode);
var output = outWriter.ToString();
Assert.Empty(output);
CleanupAllGeneratedFiles(srcFile.Path);
}
[Fact]
public void GlobalAnalyzerConfigsAllowedInSameDir()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("test.cs").WriteAllText(@"
class C
{
int _f;
}");
var configText = @"
is_global = true
";
var analyzerConfig1 = dir.CreateFile("analyzerconfig1").WriteAllText(configText);
var analyzerConfig2 = dir.CreateFile("analyzerconfig2").WriteAllText(configText);
var cmd = CreateCSharpCompiler(null, dir.Path, new[] {
"/nologo",
"/t:library",
"/preferreduilang:en",
"/analyzerconfig:" + analyzerConfig1.Path,
"/analyzerconfig:" + analyzerConfig2.Path,
src.Path
});
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = cmd.Run(outWriter);
Assert.Equal(0, exitCode);
}
[Fact]
public void GlobalAnalyzerConfigMultipleSetKeys()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
}");
var analyzerConfigFile = dir.CreateFile(".globalconfig");
var analyzerConfig = analyzerConfigFile.WriteAllText(@"
is_global = true
global_level = 100
option1 = abc");
var analyzerConfigFile2 = dir.CreateFile(".globalconfig2");
var analyzerConfig2 = analyzerConfigFile2.WriteAllText(@"
is_global = true
global_level = 100
option1 = def");
var output = VerifyOutput(dir, src, additionalFlags: new[] { "/analyzerconfig:" + analyzerConfig.Path + "," + analyzerConfig2.Path }, expectedWarningCount: 1, includeCurrentAssemblyAsAnalyzerReference: false);
// warning MultipleGlobalAnalyzerKeys: Multiple global analyzer config files set the same key 'option1' in section 'Global Section'. It has been unset. Key was set by the following files: ...
Assert.Contains("MultipleGlobalAnalyzerKeys:", output, StringComparison.Ordinal);
Assert.Contains("'option1'", output, StringComparison.Ordinal);
Assert.Contains("'Global Section'", output, StringComparison.Ordinal);
analyzerConfig = analyzerConfigFile.WriteAllText(@"
is_global = true
global_level = 100
[/file.cs]
option1 = abc");
analyzerConfig2 = analyzerConfigFile2.WriteAllText(@"
is_global = true
global_level = 100
[/file.cs]
option1 = def");
output = VerifyOutput(dir, src, additionalFlags: new[] { "/analyzerconfig:" + analyzerConfig.Path + "," + analyzerConfig2.Path }, expectedWarningCount: 1, includeCurrentAssemblyAsAnalyzerReference: false);
// warning MultipleGlobalAnalyzerKeys: Multiple global analyzer config files set the same key 'option1' in section 'file.cs'. It has been unset. Key was set by the following files: ...
Assert.Contains("MultipleGlobalAnalyzerKeys:", output, StringComparison.Ordinal);
Assert.Contains("'option1'", output, StringComparison.Ordinal);
Assert.Contains("'/file.cs'", output, StringComparison.Ordinal);
}
[Fact]
public void GlobalAnalyzerConfigWithOptions()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("test.cs").WriteAllText(@"
class C
{
}");
var additionalFile = dir.CreateFile("file.txt");
var analyzerConfig = dir.CreateFile(".editorconfig").WriteAllText(@"
[*.cs]
key1 = value1
[*.txt]
key2 = value2");
var globalConfig = dir.CreateFile(".globalconfig").WriteAllText(@"
is_global = true
key3 = value3");
var cmd = CreateCSharpCompiler(null, dir.Path, new[] {
"/nologo",
"/t:library",
"/analyzerconfig:" + analyzerConfig.Path,
"/analyzerconfig:" + globalConfig.Path,
"/analyzer:" + Assembly.GetExecutingAssembly().Location,
"/nowarn:8032,Warning01",
"/additionalfile:" + additionalFile.Path,
src.Path });
var outWriter = new StringWriter(CultureInfo.InvariantCulture);
var exitCode = cmd.Run(outWriter);
Assert.Equal("", outWriter.ToString());
Assert.Equal(0, exitCode);
var comp = cmd.Compilation;
var tree = comp.SyntaxTrees.Single();
var provider = cmd.AnalyzerOptions.AnalyzerConfigOptionsProvider;
var options = provider.GetOptions(tree);
Assert.NotNull(options);
Assert.True(options.TryGetValue("key1", out string val));
Assert.Equal("value1", val);
Assert.False(options.TryGetValue("key2", out _));
Assert.True(options.TryGetValue("key3", out val));
Assert.Equal("value3", val);
options = provider.GetOptions(cmd.AnalyzerOptions.AdditionalFiles.Single());
Assert.NotNull(options);
Assert.False(options.TryGetValue("key1", out _));
Assert.True(options.TryGetValue("key2", out val));
Assert.Equal("value2", val);
Assert.True(options.TryGetValue("key3", out val));
Assert.Equal("value3", val);
options = provider.GlobalOptions;
Assert.NotNull(options);
Assert.False(options.TryGetValue("key1", out _));
Assert.False(options.TryGetValue("key2", out _));
Assert.True(options.TryGetValue("key3", out val));
Assert.Equal("value3", val);
}
[Fact]
[WorkItem(44087, "https://github.com/dotnet/roslyn/issues/44804")]
public void GlobalAnalyzerConfigDiagnosticOptionsCanBeOverridenByCommandLine()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
void M()
{
label1:;
}
}");
var globalConfig = dir.CreateFile(".globalconfig").WriteAllText(@"
is_global = true
dotnet_diagnostic.CS0164.severity = error;
");
var analyzerConfig = dir.CreateFile(".editorconfig").WriteAllText(@"
[*.cs]
dotnet_diagnostic.CS0164.severity = warning;
");
var none = Array.Empty<TempFile>();
var globalOnly = new[] { globalConfig };
var globalAndSpecific = new[] { globalConfig, analyzerConfig };
// by default a warning, which can be suppressed via cmdline
verify(configs: none, expectedWarnings: 1);
verify(configs: none, noWarn: "CS0164", expectedWarnings: 0);
// the global analyzer config ups the warning to an error, but the cmdline setting overrides it
verify(configs: globalOnly, expectedErrors: 1);
verify(configs: globalOnly, noWarn: "CS0164", expectedWarnings: 0);
verify(configs: globalOnly, noWarn: "164", expectedWarnings: 0); // cmdline can be shortened, but still works
// the editor config downgrades the error back to warning, but the cmdline setting overrides it
verify(configs: globalAndSpecific, expectedWarnings: 1);
verify(configs: globalAndSpecific, noWarn: "CS0164", expectedWarnings: 0);
void verify(TempFile[] configs, int expectedWarnings = 0, int expectedErrors = 0, string noWarn = "0")
=> VerifyOutput(dir, src,
expectedErrorCount: expectedErrors,
expectedWarningCount: expectedWarnings,
includeCurrentAssemblyAsAnalyzerReference: false,
analyzers: null,
additionalFlags: configs.SelectAsArray(c => "/analyzerconfig:" + c.Path)
.Add("/noWarn:" + noWarn).ToArray());
}
[Fact]
[WorkItem(44087, "https://github.com/dotnet/roslyn/issues/44804")]
public void GlobalAnalyzerConfigSpecificDiagnosticOptionsOverrideGeneralCommandLineOptions()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
void M()
{
label1:;
}
}");
var globalConfig = dir.CreateFile(".globalconfig").WriteAllText($@"
is_global = true
dotnet_diagnostic.CS0164.severity = none;
");
VerifyOutput(dir, src, additionalFlags: new[] { "/warnaserror+", "/analyzerconfig:" + globalConfig.Path }, includeCurrentAssemblyAsAnalyzerReference: false);
}
[Theory, CombinatorialData]
[WorkItem(43051, "https://github.com/dotnet/roslyn/issues/43051")]
public void WarnAsErrorIsRespectedForForWarningsConfiguredInRulesetOrGlobalConfig(bool useGlobalConfig)
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
void M()
{
label1:;
}
}");
var additionalFlags = new[] { "/warnaserror+" };
if (useGlobalConfig)
{
var globalConfig = dir.CreateFile(".globalconfig").WriteAllText($@"
is_global = true
dotnet_diagnostic.CS0164.severity = warning;
");
additionalFlags = additionalFlags.Append("/analyzerconfig:" + globalConfig.Path).ToArray();
}
else
{
string ruleSetSource = @"<?xml version=""1.0"" encoding=""utf-8""?>
<RuleSet Name=""Ruleset1"" Description=""Test"" ToolsVersion=""15.0"">
<Rules AnalyzerId=""Compiler"" RuleNamespace=""Compiler"">
<Rule Id=""CS0164"" Action=""Warning"" />
</Rules>
</RuleSet>
";
_ = dir.CreateFile("Rules.ruleset").WriteAllText(ruleSetSource);
additionalFlags = additionalFlags.Append("/ruleset:Rules.ruleset").ToArray();
}
VerifyOutput(dir, src, additionalFlags: additionalFlags, expectedErrorCount: 1, includeCurrentAssemblyAsAnalyzerReference: false);
}
[Fact]
[WorkItem(44087, "https://github.com/dotnet/roslyn/issues/44804")]
public void GlobalAnalyzerConfigSectionsDoNotOverrideCommandLine()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(@"
class C
{
void M()
{
label1:;
}
}");
var globalConfig = dir.CreateFile(".globalconfig").WriteAllText($@"
is_global = true
[{PathUtilities.NormalizeWithForwardSlash(src.Path)}]
dotnet_diagnostic.CS0164.severity = error;
");
VerifyOutput(dir, src, additionalFlags: new[] { "/nowarn:0164", "/analyzerconfig:" + globalConfig.Path }, expectedErrorCount: 0, includeCurrentAssemblyAsAnalyzerReference: false);
}
[Fact]
[WorkItem(44087, "https://github.com/dotnet/roslyn/issues/44804")]
public void GlobalAnalyzerConfigCanSetDiagnosticWithNoLocation()
{
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("test.cs").WriteAllText(@"
class C
{
}");
var globalConfig = dir.CreateFile(".globalconfig").WriteAllText(@"
is_global = true
dotnet_diagnostic.Warning01.severity = error;
");
VerifyOutput(dir, src, additionalFlags: new[] { "/analyzerconfig:" + globalConfig.Path }, expectedErrorCount: 1, includeCurrentAssemblyAsAnalyzerReference: false, analyzers: new WarningDiagnosticAnalyzer());
VerifyOutput(dir, src, additionalFlags: new[] { "/nowarn:Warning01", "/analyzerconfig:" + globalConfig.Path }, includeCurrentAssemblyAsAnalyzerReference: false, analyzers: new WarningDiagnosticAnalyzer());
}
[Theory, CombinatorialData]
public void TestAdditionalFileAnalyzer(bool registerFromInitialize)
{
var srcDirectory = Temp.CreateDirectory();
var source = "class C { }";
var srcFile = srcDirectory.CreateFile("a.cs");
srcFile.WriteAllText(source);
var additionalText = "Additional Text";
var additionalFile = srcDirectory.CreateFile("b.txt");
additionalFile.WriteAllText(additionalText);
var diagnosticSpan = new TextSpan(2, 2);
var analyzer = new AdditionalFileAnalyzer(registerFromInitialize, diagnosticSpan);
var output = VerifyOutput(srcDirectory, srcFile, expectedWarningCount: 1, includeCurrentAssemblyAsAnalyzerReference: false,
additionalFlags: new[] { "/additionalfile:" + additionalFile.Path },
analyzers: analyzer);
Assert.Contains("b.txt(1,3): warning ID0001", output, StringComparison.Ordinal);
CleanupAllGeneratedFiles(srcDirectory.Path);
}
[Theory]
// "/warnaserror" tests
[InlineData(/*analyzerConfigSeverity*/"warning", "/warnaserror", /*expectError*/true, /*expectWarning*/false)]
[InlineData(/*analyzerConfigSeverity*/"error", "/warnaserror", /*expectError*/true, /*expectWarning*/false)]
[InlineData(/*analyzerConfigSeverity*/null, "/warnaserror", /*expectError*/true, /*expectWarning*/false)]
// "/warnaserror:CS0169" tests
[InlineData(/*analyzerConfigSeverity*/"warning", "/warnaserror:CS0169", /*expectError*/true, /*expectWarning*/false)]
[InlineData(/*analyzerConfigSeverity*/"error", "/warnaserror:CS0169", /*expectError*/true, /*expectWarning*/false)]
[InlineData(/*analyzerConfigSeverity*/null, "/warnaserror:CS0169", /*expectError*/true, /*expectWarning*/false)]
// "/nowarn" tests
[InlineData(/*analyzerConfigSeverity*/"warning", "/nowarn:CS0169", /*expectError*/false, /*expectWarning*/false)]
[InlineData(/*analyzerConfigSeverity*/"error", "/nowarn:CS0169", /*expectError*/false, /*expectWarning*/false)]
[InlineData(/*analyzerConfigSeverity*/null, "/nowarn:CS0169", /*expectError*/false, /*expectWarning*/false)]
// Neither "/nowarn" nor "/warnaserror" tests
[InlineData(/*analyzerConfigSeverity*/"warning", /*additionalArg*/null, /*expectError*/false, /*expectWarning*/true)]
[InlineData(/*analyzerConfigSeverity*/"error", /*additionalArg*/null, /*expectError*/true, /*expectWarning*/false)]
[InlineData(/*analyzerConfigSeverity*/null, /*additionalArg*/null, /*expectError*/false, /*expectWarning*/true)]
[WorkItem(43051, "https://github.com/dotnet/roslyn/issues/43051")]
public void TestCompilationOptionsOverrideAnalyzerConfig_CompilerWarning(string analyzerConfigSeverity, string additionalArg, bool expectError, bool expectWarning)
{
var src = @"
class C
{
int _f; // CS0169: unused field
}";
TestCompilationOptionsOverrideAnalyzerConfigCore(src, diagnosticId: "CS0169", analyzerConfigSeverity, additionalArg, expectError, expectWarning);
}
[Theory]
// "/warnaserror" tests
[InlineData(/*analyzerConfigSeverity*/"warning", "/warnaserror", /*expectError*/true, /*expectWarning*/false)]
[InlineData(/*analyzerConfigSeverity*/"error", "/warnaserror", /*expectError*/true, /*expectWarning*/false)]
[InlineData(/*analyzerConfigSeverity*/null, "/warnaserror", /*expectError*/true, /*expectWarning*/false)]
// "/warnaserror:DiagnosticId" tests
[InlineData(/*analyzerConfigSeverity*/"warning", "/warnaserror:" + CompilationAnalyzerWithSeverity.DiagnosticId, /*expectError*/true, /*expectWarning*/false)]
[InlineData(/*analyzerConfigSeverity*/"error", "/warnaserror:" + CompilationAnalyzerWithSeverity.DiagnosticId, /*expectError*/true, /*expectWarning*/false)]
[InlineData(/*analyzerConfigSeverity*/null, "/warnaserror:" + CompilationAnalyzerWithSeverity.DiagnosticId, /*expectError*/true, /*expectWarning*/false)]
// "/nowarn" tests
[InlineData(/*analyzerConfigSeverity*/"warning", "/nowarn:" + CompilationAnalyzerWithSeverity.DiagnosticId, /*expectError*/false, /*expectWarning*/false)]
[InlineData(/*analyzerConfigSeverity*/"error", "/nowarn:" + CompilationAnalyzerWithSeverity.DiagnosticId, /*expectError*/false, /*expectWarning*/false)]
[InlineData(/*analyzerConfigSeverity*/null, "/nowarn:" + CompilationAnalyzerWithSeverity.DiagnosticId, /*expectError*/false, /*expectWarning*/false)]
// Neither "/nowarn" nor "/warnaserror" tests
[InlineData(/*analyzerConfigSeverity*/"warning", /*additionalArg*/null, /*expectError*/false, /*expectWarning*/true)]
[InlineData(/*analyzerConfigSeverity*/"error", /*additionalArg*/null, /*expectError*/true, /*expectWarning*/false)]
[InlineData(/*analyzerConfigSeverity*/null, /*additionalArg*/null, /*expectError*/false, /*expectWarning*/true)]
[WorkItem(43051, "https://github.com/dotnet/roslyn/issues/43051")]
public void TestCompilationOptionsOverrideAnalyzerConfig_AnalyzerWarning(string analyzerConfigSeverity, string additionalArg, bool expectError, bool expectWarning)
{
var analyzer = new CompilationAnalyzerWithSeverity(DiagnosticSeverity.Warning, configurable: true);
var src = @"class C { }";
TestCompilationOptionsOverrideAnalyzerConfigCore(src, CompilationAnalyzerWithSeverity.DiagnosticId, analyzerConfigSeverity, additionalArg, expectError, expectWarning, analyzer);
}
private void TestCompilationOptionsOverrideAnalyzerConfigCore(
string source,
string diagnosticId,
string analyzerConfigSeverity,
string additionalArg,
bool expectError,
bool expectWarning,
params DiagnosticAnalyzer[] analyzers)
{
Assert.True(!expectError || !expectWarning);
var dir = Temp.CreateDirectory();
var src = dir.CreateFile("temp.cs").WriteAllText(source);
var additionalArgs = Array.Empty<string>();
if (analyzerConfigSeverity != null)
{
var analyzerConfig = dir.CreateFile(".editorconfig").WriteAllText($@"
[*.cs]
dotnet_diagnostic.{diagnosticId}.severity = {analyzerConfigSeverity}");
additionalArgs = additionalArgs.Append("/analyzerconfig:" + analyzerConfig.Path).ToArray();
}
if (!string.IsNullOrEmpty(additionalArg))
{
additionalArgs = additionalArgs.Append(additionalArg);
}
var output = VerifyOutput(dir, src, includeCurrentAssemblyAsAnalyzerReference: false,
additionalArgs,
expectedErrorCount: expectError ? 1 : 0,
expectedWarningCount: expectWarning ? 1 : 0,
analyzers: analyzers);
if (expectError)
{
Assert.Contains($"error {diagnosticId}", output);
}
else if (expectWarning)
{
Assert.Contains($"warning {diagnosticId}", output);
}
else
{
Assert.DoesNotContain(diagnosticId, output);
}
}
[ConditionalFact(typeof(CoreClrOnly), Reason = "Can't load a coreclr targeting generator on net framework / mono")]
public void TestGeneratorsCantTargetNetFramework()
{
var directory = Temp.CreateDirectory();
var src = directory.CreateFile("test.cs").WriteAllText(@"
class C
{
}");
// core
var coreGenerator = emitGenerator(".NETCoreApp,Version=v5.0");
VerifyOutput(directory, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/analyzer:" + coreGenerator });
// netstandard
var nsGenerator = emitGenerator(".NETStandard,Version=v2.0");
VerifyOutput(directory, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/analyzer:" + nsGenerator });
// no target
var ntGenerator = emitGenerator(targetFramework: null);
VerifyOutput(directory, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/analyzer:" + ntGenerator });
// framework
var frameworkGenerator = emitGenerator(".NETFramework,Version=v4.7.2");
var output = VerifyOutput(directory, src, expectedWarningCount: 2, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/analyzer:" + frameworkGenerator });
Assert.Contains("CS8850", output); // ref's net fx
Assert.Contains("CS8033", output); // no analyzers in assembly
// framework, suppressed
output = VerifyOutput(directory, src, expectedWarningCount: 1, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/nowarn:CS8850", "/analyzer:" + frameworkGenerator });
Assert.Contains("CS8033", output);
VerifyOutput(directory, src, includeCurrentAssemblyAsAnalyzerReference: false, additionalFlags: new[] { "/nowarn:CS8850,CS8033", "/analyzer:" + frameworkGenerator });
string emitGenerator(string targetFramework)
{
string targetFrameworkAttributeText = targetFramework is object
? $"[assembly: System.Runtime.Versioning.TargetFramework(\"{targetFramework}\")]"
: string.Empty;
string generatorSource = $@"
using Microsoft.CodeAnalysis;
{targetFrameworkAttributeText}
[Generator]
public class Generator : ISourceGenerator
{{
public void Execute(GeneratorExecutionContext context) {{ }}
public void Initialize(GeneratorInitializationContext context) {{ }}
}}";
var directory = Temp.CreateDirectory();
var generatorPath = Path.Combine(directory.Path, "generator.dll");
var compilation = CSharpCompilation.Create($"generator",
new[] { CSharpSyntaxTree.ParseText(generatorSource) },
TargetFrameworkUtil.GetReferences(TargetFramework.Standard, new[] { MetadataReference.CreateFromAssemblyInternal(typeof(ISourceGenerator).Assembly) }),
new CSharpCompilationOptions(OutputKind.DynamicallyLinkedLibrary));
compilation.VerifyDiagnostics();
var result = compilation.Emit(generatorPath);
Assert.True(result.Success);
return generatorPath;
}
}
[Theory]
[InlineData("a.txt", "b.txt", 2)]
[InlineData("a.txt", "a.txt", 1)]
[InlineData("abc/a.txt", "def/a.txt", 2)]
[InlineData("abc/a.txt", "abc/a.txt", 1)]
[InlineData("abc/a.txt", "abc/../a.txt", 2)]
[InlineData("abc/a.txt", "abc/./a.txt", 1)]
[InlineData("abc/a.txt", "abc/../abc/a.txt", 1)]
[InlineData("abc/a.txt", "abc/.././abc/a.txt", 1)]
[InlineData("abc/a.txt", "./abc/a.txt", 1)]
[InlineData("abc/a.txt", "../abc/../abc/a.txt", 2)]
[InlineData("abc/a.txt", "./abc/../abc/a.txt", 1)]
[InlineData("../abc/a.txt", "../abc/../abc/a.txt", 1)]
[InlineData("../abc/a.txt", "../abc/a.txt", 1)]
[InlineData("./abc/a.txt", "abc/a.txt", 1)]
public void TestDuplicateAdditionalFiles(string additionalFilePath1, string additionalFilePath2, int expectedCount)
{
var srcDirectory = Temp.CreateDirectory();
var srcFile = srcDirectory.CreateFile("a.cs").WriteAllText("class C { }");
// make sure any parent or sub dirs exist too
Directory.CreateDirectory(Path.GetDirectoryName(Path.Combine(srcDirectory.Path, additionalFilePath1)));
Directory.CreateDirectory(Path.GetDirectoryName(Path.Combine(srcDirectory.Path, additionalFilePath2)));
var additionalFile1 = srcDirectory.CreateFile(additionalFilePath1);
var additionalFile2 = expectedCount == 2 ? srcDirectory.CreateFile(additionalFilePath2) : null;
string path1 = additionalFile1.Path;
string path2 = additionalFile2?.Path ?? Path.Combine(srcDirectory.Path, additionalFilePath2);
int count = 0;
var generator = new PipelineCallbackGenerator(ctx =>
{
ctx.RegisterSourceOutput(ctx.AdditionalTextsProvider, (spc, t) =>
{
count++;
});
});
var output = VerifyOutput(srcDirectory, srcFile, includeCurrentAssemblyAsAnalyzerReference: false,
additionalFlags: new[] { "/additionalfile:" + path1, "/additionalfile:" + path2 },
generators: new[] { generator.AsSourceGenerator() });
Assert.Equal(expectedCount, count);
CleanupAllGeneratedFiles(srcDirectory.Path);
}
[ConditionalTheory(typeof(WindowsOnly))]
[InlineData("abc/a.txt", "abc\\a.txt", 1)]
[InlineData("abc\\a.txt", "abc\\a.txt", 1)]
[InlineData("abc/a.txt", "abc\\..\\a.txt", 2)]
[InlineData("abc/a.txt", "abc\\..\\abc\\a.txt", 1)]
[InlineData("abc/a.txt", "../abc\\../abc\\a.txt", 2)]
[InlineData("abc/a.txt", "./abc\\../abc\\a.txt", 1)]
[InlineData("../abc/a.txt", "../abc\\../abc\\a.txt", 1)]
[InlineData("a.txt", "A.txt", 1)]
[InlineData("abc/a.txt", "ABC\\a.txt", 1)]
[InlineData("abc/a.txt", "ABC\\A.txt", 1)]
public void TestDuplicateAdditionalFiles_Windows(string additionalFilePath1, string additionalFilePath2, int expectedCount) => TestDuplicateAdditionalFiles(additionalFilePath1, additionalFilePath2, expectedCount);
[ConditionalTheory(typeof(LinuxOnly))]
[InlineData("a.txt", "A.txt", 2)]
[InlineData("abc/a.txt", "abc/A.txt", 2)]
[InlineData("abc/a.txt", "ABC/a.txt", 2)]
[InlineData("abc/a.txt", "./../abc/A.txt", 2)]
[InlineData("abc/a.txt", "./../ABC/a.txt", 2)]
public void TestDuplicateAdditionalFiles_Linux(string additionalFilePath1, string additionalFilePath2, int expectedCount) => TestDuplicateAdditionalFiles(additionalFilePath1, additionalFilePath2, expectedCount);
}
[DiagnosticAnalyzer(LanguageNames.CSharp, LanguageNames.VisualBasic)]
internal abstract class CompilationStartedAnalyzer : DiagnosticAnalyzer
{
public abstract override ImmutableArray<DiagnosticDescriptor> SupportedDiagnostics { get; }
public abstract void CreateAnalyzerWithinCompilation(CompilationStartAnalysisContext context);
public override void Initialize(AnalysisContext context)
{
context.RegisterCompilationStartAction(CreateAnalyzerWithinCompilation);
}
}
[DiagnosticAnalyzer(LanguageNames.CSharp, LanguageNames.VisualBasic)]
internal class HiddenDiagnosticAnalyzer : CompilationStartedAnalyzer
{
internal static readonly DiagnosticDescriptor Hidden01 = new DiagnosticDescriptor("Hidden01", "", "Throwing a diagnostic for #region", "", DiagnosticSeverity.Hidden, isEnabledByDefault: true);
internal static readonly DiagnosticDescriptor Hidden02 = new DiagnosticDescriptor("Hidden02", "", "Throwing a diagnostic for something else", "", DiagnosticSeverity.Hidden, isEnabledByDefault: true);
public override ImmutableArray<DiagnosticDescriptor> SupportedDiagnostics
{
get
{
return ImmutableArray.Create(Hidden01, Hidden02);
}
}
private void AnalyzeNode(SyntaxNodeAnalysisContext context)
{
context.ReportDiagnostic(Diagnostic.Create(Hidden01, context.Node.GetLocation()));
}
public override void CreateAnalyzerWithinCompilation(CompilationStartAnalysisContext context)
{
context.RegisterSyntaxNodeAction(AnalyzeNode, SyntaxKind.RegionDirectiveTrivia);
}
}
[DiagnosticAnalyzer(LanguageNames.CSharp, LanguageNames.VisualBasic)]
internal class InfoDiagnosticAnalyzer : CompilationStartedAnalyzer
{
internal static readonly DiagnosticDescriptor Info01 = new DiagnosticDescriptor("Info01", "", "Throwing a diagnostic for #pragma restore", "", DiagnosticSeverity.Info, isEnabledByDefault: true);
public override ImmutableArray<DiagnosticDescriptor> SupportedDiagnostics
{
get
{
return ImmutableArray.Create(Info01);
}
}
private void AnalyzeNode(SyntaxNodeAnalysisContext context)
{
if ((context.Node as PragmaWarningDirectiveTriviaSyntax).DisableOrRestoreKeyword.IsKind(SyntaxKind.RestoreKeyword))
{
context.ReportDiagnostic(Diagnostic.Create(Info01, context.Node.GetLocation()));
}
}
public override void CreateAnalyzerWithinCompilation(CompilationStartAnalysisContext context)
{
context.RegisterSyntaxNodeAction(AnalyzeNode, SyntaxKind.PragmaWarningDirectiveTrivia);
}
}
[DiagnosticAnalyzer(LanguageNames.CSharp, LanguageNames.VisualBasic)]
internal class WarningDiagnosticAnalyzer : CompilationStartedAnalyzer
{
internal static readonly DiagnosticDescriptor Warning01 = new DiagnosticDescriptor("Warning01", "", "Throwing a diagnostic for types declared", "", DiagnosticSeverity.Warning, isEnabledByDefault: true);
public override ImmutableArray<DiagnosticDescriptor> SupportedDiagnostics
{
get
{
return ImmutableArray.Create(Warning01);
}
}
public override void CreateAnalyzerWithinCompilation(CompilationStartAnalysisContext context)
{
context.RegisterSymbolAction(
(symbolContext) =>
{
symbolContext.ReportDiagnostic(Diagnostic.Create(Warning01, symbolContext.Symbol.Locations.First()));
},
SymbolKind.NamedType);
}
}
[DiagnosticAnalyzer(LanguageNames.CSharp, LanguageNames.VisualBasic)]
internal class ErrorDiagnosticAnalyzer : CompilationStartedAnalyzer
{
internal static readonly DiagnosticDescriptor Error01 = new DiagnosticDescriptor("Error01", "", "Throwing a diagnostic for #pragma disable", "", DiagnosticSeverity.Error, isEnabledByDefault: true);
internal static readonly DiagnosticDescriptor Error02 = new DiagnosticDescriptor("Error02", "", "Throwing a diagnostic for something else", "", DiagnosticSeverity.Error, isEnabledByDefault: true);
public override ImmutableArray<DiagnosticDescriptor> SupportedDiagnostics
{
get
{
return ImmutableArray.Create(Error01, Error02);
}
}
public override void CreateAnalyzerWithinCompilation(CompilationStartAnalysisContext context)
{
context.RegisterSyntaxNodeAction(
(nodeContext) =>
{
if ((nodeContext.Node as PragmaWarningDirectiveTriviaSyntax).DisableOrRestoreKeyword.IsKind(SyntaxKind.DisableKeyword))
{
nodeContext.ReportDiagnostic(Diagnostic.Create(Error01, nodeContext.Node.GetLocation()));
}
},
SyntaxKind.PragmaWarningDirectiveTrivia
);
}
}
}
| sharwell/roslyn | src/Compilers/CSharp/Test/CommandLine/CommandLineTests.cs | C# | mit | 730,075 |
(function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o<r.length;o++)s(r[o]);return s})({1:[function(require,module,exports){
module.exports = { "default": require("core-js/library/fn/get-iterator"), __esModule: true };
},{"core-js/library/fn/get-iterator":6}],2:[function(require,module,exports){
module.exports = { "default": require("core-js/library/fn/object/define-property"), __esModule: true };
},{"core-js/library/fn/object/define-property":7}],3:[function(require,module,exports){
module.exports = { "default": require("core-js/library/fn/object/keys"), __esModule: true };
},{"core-js/library/fn/object/keys":8}],4:[function(require,module,exports){
"use strict";
exports.__esModule = true;
exports.default = function (instance, Constructor) {
if (!(instance instanceof Constructor)) {
throw new TypeError("Cannot call a class as a function");
}
};
},{}],5:[function(require,module,exports){
"use strict";
exports.__esModule = true;
var _defineProperty = require("../core-js/object/define-property");
var _defineProperty2 = _interopRequireDefault(_defineProperty);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
exports.default = function () {
function defineProperties(target, props) {
for (var i = 0; i < props.length; i++) {
var descriptor = props[i];
descriptor.enumerable = descriptor.enumerable || false;
descriptor.configurable = true;
if ("value" in descriptor) descriptor.writable = true;
(0, _defineProperty2.default)(target, descriptor.key, descriptor);
}
}
return function (Constructor, protoProps, staticProps) {
if (protoProps) defineProperties(Constructor.prototype, protoProps);
if (staticProps) defineProperties(Constructor, staticProps);
return Constructor;
};
}();
},{"../core-js/object/define-property":2}],6:[function(require,module,exports){
require('../modules/web.dom.iterable');
require('../modules/es6.string.iterator');
module.exports = require('../modules/core.get-iterator');
},{"../modules/core.get-iterator":57,"../modules/es6.string.iterator":61,"../modules/web.dom.iterable":62}],7:[function(require,module,exports){
require('../../modules/es6.object.define-property');
var $Object = require('../../modules/_core').Object;
module.exports = function defineProperty(it, key, desc){
return $Object.defineProperty(it, key, desc);
};
},{"../../modules/_core":15,"../../modules/es6.object.define-property":59}],8:[function(require,module,exports){
require('../../modules/es6.object.keys');
module.exports = require('../../modules/_core').Object.keys;
},{"../../modules/_core":15,"../../modules/es6.object.keys":60}],9:[function(require,module,exports){
module.exports = function(it){
if(typeof it != 'function')throw TypeError(it + ' is not a function!');
return it;
};
},{}],10:[function(require,module,exports){
module.exports = function(){ /* empty */ };
},{}],11:[function(require,module,exports){
var isObject = require('./_is-object');
module.exports = function(it){
if(!isObject(it))throw TypeError(it + ' is not an object!');
return it;
};
},{"./_is-object":29}],12:[function(require,module,exports){
// false -> Array#indexOf
// true -> Array#includes
var toIObject = require('./_to-iobject')
, toLength = require('./_to-length')
, toIndex = require('./_to-index');
module.exports = function(IS_INCLUDES){
return function($this, el, fromIndex){
var O = toIObject($this)
, length = toLength(O.length)
, index = toIndex(fromIndex, length)
, value;
// Array#includes uses SameValueZero equality algorithm
if(IS_INCLUDES && el != el)while(length > index){
value = O[index++];
if(value != value)return true;
// Array#toIndex ignores holes, Array#includes - not
} else for(;length > index; index++)if(IS_INCLUDES || index in O){
if(O[index] === el)return IS_INCLUDES || index || 0;
} return !IS_INCLUDES && -1;
};
};
},{"./_to-index":48,"./_to-iobject":50,"./_to-length":51}],13:[function(require,module,exports){
// getting tag from 19.1.3.6 Object.prototype.toString()
var cof = require('./_cof')
, TAG = require('./_wks')('toStringTag')
// ES3 wrong here
, ARG = cof(function(){ return arguments; }()) == 'Arguments';
// fallback for IE11 Script Access Denied error
var tryGet = function(it, key){
try {
return it[key];
} catch(e){ /* empty */ }
};
module.exports = function(it){
var O, T, B;
return it === undefined ? 'Undefined' : it === null ? 'Null'
// @@toStringTag case
: typeof (T = tryGet(O = Object(it), TAG)) == 'string' ? T
// builtinTag case
: ARG ? cof(O)
// ES3 arguments fallback
: (B = cof(O)) == 'Object' && typeof O.callee == 'function' ? 'Arguments' : B;
};
},{"./_cof":14,"./_wks":55}],14:[function(require,module,exports){
var toString = {}.toString;
module.exports = function(it){
return toString.call(it).slice(8, -1);
};
},{}],15:[function(require,module,exports){
var core = module.exports = {version: '2.4.0'};
if(typeof __e == 'number')__e = core; // eslint-disable-line no-undef
},{}],16:[function(require,module,exports){
// optional / simple context binding
var aFunction = require('./_a-function');
module.exports = function(fn, that, length){
aFunction(fn);
if(that === undefined)return fn;
switch(length){
case 1: return function(a){
return fn.call(that, a);
};
case 2: return function(a, b){
return fn.call(that, a, b);
};
case 3: return function(a, b, c){
return fn.call(that, a, b, c);
};
}
return function(/* ...args */){
return fn.apply(that, arguments);
};
};
},{"./_a-function":9}],17:[function(require,module,exports){
// 7.2.1 RequireObjectCoercible(argument)
module.exports = function(it){
if(it == undefined)throw TypeError("Can't call method on " + it);
return it;
};
},{}],18:[function(require,module,exports){
// Thank's IE8 for his funny defineProperty
module.exports = !require('./_fails')(function(){
return Object.defineProperty({}, 'a', {get: function(){ return 7; }}).a != 7;
});
},{"./_fails":22}],19:[function(require,module,exports){
var isObject = require('./_is-object')
, document = require('./_global').document
// in old IE typeof document.createElement is 'object'
, is = isObject(document) && isObject(document.createElement);
module.exports = function(it){
return is ? document.createElement(it) : {};
};
},{"./_global":23,"./_is-object":29}],20:[function(require,module,exports){
// IE 8- don't enum bug keys
module.exports = (
'constructor,hasOwnProperty,isPrototypeOf,propertyIsEnumerable,toLocaleString,toString,valueOf'
).split(',');
},{}],21:[function(require,module,exports){
var global = require('./_global')
, core = require('./_core')
, ctx = require('./_ctx')
, hide = require('./_hide')
, PROTOTYPE = 'prototype';
var $export = function(type, name, source){
var IS_FORCED = type & $export.F
, IS_GLOBAL = type & $export.G
, IS_STATIC = type & $export.S
, IS_PROTO = type & $export.P
, IS_BIND = type & $export.B
, IS_WRAP = type & $export.W
, exports = IS_GLOBAL ? core : core[name] || (core[name] = {})
, expProto = exports[PROTOTYPE]
, target = IS_GLOBAL ? global : IS_STATIC ? global[name] : (global[name] || {})[PROTOTYPE]
, key, own, out;
if(IS_GLOBAL)source = name;
for(key in source){
// contains in native
own = !IS_FORCED && target && target[key] !== undefined;
if(own && key in exports)continue;
// export native or passed
out = own ? target[key] : source[key];
// prevent global pollution for namespaces
exports[key] = IS_GLOBAL && typeof target[key] != 'function' ? source[key]
// bind timers to global for call from export context
: IS_BIND && own ? ctx(out, global)
// wrap global constructors for prevent change them in library
: IS_WRAP && target[key] == out ? (function(C){
var F = function(a, b, c){
if(this instanceof C){
switch(arguments.length){
case 0: return new C;
case 1: return new C(a);
case 2: return new C(a, b);
} return new C(a, b, c);
} return C.apply(this, arguments);
};
F[PROTOTYPE] = C[PROTOTYPE];
return F;
// make static versions for prototype methods
})(out) : IS_PROTO && typeof out == 'function' ? ctx(Function.call, out) : out;
// export proto methods to core.%CONSTRUCTOR%.methods.%NAME%
if(IS_PROTO){
(exports.virtual || (exports.virtual = {}))[key] = out;
// export proto methods to core.%CONSTRUCTOR%.prototype.%NAME%
if(type & $export.R && expProto && !expProto[key])hide(expProto, key, out);
}
}
};
// type bitmap
$export.F = 1; // forced
$export.G = 2; // global
$export.S = 4; // static
$export.P = 8; // proto
$export.B = 16; // bind
$export.W = 32; // wrap
$export.U = 64; // safe
$export.R = 128; // real proto method for `library`
module.exports = $export;
},{"./_core":15,"./_ctx":16,"./_global":23,"./_hide":25}],22:[function(require,module,exports){
module.exports = function(exec){
try {
return !!exec();
} catch(e){
return true;
}
};
},{}],23:[function(require,module,exports){
// https://github.com/zloirock/core-js/issues/86#issuecomment-115759028
var global = module.exports = typeof window != 'undefined' && window.Math == Math
? window : typeof self != 'undefined' && self.Math == Math ? self : Function('return this')();
if(typeof __g == 'number')__g = global; // eslint-disable-line no-undef
},{}],24:[function(require,module,exports){
var hasOwnProperty = {}.hasOwnProperty;
module.exports = function(it, key){
return hasOwnProperty.call(it, key);
};
},{}],25:[function(require,module,exports){
var dP = require('./_object-dp')
, createDesc = require('./_property-desc');
module.exports = require('./_descriptors') ? function(object, key, value){
return dP.f(object, key, createDesc(1, value));
} : function(object, key, value){
object[key] = value;
return object;
};
},{"./_descriptors":18,"./_object-dp":36,"./_property-desc":42}],26:[function(require,module,exports){
module.exports = require('./_global').document && document.documentElement;
},{"./_global":23}],27:[function(require,module,exports){
module.exports = !require('./_descriptors') && !require('./_fails')(function(){
return Object.defineProperty(require('./_dom-create')('div'), 'a', {get: function(){ return 7; }}).a != 7;
});
},{"./_descriptors":18,"./_dom-create":19,"./_fails":22}],28:[function(require,module,exports){
// fallback for non-array-like ES3 and non-enumerable old V8 strings
var cof = require('./_cof');
module.exports = Object('z').propertyIsEnumerable(0) ? Object : function(it){
return cof(it) == 'String' ? it.split('') : Object(it);
};
},{"./_cof":14}],29:[function(require,module,exports){
module.exports = function(it){
return typeof it === 'object' ? it !== null : typeof it === 'function';
};
},{}],30:[function(require,module,exports){
'use strict';
var create = require('./_object-create')
, descriptor = require('./_property-desc')
, setToStringTag = require('./_set-to-string-tag')
, IteratorPrototype = {};
// 25.1.2.1.1 %IteratorPrototype%[@@iterator]()
require('./_hide')(IteratorPrototype, require('./_wks')('iterator'), function(){ return this; });
module.exports = function(Constructor, NAME, next){
Constructor.prototype = create(IteratorPrototype, {next: descriptor(1, next)});
setToStringTag(Constructor, NAME + ' Iterator');
};
},{"./_hide":25,"./_object-create":35,"./_property-desc":42,"./_set-to-string-tag":44,"./_wks":55}],31:[function(require,module,exports){
'use strict';
var LIBRARY = require('./_library')
, $export = require('./_export')
, redefine = require('./_redefine')
, hide = require('./_hide')
, has = require('./_has')
, Iterators = require('./_iterators')
, $iterCreate = require('./_iter-create')
, setToStringTag = require('./_set-to-string-tag')
, getPrototypeOf = require('./_object-gpo')
, ITERATOR = require('./_wks')('iterator')
, BUGGY = !([].keys && 'next' in [].keys()) // Safari has buggy iterators w/o `next`
, FF_ITERATOR = '@@iterator'
, KEYS = 'keys'
, VALUES = 'values';
var returnThis = function(){ return this; };
module.exports = function(Base, NAME, Constructor, next, DEFAULT, IS_SET, FORCED){
$iterCreate(Constructor, NAME, next);
var getMethod = function(kind){
if(!BUGGY && kind in proto)return proto[kind];
switch(kind){
case KEYS: return function keys(){ return new Constructor(this, kind); };
case VALUES: return function values(){ return new Constructor(this, kind); };
} return function entries(){ return new Constructor(this, kind); };
};
var TAG = NAME + ' Iterator'
, DEF_VALUES = DEFAULT == VALUES
, VALUES_BUG = false
, proto = Base.prototype
, $native = proto[ITERATOR] || proto[FF_ITERATOR] || DEFAULT && proto[DEFAULT]
, $default = $native || getMethod(DEFAULT)
, $entries = DEFAULT ? !DEF_VALUES ? $default : getMethod('entries') : undefined
, $anyNative = NAME == 'Array' ? proto.entries || $native : $native
, methods, key, IteratorPrototype;
// Fix native
if($anyNative){
IteratorPrototype = getPrototypeOf($anyNative.call(new Base));
if(IteratorPrototype !== Object.prototype){
// Set @@toStringTag to native iterators
setToStringTag(IteratorPrototype, TAG, true);
// fix for some old engines
if(!LIBRARY && !has(IteratorPrototype, ITERATOR))hide(IteratorPrototype, ITERATOR, returnThis);
}
}
// fix Array#{values, @@iterator}.name in V8 / FF
if(DEF_VALUES && $native && $native.name !== VALUES){
VALUES_BUG = true;
$default = function values(){ return $native.call(this); };
}
// Define iterator
if((!LIBRARY || FORCED) && (BUGGY || VALUES_BUG || !proto[ITERATOR])){
hide(proto, ITERATOR, $default);
}
// Plug for library
Iterators[NAME] = $default;
Iterators[TAG] = returnThis;
if(DEFAULT){
methods = {
values: DEF_VALUES ? $default : getMethod(VALUES),
keys: IS_SET ? $default : getMethod(KEYS),
entries: $entries
};
if(FORCED)for(key in methods){
if(!(key in proto))redefine(proto, key, methods[key]);
} else $export($export.P + $export.F * (BUGGY || VALUES_BUG), NAME, methods);
}
return methods;
};
},{"./_export":21,"./_has":24,"./_hide":25,"./_iter-create":30,"./_iterators":33,"./_library":34,"./_object-gpo":38,"./_redefine":43,"./_set-to-string-tag":44,"./_wks":55}],32:[function(require,module,exports){
module.exports = function(done, value){
return {value: value, done: !!done};
};
},{}],33:[function(require,module,exports){
module.exports = {};
},{}],34:[function(require,module,exports){
module.exports = true;
},{}],35:[function(require,module,exports){
// 19.1.2.2 / 15.2.3.5 Object.create(O [, Properties])
var anObject = require('./_an-object')
, dPs = require('./_object-dps')
, enumBugKeys = require('./_enum-bug-keys')
, IE_PROTO = require('./_shared-key')('IE_PROTO')
, Empty = function(){ /* empty */ }
, PROTOTYPE = 'prototype';
// Create object with fake `null` prototype: use iframe Object with cleared prototype
var createDict = function(){
// Thrash, waste and sodomy: IE GC bug
var iframe = require('./_dom-create')('iframe')
, i = enumBugKeys.length
, lt = '<'
, gt = '>'
, iframeDocument;
iframe.style.display = 'none';
require('./_html').appendChild(iframe);
iframe.src = 'javascript:'; // eslint-disable-line no-script-url
// createDict = iframe.contentWindow.Object;
// html.removeChild(iframe);
iframeDocument = iframe.contentWindow.document;
iframeDocument.open();
iframeDocument.write(lt + 'script' + gt + 'document.F=Object' + lt + '/script' + gt);
iframeDocument.close();
createDict = iframeDocument.F;
while(i--)delete createDict[PROTOTYPE][enumBugKeys[i]];
return createDict();
};
module.exports = Object.create || function create(O, Properties){
var result;
if(O !== null){
Empty[PROTOTYPE] = anObject(O);
result = new Empty;
Empty[PROTOTYPE] = null;
// add "__proto__" for Object.getPrototypeOf polyfill
result[IE_PROTO] = O;
} else result = createDict();
return Properties === undefined ? result : dPs(result, Properties);
};
},{"./_an-object":11,"./_dom-create":19,"./_enum-bug-keys":20,"./_html":26,"./_object-dps":37,"./_shared-key":45}],36:[function(require,module,exports){
var anObject = require('./_an-object')
, IE8_DOM_DEFINE = require('./_ie8-dom-define')
, toPrimitive = require('./_to-primitive')
, dP = Object.defineProperty;
exports.f = require('./_descriptors') ? Object.defineProperty : function defineProperty(O, P, Attributes){
anObject(O);
P = toPrimitive(P, true);
anObject(Attributes);
if(IE8_DOM_DEFINE)try {
return dP(O, P, Attributes);
} catch(e){ /* empty */ }
if('get' in Attributes || 'set' in Attributes)throw TypeError('Accessors not supported!');
if('value' in Attributes)O[P] = Attributes.value;
return O;
};
},{"./_an-object":11,"./_descriptors":18,"./_ie8-dom-define":27,"./_to-primitive":53}],37:[function(require,module,exports){
var dP = require('./_object-dp')
, anObject = require('./_an-object')
, getKeys = require('./_object-keys');
module.exports = require('./_descriptors') ? Object.defineProperties : function defineProperties(O, Properties){
anObject(O);
var keys = getKeys(Properties)
, length = keys.length
, i = 0
, P;
while(length > i)dP.f(O, P = keys[i++], Properties[P]);
return O;
};
},{"./_an-object":11,"./_descriptors":18,"./_object-dp":36,"./_object-keys":40}],38:[function(require,module,exports){
// 19.1.2.9 / 15.2.3.2 Object.getPrototypeOf(O)
var has = require('./_has')
, toObject = require('./_to-object')
, IE_PROTO = require('./_shared-key')('IE_PROTO')
, ObjectProto = Object.prototype;
module.exports = Object.getPrototypeOf || function(O){
O = toObject(O);
if(has(O, IE_PROTO))return O[IE_PROTO];
if(typeof O.constructor == 'function' && O instanceof O.constructor){
return O.constructor.prototype;
} return O instanceof Object ? ObjectProto : null;
};
},{"./_has":24,"./_shared-key":45,"./_to-object":52}],39:[function(require,module,exports){
var has = require('./_has')
, toIObject = require('./_to-iobject')
, arrayIndexOf = require('./_array-includes')(false)
, IE_PROTO = require('./_shared-key')('IE_PROTO');
module.exports = function(object, names){
var O = toIObject(object)
, i = 0
, result = []
, key;
for(key in O)if(key != IE_PROTO)has(O, key) && result.push(key);
// Don't enum bug & hidden keys
while(names.length > i)if(has(O, key = names[i++])){
~arrayIndexOf(result, key) || result.push(key);
}
return result;
};
},{"./_array-includes":12,"./_has":24,"./_shared-key":45,"./_to-iobject":50}],40:[function(require,module,exports){
// 19.1.2.14 / 15.2.3.14 Object.keys(O)
var $keys = require('./_object-keys-internal')
, enumBugKeys = require('./_enum-bug-keys');
module.exports = Object.keys || function keys(O){
return $keys(O, enumBugKeys);
};
},{"./_enum-bug-keys":20,"./_object-keys-internal":39}],41:[function(require,module,exports){
// most Object methods by ES6 should accept primitives
var $export = require('./_export')
, core = require('./_core')
, fails = require('./_fails');
module.exports = function(KEY, exec){
var fn = (core.Object || {})[KEY] || Object[KEY]
, exp = {};
exp[KEY] = exec(fn);
$export($export.S + $export.F * fails(function(){ fn(1); }), 'Object', exp);
};
},{"./_core":15,"./_export":21,"./_fails":22}],42:[function(require,module,exports){
module.exports = function(bitmap, value){
return {
enumerable : !(bitmap & 1),
configurable: !(bitmap & 2),
writable : !(bitmap & 4),
value : value
};
};
},{}],43:[function(require,module,exports){
module.exports = require('./_hide');
},{"./_hide":25}],44:[function(require,module,exports){
var def = require('./_object-dp').f
, has = require('./_has')
, TAG = require('./_wks')('toStringTag');
module.exports = function(it, tag, stat){
if(it && !has(it = stat ? it : it.prototype, TAG))def(it, TAG, {configurable: true, value: tag});
};
},{"./_has":24,"./_object-dp":36,"./_wks":55}],45:[function(require,module,exports){
var shared = require('./_shared')('keys')
, uid = require('./_uid');
module.exports = function(key){
return shared[key] || (shared[key] = uid(key));
};
},{"./_shared":46,"./_uid":54}],46:[function(require,module,exports){
var global = require('./_global')
, SHARED = '__core-js_shared__'
, store = global[SHARED] || (global[SHARED] = {});
module.exports = function(key){
return store[key] || (store[key] = {});
};
},{"./_global":23}],47:[function(require,module,exports){
var toInteger = require('./_to-integer')
, defined = require('./_defined');
// true -> String#at
// false -> String#codePointAt
module.exports = function(TO_STRING){
return function(that, pos){
var s = String(defined(that))
, i = toInteger(pos)
, l = s.length
, a, b;
if(i < 0 || i >= l)return TO_STRING ? '' : undefined;
a = s.charCodeAt(i);
return a < 0xd800 || a > 0xdbff || i + 1 === l || (b = s.charCodeAt(i + 1)) < 0xdc00 || b > 0xdfff
? TO_STRING ? s.charAt(i) : a
: TO_STRING ? s.slice(i, i + 2) : (a - 0xd800 << 10) + (b - 0xdc00) + 0x10000;
};
};
},{"./_defined":17,"./_to-integer":49}],48:[function(require,module,exports){
var toInteger = require('./_to-integer')
, max = Math.max
, min = Math.min;
module.exports = function(index, length){
index = toInteger(index);
return index < 0 ? max(index + length, 0) : min(index, length);
};
},{"./_to-integer":49}],49:[function(require,module,exports){
// 7.1.4 ToInteger
var ceil = Math.ceil
, floor = Math.floor;
module.exports = function(it){
return isNaN(it = +it) ? 0 : (it > 0 ? floor : ceil)(it);
};
},{}],50:[function(require,module,exports){
// to indexed object, toObject with fallback for non-array-like ES3 strings
var IObject = require('./_iobject')
, defined = require('./_defined');
module.exports = function(it){
return IObject(defined(it));
};
},{"./_defined":17,"./_iobject":28}],51:[function(require,module,exports){
// 7.1.15 ToLength
var toInteger = require('./_to-integer')
, min = Math.min;
module.exports = function(it){
return it > 0 ? min(toInteger(it), 0x1fffffffffffff) : 0; // pow(2, 53) - 1 == 9007199254740991
};
},{"./_to-integer":49}],52:[function(require,module,exports){
// 7.1.13 ToObject(argument)
var defined = require('./_defined');
module.exports = function(it){
return Object(defined(it));
};
},{"./_defined":17}],53:[function(require,module,exports){
// 7.1.1 ToPrimitive(input [, PreferredType])
var isObject = require('./_is-object');
// instead of the ES6 spec version, we didn't implement @@toPrimitive case
// and the second argument - flag - preferred type is a string
module.exports = function(it, S){
if(!isObject(it))return it;
var fn, val;
if(S && typeof (fn = it.toString) == 'function' && !isObject(val = fn.call(it)))return val;
if(typeof (fn = it.valueOf) == 'function' && !isObject(val = fn.call(it)))return val;
if(!S && typeof (fn = it.toString) == 'function' && !isObject(val = fn.call(it)))return val;
throw TypeError("Can't convert object to primitive value");
};
},{"./_is-object":29}],54:[function(require,module,exports){
var id = 0
, px = Math.random();
module.exports = function(key){
return 'Symbol('.concat(key === undefined ? '' : key, ')_', (++id + px).toString(36));
};
},{}],55:[function(require,module,exports){
var store = require('./_shared')('wks')
, uid = require('./_uid')
, Symbol = require('./_global').Symbol
, USE_SYMBOL = typeof Symbol == 'function';
var $exports = module.exports = function(name){
return store[name] || (store[name] =
USE_SYMBOL && Symbol[name] || (USE_SYMBOL ? Symbol : uid)('Symbol.' + name));
};
$exports.store = store;
},{"./_global":23,"./_shared":46,"./_uid":54}],56:[function(require,module,exports){
var classof = require('./_classof')
, ITERATOR = require('./_wks')('iterator')
, Iterators = require('./_iterators');
module.exports = require('./_core').getIteratorMethod = function(it){
if(it != undefined)return it[ITERATOR]
|| it['@@iterator']
|| Iterators[classof(it)];
};
},{"./_classof":13,"./_core":15,"./_iterators":33,"./_wks":55}],57:[function(require,module,exports){
var anObject = require('./_an-object')
, get = require('./core.get-iterator-method');
module.exports = require('./_core').getIterator = function(it){
var iterFn = get(it);
if(typeof iterFn != 'function')throw TypeError(it + ' is not iterable!');
return anObject(iterFn.call(it));
};
},{"./_an-object":11,"./_core":15,"./core.get-iterator-method":56}],58:[function(require,module,exports){
'use strict';
var addToUnscopables = require('./_add-to-unscopables')
, step = require('./_iter-step')
, Iterators = require('./_iterators')
, toIObject = require('./_to-iobject');
// 22.1.3.4 Array.prototype.entries()
// 22.1.3.13 Array.prototype.keys()
// 22.1.3.29 Array.prototype.values()
// 22.1.3.30 Array.prototype[@@iterator]()
module.exports = require('./_iter-define')(Array, 'Array', function(iterated, kind){
this._t = toIObject(iterated); // target
this._i = 0; // next index
this._k = kind; // kind
// 22.1.5.2.1 %ArrayIteratorPrototype%.next()
}, function(){
var O = this._t
, kind = this._k
, index = this._i++;
if(!O || index >= O.length){
this._t = undefined;
return step(1);
}
if(kind == 'keys' )return step(0, index);
if(kind == 'values')return step(0, O[index]);
return step(0, [index, O[index]]);
}, 'values');
// argumentsList[@@iterator] is %ArrayProto_values% (9.4.4.6, 9.4.4.7)
Iterators.Arguments = Iterators.Array;
addToUnscopables('keys');
addToUnscopables('values');
addToUnscopables('entries');
},{"./_add-to-unscopables":10,"./_iter-define":31,"./_iter-step":32,"./_iterators":33,"./_to-iobject":50}],59:[function(require,module,exports){
var $export = require('./_export');
// 19.1.2.4 / 15.2.3.6 Object.defineProperty(O, P, Attributes)
$export($export.S + $export.F * !require('./_descriptors'), 'Object', {defineProperty: require('./_object-dp').f});
},{"./_descriptors":18,"./_export":21,"./_object-dp":36}],60:[function(require,module,exports){
// 19.1.2.14 Object.keys(O)
var toObject = require('./_to-object')
, $keys = require('./_object-keys');
require('./_object-sap')('keys', function(){
return function keys(it){
return $keys(toObject(it));
};
});
},{"./_object-keys":40,"./_object-sap":41,"./_to-object":52}],61:[function(require,module,exports){
'use strict';
var $at = require('./_string-at')(true);
// 21.1.3.27 String.prototype[@@iterator]()
require('./_iter-define')(String, 'String', function(iterated){
this._t = String(iterated); // target
this._i = 0; // next index
// 21.1.5.2.1 %StringIteratorPrototype%.next()
}, function(){
var O = this._t
, index = this._i
, point;
if(index >= O.length)return {value: undefined, done: true};
point = $at(O, index);
this._i += point.length;
return {value: point, done: false};
});
},{"./_iter-define":31,"./_string-at":47}],62:[function(require,module,exports){
require('./es6.array.iterator');
var global = require('./_global')
, hide = require('./_hide')
, Iterators = require('./_iterators')
, TO_STRING_TAG = require('./_wks')('toStringTag');
for(var collections = ['NodeList', 'DOMTokenList', 'MediaList', 'StyleSheetList', 'CSSRuleList'], i = 0; i < 5; i++){
var NAME = collections[i]
, Collection = global[NAME]
, proto = Collection && Collection.prototype;
if(proto && !proto[TO_STRING_TAG])hide(proto, TO_STRING_TAG, NAME);
Iterators[NAME] = Iterators.Array;
}
},{"./_global":23,"./_hide":25,"./_iterators":33,"./_wks":55,"./es6.array.iterator":58}],63:[function(require,module,exports){
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
var _keys = require("babel-runtime/core-js/object/keys");
var _keys2 = _interopRequireDefault(_keys);
var _getIterator2 = require("babel-runtime/core-js/get-iterator");
var _getIterator3 = _interopRequireDefault(_getIterator2);
var _classCallCheck2 = require("babel-runtime/helpers/classCallCheck");
var _classCallCheck3 = _interopRequireDefault(_classCallCheck2);
var _createClass2 = require("babel-runtime/helpers/createClass");
var _createClass3 = _interopRequireDefault(_createClass2);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
/**
* Emitter
*/
var Emitter = function () {
function Emitter() {
(0, _classCallCheck3.default)(this, Emitter);
this._data = {};
}
(0, _createClass3.default)(Emitter, [{
key: "on",
value: function on(name, data) {
if (!name) return;
if (!this._data[name]) {
this._data[name] = {};
}
this._data[name] = data;
}
}, {
key: "emit",
value: function emit(name, method, context) {
if (!name || !method) return;
this._data[name].run(method, context);
}
}, {
key: "broadcast",
value: function broadcast(method, context) {
if (!method) return;
var _iteratorNormalCompletion = true;
var _didIteratorError = false;
var _iteratorError = undefined;
try {
for (var _iterator = (0, _getIterator3.default)((0, _keys2.default)(this._data)), _step; !(_iteratorNormalCompletion = (_step = _iterator.next()).done); _iteratorNormalCompletion = true) {
var name = _step.value;
this._data[name].run(method, context);
}
} catch (err) {
_didIteratorError = true;
_iteratorError = err;
} finally {
try {
if (!_iteratorNormalCompletion && _iterator.return) {
_iterator.return();
}
} finally {
if (_didIteratorError) {
throw _iteratorError;
}
}
}
}
}, {
key: "keys",
value: function keys() {
return (0, _keys2.default)(this._data);
}
}, {
key: "is",
value: function is(name) {
return !!this._data[name];
}
}, {
key: "get",
value: function get(name) {
return this._data[name];
}
}]);
return Emitter;
}();
exports.default = Emitter;
},{"babel-runtime/core-js/get-iterator":1,"babel-runtime/core-js/object/keys":3,"babel-runtime/helpers/classCallCheck":4,"babel-runtime/helpers/createClass":5}],64:[function(require,module,exports){
'use strict';
Object.defineProperty(exports, "__esModule", {
value: true
});
var _keys = require('babel-runtime/core-js/object/keys');
var _keys2 = _interopRequireDefault(_keys);
var _getIterator2 = require('babel-runtime/core-js/get-iterator');
var _getIterator3 = _interopRequireDefault(_getIterator2);
var _classCallCheck2 = require('babel-runtime/helpers/classCallCheck');
var _classCallCheck3 = _interopRequireDefault(_classCallCheck2);
var _createClass2 = require('babel-runtime/helpers/createClass');
var _createClass3 = _interopRequireDefault(_createClass2);
var _Emitter = require('../class/_Emitter');
var _Emitter2 = _interopRequireDefault(_Emitter);
var _Subscriber = require('../class/_Subscriber');
var _Subscriber2 = _interopRequireDefault(_Subscriber);
var _RequestAnim = require('../class/_RequestAnim');
var _RequestAnim2 = _interopRequireDefault(_RequestAnim);
var _calc = require('../module/_calc');
var _calc2 = _interopRequireDefault(_calc);
var _help = require('../module/_help');
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
var Flyby = function () {
function Flyby(options) {
(0, _classCallCheck3.default)(this, Flyby);
this.enabled = false;
this.mode = '';
this.painting = false;
this.startX = 0;
this.startY = 0;
this.ghostX = 0;
this.ghostY = 0;
this.currentX = 0;
this.currentY = 0;
this.distanceX = 0;
this.distanceY = 0;
this.distanceAngle = 0;
this.invertX = 0;
this.invertY = 0;
this.originX = 0;
this.originY = 0;
this.between = 0;
this.betweenX = 0;
this.betweenY = 0;
this.betweenAngle = 0;
this.previousX = 0;
this.previousY = 0;
this.direction = '';
this.directionX = '';
this.directionY = '';
this.targetElement = null; // <event.target>
this.startTime = 0; // timestamp by OnStart
this.elapsed = 0; // <endTime> - <startTime>
this.ghostTime = 0; // previously <startTime>
this.onStart = this.onStart.bind(this);
this.onMove = this.onMove.bind(this);
this.onEnd = this.onEnd.bind(this);
this.update = this.update.bind(this);
// In this element, that enabled to mouse and touch events.
this.rangeElement = options.rangeElement || document;
this._addEventListeners();
// Browser painting update
// call requestAnimationFrame
this.paint = new _RequestAnim2.default();
this.paint.tick = this.update;
this.first = false;
this.pinchOnDesktop = false;
this.flickTime = 250;
// event emitter
this.emitter = new _Emitter2.default();
this._initSubscribe(options.subscribers);
// emit configuration
this.emitter.broadcast('onConfig', this);
this.subscriber = null;
}
(0, _createClass3.default)(Flyby, [{
key: 'onStart',
value: function onStart(event) {
// set the flags to initialize
this.enabled = true;
this.painting = true;
this.touched = event.touches ? true : false;
this.first = true;
this.pinchOnDesktop = false;
// set the position to initalize
this.startTime = Date.now();
this.startX = (0, _help.pageX)(event);
this.startY = (0, _help.pageY)(event);
this.distanceX = 0;
this.distanceY = 0;
this.distanceAngle = 0;
this.distance = 0;
this.currentX = this.startX;
this.currentY = this.startY;
this.invertX = this.startX;
this.invertY = this.startY;
this.previousX = this.startX;
this.previousY = this.startY;
this.between = 0;
this.betweenX = 0;
this.betweenY = 0;
this.betweenAngle = 0;
this.direction = '';
this.directionX = '';
this.directionY = '';
this.targetElement = event.target;
if (!this.touched) {
if (this.startTime - this.ghostTime < 750) {
this.pinchOnDesktop = true;
}
}
// find a target & subscriber
this._find(event.target);
// subscribe
this._emit('onStart');
// paint
this.paint.cancel();
this.paint.play();
this.ghostTime = this.startTime;
event.preventDefault();
}
}, {
key: 'onMove',
value: function onMove(event) {
if (!this.enabled) return;
if ((0, _help.hasTouches)(event, 2) || this.pinchOnDesktop) {
this.mode = 'pinch';
} else {
this.mode = 'swipe';
}
this.currentX = (0, _help.pageX)(event);
this.currentY = (0, _help.pageY)(event);
if (this.mode === 'pinch') {
this._invert(event);
this._between();
}
this._distance();
this.originX = this.currentX - parseInt(this.betweenX / 2, 10);
this.originY = this.currentY - parseInt(this.betweenY / 2, 10);
this._direction();
this.targetElement = event.target;
// check ignore list to subscribe
if (!this._inIgnore(this.mode)) {
if (this.first) {
// do of only once on `onMove` method.
this.direction = _calc2.default.which(this.distanceAngle);
}
if (this.mode !== 'swipe' || !this._inIgnore(this.direction)) {
if (this.first) {
this._emit('onOnce');
} else {
this._emit('on' + (0, _help.camel)(this.mode));
}
event.preventDefault();
}
}
// to next step
this.first = false;
this.previousX = this.currentX;
this.previousY = this.currentY;
}
}, {
key: 'onEnd',
value: function onEnd(event) {
this.enabled = false;
this.touched = false;
this.elapsed = Date.now() - this.startTime;
this.ghostX = this.startX;
this.ghostY = this.startY;
this.targetElement = event.target;
if (this._isFlick(this.elapsed)) {
this._emit('onFlick');
}
// element & subscriber
this._emit('onEnd');
}
/**
* update
* Call in requestAnimationFrame
* Related Paiting Dom is here.
*/
}, {
key: 'update',
value: function update() {
if (!this.painting) return;
this.paint.play();
// subscribe
this._emit('onUpdate');
}
/**
* invert
* The posision is another one that is B.
* @private
* @param {Object} event<EventObject>
*/
}, {
key: '_invert',
value: function _invert(event) {
if (this.touched && event.touches[1]) {
this.currentX = event.touches[0].pageX;
this.currentY = event.touches[0].pageY;
this.invertX = event.touches[1].pageX;
this.invertY = event.touches[1].pageY;
} else {
this.invertX = this.ghostX;
this.invertY = this.ghostY;
}
}
/**
* distance
* @private
*/
}, {
key: '_distance',
value: function _distance() {
this.distanceX = this.currentX - this.startX;
this.distanceY = this.currentY - this.startY;
this.distance = _calc2.default.diagonal(this.distanceX, this.distanceY);
this.distanceAngle = _calc2.default.angle(this.distanceY * -1, this.distanceX, true);
}
/**
* between
* Distance of between A and B
* @private
*/
}, {
key: '_between',
value: function _between() {
this.betweenX = this.currentX - this.invertX;
this.betweenY = this.currentY - this.invertY;
this.between = _calc2.default.diagonal(this.betweenX, this.betweenY);
this.betweenAngle = _calc2.default.angle(this.betweenY * -1, this.betweenX, true);
}
/**
* direction
* @private
*/
}, {
key: '_direction',
value: function _direction() {
if (this.currentX > this.previousX) {
this.directionX = 'to right';
} else if (this.currentX < this.previousX) {
this.directionX = 'to left';
}
if (this.currentY > this.previousY) {
this.directionY = 'to bottom';
} else if (this.currentY < this.previousY) {
this.directionY = 'to top';
}
}
/**
* emit
* @private
* @param {String} suffix
* 指定された文字列をキャメルケースに変換し, on と結合してメソッド名にする
* subscriber 内のメソッドを実行する
*/
}, {
key: '_emit',
value: function _emit(method) {
if (!method || !this.subscriber) return;
this.emitter.emit(this.subscriber.selector, method, this);
}
/**
* find
* @private
* @param {Object} node<HTMLElement>
* 今回実行するべき subscriber を探します
*/
}, {
key: '_find',
value: function _find(el) {
var found = false;
this.subscriber = null;
// nodeTree を上方向に探索します
while (el && !found) {
if (el === (0, _help.documentElement)()) {
return found;
}
var _iteratorNormalCompletion = true;
var _didIteratorError = false;
var _iteratorError = undefined;
try {
for (var _iterator = (0, _getIterator3.default)(this.emitter.keys()), _step; !(_iteratorNormalCompletion = (_step = _iterator.next()).done); _iteratorNormalCompletion = true) {
var name = _step.value;
var prefix = name.slice(0, 1);
var selector = name.substr(1);
if ((0, _help.matchElement)(el, selector)) {
if (this.emitter.is(name)) {
this.subscriber = this.emitter.get(name);
this.subscriber.el = el;
found = true;
return found;
}
}
}
} catch (err) {
_didIteratorError = true;
_iteratorError = err;
} finally {
try {
if (!_iteratorNormalCompletion && _iterator.return) {
_iterator.return();
}
} finally {
if (_didIteratorError) {
throw _iteratorError;
}
}
}
el = el.parentNode;
}
return found;
}
/**
* initSubscribe
* @private
*/
}, {
key: '_initSubscribe',
value: function _initSubscribe(subscribers) {
if (subscribers) {
var _iteratorNormalCompletion2 = true;
var _didIteratorError2 = false;
var _iteratorError2 = undefined;
try {
for (var _iterator2 = (0, _getIterator3.default)((0, _keys2.default)(subscribers)), _step2; !(_iteratorNormalCompletion2 = (_step2 = _iterator2.next()).done); _iteratorNormalCompletion2 = true) {
var name = _step2.value;
this.emitter.on(name, new _Subscriber2.default(name, subscribers[name]));
}
} catch (err) {
_didIteratorError2 = true;
_iteratorError2 = err;
} finally {
try {
if (!_iteratorNormalCompletion2 && _iterator2.return) {
_iterator2.return();
}
} finally {
if (_didIteratorError2) {
throw _iteratorError2;
}
}
}
}
}
/**
* The `str` includes in ignore list.
* @param {String} str
* @return {Boolean}
*/
}, {
key: '_inIgnore',
value: function _inIgnore(str) {
return this.subscriber.ignore.includes(str);
}
/**
* is Flick?
* @return {Boolean}
*/
}, {
key: '_isFlick',
value: function _isFlick(elapsed) {
return elapsed < this.flickTime && elapsed > 50;
}
/**
* addEventListeners
* @private
*/
}, {
key: '_addEventListeners',
value: function _addEventListeners() {
this.rangeElement.addEventListener('mousedown', this.onStart);
this.rangeElement.addEventListener('mousemove', this.onMove);
this.rangeElement.addEventListener('mouseup', this.onEnd);
this.rangeElement.addEventListener('touchstart', this.onStart);
this.rangeElement.addEventListener('touchmove', this.onMove);
this.rangeElement.addEventListener('touchend', this.onEnd);
}
}]);
return Flyby;
}();
exports.default = Flyby;
},{"../class/_Emitter":63,"../class/_RequestAnim":65,"../class/_Subscriber":66,"../module/_calc":68,"../module/_help":69,"babel-runtime/core-js/get-iterator":1,"babel-runtime/core-js/object/keys":3,"babel-runtime/helpers/classCallCheck":4,"babel-runtime/helpers/createClass":5}],65:[function(require,module,exports){
"use strict";
Object.defineProperty(exports, "__esModule", {
value: true
});
var _classCallCheck2 = require("babel-runtime/helpers/classCallCheck");
var _classCallCheck3 = _interopRequireDefault(_classCallCheck2);
var _createClass2 = require("babel-runtime/helpers/createClass");
var _createClass3 = _interopRequireDefault(_createClass2);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
var requestAnimationFrame = window.requestAnimationFrame || window.mozRequestAnimationFrame || window.setTimeout;
var cancelAnimationFrame = window.cancelAnimationFrame || window.mozCancelAnimationFrame || window.clearTimeout;
window.requestAnimationFrame = requestAnimationFrame;
window.cancelAnimationFrame = cancelAnimationFrame;
var ReqestAnim = function () {
function ReqestAnim() {
(0, _classCallCheck3.default)(this, ReqestAnim);
this._id = null;
this._tick = null;
}
(0, _createClass3.default)(ReqestAnim, [{
key: "play",
value: function play(callback) {
var _this = this;
this.id = requestAnimationFrame(function () {
if (callback) callback();
_this._tick();
});
}
}, {
key: "cancel",
value: function cancel() {
if (this._id != null) {
cancelAnimationFrame(this._id);
}
}
}, {
key: "id",
set: function set(id) {
this._id = id;
},
get: function get() {
return this._id;
}
}, {
key: "tick",
set: function set(callback) {
this._tick = callback;
}
}]);
return ReqestAnim;
}();
exports.default = ReqestAnim;
},{"babel-runtime/helpers/classCallCheck":4,"babel-runtime/helpers/createClass":5}],66:[function(require,module,exports){
'use strict';
Object.defineProperty(exports, "__esModule", {
value: true
});
var _keys = require('babel-runtime/core-js/object/keys');
var _keys2 = _interopRequireDefault(_keys);
var _classCallCheck2 = require('babel-runtime/helpers/classCallCheck');
var _classCallCheck3 = _interopRequireDefault(_classCallCheck2);
var _createClass2 = require('babel-runtime/helpers/createClass');
var _createClass3 = _interopRequireDefault(_createClass2);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
/**
* Target
* @member {String} _name
* @member {HTMLElement} _el
* @member {Object} _methods
* @member {Array} _ignore
*/
var Subscriber = function () {
function Subscriber(name, body) {
(0, _classCallCheck3.default)(this, Subscriber);
this._name = '';
this._selector = '';
this._element = null;
this._methods = this._defaultMethods();
this._ignore = [];
this._scope = {};
this.name = name.replace(/^[.#]/, '');
this.selector = name;
this.ignore = body.ignore;
this.methods = body;
}
(0, _createClass3.default)(Subscriber, [{
key: 'run',
value: function run(methodName, context) {
if (this._methods[methodName]) {
this._methods[methodName].bind(context)(this._scope, this._elementement);
}
}
}, {
key: '_defaultMethods',
value: function _defaultMethods() {
var noop = function noop() {};
var methods = ['Config', 'Start', 'Once', 'Swipe', 'Pinch', 'Flick', 'End', 'Update'];
return function (res) {
methods.forEach(function (name) {
var key = ['on', name].join('');
res[key] = noop;
});
return res;
}({});
}
}, {
key: 'name',
set: function set(str) {
this._name = str;
},
get: function get() {
return this._name;
}
}, {
key: 'selector',
set: function set(str) {
this._selector = str;
},
get: function get() {
return this._selector;
}
}, {
key: 'methods',
set: function set(obj) {
var _this = this;
(0, _keys2.default)(obj).forEach(function (key) {
if (typeof obj[key] === 'function') {
_this._methods[key] = obj[key];
}
});
},
get: function get() {
return this._methods;
}
}, {
key: 'ignore',
set: function set(array) {
this._ignore = array || [];
},
get: function get() {
return this._ignore;
}
}, {
key: 'el',
set: function set(element) {
this._element = element;
},
get: function get() {
return this._element;
}
}]);
return Subscriber;
}();
exports.default = Subscriber;
},{"babel-runtime/core-js/object/keys":3,"babel-runtime/helpers/classCallCheck":4,"babel-runtime/helpers/createClass":5}],67:[function(require,module,exports){
'use strict';
Object.defineProperty(exports, "__esModule", {
value: true
});
var _Flyby = require('./class/_Flyby');
var _Flyby2 = _interopRequireDefault(_Flyby);
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
exports.default = _Flyby2.default; /**
* NOTE: Positions declarations
* Start - 始点 <x, y>
* End - 終点 <x, y> **不要?**
* Current - 現在地 <x, y>
* Distance - 始点から現在地への距離 <x, y> [swipe]
* Invert - 2つ目の始点 <x, y> [pinch]
* Between - Start と Invert の距離 <d> [pinch]
* Origin - Start と Invert の開始時の中間地点 <x, y> [pinch]
* Offset - 対象ノードの座標 <x, y> **外側でやる**
*/
/**
* NOTE: API methods
* onConfig(scope)
* onStart(scope, element)
* onEnd(scope, element)
* onFirstStep(scope, element)
* onPinch(scope, element)
* onSwipe(scope, element)
* onFlick(scope, element)
* onUpdate(scope, element)
* ignore [x, y, swipe, pinch]
*/
},{"./class/_Flyby":64}],68:[function(require,module,exports){
'use strict';
Object.defineProperty(exports, "__esModule", {
value: true
});
var _help = require('./_help');
exports.default = function () {
return { degree: degree, radian: radian, angle: angle, diagonal: diagonal, which: which };
}();
/**
* diagonal
* @param {Number} x
* @param {Number} y
* @return {Number} d
*/
function diagonal(x, y) {
return parseInt((0, _help.sqrt)((0, _help.pow)(x, 2) + (0, _help.pow)(y, 2)));
}
/**
* Wrapped `Math.atan2`
* @private
* @param {Number} y
* @param {Number} x
*/
function angle(y, x) {
var toDegree = arguments.length <= 2 || arguments[2] === undefined ? false : arguments[2];
if (toDegree) {
return degree((0, _help.atan2)(y, x));
}
return (0, _help.atan2)(y, x);
}
/**
* which
* swipe の軸の向きを検出します
* @return {String}
*/
function which(angle) {
angle = (0, _help.abs)(angle);
if (angle < 180 + 5 && angle > 180 - 5 || angle < 10) {
return 'x';
}
return 'y';
}
/**
* degree
* The radian convert to degree.
* @private
* @param {Number} radian
* @param {Number} degree
*/
function degree(radian) {
return radian * 180 / _help.PI;
}
/**
* radian
* The degree convert to radian
* @private
* @param {Number} degree
* @return {Number} radian
*/
function radian(degree) {
return degree * _help.PI / 180;
}
},{"./_help":69}],69:[function(require,module,exports){
'use strict';
Object.defineProperty(exports, "__esModule", {
value: true
});
exports.camel = camel;
exports.hasClass = hasClass;
exports.hasId = hasId;
exports.matchElement = matchElement;
exports.documentElement = documentElement;
exports.pageX = pageX;
exports.pageY = pageY;
exports.touches = touches;
exports.hasTouches = hasTouches;
exports.noop = noop;
/**
* help.js
*/
var PI = Math.PI;
var sqrt = Math.sqrt;
var atan2 = Math.atan2;
var abs = Math.abs;
var pow = Math.pow;
exports.PI = PI;
exports.sqrt = sqrt;
exports.atan2 = atan2;
exports.abs = abs;
exports.pow = pow;
function camel(str) {
return [str.substr(0, 1).toUpperCase(), str.substr(1)].join('');
}
function hasClass(el, selector) {
return el.classList.contains(selector);
}
function hasId(el, selector) {
return el.getAttribute('id') === selector;
}
function matchElement(el, selector) {
return hasClass(el, selector) || hasId(el, selector);
}
function documentElement() {
return document.documentElement;
}
/**
* pageX
* @private
* @param {Object} event<EventObject>
*/
function pageX(event) {
if (event.pageX != null) {
return event.pageX;
}
return event.touches[0].pageX;
}
/**
* pageY
* @private
* @param {Object} event<EventObject>
*/
function pageY(event) {
if (event.pageY != null) {
return event.pageY;
}
return event.touches[0].pageY;
}
/**
* touches
* @param {Object} event<EventObject>
* @return {Boolean}
* Event オブジェクトから touches 抽出して返す
*/
function touches(event) {
return event.originalEvent ? event.originalEvent.touches : event.touches;
}
/**
* hasTouches
* @private
* @param {Object} event<EventObject>
* @param {Number} length
* @return {Boolean}
*/
function hasTouches(event, length) {
var _touches = touches(event);
if (_touches != null && _touches.length === length) {
return true;
}
return false;
}
/**
* No operation
*/
function noop() {
return;
}
},{}]},{},[67]);
| pixel-metal/flyby.js | dist/flyby.js | JavaScript | mit | 53,276 |
from .stats_view_base import StatsViewSwagger, StatsViewSwaggerKeyRequired
from .stats_util_dataverses import StatsMakerDataverses
class DataverseCountByMonthView(StatsViewSwaggerKeyRequired):
"""API View - Dataverse counts by Month."""
# Define the swagger attributes
# Note: api_path must match the path in urls.py
#
api_path = '/dataverses/count/monthly'
summary = ('Number of published Dataverses by'
' the month they were created*. (*'
' Not month published)')
description = ('Returns a list of counts and'
' cumulative counts of all Dataverses added in a month')
description_200 = 'A list of Dataverse counts by month'
param_names = StatsViewSwagger.PARAM_DV_API_KEY +\
StatsViewSwagger.BASIC_DATE_PARAMS +\
StatsViewSwagger.PUBLISH_PARAMS +\
StatsViewSwagger.PRETTY_JSON_PARAM +\
StatsViewSwagger.PARAM_AS_CSV
tags = [StatsViewSwagger.TAG_DATAVERSES]
def get_stats_result(self, request):
"""Return the StatsResult object for this statistic"""
stats_datasets = StatsMakerDataverses(**request.GET.dict())
pub_state = self.get_pub_state(request)
if pub_state == self.PUB_STATE_ALL:
stats_result = stats_datasets.get_dataverse_counts_by_month()
elif pub_state == self.PUB_STATE_UNPUBLISHED:
stats_result = stats_datasets.get_dataverse_counts_by_month_unpublished()
else:
stats_result = stats_datasets.get_dataverse_counts_by_month_published()
return stats_result
class DataverseTotalCounts(StatsViewSwaggerKeyRequired):
"""API View - Total count of all Dataverses"""
# Define the swagger attributes
# Note: api_path must match the path in urls.py
#
api_path = '/dataverses/count'
summary = ('Simple count of published Dataverses')
description = ('Returns number of published Dataverses')
description_200 = 'Number of published Dataverses'
param_names = StatsViewSwagger.PARAM_DV_API_KEY + StatsViewSwagger.PUBLISH_PARAMS + StatsViewSwagger.PRETTY_JSON_PARAM
tags = [StatsViewSwagger.TAG_DATAVERSES]
result_name = StatsViewSwagger.RESULT_NAME_TOTAL_COUNT
def get_stats_result(self, request):
"""Return the StatsResult object for this statistic"""
stats_datasets = StatsMakerDataverses(**request.GET.dict())
pub_state = self.get_pub_state(request)
if pub_state == self.PUB_STATE_ALL:
stats_result = stats_datasets.get_dataverse_count()
elif pub_state == self.PUB_STATE_UNPUBLISHED:
stats_result = stats_datasets.get_dataverse_count_unpublished()
else:
stats_result = stats_datasets.get_dataverse_count_published()
return stats_result
class DataverseAffiliationCounts(StatsViewSwaggerKeyRequired):
"""API View - Number of Dataverses by Affiliation"""
# Define the swagger attributes
# Note: api_path must match the path in urls.py
#
api_path = '/dataverses/count/by-affiliation'
summary = ('Number of Dataverses by Affiliation')
description = ('Number of Dataverses by Affiliation.')
description_200 = 'Number of published Dataverses by Affiliation.'
param_names = StatsViewSwagger.PARAM_DV_API_KEY\
+ StatsViewSwagger.PUBLISH_PARAMS\
+ StatsViewSwagger.PRETTY_JSON_PARAM\
+ StatsViewSwagger.PARAM_AS_CSV
result_name = StatsViewSwagger.RESULT_NAME_AFFILIATION_COUNTS
tags = [StatsViewSwagger.TAG_DATAVERSES]
def get_stats_result(self, request):
"""Return the StatsResult object for this statistic"""
stats_datasets = StatsMakerDataverses(**request.GET.dict())
pub_state = self.get_pub_state(request)
if pub_state == self.PUB_STATE_ALL:
stats_result = stats_datasets.get_dataverse_affiliation_counts()
elif pub_state == self.PUB_STATE_UNPUBLISHED:
stats_result = stats_datasets.get_dataverse_affiliation_counts_unpublished()
else:
stats_result = stats_datasets.get_dataverse_affiliation_counts_published()
return stats_result
class DataverseTypeCounts(StatsViewSwaggerKeyRequired):
# Define the swagger attributes
# Note: api_path must match the path in urls.py
#
api_path = '/dataverses/count/by-type'
summary = ('Number of Dataverses by Type')
description = ('Number of Dataverses by Type.')
description_200 = 'Number of published Dataverses by Type.'
param_names = StatsViewSwagger.PARAM_DV_API_KEY + StatsViewSwagger.PUBLISH_PARAMS +\
StatsViewSwagger.PRETTY_JSON_PARAM +\
StatsViewSwagger.DV_TYPE_UNCATEGORIZED_PARAM +\
StatsViewSwagger.PARAM_AS_CSV
result_name = StatsViewSwagger.RESULT_NAME_DATAVERSE_TYPE_COUNTS
tags = [StatsViewSwagger.TAG_DATAVERSES]
def is_show_uncategorized(self, request):
"""Return the result of the "?show_uncategorized" query string param"""
show_uncategorized = request.GET.get('show_uncategorized', False)
if show_uncategorized is True or show_uncategorized == 'true':
return True
return False
def get_stats_result(self, request):
"""Return the StatsResult object for this statistic"""
stats_datasets = StatsMakerDataverses(**request.GET.dict())
if self.is_show_uncategorized(request):
exclude_uncategorized = False
else:
exclude_uncategorized = True
pub_state = self.get_pub_state(request)
if pub_state == self.PUB_STATE_ALL:
stats_result = stats_datasets.get_dataverse_counts_by_type(exclude_uncategorized)
elif pub_state == self.PUB_STATE_UNPUBLISHED:
stats_result = stats_datasets.get_dataverse_counts_by_type_unpublished(exclude_uncategorized)
else:
stats_result = stats_datasets.get_dataverse_counts_by_type_published(exclude_uncategorized)
return stats_result
| IQSS/miniverse | dv_apps/metrics/stats_views_dataverses.py | Python | mit | 6,085 |
//
// Generated by class-dump 3.5 (64 bit).
//
// class-dump is Copyright (C) 1997-1998, 2000-2001, 2004-2013 by Steve Nygard.
//
#import "CDStructures.h"
#import <IDEKit/IDENavigableItemDomainProvider.h>
@interface IDENavigableItemSymbolsDomainProvider : IDENavigableItemDomainProvider
{
}
+ (id)domainObjectForWorkspace:(id)arg1;
@end
| kolinkrewinkel/Multiplex | Multiplex/IDEHeaders/IDEHeaders/IDEKit/IDENavigableItemSymbolsDomainProvider.h | C | mit | 350 |
/*
* Code used in the "Software Engineering" course.
*
* Copyright 2017 by Claudio Cusano (claudio.cusano@unipv.it)
* Dept of Electrical, Computer and Biomedical Engineering,
* University of Pavia.
*/
package goldrush;
/**
* @author Reina Michele cl418656
* @author Bonissone Davidecl427113
*/
public class BoniMichele extends GoldDigger{ //
int t=0;
int j=99;
@Override
public int chooseDiggingSite(int[] distances) {
for (int i=0; i<distances.length; i++){
if (t==0){
if (distances[i]==140) {
j=i;
t++;
}
}
else if (t<3) {
if (distances[i]== 30) {
j=i;
t=0;
}
}
else {
if (distances[i]== 200) {
j=i;
t=0;
}
}
}
return j;
}
}
| IngSW-unipv/GoldRush | GoldRush/src/goldrush/BoniMichele.java | Java | mit | 932 |
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Input;
namespace Paradix
{
public sealed class KeyboardController : IController
{
// TODO : List of keys UP / DOWN / PRESSED / RELEASED
public PlayerIndex Player { get; set; } = PlayerIndex.One;
public KeyboardState CurrentState { get; private set; }
public KeyboardState PreviousState { get; private set; }
public KeyboardController (PlayerIndex player = PlayerIndex.One)
{
Player = player;
CurrentState = Keyboard.GetState (Player);
PreviousState = CurrentState;
}
public bool IsKeyDown (Keys key)
{
return CurrentState.IsKeyDown (key);
}
public bool IsKeyUp (Keys key)
{
return CurrentState.IsKeyUp (key);
}
public bool IsKeyPressed (Keys key)
{
return CurrentState.IsKeyDown (key) && PreviousState.IsKeyUp (key);
}
public bool IsKeyReleased (Keys key)
{
return PreviousState.IsKeyDown (key) && CurrentState.IsKeyUp (key);
}
public void Flush (GameTime gameTime)
{
PreviousState = CurrentState;
CurrentState = Keyboard.GetState (Player);
}
}
} | NySwann/Paradix | Paradix.Engine/Input/KeyboardController.cs | C# | mit | 1,092 |
module.exports = {
parserOptions: {
sourceType: 'script',
},
};
| jpikl/cfxnes | core/bin/.eslintrc.js | JavaScript | mit | 72 |
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package com.docuware.dev.Extensions;
import java.io.Closeable;
import java.io.InputStream;
/**
*
* @author Patrick
*/
public class EasyCheckoutResult implements Closeable {
private String EncodedFileName;
public String getEncodedFileName() {
return EncodedFileName;
}
void setEncodedFileName(String data) {
EncodedFileName = data;
}
private DeserializedHttpResponseGen<InputStream> response;
public DeserializedHttpResponseGen<InputStream> getResponse() {
return response;
}
void setResponse(DeserializedHttpResponseGen<InputStream> data) {
response = data;
}
@Override
public void close() {
this.response.close();
}
}
| DocuWare/PlatformJavaClient | src/com/docuware/dev/Extensions/EasyCheckoutResult.java | Java | mit | 949 |
using System.Reflection;
using System.Runtime.CompilerServices;
using System.Runtime.InteropServices;
// General Information about an assembly is controlled through the following
// set of attributes. Change these attribute values to modify the information
// associated with an assembly.
[assembly: AssemblyTitle("BB.Poker.WinFormsClient")]
[assembly: AssemblyDescription("")]
[assembly: AssemblyConfiguration("")]
[assembly: AssemblyCompany("")]
[assembly: AssemblyProduct("BB.Poker.WinFormsClient")]
[assembly: AssemblyCopyright("Copyright © 2012")]
[assembly: AssemblyTrademark("")]
[assembly: AssemblyCulture("")]
// Setting ComVisible to false makes the types in this assembly not visible
// to COM components. If you need to access a type in this assembly from
// COM, set the ComVisible attribute to true on that type.
[assembly: ComVisible(false)]
// The following GUID is for the ID of the typelib if this project is exposed to COM
[assembly: Guid("9703352c-1cab-4c2a-bbc9-183b9245edc6")]
// Version information for an assembly consists of the following four values:
//
// Major Version
// Minor Version
// Build Number
// Revision
//
// You can specify all the values or you can default the Build and Revision Numbers
// by using the '*' as shown below:
// [assembly: AssemblyVersion("1.0.*")]
[assembly: AssemblyVersion("1.0.0.0")]
[assembly: AssemblyFileVersion("1.0.0.0")]
| bberak/PokerDotNet | BB.Poker.WinFormsClient/Properties/AssemblyInfo.cs | C# | mit | 1,422 |
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CharacterModelLib.Models
{
public class CharacterProject : NotifyableBase
{
public CharacterProject()
{
characterCollection = new ObservableCollection<Character>();
}
void characterCollection_CollectionChanged(object sender, System.Collections.Specialized.NotifyCollectionChangedEventArgs e)
{
RaisePropertyChanged("CharacterCollection");
}
Character selectedCharacter;
public Character SelectedCharacter
{
get { return selectedCharacter; }
set
{
if (selectedCharacter != null)
{
selectedCharacter.PropertyChanged -= selectedCharacter_PropertyChanged;
}
selectedCharacter = value;
if (selectedCharacter != null)
{
selectedCharacter.PropertyChanged += selectedCharacter_PropertyChanged;
}
RaisePropertyChanged("SelectedCharacter");
RaisePropertyChanged("NextCharacter");
RaisePropertyChanged("PreviousCharacter");
}
}
public Character NextCharacter
{
get
{
if (selectedCharacter == null)
{
return CharacterCollection[0];
}
int index = CharacterCollection.IndexOf(selectedCharacter);
if (index >= CharacterCollection.Count - 1)
{
return CharacterCollection[0];
}
else
{
return CharacterCollection[index + 1];
}
}
}
public Character PreviousCharacter
{
get
{
if (selectedCharacter == null)
{
return CharacterCollection[CharacterCollection.Count - 1];
}
int index = CharacterCollection.IndexOf(selectedCharacter);
if (index <= 0)
{
return CharacterCollection[CharacterCollection.Count - 1];
}
else
{
return CharacterCollection[index - 1];
}
}
}
private void selectedCharacter_PropertyChanged(object sender, System.ComponentModel.PropertyChangedEventArgs e)
{
RaisePropertyChanged("SelectedCharacter." + e.PropertyName);
//RaisePropertyChanged(e.PropertyName);
//RaisePropertyChanged("SelectedCharacter");
}
private ObservableCollection<Character> characterCollection;
public ObservableCollection<Character> CharacterCollection
{
get { return characterCollection; }
set
{
if (characterCollection != null)
{
characterCollection.CollectionChanged -= characterCollection_CollectionChanged;
}
characterCollection = value;
if (characterCollection != null)
{
characterCollection.CollectionChanged += characterCollection_CollectionChanged;
}
RaisePropertyChanged("CharacterCollection");
}
}
private string name;
public string Name
{
get { return name; }
set
{
name = value;
RaisePropertyChanged("Name");
}
}
private string projectPath;
public string ProjectPath
{
get { return projectPath; }
set
{
projectPath = value;
RaisePropertyChanged("ProjectPath");
}
}
public override string ToString()
{
return base.ToString() + ": Name=" + Name;
}
public override bool Equals(object obj)
{
if (obj.GetType() == this.GetType())
{
return ((obj as CharacterProject).Name == this.Name);
}
return false;
}
public override int GetHashCode()
{
return base.GetHashCode();
}
}
}
| Salem5/CharacterEditor | CharacterModelLib/Models/CharacterProject.cs | C# | mit | 4,559 |
import uuid
from django.db import models
from django.conf import settings
from django.contrib.auth.models import AbstractUser
from django.contrib.auth.models import BaseUserManager
from django.utils import timezone
from accelerator_abstract.models import BaseUserRole
from accelerator_abstract.models.base_base_profile import EXPERT_USER_TYPE
MAX_USERNAME_LENGTH = 30
class UserManager(BaseUserManager):
use_in_migrations = True
def _create_user(self, email, password,
is_staff, is_superuser, **extra_fields):
"""
Creates and saves an User with the given email and password.
"""
now = timezone.now()
if not email:
raise ValueError('An email address must be provided.')
email = self.normalize_email(email)
if "is_active" not in extra_fields:
extra_fields["is_active"] = True
if "username" not in extra_fields:
# For now we need to have a unique id that is at
# most 30 characters long. Using uuid and truncating.
# Ideally username goes away entirely at some point
# since we're really using email. If we have to keep
# username for some reason then we could switch over
# to a string version of the pk which is guaranteed
# be unique.
extra_fields["username"] = str(uuid.uuid4())[:MAX_USERNAME_LENGTH]
user = self.model(email=email,
is_staff=is_staff,
is_superuser=is_superuser,
last_login=None,
date_joined=now,
**extra_fields)
user.set_password(password)
user.save(using=self._db)
return user
def create_user(self, email=None, password=None, **extra_fields):
return self._create_user(email, password, False, False,
**extra_fields)
def create_superuser(self, email, password, **extra_fields):
return self._create_user(email, password, True, True,
**extra_fields)
class User(AbstractUser):
# Override the parent email field to add uniqueness constraint
email = models.EmailField(blank=True, unique=True)
objects = UserManager()
class Meta:
db_table = 'auth_user'
managed = settings.ACCELERATOR_MODELS_ARE_MANAGED
def __init__(self, *args, **kwargs):
super(User, self).__init__(*args, **kwargs)
self.startup = None
self.team_member = None
self.profile = None
self.user_finalist_roles = None
class AuthenticationException(Exception):
pass
def __str__(self):
return self.email
def full_name(self):
fn = self.first_name
ln = self.last_name
if fn and ln:
name = u"%s %s" % (fn, ln)
else:
name = str(self.email)
return name
def user_phone(self):
return self._get_profile().phone
def image_url(self):
return self._get_profile().image_url()
def team_member_id(self):
return self.team_member.id if self._get_member() else ''
def user_title(self):
return self._get_title_and_company()['title']
def user_twitter_handle(self):
return self._get_profile().twitter_handle
def user_linked_in_url(self):
return self._get_profile().linked_in_url
def user_facebook_url(self):
return self._get_profile().facebook_url
def user_personal_website_url(self):
return self._get_profile().personal_website_url
def type(self):
return self._get_profile().user_type
def startup_name(self):
return self._get_title_and_company()['company']
def _get_title_and_company(self):
if self._is_expert() and self._has_expert_details():
profile = self._get_profile()
title = profile.title
company = profile.company
return {
"title": title,
"company": company
}
self._get_member()
title = self.team_member.title if self.team_member else ""
company = self.startup.name if self._get_startup() else None
return {
"title": title,
"company": company
}
def _has_expert_details(self):
if self._is_expert():
profile = self._get_profile()
return True if profile.title or profile.company else False
def startup_industry(self):
return self.startup.primary_industry if self._get_startup() else None
def top_level_startup_industry(self):
industry = (
self.startup.primary_industry if self._get_startup() else None)
return industry.parent if industry and industry.parent else industry
def startup_status_names(self):
if self._get_startup():
return [startup_status.program_startup_status.startup_status
for startup_status in self.startup.startupstatus_set.all()]
def finalist_user_roles(self):
if not self.user_finalist_roles:
finalist_roles = BaseUserRole.FINALIST_USER_ROLES
self.user_finalist_roles = self.programrolegrant_set.filter(
program_role__user_role__name__in=finalist_roles
).values_list('program_role__name', flat=True).distinct()
return list(self.user_finalist_roles)
def program(self):
return self.startup.current_program() if self._get_startup() else None
def location(self):
program = self.program()
return program.program_family.name if program else None
def year(self):
program = self.program()
return program.start_date.year if program else None
def is_team_member(self):
return True if self._get_member() else False
def _get_startup(self):
if not self.startup:
self._get_member()
if self.team_member:
self.startup = self.team_member.startup
return self.startup
def _get_member(self):
if not self.team_member:
self.team_member = self.startupteammember_set.last()
return self.team_member
def _get_profile(self):
if self.profile:
return self.profile
self.profile = self.get_profile()
return self.profile
def has_a_finalist_role(self):
return len(self.finalist_user_roles()) > 0
def _is_expert(self):
profile = self._get_profile()
return profile.user_type == EXPERT_USER_TYPE.lower()
| masschallenge/django-accelerator | simpleuser/models.py | Python | mit | 6,632 |
from setuptools import setup, find_packages
from codecs import open
import os
def read(*paths):
"""Build a file path from *paths* and return the contents."""
with open(os.path.join(*paths), 'r') as f:
return f.read()
setup(
name='transposer',
version='0.0.3',
description='Transposes columns and rows in delimited text files',
long_description=(read('README.rst')),
url='https://github.com/keithhamilton/transposer',
author='Keith Hamilton',
maintainer='Keith Hamilton',
maintainer_email='the.keith.hamilton@gmail.com',
license='BSD License',
classifiers=[
'Development Status :: 4 - Beta',
'Intended Audience :: Developers',
'License :: OSI Approved :: MIT License',
'Programming Language :: Python',
'Programming Language :: Python :: 2.6',
'Programming Language :: Python :: 2.7',
'Topic :: Office/Business'
],
keywords='text, csv, tab-delimited, delimited, excel, sheet, spreadsheet',
packages=find_packages(exclude=['contrib', 'docs', 'test*', 'bin', 'include', 'lib', '.idea']),
install_requires=[],
package_data={},
data_files=[],
entry_points={
'console_scripts': [
'transposer=transposer.script.console_script:main'
]
}
)
| keithhamilton/transposer | setup.py | Python | mit | 1,307 |
<?php
/*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
* "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
* LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
* A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
* OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
* LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
* This software consists of voluntary contributions made by many individuals
* and is licensed under the MIT license. For more information, see
* <http://www.doctrine-project.org>.
*/
namespace Doctrine\ODM\MongoDB\Mapping\Annotations;
use Doctrine\Common\Annotations\Annotation;
abstract class AbstractDocument extends Annotation
{
}
| CaoPhiHung/CRM | vendor/doctrine/mongodb-odm/lib/Doctrine/ODM/MongoDB/Mapping/Annotations/AbstractDocument.php | PHP | mit | 1,168 |
//
// Generated by class-dump 3.5 (64 bit).
//
// class-dump is Copyright (C) 1997-1998, 2000-2001, 2004-2013 by Steve Nygard.
//
#import "NSObject.h"
@class KFContact;
@protocol IKFContactExt <NSObject>
@optional
- (void)onKFContactHeadImgUpdate:(KFContact *)arg1;
- (void)onModifyKFContact:(KFContact *)arg1;
@end
| walkdianzi/DashengHook | WeChat-Headers/IKFContactExt-Protocol.h | C | mit | 329 |
package lv.emes.libraries.utilities.validation;
/**
* Actions for error that occur in validation process.
*
* @author eMeS
* @version 1.2.
*/
public interface MS_ValidationError<T> {
MS_ValidationError withErrorMessageFormingAction(IFuncFormValidationErrorMessage action);
/**
* Returns message of validation error using pre-defined method to form message.
* @return formatted message describing essence of this particular validation error.
*/
String getMessage();
Integer getNumber();
T getObject();
/**
* @param object an object to validate.
* @return reference to validation error itself.
*/
MS_ValidationError withObject(T object);
}
| LV-eMeS/eMeS_Libraries | src/main/java/lv/emes/libraries/utilities/validation/MS_ValidationError.java | Java | mit | 706 |
#ifndef _absnodeaction_h_
#define _absnodeaction_h_
#include "cocos2d.h"
using namespace cocos2d;
#include <string>
#include <iostream>
#include <vector>
using namespace std;
namespace uilib
{
class TouchNode;
enum EaseType
{
EaseNone,
EaseIn,
EaseOut,
EaseInOut,
EaseExponentialIn,
EaseExponentialOut,
EaseExponentialInOut,
EaseSineIn,
EaseSineOut,
EaseSineInOut,
EaseElastic,
EaseElasticIn,
EaseElasticOut,
EaseElasticInOut,
EaseBounce,
EaseBounceIn,
EaseBounceOut,
EaseBounceInOut,
EaseBackIn,
EaseBackOut,
EaseBackInOut
};
enum MoveInType{
HorizontalRightIn,
HorizontalLeftIn,
HorizontalBothIn,
VerticalTopIn,
VerticalBottomIn,
VerticalBothin,
ScaleIn,
ScaleXIn,
ScaleYIn,
SwayIn,
RotateIn,
BlinkIn,
ReelIn,
FireIn,
DropScaleIn
};
enum MoveOutType{
HorizontalRightOut,
HorizontalLeftOut,
HorizontalBothOut,
VerticalTopOut,
VerticalBottomOut,
VerticalBothOut,
ScaleOut,
ScaleXOut,
ScaleYOut,
SwayOut,
RotateOut,
BlinkOut,
ReelOut,
FireOut
};
enum RunningEffectType
{
ShineEffect,
SwayEffect,
ScaleEffect,
AnimEffect
};
class BasNodeEffectAction : public CCNode
{
public:
BasNodeEffectAction();
virtual ~BasNodeEffectAction();
virtual void finished();
virtual void doAction(TouchNode *node,bool enable) = 0;
inline void setEaseType(EaseType type) { m_easeType = type;}
inline EaseType getEaseType() { return m_easeType;}
inline void setActionTime(float time) { m_actionTime = time;}
inline float getActionTime() { return m_actionTime;}
inline void setStartTime(float time) { m_startTime = time;}
inline float getStartTime() { return m_startTime;}
void setFinishCB(CCNode *listener,SEL_CallFuncN func);
inline bool isRunning() { return m_running;}
protected:
EaseType m_easeType;
float m_actionTime;
float m_startTime;
protected:
bool m_running;
protected:
SEL_CallFuncN m_finishFunc;
CCNode *m_listener;
};
class BasNodeAction : public CCNode
{
public:
BasNodeAction();
virtual ~BasNodeAction();
virtual void finished();
virtual void doAction(const std::vector<TouchNode*> &nodes) = 0;
void setDelayTime(float delay) { m_delayTime = delay;}
float getDelayTime() { return m_delayTime;}
inline void setEaseType(EaseType type) { m_easeType = type;}
inline EaseType getEaseType() { return m_easeType;}
inline void setMoveInType(MoveInType type) { m_inType = type;}
inline MoveInType getMoveInType() { return m_inType;}
inline void setMoveOutType(MoveOutType type) { m_outType = type;}
inline MoveOutType getMoveOutType() { return m_outType;}
inline void setActionTime(float time) { m_actionTime = time;}
inline float getActionTime() { return m_actionTime;}
inline void setStartTime(float time) { m_startTime = time;}
inline float getStartTime() { return m_startTime;}
inline void setRate(float rate) { m_rate = rate;}
inline float getRate() { return m_rate;}
void setFinishCB(CCNode *listener,SEL_CallFuncN func,CCNode *actionNode = 0);
protected:
CCActionEase *createEaseAction();
protected:
int m_actionRunNum;
float m_delayTime;
EaseType m_easeType;
MoveInType m_inType;
MoveOutType m_outType;
float m_actionTime;
float m_rate;
float m_startTime;
protected:
SEL_CallFuncN m_finishFunc;
CCNode *m_listener;
CCNode *m_actionNode;
};
class UiNodeActionFactory
{
UiNodeActionFactory();
~UiNodeActionFactory();
static UiNodeActionFactory *m_instance;
public:
static UiNodeActionFactory *getInstance();
BasNodeAction *getMoveActionByName(const std::string &name);
BasNodeEffectAction *getEffectActionByName(const std::string &name);
protected:
};
}
#endif
| zhwsh00/DirectFire-android | directfire_github/trunk/uilib/actions/absnodeaction.h | C | mit | 3,894 |
window.ImageViewer = function(url, alt, title){
var img = $('<img />').attr('src', url).attr('alt', title).css({
display: 'inline-block',
'max-width': '90vw',
'max-height': '90vh'
});
var a = $('<a></a>').attr('target', '_blank')
.attr('title', title)
.attr('href', url)
.css({
display: 'inline-block',
height: '100%'
})
.append(img);
var close_it = function(){
overlay.remove();
container.remove();
};
var closeBtn = $('<a class="icon-remove-sign"></a>').css({
color: 'red',
'font-size': 'x-large',
'margin-left': '-0.1em'
}).bind('click', close_it);
var closeWrapper = $('<div></div>').css({
height: '100%',
width: '2em',
'text-align': 'left',
'display': 'inline-block',
'vertical-algin': 'top',
'margin-top': '-0.6em',
'float': 'right'
}).append(closeBtn);
var container = $('<div></div>').append(
$('<div></div>').css({
margin: '5vh 1vw',
display: 'inline-block',
'vertical-align': 'top'
}).append(a).append(closeWrapper))
.css({
'z-index': 30000000,
'position': 'fixed',
'padding': 0,
'margin': 0,
'width': '100vw',
'height': '100vh',
'top': 0,
'left': 0,
'text-align': 'center',
'cursor': 'default',
'vertical-align': 'middle'
})
.bind('click',close_it)
.appendTo('body');
var overlay = $('<div class="blockUI blockMsg blockPage">').css({
'z-index': 9999,
'position': 'fixed',
padding: 0,
margin: 0,
width: '100vw',
height: '100vh',
top: '0vh',
left: '0vw',
'text-align': 'center',
'cursor': 'default',
'vertical-align': 'middle',
'background-color': 'gray',
'opacity': '0.4'
}).bind('click', close_it).appendTo('body');
this.close = close_it;
return this;
} | clazz/clazz.github.io | js/image-viewer.js | JavaScript | mit | 2,216 |
---
layout: post.html
title: "Fundraising for PyLadies for PyCon 2015"
tag: [PyCon]
author: Lynn Root
author_link: http://twitter.com/roguelynn
---
**TL;DR**: [Donate](#ways-to-donate) to PyLadies for PyCon!
It's that time again! With [PyCon 2015][0] planning in high gear, PyLadies is revving up to raise funds to help women attend the biggest Python conference of the year.
Last year, we raised **$40,000**. We're hoping to do that again to help women attend PyCon. Here's the breakdown:
### Our numbers
* In addition to registration, it will take about $500-1000 per woman in North America to attend PyCon 2015 in Montreal
* In addition to registration, it will take about $1000-2000 per woman outside of North America to attend PyCon 2015 in Montreal
### Why PyLadies? Our effect on the community
_(percentages are not at all accurate, and are roughly estimated based on guessing names-to-gender scripts):_
* PyCon 2011 had less than 10% women attendees (speaker % unknown)
* PyLadies started in late 2011
* PyCon 2012 had about 11% women attendees, and about the same in speakers.
* This included giving PyLadies and [Women Who Code][8] booths to help promote our messages
* At the time of PyCon 2014, PyLadies had over [35 chapters][7], doing stuff like:
* hosts events like learning python, learning git/github,
* providing space for study groups,
* enriching members with speaker series (like Guido van Rossum!),
* events to help think up of talks to submit for PyCon (as well as DjangoCon),
* hold events for folks to practice their talk, etc
* PyLadies work showed:
* PyCon 2013 had over 20% women attendees, and about 22% women speakers.
* PyCon 2014 had over 30% women attendees, and about a third of the speakers were women.
* No overhead - we're all volunteers! ;-)
* Lastly, donations tax deductible since PyLadies is under the financial enclave of the Python Software Foundation
### What we're actively doing:
* We're using all of the $12k that PyLadies raised at last year's PyCon auction to increase the aid pool - something that we were hoping to actually use towards growing PyLadies locations (but it's okay! $10k for more women friends at PyCon!)
* We're bugging companies and individuals do donate (hence this blog post!).
### What you will get in return
* Unless you explicitly choose to be anonymous (though the [online donation site][5] or in private discussions), we will profusely thank you via our public channels (Twitter, this blog, and at our PyCon booth)
* Provide a detailed write-up and financial introspection of how many women we've helped because of this campaign
* Visibility! It shows the PyCon/Python community that your company/you are serious about women in tech - which can directly benefit sponsors with increased hiring pool of female Pythonistas.
### Ways to donate
All of the following methods are tax-deductible with funds going to the [Python Software Foundation][1], a 501(c)3 charitable organization.
* Through our [donation page][4] (ideal for individuals)
* Email me at [lynn@lynnroot.com][2] (ideal for companies or anyone needing a proper invoice, or individualized solutions)
* Become a [sponsor][6] for PyCon (this is more indirect and less potent, but overall helpful to PyCon)
More information is available on the [PyCon Financial Aid][5] site if you would like to apply for financial aid for PyCon, whether you are a PyLady or PyGent.
[0]: http://us.pycon.org/2015
[1]: http://python.org/psf
[2]: mailto:lynn@lynnroot.com?subject=PyLadies%20Donation
[3]: http://pyladies.com/blog
[4]: https://psfmember.org/civicrm/contribute/transact?reset=1&id=6
[5]: https://us.pycon.org/2014/assistance/
[6]: https://us.pycon.org/2014/sponsors/prospectus/
[7]: https://github.com/pyladies/pyladies/tree/master/www/locations
[8]: http://www.meetup.com/women-who-code-sf
[9]: http://www.marketwired.com/press-release/-1771597.htm
| pyladies/pyladies-theme | _posts/2014-11-02-fundraising-for-pycon-2015.md | Markdown | mit | 3,931 |
// Copyright (c) 2017-2017 The Bitcoin Core developers
// Distributed under the MIT software license, see the accompanying
// file COPYING or http://www.opensource.org/licenses/mit-license.php.
#ifndef BITCOIN_CONSENSUS_TX_VERIFY_H
#define BITCOIN_CONSENSUS_TX_VERIFY_H
#include "amount.h"
#include <stdint.h>
#include <vector>
class CBlockIndex;
class CCoinsViewCache;
class CTransaction;
class CValidationState;
/** Transaction validation functions */
/** Context-independent validity checks */
bool CheckTransaction(const CTransaction& tx, CValidationState& state);
namespace Consensus {
/**
* Check whether all inputs of this transaction are valid (no double spends and amounts)
* This does not modify the UTXO set. This does not check scripts and sigs.
* @param[out] txfee Set to the transaction fee if successful.
* Preconditions: tx.IsCoinBase() is false.
*/
bool CheckTxInputs(const CTransaction& tx, CValidationState& state, const CCoinsViewCache& inputs, int nSpendHeight, CAmount& txfee);
} // namespace Consensus
/** Auxiliary functions for transaction validation (ideally should not be exposed) */
/**
* Count ECDSA signature operations the old-fashioned (pre-0.6) way
* @return number of sigops this transaction's outputs will produce when spent
* @see CTransaction::FetchInputs
*/
unsigned int GetLegacySigOpCount(const CTransaction& tx);
/**
* Count ECDSA signature operations in pay-to-script-hash inputs.
*
* @param[in] mapInputs Map of previous transactions that have outputs we're spending
* @return maximum number of sigops required to validate this transaction's inputs
* @see CTransaction::FetchInputs
*/
unsigned int GetP2SHSigOpCount(const CTransaction& tx, const CCoinsViewCache& mapInputs);
/**
* Count total signature operations for a transaction.
* @param[in] tx Transaction for which we are counting sigops
* @param[in] inputs Map of previous transactions that have outputs we're spending
* @param[out] flags Script verification flags
* @return Total signature operation count for a tx
*/
unsigned int GetTransactionSigOpCount(const CTransaction& tx, const CCoinsViewCache& inputs, int flags);
/**
* Check if transaction is final and can be included in a block with the
* specified height and time. Consensus critical.
*/
bool IsFinalTx(const CTransaction &tx, int nBlockHeight, int64_t nBlockTime);
/**
* Calculates the block height and previous block's median time past at
* which the transaction will be considered final in the context of BIP 68.
* Also removes from the vector of input heights any entries which did not
* correspond to sequence locked inputs as they do not affect the calculation.
*/
std::pair<int, int64_t> CalculateSequenceLocks(const CTransaction &tx, int flags, std::vector<int>* prevHeights, const CBlockIndex& block);
bool EvaluateSequenceLocks(const CBlockIndex& block, std::pair<int, int64_t> lockPair);
/**
* Check if transaction is final per BIP 68 sequence numbers and can be included in a block.
* Consensus critical. Takes as input a list of heights at which tx's inputs (in order) confirmed.
*/
bool SequenceLocks(const CTransaction &tx, int flags, std::vector<int>* prevHeights, const CBlockIndex& block);
#endif // BITCOIN_CONSENSUS_TX_VERIFY_H
| nmarley/dash | src/consensus/tx_verify.h | C | mit | 3,264 |
#include <compiler.h>
#if defined(CPUCORE_IA32) && defined(SUPPORT_MEMDBG32)
#include <common/strres.h>
#include <cpucore.h>
#include <pccore.h>
#include <io/iocore.h>
#include <generic/memdbg32.h>
#define MEMDBG32_MAXMEM 16
#define MEMDBG32_DATAPERLINE 128
#define MEMDBG32_LEFTMARGIN 8
typedef struct {
UINT mode;
int width;
int height;
int bpp;
CMNPAL pal[MEMDBG32_PALS];
} MEMDBG32;
static MEMDBG32 memdbg32;
static const char _mode0[] = "Real Mode";
static const char _mode1[] = "Protected Mode";
static const char _mode2[] = "Virtual86";
static const char *modestr[3] = {_mode0, _mode1, _mode2};
static const RGB32 md32pal[MEMDBG32_PALS] = {
RGB32D(0x33, 0x33, 0x33),
RGB32D(0x00, 0x00, 0x00),
RGB32D(0xff, 0xaa, 0x00),
RGB32D(0xff, 0x00, 0x00),
RGB32D(0x11, 0x88, 0x11),
RGB32D(0x00, 0xff, 0x00),
RGB32D(0xff, 0xff, 0xff)};
void memdbg32_initialize(void) {
ZeroMemory(&memdbg32, sizeof(memdbg32));
memdbg32.width = (MEMDBG32_BLOCKW * MEMDBG32_DATAPERLINE) + MEMDBG32_LEFTMARGIN;
memdbg32.height = (MEMDBG32_BLOCKH * 2 * MEMDBG32_MAXMEM) + 8;
}
void memdbg32_getsize(int *width, int *height) {
if (width) {
*width = memdbg32.width;
}
if (height) {
*height = memdbg32.height;
}
}
REG8 memdbg32_process(void) {
return(MEMDBG32_FLAGDRAW);
}
BOOL memdbg32_paint(CMNVRAM *vram, CMNPALCNV cnv, BOOL redraw) {
UINT mode;
UINT8 use[MEMDBG32_MAXMEM*MEMDBG32_DATAPERLINE*2 + 256];
UINT32 pd[1024];
UINT pdmax;
UINT i, j;
UINT32 pde;
UINT32 pdea;
UINT32 pte;
char str[4];
mode = 0;
if (CPU_STAT_PM) {
mode = 1;
}
if (CPU_STAT_VM86) {
mode = 2;
}
if (memdbg32.mode != mode) {
memdbg32.mode = mode;
redraw = TRUE;
}
if ((!redraw) && (!CPU_STAT_PAGING)) {
return(FALSE);
}
if (vram == NULL) {
return(FALSE);
}
if ((memdbg32.bpp != vram->bpp) || (redraw)) {
if (cnv == NULL) {
return(FALSE);
}
(*cnv)(memdbg32.pal, md32pal, MEMDBG32_PALS, vram->bpp);
memdbg32.bpp = vram->bpp;
}
cmndraw_fill(vram, 0, 0, memdbg32.width, memdbg32.height,
memdbg32.pal[MEMDBG32_PALBDR]);
ZeroMemory(use, sizeof(use));
if (CPU_STAT_PAGING) {
pdmax = 0;
for (i=0; i<1024; i++) {
pde = cpu_memoryread_d(CPU_STAT_PDE_BASE + (i * 4));
if (pde & CPU_PDE_PRESENT) {
for (j=0; j<pdmax; j++) {
if (!((pde ^ pd[j]) & CPU_PDE_BASEADDR_MASK)) {
break;
}
}
if (j < pdmax) {
pd[j] |= pde & CPU_PDE_ACCESS;
}
else {
pd[pdmax++] = pde;
}
}
}
for (i=0; i<pdmax; i++) {
pde = pd[i];
pdea = pde & CPU_PDE_BASEADDR_MASK;
for (j=0; j<1024; j++) {
pte = cpu_memoryread_d(pdea + (j * 4));
if ((pte & CPU_PTE_PRESENT) && (pte < 0x1000000/16*MEMDBG32_MAXMEM/128*MEMDBG32_DATAPERLINE)) {
if ((pde & CPU_PDE_ACCESS) && (pte & CPU_PTE_ACCESS)) {
use[pte >> 12] = MEMDBG32_PALPAGE1;
}
else if (!use[pte >> 12]) {
use[pte >> 12] = MEMDBG32_PALPAGE0;
}
}
}
}
}
else {
FillMemory(use, 256, MEMDBG32_PALREAL);
FillMemory(use + (0xfa0000 >> 12), (0x60000 >> 12), MEMDBG32_PALREAL);
if ((CPU_STAT_PM) && (pccore.extmem)) {
FillMemory(use + 256, MIN(MEMDBG32_DATAPERLINE * 2 * pccore.extmem, sizeof(use) - 256), MEMDBG32_PALPM);
}
}
for (i=0; i<MEMDBG32_MAXMEM*2; i++) {
for (j=0; j<MEMDBG32_DATAPERLINE; j++) {
cmndraw_fill(vram, MEMDBG32_LEFTMARGIN + j * MEMDBG32_BLOCKW, i * MEMDBG32_BLOCKH,
MEMDBG32_BLOCKW - 1, MEMDBG32_BLOCKH - 1,
memdbg32.pal[use[(i * MEMDBG32_DATAPERLINE) + j]]);
}
}
for (i=0; i<MEMDBG32_MAXMEM; i++) {
SPRINTF(str, "%x", i);
cmddraw_text8(vram, 0, i * MEMDBG32_BLOCKH * 2, str,
memdbg32.pal[MEMDBG32_PALTXT]);
}
cmddraw_text8(vram, 0, memdbg32.height - 8, modestr[mode],
memdbg32.pal[MEMDBG32_PALTXT]);
return(TRUE);
}
#endif
| AZO234/NP2kai | generic/memdbg32.c | C | mit | 3,962 |
//
// opjlib.h
// opjlib
//
// Created by Rich Stoner on 11/26/13.
// Copyright (c) 2013 WholeSlide. All rights reserved.
//
#import <Foundation/Foundation.h>
@interface opjlib : NSObject
@end
| richstoner/openjpeg-framework-ios | opjlib/opjlib.h | C | mit | 200 |
# -*- coding: utf-8 -*-
# Generated by Django 1.11 on 2017-11-01 20:02
from __future__ import unicode_literals
from django.db import migrations, models
import django.db.models.deletion
class Migration(migrations.Migration):
initial = True
dependencies = [
('phone_numbers', '0001_initial'),
('sims', '0001_initial'),
]
operations = [
migrations.AddField(
model_name='phonenumber',
name='related_sim',
field=models.ForeignKey(null=True, on_delete=django.db.models.deletion.CASCADE, related_name='phone_numbers', to='sims.Sim'),
),
]
| RobSpectre/garfield | garfield/phone_numbers/migrations/0002_phonenumber_related_sim.py | Python | mit | 626 |
package ch.spacebase.openclassic.api;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.UnsupportedEncodingException;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.ProtocolException;
import java.net.URL;
import java.net.URLEncoder;
import java.security.SecureRandom;
import java.util.HashMap;
import java.util.Map;
import ch.spacebase.openclassic.api.util.Constants;
/**
* Manages the server's web heartbeats.
*/
public final class HeartbeatManager {
private static final long salt = new SecureRandom().nextLong();
private static final Map<String, Runnable> customBeats = new HashMap<String, Runnable>();
private static String url = "";
/**
* Gets the server's current salt.
* @return The server's salt.
*/
public static long getSalt() {
return salt;
}
/**
* Gets the server's minecraft.net url.
* @return The url.
*/
public static String getURL() {
return url;
}
/**
* Sets the server's known minecraft.net url.
* @param url The url.
*/
public static void setURL(String url) {
HeartbeatManager.url = url;
}
/**
* Triggers a heartbeat.
*/
public static void beat() {
mineBeat();
womBeat();
for(String id : customBeats.keySet()) {
try {
customBeats.get(id).run();
} catch(Exception e) {
OpenClassic.getLogger().severe("Exception while running a custom heartbeat with the ID \"" + id + "\"!");
e.printStackTrace();
}
}
}
/**
* Adds a custom heartbeat to run when {@link beat()} is called.
* @param id ID of the custom heartbeat.
* @param run Runnable to call when beating.
*/
public static void addBeat(String id, Runnable run) {
customBeats.put(id, run);
}
/**
* Removes a custom heartbeat.
* @param id ID of the heartbeat.
*/
public static void removeBeat(String id) {
customBeats.remove(id);
}
/**
* Clears the custom heartbeat list.
*/
public static void clearBeats() {
customBeats.clear();
}
private static void mineBeat() {
URL url = null;
try {
url = new URL("https://minecraft.net/heartbeat.jsp?port=" + OpenClassic.getServer().getPort() + "&max=" + OpenClassic.getServer().getMaxPlayers() + "&name=" + URLEncoder.encode(Color.stripColor(OpenClassic.getServer().getServerName()), "UTF-8") + "&public=" + OpenClassic.getServer().isPublic() + "&version=" + Constants.PROTOCOL_VERSION + "&salt=" + salt + "&users=" + OpenClassic.getServer().getPlayers().size());
} catch(MalformedURLException e) {
OpenClassic.getLogger().severe("Malformed URL while attempting minecraft.net heartbeat?");
return;
} catch(UnsupportedEncodingException e) {
OpenClassic.getLogger().severe("UTF-8 URL encoding is unsupported on your system.");
return;
}
HttpURLConnection conn = null;
try {
conn = (HttpURLConnection) url.openConnection();
try {
conn.setRequestMethod("GET");
} catch (ProtocolException e) {
OpenClassic.getLogger().severe("Exception while performing minecraft.net heartbeat: Connection doesn't support GET...?");
return;
}
conn.setDoOutput(false);
conn.setDoInput(true);
conn.setUseCaches(false);
conn.setAllowUserInteraction(false);
conn.setRequestProperty("Content-type", "text/xml; charset=" + "UTF-8");
InputStream input = conn.getInputStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(input));
String result = reader.readLine();
reader.close();
input.close();
if(!HeartbeatManager.url.equals(result)) {
HeartbeatManager.url = result;
OpenClassic.getLogger().info(Color.GREEN + "The server's URL is now \"" + getURL() + "\".");
try {
File file = new File(OpenClassic.getGame().getDirectory(), "server-address.txt");
if(!file.exists()) file.createNewFile();
BufferedWriter writer = new BufferedWriter(new FileWriter(file));
writer.write(result);
writer.close();
} catch(IOException e) {
OpenClassic.getLogger().severe("Failed to save server address!");
e.printStackTrace();
}
}
} catch (IOException e) {
OpenClassic.getLogger().severe("Exception while performing minecraft.net heartbeat!");
e.printStackTrace();
} finally {
if (conn != null) conn.disconnect();
}
}
private static void womBeat() {
URL url = null;
try {
url = new URL("http://direct.worldofminecraft.com/hb.php?port=" + OpenClassic.getServer().getPort() + "&max=" + OpenClassic.getServer().getMaxPlayers() + "&name=" + URLEncoder.encode(Color.stripColor(OpenClassic.getServer().getServerName()), "UTF-8") + "&public=" + OpenClassic.getServer().isPublic() + "&version=" + Constants.PROTOCOL_VERSION + "&salt=" + salt + "&users=" + OpenClassic.getServer().getPlayers().size() + "&noforward=1");
} catch(MalformedURLException e) {
OpenClassic.getLogger().severe("Malformed URL while attempting WOM heartbeat?");
return;
} catch(UnsupportedEncodingException e) {
OpenClassic.getLogger().severe("UTF-8 URL encoding is unsupported on your system.");
return;
}
HttpURLConnection conn = null;
try {
conn = (HttpURLConnection) url.openConnection();
conn.setDoOutput(false);
conn.setDoInput(false);
conn.setUseCaches(false);
conn.setAllowUserInteraction(false);
conn.setRequestProperty("Content-type", "text/xml; charset=" + "UTF-8");
} catch (IOException e) {
OpenClassic.getLogger().severe("Exception while performing WOM heartbeat!");
e.printStackTrace();
} finally {
if (conn != null) conn.disconnect();
}
}
}
| good2000mo/OpenClassicAPI | src/main/java/ch/spacebase/openclassic/api/HeartbeatManager.java | Java | mit | 5,720 |
#! /bin/bash
BASHRC="$HOME/.bashrc"
echo "# java setting" >> $BASHRC
echo "export JAVA_HOME=\$(/usr/libexec/java_home)" >> $BASHRC
echo "" >> $BASHRC
| terracotta-ko/BashScripts | installScript/java_config.sh | Shell | mit | 152 |
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ParkingRampSimulator
{
public class ParkingRamp : ParkingConstruct
{
[Newtonsoft.Json.JsonIgnore]
public List<ParkingFloor> Floors { get; private set; }
public override bool IsFull
{
get { return OpenLocations < 1; }
}
public override int OpenLocations
{
get
{
return Floors.Sum(r => r.OpenLocations) - InQueue.Count;
}
}
public override int TotalLocations
{
get
{
return Floors.Sum(r => r.TotalLocations);
}
}
public ParkingRamp(ParkingConstruct parent, string name, int floorCount, int locationCount)
: base(parent, name)
{
Name = name;
Floors = new List<ParkingFloor>();
for (int i = 0; i < floorCount; i++)
{
Floors.Add(new ParkingFloor(this, string.Format("{0}-{1}", Name, i.ToString()), locationCount));
}
}
public override void Tick()
{
var openCount = Floors.Count(r => !r.IsFull);
if (openCount > 0)
{
var gateCapacity = (int)(Simulator.Interval.TotalSeconds / 10.0);
for (int i = 0; i < gateCapacity; i++)
{
var floorsWithRoom = Floors.Where(r => !r.IsFull).ToList();
if (InQueue.Count > 0 && floorsWithRoom.Count > 0)
{
var floor = Simulator.Random.Next(floorsWithRoom.Count);
floorsWithRoom[floor].InQueue.Enqueue(InQueue.Dequeue());
}
}
}
foreach (var item in Floors)
item.Tick();
base.Tick();
while (OutQueue.Count > 0)
Parent.OutQueue.Enqueue(OutQueue.Dequeue());
}
}
}
| rockfordlhotka/DistributedComputingDemo | src/ParkingSim/ParkingRampSimulator/ParkingRamp.cs | C# | mit | 2,080 |
package org.gojul.gojulutils.data;
/**
* Class {@code GojulPair} is a simple stupid pair class. This class is notably necessary
* when emulating JOIN in database and such a class does not exist natively in the JDK.
* This object is immutable as long as the object it contains are immutable. Since
* this object is not serializable it should not be stored in objects which could be serialized,
* especially Java HttpSession objects.
*
* @param <S> the type of the first object of the pair.
* @param <T> the type of the second object of the pair.
* @author jaubin
*/
public final class GojulPair<S, T> {
private final S first;
private final T second;
/**
* Constructor. Both parameters are nullable. Note that this constructor
* does not perform any defensive copy as it is not possible there.
*
* @param first the first object.
* @param second the second object.
*/
public GojulPair(final S first, final T second) {
this.first = first;
this.second = second;
}
/**
* Return the first object.
*
* @return the first object.
*/
public S getFirst() {
return first;
}
/**
* Return the second object.
*
* @return the second object.
*/
public T getSecond() {
return second;
}
/**
* {@inheritDoc}
*/
@Override
public boolean equals(final Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
GojulPair<?, ?> pair = (GojulPair<?, ?>) o;
if (getFirst() != null ? !getFirst().equals(pair.getFirst()) : pair.getFirst() != null) return false;
return getSecond() != null ? getSecond().equals(pair.getSecond()) : pair.getSecond() == null;
}
/**
* {@inheritDoc}
*/
@Override
public int hashCode() {
int result = getFirst() != null ? getFirst().hashCode() : 0;
result = 31 * result + (getSecond() != null ? getSecond().hashCode() : 0);
return result;
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
return "GojulPair{" +
"first=" + first +
", second=" + second +
'}';
}
}
| jaubin/gojulutils | src/main/java/org/gojul/gojulutils/data/GojulPair.java | Java | mit | 2,277 |
using System.Collections.Generic;
using System.Diagnostics.Contracts;
using Microsoft.CodeAnalysis;
namespace ErrorProne.NET.Extensions
{
public static class SyntaxNodeExtensions
{
public static IEnumerable<SyntaxNode> EnumerateParents(this SyntaxNode node)
{
Contract.Requires(node != null);
while (node.Parent != null)
{
yield return node.Parent;
node = node.Parent;
}
}
}
} | SergeyTeplyakov/ErrorProne.NET | src/ErrorProne.NET/ErrorProne.NET/Extensions/SyntaxNodeExtensions.cs | C# | mit | 496 |
/*--------------------------------------------
Created by Sina on 06/05/13.
Copyright (c) 2013 MIT. All rights reserved.
--------------------------------------------*/
#include "elements.h"
#include "mpi_compat.h"
#include "gcmc.h"
#include "memory.h"
#include "random.h"
#include "neighbor.h"
#include "ff_md.h"
#include "MAPP.h"
#include "atoms_md.h"
#include "comm.h"
#include "dynamic_md.h"
using namespace MAPP_NS;
/*--------------------------------------------
constructor
--------------------------------------------*/
GCMC::GCMC(AtomsMD*& __atoms,ForceFieldMD*&__ff,DynamicMD*& __dynamic,elem_type __gas_type,type0 __mu,type0 __T,int seed):
gas_type(__gas_type),
T(__T),
mu(__mu),
natms_lcl(__atoms->natms_lcl),
natms_ph(__atoms->natms_ph),
cut_sq(__ff->cut_sq),
s_lo(__atoms->comm.s_lo),
s_hi(__atoms->comm.s_hi),
dynamic(__dynamic),
world(__atoms->comm.world),
atoms(__atoms),
ff(__ff),
ielem(gas_type)
{
random=new Random(seed);
s_trials=new type0*[__dim__];
*s_trials=NULL;
del_ids=NULL;
del_ids_sz=del_ids_cpcty=0;
vars=lcl_vars=NULL;
}
/*--------------------------------------------
destructor
--------------------------------------------*/
GCMC::~GCMC()
{
delete [] del_ids;
delete [] s_trials;
delete random;
}
/*--------------------------------------------
--------------------------------------------*/
void GCMC::add_del_id(int* new_ids,int no)
{
if(del_ids_sz+no>del_ids_cpcty)
{
int* del_ids_=new int[del_ids_sz+no];
memcpy(del_ids_,del_ids,del_ids_sz*sizeof(int));
del_ids_cpcty=del_ids_sz+no;
delete [] del_ids;
del_ids=del_ids_;
}
memcpy(del_ids+del_ids_sz,new_ids,sizeof(int)*no);
del_ids_sz+=no;
}
/*--------------------------------------------
--------------------------------------------*/
int GCMC::get_new_id()
{
if(del_ids_sz)
{
del_ids_sz--;
return del_ids[del_ids_sz];
}
else
{
max_id++;
return max_id;
}
}
/*--------------------------------------------
--------------------------------------------*/
void GCMC::init()
{
cut=ff->cut[ielem][0];
for(size_t i=1;i<atoms->elements.nelems;i++)
cut=MAX(cut,ff->cut[ielem][i]);
gas_mass=atoms->elements.masses[gas_type];
kbT=atoms->kB*T;
beta=1.0/kbT;
lambda=atoms->hP/sqrt(2.0*M_PI*kbT*gas_mass);
sigma=sqrt(kbT/gas_mass);
z_fac=1.0;
for(int i=0;i<__dim__;i++) z_fac/=lambda;
z_fac*=exp(beta*mu);
vol=1.0;
for(int i=0;i<__dim__;i++)vol*=atoms->H[i][i];
id_type max_id_=0;
id_type* id=atoms->id->begin();
for(int i=0;i<natms_lcl;i++)
max_id_=MAX(id[i],max_id_);
MPI_Allreduce(&max_id_,&max_id,1,Vec<id_type>::MPI_T,MPI_MAX,world);
for(int i=0;i<del_ids_sz;i++)
max_id=MAX(max_id,del_ids[i]);
ngas_lcl=0;
elem_type* elem=atoms->elem->begin();
for(int i=0;i<natms_lcl;i++)
if(elem[i]==gas_type) ngas_lcl++;
MPI_Allreduce(&ngas_lcl,&ngas,1,MPI_INT,MPI_SUM,world);
}
/*--------------------------------------------
--------------------------------------------*/
void GCMC::fin()
{
}
/*--------------------------------------------
--------------------------------------------*/
void GCMC::box_setup()
{
int sz=0;
max_ntrial_atms=1;
for(int i=0;i<__dim__;i++)
{
type0 tmp=0.0;
for(int j=i;j<__dim__;j++)
tmp+=atoms->B[j][i]*atoms->B[j][i];
cut_s[i]=sqrt(tmp)*cut;
s_lo_ph[i]=s_lo[i]-cut_s[i];
s_hi_ph[i]=s_hi[i]+cut_s[i];
nimages_per_dim[i][0]=static_cast<int>(floor(s_hi_ph[i]));
nimages_per_dim[i][1]=-static_cast<int>(floor(s_lo_ph[i]));
max_ntrial_atms*=1+nimages_per_dim[i][0]+nimages_per_dim[i][1];
sz+=1+nimages_per_dim[i][0]+nimages_per_dim[i][1];
}
*s_trials=new type0[sz];
for(int i=1;i<__dim__;i++)
s_trials[i]=s_trials[i-1]+1+nimages_per_dim[i-1][0]+nimages_per_dim[i-1][1];
}
/*--------------------------------------------
--------------------------------------------*/
void GCMC::box_dismantle()
{
delete [] *s_trials;
*s_trials=NULL;
}
| sinamoeini/mapp4py | src/gcmc.cpp | C++ | mit | 4,193 |
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
var app = {
// Application Constructor
initialize: function() {
this.bindEvents();
},
// Bind Event Listeners
//
// Bind any events that are required on startup. Common events are:
// 'load', 'deviceready', 'offline', and 'online'.
bindEvents: function() {
document.addEventListener('deviceready', this.onDeviceReady, false);
},
// deviceready Event Handler
//
// The scope of 'this' is the event. In order to call the 'receivedEvent'
// function, we must explicitly call 'app.receivedEvent(...);'
onDeviceReady: function() {
app.receivedEvent('deviceready');
},
// Update DOM on a Received Event
receivedEvent: function(id) {
}
};
app.initialize();
| ttosi/moodbeam | mobile/www/js/index.js | JavaScript | mit | 1,553 |
#include <ESP8266WiFi.h>
#include <PubSubClient.h>
#include "mqtt.h"
// Connect to MQTT and set up subscriptions based on configuration
void MQTT::connect() {
// Connect to broker
this->mqttClient.setServer(this->host, this->port);
mqttClient.connect(this->clientId);
Serial.print("Connected to MQTT, with server: ");
Serial.print(this->host);
Serial.print(", port: ");
Serial.print(this->port);
Serial.print(", with client ID: ");
Serial.println(this->clientId);
// Send ping
ping();
}
// Send ping to MQTT broker
void MQTT::ping() {
char payload[50];
sprintf(payload, "%s ok", this->clientId);
this->mqttClient.publish("/status", payload);
Serial.println("Sent ping to broker");
}
| capmake/robot-firmware | src/mqtt.cpp | C++ | mit | 745 |
using System.Collections.Generic;
namespace SmartMeter.Business.Interface.Mapper {
public interface IMapTelegram {
Persistence.Interface.ITelegram Map(ITelegram businessTelegram);
Business.Interface.ITelegram Map(Persistence.Interface.ITelegram persistenceTelegram);
IEnumerable<ITelegram> Map(IEnumerable<Persistence.Interface.ITelegram> persistenceTelegrams);
}
} | jeroen-corsius/smart-meter | SmartMeter.Business.Interface/Mapper/IMapTelegram.cs | C# | mit | 393 |
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>NTU D3js HW01</title>
<link rel="stylesheet" type="text/css" href="main.css">
<script src="https://d3js.org/d3.v3.min.js"></script>
</head>
<body>
<!-- page 4 -->
關 關 雎 鳩<br>
在 河 之 洲<br>
<!-- 註 解 此 行 -->
窈 窕 淑 女<br>
君 子 好 逑<br><br>
詩.周南.關睢
<!-- page 8 -->
<div>
<hr>
<h1>小王子</h1>
<p>然後他又回到狐狸那裡。</p>
<p>「再見了!」小王子說。</p>
<p>「再見了!」狐狸說。</p>
<blockquote>
「我的秘密很簡單:<strong>唯有心才能看得清楚,眼睛是看不到真正重要的東西的</strong>。」
</blockquote>
<p>小王子複述一遍:「真正的東西不是用眼睛可以看得到的。」</p>
</div>
<hr>
<!-- page 13 -->
<table border="1">
<tr>
<th colspan = "4">直轄市長辦公地址</th>
</tr>
<tr>
<th>直轄市</th>
<th>職稱</th>
<th>姓名</th>
<th>辦公地址</th>
</tr>
<tr>
<td>臺北市</td>
<td rowspan = "6">市長</td>
<td>柯文哲</td>
<td>臺北市信義區西村里市府路1號</td>
</tr>
<tr>
<td>高雄市</td>
<td>陳菊</td>
<td>高雄市苓雅區晴朗里四維3路2號</td>
</tr>
<tr>
<td>新北市</td>
<td>朱立倫</td>
<td>新北市板橋區中山路1段161號</td>
</tr>
<tr>
<td>臺中市</td>
<td>林佳龍</td>
<td>臺中市西屯區臺灣大道3段99號</td>
</tr>
<tr>
<td>臺南市</td>
<td>賴清德</td>
<td>臺南市安平區永華路2段6號</td>
</tr>
<tr>
<td>桃園市</td>
<td>鄭文燦</td>
<td>桃園市桃園區光興里縣府路1號</td>
</tr>
</table>
<hr>
<!-- page 17 -->
<a href="http://www.google.com/doodles/evidence-of-water-found-on-mars" target = "_new">
<img src="http://www.google.com/logos/doodles/2015/evidence-of-water-found-on-mars-5652760466817024.2-hp2x.gif" alt="在火星上發現水存在的證據" width = "400">
</a>
<hr>
<!--02 page 58 -->
<h1>一個1911~2016之間的數字</h1>
<p id='num1'></p>
<input type="button" onclick="launch()" value="啟動">
<script>
function launch(){
var number = Math.floor(Math.random()*106)+1911;
d3.select('#num1').text(number);
}
</script>
</body>
</html> | bill9800/NTU_D3js | HW01/index.html | HTML | mit | 2,618 |
define("helios/Helios-Debugger", ["amber/boot", "amber_core/Kernel-Objects", "helios/Helios-Core", "helios/Helios-Workspace"], function($boot){
var smalltalk=$boot.vm,nil=$boot.nil,_st=$boot.asReceiver,globals=$boot.globals;
smalltalk.addPackage('Helios-Debugger');
smalltalk.packages["Helios-Debugger"].transport = {"type":"amd","amdNamespace":"helios"};
smalltalk.addClass('HLContextInspectorDecorator', globals.Object, ['context'], 'Helios-Debugger');
smalltalk.addMethod(
smalltalk.method({
selector: "context",
protocol: 'accessing',
fn: function (){
var self=this;
var $1;
$1=self["@context"];
return $1;
},
args: [],
source: "context\x0a\x09^ context",
messageSends: [],
referencedClasses: []
}),
globals.HLContextInspectorDecorator);
smalltalk.addMethod(
smalltalk.method({
selector: "evaluate:on:",
protocol: 'evaluating',
fn: function (aString,anEvaluator){
var self=this;
return smalltalk.withContext(function($ctx1) {
var $1;
$1=_st(self._context())._evaluate_on_(aString,anEvaluator);
return $1;
}, function($ctx1) {$ctx1.fill(self,"evaluate:on:",{aString:aString,anEvaluator:anEvaluator},globals.HLContextInspectorDecorator)})},
args: ["aString", "anEvaluator"],
source: "evaluate: aString on: anEvaluator\x0a\x09^ self context evaluate: aString on: anEvaluator",
messageSends: ["evaluate:on:", "context"],
referencedClasses: []
}),
globals.HLContextInspectorDecorator);
smalltalk.addMethod(
smalltalk.method({
selector: "initializeFromContext:",
protocol: 'initialization',
fn: function (aContext){
var self=this;
self["@context"]=aContext;
return self},
args: ["aContext"],
source: "initializeFromContext: aContext\x0a\x09context := aContext",
messageSends: [],
referencedClasses: []
}),
globals.HLContextInspectorDecorator);
smalltalk.addMethod(
smalltalk.method({
selector: "inspectOn:",
protocol: 'inspecting',
fn: function (anInspector){
var self=this;
var variables,inspectedContext;
function $Dictionary(){return globals.Dictionary||(typeof Dictionary=="undefined"?nil:Dictionary)}
return smalltalk.withContext(function($ctx1) {
var $1,$2,$3,$4,$receiver;
variables=_st($Dictionary())._new();
inspectedContext=self._context();
$1=variables;
$2=_st(inspectedContext)._locals();
$ctx1.sendIdx["locals"]=1;
_st($1)._addAll_($2);
$ctx1.sendIdx["addAll:"]=1;
_st((function(){
return smalltalk.withContext(function($ctx2) {
return _st(_st(inspectedContext)._notNil())._and_((function(){
return smalltalk.withContext(function($ctx3) {
return _st(inspectedContext)._isBlockContext();
}, function($ctx3) {$ctx3.fillBlock({},$ctx2,2)})}));
}, function($ctx2) {$ctx2.fillBlock({},$ctx1,1)})}))._whileTrue_((function(){
return smalltalk.withContext(function($ctx2) {
inspectedContext=_st(inspectedContext)._outerContext();
inspectedContext;
$3=inspectedContext;
if(($receiver = $3) == null || $receiver.isNil){
return $3;
} else {
return _st(variables)._addAll_(_st(inspectedContext)._locals());
};
}, function($ctx2) {$ctx2.fillBlock({},$ctx1,3)})}));
_st(anInspector)._setLabel_("Context");
$4=_st(anInspector)._setVariables_(variables);
return self}, function($ctx1) {$ctx1.fill(self,"inspectOn:",{anInspector:anInspector,variables:variables,inspectedContext:inspectedContext},globals.HLContextInspectorDecorator)})},
args: ["anInspector"],
source: "inspectOn: anInspector\x0a\x09| variables inspectedContext |\x0a\x09\x0a\x09variables := Dictionary new.\x0a\x09inspectedContext := self context.\x0a\x09\x0a\x09variables addAll: inspectedContext locals.\x0a\x09\x0a\x09[ inspectedContext notNil and: [ inspectedContext isBlockContext ] ] whileTrue: [\x0a\x09\x09inspectedContext := inspectedContext outerContext.\x0a\x09\x09inspectedContext ifNotNil: [\x0a\x09\x09\x09variables addAll: inspectedContext locals ] ].\x0a\x09\x0a\x09anInspector\x0a\x09\x09setLabel: 'Context';\x0a\x09\x09setVariables: variables",
messageSends: ["new", "context", "addAll:", "locals", "whileTrue:", "and:", "notNil", "isBlockContext", "outerContext", "ifNotNil:", "setLabel:", "setVariables:"],
referencedClasses: ["Dictionary"]
}),
globals.HLContextInspectorDecorator);
smalltalk.addMethod(
smalltalk.method({
selector: "on:",
protocol: 'instance creation',
fn: function (aContext){
var self=this;
return smalltalk.withContext(function($ctx1) {
var $2,$3,$1;
$2=self._new();
_st($2)._initializeFromContext_(aContext);
$3=_st($2)._yourself();
$1=$3;
return $1;
}, function($ctx1) {$ctx1.fill(self,"on:",{aContext:aContext},globals.HLContextInspectorDecorator.klass)})},
args: ["aContext"],
source: "on: aContext\x0a\x09^ self new\x0a\x09\x09initializeFromContext: aContext;\x0a\x09\x09yourself",
messageSends: ["initializeFromContext:", "new", "yourself"],
referencedClasses: []
}),
globals.HLContextInspectorDecorator.klass);
smalltalk.addClass('HLDebugger', globals.HLFocusableWidget, ['model', 'stackListWidget', 'codeWidget', 'inspectorWidget'], 'Helios-Debugger');
globals.HLDebugger.comment="I am the main widget for the Helios debugger.";
smalltalk.addMethod(
smalltalk.method({
selector: "codeWidget",
protocol: 'widgets',
fn: function (){
var self=this;
function $HLDebuggerCodeWidget(){return globals.HLDebuggerCodeWidget||(typeof HLDebuggerCodeWidget=="undefined"?nil:HLDebuggerCodeWidget)}
function $HLDebuggerCodeModel(){return globals.HLDebuggerCodeModel||(typeof HLDebuggerCodeModel=="undefined"?nil:HLDebuggerCodeModel)}
return smalltalk.withContext(function($ctx1) {
var $2,$3,$4,$6,$7,$8,$9,$5,$10,$1,$receiver;
$2=self["@codeWidget"];
if(($receiver = $2) == null || $receiver.isNil){
$3=_st($HLDebuggerCodeWidget())._new();
$ctx1.sendIdx["new"]=1;
$4=$3;
$6=_st($HLDebuggerCodeModel())._new();
$7=$6;
$8=self._model();
$ctx1.sendIdx["model"]=1;
_st($7)._debuggerModel_($8);
$9=_st($6)._yourself();
$ctx1.sendIdx["yourself"]=1;
$5=$9;
_st($4)._model_($5);
_st($3)._browserModel_(self._model());
$10=_st($3)._yourself();
self["@codeWidget"]=$10;
$1=self["@codeWidget"];
} else {
$1=$2;
};
return $1;
}, function($ctx1) {$ctx1.fill(self,"codeWidget",{},globals.HLDebugger)})},
args: [],
source: "codeWidget\x0a\x09^ codeWidget ifNil: [ codeWidget := HLDebuggerCodeWidget new\x0a\x09\x09model: (HLDebuggerCodeModel new\x0a\x09\x09\x09debuggerModel: self model;\x0a\x09\x09\x09yourself);\x0a\x09\x09browserModel: self model;\x0a\x09\x09yourself ]",
messageSends: ["ifNil:", "model:", "new", "debuggerModel:", "model", "yourself", "browserModel:"],
referencedClasses: ["HLDebuggerCodeWidget", "HLDebuggerCodeModel"]
}),
globals.HLDebugger);
smalltalk.addMethod(
smalltalk.method({
selector: "cssClass",
protocol: 'accessing',
fn: function (){
var self=this;
return smalltalk.withContext(function($ctx1) {
var $2,$1;
$2=($ctx1.supercall = true, globals.HLDebugger.superclass.fn.prototype._cssClass.apply(_st(self), []));
$ctx1.supercall = false;
$1=_st($2).__comma(" hl_debugger");
return $1;
}, function($ctx1) {$ctx1.fill(self,"cssClass",{},globals.HLDebugger)})},
args: [],
source: "cssClass\x0a\x09^ super cssClass, ' hl_debugger'",
messageSends: [",", "cssClass"],
referencedClasses: []
}),
globals.HLDebugger);
smalltalk.addMethod(
smalltalk.method({
selector: "focus",
protocol: 'actions',
fn: function (){
var self=this;
return smalltalk.withContext(function($ctx1) {
_st(self._stackListWidget())._focus();
return self}, function($ctx1) {$ctx1.fill(self,"focus",{},globals.HLDebugger)})},
args: [],
source: "focus\x0a\x09self stackListWidget focus",
messageSends: ["focus", "stackListWidget"],
referencedClasses: []
}),
globals.HLDebugger);
smalltalk.addMethod(
smalltalk.method({
selector: "initializeFromError:",
protocol: 'initialization',
fn: function (anError){
var self=this;
function $HLDebuggerModel(){return globals.HLDebuggerModel||(typeof HLDebuggerModel=="undefined"?nil:HLDebuggerModel)}
return smalltalk.withContext(function($ctx1) {
self["@model"]=_st($HLDebuggerModel())._on_(anError);
self._observeModel();
return self}, function($ctx1) {$ctx1.fill(self,"initializeFromError:",{anError:anError},globals.HLDebugger)})},
args: ["anError"],
source: "initializeFromError: anError\x0a\x09model := HLDebuggerModel on: anError.\x0a\x09self observeModel",
messageSends: ["on:", "observeModel"],
referencedClasses: ["HLDebuggerModel"]
}),
globals.HLDebugger);
smalltalk.addMethod(
smalltalk.method({
selector: "inspectorWidget",
protocol: 'widgets',
fn: function (){
var self=this;
function $HLInspectorWidget(){return globals.HLInspectorWidget||(typeof HLInspectorWidget=="undefined"?nil:HLInspectorWidget)}
return smalltalk.withContext(function($ctx1) {
var $2,$1,$receiver;
$2=self["@inspectorWidget"];
if(($receiver = $2) == null || $receiver.isNil){
self["@inspectorWidget"]=_st($HLInspectorWidget())._new();
$1=self["@inspectorWidget"];
} else {
$1=$2;
};
return $1;
}, function($ctx1) {$ctx1.fill(self,"inspectorWidget",{},globals.HLDebugger)})},
args: [],
source: "inspectorWidget\x0a\x09^ inspectorWidget ifNil: [ \x0a\x09\x09inspectorWidget := HLInspectorWidget new ]",
messageSends: ["ifNil:", "new"],
referencedClasses: ["HLInspectorWidget"]
}),
globals.HLDebugger);
smalltalk.addMethod(
smalltalk.method({
selector: "model",
protocol: 'accessing',
fn: function (){
var self=this;
function $HLDebuggerModel(){return globals.HLDebuggerModel||(typeof HLDebuggerModel=="undefined"?nil:HLDebuggerModel)}
return smalltalk.withContext(function($ctx1) {
var $2,$1,$receiver;
$2=self["@model"];
if(($receiver = $2) == null || $receiver.isNil){
self["@model"]=_st($HLDebuggerModel())._new();
$1=self["@model"];
} else {
$1=$2;
};
return $1;
}, function($ctx1) {$ctx1.fill(self,"model",{},globals.HLDebugger)})},
args: [],
source: "model\x0a\x09^ model ifNil: [ model := HLDebuggerModel new ]",
messageSends: ["ifNil:", "new"],
referencedClasses: ["HLDebuggerModel"]
}),
globals.HLDebugger);
smalltalk.addMethod(
smalltalk.method({
selector: "observeModel",
protocol: 'actions',
fn: function (){
var self=this;
function $HLDebuggerContextSelected(){return globals.HLDebuggerContextSelected||(typeof HLDebuggerContextSelected=="undefined"?nil:HLDebuggerContextSelected)}
function $HLDebuggerStepped(){return globals.HLDebuggerStepped||(typeof HLDebuggerStepped=="undefined"?nil:HLDebuggerStepped)}
function $HLDebuggerProceeded(){return globals.HLDebuggerProceeded||(typeof HLDebuggerProceeded=="undefined"?nil:HLDebuggerProceeded)}
return smalltalk.withContext(function($ctx1) {
var $1,$2;
$1=_st(self._model())._announcer();
_st($1)._on_send_to_($HLDebuggerContextSelected(),"onContextSelected:",self);
$ctx1.sendIdx["on:send:to:"]=1;
_st($1)._on_send_to_($HLDebuggerStepped(),"onDebuggerStepped:",self);
$ctx1.sendIdx["on:send:to:"]=2;
$2=_st($1)._on_send_to_($HLDebuggerProceeded(),"onDebuggerProceeded",self);
return self}, function($ctx1) {$ctx1.fill(self,"observeModel",{},globals.HLDebugger)})},
args: [],
source: "observeModel\x0a\x09self model announcer \x0a\x09\x09on: HLDebuggerContextSelected\x0a\x09\x09send: #onContextSelected:\x0a\x09\x09to: self;\x0a\x09\x09\x0a\x09\x09on: HLDebuggerStepped\x0a\x09\x09send: #onDebuggerStepped:\x0a\x09\x09to: self;\x0a\x09\x09\x0a\x09\x09on: HLDebuggerProceeded\x0a\x09\x09send: #onDebuggerProceeded\x0a\x09\x09to: self",
messageSends: ["on:send:to:", "announcer", "model"],
referencedClasses: ["HLDebuggerContextSelected", "HLDebuggerStepped", "HLDebuggerProceeded"]
}),
globals.HLDebugger);
smalltalk.addMethod(
smalltalk.method({
selector: "onContextSelected:",
protocol: 'reactions',
fn: function (anAnnouncement){
var self=this;
function $HLContextInspectorDecorator(){return globals.HLContextInspectorDecorator||(typeof HLContextInspectorDecorator=="undefined"?nil:HLContextInspectorDecorator)}
return smalltalk.withContext(function($ctx1) {
_st(self._inspectorWidget())._inspect_(_st($HLContextInspectorDecorator())._on_(_st(anAnnouncement)._context()));
return self}, function($ctx1) {$ctx1.fill(self,"onContextSelected:",{anAnnouncement:anAnnouncement},globals.HLDebugger)})},
args: ["anAnnouncement"],
source: "onContextSelected: anAnnouncement\x0a\x09self inspectorWidget inspect: (HLContextInspectorDecorator on: anAnnouncement context)",
messageSends: ["inspect:", "inspectorWidget", "on:", "context"],
referencedClasses: ["HLContextInspectorDecorator"]
}),
globals.HLDebugger);
smalltalk.addMethod(
smalltalk.method({
selector: "onDebuggerProceeded",
protocol: 'reactions',
fn: function (){
var self=this;
return smalltalk.withContext(function($ctx1) {
self._removeTab();
return self}, function($ctx1) {$ctx1.fill(self,"onDebuggerProceeded",{},globals.HLDebugger)})},
args: [],
source: "onDebuggerProceeded\x0a\x09self removeTab",
messageSends: ["removeTab"],
referencedClasses: []
}),
globals.HLDebugger);
smalltalk.addMethod(
smalltalk.method({
selector: "onDebuggerStepped:",
protocol: 'reactions',
fn: function (anAnnouncement){
var self=this;
function $HLContextInspectorDecorator(){return globals.HLContextInspectorDecorator||(typeof HLContextInspectorDecorator=="undefined"?nil:HLContextInspectorDecorator)}
return smalltalk.withContext(function($ctx1) {
var $1;
$1=_st(self._model())._atEnd();
if(smalltalk.assert($1)){
self._removeTab();
};
_st(self._inspectorWidget())._inspect_(_st($HLContextInspectorDecorator())._on_(_st(anAnnouncement)._context()));
_st(self._stackListWidget())._refresh();
return self}, function($ctx1) {$ctx1.fill(self,"onDebuggerStepped:",{anAnnouncement:anAnnouncement},globals.HLDebugger)})},
args: ["anAnnouncement"],
source: "onDebuggerStepped: anAnnouncement\x0a\x09self model atEnd ifTrue: [ self removeTab ].\x0a\x09\x0a\x09self inspectorWidget inspect: (HLContextInspectorDecorator on: anAnnouncement context).\x0a\x09self stackListWidget refresh",
messageSends: ["ifTrue:", "atEnd", "model", "removeTab", "inspect:", "inspectorWidget", "on:", "context", "refresh", "stackListWidget"],
referencedClasses: ["HLContextInspectorDecorator"]
}),
globals.HLDebugger);
smalltalk.addMethod(
smalltalk.method({
selector: "registerBindingsOn:",
protocol: 'keybindings',
fn: function (aBindingGroup){
var self=this;
function $HLToolCommand(){return globals.HLToolCommand||(typeof HLToolCommand=="undefined"?nil:HLToolCommand)}
return smalltalk.withContext(function($ctx1) {
_st($HLToolCommand())._registerConcreteClassesOn_for_(aBindingGroup,self._model());
return self}, function($ctx1) {$ctx1.fill(self,"registerBindingsOn:",{aBindingGroup:aBindingGroup},globals.HLDebugger)})},
args: ["aBindingGroup"],
source: "registerBindingsOn: aBindingGroup\x0a\x09HLToolCommand \x0a\x09\x09registerConcreteClassesOn: aBindingGroup \x0a\x09\x09for: self model",
messageSends: ["registerConcreteClassesOn:for:", "model"],
referencedClasses: ["HLToolCommand"]
}),
globals.HLDebugger);
smalltalk.addMethod(
smalltalk.method({
selector: "renderContentOn:",
protocol: 'rendering',
fn: function (html){
var self=this;
function $HLContainer(){return globals.HLContainer||(typeof HLContainer=="undefined"?nil:HLContainer)}
function $HLVerticalSplitter(){return globals.HLVerticalSplitter||(typeof HLVerticalSplitter=="undefined"?nil:HLVerticalSplitter)}
function $HLHorizontalSplitter(){return globals.HLHorizontalSplitter||(typeof HLHorizontalSplitter=="undefined"?nil:HLHorizontalSplitter)}
return smalltalk.withContext(function($ctx1) {
var $2,$1;
self._renderHeadOn_(html);
$2=_st($HLVerticalSplitter())._with_with_(self._codeWidget(),_st($HLHorizontalSplitter())._with_with_(self._stackListWidget(),self._inspectorWidget()));
$ctx1.sendIdx["with:with:"]=1;
$1=_st($HLContainer())._with_($2);
_st(html)._with_($1);
$ctx1.sendIdx["with:"]=1;
return self}, function($ctx1) {$ctx1.fill(self,"renderContentOn:",{html:html},globals.HLDebugger)})},
args: ["html"],
source: "renderContentOn: html\x0a\x09self renderHeadOn: html.\x0a\x09html with: (HLContainer with: (HLVerticalSplitter\x0a\x09\x09with: self codeWidget\x0a\x09\x09with: (HLHorizontalSplitter\x0a\x09\x09\x09with: self stackListWidget\x0a\x09\x09\x09with: self inspectorWidget)))",
messageSends: ["renderHeadOn:", "with:", "with:with:", "codeWidget", "stackListWidget", "inspectorWidget"],
referencedClasses: ["HLContainer", "HLVerticalSplitter", "HLHorizontalSplitter"]
}),
globals.HLDebugger);
smalltalk.addMethod(
smalltalk.method({
selector: "renderHeadOn:",
protocol: 'rendering',
fn: function (html){
var self=this;
return smalltalk.withContext(function($ctx1) {
var $1,$2;
$1=_st(html)._div();
_st($1)._class_("head");
$2=_st($1)._with_((function(){
return smalltalk.withContext(function($ctx2) {
return _st(_st(html)._h2())._with_(_st(_st(self._model())._error())._messageText());
}, function($ctx2) {$ctx2.fillBlock({},$ctx1,1)})}));
$ctx1.sendIdx["with:"]=1;
return self}, function($ctx1) {$ctx1.fill(self,"renderHeadOn:",{html:html},globals.HLDebugger)})},
args: ["html"],
source: "renderHeadOn: html\x0a\x09html div \x0a\x09\x09class: 'head'; \x0a\x09\x09with: [ html h2 with: self model error messageText ]",
messageSends: ["class:", "div", "with:", "h2", "messageText", "error", "model"],
referencedClasses: []
}),
globals.HLDebugger);
smalltalk.addMethod(
smalltalk.method({
selector: "stackListWidget",
protocol: 'widgets',
fn: function (){
var self=this;
function $HLStackListWidget(){return globals.HLStackListWidget||(typeof HLStackListWidget=="undefined"?nil:HLStackListWidget)}
return smalltalk.withContext(function($ctx1) {
var $2,$3,$4,$1,$receiver;
$2=self["@stackListWidget"];
if(($receiver = $2) == null || $receiver.isNil){
$3=_st($HLStackListWidget())._on_(self._model());
_st($3)._next_(self._codeWidget());
$4=_st($3)._yourself();
self["@stackListWidget"]=$4;
$1=self["@stackListWidget"];
} else {
$1=$2;
};
return $1;
}, function($ctx1) {$ctx1.fill(self,"stackListWidget",{},globals.HLDebugger)})},
args: [],
source: "stackListWidget\x0a\x09^ stackListWidget ifNil: [ \x0a\x09\x09stackListWidget := (HLStackListWidget on: self model)\x0a\x09\x09\x09next: self codeWidget;\x0a\x09\x09\x09yourself ]",
messageSends: ["ifNil:", "next:", "on:", "model", "codeWidget", "yourself"],
referencedClasses: ["HLStackListWidget"]
}),
globals.HLDebugger);
smalltalk.addMethod(
smalltalk.method({
selector: "unregister",
protocol: 'actions',
fn: function (){
var self=this;
return smalltalk.withContext(function($ctx1) {
($ctx1.supercall = true, globals.HLDebugger.superclass.fn.prototype._unregister.apply(_st(self), []));
$ctx1.supercall = false;
$ctx1.sendIdx["unregister"]=1;
_st(self._inspectorWidget())._unregister();
return self}, function($ctx1) {$ctx1.fill(self,"unregister",{},globals.HLDebugger)})},
args: [],
source: "unregister\x0a\x09super unregister.\x0a\x09self inspectorWidget unregister",
messageSends: ["unregister", "inspectorWidget"],
referencedClasses: []
}),
globals.HLDebugger);
smalltalk.addMethod(
smalltalk.method({
selector: "on:",
protocol: 'instance creation',
fn: function (anError){
var self=this;
return smalltalk.withContext(function($ctx1) {
var $2,$3,$1;
$2=self._new();
_st($2)._initializeFromError_(anError);
$3=_st($2)._yourself();
$1=$3;
return $1;
}, function($ctx1) {$ctx1.fill(self,"on:",{anError:anError},globals.HLDebugger.klass)})},
args: ["anError"],
source: "on: anError\x0a\x09^ self new\x0a\x09\x09initializeFromError: anError;\x0a\x09\x09yourself",
messageSends: ["initializeFromError:", "new", "yourself"],
referencedClasses: []
}),
globals.HLDebugger.klass);
smalltalk.addMethod(
smalltalk.method({
selector: "tabClass",
protocol: 'accessing',
fn: function (){
var self=this;
return "debugger";
},
args: [],
source: "tabClass\x0a\x09^ 'debugger'",
messageSends: [],
referencedClasses: []
}),
globals.HLDebugger.klass);
smalltalk.addMethod(
smalltalk.method({
selector: "tabLabel",
protocol: 'accessing',
fn: function (){
var self=this;
return "Debugger";
},
args: [],
source: "tabLabel\x0a\x09^ 'Debugger'",
messageSends: [],
referencedClasses: []
}),
globals.HLDebugger.klass);
smalltalk.addClass('HLDebuggerCodeModel', globals.HLCodeModel, ['debuggerModel'], 'Helios-Debugger');
smalltalk.addMethod(
smalltalk.method({
selector: "debuggerModel",
protocol: 'accessing',
fn: function (){
var self=this;
var $1;
$1=self["@debuggerModel"];
return $1;
},
args: [],
source: "debuggerModel\x0a\x09^ debuggerModel",
messageSends: [],
referencedClasses: []
}),
globals.HLDebuggerCodeModel);
smalltalk.addMethod(
smalltalk.method({
selector: "debuggerModel:",
protocol: 'accessing',
fn: function (anObject){
var self=this;
self["@debuggerModel"]=anObject;
return self},
args: ["anObject"],
source: "debuggerModel: anObject\x0a\x09debuggerModel := anObject",
messageSends: [],
referencedClasses: []
}),
globals.HLDebuggerCodeModel);
smalltalk.addMethod(
smalltalk.method({
selector: "doIt:",
protocol: 'actions',
fn: function (aString){
var self=this;
function $ErrorHandler(){return globals.ErrorHandler||(typeof ErrorHandler=="undefined"?nil:ErrorHandler)}
return smalltalk.withContext(function($ctx1) {
var $1;
$1=_st((function(){
return smalltalk.withContext(function($ctx2) {
return _st(self._debuggerModel())._evaluate_(aString);
}, function($ctx2) {$ctx2.fillBlock({},$ctx1,1)})}))._tryCatch_((function(e){
return smalltalk.withContext(function($ctx2) {
_st($ErrorHandler())._handleError_(e);
return nil;
}, function($ctx2) {$ctx2.fillBlock({e:e},$ctx1,2)})}));
return $1;
}, function($ctx1) {$ctx1.fill(self,"doIt:",{aString:aString},globals.HLDebuggerCodeModel)})},
args: ["aString"],
source: "doIt: aString\x0a\x09^ [ self debuggerModel evaluate: aString ]\x0a\x09\x09tryCatch: [ :e | \x0a\x09\x09\x09ErrorHandler handleError: e.\x0a\x09\x09\x09nil ]",
messageSends: ["tryCatch:", "evaluate:", "debuggerModel", "handleError:"],
referencedClasses: ["ErrorHandler"]
}),
globals.HLDebuggerCodeModel);
smalltalk.addClass('HLDebuggerCodeWidget', globals.HLBrowserCodeWidget, [], 'Helios-Debugger');
smalltalk.addMethod(
smalltalk.method({
selector: "addStopAt:",
protocol: 'actions',
fn: function (anInteger){
var self=this;
return smalltalk.withContext(function($ctx1) {
_st(self["@editor"])._setGutterMarker_gutter_value_(anInteger,"stops",_st(_st("<div class=\x22stop\x22></stop>"._asJQuery())._toArray())._first());
return self}, function($ctx1) {$ctx1.fill(self,"addStopAt:",{anInteger:anInteger},globals.HLDebuggerCodeWidget)})},
args: ["anInteger"],
source: "addStopAt: anInteger\x0a\x09editor\x0a\x09\x09setGutterMarker: anInteger\x0a\x09\x09gutter: 'stops'\x0a\x09\x09value: '<div class=\x22stop\x22></stop>' asJQuery toArray first",
messageSends: ["setGutterMarker:gutter:value:", "first", "toArray", "asJQuery"],
referencedClasses: []
}),
globals.HLDebuggerCodeWidget);
smalltalk.addMethod(
smalltalk.method({
selector: "clearHighlight",
protocol: 'actions',
fn: function (){
var self=this;
return smalltalk.withContext(function($ctx1) {
_st(self._editor())._clearGutter_("stops");
return self}, function($ctx1) {$ctx1.fill(self,"clearHighlight",{},globals.HLDebuggerCodeWidget)})},
args: [],
source: "clearHighlight\x0a\x09self editor clearGutter: 'stops'",
messageSends: ["clearGutter:", "editor"],
referencedClasses: []
}),
globals.HLDebuggerCodeWidget);
smalltalk.addMethod(
smalltalk.method({
selector: "contents:",
protocol: 'accessing',
fn: function (aString){
var self=this;
return smalltalk.withContext(function($ctx1) {
self._clearHighlight();
($ctx1.supercall = true, globals.HLDebuggerCodeWidget.superclass.fn.prototype._contents_.apply(_st(self), [aString]));
$ctx1.supercall = false;
return self}, function($ctx1) {$ctx1.fill(self,"contents:",{aString:aString},globals.HLDebuggerCodeWidget)})},
args: ["aString"],
source: "contents: aString\x0a\x09self clearHighlight.\x0a\x09super contents: aString",
messageSends: ["clearHighlight", "contents:"],
referencedClasses: []
}),
globals.HLDebuggerCodeWidget);
smalltalk.addMethod(
smalltalk.method({
selector: "editorOptions",
protocol: 'accessing',
fn: function (){
var self=this;
return smalltalk.withContext(function($ctx1) {
var $2,$3,$1;
$2=($ctx1.supercall = true, globals.HLDebuggerCodeWidget.superclass.fn.prototype._editorOptions.apply(_st(self), []));
$ctx1.supercall = false;
_st($2)._at_put_("gutters",["CodeMirror-linenumbers", "stops"]);
$3=_st($2)._yourself();
$1=$3;
return $1;
}, function($ctx1) {$ctx1.fill(self,"editorOptions",{},globals.HLDebuggerCodeWidget)})},
args: [],
source: "editorOptions\x0a\x09^ super editorOptions\x0a\x09\x09at: 'gutters' put: #('CodeMirror-linenumbers' 'stops');\x0a\x09\x09yourself",
messageSends: ["at:put:", "editorOptions", "yourself"],
referencedClasses: []
}),
globals.HLDebuggerCodeWidget);
smalltalk.addMethod(
smalltalk.method({
selector: "highlight",
protocol: 'actions',
fn: function (){
var self=this;
return smalltalk.withContext(function($ctx1) {
var $1,$receiver;
$1=_st(self._browserModel())._nextNode();
if(($receiver = $1) == null || $receiver.isNil){
$1;
} else {
var node;
node=$receiver;
self._highlightNode_(node);
};
return self}, function($ctx1) {$ctx1.fill(self,"highlight",{},globals.HLDebuggerCodeWidget)})},
args: [],
source: "highlight\x0a\x09self browserModel nextNode ifNotNil: [ :node |\x0a\x09\x09self highlightNode: node ]",
messageSends: ["ifNotNil:", "nextNode", "browserModel", "highlightNode:"],
referencedClasses: []
}),
globals.HLDebuggerCodeWidget);
smalltalk.addMethod(
smalltalk.method({
selector: "highlightNode:",
protocol: 'actions',
fn: function (aNode){
var self=this;
var token;
return smalltalk.withContext(function($ctx1) {
var $4,$3,$2,$1,$5,$9,$8,$7,$11,$10,$6,$15,$14,$13,$12,$receiver;
if(($receiver = aNode) == null || $receiver.isNil){
aNode;
} else {
self._clearHighlight();
$4=_st(aNode)._positionStart();
$ctx1.sendIdx["positionStart"]=1;
$3=_st($4)._x();
$ctx1.sendIdx["x"]=1;
$2=_st($3).__minus((1));
$ctx1.sendIdx["-"]=1;
$1=self._addStopAt_($2);
$1;
$5=self._editor();
$9=_st(aNode)._positionStart();
$ctx1.sendIdx["positionStart"]=2;
$8=_st($9)._x();
$ctx1.sendIdx["x"]=2;
$7=_st($8).__minus((1));
$ctx1.sendIdx["-"]=2;
$11=_st(_st(aNode)._positionStart())._y();
$ctx1.sendIdx["y"]=1;
$10=_st($11).__minus((1));
$ctx1.sendIdx["-"]=3;
$6=globals.HashedCollection._newFromPairs_(["line",$7,"ch",$10]);
$15=_st(aNode)._positionEnd();
$ctx1.sendIdx["positionEnd"]=1;
$14=_st($15)._x();
$13=_st($14).__minus((1));
$12=globals.HashedCollection._newFromPairs_(["line",$13,"ch",_st(_st(aNode)._positionEnd())._y()]);
_st($5)._setSelection_to_($6,$12);
};
return self}, function($ctx1) {$ctx1.fill(self,"highlightNode:",{aNode:aNode,token:token},globals.HLDebuggerCodeWidget)})},
args: ["aNode"],
source: "highlightNode: aNode\x0a\x09| token |\x0a\x09\x0a\x09aNode ifNotNil: [\x0a\x09\x09self\x0a\x09\x09\x09clearHighlight;\x0a\x09\x09\x09addStopAt: aNode positionStart x - 1.\x0a\x0a\x09\x09self editor \x0a\x09\x09\x09setSelection: #{ 'line' -> (aNode positionStart x - 1). 'ch' -> (aNode positionStart y - 1) }\x0a\x09\x09\x09to: #{ 'line' -> (aNode positionEnd x - 1). 'ch' -> (aNode positionEnd y) } ]",
messageSends: ["ifNotNil:", "clearHighlight", "addStopAt:", "-", "x", "positionStart", "setSelection:to:", "editor", "y", "positionEnd"],
referencedClasses: []
}),
globals.HLDebuggerCodeWidget);
smalltalk.addMethod(
smalltalk.method({
selector: "observeBrowserModel",
protocol: 'actions',
fn: function (){
var self=this;
function $HLDebuggerContextSelected(){return globals.HLDebuggerContextSelected||(typeof HLDebuggerContextSelected=="undefined"?nil:HLDebuggerContextSelected)}
function $HLDebuggerStepped(){return globals.HLDebuggerStepped||(typeof HLDebuggerStepped=="undefined"?nil:HLDebuggerStepped)}
function $HLDebuggerWhere(){return globals.HLDebuggerWhere||(typeof HLDebuggerWhere=="undefined"?nil:HLDebuggerWhere)}
return smalltalk.withContext(function($ctx1) {
var $2,$1,$4,$3;
($ctx1.supercall = true, globals.HLDebuggerCodeWidget.superclass.fn.prototype._observeBrowserModel.apply(_st(self), []));
$ctx1.supercall = false;
$2=self._browserModel();
$ctx1.sendIdx["browserModel"]=1;
$1=_st($2)._announcer();
$ctx1.sendIdx["announcer"]=1;
_st($1)._on_send_to_($HLDebuggerContextSelected(),"onContextSelected",self);
$ctx1.sendIdx["on:send:to:"]=1;
$4=self._browserModel();
$ctx1.sendIdx["browserModel"]=2;
$3=_st($4)._announcer();
$ctx1.sendIdx["announcer"]=2;
_st($3)._on_send_to_($HLDebuggerStepped(),"onContextSelected",self);
$ctx1.sendIdx["on:send:to:"]=2;
_st(_st(self._browserModel())._announcer())._on_send_to_($HLDebuggerWhere(),"onContextSelected",self);
return self}, function($ctx1) {$ctx1.fill(self,"observeBrowserModel",{},globals.HLDebuggerCodeWidget)})},
args: [],
source: "observeBrowserModel\x0a\x09super observeBrowserModel.\x0a\x09\x0a\x09self browserModel announcer \x0a\x09\x09on: HLDebuggerContextSelected\x0a\x09\x09send: #onContextSelected\x0a\x09\x09to: self.\x0a\x09\x0a\x09self browserModel announcer \x0a\x09\x09on: HLDebuggerStepped\x0a\x09\x09send: #onContextSelected\x0a\x09\x09to: self.\x0a\x09\x0a\x09self browserModel announcer \x0a\x09\x09on: HLDebuggerWhere\x0a\x09\x09send: #onContextSelected\x0a\x09\x09to: self",
messageSends: ["observeBrowserModel", "on:send:to:", "announcer", "browserModel"],
referencedClasses: ["HLDebuggerContextSelected", "HLDebuggerStepped", "HLDebuggerWhere"]
}),
globals.HLDebuggerCodeWidget);
smalltalk.addMethod(
smalltalk.method({
selector: "onContextSelected",
protocol: 'reactions',
fn: function (){
var self=this;
return smalltalk.withContext(function($ctx1) {
self._highlight();
return self}, function($ctx1) {$ctx1.fill(self,"onContextSelected",{},globals.HLDebuggerCodeWidget)})},
args: [],
source: "onContextSelected\x0a\x09self highlight",
messageSends: ["highlight"],
referencedClasses: []
}),
globals.HLDebuggerCodeWidget);
smalltalk.addMethod(
smalltalk.method({
selector: "renderOn:",
protocol: 'rendering',
fn: function (html){
var self=this;
return smalltalk.withContext(function($ctx1) {
($ctx1.supercall = true, globals.HLDebuggerCodeWidget.superclass.fn.prototype._renderOn_.apply(_st(self), [html]));
$ctx1.supercall = false;
self._contents_(_st(_st(self._browserModel())._selectedMethod())._source());
return self}, function($ctx1) {$ctx1.fill(self,"renderOn:",{html:html},globals.HLDebuggerCodeWidget)})},
args: ["html"],
source: "renderOn: html\x0a\x09super renderOn: html.\x0a\x09self contents: self browserModel selectedMethod source",
messageSends: ["renderOn:", "contents:", "source", "selectedMethod", "browserModel"],
referencedClasses: []
}),
globals.HLDebuggerCodeWidget);
smalltalk.addClass('HLDebuggerModel', globals.HLToolModel, ['rootContext', 'debugger', 'error'], 'Helios-Debugger');
globals.HLDebuggerModel.comment="I am a model for debugging Amber code in Helios.\x0a\x0aMy instances hold a reference to an `ASTDebugger` instance, itself referencing the current `context`. The context should be the root of the context stack.";
smalltalk.addMethod(
smalltalk.method({
selector: "atEnd",
protocol: 'testing',
fn: function (){
var self=this;
return smalltalk.withContext(function($ctx1) {
var $1;
$1=_st(self._debugger())._atEnd();
return $1;
}, function($ctx1) {$ctx1.fill(self,"atEnd",{},globals.HLDebuggerModel)})},
args: [],
source: "atEnd\x0a\x09^ self debugger atEnd",
messageSends: ["atEnd", "debugger"],
referencedClasses: []
}),
globals.HLDebuggerModel);
smalltalk.addMethod(
smalltalk.method({
selector: "contexts",
protocol: 'accessing',
fn: function (){
var self=this;
var contexts,context;
function $OrderedCollection(){return globals.OrderedCollection||(typeof OrderedCollection=="undefined"?nil:OrderedCollection)}
return smalltalk.withContext(function($ctx1) {
var $1;
contexts=_st($OrderedCollection())._new();
context=self._rootContext();
_st((function(){
return smalltalk.withContext(function($ctx2) {
return _st(context)._notNil();
}, function($ctx2) {$ctx2.fillBlock({},$ctx1,1)})}))._whileTrue_((function(){
return smalltalk.withContext(function($ctx2) {
_st(contexts)._add_(context);
context=_st(context)._outerContext();
return context;
}, function($ctx2) {$ctx2.fillBlock({},$ctx1,2)})}));
$1=contexts;
return $1;
}, function($ctx1) {$ctx1.fill(self,"contexts",{contexts:contexts,context:context},globals.HLDebuggerModel)})},
args: [],
source: "contexts\x0a\x09| contexts context |\x0a\x09\x0a\x09contexts := OrderedCollection new.\x0a\x09context := self rootContext.\x0a\x09\x0a\x09[ context notNil ] whileTrue: [\x0a\x09\x09contexts add: context.\x0a\x09\x09context := context outerContext ].\x0a\x09\x09\x0a\x09^ contexts",
messageSends: ["new", "rootContext", "whileTrue:", "notNil", "add:", "outerContext"],
referencedClasses: ["OrderedCollection"]
}),
globals.HLDebuggerModel);
smalltalk.addMethod(
smalltalk.method({
selector: "currentContext",
protocol: 'accessing',
fn: function (){
var self=this;
return smalltalk.withContext(function($ctx1) {
var $1;
$1=_st(self._debugger())._context();
return $1;
}, function($ctx1) {$ctx1.fill(self,"currentContext",{},globals.HLDebuggerModel)})},
args: [],
source: "currentContext\x0a\x09^ self debugger context",
messageSends: ["context", "debugger"],
referencedClasses: []
}),
globals.HLDebuggerModel);
smalltalk.addMethod(
smalltalk.method({
selector: "currentContext:",
protocol: 'accessing',
fn: function (aContext){
var self=this;
function $HLDebuggerContextSelected(){return globals.HLDebuggerContextSelected||(typeof HLDebuggerContextSelected=="undefined"?nil:HLDebuggerContextSelected)}
return smalltalk.withContext(function($ctx1) {
var $1,$2;
self._withChangesDo_((function(){
return smalltalk.withContext(function($ctx2) {
self._selectedMethod_(_st(aContext)._method());
_st(self._debugger())._context_(aContext);
$ctx2.sendIdx["context:"]=1;
$1=_st($HLDebuggerContextSelected())._new();
_st($1)._context_(aContext);
$2=_st($1)._yourself();
return _st(self._announcer())._announce_($2);
}, function($ctx2) {$ctx2.fillBlock({},$ctx1,1)})}));
return self}, function($ctx1) {$ctx1.fill(self,"currentContext:",{aContext:aContext},globals.HLDebuggerModel)})},
args: ["aContext"],
source: "currentContext: aContext\x0a\x09self withChangesDo: [ \x0a\x09\x09self selectedMethod: aContext method.\x0a\x09\x09self debugger context: aContext.\x0a\x09\x09self announcer announce: (HLDebuggerContextSelected new\x0a\x09\x09\x09context: aContext;\x0a\x09\x09\x09yourself) ]",
messageSends: ["withChangesDo:", "selectedMethod:", "method", "context:", "debugger", "announce:", "announcer", "new", "yourself"],
referencedClasses: ["HLDebuggerContextSelected"]
}),
globals.HLDebuggerModel);
smalltalk.addMethod(
smalltalk.method({
selector: "debugger",
protocol: 'accessing',
fn: function (){
var self=this;
function $ASTDebugger(){return globals.ASTDebugger||(typeof ASTDebugger=="undefined"?nil:ASTDebugger)}
return smalltalk.withContext(function($ctx1) {
var $2,$1,$receiver;
$2=self["@debugger"];
if(($receiver = $2) == null || $receiver.isNil){
self["@debugger"]=_st($ASTDebugger())._new();
$1=self["@debugger"];
} else {
$1=$2;
};
return $1;
}, function($ctx1) {$ctx1.fill(self,"debugger",{},globals.HLDebuggerModel)})},
args: [],
source: "debugger\x0a\x09^ debugger ifNil: [ debugger := ASTDebugger new ]",
messageSends: ["ifNil:", "new"],
referencedClasses: ["ASTDebugger"]
}),
globals.HLDebuggerModel);
smalltalk.addMethod(
smalltalk.method({
selector: "error",
protocol: 'accessing',
fn: function (){
var self=this;
var $1;
$1=self["@error"];
return $1;
},
args: [],
source: "error\x0a\x09^ error",
messageSends: [],
referencedClasses: []
}),
globals.HLDebuggerModel);
smalltalk.addMethod(
smalltalk.method({
selector: "evaluate:",
protocol: 'evaluating',
fn: function (aString){
var self=this;
return smalltalk.withContext(function($ctx1) {
var $1;
$1=_st(self._environment())._evaluate_for_(aString,self._currentContext());
return $1;
}, function($ctx1) {$ctx1.fill(self,"evaluate:",{aString:aString},globals.HLDebuggerModel)})},
args: ["aString"],
source: "evaluate: aString\x0a\x09^ self environment \x0a\x09\x09evaluate: aString \x0a\x09\x09for: self currentContext",
messageSends: ["evaluate:for:", "environment", "currentContext"],
referencedClasses: []
}),
globals.HLDebuggerModel);
smalltalk.addMethod(
smalltalk.method({
selector: "flushInnerContexts",
protocol: 'private',
fn: function (){
var self=this;
return smalltalk.withContext(function($ctx1) {
var $1;
$1=self._currentContext();
$ctx1.sendIdx["currentContext"]=1;
_st($1)._innerContext_(nil);
self["@rootContext"]=self._currentContext();
self._initializeContexts();
return self}, function($ctx1) {$ctx1.fill(self,"flushInnerContexts",{},globals.HLDebuggerModel)})},
args: [],
source: "flushInnerContexts\x0a\x09\x22When stepping, the inner contexts are not relevent anymore,\x0a\x09and can be flushed\x22\x0a\x09\x0a\x09self currentContext innerContext: nil.\x0a\x09rootContext := self currentContext.\x0a\x09self initializeContexts",
messageSends: ["innerContext:", "currentContext", "initializeContexts"],
referencedClasses: []
}),
globals.HLDebuggerModel);
smalltalk.addMethod(
smalltalk.method({
selector: "initializeFromError:",
protocol: 'initialization',
fn: function (anError){
var self=this;
var errorContext;
function $AIContext(){return globals.AIContext||(typeof AIContext=="undefined"?nil:AIContext)}
return smalltalk.withContext(function($ctx1) {
self["@error"]=anError;
errorContext=_st($AIContext())._fromMethodContext_(_st(self["@error"])._context());
self["@rootContext"]=_st(self["@error"])._signalerContextFrom_(errorContext);
self._selectedMethod_(_st(self["@rootContext"])._method());
return self}, function($ctx1) {$ctx1.fill(self,"initializeFromError:",{anError:anError,errorContext:errorContext},globals.HLDebuggerModel)})},
args: ["anError"],
source: "initializeFromError: anError\x0a\x09| errorContext |\x0a\x09\x0a\x09error := anError.\x0a\x09errorContext := (AIContext fromMethodContext: error context).\x0a\x09rootContext := error signalerContextFrom: errorContext.\x0a\x09self selectedMethod: rootContext method",
messageSends: ["fromMethodContext:", "context", "signalerContextFrom:", "selectedMethod:", "method"],
referencedClasses: ["AIContext"]
}),
globals.HLDebuggerModel);
smalltalk.addMethod(
smalltalk.method({
selector: "nextNode",
protocol: 'accessing',
fn: function (){
var self=this;
return smalltalk.withContext(function($ctx1) {
var $1;
$1=_st(self._debugger())._node();
return $1;
}, function($ctx1) {$ctx1.fill(self,"nextNode",{},globals.HLDebuggerModel)})},
args: [],
source: "nextNode\x0a\x09^ self debugger node",
messageSends: ["node", "debugger"],
referencedClasses: []
}),
globals.HLDebuggerModel);
smalltalk.addMethod(
smalltalk.method({
selector: "onStep",
protocol: 'reactions',
fn: function (){
var self=this;
function $HLDebuggerContextSelected(){return globals.HLDebuggerContextSelected||(typeof HLDebuggerContextSelected=="undefined"?nil:HLDebuggerContextSelected)}
return smalltalk.withContext(function($ctx1) {
var $2,$1,$3,$4;
self["@rootContext"]=self._currentContext();
$ctx1.sendIdx["currentContext"]=1;
$2=self._currentContext();
$ctx1.sendIdx["currentContext"]=2;
$1=_st($2)._method();
self._selectedMethod_($1);
$3=_st($HLDebuggerContextSelected())._new();
_st($3)._context_(self._currentContext());
$4=_st($3)._yourself();
_st(self._announcer())._announce_($4);
return self}, function($ctx1) {$ctx1.fill(self,"onStep",{},globals.HLDebuggerModel)})},
args: [],
source: "onStep\x0a\x09rootContext := self currentContext.\x0a\x09\x0a\x09\x22Force a refresh of the context list and code widget\x22\x0a\x09self selectedMethod: self currentContext method.\x0a\x09self announcer announce: (HLDebuggerContextSelected new\x0a\x09\x09context: self currentContext;\x0a\x09\x09yourself)",
messageSends: ["currentContext", "selectedMethod:", "method", "announce:", "announcer", "context:", "new", "yourself"],
referencedClasses: ["HLDebuggerContextSelected"]
}),
globals.HLDebuggerModel);
smalltalk.addMethod(
smalltalk.method({
selector: "proceed",
protocol: 'actions',
fn: function (){
var self=this;
function $HLDebuggerProceeded(){return globals.HLDebuggerProceeded||(typeof HLDebuggerProceeded=="undefined"?nil:HLDebuggerProceeded)}
return smalltalk.withContext(function($ctx1) {
_st(self._debugger())._proceed();
_st(self._announcer())._announce_(_st($HLDebuggerProceeded())._new());
return self}, function($ctx1) {$ctx1.fill(self,"proceed",{},globals.HLDebuggerModel)})},
args: [],
source: "proceed\x0a\x09self debugger proceed.\x0a\x09\x0a\x09self announcer announce: HLDebuggerProceeded new",
messageSends: ["proceed", "debugger", "announce:", "announcer", "new"],
referencedClasses: ["HLDebuggerProceeded"]
}),
globals.HLDebuggerModel);
smalltalk.addMethod(
smalltalk.method({
selector: "restart",
protocol: 'actions',
fn: function (){
var self=this;
function $HLDebuggerStepped(){return globals.HLDebuggerStepped||(typeof HLDebuggerStepped=="undefined"?nil:HLDebuggerStepped)}
return smalltalk.withContext(function($ctx1) {
var $1,$2;
_st(self._debugger())._restart();
self._onStep();
$1=_st($HLDebuggerStepped())._new();
_st($1)._context_(self._currentContext());
$2=_st($1)._yourself();
_st(self._announcer())._announce_($2);
return self}, function($ctx1) {$ctx1.fill(self,"restart",{},globals.HLDebuggerModel)})},
args: [],
source: "restart\x0a\x09self debugger restart.\x0a\x09self onStep.\x0a\x09\x0a\x09self announcer announce: (HLDebuggerStepped new\x0a\x09\x09context: self currentContext;\x0a\x09\x09yourself)",
messageSends: ["restart", "debugger", "onStep", "announce:", "announcer", "context:", "new", "currentContext", "yourself"],
referencedClasses: ["HLDebuggerStepped"]
}),
globals.HLDebuggerModel);
smalltalk.addMethod(
smalltalk.method({
selector: "rootContext",
protocol: 'accessing',
fn: function (){
var self=this;
var $1;
$1=self["@rootContext"];
return $1;
},
args: [],
source: "rootContext\x0a\x09^ rootContext",
messageSends: [],
referencedClasses: []
}),
globals.HLDebuggerModel);
smalltalk.addMethod(
smalltalk.method({
selector: "stepOver",
protocol: 'actions',
fn: function (){
var self=this;
function $HLDebuggerStepped(){return globals.HLDebuggerStepped||(typeof HLDebuggerStepped=="undefined"?nil:HLDebuggerStepped)}
return smalltalk.withContext(function($ctx1) {
var $1,$2;
_st(self._debugger())._stepOver();
self._onStep();
$1=_st($HLDebuggerStepped())._new();
_st($1)._context_(self._currentContext());
$2=_st($1)._yourself();
_st(self._announcer())._announce_($2);
return self}, function($ctx1) {$ctx1.fill(self,"stepOver",{},globals.HLDebuggerModel)})},
args: [],
source: "stepOver\x0a\x09self debugger stepOver.\x0a\x09self onStep.\x0a\x09\x0a\x09self announcer announce: (HLDebuggerStepped new\x0a\x09\x09context: self currentContext;\x0a\x09\x09yourself)",
messageSends: ["stepOver", "debugger", "onStep", "announce:", "announcer", "context:", "new", "currentContext", "yourself"],
referencedClasses: ["HLDebuggerStepped"]
}),
globals.HLDebuggerModel);
smalltalk.addMethod(
smalltalk.method({
selector: "where",
protocol: 'actions',
fn: function (){
var self=this;
function $HLDebuggerWhere(){return globals.HLDebuggerWhere||(typeof HLDebuggerWhere=="undefined"?nil:HLDebuggerWhere)}
return smalltalk.withContext(function($ctx1) {
_st(self._announcer())._announce_(_st($HLDebuggerWhere())._new());
return self}, function($ctx1) {$ctx1.fill(self,"where",{},globals.HLDebuggerModel)})},
args: [],
source: "where\x0a\x09self announcer announce: HLDebuggerWhere new",
messageSends: ["announce:", "announcer", "new"],
referencedClasses: ["HLDebuggerWhere"]
}),
globals.HLDebuggerModel);
smalltalk.addMethod(
smalltalk.method({
selector: "on:",
protocol: 'instance creation',
fn: function (anError){
var self=this;
return smalltalk.withContext(function($ctx1) {
var $2,$3,$1;
$2=self._new();
_st($2)._initializeFromError_(anError);
$3=_st($2)._yourself();
$1=$3;
return $1;
}, function($ctx1) {$ctx1.fill(self,"on:",{anError:anError},globals.HLDebuggerModel.klass)})},
args: ["anError"],
source: "on: anError\x0a\x09^ self new\x0a\x09\x09initializeFromError: anError;\x0a\x09\x09yourself",
messageSends: ["initializeFromError:", "new", "yourself"],
referencedClasses: []
}),
globals.HLDebuggerModel.klass);
smalltalk.addClass('HLErrorHandler', globals.Object, [], 'Helios-Debugger');
smalltalk.addMethod(
smalltalk.method({
selector: "confirmDebugError:",
protocol: 'error handling',
fn: function (anError){
var self=this;
function $HLConfirmationWidget(){return globals.HLConfirmationWidget||(typeof HLConfirmationWidget=="undefined"?nil:HLConfirmationWidget)}
return smalltalk.withContext(function($ctx1) {
var $1,$2;
$1=_st($HLConfirmationWidget())._new();
_st($1)._confirmationString_(_st(anError)._messageText());
_st($1)._actionBlock_((function(){
return smalltalk.withContext(function($ctx2) {
return self._debugError_(anError);
}, function($ctx2) {$ctx2.fillBlock({},$ctx1,1)})}));
_st($1)._cancelButtonLabel_("Abandon");
_st($1)._confirmButtonLabel_("Debug");
$2=_st($1)._show();
return self}, function($ctx1) {$ctx1.fill(self,"confirmDebugError:",{anError:anError},globals.HLErrorHandler)})},
args: ["anError"],
source: "confirmDebugError: anError\x0a\x09HLConfirmationWidget new\x0a\x09\x09confirmationString: anError messageText;\x0a\x09\x09actionBlock: [ self debugError: anError ];\x0a\x09\x09cancelButtonLabel: 'Abandon';\x0a\x09\x09confirmButtonLabel: 'Debug';\x0a\x09\x09show",
messageSends: ["confirmationString:", "new", "messageText", "actionBlock:", "debugError:", "cancelButtonLabel:", "confirmButtonLabel:", "show"],
referencedClasses: ["HLConfirmationWidget"]
}),
globals.HLErrorHandler);
smalltalk.addMethod(
smalltalk.method({
selector: "debugError:",
protocol: 'error handling',
fn: function (anError){
var self=this;
function $HLDebugger(){return globals.HLDebugger||(typeof HLDebugger=="undefined"?nil:HLDebugger)}
function $Error(){return globals.Error||(typeof Error=="undefined"?nil:Error)}
function $ConsoleErrorHandler(){return globals.ConsoleErrorHandler||(typeof ConsoleErrorHandler=="undefined"?nil:ConsoleErrorHandler)}
return smalltalk.withContext(function($ctx1) {
_st((function(){
return smalltalk.withContext(function($ctx2) {
return _st(_st($HLDebugger())._on_(anError))._openAsTab();
}, function($ctx2) {$ctx2.fillBlock({},$ctx1,1)})}))._on_do_($Error(),(function(error){
return smalltalk.withContext(function($ctx2) {
return _st(_st($ConsoleErrorHandler())._new())._handleError_(error);
}, function($ctx2) {$ctx2.fillBlock({error:error},$ctx1,2)})}));
return self}, function($ctx1) {$ctx1.fill(self,"debugError:",{anError:anError},globals.HLErrorHandler)})},
args: ["anError"],
source: "debugError: anError\x0a\x0a\x09[ \x0a\x09\x09(HLDebugger on: anError) openAsTab \x0a\x09] \x0a\x09\x09on: Error \x0a\x09\x09do: [ :error | ConsoleErrorHandler new handleError: error ]",
messageSends: ["on:do:", "openAsTab", "on:", "handleError:", "new"],
referencedClasses: ["HLDebugger", "Error", "ConsoleErrorHandler"]
}),
globals.HLErrorHandler);
smalltalk.addMethod(
smalltalk.method({
selector: "handleError:",
protocol: 'error handling',
fn: function (anError){
var self=this;
return smalltalk.withContext(function($ctx1) {
self._confirmDebugError_(anError);
return self}, function($ctx1) {$ctx1.fill(self,"handleError:",{anError:anError},globals.HLErrorHandler)})},
args: ["anError"],
source: "handleError: anError\x0a\x09self confirmDebugError: anError",
messageSends: ["confirmDebugError:"],
referencedClasses: []
}),
globals.HLErrorHandler);
smalltalk.addMethod(
smalltalk.method({
selector: "onErrorHandled",
protocol: 'error handling',
fn: function (){
var self=this;
function $HLProgressWidget(){return globals.HLProgressWidget||(typeof HLProgressWidget=="undefined"?nil:HLProgressWidget)}
return smalltalk.withContext(function($ctx1) {
var $1,$2;
$1=_st($HLProgressWidget())._default();
_st($1)._flush();
$2=_st($1)._remove();
return self}, function($ctx1) {$ctx1.fill(self,"onErrorHandled",{},globals.HLErrorHandler)})},
args: [],
source: "onErrorHandled\x0a\x09\x22when an error is handled, we need to make sure that\x0a\x09any progress bar widget gets removed. Because HLProgressBarWidget is asynchronous,\x0a\x09it has to be done here.\x22\x0a\x09\x0a\x09HLProgressWidget default \x0a\x09\x09flush; \x0a\x09\x09remove",
messageSends: ["flush", "default", "remove"],
referencedClasses: ["HLProgressWidget"]
}),
globals.HLErrorHandler);
smalltalk.addClass('HLStackListWidget', globals.HLToolListWidget, [], 'Helios-Debugger');
smalltalk.addMethod(
smalltalk.method({
selector: "items",
protocol: 'accessing',
fn: function (){
var self=this;
return smalltalk.withContext(function($ctx1) {
var $1;
$1=_st(self._model())._contexts();
return $1;
}, function($ctx1) {$ctx1.fill(self,"items",{},globals.HLStackListWidget)})},
args: [],
source: "items\x0a\x09^ self model contexts",
messageSends: ["contexts", "model"],
referencedClasses: []
}),
globals.HLStackListWidget);
smalltalk.addMethod(
smalltalk.method({
selector: "label",
protocol: 'accessing',
fn: function (){
var self=this;
return "Call stack";
},
args: [],
source: "label\x0a\x09^ 'Call stack'",
messageSends: [],
referencedClasses: []
}),
globals.HLStackListWidget);
smalltalk.addMethod(
smalltalk.method({
selector: "observeModel",
protocol: 'actions',
fn: function (){
var self=this;
function $HLDebuggerStepped(){return globals.HLDebuggerStepped||(typeof HLDebuggerStepped=="undefined"?nil:HLDebuggerStepped)}
return smalltalk.withContext(function($ctx1) {
($ctx1.supercall = true, globals.HLStackListWidget.superclass.fn.prototype._observeModel.apply(_st(self), []));
$ctx1.supercall = false;
_st(_st(self._model())._announcer())._on_send_to_($HLDebuggerStepped(),"onDebuggerStepped:",self);
return self}, function($ctx1) {$ctx1.fill(self,"observeModel",{},globals.HLStackListWidget)})},
args: [],
source: "observeModel\x0a\x09super observeModel.\x0a\x09\x0a\x09self model announcer \x0a\x09\x09on: HLDebuggerStepped\x0a\x09\x09send: #onDebuggerStepped:\x0a\x09\x09to: self",
messageSends: ["observeModel", "on:send:to:", "announcer", "model"],
referencedClasses: ["HLDebuggerStepped"]
}),
globals.HLStackListWidget);
smalltalk.addMethod(
smalltalk.method({
selector: "onDebuggerStepped:",
protocol: 'reactions',
fn: function (anAnnouncement){
var self=this;
return smalltalk.withContext(function($ctx1) {
self["@items"]=nil;
self._refresh();
return self}, function($ctx1) {$ctx1.fill(self,"onDebuggerStepped:",{anAnnouncement:anAnnouncement},globals.HLStackListWidget)})},
args: ["anAnnouncement"],
source: "onDebuggerStepped: anAnnouncement\x0a\x09items := nil.\x0a\x09self refresh",
messageSends: ["refresh"],
referencedClasses: []
}),
globals.HLStackListWidget);
smalltalk.addMethod(
smalltalk.method({
selector: "proceed",
protocol: 'actions',
fn: function (){
var self=this;
return smalltalk.withContext(function($ctx1) {
_st(self._model())._proceed();
return self}, function($ctx1) {$ctx1.fill(self,"proceed",{},globals.HLStackListWidget)})},
args: [],
source: "proceed\x0a\x09self model proceed",
messageSends: ["proceed", "model"],
referencedClasses: []
}),
globals.HLStackListWidget);
smalltalk.addMethod(
smalltalk.method({
selector: "renderButtonsOn:",
protocol: 'rendering',
fn: function (html){
var self=this;
return smalltalk.withContext(function($ctx1) {
var $1,$3,$4,$5,$6,$7,$8,$9,$10,$2;
$1=_st(html)._div();
_st($1)._class_("debugger_bar");
$ctx1.sendIdx["class:"]=1;
$2=_st($1)._with_((function(){
return smalltalk.withContext(function($ctx2) {
$3=_st(html)._button();
$ctx2.sendIdx["button"]=1;
_st($3)._class_("btn restart");
$ctx2.sendIdx["class:"]=2;
_st($3)._with_("Restart");
$ctx2.sendIdx["with:"]=2;
$4=_st($3)._onClick_((function(){
return smalltalk.withContext(function($ctx3) {
return self._restart();
}, function($ctx3) {$ctx3.fillBlock({},$ctx2,2)})}));
$ctx2.sendIdx["onClick:"]=1;
$4;
$5=_st(html)._button();
$ctx2.sendIdx["button"]=2;
_st($5)._class_("btn where");
$ctx2.sendIdx["class:"]=3;
_st($5)._with_("Where");
$ctx2.sendIdx["with:"]=3;
$6=_st($5)._onClick_((function(){
return smalltalk.withContext(function($ctx3) {
return self._where();
}, function($ctx3) {$ctx3.fillBlock({},$ctx2,3)})}));
$ctx2.sendIdx["onClick:"]=2;
$6;
$7=_st(html)._button();
$ctx2.sendIdx["button"]=3;
_st($7)._class_("btn stepOver");
$ctx2.sendIdx["class:"]=4;
_st($7)._with_("Step over");
$ctx2.sendIdx["with:"]=4;
$8=_st($7)._onClick_((function(){
return smalltalk.withContext(function($ctx3) {
return self._stepOver();
}, function($ctx3) {$ctx3.fillBlock({},$ctx2,4)})}));
$ctx2.sendIdx["onClick:"]=3;
$8;
$9=_st(html)._button();
_st($9)._class_("btn proceed");
_st($9)._with_("Proceed");
$10=_st($9)._onClick_((function(){
return smalltalk.withContext(function($ctx3) {
return self._proceed();
}, function($ctx3) {$ctx3.fillBlock({},$ctx2,5)})}));
return $10;
}, function($ctx2) {$ctx2.fillBlock({},$ctx1,1)})}));
$ctx1.sendIdx["with:"]=1;
return self}, function($ctx1) {$ctx1.fill(self,"renderButtonsOn:",{html:html},globals.HLStackListWidget)})},
args: ["html"],
source: "renderButtonsOn: html\x0a\x09html div \x0a\x09\x09class: 'debugger_bar'; \x0a\x09\x09with: [\x0a\x09\x09\x09html button \x0a\x09\x09\x09\x09class: 'btn restart';\x0a\x09\x09\x09\x09with: 'Restart';\x0a\x09\x09\x09\x09onClick: [ self restart ].\x0a\x09\x09\x09html button \x0a\x09\x09\x09\x09class: 'btn where';\x0a\x09\x09\x09\x09with: 'Where';\x0a\x09\x09\x09\x09onClick: [ self where ].\x0a\x09\x09\x09html button \x0a\x09\x09\x09\x09class: 'btn stepOver';\x0a\x09\x09\x09\x09with: 'Step over';\x0a\x09\x09\x09\x09onClick: [ self stepOver ].\x0a\x09\x09\x09html button \x0a\x09\x09\x09\x09class: 'btn proceed';\x0a\x09\x09\x09\x09with: 'Proceed';\x0a\x09\x09\x09\x09onClick: [ self proceed ] ]",
messageSends: ["class:", "div", "with:", "button", "onClick:", "restart", "where", "stepOver", "proceed"],
referencedClasses: []
}),
globals.HLStackListWidget);
smalltalk.addMethod(
smalltalk.method({
selector: "restart",
protocol: 'actions',
fn: function (){
var self=this;
return smalltalk.withContext(function($ctx1) {
_st(self._model())._restart();
return self}, function($ctx1) {$ctx1.fill(self,"restart",{},globals.HLStackListWidget)})},
args: [],
source: "restart\x0a\x09self model restart",
messageSends: ["restart", "model"],
referencedClasses: []
}),
globals.HLStackListWidget);
smalltalk.addMethod(
smalltalk.method({
selector: "selectItem:",
protocol: 'actions',
fn: function (aContext){
var self=this;
return smalltalk.withContext(function($ctx1) {
_st(self._model())._currentContext_(aContext);
($ctx1.supercall = true, globals.HLStackListWidget.superclass.fn.prototype._selectItem_.apply(_st(self), [aContext]));
$ctx1.supercall = false;
return self}, function($ctx1) {$ctx1.fill(self,"selectItem:",{aContext:aContext},globals.HLStackListWidget)})},
args: ["aContext"],
source: "selectItem: aContext\x0a \x09self model currentContext: aContext.\x0a\x09super selectItem: aContext",
messageSends: ["currentContext:", "model", "selectItem:"],
referencedClasses: []
}),
globals.HLStackListWidget);
smalltalk.addMethod(
smalltalk.method({
selector: "selectedItem",
protocol: 'actions',
fn: function (){
var self=this;
return smalltalk.withContext(function($ctx1) {
var $1;
$1=_st(self._model())._currentContext();
return $1;
}, function($ctx1) {$ctx1.fill(self,"selectedItem",{},globals.HLStackListWidget)})},
args: [],
source: "selectedItem\x0a \x09^ self model currentContext",
messageSends: ["currentContext", "model"],
referencedClasses: []
}),
globals.HLStackListWidget);
smalltalk.addMethod(
smalltalk.method({
selector: "stepOver",
protocol: 'actions',
fn: function (){
var self=this;
return smalltalk.withContext(function($ctx1) {
_st(self._model())._stepOver();
return self}, function($ctx1) {$ctx1.fill(self,"stepOver",{},globals.HLStackListWidget)})},
args: [],
source: "stepOver\x0a\x09self model stepOver",
messageSends: ["stepOver", "model"],
referencedClasses: []
}),
globals.HLStackListWidget);
smalltalk.addMethod(
smalltalk.method({
selector: "where",
protocol: 'actions',
fn: function (){
var self=this;
return smalltalk.withContext(function($ctx1) {
_st(self._model())._where();
return self}, function($ctx1) {$ctx1.fill(self,"where",{},globals.HLStackListWidget)})},
args: [],
source: "where\x0a\x09self model where",
messageSends: ["where", "model"],
referencedClasses: []
}),
globals.HLStackListWidget);
});
| Alexander-Remizov/SVG-Amber-Tools | bower_components/amber/support/helios/src/Helios-Debugger.js | JavaScript | mit | 56,069 |
MIME-Version: 1.0
Server: CERN/3.0
Date: Monday, 16-Dec-96 23:33:46 GMT
Content-Type: text/html
Content-Length: 3390
Last-Modified: Tuesday, 20-Feb-96 22:21:50 GMT
<html>
<head>
<title> CodeWarrior for CS100 </title>
</head>
<body>
<h2> Setting Up CodeWarrior for CS100 </h2>
CodeWarrior can be run on your own personal Macintosh. Copies of
CodeWarrior 8 can be purchased from the Campus Store. The CodeWarrior
installed in the CIT labs is just like the one you would install on
your Mac, except for a few things we have added -- the CSLib library
and the CS100 Basic project stationery. <p>
<strong>Note:</strong> These additions were built using CodeWarrior
version 8, so it is possible that they will not work with earlier
versions of CodeWarrior (although we have heard from people that they
do). <p>
Once you have retrieved the CS100 Additions folder, install the
additions as follows:
<ul>
<li> Open the CS100 Additions folder. Inside it there is a SimpleText
file containing instructions similar to these. There are two folders
called INTO MacOS Support and INTO (Project Stationery). The folder
structure in the CS100 Additions folder is meant to mirror the folder
structure inside the Metrowerks CodeWarrior folder of your copy of
CodeWarrior, to make it easy to follow these instructions. <p>
<li> Open your CodeWarrior8 or CW 8 Gold folder. Open the Metrowerks
CodeWarrior folder inside it. <p>
<li> Open the INTO MacOS Support folder in the CS100 additions. Move
the CS100 Support folder into the MacOS Support folder in your
Metrowerks CodeWarrior folder. <p>
<li> Open the INTO (Project Stationery) folder. Move the CS100 Basic
68K.mu file into the (Project Stationery) folder in your Metrowerks
CodeWarrior folder. <p>
<li> Open the INTO Proj. Stat. Support folder in the INTO (Project
Stationery) folder. Move the <replace me CS100>.c file into the
Project Stationery Support folder in the (Project Stationery) folder
of your CodeWarrior. <p>
</ul>
When you open a new project with CodeWarrior, you should now be able
to select the CS100 Basic 68K stationery. This will include the CSLib
library, which should also now be available to you.
<hr>
Click here to get the CS100 Additions folder. <p>
<a href =
"ftp://ftp.cs.cornell.edu/pub/courses/cs100-s96/CS100Additions.sea.hqx">
CS100 Additions Folder </a>
<hr>
<h3>Other Machines</h3>
If you have a copy of CodeWarrior for a computer other than the Mac,
you may still be able to set up the CS100 environment. However, the
course staff will not be able support this.
<ol>
<li> Build the CSLib library. Get the source code for by anonymous
FTP from Addison-Wesley. Follow the instructions on page 670 in the
Roberts textbook (Appendix B, Library Sources). Compile the source
code on your machine using CodeWarrior. (To create the CSLib library
for CS100 we only compiled the <code>genlib</code>,
<code>simpio</code>, <code>string</code>, <code>random</code>, and
<code>exception</code> parts of the library; we left out the graphics
stuff. If everything seems to work for your machine, feel free to
compile all of it.) Put the compiled library and the library header
files into the support directory for your CodeWarrior. <p>
<li> Make the CS100 Basic project stationery. Our project stationery
is based on the ANSI project stationery, with the CSLib library added.
Put your project stationery in the project stationery directory of
your CodeWarrior.
</ol>
</body>
<hr>
<address>
CS100 Spring 1996 <br>
pierce@cs.cornell.edu
</address>
</html>
| ML-SWAT/Web2KnowledgeBase | webkb/other/cornell/http:^^www.cs.cornell.edu^Info^Courses^Spring-96^CS100^info^codewarrior.html | HTML | mit | 3,562 |
def calc():
h, l = input().split(' ')
mapa = []
for i_row in range(int(h)):
mapa.append(input().split(' '))
maior_num = 0
for row in mapa:
for col in row:
n = int(col)
if (n > maior_num):
maior_num = n
qtd = [0 for i in range(maior_num + 1)]
for row in mapa:
for col in row:
n = int(col)
qtd[n] = qtd[n] + 1
menor = 1
for i in range(1, len(qtd)):
if (qtd[i] <= qtd[menor]):
menor = i
print(menor)
calc()
| DestructHub/bcs-contest | 2016/Main/L/Python/solution_1_wrong.py | Python | mit | 471 |
package com.stulsoft.ysps.pduration
import scala.concurrent.duration._
/**
* @author Yuriy Stul.
* Created on 9/15/2016.
*/
object PDuration {
def main(args: Array[String]): Unit = {
println("==>main")
var fiveSec = 5.seconds
println(s"fiveSec=$fiveSec")
fiveSec = 15.seconds
println(s"fiveSec=$fiveSec")
println("<==main")
}
}
| ysden123/ysps | src/main/scala/com/stulsoft/ysps/pduration/PDuration.scala | Scala | mit | 390 |
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QApplication>
#include <QMainWindow>
#include <QTextEdit>
#include <QMenu>
#include <QMenuBar>
#include <QAction>
#include <QDialog>
#include <QDesktopWidget>
#include <QMdiArea>
#include <QMdiSubWindow>
#include <QDockWidget>
#include <QTreeWidget>
#include <QProcess>
#include <QTimer>
#include <vector>
#include <cassert>
#include "CodeArea.h"
#include "Console.h"
#include "FnSelectDialog.h"
#include "Outline.h"
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow();
QSize sizeHint() const;
void flattenedOutline(FlattenedOutline *outline) const;
signals:
public slots:
void fnSelect();
void toggleSimple();
void openFileDialog();
void saveFile();
void saveFileAsDialog();
void setActiveCodeArea(QMdiSubWindow *area);
void runProgram();
void runPythonParser();
void updateConsoleFromProcess();
void updateCodeOutlineFromProcess(int exitCode, QProcess::ExitStatus exitStatus);
void jumpToFunction(QTreeWidgetItem* item, int column);
private:
QMdiArea *mainArea;
// process
QProcess *programProcess;
QProcess *pythonParserProcess;
// console
Console *console;
QDockWidget *consoleDock;
// editor
CodeArea *activeCodeArea;
std::vector<CodeArea*> codeAreas;
FnSelectDialog *fnSelectDialog;
// code outline
QTreeWidget *codeOutline;
QDockWidget *codeOutlineDock;
std::vector<OutlineClass> outlineClasses;
QTreeWidgetItem *functionsHeader;
// timer
QTimer *parseTimer;
// console actions
QAction *runProgramAction;
// menus
QMenu *fileMenu;
QMenu *helpMenu;
// menu actions
QAction *openFileAction;
QAction *saveFileAction;
QAction *saveFileAsAction;
// code actions
QAction *fnSelectAction;
// ui actions
QAction *toggleSimpleAction;
bool usingSimple;
// project actions
QAction *addFileToProjectAction;
QAction *removeFileFromProjectAction;
// git actions
QAction *openGitDialogAction;
// program actions
QAction *exitProgramAction;
// meta
QString lastUsedDirectory;
};
#endif
| mdenchev/IDL | src/MainWindow.h | C | mit | 2,202 |
/**
* Error for services to through when they encounter a problem with the request.
* Distinguishes between a bad service request and a general error
*/
function ServiceError(message) {
this.name = "ServiceError";
this.message = (message || "");
}
ServiceError.prototype = Object.create(Error.prototype, {
constructor: {value: ServiceError}
});
/**
* Error for when an item is not found
*/
function NotFoundError(message) {
this.name = "NotFoundError";
this.message = (message || "Not found");
}
NotFoundError.prototype = Object.create(ServiceError.prototype, {
constructor: { value: NotFoundError}
});
exports.ServiceError = ServiceError;
exports.NotFoundError = NotFoundError; | James-Tolley/Manny.js | src/services/errors.js | JavaScript | mit | 692 |
require File.dirname(__FILE__) + '/spec'
class Object
class << self
# Lookup missing generators using const_missing. This allows any
# generator to reference another without having to know its location:
# RubyGems, ~/.rubigen/generators, and APP_ROOT/generators.
def lookup_missing_generator(class_id)
if md = /(.+)Generator$/.match(class_id.to_s)
name = md.captures.first.demodulize.underscore
RubiGen::Base.active.lookup(name).klass
else
const_missing_before_generators(class_id)
end
end
unless respond_to?(:const_missing_before_generators)
alias_method :const_missing_before_generators, :const_missing
alias_method :const_missing, :lookup_missing_generator
end
end
end
# User home directory lookup adapted from RubyGems.
def Dir.user_home
if ENV['HOME']
ENV['HOME']
elsif ENV['USERPROFILE']
ENV['USERPROFILE']
elsif ENV['HOMEDRIVE'] and ENV['HOMEPATH']
"#{ENV['HOMEDRIVE']}:#{ENV['HOMEPATH']}"
else
File.expand_path '~'
end
end
module RubiGen
# Generator lookup is managed by a list of sources which return specs
# describing where to find and how to create generators. This module
# provides class methods for manipulating the source list and looking up
# generator specs, and an #instance wrapper for quickly instantiating
# generators by name.
#
# A spec is not a generator: it's a description of where to find
# the generator and how to create it. A source is anything that
# yields generators from #each. PathSource and GemGeneratorSource are provided.
module Lookup
def self.included(base)
base.extend(ClassMethods)
# base.use_component_sources! # TODO is this required since it has no scope/source context
end
# Convenience method to instantiate another generator.
def instance(generator_name, args, runtime_options = {})
self.class.active.instance(generator_name, args, runtime_options)
end
module ClassMethods
# The list of sources where we look, in order, for generators.
def sources
if read_inheritable_attribute(:sources).blank?
if superclass == RubiGen::Base
superclass_sources = superclass.sources
diff = superclass_sources.inject([]) do |mem, source|
found = false
application_sources.each { |app_source| found ||= true if app_source == source}
mem << source unless found
mem
end
write_inheritable_attribute(:sources, diff)
end
active.use_component_sources! if read_inheritable_attribute(:sources).blank?
end
read_inheritable_attribute(:sources)
end
# Add a source to the end of the list.
def append_sources(*args)
sources.concat(args.flatten)
invalidate_cache!
end
# Add a source to the beginning of the list.
def prepend_sources(*args)
sources = self.sources
reset_sources
write_inheritable_array(:sources, args.flatten + sources)
invalidate_cache!
end
# Reset the source list.
def reset_sources
write_inheritable_attribute(:sources, [])
invalidate_cache!
end
# Use application generators (app, ?).
def use_application_sources!(*filters)
reset_sources
write_inheritable_attribute(:sources, application_sources(filters))
end
def application_sources(filters = [])
filters.unshift 'app'
app_sources = []
app_sources << PathSource.new(:builtin, File.join(File.dirname(__FILE__), %w[.. .. app_generators]))
app_sources << filtered_sources(filters)
app_sources.flatten
end
# Use component generators (test_unit, etc).
# 1. Current application. If APP_ROOT is defined we know we're
# generating in the context of this application, so search
# APP_ROOT/generators.
# 2. User home directory. Search ~/.rubigen/generators.
# 3. RubyGems. Search for gems containing /{scope}_generators folder.
# 4. Builtins. None currently.
#
# Search can be filtered by passing one or more prefixes.
# e.g. use_component_sources!(:rubygems) means it will also search in the following
# folders:
# 5. User home directory. Search ~/.rubigen/rubygems_generators.
# 6. RubyGems. Search for gems containing /rubygems_generators folder.
def use_component_sources!(*filters)
reset_sources
new_sources = []
if defined? ::APP_ROOT
new_sources << PathSource.new(:root, "#{::APP_ROOT}/generators")
new_sources << PathSource.new(:vendor, "#{::APP_ROOT}/vendor/generators")
new_sources << PathSource.new(:plugins, "#{::APP_ROOT}/vendor/plugins/*/**/generators")
end
new_sources << filtered_sources(filters)
write_inheritable_attribute(:sources, new_sources.flatten)
end
def filtered_sources(filters)
new_sources = []
new_sources << PathFilteredSource.new(:user, "#{Dir.user_home}/.rubigen/", *filters)
if Object.const_defined?(:Gem)
new_sources << GemPathSource.new(*filters)
end
new_sources
end
# Lookup knows how to find generators' Specs from a list of Sources.
# Searches the sources, in order, for the first matching name.
def lookup(generator_name)
@found ||= {}
generator_name = generator_name.to_s.downcase
@found[generator_name] ||= cache.find { |spec| spec.name == generator_name }
unless @found[generator_name]
chars = generator_name.scan(/./).map{|c|"#{c}.*?"}
rx = /^#{chars}$/
gns = cache.select {|spec| spec.name =~ rx }
@found[generator_name] ||= gns.first if gns.length == 1
raise GeneratorError, "Pattern '#{generator_name}' matches more than one generator: #{gns.map{|sp|sp.name}.join(', ')}" if gns.length > 1
end
@found[generator_name] or raise GeneratorError, "Couldn't find '#{generator_name}' generator"
end
# Convenience method to lookup and instantiate a generator.
def instance(generator_name, args = [], runtime_options = {})
active.lookup(generator_name).klass.new(args, full_options(runtime_options))
end
private
# Lookup and cache every generator from the source list.
def cache
@cache ||= sources.inject([]) { |cache, source| cache + source.to_a }
end
# Clear the cache whenever the source list changes.
def invalidate_cache!
@cache = nil
end
end
end
# Sources enumerate (yield from #each) generator specs which describe
# where to find and how to create generators. Enumerable is mixed in so,
# for example, source.collect will retrieve every generator.
# Sources may be assigned a label to distinguish them.
class Source
include Enumerable
attr_reader :label
def initialize(label)
@label = label
end
# The each method must be implemented in subclasses.
# The base implementation raises an error.
def each
raise NotImplementedError
end
# Return a convenient sorted list of all generator names.
def names(filter = nil)
inject([]) do |mem, spec|
case filter
when :visible
mem << spec.name if spec.visible?
end
mem
end.sort
end
end
# PathSource looks for generators in a filesystem directory.
class PathSource < Source
attr_reader :path
def initialize(label, path)
super label
@path = File.expand_path path
end
# Yield each eligible subdirectory.
def each
Dir["#{path}/[a-z]*"].each do |dir|
if File.directory?(dir)
yield Spec.new(File.basename(dir), dir, label)
end
end
end
def ==(source)
self.class == source.class && path == source.path
end
end
class PathFilteredSource < PathSource
attr_reader :filters
def initialize(label, path, *filters)
super label, File.join(path, "#{filter_str(filters)}generators")
end
def filter_str(filters)
@filters = filters.first.is_a?(Array) ? filters.first : filters
return "" if @filters.blank?
filter_str = @filters.map {|filter| "#{filter}_"}.join(",")
filter_str += ","
"{#{filter_str}}"
end
def ==(source)
self.class == source.class && path == source.path && filters == source.filters && label == source.label
end
end
class AbstractGemSource < Source
def initialize
super :RubyGems
end
end
# GemPathSource looks for generators within any RubyGem's /{filter_}generators/**/<generator_name>_generator.rb file.
class GemPathSource < AbstractGemSource
attr_accessor :filters
def initialize(*filters)
super()
@filters = filters
end
# Yield each generator within rails_generator subdirectories.
def each
generator_full_paths.each do |generator|
yield Spec.new(File.basename(generator).sub(/_generator.rb$/, ''), File.dirname(generator), label)
end
end
def ==(source)
self.class == source.class && filters == source.filters
end
private
def generator_full_paths
@generator_full_paths ||=
Gem::cache.inject({}) do |latest, name_gem|
name, gem = name_gem
hem = latest[gem.name]
latest[gem.name] = gem if hem.nil? or gem.version > hem.version
latest
end.values.inject([]) do |mem, gem|
Dir[gem.full_gem_path + "/#{filter_str}generators/**/*_generator.rb"].each do |generator|
mem << generator
end
mem
end.reverse
end
def filter_str
@filters = filters.first.is_a?(Array) ? filters.first : filters
return "" if filters.blank?
filter_str = filters.map {|filter| "#{filter}_"}.join(",")
filter_str += ","
"{#{filter_str}}"
end
end
end
| cowboyd/rubigen | lib/rubigen/lookup.rb | Ruby | mit | 10,194 |
---
layout: post_n
title: 《Rust权威指南》笔记
date: 2021-11-20
categories:
- Reading
description: 陆陆续续将《Rust权威指南》看完了,文中的例子全部按自己的理解重新实现了一遍。回望来途,可谓困难坎坷,步履艰辛;即是如此,也收获满满。Rust语言的学习给我耳目一新的感觉,上一次对学习语言有这种感觉还是在Haskell的学习中。他完全颠覆我理解的语言设计,在除了垃圾回收语言和内存自主控制语言,竟然还有如此方式来保证内存安全。震撼的同时,也感觉整体学习非常吃力,学习曲线异常陡峭。虽然把整本书都看完了,但还有非常多的细节似懂非懂,也无法完全不参照例子自行写出相对复杂的程序;一些语言中很容易实现的代码在Rust中也无法自行实现出来。所以目前只是第一阶段,即入门,以此为记。后面需要通过开源项目学习练手,通过了解常用的写法去深刻体验Rust的设计精髓。
image: /assets/images/rust_programer_cover.jpeg
image-sm: /assets/images/rust_programer_cover.jpeg
---
* ignore but need
{:toc}
## 前言
陆陆续续将《Rust权威指南》(即英文版的[《The Rust Programming Language》](https://doc.rust-lang.org/book/))看完了,文中的例子全部按自己的理解重新实现了一遍(代码在[github](https://github.com/xiaochai/batman/tree/master/RustProject)上)。回望来途,可谓困难坎坷,步履艰辛;即是如此,也收获满满。
Rust语言的学习给我耳目一新的感觉,上一次对学习语言有这种感觉还是在Haskell的学习中。他完全颠覆我理解的语言设计,在除了垃圾回收语言和内存自主控制语言,竟然还有如此方式来保证内存安全。震撼的同时,也感觉整体学习非常吃力,学习曲线异常陡峭。虽然把整本书都看完了,但还有非常多的细节似懂非懂,也无法完全不参照例子自行写出相对复杂的程序;一些语言中很容易实现的代码在Rust中也无法自行实现出来。所以目前只是第一阶段,即入门,以此为记。后面需要通过开源项目学习练手,通过了解常用的写法去深刻体验Rust的设计精髓。
## 学习与参考资料汇总
| 标题 | 说明|链接 |
| ------------- |:----||:-------------|
|The Rust Reference| 官方文档,也是本文的英文版 |[https://doc.rust-lang.org/reference/introduction.html](https://doc.rust-lang.org/reference/introduction.html) |
|Rust Primer |gitlab上的学习笔记|[https://hardocs.com/d/rustprimer/](https://hardocs.com/d/rustprimer/) |
|Rust Magazine |Rust月刊|[https://rustmagazine.github.io/rust_magazine_2021/chapter_1/rustc_part1.html](https://rustmagazine.github.io/rust_magazine_2021/chapter_1/rustc_part1.html) |
|Rust数据内存布局| 内存布局|[https://juejin.cn/post/6987960007245430797](https://juejin.cn/post/6987960007245430797) |
|The Rustonomicon |官方文档,说明一些语言的灰暗角落|[https://doc.rust-lang.org/nomicon/intro.html#the-rustonomicon](https://doc.rust-lang.org/nomicon/intro.html#the-rustonomicon) |
|The Unstable Book|官方文档,不稳定特性说明 |[https://doc.rust-lang.org/beta/unstable-book/the-unstable-book.html](https://doc.rust-lang.org/beta/unstable-book/the-unstable-book.html) |
|Std Lib Document|标准库文档|[https://doc.rust-lang.org/std/](https://doc.rust-lang.org/std/)|
## 安装与示例
如[官网](https://www.rust-lang.org/tools/install)所说,运行以下命令即可安装:
```bash
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
```
Rust相关的工具会被安装到```/Users/bytedance/.cargo/bin```这个目录下,如果没有把这个目录加到PATH下,添加即可使用。
### 创建Hello World程序
位于hello_world/main.rs
```rust
fn main(){
println!("Hello world");
}
```
编译运行:
```
➜ hello_world git:(master) ✗ rustc main.rs
➜ hello_world git:(master) ✗ ./main
Hello world
```
我们注意到以上程序的几点,细节在后续章节中说明:缩进以4个空格为准;println!为宏,普通函数调用不需要```!```;你会发现去掉println最后的分号也能运行。
### 使用cargo
Cargo是Rust工具链中内置的构建系统及包管理器,常见的命令汇总如下:
| 命令 | 说明 |
| ------------- |:-------------|
| cargo new \<path\> | 创建一个新的cargo项目,会生成Cargo.toml和src/main.rs|
| cargo new \-\-lib \<path\> | 创建一个新的库项目,会生成Cargo.toml和src/lib.rs|
| cargo build |编译本项目,生成的可执行文件位于./target/debug/下;<br/>如果添加\-\-release,则会生成到./target/release/下;<br/>\-\-release参数将花费更多的时间来编译以优化代码,一般用于发布生产环境时使用 |
| cargo run | 编译并运行本项目,也支持\-\-release参数,常用于运行压测; <br/>-p 用于工作空间下有多个二进制包时指定运行哪个包 |
| cargo run \-\-bin \<target\> | 编译并运行指定的bin文件,一般位于src/bin目录下,target不带.rs后缀 |
| cargo check | 仅检查是否通过编译,由于不生成二进制文件,速度快于cargo build |
| cargo doc | 在当前项目的target/doc目录生成使用到的库的文档,可以使用\-\-open选项直接打开浏览器 |
| cargo update | 忽略Cargo.lock文件中的版本信息,并更新为语义化版本所表示的最新版本,用于升级依赖包 |
| cargo test | 运行测试用例,默认情况下是多个测试case并行运行;<br/> cargo test接收两种参数,第一种传递给cargo test使用,第二种是传递给编译出来的测试二进制使用的;<br/>这两种参数中间使用\-\-分开;<br/>例如 cargo test -q tests::it_works -- --test-threads=1;<br/> 这一命令,会以安静模式(-q)运行tests::it_works下的测试,并且只使用一个线程串行运行(--test-threads=1) |
|cargo publish | 发布项目到crate.io上,添加\-\-allow-dirty可以跳过本地git未提交的错误|
|cargo yank \-\-vers 0.0.1| 撤回某个版本,添加\-\-undo取消撤回操作|
Cargo.toml说明:
| 段名 | 说明 |
| ------------- |:-------------|
| package | 本包(crate)的信息说明|
| dependencies |依赖的外部包,版本是语义化的版本,用于update时判断最新的可用版本 |
| profile.dev | 在非\-\-release模式下的编译参数,例如opt-level优化等级配置等,覆盖默认值,要省略 |
|profile.release| 在\-\-release模式下的编译参数,覆盖默认值,可省略
crate是Rust中最小的编译单元,package是单个或多个crate的集合;crate和package都可以被叫作包,因为单个crate也是一个package,但package通常倾向于多个crate的组合。
Rust中的包(crate)代表了一系列源代码文件的集合。用于生成可执行程序的称为二进制包(binary crate),而用于复用功能的称为库包(library crate,代码包),例如rand库等。
创建项目:(hello_cargo项目)
```
➜ RustProject git:(master) ✗ cargo new hello_cargo
Created binary (application) `hello_cargo` package
➜ RustProject git:(master) ✗ cd hello_cargo
➜ hello_cargo git:(master) ✗ tree .
.
├── Cargo.toml
└── src
└── main.rs
1 directory, 2 files
```
Cargo.toml
```ini
[package]
name = "hello_cargo"
version = "0.1.0"
edition = "2018"
# See more keys and their definitions at https://doc.rust-lang.org/cargo/reference/manifest.html
[dependencies]
rand = "0.8.0" # 手动添加的,默认没有这一行,用于说明
```
src/main.rs
```
fn main() {
println!("Hello, world!");
}
```
运行:
```
➜ hello_cargo git:(master) ✗ cargo build
Compiling hello_cargo v0.1.0 (/Users/bytedance/xiaochai/batman/RustProject/hello_cargo)
Finished dev [unoptimized + debuginfo] target(s) in 1.22s
➜ hello_cargo git:(master) ✗ ./target/debug/hello_cargo
Hello, world!
➜ hello_cargo git:(master) ✗ cargo run
Compiling hello_cargo v0.1.0 (/Users/bytedance/xiaochai/batman/RustProject/hello_cargo)
Finished dev [unoptimized + debuginfo] target(s) in 0.27s
Running `target/debug/hello_cargo`
Hello, world
```
## 猜数字例子
位于guessing_game中
```rust
// 标准库中定义的比较结果的枚举
use std::cmp::Ordering;
// 使用use语句进行包导入;rust默认会预导入(prelude)一部分常用的类型,而std::io不在此范围,需要使用use语句
use std::io;
// 首先使用了rand包,需要在Cargo.toml中添加rand = "0.8.0"
// 在run或者build的时候,会根据crates.io上的最新版本、依赖关系下载所需要的包
// rand::Rng为trait(后面解析),gen_range定义于此Trait中
// 如果不导入,调用gen_range将报错,因为ThreadRng的对应实现定义于Rng trait中
use rand::Rng;
fn main() {
// rand::thread_rng()将返回位于本地线程空间的随机数生成器ThreadRng,实现了rand::Rng这一trait
// gen_range的参数签名在0.7.0的包和0.8.x的包上不一样,在旧版中支持两个参数,而新版本中只支持一个参数
// 1..101的用法后面介绍,这一行表示生成[1,101)的随机数
let secret_num = rand::thread_rng().gen_range(1..101);
println!("secret number is {}", secret_num);
// 死循环
loop {
// let关键字用于创建变量
// 默认变量都是不可变的,使用mut关键字修饰可以使变量可变
// String是标准库中的字符串类型,内部我问个他UTF-8编码并可动态扩展
// new是String的一个关联函数(静态方法),用于创建一个空的字段串
let mut guess = String::new();
println!("Guess the number!\nPlease input your guess:");
// std::io::stdin()会返回标准输入的句柄
// 参数&mut guess表示read_line接收一个可变字符串的引用(后面介绍),将读取到的值存入其中
// read_line返回io::Result枚举类型,有Ok和Err两个变体(枚举类型的值列表称为变体)
// 返回Ok时表示正常并通过expect提取附带的值(字节数);返回Err时expect将中断程序,并将参数显示出来
// 不带expect时也能通过编译,但会收到Result没有被处理的警告(warning: unused `Result` that must be used)
io::stdin().read_line(&mut guess).expect("Failed to read line");
// Rust中允许使用同名新变量来隐藏(shadow)旧值
// guess:u32是明确guess的类型,以此来使得让编译器推到出parse要返回包含u32的值
// parse的返回值是一个Result枚举,有Ok和Err两个变体(枚举值),用match来判断两种情况
let guess: u32 = match guess.trim().parse() {
Ok(num) => num,
Err(e) => {
println!("Please type a number!");
// continue回到loop开头继续
continue;
}
};
// {}为println!的占位符,第1个花括号表示格式化字符串后的第一个参数的值,以此类推
println!("You guessed:{}", guess);
// 模式匹配,由match表达式和多个分支组成,Rust可以保证使用match时不会漏掉任何一种情况
// Rust会将secret_num也推导成u32与guess比较
match guess.cmp(&secret_num) {
Ordering::Less => println!("Too small!"),
Ordering::Equal => {
println!("You WIN!");
// 退出循环
break;
}
Ordering::Greater => { println!("Too big!") }
}
}
}
```
## 通用编程概念
本章节例子位于example/src/bin/main.rs中。
### 变量与可变性
变量默认是不可变的,这意味着一但赋值,再也无法改变:
```rust
let x: i32;
x = 5;
let y: i32 = 10;
// 以下行报错:Cannot assign twice to immutable variable [E0384]
// y=9
```
可以变量名前添加mut使得此变量可变:
```rust
let mut x = 10;
x = 100;
x = 1000;
```
常量使用const修饰,名称全部大写,并用下划线分隔;常量可以是全局的(例如main函数之外),也可以是局部的;常量必须显示指定类型:
```rust
const MAX_SCORE: i32 = 199;
fn main() {
// some other code
const MY_SCORE: i32 = 200;
const MAX_SCORE: i32 = 299;
// 200,299
println!("{},{} ", MY_SCORE, MAX_SCORE);
}
```
隐藏变量是指使用相同名称来定义变量,重新定义后,之前的变量值和类型被隐藏了:
```rust
let space = " ";
let space = space.len();
```
### 数据类型
Rust是一门静态语言,所以变量在编译时就确定了其数据类型。Rust的数据类型分为标量类型(scalar)和复合类型(compound)。
一般情况下,在编译器可以推断出类型的场景中,可以省略类型标注,但在无法推断的情况下,就必须显示的声明类型了。
```rust
// 以下报错:type annotations needed
// let k = ("32").parse().expect("not ok");
let k: i32 = ("32").parse().expect("not ok");
```
标量类型是单个值类型的统称,有4种标量类型:整数、浮点数、布尔值及字符。
整数类型:i8、u8、i16、u16、i32、u32、isize、usize;以上除了isize和usize所占的字节数是根据平台(32位/64位)来确定的,其它的的类型都有明确的大小。
整数类型的默认推导是i32:
```rust
// 整数类型字面量
// 10进制,可以用下划分分隔
let x: u32 = 98_000;
let x: u32 = 0xff; // 十六进制
let x: u32 = 0o77; // 八进制
let x: u32 = 0b1111_0000; // 二进制
let x = b'A'; // u8
```
对于整数溢出,编译期会尽可能的检查溢出可能,如果检测到,则直接编译报错。
如果使用debug编译,则在运行时发生溢出时,会触发panic;如果是release,则会执行数值环绕,从最小的重新开始记录。
```rust
// 整数溢出
let x: u8 = 252;
let y: u8 = ("32").parse().expect("not ok");
// 以下行在debug模式下,会在运行时报错
// thread 'main' panicked at 'attempt to add with overflow', src/main.rs:41:26
// note: run with `RUST_BACKTRACE=1` environment variable to display a backtrace
// 在release模式下,则不会报错,输出28
println!("{},see!", x + y);
```
浮点数类型:f32,f64;默认推导为f64。
数值运算:加(+)、减(-)、乘(x)、除(/)、取余(%);只有相同类型间才能进行操作:
```rust
// 类型不一样,无法操作
// let x:u8 = 10;
// let y:u32 = 100;
// let z = y+x;
```
布尔值,只拥有true和false,占据一个字节大小,常用于if的判断:
```rust
let x:bool = false;
```
字符类型,单引号指定,是Unicode标量值,占4个字符:
```rust
// 字符类型
let x = 'c';
let y = '李';
let z:char = '李';
```
将多个不同类型的值合成一个类型,称为复合类型;Rust有两种内置复合类型:元组(tunple)和数组(array);
元组类型,在括号内放置一系列以逗号分隔的值,即可组成元组:
```rust
// 元组
// 也可以省略类型,让编译器推断
let x:(i64,f64,char) = (2,3.4, 'c');
// 使用模式匹配来解构元组
let (a,b,c) = x;
// 通过点来访问元组
println!("{},{},{}", x.0, x.1, x.2);
```
数组类型:
每一个元素必须类型一致,数组大小不可改变,在栈上分配:
```rust
// 数组
// 声明一个长度为5,元素为int32类型的数组;类型[i32;5]也可以省略,由编译器推断
let x: [i32; 5] = [1, 2, 3, 4, 5];
// 定义了一个由7个1组成的数组,即[1,1,1,1,1,1,1]
let y = [1; 7];
// 通过下标来访问,从0开始;以下输出3,1;下标的类型是usize的
println!("{},{}", x[2], y[6]);
// 越界时将发生严重错误
// 编译时报错:index out of bounds: the length is 5 but the index is 10
// 如果下标是运行时才确定的值,则这块的报错将在运行时报错
// let k:usize = "10".parse().expect("not a number");
// println!("{}", x[k]);
```
### 函数
函数的命名由下划线分隔的多个小写单词组成:
```rust
fn my_sum(x: i32, y: i32) -> i32 {
// 可以直接是return,也可以直接写表达式x+y,函数中将最后一个表达式的值做为返回值
return x + y;
}
```
语句和表达式:
语句指执行操作,但不返回值的指令;表达式指进行计算并且产生结果的指令。
表达式是语句的一部分;字面量、函数调用、宏调用、新作用域的花括号都是表达式。
```rust
// 语句,没有返回值
let x = 6;
// 所以不能将语句赋值给变量
// let y = (let x = 7);
// 以下也不行
// let a = b = 6;
// 花括号的代码块也是表达式,以下表达式的值为11
let x = {
let y = 10;
// 注意这一行不能有分号,添加了分号后,这个代码块的值就是空元组了()
y+1
};
```
### 控制流
if的条件表达式必须是bool值;if表达式可以用于赋值:
```rust
// if的用法,多分支判断,条件表达式的值只能是bool类型
let num = 100;
if num % 4 == 0 {
println!("number is divisible by 4")
} else if num % 3 == 0 {
println!("number is divisible by 3")
} else {
println!("number is not divisible by 3,4")
}
// if为表达式,所以可以使用if来赋值
// 此处要注意各个分支返回的数据类型要一样,否则编译期直接报错
let condition = true;
let x = if condition {
10
}else {
20
};
```
loop 循环:
```rust
// loop表达式的值,可以从break返回
let mut i = 1;
let count = loop{
i+=1;
if i > 10 {
break i
}
// 两个break的值类型要一样,所以以下这一行无法通过编译
// break 'a'
};
```
while表达式的值一直都是空值:
```rust
// while表达式的值一直是空值,如果使用break,后面不能跟返回值
let mut i = 1;
let count:() = while i > 10 {
i += 1;
};
```
for:
```rust
// y是一个Range<i32>类型,本身是一个迭代器;值为1,2(不包括3)
// rev是从后往前遍历,所以这块打印的是2,1,
let y = 1..3;
for e in y.rev() {
print!("{},", e)
}
```
结尾习题:
```rust
// 摄氏温度与华氏温度的相互转换
// println!("celsius_2_fahrenheit(1):expect{}, actual:{}", 33.8, celsius_2_fahrenheit(1.0));
fn celsius_2_fahrenheit(celsius: f64) -> f64 {
celsius * 1.8 + 32.0
}
// 生成一个n阶的斐波那契数列
// println!("fibonacci(10):expect{}, actual:{}", 34, fibonacci(10));
fn fibonacci(n: i64) -> i64 {
if n == 1 {
0
} else if n == 2 {
1
} else {
fibonacci(n - 1) + fibonacci(n - 2)
}
}
// 打印圣诞颂歌The Twelve Days of Christmas的歌词,并利用循环处理其中重复的内容。
// 太长了,改成The Six Days of Christmas
// the_six_days_of_christmas();
fn the_six_days_of_christmas() {
let num_map = ["first", "second", "third", "forth", "fifth", "sixth"];
let gifts = ["a partridge in a pear tree",
"two turtle doves",
"three French hens",
"four calling birds",
"five golden rings",
"six geese a-laying",
];
for i in 0..6 {
print!("On the {} day of Christmas, my true love sent to me:", num_map[i]);
let mut j = i;
while j > 0 {
print!("{},", gifts[j]);
j -= 1;
}
// 最后一个礼物不需要逗号
println!("{}.", gifts[0]);
}
}
```
## 所有权
不同语言管理内存的方式:
* Java:通过垃圾回收来管理内存;
* C/C++:开发者手动地分配和释放;
* Rust:通过应用所有权系统规则,在编译期间检查,来保证内容安全。
所有权系统使得Rust在没有垃圾回收的情况下,保证内存安全。其所有权规则包含以下三个规则:
1. <strong>Rust中每一个值都有一个变量作为他的所有者;</strong>
2. <strong>在同一个时间内,值有且仅有一个所有者;</strong>
3. <strong>当所有者离开他的作用域时,他所持有的值将被释放。</strong>
使用域的概念与其它语言类似,不再赘诉。
以下例子位于example/src/bin/main.rs中。
### String类型介绍
字符串的字面量是编译进二进制文件中,但运行时可动态变化的字符串类型则需要存储到堆上。
```rust
// 字符串类型在堆上分配
// String::from方法,使用字符串字面量来创建String类型,这里s必须是可变的
let mut s = String::from("Hello");
s.push_str(", world");
println!("{}", s)
```
下面以例子的方式来说明所有权的动作规则:
```rust
let s1 = String::from("Hello");
let s2 = s1;
println!("{}", s1)
```
String类型由两大部分组成,存储于栈上的元信息(ptr, len, cap),即指向堆上的指针,字符串长度,分配容量;而实际的内容保存在ptr所指的堆上,如下图:

将s1赋值给s2时,由于Rust不会对堆上值也进行拷贝,只会将栈上的元数据进行拷贝,所以目前的状态有可能是s1,s2所指向的String元数据中的指针都指向了同一片堆区域。

在s1和s2变量离开作用域后,Rust会自动执行对应类型上的drop方法,释放对应的内存,问题就产生了,这将产生二次释放问题。
为了解决这个问题,Rust在种情况下,会将s1的变得无效,这就是之前例子中第三行无法通过编译的原因。
这种只有拷贝了栈上的数据而没有拷贝堆上的数据的浅拷贝,在Rust中称为移动(move),上面例子中,s1被移动到s2了
Rust永远不会自动地创建数据的深度拷贝。因此在Rust中,任何自动的赋值操作都可以被视为高效的。
如果确实需要使用深拷贝,可以使用clone函数,如下例子可以通过编译
```rust
// 对s1进行深拷贝,即将堆上的内容也进行了拷贝
let s1 = String::from("Hello");
let s2 = s1.clone();
println!("{}, {}", s1, s2);
```
对于完全存储在栈上的数据,赋值本身已经将全部数据都拷贝,所以不用调用clone方法。**在Rust中,实现了Copy trait的类型,都可以在将变量赋值给其它变量时原变量保持可用**。
所有的标量类型都实现了Copy trait;元组或者数组中包含的元素是Copy的,则元组或者数组就是Copy的:
```rust
// 如果这个元组加了String类型,如下,则不能通过编译
let x = (1,2,3.0);
// let x = (1, 2, 3.0, String::from("Hello"));
let y = x;
println!("{}", x.1);
let x = [1, 2, 3];
let y = x;
println!("{}", x[1]);
```
**注意Copy和Drop是互斥的,如果一个类型本身或者成员变量实现了Copy,则这个类型就无法实现Drop**。
### 函数与所有权
函数参数与赋值是一样的,返回值也是一样的:
```rust
fn take_owner(s: String) {}
let s = String::from("hello");
take_owner(s);
// 将发生编译错误,因为s的所有权移进了take_owner中
// println!("{}", s);
fn take_owner_and_return(s: String)->String{
// 函数又将s的所有权转移到返回值,所以s不会被drop掉
return s;
}
let s1 = String::from("hellow");
let s2 = s1;// 到这里,s1已经失效
let s3 = take_owner_and_return(s2); // 到这里s2已经失效
// 这里只有s3可用,其它的s1,s2失效
```
### 引用与借用
```rust
// 引用传递时,并不取得所有权,但可以使用值
fn get_len(s: &String) -> usize {
// s是一个不可变引用,所以无法对s的值进行改变
// 以下无法通过编译
// s.push_str(", world");
return s.len();
}
let s1 = String::from("hello");
// s2为可变引用,默认的引用不可变
let mut s2: &String = &s1;
let mut s3 = String::from("hello");
// 注意s2为可变引用的意思是可以改变s2引用到哪个值,而不能改变s2引用的值
// 以下无法通过编译, 报错: cannot borrow `*s2` as mutable, as it is behind a `&` reference
// s2.push_str(", world");
// 因为两行可以运行,因为s2是可变引用,而且s3是可变String
s2 = &mut s3;
// 虽然s2引用了可变的s3,但由于s2的类型是&String不是&mut String,所以到下这一行还是无法通过编译
// s2.push_str(", world");
// 获取s1的长度,而不取得s1的所有权
let size = get_len(&s1);
{
let s4 = String::from("you");
// 由于s4的生命周期比s2短,所以s2无法引用s4,这避免了悬垂指针的出现
// s2 = &s4;
}
// 在这里s1还是能用
println!("{},{},{},{}", s1, s2, size, s1);
```
通过引用,可以在不取得所有权的情况下,使用对应值。当引用离开作用域时,由于不持有所有权,所以也不会释放所指向的值。
引用分成可变引用和不可变引用,但这块的可变是指可以改变指向哪个值,而无法改变值本身,注意以下两组的区别:
```rust
let mut s:String = String::from("hello");
let s1:&mut String = &mut s;
s1.push_str(",world");
let mut s:String = String::from("hello");
let mut s2:&String = &mut s;
// 以下无法通过编译,因为s2是&String不可变类型
s2.push_str(", world");
```
另外,只能申明一个可变引用,如果某个变量已经被可变引用了,也不允许再被不可变引用。这可以很好的避免数据竞争:
```rust
let mut s = String::from("hello");
let s1 = &mut s;
// cannot borrow `s` as mutable more than once at a time
// let s2 = &mut s;
// cannot borrow `s` as immutable because it is also borrowed as mutable
// let s3 = &s;
println!("{},{}, {}", s1, s2, s3);
```
总结出来以下规则:
1. **在任何一段给定的时间里,要么只能拥有一个可变引用,要么只能拥有任意数量的不可变引用**。
2. **引用总是有效的**。
### 切片
```rust
// 字符串切片,类似与go,获取[begin,end)之间的内容,注意end最大是字符串的长度,超过后会报错
let s = String::from("0123456789");
// 以下两个hello和world等价
let hello = &s[0..5];
let hello: &str = &s[..5];
let world = &s[5..10];
let world = &s[5..];
// 01234,56789
println!("{},{}", hello, world);
// 获取第一个单词,返回字符串切片
// 这里的函数参数也可以使用&str,可以更通用
fn first_world(s: &String) -> &str {
// s.as_bytes()将字符串转成字节数组&[u8]
// iter返回迭代器,enumerate将每一个元素按元组的形式返回
for (i, &item) in s.as_bytes().iter().enumerate() {
// 判断是空格,直接返回切片
if item == b' ' {
return &s[0..i];
}
}
&s[..] // 使用&s也可以
}
// 输出hello
println!("{}", first_world(&String::from("hello world")));
let mut s = String::from("0123456789");
let t = first_world(&s);
// 以下无法通过编译,因为s已经是不可变引用了,s.clear又使用了可变引用
// cannot borrow `s` as mutable because it is also borrowed as immutable
// s.clear();
println!("{},{}", s, t);
```
字符串字面量就是字符串切片,切片包括指向值的指针和长度。
其它类型的切片:
```rust
// 其它切片
let ia = [1, 2, 3, 4, 5, 6];
let sia: &[i32] = &ia[1..3];
```
## 结构体
结构体的一些规则:(以下例子位于example/src/bin/main.rs中)
1. 一个结构体的实例是可变的,则这个结构体的所有成员变量都是可变的;
2. 在创建结构体实例时,如果变量名与字段名同名时,可以省略字段名,直接写变量名;
3. 可以使用```..old```这种语法来快速从old创建一个只有部分值改变的新变量;
4. 支持不带字段名的元组结构体;
5. 支持空结构体;
6. 如果结构体的成员是引用时,需要带上生命周期的标识。
```rust
// 结构体定义
struct User {
username: String,
email: String,
sign_in_count: u64,
active: bool,
}
// 创建实例
let mut user1 = User {
username: String::from("xiaochai"),
email: "soso2501@mgail.comxxx".to_string(),
sign_in_count: 1,
active: true,
};
println!("{}", user1.email); // soso2501@mgail.comxxx
// 访问和修改,注意一旦实例可变,则实例的所有成员都可变
user1.email = String::from("soso2501@mgail.com");
println!("{}", user1.email); // soso2501@mgail.com
fn build_user(email: String, username: String) -> User {
User {
username, // 由于变量名了字段同名,所以可以省略掉字段名
email,
sign_in_count: 1,
active: true,
}
}
let mut user1 = build_user("soso2501@mgail.com".to_string(), "xiaochai".to_string());
let mut user2 = User {
username: "xiaochai2".to_string(),
// 可以使用以下语法从user1复制剩下的字段
..user1
};
user1.email = "sosoxm@163.com".to_string();
// soso2501@mgail.com,sosoxm@163.com
println!("{},{}", user2.email, user1.email);
// 元组结构体
// 当成员变量没有名字时,结构体与元组类似,称为元组结构体
struct Point(u32, u32, u32);
let origin = Point(0, 0, 0);
// 可以使用数字下标来访问
println!("{},{},{}", origin.0, origin.1, origin.2);
// 也可以通过模式匹配来结构
let Point(x, y, z) = origin;
println!("{},{},{}", x, y, z);
// 空结构体,一般用于trait
struct Empty{}
// 如果结构体的成员是引用时,需要带上生命周期的标识
struct User2<'a> {
username: &'a str,
}
```
### 实例
结构体的应用举例:
1. 通过结构体来更清晰表达字段含义;
2. 使用注解来快速实现trait,使得可以在println!中使用```{:?}```和`{:#?}`来输出自定义结构体;
3. 使用impl关键字为结构体实现方法;
4. 方法第一个参数如果是self,可以是获得所有权(self),也可以是借用(&self),还可以是可变的(&mut self或者是mut self);
5. 同一个结构体,可以写多个impl,但不能多次定义同一个方法,即使参数不一样也不行;
6. 如果方法的第一个参数不为self,则称为关联函数,类似与静态方法。
```rust
// 使用结构的例子,说明trait的使用
#[derive(Debug)] // 添加注解来派生Debug trait
struct Rectangle {
width: u32,
height: u32,
}
fn area(rect: &Rectangle) -> u32 {
rect.width * rect.height
}
let rect1 = Rectangle { width: 10, height: 20 };
// {:?}需要结构体实现Debug这一trait,也可以使用{:#?}来分行打印
// the area of rectangle Rectangle { width: 10, height: 20 } is 200
println!("the area of rectangle {:?} is {}", rect1, area(&rect1))
// 为结构体定义方法
impl Rectangle {
// 方法的第一个参数永远是self
fn area(&self) -> u32 {
self.height * self.width
}
}
println!("the area of rectangle {:?} is {}", rect1, rect1.area());
impl Rectangle {
// 以下无法通过编译,因为area重复定义了
// fn area(&self, i: i32) -> u32 {
// self.height * self.width
// }
fn can_hold(&self, rect2: &Rectangle) -> bool {
self.width > rect2.width && self.height > rect2.height
}
// 关联函数
fn new(width: u32, height: u32) -> Rectangle {
Rectangle {
width,
height,
}
}
}
println!("the area of rectangle {:?} is {}", Rectangle::new(3, 2), Rectangle::new(2, 3).area());
```
## 枚举
1. 枚举使用enum关键字定义,每一个枚举值称之为变体(variant);
2. 每一个变体可以有不同的数据类型和数量的数据关联;
3. 枚举相校与结构体不同的地方在于,如果对变体的不数数据定义各自的结构,则他们属于不同类型,而使用枚举则可以使用一种类型来描述不同的数据结构;
4. 同样可以为枚举实现方法;
5. 使用match模式匹配处理每一种变体,必须处理所有的变体,否则编译不通过;当然可以使用通配符来匹配任意值/类型;
6. match还可以绑定匹配对应的部分值。
以下例子位于example/src/bin/main.rs中:
```rust
enum IPAddr {
// 变体中可以保存数值
IPV4(u32, u32, u32, u32),
IPV6(String),
}
// 可以为枚举定义函数
impl IPAddr {
fn print(&self) {
// 使用match来处理每一种变体,注意需要处理所有变体,否则编译保险错
match self {
// 模式匹配可以直接解构变体内的数值
IPAddr::IPV4(u1, u2, u3, u4) =>
println!("{}.{}.{}.{}", u1, u2, u3, u4),
IPAddr::IPV6(s) => println!("{}", s),
}
}
}
let ipv4 = IPAddr::IPV4(1, 2, 3, 4);
let ipv6 = IPAddr::IPV6("::1".to_string());
ipv4.print();
ipv6.print();
```
预导入库中的非常常用的Option类型,用在需要表示空值的场景,其定义为(省略了注解):
```rust
pub enum Option<T> {
None,
Some(T),
}
```
这里的\<T\>为泛型参数,None表示空值,Some表示有值,并持有值:
```rust
// 标准库中的Option类型
// 为一个Option值加1
fn plus_one(c: Option<i32>) -> Option<i32> {
match c {
None => None,
Some(i) => Some(i + 1)
}
}
let five = plus_one(Some(4));
let none = plus_one(None);
match five {
None => println!("none"),
Some(i) => println!("val is {}", i)
};
match none {
None => println!("none"),
Some(i) => println!("val is {}", i)
};
// 必须匹配每一个可能的值
let c = 2;
match c {
1 => println!("is 1"),
2 => println!("is 2"),
// 如果没有以下这一行,则编译报错
// non-exhaustive patterns: `i32::MIN..=0_i32` and `3_i32..=i32::MAX` not covered
_ => println!("other")
}
```
在只关心某一种匹配而忽略其它匹配的时候,可以使用if let来简化代码:
```rust
// 使用if let来简化处理
let five: Option<i32> = Some(5);
if let Some(i) = five {
println!("has value {}", i)
}
```
## 包管理
* 包(package:一个用于构建、测试并分享单元包的Cargo功能;
* 单元包(crate):一个用于生成库或可执行文件的树形模块结构;
* 模块(module)及use关键字:它们被用于控制文件结构、作用域及路径的私有性;
* 路径(path):一种用于命名条目的方法,这些条目包括结构体、函数和模块等。
以下为关于包的一些定义和规则:
* 用于生成可执行程序的的单元包称为二进制单元包;
* 用于生成库的单元包称为库单元包;
* 一个包至少要有一个单元包,并且最多只能包含一个库单元包,但可以包含多个二进制单元包;
* Rust编译时所使用的入口文件被称为根节点,例如src/main.rs;
* src/main.rs和src/lib.rs做为默认的二进制单元包和库单元包的根节点,无需在cargo.toml中指定;
* mod关键字可以定义模块,模块内可以嵌套定义子模块;
* 可以使用两模式定位到模块内的函数/枚举等:1. 使用单元包名或字面量crate从根节点开始的绝对路径;2. 使用单元包名或字面量crate从根节点开始的绝对路径;
* Rust中所有的条目包括函数、方法、结构体、枚举、模块、常量默认都是私有的;
* 对于模块来说,父级模块无法使用其子模块中的私有的条目,而子模块可以使用所有祖先模块中的条目;
* 公开的结构体其成员默认还是私有的,公开枚举时,其变体自动变成公开。
以下例子位于restaurant项目中:
```rust
// 定义模块,可以嵌套子模块
mod front_of_house {
pub mod hosting {
pub fn add_to_waitlist() {}
pub fn seat_at_table() {}
}
pub mod serving {
fn take_order() {}
fn serve_order() {}
fn take_payment() {}
mod back_of_house {
fn fix_incorrect_order() {
// 使用super关键字引用父模块的,由于子模块可以使用父模块的所有条目,包括私有
super::serve_order();
cook_order();
}
fn cook_order() {}
pub struct Breakfast {
pub toast: String,
// 虽然结构体是公有的,但字段默认还是私有的,需要用pub指定
season_fruit: String,
}
impl Breakfast {
pub fn summer(toast: &str) -> Breakfast {
Breakfast {
toast: String::from(toast),
season_fruit: String::from("peaches"),
}
}
}
pub enum Appetizer {
// 以下两个变体是公开的
Soup,
Salad,
}
}
}
}
pub fn eat_at_restaurant() {
// 以下两种调用是等价的
// 使用绝对路径,从crate关键字(即根节点)开始
crate::front_of_house::hosting::add_to_waitlist();
// 使用相对路径,从当前模块开始
front_of_house::hosting::add_to_waitlist();
}
```
* 使用use关键字可以简化引用路径;
* 使用as关键字使用新的名称,可以解决重名的问题;
* 使用pub use重导出名称,使用pub use的名称不仅在本作用域内可以使用,外部也可以通过引入本作用域来调用到pub use导出的包。可以用于重新组织包结构;
* 使用外部包与使用std包类似,只是需要在cargo.toml文件中的dependencies小节中添加包名以及对应的版本;
* 如果要导入一个树型包中的几个包,可以使用嵌套的语法来减少use语句的使用。
```rust
// 使用use关键字可以简化路径
// 以下两行等价,self关键字可能在后续版本中去掉
use front_of_house::hosting;
// use self::front_of_house::hosting;
pub fn eat_at_restaurant2() {
hosting::add_to_waitlist();
}
// 为防止重名,使用as来重命名
use std::fmt::Result;
use std::io::Result as IOResult;
// 等价于导入std::cmp::Ordering和std::io这两个包
use std::{cmp::Ordering, fs};
// 等价于导入std::io和std::io::Write这两个包
use std::io::{self, Write};
// 导入std::collections下的所有包,一般不推荐,容易造成命名冲突
use std::collections::*;
pub fn test(){
Result::Ok(());
IOResult::Ok("FD");
}
```
可以将模块的层级关系使用目录关系来组织起来,例如原例子中在libs下面创建如下关系的包:
```rust
mod front_of_house {
pub mod hosting {
pub fn add_to_waitlist() {}
pub fn seat_at_table() {}
}
```
可以将front_of_house移到front_of_house.rs中,则以下两个文件变成:
```rust
// 以下是位于lib.rs
mod front_of_house;
```
```rust
// 以下位于front_of_house.rs中
pub mod hosting {
pub fn add_to_waitlist() {}
pub fn seat_at_table() {}
}
```
也可以将front_of_house.rs再拆分:
```rust
// libs.rs
mod front_of_house;
```
```rust
// front_of_house.rs
mod hosting;
```
```rust
// front_of_house/hosting.rs
pub fn add_to_waitlist() {}
pub fn seat_at_table() {}
```
## 集合
以下例子位于example/src/bin/collection.rs中:
### 动态数组
* 动态数组Vec是范型,在无法推断类型时,必须显示指定类型;
* 使用vec!宏可以快速构建有初始值的数组;
* 数组中的元素会在动态数组销毁时跟着销毁;
* 数组元素的引用会对整个数组造成影响,即不可以在有只读引用的情况下push元素;
* 结合枚举,可以间接地在Vec中存储多种不同的类型。
```rust
// 创建一个动态数组
let v: Vec<i32> = Vec::new();
// 使用vec!宏来快速创建一个带有初始值的数组
let v = vec![1, 2, 3];
// 必须是mut的动态数组才能push进数据
let mut v: Vec<i32> = Vec::new();
// 往动态数组里添加数据
v.push(5);
v.push(6);
// 获取数组元素的引用,如果数组越界将发生panic
let e1: &i32 = &v[0];
// 返回Option<&T>类型,如果不越界将返回Option
let e2: Option<&i32> = v.get(0);
// 注意数组元素的引用会对整个数组造成影响,这就导致了在只读引用存在的情况下无法往数组中push元素
// 以下行无法通过编译:cannot borrow `v` as mutable because it is also borrowed as immutable
// v.push(7);
println!("{}", e1);
// 使用for来遍历所有元素,这里的i为&i32
for i in &v {
// 5 6
println!("{}", i)
}
// 这里的i值为&mut i32
for i in &mut v {
// 将i解引用,指向对应的值,并修改
*i *= 2;
}
for i in &v {
// 10 12
println!("{}", i)
}
```
### 字符串
* 字符串本身是基于字节的集合,通过功能性的方法将字节解析为文本;
* Rust语言核心部分只有一种字符串类型,即字符串切片str,通常以引用形式出现(&str),它是指向存储在别处的一些UTF8编码字符串的引用,例如字符串字面量;
* String类型定义在标准库中,不是语言核心的一部分,提供UTF8编码;
* 标准库还提供了OsString,OsStr,CString和CStr,一般以Str结尾的是借用版本,String结尾是所有权版本。这些类型提供了不同的编码或者不同内存布局的字符串类型;
* String类型实际使用Vec\<u8\>进行封装;
* 为了避免出现多字节情景下你拿到半个字符,所以Rust不允许使用下标访问获取字符串的字符。而是通过特定的功能函数指定对字节,字符,字形簇进行处理;
* 但是Rust却允许字符串切片的使用,但使用时要格外小心,因为如果截取的范围不是有效的字符串,将发生panic。
```rust
// 字符串字面量是&str类型
let c: &str = "ab";
println!("{}", c);
// 创建一个新空字符串
let s: String = String::new();
// 从字面量创建一个字符串的两种方法,String::from的静态方法,&str的to_string方法
let s = String::from("hello");
let s = "hello".to_string();
// 修改字符串
let mut s = String::from("abc");
// 往后添加字符串,push_str的参数是引用的形式&str
s.push_str("def");
// 插入单个字符,单个字符使用单引号
s.push('g');
// 使用+号拼接字符串,加号的左边是String类型,右边是&str类型,左边的变量的所有权将被加号获取而不再有效
let s1 = String::from("hello");
let s2 = String::from(", world");
// s1 的所有权将被转移,不再可用,而s2由于使用引用,所以可以继续使用
// 加号的第二个签名是&str,而我们传入的是&String也是合法的,因为Rust使用使用解引用强制转换的技术,将&s2转化为&s2[..]
// &s2[..] 是&str类型
let s = s1 + &s2;
println!("{},{}", s, s2);
let k:&str = &s[2..];
// 使用format!宏来拼接字符串,format!不会夺取任何参数的所有权
let s = format!("{}, {}! {}.", "hello", "world", "lee");
println!("{}", s);
// String采用utf-8编码,所以一个中文占用3个字节;以下输出3
println!("{}", "我".to_string().len());
// 虽然字符串不允许使用下标直接访问,但可以使用切片获取某个范围的字符串
println!("{}", &s[0..1]);
let s = "我是中国人".to_string();
// 需要注意如果切片的范围不是一个合法的字符串,则会直接panic
// 以下将发生运行时panic:thread 'main' panicked at 'byte index 1 is not a char boundary; it is inside '我' (bytes 0..3) of `我`', src/main.rs:69:21
// println!("{}", &s[0..1]);
// 使用chars函数,可以获取根据编码获取字符串中的字符值
for i in s.chars(){
println!("{}", i);
}
// 与此相对,使用bytes,则获取每一个字节的内容
for i in s.bytes(){
println!("{}", i);
}
```
### HashMap
HashMap并没有在预加载库中,所以需要使用`use std::collections::HashMap;`进行导入。
HashMap如果键和值实现了CopyTrait,则会复制一份。如果持有所有权的类型,则会将所有权转移到HashMap中。如果是引用类型,则不会取得所有权,由生命周期保证引用的有效性。
```rust
use std::collections::HashMap;
// 初始化一个hashmap,不指定类型,编译器可以从h.insert里推断出类型来为HashMap<&str,i32>
let mut h = HashMap::new();
h.insert("lee", 1220);
// 使用Vec来构建HashMap
let teams = vec![String::from("blue"), String::from("yellow")];
let scores = vec![10, 50];
// h的类型声明是必须的,因为collect可以返回多种类型,需要明确这里需要返回的类型,但是泛型可以使用_代替,由编译器来推断
// h的类型为HashMap<&String, &i32>
let mut h: HashMap<_, _> = teams.iter().zip(scores.iter()).collect();
// 获取HashMap中的值,注意这里的值是&String,不能直接使用&str
let blue = String::from("blue");
// get函数获取的值为Option,如果不存在,则返回None
// get取得的结果是value的引用值,在这个场景中为&&i32
let blue_team_score = match h.get(&blue) {
// 由于值是&&i32,所以需要两次解引用成i32值,否则与None的返回值不匹配
Some(i) => **i,
None => 0,
};
println!("{}", blue_team_score);
// 使用for循环获取HashMap里的值,由于使用&h,所以这里的key为&&String,value为&&i32
for (key, value) in &h {
println!("key:{}, value:{}", key, value);
}
let k = String::from("blue");
// 更新值,如果是直接覆盖,使用insert即可
h.insert(&k, &20);
// 通过entry函数返回Entry枚举类型,其or_insert方法可以判断值是否存在,不存在则插入,存在则不处理
// 其返回HashMap中value的可变引用,在此为&mut &i32,可以对其进行修改
let e = h.entry(&k).or_insert(&30);
*e = &11;
// {"blue": 11, "yellow": 50}
println!("{:?}", h);
// 例子,查看一个字符串中每一个字符出现的次数
let text = "hello world hello lee ok";
let mut map = HashMap::new();
for i in text.split_whitespace() {
let count = map.entry(i).or_insert(0);
*count += 1;
}
// {"ok": 1, "world": 1, "lee": 1, "hello": 2}
println!("{:?}", map);
```
## 错误处理
* 不可恢复错误:使用panic!宏,其参数与println!类似,支持占位符;
* 访问Vec越界也会产生panic;
* 在cargo.toml中的profile.release节添加`panic= 'abort'`来减少bin文件的大小(因为减少了栈展开所需要的信息);
* 添加`RUST_BACKTRACE=1`可以输出更加详细的panic信息,例如`cargo run --bin error --release`;
* 如果是可恢复错误,使用Result<T, E>做为返回值来处理包含正常情况和异常情况,正常情况下返回Ok(T),异常时返回Err(E)。
```rust
enum Result<T, E>{
Ok(T),
Err(E),
}
```
以下例子位于example/src/bin/error.rs:
```rust
let file_name = "Cargo.toml";
// 对于有可能出错的函数可以返回Result,Ok表示正常返回,Err表示异常
let mut f = match File::open(file_name) {
Ok(file) => file,
Err(e) => panic!("open file error:{:?}", e),
};
// 读取文件的内容,并输出
let mut c = String::new();
f.read_to_string(&mut c);
println!("{}", c);
let file_name = "hello.txt";
let f = match File::open(file_name) {
Ok(file) => file,
// 使用e.kind()为区别不一样的类型
Err(e) => match e.kind() {
// 不存在的时候就创建,返回成功创建的句柄
ErrorKind::NotFound => match File::create(file_name) {
Ok(file) => file,
Err(e) => panic!("create file error:{:?}", e),
},
// 其它错误统一命中这个分支,报错panic
other_error => panic!("open file error:{:?}", other_error),
}
};
// 使用Result.unwrap()函数来快速获取Ok的值,如果是Err,则直接panic
let f: File = File::open(file_name).unwrap();
// 与unwrap一样,只是传入了一个字符串做为panic时的信息
let f: File = File::open(file_name).expect("Fail to open file");
// 传播错误
// 以下函数的功能等同于std::fs::read_to_string(file_name)
fn get_content_by_file_name(name: &str) -> Result<String, io::Error> {
let mut f = match File::open(name) {
Ok(file) => file,
Err(e) => return Err(e),
};
// 读取文件的内容,并输出
let mut c = String::new();
return match f.read_to_string(&mut c) {
Ok(_) => Ok(c),
Err(e) => Err(e),
};
}
// 使用?运算符简化写法
// 注意使用?运算符与match不一样的地方是在Err类型不匹配的时候,会自动调用from函数进行隐式转换(需要实现From trait)
fn get_content_by_file_name2(name: &str) -> Result<String, io::Error> {
// 如果需要将Result的Err返回,则在最后使用?表达式来达到目的
let mut f = File::open(name)?;
// 读取文件的内容,并输出
let mut c = String::new();
f.read_to_string(&mut c)?;
Ok(c)
}
// 使用链式调用更加简化写法
fn get_content_by_file_name3(name: &str) -> Result<String, io::Error> {
let mut c = String::new();
File::open(name)?.read_to_string(&mut c)?;
Ok(c)
}
```
## 泛型、Trait、生命周期
### 泛型
泛型代码的性能问题:Rust在编译期间将泛型代码单态化(monomorphization),即将泛型代码根据调用时的类型生成对应的代码。所以不会对运行时造成性能影响。例如 Option\<T\>类型在应用到i32和i64上时,生成了以下两种类型Option_i32,Option_i64。
以下例子位于example/src/bin/generic.rs:
```rust
fn main() {
// 在方法中使用泛型,在函数名称后添加尖括号,并在括号中添加类型说明,
// <T:PartialOrd + Copy>表示这个类型必须实现PartialOrd和Copy这两个Trait
// 参数为对应类型的数组切片,返回对应类型的值
// 由于对元素使用了大于比较计算符,所以类型T必须实现std::cmp::PartialOrd
// 由于需要从list[0]中取出数据,所以需要实现Copy;也可以使用引用来处理
fn largest<T: PartialOrd + Copy>(list: &[T]) -> T {
let mut max = list[0];
for &item in list.iter() {
if item > max {
max = item
}
}
max
}
// 使用引用的版本,不需要实现Copy
fn largest2<T: PartialOrd>(list: &[T]) -> &T {
let mut max = &list[0];
for item in list.iter() {
if *item > *max {
max = item
}
}
max
}
println!(
"{},{},{}, {}",
largest(&[1, 2, 3, 4, 5, 6, 9, 3, 4, 6]),
largest(&[1.0, 3.0, 1.1, 5.5, -1.0, -2.4]),
// 动态数组可以转化为数组切片
largest(&vec![1, 2, 3, 4, 5, 4, 3, 2, 1]),
largest(&vec!['a', 'b', 'e', 'd', 'k', 'i', 'g']),
);
// 在结构体中使用泛型,在结构体名之后使用尖括号来声明
struct Point<T> {
x: T,
y: T,
}
impl<T> Point<T> {
fn x(&self) -> &T {
&self.x
}
// 在泛型的结构里定义泛型的方法
fn other<U>(&self, other: Point<U>) -> Point<U> {
other
}
}
// 在枚举中使用泛型,我们之前看到的Option和Result都有使用,不再举例
enum Option<T> {
Some(T),
None,
}
enum Result<T, E> {
Ok(T),
Err(E),
}
}
```
### Trait
* trait是指某些特定类型拥有的,而且可以被其它类型所共享的功能集合,类似于其它语言的interface。
* **实现trait的代码要么位于trait定义的包中,要么位于结构体定义的包中,而不能在这两个包外的其它包中,这个规则称之为孤儿规则,是程序一致性的组成部分**。
以下例子位于example/src/bin/trait.rs:
```rust
// 定义多种文章共有的摘要功能trait
pub trait Summary {
fn summarize(&self) -> String;
// trait也可以提供一个默认实现,这样实现了这一trait的结构体,如果没有提供实现,则以默认实现为准
fn summarize2(&self) -> String {
String::from("Read more")
}
}
pub struct NewsArticle {
pub headline: String,
pub location: String,
pub author: String,
pub content: String,
}
// 为某一个结构实现trait,使用关键字impl和for
// 实现的trait跟普通函数一样,可以被调用
impl Summary for NewsArticle {
fn summarize(&self) -> String {
format!("{},{},{}", self.headline, self.author, self.location)
}
}
pub struct Tweet {
pub username: String,
pub content: String,
pub reply: bool,
pub retweet: bool,
}
impl Summary for Tweet {
fn summarize(&self) -> String {
format!("{},{}", self.username, self.content)
}
// 重载了默认实现,这样就无法调用到默认实现了
fn summarize2(&self) -> String {
format!("summarize2....")
}
}
let tweet = Tweet {
username: "lee".to_string(),
content: "content".to_string(),
reply: false,
retweet: false,
};
// trait实现的函数,可以像普通函数一样调用,summarize2调用则是默认的实现
println!("tweet: {}, summary2:{}", tweet.summarize(), tweet.summarize2());
// 将trait作为参数
// 这个函数接收实现了Summary trait的结构体类型,在这里可传入Tweet和NewsArticle
pub fn notify<T: Summary>(item: &T) {
println!("breaking news1:{}", item.summarize())
}
notify(&tweet);
// 可以使用impl形式的语法糖来简化写法,与之前的一致
// 是否简化也区别于实际场景,例如多个函数使用同一个约束时,使用泛型表达式则更加方便
pub fn notify2(item: &impl Summary) {
println!("breaking news2:{}", item.summarize())
}
notify2(&tweet);
// 使用多个约束时使用+号来处理,这里的item必须实现Display和Summary两个trait
pub fn notify3<T: Display + Summary>(item: T) {}
// 在复杂情况下使用where语句可以使得函数签名更清晰,以下两种方式是等价的
fn some_func<T: Display + Clone, U: Clone + Debug>(t: T, u: U) -> i32 { 1 }
fn some_func2<T, U>(t: T, u: U) -> i32 where T: Display + Clone, U: Clone + Debug { 1 }
// 可以返回可以使用impl形式,但只能返回一中类型,要么是Tweet,要么是NewsArticle,不能在不同的分支返回两种类型
fn return_summarizable() -> impl Summary {
Tweet {
username: "".to_string(),
content: "".to_string(),
reply: false,
retweet: false,
}
}
// 以下无法通过编译,对于泛型,需要深入研究一下机制,为什么以下函数无法通过编译
// fn return_summarizable2<T: Summary>(item:T) -> T {
// Tweet {
// username: "".to_string(),
// content: "".to_string(),
// reply: false,
// retweet: false,
// }
// }
// 使用trait约束来有条件地实现方法
struct Point<T> {
x: T,
y: T,
}
// 为所有类型的T的Point实现new方法
impl<T> Point<T> {
// 大写的Self与小写的self区别
fn new(x: T, y: T) -> Self {
Point { x, y }
}
}
// 只为实现了PartialOrd和Display的Point实现cmp方法
impl<T: PartialOrd + Display> Point<T> {
fn cmp(&self) {
if self.x > self.y {
println!("x>y")
} else {
println!("x<=y")
}
}
}
// 也可以使用一个trait约束来实现另外一个trait,称之为覆盖实现(blanket implementation)
// 例如以下的例子,为了实现了Display的类型实现Summary方法
impl<T: Display> Summary for T {
fn summarize(&self) -> String {
format!("read more:{}", self)
}
}
// 以下例子无法通过编译,因为Display不在此包中,T这一也不在此包中,受孤儿规则限制,将报错
// 报错:Only traits defined in the current crate can be implemented for arbitrary types [E0117]
// impl<T: Summary> Display for T {
// fn fmt(&self, f: &mut core::fmt::Formatter<'_>) -> core::fmt::Result {
// write!(f, "({}, {})", self.x, self.y)
// }
// }
// 因为上面为实现Display的类型实现了Summary,而i32实现了Display,所以i32实现了Summary
// 输出read more:2
println!("{}", 2.summarize());
```
### 生命周期
* 生命周期大部分情况下可以推导出来,当无法推导时,就必须手动标注生命周期;
* 生命周期最主要的目的是避免悬垂引用,进而避免程序引用到非预期的数据;
* Rust中不允许空值的存在;
* 借用检查器(borrow checker):用于检查各个变量的生命周期长短,以判断引用是否合法。
生命周期的标注:
* 生命周期的标注以单引号开始,后跟小写字母(通常情况下),通常非常简短,例如```'a```;
* 标注跟在&之后,并使用空格与引用类型区分开,例如`&'a i32`、`&'a mut i32`等。
每一个引用都有生命周期,而函数在满足一定条件下,可以省略生命周期声明,称为生命周期省略规则。
使用以下三条规则计算出生命周期后,如果仍然有无法计算出生命周期的引用时,则编译出错:
1. 每一个引用参数都有自己的生命周期,这一条用于计算输入生命周期;
2. 只存在一个输入生命周期时,这个生命周期将赋值给所有的输出生命周期参数,这一条用于计算输出生命周期;
3. 当拥有多个输入生命周期参数,而其中一个是&self或&mut self时,self的生命周期会被赋予给所有的输出生命周期参数。这条规则使方法更加易于阅读和编写,因为它省略了一些不必要的符号。
以下例子位于example/src/bin/live_time.rs:
```rust
let mut r = &2;
{
let x = 5;
// 以下无法通过编译,因为x在内部作用域内,而r在main作用域,r的生命周期大于x,无法使用x的引用。
// `x` does not live long enough
// r = &x
}
println!("{}", r);
// 如果不加生命周期标注的话,无法确定返回值的生命周期,无法通过编译
// 由于x,y两个参数都用于做为引用返回,所以x,y必须都要标明生命周期
// 这里的'a会被具体化为x,y的生命周期中重叠的那一部分
fn longest<'a>(x: &'a str, y: &'a str) -> &'a str {
if x.len() > y.len() {
x
} else {
y
}
}
let s1 = String::from("s11");
let mut s4 = "";
{
let s2 = String::from("s2");
// longest返回值的生命周期是s1和s2中生命周期较短的那个,即s2的生命周期
// s3的生命周期与s2一样,所以能通过编译
let s3 = longest(&s1, &s2);
println!("{}", s3);
// 以下无法通过编译,因为s4的生命周期大于s2
// s4 = longest(&s1, &s2);
}
println!("{}", s4);
// 以下这个函数不满足生命周期省略规则,所以必须手动标注
// fn longest(x:&str, y:&str)->&str{}
// 以下函数应用规则1和规则2后,所有的输入输出引用参数周期都确定,所以可以省略
// fn first_word(x:&str)->&str{}
// 结构体中引用字段的生命周期标
struct ImportantExcerpt<'a> {
part: &'a str,
}
impl<'a> ImportantExcerpt<'a> {
fn level(&self) -> i32 {
2
}
// 应用第一条规则和第三条规则,可以得出正确的生命周期,所以以下这个可以周期可以省略
fn announce_and_return_part(&self, announcement: &str) -> &str {
self.part
}
// 应用第一条和第三条规则,得出的生命周期不正确,所以在没有生命周期房间里时编译报错
fn display_part_and_return<'b>(&self, announcement: &'b str) -> &'b str {
announcement
}
}
// 'static是固定写法,表示生命周期是整个程序的执行周期
// 所有字符串字面量都拥有'static生命周期
let s: &'static str = "abc";
use std::fmt::Display;
// 同时使用生命周期,泛型,trait约束的例子
// 生命周期必须在泛型类型声明之前,也可以使用where进行trait约束
// 生命周期也是泛型的一种??
fn longest_with_announcement<'a, T:Display>(x: &'a str, y: &'a str, anno: T) -> &'a str {
println!("{}", anno);
if x.len() > y.len() {
x
} else {
y
}
}
```
## 编写自动化测试
Rust中的单元测试一般与要测试的代码放在同一个文件中,使用的模块名称为tests,通过```#[cfg(test)]```来标记,让编译器只在cargo test时才编译和运行这一部分代码,在cargo build等场景会剔除这些代码。
在函数上使用```#[test]```称为属性(attribute),与C#的attribute以及Java的annotation一样,用于给编译器提供更多的信息。
cargo test参数说明之\-\-之后的参数:
* \-\-test-threads=1:运行的并发数,默认是多个case并行执行,可以设置成1为串行执行;
* \-\-nocapture:将测试用例中打印出来内容输出显示出来。默认情况下这些内容会被捕获并丢弃;
* \-\-ignored:专门运行被```#[ignore]```标记的case。
cargo test参数说明之\-\-之前的参数:
* \-q:静默情况下运行测试用例,输出的信息比较有限;
* \-\-test file_name:用于指定运行集成测试的文件,注意不用跟.rs后缀名。
cargo test允许我们指定需要运行的用例名,例如cargo test adder,则所有case名中包含有adder的case都会被运行(例如:tests::adder_test),这种方法只能指定一个匹配字符串,无法指定多个。
在```#[test]```标记之后,跟上```#[ignore]```的测试函数,默认情况下不会运行,只有使用\-\-ignored时,才会专门运行这些case,例如```cargo test -- --ignored```。
本单元的例子位于adder项目中:
```rust
//! # Adder
//!
//! 测试做为包的注释,包含有一些小组件
use std::ops::Add;
/// 定义一个泛型加法
///
/// # Example
///
/// 例子,一般是测试用例
/// ```
/// assert_eq!(5, adder::adder(3,2));
/// assert_eq!(9.8, adder::adder(3.3, 6.5));
/// ```
/// # Panics
///
/// 可能Panic的场景
///
/// # Errors
/// 如果返回Result时,这里写明Error返回的场景,以及返回的Error值
///
/// # Safety
///
/// 当使用了unsafe关键字时,这里可以说明使用unsafe的原因,以及调用者的注意事项
///
pub fn adder<T: Add + Add<Output=T>>(a: T, b: T) -> T {
return a + b;
}
#[cfg(test)]
mod tests {
// 引入所有父模块的所有包,这样可以直接使用adder方法,否则adder需要指定完整路径才能使用
use super::*;
use std::fs::File;
use std::io::Read;
// 标记以下函数是一个测试函数,如果没有这个标记,cargo test的时候不会运行
#[test]
fn it_works() {
// 相等断言
assert_eq!(2 + 2, 4);
}
#[test]
fn adder_test() {
// 注意使用了assert_eq和assert_ne这两个断言时,两个参数必须实现了PartialEq和Debug这两个trait,一个用于判断相等,一个用于出错时输出信息
// 以下两个写法等价,推荐使用第一个写法,因为在失败时可以打印出两个参数的值,方便分析原因
assert_eq!(adder(2, 2), 4);
assert!(adder(2, 2) == 4); // assert!接收一个bool类型的参数,只有为true时测试才通过
// assert_ne!用于断言两个值不相等
assert_ne!(adder(2.0, 3.0), 6.0);
// 这些断言在必要参数之后的信息都会被用于format!输出额外的信息
assert_eq!(adder(3, 4), 7, "{}+{} is not equal 7", 3, 4);
}
#[test]
// should_panic属性用于表示这个函数会发生panic
// 参数expected会比较panic的信息是否与此相匹配,注意中人包含expected的内容即可
#[should_panic(expected = "panicked")]
fn check_panic() {
panic!("i panicked")
}
#[test]
// 使用Result做为测试用例的返回值时,只要有Err返回,就测试失败
// 这种情况下可以使用?表达式来简化测试用例的编写
fn use_result() -> Result<(), std::io::Error> {
let mut f = File::open("./cargo.toml")?;
let mut s = String::new();
let _ = f.read_to_string(&mut s)?;
return Ok(());
}
#[test]
#[ignore] // 默认情况下忽略此case,可以通过--ignored来专门运行这种case
fn long_time_test() {}
}
```
集成测试:
* 在src同级别建立tests目录,在这个目录下可以创建任意的集成测试用例;tests目录只在cargo test时进行编译和执行,其它情况会忽略;
* tests目录下每一个文件都是独立包名,所以不用担心测试函数会重名;
* 可以在tests目录下建立子目录,做为公共使用的部分,或者隐藏测试中的细节等等,子目录中的函数也可以标记为```#[test]```,但他只在tests目录下的文件引用到时执行;
* 如果将子目录做为公共部分使用,一般不在里面设置测试用例,因为如果多个测试模块引用的话,这个case将被运行多次;
* 无法在集成测试中引用main.rs,所以一般我们将复杂的操作移到lib.rs中,而main.rs中只保留简单的胶水代码逻辑。
```rust
// src/tests/integation.rs
#[test]
fn adder_test() {
assert_eq!(adder::adder(9.0, 10.0), 19.0);
}
#[test]
fn my_note() {
assert_eq!(adder::adder(9.0, 10.0), 19.0);
}
mod sub;
```
```rust
// src/tests/sub/mod.rs
#[test]
pub fn setup() {}
```
## minigrep例子
* `std::env::args()`获取命令行参数,格式与其它语言一样;
* `std::process::exit(1)`退出进程,这个函数签名为`pub fn exit(code: i32) -> !`,`!`表示从来不会返回;
* `std::fs::read_to_string()`读取文件内容;
* `std::env::var("CASE_INSENSITIVE")`获取环境变量值;
* `eprintln!`将错误信息打印到标准错误输出。
代码位于minigrep项目中:
```rust
// main.rs
use minigrep::*;
// main函数里的代码无法进行单元测试和集成测试,所以main函数这块保持简单,把逻辑移到lib.rs下
fn main() {
// std::env::args()返回的是Args实现了Iterator,使用collect转化为Vec<String>
// 因为collect返回的也是泛型,编译器无法自动推断需要返回的类型,所以给args的类型标注不可省略
let args: Vec<String> = std::env::args().collect();
// Result结构的unwrap_or_else方法接收一个函数来做错误处理
// 处理函数包含一个闭包参数,使用竖线包起来,获取到的值为Result的E中的值
// 处理函数必须返回Result的T值(正确的值),但std::process::exit(1)直接退出进程,所以可以编译通过
// exit的返回值为->!,表示从不会返回:pub fn exit(code: i32) -> !
let config = Config::new(&args).unwrap_or_else(|err| {
// 使用eprintln!将错误信息输出到标准错误输出
eprintln!("Problem parsing arguments: {}", err);
std::process::exit(1);
});
// // 使用迭代器的版本
// let config = Config::new2(std::env::args()).unwrap_or_else(|err| {
// eprintln!("Problem parsing arguments: {}", err);
// std::process::exit(1);
// });
// 使用if let语法,只处理关心的变体
if let Err(e) = run(&config) {
eprintln!("Application error: {}", e);
std::process::exit(1);
}
}
```
```rust
// lib.rs
// 返回Result中的E为Box<dyn std::error::Error>,表示为实现了Error这一trait的类型
// 具体的类型需要在具体的场景中才能确定,这里的dyn关键字也说明了是一个动态的类型
pub fn run(config: &Config) -> Result<(), Box<dyn std::error::Error>> {
let contents = std::fs::read_to_string(&config.filename)?;
let result = if config.case_sensitive {
search(&config.query, &contents)
} else {
search_case_insensitive(&config.query, &contents)
};
for l in result {
println!("{}", l);
}
Ok(())
}
pub struct Config {
query: String,
filename: String,
case_sensitive: bool,
}
impl Config {
// 使用命令行参数来构造Config结构,返回Result结构,在出错时返回str
// 返回值中的str中声明了生命周期为全局的,因为字面量常量可以是全局的,此处不设置其实也没有问题,生命周期与输入一致
pub fn new(args: &[String]) -> Result<Config, &'static str> {
if args.len() < 3 {
return Err("not enough arguments!");
}
let query = args[1].clone();
let filename = args[2].clone();
// 读取环境变量来确定是否需要大小写敏感,读取到无error则case_sensitive=false,不区分大小写,如下
// CASE_INSENSITIVE=1 cargo run Nobody poem.txt
let case_sensitive = std::env::var("CASE_INSENSITIVE").is_err();
// 变量与结构体字段重名时,可以使用此种方式快速构建
Ok(Config { query, filename, case_sensitive })
}
// 使用迭代器版本
pub fn new2(mut args: std::env::Args) -> Result<Config, &'static str> {
args.next();
let query = match args.next() {
Some(q) => q,
None => return Err("no query")
};
let filename = match args.next() {
Some(f) => f,
None => return Err("no filename")
};
let case_sensitive = std::env::var("CASE_INSENSITIVE").is_err();
Ok(Config { query, filename, case_sensitive })
}
}
// 这块需要指定生命周期,表明Vec返回的&str,与contents的生命周期绑定
// 如果contents失败了,那么返回值也将没有意义了
fn search<'a>(query: &str, contents: &'a str) -> Vec<&'a str> {
let mut ret: Vec<&str> = Vec::new();
for line in contents.lines() {
if line.contains(query) {
ret.push(line)
}
}
ret
// 使用迭代器版本
// contents.lines().filter(|line| line.contains(query)).collect()
}
fn search_case_insensitive<'a>(query: &str, contents: &'a str) -> Vec<&'a str> {
let mut ret: Vec<&str> = Vec::new();
let q = query.to_lowercase();
for l in contents.lines() {
if l.to_lowercase().contains(&q) {
ret.push(l)
}
}
ret
}
#[cfg(test)]
mod tests {
use super::*;
#[test]
fn one_result() {
let query = "duct";
let contents = "\
Rust:
safe, fast, productive.
Pick three.
";
assert_eq!(
vec!["safe, fast, productive."],
search(query, contents),
);
}
#[test]
fn case_insensitive() {
let query = "rust";
let contents = "\
Rust:
safe, fast, productive.
Pick three.
";
assert_eq!(
vec!["Rust:"],
search_case_insensitive(query, contents),
);
}
}
```
## 函数式语言特性:迭代器与闭包
### 闭包
与闭包相关的三种trait: Fn, FnMut, FnOnce,所有闭包至少实现其中的一个。
这三个trait代表了函数接收参数的三种方式:不可变引用(Fn),可变引用(FnMut),获取所有权(FnOnce)。
rust自动从闭包的定义中推导出其所实现的trait。
所有函数都实现了这三个trait,所以要求传闭包的地方都可以传函数。
这三个trait的区别:
* 实现了FnOnce的闭包可以从环境中获取变量的所有权,所以这个类型的闭包只能被调用一次;
* 实现了FnMut的闭包从环境中可变地借用值;
* 实现了Fn的闭包从环境中不可变地借用值;
* 所有闭包都实现了FnOnce,实现了Fn的闭包也实现了FnMut。
以下例子位于example/src/bin/functional.rs中:
```rust
fn main() {
// 闭包当作匿名函数的一般写法,参数和返回值的类型,由编译器推断出来
let add_one = |x| {
x + 1
};
// 如果无法推断,则需要写成这种详细的形式,与函数定义非常类似
let add_one2 = |x: i32| -> i32{
x + 1
};
// 在匿名函数没有捕获环境变量的情况下,与函数一样
fn add_one_fn(x: i32) -> i32 {
x + 1
}
fn use_fn(x: i32, f: fn(i32) -> i32) -> i32 {
f(x)
}
// 输出2,2,2
// 可见无捕获上下文的闭包与函数一样
println!("{},{},{}", use_fn(1, add_one), use_fn(1, add_one2), use_fn(1, add_one_fn));
// 如果捕获了环境变量,则闭包与函数就不能通用了
let k = 1;
let add_one_closure = |x: i32| {
x + k + 1
};
// 这里报错,expected fn pointer, found closure
// closures can only be coerced to `fn` types if they do not capture any variables
// println!("{}", use_fn(1, add_one_closure));
// 函数无法捕获上下文:can't capture dynamic environment in a fn item
// use the `|| { ... }` closure form instead
// fn f_error(x: i32) -> i32 {
// x + k + 1;
// }
// 以下正常运行,输出12
println!("{}", add_one_closure(10));
// 多个参数时,使用逗号隔离开
let sum = |x, y| {
x + y
};
// 如果只有一行,可以省略掉大括号
let sum2 = |x, y| x + y;
println!("{},{}", sum(1, 2), sum2(2, 3));
// 在不指定类型的闭包,只能推断出一种类型
let closure = |x| x;
println!("{}", closure(10));
// 以下将报错,办为之前的调用已经推断closure为(i32)->i32的闭包,不能再使用&str类型了
// expected integer, found `&str`
// println!("{}", closure("10"));
// 与闭包相关的三种trait: Fn, FnMut, FnOnce,所有闭包至少实现其中的一个
// 这三个trait代表了函数接收参数的三种方式:不可变引用(Fn),可变引用(FnMut),获取所有权(FnOnce)
// rust自动从闭包的定义中推导出其所实现的trait
// 所有函数都实现了这三个trait,所以要求传闭包的地方都可以传函数
// 这三个trait的区别:
// 实现了FnOnce的闭包可以从环境中获取变量的所有权,所以这个类型的闭包只能被调用一次
// 实现了FnMut的闭包从环境中可变地借用值
// 实现了Fn的闭包从环境中不可变地借用值
// 所有闭包都实现了FnOnce,实现了Fn的闭包也实现了FnMut
// 接收Fn trait做为参数的函数
fn do_1<T: Fn(i32) -> i32>(x: i32, f: T) -> i32 {
f(x)
}
let k = vec![1, 2];
let c_1 = |x| x + k[0] + 1;
fn f_1(x: i32) -> i32 { x + 1 }
//
// 输出5,3
println!("{},{}", do_1(1, c_1), do_1(1, f_1));
println!("{}", c_1(1));
// 使用move关键可以获取变量的所有权
let c_1 = move |x| x + k[0] + 1;
// ???很奇怪的现象,如果加上下面这一行,则后面的两次调用将报错: borrow of moved value: `c_1`
// 但如果没有下面这一行,则后面的两次调用则可以通过
// do_1(1, c_1);
println!("{}", c_1(1));
println!("{}", c_1(1));
// FnOnce的例子
fn do_2<T: Fn() -> String>(f: T) {
f();
}
let a = "aa".to_string();
// c_2因为返回了a变量,而String没有实现Copy trait,所以相当于c_2获取了a的所有权并返回了a,所以c_2只实现了FnOnce trait,无法被传递到Fn作为参数的函数中
let c_2 = || a;
// 以下一行将报错: this closure implements `FnOnce`, not `Fn`
// do_2(c_2);
fn do_3<T: FnOnce() -> String>(f: T) {
f();
}
// 运行正常
do_3(c_2);
// 以下编译报错: use of moved value: `c_2`
// 因为c_2是FnOnce没有实现Copy,所以无法被使用两次
// do_3(c_2);
// FnMut的例子
let mut k = 3;
let mut c_3 = || {
k = k + 1;
k
};
println!("{}", c_3());
// 以下将无法通过编译,因为c_3使用k的可变引用,不能再拥有k的其它引用了
// println!("{},{}", k, c_3());
}
```
### 迭代器
比起使用for循环的原始实现,Rust更倾向于使用迭代器风格;迭代器可以让开发者专注于高层的业务逻辑,而不必陷入编写循环、维护中间变量这些具体的细节中。通过高层抽象去消除一些惯例化的模板代码,也可以让代码的重点逻辑(例如filter方法的过滤条件)更加突出。
**迭代器是Rust语言中的一种零开销抽象(zero-cost abstraction)**,这个词意味着我们在使用这些抽象时不会引入额外的运行时开销。
以下例子位于example/src/bin/iterator.rs中:
```rust
fn main() {
// 迭代器允许你依次为序列中的每一个元素执行某些任务
// 迭代器是惰性的,除非主动调用方法来消耗,否则不会有任何作用
// 迭代器实现了Iterator 这一trait,Iterator有多个方法,但大多都提供了默认的实现
// 要实现Iterator只需要实现next方法即可
trait Iterator1 {
// 关联类型,用于存储next返回值的类型
type Item;
// Self::Item表示返回的Option存储的是Item的类型
// next返回被包裹在Some中的元素,在遍历结束时返回None
fn next(&mut self) -> Option<Self::Item>;
// 省略默认实现的方法
// ...
}
let mut v = vec![1, 2, 3];
// 必须将iter标识成mut的,因为调用next会改变iter内部的状态
// iter()方法返回的是一个不可变引用迭代器,next的返回值是数组中元素的不可变引用
let mut iter = v.iter();
// 1,2,3,true
println!("{},{},{},{}", iter.next().unwrap(), iter.next().unwrap(), iter.next().unwrap(), iter.next() == None);
// 返回可变引用
for i in v.iter_mut() {
*i += 1;
}
// [2,3,4]
println!("{:?}", v);
// 使用into_iter获取其所有权,后续v不再可用
println!("{}", v.into_iter().next().unwrap());
// 迭代适配器,以及与闭包共同实现
let v = vec![1, 2, 3, 4, 5, 6, 7, 8, 9];
let add2: Vec<i32> = v.iter().map(|x| x + 2).collect();
let min = 5;
// 这里使用filter的给到的闭包参数为&&x,要么使用**x解引用,要么在参数中使用&&x来表示x是一个i32
let big: Vec<&i32> = v.iter().filter(|&&x| x + 2 > min).collect();
// [3, 4, 5, 6, 7, 8, 9, 10, 11],[6, 7, 8, 9]
println!("{:?},{:?}", add2, big);
// 创建自定义的迭代器
struct Counter {
count: i32,
}
impl Counter {
fn new() -> Counter {
Counter { count: 0 }
}
}
impl std::iter::Iterator for Counter {
type Item = i32;
fn next(&mut self) -> Option<Self::Item> {
self.count += 1;
if self.count > 5 {
None
} else {
Some(self.count)
}
}
}
for i in Counter::new() {
println!("{}", i)
}
// 一些迭代器复杂的用法
// Iterator这个trait有很多方法的默认实现,依赖于next方法,我们可以直接使用完成复杂的功能
// 以下有一个特殊的语法,在方法后面添加::<typeHint>,可以给编译器提示返回的值类型为typeHint,用于无法推断类型的场景时使用
// Counter::new() is [1, 2, 3, 4, 5]
// Counter::new().skip(1) is [2, 3, 4, 5]
// Counter::new().zip(Counter::new().skip(1)) is [(1, 2), (2, 3), (3, 4), (4, 5)]
// .map(|(a,b)| a*b) is [2, 6, 12, 20]
// .filter(|x| x % 3 == 0) [6, 12]
// .sum() is 18
let c: i32 = Counter::new().zip(Counter::new().skip(1))
.map(|(a, b)| a * b)
.filter(|x| x % 3 == 0)
.sum();
println!("Counter::new() is {:?}", Counter::new().collect::<Vec<i32>>());
println!("Counter::new().skip(1) is {:?}", Counter::new().skip(1).collect::<Vec<i32>>());
println!("Counter::new().zip(Counter::new().skip(1)) is {:?}", Counter::new().zip(Counter::new().skip(1)).collect::<Vec<(i32, i32)>>());
println!(" .map(|(a,b)| a*b) is {:?}", Counter::new().zip(Counter::new().skip(1)).map(|(a, b)| a * b).collect::<Vec<i32>>());
println!(" .filter(|x| x % 3 == 0) {:?}", Counter::new().zip(Counter::new().skip(1)).map(|(a, b)| a * b).filter(|x| x % 3 == 0).collect::<Vec<i32>>());
println!(" .sum() is {:?}", c);
}
```
## 进一步认识Cargo及crates.io
Rust中的发布配置是一系列定义好的配置方案,例如cargo build时使用dev配置方案,添加`--release`后,使用release配置方案。
在Cargo.toml中添加`[profile.dev]`和`[profile.release]`配置段可以对这两个方案进行配置。
在没有配置时,有一套默认的配置,当自定义了配置时,会使用自定义配置的子集覆盖默认的配置。
提到的配置:
* `opt-level=1`:编译优化程序,0~3优化递增,release默认是3,dev为0。
### 文档注释
Rust中使用三斜线做为文档注释,支持markdown语法;被包在markdown里的代码片段会被当成测试case,在运行cargo test的时候运行。
```rust
// 位于adder项目中
/// 定义一个泛型加法
///
/// # Example
///
/// 例子,一般是测试用例
/// ```
/// assert_eq!(5, adder::adder(3,2));
/// assert_eq!(9.8, adder::adder(3.3, 6.5));
/// ```
/// # Panics
///
/// 可能Panic的场景
///
/// # Errors
/// 如果返回Result时,这里写明Error返回的场景,以及返回的Error值
///
/// # Safety
///
/// 当使用了unsafe关键字时,这里可以说明使用unsafe的原因,以及调用者的注意事项
///
pub fn adder<T: Add + Add<Output=T>>(a: T, b: T) -> T {
return a + b;
}
```
可以使用```cargo doc --open```来打开文档查看。
使用```//!```开头的注释,不像```///```为紧跟的代码提供注释,而是为整个包,或者整个模块提供注释; 这种类型的注释,只能放在文件最开头。
### 使用pub use重新组织结构
```rust
// example/src/lib.rs
//! # Art
//!
//! 测试用于艺术建模的库
// 使用pub use 重新组织API结构
pub use crate::kinds::PrimaryColor;
pub use crate::kinds::SecondaryColor;
pub use crate::utils::mixed;
pub mod kinds {
pub enum PrimaryColor {
Red,
Yellow,
Blue,
}
pub enum SecondaryColor {
Orange,
Green,
Purple,
}
}
pub mod utils{
use crate::kinds::*;
pub fn mixed(c1:PrimaryColor, c2:PrimaryColor) -> SecondaryColor{
SecondaryColor::Orange
}
}
```
```rust
// example/src/bin/pub_use_example.rs
// use example::kinds::PrimaryColor;
// use example::utils::mixed;
// 使用pub use重新导出包结构后,就可以使用直接使用一级目录的结构,不用关心原包中的结构了
use example::PrimaryColor;
use example::mixed;
fn main(){
let red = PrimaryColor::Red;
let yellow = PrimaryColor::Yellow;
mixed(red, yellow);
}
```
### 使用crates.io分享代码
创建步骤:
1. 使用github账号登录[https://crates.io/](https://crates.io/);
2. 在账号设置中绑定邮箱,并验证;
3. 在账号设置中生成New Token;
4. 在本地使用cargo login xxxx(your token)来保存token到本地(~/.cargo/credentials);
5. 在项目中填写足够的信息(Cargo.toml):
```ini
[package]
name = "guessing_game_xiaochai"
version = "0.1.0"
authors = "[xiaochai<soso2501@gmail.com>]"
edition = "2018"
description = "a game demo"
license = "MIT"
```
6. 在命令行中运行cargo publish,此时,会将代码提交到远程:https://crates.io/crates/guessing_game_xiaochai。如果本地是git,并且有未提交的文件,将会生成错误,可以使用``` cargo publish --allow-dirty```忽略git信息。<br/>
更新版本:
1. 修改Cargo.toml,增加版本号;
2. 运行```cargo publish```即可提交新版本。
撤回某个版本:
* ```cargo yank --vers 0.1.0```可以撤回某个版本,虽然还能在crate.io上看到对应版本,但新的项目无法引用这个版本了;
* 使用```cargo yank --vers 0.1.0 --undo```可以取消撤回操作。
如果你分享的是一个二进制包,可以使用`cargo install guessing_game_xiaochai`来下载安装,会安装到~/.cargo/bin目录下。
### 工作空间
同一个工作空间下的项目使用同一个Cargo.lock文件,使用以下步骤新建一个工作空间:
1. 新起目录,例如workspace_example,cd workspace_example;
2. 创建Cargo.toml文件,内容为:
```ini
[workspace]
members = [
"adder"
]
```
3. 在目录下使用cargo new来创建adder二进制包;
4. 此时不管在workspace_example目录下运行cargo run 还是在workspace_example/adder下,最终的target都会在workspace_example/target目录下;换种说法,adder项目目前已经不是一个独立的项目了,要么1. 在workspace_example/Cargo.toml添加exclude来排除adder项目;2. 或者是在workspace_example/adder/Cargo.toml中添加空的[workspace]段,这样才能将adder单独编译:
```ini
[workspace]
members = [
]
exclude = [
"adder",
]
```
5. 添加add-one这个代码包```cargo new add-one --lib```;
6. adder的二进制文件需要依赖adder-one包,需要在adder/Cargo.toml的dependencies段添加```add-one = { path = "../add-one" }```;
7. 在main函数中使用。
```rust
fn main() {
println!("Hello, world!");
println!("{}", add_one::add_one(10));
}
```
8. 在workspace_example下运行```cargo run```,则运行了adder包中的二进制文件;如果一个工作空间下有多个二进制项目的话,可以使用\-p参数来指定项目
```cargo run -p adder```
### 扩展cargo
如果在$PATH中包含有cargo-something的可执行文件,那么可以使用cargo something来运行此文件。
例如刚安装的guessing_game_xiaochai程序:
```shell
➜ workspace_example git:(master) ✗ echo $PATH
/usr/local/bin:/usr/bin:/bin:/usr/sbin:/Users/bytedance/.cargo/bin
➜ workspace_example git:(master) ✗ mv ~/.cargo/bin/guessing_game_xiaochai ~/.cargo/bin/cargo-guessing_game_xiaochai
➜ workspace_example git:(master) ✗ cargo guessing_game_xiaochai
secret number is 86
Guess the number!
Please input your guess:
...
```
## 智能指针
* 指针是指包含内存地址的变量;
* 引用是最常用的指针,没有额外的开销;
* 智能指针是一些数据结构,他们的功能类似于指针,但拥有额外的元数据和附加功能;
* 引用只是借用数据指针,而智能指针一般拥有数据所有权;
* String和`Vec<T>`是智能指针,他们拥有一片内存区域并允许进行操作,他们还拥有元数据(如容量),并提供额外的功能或保障;
* 智能指针一般使用结构体来实现,需要实现Drop和Deref两个trait:
> Deref trait使得此结构体的实例可以拥有与引用一致的行为;<br/>
> Drop trait使得在离开作用域时运行一段自定义代码。
常用智能指针:
* `Box<T>`: 可用于在堆上分配值;
* `Rc<T>`: 允许多重所有权的引用计数智能指针;
* `Ref<T>`,`RefMut<T>`:可以通过`RefCell<T>`访问,是一种可以在运行时而非编译时执行借用规则的类型。
内部可变性:不可变对象可以暴露出能够改变内部值的API。
规避循环引用导致的内存泄露。
### 使用`Box<T>`在堆上分配内存
使用场景:
* 在需要固定尺寸变量的上下文中使用编译期无法确定大小的类型;
* 需要传递大量数据的所有权,但又不希望对数据进行复制;
* 当希望使用实现了某个trait的类型,而又不关心具体类型时。
基本使用方法:
1. 使用Box::new(T)在堆上空间,将T保存于这一空间内,并返回指向堆上这一空间的指针;
2. 在变量前加*(解引用运算符)来获取堆上真正的值,称为解引用。
以下代码位于example/src/bin/smart_pointer.rs中:
```rust
// Box的基本使用方法
// a和b都是Box<i32>类型,与i32不同的是,Box<i32>类似于指向堆上两个i32类型的指针
let a = Box::new(128);
let b = Box::new(256);
// 所以a和b无法直接进行操作,因为Box实现了Deref这一trait,所以可以使用*来解引用
let c: i64 = *a + *b;
println!("{}", c); // 384
```
定义递归类型时需要使用Box,因为不能在编译期确定内存空间的大小
```rust
// 递归类型必须使用Box
enum List {
Cons(i32, Box<List>),
// 使用Cons(i32, List)将无法通过编译:recursive type `List` has infinite size
Nil,
}
use List::{Nil, Cons};
let list = Cons(
1,
Box::new(Cons(
2,
Box::new(Cons(
3, Box::new(
Nil
),
)),
)),
);
fn print_list(l: List) {
match l {
Cons(i, nl) => {
print!("{}=>", i);
print_list(*nl);
}
Nil => {
println!("end");
}
}
}
print_list(list);
```
### 自定义智能指针
```rust
// 自定义类似于Box的智能指针(但数据存在在栈上)
struct MyBox<T> (T); // 元组结构体
impl<T> MyBox<T> {
// 提供一个参数,并将此存入结构体中
fn new(x: T) -> MyBox<T> {
MyBox(x)
}
}
```
涉及到两个trait: Deref 和Drop。
#### Deref
Deref解引用trait,一个类型实现了Deref trait时,可以使用解引用运算符:
```rust
// 为MyBox实现Deref,这样就可以使用解引用运算符
impl<T> Deref for MyBox<T> {
// 关联类型
type Target = T;
// 也可以写成fn deref(&self) -> &T {
fn deref(&self) -> &Self::Target {
// 以下等价于self.0
match self {
MyBox(i) => i
}
}
}
let a = MyBox(10);
// 这里的*a类似于*(a.deref()),称之为隐式展开
println!("{}", *a + 10)
```
隐式解引用转换:当函数参数为某个类型的引用时,如果传入的参数与此不匹配,则编译器会自动进行解引用转换,直到类型满足要求。
解可变引用:实现DerefMut trait,要实现这一trait,必须首先实现Deref trait。
可变性转换有三条:
1. 当T: Deref<Target=U>时,允许&T转换为&U;
2. 当T: DerefMut<Target=U>时,允许&mut T转换为&mut U;
3. 当T: Deref<Target=U>时,允许&mut T转换为&U;
这三条规则不会破坏借用规则。
```rust
// 隐式解引用转换
fn hello(name: &str) {
println!("hello, {}", name);
}
let mb: MyBox<String> = MyBox::new("lee".to_string());
// 正常的写法如下,其发生的步骤1. 需要将MyBox解引用转化为String,2. 使用[..]将String转化为切片&str,这样才符合参数要求
hello(&(*mb)[..]);
// 使用自动解引用也能满足这个要求,mb实现了Deref,所以可以获取到String,String也实现了deref,其返回为&str,所以编译器进行了两次解引用转换
// 以上是编译期完成,不会有任何运行时开销
hello(&mb);
// 实现可变解引用运算符,实现DerefMut之前必须实现Deref
impl<T> DerefMut for MyBox<T> {
fn deref_mut(&mut self) -> &mut Self::Target {
&mut self.0
}
}
// 满足自动解引用的三条规则
// 当T: Deref<Target=U>时,允许&T转换为&U。
// 当T: DerefMut<Target=U>时,允许&mut T转换为&mut U。
// 当T: Deref<Target=U>时,允许&mut T转换为&U。
fn hello_exp(name: &mut String) -> &mut String {
name.push_str(", hello!");
name
}
let mut b = Box::new("abc".to_string());
// 当T: DerefMut<Target=U>时,允许&mut T转换为&mut U,与&mut *b是一样的
let c = hello_exp(&mut b);
println!("{}", c);
// 当T: Deref<Target=U>时,允许&mut T转换为&U。
hello(&mut b);
```
#### Drop
Drop trait允许我们在离开变量作用域时执行某些自定义的操作,例如释放文件和网络连接等。
`Box<T>`类型通过Drop释放指向堆上的内存。
drop的执行顺序与变量的创建顺序相反。
无法禁用drop的功能,也无法手动调用Drop的drop方法,但可以使用std::mem::drop来提前清理某个值,这个函数在预导入模块中,所以可以直接使用drop来调用。
```rust
// 实现Drop的例子
{
struct TestDropStruct {
data:String,
}
impl Drop for TestDropStruct{
fn drop(&mut self) {
println!("{}", self.data)
}
}
let _a = TestDropStruct{data:"first object".to_string()};
let _b = TestDropStruct{data:"second object".to_string()};
let _c = TestDropStruct{data:"third object".to_string()};
println!("main end");
// 手动使用std::mem::drop函数来提前清理_c的值
drop(_a);
// 以上输出顺序为
// main end
// first object
// third object
// second object
// 对于_b,_c来说,丢弃顺序与创建顺序相反,所以_c先调用drop函数
// _a则是由于手动释放导致先执行
}
```
### `Rc<T>`基于引用计数的智能指针
`Rc<T>`只能用于单线程中,用于那些在编译期无法确认哪个部分会最后释放的场景。
`RC<T>`只能持有不可变的引用,否则会打破引用规则。
```rust
{
// 测试引用计数智能指针Rc<T>类型
enum RcList {
RcCons(i32, Rc<RcList>),
RcNil,
}
use RcList::*;
// 新建一个引用记录类型使用Rc::new来创建,此处a为Rc<RcList>类型
let a = Rc::new( RcCons(3, Rc::new(
RcCons(4, Rc::new(RcNil)),
)));
{
// Rc::clone全程参数对应的引用计数增加1
let _b = RcCons(1, Rc::clone(&a));
let _c = RcCons(2, Rc::clone(&a));
// 目前有a,_b,_c三个变量引用a,所以a的引用计数为3
// 除了strong_count,还有week_count,用于避免循环引用
println!("{}", Rc::strong_count(&a))
}
// _b,_c离开作用域,减少了引用计数,但a还在,所以这块的数量是1
println!("{}", Rc::strong_count(&a))
}
```
### `RefCell<T>`和内部可变性模式
内部可变性是Rust的设计模式之一,它允许你在只持有不可变引用的情况下修改数据。这在现有的借用规则下是禁止的,所以此类数据结构借用了unsafe代码来绕过可变性和借用规则的限制。
`RefCell<T>`是使用了内部可变性模式的类型。
与`Rc<T>`不同,`RefCell<T>`持有数据的维一所有权。而与`Box<T>`不同,`RefCell<T>`会在运行时检查借用规则,而非在编译时期,如果运行时发现不满足规则 ,则直接panic。所以`RefCell<T>`类型由开发者来保证借用规则 ,则不是编译器。
此类型只能用于单线程中。
`Box<T>`,`Rc<T>`,`RefCell<T>`三者的区别:
1. `Rc<T>`允许一份数据有多个所有者,而`Box<T>`和R`efCell<T>`都只有一个所有者;
2. `Box<T>`允许在编译时检查的可变或不可变借用,`Rc<T>`仅允许编译时检查的不可变借用,`RefCell<T>`允许运行时检查的可变或不可变借用;
3. 由于`RefCell<T>`允许我们在运行时检查可变借用,所以即便`RefCell<T>`本身是不可变的,我们仍然能够更改其中存储的值。
```rust
{
// 假设有一个消息trait,需要实现send方法,而这一方法传递self的不可变引用
pub trait Messenger {
fn send(&self, msg: &str);
}
// 但是在测试的时候,我们使用Mock类来模拟Messenger的send的时候,需要把消息存储下来
// 这样就可以验证存下来的消息是否符合预期,但传入不可变的self阻止了这种做法
// 此时就需要使用RefCell来实现
struct Mock {
message: RefCell<Vec<String>>,
}
impl Mock{
fn new()->Mock{
Mock{
// 使用RefCell::new来创建新的RefCell
message:RefCell::new(vec![])
}
}
}
impl Messenger for Mock {
fn send(&self, msg: &str) {
// 如果这里的self.message是普通的Vec<String>,则无法执行push方法
// 而使用RefCell的borrow_mut来绕过此限制,得到可变引用
self.message.borrow_mut().push(String::from(msg))
}
}
let m = Mock::new();
m.send("message1");
m.send("message2");
// ["message1", "message2"]
println!("{:?}", m.message.borrow());
// 以下两行可以通过编译,但在运行时报错:thread 'main' panicked at 'already borrowed: BorrowMutError'
// 这是因为a是不可变引用,在已经持有不可变引用的情况下,又搞出了一个可变的引用,破坏了借用规则 ,所以panic
// let a = m.message.borrow();
// let mut b = m.message.borrow_mut();
}
```
结合使用`Rc<T>`和`RefCell<T>`来实现某个数据有多个所有者,并且都可以对数据进行修改。
```rust
{
#[derive(Debug)]
enum RcRefCellList {
RcRefCellCons(Rc<RefCell<i32>>, Rc<RcRefCellList>),
RcRefCellNil,
}
use RcRefCellList::*;
let val = Rc::new(RefCell::new(5));
let a = Rc::new(RcRefCellCons(Rc::clone(&val), Rc::new(RcRefCellNil)));
let b = RcRefCellCons(Rc::new(RefCell::new(6)), Rc::clone(&a));
let c = RcRefCellCons(Rc::new(RefCell::new(6)), Rc::clone(&a));
// 这块改动将a,b和c以及val都修改了
*val.borrow_mut() = 10;
// a:RcRefCellCons(RefCell { value: 10 }, RcRefCellNil)
// b:RcRefCellCons(RefCell { value: 6 }, RcRefCellCons(RefCell { value: 10 }, RcRefCellNil))
// c:RcRefCellCons(RefCell { value: 6 }, RcRefCellCons(RefCell { value: 10 }, RcRefCellNil))
println!("a:{:?}", a);
println!("b:{:?}", b);
println!("c:{:?}", c);
}
```
Rust不保证在编译器彻底防止内存泄露。我们可以创建出一个环状引用使得引用计数不会减到0,对应的值不会被丢弃,从而造成内存泄露。
```rust
{
// 创建出循环引用
// 定义一个链接,为了方便改动,这次的链接使用ReCell来处理
enum RefCellRcList {
RefCellRcCons(i32, RefCell<Rc<RefCellRcList>>),
RefCellRcNil,
}
use RefCellRcList::*;
// 定义一个a,指向b
// a -> 5 -> Nil
let a = Rc::new(
RefCellRcCons(5, RefCell::new(Rc::new(RefCellRcNil))),
);
println!("reference count:a:{}", Rc::strong_count(&a));
// b -> 10 -> a
let b = Rc::new(
RefCellRcCons(10, RefCell::new(Rc::clone(&a))),
);
println!("reference count:a:{}, b:{}", Rc::strong_count(&a), Rc::strong_count(&b));
// 将a -> b;最终变成a->b->10->a
if let RefCellRcCons(i, r) = a.borrow() {
*r.borrow_mut() = Rc::clone(&b);
};
println!("reference count:a:{}, b:{}", Rc::strong_count(&a), Rc::strong_count(&b));
// reference count:a:1
// reference count:a:2, b:1
// reference count:a:2, b:2
// 此时a和b的引用计数都是2,在结束时,先释放b,将b的引用计数减1,但此时b已经无法将引用计数减成0了,所以无法释放
}
```
可以合理使用弱引用`Weak<T>`来规避这个问题,Rust在回收内存时不需要强制弱引用减成0。
与Rc::clone()类似,使用Rc::downgrade()获取`Rc<T>`的弱引用,获取对应弱引用的值时,使用对应弱引用的upgrade()方法,注意这个方法可能返回None。
```rust
{
// 使用Weak<T>创建树结构,子节点指向父节点使用弱引用
#[derive(Debug)]
struct Node {
val: i32,
// 多个子节点使用Vec来保存子节点的强引用,RefCell方便修改对应的值
children: RefCell<Vec<Rc<Node>>>,
// 父节点点使用弱引用
parent: RefCell<Weak<Node>>,
}
let leaf = Rc::new(Node {
val: 10,
children: RefCell::new(vec![]),
// Weak::new()创建出一个空的弱引用
parent: RefCell::new(Weak::new()),
});
// 弱引用获取时,由于不确定值是否回收,所以使用upgrade()时会返回Option<T>,如果已经回收或者没有值,返回None
// 以下返回None
println!("{:?}", leaf.parent.borrow().upgrade());
let branch = Rc::new(Node {
val: 5,
children: RefCell::new(vec![Rc::clone(&leaf)]),
parent: RefCell::new(Weak::new()),
});
*(leaf.parent.borrow_mut()) = Rc::downgrade(&branch);
println!("leaf's parent:{:?}", leaf.parent.borrow().upgrade());
println!("branch's parent:{:?}", branch.parent.borrow().upgrade());
{
// 在另外一个作用域中添加branch的parent
let new_branch = Rc::new(Node {
val: 6,
children: RefCell::new(vec![Rc::clone(&branch)]),
parent: RefCell::new(Weak::new()),
});
*branch.parent.borrow_mut() = Rc::downgrade(&new_branch);
println!("branch's parent in new area:{:?}", branch.parent.borrow().upgrade());
}
// 由于new_branch离开了作用域,所以被销毁,这块拿到的是None
println!("branch's parent out area:{:?}", branch.parent.borrow().upgrade());
}
```
## 线程
使用std::thread::spawn()向参数中传入一个闭包函数来支持多线程运行。
spawn返回线程句柄,其join方法会阻塞等待线程执行完成。
在闭包中使用move关键字可使得闭包函数获取变量的所有权。
此章节例子位于example/src/bin/thread.rs中:
```rust
let v = vec![1,2,3];
// 使用thread::spawn启动一个线程,rust原生不支持协程,但有其它库提供支持
// spawn的参数是一个无参数闭包或者函数,会在另外一个线程中运行这个函数的代码
// 返回一个Handler,可以调用其join函数等待线程执行完成
// 如果在闭包中使用环境中的值,需要将其所有权move到新线程中
// 否则报错closure may outlive the current function, but it borrows `v`, which is owned by the current function
let t = std::thread::spawn(move || {
for i in 1..10 {
println!("in thread {}", i + v[0]);
std::thread::sleep(std::time::Duration::from_millis(1));
}
});
for i in 1..5 {
println!("out thread {}", i);
std::thread::sleep(std::time::Duration::from_millis(1));
}
// 由于v的所有权已经被移入新线程了,所以这里就无法再使用v了
// println!("v:{:?}", v);
// 使用JoinHandler的join方法来等待线程执行完成
// 返回的是Result<T, E>类型
t.join().unwrap()
```
### 使用通道来传递消息
使用std::sync::mpsc::channel()创建一个通道,获得一个发送端和一个接收端。
发送端可以使用send来发送消息,接收端使用recv和try_recv来阻塞(非阻塞)地接收消息。
接收端还可以被当成有迭代器来处理接收到的消息。
使用std::sync::mpsc::Sender::clone()方法可以复制发送端,从而由多个发送源发送消息。
```rust
// 使用通道(channel)在线程中传递信息
// mpsc是multiple producer,single consumer的缩写,顾名思义,只能有一个消费者,但可以多个发送方
let (tx, rx) = std::sync::mpsc::channel();
std::thread::spawn(move || {
let hi = "hi".to_string();
tx.send(hi).unwrap();
// send会将取得没有实现Copy trait的所有权,所以以下语句将报错
// println!("send {}", hi);
});
// recv会阻塞线程,直到收到消息
// 可以使用try_recv来非阻塞地试探是否有消息可以接收,没有消息时将返回Err
println!("{}", rx.recv().unwrap());
let (tx, rx) = std::sync::mpsc::channel();
// mpsc支持多个发送方,可以通过clone方法来创建出多个发送端来
let tx_2 = std::sync::mpsc::Sender::clone(&tx);
std::thread::spawn(move || {
for _i in 1..5 {
tx.send("hi".to_string());
std::thread::sleep(std::time::Duration::from_millis(100))
}
});
std::thread::spawn(move || {
for _i in 1..10 {
tx_2.send("there".to_string());
std::thread::sleep(std::time::Duration::from_millis(100))
}
});
// 将rx当成迭代器来遍历收到的数据
// 由于tx在线程退出时执行了Drop,整个通道就关闭了,所以rx的迭代就会结束了
for m in rx {
println!("got:{}", m)
}
```
### 使用共享状态来实现消息传递
使用通道类似于单一所有权,使用共享状态类似于多重所有权。
并发原语(互斥体/mutex)一次只允许一个线程访问数据:
1. 必须在使用数据前尝试获取锁;
2. 必须在使用完互斥体守护的数据后释放锁,这样其他线程才能继续完成获取锁的操作。
使用std::sync::Mutex::new(T)创建一个互斥量,保存着一个T值。
lock返回一个智能指针,在离开作用域时自动解锁。
```rust
// mutex的使用
// 使用new函数创建互斥量,存储一个i32的值
let m = std::sync::Mutex::new(10);
{
// 使用lock返回一个Result,正常时为MutexGuard是一个智能指针
let mut i = m.lock().unwrap();
// 修改值为20
*i = 20;
// 在这个作用域的结尾,智能指针执行了drop方法,将m解锁了
}
// m现在的值为20了
// Mutex { data: 20, poisoned: false, .. }
println!("{:?}", m);
```
在并发场景需要使用Mutex,需要配合引用计数智能指针使用。
`Rc<T>`无法用于并发场景,需要使用`Arc<T>`类型,并发场景下的引用计数智能指针。
多线程模式下两个重要的trait:Sync和Send。Sync和Send这两个trait是内嵌于语言实现的。
Send trait表示可以在线程间安全地转移所有权,Sync trait表示此类型可以安全地被多个线程引用。
除了裸指针外,所有基础类型都实现了Send trait,任何有Send类型组成的复合类型也都实现了Send;如果类型T是Send类型,则&T满足Sync约束。
Send和Sync属于标签trait(marker trait),没有可供实现的方法,手动实现需要使用不安全的语言特性。
自动实现了Send和Sync的语法特性称之为自动trait(auto trait),与此相对,如果不想使某个类型自动实现trait,则可以使用negative impl语法来处理,例如:
```rust
impl<T: ?Sized> !Send for MutexGuard<'_, T> {}
```
`Rc<T>`无法在多个线程场景下安全地更新引用计数值,所以Rc只设计用于单线程的场景,没有实现Send trait和Sync trait。
```rust
// 并发场景下使用mutex
use std::sync::{Mutex, Arc};
use std::thread;
// Arc<T>是并发场景下的Rc<T>
// 使用Rc<T>会报错:`Rc<Mutex<i32>>` cannot be sent between threads safely
// the trait `Send` is not implemented for `Rc<Mutex<i32>>`
let counter = Arc::new(Mutex::new(0));
// let countet1 = Rc::new(Mutex::new(0));
let mut handlers = vec![];
for i in 0..30 {
let c = counter.clone();
// let c2 = countet1.clone();
let h = thread::spawn(move || {
let mut t = c.lock().unwrap();
// c2.lock().unwrap();
*t += 1;
});
handlers.push(h);
}
for h in handlers {
h.join().unwrap();
}
println!("{:?}", counter)
```
## 面向对象的语言特性
对象包含数据和行为:Rust中提供了struct和enum,可以使用impl来对这些数据实现操作方法;
封装细节:Rust提供了数据字段的公私有性;对于私有的属性,外部只能通过封装好的方法进行访问;另外只要公共的对外接口不变,内部的实现细节变动不影响外部使用方;
继承:Rust没有继承,但使用继承所能达到的目的,Rust可以通过其它方式来实现:
> 代码复用:通过trait的默认实现可以共享代码;<br/>
> 多态:如下,通过```Box<dyn Trait>```来实现。
### 实现多态
使用```Box<dyn Trait>```可以实现多态的能力:
```rust
// 定义一个含有draw方法的trait
trait Draw{
fn draw(&self);
}
// 以下两个类型,都实现了Draw
struct Button();
impl Draw for Button{
fn draw(&self) {
println!("drawing Button")
}
}
struct TextField();
impl Draw for TextField{
fn draw(&self) {
println!("drawing TextField")
}
}
// 使用vec来保存实现了Draw trait的变量
// Box<dyn Draw>表示实现了Draw trait的对象
// 与泛型不同,这种方式使得在component里可以存储任意多种类型,只要实现了Draw即可
let mut components:Vec<Box<dyn Draw>> = Vec::new();
// 可以将实现了Draw的类型push进components
components.push(Box::new(Button{}));
components.push(Box::new(TextField{}));
// 并且可以遍历调用draw方法,而不需要关心存储的具体类型是什么
for c in components{
c.draw();
}
```
泛型中使用的trait约束,会在编译期间展开成具体的类型,称之为静态派发。
而在使用trait对象时,编译期无法确定使用哪种具体类型,需要生成一些额外代码在运行期间查找应该调用的方法,这些称之为动态派发。
动态派发会产生一些运行时开销,例如对于trait对象来说,Rust会根据对象内部的指针来确定调用哪个方法,而动态派发还会阻止编译器内联代码,使得一些优化操作无法进行。但也带来了灵活性,需要根据具体场景确定使用哪种方式。
### 对象安全
只有满足对象安全的trait才能转成trait对象;如果一个trait中定义的所有方法满足以下两条规则,则它就是对象安全的:
1. 方法中不能返回Self类型。Self方法是当前实现此trait的类型别名,如果trait返回了Self,则rust无法确定具体会返回什么类型。例如Clone trait。
2. 方法中不包含任何泛型参数。泛型参数会被展开成具体的类型,这些具体类型会被视作当前类型的一部分。由于trait对象忘记了类型信息,所以我们无法确定被填入泛型参数处的类型究竟是哪一个。
如果使用了类型不安全的trait,会在编译时出错:
```rust
// 不满足对象安全的trait不能转化为trait对象
// `Clone` cannot be made into an object
// note: the trait cannot be made into an object because it requires `Self: Sized`
// note: for a trait to be "object safe" it needs to allow building a vtable to allow the call to be resolvable dynamically;
// let wrong:Vec<Box<dyn Clone>> = vec![];
```
使用Rust实现状态模式的例子:
```rust
// 实现状态模式
// Post表示发布文章的流程,从草稿到审核再到发布
fn main() {
let mut post = Post::new();
post.add_text("I'm greate");
println!("after add_text:{}", post.content());
post.request_review();
println!("after request_review:{}", post.content());
post.approve();
println!("after approve:{}", post.content());
}
struct Post {
// 状态机:草稿->审核->发布
// 为什么要用Option呢?如果不用Option在处理所有权的时候会比较麻烦,在所有权处理的时候需要注意
state: Option<Box<dyn State>>,
content: String,
}
impl Post {
fn new() -> Post {
Post {
// 默认是草稿的状态
state: Some(Box::new(Draft {})),
content: String::new(),
}
}
fn add_text(&mut self, text: &str) {
self.content.push_str(text)
}
fn request_review(&mut self){
// 将这些操作都代理给state,这些处理会导致新的状态,替换原来旧的状态
// Option::take()获取变量的所有权,在这里把state的所有权取出,因为马上就要使用新的state来替换进去了
if let Some(s) = self.state.take(){
self.state = Some(s.request_review())
}
}
fn approve(&mut self){
if let Some(s) = self.state.take(){
self.state = Some(s.approve())
}
}
fn content(&self)-> &str{
self.state.as_ref().unwrap().content(self)
}
}
trait State {
fn request_review(self: Box<Self>) -> Box<dyn State>;
fn approve(self: Box<Self>) -> Box<dyn State>;
fn content<'a>(&self, post:&'a Post)->&'a str{
""
}
}
struct Draft {}
impl State for Draft {
fn request_review(self: Box<Self>) -> Box<dyn State> {
Box::new(PendingReview{})
}
fn approve(self: Box<Self>) -> Box<dyn State> {
self
}
}
struct PendingReview {}
impl State for PendingReview {
fn request_review(self: Box<Self>) -> Box<dyn State> {
self
}
fn approve(self: Box<Self>) -> Box<dyn State> {
Box::new(Publish{})
}
}
struct Publish {}
impl State for Publish {
fn request_review(self: Box<Self>) -> Box<dyn State> {
self
}
fn approve(self: Box<Self>) -> Box<dyn State> {
self
}
fn content<'a>(&self, post:&'a Post)->&'a str{
&post.content
}
}
```
## 匹配模式
模式匹配分成可失败匹配(refutable)和不可失败匹配(irrefutable):
* 不可失败匹配是指可以匹配任何输入值的场景,例如let x = 5;
* 可失败是指有可能出现某些值不匹配导致匹配失败的,例如let Some(x) = a; 如果a为None时,这个匹配就不成功了。
for、let、函数参数只接收不可失败的匹配,如果下代码将报错:
```rust
// let只接收不可失败的匹配,所以下将编译报错
// refutable pattern in local binding: `None` not covered
// `let` bindings require an "irrefutable pattern", like a `struct` or an `enum` with only one variant
// let Some(x) = v.iter().next();
```
使用匹配模式的场景
| 模式匹配场景 | 可失败or不可失败 |
| ------------- |:-------------| :----------|
|match |除了最后一个模式,其它模式是可失败的模式,最后一个可以是可失败的也可以是不可失败的|
|if let | 可失败,如果使用不可失败将收到warning|
|while let |可失败,如果使用不可失败将收到warning|
|for |不可失败, 这里的不可失败是指Some里面的值,而不包含Some匹配|
|let | 不可失败|
|函数参数 |不可失败|
匹配的类型
| 模式匹配场景 | 举例 |
| ------------- |:-------------|
|字面量匹配| match m{<br/>1 => {}<br>...|
|变量名匹配| match m{<br/>x => {x}<br>...|
|使用\|的多重模式| match m{<br/>1\|2 => {}<br>...|
|使用..=来匹配区间,注意...已经不推荐使用| match m{<br/>1..=2 => {}<br>...|
| 解构结构体匹配| if let Point { x, y } = p |
| 解构枚举匹配| if let Some(c) = favorite_color { |
| 解构嵌套的枚举和结构体匹配| let ((feat, inches), Point { x, y }) = ((3, 10), Point { x: 3, y: -10 });|
| 解析结构体和元组匹配| let ((feat, inches), Point { x, y }) = ((3, 10), Point { x: 3, y: -10 }); |
|忽略模式匹配中的某些值| let (x, _, z) = (1, 2, 3); |
|使用..来忽略值的剩余部分| let (_first, .., _last) = (1, 2, 3, 4, 5, 6, 7, 8, 9); |
|使用匹配守卫(match guard)添加额外条件| match c {<br/> Some(x) if x > 6 => {}<br/>...|
|@绑定| if let Some(i @ 1..=5) = c { |
以下例子位于example/src/bin/pattern.rs中:
```rust
// match匹配模式
// 必须穷尽所有的可能性;为了满足这一条件,可以使用两种方式
// 1. 最后一个分支使用全匹配模式,可以使用一个变量名来处理
// 2. 使用下划线这一特殊的模式来匹配所有值
let m = 5;
match m {
// 字面量匹配
1 => println!("is 1"),
// 变量名匹配
x => println!("is not 1, is {}", x)
}
match m {
// 匹配区间值
// 在老的版本中1..=5的语法也写作1...5,但最新的rust 2021只能使用1..=5,表示1~5包含1和5
// 也可以使用|来表示,等价于1|2|3|4|5,但写起来更简单,字符类型也可以使用,例如'a'..='k'
1..=5 => println!("between 1~5"),
_ => println!("others")
}
// if let条件表达式
// if let表达式只匹配match中的一种情况
// 可以与if, else if, else, else if let等混合使用,以下是一个例子
let favorite_color: Option<&str> = None;
let is_friday = false;
let age: Result<u8, _> = "34".parse();
if let Some(c) = favorite_color {
println!("using your favorite color: {}", c);
} else if is_friday {
println!("using friday color: red");
} else if let Ok(a) = age {
println!("your age {} colour: blue", a);
} else {
println!("default color: black");
}
// while let 条件循环
// 与if let类似,当匹配不上时,结束循环
let v = vec![1, 2, 3, 4, 5, 6, 7, 8, 9];
let mut i = v.iter();
while let Some(elem) = i.next() {
// 123456789
print!("{}", elem)
}
println!();
let mut i = v.iter();
// 任何能用于match的分支都能用于其它的匹配模式
while let Some(1..=5) = i.next() {
// aaaaa
print!("a")
}
println!();
// for循环
// for循环自动把Some给解构了,并且自动调用了迭代器的next函数
for (i, v) in v.iter().enumerate() {
print!("({},{})", i, v);
}
println!();
// 以上与以下等价
let mut it = v.iter().enumerate();
while let Some((i, v)) = it.next() {
print!("({},{})", i, v);
}
println!();
// let语句
// 我们日常使用的let语句也是使用了模式匹配
let (x, y, z) = (1, 2, 3);
println!("x:{}, y:{}, z{}", x, y, z);
// 函数参数也使用了模式匹配
// 如下例子的函数参数类似于let &(x,y) = &p
fn print_point(&(x, y): &(i32, i32)) {
println!("point({},{})", x, y);
}
let p = (3, 4);
print_point(&p); // point(3,4)
// 可失败模式匹配和不可失败模式匹配
// let, for, 函数参数必须是不可失败的模式匹配,在不可失败的模式匹配中使用可失败的模式,将发生编译报错
// let只接收不可失败的匹配,所以下将编译报错
// refutable pattern in local binding: `None` not covered
// `let` bindings require an "irrefutable pattern", like a `struct` or an `enum` with only one variant
// let Some(x) = v.iter().next();
// 在接收可失败的模式中使用不可失败模式,将收到编译器警告
// irrefutable `if let` pattern
// this pattern will always match, so the `if let` is useless
if let x = 5 {
println!("no meaning:{}", x)
}
let p = Point { x: 10, y: 10 };
if let Point { x, y } = p {
println!("pppp({}, {})", x, y);
}
// 使用解构来分解值
// 可以使用模式来分解结构体、枚举、元组、引用
// 解构结构体
struct Point {
x: i32,
y: i32,
}
let p = Point { x: 10, y: 2 };
// 指定字段值赋于的变量名a和b
let Point { x: a, y: b } = p;
println!("a:{}, b:{}", a, b); // a:10, b:2
// 如果字段名与变量名一致时,可以简写成如下
let Point { x, y } = p;
println!("x:{}, x:{}", x, y); // x:10, x:2
// 也可以使用match让某个字段指定某个值来匹配
match p {
Point { x: 0, y } => { println!("point at y axial, y:{}", y) }
Point { x, y: 0 } => { println!("point at x axial, x:{}", x) }
Point { x, y } => { println!("point at space:({}, {})", x, y) }
}
// 解构枚举之前看到的Option已经使用过多次了
// 这里看一下解构嵌套的结构体和枚举
let p = Some(Point { x: 10, y: 20 });
if let Some(Point { x, y }) = p {
println!("x:{}, x:{}", x, y); // x:10, x:20
}
// 解析结构体和元组
let ((feat, inches), Point { x, y }) = ((3, 10), Point { x: 3, y: -10 });
println!("{},{},{},{}", feat, inches, x, y); // 3,10,3,-10
// 使用_来忽略某些值
// 忽略第一个参数
fn foo(_: i32, y: i32) {
println!("{}", y);
}
// 忽略元组中的第二个元素
let (x, _, z) = (1, 2, 3);
println!("x:{}, z:{}", x, z);
// 使用下划线开头的变量来防止编译器未使用的报警
// 以下如果不使用_,则会有警告:unused variable: `x`
// 注意,你还是可以使用下划线开头的变量的 println!("{}", _x);
let _x = 10;
// 下划线开头的变量依然会绑定值,这与纯下划线忽略值不一样
let s = Some("hello".to_string());
// 这里的_如果替换成_k,则最后打印s将报错,因为此时s中的值的所有权已经移到_k中了
// 而_不会绑定值,所以还可以使用s
if let Some(_) = s {}
println!("{:?}", s);
// 使用..来忽略值的值的剩余部分
// 只匹配x的值,忽略剩下部分的字段
let Point { x: _x, .. } = Point { x: 10, y: 10 };
// 匹配第一个和最后一个,分别是1和9
let (_first, .., _last) = (1, 2, 3, 4, 5, 6, 7, 8, 9);
// 如果编译器无法确认匹配,即..产生歧义的匹配时,将报错
// can only be used once per tuple pattern
// let (.., middle, ..) = (1,2,3,4,5,6,7,8,9);
// 使用匹配守卫额外添加条件
let c = Some(10);
match c {
// 在匹配分支后面添加if表达来额外限定匹配条件
Some(x) if x > 6 => {}
Some(_) => {}
None => {}
}
// 使用@绑定
// 用于像范围匹配这种条件的情况下,将匹配到的值赋值到变量
let c = Some(2);
if let Some(i @ 1..=5) = c {
println!("c is between 1~5:{}", i);
}
```
## 高级特性
### 不安全rust
不安全超能力(unsafe superpower):
1. 解引用裸指针;
2. 调用不安全的函数或者方法;
3. 访问或者修改可变的静态变量;
4. 实现不安全的trait。
#### 裸指针
裸指针要么可变(\*mut T),要么不可变(\*const T),不可变意味着不能对解引用后的指针直接赋值
与引用和智能指针的区别:
1. 允许忽略借用规则,即可以同时拥有同一内存地址的可变和不可变指针,或者拥有多个同一内存地址的可变指针;
2. 不能保证自己总是指向了有效的内存地址;
3. 允许为空;
4. 没有实现任何自动清理机制。
优势:
1. 更好的性能;
2. 与其它语言、硬件交互的能力。
以下例子位于example/src/bin/unsafe.rs中:
```rust
// 解引用裸指针
let mut num = 5;
// 如果只是创建裸针针,并不需要unsafe关键字
// 使用as关键来转化类型,以下两个指针都是从有效引用创建的,所以是有效的
let r1 = &num as *const i32;
let r2 = &mut num as *mut i32;
// 从任意地址创建指针,这里的r3可能就不是一个可用的指针
let t = 0x423243242usize;
let _r3 = t as *const i32;
// 如果需要解引用裸指针,则要将代码包在unsafe里
unsafe {
// 只有可变的裸指针才能赋值,不能对*r1进行赋值
// 其实以下如果不是裸指针,已经破坏了引用规则,同一个地址的可变和不可变引用,但在裸指针中不受此限制
*r2 = 10;
println!("r1 val:{}", *r1); // r1 val:10
// 以下由于指到了不合法的内存地址,直接段错误: segmentation fault
// println!("r3 val:{}", *_r3);
}
```
裸指针一个主要用途是与C代码接口进行交互。
不安全的方法与普通方法一样,只是在fn之前添加unsafe关键字,在不安全的函数中不需要使用unsafe关键字就可以使用不安全的特性。
```rust
// 使用不安全的函数
// 不安全的方法与普通方法一样,只是在fn之前添加unsafe关键字
// 在不安全的函数体内使用不安全特性就不需要包裹unsafe了
unsafe fn dangerous() {}
// 不安全的方法需要包裹在unsafe中调用
unsafe {
dangerous();
}
```
使用不安全特性的函数不一定都是不安全的函数,可以基于不安全的代码创建一个安全的抽象,例如标准库中的split_at_mut方法:
```rust
pub fn split_at_mut(&mut self, mid: usize) -> (&mut [T], &mut [T]) {
assert!(mid <= self.len());
// SAFETY: `[ptr; mid]` and `[mid; len]` are inside `self`, which
// fulfills the requirements of `from_raw_parts_mut`.
unsafe { self.split_at_mut_unchecked(mid) }
}
```
这个函数无法仅仅使用安全的Rust来实现,因为返回的两个切片都是self的一部分,造成两个可变引用的存在。
我们尝试通过实现简化的版本来验证一下。
```rust
// 尝试实现自己的简化版本split_at_mut,使用了不安全特性
fn split_at_mut(v: &mut [i32], mid: usize) -> (&mut [i32], &mut [i32]) {
assert!(mid <= v.len());
// 仅仅使用安全的Rust,会报两个可变指针的错误,而我们能保证这两个可变指针其实指向的是切片非重合的两部分
// (&mut v[..mid], &mut v[mid..])
// 所以可以使用unsafe特性来实现
// 获取指向切片的可变裸指针
let p = v.as_mut_ptr();
unsafe {
// 以下的from_raw_parts_mut函数是unsafe的,所以这块要包含在unsafe中
(core::slice::from_raw_parts_mut(p, mid),
core::slice::from_raw_parts_mut(p.offset(mid as isize), v.len() - mid)
)
}
}
let mut v = vec![1, 2, 3, 4, 5];
let (a, b) = split_at_mut(&mut v[..], 3);
println!("{:?}, {:?}", a, b);
```
使用extern关键字可以引入其它语言的函数,所有通过extern引入的函数都是不安全的,需要使用unsafe关键字。
```rust
extern "C"{
fn abs(input:i32)->i32;
}
unsafe {
println!("{}", abs(-20));
}
```
#### 访问或者修改可变的静态变量
静态变量,也称为全局变量。
不可变的静态变量与常量类似,静态变量的值在内存中拥有固定的地址,使用它的值总是会访问到同样的数据。与之相反的是,常量则允许在任何被使用到的时候复制其数据。
静态变量还可以是可变的,但访问或者修改可变的静态变量,都是unsafe的操作。这是因为Rust无法保证全局变量的修改和访问是否有发生竞争。
```rust
// 可变静态变量
static mut COUNTER:u32 = 10;
// 访问和修改可变静态变量需要使用unsafe特性
fn incr_counter(){
unsafe {
COUNTER += 1;
}
}
fn get_counter() -> u32{
unsafe {
COUNTER
}
}
fn main(){
incr_counter();
println!("{}", get_counter()); // 11
}
```
#### 使用不安全的trait
当trait中存在至少一个拥有编译器无法校验的不安全因素时,这个trait也需要声明为unsafe。
实现这个unsafe trait的impl需要添加unsafe声明。
```rust
// 不安全的trait
unsafe trait Foo {}
unsafe impl Foo for i32 {}
// 在并发章节说明的Send和Sync这两个trait就是不安全的trait,要为某个不安全的类型实现trait,需要使用unsafe关键字
struct Point {
x: i32,
y: i32,
}
unsafe impl Send for Point {}
unsafe impl Sync for Point {}
```
### 高级trait
#### 在trait定义中使用关联类型指定占位类型
例如Iterator trait,使用关联类型定义了Item,这个Item类型做为next的返回值。
实现Iterator的时候,需要将Item赋值到对应的类型,这样next就能返回此类型。
与泛型不同的是,如果Iterator是泛型,那么我们需要实现某个特定版本的泛型,例如实现`Iterator<i32>`这种泛型,调用的时候也要显示地指定对应的类型。
```rust
// 标准库中Iterator迭代器trait的声明,内有关联类型Item
// next返回的类型是Option<Self::Item>类型,即返回Item指定的类型
pub trait Iterator {
type Item;
fn next(&mut self) -> Option<Self::Item>;
}
// 定义一个无限计数器,将关联类型指定成i32,返回一个Some(i32)
struct Counter(i32);
impl Iterator for Counter {
type Item = i32;
fn next(&mut self) -> Option<Self::Item> {
self.0 += 1;
Some(self.0)
}
}
// 如果使用泛型来实现就显得很奇怪
pub trait Iterator2<T> {
fn next(&mut self) -> Option<T>;
}
// 在指定实现泛型trait时,需要显示指定实现的版本是什么
// 如果有多个类型的实现,在调用的时候还要指定调用的是哪个版本的实现
// 而使用关联类型则可以保证只有一种实现
impl Iterator2<i32> for Counter {
fn next(&mut self) -> Option<i32> {
self.0 += 1;
Some(self.0)
}
}
```
#### 默认泛型参数
即在实现泛型的trait时,不指定实现的类型,此时默认泛型参数发挥作用,实现的这个默认类型。
这个功能经常用于运算符重载,Rust提供了有限的运算符重载功能。
这个功能还能让在原来的实现上添加新的类型而不用修改原来的代码实现,只需要在这个新的泛型类型上添加默认值即可。
```rust
// 默认泛型参数
// 公共库中的Add trait实现加法运算符的重载,默认类型与被加数(Right-hand side)相同(Rhs = Self)
pub trait Add<Rhs = Self> {
type Output;
fn add(self, rhs: Rhs) -> Self::Output;
}
#[derive(Debug, PartialEq)]
struct Point(i32, i32);
// 为Point实现了Add trait,没有指定泛型的类型,默认是Self,即Point类型
// 等价于impl std::ops::Add<Point> for Point
impl std::ops::Add for Point {
type Output = Point;
fn add(self, rhs: Point) -> Self::Output {
Point(self.0 + rhs.0, self.1 + rhs.1)
}
}
assert_eq!(Point(1, 2) + Point(2, 3), Point(3, 5));
// 为非默认类型(i32)实现Add操作
impl std::ops::Add<i32> for Point {
type Output = Point;
fn add(self, rhs: i32) -> Self::Output {
Point(self.0 + rhs, self.1 + rhs)
}
}
assert_eq!(Point(1, 2) + 2, Point(3, 4));
```
#### 完全限定语法
两个trait可以定义同名的方法,而且一个类型可以同时实现这两个trait,但当调用方法时,就有可能出现冲突,编译器报错。
此时可以使用完全限定语法来处理,例子如下:
```rust
// 有两个同名函数的trait,以及同时实现这两个trait的类型在调用时发生的歧义调用
trait Pilot {
fn fly(&self) {
println!("Engine start!")
}
fn name(){
println!("Pilot")
}
}
trait Wizard {
fn fly(&self) {
println!("Up!")
}
fn name(){
println!("Wizard")
}
}
struct Human {}
impl Pilot for Human {}
impl Wizard for Human {}
impl Human {
fn fly(&self) {
println!("Dreaming!")
}
fn name(){
println!("Human")
}
}
// Human实现了Wizard的fly、Pilot的fly、以及自己也有fly方法
let h = Human {};
// 此时在调用fly时,会直接使用类型定义的方法
// 如果Human没有实现自己的fly,此时将出现编译错误:multiple `fly` found
h.fly(); // Dreaming!
// 可以使用全限定的语法来指定调用哪一个fly方法
Human::fly(&h); // Dreaming!
Wizard::fly(&h); // Up!
Pilot::fly(&h); //Engine start!
// 对于没有self参数的函数冲突,原来的办法无能为力
// 以下报错:cannot infer type
// note: cannot satisfy `_: Wizard`
// Wizard::name();
// Human可以直接调用,输出Human的方法内容
Human::name(); // Human
// trait不能直接调用其函数,需要使用以下的限定语法
<Human as Pilot>::name(); // Pilot
<Human as Wizard>::name(); // Wizard
```
#### 超trait
当一个trait功能依赖于另外一个trait时,被依赖的这个trait称为当前trait的超trait(super trait)。
```rust
// 超trait,依赖于另外一个trait的trait
trait Animal {
fn class(&self){}
}
// 依赖于类型实现了Animal,因为需要调用其class方法
trait Cat:Animal{
fn miao(&self){
self.class()
}
}
struct Persian{}
// Persian必须实现了Animal,才能实现Cat
impl Animal for Persian{}
impl Cat for Persian{}
```
#### newtype模式
newtype模式是指将一个类型使用struct的元组模式封装一下成为一个新类型,这个类型只有一个字段,这种类型称之为瘦封闭(thin wrapper)。
在Rust中,这一模式不会导致运行时开销,编译器在在编译阶段优化掉。
可以使用这一模式绕过为类型实现trait的孤儿规则,即可以在trait、类型包之外的其它包中为类型实现trait。
但其实并没有真正地为类型实现了对应的trait,毕竟进行了封装,但可以使用Deref来实现与原来类型一样的效果。
```rust
// newtype模式
// 绕过孤儿规则,为Vec<String>实现Display trait
struct Wrapper<'a>(Vec<&'a str>);
impl<'a> std::fmt::Display for Wrapper<'a> {
fn fmt(&self, f: &mut Formatter<'_>) -> std::fmt::Result {
write!(f, "[{}]", self.0.join(","))
}
}
let w = Wrapper(vec!["i", "am", "supper", "man"]);
println!("{}", w); // [i,am,supper,man]
// 但是封装类型并没有实现所有Vec<&str>的能力,比如len函数,w.len()将报错,因为没有实现
// 此时可以使用Deref解引用trait以及自动解引用的性质来实现Wrapper与Vec<&str>更一致
impl<'a> std::ops::Deref for Wrapper<'a> {
type Target = Vec<&'a str>;
fn deref(&self) -> &Self::Target {
&self.0
}
}
println!("len:{}", w.len());
```
### 高级类型
* newtype模式实现的类型,可以隐藏原始类型的一些实现,提供封装抽象能力。并且新的类型与原来的类型为两种不同的类型,编译器检查为这两种类型不能混用提供了检查。
* 而类型别名,则与newtype不同,他只是原类型的别名,两者等价可以混用,一般用于简化类型的书写,例如io库中的Result。
```rust
pub type Result<T> = result::Result<T, Error>;
```
* 永不返回的Never类型(!);一般用于在match中处理异常等情况,例如exit(),panic!等都是返回Never类型。
```rust
pub fn exit(code: i32) -> ! {
crate::sys_common::rt::cleanup();
crate::sys::os::exit(code)
}
```
### 动态大小类型与Size trait
str是动态大小类型,只能在运行时确认类型的大小。
我们无法创建动态大小类型的变量,也无法使用其作为函数参数,这种类型需要放在某种指针的后面,例如&str。
Rust提供了一个特殊的Size trait,编译时可确定大小的类型自动实现了Size trait,另外Rust为泛型类型隐式地添加了Size约束,也就是说泛型默认只能用于编译期可确定大小的类型。但可以显示地使用?Size来解除限制。
```rust
// 解除了Size的限制,并且参数需要使用&T,因为在编译期无法确定大小的类型,必须使用某种指针来引用。
fn generic<T:?Size> (t:&T){}
```
### 高级函数与闭包
```rust
// 我们之前试过使用函数指针,闭包的Fn, FnMut, FnOnce这些trait来实现参数传递
// 除此之外,还可以返回函数类型和trait类型做为函数的返回值
// 以下函数,以函数指针做为返回值
fn return_fn() -> fn(i32) -> i32 {
|x| x + 100
}
let t = return_fn();
println!("{}", t(10)); // 110
// 以下函数,返回实现了Fn trait的函数指针或者是闭包
fn return_fn_trait() -> Box<dyn Fn(i32) -> i32> {
Box::new(|x| x + 100 )
}
let t= return_fn_trait();
println!("{}", t(10));
```
## server服务程序的例子
以下例子实现了线程池、优雅退出等特性,但比较粗糙。
```rust
// lib.rs
use std::sync::{Arc, mpsc, Mutex};
use std::sync::mpsc::{Receiver, Sender};
use std::thread;
// 封闭的线程运行环境
pub struct Worker {
id: usize,
// 为什么要用Option,因为drop的时候,需要获取到thread的所有权
// 使用take获取所有权之后,此处的值就是None了
thread: Option<thread::JoinHandle<()>>,
}
impl Worker {
fn new(id: usize, receiver: Arc<Mutex<Receiver<Message>>>) -> Worker {
// 每一个worker会起一个线程来接收对应的消息
let thread = thread::spawn(move || {
loop {
// receiver需要lock,这里在let语句结束之后,这个receive已经unlock了
// 因为如果MutexGuard不再使用,则将drop掉
let message = receiver.lock().unwrap().recv().unwrap();
// 换成以下两句,则这个l走到job完成后才释放unlock,不满足要求
// let l = receiver.lock().unwrap();
// let message = l.recv().unwrap();
match message {
Message::Terminal => {
break;
}
Message::NewJob(job) => {
print!("{} is doing the job:", id);
job();
}
}
}
});
let thread = Some(thread);
Worker { id, thread }
}
}
// 一个任务即一个闭包函数,因为需要用于在不同的线程之间传递,所以需要实现Send
pub type Job = Box<dyn FnOnce() + Send>;
// 用于发送任务用的Message,有两种类型
// 一种是Terminal结束线程运行,另外一种是NewJob消息,即新的任务
pub enum Message {
Terminal,
NewJob(Job),
}
// 线程池,包含有发送Message用的sender,以及运行的Worker
pub struct ThreadPool {
sender: Sender<Message>,
workers: Vec<Worker>,
}
impl ThreadPool {
/// 创建一个指定线程数的线程池
///
/// # Panics
///
/// `new`函数会在参数为0时panic
pub fn new(s: usize) -> ThreadPool {
assert!(s > 0);
// 使用mpsc::channel来与给多个线程传递信息,但由于不能有多个消费者(即Receiver没有实现Sync)
let (sender, receiver) = mpsc::channel();
// with_capacity可以用于预分配对应大小的动态数组,减少动态扩容
let mut workers = Vec::with_capacity(s);
// receiver没有实现Sync,需要使用Mutex包裹起来实现Sync,另外需要在多个线程中使用,必须使用Arc引用计数
let receiver = Arc::new(Mutex::new(receiver));
for id in 0..s {
// 每一个线程就是一个worker,保存于workers中
workers.push(Worker::new(id, receiver.clone()));
}
ThreadPool { sender, workers }
}
pub fn execute<T>(&self, f: T) where T: FnOnce() + Send + 'static {
self.sender.send(Message::NewJob(Box::new(f))).unwrap();
}
}
impl Drop for ThreadPool {
fn drop(&mut self) {
for _ in &mut self.workers {
println!("send terminal to workers");
self.sender.send(Message::Terminal).unwrap();
}
for w in &mut self.workers {
// 因为join需要获取所有权,所以这块使用了take
if let Some(t) = w.thread.take() {
t.join().unwrap();
println!("No. {} thread has terminal!", w.id);
}
}
}
}
```
```rust
// main.rs
use std::io::{Read, Write};
use std::net::TcpListener;
use std::sync::mpsc;
use std::thread;
use std::time::Duration;
use signal_hook::consts::SIGINT;
use signal_hook::iterator::Signals;
use server::ThreadPool;
fn main() {
// 起一个线程数量为4的线程池
let thread_pool = ThreadPool::new(2);
// 起一个线程单独处理信号,收到信号时,需要将信息传递给accept来结束接收连接,所以这里加了一个channel
// 信号处理使用signal_hook包来处理
let (terminal_sender, terminal_receiver) = mpsc::channel();
let sig = thread::spawn(move || {
let mut signal = Signals::new(&[SIGINT]).unwrap();
for sig in signal.forever() {
println!("rec sig:{}", sig);
terminal_sender.send(()).unwrap();
break;
}
});
// 将listener放在单独的作用域里,这样当信号发出时,第一时间结束监听端口
// 这样新的连接就不会打进来了,但旧的请求还是能被正常处理
{
// 使用TcpListener::bind让程序监听端口;
// bind接收泛型参数,只要实现了ToSocketAddrs即可,例如String类型,不管是&str还是String都行
let listener = TcpListener::bind("localhost:8888".to_string()).unwrap();
// TcpListener的incoming返回迭代器,产生std::io::Result<TcpStream>类型
// 也可以使用accept函数,将其放在loop中,其返回Result<(TcpStream, SocketAddr)>类型
for stream in listener.incoming() {
// 1. 最原始每一个请求阻塞处理
// handler_connection(stream.unwrap());
// 2. 每一个请求都启动一个线程来执行,但这样很快就会耗尽机器资源,容易被ddos攻击
// thread::spawn(move || {
// handler_connection(stream.unwrap());
// });
// 3. 使用线程池来处理只保留有限的线程数来处理
thread_pool.execute(move || {
handler_connection(stream.unwrap());
});
// 由于把这个接收函数放在了接收任务时,所以这块有一个限制就是如果没有请求就无法运行到此处
// 暂时没有想到很好的办法来处理这个
if let Ok(_) = terminal_receiver.try_recv() {
println!("get terminal sig!");
break;
}
}
}
sig.join().unwrap();
// 退出此作用域时,会调用thread_pool的drop函数来处理完请求
println!("main end!");
}
// 处理每一个请求
fn handler_connection(mut stream: std::net::TcpStream) {
let mut buffer = [0; 1000];
let len = stream.read(&mut buffer).unwrap();
let content = String::from_utf8_lossy(&buffer[0..10]);
println!("receive a connection: remoteAddr {:?}, receive data len:{}, content:{:?}", stream.peer_addr().unwrap(), len, content);
let index = b"GET / HTTP/1.1\r\n";
let sleep = b"GET /sleep HTTP/1.1\r\n";
if buffer.starts_with(index) {
let response = "HTTP/1.1 200 OK\r\n\r\nOK";
stream.write(response.as_bytes()).unwrap();
} else if buffer.starts_with(sleep) {
thread::sleep(Duration::from_secs(10));
stream.write(b"HTTP/1.1 200 OK\r\n\r\nsleep").unwrap();
} else {
stream.write(b"HTTP/1.1 404 NOT FOUND\r\n\r\nnot found").unwrap();
}
}
```
<style>
center{
font-size: 0.7em;
margin-top: -20px;
margin-bottom: 15px;
}
table{
font-size:0.7em;
margin-bottom: 29px;
border: 2px solid #2b5fa8;
}
table th {
background: #cef;
padding: 5px;
}
.post ul {
font-size: 0.75em;
list-style-type: disc;
padding-left: 16px;
}
td{
padding: 8px;
}
td.special-title{
color: #a30623;
}
table .field{
background-color:#cef
}
table .allowed{
background-color:#cfe
}
table .required{
background-color:#cfa
}
table .empty{
background-color:#fbb
}
.postn ul {
font-size: 0.75em;
list-style-type: disc;
padding-left: 16px;
margin-top:0px;
}
</style>
| xiaochai/xiaochai.github.io | _posts/2021-11-20-the-rust-programming-language.markdown | Markdown | mit | 148,083 |
import Ember from "ember";
export default Ember.Route.extend({
model: function() {
return this.store.query('answer', {correct: true});
}
});
| dpatz/scroll-of-heroes-client | app/routes/application.js | JavaScript | mit | 150 |
<?php
/**
* This file is part of the PHPMongo package.
*
* (c) Dmytro Sokil <dmytro.sokil@gmail.com>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Sokil\Mongo\Validator;
/**
* Alphanumeric values validator
*
* @author Dmytro Sokil <dmytro.sokil@gmail.com>
*/
class AlphaNumericValidator extends \Sokil\Mongo\Validator
{
public function validateField(\Sokil\Mongo\Document $document, $fieldName, array $params)
{
if (!$document->get($fieldName)) {
return;
}
if (preg_match('/^\w+$/', $document->get($fieldName))) {
return;
}
if (!isset($params['message'])) {
$params['message'] = 'Field "' . $fieldName . '" not alpha-numeric in model ' . get_called_class();
}
$document->addError($fieldName, $this->getName(), $params['message']);
}
}
| ngohuynhngockhanh/pyBearServer | vendor/sokil/php-mongo/src/Validator/AlphaNumericValidator.php | PHP | mit | 961 |
<?php
/**
* swPagesAdmin actions.
*
* @package soleoweb
* @subpackage swPagesAdmin
* @author Your name here
* @version SVN: $Id: actions.class.php 8507 2008-04-17 17:32:20Z fabien $
*/
class baseSwBlogTagsAdminActions extends sfActions
{
public function executeIndex($request)
{
$this->sw_blog_tagList = new swBlogTagsDatagrid(
$request->getParameter('filters', array()),
array(
'page' => $request->getParameter('page'),
'per_page' => 10,
)
);
}
public function executeCreate()
{
$this->form = new swBlogTagForm();
$this->setTemplate('edit');
}
public function executeEdit($request)
{
$this->form = $this->getswBlogTagForm($request->getParameter('id'));
}
public function executeUpdate($request)
{
$this->forward404Unless($request->isMethod('post'));
$this->form = $this->getswBlogTagForm($request->getParameter('id'));
$this->form->bind($request->getParameter('sw_blog_tag'));
if ($this->form->isValid())
{
$sw_blog_tag = $this->form->save();
$this->getUser()->setFlash('notice-ok', __('notice_your_change_has_been_saved', null, 'swToolbox'));
$this->redirect('swBlogTagsAdmin/edit?id='.$sw_blog_tag['id']);
}
$this->getUser()->setFlash('notice-error', __('notice_an_error_occurred_while_saving', null, 'swToolbox'));
$this->setTemplate('edit');
}
public function executeDelete($request)
{
$this->forward404Unless($sw_blog_tag = $this->getswBlogTagById($request->getParameter('id')));
$sw_blog_tag->delete();
$this->getUser()->setFlash('notice-ok', __('notice_element_deleted', null, 'swToolbox'));
$this->redirect('swBlogTagsAdmin/index');
}
private function getswBlogTagTable()
{
return Doctrine::getTable('swBlogTag');
}
private function getswBlogTagById($id)
{
return $this->getswBlogTagTable()->find($id);
}
private function getswBlogTagForm($id)
{
$sw_blog_tag = $this->getswBlogTagById($id);
if ($sw_blog_tag instanceof swBlogTag)
{
return new swBlogTagForm($sw_blog_tag);
}
else
{
return new swBlogTagForm();
}
}
}
| rande/swBlogPlugin | modules/swBlogTagsAdmin/lib/baseSwBlogTagsAdminActions.class.php | PHP | mit | 2,201 |
#ifdef __OBJC__
#import <UIKit/UIKit.h>
#else
#ifndef FOUNDATION_EXPORT
#if defined(__cplusplus)
#define FOUNDATION_EXPORT extern "C"
#else
#define FOUNDATION_EXPORT extern
#endif
#endif
#endif
FOUNDATION_EXPORT double FlowCoreVersionNumber;
FOUNDATION_EXPORT const unsigned char FlowCoreVersionString[];
| flow-ai/flowai-swift | Example/Pods/Target Support Files/FlowCore/FlowCore-umbrella.h | C | mit | 308 |
//This file is automatically rebuilt by the Cesium build process.
/*global define*/
define(function() {
'use strict';
return "/**\n\
* Converts an RGB color to HSB (hue, saturation, brightness)\n\
* HSB <-> RGB conversion with minimal branching: {@link http://lolengine.net/blog/2013/07/27/rgb-to-hsv-in-glsl}\n\
*\n\
* @name czm_RGBToHSB\n\
* @glslFunction\n\
* \n\
* @param {vec3} rgb The color in RGB.\n\
*\n\
* @returns {vec3} The color in HSB.\n\
*\n\
* @example\n\
* vec3 hsb = czm_RGBToHSB(rgb);\n\
* hsb.z *= 0.1;\n\
* rgb = czm_HSBToRGB(hsb);\n\
*/\n\
\n\
const vec4 K_RGB2HSB = vec4(0.0, -1.0 / 3.0, 2.0 / 3.0, -1.0);\n\
\n\
vec3 czm_RGBToHSB(vec3 rgb)\n\
{\n\
vec4 p = mix(vec4(rgb.bg, K_RGB2HSB.wz), vec4(rgb.gb, K_RGB2HSB.xy), step(rgb.b, rgb.g));\n\
vec4 q = mix(vec4(p.xyw, rgb.r), vec4(rgb.r, p.yzx), step(p.x, rgb.r));\n\
\n\
float d = q.x - min(q.w, q.y);\n\
return vec3(abs(q.z + (q.w - q.y) / (6.0 * d + czm_epsilon7)), d / (q.x + czm_epsilon7), q.x);\n\
}\n\
";
}); | coderFirework/app | js/Cesium-Tiles/Source/Shaders/Builtin/Functions/RGBToHSB.js | JavaScript | mit | 1,025 |
# Intro
This is the documentation of [Weave.jl](http://github.com/mpastell/weave.jl). Weave is a scientific report generator/literate programming tool
for Julia. It resembles [Pweave](http://mpastell.com/pweave) and, Knitr
and Sweave.
**Current features**
* Noweb, markdown or script syntax for input documents.
* Execute code as terminal or "script" chunks.
* Capture Gadfly, PyPlot and Winston figures.
* Supports LaTex, Pandoc, Github markdown, MultiMarkdown, Asciidoc and reStructuredText output
* Publish markdown directly to html and pdf using Pandoc.
* Simple caching of results
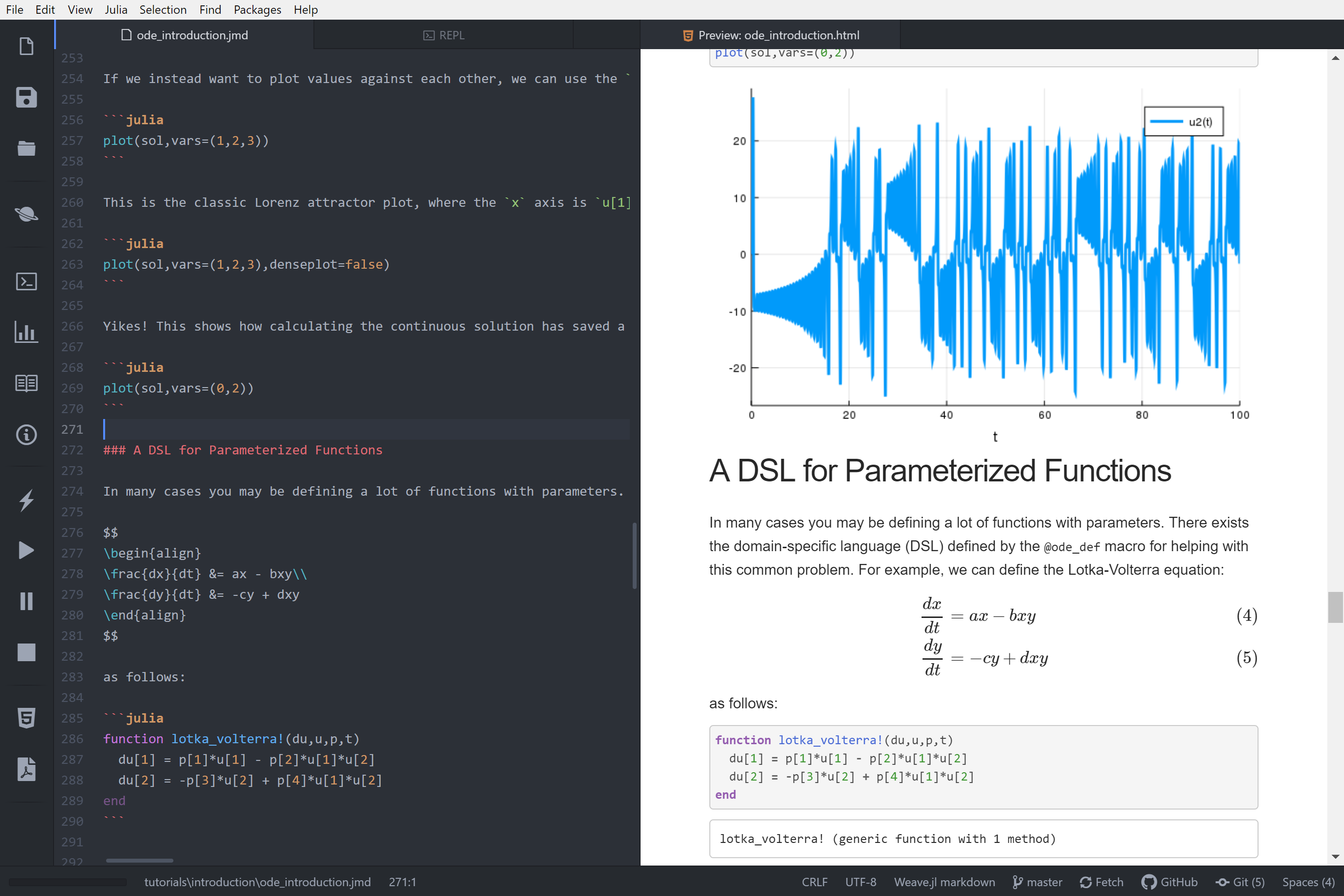
## Contents
```@contents
```
| JuliaPackageMirrors/Weave.jl | doc/src/index.md | Markdown | mit | 691 |
/**
* Demo App for TopcoatTouch
*/
$(document).ready(function() {
<% if (kitchenSink) {
if (mvc) { %>
// Create the topcoatTouch object
var tt = new TopcoatTouch({menu: [{id: 'help', name: 'Help'}, {id: 'info', name: 'Info'}, {id: 'about', name: 'About'}]});
tt.on(tt.EVENTS.MENU_ITEM_CLICKED, function(page, id) {
if (id == 'help') {
tt.goTo('help', 'slidedown', true);
} else if (id == 'about') {
tt.goTo('about', 'pop', true);
}
});
tt.createController('home');
tt.createController('about').addEvent('click', 'button', function() {
tt.goBack();
});
tt.createController('info').addEvent('click', 'button', function() {
tt.goBack();
});
tt.createController('help').addEvent('click', 'button', function() {
tt.goBack();
});
tt.createController('buttonExample', {
postrender: function($page) {
// Show a message when anyone clicks on button of the test form...
$page.find('.testForm').submit(function() {
tt.showDialog('<h3>Button Clicked</h3>');
return false;
});
}
});
tt.createController('carouselExample', {
postadd: function() {
// When the page is loaded, run the following...
// Setup iScroll..
this.carouselScroll = new IScroll('#carouselWrapper', {
scrollX: true,
scrollY: false,
momentum: false,
snap: true,
snapSpeed: 400,
keyBindings: true,
indicators: {
el: document.getElementById('carouselIndicator'),
resize: false
}
});
},
pageend: function() {
if (this.carouselScroll != null) {
this.carouselScroll.destroy();
this.carouselScroll = null;
}
}
});
tt.createController('checkRadioExample');
tt.createController('formExample');
tt.createController('galleryExample', {
postrender: function($page) {
$page.find('#changeButton').click(function() {
createPlaceHolder($page, $('#gallery-picker').data('value'));
});
createPlaceHolder($page, 'kittens');
}
});
tt.createController('waitingDialogExample', {
postadd: function() {
// Show the loading message...
$(document).on('click', '#showLoading', function() {
tt.showLoading('10 seconds');
var count = 10;
var interval = setInterval(function() {
if (--count <= 0) {
clearInterval(interval);
tt.hideLoading();
} else {
$('#topcoat-loading-message').text(count + ' seconds');
}
},1000);
});
// Show the dialog...
$(document).on('click', '#showDialog', function() {
tt.showDialog('This is a dialog', 'Example Dialog', {OK: function() { console.log('OK Pressed') }
, Cancel: function() { console.log('Cancel Pressed')}});
});
}
});
// First page we go to home... This could be done in code by setting the class to 'page page-center', but here is how to do it in code...
tt.goTo('home');
function getRandomInt(min, max) {
return Math.floor(Math.random() * (max - min + 1) + min);
}
// Create the placeholders in the gallery...
function createPlaceHolder($page, type) {
var placeHolders = { kittens : 'placekitten.com', bears: 'placebear.com', lorem: 'lorempixel.com',
bacon: 'baconmockup.com', murray: 'www.fillmurray.com'};
var gallery = '';
for (var i = 0; i < getRandomInt(50,100); i++) {
gallery += '<li class="photoClass" style="background:url(http://' + placeHolders[type] + '/' +
getRandomInt(200,300) + '/' + getRandomInt(200,300) + ') 50% 50% no-repeat"></li>';
}
$page.find('.photo-gallery').html(gallery);
tt.refreshScroll(); // Refresh the scroller
tt.scrollTo(0,0); // Move back to the top of the page...
}
<%
// End If MVC KitchenSink
} else {
// Start SingleDocument KitchenSink
%>
// Create the topcoatTouch object
var tt = new TopcoatTouch({menu: [{id: 'help', name: 'Help'}, {id: 'info', name: 'Info'}, {id: 'about', name: 'About'}]});
// First page we go to home... This could be done in code by setting the class to 'page page-center', but here is how to do it in code...
tt.goTo('home');
var carouselScroll = null;
tt.on(tt.EVENTS.MENU_ITEM_CLICKED, function(page, id) {
if (id == 'help') {
tt.goTo('help', 'slidedown', true);
} else if (id == 'info') {
tt.goTo('info', 'flip', true);
} else if (id == 'about') {
tt.goTo('about', 'pop', true);
}
});
tt.on('click', 'button', 'help about info', function() {
tt.goBack();
});
// Show the loading message...
$('#showLoading').click(function() {
tt.showLoading('10 seconds');
var count = 10;
var interval = setInterval(function() {
if (--count <= 0) {
clearInterval(interval);
tt.hideLoading();
} else {
$('#topcoat-loading-message').text(count + ' seconds');
}
},1000);
});
// Show the dialog...
$('#showDialog').click(function() {
tt.showDialog('This is a dialog', 'Example Dialog', {OK: function() { console.log('OK Pressed') }
, Cancel: function() { console.log('Cancel Pressed')}});
});
tt.on(tt.EVENTS.PAGE_START, 'carouselExample', function() {
// When the page is loaded, run the following...
// Setup iScroll..
carouselScroll = new IScroll('#carouselWrapper', {
scrollX: true,
scrollY: false,
momentum: false,
snap: true,
snapSpeed: 400,
keyBindings: true,
indicators: {
el: document.getElementById('carouselIndicator'),
resize: false
}
});
}).on(tt.EVENTS.PAGE_END, 'carouselExample', function() {
// When the page is unloaded, run the following...
if (carouselScroll != null) {
carouselScroll.destroy();
carouselScroll = null;
}
});
// Show a message when anyone clicks on button of the test form...
$('.testForm').submit(function() {
tt.showDialog('<h3>Button Clicked</h3>');
return false;
});
function getRandomInt(min, max) {
return Math.floor(Math.random() * (max - min + 1) + min);
}
// Create the placeholders in the gallery...
function createPlaceHolder(type) {
var placeHolders = { kittens : 'placekitten.com', bears: 'placebear.com', lorem: 'lorempixel.com',
bacon: 'baconmockup.com', murray: 'www.fillmurray.com'};
var gallery = '';
for (var i = 0; i < getRandomInt(50,100); i++) {
gallery += '<li class="photoClass" style="background:url(http://' + placeHolders[type] + '/' +
getRandomInt(200,300) + '/' + getRandomInt(200,300) + ') 50% 50% no-repeat"></li>';
}
$('.photo-gallery').html(gallery);
tt.refreshScroll(); // Refresh the scroller
tt.scrollTo(0,0); // Move back to the top of the page...
}
$('#gallery-picker').change(function(e, id) {
createPlaceHolder(id);
});
createPlaceHolder('kittens');
<%
}
} else {
%>
// Create the topcoatTouch object
var tt = new TopcoatTouch();
<% if (mvc) { %>
tt.createController('home');
<% } else { %>
// First page we go to home... This could be done in code by setting the class to 'page page-center', but here is how to do it in code...
<% } %>
tt.goTo('home');
<% } %>
});
| kriserickson/generator-topcoat-touch | app/templates/_app.js | JavaScript | mit | 8,221 |
use std::borrow::{Borrow, Cow};
use std::ffi::{CStr, CString};
use std::fmt;
use std::mem;
use std::ops;
use std::os::raw::c_char;
use std::ptr;
const STRING_SIZE: usize = 512;
/// This is a C String abstractions that presents a CStr like
/// interface for interop purposes but tries to be little nicer
/// by avoiding heap allocations if the string is within the
/// generous bounds (512 bytes) of the statically sized buffer.
/// Strings over this limit will be heap allocated, but the
/// interface outside of this abstraction remains the same.
pub enum CFixedString {
Local {
s: [c_char; STRING_SIZE],
len: usize,
},
Heap {
s: CString,
len: usize,
},
}
impl CFixedString {
/// Creates an empty CFixedString, this is intended to be
/// used with write! or the `fmt::Write` trait
pub fn new() -> Self {
unsafe {
CFixedString::Local {
s: mem::uninitialized(),
len: 0,
}
}
}
pub fn from_str<S: AsRef<str>>(s: S) -> Self {
Self::from(s.as_ref())
}
pub fn as_ptr(&self) -> *const c_char {
match *self {
CFixedString::Local { ref s, .. } => s.as_ptr(),
CFixedString::Heap { ref s, .. } => s.as_ptr(),
}
}
/// Returns true if the string has been heap allocated
pub fn is_allocated(&self) -> bool {
match *self {
CFixedString::Local { .. } => false,
_ => true,
}
}
/// Converts a `CFixedString` into a `Cow<str>`.
///
/// This function will calculate the length of this string (which normally
/// requires a linear amount of work to be done) and then return the
/// resulting slice as a `Cow<str>`, replacing any invalid UTF-8 sequences
/// with `U+FFFD REPLACEMENT CHARACTER`. If there are no invalid UTF-8
/// sequences, this will merely return a borrowed slice.
pub fn to_string(&self) -> Cow<str> {
String::from_utf8_lossy(&self.to_bytes())
}
pub unsafe fn as_str(&self) -> &str {
use std::slice;
use std::str;
match *self {
CFixedString::Local { ref s, len } => {
str::from_utf8_unchecked(slice::from_raw_parts(s.as_ptr() as *const u8, len))
}
CFixedString::Heap { ref s, len } => {
str::from_utf8_unchecked(slice::from_raw_parts(s.as_ptr() as *const u8, len))
}
}
}
}
impl<'a> From<&'a str> for CFixedString {
fn from(s: &'a str) -> Self {
use std::fmt::Write;
let mut string = CFixedString::new();
string.write_str(s).unwrap();
string
}
}
impl fmt::Write for CFixedString {
fn write_str(&mut self, s: &str) -> Result<(), fmt::Error> {
// use std::fmt::Write;
unsafe {
let cur_len = self.as_str().len();
match cur_len + s.len() {
len if len <= STRING_SIZE - 1 => {
match *self {
CFixedString::Local { s: ref mut ls, len: ref mut lslen } => {
let ptr = ls.as_mut_ptr() as *mut u8;
ptr::copy(s.as_ptr(), ptr.offset(cur_len as isize), s.len());
*ptr.offset(len as isize) = 0;
*lslen = len;
}
_ => unreachable!(),
}
}
len => {
let mut heapstring = String::with_capacity(len + 1);
heapstring.write_str(self.as_str()).unwrap();
heapstring.write_str(s).unwrap();
*self = CFixedString::Heap {
s: CString::new(heapstring).unwrap(),
len: len,
};
}
}
}
// Yah....we should do error handling
Ok(())
}
}
impl From<CFixedString> for String {
fn from(s: CFixedString) -> Self {
String::from_utf8_lossy(&s.to_bytes()).into_owned()
}
}
impl ops::Deref for CFixedString {
type Target = CStr;
fn deref(&self) -> &CStr {
use std::slice;
match *self {
CFixedString::Local { ref s, len } => unsafe {
mem::transmute(slice::from_raw_parts(s.as_ptr(), len + 1))
},
CFixedString::Heap { ref s, .. } => s,
}
}
}
impl Borrow<CStr> for CFixedString {
fn borrow(&self) -> &CStr {
self
}
}
impl AsRef<CStr> for CFixedString {
fn as_ref(&self) -> &CStr {
self
}
}
impl Borrow<str> for CFixedString {
fn borrow(&self) -> &str {
unsafe { self.as_str() }
}
}
impl AsRef<str> for CFixedString {
fn as_ref(&self) -> &str {
unsafe { self.as_str() }
}
}
macro_rules! format_c {
// This does not work on stable, to change the * to a + and
// have this arm be used when there are no arguments :(
// ($fmt:expr) => {
// use std::fmt::Write;
// let mut fixed = CFixedString::new();
// write!(&mut fixed, $fmt).unwrap();
// fixed
// }
($fmt:expr, $($args:tt)*) => ({
use std::fmt::Write;
let mut fixed = CFixedString::new();
write!(&mut fixed, $fmt, $($args)*).unwrap();
fixed
})
}
#[cfg(test)]
mod tests {
use super::*;
use std::fmt::Write;
fn gen_string(len: usize) -> String {
let mut out = String::with_capacity(len);
for _ in 0..len / 16 {
out.write_str("zyxvutabcdef9876").unwrap();
}
for i in 0..len % 16 {
out.write_char((i as u8 + 'A' as u8) as char).unwrap();
}
assert_eq!(out.len(), len);
out
}
#[test]
fn test_empty_handler() {
let short_string = "";
let t = CFixedString::from_str(short_string);
assert!(!t.is_allocated());
assert_eq!(&t.to_string(), short_string);
}
#[test]
fn test_short_1() {
let short_string = "test_local";
let t = CFixedString::from_str(short_string);
assert!(!t.is_allocated());
assert_eq!(&t.to_string(), short_string);
}
#[test]
fn test_short_2() {
let short_string = "test_local stoheusthsotheost";
let t = CFixedString::from_str(short_string);
assert!(!t.is_allocated());
assert_eq!(&t.to_string(), short_string);
}
#[test]
fn test_511() {
// this string (width 511) buffer should just fit
let test_511_string = gen_string(511);
let t = CFixedString::from_str(&test_511_string);
assert!(!t.is_allocated());
assert_eq!(&t.to_string(), &test_511_string);
}
#[test]
fn test_512() {
// this string (width 512) buffer should not fit
let test_512_string = gen_string(512);
let t = CFixedString::from_str(&test_512_string);
assert!(t.is_allocated());
assert_eq!(&t.to_string(), &test_512_string);
}
#[test]
fn test_513() {
// this string (width 513) buffer should not fit
let test_513_string = gen_string(513);
let t = CFixedString::from_str(&test_513_string);
assert!(t.is_allocated());
assert_eq!(&t.to_string(), &test_513_string);
}
#[test]
fn test_to_owned() {
let short = "this is an amazing string";
let t = CFixedString::from_str(short);
assert!(!t.is_allocated());
assert_eq!(&String::from(t), short);
let long = gen_string(1025);
let t = CFixedString::from_str(&long);
assert!(t.is_allocated());
assert_eq!(&String::from(t), &long);
}
#[test]
fn test_short_format() {
let mut fixed = CFixedString::new();
write!(&mut fixed, "one_{}", 1).unwrap();
write!(&mut fixed, "_two_{}", "two").unwrap();
write!(&mut fixed,
"_three_{}-{}-{:.3}",
23,
"some string data",
56.789)
.unwrap();
assert!(!fixed.is_allocated());
assert_eq!(&fixed.to_string(),
"one_1_two_two_three_23-some string data-56.789");
}
#[test]
fn test_long_format() {
let mut fixed = CFixedString::new();
let mut string = String::new();
for i in 1..30 {
let genned = gen_string(i * i);
write!(&mut fixed, "{}_{}", i, genned).unwrap();
write!(&mut string, "{}_{}", i, genned).unwrap();
}
assert!(fixed.is_allocated());
assert_eq!(&fixed.to_string(), &string);
}
// TODO: Reenable this test once the empty match arm is allowed
// by the compiler
// #[test]
// fn test_empty_fmt_macro() {
// let empty = format_c!("");
// let no_args = format_c!("there are no format args");
//
// assert!(!empty.is_allocated());
// assert_eq!(&empty.to_string(), "");
//
// assert!(!no_args.is_allocated());
// assert_eq!(&no_args.to_string(), "there are no format args");
// }
#[test]
fn test_short_fmt_macro() {
let first = 23;
let second = "#@!*()&^%_-+={}[]|\\/?><,.:;~`";
let third = u32::max_value();
let fourth = gen_string(512 - 45);
let fixed = format_c!("{}_{}_0x{:x}_{}", first, second, third, fourth);
let heaped = format!("{}_{}_0x{:x}_{}", first, second, third, fourth);
assert!(!fixed.is_allocated());
assert_eq!(&fixed.to_string(), &heaped);
}
#[test]
fn test_long_fmt_macro() {
let first = "";
let second = gen_string(510);
let third = 3;
let fourth = gen_string(513 * 8);
let fixed = format_c!("{}_{}{}{}", first, second, third, fourth);
let heaped = format!("{}_{}{}{}", first, second, third, fourth);
assert!(fixed.is_allocated());
assert_eq!(&fixed.to_string(), &heaped);
}
}
| emoon/ProDBG | api/rust/prodbg/src/cfixed_string.rs | Rust | mit | 10,053 |
const sizeOf = {
object: function () {
return function (object) {
let $start = 0
$start += 1 * object.array.length + 2
return $start
}
} ()
}
const serializer = {
all: {
object: function () {
return function (object, $buffer, $start) {
let $i = []
for ($i[0] = 0; $i[0] < object.array.length; $i[0]++) {
$buffer[$start++] = object.array[$i[0]] & 0xff
}
$buffer[$start++] = 0xd
$buffer[$start++] = 0xa
return { start: $start, serialize: null }
}
} ()
},
inc: {
object: function () {
return function (object, $step = 0, $i = []) {
let $_, $bite
return function $serialize ($buffer, $start, $end) {
for (;;) {
switch ($step) {
case 0:
$i[0] = 0
$step = 1
case 1:
$bite = 0
$_ = object.array[$i[0]]
case 2:
while ($bite != -1) {
if ($start == $end) {
$step = 2
return { start: $start, serialize: $serialize }
}
$buffer[$start++] = $_ >>> $bite * 8 & 0xff
$bite--
}
if (++$i[0] != object.array.length) {
$step = 1
continue
}
$step = 3
case 3:
if ($start == $end) {
$step = 3
return { start: $start, serialize: $serialize }
}
$buffer[$start++] = 0xd
case 4:
if ($start == $end) {
$step = 4
return { start: $start, serialize: $serialize }
}
$buffer[$start++] = 0xa
case 5:
}
break
}
return { start: $start, serialize: null }
}
}
} ()
}
}
const parser = {
all: {
object: function () {
return function ($buffer, $start) {
let $i = []
let object = {
array: []
}
$i[0] = 0
for (;;) {
if (
$buffer[$start] == 0xd &&
$buffer[$start + 1] == 0xa
) {
$start += 2
break
}
object.array[$i[0]] = $buffer[$start++]
$i[0]++
}
return object
}
} ()
},
inc: {
object: function () {
return function (object, $step = 0, $i = []) {
let $length = 0
return function $parse ($buffer, $start, $end) {
for (;;) {
switch ($step) {
case 0:
object = {
array: []
}
case 1:
$i[0] = 0
case 2:
$step = 2
if ($start == $end) {
return { start: $start, object: null, parse: $parse }
}
if ($buffer[$start] != 0xd) {
$step = 4
continue
}
$start++
$step = 3
case 3:
$step = 3
if ($start == $end) {
return { start: $start, object: null, parse: $parse }
}
if ($buffer[$start] != 0xa) {
$step = 4
$parse(Buffer.from([ 0xd ]), 0, 1)
continue
}
$start++
$step = 7
continue
case 4:
case 5:
if ($start == $end) {
$step = 5
return { start: $start, object: null, parse: $parse }
}
object.array[$i[0]] = $buffer[$start++]
case 6:
$i[0]++
$step = 2
continue
case 7:
}
return { start: $start, object: object, parse: null }
break
}
}
}
} ()
}
}
module.exports = {
sizeOf: sizeOf,
serializer: {
all: serializer.all,
inc: serializer.inc,
bff: function ($incremental) {
return {
object: function () {
return function (object) {
return function ($buffer, $start, $end) {
let $i = []
if ($end - $start < 2 + object.array.length * 1) {
return $incremental.object(object, 0, $i)($buffer, $start, $end)
}
for ($i[0] = 0; $i[0] < object.array.length; $i[0]++) {
$buffer[$start++] = object.array[$i[0]] & 0xff
}
$buffer[$start++] = 0xd
$buffer[$start++] = 0xa
return { start: $start, serialize: null }
}
}
} ()
}
} (serializer.inc)
},
parser: {
all: parser.all,
inc: parser.inc,
bff: function ($incremental) {
return {
object: function () {
return function () {
return function ($buffer, $start, $end) {
let $i = []
let object = {
array: []
}
$i[0] = 0
for (;;) {
if ($end - $start < 2) {
return $incremental.object(object, 2, $i)($buffer, $start, $end)
}
if (
$buffer[$start] == 0xd &&
$buffer[$start + 1] == 0xa
) {
$start += 2
break
}
if ($end - $start < 1) {
return $incremental.object(object, 4, $i)($buffer, $start, $end)
}
object.array[$i[0]] = $buffer[$start++]
$i[0]++
}
return { start: $start, object: object, parse: null }
}
} ()
}
}
} (parser.inc)
}
}
| bigeasy/packet | test/readme/terminated-multibyte.js | JavaScript | mit | 8,252 |
#body {
width: auto;
}
/* Coding Table */
.table_stat {
z-index: 2;
position: relative;
}
.table_bar {
height: 80%;
position: absolute;
background: #DEF; /* cornflowerblue */
z-index: -1;
top: 10%;
left: 0%;
}
#agreement_table, #tweet_table {
font-size: 10pt;
}
#agreement_table small {
opacity: 0.5;
}
.tweet_table_Text {
width: 60%;
}
#agreement_table tr:nth-child(even){
background-color: #F7F7F7;
}
.matrix-table-div {
text-align: center;
display: inline-block;
position: relative;
}
.ylabel {
top: 50%;
height: 100%;
left: 0px;
transform: translate(-100%, -50%);
text-orientation: sideways;
writing-mode: vertical-lr;
position: absolute;
}
#lMatrices table {
table-layout: fixed;
width: inherit;
}
#lMatrices td {
font-size: 12px;
width: 23px;
height: 23px;
overflow: hidden;
white-space: nowrap;
text-align: center;
border-right-style: dotted;
border-bottom-style: dotted;
border-top-style: none;
border-left-style: none;
border-width: 1px;
}
#lMatrices tr td:last-child {
border-right-style: none;
border-left-style: double;
border-left-width: 3px;
}
#lMatrices table tr:first-child, #lMatrices tr td:first-child {
font-weight: bold;
}
#codes_confusion_matrix td {
width: 30px;
height: 30px;
}
#codes_confusion_matrix tr:last-child td {
border-bottom-style: none;
border-top-style: double;
border-top-width: 3px;
}
/*
#matrices tr:hover {
background: #EEE;
}*/
.coding_header h2 {
display: inline;
margin-right: 30px;
}
.u_cell_plain {
border-right-style: none !important;
border-left-style: none !important;
border-top-style: none !important;
border-bottom-style: none !important;
}
.u_cell_pairwise {
border-right-style: dotted !important;
border-left-style: none !important;
border-top-style: none !important;
border-bottom-style: dotted !important;
}
.u_cell_coltotal {
border-right-style: dotted !important;
border-left-style: none !important;
border-top-style: double !important;
border-bottom-style: none !important;
}
.u_cell_rowtotal {
border-right-style: none !important;
border-left-style: double !important;
border-top-style: none !important;
border-bottom-style: dotted !important;
}
.u_cell_toolow {
background-color: #ff9896
}
.u_cell_toohigh {
background-color: #aec7e8
}
.u_cell_lt75 {
background-color: #dbdb8d
}
.u_cell_gt90 {
background-color: #98df8a
}
.u_cell_colorscale {
padding: 0px 5px;
margin: 0px 5px;
}
| emCOMP/visualization | src/css/annotated_tweets.css | CSS | mit | 2,629 |
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<title>Submission 944</title>
<meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1">
</head>
<body topmargin="0" leftmargin="0" marginheight="0" marginwidth="0">
<img src="gallery/submissions/944.jpg" height="400">
</body>
</html>
| heyitsgarrett/envelopecollective | gallery_submission.php-id=944.html | HTML | mit | 367 |
@charset "UTF-8";
/* グレー系 */
/* シアン系 */
/* マージン、パディング */
/* フォントサイズ */
/* 行の高さ */
/* グレー系 */
/* シアン系 */
/* マージン、パディング */
/* フォントサイズ */
/* 行の高さ */
/* line 6, /vagrant/app/assets/stylesheets/staff/container.css.scss */
div#wrapper div#container h1 {
margin: 0;
padding: 9px 6px;
font-size: 16px;
font-weight: normal;
background-color: #1a3333;
color: #eeeeee;
}
/* マージン、パディング */
/* フォントサイズ */
/* 行の高さ */
/* グレー系 */
/* シアン系 */
/* マージン、パディング */
/* フォントサイズ */
/* 行の高さ */
/* line 4, /vagrant/app/assets/stylesheets/staff/layout.css.scss */
html, body {
margin: 0;
padding: 0;
height: 100%;
}
/* line 9, /vagrant/app/assets/stylesheets/staff/layout.css.scss */
div#wrapper {
position: relative;
box-sizing: border-box;
min-height: 100%;
margin: 0 auto;
padding-bottom: 48px;
background-color: #cccccc;
}
/* line 17, /vagrant/app/assets/stylesheets/staff/layout.css.scss */
header {
padding: 6px;
background-color: #448888;
color: #fafafa;
}
/* line 21, /vagrant/app/assets/stylesheets/staff/layout.css.scss */
header span.logo-mark {
font-weight: bold;
}
/* line 25, /vagrant/app/assets/stylesheets/staff/layout.css.scss */
footer {
bottom: 0;
position: absolute;
width: 100%;
background-color: #666666;
color: #fafafa;
}
/* line 31, /vagrant/app/assets/stylesheets/staff/layout.css.scss */
footer p {
text-align: center;
padding: 6px;
margin: 0;
}
/*
*/
/*
* This is a manifest file that'll be compiled into application.css, which will include all the files
* listed below.
*
* Any CSS and SCSS file within this directory, lib/assets/stylesheets, vendor/assets/stylesheets,
* or vendor/assets/stylesheets of plugins, if any, can be referenced here using a relative path.
*
* You're free to add application-wide styles to this file and they'll appear at the bottom of the
* compiled file so the styles you add here take precedence over styles defined in any styles
* defined in the other CSS/SCSS files in this directory. It is generally better to create a new
* file per style scope.
*
*/
| amagasa99/baukis | public/assets/application-358366aec1b89d5184b3442fddc3a7d2.css | CSS | mit | 2,277 |
<?php namespace Fannan\MembersModule\Member;
use Anomaly\Streams\Platform\Database\Seeder\Seeder;
class MemberSeeder extends Seeder
{
/**
* Run the seeder.
*/
public function run()
{
//
}
}
| fannan1991/pyrocms | addons/shared/fannan/members-module/src/Member/MemberSeeder.php | PHP | mit | 227 |
import modelExtend from 'dva-model-extend'
import { create, remove, update } from '../services/user'
import * as usersService from '../services/users'
import { pageModel } from './common'
import { config } from 'utils'
const { query } = usersService
const { prefix } = config
export default modelExtend(pageModel, {
namespace: 'user',
state: {
currentItem: {},
modalVisible: false,
modalType: 'create',
selectedRowKeys: [],
isMotion: localStorage.getItem(`${prefix}userIsMotion`) === 'true',
},
subscriptions: {
setup ({ dispatch, history }) {
history.listen(location => {
if (location.pathname === '/user') {
dispatch({
type: 'query',
payload: location.query,
})
}
})
},
},
effects: {
*query ({ payload = {} }, { call, put }) {
const data = yield call(query, payload)
if (data) {
yield put({
type: 'querySuccess',
payload: {
list: data.data,
pagination: {
current: Number(payload.page) || 1,
pageSize: Number(payload.pageSize) || 10,
total: data.total,
},
},
})
}
},
*'delete' ({ payload }, { call, put, select }) {
const data = yield call(remove, { id: payload })
const { selectedRowKeys } = yield select(_ => _.user)
if (data.success) {
yield put({ type: 'updateState', payload: { selectedRowKeys: selectedRowKeys.filter(_ => _ !== payload) } })
yield put({ type: 'query' })
} else {
throw data
}
},
*'multiDelete' ({ payload }, { call, put }) {
const data = yield call(usersService.remove, payload)
if (data.success) {
yield put({ type: 'updateState', payload: { selectedRowKeys: [] } })
yield put({ type: 'query' })
} else {
throw data
}
},
*create ({ payload }, { call, put }) {
const data = yield call(create, payload)
if (data.success) {
yield put({ type: 'hideModal' })
yield put({ type: 'query' })
} else {
throw data
}
},
*update ({ payload }, { select, call, put }) {
const id = yield select(({ user }) => user.currentItem.id)
const newUser = { ...payload, id }
const data = yield call(update, newUser)
if (data.success) {
yield put({ type: 'hideModal' })
yield put({ type: 'query' })
} else {
throw data
}
},
},
reducers: {
showModal (state, { payload }) {
return { ...state, ...payload, modalVisible: true }
},
hideModal (state) {
return { ...state, modalVisible: false }
},
switchIsMotion (state) {
localStorage.setItem(`${prefix}userIsMotion`, !state.isMotion)
return { ...state, isMotion: !state.isMotion }
},
},
})
| shaohuawang2015/goldbeans-admin | src/models/user.js | JavaScript | mit | 2,907 |
#!/bin/bash
cd "$(dirname "$BASH_SOURCE")" || {
echo "Error getting script directory" >&2
exit 1
}
./hallo.command
sudo launchctl load -w /System/Library/LaunchDaemons/com.apple.metadata.mds.plist | kiuz/Osx_Terminal_shell_script_collection | enable_spotlight.command | Shell | mit | 204 |
<?php
class C_admin extends CI_Controller {
public function __construct() {
parent::__construct();
if($this->session->userdata('level') != 'admin') {
redirect('auth'); }
}
public function index() {
$data['username'] = $this->session->userdata('username');
$this->load->view('dashboard_admin', $data);
}
public function activity() {
$data['username'] = $this->session->userdata('username');
$this->load->view('activity_admin', $data);
}
public function mahasiswa() {
$data['username'] = $this->session->userdata('username');
$this->load->view('mahasiswa_admin', $data);
}
public function logout() {
$this->session->unset_userdata('username');
$this->session->unset_userdata('level');
$this->session->sess_destroy();
redirect('auth'); }
}
?>
| dinanandka/MonitoringKP_KPPL | application/controllers/c_admin.php | PHP | mit | 787 |
var blueMarker = L.AwesomeMarkers.icon({
icon: 'record',
prefix: 'glyphicon',
markerColor: 'blue'
});
var airportMarker = L.AwesomeMarkers.icon({
icon: 'plane',
prefix: 'fa',
markerColor: 'cadetblue'
});
var cityMarker = L.AwesomeMarkers.icon({
icon: 'home',
markerColor: 'red'
});
var stationMarker = L.AwesomeMarkers.icon({
icon: 'train',
prefix: 'fa',
markerColor: 'cadetblue'
});
| alemela/wiki-needs-pictures | js/icons.js | JavaScript | mit | 431 |
<?php
namespace Phossa\Cache;
interface CachePoolInterface
{
}
class CachePool implements CachePoolInterface
{
}
class TestMap {
private $cache;
public function __construct(CachePoolInterface $cache) {
$this->cache = $cache;
}
public function getCache() {
return $this->cache;
}
}
| phossa/phossa-di | tests/src/Phossa/Di/testData6.php | PHP | mit | 322 |
/**
* jspsych plugin for categorization trials with feedback and animated stimuli
* Josh de Leeuw
*
* documentation: docs.jspsych.org
**/
jsPsych.plugins["categorize-animation"] = (function() {
var plugin = {};
jsPsych.pluginAPI.registerPreload('categorize-animation', 'stimuli', 'image');
plugin.info = {
name: 'categorize-animation',
description: '',
parameters: {
stimuli: {
type: jsPsych.plugins.parameterType.IMAGE,
pretty_name: 'Stimuli',
default: undefined,
description: 'Array of paths to image files.'
},
key_answer: {
type: jsPsych.plugins.parameterType.KEYCODE,
pretty_name: 'Key answer',
default: undefined,
description: 'The key to indicate correct response'
},
choices: {
type: jsPsych.plugins.parameterType.KEYCODE,
pretty_name: 'Choices',
default: jsPsych.ALL_KEYS,
array: true,
description: 'The keys subject is allowed to press to respond to stimuli.'
},
text_answer: {
type: jsPsych.plugins.parameterType.STRING,
pretty_name: 'Text answer',
default: null,
description: 'Text to describe correct answer.'
},
correct_text: {
type: jsPsych.plugins.parameterType.STRING,
pretty_name: 'Correct text',
default: 'Correct.',
description: 'String to show when subject gives correct answer'
},
incorrect_text: {
type: jsPsych.plugins.parameterType.STRING,
pretty_name: 'Incorrect text',
default: 'Wrong.',
description: 'String to show when subject gives incorrect answer.'
},
frame_time: {
type: jsPsych.plugins.parameterType.INT,
pretty_name: 'Frame time',
default: 500,
description: 'Duration to display each image.'
},
sequence_reps: {
type: jsPsych.plugins.parameterType.INT,
pretty_name: 'Sequence repetitions',
default: 1,
description: 'How many times to display entire sequence.'
},
allow_response_before_complete: {
type: jsPsych.plugins.parameterType.BOOL,
pretty_name: 'Allow response before complete',
default: false,
description: 'If true, subject can response before the animation sequence finishes'
},
feedback_duration: {
type: jsPsych.plugins.parameterType.INT,
pretty_name: 'Feedback duration',
default: 2000,
description: 'How long to show feedback'
},
prompt: {
type: jsPsych.plugins.parameterType.STRING,
pretty_name: 'Prompt',
default: null,
description: 'Any content here will be displayed below the stimulus.'
},
render_on_canvas: {
type: jsPsych.plugins.parameterType.BOOL,
pretty_name: 'Render on canvas',
default: true,
description: 'If true, the images will be drawn onto a canvas element (prevents blank screen between consecutive images in some browsers).'+
'If false, the image will be shown via an img element.'
}
}
}
plugin.trial = function(display_element, trial) {
var animate_frame = -1;
var reps = 0;
var showAnimation = true;
var responded = false;
var timeoutSet = false;
var correct;
if (trial.render_on_canvas) {
// first clear the display element (because the render_on_canvas method appends to display_element instead of overwriting it with .innerHTML)
if (display_element.hasChildNodes()) {
// can't loop through child list because the list will be modified by .removeChild()
while (display_element.firstChild) {
display_element.removeChild(display_element.firstChild);
}
}
var canvas = document.createElement("canvas");
canvas.id = "jspsych-categorize-animation-stimulus";
canvas.style.margin = 0;
canvas.style.padding = 0;
display_element.insertBefore(canvas, null);
var ctx = canvas.getContext("2d");
if (trial.prompt !== null) {
var prompt_div = document.createElement("div");
prompt_div.id = "jspsych-categorize-animation-prompt";
prompt_div.style.visibility = "hidden";
prompt_div.innerHTML = trial.prompt;
display_element.insertBefore(prompt_div, canvas.nextElementSibling);
}
var feedback_div = document.createElement("div");
display_element.insertBefore(feedback_div, display_element.nextElementSibling);
}
// show animation
var animate_interval = setInterval(function() {
if (!trial.render_on_canvas) {
display_element.innerHTML = ''; // clear everything
}
animate_frame++;
if (animate_frame == trial.stimuli.length) {
animate_frame = 0;
reps++;
// check if reps complete //
if (trial.sequence_reps != -1 && reps >= trial.sequence_reps) {
// done with animation
showAnimation = false;
}
}
if (showAnimation) {
if (trial.render_on_canvas) {
display_element.querySelector('#jspsych-categorize-animation-stimulus').style.visibility = 'visible';
var img = new Image();
img.src = trial.stimuli[animate_frame];
canvas.height = img.naturalHeight;
canvas.width = img.naturalWidth;
ctx.drawImage(img,0,0);
} else {
display_element.innerHTML += '<img src="'+trial.stimuli[animate_frame]+'" class="jspsych-categorize-animation-stimulus"></img>';
}
}
if (!responded && trial.allow_response_before_complete) {
// in here if the user can respond before the animation is done
if (trial.prompt !== null) {
if (trial.render_on_canvas) {
prompt_div.style.visibility = "visible";
} else {
display_element.innerHTML += trial.prompt;
}
}
if (trial.render_on_canvas) {
if (!showAnimation) {
canvas.remove();
}
}
} else if (!responded) {
// in here if the user has to wait to respond until animation is done.
// if this is the case, don't show the prompt until the animation is over.
if (!showAnimation) {
if (trial.prompt !== null) {
if (trial.render_on_canvas) {
prompt_div.style.visibility = "visible";
} else {
display_element.innerHTML += trial.prompt;
}
}
if (trial.render_on_canvas) {
canvas.remove();
}
}
} else {
// user has responded if we get here.
// show feedback
var feedback_text = "";
if (correct) {
feedback_text = trial.correct_text.replace("%ANS%", trial.text_answer);
} else {
feedback_text = trial.incorrect_text.replace("%ANS%", trial.text_answer);
}
if (trial.render_on_canvas) {
if (trial.prompt !== null) {
prompt_div.remove();
}
feedback_div.innerHTML = feedback_text;
} else {
display_element.innerHTML += feedback_text;
}
// set timeout to clear feedback
if (!timeoutSet) {
timeoutSet = true;
jsPsych.pluginAPI.setTimeout(function() {
endTrial();
}, trial.feedback_duration);
}
}
}, trial.frame_time);
var keyboard_listener;
var trial_data = {};
var after_response = function(info) {
// ignore the response if animation is playing and subject
// not allowed to respond before it is complete
if (!trial.allow_response_before_complete && showAnimation) {
return false;
}
correct = false;
if (trial.key_answer == info.key) {
correct = true;
}
responded = true;
trial_data = {
"stimulus": JSON.stringify(trial.stimuli),
"rt": info.rt,
"correct": correct,
"key_press": info.key
};
jsPsych.pluginAPI.cancelKeyboardResponse(keyboard_listener);
}
keyboard_listener = jsPsych.pluginAPI.getKeyboardResponse({
callback_function: after_response,
valid_responses: trial.choices,
rt_method: 'performance',
persist: true,
allow_held_key: false
});
function endTrial() {
clearInterval(animate_interval); // stop animation!
display_element.innerHTML = ''; // clear everything
jsPsych.finishTrial(trial_data);
}
};
return plugin;
})();
| wolfgangwalther/jsPsych | plugins/jspsych-categorize-animation.js | JavaScript | mit | 8,599 |
<?php
/*
* This file is part of the Pho package.
*
* (c) Emre Sokullu <emre@phonetworks.org>
*
* For the full copyright and license information, please view the LICENSE
* file that was distributed with this source code.
*/
namespace Pho\Lib\Graph;
/**
* Holds the relationship between nodes and edges.
*
* EdgeList objects are attached to all Node objects, they are
* created at object initialization. They contain edge objects
* categorized by their direction.
*
* @see ImmutableEdgeList For a list that doesn't accept new values.
*
* @author Emre Sokullu <emre@phonetworks.org>
*/
class EdgeList
{
/**
* The node thay this edgelist belongs to
*
* @var NodeInterface
*/
private $master;
/**
* An internal pointer of outgoing nodes in [ID=>EncapsulatedEdge] format
* where ID belongs to the edge.
*
* @var array
*/
private $out = [];
/**
* An internal pointer of incoming nodes in [ID=>EncapsulatedEdge] format
* where ID belongs to the edge
*
* @var array
*/
private $in = [];
/**
* An internal pointer of incoming nodes in [ID=>[ID=>EncapsulatedEdge]] format
* where first ID belongs to the node, and second to the edge.
*
* @var array
*/
private $from = [];
/**
* An internal pointer of outgoing nodes in [ID=>[ID=>EncapsulatedEdge]] format
* where first ID belongs to the node, and second to the edge.
*
* @var array
*/
private $to = [];
/**
* Constructor
*
* For performance reasons, the constructor doesn't load the seed data
* (if available) but waits for a method to attempt to access.
*
* @param NodeInterface $node The master, the owner of this EdgeList object.
* @param array $data Initial data to seed.
*/
public function __construct(NodeInterface $node, array $data = [])
{
$this->master = $node;
$this->import($data);
}
public function delete(ID $id): void
{
$id = (string) $id;
foreach($this->from as $key=>$val) {
unset($this->from[$key][$id]);
}
foreach($this->to as $key=>$val) {
unset($this->to[$key][$id]);
}
unset($this->in[$id]);
unset($this->out[$id]);
$this->master->emit("modified");
}
/**
* Imports data from given array source
*
* @param array $data Data source.
*
* @return void
*/
public function import(array $data): void
{
if(!$this->isDataSetProperly($data)) {
return;
}
$wakeup = function (array $array): EncapsulatedEdge {
return EncapsulatedEdge::fromArray($array);
};
$this->out = array_map($wakeup, $data["out"]);
$this->in = array_map($wakeup, $data["in"]);
foreach($data["from"] as $from => $frozen) {
$this->from[$from] = array_map($wakeup, $frozen);
}
foreach($data["to"] as $to => $frozen) {
$this->to[$to] = array_map($wakeup, $frozen);
}
}
/**
* Checks if the data source for import is valid.
*
* @param array $data
*
* @return bool
*/
private function isDataSetProperly(array $data): bool
{
return (isset($data["in"]) && isset($data["out"]) && isset($data["from"]) && isset($data["to"]));
}
/**
* Retrieves this object in array format
*
* With all "in" and "out" values in simple string format.
* The "to" array can be reconstructed.
*
* @return array
*/
public function toArray(): array
{
$to_array = function (EncapsulatedEdge $encapsulated): array {
return $encapsulated->toArray();
};
$array = [];
$array["to"] = [];
foreach($this->to as $to => $encapsulated) {
$array["to"][$to] = array_map($to_array, $encapsulated);
}
$array["from"] = [];
foreach($this->from as $from => $encapsulated) {
$array["from"][$from] = array_map($to_array, $encapsulated);
}
$array["in"] = array_map($to_array, $this->in);
$array["out"] = array_map($to_array, $this->out);
return $array;
}
/**
* Adds an incoming edge to the list.
*
* The edge must be already initialized.
*
* @param EdgeInterface $edge
*
* @return void
*/
public function addIncoming(EdgeInterface $edge): void
{
$edge_encapsulated = EncapsulatedEdge::fromEdge($edge);
$this->from[(string) $edge->tail()->id()][(string) $edge->id()] = $edge_encapsulated;
$this->in[(string) $edge->id()] = $edge_encapsulated;
$this->master->emit("modified");
}
/**
* Adds an outgoing edge to the list.
*
* The edge must be already initialized.
*
* @param EdgeInterface $edge
*
* @return void
*/
public function addOutgoing(EdgeInterface $edge): void
{
$edge_encapsulated = EncapsulatedEdge::fromEdge($edge);
$this->to[(string) $edge->head()->id()][(string) $edge->id()] = $edge_encapsulated;
$this->out[(string) $edge->id()] = $edge_encapsulated;
$this->master->emit("modified");
}
/**
* Returns a list of all the edges directed towards
* this particular node.
*
* @see retrieve Used by this method to fetch objects.
*
* @param string $class The type of edge (defined in edge class) to return
*
* @return \ArrayIterator An array of EdgeInterface objects.
*/
public function in(string $class=""): \ArrayIterator
{
return $this->retrieve(Direction::in(), $class);
}
/**
* Returns a list of all the edges originating from
* this particular node.
*
* @see retrieve Used by this method to fetch objects.
*
* @param string $class The type of edge (defined in edge class) to return
*
* @return \ArrayIterator An array of EdgeInterface objects.
*/
public function out(string $class=""): \ArrayIterator
{
return $this->retrieve(Direction::out(), $class);
}
/**
* A helper method to retrieve edges.
*
* @see out A method that uses this function
* @see in A method that uses this function
*
* @param Direction $direction Lets you choose to fetch incoming or outgoing edges.
* @param string $class The type of edge (defined in edge class) to return
*
* @return \ArrayIterator An array of EdgeInterface objects.
*/
protected function retrieve(Direction $direction, string $class): \ArrayIterator
{
$d = (string) $direction;
$hydrate = function (EncapsulatedEdge $encapsulated): EdgeInterface {
if(!$encapsulated->hydrated())
return $this->master->edge($encapsulated->id());
return $encapsulated->edge();
};
$filter_classes = function (EncapsulatedEdge $encapsulated) use ($class): bool {
return in_array($class, $encapsulated->classes());
};
if(empty($class)) {
return new \ArrayIterator(
array_map($hydrate, $this->$d)
);
}
return new \ArrayIterator(
array_map($hydrate,
array_filter($this->$d, $filter_classes)
)
);
}
/**
* Returns a list of all the edges (both in and out) pertaining to
* this particular node.
*
* @param string $class The type of edge (defined in edge class) to return
*
* @return \ArrayIterator An array of EdgeInterface objects.
*/
public function all(string $class=""): \ArrayIterator
{
return new \ArrayIterator(
array_merge(
$this->in($class)->getArrayCopy(),
$this->out($class)->getArrayCopy()
)
);
}
/**
* Retrieves a list of edges from the list's owner node to the given
* target node.
*
* @param ID $node_id Target (head) node.
* @param string $class The type of edge (defined in edge class) to return
*
* @return \ArrayIterator An array of edge objects to. Returns an empty array if there is no such connections.
*/
public function to(ID $node_id, string $class=""): \ArrayIterator
{
return $this->retrieveDirected(Direction::out(), $node_id, $class);
}
/**
* Retrieves a list of edges to the list's owner node from the given
* source node.
*
* @param ID $node_id Source (tail) node.
* @param string $class The type of edge (defined in edge class) to return
*
* @return \ArrayIterator An array of edge objects from. Returns an empty array if there is no such connections.
*/
public function from(ID $node_id, string $class=""): \ArrayIterator
{
return $this->retrieveDirected(Direction::in(), $node_id, $class);
}
/**
* Retrieves a list of edges between the list's owner node and the given
* node.
*
* @param ID $node_id The other node.
* @param string $class The type of edge (defined in edge class) to return
*
* @return \ArrayIterator An array of edge objects in between. Returns an empty array if there is no such connections.
*/
public function between(ID $node_id, string $class=""): \ArrayIterator
{
return new \ArrayIterator(
array_merge(
$this->from($node_id, $class)->getArrayCopy(),
$this->to($node_id, $class)->getArrayCopy()
)
);
}
/**
* A helper method to retrieve directed edges.
*
* @see from A method that uses this function
* @see to A method that uses this function
*
* @param Direction $direction Lets you choose to fetch incoming or outgoing edges.
* @param ID $node_id Directed towards which node.
* @param string $class The type of edge (defined in edge class) to return.
*
* @return \ArrayIterator An array of EdgeInterface objects.
*/
protected function retrieveDirected(Direction $direction, ID $node_id, string $class): \ArrayIterator
{
$key = $direction->equals(Direction::in()) ? "from" : "to";
$direction = (string) $direction;
$hydrate = function (EncapsulatedEdge $encapsulated): EdgeInterface {
if(!$encapsulated->hydrated())
return $this->master->edge($encapsulated->id());
return $encapsulated->edge();
};
$filter_classes = function (EncapsulatedEdge $encapsulated) use ($class): bool {
return in_array($class, $encapsulated->classes());
};
if(!isset($this->$key[(string) $node_id])) {
return new \ArrayIterator();
}
if(empty($class)) {
return new \ArrayIterator(
array_map($hydrate, $this->$key[(string) $node_id])
);
}
return new \ArrayIterator(
array_map($hydrate, array_filter($this->$key[(string) $node_id], $filter_classes))
);
}
} | phonetworks/pho-lib-graph | src/Pho/Lib/Graph/EdgeList.php | PHP | mit | 11,362 |
package me.august.lumen.data;
import me.august.lumen.compile.resolve.data.ClassData;
import me.august.lumen.compile.resolve.lookup.DependencyManager;
import org.junit.Assert;
import org.junit.Test;
public class DataTest {
@Test
public void testClassData() {
ClassData data = ClassData.fromClass(String.class);
Assert.assertEquals(
String.class.getName(),
data.getName()
);
String[] expected = new String[]{
"java.io.Serializable", "java.lang.Comparable",
"java.lang.CharSequence"
};
Assert.assertArrayEquals(
expected,
data.getInterfaces()
);
}
@Test
public void testAssignableTo() {
DependencyManager deps = new DependencyManager();
ClassData data;
data = ClassData.fromClass(String.class);
Assert.assertTrue(
"Expected String to be assignable to String",
data.isAssignableTo("java.lang.String", deps)
);
Assert.assertTrue(
"Expected String to be assignable to Object",
data.isAssignableTo("java.lang.Object", deps)
);
Assert.assertTrue(
"Expected String to be assignable to CharSequence",
data.isAssignableTo("java.lang.CharSequence", deps)
);
data = ClassData.fromClass(Object.class);
Assert.assertFalse(
"Expected Object to not be assignable to String",
data.isAssignableTo("java.lang.String", deps)
);
data = ClassData.fromClass(CharSequence.class);
Assert.assertTrue(
"Expected CharSequence to be assignable to Object",
data.isAssignableTo("java.lang.Object", deps)
);
}
}
| augustt198/lumen | src/test/java/me/august/lumen/data/DataTest.java | Java | mit | 1,850 |
<?php
/**
* CodeBin
*
* @author CRH380A-2722 <609657831@qq.com>
* @copyright 2016 CRH380A-2722
* @license MIT
*/
/** 配置文件 */
/** 站点域名 */
define("SITEDOMAIN", "{SITE-DOMAIN}");
/** 数据库信息 */
//数据库服务器
define("DB_HOST", "{DB-HOST}");
//数据库用户名
define("DB_USER", "{DB-USER}");
//数据库密码
define("DB_PASSWORD", "{DB-PASS}");
//数据库名
define("DB_NAME", "{DB-NAME}");
//数据表前缀
define("DB_PREFIX", "{DB-PREFIX}");
//Flag: 开发者模式?(1=ON, 0=OFF)
$devMode = 0;
/**
* Gravatar镜像地址
*
* @var string $link
* @note 此处填上你的Gravatar镜像地址(不要带http://或https://)如:gravatar.duoshuo.com
* @note HTTP可用gravatar.duoshuo.com, HTTPS建议secure.gravatar.com
* @note 或者你自己设立一个镜像存储也行
* @note 一般对墙内用户来说比较有用
*/
$link = "{GRAVATAR-LINK}";
/** 好了,以下内容不要再编辑了!!*/
if (!$devMode) error_reporting(0);
define("BASEROOT", dirname(__FILE__));
define("SESSIONNAME", "CodeClipboard");
require BASEROOT . "/includes/functions.php";
session_name(SESSIONNAME);
| CRH380A-2722/Code-Clipboard | config-sample.php | PHP | mit | 1,219 |
<?php
/**
* @package Response
* @subpackage Header
*
* @author Wojtek Zalewski <wojtek@neverbland.com>
*
* @copyright Copyright (c) 2013, Wojtek Zalewski
* @license MIT
*/
namespace Wtk\Response\Header\Field;
use Wtk\Response\Header\Field\AbstractField;
use Wtk\Response\Header\Field\FieldInterface;
/**
* Basic implementation - simple Value Object for Header elements
*
* @author Wojtek Zalewski <wojtek@neverbland.com>
*/
class Field extends AbstractField implements FieldInterface
{
/**
* Field name
*
* @var string
*/
protected $name;
/**
* Field value
*
* @var mixed
*/
protected $value;
/**
*
* @param string $name
* @param mixed $value
*/
public function __construct($name, $value)
{
$this->name = $name;
/**
* @todo: is is object, than it has to
* implement toString method
* We have to check for it now.
*/
$this->value = $value;
}
/**
* Returns field name
*
* @return string
*/
public function getName()
{
return $this->name;
}
/**
* Returns header field value
*
* @return string
*/
public function getValue()
{
return $this->value;
}
}
| wotek/response-builder | src/Wtk/Response/Header/Field.php | PHP | mit | 1,319 |
---
layout: post
author: Najko Jahn
title: Max Planck Digital Library updates expenditures for articles published in the New Journal of Physics
date: 2015-12-23 15:21:29
summary:
categories: general
comments: true
---
[Max Planck Digital Library (MPDL)](https://www.mpdl.mpg.de/en/) provides [institutional funding for Open Access publications](https://www.mpdl.mpg.de/en/?id=50:open-access-publishing&catid=17:open-access) for researchers affiliated with the [Max Planck Society](http://www.mpg.de/en). MPDL makes use of central agreements with Open Access publishers. It has now updated its expenditures for Open Access articles in the [New Journal of Physics](http://iopscience.iop.org/1367-2630).
Please note that article processing charges funded locally by Max Planck Institutes are not part of this data set. The Max Planck Society has a limited input tax reduction. The refund of input VAT for APC is 20%.
## Cost data
The data set covers publication fees for 522 articles published in the New Journal of Physics by MPG researchers, which MPDL covered from 2006 until 2014. Total expenditure amounts to 497 229€ and the average fee is 953€.
### Average costs per year (in EURO)

| OpenAPC/openapc.github.io | _posts/2015-12-23-mpdl.md | Markdown | mit | 1,296 |
'use strict';
var app = angular.module('Fablab');
app.controller('GlobalPurchaseEditController', function ($scope, $location, $filter, $window,
PurchaseService, NotificationService, StaticDataService, SupplyService) {
$scope.selected = {purchase: undefined};
$scope.currency = App.CONFIG.CURRENCY;
$scope.loadPurchase = function (id) {
PurchaseService.get(id, function (data) {
$scope.purchase = data;
});
};
$scope.save = function () {
var purchaseCurrent = angular.copy($scope.purchase);
updateStock();
PurchaseService.save(purchaseCurrent, function (data) {
$scope.purchase = data;
NotificationService.notify("success", "purchase.notification.saved");
$location.path("purchases");
});
};
var updateStock = function () {
var stockInit = $scope.purchase.supply.quantityStock;
$scope.purchase.supply.quantityStock = parseFloat(stockInit) - parseFloat($scope.purchase.quantity);
var supplyCurrent = angular.copy($scope.purchase.supply);
SupplyService.save(supplyCurrent, function (data) {
$scope.purchase.supply = data;
});
};
$scope.maxMoney = function () {
return parseFloat($scope.purchase.quantity) * parseFloat($scope.purchase.supply.sellingPrice);
};
$scope.updatePrice = function () {
var interTotal = parseFloat($scope.purchase.quantity) * parseFloat($scope.purchase.supply.sellingPrice);
if ($scope.purchase.discount === undefined || !$scope.purchase.discount) {
//0.05 cts ceil
var val = $window.Math.ceil(interTotal * 20) / 20;
$scope.purchase.purchasePrice = $filter('number')(val, 2);
;
} else {
if ($scope.purchase.discountPercent) {
var discountInter = parseFloat(interTotal) * (parseFloat($scope.purchase.discount) / parseFloat(100));
var total = parseFloat(interTotal) - parseFloat(discountInter);
//0.05 cts ceil
var val = $window.Math.ceil(total * 20) / 20;
$scope.purchase.purchasePrice = $filter('number')(val, 2);
} else {
var total = parseFloat(interTotal) - parseFloat($scope.purchase.discount);
//0.05 cts ceil
var val = $window.Math.ceil(total * 20) / 20;
$scope.purchase.purchasePrice = $filter('number')(val, 2);
}
}
};
$scope.firstPercent = App.CONFIG.FIRST_PERCENT.toUpperCase() === "PERCENT";
$scope.optionsPercent = [{
name: "%",
value: true
}, {
name: App.CONFIG.CURRENCY,
value: false
}];
$scope.today = function () {
$scope.dt = new Date();
};
$scope.today();
$scope.clear = function () {
$scope.dt = null;
};
$scope.open = function ($event) {
$event.preventDefault();
$event.stopPropagation();
$scope.opened = true;
};
$scope.dateOptions = {
formatYear: 'yy',
startingDay: 1
};
$scope.formats = ['dd-MMMM-yyyy', 'yyyy/MM/dd', 'dd.MM.yyyy', 'shortDate'];
$scope.format = $scope.formats[2];
var tomorrow = new Date();
tomorrow.setDate(tomorrow.getDate() + 1);
var afterTomorrow = new Date();
afterTomorrow.setDate(tomorrow.getDate() + 2);
$scope.events =
[
{
date: tomorrow,
status: 'full'
},
{
date: afterTomorrow,
status: 'partially'
}
];
$scope.getDayClass = function (date, mode) {
if (mode === 'day') {
var dayToCheck = new Date(date).setHours(0, 0, 0, 0);
for (var i = 0; i < $scope.events.length; i++) {
var currentDay = new Date($scope.events[i].date).setHours(0, 0, 0, 0);
if (dayToCheck === currentDay) {
return $scope.events[i].status;
}
}
}
return '';
};
StaticDataService.loadSupplyStock(function (data) {
$scope.supplyStock = data;
});
StaticDataService.loadCashiers(function (data) {
$scope.cashierList = data;
});
}
);
app.controller('PurchaseNewController', function ($scope, $controller, $rootScope) {
$controller('GlobalPurchaseEditController', {$scope: $scope});
$scope.newPurchase = true;
$scope.paidDirectly = false;
$scope.purchase = {
purchaseDate: new Date(),
user: $rootScope.connectedUser.user
};
}
);
app.controller('PurchaseEditController', function ($scope, $routeParams, $controller) {
$controller('GlobalPurchaseEditController', {$scope: $scope});
$scope.newPurchase = false;
$scope.loadPurchase($routeParams.id);
}
);
| fabienvuilleumier/fb | src/main/webapp/components/purchase/purchase-edit-ctrl.js | JavaScript | mit | 4,952 |
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package ons;
import ons.util.WeightedGraph;
import org.w3c.dom.*;
/**
* The physical topology of a network refers to he physical layout of devices on
* a network, or to the way that the devices on a network are arranged and how
* they communicate with each other.
*
* @author andred
*/
public abstract class PhysicalTopology {
protected int nodes;
protected int links;
protected OXC[] nodeVector;
protected Link[] linkVector;
protected Link[][] adjMatrix;
/**
* Creates a new PhysicalTopology object. Takes the XML file containing all
* the information about the simulation environment and uses it to populate
* the PhysicalTopology object. The physical topology is basically composed
* of nodes connected by links, each supporting different wavelengths.
*
* @param xml file that contains the simulation environment information
*/
public PhysicalTopology(Element xml) {
try {
if (Simulator.verbose) {
System.out.println(xml.getAttribute("name"));
}
} catch (Throwable t) {
t.printStackTrace();
}
}
/**
* Retrieves the number of nodes in a given PhysicalTopology.
*
* @return the value of the PhysicalTopology's nodes attribute
*/
public int getNumNodes() {
return nodes;
}
/**
* Retrieves the number of links in a given PhysicalTopology.
*
* @return number of items in the PhysicalTopology's linkVector attribute
*/
public int getNumLinks() {
return linkVector.length;
}
/**
* Retrieves a specific node in the PhysicalTopology object.
*
* @param id the node's unique identifier
* @return specified node from the PhysicalTopology's nodeVector
*/
public OXC getNode(int id) {
return nodeVector[id];
}
/**
* Retrieves a specific link in the PhysicalTopology object, based on its
* unique identifier.
*
* @param linkid the link's unique identifier
* @return specified link from the PhysicalTopology's linkVector
*/
public Link getLink(int linkid) {
return linkVector[linkid];
}
/**
* Retrieves a specific link in the PhysicalTopology object, based on its
* source and destination nodes.
*
* @param src the link's source node
* @param dst the link's destination node
* @return the specified link from the PhysicalTopology's adjMatrix
*/
public Link getLink(int src, int dst) {
return adjMatrix[src][dst];
}
/**
* Retrives a given PhysicalTopology's adjancency matrix, which contains the
* links between source and destination nodes.
*
* @return the PhysicalTopology's adjMatrix
*/
public Link[][] getAdjMatrix() {
return adjMatrix;
}
/**
* Says whether exists or not a link between two given nodes.
*
* @param node1 possible link's source node
* @param node2 possible link's destination node
* @return true if the link exists in the PhysicalTopology's adjMatrix
*/
public boolean hasLink(int node1, int node2) {
if (adjMatrix[node1][node2] != null) {
return true;
} else {
return false;
}
}
/**
* Checks if a path made of links makes sense by checking its continuity
*
* @param links to be checked
* @return true if the link exists in the PhysicalTopology's adjMatrix
*/
public boolean checkLinkPath(int links[]) {
for (int i = 0; i < links.length - 1; i++) {
if (!(getLink(links[i]).dst == getLink(links[i + 1]).src)) {
return false;
}
}
return true;
}
/**
* Returns a weighted graph with vertices, edges and weights representing
* the physical network nodes, links and weights implemented by this class
* object.
*
* @return an WeightedGraph class object
*/
public WeightedGraph getWeightedGraph() {
WeightedGraph g = new WeightedGraph(nodes);
for (int i = 0; i < nodes; i++) {
for (int j = 0; j < nodes; j++) {
if (hasLink(i, j)) {
g.addEdge(i, j, getLink(i, j).getWeight());
}
}
}
return g;
}
/**
*
*
*/
public void printXpressInputFile() {
// Edges
System.out.println("EDGES: [");
for (int i = 0; i < this.getNumNodes(); i++) {
for (int j = 0; j < this.getNumNodes(); j++) {
if (this.hasLink(i, j)) {
System.out.println("(" + Integer.toString(i + 1) + " " + Integer.toString(j + 1) + ") 1");
} else {
System.out.println("(" + Integer.toString(i + 1) + " " + Integer.toString(j + 1) + ") 0");
}
}
}
System.out.println("]");
System.out.println();
// SD Pairs
System.out.println("TRAFFIC: [");
for (int i = 0; i < this.getNumNodes(); i++) {
for (int j = 0; j < this.getNumNodes(); j++) {
if (i != j) {
System.out.println("(" + Integer.toString(i + 1) + " " + Integer.toString(j + 1) + ") 1");
} else {
System.out.println("(" + Integer.toString(i + 1) + " " + Integer.toString(j + 1) + ") 0");
}
}
}
System.out.println("]");
}
/**
* Prints all nodes and links between them in the PhysicalTopology object.
*
* @return string containing the PhysicalTopology's adjMatrix values
*/
@Override
public String toString() {
String topo = "";
for (int i = 0; i < nodes; i++) {
for (int j = 0; j < nodes; j++) {
if (adjMatrix[i][j] != null) {
topo += adjMatrix[i][j].toString() + "\n\n";
}
}
}
return topo;
}
public abstract void createPhysicalLightpath(LightPath lp);
public abstract void removePhysicalLightpath(LightPath lp);
public abstract boolean canCreatePhysicalLightpath(LightPath lp);
public abstract double getBW(LightPath lp);
public abstract double getBWAvailable(LightPath lp);
public abstract boolean canAddFlow(Flow flow, LightPath lightpath);
public abstract void addFlow(Flow flow, LightPath lightpath);
public abstract void addBulkData(BulkData bulkData, LightPath lightpath);
public abstract void removeFlow(Flow flow, LightPath lightpath);
public abstract boolean canAddBulkData(BulkData bulkData, LightPath lightpath);
}
| leiasousa/MySim | src/ons/PhysicalTopology.java | Java | mit | 6,858 |
require File.join [Pluct.root, 'lib/pluct/extensions/json']
describe JSON do
it 'returns false for invalid data' do
['', ' ', 'string'].each do |s|
expect(JSON.is_json?(s)).to be_false
end
end
it 'returns true for valid data' do
expect(JSON.is_json?('{"name" : "foo"}')).to be_true
end
end | pombredanne/pluct-ruby | spec/pluct/extensions/json_spec.rb | Ruby | mit | 319 |
module VhdlTestScript
class Wait
CLOCK_LENGTH = 2
def initialize(length)
@length = length
end
def to_vhdl
"wait for #{@length * CLOCK_LENGTH} ns;"
end
def in(ports)
self
end
def origin
self
end
end
end
| tomoasleep/vhdl_test_script | lib/vhdl_test_script/wait.rb | Ruby | mit | 271 |
---
version: v1.4.13
category: API
redirect_from:
- /docs/v0.37.8/api/global-shortcut
- /docs/v0.37.7/api/global-shortcut
- /docs/v0.37.6/api/global-shortcut
- /docs/v0.37.5/api/global-shortcut
- /docs/v0.37.4/api/global-shortcut
- /docs/v0.37.3/api/global-shortcut
- /docs/v0.36.12/api/global-shortcut
- /docs/v0.37.1/api/global-shortcut
- /docs/v0.37.0/api/global-shortcut
- /docs/v0.36.11/api/global-shortcut
- /docs/v0.36.10/api/global-shortcut
- /docs/v0.36.9/api/global-shortcut
- /docs/v0.36.8/api/global-shortcut
- /docs/v0.36.7/api/global-shortcut
- /docs/v0.36.6/api/global-shortcut
- /docs/v0.36.5/api/global-shortcut
- /docs/v0.36.4/api/global-shortcut
- /docs/v0.36.3/api/global-shortcut
- /docs/v0.35.5/api/global-shortcut
- /docs/v0.36.2/api/global-shortcut
- /docs/v0.36.0/api/global-shortcut
- /docs/v0.35.4/api/global-shortcut
- /docs/v0.35.3/api/global-shortcut
- /docs/v0.35.2/api/global-shortcut
- /docs/v0.34.4/api/global-shortcut
- /docs/v0.35.1/api/global-shortcut
- /docs/v0.34.3/api/global-shortcut
- /docs/v0.34.2/api/global-shortcut
- /docs/v0.34.1/api/global-shortcut
- /docs/v0.34.0/api/global-shortcut
- /docs/v0.33.9/api/global-shortcut
- /docs/v0.33.8/api/global-shortcut
- /docs/v0.33.7/api/global-shortcut
- /docs/v0.33.6/api/global-shortcut
- /docs/v0.33.4/api/global-shortcut
- /docs/v0.33.3/api/global-shortcut
- /docs/v0.33.2/api/global-shortcut
- /docs/v0.33.1/api/global-shortcut
- /docs/v0.33.0/api/global-shortcut
- /docs/v0.32.3/api/global-shortcut
- /docs/v0.32.2/api/global-shortcut
- /docs/v0.31.2/api/global-shortcut
- /docs/v0.31.0/api/global-shortcut
- /docs/v0.30.4/api/global-shortcut
- /docs/v0.29.2/api/global-shortcut
- /docs/v0.29.1/api/global-shortcut
- /docs/v0.28.3/api/global-shortcut
- /docs/v0.28.2/api/global-shortcut
- /docs/v0.28.1/api/global-shortcut
- /docs/v0.28.0/api/global-shortcut
- /docs/v0.27.3/api/global-shortcut
- /docs/v0.27.2/api/global-shortcut
- /docs/v0.27.1/api/global-shortcut
- /docs/v0.27.0/api/global-shortcut
- /docs/v0.26.1/api/global-shortcut
- /docs/v0.26.0/api/global-shortcut
- /docs/v0.25.3/api/global-shortcut
- /docs/v0.25.2/api/global-shortcut
- /docs/v0.25.1/api/global-shortcut
- /docs/v0.25.0/api/global-shortcut
- /docs/v0.24.0/api/global-shortcut
- /docs/v0.23.0/api/global-shortcut
- /docs/v0.22.3/api/global-shortcut
- /docs/v0.22.2/api/global-shortcut
- /docs/v0.22.1/api/global-shortcut
- /docs/v0.21.3/api/global-shortcut
- /docs/v0.21.2/api/global-shortcut
- /docs/v0.21.1/api/global-shortcut
- /docs/v0.21.0/api/global-shortcut
- /docs/v0.20.8/api/global-shortcut
- /docs/v0.20.7/api/global-shortcut
- /docs/v0.20.6/api/global-shortcut
- /docs/v0.20.5/api/global-shortcut
- /docs/v0.20.4/api/global-shortcut
- /docs/v0.20.3/api/global-shortcut
- /docs/v0.20.2/api/global-shortcut
- /docs/v0.20.1/api/global-shortcut
- /docs/v0.20.0/api/global-shortcut
- /docs/vlatest/api/global-shortcut
source_url: 'https://github.com/electron/electron/blob/master/docs/api/global-shortcut.md'
title: globalShortcut
excerpt: Detect keyboard events when the application does not have keyboard focus.
sort_title: global-shortcut
---
# globalShortcut
> Detect keyboard events when the application does not have keyboard focus.
Process: [Main]({{site.baseurl}}/docs/tutorial/quick-start#main-process)
The `globalShortcut` module can register/unregister a global keyboard shortcut with the operating system so that you can customize the operations for various shortcuts.
**Note:** The shortcut is global; it will work even if the app does not have the keyboard focus. You should not use this module until the `ready` event of the app module is emitted.
```javascript
const {app, globalShortcut} = require('electron')
app.on('ready', () => {
// Register a 'CommandOrControl+X' shortcut listener.
const ret = globalShortcut.register('CommandOrControl+X', () => {
console.log('CommandOrControl+X is pressed')
})
if (!ret) {
console.log('registration failed')
}
// Check whether a shortcut is registered.
console.log(globalShortcut.isRegistered('CommandOrControl+X'))
})
app.on('will-quit', () => {
// Unregister a shortcut.
globalShortcut.unregister('CommandOrControl+X')
// Unregister all shortcuts.
globalShortcut.unregisterAll()
})
```
## Methods
The `globalShortcut` module has the following methods:
### `globalShortcut.register(accelerator, callback)`
* `accelerator` [Accelerator]({{site.baseurl}}/docs/api/accelerator)
* `callback` Function
Registers a global shortcut of `accelerator`. The `callback` is called when the registered shortcut is pressed by the user.
When the accelerator is already taken by other applications, this call will silently fail. This behavior is intended by operating systems, since they don't want applications to fight for global shortcuts.
### `globalShortcut.isRegistered(accelerator)`
* `accelerator` [Accelerator]({{site.baseurl}}/docs/api/accelerator)
Returns `Boolean` - Whether this application has registered `accelerator`.
When the accelerator is already taken by other applications, this call will still return `false`. This behavior is intended by operating systems, since they don't want applications to fight for global shortcuts.
### `globalShortcut.unregister(accelerator)`
* `accelerator` [Accelerator]({{site.baseurl}}/docs/api/accelerator)
Unregisters the global shortcut of `accelerator`.
### `globalShortcut.unregisterAll()`
Unregisters all of the global shortcuts.
| CONVIEUFO/convieufo.github.io | _docs/api/global-shortcut.md | Markdown | mit | 5,630 |
---
uid: SolidEdgeFrameworkSupport.BSplineCurve2d.GetParameterRange(System.Double@,System.Double@)
summary:
remarks:
syntax:
parameters:
- id: End
description: Specifies the end parameter of the curve.
- id: Start
description: Specifies the start parameter of the curve.
---
| SolidEdgeCommunity/docs | docfx_project/apidoc/SolidEdgeFrameworkSupport.BSplineCurve2d.GetParameterRange.md | Markdown | mit | 304 |
// Copyright 2015 The Chromium Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
#ifndef BASE_TRACE_EVENT_MEMORY_DUMP_MANAGER_H_
#define BASE_TRACE_EVENT_MEMORY_DUMP_MANAGER_H_
#include <stdint.h>
#include <map>
#include <memory>
#include <set>
#include <vector>
#include "base/atomicops.h"
#include "base/containers/hash_tables.h"
#include "base/macros.h"
#include "base/memory/ref_counted.h"
#include "base/memory/singleton.h"
#include "base/synchronization/lock.h"
#include "base/timer/timer.h"
#include "base/trace_event/memory_dump_request_args.h"
#include "base/trace_event/process_memory_dump.h"
#include "base/trace_event/trace_event.h"
namespace base {
class SingleThreadTaskRunner;
class Thread;
namespace trace_event {
class MemoryDumpManagerDelegate;
class MemoryDumpProvider;
class MemoryDumpSessionState;
// This is the interface exposed to the rest of the codebase to deal with
// memory tracing. The main entry point for clients is represented by
// RequestDumpPoint(). The extension by Un(RegisterDumpProvider).
class BASE_EXPORT MemoryDumpManager : public TraceLog::EnabledStateObserver {
public:
static const char* const kTraceCategory;
// This value is returned as the tracing id of the child processes by
// GetTracingProcessId() when tracing is not enabled.
static const uint64_t kInvalidTracingProcessId;
static MemoryDumpManager* GetInstance();
// Invoked once per process to listen to trace begin / end events.
// Initialization can happen after (Un)RegisterMemoryDumpProvider() calls
// and the MemoryDumpManager guarantees to support this.
// On the other side, the MemoryDumpManager will not be fully operational
// (i.e. will NACK any RequestGlobalMemoryDump()) until initialized.
// Arguments:
// is_coordinator: if true this MemoryDumpManager instance will act as a
// coordinator and schedule periodic dumps (if enabled via TraceConfig);
// false when the MemoryDumpManager is initialized in a slave process.
// delegate: inversion-of-control interface for embedder-specific behaviors
// (multiprocess handshaking). See the lifetime and thread-safety
// requirements in the |MemoryDumpManagerDelegate| docstring.
void Initialize(MemoryDumpManagerDelegate* delegate, bool is_coordinator);
// (Un)Registers a MemoryDumpProvider instance.
// Args:
// - mdp: the MemoryDumpProvider instance to be registered. MemoryDumpManager
// does NOT take memory ownership of |mdp|, which is expected to either
// be a singleton or unregister itself.
// - name: a friendly name (duplicates allowed). Used for debugging and
// run-time profiling of memory-infra internals. Must be a long-lived
// C string.
// - task_runner: either a SingleThreadTaskRunner or SequencedTaskRunner. All
// the calls to |mdp| will be run on the given |task_runner|. If passed
// null |mdp| should be able to handle calls on arbitrary threads.
// - options: extra optional arguments. See memory_dump_provider.h.
void RegisterDumpProvider(MemoryDumpProvider* mdp,
const char* name,
scoped_refptr<SingleThreadTaskRunner> task_runner);
void RegisterDumpProvider(MemoryDumpProvider* mdp,
const char* name,
scoped_refptr<SingleThreadTaskRunner> task_runner,
MemoryDumpProvider::Options options);
void RegisterDumpProviderWithSequencedTaskRunner(
MemoryDumpProvider* mdp,
const char* name,
scoped_refptr<SequencedTaskRunner> task_runner,
MemoryDumpProvider::Options options);
void UnregisterDumpProvider(MemoryDumpProvider* mdp);
// Unregisters an unbound dump provider and takes care about its deletion
// asynchronously. Can be used only for for dump providers with no
// task-runner affinity.
// This method takes ownership of the dump provider and guarantees that:
// - The |mdp| will be deleted at some point in the near future.
// - Its deletion will not happen concurrently with the OnMemoryDump() call.
// Note that OnMemoryDump() calls can still happen after this method returns.
void UnregisterAndDeleteDumpProviderSoon(scoped_ptr<MemoryDumpProvider> mdp);
// Requests a memory dump. The dump might happen or not depending on the
// filters and categories specified when enabling tracing.
// The optional |callback| is executed asynchronously, on an arbitrary thread,
// to notify about the completion of the global dump (i.e. after all the
// processes have dumped) and its success (true iff all the dumps were
// successful).
void RequestGlobalDump(MemoryDumpType dump_type,
MemoryDumpLevelOfDetail level_of_detail,
const MemoryDumpCallback& callback);
// Same as above (still asynchronous), but without callback.
void RequestGlobalDump(MemoryDumpType dump_type,
MemoryDumpLevelOfDetail level_of_detail);
// TraceLog::EnabledStateObserver implementation.
void OnTraceLogEnabled() override;
void OnTraceLogDisabled() override;
// Returns the MemoryDumpSessionState object, which is shared by all the
// ProcessMemoryDump and MemoryAllocatorDump instances through all the tracing
// session lifetime.
const scoped_refptr<MemoryDumpSessionState>& session_state() const {
return session_state_;
}
// Returns a unique id for identifying the processes. The id can be
// retrieved by child processes only when tracing is enabled. This is
// intended to express cross-process sharing of memory dumps on the
// child-process side, without having to know its own child process id.
uint64_t GetTracingProcessId() const;
// Returns the name for a the allocated_objects dump. Use this to declare
// suballocator dumps from other dump providers.
// It will return nullptr if there is no dump provider for the system
// allocator registered (which is currently the case for Mac OS).
const char* system_allocator_pool_name() const {
return kSystemAllocatorPoolName;
};
// When set to true, calling |RegisterMemoryDumpProvider| is a no-op.
void set_dumper_registrations_ignored_for_testing(bool ignored) {
dumper_registrations_ignored_for_testing_ = ignored;
}
private:
friend std::default_delete<MemoryDumpManager>; // For the testing instance.
friend struct DefaultSingletonTraits<MemoryDumpManager>;
friend class MemoryDumpManagerDelegate;
friend class MemoryDumpManagerTest;
// Descriptor used to hold information about registered MDPs.
// Some important considerations about lifetime of this object:
// - In nominal conditions, all the MemoryDumpProviderInfo instances live in
// the |dump_providers_| collection (% unregistration while dumping).
// - Upon each dump they (actually their scoped_refptr-s) are copied into
// the ProcessMemoryDumpAsyncState. This is to allow removal (see below).
// - When the MDP.OnMemoryDump() is invoked, the corresponding MDPInfo copy
// inside ProcessMemoryDumpAsyncState is removed.
// - In most cases, the MDPInfo is destroyed within UnregisterDumpProvider().
// - If UnregisterDumpProvider() is called while a dump is in progress, the
// MDPInfo is destroyed in SetupNextMemoryDump() or InvokeOnMemoryDump(),
// when the copy inside ProcessMemoryDumpAsyncState is erase()-d.
// - The non-const fields of MemoryDumpProviderInfo are safe to access only
// on tasks running in the |task_runner|, unless the thread has been
// destroyed.
struct MemoryDumpProviderInfo
: public RefCountedThreadSafe<MemoryDumpProviderInfo> {
// Define a total order based on the |task_runner| affinity, so that MDPs
// belonging to the same SequencedTaskRunner are adjacent in the set.
struct Comparator {
bool operator()(const scoped_refptr<MemoryDumpProviderInfo>& a,
const scoped_refptr<MemoryDumpProviderInfo>& b) const;
};
using OrderedSet =
std::set<scoped_refptr<MemoryDumpProviderInfo>, Comparator>;
MemoryDumpProviderInfo(MemoryDumpProvider* dump_provider,
const char* name,
scoped_refptr<SequencedTaskRunner> task_runner,
const MemoryDumpProvider::Options& options);
MemoryDumpProvider* const dump_provider;
// Used to transfer ownership for UnregisterAndDeleteDumpProviderSoon().
// nullptr in all other cases.
scoped_ptr<MemoryDumpProvider> owned_dump_provider;
// Human readable name, for debugging and testing. Not necessarily unique.
const char* const name;
// The task runner affinity. Can be nullptr, in which case the dump provider
// will be invoked on |dump_thread_|.
const scoped_refptr<SequencedTaskRunner> task_runner;
// The |options| arg passed to RegisterDumpProvider().
const MemoryDumpProvider::Options options;
// For fail-safe logic (auto-disable failing MDPs).
int consecutive_failures;
// Flagged either by the auto-disable logic or during unregistration.
bool disabled;
private:
friend class base::RefCountedThreadSafe<MemoryDumpProviderInfo>;
~MemoryDumpProviderInfo();
DISALLOW_COPY_AND_ASSIGN(MemoryDumpProviderInfo);
};
// Holds the state of a process memory dump that needs to be carried over
// across task runners in order to fulfil an asynchronous CreateProcessDump()
// request. At any time exactly one task runner owns a
// ProcessMemoryDumpAsyncState.
struct ProcessMemoryDumpAsyncState {
ProcessMemoryDumpAsyncState(
MemoryDumpRequestArgs req_args,
const MemoryDumpProviderInfo::OrderedSet& dump_providers,
scoped_refptr<MemoryDumpSessionState> session_state,
MemoryDumpCallback callback,
scoped_refptr<SingleThreadTaskRunner> dump_thread_task_runner);
~ProcessMemoryDumpAsyncState();
// Gets or creates the memory dump container for the given target process.
ProcessMemoryDump* GetOrCreateMemoryDumpContainerForProcess(ProcessId pid);
// A map of ProcessId -> ProcessMemoryDump, one for each target process
// being dumped from the current process. Typically each process dumps only
// for itself, unless dump providers specify a different |target_process| in
// MemoryDumpProvider::Options.
std::map<ProcessId, scoped_ptr<ProcessMemoryDump>> process_dumps;
// The arguments passed to the initial CreateProcessDump() request.
const MemoryDumpRequestArgs req_args;
// An ordered sequence of dump providers that have to be invoked to complete
// the dump. This is a copy of |dump_providers_| at the beginning of a dump
// and becomes empty at the end, when all dump providers have been invoked.
std::vector<scoped_refptr<MemoryDumpProviderInfo>> pending_dump_providers;
// The trace-global session state.
scoped_refptr<MemoryDumpSessionState> session_state;
// Callback passed to the initial call to CreateProcessDump().
MemoryDumpCallback callback;
// The |success| field that will be passed as argument to the |callback|.
bool dump_successful;
// The thread on which FinalizeDumpAndAddToTrace() (and hence |callback|)
// should be invoked. This is the thread on which the initial
// CreateProcessDump() request was called.
const scoped_refptr<SingleThreadTaskRunner> callback_task_runner;
// The thread on which unbound dump providers should be invoked.
// This is essentially |dump_thread_|.task_runner() but needs to be kept
// as a separate variable as it needs to be accessed by arbitrary dumpers'
// threads outside of the lock_ to avoid races when disabling tracing.
// It is immutable for all the duration of a tracing session.
const scoped_refptr<SingleThreadTaskRunner> dump_thread_task_runner;
private:
DISALLOW_COPY_AND_ASSIGN(ProcessMemoryDumpAsyncState);
};
static const int kMaxConsecutiveFailuresCount;
static const char* const kSystemAllocatorPoolName;
MemoryDumpManager();
~MemoryDumpManager() override;
static void SetInstanceForTesting(MemoryDumpManager* instance);
static void FinalizeDumpAndAddToTrace(
scoped_ptr<ProcessMemoryDumpAsyncState> pmd_async_state);
// Enable heap profiling if kEnableHeapProfiling is specified.
void EnableHeapProfilingIfNeeded();
// Internal, used only by MemoryDumpManagerDelegate.
// Creates a memory dump for the current process and appends it to the trace.
// |callback| will be invoked asynchronously upon completion on the same
// thread on which CreateProcessDump() was called.
void CreateProcessDump(const MemoryDumpRequestArgs& args,
const MemoryDumpCallback& callback);
// Calls InvokeOnMemoryDump() for the next MDP on the task runner specified by
// the MDP while registration. On failure to do so, skips and continues to
// next MDP.
void SetupNextMemoryDump(
scoped_ptr<ProcessMemoryDumpAsyncState> pmd_async_state);
// Invokes OnMemoryDump() of the next MDP and calls SetupNextMemoryDump() at
// the end to continue the ProcessMemoryDump. Should be called on the MDP task
// runner.
void InvokeOnMemoryDump(ProcessMemoryDumpAsyncState* owned_pmd_async_state);
// Helper for RegierDumpProvider* functions.
void RegisterDumpProviderInternal(
MemoryDumpProvider* mdp,
const char* name,
scoped_refptr<SequencedTaskRunner> task_runner,
const MemoryDumpProvider::Options& options);
// Helper for the public UnregisterDumpProvider* functions.
void UnregisterDumpProviderInternal(MemoryDumpProvider* mdp,
bool take_mdp_ownership_and_delete_async);
// An ordererd set of registered MemoryDumpProviderInfo(s), sorted by task
// runner affinity (MDPs belonging to the same task runners are adjacent).
MemoryDumpProviderInfo::OrderedSet dump_providers_;
// Shared among all the PMDs to keep state scoped to the tracing session.
scoped_refptr<MemoryDumpSessionState> session_state_;
MemoryDumpManagerDelegate* delegate_; // Not owned.
// When true, this instance is in charge of coordinating periodic dumps.
bool is_coordinator_;
// Protects from concurrent accesses to the |dump_providers_*| and |delegate_|
// to guard against disabling logging while dumping on another thread.
Lock lock_;
// Optimization to avoid attempting any memory dump (i.e. to not walk an empty
// dump_providers_enabled_ list) when tracing is not enabled.
subtle::AtomicWord memory_tracing_enabled_;
// For time-triggered periodic dumps.
RepeatingTimer periodic_dump_timer_;
// Thread used for MemoryDumpProviders which don't specify a task runner
// affinity.
scoped_ptr<Thread> dump_thread_;
// The unique id of the child process. This is created only for tracing and is
// expected to be valid only when tracing is enabled.
uint64_t tracing_process_id_;
// When true, calling |RegisterMemoryDumpProvider| is a no-op.
bool dumper_registrations_ignored_for_testing_;
// Whether new memory dump providers should be told to enable heap profiling.
bool heap_profiling_enabled_;
DISALLOW_COPY_AND_ASSIGN(MemoryDumpManager);
};
// The delegate is supposed to be long lived (read: a Singleton) and thread
// safe (i.e. should expect calls from any thread and handle thread hopping).
class BASE_EXPORT MemoryDumpManagerDelegate {
public:
virtual void RequestGlobalMemoryDump(const MemoryDumpRequestArgs& args,
const MemoryDumpCallback& callback) = 0;
// Returns tracing process id of the current process. This is used by
// MemoryDumpManager::GetTracingProcessId.
virtual uint64_t GetTracingProcessId() const = 0;
protected:
MemoryDumpManagerDelegate() {}
virtual ~MemoryDumpManagerDelegate() {}
void CreateProcessDump(const MemoryDumpRequestArgs& args,
const MemoryDumpCallback& callback) {
MemoryDumpManager::GetInstance()->CreateProcessDump(args, callback);
}
private:
DISALLOW_COPY_AND_ASSIGN(MemoryDumpManagerDelegate);
};
} // namespace trace_event
} // namespace base
#endif // BASE_TRACE_EVENT_MEMORY_DUMP_MANAGER_H_
| junhuac/MQUIC | src/base/trace_event/memory_dump_manager.h | C | mit | 16,373 |
class CreateUser < ActiveRecord::Migration
def up
create_table :users do |t|
t.integer :user_id, :limit => 8
t.string :screen_name
t.boolean :following, :default => false
t.boolean :followed, :default => false
t.boolean :unfollowed, :default => false
t.timestamp :followed_at
t.timestamp :created_at
t.timestamp :updated_at
t.boolean :keep
t.text :twitter_attributes
end
end
end
| fdv/social-penis-enlargement | migrate.rb | Ruby | mit | 452 |
/* crypto/cryptlib.c */
/* ====================================================================
* Copyright (c) 1998-2006 The OpenSSL Project. All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
*
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in
* the documentation and/or other materials provided with the
* distribution.
*
* 3. All advertising materials mentioning features or use of this
* software must display the following acknowledgment:
* "This product includes software developed by the OpenSSL Project
* for use in the OpenSSL Toolkit. (http://www.openssl.org/)"
*
* 4. The names "OpenSSL Toolkit" and "OpenSSL Project" must not be used to
* endorse or promote products derived from this software without
* prior written permission. For written permission, please contact
* openssl-core@openssl.org.
*
* 5. Products derived from this software may not be called "OpenSSL"
* nor may "OpenSSL" appear in their names without prior written
* permission of the OpenSSL Project.
*
* 6. Redistributions of any form whatsoever must retain the following
* acknowledgment:
* "This product includes software developed by the OpenSSL Project
* for use in the OpenSSL Toolkit (http://www.openssl.org/)"
*
* THIS SOFTWARE IS PROVIDED BY THE OpenSSL PROJECT ``AS IS'' AND ANY
* EXPRESSED OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE OpenSSL PROJECT OR
* ITS CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
* NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION)
* HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT,
* STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED
* OF THE POSSIBILITY OF SUCH DAMAGE.
* ====================================================================
*
* This product includes cryptographic software written by Eric Young
* (eay@cryptsoft.com). This product includes software written by Tim
* Hudson (tjh@cryptsoft.com).
*
*/
/* Copyright (C) 1995-1998 Eric Young (eay@cryptsoft.com)
* All rights reserved.
*
* This package is an SSL implementation written
* by Eric Young (eay@cryptsoft.com).
* The implementation was written so as to conform with Netscapes SSL.
*
* This library is free for commercial and non-commercial use as long as
* the following conditions are aheared to. The following conditions
* apply to all code found in this distribution, be it the RC4, RSA,
* lhash, DES, etc., code; not just the SSL code. The SSL documentation
* included with this distribution is covered by the same copyright terms
* except that the holder is Tim Hudson (tjh@cryptsoft.com).
*
* Copyright remains Eric Young's, and as such any Copyright notices in
* the code are not to be removed.
* If this package is used in a product, Eric Young should be given attribution
* as the author of the parts of the library used.
* This can be in the form of a textual message at program startup or
* in documentation (online or textual) provided with the package.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
* 1. Redistributions of source code must retain the copyright
* notice, this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* 3. All advertising materials mentioning features or use of this software
* must display the following acknowledgement:
* "This product includes cryptographic software written by
* Eric Young (eay@cryptsoft.com)"
* The word 'cryptographic' can be left out if the rouines from the library
* being used are not cryptographic related :-).
* 4. If you include any Windows specific code (or a derivative thereof) from
* the apps directory (application code) you must include an acknowledgement:
* "This product includes software written by Tim Hudson (tjh@cryptsoft.com)"
*
* THIS SOFTWARE IS PROVIDED BY ERIC YOUNG ``AS IS'' AND
* ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE AUTHOR OR CONTRIBUTORS BE LIABLE
* FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
* DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS
* OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION)
* HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT
* LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY
* OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF
* SUCH DAMAGE.
*
* The licence and distribution terms for any publically available version or
* derivative of this code cannot be changed. i.e. this code cannot simply be
* copied and put under another distribution licence
* [including the GNU Public Licence.]
*/
/* ====================================================================
* Copyright 2002 Sun Microsystems, Inc. ALL RIGHTS RESERVED.
* ECDH support in OpenSSL originally developed by
* SUN MICROSYSTEMS, INC., and contributed to the OpenSSL project.
*/
#include "internal/cryptlib.h"
#ifndef OPENSSL_NO_DEPRECATED
static unsigned long (*id_callback) (void) = 0;
#endif
static void (*threadid_callback) (CRYPTO_THREADID *) = 0;
/*
* the memset() here and in set_pointer() seem overkill, but for the sake of
* CRYPTO_THREADID_cmp() this avoids any platform silliness that might cause
* two "equal" THREADID structs to not be memcmp()-identical.
*/
void CRYPTO_THREADID_set_numeric(CRYPTO_THREADID *id, unsigned long val)
{
memset(id, 0, sizeof(*id));
id->val = val;
}
static const unsigned char hash_coeffs[] = { 3, 5, 7, 11, 13, 17, 19, 23 };
void CRYPTO_THREADID_set_pointer(CRYPTO_THREADID *id, void *ptr)
{
unsigned char *dest = (void *)&id->val;
unsigned int accum = 0;
unsigned char dnum = sizeof(id->val);
memset(id, 0, sizeof(*id));
id->ptr = ptr;
if (sizeof(id->val) >= sizeof(id->ptr)) {
/*
* 'ptr' can be embedded in 'val' without loss of uniqueness
*/
id->val = (unsigned long)id->ptr;
return;
}
/*
* hash ptr ==> val. Each byte of 'val' gets the mod-256 total of a
* linear function over the bytes in 'ptr', the co-efficients of which
* are a sequence of low-primes (hash_coeffs is an 8-element cycle) - the
* starting prime for the sequence varies for each byte of 'val' (unique
* polynomials unless pointers are >64-bit). For added spice, the totals
* accumulate rather than restarting from zero, and the index of the
* 'val' byte is added each time (position dependence). If I was a
* black-belt, I'd scan big-endian pointers in reverse to give low-order
* bits more play, but this isn't crypto and I'd prefer nobody mistake it
* as such. Plus I'm lazy.
*/
while (dnum--) {
const unsigned char *src = (void *)&id->ptr;
unsigned char snum = sizeof(id->ptr);
while (snum--)
accum += *(src++) * hash_coeffs[(snum + dnum) & 7];
accum += dnum;
*(dest++) = accum & 255;
}
}
int CRYPTO_THREADID_set_callback(void (*func) (CRYPTO_THREADID *))
{
if (threadid_callback)
return 0;
threadid_callback = func;
return 1;
}
void (*CRYPTO_THREADID_get_callback(void)) (CRYPTO_THREADID *) {
return threadid_callback;
}
void CRYPTO_THREADID_current(CRYPTO_THREADID *id)
{
if (threadid_callback) {
threadid_callback(id);
return;
}
#ifndef OPENSSL_NO_DEPRECATED
/* If the deprecated callback was set, fall back to that */
if (id_callback) {
CRYPTO_THREADID_set_numeric(id, id_callback());
return;
}
#endif
/* Else pick a backup */
#if defined(OPENSSL_SYS_WIN32)
CRYPTO_THREADID_set_numeric(id, (unsigned long)GetCurrentThreadId());
#else
/* For everything else, default to using the address of 'errno' */
CRYPTO_THREADID_set_pointer(id, (void *)&errno);
#endif
}
int CRYPTO_THREADID_cmp(const CRYPTO_THREADID *a, const CRYPTO_THREADID *b)
{
return memcmp(a, b, sizeof(*a));
}
void CRYPTO_THREADID_cpy(CRYPTO_THREADID *dest, const CRYPTO_THREADID *src)
{
memcpy(dest, src, sizeof(*src));
}
unsigned long CRYPTO_THREADID_hash(const CRYPTO_THREADID *id)
{
return id->val;
}
#ifndef OPENSSL_NO_DEPRECATED
unsigned long (*CRYPTO_get_id_callback(void)) (void) {
return (id_callback);
}
void CRYPTO_set_id_callback(unsigned long (*func) (void))
{
id_callback = func;
}
unsigned long CRYPTO_thread_id(void)
{
unsigned long ret = 0;
if (id_callback == NULL) {
# if defined(OPENSSL_SYS_WIN32)
ret = (unsigned long)GetCurrentThreadId();
# elif defined(GETPID_IS_MEANINGLESS)
ret = 1L;
# else
ret = (unsigned long)getpid();
# endif
} else
ret = id_callback();
return (ret);
}
#endif
| vbloodv/blood | extern/openssl.orig/crypto/thr_id.c | C | mit | 9,864 |
// WinProm Copyright 2015 Edward Earl
// All rights reserved.
//
// This software is distributed under a license that is described in
// the LICENSE file that accompanies it.
//
// DomapInfo_dlg.cpp : implementation file
//
#include "stdafx.h"
#include "winprom.h"
#include "DomapInfo_dlg.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#undef THIS_FILE
static char THIS_FILE[] = __FILE__;
#endif
/////////////////////////////////////////////////////////////////////////////
// CDomapInfo_dlg dialog
CDomapInfo_dlg::CDomapInfo_dlg(const Area_info& a, const Area_info& d,
const CString& n, bool m)
: CMapInfo_dlg(a,d,n,m,CDomapInfo_dlg::IDD)
{
//{{AFX_DATA_INIT(CDomapInfo_dlg)
m_ndom_0peak = 0;
m_ndom_1peak = 0;
m_ndom_peaks = 0;
m_ndom_total = 0;
m_ndom_area = 0;
//}}AFX_DATA_INIT
}
void CDomapInfo_dlg::DoDataExchange(CDataExchange* pDX)
{
CMapInfo_dlg::DoDataExchange(pDX);
//{{AFX_DATA_MAP(CDomapInfo_dlg)
DDX_Text(pDX, IDC_NDOM_0PEAK, m_ndom_0peak);
DDX_Text(pDX, IDC_NDOM_1PEAK, m_ndom_1peak);
DDX_Text(pDX, IDC_NDOM_PEAKS, m_ndom_peaks);
DDX_Text(pDX, IDC_NDOM, m_ndom_total);
DDX_Text(pDX, IDC_NDOM_AREA, m_ndom_area);
//}}AFX_DATA_MAP
}
BEGIN_MESSAGE_MAP(CDomapInfo_dlg, CMapInfo_dlg)
//{{AFX_MSG_MAP(CDomapInfo_dlg)
// NOTE: the ClassWizard will add message map macros here
//}}AFX_MSG_MAP
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
// CDomapInfo_dlg message handlers
| edwardearl/winprom | winprom/DomapInfo_dlg.cpp | C++ | mit | 1,484 |
{{<govuk_template}}
{{$head}}
{{>includes/elements_head}}
{{>includes/elements_scripts}}
{{/head}}
{{$propositionHeader}}
{{>includes/propositional_navigation4}}
{{/propositionHeader}}
{{$headerClass}}with-proposition{{/headerClass}}
{{$content}}
<script>
if(sessionStorage.condition) {
sessionStorage.removeItem('condition');
}
function start() {
window.location.href = 'condition-selection';
}
</script>
<main id="page-container" role="main">
{{>includes/phase_banner}}
<div id="notify" class="starthidden">
<div class="grid-row" style="margin-top:1.5em">
<div class="column-two-thirds">
<h1 style="margin-top:0.5em;" class="form-title heading-xlarge">
Tell DVLA about your medical condition
</h1>
<p>You can use this service to tell DVLA about your medical condition.</p>
<p>It is a legal requirement to tell DVLA of a medical condition that might affect your driving.</p>
<div style="padding-top: 0px; padding-bottom: 5px;" class="panel-indent">
<!--div class="help-notice">
<h2 class="heading-medium">Don’t worry</h2>
</div-->
<h2 class="heading-medium">Don’t worry</h2>
<p>Most drivers are allowed to continue driving.</p>
</div>
<h2 class="heading-medium">What you'll need</h2>
<ul class="list-bullet" style="margin-bottom: 0;">
<li>to be a resident in Great Britain - there is a different process in <a href="http://amdr.herokuapp.com/COA_Direct_v4/Landing_page">Northern Ireland, Isle of Man and the Channel Islands</a>
<li>your healthcare professionals' (eg GP, nurse, consultant) details</li>
</ul>
<!--details style="margin-top: 1em;">
<summary>
<span class="summary">
What you'll need
</span>
</summary>
<div class="panel-indent">
<ul class="list-bullet" style="margin-bottom: 0;">
<li>to be a resident in Great Britain - there is a different process in <a href="http://amdr.herokuapp.com/COA_Direct_v4/Landing_page">Northern Ireland, Isle of Man and the Channel Islands</a>.
<li>your personal details</li>
<li>your healthcare professionals' (e.g. GP, Nurse, Consultant) details</li>
</ul>
</div>
</details-->
<br/><br/>
<div style="">
<h2 style="display: inline;">
<img src="/public/images/verify-logo-inline.png" style="width: 100px; display: inline; float: left;"/>
<strong>GOV.UK</strong>
<br/>
VERIFY
</h2>
</div>
<br/>
<p>This service uses GOV.UK Verify to check your identity.</p>
<br/>
<a href="javascript:start();" class="button button-get-started" role="button">Start now</a>
</div>
<div class="column-third" style=";margin-bottom:0.75em">
<nav class="popular-needs">
<h3 class="heading-medium" style="border-top:10px solid #005ea5;margin-top:1em;padding-top:1em">Driving with a medical condition</h3>
<ul style="list-style-type: none;">
<li style="margin-top: 0.5em;"><a href="https://www.gov.uk/health-conditions-and-driving">Conditions that need to be reported</a></li>
<li style="margin-top: 0.5em;"><a href="https://www.gov.uk/driving-licence-categories">Types of driving licences</a></li>
<li style="margin-top: 0.5em;"><a href="https://www.gov.uk/giving-up-your-driving-licence">I want to give up my licence</a></li>
</ul>
</nav>
</div>
</div>
</div>
<div id="renewal" class="starthidden">
<div class="grid-row" style="margin-top:1.5em">
<div class="column-two-thirds">
<h1 style="margin-top:0.5em;" class="form-title heading-xlarge">
Renew your medical driving licence
</h1>
<p>Use this service to:</p>
<ul class="list-bullet">
<li>renew your medical driving licence</li>
</ul>
<p>If you need to tell DVLA about a different condition, download and complete the paper form – then post it to DVLA.</p>
<a href="javascript:start();" class="button button-get-started" role="button">Start now</a>
<h2 class="heading-medium" style="margin-top:0.75em">Other ways to apply</h2>
<h2 class="heading-small" style="margin-top:0.75em">By post</h2> Please provide the following:
<ul class="list-bullet">
<li>your full name and address</li>
<li>your driving licence number (or your date of birth if you do not know your driving licence number)</li>
<li>details about your medical condition</li>
</ul>
<p>Send to:</p>
Drivers Medical Group
<br> DVLA
<br> Swansea
<br> SA99 1TU
</div>
<div class="column-third" style=";margin-bottom:0.75em">
<nav class="popular-needs">
<h3 class="heading-medium" style="border-top:10px solid #005ea5;margin-top:1em;padding-top:1em">Driving with a medical condition</h3>
<ul style=";list-style-type: none">
<li style="margin-top: 0.5em;"><a href="https://www.gov.uk/health-conditions-and-driving">Conditions that need to be reported</a></li>
<li style="margin-top: 0.5em;"><a href="https://www.gov.uk/driving-licence-categories">Types of driving licences</a></li>
<li style="margin-top: 0.5em;"><a href="https://www.gov.uk/giving-up-your-driving-licence">I want to give up my licence</a></li>
</ul>
</nav>
</div>
</div>
</div>
<script>
show((QueryString.service == null) ? 'notify' : QueryString.service);
</script>
</main>
{{/content}}
{{$bodyEnd}}
{{/bodyEnd}}
{{/govuk_template}} | hannifd/F2D-Build | app/views/index.html | HTML | mit | 5,726 |